text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoActivityIndicator].
void main() => runApp(const CupertinoIndicatorApp());
class CupertinoIndicatorApp extends StatelessWidget {
const CupertinoIndicatorApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: CupertinoIndicatorExample(),
);
}
}
class CupertinoIndicatorExample extends StatelessWidget {
const CupertinoIndicatorExample({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('CupertinoActivityIndicator Sample'),
),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
// Cupertino activity indicator with default properties.
CupertinoActivityIndicator(),
SizedBox(height: 10),
Text('Default'),
],
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
// Cupertino activity indicator with custom radius and color.
CupertinoActivityIndicator(radius: 20.0, color: CupertinoColors.activeBlue),
SizedBox(height: 10),
Text(
'radius: 20.0\ncolor: CupertinoColors.activeBlue',
textAlign: TextAlign.center,
),
],
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
// Cupertino activity indicator with custom radius and disabled
// animation.
CupertinoActivityIndicator(radius: 20.0, animating: false),
SizedBox(height: 10),
Text(
'radius: 20.0\nanimating: false',
textAlign: TextAlign.center,
),
],
),
],
),
),
);
}
}
| flutter/examples/api/lib/cupertino/activity_indicator/cupertino_activity_indicator.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/activity_indicator/cupertino_activity_indicator.0.dart",
"repo_id": "flutter",
"token_count": 1143
} | 554 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoRadio].
void main() => runApp(const CupertinoRadioApp());
class CupertinoRadioApp extends StatelessWidget {
const CupertinoRadioApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('CupertinoRadio Example'),
),
child: SafeArea(
child: CupertinoRadioExample(),
),
),
);
}
}
enum SingingCharacter { lafayette, jefferson }
class CupertinoRadioExample extends StatefulWidget {
const CupertinoRadioExample({super.key});
@override
State<CupertinoRadioExample> createState() => _CupertinoRadioExampleState();
}
class _CupertinoRadioExampleState extends State<CupertinoRadioExample> {
SingingCharacter? _character = SingingCharacter.lafayette;
@override
Widget build(BuildContext context) {
return CupertinoListSection(
children: <Widget>[
CupertinoListTile(
title: const Text('Lafayette'),
leading: CupertinoRadio<SingingCharacter>(
value: SingingCharacter.lafayette,
groupValue: _character,
onChanged: (SingingCharacter? value) {
setState(() {
_character = value;
});
},
),
),
CupertinoListTile(
title: const Text('Thomas Jefferson'),
leading: CupertinoRadio<SingingCharacter>(
value: SingingCharacter.jefferson,
groupValue: _character,
onChanged: (SingingCharacter? value) {
setState(() {
_character = value;
});
},
),
),
],
);
}
}
| flutter/examples/api/lib/cupertino/radio/cupertino_radio.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/radio/cupertino_radio.0.dart",
"repo_id": "flutter",
"token_count": 853
} | 555 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoTextFormFieldRow].
void main() => runApp(const FormSectionApp());
class FormSectionApp extends StatelessWidget {
const FormSectionApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: FromSectionExample(),
);
}
}
class FromSectionExample extends StatelessWidget {
const FromSectionExample({super.key});
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoFormSection Sample'),
),
// Add safe area widget to place the CupertinoFormSection below the navigation bar.
child: SafeArea(
child: Form(
autovalidateMode: AutovalidateMode.always,
onChanged: () {
Form.maybeOf(primaryFocus!.context!)?.save();
},
child: CupertinoFormSection.insetGrouped(
header: const Text('SECTION 1'),
children: List<Widget>.generate(5, (int index) {
return CupertinoTextFormFieldRow(
prefix: const Text('Enter text'),
placeholder: 'Enter text',
validator: (String? value) {
if (value == null || value.isEmpty) {
return 'Please enter a value';
}
return null;
},
);
}),
),
),
),
);
}
}
| flutter/examples/api/lib/cupertino/text_form_field_row/cupertino_text_form_field_row.1.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/text_form_field_row/cupertino_text_form_field_row.1.dart",
"repo_id": "flutter",
"token_count": 742
} | 556 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverAppBar].
void main() {
runApp(const StretchableSliverAppBar());
}
class StretchableSliverAppBar extends StatefulWidget {
const StretchableSliverAppBar({super.key});
@override
State<StretchableSliverAppBar> createState() =>
_StretchableSliverAppBarState();
}
class _StretchableSliverAppBarState extends State<StretchableSliverAppBar> {
bool _stretch = true;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: CustomScrollView(
physics: const BouncingScrollPhysics(),
slivers: <Widget>[
SliverAppBar(
stretch: _stretch,
onStretchTrigger: () async {
// Triggers when stretching
},
// [stretchTriggerOffset] describes the amount of overscroll that must occur
// to trigger [onStretchTrigger]
//
// Setting [stretchTriggerOffset] to a value of 300.0 will trigger
// [onStretchTrigger] when the user has overscrolled by 300.0 pixels.
stretchTriggerOffset: 300.0,
expandedHeight: 200.0,
flexibleSpace: const FlexibleSpaceBar(
title: Text('SliverAppBar'),
background: FlutterLogo(),
),
),
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return Container(
color: index.isOdd ? Colors.white : Colors.black12,
height: 100.0,
child: Center(
child: Text('$index', textScaler: const TextScaler.linear(5.0)),
),
);
},
childCount: 20,
),
),
],
),
bottomNavigationBar: BottomAppBar(
child: Padding(
padding: const EdgeInsets.all(8),
child: OverflowBar(
overflowAlignment: OverflowBarAlignment.center,
alignment: MainAxisAlignment.center,
children: <Widget>[
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Text('stretch'),
Switch(
onChanged: (bool val) {
setState(() {
_stretch = val;
});
},
value: _stretch,
),
],
),
],
),
),
),
));
}
}
| flutter/examples/api/lib/material/app_bar/sliver_app_bar.4.dart/0 | {
"file_path": "flutter/examples/api/lib/material/app_bar/sliver_app_bar.4.dart",
"repo_id": "flutter",
"token_count": 1404
} | 557 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for using [ButtonStyleButton.iconAlignment] parameter.
void main() {
runApp(const ButtonStyleButtonIconAlignmentApp());
}
class ButtonStyleButtonIconAlignmentApp extends StatelessWidget {
const ButtonStyleButtonIconAlignmentApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: Scaffold(
body: ButtonStyleButtonIconAlignmentExample(),
),
);
}
}
class ButtonStyleButtonIconAlignmentExample extends StatefulWidget {
const ButtonStyleButtonIconAlignmentExample({super.key});
@override
State<ButtonStyleButtonIconAlignmentExample> createState() => _ButtonStyleButtonIconAlignmentExampleState();
}
class _ButtonStyleButtonIconAlignmentExampleState extends State<ButtonStyleButtonIconAlignmentExample> {
TextDirection _textDirection = TextDirection.ltr;
IconAlignment _iconAlignment = IconAlignment.start;
@override
Widget build(BuildContext context) {
return SafeArea(
child: Directionality(
key: const Key('Directionality'),
textDirection: _textDirection,
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Spacer(),
OverflowBar(
spacing: 10,
overflowSpacing: 20,
alignment: MainAxisAlignment.center,
overflowAlignment: OverflowBarAlignment.center,
children: <Widget>[
ElevatedButton.icon(
onPressed: () {},
icon: const Icon(Icons.sunny),
label: const Text('ElevatedButton'),
iconAlignment: _iconAlignment,
),
FilledButton.icon(
onPressed: () {},
icon: const Icon(Icons.beach_access),
label: const Text('FilledButton'),
iconAlignment: _iconAlignment,
),
FilledButton.tonalIcon(
onPressed: () {},
icon: const Icon(Icons.cloud),
label: const Text('FilledButton Tonal'),
iconAlignment: _iconAlignment,
),
OutlinedButton.icon(
onPressed: () {},
icon: const Icon(Icons.light),
label: const Text('OutlinedButton'),
iconAlignment: _iconAlignment,
),
TextButton.icon(
onPressed: () {},
icon: const Icon(Icons.flight_takeoff),
label: const Text('TextButton'),
iconAlignment: _iconAlignment,
),
],
),
const Spacer(),
OverflowBar(
alignment: MainAxisAlignment.spaceEvenly,
overflowAlignment: OverflowBarAlignment.center,
spacing: 10,
overflowSpacing: 10,
children: <Widget>[
Column(
children: <Widget>[
const Text('Icon alignment'),
const SizedBox(height: 10),
SegmentedButton<IconAlignment>(
onSelectionChanged: (Set<IconAlignment> value) {
setState(() {
_iconAlignment = value.first;
});
},
selected: <IconAlignment>{ _iconAlignment },
segments: IconAlignment.values.map((IconAlignment iconAlignment) {
return ButtonSegment<IconAlignment>(
value: iconAlignment,
label: Text(iconAlignment.name),
);
}).toList(),
),
],
),
Column(
children: <Widget>[
const Text('Text direction'),
const SizedBox(height: 10),
SegmentedButton<TextDirection>(
onSelectionChanged: (Set<TextDirection> value) {
setState(() {
_textDirection = value.first;
});
},
selected: <TextDirection>{ _textDirection },
segments: const <ButtonSegment<TextDirection>>[
ButtonSegment<TextDirection>(
value: TextDirection.ltr,
label: Text('LTR'),
),
ButtonSegment<TextDirection>(
value: TextDirection.rtl,
label: Text('RTL'),
),
],
),
],
),
],
),
const Spacer(),
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/button_style_button/button_style_button.icon_alignment.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/button_style_button/button_style_button.icon_alignment.0.dart",
"repo_id": "flutter",
"token_count": 3042
} | 558 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This sample demonstrates allowing a context menu to be shown in a widget
// subtree in response to user gestures.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() => runApp(const ContextMenuControllerExampleApp());
/// A builder that includes an Offset to draw the context menu at.
typedef ContextMenuBuilder = Widget Function(BuildContext context, Offset offset);
class ContextMenuControllerExampleApp extends StatefulWidget {
const ContextMenuControllerExampleApp({super.key});
@override
State<ContextMenuControllerExampleApp> createState() => _ContextMenuControllerExampleAppState();
}
class _ContextMenuControllerExampleAppState extends State<ContextMenuControllerExampleApp> {
void _showDialog(BuildContext context) {
Navigator.of(context).push(
DialogRoute<void>(
context: context,
builder: (BuildContext context) => const AlertDialog(title: Text('You clicked print!')),
),
);
}
@override
void initState() {
super.initState();
// On web, disable the browser's context menu since this example uses a custom
// Flutter-rendered context menu.
if (kIsWeb) {
BrowserContextMenu.disableContextMenu();
}
}
@override
void dispose() {
if (kIsWeb) {
BrowserContextMenu.enableContextMenu();
}
super.dispose();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Context menu outside of text'),
),
body: _ContextMenuRegion(
contextMenuBuilder: (BuildContext context, Offset offset) {
// The custom context menu will look like the default context menu
// on the current platform with a single 'Print' button.
return AdaptiveTextSelectionToolbar.buttonItems(
anchors: TextSelectionToolbarAnchors(
primaryAnchor: offset,
),
buttonItems: <ContextMenuButtonItem>[
ContextMenuButtonItem(
onPressed: () {
ContextMenuController.removeAny();
_showDialog(context);
},
label: 'Print',
),
],
);
},
// In this case this wraps a big open space in a GestureDetector in
// order to show the context menu, but it could also wrap a single
// widget like an Image to give it a context menu.
child: ListView(
children: <Widget>[
Container(height: 20.0),
const Text(
'Right click (desktop) or long press (mobile) anywhere, not just on this text, to show the custom menu.'),
],
),
),
),
);
}
}
/// Shows and hides the context menu based on user gestures.
///
/// By default, shows the menu on right clicks and long presses.
class _ContextMenuRegion extends StatefulWidget {
/// Creates an instance of [_ContextMenuRegion].
const _ContextMenuRegion({
required this.child,
required this.contextMenuBuilder,
});
/// Builds the context menu.
final ContextMenuBuilder contextMenuBuilder;
/// The child widget that will be listened to for gestures.
final Widget child;
@override
State<_ContextMenuRegion> createState() => _ContextMenuRegionState();
}
class _ContextMenuRegionState extends State<_ContextMenuRegion> {
Offset? _longPressOffset;
final ContextMenuController _contextMenuController = ContextMenuController();
static bool get _longPressEnabled {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
return true;
case TargetPlatform.macOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return false;
}
}
void _onSecondaryTapUp(TapUpDetails details) {
_show(details.globalPosition);
}
void _onTap() {
if (!_contextMenuController.isShown) {
return;
}
_hide();
}
void _onLongPressStart(LongPressStartDetails details) {
_longPressOffset = details.globalPosition;
}
void _onLongPress() {
assert(_longPressOffset != null);
_show(_longPressOffset!);
_longPressOffset = null;
}
void _show(Offset position) {
_contextMenuController.show(
context: context,
contextMenuBuilder: (BuildContext context) {
return widget.contextMenuBuilder(context, position);
},
);
}
void _hide() {
_contextMenuController.remove();
}
@override
void dispose() {
_hide();
super.dispose();
}
@override
Widget build(BuildContext context) {
return GestureDetector(
behavior: HitTestBehavior.opaque,
onSecondaryTapUp: _onSecondaryTapUp,
onTap: _onTap,
onLongPress: _longPressEnabled ? _onLongPress : null,
onLongPressStart: _longPressEnabled ? _onLongPressStart : null,
child: widget.child,
);
}
}
| flutter/examples/api/lib/material/context_menu/context_menu_controller.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/context_menu/context_menu_controller.0.dart",
"repo_id": "flutter",
"token_count": 1935
} | 559 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ExpansionTile] and [ExpansionTileController].
void main() {
runApp(const ExpansionTileControllerApp());
}
class ExpansionTileControllerApp extends StatefulWidget {
const ExpansionTileControllerApp({super.key});
@override
State<ExpansionTileControllerApp> createState() => _ExpansionTileControllerAppState();
}
class _ExpansionTileControllerAppState extends State<ExpansionTileControllerApp> {
final ExpansionTileController controller = ExpansionTileController();
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('ExpansionTileController Sample')),
body: Column(
children: <Widget>[
// A controller has been provided to the ExpansionTile because it's
// going to be accessed from a component that is not within the
// tile's BuildContext.
ExpansionTile(
controller: controller,
title: const Text('ExpansionTile with explicit controller.'),
children: <Widget>[
Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(24),
child: const Text('ExpansionTile Contents'),
),
],
),
const SizedBox(height: 8),
ElevatedButton(
child: const Text('Expand/Collapse the Tile Above'),
onPressed: () {
if (controller.isExpanded) {
controller.collapse();
} else {
controller.expand();
}
},
),
const SizedBox(height: 48),
// A controller has not been provided to the ExpansionTile because
// the automatically created one can be retrieved via the tile's BuildContext.
ExpansionTile(
title: const Text('ExpansionTile with implicit controller.'),
children: <Widget>[
Builder(
builder: (BuildContext context) {
return Container(
padding: const EdgeInsets.all(24),
alignment: Alignment.center,
child: ElevatedButton(
child: const Text('Collapse This Tile'),
onPressed: () {
return ExpansionTileController.of(context).collapse();
},
),
);
},
),
],
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/expansion_tile/expansion_tile.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/expansion_tile/expansion_tile.1.dart",
"repo_id": "flutter",
"token_count": 1359
} | 560 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Flutter code sample InputChip.
import 'package:flutter/material.dart';
void main() => runApp(const ChipApp());
class ChipApp extends StatelessWidget {
const ChipApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(colorSchemeSeed: const Color(0xff6750a4), useMaterial3: true),
home: const InputChipExample(),
);
}
}
class InputChipExample extends StatefulWidget {
const InputChipExample({super.key});
@override
State<InputChipExample> createState() => _InputChipExampleState();
}
class _InputChipExampleState extends State<InputChipExample> {
int inputs = 3;
int? selectedIndex;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('InputChip Sample'),
),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Wrap(
alignment: WrapAlignment.center,
spacing: 5.0,
children: List<Widget>.generate(
inputs,
(int index) {
return InputChip(
label: Text('Person ${index + 1}'),
selected: selectedIndex == index,
onSelected: (bool selected) {
setState(() {
if (selectedIndex == index) {
selectedIndex = null;
} else {
selectedIndex = index;
}
});
},
onDeleted: () {
setState(() {
inputs = inputs - 1;
});
},
);
},
).toList(),
),
const SizedBox(height: 10),
ElevatedButton(
onPressed: () {
setState(() {
inputs = 3;
});
},
child: const Text('Reset'),
)
],
),
),
);
}
}
| flutter/examples/api/lib/material/input_chip/input_chip.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/input_chip/input_chip.0.dart",
"repo_id": "flutter",
"token_count": 1241
} | 561 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for custom list items.
void main() => runApp(const CustomListItemApp());
class CustomListItemApp extends StatelessWidget {
const CustomListItemApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: CustomListItemExample(),
);
}
}
class CustomListItem extends StatelessWidget {
const CustomListItem({
super.key,
required this.thumbnail,
required this.title,
required this.user,
required this.viewCount,
});
final Widget thumbnail;
final String title;
final String user;
final int viewCount;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(vertical: 5.0),
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
flex: 2,
child: thumbnail,
),
Expanded(
flex: 3,
child: _VideoDescription(
title: title,
user: user,
viewCount: viewCount,
),
),
const Icon(
Icons.more_vert,
size: 16.0,
),
],
),
);
}
}
class _VideoDescription extends StatelessWidget {
const _VideoDescription({
required this.title,
required this.user,
required this.viewCount,
});
final String title;
final String user;
final int viewCount;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.fromLTRB(5.0, 0.0, 0.0, 0.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
title,
style: const TextStyle(
fontWeight: FontWeight.w500,
fontSize: 14.0,
),
),
const Padding(padding: EdgeInsets.symmetric(vertical: 2.0)),
Text(
user,
style: const TextStyle(fontSize: 10.0),
),
const Padding(padding: EdgeInsets.symmetric(vertical: 1.0)),
Text(
'$viewCount views',
style: const TextStyle(fontSize: 10.0),
),
],
),
);
}
}
class CustomListItemExample extends StatelessWidget {
const CustomListItemExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Custom List Item Sample')),
body: ListView(
padding: const EdgeInsets.all(8.0),
itemExtent: 106.0,
children: <CustomListItem>[
CustomListItem(
user: 'Flutter',
viewCount: 999000,
thumbnail: Container(
decoration: const BoxDecoration(color: Colors.blue),
),
title: 'The Flutter YouTube Channel',
),
CustomListItem(
user: 'Dash',
viewCount: 884000,
thumbnail: Container(
decoration: const BoxDecoration(color: Colors.yellow),
),
title: 'Announcing Flutter 1.0',
),
],
),
);
}
}
| flutter/examples/api/lib/material/list_tile/custom_list_item.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/list_tile/custom_list_item.0.dart",
"repo_id": "flutter",
"token_count": 1548
} | 562 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MenuAnchor].
void main() => runApp(const MenuAnchorApp());
// This is the type used by the menu below.
enum SampleItem { itemOne, itemTwo, itemThree }
class MenuAnchorApp extends StatelessWidget {
const MenuAnchorApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const MenuAnchorExample(),
);
}
}
class MenuAnchorExample extends StatefulWidget {
const MenuAnchorExample({super.key});
@override
State<MenuAnchorExample> createState() => _MenuAnchorExampleState();
}
class _MenuAnchorExampleState extends State<MenuAnchorExample> {
SampleItem? selectedMenu;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('MenuAnchorButton'),
backgroundColor: Theme.of(context).primaryColorLight,
),
body: Center(
child: MenuAnchor(
builder:
(BuildContext context, MenuController controller, Widget? child) {
return IconButton(
onPressed: () {
if (controller.isOpen) {
controller.close();
} else {
controller.open();
}
},
icon: const Icon(Icons.more_horiz),
tooltip: 'Show menu',
);},
menuChildren: List<MenuItemButton>.generate(
3,
(int index) => MenuItemButton(
onPressed: () => setState(() => selectedMenu = SampleItem.values[index]),
child: Text('Item ${index + 1}'),
),
),
),
),
);
}
}
| flutter/examples/api/lib/material/menu_anchor/menu_anchor.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/menu_anchor/menu_anchor.2.dart",
"repo_id": "flutter",
"token_count": 820
} | 563 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [PopupMenuButton].
// This is the type used by the popup menu below.
enum SampleItem { itemOne, itemTwo, itemThree }
void main() => runApp(const PopupMenuApp());
class PopupMenuApp extends StatelessWidget {
const PopupMenuApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: PopupMenuExample(),
);
}
}
class PopupMenuExample extends StatefulWidget {
const PopupMenuExample({super.key});
@override
State<PopupMenuExample> createState() => _PopupMenuExampleState();
}
class _PopupMenuExampleState extends State<PopupMenuExample> {
SampleItem? selectedItem;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('PopupMenuButton')),
body: Center(
child: PopupMenuButton<SampleItem>(
initialValue: selectedItem,
onSelected: (SampleItem item) {
setState(() {
selectedItem = item;
});
},
itemBuilder: (BuildContext context) => <PopupMenuEntry<SampleItem>>[
const PopupMenuItem<SampleItem>(
value: SampleItem.itemOne,
child: Text('Item 1'),
),
const PopupMenuItem<SampleItem>(
value: SampleItem.itemTwo,
child: Text('Item 2'),
),
const PopupMenuItem<SampleItem>(
value: SampleItem.itemThree,
child: Text('Item 3'),
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/popup_menu/popup_menu.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/popup_menu/popup_menu.0.dart",
"repo_id": "flutter",
"token_count": 730
} | 564 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
/// Flutter code sample for [RefreshIndicator].
void main() => runApp(const RefreshIndicatorExampleApp());
class RefreshIndicatorExampleApp extends StatelessWidget {
const RefreshIndicatorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
scrollBehavior: const MaterialScrollBehavior().copyWith(dragDevices: PointerDeviceKind.values.toSet()),
home: const RefreshIndicatorExample(),
);
}
}
class RefreshIndicatorExample extends StatelessWidget {
const RefreshIndicatorExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('RefreshIndicator Sample'),
),
body: RefreshIndicator(
color: Colors.white,
backgroundColor: Colors.blue,
onRefresh: () async {
// Replace this delay with the code to be executed during refresh
// and return asynchronous code
return Future<void>.delayed(const Duration(seconds: 3));
},
// This check is used to customize listening to scroll notifications
// from the widget's children.
//
// By default this is set to `notification.depth == 0`, which ensures
// the only the scroll notifications from the first scroll view are listened to.
//
// Here setting `notification.depth == 1` triggers the refresh indicator
// when overscrolling the nested scroll view.
notificationPredicate: (ScrollNotification notification) {
return notification.depth == 1;
},
child: CustomScrollView(
slivers: <Widget>[
SliverToBoxAdapter(
child: Container(
height: 100,
alignment: Alignment.center,
color: Colors.pink[100],
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'Pull down here',
style: Theme.of(context).textTheme.headlineMedium,
),
const Text("RefreshIndicator won't trigger"),
],
),
),
),
SliverToBoxAdapter(
child: Container(
color: Colors.green[100],
height: 300,
child: ListView.builder(
itemCount: 25,
itemBuilder: (BuildContext context, int index) {
return const ListTile(
title: Text('Pull down here'),
subtitle: Text('RefreshIndicator will trigger'),
);
},
),
),
),
SliverList.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index) {
return const ListTile(
title: Text('Pull down here'),
subtitle: Text("Refresh indicator won't trigger"),
);
}
)
],
),
),
);
}
}
| flutter/examples/api/lib/material/refresh_indicator/refresh_indicator.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/refresh_indicator/refresh_indicator.1.dart",
"repo_id": "flutter",
"token_count": 1569
} | 565 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ScaffoldMessengerState.showMaterialBanner].
void main() => runApp(const ShowMaterialBannerExampleApp());
class ShowMaterialBannerExampleApp extends StatelessWidget {
const ShowMaterialBannerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ScaffoldMessengerState Sample')),
body: const Center(
child: ShowMaterialBannerExample(),
),
),
);
}
}
class ShowMaterialBannerExample extends StatelessWidget {
const ShowMaterialBannerExample({super.key});
@override
Widget build(BuildContext context) {
return OutlinedButton(
onPressed: () {
ScaffoldMessenger.of(context).showMaterialBanner(
const MaterialBanner(
content: Text('This is a MaterialBanner'),
actions: <Widget>[
TextButton(
onPressed: null,
child: Text('DISMISS'),
),
],
),
);
},
child: const Text('Show MaterialBanner'),
);
}
}
| flutter/examples/api/lib/material/scaffold/scaffold_messenger_state.show_material_banner.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/scaffold/scaffold_messenger_state.show_material_banner.0.dart",
"repo_id": "flutter",
"token_count": 527
} | 566 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SelectionArea].
void main() => runApp(const SelectionAreaExampleApp());
class SelectionAreaExampleApp extends StatelessWidget {
const SelectionAreaExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SelectionArea(
child: Scaffold(
appBar: AppBar(title: const Text('SelectionArea Sample')),
body: const Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Row 1'),
Text('Row 2'),
Text('Row 3'),
],
),
),
),
),
);
}
}
| flutter/examples/api/lib/material/selection_area/selection_area.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/selection_area/selection_area.0.dart",
"repo_id": "flutter",
"token_count": 389
} | 567 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Switch.adaptive].
void main() => runApp(const SwitchApp());
class SwitchApp extends StatefulWidget {
const SwitchApp({super.key});
@override
State<SwitchApp> createState() => _SwitchAppState();
}
class _SwitchAppState extends State<SwitchApp> {
bool isMaterial = true;
bool isCustomized = false;
@override
Widget build(BuildContext context) {
final ThemeData theme = ThemeData(
platform: isMaterial ? TargetPlatform.android : TargetPlatform.iOS,
adaptations: <Adaptation<Object>>[
if (isCustomized) const _SwitchThemeAdaptation()
]
);
final ButtonStyle style = OutlinedButton.styleFrom(
fixedSize: const Size(220, 40),
);
return MaterialApp(
theme: theme,
home: Scaffold(
appBar: AppBar(title: const Text('Adaptive Switches')),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
OutlinedButton(
style: style,
onPressed: () {
setState(() {
isMaterial = !isMaterial;
});
},
child: isMaterial ? const Text('Show cupertino style') : const Text('Show material style'),
),
OutlinedButton(
style: style,
onPressed: () {
setState(() {
isCustomized = !isCustomized;
});
},
child: isCustomized ? const Text('Remove customization') : const Text('Add customization'),
),
const SizedBox(height: 20),
const SwitchWithLabel(label: 'enabled', enabled: true),
const SwitchWithLabel(label: 'disabled', enabled: false),
],
),
),
);
}
}
class SwitchWithLabel extends StatefulWidget {
const SwitchWithLabel({
super.key,
required this.enabled,
required this.label,
});
final bool enabled;
final String label;
@override
State<SwitchWithLabel> createState() => _SwitchWithLabelState();
}
class _SwitchWithLabelState extends State<SwitchWithLabel> {
bool active = true;
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Container(
width: 150,
padding: const EdgeInsets.only(right: 20),
child: Text(widget.label)
),
Switch.adaptive(
value: active,
onChanged: !widget.enabled ? null : (bool value) {
setState(() {
active = value;
});
},
),
],
);
}
}
class _SwitchThemeAdaptation extends Adaptation<SwitchThemeData> {
const _SwitchThemeAdaptation();
@override
SwitchThemeData adapt(ThemeData theme, SwitchThemeData defaultValue) {
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return defaultValue;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Colors.yellow;
}
return null; // Use the default.
}),
trackColor: const MaterialStatePropertyAll<Color>(Colors.brown),
);
}
}
}
| flutter/examples/api/lib/material/switch/switch.4.dart/0 | {
"file_path": "flutter/examples/api/lib/material/switch/switch.4.dart",
"repo_id": "flutter",
"token_count": 1566
} | 568 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() {
runApp(const ThemeDataExampleApp());
}
// This app's theme specifies an overall ColorScheme as well as overrides
// for the default configuration of FloatingActionButtons. To customize
// the appearance of other components, add additional component specific
// themes, rather than tweaking the color scheme.
//
// Creating an entire color scheme from a single seed color is a good
// way to ensure a visually appealing color palette where the default
// component colors have sufficient contrast for accessibility. Another
// good way to create an app's color scheme is to use
// ColorScheme.fromImageProvider.
//
// The color scheme reflects the platform's light or dark setting
// which is retrieved with `MediaQuery.platformBrightnessOf`. The color
// scheme's colors will be different for light and dark settings although
// they'll all be related to the seed color in both cases.
//
// Color scheme colors have been used where component defaults have
// been overridden so that the app will look good and remain accessible
// in both light and dark modes.
//
// Text styles are derived from the theme's textTheme (not the obsolete
// primaryTextTheme property) and then customized using copyWith.
// Using the _on_ version of a color scheme color as the foreground,
// as in `tertiary` and `onTertiary`, guarantees sufficient contrast
// for readability/accessibility.
class ThemeDataExampleApp extends StatelessWidget {
const ThemeDataExampleApp({ super.key });
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = ColorScheme.fromSeed(
brightness: MediaQuery.platformBrightnessOf(context),
seedColor: Colors.indigo,
);
return MaterialApp(
title: 'ThemeData Demo',
theme: ThemeData(
colorScheme: colorScheme,
floatingActionButtonTheme: FloatingActionButtonThemeData(
backgroundColor: colorScheme.tertiary,
foregroundColor: colorScheme.onTertiary,
),
),
home: const Home(),
);
}
}
class Home extends StatefulWidget {
const Home({ super.key });
@override
State<Home> createState() => _HomeState();
}
class _HomeState extends State<Home> {
int buttonPressCount = 0;
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final ColorScheme colorScheme = theme.colorScheme;
final double pointCount = 8 + (buttonPressCount % 6);
return Scaffold(
appBar: AppBar(
title: const Text('Press the + Button'),
),
// An AnimatedContainer makes the decoration changes entertaining.
body: AnimatedContainer(
duration: const Duration(milliseconds: 500),
margin: const EdgeInsets.all(32),
alignment: Alignment.center,
decoration: ShapeDecoration(
color: colorScheme.tertiaryContainer,
shape: StarBorder(
points: pointCount,
pointRounding: 0.4,
valleyRounding: 0.6,
side: BorderSide(
width: 9,
color: colorScheme.tertiary
),
),
),
child: Text(
'${pointCount.toInt()} Points',
style: theme.textTheme.headlineMedium!.copyWith(
color: colorScheme.onPrimaryContainer,
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
buttonPressCount += 1;
});
},
tooltip: "Change the shape's point count",
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/api/lib/material/theme_data/theme_data.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/theme_data/theme_data.0.dart",
"repo_id": "flutter",
"token_count": 1305
} | 569 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
/// Flutter code sample for [ScrollDirection].
void main() => runApp(const ExampleApp());
class ExampleApp extends StatelessWidget {
const ExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MyWidget(),
);
}
}
class MyWidget extends StatefulWidget {
const MyWidget({super.key});
@override
State<MyWidget> createState() => _MyWidgetState();
}
class _MyWidgetState extends State<MyWidget> {
final List<String> alphabet = <String>[
'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O',
'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z',
];
final Widget spacer = const SizedBox.square(dimension: 10);
ScrollDirection scrollDirection = ScrollDirection.idle;
AxisDirection _axisDirection = AxisDirection.down;
Widget _getArrows() {
final Widget arrow;
switch (_axisDirection) {
case AxisDirection.up:
arrow = const Icon(Icons.arrow_upward_rounded);
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.down:
arrow = const Icon(Icons.arrow_downward_rounded);
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.left:
arrow = const Icon(Icons.arrow_back_rounded);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.right:
arrow = const Icon(Icons.arrow_forward_rounded);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
}
}
void _onAxisDirectionChanged(AxisDirection? axisDirection) {
if (axisDirection != null && axisDirection != _axisDirection) {
setState(() {
// Respond to change in axis direction.
_axisDirection = axisDirection;
});
}
}
Widget _getLeading() {
return Container(
color: Colors.blue[100],
padding: const EdgeInsets.all(8.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Text(axisDirectionToAxis(_axisDirection).toString()),
spacer,
Text(_axisDirection.toString()),
spacer,
const Text('GrowthDirection.forward'),
spacer,
Text(scrollDirection.toString()),
spacer,
_getArrows(),
],
),
);
}
Widget _getRadioRow() {
return DefaultTextStyle(
style: const TextStyle(fontWeight: FontWeight.bold, color: Colors.white),
child: RadioTheme(
data: RadioThemeData(
fillColor: MaterialStateProperty.all<Color>(Colors.white),
),
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
Radio<AxisDirection>(
value: AxisDirection.up,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('up'),
spacer,
Radio<AxisDirection>(
value: AxisDirection.down,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('down'),
spacer,
Radio<AxisDirection>(
value: AxisDirection.left,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('left'),
spacer,
Radio<AxisDirection>(
value: AxisDirection.right,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('right'),
spacer,
],
),
),
),
);
}
bool _handleNotification(UserScrollNotification notification) {
if (notification.direction != scrollDirection) {
setState(() {
scrollDirection = notification.direction;
});
}
return false;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('ScrollDirections'),
bottom: PreferredSize(
preferredSize: const Size.fromHeight(50),
child: Padding(
padding: const EdgeInsets.all(8.0),
child: _getRadioRow(),
),
),
),
body: NotificationListener<UserScrollNotification>(
onNotification: _handleNotification,
// Also works for ListView.builder, which creates a SliverList for itself.
// A CustomScrollView allows multiple slivers to be composed together.
child: CustomScrollView(
// This method is available to conveniently determine if an scroll
// view is reversed by its AxisDirection.
reverse: axisDirectionIsReversed(_axisDirection),
// This method is available to conveniently convert an AxisDirection
// into its Axis.
scrollDirection: axisDirectionToAxis(_axisDirection),
slivers: <Widget>[
SliverList.builder(
itemCount: 27,
itemBuilder: (BuildContext context, int index) {
final Widget child;
if (index == 0) {
child = _getLeading();
} else {
child = Container(
color: index.isEven ? Colors.amber[100] : Colors.amberAccent,
padding: const EdgeInsets.all(8.0),
child: Center(child: Text(alphabet[index - 1])),
);
}
return Padding(
padding: const EdgeInsets.all(8.0),
child: child,
);
},
),
],
),
),
);
}
}
| flutter/examples/api/lib/rendering/scroll_direction/scroll_direction.0.dart/0 | {
"file_path": "flutter/examples/api/lib/rendering/scroll_direction/scroll_direction.0.dart",
"repo_id": "flutter",
"token_count": 2978
} | 570 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AnimatedSwitcher].
void main() => runApp(const AnimatedSwitcherExampleApp());
class AnimatedSwitcherExampleApp extends StatelessWidget {
const AnimatedSwitcherExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: AnimatedSwitcherExample(),
);
}
}
class AnimatedSwitcherExample extends StatefulWidget {
const AnimatedSwitcherExample({super.key});
@override
State<AnimatedSwitcherExample> createState() => _AnimatedSwitcherExampleState();
}
class _AnimatedSwitcherExampleState extends State<AnimatedSwitcherExample> {
int _count = 0;
@override
Widget build(BuildContext context) {
return ColoredBox(
color: Colors.white,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
AnimatedSwitcher(
duration: const Duration(milliseconds: 500),
transitionBuilder: (Widget child, Animation<double> animation) {
return ScaleTransition(scale: animation, child: child);
},
child: Text(
'$_count',
// This key causes the AnimatedSwitcher to interpret this as a "new"
// child each time the count changes, so that it will begin its animation
// when the count changes.
key: ValueKey<int>(_count),
style: Theme.of(context).textTheme.headlineMedium,
),
),
ElevatedButton(
child: const Text('Increment'),
onPressed: () {
setState(() {
_count += 1;
});
},
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/animated_switcher/animated_switcher.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/animated_switcher/animated_switcher.0.dart",
"repo_id": "flutter",
"token_count": 773
} | 571 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ClipRRect].
void main() => runApp(const ClipRRectApp());
class ClipRRectApp extends StatelessWidget {
const ClipRRectApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ClipRRect Sample')),
body: const ClipRRectExample(),
),
);
}
}
class ClipRRectExample extends StatelessWidget {
const ClipRRectExample({super.key});
@override
Widget build(BuildContext context) {
return Container(
padding: const EdgeInsets.all(40.0),
constraints: const BoxConstraints.expand(),
// Add a FittedBox to make ClipRRect sized accordingly to the image it contains
child: FittedBox(
child: ClipRRect(
borderRadius: BorderRadius.circular(40.0),
child: const _FakedImage(),
),
),
);
}
}
// A widget exposing the FlutterLogo as a 400x400 image.
//
// It can be replaced by a NetworkImage if internet connection is available, e.g. :
// const Image(
// image: NetworkImage(
// 'https://flutter.github.io/assets-for-api-docs/assets/widgets/owl.jpg'),
// );
class _FakedImage extends StatelessWidget {
const _FakedImage();
@override
Widget build(BuildContext context) {
return Container(
// Set constraints as if it were a 400x400 image
constraints: BoxConstraints.tight(const Size(400, 400)),
color: Colors.blueGrey,
child: const FlutterLogo(),
);
}
}
| flutter/examples/api/lib/widgets/basic/clip_rrect.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/clip_rrect.1.dart",
"repo_id": "flutter",
"token_count": 617
} | 572 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [WidgetBindingsObserver].
void main() => runApp(const WidgetBindingObserverExampleApp());
class WidgetBindingObserverExampleApp extends StatelessWidget {
const WidgetBindingObserverExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('WidgetBindingsObserver Sample')),
body: const WidgetBindingsObserverSample(),
),
);
}
}
class WidgetBindingsObserverSample extends StatefulWidget {
const WidgetBindingsObserverSample({super.key});
@override
State<WidgetBindingsObserverSample> createState() => _WidgetBindingsObserverSampleState();
}
class _WidgetBindingsObserverSampleState extends State<WidgetBindingsObserverSample> with WidgetsBindingObserver {
final List<AppLifecycleState> _stateHistoryList = <AppLifecycleState>[];
@override
void initState() {
super.initState();
WidgetsBinding.instance.addObserver(this);
if (WidgetsBinding.instance.lifecycleState != null) {
_stateHistoryList.add(WidgetsBinding.instance.lifecycleState!);
}
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
setState(() {
_stateHistoryList.add(state);
});
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
super.dispose();
}
@override
Widget build(BuildContext context) {
if (_stateHistoryList.isNotEmpty) {
return ListView.builder(
key: const ValueKey<String>('stateHistoryList'),
itemCount: _stateHistoryList.length,
itemBuilder: (BuildContext context, int index) {
return Text('state is: ${_stateHistoryList[index]}');
},
);
}
return const Center(child: Text('There are no AppLifecycleStates to show.'));
}
}
| flutter/examples/api/lib/widgets/binding/widget_binding_observer.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/binding/widget_binding_observer.0.dart",
"repo_id": "flutter",
"token_count": 700
} | 573 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [OrderedTraversalPolicy].
void main() => runApp(const OrderedTraversalPolicyExampleApp());
class OrderedTraversalPolicyExampleApp extends StatelessWidget {
const OrderedTraversalPolicyExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('OrderedTraversalPolicy Sample')),
body: const Center(
child: OrderedTraversalPolicyExample(),
),
),
);
}
}
class DemoButton extends StatelessWidget {
const DemoButton({
super.key,
required this.name,
this.autofocus = false,
required this.order,
});
final String name;
final bool autofocus;
final double order;
void _handleOnPressed() {
debugPrint('Button $name pressed.');
debugDumpFocusTree();
}
@override
Widget build(BuildContext context) {
return FocusTraversalOrder(
order: NumericFocusOrder(order),
child: TextButton(
autofocus: autofocus,
onPressed: () => _handleOnPressed(),
child: Text(name),
),
);
}
}
class OrderedTraversalPolicyExample extends StatelessWidget {
const OrderedTraversalPolicyExample({super.key});
@override
Widget build(BuildContext context) {
return FocusTraversalGroup(
policy: OrderedTraversalPolicy(),
child: const Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Six', order: 6),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Five', order: 5),
DemoButton(name: 'Four', order: 4),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Three', order: 3),
DemoButton(name: 'Two', order: 2),
DemoButton(name: 'One', order: 1, autofocus: true),
],
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/focus_traversal/ordered_traversal_policy.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/focus_traversal/ordered_traversal_policy.0.dart",
"repo_id": "flutter",
"token_count": 1021
} | 574 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AnimatedFractionallySizedBox].
void main() => runApp(const AnimatedFractionallySizedBoxExampleApp());
class AnimatedFractionallySizedBoxExampleApp extends StatelessWidget {
const AnimatedFractionallySizedBoxExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('AnimatedFractionallySizedBox Sample')),
body: const AnimatedFractionallySizedBoxExample(),
),
);
}
}
class AnimatedFractionallySizedBoxExample extends StatefulWidget {
const AnimatedFractionallySizedBoxExample({super.key});
@override
State<AnimatedFractionallySizedBoxExample> createState() => _AnimatedFractionallySizedBoxExampleState();
}
class _AnimatedFractionallySizedBoxExampleState extends State<AnimatedFractionallySizedBoxExample> {
bool selected = false;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () {
setState(() {
selected = !selected;
});
},
child: Center(
child: SizedBox(
width: 200,
height: 200,
child: ColoredBox(
color: Colors.red,
child: AnimatedFractionallySizedBox(
widthFactor: selected ? 0.25 : 0.75,
heightFactor: selected ? 0.75 : 0.25,
alignment: selected ? Alignment.topLeft : Alignment.bottomRight,
duration: const Duration(seconds: 1),
curve: Curves.fastOutSlowIn,
child: const ColoredBox(
color: Colors.blue,
child: FlutterLogo(size: 75),
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/implicit_animations/animated_fractionally_sized_box.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/implicit_animations/animated_fractionally_sized_box.0.dart",
"repo_id": "flutter",
"token_count": 789
} | 575 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Navigator.restorablePush].
void main() => runApp(const RestorablePushExampleApp());
class RestorablePushExampleApp extends StatelessWidget {
const RestorablePushExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: RestorablePushExample(),
);
}
}
class RestorablePushExample extends StatefulWidget {
const RestorablePushExample({super.key});
@override
State<RestorablePushExample> createState() => _RestorablePushExampleState();
}
class _RestorablePushExampleState extends State<RestorablePushExample> {
@pragma('vm:entry-point')
static Route<void> _myRouteBuilder(BuildContext context, Object? arguments) {
return MaterialPageRoute<void>(
builder: (BuildContext context) => const RestorablePushExample(),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Sample Code'),
),
floatingActionButton: FloatingActionButton(
onPressed: () => Navigator.restorablePush(context, _myRouteBuilder),
tooltip: 'Increment Counter',
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/api/lib/widgets/navigator/navigator.restorable_push.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/navigator/navigator.restorable_push.0.dart",
"repo_id": "flutter",
"token_count": 455
} | 576 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [OverlayPortal].
void main() => runApp(const OverlayPortalExampleApp());
class OverlayPortalExampleApp extends StatelessWidget {
const OverlayPortalExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('OverlayPortal Example')),
body: const Center(child: ClickableTooltipWidget()),
),
);
}
}
class ClickableTooltipWidget extends StatefulWidget {
const ClickableTooltipWidget({super.key});
@override
State<StatefulWidget> createState() => ClickableTooltipWidgetState();
}
class ClickableTooltipWidgetState extends State<ClickableTooltipWidget> {
final OverlayPortalController _tooltipController = OverlayPortalController();
@override
Widget build(BuildContext context) {
return TextButton(
onPressed: _tooltipController.toggle,
child: DefaultTextStyle(
style: DefaultTextStyle.of(context).style.copyWith(fontSize: 50),
child: OverlayPortal(
controller: _tooltipController,
overlayChildBuilder: (BuildContext context) {
return const Positioned(
right: 50,
bottom: 50,
child: ColoredBox(
color: Colors.amberAccent,
child: Text('tooltip'),
),
);
},
child: const Text('Press to show/hide tooltip'),
),
),
);
}
}
| flutter/examples/api/lib/widgets/overlay/overlay_portal.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/overlay/overlay_portal.0.dart",
"repo_id": "flutter",
"token_count": 645
} | 577 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ScrollMetricsNotification].
void main() => runApp(const ScrollMetricsDemo());
class ScrollMetricsDemo extends StatefulWidget {
const ScrollMetricsDemo({super.key});
@override
State<ScrollMetricsDemo> createState() => ScrollMetricsDemoState();
}
class ScrollMetricsDemoState extends State<ScrollMetricsDemo> {
double windowSize = 200.0;
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('ScrollMetrics Demo'),
),
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.add),
onPressed: () => setState(() {
windowSize += 10.0;
}),
),
body: NotificationListener<ScrollMetricsNotification>(
onNotification: (ScrollMetricsNotification notification) {
ScaffoldMessenger.of(notification.context).showSnackBar(
const SnackBar(
content: Text('Scroll metrics changed!'),
),
);
return false;
},
child: Scrollbar(
thumbVisibility: true,
child: SizedBox(
height: windowSize,
width: double.infinity,
child: const SingleChildScrollView(
primary: true,
child: FlutterLogo(
size: 300.0,
),
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/scroll_position/scroll_metrics_notification.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scroll_position/scroll_metrics_notification.0.dart",
"repo_id": "flutter",
"token_count": 777
} | 578 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [Shortcuts].
void main() => runApp(const ShortcutsExampleApp());
class ShortcutsExampleApp extends StatelessWidget {
const ShortcutsExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Shortcuts Sample')),
body: const Center(
child: ShortcutsExample(),
),
),
);
}
}
class Model with ChangeNotifier {
int count = 0;
void incrementBy(int amount) {
count += amount;
notifyListeners();
}
void decrementBy(int amount) {
count -= amount;
notifyListeners();
}
}
class IncrementIntent extends Intent {
const IncrementIntent(this.amount);
final int amount;
}
class DecrementIntent extends Intent {
const DecrementIntent(this.amount);
final int amount;
}
class IncrementAction extends Action<IncrementIntent> {
IncrementAction(this.model);
final Model model;
@override
void invoke(covariant IncrementIntent intent) {
model.incrementBy(intent.amount);
}
}
class DecrementAction extends Action<DecrementIntent> {
DecrementAction(this.model);
final Model model;
@override
void invoke(covariant DecrementIntent intent) {
model.decrementBy(intent.amount);
}
}
class ShortcutsExample extends StatefulWidget {
const ShortcutsExample({super.key});
@override
State<ShortcutsExample> createState() => _ShortcutsExampleState();
}
class _ShortcutsExampleState extends State<ShortcutsExample> {
Model model = Model();
@override
Widget build(BuildContext context) {
return Shortcuts(
shortcuts: <ShortcutActivator, Intent>{
LogicalKeySet(LogicalKeyboardKey.arrowUp): const IncrementIntent(2),
LogicalKeySet(LogicalKeyboardKey.arrowDown): const DecrementIntent(2),
},
child: Actions(
actions: <Type, Action<Intent>>{
IncrementIntent: IncrementAction(model),
DecrementIntent: DecrementAction(model),
},
child: Focus(
autofocus: true,
child: Column(
children: <Widget>[
const Text('Add to the counter by pressing the up arrow key'),
const Text('Subtract from the counter by pressing the down arrow key'),
ListenableBuilder(
listenable: model,
builder: (BuildContext context, Widget? child) {
return Text('count: ${model.count}');
},
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/shortcuts/shortcuts.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/shortcuts/shortcuts.1.dart",
"repo_id": "flutter",
"token_count": 1068
} | 579 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [DefaultTextStyle].
void main() => runApp(const DefaultTextStyleApp());
class DefaultTextStyleApp extends StatelessWidget {
const DefaultTextStyleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
useMaterial3: true,
brightness: Brightness.light,
colorSchemeSeed: Colors.purple,
),
home: const DefaultTextStyleExample(),
);
}
}
class DefaultTextStyleExample extends StatelessWidget {
const DefaultTextStyleExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('DefaultTextStyle.merge Sample')),
// Inherit MaterialApp text theme and override font size and font weight.
body: DefaultTextStyle.merge(
style: const TextStyle(
fontSize: 24,
fontWeight: FontWeight.bold,
),
child: const Center(
child: Text('Flutter'),
),
),
);
}
}
| flutter/examples/api/lib/widgets/text/text.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/text/text.0.dart",
"repo_id": "flutter",
"token_count": 444
} | 580 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ScaleTransition].
void main() => runApp(const ScaleTransitionExampleApp());
class ScaleTransitionExampleApp extends StatelessWidget {
const ScaleTransitionExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: ScaleTransitionExample(),
);
}
}
class ScaleTransitionExample extends StatefulWidget {
const ScaleTransitionExample({super.key});
@override
State<ScaleTransitionExample> createState() => _ScaleTransitionExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _ScaleTransitionExampleState extends State<ScaleTransitionExample> with TickerProviderStateMixin {
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true);
late final Animation<double> _animation = CurvedAnimation(
parent: _controller,
curve: Curves.fastOutSlowIn,
);
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: ScaleTransition(
scale: _animation,
child: const Padding(
padding: EdgeInsets.all(8.0),
child: FlutterLogo(size: 150.0),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/scale_transition.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/scale_transition.0.dart",
"repo_id": "flutter",
"token_count": 549
} | 581 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| flutter/examples/api/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "flutter/examples/api/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "flutter",
"token_count": 32
} | 582 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/dialog/cupertino_action_sheet.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Perform an action on CupertinoActionSheet', (WidgetTester tester) async {
const String actionText = 'Destructive Action';
await tester.pumpWidget(
const example.ActionSheetApp(),
);
// Launch the CupertinoActionSheet.
await tester.tap(find.byType(CupertinoButton));
await tester.pump();
await tester.pumpAndSettle();
expect(find.text(actionText), findsOneWidget);
// Tap on an action to close the CupertinoActionSheet.
await tester.tap(find.text(actionText));
await tester.pumpAndSettle();
expect(find.text(actionText), findsNothing);
});
}
| flutter/examples/api/test/cupertino/dialog/cupertino_action_sheet.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/dialog/cupertino_action_sheet.0_test.dart",
"repo_id": "flutter",
"token_count": 338
} | 583 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/search_field/cupertino_search_field.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('CupertinoTextField has initial text', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SearchTextFieldApp(),
);
expect(find.byType(CupertinoSearchTextField), findsOneWidget);
expect(find.text('initial text'), findsOneWidget);
await tester.tap(find.byIcon(CupertinoIcons.xmark_circle_fill));
await tester.pump();
expect(find.text('initial text'), findsNothing);
await tester.enterText(find.byType(CupertinoSearchTextField), 'photos');
await tester.pump();
expect(find.text('photos'), findsOneWidget);
});
}
| flutter/examples/api/test/cupertino/search_field/cupertino_search_field.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/search_field/cupertino_search_field.0_test.dart",
"repo_id": "flutter",
"token_count": 326
} | 584 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/animated_icon/animated_icon.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AnimatedIcon animates', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AnimatedIconApp(),
);
// Test the AnimatedIcon size.
final Size iconSize = tester.getSize(find.byType(AnimatedIcon));
expect(iconSize.width, 72.0);
expect(iconSize.height, 72.0);
// Check if AnimatedIcon is animating.
await tester.pump(const Duration(milliseconds: 500));
AnimatedIcon animatedIcon = tester.widget(find.byType(AnimatedIcon));
expect(animatedIcon.progress.value, 0.25);
// Check if animation is completed.
await tester.pump(const Duration(milliseconds: 1500));
animatedIcon = tester.widget(find.byType(AnimatedIcon));
expect(animatedIcon.progress.value, 1.0);
});
}
| flutter/examples/api/test/material/animated_icon/animated_icon.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/animated_icon/animated_icon.0_test.dart",
"repo_id": "flutter",
"token_count": 383
} | 585 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/bottom_navigation_bar/bottom_navigation_bar.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('BottomNavigationBar Updates Screen Content', (WidgetTester tester) async {
await tester.pumpWidget(
const example.BottomNavigationBarExampleApp(),
);
expect(find.widgetWithText(AppBar, 'BottomNavigationBar Sample'), findsOneWidget);
expect(find.byType(BottomNavigationBar), findsOneWidget);
expect(find.widgetWithText(Center, 'Item 0'), findsOneWidget);
await tester.scrollUntilVisible(find.widgetWithText(Center, 'Item 49'), 100);
await tester.pumpAndSettle();
expect(find.widgetWithText(Center, 'Item 49'), findsOneWidget);
await tester.tap(find.byIcon(Icons.home));
await tester.tap(find.byIcon(Icons.home));
await tester.pumpAndSettle();
final Scrollable bodyScrollView = tester.widget(find.byType(Scrollable));
expect(bodyScrollView.controller?.offset, 0.0);
await tester.tap(find.byIcon(Icons.open_in_new_rounded));
await tester.pumpAndSettle();
expect(find.byType(AlertDialog), findsOneWidget);
});
}
| flutter/examples/api/test/material/bottom_navigation_bar/bottom_navigation_bar.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/bottom_navigation_bar/bottom_navigation_bar.2_test.dart",
"repo_id": "flutter",
"token_count": 464
} | 586 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/color_scheme/color_scheme.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ColorScheme Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ColorSchemeExample(),
);
expect(find.text('Light ColorScheme'), findsOneWidget);
expect(find.text('Dark ColorScheme'), findsOneWidget);
expect(find.byType(example.ColorChip), findsNWidgets(86));
});
testWidgets('Change color seed', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ColorSchemeExample(),
);
ColoredBox coloredBox() {
return tester.widget<ColoredBox>(
find.descendant(
of: find.widgetWithText(example.ColorChip, 'primary').first,
matching: find.byType(ColoredBox)
)
);
}
expect(coloredBox().color, const Color(0xFF6750A4));
await tester.tap(find.byType(MenuAnchor));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(MenuItemButton, 'Yellow'));
await tester.pumpAndSettle();
expect(coloredBox().color, const Color(0xFF685F12));
});
}
| flutter/examples/api/test/material/color_scheme/color_scheme.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/color_scheme/color_scheme.0_test.dart",
"repo_id": "flutter",
"token_count": 510
} | 587 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/expansion_tile/expansion_tile.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ExpansionTile animation can be customized using AnimationStyle', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ExpansionTileAnimationStyleApp(),
);
double getHeight(WidgetTester tester) {
return tester.getSize(find.byType(ExpansionTile)).height;
}
expect(getHeight(tester), 58.0);
// Test the default animation style.
await tester.tap(find.text('ExpansionTile'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(getHeight(tester), closeTo(93.4, 0.1));
await tester.pumpAndSettle();
expect(getHeight(tester), 170.0);
// Tap to collapse.
await tester.tap(find.text('ExpansionTile'));
await tester.pumpAndSettle();
// Test the custom animation style.
await tester.tap(find.text('Custom'));
await tester.pumpAndSettle();
await tester.tap(find.text('ExpansionTile'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(getHeight(tester), closeTo(59.2, 0.1));
await tester.pumpAndSettle();
expect(getHeight(tester), 170.0);
// Tap to collapse.
await tester.tap(find.text('ExpansionTile'));
await tester.pumpAndSettle();
// Test the no animation style.
await tester.tap(find.text('None'));
await tester.pumpAndSettle();
await tester.tap(find.text('ExpansionTile'));
await tester.pump();
expect(getHeight(tester), 170.0);
});
}
| flutter/examples/api/test/material/expansion_tile/expansion_tile.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/expansion_tile/expansion_tile.2_test.dart",
"repo_id": "flutter",
"token_count": 668
} | 588 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/input_decorator/input_decoration.label_style_error.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('InputDecorator label uses error color', (WidgetTester tester) async {
await tester.pumpWidget(
const example.LabelStyleErrorExampleApp(),
);
final Theme theme = tester.firstWidget(find.byType(Theme));
final AnimatedDefaultTextStyle label = tester.firstWidget(find.ancestor(of: find.text('Name'), matching: find.byType(AnimatedDefaultTextStyle)));
expect(label.style.color, theme.data.colorScheme.error);
});
}
| flutter/examples/api/test/material/input_decorator/input_decoration.label_style_error.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/input_decorator/input_decoration.label_style_error.0_test.dart",
"repo_id": "flutter",
"token_count": 269
} | 589 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/material/menu_anchor/radio_menu_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can open menu', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MenuApp(),
);
await tester.tap(find.byType(TextButton));
await tester.pump();
await tester.pump();
expect(find.text('Red Background'), findsOneWidget);
expect(find.text('Green Background'), findsOneWidget);
expect(find.text('Blue Background'), findsOneWidget);
expect(find.byType(Radio<Color>), findsNWidgets(3));
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.red));
await tester.tap(find.text('Green Background'));
await tester.pumpAndSettle();
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.green));
});
testWidgets('Shortcuts work', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MenuApp(),
);
// Open the menu so we can watch state changes resulting from the shortcuts
// firing.
await tester.tap(find.byType(TextButton));
await tester.pump();
expect(find.text('Red Background'), findsOneWidget);
expect(find.text('Green Background'), findsOneWidget);
expect(find.text('Blue Background'), findsOneWidget);
expect(find.byType(Radio<Color>), findsNWidgets(3));
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.red));
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyG);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
// Need to pump twice because of the one frame delay in the notification to
// update the overlay entry.
await tester.pump();
expect(tester.widget<Radio<Color>>(find.descendant(of: find.byType(RadioMenuButton<Color>).at(0), matching: find.byType(Radio<Color>))).groupValue, equals(Colors.green));
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.green));
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyR);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
await tester.pump();
expect(tester.widget<Radio<Color>>(find.descendant(of: find.byType(RadioMenuButton<Color>).at(1), matching: find.byType(Radio<Color>))).groupValue, equals(Colors.red));
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.red));
await tester.sendKeyDownEvent(LogicalKeyboardKey.controlLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.keyB);
await tester.sendKeyUpEvent(LogicalKeyboardKey.controlLeft);
await tester.pump();
await tester.pump();
expect(tester.widget<Radio<Color>>(find.descendant(of: find.byType(RadioMenuButton<Color>).at(2), matching: find.byType(Radio<Color>))).groupValue, equals(Colors.blue));
expect(tester.widget<Container>(find.byType(Container)).color, equals(Colors.blue));
});
testWidgets('MenuAnchor is wrapped in a SafeArea', (WidgetTester tester) async {
const double safeAreaPadding = 100.0;
await tester.pumpWidget(
const MediaQuery(
data: MediaQueryData(
padding: EdgeInsets.symmetric(vertical: safeAreaPadding),
),
child: example.MenuApp(),
),
);
expect(tester.getTopLeft(find.byType(MenuAnchor)), const Offset(0.0, safeAreaPadding));
});
}
| flutter/examples/api/test/material/menu_anchor/radio_menu_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/menu_anchor/radio_menu_button.0_test.dart",
"repo_id": "flutter",
"token_count": 1337
} | 590 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/progress_indicator/circular_progress_indicator.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Finds CircularProgressIndicator', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ProgressIndicatorApp(),
);
expect(
find.bySemanticsLabel('Circular progress indicator').first,
findsOneWidget,
);
// Test if CircularProgressIndicator is animating.
expect(tester.hasRunningAnimations, isTrue);
await tester.pump(const Duration(seconds: 1));
expect(tester.hasRunningAnimations, isTrue);
// Test determinate mode button.
await tester.tap(find.byType(Switch));
await tester.pumpAndSettle();
expect(tester.hasRunningAnimations, isFalse);
await tester.tap(find.byType(Switch));
await tester.pump(const Duration(seconds: 1));
expect(tester.hasRunningAnimations, isTrue);
});
}
| flutter/examples/api/test/material/progress_indicator/circular_progress_indicator.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/progress_indicator/circular_progress_indicator.1_test.dart",
"repo_id": "flutter",
"token_count": 399
} | 591 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/scaffold/scaffold_messenger_state.show_snack_bar.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Floating SnackBar is visible', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SnackBarApp(),
);
final Finder buttonFinder = find.byType(ElevatedButton);
await tester.tap(buttonFinder.first);
// Have the SnackBar fully animate out.
await tester.pumpAndSettle();
final Finder snackBarFinder = find.byType(SnackBar);
expect(snackBarFinder, findsOneWidget);
// Grow logo to send SnackBar off screen.
await tester.tap(buttonFinder.last);
await tester.pumpAndSettle();
final AssertionError exception = tester.takeException() as AssertionError;
const String message = 'Floating SnackBar presented off screen.\n'
'A SnackBar with behavior property set to SnackBarBehavior.floating is fully '
'or partially off screen because some or all the widgets provided to '
'Scaffold.floatingActionButton, Scaffold.persistentFooterButtons and '
'Scaffold.bottomNavigationBar take up too much vertical space.\n'
'Consider constraining the size of these widgets to allow room for the SnackBar to be visible.';
expect(exception.message, message);
});
}
| flutter/examples/api/test/material/scaffold/scaffold_messenger_state.show_snack_bar.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/scaffold/scaffold_messenger_state.show_snack_bar.1_test.dart",
"repo_id": "flutter",
"token_count": 512
} | 592 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/tooltip/tooltip.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tooltip is visible when hovering over text', (WidgetTester tester) async {
const String tooltipText = 'I am a Tooltip';
await tester.pumpWidget(
const example.TooltipExampleApp(),
);
TestGesture? gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
addTearDown(() async {
if (gesture != null) {
return gesture.removePointer();
}
});
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
expect(find.text(tooltipText), findsNothing);
// Move the mouse over the text and wait for the tooltip to appear.
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(tester.getCenter(tooltip));
await tester.pump(const Duration(milliseconds: 10));
expect(find.text(tooltipText), findsOneWidget);
// Move the mouse away and wait for the tooltip to disappear.
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
await gesture.removePointer();
gesture = null;
expect(find.text(tooltipText), findsNothing);
});
}
| flutter/examples/api/test/material/tooltip/tooltip.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/tooltip/tooltip.0_test.dart",
"repo_id": "flutter",
"token_count": 523
} | 593 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/services/keyboard_key/logical_keyboard_key.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Responds to key', (WidgetTester tester) async {
await tester.pumpWidget(
const example.KeyExampleApp(),
);
await tester.tap(find.text('Click to focus'));
await tester.pumpAndSettle();
expect(find.text('Press a key'), findsOneWidget);
await tester.sendKeyEvent(LogicalKeyboardKey.keyQ);
await tester.pumpAndSettle();
expect(find.text('Pressed the "Q" key!'), findsOneWidget);
await tester.sendKeyEvent(LogicalKeyboardKey.keyB);
await tester.pumpAndSettle();
expect(find.text('Not a Q: Pressed Key B'), findsOneWidget);
});
}
| flutter/examples/api/test/services/keyboard_key/logical_keyboard_key.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/services/keyboard_key/logical_keyboard_key.0_test.dart",
"repo_id": "flutter",
"token_count": 338
} | 594 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/widgets/actions/action.action_overridable.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final _MockClipboard mockClipboard = _MockClipboard();
testWidgets('Copies text on Ctrl-C', (WidgetTester tester) async {
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, mockClipboard.handleMethodCall);
await tester.pumpWidget(const MaterialApp(
home: Scaffold(
body: Center(child: example.VerificationCodeGenerator()),
),
),
);
expect(primaryFocus, isNotNull);
expect(mockClipboard.clipboardData, isNull);
await tester.sendKeyDownEvent(LogicalKeyboardKey.control);
await tester.sendKeyDownEvent(LogicalKeyboardKey.keyC);
await tester.sendKeyUpEvent(LogicalKeyboardKey.control);
await tester.sendKeyUpEvent(LogicalKeyboardKey.keyC);
expect(mockClipboard.clipboardData?['text'], '111222333');
});
}
class _MockClipboard {
_MockClipboard();
Map<String, dynamic>? clipboardData;
Future<Object?> handleMethodCall(MethodCall methodCall) async {
switch (methodCall.method) {
case 'Clipboard.setData':
clipboardData = methodCall.arguments as Map<String, dynamic>;
return null;
}
if (methodCall.method.startsWith('Clipboard')) {
throw StateError('unrecognized method call: ${methodCall.method}');
}
return null;
}
}
| flutter/examples/api/test/widgets/actions/action.action_overridable.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/actions/action.action_overridable.0_test.dart",
"repo_id": "flutter",
"token_count": 625
} | 595 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/fitted_box.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
setUpAll(() {
HttpOverrides.global = null;
});
testWidgets('FittedBox scales the image to fill the parent container', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FittedBoxApp(),
);
final Size containerSize = tester.getSize(find.byType(Container));
expect(containerSize, const Size(300, 400));
// FittedBox should scale the image to fill the parent container.
final FittedBox fittedBox = tester.widget(find.byType(FittedBox));
expect(fittedBox.fit, BoxFit.fill);
});
}
| flutter/examples/api/test/widgets/basic/fitted_box.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/fitted_box.0_test.dart",
"repo_id": "flutter",
"token_count": 304
} | 596 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/form/form.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Form Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FormExampleApp(),
);
expect(find.widgetWithText(AppBar, 'Form Sample'), findsOneWidget);
final Finder textField = find.byType(TextField);
final Finder button = find.byType(ElevatedButton);
final TextField textFieldWidget = tester.widget<TextField>(textField);
expect(textField, findsOneWidget);
expect(button, findsOneWidget);
expect(textFieldWidget.controller?.text, '');
await tester.tap(button);
await tester.pumpAndSettle();
expect(find.text('Please enter some text'), findsOneWidget);
await tester.enterText(textField, 'Hello World');
expect(textFieldWidget.controller?.text, 'Hello World');
await tester.tap(button);
await tester.pumpAndSettle();
expect(find.text('Please enter some text'), findsNothing);
});
}
| flutter/examples/api/test/widgets/form/form.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/form/form.0_test.dart",
"repo_id": "flutter",
"token_count": 410
} | 597 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/page_view/page_view.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('PageView swipe gestures on mobile platforms', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PageViewExampleApp(),
);
// Verify that first page is shown initially.
expect(find.text('First Page'), findsOneWidget);
// Swipe to the left.
await tester.fling(find.text('First Page'), const Offset(-300.0, 0.0), 3000);
await tester.pumpAndSettle();
// Verify that the second page is shown.
expect(find.text('Second Page'), findsOneWidget);
// Swipe back to the right.
await tester.fling(find.text('Second Page'), const Offset(300.0, 0.0), 3000);
await tester.pumpAndSettle();
// Verify that first page is shown.
expect(find.text('First Page'), findsOneWidget);
}, variant: TargetPlatformVariant.mobile());
testWidgets('PageView navigation using forward/backward buttons on desktop platforms', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PageViewExampleApp(),
);
// Verify that first page is shown along with forward/backward buttons in page indicator.
expect(find.text('First Page'), findsOneWidget);
expect(find.byType(TabPageSelector), findsOneWidget);
// Tap forward button on page indicator area.
await tester.tap(find.byIcon(Icons.arrow_right_rounded));
await tester.pumpAndSettle();
// Verify that second page is shown.
expect(find.text('Second Page'), findsOneWidget);
// Verify that page indicator index is updated.
final TabPageSelector pageIndicator = tester.widget<TabPageSelector>(find.byType(TabPageSelector));
expect(pageIndicator.controller?.index, 1);
// Verify that page view index is also updated with same index to page indicator.
final PageView pageView = tester.widget<PageView>(find.byType(PageView));
expect(pageView.controller!.page, 1);
// Tap backward button on page indicator area.
await tester.tap(find.byIcon(Icons.arrow_left_rounded));
await tester.pumpAndSettle();
// Verify that first page is shown.
expect(find.text('First Page'), findsOneWidget);
// Tap backward button one more time.
await tester.tap(find.byIcon(Icons.arrow_left_rounded));
await tester.pumpAndSettle();
// Verify that first page is still shown.
expect(find.text('First Page'), findsOneWidget);
}, variant: TargetPlatformVariant.desktop());
}
| flutter/examples/api/test/widgets/page_view/page_view.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/page_view/page_view.0_test.dart",
"repo_id": "flutter",
"token_count": 879
} | 598 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_api_samples/widgets/sliver/sliver_main_axis_group.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('SliverMainAxisGroup example', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SliverMainAxisGroupExampleApp(),
);
final RenderSliverMainAxisGroup renderSliverGroup = tester.renderObject(find.byType(SliverMainAxisGroup));
expect(renderSliverGroup, isNotNull);
final RenderSliverPersistentHeader renderAppBar = tester.renderObject<RenderSliverPersistentHeader>(find.byType(SliverAppBar));
final RenderSliverList renderSliverList = tester.renderObject<RenderSliverList>(find.byType(SliverList));
final RenderSliverToBoxAdapter renderSliverAdapter = tester.renderObject<RenderSliverToBoxAdapter>(find.byType(SliverToBoxAdapter));
// renderAppBar, renderSliverList, and renderSliverAdapter1 are part of the same sliver group.
expect(renderAppBar.geometry!.scrollExtent, equals(70.0));
expect(renderSliverList.geometry!.scrollExtent, equals(100.0 * 5));
expect(renderSliverAdapter.geometry!.scrollExtent, equals(100.0));
expect(renderSliverGroup.geometry!.scrollExtent, equals(70.0 + 100.0 * 5 + 100.0));
});
}
| flutter/examples/api/test/widgets/sliver/sliver_main_axis_group.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/sliver/sliver_main_axis_group.0_test.dart",
"repo_id": "flutter",
"token_count": 493
} | 599 |
# Example of embedding Flutter using FlutterView
This project demonstrates how to embed Flutter within an iOS or Android
application. On iOS, the iOS and Flutter components are built with Xcode. On
Android, the Android and Flutter components are built with Android Studio or
Gradle.
You can read more about
[accessing platform and third-party services in Flutter](https://flutter.dev/platform-services/).
## iOS
You can open `ios/Runner.xcworkspace` in Xcode and build the project as
usual.
## Android
You can open `android/` in Android Studio and build the project as usual.
| flutter/examples/flutter_view/README.md/0 | {
"file_path": "flutter/examples/flutter_view/README.md",
"repo_id": "flutter",
"token_count": 150
} | 600 |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="15705" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" colorMatched="YES" initialViewController="FnB-1o-m6P">
<device id="retina4_7" orientation="portrait" appearance="light"/>
<dependencies>
<deployment identifier="iOS"/>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="15706"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--Main View Controller-->
<scene sceneID="nCd-Pe-nwC">
<objects>
<viewController id="FnB-1o-m6P" customClass="MainViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="MCZ-qn-U8U"/>
<viewControllerLayoutGuide type="bottom" id="T9c-61-ZEO"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="Nt5-YR-XLZ">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<subviews>
<stackView opaque="NO" contentMode="scaleToFill" misplaced="YES" axis="vertical" translatesAutoresizingMaskIntoConstraints="NO" id="Jdh-p2-Syc">
<rect key="frame" x="0.0" y="6" width="375" height="667"/>
<subviews>
<containerView opaque="NO" contentMode="scaleToFill" translatesAutoresizingMaskIntoConstraints="NO" id="G3K-Ct-amG">
<rect key="frame" x="0.0" y="0.0" width="375" height="333.5"/>
<connections>
<segue destination="yjl-pP-dW2" kind="embed" identifier="FlutterViewControllerSegue" id="zVC-ur-hSh"/>
</connections>
</containerView>
<containerView opaque="NO" contentMode="scaleToFill" translatesAutoresizingMaskIntoConstraints="NO" id="EWt-tU-lT8">
<rect key="frame" x="0.0" y="333.5" width="375" height="333.5"/>
<connections>
<segue destination="g6V-0q-Qmt" kind="embed" identifier="NativeViewControllerSegue" id="TiA-wb-9xc"/>
</connections>
</containerView>
</subviews>
<constraints>
<constraint firstItem="EWt-tU-lT8" firstAttribute="height" secondItem="G3K-Ct-amG" secondAttribute="height" id="OWV-Bz-aZ9"/>
<constraint firstItem="EWt-tU-lT8" firstAttribute="width" secondItem="G3K-Ct-amG" secondAttribute="width" id="Vni-bz-bSt"/>
</constraints>
</stackView>
</subviews>
<color key="backgroundColor" red="1" green="1" blue="1" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstItem="Jdh-p2-Syc" firstAttribute="leading" secondItem="Nt5-YR-XLZ" secondAttribute="leading" id="736-ju-cs1"/>
<constraint firstAttribute="bottom" secondItem="Jdh-p2-Syc" secondAttribute="bottom" id="NJj-PW-ivg"/>
<constraint firstAttribute="trailing" secondItem="Jdh-p2-Syc" secondAttribute="trailing" id="Rlp-q3-1xR"/>
<constraint firstItem="Jdh-p2-Syc" firstAttribute="top" secondItem="Nt5-YR-XLZ" secondAttribute="top" id="XpW-hj-pI4"/>
</constraints>
</view>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="YK3-cu-zew" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="-1858" y="211"/>
</scene>
<!--Flutter View Controller-->
<scene sceneID="QiU-td-PNw">
<objects>
<viewController id="yjl-pP-dW2" customClass="FlutterViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="GZT-2O-K6G"/>
<viewControllerLayoutGuide type="bottom" id="meP-7L-4EO"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="jhP-0W-2HS">
<rect key="frame" x="0.0" y="0.0" width="375" height="333.5"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
</view>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="nvJ-Dz-mOO" userLabel="First Responder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="-1060" y="76"/>
</scene>
<!--Native View Controller-->
<scene sceneID="W4R-Y9-Lec">
<objects>
<viewController id="g6V-0q-Qmt" customClass="NativeViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="wrm-99-E0t"/>
<viewControllerLayoutGuide type="bottom" id="H72-eC-cuN"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="Lrt-2J-Mko" userLabel="ParentView">
<rect key="frame" x="0.0" y="0.0" width="375" height="333.5"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<subviews>
<stackView opaque="NO" contentMode="scaleToFill" axis="vertical" translatesAutoresizingMaskIntoConstraints="NO" id="zVI-Xh-iNx">
<rect key="frame" x="0.0" y="0.0" width="375" height="333.5"/>
<subviews>
<view contentMode="scaleToFill" translatesAutoresizingMaskIntoConstraints="NO" id="NLS-lx-anZ" userLabel="Top">
<rect key="frame" x="0.0" y="0.0" width="375" height="263.5"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="Flutter button tapped 0 times." textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="PJ2-AA-Riy" userLabel="IncrementLabel">
<rect key="frame" x="73.5" y="121.5" width="228.5" height="20.5"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<color key="textColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<nil key="highlightedColor"/>
</label>
</subviews>
<constraints>
<constraint firstItem="PJ2-AA-Riy" firstAttribute="centerX" secondItem="NLS-lx-anZ" secondAttribute="centerX" id="H1S-ZO-f6z"/>
<constraint firstItem="PJ2-AA-Riy" firstAttribute="centerY" secondItem="NLS-lx-anZ" secondAttribute="centerY" id="ZSn-Gi-wdO"/>
</constraints>
</view>
<view contentMode="scaleToFill" restorationIdentifier="Bottom" translatesAutoresizingMaskIntoConstraints="NO" id="Qxj-hW-CeP" userLabel="Bottom">
<rect key="frame" x="0.0" y="263.5" width="375" height="70"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="iOS" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="K0h-kv-J7E">
<rect key="frame" x="20" y="14" width="47" height="36"/>
<fontDescription key="fontDescription" type="system" pointSize="30"/>
<color key="textColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<nil key="highlightedColor"/>
</label>
<button opaque="NO" contentMode="scaleToFill" contentHorizontalAlignment="center" contentVerticalAlignment="center" lineBreakMode="middleTruncation" translatesAutoresizingMaskIntoConstraints="NO" id="Jfa-Lk-nDI">
<rect key="frame" x="300" y="-5" width="55" height="55"/>
<color key="backgroundColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<constraints>
<constraint firstAttribute="height" constant="55" id="QYW-fS-Hcx"/>
<constraint firstAttribute="width" constant="55" id="zeJ-gS-6zj"/>
</constraints>
<color key="tintColor" white="1" alpha="1" colorSpace="calibratedWhite"/>
<state key="normal" image="ic_add.png"/>
<state key="disabled" image="ic_add.png"/>
<state key="selected" image="ic_add.png"/>
<state key="highlighted" image="ic_add.png"/>
<connections>
<action selector="handleIncrement:" destination="g6V-0q-Qmt" eventType="touchUpInside" id="3ie-8K-E0v"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstAttribute="bottom" secondItem="Jfa-Lk-nDI" secondAttribute="bottom" constant="20" symbolic="YES" id="Dl7-lD-YH3"/>
<constraint firstAttribute="height" constant="70" id="Hgs-wm-FFX"/>
<constraint firstAttribute="bottom" secondItem="K0h-kv-J7E" secondAttribute="bottom" constant="20" symbolic="YES" id="Iem-H7-VWN"/>
<constraint firstItem="K0h-kv-J7E" firstAttribute="leading" secondItem="Qxj-hW-CeP" secondAttribute="leading" constant="20" symbolic="YES" id="Vtt-ur-fpR"/>
<constraint firstAttribute="trailing" secondItem="Jfa-Lk-nDI" secondAttribute="trailing" constant="20" symbolic="YES" id="yCg-We-tBg"/>
</constraints>
</view>
</subviews>
</stackView>
</subviews>
<color key="backgroundColor" red="0.66666666669999997" green="0.66666666669999997" blue="0.66666666669999997" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstItem="zVI-Xh-iNx" firstAttribute="leading" secondItem="Lrt-2J-Mko" secondAttribute="leading" id="HoL-50-e9R"/>
<constraint firstAttribute="trailing" secondItem="zVI-Xh-iNx" secondAttribute="trailing" id="QMD-YW-tUG"/>
<constraint firstAttribute="bottom" secondItem="zVI-Xh-iNx" secondAttribute="bottom" id="VJM-I0-53q"/>
<constraint firstItem="zVI-Xh-iNx" firstAttribute="top" secondItem="Lrt-2J-Mko" secondAttribute="top" id="p1C-xB-mpQ"/>
</constraints>
</view>
<connections>
<outlet property="incrementLabel" destination="PJ2-AA-Riy" id="GmJ-fE-2WO"/>
</connections>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="YRn-0M-UIv" userLabel="First Responder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="-1090.4000000000001" y="489.3553223388306"/>
</scene>
</scenes>
<resources>
<image name="ic_add.png" width="24" height="24"/>
</resources>
</document>
| flutter/examples/flutter_view/ios/Runner/Base.lproj/Main.storyboard/0 | {
"file_path": "flutter/examples/flutter_view/ios/Runner/Base.lproj/Main.storyboard",
"repo_id": "flutter",
"token_count": 7700
} | 601 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/hello_world/macos/Flutter/Flutter-Debug.xcconfig/0 | {
"file_path": "flutter/examples/hello_world/macos/Flutter/Flutter-Debug.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 602 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/layers/macos/Flutter/Flutter-Release.xcconfig/0 | {
"file_path": "flutter/examples/layers/macos/Flutter/Flutter-Release.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 603 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:isolate';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
typedef OnProgressListener = void Function(double completed, double total);
typedef OnResultListener = void Function(String result);
// An encapsulation of a large amount of synchronous processing.
//
// The choice of JSON parsing here is meant as an example that might surface
// in real-world applications.
class Calculator {
Calculator({ required this.onProgressListener, required this.onResultListener, String? data })
: _data = _replicateJson(data, 10000);
final OnProgressListener onProgressListener;
final OnResultListener onResultListener;
final String _data;
// This example assumes that the number of objects to parse is known in
// advance. In a real-world situation, this might not be true; in that case,
// the app might choose to display an indeterminate progress indicator.
static const int _NUM_ITEMS = 110000;
static const int _NOTIFY_INTERVAL = 1000;
// Run the computation associated with this Calculator.
void run() {
int i = 0;
final JsonDecoder decoder = JsonDecoder(
(dynamic key, dynamic value) {
if (key is int && i++ % _NOTIFY_INTERVAL == 0) {
onProgressListener(i.toDouble(), _NUM_ITEMS.toDouble());
}
return value;
},
);
try {
final List<dynamic> result = decoder.convert(_data) as List<dynamic>;
final int n = result.length;
onResultListener('Decoded $n results');
} on FormatException catch (e, stack) {
debugPrint('Invalid JSON file: $e');
debugPrint('$stack');
}
}
static String _replicateJson(String? data, int count) {
final StringBuffer buffer = StringBuffer()..write('[');
for (int i = 0; i < count; i++) {
buffer.write(data);
if (i < count - 1) {
buffer.write(',');
}
}
buffer.write(']');
return buffer.toString();
}
}
// The current state of the calculation.
enum CalculationState {
idle,
loading,
calculating
}
// Structured message to initialize the spawned isolate.
class CalculationMessage {
CalculationMessage(this.data, this.sendPort);
String data;
SendPort sendPort;
}
// A manager for the connection to a spawned isolate.
//
// Isolates communicate with each other via ReceivePorts and SendPorts.
// This class manages these ports and maintains state related to the
// progress of the background computation.
class CalculationManager {
CalculationManager({ required this.onProgressListener, required this.onResultListener })
: _receivePort = ReceivePort() {
_receivePort.listen(_handleMessage);
}
CalculationState _state = CalculationState.idle;
CalculationState get state => _state;
bool get isRunning => _state != CalculationState.idle;
double _completed = 0.0;
double _total = 1.0;
final OnProgressListener onProgressListener;
final OnResultListener onResultListener;
// Start the background computation.
//
// Does nothing if the computation is already running.
void start() {
if (!isRunning) {
_state = CalculationState.loading;
_runCalculation();
}
}
// Stop the background computation.
//
// Kills the isolate immediately, if spawned. Does nothing if the
// computation is not running.
void stop() {
if (isRunning) {
_state = CalculationState.idle;
if (_isolate != null) {
_isolate!.kill(priority: Isolate.immediate);
_isolate = null;
_completed = 0.0;
_total = 1.0;
}
}
}
final ReceivePort _receivePort;
Isolate? _isolate;
void _runCalculation() {
// Load the JSON string. This is done in the main isolate because spawned
// isolates do not have access to the root bundle. However, the loading
// process is asynchronous, so the UI will not block while the file is
// loaded.
rootBundle.loadString('services/data.json').then<void>((String data) {
if (isRunning) {
final CalculationMessage message = CalculationMessage(data, _receivePort.sendPort);
// Spawn an isolate to JSON-parse the file contents. The JSON parsing
// is synchronous, so if done in the main isolate, the UI would block.
Isolate.spawn<CalculationMessage>(_calculate, message).then<void>((Isolate isolate) {
if (!isRunning) {
isolate.kill(priority: Isolate.immediate);
} else {
_state = CalculationState.calculating;
_isolate = isolate;
}
});
}
});
}
void _handleMessage(dynamic message) {
if (message is List<double>) {
_completed = message[0];
_total = message[1];
onProgressListener(_completed, _total);
} else if (message is String) {
_completed = 0.0;
_total = 1.0;
_isolate = null;
_state = CalculationState.idle;
onResultListener(message);
}
}
// Main entry point for the spawned isolate.
//
// This entry point must be static, and its (single) argument must match
// the message passed in Isolate.spawn above. Typically, some part of the
// message will contain a SendPort so that the spawned isolate can
// communicate back to the main isolate.
//
// Static and global variables are initialized anew in the spawned isolate,
// in a separate memory space.
static void _calculate(CalculationMessage message) {
final SendPort sender = message.sendPort;
final Calculator calculator = Calculator(
onProgressListener: (double completed, double total) {
sender.send(<double>[ completed, total ]);
},
onResultListener: sender.send,
data: message.data,
);
calculator.run();
}
}
// Main app widget.
//
// The app shows a simple UI that allows control of the background computation,
// as well as an animation to illustrate that the UI does not block while this
// computation is performed.
//
// This is a StatefulWidget in order to hold the CalculationManager and
// the AnimationController for the running animation.
class IsolateExampleWidget extends StatefulWidget {
const IsolateExampleWidget({super.key});
@override
IsolateExampleState createState() => IsolateExampleState();
}
// Main application state.
class IsolateExampleState extends State<StatefulWidget> with SingleTickerProviderStateMixin {
String _status = 'Idle';
String _label = 'Start';
String _result = ' ';
double _progress = 0.0;
late final AnimationController _animation = AnimationController(
duration: const Duration(milliseconds: 3600),
vsync: this,
)..repeat();
late final CalculationManager _calculationManager = CalculationManager(
onProgressListener: _handleProgressUpdate,
onResultListener: _handleResult,
);
@override
void dispose() {
_animation.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
RotationTransition(
turns: _animation,
child: Container(
width: 120.0,
height: 120.0,
color: const Color(0xFF882222),
),
),
Opacity(
opacity: _calculationManager.isRunning ? 1.0 : 0.0,
child: CircularProgressIndicator(
value: _progress,
),
),
Text(_status),
Center(
child: ElevatedButton(
onPressed: _handleButtonPressed,
child: Text(_label),
),
),
Text(_result),
],
),
);
}
void _handleProgressUpdate(double completed, double total) {
_updateState(' ', completed / total);
}
void _handleResult(String result) {
_updateState(result, 0.0);
}
void _handleButtonPressed() {
if (_calculationManager.isRunning) {
_calculationManager.stop();
} else {
_calculationManager.start();
}
_updateState(' ', 0.0);
}
String _getStatus(CalculationState state) {
return switch (state) {
CalculationState.loading => 'Loading...',
CalculationState.calculating => 'In Progress',
CalculationState.idle => 'Idle',
};
}
void _updateState(String result, double progress) {
setState(() {
_result = result;
_progress = progress;
_label = _calculationManager.isRunning ? 'Stop' : 'Start';
_status = _getStatus(_calculationManager.state);
});
}
}
void main() {
runApp(const MaterialApp(home: IsolateExampleWidget()));
}
| flutter/examples/layers/services/isolate.dart/0 | {
"file_path": "flutter/examples/layers/services/isolate.dart",
"repo_id": "flutter",
"token_count": 3103
} | 604 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import '../rendering/src/solid_color_box.dart';
// Solid color, RenderObject version
void addFlexChildSolidColor(RenderFlex parent, Color backgroundColor, { int flex = 0 }) {
final RenderSolidColorBox child = RenderSolidColorBox(backgroundColor);
parent.add(child);
final FlexParentData childParentData = child.parentData! as FlexParentData;
childParentData.flex = flex;
}
// Solid color, Widget version
class Rectangle extends StatelessWidget {
const Rectangle(this.color, { super.key });
final Color color;
@override
Widget build(BuildContext context) {
return Expanded(
child: Container(
color: color,
),
);
}
}
double? value;
RenderObjectToWidgetElement<RenderBox>? element;
void attachWidgetTreeToRenderTree(RenderProxyBox container) {
element = RenderObjectToWidgetAdapter<RenderBox>(
container: container,
child: Directionality(
textDirection: TextDirection.ltr,
child: SizedBox(
height: 300.0,
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
const Rectangle(Color(0xFF00FFFF)),
Material(
child: Container(
padding: const EdgeInsets.all(10.0),
margin: const EdgeInsets.all(10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
ElevatedButton(
child: const Row(
children: <Widget>[
FlutterLogo(),
Text('PRESS ME'),
],
),
onPressed: () {
value = value == null ? 0.1 : (value! + 0.1) % 1.0;
attachWidgetTreeToRenderTree(container);
},
),
CircularProgressIndicator(value: value),
],
),
),
),
const Rectangle(Color(0xFFFFFF00)),
],
),
),
),
).attachToRenderTree(WidgetsBinding.instance.buildOwner!, element);
}
Duration? timeBase;
late RenderTransform transformBox;
void rotate(Duration timeStamp) {
timeBase ??= timeStamp;
final double delta = (timeStamp - timeBase!).inMicroseconds.toDouble() / Duration.microsecondsPerSecond; // radians
transformBox.setIdentity();
transformBox.rotateZ(delta);
WidgetsBinding.instance.buildOwner!.buildScope(element!);
}
void main() {
final WidgetsBinding binding = WidgetsFlutterBinding.ensureInitialized();
final RenderProxyBox proxy = RenderProxyBox();
attachWidgetTreeToRenderTree(proxy);
final RenderFlex flexRoot = RenderFlex(direction: Axis.vertical);
addFlexChildSolidColor(flexRoot, const Color(0xFFFF00FF), flex: 1);
flexRoot.add(proxy);
addFlexChildSolidColor(flexRoot, const Color(0xFF0000FF), flex: 1);
transformBox = RenderTransform(child: flexRoot, transform: Matrix4.identity(), alignment: Alignment.center);
final RenderPadding root = RenderPadding(padding: const EdgeInsets.all(80.0), child: transformBox);
// TODO(goderbauer): Create a window if embedder doesn't provide an implicit view to draw into.
assert(binding.platformDispatcher.implicitView != null);
final RenderView view = RenderView(
view: binding.platformDispatcher.implicitView!,
child: root,
);
final PipelineOwner pipelineOwner = PipelineOwner()..rootNode = view;
binding.rootPipelineOwner.adoptChild(pipelineOwner);
binding.addRenderView(view);
view.prepareInitialFrame();
binding.addPersistentFrameCallback(rotate);
}
| flutter/examples/layers/widgets/spinning_mixed.dart/0 | {
"file_path": "flutter/examples/layers/widgets/spinning_mixed.dart",
"repo_id": "flutter",
"token_count": 1591
} | 605 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| flutter/examples/platform_channel/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "flutter/examples/platform_channel/macos/Runner/Configs/Release.xcconfig",
"repo_id": "flutter",
"token_count": 32
} | 606 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "PlatformViewController.h"
#import <Foundation/Foundation.h>
@interface PlatformViewController ()
@property(weak, nonatomic) IBOutlet UILabel* countLabel;
@end
@implementation PlatformViewController
- (void)viewDidLoad {
[super viewDidLoad];
[self updateCountLabelText];
}
- (IBAction)handleIncrement:(id)sender {
self.counter++;
[self updateCountLabelText];
}
- (IBAction)switchToFlutterView:(id)sender {
[self.delegate didUpdateCounter:self.counter];
[self dismissViewControllerAnimated:NO completion:nil];
}
- (void)updateCountLabelText {
NSString* text = [NSString stringWithFormat:@"Button tapped %d %@.", self.counter,
(self.counter == 1) ? @"time" : @"times"];
self.countLabel.text = text;
}
@end
| flutter/examples/platform_view/ios/Runner/PlatformViewController.m/0 | {
"file_path": "flutter/examples/platform_view/ios/Runner/PlatformViewController.m",
"repo_id": "flutter",
"token_count": 336
} | 607 |
# Flutter Texture
An example to show how to use custom Flutter textures.
| flutter/examples/texture/README.md/0 | {
"file_path": "flutter/examples/texture/README.md",
"repo_id": "flutter",
"token_count": 19
} | 608 |
targets:
$default:
sources:
exclude:
- "test/examples/sector_layout_test.dart"
| flutter/packages/flutter/build.yaml/0 | {
"file_path": "flutter/packages/flutter/build.yaml",
"repo_id": "flutter",
"token_count": 48
} | 609 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are from the Painting library. *
version: 1
transforms:
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'painting.dart' ]
method: 'computeMaxIntrinsicWidth'
inClass: 'TextPainter'
changes:
- kind: 'addParameter'
index: 4
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'painting.dart' ]
method: 'computeWidth'
inClass: 'TextPainter'
changes:
- kind: 'addParameter'
index: 4
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'painting.dart' ]
constructor: ''
inClass: 'TextPainter'
changes:
- kind: 'addParameter'
index: 0
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'painting.dart' ]
method: 'getTextStyle'
inClass: 'TextStyle'
changes:
- kind: 'addParameter'
index: 0
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Changes made in https://github.com/flutter/flutter/pull/121152
- title: "Rename to 'fromViewPadding'"
date: 2022-02-21
element:
uris: [ 'painting.dart' ]
constructor: 'fromWindowPadding'
inClass: 'EdgeInsets'
changes:
- kind: 'rename'
newName: 'fromViewPadding'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_painting.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_painting.yaml",
"repo_id": "flutter",
"token_count": 1534
} | 610 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The Flutter painting library.
///
/// To use, import `package:flutter/painting.dart`.
///
/// This library includes a variety of classes that wrap the Flutter
/// engine's painting API for more specialized purposes, such as painting scaled
/// images, interpolating between shadows, painting borders around boxes, etc.
///
/// In particular:
///
/// * Use the [TextPainter] class for painting text.
/// * Use [Decoration] (and more concretely [BoxDecoration]) for
/// painting boxes.
library painting;
export 'dart:ui' show PlaceholderAlignment, Shadow, TextHeightBehavior, TextLeadingDistribution;
export 'src/painting/alignment.dart';
export 'src/painting/basic_types.dart';
export 'src/painting/beveled_rectangle_border.dart';
export 'src/painting/binding.dart';
export 'src/painting/border_radius.dart';
export 'src/painting/borders.dart';
export 'src/painting/box_border.dart';
export 'src/painting/box_decoration.dart';
export 'src/painting/box_fit.dart';
export 'src/painting/box_shadow.dart';
export 'src/painting/circle_border.dart';
export 'src/painting/clip.dart';
export 'src/painting/colors.dart';
export 'src/painting/continuous_rectangle_border.dart';
export 'src/painting/debug.dart';
export 'src/painting/decoration.dart';
export 'src/painting/decoration_image.dart';
export 'src/painting/edge_insets.dart';
export 'src/painting/flutter_logo.dart';
export 'src/painting/fractional_offset.dart';
export 'src/painting/geometry.dart';
export 'src/painting/gradient.dart';
export 'src/painting/image_cache.dart';
export 'src/painting/image_decoder.dart';
export 'src/painting/image_provider.dart';
export 'src/painting/image_resolution.dart';
export 'src/painting/image_stream.dart';
export 'src/painting/inline_span.dart';
export 'src/painting/linear_border.dart';
export 'src/painting/matrix_utils.dart';
export 'src/painting/notched_shapes.dart';
export 'src/painting/oval_border.dart';
export 'src/painting/paint_utilities.dart';
export 'src/painting/placeholder_span.dart';
export 'src/painting/rounded_rectangle_border.dart';
export 'src/painting/shader_warm_up.dart';
export 'src/painting/shape_decoration.dart';
export 'src/painting/stadium_border.dart';
export 'src/painting/star_border.dart';
export 'src/painting/strut_style.dart';
export 'src/painting/text_painter.dart';
export 'src/painting/text_scaler.dart';
export 'src/painting/text_span.dart';
export 'src/painting/text_style.dart';
| flutter/packages/flutter/lib/painting.dart/0 | {
"file_path": "flutter/packages/flutter/lib/painting.dart",
"repo_id": "flutter",
"token_count": 885
} | 611 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/widgets.dart';
import 'button.dart';
import 'colors.dart';
import 'icons.dart';
import 'interface_level.dart';
import 'localizations.dart';
import 'route.dart';
import 'scrollbar.dart';
import 'theme.dart';
/// An application that uses Cupertino design.
///
/// A convenience widget that wraps a number of widgets that are commonly
/// required for an iOS-design targeting application. It builds upon a
/// [WidgetsApp] by iOS specific defaulting such as fonts and scrolling
/// physics.
///
/// The [CupertinoApp] configures the top-level [Navigator] to search for routes
/// in the following order:
///
/// 1. For the `/` route, the [home] property, if non-null, is used.
///
/// 2. Otherwise, the [routes] table is used, if it has an entry for the route.
///
/// 3. Otherwise, [onGenerateRoute] is called, if provided. It should return a
/// non-null value for any _valid_ route not handled by [home] and [routes].
///
/// 4. Finally if all else fails [onUnknownRoute] is called.
///
/// If [home], [routes], [onGenerateRoute], and [onUnknownRoute] are all null,
/// and [builder] is not null, then no [Navigator] is created.
///
/// This widget also configures the observer of the top-level [Navigator] (if
/// any) to perform [Hero] animations.
///
/// The [CupertinoApp] widget isn't a required ancestor for other Cupertino
/// widgets, but many Cupertino widgets could depend on the [CupertinoTheme]
/// widget, which the [CupertinoApp] composes. If you use Material widgets, a
/// [MaterialApp] also creates the needed dependencies for Cupertino widgets.
///
/// {@template flutter.cupertino.CupertinoApp.defaultSelectionStyle}
/// The [CupertinoApp] automatically creates a [DefaultSelectionStyle] with
/// selectionColor sets to [CupertinoThemeData.primaryColor] with 0.2 opacity
/// and cursorColor sets to [CupertinoThemeData.primaryColor].
/// {@endtemplate}
///
/// Use this widget with caution on Android since it may produce behaviors
/// Android users are not expecting such as:
///
/// * Pages will be dismissible via a back swipe.
/// * Scrolling past extremities will trigger iOS-style spring overscrolls.
/// * The San Francisco font family is unavailable on Android and can result
/// in undefined font behavior.
///
/// {@tool snippet}
/// This example shows how to create a [CupertinoApp] that disables the "debug"
/// banner with a [home] route that will be displayed when the app is launched.
///
/// 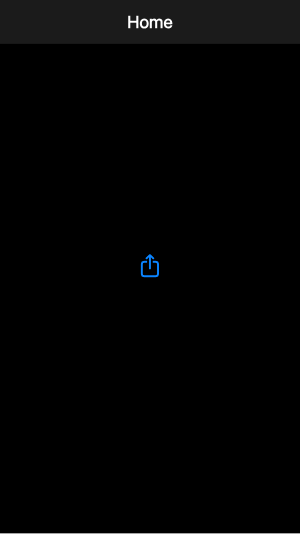
///
/// ```dart
/// const CupertinoApp(
/// home: CupertinoPageScaffold(
/// navigationBar: CupertinoNavigationBar(
/// middle: Text('Home'),
/// ),
/// child: Center(child: Icon(CupertinoIcons.share)),
/// ),
/// debugShowCheckedModeBanner: false,
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example shows how to create a [CupertinoApp] that uses the [routes]
/// `Map` to define the "home" route and an "about" route.
///
/// ```dart
/// CupertinoApp(
/// routes: <String, WidgetBuilder>{
/// '/': (BuildContext context) {
/// return const CupertinoPageScaffold(
/// navigationBar: CupertinoNavigationBar(
/// middle: Text('Home Route'),
/// ),
/// child: Center(child: Icon(CupertinoIcons.share)),
/// );
/// },
/// '/about': (BuildContext context) {
/// return const CupertinoPageScaffold(
/// navigationBar: CupertinoNavigationBar(
/// middle: Text('About Route'),
/// ),
/// child: Center(child: Icon(CupertinoIcons.share)),
/// );
/// }
/// },
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example shows how to create a [CupertinoApp] that defines a [theme] that
/// will be used for Cupertino widgets in the app.
///
/// 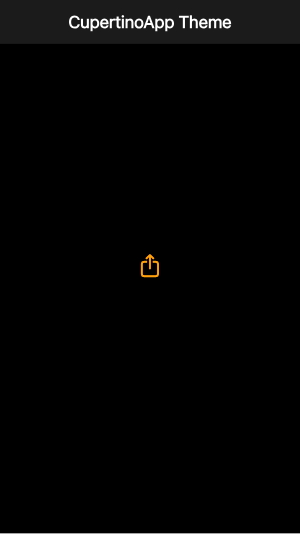
///
/// ```dart
/// const CupertinoApp(
/// theme: CupertinoThemeData(
/// brightness: Brightness.dark,
/// primaryColor: CupertinoColors.systemOrange,
/// ),
/// home: CupertinoPageScaffold(
/// navigationBar: CupertinoNavigationBar(
/// middle: Text('CupertinoApp Theme'),
/// ),
/// child: Center(child: Icon(CupertinoIcons.share)),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoPageScaffold], which provides a standard page layout default
/// with nav bars.
/// * [Navigator], which is used to manage the app's stack of pages.
/// * [CupertinoPageRoute], which defines an app page that transitions in an
/// iOS-specific way.
/// * [WidgetsApp], which defines the basic app elements but does not depend
/// on the Cupertino library.
class CupertinoApp extends StatefulWidget {
/// Creates a CupertinoApp.
///
/// At least one of [home], [routes], [onGenerateRoute], or [builder] must be
/// non-null. If only [routes] is given, it must include an entry for the
/// [Navigator.defaultRouteName] (`/`), since that is the route used when the
/// application is launched with an intent that specifies an otherwise
/// unsupported route.
///
/// This class creates an instance of [WidgetsApp].
const CupertinoApp({
super.key,
this.navigatorKey,
this.home,
this.theme,
Map<String, Widget Function(BuildContext)> this.routes = const <String, WidgetBuilder>{},
this.initialRoute,
this.onGenerateRoute,
this.onGenerateInitialRoutes,
this.onUnknownRoute,
this.onNavigationNotification,
List<NavigatorObserver> this.navigatorObservers = const <NavigatorObserver>[],
this.builder,
this.title = '',
this.onGenerateTitle,
this.color,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowCheckedModeBanner = true,
this.shortcuts,
this.actions,
this.restorationScopeId,
this.scrollBehavior,
@Deprecated(
'Remove this parameter as it is now ignored. '
'CupertinoApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
}) : routeInformationProvider = null,
routeInformationParser = null,
routerDelegate = null,
backButtonDispatcher = null,
routerConfig = null;
/// Creates a [CupertinoApp] that uses the [Router] instead of a [Navigator].
///
/// {@macro flutter.widgets.WidgetsApp.router}
const CupertinoApp.router({
super.key,
this.routeInformationProvider,
this.routeInformationParser,
this.routerDelegate,
this.backButtonDispatcher,
this.routerConfig,
this.theme,
this.builder,
this.title = '',
this.onGenerateTitle,
this.onNavigationNotification,
this.color,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowCheckedModeBanner = true,
this.shortcuts,
this.actions,
this.restorationScopeId,
this.scrollBehavior,
@Deprecated(
'Remove this parameter as it is now ignored. '
'CupertinoApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
}) : assert(routerDelegate != null || routerConfig != null),
navigatorObservers = null,
navigatorKey = null,
onGenerateRoute = null,
home = null,
onGenerateInitialRoutes = null,
onUnknownRoute = null,
routes = null,
initialRoute = null;
/// {@macro flutter.widgets.widgetsApp.navigatorKey}
final GlobalKey<NavigatorState>? navigatorKey;
/// {@macro flutter.widgets.widgetsApp.home}
final Widget? home;
/// The top-level [CupertinoTheme] styling.
///
/// A null [theme] or unspecified [theme] attributes will default to iOS
/// system values.
final CupertinoThemeData? theme;
/// The application's top-level routing table.
///
/// When a named route is pushed with [Navigator.pushNamed], the route name is
/// looked up in this map. If the name is present, the associated
/// [widgets.WidgetBuilder] is used to construct a [CupertinoPageRoute] that
/// performs an appropriate transition, including [Hero] animations, to the
/// new route.
///
/// {@macro flutter.widgets.widgetsApp.routes}
final Map<String, WidgetBuilder>? routes;
/// {@macro flutter.widgets.widgetsApp.initialRoute}
final String? initialRoute;
/// {@macro flutter.widgets.widgetsApp.onGenerateRoute}
final RouteFactory? onGenerateRoute;
/// {@macro flutter.widgets.widgetsApp.onGenerateInitialRoutes}
final InitialRouteListFactory? onGenerateInitialRoutes;
/// {@macro flutter.widgets.widgetsApp.onUnknownRoute}
final RouteFactory? onUnknownRoute;
/// {@macro flutter.widgets.widgetsApp.onNavigationNotification}
final NotificationListenerCallback<NavigationNotification>? onNavigationNotification;
/// {@macro flutter.widgets.widgetsApp.navigatorObservers}
final List<NavigatorObserver>? navigatorObservers;
/// {@macro flutter.widgets.widgetsApp.routeInformationProvider}
final RouteInformationProvider? routeInformationProvider;
/// {@macro flutter.widgets.widgetsApp.routeInformationParser}
final RouteInformationParser<Object>? routeInformationParser;
/// {@macro flutter.widgets.widgetsApp.routerDelegate}
final RouterDelegate<Object>? routerDelegate;
/// {@macro flutter.widgets.widgetsApp.backButtonDispatcher}
final BackButtonDispatcher? backButtonDispatcher;
/// {@macro flutter.widgets.widgetsApp.routerConfig}
final RouterConfig<Object>? routerConfig;
/// {@macro flutter.widgets.widgetsApp.builder}
final TransitionBuilder? builder;
/// {@macro flutter.widgets.widgetsApp.title}
///
/// This value is passed unmodified to [WidgetsApp.title].
final String title;
/// {@macro flutter.widgets.widgetsApp.onGenerateTitle}
///
/// This value is passed unmodified to [WidgetsApp.onGenerateTitle].
final GenerateAppTitle? onGenerateTitle;
/// {@macro flutter.widgets.widgetsApp.color}
final Color? color;
/// {@macro flutter.widgets.widgetsApp.locale}
final Locale? locale;
/// {@macro flutter.widgets.widgetsApp.localizationsDelegates}
final Iterable<LocalizationsDelegate<dynamic>>? localizationsDelegates;
/// {@macro flutter.widgets.widgetsApp.localeListResolutionCallback}
///
/// This callback is passed along to the [WidgetsApp] built by this widget.
final LocaleListResolutionCallback? localeListResolutionCallback;
/// {@macro flutter.widgets.LocaleResolutionCallback}
///
/// This callback is passed along to the [WidgetsApp] built by this widget.
final LocaleResolutionCallback? localeResolutionCallback;
/// {@macro flutter.widgets.widgetsApp.supportedLocales}
///
/// It is passed along unmodified to the [WidgetsApp] built by this widget.
final Iterable<Locale> supportedLocales;
/// Turns on a performance overlay.
///
/// See also:
///
/// * <https://flutter.dev/debugging/#performance-overlay>
final bool showPerformanceOverlay;
/// Turns on checkerboarding of raster cache images.
final bool checkerboardRasterCacheImages;
/// Turns on checkerboarding of layers rendered to offscreen bitmaps.
final bool checkerboardOffscreenLayers;
/// Turns on an overlay that shows the accessibility information
/// reported by the framework.
final bool showSemanticsDebugger;
/// {@macro flutter.widgets.widgetsApp.debugShowCheckedModeBanner}
final bool debugShowCheckedModeBanner;
/// {@macro flutter.widgets.widgetsApp.shortcuts}
/// {@tool snippet}
/// This example shows how to add a single shortcut for
/// [LogicalKeyboardKey.select] to the default shortcuts without needing to
/// add your own [Shortcuts] widget.
///
/// Alternatively, you could insert a [Shortcuts] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// shortcuts: <ShortcutActivator, Intent>{
/// ... WidgetsApp.defaultShortcuts,
/// const SingleActivator(LogicalKeyboardKey.select): const ActivateIntent(),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
/// {@macro flutter.widgets.widgetsApp.shortcuts.seeAlso}
final Map<ShortcutActivator, Intent>? shortcuts;
/// {@macro flutter.widgets.widgetsApp.actions}
/// {@tool snippet}
/// This example shows how to add a single action handling an
/// [ActivateAction] to the default actions without needing to
/// add your own [Actions] widget.
///
/// Alternatively, you could insert a [Actions] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// actions: <Type, Action<Intent>>{
/// ... WidgetsApp.defaultActions,
/// ActivateAction: CallbackAction<Intent>(
/// onInvoke: (Intent intent) {
/// // Do something here...
/// return null;
/// },
/// ),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
/// {@macro flutter.widgets.widgetsApp.actions.seeAlso}
final Map<Type, Action<Intent>>? actions;
/// {@macro flutter.widgets.widgetsApp.restorationScopeId}
final String? restorationScopeId;
/// {@macro flutter.material.materialApp.scrollBehavior}
///
/// When null, defaults to [CupertinoScrollBehavior].
///
/// See also:
///
/// * [ScrollConfiguration], which controls how [Scrollable] widgets behave
/// in a subtree.
final ScrollBehavior? scrollBehavior;
/// {@macro flutter.widgets.widgetsApp.useInheritedMediaQuery}
@Deprecated(
'This setting is now ignored. '
'CupertinoApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
final bool useInheritedMediaQuery;
@override
State<CupertinoApp> createState() => _CupertinoAppState();
/// The [HeroController] used for Cupertino page transitions.
///
/// Used by [CupertinoTabView] and [CupertinoApp].
static HeroController createCupertinoHeroController() =>
HeroController(); // Linear tweening.
}
/// Describes how [Scrollable] widgets behave for [CupertinoApp]s.
///
/// {@macro flutter.widgets.scrollBehavior}
///
/// Setting a [CupertinoScrollBehavior] will result in descendant [Scrollable] widgets
/// using [BouncingScrollPhysics] by default. No [GlowingOverscrollIndicator] is
/// applied when using a [CupertinoScrollBehavior] either, regardless of platform.
/// When executing on desktop platforms, a [CupertinoScrollbar] is applied to the child.
///
/// See also:
///
/// * [ScrollBehavior], the default scrolling behavior extended by this class.
class CupertinoScrollBehavior extends ScrollBehavior {
/// Creates a CupertinoScrollBehavior that uses [BouncingScrollPhysics] and
/// adds [CupertinoScrollbar]s on desktop platforms.
const CupertinoScrollBehavior();
@override
Widget buildScrollbar(BuildContext context , Widget child, ScrollableDetails details) {
// When modifying this function, consider modifying the implementation in
// the base class as well.
switch (getPlatform(context)) {
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
assert(details.controller != null);
return CupertinoScrollbar(
controller: details.controller,
child: child,
);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
return child;
}
}
@override
Widget buildOverscrollIndicator(BuildContext context, Widget child, ScrollableDetails details) {
// No overscroll indicator.
// When modifying this function, consider modifying the implementation in
// the base class as well.
return child;
}
@override
ScrollPhysics getScrollPhysics(BuildContext context) {
// When modifying this function, consider modifying the implementation in
// the base class ScrollBehavior as well.
if (getPlatform(context) == TargetPlatform.macOS) {
return const BouncingScrollPhysics(decelerationRate: ScrollDecelerationRate.fast);
}
return const BouncingScrollPhysics();
}
@override
MultitouchDragStrategy getMultitouchDragStrategy(BuildContext context) => MultitouchDragStrategy.averageBoundaryPointers;
}
class _CupertinoAppState extends State<CupertinoApp> {
late HeroController _heroController;
bool get _usesRouter => widget.routerDelegate != null || widget.routerConfig != null;
@override
void initState() {
super.initState();
_heroController = CupertinoApp.createCupertinoHeroController();
}
@override
void dispose() {
_heroController.dispose();
super.dispose();
}
// Combine the default localization for Cupertino with the ones contributed
// by the localizationsDelegates parameter, if any. Only the first delegate
// of a particular LocalizationsDelegate.type is loaded so the
// localizationsDelegate parameter can be used to override
// _CupertinoLocalizationsDelegate.
Iterable<LocalizationsDelegate<dynamic>> get _localizationsDelegates {
return <LocalizationsDelegate<dynamic>>[
if (widget.localizationsDelegates != null)
...widget.localizationsDelegates!,
DefaultCupertinoLocalizations.delegate,
];
}
Widget _inspectorSelectButtonBuilder(BuildContext context, VoidCallback onPressed) {
return CupertinoButton.filled(
padding: EdgeInsets.zero,
onPressed: onPressed,
child: const Icon(
CupertinoIcons.search,
size: 28.0,
color: CupertinoColors.white,
),
);
}
WidgetsApp _buildWidgetApp(BuildContext context) {
final CupertinoThemeData effectiveThemeData = CupertinoTheme.of(context);
final Color color = CupertinoDynamicColor.resolve(widget.color ?? effectiveThemeData.primaryColor, context);
if (_usesRouter) {
return WidgetsApp.router(
key: GlobalObjectKey(this),
routeInformationProvider: widget.routeInformationProvider,
routeInformationParser: widget.routeInformationParser,
routerDelegate: widget.routerDelegate,
routerConfig: widget.routerConfig,
backButtonDispatcher: widget.backButtonDispatcher,
onNavigationNotification: widget.onNavigationNotification,
builder: widget.builder,
title: widget.title,
onGenerateTitle: widget.onGenerateTitle,
textStyle: effectiveThemeData.textTheme.textStyle,
color: color,
locale: widget.locale,
localizationsDelegates: _localizationsDelegates,
localeResolutionCallback: widget.localeResolutionCallback,
localeListResolutionCallback: widget.localeListResolutionCallback,
supportedLocales: widget.supportedLocales,
showPerformanceOverlay: widget.showPerformanceOverlay,
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
showSemanticsDebugger: widget.showSemanticsDebugger,
debugShowCheckedModeBanner: widget.debugShowCheckedModeBanner,
inspectorSelectButtonBuilder: _inspectorSelectButtonBuilder,
shortcuts: widget.shortcuts,
actions: widget.actions,
restorationScopeId: widget.restorationScopeId,
);
}
return WidgetsApp(
key: GlobalObjectKey(this),
navigatorKey: widget.navigatorKey,
navigatorObservers: widget.navigatorObservers!,
pageRouteBuilder: <T>(RouteSettings settings, WidgetBuilder builder) {
return CupertinoPageRoute<T>(settings: settings, builder: builder);
},
home: widget.home,
routes: widget.routes!,
initialRoute: widget.initialRoute,
onGenerateRoute: widget.onGenerateRoute,
onGenerateInitialRoutes: widget.onGenerateInitialRoutes,
onUnknownRoute: widget.onUnknownRoute,
onNavigationNotification: widget.onNavigationNotification,
builder: widget.builder,
title: widget.title,
onGenerateTitle: widget.onGenerateTitle,
textStyle: effectiveThemeData.textTheme.textStyle,
color: color,
locale: widget.locale,
localizationsDelegates: _localizationsDelegates,
localeResolutionCallback: widget.localeResolutionCallback,
localeListResolutionCallback: widget.localeListResolutionCallback,
supportedLocales: widget.supportedLocales,
showPerformanceOverlay: widget.showPerformanceOverlay,
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
showSemanticsDebugger: widget.showSemanticsDebugger,
debugShowCheckedModeBanner: widget.debugShowCheckedModeBanner,
inspectorSelectButtonBuilder: _inspectorSelectButtonBuilder,
shortcuts: widget.shortcuts,
actions: widget.actions,
restorationScopeId: widget.restorationScopeId,
);
}
@override
Widget build(BuildContext context) {
final CupertinoThemeData effectiveThemeData = (widget.theme ?? const CupertinoThemeData()).resolveFrom(context);
return ScrollConfiguration(
behavior: widget.scrollBehavior ?? const CupertinoScrollBehavior(),
child: CupertinoUserInterfaceLevel(
data: CupertinoUserInterfaceLevelData.base,
child: CupertinoTheme(
data: effectiveThemeData,
child: DefaultSelectionStyle(
selectionColor: effectiveThemeData.primaryColor.withOpacity(0.2),
cursorColor: effectiveThemeData.primaryColor,
child: HeroControllerScope(
controller: _heroController,
child: Builder(
builder: _buildWidgetApp,
),
),
),
),
),
);
}
}
| flutter/packages/flutter/lib/src/cupertino/app.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/app.dart",
"repo_id": "flutter",
"token_count": 7853
} | 612 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
/// An [IconThemeData] subclass that automatically resolves its [color] when retrieved
/// using [IconTheme.of].
class CupertinoIconThemeData extends IconThemeData with Diagnosticable {
/// Creates a [CupertinoIconThemeData].
const CupertinoIconThemeData({
super.size,
super.fill,
super.weight,
super.grade,
super.opticalSize,
super.color,
super.opacity,
super.shadows,
super.applyTextScaling,
});
/// Called by [IconTheme.of] to resolve [color] against the given [BuildContext].
@override
IconThemeData resolve(BuildContext context) {
final Color? resolvedColor = CupertinoDynamicColor.maybeResolve(color, context);
return resolvedColor == color ? this : copyWith(color: resolvedColor);
}
/// Creates a copy of this icon theme but with the given fields replaced with
/// the new values.
@override
CupertinoIconThemeData copyWith({
double? size,
double? fill,
double? weight,
double? grade,
double? opticalSize,
Color? color,
double? opacity,
List<Shadow>? shadows,
bool? applyTextScaling,
}) {
return CupertinoIconThemeData(
size: size ?? this.size,
fill: fill ?? this.fill,
weight: weight ?? this.weight,
grade: grade ?? this.grade,
opticalSize: opticalSize ?? this.opticalSize,
color: color ?? this.color,
opacity: opacity ?? this.opacity,
shadows: shadows ?? this.shadows,
applyTextScaling: applyTextScaling ?? this.applyTextScaling,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(createCupertinoColorProperty('color', color, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/cupertino/icon_theme_data.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/icon_theme_data.dart",
"repo_id": "flutter",
"token_count": 665
} | 613 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'theme.dart';
import 'thumb_painter.dart';
// Examples can assume:
// int _cupertinoSliderValue = 1;
// void setState(VoidCallback fn) { }
/// An iOS-style slider.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ufb4gIPDmEs}
///
/// Used to select from a range of values.
///
/// A slider can be used to select from either a continuous or a discrete set of
/// values. The default is use a continuous range of values from [min] to [max].
/// To use discrete values, use a non-null value for [divisions], which
/// indicates the number of discrete intervals. For example, if [min] is 0.0 and
/// [max] is 50.0 and [divisions] is 5, then the slider can take on the values
/// discrete values 0.0, 10.0, 20.0, 30.0, 40.0, and 50.0.
///
/// The slider itself does not maintain any state. Instead, when the state of
/// the slider changes, the widget calls the [onChanged] callback. Most widgets
/// that use a slider will listen for the [onChanged] callback and rebuild the
/// slider with a new [value] to update the visual appearance of the slider.
///
/// {@tool dartpad}
/// This example shows how to show the current slider value as it changes.
///
/// ** See code in examples/api/lib/cupertino/slider/cupertino_slider.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * <https://developer.apple.com/design/human-interface-guidelines/sliders/>
class CupertinoSlider extends StatefulWidget {
/// Creates an iOS-style slider.
///
/// The slider itself does not maintain any state. Instead, when the state of
/// the slider changes, the widget calls the [onChanged] callback. Most widgets
/// that use a slider will listen for the [onChanged] callback and rebuild the
/// slider with a new [value] to update the visual appearance of the slider.
///
/// * [value] determines currently selected value for this slider.
/// * [onChanged] is called when the user selects a new value for the slider.
/// * [onChangeStart] is called when the user starts to select a new value for
/// the slider.
/// * [onChangeEnd] is called when the user is done selecting a new value for
/// the slider.
const CupertinoSlider({
super.key,
required this.value,
required this.onChanged,
this.onChangeStart,
this.onChangeEnd,
this.min = 0.0,
this.max = 1.0,
this.divisions,
this.activeColor,
this.thumbColor = CupertinoColors.white,
}) : assert(value >= min && value <= max),
assert(divisions == null || divisions > 0);
/// The currently selected value for this slider.
///
/// The slider's thumb is drawn at a position that corresponds to this value.
final double value;
/// Called when the user selects a new value for the slider.
///
/// The slider passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the slider with the new
/// value.
///
/// If null, the slider will be displayed as disabled.
///
/// The callback provided to onChanged should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// ```dart
/// CupertinoSlider(
/// value: _cupertinoSliderValue.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// onChanged: (double newValue) {
/// setState(() {
/// _cupertinoSliderValue = newValue.round();
/// });
/// },
/// )
/// ```
///
/// See also:
///
/// * [onChangeStart] for a callback that is called when the user starts
/// changing the value.
/// * [onChangeEnd] for a callback that is called when the user stops
/// changing the value.
final ValueChanged<double>? onChanged;
/// Called when the user starts selecting a new value for the slider.
///
/// This callback shouldn't be used to update the slider [value] (use
/// [onChanged] for that), but rather to be notified when the user has started
/// selecting a new value by starting a drag.
///
/// The value passed will be the last [value] that the slider had before the
/// change began.
///
/// {@tool snippet}
///
/// ```dart
/// CupertinoSlider(
/// value: _cupertinoSliderValue.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// onChanged: (double newValue) {
/// setState(() {
/// _cupertinoSliderValue = newValue.round();
/// });
/// },
/// onChangeStart: (double startValue) {
/// print('Started change at $startValue');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeEnd] for a callback that is called when the value change is
/// complete.
final ValueChanged<double>? onChangeStart;
/// Called when the user is done selecting a new value for the slider.
///
/// This callback shouldn't be used to update the slider [value] (use
/// [onChanged] for that), but rather to know when the user has completed
/// selecting a new [value] by ending a drag.
///
/// {@tool snippet}
///
/// ```dart
/// CupertinoSlider(
/// value: _cupertinoSliderValue.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// onChanged: (double newValue) {
/// setState(() {
/// _cupertinoSliderValue = newValue.round();
/// });
/// },
/// onChangeEnd: (double newValue) {
/// print('Ended change on $newValue');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeStart] for a callback that is called when a value change
/// begins.
final ValueChanged<double>? onChangeEnd;
/// The minimum value the user can select.
///
/// Defaults to 0.0.
final double min;
/// The maximum value the user can select.
///
/// Defaults to 1.0.
final double max;
/// The number of discrete divisions.
///
/// If null, the slider is continuous.
final int? divisions;
/// The color to use for the portion of the slider that has been selected.
///
/// Defaults to the [CupertinoTheme]'s primary color if null.
final Color? activeColor;
/// The color to use for the thumb of the slider.
///
/// Defaults to [CupertinoColors.white].
final Color thumbColor;
@override
State<CupertinoSlider> createState() => _CupertinoSliderState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('value', value));
properties.add(DoubleProperty('min', min));
properties.add(DoubleProperty('max', max));
}
}
class _CupertinoSliderState extends State<CupertinoSlider> with TickerProviderStateMixin {
void _handleChanged(double value) {
assert(widget.onChanged != null);
final double lerpValue = lerpDouble(widget.min, widget.max, value)!;
if (lerpValue != widget.value) {
widget.onChanged!(lerpValue);
}
}
void _handleDragStart(double value) {
assert(widget.onChangeStart != null);
widget.onChangeStart!(lerpDouble(widget.min, widget.max, value)!);
}
void _handleDragEnd(double value) {
assert(widget.onChangeEnd != null);
widget.onChangeEnd!(lerpDouble(widget.min, widget.max, value)!);
}
@override
Widget build(BuildContext context) {
return _CupertinoSliderRenderObjectWidget(
value: (widget.value - widget.min) / (widget.max - widget.min),
divisions: widget.divisions,
activeColor: CupertinoDynamicColor.resolve(
widget.activeColor ?? CupertinoTheme.of(context).primaryColor,
context,
),
thumbColor: widget.thumbColor,
onChanged: widget.onChanged != null ? _handleChanged : null,
onChangeStart: widget.onChangeStart != null ? _handleDragStart : null,
onChangeEnd: widget.onChangeEnd != null ? _handleDragEnd : null,
vsync: this,
);
}
}
class _CupertinoSliderRenderObjectWidget extends LeafRenderObjectWidget {
const _CupertinoSliderRenderObjectWidget({
required this.value,
this.divisions,
required this.activeColor,
required this.thumbColor,
this.onChanged,
this.onChangeStart,
this.onChangeEnd,
required this.vsync,
});
final double value;
final int? divisions;
final Color activeColor;
final Color thumbColor;
final ValueChanged<double>? onChanged;
final ValueChanged<double>? onChangeStart;
final ValueChanged<double>? onChangeEnd;
final TickerProvider vsync;
@override
_RenderCupertinoSlider createRenderObject(BuildContext context) {
assert(debugCheckHasDirectionality(context));
return _RenderCupertinoSlider(
value: value,
divisions: divisions,
activeColor: activeColor,
thumbColor: CupertinoDynamicColor.resolve(thumbColor, context),
trackColor: CupertinoDynamicColor.resolve(CupertinoColors.systemFill, context),
onChanged: onChanged,
onChangeStart: onChangeStart,
onChangeEnd: onChangeEnd,
vsync: vsync,
textDirection: Directionality.of(context),
cursor: kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
);
}
@override
void updateRenderObject(BuildContext context, _RenderCupertinoSlider renderObject) {
assert(debugCheckHasDirectionality(context));
renderObject
..value = value
..divisions = divisions
..activeColor = activeColor
..thumbColor = CupertinoDynamicColor.resolve(thumbColor, context)
..trackColor = CupertinoDynamicColor.resolve(CupertinoColors.systemFill, context)
..onChanged = onChanged
..onChangeStart = onChangeStart
..onChangeEnd = onChangeEnd
..textDirection = Directionality.of(context);
// Ticker provider cannot change since there's a 1:1 relationship between
// the _SliderRenderObjectWidget object and the _SliderState object.
}
}
const double _kPadding = 8.0;
const double _kSliderHeight = 2.0 * (CupertinoThumbPainter.radius + _kPadding);
const double _kSliderWidth = 176.0; // Matches Material Design slider.
const Duration _kDiscreteTransitionDuration = Duration(milliseconds: 500);
const double _kAdjustmentUnit = 0.1; // Matches iOS implementation of material slider.
class _RenderCupertinoSlider extends RenderConstrainedBox implements MouseTrackerAnnotation {
_RenderCupertinoSlider({
required double value,
int? divisions,
required Color activeColor,
required Color thumbColor,
required Color trackColor,
ValueChanged<double>? onChanged,
this.onChangeStart,
this.onChangeEnd,
required TickerProvider vsync,
required TextDirection textDirection,
MouseCursor cursor = MouseCursor.defer,
}) : assert(value >= 0.0 && value <= 1.0),
_cursor = cursor,
_value = value,
_divisions = divisions,
_activeColor = activeColor,
_thumbColor = thumbColor,
_trackColor = trackColor,
_onChanged = onChanged,
_textDirection = textDirection,
super(additionalConstraints: const BoxConstraints.tightFor(width: _kSliderWidth, height: _kSliderHeight)) {
_drag = HorizontalDragGestureRecognizer()
..onStart = _handleDragStart
..onUpdate = _handleDragUpdate
..onEnd = _handleDragEnd;
_position = AnimationController(
value: value,
duration: _kDiscreteTransitionDuration,
vsync: vsync,
)..addListener(markNeedsPaint);
}
double get value => _value;
double _value;
set value(double newValue) {
assert(newValue >= 0.0 && newValue <= 1.0);
if (newValue == _value) {
return;
}
_value = newValue;
if (divisions != null) {
_position.animateTo(newValue, curve: Curves.fastOutSlowIn);
} else {
_position.value = newValue;
}
markNeedsSemanticsUpdate();
}
int? get divisions => _divisions;
int? _divisions;
set divisions(int? value) {
if (value == _divisions) {
return;
}
_divisions = value;
markNeedsPaint();
}
Color get activeColor => _activeColor;
Color _activeColor;
set activeColor(Color value) {
if (value == _activeColor) {
return;
}
_activeColor = value;
markNeedsPaint();
}
Color get thumbColor => _thumbColor;
Color _thumbColor;
set thumbColor(Color value) {
if (value == _thumbColor) {
return;
}
_thumbColor = value;
markNeedsPaint();
}
Color get trackColor => _trackColor;
Color _trackColor;
set trackColor(Color value) {
if (value == _trackColor) {
return;
}
_trackColor = value;
markNeedsPaint();
}
ValueChanged<double>? get onChanged => _onChanged;
ValueChanged<double>? _onChanged;
set onChanged(ValueChanged<double>? value) {
if (value == _onChanged) {
return;
}
final bool wasInteractive = isInteractive;
_onChanged = value;
if (wasInteractive != isInteractive) {
markNeedsSemanticsUpdate();
}
}
ValueChanged<double>? onChangeStart;
ValueChanged<double>? onChangeEnd;
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
markNeedsPaint();
}
late AnimationController _position;
late HorizontalDragGestureRecognizer _drag;
double _currentDragValue = 0.0;
double get _discretizedCurrentDragValue {
double dragValue = clampDouble(_currentDragValue, 0.0, 1.0);
if (divisions != null) {
dragValue = (dragValue * divisions!).round() / divisions!;
}
return dragValue;
}
double get _trackLeft => _kPadding;
double get _trackRight => size.width - _kPadding;
double get _thumbCenter {
final double visualPosition = switch (textDirection) {
TextDirection.rtl => 1.0 - _value,
TextDirection.ltr => _value,
};
return lerpDouble(_trackLeft + CupertinoThumbPainter.radius, _trackRight - CupertinoThumbPainter.radius, visualPosition)!;
}
bool get isInteractive => onChanged != null;
void _handleDragStart(DragStartDetails details) => _startInteraction(details.globalPosition);
void _handleDragUpdate(DragUpdateDetails details) {
if (isInteractive) {
final double extent = math.max(_kPadding, size.width - 2.0 * (_kPadding + CupertinoThumbPainter.radius));
final double valueDelta = details.primaryDelta! / extent;
_currentDragValue += switch (textDirection) {
TextDirection.rtl => -valueDelta,
TextDirection.ltr => valueDelta,
};
onChanged!(_discretizedCurrentDragValue);
}
}
void _handleDragEnd(DragEndDetails details) => _endInteraction();
void _startInteraction(Offset globalPosition) {
if (isInteractive) {
onChangeStart?.call(_discretizedCurrentDragValue);
_currentDragValue = _value;
onChanged!(_discretizedCurrentDragValue);
}
}
void _endInteraction() {
onChangeEnd?.call(_discretizedCurrentDragValue);
_currentDragValue = 0.0;
}
@override
bool hitTestSelf(Offset position) {
return (position.dx - _thumbCenter).abs() < CupertinoThumbPainter.radius + _kPadding;
}
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
assert(debugHandleEvent(event, entry));
if (event is PointerDownEvent && isInteractive) {
_drag.addPointer(event);
}
}
@override
void paint(PaintingContext context, Offset offset) {
final (double visualPosition, Color leftColor, Color rightColor) = switch (textDirection) {
TextDirection.rtl => (1.0 - _position.value, _activeColor, trackColor),
TextDirection.ltr => (_position.value, trackColor, _activeColor),
};
final double trackCenter = offset.dy + size.height / 2.0;
final double trackLeft = offset.dx + _trackLeft;
final double trackTop = trackCenter - 1.0;
final double trackBottom = trackCenter + 1.0;
final double trackRight = offset.dx + _trackRight;
final double trackActive = offset.dx + _thumbCenter;
final Canvas canvas = context.canvas;
if (visualPosition > 0.0) {
final Paint paint = Paint()..color = rightColor;
canvas.drawRRect(RRect.fromLTRBXY(trackLeft, trackTop, trackActive, trackBottom, 1.0, 1.0), paint);
}
if (visualPosition < 1.0) {
final Paint paint = Paint()..color = leftColor;
canvas.drawRRect(RRect.fromLTRBXY(trackActive, trackTop, trackRight, trackBottom, 1.0, 1.0), paint);
}
final Offset thumbCenter = Offset(trackActive, trackCenter);
CupertinoThumbPainter(color: thumbColor).paint(canvas, Rect.fromCircle(center: thumbCenter, radius: CupertinoThumbPainter.radius));
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.isSemanticBoundary = isInteractive;
config.isSlider = true;
if (isInteractive) {
config.textDirection = textDirection;
config.onIncrease = _increaseAction;
config.onDecrease = _decreaseAction;
config.value = '${(value * 100).round()}%';
config.increasedValue = '${(clampDouble(value + _semanticActionUnit, 0.0, 1.0) * 100).round()}%';
config.decreasedValue = '${(clampDouble(value - _semanticActionUnit, 0.0, 1.0) * 100).round()}%';
}
}
double get _semanticActionUnit => divisions != null ? 1.0 / divisions! : _kAdjustmentUnit;
void _increaseAction() {
if (isInteractive) {
onChanged!(clampDouble(value + _semanticActionUnit, 0.0, 1.0));
}
}
void _decreaseAction() {
if (isInteractive) {
onChanged!(clampDouble(value - _semanticActionUnit, 0.0, 1.0));
}
}
@override
MouseCursor get cursor => _cursor;
MouseCursor _cursor;
set cursor(MouseCursor value) {
if (_cursor != value) {
_cursor = value;
// A repaint is needed in order to trigger a device update of
// [MouseTracker] so that this new value can be found.
markNeedsPaint();
}
}
@override
PointerEnterEventListener? onEnter;
PointerHoverEventListener? onHover;
@override
PointerExitEventListener? onExit;
@override
bool get validForMouseTracker => false;
@override
void dispose() {
_drag.dispose();
_position.dispose();
super.dispose();
}
}
| flutter/packages/flutter/lib/src/cupertino/slider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/slider.dart",
"repo_id": "flutter",
"token_count": 6451
} | 614 |
The rule for packages in this directory is that they can depend on
nothing but core Dart packages. They can't depend on `dart:ui`, they
can't depend on any `package:`, and they can't depend on anything
outside this directory.
Currently they do depend on dart:ui, but only for `VoidCallback` and
`clampDouble` (and maybe one day `lerpDouble`), which are all intended
to be moved out of `dart:ui` and into `dart:core`.
There is currently also an unfortunate dependency on the platform
dispatcher logic (SingletonFlutterWindow, Brightness,
PlatformDispatcher, window), though that should probably move to the
'services' library.
See also:
* https://github.com/dart-lang/sdk/issues/25217
| flutter/packages/flutter/lib/src/foundation/README.md/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/README.md",
"repo_id": "flutter",
"token_count": 190
} | 615 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file implements debugPrint in terms of print, so avoiding
// calling "print" is sort of a non-starter here...
// ignore_for_file: avoid_print
import 'dart:async';
import 'dart:collection';
/// Signature for [debugPrint] implementations.
///
/// If a [wrapWidth] is provided, each line of the [message] is word-wrapped to
/// that width. (Lines may be separated by newline characters, as in '\n'.)
///
/// By default, this function very crudely attempts to throttle the rate at
/// which messages are sent to avoid data loss on Android. This means that
/// interleaving calls to this function (directly or indirectly via, e.g.,
/// [debugDumpRenderTree] or [debugDumpApp]) and to the Dart [print] method can
/// result in out-of-order messages in the logs.
///
/// The implementation of this function can be replaced by setting the
/// [debugPrint] variable to a new implementation that matches the
/// [DebugPrintCallback] signature. For example, flutter_test does this.
///
/// The default value is [debugPrintThrottled]. For a version that acts
/// identically but does not throttle, use [debugPrintSynchronously].
typedef DebugPrintCallback = void Function(String? message, { int? wrapWidth });
/// Prints a message to the console, which you can access using the "flutter"
/// tool's "logs" command ("flutter logs").
///
/// The [debugPrint] function logs to console even in [release mode](https://docs.flutter.dev/testing/build-modes#release).
/// As per convention, calls to [debugPrint] should be within a debug mode check or an assert:
/// ```dart
/// if (kDebugMode) {
/// debugPrint('A useful message');
/// }
/// ```
///
/// See also:
///
/// * [DebugPrintCallback], for function parameters and usage details.
/// * [debugPrintThrottled], the default implementation.
DebugPrintCallback debugPrint = debugPrintThrottled;
/// Alternative implementation of [debugPrint] that does not throttle.
/// Used by tests.
void debugPrintSynchronously(String? message, { int? wrapWidth }) {
if (message != null && wrapWidth != null) {
print(message.split('\n').expand<String>((String line) => debugWordWrap(line, wrapWidth)).join('\n'));
} else {
print(message);
}
}
/// Implementation of [debugPrint] that throttles messages. This avoids dropping
/// messages on platforms that rate-limit their logging (for example, Android).
///
/// If `wrapWidth` is not null, the message is wrapped using [debugWordWrap].
void debugPrintThrottled(String? message, { int? wrapWidth }) {
final List<String> messageLines = message?.split('\n') ?? <String>['null'];
if (wrapWidth != null) {
_debugPrintBuffer.addAll(messageLines.expand<String>((String line) => debugWordWrap(line, wrapWidth)));
} else {
_debugPrintBuffer.addAll(messageLines);
}
if (!_debugPrintScheduled) {
_debugPrintTask();
}
}
int _debugPrintedCharacters = 0;
const int _kDebugPrintCapacity = 12 * 1024;
const Duration _kDebugPrintPauseTime = Duration(seconds: 1);
final Queue<String> _debugPrintBuffer = Queue<String>();
final Stopwatch _debugPrintStopwatch = Stopwatch(); // flutter_ignore: stopwatch (see analyze.dart)
// Ignore context: This is not used in tests, only for throttled logging.
Completer<void>? _debugPrintCompleter;
bool _debugPrintScheduled = false;
void _debugPrintTask() {
_debugPrintScheduled = false;
if (_debugPrintStopwatch.elapsed > _kDebugPrintPauseTime) {
_debugPrintStopwatch.stop();
_debugPrintStopwatch.reset();
_debugPrintedCharacters = 0;
}
while (_debugPrintedCharacters < _kDebugPrintCapacity && _debugPrintBuffer.isNotEmpty) {
final String line = _debugPrintBuffer.removeFirst();
_debugPrintedCharacters += line.length; // TODO(ianh): Use the UTF-8 byte length instead
print(line);
}
if (_debugPrintBuffer.isNotEmpty) {
_debugPrintScheduled = true;
_debugPrintedCharacters = 0;
Timer(_kDebugPrintPauseTime, _debugPrintTask);
_debugPrintCompleter ??= Completer<void>();
} else {
_debugPrintStopwatch.start();
_debugPrintCompleter?.complete();
_debugPrintCompleter = null;
}
}
/// A Future that resolves when there is no longer any buffered content being
/// printed by [debugPrintThrottled] (which is the default implementation for
/// [debugPrint], which is used to report errors to the console).
Future<void> get debugPrintDone => _debugPrintCompleter?.future ?? Future<void>.value();
final RegExp _indentPattern = RegExp('^ *(?:[-+*] |[0-9]+[.):] )?');
enum _WordWrapParseMode { inSpace, inWord, atBreak }
/// Wraps the given string at the given width.
///
/// The `message` should not contain newlines (`\n`, U+000A). Strings that may
/// contain newlines should be [String.split] before being wrapped.
///
/// Wrapping occurs at space characters (U+0020). Lines that start with an
/// octothorpe ("#", U+0023) are not wrapped (so for example, Dart stack traces
/// won't be wrapped).
///
/// Subsequent lines attempt to duplicate the indentation of the first line, for
/// example if the first line starts with multiple spaces. In addition, if a
/// `wrapIndent` argument is provided, each line after the first is prefixed by
/// that string.
///
/// This is not suitable for use with arbitrary Unicode text. For example, it
/// doesn't implement UAX #14, can't handle ideographic text, doesn't hyphenate,
/// and so forth. It is only intended for formatting error messages.
///
/// The default [debugPrint] implementation uses this for its line wrapping.
Iterable<String> debugWordWrap(String message, int width, { String wrapIndent = '' }) {
if (message.length < width || message.trimLeft()[0] == '#') {
return <String>[message];
}
final List<String> wrapped = <String>[];
final Match prefixMatch = _indentPattern.matchAsPrefix(message)!;
final String prefix = wrapIndent + ' ' * prefixMatch.group(0)!.length;
int start = 0;
int startForLengthCalculations = 0;
bool addPrefix = false;
int index = prefix.length;
_WordWrapParseMode mode = _WordWrapParseMode.inSpace;
late int lastWordStart;
int? lastWordEnd;
while (true) {
switch (mode) {
case _WordWrapParseMode.inSpace: // at start of break point (or start of line); can't break until next break
while ((index < message.length) && (message[index] == ' ')) {
index += 1;
}
lastWordStart = index;
mode = _WordWrapParseMode.inWord;
case _WordWrapParseMode.inWord: // looking for a good break point
while ((index < message.length) && (message[index] != ' ')) {
index += 1;
}
mode = _WordWrapParseMode.atBreak;
case _WordWrapParseMode.atBreak: // at start of break point
if ((index - startForLengthCalculations > width) || (index == message.length)) {
// we are over the width line, so break
if ((index - startForLengthCalculations <= width) || (lastWordEnd == null)) {
// we should use this point, because either it doesn't actually go over the
// end (last line), or it does, but there was no earlier break point
lastWordEnd = index;
}
if (addPrefix) {
wrapped.add(prefix + message.substring(start, lastWordEnd));
} else {
wrapped.add(message.substring(start, lastWordEnd));
addPrefix = true;
}
if (lastWordEnd >= message.length) {
return wrapped;
}
// just yielded a line
if (lastWordEnd == index) {
// we broke at current position
// eat all the spaces, then set our start point
while ((index < message.length) && (message[index] == ' ')) {
index += 1;
}
start = index;
mode = _WordWrapParseMode.inWord;
} else {
// we broke at the previous break point, and we're at the start of a new one
assert(lastWordStart > lastWordEnd);
start = lastWordStart;
mode = _WordWrapParseMode.atBreak;
}
startForLengthCalculations = start - prefix.length;
assert(addPrefix);
lastWordEnd = null;
} else {
// save this break point, we're not yet over the line width
lastWordEnd = index;
// skip to the end of this break point
mode = _WordWrapParseMode.inSpace;
}
}
}
}
| flutter/packages/flutter/lib/src/foundation/print.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/print.dart",
"repo_id": "flutter",
"token_count": 2893
} | 616 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show clampDouble;
import 'events.dart';
import 'recognizer.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
export 'events.dart' show PointerDownEvent, PointerEvent;
enum _ForceState {
// No pointer has touched down and the detector is ready for a pointer down to occur.
ready,
// A pointer has touched down, but a force press gesture has not yet been detected.
possible,
// A pointer is down and a force press gesture has been detected. However, if
// the ForcePressGestureRecognizer is the only recognizer in the arena, thus
// accepted as soon as the gesture state is possible, the gesture will not
// yet have started.
accepted,
// A pointer is down and the gesture has started, ie. the pressure of the pointer
// has just become greater than the ForcePressGestureRecognizer.startPressure.
started,
// A pointer is down and the pressure of the pointer has just become greater
// than the ForcePressGestureRecognizer.peakPressure. Even after a pointer
// crosses this threshold, onUpdate callbacks will still be sent.
peaked,
}
/// Details object for callbacks that use [GestureForcePressStartCallback],
/// [GestureForcePressPeakCallback], [GestureForcePressEndCallback] or
/// [GestureForcePressUpdateCallback].
///
/// See also:
///
/// * [ForcePressGestureRecognizer.onStart], [ForcePressGestureRecognizer.onPeak],
/// [ForcePressGestureRecognizer.onEnd], and [ForcePressGestureRecognizer.onUpdate]
/// which use [ForcePressDetails].
class ForcePressDetails {
/// Creates details for a [GestureForcePressStartCallback],
/// [GestureForcePressPeakCallback] or [GestureForcePressEndCallback].
ForcePressDetails({
required this.globalPosition,
Offset? localPosition,
required this.pressure,
}) : localPosition = localPosition ?? globalPosition;
/// The global position at which the function was called.
final Offset globalPosition;
/// The local position at which the function was called.
final Offset localPosition;
/// The pressure of the pointer on the screen.
final double pressure;
}
/// Signature used by a [ForcePressGestureRecognizer] for when a pointer has
/// pressed with at least [ForcePressGestureRecognizer.startPressure].
typedef GestureForcePressStartCallback = void Function(ForcePressDetails details);
/// Signature used by [ForcePressGestureRecognizer] for when a pointer that has
/// pressed with at least [ForcePressGestureRecognizer.peakPressure].
typedef GestureForcePressPeakCallback = void Function(ForcePressDetails details);
/// Signature used by [ForcePressGestureRecognizer] during the frames
/// after the triggering of a [ForcePressGestureRecognizer.onStart] callback.
typedef GestureForcePressUpdateCallback = void Function(ForcePressDetails details);
/// Signature for when the pointer that previously triggered a
/// [ForcePressGestureRecognizer.onStart] callback is no longer in contact
/// with the screen.
typedef GestureForcePressEndCallback = void Function(ForcePressDetails details);
/// Signature used by [ForcePressGestureRecognizer] for interpolating the raw
/// device pressure to a value in the range `[0, 1]` given the device's pressure
/// min and pressure max.
typedef GestureForceInterpolation = double Function(double pressureMin, double pressureMax, double pressure);
/// Recognizes a force press on devices that have force sensors.
///
/// Only the force from a single pointer is used to invoke events. A tap
/// recognizer will win against this recognizer on pointer up as long as the
/// pointer has not pressed with a force greater than
/// [ForcePressGestureRecognizer.startPressure]. A long press recognizer will
/// win when the press down time exceeds the threshold time as long as the
/// pointer's pressure was never greater than
/// [ForcePressGestureRecognizer.startPressure] in that duration.
///
/// As of November, 2018 iPhone devices of generation 6S and higher have
/// force touch functionality, with the exception of the iPhone XR. In addition,
/// a small handful of Android devices have this functionality as well.
///
/// Devices with faux screen pressure sensors like the Pixel 2 and 3 will not
/// send any force press related callbacks.
///
/// Reported pressure will always be in the range 0.0 to 1.0, where 1.0 is
/// maximum pressure and 0.0 is minimum pressure. If using a custom
/// [interpolation] callback, the pressure reported will correspond to that
/// custom curve.
class ForcePressGestureRecognizer extends OneSequenceGestureRecognizer {
/// Creates a force press gesture recognizer.
///
/// The [startPressure] defaults to 0.4, and [peakPressure] defaults to 0.85
/// where a value of 0.0 is no pressure and a value of 1.0 is maximum pressure.
///
/// The [startPressure], [peakPressure] and [interpolation] arguments must not
/// be null. The [peakPressure] argument must be greater than [startPressure].
/// The [interpolation] callback must always return a value in the range 0.0
/// to 1.0 for values of `pressure` that are between `pressureMin` and
/// `pressureMax`.
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
ForcePressGestureRecognizer({
this.startPressure = 0.4,
this.peakPressure = 0.85,
this.interpolation = _inverseLerp,
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
}) : assert(peakPressure > startPressure);
/// A pointer is in contact with the screen and has just pressed with a force
/// exceeding the [startPressure]. Consequently, if there were other gesture
/// detectors, only the force press gesture will be detected and all others
/// will be rejected.
///
/// The position of the pointer is provided in the callback's `details`
/// argument, which is a [ForcePressDetails] object.
GestureForcePressStartCallback? onStart;
/// A pointer is in contact with the screen and is either moving on the plane
/// of the screen, pressing the screen with varying forces or both
/// simultaneously.
///
/// This callback will be invoked for every pointer event after the invocation
/// of [onStart] and/or [onPeak] and before the invocation of [onEnd], no
/// matter what the pressure is during this time period. The position and
/// pressure of the pointer is provided in the callback's `details` argument,
/// which is a [ForcePressDetails] object.
GestureForcePressUpdateCallback? onUpdate;
/// A pointer is in contact with the screen and has just pressed with a force
/// exceeding the [peakPressure]. This is an arbitrary second level action
/// threshold and isn't necessarily the maximum possible device pressure
/// (which is 1.0).
///
/// The position of the pointer is provided in the callback's `details`
/// argument, which is a [ForcePressDetails] object.
GestureForcePressPeakCallback? onPeak;
/// A pointer is no longer in contact with the screen.
///
/// The position of the pointer is provided in the callback's `details`
/// argument, which is a [ForcePressDetails] object.
GestureForcePressEndCallback? onEnd;
/// The pressure of the press required to initiate a force press.
///
/// A value of 0.0 is no pressure, and 1.0 is maximum pressure.
final double startPressure;
/// The pressure of the press required to peak a force press.
///
/// A value of 0.0 is no pressure, and 1.0 is maximum pressure. This value
/// must be greater than [startPressure].
final double peakPressure;
/// The function used to convert the raw device pressure values into a value
/// in the range 0.0 to 1.0.
///
/// The function takes in the device's minimum, maximum and raw touch pressure
/// and returns a value in the range 0.0 to 1.0 denoting the interpolated
/// touch pressure.
///
/// This function must always return values in the range 0.0 to 1.0 given a
/// pressure that is between the minimum and maximum pressures. It may return
/// `double.NaN` for values that it does not want to support.
///
/// By default, the function is a linear interpolation; however, changing the
/// function could be useful to accommodate variations in the way different
/// devices respond to pressure, or to change how animations from pressure
/// feedback are rendered.
///
/// For example, an ease-in curve can be used to determine the interpolated
/// value:
///
/// ```dart
/// double interpolateWithEasing(double min, double max, double t) {
/// final double lerp = (t - min) / (max - min);
/// return Curves.easeIn.transform(lerp);
/// }
/// ```
final GestureForceInterpolation interpolation;
late OffsetPair _lastPosition;
late double _lastPressure;
_ForceState _state = _ForceState.ready;
@override
void addAllowedPointer(PointerDownEvent event) {
// If the device has a maximum pressure of less than or equal to 1, it
// doesn't have touch pressure sensing capabilities. Do not participate
// in the gesture arena.
if (event.pressureMax <= 1.0) {
resolve(GestureDisposition.rejected);
} else {
super.addAllowedPointer(event);
if (_state == _ForceState.ready) {
_state = _ForceState.possible;
_lastPosition = OffsetPair.fromEventPosition(event);
}
}
}
@override
void handleEvent(PointerEvent event) {
assert(_state != _ForceState.ready);
// A static pointer with changes in pressure creates PointerMoveEvent events.
if (event is PointerMoveEvent || event is PointerDownEvent) {
final double pressure = interpolation(event.pressureMin, event.pressureMax, event.pressure);
assert(
(pressure >= 0.0 && pressure <= 1.0) || // Interpolated pressure must be between 1.0 and 0.0...
pressure.isNaN, // and interpolation may return NaN for values it doesn't want to support...
);
_lastPosition = OffsetPair.fromEventPosition(event);
_lastPressure = pressure;
if (_state == _ForceState.possible) {
if (pressure > startPressure) {
_state = _ForceState.started;
resolve(GestureDisposition.accepted);
} else if (event.delta.distanceSquared > computeHitSlop(event.kind, gestureSettings)) {
resolve(GestureDisposition.rejected);
}
}
// In case this is the only gesture detector we still don't want to start
// the gesture until the pressure is greater than the startPressure.
if (pressure > startPressure && _state == _ForceState.accepted) {
_state = _ForceState.started;
if (onStart != null) {
invokeCallback<void>('onStart', () => onStart!(ForcePressDetails(
pressure: pressure,
globalPosition: _lastPosition.global,
localPosition: _lastPosition.local,
)));
}
}
if (onPeak != null && pressure > peakPressure &&
(_state == _ForceState.started)) {
_state = _ForceState.peaked;
if (onPeak != null) {
invokeCallback<void>('onPeak', () => onPeak!(ForcePressDetails(
pressure: pressure,
globalPosition: event.position,
localPosition: event.localPosition,
)));
}
}
if (onUpdate != null && !pressure.isNaN &&
(_state == _ForceState.started || _state == _ForceState.peaked)) {
if (onUpdate != null) {
invokeCallback<void>('onUpdate', () => onUpdate!(ForcePressDetails(
pressure: pressure,
globalPosition: event.position,
localPosition: event.localPosition,
)));
}
}
}
stopTrackingIfPointerNoLongerDown(event);
}
@override
void acceptGesture(int pointer) {
if (_state == _ForceState.possible) {
_state = _ForceState.accepted;
}
if (onStart != null && _state == _ForceState.started) {
invokeCallback<void>('onStart', () => onStart!(ForcePressDetails(
pressure: _lastPressure,
globalPosition: _lastPosition.global,
localPosition: _lastPosition.local,
)));
}
}
@override
void didStopTrackingLastPointer(int pointer) {
final bool wasAccepted = _state == _ForceState.started || _state == _ForceState.peaked;
if (_state == _ForceState.possible) {
resolve(GestureDisposition.rejected);
return;
}
if (wasAccepted && onEnd != null) {
if (onEnd != null) {
invokeCallback<void>('onEnd', () => onEnd!(ForcePressDetails(
pressure: 0.0,
globalPosition: _lastPosition.global,
localPosition: _lastPosition.local,
)));
}
}
_state = _ForceState.ready;
}
@override
void rejectGesture(int pointer) {
stopTrackingPointer(pointer);
didStopTrackingLastPointer(pointer);
}
static double _inverseLerp(double min, double max, double t) {
assert(min <= max);
double value = (t - min) / (max - min);
// If the device incorrectly reports a pressure outside of pressureMin
// and pressureMax, we still want this recognizer to respond normally.
if (!value.isNaN) {
value = clampDouble(value, 0.0, 1.0);
}
return value;
}
@override
String get debugDescription => 'force press';
}
| flutter/packages/flutter/lib/src/gestures/force_press.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/force_press.dart",
"repo_id": "flutter",
"token_count": 4173
} | 617 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'binding.dart';
import 'events.dart';
import 'lsq_solver.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
/// A velocity in two dimensions.
@immutable
class Velocity {
/// Creates a [Velocity].
const Velocity({
required this.pixelsPerSecond,
});
/// A velocity that isn't moving at all.
static const Velocity zero = Velocity(pixelsPerSecond: Offset.zero);
/// The number of pixels per second of velocity in the x and y directions.
final Offset pixelsPerSecond;
/// Return the negation of a velocity.
Velocity operator -() => Velocity(pixelsPerSecond: -pixelsPerSecond);
/// Return the difference of two velocities.
Velocity operator -(Velocity other) {
return Velocity(pixelsPerSecond: pixelsPerSecond - other.pixelsPerSecond);
}
/// Return the sum of two velocities.
Velocity operator +(Velocity other) {
return Velocity(pixelsPerSecond: pixelsPerSecond + other.pixelsPerSecond);
}
/// Return a velocity whose magnitude has been clamped to [minValue]
/// and [maxValue].
///
/// If the magnitude of this Velocity is less than minValue then return a new
/// Velocity with the same direction and with magnitude [minValue]. Similarly,
/// if the magnitude of this Velocity is greater than maxValue then return a
/// new Velocity with the same direction and magnitude [maxValue].
///
/// If the magnitude of this Velocity is within the specified bounds then
/// just return this.
Velocity clampMagnitude(double minValue, double maxValue) {
assert(minValue >= 0.0);
assert(maxValue >= 0.0 && maxValue >= minValue);
final double valueSquared = pixelsPerSecond.distanceSquared;
if (valueSquared > maxValue * maxValue) {
return Velocity(pixelsPerSecond: (pixelsPerSecond / pixelsPerSecond.distance) * maxValue);
}
if (valueSquared < minValue * minValue) {
return Velocity(pixelsPerSecond: (pixelsPerSecond / pixelsPerSecond.distance) * minValue);
}
return this;
}
@override
bool operator ==(Object other) {
return other is Velocity
&& other.pixelsPerSecond == pixelsPerSecond;
}
@override
int get hashCode => pixelsPerSecond.hashCode;
@override
String toString() => 'Velocity(${pixelsPerSecond.dx.toStringAsFixed(1)}, ${pixelsPerSecond.dy.toStringAsFixed(1)})';
}
/// A two dimensional velocity estimate.
///
/// VelocityEstimates are computed by [VelocityTracker.getVelocityEstimate]. An
/// estimate's [confidence] measures how well the velocity tracker's position
/// data fit a straight line, [duration] is the time that elapsed between the
/// first and last position sample used to compute the velocity, and [offset]
/// is similarly the difference between the first and last positions.
///
/// See also:
///
/// * [VelocityTracker], which computes [VelocityEstimate]s.
/// * [Velocity], which encapsulates (just) a velocity vector and provides some
/// useful velocity operations.
class VelocityEstimate {
/// Creates a dimensional velocity estimate.
const VelocityEstimate({
required this.pixelsPerSecond,
required this.confidence,
required this.duration,
required this.offset,
});
/// The number of pixels per second of velocity in the x and y directions.
final Offset pixelsPerSecond;
/// A value between 0.0 and 1.0 that indicates how well [VelocityTracker]
/// was able to fit a straight line to its position data.
///
/// The value of this property is 1.0 for a perfect fit, 0.0 for a poor fit.
final double confidence;
/// The time that elapsed between the first and last position sample used
/// to compute [pixelsPerSecond].
final Duration duration;
/// The difference between the first and last position sample used
/// to compute [pixelsPerSecond].
final Offset offset;
@override
String toString() => 'VelocityEstimate(${pixelsPerSecond.dx.toStringAsFixed(1)}, ${pixelsPerSecond.dy.toStringAsFixed(1)}; offset: $offset, duration: $duration, confidence: ${confidence.toStringAsFixed(1)})';
}
class _PointAtTime {
const _PointAtTime(this.point, this.time);
final Duration time;
final Offset point;
@override
String toString() => '_PointAtTime($point at $time)';
}
/// Computes a pointer's velocity based on data from [PointerMoveEvent]s.
///
/// The input data is provided by calling [addPosition]. Adding data is cheap.
///
/// To obtain a velocity, call [getVelocity] or [getVelocityEstimate]. This will
/// compute the velocity based on the data added so far. Only call these when
/// you need to use the velocity, as they are comparatively expensive.
///
/// The quality of the velocity estimation will be better if more data points
/// have been received.
class VelocityTracker {
/// Create a new velocity tracker for a pointer [kind].
VelocityTracker.withKind(this.kind);
static const int _assumePointerMoveStoppedMilliseconds = 40;
static const int _historySize = 20;
static const int _horizonMilliseconds = 100;
static const int _minSampleSize = 3;
/// The kind of pointer this tracker is for.
final PointerDeviceKind kind;
// Time difference since the last sample was added
Stopwatch get _sinceLastSample {
_stopwatch ??= GestureBinding.instance.samplingClock.stopwatch();
return _stopwatch!;
}
Stopwatch? _stopwatch;
// Circular buffer; current sample at _index.
final List<_PointAtTime?> _samples = List<_PointAtTime?>.filled(_historySize, null);
int _index = 0;
/// Adds a position as the given time to the tracker.
void addPosition(Duration time, Offset position) {
_sinceLastSample.start();
_sinceLastSample.reset();
_index += 1;
if (_index == _historySize) {
_index = 0;
}
_samples[_index] = _PointAtTime(position, time);
}
/// Returns an estimate of the velocity of the object being tracked by the
/// tracker given the current information available to the tracker.
///
/// Information is added using [addPosition].
///
/// Returns null if there is no data on which to base an estimate.
VelocityEstimate? getVelocityEstimate() {
// Has user recently moved since last sample?
if (_sinceLastSample.elapsedMilliseconds > _assumePointerMoveStoppedMilliseconds) {
return const VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 1.0,
duration: Duration.zero,
offset: Offset.zero,
);
}
final List<double> x = <double>[];
final List<double> y = <double>[];
final List<double> w = <double>[];
final List<double> time = <double>[];
int sampleCount = 0;
int index = _index;
final _PointAtTime? newestSample = _samples[index];
if (newestSample == null) {
return null;
}
_PointAtTime previousSample = newestSample;
_PointAtTime oldestSample = newestSample;
// Starting with the most recent PointAtTime sample, iterate backwards while
// the samples represent continuous motion.
do {
final _PointAtTime? sample = _samples[index];
if (sample == null) {
break;
}
final double age = (newestSample.time - sample.time).inMicroseconds.toDouble() / 1000;
final double delta = (sample.time - previousSample.time).inMicroseconds.abs().toDouble() / 1000;
previousSample = sample;
if (age > _horizonMilliseconds || delta > _assumePointerMoveStoppedMilliseconds) {
break;
}
oldestSample = sample;
final Offset position = sample.point;
x.add(position.dx);
y.add(position.dy);
w.add(1.0);
time.add(-age);
index = (index == 0 ? _historySize : index) - 1;
sampleCount += 1;
} while (sampleCount < _historySize);
if (sampleCount >= _minSampleSize) {
final LeastSquaresSolver xSolver = LeastSquaresSolver(time, x, w);
final PolynomialFit? xFit = xSolver.solve(2);
if (xFit != null) {
final LeastSquaresSolver ySolver = LeastSquaresSolver(time, y, w);
final PolynomialFit? yFit = ySolver.solve(2);
if (yFit != null) {
return VelocityEstimate( // convert from pixels/ms to pixels/s
pixelsPerSecond: Offset(xFit.coefficients[1] * 1000, yFit.coefficients[1] * 1000),
confidence: xFit.confidence * yFit.confidence,
duration: newestSample.time - oldestSample.time,
offset: newestSample.point - oldestSample.point,
);
}
}
}
// We're unable to make a velocity estimate but we did have at least one
// valid pointer position.
return VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 1.0,
duration: newestSample.time - oldestSample.time,
offset: newestSample.point - oldestSample.point,
);
}
/// Computes the velocity of the pointer at the time of the last
/// provided data point.
///
/// This can be expensive. Only call this when you need the velocity.
///
/// Returns [Velocity.zero] if there is no data from which to compute an
/// estimate or if the estimated velocity is zero.
Velocity getVelocity() {
final VelocityEstimate? estimate = getVelocityEstimate();
if (estimate == null || estimate.pixelsPerSecond == Offset.zero) {
return Velocity.zero;
}
return Velocity(pixelsPerSecond: estimate.pixelsPerSecond);
}
}
/// A [VelocityTracker] subclass that provides a close approximation of iOS
/// scroll view's velocity estimation strategy.
///
/// The estimated velocity reported by this class is a close approximation of
/// the velocity an iOS scroll view would report with the same
/// [PointerMoveEvent]s, when the touch that initiates a fling is released.
///
/// This class differs from the [VelocityTracker] class in that it uses weighted
/// average of the latest few velocity samples of the tracked pointer, instead
/// of doing a linear regression on a relatively large amount of data points, to
/// estimate the velocity of the tracked pointer. Adding data points and
/// estimating the velocity are both cheap.
///
/// To obtain a velocity, call [getVelocity] or [getVelocityEstimate]. The
/// estimated velocity is typically used as the initial flinging velocity of a
/// `Scrollable`, when its drag gesture ends.
///
/// See also:
///
/// * [scrollViewWillEndDragging(_:withVelocity:targetContentOffset:)](https://developer.apple.com/documentation/uikit/uiscrollviewdelegate/1619385-scrollviewwillenddragging),
/// the iOS method that reports the fling velocity when the touch is released.
class IOSScrollViewFlingVelocityTracker extends VelocityTracker {
/// Create a new IOSScrollViewFlingVelocityTracker.
IOSScrollViewFlingVelocityTracker(super.kind) : super.withKind();
/// The velocity estimation uses at most 4 `_PointAtTime` samples. The extra
/// samples are there to make the `VelocityEstimate.offset` sufficiently large
/// to be recognized as a fling. See
/// `VerticalDragGestureRecognizer.isFlingGesture`.
static const int _sampleSize = 20;
final List<_PointAtTime?> _touchSamples = List<_PointAtTime?>.filled(_sampleSize, null);
@override
void addPosition(Duration time, Offset position) {
_sinceLastSample.start();
_sinceLastSample.reset();
assert(() {
final _PointAtTime? previousPoint = _touchSamples[_index];
if (previousPoint == null || previousPoint.time <= time) {
return true;
}
throw FlutterError(
'The position being added ($position) has a smaller timestamp ($time) '
'than its predecessor: $previousPoint.',
);
}());
_index = (_index + 1) % _sampleSize;
_touchSamples[_index] = _PointAtTime(position, time);
}
// Computes the velocity using 2 adjacent points in history. When index = 0,
// it uses the latest point recorded and the point recorded immediately before
// it. The smaller index is, the earlier in history the points used are.
Offset _previousVelocityAt(int index) {
final int endIndex = (_index + index) % _sampleSize;
final int startIndex = (_index + index - 1) % _sampleSize;
final _PointAtTime? end = _touchSamples[endIndex];
final _PointAtTime? start = _touchSamples[startIndex];
if (end == null || start == null) {
return Offset.zero;
}
final int dt = (end.time - start.time).inMicroseconds;
assert(dt >= 0);
return dt > 0
// Convert dt to milliseconds to preserve floating point precision.
? (end.point - start.point) * 1000 / (dt.toDouble() / 1000)
: Offset.zero;
}
@override
VelocityEstimate getVelocityEstimate() {
// Has user recently moved since last sample?
if (_sinceLastSample.elapsedMilliseconds > VelocityTracker._assumePointerMoveStoppedMilliseconds) {
return const VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 1.0,
duration: Duration.zero,
offset: Offset.zero,
);
}
// The velocity estimated using this expression is an approximation of the
// scroll velocity of an iOS scroll view at the moment the user touch was
// released, not the final velocity of the iOS pan gesture recognizer
// installed on the scroll view would report. Typically in an iOS scroll
// view the velocity values are different between the two, because the
// scroll view usually slows down when the touch is released.
final Offset estimatedVelocity = _previousVelocityAt(-2) * 0.6
+ _previousVelocityAt(-1) * 0.35
+ _previousVelocityAt(0) * 0.05;
final _PointAtTime? newestSample = _touchSamples[_index];
_PointAtTime? oldestNonNullSample;
for (int i = 1; i <= _sampleSize; i += 1) {
oldestNonNullSample = _touchSamples[(_index + i) % _sampleSize];
if (oldestNonNullSample != null) {
break;
}
}
if (oldestNonNullSample == null || newestSample == null) {
assert(false, 'There must be at least 1 point in _touchSamples: $_touchSamples');
return const VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 0.0,
duration: Duration.zero,
offset: Offset.zero,
);
} else {
return VelocityEstimate(
pixelsPerSecond: estimatedVelocity,
confidence: 1.0,
duration: newestSample.time - oldestNonNullSample.time,
offset: newestSample.point - oldestNonNullSample.point,
);
}
}
}
/// A [VelocityTracker] subclass that provides a close approximation of macOS
/// scroll view's velocity estimation strategy.
///
/// The estimated velocity reported by this class is a close approximation of
/// the velocity a macOS scroll view would report with the same
/// [PointerMoveEvent]s, when the touch that initiates a fling is released.
///
/// This class differs from the [VelocityTracker] class in that it uses weighted
/// average of the latest few velocity samples of the tracked pointer, instead
/// of doing a linear regression on a relatively large amount of data points, to
/// estimate the velocity of the tracked pointer. Adding data points and
/// estimating the velocity are both cheap.
///
/// To obtain a velocity, call [getVelocity] or [getVelocityEstimate]. The
/// estimated velocity is typically used as the initial flinging velocity of a
/// `Scrollable`, when its drag gesture ends.
class MacOSScrollViewFlingVelocityTracker extends IOSScrollViewFlingVelocityTracker {
/// Create a new MacOSScrollViewFlingVelocityTracker.
MacOSScrollViewFlingVelocityTracker(super.kind);
@override
VelocityEstimate getVelocityEstimate() {
// Has user recently moved since last sample?
if (_sinceLastSample.elapsedMilliseconds > VelocityTracker._assumePointerMoveStoppedMilliseconds) {
return const VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 1.0,
duration: Duration.zero,
offset: Offset.zero,
);
}
// The velocity estimated using this expression is an approximation of the
// scroll velocity of a macOS scroll view at the moment the user touch was
// released.
final Offset estimatedVelocity = _previousVelocityAt(-2) * 0.15
+ _previousVelocityAt(-1) * 0.65
+ _previousVelocityAt(0) * 0.2;
final _PointAtTime? newestSample = _touchSamples[_index];
_PointAtTime? oldestNonNullSample;
for (int i = 1; i <= IOSScrollViewFlingVelocityTracker._sampleSize; i += 1) {
oldestNonNullSample = _touchSamples[(_index + i) % IOSScrollViewFlingVelocityTracker._sampleSize];
if (oldestNonNullSample != null) {
break;
}
}
if (oldestNonNullSample == null || newestSample == null) {
assert(false, 'There must be at least 1 point in _touchSamples: $_touchSamples');
return const VelocityEstimate(
pixelsPerSecond: Offset.zero,
confidence: 0.0,
duration: Duration.zero,
offset: Offset.zero,
);
} else {
return VelocityEstimate(
pixelsPerSecond: estimatedVelocity,
confidence: 1.0,
duration: newestSample.time - oldestNonNullSample.time,
offset: newestSample.point - oldestNonNullSample.point,
);
}
}
}
| flutter/packages/flutter/lib/src/gestures/velocity_tracker.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/velocity_tracker.dart",
"repo_id": "flutter",
"token_count": 5649
} | 618 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$menu_arrow = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 26.0),
Offset(5.976562557689849, 25.638185989482512),
Offset(5.951781669661045, 24.367972149512962),
Offset(6.172793116155802, 21.823631861702058),
Offset(7.363587976838016, 17.665129222832853),
Offset(11.400806749308899, 11.800457098273661),
Offset(17.41878573585796, 8.03287301910486),
Offset(24.257523532175192, 6.996159828679087),
Offset(29.90338248135665, 8.291042849526),
Offset(33.76252909490214, 10.56619705548221),
Offset(36.23501636298456, 12.973675163618006),
Offset(37.77053540180521, 15.158665125787222),
Offset(38.70420448893307, 17.008159945496722),
Offset(39.260392038988186, 18.5104805430827),
Offset(39.58393261852967, 19.691668944482075),
Offset(39.766765502294305, 20.58840471665747),
Offset(39.866421084642994, 21.237322746452932),
Offset(39.91802804639694, 21.671102155152063),
Offset(39.94204075298555, 21.917555098992118),
Offset(39.94920417650143, 21.999827480806236),
Offset(39.94921875, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 26.0),
Offset(5.976562557689849, 25.638185989482512),
Offset(5.951781669661045, 24.367972149512962),
Offset(6.172793116155802, 21.823631861702058),
Offset(7.363587976838016, 17.665129222832853),
Offset(11.400806749308899, 11.800457098273661),
Offset(17.41878573585796, 8.03287301910486),
Offset(24.257523532175192, 6.996159828679087),
Offset(29.90338248135665, 8.291042849526),
Offset(33.76252909490214, 10.56619705548221),
Offset(36.23501636298456, 12.973675163618006),
Offset(37.77053540180521, 15.158665125787222),
Offset(38.70420448893307, 17.008159945496722),
Offset(39.260392038988186, 18.5104805430827),
Offset(39.58393261852967, 19.691668944482075),
Offset(39.766765502294305, 20.58840471665747),
Offset(39.866421084642994, 21.237322746452932),
Offset(39.91802804639694, 21.671102155152063),
Offset(39.94204075298555, 21.917555098992118),
Offset(39.94920417650143, 21.999827480806236),
Offset(39.94921875, 22.0),
],
<Offset>[
Offset(42.0, 26.0),
Offset(41.91421333157091, 26.360426629492423),
Offset(41.55655262500356, 27.60382930516768),
Offset(40.57766190556539, 29.99090297157744),
Offset(38.19401046368096, 33.57567286235671),
Offset(32.70215654116029, 37.756226919427284),
Offset(26.22621984436523, 39.26167875408963),
Offset(20.102351173097617, 38.04803275423973),
Offset(15.903199608216863, 35.25316524725598),
Offset(13.57741782841064, 32.27000071222682),
Offset(12.442030802775209, 29.665215617986277),
Offset(11.981806515947115, 27.560177578292762),
Offset(11.879421136842055, 25.918712565594948),
Offset(11.95091483982305, 24.66543021784112),
Offset(12.092167805674123, 23.72603017548901),
Offset(12.245452640806768, 23.03857447590349),
Offset(12.379956070248545, 22.554583229506296),
Offset(12.480582865035407, 22.237279988168645),
Offset(12.541514124262473, 22.059212079933666),
Offset(12.562455771803593, 22.000123717314214),
Offset(12.562499999999996, 22.000000000000004),
],
<Offset>[
Offset(42.0, 26.0),
Offset(41.91421333157091, 26.360426629492423),
Offset(41.55655262500356, 27.60382930516768),
Offset(40.57766190556539, 29.99090297157744),
Offset(38.19401046368096, 33.57567286235671),
Offset(32.70215654116029, 37.756226919427284),
Offset(26.22621984436523, 39.26167875408963),
Offset(20.102351173097617, 38.04803275423973),
Offset(15.903199608216863, 35.25316524725598),
Offset(13.57741782841064, 32.27000071222682),
Offset(12.442030802775209, 29.665215617986277),
Offset(11.981806515947115, 27.560177578292762),
Offset(11.879421136842055, 25.918712565594948),
Offset(11.95091483982305, 24.66543021784112),
Offset(12.092167805674123, 23.72603017548901),
Offset(12.245452640806768, 23.03857447590349),
Offset(12.379956070248545, 22.554583229506296),
Offset(12.480582865035407, 22.237279988168645),
Offset(12.541514124262473, 22.059212079933666),
Offset(12.562455771803593, 22.000123717314214),
Offset(12.562499999999996, 22.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 26.0),
Offset(41.91421333157091, 26.360426629492423),
Offset(41.55655262500356, 27.60382930516768),
Offset(40.57766190556539, 29.99090297157744),
Offset(38.19401046368096, 33.57567286235671),
Offset(32.70215654116029, 37.756226919427284),
Offset(26.22621984436523, 39.26167875408963),
Offset(20.102351173097617, 38.04803275423973),
Offset(15.903199608216863, 35.25316524725598),
Offset(13.57741782841064, 32.27000071222682),
Offset(12.442030802775209, 29.665215617986277),
Offset(11.981806515947115, 27.560177578292762),
Offset(11.879421136842055, 25.918712565594948),
Offset(11.95091483982305, 24.66543021784112),
Offset(12.092167805674123, 23.72603017548901),
Offset(12.245452640806768, 23.03857447590349),
Offset(12.379956070248545, 22.554583229506296),
Offset(12.480582865035407, 22.237279988168645),
Offset(12.541514124262473, 22.059212079933666),
Offset(12.562455771803593, 22.000123717314214),
Offset(12.562499999999996, 22.000000000000004),
],
<Offset>[
Offset(42.0, 22.0),
Offset(41.99458528858859, 22.361234167441474),
Offset(41.91859127809106, 23.620246996030513),
Offset(41.501535596836376, 26.09905798461081),
Offset(40.02840620381446, 30.021099432452637),
Offset(35.79419835461124, 35.2186537827727),
Offset(30.076040790179817, 38.175916954629336),
Offset(24.067012730992623, 38.57855959743385),
Offset(19.453150566288006, 37.096490556388844),
Offset(16.506465839286186, 34.99409280868502),
Offset(14.73924581501028, 32.939784778587686),
Offset(13.715334530064114, 31.165018854170466),
Offset(13.140377980959201, 29.714761542791386),
Offset(12.83036672005031, 28.56755327976071),
Offset(12.672939622830032, 27.683643609921106),
Offset(12.600162038813565, 27.02281609043513),
Offset(12.571432188039635, 26.54999771317575),
Offset(12.56310619400641, 26.23642863509033),
Offset(12.562193301685781, 26.059158626029138),
Offset(12.562499038934627, 26.000123717080207),
Offset(12.562499999999996, 26.000000000000004),
],
<Offset>[
Offset(42.0, 22.0),
Offset(41.99458528858859, 22.361234167441474),
Offset(41.91859127809106, 23.620246996030513),
Offset(41.501535596836376, 26.09905798461081),
Offset(40.02840620381446, 30.021099432452637),
Offset(35.79419835461124, 35.2186537827727),
Offset(30.076040790179817, 38.175916954629336),
Offset(24.067012730992623, 38.57855959743385),
Offset(19.453150566288006, 37.096490556388844),
Offset(16.506465839286186, 34.99409280868502),
Offset(14.73924581501028, 32.939784778587686),
Offset(13.715334530064114, 31.165018854170466),
Offset(13.140377980959201, 29.714761542791386),
Offset(12.83036672005031, 28.56755327976071),
Offset(12.672939622830032, 27.683643609921106),
Offset(12.600162038813565, 27.02281609043513),
Offset(12.571432188039635, 26.54999771317575),
Offset(12.56310619400641, 26.23642863509033),
Offset(12.562193301685781, 26.059158626029138),
Offset(12.562499038934627, 26.000123717080207),
Offset(12.562499999999996, 26.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 22.0),
Offset(41.99458528858859, 22.361234167441474),
Offset(41.91859127809106, 23.620246996030513),
Offset(41.501535596836376, 26.09905798461081),
Offset(40.02840620381446, 30.021099432452637),
Offset(35.79419835461124, 35.2186537827727),
Offset(30.076040790179817, 38.175916954629336),
Offset(24.067012730992623, 38.57855959743385),
Offset(19.453150566288006, 37.096490556388844),
Offset(16.506465839286186, 34.99409280868502),
Offset(14.73924581501028, 32.939784778587686),
Offset(13.715334530064114, 31.165018854170466),
Offset(13.140377980959201, 29.714761542791386),
Offset(12.83036672005031, 28.56755327976071),
Offset(12.672939622830032, 27.683643609921106),
Offset(12.600162038813565, 27.02281609043513),
Offset(12.571432188039635, 26.54999771317575),
Offset(12.56310619400641, 26.23642863509033),
Offset(12.562193301685781, 26.059158626029138),
Offset(12.562499038934627, 26.000123717080207),
Offset(12.562499999999996, 26.000000000000004),
],
<Offset>[
Offset(6.0, 22.0),
Offset(6.056934514707525, 21.63899352743156),
Offset(6.3138203227485405, 20.384389840375796),
Offset(7.096666807426793, 17.931786874735423),
Offset(9.197983716971518, 14.110555792928775),
Offset(14.492848562759846, 9.262883961619078),
Offset(21.26860668167255, 6.947111219644562),
Offset(28.222185090070198, 7.526686671873211),
Offset(33.453333439427794, 10.134368158658866),
Offset(36.69157710577769, 13.290289151940406),
Offset(38.53223137521963, 16.248244324219414),
Offset(39.50406341592221, 18.763506401664923),
Offset(39.965161333050226, 20.80420892269316),
Offset(40.139843919215444, 22.41260360500229),
Offset(40.164704435685586, 23.649282378914172),
Offset(40.1214749003011, 24.572646331189105),
Offset(40.057897202434084, 25.232737230122385),
Offset(40.00055137536795, 25.670250802073745),
Offset(39.96271993040885, 25.917501645087587),
Offset(39.949247443632466, 25.99982748057223),
Offset(39.94921875, 26.0),
],
<Offset>[
Offset(6.0, 22.0),
Offset(6.056934514707525, 21.63899352743156),
Offset(6.3138203227485405, 20.384389840375796),
Offset(7.096666807426793, 17.931786874735423),
Offset(9.197983716971518, 14.110555792928775),
Offset(14.492848562759846, 9.262883961619078),
Offset(21.26860668167255, 6.947111219644562),
Offset(28.222185090070198, 7.526686671873211),
Offset(33.453333439427794, 10.134368158658866),
Offset(36.69157710577769, 13.290289151940406),
Offset(38.53223137521963, 16.248244324219414),
Offset(39.50406341592221, 18.763506401664923),
Offset(39.965161333050226, 20.80420892269316),
Offset(40.139843919215444, 22.41260360500229),
Offset(40.164704435685586, 23.649282378914172),
Offset(40.1214749003011, 24.572646331189105),
Offset(40.057897202434084, 25.232737230122385),
Offset(40.00055137536795, 25.670250802073745),
Offset(39.96271993040885, 25.917501645087587),
Offset(39.949247443632466, 25.99982748057223),
Offset(39.94921875, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 22.0),
Offset(6.056934514707525, 21.63899352743156),
Offset(6.3138203227485405, 20.384389840375796),
Offset(7.096666807426793, 17.931786874735423),
Offset(9.197983716971518, 14.110555792928775),
Offset(14.492848562759846, 9.262883961619078),
Offset(21.26860668167255, 6.947111219644562),
Offset(28.222185090070198, 7.526686671873211),
Offset(33.453333439427794, 10.134368158658866),
Offset(36.69157710577769, 13.290289151940406),
Offset(38.53223137521963, 16.248244324219414),
Offset(39.50406341592221, 18.763506401664923),
Offset(39.965161333050226, 20.80420892269316),
Offset(40.139843919215444, 22.41260360500229),
Offset(40.164704435685586, 23.649282378914172),
Offset(40.1214749003011, 24.572646331189105),
Offset(40.057897202434084, 25.232737230122385),
Offset(40.00055137536795, 25.670250802073745),
Offset(39.96271993040885, 25.917501645087587),
Offset(39.949247443632466, 25.99982748057223),
Offset(39.94921875, 26.0),
],
<Offset>[
Offset(6.0, 26.0),
Offset(5.976562557689849, 25.638185989482512),
Offset(5.951781669661045, 24.367972149512962),
Offset(6.172793116155802, 21.823631861702058),
Offset(7.363587976838016, 17.665129222832853),
Offset(11.400806749308899, 11.800457098273661),
Offset(17.41878573585796, 8.03287301910486),
Offset(24.257523532175192, 6.996159828679087),
Offset(29.90338248135665, 8.291042849526),
Offset(33.76252909490214, 10.56619705548221),
Offset(36.23501636298456, 12.973675163618006),
Offset(37.77053540180521, 15.158665125787222),
Offset(38.70420448893307, 17.008159945496722),
Offset(39.260392038988186, 18.5104805430827),
Offset(39.58393261852967, 19.691668944482075),
Offset(39.766765502294305, 20.58840471665747),
Offset(39.866421084642994, 21.237322746452932),
Offset(39.91802804639694, 21.671102155152063),
Offset(39.94204075298555, 21.917555098992118),
Offset(39.94920417650143, 21.999827480806236),
Offset(39.94921875, 22.0),
],
<Offset>[
Offset(6.0, 26.0),
Offset(5.976562557689849, 25.638185989482512),
Offset(5.951781669661045, 24.367972149512962),
Offset(6.172793116155802, 21.823631861702058),
Offset(7.363587976838016, 17.665129222832853),
Offset(11.400806749308899, 11.800457098273661),
Offset(17.41878573585796, 8.03287301910486),
Offset(24.257523532175192, 6.996159828679087),
Offset(29.90338248135665, 8.291042849526),
Offset(33.76252909490214, 10.56619705548221),
Offset(36.23501636298456, 12.973675163618006),
Offset(37.77053540180521, 15.158665125787222),
Offset(38.70420448893307, 17.008159945496722),
Offset(39.260392038988186, 18.5104805430827),
Offset(39.58393261852967, 19.691668944482075),
Offset(39.766765502294305, 20.58840471665747),
Offset(39.866421084642994, 21.237322746452932),
Offset(39.91802804639694, 21.671102155152063),
Offset(39.94204075298555, 21.917555098992118),
Offset(39.94920417650143, 21.999827480806236),
Offset(39.94921875, 22.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 36.0),
Offset(5.8396336833594695, 35.66398057820908),
Offset(5.329309336374063, 34.47365089829387),
Offset(4.546341863759643, 32.03857491308836),
Offset(3.9472816617934896, 27.893335303194206),
Offset(4.788314785722232, 21.470485758169694),
Offset(7.406922551234356, 16.186721598040453),
Offset(10.987511722222681, 12.449414121983239),
Offset(14.290737577882037, 10.382465570533384),
Offset(16.84152025666389, 9.340052761292668),
Offset(18.753361861843203, 8.79207829497377),
Offset(20.19495897321279, 8.483469022255434),
Offset(21.293826339887335, 8.297708512391797),
Offset(22.135385178177998, 8.180000583359465),
Offset(22.776244370552647, 8.102975309903787),
Offset(23.25488929254563, 8.051973096906334),
Offset(23.598629725699347, 8.018606137477462),
Offset(23.827700643867974, 7.99783596371886),
Offset(23.95771797811348, 7.986559676107813),
Offset(24.001111438945117, 7.982878122631195),
Offset(24.001202429357242, 7.98287044589657),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 36.0),
Offset(5.8396336833594695, 35.66398057820908),
Offset(5.329309336374063, 34.47365089829387),
Offset(4.546341863759643, 32.03857491308836),
Offset(3.9472816617934896, 27.893335303194206),
Offset(4.788314785722232, 21.470485758169694),
Offset(7.406922551234356, 16.186721598040453),
Offset(10.987511722222681, 12.449414121983239),
Offset(14.290737577882037, 10.382465570533384),
Offset(16.84152025666389, 9.340052761292668),
Offset(18.753361861843203, 8.79207829497377),
Offset(20.19495897321279, 8.483469022255434),
Offset(21.293826339887335, 8.297708512391797),
Offset(22.135385178177998, 8.180000583359465),
Offset(22.776244370552647, 8.102975309903787),
Offset(23.25488929254563, 8.051973096906334),
Offset(23.598629725699347, 8.018606137477462),
Offset(23.827700643867974, 7.99783596371886),
Offset(23.95771797811348, 7.986559676107813),
Offset(24.001111438945117, 7.982878122631195),
Offset(24.001202429357242, 7.98287044589657),
],
<Offset>[
Offset(42.0, 36.0),
Offset(41.7493389152824, 36.20520796529164),
Offset(40.85819701033384, 36.89246335931071),
Offset(39.01294315759756, 38.1256246432051),
Offset(35.758514239960064, 39.76970128020763),
Offset(30.180134511403956, 41.28645636464381),
Offset(24.56603417073137, 41.32925393403815),
Offset(19.271926095830622, 39.91690773672663),
Offset(15.201959304751512, 37.5726832793895),
Offset(12.456295622648877, 35.01429311055303),
Offset(10.686459838185314, 32.608514843335385),
Offset(9.579921816288039, 30.502293804851334),
Offset(8.90802993167501, 28.734147272525124),
Offset(8.513791284564158, 27.294928344333726),
Offset(8.292240475325507, 26.156988797411067),
Offset(8.174465865426919, 25.287693028463128),
Offset(8.11616441641861, 24.655137447505503),
Offset(8.089821190085125, 24.230473791307258),
Offset(8.079382709319852, 23.988506993748523),
Offset(8.076631388780909, 23.907616552409003),
Offset(8.076626005900048, 23.907446869353766),
],
<Offset>[
Offset(42.0, 36.0),
Offset(41.7493389152824, 36.20520796529164),
Offset(40.85819701033384, 36.89246335931071),
Offset(39.01294315759756, 38.1256246432051),
Offset(35.758514239960064, 39.76970128020763),
Offset(30.180134511403956, 41.28645636464381),
Offset(24.56603417073137, 41.32925393403815),
Offset(19.271926095830622, 39.91690773672663),
Offset(15.201959304751512, 37.5726832793895),
Offset(12.456295622648877, 35.01429311055303),
Offset(10.686459838185314, 32.608514843335385),
Offset(9.579921816288039, 30.502293804851334),
Offset(8.90802993167501, 28.734147272525124),
Offset(8.513791284564158, 27.294928344333726),
Offset(8.292240475325507, 26.156988797411067),
Offset(8.174465865426919, 25.287693028463128),
Offset(8.11616441641861, 24.655137447505503),
Offset(8.089821190085125, 24.230473791307258),
Offset(8.079382709319852, 23.988506993748523),
Offset(8.076631388780909, 23.907616552409003),
Offset(8.076626005900048, 23.907446869353766),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 36.0),
Offset(41.7493389152824, 36.20520796529164),
Offset(40.85819701033384, 36.89246335931071),
Offset(39.01294315759756, 38.1256246432051),
Offset(35.758514239960064, 39.76970128020763),
Offset(30.180134511403956, 41.28645636464381),
Offset(24.56603417073137, 41.32925393403815),
Offset(19.271926095830622, 39.91690773672663),
Offset(15.201959304751512, 37.5726832793895),
Offset(12.456295622648877, 35.01429311055303),
Offset(10.686459838185314, 32.608514843335385),
Offset(9.579921816288039, 30.502293804851334),
Offset(8.90802993167501, 28.734147272525124),
Offset(8.513791284564158, 27.294928344333726),
Offset(8.292240475325507, 26.156988797411067),
Offset(8.174465865426919, 25.287693028463128),
Offset(8.11616441641861, 24.655137447505503),
Offset(8.089821190085125, 24.230473791307258),
Offset(8.079382709319852, 23.988506993748523),
Offset(8.076631388780909, 23.907616552409003),
Offset(8.076626005900048, 23.907446869353766),
],
<Offset>[
Offset(42.0, 32.0),
Offset(41.803966700752746, 32.205577011286266),
Offset(41.104447603276626, 32.89996903899956),
Offset(39.64402995767152, 34.17517788052204),
Offset(37.031973302731046, 35.97545970343111),
Offset(32.44508133022271, 37.98012671725157),
Offset(27.6644042246058, 38.77327245743646),
Offset(22.963108117227325, 38.302914175295534),
Offset(19.18039906547299, 36.862333955479784),
Offset(16.509090720567585, 35.04434211490934),
Offset(14.703380298498667, 33.21759365821649),
Offset(13.512146444284534, 31.556733263561572),
Offset(12.740174664860898, 30.12862517729895),
Offset(12.248059307884624, 28.947244716051806),
Offset(11.939734974297815, 28.002595790430043),
Offset(11.750425410476474, 27.27521551305395),
Offset(11.637314290474384, 26.742992599694542),
Offset(11.572897732210654, 26.384358993735816),
Offset(11.54031155133882, 26.17955109507089),
Offset(11.530083003283234, 26.111009046369567),
Offset(11.530061897030713, 26.110865227715482),
],
<Offset>[
Offset(42.0, 32.0),
Offset(41.803966700752746, 32.205577011286266),
Offset(41.104447603276626, 32.89996903899956),
Offset(39.64402995767152, 34.17517788052204),
Offset(37.031973302731046, 35.97545970343111),
Offset(32.44508133022271, 37.98012671725157),
Offset(27.6644042246058, 38.77327245743646),
Offset(22.963108117227325, 38.302914175295534),
Offset(19.18039906547299, 36.862333955479784),
Offset(16.509090720567585, 35.04434211490934),
Offset(14.703380298498667, 33.21759365821649),
Offset(13.512146444284534, 31.556733263561572),
Offset(12.740174664860898, 30.12862517729895),
Offset(12.248059307884624, 28.947244716051806),
Offset(11.939734974297815, 28.002595790430043),
Offset(11.750425410476474, 27.27521551305395),
Offset(11.637314290474384, 26.742992599694542),
Offset(11.572897732210654, 26.384358993735816),
Offset(11.54031155133882, 26.17955109507089),
Offset(11.530083003283234, 26.111009046369567),
Offset(11.530061897030713, 26.110865227715482),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 32.0),
Offset(41.803966700752746, 32.205577011286266),
Offset(41.104447603276626, 32.89996903899956),
Offset(39.64402995767152, 34.17517788052204),
Offset(37.031973302731046, 35.97545970343111),
Offset(32.44508133022271, 37.98012671725157),
Offset(27.6644042246058, 38.77327245743646),
Offset(22.963108117227325, 38.302914175295534),
Offset(19.18039906547299, 36.862333955479784),
Offset(16.509090720567585, 35.04434211490934),
Offset(14.703380298498667, 33.21759365821649),
Offset(13.512146444284534, 31.556733263561572),
Offset(12.740174664860898, 30.12862517729895),
Offset(12.248059307884624, 28.947244716051806),
Offset(11.939734974297815, 28.002595790430043),
Offset(11.750425410476474, 27.27521551305395),
Offset(11.637314290474384, 26.742992599694542),
Offset(11.572897732210654, 26.384358993735816),
Offset(11.54031155133882, 26.17955109507089),
Offset(11.530083003283234, 26.111009046369567),
Offset(11.530061897030713, 26.110865227715482),
],
<Offset>[
Offset(6.0, 32.0),
Offset(5.899914425897517, 31.66443482499171),
Offset(5.601001082666045, 30.482888615847468),
Offset(5.242005036683729, 28.09953280239226),
Offset(5.346316156571252, 24.145975901906155),
Offset(7.249241148069178, 18.317100047682345),
Offset(10.710823881370487, 13.931896549234073),
Offset(14.817117889097364, 11.294374466111893),
Offset(18.288493245756, 10.248489378687303),
Offset(20.784419638077317, 10.013509863155594),
Offset(22.541938014255397, 10.075312777589325),
Offset(23.798109358346892, 10.220508832423288),
Offset(24.71461203122786, 10.370924674281323),
Offset(25.392890381083, 10.501349297587215),
Offset(25.896277759611298, 10.60605174724228),
Offset(26.265268043339944, 10.685909272436422),
Offset(26.526795349038366, 10.74364670273436),
Offset(26.699555102368272, 10.782158496973931),
Offset(26.79709065296033, 10.80399872839147),
Offset(26.829561509459538, 10.811282301423006),
Offset(26.829629554119695, 10.811297570626497),
],
<Offset>[
Offset(6.0, 32.0),
Offset(5.899914425897517, 31.66443482499171),
Offset(5.601001082666045, 30.482888615847468),
Offset(5.242005036683729, 28.09953280239226),
Offset(5.346316156571252, 24.145975901906155),
Offset(7.249241148069178, 18.317100047682345),
Offset(10.710823881370487, 13.931896549234073),
Offset(14.817117889097364, 11.294374466111893),
Offset(18.288493245756, 10.248489378687303),
Offset(20.784419638077317, 10.013509863155594),
Offset(22.541938014255397, 10.075312777589325),
Offset(23.798109358346892, 10.220508832423288),
Offset(24.71461203122786, 10.370924674281323),
Offset(25.392890381083, 10.501349297587215),
Offset(25.896277759611298, 10.60605174724228),
Offset(26.265268043339944, 10.685909272436422),
Offset(26.526795349038366, 10.74364670273436),
Offset(26.699555102368272, 10.782158496973931),
Offset(26.79709065296033, 10.80399872839147),
Offset(26.829561509459538, 10.811282301423006),
Offset(26.829629554119695, 10.811297570626497),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 32.0),
Offset(5.899914425897517, 31.66443482499171),
Offset(5.601001082666045, 30.482888615847468),
Offset(5.242005036683729, 28.09953280239226),
Offset(5.346316156571252, 24.145975901906155),
Offset(7.249241148069178, 18.317100047682345),
Offset(10.710823881370487, 13.931896549234073),
Offset(14.817117889097364, 11.294374466111893),
Offset(18.288493245756, 10.248489378687303),
Offset(20.784419638077317, 10.013509863155594),
Offset(22.541938014255397, 10.075312777589325),
Offset(23.798109358346892, 10.220508832423288),
Offset(24.71461203122786, 10.370924674281323),
Offset(25.392890381083, 10.501349297587215),
Offset(25.896277759611298, 10.60605174724228),
Offset(26.265268043339944, 10.685909272436422),
Offset(26.526795349038366, 10.74364670273436),
Offset(26.699555102368272, 10.782158496973931),
Offset(26.79709065296033, 10.80399872839147),
Offset(26.829561509459538, 10.811282301423006),
Offset(26.829629554119695, 10.811297570626497),
],
<Offset>[
Offset(6.0, 36.0),
Offset(5.839633683308566, 35.66398057820831),
Offset(5.329309336323984, 34.47365089829046),
Offset(4.546341863735712, 32.03857491308413),
Offset(3.947281661825336, 27.893335303206097),
Offset(4.788314785746671, 21.47048575818877),
Offset(7.406922551270995, 16.18672159809414),
Offset(10.98751172223972, 12.449414122039723),
Offset(14.290737577881032, 10.382465570503403),
Offset(16.841520256655304, 9.340052761342939),
Offset(18.753361861827802, 8.792078295019234),
Offset(20.194958973207576, 8.483469022266245),
Offset(21.293826339889407, 8.297708512388375),
Offset(22.13538517817335, 8.180000583365981),
Offset(22.776244370563283, 8.102975309890528),
Offset(23.25488929251534, 8.051973096940955),
Offset(23.598629725644848, 8.018606137536025),
Offset(23.82770064384222, 7.997835963745423),
Offset(23.957717978081078, 7.986559676140466),
Offset(24.001111438940168, 7.982878122636148),
Offset(24.001202429373503, 7.982870445880305),
],
<Offset>[
Offset(6.0, 36.0),
Offset(5.839633683308566, 35.66398057820831),
Offset(5.329309336323984, 34.47365089829046),
Offset(4.546341863735712, 32.03857491308413),
Offset(3.947281661825336, 27.893335303206097),
Offset(4.788314785746671, 21.47048575818877),
Offset(7.406922551270995, 16.18672159809414),
Offset(10.98751172223972, 12.449414122039723),
Offset(14.290737577881032, 10.382465570503403),
Offset(16.841520256655304, 9.340052761342939),
Offset(18.753361861827802, 8.792078295019234),
Offset(20.194958973207576, 8.483469022266245),
Offset(21.293826339889407, 8.297708512388375),
Offset(22.13538517817335, 8.180000583365981),
Offset(22.776244370563283, 8.102975309890528),
Offset(23.25488929251534, 8.051973096940955),
Offset(23.598629725644848, 8.018606137536025),
Offset(23.82770064384222, 7.997835963745423),
Offset(23.957717978081078, 7.986559676140466),
Offset(24.001111438940168, 7.982878122636148),
Offset(24.001202429373503, 7.982870445880305),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 16.0),
Offset(6.222470088677106, 15.614531066984553),
Offset(7.071161725316092, 14.306422712262563),
Offset(9.085869786142727, 11.907139949336411),
Offset(13.311519331212619, 8.711520321213257),
Offset(21.694206315186374, 6.462423500731354),
Offset(30.07031570748504, 8.471955170698632),
Offset(36.20036889900587, 14.155750775196541),
Offset(38.533897479983715, 20.76099122996903),
Offset(38.182626701431914, 26.194302454359914),
Offset(36.59711302702814, 30.110286603895076),
Offset(34.63761335058528, 32.76106836363335),
Offset(32.7272901891386, 34.4927008221791),
Offset(31.04869117038896, 35.596105690451935),
Offset(29.664526028757855, 36.28441549314729),
Offset(28.581655311555835, 36.70452225851578),
Offset(27.782897949107628, 36.95396775456513),
Offset(27.242531133855476, 37.09522522130338),
Offset(26.933380541033216, 37.166375518103024),
Offset(26.82984682779076, 37.188656481991416),
Offset(26.829629554103434, 37.18870242935725),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 16.0),
Offset(6.222470088677106, 15.614531066984553),
Offset(7.071161725316092, 14.306422712262563),
Offset(9.085869786142727, 11.907139949336411),
Offset(13.311519331212619, 8.711520321213257),
Offset(21.694206315186374, 6.462423500731354),
Offset(30.07031570748504, 8.471955170698632),
Offset(36.20036889900587, 14.155750775196541),
Offset(38.533897479983715, 20.76099122996903),
Offset(38.182626701431914, 26.194302454359914),
Offset(36.59711302702814, 30.110286603895076),
Offset(34.63761335058528, 32.76106836363335),
Offset(32.7272901891386, 34.4927008221791),
Offset(31.04869117038896, 35.596105690451935),
Offset(29.664526028757855, 36.28441549314729),
Offset(28.581655311555835, 36.70452225851578),
Offset(27.782897949107628, 36.95396775456513),
Offset(27.242531133855476, 37.09522522130338),
Offset(26.933380541033216, 37.166375518103024),
Offset(26.82984682779076, 37.188656481991416),
Offset(26.829629554103434, 37.18870242935725),
],
<Offset>[
Offset(42.0, 16.0),
Offset(42.119273441095075, 16.516374018071716),
Offset(42.428662704565184, 18.32937541467259),
Offset(42.54812490043565, 21.94159775950881),
Offset(41.3111285319893, 27.683594454682137),
Offset(36.06395079582478, 35.01020271691918),
Offset(28.59459512599702, 38.51093769070532),
Offset(21.239886122259133, 38.07233071493643),
Offset(16.251628495692138, 35.34156866251391),
Offset(13.527101819238178, 32.27103394597236),
Offset(12.16858814546228, 29.604397296366464),
Offset(11.548946515009288, 27.474331231158473),
Offset(11.311114637013635, 25.826563435488687),
Offset(11.262012546535352, 24.572239162454554),
Offset(11.298221100690522, 23.63118177535833),
Offset(11.364474416879979, 22.940254245947138),
Offset(11.431638843687892, 22.451805922237554),
Offset(11.485090012547001, 22.130328573710905),
Offset(11.518417313485447, 21.949395273355513),
Offset(11.530012405933167, 21.889264075838188),
Offset(11.53003696527787, 21.889138124802937),
],
<Offset>[
Offset(42.0, 16.0),
Offset(42.119273441095075, 16.516374018071716),
Offset(42.428662704565184, 18.32937541467259),
Offset(42.54812490043565, 21.94159775950881),
Offset(41.3111285319893, 27.683594454682137),
Offset(36.06395079582478, 35.01020271691918),
Offset(28.59459512599702, 38.51093769070532),
Offset(21.239886122259133, 38.07233071493643),
Offset(16.251628495692138, 35.34156866251391),
Offset(13.527101819238178, 32.27103394597236),
Offset(12.16858814546228, 29.604397296366464),
Offset(11.548946515009288, 27.474331231158473),
Offset(11.311114637013635, 25.826563435488687),
Offset(11.262012546535352, 24.572239162454554),
Offset(11.298221100690522, 23.63118177535833),
Offset(11.364474416879979, 22.940254245947138),
Offset(11.431638843687892, 22.451805922237554),
Offset(11.485090012547001, 22.130328573710905),
Offset(11.518417313485447, 21.949395273355513),
Offset(11.530012405933167, 21.889264075838188),
Offset(11.53003696527787, 21.889138124802937),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 16.0),
Offset(42.119273441095075, 16.516374018071716),
Offset(42.428662704565184, 18.32937541467259),
Offset(42.54812490043565, 21.94159775950881),
Offset(41.3111285319893, 27.683594454682137),
Offset(36.06395079582478, 35.01020271691918),
Offset(28.59459512599702, 38.51093769070532),
Offset(21.239886122259133, 38.07233071493643),
Offset(16.251628495692138, 35.34156866251391),
Offset(13.527101819238178, 32.27103394597236),
Offset(12.16858814546228, 29.604397296366464),
Offset(11.548946515009288, 27.474331231158473),
Offset(11.311114637013635, 25.826563435488687),
Offset(11.262012546535352, 24.572239162454554),
Offset(11.298221100690522, 23.63118177535833),
Offset(11.364474416879979, 22.940254245947138),
Offset(11.431638843687892, 22.451805922237554),
Offset(11.485090012547001, 22.130328573710905),
Offset(11.518417313485447, 21.949395273355513),
Offset(11.530012405933167, 21.889264075838188),
Offset(11.53003696527787, 21.889138124802937),
],
<Offset>[
Offset(42.0, 12.0),
Offset(42.22538630246601, 12.517777761542249),
Offset(42.90619853384615, 14.357900907446863),
Offset(43.759884509852945, 18.128995147835514),
Offset(43.66585885175813, 24.44736028078141),
Offset(39.74861752085834, 33.43380529842439),
Offset(32.57188683977151, 39.07136996422343),
Offset(24.376857043988256, 40.600018479197814),
Offset(17.959269400168804, 39.004426856660785),
Offset(13.850567169499653, 36.311009998593796),
Offset(11.374155956344177, 33.58880277176081),
Offset(9.917496515696001, 31.204288894581083),
Offset(9.07498759074148, 29.236785710939074),
Offset(8.597571742452605, 27.666692096657314),
Offset(8.334783321442917, 26.44693980672826),
Offset(8.195874559699876, 25.52824222288586),
Offset(8.126295299747222, 24.866824239052814),
Offset(8.093843447379264, 24.426077640310794),
Offset(8.080338503727083, 24.17611706018137),
Offset(8.076619249177135, 24.092742069165425),
Offset(8.07661186374038, 24.09256727275783),
],
<Offset>[
Offset(42.0, 12.0),
Offset(42.22538630246601, 12.517777761542249),
Offset(42.90619853384615, 14.357900907446863),
Offset(43.759884509852945, 18.128995147835514),
Offset(43.66585885175813, 24.44736028078141),
Offset(39.74861752085834, 33.43380529842439),
Offset(32.57188683977151, 39.07136996422343),
Offset(24.376857043988256, 40.600018479197814),
Offset(17.959269400168804, 39.004426856660785),
Offset(13.850567169499653, 36.311009998593796),
Offset(11.374155956344177, 33.58880277176081),
Offset(9.917496515696001, 31.204288894581083),
Offset(9.07498759074148, 29.236785710939074),
Offset(8.597571742452605, 27.666692096657314),
Offset(8.334783321442917, 26.44693980672826),
Offset(8.195874559699876, 25.52824222288586),
Offset(8.126295299747222, 24.866824239052814),
Offset(8.093843447379264, 24.426077640310794),
Offset(8.080338503727083, 24.17611706018137),
Offset(8.076619249177135, 24.092742069165425),
Offset(8.07661186374038, 24.09256727275783),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 12.0),
Offset(42.22538630246601, 12.517777761542249),
Offset(42.90619853384615, 14.357900907446863),
Offset(43.759884509852945, 18.128995147835514),
Offset(43.66585885175813, 24.44736028078141),
Offset(39.74861752085834, 33.43380529842439),
Offset(32.57188683977151, 39.07136996422343),
Offset(24.376857043988256, 40.600018479197814),
Offset(17.959269400168804, 39.004426856660785),
Offset(13.850567169499653, 36.311009998593796),
Offset(11.374155956344177, 33.58880277176081),
Offset(9.917496515696001, 31.204288894581083),
Offset(9.07498759074148, 29.236785710939074),
Offset(8.597571742452605, 27.666692096657314),
Offset(8.334783321442917, 26.44693980672826),
Offset(8.195874559699876, 25.52824222288586),
Offset(8.126295299747222, 24.866824239052814),
Offset(8.093843447379264, 24.426077640310794),
Offset(8.080338503727083, 24.17611706018137),
Offset(8.076619249177135, 24.092742069165425),
Offset(8.07661186374038, 24.09256727275783),
],
<Offset>[
Offset(6.0, 12.0),
Offset(6.3229312318803075, 11.61579282114921),
Offset(7.523361420980265, 10.332065476778915),
Offset(10.234818160108134, 8.075701885898315),
Offset(15.555284551985588, 5.400098023461183),
Offset(25.267103519984172, 4.663978182144188),
Offset(34.065497532306516, 8.668225867992323),
Offset(39.59155761731576, 16.27703318845691),
Offset(40.72409454498984, 24.108085016590273),
Offset(39.139841854472834, 30.0780814324673),
Offset(36.514293313228855, 34.10942912386185),
Offset(33.744815583253256, 36.6601595585975),
Offset(31.226861893018718, 38.20062678263231),
Offset(29.10189988007002, 39.09038725780428),
Offset(27.3951953205187, 39.57837027981981),
Offset(26.083922435637483, 39.82883505984612),
Offset(25.128742795932077, 39.94653528477588),
Offset(24.487982707377697, 39.99564983955995),
Offset(24.123290412440365, 40.013021521592925),
Offset(24.001457946431486, 40.017121849607435),
Offset(24.001202429333205, 40.017129554079396),
],
<Offset>[
Offset(6.0, 12.0),
Offset(6.3229312318803075, 11.61579282114921),
Offset(7.523361420980265, 10.332065476778915),
Offset(10.234818160108134, 8.075701885898315),
Offset(15.555284551985588, 5.400098023461183),
Offset(25.267103519984172, 4.663978182144188),
Offset(34.065497532306516, 8.668225867992323),
Offset(39.59155761731576, 16.27703318845691),
Offset(40.72409454498984, 24.108085016590273),
Offset(39.139841854472834, 30.0780814324673),
Offset(36.514293313228855, 34.10942912386185),
Offset(33.744815583253256, 36.6601595585975),
Offset(31.226861893018718, 38.20062678263231),
Offset(29.10189988007002, 39.09038725780428),
Offset(27.3951953205187, 39.57837027981981),
Offset(26.083922435637483, 39.82883505984612),
Offset(25.128742795932077, 39.94653528477588),
Offset(24.487982707377697, 39.99564983955995),
Offset(24.123290412440365, 40.013021521592925),
Offset(24.001457946431486, 40.017121849607435),
Offset(24.001202429333205, 40.017129554079396),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 12.0),
Offset(6.3229312318803075, 11.61579282114921),
Offset(7.523361420980265, 10.332065476778915),
Offset(10.234818160108134, 8.075701885898315),
Offset(15.555284551985588, 5.400098023461183),
Offset(25.267103519984172, 4.663978182144188),
Offset(34.065497532306516, 8.668225867992323),
Offset(39.59155761731576, 16.27703318845691),
Offset(40.72409454498984, 24.108085016590273),
Offset(39.139841854472834, 30.0780814324673),
Offset(36.514293313228855, 34.10942912386185),
Offset(33.744815583253256, 36.6601595585975),
Offset(31.226861893018718, 38.20062678263231),
Offset(29.10189988007002, 39.09038725780428),
Offset(27.3951953205187, 39.57837027981981),
Offset(26.083922435637483, 39.82883505984612),
Offset(25.128742795932077, 39.94653528477588),
Offset(24.487982707377697, 39.99564983955995),
Offset(24.123290412440365, 40.013021521592925),
Offset(24.001457946431486, 40.017121849607435),
Offset(24.001202429333205, 40.017129554079396),
],
<Offset>[
Offset(6.0, 16.0),
Offset(6.22247008872931, 15.614531066985863),
Offset(7.071161725356028, 14.306422712267109),
Offset(9.085869786222908, 11.907139949360454),
Offset(13.311519331206826, 8.711520321209331),
Offset(21.69420631520211, 6.462423500762615),
Offset(30.070315707485825, 8.471955170682651),
Offset(36.20036889903345, 14.155750775152455),
Offset(38.53389748002304, 20.760991229943293),
Offset(38.18262670145813, 26.194302454353455),
Offset(36.597113027065134, 30.110286603895844),
Offset(34.63761335066132, 32.761068363650764),
Offset(32.72729018913396, 34.49270082217723),
Offset(31.048691170407302, 35.59610569046216),
Offset(29.66452602881138, 36.28441549318417),
Offset(28.58165531160348, 36.70452225855387),
Offset(27.78289794916673, 36.95396775461755),
Offset(27.24253113386635, 37.09522522131371),
Offset(26.933380541051008, 37.16637551812059),
Offset(26.829846827821875, 37.18865648202253),
Offset(26.829629554079393, 37.188702429333205),
],
<Offset>[
Offset(6.0, 16.0),
Offset(6.22247008872931, 15.614531066985863),
Offset(7.071161725356028, 14.306422712267109),
Offset(9.085869786222908, 11.907139949360454),
Offset(13.311519331206826, 8.711520321209331),
Offset(21.69420631520211, 6.462423500762615),
Offset(30.070315707485825, 8.471955170682651),
Offset(36.20036889903345, 14.155750775152455),
Offset(38.53389748002304, 20.760991229943293),
Offset(38.18262670145813, 26.194302454353455),
Offset(36.597113027065134, 30.110286603895844),
Offset(34.63761335066132, 32.761068363650764),
Offset(32.72729018913396, 34.49270082217723),
Offset(31.048691170407302, 35.59610569046216),
Offset(29.66452602881138, 36.28441549318417),
Offset(28.58165531160348, 36.70452225855387),
Offset(27.78289794916673, 36.95396775461755),
Offset(27.24253113386635, 37.09522522131371),
Offset(26.933380541051008, 37.16637551812059),
Offset(26.829846827821875, 37.18865648202253),
Offset(26.829629554079393, 37.188702429333205),
],
),
_PathClose(
),
],
),
],
matchTextDirection: true,
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/menu_arrow.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/menu_arrow.g.dart",
"repo_id": "flutter",
"token_count": 29543
} | 619 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the visual properties of [MaterialBanner] widgets.
///
/// Descendant widgets obtain the current [MaterialBannerThemeData] object using
/// `MaterialBannerTheme.of(context)`. Instances of [MaterialBannerThemeData]
/// can be customized with [MaterialBannerThemeData.copyWith].
///
/// Typically a [MaterialBannerThemeData] is specified as part of the overall
/// [Theme] with [ThemeData.bannerTheme].
///
/// All [MaterialBannerThemeData] properties are `null` by default. When null,
/// the [MaterialBanner] will provide its own defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class MaterialBannerThemeData with Diagnosticable {
/// Creates a theme that can be used for [MaterialBannerTheme] or
/// [ThemeData.bannerTheme].
const MaterialBannerThemeData({
this.backgroundColor,
this.surfaceTintColor,
this.shadowColor,
this.dividerColor,
this.contentTextStyle,
this.elevation,
this.padding,
this.leadingPadding,
});
/// The background color of a [MaterialBanner].
final Color? backgroundColor;
/// Overrides the default value of [MaterialBanner.surfaceTintColor].
final Color? surfaceTintColor;
/// Overrides the default value of [MaterialBanner.shadowColor].
final Color? shadowColor;
/// Overrides the default value of [MaterialBanner.dividerColor].
final Color? dividerColor;
/// Used to configure the [DefaultTextStyle] for the [MaterialBanner.content]
/// widget.
final TextStyle? contentTextStyle;
/// Default value for [MaterialBanner.elevation].
//
// If null, MaterialBanner uses a default of 0.0.
final double? elevation;
/// The amount of space by which to inset [MaterialBanner.content].
final EdgeInsetsGeometry? padding;
/// The amount of space by which to inset [MaterialBanner.leading].
final EdgeInsetsGeometry? leadingPadding;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
MaterialBannerThemeData copyWith({
Color? backgroundColor,
Color? surfaceTintColor,
Color? shadowColor,
Color? dividerColor,
TextStyle? contentTextStyle,
double? elevation,
EdgeInsetsGeometry? padding,
EdgeInsetsGeometry? leadingPadding,
}) {
return MaterialBannerThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
shadowColor: shadowColor ?? this.shadowColor,
dividerColor: dividerColor ?? this.dividerColor,
contentTextStyle: contentTextStyle ?? this.contentTextStyle,
elevation: elevation ?? this.elevation,
padding: padding ?? this.padding,
leadingPadding: leadingPadding ?? this.leadingPadding,
);
}
/// Linearly interpolate between two Banner themes.
///
/// {@macro dart.ui.shadow.lerp}
static MaterialBannerThemeData lerp(MaterialBannerThemeData? a, MaterialBannerThemeData? b, double t) {
return MaterialBannerThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
dividerColor: Color.lerp(a?.dividerColor, b?.dividerColor, t),
contentTextStyle: TextStyle.lerp(a?.contentTextStyle, b?.contentTextStyle, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
padding: EdgeInsetsGeometry.lerp(a?.padding, b?.padding, t),
leadingPadding: EdgeInsetsGeometry.lerp(a?.leadingPadding, b?.leadingPadding, t),
);
}
@override
int get hashCode => Object.hash(
backgroundColor,
surfaceTintColor,
shadowColor,
dividerColor,
contentTextStyle,
elevation,
padding,
leadingPadding,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is MaterialBannerThemeData
&& other.backgroundColor == backgroundColor
&& other.surfaceTintColor == surfaceTintColor
&& other.shadowColor == shadowColor
&& other.dividerColor == dividerColor
&& other.contentTextStyle == contentTextStyle
&& other.elevation == elevation
&& other.padding == padding
&& other.leadingPadding == leadingPadding;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('dividerColor', dividerColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('contentTextStyle', contentTextStyle, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('leadingPadding', leadingPadding, defaultValue: null));
}
}
/// An inherited widget that defines the configuration for
/// [MaterialBanner]s in this widget's subtree.
///
/// Values specified here are used for [MaterialBanner] properties that are not
/// given an explicit non-null value.
class MaterialBannerTheme extends InheritedTheme {
/// Creates a banner theme that controls the configurations for
/// [MaterialBanner]s in its widget subtree.
const MaterialBannerTheme({
super.key,
this.data,
required super.child,
});
/// The properties for descendant [MaterialBanner] widgets.
final MaterialBannerThemeData? data;
/// The closest instance of this class's [data] value that encloses the given
/// context.
///
/// If there is no ancestor, it returns [ThemeData.bannerTheme]. Applications
/// can assume that the returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// MaterialBannerThemeData theme = MaterialBannerTheme.of(context);
/// ```
static MaterialBannerThemeData of(BuildContext context) {
final MaterialBannerTheme? bannerTheme = context.dependOnInheritedWidgetOfExactType<MaterialBannerTheme>();
return bannerTheme?.data ?? Theme.of(context).bannerTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return MaterialBannerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(MaterialBannerTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/banner_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/banner_theme.dart",
"repo_id": "flutter",
"token_count": 2218
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'checkbox_theme.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'toggleable.dart';
// Examples can assume:
// bool _throwShotAway = false;
// late StateSetter setState;
enum _CheckboxType { material, adaptive }
/// A Material Design checkbox.
///
/// The checkbox itself does not maintain any state. Instead, when the state of
/// the checkbox changes, the widget calls the [onChanged] callback. Most
/// widgets that use a checkbox will listen for the [onChanged] callback and
/// rebuild the checkbox with a new [value] to update the visual appearance of
/// the checkbox.
///
/// The checkbox can optionally display three values - true, false, and null -
/// if [tristate] is true. When [value] is null a dash is displayed. By default
/// [tristate] is false and the checkbox's [value] must be true or false.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// {@tool dartpad}
/// This example shows how you can override the default theme of
/// a [Checkbox] with a [MaterialStateProperty].
/// In this example, the checkbox's color will be `Colors.blue` when the [Checkbox]
/// is being pressed, hovered, or focused. Otherwise, the checkbox's color will
/// be `Colors.red`.
///
/// ** See code in examples/api/lib/material/checkbox/checkbox.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows what the checkbox error state looks like.
///
/// ** See code in examples/api/lib/material/checkbox/checkbox.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CheckboxListTile], which combines this widget with a [ListTile] so that
/// you can give the checkbox a label.
/// * [Switch], a widget with semantics similar to [Checkbox].
/// * [Radio], for selecting among a set of explicit values.
/// * [Slider], for selecting a value in a range.
/// * <https://material.io/design/components/selection-controls.html#checkboxes>
/// * <https://material.io/design/components/lists.html#types>
class Checkbox extends StatefulWidget {
/// Creates a Material Design checkbox.
///
/// The checkbox itself does not maintain any state. Instead, when the state of
/// the checkbox changes, the widget calls the [onChanged] callback. Most
/// widgets that use a checkbox will listen for the [onChanged] callback and
/// rebuild the checkbox with a new [value] to update the visual appearance of
/// the checkbox.
///
/// The following arguments are required:
///
/// * [value], which determines whether the checkbox is checked. The [value]
/// can only be null if [tristate] is true.
/// * [onChanged], which is called when the value of the checkbox should
/// change. It can be set to null to disable the checkbox.
const Checkbox({
super.key,
required this.value,
this.tristate = false,
required this.onChanged,
this.mouseCursor,
this.activeColor,
this.fillColor,
this.checkColor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
this.shape,
this.side,
this.isError = false,
this.semanticLabel,
}) : _checkboxType = _CheckboxType.material,
assert(tristate || value != null);
/// Creates an adaptive [Checkbox] based on whether the target platform is iOS
/// or macOS, following Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// On iOS and macOS, this constructor creates a [CupertinoCheckbox], which has
/// matching functionality and presentation as Material checkboxes, and are the
/// graphics expected on iOS. On other platforms, this creates a Material
/// design [Checkbox].
///
/// If a [CupertinoCheckbox] is created, the following parameters are ignored:
/// [mouseCursor], [fillColor], [hoverColor], [overlayColor], [splashRadius],
/// [materialTapTargetSize], [visualDensity], [isError]. However, [shape] and
/// [side] will still affect the [CupertinoCheckbox] and should be handled if
/// native fidelity is important.
///
/// The target platform is based on the current [Theme]: [ThemeData.platform].
const Checkbox.adaptive({
super.key,
required this.value,
this.tristate = false,
required this.onChanged,
this.mouseCursor,
this.activeColor,
this.fillColor,
this.checkColor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
this.shape,
this.side,
this.isError = false,
this.semanticLabel,
}) : _checkboxType = _CheckboxType.adaptive,
assert(tristate || value != null);
/// Whether this checkbox is checked.
///
/// When [tristate] is true, a value of null corresponds to the mixed state.
/// When [tristate] is false, this value must not be null.
final bool? value;
/// Called when the value of the checkbox should change.
///
/// The checkbox passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the checkbox with the new
/// value.
///
/// If this callback is null, the checkbox will be displayed as disabled
/// and will not respond to input gestures.
///
/// When the checkbox is tapped, if [tristate] is false (the default) then
/// the [onChanged] callback will be applied to `!value`. If [tristate] is
/// true this callback cycle from false to true to null.
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// ```dart
/// Checkbox(
/// value: _throwShotAway,
/// onChanged: (bool? newValue) {
/// setState(() {
/// _throwShotAway = newValue!;
/// });
/// },
/// )
/// ```
final ValueChanged<bool?>? onChanged;
/// {@template flutter.material.checkbox.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// When [value] is null and [tristate] is true, [MaterialState.selected] is
/// included as a state.
///
/// If null, then the value of [CheckboxThemeData.mouseCursor] is used. If
/// that is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], a [MouseCursor] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept
/// either a [MouseCursor] or a [MaterialStateProperty<MouseCursor>].
final MouseCursor? mouseCursor;
/// The color to use when this checkbox is checked.
///
/// Defaults to [ColorScheme.secondary].
///
/// If [fillColor] returns a non-null color in the [MaterialState.selected]
/// state, it will be used instead of this color.
final Color? activeColor;
/// {@template flutter.material.checkbox.fillColor}
/// The color that fills the checkbox, in all [MaterialState]s.
///
/// Resolves in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [fillColor] based on the current [MaterialState]
/// of the [Checkbox], providing a different [Color] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Checkbox(
/// value: true,
/// onChanged: (_){},
/// fillColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return Colors.orange.withOpacity(.32);
/// }
/// return Colors.orange;
/// })
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// If null, then the value of [activeColor] is used in the selected
/// state. If that is also null, the value of [CheckboxThemeData.fillColor]
/// is used. If that is also null, then [ThemeData.disabledColor] is used in
/// the disabled state, [ColorScheme.secondary] is used in the
/// selected state, and [ThemeData.unselectedWidgetColor] is used in the
/// default state.
final MaterialStateProperty<Color?>? fillColor;
/// {@template flutter.material.checkbox.checkColor}
/// The color to use for the check icon when this checkbox is checked.
/// {@endtemplate}
///
/// If null, then the value of [CheckboxThemeData.checkColor] is used. If
/// that is also null, then Color(0xFFFFFFFF) is used.
final Color? checkColor;
/// If true the checkbox's [value] can be true, false, or null.
///
/// [Checkbox] displays a dash when its value is null.
///
/// When a tri-state checkbox ([tristate] is true) is tapped, its [onChanged]
/// callback will be applied to true if the current value is false, to null if
/// value is true, and to false if value is null (i.e. it cycles through false
/// => true => null => false when tapped).
///
/// If tristate is false (the default), [value] must not be null.
final bool tristate;
/// {@template flutter.material.checkbox.materialTapTargetSize}
/// Configures the minimum size of the tap target.
/// {@endtemplate}
///
/// If null, then the value of [CheckboxThemeData.materialTapTargetSize] is
/// used. If that is also null, then the value of
/// [ThemeData.materialTapTargetSize] is used.
///
/// See also:
///
/// * [MaterialTapTargetSize], for a description of how this affects tap targets.
final MaterialTapTargetSize? materialTapTargetSize;
/// {@template flutter.material.checkbox.visualDensity}
/// Defines how compact the checkbox's layout will be.
/// {@endtemplate}
///
/// {@macro flutter.material.themedata.visualDensity}
///
/// If null, then the value of [CheckboxThemeData.visualDensity] is used. If
/// that is also null, then the value of [ThemeData.visualDensity] is used.
///
/// See also:
///
/// * [ThemeData.visualDensity], which specifies the [visualDensity] for all
/// widgets within a [Theme].
final VisualDensity? visualDensity;
/// The color for the checkbox's [Material] when it has the input focus.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.focused]
/// state, it will be used instead.
///
/// If null, then the value of [CheckboxThemeData.overlayColor] is used in the
/// focused state. If that is also null, then the value of
/// [ThemeData.focusColor] is used.
final Color? focusColor;
/// {@template flutter.material.checkbox.hoverColor}
/// The color for the checkbox's [Material] when a pointer is hovering over it.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.hovered]
/// state, it will be used instead.
/// {@endtemplate}
///
/// If null, then the value of [CheckboxThemeData.overlayColor] is used in the
/// hovered state. If that is also null, then the value of
/// [ThemeData.hoverColor] is used.
final Color? hoverColor;
/// {@template flutter.material.checkbox.overlayColor}
/// The color for the checkbox's [Material].
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// {@endtemplate}
///
/// If null, then the value of [activeColor] with alpha
/// [kRadialReactionAlpha], [focusColor] and [hoverColor] is used in the
/// pressed, focused and hovered state. If that is also null,
/// the value of [CheckboxThemeData.overlayColor] is used. If that is
/// also null, then the value of [ColorScheme.secondary] with alpha
/// [kRadialReactionAlpha], [ThemeData.focusColor] and [ThemeData.hoverColor]
/// is used in the pressed, focused and hovered state.
final MaterialStateProperty<Color?>? overlayColor;
/// {@template flutter.material.checkbox.splashRadius}
/// The splash radius of the circular [Material] ink response.
/// {@endtemplate}
///
/// If null, then the value of [CheckboxThemeData.splashRadius] is used. If
/// that is also null, then [kRadialReactionRadius] is used.
final double? splashRadius;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@template flutter.material.checkbox.shape}
/// The shape of the checkbox's [Material].
/// {@endtemplate}
///
/// If this property is null then [CheckboxThemeData.shape] of [ThemeData.checkboxTheme]
/// is used. If that's null then the shape will be a [RoundedRectangleBorder]
/// with a circular corner radius of 1.0 in Material 2, and 2.0 in Material 3.
final OutlinedBorder? shape;
/// {@template flutter.material.checkbox.side}
/// The color and width of the checkbox's border.
///
/// This property can be a [MaterialStateBorderSide] that can
/// specify different border color and widths depending on the
/// checkbox's state.
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// * [MaterialState.error].
///
/// If this property is not a [MaterialStateBorderSide] and it is
/// non-null, then it is only rendered when the checkbox's value is
/// false. The difference in interpretation is for backwards
/// compatibility.
/// {@endtemplate}
///
/// If this property is null, then [CheckboxThemeData.side] of
/// [ThemeData.checkboxTheme] is used. If that is also null, then the side
/// will be width 2.
final BorderSide? side;
/// {@template flutter.material.checkbox.isError}
/// True if this checkbox wants to show an error state.
///
/// The checkbox will have different default container color and check color when
/// this is true. This is only used when [ThemeData.useMaterial3] is set to true.
/// {@endtemplate}
///
/// Defaults to false.
final bool isError;
/// {@template flutter.material.checkbox.semanticLabel}
/// The semantic label for the checkbox that will be announced by screen readers.
///
/// This is announced in accessibility modes (e.g TalkBack/VoiceOver).
///
/// This label does not show in the UI.
/// {@endtemplate}
final String? semanticLabel;
/// The width of a checkbox widget.
static const double width = 18.0;
final _CheckboxType _checkboxType;
@override
State<Checkbox> createState() => _CheckboxState();
}
class _CheckboxState extends State<Checkbox> with TickerProviderStateMixin, ToggleableStateMixin {
final _CheckboxPainter _painter = _CheckboxPainter();
bool? _previousValue;
@override
void initState() {
super.initState();
_previousValue = widget.value;
}
@override
void didUpdateWidget(Checkbox oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.value != widget.value) {
_previousValue = oldWidget.value;
animateToValue();
}
}
@override
void dispose() {
_painter.dispose();
super.dispose();
}
@override
ValueChanged<bool?>? get onChanged => widget.onChanged;
@override
bool get tristate => widget.tristate;
@override
bool? get value => widget.value;
MaterialStateProperty<Color?> get _widgetFillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return null;
}
if (states.contains(MaterialState.selected)) {
return widget.activeColor;
}
return null;
});
}
BorderSide? _resolveSide(BorderSide? side, Set<MaterialState> states) {
if (side is MaterialStateBorderSide) {
return MaterialStateProperty.resolveAs<BorderSide?>(side, states);
}
if (!states.contains(MaterialState.selected)) {
return side;
}
return null;
}
@override
Widget build(BuildContext context) {
switch (widget._checkboxType) {
case _CheckboxType.material:
break;
case _CheckboxType.adaptive:
final ThemeData theme = Theme.of(context);
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return CupertinoCheckbox(
value: value,
tristate: tristate,
onChanged: onChanged,
activeColor: widget.activeColor,
checkColor: widget.checkColor,
focusColor: widget.focusColor,
focusNode: widget.focusNode,
autofocus: widget.autofocus,
side: widget.side,
shape: widget.shape,
);
}
}
assert(debugCheckHasMaterial(context));
final CheckboxThemeData checkboxTheme = CheckboxTheme.of(context);
final CheckboxThemeData defaults = Theme.of(context).useMaterial3
? _CheckboxDefaultsM3(context)
: _CheckboxDefaultsM2(context);
final MaterialTapTargetSize effectiveMaterialTapTargetSize = widget.materialTapTargetSize
?? checkboxTheme.materialTapTargetSize
?? defaults.materialTapTargetSize!;
final VisualDensity effectiveVisualDensity = widget.visualDensity
?? checkboxTheme.visualDensity
?? defaults.visualDensity!;
Size size = switch (effectiveMaterialTapTargetSize) {
MaterialTapTargetSize.padded => const Size(kMinInteractiveDimension, kMinInteractiveDimension),
MaterialTapTargetSize.shrinkWrap => const Size(kMinInteractiveDimension - 8.0, kMinInteractiveDimension - 8.0),
};
size += effectiveVisualDensity.baseSizeAdjustment;
final MaterialStateProperty<MouseCursor> effectiveMouseCursor = MaterialStateProperty.resolveWith<MouseCursor>((Set<MaterialState> states) {
return MaterialStateProperty.resolveAs<MouseCursor?>(widget.mouseCursor, states)
?? checkboxTheme.mouseCursor?.resolve(states)
?? MaterialStateMouseCursor.clickable.resolve(states);
});
// Colors need to be resolved in selected and non selected states separately
final Set<MaterialState> activeStates = states..add(MaterialState.selected);
final Set<MaterialState> inactiveStates = states..remove(MaterialState.selected);
if (widget.isError) {
activeStates.add(MaterialState.error);
inactiveStates.add(MaterialState.error);
}
final Color? activeColor = widget.fillColor?.resolve(activeStates)
?? _widgetFillColor.resolve(activeStates)
?? checkboxTheme.fillColor?.resolve(activeStates);
final Color effectiveActiveColor = activeColor
?? defaults.fillColor!.resolve(activeStates)!;
final Color? inactiveColor = widget.fillColor?.resolve(inactiveStates)
?? _widgetFillColor.resolve(inactiveStates)
?? checkboxTheme.fillColor?.resolve(inactiveStates);
final Color effectiveInactiveColor = inactiveColor
?? defaults.fillColor!.resolve(inactiveStates)!;
final BorderSide activeSide = _resolveSide(widget.side, activeStates)
?? _resolveSide(checkboxTheme.side, activeStates)
?? _resolveSide(defaults.side, activeStates)!;
final BorderSide inactiveSide = _resolveSide(widget.side, inactiveStates)
?? _resolveSide(checkboxTheme.side, inactiveStates)
?? _resolveSide(defaults.side, inactiveStates)!;
final Set<MaterialState> focusedStates = states..add(MaterialState.focused);
if (widget.isError) {
focusedStates.add(MaterialState.error);
}
Color effectiveFocusOverlayColor = widget.overlayColor?.resolve(focusedStates)
?? widget.focusColor
?? checkboxTheme.overlayColor?.resolve(focusedStates)
?? defaults.overlayColor!.resolve(focusedStates)!;
final Set<MaterialState> hoveredStates = states..add(MaterialState.hovered);
if (widget.isError) {
hoveredStates.add(MaterialState.error);
}
Color effectiveHoverOverlayColor = widget.overlayColor?.resolve(hoveredStates)
?? widget.hoverColor
?? checkboxTheme.overlayColor?.resolve(hoveredStates)
?? defaults.overlayColor!.resolve(hoveredStates)!;
final Set<MaterialState> activePressedStates = activeStates..add(MaterialState.pressed);
final Color effectiveActivePressedOverlayColor = widget.overlayColor?.resolve(activePressedStates)
?? checkboxTheme.overlayColor?.resolve(activePressedStates)
?? activeColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(activePressedStates)!;
final Set<MaterialState> inactivePressedStates = inactiveStates..add(MaterialState.pressed);
final Color effectiveInactivePressedOverlayColor = widget.overlayColor?.resolve(inactivePressedStates)
?? checkboxTheme.overlayColor?.resolve(inactivePressedStates)
?? inactiveColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(inactivePressedStates)!;
if (downPosition != null) {
effectiveHoverOverlayColor = states.contains(MaterialState.selected)
? effectiveActivePressedOverlayColor
: effectiveInactivePressedOverlayColor;
effectiveFocusOverlayColor = states.contains(MaterialState.selected)
? effectiveActivePressedOverlayColor
: effectiveInactivePressedOverlayColor;
}
final Set<MaterialState> checkStates = widget.isError ? (states..add(MaterialState.error)) : states;
final Color effectiveCheckColor = widget.checkColor
?? checkboxTheme.checkColor?.resolve(checkStates)
?? defaults.checkColor!.resolve(checkStates)!;
final double effectiveSplashRadius = widget.splashRadius
?? checkboxTheme.splashRadius
?? defaults.splashRadius!;
return Semantics(
label: widget.semanticLabel,
checked: widget.value ?? false,
mixed: widget.tristate ? widget.value == null : null,
child: buildToggleable(
mouseCursor: effectiveMouseCursor,
focusNode: widget.focusNode,
autofocus: widget.autofocus,
size: size,
painter: _painter
..position = position
..reaction = reaction
..reactionFocusFade = reactionFocusFade
..reactionHoverFade = reactionHoverFade
..inactiveReactionColor = effectiveInactivePressedOverlayColor
..reactionColor = effectiveActivePressedOverlayColor
..hoverColor = effectiveHoverOverlayColor
..focusColor = effectiveFocusOverlayColor
..splashRadius = effectiveSplashRadius
..downPosition = downPosition
..isFocused = states.contains(MaterialState.focused)
..isHovered = states.contains(MaterialState.hovered)
..activeColor = effectiveActiveColor
..inactiveColor = effectiveInactiveColor
..checkColor = effectiveCheckColor
..value = value
..previousValue = _previousValue
..shape = widget.shape ?? checkboxTheme.shape ?? defaults.shape!
..activeSide = activeSide
..inactiveSide = inactiveSide,
),
);
}
}
const double _kEdgeSize = Checkbox.width;
const double _kStrokeWidth = 2.0;
class _CheckboxPainter extends ToggleablePainter {
Color get checkColor => _checkColor!;
Color? _checkColor;
set checkColor(Color value) {
if (_checkColor == value) {
return;
}
_checkColor = value;
notifyListeners();
}
bool? get value => _value;
bool? _value;
set value(bool? value) {
if (_value == value) {
return;
}
_value = value;
notifyListeners();
}
bool? get previousValue => _previousValue;
bool? _previousValue;
set previousValue(bool? value) {
if (_previousValue == value) {
return;
}
_previousValue = value;
notifyListeners();
}
OutlinedBorder get shape => _shape!;
OutlinedBorder? _shape;
set shape(OutlinedBorder value) {
if (_shape == value) {
return;
}
_shape = value;
notifyListeners();
}
BorderSide get activeSide => _activeSide!;
BorderSide? _activeSide;
set activeSide(BorderSide value) {
if (_activeSide == value) {
return;
}
_activeSide = value;
notifyListeners();
}
BorderSide get inactiveSide => _inactiveSide!;
BorderSide? _inactiveSide;
set inactiveSide(BorderSide value) {
if (_inactiveSide == value) {
return;
}
_inactiveSide = value;
notifyListeners();
}
// The square outer bounds of the checkbox at t, with the specified origin.
// At t == 0.0, the outer rect's size is _kEdgeSize (Checkbox.width)
// At t == 0.5, .. is _kEdgeSize - _kStrokeWidth
// At t == 1.0, .. is _kEdgeSize
Rect _outerRectAt(Offset origin, double t) {
final double inset = 1.0 - (t - 0.5).abs() * 2.0;
final double size = _kEdgeSize - inset * _kStrokeWidth;
final Rect rect = Rect.fromLTWH(origin.dx + inset, origin.dy + inset, size, size);
return rect;
}
// The checkbox's fill color
Color _colorAt(double t) {
// As t goes from 0.0 to 0.25, animate from the inactiveColor to activeColor.
return t >= 0.25 ? activeColor : Color.lerp(inactiveColor, activeColor, t * 4.0)!;
}
// White stroke used to paint the check and dash.
Paint _createStrokePaint() {
return Paint()
..color = checkColor
..style = PaintingStyle.stroke
..strokeWidth = _kStrokeWidth;
}
void _drawBox(Canvas canvas, Rect outer, Paint paint, BorderSide? side) {
canvas.drawPath(shape.getOuterPath(outer), paint);
if (side != null) {
shape.copyWith(side: side).paint(canvas, outer);
}
}
void _drawCheck(Canvas canvas, Offset origin, double t, Paint paint) {
assert(t >= 0.0 && t <= 1.0);
// As t goes from 0.0 to 1.0, animate the two check mark strokes from the
// short side to the long side.
final Path path = Path();
const Offset start = Offset(_kEdgeSize * 0.15, _kEdgeSize * 0.45);
const Offset mid = Offset(_kEdgeSize * 0.4, _kEdgeSize * 0.7);
const Offset end = Offset(_kEdgeSize * 0.85, _kEdgeSize * 0.25);
if (t < 0.5) {
final double strokeT = t * 2.0;
final Offset drawMid = Offset.lerp(start, mid, strokeT)!;
path.moveTo(origin.dx + start.dx, origin.dy + start.dy);
path.lineTo(origin.dx + drawMid.dx, origin.dy + drawMid.dy);
} else {
final double strokeT = (t - 0.5) * 2.0;
final Offset drawEnd = Offset.lerp(mid, end, strokeT)!;
path.moveTo(origin.dx + start.dx, origin.dy + start.dy);
path.lineTo(origin.dx + mid.dx, origin.dy + mid.dy);
path.lineTo(origin.dx + drawEnd.dx, origin.dy + drawEnd.dy);
}
canvas.drawPath(path, paint);
}
void _drawDash(Canvas canvas, Offset origin, double t, Paint paint) {
assert(t >= 0.0 && t <= 1.0);
// As t goes from 0.0 to 1.0, animate the horizontal line from the
// mid point outwards.
const Offset start = Offset(_kEdgeSize * 0.2, _kEdgeSize * 0.5);
const Offset mid = Offset(_kEdgeSize * 0.5, _kEdgeSize * 0.5);
const Offset end = Offset(_kEdgeSize * 0.8, _kEdgeSize * 0.5);
final Offset drawStart = Offset.lerp(start, mid, 1.0 - t)!;
final Offset drawEnd = Offset.lerp(mid, end, t)!;
canvas.drawLine(origin + drawStart, origin + drawEnd, paint);
}
@override
void paint(Canvas canvas, Size size) {
paintRadialReaction(canvas: canvas, origin: size.center(Offset.zero));
final Paint strokePaint = _createStrokePaint();
final Offset origin = size / 2.0 - const Size.square(_kEdgeSize) / 2.0 as Offset;
final AnimationStatus status = position.status;
final double tNormalized = status == AnimationStatus.forward || status == AnimationStatus.completed
? position.value
: 1.0 - position.value;
// Four cases: false to null, false to true, null to false, true to false
if (previousValue == false || value == false) {
final double t = value == false ? 1.0 - tNormalized : tNormalized;
final Rect outer = _outerRectAt(origin, t);
final Paint paint = Paint()..color = _colorAt(t);
if (t <= 0.5) {
final BorderSide border = BorderSide.lerp(inactiveSide, activeSide, t);
_drawBox(canvas, outer, paint, border);
} else {
_drawBox(canvas, outer, paint, activeSide);
final double tShrink = (t - 0.5) * 2.0;
if (previousValue == null || value == null) {
_drawDash(canvas, origin, tShrink, strokePaint);
} else {
_drawCheck(canvas, origin, tShrink, strokePaint);
}
}
} else { // Two cases: null to true, true to null
final Rect outer = _outerRectAt(origin, 1.0);
final Paint paint = Paint() ..color = _colorAt(1.0);
_drawBox(canvas, outer, paint, activeSide);
if (tNormalized <= 0.5) {
final double tShrink = 1.0 - tNormalized * 2.0;
if (previousValue ?? false) {
_drawCheck(canvas, origin, tShrink, strokePaint);
} else {
_drawDash(canvas, origin, tShrink, strokePaint);
}
} else {
final double tExpand = (tNormalized - 0.5) * 2.0;
if (value ?? false) {
_drawCheck(canvas, origin, tExpand, strokePaint);
} else {
_drawDash(canvas, origin, tExpand, strokePaint);
}
}
}
}
}
// Hand coded defaults based on Material Design 2.
class _CheckboxDefaultsM2 extends CheckboxThemeData {
_CheckboxDefaultsM2(BuildContext context)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme;
final ThemeData _theme;
final ColorScheme _colors;
@override
MaterialStateBorderSide? get side {
return MaterialStateBorderSide.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 2.0, color: Colors.transparent);
}
return BorderSide(width: 2.0, color: _theme.disabledColor);
}
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 2.0, color: Colors.transparent);
}
return BorderSide(width: 2.0, color: _theme.unselectedWidgetColor);
});
}
@override
MaterialStateProperty<Color> get fillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _theme.disabledColor;
}
return Colors.transparent;
}
if (states.contains(MaterialState.selected)) {
return _colors.secondary;
}
return Colors.transparent;
});
}
@override
MaterialStateProperty<Color> get checkColor {
return MaterialStateProperty.all<Color>(const Color(0xFFFFFFFF));
}
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return fillColor.resolve(states).withAlpha(kRadialReactionAlpha);
}
if (states.contains(MaterialState.hovered)) {
return _theme.hoverColor;
}
if (states.contains(MaterialState.focused)) {
return _theme.focusColor;
}
return Colors.transparent;
});
}
@override
double get splashRadius => kRadialReactionRadius;
@override
MaterialTapTargetSize get materialTapTargetSize => _theme.materialTapTargetSize;
@override
VisualDensity get visualDensity => _theme.visualDensity;
@override
OutlinedBorder get shape => const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(1.0)),
);
}
// BEGIN GENERATED TOKEN PROPERTIES - Checkbox
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _CheckboxDefaultsM3 extends CheckboxThemeData {
_CheckboxDefaultsM3(BuildContext context)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme;
final ThemeData _theme;
final ColorScheme _colors;
@override
MaterialStateBorderSide? get side {
return MaterialStateBorderSide.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 2.0, color: Colors.transparent);
}
return BorderSide(width: 2.0, color: _colors.onSurface.withOpacity(0.38));
}
if (states.contains(MaterialState.selected)) {
return const BorderSide(width: 0.0, color: Colors.transparent);
}
if (states.contains(MaterialState.error)) {
return BorderSide(width: 2.0, color: _colors.error);
}
if (states.contains(MaterialState.pressed)) {
return BorderSide(width: 2.0, color: _colors.onSurface);
}
if (states.contains(MaterialState.hovered)) {
return BorderSide(width: 2.0, color: _colors.onSurface);
}
if (states.contains(MaterialState.focused)) {
return BorderSide(width: 2.0, color: _colors.onSurface);
}
return BorderSide(width: 2.0, color: _colors.onSurfaceVariant);
});
}
@override
MaterialStateProperty<Color> get fillColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.onSurface.withOpacity(0.38);
}
return Colors.transparent;
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.error)) {
return _colors.error;
}
return _colors.primary;
}
return Colors.transparent;
});
}
@override
MaterialStateProperty<Color> get checkColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.surface;
}
return Colors.transparent; // No icons available when the checkbox is unselected.
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.error)) {
return _colors.onError;
}
return _colors.onPrimary;
}
return Colors.transparent; // No icons available when the checkbox is unselected.
});
}
@override
MaterialStateProperty<Color> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.error)) {
if (states.contains(MaterialState.pressed)) {
return _colors.error.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.error.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.error.withOpacity(0.1);
}
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return Colors.transparent;
}
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return Colors.transparent;
});
}
@override
double get splashRadius => 40.0 / 2;
@override
MaterialTapTargetSize get materialTapTargetSize => _theme.materialTapTargetSize;
@override
VisualDensity get visualDensity => _theme.visualDensity;
@override
OutlinedBorder get shape => const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(2.0)),
);
}
// END GENERATED TOKEN PROPERTIES - Checkbox
| flutter/packages/flutter/lib/src/material/checkbox.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/checkbox.dart",
"repo_id": "flutter",
"token_count": 13054
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'input_decorator.dart';
import 'material_state.dart';
import 'text_button.dart';
import 'text_theme.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Overrides the default values of visual properties for descendant
/// [DatePickerDialog] widgets.
///
/// Descendant widgets obtain the current [DatePickerThemeData] object with
/// [DatePickerTheme.of]. Instances of [DatePickerTheme] can
/// be customized with [DatePickerThemeData.copyWith].
///
/// Typically a [DatePickerTheme] is specified as part of the overall
/// [Theme] with [ThemeData.datePickerTheme].
///
/// All [DatePickerThemeData] properties are null by default. When null,
/// the [DatePickerDialog] computes its own default values, typically based on
/// the overall theme's [ThemeData.colorScheme], [ThemeData.textTheme], and
/// [ThemeData.iconTheme].
@immutable
class DatePickerThemeData with Diagnosticable {
/// Creates a [DatePickerThemeData] that can be used to override default properties
/// in a [DatePickerTheme] widget.
const DatePickerThemeData({
this.backgroundColor,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.shape,
this.headerBackgroundColor,
this.headerForegroundColor,
this.headerHeadlineStyle,
this.headerHelpStyle,
this.weekdayStyle,
this.dayStyle,
this.dayForegroundColor,
this.dayBackgroundColor,
this.dayOverlayColor,
this.dayShape,
this.todayForegroundColor,
this.todayBackgroundColor,
this.todayBorder,
this.yearStyle,
this.yearForegroundColor,
this.yearBackgroundColor,
this.yearOverlayColor,
this.rangePickerBackgroundColor,
this.rangePickerElevation,
this.rangePickerShadowColor,
this.rangePickerSurfaceTintColor,
this.rangePickerShape,
this.rangePickerHeaderBackgroundColor,
this.rangePickerHeaderForegroundColor,
this.rangePickerHeaderHeadlineStyle,
this.rangePickerHeaderHelpStyle,
this.rangeSelectionBackgroundColor,
this.rangeSelectionOverlayColor,
this.dividerColor,
this.inputDecorationTheme,
this.cancelButtonStyle,
this.confirmButtonStyle,
});
/// Overrides the default value of [Dialog.backgroundColor].
final Color? backgroundColor;
/// Overrides the default value of [Dialog.elevation].
///
/// See also:
/// [Material.elevation], which explains how elevation is related to a component's shadow.
final double? elevation;
/// Overrides the default value of [Dialog.shadowColor].
///
/// See also:
/// [Material.shadowColor], which explains how the shadow is rendered.
final Color? shadowColor;
/// Overrides the default value of [Dialog.surfaceTintColor].
///
/// See also:
/// [Material.surfaceTintColor], which explains how this color is related to
/// [elevation] and [backgroundColor].
final Color? surfaceTintColor;
/// Overrides the default value of [Dialog.shape].
///
/// If [elevation] is greater than zero then a shadow is shown and the shadow's
/// shape mirrors the shape of the dialog.
final ShapeBorder? shape;
/// Overrides the header's default background fill color.
///
/// The dialog's header displays the currently selected date.
final Color? headerBackgroundColor;
/// Overrides the header's default color used for text labels and icons.
///
/// The dialog's header displays the currently selected date.
///
/// This is used instead of the [TextStyle.color] property of [headerHeadlineStyle]
/// and [headerHelpStyle].
final Color? headerForegroundColor;
/// Overrides the header's default headline text style.
///
/// The dialog's header displays the currently selected date.
///
/// The [TextStyle.color] of the [headerHeadlineStyle] is not used,
/// [headerForegroundColor] is used instead.
final TextStyle? headerHeadlineStyle;
/// Overrides the header's default help text style.
///
/// The help text (also referred to as "supporting text" in the Material
/// spec) is usually a prompt to the user at the top of the header
/// (i.e. 'Select date').
///
/// The [TextStyle.color] of the [headerHelpStyle] is not used,
/// [headerForegroundColor] is used instead.
///
/// See also:
/// [DatePickerDialog.helpText], which specifies the help text.
final TextStyle? headerHelpStyle;
/// Overrides the default text style used for the row of weekday
/// labels at the top of the date picker grid.
final TextStyle? weekdayStyle;
/// Overrides the default text style used for each individual day
/// label in the grid of the date picker.
///
/// The [TextStyle.color] of the [dayStyle] is not used,
/// [dayForegroundColor] is used instead.
final TextStyle? dayStyle;
/// Overrides the default color used to paint the day labels in the
/// grid of the date picker.
///
/// This will be used instead of the color provided in [dayStyle].
final MaterialStateProperty<Color?>? dayForegroundColor;
/// Overrides the default color used to paint the background of the
/// day labels in the grid of the date picker.
final MaterialStateProperty<Color?>? dayBackgroundColor;
/// Overrides the default highlight color that's typically used to
/// indicate that a day in the grid is focused, hovered, or pressed.
final MaterialStateProperty<Color?>? dayOverlayColor;
/// Overrides the default shape used to paint the shape decoration of the
/// day labels in the grid of the date picker.
///
/// If the selected day is the current day, the provided shape with the
/// value of [todayBackgroundColor] is used to paint the shape decoration of
/// the day label and the value of [todayBorder] and [todayForegroundColor] is
/// used to paint the border.
///
/// If the selected day is not the current day, the provided shape with the
/// value of [dayBackgroundColor] is used to paint the shape decoration of
/// the day label.
///
/// {@tool dartpad}
/// This sample demonstrates how to customize the day selector shape decoration
/// using the [dayShape], [todayForegroundColor], [todayBackgroundColor], and
/// [todayBorder] properties.
///
/// ** See code in examples/api/lib/material/date_picker/date_picker_theme_day_shape.0.dart **
/// {@end-tool}
final MaterialStateProperty<OutlinedBorder?>? dayShape;
/// Overrides the default color used to paint the
/// [DatePickerDialog.currentDate] label in the grid of the dialog's
/// [CalendarDatePicker] and the corresponding year in the dialog's
/// [YearPicker].
///
/// This will be used instead of the [TextStyle.color] provided in [dayStyle].
///
/// {@tool dartpad}
/// This sample demonstrates how to customize the day selector shape decoration
/// using the [dayShape], [todayForegroundColor], [todayBackgroundColor], and
/// [todayBorder] properties.
///
/// ** See code in examples/api/lib/material/date_picker/date_picker_theme_day_shape.0.dart **
/// {@end-tool}
final MaterialStateProperty<Color?>? todayForegroundColor;
/// Overrides the default color used to paint the background of the
/// [DatePickerDialog.currentDate] label in the grid of the date picker.
final MaterialStateProperty<Color?>? todayBackgroundColor;
/// Overrides the border used to paint the
/// [DatePickerDialog.currentDate] label in the grid of the date
/// picker.
///
/// The border side's [BorderSide.color] is not used,
/// [todayForegroundColor] is used instead.
///
/// {@tool dartpad}
/// This sample demonstrates how to customize the day selector shape decoration
/// using the [dayShape], [todayForegroundColor], [todayBackgroundColor], and
/// [todayBorder] properties.
///
/// ** See code in examples/api/lib/material/date_picker/date_picker_theme_day_shape.0.dart **
/// {@end-tool}
final BorderSide? todayBorder;
/// Overrides the default text style used to paint each of the year
/// entries in the year selector of the date picker.
///
/// The [TextStyle.color] of the [yearStyle] is not used,
/// [yearForegroundColor] is used instead.
final TextStyle? yearStyle;
/// Overrides the default color used to paint the year labels in the year
/// selector of the date picker.
///
/// This will be used instead of the color provided in [yearStyle].
final MaterialStateProperty<Color?>? yearForegroundColor;
/// Overrides the default color used to paint the background of the
/// year labels in the year selector of the of the date picker.
final MaterialStateProperty<Color?>? yearBackgroundColor;
/// Overrides the default highlight color that's typically used to
/// indicate that a year in the year selector is focused, hovered,
/// or pressed.
final MaterialStateProperty<Color?>? yearOverlayColor;
/// Overrides the default [Scaffold.backgroundColor] for
/// [DateRangePickerDialog].
final Color? rangePickerBackgroundColor;
/// Overrides the default elevation of the full screen
/// [DateRangePickerDialog].
///
/// See also:
/// [Material.elevation], which explains how elevation is related to a component's shadow.
final double? rangePickerElevation;
/// Overrides the color of the shadow painted below a full screen
/// [DateRangePickerDialog].
///
/// See also:
/// [Material.shadowColor], which explains how the shadow is rendered.
final Color? rangePickerShadowColor;
/// Overrides the default color of the surface tint overlay applied
/// to the [backgroundColor] of a full screen
/// [DateRangePickerDialog]'s to indicate elevation.
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// See also:
/// [Material.surfaceTintColor], which explains how this color is related to
/// [elevation].
final Color? rangePickerSurfaceTintColor;
/// Overrides the default overall shape of a full screen
/// [DateRangePickerDialog].
///
/// If [elevation] is greater than zero then a shadow is shown and the shadow's
/// shape mirrors the shape of the dialog.
///
/// [Material.surfaceTintColor], which explains how this color is related to
/// [elevation].
final ShapeBorder? rangePickerShape;
/// Overrides the default background fill color for [DateRangePickerDialog].
///
/// The dialog's header displays the currently selected date range.
final Color? rangePickerHeaderBackgroundColor;
/// Overrides the default color used for text labels and icons in
/// the header of a full screen [DateRangePickerDialog]
///
/// The dialog's header displays the currently selected date range.
///
/// This is used instead of any colors provided by
/// [rangePickerHeaderHeadlineStyle] or [rangePickerHeaderHelpStyle].
final Color? rangePickerHeaderForegroundColor;
/// Overrides the default text style used for the headline text in
/// the header of a full screen [DateRangePickerDialog].
///
/// The dialog's header displays the currently selected date range.
///
/// The [TextStyle.color] of [rangePickerHeaderHeadlineStyle] is not used,
/// [rangePickerHeaderForegroundColor] is used instead.
final TextStyle? rangePickerHeaderHeadlineStyle;
/// Overrides the default text style used for the help text of the
/// header of a full screen [DateRangePickerDialog].
///
/// The help text (also referred to as "supporting text" in the Material
/// spec) is usually a prompt to the user at the top of the header
/// (i.e. 'Select date').
///
/// The [TextStyle.color] of the [rangePickerHeaderHelpStyle] is not used,
/// [rangePickerHeaderForegroundColor] is used instead.
///
/// See also:
/// [DateRangePickerDialog.helpText], which specifies the help text.
final TextStyle? rangePickerHeaderHelpStyle;
/// Overrides the default background color used to paint days
/// selected between the start and end dates in a
/// [DateRangePickerDialog].
final Color? rangeSelectionBackgroundColor;
/// Overrides the default highlight color that's typically used to
/// indicate that a date in the selected range of a
/// [DateRangePickerDialog] is focused, hovered, or pressed.
final MaterialStateProperty<Color?>? rangeSelectionOverlayColor;
/// Overrides the default color used to paint the horizontal divider
/// below the header text when dialog is in portrait orientation
/// and vertical divider when the dialog is in landscape orientation.
final Color? dividerColor;
/// Overrides the [InputDatePickerFormField]'s input decoration theme.
/// If this is null, [ThemeData.inputDecorationTheme] is used instead.
final InputDecorationTheme? inputDecorationTheme;
/// Overrides the default style of the cancel button of a [DatePickerDialog].
final ButtonStyle? cancelButtonStyle;
/// Overrides the default style of the confirm (OK) button of a [DatePickerDialog].
final ButtonStyle? confirmButtonStyle;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
DatePickerThemeData copyWith({
Color? backgroundColor,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
ShapeBorder? shape,
Color? headerBackgroundColor,
Color? headerForegroundColor,
TextStyle? headerHeadlineStyle,
TextStyle? headerHelpStyle,
TextStyle? weekdayStyle,
TextStyle? dayStyle,
MaterialStateProperty<Color?>? dayForegroundColor,
MaterialStateProperty<Color?>? dayBackgroundColor,
MaterialStateProperty<Color?>? dayOverlayColor,
MaterialStateProperty<OutlinedBorder?>? dayShape,
MaterialStateProperty<Color?>? todayForegroundColor,
MaterialStateProperty<Color?>? todayBackgroundColor,
BorderSide? todayBorder,
TextStyle? yearStyle,
MaterialStateProperty<Color?>? yearForegroundColor,
MaterialStateProperty<Color?>? yearBackgroundColor,
MaterialStateProperty<Color?>? yearOverlayColor,
Color? rangePickerBackgroundColor,
double? rangePickerElevation,
Color? rangePickerShadowColor,
Color? rangePickerSurfaceTintColor,
ShapeBorder? rangePickerShape,
Color? rangePickerHeaderBackgroundColor,
Color? rangePickerHeaderForegroundColor,
TextStyle? rangePickerHeaderHeadlineStyle,
TextStyle? rangePickerHeaderHelpStyle,
Color? rangeSelectionBackgroundColor,
MaterialStateProperty<Color?>? rangeSelectionOverlayColor,
Color? dividerColor,
InputDecorationTheme? inputDecorationTheme,
ButtonStyle? cancelButtonStyle,
ButtonStyle? confirmButtonStyle,
}) {
return DatePickerThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
elevation: elevation ?? this.elevation,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
shape: shape ?? this.shape,
headerBackgroundColor: headerBackgroundColor ?? this.headerBackgroundColor,
headerForegroundColor: headerForegroundColor ?? this.headerForegroundColor,
headerHeadlineStyle: headerHeadlineStyle ?? this.headerHeadlineStyle,
headerHelpStyle: headerHelpStyle ?? this.headerHelpStyle,
weekdayStyle: weekdayStyle ?? this.weekdayStyle,
dayStyle: dayStyle ?? this.dayStyle,
dayForegroundColor: dayForegroundColor ?? this.dayForegroundColor,
dayBackgroundColor: dayBackgroundColor ?? this.dayBackgroundColor,
dayOverlayColor: dayOverlayColor ?? this.dayOverlayColor,
dayShape: dayShape ?? this.dayShape,
todayForegroundColor: todayForegroundColor ?? this.todayForegroundColor,
todayBackgroundColor: todayBackgroundColor ?? this.todayBackgroundColor,
todayBorder: todayBorder ?? this.todayBorder,
yearStyle: yearStyle ?? this.yearStyle,
yearForegroundColor: yearForegroundColor ?? this.yearForegroundColor,
yearBackgroundColor: yearBackgroundColor ?? this.yearBackgroundColor,
yearOverlayColor: yearOverlayColor ?? this.yearOverlayColor,
rangePickerBackgroundColor: rangePickerBackgroundColor ?? this.rangePickerBackgroundColor,
rangePickerElevation: rangePickerElevation ?? this.rangePickerElevation,
rangePickerShadowColor: rangePickerShadowColor ?? this.rangePickerShadowColor,
rangePickerSurfaceTintColor: rangePickerSurfaceTintColor ?? this.rangePickerSurfaceTintColor,
rangePickerShape: rangePickerShape ?? this.rangePickerShape,
rangePickerHeaderBackgroundColor: rangePickerHeaderBackgroundColor ?? this.rangePickerHeaderBackgroundColor,
rangePickerHeaderForegroundColor: rangePickerHeaderForegroundColor ?? this.rangePickerHeaderForegroundColor,
rangePickerHeaderHeadlineStyle: rangePickerHeaderHeadlineStyle ?? this.rangePickerHeaderHeadlineStyle,
rangePickerHeaderHelpStyle: rangePickerHeaderHelpStyle ?? this.rangePickerHeaderHelpStyle,
rangeSelectionBackgroundColor: rangeSelectionBackgroundColor ?? this.rangeSelectionBackgroundColor,
rangeSelectionOverlayColor: rangeSelectionOverlayColor ?? this.rangeSelectionOverlayColor,
dividerColor: dividerColor ?? this.dividerColor,
inputDecorationTheme: inputDecorationTheme ?? this.inputDecorationTheme,
cancelButtonStyle: cancelButtonStyle ?? this.cancelButtonStyle,
confirmButtonStyle: confirmButtonStyle ?? this.confirmButtonStyle,
);
}
/// Linearly interpolates between two [DatePickerThemeData].
static DatePickerThemeData lerp(DatePickerThemeData? a, DatePickerThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return DatePickerThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
shape: ShapeBorder.lerp(a?.shape, b?.shape, t),
headerBackgroundColor: Color.lerp(a?.headerBackgroundColor, b?.headerBackgroundColor, t),
headerForegroundColor: Color.lerp(a?.headerForegroundColor, b?.headerForegroundColor, t),
headerHeadlineStyle: TextStyle.lerp(a?.headerHeadlineStyle, b?.headerHeadlineStyle, t),
headerHelpStyle: TextStyle.lerp(a?.headerHelpStyle, b?.headerHelpStyle, t),
weekdayStyle: TextStyle.lerp(a?.weekdayStyle, b?.weekdayStyle, t),
dayStyle: TextStyle.lerp(a?.dayStyle, b?.dayStyle, t),
dayForegroundColor: MaterialStateProperty.lerp<Color?>(a?.dayForegroundColor, b?.dayForegroundColor, t, Color.lerp),
dayBackgroundColor: MaterialStateProperty.lerp<Color?>(a?.dayBackgroundColor, b?.dayBackgroundColor, t, Color.lerp),
dayOverlayColor: MaterialStateProperty.lerp<Color?>(a?.dayOverlayColor, b?.dayOverlayColor, t, Color.lerp),
dayShape: MaterialStateProperty.lerp<OutlinedBorder?>(a?.dayShape, b?.dayShape, t, OutlinedBorder.lerp),
todayForegroundColor: MaterialStateProperty.lerp<Color?>(a?.todayForegroundColor, b?.todayForegroundColor, t, Color.lerp),
todayBackgroundColor: MaterialStateProperty.lerp<Color?>(a?.todayBackgroundColor, b?.todayBackgroundColor, t, Color.lerp),
todayBorder: _lerpBorderSide(a?.todayBorder, b?.todayBorder, t),
yearStyle: TextStyle.lerp(a?.yearStyle, b?.yearStyle, t),
yearForegroundColor: MaterialStateProperty.lerp<Color?>(a?.yearForegroundColor, b?.yearForegroundColor, t, Color.lerp),
yearBackgroundColor: MaterialStateProperty.lerp<Color?>(a?.yearBackgroundColor, b?.yearBackgroundColor, t, Color.lerp),
yearOverlayColor: MaterialStateProperty.lerp<Color?>(a?.yearOverlayColor, b?.yearOverlayColor, t, Color.lerp),
rangePickerBackgroundColor: Color.lerp(a?.rangePickerBackgroundColor, b?.rangePickerBackgroundColor, t),
rangePickerElevation: lerpDouble(a?.rangePickerElevation, b?.rangePickerElevation, t),
rangePickerShadowColor: Color.lerp(a?.rangePickerShadowColor, b?.rangePickerShadowColor, t),
rangePickerSurfaceTintColor: Color.lerp(a?.rangePickerSurfaceTintColor, b?.rangePickerSurfaceTintColor, t),
rangePickerShape: ShapeBorder.lerp(a?.rangePickerShape, b?.rangePickerShape, t),
rangePickerHeaderBackgroundColor: Color.lerp(a?.rangePickerHeaderBackgroundColor, b?.rangePickerHeaderBackgroundColor, t),
rangePickerHeaderForegroundColor: Color.lerp(a?.rangePickerHeaderForegroundColor, b?.rangePickerHeaderForegroundColor, t),
rangePickerHeaderHeadlineStyle: TextStyle.lerp(a?.rangePickerHeaderHeadlineStyle, b?.rangePickerHeaderHeadlineStyle, t),
rangePickerHeaderHelpStyle: TextStyle.lerp(a?.rangePickerHeaderHelpStyle, b?.rangePickerHeaderHelpStyle, t),
rangeSelectionBackgroundColor: Color.lerp(a?.rangeSelectionBackgroundColor, b?.rangeSelectionBackgroundColor, t),
rangeSelectionOverlayColor: MaterialStateProperty.lerp<Color?>(a?.rangeSelectionOverlayColor, b?.rangeSelectionOverlayColor, t, Color.lerp),
dividerColor: Color.lerp(a?.dividerColor, b?.dividerColor, t),
inputDecorationTheme: t < 0.5 ? a?.inputDecorationTheme : b?.inputDecorationTheme,
cancelButtonStyle: ButtonStyle.lerp(a?.cancelButtonStyle, b?.cancelButtonStyle, t),
confirmButtonStyle: ButtonStyle.lerp(a?.confirmButtonStyle, b?.confirmButtonStyle, t),
);
}
static BorderSide? _lerpBorderSide(BorderSide? a, BorderSide? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return BorderSide.lerp(BorderSide(width: 0, color: b!.color.withAlpha(0)), b, t);
}
return BorderSide.lerp(a, BorderSide(width: 0, color: a.color.withAlpha(0)), t);
}
@override
int get hashCode => Object.hashAll(<Object?>[
backgroundColor,
elevation,
shadowColor,
surfaceTintColor,
shape,
headerBackgroundColor,
headerForegroundColor,
headerHeadlineStyle,
headerHelpStyle,
weekdayStyle,
dayStyle,
dayForegroundColor,
dayBackgroundColor,
dayOverlayColor,
dayShape,
todayForegroundColor,
todayBackgroundColor,
todayBorder,
yearStyle,
yearForegroundColor,
yearBackgroundColor,
yearOverlayColor,
rangePickerBackgroundColor,
rangePickerElevation,
rangePickerShadowColor,
rangePickerSurfaceTintColor,
rangePickerShape,
rangePickerHeaderBackgroundColor,
rangePickerHeaderForegroundColor,
rangePickerHeaderHeadlineStyle,
rangePickerHeaderHelpStyle,
rangeSelectionBackgroundColor,
rangeSelectionOverlayColor,
dividerColor,
inputDecorationTheme,
cancelButtonStyle,
confirmButtonStyle,
]);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
return other is DatePickerThemeData
&& other.backgroundColor == backgroundColor
&& other.elevation == elevation
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.shape == shape
&& other.headerBackgroundColor == headerBackgroundColor
&& other.headerForegroundColor == headerForegroundColor
&& other.headerHeadlineStyle == headerHeadlineStyle
&& other.headerHelpStyle == headerHelpStyle
&& other.weekdayStyle == weekdayStyle
&& other.dayStyle == dayStyle
&& other.dayForegroundColor == dayForegroundColor
&& other.dayBackgroundColor == dayBackgroundColor
&& other.dayOverlayColor == dayOverlayColor
&& other.dayShape == dayShape
&& other.todayForegroundColor == todayForegroundColor
&& other.todayBackgroundColor == todayBackgroundColor
&& other.todayBorder == todayBorder
&& other.yearStyle == yearStyle
&& other.yearForegroundColor == yearForegroundColor
&& other.yearBackgroundColor == yearBackgroundColor
&& other.yearOverlayColor == yearOverlayColor
&& other.rangePickerBackgroundColor == rangePickerBackgroundColor
&& other.rangePickerElevation == rangePickerElevation
&& other.rangePickerShadowColor == rangePickerShadowColor
&& other.rangePickerSurfaceTintColor == rangePickerSurfaceTintColor
&& other.rangePickerShape == rangePickerShape
&& other.rangePickerHeaderBackgroundColor == rangePickerHeaderBackgroundColor
&& other.rangePickerHeaderForegroundColor == rangePickerHeaderForegroundColor
&& other.rangePickerHeaderHeadlineStyle == rangePickerHeaderHeadlineStyle
&& other.rangePickerHeaderHelpStyle == rangePickerHeaderHelpStyle
&& other.rangeSelectionBackgroundColor == rangeSelectionBackgroundColor
&& other.rangeSelectionOverlayColor == rangeSelectionOverlayColor
&& other.dividerColor == dividerColor
&& other.inputDecorationTheme == inputDecorationTheme
&& other.cancelButtonStyle == cancelButtonStyle
&& other.confirmButtonStyle == confirmButtonStyle;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(ColorProperty('headerBackgroundColor', headerBackgroundColor, defaultValue: null));
properties.add(ColorProperty('headerForegroundColor', headerForegroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('headerHeadlineStyle', headerHeadlineStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('headerHelpStyle', headerHelpStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('weekDayStyle', weekdayStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('dayStyle', dayStyle, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('dayForegroundColor', dayForegroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('dayBackgroundColor', dayBackgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('dayOverlayColor', dayOverlayColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<OutlinedBorder?>>('dayShape', dayShape, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('todayForegroundColor', todayForegroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('todayBackgroundColor', todayBackgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<BorderSide?>('todayBorder', todayBorder, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('yearStyle', yearStyle, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('yearForegroundColor', yearForegroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('yearBackgroundColor', yearBackgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('yearOverlayColor', yearOverlayColor, defaultValue: null));
properties.add(ColorProperty('rangePickerBackgroundColor', rangePickerBackgroundColor, defaultValue: null));
properties.add(DoubleProperty('rangePickerElevation', rangePickerElevation, defaultValue: null));
properties.add(ColorProperty('rangePickerShadowColor', rangePickerShadowColor, defaultValue: null));
properties.add(ColorProperty('rangePickerSurfaceTintColor', rangePickerSurfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('rangePickerShape', rangePickerShape, defaultValue: null));
properties.add(ColorProperty('rangePickerHeaderBackgroundColor', rangePickerHeaderBackgroundColor, defaultValue: null));
properties.add(ColorProperty('rangePickerHeaderForegroundColor', rangePickerHeaderForegroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('rangePickerHeaderHeadlineStyle', rangePickerHeaderHeadlineStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('rangePickerHeaderHelpStyle', rangePickerHeaderHelpStyle, defaultValue: null));
properties.add(ColorProperty('rangeSelectionBackgroundColor', rangeSelectionBackgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('rangeSelectionOverlayColor', rangeSelectionOverlayColor, defaultValue: null));
properties.add(ColorProperty('dividerColor', dividerColor, defaultValue: null));
properties.add(DiagnosticsProperty<InputDecorationTheme>('inputDecorationTheme', inputDecorationTheme, defaultValue: null));
properties.add(DiagnosticsProperty<ButtonStyle>('cancelButtonStyle', cancelButtonStyle, defaultValue: null));
properties.add(DiagnosticsProperty<ButtonStyle>('confirmButtonStyle', confirmButtonStyle, defaultValue: null));
}
}
/// An inherited widget that defines the visual properties for
/// [DatePickerDialog]s in this widget's subtree.
///
/// Values specified here are used for [DatePickerDialog] properties that are not
/// given an explicit non-null value.
class DatePickerTheme extends InheritedTheme {
/// Creates a [DatePickerTheme] that controls visual parameters for
/// descendent [DatePickerDialog]s.
const DatePickerTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the visual properties used by descendant [DatePickerDialog]
/// widgets.
final DatePickerThemeData data;
/// The [data] from the closest instance of this class that encloses the given
/// context.
///
/// If there is no [DatePickerTheme] in scope, this will return
/// [ThemeData.datePickerTheme] from the ambient [Theme].
///
/// Typical usage is as follows:
///
/// ```dart
/// DatePickerThemeData theme = DatePickerTheme.of(context);
/// ```
///
/// See also:
///
/// * [maybeOf], which returns null if it doesn't find a
/// [DatePickerTheme] ancestor.
/// * [defaults], which will return the default properties used when no
/// other [DatePickerTheme] has been provided.
static DatePickerThemeData of(BuildContext context) {
return maybeOf(context) ?? Theme.of(context).datePickerTheme;
}
/// The data from the closest instance of this class that encloses the given
/// context, if any.
///
/// Use this function if you want to allow situations where no
/// [DatePickerTheme] is in scope. Prefer using [DatePickerTheme.of]
/// in situations where a [DatePickerThemeData] is expected to be
/// non-null.
///
/// If there is no [DatePickerTheme] in scope, then this function will
/// return null.
///
/// Typical usage is as follows:
///
/// ```dart
/// DatePickerThemeData? theme = DatePickerTheme.maybeOf(context);
/// if (theme == null) {
/// // Do something else instead.
/// }
/// ```
///
/// See also:
///
/// * [of], which will return [ThemeData.datePickerTheme] if it doesn't
/// find a [DatePickerTheme] ancestor, instead of returning null.
/// * [defaults], which will return the default properties used when no
/// other [DatePickerTheme] has been provided.
static DatePickerThemeData? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<DatePickerTheme>()?.data;
}
/// A DatePickerThemeData used as the default properties for date pickers.
///
/// This is only used for properties not already specified in the ambient
/// [DatePickerTheme.of].
///
/// See also:
///
/// * [of], which will return [ThemeData.datePickerTheme] if it doesn't
/// find a [DatePickerTheme] ancestor, instead of returning null.
/// * [maybeOf], which returns null if it doesn't find a
/// [DatePickerTheme] ancestor.
static DatePickerThemeData defaults(BuildContext context) {
return Theme.of(context).useMaterial3
? _DatePickerDefaultsM3(context)
: _DatePickerDefaultsM2(context);
}
@override
Widget wrap(BuildContext context, Widget child) {
return DatePickerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(DatePickerTheme oldWidget) => data != oldWidget.data;
}
// Hand coded defaults based on Material Design 2.
class _DatePickerDefaultsM2 extends DatePickerThemeData {
_DatePickerDefaultsM2(this.context)
: super(
elevation: 24.0,
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))),
dayShape: const MaterialStatePropertyAll<OutlinedBorder>(CircleBorder()),
rangePickerElevation: 0.0,
rangePickerShape: const RoundedRectangleBorder(),
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
late final bool _isDark = _colors.brightness == Brightness.dark;
@override
Color? get headerBackgroundColor => _isDark ? _colors.surface : _colors.primary;
@override
ButtonStyle get cancelButtonStyle {
return TextButton.styleFrom();
}
@override
ButtonStyle get confirmButtonStyle {
return TextButton.styleFrom();
}
@override
Color? get headerForegroundColor => _isDark ? _colors.onSurface : _colors.onPrimary;
@override
TextStyle? get headerHeadlineStyle => _textTheme.headlineSmall;
@override
TextStyle? get headerHelpStyle => _textTheme.labelSmall;
@override
TextStyle? get weekdayStyle => _textTheme.bodySmall?.apply(
color: _colors.onSurface.withOpacity(0.60),
);
@override
TextStyle? get dayStyle => _textTheme.bodySmall;
@override
MaterialStateProperty<Color?>? get dayForegroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
} else if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.onSurface;
});
@override
MaterialStateProperty<Color?>? get dayBackgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.primary;
}
return null;
});
@override
MaterialStateProperty<Color?>? get dayOverlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.38);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.12);
}
} else {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.12);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.12);
}
}
return null;
});
@override
MaterialStateProperty<Color?>? get todayForegroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
} else if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.primary;
});
@override
MaterialStateProperty<Color?>? get todayBackgroundColor => dayBackgroundColor;
@override
BorderSide? get todayBorder => BorderSide(color: _colors.primary);
@override
TextStyle? get yearStyle => _textTheme.bodyLarge;
@override
Color? get rangePickerBackgroundColor => _colors.surface;
@override
Color? get rangePickerShadowColor => Colors.transparent;
@override
Color? get rangePickerSurfaceTintColor => Colors.transparent;
@override
Color? get rangePickerHeaderBackgroundColor => _isDark ? _colors.surface : _colors.primary;
@override
Color? get rangePickerHeaderForegroundColor => _isDark ? _colors.onSurface : _colors.onPrimary;
@override
TextStyle? get rangePickerHeaderHeadlineStyle => _textTheme.headlineSmall;
@override
TextStyle? get rangePickerHeaderHelpStyle => _textTheme.labelSmall;
@override
Color? get rangeSelectionBackgroundColor => _colors.primary.withOpacity(0.12);
@override
MaterialStateProperty<Color?>? get rangeSelectionOverlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.38);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.12);
}
} else {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.12);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.12);
}
}
return null;
});
}
// BEGIN GENERATED TOKEN PROPERTIES - DatePicker
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _DatePickerDefaultsM3 extends DatePickerThemeData {
_DatePickerDefaultsM3(this.context)
: super(
elevation: 6.0,
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(28.0))),
// TODO(tahatesser): Update this to use token when gen_defaults
// supports `CircleBorder` for fully rounded corners.
dayShape: const MaterialStatePropertyAll<OutlinedBorder>(CircleBorder()),
rangePickerElevation: 0.0,
rangePickerShape: const RoundedRectangleBorder(),
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
@override
Color? get backgroundColor => _colors.surfaceContainerHigh;
@override
ButtonStyle get cancelButtonStyle {
return TextButton.styleFrom();
}
@override
ButtonStyle get confirmButtonStyle {
return TextButton.styleFrom();
}
@override
Color? get shadowColor => Colors.transparent;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
Color? get headerBackgroundColor => Colors.transparent;
@override
Color? get headerForegroundColor => _colors.onSurfaceVariant;
@override
TextStyle? get headerHeadlineStyle => _textTheme.headlineLarge;
@override
TextStyle? get headerHelpStyle => _textTheme.labelLarge;
@override
TextStyle? get weekdayStyle => _textTheme.bodyLarge?.apply(
color: _colors.onSurface,
);
@override
TextStyle? get dayStyle => _textTheme.bodyLarge;
@override
MaterialStateProperty<Color?>? get dayForegroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
} else if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.onSurface;
});
@override
MaterialStateProperty<Color?>? get dayBackgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.primary;
}
return null;
});
@override
MaterialStateProperty<Color?>? get dayOverlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.1);
}
} else {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
}
return null;
});
@override
MaterialStateProperty<Color?>? get todayForegroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
} else if (states.contains(MaterialState.disabled)) {
return _colors.primary.withOpacity(0.38);
}
return _colors.primary;
});
@override
MaterialStateProperty<Color?>? get todayBackgroundColor => dayBackgroundColor;
@override
BorderSide? get todayBorder => BorderSide(color: _colors.primary);
@override
TextStyle? get yearStyle => _textTheme.bodyLarge;
@override
MaterialStateProperty<Color?>? get yearForegroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
} else if (states.contains(MaterialState.disabled)) {
return _colors.onSurfaceVariant.withOpacity(0.38);
}
return _colors.onSurfaceVariant;
});
@override
MaterialStateProperty<Color?>? get yearBackgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _colors.primary;
}
return null;
});
@override
MaterialStateProperty<Color?>? get yearOverlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.1);
}
} else {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
}
return null;
});
@override
Color? get rangePickerShadowColor => Colors.transparent;
@override
Color? get rangePickerSurfaceTintColor => Colors.transparent;
@override
Color? get rangeSelectionBackgroundColor => _colors.secondaryContainer;
@override
MaterialStateProperty<Color?>? get rangeSelectionOverlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimaryContainer.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimaryContainer.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimaryContainer.withOpacity(0.1);
}
return null;
});
@override
Color? get rangePickerHeaderBackgroundColor => Colors.transparent;
@override
Color? get rangePickerHeaderForegroundColor => _colors.onSurfaceVariant;
@override
TextStyle? get rangePickerHeaderHeadlineStyle => _textTheme.titleLarge;
@override
TextStyle? get rangePickerHeaderHelpStyle => _textTheme.titleSmall;
}
// END GENERATED TOKEN PROPERTIES - DatePicker
| flutter/packages/flutter/lib/src/material/date_picker_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/date_picker_theme.dart",
"repo_id": "flutter",
"token_count": 14698
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// A [ButtonStyle] that overrides the default appearance of
/// [ElevatedButton]s when it's used with [ElevatedButtonTheme] or with the
/// overall [Theme]'s [ThemeData.elevatedButtonTheme].
///
/// The [style]'s properties override [ElevatedButton]'s default style,
/// i.e. the [ButtonStyle] returned by [ElevatedButton.defaultStyleOf]. Only
/// the style's non-null property values or resolved non-null
/// [MaterialStateProperty] values are used.
///
/// See also:
///
/// * [ElevatedButtonTheme], the theme which is configured with this class.
/// * [ElevatedButton.defaultStyleOf], which returns the default [ButtonStyle]
/// for text buttons.
/// * [ElevatedButton.styleFrom], which converts simple values into a
/// [ButtonStyle] that's consistent with [ElevatedButton]'s defaults.
/// * [MaterialStateProperty.resolve], "resolve" a material state property
/// to a simple value based on a set of [MaterialState]s.
/// * [ThemeData.elevatedButtonTheme], which can be used to override the default
/// [ButtonStyle] for [ElevatedButton]s below the overall [Theme].
@immutable
class ElevatedButtonThemeData with Diagnosticable {
/// Creates an [ElevatedButtonThemeData].
///
/// The [style] may be null.
const ElevatedButtonThemeData({ this.style });
/// Overrides for [ElevatedButton]'s default style.
///
/// Non-null properties or non-null resolved [MaterialStateProperty]
/// values override the [ButtonStyle] returned by
/// [ElevatedButton.defaultStyleOf].
///
/// If [style] is null, then this theme doesn't override anything.
final ButtonStyle? style;
/// Linearly interpolate between two elevated button themes.
static ElevatedButtonThemeData? lerp(ElevatedButtonThemeData? a, ElevatedButtonThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return ElevatedButtonThemeData(
style: ButtonStyle.lerp(a?.style, b?.style, t),
);
}
@override
int get hashCode => style.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ElevatedButtonThemeData && other.style == style;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ButtonStyle>('style', style, defaultValue: null));
}
}
/// Overrides the default [ButtonStyle] of its [ElevatedButton] descendants.
///
/// See also:
///
/// * [ElevatedButtonThemeData], which is used to configure this theme.
/// * [ElevatedButton.defaultStyleOf], which returns the default [ButtonStyle]
/// for elevated buttons.
/// * [ElevatedButton.styleFrom], which converts simple values into a
/// [ButtonStyle] that's consistent with [ElevatedButton]'s defaults.
/// * [ThemeData.elevatedButtonTheme], which can be used to override the default
/// [ButtonStyle] for [ElevatedButton]s below the overall [Theme].
class ElevatedButtonTheme extends InheritedTheme {
/// Create a [ElevatedButtonTheme].
const ElevatedButtonTheme({
super.key,
required this.data,
required super.child,
});
/// The configuration of this theme.
final ElevatedButtonThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [ElevatedButtonTheme] widget, then
/// [ThemeData.elevatedButtonTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// ElevatedButtonThemeData theme = ElevatedButtonTheme.of(context);
/// ```
static ElevatedButtonThemeData of(BuildContext context) {
final ElevatedButtonTheme? buttonTheme = context.dependOnInheritedWidgetOfExactType<ElevatedButtonTheme>();
return buttonTheme?.data ?? Theme.of(context).elevatedButtonTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ElevatedButtonTheme(data: data, child: child);
}
@override
bool updateShouldNotify(ElevatedButtonTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/elevated_button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/elevated_button_theme.dart",
"repo_id": "flutter",
"token_count": 1353
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'theme.dart';
/// A header used in a Material Design [GridTile].
///
/// Typically used to add a one or two line header or footer on a [GridTile].
///
/// For a one-line header, include a [title] widget. To add a second line, also
/// include a [subtitle] widget. Use [leading] or [trailing] to add an icon.
///
/// See also:
///
/// * [GridTile]
/// * <https://material.io/design/components/image-lists.html#anatomy>
class GridTileBar extends StatelessWidget {
/// Creates a grid tile bar.
///
/// Typically used to with [GridTile].
const GridTileBar({
super.key,
this.backgroundColor,
this.leading,
this.title,
this.subtitle,
this.trailing,
});
/// The color to paint behind the child widgets.
///
/// Defaults to transparent.
final Color? backgroundColor;
/// A widget to display before the title.
///
/// Typically an [Icon] or an [IconButton] widget.
final Widget? leading;
/// The primary content of the list item.
///
/// Typically a [Text] widget.
final Widget? title;
/// Additional content displayed below the title.
///
/// Typically a [Text] widget.
final Widget? subtitle;
/// A widget to display after the title.
///
/// Typically an [Icon] or an [IconButton] widget.
final Widget? trailing;
@override
Widget build(BuildContext context) {
BoxDecoration? decoration;
if (backgroundColor != null) {
decoration = BoxDecoration(color: backgroundColor);
}
final EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(
start: leading != null ? 8.0 : 16.0,
end: trailing != null ? 8.0 : 16.0,
);
final ThemeData darkTheme = ThemeData.dark();
return Container(
padding: padding,
decoration: decoration,
height: (title != null && subtitle != null) ? 68.0 : 48.0,
child: Theme(
data: darkTheme,
child: IconTheme.merge(
data: const IconThemeData(color: Colors.white),
child: Row(
children: <Widget>[
if (leading != null)
Padding(padding: const EdgeInsetsDirectional.only(end: 8.0), child: leading),
if (title != null && subtitle != null)
Expanded(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
DefaultTextStyle(
style: darkTheme.textTheme.titleMedium!,
softWrap: false,
overflow: TextOverflow.ellipsis,
child: title!,
),
DefaultTextStyle(
style: darkTheme.textTheme.bodySmall!,
softWrap: false,
overflow: TextOverflow.ellipsis,
child: subtitle!,
),
],
),
)
else if (title != null || subtitle != null)
Expanded(
child: DefaultTextStyle(
style: darkTheme.textTheme.titleMedium!,
softWrap: false,
overflow: TextOverflow.ellipsis,
child: title ?? subtitle!,
),
),
if (trailing != null)
Padding(padding: const EdgeInsetsDirectional.only(start: 8.0), child: trailing),
],
),
),
),
);
}
}
| flutter/packages/flutter/lib/src/material/grid_tile_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/grid_tile_bar.dart",
"repo_id": "flutter",
"token_count": 1713
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
/// {@template widgets.material.magnifier.magnifier}
/// A [Magnifier] positioned by rules dictated by the native Android magnifier.
/// {@endtemplate}
///
/// {@template widgets.material.magnifier.positionRules}
/// Positions itself based on [magnifierInfo]. Specifically, follows the
/// following rules:
/// - Tracks the gesture's x coordinate, but clamped to the beginning and end of the
/// currently editing line.
/// - Focal point may never contain anything out of bounds.
/// - Never goes out of bounds vertically; offset until the entire magnifier is in the screen. The
/// focal point, regardless of this transformation, always points to the touch y coordinate.
/// - If just jumped between lines (prevY != currentY) then animate for duration
/// [jumpBetweenLinesAnimationDuration].
/// {@endtemplate}
class TextMagnifier extends StatefulWidget {
/// {@macro widgets.material.magnifier.magnifier}
///
/// {@template widgets.material.magnifier.androidDisclaimer}
/// These constants and default parameters were taken from the
/// Android 12 source code where directly transferable, and eyeballed on
/// a Pixel 6 running Android 12 otherwise.
/// {@endtemplate}
///
/// {@macro widgets.material.magnifier.positionRules}
const TextMagnifier({
super.key,
required this.magnifierInfo,
});
/// A [TextMagnifierConfiguration] that returns a [CupertinoTextMagnifier] on iOS,
/// [TextMagnifier] on Android, and null on all other platforms, and shows the editing handles
/// only on iOS.
static TextMagnifierConfiguration adaptiveMagnifierConfiguration = TextMagnifierConfiguration(
shouldDisplayHandlesInMagnifier: defaultTargetPlatform == TargetPlatform.iOS,
magnifierBuilder: (
BuildContext context,
MagnifierController controller,
ValueNotifier<MagnifierInfo> magnifierInfo,
) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
return CupertinoTextMagnifier(
controller: controller,
magnifierInfo: magnifierInfo,
);
case TargetPlatform.android:
return TextMagnifier(
magnifierInfo: magnifierInfo,
);
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return null;
}
}
);
/// The duration that the position is animated if [TextMagnifier] just switched
/// between lines.
@visibleForTesting
static const Duration jumpBetweenLinesAnimationDuration =
Duration(milliseconds: 70);
/// [TextMagnifier] positions itself based on [magnifierInfo].
///
/// {@macro widgets.material.magnifier.positionRules}
final ValueNotifier<MagnifierInfo>
magnifierInfo;
@override
State<TextMagnifier> createState() => _TextMagnifierState();
}
class _TextMagnifierState extends State<TextMagnifier> {
// Should _only_ be null on construction. This is because of the animation logic.
//
// Animations are added when `last_build_y != current_build_y`. This condition
// is true on the initial render, which would mean that the initial
// build would be animated - this is undesired. Thus, this is null for the
// first frame and the condition becomes `magnifierPosition != null && last_build_y != this_build_y`.
Offset? _magnifierPosition;
// A timer that unsets itself after an animation duration.
// If the timer exists, then the magnifier animates its position -
// if this timer does not exist, the magnifier tracks the gesture (with respect
// to the positioning rules) directly.
Timer? _positionShouldBeAnimatedTimer;
bool get _positionShouldBeAnimated => _positionShouldBeAnimatedTimer != null;
Offset _extraFocalPointOffset = Offset.zero;
@override
void initState() {
super.initState();
widget.magnifierInfo
.addListener(_determineMagnifierPositionAndFocalPoint);
}
@override
void dispose() {
widget.magnifierInfo
.removeListener(_determineMagnifierPositionAndFocalPoint);
_positionShouldBeAnimatedTimer?.cancel();
super.dispose();
}
@override
void didChangeDependencies() {
_determineMagnifierPositionAndFocalPoint();
super.didChangeDependencies();
}
@override
void didUpdateWidget(TextMagnifier oldWidget) {
if (oldWidget.magnifierInfo != widget.magnifierInfo) {
oldWidget.magnifierInfo.removeListener(_determineMagnifierPositionAndFocalPoint);
widget.magnifierInfo.addListener(_determineMagnifierPositionAndFocalPoint);
}
super.didUpdateWidget(oldWidget);
}
/// {@macro widgets.material.magnifier.positionRules}
void _determineMagnifierPositionAndFocalPoint() {
final MagnifierInfo selectionInfo =
widget.magnifierInfo.value;
final Rect screenRect = Offset.zero & MediaQuery.sizeOf(context);
// Since by default we draw at the top left corner, this offset
// shifts the magnifier so we draw at the center, and then also includes
// the "above touch point" shift.
final Offset basicMagnifierOffset = Offset(
Magnifier.kDefaultMagnifierSize.width / 2,
Magnifier.kDefaultMagnifierSize.height +
Magnifier.kStandardVerticalFocalPointShift);
// Since the magnifier should not go past the edges of the line,
// but must track the gesture otherwise, constrain the X of the magnifier
// to always stay between line start and end.
final double magnifierX = clampDouble(
selectionInfo.globalGesturePosition.dx,
selectionInfo.currentLineBoundaries.left,
selectionInfo.currentLineBoundaries.right);
// Place the magnifier at the previously calculated X, and the Y should be
// exactly at the center of the handle.
final Rect unadjustedMagnifierRect =
Offset(magnifierX, selectionInfo.caretRect.center.dy) - basicMagnifierOffset &
Magnifier.kDefaultMagnifierSize;
// Shift the magnifier so that, if we are ever out of the screen, we become in bounds.
// This probably won't have much of an effect on the X, since it is already bound
// to the currentLineBoundaries, but will shift vertically if the magnifier is out of bounds.
final Rect screenBoundsAdjustedMagnifierRect =
MagnifierController.shiftWithinBounds(
bounds: screenRect, rect: unadjustedMagnifierRect);
// Done with the magnifier position!
final Offset finalMagnifierPosition = screenBoundsAdjustedMagnifierRect.topLeft;
// The insets, from either edge, that the focal point should not point
// past lest the magnifier displays something out of bounds.
final double horizontalMaxFocalPointEdgeInsets =
(Magnifier.kDefaultMagnifierSize.width / 2) / Magnifier._magnification;
// Adjust the focal point horizontally such that none of the magnifier
// ever points to anything out of bounds.
final double newGlobalFocalPointX;
// If the text field is so narrow that we must show out of bounds,
// then settle for pointing to the center all the time.
if (selectionInfo.fieldBounds.width <
horizontalMaxFocalPointEdgeInsets * 2) {
newGlobalFocalPointX = selectionInfo.fieldBounds.center.dx;
} else {
// Otherwise, we can clamp the focal point to always point in bounds.
newGlobalFocalPointX = clampDouble(
screenBoundsAdjustedMagnifierRect.center.dx,
selectionInfo.fieldBounds.left + horizontalMaxFocalPointEdgeInsets,
selectionInfo.fieldBounds.right - horizontalMaxFocalPointEdgeInsets);
}
// Since the previous value is now a global offset (i.e. `newGlobalFocalPoint`
// is now a global offset), we must subtract the magnifier's global offset
// to obtain the relative shift in the focal point.
final double newRelativeFocalPointX =
newGlobalFocalPointX - screenBoundsAdjustedMagnifierRect.center.dx;
// The Y component means that if we are pressed up against the top of the screen,
// then we should adjust the focal point such that it now points to how far we moved
// the magnifier. screenBoundsAdjustedMagnifierRect.top == unadjustedMagnifierRect.top for most cases,
// but when pressed up against the top of the screen, we adjust the focal point by
// the amount that we shifted from our "natural" position.
final Offset focalPointAdjustmentForScreenBoundsAdjustment = Offset(
newRelativeFocalPointX,
unadjustedMagnifierRect.top - screenBoundsAdjustedMagnifierRect.top,
);
Timer? positionShouldBeAnimated = _positionShouldBeAnimatedTimer;
if (_magnifierPosition != null && finalMagnifierPosition.dy != _magnifierPosition!.dy) {
if (_positionShouldBeAnimatedTimer != null &&
_positionShouldBeAnimatedTimer!.isActive) {
_positionShouldBeAnimatedTimer!.cancel();
}
// Create a timer that deletes itself when the timer is complete.
// This is `mounted` safe, since the timer is canceled in `dispose`.
positionShouldBeAnimated = Timer(
TextMagnifier.jumpBetweenLinesAnimationDuration,
() => setState(() {
_positionShouldBeAnimatedTimer = null;
}));
}
setState(() {
_magnifierPosition = finalMagnifierPosition;
_positionShouldBeAnimatedTimer = positionShouldBeAnimated;
_extraFocalPointOffset = focalPointAdjustmentForScreenBoundsAdjustment;
});
}
@override
Widget build(BuildContext context) {
assert(_magnifierPosition != null,
'Magnifier position should only be null before the first build.');
return AnimatedPositioned(
top: _magnifierPosition!.dy,
left: _magnifierPosition!.dx,
// Material magnifier typically does not animate, unless we jump between lines,
// in which case we animate between lines.
duration: _positionShouldBeAnimated
? TextMagnifier.jumpBetweenLinesAnimationDuration
: Duration.zero,
child: Magnifier(
additionalFocalPointOffset: _extraFocalPointOffset,
),
);
}
}
/// A Material styled magnifying glass.
///
/// {@macro flutter.widgets.magnifier.intro}
///
/// This widget focuses on mimicking the _style_ of the magnifier on material. For a
/// widget that is focused on mimicking the behavior of a material magnifier, see [TextMagnifier].
class Magnifier extends StatelessWidget {
/// Creates a [RawMagnifier] in the Material style.
///
/// {@macro widgets.material.magnifier.androidDisclaimer}
const Magnifier({
super.key,
this.additionalFocalPointOffset = Offset.zero,
this.borderRadius = const BorderRadius.all(Radius.circular(_borderRadius)),
this.filmColor = const Color.fromARGB(8, 158, 158, 158),
this.shadows = const <BoxShadow>[
BoxShadow(
blurRadius: 1.5,
offset: Offset(0, 2),
spreadRadius: 0.75,
color: Color.fromARGB(25, 0, 0, 0))
],
this.size = Magnifier.kDefaultMagnifierSize,
});
/// The default size of this [Magnifier].
///
/// The size of the magnifier may be modified through the constructor;
/// [kDefaultMagnifierSize] is extracted from the default parameter of
/// [Magnifier]'s constructor so that positioners may depend on it.
@visibleForTesting
static const Size kDefaultMagnifierSize = Size(77.37, 37.9);
/// The vertical distance that the magnifier should be above the focal point.
///
/// [kStandardVerticalFocalPointShift] is an unmodifiable constant so that positioning of this
/// [Magnifier] can be done with a guaranteed size, as opposed to an estimate.
@visibleForTesting
static const double kStandardVerticalFocalPointShift = 22;
static const double _borderRadius = 40;
static const double _magnification = 1.25;
/// Any additional offset the focal point requires to "point"
/// to the correct place.
///
/// This is useful for instances where the magnifier is not pointing to something
/// directly below it.
final Offset additionalFocalPointOffset;
/// The border radius for this magnifier.
final BorderRadius borderRadius;
/// The color to tint the image in this [Magnifier].
///
/// On native Android, there is a almost transparent gray tint to the
/// magnifier, in order to better distinguish the contents of the lens from
/// the background.
final Color filmColor;
/// The shadows for this [Magnifier].
final List<BoxShadow> shadows;
/// The [Size] of this [Magnifier].
///
/// This size does not include the border.
final Size size;
@override
Widget build(BuildContext context) {
return RawMagnifier(
decoration: MagnifierDecoration(
shape: RoundedRectangleBorder(borderRadius: borderRadius),
shadows: shadows,
),
magnificationScale: _magnification,
focalPointOffset: additionalFocalPointOffset +
Offset(0, kStandardVerticalFocalPointShift + kDefaultMagnifierSize.height / 2),
size: size,
child: ColoredBox(
color: filmColor,
),
);
}
}
| flutter/packages/flutter/lib/src/material/magnifier.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/magnifier.dart",
"repo_id": "flutter",
"token_count": 4235
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'material_state.dart';
import 'navigation_drawer.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [NavigationDrawer]
/// widgets.
///
/// Descendant widgets obtain the current [NavigationDrawerThemeData] object
/// using `NavigationDrawerTheme.of(context)`. Instances of
/// [NavigationDrawerThemeData] can be customized with
/// [NavigationDrawerThemeData.copyWith].
///
/// Typically a [NavigationDrawerThemeData] is specified as part of the
/// overall [Theme] with [ThemeData.navigationDrawerTheme]. Alternatively, a
/// [NavigationDrawerTheme] inherited widget can be used to theme [NavigationDrawer]s
/// in a subtree of widgets.
///
/// All [NavigationDrawerThemeData] properties are `null` by default.
/// When null, the [NavigationDrawer] will provide its own defaults based on the
/// overall [Theme]'s textTheme and colorScheme. See the individual
/// [NavigationDrawer] properties for details.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class NavigationDrawerThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.navigationDrawerTheme] and
/// [NavigationDrawerTheme].
const NavigationDrawerThemeData({
this.tileHeight,
this.backgroundColor,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.indicatorColor,
this.indicatorShape,
this.indicatorSize,
this.labelTextStyle,
this.iconTheme,
});
/// Overrides the default height of [NavigationDrawerDestination].
final double? tileHeight;
/// Overrides the default value of [NavigationDrawer.backgroundColor].
final Color? backgroundColor;
/// Overrides the default value of [NavigationDrawer.elevation].
final double? elevation;
/// Overrides the default value of [NavigationDrawer.shadowColor].
final Color? shadowColor;
/// Overrides the default value of [NavigationDrawer.surfaceTintColor].
final Color? surfaceTintColor;
/// Overrides the default value of [NavigationDrawer]'s selection indicator.
final Color? indicatorColor;
/// Overrides the default shape of the [NavigationDrawer]'s selection indicator.
final ShapeBorder? indicatorShape;
/// Overrides the default size of the [NavigationDrawer]'s selection indicator.
final Size? indicatorSize;
/// The style to merge with the default text style for
/// [NavigationDestination] labels.
///
/// You can use this to specify a different style when the label is selected.
final MaterialStateProperty<TextStyle?>? labelTextStyle;
/// The theme to merge with the default icon theme for
/// [NavigationDestination] icons.
///
/// You can use this to specify a different icon theme when the icon is
/// selected.
final MaterialStateProperty<IconThemeData?>? iconTheme;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
NavigationDrawerThemeData copyWith({
double? tileHeight,
Color? backgroundColor,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
Color? indicatorColor,
ShapeBorder? indicatorShape,
Size? indicatorSize,
MaterialStateProperty<TextStyle?>? labelTextStyle,
MaterialStateProperty<IconThemeData?>? iconTheme,
}) {
return NavigationDrawerThemeData(
tileHeight: tileHeight ?? this.tileHeight,
backgroundColor: backgroundColor ?? this.backgroundColor,
elevation: elevation ?? this.elevation,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
indicatorColor: indicatorColor ?? this.indicatorColor,
indicatorShape: indicatorShape ?? this.indicatorShape,
indicatorSize: indicatorSize ?? this.indicatorSize,
labelTextStyle: labelTextStyle ?? this.labelTextStyle,
iconTheme: iconTheme ?? this.iconTheme,
);
}
/// Linearly interpolate between two navigation rail themes.
///
/// If both arguments are null then null is returned.
///
/// {@macro dart.ui.shadow.lerp}
static NavigationDrawerThemeData? lerp(NavigationDrawerThemeData? a, NavigationDrawerThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return NavigationDrawerThemeData(
tileHeight: lerpDouble(a?.tileHeight, b?.tileHeight, t),
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
indicatorColor: Color.lerp(a?.indicatorColor, b?.indicatorColor, t),
indicatorShape: ShapeBorder.lerp(a?.indicatorShape, b?.indicatorShape, t),
indicatorSize: Size.lerp(a?.indicatorSize, a?.indicatorSize, t),
labelTextStyle: MaterialStateProperty.lerp<TextStyle?>(
a?.labelTextStyle, b?.labelTextStyle, t, TextStyle.lerp),
iconTheme: MaterialStateProperty.lerp<IconThemeData?>(
a?.iconTheme, b?.iconTheme, t, IconThemeData.lerp),
);
}
@override
int get hashCode => Object.hash(
tileHeight,
backgroundColor,
elevation,
shadowColor,
surfaceTintColor,
indicatorColor,
indicatorShape,
indicatorSize,
labelTextStyle,
iconTheme,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is NavigationDrawerThemeData &&
other.tileHeight == tileHeight &&
other.backgroundColor == backgroundColor &&
other.elevation == elevation &&
other.shadowColor == shadowColor &&
other.surfaceTintColor == surfaceTintColor &&
other.indicatorColor == indicatorColor &&
other.indicatorShape == indicatorShape &&
other.indicatorSize == indicatorSize &&
other.labelTextStyle == labelTextStyle &&
other.iconTheme == iconTheme;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties
.add(DoubleProperty('tileHeight', tileHeight, defaultValue: null));
properties.add(
ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor,
defaultValue: null));
properties.add(
ColorProperty('indicatorColor', indicatorColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>(
'indicatorShape', indicatorShape,
defaultValue: null));
properties.add(DiagnosticsProperty<Size>('indicatorSize', indicatorSize,
defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<TextStyle?>>(
'labelTextStyle', labelTextStyle,
defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<IconThemeData?>>(
'iconTheme', iconTheme,
defaultValue: null));
}
}
/// An inherited widget that defines visual properties for [NavigationDrawer]s and
/// [NavigationDestination]s in this widget's subtree.
///
/// Values specified here are used for [NavigationDrawer] properties that are not
/// given an explicit non-null value.
///
/// See also:
///
/// * [ThemeData.navigationDrawerTheme], which describes the
/// [NavigationDrawerThemeData] in the overall theme for the application.
class NavigationDrawerTheme extends InheritedTheme {
/// Creates a navigation rail theme that controls the
/// [NavigationDrawerThemeData] properties for a [NavigationDrawer].
const NavigationDrawerTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the background color, label text style, icon theme, and label
/// type values for descendant [NavigationDrawer] widgets.
final NavigationDrawerThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [NavigationDrawerTheme] widget, then
/// [ThemeData.navigationDrawerTheme] is used.
static NavigationDrawerThemeData of(BuildContext context) {
final NavigationDrawerTheme? navigationDrawerTheme =
context.dependOnInheritedWidgetOfExactType<NavigationDrawerTheme>();
return navigationDrawerTheme?.data ??
Theme.of(context).navigationDrawerTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return NavigationDrawerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(NavigationDrawerTheme oldWidget) =>
data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/navigation_drawer_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/navigation_drawer_theme.dart",
"repo_id": "flutter",
"token_count": 2974
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart' show timeDilation;
import 'package:flutter/widgets.dart';
import 'constants.dart';
import 'debug.dart';
import 'material_state.dart';
import 'slider_theme.dart';
import 'theme.dart';
// Examples can assume:
// RangeValues _rangeValues = const RangeValues(0.3, 0.7);
// RangeValues _dollarsRange = const RangeValues(50, 100);
// void setState(VoidCallback fn) { }
/// [RangeSlider] uses this callback to paint the value indicator on the overlay.
/// Since the value indicator is painted on the Overlay; this method paints the
/// value indicator in a [RenderBox] that appears in the [Overlay].
typedef PaintRangeValueIndicator = void Function(PaintingContext context, Offset offset);
/// A Material Design range slider.
///
/// Used to select a range from a range of values.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ufb4gIPDmEs}
///
/// {@tool dartpad}
/// 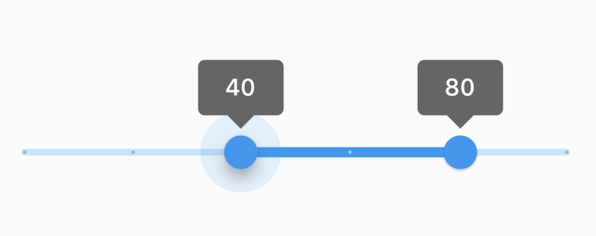
///
/// This range values are in intervals of 20 because the Range Slider has 5
/// divisions, from 0 to 100. This means are values are split between 0, 20, 40,
/// 60, 80, and 100. The range values are initialized with 40 and 80 in this demo.
///
/// ** See code in examples/api/lib/material/range_slider/range_slider.0.dart **
/// {@end-tool}
///
/// A range slider can be used to select from either a continuous or a discrete
/// set of values. The default is to use a continuous range of values from [min]
/// to [max]. To use discrete values, use a non-null value for [divisions], which
/// indicates the number of discrete intervals. For example, if [min] is 0.0 and
/// [max] is 50.0 and [divisions] is 5, then the slider can take on the
/// discrete values 0.0, 10.0, 20.0, 30.0, 40.0, and 50.0.
///
/// The terms for the parts of a slider are:
///
/// * The "thumbs", which are the shapes that slide horizontally when the user
/// drags them to change the selected range.
/// * The "track", which is the horizontal line that the thumbs can be dragged
/// along.
/// * The "tick marks", which mark the discrete values of a discrete slider.
/// * The "overlay", which is a highlight that's drawn over a thumb in response
/// to a user tap-down gesture.
/// * The "value indicators", which are the shapes that pop up when the user
/// is dragging a thumb to show the value being selected.
/// * The "active" segment of the slider is the segment between the two thumbs.
/// * The "inactive" slider segments are the two track intervals outside of the
/// slider's thumbs.
///
/// The range slider will be disabled if [onChanged] is null or if the range
/// given by [min]..[max] is empty (i.e. if [min] is equal to [max]).
///
/// The range slider widget itself does not maintain any state. Instead, when
/// the state of the slider changes, the widget calls the [onChanged] callback.
/// Most widgets that use a range slider will listen for the [onChanged] callback
/// and rebuild the slider with new [values] to update the visual appearance of
/// the slider. To know when the value starts to change, or when it is done
/// changing, set the optional callbacks [onChangeStart] and/or [onChangeEnd].
///
/// By default, a slider will be as wide as possible, centered vertically. When
/// given unbounded constraints, it will attempt to make the track 144 pixels
/// wide (including margins on each side) and will shrink-wrap vertically.
///
/// Requires one of its ancestors to be a [Material] widget. This is typically
/// provided by a [Scaffold] widget.
///
/// Requires one of its ancestors to be a [MediaQuery] widget. Typically, a
/// [MediaQuery] widget is introduced by the [MaterialApp] or [WidgetsApp]
/// widget at the top of your application widget tree.
///
/// To determine how it should be displayed (e.g. colors, thumb shape, etc.),
/// a slider uses the [SliderThemeData] available from either a [SliderTheme]
/// widget, or the [ThemeData.sliderTheme] inside a [Theme] widget above it in
/// the widget tree. You can also override some of the colors with the
/// [activeColor] and [inactiveColor] properties, although more fine-grained
/// control of the colors, and other visual properties is achieved using a
/// [SliderThemeData].
///
/// See also:
///
/// * [SliderTheme] and [SliderThemeData] for information about controlling
/// the visual appearance of the slider.
/// * [Slider], for a single-valued slider.
/// * [Radio], for selecting among a set of explicit values.
/// * [Checkbox] and [Switch], for toggling a particular value on or off.
/// * <https://material.io/design/components/sliders.html>
/// * [MediaQuery], from which the text scale factor is obtained.
class RangeSlider extends StatefulWidget {
/// Creates a Material Design range slider.
///
/// The range slider widget itself does not maintain any state. Instead, when
/// the state of the slider changes, the widget calls the [onChanged]
/// callback. Most widgets that use a range slider will listen for the
/// [onChanged] callback and rebuild the slider with new [values] to update
/// the visual appearance of the slider. To know when the value starts to
/// change, or when it is done changing, set the optional callbacks
/// [onChangeStart] and/or [onChangeEnd].
///
/// * [values], which determines currently selected values for this range
/// slider.
/// * [onChanged], which is called while the user is selecting a new value for
/// the range slider.
/// * [onChangeStart], which is called when the user starts to select a new
/// value for the range slider.
/// * [onChangeEnd], which is called when the user is done selecting a new
/// value for the range slider.
///
/// You can override some of the colors with the [activeColor] and
/// [inactiveColor] properties, although more fine-grained control of the
/// appearance is achieved using a [SliderThemeData].
///
/// The [min] must be less than or equal to the [max].
///
/// The [RangeValues.start] attribute of the [values] parameter must be less
/// than or equal to its [RangeValues.end] attribute. The [RangeValues.start]
/// and [RangeValues.end] attributes of the [values] parameter must be greater
/// than or equal to the [min] parameter and less than or equal to the [max]
/// parameter.
///
/// The [divisions] parameter must be null or greater than zero.
RangeSlider({
super.key,
required this.values,
required this.onChanged,
this.onChangeStart,
this.onChangeEnd,
this.min = 0.0,
this.max = 1.0,
this.divisions,
this.labels,
this.activeColor,
this.inactiveColor,
this.overlayColor,
this.mouseCursor,
this.semanticFormatterCallback,
}) : assert(min <= max),
assert(values.start <= values.end),
assert(values.start >= min && values.start <= max),
assert(values.end >= min && values.end <= max),
assert(divisions == null || divisions > 0);
/// The currently selected values for this range slider.
///
/// The slider's thumbs are drawn at horizontal positions that corresponds to
/// these values.
final RangeValues values;
/// Called when the user is selecting a new value for the slider by dragging.
///
/// The slider passes the new values to the callback but does not actually
/// change state until the parent widget rebuilds the slider with the new
/// values.
///
/// If null, the slider will be displayed as disabled.
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// {@tool snippet}
///
/// ```dart
/// RangeSlider(
/// values: _rangeValues,
/// min: 1.0,
/// max: 10.0,
/// onChanged: (RangeValues newValues) {
/// setState(() {
/// _rangeValues = newValues;
/// });
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeStart], which is called when the user starts changing the
/// values.
/// * [onChangeEnd], which is called when the user stops changing the values.
final ValueChanged<RangeValues>? onChanged;
/// Called when the user starts selecting new values for the slider.
///
/// This callback shouldn't be used to update the slider [values] (use
/// [onChanged] for that). Rather, it should be used to be notified when the
/// user has started selecting a new value by starting a drag or with a tap.
///
/// The values passed will be the last [values] that the slider had before the
/// change began.
///
/// {@tool snippet}
///
/// ```dart
/// RangeSlider(
/// values: _rangeValues,
/// min: 1.0,
/// max: 10.0,
/// onChanged: (RangeValues newValues) {
/// setState(() {
/// _rangeValues = newValues;
/// });
/// },
/// onChangeStart: (RangeValues startValues) {
/// print('Started change at $startValues');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeEnd] for a callback that is called when the value change is
/// complete.
final ValueChanged<RangeValues>? onChangeStart;
/// Called when the user is done selecting new values for the slider.
///
/// This differs from [onChanged] because it is only called once at the end
/// of the interaction, while [onChanged] is called as the value is getting
/// updated within the interaction.
///
/// This callback shouldn't be used to update the slider [values] (use
/// [onChanged] for that). Rather, it should be used to know when the user has
/// completed selecting a new [values] by ending a drag or a click.
///
/// {@tool snippet}
///
/// ```dart
/// RangeSlider(
/// values: _rangeValues,
/// min: 1.0,
/// max: 10.0,
/// onChanged: (RangeValues newValues) {
/// setState(() {
/// _rangeValues = newValues;
/// });
/// },
/// onChangeEnd: (RangeValues endValues) {
/// print('Ended change at $endValues');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeStart] for a callback that is called when a value change
/// begins.
final ValueChanged<RangeValues>? onChangeEnd;
/// The minimum value the user can select.
///
/// Defaults to 0.0. Must be less than or equal to [max].
///
/// If the [max] is equal to the [min], then the slider is disabled.
final double min;
/// The maximum value the user can select.
///
/// Defaults to 1.0. Must be greater than or equal to [min].
///
/// If the [max] is equal to the [min], then the slider is disabled.
final double max;
/// The number of discrete divisions.
///
/// Typically used with [labels] to show the current discrete values.
///
/// If null, the slider is continuous.
final int? divisions;
/// Labels to show as text in the [SliderThemeData.rangeValueIndicatorShape]
/// when the slider is active and [SliderThemeData.showValueIndicator]
/// is satisfied.
///
/// There are two labels: one for the start thumb and one for the end thumb.
///
/// Each label is rendered using the active [ThemeData]'s
/// [TextTheme.bodyLarge] text style, with the theme data's
/// [ColorScheme.onPrimary] color. The label's text style can be overridden
/// with [SliderThemeData.valueIndicatorTextStyle].
///
/// If null, then the value indicator will not be displayed.
///
/// See also:
///
/// * [RangeSliderValueIndicatorShape] for how to create a custom value
/// indicator shape.
final RangeLabels? labels;
/// The color of the track's active segment, i.e. the span of track between
/// the thumbs.
///
/// Defaults to [ColorScheme.primary].
///
/// Using a [SliderTheme] gives more fine-grained control over the
/// appearance of various components of the slider.
final Color? activeColor;
/// The color of the track's inactive segments, i.e. the span of tracks
/// between the min and the start thumb, and the end thumb and the max.
///
/// Defaults to [ColorScheme.primary] with 24% opacity.
///
/// Using a [SliderTheme] gives more fine-grained control over the
/// appearance of various components of the slider.
final Color? inactiveColor;
/// The highlight color that's typically used to indicate that
/// the range slider thumb is hovered or dragged.
///
/// If this property is null, [RangeSlider] will use [activeColor] with
/// an opacity of 0.12. If null, [SliderThemeData.overlayColor]
/// will be used, otherwise defaults to [ColorScheme.primary] with
/// an opacity of 0.12.
final MaterialStateProperty<Color?>? overlayColor;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If null, then the value of [SliderThemeData.mouseCursor] is used. If that
/// is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], which can be used to create a [MouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// The callback used to create a semantic value from the slider's values.
///
/// Defaults to formatting values as a percentage.
///
/// This is used by accessibility frameworks like TalkBack on Android to
/// inform users what the currently selected value is with more context.
///
/// {@tool snippet}
///
/// In the example below, a slider for currency values is configured to
/// announce a value with a currency label.
///
/// ```dart
/// RangeSlider(
/// values: _dollarsRange,
/// min: 20.0,
/// max: 330.0,
/// onChanged: (RangeValues newValues) {
/// setState(() {
/// _dollarsRange = newValues;
/// });
/// },
/// semanticFormatterCallback: (double newValue) {
/// return '${newValue.round()} dollars';
/// }
/// )
/// ```
/// {@end-tool}
final SemanticFormatterCallback? semanticFormatterCallback;
// Touch width for the tap boundary of the slider thumbs.
static const double _minTouchTargetWidth = kMinInteractiveDimension;
@override
State<RangeSlider> createState() => _RangeSliderState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('valueStart', values.start));
properties.add(DoubleProperty('valueEnd', values.end));
properties.add(ObjectFlagProperty<ValueChanged<RangeValues>>('onChanged', onChanged, ifNull: 'disabled'));
properties.add(ObjectFlagProperty<ValueChanged<RangeValues>>.has('onChangeStart', onChangeStart));
properties.add(ObjectFlagProperty<ValueChanged<RangeValues>>.has('onChangeEnd', onChangeEnd));
properties.add(DoubleProperty('min', min));
properties.add(DoubleProperty('max', max));
properties.add(IntProperty('divisions', divisions));
properties.add(StringProperty('labelStart', labels?.start));
properties.add(StringProperty('labelEnd', labels?.end));
properties.add(ColorProperty('activeColor', activeColor));
properties.add(ColorProperty('inactiveColor', inactiveColor));
properties.add(ObjectFlagProperty<ValueChanged<double>>.has('semanticFormatterCallback', semanticFormatterCallback));
}
}
class _RangeSliderState extends State<RangeSlider> with TickerProviderStateMixin {
static const Duration enableAnimationDuration = Duration(milliseconds: 75);
static const Duration valueIndicatorAnimationDuration = Duration(milliseconds: 100);
// Animation controller that is run when the overlay (a.k.a radial reaction)
// changes visibility in response to user interaction.
late AnimationController overlayController;
// Animation controller that is run when the value indicators change visibility.
late AnimationController valueIndicatorController;
// Animation controller that is run when enabling/disabling the slider.
late AnimationController enableController;
// Animation controllers that are run when transitioning between one value
// and the next on a discrete slider.
late AnimationController startPositionController;
late AnimationController endPositionController;
Timer? interactionTimer;
// Value Indicator paint Animation that appears on the Overlay.
PaintRangeValueIndicator? paintTopValueIndicator;
PaintRangeValueIndicator? paintBottomValueIndicator;
bool get _enabled => widget.onChanged != null;
bool _dragging = false;
bool _hovering = false;
void _handleHoverChanged(bool hovering) {
if (hovering != _hovering) {
setState(() { _hovering = hovering; });
}
}
@override
void initState() {
super.initState();
overlayController = AnimationController(
duration: kRadialReactionDuration,
vsync: this,
);
valueIndicatorController = AnimationController(
duration: valueIndicatorAnimationDuration,
vsync: this,
);
enableController = AnimationController(
duration: enableAnimationDuration,
vsync: this,
value: _enabled ? 1.0 : 0.0,
);
startPositionController = AnimationController(
duration: Duration.zero,
vsync: this,
value: _unlerp(widget.values.start),
);
endPositionController = AnimationController(
duration: Duration.zero,
vsync: this,
value: _unlerp(widget.values.end),
);
}
@override
void didUpdateWidget(RangeSlider oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.onChanged == widget.onChanged) {
return;
}
final bool wasEnabled = oldWidget.onChanged != null;
final bool isEnabled = _enabled;
if (wasEnabled != isEnabled) {
if (isEnabled) {
enableController.forward();
} else {
enableController.reverse();
}
}
}
@override
void dispose() {
interactionTimer?.cancel();
overlayController.dispose();
valueIndicatorController.dispose();
enableController.dispose();
startPositionController.dispose();
endPositionController.dispose();
overlayEntry?.remove();
overlayEntry?.dispose();
overlayEntry = null;
super.dispose();
}
void _handleChanged(RangeValues values) {
assert(_enabled);
final RangeValues lerpValues = _lerpRangeValues(values);
if (lerpValues != widget.values) {
widget.onChanged!(lerpValues);
}
}
void _handleDragStart(RangeValues values) {
assert(widget.onChangeStart != null);
_dragging = true;
widget.onChangeStart!(_lerpRangeValues(values));
}
void _handleDragEnd(RangeValues values) {
assert(widget.onChangeEnd != null);
_dragging = false;
widget.onChangeEnd!(_lerpRangeValues(values));
}
// Returns a number between min and max, proportional to value, which must
// be between 0.0 and 1.0.
double _lerp(double value) => ui.lerpDouble(widget.min, widget.max, value)!;
// Returns a new range value with the start and end lerped.
RangeValues _lerpRangeValues(RangeValues values) {
return RangeValues(_lerp(values.start), _lerp(values.end));
}
// Returns a number between 0.0 and 1.0, given a value between min and max.
double _unlerp(double value) {
assert(value <= widget.max);
assert(value >= widget.min);
return widget.max > widget.min ? (value - widget.min) / (widget.max - widget.min) : 0.0;
}
// Returns a new range value with the start and end unlerped.
RangeValues _unlerpRangeValues(RangeValues values) {
return RangeValues(_unlerp(values.start), _unlerp(values.end));
}
// Finds closest thumb. If the thumbs are close to each other, no thumb is
// immediately selected while the drag displacement is zero. If the first
// non-zero displacement is negative, then the left thumb is selected, and if its
// positive, then the right thumb is selected.
Thumb? _defaultRangeThumbSelector(
TextDirection textDirection,
RangeValues values,
double tapValue,
Size thumbSize,
Size trackSize,
double dx, // The horizontal delta or displacement of the drag update.
) {
final double touchRadius = math.max(thumbSize.width, RangeSlider._minTouchTargetWidth) / 2;
final bool inStartTouchTarget = (tapValue - values.start).abs() * trackSize.width < touchRadius;
final bool inEndTouchTarget = (tapValue - values.end).abs() * trackSize.width < touchRadius;
// Use dx if the thumb touch targets overlap. If dx is 0 and the drag
// position is in both touch targets, no thumb is selected because it is
// ambiguous to which thumb should be selected. If the dx is non-zero, the
// thumb selection is determined by the direction of the dx. The left thumb
// is chosen for negative dx, and the right thumb is chosen for positive dx.
if (inStartTouchTarget && inEndTouchTarget) {
final (bool towardsStart, bool towardsEnd) = switch (textDirection) {
TextDirection.ltr => (dx < 0, dx > 0),
TextDirection.rtl => (dx > 0, dx < 0),
};
if (towardsStart) {
return Thumb.start;
}
if (towardsEnd) {
return Thumb.end;
}
} else {
// Snap position on the track if its in the inactive range.
if (tapValue < values.start || inStartTouchTarget) {
return Thumb.start;
}
if (tapValue > values.end || inEndTouchTarget) {
return Thumb.end;
}
}
return null;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMediaQuery(context));
final ThemeData theme = Theme.of(context);
SliderThemeData sliderTheme = SliderTheme.of(context);
// If the widget has active or inactive colors specified, then we plug them
// in to the slider theme as best we can. If the developer wants more
// control than that, then they need to use a SliderTheme. The default
// colors come from the ThemeData.colorScheme. These colors, along with
// the default shapes and text styles are aligned to the Material
// Guidelines.
const double defaultTrackHeight = 4;
const RangeSliderTrackShape defaultTrackShape = RoundedRectRangeSliderTrackShape();
const RangeSliderTickMarkShape defaultTickMarkShape = RoundRangeSliderTickMarkShape();
const SliderComponentShape defaultOverlayShape = RoundSliderOverlayShape();
const RangeSliderThumbShape defaultThumbShape = RoundRangeSliderThumbShape();
const RangeSliderValueIndicatorShape defaultValueIndicatorShape = RectangularRangeSliderValueIndicatorShape();
const ShowValueIndicator defaultShowValueIndicator = ShowValueIndicator.onlyForDiscrete;
const double defaultMinThumbSeparation = 8;
final Set<MaterialState> states = <MaterialState>{
if (!_enabled) MaterialState.disabled,
if (_hovering) MaterialState.hovered,
if (_dragging) MaterialState.dragged,
};
// The value indicator's color is not the same as the thumb and active track
// (which can be defined by activeColor) if the
// RectangularSliderValueIndicatorShape is used. In all other cases, the
// value indicator is assumed to be the same as the active color.
final RangeSliderValueIndicatorShape valueIndicatorShape = sliderTheme.rangeValueIndicatorShape ?? defaultValueIndicatorShape;
final Color valueIndicatorColor;
if (valueIndicatorShape is RectangularRangeSliderValueIndicatorShape) {
valueIndicatorColor = sliderTheme.valueIndicatorColor ?? Color.alphaBlend(theme.colorScheme.onSurface.withOpacity(0.60), theme.colorScheme.surface.withOpacity(0.90));
} else {
valueIndicatorColor = widget.activeColor ?? sliderTheme.valueIndicatorColor ?? theme.colorScheme.primary;
}
Color? effectiveOverlayColor() {
return widget.overlayColor?.resolve(states)
?? widget.activeColor?.withOpacity(0.12)
?? MaterialStateProperty.resolveAs<Color?>(sliderTheme.overlayColor, states)
?? theme.colorScheme.primary.withOpacity(0.12);
}
sliderTheme = sliderTheme.copyWith(
trackHeight: sliderTheme.trackHeight ?? defaultTrackHeight,
activeTrackColor: widget.activeColor ?? sliderTheme.activeTrackColor ?? theme.colorScheme.primary,
inactiveTrackColor: widget.inactiveColor ?? sliderTheme.inactiveTrackColor ?? theme.colorScheme.primary.withOpacity(0.24),
disabledActiveTrackColor: sliderTheme.disabledActiveTrackColor ?? theme.colorScheme.onSurface.withOpacity(0.32),
disabledInactiveTrackColor: sliderTheme.disabledInactiveTrackColor ?? theme.colorScheme.onSurface.withOpacity(0.12),
activeTickMarkColor: widget.inactiveColor ?? sliderTheme.activeTickMarkColor ?? theme.colorScheme.onPrimary.withOpacity(0.54),
inactiveTickMarkColor: widget.activeColor ?? sliderTheme.inactiveTickMarkColor ?? theme.colorScheme.primary.withOpacity(0.54),
disabledActiveTickMarkColor: sliderTheme.disabledActiveTickMarkColor ?? theme.colorScheme.onPrimary.withOpacity(0.12),
disabledInactiveTickMarkColor: sliderTheme.disabledInactiveTickMarkColor ?? theme.colorScheme.onSurface.withOpacity(0.12),
thumbColor: widget.activeColor ?? sliderTheme.thumbColor ?? theme.colorScheme.primary,
overlappingShapeStrokeColor: sliderTheme.overlappingShapeStrokeColor ?? theme.colorScheme.surface,
disabledThumbColor: sliderTheme.disabledThumbColor ?? Color.alphaBlend(theme.colorScheme.onSurface.withOpacity(.38), theme.colorScheme.surface),
overlayColor: effectiveOverlayColor(),
valueIndicatorColor: valueIndicatorColor,
rangeTrackShape: sliderTheme.rangeTrackShape ?? defaultTrackShape,
rangeTickMarkShape: sliderTheme.rangeTickMarkShape ?? defaultTickMarkShape,
rangeThumbShape: sliderTheme.rangeThumbShape ?? defaultThumbShape,
overlayShape: sliderTheme.overlayShape ?? defaultOverlayShape,
rangeValueIndicatorShape: valueIndicatorShape,
showValueIndicator: sliderTheme.showValueIndicator ?? defaultShowValueIndicator,
valueIndicatorTextStyle: sliderTheme.valueIndicatorTextStyle ?? theme.textTheme.bodyLarge!.copyWith(
color: theme.colorScheme.onPrimary,
),
minThumbSeparation: sliderTheme.minThumbSeparation ?? defaultMinThumbSeparation,
thumbSelector: sliderTheme.thumbSelector ?? _defaultRangeThumbSelector,
);
final MouseCursor effectiveMouseCursor = widget.mouseCursor?.resolve(states)
?? sliderTheme.mouseCursor?.resolve(states)
?? MaterialStateMouseCursor.clickable.resolve(states);
// This size is used as the max bounds for the painting of the value
// indicators. It must be kept in sync with the function with the same name
// in slider.dart.
Size screenSize() => MediaQuery.sizeOf(context);
final double fontSize = sliderTheme.valueIndicatorTextStyle?.fontSize ?? kDefaultFontSize;
final double fontSizeToScale = fontSize == 0.0 ? kDefaultFontSize : fontSize;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(fontSizeToScale) / fontSizeToScale;
return FocusableActionDetector(
enabled: _enabled,
onShowHoverHighlight: _handleHoverChanged,
includeFocusSemantics: false,
mouseCursor: effectiveMouseCursor,
child: CompositedTransformTarget(
link: _layerLink,
child: _RangeSliderRenderObjectWidget(
values: _unlerpRangeValues(widget.values),
divisions: widget.divisions,
labels: widget.labels,
sliderTheme: sliderTheme,
textScaleFactor: effectiveTextScale,
screenSize: screenSize(),
onChanged: _enabled && (widget.max > widget.min) ? _handleChanged : null,
onChangeStart: widget.onChangeStart != null ? _handleDragStart : null,
onChangeEnd: widget.onChangeEnd != null ? _handleDragEnd : null,
state: this,
semanticFormatterCallback: widget.semanticFormatterCallback,
hovering: _hovering,
),
),
);
}
final LayerLink _layerLink = LayerLink();
OverlayEntry? overlayEntry;
void showValueIndicator() {
if (overlayEntry == null) {
overlayEntry = OverlayEntry(
builder: (BuildContext context) {
return CompositedTransformFollower(
link: _layerLink,
child: _ValueIndicatorRenderObjectWidget(
state: this,
),
);
},
);
Overlay.of(context, debugRequiredFor: widget).insert(overlayEntry!);
}
}
}
class _RangeSliderRenderObjectWidget extends LeafRenderObjectWidget {
const _RangeSliderRenderObjectWidget({
required this.values,
required this.divisions,
required this.labels,
required this.sliderTheme,
required this.textScaleFactor,
required this.screenSize,
required this.onChanged,
required this.onChangeStart,
required this.onChangeEnd,
required this.state,
required this.semanticFormatterCallback,
required this.hovering,
});
final RangeValues values;
final int? divisions;
final RangeLabels? labels;
final SliderThemeData sliderTheme;
final double textScaleFactor;
final Size screenSize;
final ValueChanged<RangeValues>? onChanged;
final ValueChanged<RangeValues>? onChangeStart;
final ValueChanged<RangeValues>? onChangeEnd;
final SemanticFormatterCallback? semanticFormatterCallback;
final _RangeSliderState state;
final bool hovering;
@override
_RenderRangeSlider createRenderObject(BuildContext context) {
return _RenderRangeSlider(
values: values,
divisions: divisions,
labels: labels,
sliderTheme: sliderTheme,
theme: Theme.of(context),
textScaleFactor: textScaleFactor,
screenSize: screenSize,
onChanged: onChanged,
onChangeStart: onChangeStart,
onChangeEnd: onChangeEnd,
state: state,
textDirection: Directionality.of(context),
semanticFormatterCallback: semanticFormatterCallback,
platform: Theme.of(context).platform,
hovering: hovering,
gestureSettings: MediaQuery.gestureSettingsOf(context),
);
}
@override
void updateRenderObject(BuildContext context, _RenderRangeSlider renderObject) {
renderObject
// We should update the `divisions` ahead of `values`, because the `values`
// setter dependent on the `divisions`.
..divisions = divisions
..values = values
..labels = labels
..sliderTheme = sliderTheme
..theme = Theme.of(context)
..textScaleFactor = textScaleFactor
..screenSize = screenSize
..onChanged = onChanged
..onChangeStart = onChangeStart
..onChangeEnd = onChangeEnd
..textDirection = Directionality.of(context)
..semanticFormatterCallback = semanticFormatterCallback
..platform = Theme.of(context).platform
..hovering = hovering
..gestureSettings = MediaQuery.gestureSettingsOf(context);
}
}
class _RenderRangeSlider extends RenderBox with RelayoutWhenSystemFontsChangeMixin {
_RenderRangeSlider({
required RangeValues values,
required int? divisions,
required RangeLabels? labels,
required SliderThemeData sliderTheme,
required ThemeData? theme,
required double textScaleFactor,
required Size screenSize,
required TargetPlatform platform,
required ValueChanged<RangeValues>? onChanged,
required SemanticFormatterCallback? semanticFormatterCallback,
required this.onChangeStart,
required this.onChangeEnd,
required _RangeSliderState state,
required TextDirection textDirection,
required bool hovering,
required DeviceGestureSettings gestureSettings,
}) : assert(values.start >= 0.0 && values.start <= 1.0),
assert(values.end >= 0.0 && values.end <= 1.0),
_platform = platform,
_semanticFormatterCallback = semanticFormatterCallback,
_labels = labels,
_values = values,
_divisions = divisions,
_sliderTheme = sliderTheme,
_theme = theme,
_textScaleFactor = textScaleFactor,
_screenSize = screenSize,
_onChanged = onChanged,
_state = state,
_textDirection = textDirection,
_hovering = hovering {
_updateLabelPainters();
final GestureArenaTeam team = GestureArenaTeam();
_drag = HorizontalDragGestureRecognizer()
..team = team
..onStart = _handleDragStart
..onUpdate = _handleDragUpdate
..onEnd = _handleDragEnd
..onCancel = _handleDragCancel
..gestureSettings = gestureSettings;
_tap = TapGestureRecognizer()
..team = team
..onTapDown = _handleTapDown
..onTapUp = _handleTapUp
..gestureSettings = gestureSettings;
_overlayAnimation = CurvedAnimation(
parent: _state.overlayController,
curve: Curves.fastOutSlowIn,
);
_valueIndicatorAnimation = CurvedAnimation(
parent: _state.valueIndicatorController,
curve: Curves.fastOutSlowIn,
)..addStatusListener((AnimationStatus status) {
if (status == AnimationStatus.dismissed) {
_state.overlayEntry?.remove();
_state.overlayEntry?.dispose();
_state.overlayEntry = null;
}
});
_enableAnimation = CurvedAnimation(
parent: _state.enableController,
curve: Curves.easeInOut,
);
}
// Keep track of the last selected thumb so they can be drawn in the
// right order.
Thumb? _lastThumbSelection;
static const Duration _positionAnimationDuration = Duration(milliseconds: 75);
// This value is the touch target, 48, multiplied by 3.
static const double _minPreferredTrackWidth = 144.0;
// Compute the largest width and height needed to paint the slider shapes,
// other than the track shape. It is assumed that these shapes are vertically
// centered on the track.
double get _maxSliderPartWidth => _sliderPartSizes.map((Size size) => size.width).reduce(math.max);
double get _maxSliderPartHeight => _sliderPartSizes.map((Size size) => size.height).reduce(math.max);
List<Size> get _sliderPartSizes => <Size>[
_sliderTheme.overlayShape!.getPreferredSize(isEnabled, isDiscrete),
_sliderTheme.rangeThumbShape!.getPreferredSize(isEnabled, isDiscrete),
_sliderTheme.rangeTickMarkShape!.getPreferredSize(isEnabled: isEnabled, sliderTheme: sliderTheme),
];
double? get _minPreferredTrackHeight => _sliderTheme.trackHeight;
// This rect is used in gesture calculations, where the gesture coordinates
// are relative to the sliders origin. Therefore, the offset is passed as
// (0,0).
Rect get _trackRect => _sliderTheme.rangeTrackShape!.getPreferredRect(
parentBox: this,
sliderTheme: _sliderTheme,
isDiscrete: false,
);
static const Duration _minimumInteractionTime = Duration(milliseconds: 500);
final _RangeSliderState _state;
late CurvedAnimation _overlayAnimation;
late CurvedAnimation _valueIndicatorAnimation;
late CurvedAnimation _enableAnimation;
final TextPainter _startLabelPainter = TextPainter();
final TextPainter _endLabelPainter = TextPainter();
late HorizontalDragGestureRecognizer _drag;
late TapGestureRecognizer _tap;
bool _active = false;
late RangeValues _newValues;
Offset _startThumbCenter = Offset.zero;
Offset _endThumbCenter = Offset.zero;
Rect? overlayStartRect;
Rect? overlayEndRect;
bool get isEnabled => onChanged != null;
bool get isDiscrete => divisions != null && divisions! > 0;
double get _minThumbSeparationValue => isDiscrete ? 0 : sliderTheme.minThumbSeparation! / _trackRect.width;
RangeValues get values => _values;
RangeValues _values;
set values(RangeValues newValues) {
assert(newValues.start >= 0.0 && newValues.start <= 1.0);
assert(newValues.end >= 0.0 && newValues.end <= 1.0);
assert(newValues.start <= newValues.end);
final RangeValues convertedValues = isDiscrete ? _discretizeRangeValues(newValues) : newValues;
if (convertedValues == _values) {
return;
}
_values = convertedValues;
if (isDiscrete) {
// Reset the duration to match the distance that we're traveling, so that
// whatever the distance, we still do it in _positionAnimationDuration,
// and if we get re-targeted in the middle, it still takes that long to
// get to the new location.
final double startDistance = (_values.start - _state.startPositionController.value).abs();
_state.startPositionController.duration = startDistance != 0.0 ? _positionAnimationDuration * (1.0 / startDistance) : Duration.zero;
_state.startPositionController.animateTo(_values.start, curve: Curves.easeInOut);
final double endDistance = (_values.end - _state.endPositionController.value).abs();
_state.endPositionController.duration = endDistance != 0.0 ? _positionAnimationDuration * (1.0 / endDistance) : Duration.zero;
_state.endPositionController.animateTo(_values.end, curve: Curves.easeInOut);
} else {
_state.startPositionController.value = convertedValues.start;
_state.endPositionController.value = convertedValues.end;
}
markNeedsSemanticsUpdate();
}
TargetPlatform _platform;
TargetPlatform get platform => _platform;
set platform(TargetPlatform value) {
if (_platform == value) {
return;
}
_platform = value;
markNeedsSemanticsUpdate();
}
DeviceGestureSettings? get gestureSettings => _drag.gestureSettings;
set gestureSettings(DeviceGestureSettings? gestureSettings) {
_drag.gestureSettings = gestureSettings;
_tap.gestureSettings = gestureSettings;
}
SemanticFormatterCallback? _semanticFormatterCallback;
SemanticFormatterCallback? get semanticFormatterCallback => _semanticFormatterCallback;
set semanticFormatterCallback(SemanticFormatterCallback? value) {
if (_semanticFormatterCallback == value) {
return;
}
_semanticFormatterCallback = value;
markNeedsSemanticsUpdate();
}
int? get divisions => _divisions;
int? _divisions;
set divisions(int? value) {
if (value == _divisions) {
return;
}
_divisions = value;
markNeedsPaint();
}
RangeLabels? get labels => _labels;
RangeLabels? _labels;
set labels(RangeLabels? labels) {
if (labels == _labels) {
return;
}
_labels = labels;
_updateLabelPainters();
}
SliderThemeData get sliderTheme => _sliderTheme;
SliderThemeData _sliderTheme;
set sliderTheme(SliderThemeData value) {
if (value == _sliderTheme) {
return;
}
_sliderTheme = value;
markNeedsPaint();
}
ThemeData? get theme => _theme;
ThemeData? _theme;
set theme(ThemeData? value) {
if (value == _theme) {
return;
}
_theme = value;
markNeedsPaint();
}
double get textScaleFactor => _textScaleFactor;
double _textScaleFactor;
set textScaleFactor(double value) {
if (value == _textScaleFactor) {
return;
}
_textScaleFactor = value;
_updateLabelPainters();
}
Size get screenSize => _screenSize;
Size _screenSize;
set screenSize(Size value) {
if (value == screenSize) {
return;
}
_screenSize = value;
markNeedsPaint();
}
ValueChanged<RangeValues>? get onChanged => _onChanged;
ValueChanged<RangeValues>? _onChanged;
set onChanged(ValueChanged<RangeValues>? value) {
if (value == _onChanged) {
return;
}
final bool wasEnabled = isEnabled;
_onChanged = value;
if (wasEnabled != isEnabled) {
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
ValueChanged<RangeValues>? onChangeStart;
ValueChanged<RangeValues>? onChangeEnd;
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (value == _textDirection) {
return;
}
_textDirection = value;
_updateLabelPainters();
}
/// True if this slider is being hovered over by a pointer.
bool get hovering => _hovering;
bool _hovering;
set hovering(bool value) {
if (value == _hovering) {
return;
}
_hovering = value;
_updateForHover(_hovering);
}
/// True if the slider is interactive and the start thumb is being
/// hovered over by a pointer.
bool _hoveringStartThumb = false;
bool get hoveringStartThumb => _hoveringStartThumb;
set hoveringStartThumb(bool value) {
if (value == _hoveringStartThumb) {
return;
}
_hoveringStartThumb = value;
_updateForHover(_hovering);
}
/// True if the slider is interactive and the end thumb is being
/// hovered over by a pointer.
bool _hoveringEndThumb = false;
bool get hoveringEndThumb => _hoveringEndThumb;
set hoveringEndThumb(bool value) {
if (value == _hoveringEndThumb) {
return;
}
_hoveringEndThumb = value;
_updateForHover(_hovering);
}
void _updateForHover(bool hovered) {
// Only show overlay when pointer is hovering the thumb.
if (hovered && (hoveringStartThumb || hoveringEndThumb)) {
_state.overlayController.forward();
} else {
_state.overlayController.reverse();
}
}
bool get showValueIndicator {
return switch (_sliderTheme.showValueIndicator!) {
ShowValueIndicator.onlyForDiscrete => isDiscrete,
ShowValueIndicator.onlyForContinuous => !isDiscrete,
ShowValueIndicator.always => true,
ShowValueIndicator.never => false,
};
}
Size get _thumbSize => _sliderTheme.rangeThumbShape!.getPreferredSize(isEnabled, isDiscrete);
double get _adjustmentUnit {
switch (_platform) {
case TargetPlatform.iOS:
// Matches iOS implementation of material slider.
return 0.1;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
// Matches Android implementation of material slider.
return 0.05;
}
}
void _updateLabelPainters() {
_updateLabelPainter(Thumb.start);
_updateLabelPainter(Thumb.end);
}
void _updateLabelPainter(Thumb thumb) {
final RangeLabels? labels = this.labels;
if (labels == null) {
return;
}
final (String text, TextPainter labelPainter) = switch (thumb) {
Thumb.start => (labels.start, _startLabelPainter),
Thumb.end => (labels.end, _endLabelPainter),
};
labelPainter
..text = TextSpan(
style: _sliderTheme.valueIndicatorTextStyle,
text: text,
)
..textDirection = textDirection
..textScaleFactor = textScaleFactor
..layout();
// Changing the textDirection can result in the layout changing, because the
// bidi algorithm might line up the glyphs differently which can result in
// different ligatures, different shapes, etc. So we always markNeedsLayout.
markNeedsLayout();
}
@override
void systemFontsDidChange() {
super.systemFontsDidChange();
_startLabelPainter.markNeedsLayout();
_endLabelPainter.markNeedsLayout();
_updateLabelPainters();
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_overlayAnimation.addListener(markNeedsPaint);
_valueIndicatorAnimation.addListener(markNeedsPaint);
_enableAnimation.addListener(markNeedsPaint);
_state.startPositionController.addListener(markNeedsPaint);
_state.endPositionController.addListener(markNeedsPaint);
}
@override
void detach() {
_overlayAnimation.removeListener(markNeedsPaint);
_valueIndicatorAnimation.removeListener(markNeedsPaint);
_enableAnimation.removeListener(markNeedsPaint);
_state.startPositionController.removeListener(markNeedsPaint);
_state.endPositionController.removeListener(markNeedsPaint);
super.detach();
}
@override
void dispose() {
_drag.dispose();
_tap.dispose();
_startLabelPainter.dispose();
_endLabelPainter.dispose();
_enableAnimation.dispose();
_valueIndicatorAnimation.dispose();
_overlayAnimation.dispose();
super.dispose();
}
double _getValueFromVisualPosition(double visualPosition) {
return switch (textDirection) {
TextDirection.rtl => 1.0 - visualPosition,
TextDirection.ltr => visualPosition,
};
}
double _getValueFromGlobalPosition(Offset globalPosition) {
final double visualPosition = (globalToLocal(globalPosition).dx - _trackRect.left) / _trackRect.width;
return _getValueFromVisualPosition(visualPosition);
}
double _discretize(double value) {
double result = clampDouble(value, 0.0, 1.0);
if (isDiscrete) {
result = (result * divisions!).round() / divisions!;
}
return result;
}
RangeValues _discretizeRangeValues(RangeValues values) {
return RangeValues(_discretize(values.start), _discretize(values.end));
}
void _startInteraction(Offset globalPosition) {
if (_active) {
return;
}
_state.showValueIndicator();
final double tapValue = clampDouble(_getValueFromGlobalPosition(globalPosition), 0.0, 1.0);
_lastThumbSelection = sliderTheme.thumbSelector!(textDirection, values, tapValue, _thumbSize, size, 0);
if (_lastThumbSelection != null) {
_active = true;
// We supply the *current* values as the start locations, so that if we have
// a tap, it consists of a call to onChangeStart with the previous value and
// a call to onChangeEnd with the new value.
final RangeValues currentValues = _discretizeRangeValues(values);
if (_lastThumbSelection == Thumb.start) {
_newValues = RangeValues(tapValue, currentValues.end);
} else if (_lastThumbSelection == Thumb.end) {
_newValues = RangeValues(currentValues.start, tapValue);
}
_updateLabelPainter(_lastThumbSelection!);
onChangeStart?.call(currentValues);
onChanged!(_discretizeRangeValues(_newValues));
_state.overlayController.forward();
if (showValueIndicator) {
_state.valueIndicatorController.forward();
_state.interactionTimer?.cancel();
_state.interactionTimer =
Timer(_minimumInteractionTime * timeDilation, () {
_state.interactionTimer = null;
if (!_active && _state.valueIndicatorController.status == AnimationStatus.completed) {
_state.valueIndicatorController.reverse();
}
});
}
}
}
void _handleDragUpdate(DragUpdateDetails details) {
if (!_state.mounted) {
return;
}
final double dragValue = _getValueFromGlobalPosition(details.globalPosition);
// If no selection has been made yet, test for thumb selection again now
// that the value of dx can be non-zero. If this is the first selection of
// the interaction, then onChangeStart must be called.
bool shouldCallOnChangeStart = false;
if (_lastThumbSelection == null) {
_lastThumbSelection = sliderTheme.thumbSelector!(textDirection, values, dragValue, _thumbSize, size, details.delta.dx);
if (_lastThumbSelection != null) {
shouldCallOnChangeStart = true;
_active = true;
_state.overlayController.forward();
if (showValueIndicator) {
_state.valueIndicatorController.forward();
}
}
}
if (isEnabled && _lastThumbSelection != null) {
final RangeValues currentValues = _discretizeRangeValues(values);
if (onChangeStart != null && shouldCallOnChangeStart) {
onChangeStart!(currentValues);
}
final double currentDragValue = _discretize(dragValue);
if (_lastThumbSelection == Thumb.start) {
_newValues = RangeValues(math.min(currentDragValue, currentValues.end - _minThumbSeparationValue), currentValues.end);
} else if (_lastThumbSelection == Thumb.end) {
_newValues = RangeValues(currentValues.start, math.max(currentDragValue, currentValues.start + _minThumbSeparationValue));
}
onChanged!(_newValues);
}
}
void _endInteraction() {
if (!_state.mounted) {
return;
}
if (showValueIndicator && _state.interactionTimer == null) {
_state.valueIndicatorController.reverse();
}
if (_active && _state.mounted && _lastThumbSelection != null) {
final RangeValues discreteValues = _discretizeRangeValues(_newValues);
onChangeEnd?.call(discreteValues);
_active = false;
}
_state.overlayController.reverse();
}
void _handleDragStart(DragStartDetails details) {
_startInteraction(details.globalPosition);
}
void _handleDragEnd(DragEndDetails details) {
_endInteraction();
}
void _handleDragCancel() {
_endInteraction();
}
void _handleTapDown(TapDownDetails details) {
_startInteraction(details.globalPosition);
}
void _handleTapUp(TapUpDetails details) {
_endInteraction();
}
@override
bool hitTestSelf(Offset position) => true;
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
assert(debugHandleEvent(event, entry));
if (event is PointerDownEvent && isEnabled) {
// We need to add the drag first so that it has priority.
_drag.addPointer(event);
_tap.addPointer(event);
}
if (isEnabled) {
if (overlayStartRect != null) {
hoveringStartThumb = overlayStartRect!.contains(event.localPosition);
}
if (overlayEndRect != null) {
hoveringEndThumb = overlayEndRect!.contains(event.localPosition);
}
}
}
@override
double computeMinIntrinsicWidth(double height) => _minPreferredTrackWidth + _maxSliderPartWidth;
@override
double computeMaxIntrinsicWidth(double height) => _minPreferredTrackWidth + _maxSliderPartWidth;
@override
double computeMinIntrinsicHeight(double width) => math.max(_minPreferredTrackHeight!, _maxSliderPartHeight);
@override
double computeMaxIntrinsicHeight(double width) => math.max(_minPreferredTrackHeight!, _maxSliderPartHeight);
@override
bool get sizedByParent => true;
@override
Size computeDryLayout(BoxConstraints constraints) {
return Size(
constraints.hasBoundedWidth ? constraints.maxWidth : _minPreferredTrackWidth + _maxSliderPartWidth,
constraints.hasBoundedHeight ? constraints.maxHeight : math.max(_minPreferredTrackHeight!, _maxSliderPartHeight),
);
}
@override
void paint(PaintingContext context, Offset offset) {
final double startValue = _state.startPositionController.value;
final double endValue = _state.endPositionController.value;
// The visual position is the position of the thumb from 0 to 1 from left
// to right. In left to right, this is the same as the value, but it is
// reversed for right to left text.
final (double startVisualPosition, double endVisualPosition) = switch (textDirection) {
TextDirection.rtl => (1.0 - startValue, 1.0 - endValue),
TextDirection.ltr => (startValue, endValue),
};
final Rect trackRect = _sliderTheme.rangeTrackShape!.getPreferredRect(
parentBox: this,
offset: offset,
sliderTheme: _sliderTheme,
isDiscrete: isDiscrete,
);
_startThumbCenter = Offset(trackRect.left + startVisualPosition * trackRect.width, trackRect.center.dy);
_endThumbCenter = Offset(trackRect.left + endVisualPosition * trackRect.width, trackRect.center.dy);
if (isEnabled) {
final Size overlaySize = sliderTheme.overlayShape!.getPreferredSize(isEnabled, false);
overlayStartRect = Rect.fromCircle(center: _startThumbCenter, radius: overlaySize.width / 2.0);
overlayEndRect = Rect.fromCircle(center: _endThumbCenter, radius: overlaySize.width / 2.0);
}
_sliderTheme.rangeTrackShape!.paint(
context,
offset,
parentBox: this,
sliderTheme: _sliderTheme,
enableAnimation: _enableAnimation,
textDirection: _textDirection,
startThumbCenter: _startThumbCenter,
endThumbCenter: _endThumbCenter,
isDiscrete: isDiscrete,
isEnabled: isEnabled,
);
final bool startThumbSelected = _lastThumbSelection == Thumb.start;
final bool endThumbSelected = _lastThumbSelection == Thumb.end;
final Size resolvedscreenSize = screenSize.isEmpty ? size : screenSize;
if (!_overlayAnimation.isDismissed) {
if (startThumbSelected || hoveringStartThumb) {
_sliderTheme.overlayShape!.paint(
context,
_startThumbCenter,
activationAnimation: _overlayAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
labelPainter: _startLabelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
value: startValue,
textScaleFactor: _textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
}
if (endThumbSelected || hoveringEndThumb) {
_sliderTheme.overlayShape!.paint(
context,
_endThumbCenter,
activationAnimation: _overlayAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
labelPainter: _endLabelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
value: endValue,
textScaleFactor: _textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
}
}
if (isDiscrete) {
final double tickMarkWidth = _sliderTheme.rangeTickMarkShape!.getPreferredSize(
isEnabled: isEnabled,
sliderTheme: _sliderTheme,
).width;
final double padding = trackRect.height;
final double adjustedTrackWidth = trackRect.width - padding;
// If the tick marks would be too dense, don't bother painting them.
if (adjustedTrackWidth / divisions! >= 3.0 * tickMarkWidth) {
final double dy = trackRect.center.dy;
for (int i = 0; i <= divisions!; i++) {
final double value = i / divisions!;
// The ticks are mapped to be within the track, so the tick mark width
// must be subtracted from the track width.
final double dx = trackRect.left + value * adjustedTrackWidth + padding / 2;
final Offset tickMarkOffset = Offset(dx, dy);
_sliderTheme.rangeTickMarkShape!.paint(
context,
tickMarkOffset,
parentBox: this,
sliderTheme: _sliderTheme,
enableAnimation: _enableAnimation,
textDirection: _textDirection,
startThumbCenter: _startThumbCenter,
endThumbCenter: _endThumbCenter,
isEnabled: isEnabled,
);
}
}
}
final double thumbDelta = (_endThumbCenter.dx - _startThumbCenter.dx).abs();
final bool isLastThumbStart = _lastThumbSelection == Thumb.start;
final Thumb bottomThumb = isLastThumbStart ? Thumb.end : Thumb.start;
final Thumb topThumb = isLastThumbStart ? Thumb.start : Thumb.end;
final Offset bottomThumbCenter = isLastThumbStart ? _endThumbCenter : _startThumbCenter;
final Offset topThumbCenter = isLastThumbStart ? _startThumbCenter : _endThumbCenter;
final TextPainter bottomLabelPainter = isLastThumbStart ? _endLabelPainter : _startLabelPainter;
final TextPainter topLabelPainter = isLastThumbStart ? _startLabelPainter : _endLabelPainter;
final double bottomValue = isLastThumbStart ? endValue : startValue;
final double topValue = isLastThumbStart ? startValue : endValue;
final bool shouldPaintValueIndicators = isEnabled && labels != null && !_valueIndicatorAnimation.isDismissed && showValueIndicator;
if (shouldPaintValueIndicators) {
_state.paintBottomValueIndicator = (PaintingContext context, Offset offset) {
if (attached) {
_sliderTheme.rangeValueIndicatorShape!.paint(
context,
bottomThumbCenter,
activationAnimation: _valueIndicatorAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
isOnTop: false,
labelPainter: bottomLabelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
thumb: bottomThumb,
value: bottomValue,
textScaleFactor: textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
}
};
}
_sliderTheme.rangeThumbShape!.paint(
context,
bottomThumbCenter,
activationAnimation: _valueIndicatorAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
isOnTop: false,
textDirection: textDirection,
sliderTheme: _sliderTheme,
thumb: bottomThumb,
isPressed: bottomThumb == Thumb.start ? startThumbSelected : endThumbSelected,
);
if (shouldPaintValueIndicators) {
final double startOffset = sliderTheme.rangeValueIndicatorShape!.getHorizontalShift(
parentBox: this,
center: _startThumbCenter,
labelPainter: _startLabelPainter,
activationAnimation: _valueIndicatorAnimation,
textScaleFactor: textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
final double endOffset = sliderTheme.rangeValueIndicatorShape!.getHorizontalShift(
parentBox: this,
center: _endThumbCenter,
labelPainter: _endLabelPainter,
activationAnimation: _valueIndicatorAnimation,
textScaleFactor: textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
final double startHalfWidth = sliderTheme.rangeValueIndicatorShape!.getPreferredSize(
isEnabled,
isDiscrete,
labelPainter: _startLabelPainter,
textScaleFactor: textScaleFactor,
).width / 2;
final double endHalfWidth = sliderTheme.rangeValueIndicatorShape!.getPreferredSize(
isEnabled,
isDiscrete,
labelPainter: _endLabelPainter,
textScaleFactor: textScaleFactor,
).width / 2;
final double innerOverflow = startHalfWidth + endHalfWidth + switch (textDirection) {
TextDirection.ltr => startOffset - endOffset,
TextDirection.rtl => endOffset - startOffset,
};
_state.paintTopValueIndicator = (PaintingContext context, Offset offset) {
if (attached) {
_sliderTheme.rangeValueIndicatorShape!.paint(
context,
topThumbCenter,
activationAnimation: _valueIndicatorAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
isOnTop: thumbDelta < innerOverflow,
labelPainter: topLabelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
thumb: topThumb,
value: topValue,
textScaleFactor: textScaleFactor,
sizeWithOverflow: resolvedscreenSize,
);
}
};
}
_sliderTheme.rangeThumbShape!.paint(
context,
topThumbCenter,
activationAnimation: _overlayAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
isOnTop: thumbDelta < sliderTheme.rangeThumbShape!.getPreferredSize(isEnabled, isDiscrete).width,
textDirection: textDirection,
sliderTheme: _sliderTheme,
thumb: topThumb,
isPressed: topThumb == Thumb.start ? startThumbSelected : endThumbSelected,
);
}
/// Describe the semantics of the start thumb.
SemanticsNode? _startSemanticsNode;
/// Describe the semantics of the end thumb.
SemanticsNode? _endSemanticsNode;
// Create the semantics configuration for a single value.
SemanticsConfiguration _createSemanticsConfiguration(
double value,
double increasedValue,
double decreasedValue,
VoidCallback increaseAction,
VoidCallback decreaseAction,
) {
final SemanticsConfiguration config = SemanticsConfiguration();
config.isEnabled = isEnabled;
config.textDirection = textDirection;
config.isSlider = true;
if (isEnabled) {
config.onIncrease = increaseAction;
config.onDecrease = decreaseAction;
}
if (semanticFormatterCallback != null) {
config.value = semanticFormatterCallback!(_state._lerp(value));
config.increasedValue = semanticFormatterCallback!(_state._lerp(increasedValue));
config.decreasedValue = semanticFormatterCallback!(_state._lerp(decreasedValue));
} else {
config.value = '${(value * 100).round()}%';
config.increasedValue = '${(increasedValue * 100).round()}%';
config.decreasedValue = '${(decreasedValue * 100).round()}%';
}
return config;
}
@override
void assembleSemanticsNode(
SemanticsNode node,
SemanticsConfiguration config,
Iterable<SemanticsNode> children,
) {
assert(children.isEmpty);
final SemanticsConfiguration startSemanticsConfiguration = _createSemanticsConfiguration(
values.start,
_increasedStartValue,
_decreasedStartValue,
_increaseStartAction,
_decreaseStartAction,
);
final SemanticsConfiguration endSemanticsConfiguration = _createSemanticsConfiguration(
values.end,
_increasedEndValue,
_decreasedEndValue,
_increaseEndAction,
_decreaseEndAction,
);
// Split the semantics node area between the start and end nodes.
final Rect leftRect = Rect.fromCenter(
center: _startThumbCenter,
width: kMinInteractiveDimension,
height: kMinInteractiveDimension,
);
final Rect rightRect = Rect.fromCenter(
center: _endThumbCenter,
width: kMinInteractiveDimension,
height: kMinInteractiveDimension,
);
_startSemanticsNode ??= SemanticsNode();
_endSemanticsNode ??= SemanticsNode();
switch (textDirection) {
case TextDirection.ltr:
_startSemanticsNode!.rect = leftRect;
_endSemanticsNode!.rect = rightRect;
case TextDirection.rtl:
_startSemanticsNode!.rect = rightRect;
_endSemanticsNode!.rect = leftRect;
}
_startSemanticsNode!.updateWith(config: startSemanticsConfiguration);
_endSemanticsNode!.updateWith(config: endSemanticsConfiguration);
final List<SemanticsNode> finalChildren = <SemanticsNode>[
_startSemanticsNode!,
_endSemanticsNode!,
];
node.updateWith(config: config, childrenInInversePaintOrder: finalChildren);
}
@override
void clearSemantics() {
super.clearSemantics();
_startSemanticsNode = null;
_endSemanticsNode = null;
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.isSemanticBoundary = true;
}
double get _semanticActionUnit => divisions != null ? 1.0 / divisions! : _adjustmentUnit;
void _increaseStartAction() {
if (isEnabled) {
onChanged!(RangeValues(_increasedStartValue, values.end));
}
}
void _decreaseStartAction() {
if (isEnabled) {
onChanged!(RangeValues(_decreasedStartValue, values.end));
}
}
void _increaseEndAction() {
if (isEnabled) {
onChanged!(RangeValues(values.start, _increasedEndValue));
}
}
void _decreaseEndAction() {
if (isEnabled) {
onChanged!(RangeValues(values.start, _decreasedEndValue));
}
}
double get _increasedStartValue {
// Due to floating-point operations, this value can actually be greater than
// expected (e.g. 0.4 + 0.2 = 0.600000000001), so we limit to 2 decimal points.
final double increasedStartValue = double.parse((values.start + _semanticActionUnit).toStringAsFixed(2));
return increasedStartValue <= values.end - _minThumbSeparationValue ? increasedStartValue : values.start;
}
double get _decreasedStartValue {
return clampDouble(values.start - _semanticActionUnit, 0.0, 1.0);
}
double get _increasedEndValue {
return clampDouble(values.end + _semanticActionUnit, 0.0, 1.0);
}
double get _decreasedEndValue {
final double decreasedEndValue = values.end - _semanticActionUnit;
return decreasedEndValue >= values.start + _minThumbSeparationValue ? decreasedEndValue : values.end;
}
}
class _ValueIndicatorRenderObjectWidget extends LeafRenderObjectWidget {
const _ValueIndicatorRenderObjectWidget({
required this.state,
});
final _RangeSliderState state;
@override
_RenderValueIndicator createRenderObject(BuildContext context) {
return _RenderValueIndicator(
state: state,
);
}
@override
void updateRenderObject(BuildContext context, _RenderValueIndicator renderObject) {
renderObject._state = state;
}
}
class _RenderValueIndicator extends RenderBox with RelayoutWhenSystemFontsChangeMixin {
_RenderValueIndicator({
required _RangeSliderState state,
}) :_state = state {
_valueIndicatorAnimation = CurvedAnimation(
parent: _state.valueIndicatorController,
curve: Curves.fastOutSlowIn,
);
}
late Animation<double> _valueIndicatorAnimation;
late _RangeSliderState _state;
@override
bool get sizedByParent => true;
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_valueIndicatorAnimation.addListener(markNeedsPaint);
_state.startPositionController.addListener(markNeedsPaint);
_state.endPositionController.addListener(markNeedsPaint);
}
@override
void detach() {
_valueIndicatorAnimation.removeListener(markNeedsPaint);
_state.startPositionController.removeListener(markNeedsPaint);
_state.endPositionController.removeListener(markNeedsPaint);
super.detach();
}
@override
void paint(PaintingContext context, Offset offset) {
_state.paintBottomValueIndicator?.call(context, offset);
_state.paintTopValueIndicator?.call(context, offset);
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return constraints.smallest;
}
}
| flutter/packages/flutter/lib/src/material/range_slider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/range_slider.dart",
"repo_id": "flutter",
"token_count": 22537
} | 627 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart' show timeDilation;
import 'package:flutter/services.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'material.dart';
import 'material_state.dart';
import 'slider_theme.dart';
import 'theme.dart';
// Examples can assume:
// int _dollars = 0;
// int _duelCommandment = 1;
// void setState(VoidCallback fn) { }
/// [Slider] uses this callback to paint the value indicator on the overlay.
///
/// Since the value indicator is painted on the Overlay; this method paints the
/// value indicator in a [RenderBox] that appears in the [Overlay].
typedef PaintValueIndicator = void Function(PaintingContext context, Offset offset);
enum _SliderType { material, adaptive }
/// Possible ways for a user to interact with a [Slider].
enum SliderInteraction {
/// Allows the user to interact with a [Slider] by tapping or sliding anywhere
/// on the track.
///
/// Essentially all possible interactions are allowed.
///
/// This is different from [SliderInteraction.slideOnly] as when you try
/// to slide anywhere other than the thumb, the thumb will move to the first
/// point of contact.
tapAndSlide,
/// Allows the user to interact with a [Slider] by only tapping anywhere on
/// the track.
///
/// Sliding interaction is ignored.
tapOnly,
/// Allows the user to interact with a [Slider] only by sliding anywhere on
/// the track.
///
/// Tapping interaction is ignored.
slideOnly,
/// Allows the user to interact with a [Slider] only by sliding the thumb.
///
/// Tapping and sliding interactions on the track are ignored.
slideThumb;
}
/// A Material Design slider.
///
/// Used to select from a range of values.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ufb4gIPDmEs}
///
/// {@tool dartpad}
/// 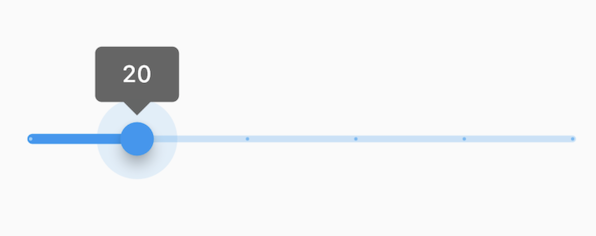
///
/// The Sliders value is part of the Stateful widget subclass to change the value
/// setState was called.
///
/// ** See code in examples/api/lib/material/slider/slider.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of a [Slider] using [ThemeData.useMaterial3] flag,
/// as described in: https://m3.material.io/components/sliders/overview.
///
/// ** See code in examples/api/lib/material/slider/slider.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows a [Slider] widget using the [Slider.secondaryTrackValue]
/// to show a secondary track in the slider.
///
/// ** See code in examples/api/lib/material/slider/slider.2.dart **
/// {@end-tool}
///
/// A slider can be used to select from either a continuous or a discrete set of
/// values. The default is to use a continuous range of values from [min] to
/// [max]. To use discrete values, use a non-null value for [divisions], which
/// indicates the number of discrete intervals. For example, if [min] is 0.0 and
/// [max] is 50.0 and [divisions] is 5, then the slider can take on the
/// discrete values 0.0, 10.0, 20.0, 30.0, 40.0, and 50.0.
///
/// The terms for the parts of a slider are:
///
/// * The "thumb", which is a shape that slides horizontally when the user
/// drags it.
/// * The "track", which is the line that the slider thumb slides along.
/// * The "value indicator", which is a shape that pops up when the user
/// is dragging the thumb to indicate the value being selected.
/// * The "active" side of the slider is the side between the thumb and the
/// minimum value.
/// * The "inactive" side of the slider is the side between the thumb and the
/// maximum value.
///
/// The slider will be disabled if [onChanged] is null or if the range given by
/// [min]..[max] is empty (i.e. if [min] is equal to [max]).
///
/// The slider widget itself does not maintain any state. Instead, when the state
/// of the slider changes, the widget calls the [onChanged] callback. Most
/// widgets that use a slider will listen for the [onChanged] callback and
/// rebuild the slider with a new [value] to update the visual appearance of the
/// slider. To know when the value starts to change, or when it is done
/// changing, set the optional callbacks [onChangeStart] and/or [onChangeEnd].
///
/// By default, a slider will be as wide as possible, centered vertically. When
/// given unbounded constraints, it will attempt to make the track 144 pixels
/// wide (with margins on each side) and will shrink-wrap vertically.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// Requires one of its ancestors to be a [MediaQuery] widget. Typically, these
/// are introduced by the [MaterialApp] or [WidgetsApp] widget at the top of
/// your application widget tree.
///
/// To determine how it should be displayed (e.g. colors, thumb shape, etc.),
/// a slider uses the [SliderThemeData] available from either a [SliderTheme]
/// widget or the [ThemeData.sliderTheme] a [Theme] widget above it in the
/// widget tree. You can also override some of the colors with the [activeColor]
/// and [inactiveColor] properties, although more fine-grained control of the
/// look is achieved using a [SliderThemeData].
///
/// See also:
///
/// * [SliderTheme] and [SliderThemeData] for information about controlling
/// the visual appearance of the slider.
/// * [Radio], for selecting among a set of explicit values.
/// * [Checkbox] and [Switch], for toggling a particular value on or off.
/// * <https://material.io/design/components/sliders.html>
/// * [MediaQuery], from which the text scale factor is obtained.
class Slider extends StatefulWidget {
/// Creates a Material Design slider.
///
/// The slider itself does not maintain any state. Instead, when the state of
/// the slider changes, the widget calls the [onChanged] callback. Most
/// widgets that use a slider will listen for the [onChanged] callback and
/// rebuild the slider with a new [value] to update the visual appearance of
/// the slider.
///
/// * [value] determines currently selected value for this slider.
/// * [onChanged] is called while the user is selecting a new value for the
/// slider.
/// * [onChangeStart] is called when the user starts to select a new value for
/// the slider.
/// * [onChangeEnd] is called when the user is done selecting a new value for
/// the slider.
///
/// You can override some of the colors with the [activeColor] and
/// [inactiveColor] properties, although more fine-grained control of the
/// appearance is achieved using a [SliderThemeData].
const Slider({
super.key,
required this.value,
this.secondaryTrackValue,
required this.onChanged,
this.onChangeStart,
this.onChangeEnd,
this.min = 0.0,
this.max = 1.0,
this.divisions,
this.label,
this.activeColor,
this.inactiveColor,
this.secondaryActiveColor,
this.thumbColor,
this.overlayColor,
this.mouseCursor,
this.semanticFormatterCallback,
this.focusNode,
this.autofocus = false,
this.allowedInteraction,
}) : _sliderType = _SliderType.material,
assert(min <= max),
assert(value >= min && value <= max,
'Value $value is not between minimum $min and maximum $max'),
assert(secondaryTrackValue == null || (secondaryTrackValue >= min && secondaryTrackValue <= max),
'SecondaryValue $secondaryTrackValue is not between $min and $max'),
assert(divisions == null || divisions > 0);
/// Creates an adaptive [Slider] based on the target platform, following
/// Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// Creates a [CupertinoSlider] if the target platform is iOS or macOS, creates a
/// Material Design slider otherwise.
///
/// If a [CupertinoSlider] is created, the following parameters are ignored:
/// [secondaryTrackValue], [label], [inactiveColor], [secondaryActiveColor],
/// [semanticFormatterCallback].
///
/// The target platform is based on the current [Theme]: [ThemeData.platform].
const Slider.adaptive({
super.key,
required this.value,
this.secondaryTrackValue,
required this.onChanged,
this.onChangeStart,
this.onChangeEnd,
this.min = 0.0,
this.max = 1.0,
this.divisions,
this.label,
this.mouseCursor,
this.activeColor,
this.inactiveColor,
this.secondaryActiveColor,
this.thumbColor,
this.overlayColor,
this.semanticFormatterCallback,
this.focusNode,
this.autofocus = false,
this.allowedInteraction,
}) : _sliderType = _SliderType.adaptive,
assert(min <= max),
assert(value >= min && value <= max,
'Value $value is not between minimum $min and maximum $max'),
assert(secondaryTrackValue == null || (secondaryTrackValue >= min && secondaryTrackValue <= max),
'SecondaryValue $secondaryTrackValue is not between $min and $max'),
assert(divisions == null || divisions > 0);
/// The currently selected value for this slider.
///
/// The slider's thumb is drawn at a position that corresponds to this value.
final double value;
/// The secondary track value for this slider.
///
/// If not null, a secondary track using [Slider.secondaryActiveColor] color
/// is drawn between the thumb and this value, over the inactive track.
///
/// If less than [Slider.value], then the secondary track is not shown.
///
/// It can be ideal for media scenarios such as showing the buffering progress
/// while the [Slider.value] shows the play progress.
final double? secondaryTrackValue;
/// Called during a drag when the user is selecting a new value for the slider
/// by dragging.
///
/// The slider passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the slider with the new
/// value.
///
/// If null, the slider will be displayed as disabled.
///
/// The callback provided to onChanged should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// {@tool snippet}
///
/// ```dart
/// Slider(
/// value: _duelCommandment.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// label: '$_duelCommandment',
/// onChanged: (double newValue) {
/// setState(() {
/// _duelCommandment = newValue.round();
/// });
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeStart] for a callback that is called when the user starts
/// changing the value.
/// * [onChangeEnd] for a callback that is called when the user stops
/// changing the value.
final ValueChanged<double>? onChanged;
/// Called when the user starts selecting a new value for the slider.
///
/// This callback shouldn't be used to update the slider [value] (use
/// [onChanged] for that), but rather to be notified when the user has started
/// selecting a new value by starting a drag or with a tap.
///
/// The value passed will be the last [value] that the slider had before the
/// change began.
///
/// {@tool snippet}
///
/// ```dart
/// Slider(
/// value: _duelCommandment.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// label: '$_duelCommandment',
/// onChanged: (double newValue) {
/// setState(() {
/// _duelCommandment = newValue.round();
/// });
/// },
/// onChangeStart: (double startValue) {
/// print('Started change at $startValue');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeEnd] for a callback that is called when the value change is
/// complete.
final ValueChanged<double>? onChangeStart;
/// Called when the user is done selecting a new value for the slider.
///
/// This callback shouldn't be used to update the slider [value] (use
/// [onChanged] for that), but rather to know when the user has completed
/// selecting a new [value] by ending a drag or a click.
///
/// {@tool snippet}
///
/// ```dart
/// Slider(
/// value: _duelCommandment.toDouble(),
/// min: 1.0,
/// max: 10.0,
/// divisions: 10,
/// label: '$_duelCommandment',
/// onChanged: (double newValue) {
/// setState(() {
/// _duelCommandment = newValue.round();
/// });
/// },
/// onChangeEnd: (double newValue) {
/// print('Ended change on $newValue');
/// },
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [onChangeStart] for a callback that is called when a value change
/// begins.
final ValueChanged<double>? onChangeEnd;
/// The minimum value the user can select.
///
/// Defaults to 0.0. Must be less than or equal to [max].
///
/// If the [max] is equal to the [min], then the slider is disabled.
final double min;
/// The maximum value the user can select.
///
/// Defaults to 1.0. Must be greater than or equal to [min].
///
/// If the [max] is equal to the [min], then the slider is disabled.
final double max;
/// The number of discrete divisions.
///
/// Typically used with [label] to show the current discrete value.
///
/// If null, the slider is continuous.
final int? divisions;
/// A label to show above the slider when the slider is active and
/// [SliderThemeData.showValueIndicator] is satisfied.
///
/// It is used to display the value of a discrete slider, and it is displayed
/// as part of the value indicator shape.
///
/// The label is rendered using the active [ThemeData]'s [TextTheme.bodyLarge]
/// text style, with the theme data's [ColorScheme.onPrimary] color. The
/// label's text style can be overridden with
/// [SliderThemeData.valueIndicatorTextStyle].
///
/// If null, then the value indicator will not be displayed.
///
/// Ignored if this slider is created with [Slider.adaptive].
///
/// See also:
///
/// * [SliderComponentShape] for how to create a custom value indicator
/// shape.
final String? label;
/// The color to use for the portion of the slider track that is active.
///
/// The "active" side of the slider is the side between the thumb and the
/// minimum value.
///
/// If null, [SliderThemeData.activeTrackColor] of the ambient
/// [SliderTheme] is used. If that is null, [ColorScheme.primary] of the
/// surrounding [ThemeData] is used.
///
/// Using a [SliderTheme] gives much more fine-grained control over the
/// appearance of various components of the slider.
final Color? activeColor;
/// The color for the inactive portion of the slider track.
///
/// The "inactive" side of the slider is the side between the thumb and the
/// maximum value.
///
/// If null, [SliderThemeData.inactiveTrackColor] of the ambient [SliderTheme]
/// is used. If that is null and [ThemeData.useMaterial3] is true,
/// [ColorScheme.surfaceContainerHighest] will be used, otherwise [ColorScheme.primary]
/// with an opacity of 0.24 will be used.
///
/// Using a [SliderTheme] gives much more fine-grained control over the
/// appearance of various components of the slider.
///
/// Ignored if this slider is created with [Slider.adaptive].
final Color? inactiveColor;
/// The color to use for the portion of the slider track between the thumb and
/// the [Slider.secondaryTrackValue].
///
/// Defaults to the [SliderThemeData.secondaryActiveTrackColor] of the current
/// [SliderTheme].
///
/// If that is also null, defaults to [ColorScheme.primary] with an
/// opacity of 0.54.
///
/// Using a [SliderTheme] gives much more fine-grained control over the
/// appearance of various components of the slider.
///
/// Ignored if this slider is created with [Slider.adaptive].
final Color? secondaryActiveColor;
/// The color of the thumb.
///
/// If this color is null, [Slider] will use [activeColor], If [activeColor]
/// is also null, [Slider] will use [SliderThemeData.thumbColor].
///
/// If that is also null, defaults to [ColorScheme.primary].
///
/// * [CupertinoSlider] will have a white thumb
/// (like the native default iOS slider).
final Color? thumbColor;
/// The highlight color that's typically used to indicate that
/// the slider thumb is focused, hovered, or dragged.
///
/// If this property is null, [Slider] will use [activeColor] with
/// an opacity of 0.12, If null, [SliderThemeData.overlayColor]
/// will be used.
///
/// If that is also null, If [ThemeData.useMaterial3] is true,
/// Slider will use [ColorScheme.primary] with an opacity of 0.08 when
/// slider thumb is hovered and with an opacity of 0.1 when slider thumb
/// is focused or dragged, If [ThemeData.useMaterial3] is false, defaults
/// to [ColorScheme.primary] with an opacity of 0.12.
final MaterialStateProperty<Color?>? overlayColor;
/// {@template flutter.material.slider.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.dragged].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// If null, then the value of [SliderThemeData.mouseCursor] is used. If that
/// is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], which can be used to create a [MouseCursor]
/// that is also a [MaterialStateProperty<MouseCursor>].
final MouseCursor? mouseCursor;
/// The callback used to create a semantic value from a slider value.
///
/// Defaults to formatting values as a percentage.
///
/// This is used by accessibility frameworks like TalkBack on Android to
/// inform users what the currently selected value is with more context.
///
/// {@tool snippet}
///
/// In the example below, a slider for currency values is configured to
/// announce a value with a currency label.
///
/// ```dart
/// Slider(
/// value: _dollars.toDouble(),
/// min: 20.0,
/// max: 330.0,
/// label: '$_dollars dollars',
/// onChanged: (double newValue) {
/// setState(() {
/// _dollars = newValue.round();
/// });
/// },
/// semanticFormatterCallback: (double newValue) {
/// return '${newValue.round()} dollars';
/// }
/// )
/// ```
/// {@end-tool}
///
/// Ignored if this slider is created with [Slider.adaptive]
final SemanticFormatterCallback? semanticFormatterCallback;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// Allowed way for the user to interact with the [Slider].
///
/// For example, if this is set to [SliderInteraction.tapOnly], the user can
/// interact with the slider only by tapping anywhere on the track. Sliding
/// will have no effect.
///
/// Defaults to [SliderInteraction.tapAndSlide].
final SliderInteraction? allowedInteraction;
final _SliderType _sliderType ;
@override
State<Slider> createState() => _SliderState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('value', value));
properties.add(DoubleProperty('secondaryTrackValue', secondaryTrackValue));
properties.add(ObjectFlagProperty<ValueChanged<double>>('onChanged', onChanged, ifNull: 'disabled'));
properties.add(ObjectFlagProperty<ValueChanged<double>>.has('onChangeStart', onChangeStart));
properties.add(ObjectFlagProperty<ValueChanged<double>>.has('onChangeEnd', onChangeEnd));
properties.add(DoubleProperty('min', min));
properties.add(DoubleProperty('max', max));
properties.add(IntProperty('divisions', divisions));
properties.add(StringProperty('label', label));
properties.add(ColorProperty('activeColor', activeColor));
properties.add(ColorProperty('inactiveColor', inactiveColor));
properties.add(ColorProperty('secondaryActiveColor', secondaryActiveColor));
properties.add(ObjectFlagProperty<ValueChanged<double>>.has('semanticFormatterCallback', semanticFormatterCallback));
properties.add(ObjectFlagProperty<FocusNode>.has('focusNode', focusNode));
properties.add(FlagProperty('autofocus', value: autofocus, ifTrue: 'autofocus'));
}
}
class _SliderState extends State<Slider> with TickerProviderStateMixin {
static const Duration enableAnimationDuration = Duration(milliseconds: 75);
static const Duration valueIndicatorAnimationDuration = Duration(milliseconds: 100);
// Animation controller that is run when the overlay (a.k.a radial reaction)
// is shown in response to user interaction.
late AnimationController overlayController;
// Animation controller that is run when the value indicator is being shown
// or hidden.
late AnimationController valueIndicatorController;
// Animation controller that is run when enabling/disabling the slider.
late AnimationController enableController;
// Animation controller that is run when transitioning between one value
// and the next on a discrete slider.
late AnimationController positionController;
Timer? interactionTimer;
final GlobalKey _renderObjectKey = GlobalKey();
// Keyboard mapping for a focused slider.
static const Map<ShortcutActivator, Intent> _traditionalNavShortcutMap = <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.arrowUp): _AdjustSliderIntent.up(),
SingleActivator(LogicalKeyboardKey.arrowDown): _AdjustSliderIntent.down(),
SingleActivator(LogicalKeyboardKey.arrowLeft): _AdjustSliderIntent.left(),
SingleActivator(LogicalKeyboardKey.arrowRight): _AdjustSliderIntent.right(),
};
// Keyboard mapping for a focused slider when using directional navigation.
// The vertical inputs are not handled to allow navigating out of the slider.
static const Map<ShortcutActivator, Intent> _directionalNavShortcutMap = <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.arrowLeft): _AdjustSliderIntent.left(),
SingleActivator(LogicalKeyboardKey.arrowRight): _AdjustSliderIntent.right(),
};
// Action mapping for a focused slider.
late Map<Type, Action<Intent>> _actionMap;
bool get _enabled => widget.onChanged != null;
// Value Indicator Animation that appears on the Overlay.
PaintValueIndicator? paintValueIndicator;
bool _dragging = false;
// For discrete sliders, _handleChanged might receive the same value
// multiple times. To avoid calling widget.onChanged repeatedly, the
// value from _handleChanged is temporarily saved here.
double? _currentChangedValue;
FocusNode? _focusNode;
FocusNode get focusNode => widget.focusNode ?? _focusNode!;
@override
void initState() {
super.initState();
overlayController = AnimationController(
duration: kRadialReactionDuration,
vsync: this,
);
valueIndicatorController = AnimationController(
duration: valueIndicatorAnimationDuration,
vsync: this,
);
enableController = AnimationController(
duration: enableAnimationDuration,
vsync: this,
);
positionController = AnimationController(
duration: Duration.zero,
vsync: this,
);
enableController.value = widget.onChanged != null ? 1.0 : 0.0;
positionController.value = _convert(widget.value);
_actionMap = <Type, Action<Intent>>{
_AdjustSliderIntent: CallbackAction<_AdjustSliderIntent>(
onInvoke: _actionHandler,
),
};
if (widget.focusNode == null) {
// Only create a new node if the widget doesn't have one.
_focusNode ??= FocusNode();
}
}
@override
void dispose() {
interactionTimer?.cancel();
overlayController.dispose();
valueIndicatorController.dispose();
enableController.dispose();
positionController.dispose();
overlayEntry?.remove();
overlayEntry?.dispose();
overlayEntry = null;
_focusNode?.dispose();
super.dispose();
}
void _handleChanged(double value) {
assert(widget.onChanged != null);
final double lerpValue = _lerp(value);
if (_currentChangedValue != lerpValue) {
_currentChangedValue = lerpValue;
if (_currentChangedValue != widget.value) {
widget.onChanged!(_currentChangedValue!);
}
}
}
void _handleDragStart(double value) {
_dragging = true;
widget.onChangeStart?.call(_lerp(value));
}
void _handleDragEnd(double value) {
_dragging = false;
_currentChangedValue = null;
widget.onChangeEnd?.call(_lerp(value));
}
void _actionHandler(_AdjustSliderIntent intent) {
final _RenderSlider renderSlider = _renderObjectKey.currentContext!.findRenderObject()! as _RenderSlider;
final TextDirection textDirection = Directionality.of(_renderObjectKey.currentContext!);
switch (intent.type) {
case _SliderAdjustmentType.right:
switch (textDirection) {
case TextDirection.rtl:
renderSlider.decreaseAction();
case TextDirection.ltr:
renderSlider.increaseAction();
}
case _SliderAdjustmentType.left:
switch (textDirection) {
case TextDirection.rtl:
renderSlider.increaseAction();
case TextDirection.ltr:
renderSlider.decreaseAction();
}
case _SliderAdjustmentType.up:
renderSlider.increaseAction();
case _SliderAdjustmentType.down:
renderSlider.decreaseAction();
}
}
bool _focused = false;
void _handleFocusHighlightChanged(bool focused) {
if (focused != _focused) {
setState(() { _focused = focused; });
}
}
bool _hovering = false;
void _handleHoverChanged(bool hovering) {
if (hovering != _hovering) {
setState(() { _hovering = hovering; });
}
}
// Returns a number between min and max, proportional to value, which must
// be between 0.0 and 1.0.
double _lerp(double value) {
assert(value >= 0.0);
assert(value <= 1.0);
return value * (widget.max - widget.min) + widget.min;
}
double _discretize(double value) {
assert(widget.divisions != null);
assert(value >= 0.0 && value <= 1.0);
final int divisions = widget.divisions!;
return (value * divisions).round() / divisions;
}
double _convert(double value) {
double ret = _unlerp(value);
if (widget.divisions != null) {
ret = _discretize(ret);
}
return ret;
}
// Returns a number between 0.0 and 1.0, given a value between min and max.
double _unlerp(double value) {
assert(value <= widget.max);
assert(value >= widget.min);
return widget.max > widget.min ? (value - widget.min) / (widget.max - widget.min) : 0.0;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMediaQuery(context));
switch (widget._sliderType) {
case _SliderType.material:
return _buildMaterialSlider(context);
case _SliderType.adaptive: {
final ThemeData theme = Theme.of(context);
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return _buildMaterialSlider(context);
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return _buildCupertinoSlider(context);
}
}
}
}
Widget _buildMaterialSlider(BuildContext context) {
final ThemeData theme = Theme.of(context);
SliderThemeData sliderTheme = SliderTheme.of(context);
final SliderThemeData defaults = theme.useMaterial3 ? _SliderDefaultsM3(context) : _SliderDefaultsM2(context);
// If the widget has active or inactive colors specified, then we plug them
// in to the slider theme as best we can. If the developer wants more
// control than that, then they need to use a SliderTheme. The default
// colors come from the ThemeData.colorScheme. These colors, along with
// the default shapes and text styles are aligned to the Material
// Guidelines.
const SliderTrackShape defaultTrackShape = RoundedRectSliderTrackShape();
const SliderTickMarkShape defaultTickMarkShape = RoundSliderTickMarkShape();
const SliderComponentShape defaultOverlayShape = RoundSliderOverlayShape();
const SliderComponentShape defaultThumbShape = RoundSliderThumbShape();
final SliderComponentShape defaultValueIndicatorShape = defaults.valueIndicatorShape!;
const ShowValueIndicator defaultShowValueIndicator = ShowValueIndicator.onlyForDiscrete;
const SliderInteraction defaultAllowedInteraction = SliderInteraction.tapAndSlide;
final Set<MaterialState> states = <MaterialState>{
if (!_enabled) MaterialState.disabled,
if (_hovering) MaterialState.hovered,
if (_focused) MaterialState.focused,
if (_dragging) MaterialState.dragged,
};
// The value indicator's color is not the same as the thumb and active track
// (which can be defined by activeColor) if the
// RectangularSliderValueIndicatorShape is used. In all other cases, the
// value indicator is assumed to be the same as the active color.
final SliderComponentShape valueIndicatorShape = sliderTheme.valueIndicatorShape ?? defaultValueIndicatorShape;
final Color valueIndicatorColor;
if (valueIndicatorShape is RectangularSliderValueIndicatorShape) {
valueIndicatorColor = sliderTheme.valueIndicatorColor ?? Color.alphaBlend(theme.colorScheme.onSurface.withOpacity(0.60), theme.colorScheme.surface.withOpacity(0.90));
} else {
valueIndicatorColor = widget.activeColor ?? sliderTheme.valueIndicatorColor ?? theme.colorScheme.primary;
}
Color? effectiveOverlayColor() {
return widget.overlayColor?.resolve(states)
?? widget.activeColor?.withOpacity(0.12)
?? MaterialStateProperty.resolveAs<Color?>(sliderTheme.overlayColor, states)
?? MaterialStateProperty.resolveAs<Color?>(defaults.overlayColor, states);
}
sliderTheme = sliderTheme.copyWith(
trackHeight: sliderTheme.trackHeight ?? defaults.trackHeight,
activeTrackColor: widget.activeColor ?? sliderTheme.activeTrackColor ?? defaults.activeTrackColor,
inactiveTrackColor: widget.inactiveColor ?? sliderTheme.inactiveTrackColor ?? defaults.inactiveTrackColor,
secondaryActiveTrackColor: widget.secondaryActiveColor ?? sliderTheme.secondaryActiveTrackColor ?? defaults.secondaryActiveTrackColor,
disabledActiveTrackColor: sliderTheme.disabledActiveTrackColor ?? defaults.disabledActiveTrackColor,
disabledInactiveTrackColor: sliderTheme.disabledInactiveTrackColor ?? defaults.disabledInactiveTrackColor,
disabledSecondaryActiveTrackColor: sliderTheme.disabledSecondaryActiveTrackColor ?? defaults.disabledSecondaryActiveTrackColor,
activeTickMarkColor: widget.inactiveColor ?? sliderTheme.activeTickMarkColor ?? defaults.activeTickMarkColor,
inactiveTickMarkColor: widget.activeColor ?? sliderTheme.inactiveTickMarkColor ?? defaults.inactiveTickMarkColor,
disabledActiveTickMarkColor: sliderTheme.disabledActiveTickMarkColor ?? defaults.disabledActiveTickMarkColor,
disabledInactiveTickMarkColor: sliderTheme.disabledInactiveTickMarkColor ?? defaults.disabledInactiveTickMarkColor,
thumbColor: widget.thumbColor ?? widget.activeColor ?? sliderTheme.thumbColor ?? defaults.thumbColor,
disabledThumbColor: sliderTheme.disabledThumbColor ?? defaults.disabledThumbColor,
overlayColor: effectiveOverlayColor(),
valueIndicatorColor: valueIndicatorColor,
trackShape: sliderTheme.trackShape ?? defaultTrackShape,
tickMarkShape: sliderTheme.tickMarkShape ?? defaultTickMarkShape,
thumbShape: sliderTheme.thumbShape ?? defaultThumbShape,
overlayShape: sliderTheme.overlayShape ?? defaultOverlayShape,
valueIndicatorShape: valueIndicatorShape,
showValueIndicator: sliderTheme.showValueIndicator ?? defaultShowValueIndicator,
valueIndicatorTextStyle: sliderTheme.valueIndicatorTextStyle ?? defaults.valueIndicatorTextStyle,
);
final MouseCursor effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor?>(widget.mouseCursor, states)
?? sliderTheme.mouseCursor?.resolve(states)
?? MaterialStateMouseCursor.clickable.resolve(states);
final SliderInteraction effectiveAllowedInteraction = widget.allowedInteraction
?? sliderTheme.allowedInteraction
?? defaultAllowedInteraction;
// This size is used as the max bounds for the painting of the value
// indicators It must be kept in sync with the function with the same name
// in range_slider.dart.
Size screenSize() => MediaQuery.sizeOf(context);
VoidCallback? handleDidGainAccessibilityFocus;
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.macOS:
break;
case TargetPlatform.windows:
handleDidGainAccessibilityFocus = () {
// Automatically activate the slider when it receives a11y focus.
if (!focusNode.hasFocus && focusNode.canRequestFocus) {
focusNode.requestFocus();
}
};
}
final Map<ShortcutActivator, Intent> shortcutMap = switch (MediaQuery.navigationModeOf(context)) {
NavigationMode.directional => _directionalNavShortcutMap,
NavigationMode.traditional => _traditionalNavShortcutMap,
};
final double fontSize = sliderTheme.valueIndicatorTextStyle?.fontSize ?? kDefaultFontSize;
final double fontSizeToScale = fontSize == 0.0 ? kDefaultFontSize : fontSize;
final TextScaler textScaler = theme.useMaterial3
// TODO(tahatesser): This is an eye-balled value.
// This needs to be updated when accessibility
// guidelines are available on the material specs page
// https://m3.material.io/components/sliders/accessibility.
? MediaQuery.textScalerOf(context).clamp(maxScaleFactor: 1.3)
: MediaQuery.textScalerOf(context);
final double effectiveTextScale = textScaler.scale(fontSizeToScale) / fontSizeToScale;
return Semantics(
container: true,
slider: true,
onDidGainAccessibilityFocus: handleDidGainAccessibilityFocus,
child: FocusableActionDetector(
actions: _actionMap,
shortcuts: shortcutMap,
focusNode: focusNode,
autofocus: widget.autofocus,
enabled: _enabled,
onShowFocusHighlight: _handleFocusHighlightChanged,
onShowHoverHighlight: _handleHoverChanged,
mouseCursor: effectiveMouseCursor,
child: CompositedTransformTarget(
link: _layerLink,
child: _SliderRenderObjectWidget(
key: _renderObjectKey,
value: _convert(widget.value),
secondaryTrackValue: (widget.secondaryTrackValue != null) ? _convert(widget.secondaryTrackValue!) : null,
divisions: widget.divisions,
label: widget.label,
sliderTheme: sliderTheme,
textScaleFactor: effectiveTextScale,
screenSize: screenSize(),
onChanged: (widget.onChanged != null) && (widget.max > widget.min) ? _handleChanged : null,
onChangeStart: _handleDragStart,
onChangeEnd: _handleDragEnd,
state: this,
semanticFormatterCallback: widget.semanticFormatterCallback,
hasFocus: _focused,
hovering: _hovering,
allowedInteraction: effectiveAllowedInteraction,
),
),
),
);
}
Widget _buildCupertinoSlider(BuildContext context) {
// The render box of a slider has a fixed height but takes up the available
// width. Wrapping the [CupertinoSlider] in this manner will help maintain
// the same size.
return SizedBox(
width: double.infinity,
child: CupertinoSlider(
value: widget.value,
onChanged: widget.onChanged,
onChangeStart: widget.onChangeStart,
onChangeEnd: widget.onChangeEnd,
min: widget.min,
max: widget.max,
divisions: widget.divisions,
activeColor: widget.activeColor,
thumbColor: widget.thumbColor ?? CupertinoColors.white,
),
);
}
final LayerLink _layerLink = LayerLink();
OverlayEntry? overlayEntry;
void showValueIndicator() {
if (overlayEntry == null) {
overlayEntry = OverlayEntry(
builder: (BuildContext context) {
return CompositedTransformFollower(
link: _layerLink,
child: _ValueIndicatorRenderObjectWidget(
state: this,
),
);
},
);
Overlay.of(context, debugRequiredFor: widget).insert(overlayEntry!);
}
}
}
class _SliderRenderObjectWidget extends LeafRenderObjectWidget {
const _SliderRenderObjectWidget({
super.key,
required this.value,
required this.secondaryTrackValue,
required this.divisions,
required this.label,
required this.sliderTheme,
required this.textScaleFactor,
required this.screenSize,
required this.onChanged,
required this.onChangeStart,
required this.onChangeEnd,
required this.state,
required this.semanticFormatterCallback,
required this.hasFocus,
required this.hovering,
required this.allowedInteraction,
});
final double value;
final double? secondaryTrackValue;
final int? divisions;
final String? label;
final SliderThemeData sliderTheme;
final double textScaleFactor;
final Size screenSize;
final ValueChanged<double>? onChanged;
final ValueChanged<double>? onChangeStart;
final ValueChanged<double>? onChangeEnd;
final SemanticFormatterCallback? semanticFormatterCallback;
final _SliderState state;
final bool hasFocus;
final bool hovering;
final SliderInteraction allowedInteraction;
@override
_RenderSlider createRenderObject(BuildContext context) {
return _RenderSlider(
value: value,
secondaryTrackValue: secondaryTrackValue,
divisions: divisions,
label: label,
sliderTheme: sliderTheme,
textScaleFactor: textScaleFactor,
screenSize: screenSize,
onChanged: onChanged,
onChangeStart: onChangeStart,
onChangeEnd: onChangeEnd,
state: state,
textDirection: Directionality.of(context),
semanticFormatterCallback: semanticFormatterCallback,
platform: Theme.of(context).platform,
hasFocus: hasFocus,
hovering: hovering,
gestureSettings: MediaQuery.gestureSettingsOf(context),
allowedInteraction: allowedInteraction,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSlider renderObject) {
renderObject
// We should update the `divisions` ahead of `value`, because the `value`
// setter dependent on the `divisions`.
..divisions = divisions
..value = value
..secondaryTrackValue = secondaryTrackValue
..label = label
..sliderTheme = sliderTheme
..textScaleFactor = textScaleFactor
..screenSize = screenSize
..onChanged = onChanged
..onChangeStart = onChangeStart
..onChangeEnd = onChangeEnd
..textDirection = Directionality.of(context)
..semanticFormatterCallback = semanticFormatterCallback
..platform = Theme.of(context).platform
..hasFocus = hasFocus
..hovering = hovering
..gestureSettings = MediaQuery.gestureSettingsOf(context)
..allowedInteraction = allowedInteraction;
// Ticker provider cannot change since there's a 1:1 relationship between
// the _SliderRenderObjectWidget object and the _SliderState object.
}
}
class _RenderSlider extends RenderBox with RelayoutWhenSystemFontsChangeMixin {
_RenderSlider({
required double value,
required double? secondaryTrackValue,
required int? divisions,
required String? label,
required SliderThemeData sliderTheme,
required double textScaleFactor,
required Size screenSize,
required TargetPlatform platform,
required ValueChanged<double>? onChanged,
required SemanticFormatterCallback? semanticFormatterCallback,
required this.onChangeStart,
required this.onChangeEnd,
required _SliderState state,
required TextDirection textDirection,
required bool hasFocus,
required bool hovering,
required DeviceGestureSettings gestureSettings,
required SliderInteraction allowedInteraction,
}) : assert(value >= 0.0 && value <= 1.0),
assert(secondaryTrackValue == null || (secondaryTrackValue >= 0.0 && secondaryTrackValue <= 1.0)),
_platform = platform,
_semanticFormatterCallback = semanticFormatterCallback,
_label = label,
_value = value,
_secondaryTrackValue = secondaryTrackValue,
_divisions = divisions,
_sliderTheme = sliderTheme,
_textScaleFactor = textScaleFactor,
_screenSize = screenSize,
_onChanged = onChanged,
_state = state,
_textDirection = textDirection,
_hasFocus = hasFocus,
_hovering = hovering,
_allowedInteraction = allowedInteraction {
_updateLabelPainter();
final GestureArenaTeam team = GestureArenaTeam();
_drag = HorizontalDragGestureRecognizer()
..team = team
..onStart = _handleDragStart
..onUpdate = _handleDragUpdate
..onEnd = _handleDragEnd
..onCancel = _endInteraction
..gestureSettings = gestureSettings;
_tap = TapGestureRecognizer()
..team = team
..onTapDown = _handleTapDown
..onTapUp = _handleTapUp
..gestureSettings = gestureSettings;
_overlayAnimation = CurvedAnimation(
parent: _state.overlayController,
curve: Curves.fastOutSlowIn,
);
_valueIndicatorAnimation = CurvedAnimation(
parent: _state.valueIndicatorController,
curve: Curves.fastOutSlowIn,
)..addStatusListener((AnimationStatus status) {
if (status == AnimationStatus.dismissed) {
_state.overlayEntry?.remove();
_state.overlayEntry?.dispose();
_state.overlayEntry = null;
}
});
_enableAnimation = CurvedAnimation(
parent: _state.enableController,
curve: Curves.easeInOut,
);
}
static const Duration _positionAnimationDuration = Duration(milliseconds: 75);
static const Duration _minimumInteractionTime = Duration(milliseconds: 500);
// This value is the touch target, 48, multiplied by 3.
static const double _minPreferredTrackWidth = 144.0;
// Compute the largest width and height needed to paint the slider shapes,
// other than the track shape. It is assumed that these shapes are vertically
// centered on the track.
double get _maxSliderPartWidth => _sliderPartSizes.map((Size size) => size.width).reduce(math.max);
double get _maxSliderPartHeight => _sliderPartSizes.map((Size size) => size.height).reduce(math.max);
List<Size> get _sliderPartSizes => <Size>[
_sliderTheme.overlayShape!.getPreferredSize(isInteractive, isDiscrete),
_sliderTheme.thumbShape!.getPreferredSize(isInteractive, isDiscrete),
_sliderTheme.tickMarkShape!.getPreferredSize(isEnabled: isInteractive, sliderTheme: sliderTheme),
];
double get _minPreferredTrackHeight => _sliderTheme.trackHeight!;
final _SliderState _state;
late Animation<double> _overlayAnimation;
late Animation<double> _valueIndicatorAnimation;
late Animation<double> _enableAnimation;
final TextPainter _labelPainter = TextPainter();
late HorizontalDragGestureRecognizer _drag;
late TapGestureRecognizer _tap;
bool _active = false;
double _currentDragValue = 0.0;
Rect? overlayRect;
// This rect is used in gesture calculations, where the gesture coordinates
// are relative to the sliders origin. Therefore, the offset is passed as
// (0,0).
Rect get _trackRect => _sliderTheme.trackShape!.getPreferredRect(
parentBox: this,
sliderTheme: _sliderTheme,
isDiscrete: false,
);
bool get isInteractive => onChanged != null;
bool get isDiscrete => divisions != null && divisions! > 0;
double get value => _value;
double _value;
set value(double newValue) {
assert(newValue >= 0.0 && newValue <= 1.0);
final double convertedValue = isDiscrete ? _discretize(newValue) : newValue;
if (convertedValue == _value) {
return;
}
_value = convertedValue;
if (isDiscrete) {
// Reset the duration to match the distance that we're traveling, so that
// whatever the distance, we still do it in _positionAnimationDuration,
// and if we get re-targeted in the middle, it still takes that long to
// get to the new location.
final double distance = (_value - _state.positionController.value).abs();
_state.positionController.duration = distance != 0.0
? _positionAnimationDuration * (1.0 / distance)
: Duration.zero;
_state.positionController.animateTo(convertedValue, curve: Curves.easeInOut);
} else {
_state.positionController.value = convertedValue;
}
markNeedsSemanticsUpdate();
}
double? get secondaryTrackValue => _secondaryTrackValue;
double? _secondaryTrackValue;
set secondaryTrackValue(double? newValue) {
assert(newValue == null || (newValue >= 0.0 && newValue <= 1.0));
if (newValue == _secondaryTrackValue) {
return;
}
_secondaryTrackValue = newValue;
markNeedsSemanticsUpdate();
}
DeviceGestureSettings? get gestureSettings => _drag.gestureSettings;
set gestureSettings(DeviceGestureSettings? gestureSettings) {
_drag.gestureSettings = gestureSettings;
_tap.gestureSettings = gestureSettings;
}
TargetPlatform _platform;
TargetPlatform get platform => _platform;
set platform(TargetPlatform value) {
if (_platform == value) {
return;
}
_platform = value;
markNeedsSemanticsUpdate();
}
SemanticFormatterCallback? _semanticFormatterCallback;
SemanticFormatterCallback? get semanticFormatterCallback => _semanticFormatterCallback;
set semanticFormatterCallback(SemanticFormatterCallback? value) {
if (_semanticFormatterCallback == value) {
return;
}
_semanticFormatterCallback = value;
markNeedsSemanticsUpdate();
}
int? get divisions => _divisions;
int? _divisions;
set divisions(int? value) {
if (value == _divisions) {
return;
}
_divisions = value;
markNeedsPaint();
}
String? get label => _label;
String? _label;
set label(String? value) {
if (value == _label) {
return;
}
_label = value;
_updateLabelPainter();
}
SliderThemeData get sliderTheme => _sliderTheme;
SliderThemeData _sliderTheme;
set sliderTheme(SliderThemeData value) {
if (value == _sliderTheme) {
return;
}
_sliderTheme = value;
_updateLabelPainter();
}
double get textScaleFactor => _textScaleFactor;
double _textScaleFactor;
set textScaleFactor(double value) {
if (value == _textScaleFactor) {
return;
}
_textScaleFactor = value;
_updateLabelPainter();
}
Size get screenSize => _screenSize;
Size _screenSize;
set screenSize(Size value) {
if (value == _screenSize) {
return;
}
_screenSize = value;
markNeedsPaint();
}
ValueChanged<double>? get onChanged => _onChanged;
ValueChanged<double>? _onChanged;
set onChanged(ValueChanged<double>? value) {
if (value == _onChanged) {
return;
}
final bool wasInteractive = isInteractive;
_onChanged = value;
if (wasInteractive != isInteractive) {
if (isInteractive) {
_state.enableController.forward();
} else {
_state.enableController.reverse();
}
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
ValueChanged<double>? onChangeStart;
ValueChanged<double>? onChangeEnd;
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (value == _textDirection) {
return;
}
_textDirection = value;
_updateLabelPainter();
}
/// True if this slider has the input focus.
bool get hasFocus => _hasFocus;
bool _hasFocus;
set hasFocus(bool value) {
if (value == _hasFocus) {
return;
}
_hasFocus = value;
_updateForFocus(_hasFocus);
markNeedsSemanticsUpdate();
}
/// True if this slider is being hovered over by a pointer.
bool get hovering => _hovering;
bool _hovering;
set hovering(bool value) {
if (value == _hovering) {
return;
}
_hovering = value;
_updateForHover(_hovering);
}
/// True if the slider is interactive and the slider thumb is being
/// hovered over by a pointer.
bool _hoveringThumb = false;
bool get hoveringThumb => _hoveringThumb;
set hoveringThumb(bool value) {
if (value == _hoveringThumb) {
return;
}
_hoveringThumb = value;
_updateForHover(_hovering);
}
SliderInteraction _allowedInteraction;
SliderInteraction get allowedInteraction => _allowedInteraction;
set allowedInteraction(SliderInteraction value) {
if (value == _allowedInteraction) {
return;
}
_allowedInteraction = value;
markNeedsSemanticsUpdate();
}
void _updateForFocus(bool focused) {
if (focused) {
_state.overlayController.forward();
if (showValueIndicator) {
_state.valueIndicatorController.forward();
}
} else {
_state.overlayController.reverse();
if (showValueIndicator) {
_state.valueIndicatorController.reverse();
}
}
}
void _updateForHover(bool hovered) {
// Only show overlay when pointer is hovering the thumb.
if (hovered && hoveringThumb) {
_state.overlayController.forward();
} else {
// Only remove overlay when Slider is inactive and unfocused.
if (!_active && !hasFocus) {
_state.overlayController.reverse();
}
}
}
bool get showValueIndicator {
return switch (_sliderTheme.showValueIndicator!) {
ShowValueIndicator.onlyForDiscrete => isDiscrete,
ShowValueIndicator.onlyForContinuous => !isDiscrete,
ShowValueIndicator.always => true,
ShowValueIndicator.never => false,
};
}
double get _adjustmentUnit {
switch (_platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
// Matches iOS implementation of material slider.
return 0.1;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
// Matches Android implementation of material slider.
return 0.05;
}
}
void _updateLabelPainter() {
if (label != null) {
_labelPainter
..text = TextSpan(
style: _sliderTheme.valueIndicatorTextStyle,
text: label,
)
..textDirection = textDirection
..textScaleFactor = textScaleFactor
..layout();
} else {
_labelPainter.text = null;
}
// Changing the textDirection can result in the layout changing, because the
// bidi algorithm might line up the glyphs differently which can result in
// different ligatures, different shapes, etc. So we always markNeedsLayout.
markNeedsLayout();
}
@override
void systemFontsDidChange() {
super.systemFontsDidChange();
_labelPainter.markNeedsLayout();
_updateLabelPainter();
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_overlayAnimation.addListener(markNeedsPaint);
_valueIndicatorAnimation.addListener(markNeedsPaint);
_enableAnimation.addListener(markNeedsPaint);
_state.positionController.addListener(markNeedsPaint);
}
@override
void detach() {
_overlayAnimation.removeListener(markNeedsPaint);
_valueIndicatorAnimation.removeListener(markNeedsPaint);
_enableAnimation.removeListener(markNeedsPaint);
_state.positionController.removeListener(markNeedsPaint);
super.detach();
}
@override
void dispose() {
_drag.dispose();
_tap.dispose();
_labelPainter.dispose();
super.dispose();
}
double _getValueFromVisualPosition(double visualPosition) {
return switch (textDirection) {
TextDirection.rtl => 1.0 - visualPosition,
TextDirection.ltr => visualPosition,
};
}
double _getValueFromGlobalPosition(Offset globalPosition) {
final double visualPosition = (globalToLocal(globalPosition).dx - _trackRect.left) / _trackRect.width;
return _getValueFromVisualPosition(visualPosition);
}
double _discretize(double value) {
double result = clampDouble(value, 0.0, 1.0);
if (isDiscrete) {
result = (result * divisions!).round() / divisions!;
}
return result;
}
void _startInteraction(Offset globalPosition) {
if (!_state.mounted) {
return;
}
_state.showValueIndicator();
if (!_active && isInteractive) {
switch (allowedInteraction) {
case SliderInteraction.tapAndSlide:
case SliderInteraction.tapOnly:
_active = true;
_currentDragValue = _getValueFromGlobalPosition(globalPosition);
case SliderInteraction.slideThumb:
if (_isPointerOnOverlay(globalPosition)) {
_active = true;
_currentDragValue = value;
}
case SliderInteraction.slideOnly:
onChangeStart?.call(_discretize(value));
}
if (_active) {
// We supply the *current* value as the start location, so that if we have
// a tap, it consists of a call to onChangeStart with the previous value and
// a call to onChangeEnd with the new value.
onChangeStart?.call(_discretize(value));
onChanged!(_discretize(_currentDragValue));
_state.overlayController.forward();
if (showValueIndicator) {
_state.valueIndicatorController.forward();
_state.interactionTimer?.cancel();
_state.interactionTimer = Timer(_minimumInteractionTime * timeDilation, () {
_state.interactionTimer = null;
if (!_active && _state.valueIndicatorController.status == AnimationStatus.completed) {
_state.valueIndicatorController.reverse();
}
});
}
}
}
}
void _endInteraction() {
if (!_state.mounted) {
return;
}
if (_active && _state.mounted) {
onChangeEnd?.call(_discretize(_currentDragValue));
_active = false;
_currentDragValue = 0.0;
_state.overlayController.reverse();
if (showValueIndicator && _state.interactionTimer == null) {
_state.valueIndicatorController.reverse();
}
}
}
void _handleDragStart(DragStartDetails details) {
_startInteraction(details.globalPosition);
}
void _handleDragUpdate(DragUpdateDetails details) {
if (!_state.mounted) {
return;
}
// for slide only, there is no start interaction trigger, so _active
// will be false and needs to be made true.
if (!_active && allowedInteraction == SliderInteraction.slideOnly) {
_active = true;
_currentDragValue = value;
}
switch (allowedInteraction) {
case SliderInteraction.tapAndSlide:
case SliderInteraction.slideOnly:
case SliderInteraction.slideThumb:
if (_active && isInteractive) {
final double valueDelta = details.primaryDelta! / _trackRect.width;
_currentDragValue += switch (textDirection) {
TextDirection.rtl => -valueDelta,
TextDirection.ltr => valueDelta,
};
onChanged!(_discretize(_currentDragValue));
}
case SliderInteraction.tapOnly:
// cannot slide (drag) as its tapOnly.
break;
}
}
void _handleDragEnd(DragEndDetails details) {
_endInteraction();
}
void _handleTapDown(TapDownDetails details) {
_startInteraction(details.globalPosition);
}
void _handleTapUp(TapUpDetails details) {
_endInteraction();
}
bool _isPointerOnOverlay(Offset globalPosition) {
return overlayRect!.contains(globalToLocal(globalPosition));
}
@override
bool hitTestSelf(Offset position) => true;
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
if (!_state.mounted) {
return;
}
assert(debugHandleEvent(event, entry));
if (event is PointerDownEvent && isInteractive) {
// We need to add the drag first so that it has priority.
_drag.addPointer(event);
_tap.addPointer(event);
}
if (isInteractive && overlayRect != null) {
hoveringThumb = overlayRect!.contains(event.localPosition);
}
}
@override
double computeMinIntrinsicWidth(double height) => _minPreferredTrackWidth + _maxSliderPartWidth;
@override
double computeMaxIntrinsicWidth(double height) => _minPreferredTrackWidth + _maxSliderPartWidth;
@override
double computeMinIntrinsicHeight(double width) => math.max(_minPreferredTrackHeight, _maxSliderPartHeight);
@override
double computeMaxIntrinsicHeight(double width) => math.max(_minPreferredTrackHeight, _maxSliderPartHeight);
@override
bool get sizedByParent => true;
@override
Size computeDryLayout(BoxConstraints constraints) {
return Size(
constraints.hasBoundedWidth ? constraints.maxWidth : _minPreferredTrackWidth + _maxSliderPartWidth,
constraints.hasBoundedHeight ? constraints.maxHeight : math.max(_minPreferredTrackHeight, _maxSliderPartHeight),
);
}
@override
void paint(PaintingContext context, Offset offset) {
final double controllerValue = _state.positionController.value;
// The visual position is the position of the thumb from 0 to 1 from left
// to right. In left to right, this is the same as the value, but it is
// reversed for right to left text.
final (double visualPosition, double? secondaryVisualPosition) = switch (textDirection) {
TextDirection.rtl when _secondaryTrackValue == null => (1.0 - controllerValue, null),
TextDirection.rtl => (1.0 - controllerValue, 1.0 - _secondaryTrackValue!),
TextDirection.ltr => (controllerValue, _secondaryTrackValue),
};
final Rect trackRect = _sliderTheme.trackShape!.getPreferredRect(
parentBox: this,
offset: offset,
sliderTheme: _sliderTheme,
isDiscrete: isDiscrete,
);
final Offset thumbCenter = Offset(trackRect.left + visualPosition * trackRect.width, trackRect.center.dy);
if (isInteractive) {
final Size overlaySize = sliderTheme.overlayShape!.getPreferredSize(isInteractive, false);
overlayRect = Rect.fromCircle(center: thumbCenter, radius: overlaySize.width / 2.0);
}
final Offset? secondaryOffset = (secondaryVisualPosition != null) ? Offset(trackRect.left + secondaryVisualPosition * trackRect.width, trackRect.center.dy) : null;
_sliderTheme.trackShape!.paint(
context,
offset,
parentBox: this,
sliderTheme: _sliderTheme,
enableAnimation: _enableAnimation,
textDirection: _textDirection,
thumbCenter: thumbCenter,
secondaryOffset: secondaryOffset,
isDiscrete: isDiscrete,
isEnabled: isInteractive,
);
if (!_overlayAnimation.isDismissed) {
_sliderTheme.overlayShape!.paint(
context,
thumbCenter,
activationAnimation: _overlayAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
labelPainter: _labelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
value: _value,
textScaleFactor: _textScaleFactor,
sizeWithOverflow: screenSize.isEmpty ? size : screenSize,
);
}
if (isDiscrete) {
final double tickMarkWidth = _sliderTheme.tickMarkShape!.getPreferredSize(
isEnabled: isInteractive,
sliderTheme: _sliderTheme,
).width;
final double padding = trackRect.height;
final double adjustedTrackWidth = trackRect.width - padding;
// If the tick marks would be too dense, don't bother painting them.
if (adjustedTrackWidth / divisions! >= 3.0 * tickMarkWidth) {
final double dy = trackRect.center.dy;
for (int i = 0; i <= divisions!; i++) {
final double value = i / divisions!;
// The ticks are mapped to be within the track, so the tick mark width
// must be subtracted from the track width.
final double dx = trackRect.left + value * adjustedTrackWidth + padding / 2;
final Offset tickMarkOffset = Offset(dx, dy);
_sliderTheme.tickMarkShape!.paint(
context,
tickMarkOffset,
parentBox: this,
sliderTheme: _sliderTheme,
enableAnimation: _enableAnimation,
textDirection: _textDirection,
thumbCenter: thumbCenter,
isEnabled: isInteractive,
);
}
}
}
if (isInteractive && label != null && !_valueIndicatorAnimation.isDismissed) {
if (showValueIndicator) {
_state.paintValueIndicator = (PaintingContext context, Offset offset) {
if (attached) {
_sliderTheme.valueIndicatorShape!.paint(
context,
offset + thumbCenter,
activationAnimation: _valueIndicatorAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
labelPainter: _labelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
value: _value,
textScaleFactor: textScaleFactor,
sizeWithOverflow: screenSize.isEmpty ? size : screenSize,
);
}
};
}
}
_sliderTheme.thumbShape!.paint(
context,
thumbCenter,
activationAnimation: _overlayAnimation,
enableAnimation: _enableAnimation,
isDiscrete: isDiscrete,
labelPainter: _labelPainter,
parentBox: this,
sliderTheme: _sliderTheme,
textDirection: _textDirection,
value: _value,
textScaleFactor: textScaleFactor,
sizeWithOverflow: screenSize.isEmpty ? size : screenSize,
);
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
// The Slider widget has its own Focus widget with semantics information,
// and we want that semantics node to collect the semantics information here
// so that it's all in the same node: otherwise Talkback sees that the node
// has focusable children, and it won't focus the Slider's Focus widget
// because it thinks the Focus widget's node doesn't have anything to say
// (which it doesn't, but this child does). Aggregating the semantic
// information into one node means that Talkback will recognize that it has
// something to say and focus it when it receives keyboard focus.
// (See https://github.com/flutter/flutter/issues/57038 for context).
config.isSemanticBoundary = false;
config.isEnabled = isInteractive;
config.textDirection = textDirection;
if (isInteractive) {
config.onIncrease = increaseAction;
config.onDecrease = decreaseAction;
}
if (semanticFormatterCallback != null) {
config.value = semanticFormatterCallback!(_state._lerp(value));
config.increasedValue = semanticFormatterCallback!(_state._lerp(clampDouble(value + _semanticActionUnit, 0.0, 1.0)));
config.decreasedValue = semanticFormatterCallback!(_state._lerp(clampDouble(value - _semanticActionUnit, 0.0, 1.0)));
} else {
config.value = '${(value * 100).round()}%';
config.increasedValue = '${(clampDouble(value + _semanticActionUnit, 0.0, 1.0) * 100).round()}%';
config.decreasedValue = '${(clampDouble(value - _semanticActionUnit, 0.0, 1.0) * 100).round()}%';
}
}
double get _semanticActionUnit => divisions != null ? 1.0 / divisions! : _adjustmentUnit;
void increaseAction() {
if (isInteractive) {
onChangeStart!(currentValue);
final double increase = increaseValue();
onChanged!(increase);
onChangeEnd!(increase);
}
}
void decreaseAction() {
if (isInteractive) {
onChangeStart!(currentValue);
final double decrease = decreaseValue();
onChanged!(decrease);
onChangeEnd!(decrease);
}
}
double get currentValue {
return clampDouble(value, 0.0, 1.0);
}
double increaseValue() {
return clampDouble(value + _semanticActionUnit, 0.0, 1.0);
}
double decreaseValue() {
return clampDouble(value - _semanticActionUnit, 0.0, 1.0);
}
}
class _AdjustSliderIntent extends Intent {
const _AdjustSliderIntent({
required this.type,
});
const _AdjustSliderIntent.right() : type = _SliderAdjustmentType.right;
const _AdjustSliderIntent.left() : type = _SliderAdjustmentType.left;
const _AdjustSliderIntent.up() : type = _SliderAdjustmentType.up;
const _AdjustSliderIntent.down() : type = _SliderAdjustmentType.down;
final _SliderAdjustmentType type;
}
enum _SliderAdjustmentType {
right,
left,
up,
down,
}
class _ValueIndicatorRenderObjectWidget extends LeafRenderObjectWidget {
const _ValueIndicatorRenderObjectWidget({
required this.state,
});
final _SliderState state;
@override
_RenderValueIndicator createRenderObject(BuildContext context) {
return _RenderValueIndicator(
state: state,
);
}
@override
void updateRenderObject(BuildContext context, _RenderValueIndicator renderObject) {
renderObject._state = state;
}
}
class _RenderValueIndicator extends RenderBox with RelayoutWhenSystemFontsChangeMixin {
_RenderValueIndicator({
required _SliderState state,
}) : _state = state {
_valueIndicatorAnimation = CurvedAnimation(
parent: _state.valueIndicatorController,
curve: Curves.fastOutSlowIn,
);
}
late Animation<double> _valueIndicatorAnimation;
_SliderState _state;
@override
bool get sizedByParent => true;
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_valueIndicatorAnimation.addListener(markNeedsPaint);
_state.positionController.addListener(markNeedsPaint);
}
@override
void detach() {
_valueIndicatorAnimation.removeListener(markNeedsPaint);
_state.positionController.removeListener(markNeedsPaint);
super.detach();
}
@override
void paint(PaintingContext context, Offset offset) {
_state.paintValueIndicator?.call(context, offset);
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return constraints.smallest;
}
}
class _SliderDefaultsM2 extends SliderThemeData {
_SliderDefaultsM2(this.context)
: _colors = Theme.of(context).colorScheme,
super(trackHeight: 4.0);
final BuildContext context;
final ColorScheme _colors;
@override
Color? get activeTrackColor => _colors.primary;
@override
Color? get inactiveTrackColor => _colors.primary.withOpacity(0.24);
@override
Color? get secondaryActiveTrackColor => _colors.primary.withOpacity(0.54);
@override
Color? get disabledActiveTrackColor => _colors.onSurface.withOpacity(0.32);
@override
Color? get disabledInactiveTrackColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get disabledSecondaryActiveTrackColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get activeTickMarkColor => _colors.onPrimary.withOpacity(0.54);
@override
Color? get inactiveTickMarkColor => _colors.primary.withOpacity(0.54);
@override
Color? get disabledActiveTickMarkColor => _colors.onPrimary.withOpacity(0.12);
@override
Color? get disabledInactiveTickMarkColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get thumbColor => _colors.primary;
@override
Color? get disabledThumbColor => Color.alphaBlend(_colors.onSurface.withOpacity(.38), _colors.surface);
@override
Color? get overlayColor => _colors.primary.withOpacity(0.12);
@override
TextStyle? get valueIndicatorTextStyle => Theme.of(context).textTheme.bodyLarge!.copyWith(
color: _colors.onPrimary,
);
@override
SliderComponentShape? get valueIndicatorShape => const RectangularSliderValueIndicatorShape();
}
// BEGIN GENERATED TOKEN PROPERTIES - Slider
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _SliderDefaultsM3 extends SliderThemeData {
_SliderDefaultsM3(this.context)
: super(trackHeight: 4.0);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color? get activeTrackColor => _colors.primary;
@override
Color? get inactiveTrackColor => _colors.surfaceContainerHighest;
@override
Color? get secondaryActiveTrackColor => _colors.primary.withOpacity(0.54);
@override
Color? get disabledActiveTrackColor => _colors.onSurface.withOpacity(0.38);
@override
Color? get disabledInactiveTrackColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get disabledSecondaryActiveTrackColor => _colors.onSurface.withOpacity(0.12);
@override
Color? get activeTickMarkColor => _colors.onPrimary.withOpacity(0.38);
@override
Color? get inactiveTickMarkColor => _colors.onSurfaceVariant.withOpacity(0.38);
@override
Color? get disabledActiveTickMarkColor => _colors.onSurface.withOpacity(0.38);
@override
Color? get disabledInactiveTickMarkColor => _colors.onSurface.withOpacity(0.38);
@override
Color? get thumbColor => _colors.primary;
@override
Color? get disabledThumbColor => Color.alphaBlend(_colors.onSurface.withOpacity(0.38), _colors.surface);
@override
Color? get overlayColor => MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.dragged)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return Colors.transparent;
});
@override
TextStyle? get valueIndicatorTextStyle => Theme.of(context).textTheme.labelMedium!.copyWith(
color: _colors.onPrimary,
);
@override
SliderComponentShape? get valueIndicatorShape => const DropSliderValueIndicatorShape();
}
// END GENERATED TOKEN PROPERTIES - Slider
| flutter/packages/flutter/lib/src/material/slider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/slider.dart",
"repo_id": "flutter",
"token_count": 24274
} | 628 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'adaptive_text_selection_toolbar.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'debug.dart';
import 'desktop_text_selection.dart';
import 'feedback.dart';
import 'input_decorator.dart';
import 'magnifier.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'selectable_text.dart' show iOSHorizontalOffset;
import 'spell_check_suggestions_toolbar.dart';
import 'text_selection.dart';
import 'theme.dart';
export 'package:flutter/services.dart' show SmartDashesType, SmartQuotesType, TextCapitalization, TextInputAction, TextInputType;
// Examples can assume:
// late BuildContext context;
// late FocusNode myFocusNode;
/// Signature for the [TextField.buildCounter] callback.
typedef InputCounterWidgetBuilder = Widget? Function(
/// The build context for the TextField.
BuildContext context, {
/// The length of the string currently in the input.
required int currentLength,
/// The maximum string length that can be entered into the TextField.
required int? maxLength,
/// Whether or not the TextField is currently focused. Mainly provided for
/// the [liveRegion] parameter in the [Semantics] widget for accessibility.
required bool isFocused,
});
class _TextFieldSelectionGestureDetectorBuilder extends TextSelectionGestureDetectorBuilder {
_TextFieldSelectionGestureDetectorBuilder({
required _TextFieldState state,
}) : _state = state,
super(delegate: state);
final _TextFieldState _state;
@override
void onForcePressStart(ForcePressDetails details) {
super.onForcePressStart(details);
if (delegate.selectionEnabled && shouldShowSelectionToolbar) {
editableText.showToolbar();
}
}
@override
void onForcePressEnd(ForcePressDetails details) {
// Not required.
}
@override
bool get onUserTapAlwaysCalled => _state.widget.onTapAlwaysCalled;
@override
void onUserTap() {
_state.widget.onTap?.call();
}
@override
void onSingleLongTapStart(LongPressStartDetails details) {
super.onSingleLongTapStart(details);
if (delegate.selectionEnabled) {
switch (Theme.of(_state.context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
break;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
Feedback.forLongPress(_state.context);
}
}
}
}
/// A Material Design text field.
///
/// A text field lets the user enter text, either with hardware keyboard or with
/// an onscreen keyboard.
///
/// The text field calls the [onChanged] callback whenever the user changes the
/// text in the field. If the user indicates that they are done typing in the
/// field (e.g., by pressing a button on the soft keyboard), the text field
/// calls the [onSubmitted] callback.
///
/// To control the text that is displayed in the text field, use the
/// [controller]. For example, to set the initial value of the text field, use
/// a [controller] that already contains some text. The [controller] can also
/// control the selection and composing region (and to observe changes to the
/// text, selection, and composing region).
///
/// By default, a text field has a [decoration] that draws a divider below the
/// text field. You can use the [decoration] property to control the decoration,
/// for example by adding a label or an icon. If you set the [decoration]
/// property to null, the decoration will be removed entirely, including the
/// extra padding introduced by the decoration to save space for the labels.
///
/// If [decoration] is non-null (which is the default), the text field requires
/// one of its ancestors to be a [Material] widget.
///
/// To integrate the [TextField] into a [Form] with other [FormField] widgets,
/// consider using [TextFormField].
///
/// {@template flutter.material.textfield.wantKeepAlive}
/// When the widget has focus, it will prevent itself from disposing via its
/// underlying [EditableText]'s [AutomaticKeepAliveClientMixin.wantKeepAlive] in
/// order to avoid losing the selection. Removing the focus will allow it to be
/// disposed.
/// {@endtemplate}
///
/// Remember to call [TextEditingController.dispose] of the [TextEditingController]
/// when it is no longer needed. This will ensure we discard any resources used
/// by the object.
///
/// ## Obscured Input
///
/// {@tool dartpad}
/// This example shows how to create a [TextField] that will obscure input. The
/// [InputDecoration] surrounds the field in a border using [OutlineInputBorder]
/// and adds a label.
///
/// ** See code in examples/api/lib/material/text_field/text_field.0.dart **
/// {@end-tool}
///
/// ## Reading values
///
/// A common way to read a value from a TextField is to use the [onSubmitted]
/// callback. This callback is applied to the text field's current value when
/// the user finishes editing.
///
/// {@tool dartpad}
/// This sample shows how to get a value from a TextField via the [onSubmitted]
/// callback.
///
/// ** See code in examples/api/lib/material/text_field/text_field.1.dart **
/// {@end-tool}
///
/// {@macro flutter.widgets.EditableText.lifeCycle}
///
/// For most applications the [onSubmitted] callback will be sufficient for
/// reacting to user input.
///
/// The [onEditingComplete] callback also runs when the user finishes editing.
/// It's different from [onSubmitted] because it has a default value which
/// updates the text controller and yields the keyboard focus. Applications that
/// require different behavior can override the default [onEditingComplete]
/// callback.
///
/// Keep in mind you can also always read the current string from a TextField's
/// [TextEditingController] using [TextEditingController.text].
///
/// ## Handling emojis and other complex characters
/// {@macro flutter.widgets.EditableText.onChanged}
///
/// In the live Dartpad example above, try typing the emoji 👨👩👦
/// into the field and submitting. Because the example code measures the length
/// with `value.characters.length`, the emoji is correctly counted as a single
/// character.
///
/// {@macro flutter.widgets.editableText.showCaretOnScreen}
///
/// {@macro flutter.widgets.editableText.accessibility}
///
/// {@tool dartpad}
/// This sample shows how to style a text field to match a filled or outlined
/// Material Design 3 text field.
///
/// ** See code in examples/api/lib/material/text_field/text_field.2.dart **
/// {@end-tool}
///
/// ## Scrolling Considerations
///
/// If this [TextField] is not a descendant of [Scaffold] and is being used
/// within a [Scrollable] or nested [Scrollable]s, consider placing a
/// [ScrollNotificationObserver] above the root [Scrollable] that contains this
/// [TextField] to ensure proper scroll coordination for [TextField] and its
/// components like [TextSelectionOverlay].
///
/// See also:
///
/// * [TextFormField], which integrates with the [Form] widget.
/// * [InputDecorator], which shows the labels and other visual elements that
/// surround the actual text editing widget.
/// * [EditableText], which is the raw text editing control at the heart of a
/// [TextField]. The [EditableText] widget is rarely used directly unless
/// you are implementing an entirely different design language, such as
/// Cupertino.
/// * <https://material.io/design/components/text-fields.html>
/// * Cookbook: [Create and style a text field](https://flutter.dev/docs/cookbook/forms/text-input)
/// * Cookbook: [Handle changes to a text field](https://flutter.dev/docs/cookbook/forms/text-field-changes)
/// * Cookbook: [Retrieve the value of a text field](https://flutter.dev/docs/cookbook/forms/retrieve-input)
/// * Cookbook: [Focus and text fields](https://flutter.dev/docs/cookbook/forms/focus)
class TextField extends StatefulWidget {
/// Creates a Material Design text field.
///
/// If [decoration] is non-null (which is the default), the text field requires
/// one of its ancestors to be a [Material] widget.
///
/// To remove the decoration entirely (including the extra padding introduced
/// by the decoration to save space for the labels), set the [decoration] to
/// null.
///
/// The [maxLines] property can be set to null to remove the restriction on
/// the number of lines. By default, it is one, meaning this is a single-line
/// text field. [maxLines] must not be zero.
///
/// The [maxLength] property is set to null by default, which means the
/// number of characters allowed in the text field is not restricted. If
/// [maxLength] is set a character counter will be displayed below the
/// field showing how many characters have been entered. If the value is
/// set to a positive integer it will also display the maximum allowed
/// number of characters to be entered. If the value is set to
/// [TextField.noMaxLength] then only the current length is displayed.
///
/// After [maxLength] characters have been input, additional input
/// is ignored, unless [maxLengthEnforcement] is set to
/// [MaxLengthEnforcement.none].
/// The text field enforces the length with a [LengthLimitingTextInputFormatter],
/// which is evaluated after the supplied [inputFormatters], if any.
/// The [maxLength] value must be either null or greater than zero.
///
/// If [maxLengthEnforcement] is set to [MaxLengthEnforcement.none], then more
/// than [maxLength] characters may be entered, and the error counter and
/// divider will switch to the [decoration].errorStyle when the limit is
/// exceeded.
///
/// The text cursor is not shown if [showCursor] is false or if [showCursor]
/// is null (the default) and [readOnly] is true.
///
/// The [selectionHeightStyle] and [selectionWidthStyle] properties allow
/// changing the shape of the selection highlighting. These properties default
/// to [ui.BoxHeightStyle.tight] and [ui.BoxWidthStyle.tight], respectively.
///
/// See also:
///
/// * [maxLength], which discusses the precise meaning of "number of
/// characters" and how it may differ from the intuitive meaning.
const TextField({
super.key,
this.controller,
this.focusNode,
this.undoController,
this.decoration = const InputDecoration(),
TextInputType? keyboardType,
this.textInputAction,
this.textCapitalization = TextCapitalization.none,
this.style,
this.strutStyle,
this.textAlign = TextAlign.start,
this.textAlignVertical,
this.textDirection,
this.readOnly = false,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
this.toolbarOptions,
this.showCursor,
this.autofocus = false,
this.statesController,
this.obscuringCharacter = '•',
this.obscureText = false,
this.autocorrect = true,
SmartDashesType? smartDashesType,
SmartQuotesType? smartQuotesType,
this.enableSuggestions = true,
this.maxLines = 1,
this.minLines,
this.expands = false,
this.maxLength,
this.maxLengthEnforcement,
this.onChanged,
this.onEditingComplete,
this.onSubmitted,
this.onAppPrivateCommand,
this.inputFormatters,
this.enabled,
this.ignorePointers,
this.cursorWidth = 2.0,
this.cursorHeight,
this.cursorRadius,
this.cursorOpacityAnimates,
this.cursorColor,
this.cursorErrorColor,
this.selectionHeightStyle = ui.BoxHeightStyle.tight,
this.selectionWidthStyle = ui.BoxWidthStyle.tight,
this.keyboardAppearance,
this.scrollPadding = const EdgeInsets.all(20.0),
this.dragStartBehavior = DragStartBehavior.start,
bool? enableInteractiveSelection,
this.selectionControls,
this.onTap,
this.onTapAlwaysCalled = false,
this.onTapOutside,
this.mouseCursor,
this.buildCounter,
this.scrollController,
this.scrollPhysics,
this.autofillHints = const <String>[],
this.contentInsertionConfiguration,
this.clipBehavior = Clip.hardEdge,
this.restorationId,
this.scribbleEnabled = true,
this.enableIMEPersonalizedLearning = true,
this.contextMenuBuilder = _defaultContextMenuBuilder,
this.canRequestFocus = true,
this.spellCheckConfiguration,
this.magnifierConfiguration,
}) : assert(obscuringCharacter.length == 1),
smartDashesType = smartDashesType ?? (obscureText ? SmartDashesType.disabled : SmartDashesType.enabled),
smartQuotesType = smartQuotesType ?? (obscureText ? SmartQuotesType.disabled : SmartQuotesType.enabled),
assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
!expands || (maxLines == null && minLines == null),
'minLines and maxLines must be null when expands is true.',
),
assert(!obscureText || maxLines == 1, 'Obscured fields cannot be multiline.'),
assert(maxLength == null || maxLength == TextField.noMaxLength || maxLength > 0),
// Assert the following instead of setting it directly to avoid surprising the user by silently changing the value they set.
assert(
!identical(textInputAction, TextInputAction.newline) ||
maxLines == 1 ||
!identical(keyboardType, TextInputType.text),
'Use keyboardType TextInputType.multiline when using TextInputAction.newline on a multiline TextField.',
),
keyboardType = keyboardType ?? (maxLines == 1 ? TextInputType.text : TextInputType.multiline),
enableInteractiveSelection = enableInteractiveSelection ?? (!readOnly || !obscureText);
/// The configuration for the magnifier of this text field.
///
/// By default, builds a [CupertinoTextMagnifier] on iOS and [TextMagnifier]
/// on Android, and builds nothing on all other platforms. To suppress the
/// magnifier, consider passing [TextMagnifierConfiguration.disabled].
///
/// {@macro flutter.widgets.magnifier.intro}
///
/// {@tool dartpad}
/// This sample demonstrates how to customize the magnifier that this text field uses.
///
/// ** See code in examples/api/lib/widgets/text_magnifier/text_magnifier.0.dart **
/// {@end-tool}
final TextMagnifierConfiguration? magnifierConfiguration;
/// Controls the text being edited.
///
/// If null, this widget will create its own [TextEditingController].
final TextEditingController? controller;
/// Defines the keyboard focus for this widget.
///
/// The [focusNode] is a long-lived object that's typically managed by a
/// [StatefulWidget] parent. See [FocusNode] for more information.
///
/// To give the keyboard focus to this widget, provide a [focusNode] and then
/// use the current [FocusScope] to request the focus:
///
/// ```dart
/// FocusScope.of(context).requestFocus(myFocusNode);
/// ```
///
/// This happens automatically when the widget is tapped.
///
/// To be notified when the widget gains or loses the focus, add a listener
/// to the [focusNode]:
///
/// ```dart
/// myFocusNode.addListener(() { print(myFocusNode.hasFocus); });
/// ```
///
/// If null, this widget will create its own [FocusNode].
///
/// ## Keyboard
///
/// Requesting the focus will typically cause the keyboard to be shown
/// if it's not showing already.
///
/// On Android, the user can hide the keyboard - without changing the focus -
/// with the system back button. They can restore the keyboard's visibility
/// by tapping on a text field. The user might hide the keyboard and
/// switch to a physical keyboard, or they might just need to get it
/// out of the way for a moment, to expose something it's
/// obscuring. In this case requesting the focus again will not
/// cause the focus to change, and will not make the keyboard visible.
///
/// This widget builds an [EditableText] and will ensure that the keyboard is
/// showing when it is tapped by calling [EditableTextState.requestKeyboard()].
final FocusNode? focusNode;
/// The decoration to show around the text field.
///
/// By default, draws a horizontal line under the text field but can be
/// configured to show an icon, label, hint text, and error text.
///
/// Specify null to remove the decoration entirely (including the
/// extra padding introduced by the decoration to save space for the labels).
final InputDecoration? decoration;
/// {@macro flutter.widgets.editableText.keyboardType}
final TextInputType keyboardType;
/// {@template flutter.widgets.TextField.textInputAction}
/// The type of action button to use for the keyboard.
///
/// Defaults to [TextInputAction.newline] if [keyboardType] is
/// [TextInputType.multiline] and [TextInputAction.done] otherwise.
/// {@endtemplate}
final TextInputAction? textInputAction;
/// {@macro flutter.widgets.editableText.textCapitalization}
final TextCapitalization textCapitalization;
/// The style to use for the text being edited.
///
/// This text style is also used as the base style for the [decoration].
///
/// If null, [TextTheme.bodyLarge] will be used. When the text field is disabled,
/// [TextTheme.bodyLarge] with an opacity of 0.38 will be used instead.
///
/// If null and [ThemeData.useMaterial3] is false, [TextTheme.titleMedium] will
/// be used. When the text field is disabled, [TextTheme.titleMedium] with
/// [ThemeData.disabledColor] will be used instead.
final TextStyle? style;
/// {@macro flutter.widgets.editableText.strutStyle}
final StrutStyle? strutStyle;
/// {@macro flutter.widgets.editableText.textAlign}
final TextAlign textAlign;
/// {@macro flutter.material.InputDecorator.textAlignVertical}
final TextAlignVertical? textAlignVertical;
/// {@macro flutter.widgets.editableText.textDirection}
final TextDirection? textDirection;
/// {@macro flutter.widgets.editableText.autofocus}
final bool autofocus;
/// Represents the interactive "state" of this widget in terms of a set of
/// [MaterialState]s, including [MaterialState.disabled], [MaterialState.hovered],
/// [MaterialState.error], and [MaterialState.focused].
///
/// Classes based on this one can provide their own
/// [MaterialStatesController] to which they've added listeners.
/// They can also update the controller's [MaterialStatesController.value]
/// however, this may only be done when it's safe to call
/// [State.setState], like in an event handler.
///
/// The controller's [MaterialStatesController.value] represents the set of
/// states that a widget's visual properties, typically [MaterialStateProperty]
/// values, are resolved against. It is _not_ the intrinsic state of the widget.
/// The widget is responsible for ensuring that the controller's
/// [MaterialStatesController.value] tracks its intrinsic state. For example
/// one cannot request the keyboard focus for a widget by adding [MaterialState.focused]
/// to its controller. When the widget gains the or loses the focus it will
/// [MaterialStatesController.update] its controller's [MaterialStatesController.value]
/// and notify listeners of the change.
final MaterialStatesController? statesController;
/// {@macro flutter.widgets.editableText.obscuringCharacter}
final String obscuringCharacter;
/// {@macro flutter.widgets.editableText.obscureText}
final bool obscureText;
/// {@macro flutter.widgets.editableText.autocorrect}
final bool autocorrect;
/// {@macro flutter.services.TextInputConfiguration.smartDashesType}
final SmartDashesType smartDashesType;
/// {@macro flutter.services.TextInputConfiguration.smartQuotesType}
final SmartQuotesType smartQuotesType;
/// {@macro flutter.services.TextInputConfiguration.enableSuggestions}
final bool enableSuggestions;
/// {@macro flutter.widgets.editableText.maxLines}
/// * [expands], which determines whether the field should fill the height of
/// its parent.
final int? maxLines;
/// {@macro flutter.widgets.editableText.minLines}
/// * [expands], which determines whether the field should fill the height of
/// its parent.
final int? minLines;
/// {@macro flutter.widgets.editableText.expands}
final bool expands;
/// {@macro flutter.widgets.editableText.readOnly}
final bool readOnly;
/// Configuration of toolbar options.
///
/// If not set, select all and paste will default to be enabled. Copy and cut
/// will be disabled if [obscureText] is true. If [readOnly] is true,
/// paste and cut will be disabled regardless.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
final ToolbarOptions? toolbarOptions;
/// {@macro flutter.widgets.editableText.showCursor}
final bool? showCursor;
/// If [maxLength] is set to this value, only the "current input length"
/// part of the character counter is shown.
static const int noMaxLength = -1;
/// The maximum number of characters (Unicode grapheme clusters) to allow in
/// the text field.
///
/// If set, a character counter will be displayed below the
/// field showing how many characters have been entered. If set to a number
/// greater than 0, it will also display the maximum number allowed. If set
/// to [TextField.noMaxLength] then only the current character count is displayed.
///
/// After [maxLength] characters have been input, additional input
/// is ignored, unless [maxLengthEnforcement] is set to
/// [MaxLengthEnforcement.none].
///
/// The text field enforces the length with a [LengthLimitingTextInputFormatter],
/// which is evaluated after the supplied [inputFormatters], if any.
///
/// This value must be either null, [TextField.noMaxLength], or greater than 0.
/// If null (the default) then there is no limit to the number of characters
/// that can be entered. If set to [TextField.noMaxLength], then no limit will
/// be enforced, but the number of characters entered will still be displayed.
///
/// Whitespace characters (e.g. newline, space, tab) are included in the
/// character count.
///
/// If [maxLengthEnforcement] is [MaxLengthEnforcement.none], then more than
/// [maxLength] characters may be entered, but the error counter and divider
/// will switch to the [decoration]'s [InputDecoration.errorStyle] when the
/// limit is exceeded.
///
/// {@macro flutter.services.lengthLimitingTextInputFormatter.maxLength}
final int? maxLength;
/// Determines how the [maxLength] limit should be enforced.
///
/// {@macro flutter.services.textFormatter.effectiveMaxLengthEnforcement}
///
/// {@macro flutter.services.textFormatter.maxLengthEnforcement}
final MaxLengthEnforcement? maxLengthEnforcement;
/// {@macro flutter.widgets.editableText.onChanged}
///
/// See also:
///
/// * [inputFormatters], which are called before [onChanged]
/// runs and can validate and change ("format") the input value.
/// * [onEditingComplete], [onSubmitted]:
/// which are more specialized input change notifications.
final ValueChanged<String>? onChanged;
/// {@macro flutter.widgets.editableText.onEditingComplete}
final VoidCallback? onEditingComplete;
/// {@macro flutter.widgets.editableText.onSubmitted}
///
/// See also:
///
/// * [TextInputAction.next] and [TextInputAction.previous], which
/// automatically shift the focus to the next/previous focusable item when
/// the user is done editing.
final ValueChanged<String>? onSubmitted;
/// {@macro flutter.widgets.editableText.onAppPrivateCommand}
final AppPrivateCommandCallback? onAppPrivateCommand;
/// {@macro flutter.widgets.editableText.inputFormatters}
final List<TextInputFormatter>? inputFormatters;
/// If false the text field is "disabled": it ignores taps and its
/// [decoration] is rendered in grey.
///
/// If non-null this property overrides the [decoration]'s
/// [InputDecoration.enabled] property.
final bool? enabled;
/// Determines whether this widget ignores pointer events.
///
/// Defaults to null, and when null, does nothing.
final bool? ignorePointers;
/// {@macro flutter.widgets.editableText.cursorWidth}
final double cursorWidth;
/// {@macro flutter.widgets.editableText.cursorHeight}
final double? cursorHeight;
/// {@macro flutter.widgets.editableText.cursorRadius}
final Radius? cursorRadius;
/// {@macro flutter.widgets.editableText.cursorOpacityAnimates}
final bool? cursorOpacityAnimates;
/// The color of the cursor.
///
/// The cursor indicates the current location of text insertion point in
/// the field.
///
/// If this is null it will default to the ambient
/// [DefaultSelectionStyle.cursorColor]. If that is null, and the
/// [ThemeData.platform] is [TargetPlatform.iOS] or [TargetPlatform.macOS]
/// it will use [CupertinoThemeData.primaryColor]. Otherwise it will use
/// the value of [ColorScheme.primary] of [ThemeData.colorScheme].
final Color? cursorColor;
/// The color of the cursor when the [InputDecorator] is showing an error.
///
/// If this is null it will default to [TextStyle.color] of
/// [InputDecoration.errorStyle]. If that is null, it will use
/// [ColorScheme.error] of [ThemeData.colorScheme].
final Color? cursorErrorColor;
/// Controls how tall the selection highlight boxes are computed to be.
///
/// See [ui.BoxHeightStyle] for details on available styles.
final ui.BoxHeightStyle selectionHeightStyle;
/// Controls how wide the selection highlight boxes are computed to be.
///
/// See [ui.BoxWidthStyle] for details on available styles.
final ui.BoxWidthStyle selectionWidthStyle;
/// The appearance of the keyboard.
///
/// This setting is only honored on iOS devices.
///
/// If unset, defaults to [ThemeData.brightness].
final Brightness? keyboardAppearance;
/// {@macro flutter.widgets.editableText.scrollPadding}
final EdgeInsets scrollPadding;
/// {@macro flutter.widgets.editableText.enableInteractiveSelection}
final bool enableInteractiveSelection;
/// {@macro flutter.widgets.editableText.selectionControls}
final TextSelectionControls? selectionControls;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@macro flutter.widgets.editableText.selectionEnabled}
bool get selectionEnabled => enableInteractiveSelection;
/// {@template flutter.material.textfield.onTap}
/// Called for the first tap in a series of taps.
///
/// The text field builds a [GestureDetector] to handle input events like tap,
/// to trigger focus requests, to move the caret, adjust the selection, etc.
/// Handling some of those events by wrapping the text field with a competing
/// GestureDetector is problematic.
///
/// To unconditionally handle taps, without interfering with the text field's
/// internal gesture detector, provide this callback.
///
/// If the text field is created with [enabled] false, taps will not be
/// recognized.
///
/// To be notified when the text field gains or loses the focus, provide a
/// [focusNode] and add a listener to that.
///
/// To listen to arbitrary pointer events without competing with the
/// text field's internal gesture detector, use a [Listener].
/// {@endtemplate}
///
/// If [onTapAlwaysCalled] is enabled, this will also be called for consecutive
/// taps.
final GestureTapCallback? onTap;
/// Whether [onTap] should be called for every tap.
///
/// Defaults to false, so [onTap] is only called for each distinct tap. When
/// enabled, [onTap] is called for every tap including consecutive taps.
final bool onTapAlwaysCalled;
/// {@macro flutter.widgets.editableText.onTapOutside}
///
/// {@tool dartpad}
/// This example shows how to use a `TextFieldTapRegion` to wrap a set of
/// "spinner" buttons that increment and decrement a value in the [TextField]
/// without causing the text field to lose keyboard focus.
///
/// This example includes a generic `SpinnerField<T>` class that you can copy
/// into your own project and customize.
///
/// ** See code in examples/api/lib/widgets/tap_region/text_field_tap_region.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TapRegion] for how the region group is determined.
final TapRegionCallback? onTapOutside;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.error].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// If this property is null, [MaterialStateMouseCursor.textable] will be used.
///
/// The [mouseCursor] is the only property of [TextField] that controls the
/// appearance of the mouse pointer. All other properties related to "cursor"
/// stand for the text cursor, which is usually a blinking vertical line at
/// the editing position.
final MouseCursor? mouseCursor;
/// Callback that generates a custom [InputDecoration.counter] widget.
///
/// See [InputCounterWidgetBuilder] for an explanation of the passed in
/// arguments. The returned widget will be placed below the line in place of
/// the default widget built when [InputDecoration.counterText] is specified.
///
/// The returned widget will be wrapped in a [Semantics] widget for
/// accessibility, but it also needs to be accessible itself. For example,
/// if returning a Text widget, set the [Text.semanticsLabel] property.
///
/// {@tool snippet}
/// ```dart
/// Widget counter(
/// BuildContext context,
/// {
/// required int currentLength,
/// required int? maxLength,
/// required bool isFocused,
/// }
/// ) {
/// return Text(
/// '$currentLength of $maxLength characters',
/// semanticsLabel: 'character count',
/// );
/// }
/// ```
/// {@end-tool}
///
/// If buildCounter returns null, then no counter and no Semantics widget will
/// be created at all.
final InputCounterWidgetBuilder? buildCounter;
/// {@macro flutter.widgets.editableText.scrollPhysics}
final ScrollPhysics? scrollPhysics;
/// {@macro flutter.widgets.editableText.scrollController}
final ScrollController? scrollController;
/// {@macro flutter.widgets.editableText.autofillHints}
/// {@macro flutter.services.AutofillConfiguration.autofillHints}
final Iterable<String>? autofillHints;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// {@template flutter.material.textfield.restorationId}
/// Restoration ID to save and restore the state of the text field.
///
/// If non-null, the text field will persist and restore its current scroll
/// offset and - if no [controller] has been provided - the content of the
/// text field. If a [controller] has been provided, it is the responsibility
/// of the owner of that controller to persist and restore it, e.g. by using
/// a [RestorableTextEditingController].
///
/// The state of this widget is persisted in a [RestorationBucket] claimed
/// from the surrounding [RestorationScope] using the provided restoration ID.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
/// {@endtemplate}
final String? restorationId;
/// {@macro flutter.widgets.editableText.scribbleEnabled}
final bool scribbleEnabled;
/// {@macro flutter.services.TextInputConfiguration.enableIMEPersonalizedLearning}
final bool enableIMEPersonalizedLearning;
/// {@macro flutter.widgets.editableText.contentInsertionConfiguration}
final ContentInsertionConfiguration? contentInsertionConfiguration;
/// {@macro flutter.widgets.EditableText.contextMenuBuilder}
///
/// If not provided, will build a default menu based on the platform.
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar], which is built by default.
final EditableTextContextMenuBuilder? contextMenuBuilder;
/// Determine whether this text field can request the primary focus.
///
/// Defaults to true. If false, the text field will not request focus
/// when tapped, or when its context menu is displayed. If false it will not
/// be possible to move the focus to the text field with tab key.
final bool canRequestFocus;
/// {@macro flutter.widgets.undoHistory.controller}
final UndoHistoryController? undoController;
static Widget _defaultContextMenuBuilder(BuildContext context, EditableTextState editableTextState) {
return AdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
}
/// {@macro flutter.widgets.EditableText.spellCheckConfiguration}
///
/// If [SpellCheckConfiguration.misspelledTextStyle] is not specified in this
/// configuration, then [materialMisspelledTextStyle] is used by default.
final SpellCheckConfiguration? spellCheckConfiguration;
/// The [TextStyle] used to indicate misspelled words in the Material style.
///
/// See also:
/// * [SpellCheckConfiguration.misspelledTextStyle], the style configured to
/// mark misspelled words with.
/// * [CupertinoTextField.cupertinoMisspelledTextStyle], the style configured
/// to mark misspelled words with in the Cupertino style.
static const TextStyle materialMisspelledTextStyle =
TextStyle(
decoration: TextDecoration.underline,
decorationColor: Colors.red,
decorationStyle: TextDecorationStyle.wavy,
);
/// Default builder for [TextField]'s spell check suggestions toolbar.
///
/// On Apple platforms, builds an iOS-style toolbar. Everywhere else, builds
/// an Android-style toolbar.
///
/// See also:
/// * [spellCheckConfiguration], where this is typically specified for
/// [TextField].
/// * [SpellCheckConfiguration.spellCheckSuggestionsToolbarBuilder], the
/// parameter for which this is the default value for [TextField].
/// * [CupertinoTextField.defaultSpellCheckSuggestionsToolbarBuilder], which
/// is like this but specifies the default for [CupertinoTextField].
@visibleForTesting
static Widget defaultSpellCheckSuggestionsToolbarBuilder(
BuildContext context,
EditableTextState editableTextState,
) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return CupertinoSpellCheckSuggestionsToolbar.editableText(
editableTextState: editableTextState,
);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return SpellCheckSuggestionsToolbar.editableText(
editableTextState: editableTextState,
);
}
}
/// Returns a new [SpellCheckConfiguration] where the given configuration has
/// had any missing values replaced with their defaults for the Android
/// platform.
static SpellCheckConfiguration inferAndroidSpellCheckConfiguration(
SpellCheckConfiguration? configuration,
) {
if (configuration == null
|| configuration == const SpellCheckConfiguration.disabled()) {
return const SpellCheckConfiguration.disabled();
}
return configuration.copyWith(
misspelledTextStyle: configuration.misspelledTextStyle
?? TextField.materialMisspelledTextStyle,
spellCheckSuggestionsToolbarBuilder:
configuration.spellCheckSuggestionsToolbarBuilder
?? TextField.defaultSpellCheckSuggestionsToolbarBuilder,
);
}
@override
State<TextField> createState() => _TextFieldState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<TextEditingController>('controller', controller, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode, defaultValue: null));
properties.add(DiagnosticsProperty<UndoHistoryController>('undoController', undoController, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('enabled', enabled, defaultValue: null));
properties.add(DiagnosticsProperty<InputDecoration>('decoration', decoration, defaultValue: const InputDecoration()));
properties.add(DiagnosticsProperty<TextInputType>('keyboardType', keyboardType, defaultValue: TextInputType.text));
properties.add(DiagnosticsProperty<TextStyle>('style', style, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('autofocus', autofocus, defaultValue: false));
properties.add(DiagnosticsProperty<String>('obscuringCharacter', obscuringCharacter, defaultValue: '•'));
properties.add(DiagnosticsProperty<bool>('obscureText', obscureText, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('autocorrect', autocorrect, defaultValue: true));
properties.add(EnumProperty<SmartDashesType>('smartDashesType', smartDashesType, defaultValue: obscureText ? SmartDashesType.disabled : SmartDashesType.enabled));
properties.add(EnumProperty<SmartQuotesType>('smartQuotesType', smartQuotesType, defaultValue: obscureText ? SmartQuotesType.disabled : SmartQuotesType.enabled));
properties.add(DiagnosticsProperty<bool>('enableSuggestions', enableSuggestions, defaultValue: true));
properties.add(IntProperty('maxLines', maxLines, defaultValue: 1));
properties.add(IntProperty('minLines', minLines, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('expands', expands, defaultValue: false));
properties.add(IntProperty('maxLength', maxLength, defaultValue: null));
properties.add(EnumProperty<MaxLengthEnforcement>('maxLengthEnforcement', maxLengthEnforcement, defaultValue: null));
properties.add(EnumProperty<TextInputAction>('textInputAction', textInputAction, defaultValue: null));
properties.add(EnumProperty<TextCapitalization>('textCapitalization', textCapitalization, defaultValue: TextCapitalization.none));
properties.add(EnumProperty<TextAlign>('textAlign', textAlign, defaultValue: TextAlign.start));
properties.add(DiagnosticsProperty<TextAlignVertical>('textAlignVertical', textAlignVertical, defaultValue: null));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(DoubleProperty('cursorWidth', cursorWidth, defaultValue: 2.0));
properties.add(DoubleProperty('cursorHeight', cursorHeight, defaultValue: null));
properties.add(DiagnosticsProperty<Radius>('cursorRadius', cursorRadius, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('cursorOpacityAnimates', cursorOpacityAnimates, defaultValue: null));
properties.add(ColorProperty('cursorColor', cursorColor, defaultValue: null));
properties.add(ColorProperty('cursorErrorColor', cursorErrorColor, defaultValue: null));
properties.add(DiagnosticsProperty<Brightness>('keyboardAppearance', keyboardAppearance, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('scrollPadding', scrollPadding, defaultValue: const EdgeInsets.all(20.0)));
properties.add(FlagProperty('selectionEnabled', value: selectionEnabled, defaultValue: true, ifFalse: 'selection disabled'));
properties.add(DiagnosticsProperty<TextSelectionControls>('selectionControls', selectionControls, defaultValue: null));
properties.add(DiagnosticsProperty<ScrollController>('scrollController', scrollController, defaultValue: null));
properties.add(DiagnosticsProperty<ScrollPhysics>('scrollPhysics', scrollPhysics, defaultValue: null));
properties.add(DiagnosticsProperty<Clip>('clipBehavior', clipBehavior, defaultValue: Clip.hardEdge));
properties.add(DiagnosticsProperty<bool>('scribbleEnabled', scribbleEnabled, defaultValue: true));
properties.add(DiagnosticsProperty<bool>('enableIMEPersonalizedLearning', enableIMEPersonalizedLearning, defaultValue: true));
properties.add(DiagnosticsProperty<SpellCheckConfiguration>('spellCheckConfiguration', spellCheckConfiguration, defaultValue: null));
properties.add(DiagnosticsProperty<List<String>>('contentCommitMimeTypes', contentInsertionConfiguration?.allowedMimeTypes ?? const <String>[], defaultValue: contentInsertionConfiguration == null ? const <String>[] : kDefaultContentInsertionMimeTypes));
}
}
class _TextFieldState extends State<TextField> with RestorationMixin implements TextSelectionGestureDetectorBuilderDelegate, AutofillClient {
RestorableTextEditingController? _controller;
TextEditingController get _effectiveController => widget.controller ?? _controller!.value;
FocusNode? _focusNode;
FocusNode get _effectiveFocusNode => widget.focusNode ?? (_focusNode ??= FocusNode());
MaxLengthEnforcement get _effectiveMaxLengthEnforcement => widget.maxLengthEnforcement
?? LengthLimitingTextInputFormatter.getDefaultMaxLengthEnforcement(Theme.of(context).platform);
bool _isHovering = false;
bool get needsCounter => widget.maxLength != null
&& widget.decoration != null
&& widget.decoration!.counterText == null;
bool _showSelectionHandles = false;
late _TextFieldSelectionGestureDetectorBuilder _selectionGestureDetectorBuilder;
// API for TextSelectionGestureDetectorBuilderDelegate.
@override
late bool forcePressEnabled;
@override
final GlobalKey<EditableTextState> editableTextKey = GlobalKey<EditableTextState>();
@override
bool get selectionEnabled => widget.selectionEnabled && _isEnabled;
// End of API for TextSelectionGestureDetectorBuilderDelegate.
bool get _isEnabled => widget.enabled ?? widget.decoration?.enabled ?? true;
int get _currentLength => _effectiveController.value.text.characters.length;
bool get _hasIntrinsicError => widget.maxLength != null &&
widget.maxLength! > 0 &&
(widget.controller == null ?
!restorePending && _effectiveController.value.text.characters.length > widget.maxLength! :
_effectiveController.value.text.characters.length > widget.maxLength!);
bool get _hasError => widget.decoration?.errorText != null || widget.decoration?.error != null || _hasIntrinsicError;
Color get _errorColor => widget.cursorErrorColor ?? _getEffectiveDecoration().errorStyle?.color ?? Theme.of(context).colorScheme.error;
InputDecoration _getEffectiveDecoration() {
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final ThemeData themeData = Theme.of(context);
final InputDecoration effectiveDecoration = (widget.decoration ?? const InputDecoration())
.applyDefaults(themeData.inputDecorationTheme)
.copyWith(
enabled: _isEnabled,
hintMaxLines: widget.decoration?.hintMaxLines ?? widget.maxLines,
);
// No need to build anything if counter or counterText were given directly.
if (effectiveDecoration.counter != null || effectiveDecoration.counterText != null) {
return effectiveDecoration;
}
// If buildCounter was provided, use it to generate a counter widget.
Widget? counter;
final int currentLength = _currentLength;
if (effectiveDecoration.counter == null
&& effectiveDecoration.counterText == null
&& widget.buildCounter != null) {
final bool isFocused = _effectiveFocusNode.hasFocus;
final Widget? builtCounter = widget.buildCounter!(
context,
currentLength: currentLength,
maxLength: widget.maxLength,
isFocused: isFocused,
);
// If buildCounter returns null, don't add a counter widget to the field.
if (builtCounter != null) {
counter = Semantics(
container: true,
liveRegion: isFocused,
child: builtCounter,
);
}
return effectiveDecoration.copyWith(counter: counter);
}
if (widget.maxLength == null) {
return effectiveDecoration;
} // No counter widget
String counterText = '$currentLength';
String semanticCounterText = '';
// Handle a real maxLength (positive number)
if (widget.maxLength! > 0) {
// Show the maxLength in the counter
counterText += '/${widget.maxLength}';
final int remaining = (widget.maxLength! - currentLength).clamp(0, widget.maxLength!);
semanticCounterText = localizations.remainingTextFieldCharacterCount(remaining);
}
if (_hasIntrinsicError) {
return effectiveDecoration.copyWith(
errorText: effectiveDecoration.errorText ?? '',
counterStyle: effectiveDecoration.errorStyle
?? (themeData.useMaterial3 ? _m3CounterErrorStyle(context): _m2CounterErrorStyle(context)),
counterText: counterText,
semanticCounterText: semanticCounterText,
);
}
return effectiveDecoration.copyWith(
counterText: counterText,
semanticCounterText: semanticCounterText,
);
}
@override
void initState() {
super.initState();
_selectionGestureDetectorBuilder = _TextFieldSelectionGestureDetectorBuilder(state: this);
if (widget.controller == null) {
_createLocalController();
}
_effectiveFocusNode.canRequestFocus = widget.canRequestFocus && _isEnabled;
_effectiveFocusNode.addListener(_handleFocusChanged);
_initStatesController();
}
bool get _canRequestFocus {
final NavigationMode mode = MediaQuery.maybeNavigationModeOf(context) ?? NavigationMode.traditional;
return switch (mode) {
NavigationMode.traditional => widget.canRequestFocus && _isEnabled,
NavigationMode.directional => true,
};
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_effectiveFocusNode.canRequestFocus = _canRequestFocus;
}
@override
void didUpdateWidget(TextField oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.controller == null && oldWidget.controller != null) {
_createLocalController(oldWidget.controller!.value);
} else if (widget.controller != null && oldWidget.controller == null) {
unregisterFromRestoration(_controller!);
_controller!.dispose();
_controller = null;
}
if (widget.focusNode != oldWidget.focusNode) {
(oldWidget.focusNode ?? _focusNode)?.removeListener(_handleFocusChanged);
(widget.focusNode ?? _focusNode)?.addListener(_handleFocusChanged);
}
_effectiveFocusNode.canRequestFocus = _canRequestFocus;
if (_effectiveFocusNode.hasFocus && widget.readOnly != oldWidget.readOnly && _isEnabled) {
if (_effectiveController.selection.isCollapsed) {
_showSelectionHandles = !widget.readOnly;
}
}
if (widget.statesController == oldWidget.statesController) {
_statesController.update(MaterialState.disabled, !_isEnabled);
_statesController.update(MaterialState.hovered, _isHovering);
_statesController.update(MaterialState.focused, _effectiveFocusNode.hasFocus);
_statesController.update(MaterialState.error, _hasError);
} else {
oldWidget.statesController?.removeListener(_handleStatesControllerChange);
if (widget.statesController != null) {
_internalStatesController?.dispose();
_internalStatesController = null;
}
_initStatesController();
}
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
if (_controller != null) {
_registerController();
}
}
void _registerController() {
assert(_controller != null);
registerForRestoration(_controller!, 'controller');
}
void _createLocalController([TextEditingValue? value]) {
assert(_controller == null);
_controller = value == null
? RestorableTextEditingController()
: RestorableTextEditingController.fromValue(value);
if (!restorePending) {
_registerController();
}
}
@override
String? get restorationId => widget.restorationId;
@override
void dispose() {
_effectiveFocusNode.removeListener(_handleFocusChanged);
_focusNode?.dispose();
_controller?.dispose();
_statesController.removeListener(_handleStatesControllerChange);
_internalStatesController?.dispose();
super.dispose();
}
EditableTextState? get _editableText => editableTextKey.currentState;
void _requestKeyboard() {
_editableText?.requestKeyboard();
}
bool _shouldShowSelectionHandles(SelectionChangedCause? cause) {
// When the text field is activated by something that doesn't trigger the
// selection overlay, we shouldn't show the handles either.
if (!_selectionGestureDetectorBuilder.shouldShowSelectionToolbar) {
return false;
}
if (cause == SelectionChangedCause.keyboard) {
return false;
}
if (widget.readOnly && _effectiveController.selection.isCollapsed) {
return false;
}
if (!_isEnabled) {
return false;
}
if (cause == SelectionChangedCause.longPress || cause == SelectionChangedCause.scribble) {
return true;
}
if (_effectiveController.text.isNotEmpty) {
return true;
}
return false;
}
void _handleFocusChanged() {
setState(() {
// Rebuild the widget on focus change to show/hide the text selection
// highlight.
});
_statesController.update(MaterialState.focused, _effectiveFocusNode.hasFocus);
}
void _handleSelectionChanged(TextSelection selection, SelectionChangedCause? cause) {
final bool willShowSelectionHandles = _shouldShowSelectionHandles(cause);
if (willShowSelectionHandles != _showSelectionHandles) {
setState(() {
_showSelectionHandles = willShowSelectionHandles;
});
}
switch (Theme.of(context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
case TargetPlatform.fuchsia:
case TargetPlatform.android:
if (cause == SelectionChangedCause.longPress) {
_editableText?.bringIntoView(selection.extent);
}
}
switch (Theme.of(context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
case TargetPlatform.android:
break;
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
if (cause == SelectionChangedCause.drag) {
_editableText?.hideToolbar();
}
}
}
/// Toggle the toolbar when a selection handle is tapped.
void _handleSelectionHandleTapped() {
if (_effectiveController.selection.isCollapsed) {
_editableText!.toggleToolbar();
}
}
void _handleHover(bool hovering) {
if (hovering != _isHovering) {
setState(() {
_isHovering = hovering;
});
_statesController.update(MaterialState.hovered, _isHovering);
}
}
// Material states controller.
MaterialStatesController? _internalStatesController;
void _handleStatesControllerChange() {
// Force a rebuild to resolve MaterialStateProperty properties.
setState(() { });
}
MaterialStatesController get _statesController => widget.statesController ?? _internalStatesController!;
void _initStatesController() {
if (widget.statesController == null) {
_internalStatesController = MaterialStatesController();
}
_statesController.update(MaterialState.disabled, !_isEnabled);
_statesController.update(MaterialState.hovered, _isHovering);
_statesController.update(MaterialState.focused, _effectiveFocusNode.hasFocus);
_statesController.update(MaterialState.error, _hasError);
_statesController.addListener(_handleStatesControllerChange);
}
// AutofillClient implementation start.
@override
String get autofillId => _editableText!.autofillId;
@override
void autofill(TextEditingValue newEditingValue) => _editableText!.autofill(newEditingValue);
@override
TextInputConfiguration get textInputConfiguration {
final List<String>? autofillHints = widget.autofillHints?.toList(growable: false);
final AutofillConfiguration autofillConfiguration = autofillHints != null
? AutofillConfiguration(
uniqueIdentifier: autofillId,
autofillHints: autofillHints,
currentEditingValue: _effectiveController.value,
hintText: (widget.decoration ?? const InputDecoration()).hintText,
)
: AutofillConfiguration.disabled;
return _editableText!.textInputConfiguration.copyWith(autofillConfiguration: autofillConfiguration);
}
// AutofillClient implementation end.
TextStyle _getInputStyleForState(TextStyle style) {
final ThemeData theme = Theme.of(context);
final TextStyle stateStyle = MaterialStateProperty.resolveAs(theme.useMaterial3 ? _m3StateInputStyle(context)! : _m2StateInputStyle(context)!, _statesController.value);
final TextStyle providedStyle = MaterialStateProperty.resolveAs(style, _statesController.value);
return providedStyle.merge(stateStyle);
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMaterialLocalizations(context));
assert(debugCheckHasDirectionality(context));
assert(
!(widget.style != null && !widget.style!.inherit &&
(widget.style!.fontSize == null || widget.style!.textBaseline == null)),
'inherit false style must supply fontSize and textBaseline',
);
final ThemeData theme = Theme.of(context);
final DefaultSelectionStyle selectionStyle = DefaultSelectionStyle.of(context);
final TextStyle? providedStyle = MaterialStateProperty.resolveAs(widget.style, _statesController.value);
final TextStyle style = _getInputStyleForState(theme.useMaterial3 ? _m3InputStyle(context) : theme.textTheme.titleMedium!).merge(providedStyle);
final Brightness keyboardAppearance = widget.keyboardAppearance ?? theme.brightness;
final TextEditingController controller = _effectiveController;
final FocusNode focusNode = _effectiveFocusNode;
final List<TextInputFormatter> formatters = <TextInputFormatter>[
...?widget.inputFormatters,
if (widget.maxLength != null)
LengthLimitingTextInputFormatter(
widget.maxLength,
maxLengthEnforcement: _effectiveMaxLengthEnforcement,
),
];
// Set configuration as disabled if not otherwise specified. If specified,
// ensure that configuration uses the correct style for misspelled words for
// the current platform, unless a custom style is specified.
final SpellCheckConfiguration spellCheckConfiguration;
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
spellCheckConfiguration =
CupertinoTextField.inferIOSSpellCheckConfiguration(
widget.spellCheckConfiguration,
);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
spellCheckConfiguration = TextField.inferAndroidSpellCheckConfiguration(
widget.spellCheckConfiguration,
);
}
TextSelectionControls? textSelectionControls = widget.selectionControls;
final bool paintCursorAboveText;
bool? cursorOpacityAnimates = widget.cursorOpacityAnimates;
Offset? cursorOffset;
final Color cursorColor;
final Color selectionColor;
Color? autocorrectionTextRectColor;
Radius? cursorRadius = widget.cursorRadius;
VoidCallback? handleDidGainAccessibilityFocus;
VoidCallback? handleDidLoseAccessibilityFocus;
switch (theme.platform) {
case TargetPlatform.iOS:
final CupertinoThemeData cupertinoTheme = CupertinoTheme.of(context);
forcePressEnabled = true;
textSelectionControls ??= cupertinoTextSelectionHandleControls;
paintCursorAboveText = true;
cursorOpacityAnimates ??= true;
cursorColor = _hasError ? _errorColor : widget.cursorColor ?? selectionStyle.cursorColor ?? cupertinoTheme.primaryColor;
selectionColor = selectionStyle.selectionColor ?? cupertinoTheme.primaryColor.withOpacity(0.40);
cursorRadius ??= const Radius.circular(2.0);
cursorOffset = Offset(iOSHorizontalOffset / MediaQuery.devicePixelRatioOf(context), 0);
autocorrectionTextRectColor = selectionColor;
case TargetPlatform.macOS:
final CupertinoThemeData cupertinoTheme = CupertinoTheme.of(context);
forcePressEnabled = false;
textSelectionControls ??= cupertinoDesktopTextSelectionHandleControls;
paintCursorAboveText = true;
cursorOpacityAnimates ??= false;
cursorColor = _hasError ? _errorColor : widget.cursorColor ?? selectionStyle.cursorColor ?? cupertinoTheme.primaryColor;
selectionColor = selectionStyle.selectionColor ?? cupertinoTheme.primaryColor.withOpacity(0.40);
cursorRadius ??= const Radius.circular(2.0);
cursorOffset = Offset(iOSHorizontalOffset / MediaQuery.devicePixelRatioOf(context), 0);
handleDidGainAccessibilityFocus = () {
// Automatically activate the TextField when it receives accessibility focus.
if (!_effectiveFocusNode.hasFocus && _effectiveFocusNode.canRequestFocus) {
_effectiveFocusNode.requestFocus();
}
};
handleDidLoseAccessibilityFocus = () {
_effectiveFocusNode.unfocus();
};
case TargetPlatform.android:
case TargetPlatform.fuchsia:
forcePressEnabled = false;
textSelectionControls ??= materialTextSelectionHandleControls;
paintCursorAboveText = false;
cursorOpacityAnimates ??= false;
cursorColor = _hasError ? _errorColor : widget.cursorColor ?? selectionStyle.cursorColor ?? theme.colorScheme.primary;
selectionColor = selectionStyle.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
case TargetPlatform.linux:
forcePressEnabled = false;
textSelectionControls ??= desktopTextSelectionHandleControls;
paintCursorAboveText = false;
cursorOpacityAnimates ??= false;
cursorColor = _hasError ? _errorColor : widget.cursorColor ?? selectionStyle.cursorColor ?? theme.colorScheme.primary;
selectionColor = selectionStyle.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
handleDidGainAccessibilityFocus = () {
// Automatically activate the TextField when it receives accessibility focus.
if (!_effectiveFocusNode.hasFocus && _effectiveFocusNode.canRequestFocus) {
_effectiveFocusNode.requestFocus();
}
};
handleDidLoseAccessibilityFocus = () {
_effectiveFocusNode.unfocus();
};
case TargetPlatform.windows:
forcePressEnabled = false;
textSelectionControls ??= desktopTextSelectionHandleControls;
paintCursorAboveText = false;
cursorOpacityAnimates ??= false;
cursorColor = _hasError ? _errorColor : widget.cursorColor ?? selectionStyle.cursorColor ?? theme.colorScheme.primary;
selectionColor = selectionStyle.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
handleDidGainAccessibilityFocus = () {
// Automatically activate the TextField when it receives accessibility focus.
if (!_effectiveFocusNode.hasFocus && _effectiveFocusNode.canRequestFocus) {
_effectiveFocusNode.requestFocus();
}
};
handleDidLoseAccessibilityFocus = () {
_effectiveFocusNode.unfocus();
};
}
Widget child = RepaintBoundary(
child: UnmanagedRestorationScope(
bucket: bucket,
child: EditableText(
key: editableTextKey,
readOnly: widget.readOnly || !_isEnabled,
toolbarOptions: widget.toolbarOptions,
showCursor: widget.showCursor,
showSelectionHandles: _showSelectionHandles,
controller: controller,
focusNode: focusNode,
undoController: widget.undoController,
keyboardType: widget.keyboardType,
textInputAction: widget.textInputAction,
textCapitalization: widget.textCapitalization,
style: style,
strutStyle: widget.strutStyle,
textAlign: widget.textAlign,
textDirection: widget.textDirection,
autofocus: widget.autofocus,
obscuringCharacter: widget.obscuringCharacter,
obscureText: widget.obscureText,
autocorrect: widget.autocorrect,
smartDashesType: widget.smartDashesType,
smartQuotesType: widget.smartQuotesType,
enableSuggestions: widget.enableSuggestions,
maxLines: widget.maxLines,
minLines: widget.minLines,
expands: widget.expands,
// Only show the selection highlight when the text field is focused.
selectionColor: focusNode.hasFocus ? selectionColor : null,
selectionControls: widget.selectionEnabled ? textSelectionControls : null,
onChanged: widget.onChanged,
onSelectionChanged: _handleSelectionChanged,
onEditingComplete: widget.onEditingComplete,
onSubmitted: widget.onSubmitted,
onAppPrivateCommand: widget.onAppPrivateCommand,
onSelectionHandleTapped: _handleSelectionHandleTapped,
onTapOutside: widget.onTapOutside,
inputFormatters: formatters,
rendererIgnoresPointer: true,
mouseCursor: MouseCursor.defer, // TextField will handle the cursor
cursorWidth: widget.cursorWidth,
cursorHeight: widget.cursorHeight,
cursorRadius: cursorRadius,
cursorColor: cursorColor,
selectionHeightStyle: widget.selectionHeightStyle,
selectionWidthStyle: widget.selectionWidthStyle,
cursorOpacityAnimates: cursorOpacityAnimates,
cursorOffset: cursorOffset,
paintCursorAboveText: paintCursorAboveText,
backgroundCursorColor: CupertinoColors.inactiveGray,
scrollPadding: widget.scrollPadding,
keyboardAppearance: keyboardAppearance,
enableInteractiveSelection: widget.enableInteractiveSelection,
dragStartBehavior: widget.dragStartBehavior,
scrollController: widget.scrollController,
scrollPhysics: widget.scrollPhysics,
autofillClient: this,
autocorrectionTextRectColor: autocorrectionTextRectColor,
clipBehavior: widget.clipBehavior,
restorationId: 'editable',
scribbleEnabled: widget.scribbleEnabled,
enableIMEPersonalizedLearning: widget.enableIMEPersonalizedLearning,
contentInsertionConfiguration: widget.contentInsertionConfiguration,
contextMenuBuilder: widget.contextMenuBuilder,
spellCheckConfiguration: spellCheckConfiguration,
magnifierConfiguration: widget.magnifierConfiguration ?? TextMagnifier.adaptiveMagnifierConfiguration,
),
),
);
if (widget.decoration != null) {
child = AnimatedBuilder(
animation: Listenable.merge(<Listenable>[ focusNode, controller ]),
builder: (BuildContext context, Widget? child) {
return InputDecorator(
decoration: _getEffectiveDecoration(),
baseStyle: widget.style,
textAlign: widget.textAlign,
textAlignVertical: widget.textAlignVertical,
isHovering: _isHovering,
isFocused: focusNode.hasFocus,
isEmpty: controller.value.text.isEmpty,
expands: widget.expands,
child: child,
);
},
child: child,
);
}
final MouseCursor effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor>(
widget.mouseCursor ?? MaterialStateMouseCursor.textable,
_statesController.value,
);
final int? semanticsMaxValueLength;
if (_effectiveMaxLengthEnforcement != MaxLengthEnforcement.none &&
widget.maxLength != null &&
widget.maxLength! > 0) {
semanticsMaxValueLength = widget.maxLength;
} else {
semanticsMaxValueLength = null;
}
return MouseRegion(
cursor: effectiveMouseCursor,
onEnter: (PointerEnterEvent event) => _handleHover(true),
onExit: (PointerExitEvent event) => _handleHover(false),
child: TextFieldTapRegion(
child: IgnorePointer(
ignoring: widget.ignorePointers ?? !_isEnabled,
child: AnimatedBuilder(
animation: controller, // changes the _currentLength
builder: (BuildContext context, Widget? child) {
return Semantics(
enabled: _isEnabled,
maxValueLength: semanticsMaxValueLength,
currentValueLength: _currentLength,
onTap: widget.readOnly ? null : () {
if (!_effectiveController.selection.isValid) {
_effectiveController.selection = TextSelection.collapsed(offset: _effectiveController.text.length);
}
_requestKeyboard();
},
onDidGainAccessibilityFocus: handleDidGainAccessibilityFocus,
onDidLoseAccessibilityFocus: handleDidLoseAccessibilityFocus,
child: child,
);
},
child: _selectionGestureDetectorBuilder.buildGestureDetector(
behavior: HitTestBehavior.translucent,
child: child,
),
),
),
),
);
}
}
TextStyle? _m2StateInputStyle(BuildContext context) => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
final ThemeData theme = Theme.of(context);
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: theme.disabledColor);
}
return TextStyle(color: theme.textTheme.titleMedium?.color);
});
TextStyle _m2CounterErrorStyle(BuildContext context) =>
Theme.of(context).textTheme.bodySmall!.copyWith(color: Theme.of(context).colorScheme.error);
// BEGIN GENERATED TOKEN PROPERTIES - TextField
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
TextStyle? _m3StateInputStyle(BuildContext context) => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: Theme.of(context).textTheme.bodyLarge!.color?.withOpacity(0.38));
}
return TextStyle(color: Theme.of(context).textTheme.bodyLarge!.color);
});
TextStyle _m3InputStyle(BuildContext context) => Theme.of(context).textTheme.bodyLarge!;
TextStyle _m3CounterErrorStyle(BuildContext context) =>
Theme.of(context).textTheme.bodySmall!.copyWith(color: Theme.of(context).colorScheme.error);
// END GENERATED TOKEN PROPERTIES - TextField
| flutter/packages/flutter/lib/src/material/text_field.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/text_field.dart",
"repo_id": "flutter",
"token_count": 21589
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the visual properties of [Tooltip] widgets, a tooltip theme.
///
/// Each property of [TooltipThemeData] corresponds to a property of [Tooltip],
/// and describes the value to use when the [Tooltip] property is
/// not given an explicit non-null value.
///
/// Use this class to configure a [TooltipTheme] widget, or to set the
/// [ThemeData.tooltipTheme] for a [Theme] widget or [MaterialApp.theme].
///
/// To obtain the current ambient tooltip theme, use [TooltipTheme.of].
///
/// See also:
///
/// * [TooltipTheme], a widget which overrides the tooltip theme for a subtree.
/// * [ThemeData.tooltipTheme], which specifies a tooltip theme as part of
/// an overall theme.
/// * [MaterialApp.theme], which specifies a theme for the whole application.
@immutable
class TooltipThemeData with Diagnosticable {
/// Creates the set of properties used to configure [Tooltip]s.
const TooltipThemeData({
this.height,
this.padding,
this.margin,
this.verticalOffset,
this.preferBelow,
this.excludeFromSemantics,
this.decoration,
this.textStyle,
this.textAlign,
this.waitDuration,
this.showDuration,
this.exitDuration,
this.triggerMode,
this.enableFeedback,
});
/// The height of [Tooltip.child].
final double? height;
/// If provided, the amount of space by which to inset [Tooltip.child].
final EdgeInsetsGeometry? padding;
/// If provided, the amount of empty space to surround the [Tooltip].
final EdgeInsetsGeometry? margin;
/// The vertical gap between the widget and the displayed tooltip.
///
/// When [preferBelow] is set to true and tooltips have sufficient space to
/// display themselves, this property defines how much vertical space
/// tooltips will position themselves under their corresponding widgets.
/// Otherwise, tooltips will position themselves above their corresponding
/// widgets with the given offset.
final double? verticalOffset;
/// Whether the tooltip is displayed below its widget by default.
///
/// If there is insufficient space to display the tooltip in the preferred
/// direction, the tooltip will be displayed in the opposite direction.
///
/// Applying `false` for the entire app is recommended
/// to avoid having a finger or cursor hide a tooltip.
final bool? preferBelow;
/// Whether the [Tooltip.message] should be excluded from the semantics
/// tree.
///
/// By default, [Tooltip]s will add a [Semantics] label that is set to
/// [Tooltip.message]. Set this property to true if the app is going to
/// provide its own custom semantics label.
final bool? excludeFromSemantics;
/// The [Tooltip]'s shape and background color.
final Decoration? decoration;
/// The style to use for the message of [Tooltip]s.
final TextStyle? textStyle;
/// The [TextAlign] to use for the message of [Tooltip]s.
final TextAlign? textAlign;
/// The length of time that a pointer must hover over a tooltip's widget
/// before the tooltip will be shown.
final Duration? waitDuration;
/// The length of time that the tooltip will be shown once it has appeared.
final Duration? showDuration;
/// The length of time that a pointer must have stopped hovering over a
/// tooltip's widget before the tooltip will be hidden.
final Duration? exitDuration;
/// The [TooltipTriggerMode] that will show the tooltip.
final TooltipTriggerMode? triggerMode;
/// Whether the tooltip should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// This value is used if [Tooltip.enableFeedback] is null.
/// If this value is null, the default is true.
///
/// See also:
///
/// * [Feedback], for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
TooltipThemeData copyWith({
double? height,
EdgeInsetsGeometry? padding,
EdgeInsetsGeometry? margin,
double? verticalOffset,
bool? preferBelow,
bool? excludeFromSemantics,
Decoration? decoration,
TextStyle? textStyle,
TextAlign? textAlign,
Duration? waitDuration,
Duration? showDuration,
Duration? exitDuration,
TooltipTriggerMode? triggerMode,
bool? enableFeedback,
}) {
return TooltipThemeData(
height: height ?? this.height,
padding: padding ?? this.padding,
margin: margin ?? this.margin,
verticalOffset: verticalOffset ?? this.verticalOffset,
preferBelow: preferBelow ?? this.preferBelow,
excludeFromSemantics: excludeFromSemantics ?? this.excludeFromSemantics,
decoration: decoration ?? this.decoration,
textStyle: textStyle ?? this.textStyle,
textAlign: textAlign ?? this.textAlign,
waitDuration: waitDuration ?? this.waitDuration,
showDuration: showDuration ?? this.showDuration,
triggerMode: triggerMode ?? this.triggerMode,
enableFeedback: enableFeedback ?? this.enableFeedback,
);
}
/// Linearly interpolate between two tooltip themes.
///
/// If both arguments are null, then null is returned.
///
/// {@macro dart.ui.shadow.lerp}
static TooltipThemeData? lerp(TooltipThemeData? a, TooltipThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return TooltipThemeData(
height: lerpDouble(a?.height, b?.height, t),
padding: EdgeInsetsGeometry.lerp(a?.padding, b?.padding, t),
margin: EdgeInsetsGeometry.lerp(a?.margin, b?.margin, t),
verticalOffset: lerpDouble(a?.verticalOffset, b?.verticalOffset, t),
preferBelow: t < 0.5 ? a?.preferBelow: b?.preferBelow,
excludeFromSemantics: t < 0.5 ? a?.excludeFromSemantics : b?.excludeFromSemantics,
decoration: Decoration.lerp(a?.decoration, b?.decoration, t),
textStyle: TextStyle.lerp(a?.textStyle, b?.textStyle, t),
textAlign: t < 0.5 ? a?.textAlign: b?.textAlign,
);
}
@override
int get hashCode => Object.hash(
height,
padding,
margin,
verticalOffset,
preferBelow,
excludeFromSemantics,
decoration,
textStyle,
textAlign,
waitDuration,
showDuration,
exitDuration,
triggerMode,
enableFeedback,
);
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is TooltipThemeData
&& other.height == height
&& other.padding == padding
&& other.margin == margin
&& other.verticalOffset == verticalOffset
&& other.preferBelow == preferBelow
&& other.excludeFromSemantics == excludeFromSemantics
&& other.decoration == decoration
&& other.textStyle == textStyle
&& other.textAlign == textAlign
&& other.waitDuration == waitDuration
&& other.showDuration == showDuration
&& other.exitDuration == exitDuration
&& other.triggerMode == triggerMode
&& other.enableFeedback == enableFeedback;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('height', height, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('margin', margin, defaultValue: null));
properties.add(DoubleProperty('vertical offset', verticalOffset, defaultValue: null));
properties.add(FlagProperty('position', value: preferBelow, ifTrue: 'below', ifFalse: 'above', showName: true));
properties.add(FlagProperty('semantics', value: excludeFromSemantics, ifTrue: 'excluded', showName: true));
properties.add(DiagnosticsProperty<Decoration>('decoration', decoration, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('textStyle', textStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextAlign>('textAlign', textAlign, defaultValue: null));
properties.add(DiagnosticsProperty<Duration>('wait duration', waitDuration, defaultValue: null));
properties.add(DiagnosticsProperty<Duration>('show duration', showDuration, defaultValue: null));
properties.add(DiagnosticsProperty<Duration>('exit duration', exitDuration, defaultValue: null));
properties.add(DiagnosticsProperty<TooltipTriggerMode>('triggerMode', triggerMode, defaultValue: null));
properties.add(FlagProperty('enableFeedback', value: enableFeedback, ifTrue: 'true', showName: true));
}
}
/// Applies a tooltip theme to descendant [Tooltip] widgets.
///
/// A tooltip theme describes the values to use for [Tooltip] properties
/// that are not given an explicit non-null value.
///
/// Descendant widgets obtain the ambient tooltip theme, a [TooltipThemeData],
/// using [TooltipTheme.of].
///
/// {@tool snippet}
///
/// Here is an example of a tooltip theme that applies a blue foreground
/// with non-rounded corners.
///
/// ```dart
/// TooltipTheme(
/// data: TooltipThemeData(
/// decoration: BoxDecoration(
/// color: Colors.blue.withOpacity(0.9),
/// borderRadius: BorderRadius.zero,
/// ),
/// ),
/// child: Tooltip(
/// message: 'Example tooltip',
/// child: IconButton(
/// iconSize: 36.0,
/// icon: const Icon(Icons.touch_app),
/// onPressed: () {},
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [TooltipThemeData], which describes the actual configuration of a
/// tooltip theme.
/// * [TooltipVisibility], which can be used to visually disable descendant [Tooltip]s.
class TooltipTheme extends InheritedTheme {
/// Creates a tooltip theme that controls the configurations for
/// [Tooltip].
const TooltipTheme({
super.key,
required this.data,
required super.child,
});
/// The properties for descendant [Tooltip] widgets.
final TooltipThemeData data;
/// Returns the ambient tooltip theme.
///
/// The result comes from the closest [TooltipTheme] ancestor if any,
/// and otherwise from [Theme.of] and [ThemeData.tooltipTheme].
///
/// When a widget uses this method, it is automatically rebuilt if the
/// tooltip theme later changes, so that the changes can be applied.
///
/// Typical usage is as follows:
///
/// ```dart
/// TooltipThemeData theme = TooltipTheme.of(context);
/// ```
static TooltipThemeData of(BuildContext context) {
final TooltipTheme? tooltipTheme = context.dependOnInheritedWidgetOfExactType<TooltipTheme>();
return tooltipTheme?.data ?? Theme.of(context).tooltipTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return TooltipTheme(data: data, child: child);
}
@override
bool updateShouldNotify(TooltipTheme oldWidget) => data != oldWidget.data;
}
/// The method of interaction that will trigger a tooltip.
/// Used in [Tooltip.triggerMode] and [TooltipThemeData.triggerMode].
///
/// On desktop, a tooltip will be shown as soon as a pointer hovers over
/// the widget, regardless of the value of [Tooltip.triggerMode].
///
/// See also:
///
/// * [Tooltip.waitDuration], which defines the length of time that
/// a pointer must hover over a tooltip's widget before the tooltip
/// will be shown.
enum TooltipTriggerMode {
/// Tooltip will only be shown by calling `ensureTooltipVisible`.
manual,
/// Tooltip will be shown after a long press.
///
/// See also:
///
/// * [GestureDetector.onLongPress], the event that is used for trigger.
/// * [Feedback.forLongPress], the feedback method called when feedback is enabled.
longPress,
/// Tooltip will be shown after a single tap.
///
/// See also:
///
/// * [GestureDetector.onTap], the event that is used for trigger.
/// * [Feedback.forTap], the feedback method called when feedback is enabled.
tap,
}
| flutter/packages/flutter/lib/src/material/tooltip_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/tooltip_theme.dart",
"repo_id": "flutter",
"token_count": 3887
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'borders.dart';
/// A border that fits a circle within the available space.
///
/// Typically used with [ShapeDecoration] to draw a circle.
///
/// The [dimensions] assume that the border is being used in a square space.
/// When applied to a rectangular space, the border paints in the center of the
/// rectangle.
///
/// The [eccentricity] parameter describes how much a circle will deform to
/// fit the rectangle it is a border for. A value of zero implies no
/// deformation (a circle touching at least two sides of the rectangle), a
/// value of one implies full deformation (an oval touching all sides of the
/// rectangle).
///
/// See also:
///
/// * [OvalBorder], which draws a Circle touching all the edges of the box.
/// * [BorderSide], which is used to describe each side of the box.
/// * [Border], which, when used with [BoxDecoration], can also describe a circle.
class CircleBorder extends OutlinedBorder {
/// Create a circle border.
const CircleBorder({ super.side, this.eccentricity = 0.0 })
: assert(eccentricity >= 0.0, 'The eccentricity argument $eccentricity is not greater than or equal to zero.'),
assert(eccentricity <= 1.0, 'The eccentricity argument $eccentricity is not less than or equal to one.');
/// Defines the ratio (0.0-1.0) from which the border will deform
/// to fit a rectangle.
/// When 0.0, it draws a circle touching at least two sides of the rectangle.
/// When 1.0, it draws an oval touching all sides of the rectangle.
final double eccentricity;
@override
ShapeBorder scale(double t) => CircleBorder(side: side.scale(t), eccentricity: eccentricity);
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is CircleBorder) {
return CircleBorder(
side: BorderSide.lerp(a.side, side, t),
eccentricity: clampDouble(ui.lerpDouble(a.eccentricity, eccentricity, t)!, 0.0, 1.0),
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is CircleBorder) {
return CircleBorder(
side: BorderSide.lerp(side, b.side, t),
eccentricity: clampDouble(ui.lerpDouble(eccentricity, b.eccentricity, t)!, 0.0, 1.0),
);
}
return super.lerpTo(b, t);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
return Path()..addOval(_adjustRect(rect).deflate(side.strokeInset));
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return Path()..addOval(_adjustRect(rect));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
if (eccentricity == 0.0) {
canvas.drawCircle(rect.center, rect.shortestSide / 2.0, paint);
} else {
canvas.drawOval(_adjustRect(rect), paint);
}
}
@override
bool get preferPaintInterior => true;
@override
CircleBorder copyWith({ BorderSide? side, double? eccentricity }) {
return CircleBorder(side: side ?? this.side, eccentricity: eccentricity ?? this.eccentricity);
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
if (eccentricity == 0.0) {
canvas.drawCircle(rect.center, (rect.shortestSide + side.strokeOffset) / 2, side.toPaint());
} else {
final Rect borderRect = _adjustRect(rect);
canvas.drawOval(borderRect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
}
Rect _adjustRect(Rect rect) {
if (eccentricity == 0.0 || rect.width == rect.height) {
return Rect.fromCircle(center: rect.center, radius: rect.shortestSide / 2.0);
}
if (rect.width < rect.height) {
final double delta = (1.0 - eccentricity) * (rect.height - rect.width) / 2.0;
return Rect.fromLTRB(
rect.left,
rect.top + delta,
rect.right,
rect.bottom - delta,
);
} else {
final double delta = (1.0 - eccentricity) * (rect.width - rect.height) / 2.0;
return Rect.fromLTRB(
rect.left + delta,
rect.top,
rect.right - delta,
rect.bottom,
);
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is CircleBorder
&& other.side == side
&& other.eccentricity == eccentricity;
}
@override
int get hashCode => Object.hash(side, eccentricity);
@override
String toString() {
if (eccentricity != 0.0) {
return '${objectRuntimeType(this, 'CircleBorder')}($side, eccentricity: $eccentricity)';
}
return '${objectRuntimeType(this, 'CircleBorder')}($side)';
}
}
| flutter/packages/flutter/lib/src/painting/circle_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/circle_border.dart",
"repo_id": "flutter",
"token_count": 1840
} | 631 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui' as ui show Codec, FrameInfo, Image;
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
const String _flutterPaintingLibrary = 'package:flutter/painting.dart';
/// A [dart:ui.Image] object with its corresponding scale.
///
/// ImageInfo objects are used by [ImageStream] objects to represent the
/// actual data of the image once it has been obtained.
///
/// The disposing contract for [ImageInfo] (as well as for [ui.Image])
/// is different from traditional one, where
/// an object should dispose a member if the object created the member.
/// Instead, the disposal contract is as follows:
///
/// * [ImageInfo] disposes [image], even if it is received as a constructor argument.
/// * [ImageInfo] is expected to be disposed not by the object, that created it,
/// but by the object that owns reference to it.
/// * It is expected that only one object owns reference to [ImageInfo] object.
///
/// Safety tips:
///
/// * To share the [ImageInfo] or [ui.Image] between objects, use the [clone] method,
/// which will not clone the entire underlying image, but only reference to it and information about it.
/// * After passing a [ui.Image] or [ImageInfo] reference to another object,
/// release the reference.
@immutable
class ImageInfo {
/// Creates an [ImageInfo] object for the given [image] and [scale].
///
/// The [debugLabel] may be used to identify the source of this image.
///
/// See details for disposing contract in the class description.
ImageInfo({ required this.image, this.scale = 1.0, this.debugLabel }) {
if (kFlutterMemoryAllocationsEnabled) {
MemoryAllocations.instance.dispatchObjectCreated(
library: _flutterPaintingLibrary,
className: '$ImageInfo',
object: this,
);
}
}
/// Creates an [ImageInfo] with a cloned [image].
///
/// This method must be used in cases where a client holding an [ImageInfo]
/// needs to share the image info object with another client and will still
/// need to access the underlying image data at some later point, e.g. to
/// share it again with another client.
///
/// See details for disposing contract in the class description.
///
/// See also:
///
/// * [Image.clone], which describes how and why to clone images.
ImageInfo clone() {
return ImageInfo(
image: image.clone(),
scale: scale,
debugLabel: debugLabel,
);
}
/// Whether this [ImageInfo] is a [clone] of the `other`.
///
/// This method is a convenience wrapper for [Image.isCloneOf], and is useful
/// for clients that are trying to determine whether new layout or painting
/// logic is required when receiving a new image reference.
///
/// {@tool snippet}
///
/// The following sample shows how to appropriately check whether the
/// [ImageInfo] reference refers to new image data or not (in this case in a
/// setter).
///
/// ```dart
/// ImageInfo? get imageInfo => _imageInfo;
/// ImageInfo? _imageInfo;
/// set imageInfo (ImageInfo? value) {
/// // If the image reference is exactly the same, do nothing.
/// if (value == _imageInfo) {
/// return;
/// }
/// // If it is a clone of the current reference, we must dispose of it and
/// // can do so immediately. Since the underlying image has not changed,
/// // We don't have any additional work to do here.
/// if (value != null && _imageInfo != null && value.isCloneOf(_imageInfo!)) {
/// value.dispose();
/// return;
/// }
/// // It is a new image. Dispose of the old one and take a reference
/// // to the new one.
/// _imageInfo?.dispose();
/// _imageInfo = value;
/// // Perform work to determine size, paint the image, etc.
/// // ...
/// }
/// ```
/// {@end-tool}
bool isCloneOf(ImageInfo other) {
return other.image.isCloneOf(image)
&& scale == scale
&& other.debugLabel == debugLabel;
}
/// The raw image pixels.
///
/// This is the object to pass to the [Canvas.drawImage],
/// [Canvas.drawImageRect], or [Canvas.drawImageNine] methods when painting
/// the image.
final ui.Image image;
/// The size of raw image pixels in bytes.
int get sizeBytes => image.height * image.width * 4;
/// The linear scale factor for drawing this image at its intended size.
///
/// The scale factor applies to the width and the height.
///
/// {@template flutter.painting.imageInfo.scale}
/// For example, if this is 2.0, it means that there are four image pixels for
/// every one logical pixel, and the image's actual width and height (as given
/// by the [dart:ui.Image.width] and [dart:ui.Image.height] properties) are
/// double the height and width that should be used when painting the image
/// (e.g. in the arguments given to [Canvas.drawImage]).
/// {@endtemplate}
final double scale;
/// A string used for debugging purposes to identify the source of this image.
final String? debugLabel;
/// Disposes of this object.
///
/// Once this method has been called, the object should not be used anymore,
/// and no clones of it or the image it contains can be made.
void dispose() {
assert((image.debugGetOpenHandleStackTraces()?.length ?? 1) > 0);
if (kFlutterMemoryAllocationsEnabled) {
MemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
image.dispose();
}
@override
String toString() => '${debugLabel != null ? '$debugLabel ' : ''}$image @ ${debugFormatDouble(scale)}x';
@override
int get hashCode => Object.hash(image, scale, debugLabel);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ImageInfo
&& other.image == image
&& other.scale == scale
&& other.debugLabel == debugLabel;
}
}
/// Interface for receiving notifications about the loading of an image.
///
/// This class overrides [operator ==] and [hashCode] to compare the individual
/// callbacks in the listener, meaning that if you add an instance of this class
/// as a listener (e.g. via [ImageStream.addListener]), you can instantiate a
/// _different_ instance of this class when you remove the listener, and the
/// listener will be properly removed as long as all associated callbacks are
/// equal.
///
/// Used by [ImageStream] and [ImageStreamCompleter].
@immutable
class ImageStreamListener {
/// Creates a new [ImageStreamListener].
const ImageStreamListener(
this.onImage, {
this.onChunk,
this.onError,
});
/// Callback for getting notified that an image is available.
///
/// This callback may fire multiple times (e.g. if the [ImageStreamCompleter]
/// that drives the notifications fires multiple times). An example of such a
/// case would be an image with multiple frames within it (such as an animated
/// GIF).
///
/// For more information on how to interpret the parameters to the callback,
/// see the documentation on [ImageListener].
///
/// See also:
///
/// * [onError], which will be called instead of [onImage] if an error occurs
/// during loading.
final ImageListener onImage;
/// Callback for getting notified when a chunk of bytes has been received
/// during the loading of the image.
///
/// This callback may fire many times (e.g. when used with a [NetworkImage],
/// where the image bytes are loaded incrementally over the wire) or not at
/// all (e.g. when used with a [MemoryImage], where the image bytes are
/// already available in memory).
///
/// This callback may also continue to fire after the [onImage] callback has
/// fired (e.g. for multi-frame images that continue to load after the first
/// frame is available).
final ImageChunkListener? onChunk;
/// Callback for getting notified when an error occurs while loading an image.
///
/// If an error occurs during loading, [onError] will be called instead of
/// [onImage].
///
/// If [onError] is called and does not throw, then the error is considered to
/// be handled. An error handler can explicitly rethrow the exception reported
/// to it to safely indicate that it did not handle the exception.
///
/// If an image stream has no listeners that handled the error when the error
/// was first encountered, then the error is reported using
/// [FlutterError.reportError], with the [FlutterErrorDetails.silent] flag set
/// to true.
final ImageErrorListener? onError;
@override
int get hashCode => Object.hash(onImage, onChunk, onError);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ImageStreamListener
&& other.onImage == onImage
&& other.onChunk == onChunk
&& other.onError == onError;
}
}
/// Signature for callbacks reporting that an image is available.
///
/// Used in [ImageStreamListener].
///
/// The `image` argument contains information about the image to be rendered.
/// The implementer of [ImageStreamListener.onImage] is expected to call dispose
/// on the [ui.Image] it receives.
///
/// The `synchronousCall` argument is true if the listener is being invoked
/// during the call to `addListener`. This can be useful if, for example,
/// [ImageStream.addListener] is invoked during a frame, so that a new rendering
/// frame is requested if the call was asynchronous (after the current frame)
/// and no rendering frame is requested if the call was synchronous (within the
/// same stack frame as the call to [ImageStream.addListener]).
typedef ImageListener = void Function(ImageInfo image, bool synchronousCall);
/// Signature for listening to [ImageChunkEvent] events.
///
/// Used in [ImageStreamListener].
typedef ImageChunkListener = void Function(ImageChunkEvent event);
/// Signature for reporting errors when resolving images.
///
/// Used in [ImageStreamListener], as well as by [ImageCache.putIfAbsent] and
/// [precacheImage], to report errors.
typedef ImageErrorListener = void Function(Object exception, StackTrace? stackTrace);
/// An immutable notification of image bytes that have been incrementally loaded.
///
/// Chunk events represent progress notifications while an image is being
/// loaded (e.g. from disk or over the network).
///
/// See also:
///
/// * [ImageChunkListener], the means by which callers get notified of
/// these events.
@immutable
class ImageChunkEvent with Diagnosticable {
/// Creates a new chunk event.
const ImageChunkEvent({
required this.cumulativeBytesLoaded,
required this.expectedTotalBytes,
}) : assert(cumulativeBytesLoaded >= 0),
assert(expectedTotalBytes == null || expectedTotalBytes >= 0);
/// The number of bytes that have been received across the wire thus far.
final int cumulativeBytesLoaded;
/// The expected number of bytes that need to be received to finish loading
/// the image.
///
/// This value is not necessarily equal to the expected _size_ of the image
/// in bytes, as the bytes required to load the image may be compressed.
///
/// This value will be null if the number is not known in advance.
///
/// When this value is null, the chunk event may still be useful as an
/// indication that data is loading (and how much), but it cannot represent a
/// loading completion percentage.
final int? expectedTotalBytes;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('cumulativeBytesLoaded', cumulativeBytesLoaded));
properties.add(IntProperty('expectedTotalBytes', expectedTotalBytes));
}
}
/// A handle to an image resource.
///
/// ImageStream represents a handle to a [dart:ui.Image] object and its scale
/// (together represented by an [ImageInfo] object). The underlying image object
/// might change over time, either because the image is animating or because the
/// underlying image resource was mutated.
///
/// ImageStream objects can also represent an image that hasn't finished
/// loading.
///
/// ImageStream objects are backed by [ImageStreamCompleter] objects.
///
/// The [ImageCache] will consider an image to be live until the listener count
/// drops to zero after adding at least one listener. The
/// [ImageStreamCompleter.addOnLastListenerRemovedCallback] method is used for
/// tracking this information.
///
/// See also:
///
/// * [ImageProvider], which has an example that includes the use of an
/// [ImageStream] in a [Widget].
class ImageStream with Diagnosticable {
/// Create an initially unbound image stream.
///
/// Once an [ImageStreamCompleter] is available, call [setCompleter].
ImageStream();
/// The completer that has been assigned to this image stream.
///
/// Generally there is no need to deal with the completer directly.
ImageStreamCompleter? get completer => _completer;
ImageStreamCompleter? _completer;
List<ImageStreamListener>? _listeners;
/// Assigns a particular [ImageStreamCompleter] to this [ImageStream].
///
/// This is usually done automatically by the [ImageProvider] that created the
/// [ImageStream].
///
/// This method can only be called once per stream. To have an [ImageStream]
/// represent multiple images over time, assign it a completer that
/// completes several images in succession.
void setCompleter(ImageStreamCompleter value) {
assert(_completer == null);
_completer = value;
if (_listeners != null) {
final List<ImageStreamListener> initialListeners = _listeners!;
_listeners = null;
_completer!._addingInitialListeners = true;
initialListeners.forEach(_completer!.addListener);
_completer!._addingInitialListeners = false;
}
}
/// Adds a listener callback that is called whenever a new concrete [ImageInfo]
/// object is available. If a concrete image is already available, this object
/// will call the listener synchronously.
///
/// If the assigned [completer] completes multiple images over its lifetime,
/// this listener will fire multiple times.
///
/// {@template flutter.painting.imageStream.addListener}
/// The listener will be passed a flag indicating whether a synchronous call
/// occurred. If the listener is added within a render object paint function,
/// then use this flag to avoid calling [RenderObject.markNeedsPaint] during
/// a paint.
///
/// If a duplicate `listener` is registered N times, then it will be called N
/// times when the image stream completes (whether because a new image is
/// available or because an error occurs). Likewise, to remove all instances
/// of the listener, [removeListener] would need to called N times as well.
///
/// When a `listener` receives an [ImageInfo] object, the `listener` is
/// responsible for disposing of the [ImageInfo.image].
/// {@endtemplate}
void addListener(ImageStreamListener listener) {
if (_completer != null) {
return _completer!.addListener(listener);
}
_listeners ??= <ImageStreamListener>[];
_listeners!.add(listener);
}
/// Stops listening for events from this stream's [ImageStreamCompleter].
///
/// If [listener] has been added multiple times, this removes the _first_
/// instance of the listener.
void removeListener(ImageStreamListener listener) {
if (_completer != null) {
return _completer!.removeListener(listener);
}
assert(_listeners != null);
for (int i = 0; i < _listeners!.length; i += 1) {
if (_listeners![i] == listener) {
_listeners!.removeAt(i);
break;
}
}
}
/// Returns an object which can be used with `==` to determine if this
/// [ImageStream] shares the same listeners list as another [ImageStream].
///
/// This can be used to avoid un-registering and re-registering listeners
/// after calling [ImageProvider.resolve] on a new, but possibly equivalent,
/// [ImageProvider].
///
/// The key may change once in the lifetime of the object. When it changes, it
/// will go from being different than other [ImageStream]'s keys to
/// potentially being the same as others'. No notification is sent when this
/// happens.
Object get key => _completer ?? this;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ObjectFlagProperty<ImageStreamCompleter>(
'completer',
_completer,
ifPresent: _completer?.toStringShort(),
ifNull: 'unresolved',
));
properties.add(ObjectFlagProperty<List<ImageStreamListener>>(
'listeners',
_listeners,
ifPresent: '${_listeners?.length} listener${_listeners?.length == 1 ? "" : "s" }',
ifNull: 'no listeners',
level: _completer != null ? DiagnosticLevel.hidden : DiagnosticLevel.info,
));
_completer?.debugFillProperties(properties);
}
}
/// An opaque handle that keeps an [ImageStreamCompleter] alive even if it has
/// lost its last listener.
///
/// To create a handle, use [ImageStreamCompleter.keepAlive].
///
/// Such handles are useful when an image cache needs to keep a completer alive
/// but does not actually have a listener subscribed, or when a widget that
/// displays an image needs to temporarily unsubscribe from the completer but
/// may re-subscribe in the future, for example when the [TickerMode] changes.
class ImageStreamCompleterHandle {
ImageStreamCompleterHandle._(ImageStreamCompleter this._completer) {
_completer!._keepAliveHandles += 1;
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterPaintingLibrary,
className: '$ImageStreamCompleterHandle',
object: this,
);
}
}
ImageStreamCompleter? _completer;
/// Call this method to signal the [ImageStreamCompleter] that it can now be
/// disposed when its last listener drops.
///
/// This method must only be called once per object.
void dispose() {
assert(_completer != null);
assert(_completer!._keepAliveHandles > 0);
assert(!_completer!._disposed);
_completer!._keepAliveHandles -= 1;
_completer!._maybeDispose();
_completer = null;
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
}
}
/// Base class for those that manage the loading of [dart:ui.Image] objects for
/// [ImageStream]s.
///
/// [ImageStreamListener] objects are rarely constructed directly. Generally, an
/// [ImageProvider] subclass will return an [ImageStream] and automatically
/// configure it with the right [ImageStreamCompleter] when possible.
abstract class ImageStreamCompleter with Diagnosticable {
final List<ImageStreamListener> _listeners = <ImageStreamListener>[];
final List<ImageErrorListener> _ephemeralErrorListeners = <ImageErrorListener>[];
ImageInfo? _currentImage;
FlutterErrorDetails? _currentError;
/// A string identifying the source of the underlying image.
String? debugLabel;
/// Whether any listeners are currently registered.
///
/// Clients should not depend on this value for their behavior, because having
/// one listener's logic change when another listener happens to start or stop
/// listening will lead to extremely hard-to-track bugs. Subclasses might use
/// this information to determine whether to do any work when there are no
/// listeners, however; for example, [MultiFrameImageStreamCompleter] uses it
/// to determine when to iterate through frames of an animated image.
///
/// Typically this is used by overriding [addListener], checking if
/// [hasListeners] is false before calling `super.addListener()`, and if so,
/// starting whatever work is needed to determine when to notify listeners;
/// and similarly, by overriding [removeListener], checking if [hasListeners]
/// is false after calling `super.removeListener()`, and if so, stopping that
/// same work.
///
/// The ephemeral error listeners (added through [addEphemeralErrorListener])
/// will not be taken into consideration in this property.
@protected
@visibleForTesting
bool get hasListeners => _listeners.isNotEmpty;
/// We must avoid disposing a completer if it has never had a listener, even
/// if all [keepAlive] handles get disposed.
bool _hadAtLeastOneListener = false;
/// Whether the future listeners added to this completer are initial listeners.
///
/// This can be set to true when an [ImageStream] adds its initial listeners to
/// this completer. This ultimately controls the synchronousCall parameter for
/// the listener callbacks. When adding cached listeners to a completer,
/// [_addingInitialListeners] can be set to false to indicate to the listeners
/// that they are being called asynchronously.
bool _addingInitialListeners = false;
/// Adds a listener callback that is called whenever a new concrete [ImageInfo]
/// object is available or an error is reported. If a concrete image is
/// already available, or if an error has been already reported, this object
/// will notify the listener synchronously.
///
/// If the [ImageStreamCompleter] completes multiple images over its lifetime,
/// this listener's [ImageStreamListener.onImage] will fire multiple times.
///
/// {@macro flutter.painting.imageStream.addListener}
///
/// See also:
///
/// * [addEphemeralErrorListener], which adds an error listener that is
/// automatically removed after first image load or error.
void addListener(ImageStreamListener listener) {
_checkDisposed();
_hadAtLeastOneListener = true;
_listeners.add(listener);
if (_currentImage != null) {
try {
listener.onImage(_currentImage!.clone(), !_addingInitialListeners);
} catch (exception, stack) {
reportError(
context: ErrorDescription('by a synchronously-called image listener'),
exception: exception,
stack: stack,
);
}
}
if (_currentError != null && listener.onError != null) {
try {
listener.onError!(_currentError!.exception, _currentError!.stack);
} catch (newException, newStack) {
if (newException != _currentError!.exception) {
FlutterError.reportError(
FlutterErrorDetails(
exception: newException,
library: 'image resource service',
context: ErrorDescription('by a synchronously-called image error listener'),
stack: newStack,
),
);
}
}
}
}
/// Adds an error listener callback that is called when the first error is reported.
///
/// The callback will be removed automatically after the first successful
/// image load or the first error - that is why it is called "ephemeral".
///
/// If a concrete image is already available, the listener will be discarded
/// synchronously. If an error has been already reported, the listener
/// will be notified synchronously.
///
/// The presence of a listener will affect neither the lifecycle of this object
/// nor what [hasListeners] reports.
///
/// It is different from [addListener] in a few points: Firstly, this one only
/// listens to errors, while [addListener] listens to all kinds of events.
/// Secondly, this listener will be automatically removed according to the
/// rules mentioned above, while [addListener] will need manual removal.
/// Thirdly, this listener will not affect how this object is disposed, while
/// any non-removed listener added via [addListener] will forbid this object
/// from disposal.
///
/// When you want to know full information and full control, use [addListener].
/// When you only want to get notified for an error ephemerally, use this function.
///
/// See also:
///
/// * [addListener], which adds a full-featured listener and needs manual
/// removal.
void addEphemeralErrorListener(ImageErrorListener listener) {
_checkDisposed();
if (_currentError != null) {
// immediately fire the listener, and no need to add to _ephemeralErrorListeners
try {
listener(_currentError!.exception, _currentError!.stack);
} catch (newException, newStack) {
if (newException != _currentError!.exception) {
FlutterError.reportError(
FlutterErrorDetails(
exception: newException,
library: 'image resource service',
context: ErrorDescription('by a synchronously-called image error listener'),
stack: newStack,
),
);
}
}
} else if (_currentImage == null) {
// add to _ephemeralErrorListeners to wait for the error,
// only if no image has been loaded
_ephemeralErrorListeners.add(listener);
}
}
int _keepAliveHandles = 0;
/// Creates an [ImageStreamCompleterHandle] that will prevent this stream from
/// being disposed at least until the handle is disposed.
///
/// Such handles are useful when an image cache needs to keep a completer
/// alive but does not itself have a listener subscribed, or when a widget
/// that displays an image needs to temporarily unsubscribe from the completer
/// but may re-subscribe in the future, for example when the [TickerMode]
/// changes.
ImageStreamCompleterHandle keepAlive() {
_checkDisposed();
return ImageStreamCompleterHandle._(this);
}
/// Stops the specified [listener] from receiving image stream events.
///
/// If [listener] has been added multiple times, this removes the _first_
/// instance of the listener.
///
/// Once all listeners have been removed and all [keepAlive] handles have been
/// disposed, this image stream is no longer usable.
void removeListener(ImageStreamListener listener) {
_checkDisposed();
for (int i = 0; i < _listeners.length; i += 1) {
if (_listeners[i] == listener) {
_listeners.removeAt(i);
break;
}
}
if (_listeners.isEmpty) {
final List<VoidCallback> callbacks = _onLastListenerRemovedCallbacks.toList();
for (final VoidCallback callback in callbacks) {
callback();
}
_onLastListenerRemovedCallbacks.clear();
_maybeDispose();
}
}
bool _disposed = false;
@mustCallSuper
void _maybeDispose() {
if (!_hadAtLeastOneListener || _disposed || _listeners.isNotEmpty || _keepAliveHandles != 0) {
return;
}
_ephemeralErrorListeners.clear();
_currentImage?.dispose();
_currentImage = null;
_disposed = true;
}
void _checkDisposed() {
if (_disposed) {
throw StateError(
'Stream has been disposed.\n'
'An ImageStream is considered disposed once at least one listener has '
'been added and subsequently all listeners have been removed and no '
'handles are outstanding from the keepAlive method.\n'
'To resolve this error, maintain at least one listener on the stream, '
'or create an ImageStreamCompleterHandle from the keepAlive '
'method, or create a new stream for the image.',
);
}
}
final List<VoidCallback> _onLastListenerRemovedCallbacks = <VoidCallback>[];
/// Adds a callback to call when [removeListener] results in an empty
/// list of listeners and there are no [keepAlive] handles outstanding.
///
/// This callback will never fire if [removeListener] is never called.
void addOnLastListenerRemovedCallback(VoidCallback callback) {
_checkDisposed();
_onLastListenerRemovedCallbacks.add(callback);
}
/// Removes a callback previously supplied to
/// [addOnLastListenerRemovedCallback].
void removeOnLastListenerRemovedCallback(VoidCallback callback) {
_checkDisposed();
_onLastListenerRemovedCallbacks.remove(callback);
}
/// Calls all the registered listeners to notify them of a new image.
@protected
@pragma('vm:notify-debugger-on-exception')
void setImage(ImageInfo image) {
_checkDisposed();
_currentImage?.dispose();
_currentImage = image;
_ephemeralErrorListeners.clear();
if (_listeners.isEmpty) {
return;
}
// Make a copy to allow for concurrent modification.
final List<ImageStreamListener> localListeners =
List<ImageStreamListener>.of(_listeners);
for (final ImageStreamListener listener in localListeners) {
try {
listener.onImage(image.clone(), false);
} catch (exception, stack) {
reportError(
context: ErrorDescription('by an image listener'),
exception: exception,
stack: stack,
);
}
}
}
/// Calls all the registered error listeners to notify them of an error that
/// occurred while resolving the image.
///
/// If no error listeners (listeners with an [ImageStreamListener.onError]
/// specified) are attached, or if the handlers all rethrow the exception
/// verbatim (with `throw exception`), a [FlutterError] will be reported using
/// [FlutterError.reportError].
///
/// The `context` should be a string describing where the error was caught, in
/// a form that will make sense in English when following the word "thrown",
/// as in "thrown while obtaining the image from the network" (for the context
/// "while obtaining the image from the network").
///
/// The `exception` is the error being reported; the `stack` is the
/// [StackTrace] associated with the exception.
///
/// The `informationCollector` is a callback (of type [InformationCollector])
/// that is called when the exception is used by [FlutterError.reportError].
/// It is used to obtain further details to include in the logs, which may be
/// expensive to collect, and thus should only be collected if the error is to
/// be logged in the first place.
///
/// The `silent` argument causes the exception to not be reported to the logs
/// in release builds, if passed to [FlutterError.reportError]. (It is still
/// sent to error handlers.) It should be set to true if the error is one that
/// is expected to be encountered in release builds, for example network
/// errors. That way, logs on end-user devices will not have spurious
/// messages, but errors during development will still be reported.
///
/// See [FlutterErrorDetails] for further details on these values.
@pragma('vm:notify-debugger-on-exception')
void reportError({
DiagnosticsNode? context,
required Object exception,
StackTrace? stack,
InformationCollector? informationCollector,
bool silent = false,
}) {
_currentError = FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'image resource service',
context: context,
informationCollector: informationCollector,
silent: silent,
);
// Make a copy to allow for concurrent modification.
final List<ImageErrorListener> localErrorListeners = <ImageErrorListener>[
..._listeners
.map<ImageErrorListener?>((ImageStreamListener listener) => listener.onError)
.whereType<ImageErrorListener>(),
..._ephemeralErrorListeners,
];
_ephemeralErrorListeners.clear();
bool handled = false;
for (final ImageErrorListener errorListener in localErrorListeners) {
try {
errorListener(exception, stack);
handled = true;
} catch (newException, newStack) {
if (newException != exception) {
FlutterError.reportError(
FlutterErrorDetails(
context: ErrorDescription('when reporting an error to an image listener'),
library: 'image resource service',
exception: newException,
stack: newStack,
),
);
}
}
}
if (!handled) {
FlutterError.reportError(_currentError!);
}
}
/// Calls all the registered [ImageChunkListener]s (listeners with an
/// [ImageStreamListener.onChunk] specified) to notify them of a new
/// [ImageChunkEvent].
@protected
void reportImageChunkEvent(ImageChunkEvent event) {
_checkDisposed();
if (hasListeners) {
// Make a copy to allow for concurrent modification.
final List<ImageChunkListener> localListeners = _listeners
.map<ImageChunkListener?>((ImageStreamListener listener) => listener.onChunk)
.whereType<ImageChunkListener>()
.toList();
for (final ImageChunkListener listener in localListeners) {
listener(event);
}
}
}
/// Accumulates a list of strings describing the object's state. Subclasses
/// should override this to have their information included in [toString].
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<ImageInfo>('current', _currentImage, ifNull: 'unresolved', showName: false));
description.add(ObjectFlagProperty<List<ImageStreamListener>>(
'listeners',
_listeners,
ifPresent: '${_listeners.length} listener${_listeners.length == 1 ? "" : "s" }',
));
description.add(ObjectFlagProperty<List<ImageErrorListener>>(
'ephemeralErrorListeners',
_ephemeralErrorListeners,
ifPresent: '${_ephemeralErrorListeners.length} ephemeralErrorListener${_ephemeralErrorListeners.length == 1 ? "" : "s" }',
));
description.add(FlagProperty('disposed', value: _disposed, ifTrue: '<disposed>'));
}
}
/// Manages the loading of [dart:ui.Image] objects for static [ImageStream]s (those
/// with only one frame).
class OneFrameImageStreamCompleter extends ImageStreamCompleter {
/// Creates a manager for one-frame [ImageStream]s.
///
/// The image resource awaits the given [Future]. When the future resolves,
/// it notifies the [ImageListener]s that have been registered with
/// [addListener].
///
/// The [InformationCollector], if provided, is invoked if the given [Future]
/// resolves with an error, and can be used to supplement the reported error
/// message (for example, giving the image's URL).
///
/// Errors are reported using [FlutterError.reportError] with the `silent`
/// argument on [FlutterErrorDetails] set to true, meaning that by default the
/// message is only dumped to the console in debug mode (see [
/// FlutterErrorDetails]).
OneFrameImageStreamCompleter(Future<ImageInfo> image, { InformationCollector? informationCollector }) {
image.then<void>(setImage, onError: (Object error, StackTrace stack) {
reportError(
context: ErrorDescription('resolving a single-frame image stream'),
exception: error,
stack: stack,
informationCollector: informationCollector,
silent: true,
);
});
}
}
/// Manages the decoding and scheduling of image frames.
///
/// New frames will only be emitted while there are registered listeners to the
/// stream (registered with [addListener]).
///
/// This class deals with 2 types of frames:
///
/// * image frames - image frames of an animated image.
/// * app frames - frames that the flutter engine is drawing to the screen to
/// show the app GUI.
///
/// For single frame images the stream will only complete once.
///
/// For animated images, this class eagerly decodes the next image frame,
/// and notifies the listeners that a new frame is ready on the first app frame
/// that is scheduled after the image frame duration has passed.
///
/// Scheduling new timers only from scheduled app frames, makes sure we pause
/// the animation when the app is not visible (as new app frames will not be
/// scheduled).
///
/// See the following timeline example:
///
/// | Time | Event | Comment |
/// |------|--------------------------------------------|---------------------------|
/// | t1 | App frame scheduled (image frame A posted) | |
/// | t2 | App frame scheduled | |
/// | t3 | App frame scheduled | |
/// | t4 | Image frame B decoded | |
/// | t5 | App frame scheduled | t5 - t1 < frameB_duration |
/// | t6 | App frame scheduled (image frame B posted) | t6 - t1 > frameB_duration |
///
class MultiFrameImageStreamCompleter extends ImageStreamCompleter {
/// Creates a image stream completer.
///
/// Immediately starts decoding the first image frame when the codec is ready.
///
/// The `codec` parameter is a future for an initialized [ui.Codec] that will
/// be used to decode the image.
///
/// The `scale` parameter is the linear scale factor for drawing this frames
/// of this image at their intended size.
///
/// The `tag` parameter is passed on to created [ImageInfo] objects to
/// help identify the source of the image.
///
/// The `chunkEvents` parameter is an optional stream of notifications about
/// the loading progress of the image. If this stream is provided, the events
/// produced by the stream will be delivered to registered [ImageChunkListener]s
/// (see [addListener]).
MultiFrameImageStreamCompleter({
required Future<ui.Codec> codec,
required double scale,
String? debugLabel,
Stream<ImageChunkEvent>? chunkEvents,
InformationCollector? informationCollector,
}) : _informationCollector = informationCollector,
_scale = scale {
this.debugLabel = debugLabel;
codec.then<void>(_handleCodecReady, onError: (Object error, StackTrace stack) {
reportError(
context: ErrorDescription('resolving an image codec'),
exception: error,
stack: stack,
informationCollector: informationCollector,
silent: true,
);
});
if (chunkEvents != null) {
_chunkSubscription = chunkEvents.listen(reportImageChunkEvent,
onError: (Object error, StackTrace stack) {
reportError(
context: ErrorDescription('loading an image'),
exception: error,
stack: stack,
informationCollector: informationCollector,
silent: true,
);
},
);
}
}
StreamSubscription<ImageChunkEvent>? _chunkSubscription;
ui.Codec? _codec;
final double _scale;
final InformationCollector? _informationCollector;
ui.FrameInfo? _nextFrame;
// When the current was first shown.
late Duration _shownTimestamp;
// The requested duration for the current frame;
Duration? _frameDuration;
// How many frames have been emitted so far.
int _framesEmitted = 0;
Timer? _timer;
// Used to guard against registering multiple _handleAppFrame callbacks for the same frame.
bool _frameCallbackScheduled = false;
void _handleCodecReady(ui.Codec codec) {
_codec = codec;
assert(_codec != null);
if (hasListeners) {
_decodeNextFrameAndSchedule();
}
}
void _handleAppFrame(Duration timestamp) {
_frameCallbackScheduled = false;
if (!hasListeners) {
return;
}
assert(_nextFrame != null);
if (_isFirstFrame() || _hasFrameDurationPassed(timestamp)) {
_emitFrame(ImageInfo(
image: _nextFrame!.image.clone(),
scale: _scale,
debugLabel: debugLabel,
));
_shownTimestamp = timestamp;
_frameDuration = _nextFrame!.duration;
_nextFrame!.image.dispose();
_nextFrame = null;
final int completedCycles = _framesEmitted ~/ _codec!.frameCount;
if (_codec!.repetitionCount == -1 || completedCycles <= _codec!.repetitionCount) {
_decodeNextFrameAndSchedule();
}
return;
}
final Duration delay = _frameDuration! - (timestamp - _shownTimestamp);
_timer = Timer(delay * timeDilation, () {
_scheduleAppFrame();
});
}
bool _isFirstFrame() {
return _frameDuration == null;
}
bool _hasFrameDurationPassed(Duration timestamp) {
return timestamp - _shownTimestamp >= _frameDuration!;
}
Future<void> _decodeNextFrameAndSchedule() async {
// This will be null if we gave it away. If not, it's still ours and it
// must be disposed of.
_nextFrame?.image.dispose();
_nextFrame = null;
try {
_nextFrame = await _codec!.getNextFrame();
} catch (exception, stack) {
reportError(
context: ErrorDescription('resolving an image frame'),
exception: exception,
stack: stack,
informationCollector: _informationCollector,
silent: true,
);
return;
}
if (_codec!.frameCount == 1) {
// ImageStreamCompleter listeners removed while waiting for next frame to
// be decoded.
// There's no reason to emit the frame without active listeners.
if (!hasListeners) {
return;
}
// This is not an animated image, just return it and don't schedule more
// frames.
_emitFrame(ImageInfo(
image: _nextFrame!.image.clone(),
scale: _scale,
debugLabel: debugLabel,
));
_nextFrame!.image.dispose();
_nextFrame = null;
return;
}
_scheduleAppFrame();
}
void _scheduleAppFrame() {
if (_frameCallbackScheduled) {
return;
}
_frameCallbackScheduled = true;
SchedulerBinding.instance.scheduleFrameCallback(_handleAppFrame);
}
void _emitFrame(ImageInfo imageInfo) {
setImage(imageInfo);
_framesEmitted += 1;
}
@override
void addListener(ImageStreamListener listener) {
if (!hasListeners && _codec != null && (_currentImage == null || _codec!.frameCount > 1)) {
_decodeNextFrameAndSchedule();
}
super.addListener(listener);
}
@override
void removeListener(ImageStreamListener listener) {
super.removeListener(listener);
if (!hasListeners) {
_timer?.cancel();
_timer = null;
}
}
@override
void _maybeDispose() {
super._maybeDispose();
if (_disposed) {
_chunkSubscription?.onData(null);
_chunkSubscription?.cancel();
_chunkSubscription = null;
}
}
}
| flutter/packages/flutter/lib/src/painting/image_stream.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/image_stream.dart",
"repo_id": "flutter",
"token_count": 13416
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show Locale, LocaleStringAttribute, ParagraphBuilder, SpellOutStringAttribute, StringAttribute;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/services.dart';
import 'basic_types.dart';
import 'inline_span.dart';
import 'text_painter.dart';
import 'text_scaler.dart';
// Examples can assume:
// late TextSpan myTextSpan;
/// An immutable span of text.
///
/// A [TextSpan] object can be styled using its [style] property. The style will
/// be applied to the [text] and the [children].
///
/// A [TextSpan] object can just have plain text, or it can have children
/// [TextSpan] objects with their own styles that (possibly only partially)
/// override the [style] of this object. If a [TextSpan] has both [text] and
/// [children], then the [text] is treated as if it was an un-styled [TextSpan]
/// at the start of the [children] list. Leaving the [TextSpan.text] field null
/// results in the [TextSpan] acting as an empty node in the [InlineSpan] tree
/// with a list of children.
///
/// To paint a [TextSpan] on a [Canvas], use a [TextPainter]. To display a text
/// span in a widget, use a [RichText]. For text with a single style, consider
/// using the [Text] widget.
///
/// {@tool snippet}
///
/// The text "Hello world!", in black:
///
/// ```dart
/// const TextSpan(
/// text: 'Hello world!',
/// style: TextStyle(color: Colors.black),
/// )
/// ```
/// {@end-tool}
///
/// _There is some more detailed sample code in the documentation for the
/// [recognizer] property._
///
/// The [TextSpan.text] will be used as the semantics label unless overridden
/// by the [TextSpan.semanticsLabel] property. Any [PlaceholderSpan]s in the
/// [TextSpan.children] list will separate the text before and after it into two
/// semantics nodes.
///
/// See also:
///
/// * [WidgetSpan], a leaf node that represents an embedded inline widget in an
/// [InlineSpan] tree. Specify a widget within the [children] list by
/// wrapping the widget with a [WidgetSpan]. The widget will be laid out
/// inline within the paragraph.
/// * [Text], a widget for showing uniformly-styled text.
/// * [RichText], a widget for finer control of text rendering.
/// * [TextPainter], a class for painting [TextSpan] objects on a [Canvas].
@immutable
class TextSpan extends InlineSpan implements HitTestTarget, MouseTrackerAnnotation {
/// Creates a [TextSpan] with the given values.
///
/// For the object to be useful, at least one of [text] or
/// [children] should be set.
const TextSpan({
this.text,
this.children,
super.style,
this.recognizer,
MouseCursor? mouseCursor,
this.onEnter,
this.onExit,
this.semanticsLabel,
this.locale,
this.spellOut,
}) : mouseCursor = mouseCursor ??
(recognizer == null ? MouseCursor.defer : SystemMouseCursors.click),
assert(!(text == null && semanticsLabel != null));
/// The text contained in this span.
///
/// If both [text] and [children] are non-null, the text will precede the
/// children.
///
/// This getter does not include the contents of its children.
final String? text;
/// Additional spans to include as children.
///
/// If both [text] and [children] are non-null, the text will precede the
/// children.
///
/// Modifying the list after the [TextSpan] has been created is not supported
/// and may have unexpected results.
///
/// The list must not contain any nulls.
final List<InlineSpan>? children;
/// A gesture recognizer that will receive events that hit this span.
///
/// [InlineSpan] itself does not implement hit testing or event dispatch. The
/// object that manages the [InlineSpan] painting is also responsible for
/// dispatching events. In the rendering library, that is the
/// [RenderParagraph] object, which corresponds to the [RichText] widget in
/// the widgets layer; these objects do not bubble events in [InlineSpan]s,
/// so a [recognizer] is only effective for events that directly hit the
/// [text] of that [InlineSpan], not any of its [children].
///
/// [InlineSpan] also does not manage the lifetime of the gesture recognizer.
/// The code that owns the [GestureRecognizer] object must call
/// [GestureRecognizer.dispose] when the [InlineSpan] object is no longer
/// used.
///
/// {@tool snippet}
///
/// This example shows how to manage the lifetime of a gesture recognizer
/// provided to an [InlineSpan] object. It defines a `BuzzingText` widget
/// which uses the [HapticFeedback] class to vibrate the device when the user
/// long-presses the "find the" span, which is underlined in wavy green. The
/// hit-testing is handled by the [RichText] widget. It also changes the
/// hovering mouse cursor to `precise`.
///
/// ```dart
/// class BuzzingText extends StatefulWidget {
/// const BuzzingText({super.key});
///
/// @override
/// State<BuzzingText> createState() => _BuzzingTextState();
/// }
///
/// class _BuzzingTextState extends State<BuzzingText> {
/// late LongPressGestureRecognizer _longPressRecognizer;
///
/// @override
/// void initState() {
/// super.initState();
/// _longPressRecognizer = LongPressGestureRecognizer()
/// ..onLongPress = _handlePress;
/// }
///
/// @override
/// void dispose() {
/// _longPressRecognizer.dispose();
/// super.dispose();
/// }
///
/// void _handlePress() {
/// HapticFeedback.vibrate();
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Text.rich(
/// TextSpan(
/// text: 'Can you ',
/// style: const TextStyle(color: Colors.black),
/// children: <InlineSpan>[
/// TextSpan(
/// text: 'find the',
/// style: const TextStyle(
/// color: Colors.green,
/// decoration: TextDecoration.underline,
/// decorationStyle: TextDecorationStyle.wavy,
/// ),
/// recognizer: _longPressRecognizer,
/// mouseCursor: SystemMouseCursors.precise,
/// ),
/// const TextSpan(
/// text: ' secret?',
/// ),
/// ],
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
final GestureRecognizer? recognizer;
/// Mouse cursor when the mouse hovers over this span.
///
/// The default value is [SystemMouseCursors.click] if [recognizer] is not
/// null, or [MouseCursor.defer] otherwise.
///
/// [TextSpan] itself does not implement hit testing or cursor changing.
/// The object that manages the [TextSpan] painting is responsible
/// to return the [TextSpan] in its hit test, as well as providing the
/// correct mouse cursor when the [TextSpan]'s mouse cursor is
/// [MouseCursor.defer].
final MouseCursor mouseCursor;
@override
final PointerEnterEventListener? onEnter;
@override
final PointerExitEventListener? onExit;
/// Returns the value of [mouseCursor].
///
/// This field, required by [MouseTrackerAnnotation], is hidden publicly to
/// avoid the confusion as a text cursor.
@protected
@override
MouseCursor get cursor => mouseCursor;
/// An alternative semantics label for this [TextSpan].
///
/// If present, the semantics of this span will contain this value instead
/// of the actual text.
///
/// This is useful for replacing abbreviations or shorthands with the full
/// text value:
///
/// ```dart
/// const TextSpan(text: r'$$', semanticsLabel: 'Double dollars')
/// ```
final String? semanticsLabel;
/// The language of the text in this span and its span children.
///
/// Setting the locale of this text span affects the way that assistive
/// technologies, such as VoiceOver or TalkBack, pronounce the text.
///
/// If this span contains other text span children, they also inherit the
/// locale from this span unless explicitly set to different locales.
final ui.Locale? locale;
/// Whether the assistive technologies should spell out this text character
/// by character.
///
/// If the text is 'hello world', setting this to true causes the assistive
/// technologies, such as VoiceOver or TalkBack, to pronounce
/// 'h-e-l-l-o-space-w-o-r-l-d' instead of complete words. This is useful for
/// texts, such as passwords or verification codes.
///
/// If this span contains other text span children, they also inherit the
/// property from this span unless explicitly set.
///
/// If the property is not set, this text span inherits the spell out setting
/// from its parent. If this text span does not have a parent or the parent
/// does not have a spell out setting, this text span does not spell out the
/// text by default.
final bool? spellOut;
@override
bool get validForMouseTracker => true;
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
if (event is PointerDownEvent) {
recognizer?.addPointer(event);
}
}
/// Apply the [style], [text], and [children] of this object to the
/// given [ParagraphBuilder], from which a [Paragraph] can be obtained.
/// [Paragraph] objects can be drawn on [Canvas] objects.
///
/// Rather than using this directly, it's simpler to use the
/// [TextPainter] class to paint [TextSpan] objects onto [Canvas]
/// objects.
@override
void build(
ui.ParagraphBuilder builder, {
TextScaler textScaler = TextScaler.noScaling,
List<PlaceholderDimensions>? dimensions,
}) {
assert(debugAssertIsValid());
final bool hasStyle = style != null;
if (hasStyle) {
builder.pushStyle(style!.getTextStyle(textScaler: textScaler));
}
if (text != null) {
try {
builder.addText(text!);
} on ArgumentError catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'painting library',
context: ErrorDescription('while building a TextSpan'),
silent: true,
));
// Use a Unicode replacement character as a substitute for invalid text.
builder.addText('\uFFFD');
}
}
final List<InlineSpan>? children = this.children;
if (children != null) {
for (final InlineSpan child in children) {
child.build(
builder,
textScaler: textScaler,
dimensions: dimensions,
);
}
}
if (hasStyle) {
builder.pop();
}
}
/// Walks this [TextSpan] and its descendants in pre-order and calls [visitor]
/// for each span that has text.
///
/// When `visitor` returns true, the walk will continue. When `visitor`
/// returns false, then the walk will end.
@override
bool visitChildren(InlineSpanVisitor visitor) {
if (text != null && !visitor(this)) {
return false;
}
final List<InlineSpan>? children = this.children;
if (children != null) {
for (final InlineSpan child in children) {
if (!child.visitChildren(visitor)) {
return false;
}
}
}
return true;
}
@override
bool visitDirectChildren(InlineSpanVisitor visitor) {
final List<InlineSpan>? children = this.children;
if (children != null) {
for (final InlineSpan child in children) {
if (!visitor(child)) {
return false;
}
}
}
return true;
}
/// Returns the text span that contains the given position in the text.
@override
InlineSpan? getSpanForPositionVisitor(TextPosition position, Accumulator offset) {
final String? text = this.text;
if (text == null || text.isEmpty) {
return null;
}
final TextAffinity affinity = position.affinity;
final int targetOffset = position.offset;
final int endOffset = offset.value + text.length;
if (offset.value == targetOffset && affinity == TextAffinity.downstream ||
offset.value < targetOffset && targetOffset < endOffset ||
endOffset == targetOffset && affinity == TextAffinity.upstream) {
return this;
}
offset.increment(text.length);
return null;
}
@override
void computeToPlainText(
StringBuffer buffer, {
bool includeSemanticsLabels = true,
bool includePlaceholders = true,
}) {
assert(debugAssertIsValid());
if (semanticsLabel != null && includeSemanticsLabels) {
buffer.write(semanticsLabel);
} else if (text != null) {
buffer.write(text);
}
if (children != null) {
for (final InlineSpan child in children!) {
child.computeToPlainText(buffer,
includeSemanticsLabels: includeSemanticsLabels,
includePlaceholders: includePlaceholders,
);
}
}
}
@override
void computeSemanticsInformation(
List<InlineSpanSemanticsInformation> collector, {
ui.Locale? inheritedLocale,
bool inheritedSpellOut = false,
}) {
assert(debugAssertIsValid());
final ui.Locale? effectiveLocale = locale ?? inheritedLocale;
final bool effectiveSpellOut = spellOut ?? inheritedSpellOut;
if (text != null) {
final int textLength = semanticsLabel?.length ?? text!.length;
collector.add(InlineSpanSemanticsInformation(
text!,
stringAttributes: <ui.StringAttribute>[
if (effectiveSpellOut && textLength > 0)
ui.SpellOutStringAttribute(range: TextRange(start: 0, end: textLength)),
if (effectiveLocale != null && textLength > 0)
ui.LocaleStringAttribute(locale: effectiveLocale, range: TextRange(start: 0, end: textLength)),
],
semanticsLabel: semanticsLabel,
recognizer: recognizer,
));
}
final List<InlineSpan>? children = this.children;
if (children != null) {
for (final InlineSpan child in children) {
if (child is TextSpan) {
child.computeSemanticsInformation(
collector,
inheritedLocale: effectiveLocale,
inheritedSpellOut: effectiveSpellOut,
);
} else {
child.computeSemanticsInformation(collector);
}
}
}
}
@override
int? codeUnitAtVisitor(int index, Accumulator offset) {
final String? text = this.text;
if (text == null) {
return null;
}
final int localOffset = index - offset.value;
assert(localOffset >= 0);
offset.increment(text.length);
return localOffset < text.length ? text.codeUnitAt(localOffset) : null;
}
/// In debug mode, throws an exception if the object is not in a valid
/// configuration. Otherwise, returns true.
///
/// This is intended to be used as follows:
///
/// ```dart
/// assert(myTextSpan.debugAssertIsValid());
/// ```
@override
bool debugAssertIsValid() {
assert(() {
if (children != null) {
for (final InlineSpan child in children!) {
assert(child.debugAssertIsValid());
}
}
return true;
}());
return super.debugAssertIsValid();
}
@override
RenderComparison compareTo(InlineSpan other) {
if (identical(this, other)) {
return RenderComparison.identical;
}
if (other.runtimeType != runtimeType) {
return RenderComparison.layout;
}
final TextSpan textSpan = other as TextSpan;
if (textSpan.text != text ||
children?.length != textSpan.children?.length ||
(style == null) != (textSpan.style == null)) {
return RenderComparison.layout;
}
RenderComparison result = recognizer == textSpan.recognizer ?
RenderComparison.identical :
RenderComparison.metadata;
if (style != null) {
final RenderComparison candidate = style!.compareTo(textSpan.style!);
if (candidate.index > result.index) {
result = candidate;
}
if (result == RenderComparison.layout) {
return result;
}
}
if (children != null) {
for (int index = 0; index < children!.length; index += 1) {
final RenderComparison candidate = children![index].compareTo(textSpan.children![index]);
if (candidate.index > result.index) {
result = candidate;
}
if (result == RenderComparison.layout) {
return result;
}
}
}
return result;
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
if (super != other) {
return false;
}
return other is TextSpan
&& other.text == text
&& other.recognizer == recognizer
&& other.semanticsLabel == semanticsLabel
&& onEnter == other.onEnter
&& onExit == other.onExit
&& mouseCursor == other.mouseCursor
&& listEquals<InlineSpan>(other.children, children);
}
@override
int get hashCode => Object.hash(
super.hashCode,
text,
recognizer,
semanticsLabel,
onEnter,
onExit,
mouseCursor,
children == null ? null : Object.hashAll(children!),
);
@override
String toStringShort() => objectRuntimeType(this, 'TextSpan');
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(
StringProperty(
'text',
text,
showName: false,
defaultValue: null,
),
);
if (style == null && text == null && children == null) {
properties.add(DiagnosticsNode.message('(empty)'));
}
properties.add(DiagnosticsProperty<GestureRecognizer>(
'recognizer', recognizer,
description: recognizer?.runtimeType.toString(),
defaultValue: null,
));
properties.add(FlagsSummary<Function?>(
'callbacks',
<String, Function?> {
'enter': onEnter,
'exit': onExit,
},
));
properties.add(DiagnosticsProperty<MouseCursor>('mouseCursor', cursor, defaultValue: MouseCursor.defer));
if (semanticsLabel != null) {
properties.add(StringProperty('semanticsLabel', semanticsLabel));
}
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return children?.map<DiagnosticsNode>((InlineSpan child) {
return child.toDiagnosticsNode();
}).toList() ?? const <DiagnosticsNode>[];
}
}
| flutter/packages/flutter/lib/src/painting/text_span.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/text_span.dart",
"repo_id": "flutter",
"token_count": 6600
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'object.dart';
import 'proxy_box.dart';
import 'proxy_sliver.dart';
import 'sliver.dart';
/// Paints a [Decoration] either before or after its child paints.
///
/// If the child has infinite scroll extent, then the [Decoration] paints itself up to the
/// bottom cache extent.
class RenderDecoratedSliver extends RenderProxySliver {
/// Creates a decorated sliver.
///
/// The [decoration], [position], and [configuration] arguments must not be
/// null. By default the decoration paints behind the child.
///
/// The [ImageConfiguration] will be passed to the decoration (with the size
/// filled in) to let it resolve images.
RenderDecoratedSliver({
required Decoration decoration,
DecorationPosition position = DecorationPosition.background,
ImageConfiguration configuration = ImageConfiguration.empty,
}) : _decoration = decoration,
_position = position,
_configuration = configuration;
/// What decoration to paint.
///
/// Commonly a [BoxDecoration].
Decoration get decoration => _decoration;
Decoration _decoration;
set decoration(Decoration value) {
if (value == decoration) {
return;
}
_decoration = value;
_painter?.dispose();
_painter = decoration.createBoxPainter(markNeedsPaint);
markNeedsPaint();
}
/// Whether to paint the box decoration behind or in front of the child.
DecorationPosition get position => _position;
DecorationPosition _position;
set position(DecorationPosition value) {
if (value == position) {
return;
}
_position = value;
markNeedsPaint();
}
/// The settings to pass to the decoration when painting, so that it can
/// resolve images appropriately. See [ImageProvider.resolve] and
/// [BoxPainter.paint].
///
/// The [ImageConfiguration.textDirection] field is also used by
/// direction-sensitive [Decoration]s for painting and hit-testing.
ImageConfiguration get configuration => _configuration;
ImageConfiguration _configuration;
set configuration(ImageConfiguration value) {
if (value == configuration) {
return;
}
_configuration = value;
markNeedsPaint();
}
BoxPainter? _painter;
@override
void attach(covariant PipelineOwner owner) {
_painter = decoration.createBoxPainter(markNeedsPaint);
super.attach(owner);
}
@override
void detach() {
_painter?.dispose();
_painter = null;
super.detach();
}
@override
void dispose() {
_painter?.dispose();
_painter = null;
super.dispose();
}
@override
void paint(PaintingContext context, Offset offset) {
if (child == null || !child!.geometry!.visible) {
return;
}
// In the case where the child sliver has infinite scroll extent, the decoration
// should only extend down to the bottom cache extent.
final double cappedMainAxisExtent = child!.geometry!.scrollExtent.isInfinite
? constraints.scrollOffset + child!.geometry!.cacheExtent + constraints.cacheOrigin
: child!.geometry!.scrollExtent;
final (Size childSize, Offset scrollOffset) = switch (constraints.axis) {
Axis.horizontal => (Size(cappedMainAxisExtent, constraints.crossAxisExtent), Offset(-constraints.scrollOffset, 0.0)),
Axis.vertical => (Size(constraints.crossAxisExtent, cappedMainAxisExtent), Offset(0.0, -constraints.scrollOffset)),
};
offset += (child!.parentData! as SliverPhysicalParentData).paintOffset;
void paintDecoration() => _painter!.paint(context.canvas, offset + scrollOffset, configuration.copyWith(size: childSize));
switch (position) {
case DecorationPosition.background:
paintDecoration();
context.paintChild(child!, offset);
case DecorationPosition.foreground:
context.paintChild(child!, offset);
paintDecoration();
}
}
}
| flutter/packages/flutter/lib/src/rendering/decorated_sliver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/decorated_sliver.dart",
"repo_id": "flutter",
"token_count": 1278
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'dart:ui' as ui show Color;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/semantics.dart';
import 'layer.dart';
import 'object.dart';
import 'proxy_box.dart';
import 'sliver.dart';
/// A base class for sliver render objects that resemble their children.
///
/// A proxy sliver has a single child and mimics all the properties of
/// that child by calling through to the child for each function in the render
/// sliver protocol. For example, a proxy sliver determines its geometry by
/// asking its sliver child to layout with the same constraints and then
/// matching the geometry.
///
/// A proxy sliver isn't useful on its own because you might as well just
/// replace the proxy sliver with its child. However, RenderProxySliver is a
/// useful base class for render objects that wish to mimic most, but not all,
/// of the properties of their sliver child.
///
/// See also:
///
/// * [RenderProxyBox], a base class for render boxes that resemble their
/// children.
abstract class RenderProxySliver extends RenderSliver with RenderObjectWithChildMixin<RenderSliver> {
/// Creates a proxy render sliver.
///
/// Proxy render slivers aren't created directly because they proxy
/// the render sliver protocol to their sliver [child]. Instead, use one of
/// the subclasses.
RenderProxySliver([RenderSliver? child]) {
this.child = child;
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverPhysicalParentData) {
child.parentData = SliverPhysicalParentData();
}
}
@override
void performLayout() {
assert(child != null);
child!.layout(constraints, parentUsesSize: true);
geometry = child!.geometry;
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null) {
context.paintChild(child!, offset);
}
}
@override
bool hitTestChildren(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
return child != null
&& child!.geometry!.hitTestExtent > 0
&& child!.hitTest(
result,
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
);
}
@override
double childMainAxisPosition(RenderSliver child) {
assert(child == this.child);
return 0.0;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.applyPaintTransform(transform);
}
}
/// Makes its sliver child partially transparent.
///
/// This class paints its sliver child into an intermediate buffer and then
/// blends the sliver child back into the scene, partially transparent.
///
/// For values of opacity other than 0.0 and 1.0, this class is relatively
/// expensive, because it requires painting the sliver child into an intermediate
/// buffer. For the value 0.0, the sliver child is not painted at all.
/// For the value 1.0, the sliver child is painted immediately without an
/// intermediate buffer.
class RenderSliverOpacity extends RenderProxySliver {
/// Creates a partially transparent render object.
///
/// The [opacity] argument must be between 0.0 and 1.0, inclusive.
RenderSliverOpacity({
double opacity = 1.0,
bool alwaysIncludeSemantics = false,
RenderSliver? sliver,
}) : assert(opacity >= 0.0 && opacity <= 1.0),
_opacity = opacity,
_alwaysIncludeSemantics = alwaysIncludeSemantics,
_alpha = ui.Color.getAlphaFromOpacity(opacity) {
child = sliver;
}
@override
bool get alwaysNeedsCompositing => child != null && (_alpha > 0);
int _alpha;
/// The fraction to scale the child's alpha value.
///
/// An opacity of one is fully opaque. An opacity of zero is fully transparent
/// (i.e. invisible).
///
/// Values one and zero are painted with a fast path. Other values require
/// painting the child into an intermediate buffer, which is expensive.
double get opacity => _opacity;
double _opacity;
set opacity(double value) {
assert(value >= 0.0 && value <= 1.0);
if (_opacity == value) {
return;
}
final bool didNeedCompositing = alwaysNeedsCompositing;
final bool wasVisible = _alpha != 0;
_opacity = value;
_alpha = ui.Color.getAlphaFromOpacity(_opacity);
if (didNeedCompositing != alwaysNeedsCompositing) {
markNeedsCompositingBitsUpdate();
}
markNeedsPaint();
if (wasVisible != (_alpha != 0) && !alwaysIncludeSemantics) {
markNeedsSemanticsUpdate();
}
}
/// Whether child semantics are included regardless of the opacity.
///
/// If false, semantics are excluded when [opacity] is 0.0.
///
/// Defaults to false.
bool get alwaysIncludeSemantics => _alwaysIncludeSemantics;
bool _alwaysIncludeSemantics;
set alwaysIncludeSemantics(bool value) {
if (value == _alwaysIncludeSemantics) {
return;
}
_alwaysIncludeSemantics = value;
markNeedsSemanticsUpdate();
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null && child!.geometry!.visible) {
if (_alpha == 0) {
// No need to keep the layer. We'll create a new one if necessary.
layer = null;
return;
}
assert(needsCompositing);
layer = context.pushOpacity(
offset,
_alpha,
super.paint,
oldLayer: layer as OpacityLayer?,
);
assert(() {
layer!.debugCreator = debugCreator;
return true;
}());
}
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (child != null && (_alpha != 0 || alwaysIncludeSemantics)) {
visitor(child!);
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('opacity', opacity));
properties.add(FlagProperty('alwaysIncludeSemantics', value: alwaysIncludeSemantics, ifTrue: 'alwaysIncludeSemantics'));
}
}
/// A render object that is invisible during hit testing.
///
/// When [ignoring] is true, this render object (and its subtree) is invisible
/// to hit testing. It still consumes space during layout and paints its sliver
/// child as usual. It just cannot be the target of located events, because its
/// render object returns false from [hitTest].
///
/// ## Semantics
///
/// Using this class may also affect how the semantics subtree underneath is
/// collected.
///
/// {@macro flutter.widgets.IgnorePointer.semantics}
///
/// {@macro flutter.widgets.IgnorePointer.ignoringSemantics}
class RenderSliverIgnorePointer extends RenderProxySliver {
/// Creates a render object that is invisible to hit testing.
RenderSliverIgnorePointer({
RenderSliver? sliver,
bool ignoring = true,
@Deprecated(
'Create a custom sliver ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
bool? ignoringSemantics,
}) : _ignoring = ignoring,
_ignoringSemantics = ignoringSemantics {
child = sliver;
}
/// Whether this render object is ignored during hit testing.
///
/// Regardless of whether this render object is ignored during hit testing, it
/// will still consume space during layout and be visible during painting.
///
/// {@macro flutter.widgets.IgnorePointer.semantics}
bool get ignoring => _ignoring;
bool _ignoring;
set ignoring(bool value) {
if (value == _ignoring) {
return;
}
_ignoring = value;
if (ignoringSemantics == null) {
markNeedsSemanticsUpdate();
}
}
/// Whether the semantics of this render object is ignored when compiling the
/// semantics tree.
///
/// {@macro flutter.widgets.IgnorePointer.ignoringSemantics}
@Deprecated(
'Create a custom sliver ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
bool? get ignoringSemantics => _ignoringSemantics;
bool? _ignoringSemantics;
set ignoringSemantics(bool? value) {
if (value == _ignoringSemantics) {
return;
}
_ignoringSemantics = value;
markNeedsSemanticsUpdate();
}
@override
bool hitTest(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
return !ignoring
&& super.hitTest(
result,
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
);
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (_ignoringSemantics ?? false) {
return;
}
super.visitChildrenForSemantics(visitor);
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
// Do not block user interactions if _ignoringSemantics is false; otherwise,
// delegate to absorbing
config.isBlockingUserActions = ignoring && (_ignoringSemantics ?? true);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('ignoring', ignoring));
properties.add(
DiagnosticsProperty<bool>(
'ignoringSemantics',
ignoringSemantics,
description: ignoringSemantics == null ? null : 'implicitly $ignoringSemantics',
),
);
}
}
/// Lays the sliver child out as if it was in the tree, but without painting
/// anything, without making the sliver child available for hit testing, and
/// without taking any room in the parent.
class RenderSliverOffstage extends RenderProxySliver {
/// Creates an offstage render object.
RenderSliverOffstage({
bool offstage = true,
RenderSliver? sliver,
}) : _offstage = offstage {
child = sliver;
}
/// Whether the sliver child is hidden from the rest of the tree.
///
/// If true, the sliver child is laid out as if it was in the tree, but
/// without painting anything, without making the sliver child available for
/// hit testing, and without taking any room in the parent.
///
/// If false, the sliver child is included in the tree as normal.
bool get offstage => _offstage;
bool _offstage;
set offstage(bool value) {
if (value == _offstage) {
return;
}
_offstage = value;
markNeedsLayoutForSizedByParentChange();
}
@override
void performLayout() {
assert(child != null);
child!.layout(constraints, parentUsesSize: true);
if (!offstage) {
geometry = child!.geometry;
} else {
geometry = SliverGeometry.zero;
}
}
@override
bool hitTest(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
return !offstage && super.hitTest(
result,
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
);
}
@override
bool hitTestChildren(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
return !offstage
&& child != null
&& child!.geometry!.hitTestExtent > 0
&& child!.hitTest(
result,
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
);
}
@override
void paint(PaintingContext context, Offset offset) {
if (offstage) {
return;
}
context.paintChild(child!, offset);
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (offstage) {
return;
}
super.visitChildrenForSemantics(visitor);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('offstage', offstage));
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
if (child == null) {
return <DiagnosticsNode>[];
}
return <DiagnosticsNode>[
child!.toDiagnosticsNode(
name: 'child',
style: offstage ? DiagnosticsTreeStyle.offstage : DiagnosticsTreeStyle.sparse,
),
];
}
}
/// Makes its sliver child partially transparent, driven from an [Animation].
///
/// This is a variant of [RenderSliverOpacity] that uses an [Animation<double>]
/// rather than a [double] to control the opacity.
class RenderSliverAnimatedOpacity extends RenderProxySliver with RenderAnimatedOpacityMixin<RenderSliver> {
/// Creates a partially transparent render object.
RenderSliverAnimatedOpacity({
required Animation<double> opacity,
bool alwaysIncludeSemantics = false,
RenderSliver? sliver,
}) {
this.opacity = opacity;
this.alwaysIncludeSemantics = alwaysIncludeSemantics;
child = sliver;
}
}
/// Applies a cross-axis constraint to its sliver child.
///
/// This render object takes a [maxExtent] parameter and uses the smaller of
/// [maxExtent] and the parent's [SliverConstraints.crossAxisExtent] as the
/// cross axis extent of the [SliverConstraints] passed to the sliver child.
class RenderSliverConstrainedCrossAxis extends RenderProxySliver {
/// Creates a render object that constrains the cross axis extent of its sliver child.
///
/// The [maxExtent] parameter must be nonnegative.
RenderSliverConstrainedCrossAxis({
required double maxExtent
}) : _maxExtent = maxExtent,
assert(maxExtent >= 0.0);
/// The cross axis extent to apply to the sliver child.
///
/// This value must be nonnegative.
double get maxExtent => _maxExtent;
double _maxExtent;
set maxExtent(double value) {
if (_maxExtent == value) {
return;
}
_maxExtent = value;
markNeedsLayout();
}
@override
void performLayout() {
assert(child != null);
assert(maxExtent >= 0.0);
child!.layout(
constraints.copyWith(crossAxisExtent: min(_maxExtent, constraints.crossAxisExtent)),
parentUsesSize: true,
);
final SliverGeometry childLayoutGeometry = child!.geometry!;
geometry = childLayoutGeometry.copyWith(crossAxisExtent: min(_maxExtent, constraints.crossAxisExtent));
}
}
| flutter/packages/flutter/lib/src/rendering/proxy_sliver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/proxy_sliver.dart",
"repo_id": "flutter",
"token_count": 4706
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart' hide Border;
/// Border specification for [Table] widgets.
///
/// This is like [Border], with the addition of two sides: the inner horizontal
/// borders between rows and the inner vertical borders between columns.
///
/// The sides are represented by [BorderSide] objects.
@immutable
class TableBorder {
/// Creates a border for a table.
///
/// All the sides of the border default to [BorderSide.none].
const TableBorder({
this.top = BorderSide.none,
this.right = BorderSide.none,
this.bottom = BorderSide.none,
this.left = BorderSide.none,
this.horizontalInside = BorderSide.none,
this.verticalInside = BorderSide.none,
this.borderRadius = BorderRadius.zero,
});
/// A uniform border with all sides the same color and width.
///
/// The sides default to black solid borders, one logical pixel wide.
factory TableBorder.all({
Color color = const Color(0xFF000000),
double width = 1.0,
BorderStyle style = BorderStyle.solid,
BorderRadius borderRadius = BorderRadius.zero,
}) {
final BorderSide side = BorderSide(color: color, width: width, style: style);
return TableBorder(top: side, right: side, bottom: side, left: side, horizontalInside: side, verticalInside: side, borderRadius: borderRadius);
}
/// Creates a border for a table where all the interior sides use the same
/// styling and all the exterior sides use the same styling.
const TableBorder.symmetric({
BorderSide inside = BorderSide.none,
BorderSide outside = BorderSide.none,
this.borderRadius = BorderRadius.zero,
}) : top = outside,
right = outside,
bottom = outside,
left = outside,
horizontalInside = inside,
verticalInside = inside;
/// The top side of this border.
final BorderSide top;
/// The right side of this border.
final BorderSide right;
/// The bottom side of this border.
final BorderSide bottom;
/// The left side of this border.
final BorderSide left;
/// The horizontal interior sides of this border.
final BorderSide horizontalInside;
/// The vertical interior sides of this border.
final BorderSide verticalInside;
/// The [BorderRadius] to use when painting the corners of this border.
///
/// It is also applied to [DataTable]'s [Material].
final BorderRadius borderRadius;
/// The widths of the sides of this border represented as an [EdgeInsets].
///
/// This can be used, for example, with a [Padding] widget to inset a box by
/// the size of these borders.
EdgeInsets get dimensions {
return EdgeInsets.fromLTRB(left.width, top.width, right.width, bottom.width);
}
/// Whether all the sides of the border (outside and inside) are identical.
/// Uniform borders are typically more efficient to paint.
bool get isUniform {
final Color topColor = top.color;
if (right.color != topColor ||
bottom.color != topColor ||
left.color != topColor ||
horizontalInside.color != topColor ||
verticalInside.color != topColor) {
return false;
}
final double topWidth = top.width;
if (right.width != topWidth ||
bottom.width != topWidth ||
left.width != topWidth ||
horizontalInside.width != topWidth ||
verticalInside.width != topWidth) {
return false;
}
final BorderStyle topStyle = top.style;
if (right.style != topStyle ||
bottom.style != topStyle ||
left.style != topStyle ||
horizontalInside.style != topStyle ||
verticalInside.style != topStyle) {
return false;
}
return true;
}
/// Creates a copy of this border but with the widths scaled by the factor `t`.
///
/// The `t` argument represents the multiplicand, or the position on the
/// timeline for an interpolation from nothing to `this`, with 0.0 meaning
/// that the object returned should be the nil variant of this object, 1.0
/// meaning that no change should be applied, returning `this` (or something
/// equivalent to `this`), and other values meaning that the object should be
/// multiplied by `t`. Negative values are treated like zero.
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// See also:
///
/// * [BorderSide.scale], which is used to implement this method.
TableBorder scale(double t) {
return TableBorder(
top: top.scale(t),
right: right.scale(t),
bottom: bottom.scale(t),
left: left.scale(t),
horizontalInside: horizontalInside.scale(t),
verticalInside: verticalInside.scale(t),
);
}
/// Linearly interpolate between two table borders.
///
/// If a border is null, it is treated as having only [BorderSide.none]
/// borders.
///
/// {@macro dart.ui.shadow.lerp}
static TableBorder? lerp(TableBorder? a, TableBorder? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
return TableBorder(
top: BorderSide.lerp(a.top, b.top, t),
right: BorderSide.lerp(a.right, b.right, t),
bottom: BorderSide.lerp(a.bottom, b.bottom, t),
left: BorderSide.lerp(a.left, b.left, t),
horizontalInside: BorderSide.lerp(a.horizontalInside, b.horizontalInside, t),
verticalInside: BorderSide.lerp(a.verticalInside, b.verticalInside, t),
);
}
/// Paints the border around the given [Rect] on the given [Canvas], with the
/// given rows and columns.
///
/// Uniform borders are more efficient to paint than more complex borders.
///
/// The `rows` argument specifies the vertical positions between the rows,
/// relative to the given rectangle. For example, if the table contained two
/// rows of height 100.0 each, then `rows` would contain a single value,
/// 100.0, which is the vertical position between the two rows (relative to
/// the top edge of `rect`).
///
/// The `columns` argument specifies the horizontal positions between the
/// columns, relative to the given rectangle. For example, if the table
/// contained two columns of height 100.0 each, then `columns` would contain a
/// single value, 100.0, which is the vertical position between the two
/// columns (relative to the left edge of `rect`).
///
/// The [verticalInside] border is only drawn if there are at least two
/// columns. The [horizontalInside] border is only drawn if there are at least
/// two rows. The horizontal borders are drawn after the vertical borders.
///
/// The outer borders (in the order [top], [right], [bottom], [left], with
/// [left] above the others) are painted after the inner borders.
///
/// The paint order is particularly notable in the case of
/// partially-transparent borders.
void paint(
Canvas canvas,
Rect rect, {
required Iterable<double> rows,
required Iterable<double> columns,
}) {
// properties can't be null
// arguments can't be null
assert(rows.isEmpty || (rows.first >= 0.0 && rows.last <= rect.height));
assert(columns.isEmpty || (columns.first >= 0.0 && columns.last <= rect.width));
if (columns.isNotEmpty || rows.isNotEmpty) {
final Paint paint = Paint();
final Path path = Path();
if (columns.isNotEmpty) {
switch (verticalInside.style) {
case BorderStyle.solid:
paint
..color = verticalInside.color
..strokeWidth = verticalInside.width
..style = PaintingStyle.stroke;
path.reset();
for (final double x in columns) {
path.moveTo(rect.left + x, rect.top);
path.lineTo(rect.left + x, rect.bottom);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
}
if (rows.isNotEmpty) {
switch (horizontalInside.style) {
case BorderStyle.solid:
paint
..color = horizontalInside.color
..strokeWidth = horizontalInside.width
..style = PaintingStyle.stroke;
path.reset();
for (final double y in rows) {
path.moveTo(rect.left, rect.top + y);
path.lineTo(rect.right, rect.top + y);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
}
}
if (!isUniform || borderRadius == BorderRadius.zero) {
paintBorder(canvas, rect, top: top, right: right, bottom: bottom, left: left);
} else {
final RRect outer = borderRadius.toRRect(rect);
final RRect inner = outer.deflate(top.width);
final Paint paint = Paint()..color = top.color;
canvas.drawDRRect(outer, inner, paint);
}
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is TableBorder
&& other.top == top
&& other.right == right
&& other.bottom == bottom
&& other.left == left
&& other.horizontalInside == horizontalInside
&& other.verticalInside == verticalInside
&& other.borderRadius == borderRadius;
}
@override
int get hashCode => Object.hash(top, right, bottom, left, horizontalInside, verticalInside, borderRadius);
@override
String toString() => 'TableBorder($top, $right, $bottom, $left, $horizontalInside, $verticalInside, $borderRadius)';
}
| flutter/packages/flutter/lib/src/rendering/table_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/table_border.dart",
"repo_id": "flutter",
"token_count": 3406
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show TextDirection;
import 'package:flutter/services.dart' show SystemChannels;
import 'semantics_event.dart' show AnnounceSemanticsEvent, Assertiveness, TooltipSemanticsEvent;
export 'dart:ui' show TextDirection;
/// Allows access to the platform's accessibility services.
///
/// Events sent by this service are handled by the platform-specific
/// accessibility bridge in Flutter's engine.
///
/// When possible, prefer using mechanisms like [Semantics] to implicitly
/// trigger announcements over using this event.
abstract final class SemanticsService {
/// Sends a semantic announcement.
///
/// This should be used for announcement that are not seamlessly announced by
/// the system as a result of a UI state change.
///
/// For example a camera application can use this method to make accessibility
/// announcements regarding objects in the viewfinder.
///
/// The assertiveness level of the announcement is determined by [assertiveness].
/// Currently, this is only supported by the web engine and has no effect on
/// other platforms. The default mode is [Assertiveness.polite].
static Future<void> announce(String message, TextDirection textDirection, {Assertiveness assertiveness = Assertiveness.polite}) async {
final AnnounceSemanticsEvent event = AnnounceSemanticsEvent(message, textDirection, assertiveness: assertiveness);
await SystemChannels.accessibility.send(event.toMap());
}
/// Sends a semantic announcement of a tooltip.
///
/// Currently only honored on Android. The contents of [message] will be
/// read by TalkBack.
static Future<void> tooltip(String message) async {
final TooltipSemanticsEvent event = TooltipSemanticsEvent(message);
await SystemChannels.accessibility.send(event.toMap());
}
}
| flutter/packages/flutter/lib/src/semantics/semantics_service.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/semantics/semantics_service.dart",
"repo_id": "flutter",
"token_count": 498
} | 637 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
/// A class representing rich content (such as a PNG image) inserted via the
/// system input method.
///
/// The following data is represented in this class:
/// - MIME Type
/// - Bytes
/// - URI
@immutable
class KeyboardInsertedContent {
/// Creates an object to represent content that is inserted from the virtual
/// keyboard.
///
/// The mime type and URI will always be provided, but the bytedata may be null.
const KeyboardInsertedContent({required this.mimeType, required this.uri, this.data});
/// Converts JSON received from the Flutter Engine into the Dart class.
KeyboardInsertedContent.fromJson(Map<String, dynamic> metadata):
mimeType = metadata['mimeType'] as String,
uri = metadata['uri'] as String,
data = metadata['data'] != null
? Uint8List.fromList(List<int>.from(metadata['data'] as Iterable<dynamic>))
: null;
/// The mime type of the inserted content.
final String mimeType;
/// The URI (location) of the inserted content, usually a "content://" URI.
final String uri;
/// The bytedata of the inserted content.
final Uint8List? data;
/// Convenience getter to check if bytedata is available for the inserted content.
bool get hasData => data?.isNotEmpty ?? false;
@override
String toString() => '${objectRuntimeType(this, 'KeyboardInsertedContent')}($mimeType, $uri, $data)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is KeyboardInsertedContent
&& other.mimeType == mimeType
&& other.uri == uri
&& other.data == data;
}
@override
int get hashCode => Object.hash(mimeType, uri, data);
}
| flutter/packages/flutter/lib/src/services/keyboard_inserted_content.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/keyboard_inserted_content.dart",
"repo_id": "flutter",
"token_count": 607
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'keyboard_maps.g.dart';
import 'raw_keyboard.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'keyboard_key.g.dart' show LogicalKeyboardKey, PhysicalKeyboardKey;
/// Convert a UTF32 rune to its lower case.
int runeToLowerCase(int rune) {
// Assume only Basic Multilingual Plane runes have lower and upper cases.
// For other characters, return them as is.
const int utf16BmpUpperBound = 0xD7FF;
if (rune > utf16BmpUpperBound) {
return rune;
}
return String.fromCharCode(rune).toLowerCase().codeUnitAt(0);
}
/// Platform-specific key event data for macOS.
///
/// This class is deprecated and will be removed. Platform specific key event
/// data will no longer be available. See [KeyEvent] for what is available.
///
/// This object contains information about key events obtained from macOS's
/// `NSEvent` interface.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyEventDataMacOs extends RawKeyEventData {
/// Creates a key event data structure specific for macOS.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEventDataMacOs({
this.characters = '',
this.charactersIgnoringModifiers = '',
this.keyCode = 0,
this.modifiers = 0,
this.specifiedLogicalKey,
});
/// The Unicode characters associated with a key-up or key-down event.
///
/// See also:
///
/// * [Apple's NSEvent documentation](https://developer.apple.com/documentation/appkit/nsevent/1534183-characters?language=objc)
final String characters;
/// The characters generated by a key event as if no modifier key (except for
/// Shift) applies.
///
/// See also:
///
/// * [Apple's NSEvent documentation](https://developer.apple.com/documentation/appkit/nsevent/1524605-charactersignoringmodifiers?language=objc)
final String charactersIgnoringModifiers;
/// The virtual key code for the keyboard key associated with a key event.
///
/// See also:
///
/// * [Apple's NSEvent documentation](https://developer.apple.com/documentation/appkit/nsevent/1534513-keycode?language=objc)
final int keyCode;
/// A mask of the current modifiers using the values in Modifier Flags.
///
/// See also:
///
/// * [Apple's NSEvent documentation](https://developer.apple.com/documentation/appkit/nsevent/1535211-modifierflags?language=objc)
final int modifiers;
/// A logical key specified by the embedding that should be used instead of
/// deriving from raw data.
///
/// The macOS embedding detects the keyboard layout and maps some keys to
/// logical keys in a way that can not be derived from per-key information.
///
/// This is not part of the native macOS key event.
final int? specifiedLogicalKey;
@override
String get keyLabel => charactersIgnoringModifiers;
@override
PhysicalKeyboardKey get physicalKey => kMacOsToPhysicalKey[keyCode] ?? PhysicalKeyboardKey(LogicalKeyboardKey.windowsPlane + keyCode);
@override
LogicalKeyboardKey get logicalKey {
if (specifiedLogicalKey != null) {
final int key = specifiedLogicalKey!;
return LogicalKeyboardKey.findKeyByKeyId(key) ?? LogicalKeyboardKey(key);
}
// Look to see if the keyCode is a printable number pad key, so that a
// difference between regular keys (e.g. "=") and the number pad version
// (e.g. the "=" on the number pad) can be determined.
final LogicalKeyboardKey? numPadKey = kMacOsNumPadMap[keyCode];
if (numPadKey != null) {
return numPadKey;
}
// Keys that can't be derived with characterIgnoringModifiers will be
// derived from their key codes using this map.
final LogicalKeyboardKey? knownKey = kMacOsToLogicalKey[keyCode];
if (knownKey != null) {
return knownKey;
}
// If this key is a single printable character, generate the
// LogicalKeyboardKey from its Unicode value. Control keys such as ESC,
// CTRL, and SHIFT are not printable. HOME, DEL, arrow keys, and function
// keys are considered modifier function keys, which generate invalid
// Unicode scalar values. Multi-char characters are also discarded.
int? character;
if (keyLabel.isNotEmpty) {
final List<int> codePoints = keyLabel.runes.toList();
if (codePoints.length == 1 &&
// Ideally we should test whether `codePoints[0]` is in the range.
// Since LogicalKeyboardKey.isControlCharacter and _isUnprintableKey
// only tests BMP, it is fine to test keyLabel instead.
!LogicalKeyboardKey.isControlCharacter(keyLabel) &&
!_isUnprintableKey(keyLabel)) {
character = runeToLowerCase(codePoints[0]);
}
}
if (character != null) {
final int keyId = LogicalKeyboardKey.unicodePlane | (character & LogicalKeyboardKey.valueMask);
return LogicalKeyboardKey.findKeyByKeyId(keyId) ?? LogicalKeyboardKey(keyId);
}
// This is a non-printable key that we don't know about, so we mint a new
// code.
return LogicalKeyboardKey(keyCode | LogicalKeyboardKey.macosPlane);
}
bool _isLeftRightModifierPressed(KeyboardSide side, int anyMask, int leftMask, int rightMask) {
if (modifiers & anyMask == 0) {
return false;
}
if (modifiers & (leftMask | rightMask | anyMask) == anyMask) {
// If only the "anyMask" bit is set, then we respond true for requests of
// whether either left or right is pressed. Handles the case where macOS
// supplies just the "either" modifier flag, but not the left/right flag.
// (e.g. modifierShift but not modifierLeftShift).
return true;
}
return switch (side) {
KeyboardSide.any => true,
KeyboardSide.all => modifiers & leftMask != 0 && modifiers & rightMask != 0,
KeyboardSide.left => modifiers & leftMask != 0,
KeyboardSide.right => modifiers & rightMask != 0,
};
}
@override
bool isModifierPressed(ModifierKey key, {KeyboardSide side = KeyboardSide.any}) {
final int independentModifier = modifiers & deviceIndependentMask;
final bool result;
switch (key) {
case ModifierKey.controlModifier:
result = _isLeftRightModifierPressed(side, independentModifier & modifierControl, modifierLeftControl, modifierRightControl);
case ModifierKey.shiftModifier:
result = _isLeftRightModifierPressed(side, independentModifier & modifierShift, modifierLeftShift, modifierRightShift);
case ModifierKey.altModifier:
result = _isLeftRightModifierPressed(side, independentModifier & modifierOption, modifierLeftOption, modifierRightOption);
case ModifierKey.metaModifier:
result = _isLeftRightModifierPressed(side, independentModifier & modifierCommand, modifierLeftCommand, modifierRightCommand);
case ModifierKey.capsLockModifier:
result = independentModifier & modifierCapsLock != 0;
// On macOS, the function modifier bit is set for any function key, like F1,
// F2, etc., but the meaning of ModifierKey.modifierFunction in Flutter is
// that of the Fn modifier key, so there's no good way to emulate that on
// macOS.
case ModifierKey.functionModifier:
case ModifierKey.numLockModifier:
case ModifierKey.symbolModifier:
case ModifierKey.scrollLockModifier:
// These modifier masks are not used in macOS keyboards.
result = false;
}
assert(!result || getModifierSide(key) != null, "$runtimeType thinks that a modifier is pressed, but can't figure out what side it's on.");
return result;
}
@override
KeyboardSide? getModifierSide(ModifierKey key) {
KeyboardSide? findSide(int anyMask, int leftMask, int rightMask) {
final int combinedMask = leftMask | rightMask;
final int combined = modifiers & combinedMask;
if (combined == leftMask) {
return KeyboardSide.left;
} else if (combined == rightMask) {
return KeyboardSide.right;
} else if (combined == combinedMask || modifiers & (combinedMask | anyMask) == anyMask) {
// Handles the case where macOS supplies just the "either" modifier
// flag, but not the left/right flag. (e.g. modifierShift but not
// modifierLeftShift), or if left and right flags are provided, but not
// the "either" modifier flag.
return KeyboardSide.all;
}
return null;
}
switch (key) {
case ModifierKey.controlModifier:
return findSide(modifierControl, modifierLeftControl, modifierRightControl);
case ModifierKey.shiftModifier:
return findSide(modifierShift, modifierLeftShift, modifierRightShift);
case ModifierKey.altModifier:
return findSide(modifierOption, modifierLeftOption, modifierRightOption);
case ModifierKey.metaModifier:
return findSide(modifierCommand, modifierLeftCommand, modifierRightCommand);
case ModifierKey.capsLockModifier:
case ModifierKey.numLockModifier:
case ModifierKey.scrollLockModifier:
case ModifierKey.functionModifier:
case ModifierKey.symbolModifier:
return KeyboardSide.all;
}
}
@override
bool shouldDispatchEvent() {
// On macOS laptop keyboards, the fn key is used to generate home/end and
// f1-f12, but it ALSO generates a separate down/up event for the fn key
// itself. Other platforms hide the fn key, and just produce the key that
// it is combined with, so to keep it possible to write cross platform
// code that looks at which keys are pressed, the fn key is ignored on
// macOS.
return logicalKey != LogicalKeyboardKey.fn;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('characters', characters));
properties.add(DiagnosticsProperty<String>('charactersIgnoringModifiers', charactersIgnoringModifiers));
properties.add(DiagnosticsProperty<int>('keyCode', keyCode));
properties.add(DiagnosticsProperty<int>('modifiers', modifiers));
properties.add(DiagnosticsProperty<int?>('specifiedLogicalKey', specifiedLogicalKey, defaultValue: null));
}
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is RawKeyEventDataMacOs
&& other.characters == characters
&& other.charactersIgnoringModifiers == charactersIgnoringModifiers
&& other.keyCode == keyCode
&& other.modifiers == modifiers;
}
@override
int get hashCode => Object.hash(
characters,
charactersIgnoringModifiers,
keyCode,
modifiers,
);
/// Returns true if the given label represents an unprintable key.
///
/// Examples of unprintable keys are "NSUpArrowFunctionKey = 0xF700"
/// or "NSHomeFunctionKey = 0xF729".
///
/// See <https://developer.apple.com/documentation/appkit/1535851-function-key_unicodes?language=objc> for more
/// information.
///
/// Used by [RawKeyEvent] subclasses to help construct IDs.
static bool _isUnprintableKey(String label) {
if (label.length != 1) {
return false;
}
final int codeUnit = label.codeUnitAt(0);
return codeUnit >= 0xF700 && codeUnit <= 0xF8FF;
}
// Modifier key masks. See Apple's NSEvent documentation
// https://developer.apple.com/documentation/appkit/nseventmodifierflags?language=objc
// https://opensource.apple.com/source/IOHIDFamily/IOHIDFamily-86/IOHIDSystem/IOKit/hidsystem/IOLLEvent.h.auto.html
/// This mask is used to check the [modifiers] field to test whether the CAPS
/// LOCK modifier key is on.
///
/// {@template flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
/// Use this value if you need to decode the [modifiers] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
/// {@endtemplate}
static const int modifierCapsLock = 0x10000;
/// This mask is used to check the [modifiers] field to test whether one of the
/// SHIFT modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierShift = 0x20000;
/// This mask is used to check the [modifiers] field to test whether the left
/// SHIFT modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierLeftShift = 0x02;
/// This mask is used to check the [modifiers] field to test whether the right
/// SHIFT modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierRightShift = 0x04;
/// This mask is used to check the [modifiers] field to test whether one of the
/// CTRL modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierControl = 0x40000;
/// This mask is used to check the [modifiers] field to test whether the left
/// CTRL modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierLeftControl = 0x01;
/// This mask is used to check the [modifiers] field to test whether the right
/// CTRL modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierRightControl = 0x2000;
/// This mask is used to check the [modifiers] field to test whether one of the
/// ALT modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierOption = 0x80000;
/// This mask is used to check the [modifiers] field to test whether the left
/// ALT modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierLeftOption = 0x20;
/// This mask is used to check the [modifiers] field to test whether the right
/// ALT modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierRightOption = 0x40;
/// This mask is used to check the [modifiers] field to test whether one of the
/// CMD modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierCommand = 0x100000;
/// This mask is used to check the [modifiers] field to test whether the left
/// CMD modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierLeftCommand = 0x08;
/// This mask is used to check the [modifiers] field to test whether the right
/// CMD modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierRightCommand = 0x10;
/// This mask is used to check the [modifiers] field to test whether any key in
/// the numeric keypad is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierNumericPad = 0x200000;
/// This mask is used to check the [modifiers] field to test whether the
/// HELP modifier key is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierHelp = 0x400000;
/// This mask is used to check the [modifiers] field to test whether one of the
/// FUNCTION modifier keys is pressed.
///
/// {@macro flutter.services.RawKeyEventDataMacOs.modifierCapsLock}
static const int modifierFunction = 0x800000;
/// Used to retrieve only the device-independent modifier flags, allowing
/// applications to mask off the device-dependent modifier flags, including
/// event coalescing information.
static const int deviceIndependentMask = 0xffff0000;
}
| flutter/packages/flutter/lib/src/services/raw_keyboard_macos.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/raw_keyboard_macos.dart",
"repo_id": "flutter",
"token_count": 5227
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import '../../services.dart';
/// The direction in which an undo action should be performed, whether undo or redo.
enum UndoDirection {
/// Perform an undo action.
undo,
/// Perform a redo action.
redo
}
/// A low-level interface to the system's undo manager.
///
/// To receive events from the system undo manager, create an
/// [UndoManagerClient] and set it as the [client] on [UndoManager].
///
/// The [setUndoState] method can be used to update the system's undo manager
/// using the [canUndo] and [canRedo] parameters.
///
/// When the system undo or redo button is tapped, the current
/// [UndoManagerClient] will receive [UndoManagerClient.handlePlatformUndo]
/// with an [UndoDirection] representing whether the event is "undo" or "redo".
///
/// Currently, only iOS has an UndoManagerPlugin implemented on the engine side.
/// On iOS, this can be used to listen to the keyboard undo/redo buttons and the
/// undo/redo gestures.
///
/// See also:
///
/// * [NSUndoManager](https://developer.apple.com/documentation/foundation/nsundomanager)
class UndoManager {
UndoManager._() {
_channel = SystemChannels.undoManager;
_channel.setMethodCallHandler(_handleUndoManagerInvocation);
}
/// Set the [MethodChannel] used to communicate with the system's undo manager.
///
/// This is only meant for testing within the Flutter SDK. Changing this
/// will break the ability to set the undo status or receive undo and redo
/// events from the system. This has no effect if asserts are disabled.
@visibleForTesting
static void setChannel(MethodChannel newChannel) {
assert(() {
_instance._channel = newChannel..setMethodCallHandler(_instance._handleUndoManagerInvocation);
return true;
}());
}
static final UndoManager _instance = UndoManager._();
/// Receive undo and redo events from the system's [UndoManager].
///
/// Setting the [client] will cause [UndoManagerClient.handlePlatformUndo]
/// to be called when a system undo or redo is triggered, such as by tapping
/// the undo/redo keyboard buttons or using the 3-finger swipe gestures.
static set client(UndoManagerClient? client) {
_instance._currentClient = client;
}
/// Return the current [UndoManagerClient].
static UndoManagerClient? get client => _instance._currentClient;
/// Set the current state of the system UndoManager. [canUndo] and [canRedo]
/// control the respective "undo" and "redo" buttons of the system UndoManager.
static void setUndoState({bool canUndo = false, bool canRedo = false}) {
_instance._setUndoState(canUndo: canUndo, canRedo: canRedo);
}
late MethodChannel _channel;
UndoManagerClient? _currentClient;
Future<dynamic> _handleUndoManagerInvocation(MethodCall methodCall) async {
final String method = methodCall.method;
final List<dynamic> args = methodCall.arguments as List<dynamic>;
if (method == 'UndoManagerClient.handleUndo') {
assert(_currentClient != null, 'There must be a current UndoManagerClient.');
_currentClient!.handlePlatformUndo(_toUndoDirection(args[0] as String));
return;
}
throw MissingPluginException();
}
void _setUndoState({bool canUndo = false, bool canRedo = false}) {
_channel.invokeMethod<void>(
'UndoManager.setUndoState',
<String, bool>{'canUndo': canUndo, 'canRedo': canRedo}
);
}
UndoDirection _toUndoDirection(String direction) {
return switch (direction) {
'undo' => UndoDirection.undo,
'redo' => UndoDirection.redo,
_ => throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Unknown undo direction: $direction')]),
};
}
}
/// An interface to receive events from a native UndoManager.
mixin UndoManagerClient {
/// Requests that the client perform an undo or redo operation.
///
/// Currently only used on iOS 9+ when the undo or redo methods are invoked
/// by the platform. For example, when using three-finger swipe gestures,
/// the iPad keyboard, or voice control.
void handlePlatformUndo(UndoDirection direction);
/// Reverts the value on the stack to the previous value.
void undo();
/// Updates the value on the stack to the next value.
void redo();
/// Will be true if there are past values on the stack.
bool get canUndo;
/// Will be true if there are future values on the stack.
bool get canRedo;
}
| flutter/packages/flutter/lib/src/services/undo_manager.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/undo_manager.dart",
"repo_id": "flutter",
"token_count": 1368
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/services.dart';
import 'actions.dart';
import 'basic.dart';
import 'container.dart';
import 'editable_text.dart';
import 'focus_manager.dart';
import 'framework.dart';
import 'inherited_notifier.dart';
import 'overlay.dart';
import 'shortcuts.dart';
import 'tap_region.dart';
// Examples can assume:
// late BuildContext context;
/// The type of the [RawAutocomplete] callback which computes the list of
/// optional completions for the widget's field, based on the text the user has
/// entered so far.
///
/// See also:
///
/// * [RawAutocomplete.optionsBuilder], which is of this type.
typedef AutocompleteOptionsBuilder<T extends Object> = FutureOr<Iterable<T>> Function(TextEditingValue textEditingValue);
/// The type of the callback used by the [RawAutocomplete] widget to indicate
/// that the user has selected an option.
///
/// See also:
///
/// * [RawAutocomplete.onSelected], which is of this type.
typedef AutocompleteOnSelected<T extends Object> = void Function(T option);
/// The type of the [RawAutocomplete] callback which returns a [Widget] that
/// displays the specified [options] and calls [onSelected] if the user
/// selects an option.
///
/// The returned widget from this callback will be wrapped in an
/// [AutocompleteHighlightedOption] inherited widget. This will allow
/// this callback to determine which option is currently highlighted for
/// keyboard navigation.
///
/// See also:
///
/// * [RawAutocomplete.optionsViewBuilder], which is of this type.
typedef AutocompleteOptionsViewBuilder<T extends Object> = Widget Function(
BuildContext context,
AutocompleteOnSelected<T> onSelected,
Iterable<T> options,
);
/// The type of the Autocomplete callback which returns the widget that
/// contains the input [TextField] or [TextFormField].
///
/// See also:
///
/// * [RawAutocomplete.fieldViewBuilder], which is of this type.
typedef AutocompleteFieldViewBuilder = Widget Function(
BuildContext context,
TextEditingController textEditingController,
FocusNode focusNode,
VoidCallback onFieldSubmitted,
);
/// The type of the [RawAutocomplete] callback that converts an option value to
/// a string which can be displayed in the widget's options menu.
///
/// See also:
///
/// * [RawAutocomplete.displayStringForOption], which is of this type.
typedef AutocompleteOptionToString<T extends Object> = String Function(T option);
/// A direction in which to open the options-view overlay.
///
/// See also:
///
/// * [RawAutocomplete.optionsViewOpenDirection], which is of this type.
/// * [RawAutocomplete.optionsViewBuilder] to specify how to build the
/// selectable-options widget.
/// * [RawAutocomplete.fieldViewBuilder] to optionally specify how to build the
/// corresponding field widget.
enum OptionsViewOpenDirection {
/// Open upward.
///
/// The bottom edge of the options view will align with the top edge
/// of the text field built by [RawAutocomplete.fieldViewBuilder].
up,
/// Open downward.
///
/// The top edge of the options view will align with the bottom edge
/// of the text field built by [RawAutocomplete.fieldViewBuilder].
down,
}
// TODO(justinmc): Mention AutocompleteCupertino when it is implemented.
/// {@template flutter.widgets.RawAutocomplete.RawAutocomplete}
/// A widget for helping the user make a selection by entering some text and
/// choosing from among a list of options.
///
/// The user's text input is received in a field built with the
/// [fieldViewBuilder] parameter. The options to be displayed are determined
/// using [optionsBuilder] and rendered with [optionsViewBuilder].
/// {@endtemplate}
///
/// This is a core framework widget with very basic UI.
///
/// {@tool dartpad}
/// This example shows how to create a very basic autocomplete widget using the
/// [fieldViewBuilder] and [optionsViewBuilder] parameters.
///
/// ** See code in examples/api/lib/widgets/autocomplete/raw_autocomplete.0.dart **
/// {@end-tool}
///
/// The type parameter T represents the type of the options. Most commonly this
/// is a String, as in the example above. However, it's also possible to use
/// another type with a `toString` method, or a custom [displayStringForOption].
/// Options will be compared using `==`, so it may be beneficial to override
/// [Object.==] and [Object.hashCode] for custom types.
///
/// {@tool dartpad}
/// This example is similar to the previous example, but it uses a custom T data
/// type instead of directly using String.
///
/// ** See code in examples/api/lib/widgets/autocomplete/raw_autocomplete.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows the use of RawAutocomplete in a form.
///
/// ** See code in examples/api/lib/widgets/autocomplete/raw_autocomplete.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Autocomplete], which is a Material-styled implementation that is based
/// on RawAutocomplete.
class RawAutocomplete<T extends Object> extends StatefulWidget {
/// Create an instance of RawAutocomplete.
///
/// [displayStringForOption], [optionsBuilder] and [optionsViewBuilder] must
/// not be null.
const RawAutocomplete({
super.key,
required this.optionsViewBuilder,
required this.optionsBuilder,
this.optionsViewOpenDirection = OptionsViewOpenDirection.down,
this.displayStringForOption = defaultStringForOption,
this.fieldViewBuilder,
this.focusNode,
this.onSelected,
this.textEditingController,
this.initialValue,
}) : assert(
fieldViewBuilder != null
|| (key != null && focusNode != null && textEditingController != null),
'Pass in a fieldViewBuilder, or otherwise create a separate field and pass in the FocusNode, TextEditingController, and a key. Use the key with RawAutocomplete.onFieldSubmitted.',
),
assert((focusNode == null) == (textEditingController == null)),
assert(
!(textEditingController != null && initialValue != null),
'textEditingController and initialValue cannot be simultaneously defined.',
);
/// {@template flutter.widgets.RawAutocomplete.fieldViewBuilder}
/// Builds the field whose input is used to get the options.
///
/// Pass the provided [TextEditingController] to the field built here so that
/// RawAutocomplete can listen for changes.
/// {@endtemplate}
///
/// If this parameter is null, then a [SizedBox.shrink] is built instead.
/// For how that pattern can be useful, see [textEditingController].
final AutocompleteFieldViewBuilder? fieldViewBuilder;
/// The [FocusNode] that is used for the text field.
///
/// {@template flutter.widgets.RawAutocomplete.split}
/// The main purpose of this parameter is to allow the use of a separate text
/// field located in another part of the widget tree instead of the text
/// field built by [fieldViewBuilder]. For example, it may be desirable to
/// place the text field in the AppBar and the options below in the main body.
///
/// When following this pattern, [fieldViewBuilder] can be omitted,
/// so that a text field is not drawn where it would normally be.
/// A separate text field can be created elsewhere, and a
/// FocusNode and TextEditingController can be passed both to that text field
/// and to RawAutocomplete.
///
/// {@tool dartpad}
/// This examples shows how to create an autocomplete widget with the text
/// field in the AppBar and the results in the main body of the app.
///
/// ** See code in examples/api/lib/widgets/autocomplete/raw_autocomplete.focus_node.0.dart **
/// {@end-tool}
/// {@endtemplate}
///
/// If this parameter is not null, then [textEditingController] must also be
/// not null.
final FocusNode? focusNode;
/// {@template flutter.widgets.RawAutocomplete.optionsViewBuilder}
/// Builds the selectable options widgets from a list of options objects.
///
/// The options are displayed floating below or above the field using a
/// [CompositedTransformFollower] inside of an [Overlay], not at the same
/// place in the widget tree as [RawAutocomplete]. To control whether it opens
/// upward or downward, use [optionsViewOpenDirection].
///
/// In order to track which item is highlighted by keyboard navigation, the
/// resulting options will be wrapped in an inherited
/// [AutocompleteHighlightedOption] widget.
/// Inside this callback, the index of the highlighted option can be obtained
/// from [AutocompleteHighlightedOption.of] to display the highlighted option
/// with a visual highlight to indicate it will be the option selected from
/// the keyboard.
///
/// {@endtemplate}
final AutocompleteOptionsViewBuilder<T> optionsViewBuilder;
/// {@template flutter.widgets.RawAutocomplete.optionsViewOpenDirection}
/// The direction in which to open the options-view overlay.
///
/// Defaults to [OptionsViewOpenDirection.down].
/// {@endtemplate}
final OptionsViewOpenDirection optionsViewOpenDirection;
/// {@template flutter.widgets.RawAutocomplete.displayStringForOption}
/// Returns the string to display in the field when the option is selected.
///
/// This is useful when using a custom T type and the string to display is
/// different than the string to search by.
///
/// If not provided, will use `option.toString()`.
/// {@endtemplate}
final AutocompleteOptionToString<T> displayStringForOption;
/// {@template flutter.widgets.RawAutocomplete.onSelected}
/// Called when an option is selected by the user.
/// {@endtemplate}
final AutocompleteOnSelected<T>? onSelected;
/// {@template flutter.widgets.RawAutocomplete.optionsBuilder}
/// A function that returns the current selectable options objects given the
/// current TextEditingValue.
/// {@endtemplate}
final AutocompleteOptionsBuilder<T> optionsBuilder;
/// The [TextEditingController] that is used for the text field.
///
/// {@macro flutter.widgets.RawAutocomplete.split}
///
/// If this parameter is not null, then [focusNode] must also be not null.
final TextEditingController? textEditingController;
/// {@template flutter.widgets.RawAutocomplete.initialValue}
/// The initial value to use for the text field.
/// {@endtemplate}
///
/// Setting the initial value does not notify [textEditingController]'s
/// listeners, and thus will not cause the options UI to appear.
///
/// This parameter is ignored if [textEditingController] is defined.
final TextEditingValue? initialValue;
/// Calls [AutocompleteFieldViewBuilder]'s onFieldSubmitted callback for the
/// RawAutocomplete widget indicated by the given [GlobalKey].
///
/// This is not typically used unless a custom field is implemented instead of
/// using [fieldViewBuilder]. In the typical case, the onFieldSubmitted
/// callback is passed via the [AutocompleteFieldViewBuilder] signature. When
/// not using fieldViewBuilder, the same callback can be called by using this
/// static method.
///
/// See also:
///
/// * [focusNode] and [textEditingController], which contain a code example
/// showing how to create a separate field outside of fieldViewBuilder.
static void onFieldSubmitted<T extends Object>(GlobalKey key) {
final _RawAutocompleteState<T> rawAutocomplete = key.currentState! as _RawAutocompleteState<T>;
rawAutocomplete._onFieldSubmitted();
}
/// The default way to convert an option to a string in
/// [displayStringForOption].
///
/// Uses the `toString` method of the given `option`.
static String defaultStringForOption(Object? option) {
return option.toString();
}
@override
State<RawAutocomplete<T>> createState() => _RawAutocompleteState<T>();
}
class _RawAutocompleteState<T extends Object> extends State<RawAutocomplete<T>> {
final GlobalKey _fieldKey = GlobalKey();
final LayerLink _optionsLayerLink = LayerLink();
final OverlayPortalController _optionsViewController = OverlayPortalController(debugLabel: '_RawAutocompleteState');
TextEditingController? _internalTextEditingController;
TextEditingController get _textEditingController {
return widget.textEditingController
?? (_internalTextEditingController ??= TextEditingController()..addListener(_onChangedField));
}
FocusNode? _internalFocusNode;
FocusNode get _focusNode {
return widget.focusNode
?? (_internalFocusNode ??= FocusNode()..addListener(_updateOptionsViewVisibility));
}
late final Map<Type, CallbackAction<Intent>> _actionMap = <Type, CallbackAction<Intent>>{
AutocompletePreviousOptionIntent: _AutocompleteCallbackAction<AutocompletePreviousOptionIntent>(
onInvoke: _highlightPreviousOption,
isEnabledCallback: () => _canShowOptionsView,
),
AutocompleteNextOptionIntent: _AutocompleteCallbackAction<AutocompleteNextOptionIntent>(
onInvoke: _highlightNextOption,
isEnabledCallback: () => _canShowOptionsView,
),
DismissIntent: CallbackAction<DismissIntent>(onInvoke: _hideOptions),
};
Iterable<T> _options = Iterable<T>.empty();
T? _selection;
// Set the initial value to null so when this widget gets focused for the first
// time it will try to run the options view builder.
String? _lastFieldText;
final ValueNotifier<int> _highlightedOptionIndex = ValueNotifier<int>(0);
static const Map<ShortcutActivator, Intent> _shortcuts = <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.arrowUp): AutocompletePreviousOptionIntent(),
SingleActivator(LogicalKeyboardKey.arrowDown): AutocompleteNextOptionIntent(),
};
bool get _canShowOptionsView => _focusNode.hasFocus && _selection == null && _options.isNotEmpty;
void _updateOptionsViewVisibility() {
if (_canShowOptionsView) {
_optionsViewController.show();
} else {
_optionsViewController.hide();
}
}
// Called when _textEditingController changes.
Future<void> _onChangedField() async {
final TextEditingValue value = _textEditingController.value;
final Iterable<T> options = await widget.optionsBuilder(value);
_options = options;
_updateHighlight(_highlightedOptionIndex.value);
final T? selection = _selection;
if (selection != null && value.text != widget.displayStringForOption(selection)) {
_selection = null;
}
// Make sure the options are no longer hidden if the content of the field
// changes (ignore selection changes).
if (value.text != _lastFieldText) {
_lastFieldText = value.text;
_updateOptionsViewVisibility();
}
}
// Called from fieldViewBuilder when the user submits the field.
void _onFieldSubmitted() {
if (_optionsViewController.isShowing) {
_select(_options.elementAt(_highlightedOptionIndex.value));
}
}
// Select the given option and update the widget.
void _select(T nextSelection) {
if (nextSelection == _selection) {
return;
}
_selection = nextSelection;
final String selectionString = widget.displayStringForOption(nextSelection);
_textEditingController.value = TextEditingValue(
selection: TextSelection.collapsed(offset: selectionString.length),
text: selectionString,
);
widget.onSelected?.call(nextSelection);
_updateOptionsViewVisibility();
}
void _updateHighlight(int newIndex) {
_highlightedOptionIndex.value = _options.isEmpty ? 0 : newIndex % _options.length;
}
void _highlightPreviousOption(AutocompletePreviousOptionIntent intent) {
assert(_canShowOptionsView);
_updateOptionsViewVisibility();
assert(_optionsViewController.isShowing);
_updateHighlight(_highlightedOptionIndex.value - 1);
}
void _highlightNextOption(AutocompleteNextOptionIntent intent) {
assert(_canShowOptionsView);
_updateOptionsViewVisibility();
assert(_optionsViewController.isShowing);
_updateHighlight(_highlightedOptionIndex.value + 1);
}
Object? _hideOptions(DismissIntent intent) {
if (_optionsViewController.isShowing) {
_optionsViewController.hide();
return null;
} else {
return Actions.invoke(context, intent);
}
}
Widget _buildOptionsView(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
final Alignment followerAlignment = switch (widget.optionsViewOpenDirection) {
OptionsViewOpenDirection.up => AlignmentDirectional.bottomStart,
OptionsViewOpenDirection.down => AlignmentDirectional.topStart,
}.resolve(textDirection);
final Alignment targetAnchor = switch (widget.optionsViewOpenDirection) {
OptionsViewOpenDirection.up => AlignmentDirectional.topStart,
OptionsViewOpenDirection.down => AlignmentDirectional.bottomStart,
}.resolve(textDirection);
return CompositedTransformFollower(
link: _optionsLayerLink,
showWhenUnlinked: false,
targetAnchor: targetAnchor,
followerAnchor: followerAlignment,
child: TextFieldTapRegion(
child: AutocompleteHighlightedOption(
highlightIndexNotifier: _highlightedOptionIndex,
child: Builder(
builder: (BuildContext context) => widget.optionsViewBuilder(context, _select, _options),
),
),
),
);
}
@override
void initState() {
super.initState();
final TextEditingController initialController = widget.textEditingController
?? (_internalTextEditingController = TextEditingController.fromValue(widget.initialValue));
initialController.addListener(_onChangedField);
widget.focusNode?.addListener(_updateOptionsViewVisibility);
}
@override
void didUpdateWidget(RawAutocomplete<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (!identical(oldWidget.textEditingController, widget.textEditingController)) {
oldWidget.textEditingController?.removeListener(_onChangedField);
if (oldWidget.textEditingController == null) {
_internalTextEditingController?.dispose();
_internalTextEditingController = null;
}
widget.textEditingController?.addListener(_onChangedField);
}
if (!identical(oldWidget.focusNode, widget.focusNode)) {
oldWidget.focusNode?.removeListener(_updateOptionsViewVisibility);
if (oldWidget.focusNode == null) {
_internalFocusNode?.dispose();
_internalFocusNode = null;
}
widget.focusNode?.addListener(_updateOptionsViewVisibility);
}
}
@override
void dispose() {
widget.textEditingController?.removeListener(_onChangedField);
_internalTextEditingController?.dispose();
widget.focusNode?.removeListener(_updateOptionsViewVisibility);
_internalFocusNode?.dispose();
_highlightedOptionIndex.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final Widget fieldView = widget.fieldViewBuilder?.call(context, _textEditingController, _focusNode, _onFieldSubmitted)
?? const SizedBox.shrink();
return OverlayPortal.targetsRootOverlay(
controller: _optionsViewController,
overlayChildBuilder: _buildOptionsView,
child: TextFieldTapRegion(
child: Container(
key: _fieldKey,
child: Shortcuts(
shortcuts: _shortcuts,
child: Actions(
actions: _actionMap,
child: CompositedTransformTarget(
link: _optionsLayerLink,
child: fieldView,
),
),
),
),
),
);
}
}
class _AutocompleteCallbackAction<T extends Intent> extends CallbackAction<T> {
_AutocompleteCallbackAction({
required super.onInvoke,
required this.isEnabledCallback,
});
// The enabled state determines whether the action will consume the
// key shortcut or let it continue on to the underlying text field.
// They should only be enabled when the options are showing so shortcuts
// can be used to navigate them.
final bool Function() isEnabledCallback;
@override
bool isEnabled(covariant T intent) => isEnabledCallback();
@override
bool consumesKey(covariant T intent) => isEnabled(intent);
}
/// An [Intent] to highlight the previous option in the autocomplete list.
class AutocompletePreviousOptionIntent extends Intent {
/// Creates an instance of AutocompletePreviousOptionIntent.
const AutocompletePreviousOptionIntent();
}
/// An [Intent] to highlight the next option in the autocomplete list.
class AutocompleteNextOptionIntent extends Intent {
/// Creates an instance of AutocompleteNextOptionIntent.
const AutocompleteNextOptionIntent();
}
/// An inherited widget used to indicate which autocomplete option should be
/// highlighted for keyboard navigation.
///
/// The `RawAutoComplete` widget will wrap the options view generated by the
/// `optionsViewBuilder` with this widget to provide the highlighted option's
/// index to the builder.
///
/// In the builder callback the index of the highlighted option can be obtained
/// by using the static [of] method:
///
/// ```dart
/// int highlightedIndex = AutocompleteHighlightedOption.of(context);
/// ```
///
/// which can then be used to tell which option should be given a visual
/// indication that will be the option selected with the keyboard.
class AutocompleteHighlightedOption extends InheritedNotifier<ValueNotifier<int>> {
/// Create an instance of AutocompleteHighlightedOption inherited widget.
const AutocompleteHighlightedOption({
super.key,
required ValueNotifier<int> highlightIndexNotifier,
required super.child,
}) : super(notifier: highlightIndexNotifier);
/// Returns the index of the highlighted option from the closest
/// [AutocompleteHighlightedOption] ancestor.
///
/// If there is no ancestor, it returns 0.
///
/// Typical usage is as follows:
///
/// ```dart
/// int highlightedIndex = AutocompleteHighlightedOption.of(context);
/// ```
static int of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<AutocompleteHighlightedOption>()?.notifier?.value ?? 0;
}
}
| flutter/packages/flutter/lib/src/widgets/autocomplete.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/autocomplete.dart",
"repo_id": "flutter",
"token_count": 6785
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
/// Positions the toolbar at [anchor] if it fits, otherwise moves it so that it
/// just fits fully on-screen.
///
/// See also:
///
/// * [desktopTextSelectionControls], which uses this to position
/// itself.
/// * [cupertinoDesktopTextSelectionControls], which uses this to position
/// itself.
/// * [TextSelectionToolbarLayoutDelegate], which does a similar layout for
/// the mobile text selection toolbars.
class DesktopTextSelectionToolbarLayoutDelegate extends SingleChildLayoutDelegate {
/// Creates an instance of TextSelectionToolbarLayoutDelegate.
DesktopTextSelectionToolbarLayoutDelegate({
required this.anchor,
});
/// The point at which to render the menu, if possible.
///
/// Should be provided in local coordinates.
final Offset anchor;
@override
BoxConstraints getConstraintsForChild(BoxConstraints constraints) {
return constraints.loosen();
}
@override
Offset getPositionForChild(Size size, Size childSize) {
final Offset overhang = Offset(
anchor.dx + childSize.width - size.width,
anchor.dy + childSize.height - size.height,
);
return Offset(
overhang.dx > 0.0 ? anchor.dx - overhang.dx : anchor.dx,
overhang.dy > 0.0 ? anchor.dy - overhang.dy : anchor.dy,
);
}
@override
bool shouldRelayout(DesktopTextSelectionToolbarLayoutDelegate oldDelegate) {
return anchor != oldDelegate.anchor;
}
}
| flutter/packages/flutter/lib/src/widgets/desktop_text_selection_toolbar_layout_delegate.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/desktop_text_selection_toolbar_layout_delegate.dart",
"repo_id": "flutter",
"token_count": 514
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic.dart';
import 'binding.dart';
import 'framework.dart';
import 'implicit_animations.dart';
import 'media_query.dart';
import 'navigator.dart';
import 'overlay.dart';
import 'pages.dart';
import 'routes.dart';
import 'ticker_provider.dart' show TickerMode;
import 'transitions.dart';
/// Signature for a function that takes two [Rect] instances and returns a
/// [RectTween] that transitions between them.
///
/// This is typically used with a [HeroController] to provide an animation for
/// [Hero] positions that looks nicer than a linear movement. For example, see
/// [MaterialRectArcTween].
typedef CreateRectTween = Tween<Rect?> Function(Rect? begin, Rect? end);
/// Signature for a function that builds a [Hero] placeholder widget given a
/// child and a [Size].
///
/// The child can optionally be part of the returned widget tree. The returned
/// widget should typically be constrained to [heroSize], if it doesn't do so
/// implicitly.
///
/// See also:
///
/// * [TransitionBuilder], which is similar but only takes a [BuildContext]
/// and a child widget.
typedef HeroPlaceholderBuilder = Widget Function(
BuildContext context,
Size heroSize,
Widget child,
);
/// A function that lets [Hero]es self supply a [Widget] that is shown during the
/// hero's flight from one route to another instead of default (which is to
/// show the destination route's instance of the Hero).
typedef HeroFlightShuttleBuilder = Widget Function(
BuildContext flightContext,
Animation<double> animation,
HeroFlightDirection flightDirection,
BuildContext fromHeroContext,
BuildContext toHeroContext,
);
typedef _OnFlightEnded = void Function(_HeroFlight flight);
/// Direction of the hero's flight based on the navigation operation.
enum HeroFlightDirection {
/// A flight triggered by a route push.
///
/// The animation goes from 0 to 1.
///
/// If no custom [HeroFlightShuttleBuilder] is supplied, the top route's
/// [Hero] child is shown in flight.
push,
/// A flight triggered by a route pop.
///
/// The animation goes from 1 to 0.
///
/// If no custom [HeroFlightShuttleBuilder] is supplied, the bottom route's
/// [Hero] child is shown in flight.
pop,
}
/// A widget that marks its child as being a candidate for
/// [hero animations](https://flutter.dev/docs/development/ui/animations/hero-animations).
///
/// When a [PageRoute] is pushed or popped with the [Navigator], the entire
/// screen's content is replaced. An old route disappears and a new route
/// appears. If there's a common visual feature on both routes then it can
/// be helpful for orienting the user for the feature to physically move from
/// one page to the other during the routes' transition. Such an animation
/// is called a *hero animation*. The hero widgets "fly" in the Navigator's
/// overlay during the transition and while they're in-flight they're, by
/// default, not shown in their original locations in the old and new routes.
///
/// To label a widget as such a feature, wrap it in a [Hero] widget. When
/// navigation happens, the [Hero] widgets on each route are identified
/// by the [HeroController]. For each pair of [Hero] widgets that have the
/// same tag, a hero animation is triggered.
///
/// If a [Hero] is already in flight when navigation occurs, its
/// flight animation will be redirected to its new destination. The
/// widget shown in-flight during the transition is, by default, the
/// destination route's [Hero]'s child.
///
/// For a Hero animation to trigger, the Hero has to exist on the very first
/// frame of the new page's animation.
///
/// Routes must not contain more than one [Hero] for each [tag].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=Be9UH1kXFDw}
///
/// {@tool dartpad}
/// This sample shows a [Hero] used within a [ListTile].
///
/// Tapping on the Hero-wrapped rectangle triggers a hero
/// animation as a new [MaterialPageRoute] is pushed. Both the size
/// and location of the rectangle animates.
///
/// Both widgets use the same [Hero.tag].
///
/// The Hero widget uses the matching tags to identify and execute this
/// animation.
///
/// ** See code in examples/api/lib/widgets/heroes/hero.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows [Hero] flight animations using default tween
/// and custom rect tween.
///
/// ** See code in examples/api/lib/widgets/heroes/hero.1.dart **
/// {@end-tool}
///
/// ## Discussion
///
/// Heroes and the [Navigator]'s [Overlay] [Stack] must be axis-aligned for
/// all this to work. The top left and bottom right coordinates of each animated
/// Hero will be converted to global coordinates and then from there converted
/// to that [Stack]'s coordinate space, and the entire Hero subtree will, for
/// the duration of the animation, be lifted out of its original place, and
/// positioned on that stack. If the [Hero] isn't axis aligned, this is going to
/// fail in a rather ugly fashion. Don't rotate your heroes!
///
/// To make the animations look good, it's critical that the widget tree for the
/// hero in both locations be essentially identical. The widget of the *target*
/// is, by default, used to do the transition: when going from route A to route
/// B, route B's hero's widget is placed over route A's hero's widget. Additionally,
/// if the [Hero] subtree changes appearance based on an [InheritedWidget] (such
/// as [MediaQuery] or [Theme]), then the hero animation may have discontinuity
/// at the start or the end of the animation because route A and route B provides
/// different such [InheritedWidget]s. Consider providing a custom [flightShuttleBuilder]
/// to ensure smooth transitions. The default [flightShuttleBuilder] interpolates
/// [MediaQuery]'s paddings. If your [Hero] widget uses custom [InheritedWidget]s
/// and displays a discontinuity in the animation, try to provide custom in-flight
/// transition using [flightShuttleBuilder].
///
/// By default, both route A and route B's heroes are hidden while the
/// transitioning widget is animating in-flight above the 2 routes.
/// [placeholderBuilder] can be used to show a custom widget in their place
/// instead once the transition has taken flight.
///
/// During the transition, the transition widget is animated to route B's hero's
/// position, and then the widget is inserted into route B. When going back from
/// B to A, route A's hero's widget is, by default, placed over where route B's
/// hero's widget was, and then the animation goes the other way.
///
/// ### Nested Navigators
///
/// If either or both routes contain nested [Navigator]s, only [Hero]es
/// contained in the top-most routes (as defined by [Route.isCurrent]) *of those
/// nested [Navigator]s* are considered for animation. Just like in the
/// non-nested case the top-most routes containing these [Hero]es in the nested
/// [Navigator]s have to be [PageRoute]s.
///
/// ## Parts of a Hero Transition
///
/// 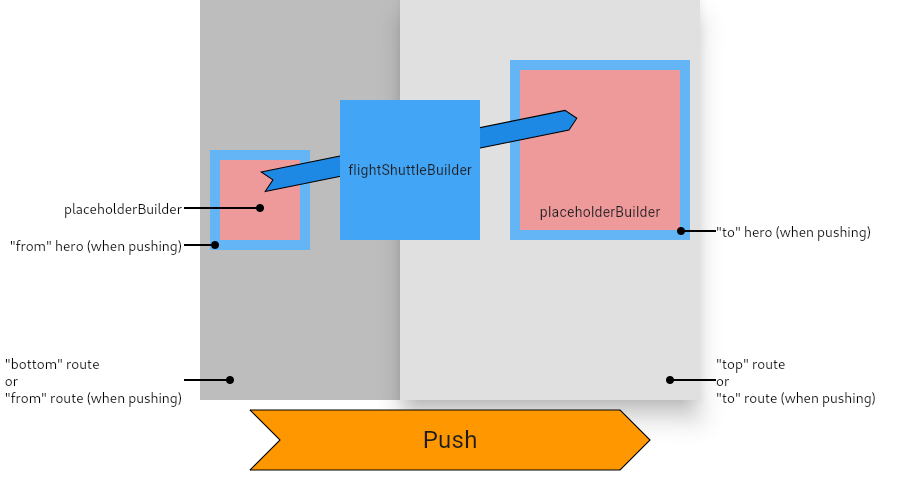
class Hero extends StatefulWidget {
/// Create a hero.
///
/// The [child] parameter and all of the its descendants must not be [Hero]es.
const Hero({
super.key,
required this.tag,
this.createRectTween,
this.flightShuttleBuilder,
this.placeholderBuilder,
this.transitionOnUserGestures = false,
required this.child,
});
/// The identifier for this particular hero. If the tag of this hero matches
/// the tag of a hero on a [PageRoute] that we're navigating to or from, then
/// a hero animation will be triggered.
final Object tag;
/// Defines how the destination hero's bounds change as it flies from the starting
/// route to the destination route.
///
/// A hero flight begins with the destination hero's [child] aligned with the
/// starting hero's child. The [Tween<Rect>] returned by this callback is used
/// to compute the hero's bounds as the flight animation's value goes from 0.0
/// to 1.0.
///
/// If this property is null, the default, then the value of
/// [HeroController.createRectTween] is used. The [HeroController] created by
/// [MaterialApp] creates a [MaterialRectArcTween].
final CreateRectTween? createRectTween;
/// The widget subtree that will "fly" from one route to another during a
/// [Navigator] push or pop transition.
///
/// The appearance of this subtree should be similar to the appearance of
/// the subtrees of any other heroes in the application with the same [tag].
/// Changes in scale and aspect ratio work well in hero animations, changes
/// in layout or composition do not.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Optional override to supply a widget that's shown during the hero's flight.
///
/// This in-flight widget can depend on the route transition's animation as
/// well as the incoming and outgoing routes' [Hero] descendants' widgets and
/// layout.
///
/// When both the source and destination [Hero]es provide a [flightShuttleBuilder],
/// the destination's [flightShuttleBuilder] takes precedence.
///
/// If none is provided, the destination route's Hero child is shown in-flight
/// by default.
///
/// ## Limitations
///
/// If a widget built by [flightShuttleBuilder] takes part in a [Navigator]
/// push transition, that widget or its descendants must not have any
/// [GlobalKey] that is used in the source Hero's descendant widgets. That is
/// because both subtrees will be included in the widget tree during the Hero
/// flight animation, and [GlobalKey]s must be unique across the entire widget
/// tree.
///
/// If the said [GlobalKey] is essential to your application, consider providing
/// a custom [placeholderBuilder] for the source Hero, to avoid the [GlobalKey]
/// collision, such as a builder that builds an empty [SizedBox], keeping the
/// Hero [child]'s original size.
final HeroFlightShuttleBuilder? flightShuttleBuilder;
/// Placeholder widget left in place as the Hero's [child] once the flight takes
/// off.
///
/// By default the placeholder widget is an empty [SizedBox] keeping the Hero
/// child's original size, unless this Hero is a source Hero of a [Navigator]
/// push transition, in which case [child] will be a descendant of the placeholder
/// and will be kept [Offstage] during the Hero's flight.
final HeroPlaceholderBuilder? placeholderBuilder;
/// Whether to perform the hero transition if the [PageRoute] transition was
/// triggered by a user gesture, such as a back swipe on iOS.
///
/// If [Hero]es with the same [tag] on both the from and the to routes have
/// [transitionOnUserGestures] set to true, a back swipe gesture will
/// trigger the same hero animation as a programmatically triggered push or
/// pop.
///
/// The route being popped to or the bottom route must also have
/// [PageRoute.maintainState] set to true for a gesture triggered hero
/// transition to work.
///
/// Defaults to false.
final bool transitionOnUserGestures;
// Returns a map of all of the heroes in `context` indexed by hero tag that
// should be considered for animation when `navigator` transitions from one
// PageRoute to another.
static Map<Object, _HeroState> _allHeroesFor(
BuildContext context,
bool isUserGestureTransition,
NavigatorState navigator,
) {
final Map<Object, _HeroState> result = <Object, _HeroState>{};
void inviteHero(StatefulElement hero, Object tag) {
assert(() {
if (result.containsKey(tag)) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('There are multiple heroes that share the same tag within a subtree.'),
ErrorDescription(
'Within each subtree for which heroes are to be animated (i.e. a PageRoute subtree), '
'each Hero must have a unique non-null tag.\n'
'In this case, multiple heroes had the following tag: $tag',
),
DiagnosticsProperty<StatefulElement>('Here is the subtree for one of the offending heroes', hero, linePrefix: '# ', style: DiagnosticsTreeStyle.dense),
]);
}
return true;
}());
final Hero heroWidget = hero.widget as Hero;
final _HeroState heroState = hero.state as _HeroState;
if (!isUserGestureTransition || heroWidget.transitionOnUserGestures) {
result[tag] = heroState;
} else {
// If transition is not allowed, we need to make sure hero is not hidden.
// A hero can be hidden previously due to hero transition.
heroState.endFlight();
}
}
void visitor(Element element) {
final Widget widget = element.widget;
if (widget is Hero) {
final StatefulElement hero = element as StatefulElement;
final Object tag = widget.tag;
if (Navigator.of(hero) == navigator) {
inviteHero(hero, tag);
} else {
// The nearest navigator to the Hero is not the Navigator that is
// currently transitioning from one route to another. This means
// the Hero is inside a nested Navigator and should only be
// considered for animation if it is part of the top-most route in
// that nested Navigator and if that route is also a PageRoute.
final ModalRoute<Object?>? heroRoute = ModalRoute.of(hero);
if (heroRoute != null && heroRoute is PageRoute && heroRoute.isCurrent) {
inviteHero(hero, tag);
}
}
} else if (widget is HeroMode && !widget.enabled) {
return;
}
element.visitChildren(visitor);
}
context.visitChildElements(visitor);
return result;
}
@override
State<Hero> createState() => _HeroState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Object>('tag', tag));
}
}
/// The [Hero] widget displays different content based on whether it is in an
/// animated transition ("flight"), from/to another [Hero] with the same tag:
/// * When [startFlight] is called, the real content of this [Hero] will be
/// replaced by a "placeholder" widget.
/// * When the flight ends, the "toHero"'s [endFlight] method must be called
/// by the hero controller, so the real content of that [Hero] becomes
/// visible again when the animation completes.
class _HeroState extends State<Hero> {
final GlobalKey _key = GlobalKey();
Size? _placeholderSize;
// Whether the placeholder widget should wrap the hero's child widget as its
// own child, when `_placeholderSize` is non-null (i.e. the hero is currently
// in its flight animation). See `startFlight`.
bool _shouldIncludeChild = true;
// The `shouldIncludeChildInPlaceholder` flag dictates if the child widget of
// this hero should be included in the placeholder widget as a descendant.
//
// When a new hero flight animation takes place, a placeholder widget
// needs to be built to replace the original hero widget. When
// `shouldIncludeChildInPlaceholder` is set to true and `widget.placeholderBuilder`
// is null, the placeholder widget will include the original hero's child
// widget as a descendant, allowing the original element tree to be preserved.
//
// It is typically set to true for the *from* hero in a push transition,
// and false otherwise.
void startFlight({ bool shouldIncludedChildInPlaceholder = false }) {
_shouldIncludeChild = shouldIncludedChildInPlaceholder;
assert(mounted);
final RenderBox box = context.findRenderObject()! as RenderBox;
assert(box.hasSize);
setState(() {
_placeholderSize = box.size;
});
}
// When `keepPlaceholder` is true, the placeholder will continue to be shown
// after the flight ends. Otherwise the child of the Hero will become visible
// and its TickerMode will be re-enabled.
//
// This method can be safely called even when this [Hero] is currently not in
// a flight.
void endFlight({ bool keepPlaceholder = false }) {
if (keepPlaceholder || _placeholderSize == null) {
return;
}
_placeholderSize = null;
if (mounted) {
// Tell the widget to rebuild if it's mounted. _placeholderSize has already
// been updated.
setState(() {});
}
}
@override
Widget build(BuildContext context) {
assert(
context.findAncestorWidgetOfExactType<Hero>() == null,
'A Hero widget cannot be the descendant of another Hero widget.',
);
final bool showPlaceholder = _placeholderSize != null;
if (showPlaceholder && widget.placeholderBuilder != null) {
return widget.placeholderBuilder!(context, _placeholderSize!, widget.child);
}
if (showPlaceholder && !_shouldIncludeChild) {
return SizedBox(
width: _placeholderSize!.width,
height: _placeholderSize!.height,
);
}
return SizedBox(
width: _placeholderSize?.width,
height: _placeholderSize?.height,
child: Offstage(
offstage: showPlaceholder,
child: TickerMode(
enabled: !showPlaceholder,
child: KeyedSubtree(key: _key, child: widget.child),
),
),
);
}
}
// Everything known about a hero flight that's to be started or diverted.
@immutable
class _HeroFlightManifest {
_HeroFlightManifest({
required this.type,
required this.overlay,
required this.navigatorSize,
required this.fromRoute,
required this.toRoute,
required this.fromHero,
required this.toHero,
required this.createRectTween,
required this.shuttleBuilder,
required this.isUserGestureTransition,
required this.isDiverted,
}) : assert(fromHero.widget.tag == toHero.widget.tag);
final HeroFlightDirection type;
final OverlayState overlay;
final Size navigatorSize;
final PageRoute<dynamic> fromRoute;
final PageRoute<dynamic> toRoute;
final _HeroState fromHero;
final _HeroState toHero;
final CreateRectTween? createRectTween;
final HeroFlightShuttleBuilder shuttleBuilder;
final bool isUserGestureTransition;
final bool isDiverted;
Object get tag => fromHero.widget.tag;
Animation<double> get animation {
return CurvedAnimation(
parent: (type == HeroFlightDirection.push) ? toRoute.animation! : fromRoute.animation!,
curve: Curves.fastOutSlowIn,
reverseCurve: isDiverted ? null : Curves.fastOutSlowIn.flipped,
);
}
Tween<Rect?> createHeroRectTween({ required Rect? begin, required Rect? end }) {
final CreateRectTween? createRectTween = toHero.widget.createRectTween ?? this.createRectTween;
return createRectTween?.call(begin, end) ?? RectTween(begin: begin, end: end);
}
// The bounding box for `context`'s render object, in `ancestorContext`'s
// render object's coordinate space.
static Rect _boundingBoxFor(BuildContext context, BuildContext? ancestorContext) {
assert(ancestorContext != null);
final RenderBox box = context.findRenderObject()! as RenderBox;
assert(box.hasSize && box.size.isFinite);
return MatrixUtils.transformRect(
box.getTransformTo(ancestorContext?.findRenderObject()),
Offset.zero & box.size,
);
}
/// The bounding box of [fromHero], in [fromRoute]'s coordinate space.
///
/// This property should only be accessed in [_HeroFlight.start].
late final Rect fromHeroLocation = _boundingBoxFor(fromHero.context, fromRoute.subtreeContext);
/// The bounding box of [toHero], in [toRoute]'s coordinate space.
///
/// This property should only be accessed in [_HeroFlight.start] or
/// [_HeroFlight.divert].
late final Rect toHeroLocation = _boundingBoxFor(toHero.context, toRoute.subtreeContext);
/// Whether this [_HeroFlightManifest] is valid and can be used to start or
/// divert a [_HeroFlight].
///
/// When starting or diverting a [_HeroFlight] with a brand new
/// [_HeroFlightManifest], this flag must be checked to ensure the [RectTween]
/// the [_HeroFlightManifest] produces does not contain coordinates that have
/// [double.infinity] or [double.nan].
late final bool isValid = toHeroLocation.isFinite && (isDiverted || fromHeroLocation.isFinite);
@override
String toString() {
return '_HeroFlightManifest($type tag: $tag from route: ${fromRoute.settings} '
'to route: ${toRoute.settings} with hero: $fromHero to $toHero)${isValid ? '' : ', INVALID'}';
}
}
// Builds the in-flight hero widget.
class _HeroFlight {
_HeroFlight(this.onFlightEnded) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$_HeroFlight',
object: this,
);
}
_proxyAnimation = ProxyAnimation()..addStatusListener(_handleAnimationUpdate);
}
final _OnFlightEnded onFlightEnded;
late Tween<Rect?> heroRectTween;
Widget? shuttle;
Animation<double> _heroOpacity = kAlwaysCompleteAnimation;
late ProxyAnimation _proxyAnimation;
// The manifest will be available once `start` is called, throughout the
// flight's lifecycle.
late _HeroFlightManifest manifest;
OverlayEntry? overlayEntry;
bool _aborted = false;
static final Animatable<double> _reverseTween = Tween<double>(begin: 1.0, end: 0.0);
// The OverlayEntry WidgetBuilder callback for the hero's overlay.
Widget _buildOverlay(BuildContext context) {
shuttle ??= manifest.shuttleBuilder(
context,
manifest.animation,
manifest.type,
manifest.fromHero.context,
manifest.toHero.context,
);
assert(shuttle != null);
return AnimatedBuilder(
animation: _proxyAnimation,
child: shuttle,
builder: (BuildContext context, Widget? child) {
final Rect rect = heroRectTween.evaluate(_proxyAnimation)!;
final RelativeRect offsets = RelativeRect.fromSize(rect, manifest.navigatorSize);
return Positioned(
top: offsets.top,
right: offsets.right,
bottom: offsets.bottom,
left: offsets.left,
child: IgnorePointer(
child: FadeTransition(
opacity: _heroOpacity,
child: child,
),
),
);
},
);
}
void _performAnimationUpdate(AnimationStatus status) {
if (status == AnimationStatus.completed || status == AnimationStatus.dismissed) {
_proxyAnimation.parent = null;
assert(overlayEntry != null);
overlayEntry!.remove();
overlayEntry!.dispose();
overlayEntry = null;
// We want to keep the hero underneath the current page hidden. If
// [AnimationStatus.completed], toHero will be the one on top and we keep
// fromHero hidden. If [AnimationStatus.dismissed], the animation is
// triggered but canceled before it finishes. In this case, we keep toHero
// hidden instead.
manifest.fromHero.endFlight(keepPlaceholder: status == AnimationStatus.completed);
manifest.toHero.endFlight(keepPlaceholder: status == AnimationStatus.dismissed);
onFlightEnded(this);
_proxyAnimation.removeListener(onTick);
}
}
bool _scheduledPerformAnimationUpdate = false;
void _handleAnimationUpdate(AnimationStatus status) {
// The animation will not finish until the user lifts their finger, so we
// should suppress the status update if the gesture is in progress, and
// delay it until the finger is lifted.
if (manifest.fromRoute.navigator?.userGestureInProgress != true) {
_performAnimationUpdate(status);
return;
}
if (_scheduledPerformAnimationUpdate) {
return;
}
// The `navigator` must be non-null here, or the first if clause above would
// have returned from this method.
final NavigatorState navigator = manifest.fromRoute.navigator!;
void delayedPerformAnimationUpdate() {
assert(!navigator.userGestureInProgress);
assert(_scheduledPerformAnimationUpdate);
_scheduledPerformAnimationUpdate = false;
navigator.userGestureInProgressNotifier.removeListener(delayedPerformAnimationUpdate);
_performAnimationUpdate(_proxyAnimation.status);
}
assert(navigator.userGestureInProgress);
_scheduledPerformAnimationUpdate = true;
navigator.userGestureInProgressNotifier.addListener(delayedPerformAnimationUpdate);
}
/// Releases resources.
@mustCallSuper
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
if (overlayEntry != null) {
overlayEntry!.remove();
overlayEntry!.dispose();
overlayEntry = null;
_proxyAnimation.parent = null;
_proxyAnimation.removeListener(onTick);
_proxyAnimation.removeStatusListener(_handleAnimationUpdate);
}
}
void onTick() {
final RenderBox? toHeroBox = (!_aborted && manifest.toHero.mounted)
? manifest.toHero.context.findRenderObject() as RenderBox?
: null;
// Try to find the new origin of the toHero, if the flight isn't aborted.
final Offset? toHeroOrigin = toHeroBox != null && toHeroBox.attached && toHeroBox.hasSize
? toHeroBox.localToGlobal(Offset.zero, ancestor: manifest.toRoute.subtreeContext?.findRenderObject() as RenderBox?)
: null;
if (toHeroOrigin != null && toHeroOrigin.isFinite) {
// If the new origin of toHero is available and also paintable, try to
// update heroRectTween with it.
if (toHeroOrigin != heroRectTween.end!.topLeft) {
final Rect heroRectEnd = toHeroOrigin & heroRectTween.end!.size;
heroRectTween = manifest.createHeroRectTween(begin: heroRectTween.begin, end: heroRectEnd);
}
} else if (_heroOpacity.isCompleted) {
// The toHero no longer exists or it's no longer the flight's destination.
// Continue flying while fading out.
_heroOpacity = _proxyAnimation.drive(
_reverseTween.chain(CurveTween(curve: Interval(_proxyAnimation.value, 1.0))),
);
}
// Update _aborted for the next animation tick.
_aborted = toHeroOrigin == null || !toHeroOrigin.isFinite;
}
// The simple case: we're either starting a push or a pop animation.
void start(_HeroFlightManifest initialManifest) {
assert(!_aborted);
assert(() {
final Animation<double> initial = initialManifest.animation;
final HeroFlightDirection type = initialManifest.type;
switch (type) {
case HeroFlightDirection.pop:
return initial.value == 1.0 && initialManifest.isUserGestureTransition
// During user gesture transitions, the animation controller isn't
// driving the reverse transition, but should still be in a previously
// completed stage with the initial value at 1.0.
? initial.status == AnimationStatus.completed
: initial.status == AnimationStatus.reverse;
case HeroFlightDirection.push:
return initial.value == 0.0 && initial.status == AnimationStatus.forward;
}
}());
manifest = initialManifest;
final bool shouldIncludeChildInPlaceholder;
switch (manifest.type) {
case HeroFlightDirection.pop:
_proxyAnimation.parent = ReverseAnimation(manifest.animation);
shouldIncludeChildInPlaceholder = false;
case HeroFlightDirection.push:
_proxyAnimation.parent = manifest.animation;
shouldIncludeChildInPlaceholder = true;
}
heroRectTween = manifest.createHeroRectTween(begin: manifest.fromHeroLocation, end: manifest.toHeroLocation);
manifest.fromHero.startFlight(shouldIncludedChildInPlaceholder: shouldIncludeChildInPlaceholder);
manifest.toHero.startFlight();
manifest.overlay.insert(overlayEntry = OverlayEntry(builder: _buildOverlay));
_proxyAnimation.addListener(onTick);
}
// While this flight's hero was in transition a push or a pop occurred for
// routes with the same hero. Redirect the in-flight hero to the new toRoute.
void divert(_HeroFlightManifest newManifest) {
assert(manifest.tag == newManifest.tag);
if (manifest.type == HeroFlightDirection.push && newManifest.type == HeroFlightDirection.pop) {
// A push flight was interrupted by a pop.
assert(newManifest.animation.status == AnimationStatus.reverse);
assert(manifest.fromHero == newManifest.toHero);
assert(manifest.toHero == newManifest.fromHero);
assert(manifest.fromRoute == newManifest.toRoute);
assert(manifest.toRoute == newManifest.fromRoute);
// The same heroRect tween is used in reverse, rather than creating
// a new heroRect with _doCreateRectTween(heroRect.end, heroRect.begin).
// That's because tweens like MaterialRectArcTween may create a different
// path for swapped begin and end parameters. We want the pop flight
// path to be the same (in reverse) as the push flight path.
_proxyAnimation.parent = ReverseAnimation(newManifest.animation);
heroRectTween = ReverseTween<Rect?>(heroRectTween);
} else if (manifest.type == HeroFlightDirection.pop && newManifest.type == HeroFlightDirection.push) {
// A pop flight was interrupted by a push.
assert(newManifest.animation.status == AnimationStatus.forward);
assert(manifest.toHero == newManifest.fromHero);
assert(manifest.toRoute == newManifest.fromRoute);
_proxyAnimation.parent = newManifest.animation.drive(
Tween<double>(
begin: manifest.animation.value,
end: 1.0,
),
);
if (manifest.fromHero != newManifest.toHero) {
manifest.fromHero.endFlight(keepPlaceholder: true);
newManifest.toHero.startFlight();
heroRectTween = manifest.createHeroRectTween(begin: heroRectTween.end, end: newManifest.toHeroLocation);
} else {
// TODO(hansmuller): Use ReverseTween here per github.com/flutter/flutter/pull/12203.
heroRectTween = manifest.createHeroRectTween(begin: heroRectTween.end, end: heroRectTween.begin);
}
} else {
// A push or a pop flight is heading to a new route, i.e.
// manifest.type == _HeroFlightType.push && newManifest.type == _HeroFlightType.push ||
// manifest.type == _HeroFlightType.pop && newManifest.type == _HeroFlightType.pop
assert(manifest.fromHero != newManifest.fromHero);
assert(manifest.toHero != newManifest.toHero);
heroRectTween = manifest.createHeroRectTween(
begin: heroRectTween.evaluate(_proxyAnimation),
end: newManifest.toHeroLocation,
);
shuttle = null;
if (newManifest.type == HeroFlightDirection.pop) {
_proxyAnimation.parent = ReverseAnimation(newManifest.animation);
} else {
_proxyAnimation.parent = newManifest.animation;
}
manifest.fromHero.endFlight(keepPlaceholder: true);
manifest.toHero.endFlight(keepPlaceholder: true);
// Let the heroes in each of the routes rebuild with their placeholders.
newManifest.fromHero.startFlight(shouldIncludedChildInPlaceholder: newManifest.type == HeroFlightDirection.push);
newManifest.toHero.startFlight();
// Let the transition overlay on top of the routes also rebuild since
// we cleared the old shuttle.
overlayEntry!.markNeedsBuild();
}
manifest = newManifest;
}
void abort() {
_aborted = true;
}
@override
String toString() {
final RouteSettings from = manifest.fromRoute.settings;
final RouteSettings to = manifest.toRoute.settings;
final Object tag = manifest.tag;
return 'HeroFlight(for: $tag, from: $from, to: $to ${_proxyAnimation.parent})';
}
}
/// A [Navigator] observer that manages [Hero] transitions.
///
/// An instance of [HeroController] should be used in [Navigator.observers].
/// This is done automatically by [MaterialApp].
class HeroController extends NavigatorObserver {
/// Creates a hero controller with the given [RectTween] constructor if any.
///
/// The [createRectTween] argument is optional. If null, the controller uses a
/// linear [Tween<Rect>].
HeroController({ this.createRectTween }) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$HeroController',
object: this,
);
}
}
/// Used to create [RectTween]s that interpolate the position of heroes in flight.
///
/// If null, the controller uses a linear [RectTween].
final CreateRectTween? createRectTween;
// All of the heroes that are currently in the overlay and in motion.
// Indexed by the hero tag.
final Map<Object, _HeroFlight> _flights = <Object, _HeroFlight>{};
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
assert(navigator != null);
_maybeStartHeroTransition(previousRoute, route, HeroFlightDirection.push, false);
}
@override
void didPop(Route<dynamic> route, Route<dynamic>? previousRoute) {
assert(navigator != null);
// Don't trigger another flight when a pop is committed as a user gesture
// back swipe is snapped.
if (!navigator!.userGestureInProgress) {
_maybeStartHeroTransition(route, previousRoute, HeroFlightDirection.pop, false);
}
}
@override
void didReplace({ Route<dynamic>? newRoute, Route<dynamic>? oldRoute }) {
assert(navigator != null);
if (newRoute?.isCurrent ?? false) {
// Only run hero animations if the top-most route got replaced.
_maybeStartHeroTransition(oldRoute, newRoute, HeroFlightDirection.push, false);
}
}
@override
void didStartUserGesture(Route<dynamic> route, Route<dynamic>? previousRoute) {
assert(navigator != null);
_maybeStartHeroTransition(route, previousRoute, HeroFlightDirection.pop, true);
}
@override
void didStopUserGesture() {
if (navigator!.userGestureInProgress) {
return;
}
// When the user gesture ends, if the user horizontal drag gesture initiated
// the flight (i.e. the back swipe) didn't move towards the pop direction at
// all, the animation will not play and thus the status update callback
// _handleAnimationUpdate will never be called when the gesture finishes. In
// this case the initiated flight needs to be manually invalidated.
bool isInvalidFlight(_HeroFlight flight) {
return flight.manifest.isUserGestureTransition
&& flight.manifest.type == HeroFlightDirection.pop
&& flight._proxyAnimation.isDismissed;
}
final List<_HeroFlight> invalidFlights = _flights.values
.where(isInvalidFlight)
.toList(growable: false);
// Treat these invalidated flights as dismissed. Calling _handleAnimationUpdate
// will also remove the flight from _flights.
for (final _HeroFlight flight in invalidFlights) {
flight._handleAnimationUpdate(AnimationStatus.dismissed);
}
}
// If we're transitioning between different page routes, start a hero transition
// after the toRoute has been laid out with its animation's value at 1.0.
void _maybeStartHeroTransition(
Route<dynamic>? fromRoute,
Route<dynamic>? toRoute,
HeroFlightDirection flightType,
bool isUserGestureTransition,
) {
if (toRoute == fromRoute ||
toRoute is! PageRoute<dynamic> ||
fromRoute is! PageRoute<dynamic>) {
return;
}
final PageRoute<dynamic> from = fromRoute;
final PageRoute<dynamic> to = toRoute;
// A user gesture may have already completed the pop, or we might be the initial route
switch (flightType) {
case HeroFlightDirection.pop:
if (from.animation!.value == 0.0) {
return;
}
case HeroFlightDirection.push:
if (to.animation!.value == 1.0) {
return;
}
}
// For pop transitions driven by a user gesture: if the "to" page has
// maintainState = true, then the hero's final dimensions can be measured
// immediately because their page's layout is still valid.
if (isUserGestureTransition && flightType == HeroFlightDirection.pop && to.maintainState) {
_startHeroTransition(from, to, flightType, isUserGestureTransition);
} else {
// Otherwise, delay measuring until the end of the next frame to allow
// the 'to' route to build and layout.
// Putting a route offstage changes its animation value to 1.0. Once this
// frame completes, we'll know where the heroes in the `to` route are
// going to end up, and the `to` route will go back onstage.
to.offstage = to.animation!.value == 0.0;
WidgetsBinding.instance.addPostFrameCallback((Duration value) {
if (from.navigator == null || to.navigator == null) {
return;
}
_startHeroTransition(from, to, flightType, isUserGestureTransition);
}, debugLabel: 'HeroController.startTransition');
}
}
// Find the matching pairs of heroes in from and to and either start or a new
// hero flight, or divert an existing one.
void _startHeroTransition(
PageRoute<dynamic> from,
PageRoute<dynamic> to,
HeroFlightDirection flightType,
bool isUserGestureTransition,
) {
// If the `to` route was offstage, then we're implicitly restoring its
// animation value back to what it was before it was "moved" offstage.
to.offstage = false;
final NavigatorState? navigator = this.navigator;
final OverlayState? overlay = navigator?.overlay;
// If the navigator or the overlay was removed before this end-of-frame
// callback was called, then don't actually start a transition, and we don't
// have to worry about any Hero widget we might have hidden in a previous
// flight, or ongoing flights.
if (navigator == null || overlay == null) {
return;
}
final RenderObject? navigatorRenderObject = navigator.context.findRenderObject();
if (navigatorRenderObject is! RenderBox) {
assert(false, 'Navigator $navigator has an invalid RenderObject type ${navigatorRenderObject.runtimeType}.');
return;
}
assert(navigatorRenderObject.hasSize);
// At this point, the toHeroes may have been built and laid out for the first time.
//
// If `fromSubtreeContext` is null, call endFlight on all toHeroes, for good measure.
// If `toSubtreeContext` is null abort existingFlights.
final BuildContext? fromSubtreeContext = from.subtreeContext;
final Map<Object, _HeroState> fromHeroes = fromSubtreeContext != null
? Hero._allHeroesFor(fromSubtreeContext, isUserGestureTransition, navigator)
: const <Object, _HeroState>{};
final BuildContext? toSubtreeContext = to.subtreeContext;
final Map<Object, _HeroState> toHeroes = toSubtreeContext != null
? Hero._allHeroesFor(toSubtreeContext, isUserGestureTransition, navigator)
: const <Object, _HeroState>{};
for (final MapEntry<Object, _HeroState> fromHeroEntry in fromHeroes.entries) {
final Object tag = fromHeroEntry.key;
final _HeroState fromHero = fromHeroEntry.value;
final _HeroState? toHero = toHeroes[tag];
final _HeroFlight? existingFlight = _flights[tag];
final _HeroFlightManifest? manifest = toHero == null
? null
: _HeroFlightManifest(
type: flightType,
overlay: overlay,
navigatorSize: navigatorRenderObject.size,
fromRoute: from,
toRoute: to,
fromHero: fromHero,
toHero: toHero,
createRectTween: createRectTween,
shuttleBuilder: toHero.widget.flightShuttleBuilder
?? fromHero.widget.flightShuttleBuilder
?? _defaultHeroFlightShuttleBuilder,
isUserGestureTransition: isUserGestureTransition,
isDiverted: existingFlight != null,
);
// Only proceed with a valid manifest. Otherwise abort the existing
// flight, and call endFlight when this for loop finishes.
if (manifest != null && manifest.isValid) {
toHeroes.remove(tag);
if (existingFlight != null) {
existingFlight.divert(manifest);
} else {
_flights[tag] = _HeroFlight(_handleFlightEnded)..start(manifest);
}
} else {
existingFlight?.abort();
}
}
// The remaining entries in toHeroes are those failed to participate in a
// new flight (for not having a valid manifest).
//
// This can happen in a route pop transition when a fromHero is no longer
// mounted, or kept alive by the [KeepAlive] mechanism but no longer visible.
// TODO(LongCatIsLooong): resume aborted flights: https://github.com/flutter/flutter/issues/72947
for (final _HeroState toHero in toHeroes.values) {
toHero.endFlight();
}
}
void _handleFlightEnded(_HeroFlight flight) {
_flights.remove(flight.manifest.tag)?.dispose();
}
Widget _defaultHeroFlightShuttleBuilder(
BuildContext flightContext,
Animation<double> animation,
HeroFlightDirection flightDirection,
BuildContext fromHeroContext,
BuildContext toHeroContext,
) {
final Hero toHero = toHeroContext.widget as Hero;
final MediaQueryData? toMediaQueryData = MediaQuery.maybeOf(toHeroContext);
final MediaQueryData? fromMediaQueryData = MediaQuery.maybeOf(fromHeroContext);
if (toMediaQueryData == null || fromMediaQueryData == null) {
return toHero.child;
}
final EdgeInsets fromHeroPadding = fromMediaQueryData.padding;
final EdgeInsets toHeroPadding = toMediaQueryData.padding;
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return MediaQuery(
data: toMediaQueryData.copyWith(
padding: (flightDirection == HeroFlightDirection.push)
? EdgeInsetsTween(
begin: fromHeroPadding,
end: toHeroPadding,
).evaluate(animation)
: EdgeInsetsTween(
begin: toHeroPadding,
end: fromHeroPadding,
).evaluate(animation),
),
child: toHero.child);
},
);
}
/// Releases resources.
@mustCallSuper
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
for (final _HeroFlight flight in _flights.values) {
flight.dispose();
}
}
}
/// Enables or disables [Hero]es in the widget subtree.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=AaIASk2u1C0}
///
/// When [enabled] is false, all [Hero] widgets in this subtree will not be
/// involved in hero animations.
///
/// When [enabled] is true (the default), [Hero] widgets may be involved in
/// hero animations, as usual.
class HeroMode extends StatelessWidget {
/// Creates a widget that enables or disables [Hero]es.
const HeroMode({
super.key,
required this.child,
this.enabled = true,
});
/// The subtree to place inside the [HeroMode].
final Widget child;
/// Whether or not [Hero]es are enabled in this subtree.
///
/// If this property is false, the [Hero]es in this subtree will not animate
/// on route changes. Otherwise, they will animate as usual.
///
/// Defaults to true.
final bool enabled;
@override
Widget build(BuildContext context) => child;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('mode', value: enabled, ifTrue: 'enabled', ifFalse: 'disabled', showName: true));
}
}
| flutter/packages/flutter/lib/src/widgets/heroes.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/heroes.dart",
"repo_id": "flutter",
"token_count": 14555
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
// Examples can assume:
// class Intl { Intl._(); static String message(String s, { String? name, String? locale }) => ''; }
// Future<void> initializeMessages(String locale) => Future<void>.value();
// late BuildContext context;
// class Foo { }
// const Widget myWidget = Placeholder();
// Used by loadAll() to record LocalizationsDelegate.load() futures we're
// waiting for.
class _Pending {
_Pending(this.delegate, this.futureValue);
final LocalizationsDelegate<dynamic> delegate;
final Future<dynamic> futureValue;
}
// A utility function used by Localizations to generate one future
// that completes when all of the LocalizationsDelegate.load() futures
// complete. The returned map is indexed by each delegate's type.
//
// The input future values must have distinct types.
//
// The returned Future<Map> will resolve when all of the input map's
// future values have resolved. If all of the input map's values are
// SynchronousFutures then a SynchronousFuture will be returned
// immediately.
//
// This is more complicated than just applying Future.wait to input
// because some of the input.values may be SynchronousFutures. We don't want
// to Future.wait for the synchronous futures.
Future<Map<Type, dynamic>> _loadAll(Locale locale, Iterable<LocalizationsDelegate<dynamic>> allDelegates) {
final Map<Type, dynamic> output = <Type, dynamic>{};
List<_Pending>? pendingList;
// Only load the first delegate for each delegate type that supports
// locale.languageCode.
final Set<Type> types = <Type>{};
final List<LocalizationsDelegate<dynamic>> delegates = <LocalizationsDelegate<dynamic>>[];
for (final LocalizationsDelegate<dynamic> delegate in allDelegates) {
if (!types.contains(delegate.type) && delegate.isSupported(locale)) {
types.add(delegate.type);
delegates.add(delegate);
}
}
for (final LocalizationsDelegate<dynamic> delegate in delegates) {
final Future<dynamic> inputValue = delegate.load(locale);
dynamic completedValue;
final Future<dynamic> futureValue = inputValue.then<dynamic>((dynamic value) {
return completedValue = value;
});
if (completedValue != null) { // inputValue was a SynchronousFuture
final Type type = delegate.type;
assert(!output.containsKey(type));
output[type] = completedValue;
} else {
pendingList ??= <_Pending>[];
pendingList.add(_Pending(delegate, futureValue));
}
}
// All of the delegate.load() values were synchronous futures, we're done.
if (pendingList == null) {
return SynchronousFuture<Map<Type, dynamic>>(output);
}
// Some of delegate.load() values were asynchronous futures. Wait for them.
return Future.wait<dynamic>(pendingList.map<Future<dynamic>>((_Pending p) => p.futureValue))
.then<Map<Type, dynamic>>((List<dynamic> values) {
assert(values.length == pendingList!.length);
for (int i = 0; i < values.length; i += 1) {
final Type type = pendingList![i].delegate.type;
assert(!output.containsKey(type));
output[type] = values[i];
}
return output;
});
}
/// A factory for a set of localized resources of type `T`, to be loaded by a
/// [Localizations] widget.
///
/// Typical applications have one [Localizations] widget which is created by the
/// [WidgetsApp] and configured with the app's `localizationsDelegates`
/// parameter (a list of delegates). The delegate's [type] is used to identify
/// the object created by an individual delegate's [load] method.
///
/// An example of a class used as the value of `T` here would be
/// [MaterialLocalizations].
abstract class LocalizationsDelegate<T> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const LocalizationsDelegate();
/// Whether resources for the given locale can be loaded by this delegate.
///
/// Return true if the instance of `T` loaded by this delegate's [load]
/// method supports the given `locale`'s language.
bool isSupported(Locale locale);
/// Start loading the resources for `locale`. The returned future completes
/// when the resources have finished loading.
///
/// It's assumed that this method will return an object that contains a
/// collection of related string resources (typically defined with one method
/// per resource). The object will be retrieved with [Localizations.of].
Future<T> load(Locale locale);
/// Returns true if the resources for this delegate should be loaded
/// again by calling the [load] method.
///
/// This method is called whenever its [Localizations] widget is
/// rebuilt. If it returns true then dependent widgets will be rebuilt
/// after [load] has completed.
bool shouldReload(covariant LocalizationsDelegate<T> old);
/// The type of the object returned by the [load] method, T by default.
///
/// This type is used to retrieve the object "loaded" by this
/// [LocalizationsDelegate] from the [Localizations] inherited widget.
/// For example the object loaded by `LocalizationsDelegate<Foo>` would
/// be retrieved with:
///
/// ```dart
/// Foo foo = Localizations.of<Foo>(context, Foo)!;
/// ```
///
/// It's rarely necessary to override this getter.
Type get type => T;
@override
String toString() => '${objectRuntimeType(this, 'LocalizationsDelegate')}[$type]';
}
/// Interface for localized resource values for the lowest levels of the Flutter
/// framework.
///
/// This class also maps locales to a specific [Directionality] using the
/// [textDirection] property.
///
/// See also:
///
/// * [DefaultWidgetsLocalizations], which implements this interface and
/// supports a variety of locales.
abstract class WidgetsLocalizations {
/// The reading direction for text in this locale.
TextDirection get textDirection;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list to the start of the list.
String get reorderItemToStart;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list to the end of the list.
String get reorderItemToEnd;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list one space up the list.
String get reorderItemUp;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list one space down the list.
String get reorderItemDown;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list one space left in the list.
String get reorderItemLeft;
/// The semantics label used for [SliverReorderableList] to reorder an item in the
/// list one space right in the list.
String get reorderItemRight;
/// The `WidgetsLocalizations` from the closest [Localizations] instance
/// that encloses the given context.
///
/// This method is just a convenient shorthand for:
/// `Localizations.of<WidgetsLocalizations>(context, WidgetsLocalizations)!`.
///
/// References to the localized resources defined by this class are typically
/// written in terms of this method. For example:
///
/// ```dart
/// textDirection: WidgetsLocalizations.of(context).textDirection,
/// ```
static WidgetsLocalizations of(BuildContext context) {
assert(debugCheckHasWidgetsLocalizations(context));
return Localizations.of<WidgetsLocalizations>(context, WidgetsLocalizations)!;
}
}
class _WidgetsLocalizationsDelegate extends LocalizationsDelegate<WidgetsLocalizations> {
const _WidgetsLocalizationsDelegate();
// This is convenient simplification. It would be more correct test if the locale's
// text-direction is LTR.
@override
bool isSupported(Locale locale) => true;
@override
Future<WidgetsLocalizations> load(Locale locale) => DefaultWidgetsLocalizations.load(locale);
@override
bool shouldReload(_WidgetsLocalizationsDelegate old) => false;
@override
String toString() => 'DefaultWidgetsLocalizations.delegate(en_US)';
}
/// US English localizations for the widgets library.
///
/// See also:
///
/// * [GlobalWidgetsLocalizations], which provides widgets localizations for
/// many languages.
/// * [WidgetsApp.localizationsDelegates], which automatically includes
/// [DefaultWidgetsLocalizations.delegate] by default.
class DefaultWidgetsLocalizations implements WidgetsLocalizations {
/// Construct an object that defines the localized values for the widgets
/// library for US English (only).
///
/// [LocalizationsDelegate] implementations typically call the static [load]
const DefaultWidgetsLocalizations();
@override
String get reorderItemUp => 'Move up';
@override
String get reorderItemDown => 'Move down';
@override
String get reorderItemLeft => 'Move left';
@override
String get reorderItemRight => 'Move right';
@override
String get reorderItemToEnd => 'Move to the end';
@override
String get reorderItemToStart => 'Move to the start';
@override
TextDirection get textDirection => TextDirection.ltr;
/// Creates an object that provides US English resource values for the
/// lowest levels of the widgets library.
///
/// The [locale] parameter is ignored.
///
/// This method is typically used to create a [LocalizationsDelegate].
/// The [WidgetsApp] does so by default.
static Future<WidgetsLocalizations> load(Locale locale) {
return SynchronousFuture<WidgetsLocalizations>(const DefaultWidgetsLocalizations());
}
/// A [LocalizationsDelegate] that uses [DefaultWidgetsLocalizations.load]
/// to create an instance of this class.
///
/// [WidgetsApp] automatically adds this value to [WidgetsApp.localizationsDelegates].
static const LocalizationsDelegate<WidgetsLocalizations> delegate = _WidgetsLocalizationsDelegate();
}
class _LocalizationsScope extends InheritedWidget {
const _LocalizationsScope({
super.key,
required this.locale,
required this.localizationsState,
required this.typeToResources,
required super.child,
});
final Locale locale;
final _LocalizationsState localizationsState;
final Map<Type, dynamic> typeToResources;
@override
bool updateShouldNotify(_LocalizationsScope old) {
return typeToResources != old.typeToResources;
}
}
/// Defines the [Locale] for its `child` and the localized resources that the
/// child depends on.
///
/// ## Defining localized resources
///
/// {@tool snippet}
///
/// This following class is defined in terms of the
/// [Dart `intl` package](https://github.com/dart-lang/intl). Using the `intl`
/// package isn't required.
///
/// ```dart
/// class MyLocalizations {
/// MyLocalizations(this.locale);
///
/// final Locale locale;
///
/// static Future<MyLocalizations> load(Locale locale) {
/// return initializeMessages(locale.toString())
/// .then((void _) {
/// return MyLocalizations(locale);
/// });
/// }
///
/// static MyLocalizations of(BuildContext context) {
/// return Localizations.of<MyLocalizations>(context, MyLocalizations)!;
/// }
///
/// String title() => Intl.message('<title>', name: 'title', locale: locale.toString());
/// // ... more Intl.message() methods like title()
/// }
/// ```
/// {@end-tool}
/// A class based on the `intl` package imports a generated message catalog that provides
/// the `initializeMessages()` function and the per-locale backing store for `Intl.message()`.
/// The message catalog is produced by an `intl` tool that analyzes the source code for
/// classes that contain `Intl.message()` calls. In this case that would just be the
/// `MyLocalizations` class.
///
/// One could choose another approach for loading localized resources and looking them up while
/// still conforming to the structure of this example.
///
/// ## Loading localized resources
///
/// Localized resources are loaded by the list of [LocalizationsDelegate]
/// `delegates`. Each delegate is essentially a factory for a collection
/// of localized resources. There are multiple delegates because there are
/// multiple sources for localizations within an app.
///
/// Delegates are typically simple subclasses of [LocalizationsDelegate] that
/// override [LocalizationsDelegate.load]. For example a delegate for the
/// `MyLocalizations` class defined above would be:
///
/// ```dart
/// // continuing from previous example...
/// class _MyDelegate extends LocalizationsDelegate<MyLocalizations> {
/// @override
/// Future<MyLocalizations> load(Locale locale) => MyLocalizations.load(locale);
///
/// @override
/// bool isSupported(Locale locale) {
/// // in a real implementation this would only return true for
/// // locales that are definitely supported.
/// return true;
/// }
///
/// @override
/// bool shouldReload(_MyDelegate old) => false;
/// }
/// ```
///
/// Each delegate can be viewed as a factory for objects that encapsulate a set
/// of localized resources. These objects are retrieved with
/// by runtime type with [Localizations.of].
///
/// The [WidgetsApp] class creates a [Localizations] widget so most apps
/// will not need to create one. The widget app's [Localizations] delegates can
/// be initialized with [WidgetsApp.localizationsDelegates]. The [MaterialApp]
/// class also provides a `localizationsDelegates` parameter that's just
/// passed along to the [WidgetsApp].
///
/// ## Obtaining localized resources for use in user interfaces
///
/// Apps should retrieve collections of localized resources with
/// `Localizations.of<MyLocalizations>(context, MyLocalizations)`,
/// where MyLocalizations is an app specific class defines one function per
/// resource. This is conventionally done by a static `.of` method on the
/// custom localized resource class (`MyLocalizations` in the example above).
///
/// For example, using the `MyLocalizations` class defined above, one would
/// lookup a localized title string like this:
///
/// ```dart
/// // continuing from previous example...
/// MyLocalizations.of(context).title()
/// ```
///
/// If [Localizations] were to be rebuilt with a new `locale` then
/// the widget subtree that corresponds to [BuildContext] `context` would
/// be rebuilt after the corresponding resources had been loaded.
///
/// This class is effectively an [InheritedWidget]. If it's rebuilt with
/// a new `locale` or a different list of delegates or any of its
/// delegates' [LocalizationsDelegate.shouldReload()] methods returns true,
/// then widgets that have created a dependency by calling
/// `Localizations.of(context)` will be rebuilt after the resources
/// for the new locale have been loaded.
///
/// The [Localizations] widget also instantiates [Directionality] in order to
/// support the appropriate [Directionality.textDirection] of the localized
/// resources.
class Localizations extends StatefulWidget {
/// Create a widget from which localizations (like translated strings) can be obtained.
Localizations({
super.key,
required this.locale,
required this.delegates,
this.child,
}) : assert(delegates.any((LocalizationsDelegate<dynamic> delegate) => delegate is LocalizationsDelegate<WidgetsLocalizations>));
/// Overrides the inherited [Locale] or [LocalizationsDelegate]s for `child`.
///
/// This factory constructor is used for the (usually rare) situation where part
/// of an app should be localized for a different locale than the one defined
/// for the device, or if its localizations should come from a different list
/// of [LocalizationsDelegate]s than the list defined by
/// [WidgetsApp.localizationsDelegates].
///
/// For example you could specify that `myWidget` was only to be localized for
/// the US English locale:
///
/// ```dart
/// Widget build(BuildContext context) {
/// return Localizations.override(
/// context: context,
/// locale: const Locale('en', 'US'),
/// child: myWidget,
/// );
/// }
/// ```
///
/// The `locale` and `delegates` parameters default to the [Localizations.locale]
/// and [Localizations.delegates] values from the nearest [Localizations] ancestor.
///
/// To override the [Localizations.locale] or [Localizations.delegates] for an
/// entire app, specify [WidgetsApp.locale] or [WidgetsApp.localizationsDelegates]
/// (or specify the same parameters for [MaterialApp]).
factory Localizations.override({
Key? key,
required BuildContext context,
Locale? locale,
List<LocalizationsDelegate<dynamic>>? delegates,
Widget? child,
}) {
final List<LocalizationsDelegate<dynamic>> mergedDelegates = Localizations._delegatesOf(context);
if (delegates != null) {
mergedDelegates.insertAll(0, delegates);
}
return Localizations(
key: key,
locale: locale ?? Localizations.localeOf(context),
delegates: mergedDelegates,
child: child,
);
}
/// The resources returned by [Localizations.of] will be specific to this locale.
final Locale locale;
/// This list collectively defines the localized resources objects that can
/// be retrieved with [Localizations.of].
final List<LocalizationsDelegate<dynamic>> delegates;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The locale of the Localizations widget for the widget tree that
/// corresponds to [BuildContext] `context`.
///
/// If no [Localizations] widget is in scope then the [Localizations.localeOf]
/// method will throw an exception.
static Locale localeOf(BuildContext context) {
final _LocalizationsScope? scope = context.dependOnInheritedWidgetOfExactType<_LocalizationsScope>();
assert(() {
if (scope == null) {
throw FlutterError(
'Requested the Locale of a context that does not include a Localizations ancestor.\n'
'To request the Locale, the context used to retrieve the Localizations widget must '
'be that of a widget that is a descendant of a Localizations widget.',
);
}
if (scope.localizationsState.locale == null) {
throw FlutterError(
'Localizations.localeOf found a Localizations widget that had a unexpected null locale.\n',
);
}
return true;
}());
return scope!.localizationsState.locale!;
}
/// The locale of the Localizations widget for the widget tree that
/// corresponds to [BuildContext] `context`.
///
/// If no [Localizations] widget is in scope then this function will return
/// null.
static Locale? maybeLocaleOf(BuildContext context) {
final _LocalizationsScope? scope = context.dependOnInheritedWidgetOfExactType<_LocalizationsScope>();
return scope?.localizationsState.locale;
}
// There doesn't appear to be a need to make this public. See the
// Localizations.override factory constructor.
static List<LocalizationsDelegate<dynamic>> _delegatesOf(BuildContext context) {
final _LocalizationsScope? scope = context.dependOnInheritedWidgetOfExactType<_LocalizationsScope>();
assert(scope != null, 'a Localizations ancestor was not found');
return List<LocalizationsDelegate<dynamic>>.of(scope!.localizationsState.widget.delegates);
}
/// Returns the localized resources object of the given `type` for the widget
/// tree that corresponds to the given `context`.
///
/// Returns null if no resources object of the given `type` exists within
/// the given `context`.
///
/// This method is typically used by a static factory method on the `type`
/// class. For example Flutter's MaterialLocalizations class looks up Material
/// resources with a method defined like this:
///
/// ```dart
/// static MaterialLocalizations of(BuildContext context) {
/// return Localizations.of<MaterialLocalizations>(context, MaterialLocalizations)!;
/// }
/// ```
static T? of<T>(BuildContext context, Type type) {
final _LocalizationsScope? scope = context.dependOnInheritedWidgetOfExactType<_LocalizationsScope>();
return scope?.localizationsState.resourcesFor<T?>(type);
}
@override
State<Localizations> createState() => _LocalizationsState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Locale>('locale', locale));
properties.add(IterableProperty<LocalizationsDelegate<dynamic>>('delegates', delegates));
}
}
class _LocalizationsState extends State<Localizations> {
final GlobalKey _localizedResourcesScopeKey = GlobalKey();
Map<Type, dynamic> _typeToResources = <Type, dynamic>{};
Locale? get locale => _locale;
Locale? _locale;
@override
void initState() {
super.initState();
load(widget.locale);
}
bool _anyDelegatesShouldReload(Localizations old) {
if (widget.delegates.length != old.delegates.length) {
return true;
}
final List<LocalizationsDelegate<dynamic>> delegates = widget.delegates.toList();
final List<LocalizationsDelegate<dynamic>> oldDelegates = old.delegates.toList();
for (int i = 0; i < delegates.length; i += 1) {
final LocalizationsDelegate<dynamic> delegate = delegates[i];
final LocalizationsDelegate<dynamic> oldDelegate = oldDelegates[i];
if (delegate.runtimeType != oldDelegate.runtimeType || delegate.shouldReload(oldDelegate)) {
return true;
}
}
return false;
}
@override
void didUpdateWidget(Localizations old) {
super.didUpdateWidget(old);
if (widget.locale != old.locale || (_anyDelegatesShouldReload(old))) {
load(widget.locale);
}
}
void load(Locale locale) {
final Iterable<LocalizationsDelegate<dynamic>> delegates = widget.delegates;
if (delegates.isEmpty) {
_locale = locale;
return;
}
Map<Type, dynamic>? typeToResources;
final Future<Map<Type, dynamic>> typeToResourcesFuture = _loadAll(locale, delegates)
.then<Map<Type, dynamic>>((Map<Type, dynamic> value) {
return typeToResources = value;
});
if (typeToResources != null) {
// All of the delegates' resources loaded synchronously.
_typeToResources = typeToResources!;
_locale = locale;
} else {
// - Don't rebuild the dependent widgets until the resources for the new locale
// have finished loading. Until then the old locale will continue to be used.
// - If we're running at app startup time then defer reporting the first
// "useful" frame until after the async load has completed.
RendererBinding.instance.deferFirstFrame();
typeToResourcesFuture.then<void>((Map<Type, dynamic> value) {
if (mounted) {
setState(() {
_typeToResources = value;
_locale = locale;
});
}
RendererBinding.instance.allowFirstFrame();
});
}
}
T resourcesFor<T>(Type type) {
final T resources = _typeToResources[type] as T;
return resources;
}
TextDirection get _textDirection {
final WidgetsLocalizations resources = _typeToResources[WidgetsLocalizations] as WidgetsLocalizations;
return resources.textDirection;
}
@override
Widget build(BuildContext context) {
if (_locale == null) {
return const SizedBox.shrink();
}
return Semantics(
textDirection: _textDirection,
child: _LocalizationsScope(
key: _localizedResourcesScopeKey,
locale: _locale!,
localizationsState: this,
typeToResources: _typeToResources,
child: Directionality(
textDirection: _textDirection,
child: widget.child!,
),
),
);
}
}
| flutter/packages/flutter/lib/src/widgets/localizations.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/localizations.dart",
"repo_id": "flutter",
"token_count": 7166
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'basic.dart';
import 'framework.dart';
import 'routes.dart';
/// A modal route that replaces the entire screen.
///
/// The [PageRouteBuilder] subclass provides a way to create a [PageRoute] using
/// callbacks rather than by defining a new class via subclassing.
///
/// If `barrierDismissible` is true, then pressing the escape key on the keyboard
/// will cause the current route to be popped with null as the value.
///
/// See also:
///
/// * [Route], which documents the meaning of the `T` generic type argument.
abstract class PageRoute<T> extends ModalRoute<T> {
/// Creates a modal route that replaces the entire screen.
PageRoute({
super.settings,
this.fullscreenDialog = false,
this.allowSnapshotting = true,
bool barrierDismissible = false,
}) : _barrierDismissible = barrierDismissible;
/// {@template flutter.widgets.PageRoute.fullscreenDialog}
/// Whether this page route is a full-screen dialog.
///
/// In Material and Cupertino, being fullscreen has the effects of making
/// the app bars have a close button instead of a back button. On
/// iOS, dialogs transitions animate differently and are also not closeable
/// with the back swipe gesture.
/// {@endtemplate}
final bool fullscreenDialog;
@override
final bool allowSnapshotting;
@override
bool get opaque => true;
@override
bool get barrierDismissible => _barrierDismissible;
final bool _barrierDismissible;
@override
bool canTransitionTo(TransitionRoute<dynamic> nextRoute) => nextRoute is PageRoute;
@override
bool canTransitionFrom(TransitionRoute<dynamic> previousRoute) => previousRoute is PageRoute;
}
Widget _defaultTransitionsBuilder(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) {
return child;
}
/// A utility class for defining one-off page routes in terms of callbacks.
///
/// Callers must define the [pageBuilder] function which creates the route's
/// primary contents. To add transitions define the [transitionsBuilder] function.
///
/// The `T` generic type argument corresponds to the type argument of the
/// created [Route] objects.
///
/// See also:
///
/// * [Route], which documents the meaning of the `T` generic type argument.
class PageRouteBuilder<T> extends PageRoute<T> {
/// Creates a route that delegates to builder callbacks.
PageRouteBuilder({
super.settings,
required this.pageBuilder,
this.transitionsBuilder = _defaultTransitionsBuilder,
this.transitionDuration = const Duration(milliseconds: 300),
this.reverseTransitionDuration = const Duration(milliseconds: 300),
this.opaque = true,
this.barrierDismissible = false,
this.barrierColor,
this.barrierLabel,
this.maintainState = true,
super.fullscreenDialog,
super.allowSnapshotting = true,
});
/// {@template flutter.widgets.pageRouteBuilder.pageBuilder}
/// Used build the route's primary contents.
///
/// See [ModalRoute.buildPage] for complete definition of the parameters.
/// {@endtemplate}
final RoutePageBuilder pageBuilder;
/// {@template flutter.widgets.pageRouteBuilder.transitionsBuilder}
/// Used to build the route's transitions.
///
/// See [ModalRoute.buildTransitions] for complete definition of the parameters.
/// {@endtemplate}
///
/// The default transition is a jump cut (i.e. no animation).
final RouteTransitionsBuilder transitionsBuilder;
@override
final Duration transitionDuration;
@override
final Duration reverseTransitionDuration;
@override
final bool opaque;
@override
final bool barrierDismissible;
@override
final Color? barrierColor;
@override
final String? barrierLabel;
@override
final bool maintainState;
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
return pageBuilder(context, animation, secondaryAnimation);
}
@override
Widget buildTransitions(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation, Widget child) {
return transitionsBuilder(context, animation, secondaryAnimation, child);
}
}
| flutter/packages/flutter/lib/src/widgets/pages.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/pages.dart",
"repo_id": "flutter",
"token_count": 1221
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'scroll_metrics.dart';
import 'scroll_notification.dart';
/// A backend for a [ScrollActivity].
///
/// Used by subclasses of [ScrollActivity] to manipulate the scroll view that
/// they are acting upon.
///
/// See also:
///
/// * [ScrollActivity], which uses this class as its delegate.
/// * [ScrollPositionWithSingleContext], the main implementation of this interface.
abstract class ScrollActivityDelegate {
/// The direction in which the scroll view scrolls.
AxisDirection get axisDirection;
/// Update the scroll position to the given pixel value.
///
/// Returns the overscroll, if any. See [ScrollPosition.setPixels] for more
/// information.
double setPixels(double pixels);
/// Updates the scroll position by the given amount.
///
/// Appropriate for when the user is directly manipulating the scroll
/// position, for example by dragging the scroll view. Typically applies
/// [ScrollPhysics.applyPhysicsToUserOffset] and other transformations that
/// are appropriate for user-driving scrolling.
void applyUserOffset(double delta);
/// Terminate the current activity and start an idle activity.
void goIdle();
/// Terminate the current activity and start a ballistic activity with the
/// given velocity.
void goBallistic(double velocity);
}
/// Base class for scrolling activities like dragging and flinging.
///
/// See also:
///
/// * [ScrollPosition], which uses [ScrollActivity] objects to manage the
/// [ScrollPosition] of a [Scrollable].
abstract class ScrollActivity {
/// Initializes [delegate] for subclasses.
ScrollActivity(this._delegate) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$ScrollActivity',
object: this,
);
}
}
/// The delegate that this activity will use to actuate the scroll view.
ScrollActivityDelegate get delegate => _delegate;
ScrollActivityDelegate _delegate;
bool _isDisposed = false;
/// Updates the activity's link to the [ScrollActivityDelegate].
///
/// This should only be called when an activity is being moved from a defunct
/// (or about-to-be defunct) [ScrollActivityDelegate] object to a new one.
void updateDelegate(ScrollActivityDelegate value) {
assert(_delegate != value);
_delegate = value;
}
/// Called by the [ScrollActivityDelegate] when it has changed type (for
/// example, when changing from an Android-style scroll position to an
/// iOS-style scroll position). If this activity can differ between the two
/// modes, then it should tell the position to restart that activity
/// appropriately.
///
/// For example, [BallisticScrollActivity]'s implementation calls
/// [ScrollActivityDelegate.goBallistic].
void resetActivity() { }
/// Dispatch a [ScrollStartNotification] with the given metrics.
void dispatchScrollStartNotification(ScrollMetrics metrics, BuildContext? context) {
ScrollStartNotification(metrics: metrics, context: context).dispatch(context);
}
/// Dispatch a [ScrollUpdateNotification] with the given metrics and scroll delta.
void dispatchScrollUpdateNotification(ScrollMetrics metrics, BuildContext context, double scrollDelta) {
ScrollUpdateNotification(metrics: metrics, context: context, scrollDelta: scrollDelta).dispatch(context);
}
/// Dispatch an [OverscrollNotification] with the given metrics and overscroll.
void dispatchOverscrollNotification(ScrollMetrics metrics, BuildContext context, double overscroll) {
OverscrollNotification(metrics: metrics, context: context, overscroll: overscroll).dispatch(context);
}
/// Dispatch a [ScrollEndNotification] with the given metrics and overscroll.
void dispatchScrollEndNotification(ScrollMetrics metrics, BuildContext context) {
ScrollEndNotification(metrics: metrics, context: context).dispatch(context);
}
/// Called when the scroll view that is performing this activity changes its metrics.
void applyNewDimensions() { }
/// Whether the scroll view should ignore pointer events while performing this
/// activity.
///
/// See also:
///
/// * [isScrolling], which describes whether the activity is considered
/// to represent user interaction or not.
bool get shouldIgnorePointer;
/// Whether performing this activity constitutes scrolling.
///
/// Used, for example, to determine whether the user scroll
/// direction (see [ScrollPosition.userScrollDirection]) is
/// [ScrollDirection.idle].
///
/// See also:
///
/// * [shouldIgnorePointer], which controls whether pointer events
/// are allowed while the activity is live.
/// * [UserScrollNotification], which exposes this status.
bool get isScrolling;
/// If applicable, the velocity at which the scroll offset is currently
/// independently changing (i.e. without external stimuli such as a dragging
/// gestures) in logical pixels per second for this activity.
double get velocity;
/// Called when the scroll view stops performing this activity.
@mustCallSuper
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_isDisposed = true;
}
@override
String toString() => describeIdentity(this);
}
/// A scroll activity that does nothing.
///
/// When a scroll view is not scrolling, it is performing the idle activity.
///
/// If the [Scrollable] changes dimensions, this activity triggers a ballistic
/// activity to restore the view.
class IdleScrollActivity extends ScrollActivity {
/// Creates a scroll activity that does nothing.
IdleScrollActivity(super.delegate);
@override
void applyNewDimensions() {
delegate.goBallistic(0.0);
}
@override
bool get shouldIgnorePointer => false;
@override
bool get isScrolling => false;
@override
double get velocity => 0.0;
}
/// Interface for holding a [Scrollable] stationary.
///
/// An object that implements this interface is returned by
/// [ScrollPosition.hold]. It holds the scrollable stationary until an activity
/// is started or the [cancel] method is called.
abstract class ScrollHoldController {
/// Release the [Scrollable], potentially letting it go ballistic if
/// necessary.
void cancel();
}
/// A scroll activity that does nothing but can be released to resume
/// normal idle behavior.
///
/// This is used while the user is touching the [Scrollable] but before the
/// touch has become a [Drag].
///
/// For the purposes of [ScrollNotification]s, this activity does not constitute
/// scrolling, and does not prevent the user from interacting with the contents
/// of the [Scrollable] (unlike when a drag has begun or there is a scroll
/// animation underway).
class HoldScrollActivity extends ScrollActivity implements ScrollHoldController {
/// Creates a scroll activity that does nothing.
HoldScrollActivity({
required ScrollActivityDelegate delegate,
this.onHoldCanceled,
}) : super(delegate);
/// Called when [dispose] is called.
final VoidCallback? onHoldCanceled;
@override
bool get shouldIgnorePointer => false;
@override
bool get isScrolling => false;
@override
double get velocity => 0.0;
@override
void cancel() {
delegate.goBallistic(0.0);
}
@override
void dispose() {
onHoldCanceled?.call();
super.dispose();
}
}
/// Scrolls a scroll view as the user drags their finger across the screen.
///
/// See also:
///
/// * [DragScrollActivity], which is the activity the scroll view performs
/// while a drag is underway.
class ScrollDragController implements Drag {
/// Creates an object that scrolls a scroll view as the user drags their
/// finger across the screen.
ScrollDragController({
required ScrollActivityDelegate delegate,
required DragStartDetails details,
this.onDragCanceled,
this.carriedVelocity,
this.motionStartDistanceThreshold,
}) : assert(
motionStartDistanceThreshold == null || motionStartDistanceThreshold > 0.0,
'motionStartDistanceThreshold must be a positive number or null',
),
_delegate = delegate,
_lastDetails = details,
_retainMomentum = carriedVelocity != null && carriedVelocity != 0.0,
_lastNonStationaryTimestamp = details.sourceTimeStamp,
_kind = details.kind,
_offsetSinceLastStop = motionStartDistanceThreshold == null ? null : 0.0 {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$ScrollDragController',
object: this,
);
}
}
/// The object that will actuate the scroll view as the user drags.
ScrollActivityDelegate get delegate => _delegate;
ScrollActivityDelegate _delegate;
/// Called when [dispose] is called.
final VoidCallback? onDragCanceled;
/// Velocity that was present from a previous [ScrollActivity] when this drag
/// began.
final double? carriedVelocity;
/// Amount of pixels in either direction the drag has to move by to start
/// scroll movement again after each time scrolling came to a stop.
final double? motionStartDistanceThreshold;
Duration? _lastNonStationaryTimestamp;
bool _retainMomentum;
/// Null if already in motion or has no [motionStartDistanceThreshold].
double? _offsetSinceLastStop;
/// Maximum amount of time interval the drag can have consecutive stationary
/// pointer update events before losing the momentum carried from a previous
/// scroll activity.
static const Duration momentumRetainStationaryDurationThreshold =
Duration(milliseconds: 20);
/// The minimum amount of velocity needed to apply the [carriedVelocity] at
/// the end of a drag. Expressed as a factor. For example with a
/// [carriedVelocity] of 2000, we will need a velocity of at least 1000 to
/// apply the [carriedVelocity] as well. If the velocity does not meet the
/// threshold, the [carriedVelocity] is lost. Decided by fair eyeballing
/// with the scroll_overlay platform test.
static const double momentumRetainVelocityThresholdFactor = 0.5;
/// Maximum amount of time interval the drag can have consecutive stationary
/// pointer update events before needing to break the
/// [motionStartDistanceThreshold] to start motion again.
static const Duration motionStoppedDurationThreshold =
Duration(milliseconds: 50);
/// The drag distance past which, a [motionStartDistanceThreshold] breaking
/// drag is considered a deliberate fling.
static const double _bigThresholdBreakDistance = 24.0;
bool get _reversed => axisDirectionIsReversed(delegate.axisDirection);
/// Updates the controller's link to the [ScrollActivityDelegate].
///
/// This should only be called when a controller is being moved from a defunct
/// (or about-to-be defunct) [ScrollActivityDelegate] object to a new one.
void updateDelegate(ScrollActivityDelegate value) {
assert(_delegate != value);
_delegate = value;
}
/// Determines whether to lose the existing incoming velocity when starting
/// the drag.
void _maybeLoseMomentum(double offset, Duration? timestamp) {
if (_retainMomentum &&
offset == 0.0 &&
(timestamp == null || // If drag event has no timestamp, we lose momentum.
timestamp - _lastNonStationaryTimestamp! > momentumRetainStationaryDurationThreshold)) {
// If pointer is stationary for too long, we lose momentum.
_retainMomentum = false;
}
}
/// If a motion start threshold exists, determine whether the threshold needs
/// to be broken to scroll. Also possibly apply an offset adjustment when
/// threshold is first broken.
///
/// Returns `0.0` when stationary or within threshold. Returns `offset`
/// transparently when already in motion.
double _adjustForScrollStartThreshold(double offset, Duration? timestamp) {
if (timestamp == null) {
// If we can't track time, we can't apply thresholds.
// May be null for proxied drags like via accessibility.
return offset;
}
if (offset == 0.0) {
if (motionStartDistanceThreshold != null &&
_offsetSinceLastStop == null &&
timestamp - _lastNonStationaryTimestamp! > motionStoppedDurationThreshold) {
// Enforce a new threshold.
_offsetSinceLastStop = 0.0;
}
// Not moving can't break threshold.
return 0.0;
} else {
if (_offsetSinceLastStop == null) {
// Already in motion or no threshold behavior configured such as for
// Android. Allow transparent offset transmission.
return offset;
} else {
_offsetSinceLastStop = _offsetSinceLastStop! + offset;
if (_offsetSinceLastStop!.abs() > motionStartDistanceThreshold!) {
// Threshold broken.
_offsetSinceLastStop = null;
if (offset.abs() > _bigThresholdBreakDistance) {
// This is heuristically a very deliberate fling. Leave the motion
// unaffected.
return offset;
} else {
// This is a normal speed threshold break.
return math.min(
// Ease into the motion when the threshold is initially broken
// to avoid a visible jump.
motionStartDistanceThreshold! / 3.0,
offset.abs(),
) * offset.sign;
}
} else {
return 0.0;
}
}
}
}
@override
void update(DragUpdateDetails details) {
assert(details.primaryDelta != null);
_lastDetails = details;
double offset = details.primaryDelta!;
if (offset != 0.0) {
_lastNonStationaryTimestamp = details.sourceTimeStamp;
}
// By default, iOS platforms carries momentum and has a start threshold
// (configured in [BouncingScrollPhysics]). The 2 operations below are
// no-ops on Android.
_maybeLoseMomentum(offset, details.sourceTimeStamp);
offset = _adjustForScrollStartThreshold(offset, details.sourceTimeStamp);
if (offset == 0.0) {
return;
}
if (_reversed) {
offset = -offset;
}
delegate.applyUserOffset(offset);
}
@override
void end(DragEndDetails details) {
assert(details.primaryVelocity != null);
// We negate the velocity here because if the touch is moving downwards,
// the scroll has to move upwards. It's the same reason that update()
// above negates the delta before applying it to the scroll offset.
double velocity = -details.primaryVelocity!;
if (_reversed) {
velocity = -velocity;
}
_lastDetails = details;
if (_retainMomentum) {
// Build momentum only if dragging in the same direction.
final bool isFlingingInSameDirection = velocity.sign == carriedVelocity!.sign;
// Build momentum only if the velocity of the last drag was not
// substantially lower than the carried momentum.
final bool isVelocityNotSubstantiallyLessThanCarriedMomentum =
velocity.abs() > carriedVelocity!.abs() * momentumRetainVelocityThresholdFactor;
if (isFlingingInSameDirection && isVelocityNotSubstantiallyLessThanCarriedMomentum) {
velocity += carriedVelocity!;
}
}
delegate.goBallistic(velocity);
}
@override
void cancel() {
delegate.goBallistic(0.0);
}
/// Called by the delegate when it is no longer sending events to this object.
@mustCallSuper
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_lastDetails = null;
onDragCanceled?.call();
}
/// The type of input device driving the drag.
final PointerDeviceKind? _kind;
/// The most recently observed [DragStartDetails], [DragUpdateDetails], or
/// [DragEndDetails] object.
dynamic get lastDetails => _lastDetails;
dynamic _lastDetails;
@override
String toString() => describeIdentity(this);
}
/// The activity a scroll view performs when the user drags their finger
/// across the screen.
///
/// See also:
///
/// * [ScrollDragController], which listens to the [Drag] and actually scrolls
/// the scroll view.
class DragScrollActivity extends ScrollActivity {
/// Creates an activity for when the user drags their finger across the
/// screen.
DragScrollActivity(
super.delegate,
ScrollDragController controller,
) : _controller = controller;
ScrollDragController? _controller;
@override
void dispatchScrollStartNotification(ScrollMetrics metrics, BuildContext? context) {
final dynamic lastDetails = _controller!.lastDetails;
assert(lastDetails is DragStartDetails);
ScrollStartNotification(metrics: metrics, context: context, dragDetails: lastDetails as DragStartDetails).dispatch(context);
}
@override
void dispatchScrollUpdateNotification(ScrollMetrics metrics, BuildContext context, double scrollDelta) {
final dynamic lastDetails = _controller!.lastDetails;
assert(lastDetails is DragUpdateDetails);
ScrollUpdateNotification(metrics: metrics, context: context, scrollDelta: scrollDelta, dragDetails: lastDetails as DragUpdateDetails).dispatch(context);
}
@override
void dispatchOverscrollNotification(ScrollMetrics metrics, BuildContext context, double overscroll) {
final dynamic lastDetails = _controller!.lastDetails;
assert(lastDetails is DragUpdateDetails);
OverscrollNotification(metrics: metrics, context: context, overscroll: overscroll, dragDetails: lastDetails as DragUpdateDetails).dispatch(context);
}
@override
void dispatchScrollEndNotification(ScrollMetrics metrics, BuildContext context) {
// We might not have DragEndDetails yet if we're being called from beginActivity.
final dynamic lastDetails = _controller!.lastDetails;
ScrollEndNotification(
metrics: metrics,
context: context,
dragDetails: lastDetails is DragEndDetails ? lastDetails : null,
).dispatch(context);
}
@override
bool get shouldIgnorePointer => _controller?._kind != PointerDeviceKind.trackpad;
@override
bool get isScrolling => true;
// DragScrollActivity is not independently changing velocity yet
// until the drag is ended.
@override
double get velocity => 0.0;
@override
void dispose() {
_controller = null;
super.dispose();
}
@override
String toString() {
return '${describeIdentity(this)}($_controller)';
}
}
/// An activity that animates a scroll view based on a physics [Simulation].
///
/// A [BallisticScrollActivity] is typically used when the user lifts their
/// finger off the screen to continue the scrolling gesture with the current velocity.
///
/// [BallisticScrollActivity] is also used to restore a scroll view to a valid
/// scroll offset when the geometry of the scroll view changes. In these
/// situations, the [Simulation] typically starts with a zero velocity.
///
/// See also:
///
/// * [DrivenScrollActivity], which animates a scroll view based on a set of
/// animation parameters.
class BallisticScrollActivity extends ScrollActivity {
/// Creates an activity that animates a scroll view based on a [simulation].
BallisticScrollActivity(
super.delegate,
Simulation simulation,
TickerProvider vsync,
this.shouldIgnorePointer,
) {
_controller = AnimationController.unbounded(
debugLabel: kDebugMode ? objectRuntimeType(this, 'BallisticScrollActivity') : null,
vsync: vsync,
)
..addListener(_tick)
..animateWith(simulation)
.whenComplete(_end); // won't trigger if we dispose _controller before it completes.
}
late AnimationController _controller;
@override
void resetActivity() {
delegate.goBallistic(velocity);
}
@override
void applyNewDimensions() {
delegate.goBallistic(velocity);
}
void _tick() {
if (!applyMoveTo(_controller.value)) {
delegate.goIdle();
}
}
/// Move the position to the given location.
///
/// If the new position was fully applied, returns true. If there was any
/// overflow, returns false.
///
/// The default implementation calls [ScrollActivityDelegate.setPixels]
/// and returns true if the overflow was zero.
@protected
bool applyMoveTo(double value) {
return delegate.setPixels(value).abs() < precisionErrorTolerance;
}
void _end() {
// Check if the activity was disposed before going ballistic because _end might be called
// if _controller is disposed just after completion.
if (!_isDisposed) {
delegate.goBallistic(0.0);
}
}
@override
void dispatchOverscrollNotification(ScrollMetrics metrics, BuildContext context, double overscroll) {
OverscrollNotification(metrics: metrics, context: context, overscroll: overscroll, velocity: velocity).dispatch(context);
}
@override
final bool shouldIgnorePointer;
@override
bool get isScrolling => true;
@override
double get velocity => _controller.velocity;
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
String toString() {
return '${describeIdentity(this)}($_controller)';
}
}
/// An activity that animates a scroll view based on animation parameters.
///
/// For example, a [DrivenScrollActivity] is used to implement
/// [ScrollController.animateTo].
///
/// See also:
///
/// * [BallisticScrollActivity], which animates a scroll view based on a
/// physics [Simulation].
class DrivenScrollActivity extends ScrollActivity {
/// Creates an activity that animates a scroll view based on animation
/// parameters.
DrivenScrollActivity(
super.delegate, {
required double from,
required double to,
required Duration duration,
required Curve curve,
required TickerProvider vsync,
}) : assert(duration > Duration.zero) {
_completer = Completer<void>();
_controller = AnimationController.unbounded(
value: from,
debugLabel: objectRuntimeType(this, 'DrivenScrollActivity'),
vsync: vsync,
)
..addListener(_tick)
..animateTo(to, duration: duration, curve: curve)
.whenComplete(_end); // won't trigger if we dispose _controller before it completes.
}
late final Completer<void> _completer;
late final AnimationController _controller;
/// A [Future] that completes when the activity stops.
///
/// For example, this [Future] will complete if the animation reaches the end
/// or if the user interacts with the scroll view in way that causes the
/// animation to stop before it reaches the end.
Future<void> get done => _completer.future;
void _tick() {
if (delegate.setPixels(_controller.value) != 0.0) {
delegate.goIdle();
}
}
void _end() {
// Check if the activity was disposed before going ballistic because _end might be called
// if _controller is disposed just after completion.
if (!_isDisposed) {
delegate.goBallistic(velocity);
}
}
@override
void dispatchOverscrollNotification(ScrollMetrics metrics, BuildContext context, double overscroll) {
OverscrollNotification(metrics: metrics, context: context, overscroll: overscroll, velocity: velocity).dispatch(context);
}
@override
bool get shouldIgnorePointer => true;
@override
bool get isScrolling => true;
@override
double get velocity => _controller.velocity;
@override
void dispose() {
_completer.complete();
_controller.dispose();
super.dispose();
}
@override
String toString() {
return '${describeIdentity(this)}($_controller)';
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_activity.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_activity.dart",
"repo_id": "flutter",
"token_count": 7334
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'binding.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'media_query.dart';
import 'notification_listener.dart';
import 'primary_scroll_controller.dart';
import 'scroll_configuration.dart';
import 'scroll_controller.dart';
import 'scroll_metrics.dart';
import 'scroll_notification.dart';
import 'scroll_position.dart';
import 'scrollable.dart';
import 'scrollable_helpers.dart';
import 'ticker_provider.dart';
const double _kMinThumbExtent = 18.0;
const double _kMinInteractiveSize = 48.0;
const double _kScrollbarThickness = 6.0;
const Duration _kScrollbarFadeDuration = Duration(milliseconds: 300);
const Duration _kScrollbarTimeToFade = Duration(milliseconds: 600);
/// An orientation along either the horizontal or vertical [Axis].
enum ScrollbarOrientation {
/// Place towards the left of the screen.
left,
/// Place towards the right of the screen.
right,
/// Place on top of the screen.
top,
/// Place on the bottom of the screen.
bottom,
}
/// Paints a scrollbar's track and thumb.
///
/// The size of the scrollbar along its scroll direction is typically
/// proportional to the percentage of content completely visible on screen,
/// as long as its size isn't less than [minLength] and it isn't overscrolling.
///
/// Unlike [CustomPainter]s that subclasses [CustomPainter] and only repaint
/// when [shouldRepaint] returns true (which requires this [CustomPainter] to
/// be rebuilt), this painter has the added optimization of repainting and not
/// rebuilding when:
///
/// * the scroll position changes; and
/// * when the scrollbar fades away.
///
/// Calling [update] with the new [ScrollMetrics] will repaint the new scrollbar
/// position.
///
/// Updating the value on the provided [fadeoutOpacityAnimation] will repaint
/// with the new opacity.
///
/// You must call [dispose] on this [ScrollbarPainter] when it's no longer used.
///
/// See also:
///
/// * [Scrollbar] for a widget showing a scrollbar around a [Scrollable] in the
/// Material Design style.
/// * [CupertinoScrollbar] for a widget showing a scrollbar around a
/// [Scrollable] in the iOS style.
class ScrollbarPainter extends ChangeNotifier implements CustomPainter {
/// Creates a scrollbar with customizations given by construction arguments.
ScrollbarPainter({
required Color color,
required this.fadeoutOpacityAnimation,
Color trackColor = const Color(0x00000000),
Color trackBorderColor = const Color(0x00000000),
TextDirection? textDirection,
double thickness = _kScrollbarThickness,
EdgeInsets padding = EdgeInsets.zero,
double mainAxisMargin = 0.0,
double crossAxisMargin = 0.0,
Radius? radius,
Radius? trackRadius,
OutlinedBorder? shape,
double minLength = _kMinThumbExtent,
double? minOverscrollLength,
ScrollbarOrientation? scrollbarOrientation,
bool ignorePointer = false,
}) : assert(radius == null || shape == null),
assert(minLength >= 0),
assert(minOverscrollLength == null || minOverscrollLength <= minLength),
assert(minOverscrollLength == null || minOverscrollLength >= 0),
assert(padding.isNonNegative),
_color = color,
_textDirection = textDirection,
_thickness = thickness,
_radius = radius,
_shape = shape,
_padding = padding,
_mainAxisMargin = mainAxisMargin,
_crossAxisMargin = crossAxisMargin,
_minLength = minLength,
_trackColor = trackColor,
_trackBorderColor = trackBorderColor,
_trackRadius = trackRadius,
_scrollbarOrientation = scrollbarOrientation,
_minOverscrollLength = minOverscrollLength ?? minLength,
_ignorePointer = ignorePointer {
fadeoutOpacityAnimation.addListener(notifyListeners);
}
/// [Color] of the thumb. Mustn't be null.
Color get color => _color;
Color _color;
set color(Color value) {
if (color == value) {
return;
}
_color = value;
notifyListeners();
}
/// [Color] of the track. Mustn't be null.
Color get trackColor => _trackColor;
Color _trackColor;
set trackColor(Color value) {
if (trackColor == value) {
return;
}
_trackColor = value;
notifyListeners();
}
/// [Color] of the track border. Mustn't be null.
Color get trackBorderColor => _trackBorderColor;
Color _trackBorderColor;
set trackBorderColor(Color value) {
if (trackBorderColor == value) {
return;
}
_trackBorderColor = value;
notifyListeners();
}
/// [Radius] of corners of the Scrollbar's track.
///
/// Scrollbar's track will be rectangular if [trackRadius] is null.
Radius? get trackRadius => _trackRadius;
Radius? _trackRadius;
set trackRadius(Radius? value) {
if (trackRadius == value) {
return;
}
_trackRadius = value;
notifyListeners();
}
/// [TextDirection] of the [BuildContext] which dictates the side of the
/// screen the scrollbar appears in (the trailing side). Must be set prior to
/// calling paint.
TextDirection? get textDirection => _textDirection;
TextDirection? _textDirection;
set textDirection(TextDirection? value) {
assert(value != null);
if (textDirection == value) {
return;
}
_textDirection = value;
notifyListeners();
}
/// Thickness of the scrollbar in its cross-axis in logical pixels. Mustn't be null.
double get thickness => _thickness;
double _thickness;
set thickness(double value) {
if (thickness == value) {
return;
}
_thickness = value;
notifyListeners();
}
/// An opacity [Animation] that dictates the opacity of the thumb.
/// Changes in value of this [Listenable] will automatically trigger repaints.
/// Mustn't be null.
final Animation<double> fadeoutOpacityAnimation;
/// Distance from the scrollbar thumb's start and end to the edge of the
/// viewport in logical pixels. It affects the amount of available paint area.
///
/// The scrollbar track consumes this space.
///
/// Mustn't be null and defaults to 0.
double get mainAxisMargin => _mainAxisMargin;
double _mainAxisMargin;
set mainAxisMargin(double value) {
if (mainAxisMargin == value) {
return;
}
_mainAxisMargin = value;
notifyListeners();
}
/// Distance from the scrollbar thumb to the nearest cross axis edge
/// in logical pixels.
///
/// The scrollbar track consumes this space.
///
/// Defaults to zero.
double get crossAxisMargin => _crossAxisMargin;
double _crossAxisMargin;
set crossAxisMargin(double value) {
if (crossAxisMargin == value) {
return;
}
_crossAxisMargin = value;
notifyListeners();
}
/// [Radius] of corners if the scrollbar should have rounded corners.
///
/// Scrollbar will be rectangular if [radius] is null.
Radius? get radius => _radius;
Radius? _radius;
set radius(Radius? value) {
assert(shape == null || value == null);
if (radius == value) {
return;
}
_radius = value;
notifyListeners();
}
/// The [OutlinedBorder] of the scrollbar's thumb.
///
/// Only one of [radius] and [shape] may be specified. For a rounded rectangle,
/// it's simplest to just specify [radius]. By default, the scrollbar thumb's
/// shape is a simple rectangle.
///
/// If [shape] is specified, the thumb will take the shape of the passed
/// [OutlinedBorder] and fill itself with [color] (or grey if it
/// is unspecified).
///
OutlinedBorder? get shape => _shape;
OutlinedBorder? _shape;
set shape(OutlinedBorder? value){
assert(radius == null || value == null);
if (shape == value) {
return;
}
_shape = value;
notifyListeners();
}
/// The amount of space by which to inset the scrollbar's start and end, as
/// well as its side to the nearest edge, in logical pixels.
///
/// This is typically set to the current [MediaQueryData.padding] to avoid
/// partial obstructions such as display notches. If you only want additional
/// margins around the scrollbar, see [mainAxisMargin].
///
/// Defaults to [EdgeInsets.zero]. Offsets from all four directions must be
/// greater than or equal to zero.
EdgeInsets get padding => _padding;
EdgeInsets _padding;
set padding(EdgeInsets value) {
if (padding == value) {
return;
}
_padding = value;
notifyListeners();
}
/// The preferred smallest size the scrollbar thumb can shrink to when the total
/// scrollable extent is large, the current visible viewport is small, and the
/// viewport is not overscrolled.
///
/// The size of the scrollbar may shrink to a smaller size than [minLength] to
/// fit in the available paint area. E.g., when [minLength] is
/// `double.infinity`, it will not be respected if
/// [ScrollMetrics.viewportDimension] and [mainAxisMargin] are finite.
///
/// Mustn't be null and the value has to be greater or equal to
/// [minOverscrollLength], which in turn is >= 0. Defaults to 18.0.
double get minLength => _minLength;
double _minLength;
set minLength(double value) {
if (minLength == value) {
return;
}
_minLength = value;
notifyListeners();
}
/// The preferred smallest size the scrollbar thumb can shrink to when viewport is
/// overscrolled.
///
/// When overscrolling, the size of the scrollbar may shrink to a smaller size
/// than [minOverscrollLength] to fit in the available paint area. E.g., when
/// [minOverscrollLength] is `double.infinity`, it will not be respected if
/// the [ScrollMetrics.viewportDimension] and [mainAxisMargin] are finite.
///
/// The value is less than or equal to [minLength] and greater than or equal to 0.
/// When null, it will default to the value of [minLength].
double get minOverscrollLength => _minOverscrollLength;
double _minOverscrollLength;
set minOverscrollLength(double value) {
if (minOverscrollLength == value) {
return;
}
_minOverscrollLength = value;
notifyListeners();
}
/// {@template flutter.widgets.Scrollbar.scrollbarOrientation}
/// Dictates the orientation of the scrollbar.
///
/// [ScrollbarOrientation.top] places the scrollbar on top of the screen.
/// [ScrollbarOrientation.bottom] places the scrollbar on the bottom of the screen.
/// [ScrollbarOrientation.left] places the scrollbar on the left of the screen.
/// [ScrollbarOrientation.right] places the scrollbar on the right of the screen.
///
/// [ScrollbarOrientation.top] and [ScrollbarOrientation.bottom] can only be
/// used with a vertical scroll.
/// [ScrollbarOrientation.left] and [ScrollbarOrientation.right] can only be
/// used with a horizontal scroll.
///
/// For a vertical scroll the orientation defaults to
/// [ScrollbarOrientation.right] for [TextDirection.ltr] and
/// [ScrollbarOrientation.left] for [TextDirection.rtl].
/// For a horizontal scroll the orientation defaults to [ScrollbarOrientation.bottom].
/// {@endtemplate}
ScrollbarOrientation? get scrollbarOrientation => _scrollbarOrientation;
ScrollbarOrientation? _scrollbarOrientation;
set scrollbarOrientation(ScrollbarOrientation? value) {
if (scrollbarOrientation == value) {
return;
}
_scrollbarOrientation = value;
notifyListeners();
}
/// Whether the painter will be ignored during hit testing.
bool get ignorePointer => _ignorePointer;
bool _ignorePointer;
set ignorePointer(bool value) {
if (ignorePointer == value) {
return;
}
_ignorePointer = value;
notifyListeners();
}
// - Scrollbar Details
Rect? _trackRect;
// The full painted length of the track
double get _trackExtent => _lastMetrics!.viewportDimension - _totalTrackMainAxisOffsets;
// The full length of the track that the thumb can travel
double get _traversableTrackExtent => _trackExtent - (2 * mainAxisMargin);
// Track Offsets
// The track is offset by only padding.
double get _totalTrackMainAxisOffsets => _isVertical ? padding.vertical : padding.horizontal;
double get _leadingTrackMainAxisOffset {
return switch (_resolvedOrientation) {
ScrollbarOrientation.left || ScrollbarOrientation.right => padding.top,
ScrollbarOrientation.top || ScrollbarOrientation.bottom => padding.left,
};
}
Rect? _thumbRect;
// The current scroll position + _leadingThumbMainAxisOffset
late double _thumbOffset;
// The fraction visible in relation to the traversable length of the track.
late double _thumbExtent;
// Thumb Offsets
// The thumb is offset by padding and margins.
double get _leadingThumbMainAxisOffset => _leadingTrackMainAxisOffset + mainAxisMargin;
void _setThumbExtent() {
// Thumb extent reflects fraction of content visible, as long as this
// isn't less than the absolute minimum size.
// _totalContentExtent >= viewportDimension, so (_totalContentExtent - _mainAxisPadding) > 0
final double fractionVisible = clampDouble(
(_lastMetrics!.extentInside - _totalTrackMainAxisOffsets)
/ (_totalContentExtent - _totalTrackMainAxisOffsets),
0.0,
1.0,
);
final double thumbExtent = math.max(
math.min(_traversableTrackExtent, minOverscrollLength),
_traversableTrackExtent * fractionVisible,
);
final double fractionOverscrolled = 1.0 - _lastMetrics!.extentInside / _lastMetrics!.viewportDimension;
final double safeMinLength = math.min(minLength, _traversableTrackExtent);
final double newMinLength = (_beforeExtent > 0 && _afterExtent > 0)
// Thumb extent is no smaller than minLength if scrolling normally.
? safeMinLength
// User is overscrolling. Thumb extent can be less than minLength
// but no smaller than minOverscrollLength. We can't use the
// fractionVisible to produce intermediate values between minLength and
// minOverscrollLength when the user is transitioning from regular
// scrolling to overscrolling, so we instead use the percentage of the
// content that is still in the viewport to determine the size of the
// thumb. iOS behavior appears to have the thumb reach its minimum size
// with ~20% of overscroll. We map the percentage of minLength from
// [0.8, 1.0] to [0.0, 1.0], so 0% to 20% of overscroll will produce
// values for the thumb that range between minLength and the smallest
// possible value, minOverscrollLength.
: safeMinLength * (1.0 - clampDouble(fractionOverscrolled, 0.0, 0.2) / 0.2);
// The `thumbExtent` should be no greater than `trackSize`, otherwise
// the scrollbar may scroll towards the wrong direction.
_thumbExtent = clampDouble(thumbExtent, newMinLength, _traversableTrackExtent);
}
// - Scrollable Details
ScrollMetrics? _lastMetrics;
bool get _lastMetricsAreScrollable => _lastMetrics!.minScrollExtent != _lastMetrics!.maxScrollExtent;
AxisDirection? _lastAxisDirection;
bool get _isVertical => _lastAxisDirection == AxisDirection.down || _lastAxisDirection == AxisDirection.up;
bool get _isReversed => _lastAxisDirection == AxisDirection.up || _lastAxisDirection == AxisDirection.left;
// The amount of scroll distance before and after the current position.
double get _beforeExtent => _isReversed ? _lastMetrics!.extentAfter : _lastMetrics!.extentBefore;
double get _afterExtent => _isReversed ? _lastMetrics!.extentBefore : _lastMetrics!.extentAfter;
// The total size of the scrollable content.
double get _totalContentExtent {
return _lastMetrics!.maxScrollExtent
- _lastMetrics!.minScrollExtent
+ _lastMetrics!.viewportDimension;
}
ScrollbarOrientation get _resolvedOrientation {
if (scrollbarOrientation == null) {
if (_isVertical) {
return textDirection == TextDirection.ltr
? ScrollbarOrientation.right
: ScrollbarOrientation.left;
}
return ScrollbarOrientation.bottom;
}
return scrollbarOrientation!;
}
void _debugAssertIsValidOrientation(ScrollbarOrientation orientation) {
assert(
() {
bool isVerticalOrientation(ScrollbarOrientation orientation) =>
orientation == ScrollbarOrientation.left
|| orientation == ScrollbarOrientation.right;
return (_isVertical && isVerticalOrientation(orientation))
|| (!_isVertical && !isVerticalOrientation(orientation));
}(),
'The given ScrollbarOrientation: $orientation is incompatible with the '
'current AxisDirection: $_lastAxisDirection.'
);
}
// - Updating
/// Update with new [ScrollMetrics]. If the metrics change, the scrollbar will
/// show and redraw itself based on these new metrics.
///
/// The scrollbar will remain on screen.
void update(
ScrollMetrics metrics,
AxisDirection axisDirection,
) {
if (_lastMetrics != null &&
_lastMetrics!.extentBefore == metrics.extentBefore &&
_lastMetrics!.extentInside == metrics.extentInside &&
_lastMetrics!.extentAfter == metrics.extentAfter &&
_lastAxisDirection == axisDirection) {
return;
}
final ScrollMetrics? oldMetrics = _lastMetrics;
_lastMetrics = metrics;
_lastAxisDirection = axisDirection;
bool needPaint(ScrollMetrics? metrics) => metrics != null && metrics.maxScrollExtent > metrics.minScrollExtent;
if (!needPaint(oldMetrics) && !needPaint(metrics)) {
return;
}
notifyListeners();
}
/// Update and redraw with new scrollbar thickness and radius.
void updateThickness(double nextThickness, Radius nextRadius) {
thickness = nextThickness;
radius = nextRadius;
}
// - Painting
Paint get _paintThumb {
return Paint()
..color = color.withOpacity(color.opacity * fadeoutOpacityAnimation.value);
}
Paint _paintTrack({ bool isBorder = false }) {
if (isBorder) {
return Paint()
..color = trackBorderColor.withOpacity(trackBorderColor.opacity * fadeoutOpacityAnimation.value)
..style = PaintingStyle.stroke
..strokeWidth = 1.0;
}
return Paint()
..color = trackColor.withOpacity(trackColor.opacity * fadeoutOpacityAnimation.value);
}
void _paintScrollbar(Canvas canvas, Size size) {
assert(
textDirection != null,
'A TextDirection must be provided before a Scrollbar can be painted.',
);
final double x, y;
final Size thumbSize, trackSize;
final Offset trackOffset, borderStart, borderEnd;
_debugAssertIsValidOrientation(_resolvedOrientation);
switch (_resolvedOrientation) {
case ScrollbarOrientation.left:
thumbSize = Size(thickness, _thumbExtent);
trackSize = Size(thickness + 2 * crossAxisMargin, _trackExtent);
x = crossAxisMargin + padding.left;
y = _thumbOffset;
trackOffset = Offset(x - crossAxisMargin, _leadingTrackMainAxisOffset);
borderStart = trackOffset + Offset(trackSize.width, 0.0);
borderEnd = Offset(trackOffset.dx + trackSize.width, trackOffset.dy + _trackExtent);
case ScrollbarOrientation.right:
thumbSize = Size(thickness, _thumbExtent);
trackSize = Size(thickness + 2 * crossAxisMargin, _trackExtent);
x = size.width - thickness - crossAxisMargin - padding.right;
y = _thumbOffset;
trackOffset = Offset(x - crossAxisMargin, _leadingTrackMainAxisOffset);
borderStart = trackOffset;
borderEnd = Offset(trackOffset.dx, trackOffset.dy + _trackExtent);
case ScrollbarOrientation.top:
thumbSize = Size(_thumbExtent, thickness);
trackSize = Size(_trackExtent, thickness + 2 * crossAxisMargin);
x = _thumbOffset;
y = crossAxisMargin + padding.top;
trackOffset = Offset(_leadingTrackMainAxisOffset, y - crossAxisMargin);
borderStart = trackOffset + Offset(0.0, trackSize.height);
borderEnd = Offset(trackOffset.dx + _trackExtent, trackOffset.dy + trackSize.height);
case ScrollbarOrientation.bottom:
thumbSize = Size(_thumbExtent, thickness);
trackSize = Size(_trackExtent, thickness + 2 * crossAxisMargin);
x = _thumbOffset;
y = size.height - thickness - crossAxisMargin - padding.bottom;
trackOffset = Offset(_leadingTrackMainAxisOffset, y - crossAxisMargin);
borderStart = trackOffset;
borderEnd = Offset(trackOffset.dx + _trackExtent, trackOffset.dy);
}
// Whether we paint or not, calculating these rects allows us to hit test
// when the scrollbar is transparent.
_trackRect = trackOffset & trackSize;
_thumbRect = Offset(x, y) & thumbSize;
// Paint if the opacity dictates visibility
if (fadeoutOpacityAnimation.value != 0.0) {
// Track
if (trackRadius == null) {
canvas.drawRect(_trackRect!, _paintTrack());
} else {
canvas.drawRRect(RRect.fromRectAndRadius(_trackRect!, trackRadius!), _paintTrack());
}
// Track Border
canvas.drawLine(borderStart, borderEnd, _paintTrack(isBorder: true));
if (radius != null) {
// Rounded rect thumb
canvas.drawRRect(RRect.fromRectAndRadius(_thumbRect!, radius!), _paintThumb);
return;
}
if (shape == null) {
// Square thumb
canvas.drawRect(_thumbRect!, _paintThumb);
return;
}
// Custom-shaped thumb
final Path outerPath = shape!.getOuterPath(_thumbRect!);
canvas.drawPath(outerPath, _paintThumb);
shape!.paint(canvas, _thumbRect!);
}
}
@override
void paint(Canvas canvas, Size size) {
if (_lastAxisDirection == null
|| _lastMetrics == null
|| _lastMetrics!.maxScrollExtent <= _lastMetrics!.minScrollExtent) {
return;
}
// Skip painting if there's not enough space.
if (_traversableTrackExtent <= 0) {
return;
}
// Do not paint a scrollbar if the scroll view is infinitely long.
// TODO(Piinks): Special handling for infinite scroll views,
// https://github.com/flutter/flutter/issues/41434
if (_lastMetrics!.maxScrollExtent.isInfinite) {
return;
}
_setThumbExtent();
final double thumbPositionOffset = _getScrollToTrack(_lastMetrics!, _thumbExtent);
_thumbOffset = thumbPositionOffset + _leadingThumbMainAxisOffset;
return _paintScrollbar(canvas, size);
}
// - Scroll Position Conversion
/// Convert between a thumb track position and the corresponding scroll
/// position.
///
/// The `thumbOffsetLocal` argument is a position in the thumb track.
double getTrackToScroll(double thumbOffsetLocal) {
final double scrollableExtent = _lastMetrics!.maxScrollExtent - _lastMetrics!.minScrollExtent;
final double thumbMovableExtent = _traversableTrackExtent - _thumbExtent;
return scrollableExtent * thumbOffsetLocal / thumbMovableExtent;
}
/// The thumb's corresponding scroll offset in the track.
double getThumbScrollOffset() {
final double scrollableExtent = _lastMetrics!.maxScrollExtent - _lastMetrics!.minScrollExtent;
final double fractionPast = (scrollableExtent > 0)
? clampDouble(_lastMetrics!.pixels / scrollableExtent, 0.0, 1.0)
: 0;
return fractionPast * (_traversableTrackExtent - _thumbExtent);
}
// Converts between a scroll position and the corresponding position in the
// thumb track.
double _getScrollToTrack(ScrollMetrics metrics, double thumbExtent) {
final double scrollableExtent = metrics.maxScrollExtent - metrics.minScrollExtent;
final double fractionPast = (scrollableExtent > 0)
? clampDouble((metrics.pixels - metrics.minScrollExtent) / scrollableExtent, 0.0, 1.0)
: 0;
return (_isReversed ? 1 - fractionPast : fractionPast) * (_traversableTrackExtent - thumbExtent);
}
// - Hit Testing
@override
bool? hitTest(Offset? position) {
// There is nothing painted to hit.
if (_thumbRect == null) {
return null;
}
// Interaction disabled.
if (ignorePointer
// The thumb is not able to be hit when transparent.
|| fadeoutOpacityAnimation.value == 0.0
// Not scrollable
|| !_lastMetricsAreScrollable) {
return false;
}
return _trackRect!.contains(position!);
}
/// Same as hitTest, but includes some padding when the [PointerEvent] is
/// caused by [PointerDeviceKind.touch] to make sure that the region
/// isn't too small to be interacted with by the user.
///
/// The hit test area for hovering with [PointerDeviceKind.mouse] over the
/// scrollbar also uses this extra padding. This is to make it easier to
/// interact with the scrollbar by presenting it to the mouse for interaction
/// based on proximity. When `forHover` is true, the larger hit test area will
/// be used.
bool hitTestInteractive(Offset position, PointerDeviceKind kind, { bool forHover = false }) {
if (_trackRect == null) {
// We have not computed the scrollbar position yet.
return false;
}
if (ignorePointer) {
return false;
}
if (!_lastMetricsAreScrollable) {
return false;
}
final Rect interactiveRect = _trackRect!;
final Rect paddedRect = interactiveRect.expandToInclude(
Rect.fromCircle(center: _thumbRect!.center, radius: _kMinInteractiveSize / 2),
);
// The scrollbar is not able to be hit when transparent - except when
// hovering with a mouse. This should bring the scrollbar into view so the
// mouse can interact with it.
if (fadeoutOpacityAnimation.value == 0.0) {
if (forHover && kind == PointerDeviceKind.mouse) {
return paddedRect.contains(position);
}
return false;
}
switch (kind) {
case PointerDeviceKind.touch:
case PointerDeviceKind.trackpad:
return paddedRect.contains(position);
case PointerDeviceKind.mouse:
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.unknown:
return interactiveRect.contains(position);
}
}
/// Same as hitTestInteractive, but excludes the track portion of the scrollbar.
/// Used to evaluate interactions with only the scrollbar thumb.
bool hitTestOnlyThumbInteractive(Offset position, PointerDeviceKind kind) {
if (_thumbRect == null) {
return false;
}
if (ignorePointer) {
return false;
}
// The thumb is not able to be hit when transparent.
if (fadeoutOpacityAnimation.value == 0.0) {
return false;
}
if (!_lastMetricsAreScrollable) {
return false;
}
switch (kind) {
case PointerDeviceKind.touch:
case PointerDeviceKind.trackpad:
final Rect touchThumbRect = _thumbRect!.expandToInclude(
Rect.fromCircle(center: _thumbRect!.center, radius: _kMinInteractiveSize / 2),
);
return touchThumbRect.contains(position);
case PointerDeviceKind.mouse:
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.unknown:
return _thumbRect!.contains(position);
}
}
@override
bool shouldRepaint(ScrollbarPainter oldDelegate) {
// Should repaint if any properties changed.
return color != oldDelegate.color
|| trackColor != oldDelegate.trackColor
|| trackBorderColor != oldDelegate.trackBorderColor
|| textDirection != oldDelegate.textDirection
|| thickness != oldDelegate.thickness
|| fadeoutOpacityAnimation != oldDelegate.fadeoutOpacityAnimation
|| mainAxisMargin != oldDelegate.mainAxisMargin
|| crossAxisMargin != oldDelegate.crossAxisMargin
|| radius != oldDelegate.radius
|| trackRadius != oldDelegate.trackRadius
|| shape != oldDelegate.shape
|| padding != oldDelegate.padding
|| minLength != oldDelegate.minLength
|| minOverscrollLength != oldDelegate.minOverscrollLength
|| scrollbarOrientation != oldDelegate.scrollbarOrientation
|| ignorePointer != oldDelegate.ignorePointer;
}
@override
bool shouldRebuildSemantics(CustomPainter oldDelegate) => false;
@override
SemanticsBuilderCallback? get semanticsBuilder => null;
@override
String toString() => describeIdentity(this);
@override
void dispose() {
fadeoutOpacityAnimation.removeListener(notifyListeners);
super.dispose();
}
}
/// An extendable base class for building scrollbars that fade in and out.
///
/// To add a scrollbar to a [ScrollView], like a [ListView] or a
/// [CustomScrollView], wrap the scroll view widget in a [RawScrollbar] widget.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=DbkIQSvwnZc}
///
/// {@template flutter.widgets.Scrollbar}
/// A scrollbar thumb indicates which portion of a [ScrollView] is actually
/// visible.
///
/// By default, the thumb will fade in and out as the child scroll view
/// scrolls. When [thumbVisibility] is true, the scrollbar thumb will remain
/// visible without the fade animation. This requires that the [ScrollController]
/// associated with the Scrollable widget is provided to [controller], or that
/// the [PrimaryScrollController] is being used by that Scrollable widget.
///
/// If the scrollbar is wrapped around multiple [ScrollView]s, it only responds to
/// the nearest ScrollView and shows the corresponding scrollbar thumb by default.
/// The [notificationPredicate] allows the ability to customize which
/// [ScrollNotification]s the Scrollbar should listen to.
///
/// If the child [ScrollView] is infinitely long, the [RawScrollbar] will not be
/// painted. In this case, the scrollbar cannot accurately represent the
/// relative location of the visible area, or calculate the accurate delta to
/// apply when dragging on the thumb or tapping on the track.
///
/// ### Interaction
///
/// Scrollbars are interactive and can use the [PrimaryScrollController] if
/// a [controller] is not set. Interactive Scrollbar thumbs can be dragged along
/// the main axis of the [ScrollView] to change the [ScrollPosition]. Tapping
/// along the track exclusive of the thumb will trigger a
/// [ScrollIncrementType.page] based on the relative position to the thumb.
///
/// When using the [PrimaryScrollController], it must not be attached to more
/// than one [ScrollPosition]. [ScrollView]s that have not been provided a
/// [ScrollController] and have a [ScrollView.scrollDirection] of
/// [Axis.vertical] will automatically attach their ScrollPosition to the
/// PrimaryScrollController. Provide a unique ScrollController to each
/// [Scrollable] in this case to prevent having multiple ScrollPositions
/// attached to the PrimaryScrollController.
///
/// {@tool dartpad}
/// This sample shows an app with two scrollables in the same route. Since by
/// default, there is one [PrimaryScrollController] per route, and they both have a
/// scroll direction of [Axis.vertical], they would both try to attach to that
/// controller on mobile platforms. The [Scrollbar] cannot support multiple
/// positions attached to the same controller, so one [ListView], and its
/// [Scrollbar] have been provided a unique [ScrollController]. Desktop
/// platforms do not automatically attach to the PrimaryScrollController,
/// requiring [ScrollView.primary] to be true instead in order to use the
/// PrimaryScrollController.
///
/// Alternatively, a new PrimaryScrollController could be created above one of
/// the [ListView]s.
///
/// ** See code in examples/api/lib/widgets/scrollbar/raw_scrollbar.0.dart **
/// {@end-tool}
///
/// ### Automatic Scrollbars on Desktop Platforms
///
/// Scrollbars are added to most [Scrollable] widgets by default on
/// [TargetPlatformVariant.desktop] platforms. This is done through
/// [ScrollBehavior.buildScrollbar] as part of an app's
/// [ScrollConfiguration]. Scrollables that do not use the
/// [PrimaryScrollController] or have a [ScrollController] provided to them
/// will receive a unique ScrollController for use with the Scrollbar. In this
/// case, only one Scrollable can be using the PrimaryScrollController, unless
/// [interactive] is false. To prevent [Axis.vertical] Scrollables from using
/// the PrimaryScrollController, set [ScrollView.primary] to false. Scrollable
/// widgets that do not have automatically applied Scrollbars include
///
/// * [EditableText]
/// * [ListWheelScrollView]
/// * [PageView]
/// * [NestedScrollView]
/// * [DropdownButton]
///
/// Default Scrollbars can be disabled for the whole app by setting a
/// [ScrollBehavior] with `scrollbars` set to false.
///
/// {@tool snippet}
/// ```dart
/// MaterialApp(
/// scrollBehavior: const MaterialScrollBehavior()
/// .copyWith(scrollbars: false),
/// home: Scaffold(
/// appBar: AppBar(title: const Text('Home')),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows how to disable the default Scrollbar for a [Scrollable]
/// widget to avoid duplicate Scrollbars when running on desktop platforms.
///
/// ** See code in examples/api/lib/widgets/scrollbar/raw_scrollbar.desktop.0.dart **
/// {@end-tool}
/// {@endtemplate}
///
/// {@tool dartpad}
/// This sample shows a [RawScrollbar] that executes a fade animation as
/// scrolling occurs. The RawScrollbar will fade into view as the user scrolls,
/// and fade out when scrolling stops. The [GridView] uses the
/// [PrimaryScrollController] since it has an [Axis.vertical] scroll direction
/// and has not been provided a [ScrollController].
///
/// ** See code in examples/api/lib/widgets/scrollbar/raw_scrollbar.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// When `thumbVisibility` is true, the scrollbar thumb will remain visible without
/// the fade animation. This requires that a [ScrollController] is provided to
/// `controller` for both the [RawScrollbar] and the [GridView].
/// Alternatively, the [PrimaryScrollController] can be used automatically so long
/// as it is attached to the singular [ScrollPosition] associated with the GridView.
///
/// ** See code in examples/api/lib/widgets/scrollbar/raw_scrollbar.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListView], which displays a linear, scrollable list of children.
/// * [GridView], which displays a 2 dimensional, scrollable array of children.
class RawScrollbar extends StatefulWidget {
/// Creates a basic raw scrollbar that wraps the given [child].
///
/// The [child], or a descendant of the [child], should be a source of
/// [ScrollNotification] notifications, typically a [Scrollable] widget.
const RawScrollbar({
super.key,
required this.child,
this.controller,
this.thumbVisibility,
this.shape,
this.radius,
this.thickness,
this.thumbColor,
this.minThumbLength = _kMinThumbExtent,
this.minOverscrollLength,
this.trackVisibility,
this.trackRadius,
this.trackColor,
this.trackBorderColor,
this.fadeDuration = _kScrollbarFadeDuration,
this.timeToFade = _kScrollbarTimeToFade,
this.pressDuration = Duration.zero,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.interactive,
this.scrollbarOrientation,
this.mainAxisMargin = 0.0,
this.crossAxisMargin = 0.0,
this.padding,
}) : assert(
!(thumbVisibility == false && (trackVisibility ?? false)),
'A scrollbar track cannot be drawn without a scrollbar thumb.',
),
assert(minThumbLength >= 0),
assert(minOverscrollLength == null || minOverscrollLength <= minThumbLength),
assert(minOverscrollLength == null || minOverscrollLength >= 0),
assert(radius == null || shape == null);
/// {@template flutter.widgets.Scrollbar.child}
/// The widget below this widget in the tree.
///
/// The scrollbar will be stacked on top of this child. This child (and its
/// subtree) should include a source of [ScrollNotification] notifications.
/// Typically a [Scrollbar] is created on desktop platforms by a
/// [ScrollBehavior.buildScrollbar] method, in which case the child is usually
/// the one provided as an argument to that method.
///
/// Typically a [ListView] or [CustomScrollView].
/// {@endtemplate}
final Widget child;
/// {@template flutter.widgets.Scrollbar.controller}
/// The [ScrollController] used to implement Scrollbar dragging.
///
/// If nothing is passed to controller, the default behavior is to automatically
/// enable scrollbar dragging on the nearest ScrollController using
/// [PrimaryScrollController.of].
///
/// If a ScrollController is passed, then dragging on the scrollbar thumb will
/// update the [ScrollPosition] attached to the controller. A stateful ancestor
/// of this widget needs to manage the ScrollController and either pass it to
/// a scrollable descendant or use a PrimaryScrollController to share it.
///
/// {@tool snippet}
/// Here is an example of using the [controller] attribute to enable
/// scrollbar dragging for multiple independent ListViews:
///
/// ```dart
/// // (e.g. in a stateful widget)
///
/// final ScrollController controllerOne = ScrollController();
/// final ScrollController controllerTwo = ScrollController();
///
/// @override
/// Widget build(BuildContext context) {
/// return Column(
/// children: <Widget>[
/// SizedBox(
/// height: 200,
/// child: CupertinoScrollbar(
/// controller: controllerOne,
/// child: ListView.builder(
/// controller: controllerOne,
/// itemCount: 120,
/// itemBuilder: (BuildContext context, int index) => Text('item $index'),
/// ),
/// ),
/// ),
/// SizedBox(
/// height: 200,
/// child: CupertinoScrollbar(
/// controller: controllerTwo,
/// child: ListView.builder(
/// controller: controllerTwo,
/// itemCount: 120,
/// itemBuilder: (BuildContext context, int index) => Text('list 2 item $index'),
/// ),
/// ),
/// ),
/// ],
/// );
/// }
/// ```
/// {@end-tool}
/// {@endtemplate}
final ScrollController? controller;
/// {@template flutter.widgets.Scrollbar.thumbVisibility}
/// Indicates that the scrollbar thumb should be visible, even when a scroll
/// is not underway.
///
/// When false, the scrollbar will be shown during scrolling
/// and will fade out otherwise.
///
/// When true, the scrollbar will always be visible and never fade out. This
/// requires that the Scrollbar can access the [ScrollController] of the
/// associated Scrollable widget. This can either be the provided [controller],
/// or the [PrimaryScrollController] of the current context.
///
/// * When providing a controller, the same ScrollController must also be
/// provided to the associated Scrollable widget.
/// * The [PrimaryScrollController] is used by default for a [ScrollView]
/// that has not been provided a [ScrollController] and that has a
/// [ScrollView.scrollDirection] of [Axis.vertical]. This automatic
/// behavior does not apply to those with [Axis.horizontal]. To explicitly
/// use the PrimaryScrollController, set [ScrollView.primary] to true.
///
/// Defaults to false when null.
///
/// {@tool snippet}
///
/// ```dart
/// // (e.g. in a stateful widget)
///
/// final ScrollController controllerOne = ScrollController();
/// final ScrollController controllerTwo = ScrollController();
///
/// @override
/// Widget build(BuildContext context) {
/// return Column(
/// children: <Widget>[
/// SizedBox(
/// height: 200,
/// child: Scrollbar(
/// thumbVisibility: true,
/// controller: controllerOne,
/// child: ListView.builder(
/// controller: controllerOne,
/// itemCount: 120,
/// itemBuilder: (BuildContext context, int index) {
/// return Text('item $index');
/// },
/// ),
/// ),
/// ),
/// SizedBox(
/// height: 200,
/// child: CupertinoScrollbar(
/// thumbVisibility: true,
/// controller: controllerTwo,
/// child: SingleChildScrollView(
/// controller: controllerTwo,
/// child: const SizedBox(
/// height: 2000,
/// width: 500,
/// child: Placeholder(),
/// ),
/// ),
/// ),
/// ),
/// ],
/// );
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [RawScrollbarState.showScrollbar], an overridable getter which uses
/// this value to override the default behavior.
/// * [ScrollView.primary], which indicates whether the ScrollView is the primary
/// scroll view associated with the parent [PrimaryScrollController].
/// * [PrimaryScrollController], which associates a [ScrollController] with
/// a subtree.
/// {@endtemplate}
///
/// Subclass [Scrollbar] can hide and show the scrollbar thumb in response to
/// [MaterialState]s by using [ScrollbarThemeData.thumbVisibility].
final bool? thumbVisibility;
/// The [OutlinedBorder] of the scrollbar's thumb.
///
/// Only one of [radius] and [shape] may be specified. For a rounded rectangle,
/// it's simplest to just specify [radius]. By default, the scrollbar thumb's
/// shape is a simple rectangle.
///
/// If [shape] is specified, the thumb will take the shape of the passed
/// [OutlinedBorder] and fill itself with [thumbColor] (or grey if it
/// is unspecified).
///
/// {@tool dartpad}
/// This is an example of using a [StadiumBorder] for drawing the [shape] of the
/// thumb in a [RawScrollbar].
///
/// ** See code in examples/api/lib/widgets/scrollbar/raw_scrollbar.shape.0.dart **
/// {@end-tool}
final OutlinedBorder? shape;
/// The [Radius] of the scrollbar thumb's rounded rectangle corners.
///
/// Scrollbar will be rectangular if [radius] is null, which is the default
/// behavior.
final Radius? radius;
/// The thickness of the scrollbar in the cross axis of the scrollable.
///
/// If null, will default to 6.0 pixels.
final double? thickness;
/// The color of the scrollbar thumb.
///
/// If null, defaults to Color(0x66BCBCBC).
final Color? thumbColor;
/// The preferred smallest size the scrollbar thumb can shrink to when the total
/// scrollable extent is large, the current visible viewport is small, and the
/// viewport is not overscrolled.
///
/// The size of the scrollbar's thumb may shrink to a smaller size than [minThumbLength]
/// to fit in the available paint area (e.g., when [minThumbLength] is greater
/// than [ScrollMetrics.viewportDimension] and [mainAxisMargin] combined).
///
/// Mustn't be null and the value has to be greater or equal to
/// [minOverscrollLength], which in turn is >= 0. Defaults to 18.0.
final double minThumbLength;
/// The preferred smallest size the scrollbar thumb can shrink to when viewport is
/// overscrolled.
///
/// When overscrolling, the size of the scrollbar's thumb may shrink to a smaller size
/// than [minOverscrollLength] to fit in the available paint area (e.g., when
/// [minOverscrollLength] is greater than [ScrollMetrics.viewportDimension] and
/// [mainAxisMargin] combined).
///
/// Overscrolling can be made possible by setting the `physics` property
/// of the `child` Widget to a `BouncingScrollPhysics`, which is a special
/// `ScrollPhysics` that allows overscrolling.
///
/// The value is less than or equal to [minThumbLength] and greater than or equal to 0.
/// When null, it will default to the value of [minThumbLength].
final double? minOverscrollLength;
/// {@template flutter.widgets.Scrollbar.trackVisibility}
/// Indicates that the scrollbar track should be visible.
///
/// When true, the scrollbar track will always be visible so long as the thumb
/// is visible. If the scrollbar thumb is not visible, the track will not be
/// visible either.
///
/// Defaults to false when null.
/// {@endtemplate}
///
/// Subclass [Scrollbar] can hide and show the scrollbar thumb in response to
/// [MaterialState]s by using [ScrollbarThemeData.trackVisibility].
final bool? trackVisibility;
/// The [Radius] of the scrollbar track's rounded rectangle corners.
///
/// Scrollbar's track will be rectangular if [trackRadius] is null, which is
/// the default behavior.
final Radius? trackRadius;
/// The color of the scrollbar track.
///
/// The scrollbar track will only be visible when [trackVisibility] and
/// [thumbVisibility] are true.
///
/// If null, defaults to Color(0x08000000).
final Color? trackColor;
/// The color of the scrollbar track's border.
///
/// The scrollbar track will only be visible when [trackVisibility] and
/// [thumbVisibility] are true.
///
/// If null, defaults to Color(0x1a000000).
final Color? trackBorderColor;
/// The [Duration] of the fade animation.
///
/// Defaults to a [Duration] of 300 milliseconds.
final Duration fadeDuration;
/// The [Duration] of time until the fade animation begins.
///
/// Defaults to a [Duration] of 600 milliseconds.
final Duration timeToFade;
/// The [Duration] of time that a LongPress will trigger the drag gesture of
/// the scrollbar thumb.
///
/// Defaults to [Duration.zero].
final Duration pressDuration;
/// {@template flutter.widgets.Scrollbar.notificationPredicate}
/// A check that specifies whether a [ScrollNotification] should be
/// handled by this widget.
///
/// By default, checks whether `notification.depth == 0`. That means if the
/// scrollbar is wrapped around multiple [ScrollView]s, it only responds to the
/// nearest scrollView and shows the corresponding scrollbar thumb.
/// {@endtemplate}
final ScrollNotificationPredicate notificationPredicate;
/// {@template flutter.widgets.Scrollbar.interactive}
/// Whether the Scrollbar should be interactive and respond to dragging on the
/// thumb, or tapping in the track area.
///
/// Does not apply to the [CupertinoScrollbar], which is always interactive to
/// match native behavior. On Android, the scrollbar is not interactive by
/// default.
///
/// When false, the scrollbar will not respond to gesture or hover events,
/// and will allow to click through it.
///
/// Defaults to true when null, unless on Android, which will default to false
/// when null.
///
/// See also:
///
/// * [RawScrollbarState.enableGestures], an overridable getter which uses
/// this value to override the default behavior.
/// {@endtemplate}
final bool? interactive;
/// {@macro flutter.widgets.Scrollbar.scrollbarOrientation}
final ScrollbarOrientation? scrollbarOrientation;
/// Distance from the scrollbar thumb's start or end to the nearest edge of
/// the viewport in logical pixels. It affects the amount of available
/// paint area.
///
/// The scrollbar track consumes this space.
///
/// Mustn't be null and defaults to 0.
final double mainAxisMargin;
/// Distance from the scrollbar thumb's side to the nearest cross axis edge
/// in logical pixels.
///
/// The scrollbar track consumes this space.
///
/// Defaults to zero.
final double crossAxisMargin;
/// The insets by which the scrollbar thumb and track should be padded.
///
/// When null, the inherited [MediaQueryData.padding] is used.
///
/// Defaults to null.
final EdgeInsets? padding;
@override
RawScrollbarState<RawScrollbar> createState() => RawScrollbarState<RawScrollbar>();
}
/// The state for a [RawScrollbar] widget, also shared by the [Scrollbar] and
/// [CupertinoScrollbar] widgets.
///
/// Controls the animation that fades a scrollbar's thumb in and out of view.
///
/// Provides defaults gestures for dragging the scrollbar thumb and tapping on the
/// scrollbar track.
class RawScrollbarState<T extends RawScrollbar> extends State<T> with TickerProviderStateMixin<T> {
Offset? _startDragScrollbarAxisOffset;
Offset? _lastDragUpdateOffset;
double? _startDragThumbOffset;
ScrollController? _cachedController;
Timer? _fadeoutTimer;
late AnimationController _fadeoutAnimationController;
late Animation<double> _fadeoutOpacityAnimation;
final GlobalKey _scrollbarPainterKey = GlobalKey();
bool _hoverIsActive = false;
bool _thumbDragging = false;
ScrollController? get _effectiveScrollController => widget.controller ?? PrimaryScrollController.maybeOf(context);
/// Used to paint the scrollbar.
///
/// Can be customized by subclasses to change scrollbar behavior by overriding
/// [updateScrollbarPainter].
@protected
late final ScrollbarPainter scrollbarPainter;
/// Overridable getter to indicate that the scrollbar should be visible, even
/// when a scroll is not underway.
///
/// Subclasses can override this getter to make its value depend on an inherited
/// theme.
///
/// Defaults to false when [RawScrollbar.thumbVisibility] is null.
@protected
bool get showScrollbar => widget.thumbVisibility ?? false;
bool get _showTrack => showScrollbar && (widget.trackVisibility ?? false);
/// Overridable getter to indicate is gestures should be enabled on the
/// scrollbar.
///
/// When false, the scrollbar will not respond to gesture or hover events,
/// and will allow to click through it.
///
/// Subclasses can override this getter to make its value depend on an inherited
/// theme.
///
/// Defaults to true when [RawScrollbar.interactive] is null.
///
/// See also:
///
/// * [RawScrollbar.interactive], which overrides the default behavior.
@protected
bool get enableGestures => widget.interactive ?? true;
@override
void initState() {
super.initState();
_fadeoutAnimationController = AnimationController(
vsync: this,
duration: widget.fadeDuration,
)..addStatusListener(_validateInteractions);
_fadeoutOpacityAnimation = CurvedAnimation(
parent: _fadeoutAnimationController,
curve: Curves.fastOutSlowIn,
);
scrollbarPainter = ScrollbarPainter(
color: widget.thumbColor ?? const Color(0x66BCBCBC),
fadeoutOpacityAnimation: _fadeoutOpacityAnimation,
thickness: widget.thickness ?? _kScrollbarThickness,
radius: widget.radius,
trackRadius: widget.trackRadius,
scrollbarOrientation: widget.scrollbarOrientation,
mainAxisMargin: widget.mainAxisMargin,
shape: widget.shape,
crossAxisMargin: widget.crossAxisMargin,
minLength: widget.minThumbLength,
minOverscrollLength: widget.minOverscrollLength ?? widget.minThumbLength,
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
assert(_debugScheduleCheckHasValidScrollPosition());
}
bool _debugScheduleCheckHasValidScrollPosition() {
if (!showScrollbar) {
return true;
}
WidgetsBinding.instance.addPostFrameCallback((Duration duration) {
assert(_debugCheckHasValidScrollPosition());
}, debugLabel: 'RawScrollbar.checkScrollPosition');
return true;
}
void _validateInteractions(AnimationStatus status) {
if (status == AnimationStatus.dismissed) {
assert(_fadeoutOpacityAnimation.value == 0.0);
// We do not check for a valid scroll position if the scrollbar is not
// visible, because it cannot be interacted with.
} else if (_effectiveScrollController != null && enableGestures) {
// Interactive scrollbars need to be properly configured. If it is visible
// for interaction, ensure we are set up properly.
assert(_debugCheckHasValidScrollPosition());
}
}
bool _debugCheckHasValidScrollPosition() {
if (!mounted) {
return true;
}
final ScrollController? scrollController = _effectiveScrollController;
final bool tryPrimary = widget.controller == null;
final String controllerForError = tryPrimary
? 'PrimaryScrollController'
: 'provided ScrollController';
String when = '';
if (widget.thumbVisibility ?? false) {
when = 'Scrollbar.thumbVisibility is true';
} else if (enableGestures) {
when = 'the scrollbar is interactive';
} else {
when = 'using the Scrollbar';
}
assert(
scrollController != null,
'A ScrollController is required when $when. '
'${tryPrimary ? 'The Scrollbar was not provided a ScrollController, '
'and attempted to use the PrimaryScrollController, but none was found.' :''}',
);
assert (() {
if (!scrollController!.hasClients) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
"The Scrollbar's ScrollController has no ScrollPosition attached.",
),
ErrorDescription(
'A Scrollbar cannot be painted without a ScrollPosition. ',
),
ErrorHint(
'The Scrollbar attempted to use the $controllerForError. This '
'ScrollController should be associated with the ScrollView that '
'the Scrollbar is being applied to.',
),
if (tryPrimary) ...<ErrorHint>[
ErrorHint(
'If a ScrollController has not been provided, the '
'PrimaryScrollController is used by default on mobile platforms '
'for ScrollViews with an Axis.vertical scroll direction.',
),
ErrorHint(
'To use the PrimaryScrollController explicitly, '
'set ScrollView.primary to true on the Scrollable widget.',
),
]
else
ErrorHint(
'When providing your own ScrollController, ensure both the '
'Scrollbar and the Scrollable widget use the same one.',
),
]);
}
return true;
}());
assert (() {
try {
scrollController!.position;
} catch (error) {
if (scrollController == null || scrollController.positions.length <= 1) {
rethrow;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The $controllerForError is attached to more than one ScrollPosition.',
),
ErrorDescription(
'The Scrollbar requires a single ScrollPosition in order to be painted.',
),
ErrorHint(
'When $when, the associated ScrollController must only have one '
'ScrollPosition attached.',
),
if (tryPrimary) ...<ErrorHint>[
ErrorHint(
'If a ScrollController has not been provided, the '
'PrimaryScrollController is used by default on mobile platforms '
'for ScrollViews with an Axis.vertical scroll direction.'
),
ErrorHint(
'More than one ScrollView may have tried to use the '
'PrimaryScrollController of the current context. '
'ScrollView.primary can override this behavior.'
),
]
else
ErrorHint(
'The provided ScrollController cannot be shared by multiple '
'ScrollView widgets.'
),
]);
}
return true;
}());
return true;
}
/// This method is responsible for configuring the [scrollbarPainter]
/// according to the [widget]'s properties and any inherited widgets the
/// painter depends on, like [Directionality] and [MediaQuery].
///
/// Subclasses can override to configure the [scrollbarPainter].
@protected
void updateScrollbarPainter() {
scrollbarPainter
..color = widget.thumbColor ?? const Color(0x66BCBCBC)
..trackRadius = widget.trackRadius
..trackColor = _showTrack
? widget.trackColor ?? const Color(0x08000000)
: const Color(0x00000000)
..trackBorderColor = _showTrack
? widget.trackBorderColor ?? const Color(0x1a000000)
: const Color(0x00000000)
..textDirection = Directionality.of(context)
..thickness = widget.thickness ?? _kScrollbarThickness
..radius = widget.radius
..padding = widget.padding ?? MediaQuery.paddingOf(context)
..scrollbarOrientation = widget.scrollbarOrientation
..mainAxisMargin = widget.mainAxisMargin
..shape = widget.shape
..crossAxisMargin = widget.crossAxisMargin
..minLength = widget.minThumbLength
..minOverscrollLength = widget.minOverscrollLength ?? widget.minThumbLength
..ignorePointer = !enableGestures;
}
@override
void didUpdateWidget(T oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.thumbVisibility != oldWidget.thumbVisibility) {
if (widget.thumbVisibility ?? false) {
assert(_debugScheduleCheckHasValidScrollPosition());
_fadeoutTimer?.cancel();
_fadeoutAnimationController.animateTo(1.0);
} else {
_fadeoutAnimationController.reverse();
}
}
}
void _updateScrollPosition(Offset updatedOffset) {
assert(_cachedController != null);
assert(_startDragScrollbarAxisOffset != null);
assert(_lastDragUpdateOffset != null);
assert(_startDragThumbOffset != null);
final ScrollPosition position = _cachedController!.position;
late double primaryDeltaFromDragStart;
late double primaryDeltaFromLastDragUpdate;
switch (position.axisDirection) {
case AxisDirection.up:
primaryDeltaFromDragStart = _startDragScrollbarAxisOffset!.dy - updatedOffset.dy;
primaryDeltaFromLastDragUpdate = _lastDragUpdateOffset!.dy - updatedOffset.dy;
case AxisDirection.right:
primaryDeltaFromDragStart = updatedOffset.dx -_startDragScrollbarAxisOffset!.dx;
primaryDeltaFromLastDragUpdate = updatedOffset.dx -_lastDragUpdateOffset!.dx;
case AxisDirection.down:
primaryDeltaFromDragStart = updatedOffset.dy -_startDragScrollbarAxisOffset!.dy;
primaryDeltaFromLastDragUpdate = updatedOffset.dy -_lastDragUpdateOffset!.dy;
case AxisDirection.left:
primaryDeltaFromDragStart = _startDragScrollbarAxisOffset!.dx - updatedOffset.dx;
primaryDeltaFromLastDragUpdate = _lastDragUpdateOffset!.dx - updatedOffset.dx;
}
// Convert primaryDelta, the amount that the scrollbar moved since the last
// time when drag started or last updated, into the coordinate space of the scroll
// position, and jump to that position.
double scrollOffsetGlobal = scrollbarPainter.getTrackToScroll(primaryDeltaFromDragStart + _startDragThumbOffset!);
if (primaryDeltaFromDragStart > 0 && scrollOffsetGlobal < position.pixels
|| primaryDeltaFromDragStart < 0 && scrollOffsetGlobal > position.pixels) {
// Adjust the position value if the scrolling direction conflicts with
// the dragging direction due to scroll metrics shrink.
scrollOffsetGlobal = position.pixels + scrollbarPainter.getTrackToScroll(primaryDeltaFromLastDragUpdate);
}
if (scrollOffsetGlobal != position.pixels) {
// Ensure we don't drag into overscroll if the physics do not allow it.
final double physicsAdjustment = position.physics.applyBoundaryConditions(position, scrollOffsetGlobal);
double newPosition = scrollOffsetGlobal - physicsAdjustment;
// The physics may allow overscroll when actually *scrolling*, but
// dragging on the scrollbar does not always allow us to enter overscroll.
switch (ScrollConfiguration.of(context).getPlatform(context)) {
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
newPosition = clampDouble(newPosition, position.minScrollExtent, position.maxScrollExtent);
case TargetPlatform.iOS:
case TargetPlatform.android:
// We can only drag the scrollbar into overscroll on mobile
// platforms, and only then if the physics allow it.
break;
}
position.jumpTo(newPosition);
}
}
void _maybeStartFadeoutTimer() {
if (!showScrollbar) {
_fadeoutTimer?.cancel();
_fadeoutTimer = Timer(widget.timeToFade, () {
_fadeoutAnimationController.reverse();
_fadeoutTimer = null;
});
}
}
/// Returns the [Axis] of the child scroll view, or null if the
/// current scroll controller does not have any attached positions.
@protected
Axis? getScrollbarDirection() {
assert(_cachedController != null);
if (_cachedController!.hasClients) {
return _cachedController!.position.axis;
}
return null;
}
/// Handler called when a press on the scrollbar thumb has been recognized.
///
/// Cancels the [Timer] associated with the fade animation of the scrollbar.
@protected
@mustCallSuper
void handleThumbPress() {
assert(_debugCheckHasValidScrollPosition());
if (getScrollbarDirection() == null) {
return;
}
_fadeoutTimer?.cancel();
}
/// Handler called when a long press gesture has started.
///
/// Begins the fade out animation and initializes dragging the scrollbar thumb.
@protected
@mustCallSuper
void handleThumbPressStart(Offset localPosition) {
assert(_debugCheckHasValidScrollPosition());
_cachedController = _effectiveScrollController;
final Axis? direction = getScrollbarDirection();
if (direction == null) {
return;
}
_fadeoutTimer?.cancel();
_fadeoutAnimationController.forward();
_startDragScrollbarAxisOffset = localPosition;
_lastDragUpdateOffset = localPosition;
_startDragThumbOffset = scrollbarPainter.getThumbScrollOffset();
_thumbDragging = true;
}
/// Handler called when a currently active long press gesture moves.
///
/// Updates the position of the child scrollable.
@protected
@mustCallSuper
void handleThumbPressUpdate(Offset localPosition) {
assert(_debugCheckHasValidScrollPosition());
if (_lastDragUpdateOffset == localPosition) {
return;
}
final ScrollPosition position = _cachedController!.position;
if (!position.physics.shouldAcceptUserOffset(position)) {
return;
}
final Axis? direction = getScrollbarDirection();
if (direction == null) {
return;
}
_updateScrollPosition(localPosition);
_lastDragUpdateOffset = localPosition;
}
/// Handler called when a long press has ended.
@protected
@mustCallSuper
void handleThumbPressEnd(Offset localPosition, Velocity velocity) {
assert(_debugCheckHasValidScrollPosition());
_thumbDragging = false;
final Axis? direction = getScrollbarDirection();
if (direction == null) {
return;
}
_maybeStartFadeoutTimer();
_startDragScrollbarAxisOffset = null;
_lastDragUpdateOffset = null;
_startDragThumbOffset = null;
_cachedController = null;
}
void _handleTrackTapDown(TapDownDetails details) {
// The Scrollbar should page towards the position of the tap on the track.
assert(_debugCheckHasValidScrollPosition());
_cachedController = _effectiveScrollController;
final ScrollPosition position = _cachedController!.position;
if (!position.physics.shouldAcceptUserOffset(position)) {
return;
}
// Determines the scroll direction.
final AxisDirection scrollDirection;
switch (position.axisDirection) {
case AxisDirection.up:
case AxisDirection.down:
if (details.localPosition.dy > scrollbarPainter._thumbOffset) {
scrollDirection = AxisDirection.down;
} else {
scrollDirection = AxisDirection.up;
}
case AxisDirection.left:
case AxisDirection.right:
if (details.localPosition.dx > scrollbarPainter._thumbOffset) {
scrollDirection = AxisDirection.right;
} else {
scrollDirection = AxisDirection.left;
}
}
final ScrollableState? state = Scrollable.maybeOf(position.context.notificationContext!);
final ScrollIntent intent = ScrollIntent(direction: scrollDirection, type: ScrollIncrementType.page);
assert(state != null);
final double scrollIncrement = ScrollAction.getDirectionalIncrement(state!, intent);
_cachedController!.position.moveTo(
_cachedController!.position.pixels + scrollIncrement,
duration: const Duration(milliseconds: 100),
curve: Curves.easeInOut,
);
}
// ScrollController takes precedence over ScrollNotification
bool _shouldUpdatePainter(Axis notificationAxis) {
final ScrollController? scrollController = _effectiveScrollController;
// Only update the painter of this scrollbar if the notification
// metrics do not conflict with the information we have from the scroll
// controller.
// We do not have a scroll controller dictating axis.
if (scrollController == null) {
return true;
}
// Has more than one attached positions.
if (scrollController.positions.length > 1) {
return false;
}
return
// The scroll controller is not attached to a position.
!scrollController.hasClients
// The notification matches the scroll controller's axis.
|| scrollController.position.axis == notificationAxis;
}
bool _handleScrollMetricsNotification(ScrollMetricsNotification notification) {
if (!widget.notificationPredicate(notification.asScrollUpdate())) {
return false;
}
if (showScrollbar) {
if (_fadeoutAnimationController.status != AnimationStatus.forward &&
_fadeoutAnimationController.status != AnimationStatus.completed) {
_fadeoutAnimationController.forward();
}
}
final ScrollMetrics metrics = notification.metrics;
if (_shouldUpdatePainter(metrics.axis)) {
scrollbarPainter.update(metrics, metrics.axisDirection);
}
return false;
}
bool _handleScrollNotification(ScrollNotification notification) {
if (!widget.notificationPredicate(notification)) {
return false;
}
final ScrollMetrics metrics = notification.metrics;
if (metrics.maxScrollExtent <= metrics.minScrollExtent) {
// Hide the bar when the Scrollable widget has no space to scroll.
if (_fadeoutAnimationController.status != AnimationStatus.dismissed &&
_fadeoutAnimationController.status != AnimationStatus.reverse) {
_fadeoutAnimationController.reverse();
}
if (_shouldUpdatePainter(metrics.axis)) {
scrollbarPainter.update(metrics, metrics.axisDirection);
}
return false;
}
if (notification is ScrollUpdateNotification ||
notification is OverscrollNotification) {
// Any movements always makes the scrollbar start showing up.
if (_fadeoutAnimationController.status != AnimationStatus.forward &&
_fadeoutAnimationController.status != AnimationStatus.completed) {
_fadeoutAnimationController.forward();
}
_fadeoutTimer?.cancel();
if (_shouldUpdatePainter(metrics.axis)) {
scrollbarPainter.update(metrics, metrics.axisDirection);
}
} else if (notification is ScrollEndNotification) {
if (_startDragScrollbarAxisOffset == null) {
_maybeStartFadeoutTimer();
}
}
return false;
}
Map<Type, GestureRecognizerFactory> get _gestures {
final Map<Type, GestureRecognizerFactory> gestures = <Type, GestureRecognizerFactory>{};
if (_effectiveScrollController == null || !enableGestures) {
return gestures;
}
gestures[_ThumbPressGestureRecognizer] =
GestureRecognizerFactoryWithHandlers<_ThumbPressGestureRecognizer>(
() => _ThumbPressGestureRecognizer(
debugOwner: this,
customPaintKey: _scrollbarPainterKey,
duration: widget.pressDuration,
),
(_ThumbPressGestureRecognizer instance) {
instance.onLongPress = handleThumbPress;
instance.onLongPressStart = (LongPressStartDetails details) => handleThumbPressStart(details.localPosition);
instance.onLongPressMoveUpdate = (LongPressMoveUpdateDetails details) => handleThumbPressUpdate(details.localPosition);
instance.onLongPressEnd = (LongPressEndDetails details) => handleThumbPressEnd(details.localPosition, details.velocity);
},
);
gestures[_TrackTapGestureRecognizer] =
GestureRecognizerFactoryWithHandlers<_TrackTapGestureRecognizer>(
() => _TrackTapGestureRecognizer(
debugOwner: this,
customPaintKey: _scrollbarPainterKey,
),
(_TrackTapGestureRecognizer instance) {
instance.onTapDown = _handleTrackTapDown;
},
);
return gestures;
}
/// Returns true if the provided [Offset] is located over the track of the
/// [RawScrollbar].
///
/// Excludes the [RawScrollbar] thumb.
@protected
bool isPointerOverTrack(Offset position, PointerDeviceKind kind) {
if (_scrollbarPainterKey.currentContext == null) {
return false;
}
final Offset localOffset = _getLocalOffset(_scrollbarPainterKey, position);
return scrollbarPainter.hitTestInteractive(localOffset, kind)
&& !scrollbarPainter.hitTestOnlyThumbInteractive(localOffset, kind);
}
/// Returns true if the provided [Offset] is located over the thumb of the
/// [RawScrollbar].
@protected
bool isPointerOverThumb(Offset position, PointerDeviceKind kind) {
if (_scrollbarPainterKey.currentContext == null) {
return false;
}
final Offset localOffset = _getLocalOffset(_scrollbarPainterKey, position);
return scrollbarPainter.hitTestOnlyThumbInteractive(localOffset, kind);
}
/// Returns true if the provided [Offset] is located over the track or thumb
/// of the [RawScrollbar].
///
/// The hit test area for mouse hovering over the scrollbar is larger than
/// regular hit testing. This is to make it easier to interact with the
/// scrollbar and present it to the mouse for interaction based on proximity.
/// When `forHover` is true, the larger hit test area will be used.
@protected
bool isPointerOverScrollbar(Offset position, PointerDeviceKind kind, { bool forHover = false }) {
if (_scrollbarPainterKey.currentContext == null) {
return false;
}
final Offset localOffset = _getLocalOffset(_scrollbarPainterKey, position);
return scrollbarPainter.hitTestInteractive(localOffset, kind, forHover: true);
}
/// Cancels the fade out animation so the scrollbar will remain visible for
/// interaction.
///
/// Can be overridden by subclasses to respond to a [PointerHoverEvent].
///
/// Helper methods [isPointerOverScrollbar], [isPointerOverThumb], and
/// [isPointerOverTrack] can be used to determine the location of the pointer
/// relative to the painter scrollbar elements.
@protected
@mustCallSuper
void handleHover(PointerHoverEvent event) {
// Check if the position of the pointer falls over the painted scrollbar
if (isPointerOverScrollbar(event.position, event.kind, forHover: true)) {
_hoverIsActive = true;
// Bring the scrollbar back into view if it has faded or started to fade
// away.
_fadeoutAnimationController.forward();
_fadeoutTimer?.cancel();
} else if (_hoverIsActive) {
// Pointer is not over painted scrollbar.
_hoverIsActive = false;
_maybeStartFadeoutTimer();
}
}
/// Initiates the fade out animation.
///
/// Can be overridden by subclasses to respond to a [PointerExitEvent].
@protected
@mustCallSuper
void handleHoverExit(PointerExitEvent event) {
_hoverIsActive = false;
_maybeStartFadeoutTimer();
}
// Returns the delta that should result from applying [event] with axis and
// direction taken into account.
double _pointerSignalEventDelta(PointerScrollEvent event) {
assert(_cachedController != null);
double delta = _cachedController!.position.axis == Axis.horizontal
? event.scrollDelta.dx
: event.scrollDelta.dy;
if (axisDirectionIsReversed(_cachedController!.position.axisDirection)) {
delta *= -1;
}
return delta;
}
// Returns the offset that should result from applying [event] to the current
// position, taking min/max scroll extent into account.
double _targetScrollOffsetForPointerScroll(double delta) {
assert(_cachedController != null);
return math.min(
math.max(_cachedController!.position.pixels + delta, _cachedController!.position.minScrollExtent),
_cachedController!.position.maxScrollExtent,
);
}
void _handlePointerScroll(PointerEvent event) {
assert(event is PointerScrollEvent);
_cachedController = _effectiveScrollController;
final double delta = _pointerSignalEventDelta(event as PointerScrollEvent);
final double targetScrollOffset = _targetScrollOffsetForPointerScroll(delta);
if (delta != 0.0 && targetScrollOffset != _cachedController!.position.pixels) {
_cachedController!.position.pointerScroll(delta);
}
}
void _receivedPointerSignal(PointerSignalEvent event) {
_cachedController = _effectiveScrollController;
// Only try to scroll if the bar absorb the hit test.
if ((scrollbarPainter.hitTest(event.localPosition) ?? false) &&
_cachedController != null &&
_cachedController!.hasClients &&
(!_thumbDragging || kIsWeb)) {
final ScrollPosition position = _cachedController!.position;
if (event is PointerScrollEvent) {
if (!position.physics.shouldAcceptUserOffset(position)) {
return;
}
final double delta = _pointerSignalEventDelta(event);
final double targetScrollOffset = _targetScrollOffsetForPointerScroll(delta);
if (delta != 0.0 && targetScrollOffset != position.pixels) {
GestureBinding.instance.pointerSignalResolver.register(event, _handlePointerScroll);
}
} else if (event is PointerScrollInertiaCancelEvent) {
position.jumpTo(position.pixels);
// Don't use the pointer signal resolver, all hit-tested scrollables should stop.
}
}
}
@override
void dispose() {
_fadeoutAnimationController.dispose();
_fadeoutTimer?.cancel();
scrollbarPainter.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
updateScrollbarPainter();
return NotificationListener<ScrollMetricsNotification>(
onNotification: _handleScrollMetricsNotification,
child: NotificationListener<ScrollNotification>(
onNotification: _handleScrollNotification,
child: RepaintBoundary(
child: Listener(
onPointerSignal: _receivedPointerSignal,
child: RawGestureDetector(
gestures: _gestures,
child: MouseRegion(
onExit: (PointerExitEvent event) {
switch (event.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
if (enableGestures) {
handleHoverExit(event);
}
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.unknown:
case PointerDeviceKind.touch:
break;
}
},
onHover: (PointerHoverEvent event) {
switch (event.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
if (enableGestures) {
handleHover(event);
}
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.unknown:
case PointerDeviceKind.touch:
break;
}
},
child: CustomPaint(
key: _scrollbarPainterKey,
foregroundPainter: scrollbarPainter,
child: RepaintBoundary(child: widget.child),
),
),
),
),
),
),
);
}
}
// A long press gesture detector that only responds to events on the scrollbar's
// thumb and ignores everything else.
class _ThumbPressGestureRecognizer extends LongPressGestureRecognizer {
_ThumbPressGestureRecognizer({
required Object super.debugOwner,
required GlobalKey customPaintKey,
required super.duration,
}) : _customPaintKey = customPaintKey;
final GlobalKey _customPaintKey;
@override
bool isPointerAllowed(PointerDownEvent event) {
if (!_hitTestInteractive(_customPaintKey, event.position, event.kind)) {
return false;
}
return super.isPointerAllowed(event);
}
bool _hitTestInteractive(GlobalKey customPaintKey, Offset offset, PointerDeviceKind kind) {
if (customPaintKey.currentContext == null) {
return false;
}
final CustomPaint customPaint = customPaintKey.currentContext!.widget as CustomPaint;
final ScrollbarPainter painter = customPaint.foregroundPainter! as ScrollbarPainter;
final Offset localOffset = _getLocalOffset(customPaintKey, offset);
return painter.hitTestOnlyThumbInteractive(localOffset, kind);
}
}
// A tap gesture detector that only responds to events on the scrollbar's
// track and ignores everything else, including the thumb.
class _TrackTapGestureRecognizer extends TapGestureRecognizer {
_TrackTapGestureRecognizer({
required super.debugOwner,
required GlobalKey customPaintKey,
}) : _customPaintKey = customPaintKey;
final GlobalKey _customPaintKey;
@override
bool isPointerAllowed(PointerDownEvent event) {
if (!_hitTestInteractive(_customPaintKey, event.position, event.kind)) {
return false;
}
return super.isPointerAllowed(event);
}
bool _hitTestInteractive(GlobalKey customPaintKey, Offset offset, PointerDeviceKind kind) {
if (customPaintKey.currentContext == null) {
return false;
}
final CustomPaint customPaint = customPaintKey.currentContext!.widget as CustomPaint;
final ScrollbarPainter painter = customPaint.foregroundPainter! as ScrollbarPainter;
final Offset localOffset = _getLocalOffset(customPaintKey, offset);
// We only receive track taps that are not on the thumb.
return painter.hitTestInteractive(localOffset, kind) && !painter.hitTestOnlyThumbInteractive(localOffset, kind);
}
}
Offset _getLocalOffset(GlobalKey scrollbarPainterKey, Offset position) {
final RenderBox renderBox = scrollbarPainterKey.currentContext!.findRenderObject()! as RenderBox;
return renderBox.globalToLocal(position);
}
| flutter/packages/flutter/lib/src/widgets/scrollbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scrollbar.dart",
"repo_id": "flutter",
"token_count": 26717
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
import 'media_query.dart';
/// Controls how the [SnapshotWidget] paints its child.
enum SnapshotMode {
/// The child is snapshotted, but only if all descendants can be snapshotted.
///
/// If there is a platform view in the children of a snapshot widget, the
/// snapshot will not be used and the child will be rendered using
/// [SnapshotPainter.paint]. This uses an un-snapshotted child and by default
/// paints it with no additional modification.
permissive,
/// An error is thrown if the child cannot be snapshotted.
///
/// This setting is the default state of the [SnapshotWidget].
normal,
/// The child is snapshotted and any child platform views are ignored.
///
/// This mode can be useful if there is a platform view descendant that does
/// not need to be included in the snapshot.
forced,
}
/// A controller for the [SnapshotWidget] that controls when the child image is displayed
/// and when to regenerated the child image.
///
/// When the value of [allowSnapshotting] is true, the [SnapshotWidget] will paint the child
/// widgets based on the [SnapshotMode] of the snapshot widget.
///
/// The controller notifies its listeners when the value of [allowSnapshotting] changes
/// or when [clear] is called.
///
/// To force [SnapshotWidget] to recreate the child image, call [clear].
class SnapshotController extends ChangeNotifier {
/// Create a new [SnapshotController].
///
/// By default, [allowSnapshotting] is `false` and cannot be `null`.
SnapshotController({
bool allowSnapshotting = false,
}) : _allowSnapshotting = allowSnapshotting;
/// Reset the snapshot held by any listening [SnapshotWidget].
///
/// This has no effect if [allowSnapshotting] is `false`.
void clear() {
notifyListeners();
}
/// Whether a snapshot of this child widget is painted in its place.
bool get allowSnapshotting => _allowSnapshotting;
bool _allowSnapshotting;
set allowSnapshotting(bool value) {
if (value == allowSnapshotting) {
return;
}
_allowSnapshotting = value;
notifyListeners();
}
}
/// A widget that can replace its child with a snapshotted version of the child.
///
/// A snapshot is a frozen texture-backed representation of all child pictures
/// and layers stored as a [ui.Image].
///
/// This widget is useful for performing short animations that would otherwise
/// be expensive or that cannot rely on raster caching. For example, scale and
/// skew animations are often expensive to perform on complex children, as are
/// blurs. For a short animation, a widget that contains these expensive effects
/// can be replaced with a snapshot of itself and manipulated instead.
///
/// For example, the Android Q [ZoomPageTransitionsBuilder] uses a snapshot widget
/// for the forward and entering route to avoid the expensive scale animation.
/// This also has the effect of briefly pausing any animations on the page.
///
/// Generally, this widget should not be used in places where users expect the
/// child widget to continue animating or to be responsive, such as an unbounded
/// animation.
///
/// Caveats:
///
/// * The contents of platform views cannot be captured by a snapshot
/// widget. If a platform view is encountered, then the snapshot widget will
/// determine how to render its children based on the [SnapshotMode]. This
/// defaults to [SnapshotMode.normal] which will throw an exception if a
/// platform view is encountered.
///
/// * The snapshotting functionality of this widget is not supported on the HTML
/// backend of Flutter for the Web. Setting [SnapshotController.allowSnapshotting] to true
/// may cause an error to be thrown. On the CanvasKit backend of Flutter, the
/// performance of using this widget may regress performance due to the fact
/// that both the UI and engine share a single thread.
class SnapshotWidget extends SingleChildRenderObjectWidget {
/// Create a new [SnapshotWidget].
///
/// The [controller] and [child] arguments are required.
const SnapshotWidget({
super.key,
this.mode = SnapshotMode.normal,
this.painter = const _DefaultSnapshotPainter(),
this.autoresize = false,
required this.controller,
required super.child
});
/// The controller that determines when to display the children as a snapshot.
final SnapshotController controller;
/// Configuration that controls how the snapshot widget decides to paint its children.
///
/// Defaults to [SnapshotMode.normal], which throws an error when a platform view
/// or texture view is encountered.
///
/// See [SnapshotMode] for more information.
final SnapshotMode mode;
/// Whether or not changes in render object size should automatically re-create
/// the snapshot.
///
/// Defaults to false.
final bool autoresize;
/// The painter used to paint the child snapshot or child widgets.
final SnapshotPainter painter;
@override
RenderObject createRenderObject(BuildContext context) {
debugCheckHasMediaQuery(context);
return _RenderSnapshotWidget(
controller: controller,
mode: mode,
devicePixelRatio: MediaQuery.devicePixelRatioOf(context),
painter: painter,
autoresize: autoresize,
);
}
@override
void updateRenderObject(BuildContext context, covariant RenderObject renderObject) {
debugCheckHasMediaQuery(context);
(renderObject as _RenderSnapshotWidget)
..controller = controller
..mode = mode
..devicePixelRatio = MediaQuery.devicePixelRatioOf(context)
..painter = painter
..autoresize = autoresize;
}
}
// A render object that conditionally converts its child into a [ui.Image]
// and then paints it in place of the child.
class _RenderSnapshotWidget extends RenderProxyBox {
// Create a new [_RenderSnapshotWidget].
_RenderSnapshotWidget({
required double devicePixelRatio,
required SnapshotController controller,
required SnapshotMode mode,
required SnapshotPainter painter,
required bool autoresize,
}) : _devicePixelRatio = devicePixelRatio,
_controller = controller,
_mode = mode,
_painter = painter,
_autoresize = autoresize;
/// The device pixel ratio used to create the child image.
double get devicePixelRatio => _devicePixelRatio;
double _devicePixelRatio;
set devicePixelRatio(double value) {
if (value == devicePixelRatio) {
return;
}
_devicePixelRatio = value;
if (_childRaster == null) {
return;
} else {
_childRaster?.dispose();
_childRaster = null;
markNeedsPaint();
}
}
/// The painter used to paint the child snapshot or child widgets.
SnapshotPainter get painter => _painter;
SnapshotPainter _painter;
set painter(SnapshotPainter value) {
if (value == painter) {
return;
}
final SnapshotPainter oldPainter = painter;
oldPainter.removeListener(markNeedsPaint);
_painter = value;
if (oldPainter.runtimeType != painter.runtimeType ||
painter.shouldRepaint(oldPainter)) {
markNeedsPaint();
}
if (attached) {
painter.addListener(markNeedsPaint);
}
}
/// A controller that determines whether to paint the child normally or to
/// paint a snapshotted version of that child.
SnapshotController get controller => _controller;
SnapshotController _controller;
set controller(SnapshotController value) {
if (value == controller) {
return;
}
controller.removeListener(_onRasterValueChanged);
final bool oldValue = controller.allowSnapshotting;
_controller = value;
if (attached) {
controller.addListener(_onRasterValueChanged);
if (oldValue != controller.allowSnapshotting) {
_onRasterValueChanged();
}
}
}
/// How the snapshot widget will handle platform views in child layers.
SnapshotMode get mode => _mode;
SnapshotMode _mode;
set mode(SnapshotMode value) {
if (value == _mode) {
return;
}
_mode = value;
markNeedsPaint();
}
/// Whether or not changes in render object size should automatically re-rasterize.
bool get autoresize => _autoresize;
bool _autoresize;
set autoresize(bool value) {
if (value == autoresize) {
return;
}
_autoresize = value;
markNeedsPaint();
}
ui.Image? _childRaster;
Size? _childRasterSize;
// Set to true if the snapshot mode was not forced and a platform view
// was encountered while attempting to snapshot the child.
bool _disableSnapshotAttempt = false;
@override
void attach(covariant PipelineOwner owner) {
controller.addListener(_onRasterValueChanged);
painter.addListener(markNeedsPaint);
super.attach(owner);
}
@override
void detach() {
_disableSnapshotAttempt = false;
controller.removeListener(_onRasterValueChanged);
painter.removeListener(markNeedsPaint);
_childRaster?.dispose();
_childRaster = null;
_childRasterSize = null;
super.detach();
}
@override
void dispose() {
controller.removeListener(_onRasterValueChanged);
painter.removeListener(markNeedsPaint);
_childRaster?.dispose();
_childRaster = null;
_childRasterSize = null;
super.dispose();
}
void _onRasterValueChanged() {
_disableSnapshotAttempt = false;
_childRaster?.dispose();
_childRaster = null;
_childRasterSize = null;
markNeedsPaint();
}
// Paint [child] with this painting context, then convert to a raster and detach all
// children from this layer.
ui.Image? _paintAndDetachToImage() {
final OffsetLayer offsetLayer = OffsetLayer();
final PaintingContext context = PaintingContext(offsetLayer, Offset.zero & size);
super.paint(context, Offset.zero);
// This ignore is here because this method is protected by the `PaintingContext`. Adding a new
// method that performs the work of `_paintAndDetachToImage` would avoid the need for this, but
// that would conflict with our goals of minimizing painting context.
// ignore: invalid_use_of_protected_member
context.stopRecordingIfNeeded();
if (mode != SnapshotMode.forced && !offsetLayer.supportsRasterization()) {
offsetLayer.dispose();
if (mode == SnapshotMode.normal) {
throw FlutterError('SnapshotWidget used with a child that contains a PlatformView.');
}
_disableSnapshotAttempt = true;
return null;
}
final ui.Image image = offsetLayer.toImageSync(Offset.zero & size, pixelRatio: devicePixelRatio);
offsetLayer.dispose();
_lastCachedSize = size;
return image;
}
Size? _lastCachedSize;
@override
void paint(PaintingContext context, Offset offset) {
if (size.isEmpty) {
_childRaster?.dispose();
_childRaster = null;
_childRasterSize = null;
return;
}
if (!controller.allowSnapshotting || _disableSnapshotAttempt) {
_childRaster?.dispose();
_childRaster = null;
_childRasterSize = null;
painter.paint(context, offset, size, super.paint);
return;
}
if (autoresize && size != _lastCachedSize && _lastCachedSize != null) {
_childRaster?.dispose();
_childRaster = null;
}
if (_childRaster == null) {
_childRaster = _paintAndDetachToImage();
_childRasterSize = size * devicePixelRatio;
}
if (_childRaster == null) {
painter.paint(context, offset, size, super.paint);
} else {
painter.paintSnapshot(context, offset, size, _childRaster!, _childRasterSize!, devicePixelRatio);
}
}
}
/// A painter used to paint either a snapshot or the child widgets that
/// would be a snapshot.
///
/// The painter can call [notifyListeners] to have the [SnapshotWidget]
/// re-paint (re-using the same raster). This allows animations to be performed
/// without re-snapshotting of children. For certain scale or perspective changing
/// transforms, such as a rotation, this can be significantly faster than performing
/// the same animation at the widget level.
///
/// By default, the [SnapshotWidget] includes a delegate that draws the child raster
/// exactly as the child widgets would have been drawn. Nevertheless, this can
/// also be used to efficiently transform the child raster and apply complex paint
/// effects.
///
/// {@tool snippet}
///
/// The following method shows how to efficiently rotate the child raster.
///
/// ```dart
/// void paint(PaintingContext context, Offset offset, Size size, ui.Image image, double pixelRatio) {
/// const double radians = 0.5; // Could be driven by an animation.
/// final Matrix4 transform = Matrix4.rotationZ(radians);
/// context.canvas.transform(transform.storage);
/// final Rect src = Rect.fromLTWH(0, 0, image.width.toDouble(), image.height.toDouble());
/// final Rect dst = Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height);
/// final Paint paint = Paint()
/// ..filterQuality = FilterQuality.low;
/// context.canvas.drawImageRect(image, src, dst, paint);
/// }
/// ```
/// {@end-tool}
abstract class SnapshotPainter extends ChangeNotifier {
/// Creates an instance of [SnapshotPainter].
SnapshotPainter() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// Called whenever the [image] that represents a [SnapshotWidget]s child should be painted.
///
/// The image is rasterized at the physical pixel resolution and should be scaled down by
/// [pixelRatio] to account for device independent pixels.
///
/// {@tool snippet}
///
/// The following method shows how the default implementation of the delegate used by the
/// [SnapshotPainter] paints the snapshot. This must account for the fact that the image
/// width and height will be given in physical pixels, while the image must be painted with
/// device independent pixels. That is, the width and height of the image is the widget and
/// height of the provided `size`, multiplied by the `pixelRatio`. In addition, the actual
/// size of the scene captured by the `image` is not `image.width` or `image.height`, but
/// indeed `sourceSize`, because the former is a rounded inaccurate integer:
///
/// ```dart
/// void paint(PaintingContext context, Offset offset, Size size, ui.Image image, Size sourceSize, double pixelRatio) {
/// final Rect src = Rect.fromLTWH(0, 0, sourceSize.width, sourceSize.height);
/// final Rect dst = Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height);
/// final Paint paint = Paint()
/// ..filterQuality = FilterQuality.low;
/// context.canvas.drawImageRect(image, src, dst, paint);
/// }
/// ```
/// {@end-tool}
void paintSnapshot(PaintingContext context, Offset offset, Size size, ui.Image image, Size sourceSize, double pixelRatio);
/// Paint the child via [painter], applying any effects that would have been painted
/// in [SnapshotPainter.paintSnapshot].
///
/// This method is called when snapshotting is disabled, or when [SnapshotMode.permissive]
/// is used and a child platform view prevents snapshotting.
///
/// The [offset] and [size] are the location and dimensions of the render object.
void paint(PaintingContext context, Offset offset, Size size, PaintingContextCallback painter);
/// Called whenever a new instance of the snapshot widget delegate class is
/// provided to the [SnapshotWidget] object, or any time that a new
/// [SnapshotPainter] object is created with a new instance of the
/// delegate class (which amounts to the same thing, because the latter is
/// implemented in terms of the former).
///
/// If the new instance represents different information than the old
/// instance, then the method should return true, otherwise it should return
/// false.
///
/// If the method returns false, then the [paint] call might be optimized
/// away.
///
/// It's possible that the [paint] method will get called even if
/// [shouldRepaint] returns false (e.g. if an ancestor or descendant needed to
/// be repainted). It's also possible that the [paint] method will get called
/// without [shouldRepaint] being called at all (e.g. if the box changes
/// size).
///
/// Changing the delegate will not cause the child image retained by the
/// [SnapshotWidget] to be updated. Instead, [SnapshotController.clear] can
/// be used to force the generation of a new image.
///
/// The `oldPainter` argument will never be null.
bool shouldRepaint(covariant SnapshotPainter oldPainter);
}
class _DefaultSnapshotPainter implements SnapshotPainter {
const _DefaultSnapshotPainter();
@override
void addListener(ui.VoidCallback listener) { }
@override
void dispose() { }
@override
bool get hasListeners => false;
@override
void notifyListeners() { }
@override
void paint(PaintingContext context, ui.Offset offset, ui.Size size, PaintingContextCallback painter) {
painter(context, offset);
}
@override
void paintSnapshot(PaintingContext context, ui.Offset offset, ui.Size size, ui.Image image, Size sourceSize, double pixelRatio) {
final Rect src = Rect.fromLTWH(0, 0, sourceSize.width, sourceSize.height);
final Rect dst = Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height);
final Paint paint = Paint()
..filterQuality = FilterQuality.low;
context.canvas.drawImageRect(image, src, dst, paint);
}
@override
void removeListener(ui.VoidCallback listener) { }
@override
bool shouldRepaint(covariant _DefaultSnapshotPainter oldPainter) => false;
}
| flutter/packages/flutter/lib/src/widgets/snapshot_widget.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/snapshot_widget.dart",
"repo_id": "flutter",
"token_count": 5418
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'notification_listener.dart';
import 'primary_scroll_controller.dart';
import 'scroll_controller.dart';
import 'scroll_delegate.dart';
import 'scroll_notification.dart';
import 'scroll_physics.dart';
import 'scroll_view.dart';
import 'scrollable.dart';
import 'scrollable_helpers.dart';
import 'two_dimensional_viewport.dart';
/// A widget that combines a [TwoDimensionalScrollable] and a
/// [TwoDimensionalViewport] to create an interactive scrolling pane of content
/// in both vertical and horizontal dimensions.
///
/// A two-way scrollable widget consist of three pieces:
///
/// 1. A [TwoDimensionalScrollable] widget, which listens for various user
/// gestures and implements the interaction design for scrolling.
/// 2. A [TwoDimensionalViewport] widget, which implements the visual design
/// for scrolling by displaying only a portion
/// of the widgets inside the scroll view.
/// 3. A [TwoDimensionalChildDelegate], which provides the children visible in
/// the scroll view.
///
/// [TwoDimensionalScrollView] helps orchestrate these pieces by creating the
/// [TwoDimensionalScrollable] and deferring to its subclass to implement
/// [buildViewport], which builds a subclass of [TwoDimensionalViewport]. The
/// [TwoDimensionalChildDelegate] is provided by the [delegate] parameter.
///
/// A [TwoDimensionalScrollView] has two different [ScrollPosition]s, one for
/// each [Axis]. This means that there are also two unique [ScrollController]s
/// for these positions. To provide a ScrollController to access the
/// ScrollPosition, use the [ScrollableDetails.controller] property of the
/// associated axis that is provided to this scroll view.
abstract class TwoDimensionalScrollView extends StatelessWidget {
/// Creates a widget that scrolls in both dimensions.
///
/// The [primary] argument is associated with the [mainAxis]. The main axis
/// [ScrollableDetails.controller] must be null if [primary] is configured for
/// that axis. If [primary] is true, the nearest [PrimaryScrollController]
/// surrounding the widget is attached to the scroll position of that axis.
const TwoDimensionalScrollView({
super.key,
this.primary,
this.mainAxis = Axis.vertical,
this.verticalDetails = const ScrollableDetails.vertical(),
this.horizontalDetails = const ScrollableDetails.horizontal(),
required this.delegate,
this.cacheExtent,
this.diagonalDragBehavior = DiagonalDragBehavior.none,
this.dragStartBehavior = DragStartBehavior.start,
this.keyboardDismissBehavior = ScrollViewKeyboardDismissBehavior.manual,
this.clipBehavior = Clip.hardEdge,
});
/// A delegate that provides the children for the [TwoDimensionalScrollView].
final TwoDimensionalChildDelegate delegate;
/// {@macro flutter.rendering.RenderViewportBase.cacheExtent}
final double? cacheExtent;
/// Whether scrolling gestures should lock to one axes, allow free movement
/// in both axes, or be evaluated on a weighted scale.
///
/// Defaults to [DiagonalDragBehavior.none], locking axes to receive input one
/// at a time.
final DiagonalDragBehavior diagonalDragBehavior;
/// {@macro flutter.widgets.scroll_view.primary}
final bool? primary;
/// The main axis of the two.
///
/// Used to determine how to apply [primary] when true.
///
/// This value should also be provided to the subclass of
/// [TwoDimensionalViewport], where it is used to determine paint order of
/// children.
final Axis mainAxis;
/// The configuration of the vertical Scrollable.
///
/// These [ScrollableDetails] can be used to set the [AxisDirection],
/// [ScrollController], [ScrollPhysics] and more for the vertical axis.
final ScrollableDetails verticalDetails;
/// The configuration of the horizontal Scrollable.
///
/// These [ScrollableDetails] can be used to set the [AxisDirection],
/// [ScrollController], [ScrollPhysics] and more for the horizontal axis.
final ScrollableDetails horizontalDetails;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@macro flutter.widgets.scroll_view.keyboardDismissBehavior}
final ScrollViewKeyboardDismissBehavior keyboardDismissBehavior;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// Build the two dimensional viewport.
///
/// Subclasses may override this method to change how the viewport is built,
/// likely a subclass of [TwoDimensionalViewport].
///
/// The `verticalOffset` and `horizontalOffset` arguments are the values
/// obtained from [TwoDimensionalScrollable.viewportBuilder].
Widget buildViewport(
BuildContext context,
ViewportOffset verticalOffset,
ViewportOffset horizontalOffset,
);
@override
Widget build(BuildContext context) {
assert(
axisDirectionToAxis(verticalDetails.direction) == Axis.vertical,
'TwoDimensionalScrollView.verticalDetails are not Axis.vertical.'
);
assert(
axisDirectionToAxis(horizontalDetails.direction) == Axis.horizontal,
'TwoDimensionalScrollView.horizontalDetails are not Axis.horizontal.'
);
ScrollableDetails mainAxisDetails = switch (mainAxis) {
Axis.vertical => verticalDetails,
Axis.horizontal => horizontalDetails,
};
final bool effectivePrimary = primary
?? mainAxisDetails.controller == null && PrimaryScrollController.shouldInherit(
context,
mainAxis,
);
if (effectivePrimary) {
// Using PrimaryScrollController for mainAxis.
assert(
mainAxisDetails.controller == null,
'TwoDimensionalScrollView.primary was explicitly set to true, but a '
'ScrollController was provided in the ScrollableDetails of the '
'TwoDimensionalScrollView.mainAxis.'
);
mainAxisDetails = mainAxisDetails.copyWith(
controller: PrimaryScrollController.of(context),
);
}
final TwoDimensionalScrollable scrollable = TwoDimensionalScrollable(
horizontalDetails : switch (mainAxis) {
Axis.horizontal => mainAxisDetails,
Axis.vertical => horizontalDetails,
},
verticalDetails: switch (mainAxis) {
Axis.vertical => mainAxisDetails,
Axis.horizontal => verticalDetails,
},
diagonalDragBehavior: diagonalDragBehavior,
viewportBuilder: buildViewport,
dragStartBehavior: dragStartBehavior,
);
final Widget scrollableResult = effectivePrimary
// Further descendant ScrollViews will not inherit the same PrimaryScrollController
? PrimaryScrollController.none(child: scrollable)
: scrollable;
if (keyboardDismissBehavior == ScrollViewKeyboardDismissBehavior.onDrag) {
return NotificationListener<ScrollUpdateNotification>(
child: scrollableResult,
onNotification: (ScrollUpdateNotification notification) {
final FocusScopeNode focusScope = FocusScope.of(context);
if (notification.dragDetails != null && focusScope.hasFocus) {
focusScope.unfocus();
}
return false;
},
);
}
return scrollableResult;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<Axis>('mainAxis', mainAxis));
properties.add(EnumProperty<DiagonalDragBehavior>('diagonalDragBehavior', diagonalDragBehavior));
properties.add(FlagProperty('primary', value: primary, ifTrue: 'using primary controller', showName: true));
properties.add(DiagnosticsProperty<ScrollableDetails>('verticalDetails', verticalDetails, showName: false));
properties.add(DiagnosticsProperty<ScrollableDetails>('horizontalDetails', horizontalDetails, showName: false));
}
}
| flutter/packages/flutter/lib/src/widgets/two_dimensional_scroll_view.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/two_dimensional_scroll_view.dart",
"repo_id": "flutter",
"token_count": 2490
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/cupertino.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Activity indicator animate property works', (WidgetTester tester) async {
await tester.pumpWidget(buildCupertinoActivityIndicator());
expect(SchedulerBinding.instance.transientCallbackCount, equals(1));
await tester.pumpWidget(buildCupertinoActivityIndicator(false));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.pumpWidget(Container());
await tester.pumpWidget(buildCupertinoActivityIndicator(false));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.pumpWidget(buildCupertinoActivityIndicator());
expect(SchedulerBinding.instance.transientCallbackCount, equals(1));
});
testWidgets('Activity indicator dark mode', (WidgetTester tester) async {
final Key key = UniqueKey();
await tester.pumpWidget(
Center(
child: MediaQuery(
data: const MediaQueryData(),
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.white,
child: CupertinoActivityIndicator(
animating: false,
radius: 35,
),
),
),
),
),
);
await expectLater(
find.byKey(key),
matchesGoldenFile('activityIndicator.paused.light.png'),
);
await tester.pumpWidget(
Center(
child: MediaQuery(
data: const MediaQueryData(platformBrightness: Brightness.dark),
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.black,
child: CupertinoActivityIndicator(
animating: false,
radius: 35,
),
),
),
),
),
);
await expectLater(
find.byKey(key),
matchesGoldenFile('activityIndicator.paused.dark.png'),
);
});
testWidgets('Activity indicator 0% in progress', (WidgetTester tester) async {
final Key key = UniqueKey();
await tester.pumpWidget(
Center(
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.white,
child: CupertinoActivityIndicator.partiallyRevealed(
progress: 0,
),
),
),
),
);
await expectLater(
find.byKey(key),
matchesGoldenFile('activityIndicator.inprogress.0.0.png'),
);
});
testWidgets('Activity indicator 30% in progress', (WidgetTester tester) async {
final Key key = UniqueKey();
await tester.pumpWidget(
Center(
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.white,
child: CupertinoActivityIndicator.partiallyRevealed(
progress: 0.5,
),
),
),
),
);
await expectLater(
find.byKey(key),
matchesGoldenFile('activityIndicator.inprogress.0.3.png'),
);
});
testWidgets('Activity indicator 100% in progress', (WidgetTester tester) async {
final Key key = UniqueKey();
await tester.pumpWidget(
Center(
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.white,
child: CupertinoActivityIndicator.partiallyRevealed(),
),
),
),
);
await expectLater(
find.byKey(key),
matchesGoldenFile('activityIndicator.inprogress.1.0.png'),
);
});
// Regression test for https://github.com/flutter/flutter/issues/41345.
testWidgets('has the correct corner radius', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoActivityIndicator(animating: false, radius: 100),
);
// An earlier implementation for the activity indicator started drawing
// the ticks at 9 o'clock, however, in order to support partially revealed
// indicator (https://github.com/flutter/flutter/issues/29159), the
// first tick was changed to be at 12 o'clock.
expect(
find.byType(CupertinoActivityIndicator),
paints
..rrect(rrect: const RRect.fromLTRBXY(-10, -100 / 3, 10, -100, 10, 10)),
);
});
testWidgets('Can specify color', (WidgetTester tester) async {
final Key key = UniqueKey();
await tester.pumpWidget(
Center(
child: RepaintBoundary(
key: key,
child: const ColoredBox(
color: CupertinoColors.white,
child: CupertinoActivityIndicator(
animating: false,
color: Color(0xFF5D3FD3),
radius: 100,
),
),
),
),
);
expect(
find.byType(CupertinoActivityIndicator),
paints
..rrect(rrect: const RRect.fromLTRBXY(-10, -100 / 3, 10, -100, 10, 10),
color: const Color(0x935d3fd3)),
);
});
}
Widget buildCupertinoActivityIndicator([bool? animating]) {
return MediaQuery(
data: const MediaQueryData(),
child: CupertinoActivityIndicator(
animating: animating ?? true,
),
);
}
| flutter/packages/flutter/test/cupertino/activity_indicator_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/activity_indicator_test.dart",
"repo_id": "flutter",
"token_count": 2430
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('IconTheme.of works', (WidgetTester tester) async {
const IconThemeData data = IconThemeData(
size: 16.0,
fill: 0.0,
weight: 400.0,
grade: 0.0,
opticalSize: 48.0,
color: Color(0xAAAAAAAA),
opacity: 0.5,
applyTextScaling: true,
);
late IconThemeData retrieved;
await tester.pumpWidget(
IconTheme(data: data, child: Builder(builder: (BuildContext context) {
retrieved = IconTheme.of(context);
return const SizedBox();
})),
);
expect(retrieved, data);
await tester.pumpWidget(
IconTheme(
data: const CupertinoIconThemeData(color: CupertinoColors.systemBlue),
child: MediaQuery(
data: const MediaQueryData(platformBrightness: Brightness.dark),
child: Builder(builder: (BuildContext context) {
retrieved = IconTheme.of(context);
return const SizedBox();
},
),
),
),
);
expect(retrieved.color, isSameColorAs(CupertinoColors.systemBlue.darkColor));
});
}
| flutter/packages/flutter/test/cupertino/icon_theme_data_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/icon_theme_data_test.dart",
"repo_id": "flutter",
"token_count": 556
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
const CupertinoDynamicColor _kScrollbarColor = CupertinoDynamicColor.withBrightness(
color: Color(0x59000000),
darkColor: Color(0x80FFFFFF),
);
void main() {
const Duration kScrollbarTimeToFade = Duration(milliseconds: 1200);
const Duration kScrollbarFadeDuration = Duration(milliseconds: 250);
const Duration kScrollbarResizeDuration = Duration(milliseconds: 100);
const Duration kLongPressDuration = Duration(milliseconds: 100);
testWidgets('Scrollbar never goes away until finger lift', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: CupertinoScrollbar(
child: SingleChildScrollView(child: SizedBox(width: 4000.0, height: 4000.0)),
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -10.0));
await tester.pump();
// Scrollbar fully showing
await tester.pump(const Duration(milliseconds: 500));
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
await tester.pump(const Duration(seconds: 3));
await tester.pump(const Duration(seconds: 3));
// Still there.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
await gesture.up();
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration * 0.5);
// Opacity going down now.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color.withAlpha(69),
));
});
testWidgets('Scrollbar dark mode', (WidgetTester tester) async {
Brightness brightness = Brightness.light;
late StateSetter setState;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return MediaQuery(
data: MediaQueryData(platformBrightness: brightness),
child: const CupertinoScrollbar(
child: SingleChildScrollView(child: SizedBox(width: 4000.0, height: 4000.0)),
),
);
},
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, 10.0));
await tester.pump();
// Scrollbar fully showing
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
setState(() { brightness = Brightness.dark; });
await tester.pump();
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.darkColor,
));
});
testWidgets('Scrollbar thumb can be dragged with long press', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: const CupertinoScrollbar(
child: SingleChildScrollView(child: SizedBox(width: 4000.0, height: 4000.0)),
),
),
),
),
);
expect(scrollController.offset, 0.0);
// Scroll a bit.
const double scrollAmount = 10.0;
final TestGesture scrollGesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
// Scroll down by swiping up.
await scrollGesture.moveBy(const Offset(0.0, -scrollAmount));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Scrollbar thumb is fully showing and scroll offset has moved by
// scrollAmount.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
expect(scrollController.offset, scrollAmount);
await scrollGesture.up();
await tester.pump();
int hapticFeedbackCalls = 0;
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'HapticFeedback.vibrate') {
hapticFeedbackCalls += 1;
}
return null;
});
// Long press on the scrollbar thumb and expect a vibration after it resizes.
expect(hapticFeedbackCalls, 0);
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(796.0, 50.0));
await tester.pump(kLongPressDuration);
expect(hapticFeedbackCalls, 0);
await tester.pump(kScrollbarResizeDuration);
// Allow the haptic feedback some slack.
await tester.pump(const Duration(milliseconds: 1));
expect(hapticFeedbackCalls, 1);
// Drag the thumb down to scroll down.
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pump(const Duration(milliseconds: 100));
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
// The scrollbar thumb is still fully visible.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
// Let the thumb fade out so all timers have resolved.
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration);
});
testWidgets('Scrollbar thumb can be dragged with long press - reverse', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: const CupertinoScrollbar(
child: SingleChildScrollView(
reverse: true,
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
),
);
expect(scrollController.offset, 0.0);
// Scroll a bit.
const double scrollAmount = 10.0;
final TestGesture scrollGesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
// Scroll up by swiping down.
await scrollGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Scrollbar thumb is fully showing and scroll offset has moved by
// scrollAmount.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
expect(scrollController.offset, scrollAmount);
await scrollGesture.up();
await tester.pump();
int hapticFeedbackCalls = 0;
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'HapticFeedback.vibrate') {
hapticFeedbackCalls += 1;
}
return null;
});
// Long press on the scrollbar thumb and expect a vibration after it resizes.
expect(hapticFeedbackCalls, 0);
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(796.0, 550.0));
await tester.pump(kLongPressDuration);
expect(hapticFeedbackCalls, 0);
await tester.pump(kScrollbarResizeDuration);
// Allow the haptic feedback some slack.
await tester.pump(const Duration(milliseconds: 1));
expect(hapticFeedbackCalls, 1);
// Drag the thumb up to scroll up.
await dragScrollbarGesture.moveBy(const Offset(0.0, -scrollAmount));
await tester.pump(const Duration(milliseconds: 100));
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
// The scrollbar thumb is still fully visible.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
// Let the thumb fade out so all timers have resolved.
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration);
});
testWidgets('Scrollbar changes thickness and radius when dragged', (WidgetTester tester) async {
const double thickness = 20;
const double thicknessWhileDragging = 40;
const double radius = 10;
const double radiusWhileDragging = 20;
const double inset = 3;
const double scaleFactor = 2;
final Size screenSize = tester.view.physicalSize / tester.view.devicePixelRatio;
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: scrollController,
child: CupertinoScrollbar(
thickness: thickness,
thicknessWhileDragging: thicknessWhileDragging,
radius: const Radius.circular(radius),
radiusWhileDragging: const Radius.circular(radiusWhileDragging),
child: SingleChildScrollView(
child: SizedBox(
width: screenSize.width * scaleFactor,
height: screenSize.height * scaleFactor,
),
),
),
),
),
),
);
expect(scrollController.offset, 0.0);
// Scroll a bit to cause the scrollbar thumb to be shown;
// undo the scroll to put the thumb back at the top.
const double scrollAmount = 10.0;
final TestGesture scrollGesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await scrollGesture.moveBy(const Offset(0.0, -scrollAmount));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
await scrollGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pump();
await scrollGesture.up();
await tester.pump();
// Long press on the scrollbar thumb and expect it to grow
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(780.0, 50.0));
await tester.pump(kLongPressDuration);
expect(find.byType(CupertinoScrollbar), paints..rrect(
rrect: RRect.fromRectAndRadius(
Rect.fromLTWH(
screenSize.width - inset - thickness,
inset,
thickness,
(screenSize.height - 2 * inset) / scaleFactor,
),
const Radius.circular(radius),
),
));
await tester.pump(kScrollbarResizeDuration ~/ 2);
const double midpointThickness = (thickness + thicknessWhileDragging) / 2;
const double midpointRadius = (radius + radiusWhileDragging) / 2;
expect(find.byType(CupertinoScrollbar), paints..rrect(
rrect: RRect.fromRectAndRadius(
Rect.fromLTWH(
screenSize.width - inset - midpointThickness,
inset,
midpointThickness,
(screenSize.height - 2 * inset) / scaleFactor,
),
const Radius.circular(midpointRadius),
),
));
await tester.pump(kScrollbarResizeDuration ~/ 2);
expect(find.byType(CupertinoScrollbar), paints..rrect(
rrect: RRect.fromRectAndRadius(
Rect.fromLTWH(
screenSize.width - inset - thicknessWhileDragging,
inset,
thicknessWhileDragging,
(screenSize.height - 2 * inset) / scaleFactor,
),
const Radius.circular(radiusWhileDragging),
),
));
// Let the thumb fade out so all timers have resolved.
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration);
});
testWidgets('When thumbVisibility is true, must pass a controller or find PrimaryScrollController', (WidgetTester tester) async {
Widget viewWithScroll() {
return const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: CupertinoScrollbar(
thumbVisibility: true,
child: SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
final AssertionError exception = tester.takeException() as AssertionError;
expect(exception, isAssertionError);
},
);
testWidgets('When thumbVisibility is true, must pass a controller or find PrimaryScrollController that is attached to a scroll view', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
controller: controller,
thumbVisibility: true,
child: const SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
);
}
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
await tester.pumpWidget(viewWithScroll());
expect(error, isNotNull);
FlutterError.onError = handler;
},
);
testWidgets('When thumbVisibility is true, must pass a controller or find PrimaryScrollController', (WidgetTester tester) async {
Widget viewWithScroll() {
return const Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData(),
child: CupertinoScrollbar(
thumbVisibility: true,
child: SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
final AssertionError exception = tester.takeException() as AssertionError;
expect(exception, isAssertionError);
},
);
testWidgets('When thumbVisibility is true, must pass a controller or find PrimaryScrollController that is attached to a scroll view', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
controller: controller,
thumbVisibility: true,
child: const SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
);
}
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
await tester.pumpWidget(viewWithScroll());
expect(error, isNotNull);
FlutterError.onError = handler;
},
);
testWidgets('On first render with thumbVisibility: true, the thumb shows with PrimaryScrollController', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: controller,
child: Builder(
builder: (BuildContext context) {
return const CupertinoScrollbar(
thumbVisibility: true,
child: SingleChildScrollView(
primary: true,
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
);
},
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rect());
},
);
testWidgets('On first render with thumbVisibility: true, the thumb shows', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: controller,
child: CupertinoScrollbar(
thumbVisibility: true,
controller: controller,
child: const SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
// The scrollbar measures its size on the first frame
// and renders starting in the second,
//
// so pumpAndSettle a frame to allow it to appear.
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rrect());
});
testWidgets('On first render with thumbVisibility: true, the thumb shows with PrimaryScrollController', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: controller,
child: Builder(
builder: (BuildContext context) {
return const CupertinoScrollbar(
thumbVisibility: true,
child: SingleChildScrollView(
primary: true,
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
);
},
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rect());
},
);
testWidgets('On first render with thumbVisibility: true, the thumb shows', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: controller,
child: CupertinoScrollbar(
thumbVisibility: true,
controller: controller,
child: const SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
// The scrollbar measures its size on the first frame
// and renders starting in the second,
//
// so pumpAndSettle a frame to allow it to appear.
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rrect());
});
testWidgets('On first render with thumbVisibility: false, the thumb is hidden', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
Widget viewWithScroll() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: controller,
child: CupertinoScrollbar(
controller: controller,
child: const SingleChildScrollView(
child: SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
),
),
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), isNot(paints..rect()));
});
testWidgets('With thumbVisibility: true, fling a scroll. While it is still scrolling, set thumbVisibility: false. The thumb should not fade out until the scrolling stops.', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
bool thumbVisibility = true;
Widget viewWithScroll() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: Stack(
children: <Widget>[
CupertinoScrollbar(
thumbVisibility: thumbVisibility,
controller: controller,
child: SingleChildScrollView(
controller: controller,
child: const SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
Positioned(
bottom: 10,
child: CupertinoButton(
onPressed: () {
setState(() {
thumbVisibility = !thumbVisibility;
});
},
child: const Text('change thumbVisibility'),
),
),
],
),
),
);
},
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
await tester.fling(
find.byType(SingleChildScrollView),
const Offset(0.0, -10.0),
10,
);
expect(find.byType(CupertinoScrollbar), paints..rrect());
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), isNot(paints..rrect()));
},
);
testWidgets(
'With thumbVisibility: false, set thumbVisibility: true. The thumb should be always shown directly',
(WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
bool thumbVisibility = false;
Widget viewWithScroll() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: Stack(
children: <Widget>[
CupertinoScrollbar(
thumbVisibility: thumbVisibility,
controller: controller,
child: SingleChildScrollView(
controller: controller,
child: const SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
Positioned(
bottom: 10,
child: CupertinoButton(
onPressed: () {
setState(() {
thumbVisibility = !thumbVisibility;
});
},
child: const Text('change thumbVisibility'),
),
),
],
),
),
);
},
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), isNot(paints..rrect()));
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rrect());
},
);
testWidgets(
'With thumbVisibility: false, fling a scroll. While it is still scrolling, set thumbVisibility: true. '
'The thumb should not fade even after the scrolling stops',
(WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
bool thumbVisibility = false;
Widget viewWithScroll() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: Stack(
children: <Widget>[
CupertinoScrollbar(
thumbVisibility: thumbVisibility,
controller: controller,
child: SingleChildScrollView(
controller: controller,
child: const SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
Positioned(
bottom: 10,
child: CupertinoButton(
onPressed: () {
setState(() {
thumbVisibility = !thumbVisibility;
});
},
child: const Text('change thumbVisibility'),
),
),
],
),
),
);
},
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), isNot(paints..rrect()));
await tester.fling(
find.byType(SingleChildScrollView),
const Offset(0.0, -10.0),
10,
);
expect(find.byType(CupertinoScrollbar), paints..rrect());
await tester.tap(find.byType(CupertinoButton));
await tester.pump();
expect(find.byType(CupertinoScrollbar), paints..rrect());
// Wait for the timer delay to expire.
await tester.pump(const Duration(milliseconds: 600)); // kScrollbarTimeToFade
await tester.pumpAndSettle();
// Scrollbar thumb is showing after scroll finishes and timer ends.
expect(find.byType(CupertinoScrollbar), paints..rrect());
},
);
testWidgets(
'Toggling thumbVisibility while not scrolling fades the thumb in/out. '
'This works even when you have never scrolled at all yet',
(WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
bool thumbVisibility = true;
Widget viewWithScroll() {
return StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: Stack(
children: <Widget>[
CupertinoScrollbar(
thumbVisibility: thumbVisibility,
controller: controller,
child: SingleChildScrollView(
controller: controller,
child: const SizedBox(
width: 4000.0,
height: 4000.0,
),
),
),
Positioned(
bottom: 10,
child: CupertinoButton(
onPressed: () {
setState(() {
thumbVisibility = !thumbVisibility;
});
},
child: const Text('change thumbVisibility'),
),
),
],
),
),
);
},
);
}
await tester.pumpWidget(viewWithScroll());
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), paints..rrect());
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(find.byType(CupertinoScrollbar), isNot(paints..rrect()));
},
);
testWidgets('Scrollbar thumb can be dragged with long press - horizontal axis', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
scrollDirection: Axis.horizontal,
child: const SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
expect(scrollController.offset, 0.0);
// Scroll a bit.
const double scrollAmount = 10.0;
final TestGesture scrollGesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
// Scroll right by swiping left.
await scrollGesture.moveBy(const Offset(-scrollAmount, 0.0));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Scrollbar thumb is fully showing and scroll offset has moved by
// scrollAmount.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
expect(scrollController.offset, scrollAmount);
await scrollGesture.up();
await tester.pump();
int hapticFeedbackCalls = 0;
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'HapticFeedback.vibrate') {
hapticFeedbackCalls += 1;
}
return null;
});
// Long press on the scrollbar thumb and expect a vibration after it resizes.
expect(hapticFeedbackCalls, 0);
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(50.0, 596.0));
await tester.pump(kLongPressDuration);
expect(hapticFeedbackCalls, 0);
await tester.pump(kScrollbarResizeDuration);
// Allow the haptic feedback some slack.
await tester.pump(const Duration(milliseconds: 1));
expect(hapticFeedbackCalls, 1);
// Drag the thumb down to scroll back to the left.
await dragScrollbarGesture.moveBy(const Offset(scrollAmount, 0.0));
await tester.pump(const Duration(milliseconds: 100));
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
// The scrollbar thumb is still fully visible.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
// Let the thumb fade out so all timers have resolved.
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration);
});
testWidgets('Scrollbar thumb can be dragged with long press - horizontal axis, reverse', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
controller: scrollController,
child: SingleChildScrollView(
reverse: true,
controller: scrollController,
scrollDirection: Axis.horizontal,
child: const SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
expect(scrollController.offset, 0.0);
// Scroll a bit.
const double scrollAmount = 10.0;
final TestGesture scrollGesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
// Scroll right by swiping right.
await scrollGesture.moveBy(const Offset(scrollAmount, 0.0));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Scrollbar thumb is fully showing and scroll offset has moved by
// scrollAmount.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
expect(scrollController.offset, scrollAmount);
await scrollGesture.up();
await tester.pump();
int hapticFeedbackCalls = 0;
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'HapticFeedback.vibrate') {
hapticFeedbackCalls += 1;
}
return null;
});
// Long press on the scrollbar thumb and expect a vibration after it resizes.
expect(hapticFeedbackCalls, 0);
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(750.0, 596.0));
await tester.pump(kLongPressDuration);
expect(hapticFeedbackCalls, 0);
await tester.pump(kScrollbarResizeDuration);
// Allow the haptic feedback some slack.
await tester.pump(const Duration(milliseconds: 1));
expect(hapticFeedbackCalls, 1);
// Drag the thumb to scroll back to the right.
await dragScrollbarGesture.moveBy(const Offset(-scrollAmount, 0.0));
await tester.pump(const Duration(milliseconds: 100));
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
// The view has scrolled more than it would have by a swipe gesture of the
// same distance.
expect(scrollController.offset, greaterThan(scrollAmount * 2));
// The scrollbar thumb is still fully visible.
expect(find.byType(CupertinoScrollbar), paints..rrect(
color: _kScrollbarColor.color,
));
// Let the thumb fade out so all timers have resolved.
await tester.pump(kScrollbarTimeToFade);
await tester.pump(kScrollbarFadeDuration);
});
testWidgets('Tapping the track area pages the Scroll View', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
thumbVisibility: true,
controller: scrollController,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(width: 1000.0, height: 1000.0),
),
),
),
),
);
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
color: _kScrollbarColor.color,
rrect: RRect.fromLTRBR(794.0, 3.0, 797.0, 359.4, const Radius.circular(1.5)),
),
);
// Tap on the track area below the thumb.
await tester.tapAt(const Offset(796.0, 550.0));
await tester.pumpAndSettle();
expect(scrollController.offset, 400.0);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
color: _kScrollbarColor.color,
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(794.0, 240.6, 797.0, 597.0),
const Radius.circular(1.5),
),
),
);
// Tap on the track area above the thumb.
await tester.tapAt(const Offset(796.0, 50.0));
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
color: _kScrollbarColor.color,
rrect: RRect.fromLTRBR(794.0, 3.0, 797.0, 359.4, const Radius.circular(1.5)),
),
);
});
testWidgets('Throw if interactive with the bar when no position attached', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoScrollbar(
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(
height: 1000.0,
width: 1000.0,
),
),
),
),
),
);
await tester.pumpAndSettle();
final ScrollPosition position = scrollController.position;
scrollController.detach(position);
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
// long press the thumb
await tester.startGesture(const Offset(796.0, 50.0));
await tester.pump(kLongPressDuration);
expect(error, isNotNull);
scrollController.attach(position);
FlutterError.onError = handler;
});
testWidgets('Interactive scrollbars should have a valid scroll controller', (WidgetTester tester) async {
final ScrollController primaryScrollController = ScrollController();
addTearDown(primaryScrollController.dispose);
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: PrimaryScrollController(
controller: primaryScrollController,
child: CupertinoScrollbar(
child: SingleChildScrollView(
controller: scrollController,
child: const SizedBox(
height: 1000.0,
width: 1000.0,
),
),
),
),
),
),
);
await tester.pumpAndSettle();
AssertionError? exception = tester.takeException() as AssertionError?;
// The scrollbar is not visible and cannot be interacted with, so no assertion.
expect(exception, isNull);
// Scroll to trigger the scrollbar to come into view.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(SingleChildScrollView)));
await gesture.moveBy(const Offset(0.0, -20.0));
exception = tester.takeException() as AssertionError;
expect(exception, isAssertionError);
expect(
exception.message,
contains("The Scrollbar's ScrollController has no ScrollPosition attached."),
);
});
testWidgets('Simultaneous dragging and pointer scrolling does not cause a crash', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/70105
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: PrimaryScrollController(
controller: scrollController,
child: CupertinoScrollbar(
thumbVisibility: true,
controller: scrollController,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
const double scrollAmount = 10.0;
await tester.pumpAndSettle();
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(794.0, 3.0, 797.0, 92.1),
const Radius.circular(1.5),
),
color: _kScrollbarColor.color,
),
);
final TestGesture dragScrollbarGesture = await tester.startGesture(const Offset(796.0, 50.0));
await tester.pump(kLongPressDuration);
await tester.pump(kScrollbarResizeDuration);
// Drag the thumb down to scroll down.
await dragScrollbarGesture.moveBy(const Offset(0.0, scrollAmount));
await tester.pumpAndSettle();
expect(scrollController.offset, greaterThan(10.0));
final double previousOffset = scrollController.offset;
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(789.0, 13.0, 797.0, 102.1),
const Radius.circular(4.0),
),
color: _kScrollbarColor.color,
),
);
// Execute a pointer scroll while dragging (drag gesture has not come up yet)
final TestPointer pointer = TestPointer(1, ui.PointerDeviceKind.mouse);
pointer.hover(const Offset(793.0, 15.0));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, 20.0)));
await tester.pumpAndSettle();
if (!kIsWeb) {
// Scrolling while holding the drag on the scrollbar and still hovered over
// the scrollbar should not have changed the scroll offset.
expect(pointer.location, const Offset(793.0, 15.0));
expect(scrollController.offset, previousOffset);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(789.0, 13.0, 797.0, 102.1),
const Radius.circular(4.0),
),
color: _kScrollbarColor.color,
),
);
} else {
expect(pointer.location, const Offset(793.0, 15.0));
expect(scrollController.offset, previousOffset + 20.0);
}
// Drag is still being held, move pointer to be hovering over another area
// of the scrollable (not over the scrollbar) and execute another pointer scroll
pointer.hover(tester.getCenter(find.byType(SingleChildScrollView)));
await tester.sendEventToBinding(pointer.scroll(const Offset(0.0, -90.0)));
await tester.pumpAndSettle();
// Scrolling while holding the drag on the scrollbar changed the offset
expect(pointer.location, const Offset(400.0, 300.0));
expect(scrollController.offset, 0.0);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(789.0, 3.0, 797.0, 92.1),
const Radius.circular(4.0),
),
color: _kScrollbarColor.color,
),
);
await dragScrollbarGesture.up();
await tester.pumpAndSettle();
expect(scrollController.offset, 0.0);
expect(
find.byType(CupertinoScrollbar),
paints..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(794.0, 3.0, 797.0, 92.1),
const Radius.circular(1.5),
),
color: _kScrollbarColor.color,
),
);
});
testWidgets('CupertinoScrollbar scrollOrientation works correctly', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: PrimaryScrollController(
controller: scrollController,
child: CupertinoScrollbar(
thumbVisibility: true,
controller: scrollController,
scrollbarOrientation: ScrollbarOrientation.left,
child: const SingleChildScrollView(
child: SizedBox(width: 4000.0, height: 4000.0),
),
),
),
),
);
await tester.pumpAndSettle();
expect(
find.byType(CupertinoScrollbar),
paints
..rect(
rect: const Rect.fromLTRB(0.0, 0.0, 9.0, 600.0),
)
..line(
p1: const Offset(9.0, 0.0),
p2: const Offset(9.0, 600.0),
strokeWidth: 1.0,
)
..rrect(
rrect: RRect.fromRectAndRadius(const Rect.fromLTRB(3.0, 3.0, 6.0, 92.1), const Radius.circular(1.5)),
color: _kScrollbarColor.color,
),
);
});
}
| flutter/packages/flutter/test/cupertino/scrollbar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/scrollbar_test.dart",
"repo_id": "flutter",
"token_count": 20509
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('CupertinoTextTheme matches Apple Design resources', () {
// Check the default cupertino text theme against the style values
// Values derived from https://developer.apple.com/design/resources/.
const CupertinoTextThemeData theme = CupertinoTextThemeData();
const FontWeight normal = FontWeight.normal;
const FontWeight regular = FontWeight.w400;
const FontWeight medium = FontWeight.w500;
const FontWeight semiBold = FontWeight.w600;
const FontWeight bold = FontWeight.w700;
// TextStyle 17 -0.41
expect(theme.textStyle.fontSize, 17);
expect(theme.textStyle.fontFamily, 'CupertinoSystemText');
expect(theme.textStyle.letterSpacing, -0.41);
expect(theme.textStyle.fontWeight, null);
// ActionTextStyle 17 -0.41
expect(theme.actionTextStyle.fontSize, 17);
expect(theme.actionTextStyle.fontFamily, 'CupertinoSystemText');
expect(theme.actionTextStyle.letterSpacing, -0.41);
expect(theme.actionTextStyle.fontWeight, null);
// TextStyle 17 -0.41
expect(theme.tabLabelTextStyle.fontSize, 10);
expect(theme.tabLabelTextStyle.fontFamily, 'CupertinoSystemText');
expect(theme.tabLabelTextStyle.letterSpacing, -0.24);
expect(theme.tabLabelTextStyle.fontWeight, medium);
// NavTitle SemiBold 17 -0.41
expect(theme.navTitleTextStyle.fontSize, 17);
expect(theme.navTitleTextStyle.fontFamily, 'CupertinoSystemText');
expect(theme.navTitleTextStyle.letterSpacing, -0.41);
expect(theme.navTitleTextStyle.fontWeight, semiBold);
// NavLargeTitle Bold 34 0.41
expect(theme.navLargeTitleTextStyle.fontSize, 34);
expect(theme.navLargeTitleTextStyle.fontFamily, 'CupertinoSystemDisplay');
expect(theme.navLargeTitleTextStyle.letterSpacing, 0.38);
expect(theme.navLargeTitleTextStyle.fontWeight, bold);
// Picker Regular 21 -0.6
expect(theme.pickerTextStyle.fontSize, 21);
expect(theme.pickerTextStyle.fontFamily, 'CupertinoSystemDisplay');
expect(theme.pickerTextStyle.letterSpacing, -0.6);
expect(theme.pickerTextStyle.fontWeight, regular);
// DateTimePicker Normal 21
expect(theme.dateTimePickerTextStyle.fontSize, 21);
expect(theme.dateTimePickerTextStyle.fontFamily, 'CupertinoSystemDisplay');
expect(theme.dateTimePickerTextStyle.letterSpacing, 0.4);
expect(theme.dateTimePickerTextStyle.fontWeight, normal);
});
}
| flutter/packages/flutter/test/cupertino/text_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/text_theme_test.dart",
"repo_id": "flutter",
"token_count": 892
} | 653 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.