text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
// Flutter code sample for [DropdownMenu]s. The first dropdown menu
// has the default outlined border and demos using the
// [DropdownMenuEntry] style parameter to customize its appearance.
// The second dropdown menu customizes the appearance of the dropdown
// menu's text field with its [InputDecorationTheme] parameter.
void main() {
runApp(const DropdownMenuExample());
}
// DropdownMenuEntry labels and values for the first dropdown menu.
enum ColorLabel {
blue('Blue', Colors.blue),
pink('Pink', Colors.pink),
green('Green', Colors.green),
yellow('Orange', Colors.orange),
grey('Grey', Colors.grey);
const ColorLabel(this.label, this.color);
final String label;
final Color color;
}
// DropdownMenuEntry labels and values for the second dropdown menu.
enum IconLabel {
smile('Smile', Icons.sentiment_satisfied_outlined),
cloud(
'Cloud',
Icons.cloud_outlined,
),
brush('Brush', Icons.brush_outlined),
heart('Heart', Icons.favorite);
const IconLabel(this.label, this.icon);
final String label;
final IconData icon;
}
class DropdownMenuExample extends StatefulWidget {
const DropdownMenuExample({super.key});
@override
State<DropdownMenuExample> createState() => _DropdownMenuExampleState();
}
class _DropdownMenuExampleState extends State<DropdownMenuExample> {
final TextEditingController colorController = TextEditingController();
final TextEditingController iconController = TextEditingController();
ColorLabel? selectedColor;
IconLabel? selectedIcon;
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
useMaterial3: true,
colorSchemeSeed: Colors.green,
),
home: Scaffold(
body: SafeArea(
child: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.symmetric(vertical: 20),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DropdownMenu<ColorLabel>(
initialSelection: ColorLabel.green,
controller: colorController,
// requestFocusOnTap is enabled/disabled by platforms when it is null.
// On mobile platforms, this is false by default. Setting this to true will
// trigger focus request on the text field and virtual keyboard will appear
// afterward. On desktop platforms however, this defaults to true.
requestFocusOnTap: true,
label: const Text('Color'),
onSelected: (ColorLabel? color) {
setState(() {
selectedColor = color;
});
},
dropdownMenuEntries: ColorLabel.values.map<DropdownMenuEntry<ColorLabel>>(
(ColorLabel color) {
return DropdownMenuEntry<ColorLabel>(
value: color,
label: color.label,
enabled: color.label != 'Grey',
style: MenuItemButton.styleFrom(
foregroundColor: color.color,
),
);
}
).toList(),
),
const SizedBox(width: 24),
DropdownMenu<IconLabel>(
controller: iconController,
enableFilter: true,
requestFocusOnTap: true,
leadingIcon: const Icon(Icons.search),
label: const Text('Icon'),
inputDecorationTheme: const InputDecorationTheme(
filled: true,
contentPadding: EdgeInsets.symmetric(vertical: 5.0),
),
onSelected: (IconLabel? icon) {
setState(() {
selectedIcon = icon;
});
},
dropdownMenuEntries: IconLabel.values.map<DropdownMenuEntry<IconLabel>>(
(IconLabel icon) {
return DropdownMenuEntry<IconLabel>(
value: icon,
label: icon.label,
leadingIcon: Icon(icon.icon),
);
},
).toList(),
),
],
),
),
if (selectedColor != null && selectedIcon != null)
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('You selected a ${selectedColor?.label} ${selectedIcon?.label}'),
Padding(
padding: const EdgeInsets.symmetric(horizontal: 5),
child: Icon(
selectedIcon?.icon,
color: selectedColor?.color,
),
)
],
)
else
const Text('Please select a color and an icon.')
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/dropdown_menu/dropdown_menu.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/dropdown_menu/dropdown_menu.0.dart",
"repo_id": "flutter",
"token_count": 2880
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [IconButton].
void main() => runApp(const IconButtonExampleApp());
class IconButtonExampleApp extends StatelessWidget {
const IconButtonExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('IconButton Sample')),
body: const Center(
child: IconButtonExample(),
),
),
);
}
}
double _volume = 0.0;
class IconButtonExample extends StatefulWidget {
const IconButtonExample({super.key});
@override
State<IconButtonExample> createState() => _IconButtonExampleState();
}
class _IconButtonExampleState extends State<IconButtonExample> {
@override
Widget build(BuildContext context) {
return Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
IconButton(
icon: const Icon(Icons.volume_up),
tooltip: 'Increase volume by 10',
onPressed: () {
setState(() {
_volume += 10;
});
},
),
Text('Volume : $_volume'),
],
);
}
}
| flutter/examples/api/lib/material/icon_button/icon_button.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/icon_button/icon_button.0.dart",
"repo_id": "flutter",
"token_count": 514
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InputDecorator].
void main() => runApp(const LabelStyleErrorExampleApp());
class LabelStyleErrorExampleApp extends StatelessWidget {
const LabelStyleErrorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('InputDecorator Sample')),
body: const Center(
child: InputDecoratorExample(),
),
),
);
}
}
class InputDecoratorExample extends StatelessWidget {
const InputDecoratorExample({super.key});
@override
Widget build(BuildContext context) {
return TextFormField(
decoration: InputDecoration(
border: const OutlineInputBorder(),
labelText: 'Name',
// The MaterialStateProperty's value is a text style that is orange
// by default, but the theme's error color if the input decorator
// is in its error state.
labelStyle: MaterialStateTextStyle.resolveWith(
(Set<MaterialState> states) {
final Color color =
states.contains(MaterialState.error) ? Theme.of(context).colorScheme.error : Colors.orange;
return TextStyle(color: color, letterSpacing: 1.3);
},
),
),
validator: (String? value) {
if (value == null || value == '') {
return 'Enter name';
}
return null;
},
autovalidateMode: AutovalidateMode.always,
);
}
}
| flutter/examples/api/lib/material/input_decorator/input_decoration.label_style_error.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/input_decorator/input_decoration.label_style_error.0.dart",
"repo_id": "flutter",
"token_count": 657
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MaterialStateMouseCursor].
void main() => runApp(const MaterialStateMouseCursorExampleApp());
class MaterialStateMouseCursorExampleApp extends StatelessWidget {
const MaterialStateMouseCursorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('MaterialStateMouseCursor Sample')),
body: const Center(
child: MaterialStateMouseCursorExample(),
),
),
);
}
}
class ListTileCursor extends MaterialStateMouseCursor {
const ListTileCursor();
@override
MouseCursor resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.forbidden;
}
return SystemMouseCursors.click;
}
@override
String get debugDescription => 'ListTileCursor()';
}
class MaterialStateMouseCursorExample extends StatelessWidget {
const MaterialStateMouseCursorExample({super.key});
@override
Widget build(BuildContext context) {
return const ListTile(
title: Text('Disabled ListTile'),
enabled: false,
mouseCursor: ListTileCursor(),
);
}
}
| flutter/examples/api/lib/material/material_state/material_state_mouse_cursor.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/material_state/material_state_mouse_cursor.0.dart",
"repo_id": "flutter",
"token_count": 459
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/material.dart';
/// Flutter code sample for [NavigationRail.extendedAnimation].
void main() => runApp(const ExtendedAnimationExampleApp());
class ExtendedAnimationExampleApp extends StatelessWidget {
const ExtendedAnimationExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: Scaffold(
body: MyNavigationRail(),
),
);
}
}
class MyNavigationRail extends StatefulWidget {
const MyNavigationRail({super.key});
@override
State<MyNavigationRail> createState() => _MyNavigationRailState();
}
class _MyNavigationRailState extends State<MyNavigationRail> {
int _selectedIndex = 0;
bool _extended = false;
@override
Widget build(BuildContext context) {
return Row(
children: <Widget>[
NavigationRail(
selectedIndex: _selectedIndex,
extended: _extended,
leading: MyNavigationRailFab(onPressed: () {
setState(() {
_extended = !_extended;
});
}),
onDestinationSelected: (int index) {
setState(() {
_selectedIndex = index;
});
},
labelType: NavigationRailLabelType.none,
destinations: const <NavigationRailDestination>[
NavigationRailDestination(
icon: Icon(Icons.favorite_border),
selectedIcon: Icon(Icons.favorite),
label: Text('First'),
),
NavigationRailDestination(
icon: Icon(Icons.bookmark_border),
selectedIcon: Icon(Icons.book),
label: Text('Second'),
),
NavigationRailDestination(
icon: Icon(Icons.star_border),
selectedIcon: Icon(Icons.star),
label: Text('Third'),
),
],
),
const VerticalDivider(thickness: 1, width: 1),
// This is the main content.
Expanded(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Tap on FloatingActionButton to expand'),
const SizedBox(height: 20),
Text('selectedIndex: $_selectedIndex'),
],
),
),
),
],
);
}
}
class MyNavigationRailFab extends StatelessWidget {
const MyNavigationRailFab({super.key, this.onPressed});
final VoidCallback? onPressed;
@override
Widget build(BuildContext context) {
final Animation<double> animation = NavigationRail.extendedAnimation(context);
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
// The extended fab has a shorter height than the regular fab.
return Container(
height: 56,
padding: EdgeInsets.symmetric(
vertical: lerpDouble(0, 6, animation.value)!,
),
child: animation.value == 0
? FloatingActionButton(
onPressed: onPressed,
child: const Icon(Icons.add),
)
: Align(
alignment: AlignmentDirectional.centerStart,
widthFactor: animation.value,
child: Padding(
padding: const EdgeInsetsDirectional.only(start: 8),
child: FloatingActionButton.extended(
icon: const Icon(Icons.add),
label: const Text('CREATE'),
onPressed: onPressed,
),
),
),
);
},
);
}
}
| flutter/examples/api/lib/material/navigation_rail/navigation_rail.extended_animation.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/navigation_rail/navigation_rail.extended_animation.0.dart",
"repo_id": "flutter",
"token_count": 1813
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
/// Flutter code sample for custom labeled radio.
void main() => runApp(const LabeledRadioApp());
class LabeledRadioApp extends StatelessWidget {
const LabeledRadioApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('Custom Labeled Radio Sample')),
body: const LabeledRadioExample(),
),
);
}
}
class LinkedLabelRadio extends StatelessWidget {
const LinkedLabelRadio({
super.key,
required this.label,
required this.padding,
required this.groupValue,
required this.value,
required this.onChanged,
});
final String label;
final EdgeInsets padding;
final bool groupValue;
final bool value;
final ValueChanged<bool> onChanged;
@override
Widget build(BuildContext context) {
return Padding(
padding: padding,
child: Row(
children: <Widget>[
Radio<bool>(
groupValue: groupValue,
value: value,
onChanged: (bool? newValue) {
onChanged(newValue!);
},
),
RichText(
text: TextSpan(
text: label,
style: TextStyle(
color: Theme.of(context).colorScheme.primary,
decoration: TextDecoration.underline,
),
recognizer: TapGestureRecognizer()
..onTap = () {
debugPrint('Label has been tapped.');
},
),
),
],
),
);
}
}
class LabeledRadioExample extends StatefulWidget {
const LabeledRadioExample({super.key});
@override
State<LabeledRadioExample> createState() => _LabeledRadioExampleState();
}
class _LabeledRadioExampleState extends State<LabeledRadioExample> {
bool _isRadioSelected = false;
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
LinkedLabelRadio(
label: 'First tappable label text',
padding: const EdgeInsets.symmetric(horizontal: 5.0),
value: true,
groupValue: _isRadioSelected,
onChanged: (bool newValue) {
setState(() {
_isRadioSelected = newValue;
});
},
),
LinkedLabelRadio(
label: 'Second tappable label text',
padding: const EdgeInsets.symmetric(horizontal: 5.0),
value: false,
groupValue: _isRadioSelected,
onChanged: (bool newValue) {
setState(() {
_isRadioSelected = newValue;
});
},
),
],
),
);
}
}
| flutter/examples/api/lib/material/radio_list_tile/custom_labeled_radio.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/radio_list_tile/custom_labeled_radio.0.dart",
"repo_id": "flutter",
"token_count": 1392
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Scaffold.drawer].
void main() => runApp(const DrawerExampleApp());
class DrawerExampleApp extends StatelessWidget {
const DrawerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: DrawerExample(),
);
}
}
class DrawerExample extends StatefulWidget {
const DrawerExample({super.key});
@override
State<DrawerExample> createState() => _DrawerExampleState();
}
class _DrawerExampleState extends State<DrawerExample> {
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
void _openDrawer() {
_scaffoldKey.currentState!.openDrawer();
}
void _closeDrawer() {
Navigator.of(context).pop();
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(title: const Text('Drawer Demo')),
body: Center(
child: ElevatedButton(
onPressed: _openDrawer,
child: const Text('Open Drawer'),
),
),
drawer: Drawer(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('This is the Drawer'),
ElevatedButton(
onPressed: _closeDrawer,
child: const Text('Close Drawer'),
),
],
),
),
),
// Disable opening the drawer with a swipe gesture.
drawerEnableOpenDragGesture: false,
);
}
}
| flutter/examples/api/lib/material/scaffold/scaffold.drawer.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/scaffold/scaffold.drawer.0.dart",
"repo_id": "flutter",
"token_count": 707
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SearchAnchor].
void main() => runApp(const SearchBarApp());
class SearchBarApp extends StatefulWidget {
const SearchBarApp({super.key});
@override
State<SearchBarApp> createState() => _SearchBarAppState();
}
class _SearchBarAppState extends State<SearchBarApp> {
final SearchController controller = SearchController();
@override
Widget build(BuildContext context) {
final ThemeData themeData = ThemeData(useMaterial3: true);
return MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(title: const Text('Search Anchor Sample')),
body: Column(
children: <Widget>[
SearchAnchor(
searchController: controller,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return List<ListTile>.generate(5, (int index) {
final String item = 'item $index';
return ListTile(
title: Text(item),
onTap: () {
setState(() {
controller.closeView(item);
});
},
);
});
}),
Center(
child: controller.text.isEmpty
? const Text('No item selected')
: Text('Selected item: ${controller.value.text}'),
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/search_anchor/search_anchor.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/search_anchor/search_anchor.2.dart",
"repo_id": "flutter",
"token_count": 986
} | 627 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [StepStyle].
void main() => runApp(const StepStyleExampleApp());
class StepStyleExampleApp extends StatelessWidget {
const StepStyleExampleApp({ super.key });
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Step Style Example')),
body: const Center(
child: StepStyleExample(),
),
),
);
}
}
class StepStyleExample extends StatefulWidget {
const StepStyleExample({ super.key });
@override
State<StepStyleExample> createState() => _StepStyleExampleState();
}
class _StepStyleExampleState extends State<StepStyleExample> {
final StepStyle _stepStyle = StepStyle(
connectorThickness: 10,
color: Colors.white,
connectorColor: Colors.red,
indexStyle: const TextStyle(
color: Colors.black,
fontSize: 20,
),
border: Border.all(
width: 2,
),
);
@override
Widget build(BuildContext context) {
return Stepper(
type: StepperType.horizontal,
stepIconHeight: 48,
stepIconWidth: 48,
stepIconMargin: EdgeInsets.zero,
steps: <Step>[
Step(
title: const SizedBox.shrink(),
content: const SizedBox.shrink(),
isActive: true,
stepStyle: _stepStyle,
),
Step(
title: const SizedBox.shrink(),
content: const SizedBox.shrink(),
isActive: true,
stepStyle: _stepStyle.copyWith(
connectorColor: Colors.orange,
gradient: const LinearGradient(
colors: <Color>[
Colors.white,
Colors.black,
],
),
),
),
Step(
title: const SizedBox.shrink(),
content: const SizedBox.shrink(),
isActive: true,
stepStyle: _stepStyle.copyWith(
connectorColor: Colors.blue,
),
),
Step(
title: const SizedBox.shrink(),
content: const SizedBox.shrink(),
isActive: true,
stepStyle: _stepStyle.merge(
StepStyle(
color: Colors.white,
indexStyle: const TextStyle(
color: Colors.black,
fontSize: 20,
),
border: Border.all(
width: 2,
),
),
),
),
],
);
}
}
| flutter/examples/api/lib/material/stepper/step_style.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/stepper/step_style.0.dart",
"repo_id": "flutter",
"token_count": 1259
} | 628 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TextButton].
void main() {
runApp(const TextButtonExampleApp());
}
class TextButtonExampleApp extends StatefulWidget {
const TextButtonExampleApp({ super.key });
@override
State<TextButtonExampleApp> createState() => _TextButtonExampleAppState();
}
class _TextButtonExampleAppState extends State<TextButtonExampleApp> {
bool darkMode = false;
@override
Widget build(BuildContext context) {
return MaterialApp(
themeMode: darkMode ? ThemeMode.dark : ThemeMode.light,
theme: ThemeData(brightness: Brightness.light),
darkTheme: ThemeData(brightness: Brightness.dark),
home: Scaffold(
body: Padding(
padding: const EdgeInsets.all(16),
child: TextButtonExample(
darkMode: darkMode,
updateDarkMode: (bool value) {
setState(() { darkMode = value; });
},
),
),
),
);
}
}
class TextButtonExample extends StatefulWidget {
const TextButtonExample({ super.key, required this.darkMode, required this.updateDarkMode });
final bool darkMode;
final ValueChanged<bool> updateDarkMode;
@override
State<TextButtonExample> createState() => _TextButtonExampleState();
}
class _TextButtonExampleState extends State<TextButtonExample> {
TextDirection textDirection = TextDirection.ltr;
ThemeMode themeMode = ThemeMode.light;
late final ScrollController scrollController;
Future<void>? currentAction;
static const Widget verticalSpacer = SizedBox(height: 16);
static const Widget horizontalSpacer = SizedBox(width: 32);
static const ImageProvider grassImage = NetworkImage(
'https://flutter.github.io/assets-for-api-docs/assets/material/text_button_grass.jpeg',
);
static const ImageProvider defaultImage = NetworkImage(
'https://flutter.github.io/assets-for-api-docs/assets/material/text_button_nhu_default.png',
);
static const ImageProvider hoveredImage = NetworkImage(
'https://flutter.github.io/assets-for-api-docs/assets/material/text_button_nhu_hovered.png',
);
static const ImageProvider pressedImage = NetworkImage(
'https://flutter.github.io/assets-for-api-docs/assets/material/text_button_nhu_pressed.png',
);
static const ImageProvider runningImage = NetworkImage(
'https://flutter.github.io/assets-for-api-docs/assets/material/text_button_nhu_end.png',
);
@override
void initState() {
scrollController = ScrollController();
super.initState();
}
@override
void dispose() {
scrollController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final ColorScheme colorScheme = theme.colorScheme;
// Adapt colors that are not part of the color scheme to
// the current dark/light mode. Used to define TextButton #7's
// gradients.
final (Color color1, Color color2, Color color3) = switch (colorScheme.brightness) {
Brightness.light => (Colors.blue.withOpacity(1.0), Colors.orange.withOpacity(1.0), Colors.yellow.withOpacity(1.0)),
Brightness.dark => (Colors.purple.withOpacity(1.0), Colors.cyan.withOpacity(1.0), Colors.yellow.withOpacity(1.0)),
};
// This gradient's appearance reflects the button's state.
// Always return a gradient decoration so that AnimatedContainer
// can interpolorate in between. Used by TextButton #7.
Decoration? statesToDecoration(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return BoxDecoration(
gradient: LinearGradient(colors: <Color>[color2, color2]), // solid fill
);
}
return BoxDecoration(
gradient: LinearGradient(
colors: switch (states.contains(MaterialState.hovered)) {
true => <Color>[color1, color2],
false => <Color>[color2, color1],
},
),
);
}
// To make this method a little easier to read, the buttons that
// appear in the two columns to the right of the demo switches
// Card are broken out below.
final List<Widget> columnOneButtons = <Widget>[
TextButton(
onPressed: () {},
child: const Text('Enabled'),
),
verticalSpacer,
const TextButton(
onPressed: null,
child: Text('Disabled'),
),
verticalSpacer,
TextButton.icon(
onPressed: () {},
icon: const Icon(Icons.access_alarm),
label: const Text('TextButton.icon #1'),
),
verticalSpacer,
// Override the foreground and background colors.
//
// In this example, and most of the ones that follow, we're using
// the TextButton.styleFrom() convenience method to create a ButtonStyle.
// The styleFrom method is a little easier because it creates
// ButtonStyle MaterialStateProperty parameters for you.
// In this case, Specifying foregroundColor overrides the text,
// icon and overlay (splash and highlight) colors a little differently
// depending on the button's state. BackgroundColor is just the background
// color for all states.
TextButton.icon(
style: TextButton.styleFrom(
foregroundColor: colorScheme.onError,
backgroundColor: colorScheme.error,
),
onPressed: () { },
icon: const Icon(Icons.access_alarm),
label: const Text('TextButton.icon #2'),
),
verticalSpacer,
// Override the button's shape and its border.
//
// In this case we've specified a shape that has border - the
// RoundedRectangleBorder's side parameter. If the styleFrom
// side parameter was also specified, or if the TextButtonTheme
// defined above included a side parameter, then that would
// override the RoundedRectangleBorder's side.
TextButton(
style: TextButton.styleFrom(
shape: RoundedRectangleBorder(
borderRadius: const BorderRadius.all(Radius.circular(8)),
side: BorderSide(
color: colorScheme.primary,
width: 5,
),
),
),
onPressed: () { },
child: const Text('TextButton #3'),
),
verticalSpacer,
// Override overlay: the ink splash and highlight colors.
//
// The styleFrom method turns the specified overlayColor
// into a value MaterialStyleProperty<Color> ButtonStyle.overlay
// value that uses opacities depending on the button's state.
// If the overlayColor was Colors.transparent, no splash
// or highlights would be shown.
TextButton(
style: TextButton.styleFrom(
overlayColor: Colors.yellow,
),
onPressed: () { },
child: const Text('TextButton #4'),
),
];
final List<Widget> columnTwoButtons = <Widget>[
// Override the foregroundBuilder: apply a ShaderMask.
//
// Apply a ShaderMask to the button's child. This kind of thing
// can be applied to one button easily enough by just wrapping the
// button's child directly. However to affect all buttons in this
// way you can specify a similar foregroundBuilder in a TextButton
// theme or the MaterialApp theme's ThemeData.textButtonTheme.
TextButton(
style: TextButton.styleFrom(
foregroundBuilder: (BuildContext context, Set<MaterialState> states, Widget? child) {
return ShaderMask(
shaderCallback: (Rect bounds) {
return LinearGradient(
begin: Alignment.bottomCenter,
end: Alignment.topCenter,
colors: <Color>[
colorScheme.primary,
colorScheme.onPrimary,
],
).createShader(bounds);
},
blendMode: BlendMode.srcATop,
child: child,
);
},
),
onPressed: () { },
child: const Text('TextButton #5'),
),
verticalSpacer,
// Override the foregroundBuilder: add an underline.
//
// Add a border around button's child. In this case the
// border only appears when the button is hovered or pressed
// (if it's pressed it's always hovered too). Not that this
// border is different than the one specified with the styleFrom
// side parameter (or the ButtonStyle.side property). The foregroundBuilder
// is applied to a widget that contains the child and has already
// included the button's padding. It is unaffected by the button's shape.
// The styleFrom side parameter controls the button's outermost border and it
// outlines the button's shape.
TextButton(
style: TextButton.styleFrom(
foregroundBuilder: (BuildContext context, Set<MaterialState> states, Widget? child) {
return DecoratedBox(
decoration: BoxDecoration(
border: states.contains(MaterialState.hovered)
? Border(bottom: BorderSide(color: colorScheme.primary))
: const Border(), // essentially "no border"
),
child: child,
);
},
),
onPressed: () { },
child: const Text('TextButton #6'),
),
verticalSpacer,
// Override the backgroundBuilder to add a state specific gradient background
// and add an outline that only appears when the button is hovered or pressed.
//
// The gradient background decoration is computed by the statesToDecoration()
// method. The gradient flips horizontally when the button is hovered (watch
// closely). Because we want the outline to only appear when the button is hovered
// we can't use the styleFrom() side parameter, because that creates the same
// outline for all states. The ButtonStyle.copyWith() method is used to add
// a MaterialState<BorderSide?> property that does the right thing.
//
// The gradient background is translucent - all of the colors have opacity 0.5 -
// so the overlay's splash and highlight colors are visible even though they're
// drawn on the Material widget that's effectively behind the background. The
// border is also translucent, so if you look carefully, you'll see that the
// background - which is part of the button's Material but is drawn on top of the
// the background gradient - shows through the border.
TextButton(
onPressed: () {},
style: TextButton.styleFrom(
overlayColor: color2,
backgroundBuilder: (BuildContext context, Set<MaterialState> states, Widget? child) {
return AnimatedContainer(
duration: const Duration(milliseconds: 500),
decoration: statesToDecoration(states),
child: child,
);
},
).copyWith(
side: MaterialStateProperty.resolveWith<BorderSide?>((Set<MaterialState> states) {
if (states.contains(MaterialState.hovered)) {
return BorderSide(width: 3, color: color3);
}
return null; // defer to the default
}),
),
child: const Text('TextButton #7'),
),
verticalSpacer,
// Override the backgroundBuilder to add a grass image background.
//
// The image is clipped to the button's shape. We've included an Ink widget
// because the background image is opaque and would otherwise obscure the splash
// and highlight overlays that are painted on the button's Material widget
// by default. They're drawn on the Ink widget instead. The foreground color
// was overridden as well because white shows up a little better on the mottled
// green background.
TextButton(
onPressed: () {},
style: TextButton.styleFrom(
foregroundColor: Colors.white,
backgroundBuilder: (BuildContext context, Set<MaterialState> states, Widget? child) {
return Ink(
decoration: const BoxDecoration(
image: DecorationImage(
image: grassImage,
fit: BoxFit.cover,
),
),
child: child,
);
},
),
child: const Text('TextButton #8'),
),
verticalSpacer,
// Override the foregroundBuilder to specify images for the button's pressed
// hovered and default states. We switch to an additional image while the
// button's callback is "running".
//
// This is an example of completely changing the default appearance of a button
// by specifying images for each state and by turning off the overlays by
// overlayColor: Colors.transparent. AnimatedContainer takes care of the
// fade in and out segues between images.
//
// This foregroundBuilder function ignores its child parameter. Unfortunately
// TextButton's child parameter is required, so we still have
// to provide one.
TextButton(
onPressed: () async {
// This is slightly complicated so that if the user presses the button
// while the current Future.delayed action is running, the currentAction
// flag is only reset to null after the _new_ action completes.
late final Future<void> thisAction;
thisAction = Future<void>.delayed(const Duration(seconds: 1), () {
if (currentAction == thisAction) {
setState(() { currentAction = null; });
}
});
setState(() { currentAction = thisAction; });
},
style: TextButton.styleFrom(
overlayColor: Colors.transparent,
foregroundBuilder: (BuildContext context, Set<WidgetState> states, Widget? child) {
late final ImageProvider image;
if (currentAction != null) {
image = runningImage;
} else if (states.contains(WidgetState.pressed)) {
image = pressedImage;
} else if (states.contains(WidgetState.hovered)) {
image = hoveredImage;
} else {
image = defaultImage;
}
return AnimatedContainer(
width: 64,
height: 64,
duration: const Duration(milliseconds: 300),
curve: Curves.fastOutSlowIn,
decoration: BoxDecoration(
image: DecorationImage(
image: image,
fit: BoxFit.contain,
),
),
);
},
),
child: const Text('This child is not used'),
),
];
return Row(
children: <Widget> [
// The dark/light and LTR/RTL switches. We use the updateDarkMode function
// provided by the parent TextButtonExampleApp to rebuild the MaterialApp
// in the appropriate dark/light ThemeMdoe. The directionality of the rest
// of the UI is controlled by the Directionality widget below, and the
// textDirection local state variable.
TextButtonExampleSwitches(
darkMode: widget.darkMode,
updateDarkMode: widget.updateDarkMode,
textDirection: textDirection,
updateRTL: (bool value) {
setState(() {
textDirection = value ? TextDirection.rtl : TextDirection.ltr;
});
},
),
horizontalSpacer,
Expanded(
child: Scrollbar(
controller: scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
controller: scrollController,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Directionality(
textDirection: textDirection,
child: Column(
children: columnOneButtons,
),
),
horizontalSpacer,
Directionality(
textDirection: textDirection,
child: Column(
children: columnTwoButtons
),
),
horizontalSpacer,
],
),
),
),
),
],
);
}
}
class TextButtonExampleSwitches extends StatelessWidget {
const TextButtonExampleSwitches({
super.key,
required this.darkMode,
required this.updateDarkMode,
required this.textDirection,
required this.updateRTL
});
final bool darkMode;
final ValueChanged<bool> updateDarkMode;
final TextDirection textDirection;
final ValueChanged<bool> updateRTL;
@override
Widget build(BuildContext context) {
return Card(
child: Padding(
padding: const EdgeInsets.all(16),
child: IntrinsicWidth(
child: Column(
children: <Widget>[
Row(
children: <Widget>[
const Expanded(child: Text('Dark Mode')),
const SizedBox(width: 4),
Switch(
value: darkMode,
onChanged: updateDarkMode,
),
],
),
const SizedBox(height: 16),
Row(
children: <Widget>[
const Expanded(child: Text('RTL Text')),
const SizedBox(width: 4),
Switch(
value: textDirection == TextDirection.rtl,
onChanged: updateRTL,
),
],
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/text_button/text_button.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/text_button/text_button.0.dart",
"repo_id": "flutter",
"token_count": 7448
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [BorderSide.strokeAlign].
void main() => runApp(const StrokeAlignApp());
class StrokeAlignApp extends StatelessWidget {
const StrokeAlignApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(home: StrokeAlignExample());
}
}
class StrokeAlignExample extends StatefulWidget {
const StrokeAlignExample({super.key});
@override
State<StrokeAlignExample> createState() => _StrokeAlignExampleState();
}
class _StrokeAlignExampleState extends State<StrokeAlignExample> with TickerProviderStateMixin {
late final AnimationController animation;
@override
void initState() {
super.initState();
animation = AnimationController(vsync: this, duration: const Duration(seconds: 1));
animation.repeat(reverse: true);
animation.addListener(_markDirty);
}
@override
void dispose() {
animation.dispose();
super.dispose();
}
void _markDirty() {
setState(() {});
}
static const double borderWidth = 10;
static const double cornerRadius = 10;
static const Color borderColor = Color(0x8000b4fc);
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
BorderedBox(
shape: StadiumBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
BorderedBox(
shape: CircleBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
BorderedBox(
shape: OvalBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
BorderedBox(
shape: BeveledRectangleBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
BorderedBox(
shape: BeveledRectangleBorder(
borderRadius: BorderRadius.circular(cornerRadius),
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
BorderedBox(
shape: RoundedRectangleBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
BorderedBox(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(cornerRadius),
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
],
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
BorderedBox(
shape: StarBorder(
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
BorderedBox(
shape: StarBorder(
pointRounding: 1,
innerRadiusRatio: 0.5,
points: 8,
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
BorderedBox(
shape: StarBorder.polygon(
sides: 6,
pointRounding: 0.5,
side: BorderSide(
color: borderColor,
width: borderWidth,
strokeAlign: (animation.value * 2) - 1,
),
),
),
],
),
],
),
),
);
}
}
class BorderedBox extends StatelessWidget {
const BorderedBox({
super.key,
required this.shape,
});
final ShapeBorder shape;
@override
Widget build(BuildContext context) {
return Container(
width: 100,
height: 50,
decoration: ShapeDecoration(
color: const Color(0xff012677),
shape: shape,
),
);
}
}
| flutter/examples/api/lib/painting/borders/border_side.stroke_align.0.dart/0 | {
"file_path": "flutter/examples/api/lib/painting/borders/border_side.stroke_align.0.dart",
"repo_id": "flutter",
"token_count": 3384
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MouseCursor].
void main() => runApp(const MouseCursorExampleApp());
class MouseCursorExampleApp extends StatelessWidget {
const MouseCursorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('MouseCursor Code Sample')),
body: const MouseCursorExample(),
),
);
}
}
class MouseCursorExample extends StatelessWidget {
const MouseCursorExample({super.key});
@override
Widget build(BuildContext context) {
return Center(
child: MouseRegion(
cursor: SystemMouseCursors.text,
child: Container(
width: 200,
height: 100,
decoration: BoxDecoration(
color: Colors.blue,
border: Border.all(color: Colors.yellow),
),
),
),
);
}
}
| flutter/examples/api/lib/services/mouse_cursor/mouse_cursor.0.dart/0 | {
"file_path": "flutter/examples/api/lib/services/mouse_cursor/mouse_cursor.0.dart",
"repo_id": "flutter",
"token_count": 416
} | 631 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Actions].
void main() => runApp(const ActionsExampleApp());
class ActionsExampleApp extends StatelessWidget {
const ActionsExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Actions Sample')),
body: const Center(
child: ActionsExample(),
),
),
);
}
}
// A simple model class that notifies listeners when it changes.
class Model {
ValueNotifier<bool> isDirty = ValueNotifier<bool>(false);
ValueNotifier<int> data = ValueNotifier<int>(0);
int save() {
if (isDirty.value) {
debugPrint('Saved Data: ${data.value}');
isDirty.value = false;
}
return data.value;
}
void setValue(int newValue) {
isDirty.value = data.value != newValue;
data.value = newValue;
}
}
class ModifyIntent extends Intent {
const ModifyIntent(this.value);
final int value;
}
// An Action that modifies the model by setting it to the value that it gets
// from the Intent passed to it when invoked.
class ModifyAction extends Action<ModifyIntent> {
ModifyAction(this.model);
final Model model;
@override
void invoke(covariant ModifyIntent intent) {
model.setValue(intent.value);
}
}
// An intent for saving data.
class SaveIntent extends Intent {
const SaveIntent();
}
// An Action that saves the data in the model it is created with.
class SaveAction extends Action<SaveIntent> {
SaveAction(this.model);
final Model model;
@override
int invoke(covariant SaveIntent intent) => model.save();
}
class SaveButton extends StatefulWidget {
const SaveButton(this.valueNotifier, {super.key});
final ValueNotifier<bool> valueNotifier;
@override
State<SaveButton> createState() => _SaveButtonState();
}
class _SaveButtonState extends State<SaveButton> {
int savedValue = 0;
@override
Widget build(BuildContext context) {
return ListenableBuilder(
listenable: widget.valueNotifier,
builder: (BuildContext context, Widget? child) {
return TextButton.icon(
icon: const Icon(Icons.save),
label: Text('$savedValue'),
style: ButtonStyle(
foregroundColor: MaterialStatePropertyAll<Color>(
widget.valueNotifier.value ? Colors.red : Colors.green,
),
),
onPressed: () {
setState(() {
savedValue = Actions.invoke(context, const SaveIntent())! as int;
});
},
);
},
);
}
}
class ActionsExample extends StatefulWidget {
const ActionsExample({super.key});
@override
State<ActionsExample> createState() => _ActionsExampleState();
}
class _ActionsExampleState extends State<ActionsExample> {
Model model = Model();
int count = 0;
@override
Widget build(BuildContext context) {
return Actions(
actions: <Type, Action<Intent>>{
ModifyIntent: ModifyAction(model),
SaveIntent: SaveAction(model),
},
child: Builder(
builder: (BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
const Spacer(),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IconButton(
icon: const Icon(Icons.exposure_plus_1),
onPressed: () {
Actions.invoke(context, ModifyIntent(++count));
},
),
ListenableBuilder(
listenable: model.data,
builder: (BuildContext context, Widget? child) {
return Padding(
padding: const EdgeInsets.all(8.0),
child: Text('${model.data.value}', style: Theme.of(context).textTheme.headlineMedium),
);
}),
IconButton(
icon: const Icon(Icons.exposure_minus_1),
onPressed: () {
Actions.invoke(context, ModifyIntent(--count));
},
),
],
),
SaveButton(model.isDirty),
const Spacer(),
],
);
},
),
);
}
}
| flutter/examples/api/lib/widgets/actions/actions.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/actions/actions.0.dart",
"repo_id": "flutter",
"token_count": 2035
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RawAutocomplete.focusNode].
void main() => runApp(const AutocompleteExampleApp());
class AutocompleteExampleApp extends StatelessWidget {
const AutocompleteExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: RawAutocompleteSplit(),
);
}
}
const List<String> _options = <String>[
'aardvark',
'bobcat',
'chameleon',
];
class RawAutocompleteSplit extends StatefulWidget {
const RawAutocompleteSplit({super.key});
@override
RawAutocompleteSplitState createState() => RawAutocompleteSplitState();
}
class RawAutocompleteSplitState extends State<RawAutocompleteSplit> {
final TextEditingController _textEditingController = TextEditingController();
final FocusNode _focusNode = FocusNode();
final GlobalKey _autocompleteKey = GlobalKey();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
// This is where the real field is being built.
title: TextFormField(
controller: _textEditingController,
focusNode: _focusNode,
decoration: const InputDecoration(
hintText: 'Split RawAutocomplete App',
),
onFieldSubmitted: (String value) {
RawAutocomplete.onFieldSubmitted<String>(_autocompleteKey);
},
),
),
body: Align(
alignment: Alignment.topLeft,
child: RawAutocomplete<String>(
key: _autocompleteKey,
focusNode: _focusNode,
textEditingController: _textEditingController,
optionsBuilder: (TextEditingValue textEditingValue) {
return _options.where((String option) {
return option.contains(textEditingValue.text.toLowerCase());
}).toList();
},
optionsViewBuilder: (
BuildContext context,
AutocompleteOnSelected<String> onSelected,
Iterable<String> options,
) {
return Material(
elevation: 4.0,
child: ListView(
children: options
.map((String option) => GestureDetector(
onTap: () {
onSelected(option);
},
child: ListTile(
title: Text(option),
),
))
.toList(),
),
);
},
),
),
);
}
}
| flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.focus_node.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.focus_node.0.dart",
"repo_id": "flutter",
"token_count": 1243
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Listener].
void main() => runApp(const ListenerApp());
class ListenerApp extends StatelessWidget {
const ListenerApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Listener Sample')),
body: const Center(
child: ListenerExample(),
),
),
);
}
}
class ListenerExample extends StatefulWidget {
const ListenerExample({super.key});
@override
State<ListenerExample> createState() => _ListenerExampleState();
}
class _ListenerExampleState extends State<ListenerExample> {
int _downCounter = 0;
int _upCounter = 0;
double x = 0.0;
double y = 0.0;
void _incrementDown(PointerEvent details) {
_updateLocation(details);
setState(() {
_downCounter++;
});
}
void _incrementUp(PointerEvent details) {
_updateLocation(details);
setState(() {
_upCounter++;
});
}
void _updateLocation(PointerEvent details) {
setState(() {
x = details.position.dx;
y = details.position.dy;
});
}
@override
Widget build(BuildContext context) {
return ConstrainedBox(
constraints: BoxConstraints.tight(const Size(300.0, 200.0)),
child: Listener(
onPointerDown: _incrementDown,
onPointerMove: _updateLocation,
onPointerUp: _incrementUp,
child: ColoredBox(
color: Colors.lightBlueAccent,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('You have pressed or released in this area this many times:'),
Text(
'$_downCounter presses\n$_upCounter releases',
style: Theme.of(context).textTheme.headlineMedium,
),
Text(
'The cursor is here: (${x.toStringAsFixed(2)}, ${y.toStringAsFixed(2)})',
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/basic/listener.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/listener.0.dart",
"repo_id": "flutter",
"token_count": 926
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [FocusNode].
void main() => runApp(const FocusNodeExampleApp());
class FocusNodeExampleApp extends StatelessWidget {
const FocusNodeExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('FocusNode Sample')),
body: const FocusNodeExample(),
),
);
}
}
class ColorfulButton extends StatefulWidget {
const ColorfulButton({super.key});
@override
State<ColorfulButton> createState() => _ColorfulButtonState();
}
class _ColorfulButtonState extends State<ColorfulButton> {
late FocusNode _node;
bool _focused = false;
late FocusAttachment _nodeAttachment;
Color _color = Colors.white;
@override
void initState() {
super.initState();
_node = FocusNode(debugLabel: 'Button');
_node.addListener(_handleFocusChange);
_nodeAttachment = _node.attach(context, onKeyEvent: _handleKeyPress);
}
void _handleFocusChange() {
if (_node.hasFocus != _focused) {
setState(() {
_focused = _node.hasFocus;
});
}
}
KeyEventResult _handleKeyPress(FocusNode node, KeyEvent event) {
if (event is KeyDownEvent) {
debugPrint('Focus node ${node.debugLabel} got key event: ${event.logicalKey}');
switch (event.logicalKey) {
case LogicalKeyboardKey.keyR:
debugPrint('Changing color to red.');
setState(() { _color = Colors.red; });
return KeyEventResult.handled;
case LogicalKeyboardKey.keyG:
debugPrint('Changing color to green.');
setState(() { _color = Colors.green; });
return KeyEventResult.handled;
case LogicalKeyboardKey.keyB:
debugPrint('Changing color to blue.');
setState(() { _color = Colors.blue; });
return KeyEventResult.handled;
}
}
return KeyEventResult.ignored;
}
@override
void dispose() {
_node.removeListener(_handleFocusChange);
// The attachment will automatically be detached in dispose().
_node.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
_nodeAttachment.reparent();
return GestureDetector(
onTap: () {
if (_focused) {
_node.unfocus();
} else {
_node.requestFocus();
}
},
child: Center(
child: Container(
width: 400,
height: 100,
color: _focused ? _color : Colors.white,
alignment: Alignment.center,
child: Text(_focused ? "I'm in color! Press R,G,B!" : 'Press to focus'),
),
),
);
}
}
class FocusNodeExample extends StatelessWidget {
const FocusNodeExample({super.key});
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return DefaultTextStyle(
style: textTheme.headlineMedium!,
child: const ColorfulButton(),
);
}
}
| flutter/examples/api/lib/widgets/focus_manager/focus_node.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/focus_manager/focus_node.0.dart",
"repo_id": "flutter",
"token_count": 1227
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Hero].
void main() => runApp(const HeroApp());
class HeroApp extends StatelessWidget {
const HeroApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: HeroExample(),
);
}
}
class HeroExample extends StatelessWidget {
const HeroExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Hero Sample')),
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
const SizedBox(height: 20.0),
ListTile(
leading: const Hero(
tag: 'hero-rectangle',
child: BoxWidget(size: Size(50.0, 50.0)),
),
onTap: () => _gotoDetailsPage(context),
title: const Text(
'Tap on the icon to view hero animation transition.',
),
),
],
),
);
}
void _gotoDetailsPage(BuildContext context) {
Navigator.of(context).push(MaterialPageRoute<void>(
builder: (BuildContext context) => Scaffold(
appBar: AppBar(
title: const Text('Second Page'),
),
body: const Center(
child: Hero(
tag: 'hero-rectangle',
child: BoxWidget(size: Size(200.0, 200.0)),
),
),
),
));
}
}
class BoxWidget extends StatelessWidget {
const BoxWidget({super.key, required this.size});
final Size size;
@override
Widget build(BuildContext context) {
return Container(
width: size.width,
height: size.height,
color: Colors.blue,
);
}
}
| flutter/examples/api/lib/widgets/heroes/hero.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/heroes/hero.0.dart",
"repo_id": "flutter",
"token_count": 794
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:vector_math/vector_math_64.dart' show Quad, Vector3;
/// Flutter code sample for [InteractiveViewer.builder].
void main() => runApp(const IVBuilderExampleApp());
class IVBuilderExampleApp extends StatelessWidget {
const IVBuilderExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('IV Builder Example'),
),
body: const _IVBuilderExample(),
),
);
}
}
class _IVBuilderExample extends StatefulWidget {
const _IVBuilderExample();
@override
State<_IVBuilderExample> createState() => _IVBuilderExampleState();
}
class _IVBuilderExampleState extends State<_IVBuilderExample> {
static const double _cellWidth = 160.0;
static const double _cellHeight = 80.0;
// Returns the axis aligned bounding box for the given Quad, which might not
// be axis aligned.
Rect axisAlignedBoundingBox(Quad quad) {
double xMin = quad.point0.x;
double xMax = quad.point0.x;
double yMin = quad.point0.y;
double yMax = quad.point0.y;
for (final Vector3 point in <Vector3>[
quad.point1,
quad.point2,
quad.point3,
]) {
if (point.x < xMin) {
xMin = point.x;
} else if (point.x > xMax) {
xMax = point.x;
}
if (point.y < yMin) {
yMin = point.y;
} else if (point.y > yMax) {
yMax = point.y;
}
}
return Rect.fromLTRB(xMin, yMin, xMax, yMax);
}
@override
Widget build(BuildContext context) {
return Center(
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return InteractiveViewer.builder(
boundaryMargin: const EdgeInsets.all(double.infinity),
builder: (BuildContext context, Quad viewport) {
return _TableBuilder(
cellWidth: _cellWidth,
cellHeight: _cellHeight,
viewport: axisAlignedBoundingBox(viewport),
builder: (BuildContext context, int row, int column) {
return Container(
height: _cellHeight,
width: _cellWidth,
color: row % 2 + column % 2 == 1 ? Colors.white : Colors.grey.withOpacity(0.1),
child: Align(
child: Text('$row x $column'),
),
);
},
);
},
);
},
),
);
}
}
typedef _CellBuilder = Widget Function(BuildContext context, int row, int column);
class _TableBuilder extends StatelessWidget {
const _TableBuilder({
required this.cellWidth,
required this.cellHeight,
required this.viewport,
required this.builder,
});
final double cellWidth;
final double cellHeight;
final Rect viewport;
final _CellBuilder builder;
@override
Widget build(BuildContext context) {
final int firstRow = (viewport.top / cellHeight).floor();
final int lastRow = (viewport.bottom / cellHeight).ceil();
final int firstCol = (viewport.left / cellWidth).floor();
final int lastCol = (viewport.right / cellWidth).ceil();
// This will create and render exactly (lastRow - firstRow) * (lastCol - firstCol) cells
return SizedBox(
// Stack needs constraints, even though we then Clip.none outside of them.
// InteractiveViewer.builder always sets constrained to false, giving infinite constraints to the child.
// See: https://api.flutter.dev/flutter/widgets/InteractiveViewer/constrained.html
width: 1,
height: 1,
child: Stack(
clipBehavior: Clip.none,
children: <Widget>[
for (int row = firstRow; row < lastRow; row++)
for (int col = firstCol; col < lastCol; col++)
Positioned(
left: col * cellWidth,
top: row * cellHeight,
child: builder(context, row, col),
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/interactive_viewer/interactive_viewer.builder.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/interactive_viewer/interactive_viewer.builder.0.dart",
"repo_id": "flutter",
"token_count": 1779
} | 637 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [NestedScrollView].
void main() => runApp(const NestedScrollViewExampleApp());
class NestedScrollViewExampleApp extends StatelessWidget {
const NestedScrollViewExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: NestedScrollViewExample(),
);
}
}
class NestedScrollViewExample extends StatelessWidget {
const NestedScrollViewExample({super.key});
@override
Widget build(BuildContext context) {
final List<String> tabs = <String>['Tab 1', 'Tab 2'];
return DefaultTabController(
length: tabs.length, // This is the number of tabs.
child: Scaffold(
body: NestedScrollView(
headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) {
// These are the slivers that show up in the "outer" scroll view.
return <Widget>[
SliverOverlapAbsorber(
// This widget takes the overlapping behavior of the SliverAppBar,
// and redirects it to the SliverOverlapInjector below. If it is
// missing, then it is possible for the nested "inner" scroll view
// below to end up under the SliverAppBar even when the inner
// scroll view thinks it has not been scrolled.
// This is not necessary if the "headerSliverBuilder" only builds
// widgets that do not overlap the next sliver.
handle: NestedScrollView.sliverOverlapAbsorberHandleFor(context),
sliver: SliverAppBar(
title: const Text('Books'), // This is the title in the app bar.
pinned: true,
expandedHeight: 150.0,
// The "forceElevated" property causes the SliverAppBar to show
// a shadow. The "innerBoxIsScrolled" parameter is true when the
// inner scroll view is scrolled beyond its "zero" point, i.e.
// when it appears to be scrolled below the SliverAppBar.
// Without this, there are cases where the shadow would appear
// or not appear inappropriately, because the SliverAppBar is
// not actually aware of the precise position of the inner
// scroll views.
forceElevated: innerBoxIsScrolled,
bottom: TabBar(
// These are the widgets to put in each tab in the tab bar.
tabs: tabs.map((String name) => Tab(text: name)).toList(),
),
),
),
];
},
body: TabBarView(
// These are the contents of the tab views, below the tabs.
children: tabs.map((String name) {
return SafeArea(
top: false,
bottom: false,
child: Builder(
// This Builder is needed to provide a BuildContext that is
// "inside" the NestedScrollView, so that
// sliverOverlapAbsorberHandleFor() can find the
// NestedScrollView.
builder: (BuildContext context) {
return CustomScrollView(
// The "controller" and "primary" members should be left
// unset, so that the NestedScrollView can control this
// inner scroll view.
// If the "controller" property is set, then this scroll
// view will not be associated with the NestedScrollView.
// The PageStorageKey should be unique to this ScrollView;
// it allows the list to remember its scroll position when
// the tab view is not on the screen.
key: PageStorageKey<String>(name),
slivers: <Widget>[
SliverOverlapInjector(
// This is the flip side of the SliverOverlapAbsorber
// above.
handle: NestedScrollView.sliverOverlapAbsorberHandleFor(context),
),
SliverPadding(
padding: const EdgeInsets.all(8.0),
// In this example, the inner scroll view has
// fixed-height list items, hence the use of
// SliverFixedExtentList. However, one could use any
// sliver widget here, e.g. SliverList or SliverGrid.
sliver: SliverFixedExtentList(
// The items in this example are fixed to 48 pixels
// high. This matches the Material Design spec for
// ListTile widgets.
itemExtent: 48.0,
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
// This builder is called for each child.
// In this example, we just number each list item.
return ListTile(
title: Text('Item $index'),
);
},
// The childCount of the SliverChildBuilderDelegate
// specifies how many children this inner list
// has. In this example, each tab has a list of
// exactly 30 items, but this is arbitrary.
childCount: 30,
),
),
),
],
);
},
),
);
}).toList(),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/nested_scroll_view/nested_scroll_view.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/nested_scroll_view/nested_scroll_view.0.dart",
"repo_id": "flutter",
"token_count": 3131
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RestorableValue].
void main() => runApp(const RestorableValueExampleApp());
class RestorableValueExampleApp extends StatelessWidget {
const RestorableValueExampleApp({super.key});
@override
Widget build(BuildContext context) {
return WidgetsApp(
title: 'RestorableValue Sample',
color: const Color(0xffffffff),
builder: (BuildContext context, Widget? child) {
return const Center(
child: RestorableValueExample(restorationId: 'main'),
);
},
);
}
}
class RestorableValueExample extends StatefulWidget {
const RestorableValueExample({super.key, this.restorationId});
final String? restorationId;
@override
State<RestorableValueExample> createState() => _RestorableValueExampleState();
}
/// RestorationProperty objects can be used because of RestorationMixin.
class _RestorableValueExampleState extends State<RestorableValueExample> with RestorationMixin {
// In this example, the restoration ID for the mixin is passed in through
// the [StatefulWidget]'s constructor.
@override
String? get restorationId => widget.restorationId;
// The current value of the answer is stored in a [RestorableProperty].
// During state restoration it is automatically restored to its old value.
// If no restoration data is available to restore the answer from, it is
// initialized to the specified default value, in this case 42.
final RestorableInt _answer = RestorableInt(42);
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
// All restorable properties must be registered with the mixin. After
// registration, the answer either has its old value restored or is
// initialized to its default value.
registerForRestoration(_answer, 'answer');
}
void _incrementAnswer() {
setState(() {
// The current value of the property can be accessed and modified via
// the value getter and setter.
_answer.value += 1;
});
}
@override
void dispose() {
// Properties must be disposed when no longer used.
_answer.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return OutlinedButton(
onPressed: _incrementAnswer,
child: Text('${_answer.value}'),
);
}
}
| flutter/examples/api/lib/widgets/restoration_properties/restorable_value.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/restoration_properties/restorable_value.0.dart",
"repo_id": "flutter",
"token_count": 752
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RawScrollbar.shape].
void main() => runApp(const ShapeExampleApp());
class ShapeExampleApp extends StatelessWidget {
const ShapeExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: ShapeExample(),
);
}
}
class ShapeExample extends StatelessWidget {
const ShapeExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: RawScrollbar(
shape: const StadiumBorder(side: BorderSide(color: Colors.brown, width: 3.0)),
thickness: 15.0,
thumbColor: Colors.blue,
thumbVisibility: true,
child: ListView(
// On mobile platforms, setting primary to true is not required, as
// the PrimaryScrollController automatically attaches to vertical
// ScrollPositions. On desktop platforms however, using the
// PrimaryScrollController requires ScrollView.primary be set.
primary: true,
physics: const BouncingScrollPhysics(),
children: List<Text>.generate(100, (int index) => Text((index * index).toString())),
),
),
);
}
}
| flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.shape.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.shape.0.dart",
"repo_id": "flutter",
"token_count": 478
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverFillRemaining].
void main() => runApp(const SliverFillRemainingExampleApp());
class SliverFillRemainingExampleApp extends StatelessWidget {
const SliverFillRemainingExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('SliverFillRemaining Sample')),
body: const SliverFillRemainingExample(),
),
);
}
}
class SliverFillRemainingExample extends StatelessWidget {
const SliverFillRemainingExample({super.key});
@override
Widget build(BuildContext context) {
return CustomScrollView(
slivers: <Widget>[
SliverToBoxAdapter(
child: Container(
color: Colors.amber[300],
height: 150.0,
),
),
SliverFillRemaining(
hasScrollBody: false,
child: Container(
color: Colors.blue[100],
child: Icon(
Icons.sentiment_very_satisfied,
size: 75,
color: Colors.blue[900],
),
),
),
],
);
}
}
| flutter/examples/api/lib/widgets/sliver_fill/sliver_fill_remaining.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/sliver_fill/sliver_fill_remaining.0.dart",
"repo_id": "flutter",
"token_count": 563
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for a [ValueNotifier] with a [ListenableBuilder].
void main() {
runApp(const ListenableBuilderExample());
}
class ListenableBuilderExample extends StatefulWidget {
const ListenableBuilderExample({super.key});
@override
State<ListenableBuilderExample> createState() => _ListenableBuilderExampleState();
}
class _ListenableBuilderExampleState extends State<ListenableBuilderExample> {
final ValueNotifier<int> _counter = ValueNotifier<int>(0);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ListenableBuilder Example')),
body: CounterBody(counterValueNotifier: _counter),
floatingActionButton: FloatingActionButton(
onPressed: () => _counter.value++,
child: const Icon(Icons.add),
),
),
);
}
}
class CounterBody extends StatelessWidget {
const CounterBody({super.key, required this.counterValueNotifier});
final ValueNotifier<int> counterValueNotifier;
@override
Widget build(BuildContext context) {
return Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Current counter value:'),
// Thanks to the ListenableBuilder, only the widget displaying the
// current count is rebuilt when counterValueNotifier notifies its
// listeners. The Text widget above and CounterBody itself aren't
// rebuilt.
ListenableBuilder(
listenable: counterValueNotifier,
builder: (BuildContext context, Widget? child) {
return Text('${counterValueNotifier.value}');
},
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/listenable_builder.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/listenable_builder.1.dart",
"repo_id": "flutter",
"token_count": 695
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/activity_indicator/cupertino_activity_indicator.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Default and customized cupertino activity indicators', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CupertinoIndicatorApp(),
);
// Cupertino activity indicator with default properties.
final Finder firstIndicator = find.byType(CupertinoActivityIndicator).at(0);
expect(tester.widget<CupertinoActivityIndicator>(firstIndicator).animating, true);
expect(tester.widget<CupertinoActivityIndicator>(firstIndicator).radius, 10.0);
// Cupertino activity indicator with custom radius and color.
final Finder secondIndicator = find.byType(CupertinoActivityIndicator).at(1);
expect(tester.widget<CupertinoActivityIndicator>(secondIndicator).animating, true);
expect(tester.widget<CupertinoActivityIndicator>(secondIndicator).radius, 20.0);
expect(tester.widget<CupertinoActivityIndicator>(secondIndicator).color, CupertinoColors.activeBlue);
// Cupertino activity indicator with custom radius and disabled animation.
final Finder thirdIndicator = find.byType(CupertinoActivityIndicator).at(2);
expect(tester.widget<CupertinoActivityIndicator>(thirdIndicator).animating, false);
expect(tester.widget<CupertinoActivityIndicator>(thirdIndicator).radius, 20.0);
});
}
| flutter/examples/api/test/cupertino/activity_indicator/cupertino_activity_indicator.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/activity_indicator/cupertino_activity_indicator.0_test.dart",
"repo_id": "flutter",
"token_count": 506
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/radio/cupertino_radio.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Has 2 CupertinoRadio widgets', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CupertinoRadioApp(),
);
expect(find.byType(CupertinoRadio<example.SingingCharacter>), findsNWidgets(2));
CupertinoRadio<example.SingingCharacter> radio = tester.widget(find.byType(CupertinoRadio<example.SingingCharacter>).first);
expect(radio.groupValue, example.SingingCharacter.lafayette);
radio = tester.widget(find.byType(CupertinoRadio<example.SingingCharacter>).last);
expect(radio.groupValue, example.SingingCharacter.lafayette);
await tester.tap(find.byType(CupertinoRadio<example.SingingCharacter>).last);
await tester.pumpAndSettle();
radio = tester.widget(find.byType(CupertinoRadio<example.SingingCharacter>).last);
expect(radio.groupValue, example.SingingCharacter.jefferson);
radio = tester.widget(find.byType(CupertinoRadio<example.SingingCharacter>).first);
expect(radio.groupValue, example.SingingCharacter.jefferson);
});
}
| flutter/examples/api/test/cupertino/radio/cupertino_radio.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/radio/cupertino_radio.0_test.dart",
"repo_id": "flutter",
"token_count": 463
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/autocomplete/autocomplete.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('can search and find options', (WidgetTester tester) async {
await tester.pumpWidget(const example.AutocompleteExampleApp());
expect(find.text('aardvark'), findsNothing);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'a');
await tester.pump();
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsOneWidget);
expect(find.text('chameleon'), findsOneWidget);
await tester.enterText(find.byType(TextFormField), 'aa');
await tester.pump();
expect(find.text('aardvark'), findsOneWidget);
expect(find.text('bobcat'), findsNothing);
expect(find.text('chameleon'), findsNothing);
});
}
| flutter/examples/api/test/material/autocomplete/autocomplete.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/autocomplete/autocomplete.0_test.dart",
"repo_id": "flutter",
"token_count": 386
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_api_samples/material/checkbox_list_tile/checkbox_list_tile.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('CheckboxListTile can be checked', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CheckboxListTileApp(),
);
CheckboxListTile checkboxListTile = tester.widget(find.byType(CheckboxListTile));
expect(checkboxListTile.value, isFalse);
await tester.tap(find.byType(CheckboxListTile));
await tester.pump();
timeDilation = 1.0;
checkboxListTile = tester.widget(find.byType(CheckboxListTile));
expect(checkboxListTile.value, isTrue);
await tester.tap(find.byType(CheckboxListTile));
await tester.pump();
checkboxListTile = tester.widget(find.byType(CheckboxListTile));
expect(checkboxListTile.value, isFalse);
});
}
| flutter/examples/api/test/material/checkbox_list_tile/checkbox_list_tile.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/checkbox_list_tile/checkbox_list_tile.0_test.dart",
"repo_id": "flutter",
"token_count": 390
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/date_picker/show_date_picker.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can show date picker', (WidgetTester tester) async {
const String datePickerTitle = 'Select date';
const String initialDate = 'Sun, Jul 25';
await tester.pumpWidget(
const example.DatePickerApp(),
);
// The date picker is not shown initially.
expect(find.text(datePickerTitle), findsNothing);
expect(find.text(initialDate), findsNothing);
// Tap the button to show the date picker.
await tester.tap(find.byType(OutlinedButton));
await tester.pumpAndSettle();
// The initial date is shown.
expect(find.text(datePickerTitle), findsOneWidget);
expect(find.text(initialDate), findsOneWidget);
// Tap another date to select it.
await tester.tap(find.text('30'));
await tester.pumpAndSettle();
// The selected date is shown.
expect(find.text(datePickerTitle), findsOneWidget);
expect(find.text('Fri, Jul 30'), findsOneWidget);
// Tap OK to confirm the selection and close the date picker.
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// The date picker is closed and the selected date is shown.
expect(find.text(datePickerTitle), findsNothing);
expect(find.text('Selected: 30/7/2021'), findsOneWidget);
});
}
| flutter/examples/api/test/material/date_picker/show_date_picker.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/date_picker/show_date_picker.0_test.dart",
"repo_id": "flutter",
"token_count": 546
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/dropdown/dropdown_button.style.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Select an item from DropdownButton', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.DropdownButtonApp(),
),
),
);
expect(find.text('One'), findsOneWidget);
expect(find.text('One', skipOffstage: false), findsNWidgets(4));
await tester.tap(find.text('One').first);
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.tap(find.text('Two'));
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
expect(find.text('Two', skipOffstage: false), findsNWidgets(4));
});
}
| flutter/examples/api/test/material/dropdown/dropdown_button.style.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/dropdown/dropdown_button.style.0_test.dart",
"repo_id": "flutter",
"token_count": 378
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/ink/ink.image_clip.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
const List<int> kTransparentImage = <int>[
0x89, 0x50, 0x4E, 0x47, 0x0D, 0x0A, 0x1A, 0x0A, 0x00, 0x00, 0x00, 0x0D, 0x49,
0x48, 0x44, 0x52, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x08, 0x06,
0x00, 0x00, 0x00, 0x1F, 0x15, 0xC4, 0x89, 0x00, 0x00, 0x00, 0x0A, 0x49, 0x44,
0x41, 0x54, 0x78, 0x9C, 0x63, 0x00, 0x01, 0x00, 0x00, 0x05, 0x00, 0x01, 0x0D,
0x0A, 0x2D, 0xB4, 0x00, 0x00, 0x00, 0x00, 0x49, 0x45, 0x4E, 0x44, 0xAE,
];
testWidgets('Ink ancestor material is not clipped', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: example.ImageClipExample(
image: MemoryImage(Uint8List.fromList(kTransparentImage)),
),
),
),
);
final Finder inkMaterialFinder = find.ancestor(of: find.byType(Ink), matching: find.byType(Material));
expect(find.ancestor(of: inkMaterialFinder, matching: find.byType(ClipRRect)), findsNothing);
});
}
| flutter/examples/api/test/material/ink/ink.image_clip.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/ink/ink.image_clip.0_test.dart",
"repo_id": "flutter",
"token_count": 646
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/list_tile/list_tile.4.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can choose different title alignments from popup menu', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ListTileApp(),
);
Offset titleOffset = tester.getTopLeft(find.text('Headline Text'));
Offset leadingOffset = tester.getTopLeft(find.byType(Checkbox));
Offset trailingOffset = tester.getTopRight(find.byIcon(Icons.adaptive.more));
// The default title alignment is threeLine.
expect(leadingOffset.dy - titleOffset.dy, 48.0);
expect(trailingOffset.dy - titleOffset.dy, 60.0);
await tester.tap(find.byIcon(Icons.adaptive.more));
await tester.pumpAndSettle();
// Change the title alignment to titleHeight.
await tester.tap(find.text('titleHeight'));
await tester.pumpAndSettle();
titleOffset = tester.getTopLeft(find.text('Headline Text'));
leadingOffset = tester.getTopLeft(find.byType(Checkbox));
trailingOffset = tester.getTopRight(find.byIcon(Icons.adaptive.more));
expect(leadingOffset.dy - titleOffset.dy, 8.0);
expect(trailingOffset.dy - titleOffset.dy, 20.0);
await tester.tap(find.byIcon(Icons.adaptive.more));
await tester.pumpAndSettle();
// Change the title alignment to bottom.
await tester.tap(find.text('bottom'));
await tester.pumpAndSettle();
titleOffset = tester.getTopLeft(find.text('Headline Text'));
leadingOffset = tester.getTopLeft(find.byType(Checkbox));
trailingOffset = tester.getTopRight(find.byIcon(Icons.adaptive.more));
expect(leadingOffset.dy - titleOffset.dy, 96.0);
expect(trailingOffset.dy - titleOffset.dy, 108.0);
});
}
| flutter/examples/api/test/material/list_tile/list_tile.4_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/list_tile/list_tile.4_test.dart",
"repo_id": "flutter",
"token_count": 691
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/page_transitions_theme/page_transitions_theme.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('MaterialApp defines a custom PageTransitionsTheme', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PageTransitionsThemeApp(),
);
final Finder homePage = find.byType(example.HomePage);
expect(homePage, findsOneWidget);
final PageTransitionsTheme theme = Theme.of(tester.element(homePage)).pageTransitionsTheme;
expect(theme.builders, isNotNull);
// Check defined page transitions builder for each platform.
for (final TargetPlatform platform in TargetPlatform.values) {
switch (platform) {
case TargetPlatform.android:
expect(theme.builders[platform], isA<ZoomPageTransitionsBuilder>());
final ZoomPageTransitionsBuilder builder = theme.builders[platform]! as ZoomPageTransitionsBuilder;
expect(builder.allowSnapshotting, isFalse);
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.fuchsia:
case TargetPlatform.windows:
expect(theme.builders[platform], isNull);
}
}
// Can navigate to the second page.
expect(find.text('To SecondPage'), findsOneWidget);
await tester.tap(find.text('To SecondPage'));
await tester.pumpAndSettle();
// Can navigate back to the home page.
expect(find.text('Back to HomePage'), findsOneWidget);
await tester.tap(find.text('Back to HomePage'));
await tester.pumpAndSettle();
expect(find.text('To SecondPage'), findsOneWidget);
});
}
| flutter/examples/api/test/material/page_transitions_theme/page_transitions_theme.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/page_transitions_theme/page_transitions_theme.1_test.dart",
"repo_id": "flutter",
"token_count": 645
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/refresh_indicator/refresh_indicator.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Trigger RefreshIndicator - Pull from top', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RefreshIndicatorExampleApp(),
);
await tester.fling(find.text('Item 1'), const Offset(0.0, 300.0), 1000.0);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
await tester.pump(const Duration(seconds: 1));
expect(tester.getCenter(find.byType(RefreshProgressIndicator)).dy, lessThan(300.0));
await tester.pumpAndSettle(); // Advance pending time
});
testWidgets('Trigger RefreshIndicator - Button', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RefreshIndicatorExampleApp(),
);
await tester.tap(find.byType(FloatingActionButton));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
await tester.pump(const Duration(seconds: 1));
expect(tester.getCenter(find.byType(RefreshProgressIndicator)).dy, lessThan(300.0));
await tester.pumpAndSettle(); // Advance pending time
});
}
| flutter/examples/api/test/material/refresh_indicator/refresh_indicator.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/refresh_indicator/refresh_indicator.0_test.dart",
"repo_id": "flutter",
"token_count": 486
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/slider/slider.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Slider shows secondary track', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SliderApp(),
);
expect(find.byType(Slider), findsNWidgets(2));
final Finder slider1Finder = find.byType(Slider).at(0);
final Finder slider2Finder = find.byType(Slider).at(1);
Slider slider1 = tester.widget(slider1Finder);
Slider slider2 = tester.widget(slider2Finder);
expect(slider1.secondaryTrackValue, slider2.value);
const double targetValue = 0.8;
final Rect rect = tester.getRect(slider2Finder);
final Offset target = Offset(rect.left + (rect.right - rect.left) * targetValue, rect.top + (rect.bottom - rect.top) / 2);
await tester.tapAt(target);
await tester.pump();
slider1 = tester.widget(slider1Finder);
slider2 = tester.widget(slider2Finder);
expect(slider1.secondaryTrackValue, closeTo(targetValue, 0.05));
expect(slider1.secondaryTrackValue, slider2.value);
});
}
| flutter/examples/api/test/material/slider/slider.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/slider/slider.2_test.dart",
"repo_id": "flutter",
"token_count": 472
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/text_button/text_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
// The app being tested loads images via HTTP which the test
// framework defeats by default.
setUpAll(() {
HttpOverrides.global = null;
});
testWidgets('TextButtonExample smoke test', (WidgetTester tester) async {
await tester.pumpWidget(const example.TextButtonExampleApp());
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'Enabled'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'Disabled'));
await tester.pumpAndSettle();
// TextButton.icon buttons are _TextButtonWithIcons rather than TextButtons.
// For the purposes of this test, just tapping in the right place is OK.
await tester.tap(find.text('TextButton.icon #1'));
await tester.pumpAndSettle();
await tester.tap(find.text('TextButton.icon #2'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #3'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #4'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #5'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #6'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #7'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(TextButton, 'TextButton #8'));
await tester.pumpAndSettle();
final Finder smileyButton = find.byType(TextButton).last;
await tester.tap(smileyButton);
await tester.pump();
String smileyButtonImageUrl() {
final AnimatedContainer container = tester.widget<AnimatedContainer>(
find.descendant(of: smileyButton, matching: find.byType(AnimatedContainer)),
);
final BoxDecoration decoration = container.decoration! as BoxDecoration;
final NetworkImage image = decoration.image!.image as NetworkImage;
return image.url;
}
// The smiley button's onPressed method changes the button image
// for one second to simulate a long action running. The button's
// image changes while the action is running.
expect(smileyButtonImageUrl().endsWith('text_button_nhu_end.png'), isTrue);
await tester.pump(const Duration(seconds: 1));
expect(smileyButtonImageUrl().endsWith('text_button_nhu_default.png'), isTrue);
// Pressing the smiley button while the one second action is
// underway starts a new one section action. The button's image
// doesn't change until the second action has finished.
await tester.tap(smileyButton);
await tester.pump(const Duration(milliseconds: 500));
expect(smileyButtonImageUrl().endsWith('text_button_nhu_end.png'), isTrue);
await tester.tap(smileyButton); // Second button press.
await tester.pump(const Duration(milliseconds: 500));
expect(smileyButtonImageUrl().endsWith('text_button_nhu_end.png'), isTrue);
await tester.pump(const Duration(milliseconds: 500));
expect(smileyButtonImageUrl().endsWith('text_button_nhu_default.png'), isTrue);
await tester.tap(find.byType(Switch).at(0)); // Dark Mode Switch
await tester.pumpAndSettle();
await tester.tap(find.byType(Switch).at(1)); // RTL Text Switch
await tester.pumpAndSettle();
});
}
| flutter/examples/api/test/material/text_button/text_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/text_button/text_button.0_test.dart",
"repo_id": "flutter",
"token_count": 1264
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/rendering/box/parent_data.0.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('parent data example', (WidgetTester tester) async {
await tester.pumpWidget(const SampleApp());
expect(tester.getTopLeft(find.byType(Headline).at(2)), const Offset(30.0, 728.0));
await tester.tap(find.byIcon(Icons.density_small));
await tester.pump();
expect(tester.getTopLeft(find.byType(Headline).at(2)), const Offset(30.0, 682.0));
});
}
| flutter/examples/api/test/rendering/box/parent_data.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/rendering/box/parent_data.0_test.dart",
"repo_id": "flutter",
"token_count": 256
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/aspect_ratio.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AspectRatio applies 2.0 aspect ratio on its child', (WidgetTester tester) async {
const Size containerSize = Size(100, 100);
await tester.pumpWidget(
const example.AspectRatioApp(),
);
final Size parentContainer = tester.getSize(find.byType(Container).first);
expect(parentContainer, containerSize);
final Size childContainer = tester.getSize(find.byType(Container).last);
expect(childContainer, Size(containerSize.height, containerSize.height / 2.0));
});
}
| flutter/examples/api/test/widgets/basic/aspect_ratio.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/aspect_ratio.1_test.dart",
"repo_id": "flutter",
"token_count": 278
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/offstage.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can off/on stage Flutter logo widget', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(home: example.OffstageApp()),
);
// The Flutter logo is off stage and not visible.
expect(find.text('Flutter logo is offstage: true'), findsOneWidget);
// Tap to get the Flutter logo size.
await tester.tap(find.text('Get Flutter Logo size'));
await tester.pumpAndSettle();
expect(find.text('Flutter Logo size is Size(150.0, 150.0)'), findsOneWidget);
// Tap to toggle the offstage value.
await tester.tap(find.text('Toggle Offstage Value'));
await tester.pumpAndSettle();
// The Flutter logo is on stage and visible.
expect(find.text('Flutter logo is offstage: false'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/basic/offstage.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/offstage.0_test.dart",
"repo_id": "flutter",
"token_count": 379
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_api_samples/widgets/layout_builder/layout_builder.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('has two containers when wide', (WidgetTester tester) async {
await tester.pumpWidget(
const example.LayoutBuilderExampleApp(),
);
final Finder containerFinder = find.byType(Container);
expect(containerFinder, findsNWidgets(2));
});
testWidgets('has one container when narrow', (WidgetTester tester) async {
await tester.pumpWidget(
ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 400),
child: const example.LayoutBuilderExampleApp(),
),
);
final Finder containerFinder = find.byType(Container);
expect(containerFinder, findsNWidgets(2));
});
}
| flutter/examples/api/test/widgets/layout_builder/layout_builder.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/layout_builder/layout_builder.0_test.dart",
"repo_id": "flutter",
"token_count": 337
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_api_samples/widgets/scroll_view/grid_view.0.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('$CustomGridLayout', (WidgetTester tester) async {
const CustomGridLayout layout = CustomGridLayout(
crossAxisCount: 2,
fullRowPeriod: 3,
dimension: 100,
);
final List<double> scrollOffsets = List<double>.generate(10, (int i) => layout.computeMaxScrollOffset(i));
expect(scrollOffsets, <double>[0.0, 0.0, 100.0, 100.0, 200.0, 300.0, 300.0, 400.0, 400.0, 500.0]);
final List<int> minOffsets = List<int>.generate(10, (int i) => layout.getMinChildIndexForScrollOffset(i * 80.0));
expect(minOffsets, <int>[0, 0, 2, 4, 5, 7, 7, 9, 10, 12]);
final List<int> maxOffsets = List<int>.generate(10, (int i) => layout.getMaxChildIndexForScrollOffset(i * 80.0));
expect(maxOffsets, <double>[1, 1, 3, 4, 6, 8, 8, 9, 11, 13]);
final List<SliverGridGeometry> offsets = List<SliverGridGeometry>.generate(20, (int i) => layout.getGeometryForChildIndex(i));
offsets.reduce((SliverGridGeometry a, SliverGridGeometry b) {
if (a.scrollOffset == b.scrollOffset) {
expect(a.crossAxisOffset, lessThan(b.crossAxisOffset));
} else {
expect(a.scrollOffset, lessThan(b.scrollOffset));
}
return b;
});
});
}
| flutter/examples/api/test/widgets/scroll_view/grid_view.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/scroll_view/grid_view.0_test.dart",
"repo_id": "flutter",
"token_count": 601
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/transitions/matrix_transition.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Shows Flutter logo inside a MatrixTransition', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MatrixTransitionExampleApp(),
);
final Finder transformFinder = find.ancestor(
of: find.byType(FlutterLogo),
matching: find.byType(MatrixTransition),
);
expect(transformFinder, findsOneWidget);
});
testWidgets('MatrixTransition animates', (WidgetTester tester) async {
await tester.pumpWidget(
const example.MatrixTransitionExampleApp(),
);
final Finder transformFinder = find.ancestor(
of: find.byType(FlutterLogo),
matching: find.byType(Transform),
);
Transform transformBox = tester.widget(transformFinder);
Matrix4 actualTransform = transformBox.transform;
// Check initial transform.
expect(actualTransform, Matrix4.fromList(<double>[
1.0, 0.0, 0.0, 0.0,
0.0, 1.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.004, 1.0,
])..transpose());
// Animate half way.
await tester.pump(const Duration(seconds: 1));
transformBox = tester.widget(transformFinder);
actualTransform = transformBox.transform;
// The transform should be updated.
expect(actualTransform, isNot(Matrix4.fromList(<double>[
1.0, 0.0, 0.0, 0.0,
0.0, 1.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.004, 1.0,
])..transpose()));
});
}
| flutter/examples/api/test/widgets/transitions/matrix_transition.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/transitions/matrix_transition.0_test.dart",
"repo_id": "flutter",
"token_count": 698
} | 660 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/flutter_view/macos/Flutter/Flutter-Debug.xcconfig/0 | {
"file_path": "flutter/examples/flutter_view/macos/Flutter/Flutter-Debug.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import Cocoa
import FlutterMacOS
/**
The window that is automatically generated when `flutter create --target=macos`
on a project. `MainFlutterWindow` uses a FlutterViewController as it's content
view controller by default.
*/
class MainFlutterWindow: NSWindow {
override func awakeFromNib() {
let windowFrame = self.frame
let storyboard = NSStoryboard(name: NSStoryboard.Name("Main"), bundle: Bundle.main)
// `flutter create --target=macos` uses this class (`self`) as an entrypoint
// for drawing on a surface. The line below intercepts that and uses
// the storyboard from `Main.storyboard`.
self.contentViewController = storyboard.instantiateController(withIdentifier: "MainViewController") as! NSViewController
self.setFrame(windowFrame, display: true)
super.awakeFromNib()
}
}
| flutter/examples/flutter_view/macos/Runner/MainFlutterWindow.swift/0 | {
"file_path": "flutter/examples/flutter_view/macos/Runner/MainFlutterWindow.swift",
"repo_id": "flutter",
"token_count": 287
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Contents of this file should be generated automatically by
// dev/tools/bin/generate_gradle_lockfiles.dart, but currently are not.
// See #141540.
pluginManagement {
val flutterSdkPath =
run {
val properties = java.util.Properties()
file("local.properties").inputStream().use { properties.load(it) }
val flutterSdkPath = properties.getProperty("flutter.sdk")
require(flutterSdkPath != null) { "flutter.sdk not set in local.properties" }
flutterSdkPath
}
includeBuild("$flutterSdkPath/packages/flutter_tools/gradle")
repositories {
google()
mavenCentral()
gradlePluginPortal()
}
}
buildscript {
dependencyLocking {
lockFile = file("${rootProject.projectDir}/buildscript-gradle.lockfile")
lockAllConfigurations()
}
}
plugins {
id("dev.flutter.flutter-plugin-loader") version "1.0.0"
id("com.android.application") version "7.4.2" apply false
id("org.jetbrains.kotlin.android") version "1.7.10" apply false
}
include(":app")
| flutter/examples/hello_world/android/settings.gradle.kts/0 | {
"file_path": "flutter/examples/hello_world/android/settings.gradle.kts",
"repo_id": "flutter",
"token_count": 482
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This example shows how to show the text 'Hello, world.' using the underlying
// render tree.
import 'package:flutter/rendering.dart';
import 'src/binding.dart';
void main() {
// We use ViewRenderingFlutterBinding to attach the render tree to the window.
ViewRenderingFlutterBinding(
// The root of our render tree is a RenderPositionedBox, which centers its
// child both vertically and horizontally.
root: RenderPositionedBox(
// We use a RenderParagraph to display the text 'Hello, world.' without
// any explicit styling.
child: RenderParagraph(
const TextSpan(text: 'Hello, world.'),
// The text is in English so we specify the text direction as
// left-to-right. If the text had been in Hebrew or Arabic, we would
// have specified right-to-left. The Flutter framework does not assume a
// particular text direction.
textDirection: TextDirection.ltr,
),
),
).scheduleFrame();
}
| flutter/examples/layers/rendering/hello_world.dart/0 | {
"file_path": "flutter/examples/layers/rendering/hello_world.dart",
"repo_id": "flutter",
"token_count": 366
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
void main() {
group('button tap test', () {
late FlutterDriver driver;
setUpAll(() async {
driver = await FlutterDriver.connect();
});
tearDownAll(() async {
driver.close();
});
test('tap on the button, verify result', () async {
final SerializableFinder batteryLevelLabel =
find.byValueKey('Battery level label');
expect(batteryLevelLabel, isNotNull);
final SerializableFinder button = find.text('Refresh');
await driver.waitFor(button);
await driver.tap(button);
String? batteryLevel;
while (batteryLevel == null || batteryLevel.contains('unknown')) {
batteryLevel = await driver.getText(batteryLevelLabel);
}
// Allow either a battery percentage or "No battery" since it will vary
// by device; either indicates that a known response came from the host
// implementation.
expect(batteryLevel.contains('%') || batteryLevel.contains('No battery'),
isTrue);
});
});
}
| flutter/examples/platform_channel/test_driver/button_tap_test.dart/0 | {
"file_path": "flutter/examples/platform_channel/test_driver/button_tap_test.dart",
"repo_id": "flutter",
"token_count": 444
} | 665 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for AppBarTheme from the Material library. *
# For fixes to
# * AppBar: fix_app_bar.yaml
# * ColorScheme: fix_color_scheme.yaml
# * Material (general): fix_material.yaml
# * SliverAppBar: fix_sliver_app_bar.yaml
# * TextTheme: fix_text_theme.yaml
# * ThemeData: fix_theme_data.yaml
# * WidgetState: fix_widget_state.yaml
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'systemOverlayStyle'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
method: 'copyWith'
inClass: 'AppBarTheme'
oneOf:
- if: "brightness == 'Brightness.dark'"
changes:
- kind: 'addParameter'
index: 14
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.light'
requiredIf: "brightness == 'Brightness.dark'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
- if: "brightness == 'Brightness.light'"
changes:
- kind: 'addParameter'
index: 14
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.dark'
requiredIf: "brightness == 'Brightness.light'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
variables:
brightness:
kind: 'fragment'
value: 'arguments[brightness]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'systemOverlayStyle'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBarTheme'
oneOf:
- if: "brightness == 'Brightness.dark'"
changes:
- kind: 'addParameter'
index: 14
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.light'
requiredIf: "brightness == 'Brightness.dark'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
- if: "brightness == 'Brightness.light'"
changes:
- kind: 'addParameter'
index: 14
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.dark'
requiredIf: "brightness == 'Brightness.light'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
variables:
brightness:
kind: 'fragment'
value: 'arguments[brightness]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'systemOverlayStyle'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
field: 'brightness'
inClass: 'AppBarTheme'
changes:
- kind: 'rename'
newName: 'systemOverlayStyle'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'toolbarTextStyle'"
date: 2021-07-12
# TODO(Piinks): Add tests when `bulkApply:false` testing is supported, https://github.com/dart-lang/sdk/issues/44639
bulkApply: false
element:
uris: [ 'material.dart' ]
field: 'textTheme'
inClass: 'AppBarTheme'
changes:
- kind: 'rename'
newName: 'toolbarTextStyle'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'titleTextStyle'"
date: 2021-07-12
# TODO(Piinks): Add tests when `bulkApply:false` testing is supported, https://github.com/dart-lang/sdk/issues/44639
bulkApply: false
element:
uris: [ 'material.dart' ]
field: 'textTheme'
inClass: 'AppBarTheme'
changes:
- kind: 'rename'
newName: 'titleTextStyle'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'toolbarTextStyle' & 'titleTextStyle'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
method: 'copyWith'
inClass: 'AppBarTheme'
changes:
- kind: 'addParameter'
index: 12
name: 'toolbarTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.bodyText2'
requiredIf: "textTheme != ''"
- kind: 'addParameter'
index: 13
name: 'titleTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.headline6'
requiredIf: "textTheme != ''"
- kind: 'removeParameter'
name: 'textTheme'
variables:
textTheme:
kind: 'fragment'
value: 'arguments[textTheme]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'toolbarTextStyle' & 'titleTextStyle'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBarTheme'
changes:
- kind: 'addParameter'
index: 13
name: 'toolbarTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.bodyText2'
requiredIf: "textTheme != ''"
- kind: 'addParameter'
index: 14
name: 'titleTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.headline6'
requiredIf: "textTheme != ''"
- kind: 'removeParameter'
name: 'textTheme'
variables:
textTheme:
kind: 'fragment'
value: 'arguments[textTheme]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'backgroundColor'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
getter: color
inClass: 'AppBarTheme'
changes:
- kind: 'rename'
newName: 'backgroundColor'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Remove 'backwardsCompatibility'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
method: 'copyWith'
inClass: 'AppBarTheme'
changes:
- kind: 'removeParameter'
name: 'backwardsCompatibility'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Remove 'backwardsCompatibility'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBarTheme'
changes:
- kind: 'removeParameter'
name: 'backwardsCompatibility'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_material/fix_app_bar_theme.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_material/fix_app_bar_theme.yaml",
"repo_id": "flutter",
"token_count": 3430
} | 666 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for ListWheelScrollView from the Widgets library. *
# For fixes to
# * Actions: fix_actions.yaml
# * BuildContext: fix_build_context.yaml
# * Element: fix_element.yaml
# * Widgets (general): fix_widgets.yaml
version: 1
transforms:
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: 'useDelegate'
inClass: 'ListWheelScrollView'
oneOf:
- if: "clipToSize == 'true'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "clipToSize == 'true'"
- kind: 'removeParameter'
name: 'clipToSize'
- if: "clipToSize == 'false'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "clipToSize == 'false'"
- kind: 'removeParameter'
name: 'clipToSize'
variables:
clipToSize:
kind: 'fragment'
value: 'arguments[clipToSize]'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
field: 'clipToSize'
inClass: 'ListWheelScrollView'
changes:
- kind: 'rename'
newName: 'clipBehavior'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
constructor: ''
inClass: 'ListWheelScrollView'
oneOf:
- if: "clipToSize == 'true'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "clipToSize == 'true'"
- kind: 'removeParameter'
name: 'clipToSize'
- if: "clipToSize == 'false'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "clipToSize == 'false'"
- kind: 'removeParameter'
name: 'clipToSize'
variables:
clipToSize:
kind: 'fragment'
value: 'arguments[clipToSize]'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_widgets/fix_list_wheel_scroll_view.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_widgets/fix_list_wheel_scroll_view.yaml",
"repo_id": "flutter",
"token_count": 1483
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'animation.dart';
import 'curves.dart';
import 'listener_helpers.dart';
export 'dart:ui' show VoidCallback;
export 'animation.dart' show Animation, AnimationStatus, AnimationStatusListener;
export 'curves.dart' show Curve;
// Examples can assume:
// late AnimationController controller;
class _AlwaysCompleteAnimation extends Animation<double> {
const _AlwaysCompleteAnimation();
@override
void addListener(VoidCallback listener) { }
@override
void removeListener(VoidCallback listener) { }
@override
void addStatusListener(AnimationStatusListener listener) { }
@override
void removeStatusListener(AnimationStatusListener listener) { }
@override
AnimationStatus get status => AnimationStatus.completed;
@override
double get value => 1.0;
@override
String toString() => 'kAlwaysCompleteAnimation';
}
/// An animation that is always complete.
///
/// Using this constant involves less overhead than building an
/// [AnimationController] with an initial value of 1.0. This is useful when an
/// API expects an animation but you don't actually want to animate anything.
const Animation<double> kAlwaysCompleteAnimation = _AlwaysCompleteAnimation();
class _AlwaysDismissedAnimation extends Animation<double> {
const _AlwaysDismissedAnimation();
@override
void addListener(VoidCallback listener) { }
@override
void removeListener(VoidCallback listener) { }
@override
void addStatusListener(AnimationStatusListener listener) { }
@override
void removeStatusListener(AnimationStatusListener listener) { }
@override
AnimationStatus get status => AnimationStatus.dismissed;
@override
double get value => 0.0;
@override
String toString() => 'kAlwaysDismissedAnimation';
}
/// An animation that is always dismissed.
///
/// Using this constant involves less overhead than building an
/// [AnimationController] with an initial value of 0.0. This is useful when an
/// API expects an animation but you don't actually want to animate anything.
const Animation<double> kAlwaysDismissedAnimation = _AlwaysDismissedAnimation();
/// An animation that is always stopped at a given value.
///
/// The [status] is always [AnimationStatus.forward].
class AlwaysStoppedAnimation<T> extends Animation<T> {
/// Creates an [AlwaysStoppedAnimation] with the given value.
///
/// Since the [value] and [status] of an [AlwaysStoppedAnimation] can never
/// change, the listeners can never be called. It is therefore safe to reuse
/// an [AlwaysStoppedAnimation] instance in multiple places. If the [value] to
/// be used is known at compile time, the constructor should be called as a
/// `const` constructor.
const AlwaysStoppedAnimation(this.value);
@override
final T value;
@override
void addListener(VoidCallback listener) { }
@override
void removeListener(VoidCallback listener) { }
@override
void addStatusListener(AnimationStatusListener listener) { }
@override
void removeStatusListener(AnimationStatusListener listener) { }
@override
AnimationStatus get status => AnimationStatus.forward;
@override
String toStringDetails() {
return '${super.toStringDetails()} $value; paused';
}
}
/// Implements most of the [Animation] interface by deferring its behavior to a
/// given [parent] Animation.
///
/// To implement an [Animation] that is driven by a parent, it is only necessary
/// to mix in this class, implement [parent], and implement `T get value`.
///
/// To define a mapping from values in the range 0..1, consider subclassing
/// [Tween] instead.
mixin AnimationWithParentMixin<T> {
/// The animation whose value this animation will proxy.
///
/// This animation must remain the same for the lifetime of this object. If
/// you wish to proxy a different animation at different times, consider using
/// [ProxyAnimation].
Animation<T> get parent;
// keep these next five dartdocs in sync with the dartdocs in Animation<T>
/// Calls the listener every time the value of the animation changes.
///
/// Listeners can be removed with [removeListener].
void addListener(VoidCallback listener) => parent.addListener(listener);
/// Stop calling the listener every time the value of the animation changes.
///
/// Listeners can be added with [addListener].
void removeListener(VoidCallback listener) => parent.removeListener(listener);
/// Calls listener every time the status of the animation changes.
///
/// Listeners can be removed with [removeStatusListener].
void addStatusListener(AnimationStatusListener listener) => parent.addStatusListener(listener);
/// Stops calling the listener every time the status of the animation changes.
///
/// Listeners can be added with [addStatusListener].
void removeStatusListener(AnimationStatusListener listener) => parent.removeStatusListener(listener);
/// The current status of this animation.
AnimationStatus get status => parent.status;
}
/// An animation that is a proxy for another animation.
///
/// A proxy animation is useful because the parent animation can be mutated. For
/// example, one object can create a proxy animation, hand the proxy to another
/// object, and then later change the animation from which the proxy receives
/// its value.
class ProxyAnimation extends Animation<double>
with AnimationLazyListenerMixin, AnimationLocalListenersMixin, AnimationLocalStatusListenersMixin {
/// Creates a proxy animation.
///
/// If the animation argument is omitted, the proxy animation will have the
/// status [AnimationStatus.dismissed] and a value of 0.0.
ProxyAnimation([Animation<double>? animation]) {
_parent = animation;
if (_parent == null) {
_status = AnimationStatus.dismissed;
_value = 0.0;
}
}
AnimationStatus? _status;
double? _value;
/// The animation whose value this animation will proxy.
///
/// This value is mutable. When mutated, the listeners on the proxy animation
/// will be transparently updated to be listening to the new parent animation.
Animation<double>? get parent => _parent;
Animation<double>? _parent;
set parent(Animation<double>? value) {
if (value == _parent) {
return;
}
if (_parent != null) {
_status = _parent!.status;
_value = _parent!.value;
if (isListening) {
didStopListening();
}
}
_parent = value;
if (_parent != null) {
if (isListening) {
didStartListening();
}
if (_value != _parent!.value) {
notifyListeners();
}
if (_status != _parent!.status) {
notifyStatusListeners(_parent!.status);
}
_status = null;
_value = null;
}
}
@override
void didStartListening() {
if (_parent != null) {
_parent!.addListener(notifyListeners);
_parent!.addStatusListener(notifyStatusListeners);
}
}
@override
void didStopListening() {
if (_parent != null) {
_parent!.removeListener(notifyListeners);
_parent!.removeStatusListener(notifyStatusListeners);
}
}
@override
AnimationStatus get status => _parent != null ? _parent!.status : _status!;
@override
double get value => _parent != null ? _parent!.value : _value!;
@override
String toString() {
if (parent == null) {
return '${objectRuntimeType(this, 'ProxyAnimation')}(null; ${super.toStringDetails()} ${value.toStringAsFixed(3)})';
}
return '$parent\u27A9${objectRuntimeType(this, 'ProxyAnimation')}';
}
}
/// An animation that is the reverse of another animation.
///
/// If the parent animation is running forward from 0.0 to 1.0, this animation
/// is running in reverse from 1.0 to 0.0.
///
/// Using a [ReverseAnimation] is different from using a [Tween] with a
/// `begin` of 1.0 and an `end` of 0.0 because the tween does not change the status
/// or direction of the animation.
///
/// See also:
///
/// * [Curve.flipped] and [FlippedCurve], which provide a similar effect but on
/// [Curve]s.
/// * [CurvedAnimation], which can take separate curves for when the animation
/// is going forward than for when it is going in reverse.
class ReverseAnimation extends Animation<double>
with AnimationLazyListenerMixin, AnimationLocalStatusListenersMixin {
/// Creates a reverse animation.
ReverseAnimation(this.parent);
/// The animation whose value and direction this animation is reversing.
final Animation<double> parent;
@override
void addListener(VoidCallback listener) {
didRegisterListener();
parent.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
parent.removeListener(listener);
didUnregisterListener();
}
@override
void didStartListening() {
parent.addStatusListener(_statusChangeHandler);
}
@override
void didStopListening() {
parent.removeStatusListener(_statusChangeHandler);
}
void _statusChangeHandler(AnimationStatus status) {
notifyStatusListeners(_reverseStatus(status));
}
@override
AnimationStatus get status => _reverseStatus(parent.status);
@override
double get value => 1.0 - parent.value;
AnimationStatus _reverseStatus(AnimationStatus status) {
return switch (status) {
AnimationStatus.forward => AnimationStatus.reverse,
AnimationStatus.reverse => AnimationStatus.forward,
AnimationStatus.completed => AnimationStatus.dismissed,
AnimationStatus.dismissed => AnimationStatus.completed,
};
}
@override
String toString() {
return '$parent\u27AA${objectRuntimeType(this, 'ReverseAnimation')}';
}
}
/// An animation that applies a curve to another animation.
///
/// [CurvedAnimation] is useful when you want to apply a non-linear [Curve] to
/// an animation object, especially if you want different curves when the
/// animation is going forward vs when it is going backward.
///
/// Depending on the given curve, the output of the [CurvedAnimation] could have
/// a wider range than its input. For example, elastic curves such as
/// [Curves.elasticIn] will significantly overshoot or undershoot the default
/// range of 0.0 to 1.0.
///
/// If you want to apply a [Curve] to a [Tween], consider using [CurveTween].
///
/// {@tool snippet}
///
/// The following code snippet shows how you can apply a curve to a linear
/// animation produced by an [AnimationController] `controller`.
///
/// ```dart
/// final Animation<double> animation = CurvedAnimation(
/// parent: controller,
/// curve: Curves.ease,
/// );
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// This second code snippet shows how to apply a different curve in the forward
/// direction than in the reverse direction. This can't be done using a
/// [CurveTween] (since [Tween]s are not aware of the animation direction when
/// they are applied).
///
/// ```dart
/// final Animation<double> animation = CurvedAnimation(
/// parent: controller,
/// curve: Curves.easeIn,
/// reverseCurve: Curves.easeOut,
/// );
/// ```
/// {@end-tool}
///
/// By default, the [reverseCurve] matches the forward [curve].
///
/// See also:
///
/// * [CurveTween], for an alternative way of expressing the first sample
/// above.
/// * [AnimationController], for examples of creating and disposing of an
/// [AnimationController].
/// * [Curve.flipped] and [FlippedCurve], which provide the reverse of a
/// [Curve].
class CurvedAnimation extends Animation<double> with AnimationWithParentMixin<double> {
/// Creates a curved animation.
CurvedAnimation({
required this.parent,
required this.curve,
this.reverseCurve,
}) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/animation.dart',
className: '$CurvedAnimation',
object: this,
);
}
_updateCurveDirection(parent.status);
parent.addStatusListener(_updateCurveDirection);
}
/// The animation to which this animation applies a curve.
@override
final Animation<double> parent;
/// The curve to use in the forward direction.
Curve curve;
/// The curve to use in the reverse direction.
///
/// If the parent animation changes direction without first reaching the
/// [AnimationStatus.completed] or [AnimationStatus.dismissed] status, the
/// [CurvedAnimation] stays on the same curve (albeit in the opposite
/// direction) to avoid visual discontinuities.
///
/// If you use a non-null [reverseCurve], you might want to hold this object
/// in a [State] object rather than recreating it each time your widget builds
/// in order to take advantage of the state in this object that avoids visual
/// discontinuities.
///
/// If this field is null, uses [curve] in both directions.
Curve? reverseCurve;
/// The direction used to select the current curve.
///
/// The curve direction is only reset when we hit the beginning or the end of
/// the timeline to avoid discontinuities in the value of any variables this
/// animation is used to animate.
AnimationStatus? _curveDirection;
/// True if this CurvedAnimation has been disposed.
bool isDisposed = false;
void _updateCurveDirection(AnimationStatus status) {
_curveDirection = switch (status) {
AnimationStatus.dismissed || AnimationStatus.completed => null,
AnimationStatus.forward || AnimationStatus.reverse => _curveDirection ?? status,
};
}
bool get _useForwardCurve {
return reverseCurve == null || (_curveDirection ?? parent.status) != AnimationStatus.reverse;
}
/// Cleans up any listeners added by this CurvedAnimation.
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
isDisposed = true;
parent.removeStatusListener(_updateCurveDirection);
}
@override
double get value {
final Curve? activeCurve = _useForwardCurve ? curve : reverseCurve;
final double t = parent.value;
if (activeCurve == null) {
return t;
}
if (t == 0.0 || t == 1.0) {
assert(() {
final double transformedValue = activeCurve.transform(t);
final double roundedTransformedValue = transformedValue.round().toDouble();
if (roundedTransformedValue != t) {
throw FlutterError(
'Invalid curve endpoint at $t.\n'
'Curves must map 0.0 to near zero and 1.0 to near one but '
'${activeCurve.runtimeType} mapped $t to $transformedValue, which '
'is near $roundedTransformedValue.',
);
}
return true;
}());
return t;
}
return activeCurve.transform(t);
}
@override
String toString() {
if (reverseCurve == null) {
return '$parent\u27A9$curve';
}
if (_useForwardCurve) {
return '$parent\u27A9$curve\u2092\u2099/$reverseCurve';
}
return '$parent\u27A9$curve/$reverseCurve\u2092\u2099';
}
}
enum _TrainHoppingMode { minimize, maximize }
/// This animation starts by proxying one animation, but when the value of that
/// animation crosses the value of the second (either because the second is
/// going in the opposite direction, or because the one overtakes the other),
/// the animation hops over to proxying the second animation.
///
/// When the [TrainHoppingAnimation] starts proxying the second animation
/// instead of the first, the [onSwitchedTrain] callback is called.
///
/// If the two animations start at the same value, then the
/// [TrainHoppingAnimation] immediately hops to the second animation, and the
/// [onSwitchedTrain] callback is not called. If only one animation is provided
/// (i.e. if the second is null), then the [TrainHoppingAnimation] just proxies
/// the first animation.
///
/// Since this object must track the two animations even when it has no
/// listeners of its own, instead of shutting down when all its listeners are
/// removed, it exposes a [dispose()] method. Call this method to shut this
/// object down.
class TrainHoppingAnimation extends Animation<double>
with AnimationEagerListenerMixin, AnimationLocalListenersMixin, AnimationLocalStatusListenersMixin {
/// Creates a train-hopping animation.
///
/// The current train argument must not be null but the next train argument
/// can be null. If the next train is null, then this object will just proxy
/// the first animation and never hop.
TrainHoppingAnimation(
Animation<double> this._currentTrain,
this._nextTrain, {
this.onSwitchedTrain,
}) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/animation.dart',
className: '$TrainHoppingAnimation',
object: this,
);
}
if (_nextTrain != null) {
if (_currentTrain!.value == _nextTrain!.value) {
_currentTrain = _nextTrain;
_nextTrain = null;
} else if (_currentTrain!.value > _nextTrain!.value) {
_mode = _TrainHoppingMode.maximize;
} else {
assert(_currentTrain!.value < _nextTrain!.value);
_mode = _TrainHoppingMode.minimize;
}
}
_currentTrain!.addStatusListener(_statusChangeHandler);
_currentTrain!.addListener(_valueChangeHandler);
_nextTrain?.addListener(_valueChangeHandler);
assert(_mode != null || _nextTrain == null);
}
/// The animation that is currently driving this animation.
///
/// The identity of this object will change from the first animation to the
/// second animation when [onSwitchedTrain] is called.
Animation<double>? get currentTrain => _currentTrain;
Animation<double>? _currentTrain;
Animation<double>? _nextTrain;
_TrainHoppingMode? _mode;
/// Called when this animation switches to be driven by the second animation.
///
/// This is not called if the two animations provided to the constructor have
/// the same value at the time of the call to the constructor. In that case,
/// the second animation is used from the start, and the first is ignored.
VoidCallback? onSwitchedTrain;
AnimationStatus? _lastStatus;
void _statusChangeHandler(AnimationStatus status) {
assert(_currentTrain != null);
if (status != _lastStatus) {
notifyListeners();
_lastStatus = status;
}
assert(_lastStatus != null);
}
@override
AnimationStatus get status => _currentTrain!.status;
double? _lastValue;
void _valueChangeHandler() {
assert(_currentTrain != null);
bool hop = false;
if (_nextTrain != null) {
assert(_mode != null);
hop = switch (_mode!) {
_TrainHoppingMode.minimize => _nextTrain!.value <= _currentTrain!.value,
_TrainHoppingMode.maximize => _nextTrain!.value >= _currentTrain!.value,
};
if (hop) {
_currentTrain!
..removeStatusListener(_statusChangeHandler)
..removeListener(_valueChangeHandler);
_currentTrain = _nextTrain;
_nextTrain = null;
_currentTrain!.addStatusListener(_statusChangeHandler);
_statusChangeHandler(_currentTrain!.status);
}
}
final double newValue = value;
if (newValue != _lastValue) {
notifyListeners();
_lastValue = newValue;
}
assert(_lastValue != null);
if (hop && onSwitchedTrain != null) {
onSwitchedTrain!();
}
}
@override
double get value => _currentTrain!.value;
/// Frees all the resources used by this performance.
/// After this is called, this object is no longer usable.
@override
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
assert(_currentTrain != null);
_currentTrain!.removeStatusListener(_statusChangeHandler);
_currentTrain!.removeListener(_valueChangeHandler);
_currentTrain = null;
_nextTrain?.removeListener(_valueChangeHandler);
_nextTrain = null;
clearListeners();
clearStatusListeners();
super.dispose();
}
@override
String toString() {
if (_nextTrain != null) {
return '$currentTrain\u27A9${objectRuntimeType(this, 'TrainHoppingAnimation')}(next: $_nextTrain)';
}
return '$currentTrain\u27A9${objectRuntimeType(this, 'TrainHoppingAnimation')}(no next)';
}
}
/// An interface for combining multiple Animations. Subclasses need only
/// implement the `value` getter to control how the child animations are
/// combined. Can be chained to combine more than 2 animations.
///
/// For example, to create an animation that is the sum of two others, subclass
/// this class and define `T get value = first.value + second.value;`
///
/// By default, the [status] of a [CompoundAnimation] is the status of the
/// [next] animation if [next] is moving, and the status of the [first]
/// animation otherwise.
abstract class CompoundAnimation<T> extends Animation<T>
with AnimationLazyListenerMixin, AnimationLocalListenersMixin, AnimationLocalStatusListenersMixin {
/// Creates a [CompoundAnimation].
///
/// Either argument can be a [CompoundAnimation] itself to combine multiple animations.
CompoundAnimation({
required this.first,
required this.next,
});
/// The first sub-animation. Its status takes precedence if neither are
/// animating.
final Animation<T> first;
/// The second sub-animation.
final Animation<T> next;
@override
void didStartListening() {
first.addListener(_maybeNotifyListeners);
first.addStatusListener(_maybeNotifyStatusListeners);
next.addListener(_maybeNotifyListeners);
next.addStatusListener(_maybeNotifyStatusListeners);
}
@override
void didStopListening() {
first.removeListener(_maybeNotifyListeners);
first.removeStatusListener(_maybeNotifyStatusListeners);
next.removeListener(_maybeNotifyListeners);
next.removeStatusListener(_maybeNotifyStatusListeners);
}
/// Gets the status of this animation based on the [first] and [next] status.
///
/// The default is that if the [next] animation is moving, use its status.
/// Otherwise, default to [first].
@override
AnimationStatus get status {
if (next.status == AnimationStatus.forward || next.status == AnimationStatus.reverse) {
return next.status;
}
return first.status;
}
@override
String toString() {
return '${objectRuntimeType(this, 'CompoundAnimation')}($first, $next)';
}
AnimationStatus? _lastStatus;
void _maybeNotifyStatusListeners(AnimationStatus _) {
if (status != _lastStatus) {
_lastStatus = status;
notifyStatusListeners(status);
}
}
T? _lastValue;
void _maybeNotifyListeners() {
if (value != _lastValue) {
_lastValue = value;
notifyListeners();
}
}
}
/// An animation of [double]s that tracks the mean of two other animations.
///
/// The [status] of this animation is the status of the `right` animation if it is
/// moving, and the `left` animation otherwise.
///
/// The [value] of this animation is the [double] that represents the mean value
/// of the values of the `left` and `right` animations.
class AnimationMean extends CompoundAnimation<double> {
/// Creates an animation that tracks the mean of two other animations.
AnimationMean({
required Animation<double> left,
required Animation<double> right,
}) : super(first: left, next: right);
@override
double get value => (first.value + next.value) / 2.0;
}
/// An animation that tracks the maximum of two other animations.
///
/// The [value] of this animation is the maximum of the values of
/// [first] and [next].
class AnimationMax<T extends num> extends CompoundAnimation<T> {
/// Creates an [AnimationMax].
///
/// Either argument can be an [AnimationMax] itself to combine multiple
/// animations.
AnimationMax(Animation<T> first, Animation<T> next) : super(first: first, next: next);
@override
T get value => math.max(first.value, next.value);
}
/// An animation that tracks the minimum of two other animations.
///
/// The [value] of this animation is the maximum of the values of
/// [first] and [next].
class AnimationMin<T extends num> extends CompoundAnimation<T> {
/// Creates an [AnimationMin].
///
/// Either argument can be an [AnimationMin] itself to combine multiple
/// animations.
AnimationMin(Animation<T> first, Animation<T> next) : super(first: first, next: next);
@override
T get value => math.min(first.value, next.value);
}
| flutter/packages/flutter/lib/src/animation/animations.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/animation/animations.dart",
"repo_id": "flutter",
"token_count": 7661
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'localizations.dart';
// Examples can assume:
// late BuildContext context;
/// Asserts that the given context has a [Localizations] ancestor that contains
/// a [CupertinoLocalizations] delegate.
///
/// To call this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasCupertinoLocalizations(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasCupertinoLocalizations(BuildContext context) {
assert(() {
if (Localizations.of<CupertinoLocalizations>(context, CupertinoLocalizations) == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No CupertinoLocalizations found.'),
ErrorDescription(
'${context.widget.runtimeType} widgets require CupertinoLocalizations '
'to be provided by a Localizations widget ancestor.',
),
ErrorDescription(
'The cupertino library uses Localizations to generate messages, '
'labels, and abbreviations.',
),
ErrorHint(
'To introduce a CupertinoLocalizations, either use a '
'CupertinoApp at the root of your application to include them '
'automatically, or add a Localization widget with a '
'CupertinoLocalizations delegate.',
),
...context.describeMissingAncestor(expectedAncestorType: CupertinoLocalizations),
]);
}
return true;
}());
return true;
}
| flutter/packages/flutter/lib/src/cupertino/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/debug.dart",
"repo_id": "flutter",
"token_count": 617
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'theme.dart';
// Eyeballed values comparing with a native picker to produce the right
// curvatures and densities.
const double _kDefaultDiameterRatio = 1.07;
const double _kDefaultPerspective = 0.003;
const double _kSqueeze = 1.45;
// Opacity fraction value that dims the wheel above and below the "magnifier"
// lens.
const double _kOverAndUnderCenterOpacity = 0.447;
/// An iOS-styled picker.
///
/// Displays its children widgets on a wheel for selection and
/// calls back when the currently selected item changes.
///
/// By default, the first child in `children` will be the initially selected child.
/// The index of a different child can be specified in [scrollController], to make
/// that child the initially selected child.
///
/// Can be used with [showCupertinoModalPopup] to display the picker modally at the
/// bottom of the screen. When calling [showCupertinoModalPopup], be sure to set
/// `semanticsDismissible` to true to enable dismissing the modal via semantics.
///
/// Sizes itself to its parent. All children are sized to the same size based
/// on [itemExtent].
///
/// By default, descendent texts are shown with [CupertinoTextThemeData.pickerTextStyle].
///
/// {@tool dartpad}
/// This example shows a [CupertinoPicker] that displays a list of fruits on a wheel for
/// selection.
///
/// ** See code in examples/api/lib/cupertino/picker/cupertino_picker.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListWheelScrollView], the generic widget backing this picker without
/// the iOS design specific chrome.
/// * <https://developer.apple.com/design/human-interface-guidelines/pickers/>
class CupertinoPicker extends StatefulWidget {
/// Creates a picker from a concrete list of children.
///
/// The [itemExtent] must be greater than zero.
///
/// The [backgroundColor] defaults to null, which disables background painting entirely.
/// (i.e. the picker is going to have a completely transparent background), to match
/// the native UIPicker and UIDatePicker. Also, if it has transparency, no gradient
/// effect will be rendered.
///
/// The [scrollController] argument can be used to specify a custom
/// [FixedExtentScrollController] for programmatically reading or changing
/// the current picker index or for selecting an initial index value.
///
/// The [looping] argument decides whether the child list loops and can be
/// scrolled infinitely. If set to true, scrolling past the end of the list
/// will loop the list back to the beginning. If set to false, the list will
/// stop scrolling when you reach the end or the beginning.
CupertinoPicker({
super.key,
this.diameterRatio = _kDefaultDiameterRatio,
this.backgroundColor,
this.offAxisFraction = 0.0,
this.useMagnifier = false,
this.magnification = 1.0,
this.scrollController,
this.squeeze = _kSqueeze,
required this.itemExtent,
required this.onSelectedItemChanged,
required List<Widget> children,
this.selectionOverlay = const CupertinoPickerDefaultSelectionOverlay(),
bool looping = false,
}) : assert(diameterRatio > 0.0, RenderListWheelViewport.diameterRatioZeroMessage),
assert(magnification > 0),
assert(itemExtent > 0),
assert(squeeze > 0),
childDelegate = looping
? ListWheelChildLoopingListDelegate(children: children)
: ListWheelChildListDelegate(children: children);
/// Creates a picker from an [IndexedWidgetBuilder] callback where the builder
/// is dynamically invoked during layout.
///
/// A child is lazily created when it starts becoming visible in the viewport.
/// All of the children provided by the builder are cached and reused, so
/// normally the builder is only called once for each index (except when
/// rebuilding - the cache is cleared).
///
/// The [childCount] argument reflects the number of children that will be
/// provided by the [itemBuilder].
/// {@macro flutter.widgets.ListWheelChildBuilderDelegate.childCount}
///
/// The [itemExtent] argument must be positive.
///
/// The [backgroundColor] defaults to null, which disables background painting entirely.
/// (i.e. the picker is going to have a completely transparent background), to match
/// the native UIPicker and UIDatePicker.
CupertinoPicker.builder({
super.key,
this.diameterRatio = _kDefaultDiameterRatio,
this.backgroundColor,
this.offAxisFraction = 0.0,
this.useMagnifier = false,
this.magnification = 1.0,
this.scrollController,
this.squeeze = _kSqueeze,
required this.itemExtent,
required this.onSelectedItemChanged,
required NullableIndexedWidgetBuilder itemBuilder,
int? childCount,
this.selectionOverlay = const CupertinoPickerDefaultSelectionOverlay(),
}) : assert(diameterRatio > 0.0, RenderListWheelViewport.diameterRatioZeroMessage),
assert(magnification > 0),
assert(itemExtent > 0),
assert(squeeze > 0),
childDelegate = ListWheelChildBuilderDelegate(builder: itemBuilder, childCount: childCount);
/// Relative ratio between this picker's height and the simulated cylinder's diameter.
///
/// Smaller values creates more pronounced curvatures in the scrollable wheel.
///
/// For more details, see [ListWheelScrollView.diameterRatio].
///
/// Defaults to 1.1 to visually mimic iOS.
final double diameterRatio;
/// Background color behind the children.
///
/// Defaults to null, which disables background painting entirely.
/// (i.e. the picker is going to have a completely transparent background), to match
/// the native UIPicker and UIDatePicker.
///
/// Any alpha value less 255 (fully opaque) will cause the removal of the
/// wheel list edge fade gradient from rendering of the widget.
final Color? backgroundColor;
/// {@macro flutter.rendering.RenderListWheelViewport.offAxisFraction}
final double offAxisFraction;
/// {@macro flutter.rendering.RenderListWheelViewport.useMagnifier}
final bool useMagnifier;
/// {@macro flutter.rendering.RenderListWheelViewport.magnification}
final double magnification;
/// A [FixedExtentScrollController] to read and control the current item, and
/// to set the initial item.
///
/// If null, an implicit one will be created internally.
final FixedExtentScrollController? scrollController;
/// {@template flutter.cupertino.picker.itemExtent}
/// The uniform height of all children.
///
/// All children will be given the [BoxConstraints] to match this exact
/// height. Must be a positive value.
/// {@endtemplate}
final double itemExtent;
/// {@macro flutter.rendering.RenderListWheelViewport.squeeze}
///
/// Defaults to `1.45` to visually mimic iOS.
final double squeeze;
/// An option callback when the currently centered item changes.
///
/// Value changes when the item closest to the center changes.
///
/// This can be called during scrolls and during ballistic flings. To get the
/// value only when the scrolling settles, use a [NotificationListener],
/// listen for [ScrollEndNotification] and read its [FixedExtentMetrics].
final ValueChanged<int>? onSelectedItemChanged;
/// A delegate that lazily instantiates children.
final ListWheelChildDelegate childDelegate;
/// A widget overlaid on the picker to highlight the currently selected entry.
///
/// The [selectionOverlay] widget drawn above the [CupertinoPicker]'s picker
/// wheel.
/// It is vertically centered in the picker and is constrained to have the
/// same height as the center row.
///
/// If unspecified, it defaults to a [CupertinoPickerDefaultSelectionOverlay]
/// which is a gray rounded rectangle overlay in iOS 14 style.
/// This property can be set to null to remove the overlay.
final Widget? selectionOverlay;
@override
State<StatefulWidget> createState() => _CupertinoPickerState();
}
class _CupertinoPickerState extends State<CupertinoPicker> {
int? _lastHapticIndex;
FixedExtentScrollController? _controller;
@override
void initState() {
super.initState();
if (widget.scrollController == null) {
_controller = FixedExtentScrollController();
}
}
@override
void didUpdateWidget(CupertinoPicker oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.scrollController != null && oldWidget.scrollController == null) {
_controller?.dispose();
_controller = null;
} else if (widget.scrollController == null && oldWidget.scrollController != null) {
assert(_controller == null);
_controller = FixedExtentScrollController();
}
}
@override
void dispose() {
_controller?.dispose();
super.dispose();
}
void _handleSelectedItemChanged(int index) {
// Only the haptic engine hardware on iOS devices would produce the
// intended effects.
final bool hasSuitableHapticHardware;
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
hasSuitableHapticHardware = true;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
hasSuitableHapticHardware = false;
}
if (hasSuitableHapticHardware && index != _lastHapticIndex) {
_lastHapticIndex = index;
HapticFeedback.selectionClick();
}
widget.onSelectedItemChanged?.call(index);
}
/// Draws the selectionOverlay.
Widget _buildSelectionOverlay(Widget selectionOverlay) {
final double height = widget.itemExtent * widget.magnification;
return IgnorePointer(
child: Center(
child: ConstrainedBox(
constraints: BoxConstraints.expand(
height: height,
),
child: selectionOverlay,
),
),
);
}
@override
Widget build(BuildContext context) {
final TextStyle textStyle = CupertinoTheme.of(context).textTheme.pickerTextStyle;
final Color? resolvedBackgroundColor = CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context);
assert(RenderListWheelViewport.defaultPerspective == _kDefaultPerspective);
final Widget result = DefaultTextStyle(
style: textStyle.copyWith(color: CupertinoDynamicColor.maybeResolve(textStyle.color, context)),
child: Stack(
children: <Widget>[
Positioned.fill(
child: _CupertinoPickerSemantics(
scrollController: widget.scrollController ?? _controller!,
child: ListWheelScrollView.useDelegate(
controller: widget.scrollController ?? _controller,
physics: const FixedExtentScrollPhysics(),
diameterRatio: widget.diameterRatio,
offAxisFraction: widget.offAxisFraction,
useMagnifier: widget.useMagnifier,
magnification: widget.magnification,
overAndUnderCenterOpacity: _kOverAndUnderCenterOpacity,
itemExtent: widget.itemExtent,
squeeze: widget.squeeze,
onSelectedItemChanged: _handleSelectedItemChanged,
childDelegate: widget.childDelegate,
),
),
),
if (widget.selectionOverlay != null)
_buildSelectionOverlay(widget.selectionOverlay!),
],
),
);
return DecoratedBox(
decoration: BoxDecoration(color: resolvedBackgroundColor),
child: result,
);
}
}
/// A default selection overlay for [CupertinoPicker]s.
///
/// It draws a gray rounded rectangle to match the picker visuals introduced in
/// iOS 14.
///
/// This widget is typically only used in [CupertinoPicker.selectionOverlay].
/// In an iOS 14 multi-column picker, the selection overlay is a single rounded
/// rectangle that spans the entire multi-column picker.
/// To achieve the same effect using [CupertinoPickerDefaultSelectionOverlay],
/// the additional margin and corner radii on the left or the right side can be
/// disabled by turning off [capStartEdge] and [capEndEdge], so this selection
/// overlay visually connects with selection overlays of adjoining
/// [CupertinoPicker]s (i.e., other "column"s).
///
/// See also:
///
/// * [CupertinoPicker], which uses this widget as its default [CupertinoPicker.selectionOverlay].
class CupertinoPickerDefaultSelectionOverlay extends StatelessWidget {
/// Creates an iOS 14 style selection overlay that highlights the magnified
/// area (or the currently selected item, depending on how you described it
/// elsewhere) of a [CupertinoPicker].
///
/// The [background] argument default value is
/// [CupertinoColors.tertiarySystemFill].
///
/// The [capStartEdge] and [capEndEdge] arguments decide whether to add a
/// default margin and use rounded corners on the left and right side of the
/// rectangular overlay, and they both default to true.
const CupertinoPickerDefaultSelectionOverlay({
super.key,
this.background = CupertinoColors.tertiarySystemFill,
this.capStartEdge = true,
this.capEndEdge = true,
});
/// Whether to use the default use rounded corners and margin on the start side.
final bool capStartEdge;
/// Whether to use the default use rounded corners and margin on the end side.
final bool capEndEdge;
/// The color to fill in the background of the [CupertinoPickerDefaultSelectionOverlay].
/// It Support for use [CupertinoDynamicColor].
///
/// Typically this should not be set to a fully opaque color, as the currently
/// selected item of the underlying [CupertinoPicker] should remain visible.
/// Defaults to [CupertinoColors.tertiarySystemFill].
final Color background;
/// Default margin of the 'SelectionOverlay'.
static const double _defaultSelectionOverlayHorizontalMargin = 9;
/// Default radius of the 'SelectionOverlay'.
static const double _defaultSelectionOverlayRadius = 8;
@override
Widget build(BuildContext context) {
const Radius radius = Radius.circular(_defaultSelectionOverlayRadius);
return Container(
margin: EdgeInsetsDirectional.only(
start: capStartEdge ? _defaultSelectionOverlayHorizontalMargin : 0,
end: capEndEdge ? _defaultSelectionOverlayHorizontalMargin : 0,
),
decoration: BoxDecoration(
borderRadius: BorderRadiusDirectional.horizontal(
start: capStartEdge ? radius : Radius.zero,
end: capEndEdge ? radius : Radius.zero,
),
color: CupertinoDynamicColor.resolve(background, context),
),
);
}
}
// Turns the scroll semantics of the ListView into a single adjustable semantics
// node. This is done by removing all of the child semantics of the scroll
// wheel and using the scroll indexes to look up the current, previous, and
// next semantic label. This label is then turned into the value of a new
// adjustable semantic node, with adjustment callbacks wired to move the
// scroll controller.
class _CupertinoPickerSemantics extends SingleChildRenderObjectWidget {
const _CupertinoPickerSemantics({
super.child,
required this.scrollController,
});
final FixedExtentScrollController scrollController;
@override
RenderObject createRenderObject(BuildContext context) {
assert(debugCheckHasDirectionality(context));
return _RenderCupertinoPickerSemantics(scrollController, Directionality.of(context));
}
@override
void updateRenderObject(BuildContext context, covariant _RenderCupertinoPickerSemantics renderObject) {
assert(debugCheckHasDirectionality(context));
renderObject
..textDirection = Directionality.of(context)
..controller = scrollController;
}
}
class _RenderCupertinoPickerSemantics extends RenderProxyBox {
_RenderCupertinoPickerSemantics(FixedExtentScrollController controller, this._textDirection) {
_updateController(null, controller);
}
FixedExtentScrollController get controller => _controller;
late FixedExtentScrollController _controller;
set controller(FixedExtentScrollController value) => _updateController(_controller, value);
// This method exists to allow controller to be non-null. It is only called with a null oldValue from constructor.
void _updateController(FixedExtentScrollController? oldValue, FixedExtentScrollController value) {
if (value == oldValue) {
return;
}
if (oldValue != null) {
oldValue.removeListener(_handleScrollUpdate);
} else {
_currentIndex = value.initialItem;
}
value.addListener(_handleScrollUpdate);
_controller = value;
}
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (textDirection == value) {
return;
}
_textDirection = value;
markNeedsSemanticsUpdate();
}
int _currentIndex = 0;
void _handleIncrease() {
controller.jumpToItem(_currentIndex + 1);
}
void _handleDecrease() {
controller.jumpToItem(_currentIndex - 1);
}
void _handleScrollUpdate() {
if (controller.selectedItem == _currentIndex) {
return;
}
_currentIndex = controller.selectedItem;
markNeedsSemanticsUpdate();
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.isSemanticBoundary = true;
config.textDirection = textDirection;
}
@override
void assembleSemanticsNode(SemanticsNode node, SemanticsConfiguration config, Iterable<SemanticsNode> children) {
if (children.isEmpty) {
return super.assembleSemanticsNode(node, config, children);
}
final SemanticsNode scrollable = children.first;
final Map<int, SemanticsNode> indexedChildren = <int, SemanticsNode>{};
scrollable.visitChildren((SemanticsNode child) {
assert(child.indexInParent != null);
indexedChildren[child.indexInParent!] = child;
return true;
});
if (indexedChildren[_currentIndex] == null) {
return node.updateWith(config: config);
}
config.value = indexedChildren[_currentIndex]!.label;
final SemanticsNode? previousChild = indexedChildren[_currentIndex - 1];
final SemanticsNode? nextChild = indexedChildren[_currentIndex + 1];
if (nextChild != null) {
config.increasedValue = nextChild.label;
config.onIncrease = _handleIncrease;
}
if (previousChild != null) {
config.decreasedValue = previousChild.label;
config.onDecrease = _handleDecrease;
}
node.updateWith(config: config);
}
@override
void dispose() {
super.dispose();
controller.removeListener(_handleScrollUpdate);
}
}
| flutter/packages/flutter/lib/src/cupertino/picker.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/picker.dart",
"repo_id": "flutter",
"token_count": 5985
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:math' as math show pi;
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart' show Brightness, clampDouble;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'text_selection_toolbar_button.dart';
import 'theme.dart';
// The radius of the toolbar RRect shape.
// Value extracted from https://developer.apple.com/design/resources/.
const Radius _kToolbarBorderRadius = Radius.circular(8.0);
// Vertical distance between the tip of the arrow and the line of text the arrow
// is pointing to. The value used here is eyeballed.
const double _kToolbarContentDistance = 8.0;
// The size of the arrow pointing to the anchor. Eyeballed value.
const Size _kToolbarArrowSize = Size(14.0, 7.0);
// Minimal padding from tip of the selection toolbar arrow to horizontal edges of the
// screen. Eyeballed value.
const double _kArrowScreenPadding = 26.0;
// The size and thickness of the chevron icon used for navigating between toolbar pages.
// Eyeballed values.
const double _kToolbarChevronSize = 10.0;
const double _kToolbarChevronThickness = 2.0;
// Color was measured from a screenshot of iOS 16.0.2
// TODO(LongCatIsLooong): https://github.com/flutter/flutter/issues/41507.
const CupertinoDynamicColor _kToolbarBackgroundColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFF6F6F6),
darkColor: Color(0xFF222222),
);
// Color was measured from a screenshot of iOS 16.0.2.
const CupertinoDynamicColor _kToolbarDividerColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFD6D6D6),
darkColor: Color(0xFF424242),
);
const CupertinoDynamicColor _kToolbarTextColor = CupertinoDynamicColor.withBrightness(
color: CupertinoColors.black,
darkColor: CupertinoColors.white,
);
const Duration _kToolbarTransitionDuration = Duration(milliseconds: 125);
/// The type for a Function that builds a toolbar's container with the given
/// child.
///
/// The anchor is provided in global coordinates.
///
/// See also:
///
/// * [CupertinoTextSelectionToolbar.toolbarBuilder], which is of this type.
/// * [TextSelectionToolbar.toolbarBuilder], which is similar, but for an
/// Material-style toolbar.
typedef CupertinoToolbarBuilder = Widget Function(
BuildContext context,
Offset anchorAbove,
Offset anchorBelow,
Widget child,
);
/// An iOS-style text selection toolbar.
///
/// Typically displays buttons for text manipulation, e.g. copying and pasting
/// text.
///
/// Tries to position itself above [anchorAbove], but if it doesn't fit, then
/// it positions itself below [anchorBelow].
///
/// If any children don't fit in the menu, an overflow menu will automatically
/// be created.
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar for the current
/// platform.
/// * [TextSelectionToolbar], which is similar, but builds an Android-style
/// toolbar.
class CupertinoTextSelectionToolbar extends StatelessWidget {
/// Creates an instance of CupertinoTextSelectionToolbar.
const CupertinoTextSelectionToolbar({
super.key,
required this.anchorAbove,
required this.anchorBelow,
required this.children,
this.toolbarBuilder = _defaultToolbarBuilder,
}) : assert(children.length > 0);
/// {@macro flutter.material.TextSelectionToolbar.anchorAbove}
final Offset anchorAbove;
/// {@macro flutter.material.TextSelectionToolbar.anchorBelow}
final Offset anchorBelow;
/// {@macro flutter.material.TextSelectionToolbar.children}
///
/// See also:
/// * [CupertinoTextSelectionToolbarButton], which builds a default
/// Cupertino-style text selection toolbar text button.
final List<Widget> children;
/// {@macro flutter.material.TextSelectionToolbar.toolbarBuilder}
///
/// The given anchor and isAbove can be used to position an arrow, as in the
/// default Cupertino toolbar.
final CupertinoToolbarBuilder toolbarBuilder;
/// Minimal padding from all edges of the selection toolbar to all edges of the
/// viewport.
///
/// See also:
///
/// * [SpellCheckSuggestionsToolbar], which uses this same value for its
/// padding from the edges of the viewport.
/// * [TextSelectionToolbar], which uses this same value as well.
static const double kToolbarScreenPadding = 8.0;
// Builds a toolbar just like the default iOS toolbar, with the right color
// background and a rounded cutout with an arrow.
static Widget _defaultToolbarBuilder(
BuildContext context,
Offset anchorAbove,
Offset anchorBelow,
Widget child,
) {
return _CupertinoTextSelectionToolbarShape(
anchorAbove: anchorAbove,
anchorBelow: anchorBelow,
shadowColor: CupertinoTheme.brightnessOf(context) == Brightness.light
? CupertinoColors.black.withOpacity(0.2)
: null,
child: ColoredBox(
color: _kToolbarBackgroundColor.resolveFrom(context),
child: child,
),
);
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
final EdgeInsets mediaQueryPadding = MediaQuery.paddingOf(context);
final double paddingAbove = mediaQueryPadding.top + kToolbarScreenPadding;
// The arrow, which points to the anchor, has some margin so it can't get
// too close to the horizontal edges of the screen.
final double leftMargin = _kArrowScreenPadding + mediaQueryPadding.left;
final double rightMargin = MediaQuery.sizeOf(context).width - mediaQueryPadding.right - _kArrowScreenPadding;
final Offset anchorAboveAdjusted = Offset(
clampDouble(anchorAbove.dx, leftMargin, rightMargin),
anchorAbove.dy - _kToolbarContentDistance - paddingAbove,
);
final Offset anchorBelowAdjusted = Offset(
clampDouble(anchorBelow.dx, leftMargin, rightMargin),
anchorBelow.dy + _kToolbarContentDistance - paddingAbove,
);
return Padding(
padding: EdgeInsets.fromLTRB(
kToolbarScreenPadding,
paddingAbove,
kToolbarScreenPadding,
kToolbarScreenPadding,
),
child: CustomSingleChildLayout(
delegate: TextSelectionToolbarLayoutDelegate(
anchorAbove: anchorAboveAdjusted,
anchorBelow: anchorBelowAdjusted,
),
child: _CupertinoTextSelectionToolbarContent(
anchorAbove: anchorAboveAdjusted,
anchorBelow: anchorBelowAdjusted,
toolbarBuilder: toolbarBuilder,
children: children,
),
),
);
}
}
// Clips the child so that it has the shape of the default iOS text selection
// toolbar, with rounded corners and an arrow pointing at the anchor.
//
// The anchor should be in global coordinates.
class _CupertinoTextSelectionToolbarShape extends SingleChildRenderObjectWidget {
const _CupertinoTextSelectionToolbarShape({
required Offset anchorAbove,
required Offset anchorBelow,
Color? shadowColor,
super.child,
}) : _anchorAbove = anchorAbove,
_anchorBelow = anchorBelow,
_shadowColor = shadowColor;
final Offset _anchorAbove;
final Offset _anchorBelow;
final Color? _shadowColor;
@override
_RenderCupertinoTextSelectionToolbarShape createRenderObject(BuildContext context) => _RenderCupertinoTextSelectionToolbarShape(
_anchorAbove,
_anchorBelow,
_shadowColor,
null,
);
@override
void updateRenderObject(BuildContext context, _RenderCupertinoTextSelectionToolbarShape renderObject) {
renderObject
..anchorAbove = _anchorAbove
..anchorBelow = _anchorBelow
..shadowColor = _shadowColor;
}
}
// Clips the child into the shape of the default iOS text selection toolbar.
//
// The shape is a rounded rectangle with a protruding arrow pointing at the
// given anchor in the direction indicated by isAbove.
//
// In order to allow the child to render itself independent of isAbove, its
// height is clipped on both the top and the bottom, leaving the arrow remaining
// on the necessary side.
class _RenderCupertinoTextSelectionToolbarShape extends RenderShiftedBox {
_RenderCupertinoTextSelectionToolbarShape(
this._anchorAbove,
this._anchorBelow,
this._shadowColor,
super.child,
);
@override
bool get isRepaintBoundary => true;
Offset get anchorAbove => _anchorAbove;
Offset _anchorAbove;
set anchorAbove(Offset value) {
if (value == _anchorAbove) {
return;
}
_anchorAbove = value;
markNeedsLayout();
}
Offset get anchorBelow => _anchorBelow;
Offset _anchorBelow;
set anchorBelow(Offset value) {
if (value == _anchorBelow) {
return;
}
_anchorBelow = value;
markNeedsLayout();
}
Color? get shadowColor => _shadowColor;
Color? _shadowColor;
set shadowColor(Color? value) {
if (value == _shadowColor) {
return;
}
_shadowColor = value;
markNeedsPaint();
}
bool get isAbove => anchorAbove.dy >= (child?.size.height ?? 0.0) - _kToolbarArrowSize.height * 2;
@override
void performLayout() {
final RenderBox? child = this.child;
if (child == null) {
return;
}
final BoxConstraints enforcedConstraint = BoxConstraints(
minWidth: _kToolbarArrowSize.width + _kToolbarBorderRadius.x * 2,
).enforce(constraints.loosen());
child.layout(enforcedConstraint, parentUsesSize: true);
// The buttons are padded on both top and bottom sufficiently to have
// the arrow clipped out of it on either side. By
// using this approach, the buttons don't need any special padding that
// depends on isAbove.
// The height of one arrow will be clipped off of the child, so adjust the
// size and position to remove that piece from the layout.
final BoxParentData childParentData = child.parentData! as BoxParentData;
childParentData.offset = Offset(
0.0,
isAbove ? -_kToolbarArrowSize.height : 0.0,
);
size = Size(
child.size.width,
child.size.height - _kToolbarArrowSize.height,
);
}
// Returns the RRect inside which the child is painted.
RRect _shapeRRect(RenderBox child) {
final Rect rect = Offset(0.0, _kToolbarArrowSize.height)
& Size(child.size.width, child.size.height - _kToolbarArrowSize.height * 2);
return RRect.fromRectAndRadius(rect, _kToolbarBorderRadius).scaleRadii();
}
// Adds the given `rrect` to the current `path`, starting from the last point
// in `path` and ends after the last corner of the rrect (closest corner to
// `startAngle` in the counterclockwise direction), without closing the path.
//
// The `startAngle` argument must be a multiple of pi / 2, with 0 being the
// positive half of the x-axis, and pi / 2 being the negative half of the
// y-axis.
//
// For instance, if `startAngle` equals pi/2 then this method draws a line
// segment to the bottom-left corner of `rrect` from the last point in `path`,
// and follows the `rrect` path clockwise until the bottom-right corner is
// added, then this method returns the mutated path without closing it.
static Path _addRRectToPath(Path path, RRect rrect, { required double startAngle }) {
const double halfPI = math.pi / 2;
assert(startAngle % halfPI == 0.0);
final Rect rect = rrect.outerRect;
final List<(Offset, Radius)> rrectCorners = <(Offset, Radius)>[
(rect.bottomRight, -rrect.brRadius),
(rect.bottomLeft, Radius.elliptical(rrect.blRadiusX, -rrect.blRadiusY)),
(rect.topLeft, rrect.tlRadius),
(rect.topRight, Radius.elliptical(-rrect.trRadiusX, rrect.trRadiusY)),
];
// Add the 4 corners to the path clockwise. Convert radians to quadrants
// to avoid fp arithmetics. The order is br -> bl -> tl -> tr if the starting
// angle is 0.
final int startQuadrantIndex = startAngle ~/ halfPI;
for (int i = startQuadrantIndex; i < rrectCorners.length + startQuadrantIndex; i += 1) {
final (Offset vertex, Radius rectCenterOffset) = rrectCorners[i % rrectCorners.length];
final Offset otherVertex = Offset(vertex.dx + 2 * rectCenterOffset.x, vertex.dy + 2 * rectCenterOffset.y);
final Rect rect = Rect.fromPoints(vertex, otherVertex);
path.arcTo(rect, halfPI * i, halfPI, false);
}
return path;
}
// The path is described in the toolbar child's coordinate system.
Path _clipPath(RenderBox child, RRect rrect) {
final Path path = Path();
// If there isn't enough width for the arrow + radii, ignore the arrow.
// Because of the constraints we gave children in performLayout, this should
// only happen if the parent isn't wide enough which should be very rare, and
// when that happens the arrow won't be too useful anyways.
if (_kToolbarBorderRadius.x * 2 + _kToolbarArrowSize.width > size.width) {
return path..addRRect(rrect);
}
final Offset localAnchor = globalToLocal(isAbove ? _anchorAbove : _anchorBelow);
final double arrowTipX = clampDouble(
localAnchor.dx,
_kToolbarBorderRadius.x + _kToolbarArrowSize.width / 2,
size.width - _kToolbarArrowSize.width / 2 - _kToolbarBorderRadius.x,
);
// Draw the path clockwise, starting from the beginning side of the arrow.
if (isAbove) {
final double arrowBaseY = child.size.height - _kToolbarArrowSize.height;
final double arrowTipY = child.size.height;
path
..moveTo(arrowTipX + _kToolbarArrowSize.width / 2, arrowBaseY) // right side of the arrow triangle
..lineTo(arrowTipX, arrowTipY) // The tip of the arrow
..lineTo(arrowTipX - _kToolbarArrowSize.width / 2, arrowBaseY); // left side of the arrow triangle
} else {
final double arrowBaseY = _kToolbarArrowSize.height;
const double arrowTipY = 0.0;
path
..moveTo(arrowTipX - _kToolbarArrowSize.width / 2, arrowBaseY) // right side of the arrow triangle
..lineTo(arrowTipX, arrowTipY) // The tip of the arrow
..lineTo(arrowTipX + _kToolbarArrowSize.width / 2, arrowBaseY); // left side of the arrow triangle
}
final double startAngle = isAbove ? math.pi / 2 : -math.pi / 2;
return _addRRectToPath(path, rrect, startAngle: startAngle)..close();
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderBox? child = this.child;
if (child == null) {
return;
}
final BoxParentData childParentData = child.parentData! as BoxParentData;
final RRect rrect = _shapeRRect(child);
final Path clipPath = _clipPath(child, rrect);
// If configured, paint the shadow beneath the shape.
if (_shadowColor != null) {
final BoxShadow boxShadow = BoxShadow(
color: _shadowColor!,
blurRadius: 15.0,
);
final RRect shadowRRect = RRect.fromLTRBR(
rrect.left,
rrect.top,
rrect.right,
rrect.bottom + _kToolbarArrowSize.height,
_kToolbarBorderRadius,
).shift(offset + childParentData.offset + boxShadow.offset);
context.canvas.drawRRect(shadowRRect, boxShadow.toPaint());
}
_clipPathLayer.layer = context.pushClipPath(
needsCompositing,
offset + childParentData.offset,
Offset.zero & child.size,
clipPath,
(PaintingContext innerContext, Offset innerOffset) => innerContext.paintChild(child, innerOffset),
oldLayer: _clipPathLayer.layer,
);
}
final LayerHandle<ClipPathLayer> _clipPathLayer = LayerHandle<ClipPathLayer>();
Paint? _debugPaint;
@override
void dispose() {
_clipPathLayer.layer = null;
super.dispose();
}
@override
void debugPaintSize(PaintingContext context, Offset offset) {
assert(() {
final RenderBox? child = this.child;
if (child == null) {
return true;
}
final ui.Paint debugPaint = _debugPaint ??= Paint()
..shader = ui.Gradient.linear(
Offset.zero,
const Offset(10.0, 10.0),
const <Color>[Color(0x00000000), Color(0xFFFF00FF), Color(0xFFFF00FF), Color(0x00000000)],
const <double>[0.25, 0.25, 0.75, 0.75],
TileMode.repeated,
)
..strokeWidth = 2.0
..style = PaintingStyle.stroke;
final BoxParentData childParentData = child.parentData! as BoxParentData;
final Path clipPath = _clipPath(child, _shapeRRect(child));
context.canvas.drawPath(clipPath.shift(offset + childParentData.offset), debugPaint);
return true;
}());
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final RenderBox? child = this.child;
if (child == null) {
return false;
}
// Positions outside of the clipped area of the child are not counted as
// hits.
final BoxParentData childParentData = child.parentData! as BoxParentData;
final Rect hitBox = Rect.fromLTWH(
childParentData.offset.dx,
childParentData.offset.dy + _kToolbarArrowSize.height,
child.size.width,
child.size.height - _kToolbarArrowSize.height * 2,
);
if (!hitBox.contains(position)) {
return false;
}
return super.hitTestChildren(result, position: position);
}
}
// A toolbar containing the given children. If they overflow the width
// available, then the menu will be paginated with the overflowing children
// displayed on subsequent pages.
//
// The anchor should be in global coordinates.
class _CupertinoTextSelectionToolbarContent extends StatefulWidget {
const _CupertinoTextSelectionToolbarContent({
required this.anchorAbove,
required this.anchorBelow,
required this.toolbarBuilder,
required this.children,
}) : assert(children.length > 0);
final Offset anchorAbove;
final Offset anchorBelow;
final List<Widget> children;
final CupertinoToolbarBuilder toolbarBuilder;
@override
_CupertinoTextSelectionToolbarContentState createState() => _CupertinoTextSelectionToolbarContentState();
}
class _CupertinoTextSelectionToolbarContentState extends State<_CupertinoTextSelectionToolbarContent> with TickerProviderStateMixin {
// Controls the fading of the buttons within the menu during page transitions.
late AnimationController _controller;
int? _nextPage;
int _page = 0;
final GlobalKey _toolbarItemsKey = GlobalKey();
void _onHorizontalDragEnd(DragEndDetails details) {
final double? velocity = details.primaryVelocity;
if (velocity != null && velocity != 0) {
if (velocity > 0) {
_handlePreviousPage();
} else {
_handleNextPage();
}
}
}
void _handleNextPage() {
final RenderBox? renderToolbar =
_toolbarItemsKey.currentContext?.findRenderObject() as RenderBox?;
if (renderToolbar is _RenderCupertinoTextSelectionToolbarItems && renderToolbar.hasNextPage) {
_controller.reverse();
_controller.addStatusListener(_statusListener);
_nextPage = _page + 1;
}
}
void _handlePreviousPage() {
final RenderBox? renderToolbar =
_toolbarItemsKey.currentContext?.findRenderObject() as RenderBox?;
if (renderToolbar is _RenderCupertinoTextSelectionToolbarItems && renderToolbar.hasPreviousPage) {
_controller.reverse();
_controller.addStatusListener(_statusListener);
_nextPage = _page - 1;
}
}
void _statusListener(AnimationStatus status) {
if (status != AnimationStatus.dismissed) {
return;
}
setState(() {
_page = _nextPage!;
_nextPage = null;
});
_controller.forward();
_controller.removeStatusListener(_statusListener);
}
@override
void initState() {
super.initState();
_controller = AnimationController(
value: 1.0,
vsync: this,
// This was eyeballed on a physical iOS device running iOS 13.
duration: _kToolbarTransitionDuration,
);
}
@override
void didUpdateWidget(_CupertinoTextSelectionToolbarContent oldWidget) {
super.didUpdateWidget(oldWidget);
// If the children are changing, the current page should be reset.
if (widget.children != oldWidget.children) {
_page = 0;
_nextPage = null;
_controller.forward();
_controller.removeStatusListener(_statusListener);
}
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final Color chevronColor = _kToolbarTextColor.resolveFrom(context);
// Wrap the children and the chevron painters in Center with widthFactor
// and heightFactor of 1.0 so _CupertinoTextSelectionToolbarItems can get
// the natural size of the buttons and then expand vertically as needed.
final Widget backButton = Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: CupertinoTextSelectionToolbarButton(
onPressed: _handlePreviousPage,
child: IgnorePointer(
child: CustomPaint(
painter: _LeftCupertinoChevronPainter(color: chevronColor),
size: const Size.square(_kToolbarChevronSize),
),
),
),
);
final Widget nextButton = Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: CupertinoTextSelectionToolbarButton(
onPressed: _handleNextPage,
child: IgnorePointer(
child: CustomPaint(
painter: _RightCupertinoChevronPainter(color: chevronColor),
size: const Size.square(_kToolbarChevronSize),
),
),
),
);
final List<Widget> children = widget.children.map((Widget child) {
return Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: child,
);
}).toList();
return widget.toolbarBuilder(context, widget.anchorAbove, widget.anchorBelow, FadeTransition(
opacity: _controller,
child: AnimatedSize(
duration: _kToolbarTransitionDuration,
curve: Curves.decelerate,
child: GestureDetector(
onHorizontalDragEnd: _onHorizontalDragEnd,
child: _CupertinoTextSelectionToolbarItems(
key: _toolbarItemsKey,
page: _page,
backButton: backButton,
dividerColor: _kToolbarDividerColor.resolveFrom(context),
dividerWidth: 1.0 / MediaQuery.devicePixelRatioOf(context),
nextButton: nextButton,
children: children,
),
),
),
));
}
}
// These classes help to test the chevrons. As _CupertinoChevronPainter must be
// private, it's possible to check the runtimeType of each chevron to know if
// they should be pointing left or right.
class _LeftCupertinoChevronPainter extends _CupertinoChevronPainter {
_LeftCupertinoChevronPainter({required super.color}) : super(isLeft: true);
}
class _RightCupertinoChevronPainter extends _CupertinoChevronPainter {
_RightCupertinoChevronPainter({required super.color}) : super(isLeft: false);
}
abstract class _CupertinoChevronPainter extends CustomPainter {
_CupertinoChevronPainter({
required this.color,
required this.isLeft,
});
final Color color;
/// If this is true the chevron will point left, else it will point right.
final bool isLeft;
@override
void paint(Canvas canvas, Size size) {
assert(size.height == size.width, 'size must have the same height and width: $size');
final double iconSize = size.height;
// The chevron is half of a square rotated 45˚, so it needs a margin of 1/4
// its size on each side to be centered horizontally.
//
// If pointing left, it means the left half of a square is being used and
// the offset is positive. If pointing right, the right half is being used
// and the offset is negative.
final Offset centerOffset = Offset(
iconSize / 4 * (isLeft ? 1 : -1),
0,
);
final Offset firstPoint = Offset(iconSize / 2, 0) + centerOffset;
final Offset middlePoint = Offset(isLeft ? 0 : iconSize, iconSize / 2) + centerOffset;
final Offset lowerPoint = Offset(iconSize / 2, iconSize) + centerOffset;
final Paint paint = Paint()
..color = color
..style = PaintingStyle.stroke
..strokeWidth = _kToolbarChevronThickness
..strokeCap = StrokeCap.round
..strokeJoin = StrokeJoin.round;
// `drawLine` is used here because it's testable. When using `drawPath`,
// there's no way to test that the chevron points to the correct side.
canvas.drawLine(firstPoint, middlePoint, paint);
canvas.drawLine(middlePoint, lowerPoint, paint);
}
@override
bool shouldRepaint(_CupertinoChevronPainter oldDelegate) =>
oldDelegate.color != color || oldDelegate.isLeft != isLeft;
}
// The custom RenderObjectWidget that, together with
// _RenderCupertinoTextSelectionToolbarItems and
// _CupertinoTextSelectionToolbarItemsElement, paginates the menu items.
class _CupertinoTextSelectionToolbarItems extends RenderObjectWidget {
_CupertinoTextSelectionToolbarItems({
super.key,
required this.page,
required this.children,
required this.backButton,
required this.dividerColor,
required this.dividerWidth,
required this.nextButton,
}) : assert(children.isNotEmpty);
final Widget backButton;
final List<Widget> children;
final Color dividerColor;
final double dividerWidth;
final Widget nextButton;
final int page;
@override
_RenderCupertinoTextSelectionToolbarItems createRenderObject(BuildContext context) {
return _RenderCupertinoTextSelectionToolbarItems(
dividerColor: dividerColor,
dividerWidth: dividerWidth,
page: page,
);
}
@override
void updateRenderObject(BuildContext context, _RenderCupertinoTextSelectionToolbarItems renderObject) {
renderObject
..page = page
..dividerColor = dividerColor
..dividerWidth = dividerWidth;
}
@override
_CupertinoTextSelectionToolbarItemsElement createElement() => _CupertinoTextSelectionToolbarItemsElement(this);
}
// The custom RenderObjectElement that helps paginate the menu items.
class _CupertinoTextSelectionToolbarItemsElement extends RenderObjectElement {
_CupertinoTextSelectionToolbarItemsElement(
_CupertinoTextSelectionToolbarItems super.widget,
);
late List<Element> _children;
final Map<_CupertinoTextSelectionToolbarItemsSlot, Element> slotToChild = <_CupertinoTextSelectionToolbarItemsSlot, Element>{};
// We keep a set of forgotten children to avoid O(n^2) work walking _children
// repeatedly to remove children.
final Set<Element> _forgottenChildren = HashSet<Element>();
@override
_RenderCupertinoTextSelectionToolbarItems get renderObject => super.renderObject as _RenderCupertinoTextSelectionToolbarItems;
void _updateRenderObject(RenderBox? child, _CupertinoTextSelectionToolbarItemsSlot slot) {
switch (slot) {
case _CupertinoTextSelectionToolbarItemsSlot.backButton:
renderObject.backButton = child;
case _CupertinoTextSelectionToolbarItemsSlot.nextButton:
renderObject.nextButton = child;
}
}
@override
void insertRenderObjectChild(RenderObject child, Object? slot) {
if (slot is _CupertinoTextSelectionToolbarItemsSlot) {
assert(child is RenderBox);
_updateRenderObject(child as RenderBox, slot);
assert(renderObject.slottedChildren.containsKey(slot));
return;
}
if (slot is IndexedSlot) {
assert(renderObject.debugValidateChild(child));
renderObject.insert(child as RenderBox, after: slot.value?.renderObject as RenderBox?);
return;
}
assert(false, 'slot must be _CupertinoTextSelectionToolbarItemsSlot or IndexedSlot');
}
// This is not reachable for children that don't have an IndexedSlot.
@override
void moveRenderObjectChild(RenderObject child, IndexedSlot<Element> oldSlot, IndexedSlot<Element> newSlot) {
assert(child.parent == renderObject);
renderObject.move(child as RenderBox, after: newSlot.value.renderObject as RenderBox?);
}
static bool _shouldPaint(Element child) {
return (child.renderObject!.parentData! as ToolbarItemsParentData).shouldPaint;
}
@override
void removeRenderObjectChild(RenderObject child, Object? slot) {
// Check if the child is in a slot.
if (slot is _CupertinoTextSelectionToolbarItemsSlot) {
assert(child is RenderBox);
assert(renderObject.slottedChildren.containsKey(slot));
_updateRenderObject(null, slot);
assert(!renderObject.slottedChildren.containsKey(slot));
return;
}
// Otherwise look for it in the list of children.
assert(slot is IndexedSlot);
assert(child.parent == renderObject);
renderObject.remove(child as RenderBox);
}
@override
void visitChildren(ElementVisitor visitor) {
slotToChild.values.forEach(visitor);
for (final Element child in _children) {
if (!_forgottenChildren.contains(child)) {
visitor(child);
}
}
}
@override
void forgetChild(Element child) {
assert(slotToChild.containsValue(child) || _children.contains(child));
assert(!_forgottenChildren.contains(child));
// Handle forgetting a child in children or in a slot.
if (slotToChild.containsKey(child.slot)) {
final _CupertinoTextSelectionToolbarItemsSlot slot = child.slot! as _CupertinoTextSelectionToolbarItemsSlot;
slotToChild.remove(slot);
} else {
_forgottenChildren.add(child);
}
super.forgetChild(child);
}
// Mount or update slotted child.
void _mountChild(Widget widget, _CupertinoTextSelectionToolbarItemsSlot slot) {
final Element? oldChild = slotToChild[slot];
final Element? newChild = updateChild(oldChild, widget, slot);
if (oldChild != null) {
slotToChild.remove(slot);
}
if (newChild != null) {
slotToChild[slot] = newChild;
}
}
@override
void mount(Element? parent, Object? newSlot) {
super.mount(parent, newSlot);
// Mount slotted children.
final _CupertinoTextSelectionToolbarItems toolbarItems = widget as _CupertinoTextSelectionToolbarItems;
_mountChild(toolbarItems.backButton, _CupertinoTextSelectionToolbarItemsSlot.backButton);
_mountChild(toolbarItems.nextButton, _CupertinoTextSelectionToolbarItemsSlot.nextButton);
// Mount list children.
_children = List<Element>.filled(toolbarItems.children.length, _NullElement.instance);
Element? previousChild;
for (int i = 0; i < _children.length; i += 1) {
final Element newChild = inflateWidget(toolbarItems.children[i], IndexedSlot<Element?>(i, previousChild));
_children[i] = newChild;
previousChild = newChild;
}
}
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
// Visit slot children.
for (final Element child in slotToChild.values) {
if (_shouldPaint(child) && !_forgottenChildren.contains(child)) {
visitor(child);
}
}
// Visit list children.
_children
.where((Element child) => !_forgottenChildren.contains(child) && _shouldPaint(child))
.forEach(visitor);
}
@override
void update(_CupertinoTextSelectionToolbarItems newWidget) {
super.update(newWidget);
assert(widget == newWidget);
// Update slotted children.
final _CupertinoTextSelectionToolbarItems toolbarItems = widget as _CupertinoTextSelectionToolbarItems;
_mountChild(toolbarItems.backButton, _CupertinoTextSelectionToolbarItemsSlot.backButton);
_mountChild(toolbarItems.nextButton, _CupertinoTextSelectionToolbarItemsSlot.nextButton);
// Update list children.
_children = updateChildren(_children, toolbarItems.children, forgottenChildren: _forgottenChildren);
_forgottenChildren.clear();
}
}
// The custom RenderBox that helps paginate the menu items.
class _RenderCupertinoTextSelectionToolbarItems extends RenderBox with ContainerRenderObjectMixin<RenderBox, ToolbarItemsParentData>, RenderBoxContainerDefaultsMixin<RenderBox, ToolbarItemsParentData> {
_RenderCupertinoTextSelectionToolbarItems({
required Color dividerColor,
required double dividerWidth,
required int page,
}) : _dividerColor = dividerColor,
_dividerWidth = dividerWidth,
_page = page,
super();
final Map<_CupertinoTextSelectionToolbarItemsSlot, RenderBox> slottedChildren = <_CupertinoTextSelectionToolbarItemsSlot, RenderBox>{};
late bool hasNextPage;
late bool hasPreviousPage;
RenderBox? _updateChild(RenderBox? oldChild, RenderBox? newChild, _CupertinoTextSelectionToolbarItemsSlot slot) {
if (oldChild != null) {
dropChild(oldChild);
slottedChildren.remove(slot);
}
if (newChild != null) {
slottedChildren[slot] = newChild;
adoptChild(newChild);
}
return newChild;
}
int _page;
int get page => _page;
set page(int value) {
if (value == _page) {
return;
}
_page = value;
markNeedsLayout();
}
Color _dividerColor;
Color get dividerColor => _dividerColor;
set dividerColor(Color value) {
if (value == _dividerColor) {
return;
}
_dividerColor = value;
markNeedsLayout();
}
double _dividerWidth;
double get dividerWidth => _dividerWidth;
set dividerWidth(double value) {
if (value == _dividerWidth) {
return;
}
_dividerWidth = value;
markNeedsLayout();
}
RenderBox? _backButton;
RenderBox? get backButton => _backButton;
set backButton(RenderBox? value) {
_backButton = _updateChild(_backButton, value, _CupertinoTextSelectionToolbarItemsSlot.backButton);
}
RenderBox? _nextButton;
RenderBox? get nextButton => _nextButton;
set nextButton(RenderBox? value) {
_nextButton = _updateChild(_nextButton, value, _CupertinoTextSelectionToolbarItemsSlot.nextButton);
}
@override
void performLayout() {
if (firstChild == null) {
size = constraints.smallest;
return;
}
// First pass: determine the height of the tallest child.
double greatestHeight = 0.0;
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final double childHeight = child.getMaxIntrinsicHeight(constraints.maxWidth);
if (childHeight > greatestHeight) {
greatestHeight = childHeight;
}
});
// Layout slotted children.
final BoxConstraints slottedConstraints = BoxConstraints(
maxWidth: constraints.maxWidth,
minHeight: greatestHeight,
maxHeight: greatestHeight,
);
_backButton!.layout(slottedConstraints, parentUsesSize: true);
_nextButton!.layout(slottedConstraints, parentUsesSize: true);
final double subsequentPageButtonsWidth = _backButton!.size.width + _nextButton!.size.width;
double currentButtonPosition = 0.0;
late double toolbarWidth; // The width of the whole widget.
int currentPage = 0;
int i = -1;
visitChildren((RenderObject renderObjectChild) {
i++;
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
childParentData.shouldPaint = false;
// Skip slotted children and children on pages after the visible page.
if (child == _backButton || child == _nextButton || currentPage > _page) {
return;
}
// If this is the last child on the first page, it's ok to fit without a forward button.
// Note childCount doesn't include slotted children which come before the list ones.
double paginationButtonsWidth = currentPage == 0
? i == childCount + 1 ? 0.0 : _nextButton!.size.width
: subsequentPageButtonsWidth;
// The width of the menu is set by the first page.
child.layout(
BoxConstraints(
maxWidth: constraints.maxWidth - paginationButtonsWidth,
minHeight: greatestHeight,
maxHeight: greatestHeight,
),
parentUsesSize: true,
);
// If this child causes the current page to overflow, move to the next
// page and relayout the child.
final double currentWidth = currentButtonPosition + paginationButtonsWidth + child.size.width;
if (currentWidth > constraints.maxWidth) {
currentPage++;
currentButtonPosition = _backButton!.size.width + dividerWidth;
paginationButtonsWidth = _backButton!.size.width + _nextButton!.size.width;
child.layout(
BoxConstraints(
maxWidth: constraints.maxWidth - paginationButtonsWidth,
minHeight: greatestHeight,
maxHeight: greatestHeight,
),
parentUsesSize: true,
);
}
childParentData.offset = Offset(currentButtonPosition, 0.0);
currentButtonPosition += child.size.width + dividerWidth;
childParentData.shouldPaint = currentPage == page;
if (currentPage == page) {
toolbarWidth = currentButtonPosition;
}
});
// It shouldn't be possible to navigate beyond the last page.
assert(page <= currentPage);
// Position page nav buttons.
if (currentPage > 0) {
final ToolbarItemsParentData nextButtonParentData = _nextButton!.parentData! as ToolbarItemsParentData;
final ToolbarItemsParentData backButtonParentData = _backButton!.parentData! as ToolbarItemsParentData;
// The forward button only shows when there's a page after this one.
if (page != currentPage) {
nextButtonParentData.offset = Offset(toolbarWidth, 0.0);
nextButtonParentData.shouldPaint = true;
toolbarWidth += nextButton!.size.width;
}
if (page > 0) {
backButtonParentData.offset = Offset.zero;
backButtonParentData.shouldPaint = true;
// No need to add the width of the back button to toolbarWidth here. It's
// already been taken care of when laying out the children to
// accommodate the back button.
}
} else {
// No divider for the next button when there's only one page.
toolbarWidth -= dividerWidth;
}
// Update previous/next page values so that we can check in the horizontal
// drag gesture callback if it's possible to navigate.
hasNextPage = page != currentPage;
hasPreviousPage = page > 0;
size = constraints.constrain(Size(toolbarWidth, greatestHeight));
}
@override
void paint(PaintingContext context, Offset offset) {
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
if (childParentData.shouldPaint) {
final Offset childOffset = childParentData.offset + offset;
context.paintChild(child, childOffset);
// backButton is a slotted child and is not in the children list, so its
// childParentData.nextSibling is null. So either when there's a
// nextSibling or when child is the backButton, draw a divider to the
// child's right.
if (childParentData.nextSibling != null || child == backButton) {
context.canvas.drawLine(
Offset(child.size.width, 0) + childOffset,
Offset(child.size.width, child.size.height) + childOffset,
Paint()..color = dividerColor,
);
}
}
});
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! ToolbarItemsParentData) {
child.parentData = ToolbarItemsParentData();
}
}
// Returns true if the single child is hit by the given position.
static bool hitTestChild(RenderBox? child, BoxHitTestResult result, { required Offset position }) {
if (child == null) {
return false;
}
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
if (!childParentData.shouldPaint) {
return false;
}
return result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child.hitTest(result, position: transformed);
},
);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
// Hit test list children.
RenderBox? child = lastChild;
while (child != null) {
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
// Don't hit test children that aren't shown.
if (!childParentData.shouldPaint) {
child = childParentData.previousSibling;
continue;
}
if (hitTestChild(child, result, position: position)) {
return true;
}
child = childParentData.previousSibling;
}
// Hit test slot children.
if (hitTestChild(backButton, result, position: position)) {
return true;
}
if (hitTestChild(nextButton, result, position: position)) {
return true;
}
return false;
}
@override
void attach(PipelineOwner owner) {
// Attach list children.
super.attach(owner);
// Attach slot children.
for (final RenderBox child in slottedChildren.values) {
child.attach(owner);
}
}
@override
void detach() {
// Detach list children.
super.detach();
// Detach slot children.
for (final RenderBox child in slottedChildren.values) {
child.detach();
}
}
@override
void redepthChildren() {
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
redepthChild(child);
});
}
@override
void visitChildren(RenderObjectVisitor visitor) {
// Visit the slotted children.
if (_backButton != null) {
visitor(_backButton!);
}
if (_nextButton != null) {
visitor(_nextButton!);
}
// Visit the list children.
super.visitChildren(visitor);
}
// Visit only the children that should be painted.
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
if (childParentData.shouldPaint) {
visitor(renderObjectChild);
}
});
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> value = <DiagnosticsNode>[];
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
if (child == backButton) {
value.add(child.toDiagnosticsNode(name: 'back button'));
} else if (child == nextButton) {
value.add(child.toDiagnosticsNode(name: 'next button'));
// List children.
} else {
value.add(child.toDiagnosticsNode(name: 'menu item'));
}
});
return value;
}
}
// The slots that can be occupied by widgets in
// _CupertinoTextSelectionToolbarItems, excluding the list of children.
enum _CupertinoTextSelectionToolbarItemsSlot {
backButton,
nextButton,
}
class _NullElement extends Element {
_NullElement() : super(const _NullWidget());
static _NullElement instance = _NullElement();
@override
bool get debugDoingBuild => throw UnimplementedError();
}
class _NullWidget extends Widget {
const _NullWidget();
@override
Element createElement() => throw UnimplementedError();
}
| flutter/packages/flutter/lib/src/cupertino/text_selection_toolbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/text_selection_toolbar.dart",
"repo_id": "flutter",
"token_count": 15274
} | 671 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:meta/meta.dart' show visibleForTesting;
/// Signature for callbacks passed to [LicenseRegistry.addLicense].
typedef LicenseEntryCollector = Stream<LicenseEntry> Function();
/// A string that represents one paragraph in a [LicenseEntry].
///
/// See [LicenseEntry.paragraphs].
class LicenseParagraph {
/// Creates a string for a license entry paragraph.
const LicenseParagraph(this.text, this.indent);
/// The text of the paragraph. Should not have any leading or trailing whitespace.
final String text;
/// How many steps of indentation the paragraph has.
///
/// * 0 means the paragraph is not indented.
/// * 1 means the paragraph is indented one unit of indentation.
/// * 2 means the paragraph is indented two units of indentation.
///
/// ...and so forth.
///
/// In addition, the special value [centeredIndent] can be used to indicate
/// that rather than being indented, the paragraph is centered.
final int indent; // can be set to centeredIndent
/// A constant that represents "centered" alignment for [indent].
static const int centeredIndent = -1;
}
/// A license that covers part of the application's software or assets, to show
/// in an interface such as the [LicensePage].
///
/// For optimal performance, [LicenseEntry] objects should only be created on
/// demand in [LicenseEntryCollector] callbacks passed to
/// [LicenseRegistry.addLicense].
abstract class LicenseEntry {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const LicenseEntry();
/// The names of the packages that this license entry applies to.
Iterable<String> get packages;
/// The paragraphs of the license, each as a [LicenseParagraph] consisting of
/// a string and some formatting information. Paragraphs can include newline
/// characters, but this is discouraged as it results in ugliness.
Iterable<LicenseParagraph> get paragraphs;
}
enum _LicenseEntryWithLineBreaksParserState {
beforeParagraph,
inParagraph,
}
/// Variant of [LicenseEntry] for licenses that separate paragraphs with blank
/// lines and that hard-wrap text within paragraphs. Lines that begin with one
/// or more space characters are also assumed to introduce new paragraphs,
/// unless they start with the same number of spaces as the previous line, in
/// which case it's assumed they are a continuation of an indented paragraph.
///
/// {@tool snippet}
///
/// For example, the BSD license in this format could be encoded as follows:
///
/// ```dart
/// void initMyLibrary() {
/// LicenseRegistry.addLicense(() => Stream<LicenseEntry>.value(
/// const LicenseEntryWithLineBreaks(<String>['my_library'], '''
/// Copyright 2016 The Sample Authors. All rights reserved.
///
/// Redistribution and use in source and binary forms, with or without
/// modification, are permitted provided that the following conditions are
/// met:
///
/// * Redistributions of source code must retain the above copyright
/// notice, this list of conditions and the following disclaimer.
/// * Redistributions in binary form must reproduce the above
/// copyright notice, this list of conditions and the following disclaimer
/// in the documentation and/or other materials provided with the
/// distribution.
/// * Neither the name of Example Inc. nor the names of its
/// contributors may be used to endorse or promote products derived from
/// this software without specific prior written permission.
///
/// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
/// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
/// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
/// A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
/// OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
/// SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
/// LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
/// DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
/// THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
/// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
/// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.''',
/// ),
/// ));
/// }
/// ```
/// {@end-tool}
///
/// This would result in a license with six [paragraphs], the third, fourth, and
/// fifth being indented one level.
///
/// ## Performance considerations
///
/// Computing the paragraphs is relatively expensive. Doing the work for one
/// license per frame is reasonable; doing more at the same time is ill-advised.
/// Consider doing all the work at once using [compute] to move the work to
/// another thread, or spreading the work across multiple frames using
/// [SchedulerBinding.scheduleTask].
class LicenseEntryWithLineBreaks extends LicenseEntry {
/// Create a license entry for a license whose text is hard-wrapped within
/// paragraphs and has paragraph breaks denoted by blank lines or with
/// indented text.
const LicenseEntryWithLineBreaks(this.packages, this.text);
@override
final List<String> packages;
/// The text of the license.
///
/// The text will be split into paragraphs according to the following
/// conventions:
///
/// * Lines starting with a different number of space characters than the
/// previous line start a new paragraph, with those spaces removed.
/// * Blank lines start a new paragraph.
/// * Other line breaks are replaced by a single space character.
/// * Leading spaces on a line are removed.
///
/// For each paragraph, the algorithm attempts (using some rough heuristics)
/// to identify how indented the paragraph is, or whether it is centered.
final String text;
@override
Iterable<LicenseParagraph> get paragraphs {
int lineStart = 0;
int currentPosition = 0;
int lastLineIndent = 0;
int currentLineIndent = 0;
int? currentParagraphIndentation;
_LicenseEntryWithLineBreaksParserState state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
final List<String> lines = <String>[];
final List<LicenseParagraph> result = <LicenseParagraph>[];
void addLine() {
assert(lineStart < currentPosition);
lines.add(text.substring(lineStart, currentPosition));
}
LicenseParagraph getParagraph() {
assert(lines.isNotEmpty);
assert(currentParagraphIndentation != null);
final LicenseParagraph result = LicenseParagraph(lines.join(' '), currentParagraphIndentation!);
assert(result.text.trimLeft() == result.text);
assert(result.text.isNotEmpty);
lines.clear();
return result;
}
while (currentPosition < text.length) {
switch (state) {
case _LicenseEntryWithLineBreaksParserState.beforeParagraph:
assert(lineStart == currentPosition);
switch (text[currentPosition]) {
case ' ':
lineStart = currentPosition + 1;
currentLineIndent += 1;
state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
case '\t':
lineStart = currentPosition + 1;
currentLineIndent += 8;
state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
case '\r':
case '\n':
case '\f':
if (lines.isNotEmpty) {
result.add(getParagraph());
}
if (text[currentPosition] == '\r' && currentPosition < text.length - 1
&& text[currentPosition + 1] == '\n') {
currentPosition += 1;
}
lastLineIndent = 0;
currentLineIndent = 0;
currentParagraphIndentation = null;
lineStart = currentPosition + 1;
state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
case '[':
// This is a bit of a hack for the LGPL 2.1, which does something like this:
//
// [this is a
// single paragraph]
//
// ...near the top.
currentLineIndent += 1;
continue startParagraph;
startParagraph:
default:
if (lines.isNotEmpty && currentLineIndent > lastLineIndent) {
result.add(getParagraph());
currentParagraphIndentation = null;
}
// The following is a wild heuristic for guessing the indentation level.
// It happens to work for common variants of the BSD and LGPL licenses.
if (currentParagraphIndentation == null) {
if (currentLineIndent > 10) {
currentParagraphIndentation = LicenseParagraph.centeredIndent;
} else {
currentParagraphIndentation = currentLineIndent ~/ 3;
}
}
state = _LicenseEntryWithLineBreaksParserState.inParagraph;
}
case _LicenseEntryWithLineBreaksParserState.inParagraph:
switch (text[currentPosition]) {
case '\n':
addLine();
lastLineIndent = currentLineIndent;
currentLineIndent = 0;
lineStart = currentPosition + 1;
state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
case '\f':
addLine();
result.add(getParagraph());
lastLineIndent = 0;
currentLineIndent = 0;
currentParagraphIndentation = null;
lineStart = currentPosition + 1;
state = _LicenseEntryWithLineBreaksParserState.beforeParagraph;
default:
state = _LicenseEntryWithLineBreaksParserState.inParagraph;
}
}
currentPosition += 1;
}
switch (state) {
case _LicenseEntryWithLineBreaksParserState.beforeParagraph:
if (lines.isNotEmpty) {
result.add(getParagraph());
}
case _LicenseEntryWithLineBreaksParserState.inParagraph:
addLine();
result.add(getParagraph());
}
return result;
}
}
/// A registry for packages to add licenses to, so that they can be displayed
/// together in an interface such as the [LicensePage].
///
/// Packages can register their licenses using [addLicense]. User interfaces
/// that wish to show all the licenses can obtain them by calling [licenses].
///
/// The flutter tool will automatically collect the contents of all the LICENSE
/// files found at the root of each package into a single LICENSE file in the
/// default asset bundle. Each license in that file is separated from the next
/// by a line of eighty hyphens (`-`), and begins with a list of package names
/// that the license applies to, one to a line, separated from the next by a
/// blank line. The `services` package registers a license collector that splits
/// that file and adds each entry to the registry.
///
/// The LICENSE files in each package can either consist of a single license, or
/// can be in the format described above. In the latter case, each component
/// license and list of package names is merged independently.
///
/// See also:
///
/// * [showAboutDialog], which shows a Material-style dialog with information
/// about the application, including a button that shows a [LicensePage] that
/// uses this API to select licenses to show.
/// * [AboutListTile], which is a widget that can be added to a [Drawer]. When
/// tapped it calls [showAboutDialog].
abstract final class LicenseRegistry {
static List<LicenseEntryCollector>? _collectors;
/// Adds licenses to the registry.
///
/// To avoid actually manipulating the licenses unless strictly necessary,
/// licenses are added by adding a closure that returns a list of
/// [LicenseEntry] objects. The closure is only called if [licenses] is itself
/// called; in normal operation, if the user does not request to see the
/// licenses, the closure will not be called.
static void addLicense(LicenseEntryCollector collector) {
_collectors ??= <LicenseEntryCollector>[];
_collectors!.add(collector);
}
/// Returns the licenses that have been registered.
///
/// Generating the list of licenses is expensive.
static Stream<LicenseEntry> get licenses {
if (_collectors == null) {
return const Stream<LicenseEntry>.empty();
}
late final StreamController<LicenseEntry> controller;
controller = StreamController<LicenseEntry>(
onListen: () async {
for (final LicenseEntryCollector collector in _collectors!) {
await controller.addStream(collector());
}
await controller.close();
},
);
return controller.stream;
}
/// Resets the internal state of [LicenseRegistry]. Intended for use in
/// testing.
@visibleForTesting
static void reset() {
_collectors = null;
}
}
| flutter/packages/flutter/lib/src/foundation/licenses.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/licenses.dart",
"repo_id": "flutter",
"token_count": 4347
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Modeled after Android's ViewConfiguration:
// https://github.com/android/platform_frameworks_base/blob/master/core/java/android/view/ViewConfiguration.java
/// The time that must elapse before a tap gesture sends onTapDown, if there's
/// any doubt that the gesture is a tap.
const Duration kPressTimeout = Duration(milliseconds: 100);
/// Maximum length of time between a tap down and a tap up for the gesture to be
/// considered a tap. (Currently not honored by the TapGestureRecognizer.)
// TODO(ianh): Remove this, or implement a hover-tap gesture recognizer which
// uses this.
const Duration kHoverTapTimeout = Duration(milliseconds: 150);
/// Maximum distance between the down and up pointers for a tap. (Currently not
/// honored by the [TapGestureRecognizer]; [PrimaryPointerGestureRecognizer],
/// which TapGestureRecognizer inherits from, uses [kTouchSlop].)
// TODO(ianh): Remove this or implement it correctly.
const double kHoverTapSlop = 20.0; // Logical pixels
/// The time before a long press gesture attempts to win.
const Duration kLongPressTimeout = Duration(milliseconds: 500);
/// The maximum time from the start of the first tap to the start of the second
/// tap in a double-tap gesture.
// In Android, this is actually the time from the first's up event
// to the second's down event, according to the ViewConfiguration docs.
const Duration kDoubleTapTimeout = Duration(milliseconds: 300);
/// The minimum time from the end of the first tap to the start of the second
/// tap in a double-tap gesture.
const Duration kDoubleTapMinTime = Duration(milliseconds: 40);
/// The maximum distance that the first touch in a double-tap gesture can travel
/// before deciding that it is not part of a double-tap gesture.
/// DoubleTapGestureRecognizer also restricts the second touch to this distance.
const double kDoubleTapTouchSlop = kTouchSlop; // Logical pixels
/// Distance between the initial position of the first touch and the start
/// position of a potential second touch for the second touch to be considered
/// the second touch of a double-tap gesture.
const double kDoubleTapSlop = 100.0; // Logical pixels
/// The time for which zoom controls (e.g. in a map interface) are to be
/// displayed on the screen, from the moment they were last requested.
const Duration kZoomControlsTimeout = Duration(milliseconds: 3000);
/// The distance a touch has to travel for the framework to be confident that
/// the gesture is a scroll gesture, or, inversely, the maximum distance that a
/// touch can travel before the framework becomes confident that it is not a
/// tap.
///
/// A total delta less than or equal to [kTouchSlop] is not considered to be a
/// drag, whereas if the delta is greater than [kTouchSlop] it is considered to
/// be a drag.
// This value was empirically derived. We started at 8.0 and increased it to
// 18.0 after getting complaints that it was too difficult to hit targets.
const double kTouchSlop = 18.0; // Logical pixels
/// The distance a touch has to travel for the framework to be confident that
/// the gesture is a paging gesture. (Currently not used, because paging uses a
/// regular drag gesture, which uses kTouchSlop.)
// TODO(ianh): Create variants of HorizontalDragGestureRecognizer et al for
// paging, which use this constant.
const double kPagingTouchSlop = kTouchSlop * 2.0; // Logical pixels
/// The distance a touch has to travel for the framework to be confident that
/// the gesture is a panning gesture.
const double kPanSlop = kTouchSlop * 2.0; // Logical pixels
/// The distance a touch has to travel for the framework to be confident that
/// the gesture is a scale gesture.
const double kScaleSlop = kTouchSlop; // Logical pixels
/// The margin around a dialog, popup menu, or other window-like widget inside
/// which we do not consider a tap to dismiss the widget. (Not currently used.)
// TODO(ianh): Make ModalBarrier support this.
const double kWindowTouchSlop = 16.0; // Logical pixels
/// The minimum velocity for a touch to consider that touch to trigger a fling
/// gesture.
// TODO(ianh): Make sure nobody has their own version of this.
const double kMinFlingVelocity = 50.0; // Logical pixels / second
// const Velocity kMinFlingVelocity = const Velocity(pixelsPerSecond: 50.0);
/// Drag gesture fling velocities are clipped to this value.
// TODO(ianh): Make sure nobody has their own version of this.
const double kMaxFlingVelocity = 8000.0; // Logical pixels / second
/// The maximum time from the start of the first tap to the start of the second
/// tap in a jump-tap gesture.
// TODO(ianh): Implement jump-tap gestures.
const Duration kJumpTapTimeout = Duration(milliseconds: 500);
/// Like [kTouchSlop], but for more precise pointers like mice and trackpads.
const double kPrecisePointerHitSlop = 1.0; // Logical pixels;
/// Like [kPanSlop], but for more precise pointers like mice and trackpads.
const double kPrecisePointerPanSlop = kPrecisePointerHitSlop * 2.0; // Logical pixels
/// Like [kScaleSlop], but for more precise pointers like mice and trackpads.
const double kPrecisePointerScaleSlop = kPrecisePointerHitSlop; // Logical pixels
| flutter/packages/flutter/lib/src/gestures/constants.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/constants.dart",
"repo_id": "flutter",
"token_count": 1416
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'events.dart';
export 'events.dart' show PointerSignalEvent;
/// The callback to register with a [PointerSignalResolver] to express
/// interest in a pointer signal event.
typedef PointerSignalResolvedCallback = void Function(PointerSignalEvent event);
bool _isSameEvent(PointerSignalEvent event1, PointerSignalEvent event2) {
return (event1.original ?? event1) == (event2.original ?? event2);
}
/// Mediates disputes over which listener should handle pointer signal events
/// when multiple listeners wish to handle those events.
///
/// Pointer signals (such as [PointerScrollEvent]) are immediate, so unlike
/// events that participate in the gesture arena, pointer signals always
/// resolve at the end of event dispatch. Yet if objects interested in handling
/// these signal events were to handle them directly, it would cause issues
/// such as multiple [Scrollable] widgets in the widget hierarchy responding
/// to the same mouse wheel event. Using this class, these events will only
/// be dispatched to the first registered handler, which will in turn
/// correspond to the widget that's deepest in the widget hierarchy.
///
/// To use this class, objects should register their event handler like so:
///
/// ```dart
/// void handleSignalEvent(PointerSignalEvent event) {
/// GestureBinding.instance.pointerSignalResolver.register(event, (PointerSignalEvent event) {
/// // handle the event...
/// });
/// }
/// ```
///
/// {@tool dartpad}
/// Here is an example that demonstrates the effect of not using the resolver
/// versus using it.
///
/// When this example is set to _not_ use the resolver, then triggering the
/// mouse wheel over the outer box will cause only the outer box to change
/// color, but triggering the mouse wheel over the inner box will cause _both_
/// the outer and the inner boxes to change color (because they're both
/// receiving the event).
///
/// When this example is set to _use_ the resolver, then only the box located
/// directly under the cursor will change color when the mouse wheel is
/// triggered.
///
/// ** See code in examples/api/lib/gestures/pointer_signal_resolver/pointer_signal_resolver.0.dart **
/// {@end-tool}
class PointerSignalResolver {
PointerSignalResolvedCallback? _firstRegisteredCallback;
PointerSignalEvent? _currentEvent;
/// Registers interest in handling [event].
///
/// This method may be called multiple times (typically from different parts
/// of the widget hierarchy) for the same `event`, with different `callback`s,
/// as the event is being dispatched across the tree. Once the dispatching is
/// complete, the [GestureBinding] calls [resolve], and the first registered
/// callback is called.
///
/// The `callback` is invoked with one argument, the `event`.
///
/// Once the [register] method has been called with a particular `event`, it
/// must not be called for other `event`s until after [resolve] has been
/// called. Only one event disambiguation can be in flight at a time. In
/// normal use this is achieved by only registering callbacks for an event as
/// it is actively being dispatched (for example, in
/// [Listener.onPointerSignal]).
///
/// See the documentation for the [PointerSignalResolver] class for an example
/// of using this method.
void register(PointerSignalEvent event, PointerSignalResolvedCallback callback) {
assert(_currentEvent == null || _isSameEvent(_currentEvent!, event));
if (_firstRegisteredCallback != null) {
return;
}
_currentEvent = event;
_firstRegisteredCallback = callback;
}
/// Resolves the event, calling the first registered callback if there was
/// one.
///
/// This is called by the [GestureBinding] after the framework has finished
/// dispatching the pointer signal event.
@pragma('vm:notify-debugger-on-exception')
void resolve(PointerSignalEvent event) {
if (_firstRegisteredCallback == null) {
assert(_currentEvent == null);
return;
}
assert(_isSameEvent(_currentEvent!, event));
try {
_firstRegisteredCallback!(_currentEvent!);
} catch (exception, stack) {
InformationCollector? collector;
assert(() {
collector = () => <DiagnosticsNode>[
DiagnosticsProperty<PointerSignalEvent>('Event', event, style: DiagnosticsTreeStyle.errorProperty),
];
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'gesture library',
context: ErrorDescription('while resolving a PointerSignalEvent'),
informationCollector: collector,
));
}
_firstRegisteredCallback = null;
_currentEvent = null;
}
}
| flutter/packages/flutter/lib/src/gestures/pointer_signal_resolver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/pointer_signal_resolver.dart",
"repo_id": "flutter",
"token_count": 1412
} | 674 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$add_event = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.190476190476,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(18.192636718749995, 24.891542968750002),
Offset(18.175135177837678, 24.768938846121223),
Offset(18.131762833609578, 24.290016731469834),
Offset(18.189398131863207, 23.129814344209493),
Offset(19.14691116775322, 20.688226165351878),
Offset(22.277081692306755, 18.381965826572245),
Offset(25.153850173498547, 18.208885778660576),
Offset(26.987657743646544, 18.810016196193768),
Offset(28.229748817157525, 19.528141401396265),
Offset(29.223774647225785, 20.19509758103034),
Offset(30.007739613111152, 20.830494962200966),
Offset(30.626964119061235, 21.41670003990219),
Offset(31.072971936868854, 21.941254300113602),
Offset(31.381936148538408, 22.388826086603757),
Offset(31.595631995844528, 22.75496752966676),
Offset(31.74362009537025, 23.04237991891895),
Offset(31.845995957897536, 23.25665695166987),
Offset(31.916063614634442, 23.404284579714346),
Offset(31.962512649081546, 23.491750839098724),
Offset(31.99086595053382, 23.525019467195833),
Offset(32.00558109744533, 23.528032986218715),
Offset(32.01015625, 23.52890625),
],
),
_PathCubicTo(
<Offset>[
Offset(18.192636718749995, 24.891542968750002),
Offset(18.175135177837678, 24.768938846121223),
Offset(18.131762833609578, 24.290016731469834),
Offset(18.189398131863207, 23.129814344209493),
Offset(19.14691116775322, 20.688226165351878),
Offset(22.277081692306755, 18.381965826572245),
Offset(25.153850173498547, 18.208885778660576),
Offset(26.987657743646544, 18.810016196193768),
Offset(28.229748817157525, 19.528141401396265),
Offset(29.223774647225785, 20.19509758103034),
Offset(30.007739613111152, 20.830494962200966),
Offset(30.626964119061235, 21.41670003990219),
Offset(31.072971936868854, 21.941254300113602),
Offset(31.381936148538408, 22.388826086603757),
Offset(31.595631995844528, 22.75496752966676),
Offset(31.74362009537025, 23.04237991891895),
Offset(31.845995957897536, 23.25665695166987),
Offset(31.916063614634442, 23.404284579714346),
Offset(31.962512649081546, 23.491750839098724),
Offset(31.99086595053382, 23.525019467195833),
Offset(32.00558109744533, 23.528032986218715),
Offset(32.01015625, 23.52890625),
],
<Offset>[
Offset(23.992636718749996, 24.891542968750002),
Offset(23.97384664920713, 24.89118970218503),
Offset(23.901054413892187, 24.886065942257186),
Offset(23.728661104347875, 24.849281041083934),
Offset(23.386158286870817, 24.64660763547994),
Offset(23.116959480006564, 24.121768211366486),
Offset(23.169707830342585, 23.691656086157646),
Offset(23.322360298939, 23.45580690452118),
Offset(23.48231873652916, 23.34351072659664),
Offset(23.629082531765302, 23.319033616339574),
Offset(23.74394094991015, 23.337067196646014),
Offset(23.829253355825543, 23.374789989582887),
Offset(23.889084353557546, 23.411693680396755),
Offset(23.930803165274646, 23.442370323371723),
Offset(23.960074268764437, 23.466992025443258),
Offset(23.980512394929132, 23.486331772113996),
Offset(23.994442088149636, 23.50119509189468),
Offset(24.003391328945682, 23.512265882811025),
Offset(24.008386840165617, 23.520115789343254),
Offset(24.010119497846063, 23.52522637938814),
Offset(24.010150449065325, 23.528032986218715),
Offset(24.01015625, 23.52890625),
],
<Offset>[
Offset(23.992636718749996, 24.891542968750002),
Offset(23.97384664920713, 24.89118970218503),
Offset(23.901054413892187, 24.886065942257186),
Offset(23.728661104347875, 24.849281041083934),
Offset(23.386158286870817, 24.64660763547994),
Offset(23.116959480006564, 24.121768211366486),
Offset(23.169707830342585, 23.691656086157646),
Offset(23.322360298939, 23.45580690452118),
Offset(23.48231873652916, 23.34351072659664),
Offset(23.629082531765302, 23.319033616339574),
Offset(23.74394094991015, 23.337067196646014),
Offset(23.829253355825543, 23.374789989582887),
Offset(23.889084353557546, 23.411693680396755),
Offset(23.930803165274646, 23.442370323371723),
Offset(23.960074268764437, 23.466992025443258),
Offset(23.980512394929132, 23.486331772113996),
Offset(23.994442088149636, 23.50119509189468),
Offset(24.003391328945682, 23.512265882811025),
Offset(24.008386840165617, 23.520115789343254),
Offset(24.010119497846063, 23.52522637938814),
Offset(24.010150449065325, 23.528032986218715),
Offset(24.01015625, 23.52890625),
],
),
_PathCubicTo(
<Offset>[
Offset(23.992636718749996, 24.891542968750002),
Offset(23.97384664920713, 24.89118970218503),
Offset(23.901054413892187, 24.886065942257186),
Offset(23.728661104347875, 24.849281041083934),
Offset(23.386158286870817, 24.64660763547994),
Offset(23.116959480006564, 24.121768211366486),
Offset(23.169707830342585, 23.691656086157646),
Offset(23.322360298939, 23.45580690452118),
Offset(23.48231873652916, 23.34351072659664),
Offset(23.629082531765302, 23.319033616339574),
Offset(23.74394094991015, 23.337067196646014),
Offset(23.829253355825543, 23.374789989582887),
Offset(23.889084353557546, 23.411693680396755),
Offset(23.930803165274646, 23.442370323371723),
Offset(23.960074268764437, 23.466992025443258),
Offset(23.980512394929132, 23.486331772113996),
Offset(23.994442088149636, 23.50119509189468),
Offset(24.003391328945682, 23.512265882811025),
Offset(24.008386840165617, 23.520115789343254),
Offset(24.010119497846063, 23.52522637938814),
Offset(24.010150449065325, 23.528032986218715),
Offset(24.01015625, 23.52890625),
],
<Offset>[
Offset(23.992636718749996, 19.091542968750005),
Offset(24.096097505270937, 19.09247823081558),
Offset(24.497103624679536, 19.11677436197458),
Offset(25.448127801222316, 19.310018068599263),
Offset(27.344539756998877, 20.407360516362342),
Offset(28.85676186480081, 23.281890423666677),
Offset(28.652478137839655, 25.675798429313613),
Offset(27.968151007266407, 27.121104349228723),
Offset(27.29768806172953, 28.090940807225007),
Offset(26.753018567074538, 28.913725731800056),
Offset(26.250513184355196, 29.600865859847012),
Offset(25.78734330550624, 30.17250075281858),
Offset(25.359523733840703, 30.595581263708063),
Offset(24.984347402042612, 30.89350330663548),
Offset(24.672098764540934, 31.102549752523352),
Offset(24.424464248124174, 31.249439472555114),
Offset(24.238980228374444, 31.352748961642575),
Offset(24.11137263204236, 31.424938168499786),
Offset(24.036751790410147, 31.47424159825918),
Offset(24.010326410038374, 31.505972832075898),
Offset(24.010150449065325, 31.523463634598716),
Offset(24.01015625, 31.52890625),
],
<Offset>[
Offset(23.992636718749996, 19.091542968750005),
Offset(24.096097505270937, 19.09247823081558),
Offset(24.497103624679536, 19.11677436197458),
Offset(25.448127801222316, 19.310018068599263),
Offset(27.344539756998877, 20.407360516362342),
Offset(28.85676186480081, 23.281890423666677),
Offset(28.652478137839655, 25.675798429313613),
Offset(27.968151007266407, 27.121104349228723),
Offset(27.29768806172953, 28.090940807225007),
Offset(26.753018567074538, 28.913725731800056),
Offset(26.250513184355196, 29.600865859847012),
Offset(25.78734330550624, 30.17250075281858),
Offset(25.359523733840703, 30.595581263708063),
Offset(24.984347402042612, 30.89350330663548),
Offset(24.672098764540934, 31.102549752523352),
Offset(24.424464248124174, 31.249439472555114),
Offset(24.238980228374444, 31.352748961642575),
Offset(24.11137263204236, 31.424938168499786),
Offset(24.036751790410147, 31.47424159825918),
Offset(24.010326410038374, 31.505972832075898),
Offset(24.010150449065325, 31.523463634598716),
Offset(24.01015625, 31.52890625),
],
),
_PathCubicTo(
<Offset>[
Offset(23.992636718749996, 19.091542968750005),
Offset(24.096097505270937, 19.09247823081558),
Offset(24.497103624679536, 19.11677436197458),
Offset(25.448127801222316, 19.310018068599263),
Offset(27.344539756998877, 20.407360516362342),
Offset(28.85676186480081, 23.281890423666677),
Offset(28.652478137839655, 25.675798429313613),
Offset(27.968151007266407, 27.121104349228723),
Offset(27.29768806172953, 28.090940807225007),
Offset(26.753018567074538, 28.913725731800056),
Offset(26.250513184355196, 29.600865859847012),
Offset(25.78734330550624, 30.17250075281858),
Offset(25.359523733840703, 30.595581263708063),
Offset(24.984347402042612, 30.89350330663548),
Offset(24.672098764540934, 31.102549752523352),
Offset(24.424464248124174, 31.249439472555114),
Offset(24.238980228374444, 31.352748961642575),
Offset(24.11137263204236, 31.424938168499786),
Offset(24.036751790410147, 31.47424159825918),
Offset(24.010326410038374, 31.505972832075898),
Offset(24.010150449065325, 31.523463634598716),
Offset(24.01015625, 31.52890625),
],
<Offset>[
Offset(18.19263671875, 19.091542968750005),
Offset(18.297386033901486, 18.970227374751772),
Offset(18.72781204439693, 18.52072515118723),
Offset(19.90886482873765, 17.59055137172482),
Offset(23.10529263788128, 16.448979046234285),
Offset(28.016884077101, 17.542088038872436),
Offset(30.636620480995617, 20.19302812181654),
Offset(31.63344845197395, 22.475313640901312),
Offset(32.0451181423579, 24.275571482024635),
Offset(32.34771068253502, 25.78978969649082),
Offset(32.5143118475562, 27.094293625401967),
Offset(32.58505406874193, 28.21441080313788),
Offset(32.54341131715201, 29.12514188342491),
Offset(32.43548038530638, 29.83995906986752),
Offset(32.307656491621024, 30.39052525674685),
Offset(32.18757194856529, 30.80548761936007),
Offset(32.09053409812234, 31.10821082141777),
Offset(32.024044917731125, 31.316956865403107),
Offset(31.990877599326076, 31.44587664801465),
Offset(31.991072862726128, 31.505765919883586),
Offset(32.00558109744533, 31.523463634598716),
Offset(32.01015625, 31.52890625),
],
<Offset>[
Offset(18.19263671875, 19.091542968750005),
Offset(18.297386033901486, 18.970227374751772),
Offset(18.72781204439693, 18.52072515118723),
Offset(19.90886482873765, 17.59055137172482),
Offset(23.10529263788128, 16.448979046234285),
Offset(28.016884077101, 17.542088038872436),
Offset(30.636620480995617, 20.19302812181654),
Offset(31.63344845197395, 22.475313640901312),
Offset(32.0451181423579, 24.275571482024635),
Offset(32.34771068253502, 25.78978969649082),
Offset(32.5143118475562, 27.094293625401967),
Offset(32.58505406874193, 28.21441080313788),
Offset(32.54341131715201, 29.12514188342491),
Offset(32.43548038530638, 29.83995906986752),
Offset(32.307656491621024, 30.39052525674685),
Offset(32.18757194856529, 30.80548761936007),
Offset(32.09053409812234, 31.10821082141777),
Offset(32.024044917731125, 31.316956865403107),
Offset(31.990877599326076, 31.44587664801465),
Offset(31.991072862726128, 31.505765919883586),
Offset(32.00558109744533, 31.523463634598716),
Offset(32.01015625, 31.52890625),
],
),
_PathCubicTo(
<Offset>[
Offset(18.19263671875, 19.091542968750005),
Offset(18.297386033901486, 18.970227374751772),
Offset(18.72781204439693, 18.52072515118723),
Offset(19.90886482873765, 17.59055137172482),
Offset(23.10529263788128, 16.448979046234285),
Offset(28.016884077101, 17.542088038872436),
Offset(30.636620480995617, 20.19302812181654),
Offset(31.63344845197395, 22.475313640901312),
Offset(32.0451181423579, 24.275571482024635),
Offset(32.34771068253502, 25.78978969649082),
Offset(32.5143118475562, 27.094293625401967),
Offset(32.58505406874193, 28.21441080313788),
Offset(32.54341131715201, 29.12514188342491),
Offset(32.43548038530638, 29.83995906986752),
Offset(32.307656491621024, 30.39052525674685),
Offset(32.18757194856529, 30.80548761936007),
Offset(32.09053409812234, 31.10821082141777),
Offset(32.024044917731125, 31.316956865403107),
Offset(31.990877599326076, 31.44587664801465),
Offset(31.991072862726128, 31.505765919883586),
Offset(32.00558109744533, 31.523463634598716),
Offset(32.01015625, 31.52890625),
],
<Offset>[
Offset(18.192636718749995, 24.891542968750002),
Offset(18.175135177837678, 24.768938846121223),
Offset(18.131762833609578, 24.290016731469834),
Offset(18.189398131863207, 23.129814344209493),
Offset(19.14691116775322, 20.688226165351878),
Offset(22.277081692306755, 18.381965826572245),
Offset(25.153850173498547, 18.208885778660576),
Offset(26.987657743646544, 18.810016196193768),
Offset(28.229748817157525, 19.528141401396265),
Offset(29.223774647225785, 20.19509758103034),
Offset(30.007739613111152, 20.830494962200966),
Offset(30.626964119061235, 21.41670003990219),
Offset(31.072971936868854, 21.941254300113602),
Offset(31.381936148538408, 22.388826086603757),
Offset(31.595631995844528, 22.75496752966676),
Offset(31.74362009537025, 23.04237991891895),
Offset(31.845995957897536, 23.25665695166987),
Offset(31.916063614634442, 23.404284579714346),
Offset(31.962512649081546, 23.491750839098724),
Offset(31.99086595053382, 23.525019467195833),
Offset(32.00558109744533, 23.528032986218715),
Offset(32.01015625, 23.52890625),
],
<Offset>[
Offset(18.192636718749995, 24.891542968750002),
Offset(18.175135177837678, 24.768938846121223),
Offset(18.131762833609578, 24.290016731469834),
Offset(18.189398131863207, 23.129814344209493),
Offset(19.14691116775322, 20.688226165351878),
Offset(22.277081692306755, 18.381965826572245),
Offset(25.153850173498547, 18.208885778660576),
Offset(26.987657743646544, 18.810016196193768),
Offset(28.229748817157525, 19.528141401396265),
Offset(29.223774647225785, 20.19509758103034),
Offset(30.007739613111152, 20.830494962200966),
Offset(30.626964119061235, 21.41670003990219),
Offset(31.072971936868854, 21.941254300113602),
Offset(31.381936148538408, 22.388826086603757),
Offset(31.595631995844528, 22.75496752966676),
Offset(31.74362009537025, 23.04237991891895),
Offset(31.845995957897536, 23.25665695166987),
Offset(31.916063614634442, 23.404284579714346),
Offset(31.962512649081546, 23.491750839098724),
Offset(31.99086595053382, 23.525019467195833),
Offset(32.00558109744533, 23.528032986218715),
Offset(32.01015625, 23.52890625),
],
),
_PathClose(
),
_PathMoveTo(
<Offset>[
Offset(19.359999999999996, 37.6),
Offset(19.07437364316861, 37.499177937670424),
Offset(17.986934103582882, 37.051154681687954),
Offset(15.536736677617021, 35.61304326763691),
Offset(11.326880236819443, 30.7735984135526),
Offset(9.869566263871294, 21.377480537283333),
Offset(12.74113193535829, 14.964919748422403),
Offset(16.0704913221344, 11.713990403514046),
Offset(18.914329388866914, 9.893901895554396),
Offset(21.25283585091745, 8.565246523821894),
Offset(23.25484407901928, 7.610299138856277),
Offset(24.968395165544187, 6.916249550797959),
Offset(26.405174995011652, 6.49649745011436),
Offset(27.573815336148815, 6.274596001300022),
Offset(28.49870054201158, 6.167904420613963),
Offset(29.208394245687987, 6.121885514098405),
Offset(29.729906624130507, 6.1022310660712105),
Offset(30.08688450541718, 6.08845659062094),
Offset(30.299438656814726, 6.069069138689281),
Offset(30.384131484923635, 6.038341565542824),
Offset(30.396344518703998, 6.009138703240001),
Offset(30.4, 6.0),
],
),
_PathCubicTo(
<Offset>[
Offset(19.359999999999996, 37.6),
Offset(19.07437364316861, 37.499177937670424),
Offset(17.986934103582882, 37.051154681687954),
Offset(15.536736677617021, 35.61304326763691),
Offset(11.326880236819443, 30.7735984135526),
Offset(9.869566263871294, 21.377480537283333),
Offset(12.74113193535829, 14.964919748422403),
Offset(16.0704913221344, 11.713990403514046),
Offset(18.914329388866914, 9.893901895554396),
Offset(21.25283585091745, 8.565246523821894),
Offset(23.25484407901928, 7.610299138856277),
Offset(24.968395165544187, 6.916249550797959),
Offset(26.405174995011652, 6.49649745011436),
Offset(27.573815336148815, 6.274596001300022),
Offset(28.49870054201158, 6.167904420613963),
Offset(29.208394245687987, 6.121885514098405),
Offset(29.729906624130507, 6.1022310660712105),
Offset(30.08688450541718, 6.08845659062094),
Offset(30.299438656814726, 6.069069138689281),
Offset(30.384131484923635, 6.038341565542824),
Offset(30.396344518703998, 6.009138703240001),
Offset(30.4, 6.0),
],
<Offset>[
Offset(19.359999999999996, 35.28),
Offset(19.123273985594132, 35.179693349122644),
Offset(18.225353787897824, 34.74343804957491),
Offset(16.224523356366795, 33.397338078643045),
Offset(12.910232824870667, 29.077899565905565),
Offset(12.165487217788991, 21.04152942220341),
Offset(14.934240058357119, 15.758576685684787),
Offset(17.928807605465366, 13.180109381397063),
Offset(20.440477118947065, 11.792873927805745),
Offset(22.502410265041142, 10.803123370006087),
Offset(24.2574729727973, 10.115818604136678),
Offset(25.751631145416468, 9.635333856092236),
Offset(26.993350747124914, 9.370052483438883),
Offset(27.995233030856, 9.255049194605526),
Offset(28.78351034032218, 9.222127511445999),
Offset(29.385974986966005, 9.227128594274852),
Offset(29.82772188022043, 9.24285261397037),
Offset(30.130077026655854, 9.253525504896444),
Offset(30.310784636912537, 9.250719462255653),
Offset(30.384214249800557, 9.230640146617926),
Offset(30.396344518703998, 9.207310962592),
Offset(30.4, 9.2),
],
<Offset>[
Offset(19.359999999999996, 35.28),
Offset(19.123273985594132, 35.179693349122644),
Offset(18.225353787897824, 34.74343804957491),
Offset(16.224523356366795, 33.397338078643045),
Offset(12.910232824870667, 29.077899565905565),
Offset(12.165487217788991, 21.04152942220341),
Offset(14.934240058357119, 15.758576685684787),
Offset(17.928807605465366, 13.180109381397063),
Offset(20.440477118947065, 11.792873927805745),
Offset(22.502410265041142, 10.803123370006087),
Offset(24.2574729727973, 10.115818604136678),
Offset(25.751631145416468, 9.635333856092236),
Offset(26.993350747124914, 9.370052483438883),
Offset(27.995233030856, 9.255049194605526),
Offset(28.78351034032218, 9.222127511445999),
Offset(29.385974986966005, 9.227128594274852),
Offset(29.82772188022043, 9.24285261397037),
Offset(30.130077026655854, 9.253525504896444),
Offset(30.310784636912537, 9.250719462255653),
Offset(30.384214249800557, 9.230640146617926),
Offset(30.396344518703998, 9.207310962592),
Offset(30.4, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(19.359999999999996, 35.28),
Offset(19.123273985594132, 35.179693349122644),
Offset(18.225353787897824, 34.74343804957491),
Offset(16.224523356366795, 33.397338078643045),
Offset(12.910232824870667, 29.077899565905565),
Offset(12.165487217788991, 21.04152942220341),
Offset(14.934240058357119, 15.758576685684787),
Offset(17.928807605465366, 13.180109381397063),
Offset(20.440477118947065, 11.792873927805745),
Offset(22.502410265041142, 10.803123370006087),
Offset(24.2574729727973, 10.115818604136678),
Offset(25.751631145416468, 9.635333856092236),
Offset(26.993350747124914, 9.370052483438883),
Offset(27.995233030856, 9.255049194605526),
Offset(28.78351034032218, 9.222127511445999),
Offset(29.385974986966005, 9.227128594274852),
Offset(29.82772188022043, 9.24285261397037),
Offset(30.130077026655854, 9.253525504896444),
Offset(30.310784636912537, 9.250719462255653),
Offset(30.384214249800557, 9.230640146617926),
Offset(30.396344518703998, 9.207310962592),
Offset(30.4, 9.2),
],
<Offset>[
Offset(28.639999999999993, 35.28),
Offset(28.401212339785253, 35.37529471882475),
Offset(27.45622031634999, 35.69711678683468),
Offset(25.08734411234227, 36.14848479364215),
Offset(19.693028215458817, 35.41130991811046),
Offset(13.509291678108683, 30.2252132378742),
Offset(11.759612309307578, 24.5310091776801),
Offset(12.064331693933298, 20.613374514720917),
Offset(12.844588989941675, 17.897464848126344),
Offset(13.550902880304374, 15.801421026500861),
Offset(14.235395111675697, 14.126334179248751),
Offset(14.875293924239358, 12.768277775581351),
Offset(15.499130613826821, 11.722755491891931),
Offset(16.07342025763398, 10.94071997343427),
Offset(16.566617976994035, 10.361366704688399),
Offset(16.965002666260215, 9.937451559386922),
Offset(17.26523568862379, 9.63411363833006),
Offset(17.469801369553835, 9.426295589851133),
Offset(17.584183342647055, 9.296103382646901),
Offset(17.61501992550015, 9.23097120612562),
Offset(17.603655481296002, 9.207310962592),
Offset(17.6, 9.2),
],
<Offset>[
Offset(28.639999999999993, 35.28),
Offset(28.401212339785253, 35.37529471882475),
Offset(27.45622031634999, 35.69711678683468),
Offset(25.08734411234227, 36.14848479364215),
Offset(19.693028215458817, 35.41130991811046),
Offset(13.509291678108683, 30.2252132378742),
Offset(11.759612309307578, 24.5310091776801),
Offset(12.064331693933298, 20.613374514720917),
Offset(12.844588989941675, 17.897464848126344),
Offset(13.550902880304374, 15.801421026500861),
Offset(14.235395111675697, 14.126334179248751),
Offset(14.875293924239358, 12.768277775581351),
Offset(15.499130613826821, 11.722755491891931),
Offset(16.07342025763398, 10.94071997343427),
Offset(16.566617976994035, 10.361366704688399),
Offset(16.965002666260215, 9.937451559386922),
Offset(17.26523568862379, 9.63411363833006),
Offset(17.469801369553835, 9.426295589851133),
Offset(17.584183342647055, 9.296103382646901),
Offset(17.61501992550015, 9.23097120612562),
Offset(17.603655481296002, 9.207310962592),
Offset(17.6, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(28.639999999999993, 35.28),
Offset(28.401212339785253, 35.37529471882475),
Offset(27.45622031634999, 35.69711678683468),
Offset(25.08734411234227, 36.14848479364215),
Offset(19.693028215458817, 35.41130991811046),
Offset(13.509291678108683, 30.2252132378742),
Offset(11.759612309307578, 24.5310091776801),
Offset(12.064331693933298, 20.613374514720917),
Offset(12.844588989941675, 17.897464848126344),
Offset(13.550902880304374, 15.801421026500861),
Offset(14.235395111675697, 14.126334179248751),
Offset(14.875293924239358, 12.768277775581351),
Offset(15.499130613826821, 11.722755491891931),
Offset(16.07342025763398, 10.94071997343427),
Offset(16.566617976994035, 10.361366704688399),
Offset(16.965002666260215, 9.937451559386922),
Offset(17.26523568862379, 9.63411363833006),
Offset(17.469801369553835, 9.426295589851133),
Offset(17.584183342647055, 9.296103382646901),
Offset(17.61501992550015, 9.23097120612562),
Offset(17.603655481296002, 9.207310962592),
Offset(17.6, 9.2),
],
<Offset>[
Offset(28.639999999999993, 37.6),
Offset(28.35231199735973, 37.69477930737253),
Offset(27.21780063203505, 38.00483341894772),
Offset(24.39955743359249, 38.36418998263601),
Offset(18.109675627407594, 37.1070087657575),
Offset(11.213370724190986, 30.561164352954123),
Offset(9.566504186308748, 23.737352240417717),
Offset(10.206015410602333, 19.1472555368379),
Offset(11.318441259861526, 15.998492815874997),
Offset(12.301328466180678, 13.563544180316669),
Offset(13.232766217897677, 11.62081471396835),
Offset(14.092057944367081, 10.049193470287074),
Offset(14.91095486171356, 8.849200458567408),
Offset(15.652002562926794, 7.960266780128766),
Offset(16.281808178683434, 7.307143613856363),
Offset(16.787421924982198, 6.832208479210475),
Offset(17.167420432533866, 6.4934920904309),
Offset(17.426608848315166, 6.261226675575628),
Offset(17.572837362549244, 6.11445305908053),
Offset(17.614937160623228, 6.038672625050518),
Offset(17.603655481296002, 6.009138703240001),
Offset(17.6, 6.0),
],
<Offset>[
Offset(28.639999999999993, 37.6),
Offset(28.35231199735973, 37.69477930737253),
Offset(27.21780063203505, 38.00483341894772),
Offset(24.39955743359249, 38.36418998263601),
Offset(18.109675627407594, 37.1070087657575),
Offset(11.213370724190986, 30.561164352954123),
Offset(9.566504186308748, 23.737352240417717),
Offset(10.206015410602333, 19.1472555368379),
Offset(11.318441259861526, 15.998492815874997),
Offset(12.301328466180678, 13.563544180316669),
Offset(13.232766217897677, 11.62081471396835),
Offset(14.092057944367081, 10.049193470287074),
Offset(14.91095486171356, 8.849200458567408),
Offset(15.652002562926794, 7.960266780128766),
Offset(16.281808178683434, 7.307143613856363),
Offset(16.787421924982198, 6.832208479210475),
Offset(17.167420432533866, 6.4934920904309),
Offset(17.426608848315166, 6.261226675575628),
Offset(17.572837362549244, 6.11445305908053),
Offset(17.614937160623228, 6.038672625050518),
Offset(17.603655481296002, 6.009138703240001),
Offset(17.6, 6.0),
],
),
_PathCubicTo(
<Offset>[
Offset(28.639999999999993, 37.6),
Offset(28.35231199735973, 37.69477930737253),
Offset(27.21780063203505, 38.00483341894772),
Offset(24.39955743359249, 38.36418998263601),
Offset(18.109675627407594, 37.1070087657575),
Offset(11.213370724190986, 30.561164352954123),
Offset(9.566504186308748, 23.737352240417717),
Offset(10.206015410602333, 19.1472555368379),
Offset(11.318441259861526, 15.998492815874997),
Offset(12.301328466180678, 13.563544180316669),
Offset(13.232766217897677, 11.62081471396835),
Offset(14.092057944367081, 10.049193470287074),
Offset(14.91095486171356, 8.849200458567408),
Offset(15.652002562926794, 7.960266780128766),
Offset(16.281808178683434, 7.307143613856363),
Offset(16.787421924982198, 6.832208479210475),
Offset(17.167420432533866, 6.4934920904309),
Offset(17.426608848315166, 6.261226675575628),
Offset(17.572837362549244, 6.11445305908053),
Offset(17.614937160623228, 6.038672625050518),
Offset(17.603655481296002, 6.009138703240001),
Offset(17.6, 6.0),
],
<Offset>[
Offset(30.959999999999994, 37.6),
Offset(30.67179658590751, 37.74367964979805),
Offset(29.525517264148096, 38.24325310326266),
Offset(26.61526262258636, 39.051976661385794),
Offset(19.80537447505463, 38.69036135380872),
Offset(11.54932183927091, 32.857085306871824),
Offset(8.772847249046361, 25.930460363416543),
Offset(8.739896432719314, 21.005571820168864),
Offset(9.419469227610179, 17.524640545955144),
Offset(10.063451619996487, 14.813118594440363),
Offset(10.727246752617276, 12.62344360774637),
Offset(11.372973639072804, 10.832429450159353),
Offset(12.037399828389036, 9.43737621068067),
Offset(12.671549369621289, 8.381684474835954),
Offset(13.227585087851399, 7.591953412166962),
Offset(13.68217884480575, 7.009789220488495),
Offset(14.026798884634708, 6.591307346520823),
Offset(14.26153993403966, 6.304419196814299),
Offset(14.391187038982872, 6.125799039178341),
Offset(14.422638579548124, 6.0387553899274415),
Offset(14.405483221944, 6.009138703240001),
Offset(14.399999999999999, 6.0),
],
<Offset>[
Offset(30.959999999999994, 37.6),
Offset(30.67179658590751, 37.74367964979805),
Offset(29.525517264148096, 38.24325310326266),
Offset(26.61526262258636, 39.051976661385794),
Offset(19.80537447505463, 38.69036135380872),
Offset(11.54932183927091, 32.857085306871824),
Offset(8.772847249046361, 25.930460363416543),
Offset(8.739896432719314, 21.005571820168864),
Offset(9.419469227610179, 17.524640545955144),
Offset(10.063451619996487, 14.813118594440363),
Offset(10.727246752617276, 12.62344360774637),
Offset(11.372973639072804, 10.832429450159353),
Offset(12.037399828389036, 9.43737621068067),
Offset(12.671549369621289, 8.381684474835954),
Offset(13.227585087851399, 7.591953412166962),
Offset(13.68217884480575, 7.009789220488495),
Offset(14.026798884634708, 6.591307346520823),
Offset(14.26153993403966, 6.304419196814299),
Offset(14.391187038982872, 6.125799039178341),
Offset(14.422638579548124, 6.0387553899274415),
Offset(14.405483221944, 6.009138703240001),
Offset(14.399999999999999, 6.0),
],
),
_PathCubicTo(
<Offset>[
Offset(30.959999999999994, 37.6),
Offset(30.67179658590751, 37.74367964979805),
Offset(29.525517264148096, 38.24325310326266),
Offset(26.61526262258636, 39.051976661385794),
Offset(19.80537447505463, 38.69036135380872),
Offset(11.54932183927091, 32.857085306871824),
Offset(8.772847249046361, 25.930460363416543),
Offset(8.739896432719314, 21.005571820168864),
Offset(9.419469227610179, 17.524640545955144),
Offset(10.063451619996487, 14.813118594440363),
Offset(10.727246752617276, 12.62344360774637),
Offset(11.372973639072804, 10.832429450159353),
Offset(12.037399828389036, 9.43737621068067),
Offset(12.671549369621289, 8.381684474835954),
Offset(13.227585087851399, 7.591953412166962),
Offset(13.68217884480575, 7.009789220488495),
Offset(14.026798884634708, 6.591307346520823),
Offset(14.26153993403966, 6.304419196814299),
Offset(14.391187038982872, 6.125799039178341),
Offset(14.422638579548124, 6.0387553899274415),
Offset(14.405483221944, 6.009138703240001),
Offset(14.399999999999999, 6.0),
],
<Offset>[
Offset(30.959999999999994, 35.28),
Offset(30.720696928333034, 35.424195061250266),
Offset(29.763936948463037, 35.93553647114962),
Offset(27.303049301336138, 36.83627147239193),
Offset(21.388727063105854, 36.99466250616168),
Offset(13.845242793188607, 32.521134191791894),
Offset(10.96595537204519, 26.72411730067893),
Offset(10.598212716050279, 22.47169079805188),
Offset(10.945616957690326, 19.423612578206495),
Offset(11.313026034120181, 17.050995440624554),
Offset(11.729875646395296, 15.128963073026771),
Offset(12.156209618945082, 13.55151375545363),
Offset(12.625575580502298, 12.310931244005193),
Offset(13.092967064328475, 11.362137668141457),
Offset(13.512394886161998, 10.646176502998998),
Offset(13.859759586083767, 10.115032300664941),
Offset(14.124614140724631, 9.731928894419983),
Offset(14.304732455278332, 9.469488111089804),
Offset(14.402533019080684, 9.307449362744713),
Offset(14.422721344425048, 9.231053971002543),
Offset(14.405483221944, 9.207310962592),
Offset(14.399999999999999, 9.2),
],
<Offset>[
Offset(30.959999999999994, 35.28),
Offset(30.720696928333034, 35.424195061250266),
Offset(29.763936948463037, 35.93553647114962),
Offset(27.303049301336138, 36.83627147239193),
Offset(21.388727063105854, 36.99466250616168),
Offset(13.845242793188607, 32.521134191791894),
Offset(10.96595537204519, 26.72411730067893),
Offset(10.598212716050279, 22.47169079805188),
Offset(10.945616957690326, 19.423612578206495),
Offset(11.313026034120181, 17.050995440624554),
Offset(11.729875646395296, 15.128963073026771),
Offset(12.156209618945082, 13.55151375545363),
Offset(12.625575580502298, 12.310931244005193),
Offset(13.092967064328475, 11.362137668141457),
Offset(13.512394886161998, 10.646176502998998),
Offset(13.859759586083767, 10.115032300664941),
Offset(14.124614140724631, 9.731928894419983),
Offset(14.304732455278332, 9.469488111089804),
Offset(14.402533019080684, 9.307449362744713),
Offset(14.422721344425048, 9.231053971002543),
Offset(14.405483221944, 9.207310962592),
Offset(14.399999999999999, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(30.959999999999994, 35.28),
Offset(30.720696928333034, 35.424195061250266),
Offset(29.763936948463037, 35.93553647114962),
Offset(27.303049301336138, 36.83627147239193),
Offset(21.388727063105854, 36.99466250616168),
Offset(13.845242793188607, 32.521134191791894),
Offset(10.96595537204519, 26.72411730067893),
Offset(10.598212716050279, 22.47169079805188),
Offset(10.945616957690326, 19.423612578206495),
Offset(11.313026034120181, 17.050995440624554),
Offset(11.729875646395296, 15.128963073026771),
Offset(12.156209618945082, 13.55151375545363),
Offset(12.625575580502298, 12.310931244005193),
Offset(13.092967064328475, 11.362137668141457),
Offset(13.512394886161998, 10.646176502998998),
Offset(13.859759586083767, 10.115032300664941),
Offset(14.124614140724631, 9.731928894419983),
Offset(14.304732455278332, 9.469488111089804),
Offset(14.402533019080684, 9.307449362744713),
Offset(14.422721344425048, 9.231053971002543),
Offset(14.405483221944, 9.207310962592),
Offset(14.399999999999999, 9.2),
],
<Offset>[
Offset(32.11999999999999, 35.28),
Offset(31.880439222606924, 35.44864523246303),
Offset(30.917795264519558, 36.05474631330709),
Offset(28.41090189583307, 37.180164811766815),
Offset(22.236576486929373, 37.786338800187295),
Offset(14.013218350728568, 33.669094668750745),
Offset(10.569126903413999, 27.820671362178345),
Offset(9.86515322710877, 23.400848939717363),
Offset(9.996130941564655, 20.18668644324657),
Offset(10.194087611028085, 17.675782647686404),
Offset(10.477115913755096, 15.63027751991578),
Offset(10.796667466297944, 13.94313174538977),
Offset(11.188798063840036, 12.605019120061824),
Offset(11.602740467675723, 11.57284651549505),
Offset(11.98528334074598, 10.788581402154296),
Offset(12.307138045995544, 10.20382267130395),
Offset(12.55430336677505, 9.780836522464943),
Offset(12.72219799814058, 9.49108437170914),
Offset(12.811707857297497, 9.313122352793618),
Offset(12.826572053887496, 9.231095353441006),
Offset(12.806397092268, 9.207310962592),
Offset(12.799999999999999, 9.2),
],
<Offset>[
Offset(32.11999999999999, 35.28),
Offset(31.880439222606924, 35.44864523246303),
Offset(30.917795264519558, 36.05474631330709),
Offset(28.41090189583307, 37.180164811766815),
Offset(22.236576486929373, 37.786338800187295),
Offset(14.013218350728568, 33.669094668750745),
Offset(10.569126903413999, 27.820671362178345),
Offset(9.86515322710877, 23.400848939717363),
Offset(9.996130941564655, 20.18668644324657),
Offset(10.194087611028085, 17.675782647686404),
Offset(10.477115913755096, 15.63027751991578),
Offset(10.796667466297944, 13.94313174538977),
Offset(11.188798063840036, 12.605019120061824),
Offset(11.602740467675723, 11.57284651549505),
Offset(11.98528334074598, 10.788581402154296),
Offset(12.307138045995544, 10.20382267130395),
Offset(12.55430336677505, 9.780836522464943),
Offset(12.72219799814058, 9.49108437170914),
Offset(12.811707857297497, 9.313122352793618),
Offset(12.826572053887496, 9.231095353441006),
Offset(12.806397092268, 9.207310962592),
Offset(12.799999999999999, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(33.40180389404459, 35.28),
Offset(33.161958350959075, 35.47566275373077),
Offset(32.19281257718938, 36.186473589070786),
Offset(29.635082731741335, 37.56016810620365),
Offset(23.17345294642977, 38.66114376269145),
Offset(14.198831905693254, 34.93759484941898),
Offset(10.130630113449154, 29.032367281195906),
Offset(9.055120030995347, 24.427571805381206),
Offset(8.946945706382945, 21.029885625705216),
Offset(8.957656897307762, 18.366174608859787),
Offset(9.09281220375468, 16.18423166660815),
Offset(9.294368823728218, 14.37587093890534),
Offset(9.601154084759504, 12.929987210338286),
Offset(9.95603507578061, 11.805680499156674),
Offset(10.297819956647084, 10.945939293764894),
Offset(10.591486032148467, 10.301936328923558),
Offset(10.819104690129567, 9.834879615634355),
Offset(10.973492110538054, 9.51494831219075),
Offset(11.053840713230413, 9.319391025841519),
Offset(11.062821729674083, 9.231141081174425),
Offset(11.039401550947822, 9.207310962592),
Offset(11.031994628903998, 9.2),
],
<Offset>[
Offset(34.428397521973196, 34.24180389404461),
Offset(34.21020673189347, 34.4593355607496),
Offset(33.32066325711653, 35.25927432509759),
Offset(30.923309576566957, 36.87298746940196),
Offset(24.632342654683864, 38.60295054594897),
Offset(15.374910124648133, 35.80319600547601),
Offset(10.760851302536071, 30.35797174936804),
Offset(9.23795982776596, 25.90595743850577),
Offset(8.789604345416578, 22.554988601644673),
Offset(8.52658499233495, 19.920553987514175),
Offset(8.43280146906967, 17.749107953581934),
Offset(8.441678273181578, 15.93923637150114),
Offset(8.59282153680617, 14.476164419419169),
Offset(8.82577643484907, 13.325904473016532),
Offset(9.073786534329603, 12.438726553996867),
Offset(9.296891581635101, 11.770106385528296),
Offset(9.473160444080882, 11.283585469050152),
Offset(9.592286389851099, 10.950423910880021),
Offset(9.651046490767305, 10.748194770371416),
Offset(9.650275412603275, 10.659725961266831),
Offset(9.624219110228523, 10.638487680623824),
Offset(9.616003417967999, 10.631994628904),
],
<Offset>[
Offset(34.428397521973196, 32.96),
Offset(34.23722425316121, 33.17781643239746),
Offset(33.45239053288022, 33.984257012427776),
Offset(31.303312871003797, 35.648806633493706),
Offset(25.507147617188025, 37.666074086448575),
Offset(16.643410305316365, 35.61758245051132),
Offset(11.972547221553633, 30.79646853933288),
Offset(10.264682693429805, 26.71599063461919),
Offset(9.632803527875225, 23.60417383682638),
Offset(9.216976953508334, 21.156984701234496),
Offset(8.98675561576204, 19.133411663582347),
Offset(8.87441746669715, 17.441535014070865),
Offset(8.917789627082632, 16.063808398499702),
Offset(9.058610418510696, 14.972609864911643),
Offset(9.2311444259402, 14.126189938095761),
Offset(9.395005239254708, 13.48575839937537),
Offset(9.527203537250292, 13.018784145695633),
Offset(9.616150330332708, 12.699129798482545),
Offset(9.657315163815205, 12.5060619144385),
Offset(9.650321140336693, 12.423476285480243),
Offset(9.624219110228523, 12.405483221944),
Offset(9.616003417967999, 12.399999999999999),
],
),
_PathCubicTo(
<Offset>[
Offset(34.428397521973196, 32.96),
Offset(34.23722425316121, 33.17781643239746),
Offset(33.45239053288022, 33.984257012427776),
Offset(31.303312871003797, 35.648806633493706),
Offset(25.507147617188025, 37.666074086448575),
Offset(16.643410305316365, 35.61758245051132),
Offset(11.972547221553633, 30.79646853933288),
Offset(10.264682693429805, 26.71599063461919),
Offset(9.632803527875225, 23.60417383682638),
Offset(9.216976953508334, 21.156984701234496),
Offset(8.98675561576204, 19.133411663582347),
Offset(8.87441746669715, 17.441535014070865),
Offset(8.917789627082632, 16.063808398499702),
Offset(9.058610418510696, 14.972609864911643),
Offset(9.2311444259402, 14.126189938095761),
Offset(9.395005239254708, 13.48575839937537),
Offset(9.527203537250292, 13.018784145695633),
Offset(9.616150330332708, 12.699129798482545),
Offset(9.657315163815205, 12.5060619144385),
Offset(9.650321140336693, 12.423476285480243),
Offset(9.624219110228523, 12.405483221944),
Offset(9.616003417967999, 12.399999999999999),
],
<Offset>[
Offset(34.440000000000516, 16.720000000000006),
Offset(34.591126550559416, 16.94166886650632),
Offset(35.132869371152644, 17.831432940717747),
Offset(36.128900514825645, 20.142309978565798),
Offset(36.59909603898658, 25.80410060706257),
Offset(32.71653709715015, 33.277406702029566),
Offset(27.320334950142062, 36.36303498327675),
Offset(23.26556451587313, 36.98811704611288),
Offset(20.306340749954074, 36.90461043133784),
Offset(17.95280607783294, 36.82837183128392),
Offset(15.99262759869828, 36.67706213593723),
Offset(14.343470999981285, 36.47904216761644),
Offset(13.020649047420964, 36.181635138771405),
Offset(11.99362883202704, 35.83788975664637),
Offset(11.209538636398053, 35.50717592712125),
Offset(10.622540896042542, 35.22334805399359),
Offset(10.196203867594564, 35.003624161748164),
Offset(9.902669253773741, 34.854828207151854),
Offset(9.720825374512906, 34.7776709214224),
Offset(9.634935591827066, 34.76956676691874),
Offset(9.608224832915282, 34.792689037407996),
Offset(9.59999999999928, 34.8),
],
<Offset>[
Offset(34.440000000000516, 16.720000000000006),
Offset(34.591126550559416, 16.94166886650632),
Offset(35.132869371152644, 17.831432940717747),
Offset(36.128900514825645, 20.142309978565798),
Offset(36.59909603898658, 25.80410060706257),
Offset(32.71653709715015, 33.277406702029566),
Offset(27.320334950142062, 36.36303498327675),
Offset(23.26556451587313, 36.98811704611288),
Offset(20.306340749954074, 36.90461043133784),
Offset(17.95280607783294, 36.82837183128392),
Offset(15.99262759869828, 36.67706213593723),
Offset(14.343470999981285, 36.47904216761644),
Offset(13.020649047420964, 36.181635138771405),
Offset(11.99362883202704, 35.83788975664637),
Offset(11.209538636398053, 35.50717592712125),
Offset(10.622540896042542, 35.22334805399359),
Offset(10.196203867594564, 35.003624161748164),
Offset(9.902669253773741, 34.854828207151854),
Offset(9.720825374512906, 34.7776709214224),
Offset(9.634935591827066, 34.76956676691874),
Offset(9.608224832915282, 34.792689037407996),
Offset(9.59999999999928, 34.8),
],
),
_PathCubicTo(
<Offset>[
Offset(34.440000000000516, 15.438196105955406),
Offset(34.61814407182715, 15.660149738154173),
Offset(35.264596646916345, 16.556415628047922),
Offset(36.50890380926249, 18.918129142657538),
Offset(37.473901001490745, 24.867224147562176),
Offset(33.98503727781838, 33.091793147064884),
Offset(28.532030869159623, 36.80153177324159),
Offset(24.292287381536976, 37.798150242226306),
Offset(21.149539932412726, 37.95379566651955),
Offset(18.643198039006325, 38.06480254500424),
Offset(16.546581745390647, 38.061365845937644),
Offset(14.776210193496857, 37.98134081018617),
Offset(13.345617137697426, 37.76927911785194),
Offset(12.226462815688665, 37.48459514854148),
Offset(11.366896528008649, 37.19463931122015),
Offset(10.72065455366215, 36.93900006784067),
Offset(10.250246960763974, 36.73882283839365),
Offset(9.92653319425535, 36.60353409475438),
Offset(9.727094047560806, 36.53553806548948),
Offset(9.634981319560485, 36.53331709113216),
Offset(9.608224832915282, 36.55968457872818),
Offset(9.59999999999928, 36.568005371096),
],
<Offset>[
Offset(33.401803894045116, 14.400000000000006),
Offset(33.602061432789306, 14.600301456800754),
Offset(34.33858973602437, 15.417023900053458),
Offset(35.825162840489824, 17.618821405258995),
Offset(37.42362623889116, 23.39985413386847),
Offset(34.862120490952606, 31.914034813700184),
Offset(29.868603220438434, 36.17527971655787),
Offset(25.77996658097369, 37.62264260632878),
Offset(22.682275277103862, 38.12063391596769),
Offset(20.203826624420508, 38.5070662245178),
Offset(18.116472247756285, 38.73390685413197),
Offset(16.343492642578116, 38.847629686554015),
Offset(14.894735853778217, 38.79198251025913),
Offset(13.748794328144617, 38.62975923890631),
Offset(12.861108141441791, 38.43394711125329),
Offset(12.18971270364993, 38.249124050511625),
Offset(11.699441994938162, 38.10047354672682),
Offset(11.362224801685391, 38.0005685406703),
Offset(11.155954534110002, 37.954243937938855),
Offset(11.063566613565678, 37.96182831085034),
Offset(11.039401550947105, 37.99086129676),
Offset(11.031994628903279, 38.0),
],
<Offset>[
Offset(32.120000000000516, 14.400000000000006),
Offset(32.32054230443716, 14.573283935533016),
Offset(33.06357242335454, 15.285296624289762),
Offset(34.60098200458155, 17.238818110822155),
Offset(36.48674977939076, 22.52504917136431),
Offset(34.67650693598792, 30.64553463303195),
Offset(30.30710001040328, 34.96358379754031),
Offset(26.58999977708711, 36.59591974066494),
Offset(23.731460512285572, 37.27743473350904),
Offset(21.440257338140828, 37.81667426334442),
Offset(19.500775957756698, 38.17995270743961),
Offset(17.84579128514784, 38.41489049303844),
Offset(16.482379832858747, 38.46701441998266),
Offset(15.39549972003973, 38.39692525524468),
Offset(14.548571525540689, 38.27658921964269),
Offset(13.905364717497006, 38.15101039289202),
Offset(13.434640671583647, 38.046430453557406),
Offset(13.110930689287915, 37.97670460018869),
Offset(12.913821678177088, 37.94797526489096),
Offset(12.827316937779091, 37.96178258311692),
Offset(12.806397092267282, 37.99086129676),
Offset(12.79999999999928, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(32.120000000000516, 14.400000000000006),
Offset(32.32054230443716, 14.573283935533016),
Offset(33.06357242335454, 15.285296624289762),
Offset(34.60098200458155, 17.238818110822155),
Offset(36.48674977939076, 22.52504917136431),
Offset(34.67650693598792, 30.64553463303195),
Offset(30.30710001040328, 34.96358379754031),
Offset(26.58999977708711, 36.59591974066494),
Offset(23.731460512285572, 37.27743473350904),
Offset(21.440257338140828, 37.81667426334442),
Offset(19.500775957756698, 38.17995270743961),
Offset(17.84579128514784, 38.41489049303844),
Offset(16.482379832858747, 38.46701441998266),
Offset(15.39549972003973, 38.39692525524468),
Offset(14.548571525540689, 38.27658921964269),
Offset(13.905364717497006, 38.15101039289202),
Offset(13.434640671583647, 38.046430453557406),
Offset(13.110930689287915, 37.97670460018869),
Offset(12.913821678177088, 37.94797526489096),
Offset(12.827316937779091, 37.96178258311692),
Offset(12.806397092267282, 37.99086129676),
Offset(12.79999999999928, 38.0),
],
<Offset>[
Offset(15.88000000000052, 14.400000000000002),
Offset(16.0841501846027, 14.23098153855435),
Offset(16.909555998563246, 13.616358834085176),
Offset(19.091045681624482, 12.424311359573721),
Offset(24.616857845861503, 11.441581055005743),
Offset(32.32484913042846, 14.574087955608066),
Offset(35.862698571239974, 19.611826936548507),
Offset(36.85283262226824, 23.587705757348193),
Offset(37.024264738045005, 26.59440062294799),
Offset(37.105395261430175, 29.069653364478558),
Offset(37.039412214719505, 31.16155045099348),
Offset(36.87938142220778, 32.932238633932485),
Offset(36.59726506613041, 34.34978415518983),
Offset(36.25867207317826, 35.44700139229438),
Offset(35.92813316136494, 36.28292063146849),
Offset(35.64206627873214, 36.90794520394589),
Offset(35.41899150687777, 37.36172366092795),
Offset(35.26641308921645, 37.674356951517986),
Offset(35.185373943141684, 37.868553404206274),
Offset(35.1734070053048, 37.961203228978455),
Offset(35.19360290773128, 37.99086129676),
Offset(35.19999999999928, 38.0),
],
<Offset>[
Offset(15.88000000000052, 14.400000000000002),
Offset(16.0841501846027, 14.23098153855435),
Offset(16.909555998563246, 13.616358834085176),
Offset(19.091045681624482, 12.424311359573721),
Offset(24.616857845861503, 11.441581055005743),
Offset(32.32484913042846, 14.574087955608066),
Offset(35.862698571239974, 19.611826936548507),
Offset(36.85283262226824, 23.587705757348193),
Offset(37.024264738045005, 26.59440062294799),
Offset(37.105395261430175, 29.069653364478558),
Offset(37.039412214719505, 31.16155045099348),
Offset(36.87938142220778, 32.932238633932485),
Offset(36.59726506613041, 34.34978415518983),
Offset(36.25867207317826, 35.44700139229438),
Offset(35.92813316136494, 36.28292063146849),
Offset(35.64206627873214, 36.90794520394589),
Offset(35.41899150687777, 37.36172366092795),
Offset(35.26641308921645, 37.674356951517986),
Offset(35.185373943141684, 37.868553404206274),
Offset(35.1734070053048, 37.961203228978455),
Offset(35.19360290773128, 37.99086129676),
Offset(35.19999999999928, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(14.59819610595592, 14.400000000000002),
Offset(14.802631056250549, 14.203964017286612),
Offset(15.634538685893425, 13.484631558321478),
Offset(17.866864845716222, 12.044308065136882),
Offset(23.679981386361106, 10.56677609250158),
Offset(32.13923557546377, 13.305587774939834),
Offset(36.30119536120482, 18.400131017530946),
Offset(37.66286581838166, 22.560982891684347),
Offset(38.073449973226715, 25.751201440489343),
Offset(38.3418259751505, 28.379261403305176),
Offset(38.423715924719914, 30.60759630430111),
Offset(38.381680064777505, 32.49949944041691),
Offset(38.184909045210944, 34.02481606491337),
Offset(37.90537746507338, 35.21416740863276),
Offset(37.615596545463845, 36.1255627398579),
Offset(37.35771829257922, 36.80983154632628),
Offset(37.15419018352325, 37.30768056775854),
Offset(37.01511897681897, 37.650493011036374),
Offset(36.94324108720877, 37.862284731158375),
Offset(36.937157329518215, 37.96115750124504),
Offset(36.96059844905146, 37.99086129676),
Offset(36.96800537109528, 38.0),
],
<Offset>[
Offset(13.560000000000521, 15.438196105955402),
Offset(13.74278277489713, 15.22004665632446),
Offset(14.49514695789896, 14.410638469213454),
Offset(16.56755710831768, 12.72804903390955),
Offset(22.212611372667403, 10.617050855101162),
Offset(30.96147724209907, 12.428504561805607),
Offset(35.67494330452109, 17.06355866625214),
Offset(37.48735818248413, 21.073303692247634),
Offset(38.24028822267485, 24.218466095798203),
Offset(38.78408965466406, 26.818632817890993),
Offset(39.09625693291425, 29.037705801935473),
Offset(39.24796894114535, 30.932216991335657),
Offset(39.20761243761814, 32.475697348832576),
Offset(39.05054155543821, 33.691835896176805),
Offset(38.854904345496976, 34.631351126424754),
Offset(38.66784227525018, 35.340773396338506),
Offset(38.51584089185641, 35.85848553358435),
Offset(38.41215342273489, 36.21480140360634),
Offset(38.36194695965814, 36.43342424460918),
Offset(38.3656685492364, 36.532572207239845),
Offset(38.39177516708328, 36.55968457872818),
Offset(38.39999999999928, 36.568005371096),
],
<Offset>[
Offset(13.560000000000521, 16.720000000000002),
Offset(13.715765253629392, 16.501565784676608),
Offset(14.363419682135264, 15.685655781883277),
Offset(16.18755381388084, 13.952229869817812),
Offset(21.337806410163243, 11.553927314601557),
Offset(29.692977061430838, 12.614118116770292),
Offset(34.463247385503536, 16.625061876287294),
Offset(36.46063531682029, 20.26327049613421),
Offset(37.3970890402162, 23.169280860616492),
Offset(38.093697693490675, 25.582202104170673),
Offset(38.54230278622188, 27.65340209193506),
Offset(38.81522974762978, 29.42991834876593),
Offset(38.882644347341675, 30.888053369752043),
Offset(38.81770757177658, 32.045130504281694),
Offset(38.69754645388638, 32.943887742325856),
Offset(38.56972861763057, 33.62512138249143),
Offset(38.461797798687, 34.12328685693886),
Offset(38.38828948225328, 34.46609551600381),
Offset(38.35567828661024, 34.67555710054209),
Offset(38.36562282150298, 34.76882188302643),
Offset(38.39177516708328, 34.792689037407996),
Offset(38.39999999999928, 34.8),
],
),
_PathCubicTo(
<Offset>[
Offset(13.560000000000521, 16.720000000000002),
Offset(13.715765253629392, 16.501565784676608),
Offset(14.363419682135264, 15.685655781883277),
Offset(16.18755381388084, 13.952229869817812),
Offset(21.337806410163243, 11.553927314601557),
Offset(29.692977061430838, 12.614118116770292),
Offset(34.463247385503536, 16.625061876287294),
Offset(36.46063531682029, 20.26327049613421),
Offset(37.3970890402162, 23.169280860616492),
Offset(38.093697693490675, 25.582202104170673),
Offset(38.54230278622188, 27.65340209193506),
Offset(38.81522974762978, 29.42991834876593),
Offset(38.882644347341675, 30.888053369752043),
Offset(38.81770757177658, 32.045130504281694),
Offset(38.69754645388638, 32.943887742325856),
Offset(38.56972861763057, 33.62512138249143),
Offset(38.461797798687, 34.12328685693886),
Offset(38.38828948225328, 34.46609551600381),
Offset(38.35567828661024, 34.67555710054209),
Offset(38.36562282150298, 34.76882188302643),
Offset(38.39177516708328, 34.792689037407996),
Offset(38.39999999999928, 34.8),
],
<Offset>[
Offset(13.56000000000052, 32.96),
Offset(13.373462856650727, 32.73795790451107),
Offset(12.694481891930677, 31.839672206674575),
Offset(11.373047062632402, 29.462166192774887),
Offset(10.254338293804675, 23.423819248130823),
Offset(13.621530384006954, 14.965775922329756),
Offset(19.111490524511733, 11.069463315450594),
Offset(23.452421333503544, 10.000437650953089),
Offset(26.714054929655155, 9.876476634857061),
Offset(29.346676794624816, 9.917064180881326),
Offset(31.523900529775755, 10.114765834972257),
Offset(33.33257788852383, 10.396328211705992),
Offset(34.76541408254884, 10.773168136480383),
Offset(35.86778370882628, 11.181958151143158),
Offset(36.70387786571219, 11.5643261065016),
Offset(37.32666342868444, 11.888419821256292),
Offset(37.777091006057546, 12.138936021644747),
Offset(38.085941833582574, 12.31061311607528),
Offset(38.27625642592556, 12.404004835577497),
Offset(38.365043467364515, 12.422731815500718),
Offset(38.39177516708328, 12.405483221944),
Offset(38.39999999999928, 12.399999999999999),
],
<Offset>[
Offset(13.56000000000052, 32.96),
Offset(13.373462856650727, 32.73795790451107),
Offset(12.694481891930677, 31.839672206674575),
Offset(11.373047062632402, 29.462166192774887),
Offset(10.254338293804675, 23.423819248130823),
Offset(13.621530384006954, 14.965775922329756),
Offset(19.111490524511733, 11.069463315450594),
Offset(23.452421333503544, 10.000437650953089),
Offset(26.714054929655155, 9.876476634857061),
Offset(29.346676794624816, 9.917064180881326),
Offset(31.523900529775755, 10.114765834972257),
Offset(33.33257788852383, 10.396328211705992),
Offset(34.76541408254884, 10.773168136480383),
Offset(35.86778370882628, 11.181958151143158),
Offset(36.70387786571219, 11.5643261065016),
Offset(37.32666342868444, 11.888419821256292),
Offset(37.777091006057546, 12.138936021644747),
Offset(38.085941833582574, 12.31061311607528),
Offset(38.27625642592556, 12.404004835577497),
Offset(38.365043467364515, 12.422731815500718),
Offset(38.39177516708328, 12.405483221944),
Offset(38.39999999999928, 12.399999999999999),
],
),
_PathCubicTo(
<Offset>[
Offset(13.560000000000517, 34.2418038940446),
Offset(13.34644533538299, 34.01947703286322),
Offset(12.56275461616698, 33.114689519344395),
Offset(10.993043768195564, 30.686347028683148),
Offset(9.379533331300514, 24.360695707631216),
Offset(12.353030203338722, 15.151389477294442),
Offset(17.899794605494172, 10.630966525485752),
Offset(22.425698467839702, 9.190404454839666),
Offset(25.870855747196508, 8.827291399675353),
Offset(28.65628483345143, 8.680633467161003),
Offset(30.969946383083386, 8.730462124971844),
Offset(32.899838695008256, 8.894029569136269),
Offset(34.44044599227237, 9.18552415739985),
Offset(35.63494972516465, 9.535252759248047),
Offset(36.546519974101585, 9.876862722402706),
Offset(37.228549771064834, 10.172767807409217),
Offset(37.723047912888134, 10.403737344999264),
Offset(38.06207789310096, 10.561907228472755),
Offset(38.26998775287766, 10.646137691510413),
Offset(38.3649977396311, 10.658981491287307),
Offset(38.39177516708328, 10.638487680623824),
Offset(38.39999999999928, 10.631994628904),
],
<Offset>[
Offset(14.598196105955916, 35.28),
Offset(14.362527974420836, 35.07932531421663),
Offset(13.488761527058955, 34.25408124733886),
Offset(11.676784736968232, 31.985654766081694),
Offset(9.429808093900096, 25.828065721324922),
Offset(11.475946990204495, 16.329147810659144),
Offset(16.56322225421536, 11.257218582169475),
Offset(20.938019268402986, 9.365912090737192),
Offset(24.338120402505368, 8.660453150227216),
Offset(27.09565624803725, 8.238369787647443),
Offset(29.40005588071775, 8.057921116777507),
Offset(31.332556245926995, 8.027740692768422),
Offset(32.89132727619159, 8.16282076499266),
Offset(34.112618212708696, 8.390088668883216),
Offset(35.05230836066845, 8.637554922369567),
Offset(35.75949162107706, 8.862643824738257),
Offset(36.273852878713946, 9.042086636666099),
Offset(36.626386285670925, 9.164872782556836),
Offset(36.84112726632846, 9.227431819061039),
Offset(36.936412445625905, 9.230470271569121),
Offset(36.96059844905146, 9.207310962592),
Offset(36.96800537109528, 9.2),
],
<Offset>[
Offset(15.880000000000516, 35.28),
Offset(15.644047102772983, 35.10634283548437),
Offset(14.763778839728777, 34.385808523102554),
Offset(12.900965572876494, 32.365658060518534),
Offset(10.36668455340049, 26.702870683829083),
Offset(11.66156054516918, 17.597647991327378),
Offset(16.12472546425052, 12.468914501187037),
Offset(20.127986072289563, 10.392634956401036),
Offset(23.288935167323658, 9.503652332685864),
Offset(25.859225534316927, 8.928761748820826),
Offset(28.015752170717334, 8.611875263469875),
Offset(29.83025760335727, 8.460479886283995),
Offset(31.303683297111053, 8.487788855269121),
Offset(32.465912820813585, 8.62292265254484),
Offset(33.36484497656955, 8.794912813980165),
Offset(34.04383960722998, 8.960757482357865),
Offset(34.538654202068464, 9.096129729835509),
Offset(34.8776803980684, 9.188736723038446),
Offset(35.08326012226138, 9.233700492108937),
Offset(35.17266212141249, 9.23051599930254),
Offset(35.19360290773128, 9.207310962592),
Offset(35.19999999999928, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(15.880000000000516, 35.28),
Offset(15.644047102772983, 35.10634283548437),
Offset(14.763778839728777, 34.385808523102554),
Offset(12.900965572876494, 32.365658060518534),
Offset(10.36668455340049, 26.702870683829083),
Offset(11.66156054516918, 17.597647991327378),
Offset(16.12472546425052, 12.468914501187037),
Offset(20.127986072289563, 10.392634956401036),
Offset(23.288935167323658, 9.503652332685864),
Offset(25.859225534316927, 8.928761748820826),
Offset(28.015752170717334, 8.611875263469875),
Offset(29.83025760335727, 8.460479886283995),
Offset(31.303683297111053, 8.487788855269121),
Offset(32.465912820813585, 8.62292265254484),
Offset(33.36484497656955, 8.794912813980165),
Offset(34.04383960722998, 8.960757482357865),
Offset(34.538654202068464, 9.096129729835509),
Offset(34.8776803980684, 9.188736723038446),
Offset(35.08326012226138, 9.233700492108937),
Offset(35.17266212141249, 9.23051599930254),
Offset(35.19360290773128, 9.207310962592),
Offset(35.19999999999928, 9.2),
],
<Offset>[
Offset(17.040000000000518, 35.28),
Offset(16.803789397046874, 35.13079300669713),
Offset(15.9176371557853, 34.50501836526003),
Offset(14.008818167373427, 32.70955139989342),
Offset(11.21453397722401, 27.494546977854696),
Offset(11.829536102709142, 18.74560846828623),
Offset(15.727896995619327, 13.565468562686451),
Offset(19.394926583348056, 11.321793098066518),
Offset(22.339449151197982, 10.266726197725939),
Offset(24.74028711122483, 9.553548955882674),
Offset(26.762992438077134, 9.113189710358885),
Offset(28.47071545071013, 8.852097876220133),
Offset(29.86690578044879, 8.781876731325752),
Offset(30.975686224160835, 8.833631499898434),
Offset(31.83773343115353, 8.937317713135464),
Offset(32.49121806714176, 9.049547852996874),
Offset(32.968343428118885, 9.14503735788047),
Offset(33.295145940930645, 9.210332983657782),
Offset(33.49243496047819, 9.239373482157845),
Offset(33.57651283087494, 9.230557381741002),
Offset(33.59451677805528, 9.207310962592),
Offset(33.599999999999284, 9.2),
],
<Offset>[
Offset(17.040000000000518, 35.28),
Offset(16.803789397046874, 35.13079300669713),
Offset(15.9176371557853, 34.50501836526003),
Offset(14.008818167373427, 32.70955139989342),
Offset(11.21453397722401, 27.494546977854696),
Offset(11.829536102709142, 18.74560846828623),
Offset(15.727896995619327, 13.565468562686451),
Offset(19.394926583348056, 11.321793098066518),
Offset(22.339449151197982, 10.266726197725939),
Offset(24.74028711122483, 9.553548955882674),
Offset(26.762992438077134, 9.113189710358885),
Offset(28.47071545071013, 8.852097876220133),
Offset(29.86690578044879, 8.781876731325752),
Offset(30.975686224160835, 8.833631499898434),
Offset(31.83773343115353, 8.937317713135464),
Offset(32.49121806714176, 9.049547852996874),
Offset(32.968343428118885, 9.14503735788047),
Offset(33.295145940930645, 9.210332983657782),
Offset(33.49243496047819, 9.239373482157845),
Offset(33.57651283087494, 9.230557381741002),
Offset(33.59451677805528, 9.207310962592),
Offset(33.599999999999284, 9.2),
],
),
_PathCubicTo(
<Offset>[
Offset(17.040000000000518, 35.28),
Offset(16.803789397046874, 35.13079300669713),
Offset(15.9176371557853, 34.50501836526003),
Offset(14.008818167373427, 32.70955139989342),
Offset(11.21453397722401, 27.494546977854696),
Offset(11.829536102709142, 18.74560846828623),
Offset(15.727896995619327, 13.565468562686451),
Offset(19.394926583348056, 11.321793098066518),
Offset(22.339449151197982, 10.266726197725939),
Offset(24.74028711122483, 9.553548955882674),
Offset(26.762992438077134, 9.113189710358885),
Offset(28.47071545071013, 8.852097876220133),
Offset(29.86690578044879, 8.781876731325752),
Offset(30.975686224160835, 8.833631499898434),
Offset(31.83773343115353, 8.937317713135464),
Offset(32.49121806714176, 9.049547852996874),
Offset(32.968343428118885, 9.14503735788047),
Offset(33.295145940930645, 9.210332983657782),
Offset(33.49243496047819, 9.239373482157845),
Offset(33.57651283087494, 9.230557381741002),
Offset(33.59451677805528, 9.207310962592),
Offset(33.599999999999284, 9.2),
],
<Offset>[
Offset(17.040000000000518, 37.6),
Offset(16.75488905462135, 37.45027759524491),
Offset(15.67921747147036, 36.81273499737307),
Offset(13.321031488623651, 34.92525658888729),
Offset(9.631181389172786, 29.190245825501734),
Offset(9.533615148791444, 19.08155958336615),
Offset(13.534788872620497, 12.771811625424068),
Offset(17.53661030001709, 9.855674120183501),
Offset(20.813301421117835, 8.36775416547459),
Offset(23.490712697101138, 7.315672109698481),
Offset(25.760363544299118, 6.607670245078484),
Offset(27.687479470837854, 6.133013570925856),
Offset(29.27873002833553, 5.908321698001231),
Offset(30.554268529453648, 5.85317830659293),
Offset(31.55292363284293, 5.883094622303428),
Offset(32.31363732586374, 5.944304772820427),
Offset(32.87052817202896, 6.00441580998131),
Offset(33.25195341969197, 6.045264069382277),
Offset(33.481088980380385, 6.0577231585914735),
Offset(33.57643006599802, 6.038258800665901),
Offset(33.59451677805528, 6.009138703240001),
Offset(33.599999999999284, 6.0),
],
<Offset>[
Offset(17.040000000000518, 37.6),
Offset(16.75488905462135, 37.45027759524491),
Offset(15.67921747147036, 36.81273499737307),
Offset(13.321031488623651, 34.92525658888729),
Offset(9.631181389172786, 29.190245825501734),
Offset(9.533615148791444, 19.08155958336615),
Offset(13.534788872620497, 12.771811625424068),
Offset(17.53661030001709, 9.855674120183501),
Offset(20.813301421117835, 8.36775416547459),
Offset(23.490712697101138, 7.315672109698481),
Offset(25.760363544299118, 6.607670245078484),
Offset(27.687479470837854, 6.133013570925856),
Offset(29.27873002833553, 5.908321698001231),
Offset(30.554268529453648, 5.85317830659293),
Offset(31.55292363284293, 5.883094622303428),
Offset(32.31363732586374, 5.944304772820427),
Offset(32.87052817202896, 6.00441580998131),
Offset(33.25195341969197, 6.045264069382277),
Offset(33.481088980380385, 6.0577231585914735),
Offset(33.57643006599802, 6.038258800665901),
Offset(33.59451677805528, 6.009138703240001),
Offset(33.599999999999284, 6.0),
],
),
_PathCubicTo(
<Offset>[
Offset(17.040000000000518, 37.6),
Offset(16.75488905462135, 37.45027759524491),
Offset(15.67921747147036, 36.81273499737307),
Offset(13.321031488623651, 34.92525658888729),
Offset(9.631181389172786, 29.190245825501734),
Offset(9.533615148791444, 19.08155958336615),
Offset(13.534788872620497, 12.771811625424068),
Offset(17.53661030001709, 9.855674120183501),
Offset(20.813301421117835, 8.36775416547459),
Offset(23.490712697101138, 7.315672109698481),
Offset(25.760363544299118, 6.607670245078484),
Offset(27.687479470837854, 6.133013570925856),
Offset(29.27873002833553, 5.908321698001231),
Offset(30.554268529453648, 5.85317830659293),
Offset(31.55292363284293, 5.883094622303428),
Offset(32.31363732586374, 5.944304772820427),
Offset(32.87052817202896, 6.00441580998131),
Offset(33.25195341969197, 6.045264069382277),
Offset(33.481088980380385, 6.0577231585914735),
Offset(33.57643006599802, 6.038258800665901),
Offset(33.59451677805528, 6.009138703240001),
Offset(33.599999999999284, 6.0),
],
<Offset>[
Offset(19.360000000000518, 37.6),
Offset(19.07437364316913, 37.49917793767044),
Offset(17.9869341035834, 37.05115468168801),
Offset(15.536736677617519, 35.61304326763707),
Offset(11.326880236819823, 30.773598413552957),
Offset(9.869566263871368, 21.377480537283848),
Offset(12.741131935358112, 14.964919748422895),
Offset(16.070491322134075, 11.713990403514464),
Offset(18.914329388866488, 9.893901895554741),
Offset(21.252835850916945, 8.565246523822175),
Offset(23.254844079018717, 7.610299138856501),
Offset(24.968395165543576, 6.916249550798135),
Offset(26.405174995011006, 6.496497450114491),
Offset(27.573815336148144, 6.274596001300116),
Offset(28.498700542010894, 6.167904420614027),
Offset(29.208394245687288, 6.1218855140984445),
Offset(29.7299066241298, 6.102231066071232),
Offset(30.08688450541647, 6.08845659062095),
Offset(30.29943865681401, 6.069069138689285),
Offset(30.384131484922918, 6.038341565542824),
Offset(30.39634451870328, 6.009138703240001),
Offset(30.39999999999928, 6.0),
],
<Offset>[
Offset(19.360000000000518, 37.6),
Offset(19.07437364316913, 37.49917793767044),
Offset(17.9869341035834, 37.05115468168801),
Offset(15.536736677617519, 35.61304326763707),
Offset(11.326880236819823, 30.773598413552957),
Offset(9.869566263871368, 21.377480537283848),
Offset(12.741131935358112, 14.964919748422895),
Offset(16.070491322134075, 11.713990403514464),
Offset(18.914329388866488, 9.893901895554741),
Offset(21.252835850916945, 8.565246523822175),
Offset(23.254844079018717, 7.610299138856501),
Offset(24.968395165543576, 6.916249550798135),
Offset(26.405174995011006, 6.496497450114491),
Offset(27.573815336148144, 6.274596001300116),
Offset(28.498700542010894, 6.167904420614027),
Offset(29.208394245687288, 6.1218855140984445),
Offset(29.7299066241298, 6.102231066071232),
Offset(30.08688450541647, 6.08845659062095),
Offset(30.29943865681401, 6.069069138689285),
Offset(30.384131484922918, 6.038341565542824),
Offset(30.39634451870328, 6.009138703240001),
Offset(30.39999999999928, 6.0),
],
),
_PathClose(
),
_PathMoveTo(
<Offset>[
Offset(15.879999999999999, 16.720000000000002),
Offset(16.035249842176654, 16.55046612710212),
Offset(16.671136314247786, 15.924075466198163),
Offset(18.403259002874208, 14.640016548567434),
Offset(23.0335052578099, 13.137279902652425),
Offset(30.028928176510686, 14.910039070687475),
Offset(33.66959044824132, 18.81816999928563),
Offset(34.9945163389376, 22.121586779464756),
Offset(35.49811700796528, 24.6954285906963),
Offset(35.85582084730699, 26.831776518294085),
Offset(36.03678332094205, 28.656030985712853),
Offset(36.09614544233611, 30.213154328638034),
Offset(36.0090893140178, 31.476229121865174),
Offset(35.83725437847175, 32.46654819898878),
Offset(35.64332336305503, 33.22869754063639),
Offset(35.46448553745482, 33.80270212376941),
Offset(35.32117625078855, 34.22110211302876),
Offset(35.22322056797849, 34.50928803724247),
Offset(35.17402796304459, 34.6869030806399),
Offset(35.1733242404286, 34.768904647903355),
Offset(35.193602907732, 34.792689037407996),
Offset(35.2, 34.8),
],
),
_PathCubicTo(
<Offset>[
Offset(15.879999999999999, 16.720000000000002),
Offset(16.035249842176654, 16.55046612710212),
Offset(16.671136314247786, 15.924075466198163),
Offset(18.403259002874208, 14.640016548567434),
Offset(23.0335052578099, 13.137279902652425),
Offset(30.028928176510686, 14.910039070687475),
Offset(33.66959044824132, 18.81816999928563),
Offset(34.9945163389376, 22.121586779464756),
Offset(35.49811700796528, 24.6954285906963),
Offset(35.85582084730699, 26.831776518294085),
Offset(36.03678332094205, 28.656030985712853),
Offset(36.09614544233611, 30.213154328638034),
Offset(36.0090893140178, 31.476229121865174),
Offset(35.83725437847175, 32.46654819898878),
Offset(35.64332336305503, 33.22869754063639),
Offset(35.46448553745482, 33.80270212376941),
Offset(35.32117625078855, 34.22110211302876),
Offset(35.22322056797849, 34.50928803724247),
Offset(35.17402796304459, 34.6869030806399),
Offset(35.1733242404286, 34.768904647903355),
Offset(35.193602907732, 34.792689037407996),
Offset(35.2, 34.8),
],
<Offset>[
Offset(32.12, 16.720000000000006),
Offset(32.27164196201112, 16.892768524080786),
Offset(32.82515273903908, 17.59301325640275),
Offset(33.91319532583128, 19.454523299815868),
Offset(34.90339719133917, 24.22074801901099),
Offset(32.380585982070144, 30.981485748111357),
Offset(28.113991887404627, 34.16992686027743),
Offset(24.731683493756478, 35.1298007627815),
Offset(22.20531278220585, 35.37846270125735),
Offset(20.190682924017636, 35.57879741715995),
Offset(18.498147063979243, 35.67443324215898),
Offset(17.062555305276174, 35.69580618774398),
Offset(15.894204080746132, 35.59345938665801),
Offset(14.974082025333214, 35.41647206193909),
Offset(14.263761727230776, 35.22236612881059),
Offset(13.727783976219687, 35.045767312715526),
Offset(13.33682541549443, 34.90580890565822),
Offset(13.067738168049956, 34.811635685913174),
Offset(12.902475698079991, 34.766324941324584),
Offset(12.827234172902884, 34.76948400204182),
Offset(12.806397092268, 34.792689037407996),
Offset(12.799999999999999, 34.8),
],
<Offset>[
Offset(32.12, 16.720000000000006),
Offset(32.27164196201112, 16.892768524080786),
Offset(32.82515273903908, 17.59301325640275),
Offset(33.91319532583128, 19.454523299815868),
Offset(34.90339719133917, 24.22074801901099),
Offset(32.380585982070144, 30.981485748111357),
Offset(28.113991887404627, 34.16992686027743),
Offset(24.731683493756478, 35.1298007627815),
Offset(22.20531278220585, 35.37846270125735),
Offset(20.190682924017636, 35.57879741715995),
Offset(18.498147063979243, 35.67443324215898),
Offset(17.062555305276174, 35.69580618774398),
Offset(15.894204080746132, 35.59345938665801),
Offset(14.974082025333214, 35.41647206193909),
Offset(14.263761727230776, 35.22236612881059),
Offset(13.727783976219687, 35.045767312715526),
Offset(13.33682541549443, 34.90580890565822),
Offset(13.067738168049956, 34.811635685913174),
Offset(12.902475698079991, 34.766324941324584),
Offset(12.827234172902884, 34.76948400204182),
Offset(12.806397092268, 34.792689037407996),
Offset(12.799999999999999, 34.8),
],
),
_PathCubicTo(
<Offset>[
Offset(32.12, 16.720000000000006),
Offset(32.27164196201112, 16.892768524080786),
Offset(32.82515273903908, 17.59301325640275),
Offset(33.91319532583128, 19.454523299815868),
Offset(34.90339719133917, 24.22074801901099),
Offset(32.380585982070144, 30.981485748111357),
Offset(28.113991887404627, 34.16992686027743),
Offset(24.731683493756478, 35.1298007627815),
Offset(22.20531278220585, 35.37846270125735),
Offset(20.190682924017636, 35.57879741715995),
Offset(18.498147063979243, 35.67443324215898),
Offset(17.062555305276174, 35.69580618774398),
Offset(15.894204080746132, 35.59345938665801),
Offset(14.974082025333214, 35.41647206193909),
Offset(14.263761727230776, 35.22236612881059),
Offset(13.727783976219687, 35.045767312715526),
Offset(13.33682541549443, 34.90580890565822),
Offset(13.067738168049956, 34.811635685913174),
Offset(12.902475698079991, 34.766324941324584),
Offset(12.827234172902884, 34.76948400204182),
Offset(12.806397092268, 34.792689037407996),
Offset(12.799999999999999, 34.8),
],
<Offset>[
Offset(32.12, 29.480000000000004),
Offset(32.00269007867074, 29.64993376109358),
Offset(31.51384447530691, 30.285454733024483),
Offset(30.130368592707512, 31.640901839282144),
Offset(26.194957957057433, 33.5470916810697),
Offset(19.753020735522814, 32.82921688105094),
Offset(16.051897210911072, 29.804813705334308),
Offset(14.51094393543618, 27.066146384424904),
Offset(13.811500266765027, 24.934116523874938),
Offset(13.31802364633732, 23.270474763146886),
Offset(12.983688148200141, 21.89407618311678),
Offset(12.754757415978641, 20.740842508625462),
Offset(12.65923744412319, 19.788906703373133),
Offset(12.656284704443689, 19.023979498758813),
Offset(12.697307836522478, 18.424139129234387),
Offset(12.751089899190589, 17.966930371745068),
Offset(12.798841506999857, 17.632390392212844),
Offset(12.83017930123726, 17.403756657397903),
Offset(12.840072807542027, 17.267248161709546),
Offset(12.826778966079804, 17.21184180612876),
Offset(12.806397092268, 17.202741610971998),
Offset(12.799999999999999, 17.2),
],
<Offset>[
Offset(32.12, 29.480000000000004),
Offset(32.00269007867074, 29.64993376109358),
Offset(31.51384447530691, 30.285454733024483),
Offset(30.130368592707512, 31.640901839282144),
Offset(26.194957957057433, 33.5470916810697),
Offset(19.753020735522814, 32.82921688105094),
Offset(16.051897210911072, 29.804813705334308),
Offset(14.51094393543618, 27.066146384424904),
Offset(13.811500266765027, 24.934116523874938),
Offset(13.31802364633732, 23.270474763146886),
Offset(12.983688148200141, 21.89407618311678),
Offset(12.754757415978641, 20.740842508625462),
Offset(12.65923744412319, 19.788906703373133),
Offset(12.656284704443689, 19.023979498758813),
Offset(12.697307836522478, 18.424139129234387),
Offset(12.751089899190589, 17.966930371745068),
Offset(12.798841506999857, 17.632390392212844),
Offset(12.83017930123726, 17.403756657397903),
Offset(12.840072807542027, 17.267248161709546),
Offset(12.826778966079804, 17.21184180612876),
Offset(12.806397092268, 17.202741610971998),
Offset(12.799999999999999, 17.2),
],
),
_PathCubicTo(
<Offset>[
Offset(32.12, 29.480000000000004),
Offset(32.00269007867074, 29.64993376109358),
Offset(31.51384447530691, 30.285454733024483),
Offset(30.130368592707512, 31.640901839282144),
Offset(26.194957957057433, 33.5470916810697),
Offset(19.753020735522814, 32.82921688105094),
Offset(16.051897210911072, 29.804813705334308),
Offset(14.51094393543618, 27.066146384424904),
Offset(13.811500266765027, 24.934116523874938),
Offset(13.31802364633732, 23.270474763146886),
Offset(12.983688148200141, 21.89407618311678),
Offset(12.754757415978641, 20.740842508625462),
Offset(12.65923744412319, 19.788906703373133),
Offset(12.656284704443689, 19.023979498758813),
Offset(12.697307836522478, 18.424139129234387),
Offset(12.751089899190589, 17.966930371745068),
Offset(12.798841506999857, 17.632390392212844),
Offset(12.83017930123726, 17.403756657397903),
Offset(12.840072807542027, 17.267248161709546),
Offset(12.826778966079804, 17.21184180612876),
Offset(12.806397092268, 17.202741610971998),
Offset(12.799999999999999, 17.2),
],
<Offset>[
Offset(15.879999999999997, 29.480000000000004),
Offset(15.766297958836272, 29.30763136411491),
Offset(15.359828050515612, 28.6165169428199),
Offset(14.620432269750436, 26.826395088033706),
Offset(14.325066023528167, 22.463623564711135),
Offset(17.401362929963348, 16.757770203627054),
Offset(21.607495771747768, 14.453056844342509),
Offset(24.7737767806173, 14.057932401108161),
Offset(27.10430449252446, 14.251082413313888),
Offset(28.983161569626667, 14.523453864281027),
Offset(30.522324405162944, 14.87567392667065),
Offset(31.78834755303858, 15.258190649519511),
Offset(32.77412267739486, 15.671676438580297),
Offset(33.51945705758222, 16.074055635808506),
Offset(34.076869472346736, 16.430470541060192),
Offset(34.487791460425726, 16.723865182798942),
Offset(34.78319234229397, 16.947683599583385),
Offset(34.98566170116579, 17.1014090087272),
Offset(35.11162507250663, 17.187826301024863),
Offset(35.17286903360552, 17.211262451990294),
Offset(35.193602907732, 17.202741610971998),
Offset(35.2, 17.2),
],
<Offset>[
Offset(15.879999999999997, 29.480000000000004),
Offset(15.766297958836272, 29.30763136411491),
Offset(15.359828050515612, 28.6165169428199),
Offset(14.620432269750436, 26.826395088033706),
Offset(14.325066023528167, 22.463623564711135),
Offset(17.401362929963348, 16.757770203627054),
Offset(21.607495771747768, 14.453056844342509),
Offset(24.7737767806173, 14.057932401108161),
Offset(27.10430449252446, 14.251082413313888),
Offset(28.983161569626667, 14.523453864281027),
Offset(30.522324405162944, 14.87567392667065),
Offset(31.78834755303858, 15.258190649519511),
Offset(32.77412267739486, 15.671676438580297),
Offset(33.51945705758222, 16.074055635808506),
Offset(34.076869472346736, 16.430470541060192),
Offset(34.487791460425726, 16.723865182798942),
Offset(34.78319234229397, 16.947683599583385),
Offset(34.98566170116579, 17.1014090087272),
Offset(35.11162507250663, 17.187826301024863),
Offset(35.17286903360552, 17.211262451990294),
Offset(35.193602907732, 17.202741610971998),
Offset(35.2, 17.2),
],
),
_PathCubicTo(
<Offset>[
Offset(15.879999999999997, 29.480000000000004),
Offset(15.766297958836272, 29.30763136411491),
Offset(15.359828050515612, 28.6165169428199),
Offset(14.620432269750436, 26.826395088033706),
Offset(14.325066023528167, 22.463623564711135),
Offset(17.401362929963348, 16.757770203627054),
Offset(21.607495771747768, 14.453056844342509),
Offset(24.7737767806173, 14.057932401108161),
Offset(27.10430449252446, 14.251082413313888),
Offset(28.983161569626667, 14.523453864281027),
Offset(30.522324405162944, 14.87567392667065),
Offset(31.78834755303858, 15.258190649519511),
Offset(32.77412267739486, 15.671676438580297),
Offset(33.51945705758222, 16.074055635808506),
Offset(34.076869472346736, 16.430470541060192),
Offset(34.487791460425726, 16.723865182798942),
Offset(34.78319234229397, 16.947683599583385),
Offset(34.98566170116579, 17.1014090087272),
Offset(35.11162507250663, 17.187826301024863),
Offset(35.17286903360552, 17.211262451990294),
Offset(35.193602907732, 17.202741610971998),
Offset(35.2, 17.2),
],
<Offset>[
Offset(15.879999999999999, 16.720000000000002),
Offset(16.035249842176654, 16.55046612710212),
Offset(16.671136314247786, 15.924075466198163),
Offset(18.403259002874208, 14.640016548567434),
Offset(23.0335052578099, 13.137279902652425),
Offset(30.028928176510686, 14.910039070687475),
Offset(33.66959044824132, 18.81816999928563),
Offset(34.9945163389376, 22.121586779464756),
Offset(35.49811700796528, 24.6954285906963),
Offset(35.85582084730699, 26.831776518294085),
Offset(36.03678332094205, 28.656030985712853),
Offset(36.09614544233611, 30.213154328638034),
Offset(36.0090893140178, 31.476229121865174),
Offset(35.83725437847175, 32.46654819898878),
Offset(35.64332336305503, 33.22869754063639),
Offset(35.46448553745482, 33.80270212376941),
Offset(35.32117625078855, 34.22110211302876),
Offset(35.22322056797849, 34.50928803724247),
Offset(35.17402796304459, 34.6869030806399),
Offset(35.1733242404286, 34.768904647903355),
Offset(35.193602907732, 34.792689037407996),
Offset(35.2, 34.8),
],
<Offset>[
Offset(15.879999999999999, 16.720000000000002),
Offset(16.035249842176654, 16.55046612710212),
Offset(16.671136314247786, 15.924075466198163),
Offset(18.403259002874208, 14.640016548567434),
Offset(23.0335052578099, 13.137279902652425),
Offset(30.028928176510686, 14.910039070687475),
Offset(33.66959044824132, 18.81816999928563),
Offset(34.9945163389376, 22.121586779464756),
Offset(35.49811700796528, 24.6954285906963),
Offset(35.85582084730699, 26.831776518294085),
Offset(36.03678332094205, 28.656030985712853),
Offset(36.09614544233611, 30.213154328638034),
Offset(36.0090893140178, 31.476229121865174),
Offset(35.83725437847175, 32.46654819898878),
Offset(35.64332336305503, 33.22869754063639),
Offset(35.46448553745482, 33.80270212376941),
Offset(35.32117625078855, 34.22110211302876),
Offset(35.22322056797849, 34.50928803724247),
Offset(35.17402796304459, 34.6869030806399),
Offset(35.1733242404286, 34.768904647903355),
Offset(35.193602907732, 34.792689037407996),
Offset(35.2, 34.8),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
0.857142857143,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(38.0, 26.0),
Offset(37.95473429086978, 26.294643953040044),
Offset(37.72034201765203, 27.428150364066923),
Offset(36.77771521052353, 30.06052753469164),
Offset(32.86770633230866, 35.01652324483241),
Offset(24.048041351653396, 38.14205402438172),
Offset(17.355303440951563, 36.48390994993835),
Offset(13.758359800160616, 33.75237437842877),
Offset(11.83452659356501, 31.211189693616653),
Offset(10.801411310099256, 29.079099978817517),
Offset(10.259029387048772, 27.34450693137431),
Offset(9.993407713414326, 25.953297856794705),
Offset(9.883312535673422, 24.848018298461085),
Offset(9.857878923767148, 23.97971539572578),
Offset(9.874779651667918, 23.308516008091793),
Offset(9.908648574481903, 22.80257985546041),
Offset(9.94452503300979, 22.436790656274262),
Offset(9.974012714390186, 22.191221223059088),
Offset(9.992956927188366, 22.049937345519467),
Offset(9.999948151863302, 22.000362970565853),
Offset(10.0, 22.0),
Offset(10.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 26.0),
Offset(9.960954773913805, 25.704467406525104),
Offset(9.86858956111531, 24.55067141543833),
Offset(10.036445688183749, 21.759653825642616),
Offset(12.402375412430613, 15.907095458007305),
Offset(19.994105175585858, 10.437079715056495),
Offset(26.88341391070376, 10.154931411525617),
Offset(31.101309765885755, 11.770061340753355),
Offset(33.659721355964926, 13.670925340326216),
Offset(35.24849714823581, 15.428459187162975),
Offset(36.254869847648365, 16.94180157425432),
Offset(36.89940519521972, 18.202988217233095),
Offset(37.31457484218441, 19.233229943465915),
Offset(37.58211592238032, 20.059679318758054),
Offset(37.7538270521331, 20.708762934698896),
Offset(37.86297500896845, 21.20394493961294),
Offset(37.93095458455382, 21.565147979219446),
Offset(37.971405851728875, 21.809151185892528),
Offset(37.99277889315371, 21.950088087914054),
Offset(37.999948142452794, 21.99963703077851),
Offset(38.0, 22.0),
Offset(38.0, 22.0),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(10.0, 22.0),
Offset(10.045265709130224, 21.705356046959963),
Offset(10.279657982347967, 20.571849635933084),
Offset(11.222284789476467, 17.93947246530836),
Offset(15.132293667691345, 12.983476755167583),
Offset(23.951958648346604, 9.857945975618275),
Offset(30.644696559048437, 11.516090050061646),
Offset(34.24164019983938, 14.247625621571231),
Offset(36.16547340643499, 16.788810306383347),
Offset(37.19858868990075, 18.920900021182483),
Offset(37.74097061295122, 20.65549306862569),
Offset(38.006592286585665, 22.046702143205295),
Offset(38.116687464326574, 23.151981701538915),
Offset(38.142121076232854, 24.02028460427422),
Offset(38.12522034833208, 24.691483991908207),
Offset(38.09135142551809, 25.19742014453959),
Offset(38.05547496699022, 25.563209343725738),
Offset(38.025987285609816, 25.808778776940912),
Offset(38.00704307281163, 25.950062654480533),
Offset(38.0000518481367, 25.999637029434147),
Offset(38.0, 26.0),
Offset(38.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
<Offset>[
Offset(38.0, 22.0),
Offset(38.039045226086195, 22.295532593474903),
Offset(38.131410438884686, 23.449328584561677),
Offset(37.96355431181625, 26.240346174357384),
Offset(35.597624587569385, 32.092904541992695),
Offset(28.005894824414142, 37.5629202849435),
Offset(21.11658608929624, 37.84506858847438),
Offset(16.898690234114245, 36.22993865924664),
Offset(14.340278644035072, 34.329074659673786),
Offset(12.751502851764192, 32.57154081283703),
Offset(11.745130152351626, 31.05819842574568),
Offset(11.10059480478027, 29.797011782766905),
Offset(10.68542515781559, 28.766770056534085),
Offset(10.41788407761968, 27.940320681241946),
Offset(10.246172947866903, 27.291237065301104),
Offset(10.137024991031543, 26.79605506038706),
Offset(10.06904541544619, 26.434852020780554),
Offset(10.028594148271123, 26.190848814107472),
Offset(10.007221106846284, 26.049911912085946),
Offset(10.000051857547207, 26.00036296922149),
Offset(10.0, 26.0),
Offset(10.0, 26.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
0.857142857143,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 38.0),
Offset(21.70535604695996, 37.95473429086978),
Offset(20.571849635933077, 37.72034201765204),
Offset(17.93947246530836, 36.77771521052353),
Offset(12.983476755167583, 32.86770633230866),
Offset(9.857945975618275, 24.048041351653396),
Offset(11.516090050061646, 17.355303440951563),
Offset(14.247625621571231, 13.758359800160616),
Offset(16.788810306383347, 11.83452659356501),
Offset(18.920900021182486, 10.801411310099253),
Offset(20.655493068625688, 10.259029387048775),
Offset(22.04670214320529, 9.99340771341433),
Offset(23.151981701538915, 9.883312535673422),
Offset(24.02028460427422, 9.857878923767148),
Offset(24.691483991908207, 9.874779651667918),
Offset(25.197420144539585, 9.908648574481907),
Offset(25.56320934372574, 9.944525033009786),
Offset(25.808778776940912, 9.974012714390186),
Offset(25.950062654480533, 9.992956927188366),
Offset(25.999637029434147, 9.999948151863302),
Offset(26.0, 10.0),
Offset(26.0, 10.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(22.0, 10.0),
Offset(22.2955325934749, 9.960954773913809),
Offset(23.449328584561673, 9.868589561115314),
Offset(26.240346174357384, 10.036445688183749),
Offset(32.092904541992695, 12.402375412430613),
Offset(37.5629202849435, 19.994105175585858),
Offset(37.84506858847438, 26.88341391070376),
Offset(36.22993865924664, 31.101309765885755),
Offset(34.329074659673786, 33.659721355964926),
Offset(32.57154081283703, 35.24849714823581),
Offset(31.058198425745676, 36.25486984764837),
Offset(29.7970117827669, 36.89940519521973),
Offset(28.766770056534085, 37.31457484218441),
Offset(27.940320681241946, 37.58211592238032),
Offset(27.291237065301104, 37.7538270521331),
Offset(26.796055060387058, 37.862975008968455),
Offset(26.434852020780554, 37.93095458455382),
Offset(26.190848814107472, 37.971405851728875),
Offset(26.049911912085946, 37.99277889315371),
Offset(26.00036296922149, 37.999948142452794),
Offset(26.0, 38.0),
Offset(26.0, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(26.0, 10.0),
Offset(26.29464395304004, 10.045265709130227),
Offset(27.428150364066923, 10.27965798234797),
Offset(30.06052753469164, 11.222284789476467),
Offset(35.01652324483241, 15.132293667691345),
Offset(38.14205402438172, 23.951958648346604),
Offset(36.48390994993835, 30.644696559048437),
Offset(33.75237437842877, 34.24164019983938),
Offset(31.211189693616653, 36.16547340643499),
Offset(29.07909997881752, 37.198588689900745),
Offset(27.344506931374305, 37.74097061295122),
Offset(25.953297856794702, 38.00659228658567),
Offset(24.848018298461085, 38.116687464326574),
Offset(23.97971539572578, 38.142121076232854),
Offset(23.308516008091793, 38.12522034833208),
Offset(22.802579855460408, 38.09135142551809),
Offset(22.436790656274265, 38.05547496699022),
Offset(22.191221223059088, 38.025987285609816),
Offset(22.049937345519467, 38.00704307281163),
Offset(22.000362970565853, 38.0000518481367),
Offset(22.0, 38.0),
Offset(22.0, 38.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
<Offset>[
Offset(26.0, 38.0),
Offset(25.7044674065251, 38.0390452260862),
Offset(24.550671415438327, 38.13141043888469),
Offset(21.759653825642616, 37.96355431181625),
Offset(15.907095458007305, 35.597624587569385),
Offset(10.437079715056495, 28.005894824414142),
Offset(10.154931411525617, 21.11658608929624),
Offset(11.770061340753355, 16.898690234114245),
Offset(13.670925340326216, 14.340278644035072),
Offset(15.428459187162979, 12.751502851764188),
Offset(16.941801574254317, 11.74513015235163),
Offset(18.20298821723309, 11.100594804780274),
Offset(19.233229943465915, 10.68542515781559),
Offset(20.059679318758054, 10.41788407761968),
Offset(20.708762934698896, 10.246172947866903),
Offset(21.203944939612935, 10.137024991031547),
Offset(21.565147979219454, 10.069045415446187),
Offset(21.809151185892528, 10.028594148271123),
Offset(21.950088087914054, 10.007221106846284),
Offset(21.99963703077851, 10.000051857547207),
Offset(22.0, 10.0),
Offset(22.0, 10.0),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/add_event.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/add_event.g.dart",
"repo_id": "flutter",
"token_count": 97338
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
/// Overrides the default values of visual properties for descendant
/// [AppBar] widgets.
///
/// Descendant widgets obtain the current [AppBarTheme] object with
/// `AppBarTheme.of(context)`. Instances of [AppBarTheme] can be customized
/// with [AppBarTheme.copyWith].
///
/// Typically an [AppBarTheme] is specified as part of the overall [Theme] with
/// [ThemeData.appBarTheme].
///
/// All [AppBarTheme] properties are `null` by default. When null, the [AppBar]
/// compute its own default values, typically based on the overall theme's
/// [ThemeData.colorScheme], [ThemeData.textTheme], and [ThemeData.iconTheme].
@immutable
class AppBarTheme with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.appBarTheme].
const AppBarTheme({
Color? color,
Color? backgroundColor,
this.foregroundColor,
this.elevation,
this.scrolledUnderElevation,
this.shadowColor,
this.surfaceTintColor,
this.shape,
this.iconTheme,
this.actionsIconTheme,
this.centerTitle,
this.titleSpacing,
this.toolbarHeight,
this.toolbarTextStyle,
this.titleTextStyle,
this.systemOverlayStyle,
}) : assert(
color == null || backgroundColor == null,
'The color and backgroundColor parameters mean the same thing. Only specify one.',
),
backgroundColor = backgroundColor ?? color;
/// Overrides the default value of [AppBar.backgroundColor] in all
/// descendant [AppBar] widgets.
///
/// See also:
///
/// * [foregroundColor], which overrides the default value of
/// [AppBar.foregroundColor] in all descendant [AppBar] widgets.
final Color? backgroundColor;
/// Overrides the default value of [AppBar.foregroundColor] in all
/// descendant [AppBar] widgets.
///
/// See also:
///
/// * [backgroundColor], which overrides the default value of
/// [AppBar.backgroundColor] in all descendant [AppBar] widgets.
final Color? foregroundColor;
/// Overrides the default value of [AppBar.elevation] in all
/// descendant [AppBar] widgets.
final double? elevation;
/// Overrides the default value of [AppBar.scrolledUnderElevation] in all
/// descendant [AppBar] widgets.
final double? scrolledUnderElevation;
/// Overrides the default value of [AppBar.shadowColor] in all
/// descendant [AppBar] widgets.
final Color? shadowColor;
/// Overrides the default value of [AppBar.surfaceTintColor] in all
/// descendant [AppBar] widgets.
final Color? surfaceTintColor;
/// Overrides the default value of [AppBar.shape] in all
/// descendant [AppBar] widgets.
final ShapeBorder? shape;
/// Overrides the default value of [AppBar.iconTheme] in all
/// descendant [AppBar] widgets.
///
/// See also:
///
/// * [actionsIconTheme], which overrides the default value of
/// [AppBar.actionsIconTheme] in all descendant [AppBar] widgets.
/// * [foregroundColor], which overrides the default value
/// [AppBar.foregroundColor] in all descendant [AppBar] widgets.
final IconThemeData? iconTheme;
/// Overrides the default value of [AppBar.actionsIconTheme] in all
/// descendant [AppBar] widgets.
///
/// See also:
///
/// * [iconTheme], which overrides the default value of
/// [AppBar.iconTheme] in all descendant [AppBar] widgets.
/// * [foregroundColor], which overrides the default value
/// [AppBar.foregroundColor] in all descendant [AppBar] widgets.
final IconThemeData? actionsIconTheme;
/// Overrides the default value of [AppBar.centerTitle]
/// property in all descendant [AppBar] widgets.
final bool? centerTitle;
/// Overrides the default value of the obsolete [AppBar.titleSpacing]
/// property in all descendant [AppBar] widgets.
///
/// If null, [AppBar] uses default value of [NavigationToolbar.kMiddleSpacing].
final double? titleSpacing;
/// Overrides the default value of the [AppBar.toolbarHeight]
/// property in all descendant [AppBar] widgets.
///
/// See also:
///
/// * [AppBar.preferredHeightFor], which computes the overall
/// height of an AppBar widget, taking this value into account.
final double? toolbarHeight;
/// Overrides the default value of the obsolete [AppBar.toolbarTextStyle]
/// property in all descendant [AppBar] widgets.
///
/// See also:
///
/// * [titleTextStyle], which overrides the default of [AppBar.titleTextStyle]
/// in all descendant [AppBar] widgets.
final TextStyle? toolbarTextStyle;
/// Overrides the default value of [AppBar.titleTextStyle]
/// property in all descendant [AppBar] widgets.
///
/// See also:
///
/// * [toolbarTextStyle], which overrides the default of [AppBar.toolbarTextStyle]
/// in all descendant [AppBar] widgets.
final TextStyle? titleTextStyle;
/// Overrides the default value of [AppBar.systemOverlayStyle]
/// property in all descendant [AppBar] widgets.
final SystemUiOverlayStyle? systemOverlayStyle;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
AppBarTheme copyWith({
IconThemeData? actionsIconTheme,
Color? color,
Color? backgroundColor,
Color? foregroundColor,
double? elevation,
double? scrolledUnderElevation,
Color? shadowColor,
Color? surfaceTintColor,
ShapeBorder? shape,
IconThemeData? iconTheme,
bool? centerTitle,
double? titleSpacing,
double? toolbarHeight,
TextStyle? toolbarTextStyle,
TextStyle? titleTextStyle,
SystemUiOverlayStyle? systemOverlayStyle,
}) {
assert(
color == null || backgroundColor == null,
'The color and backgroundColor parameters mean the same thing. Only specify one.',
);
return AppBarTheme(
backgroundColor: backgroundColor ?? color ?? this.backgroundColor,
foregroundColor: foregroundColor ?? this.foregroundColor,
elevation: elevation ?? this.elevation,
scrolledUnderElevation: scrolledUnderElevation ?? this.scrolledUnderElevation,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
shape: shape ?? this.shape,
iconTheme: iconTheme ?? this.iconTheme,
actionsIconTheme: actionsIconTheme ?? this.actionsIconTheme,
centerTitle: centerTitle ?? this.centerTitle,
titleSpacing: titleSpacing ?? this.titleSpacing,
toolbarHeight: toolbarHeight ?? this.toolbarHeight,
toolbarTextStyle: toolbarTextStyle ?? this.toolbarTextStyle,
titleTextStyle: titleTextStyle ?? this.titleTextStyle,
systemOverlayStyle: systemOverlayStyle ?? this.systemOverlayStyle,
);
}
/// The [ThemeData.appBarTheme] property of the ambient [Theme].
static AppBarTheme of(BuildContext context) {
return Theme.of(context).appBarTheme;
}
/// Linearly interpolate between two AppBar themes.
///
/// {@macro dart.ui.shadow.lerp}
static AppBarTheme lerp(AppBarTheme? a, AppBarTheme? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return AppBarTheme(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
foregroundColor: Color.lerp(a?.foregroundColor, b?.foregroundColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
scrolledUnderElevation: lerpDouble(a?.scrolledUnderElevation, b?.scrolledUnderElevation, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
shape: ShapeBorder.lerp(a?.shape, b?.shape, t),
iconTheme: IconThemeData.lerp(a?.iconTheme, b?.iconTheme, t),
actionsIconTheme: IconThemeData.lerp(a?.actionsIconTheme, b?.actionsIconTheme, t),
centerTitle: t < 0.5 ? a?.centerTitle : b?.centerTitle,
titleSpacing: lerpDouble(a?.titleSpacing, b?.titleSpacing, t),
toolbarHeight: lerpDouble(a?.toolbarHeight, b?.toolbarHeight, t),
toolbarTextStyle: TextStyle.lerp(a?.toolbarTextStyle, b?.toolbarTextStyle, t),
titleTextStyle: TextStyle.lerp(a?.titleTextStyle, b?.titleTextStyle, t),
systemOverlayStyle: t < 0.5 ? a?.systemOverlayStyle : b?.systemOverlayStyle,
);
}
@override
int get hashCode => Object.hash(
backgroundColor,
foregroundColor,
elevation,
scrolledUnderElevation,
shadowColor,
surfaceTintColor,
shape,
iconTheme,
actionsIconTheme,
centerTitle,
titleSpacing,
toolbarHeight,
toolbarTextStyle,
titleTextStyle,
systemOverlayStyle,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is AppBarTheme
&& other.backgroundColor == backgroundColor
&& other.foregroundColor == foregroundColor
&& other.elevation == elevation
&& other.scrolledUnderElevation == scrolledUnderElevation
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.shape == shape
&& other.iconTheme == iconTheme
&& other.actionsIconTheme == actionsIconTheme
&& other.centerTitle == centerTitle
&& other.titleSpacing == titleSpacing
&& other.toolbarHeight == toolbarHeight
&& other.toolbarTextStyle == toolbarTextStyle
&& other.titleTextStyle == titleTextStyle
&& other.systemOverlayStyle == systemOverlayStyle;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('foregroundColor', foregroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<double>('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<double>('scrolledUnderElevation', scrolledUnderElevation, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<IconThemeData>('iconTheme', iconTheme, defaultValue: null));
properties.add(DiagnosticsProperty<IconThemeData>('actionsIconTheme', actionsIconTheme, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('centerTitle', centerTitle, defaultValue: null));
properties.add(DiagnosticsProperty<double>('titleSpacing', titleSpacing, defaultValue: null));
properties.add(DiagnosticsProperty<double>('toolbarHeight', toolbarHeight, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('toolbarTextStyle', toolbarTextStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('titleTextStyle', titleTextStyle, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/material/app_bar_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/app_bar_theme.dart",
"repo_id": "flutter",
"token_count": 3709
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_theme.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the visual properties of [ButtonBar] widgets.
///
/// Used by [ButtonBarTheme] to control the visual properties of [ButtonBar]
/// instances in a widget subtree.
///
/// To obtain this configuration, use [ButtonBarTheme.of] to access the closest
/// ancestor [ButtonBarTheme] of the current [BuildContext].
///
/// See also:
///
/// * [ButtonBarTheme], an [InheritedWidget] that propagates the theme down
/// its subtree.
/// * [ButtonBar], which uses this to configure itself and its children
/// button widgets.
@immutable
class ButtonBarThemeData with Diagnosticable {
/// Constructs the set of properties used to configure [ButtonBar] widgets.
///
/// Both [buttonMinWidth] and [buttonHeight] must be non-negative if they
/// are not null.
const ButtonBarThemeData({
this.alignment,
this.mainAxisSize,
this.buttonTextTheme,
this.buttonMinWidth,
this.buttonHeight,
this.buttonPadding,
this.buttonAlignedDropdown,
this.layoutBehavior,
this.overflowDirection,
}) : assert(buttonMinWidth == null || buttonMinWidth >= 0.0),
assert(buttonHeight == null || buttonHeight >= 0.0);
/// How the children should be placed along the horizontal axis.
final MainAxisAlignment? alignment;
/// How much horizontal space is available. See [Row.mainAxisSize].
final MainAxisSize? mainAxisSize;
/// Defines a [ButtonBar] button's base colors, and the defaults for
/// the button's minimum size, internal padding, and shape.
///
/// This will override the surrounding [ButtonThemeData.textTheme] setting
/// for buttons contained in the [ButtonBar].
///
/// Despite the name, this property is not a [TextTheme], its value is not a
/// collection of [TextStyle]s.
final ButtonTextTheme? buttonTextTheme;
/// The minimum width for [ButtonBar] buttons.
///
/// This will override the surrounding [ButtonThemeData.minWidth] setting
/// for buttons contained in the [ButtonBar].
///
/// The actual horizontal space allocated for a button's child is
/// at least this value less the theme's horizontal [ButtonThemeData.padding].
final double? buttonMinWidth;
/// The minimum height for [ButtonBar] buttons.
///
/// This will override the surrounding [ButtonThemeData.height] setting
/// for buttons contained in the [ButtonBar].
final double? buttonHeight;
/// Padding for a [ButtonBar] button's child (typically the button's label).
///
/// This will override the surrounding [ButtonThemeData.padding] setting
/// for buttons contained in the [ButtonBar].
final EdgeInsetsGeometry? buttonPadding;
/// If true, then a [DropdownButton] menu's width will match the [ButtonBar]
/// button's width.
///
/// If false, then the dropdown's menu will be wider than
/// its button. In either case the dropdown button will line up the leading
/// edge of the menu's value with the leading edge of the values
/// displayed by the menu items.
///
/// This will override the surrounding [ButtonThemeData.alignedDropdown] setting
/// for buttons contained in the [ButtonBar].
///
/// This property only affects [DropdownButton] contained in a [ButtonBar]
/// and its menu.
final bool? buttonAlignedDropdown;
/// Defines whether a [ButtonBar] should size itself with a minimum size
/// constraint or with padding.
final ButtonBarLayoutBehavior? layoutBehavior;
/// Defines the vertical direction of a [ButtonBar]'s children if it
/// overflows.
///
/// If the [ButtonBar]'s children do not fit into a single row, then they
/// are arranged in a column. The first action is at the top of the
/// column if this property is set to [VerticalDirection.down], since it
/// "starts" at the top and "ends" at the bottom. On the other hand,
/// the first action will be at the bottom of the column if this
/// property is set to [VerticalDirection.up], since it "starts" at the
/// bottom and "ends" at the top.
final VerticalDirection? overflowDirection;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
ButtonBarThemeData copyWith({
MainAxisAlignment? alignment,
MainAxisSize? mainAxisSize,
ButtonTextTheme? buttonTextTheme,
double? buttonMinWidth,
double? buttonHeight,
EdgeInsetsGeometry? buttonPadding,
bool? buttonAlignedDropdown,
ButtonBarLayoutBehavior? layoutBehavior,
VerticalDirection? overflowDirection,
}) {
return ButtonBarThemeData(
alignment: alignment ?? this.alignment,
mainAxisSize: mainAxisSize ?? this.mainAxisSize,
buttonTextTheme: buttonTextTheme ?? this.buttonTextTheme,
buttonMinWidth: buttonMinWidth ?? this.buttonMinWidth,
buttonHeight: buttonHeight ?? this.buttonHeight,
buttonPadding: buttonPadding ?? this.buttonPadding,
buttonAlignedDropdown: buttonAlignedDropdown ?? this.buttonAlignedDropdown,
layoutBehavior: layoutBehavior ?? this.layoutBehavior,
overflowDirection: overflowDirection ?? this.overflowDirection,
);
}
/// Linearly interpolate between two button bar themes.
///
/// If both arguments are null, then null is returned.
///
/// {@macro dart.ui.shadow.lerp}
static ButtonBarThemeData? lerp(ButtonBarThemeData? a, ButtonBarThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return ButtonBarThemeData(
alignment: t < 0.5 ? a?.alignment : b?.alignment,
mainAxisSize: t < 0.5 ? a?.mainAxisSize : b?.mainAxisSize,
buttonTextTheme: t < 0.5 ? a?.buttonTextTheme : b?.buttonTextTheme,
buttonMinWidth: lerpDouble(a?.buttonMinWidth, b?.buttonMinWidth, t),
buttonHeight: lerpDouble(a?.buttonHeight, b?.buttonHeight, t),
buttonPadding: EdgeInsetsGeometry.lerp(a?.buttonPadding, b?.buttonPadding, t),
buttonAlignedDropdown: t < 0.5 ? a?.buttonAlignedDropdown : b?.buttonAlignedDropdown,
layoutBehavior: t < 0.5 ? a?.layoutBehavior : b?.layoutBehavior,
overflowDirection: t < 0.5 ? a?.overflowDirection : b?.overflowDirection,
);
}
@override
int get hashCode => Object.hash(
alignment,
mainAxisSize,
buttonTextTheme,
buttonMinWidth,
buttonHeight,
buttonPadding,
buttonAlignedDropdown,
layoutBehavior,
overflowDirection,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ButtonBarThemeData
&& other.alignment == alignment
&& other.mainAxisSize == mainAxisSize
&& other.buttonTextTheme == buttonTextTheme
&& other.buttonMinWidth == buttonMinWidth
&& other.buttonHeight == buttonHeight
&& other.buttonPadding == buttonPadding
&& other.buttonAlignedDropdown == buttonAlignedDropdown
&& other.layoutBehavior == layoutBehavior
&& other.overflowDirection == overflowDirection;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<MainAxisAlignment>('alignment', alignment, defaultValue: null));
properties.add(DiagnosticsProperty<MainAxisSize>('mainAxisSize', mainAxisSize, defaultValue: null));
properties.add(DiagnosticsProperty<ButtonTextTheme>('textTheme', buttonTextTheme, defaultValue: null));
properties.add(DoubleProperty('minWidth', buttonMinWidth, defaultValue: null));
properties.add(DoubleProperty('height', buttonHeight, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', buttonPadding, defaultValue: null));
properties.add(FlagProperty(
'buttonAlignedDropdown',
value: buttonAlignedDropdown,
ifTrue: 'dropdown width matches button',
));
properties.add(DiagnosticsProperty<ButtonBarLayoutBehavior>('layoutBehavior', layoutBehavior, defaultValue: null));
properties.add(DiagnosticsProperty<VerticalDirection>('overflowDirection', overflowDirection, defaultValue: null));
}
}
/// Applies a button bar theme to descendant [ButtonBar] widgets.
///
/// A button bar theme describes the layout and properties for the buttons
/// contained in a [ButtonBar].
///
/// Descendant widgets obtain the current theme's [ButtonBarTheme] object using
/// [ButtonBarTheme.of]. When a widget uses [ButtonBarTheme.of], it is automatically
/// rebuilt if the theme later changes.
///
/// A button bar theme can be specified as part of the overall Material theme
/// using [ThemeData.buttonBarTheme].
///
/// See also:
///
/// * [ButtonBarThemeData], which describes the actual configuration of a button
/// bar theme.
class ButtonBarTheme extends InheritedWidget {
/// Constructs a button bar theme that configures all descendant [ButtonBar]
/// widgets.
const ButtonBarTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [ButtonBar] widgets.
final ButtonBarThemeData data;
/// Returns the configuration [data] from the closest [ButtonBarTheme]
/// ancestor. If there is no ancestor, it returns [ThemeData.buttonBarTheme].
/// Applications can assume that the returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// ButtonBarThemeData theme = ButtonBarTheme.of(context);
/// ```
static ButtonBarThemeData of(BuildContext context) {
final ButtonBarTheme? buttonBarTheme = context.dependOnInheritedWidgetOfExactType<ButtonBarTheme>();
return buttonBarTheme?.data ?? Theme.of(context).buttonBarTheme;
}
@override
bool updateShouldNotify(ButtonBarTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/button_bar_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/button_bar_theme.dart",
"repo_id": "flutter",
"token_count": 3094
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
/// The minimum dimension of any interactive region according to Material
/// guidelines.
///
/// This is used to avoid small regions that are hard for the user to interact
/// with. It applies to both dimensions of a region, so a square of size
/// kMinInteractiveDimension x kMinInteractiveDimension is the smallest
/// acceptable region that should respond to gestures.
///
/// See also:
///
/// * [kMinInteractiveDimensionCupertino]
/// * The Material spec on touch targets at <https://material.io/design/usability/accessibility.html#layout-typography>.
const double kMinInteractiveDimension = 48.0;
/// The height of the toolbar component of the [AppBar].
const double kToolbarHeight = 56.0;
/// The height of the bottom navigation bar.
const double kBottomNavigationBarHeight = 56.0;
/// The height of a tab bar containing text.
const double kTextTabBarHeight = kMinInteractiveDimension;
/// The amount of time theme change animations should last.
const Duration kThemeChangeDuration = Duration(milliseconds: 200);
/// The default radius of a circular material ink response in logical pixels.
const double kRadialReactionRadius = 20.0;
/// The amount of time a circular material ink response should take to expand to its full size.
const Duration kRadialReactionDuration = Duration(milliseconds: 100);
/// The value of the alpha channel to use when drawing a circular material ink response.
const int kRadialReactionAlpha = 0x1F;
/// The duration of the horizontal scroll animation that occurs when a tab is tapped.
const Duration kTabScrollDuration = Duration(milliseconds: 300);
/// The horizontal padding included by [Tab]s.
const EdgeInsets kTabLabelPadding = EdgeInsets.symmetric(horizontal: 16.0);
/// The padding added around material list items.
const EdgeInsets kMaterialListPadding = EdgeInsets.symmetric(vertical: 8.0);
/// The default color for [ThemeData.iconTheme] when [ThemeData.brightness] is
/// [Brightness.dark]. This color is used in [IconButton] to detect whether
/// [IconTheme.of(context).color] is the same as the default color of [ThemeData.iconTheme].
// ignore: prefer_const_constructors
final Color kDefaultIconLightColor = Color(0xFFFFFFFF);
/// The default color for [ThemeData.iconTheme] when [ThemeData.brightness] is
/// [Brightness.light]. This color is used in [IconButton] to detect whether
/// [IconTheme.of(context).color] is the same as the default color of [ThemeData.iconTheme].
// ignore: prefer_const_constructors
final Color kDefaultIconDarkColor = Color(0xDD000000);
| flutter/packages/flutter/lib/src/material/constants.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/constants.dart",
"repo_id": "flutter",
"token_count": 724
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'debug.dart';
import 'drawer_theme.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// The possible alignments of a [Drawer].
enum DrawerAlignment {
/// Denotes that the [Drawer] is at the start side of the [Scaffold].
///
/// This corresponds to the left side when the text direction is left-to-right
/// and the right side when the text direction is right-to-left.
start,
/// Denotes that the [Drawer] is at the end side of the [Scaffold].
///
/// This corresponds to the right side when the text direction is left-to-right
/// and the left side when the text direction is right-to-left.
end,
}
// TODO(eseidel): Draw width should vary based on device size:
// https://material.io/design/components/navigation-drawer.html#specs
// Mobile:
// Width = Screen width − 56 dp
// Maximum width: 320dp
// Maximum width applies only when using a left nav. When using a right nav,
// the panel can cover the full width of the screen.
// Desktop/Tablet:
// Maximum width for a left nav is 400dp.
// The right nav can vary depending on content.
const double _kWidth = 304.0;
const double _kEdgeDragWidth = 20.0;
const double _kMinFlingVelocity = 365.0;
const Duration _kBaseSettleDuration = Duration(milliseconds: 246);
/// A Material Design panel that slides in horizontally from the edge of a
/// [Scaffold] to show navigation links in an application.
///
/// There is a Material 3 version of this component, [NavigationDrawer],
/// that's preferred for applications that are configured for Material 3
/// (see [ThemeData.useMaterial3]).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=WRj86iHihgY}
///
/// Drawers are typically used with the [Scaffold.drawer] property. The child of
/// the drawer is usually a [ListView] whose first child is a [DrawerHeader]
/// that displays status information about the current user. The remaining
/// drawer children are often constructed with [ListTile]s, often concluding
/// with an [AboutListTile].
///
/// The [AppBar] automatically displays an appropriate [IconButton] to show the
/// [Drawer] when a [Drawer] is available in the [Scaffold]. The [Scaffold]
/// automatically handles the edge-swipe gesture to show the drawer.
///
/// {@animation 350 622 https://flutter.github.io/assets-for-api-docs/assets/material/drawer.mp4}
///
/// ## Updating to [NavigationDrawer]
///
/// There is a Material 3 version of this component, [NavigationDrawer],
/// that's preferred for applications that are configured for Material 3
/// (see [ThemeData.useMaterial3]). The [NavigationDrawer] widget's visual
/// are a little bit different, see the Material 3 spec at
/// <https://m3.material.io/components/navigation-drawer/overview> for
/// more details. While the [Drawer] widget can have only one child, the
/// [NavigationDrawer] widget can have a list of widgets, which typically contains
/// [NavigationDrawerDestination] widgets and/or customized widgets like headlines
/// and dividers.
///
/// {@tool dartpad}
/// This example shows how to create a [Scaffold] that contains an [AppBar] and
/// a [Drawer]. A user taps the "menu" icon in the [AppBar] to open the
/// [Drawer]. The [Drawer] displays four items: A header and three menu items.
/// The [Drawer] displays the four items using a [ListView], which allows the
/// user to scroll through the items if need be.
///
/// ** See code in examples/api/lib/material/drawer/drawer.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to migrate the above [Drawer] to a [NavigationDrawer].
///
/// ** See code in examples/api/lib/material/navigation_drawer/navigation_drawer.0.dart **
/// {@end-tool}
///
/// An open drawer may be closed with a swipe to close gesture, pressing the
/// escape key, by tapping the scrim, or by calling pop route function such as
/// [Navigator.pop]. For example a drawer item might close the drawer when tapped:
///
/// ```dart
/// ListTile(
/// leading: const Icon(Icons.change_history),
/// title: const Text('Change history'),
/// onTap: () {
/// // change app state...
/// Navigator.pop(context); // close the drawer
/// },
/// );
/// ```
///
/// See also:
///
/// * [Scaffold.drawer], where one specifies a [Drawer] so that it can be
/// shown.
/// * [Scaffold.of], to obtain the current [ScaffoldState], which manages the
/// display and animation of the drawer.
/// * [ScaffoldState.openDrawer], which displays its [Drawer], if any.
/// * <https://material.io/design/components/navigation-drawer.html>
class Drawer extends StatelessWidget {
/// Creates a Material Design drawer.
///
/// Typically used in the [Scaffold.drawer] property.
///
/// The [elevation] must be non-negative.
const Drawer({
super.key,
this.backgroundColor,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.shape,
this.width,
this.child,
this.semanticLabel,
this.clipBehavior,
}) : assert(elevation == null || elevation >= 0.0);
/// Sets the color of the [Material] that holds all of the [Drawer]'s
/// contents.
///
/// If this is null, then [DrawerThemeData.backgroundColor] is used. If that
/// is also null, then it falls back to [Material]'s default.
final Color? backgroundColor;
/// The z-coordinate at which to place this drawer relative to its parent.
///
/// This controls the size of the shadow below the drawer.
///
/// If this is null, then [DrawerThemeData.elevation] is used. If that
/// is also null, then it defaults to 16.0.
final double? elevation;
/// The color used to paint a drop shadow under the drawer's [Material],
/// which reflects the drawer's [elevation].
///
/// If null and [ThemeData.useMaterial3] is true then no drop shadow will
/// be rendered.
///
/// If null and [ThemeData.useMaterial3] is false then it will default to
/// [ThemeData.shadowColor].
///
/// See also:
/// * [Material.shadowColor], which describes how the drop shadow is painted.
/// * [elevation], which affects how the drop shadow is painted.
/// * [surfaceTintColor], which can be used to indicate elevation through
/// tinting the background color.
final Color? shadowColor;
/// The color used as a surface tint overlay on the drawer's background color,
/// which reflects the drawer's [elevation].
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// To disable this feature, set [surfaceTintColor] to [Colors.transparent].
///
/// Defaults to [Colors.transparent].
///
/// See also:
/// * [Material.surfaceTintColor], which describes how the surface tint will
/// be applied to the background color of the drawer.
/// * [elevation], which affects the opacity of the surface tint.
/// * [shadowColor], which can be used to indicate elevation through
/// a drop shadow.
final Color? surfaceTintColor;
/// The shape of the drawer.
///
/// Defines the drawer's [Material.shape].
///
/// If this is null, then [DrawerThemeData.shape] is used. If that
/// is also null, then it falls back to [Material]'s default.
final ShapeBorder? shape;
/// The width of the drawer.
///
/// If this is null, then [DrawerThemeData.width] is used. If that is also
/// null, then it falls back to the Material spec's default (304.0).
final double? width;
/// The widget below this widget in the tree.
///
/// Typically a [SliverList].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The semantic label of the drawer used by accessibility frameworks to
/// announce screen transitions when the drawer is opened and closed.
///
/// If this label is not provided, it will default to
/// [MaterialLocalizations.drawerLabel].
///
/// See also:
///
/// * [SemanticsConfiguration.namesRoute], for a description of how this
/// value is used.
final String? semanticLabel;
/// {@macro flutter.material.Material.clipBehavior}
///
/// The [clipBehavior] argument specifies how to clip the drawer's [shape].
///
/// If the drawer has a [shape], it defaults to [Clip.hardEdge]. Otherwise,
/// defaults to [Clip.none].
final Clip? clipBehavior;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final DrawerThemeData drawerTheme = DrawerTheme.of(context);
String? label = semanticLabel;
switch (Theme.of(context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
break;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
label = semanticLabel ?? MaterialLocalizations.of(context).drawerLabel;
}
final bool useMaterial3 = Theme.of(context).useMaterial3;
final bool isDrawerStart = DrawerController.maybeOf(context)?.alignment != DrawerAlignment.end;
final DrawerThemeData defaults= useMaterial3 ? _DrawerDefaultsM3(context): _DrawerDefaultsM2(context);
final ShapeBorder? effectiveShape = shape ?? (isDrawerStart
? (drawerTheme.shape ?? defaults.shape)
: (drawerTheme.endShape ?? defaults.endShape));
return Semantics(
scopesRoute: true,
namesRoute: true,
explicitChildNodes: true,
label: label,
child: ConstrainedBox(
constraints: BoxConstraints.expand(width: width ?? drawerTheme.width ?? _kWidth),
child: Material(
color: backgroundColor ?? drawerTheme.backgroundColor ?? defaults.backgroundColor,
elevation: elevation ?? drawerTheme.elevation ?? defaults.elevation!,
shadowColor: shadowColor ?? drawerTheme.shadowColor ?? defaults.shadowColor,
surfaceTintColor: surfaceTintColor ?? drawerTheme.surfaceTintColor ?? defaults.surfaceTintColor,
shape: effectiveShape,
clipBehavior: effectiveShape != null ? (clipBehavior ?? Clip.hardEdge) : Clip.none,
child: child,
),
),
);
}
}
/// Signature for the callback that's called when a [DrawerController] is
/// opened or closed.
typedef DrawerCallback = void Function(bool isOpened);
class _DrawerControllerScope extends InheritedWidget {
const _DrawerControllerScope({
required this.controller,
required super.child,
});
final DrawerController controller;
@override
bool updateShouldNotify(_DrawerControllerScope old) {
return controller != old.controller;
}
}
/// Provides interactive behavior for [Drawer] widgets.
///
/// Rarely used directly. Drawer controllers are typically created automatically
/// by [Scaffold] widgets.
///
/// The drawer controller provides the ability to open and close a drawer, either
/// via an animation or via user interaction. When closed, the drawer collapses
/// to a translucent gesture detector that can be used to listen for edge
/// swipes.
///
/// See also:
///
/// * [Drawer], a container with the default width of a drawer.
/// * [Scaffold.drawer], the [Scaffold] slot for showing a drawer.
class DrawerController extends StatefulWidget {
/// Creates a controller for a [Drawer].
///
/// Rarely used directly.
///
/// The [child] argument is typically a [Drawer].
const DrawerController({
GlobalKey? key,
required this.child,
required this.alignment,
this.isDrawerOpen = false,
this.drawerCallback,
this.dragStartBehavior = DragStartBehavior.start,
this.scrimColor,
this.edgeDragWidth,
this.enableOpenDragGesture = true,
}) : super(key: key);
/// The widget below this widget in the tree.
///
/// Typically a [Drawer].
final Widget child;
/// The alignment of the [Drawer].
///
/// This controls the direction in which the user should swipe to open and
/// close the drawer.
final DrawerAlignment alignment;
/// Optional callback that is called when a [Drawer] is opened or closed.
final DrawerCallback? drawerCallback;
/// {@template flutter.material.DrawerController.dragStartBehavior}
/// Determines the way that drag start behavior is handled.
///
/// If set to [DragStartBehavior.start], the drag behavior used for opening
/// and closing a drawer will begin at the position where the drag gesture won
/// the arena. If set to [DragStartBehavior.down] it will begin at the position
/// where a down event is first detected.
///
/// In general, setting this to [DragStartBehavior.start] will make drag
/// animation smoother and setting it to [DragStartBehavior.down] will make
/// drag behavior feel slightly more reactive.
///
/// By default, the drag start behavior is [DragStartBehavior.start].
///
/// See also:
///
/// * [DragGestureRecognizer.dragStartBehavior], which gives an example for
/// the different behaviors.
///
/// {@endtemplate}
final DragStartBehavior dragStartBehavior;
/// The color to use for the scrim that obscures the underlying content while
/// a drawer is open.
///
/// If this is null, then [DrawerThemeData.scrimColor] is used. If that
/// is also null, then it defaults to [Colors.black54].
final Color? scrimColor;
/// Determines if the [Drawer] can be opened with a drag gesture.
///
/// By default, the drag gesture is enabled.
final bool enableOpenDragGesture;
/// The width of the area within which a horizontal swipe will open the
/// drawer.
///
/// By default, the value used is 20.0 added to the padding edge of
/// `MediaQuery.paddingOf(context)` that corresponds to [alignment].
/// This ensures that the drag area for notched devices is not obscured. For
/// example, if [alignment] is set to [DrawerAlignment.start] and
/// `TextDirection.of(context)` is set to [TextDirection.ltr],
/// 20.0 will be added to `MediaQuery.paddingOf(context).left`.
final double? edgeDragWidth;
/// Whether or not the drawer is opened or closed.
///
/// This parameter is primarily used by the state restoration framework
/// to restore the drawer's animation controller to the open or closed state
/// depending on what was last saved to the target platform before the
/// application was killed.
final bool isDrawerOpen;
/// The closest instance of [DrawerController] that encloses the given
/// context, or null if none is found.
///
/// {@tool snippet} Typical usage is as follows:
///
/// ```dart
/// DrawerController? controller = DrawerController.maybeOf(context);
/// ```
/// {@end-tool}
///
/// Calling this method will create a dependency on the closest
/// [DrawerController] in the [context], if there is one.
///
/// See also:
///
/// * [DrawerController.of], which is similar to this method, but asserts
/// if no [DrawerController] ancestor is found.
static DrawerController? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<_DrawerControllerScope>()?.controller;
}
/// The closest instance of [DrawerController] that encloses the given
/// context.
///
/// If no instance is found, this method will assert in debug mode and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest
/// [DrawerController] in the [context].
///
/// {@tool snippet} Typical usage is as follows:
///
/// ```dart
/// DrawerController controller = DrawerController.of(context);
/// ```
/// {@end-tool}
static DrawerController of(BuildContext context) {
final DrawerController? controller = maybeOf(context);
assert(() {
if (controller == null) {
throw FlutterError(
'DrawerController.of() was called with a context that does not '
'contain a DrawerController widget.\n'
'No DrawerController widget ancestor could be found starting from '
'the context that was passed to DrawerController.of(). This can '
'happen because you are using a widget that looks for a DrawerController '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return controller!;
}
@override
DrawerControllerState createState() => DrawerControllerState();
}
/// State for a [DrawerController].
///
/// Typically used by a [Scaffold] to [open] and [close] the drawer.
class DrawerControllerState extends State<DrawerController> with SingleTickerProviderStateMixin {
@override
void initState() {
super.initState();
_controller = AnimationController(
value: widget.isDrawerOpen ? 1.0 : 0.0,
duration: _kBaseSettleDuration,
vsync: this,
);
_controller
..addListener(_animationChanged)
..addStatusListener(_animationStatusChanged);
}
@override
void dispose() {
_historyEntry?.remove();
_controller.dispose();
_focusScopeNode.dispose();
super.dispose();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_scrimColorTween = _buildScrimColorTween();
}
@override
void didUpdateWidget(DrawerController oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.scrimColor != oldWidget.scrimColor) {
_scrimColorTween = _buildScrimColorTween();
}
if (widget.isDrawerOpen != oldWidget.isDrawerOpen) {
switch (_controller.status) {
case AnimationStatus.completed:
case AnimationStatus.dismissed:
_controller.value = widget.isDrawerOpen ? 1.0 : 0.0;
case AnimationStatus.forward:
case AnimationStatus.reverse:
break;
}
}
}
void _animationChanged() {
setState(() {
// The animation controller's state is our build state, and it changed already.
});
}
LocalHistoryEntry? _historyEntry;
final FocusScopeNode _focusScopeNode = FocusScopeNode();
void _ensureHistoryEntry() {
if (_historyEntry == null) {
final ModalRoute<dynamic>? route = ModalRoute.of(context);
if (route != null) {
_historyEntry = LocalHistoryEntry(onRemove: _handleHistoryEntryRemoved, impliesAppBarDismissal: false);
route.addLocalHistoryEntry(_historyEntry!);
FocusScope.of(context).setFirstFocus(_focusScopeNode);
}
}
}
void _animationStatusChanged(AnimationStatus status) {
switch (status) {
case AnimationStatus.forward:
_ensureHistoryEntry();
case AnimationStatus.reverse:
_historyEntry?.remove();
_historyEntry = null;
case AnimationStatus.dismissed:
break;
case AnimationStatus.completed:
break;
}
}
void _handleHistoryEntryRemoved() {
_historyEntry = null;
close();
}
late AnimationController _controller;
void _handleDragDown(DragDownDetails details) {
_controller.stop();
_ensureHistoryEntry();
}
void _handleDragCancel() {
if (_controller.isDismissed || _controller.isAnimating) {
return;
}
if (_controller.value < 0.5) {
close();
} else {
open();
}
}
final GlobalKey _drawerKey = GlobalKey();
double get _width {
final RenderBox? box = _drawerKey.currentContext?.findRenderObject() as RenderBox?;
// return _kWidth if drawer not being shown currently
return box?.size.width ?? _kWidth;
}
bool _previouslyOpened = false;
void _move(DragUpdateDetails details) {
double delta = details.primaryDelta! / _width;
switch (widget.alignment) {
case DrawerAlignment.start:
break;
case DrawerAlignment.end:
delta = -delta;
}
switch (Directionality.of(context)) {
case TextDirection.rtl:
_controller.value -= delta;
case TextDirection.ltr:
_controller.value += delta;
}
final bool opened = _controller.value > 0.5;
if (opened != _previouslyOpened && widget.drawerCallback != null) {
widget.drawerCallback!(opened);
}
_previouslyOpened = opened;
}
void _settle(DragEndDetails details) {
if (_controller.isDismissed) {
return;
}
if (details.velocity.pixelsPerSecond.dx.abs() >= _kMinFlingVelocity) {
double visualVelocity = details.velocity.pixelsPerSecond.dx / _width;
switch (widget.alignment) {
case DrawerAlignment.start:
break;
case DrawerAlignment.end:
visualVelocity = -visualVelocity;
}
switch (Directionality.of(context)) {
case TextDirection.rtl:
_controller.fling(velocity: -visualVelocity);
widget.drawerCallback?.call(visualVelocity < 0.0);
case TextDirection.ltr:
_controller.fling(velocity: visualVelocity);
widget.drawerCallback?.call(visualVelocity > 0.0);
}
} else if (_controller.value < 0.5) {
close();
} else {
open();
}
}
/// Starts an animation to open the drawer.
///
/// Typically called by [ScaffoldState.openDrawer].
void open() {
_controller.fling();
widget.drawerCallback?.call(true);
}
/// Starts an animation to close the drawer.
void close() {
_controller.fling(velocity: -1.0);
widget.drawerCallback?.call(false);
}
late ColorTween _scrimColorTween;
final GlobalKey _gestureDetectorKey = GlobalKey();
ColorTween _buildScrimColorTween() {
return ColorTween(
begin: Colors.transparent,
end: widget.scrimColor
?? DrawerTheme.of(context).scrimColor
?? Colors.black54,
);
}
AlignmentDirectional get _drawerOuterAlignment {
return switch (widget.alignment) {
DrawerAlignment.start => AlignmentDirectional.centerStart,
DrawerAlignment.end => AlignmentDirectional.centerEnd,
};
}
AlignmentDirectional get _drawerInnerAlignment {
return switch (widget.alignment) {
DrawerAlignment.start => AlignmentDirectional.centerEnd,
DrawerAlignment.end => AlignmentDirectional.centerStart,
};
}
Widget _buildDrawer(BuildContext context) {
final bool isDesktop = switch (Theme.of(context).platform) {
TargetPlatform.android || TargetPlatform.iOS || TargetPlatform.fuchsia => false,
TargetPlatform.macOS || TargetPlatform.linux || TargetPlatform.windows => true,
};
final double dragAreaWidth = widget.edgeDragWidth
?? _kEdgeDragWidth + switch ((widget.alignment, Directionality.of(context))) {
(DrawerAlignment.start, TextDirection.ltr) => MediaQuery.paddingOf(context).left,
(DrawerAlignment.start, TextDirection.rtl) => MediaQuery.paddingOf(context).right,
(DrawerAlignment.end, TextDirection.rtl) => MediaQuery.paddingOf(context).left,
(DrawerAlignment.end, TextDirection.ltr) => MediaQuery.paddingOf(context).right,
};
if (_controller.status == AnimationStatus.dismissed) {
if (widget.enableOpenDragGesture && !isDesktop) {
return Align(
alignment: _drawerOuterAlignment,
child: GestureDetector(
key: _gestureDetectorKey,
onHorizontalDragUpdate: _move,
onHorizontalDragEnd: _settle,
behavior: HitTestBehavior.translucent,
excludeFromSemantics: true,
dragStartBehavior: widget.dragStartBehavior,
child: Container(width: dragAreaWidth),
),
);
} else {
return const SizedBox.shrink();
}
} else {
final bool platformHasBackButton;
switch (Theme.of(context).platform) {
case TargetPlatform.android:
platformHasBackButton = true;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
platformHasBackButton = false;
}
final Widget child = _DrawerControllerScope(
controller: widget,
child: RepaintBoundary(
child: Stack(
children: <Widget>[
BlockSemantics(
child: ExcludeSemantics(
// On Android, the back button is used to dismiss a modal.
excluding: platformHasBackButton,
child: GestureDetector(
onTap: close,
child: Semantics(
label: MaterialLocalizations.of(context).modalBarrierDismissLabel,
child: Container( // The drawer's "scrim"
color: _scrimColorTween.evaluate(_controller),
),
),
),
),
),
Align(
alignment: _drawerOuterAlignment,
child: Align(
alignment: _drawerInnerAlignment,
widthFactor: _controller.value,
child: RepaintBoundary(
child: FocusScope(
key: _drawerKey,
node: _focusScopeNode,
child: widget.child,
),
),
),
),
],
),
),
);
if (isDesktop) {
return child;
}
return GestureDetector(
key: _gestureDetectorKey,
onHorizontalDragDown: _handleDragDown,
onHorizontalDragUpdate: _move,
onHorizontalDragEnd: _settle,
onHorizontalDragCancel: _handleDragCancel,
excludeFromSemantics: true,
dragStartBehavior: widget.dragStartBehavior,
child: child,
);
}
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
return ListTileTheme.merge(
style: ListTileStyle.drawer,
child: _buildDrawer(context),
);
}
}
class _DrawerDefaultsM2 extends DrawerThemeData {
const _DrawerDefaultsM2(this.context)
: super(elevation: 16.0);
final BuildContext context;
@override
Color? get shadowColor => Theme.of(context).shadowColor;
}
// BEGIN GENERATED TOKEN PROPERTIES - Drawer
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _DrawerDefaultsM3 extends DrawerThemeData {
_DrawerDefaultsM3(this.context)
: super(elevation: 1.0);
final BuildContext context;
late final TextDirection direction = Directionality.of(context);
@override
Color? get backgroundColor => Theme.of(context).colorScheme.surfaceContainerLow;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
Color? get shadowColor => Colors.transparent;
// There isn't currently a token for this value, but it is shown in the spec,
// so hard coding here for now.
@override
ShapeBorder? get shape => RoundedRectangleBorder(
borderRadius: const BorderRadiusDirectional.horizontal(
end: Radius.circular(16.0),
).resolve(direction),
);
// There isn't currently a token for this value, but it is shown in the spec,
// so hard coding here for now.
@override
ShapeBorder? get endShape => RoundedRectangleBorder(
borderRadius: const BorderRadiusDirectional.horizontal(
start: Radius.circular(16.0),
).resolve(direction),
);
}
// END GENERATED TOKEN PROPERTIES - Drawer
| flutter/packages/flutter/lib/src/material/drawer.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/drawer.dart",
"repo_id": "flutter",
"token_count": 9645
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show clampDouble;
import 'package:flutter/widgets.dart';
import 'chip.dart';
import 'chip_theme.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'debug.dart';
import 'icons.dart';
import 'material_state.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
enum _ChipVariant { flat, elevated }
/// A Material Design filter chip.
///
/// Filter chips use tags or descriptive words as a way to filter content.
///
/// Filter chips are a good alternative to [Checkbox] or [Switch] widgets.
/// Unlike these alternatives, filter chips allow for clearly delineated and
/// exposed options in a compact area.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// {@tool dartpad}
/// This example shows how to use [FilterChip]s to filter through exercises.
///
/// ** See code in examples/api/lib/material/filter_chip/filter_chip.0.dart **
/// {@end-tool}
///
/// ## Material Design 3
///
/// [FilterChip] can be used for multiple select Filter chip from
/// Material Design 3. If [ThemeData.useMaterial3] is true, then [FilterChip]
/// will be styled to match the Material Design 3 specification for Filter
/// chips. Use [ChoiceChip] for single select Filter chips.
///
/// See also:
///
/// * [Chip], a chip that displays information and can be deleted.
/// * [InputChip], a chip that represents a complex piece of information, such
/// as an entity (person, place, or thing) or conversational text, in a
/// compact form.
/// * [ChoiceChip], allows a single selection from a set of options. Choice
/// chips contain related descriptive text or categories.
/// * [ActionChip], represents an action related to primary content.
/// * [CircleAvatar], which shows images or initials of people.
/// * [Wrap], A widget that displays its children in multiple horizontal or
/// vertical runs.
/// * <https://material.io/design/components/chips.html>
class FilterChip extends StatelessWidget
implements
ChipAttributes,
DeletableChipAttributes,
SelectableChipAttributes,
CheckmarkableChipAttributes,
DisabledChipAttributes {
/// Create a chip that acts like a checkbox.
///
/// The [selected], [label], [autofocus], and [clipBehavior] arguments must
/// not be null. When [onSelected] is null, the [FilterChip] will be disabled.
/// The [pressElevation] and [elevation] must be null or non-negative. Typically,
/// [pressElevation] is greater than [elevation].
const FilterChip({
super.key,
this.avatar,
required this.label,
this.labelStyle,
this.labelPadding,
this.selected = false,
required this.onSelected,
this.deleteIcon,
this.onDeleted,
this.deleteIconColor,
this.deleteButtonTooltipMessage,
this.pressElevation,
this.disabledColor,
this.selectedColor,
this.tooltip,
this.side,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.color,
this.backgroundColor,
this.padding,
this.visualDensity,
this.materialTapTargetSize,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.iconTheme,
this.selectedShadowColor,
this.showCheckmark,
this.checkmarkColor,
this.avatarBorder = const CircleBorder(),
this.avatarBoxConstraints,
this.deleteIconBoxConstraints,
}) : assert(pressElevation == null || pressElevation >= 0.0),
assert(elevation == null || elevation >= 0.0),
_chipVariant = _ChipVariant.flat;
/// Create an elevated chip that acts like a checkbox.
///
/// The [selected], [label], [autofocus], and [clipBehavior] arguments must
/// not be null. When [onSelected] is null, the [FilterChip] will be disabled.
/// The [pressElevation] and [elevation] must be null or non-negative. Typically,
/// [pressElevation] is greater than [elevation].
const FilterChip.elevated({
super.key,
this.avatar,
required this.label,
this.labelStyle,
this.labelPadding,
this.selected = false,
required this.onSelected,
this.deleteIcon,
this.onDeleted,
this.deleteIconColor,
this.deleteButtonTooltipMessage,
this.pressElevation,
this.disabledColor,
this.selectedColor,
this.tooltip,
this.side,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.color,
this.backgroundColor,
this.padding,
this.visualDensity,
this.materialTapTargetSize,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.iconTheme,
this.selectedShadowColor,
this.showCheckmark,
this.checkmarkColor,
this.avatarBorder = const CircleBorder(),
this.avatarBoxConstraints,
this.deleteIconBoxConstraints,
}) : assert(pressElevation == null || pressElevation >= 0.0),
assert(elevation == null || elevation >= 0.0),
_chipVariant = _ChipVariant.elevated;
@override
final Widget? avatar;
@override
final Widget label;
@override
final TextStyle? labelStyle;
@override
final EdgeInsetsGeometry? labelPadding;
@override
final bool selected;
@override
final ValueChanged<bool>? onSelected;
@override
final Widget? deleteIcon;
@override
final VoidCallback? onDeleted;
@override
final Color? deleteIconColor;
@override
final String? deleteButtonTooltipMessage;
@override
final double? pressElevation;
@override
final Color? disabledColor;
@override
final Color? selectedColor;
@override
final String? tooltip;
@override
final BorderSide? side;
@override
final OutlinedBorder? shape;
@override
final Clip clipBehavior;
@override
final FocusNode? focusNode;
@override
final bool autofocus;
@override
final MaterialStateProperty<Color?>? color;
@override
final Color? backgroundColor;
@override
final EdgeInsetsGeometry? padding;
@override
final VisualDensity? visualDensity;
@override
final MaterialTapTargetSize? materialTapTargetSize;
@override
final double? elevation;
@override
final Color? shadowColor;
@override
final Color? surfaceTintColor;
@override
final Color? selectedShadowColor;
@override
final bool? showCheckmark;
@override
final Color? checkmarkColor;
@override
final ShapeBorder avatarBorder;
@override
final IconThemeData? iconTheme;
@override
final BoxConstraints? avatarBoxConstraints;
@override
final BoxConstraints? deleteIconBoxConstraints;
@override
bool get isEnabled => onSelected != null;
final _ChipVariant _chipVariant;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
final ChipThemeData? defaults = Theme.of(context).useMaterial3
? _FilterChipDefaultsM3(context, isEnabled, selected, _chipVariant)
: null;
final Widget? resolvedDeleteIcon = deleteIcon
?? (Theme.of(context).useMaterial3 ? const Icon(Icons.clear, size: 18) : null);
return RawChip(
defaultProperties: defaults,
avatar: avatar,
label: label,
labelStyle: labelStyle,
labelPadding: labelPadding,
onSelected: onSelected,
deleteIcon: resolvedDeleteIcon,
onDeleted: onDeleted,
deleteIconColor: deleteIconColor,
deleteButtonTooltipMessage: deleteButtonTooltipMessage,
pressElevation: pressElevation,
selected: selected,
tooltip: tooltip,
side: side,
shape: shape,
clipBehavior: clipBehavior,
focusNode: focusNode,
autofocus: autofocus,
color: color,
backgroundColor: backgroundColor,
disabledColor: disabledColor,
selectedColor: selectedColor,
padding: padding,
visualDensity: visualDensity,
isEnabled: isEnabled,
materialTapTargetSize: materialTapTargetSize,
elevation: elevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
selectedShadowColor: selectedShadowColor,
showCheckmark: showCheckmark,
checkmarkColor: checkmarkColor,
avatarBorder: avatarBorder,
iconTheme: iconTheme,
avatarBoxConstraints: avatarBoxConstraints,
deleteIconBoxConstraints: deleteIconBoxConstraints,
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - FilterChip
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FilterChipDefaultsM3 extends ChipThemeData {
_FilterChipDefaultsM3(
this.context,
this.isEnabled,
this.isSelected,
this._chipVariant,
) : super(
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(8.0))),
showCheckmark: true,
);
final BuildContext context;
final bool isEnabled;
final bool isSelected;
final _ChipVariant _chipVariant;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
double? get elevation => _chipVariant == _ChipVariant.flat
? 0.0
: isEnabled ? 1.0 : 0.0;
@override
double? get pressElevation => 1.0;
@override
TextStyle? get labelStyle => _textTheme.labelLarge?.copyWith(
color: isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.onSurfaceVariant
: _colors.onSurface,
);
@override
MaterialStateProperty<Color?>? get color =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected) && states.contains(MaterialState.disabled)) {
return _chipVariant == _ChipVariant.flat
? _colors.onSurface.withOpacity(0.12)
: _colors.onSurface.withOpacity(0.12);
}
if (states.contains(MaterialState.disabled)) {
return _chipVariant == _ChipVariant.flat
? null
: _colors.onSurface.withOpacity(0.12);
}
if (states.contains(MaterialState.selected)) {
return _chipVariant == _ChipVariant.flat
? _colors.secondaryContainer
: _colors.secondaryContainer;
}
return _chipVariant == _ChipVariant.flat
? null
: _colors.surfaceContainerLow;
});
@override
Color? get shadowColor => _chipVariant == _ChipVariant.flat
? Colors.transparent
: _colors.shadow;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
Color? get checkmarkColor => isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.primary
: _colors.onSurface;
@override
Color? get deleteIconColor => isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.onSurfaceVariant
: _colors.onSurface;
@override
BorderSide? get side => _chipVariant == _ChipVariant.flat && !isSelected
? isEnabled
? BorderSide(color: _colors.outline)
: BorderSide(color: _colors.onSurface.withOpacity(0.12))
: const BorderSide(color: Colors.transparent);
@override
IconThemeData? get iconTheme => IconThemeData(
color: isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.primary
: _colors.onSurface,
size: 18.0,
);
@override
EdgeInsetsGeometry? get padding => const EdgeInsets.all(8.0);
/// The label padding of the chip scales with the font size specified in the
/// [labelStyle], and the system font size settings that scale font sizes
/// globally.
///
/// The chip at effective font size 14.0 starts with 8px on each side and as
/// the font size scales up to closer to 28.0, the label padding is linearly
/// interpolated from 8px to 4px. Once the label has a font size of 2 or
/// higher, label padding remains 4px.
@override
EdgeInsetsGeometry? get labelPadding {
final double fontSize = labelStyle?.fontSize ?? 14.0;
final double fontSizeRatio = MediaQuery.textScalerOf(context).scale(fontSize) / 14.0;
return EdgeInsets.lerp(
const EdgeInsets.symmetric(horizontal: 8.0),
const EdgeInsets.symmetric(horizontal: 4.0),
clampDouble(fontSizeRatio - 1.0, 0.0, 1.0),
)!;
}
}
// END GENERATED TOKEN PROPERTIES - FilterChip
| flutter/packages/flutter/lib/src/material/filter_chip.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/filter_chip.dart",
"repo_id": "flutter",
"token_count": 4392
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'debug.dart';
import 'feedback.dart';
import 'ink_highlight.dart';
import 'material.dart';
import 'material_state.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// An ink feature that displays a [color] "splash" in response to a user
/// gesture that can be confirmed or canceled.
///
/// Subclasses call [confirm] when an input gesture is recognized. For
/// example a press event might trigger an ink feature that's confirmed
/// when the corresponding up event is seen.
///
/// Subclasses call [cancel] when an input gesture is aborted before it
/// is recognized. For example a press event might trigger an ink feature
/// that's canceled when the pointer is dragged out of the reference
/// box.
///
/// The [InkWell] and [InkResponse] widgets generate instances of this
/// class.
abstract class InteractiveInkFeature extends InkFeature {
/// Creates an InteractiveInkFeature.
InteractiveInkFeature({
required super.controller,
required super.referenceBox,
required Color color,
ShapeBorder? customBorder,
super.onRemoved,
}) : _color = color,
_customBorder = customBorder;
/// Called when the user input that triggered this feature's appearance was confirmed.
///
/// Typically causes the ink to propagate faster across the material. By default this
/// method does nothing.
void confirm() { }
/// Called when the user input that triggered this feature's appearance was canceled.
///
/// Typically causes the ink to gradually disappear. By default this method does
/// nothing.
void cancel() { }
/// The ink's color.
Color get color => _color;
Color _color;
set color(Color value) {
if (value == _color) {
return;
}
_color = value;
controller.markNeedsPaint();
}
/// The ink's optional custom border.
ShapeBorder? get customBorder => _customBorder;
ShapeBorder? _customBorder;
set customBorder(ShapeBorder? value) {
if (value == _customBorder) {
return;
}
_customBorder = value;
controller.markNeedsPaint();
}
/// Draws an ink splash or ink ripple on the passed in [Canvas].
///
/// The [transform] argument is the [Matrix4] transform that typically
/// shifts the coordinate space of the canvas to the space in which
/// the ink circle is to be painted.
///
/// [center] is the [Offset] from origin of the canvas where the center
/// of the circle is drawn.
///
/// [paint] takes a [Paint] object that describes the styles used to draw the ink circle.
/// For example, [paint] can specify properties like color, strokewidth, colorFilter.
///
/// [radius] is the radius of ink circle to be drawn on canvas.
///
/// [clipCallback] is the callback used to obtain the [Rect] used for clipping the ink effect.
/// If [clipCallback] is null, no clipping is performed on the ink circle.
///
/// Clipping can happen in 3 different ways:
/// 1. If [customBorder] is provided, it is used to determine the path
/// for clipping.
/// 2. If [customBorder] is null, and [borderRadius] is provided, the canvas
/// is clipped by an [RRect] created from [clipCallback] and [borderRadius].
/// 3. If [borderRadius] is the default [BorderRadius.zero], then the [Rect] provided
/// by [clipCallback] is used for clipping.
///
/// [textDirection] is used by [customBorder] if it is non-null. This allows the [customBorder]'s path
/// to be properly defined if it was the path was expressed in terms of "start" and "end" instead of
/// "left" and "right".
///
/// For examples on how the function is used, see [InkSplash] and [InkRipple].
@protected
void paintInkCircle({
required Canvas canvas,
required Matrix4 transform,
required Paint paint,
required Offset center,
required double radius,
TextDirection? textDirection,
ShapeBorder? customBorder,
BorderRadius borderRadius = BorderRadius.zero,
RectCallback? clipCallback,
}) {
final Offset? originOffset = MatrixUtils.getAsTranslation(transform);
canvas.save();
if (originOffset == null) {
canvas.transform(transform.storage);
} else {
canvas.translate(originOffset.dx, originOffset.dy);
}
if (clipCallback != null) {
final Rect rect = clipCallback();
if (customBorder != null) {
canvas.clipPath(customBorder.getOuterPath(rect, textDirection: textDirection));
} else if (borderRadius != BorderRadius.zero) {
canvas.clipRRect(RRect.fromRectAndCorners(
rect,
topLeft: borderRadius.topLeft, topRight: borderRadius.topRight,
bottomLeft: borderRadius.bottomLeft, bottomRight: borderRadius.bottomRight,
));
} else {
canvas.clipRect(rect);
}
}
canvas.drawCircle(center, radius, paint);
canvas.restore();
}
}
/// An encapsulation of an [InteractiveInkFeature] constructor used by
/// [InkWell], [InkResponse], and [ThemeData].
///
/// Interactive ink feature implementations should provide a static const
/// `splashFactory` value that's an instance of this class. The `splashFactory`
/// can be used to configure an [InkWell], [InkResponse] or [ThemeData].
///
/// See also:
///
/// * [InkSplash.splashFactory]
/// * [InkRipple.splashFactory]
abstract class InteractiveInkFeatureFactory {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
///
/// Subclasses should provide a const constructor.
const InteractiveInkFeatureFactory();
/// The factory method.
///
/// Subclasses should override this method to return a new instance of an
/// [InteractiveInkFeature].
@factory
InteractiveInkFeature create({
required MaterialInkController controller,
required RenderBox referenceBox,
required Offset position,
required Color color,
required TextDirection textDirection,
bool containedInkWell = false,
RectCallback? rectCallback,
BorderRadius? borderRadius,
ShapeBorder? customBorder,
double? radius,
VoidCallback? onRemoved,
});
}
abstract class _ParentInkResponseState {
void markChildInkResponsePressed(_ParentInkResponseState childState, bool value);
}
class _ParentInkResponseProvider extends InheritedWidget {
const _ParentInkResponseProvider({
required this.state,
required super.child,
});
final _ParentInkResponseState state;
@override
bool updateShouldNotify(_ParentInkResponseProvider oldWidget) => state != oldWidget.state;
static _ParentInkResponseState? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<_ParentInkResponseProvider>()?.state;
}
}
typedef _GetRectCallback = RectCallback? Function(RenderBox referenceBox);
typedef _CheckContext = bool Function(BuildContext context);
/// An area of a [Material] that responds to touch. Has a configurable shape and
/// can be configured to clip splashes that extend outside its bounds or not.
///
/// For a variant of this widget that is specialized for rectangular areas that
/// always clip splashes, see [InkWell].
///
/// An [InkResponse] widget does two things when responding to a tap:
///
/// * It starts to animate a _highlight_. The shape of the highlight is
/// determined by [highlightShape]. If it is a [BoxShape.circle], the
/// default, then the highlight is a circle of fixed size centered in the
/// [InkResponse]. If it is [BoxShape.rectangle], then the highlight is a box
/// the size of the [InkResponse] itself, unless [getRectCallback] is
/// provided, in which case that callback defines the rectangle. The color of
/// the highlight is set by [highlightColor].
///
/// * Simultaneously, it starts to animate a _splash_. This is a growing circle
/// initially centered on the tap location. If this is a [containedInkWell],
/// the splash grows to the [radius] while remaining centered at the tap
/// location. Otherwise, the splash migrates to the center of the box as it
/// grows.
///
/// The following two diagrams show how [InkResponse] looks when tapped if the
/// [highlightShape] is [BoxShape.circle] (the default) and [containedInkWell]
/// is false (also the default).
///
/// The first diagram shows how it looks if the [InkResponse] is relatively
/// large:
///
/// 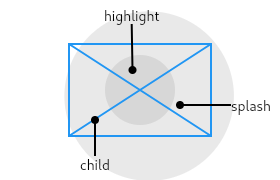
///
/// The second diagram shows how it looks if the [InkResponse] is small:
///
/// 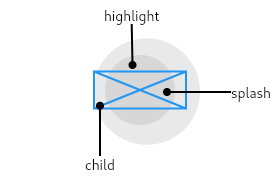
///
/// The main thing to notice from these diagrams is that the splashes happily
/// exceed the bounds of the widget (because [containedInkWell] is false).
///
/// The following diagram shows the effect when the [InkResponse] has a
/// [highlightShape] of [BoxShape.rectangle] with [containedInkWell] set to
/// true. These are the values used by [InkWell].
///
/// 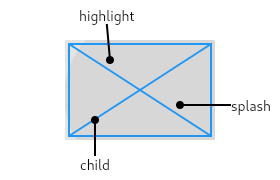
///
/// The [InkResponse] widget must have a [Material] widget as an ancestor. The
/// [Material] widget is where the ink reactions are actually painted. This
/// matches the Material Design premise wherein the [Material] is what is
/// actually reacting to touches by spreading ink.
///
/// If a Widget uses this class directly, it should include the following line
/// at the top of its build function to call [debugCheckHasMaterial]:
///
/// ```dart
/// assert(debugCheckHasMaterial(context));
/// ```
///
/// ## Troubleshooting
///
/// ### The ink splashes aren't visible!
///
/// If there is an opaque graphic, e.g. painted using a [Container], [Image], or
/// [DecoratedBox], between the [Material] widget and the [InkResponse] widget,
/// then the splash won't be visible because it will be under the opaque graphic.
/// This is because ink splashes draw on the underlying [Material] itself, as
/// if the ink was spreading inside the material.
///
/// The [Ink] widget can be used as a replacement for [Image], [Container], or
/// [DecoratedBox] to ensure that the image or decoration also paints in the
/// [Material] itself, below the ink.
///
/// If this is not possible for some reason, e.g. because you are using an
/// opaque [CustomPaint] widget, alternatively consider using a second
/// [Material] above the opaque widget but below the [InkResponse] (as an
/// ancestor to the ink response). The [MaterialType.transparency] material
/// kind can be used for this purpose.
///
/// See also:
///
/// * [GestureDetector], for listening for gestures without ink splashes.
/// * [ElevatedButton] and [TextButton], two kinds of buttons in Material Design.
/// * [IconButton], which combines [InkResponse] with an [Icon].
class InkResponse extends StatelessWidget {
/// Creates an area of a [Material] that responds to touch.
///
/// Must have an ancestor [Material] widget in which to cause ink reactions.
const InkResponse({
super.key,
this.child,
this.onTap,
this.onTapDown,
this.onTapUp,
this.onTapCancel,
this.onDoubleTap,
this.onLongPress,
this.onSecondaryTap,
this.onSecondaryTapUp,
this.onSecondaryTapDown,
this.onSecondaryTapCancel,
this.onHighlightChanged,
this.onHover,
this.mouseCursor,
this.containedInkWell = false,
this.highlightShape = BoxShape.circle,
this.radius,
this.borderRadius,
this.customBorder,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.overlayColor,
this.splashColor,
this.splashFactory,
this.enableFeedback = true,
this.excludeFromSemantics = false,
this.focusNode,
this.canRequestFocus = true,
this.onFocusChange,
this.autofocus = false,
this.statesController,
this.hoverDuration,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// Called when the user taps this part of the material.
final GestureTapCallback? onTap;
/// Called when the user taps down this part of the material.
final GestureTapDownCallback? onTapDown;
/// Called when the user releases a tap that was started on this part of the
/// material. [onTap] is called immediately after.
final GestureTapUpCallback? onTapUp;
/// Called when the user cancels a tap that was started on this part of the
/// material.
final GestureTapCallback? onTapCancel;
/// Called when the user double taps this part of the material.
final GestureTapCallback? onDoubleTap;
/// Called when the user long-presses on this part of the material.
final GestureLongPressCallback? onLongPress;
/// Called when the user taps this part of the material with a secondary button.
final GestureTapCallback? onSecondaryTap;
/// Called when the user taps down on this part of the material with a
/// secondary button.
final GestureTapDownCallback? onSecondaryTapDown;
/// Called when the user releases a secondary button tap that was started on
/// this part of the material. [onSecondaryTap] is called immediately after.
final GestureTapUpCallback? onSecondaryTapUp;
/// Called when the user cancels a secondary button tap that was started on
/// this part of the material.
final GestureTapCallback? onSecondaryTapCancel;
/// Called when this part of the material either becomes highlighted or stops
/// being highlighted.
///
/// The value passed to the callback is true if this part of the material has
/// become highlighted and false if this part of the material has stopped
/// being highlighted.
///
/// If all of [onTap], [onDoubleTap], and [onLongPress] become null while a
/// gesture is ongoing, then [onTapCancel] will be fired and
/// [onHighlightChanged] will be fired with the value false _during the
/// build_. This means, for instance, that in that scenario [State.setState]
/// cannot be called.
final ValueChanged<bool>? onHighlightChanged;
/// Called when a pointer enters or exits the ink response area.
///
/// The value passed to the callback is true if a pointer has entered this
/// part of the material and false if a pointer has exited this part of the
/// material.
final ValueChanged<bool>? onHover;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// If this property is null, [MaterialStateMouseCursor.clickable] will be used.
final MouseCursor? mouseCursor;
/// Whether this ink response should be clipped its bounds.
///
/// This flag also controls whether the splash migrates to the center of the
/// [InkResponse] or not. If [containedInkWell] is true, the splash remains
/// centered around the tap location. If it is false, the splash migrates to
/// the center of the [InkResponse] as it grows.
///
/// See also:
///
/// * [highlightShape], the shape of the focus, hover, and pressed
/// highlights.
/// * [borderRadius], which controls the corners when the box is a rectangle.
/// * [getRectCallback], which controls the size and position of the box when
/// it is a rectangle.
final bool containedInkWell;
/// The shape (e.g., circle, rectangle) to use for the highlight drawn around
/// this part of the material when pressed, hovered over, or focused.
///
/// The same shape is used for the pressed highlight (see [highlightColor]),
/// the focus highlight (see [focusColor]), and the hover highlight (see
/// [hoverColor]).
///
/// If the shape is [BoxShape.circle], then the highlight is centered on the
/// [InkResponse]. If the shape is [BoxShape.rectangle], then the highlight
/// fills the [InkResponse], or the rectangle provided by [getRectCallback] if
/// the callback is specified.
///
/// See also:
///
/// * [containedInkWell], which controls clipping behavior.
/// * [borderRadius], which controls the corners when the box is a rectangle.
/// * [highlightColor], the color of the highlight.
/// * [getRectCallback], which controls the size and position of the box when
/// it is a rectangle.
final BoxShape highlightShape;
/// The radius of the ink splash.
///
/// Splashes grow up to this size. By default, this size is determined from
/// the size of the rectangle provided by [getRectCallback], or the size of
/// the [InkResponse] itself.
///
/// See also:
///
/// * [splashColor], the color of the splash.
/// * [splashFactory], which defines the appearance of the splash.
final double? radius;
/// The border radius of the containing rectangle. This is effective only if
/// [highlightShape] is [BoxShape.rectangle].
///
/// If this is null, it is interpreted as [BorderRadius.zero].
final BorderRadius? borderRadius;
/// The custom clip border.
///
/// If this is null, the ink response will not clip its content.
final ShapeBorder? customBorder;
/// The color of the ink response when the parent widget is focused. If this
/// property is null then the focus color of the theme,
/// [ThemeData.focusColor], will be used.
///
/// See also:
///
/// * [highlightShape], the shape of the focus, hover, and pressed
/// highlights.
/// * [hoverColor], the color of the hover highlight.
/// * [splashColor], the color of the splash.
/// * [splashFactory], which defines the appearance of the splash.
final Color? focusColor;
/// The color of the ink response when a pointer is hovering over it. If this
/// property is null then the hover color of the theme,
/// [ThemeData.hoverColor], will be used.
///
/// See also:
///
/// * [highlightShape], the shape of the focus, hover, and pressed
/// highlights.
/// * [highlightColor], the color of the pressed highlight.
/// * [focusColor], the color of the focus highlight.
/// * [splashColor], the color of the splash.
/// * [splashFactory], which defines the appearance of the splash.
final Color? hoverColor;
/// The highlight color of the ink response when pressed. If this property is
/// null then the highlight color of the theme, [ThemeData.highlightColor],
/// will be used.
///
/// See also:
///
/// * [hoverColor], the color of the hover highlight.
/// * [focusColor], the color of the focus highlight.
/// * [highlightShape], the shape of the focus, hover, and pressed
/// highlights.
/// * [splashColor], the color of the splash.
/// * [splashFactory], which defines the appearance of the splash.
final Color? highlightColor;
/// Defines the ink response focus, hover, and splash colors.
///
/// This default null property can be used as an alternative to
/// [focusColor], [hoverColor], [highlightColor], and
/// [splashColor]. If non-null, it is resolved against one of
/// [MaterialState.focused], [MaterialState.hovered], and
/// [MaterialState.pressed]. It's convenient to use when the parent
/// widget can pass along its own MaterialStateProperty value for
/// the overlay color.
///
/// [MaterialState.pressed] triggers a ripple (an ink splash), per
/// the current Material Design spec. The [overlayColor] doesn't map
/// a state to [highlightColor] because a separate highlight is not
/// used by the current design guidelines. See
/// https://material.io/design/interaction/states.html#pressed
///
/// If the overlay color is null or resolves to null, then [focusColor],
/// [hoverColor], [splashColor] and their defaults are used instead.
///
/// See also:
///
/// * The Material Design specification for overlay colors and how they
/// match a component's state:
/// <https://material.io/design/interaction/states.html#anatomy>.
final MaterialStateProperty<Color?>? overlayColor;
/// The splash color of the ink response. If this property is null then the
/// splash color of the theme, [ThemeData.splashColor], will be used.
///
/// See also:
///
/// * [splashFactory], which defines the appearance of the splash.
/// * [radius], the (maximum) size of the ink splash.
/// * [highlightColor], the color of the highlight.
final Color? splashColor;
/// Defines the appearance of the splash.
///
/// Defaults to the value of the theme's splash factory: [ThemeData.splashFactory].
///
/// See also:
///
/// * [radius], the (maximum) size of the ink splash.
/// * [splashColor], the color of the splash.
/// * [highlightColor], the color of the highlight.
/// * [InkSplash.splashFactory], which defines the default splash.
/// * [InkRipple.splashFactory], which defines a splash that spreads out
/// more aggressively than the default.
final InteractiveInkFeatureFactory? splashFactory;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool enableFeedback;
/// Whether to exclude the gestures introduced by this widget from the
/// semantics tree.
///
/// For example, a long-press gesture for showing a tooltip is usually
/// excluded because the tooltip itself is included in the semantics
/// tree directly and so having a gesture to show it would result in
/// duplication of information.
final bool excludeFromSemantics;
/// {@template flutter.material.inkwell.onFocusChange}
/// Handler called when the focus changes.
///
/// Called with true if this widget's node gains focus, and false if it loses
/// focus.
/// {@endtemplate}
final ValueChanged<bool>? onFocusChange;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.canRequestFocus}
final bool canRequestFocus;
/// The rectangle to use for the highlight effect and for clipping
/// the splash effects if [containedInkWell] is true.
///
/// This method is intended to be overridden by descendants that
/// specialize [InkResponse] for unusual cases. For example,
/// [TableRowInkWell] implements this method to return the rectangle
/// corresponding to the row that the widget is in.
///
/// The default behavior returns null, which is equivalent to
/// returning the referenceBox argument's bounding box (though
/// slightly more efficient).
RectCallback? getRectCallback(RenderBox referenceBox) => null;
/// {@template flutter.material.inkwell.statesController}
/// Represents the interactive "state" of this widget in terms of
/// a set of [MaterialState]s, like [MaterialState.pressed] and
/// [MaterialState.focused].
///
/// Classes based on this one can provide their own
/// [MaterialStatesController] to which they've added listeners.
/// They can also update the controller's [MaterialStatesController.value]
/// however, this may only be done when it's safe to call
/// [State.setState], like in an event handler.
/// {@endtemplate}
final MaterialStatesController? statesController;
/// The duration of the animation that animates the hover effect.
///
/// The default is 50ms.
final Duration? hoverDuration;
@override
Widget build(BuildContext context) {
final _ParentInkResponseState? parentState = _ParentInkResponseProvider.maybeOf(context);
return _InkResponseStateWidget(
onTap: onTap,
onTapDown: onTapDown,
onTapUp: onTapUp,
onTapCancel: onTapCancel,
onDoubleTap: onDoubleTap,
onLongPress: onLongPress,
onSecondaryTap: onSecondaryTap,
onSecondaryTapUp: onSecondaryTapUp,
onSecondaryTapDown: onSecondaryTapDown,
onSecondaryTapCancel: onSecondaryTapCancel,
onHighlightChanged: onHighlightChanged,
onHover: onHover,
mouseCursor: mouseCursor,
containedInkWell: containedInkWell,
highlightShape: highlightShape,
radius: radius,
borderRadius: borderRadius,
customBorder: customBorder,
focusColor: focusColor,
hoverColor: hoverColor,
highlightColor: highlightColor,
overlayColor: overlayColor,
splashColor: splashColor,
splashFactory: splashFactory,
enableFeedback: enableFeedback,
excludeFromSemantics: excludeFromSemantics,
focusNode: focusNode,
canRequestFocus: canRequestFocus,
onFocusChange: onFocusChange,
autofocus: autofocus,
parentState: parentState,
getRectCallback: getRectCallback,
debugCheckContext: debugCheckContext,
statesController: statesController,
hoverDuration: hoverDuration,
child: child,
);
}
/// Asserts that the given context satisfies the prerequisites for
/// this class.
///
/// This method is intended to be overridden by descendants that
/// specialize [InkResponse] for unusual cases. For example,
/// [TableRowInkWell] implements this method to verify that the widget is
/// in a table.
@mustCallSuper
bool debugCheckContext(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasDirectionality(context));
return true;
}
}
class _InkResponseStateWidget extends StatefulWidget {
const _InkResponseStateWidget({
this.child,
this.onTap,
this.onTapDown,
this.onTapUp,
this.onTapCancel,
this.onDoubleTap,
this.onLongPress,
this.onSecondaryTap,
this.onSecondaryTapUp,
this.onSecondaryTapDown,
this.onSecondaryTapCancel,
this.onHighlightChanged,
this.onHover,
this.mouseCursor,
this.containedInkWell = false,
this.highlightShape = BoxShape.circle,
this.radius,
this.borderRadius,
this.customBorder,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.overlayColor,
this.splashColor,
this.splashFactory,
this.enableFeedback = true,
this.excludeFromSemantics = false,
this.focusNode,
this.canRequestFocus = true,
this.onFocusChange,
this.autofocus = false,
this.parentState,
this.getRectCallback,
required this.debugCheckContext,
this.statesController,
this.hoverDuration,
});
final Widget? child;
final GestureTapCallback? onTap;
final GestureTapDownCallback? onTapDown;
final GestureTapUpCallback? onTapUp;
final GestureTapCallback? onTapCancel;
final GestureTapCallback? onDoubleTap;
final GestureLongPressCallback? onLongPress;
final GestureTapCallback? onSecondaryTap;
final GestureTapUpCallback? onSecondaryTapUp;
final GestureTapDownCallback? onSecondaryTapDown;
final GestureTapCallback? onSecondaryTapCancel;
final ValueChanged<bool>? onHighlightChanged;
final ValueChanged<bool>? onHover;
final MouseCursor? mouseCursor;
final bool containedInkWell;
final BoxShape highlightShape;
final double? radius;
final BorderRadius? borderRadius;
final ShapeBorder? customBorder;
final Color? focusColor;
final Color? hoverColor;
final Color? highlightColor;
final MaterialStateProperty<Color?>? overlayColor;
final Color? splashColor;
final InteractiveInkFeatureFactory? splashFactory;
final bool enableFeedback;
final bool excludeFromSemantics;
final ValueChanged<bool>? onFocusChange;
final bool autofocus;
final FocusNode? focusNode;
final bool canRequestFocus;
final _ParentInkResponseState? parentState;
final _GetRectCallback? getRectCallback;
final _CheckContext debugCheckContext;
final MaterialStatesController? statesController;
final Duration? hoverDuration;
@override
_InkResponseState createState() => _InkResponseState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final List<String> gestures = <String>[
if (onTap != null) 'tap',
if (onDoubleTap != null) 'double tap',
if (onLongPress != null) 'long press',
if (onTapDown != null) 'tap down',
if (onTapUp != null) 'tap up',
if (onTapCancel != null) 'tap cancel',
if (onSecondaryTap != null) 'secondary tap',
if (onSecondaryTapUp != null) 'secondary tap up',
if (onSecondaryTapDown != null) 'secondary tap down',
if (onSecondaryTapCancel != null) 'secondary tap cancel'
];
properties.add(IterableProperty<String>('gestures', gestures, ifEmpty: '<none>'));
properties.add(DiagnosticsProperty<MouseCursor>('mouseCursor', mouseCursor));
properties.add(DiagnosticsProperty<bool>('containedInkWell', containedInkWell, level: DiagnosticLevel.fine));
properties.add(DiagnosticsProperty<BoxShape>(
'highlightShape',
highlightShape,
description: '${containedInkWell ? "clipped to " : ""}$highlightShape',
showName: false,
));
}
}
/// Used to index the allocated highlights for the different types of highlights
/// in [_InkResponseState].
enum _HighlightType {
pressed,
hover,
focus,
}
class _InkResponseState extends State<_InkResponseStateWidget>
with AutomaticKeepAliveClientMixin<_InkResponseStateWidget>
implements _ParentInkResponseState
{
Set<InteractiveInkFeature>? _splashes;
InteractiveInkFeature? _currentSplash;
bool _hovering = false;
final Map<_HighlightType, InkHighlight?> _highlights = <_HighlightType, InkHighlight?>{};
late final Map<Type, Action<Intent>> _actionMap = <Type, Action<Intent>>{
ActivateIntent: CallbackAction<ActivateIntent>(onInvoke: activateOnIntent),
ButtonActivateIntent: CallbackAction<ButtonActivateIntent>(onInvoke: activateOnIntent),
};
MaterialStatesController? internalStatesController;
bool get highlightsExist => _highlights.values.where((InkHighlight? highlight) => highlight != null).isNotEmpty;
final ObserverList<_ParentInkResponseState> _activeChildren = ObserverList<_ParentInkResponseState>();
static const Duration _activationDuration = Duration(milliseconds: 100);
Timer? _activationTimer;
@override
void markChildInkResponsePressed(_ParentInkResponseState childState, bool value) {
final bool lastAnyPressed = _anyChildInkResponsePressed;
if (value) {
_activeChildren.add(childState);
} else {
_activeChildren.remove(childState);
}
final bool nowAnyPressed = _anyChildInkResponsePressed;
if (nowAnyPressed != lastAnyPressed) {
widget.parentState?.markChildInkResponsePressed(this, nowAnyPressed);
}
}
bool get _anyChildInkResponsePressed => _activeChildren.isNotEmpty;
void activateOnIntent(Intent? intent) {
_activationTimer?.cancel();
_activationTimer = null;
_startNewSplash(context: context);
_currentSplash?.confirm();
_currentSplash = null;
if (widget.onTap != null) {
if (widget.enableFeedback) {
Feedback.forTap(context);
}
widget.onTap?.call();
}
// Delay the call to `updateHighlight` to simulate a pressed delay
// and give MaterialStatesController listeners a chance to react.
_activationTimer = Timer(_activationDuration, () {
updateHighlight(_HighlightType.pressed, value: false);
});
}
void simulateTap([Intent? intent]) {
_startNewSplash(context: context);
handleTap();
}
void simulateLongPress() {
_startNewSplash(context: context);
handleLongPress();
}
void handleStatesControllerChange() {
// Force a rebuild to resolve widget.overlayColor, widget.mouseCursor
setState(() { });
}
MaterialStatesController get statesController => widget.statesController ?? internalStatesController!;
void initStatesController() {
if (widget.statesController == null) {
internalStatesController = MaterialStatesController();
}
statesController.update(MaterialState.disabled, !enabled);
statesController.addListener(handleStatesControllerChange);
}
@override
void initState() {
super.initState();
initStatesController();
FocusManager.instance.addHighlightModeListener(handleFocusHighlightModeChange);
}
@override
void didUpdateWidget(_InkResponseStateWidget oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.statesController != oldWidget.statesController) {
oldWidget.statesController?.removeListener(handleStatesControllerChange);
if (widget.statesController != null) {
internalStatesController?.dispose();
internalStatesController = null;
}
initStatesController();
}
if (widget.radius != oldWidget.radius ||
widget.highlightShape != oldWidget.highlightShape ||
widget.borderRadius != oldWidget.borderRadius) {
final InkHighlight? hoverHighlight = _highlights[_HighlightType.hover];
if (hoverHighlight != null) {
hoverHighlight.dispose();
updateHighlight(_HighlightType.hover, value: _hovering, callOnHover: false);
}
final InkHighlight? focusHighlight = _highlights[_HighlightType.focus];
if (focusHighlight != null) {
focusHighlight.dispose();
// Do not call updateFocusHighlights() here because it is called below
}
}
if (widget.customBorder != oldWidget.customBorder) {
_updateHighlightsAndSplashes();
}
if (enabled != isWidgetEnabled(oldWidget)) {
statesController.update(MaterialState.disabled, !enabled);
if (!enabled) {
statesController.update(MaterialState.pressed, false);
// Remove the existing hover highlight immediately when enabled is false.
// Do not rely on updateHighlight or InkHighlight.deactivate to not break
// the expected lifecycle which is updating _hovering when the mouse exit.
// Manually updating _hovering here or calling InkHighlight.deactivate
// will lead to onHover not being called or call when it is not allowed.
final InkHighlight? hoverHighlight = _highlights[_HighlightType.hover];
hoverHighlight?.dispose();
}
// Don't call widget.onHover because many widgets, including the button
// widgets, apply setState to an ancestor context from onHover.
updateHighlight(_HighlightType.hover, value: _hovering, callOnHover: false);
}
updateFocusHighlights();
}
@override
void dispose() {
FocusManager.instance.removeHighlightModeListener(handleFocusHighlightModeChange);
statesController.removeListener(handleStatesControllerChange);
internalStatesController?.dispose();
_activationTimer?.cancel();
_activationTimer = null;
super.dispose();
}
@override
bool get wantKeepAlive => highlightsExist || (_splashes != null && _splashes!.isNotEmpty);
Duration getFadeDurationForType(_HighlightType type) {
switch (type) {
case _HighlightType.pressed:
return const Duration(milliseconds: 200);
case _HighlightType.hover:
case _HighlightType.focus:
return widget.hoverDuration ?? const Duration(milliseconds: 50);
}
}
void updateHighlight(_HighlightType type, { required bool value, bool callOnHover = true }) {
final InkHighlight? highlight = _highlights[type];
void handleInkRemoval() {
assert(_highlights[type] != null);
_highlights[type] = null;
updateKeepAlive();
}
switch (type) {
case _HighlightType.pressed:
statesController.update(MaterialState.pressed, value);
case _HighlightType.hover:
if (callOnHover) {
statesController.update(MaterialState.hovered, value);
}
case _HighlightType.focus:
// see handleFocusUpdate()
break;
}
if (type == _HighlightType.pressed) {
widget.parentState?.markChildInkResponsePressed(this, value);
}
if (value == (highlight != null && highlight.active)) {
return;
}
if (value) {
if (highlight == null) {
final Color resolvedOverlayColor = widget.overlayColor?.resolve(statesController.value)
?? switch (type) {
// Use the backwards compatible defaults
_HighlightType.pressed => widget.highlightColor ?? Theme.of(context).highlightColor,
_HighlightType.focus => widget.focusColor ?? Theme.of(context).focusColor,
_HighlightType.hover => widget.hoverColor ?? Theme.of(context).hoverColor,
};
final RenderBox referenceBox = context.findRenderObject()! as RenderBox;
_highlights[type] = InkHighlight(
controller: Material.of(context),
referenceBox: referenceBox,
color: enabled ? resolvedOverlayColor : resolvedOverlayColor.withAlpha(0),
shape: widget.highlightShape,
radius: widget.radius,
borderRadius: widget.borderRadius,
customBorder: widget.customBorder,
rectCallback: widget.getRectCallback!(referenceBox),
onRemoved: handleInkRemoval,
textDirection: Directionality.of(context),
fadeDuration: getFadeDurationForType(type),
);
updateKeepAlive();
} else {
highlight.activate();
}
} else {
highlight!.deactivate();
}
assert(value == (_highlights[type] != null && _highlights[type]!.active));
switch (type) {
case _HighlightType.pressed:
widget.onHighlightChanged?.call(value);
case _HighlightType.hover:
if (callOnHover) {
widget.onHover?.call(value);
}
case _HighlightType.focus:
break;
}
}
void _updateHighlightsAndSplashes() {
for (final InkHighlight? highlight in _highlights.values) {
if (highlight != null) {
highlight.customBorder = widget.customBorder;
}
}
if (_currentSplash != null) {
_currentSplash!.customBorder = widget.customBorder;
}
if (_splashes != null && _splashes!.isNotEmpty) {
for (final InteractiveInkFeature inkFeature in _splashes!) {
inkFeature.customBorder = widget.customBorder;
}
}
}
InteractiveInkFeature _createSplash(Offset globalPosition) {
final MaterialInkController inkController = Material.of(context);
final RenderBox referenceBox = context.findRenderObject()! as RenderBox;
final Offset position = referenceBox.globalToLocal(globalPosition);
final Color color = widget.overlayColor?.resolve(statesController.value) ?? widget.splashColor ?? Theme.of(context).splashColor;
final RectCallback? rectCallback = widget.containedInkWell ? widget.getRectCallback!(referenceBox) : null;
final BorderRadius? borderRadius = widget.borderRadius;
final ShapeBorder? customBorder = widget.customBorder;
InteractiveInkFeature? splash;
void onRemoved() {
if (_splashes != null) {
assert(_splashes!.contains(splash));
_splashes!.remove(splash);
if (_currentSplash == splash) {
_currentSplash = null;
}
updateKeepAlive();
} // else we're probably in deactivate()
}
splash = (widget.splashFactory ?? Theme.of(context).splashFactory).create(
controller: inkController,
referenceBox: referenceBox,
position: position,
color: color,
containedInkWell: widget.containedInkWell,
rectCallback: rectCallback,
radius: widget.radius,
borderRadius: borderRadius,
customBorder: customBorder,
onRemoved: onRemoved,
textDirection: Directionality.of(context),
);
return splash;
}
void handleFocusHighlightModeChange(FocusHighlightMode mode) {
if (!mounted) {
return;
}
setState(() {
updateFocusHighlights();
});
}
bool get _shouldShowFocus {
return switch (MediaQuery.maybeNavigationModeOf(context)) {
NavigationMode.traditional || null => enabled && _hasFocus,
NavigationMode.directional => _hasFocus,
};
}
void updateFocusHighlights() {
final bool showFocus = switch (FocusManager.instance.highlightMode) {
FocusHighlightMode.touch => false,
FocusHighlightMode.traditional => _shouldShowFocus,
};
updateHighlight(_HighlightType.focus, value: showFocus);
}
bool _hasFocus = false;
void handleFocusUpdate(bool hasFocus) {
_hasFocus = hasFocus;
// Set here rather than updateHighlight because this widget's
// (MaterialState) states include MaterialState.focused if
// the InkWell _has_ the focus, rather than if it's showing
// the focus per FocusManager.instance.highlightMode.
statesController.update(MaterialState.focused, hasFocus);
updateFocusHighlights();
widget.onFocusChange?.call(hasFocus);
}
void handleAnyTapDown(TapDownDetails details) {
if (_anyChildInkResponsePressed) {
return;
}
_startNewSplash(details: details);
}
void handleTapDown(TapDownDetails details) {
handleAnyTapDown(details);
widget.onTapDown?.call(details);
}
void handleTapUp(TapUpDetails details) {
widget.onTapUp?.call(details);
}
void handleSecondaryTapDown(TapDownDetails details) {
handleAnyTapDown(details);
widget.onSecondaryTapDown?.call(details);
}
void handleSecondaryTapUp(TapUpDetails details) {
widget.onSecondaryTapUp?.call(details);
}
void _startNewSplash({TapDownDetails? details, BuildContext? context}) {
assert(details != null || context != null);
final Offset globalPosition;
if (context != null) {
final RenderBox referenceBox = context.findRenderObject()! as RenderBox;
assert(referenceBox.hasSize, 'InkResponse must be done with layout before starting a splash.');
globalPosition = referenceBox.localToGlobal(referenceBox.paintBounds.center);
} else {
globalPosition = details!.globalPosition;
}
statesController.update(MaterialState.pressed, true); // ... before creating the splash
final InteractiveInkFeature splash = _createSplash(globalPosition);
_splashes ??= HashSet<InteractiveInkFeature>();
_splashes!.add(splash);
_currentSplash?.cancel();
_currentSplash = splash;
updateKeepAlive();
updateHighlight(_HighlightType.pressed, value: true);
}
void handleTap() {
_currentSplash?.confirm();
_currentSplash = null;
updateHighlight(_HighlightType.pressed, value: false);
if (widget.onTap != null) {
if (widget.enableFeedback) {
Feedback.forTap(context);
}
widget.onTap?.call();
}
}
void handleTapCancel() {
_currentSplash?.cancel();
_currentSplash = null;
widget.onTapCancel?.call();
updateHighlight(_HighlightType.pressed, value: false);
}
void handleDoubleTap() {
_currentSplash?.confirm();
_currentSplash = null;
updateHighlight(_HighlightType.pressed, value: false);
widget.onDoubleTap?.call();
}
void handleLongPress() {
_currentSplash?.confirm();
_currentSplash = null;
if (widget.onLongPress != null) {
if (widget.enableFeedback) {
Feedback.forLongPress(context);
}
widget.onLongPress!();
}
}
void handleSecondaryTap() {
_currentSplash?.confirm();
_currentSplash = null;
updateHighlight(_HighlightType.pressed, value: false);
widget.onSecondaryTap?.call();
}
void handleSecondaryTapCancel() {
_currentSplash?.cancel();
_currentSplash = null;
widget.onSecondaryTapCancel?.call();
updateHighlight(_HighlightType.pressed, value: false);
}
@override
void deactivate() {
if (_splashes != null) {
final Set<InteractiveInkFeature> splashes = _splashes!;
_splashes = null;
for (final InteractiveInkFeature splash in splashes) {
splash.dispose();
}
_currentSplash = null;
}
assert(_currentSplash == null);
for (final _HighlightType highlight in _highlights.keys) {
_highlights[highlight]?.dispose();
_highlights[highlight] = null;
}
widget.parentState?.markChildInkResponsePressed(this, false);
super.deactivate();
}
bool isWidgetEnabled(_InkResponseStateWidget widget) {
return _primaryButtonEnabled(widget) || _secondaryButtonEnabled(widget);
}
bool _primaryButtonEnabled(_InkResponseStateWidget widget) {
return widget.onTap != null
|| widget.onDoubleTap != null
|| widget.onLongPress != null
|| widget.onTapUp != null
|| widget.onTapDown != null;
}
bool _secondaryButtonEnabled(_InkResponseStateWidget widget) {
return widget.onSecondaryTap != null
|| widget.onSecondaryTapUp != null
|| widget.onSecondaryTapDown != null;
}
bool get enabled => isWidgetEnabled(widget);
bool get _primaryEnabled => _primaryButtonEnabled(widget);
bool get _secondaryEnabled => _secondaryButtonEnabled(widget);
void handleMouseEnter(PointerEnterEvent event) {
_hovering = true;
if (enabled) {
handleHoverChange();
}
}
void handleMouseExit(PointerExitEvent event) {
_hovering = false;
// If the exit occurs after we've been disabled, we still
// want to take down the highlights and run widget.onHover.
handleHoverChange();
}
void handleHoverChange() {
updateHighlight(_HighlightType.hover, value: _hovering);
}
bool get _canRequestFocus {
return switch (MediaQuery.maybeNavigationModeOf(context)) {
NavigationMode.traditional || null => enabled && widget.canRequestFocus,
NavigationMode.directional => true,
};
}
@override
Widget build(BuildContext context) {
assert(widget.debugCheckContext(context));
super.build(context); // See AutomaticKeepAliveClientMixin.
Color getHighlightColorForType(_HighlightType type) {
const Set<MaterialState> pressed = <MaterialState>{MaterialState.pressed};
const Set<MaterialState> focused = <MaterialState>{MaterialState.focused};
const Set<MaterialState> hovered = <MaterialState>{MaterialState.hovered};
final ThemeData theme = Theme.of(context);
return switch (type) {
// The pressed state triggers a ripple (ink splash), per the current
// Material Design spec. A separate highlight is no longer used.
// See https://material.io/design/interaction/states.html#pressed
_HighlightType.pressed => widget.overlayColor?.resolve(pressed) ?? widget.highlightColor ?? theme.highlightColor,
_HighlightType.focus => widget.overlayColor?.resolve(focused) ?? widget.focusColor ?? theme.focusColor,
_HighlightType.hover => widget.overlayColor?.resolve(hovered) ?? widget.hoverColor ?? theme.hoverColor,
};
}
for (final _HighlightType type in _highlights.keys) {
_highlights[type]?.color = getHighlightColorForType(type);
}
_currentSplash?.color = widget.overlayColor?.resolve(statesController.value) ?? widget.splashColor ?? Theme.of(context).splashColor;
final MouseCursor effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor>(
widget.mouseCursor ?? MaterialStateMouseCursor.clickable,
statesController.value,
);
return _ParentInkResponseProvider(
state: this,
child: Actions(
actions: _actionMap,
child: Focus(
focusNode: widget.focusNode,
canRequestFocus: _canRequestFocus,
onFocusChange: handleFocusUpdate,
autofocus: widget.autofocus,
child: MouseRegion(
cursor: effectiveMouseCursor,
onEnter: handleMouseEnter,
onExit: handleMouseExit,
child: DefaultSelectionStyle.merge(
mouseCursor: effectiveMouseCursor,
child: Semantics(
onTap: widget.excludeFromSemantics || widget.onTap == null ? null : simulateTap,
onLongPress: widget.excludeFromSemantics || widget.onLongPress == null ? null : simulateLongPress,
child: GestureDetector(
onTapDown: _primaryEnabled ? handleTapDown : null,
onTapUp: _primaryEnabled ? handleTapUp : null,
onTap: _primaryEnabled ? handleTap : null,
onTapCancel: _primaryEnabled ? handleTapCancel : null,
onDoubleTap: widget.onDoubleTap != null ? handleDoubleTap : null,
onLongPress: widget.onLongPress != null ? handleLongPress : null,
onSecondaryTapDown: _secondaryEnabled ? handleSecondaryTapDown : null,
onSecondaryTapUp: _secondaryEnabled ? handleSecondaryTapUp: null,
onSecondaryTap: _secondaryEnabled ? handleSecondaryTap : null,
onSecondaryTapCancel: _secondaryEnabled ? handleSecondaryTapCancel : null,
behavior: HitTestBehavior.opaque,
excludeFromSemantics: true,
child: widget.child,
),
),
),
),
),
),
);
}
}
/// A rectangular area of a [Material] that responds to touch.
///
/// For a variant of this widget that does not clip splashes, see [InkResponse].
///
/// The following diagram shows how an [InkWell] looks when tapped, when using
/// default values.
///
/// 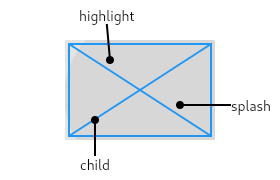
///
/// The [InkWell] widget must have a [Material] widget as an ancestor. The
/// [Material] widget is where the ink reactions are actually painted. This
/// matches the Material Design premise wherein the [Material] is what is
/// actually reacting to touches by spreading ink.
///
/// If a Widget uses this class directly, it should include the following line
/// at the top of its build function to call [debugCheckHasMaterial]:
///
/// ```dart
/// assert(debugCheckHasMaterial(context));
/// ```
///
/// ## Troubleshooting
///
/// ### The ink splashes aren't visible!
///
/// If there is an opaque graphic, e.g. painted using a [Container], [Image], or
/// [DecoratedBox], between the [Material] widget and the [InkWell] widget, then
/// the splash won't be visible because it will be under the opaque graphic.
/// This is because ink splashes draw on the underlying [Material] itself, as
/// if the ink was spreading inside the material.
///
/// The [Ink] widget can be used as a replacement for [Image], [Container], or
/// [DecoratedBox] to ensure that the image or decoration also paints in the
/// [Material] itself, below the ink.
///
/// If this is not possible for some reason, e.g. because you are using an
/// opaque [CustomPaint] widget, alternatively consider using a second
/// [Material] above the opaque widget but below the [InkWell] (as an
/// ancestor to the ink well). The [MaterialType.transparency] material
/// kind can be used for this purpose.
///
/// ### InkWell isn't clipping properly
///
/// If you want to clip an InkWell or any [Ink] widgets you need to keep in mind
/// that the [Material] that the Ink will be printed on is responsible for clipping.
/// This means you can't wrap the [Ink] widget in a clipping widget directly,
/// since this will leave the [Material] not clipped (and by extension the printed
/// [Ink] widgets as well).
///
/// An easy solution is to deliberately wrap the [Ink] widgets you want to clip
/// in a [Material], and wrap that in a clipping widget instead. See [Ink] for
/// an example.
///
/// ### The ink splashes don't track the size of an animated container
/// If the size of an InkWell's [Material] ancestor changes while the InkWell's
/// splashes are expanding, you may notice that the splashes aren't clipped
/// correctly. This can't be avoided.
///
/// An example of this situation is as follows:
///
/// {@tool dartpad}
/// Tap the container to cause it to grow. Then, tap it again and hold before
/// the widget reaches its maximum size to observe the clipped ink splash.
///
/// ** See code in examples/api/lib/material/ink_well/ink_well.0.dart **
/// {@end-tool}
///
/// An InkWell's splashes will not properly update to conform to changes if the
/// size of its underlying [Material], where the splashes are rendered, changes
/// during animation. You should avoid using InkWells within [Material] widgets
/// that are changing size.
///
/// See also:
///
/// * [GestureDetector], for listening for gestures without ink splashes.
/// * [ElevatedButton] and [TextButton], two kinds of buttons in Material Design.
/// * [InkResponse], a variant of [InkWell] that doesn't force a rectangular
/// shape on the ink reaction.
class InkWell extends InkResponse {
/// Creates an ink well.
///
/// Must have an ancestor [Material] widget in which to cause ink reactions.
const InkWell({
super.key,
super.child,
super.onTap,
super.onDoubleTap,
super.onLongPress,
super.onTapDown,
super.onTapUp,
super.onTapCancel,
super.onSecondaryTap,
super.onSecondaryTapUp,
super.onSecondaryTapDown,
super.onSecondaryTapCancel,
super.onHighlightChanged,
super.onHover,
super.mouseCursor,
super.focusColor,
super.hoverColor,
super.highlightColor,
super.overlayColor,
super.splashColor,
super.splashFactory,
super.radius,
super.borderRadius,
super.customBorder,
bool? enableFeedback = true,
super.excludeFromSemantics,
super.focusNode,
super.canRequestFocus,
super.onFocusChange,
super.autofocus,
super.statesController,
super.hoverDuration,
}) : super(
containedInkWell: true,
highlightShape: BoxShape.rectangle,
enableFeedback: enableFeedback ?? true,
);
}
| flutter/packages/flutter/lib/src/material/ink_well.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/ink_well.dart",
"repo_id": "flutter",
"token_count": 17387
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'material_state.dart';
import 'menu_anchor.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// late Widget child;
// late BuildContext context;
// late MenuStyle style;
// @immutable
// class MyAppHome extends StatelessWidget {
// const MyAppHome({super.key});
// @override
// Widget build(BuildContext context) => const SizedBox();
// }
/// The visual properties that menus have in common.
///
/// Menus created by [MenuBar] and [MenuAnchor] and their themes have a
/// [MenuStyle] property which defines the visual properties whose default
/// values are to be overridden. The default values are defined by the
/// individual menu widgets and are typically based on overall theme's
/// [ThemeData.colorScheme] and [ThemeData.textTheme].
///
/// All of the [MenuStyle] properties are null by default.
///
/// Many of the [MenuStyle] properties are [MaterialStateProperty] objects which
/// resolve to different values depending on the menu's state. For example the
/// [Color] properties are defined with `MaterialStateProperty<Color>` and can
/// resolve to different colors depending on if the menu is pressed, hovered,
/// focused, disabled, etc.
///
/// These properties can override the default value for just one state or all of
/// them. For example to create a [SubmenuButton] whose background color is the
/// color scheme’s primary color with 50% opacity, but only when the menu is
/// pressed, one could write:
///
/// ```dart
/// SubmenuButton(
/// menuStyle: MenuStyle(
/// backgroundColor: MaterialStateProperty.resolveWith<Color?>(
/// (Set<MaterialState> states) {
/// if (states.contains(MaterialState.focused)) {
/// return Theme.of(context).colorScheme.primary.withOpacity(0.5);
/// }
/// return null; // Use the component's default.
/// },
/// ),
/// ),
/// menuChildren: const <Widget>[ /* ... */ ],
/// child: const Text('Fly me to the moon'),
/// ),
/// ```
///
/// In this case the background color for all other menu states would fall back
/// to the [SubmenuButton]'s default values. To unconditionally set the menu's
/// [backgroundColor] for all states one could write:
///
/// ```dart
/// const SubmenuButton(
/// menuStyle: MenuStyle(
/// backgroundColor: MaterialStatePropertyAll<Color>(Colors.green),
/// ),
/// menuChildren: <Widget>[ /* ... */ ],
/// child: Text('Let me play among the stars'),
/// ),
/// ```
///
/// To configure all of the application's menus in the same way, specify the
/// overall theme's `menuTheme`:
///
/// ```dart
/// MaterialApp(
/// theme: ThemeData(
/// menuTheme: const MenuThemeData(
/// style: MenuStyle(backgroundColor: MaterialStatePropertyAll<Color>(Colors.red)),
/// ),
/// ),
/// home: const MyAppHome(),
/// ),
/// ```
///
/// See also:
///
/// * [MenuAnchor], a widget which hosts cascading menus.
/// * [MenuBar], a widget which defines a menu bar of buttons hosting cascading
/// menus.
/// * [MenuButtonTheme], the theme for [SubmenuButton]s and [MenuItemButton]s.
/// * [ButtonStyle], a similar configuration object for button styles.
@immutable
class MenuStyle with Diagnosticable {
/// Create a [MenuStyle].
const MenuStyle({
this.backgroundColor,
this.shadowColor,
this.surfaceTintColor,
this.elevation,
this.padding,
this.minimumSize,
this.fixedSize,
this.maximumSize,
this.side,
this.shape,
this.mouseCursor,
this.visualDensity,
this.alignment,
});
/// The menu's background fill color.
final MaterialStateProperty<Color?>? backgroundColor;
/// The shadow color of the menu's [Material].
///
/// The material's elevation shadow can be difficult to see for dark themes,
/// so by default the menu classes add a semi-transparent overlay to indicate
/// elevation. See [ThemeData.applyElevationOverlayColor].
final MaterialStateProperty<Color?>? shadowColor;
/// The surface tint color of the menu's [Material].
///
/// See [Material.surfaceTintColor] for more details.
final MaterialStateProperty<Color?>? surfaceTintColor;
/// The elevation of the menu's [Material].
final MaterialStateProperty<double?>? elevation;
/// The padding between the menu's boundary and its child.
final MaterialStateProperty<EdgeInsetsGeometry?>? padding;
/// The minimum size of the menu itself.
///
/// This value must be less than or equal to [maximumSize].
final MaterialStateProperty<Size?>? minimumSize;
/// The menu's size.
///
/// This size is still constrained by the style's [minimumSize] and
/// [maximumSize]. Fixed size dimensions whose value is [double.infinity] are
/// ignored.
///
/// To specify menus with a fixed width and the default height use `fixedSize:
/// Size.fromWidth(320)`. Similarly, to specify a fixed height and the default
/// width use `fixedSize: Size.fromHeight(100)`.
final MaterialStateProperty<Size?>? fixedSize;
/// The maximum size of the menu itself.
///
/// A [Size.infinite] or null value for this property means that the menu's
/// maximum size is not constrained.
///
/// This value must be greater than or equal to [minimumSize].
final MaterialStateProperty<Size?>? maximumSize;
/// The color and weight of the menu's outline.
///
/// This value is combined with [shape] to create a shape decorated with an
/// outline.
final MaterialStateProperty<BorderSide?>? side;
/// The shape of the menu's underlying [Material].
///
/// This shape is combined with [side] to create a shape decorated with an
/// outline.
final MaterialStateProperty<OutlinedBorder?>? shape;
/// The cursor for a mouse pointer when it enters or is hovering over this
/// menu's [InkWell].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// Defines how compact the menu's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
///
/// See also:
///
/// * [ThemeData.visualDensity], which specifies the [visualDensity] for all
/// widgets within a [Theme].
final VisualDensity? visualDensity;
/// Determines the desired alignment of the submenu when opened relative to
/// the button that opens it.
///
/// If there isn't sufficient space to open the menu with the given alignment,
/// and there's space on the other side of the button, then the alignment is
/// swapped to it's opposite (1 becomes -1, etc.), and the menu will try to
/// appear on the other side of the button. If there isn't enough space there
/// either, then the menu will be pushed as far over as necessary to display
/// as much of itself as possible, possibly overlapping the parent button.
final AlignmentGeometry? alignment;
@override
int get hashCode {
final List<Object?> values = <Object?>[
backgroundColor,
shadowColor,
surfaceTintColor,
elevation,
padding,
minimumSize,
fixedSize,
maximumSize,
side,
shape,
mouseCursor,
visualDensity,
alignment,
];
return Object.hashAll(values);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is MenuStyle
&& other.backgroundColor == backgroundColor
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.elevation == elevation
&& other.padding == padding
&& other.minimumSize == minimumSize
&& other.fixedSize == fixedSize
&& other.maximumSize == maximumSize
&& other.side == side
&& other.shape == shape
&& other.mouseCursor == mouseCursor
&& other.visualDensity == visualDensity
&& other.alignment == alignment;
}
/// Returns a copy of this MenuStyle with the given fields replaced with
/// the new values.
MenuStyle copyWith({
MaterialStateProperty<Color?>? backgroundColor,
MaterialStateProperty<Color?>? shadowColor,
MaterialStateProperty<Color?>? surfaceTintColor,
MaterialStateProperty<double?>? elevation,
MaterialStateProperty<EdgeInsetsGeometry?>? padding,
MaterialStateProperty<Size?>? minimumSize,
MaterialStateProperty<Size?>? fixedSize,
MaterialStateProperty<Size?>? maximumSize,
MaterialStateProperty<BorderSide?>? side,
MaterialStateProperty<OutlinedBorder?>? shape,
MaterialStateProperty<MouseCursor?>? mouseCursor,
VisualDensity? visualDensity,
AlignmentGeometry? alignment,
}) {
return MenuStyle(
backgroundColor: backgroundColor ?? this.backgroundColor,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
elevation: elevation ?? this.elevation,
padding: padding ?? this.padding,
minimumSize: minimumSize ?? this.minimumSize,
fixedSize: fixedSize ?? this.fixedSize,
maximumSize: maximumSize ?? this.maximumSize,
side: side ?? this.side,
shape: shape ?? this.shape,
mouseCursor: mouseCursor ?? this.mouseCursor,
visualDensity: visualDensity ?? this.visualDensity,
alignment: alignment ?? this.alignment,
);
}
/// Returns a copy of this MenuStyle where the non-null fields in [style]
/// have replaced the corresponding null fields in this MenuStyle.
///
/// In other words, [style] is used to fill in unspecified (null) fields
/// this MenuStyle.
MenuStyle merge(MenuStyle? style) {
if (style == null) {
return this;
}
return copyWith(
backgroundColor: backgroundColor ?? style.backgroundColor,
shadowColor: shadowColor ?? style.shadowColor,
surfaceTintColor: surfaceTintColor ?? style.surfaceTintColor,
elevation: elevation ?? style.elevation,
padding: padding ?? style.padding,
minimumSize: minimumSize ?? style.minimumSize,
fixedSize: fixedSize ?? style.fixedSize,
maximumSize: maximumSize ?? style.maximumSize,
side: side ?? style.side,
shape: shape ?? style.shape,
mouseCursor: mouseCursor ?? style.mouseCursor,
visualDensity: visualDensity ?? style.visualDensity,
alignment: alignment ?? style.alignment,
);
}
/// Linearly interpolate between two [MenuStyle]s.
static MenuStyle? lerp(MenuStyle? a, MenuStyle? b, double t) {
if (identical(a, b)) {
return a;
}
return MenuStyle(
backgroundColor: MaterialStateProperty.lerp<Color?>(a?.backgroundColor, b?.backgroundColor, t, Color.lerp),
shadowColor: MaterialStateProperty.lerp<Color?>(a?.shadowColor, b?.shadowColor, t, Color.lerp),
surfaceTintColor: MaterialStateProperty.lerp<Color?>(a?.surfaceTintColor, b?.surfaceTintColor, t, Color.lerp),
elevation: MaterialStateProperty.lerp<double?>(a?.elevation, b?.elevation, t, lerpDouble),
padding: MaterialStateProperty.lerp<EdgeInsetsGeometry?>(a?.padding, b?.padding, t, EdgeInsetsGeometry.lerp),
minimumSize: MaterialStateProperty.lerp<Size?>(a?.minimumSize, b?.minimumSize, t, Size.lerp),
fixedSize: MaterialStateProperty.lerp<Size?>(a?.fixedSize, b?.fixedSize, t, Size.lerp),
maximumSize: MaterialStateProperty.lerp<Size?>(a?.maximumSize, b?.maximumSize, t, Size.lerp),
side: _LerpSides(a?.side, b?.side, t),
shape: MaterialStateProperty.lerp<OutlinedBorder?>(a?.shape, b?.shape, t, OutlinedBorder.lerp),
mouseCursor: t < 0.5 ? a?.mouseCursor : b?.mouseCursor,
visualDensity: t < 0.5 ? a?.visualDensity : b?.visualDensity,
alignment: AlignmentGeometry.lerp(a?.alignment, b?.alignment, t),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('shadowColor', shadowColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<double?>>('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<EdgeInsetsGeometry?>>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Size?>>('minimumSize', minimumSize, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Size?>>('fixedSize', fixedSize, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Size?>>('maximumSize', maximumSize, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<BorderSide?>>('side', side, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<OutlinedBorder?>>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>>('mouseCursor', mouseCursor, defaultValue: null));
properties.add(DiagnosticsProperty<VisualDensity>('visualDensity', visualDensity, defaultValue: null));
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment, defaultValue: null));
}
}
/// A required helper class because [BorderSide.lerp] doesn't support passing or
/// returning null values.
class _LerpSides implements MaterialStateProperty<BorderSide?> {
const _LerpSides(this.a, this.b, this.t);
final MaterialStateProperty<BorderSide?>? a;
final MaterialStateProperty<BorderSide?>? b;
final double t;
@override
BorderSide? resolve(Set<MaterialState> states) {
final BorderSide? resolvedA = a?.resolve(states);
final BorderSide? resolvedB = b?.resolve(states);
if (resolvedA == null && resolvedB == null) {
return null;
}
if (resolvedA == null) {
return BorderSide.lerp(BorderSide(width: 0, color: resolvedB!.color.withAlpha(0)), resolvedB, t);
}
if (resolvedB == null) {
return BorderSide.lerp(resolvedA, BorderSide(width: 0, color: resolvedA.color.withAlpha(0)), t);
}
return BorderSide.lerp(resolvedA, resolvedB, t);
}
}
| flutter/packages/flutter/lib/src/material/menu_style.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/menu_style.dart",
"repo_id": "flutter",
"token_count": 4648
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'divider.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'popup_menu_theme.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'tooltip.dart';
// Examples can assume:
// enum Commands { heroAndScholar, hurricaneCame }
// late bool _heroAndScholar;
// late dynamic _selection;
// late BuildContext context;
// void setState(VoidCallback fn) { }
// enum Menu { itemOne, itemTwo, itemThree, itemFour }
const Duration _kMenuDuration = Duration(milliseconds: 300);
const double _kMenuCloseIntervalEnd = 2.0 / 3.0;
const double _kMenuDividerHeight = 16.0;
const double _kMenuMaxWidth = 5.0 * _kMenuWidthStep;
const double _kMenuMinWidth = 2.0 * _kMenuWidthStep;
const double _kMenuVerticalPadding = 8.0;
const double _kMenuWidthStep = 56.0;
const double _kMenuScreenPadding = 8.0;
/// A base class for entries in a Material Design popup menu.
///
/// The popup menu widget uses this interface to interact with the menu items.
/// To show a popup menu, use the [showMenu] function. To create a button that
/// shows a popup menu, consider using [PopupMenuButton].
///
/// The type `T` is the type of the value(s) the entry represents. All the
/// entries in a given menu must represent values with consistent types.
///
/// A [PopupMenuEntry] may represent multiple values, for example a row with
/// several icons, or a single entry, for example a menu item with an icon (see
/// [PopupMenuItem]), or no value at all (for example, [PopupMenuDivider]).
///
/// See also:
///
/// * [PopupMenuItem], a popup menu entry for a single value.
/// * [PopupMenuDivider], a popup menu entry that is just a horizontal line.
/// * [CheckedPopupMenuItem], a popup menu item with a checkmark.
/// * [showMenu], a method to dynamically show a popup menu at a given location.
/// * [PopupMenuButton], an [IconButton] that automatically shows a menu when
/// it is tapped.
abstract class PopupMenuEntry<T> extends StatefulWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const PopupMenuEntry({ super.key });
/// The amount of vertical space occupied by this entry.
///
/// This value is used at the time the [showMenu] method is called, if the
/// `initialValue` argument is provided, to determine the position of this
/// entry when aligning the selected entry over the given `position`. It is
/// otherwise ignored.
double get height;
/// Whether this entry represents a particular value.
///
/// This method is used by [showMenu], when it is called, to align the entry
/// representing the `initialValue`, if any, to the given `position`, and then
/// later is called on each entry to determine if it should be highlighted (if
/// the method returns true, the entry will have its background color set to
/// the ambient [ThemeData.highlightColor]). If `initialValue` is null, then
/// this method is not called.
///
/// If the [PopupMenuEntry] represents a single value, this should return true
/// if the argument matches that value. If it represents multiple values, it
/// should return true if the argument matches any of them.
bool represents(T? value);
}
/// A horizontal divider in a Material Design popup menu.
///
/// This widget adapts the [Divider] for use in popup menus.
///
/// See also:
///
/// * [PopupMenuItem], for the kinds of items that this widget divides.
/// * [showMenu], a method to dynamically show a popup menu at a given location.
/// * [PopupMenuButton], an [IconButton] that automatically shows a menu when
/// it is tapped.
class PopupMenuDivider extends PopupMenuEntry<Never> {
/// Creates a horizontal divider for a popup menu.
///
/// By default, the divider has a height of 16 logical pixels.
const PopupMenuDivider({ super.key, this.height = _kMenuDividerHeight });
/// The height of the divider entry.
///
/// Defaults to 16 pixels.
@override
final double height;
@override
bool represents(void value) => false;
@override
State<PopupMenuDivider> createState() => _PopupMenuDividerState();
}
class _PopupMenuDividerState extends State<PopupMenuDivider> {
@override
Widget build(BuildContext context) => Divider(height: widget.height);
}
// This widget only exists to enable _PopupMenuRoute to save the sizes of
// each menu item. The sizes are used by _PopupMenuRouteLayout to compute the
// y coordinate of the menu's origin so that the center of selected menu
// item lines up with the center of its PopupMenuButton.
class _MenuItem extends SingleChildRenderObjectWidget {
const _MenuItem({
required this.onLayout,
required super.child,
});
final ValueChanged<Size> onLayout;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderMenuItem(onLayout);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderMenuItem renderObject) {
renderObject.onLayout = onLayout;
}
}
class _RenderMenuItem extends RenderShiftedBox {
_RenderMenuItem(this.onLayout, [RenderBox? child]) : super(child);
ValueChanged<Size> onLayout;
@override
Size computeDryLayout(BoxConstraints constraints) {
return child?.getDryLayout(constraints) ?? Size.zero;
}
@override
void performLayout() {
if (child == null) {
size = Size.zero;
} else {
child!.layout(constraints, parentUsesSize: true);
size = constraints.constrain(child!.size);
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Offset.zero;
}
onLayout(size);
}
}
/// An item in a Material Design popup menu.
///
/// To show a popup menu, use the [showMenu] function. To create a button that
/// shows a popup menu, consider using [PopupMenuButton].
///
/// To show a checkmark next to a popup menu item, consider using
/// [CheckedPopupMenuItem].
///
/// Typically the [child] of a [PopupMenuItem] is a [Text] widget. More
/// elaborate menus with icons can use a [ListTile]. By default, a
/// [PopupMenuItem] is [kMinInteractiveDimension] pixels high. If you use a widget
/// with a different height, it must be specified in the [height] property.
///
/// {@tool snippet}
///
/// Here, a [Text] widget is used with a popup menu item. The `Menu` type
/// is an enum, not shown here.
///
/// ```dart
/// const PopupMenuItem<Menu>(
/// value: Menu.itemOne,
/// child: Text('Item 1'),
/// )
/// ```
/// {@end-tool}
///
/// See the example at [PopupMenuButton] for how this example could be used in a
/// complete menu, and see the example at [CheckedPopupMenuItem] for one way to
/// keep the text of [PopupMenuItem]s that use [Text] widgets in their [child]
/// slot aligned with the text of [CheckedPopupMenuItem]s or of [PopupMenuItem]
/// that use a [ListTile] in their [child] slot.
///
/// See also:
///
/// * [PopupMenuDivider], which can be used to divide items from each other.
/// * [CheckedPopupMenuItem], a variant of [PopupMenuItem] with a checkmark.
/// * [showMenu], a method to dynamically show a popup menu at a given location.
/// * [PopupMenuButton], an [IconButton] that automatically shows a menu when
/// it is tapped.
class PopupMenuItem<T> extends PopupMenuEntry<T> {
/// Creates an item for a popup menu.
///
/// By default, the item is [enabled].
const PopupMenuItem({
super.key,
this.value,
this.onTap,
this.enabled = true,
this.height = kMinInteractiveDimension,
this.padding,
this.textStyle,
this.labelTextStyle,
this.mouseCursor,
required this.child,
});
/// The value that will be returned by [showMenu] if this entry is selected.
final T? value;
/// Called when the menu item is tapped.
final VoidCallback? onTap;
/// Whether the user is permitted to select this item.
///
/// Defaults to true. If this is false, then the item will not react to
/// touches.
final bool enabled;
/// The minimum height of the menu item.
///
/// Defaults to [kMinInteractiveDimension] pixels.
@override
final double height;
/// The padding of the menu item.
///
/// The [height] property may interact with the applied padding. For example,
/// If a [height] greater than the height of the sum of the padding and [child]
/// is provided, then the padding's effect will not be visible.
///
/// If this is null and [ThemeData.useMaterial3] is true, the horizontal padding
/// defaults to 12.0 on both sides.
///
/// If this is null and [ThemeData.useMaterial3] is false, the horizontal padding
/// defaults to 16.0 on both sides.
final EdgeInsets? padding;
/// The text style of the popup menu item.
///
/// If this property is null, then [PopupMenuThemeData.textStyle] is used.
/// If [PopupMenuThemeData.textStyle] is also null, then [TextTheme.titleMedium]
/// of [ThemeData.textTheme] is used.
final TextStyle? textStyle;
/// The label style of the popup menu item.
///
/// When [ThemeData.useMaterial3] is true, this styles the text of the popup menu item.
///
/// If this property is null, then [PopupMenuThemeData.labelTextStyle] is used.
/// If [PopupMenuThemeData.labelTextStyle] is also null, then [TextTheme.labelLarge]
/// is used with the [ColorScheme.onSurface] color when popup menu item is enabled and
/// the [ColorScheme.onSurface] color with 0.38 opacity when the popup menu item is disabled.
final MaterialStateProperty<TextStyle?>? labelTextStyle;
/// {@template flutter.material.popupmenu.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// If null, then the value of [PopupMenuThemeData.mouseCursor] is used. If
/// that is also null, then [MaterialStateMouseCursor.clickable] is used.
final MouseCursor? mouseCursor;
/// The widget below this widget in the tree.
///
/// Typically a single-line [ListTile] (for menus with icons) or a [Text]. An
/// appropriate [DefaultTextStyle] is put in scope for the child. In either
/// case, the text should be short enough that it won't wrap.
final Widget? child;
@override
bool represents(T? value) => value == this.value;
@override
PopupMenuItemState<T, PopupMenuItem<T>> createState() => PopupMenuItemState<T, PopupMenuItem<T>>();
}
/// The [State] for [PopupMenuItem] subclasses.
///
/// By default this implements the basic styling and layout of Material Design
/// popup menu items.
///
/// The [buildChild] method can be overridden to adjust exactly what gets placed
/// in the menu. By default it returns [PopupMenuItem.child].
///
/// The [handleTap] method can be overridden to adjust exactly what happens when
/// the item is tapped. By default, it uses [Navigator.pop] to return the
/// [PopupMenuItem.value] from the menu route.
///
/// This class takes two type arguments. The second, `W`, is the exact type of
/// the [Widget] that is using this [State]. It must be a subclass of
/// [PopupMenuItem]. The first, `T`, must match the type argument of that widget
/// class, and is the type of values returned from this menu.
class PopupMenuItemState<T, W extends PopupMenuItem<T>> extends State<W> {
/// The menu item contents.
///
/// Used by the [build] method.
///
/// By default, this returns [PopupMenuItem.child]. Override this to put
/// something else in the menu entry.
@protected
Widget? buildChild() => widget.child;
/// The handler for when the user selects the menu item.
///
/// Used by the [InkWell] inserted by the [build] method.
///
/// By default, uses [Navigator.pop] to return the [PopupMenuItem.value] from
/// the menu route.
@protected
void handleTap() {
// Need to pop the navigator first in case onTap may push new route onto navigator.
Navigator.pop<T>(context, widget.value);
widget.onTap?.call();
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final PopupMenuThemeData popupMenuTheme = PopupMenuTheme.of(context);
final PopupMenuThemeData defaults = theme.useMaterial3 ? _PopupMenuDefaultsM3(context) : _PopupMenuDefaultsM2(context);
final Set<MaterialState> states = <MaterialState>{
if (!widget.enabled) MaterialState.disabled,
};
TextStyle style = theme.useMaterial3
? (widget.labelTextStyle?.resolve(states)
?? popupMenuTheme.labelTextStyle?.resolve(states)!
?? defaults.labelTextStyle!.resolve(states)!)
: (widget.textStyle
?? popupMenuTheme.textStyle
?? defaults.textStyle!);
if (!widget.enabled && !theme.useMaterial3) {
style = style.copyWith(color: theme.disabledColor);
}
Widget item = AnimatedDefaultTextStyle(
style: style,
duration: kThemeChangeDuration,
child: Container(
alignment: AlignmentDirectional.centerStart,
constraints: BoxConstraints(minHeight: widget.height),
padding: widget.padding ?? (theme.useMaterial3 ? _PopupMenuDefaultsM3.menuHorizontalPadding : _PopupMenuDefaultsM2.menuHorizontalPadding),
child: buildChild(),
),
);
if (!widget.enabled) {
final bool isDark = theme.brightness == Brightness.dark;
item = IconTheme.merge(
data: IconThemeData(opacity: isDark ? 0.5 : 0.38),
child: item,
);
}
return MergeSemantics(
child: Semantics(
enabled: widget.enabled,
button: true,
child: InkWell(
onTap: widget.enabled ? handleTap : null,
canRequestFocus: widget.enabled,
mouseCursor: _EffectiveMouseCursor(widget.mouseCursor, popupMenuTheme.mouseCursor),
child: ListTileTheme.merge(
contentPadding: EdgeInsets.zero,
titleTextStyle: style,
child: item,
),
),
),
);
}
}
/// An item with a checkmark in a Material Design popup menu.
///
/// To show a popup menu, use the [showMenu] function. To create a button that
/// shows a popup menu, consider using [PopupMenuButton].
///
/// A [CheckedPopupMenuItem] is kMinInteractiveDimension pixels high, which
/// matches the default minimum height of a [PopupMenuItem]. The horizontal
/// layout uses [ListTile]; the checkmark is an [Icons.done] icon, shown in the
/// [ListTile.leading] position.
///
/// {@tool snippet}
///
/// Suppose a `Commands` enum exists that lists the possible commands from a
/// particular popup menu, including `Commands.heroAndScholar` and
/// `Commands.hurricaneCame`, and further suppose that there is a
/// `_heroAndScholar` member field which is a boolean. The example below shows a
/// menu with one menu item with a checkmark that can toggle the boolean, and
/// one menu item without a checkmark for selecting the second option. (It also
/// shows a divider placed between the two menu items.)
///
/// ```dart
/// PopupMenuButton<Commands>(
/// onSelected: (Commands result) {
/// switch (result) {
/// case Commands.heroAndScholar:
/// setState(() { _heroAndScholar = !_heroAndScholar; });
/// case Commands.hurricaneCame:
/// // ...handle hurricane option
/// break;
/// // ...other items handled here
/// }
/// },
/// itemBuilder: (BuildContext context) => <PopupMenuEntry<Commands>>[
/// CheckedPopupMenuItem<Commands>(
/// checked: _heroAndScholar,
/// value: Commands.heroAndScholar,
/// child: const Text('Hero and scholar'),
/// ),
/// const PopupMenuDivider(),
/// const PopupMenuItem<Commands>(
/// value: Commands.hurricaneCame,
/// child: ListTile(leading: Icon(null), title: Text('Bring hurricane')),
/// ),
/// // ...other items listed here
/// ],
/// )
/// ```
/// {@end-tool}
///
/// In particular, observe how the second menu item uses a [ListTile] with a
/// blank [Icon] in the [ListTile.leading] position to get the same alignment as
/// the item with the checkmark.
///
/// See also:
///
/// * [PopupMenuItem], a popup menu entry for picking a command (as opposed to
/// toggling a value).
/// * [PopupMenuDivider], a popup menu entry that is just a horizontal line.
/// * [showMenu], a method to dynamically show a popup menu at a given location.
/// * [PopupMenuButton], an [IconButton] that automatically shows a menu when
/// it is tapped.
class CheckedPopupMenuItem<T> extends PopupMenuItem<T> {
/// Creates a popup menu item with a checkmark.
///
/// By default, the menu item is [enabled] but unchecked. To mark the item as
/// checked, set [checked] to true.
const CheckedPopupMenuItem({
super.key,
super.value,
this.checked = false,
super.enabled,
super.padding,
super.height,
super.labelTextStyle,
super.mouseCursor,
super.child,
super.onTap,
});
/// Whether to display a checkmark next to the menu item.
///
/// Defaults to false.
///
/// When true, an [Icons.done] checkmark is displayed.
///
/// When this popup menu item is selected, the checkmark will fade in or out
/// as appropriate to represent the implied new state.
final bool checked;
/// The widget below this widget in the tree.
///
/// Typically a [Text]. An appropriate [DefaultTextStyle] is put in scope for
/// the child. The text should be short enough that it won't wrap.
///
/// This widget is placed in the [ListTile.title] slot of a [ListTile] whose
/// [ListTile.leading] slot is an [Icons.done] icon.
@override
Widget? get child => super.child;
@override
PopupMenuItemState<T, CheckedPopupMenuItem<T>> createState() => _CheckedPopupMenuItemState<T>();
}
class _CheckedPopupMenuItemState<T> extends PopupMenuItemState<T, CheckedPopupMenuItem<T>> with SingleTickerProviderStateMixin {
static const Duration _fadeDuration = Duration(milliseconds: 150);
late AnimationController _controller;
Animation<double> get _opacity => _controller.view;
@override
void initState() {
super.initState();
_controller = AnimationController(duration: _fadeDuration, vsync: this)
..value = widget.checked ? 1.0 : 0.0
..addListener(() => setState(() { /* animation changed */ }));
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
void handleTap() {
// This fades the checkmark in or out when tapped.
if (widget.checked) {
_controller.reverse();
} else {
_controller.forward();
}
super.handleTap();
}
@override
Widget buildChild() {
final ThemeData theme = Theme.of(context);
final PopupMenuThemeData popupMenuTheme = PopupMenuTheme.of(context);
final PopupMenuThemeData defaults = theme.useMaterial3 ? _PopupMenuDefaultsM3(context) : _PopupMenuDefaultsM2(context);
final Set<MaterialState> states = <MaterialState>{
if (widget.checked) MaterialState.selected,
};
final MaterialStateProperty<TextStyle?>? effectiveLabelTextStyle = widget.labelTextStyle
?? popupMenuTheme.labelTextStyle
?? defaults.labelTextStyle;
return IgnorePointer(
child: ListTileTheme.merge(
contentPadding: EdgeInsets.zero,
child: ListTile(
enabled: widget.enabled,
titleTextStyle: effectiveLabelTextStyle?.resolve(states),
leading: FadeTransition(
opacity: _opacity,
child: Icon(_controller.isDismissed ? null : Icons.done),
),
title: widget.child,
),
),
);
}
}
class _PopupMenu<T> extends StatelessWidget {
const _PopupMenu({
super.key,
required this.itemKeys,
required this.route,
required this.semanticLabel,
this.constraints,
required this.clipBehavior,
});
final List<GlobalKey> itemKeys;
final _PopupMenuRoute<T> route;
final String? semanticLabel;
final BoxConstraints? constraints;
final Clip clipBehavior;
@override
Widget build(BuildContext context) {
final double unit = 1.0 / (route.items.length + 1.5); // 1.0 for the width and 0.5 for the last item's fade.
final List<Widget> children = <Widget>[];
final ThemeData theme = Theme.of(context);
final PopupMenuThemeData popupMenuTheme = PopupMenuTheme.of(context);
final PopupMenuThemeData defaults = theme.useMaterial3 ? _PopupMenuDefaultsM3(context) : _PopupMenuDefaultsM2(context);
for (int i = 0; i < route.items.length; i += 1) {
final double start = (i + 1) * unit;
final double end = clampDouble(start + 1.5 * unit, 0.0, 1.0);
final CurvedAnimation opacity = CurvedAnimation(
parent: route.animation!,
curve: Interval(start, end),
);
Widget item = route.items[i];
if (route.initialValue != null && route.items[i].represents(route.initialValue)) {
item = ColoredBox(
color: Theme.of(context).highlightColor,
child: item,
);
}
children.add(
_MenuItem(
onLayout: (Size size) {
route.itemSizes[i] = size;
},
child: FadeTransition(
key: itemKeys[i],
opacity: opacity,
child: item,
),
),
);
}
final CurveTween opacity = CurveTween(curve: const Interval(0.0, 1.0 / 3.0));
final CurveTween width = CurveTween(curve: Interval(0.0, unit));
final CurveTween height = CurveTween(curve: Interval(0.0, unit * route.items.length));
final Widget child = ConstrainedBox(
constraints: constraints ?? const BoxConstraints(
minWidth: _kMenuMinWidth,
maxWidth: _kMenuMaxWidth,
),
child: IntrinsicWidth(
stepWidth: _kMenuWidthStep,
child: Semantics(
scopesRoute: true,
namesRoute: true,
explicitChildNodes: true,
label: semanticLabel,
child: SingleChildScrollView(
padding: const EdgeInsets.symmetric(
vertical: _kMenuVerticalPadding,
),
child: ListBody(children: children),
),
),
),
);
return AnimatedBuilder(
animation: route.animation!,
builder: (BuildContext context, Widget? child) {
return FadeTransition(
opacity: opacity.animate(route.animation!),
child: Material(
shape: route.shape ?? popupMenuTheme.shape ?? defaults.shape,
color: route.color ?? popupMenuTheme.color ?? defaults.color,
clipBehavior: clipBehavior,
type: MaterialType.card,
elevation: route.elevation ?? popupMenuTheme.elevation ?? defaults.elevation!,
shadowColor: route.shadowColor ?? popupMenuTheme.shadowColor ?? defaults.shadowColor,
surfaceTintColor: route.surfaceTintColor ?? popupMenuTheme.surfaceTintColor ?? defaults.surfaceTintColor,
child: Align(
alignment: AlignmentDirectional.topEnd,
widthFactor: width.evaluate(route.animation!),
heightFactor: height.evaluate(route.animation!),
child: child,
),
),
);
},
child: child,
);
}
}
// Positioning of the menu on the screen.
class _PopupMenuRouteLayout extends SingleChildLayoutDelegate {
_PopupMenuRouteLayout(
this.position,
this.itemSizes,
this.selectedItemIndex,
this.textDirection,
this.padding,
this.avoidBounds,
);
// Rectangle of underlying button, relative to the overlay's dimensions.
final RelativeRect position;
// The sizes of each item are computed when the menu is laid out, and before
// the route is laid out.
List<Size?> itemSizes;
// The index of the selected item, or null if PopupMenuButton.initialValue
// was not specified.
final int? selectedItemIndex;
// Whether to prefer going to the left or to the right.
final TextDirection textDirection;
// The padding of unsafe area.
EdgeInsets padding;
// List of rectangles that we should avoid overlapping. Unusable screen area.
final Set<Rect> avoidBounds;
// We put the child wherever position specifies, so long as it will fit within
// the specified parent size padded (inset) by 8. If necessary, we adjust the
// child's position so that it fits.
@override
BoxConstraints getConstraintsForChild(BoxConstraints constraints) {
// The menu can be at most the size of the overlay minus 8.0 pixels in each
// direction.
return BoxConstraints.loose(constraints.biggest).deflate(
const EdgeInsets.all(_kMenuScreenPadding) + padding,
);
}
@override
Offset getPositionForChild(Size size, Size childSize) {
final double y = position.top;
// Find the ideal horizontal position.
// size: The size of the overlay.
// childSize: The size of the menu, when fully open, as determined by
// getConstraintsForChild.
double x;
if (position.left > position.right) {
// Menu button is closer to the right edge, so grow to the left, aligned to the right edge.
x = size.width - position.right - childSize.width;
} else if (position.left < position.right) {
// Menu button is closer to the left edge, so grow to the right, aligned to the left edge.
x = position.left;
} else {
// Menu button is equidistant from both edges, so grow in reading direction.
x = switch (textDirection) {
TextDirection.rtl => size.width - position.right - childSize.width,
TextDirection.ltr => position.left,
};
}
final Offset wantedPosition = Offset(x, y);
final Offset originCenter = position.toRect(Offset.zero & size).center;
final Iterable<Rect> subScreens = DisplayFeatureSubScreen.subScreensInBounds(Offset.zero & size, avoidBounds);
final Rect subScreen = _closestScreen(subScreens, originCenter);
return _fitInsideScreen(subScreen, childSize, wantedPosition);
}
Rect _closestScreen(Iterable<Rect> screens, Offset point) {
Rect closest = screens.first;
for (final Rect screen in screens) {
if ((screen.center - point).distance < (closest.center - point).distance) {
closest = screen;
}
}
return closest;
}
Offset _fitInsideScreen(Rect screen, Size childSize, Offset wantedPosition){
double x = wantedPosition.dx;
double y = wantedPosition.dy;
// Avoid going outside an area defined as the rectangle 8.0 pixels from the
// edge of the screen in every direction.
if (x < screen.left + _kMenuScreenPadding + padding.left) {
x = screen.left + _kMenuScreenPadding + padding.left;
} else if (x + childSize.width > screen.right - _kMenuScreenPadding - padding.right) {
x = screen.right - childSize.width - _kMenuScreenPadding - padding.right;
}
if (y < screen.top + _kMenuScreenPadding + padding.top) {
y = _kMenuScreenPadding + padding.top;
} else if (y + childSize.height > screen.bottom - _kMenuScreenPadding - padding.bottom) {
y = screen.bottom - childSize.height - _kMenuScreenPadding - padding.bottom;
}
return Offset(x,y);
}
@override
bool shouldRelayout(_PopupMenuRouteLayout oldDelegate) {
// If called when the old and new itemSizes have been initialized then
// we expect them to have the same length because there's no practical
// way to change length of the items list once the menu has been shown.
assert(itemSizes.length == oldDelegate.itemSizes.length);
return position != oldDelegate.position
|| selectedItemIndex != oldDelegate.selectedItemIndex
|| textDirection != oldDelegate.textDirection
|| !listEquals(itemSizes, oldDelegate.itemSizes)
|| padding != oldDelegate.padding
|| !setEquals(avoidBounds, oldDelegate.avoidBounds);
}
}
class _PopupMenuRoute<T> extends PopupRoute<T> {
_PopupMenuRoute({
required this.position,
required this.items,
required this.itemKeys,
this.initialValue,
this.elevation,
this.surfaceTintColor,
this.shadowColor,
required this.barrierLabel,
this.semanticLabel,
this.shape,
this.color,
required this.capturedThemes,
this.constraints,
required this.clipBehavior,
super.settings,
this.popUpAnimationStyle,
}) : itemSizes = List<Size?>.filled(items.length, null),
// Menus always cycle focus through their items irrespective of the
// focus traversal edge behavior set in the Navigator.
super(traversalEdgeBehavior: TraversalEdgeBehavior.closedLoop);
final RelativeRect position;
final List<PopupMenuEntry<T>> items;
final List<GlobalKey> itemKeys;
final List<Size?> itemSizes;
final T? initialValue;
final double? elevation;
final Color? surfaceTintColor;
final Color? shadowColor;
final String? semanticLabel;
final ShapeBorder? shape;
final Color? color;
final CapturedThemes capturedThemes;
final BoxConstraints? constraints;
final Clip clipBehavior;
final AnimationStyle? popUpAnimationStyle;
@override
Animation<double> createAnimation() {
if (popUpAnimationStyle != AnimationStyle.noAnimation) {
return CurvedAnimation(
parent: super.createAnimation(),
curve: popUpAnimationStyle?.curve ?? Curves.linear,
reverseCurve: popUpAnimationStyle?.reverseCurve ?? const Interval(0.0, _kMenuCloseIntervalEnd),
);
}
return super.createAnimation();
}
void scrollTo(int selectedItemIndex) {
SchedulerBinding.instance.addPostFrameCallback((_) {
if (itemKeys[selectedItemIndex].currentContext != null) {
Scrollable.ensureVisible(itemKeys[selectedItemIndex].currentContext!);
}
});
}
@override
Duration get transitionDuration => popUpAnimationStyle?.duration ?? _kMenuDuration;
@override
bool get barrierDismissible => true;
@override
Color? get barrierColor => null;
@override
final String barrierLabel;
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
int? selectedItemIndex;
if (initialValue != null) {
for (int index = 0; selectedItemIndex == null && index < items.length; index += 1) {
if (items[index].represents(initialValue)) {
selectedItemIndex = index;
}
}
}
if (selectedItemIndex != null) {
scrollTo(selectedItemIndex);
}
final Widget menu = _PopupMenu<T>(
route: this,
itemKeys: itemKeys,
semanticLabel: semanticLabel,
constraints: constraints,
clipBehavior: clipBehavior,
);
final MediaQueryData mediaQuery = MediaQuery.of(context);
return MediaQuery.removePadding(
context: context,
removeTop: true,
removeBottom: true,
removeLeft: true,
removeRight: true,
child: Builder(
builder: (BuildContext context) {
return CustomSingleChildLayout(
delegate: _PopupMenuRouteLayout(
position,
itemSizes,
selectedItemIndex,
Directionality.of(context),
mediaQuery.padding,
_avoidBounds(mediaQuery),
),
child: capturedThemes.wrap(menu),
);
},
),
);
}
Set<Rect> _avoidBounds(MediaQueryData mediaQuery) {
return DisplayFeatureSubScreen.avoidBounds(mediaQuery).toSet();
}
}
/// Show a popup menu that contains the `items` at `position`.
///
/// The `items` parameter must not be empty.
///
/// If `initialValue` is specified then the first item with a matching value
/// will be highlighted and the value of `position` gives the rectangle whose
/// vertical center will be aligned with the vertical center of the highlighted
/// item (when possible).
///
/// If `initialValue` is not specified then the top of the menu will be aligned
/// with the top of the `position` rectangle.
///
/// In both cases, the menu position will be adjusted if necessary to fit on the
/// screen.
///
/// Horizontally, the menu is positioned so that it grows in the direction that
/// has the most room. For example, if the `position` describes a rectangle on
/// the left edge of the screen, then the left edge of the menu is aligned with
/// the left edge of the `position`, and the menu grows to the right. If both
/// edges of the `position` are equidistant from the opposite edge of the
/// screen, then the ambient [Directionality] is used as a tie-breaker,
/// preferring to grow in the reading direction.
///
/// The positioning of the `initialValue` at the `position` is implemented by
/// iterating over the `items` to find the first whose
/// [PopupMenuEntry.represents] method returns true for `initialValue`, and then
/// summing the values of [PopupMenuEntry.height] for all the preceding widgets
/// in the list.
///
/// The `elevation` argument specifies the z-coordinate at which to place the
/// menu. The elevation defaults to 8, the appropriate elevation for popup
/// menus.
///
/// The `context` argument is used to look up the [Navigator] and [Theme] for
/// the menu. It is only used when the method is called. Its corresponding
/// widget can be safely removed from the tree before the popup menu is closed.
///
/// The `useRootNavigator` argument is used to determine whether to push the
/// menu to the [Navigator] furthest from or nearest to the given `context`. It
/// is `false` by default.
///
/// The `semanticLabel` argument is used by accessibility frameworks to
/// announce screen transitions when the menu is opened and closed. If this
/// label is not provided, it will default to
/// [MaterialLocalizations.popupMenuLabel].
///
/// The `clipBehavior` argument is used to clip the shape of the menu. Defaults to
/// [Clip.none].
///
/// See also:
///
/// * [PopupMenuItem], a popup menu entry for a single value.
/// * [PopupMenuDivider], a popup menu entry that is just a horizontal line.
/// * [CheckedPopupMenuItem], a popup menu item with a checkmark.
/// * [PopupMenuButton], which provides an [IconButton] that shows a menu by
/// calling this method automatically.
/// * [SemanticsConfiguration.namesRoute], for a description of edge triggered
/// semantics.
Future<T?> showMenu<T>({
required BuildContext context,
required RelativeRect position,
required List<PopupMenuEntry<T>> items,
T? initialValue,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
String? semanticLabel,
ShapeBorder? shape,
Color? color,
bool useRootNavigator = false,
BoxConstraints? constraints,
Clip clipBehavior = Clip.none,
RouteSettings? routeSettings,
AnimationStyle? popUpAnimationStyle,
}) {
assert(items.isNotEmpty);
assert(debugCheckHasMaterialLocalizations(context));
switch (Theme.of(context).platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
break;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
semanticLabel ??= MaterialLocalizations.of(context).popupMenuLabel;
}
final List<GlobalKey> menuItemKeys = List<GlobalKey>.generate(items.length, (int index) => GlobalKey());
final NavigatorState navigator = Navigator.of(context, rootNavigator: useRootNavigator);
return navigator.push(_PopupMenuRoute<T>(
position: position,
items: items,
itemKeys: menuItemKeys,
initialValue: initialValue,
elevation: elevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
semanticLabel: semanticLabel,
barrierLabel: MaterialLocalizations.of(context).menuDismissLabel,
shape: shape,
color: color,
capturedThemes: InheritedTheme.capture(from: context, to: navigator.context),
constraints: constraints,
clipBehavior: clipBehavior,
settings: routeSettings,
popUpAnimationStyle: popUpAnimationStyle,
));
}
/// Signature for the callback invoked when a menu item is selected. The
/// argument is the value of the [PopupMenuItem] that caused its menu to be
/// dismissed.
///
/// Used by [PopupMenuButton.onSelected].
typedef PopupMenuItemSelected<T> = void Function(T value);
/// Signature for the callback invoked when a [PopupMenuButton] is dismissed
/// without selecting an item.
///
/// Used by [PopupMenuButton.onCanceled].
typedef PopupMenuCanceled = void Function();
/// Signature used by [PopupMenuButton] to lazily construct the items shown when
/// the button is pressed.
///
/// Used by [PopupMenuButton.itemBuilder].
typedef PopupMenuItemBuilder<T> = List<PopupMenuEntry<T>> Function(BuildContext context);
/// Displays a menu when pressed and calls [onSelected] when the menu is dismissed
/// because an item was selected. The value passed to [onSelected] is the value of
/// the selected menu item.
///
/// One of [child] or [icon] may be provided, but not both. If [icon] is provided,
/// then [PopupMenuButton] behaves like an [IconButton].
///
/// If both are null, then a standard overflow icon is created (depending on the
/// platform).
///
/// ## Updating to [MenuAnchor]
///
/// There is a Material 3 component,
/// [MenuAnchor] that is preferred for applications that are configured
/// for Material 3 (see [ThemeData.useMaterial3]).
/// The [MenuAnchor] widget's visuals
/// are a little bit different, see the Material 3 spec at
/// <https://m3.material.io/components/menus/guidelines> for
/// more details.
///
/// The [MenuAnchor] widget's API is also slightly different.
/// [MenuAnchor]'s were built to be lower level interface for
/// creating menus that are displayed from an anchor.
///
/// There are a few steps you would take to migrate from
/// [PopupMenuButton] to [MenuAnchor]:
///
/// 1. Instead of using the [PopupMenuButton.itemBuilder] to build
/// a list of [PopupMenuEntry]s, you would use the [MenuAnchor.menuChildren]
/// which takes a list of [Widget]s. Usually, you would use a list of
/// [MenuItemButton]s as shown in the example below.
///
/// 2. Instead of using the [PopupMenuButton.onSelected] callback, you would
/// set individual callbacks for each of the [MenuItemButton]s using the
/// [MenuItemButton.onPressed] property.
///
/// 3. To anchor the [MenuAnchor] to a widget, you would use the [MenuAnchor.builder]
/// to return the widget of choice - usually a [TextButton] or an [IconButton].
///
/// 4. You may want to style the [MenuItemButton]s, see the [MenuItemButton]
/// documentation for details.
///
/// Use the sample below for an example of migrating from [PopupMenuButton] to
/// [MenuAnchor].
///
/// {@tool dartpad}
/// This example shows a menu with three items, selecting between an enum's
/// values and setting a `selectedMenu` field based on the selection.
///
/// ** See code in examples/api/lib/material/popup_menu/popup_menu.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to migrate the above to a [MenuAnchor].
///
/// ** See code in examples/api/lib/material/menu_anchor/menu_anchor.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of a popup menu, as described in:
/// https://m3.material.io/components/menus/overview
///
/// ** See code in examples/api/lib/material/popup_menu/popup_menu.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample showcases how to override the [PopupMenuButton] animation
/// curves and duration using [AnimationStyle].
///
/// ** See code in examples/api/lib/material/popup_menu/popup_menu.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [PopupMenuItem], a popup menu entry for a single value.
/// * [PopupMenuDivider], a popup menu entry that is just a horizontal line.
/// * [CheckedPopupMenuItem], a popup menu item with a checkmark.
/// * [showMenu], a method to dynamically show a popup menu at a given location.
class PopupMenuButton<T> extends StatefulWidget {
/// Creates a button that shows a popup menu.
const PopupMenuButton({
super.key,
required this.itemBuilder,
this.initialValue,
this.onOpened,
this.onSelected,
this.onCanceled,
this.tooltip,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.padding = const EdgeInsets.all(8.0),
this.child,
this.splashRadius,
this.icon,
this.iconSize,
this.offset = Offset.zero,
this.enabled = true,
this.shape,
this.color,
this.iconColor,
this.enableFeedback,
this.constraints,
this.position,
this.clipBehavior = Clip.none,
this.useRootNavigator = false,
this.popUpAnimationStyle,
this.routeSettings,
this.style,
}) : assert(
!(child != null && icon != null),
'You can only pass [child] or [icon], not both.',
);
/// Called when the button is pressed to create the items to show in the menu.
final PopupMenuItemBuilder<T> itemBuilder;
/// The value of the menu item, if any, that should be highlighted when the menu opens.
final T? initialValue;
/// Called when the popup menu is shown.
final VoidCallback? onOpened;
/// Called when the user selects a value from the popup menu created by this button.
///
/// If the popup menu is dismissed without selecting a value, [onCanceled] is
/// called instead.
final PopupMenuItemSelected<T>? onSelected;
/// Called when the user dismisses the popup menu without selecting an item.
///
/// If the user selects a value, [onSelected] is called instead.
final PopupMenuCanceled? onCanceled;
/// Text that describes the action that will occur when the button is pressed.
///
/// This text is displayed when the user long-presses on the button and is
/// used for accessibility.
final String? tooltip;
/// The z-coordinate at which to place the menu when open. This controls the
/// size of the shadow below the menu.
///
/// Defaults to 8, the appropriate elevation for popup menus.
final double? elevation;
/// The color used to paint the shadow below the menu.
///
/// If null then the ambient [PopupMenuThemeData.shadowColor] is used.
/// If that is null too, then the overall theme's [ThemeData.shadowColor]
/// (default black) is used.
final Color? shadowColor;
/// The color used as an overlay on [color] to indicate elevation.
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// If null, [PopupMenuThemeData.surfaceTintColor] is used. If that
/// is also null, the default value is [Colors.transparent].
///
/// See [Material.surfaceTintColor] for more details on how this
/// overlay is applied.
final Color? surfaceTintColor;
/// Matches IconButton's 8 dps padding by default. In some cases, notably where
/// this button appears as the trailing element of a list item, it's useful to be able
/// to set the padding to zero.
final EdgeInsetsGeometry padding;
/// The splash radius.
///
/// If null, default splash radius of [InkWell] or [IconButton] is used.
final double? splashRadius;
/// If provided, [child] is the widget used for this button
/// and the button will utilize an [InkWell] for taps.
final Widget? child;
/// If provided, the [icon] is used for this button
/// and the button will behave like an [IconButton].
final Widget? icon;
/// The offset is applied relative to the initial position
/// set by the [position].
///
/// When not set, the offset defaults to [Offset.zero].
final Offset offset;
/// Whether this popup menu button is interactive.
///
/// Defaults to true.
///
/// If true, the button will respond to presses by displaying the menu.
///
/// If false, the button is styled with the disabled color from the
/// current [Theme] and will not respond to presses or show the popup
/// menu and [onSelected], [onCanceled] and [itemBuilder] will not be called.
///
/// This can be useful in situations where the app needs to show the button,
/// but doesn't currently have anything to show in the menu.
final bool enabled;
/// If provided, the shape used for the menu.
///
/// If this property is null, then [PopupMenuThemeData.shape] is used.
/// If [PopupMenuThemeData.shape] is also null, then the default shape for
/// [MaterialType.card] is used. This default shape is a rectangle with
/// rounded edges of BorderRadius.circular(2.0).
final ShapeBorder? shape;
/// If provided, the background color used for the menu.
///
/// If this property is null, then [PopupMenuThemeData.color] is used.
/// If [PopupMenuThemeData.color] is also null, then
/// [ThemeData.cardColor] is used in Material 2. In Material3, defaults to
/// [ColorScheme.surfaceContainer].
final Color? color;
/// If provided, this color is used for the button icon.
///
/// If this property is null, then [PopupMenuThemeData.iconColor] is used.
/// If [PopupMenuThemeData.iconColor] is also null then defaults to
/// [IconThemeData.color].
final Color? iconColor;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// If provided, the size of the [Icon].
///
/// If this property is null, then [IconThemeData.size] is used.
/// If [IconThemeData.size] is also null, then
/// default size is 24.0 pixels.
final double? iconSize;
/// Optional size constraints for the menu.
///
/// When unspecified, defaults to:
/// ```dart
/// const BoxConstraints(
/// minWidth: 2.0 * 56.0,
/// maxWidth: 5.0 * 56.0,
/// )
/// ```
///
/// The default constraints ensure that the menu width matches maximum width
/// recommended by the Material Design guidelines.
/// Specifying this parameter enables creation of menu wider than
/// the default maximum width.
final BoxConstraints? constraints;
/// Whether the popup menu is positioned over or under the popup menu button.
///
/// [offset] is used to change the position of the popup menu relative to the
/// position set by this parameter.
///
/// If this property is `null`, then [PopupMenuThemeData.position] is used. If
/// [PopupMenuThemeData.position] is also `null`, then the position defaults
/// to [PopupMenuPosition.over] which makes the popup menu appear directly
/// over the button that was used to create it.
final PopupMenuPosition? position;
/// {@macro flutter.material.Material.clipBehavior}
///
/// The [clipBehavior] argument is used the clip shape of the menu.
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// Used to determine whether to push the menu to the [Navigator] furthest
/// from or nearest to the given `context`.
///
/// Defaults to false.
final bool useRootNavigator;
/// Used to override the default animation curves and durations of the popup
/// menu's open and close transitions.
///
/// If [AnimationStyle.curve] is provided, it will be used to override
/// the default popup animation curve. Otherwise, defaults to [Curves.linear].
///
/// If [AnimationStyle.reverseCurve] is provided, it will be used to
/// override the default popup animation reverse curve. Otherwise, defaults to
/// `Interval(0.0, 2.0 / 3.0)`.
///
/// If [AnimationStyle.duration] is provided, it will be used to override
/// the default popup animation duration. Otherwise, defaults to 300ms.
///
/// To disable the theme animation, use [AnimationStyle.noAnimation].
///
/// If this is null, then the default animation will be used.
final AnimationStyle? popUpAnimationStyle;
/// Optional route settings for the menu.
///
/// See [RouteSettings] for details.
final RouteSettings? routeSettings;
/// Customizes this icon button's appearance.
///
/// The [style] is only used for Material 3 [IconButton]s. If [ThemeData.useMaterial3]
/// is set to true, [style] is preferred for icon button customization, and any
/// parameters defined in [style] will override the same parameters in [IconButton].
///
/// Null by default.
final ButtonStyle? style;
@override
PopupMenuButtonState<T> createState() => PopupMenuButtonState<T>();
}
/// The [State] for a [PopupMenuButton].
///
/// See [showButtonMenu] for a way to programmatically open the popup menu
/// of your button state.
class PopupMenuButtonState<T> extends State<PopupMenuButton<T>> {
/// A method to show a popup menu with the items supplied to
/// [PopupMenuButton.itemBuilder] at the position of your [PopupMenuButton].
///
/// By default, it is called when the user taps the button and [PopupMenuButton.enabled]
/// is set to `true`. Moreover, you can open the button by calling the method manually.
///
/// You would access your [PopupMenuButtonState] using a [GlobalKey] and
/// show the menu of the button with `globalKey.currentState.showButtonMenu`.
void showButtonMenu() {
final PopupMenuThemeData popupMenuTheme = PopupMenuTheme.of(context);
final RenderBox button = context.findRenderObject()! as RenderBox;
final RenderBox overlay = Navigator.of(context).overlay!.context.findRenderObject()! as RenderBox;
final PopupMenuPosition popupMenuPosition = widget.position ?? popupMenuTheme.position ?? PopupMenuPosition.over;
late Offset offset;
switch (popupMenuPosition) {
case PopupMenuPosition.over:
offset = widget.offset;
case PopupMenuPosition.under:
offset = Offset(0.0, button.size.height) + widget.offset;
if (widget.child == null) {
// Remove the padding of the icon button.
offset -= Offset(0.0, widget.padding.vertical / 2);
}
}
final RelativeRect position = RelativeRect.fromRect(
Rect.fromPoints(
button.localToGlobal(offset, ancestor: overlay),
button.localToGlobal(button.size.bottomRight(Offset.zero) + offset, ancestor: overlay),
),
Offset.zero & overlay.size,
);
final List<PopupMenuEntry<T>> items = widget.itemBuilder(context);
// Only show the menu if there is something to show
if (items.isNotEmpty) {
widget.onOpened?.call();
showMenu<T?>(
context: context,
elevation: widget.elevation ?? popupMenuTheme.elevation,
shadowColor: widget.shadowColor ?? popupMenuTheme.shadowColor,
surfaceTintColor: widget.surfaceTintColor ?? popupMenuTheme.surfaceTintColor,
items: items,
initialValue: widget.initialValue,
position: position,
shape: widget.shape ?? popupMenuTheme.shape,
color: widget.color ?? popupMenuTheme.color,
constraints: widget.constraints,
clipBehavior: widget.clipBehavior,
useRootNavigator: widget.useRootNavigator,
popUpAnimationStyle: widget.popUpAnimationStyle,
routeSettings: widget.routeSettings,
)
.then<void>((T? newValue) {
if (!mounted) {
return null;
}
if (newValue == null) {
widget.onCanceled?.call();
return null;
}
widget.onSelected?.call(newValue);
});
}
}
bool get _canRequestFocus {
final NavigationMode mode = MediaQuery.maybeNavigationModeOf(context) ?? NavigationMode.traditional;
return switch (mode) {
NavigationMode.traditional => widget.enabled,
NavigationMode.directional => true,
};
}
@override
Widget build(BuildContext context) {
final IconThemeData iconTheme = IconTheme.of(context);
final PopupMenuThemeData popupMenuTheme = PopupMenuTheme.of(context);
final bool enableFeedback = widget.enableFeedback
?? PopupMenuTheme.of(context).enableFeedback
?? true;
assert(debugCheckHasMaterialLocalizations(context));
if (widget.child != null) {
return Tooltip(
message: widget.tooltip ?? MaterialLocalizations.of(context).showMenuTooltip,
child: InkWell(
onTap: widget.enabled ? showButtonMenu : null,
canRequestFocus: _canRequestFocus,
radius: widget.splashRadius,
enableFeedback: enableFeedback,
child: widget.child,
),
);
}
return IconButton(
icon: widget.icon ?? Icon(Icons.adaptive.more),
padding: widget.padding,
splashRadius: widget.splashRadius,
iconSize: widget.iconSize ?? popupMenuTheme.iconSize ?? iconTheme.size,
color: widget.iconColor ?? popupMenuTheme.iconColor ?? iconTheme.color,
tooltip: widget.tooltip ?? MaterialLocalizations.of(context).showMenuTooltip,
onPressed: widget.enabled ? showButtonMenu : null,
enableFeedback: enableFeedback,
style: widget.style,
);
}
}
// This MaterialStateProperty is passed along to the menu item's InkWell which
// resolves the property against MaterialState.disabled, MaterialState.hovered,
// MaterialState.focused.
class _EffectiveMouseCursor extends MaterialStateMouseCursor {
const _EffectiveMouseCursor(this.widgetCursor, this.themeCursor);
final MouseCursor? widgetCursor;
final MaterialStateProperty<MouseCursor?>? themeCursor;
@override
MouseCursor resolve(Set<MaterialState> states) {
return MaterialStateProperty.resolveAs<MouseCursor?>(widgetCursor, states)
?? themeCursor?.resolve(states)
?? MaterialStateMouseCursor.clickable.resolve(states);
}
@override
String get debugDescription => 'MaterialStateMouseCursor(PopupMenuItemState)';
}
class _PopupMenuDefaultsM2 extends PopupMenuThemeData {
_PopupMenuDefaultsM2(this.context)
: super(elevation: 8.0);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final TextTheme _textTheme = _theme.textTheme;
@override
TextStyle? get textStyle => _textTheme.subtitle1;
static EdgeInsets menuHorizontalPadding = const EdgeInsets.symmetric(horizontal: 16.0);
}
// BEGIN GENERATED TOKEN PROPERTIES - PopupMenu
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _PopupMenuDefaultsM3 extends PopupMenuThemeData {
_PopupMenuDefaultsM3(this.context)
: super(elevation: 3.0);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
@override MaterialStateProperty<TextStyle?>? get labelTextStyle {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
final TextStyle style = _textTheme.labelLarge!;
if (states.contains(MaterialState.disabled)) {
return style.apply(color: _colors.onSurface.withOpacity(0.38));
}
return style.apply(color: _colors.onSurface);
});
}
@override
Color? get color => _colors.surfaceContainer;
@override
Color? get shadowColor => _colors.shadow;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
ShapeBorder? get shape => const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0)));
// TODO(tahatesser): This is taken from https://m3.material.io/components/menus/specs
// Update this when the token is available.
static EdgeInsets menuHorizontalPadding = const EdgeInsets.symmetric(horizontal: 12.0);
}
// END GENERATED TOKEN PROPERTIES - PopupMenu
| flutter/packages/flutter/lib/src/material/popup_menu.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/popup_menu.dart",
"repo_id": "flutter",
"token_count": 18353
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the configuration of the search views created by the [SearchAnchor]
/// widget.
///
/// Descendant widgets obtain the current [SearchViewThemeData] object using
/// `SearchViewTheme.of(context)`.
///
/// Typically, a [SearchViewThemeData] is specified as part of the overall [Theme]
/// with [ThemeData.searchViewTheme]. Otherwise, [SearchViewTheme] can be used
/// to configure its own widget subtree.
///
/// All [SearchViewThemeData] properties are `null` by default. If any of these
/// properties are null, the search view will provide its own defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme for the application.
/// * [SearchBarThemeData], which describes the theme for the search bar itself in a
/// [SearchBar] widget.
/// * [SearchAnchor], which is used to open a search view route.
@immutable
class SearchViewThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.searchViewTheme].
const SearchViewThemeData({
this.backgroundColor,
this.elevation,
this.surfaceTintColor,
this.constraints,
this.side,
this.shape,
this.headerHeight,
this.headerTextStyle,
this.headerHintStyle,
this.dividerColor,
});
/// Overrides the default value of the [SearchAnchor.viewBackgroundColor].
final Color? backgroundColor;
/// Overrides the default value of the [SearchAnchor.viewElevation].
final double? elevation;
/// Overrides the default value of the [SearchAnchor.viewSurfaceTintColor].
final Color? surfaceTintColor;
/// Overrides the default value of the [SearchAnchor.viewSide].
final BorderSide? side;
/// Overrides the default value of the [SearchAnchor.viewShape].
final OutlinedBorder? shape;
/// Overrides the default value of the [SearchAnchor.headerHeight].
final double? headerHeight;
/// Overrides the default value for [SearchAnchor.headerTextStyle].
final TextStyle? headerTextStyle;
/// Overrides the default value for [SearchAnchor.headerHintStyle].
final TextStyle? headerHintStyle;
/// Overrides the value of size constraints for [SearchAnchor.viewConstraints].
final BoxConstraints? constraints;
/// Overrides the value of the divider color for [SearchAnchor.dividerColor].
final Color? dividerColor;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
SearchViewThemeData copyWith({
Color? backgroundColor,
double? elevation,
Color? surfaceTintColor,
BorderSide? side,
OutlinedBorder? shape,
double? headerHeight,
TextStyle? headerTextStyle,
TextStyle? headerHintStyle,
BoxConstraints? constraints,
Color? dividerColor,
}) {
return SearchViewThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
elevation: elevation ?? this.elevation,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
side: side ?? this.side,
shape: shape ?? this.shape,
headerHeight: headerHeight ?? this.headerHeight,
headerTextStyle: headerTextStyle ?? this.headerTextStyle,
headerHintStyle: headerHintStyle ?? this.headerHintStyle,
constraints: constraints ?? this.constraints,
dividerColor: dividerColor ?? this.dividerColor,
);
}
/// Linearly interpolate between two [SearchViewThemeData]s.
static SearchViewThemeData? lerp(SearchViewThemeData? a, SearchViewThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return SearchViewThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
side: _lerpSides(a?.side, b?.side, t),
shape: OutlinedBorder.lerp(a?.shape, b?.shape, t),
headerHeight: lerpDouble(a?.headerHeight, b?.headerHeight, t),
headerTextStyle: TextStyle.lerp(a?.headerTextStyle, b?.headerTextStyle, t),
headerHintStyle: TextStyle.lerp(a?.headerTextStyle, b?.headerTextStyle, t),
constraints: BoxConstraints.lerp(a?.constraints, b?.constraints, t),
dividerColor: Color.lerp(a?.dividerColor, b?.dividerColor, t),
);
}
@override
int get hashCode => Object.hash(
backgroundColor,
elevation,
surfaceTintColor,
side,
shape,
headerHeight,
headerTextStyle,
headerHintStyle,
constraints,
dividerColor,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SearchViewThemeData
&& other.backgroundColor == backgroundColor
&& other.elevation == elevation
&& other.surfaceTintColor == surfaceTintColor
&& other.side == side
&& other.shape == shape
&& other.headerHeight == headerHeight
&& other.headerTextStyle == headerTextStyle
&& other.headerHintStyle == headerHintStyle
&& other.constraints == constraints
&& other.dividerColor == dividerColor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Color?>('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<double?>('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<Color?>('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<BorderSide?>('side', side, defaultValue: null));
properties.add(DiagnosticsProperty<OutlinedBorder?>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<double?>('headerHeight', headerHeight, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle?>('headerTextStyle', headerTextStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle?>('headerHintStyle', headerHintStyle, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: null));
properties.add(DiagnosticsProperty<Color?>('dividerColor', dividerColor, defaultValue: null));
}
// Special case because BorderSide.lerp() doesn't support null arguments
static BorderSide? _lerpSides(BorderSide? a, BorderSide? b, double t) {
if (a == null || b == null) {
return null;
}
if (identical(a, b)) {
return a;
}
return BorderSide.lerp(a, b, t);
}
}
/// An inherited widget that defines the configuration in this widget's
/// descendants for search view created by the [SearchAnchor] widget.
///
/// A search view theme can be specified as part of the overall Material theme using
/// [ThemeData.searchViewTheme].
///
/// See also:
///
/// * [SearchViewThemeData], which describes the actual configuration of a search view
/// theme.
class SearchViewTheme extends InheritedTheme {
/// Creates a const theme that controls the configurations for the search view
/// created by the [SearchAnchor] widget.
const SearchViewTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [SearchAnchor] widgets.
final SearchViewThemeData data;
/// Returns the configuration [data] from the closest [SearchViewTheme] ancestor.
/// If there is no ancestor, it returns [ThemeData.searchViewTheme].
///
/// Typical usage is as follows:
///
/// ```dart
/// SearchViewThemeData theme = SearchViewTheme.of(context);
/// ```
static SearchViewThemeData of(BuildContext context) {
final SearchViewTheme? searchViewTheme = context.dependOnInheritedWidgetOfExactType<SearchViewTheme>();
return searchViewTheme?.data ?? Theme.of(context).searchViewTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return SearchViewTheme(data: data, child: child);
}
@override
bool updateShouldNotify(SearchViewTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/search_view_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/search_view_theme.dart",
"repo_id": "flutter",
"token_count": 2716
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [Switch] widgets.
///
/// Descendant widgets obtain the current [SwitchThemeData] object using
/// `SwitchTheme.of(context)`. Instances of [SwitchThemeData] can be customized
/// with [SwitchThemeData.copyWith].
///
/// Typically a [SwitchThemeData] is specified as part of the overall [Theme]
/// with [ThemeData.switchTheme].
///
/// All [SwitchThemeData] properties are `null` by default. When null, the
/// [Switch] will use the values from [ThemeData] if they exist, otherwise it
/// will provide its own defaults based on the overall [Theme]'s colorScheme.
/// See the individual [Switch] properties for details.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class SwitchThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.switchTheme].
const SwitchThemeData({
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.trackOutlineWidth,
this.materialTapTargetSize,
this.mouseCursor,
this.overlayColor,
this.splashRadius,
this.thumbIcon,
});
/// {@macro flutter.material.switch.thumbColor}
///
/// If specified, overrides the default value of [Switch.thumbColor].
final MaterialStateProperty<Color?>? thumbColor;
/// {@macro flutter.material.switch.trackColor}
///
/// If specified, overrides the default value of [Switch.trackColor].
final MaterialStateProperty<Color?>? trackColor;
/// {@macro flutter.material.switch.trackOutlineColor}
///
/// If specified, overrides the default value of [Switch.trackOutlineColor].
final MaterialStateProperty<Color?>? trackOutlineColor;
/// {@macro flutter.material.switch.trackOutlineWidth}
///
/// If specified, overrides the default value of [Switch.trackOutlineWidth].
final MaterialStateProperty<double?>? trackOutlineWidth;
/// {@macro flutter.material.switch.materialTapTargetSize}
///
/// If specified, overrides the default value of
/// [Switch.materialTapTargetSize].
final MaterialTapTargetSize? materialTapTargetSize;
/// {@macro flutter.material.switch.mouseCursor}
///
/// If specified, overrides the default value of [Switch.mouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// {@macro flutter.material.switch.overlayColor}
///
/// If specified, overrides the default value of [Switch.overlayColor].
final MaterialStateProperty<Color?>? overlayColor;
/// {@macro flutter.material.switch.splashRadius}
///
/// If specified, overrides the default value of [Switch.splashRadius].
final double? splashRadius;
/// {@macro flutter.material.switch.thumbIcon}
///
/// It is overridden by [Switch.thumbIcon].
final MaterialStateProperty<Icon?>? thumbIcon;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
SwitchThemeData copyWith({
MaterialStateProperty<Color?>? thumbColor,
MaterialStateProperty<Color?>? trackColor,
MaterialStateProperty<Color?>? trackOutlineColor,
MaterialStateProperty<double?>? trackOutlineWidth,
MaterialTapTargetSize? materialTapTargetSize,
MaterialStateProperty<MouseCursor?>? mouseCursor,
MaterialStateProperty<Color?>? overlayColor,
double? splashRadius,
MaterialStateProperty<Icon?>? thumbIcon,
}) {
return SwitchThemeData(
thumbColor: thumbColor ?? this.thumbColor,
trackColor: trackColor ?? this.trackColor,
trackOutlineColor: trackOutlineColor ?? this.trackOutlineColor,
trackOutlineWidth: trackOutlineWidth ?? this.trackOutlineWidth,
materialTapTargetSize: materialTapTargetSize ?? this.materialTapTargetSize,
mouseCursor: mouseCursor ?? this.mouseCursor,
overlayColor: overlayColor ?? this.overlayColor,
splashRadius: splashRadius ?? this.splashRadius,
thumbIcon: thumbIcon ?? this.thumbIcon,
);
}
/// Linearly interpolate between two [SwitchThemeData]s.
///
/// {@macro dart.ui.shadow.lerp}
static SwitchThemeData lerp(SwitchThemeData? a, SwitchThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return SwitchThemeData(
thumbColor: MaterialStateProperty.lerp<Color?>(a?.thumbColor, b?.thumbColor, t, Color.lerp),
trackColor: MaterialStateProperty.lerp<Color?>(a?.trackColor, b?.trackColor, t, Color.lerp),
trackOutlineColor: MaterialStateProperty.lerp<Color?>(a?.trackOutlineColor, b?.trackOutlineColor, t, Color.lerp),
trackOutlineWidth: MaterialStateProperty.lerp<double?>(a?.trackOutlineWidth, b?.trackOutlineWidth, t, lerpDouble),
materialTapTargetSize: t < 0.5 ? a?.materialTapTargetSize : b?.materialTapTargetSize,
mouseCursor: t < 0.5 ? a?.mouseCursor : b?.mouseCursor,
overlayColor: MaterialStateProperty.lerp<Color?>(a?.overlayColor, b?.overlayColor, t, Color.lerp),
splashRadius: lerpDouble(a?.splashRadius, b?.splashRadius, t),
thumbIcon: t < 0.5 ? a?.thumbIcon : b?.thumbIcon,
);
}
@override
int get hashCode => Object.hash(
thumbColor,
trackColor,
trackOutlineColor,
trackOutlineWidth,
materialTapTargetSize,
mouseCursor,
overlayColor,
splashRadius,
thumbIcon,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SwitchThemeData
&& other.thumbColor == thumbColor
&& other.trackColor == trackColor
&& other.trackOutlineColor == trackOutlineColor
&& other.trackOutlineWidth == trackOutlineWidth
&& other.materialTapTargetSize == materialTapTargetSize
&& other.mouseCursor == mouseCursor
&& other.overlayColor == overlayColor
&& other.splashRadius == splashRadius
&& other.thumbIcon == thumbIcon;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('thumbColor', thumbColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('trackColor', trackColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('trackOutlineColor', trackOutlineColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<double?>>('trackOutlineWidth', trackOutlineWidth, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialTapTargetSize>('materialTapTargetSize', materialTapTargetSize, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>>('mouseCursor', mouseCursor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('overlayColor', overlayColor, defaultValue: null));
properties.add(DoubleProperty('splashRadius', splashRadius, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Icon?>>('thumbIcon', thumbIcon, defaultValue: null));
}
}
/// Applies a switch theme to descendant [Switch] widgets.
///
/// Descendant widgets obtain the current theme's [SwitchTheme] object using
/// [SwitchTheme.of]. When a widget uses [SwitchTheme.of], it is automatically
/// rebuilt if the theme later changes.
///
/// A switch theme can be specified as part of the overall Material theme using
/// [ThemeData.switchTheme].
///
/// See also:
///
/// * [SwitchThemeData], which describes the actual configuration of a switch
/// theme.
class SwitchTheme extends InheritedWidget {
/// Constructs a switch theme that configures all descendant [Switch] widgets.
const SwitchTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [Switch] widgets.
final SwitchThemeData data;
/// Returns the configuration [data] from the closest [SwitchTheme] ancestor.
/// If there is no ancestor, it returns [ThemeData.switchTheme].
///
/// Typical usage is as follows:
///
/// ```dart
/// SwitchThemeData theme = SwitchTheme.of(context);
/// ```
static SwitchThemeData of(BuildContext context) {
final SwitchTheme? switchTheme = context.dependOnInheritedWidgetOfExactType<SwitchTheme>();
return switchTheme?.data ?? Theme.of(context).switchTheme;
}
@override
bool updateShouldNotify(SwitchTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/switch_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/switch_theme.dart",
"repo_id": "flutter",
"token_count": 2821
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'debug.dart';
import 'material_localizations.dart';
/// Whether the [TimeOfDay] is before or after noon.
enum DayPeriod {
/// Ante meridiem (before noon).
am,
/// Post meridiem (after noon).
pm,
}
/// A value representing a time during the day, independent of the date that
/// day might fall on or the time zone.
///
/// The time is represented by [hour] and [minute] pair. Once created, both
/// values cannot be changed.
///
/// You can create TimeOfDay using the constructor which requires both hour and
/// minute or using [DateTime] object.
/// Hours are specified between 0 and 23, as in a 24-hour clock.
///
/// {@tool snippet}
///
/// ```dart
/// TimeOfDay now = TimeOfDay.now();
/// const TimeOfDay releaseTime = TimeOfDay(hour: 15, minute: 0); // 3:00pm
/// TimeOfDay roomBooked = TimeOfDay.fromDateTime(DateTime.parse('2018-10-20 16:30:04Z')); // 4:30pm
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [showTimePicker], which returns this type.
/// * [MaterialLocalizations], which provides methods for formatting values of
/// this type according to the chosen [Locale].
/// * [DateTime], which represents date and time, and is subject to eras and
/// time zones.
@immutable
class TimeOfDay {
/// Creates a time of day.
///
/// The [hour] argument must be between 0 and 23, inclusive. The [minute]
/// argument must be between 0 and 59, inclusive.
const TimeOfDay({ required this.hour, required this.minute });
/// Creates a time of day based on the given time.
///
/// The [hour] is set to the time's hour and the [minute] is set to the time's
/// minute in the timezone of the given [DateTime].
TimeOfDay.fromDateTime(DateTime time)
: hour = time.hour,
minute = time.minute;
/// Creates a time of day based on the current time.
///
/// The [hour] is set to the current hour and the [minute] is set to the
/// current minute in the local time zone.
TimeOfDay.now() : this.fromDateTime(DateTime.now());
/// The number of hours in one day, i.e. 24.
static const int hoursPerDay = 24;
/// The number of hours in one day period (see also [DayPeriod]), i.e. 12.
static const int hoursPerPeriod = 12;
/// The number of minutes in one hour, i.e. 60.
static const int minutesPerHour = 60;
/// Returns a new TimeOfDay with the hour and/or minute replaced.
TimeOfDay replacing({ int? hour, int? minute }) {
assert(hour == null || (hour >= 0 && hour < hoursPerDay));
assert(minute == null || (minute >= 0 && minute < minutesPerHour));
return TimeOfDay(hour: hour ?? this.hour, minute: minute ?? this.minute);
}
/// The selected hour, in 24 hour time from 0..23.
final int hour;
/// The selected minute.
final int minute;
/// Whether this time of day is before or after noon.
DayPeriod get period => hour < hoursPerPeriod ? DayPeriod.am : DayPeriod.pm;
/// Which hour of the current period (e.g., am or pm) this time is.
///
/// For 12AM (midnight) and 12PM (noon) this returns 12.
int get hourOfPeriod => hour == 0 || hour == 12 ? 12 : hour - periodOffset;
/// The hour at which the current period starts.
int get periodOffset => period == DayPeriod.am ? 0 : hoursPerPeriod;
/// Returns the localized string representation of this time of day.
///
/// This is a shortcut for [MaterialLocalizations.formatTimeOfDay].
String format(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
assert(debugCheckHasMaterialLocalizations(context));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
return localizations.formatTimeOfDay(
this,
alwaysUse24HourFormat: MediaQuery.alwaysUse24HourFormatOf(context),
);
}
@override
bool operator ==(Object other) {
return other is TimeOfDay
&& other.hour == hour
&& other.minute == minute;
}
@override
int get hashCode => Object.hash(hour, minute);
@override
String toString() {
String addLeadingZeroIfNeeded(int value) {
if (value < 10) {
return '0$value';
}
return value.toString();
}
final String hourLabel = addLeadingZeroIfNeeded(hour);
final String minuteLabel = addLeadingZeroIfNeeded(minute);
return '$TimeOfDay($hourLabel:$minuteLabel)';
}
}
/// A [RestorableValue] that knows how to save and restore [TimeOfDay].
///
/// {@macro flutter.widgets.RestorableNum}.
class RestorableTimeOfDay extends RestorableValue<TimeOfDay> {
/// Creates a [RestorableTimeOfDay].
///
/// {@macro flutter.widgets.RestorableNum.constructor}
RestorableTimeOfDay(TimeOfDay defaultValue) : _defaultValue = defaultValue;
final TimeOfDay _defaultValue;
@override
TimeOfDay createDefaultValue() => _defaultValue;
@override
void didUpdateValue(TimeOfDay? oldValue) {
assert(debugIsSerializableForRestoration(value.hour));
assert(debugIsSerializableForRestoration(value.minute));
notifyListeners();
}
@override
TimeOfDay fromPrimitives(Object? data) {
final List<Object?> timeData = data! as List<Object?>;
return TimeOfDay(
minute: timeData[0]! as int,
hour: timeData[1]! as int,
);
}
@override
Object? toPrimitives() => <int>[value.minute, value.hour];
}
/// Determines how the time picker invoked using [showTimePicker] formats and
/// lays out the time controls.
///
/// The time picker provides layout configurations optimized for each of the
/// enum values.
enum TimeOfDayFormat {
/// Corresponds to the ICU 'HH:mm' pattern.
///
/// This format uses 24-hour two-digit zero-padded hours. Controls are always
/// laid out horizontally. Hours are separated from minutes by one colon
/// character.
HH_colon_mm,
/// Corresponds to the ICU 'HH.mm' pattern.
///
/// This format uses 24-hour two-digit zero-padded hours. Controls are always
/// laid out horizontally. Hours are separated from minutes by one dot
/// character.
HH_dot_mm,
/// Corresponds to the ICU "HH 'h' mm" pattern used in Canadian French.
///
/// This format uses 24-hour two-digit zero-padded hours. Controls are always
/// laid out horizontally. Hours are separated from minutes by letter 'h'.
frenchCanadian,
/// Corresponds to the ICU 'H:mm' pattern.
///
/// This format uses 24-hour non-padded variable-length hours. Controls are
/// always laid out horizontally. Hours are separated from minutes by one
/// colon character.
H_colon_mm,
/// Corresponds to the ICU 'h:mm a' pattern.
///
/// This format uses 12-hour non-padded variable-length hours with a day
/// period. Controls are laid out horizontally in portrait mode. In landscape
/// mode, the day period appears vertically after (consistent with the ambient
/// [TextDirection]) hour-minute indicator. Hours are separated from minutes
/// by one colon character.
h_colon_mm_space_a,
/// Corresponds to the ICU 'a h:mm' pattern.
///
/// This format uses 12-hour non-padded variable-length hours with a day
/// period. Controls are laid out horizontally in portrait mode. In landscape
/// mode, the day period appears vertically before (consistent with the
/// ambient [TextDirection]) hour-minute indicator. Hours are separated from
/// minutes by one colon character.
a_space_h_colon_mm,
}
/// Describes how hours are formatted.
enum HourFormat {
/// Zero-padded two-digit 24-hour format ranging from "00" to "23".
HH,
/// Non-padded variable-length 24-hour format ranging from "0" to "23".
H,
/// Non-padded variable-length hour in day period format ranging from "1" to
/// "12".
h,
}
/// The [HourFormat] used for the given [TimeOfDayFormat].
HourFormat hourFormat({ required TimeOfDayFormat of }) {
switch (of) {
case TimeOfDayFormat.h_colon_mm_space_a:
case TimeOfDayFormat.a_space_h_colon_mm:
return HourFormat.h;
case TimeOfDayFormat.H_colon_mm:
return HourFormat.H;
case TimeOfDayFormat.HH_dot_mm:
case TimeOfDayFormat.HH_colon_mm:
case TimeOfDayFormat.frenchCanadian:
return HourFormat.HH;
}
}
| flutter/packages/flutter/lib/src/material/time.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/time.dart",
"repo_id": "flutter",
"token_count": 2594
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart' show ServicesBinding;
import 'image_cache.dart';
import 'shader_warm_up.dart';
/// Binding for the painting library.
///
/// Hooks into the cache eviction logic to clear the image cache.
///
/// Requires the [ServicesBinding] to be mixed in earlier.
mixin PaintingBinding on BindingBase, ServicesBinding {
@override
void initInstances() {
super.initInstances();
_instance = this;
_imageCache = createImageCache();
shaderWarmUp?.execute();
}
/// The current [PaintingBinding], if one has been created.
///
/// Provides access to the features exposed by this mixin. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [runApp] or [WidgetsFlutterBinding.ensureInitialized].
static PaintingBinding get instance => BindingBase.checkInstance(_instance);
static PaintingBinding? _instance;
/// [ShaderWarmUp] instance to be executed during [initInstances].
///
/// Defaults to `null`, meaning no shader warm-up is done. Some platforms may
/// not support shader warm-up before at least one frame has been displayed.
///
/// If the application has scenes that require the compilation of complex
/// shaders, it may cause jank in the middle of an animation or interaction.
/// In that case, setting [shaderWarmUp] to a custom [ShaderWarmUp] before
/// creating the binding (usually before [runApp] for normal Flutter apps, and
/// before [enableFlutterDriverExtension] for Flutter driver tests) may help
/// if that object paints the difficult scene in its
/// [ShaderWarmUp.warmUpOnCanvas] method, as this allows Flutter to
/// pre-compile and cache the required shaders during startup.
///
/// Currently the warm-up happens synchronously on the raster thread which
/// means the rendering of the first frame on the raster thread will be
/// postponed until the warm-up is finished.
///
/// The warm up is only costly (100ms-200ms, depending on the shaders to
/// compile) during the first run after the installation or a data wipe. The
/// warm up does not block the platform thread so there should be no
/// "Application Not Responding" warning.
///
/// If this is null, no shader warm-up is executed.
///
/// See also:
///
/// * [ShaderWarmUp], the interface for implementing custom warm-up scenes.
/// * <https://flutter.dev/docs/perf/rendering/shader>
static ShaderWarmUp? shaderWarmUp;
/// The singleton that implements the Flutter framework's image cache.
///
/// The cache is used internally by [ImageProvider] and should generally not
/// be accessed directly.
///
/// The image cache is created during startup by the [createImageCache]
/// method.
ImageCache get imageCache => _imageCache;
late ImageCache _imageCache;
/// Creates the [ImageCache] singleton (accessible via [imageCache]).
///
/// This method can be overridden to provide a custom image cache.
@protected
ImageCache createImageCache() => ImageCache();
/// Calls through to [dart:ui.instantiateImageCodecFromBuffer] from [ImageCache].
///
/// The [buffer] parameter should be an [ui.ImmutableBuffer] instance which can
/// be acquired from [ui.ImmutableBuffer.fromUint8List] or [ui.ImmutableBuffer.fromAsset].
///
/// The [cacheWidth] and [cacheHeight] parameters, when specified, indicate
/// the size to decode the image to.
///
/// Both [cacheWidth] and [cacheHeight] must be positive values greater than
/// or equal to 1, or null. It is valid to specify only one of `cacheWidth`
/// and [cacheHeight] with the other remaining null, in which case the omitted
/// dimension will be scaled to maintain the aspect ratio of the original
/// dimensions. When both are null or omitted, the image will be decoded at
/// its native resolution.
///
/// The [allowUpscaling] parameter determines whether the `cacheWidth` or
/// [cacheHeight] parameters are clamped to the intrinsic width and height of
/// the original image. By default, the dimensions are clamped to avoid
/// unnecessary memory usage for images. Callers that wish to display an image
/// above its native resolution should prefer scaling the canvas the image is
/// drawn into.
@Deprecated(
'Use instantiateImageCodecWithSize instead. '
'This feature was deprecated after v3.7.0-1.4.pre.',
)
Future<ui.Codec> instantiateImageCodecFromBuffer(
ui.ImmutableBuffer buffer, {
int? cacheWidth,
int? cacheHeight,
bool allowUpscaling = false,
}) {
assert(cacheWidth == null || cacheWidth > 0);
assert(cacheHeight == null || cacheHeight > 0);
return ui.instantiateImageCodecFromBuffer(
buffer,
targetWidth: cacheWidth,
targetHeight: cacheHeight,
allowUpscaling: allowUpscaling,
);
}
/// Calls through to [dart:ui.instantiateImageCodecWithSize] from [ImageCache].
///
/// The [buffer] parameter should be an [ui.ImmutableBuffer] instance which can
/// be acquired from [ui.ImmutableBuffer.fromUint8List] or
/// [ui.ImmutableBuffer.fromAsset].
///
/// The [getTargetSize] parameter, when specified, will be invoked and passed
/// the image's intrinsic size to determine the size to decode the image to.
/// The width and the height of the size it returns must be positive values
/// greater than or equal to 1, or null. It is valid to return a [TargetImageSize]
/// that specifies only one of `width` and `height` with the other remaining
/// null, in which case the omitted dimension will be scaled to maintain the
/// aspect ratio of the original dimensions. When both are null or omitted,
/// the image will be decoded at its native resolution (as will be the case if
/// the [getTargetSize] parameter is omitted).
Future<ui.Codec> instantiateImageCodecWithSize(
ui.ImmutableBuffer buffer, {
ui.TargetImageSizeCallback? getTargetSize,
}) {
return ui.instantiateImageCodecWithSize(buffer, getTargetSize: getTargetSize);
}
@override
void evict(String asset) {
super.evict(asset);
imageCache.clear();
imageCache.clearLiveImages();
}
@override
void handleMemoryPressure() {
super.handleMemoryPressure();
imageCache.clear();
}
/// Listenable that notifies when the available fonts on the system have
/// changed.
///
/// System fonts can change when the system installs or removes new font. To
/// correctly reflect the change, it is important to relayout text related
/// widgets when this happens.
///
/// Objects that show text and/or measure text (e.g. via [TextPainter] or
/// [Paragraph]) should listen to this and redraw/remeasure.
Listenable get systemFonts => _systemFonts;
final _SystemFontsNotifier _systemFonts = _SystemFontsNotifier();
@override
Future<void> handleSystemMessage(Object systemMessage) async {
await super.handleSystemMessage(systemMessage);
final Map<String, dynamic> message = systemMessage as Map<String, dynamic>;
final String type = message['type'] as String;
switch (type) {
case 'fontsChange':
_systemFonts.notifyListeners();
}
return;
}
}
class _SystemFontsNotifier extends Listenable {
final Set<VoidCallback> _systemFontsCallbacks = <VoidCallback>{};
void notifyListeners () {
for (final VoidCallback callback in _systemFontsCallbacks) {
callback();
}
}
@override
void addListener(VoidCallback listener) {
_systemFontsCallbacks.add(listener);
}
@override
void removeListener(VoidCallback listener) {
_systemFontsCallbacks.remove(listener);
}
}
/// The singleton that implements the Flutter framework's image cache.
///
/// The cache is used internally by [ImageProvider] and should generally not be
/// accessed directly.
///
/// The image cache is created during startup by the [PaintingBinding]'s
/// [PaintingBinding.createImageCache] method.
ImageCache get imageCache => PaintingBinding.instance.imageCache;
| flutter/packages/flutter/lib/src/painting/binding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/binding.dart",
"repo_id": "flutter",
"token_count": 2360
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'alignment.dart';
import 'basic_types.dart';
/// An offset that's expressed as a fraction of a [Size].
///
/// `FractionalOffset(1.0, 0.0)` represents the top right of the [Size].
///
/// `FractionalOffset(0.0, 1.0)` represents the bottom left of the [Size].
///
/// `FractionalOffset(0.5, 2.0)` represents a point half way across the [Size],
/// below the bottom of the rectangle by the height of the [Size].
///
/// The [FractionalOffset] class specifies offsets in terms of a distance from
/// the top left, regardless of the [TextDirection].
///
/// ## Design discussion
///
/// [FractionalOffset] and [Alignment] are two different representations of the
/// same information: the location within a rectangle relative to the size of
/// the rectangle. The difference between the two classes is in the coordinate
/// system they use to represent the location.
///
/// [FractionalOffset] uses a coordinate system with an origin in the top-left
/// corner of the rectangle whereas [Alignment] uses a coordinate system with an
/// origin in the center of the rectangle.
///
/// Historically, [FractionalOffset] predates [Alignment]. When we attempted to
/// make a version of [FractionalOffset] that adapted to the [TextDirection], we
/// ran into difficulty because placing the origin in the top-left corner
/// introduced a left-to-right bias that was hard to remove.
///
/// By placing the origin in the center, [Alignment] and [AlignmentDirectional]
/// are able to use the same origin, which means we can use a linear function to
/// resolve an [AlignmentDirectional] into an [Alignment] in both
/// [TextDirection.rtl] and [TextDirection.ltr].
///
/// [Alignment] is better for most purposes than [FractionalOffset] and should
/// be used instead of [FractionalOffset]. We continue to implement
/// [FractionalOffset] to support code that predates [Alignment].
///
/// See also:
///
/// * [Alignment], which uses a coordinate system based on the center of the
/// rectangle instead of the top left corner of the rectangle.
@immutable
class FractionalOffset extends Alignment {
/// Creates a fractional offset.
const FractionalOffset(double dx, double dy)
: super(dx * 2.0 - 1.0, dy * 2.0 - 1.0);
/// Creates a fractional offset from a specific offset and size.
///
/// The returned [FractionalOffset] describes the position of the
/// [Offset] in the [Size], as a fraction of the [Size].
factory FractionalOffset.fromOffsetAndSize(Offset offset, Size size) {
return FractionalOffset(
offset.dx / size.width,
offset.dy / size.height,
);
}
/// Creates a fractional offset from a specific offset and rectangle.
///
/// The offset is assumed to be relative to the same origin as the rectangle.
///
/// If the offset is relative to the top left of the rectangle, use [
/// FractionalOffset.fromOffsetAndSize] instead, passing `rect.size`.
///
/// The returned [FractionalOffset] describes the position of the
/// [Offset] in the [Rect], as a fraction of the [Rect].
factory FractionalOffset.fromOffsetAndRect(Offset offset, Rect rect) {
return FractionalOffset.fromOffsetAndSize(
offset - rect.topLeft,
rect.size,
);
}
/// The distance fraction in the horizontal direction.
///
/// A value of 0.0 corresponds to the leftmost edge. A value of 1.0
/// corresponds to the rightmost edge. Values are not limited to that range;
/// negative values represent positions to the left of the left edge, and
/// values greater than 1.0 represent positions to the right of the right
/// edge.
double get dx => (x + 1.0) / 2.0;
/// The distance fraction in the vertical direction.
///
/// A value of 0.0 corresponds to the topmost edge. A value of 1.0 corresponds
/// to the bottommost edge. Values are not limited to that range; negative
/// values represent positions above the top, and values greater than 1.0
/// represent positions below the bottom.
double get dy => (y + 1.0) / 2.0;
/// The top left corner.
static const FractionalOffset topLeft = FractionalOffset(0.0, 0.0);
/// The center point along the top edge.
static const FractionalOffset topCenter = FractionalOffset(0.5, 0.0);
/// The top right corner.
static const FractionalOffset topRight = FractionalOffset(1.0, 0.0);
/// The center point along the left edge.
static const FractionalOffset centerLeft = FractionalOffset(0.0, 0.5);
/// The center point, both horizontally and vertically.
static const FractionalOffset center = FractionalOffset(0.5, 0.5);
/// The center point along the right edge.
static const FractionalOffset centerRight = FractionalOffset(1.0, 0.5);
/// The bottom left corner.
static const FractionalOffset bottomLeft = FractionalOffset(0.0, 1.0);
/// The center point along the bottom edge.
static const FractionalOffset bottomCenter = FractionalOffset(0.5, 1.0);
/// The bottom right corner.
static const FractionalOffset bottomRight = FractionalOffset(1.0, 1.0);
@override
Alignment operator -(Alignment other) {
if (other is! FractionalOffset) {
return super - other;
}
return FractionalOffset(dx - other.dx, dy - other.dy);
}
@override
Alignment operator +(Alignment other) {
if (other is! FractionalOffset) {
return super + other;
}
return FractionalOffset(dx + other.dx, dy + other.dy);
}
@override
FractionalOffset operator -() {
return FractionalOffset(-dx, -dy);
}
@override
FractionalOffset operator *(double other) {
return FractionalOffset(dx * other, dy * other);
}
@override
FractionalOffset operator /(double other) {
return FractionalOffset(dx / other, dy / other);
}
@override
FractionalOffset operator ~/(double other) {
return FractionalOffset((dx ~/ other).toDouble(), (dy ~/ other).toDouble());
}
@override
FractionalOffset operator %(double other) {
return FractionalOffset(dx % other, dy % other);
}
/// Linearly interpolate between two [FractionalOffset]s.
///
/// If either is null, this function interpolates from [FractionalOffset.center].
///
/// {@macro dart.ui.shadow.lerp}
static FractionalOffset? lerp(FractionalOffset? a, FractionalOffset? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return FractionalOffset(ui.lerpDouble(0.5, b!.dx, t)!, ui.lerpDouble(0.5, b.dy, t)!);
}
if (b == null) {
return FractionalOffset(ui.lerpDouble(a.dx, 0.5, t)!, ui.lerpDouble(a.dy, 0.5, t)!);
}
return FractionalOffset(ui.lerpDouble(a.dx, b.dx, t)!, ui.lerpDouble(a.dy, b.dy, t)!);
}
@override
String toString() {
return 'FractionalOffset(${dx.toStringAsFixed(1)}, '
'${dy.toStringAsFixed(1)})';
}
}
| flutter/packages/flutter/lib/src/painting/fractional_offset.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/fractional_offset.dart",
"repo_id": "flutter",
"token_count": 2212
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:developer';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'debug.dart';
/// Interface for drawing an image to warm up Skia shader compilations.
///
/// When Skia first sees a certain type of draw operation on the GPU, it needs
/// to compile the corresponding shader. The compilation can be slow (20ms-
/// 200ms). Having that time as startup latency is often better than having
/// jank in the middle of an animation.
///
/// Therefore, we use this during the [PaintingBinding.initInstances] call to
/// move common shader compilations from animation time to startup time. If
/// needed, app developers can create a custom [ShaderWarmUp] subclass and
/// hand it to [PaintingBinding.shaderWarmUp] before
/// [PaintingBinding.initInstances] is called. Usually, that can be done before
/// calling [runApp].
///
/// To determine whether a draw operation is useful for warming up shaders,
/// check whether it improves the slowest frame rasterization time. Also,
/// tracing with `flutter run --profile --trace-skia` may reveal whether there
/// is shader-compilation-related jank. If there is such jank, some long
/// `GrGLProgramBuilder::finalize` calls would appear in the middle of an
/// animation. Their parent calls, which look like `XyzOp` (e.g., `FillRecOp`,
/// `CircularRRectOp`) would suggest Xyz draw operations are causing the shaders
/// to be compiled. A useful shader warm-up draw operation would eliminate such
/// long compilation calls in the animation. To double-check the warm-up, trace
/// with `flutter run --profile --trace-skia --start-paused`. The
/// `GrGLProgramBuilder` with the associated `XyzOp` should appear during
/// startup rather than in the middle of a later animation.
///
/// This warm-up needs to be run on each individual device because the shader
/// compilation depends on the specific GPU hardware and driver a device has. It
/// can't be pre-computed during the Flutter engine compilation as the engine is
/// device-agnostic.
///
/// If no warm-up is desired (e.g., when the startup latency is crucial), set
/// [PaintingBinding.shaderWarmUp] either to a custom ShaderWarmUp with an empty
/// [warmUpOnCanvas] or null.
///
/// See also:
///
/// * [PaintingBinding.shaderWarmUp], the actual instance of [ShaderWarmUp]
/// that's used to warm up the shaders.
/// * <https://flutter.dev/docs/perf/rendering/shader>
abstract class ShaderWarmUp {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const ShaderWarmUp();
/// The size of the warm up image.
///
/// The exact size shouldn't matter much as long as all draws are onscreen.
/// 100x100 is an arbitrary small size that's easy to fit significant draw
/// calls onto.
///
/// A custom shader warm up can override this based on targeted devices.
ui.Size get size => const ui.Size(100.0, 100.0);
/// Trigger draw operations on a given canvas to warm up GPU shader
/// compilation cache.
///
/// To decide which draw operations to be added to your custom warm up
/// process, consider capturing an skp using `flutter screenshot
/// --vm-service-uri=<uri> --type=skia` and analyzing it with
/// <https://debugger.skia.org/>. Alternatively, one may run the app with
/// `flutter run --trace-skia` and then examine the raster thread in the
/// Flutter DevTools timeline to see which Skia draw operations are commonly used,
/// and which shader compilations are causing jank.
@protected
Future<void> warmUpOnCanvas(ui.Canvas canvas);
/// Construct an offscreen image of [size], and execute [warmUpOnCanvas] on a
/// canvas associated with that image.
///
/// Currently, this has no effect when [kIsWeb] is true.
Future<void> execute() async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
await warmUpOnCanvas(canvas);
final ui.Picture picture = recorder.endRecording();
assert(debugCaptureShaderWarmUpPicture(picture));
if (!kIsWeb || isCanvasKit) { // Picture.toImage is not yet implemented on the web.
TimelineTask? debugShaderWarmUpTask;
if (!kReleaseMode) {
debugShaderWarmUpTask = TimelineTask()..start('Warm-up shader');
}
try {
final ui.Image image = await picture.toImage(size.width.ceil(), size.height.ceil());
assert(debugCaptureShaderWarmUpImage(image));
image.dispose();
} finally {
if (!kReleaseMode) {
debugShaderWarmUpTask!.finish();
}
}
}
picture.dispose();
}
}
| flutter/packages/flutter/lib/src/painting/shader_warm_up.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/shader_warm_up.dart",
"repo_id": "flutter",
"token_count": 1431
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'layer.dart';
import 'object.dart';
import 'shifted_box.dart';
/// A [RenderAnimatedSize] can be in exactly one of these states.
@visibleForTesting
enum RenderAnimatedSizeState {
/// The initial state, when we do not yet know what the starting and target
/// sizes are to animate.
///
/// The next state is [stable].
start,
/// At this state the child's size is assumed to be stable and we are either
/// animating, or waiting for the child's size to change.
///
/// If the child's size changes, the state will become [changed]. Otherwise,
/// it remains [stable].
stable,
/// At this state we know that the child has changed once after being assumed
/// [stable].
///
/// The next state will be one of:
///
/// * [stable] if the child's size stabilized immediately. This is a signal
/// for the render object to begin animating the size towards the child's new
/// size.
///
/// * [unstable] if the child's size continues to change.
changed,
/// At this state the child's size is assumed to be unstable (changing each
/// frame).
///
/// Instead of chasing the child's size in this state, the render object
/// tightly tracks the child's size until it stabilizes.
///
/// The render object remains in this state until a frame where the child's
/// size remains the same as the previous frame. At that time, the next state
/// is [stable].
unstable,
}
/// A render object that animates its size to its child's size over a given
/// [duration] and with a given [curve]. If the child's size itself animates
/// (i.e. if it changes size two frames in a row, as opposed to abruptly
/// changing size in one frame then remaining that size in subsequent frames),
/// this render object sizes itself to fit the child instead of animating
/// itself.
///
/// When the child overflows the current animated size of this render object, it
/// is clipped.
class RenderAnimatedSize extends RenderAligningShiftedBox {
/// Creates a render object that animates its size to match its child.
/// The [duration] and [curve] arguments define the animation.
///
/// The [alignment] argument is used to align the child when the parent is not
/// (yet) the same size as the child.
///
/// The [duration] is required.
///
/// The [vsync] should specify a [TickerProvider] for the animation
/// controller.
///
/// The arguments [duration], [curve], [alignment], and [vsync] must
/// not be null.
RenderAnimatedSize({
required TickerProvider vsync,
required Duration duration,
Duration? reverseDuration,
Curve curve = Curves.linear,
super.alignment,
super.textDirection,
super.child,
Clip clipBehavior = Clip.hardEdge,
VoidCallback? onEnd,
}) : _vsync = vsync,
_clipBehavior = clipBehavior {
_controller = AnimationController(
vsync: vsync,
duration: duration,
reverseDuration: reverseDuration,
)..addListener(() {
if (_controller.value != _lastValue) {
markNeedsLayout();
}
});
_animation = CurvedAnimation(
parent: _controller,
curve: curve,
);
_onEnd = onEnd;
}
/// When asserts are enabled, returns the animation controller that is used
/// to drive the resizing.
///
/// Otherwise, returns null.
///
/// This getter is intended for use in framework unit tests. Applications must
/// not depend on its value.
@visibleForTesting
AnimationController? get debugController {
AnimationController? controller;
assert(() {
controller = _controller;
return true;
}());
return controller;
}
/// When asserts are enabled, returns the animation that drives the resizing.
///
/// Otherwise, returns null.
///
/// This getter is intended for use in framework unit tests. Applications must
/// not depend on its value.
@visibleForTesting
CurvedAnimation? get debugAnimation {
CurvedAnimation? animation;
assert(() {
animation = _animation;
return true;
}());
return animation;
}
late final AnimationController _controller;
late final CurvedAnimation _animation;
final SizeTween _sizeTween = SizeTween();
late bool _hasVisualOverflow;
double? _lastValue;
/// The state this size animation is in.
///
/// See [RenderAnimatedSizeState] for possible states.
@visibleForTesting
RenderAnimatedSizeState get state => _state;
RenderAnimatedSizeState _state = RenderAnimatedSizeState.start;
/// The duration of the animation.
Duration get duration => _controller.duration!;
set duration(Duration value) {
if (value == _controller.duration) {
return;
}
_controller.duration = value;
}
/// The duration of the animation when running in reverse.
Duration? get reverseDuration => _controller.reverseDuration;
set reverseDuration(Duration? value) {
if (value == _controller.reverseDuration) {
return;
}
_controller.reverseDuration = value;
}
/// The curve of the animation.
Curve get curve => _animation.curve;
set curve(Curve value) {
if (value == _animation.curve) {
return;
}
_animation.curve = value;
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
/// Whether the size is being currently animated towards the child's size.
///
/// See [RenderAnimatedSizeState] for situations when we may not be animating
/// the size.
bool get isAnimating => _controller.isAnimating;
/// The [TickerProvider] for the [AnimationController] that runs the animation.
TickerProvider get vsync => _vsync;
TickerProvider _vsync;
set vsync(TickerProvider value) {
if (value == _vsync) {
return;
}
_vsync = value;
_controller.resync(vsync);
}
/// Called every time an animation completes.
///
/// This can be useful to trigger additional actions (e.g. another animation)
/// at the end of the current animation.
VoidCallback? get onEnd => _onEnd;
VoidCallback? _onEnd;
set onEnd(VoidCallback? value) {
if (value == _onEnd) {
return;
}
_onEnd = value;
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
switch (state) {
case RenderAnimatedSizeState.start:
case RenderAnimatedSizeState.stable:
break;
case RenderAnimatedSizeState.changed:
case RenderAnimatedSizeState.unstable:
// Call markNeedsLayout in case the RenderObject isn't marked dirty
// already, to resume interrupted resizing animation.
markNeedsLayout();
}
_controller.addStatusListener(_animationStatusListener);
}
@override
void detach() {
_controller.stop();
_controller.removeStatusListener(_animationStatusListener);
super.detach();
}
Size? get _animatedSize {
return _sizeTween.evaluate(_animation);
}
@override
void performLayout() {
_lastValue = _controller.value;
_hasVisualOverflow = false;
final BoxConstraints constraints = this.constraints;
if (child == null || constraints.isTight) {
_controller.stop();
size = _sizeTween.begin = _sizeTween.end = constraints.smallest;
_state = RenderAnimatedSizeState.start;
child?.layout(constraints);
return;
}
child!.layout(constraints, parentUsesSize: true);
switch (_state) {
case RenderAnimatedSizeState.start:
_layoutStart();
case RenderAnimatedSizeState.stable:
_layoutStable();
case RenderAnimatedSizeState.changed:
_layoutChanged();
case RenderAnimatedSizeState.unstable:
_layoutUnstable();
}
size = constraints.constrain(_animatedSize!);
alignChild();
if (size.width < _sizeTween.end!.width ||
size.height < _sizeTween.end!.height) {
_hasVisualOverflow = true;
}
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (child == null || constraints.isTight) {
return constraints.smallest;
}
// This simplified version of performLayout only calculates the current
// size without modifying global state. See performLayout for comments
// explaining the rational behind the implementation.
final Size childSize = child!.getDryLayout(constraints);
switch (_state) {
case RenderAnimatedSizeState.start:
return constraints.constrain(childSize);
case RenderAnimatedSizeState.stable:
if (_sizeTween.end != childSize) {
return constraints.constrain(size);
} else if (_controller.value == _controller.upperBound) {
return constraints.constrain(childSize);
}
case RenderAnimatedSizeState.unstable:
case RenderAnimatedSizeState.changed:
if (_sizeTween.end != childSize) {
return constraints.constrain(childSize);
}
}
return constraints.constrain(_animatedSize!);
}
void _restartAnimation() {
_lastValue = 0.0;
_controller.forward(from: 0.0);
}
/// Laying out the child for the first time.
///
/// We have the initial size to animate from, but we do not have the target
/// size to animate to, so we set both ends to child's size.
void _layoutStart() {
_sizeTween.begin = _sizeTween.end = debugAdoptSize(child!.size);
_state = RenderAnimatedSizeState.stable;
}
/// At this state we're assuming the child size is stable and letting the
/// animation run its course.
///
/// If during animation the size of the child changes we restart the
/// animation.
void _layoutStable() {
if (_sizeTween.end != child!.size) {
_sizeTween.begin = size;
_sizeTween.end = debugAdoptSize(child!.size);
_restartAnimation();
_state = RenderAnimatedSizeState.changed;
} else if (_controller.value == _controller.upperBound) {
// Animation finished. Reset target sizes.
_sizeTween.begin = _sizeTween.end = debugAdoptSize(child!.size);
} else if (!_controller.isAnimating) {
_controller.forward(); // resume the animation after being detached
}
}
/// This state indicates that the size of the child changed once after being
/// considered stable.
///
/// If the child stabilizes immediately, we go back to stable state. If it
/// changes again, we match the child's size, restart animation and go to
/// unstable state.
void _layoutChanged() {
if (_sizeTween.end != child!.size) {
// Child size changed again. Match the child's size and restart animation.
_sizeTween.begin = _sizeTween.end = debugAdoptSize(child!.size);
_restartAnimation();
_state = RenderAnimatedSizeState.unstable;
} else {
// Child size stabilized.
_state = RenderAnimatedSizeState.stable;
if (!_controller.isAnimating) {
// Resume the animation after being detached.
_controller.forward();
}
}
}
/// The child's size is not stable.
///
/// Continue tracking the child's size until is stabilizes.
void _layoutUnstable() {
if (_sizeTween.end != child!.size) {
// Still unstable. Continue tracking the child.
_sizeTween.begin = _sizeTween.end = debugAdoptSize(child!.size);
_restartAnimation();
} else {
// Child size stabilized.
_controller.stop();
_state = RenderAnimatedSizeState.stable;
}
}
void _animationStatusListener(AnimationStatus status) {
switch (status) {
case AnimationStatus.completed:
_onEnd?.call();
case AnimationStatus.dismissed:
case AnimationStatus.forward:
case AnimationStatus.reverse:
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null && _hasVisualOverflow && clipBehavior != Clip.none) {
final Rect rect = Offset.zero & size;
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
rect,
super.paint,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
super.paint(context, offset);
}
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
_controller.dispose();
_animation.dispose();
super.dispose();
}
}
| flutter/packages/flutter/lib/src/rendering/animated_size.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/animated_size.dart",
"repo_id": "flutter",
"token_count": 4279
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart' show Matrix4;
import 'box.dart';
import 'layer.dart';
import 'object.dart';
import 'proxy_box.dart';
import 'viewport.dart';
import 'viewport_offset.dart';
typedef _ChildSizingFunction = double Function(RenderBox child);
/// A delegate used by [RenderListWheelViewport] to manage its children.
///
/// [RenderListWheelViewport] during layout will ask the delegate to create
/// children that are visible in the viewport and remove those that are not.
abstract class ListWheelChildManager {
/// The maximum number of children that can be provided to
/// [RenderListWheelViewport].
///
/// If non-null, the children will have index in the range
/// `[0, childCount - 1]`.
///
/// If null, then there's no explicit limits to the range of the children
/// except that it has to be contiguous. If [childExistsAt] for a certain
/// index returns false, that index is already past the limit.
int? get childCount;
/// Checks whether the delegate is able to provide a child widget at the given
/// index.
///
/// This function is not about whether the child at the given index is
/// attached to the [RenderListWheelViewport] or not.
bool childExistsAt(int index);
/// Creates a new child at the given index and updates it to the child list
/// of [RenderListWheelViewport]. If no child corresponds to `index`, then do
/// nothing.
///
/// It is possible to create children with negative indices.
void createChild(int index, { required RenderBox? after });
/// Removes the child element corresponding with the given RenderBox.
void removeChild(RenderBox child);
}
/// [ParentData] for use with [RenderListWheelViewport].
class ListWheelParentData extends ContainerBoxParentData<RenderBox> {
/// Index of this child in its parent's child list.
///
/// This must be maintained by the [ListWheelChildManager].
int? index;
/// Transform applied to this child during painting.
///
/// Can be used to find the local bounds of this child in the viewport,
/// and then use it, for example, in hit testing.
///
/// May be null if child was laid out, but not painted
/// by the parent, but normally this shouldn't happen,
/// because [RenderListWheelViewport] paints all of the
/// children it has laid out.
Matrix4? transform;
}
/// Render, onto a wheel, a bigger sequential set of objects inside this viewport.
///
/// Takes a scrollable set of fixed sized [RenderBox]es and renders them
/// sequentially from top down on a vertical scrolling axis.
///
/// It starts with the first scrollable item in the center of the main axis
/// and ends with the last scrollable item in the center of the main axis. This
/// is in contrast to typical lists that start with the first scrollable item
/// at the start of the main axis and ends with the last scrollable item at the
/// end of the main axis.
///
/// Instead of rendering its children on a flat plane, it renders them
/// as if each child is broken into its own plane and that plane is
/// perpendicularly fixed onto a cylinder which rotates along the scrolling
/// axis.
///
/// This class works in 3 coordinate systems:
///
/// 1. The **scrollable layout coordinates**. This coordinate system is used to
/// communicate with [ViewportOffset] and describes its children's abstract
/// offset from the beginning of the scrollable list at (0.0, 0.0).
///
/// The list is scrollable from the start of the first child item to the
/// start of the last child item.
///
/// Children's layout coordinates don't change as the viewport scrolls.
///
/// 2. The **untransformed plane's viewport painting coordinates**. Children are
/// not painted in this coordinate system. It's an abstract intermediary used
/// before transforming into the next cylindrical coordinate system.
///
/// This system is the **scrollable layout coordinates** translated by the
/// scroll offset such that (0.0, 0.0) is the top left corner of the
/// viewport.
///
/// Because the viewport is centered at the scrollable list's scroll offset
/// instead of starting at the scroll offset, there are paintable children
/// ~1/2 viewport length before and after the scroll offset instead of ~1
/// viewport length after the scroll offset.
///
/// Children's visibility inclusion in the viewport is determined in this
/// system regardless of the cylinder's properties such as [diameterRatio]
/// or [perspective]. In other words, a 100px long viewport will always
/// paint 10-11 visible 10px children if there are enough children in the
/// viewport.
///
/// 3. The **transformed cylindrical space viewport painting coordinates**.
/// Children from system 2 get their positions transformed into a cylindrical
/// projection matrix instead of its Cartesian offset with respect to the
/// scroll offset.
///
/// Children in this coordinate system are painted.
///
/// The wheel's size and the maximum and minimum visible angles are both
/// controlled by [diameterRatio]. Children visible in the **untransformed
/// plane's viewport painting coordinates**'s viewport will be radially
/// evenly laid out between the maximum and minimum angles determined by
/// intersecting the viewport's main axis length with a cylinder whose
/// diameter is [diameterRatio] times longer, as long as those angles are
/// between -pi/2 and pi/2.
///
/// For example, if [diameterRatio] is 2.0 and this [RenderListWheelViewport]
/// is 100.0px in the main axis, then the diameter is 200.0. And children
/// will be evenly laid out between that cylinder's -arcsin(1/2) and
/// arcsin(1/2) angles.
///
/// The cylinder's 0 degree side is always centered in the
/// [RenderListWheelViewport]. The transformation from **untransformed
/// plane's viewport painting coordinates** is also done such that the child
/// in the center of that plane will be mostly untransformed with children
/// above and below it being transformed more as the angle increases.
class RenderListWheelViewport
extends RenderBox
with ContainerRenderObjectMixin<RenderBox, ListWheelParentData>
implements RenderAbstractViewport {
/// Creates a [RenderListWheelViewport] which renders children on a wheel.
///
/// Optional arguments have reasonable defaults.
RenderListWheelViewport({
required this.childManager,
required ViewportOffset offset,
double diameterRatio = defaultDiameterRatio,
double perspective = defaultPerspective,
double offAxisFraction = 0,
bool useMagnifier = false,
double magnification = 1,
double overAndUnderCenterOpacity = 1,
required double itemExtent,
double squeeze = 1,
bool renderChildrenOutsideViewport = false,
Clip clipBehavior = Clip.none,
List<RenderBox>? children,
}) : assert(diameterRatio > 0, diameterRatioZeroMessage),
assert(perspective > 0),
assert(perspective <= 0.01, perspectiveTooHighMessage),
assert(magnification > 0),
assert(overAndUnderCenterOpacity >= 0 && overAndUnderCenterOpacity <= 1),
assert(squeeze > 0),
assert(itemExtent > 0),
assert(
!renderChildrenOutsideViewport || clipBehavior == Clip.none,
clipBehaviorAndRenderChildrenOutsideViewportConflict,
),
_offset = offset,
_diameterRatio = diameterRatio,
_perspective = perspective,
_offAxisFraction = offAxisFraction,
_useMagnifier = useMagnifier,
_magnification = magnification,
_overAndUnderCenterOpacity = overAndUnderCenterOpacity,
_itemExtent = itemExtent,
_squeeze = squeeze,
_renderChildrenOutsideViewport = renderChildrenOutsideViewport,
_clipBehavior = clipBehavior {
addAll(children);
}
/// An arbitrary but aesthetically reasonable default value for [diameterRatio].
static const double defaultDiameterRatio = 2.0;
/// An arbitrary but aesthetically reasonable default value for [perspective].
static const double defaultPerspective = 0.003;
/// An error message to show when the provided [diameterRatio] is zero.
static const String diameterRatioZeroMessage = "You can't set a diameterRatio "
'of 0 or of a negative number. It would imply a cylinder of 0 in diameter '
'in which case nothing will be drawn.';
/// An error message to show when the [perspective] value is too high.
static const String perspectiveTooHighMessage = 'A perspective too high will '
'be clipped in the z-axis and therefore not renderable. Value must be '
'between 0 and 0.01.';
/// An error message to show when [clipBehavior] and [renderChildrenOutsideViewport]
/// are set to conflicting values.
static const String clipBehaviorAndRenderChildrenOutsideViewportConflict =
'Cannot renderChildrenOutsideViewport and clip since children '
'rendered outside will be clipped anyway.';
/// The delegate that manages the children of this object.
///
/// This delegate must maintain the [ListWheelParentData.index] value.
final ListWheelChildManager childManager;
/// The associated ViewportOffset object for the viewport describing the part
/// of the content inside that's visible.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport, this value changes, which changes the content that
/// is displayed.
ViewportOffset get offset => _offset;
ViewportOffset _offset;
set offset(ViewportOffset value) {
if (value == _offset) {
return;
}
if (attached) {
_offset.removeListener(_hasScrolled);
}
_offset = value;
if (attached) {
_offset.addListener(_hasScrolled);
}
markNeedsLayout();
}
/// {@template flutter.rendering.RenderListWheelViewport.diameterRatio}
/// A ratio between the diameter of the cylinder and the viewport's size
/// in the main axis.
///
/// A value of 1 means the cylinder has the same diameter as the viewport's
/// size.
///
/// A value smaller than 1 means items at the edges of the cylinder are
/// entirely contained inside the viewport.
///
/// A value larger than 1 means angles less than ±[pi] / 2 from the
/// center of the cylinder are visible.
///
/// The same number of children will be visible in the viewport regardless of
/// the [diameterRatio]. The number of children visible is based on the
/// viewport's length along the main axis divided by the children's
/// [itemExtent]. Then the children are evenly distributed along the visible
/// angles up to ±[pi] / 2.
///
/// Just as it's impossible to stretch a paper to cover the an entire
/// half of a cylinder's surface where the cylinder has the same diameter
/// as the paper's length, choosing a [diameterRatio] smaller than [pi]
/// will leave same gaps between the children.
///
/// Defaults to an arbitrary but aesthetically reasonable number of 2.0.
///
/// Must be a positive number.
/// {@endtemplate}
double get diameterRatio => _diameterRatio;
double _diameterRatio;
set diameterRatio(double value) {
assert(
value > 0,
diameterRatioZeroMessage,
);
if (value == _diameterRatio) {
return;
}
_diameterRatio = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
/// {@template flutter.rendering.RenderListWheelViewport.perspective}
/// Perspective of the cylindrical projection.
///
/// A number between 0 and 0.01 where 0 means looking at the cylinder from
/// infinitely far with an infinitely small field of view and 1 means looking
/// at the cylinder from infinitely close with an infinitely large field of
/// view (which cannot be rendered).
///
/// Defaults to an arbitrary but aesthetically reasonable number of 0.003.
/// A larger number brings the vanishing point closer and a smaller number
/// pushes the vanishing point further.
///
/// Must be a positive number.
/// {@endtemplate}
double get perspective => _perspective;
double _perspective;
set perspective(double value) {
assert(value > 0);
assert(
value <= 0.01,
perspectiveTooHighMessage,
);
if (value == _perspective) {
return;
}
_perspective = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
/// {@template flutter.rendering.RenderListWheelViewport.offAxisFraction}
/// How much the wheel is horizontally off-center, as a fraction of its width.
/// This property creates the visual effect of looking at a vertical wheel from
/// its side where its vanishing points at the edge curves to one side instead
/// of looking at the wheel head-on.
///
/// The value is horizontal distance between the wheel's center and the vertical
/// vanishing line at the edges of the wheel, represented as a fraction of the
/// wheel's width.
///
/// The value `0.0` means the wheel is looked at head-on and its vanishing
/// line runs through the center of the wheel. Negative values means moving
/// the wheel to the left of the observer, thus the edges curve to the right.
/// Positive values means moving the wheel to the right of the observer,
/// thus the edges curve to the left.
///
/// The visual effect causes the wheel's edges to curve rather than moving
/// the center. So a value of `0.5` means the edges' vanishing line will touch
/// the wheel's size's left edge.
///
/// Defaults to `0.0`, which means looking at the wheel head-on.
/// The visual effect can be unaesthetic if this value is too far from the
/// range `[-0.5, 0.5]`.
/// {@endtemplate}
double get offAxisFraction => _offAxisFraction;
double _offAxisFraction = 0.0;
set offAxisFraction(double value) {
if (value == _offAxisFraction) {
return;
}
_offAxisFraction = value;
markNeedsPaint();
}
/// {@template flutter.rendering.RenderListWheelViewport.useMagnifier}
/// Whether to use the magnifier for the center item of the wheel.
/// {@endtemplate}
bool get useMagnifier => _useMagnifier;
bool _useMagnifier = false;
set useMagnifier(bool value) {
if (value == _useMagnifier) {
return;
}
_useMagnifier = value;
markNeedsPaint();
}
/// {@template flutter.rendering.RenderListWheelViewport.magnification}
/// The zoomed-in rate of the magnifier, if it is used.
///
/// The default value is 1.0, which will not change anything.
/// If the value is > 1.0, the center item will be zoomed in by that rate, and
/// it will also be rendered as flat, not cylindrical like the rest of the list.
/// The item will be zoomed out if magnification < 1.0.
///
/// Must be positive.
/// {@endtemplate}
double get magnification => _magnification;
double _magnification = 1.0;
set magnification(double value) {
assert(value > 0);
if (value == _magnification) {
return;
}
_magnification = value;
markNeedsPaint();
}
/// {@template flutter.rendering.RenderListWheelViewport.overAndUnderCenterOpacity}
/// The opacity value that will be applied to the wheel that appears below and
/// above the magnifier.
///
/// The default value is 1.0, which will not change anything.
///
/// Must be greater than or equal to 0, and less than or equal to 1.
/// {@endtemplate}
double get overAndUnderCenterOpacity => _overAndUnderCenterOpacity;
double _overAndUnderCenterOpacity = 1.0;
set overAndUnderCenterOpacity(double value) {
assert(value >= 0 && value <= 1);
if (value == _overAndUnderCenterOpacity) {
return;
}
_overAndUnderCenterOpacity = value;
markNeedsPaint();
}
/// {@template flutter.rendering.RenderListWheelViewport.itemExtent}
/// The size of the children along the main axis. Children [RenderBox]es will
/// be given the [BoxConstraints] of this exact size.
///
/// Must be a positive number.
/// {@endtemplate}
double get itemExtent => _itemExtent;
double _itemExtent;
set itemExtent(double value) {
assert(value > 0);
if (value == _itemExtent) {
return;
}
_itemExtent = value;
markNeedsLayout();
}
/// {@template flutter.rendering.RenderListWheelViewport.squeeze}
/// The angular compactness of the children on the wheel.
///
/// This denotes a ratio of the number of children on the wheel vs the number
/// of children that would fit on a flat list of equivalent size, assuming
/// [diameterRatio] of 1.
///
/// For instance, if this RenderListWheelViewport has a height of 100px and
/// [itemExtent] is 20px, 5 items would fit on an equivalent flat list.
/// With a [squeeze] of 1, 5 items would also be shown in the
/// RenderListWheelViewport. With a [squeeze] of 2, 10 items would be shown
/// in the RenderListWheelViewport.
///
/// Changing this value will change the number of children built and shown
/// inside the wheel.
///
/// Must be a positive number.
/// {@endtemplate}
///
/// Defaults to 1.
double get squeeze => _squeeze;
double _squeeze;
set squeeze(double value) {
assert(value > 0);
if (value == _squeeze) {
return;
}
_squeeze = value;
markNeedsLayout();
markNeedsSemanticsUpdate();
}
/// {@template flutter.rendering.RenderListWheelViewport.renderChildrenOutsideViewport}
/// Whether to paint children inside the viewport only.
///
/// If false, every child will be painted. However the [Scrollable] is still
/// the size of the viewport and detects gestures inside only.
///
/// Defaults to false. Cannot be true if [clipBehavior] is not [Clip.none]
/// since children outside the viewport will be clipped, and therefore cannot
/// render children outside the viewport.
/// {@endtemplate}
bool get renderChildrenOutsideViewport => _renderChildrenOutsideViewport;
bool _renderChildrenOutsideViewport;
set renderChildrenOutsideViewport(bool value) {
assert(
!renderChildrenOutsideViewport || clipBehavior == Clip.none,
clipBehaviorAndRenderChildrenOutsideViewportConflict,
);
if (value == _renderChildrenOutsideViewport) {
return;
}
_renderChildrenOutsideViewport = value;
markNeedsLayout();
markNeedsSemanticsUpdate();
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
void _hasScrolled() {
markNeedsLayout();
markNeedsSemanticsUpdate();
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! ListWheelParentData) {
child.parentData = ListWheelParentData();
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_offset.addListener(_hasScrolled);
}
@override
void detach() {
_offset.removeListener(_hasScrolled);
super.detach();
}
@override
bool get isRepaintBoundary => true;
/// Main axis length in the untransformed plane.
double get _viewportExtent {
assert(hasSize);
return size.height;
}
/// Main axis scroll extent in the **scrollable layout coordinates** that puts
/// the first item in the center.
double get _minEstimatedScrollExtent {
assert(hasSize);
if (childManager.childCount == null) {
return double.negativeInfinity;
}
return 0.0;
}
/// Main axis scroll extent in the **scrollable layout coordinates** that puts
/// the last item in the center.
double get _maxEstimatedScrollExtent {
assert(hasSize);
if (childManager.childCount == null) {
return double.infinity;
}
return math.max(0.0, (childManager.childCount! - 1) * _itemExtent);
}
/// Scroll extent distance in the untransformed plane between the center
/// position in the viewport and the top position in the viewport.
///
/// It's also the distance in the untransformed plane that children's painting
/// is offset by with respect to those children's [BoxParentData.offset].
double get _topScrollMarginExtent {
assert(hasSize);
// Consider adding alignment options other than center.
return -size.height / 2.0 + _itemExtent / 2.0;
}
/// Transforms a **scrollable layout coordinates**' y position to the
/// **untransformed plane's viewport painting coordinates**' y position given
/// the current scroll offset.
double _getUntransformedPaintingCoordinateY(double layoutCoordinateY) {
return layoutCoordinateY - _topScrollMarginExtent - offset.pixels;
}
/// Given the _diameterRatio, return the largest absolute angle of the item
/// at the edge of the portion of the visible cylinder.
///
/// For a _diameterRatio of 1 or less than 1 (i.e. the viewport is bigger
/// than the cylinder diameter), this value reaches and clips at pi / 2.
///
/// When the center of children passes this angle, they are no longer painted
/// if [renderChildrenOutsideViewport] is false.
double get _maxVisibleRadian {
if (_diameterRatio < 1.0) {
return math.pi / 2.0;
}
return math.asin(1.0 / _diameterRatio);
}
double _getIntrinsicCrossAxis(_ChildSizingFunction childSize) {
double extent = 0.0;
RenderBox? child = firstChild;
while (child != null) {
extent = math.max(extent, childSize(child));
child = childAfter(child);
}
return extent;
}
@override
double computeMinIntrinsicWidth(double height) {
return _getIntrinsicCrossAxis(
(RenderBox child) => child.getMinIntrinsicWidth(height),
);
}
@override
double computeMaxIntrinsicWidth(double height) {
return _getIntrinsicCrossAxis(
(RenderBox child) => child.getMaxIntrinsicWidth(height),
);
}
@override
double computeMinIntrinsicHeight(double width) {
if (childManager.childCount == null) {
return 0.0;
}
return childManager.childCount! * _itemExtent;
}
@override
double computeMaxIntrinsicHeight(double width) {
if (childManager.childCount == null) {
return 0.0;
}
return childManager.childCount! * _itemExtent;
}
@override
bool get sizedByParent => true;
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return constraints.biggest;
}
/// Gets the index of a child by looking at its [parentData].
///
/// This relies on the [childManager] maintaining [ListWheelParentData.index].
int indexOf(RenderBox child) {
final ListWheelParentData childParentData = child.parentData! as ListWheelParentData;
assert(childParentData.index != null);
return childParentData.index!;
}
/// Returns the index of the child at the given offset.
int scrollOffsetToIndex(double scrollOffset) => (scrollOffset / itemExtent).floor();
/// Returns the scroll offset of the child with the given index.
double indexToScrollOffset(int index) => index * itemExtent;
void _createChild(int index, { RenderBox? after }) {
invokeLayoutCallback<BoxConstraints>((BoxConstraints constraints) {
assert(constraints == this.constraints);
childManager.createChild(index, after: after);
});
}
void _destroyChild(RenderBox child) {
invokeLayoutCallback<BoxConstraints>((BoxConstraints constraints) {
assert(constraints == this.constraints);
childManager.removeChild(child);
});
}
void _layoutChild(RenderBox child, BoxConstraints constraints, int index) {
child.layout(constraints, parentUsesSize: true);
final ListWheelParentData childParentData = child.parentData! as ListWheelParentData;
// Centers the child horizontally.
final double crossPosition = size.width / 2.0 - child.size.width / 2.0;
childParentData.offset = Offset(crossPosition, indexToScrollOffset(index));
}
/// Performs layout based on how [childManager] provides children.
///
/// From the current scroll offset, the minimum index and maximum index that
/// is visible in the viewport can be calculated. The index range of the
/// currently active children can also be acquired by looking directly at
/// the current child list. This function has to modify the current index
/// range to match the target index range by removing children that are no
/// longer visible and creating those that are visible but not yet provided
/// by [childManager].
@override
void performLayout() {
offset.applyViewportDimension(_viewportExtent);
// Apply the content dimensions first if it has exact dimensions in case it
// changes the scroll offset which determines what should be shown. Such as
// if the child count decrease, we should correct the pixels first, otherwise,
// it may be shown blank null children.
if (childManager.childCount != null) {
offset.applyContentDimensions(_minEstimatedScrollExtent, _maxEstimatedScrollExtent);
}
// The height, in pixel, that children will be visible and might be laid out
// and painted.
double visibleHeight = size.height * _squeeze;
// If renderChildrenOutsideViewport is true, we spawn extra children by
// doubling the visibility range, those that are in the backside of the
// cylinder won't be painted anyway.
if (renderChildrenOutsideViewport) {
visibleHeight *= 2;
}
final double firstVisibleOffset =
offset.pixels + _itemExtent / 2 - visibleHeight / 2;
final double lastVisibleOffset = firstVisibleOffset + visibleHeight;
// The index range that we want to spawn children. We find indexes that
// are in the interval [firstVisibleOffset, lastVisibleOffset).
int targetFirstIndex = scrollOffsetToIndex(firstVisibleOffset);
int targetLastIndex = scrollOffsetToIndex(lastVisibleOffset);
// Because we exclude lastVisibleOffset, if there's a new child starting at
// that offset, it is removed.
if (targetLastIndex * _itemExtent == lastVisibleOffset) {
targetLastIndex--;
}
// Validates the target index range.
while (!childManager.childExistsAt(targetFirstIndex) && targetFirstIndex <= targetLastIndex) {
targetFirstIndex++;
}
while (!childManager.childExistsAt(targetLastIndex) && targetFirstIndex <= targetLastIndex) {
targetLastIndex--;
}
// If it turns out there's no children to layout, we remove old children and
// return.
if (targetFirstIndex > targetLastIndex) {
while (firstChild != null) {
_destroyChild(firstChild!);
}
return;
}
// Now there are 2 cases:
// - The target index range and our current index range have intersection:
// We shorten and extend our current child list so that the two lists
// match. Most of the time we are in this case.
// - The target list and our current child list have no intersection:
// We first remove all children and then add one child from the target
// list => this case becomes the other case.
// Case when there is no intersection.
if (childCount > 0 &&
(indexOf(firstChild!) > targetLastIndex || indexOf(lastChild!) < targetFirstIndex)) {
while (firstChild != null) {
_destroyChild(firstChild!);
}
}
final BoxConstraints childConstraints = constraints.copyWith(
minHeight: _itemExtent,
maxHeight: _itemExtent,
minWidth: 0.0,
);
// If there is no child at this stage, we add the first one that is in
// target range.
if (childCount == 0) {
_createChild(targetFirstIndex);
_layoutChild(firstChild!, childConstraints, targetFirstIndex);
}
int currentFirstIndex = indexOf(firstChild!);
int currentLastIndex = indexOf(lastChild!);
// Remove all unnecessary children by shortening the current child list, in
// both directions.
while (currentFirstIndex < targetFirstIndex) {
_destroyChild(firstChild!);
currentFirstIndex++;
}
while (currentLastIndex > targetLastIndex) {
_destroyChild(lastChild!);
currentLastIndex--;
}
// Relayout all active children.
RenderBox? child = firstChild;
int index = currentFirstIndex;
while (child != null) {
_layoutChild(child, childConstraints, index++);
child = childAfter(child);
}
// Spawning new children that are actually visible but not in child list yet.
while (currentFirstIndex > targetFirstIndex) {
_createChild(currentFirstIndex - 1);
_layoutChild(firstChild!, childConstraints, --currentFirstIndex);
}
while (currentLastIndex < targetLastIndex) {
_createChild(currentLastIndex + 1, after: lastChild);
_layoutChild(lastChild!, childConstraints, ++currentLastIndex);
}
// Applying content dimensions bases on how the childManager builds widgets:
// if it is available to provide a child just out of target range, then
// we don't know whether there's a limit yet, and set the dimension to the
// estimated value. Otherwise, we set the dimension limited to our target
// range.
final double minScrollExtent = childManager.childExistsAt(targetFirstIndex - 1)
? _minEstimatedScrollExtent
: indexToScrollOffset(targetFirstIndex);
final double maxScrollExtent = childManager.childExistsAt(targetLastIndex + 1)
? _maxEstimatedScrollExtent
: indexToScrollOffset(targetLastIndex);
offset.applyContentDimensions(minScrollExtent, maxScrollExtent);
}
bool _shouldClipAtCurrentOffset() {
final double highestUntransformedPaintY =
_getUntransformedPaintingCoordinateY(0.0);
return highestUntransformedPaintY < 0.0
|| size.height < highestUntransformedPaintY + _maxEstimatedScrollExtent + _itemExtent;
}
@override
void paint(PaintingContext context, Offset offset) {
if (childCount > 0) {
if (_shouldClipAtCurrentOffset() && clipBehavior != Clip.none) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
_paintVisibleChildren,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
_paintVisibleChildren(context, offset);
}
}
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
_childOpacityLayerHandler.layer = null;
super.dispose();
}
final LayerHandle<OpacityLayer> _childOpacityLayerHandler = LayerHandle<OpacityLayer>();
/// Paints all children visible in the current viewport.
void _paintVisibleChildren(PaintingContext context, Offset offset) {
// The magnifier cannot be turned off if the opacity is less than 1.0.
if (overAndUnderCenterOpacity >= 1) {
_paintAllChildren(context, offset);
return;
}
// In order to reduce the number of opacity layers, we first paint all
// partially opaque children, then finally paint the fully opaque children.
_childOpacityLayerHandler.layer = context.pushOpacity(offset, (overAndUnderCenterOpacity * 255).round(), (PaintingContext context, Offset offset) {
_paintAllChildren(context, offset, center: false);
});
_paintAllChildren(context, offset, center: true);
}
void _paintAllChildren(PaintingContext context, Offset offset, { bool? center }) {
RenderBox? childToPaint = firstChild;
while (childToPaint != null) {
final ListWheelParentData childParentData = childToPaint.parentData! as ListWheelParentData;
_paintTransformedChild(childToPaint, context, offset, childParentData.offset, center: center);
childToPaint = childAfter(childToPaint);
}
}
// Takes in a child with a **scrollable layout offset** and paints it in the
// **transformed cylindrical space viewport painting coordinates**.
//
// The value of `center` is passed through to _paintChildWithMagnifier only
// if the magnifier is enabled and/or opacity is < 1.0.
void _paintTransformedChild(
RenderBox child,
PaintingContext context,
Offset offset,
Offset layoutOffset, {
required bool? center,
}) {
final Offset untransformedPaintingCoordinates = offset
+ Offset(
layoutOffset.dx,
_getUntransformedPaintingCoordinateY(layoutOffset.dy),
);
// Get child's center as a fraction of the viewport's height.
final double fractionalY =
(untransformedPaintingCoordinates.dy + _itemExtent / 2.0) / size.height;
final double angle = -(fractionalY - 0.5) * 2.0 * _maxVisibleRadian / squeeze;
// Don't paint the backside of the cylinder when
// renderChildrenOutsideViewport is true. Otherwise, only children within
// suitable angles (via _first/lastVisibleLayoutOffset) reach the paint
// phase.
if (angle > math.pi / 2.0 || angle < -math.pi / 2.0 || angle.isNaN) {
return;
}
final Matrix4 transform = MatrixUtils.createCylindricalProjectionTransform(
radius: size.height * _diameterRatio / 2.0,
angle: angle,
perspective: _perspective,
);
// Offset that helps painting everything in the center (e.g. angle = 0).
final Offset offsetToCenter = Offset(
untransformedPaintingCoordinates.dx,
-_topScrollMarginExtent,
);
final bool shouldApplyOffCenterDim = overAndUnderCenterOpacity < 1;
if (useMagnifier || shouldApplyOffCenterDim) {
_paintChildWithMagnifier(context, offset, child, transform, offsetToCenter, untransformedPaintingCoordinates, center: center);
} else {
assert(center == null);
_paintChildCylindrically(context, offset, child, transform, offsetToCenter);
}
}
// Paint child with the magnifier active - the child will be rendered
// differently if it intersects with the magnifier.
//
// `center` controls how items that partially intersect the center magnifier
// are rendered. If `center` is false, items are only painted cylindrically.
// If `center` is true, only the clipped magnifier items are painted.
// If `center` is null, partially intersecting items are painted both as the
// magnifier and cylindrical item, while non-intersecting items are painted
// only cylindrically.
//
// This property is used to lift the opacity that would be applied to each
// cylindrical item into a single layer, reducing the rendering cost of the
// pickers which use this viewport.
void _paintChildWithMagnifier(
PaintingContext context,
Offset offset,
RenderBox child,
Matrix4 cylindricalTransform,
Offset offsetToCenter,
Offset untransformedPaintingCoordinates, {
required bool? center,
}) {
final double magnifierTopLinePosition =
size.height / 2 - _itemExtent * _magnification / 2;
final double magnifierBottomLinePosition =
size.height / 2 + _itemExtent * _magnification / 2;
final bool isAfterMagnifierTopLine = untransformedPaintingCoordinates.dy
>= magnifierTopLinePosition - _itemExtent * _magnification;
final bool isBeforeMagnifierBottomLine = untransformedPaintingCoordinates.dy
<= magnifierBottomLinePosition;
final Rect centerRect = Rect.fromLTWH(
0.0,
magnifierTopLinePosition,
size.width,
_itemExtent * _magnification,
);
final Rect topHalfRect = Rect.fromLTWH(
0.0,
0.0,
size.width,
magnifierTopLinePosition,
);
final Rect bottomHalfRect = Rect.fromLTWH(
0.0,
magnifierBottomLinePosition,
size.width,
magnifierTopLinePosition,
);
// Some part of the child is in the center magnifier.
final bool inCenter = isAfterMagnifierTopLine && isBeforeMagnifierBottomLine;
if ((center == null || center) && inCenter) {
// Clipping the part in the center.
context.pushClipRect(
needsCompositing,
offset,
centerRect,
(PaintingContext context, Offset offset) {
context.pushTransform(
needsCompositing,
offset,
_magnifyTransform(),
(PaintingContext context, Offset offset) {
context.paintChild(child, offset + untransformedPaintingCoordinates);
},
);
},
);
}
// Clipping the part in either the top-half or bottom-half of the wheel.
if ((center == null || !center) && inCenter) {
context.pushClipRect(
needsCompositing,
offset,
untransformedPaintingCoordinates.dy <= magnifierTopLinePosition
? topHalfRect
: bottomHalfRect,
(PaintingContext context, Offset offset) {
_paintChildCylindrically(
context,
offset,
child,
cylindricalTransform,
offsetToCenter,
);
},
);
}
if ((center == null || !center) && !inCenter) {
_paintChildCylindrically(
context,
offset,
child,
cylindricalTransform,
offsetToCenter,
);
}
}
// / Paint the child cylindrically at given offset.
void _paintChildCylindrically(
PaintingContext context,
Offset offset,
RenderBox child,
Matrix4 cylindricalTransform,
Offset offsetToCenter,
) {
final Offset paintOriginOffset = offset + offsetToCenter;
// Paint child cylindrically, without [overAndUnderCenterOpacity].
void painter(PaintingContext context, Offset offset) {
context.paintChild(
child,
// Paint everything in the center (e.g. angle = 0), then transform.
paintOriginOffset,
);
}
context.pushTransform(
needsCompositing,
offset,
_centerOriginTransform(cylindricalTransform),
// Pre-transform painting function.
painter,
);
final ListWheelParentData childParentData = child.parentData! as ListWheelParentData;
// Save the final transform that accounts both for the offset and cylindrical transform.
final Matrix4 transform = _centerOriginTransform(cylindricalTransform)
..translate(paintOriginOffset.dx, paintOriginOffset.dy);
childParentData.transform = transform;
}
/// Return the Matrix4 transformation that would zoom in content in the
/// magnified area.
Matrix4 _magnifyTransform() {
final Matrix4 magnify = Matrix4.identity();
magnify.translate(size.width * (-_offAxisFraction + 0.5), size.height / 2);
magnify.scale(_magnification, _magnification, _magnification);
magnify.translate(-size.width * (-_offAxisFraction + 0.5), -size.height / 2);
return magnify;
}
/// Apply incoming transformation with the transformation's origin at the
/// viewport's center or horizontally off to the side based on offAxisFraction.
Matrix4 _centerOriginTransform(Matrix4 originalMatrix) {
final Matrix4 result = Matrix4.identity();
final Offset centerOriginTranslation = Alignment.center.alongSize(size);
result.translate(
centerOriginTranslation.dx * (-_offAxisFraction * 2 + 1),
centerOriginTranslation.dy,
);
result.multiply(originalMatrix);
result.translate(
-centerOriginTranslation.dx * (-_offAxisFraction * 2 + 1),
-centerOriginTranslation.dy,
);
return result;
}
static bool _debugAssertValidHitTestOffsets(String context, Offset offset1, Offset offset2) {
if (offset1 != offset2) {
throw FlutterError("$context - hit test expected values didn't match: $offset1 != $offset2");
}
return true;
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
final ListWheelParentData parentData = child.parentData! as ListWheelParentData;
final Matrix4? paintTransform = parentData.transform;
if (paintTransform != null) {
transform.multiply(paintTransform);
}
}
@override
Rect? describeApproximatePaintClip(RenderObject child) {
if (_shouldClipAtCurrentOffset()) {
return Offset.zero & size;
}
return null;
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
RenderBox? child = lastChild;
while (child != null) {
final ListWheelParentData childParentData = child.parentData! as ListWheelParentData;
final Matrix4? transform = childParentData.transform;
// Skip not painted children
if (transform != null) {
final bool isHit = result.addWithPaintTransform(
transform: transform,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(() {
final Matrix4? inverted = Matrix4.tryInvert(PointerEvent.removePerspectiveTransform(transform));
if (inverted == null) {
return _debugAssertValidHitTestOffsets('Null inverted transform', transformed, position);
}
return _debugAssertValidHitTestOffsets('MatrixUtils.transformPoint', transformed, MatrixUtils.transformPoint(inverted, position));
}());
return child!.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
}
child = childParentData.previousSibling;
}
return false;
}
@override
RevealedOffset getOffsetToReveal(
RenderObject target,
double alignment, {
Rect? rect,
Axis? axis, // Unused, only Axis.vertical supported by this viewport.
}) {
// `target` is only fully revealed when in the selected/center position. Therefore,
// this method always returns the offset that shows `target` in the center position,
// which is the same offset for all `alignment` values.
rect ??= target.paintBounds;
// `child` will be the last RenderObject before the viewport when walking up from `target`.
RenderObject child = target;
while (child.parent != this) {
child = child.parent!;
}
final ListWheelParentData parentData = child.parentData! as ListWheelParentData;
final double targetOffset = parentData.offset.dy; // the so-called "centerPosition"
final Matrix4 transform = target.getTransformTo(child);
final Rect bounds = MatrixUtils.transformRect(transform, rect);
final Rect targetRect = bounds.translate(0.0, (size.height - itemExtent) / 2);
return RevealedOffset(offset: targetOffset, rect: targetRect);
}
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
if (descendant != null) {
// Shows the descendant in the selected/center position.
final RevealedOffset revealedOffset = getOffsetToReveal(descendant, 0.5, rect: rect);
if (duration == Duration.zero) {
offset.jumpTo(revealedOffset.offset);
} else {
offset.animateTo(revealedOffset.offset, duration: duration, curve: curve);
}
rect = revealedOffset.rect;
}
super.showOnScreen(
rect: rect,
duration: duration,
curve: curve,
);
}
}
| flutter/packages/flutter/lib/src/rendering/list_wheel_viewport.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/list_wheel_viewport.dart",
"repo_id": "flutter",
"token_count": 14037
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:vector_math/vector_math_64.dart';
import 'object.dart';
import 'sliver.dart';
/// A sliver that places multiple sliver children in a linear array along the cross
/// axis.
///
/// Since the extent of the viewport in the cross axis direction is finite,
/// this extent will be divided up and allocated to the children slivers.
///
/// The algorithm for dividing up the cross axis extent is as follows.
/// Every widget has a [SliverPhysicalParentData.crossAxisFlex] value associated with them.
/// First, lay out all of the slivers with flex of 0 or null, in which case the slivers themselves will
/// figure out how much cross axis extent to take up. For example, [SliverConstrainedCrossAxis]
/// is an example of a widget which sets its own flex to 0. Then [RenderSliverCrossAxisGroup] will
/// divide up the remaining space to all the remaining children proportionally
/// to each child's flex factor. By default, children of [SliverCrossAxisGroup]
/// are setup to have a flex factor of 1, but a different flex factor can be
/// specified via the [SliverCrossAxisExpanded] widgets.
class RenderSliverCrossAxisGroup extends RenderSliver with ContainerRenderObjectMixin<RenderSliver, SliverPhysicalContainerParentData> {
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverPhysicalContainerParentData) {
child.parentData = SliverPhysicalContainerParentData();
(child.parentData! as SliverPhysicalParentData).crossAxisFlex = 1;
}
}
@override
double childMainAxisPosition(RenderSliver child) => 0.0;
@override
double childCrossAxisPosition(RenderSliver child) {
final Offset paintOffset = (child.parentData! as SliverPhysicalParentData).paintOffset;
return switch (constraints.axis) {
Axis.vertical => paintOffset.dx,
Axis.horizontal => paintOffset.dy,
};
}
@override
void performLayout() {
// Iterate through each sliver.
// Get the parent's dimensions.
final double crossAxisExtent = constraints.crossAxisExtent;
assert(crossAxisExtent.isFinite);
// First, layout each child with flex == 0 or null.
int totalFlex = 0;
double remainingExtent = crossAxisExtent;
RenderSliver? child = firstChild;
while (child != null) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
final int flex = childParentData.crossAxisFlex ?? 0;
if (flex == 0) {
// If flex is 0 or null, then the child sliver must provide their own crossAxisExtent.
assert(_assertOutOfExtent(remainingExtent));
child.layout(constraints.copyWith(crossAxisExtent: remainingExtent), parentUsesSize: true);
final double? childCrossAxisExtent = child.geometry!.crossAxisExtent;
assert(childCrossAxisExtent != null);
remainingExtent = math.max(0.0, remainingExtent - childCrossAxisExtent!);
} else {
totalFlex += flex;
}
child = childAfter(child);
}
final double extentPerFlexValue = remainingExtent / totalFlex;
child = firstChild;
// At this point, all slivers with constrained cross axis should already be laid out.
// Layout the rest and keep track of the child geometry with greatest scrollExtent.
geometry = SliverGeometry.zero;
while (child != null) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
final int flex = childParentData.crossAxisFlex ?? 0;
double childExtent;
if (flex != 0) {
childExtent = extentPerFlexValue * flex;
assert(_assertOutOfExtent(childExtent));
child.layout(constraints.copyWith(
crossAxisExtent: extentPerFlexValue * flex,
), parentUsesSize: true);
} else {
childExtent = child.geometry!.crossAxisExtent!;
}
final SliverGeometry childLayoutGeometry = child.geometry!;
if (geometry!.scrollExtent < childLayoutGeometry.scrollExtent) {
geometry = childLayoutGeometry;
}
child = childAfter(child);
}
// Go back and correct any slivers using a negative paint offset if it tries
// to paint outside the bounds of the sliver group.
child = firstChild;
double offset = 0.0;
while (child != null) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
final SliverGeometry childLayoutGeometry = child.geometry!;
final double remainingExtent = geometry!.scrollExtent - constraints.scrollOffset;
final double paintCorrection = childLayoutGeometry.paintExtent > remainingExtent
? childLayoutGeometry.paintExtent - remainingExtent
: 0.0;
final double childExtent = child.geometry!.crossAxisExtent ?? extentPerFlexValue * (childParentData.crossAxisFlex ?? 0);
// Set child parent data.
childParentData.paintOffset = switch (constraints.axis) {
Axis.vertical => Offset(offset, -paintCorrection),
Axis.horizontal => Offset(-paintCorrection, offset),
};
offset += childExtent;
child = childAfter(child);
}
}
@override
void paint(PaintingContext context, Offset offset) {
RenderSliver? child = firstChild;
while (child != null) {
if (child.geometry!.visible) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
context.paintChild(child, offset + childParentData.paintOffset);
}
child = childAfter(child);
}
}
@override
void applyPaintTransform(RenderSliver child, Matrix4 transform) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.applyPaintTransform(transform);
}
@override
bool hitTestChildren(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
RenderSliver? child = lastChild;
while (child != null) {
final bool isHit = result.addWithAxisOffset(
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
paintOffset: null,
mainAxisOffset: childMainAxisPosition(child),
crossAxisOffset: childCrossAxisPosition(child),
hitTest: child.hitTest,
);
if (isHit) {
return true;
}
child = childBefore(child);
}
return false;
}
}
bool _assertOutOfExtent(double extent) {
if (extent <= 0.0) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('SliverCrossAxisGroup ran out of extent before child could be laid out.'),
ErrorDescription(
'SliverCrossAxisGroup lays out any slivers with a constrained cross '
'axis before laying out those which expand. In this case, cross axis '
'extent was used up before the next sliver could be laid out.'
),
ErrorHint(
'Make sure that the total amount of extent allocated by constrained '
'child slivers does not exceed the cross axis extent that is available '
'for the SliverCrossAxisGroup.'
),
]);
}
return true;
}
/// A sliver that places multiple sliver children in a linear array along the
/// main axis.
///
/// The layout algorithm lays out slivers one by one. If the sliver is at the top
/// of the viewport or above the top, then we pass in a nonzero [SliverConstraints.scrollOffset]
/// to inform the sliver at what point along the main axis we should start layout.
/// For the slivers that come after it, we compute the amount of space taken up so
/// far to be used as the [SliverPhysicalParentData.paintOffset] and the
/// [SliverConstraints.remainingPaintExtent] to be passed in as a constraint.
///
/// Finally, this sliver will also ensure that all child slivers are painted within
/// the total scroll extent of the group by adjusting the child's
/// [SliverPhysicalParentData.paintOffset] as necessary. This can happen for
/// slivers such as [SliverPersistentHeader] which, when pinned, positions itself
/// at the top of the [Viewport] regardless of the scroll offset.
class RenderSliverMainAxisGroup extends RenderSliver with ContainerRenderObjectMixin<RenderSliver, SliverPhysicalContainerParentData> {
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverPhysicalContainerParentData) {
child.parentData = SliverPhysicalContainerParentData();
}
}
@override
double? childScrollOffset(RenderObject child) {
assert(child.parent == this);
final GrowthDirection growthDirection = constraints.growthDirection;
switch (growthDirection) {
case GrowthDirection.forward:
double childScrollOffset = 0.0;
RenderSliver? current = childBefore(child as RenderSliver);
while (current != null) {
childScrollOffset += current.geometry!.scrollExtent;
current = childBefore(current);
}
return childScrollOffset;
case GrowthDirection.reverse:
double childScrollOffset = 0.0;
RenderSliver? current = childAfter(child as RenderSliver);
while (current != null) {
childScrollOffset -= current.geometry!.scrollExtent;
current = childAfter(current);
}
return childScrollOffset;
}
}
@override
double childMainAxisPosition(RenderSliver child) {
final Offset paintOffset = (child.parentData! as SliverPhysicalParentData).paintOffset;
return switch (constraints.axis) {
Axis.horizontal => paintOffset.dx,
Axis.vertical => paintOffset.dy,
};
}
@override
double childCrossAxisPosition(RenderSliver child) => 0.0;
@override
void performLayout() {
double offset = 0;
double maxPaintExtent = 0;
RenderSliver? child = firstChild;
while (child != null) {
final double beforeOffsetPaintExtent = calculatePaintOffset(
constraints,
from: 0.0,
to: offset,
);
child.layout(
constraints.copyWith(
scrollOffset: math.max(0.0, constraints.scrollOffset - offset),
cacheOrigin: math.min(0.0, constraints.cacheOrigin + offset),
overlap: math.max(0.0, constraints.overlap - beforeOffsetPaintExtent),
remainingPaintExtent: constraints.remainingPaintExtent - beforeOffsetPaintExtent,
remainingCacheExtent: constraints.remainingCacheExtent - calculateCacheOffset(constraints, from: 0.0, to: offset),
precedingScrollExtent: offset + constraints.precedingScrollExtent,
),
parentUsesSize: true,
);
final SliverGeometry childLayoutGeometry = child.geometry!;
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.paintOffset = switch (constraints.axis) {
Axis.vertical => Offset(0.0, beforeOffsetPaintExtent),
Axis.horizontal => Offset(beforeOffsetPaintExtent, 0.0),
};
offset += childLayoutGeometry.scrollExtent;
maxPaintExtent += child.geometry!.maxPaintExtent;
child = childAfter(child);
assert(() {
if (child != null && maxPaintExtent.isInfinite) {
throw FlutterError(
'Unreachable sliver found, you may have a sliver behind '
'a sliver with infinite extent. '
);
}
return true;
}());
}
final double totalScrollExtent = offset;
offset = 0.0;
child = firstChild;
// Second pass to correct out of bound paintOffsets.
while (child != null) {
final double beforeOffsetPaintExtent = calculatePaintOffset(
constraints,
from: 0.0,
to: offset,
);
final SliverGeometry childLayoutGeometry = child.geometry!;
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
final double remainingExtent = totalScrollExtent - constraints.scrollOffset;
if (childLayoutGeometry.paintExtent > remainingExtent) {
final double paintCorrection = childLayoutGeometry.paintExtent - remainingExtent;
childParentData.paintOffset = switch (constraints.axis) {
Axis.vertical => Offset(0.0, beforeOffsetPaintExtent - paintCorrection),
Axis.horizontal => Offset(beforeOffsetPaintExtent - paintCorrection, 0.0),
};
}
offset += child.geometry!.scrollExtent;
child = childAfter(child);
}
final double paintExtent = calculatePaintOffset(
constraints,
from: math.min(constraints.scrollOffset, 0),
to: totalScrollExtent,
);
final double cacheExtent = calculateCacheOffset(
constraints,
from: math.min(constraints.scrollOffset, 0),
to: totalScrollExtent,
);
geometry = SliverGeometry(
scrollExtent: totalScrollExtent,
paintExtent: paintExtent,
cacheExtent: cacheExtent,
maxPaintExtent: maxPaintExtent,
hasVisualOverflow: totalScrollExtent > constraints.remainingPaintExtent || constraints.scrollOffset > 0.0,
);
}
@override
void paint(PaintingContext context, Offset offset) {
RenderSliver? child = lastChild;
while (child != null) {
if (child.geometry!.visible) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
context.paintChild(child, offset + childParentData.paintOffset);
}
child = childBefore(child);
}
}
@override
void applyPaintTransform(RenderSliver child, Matrix4 transform) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.applyPaintTransform(transform);
}
@override
bool hitTestChildren(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
RenderSliver? child = firstChild;
while (child != null) {
final bool isHit = result.addWithAxisOffset(
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
paintOffset: null,
mainAxisOffset: childMainAxisPosition(child),
crossAxisOffset: childCrossAxisPosition(child),
hitTest: child.hitTest,
);
if (isHit) {
return true;
}
child = childAfter(child);
}
return false;
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
RenderSliver? child = firstChild;
while (child != null) {
if (child.geometry!.visible) {
visitor(child);
}
child = childAfter(child);
}
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_group.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_group.dart",
"repo_id": "flutter",
"token_count": 5161
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
/// A task priority, as passed to [SchedulerBinding.scheduleTask].
@immutable
class Priority {
const Priority._(this._value);
/// Integer that describes this Priority value.
int get value => _value;
final int _value;
/// A task to run after all other tasks, when no animations are running.
static const Priority idle = Priority._(0);
/// A task to run even when animations are running.
static const Priority animation = Priority._(100000);
/// A task to run even when the user is interacting with the device.
static const Priority touch = Priority._(200000);
/// Maximum offset by which to clamp relative priorities.
///
/// It is still possible to have priorities that are offset by more
/// than this amount by repeatedly taking relative offsets, but that
/// is generally discouraged.
static const int kMaxOffset = 10000;
/// Returns a priority relative to this priority.
///
/// A positive [offset] indicates a higher priority.
///
/// The parameter [offset] is clamped to ±[kMaxOffset].
Priority operator +(int offset) {
if (offset.abs() > kMaxOffset) {
// Clamp the input offset.
offset = kMaxOffset * offset.sign;
}
return Priority._(_value + offset);
}
/// Returns a priority relative to this priority.
///
/// A positive offset indicates a lower priority.
///
/// The parameter [offset] is clamped to ±[kMaxOffset].
Priority operator -(int offset) => this + (-offset);
}
| flutter/packages/flutter/lib/src/scheduler/priority.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/scheduler/priority.dart",
"repo_id": "flutter",
"token_count": 453
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'system_channels.dart';
/// Data stored on the system clipboard.
///
/// The system clipboard can contain data of various media types. This data
/// structure currently supports only plain text data, in the [text] property.
@immutable
class ClipboardData {
/// Creates data for the system clipboard.
const ClipboardData({ required String this.text });
/// Plain text variant of this clipboard data.
// This is nullable as other clipboard data variants, like images, may be
// added in the future. Currently, plain text is the only supported variant
// and this is guaranteed to be non-null.
final String? text;
}
/// Utility methods for interacting with the system's clipboard.
abstract final class Clipboard {
// Constants for common [getData] [format] types.
/// Plain text data format string.
///
/// Used with [getData].
static const String kTextPlain = 'text/plain';
/// Stores the given clipboard data on the clipboard.
static Future<void> setData(ClipboardData data) async {
await SystemChannels.platform.invokeMethod<void>(
'Clipboard.setData',
<String, dynamic>{
'text': data.text,
},
);
}
/// Retrieves data from the clipboard that matches the given format.
///
/// The `format` argument specifies the media type, such as `text/plain`, of
/// the data to obtain.
///
/// Returns a future which completes to null if the data could not be
/// obtained, and to a [ClipboardData] object if it could.
static Future<ClipboardData?> getData(String format) async {
final Map<String, dynamic>? result = await SystemChannels.platform.invokeMethod(
'Clipboard.getData',
format,
);
if (result == null) {
return null;
}
return ClipboardData(text: result['text'] as String);
}
/// Returns a future that resolves to true iff the clipboard contains string
/// data.
///
/// See also:
/// * [The iOS hasStrings method](https://developer.apple.com/documentation/uikit/uipasteboard/1829416-hasstrings?language=objc).
static Future<bool> hasStrings() async {
final Map<String, dynamic>? result = await SystemChannels.platform.invokeMethod(
'Clipboard.hasStrings',
Clipboard.kTextPlain,
);
if (result == null) {
return false;
}
return result['value'] as bool;
}
}
| flutter/packages/flutter/lib/src/services/clipboard.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/clipboard.dart",
"repo_id": "flutter",
"token_count": 781
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'message_codec.dart';
import 'system_channels.dart';
export 'dart:ui' show Offset, Size, TextDirection, VoidCallback;
export 'package:flutter/gestures.dart' show PointerEvent;
export 'message_codec.dart' show MessageCodec;
/// Converts a given point from the global coordinate system in logical pixels
/// to the local coordinate system for a box.
///
/// Used by [AndroidViewController.pointTransformer].
typedef PointTransformer = Offset Function(Offset position);
/// The [PlatformViewsRegistry] responsible for generating unique identifiers for platform views.
final PlatformViewsRegistry platformViewsRegistry = PlatformViewsRegistry._instance();
/// A registry responsible for generating unique identifier for platform views.
///
/// A Flutter application has a single [PlatformViewsRegistry] which can be accesses
/// through the [platformViewsRegistry] getter.
class PlatformViewsRegistry {
PlatformViewsRegistry._instance();
// Always non-negative. The id value -1 is used in the accessibility bridge
// to indicate the absence of a platform view.
int _nextPlatformViewId = 0;
/// Allocates a unique identifier for a platform view.
///
/// A platform view identifier can refer to a platform view that was never created,
/// a platform view that was disposed, or a platform view that is alive.
///
/// Typically a platform view identifier is passed to a platform view widget
/// which creates the platform view and manages its lifecycle.
int getNextPlatformViewId() {
// On the Android side, the interface exposed to users uses 32-bit integers.
// See https://github.com/flutter/engine/pull/39476 for more details.
// We can safely assume that a Flutter application will not require more
// than MAX_INT32 platform views during its lifetime.
const int MAX_INT32 = 0x7FFFFFFF;
assert(_nextPlatformViewId <= MAX_INT32);
return _nextPlatformViewId++;
}
}
/// Callback signature for when a platform view was created.
///
/// The `id` parameter is the platform view's unique identifier.
typedef PlatformViewCreatedCallback = void Function(int id);
/// Provides access to the platform views service.
///
/// This service allows creating and controlling platform-specific views.
class PlatformViewsService {
PlatformViewsService._() {
SystemChannels.platform_views.setMethodCallHandler(_onMethodCall);
}
static final PlatformViewsService _instance = PlatformViewsService._();
Future<void> _onMethodCall(MethodCall call) {
switch (call.method) {
case 'viewFocused':
final int id = call.arguments as int;
if (_focusCallbacks.containsKey(id)) {
_focusCallbacks[id]!();
}
default:
throw UnimplementedError("${call.method} was invoked but isn't implemented by PlatformViewsService");
}
return Future<void>.value();
}
/// Maps platform view IDs to focus callbacks.
///
/// The callbacks are invoked when the platform view asks to be focused.
final Map<int, VoidCallback> _focusCallbacks = <int, VoidCallback>{};
/// {@template flutter.services.PlatformViewsService.initAndroidView}
/// Creates a controller for a new Android view.
///
/// The `id` argument is an unused unique identifier generated with
/// [platformViewsRegistry].
///
/// The `viewType` argument is the identifier of the Android view type to be
/// created, a factory for this view type must have been registered on the
/// platform side. Platform view factories are typically registered by plugin
/// code. Plugins can register a platform view factory with
/// [PlatformViewRegistry#registerViewFactory](/javadoc/io/flutter/plugin/platform/PlatformViewRegistry.html#registerViewFactory-java.lang.String-io.flutter.plugin.platform.PlatformViewFactory-).
///
/// The `creationParams` argument will be passed as the args argument of
/// [PlatformViewFactory#create](/javadoc/io/flutter/plugin/platform/PlatformViewFactory.html#create-android.content.Context-int-java.lang.Object-)
///
/// The `creationParamsCodec` argument is the codec used to encode
/// `creationParams` before sending it to the platform side. It should match
/// the codec passed to the constructor of
/// [PlatformViewFactory](/javadoc/io/flutter/plugin/platform/PlatformViewFactory.html#PlatformViewFactory-io.flutter.plugin.common.MessageCodec-).
/// This is typically one of: [StandardMessageCodec], [JSONMessageCodec],
/// [StringCodec], or [BinaryCodec].
///
/// The `onFocus` argument is a callback that will be invoked when the Android
/// View asks to get the input focus.
///
/// The Android view will only be created after
/// [AndroidViewController.setSize] is called for the first time.
///
/// If `creationParams` is non null then `creationParamsCodec` must not be
/// null.
/// {@endtemplate}
///
/// This attempts to use the newest and most efficient platform view
/// implementation when possible. In cases where that is not supported, it
/// falls back to using Virtual Display.
static AndroidViewController initAndroidView({
required int id,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
VoidCallback? onFocus,
}) {
assert(creationParams == null || creationParamsCodec != null);
final TextureAndroidViewController controller = TextureAndroidViewController._(
viewId: id,
viewType: viewType,
layoutDirection: layoutDirection,
creationParams: creationParams,
creationParamsCodec: creationParamsCodec,
);
_instance._focusCallbacks[id] = onFocus ?? () {};
return controller;
}
/// {@macro flutter.services.PlatformViewsService.initAndroidView}
///
/// This attempts to use the newest and most efficient platform view
/// implementation when possible. In cases where that is not supported, it
/// falls back to using Hybrid Composition, which is the mode used by
/// [initExpensiveAndroidView].
static SurfaceAndroidViewController initSurfaceAndroidView({
required int id,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
VoidCallback? onFocus,
}) {
assert(creationParams == null || creationParamsCodec != null);
final SurfaceAndroidViewController controller = SurfaceAndroidViewController._(
viewId: id,
viewType: viewType,
layoutDirection: layoutDirection,
creationParams: creationParams,
creationParamsCodec: creationParamsCodec,
);
_instance._focusCallbacks[id] = onFocus ?? () {};
return controller;
}
/// {@macro flutter.services.PlatformViewsService.initAndroidView}
///
/// When this factory is used, the Android view and Flutter widgets are
/// composed at the Android view hierarchy level.
///
/// Using this method has a performance cost on devices running Android 9 or
/// earlier, or on underpowered devices. In most situations, you should use
/// [initAndroidView] or [initSurfaceAndroidView] instead.
static ExpensiveAndroidViewController initExpensiveAndroidView({
required int id,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
VoidCallback? onFocus,
}) {
final ExpensiveAndroidViewController controller = ExpensiveAndroidViewController._(
viewId: id,
viewType: viewType,
layoutDirection: layoutDirection,
creationParams: creationParams,
creationParamsCodec: creationParamsCodec,
);
_instance._focusCallbacks[id] = onFocus ?? () {};
return controller;
}
/// Factory method to create a `UiKitView`.
///
/// The `id` parameter is an unused unique identifier generated with
/// [platformViewsRegistry].
///
/// The `viewType` parameter is the identifier of the iOS view type to be
/// created, a factory for this view type must have been registered on the
/// platform side. Platform view factories are typically registered by plugin
/// code.
///
/// The `onFocus` parameter is a callback that will be invoked when the UIKit
/// view asks to get the input focus. If `creationParams` is non null then
/// `creationParamsCodec` must not be null.
///
/// See: https://docs.flutter.dev/platform-integration/ios/platform-views
static Future<UiKitViewController> initUiKitView({
required int id,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
VoidCallback? onFocus,
}) async {
assert(creationParams == null || creationParamsCodec != null);
// TODO(amirh): pass layoutDirection once the system channel supports it.
// https://github.com/flutter/flutter/issues/133682
final Map<String, dynamic> args = <String, dynamic>{
'id': id,
'viewType': viewType,
};
if (creationParams != null) {
final ByteData paramsByteData = creationParamsCodec!.encodeMessage(creationParams)!;
args['params'] = Uint8List.view(
paramsByteData.buffer,
0,
paramsByteData.lengthInBytes,
);
}
await SystemChannels.platform_views.invokeMethod<void>('create', args);
if (onFocus != null) {
_instance._focusCallbacks[id] = onFocus;
}
return UiKitViewController._(id, layoutDirection);
}
// TODO(cbracken): Write and link website docs. https://github.com/flutter/website/issues/9424.
//
/// Factory method to create an `AppKitView`.
///
/// The `id` parameter is an unused unique identifier generated with
/// [platformViewsRegistry].
///
/// The `viewType` parameter is the identifier of the iOS view type to be
/// created, a factory for this view type must have been registered on the
/// platform side. Platform view factories are typically registered by plugin
/// code.
///
/// The `onFocus` parameter is a callback that will be invoked when the UIKit
/// view asks to get the input focus. If `creationParams` is non null then
/// `creationParamsCodec` must not be null.
static Future<AppKitViewController> initAppKitView({
required int id,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
VoidCallback? onFocus,
}) async {
assert(creationParams == null || creationParamsCodec != null);
// TODO(amirh): pass layoutDirection once the system channel supports it.
// https://github.com/flutter/flutter/issues/133682
final Map<String, dynamic> args = <String, dynamic>{
'id': id,
'viewType': viewType,
};
if (creationParams != null) {
final ByteData paramsByteData = creationParamsCodec!.encodeMessage(creationParams)!;
args['params'] = Uint8List.view(
paramsByteData.buffer,
0,
paramsByteData.lengthInBytes,
);
}
await SystemChannels.platform_views.invokeMethod<void>('create', args);
if (onFocus != null) {
_instance._focusCallbacks[id] = onFocus;
}
return AppKitViewController._(id, layoutDirection);
}
}
/// Properties of an Android pointer.
///
/// A Dart version of Android's [MotionEvent.PointerProperties](https://developer.android.com/reference/android/view/MotionEvent.PointerProperties).
class AndroidPointerProperties {
/// Creates an [AndroidPointerProperties] object.
const AndroidPointerProperties({
required this.id,
required this.toolType,
});
/// See Android's [MotionEvent.PointerProperties#id](https://developer.android.com/reference/android/view/MotionEvent.PointerProperties.html#id).
final int id;
/// The type of tool used to make contact such as a finger or stylus, if known.
/// See Android's [MotionEvent.PointerProperties#toolType](https://developer.android.com/reference/android/view/MotionEvent.PointerProperties.html#toolType).
final int toolType;
/// Value for `toolType` when the tool type is unknown.
static const int kToolTypeUnknown = 0;
/// Value for `toolType` when the tool type is a finger.
static const int kToolTypeFinger = 1;
/// Value for `toolType` when the tool type is a stylus.
static const int kToolTypeStylus = 2;
/// Value for `toolType` when the tool type is a mouse.
static const int kToolTypeMouse = 3;
/// Value for `toolType` when the tool type is an eraser.
static const int kToolTypeEraser = 4;
List<int> _asList() => <int>[id, toolType];
@override
String toString() {
return '${objectRuntimeType(this, 'AndroidPointerProperties')}(id: $id, toolType: $toolType)';
}
}
/// Position information for an Android pointer.
///
/// A Dart version of Android's [MotionEvent.PointerCoords](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords).
class AndroidPointerCoords {
/// Creates an AndroidPointerCoords.
const AndroidPointerCoords({
required this.orientation,
required this.pressure,
required this.size,
required this.toolMajor,
required this.toolMinor,
required this.touchMajor,
required this.touchMinor,
required this.x,
required this.y,
});
/// The orientation of the touch area and tool area in radians clockwise from vertical.
///
/// See Android's [MotionEvent.PointerCoords#orientation](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#orientation).
final double orientation;
/// A normalized value that describes the pressure applied to the device by a finger or other tool.
///
/// See Android's [MotionEvent.PointerCoords#pressure](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#pressure).
final double pressure;
/// A normalized value that describes the approximate size of the pointer touch area in relation to the maximum detectable size of the device.
///
/// See Android's [MotionEvent.PointerCoords#size](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#size).
final double size;
/// See Android's [MotionEvent.PointerCoords#toolMajor](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#toolMajor).
final double toolMajor;
/// See Android's [MotionEvent.PointerCoords#toolMinor](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#toolMinor).
final double toolMinor;
/// See Android's [MotionEvent.PointerCoords#touchMajor](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#touchMajor).
final double touchMajor;
/// See Android's [MotionEvent.PointerCoords#touchMinor](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#touchMinor).
final double touchMinor;
/// The X component of the pointer movement.
///
/// See Android's [MotionEvent.PointerCoords#x](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#x).
final double x;
/// The Y component of the pointer movement.
///
/// See Android's [MotionEvent.PointerCoords#y](https://developer.android.com/reference/android/view/MotionEvent.PointerCoords.html#y).
final double y;
List<double> _asList() {
return <double>[
orientation,
pressure,
size,
toolMajor,
toolMinor,
touchMajor,
touchMinor,
x,
y,
];
}
@override
String toString() {
return '${objectRuntimeType(this, 'AndroidPointerCoords')}(orientation: $orientation, pressure: $pressure, size: $size, toolMajor: $toolMajor, toolMinor: $toolMinor, touchMajor: $touchMajor, touchMinor: $touchMinor, x: $x, y: $y)';
}
}
/// A Dart version of Android's [MotionEvent](https://developer.android.com/reference/android/view/MotionEvent).
///
/// This is used by [AndroidViewController] to describe pointer events that are forwarded to a platform view
/// when Flutter receives an event that it determines is to be handled by that platform view rather than by
/// another Flutter widget.
///
/// See also:
///
/// * [AndroidViewController.sendMotionEvent], which can be used to send an [AndroidMotionEvent] explicitly.
class AndroidMotionEvent {
/// Creates an AndroidMotionEvent.
AndroidMotionEvent({
required this.downTime,
required this.eventTime,
required this.action,
required this.pointerCount,
required this.pointerProperties,
required this.pointerCoords,
required this.metaState,
required this.buttonState,
required this.xPrecision,
required this.yPrecision,
required this.deviceId,
required this.edgeFlags,
required this.source,
required this.flags,
required this.motionEventId,
}) : assert(pointerProperties.length == pointerCount),
assert(pointerCoords.length == pointerCount);
/// The time (in ms) when the user originally pressed down to start a stream of position events,
/// relative to an arbitrary timeline.
///
/// See Android's [MotionEvent#getDownTime](https://developer.android.com/reference/android/view/MotionEvent.html#getDownTime()).
final int downTime;
/// The time this event occurred, relative to an arbitrary timeline.
///
/// See Android's [MotionEvent#getEventTime](https://developer.android.com/reference/android/view/MotionEvent.html#getEventTime()).
final int eventTime;
/// A value representing the kind of action being performed.
///
/// See Android's [MotionEvent#getAction](https://developer.android.com/reference/android/view/MotionEvent.html#getAction()).
final int action;
/// The number of pointers that are part of this event.
/// This must be equivalent to the length of `pointerProperties` and `pointerCoords`.
///
/// See Android's [MotionEvent#getPointerCount](https://developer.android.com/reference/android/view/MotionEvent.html#getPointerCount()).
final int pointerCount;
/// List of [AndroidPointerProperties] for each pointer that is part of this event.
final List<AndroidPointerProperties> pointerProperties;
/// List of [AndroidPointerCoords] for each pointer that is part of this event.
final List<AndroidPointerCoords> pointerCoords;
/// The state of any meta / modifier keys that were in effect when the event was generated.
///
/// See Android's [MotionEvent#getMetaState](https://developer.android.com/reference/android/view/MotionEvent.html#getMetaState()).
final int metaState;
/// The state of all buttons that are pressed such as a mouse or stylus button.
///
/// See Android's [MotionEvent#getButtonState](https://developer.android.com/reference/android/view/MotionEvent.html#getButtonState()).
final int buttonState;
/// The precision of the X coordinates being reported, in physical pixels.
///
/// See Android's [MotionEvent#getXPrecision](https://developer.android.com/reference/android/view/MotionEvent.html#getXPrecision()).
final double xPrecision;
/// The precision of the Y coordinates being reported, in physical pixels.
///
/// See Android's [MotionEvent#getYPrecision](https://developer.android.com/reference/android/view/MotionEvent.html#getYPrecision()).
final double yPrecision;
/// See Android's [MotionEvent#getDeviceId](https://developer.android.com/reference/android/view/MotionEvent.html#getDeviceId()).
final int deviceId;
/// A bit field indicating which edges, if any, were touched by this MotionEvent.
///
/// See Android's [MotionEvent#getEdgeFlags](https://developer.android.com/reference/android/view/MotionEvent.html#getEdgeFlags()).
final int edgeFlags;
/// The source of this event (e.g a touchpad or stylus).
///
/// See Android's [MotionEvent#getSource](https://developer.android.com/reference/android/view/MotionEvent.html#getSource()).
final int source;
/// See Android's [MotionEvent#getFlags](https://developer.android.com/reference/android/view/MotionEvent.html#getFlags()).
final int flags;
/// Used to identify this [MotionEvent](https://developer.android.com/reference/android/view/MotionEvent.html) uniquely in the Flutter Engine.
final int motionEventId;
List<dynamic> _asList(int viewId) {
return <dynamic>[
viewId,
downTime,
eventTime,
action,
pointerCount,
pointerProperties.map<List<int>>((AndroidPointerProperties p) => p._asList()).toList(),
pointerCoords.map<List<double>>((AndroidPointerCoords p) => p._asList()).toList(),
metaState,
buttonState,
xPrecision,
yPrecision,
deviceId,
edgeFlags,
source,
flags,
motionEventId,
];
}
@override
String toString() {
return 'AndroidPointerEvent(downTime: $downTime, eventTime: $eventTime, action: $action, pointerCount: $pointerCount, pointerProperties: $pointerProperties, pointerCoords: $pointerCoords, metaState: $metaState, buttonState: $buttonState, xPrecision: $xPrecision, yPrecision: $yPrecision, deviceId: $deviceId, edgeFlags: $edgeFlags, source: $source, flags: $flags, motionEventId: $motionEventId)';
}
}
enum _AndroidViewState {
waitingForSize,
creating,
created,
disposed,
}
// Helper for converting PointerEvents into AndroidMotionEvents.
class _AndroidMotionEventConverter {
_AndroidMotionEventConverter();
final Map<int, AndroidPointerCoords> pointerPositions =
<int, AndroidPointerCoords>{};
final Map<int, AndroidPointerProperties> pointerProperties =
<int, AndroidPointerProperties>{};
final Set<int> usedAndroidPointerIds = <int>{};
late PointTransformer pointTransformer;
int? downTimeMillis;
void handlePointerDownEvent(PointerDownEvent event) {
if (pointerProperties.isEmpty) {
downTimeMillis = event.timeStamp.inMilliseconds;
}
int androidPointerId = 0;
while (usedAndroidPointerIds.contains(androidPointerId)) {
androidPointerId++;
}
usedAndroidPointerIds.add(androidPointerId);
pointerProperties[event.pointer] = propertiesFor(event, androidPointerId);
}
void updatePointerPositions(PointerEvent event) {
final Offset position = pointTransformer(event.position);
pointerPositions[event.pointer] = AndroidPointerCoords(
orientation: event.orientation,
pressure: event.pressure,
size: event.size,
toolMajor: event.radiusMajor,
toolMinor: event.radiusMinor,
touchMajor: event.radiusMajor,
touchMinor: event.radiusMinor,
x: position.dx,
y: position.dy,
);
}
void _remove(int pointer) {
pointerPositions.remove(pointer);
usedAndroidPointerIds.remove(pointerProperties[pointer]!.id);
pointerProperties.remove(pointer);
if (pointerProperties.isEmpty) {
downTimeMillis = null;
}
}
void handlePointerUpEvent(PointerUpEvent event) {
_remove(event.pointer);
}
void handlePointerCancelEvent(PointerCancelEvent event) {
// The pointer cancel event is handled like pointer up. Normally,
// the difference is that pointer cancel doesn't perform any action,
// but in this case neither up or cancel perform any action.
_remove(event.pointer);
}
AndroidMotionEvent? toAndroidMotionEvent(PointerEvent event) {
final List<int> pointers = pointerPositions.keys.toList();
final int pointerIdx = pointers.indexOf(event.pointer);
final int numPointers = pointers.length;
// This value must match the value in engine's FlutterView.java.
// This flag indicates whether the original Android pointer events were batched together.
const int kPointerDataFlagBatched = 1;
// Android MotionEvent objects can batch information on multiple pointers.
// Flutter breaks these such batched events into multiple PointerEvent objects.
// When there are multiple active pointers we accumulate the information for all pointers
// as we get PointerEvents, and only send it to the embedded Android view when
// we see the last pointer. This way we achieve the same batching as Android.
if (event.platformData == kPointerDataFlagBatched ||
(isSinglePointerAction(event) && pointerIdx < numPointers - 1)) {
return null;
}
final int action;
if (event is PointerDownEvent) {
action = numPointers == 1
? AndroidViewController.kActionDown
: AndroidViewController.pointerAction(pointerIdx, AndroidViewController.kActionPointerDown);
} else if (event is PointerUpEvent) {
action = numPointers == 1
? AndroidViewController.kActionUp
: AndroidViewController.pointerAction(pointerIdx, AndroidViewController.kActionPointerUp);
} else if (event is PointerMoveEvent) {
action = AndroidViewController.kActionMove;
} else if (event is PointerCancelEvent) {
action = AndroidViewController.kActionCancel;
} else {
return null;
}
return AndroidMotionEvent(
downTime: downTimeMillis!,
eventTime: event.timeStamp.inMilliseconds,
action: action,
pointerCount: pointerPositions.length,
pointerProperties: pointers
.map<AndroidPointerProperties>((int i) => pointerProperties[i]!)
.toList(),
pointerCoords: pointers
.map<AndroidPointerCoords>((int i) => pointerPositions[i]!)
.toList(),
metaState: 0,
buttonState: 0,
xPrecision: 1.0,
yPrecision: 1.0,
deviceId: 0,
edgeFlags: 0,
source: _AndroidMotionEventConverter.sourceFor(event),
flags: 0,
motionEventId: event.embedderId,
);
}
static int sourceFor(PointerEvent event) {
return switch (event.kind) {
PointerDeviceKind.touch => AndroidViewController.kInputDeviceSourceTouchScreen,
PointerDeviceKind.trackpad => AndroidViewController.kInputDeviceSourceTouchPad,
PointerDeviceKind.mouse => AndroidViewController.kInputDeviceSourceMouse,
PointerDeviceKind.stylus => AndroidViewController.kInputDeviceSourceStylus,
PointerDeviceKind.invertedStylus => AndroidViewController.kInputDeviceSourceStylus,
PointerDeviceKind.unknown => AndroidViewController.kInputDeviceSourceUnknown,
};
}
AndroidPointerProperties propertiesFor(PointerEvent event, int pointerId) {
return AndroidPointerProperties(id: pointerId, toolType: switch (event.kind) {
PointerDeviceKind.touch => AndroidPointerProperties.kToolTypeFinger,
PointerDeviceKind.trackpad => AndroidPointerProperties.kToolTypeFinger,
PointerDeviceKind.mouse => AndroidPointerProperties.kToolTypeMouse,
PointerDeviceKind.stylus => AndroidPointerProperties.kToolTypeStylus,
PointerDeviceKind.invertedStylus => AndroidPointerProperties.kToolTypeEraser,
PointerDeviceKind.unknown => AndroidPointerProperties.kToolTypeUnknown,
});
}
bool isSinglePointerAction(PointerEvent event) =>
event is! PointerDownEvent && event is! PointerUpEvent;
}
class _CreationParams {
const _CreationParams(this.data, this.codec);
final dynamic data;
final MessageCodec<dynamic> codec;
}
/// Controls an Android view that is composed using a GL texture.
///
/// Typically created with [PlatformViewsService.initAndroidView].
// TODO(bparrishMines): Remove abstract methods that are not required by all subclasses.
abstract class AndroidViewController extends PlatformViewController {
AndroidViewController._({
required this.viewId,
required String viewType,
required TextDirection layoutDirection,
dynamic creationParams,
MessageCodec<dynamic>? creationParamsCodec,
}) : assert(creationParams == null || creationParamsCodec != null),
_viewType = viewType,
_layoutDirection = layoutDirection,
_creationParams = creationParams == null ? null : _CreationParams(creationParams, creationParamsCodec!);
/// Action code for when a primary pointer touched the screen.
///
/// Android's [MotionEvent.ACTION_DOWN](https://developer.android.com/reference/android/view/MotionEvent#ACTION_DOWN)
static const int kActionDown = 0;
/// Action code for when a primary pointer stopped touching the screen.
///
/// Android's [MotionEvent.ACTION_UP](https://developer.android.com/reference/android/view/MotionEvent#ACTION_UP)
static const int kActionUp = 1;
/// Action code for when the event only includes information about pointer movement.
///
/// Android's [MotionEvent.ACTION_MOVE](https://developer.android.com/reference/android/view/MotionEvent#ACTION_MOVE)
static const int kActionMove = 2;
/// Action code for when a motion event has been canceled.
///
/// Android's [MotionEvent.ACTION_CANCEL](https://developer.android.com/reference/android/view/MotionEvent#ACTION_CANCEL)
static const int kActionCancel = 3;
/// Action code for when a secondary pointer touched the screen.
///
/// Android's [MotionEvent.ACTION_POINTER_DOWN](https://developer.android.com/reference/android/view/MotionEvent#ACTION_POINTER_DOWN)
static const int kActionPointerDown = 5;
/// Action code for when a secondary pointer stopped touching the screen.
///
/// Android's [MotionEvent.ACTION_POINTER_UP](https://developer.android.com/reference/android/view/MotionEvent#ACTION_POINTER_UP)
static const int kActionPointerUp = 6;
/// Android's [View.LAYOUT_DIRECTION_LTR](https://developer.android.com/reference/android/view/View.html#LAYOUT_DIRECTION_LTR) value.
static const int kAndroidLayoutDirectionLtr = 0;
/// Android's [View.LAYOUT_DIRECTION_RTL](https://developer.android.com/reference/android/view/View.html#LAYOUT_DIRECTION_RTL) value.
static const int kAndroidLayoutDirectionRtl = 1;
/// Android's [InputDevice.SOURCE_UNKNOWN](https://developer.android.com/reference/android/view/InputDevice#SOURCE_UNKNOWN)
static const int kInputDeviceSourceUnknown = 0;
/// Android's [InputDevice.SOURCE_TOUCHSCREEN](https://developer.android.com/reference/android/view/InputDevice#SOURCE_TOUCHSCREEN)
static const int kInputDeviceSourceTouchScreen = 4098;
/// Android's [InputDevice.SOURCE_MOUSE](https://developer.android.com/reference/android/view/InputDevice#SOURCE_MOUSE)
static const int kInputDeviceSourceMouse = 8194;
/// Android's [InputDevice.SOURCE_STYLUS](https://developer.android.com/reference/android/view/InputDevice#SOURCE_STYLUS)
static const int kInputDeviceSourceStylus = 16386;
/// Android's [InputDevice.SOURCE_TOUCHPAD](https://developer.android.com/reference/android/view/InputDevice#SOURCE_TOUCHPAD)
static const int kInputDeviceSourceTouchPad = 1048584;
/// The unique identifier of the Android view controlled by this controller.
@override
final int viewId;
final String _viewType;
// Helps convert PointerEvents to AndroidMotionEvents.
final _AndroidMotionEventConverter _motionEventConverter =
_AndroidMotionEventConverter();
TextDirection _layoutDirection;
_AndroidViewState _state = _AndroidViewState.waitingForSize;
final _CreationParams? _creationParams;
final List<PlatformViewCreatedCallback> _platformViewCreatedCallbacks =
<PlatformViewCreatedCallback>[];
static int _getAndroidDirection(TextDirection direction) {
return switch (direction) {
TextDirection.ltr => kAndroidLayoutDirectionLtr,
TextDirection.rtl => kAndroidLayoutDirectionRtl,
};
}
/// Creates a masked Android MotionEvent action value for an indexed pointer.
static int pointerAction(int pointerId, int action) {
return ((pointerId << 8) & 0xff00) | (action & 0xff);
}
/// Sends the message to dispose the platform view.
Future<void> _sendDisposeMessage();
/// True if [_sendCreateMessage] can only be called with a non-null size.
bool get _createRequiresSize;
/// Sends the message to create the platform view with an initial [size].
///
/// If [_createRequiresSize] is true, `size` is non-nullable, and the call
/// should instead be deferred until the size is available.
Future<void> _sendCreateMessage({required covariant Size? size, Offset? position});
/// Sends the message to resize the platform view to [size].
Future<Size> _sendResizeMessage(Size size);
@override
bool get awaitingCreation => _state == _AndroidViewState.waitingForSize;
@override
Future<void> create({Size? size, Offset? position}) async {
assert(_state != _AndroidViewState.disposed, 'trying to create a disposed Android view');
assert(_state == _AndroidViewState.waitingForSize, 'Android view is already sized. View id: $viewId');
if (_createRequiresSize && size == null) {
// Wait for a setSize call.
return;
}
_state = _AndroidViewState.creating;
await _sendCreateMessage(size: size, position: position);
_state = _AndroidViewState.created;
for (final PlatformViewCreatedCallback callback in _platformViewCreatedCallbacks) {
callback(viewId);
}
}
/// Sizes the Android View.
///
/// [size] is the view's new size in logical pixel. It must be greater than
/// zero.
///
/// The first time a size is set triggers the creation of the Android view.
///
/// Returns the buffer size in logical pixel that backs the texture where the platform
/// view pixels are written to.
///
/// The buffer size may or may not be the same as [size].
///
/// As a result, consumers are expected to clip the texture using [size], while using
/// the return value to size the texture.
Future<Size> setSize(Size size) async {
assert(_state != _AndroidViewState.disposed, 'Android view is disposed. View id: $viewId');
if (_state == _AndroidViewState.waitingForSize) {
// Either `create` hasn't been called, or it couldn't run due to missing
// size information, so create the view now.
await create(size: size);
return size;
} else {
return _sendResizeMessage(size);
}
}
/// Sets the offset of the platform view.
///
/// [off] is the view's new offset in logical pixel.
///
/// On Android, this allows the Android native view to draw the a11y highlights in the same
/// location on the screen as the platform view widget in the Flutter framework.
Future<void> setOffset(Offset off);
/// Returns the texture entry id that the Android view is rendering into.
///
/// Returns null if the Android view has not been successfully created, if it has been
/// disposed, or if the implementation does not use textures.
int? get textureId;
/// True if the view requires native view composition rather than using a
/// texture to render.
///
/// This value may change during [create], but will not change after that
/// call's future has completed.
bool get requiresViewComposition => false;
/// Sends an Android [MotionEvent](https://developer.android.com/reference/android/view/MotionEvent)
/// to the view.
///
/// The Android MotionEvent object is created with [MotionEvent.obtain](https://developer.android.com/reference/android/view/MotionEvent.html#obtain(long,%20long,%20int,%20float,%20float,%20float,%20float,%20int,%20float,%20float,%20int,%20int)).
/// See documentation of [MotionEvent.obtain](https://developer.android.com/reference/android/view/MotionEvent.html#obtain(long,%20long,%20int,%20float,%20float,%20float,%20float,%20int,%20float,%20float,%20int,%20int))
/// for description of the parameters.
///
/// See [AndroidViewController.dispatchPointerEvent] for sending a
/// [PointerEvent].
Future<void> sendMotionEvent(AndroidMotionEvent event) async {
await SystemChannels.platform_views.invokeMethod<dynamic>(
'touch',
event._asList(viewId),
);
}
/// Converts a given point from the global coordinate system in logical pixels
/// to the local coordinate system for this box.
///
/// This is required to convert a [PointerEvent] to an [AndroidMotionEvent].
/// It is typically provided by using [RenderBox.globalToLocal].
PointTransformer get pointTransformer => _motionEventConverter.pointTransformer;
set pointTransformer(PointTransformer transformer) {
_motionEventConverter.pointTransformer = transformer;
}
/// Whether the platform view has already been created.
bool get isCreated => _state == _AndroidViewState.created;
/// Adds a callback that will get invoke after the platform view has been
/// created.
void addOnPlatformViewCreatedListener(PlatformViewCreatedCallback listener) {
assert(_state != _AndroidViewState.disposed);
_platformViewCreatedCallbacks.add(listener);
}
/// Removes a callback added with [addOnPlatformViewCreatedListener].
void removeOnPlatformViewCreatedListener(PlatformViewCreatedCallback listener) {
assert(_state != _AndroidViewState.disposed);
_platformViewCreatedCallbacks.remove(listener);
}
/// The created callbacks that are invoked after the platform view has been
/// created.
@visibleForTesting
List<PlatformViewCreatedCallback> get createdCallbacks => _platformViewCreatedCallbacks;
/// Sets the layout direction for the Android view.
Future<void> setLayoutDirection(TextDirection layoutDirection) async {
assert(
_state != _AndroidViewState.disposed,
'trying to set a layout direction for a disposed Android view. View id: $viewId',
);
if (layoutDirection == _layoutDirection) {
return;
}
_layoutDirection = layoutDirection;
// If the view was not yet created we just update _layoutDirection and return, as the new
// direction will be used in _create.
if (_state == _AndroidViewState.waitingForSize) {
return;
}
await SystemChannels.platform_views
.invokeMethod<void>('setDirection', <String, dynamic>{
'id': viewId,
'direction': _getAndroidDirection(layoutDirection),
});
}
/// Converts the [PointerEvent] and sends an Android [MotionEvent](https://developer.android.com/reference/android/view/MotionEvent)
/// to the view.
///
/// This method can only be used if a [PointTransformer] is provided to
/// [AndroidViewController.pointTransformer]. Otherwise, an [AssertionError]
/// is thrown. See [AndroidViewController.sendMotionEvent] for sending a
/// `MotionEvent` without a [PointTransformer].
///
/// The Android MotionEvent object is created with [MotionEvent.obtain](https://developer.android.com/reference/android/view/MotionEvent.html#obtain(long,%20long,%20int,%20float,%20float,%20float,%20float,%20int,%20float,%20float,%20int,%20int)).
/// See documentation of [MotionEvent.obtain](https://developer.android.com/reference/android/view/MotionEvent.html#obtain(long,%20long,%20int,%20float,%20float,%20float,%20float,%20int,%20float,%20float,%20int,%20int))
/// for description of the parameters.
@override
Future<void> dispatchPointerEvent(PointerEvent event) async {
if (event is PointerHoverEvent) {
return;
}
if (event is PointerDownEvent) {
_motionEventConverter.handlePointerDownEvent(event);
}
_motionEventConverter.updatePointerPositions(event);
final AndroidMotionEvent? androidEvent =
_motionEventConverter.toAndroidMotionEvent(event);
if (event is PointerUpEvent) {
_motionEventConverter.handlePointerUpEvent(event);
} else if (event is PointerCancelEvent) {
_motionEventConverter.handlePointerCancelEvent(event);
}
if (androidEvent != null) {
await sendMotionEvent(androidEvent);
}
}
/// Clears the focus from the Android View if it is focused.
@override
Future<void> clearFocus() {
if (_state != _AndroidViewState.created) {
return Future<void>.value();
}
return SystemChannels.platform_views.invokeMethod<void>('clearFocus', viewId);
}
/// Disposes the Android view.
///
/// The [AndroidViewController] object is unusable after calling this.
/// The identifier of the platform view cannot be reused after the view is
/// disposed.
@override
Future<void> dispose() async {
final _AndroidViewState state = _state;
_state = _AndroidViewState.disposed;
_platformViewCreatedCallbacks.clear();
PlatformViewsService._instance._focusCallbacks.remove(viewId);
if (state == _AndroidViewState.creating || state == _AndroidViewState.created) {
await _sendDisposeMessage();
}
}
}
/// Controls an Android view that is composed using a GL texture.
/// This controller is created from the [PlatformViewsService.initSurfaceAndroidView] factory,
/// and is defined for backward compatibility.
class SurfaceAndroidViewController extends AndroidViewController {
SurfaceAndroidViewController._({
required super.viewId,
required super.viewType,
required super.layoutDirection,
super.creationParams,
super.creationParamsCodec,
}) : super._();
// By default, assume the implementation will be texture-based.
_AndroidViewControllerInternals _internals = _TextureAndroidViewControllerInternals();
@override
bool get _createRequiresSize => true;
@override
Future<bool> _sendCreateMessage({required Size size, Offset? position}) async {
assert(!size.isEmpty, 'trying to create $TextureAndroidViewController without setting a valid size.');
final dynamic response = await _AndroidViewControllerInternals.sendCreateMessage(
viewId: viewId,
viewType: _viewType,
hybrid: false,
hybridFallback: true,
layoutDirection: _layoutDirection,
creationParams: _creationParams,
size: size,
position: position,
);
if (response is int) {
(_internals as _TextureAndroidViewControllerInternals).textureId = response;
} else {
// A null response indicates fallback to Hybrid Composition, so swap out
// the implementation.
_internals = _HybridAndroidViewControllerInternals();
}
return true;
}
@override
int? get textureId {
return _internals.textureId;
}
@override
bool get requiresViewComposition {
return _internals.requiresViewComposition;
}
@override
Future<void> _sendDisposeMessage() {
return _internals.sendDisposeMessage(viewId: viewId);
}
@override
Future<Size> _sendResizeMessage(Size size) {
return _internals.setSize(size, viewId: viewId, viewState: _state);
}
@override
Future<void> setOffset(Offset off) {
return _internals.setOffset(off, viewId: viewId, viewState: _state);
}
}
/// Controls an Android view that is composed using the Android view hierarchy.
/// This controller is created from the [PlatformViewsService.initExpensiveAndroidView] factory.
class ExpensiveAndroidViewController extends AndroidViewController {
ExpensiveAndroidViewController._({
required super.viewId,
required super.viewType,
required super.layoutDirection,
super.creationParams,
super.creationParamsCodec,
}) : super._();
final _AndroidViewControllerInternals _internals = _HybridAndroidViewControllerInternals();
@override
bool get _createRequiresSize => false;
@override
Future<void> _sendCreateMessage({required Size? size, Offset? position}) async {
await _AndroidViewControllerInternals.sendCreateMessage(
viewId: viewId,
viewType: _viewType,
hybrid: true,
layoutDirection: _layoutDirection,
creationParams: _creationParams,
position: position,
);
}
@override
int? get textureId {
return _internals.textureId;
}
@override
bool get requiresViewComposition {
return _internals.requiresViewComposition;
}
@override
Future<void> _sendDisposeMessage() {
return _internals.sendDisposeMessage(viewId: viewId);
}
@override
Future<Size> _sendResizeMessage(Size size) {
return _internals.setSize(size, viewId: viewId, viewState: _state);
}
@override
Future<void> setOffset(Offset off) {
return _internals.setOffset(off, viewId: viewId, viewState: _state);
}
}
/// Controls an Android view that is rendered as a texture.
/// This is typically used by [AndroidView] to display a View in the Android view hierarchy.
///
/// The platform view is created by calling [create] with an initial size.
///
/// The controller is typically created with [PlatformViewsService.initAndroidView].
class TextureAndroidViewController extends AndroidViewController {
TextureAndroidViewController._({
required super.viewId,
required super.viewType,
required super.layoutDirection,
super.creationParams,
super.creationParamsCodec,
}) : super._();
final _TextureAndroidViewControllerInternals _internals = _TextureAndroidViewControllerInternals();
@override
bool get _createRequiresSize => true;
@override
Future<void> _sendCreateMessage({required Size size, Offset? position}) async {
assert(!size.isEmpty, 'trying to create $TextureAndroidViewController without setting a valid size.');
_internals.textureId = await _AndroidViewControllerInternals.sendCreateMessage(
viewId: viewId,
viewType: _viewType,
hybrid: false,
layoutDirection: _layoutDirection,
creationParams: _creationParams,
size: size,
position: position,
) as int;
}
@override
int? get textureId {
return _internals.textureId;
}
@override
bool get requiresViewComposition {
return _internals.requiresViewComposition;
}
@override
Future<void> _sendDisposeMessage() {
return _internals.sendDisposeMessage(viewId: viewId);
}
@override
Future<Size> _sendResizeMessage(Size size) {
return _internals.setSize(size, viewId: viewId, viewState: _state);
}
@override
Future<void> setOffset(Offset off) {
return _internals.setOffset(off, viewId: viewId, viewState: _state);
}
}
// The base class for an implementation of AndroidViewController.
//
// Subclasses should correspond to different rendering modes for platform
// views, and match different mode logic on the engine side.
abstract class _AndroidViewControllerInternals {
// Sends a create message with the given parameters, and returns the result
// if any.
//
// This uses a dynamic return because depending on the mode that is selected
// on the native side, the return type is different. Callers should cast
// depending on the possible return types for their arguments.
static Future<dynamic> sendCreateMessage({
required int viewId,
required String viewType,
required TextDirection layoutDirection,
required bool hybrid,
bool hybridFallback = false,
_CreationParams? creationParams,
Size? size,
Offset? position}) {
final Map<String, dynamic> args = <String, dynamic>{
'id': viewId,
'viewType': viewType,
'direction': AndroidViewController._getAndroidDirection(layoutDirection),
if (hybrid) 'hybrid': hybrid,
if (size != null) 'width': size.width,
if (size != null) 'height': size.height,
if (hybridFallback) 'hybridFallback': hybridFallback,
if (position != null) 'left': position.dx,
if (position != null) 'top': position.dy,
};
if (creationParams != null) {
final ByteData paramsByteData = creationParams.codec.encodeMessage(creationParams.data)!;
args['params'] = Uint8List.view(
paramsByteData.buffer,
0,
paramsByteData.lengthInBytes,
);
}
return SystemChannels.platform_views.invokeMethod<dynamic>('create', args);
}
int? get textureId;
bool get requiresViewComposition;
Future<Size> setSize(
Size size, {
required int viewId,
required _AndroidViewState viewState,
});
Future<void> setOffset(
Offset offset, {
required int viewId,
required _AndroidViewState viewState,
});
Future<void> sendDisposeMessage({required int viewId});
}
// An AndroidViewController implementation for views whose contents are
// displayed via a texture rather than directly in a native view.
//
// This is used for both Virtual Display and Texture Layer Hybrid Composition.
class _TextureAndroidViewControllerInternals extends _AndroidViewControllerInternals {
_TextureAndroidViewControllerInternals();
/// The current offset of the platform view.
Offset _offset = Offset.zero;
@override
int? textureId;
@override
bool get requiresViewComposition => false;
@override
Future<Size> setSize(
Size size, {
required int viewId,
required _AndroidViewState viewState,
}) async {
assert(viewState != _AndroidViewState.waitingForSize, 'Android view must have an initial size. View id: $viewId');
assert(!size.isEmpty);
final Map<Object?, Object?>? meta = await SystemChannels.platform_views.invokeMapMethod<Object?, Object?>(
'resize',
<String, dynamic>{
'id': viewId,
'width': size.width,
'height': size.height,
},
);
assert(meta != null);
assert(meta!.containsKey('width'));
assert(meta!.containsKey('height'));
return Size(meta!['width']! as double, meta['height']! as double);
}
@override
Future<void> setOffset(
Offset offset, {
required int viewId,
required _AndroidViewState viewState,
}) async {
if (offset == _offset) {
return;
}
// Don't set the offset unless the Android view has been created.
// The implementation of this method channel throws if the Android view for this viewId
// isn't addressable.
if (viewState != _AndroidViewState.created) {
return;
}
_offset = offset;
await SystemChannels.platform_views.invokeMethod<void>(
'offset',
<String, dynamic>{
'id': viewId,
'top': offset.dy,
'left': offset.dx,
},
);
}
@override
Future<void> sendDisposeMessage({required int viewId}) {
return SystemChannels
.platform_views.invokeMethod<void>('dispose', <String, dynamic>{
'id': viewId,
'hybrid': false,
});
}
}
// An AndroidViewController implementation for views whose contents are
// displayed directly in a native view.
//
// This is used for Hybrid Composition.
class _HybridAndroidViewControllerInternals extends _AndroidViewControllerInternals {
@override
int get textureId {
throw UnimplementedError('Not supported for hybrid composition.');
}
@override
bool get requiresViewComposition => true;
@override
Future<Size> setSize(
Size size, {
required int viewId,
required _AndroidViewState viewState,
}) {
throw UnimplementedError('Not supported for hybrid composition.');
}
@override
Future<void> setOffset(
Offset offset, {
required int viewId,
required _AndroidViewState viewState,
}) {
throw UnimplementedError('Not supported for hybrid composition.');
}
@override
Future<void> sendDisposeMessage({required int viewId}) {
return SystemChannels.platform_views.invokeMethod<void>('dispose', <String, dynamic>{
'id': viewId,
'hybrid': true,
});
}
}
/// Base class for iOS and macOS view controllers.
///
/// View controllers are used to create and interact with the UIView or NSView
/// underlying a platform view.
abstract class DarwinPlatformViewController {
/// Public default for subclasses to override.
DarwinPlatformViewController(
this.id,
TextDirection layoutDirection,
) : _layoutDirection = layoutDirection;
/// The unique identifier of the iOS view controlled by this controller.
///
/// This identifier is typically generated by
/// [PlatformViewsRegistry.getNextPlatformViewId].
final int id;
bool _debugDisposed = false;
TextDirection _layoutDirection;
/// Sets the layout direction for the iOS UIView.
Future<void> setLayoutDirection(TextDirection layoutDirection) async {
assert(!_debugDisposed, 'trying to set a layout direction for a disposed iOS UIView. View id: $id');
if (layoutDirection == _layoutDirection) {
return;
}
_layoutDirection = layoutDirection;
// TODO(amirh): invoke the iOS platform views channel direction method once available.
}
/// Accept an active gesture.
///
/// When a touch sequence is happening on the embedded UIView all touch events are delayed.
/// Calling this method releases the delayed events to the embedded UIView and makes it consume
/// any following touch events for the pointers involved in the active gesture.
Future<void> acceptGesture() {
final Map<String, dynamic> args = <String, dynamic>{
'id': id,
};
return SystemChannels.platform_views.invokeMethod('acceptGesture', args);
}
/// Rejects an active gesture.
///
/// When a touch sequence is happening on the embedded UIView all touch events are delayed.
/// Calling this method drops the buffered touch events and prevents any future touch events for
/// the pointers that are part of the active touch sequence from arriving to the embedded view.
Future<void> rejectGesture() {
final Map<String, dynamic> args = <String, dynamic>{
'id': id,
};
return SystemChannels.platform_views.invokeMethod('rejectGesture', args);
}
/// Disposes the view.
///
/// The [UiKitViewController] object is unusable after calling this.
/// The `id` of the platform view cannot be reused after the view is
/// disposed.
Future<void> dispose() async {
_debugDisposed = true;
await SystemChannels.platform_views.invokeMethod<void>('dispose', id);
PlatformViewsService._instance._focusCallbacks.remove(id);
}
}
/// Controller for an iOS platform view.
///
/// View controllers create and interact with the underlying UIView.
///
/// Typically created with [PlatformViewsService.initUiKitView].
class UiKitViewController extends DarwinPlatformViewController {
UiKitViewController._(
super.id,
super.layoutDirection,
);
}
/// Controller for a macOS platform view.
class AppKitViewController extends DarwinPlatformViewController {
AppKitViewController._(
super.id,
super.layoutDirection,
);
}
/// An interface for controlling a single platform view.
///
/// Used by [PlatformViewSurface] to interface with the platform view it embeds.
abstract class PlatformViewController {
/// The viewId associated with this controller.
///
/// The viewId should always be unique and non-negative.
///
/// See also:
///
/// * [PlatformViewsRegistry], which is a helper for managing platform view IDs.
int get viewId;
/// True if [create] has not been successfully called the platform view.
///
/// This can indicate either that [create] was never called, or that [create]
/// was deferred for implementation-specific reasons.
///
/// A `false` return value does not necessarily indicate that the [Future]
/// returned by [create] has completed, only that creation has been started.
bool get awaitingCreation => false;
/// Dispatches the `event` to the platform view.
Future<void> dispatchPointerEvent(PointerEvent event);
/// Creates the platform view with the initial [size].
///
/// [size] is the view's initial size in logical pixel.
/// [size] can be omitted if the concrete implementation doesn't require an initial size
/// to create the platform view.
///
/// [position] is the view's initial position in logical pixels.
/// [position] can be omitted if the concrete implementation doesn't require
/// an initial position.
Future<void> create({Size? size, Offset? position}) async {}
/// Disposes the platform view.
///
/// The [PlatformViewController] is unusable after calling dispose.
Future<void> dispose();
/// Clears the view's focus on the platform side.
Future<void> clearFocus();
}
| flutter/packages/flutter/lib/src/services/platform_views.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/platform_views.dart",
"repo_id": "flutter",
"token_count": 17309
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'system_channels.dart';
/// A sound provided by the system.
///
/// These sounds may be played with [SystemSound.play].
enum SystemSoundType {
/// A short indication that a button was pressed.
click,
/// A short system alert sound indicating the need for user attention.
///
/// Desktop platforms are the only platforms that support a system alert
/// sound, so on mobile platforms (Android, iOS), this will be ignored. The
/// web platform does not support playing any sounds, so this will be
/// ignored on the web as well.
alert,
// If you add new values here, you also need to update the `SoundType` Java
// enum in `PlatformChannel.java`.
}
/// Provides access to the library of short system specific sounds for common
/// tasks.
abstract final class SystemSound {
/// Play the specified system sound. If that sound is not present on the
/// system, the call is ignored.
///
/// The web platform currently does not support playing sounds, so this call
/// will yield no behavior on that platform.
static Future<void> play(SystemSoundType type) async {
await SystemChannels.platform.invokeMethod<void>(
'SystemSound.play',
type.toString(),
);
}
}
| flutter/packages/flutter/lib/src/services/system_sound.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/system_sound.dart",
"repo_id": "flutter",
"token_count": 366
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic.dart';
import 'framework.dart';
import 'media_query.dart';
import 'scroll_controller.dart';
import 'scroll_delegate.dart';
import 'scroll_physics.dart';
import 'scroll_view.dart';
import 'sliver.dart';
import 'ticker_provider.dart';
/// A scrolling container that animates items when they are inserted or removed.
///
/// This widget's [AnimatedListState] can be used to dynamically insert or
/// remove items. To refer to the [AnimatedListState] either provide a
/// [GlobalKey] or use the static [of] method from an item's input callback.
///
/// This widget is similar to one created by [ListView.builder].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ZtfItHwFlZ8}
///
/// {@tool dartpad}
/// This sample application uses an [AnimatedList] to create an effect when
/// items are removed or added to the list.
///
/// ** See code in examples/api/lib/widgets/animated_list/animated_list.0.dart **
/// {@end-tool}
///
/// By default, [AnimatedList] will automatically pad the limits of the
/// list's scrollable to avoid partial obstructions indicated by
/// [MediaQuery]'s padding. To avoid this behavior, override with a
/// zero [padding] property.
///
/// {@tool snippet}
/// The following example demonstrates how to override the default top and
/// bottom padding using [MediaQuery.removePadding].
///
/// ```dart
/// Widget myWidget(BuildContext context) {
/// return MediaQuery.removePadding(
/// context: context,
/// removeTop: true,
/// removeBottom: true,
/// child: AnimatedList(
/// initialItemCount: 50,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return Card(
/// color: Colors.amber,
/// child: Center(child: Text('$index')),
/// );
/// }
/// ),
/// );
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [SliverAnimatedList], a sliver that animates items when they are inserted
/// or removed from a list.
/// * [SliverAnimatedGrid], a sliver which animates items when they are
/// inserted or removed from a grid.
/// * [AnimatedGrid], a non-sliver scrolling container that animates items when
/// they are inserted or removed in a grid.
class AnimatedList extends _AnimatedScrollView {
/// Creates a scrolling container that animates items when they are inserted
/// or removed.
const AnimatedList({
super.key,
required super.itemBuilder,
super.initialItemCount = 0,
super.scrollDirection = Axis.vertical,
super.reverse = false,
super.controller,
super.primary,
super.physics,
super.shrinkWrap = false,
super.padding,
super.clipBehavior = Clip.hardEdge,
}) : assert(initialItemCount >= 0);
/// The state from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [AnimatedList] item widgets that insert
/// or remove items in response to user input.
///
/// If no [AnimatedList] surrounds the context given, then this function will
/// assert in debug mode and throw an exception in release mode.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [maybeOf], a similar function that will return null if no
/// [AnimatedList] ancestor is found.
static AnimatedListState of(BuildContext context) {
final AnimatedListState? result = AnimatedList.maybeOf(context);
assert(() {
if (result == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('AnimatedList.of() called with a context that does not contain an AnimatedList.'),
ErrorDescription(
'No AnimatedList ancestor could be found starting from the context that was passed to AnimatedList.of().',
),
ErrorHint(
'This can happen when the context provided is from the same StatefulWidget that '
'built the AnimatedList. Please see the AnimatedList documentation for examples '
'of how to refer to an AnimatedListState object:\n'
' https://api.flutter.dev/flutter/widgets/AnimatedListState-class.html',
),
context.describeElement('The context used was'),
]);
}
return true;
}());
return result!;
}
/// The [AnimatedListState] from the closest instance of [AnimatedList] that encloses the given
/// context.
///
/// This method is typically used by [AnimatedList] item widgets that insert
/// or remove items in response to user input.
///
/// If no [AnimatedList] surrounds the context given, then this function will
/// return null.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [of], a similar function that will throw if no [AnimatedList] ancestor
/// is found.
static AnimatedListState? maybeOf(BuildContext context) {
return context.findAncestorStateOfType<AnimatedListState>();
}
@override
AnimatedListState createState() => AnimatedListState();
}
/// The [AnimatedListState] for [AnimatedList], a scrolling list container that animates items when they are
/// inserted or removed.
///
/// When an item is inserted with [insertItem] an animation begins running. The
/// animation is passed to [AnimatedList.itemBuilder] whenever the item's widget
/// is needed.
///
/// When multiple items are inserted with [insertAllItems] an animation begins running.
/// The animation is passed to [AnimatedList.itemBuilder] whenever the item's widget
/// is needed.
///
/// When an item is removed with [removeItem] its animation is reversed.
/// The removed item's animation is passed to the [removeItem] builder
/// parameter.
///
/// An app that needs to insert or remove items in response to an event
/// can refer to the [AnimatedList]'s state with a global key:
///
/// ```dart
/// // (e.g. in a stateful widget)
/// GlobalKey<AnimatedListState> listKey = GlobalKey<AnimatedListState>();
///
/// // ...
///
/// @override
/// Widget build(BuildContext context) {
/// return AnimatedList(
/// key: listKey,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return const Placeholder();
/// },
/// );
/// }
///
/// // ...
///
/// void _updateList() {
/// // adds "123" to the AnimatedList
/// listKey.currentState!.insertItem(123);
/// }
/// ```
///
/// [AnimatedList] item input handlers can also refer to their [AnimatedListState]
/// with the static [AnimatedList.of] method.
class AnimatedListState extends _AnimatedScrollViewState<AnimatedList> {
@override
Widget build(BuildContext context) {
return _wrap(
SliverAnimatedList(
key: _sliverAnimatedMultiBoxKey,
itemBuilder: widget.itemBuilder,
initialItemCount: widget.initialItemCount,
),
widget.scrollDirection,
);
}
}
/// A scrolling container that animates items when they are inserted into or removed from a grid.
/// in a grid.
///
/// This widget's [AnimatedGridState] can be used to dynamically insert or
/// remove items. To refer to the [AnimatedGridState] either provide a
/// [GlobalKey] or use the static [of] method from an item's input callback.
///
/// This widget is similar to one created by [GridView.builder].
///
/// {@tool dartpad}
/// This sample application uses an [AnimatedGrid] to create an effect when
/// items are removed or added to the grid.
///
/// ** See code in examples/api/lib/widgets/animated_grid/animated_grid.0.dart **
/// {@end-tool}
///
/// By default, [AnimatedGrid] will automatically pad the limits of the
/// grid's scrollable to avoid partial obstructions indicated by
/// [MediaQuery]'s padding. To avoid this behavior, override with a
/// zero [padding] property.
///
/// {@tool snippet}
/// The following example demonstrates how to override the default top and
/// bottom padding using [MediaQuery.removePadding].
///
/// ```dart
/// Widget myWidget(BuildContext context) {
/// return MediaQuery.removePadding(
/// context: context,
/// removeTop: true,
/// removeBottom: true,
/// child: AnimatedGrid(
/// gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
/// crossAxisCount: 3,
/// ),
/// initialItemCount: 50,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return Card(
/// color: Colors.amber,
/// child: Center(child: Text('$index')),
/// );
/// }
/// ),
/// );
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [SliverAnimatedGrid], a sliver which animates items when they are inserted
/// into or removed from a grid.
/// * [SliverAnimatedList], a sliver which animates items added and removed from
/// a list instead of a grid.
/// * [AnimatedList], which animates items added and removed from a list instead
/// of a grid.
class AnimatedGrid extends _AnimatedScrollView {
/// Creates a scrolling container that animates items when they are inserted
/// or removed.
const AnimatedGrid({
super.key,
required super.itemBuilder,
required this.gridDelegate,
super.initialItemCount = 0,
super.scrollDirection = Axis.vertical,
super.reverse = false,
super.controller,
super.primary,
super.physics,
super.padding,
super.clipBehavior = Clip.hardEdge,
}) : assert(initialItemCount >= 0);
/// {@template flutter.widgets.AnimatedGrid.gridDelegate}
/// A delegate that controls the layout of the children within the
/// [AnimatedGrid].
///
/// See also:
///
/// * [SliverGridDelegateWithFixedCrossAxisCount], which creates a layout with
/// a fixed number of tiles in the cross axis.
/// * [SliverGridDelegateWithMaxCrossAxisExtent], which creates a layout with
/// tiles that have a maximum cross-axis extent.
/// {@endtemplate}
final SliverGridDelegate gridDelegate;
/// The state from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [AnimatedGrid] item widgets that insert
/// or remove items in response to user input.
///
/// If no [AnimatedGrid] surrounds the context given, then this function will
/// assert in debug mode and throw an exception in release mode.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [maybeOf], a similar function that will return null if no
/// [AnimatedGrid] ancestor is found.
static AnimatedGridState of(BuildContext context) {
final AnimatedGridState? result = AnimatedGrid.maybeOf(context);
assert(() {
if (result == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('AnimatedGrid.of() called with a context that does not contain an AnimatedGrid.'),
ErrorDescription(
'No AnimatedGrid ancestor could be found starting from the context that was passed to AnimatedGrid.of().',
),
ErrorHint(
'This can happen when the context provided is from the same StatefulWidget that '
'built the AnimatedGrid. Please see the AnimatedGrid documentation for examples '
'of how to refer to an AnimatedGridState object:\n'
' https://api.flutter.dev/flutter/widgets/AnimatedGridState-class.html',
),
context.describeElement('The context used was'),
]);
}
return true;
}());
return result!;
}
/// The state from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [AnimatedGrid] item widgets that insert
/// or remove items in response to user input.
///
/// If no [AnimatedGrid] surrounds the context given, then this function will
/// return null.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [of], a similar function that will throw if no [AnimatedGrid] ancestor
/// is found.
static AnimatedGridState? maybeOf(BuildContext context) {
return context.findAncestorStateOfType<AnimatedGridState>();
}
@override
AnimatedGridState createState() => AnimatedGridState();
}
/// The [State] for an [AnimatedGrid] that animates items when they are
/// inserted or removed.
///
/// When an item is inserted with [insertItem] an animation begins running. The
/// animation is passed to [AnimatedGrid.itemBuilder] whenever the item's widget
/// is needed.
///
/// When an item is removed with [removeItem] its animation is reversed.
/// The removed item's animation is passed to the [removeItem] builder
/// parameter.
///
/// An app that needs to insert or remove items in response to an event
/// can refer to the [AnimatedGrid]'s state with a global key:
///
/// ```dart
/// // (e.g. in a stateful widget)
/// GlobalKey<AnimatedGridState> gridKey = GlobalKey<AnimatedGridState>();
///
/// // ...
///
/// @override
/// Widget build(BuildContext context) {
/// return AnimatedGrid(
/// key: gridKey,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return const Placeholder();
/// },
/// gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(maxCrossAxisExtent: 100.0),
/// );
/// }
///
/// // ...
///
/// void _updateGrid() {
/// // adds "123" to the AnimatedGrid
/// gridKey.currentState!.insertItem(123);
/// }
/// ```
///
/// [AnimatedGrid] item input handlers can also refer to their [AnimatedGridState]
/// with the static [AnimatedGrid.of] method.
class AnimatedGridState extends _AnimatedScrollViewState<AnimatedGrid> {
@override
Widget build(BuildContext context) {
return _wrap(
SliverAnimatedGrid(
key: _sliverAnimatedMultiBoxKey,
gridDelegate: widget.gridDelegate,
itemBuilder: widget.itemBuilder,
initialItemCount: widget.initialItemCount,
),
widget.scrollDirection,
);
}
}
abstract class _AnimatedScrollView extends StatefulWidget {
/// Creates a scrolling container that animates items when they are inserted
/// or removed.
const _AnimatedScrollView({
super.key,
required this.itemBuilder,
this.initialItemCount = 0,
this.scrollDirection = Axis.vertical,
this.reverse = false,
this.controller,
this.primary,
this.physics,
this.shrinkWrap = false,
this.padding,
this.clipBehavior = Clip.hardEdge,
}) : assert(initialItemCount >= 0);
/// {@template flutter.widgets.AnimatedScrollView.itemBuilder}
/// Called, as needed, to build children widgets.
///
/// Children are only built when they're scrolled into view.
///
/// The [AnimatedItemBuilder] index parameter indicates the item's
/// position in the scroll view. The value of the index parameter will be
/// between 0 and [initialItemCount] plus the total number of items that have
/// been inserted with [AnimatedListState.insertItem] or
/// [AnimatedGridState.insertItem] and less the total number of items that
/// have been removed with [AnimatedListState.removeItem] or
/// [AnimatedGridState.removeItem].
///
/// Implementations of this callback should assume that
/// `removeItem` removes an item immediately.
/// {@endtemplate}
final AnimatedItemBuilder itemBuilder;
/// {@template flutter.widgets.AnimatedScrollView.initialItemCount}
/// The number of items the [AnimatedList] or [AnimatedGrid] will start with.
///
/// The appearance of the initial items is not animated. They
/// are created, as needed, by [itemBuilder] with an animation parameter
/// of [kAlwaysCompleteAnimation].
/// {@endtemplate}
final int initialItemCount;
/// {@macro flutter.widgets.scroll_view.scrollDirection}
final Axis scrollDirection;
/// Whether the scroll view scrolls in the reading direction.
///
/// For example, if the reading direction is left-to-right and
/// [scrollDirection] is [Axis.horizontal], then the scroll view scrolls from
/// left to right when [reverse] is false and from right to left when
/// [reverse] is true.
///
/// Similarly, if [scrollDirection] is [Axis.vertical], then the scroll view
/// scrolls from top to bottom when [reverse] is false and from bottom to top
/// when [reverse] is true.
///
/// Defaults to false.
final bool reverse;
/// An object that can be used to control the position to which this scroll
/// view is scrolled.
///
/// Must be null if [primary] is true.
///
/// A [ScrollController] serves several purposes. It can be used to control
/// the initial scroll position (see [ScrollController.initialScrollOffset]).
/// It can be used to control whether the scroll view should automatically
/// save and restore its scroll position in the [PageStorage] (see
/// [ScrollController.keepScrollOffset]). It can be used to read the current
/// scroll position (see [ScrollController.offset]), or change it (see
/// [ScrollController.animateTo]).
final ScrollController? controller;
/// Whether this is the primary scroll view associated with the parent
/// [PrimaryScrollController].
///
/// On iOS, this identifies the scroll view that will scroll to top in
/// response to a tap in the status bar.
///
/// Defaults to true when [scrollDirection] is [Axis.vertical] and
/// [controller] is null.
final bool? primary;
/// How the scroll view should respond to user input.
///
/// For example, this determines how the scroll view continues to animate after the
/// user stops dragging the scroll view.
///
/// Defaults to matching platform conventions.
final ScrollPhysics? physics;
/// Whether the extent of the scroll view in the [scrollDirection] should be
/// determined by the contents being viewed.
///
/// If the scroll view does not shrink wrap, then the scroll view will expand
/// to the maximum allowed size in the [scrollDirection]. If the scroll view
/// has unbounded constraints in the [scrollDirection], then [shrinkWrap] must
/// be true.
///
/// Shrink wrapping the content of the scroll view is significantly more
/// expensive than expanding to the maximum allowed size because the content
/// can expand and contract during scrolling, which means the size of the
/// scroll view needs to be recomputed whenever the scroll position changes.
///
/// Defaults to false.
final bool shrinkWrap;
/// The amount of space by which to inset the children.
final EdgeInsetsGeometry? padding;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
}
abstract class _AnimatedScrollViewState<T extends _AnimatedScrollView> extends State<T> with TickerProviderStateMixin {
final GlobalKey<_SliverAnimatedMultiBoxAdaptorState<_SliverAnimatedMultiBoxAdaptor>> _sliverAnimatedMultiBoxKey = GlobalKey();
/// Insert an item at [index] and start an animation that will be passed
/// to [AnimatedGrid.itemBuilder] or [AnimatedList.itemBuilder] when the item
/// is visible.
///
/// This method's semantics are the same as Dart's [List.insert] method: it
/// increases the length of the list of items by one and shifts
/// all items at or after [index] towards the end of the list of items.
void insertItem(int index, { Duration duration = _kDuration }) {
_sliverAnimatedMultiBoxKey.currentState!.insertItem(index, duration: duration);
}
/// Insert multiple items at [index] and start an animation that will be passed
/// to [AnimatedGrid.itemBuilder] or [AnimatedList.itemBuilder] when the items
/// are visible.
void insertAllItems(int index, int length, { Duration duration = _kDuration, bool isAsync = false }) {
_sliverAnimatedMultiBoxKey.currentState!.insertAllItems(index, length, duration: duration);
}
/// Remove the item at `index` and start an animation that will be passed to
/// `builder` when the item is visible.
///
/// Items are removed immediately. After an item has been removed, its index
/// will no longer be passed to the `itemBuilder`. However, the
/// item will still appear for `duration` and during that time
/// `builder` must construct its widget as needed.
///
/// This method's semantics are the same as Dart's [List.remove] method: it
/// decreases the length of items by one and shifts all items at or before
/// `index` towards the beginning of the list of items.
///
/// See also:
///
/// * [AnimatedRemovedItemBuilder], which describes the arguments to the
/// `builder` argument.
void removeItem(int index, AnimatedRemovedItemBuilder builder, { Duration duration = _kDuration }) {
_sliverAnimatedMultiBoxKey.currentState!.removeItem(index, builder, duration: duration);
}
/// Remove all the items and start an animation that will be passed to
/// `builder` when the items are visible.
///
/// Items are removed immediately. However, the
/// items will still appear for `duration`, and during that time
/// `builder` must construct its widget as needed.
///
/// This method's semantics are the same as Dart's [List.clear] method: it
/// removes all the items in the list.
void removeAllItems(AnimatedRemovedItemBuilder builder, { Duration duration = _kDuration }) {
_sliverAnimatedMultiBoxKey.currentState!.removeAllItems(builder, duration: duration);
}
Widget _wrap(Widget sliver, Axis direction) {
EdgeInsetsGeometry? effectivePadding = widget.padding;
if (widget.padding == null) {
final MediaQueryData? mediaQuery = MediaQuery.maybeOf(context);
if (mediaQuery != null) {
// Automatically pad sliver with padding from MediaQuery.
final EdgeInsets mediaQueryHorizontalPadding =
mediaQuery.padding.copyWith(top: 0.0, bottom: 0.0);
final EdgeInsets mediaQueryVerticalPadding =
mediaQuery.padding.copyWith(left: 0.0, right: 0.0);
// Consume the main axis padding with SliverPadding.
effectivePadding = direction == Axis.vertical
? mediaQueryVerticalPadding
: mediaQueryHorizontalPadding;
// Leave behind the cross axis padding.
sliver = MediaQuery(
data: mediaQuery.copyWith(
padding: direction == Axis.vertical
? mediaQueryHorizontalPadding
: mediaQueryVerticalPadding,
),
child: sliver,
);
}
}
if (effectivePadding != null) {
sliver = SliverPadding(padding: effectivePadding, sliver: sliver);
}
return CustomScrollView(
scrollDirection: widget.scrollDirection,
reverse: widget.reverse,
controller: widget.controller,
primary: widget.primary,
physics: widget.physics,
clipBehavior: widget.clipBehavior,
shrinkWrap: widget.shrinkWrap,
slivers: <Widget>[ sliver ],
);
}
}
/// Signature for the builder callback used by [AnimatedList] & [AnimatedGrid] to
/// build their animated children.
///
/// The `context` argument is the build context where the widget will be
/// created, the `index` is the index of the item to be built, and the
/// `animation` is an [Animation] that should be used to animate an entry
/// transition for the widget that is built.
///
/// See also:
///
/// * [AnimatedRemovedItemBuilder], a builder that is for removing items with
/// animations instead of adding them.
typedef AnimatedItemBuilder = Widget Function(BuildContext context, int index, Animation<double> animation);
/// Signature for the builder callback used in [AnimatedListState.removeItem] and
/// [AnimatedGridState.removeItem] to animate their children after they have
/// been removed.
///
/// The `context` argument is the build context where the widget will be
/// created, and the `animation` is an [Animation] that should be used to
/// animate an exit transition for the widget that is built.
///
/// See also:
///
/// * [AnimatedItemBuilder], a builder that is for adding items with animations
/// instead of removing them.
typedef AnimatedRemovedItemBuilder = Widget Function(BuildContext context, Animation<double> animation);
// The default insert/remove animation duration.
const Duration _kDuration = Duration(milliseconds: 300);
// Incoming and outgoing animated items.
class _ActiveItem implements Comparable<_ActiveItem> {
_ActiveItem.incoming(this.controller, this.itemIndex) : removedItemBuilder = null;
_ActiveItem.outgoing(this.controller, this.itemIndex, this.removedItemBuilder);
_ActiveItem.index(this.itemIndex)
: controller = null,
removedItemBuilder = null;
final AnimationController? controller;
final AnimatedRemovedItemBuilder? removedItemBuilder;
int itemIndex;
@override
int compareTo(_ActiveItem other) => itemIndex - other.itemIndex;
}
/// A [SliverList] that animates items when they are inserted or removed.
///
/// This widget's [SliverAnimatedListState] can be used to dynamically insert or
/// remove items. To refer to the [SliverAnimatedListState] either provide a
/// [GlobalKey] or use the static [SliverAnimatedList.of] method from a list item's
/// input callback.
///
/// {@tool dartpad}
/// This sample application uses a [SliverAnimatedList] to create an animated
/// effect when items are removed or added to the list.
///
/// ** See code in examples/api/lib/widgets/animated_list/sliver_animated_list.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverList], which does not animate items when they are inserted or
/// removed.
/// * [AnimatedList], a non-sliver scrolling container that animates items when
/// they are inserted or removed.
/// * [SliverAnimatedGrid], a sliver which animates items when they are
/// inserted into or removed from a grid.
/// * [AnimatedGrid], a non-sliver scrolling container that animates items when
/// they are inserted into or removed from a grid.
class SliverAnimatedList extends _SliverAnimatedMultiBoxAdaptor {
/// Creates a [SliverList] that animates items when they are inserted or
/// removed.
const SliverAnimatedList({
super.key,
required super.itemBuilder,
super.findChildIndexCallback,
super.initialItemCount = 0,
}) : assert(initialItemCount >= 0);
@override
SliverAnimatedListState createState() => SliverAnimatedListState();
/// The [SliverAnimatedListState] from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [SliverAnimatedList] item widgets that
/// insert or remove items in response to user input.
///
/// If no [SliverAnimatedList] surrounds the context given, then this function
/// will assert in debug mode and throw an exception in release mode.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [maybeOf], a similar function that will return null if no
/// [SliverAnimatedList] ancestor is found.
static SliverAnimatedListState of(BuildContext context) {
final SliverAnimatedListState? result = SliverAnimatedList.maybeOf(context);
assert(() {
if (result == null) {
throw FlutterError(
'SliverAnimatedList.of() called with a context that does not contain a SliverAnimatedList.\n'
'No SliverAnimatedListState ancestor could be found starting from the '
'context that was passed to SliverAnimatedListState.of(). This can '
'happen when the context provided is from the same StatefulWidget that '
'built the AnimatedList. Please see the SliverAnimatedList documentation '
'for examples of how to refer to an AnimatedListState object: '
'https://api.flutter.dev/flutter/widgets/SliverAnimatedListState-class.html\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return result!;
}
/// The [SliverAnimatedListState] from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [SliverAnimatedList] item widgets that
/// insert or remove items in response to user input.
///
/// If no [SliverAnimatedList] surrounds the context given, then this function
/// will return null.
///
/// This method can be expensive (it walks the element tree).
///
/// This method does not create a dependency, and so will not cause rebuilding
/// when the state changes.
///
/// See also:
///
/// * [of], a similar function that will throw if no [SliverAnimatedList]
/// ancestor is found.
static SliverAnimatedListState? maybeOf(BuildContext context) {
return context.findAncestorStateOfType<SliverAnimatedListState>();
}
}
/// The state for a [SliverAnimatedList] that animates items when they are
/// inserted or removed.
///
/// When an item is inserted with [insertItem] an animation begins running. The
/// animation is passed to [SliverAnimatedList.itemBuilder] whenever the item's
/// widget is needed.
///
/// When an item is removed with [removeItem] its animation is reversed.
/// The removed item's animation is passed to the [removeItem] builder
/// parameter.
///
/// An app that needs to insert or remove items in response to an event
/// can refer to the [SliverAnimatedList]'s state with a global key:
///
/// ```dart
/// // (e.g. in a stateful widget)
/// GlobalKey<AnimatedListState> listKey = GlobalKey<AnimatedListState>();
///
/// // ...
///
/// @override
/// Widget build(BuildContext context) {
/// return AnimatedList(
/// key: listKey,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return const Placeholder();
/// },
/// );
/// }
///
/// // ...
///
/// void _updateList() {
/// // adds "123" to the AnimatedList
/// listKey.currentState!.insertItem(123);
/// }
/// ```
///
/// [SliverAnimatedList] item input handlers can also refer to their
/// [SliverAnimatedListState] with the static [SliverAnimatedList.of] method.
class SliverAnimatedListState extends _SliverAnimatedMultiBoxAdaptorState<SliverAnimatedList> {
@override
Widget build(BuildContext context) {
return SliverList(
delegate: _createDelegate(),
);
}
}
/// A [SliverGrid] that animates items when they are inserted or removed.
///
/// This widget's [SliverAnimatedGridState] can be used to dynamically insert or
/// remove items. To refer to the [SliverAnimatedGridState] either provide a
/// [GlobalKey] or use the static [SliverAnimatedGrid.of] method from an item's
/// input callback.
///
/// {@tool dartpad}
/// This sample application uses a [SliverAnimatedGrid] to create an animated
/// effect when items are removed or added to the grid.
///
/// ** See code in examples/api/lib/widgets/animated_grid/sliver_animated_grid.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedGrid], a non-sliver scrolling container that animates items when
/// they are inserted into or removed from a grid.
/// * [SliverGrid], which does not animate items when they are inserted or
/// removed from a grid.
/// * [SliverList], which displays a non-animated list of items.
/// * [SliverAnimatedList], which animates items added and removed from a list
/// instead of a grid.
class SliverAnimatedGrid extends _SliverAnimatedMultiBoxAdaptor {
/// Creates a [SliverGrid] that animates items when they are inserted or
/// removed.
const SliverAnimatedGrid({
super.key,
required super.itemBuilder,
required this.gridDelegate,
super.findChildIndexCallback,
super.initialItemCount = 0,
}) : assert(initialItemCount >= 0);
@override
SliverAnimatedGridState createState() => SliverAnimatedGridState();
/// {@macro flutter.widgets.AnimatedGrid.gridDelegate}
final SliverGridDelegate gridDelegate;
/// The state from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [SliverAnimatedGrid] item widgets that
/// insert or remove items in response to user input.
///
/// If no [SliverAnimatedGrid] surrounds the context given, then this function
/// will assert in debug mode and throw an exception in release mode.
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [maybeOf], a similar function that will return null if no
/// [SliverAnimatedGrid] ancestor is found.
static SliverAnimatedGridState of(BuildContext context) {
final SliverAnimatedGridState? result = context.findAncestorStateOfType<SliverAnimatedGridState>();
assert(() {
if (result == null) {
throw FlutterError(
'SliverAnimatedGrid.of() called with a context that does not contain a SliverAnimatedGrid.\n'
'No SliverAnimatedGridState ancestor could be found starting from the '
'context that was passed to SliverAnimatedGridState.of(). This can '
'happen when the context provided is from the same StatefulWidget that '
'built the AnimatedGrid. Please see the SliverAnimatedGrid documentation '
'for examples of how to refer to an AnimatedGridState object: '
'https://api.flutter.dev/flutter/widgets/SliverAnimatedGridState-class.html\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return result!;
}
/// The state from the closest instance of this class that encloses the given
/// context.
///
/// This method is typically used by [SliverAnimatedGrid] item widgets that
/// insert or remove items in response to user input.
///
/// If no [SliverAnimatedGrid] surrounds the context given, then this function
/// will return null.
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [of], a similar function that will throw if no [SliverAnimatedGrid]
/// ancestor is found.
static SliverAnimatedGridState? maybeOf(BuildContext context) {
return context.findAncestorStateOfType<SliverAnimatedGridState>();
}
}
/// The state for a [SliverAnimatedGrid] that animates items when they are
/// inserted or removed.
///
/// When an item is inserted with [insertItem] an animation begins running. The
/// animation is passed to [SliverAnimatedGrid.itemBuilder] whenever the item's
/// widget is needed.
///
/// When an item is removed with [removeItem] its animation is reversed.
/// The removed item's animation is passed to the [removeItem] builder
/// parameter.
///
/// An app that needs to insert or remove items in response to an event
/// can refer to the [SliverAnimatedGrid]'s state with a global key:
///
/// ```dart
/// // (e.g. in a stateful widget)
/// GlobalKey<AnimatedGridState> gridKey = GlobalKey<AnimatedGridState>();
///
/// // ...
///
/// @override
/// Widget build(BuildContext context) {
/// return AnimatedGrid(
/// key: gridKey,
/// itemBuilder: (BuildContext context, int index, Animation<double> animation) {
/// return const Placeholder();
/// },
/// gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(maxCrossAxisExtent: 100.0),
/// );
/// }
///
/// // ...
///
/// void _updateGrid() {
/// // adds "123" to the AnimatedGrid
/// gridKey.currentState!.insertItem(123);
/// }
/// ```
///
/// [SliverAnimatedGrid] item input handlers can also refer to their
/// [SliverAnimatedGridState] with the static [SliverAnimatedGrid.of] method.
class SliverAnimatedGridState extends _SliverAnimatedMultiBoxAdaptorState<SliverAnimatedGrid> {
@override
Widget build(BuildContext context) {
return SliverGrid(
gridDelegate: widget.gridDelegate,
delegate: _createDelegate(),
);
}
}
abstract class _SliverAnimatedMultiBoxAdaptor extends StatefulWidget {
/// Creates a sliver that animates items when they are inserted or removed.
const _SliverAnimatedMultiBoxAdaptor({
super.key,
required this.itemBuilder,
this.findChildIndexCallback,
this.initialItemCount = 0,
}) : assert(initialItemCount >= 0);
/// {@macro flutter.widgets.AnimatedScrollView.itemBuilder}
final AnimatedItemBuilder itemBuilder;
/// {@macro flutter.widgets.SliverChildBuilderDelegate.findChildIndexCallback}
final ChildIndexGetter? findChildIndexCallback;
/// {@macro flutter.widgets.AnimatedScrollView.initialItemCount}
final int initialItemCount;
}
abstract class _SliverAnimatedMultiBoxAdaptorState<T extends _SliverAnimatedMultiBoxAdaptor> extends State<T> with TickerProviderStateMixin {
@override
void initState() {
super.initState();
_itemsCount = widget.initialItemCount;
}
@override
void dispose() {
for (final _ActiveItem item in _incomingItems.followedBy(_outgoingItems)) {
item.controller!.dispose();
}
super.dispose();
}
final List<_ActiveItem> _incomingItems = <_ActiveItem>[];
final List<_ActiveItem> _outgoingItems = <_ActiveItem>[];
int _itemsCount = 0;
_ActiveItem? _removeActiveItemAt(List<_ActiveItem> items, int itemIndex) {
final int i = binarySearch(items, _ActiveItem.index(itemIndex));
return i == -1 ? null : items.removeAt(i);
}
_ActiveItem? _activeItemAt(List<_ActiveItem> items, int itemIndex) {
final int i = binarySearch(items, _ActiveItem.index(itemIndex));
return i == -1 ? null : items[i];
}
// The insertItem() and removeItem() index parameters are defined as if the
// removeItem() operation removed the corresponding list/grid entry
// immediately. The entry is only actually removed from the
// ListView/GridView when the remove animation finishes. The entry is added
// to _outgoingItems when removeItem is called and removed from
// _outgoingItems when the remove animation finishes.
int _indexToItemIndex(int index) {
int itemIndex = index;
for (final _ActiveItem item in _outgoingItems) {
if (item.itemIndex <= itemIndex) {
itemIndex += 1;
} else {
break;
}
}
return itemIndex;
}
int _itemIndexToIndex(int itemIndex) {
int index = itemIndex;
for (final _ActiveItem item in _outgoingItems) {
assert(item.itemIndex != itemIndex);
if (item.itemIndex < itemIndex) {
index -= 1;
} else {
break;
}
}
return index;
}
SliverChildDelegate _createDelegate() {
return SliverChildBuilderDelegate(
_itemBuilder,
childCount: _itemsCount,
findChildIndexCallback: widget.findChildIndexCallback == null
? null
: (Key key) {
final int? index = widget.findChildIndexCallback!(key);
return index != null ? _indexToItemIndex(index) : null;
},
);
}
Widget _itemBuilder(BuildContext context, int itemIndex) {
final _ActiveItem? outgoingItem = _activeItemAt(_outgoingItems, itemIndex);
if (outgoingItem != null) {
return outgoingItem.removedItemBuilder!(
context,
outgoingItem.controller!.view,
);
}
final _ActiveItem? incomingItem = _activeItemAt(_incomingItems, itemIndex);
final Animation<double> animation = incomingItem?.controller?.view ?? kAlwaysCompleteAnimation;
return widget.itemBuilder(
context,
_itemIndexToIndex(itemIndex),
animation,
);
}
/// Insert an item at [index] and start an animation that will be passed to
/// [SliverAnimatedGrid.itemBuilder] or [SliverAnimatedList.itemBuilder] when
/// the item is visible.
///
/// This method's semantics are the same as Dart's [List.insert] method: it
/// increases the length of the list of items by one and shifts
/// all items at or after [index] towards the end of the list of items.
void insertItem(int index, { Duration duration = _kDuration }) {
assert(index >= 0);
final int itemIndex = _indexToItemIndex(index);
assert(itemIndex >= 0 && itemIndex <= _itemsCount);
// Increment the incoming and outgoing item indices to account
// for the insertion.
for (final _ActiveItem item in _incomingItems) {
if (item.itemIndex >= itemIndex) {
item.itemIndex += 1;
}
}
for (final _ActiveItem item in _outgoingItems) {
if (item.itemIndex >= itemIndex) {
item.itemIndex += 1;
}
}
final AnimationController controller = AnimationController(
duration: duration,
vsync: this,
);
final _ActiveItem incomingItem = _ActiveItem.incoming(
controller,
itemIndex,
);
setState(() {
_incomingItems
..add(incomingItem)
..sort();
_itemsCount += 1;
});
controller.forward().then<void>((_) {
_removeActiveItemAt(_incomingItems, incomingItem.itemIndex)!.controller!.dispose();
});
}
/// Insert multiple items at [index] and start an animation that will be passed
/// to [AnimatedGrid.itemBuilder] or [AnimatedList.itemBuilder] when the items
/// are visible.
void insertAllItems(int index, int length, { Duration duration = _kDuration }) {
for (int i = 0; i < length; i++) {
insertItem(index + i, duration: duration);
}
}
/// Remove the item at [index] and start an animation that will be passed
/// to [builder] when the item is visible.
///
/// Items are removed immediately. After an item has been removed, its index
/// will no longer be passed to the subclass' [SliverAnimatedGrid.itemBuilder]
/// or [SliverAnimatedList.itemBuilder]. However the item will still appear
/// for [duration], and during that time [builder] must construct its widget
/// as needed.
///
/// This method's semantics are the same as Dart's [List.remove] method: it
/// decreases the length of items by one and shifts
/// all items at or before [index] towards the beginning of the list of items.
void removeItem(int index, AnimatedRemovedItemBuilder builder, { Duration duration = _kDuration }) {
assert(index >= 0);
final int itemIndex = _indexToItemIndex(index);
assert(itemIndex >= 0 && itemIndex < _itemsCount);
assert(_activeItemAt(_outgoingItems, itemIndex) == null);
final _ActiveItem? incomingItem = _removeActiveItemAt(_incomingItems, itemIndex);
final AnimationController controller =
incomingItem?.controller ?? AnimationController(duration: duration, value: 1.0, vsync: this);
final _ActiveItem outgoingItem = _ActiveItem.outgoing(controller, itemIndex, builder);
setState(() {
_outgoingItems
..add(outgoingItem)
..sort();
});
controller.reverse().then<void>((void value) {
_removeActiveItemAt(_outgoingItems, outgoingItem.itemIndex)!.controller!.dispose();
// Decrement the incoming and outgoing item indices to account
// for the removal.
for (final _ActiveItem item in _incomingItems) {
if (item.itemIndex > outgoingItem.itemIndex) {
item.itemIndex -= 1;
}
}
for (final _ActiveItem item in _outgoingItems) {
if (item.itemIndex > outgoingItem.itemIndex) {
item.itemIndex -= 1;
}
}
setState(() => _itemsCount -= 1);
});
}
/// Remove all the items and start an animation that will be passed to
/// `builder` when the items are visible.
///
/// Items are removed immediately. However, the
/// items will still appear for `duration` and during that time
/// `builder` must construct its widget as needed.
///
/// This method's semantics are the same as Dart's [List.clear] method: it
/// removes all the items in the list.
void removeAllItems(AnimatedRemovedItemBuilder builder, { Duration duration = _kDuration }) {
for (int i = _itemsCount - 1 ; i >= 0; i--) {
removeItem(i, builder, duration: duration);
}
}
}
| flutter/packages/flutter/lib/src/widgets/animated_scroll_view.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/animated_scroll_view.dart",
"repo_id": "flutter",
"token_count": 13832
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'image.dart';
// Examples can assume:
// late BuildContext context;
/// A widget that paints a [Decoration] either before or after its child paints.
///
/// [Container] insets its child by the widths of the borders; this widget does
/// not.
///
/// Commonly used with [BoxDecoration].
///
/// The [child] is not clipped. To clip a child to the shape of a particular
/// [ShapeDecoration], consider using a [ClipPath] widget.
///
/// {@tool snippet}
///
/// This sample shows a radial gradient that draws a moon on a night sky:
///
/// ```dart
/// const DecoratedBox(
/// decoration: BoxDecoration(
/// gradient: RadialGradient(
/// center: Alignment(-0.5, -0.6),
/// radius: 0.15,
/// colors: <Color>[
/// Color(0xFFEEEEEE),
/// Color(0xFF111133),
/// ],
/// stops: <double>[0.9, 1.0],
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Ink], which paints a [Decoration] on a [Material], allowing
/// [InkResponse] and [InkWell] splashes to paint over them.
/// * [DecoratedBoxTransition], the version of this class that animates on the
/// [decoration] property.
/// * [Decoration], which you can extend to provide other effects with
/// [DecoratedBox].
/// * [CustomPaint], another way to draw custom effects from the widget layer.
/// * [DecoratedSliver], which applies a [Decoration] to a sliver.
class DecoratedBox extends SingleChildRenderObjectWidget {
/// Creates a widget that paints a [Decoration].
///
/// By default the decoration paints behind the child.
const DecoratedBox({
super.key,
required this.decoration,
this.position = DecorationPosition.background,
super.child,
});
/// What decoration to paint.
///
/// Commonly a [BoxDecoration].
final Decoration decoration;
/// Whether to paint the box decoration behind or in front of the child.
final DecorationPosition position;
@override
RenderDecoratedBox createRenderObject(BuildContext context) {
return RenderDecoratedBox(
decoration: decoration,
position: position,
configuration: createLocalImageConfiguration(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderDecoratedBox renderObject) {
renderObject
..decoration = decoration
..configuration = createLocalImageConfiguration(context)
..position = position;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final String label = switch (position) {
DecorationPosition.background => 'bg',
DecorationPosition.foreground => 'fg',
};
properties.add(EnumProperty<DecorationPosition>('position', position, level: DiagnosticLevel.hidden));
properties.add(DiagnosticsProperty<Decoration>(label, decoration));
}
}
/// A convenience widget that combines common painting, positioning, and sizing
/// widgets.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=c1xLMaTUWCY}
///
/// A container first surrounds the child with [padding] (inflated by any
/// borders present in the [decoration]) and then applies additional
/// [constraints] to the padded extent (incorporating the `width` and `height`
/// as constraints, if either is non-null). The container is then surrounded by
/// additional empty space described from the [margin].
///
/// During painting, the container first applies the given [transform], then
/// paints the [decoration] to fill the padded extent, then it paints the child,
/// and finally paints the [foregroundDecoration], also filling the padded
/// extent.
///
/// Containers with no children try to be as big as possible unless the incoming
/// constraints are unbounded, in which case they try to be as small as
/// possible. Containers with children size themselves to their children. The
/// `width`, `height`, and [constraints] arguments to the constructor override
/// this.
///
/// By default, containers return false for all hit tests. If the [color]
/// property is specified, the hit testing is handled by [ColoredBox], which
/// always returns true. If the [decoration] or [foregroundDecoration] properties
/// are specified, hit testing is handled by [Decoration.hitTest].
///
/// ## Layout behavior
///
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// Since [Container] combines a number of other widgets each with their own
/// layout behavior, [Container]'s layout behavior is somewhat complicated.
///
/// Summary: [Container] tries, in order: to honor [alignment], to size itself
/// to the [child], to honor the `width`, `height`, and [constraints], to expand
/// to fit the parent, to be as small as possible.
///
/// More specifically:
///
/// If the widget has no child, no `height`, no `width`, no [constraints],
/// and the parent provides unbounded constraints, then [Container] tries to
/// size as small as possible.
///
/// If the widget has no child and no [alignment], but a `height`, `width`, or
/// [constraints] are provided, then the [Container] tries to be as small as
/// possible given the combination of those constraints and the parent's
/// constraints.
///
/// If the widget has no child, no `height`, no `width`, no [constraints], and
/// no [alignment], but the parent provides bounded constraints, then
/// [Container] expands to fit the constraints provided by the parent.
///
/// If the widget has an [alignment], and the parent provides unbounded
/// constraints, then the [Container] tries to size itself around the child.
///
/// If the widget has an [alignment], and the parent provides bounded
/// constraints, then the [Container] tries to expand to fit the parent, and
/// then positions the child within itself as per the [alignment].
///
/// Otherwise, the widget has a [child] but no `height`, no `width`, no
/// [constraints], and no [alignment], and the [Container] passes the
/// constraints from the parent to the child and sizes itself to match the
/// child.
///
/// The [margin] and [padding] properties also affect the layout, as described
/// in the documentation for those properties. (Their effects merely augment the
/// rules described above.) The [decoration] can implicitly increase the
/// [padding] (e.g. borders in a [BoxDecoration] contribute to the [padding]);
/// see [Decoration.padding].
///
/// ## Example
///
/// {@tool snippet}
/// This example shows a 48x48 amber square (placed inside a [Center] widget in
/// case the parent widget has its own opinions regarding the size that the
/// [Container] should take), with a margin so that it stays away from
/// neighboring widgets:
///
/// 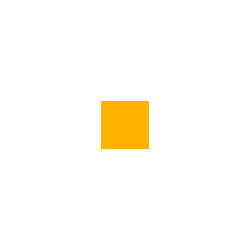
///
/// ```dart
/// Center(
/// child: Container(
/// margin: const EdgeInsets.all(10.0),
/// color: Colors.amber[600],
/// width: 48.0,
/// height: 48.0,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// This example shows how to use many of the features of [Container] at once.
/// The [constraints] are set to fit the font size plus ample headroom
/// vertically, while expanding horizontally to fit the parent. The [padding] is
/// used to make sure there is space between the contents and the text. The
/// [color] makes the box blue. The [alignment] causes the [child] to be
/// centered in the box. Finally, the [transform] applies a slight rotation to the
/// entire contraption to complete the effect.
///
/// 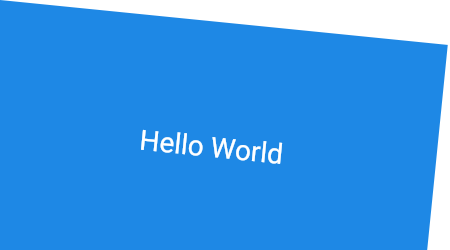
///
/// ```dart
/// Container(
/// constraints: BoxConstraints.expand(
/// height: Theme.of(context).textTheme.headlineMedium!.fontSize! * 1.1 + 200.0,
/// ),
/// padding: const EdgeInsets.all(8.0),
/// color: Colors.blue[600],
/// alignment: Alignment.center,
/// transform: Matrix4.rotationZ(0.1),
/// child: Text('Hello World',
/// style: Theme.of(context)
/// .textTheme
/// .headlineMedium!
/// .copyWith(color: Colors.white)),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedContainer], a variant that smoothly animates the properties when
/// they change.
/// * [Border], which has a sample which uses [Container] heavily.
/// * [Ink], which paints a [Decoration] on a [Material], allowing
/// [InkResponse] and [InkWell] splashes to paint over them.
/// * Cookbook: [Animate the properties of a container](https://flutter.dev/docs/cookbook/animation/animated-container)
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Container extends StatelessWidget {
/// Creates a widget that combines common painting, positioning, and sizing widgets.
///
/// The `height` and `width` values include the padding.
///
/// The `color` and `decoration` arguments cannot both be supplied, since
/// it would potentially result in the decoration drawing over the background
/// color. To supply a decoration with a color, use `decoration:
/// BoxDecoration(color: color)`.
Container({
super.key,
this.alignment,
this.padding,
this.color,
this.decoration,
this.foregroundDecoration,
double? width,
double? height,
BoxConstraints? constraints,
this.margin,
this.transform,
this.transformAlignment,
this.child,
this.clipBehavior = Clip.none,
}) : assert(margin == null || margin.isNonNegative),
assert(padding == null || padding.isNonNegative),
assert(decoration == null || decoration.debugAssertIsValid()),
assert(constraints == null || constraints.debugAssertIsValid()),
assert(decoration != null || clipBehavior == Clip.none),
assert(color == null || decoration == null,
'Cannot provide both a color and a decoration\n'
'To provide both, use "decoration: BoxDecoration(color: color)".',
),
constraints =
(width != null || height != null)
? constraints?.tighten(width: width, height: height)
?? BoxConstraints.tightFor(width: width, height: height)
: constraints;
/// The [child] contained by the container.
///
/// If null, and if the [constraints] are unbounded or also null, the
/// container will expand to fill all available space in its parent, unless
/// the parent provides unbounded constraints, in which case the container
/// will attempt to be as small as possible.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// Align the [child] within the container.
///
/// If non-null, the container will expand to fill its parent and position its
/// child within itself according to the given value. If the incoming
/// constraints are unbounded, then the child will be shrink-wrapped instead.
///
/// Ignored if [child] is null.
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry? alignment;
/// Empty space to inscribe inside the [decoration]. The [child], if any, is
/// placed inside this padding.
///
/// This padding is in addition to any padding inherent in the [decoration];
/// see [Decoration.padding].
final EdgeInsetsGeometry? padding;
/// The color to paint behind the [child].
///
/// This property should be preferred when the background is a simple color.
/// For other cases, such as gradients or images, use the [decoration]
/// property.
///
/// If the [decoration] is used, this property must be null. A background
/// color may still be painted by the [decoration] even if this property is
/// null.
final Color? color;
/// The decoration to paint behind the [child].
///
/// Use the [color] property to specify a simple solid color.
///
/// The [child] is not clipped to the decoration. To clip a child to the shape
/// of a particular [ShapeDecoration], consider using a [ClipPath] widget.
final Decoration? decoration;
/// The decoration to paint in front of the [child].
final Decoration? foregroundDecoration;
/// Additional constraints to apply to the child.
///
/// The constructor `width` and `height` arguments are combined with the
/// `constraints` argument to set this property.
///
/// The [padding] goes inside the constraints.
final BoxConstraints? constraints;
/// Empty space to surround the [decoration] and [child].
final EdgeInsetsGeometry? margin;
/// The transformation matrix to apply before painting the container.
final Matrix4? transform;
/// The alignment of the origin, relative to the size of the container, if [transform] is specified.
///
/// When [transform] is null, the value of this property is ignored.
///
/// See also:
///
/// * [Transform.alignment], which is set by this property.
final AlignmentGeometry? transformAlignment;
/// The clip behavior when [Container.decoration] is not null.
///
/// Defaults to [Clip.none]. Must be [Clip.none] if [decoration] is null.
///
/// If a clip is to be applied, the [Decoration.getClipPath] method
/// for the provided decoration must return a clip path. (This is not
/// supported by all decorations; the default implementation of that
/// method throws an [UnsupportedError].)
final Clip clipBehavior;
EdgeInsetsGeometry? get _paddingIncludingDecoration {
if (decoration == null) {
return padding;
}
final EdgeInsetsGeometry decorationPadding = decoration!.padding;
if (padding == null) {
return decorationPadding;
}
return padding!.add(decorationPadding);
}
@override
Widget build(BuildContext context) {
Widget? current = child;
if (child == null && (constraints == null || !constraints!.isTight)) {
current = LimitedBox(
maxWidth: 0.0,
maxHeight: 0.0,
child: ConstrainedBox(constraints: const BoxConstraints.expand()),
);
} else if (alignment != null) {
current = Align(alignment: alignment!, child: current);
}
final EdgeInsetsGeometry? effectivePadding = _paddingIncludingDecoration;
if (effectivePadding != null) {
current = Padding(padding: effectivePadding, child: current);
}
if (color != null) {
current = ColoredBox(color: color!, child: current);
}
if (clipBehavior != Clip.none) {
assert(decoration != null);
current = ClipPath(
clipper: _DecorationClipper(
textDirection: Directionality.maybeOf(context),
decoration: decoration!,
),
clipBehavior: clipBehavior,
child: current,
);
}
if (decoration != null) {
current = DecoratedBox(decoration: decoration!, child: current);
}
if (foregroundDecoration != null) {
current = DecoratedBox(
decoration: foregroundDecoration!,
position: DecorationPosition.foreground,
child: current,
);
}
if (constraints != null) {
current = ConstrainedBox(constraints: constraints!, child: current);
}
if (margin != null) {
current = Padding(padding: margin!, child: current);
}
if (transform != null) {
current = Transform(transform: transform!, alignment: transformAlignment, child: current);
}
return current!;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment, showName: false, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<Clip>('clipBehavior', clipBehavior, defaultValue: Clip.none));
if (color != null) {
properties.add(DiagnosticsProperty<Color>('bg', color));
} else {
properties.add(DiagnosticsProperty<Decoration>('bg', decoration, defaultValue: null));
}
properties.add(DiagnosticsProperty<Decoration>('fg', foregroundDecoration, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('margin', margin, defaultValue: null));
properties.add(ObjectFlagProperty<Matrix4>.has('transform', transform));
}
}
/// A clipper that uses [Decoration.getClipPath] to clip.
class _DecorationClipper extends CustomClipper<Path> {
_DecorationClipper({
TextDirection? textDirection,
required this.decoration,
}) : textDirection = textDirection ?? TextDirection.ltr;
final TextDirection textDirection;
final Decoration decoration;
@override
Path getClip(Size size) {
return decoration.getClipPath(Offset.zero & size, textDirection);
}
@override
bool shouldReclip(_DecorationClipper oldClipper) {
return oldClipper.decoration != decoration
|| oldClipper.textDirection != textDirection;
}
}
| flutter/packages/flutter/lib/src/widgets/container.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/container.dart",
"repo_id": "flutter",
"token_count": 5342
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/painting.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'binding.dart';
import 'focus_scope.dart';
import 'focus_traversal.dart';
import 'framework.dart';
/// Setting to true will cause extensive logging to occur when focus changes occur.
///
/// Can be used to debug focus issues: each time the focus changes, the focus
/// tree will be printed and requests for focus and other focus operations will
/// be logged.
bool debugFocusChanges = false;
// When using _focusDebug, always call it like so:
//
// assert(_focusDebug(() => 'Blah $foo'));
//
// It needs to be inside the assert in order to be removed in release mode, and
// it needs to use a closure to generate the string in order to avoid string
// interpolation when debugFocusChanges is false.
//
// It will throw a StateError if you try to call it when the app is in release
// mode.
bool _focusDebug(
String Function() messageFunc, [
Iterable<Object> Function()? detailsFunc,
]) {
if (kReleaseMode) {
throw StateError(
'_focusDebug was called in Release mode. It should always be wrapped in '
'an assert. Always call _focusDebug like so:\n'
r" assert(_focusDebug(() => 'Blah $foo'));"
);
}
if (!debugFocusChanges) {
return true;
}
debugPrint('FOCUS: ${messageFunc()}');
final Iterable<Object> details = detailsFunc?.call() ?? const <Object>[];
if (details.isNotEmpty) {
for (final Object detail in details) {
debugPrint(' $detail');
}
}
// Return true so that it can be used inside of an assert.
return true;
}
/// An enum that describes how to handle a key event handled by a
/// [FocusOnKeyCallback] or [FocusOnKeyEventCallback].
enum KeyEventResult {
/// The key event has been handled, and the event should not be propagated to
/// other key event handlers.
handled,
/// The key event has not been handled, and the event should continue to be
/// propagated to other key event handlers, even non-Flutter ones.
ignored,
/// The key event has not been handled, but the key event should not be
/// propagated to other key event handlers.
///
/// It will be returned to the platform embedding to be propagated to text
/// fields and non-Flutter key event handlers on the platform.
skipRemainingHandlers,
}
/// Combine the results returned by multiple [FocusOnKeyCallback]s or
/// [FocusOnKeyEventCallback]s.
///
/// If any callback returns [KeyEventResult.handled], the node considers the
/// message handled; otherwise, if any callback returns
/// [KeyEventResult.skipRemainingHandlers], the node skips the remaining
/// handlers without preventing the platform to handle; otherwise the node is
/// ignored.
KeyEventResult combineKeyEventResults(Iterable<KeyEventResult> results) {
bool hasSkipRemainingHandlers = false;
for (final KeyEventResult result in results) {
switch (result) {
case KeyEventResult.handled:
return KeyEventResult.handled;
case KeyEventResult.skipRemainingHandlers:
hasSkipRemainingHandlers = true;
case KeyEventResult.ignored:
break;
}
}
return hasSkipRemainingHandlers ?
KeyEventResult.skipRemainingHandlers :
KeyEventResult.ignored;
}
/// Signature of a callback used by [Focus.onKey] and [FocusScope.onKey]
/// to receive key events.
///
/// This kind of callback is deprecated and will be removed at a future date.
/// Use [FocusOnKeyEventCallback] and associated APIs instead.
///
/// The [node] is the node that received the event.
///
/// Returns a [KeyEventResult] that describes how, and whether, the key event
/// was handled.
@Deprecated(
'Use FocusOnKeyEventCallback instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
typedef FocusOnKeyCallback = KeyEventResult Function(FocusNode node, RawKeyEvent event);
/// Signature of a callback used by [Focus.onKeyEvent] and [FocusScope.onKeyEvent]
/// to receive key events.
///
/// The [node] is the node that received the event.
///
/// Returns a [KeyEventResult] that describes how, and whether, the key event
/// was handled.
typedef FocusOnKeyEventCallback = KeyEventResult Function(FocusNode node, KeyEvent event);
/// Signature of a callback used by [FocusManager.addEarlyKeyEventHandler] and
/// [FocusManager.addLateKeyEventHandler].
///
/// The `event` parameter is a [KeyEvent] that is being sent to the callback to
/// be handled.
///
/// The [KeyEventResult] return value indicates whether or not the event will
/// continue to be propagated. If the value returned is [KeyEventResult.handled]
/// or [KeyEventResult.skipRemainingHandlers], then the event will not continue
/// to be propagated.
typedef OnKeyEventCallback = KeyEventResult Function(KeyEvent event);
// Represents a pending autofocus request.
@immutable
class _Autofocus {
const _Autofocus({ required this.scope, required this.autofocusNode });
final FocusScopeNode scope;
final FocusNode autofocusNode;
// Applies the autofocus request, if the node is still attached to the
// original scope and the scope has no focused child.
//
// The widget tree is responsible for calling reparent/detach on attached
// nodes to keep their parent/manager information up-to-date, so here we can
// safely check if the scope/node involved in each autofocus request is
// still attached, and discard the ones which are no longer attached to the
// original manager.
void applyIfValid(FocusManager manager) {
final bool shouldApply = (scope.parent != null || identical(scope, manager.rootScope))
&& identical(scope._manager, manager)
&& scope.focusedChild == null
&& autofocusNode.ancestors.contains(scope);
if (shouldApply) {
assert(_focusDebug(() => 'Applying autofocus: $autofocusNode'));
autofocusNode._doRequestFocus(findFirstFocus: true);
} else {
assert(_focusDebug(() => 'Autofocus request discarded for node: $autofocusNode.'));
}
}
}
/// An attachment point for a [FocusNode].
///
/// Using a [FocusAttachment] is rarely needed, unless building something
/// akin to the [Focus] or [FocusScope] widgets from scratch.
///
/// Once created, a [FocusNode] must be attached to the widget tree by its
/// _host_ [StatefulWidget] via a [FocusAttachment] object. [FocusAttachment]s
/// are owned by the [StatefulWidget] that hosts a [FocusNode] or
/// [FocusScopeNode]. There can be multiple [FocusAttachment]s for each
/// [FocusNode], but the node will only ever be attached to one of them at a
/// time.
///
/// This attachment is created by calling [FocusNode.attach], usually from the
/// host widget's [State.initState] method. If the widget is updated to have a
/// different focus node, then the new node needs to be attached in
/// [State.didUpdateWidget], after calling [detach] on the previous
/// [FocusAttachment]. Once detached, the attachment is defunct and will no
/// longer make changes to the [FocusNode] through [reparent].
///
/// Without these attachment points, it would be possible for a focus node to
/// simultaneously be attached to more than one part of the widget tree during
/// the build stage.
class FocusAttachment {
/// A private constructor, because [FocusAttachment]s are only to be created
/// by [FocusNode.attach].
FocusAttachment._(this._node);
// The focus node that this attachment manages an attachment for. The node may
// not yet have a parent, or may have been detached from this attachment, so
// don't count on this node being in a usable state.
final FocusNode _node;
/// Returns true if the associated node is attached to this attachment.
///
/// It is possible to be attached to the widget tree, but not be placed in
/// the focus tree (i.e. to not have a parent yet in the focus tree).
bool get isAttached => _node._attachment == this;
/// Detaches the [FocusNode] this attachment point is associated with from the
/// focus tree, and disconnects it from this attachment point.
///
/// Calling [FocusNode.dispose] will also automatically detach the node.
void detach() {
assert(_focusDebug(() => 'Detaching node:', () => <Object>[_node, 'With enclosing scope ${_node.enclosingScope}']));
if (isAttached) {
if (_node.hasPrimaryFocus || (_node._manager != null && _node._manager!._markedForFocus == _node)) {
_node.unfocus(disposition: UnfocusDisposition.previouslyFocusedChild);
}
// This node is no longer in the tree, so shouldn't send notifications anymore.
_node._manager?._markDetached(_node);
_node._parent?._removeChild(_node);
_node._attachment = null;
assert(!_node.hasPrimaryFocus);
assert(_node._manager?._markedForFocus != _node);
}
assert(!isAttached);
}
/// Ensures that the [FocusNode] attached at this attachment point has the
/// proper parent node, changing it if necessary.
///
/// If given, ensures that the given [parent] node is the parent of the node
/// that is attached at this attachment point, changing it if necessary.
/// However, it is usually not necessary to supply an explicit parent, since
/// [reparent] will use [Focus.of] to determine the correct parent node for
/// the context given in [FocusNode.attach].
///
/// If [isAttached] is false, then calling this method does nothing.
///
/// Should be called whenever the associated widget is rebuilt in order to
/// maintain the focus hierarchy.
///
/// A [StatefulWidget] that hosts a [FocusNode] should call this method on the
/// node it hosts during its [State.build] or [State.didChangeDependencies]
/// methods in case the widget is moved from one location in the tree to
/// another location that has a different [FocusScope] or context.
///
/// The optional [parent] argument must be supplied when not using [Focus] and
/// [FocusScope] widgets to build the focus tree, or if there is a need to
/// supply the parent explicitly (which are both uncommon).
void reparent({FocusNode? parent}) {
if (isAttached) {
assert(_node.context != null);
parent ??= Focus.maybeOf(_node.context!, scopeOk: true);
parent ??= _node.context!.owner!.focusManager.rootScope;
parent._reparent(_node);
}
}
}
/// Describe what should happen after [FocusNode.unfocus] is called.
///
/// See also:
///
/// * [FocusNode.unfocus], which takes this as its `disposition` parameter.
enum UnfocusDisposition {
/// Focus the nearest focusable enclosing scope of this node, but do not
/// descend to locate the leaf [FocusScopeNode.focusedChild] the way
/// [previouslyFocusedChild] does.
///
/// Focusing the scope in this way clears the [FocusScopeNode.focusedChild]
/// history for the enclosing scope when it receives focus. Because of this,
/// calling a traversal method like [FocusNode.nextFocus] after unfocusing
/// will cause the [FocusTraversalPolicy] to pick the node it thinks should be
/// first in the scope.
///
/// This is the default disposition for [FocusNode.unfocus].
scope,
/// Focus the previously focused child of the nearest focusable enclosing
/// scope of this node.
///
/// If there is no previously focused child, then this is equivalent to
/// using the [scope] disposition.
///
/// Unfocusing with this disposition will cause [FocusNode.unfocus] to walk up
/// the tree to the nearest focusable enclosing scope, then start to walk down
/// the tree, looking for a focused child at its
/// [FocusScopeNode.focusedChild].
///
/// If the [FocusScopeNode.focusedChild] is a scope, then look for its
/// [FocusScopeNode.focusedChild], and so on, finding the leaf
/// [FocusScopeNode.focusedChild] that is not a scope, or, failing that, a
/// leaf scope that has no focused child.
previouslyFocusedChild,
}
/// An object that can be used by a stateful widget to obtain the keyboard focus
/// and to handle keyboard events.
///
/// _Please see the [Focus] and [FocusScope] widgets, which are utility widgets
/// that manage their own [FocusNode]s and [FocusScopeNode]s, respectively. If
/// they aren't appropriate, [FocusNode]s can be managed directly, but doing this
/// is rare._
///
/// [FocusNode]s are persistent objects that form a _focus tree_ that is a
/// representation of the widgets in the hierarchy that are interested in focus.
/// A focus node might need to be created if it is passed in from an ancestor of
/// a [Focus] widget to control the focus of the children from the ancestor, or
/// a widget might need to host one if the widget subsystem is not being used,
/// or if the [Focus] and [FocusScope] widgets provide insufficient control.
///
/// [FocusNode]s are organized into _scopes_ (see [FocusScopeNode]), which form
/// sub-trees of nodes that restrict traversal to a group of nodes. Within a
/// scope, the most recent nodes to have focus are remembered, and if a node is
/// focused and then unfocused, the previous node receives focus again.
///
/// The focus node hierarchy can be traversed using the [parent], [children],
/// [ancestors] and [descendants] accessors.
///
/// [FocusNode]s are [ChangeNotifier]s, so a listener can be registered to
/// receive a notification when the focus changes. Listeners will also be
/// notified when [skipTraversal], [canRequestFocus], [descendantsAreFocusable],
/// and [descendantsAreTraversable] properties are updated. If the [Focus] and
/// [FocusScope] widgets are being used to manage the nodes, consider
/// establishing an [InheritedWidget] dependency on them by calling [Focus.of]
/// or [FocusScope.of] instead. [FocusNode.hasFocus] can also be used to
/// establish a similar dependency, especially if all that is needed is to
/// determine whether or not the widget is focused at build time.
///
/// To see the focus tree in the debug console, call [debugDumpFocusTree]. To
/// get the focus tree as a string, call [debugDescribeFocusTree].
///
/// {@template flutter.widgets.FocusNode.lifecycle}
/// ## Lifecycle
///
/// There are several actors involved in the lifecycle of a
/// [FocusNode]/[FocusScopeNode]. They are created and disposed by their
/// _owner_, attached, detached, and re-parented using a [FocusAttachment] by
/// their _host_ (which must be owned by the [State] of a [StatefulWidget]), and
/// they are managed by the [FocusManager]. Different parts of the [FocusNode]
/// API are intended for these different actors.
///
/// [FocusNode]s (and hence [FocusScopeNode]s) are persistent objects that form
/// part of a _focus tree_ that is a sparse representation of the widgets in the
/// hierarchy that are interested in receiving keyboard events. They must be
/// managed like other persistent state, which is typically done by a
/// [StatefulWidget] that owns the node. A stateful widget that owns a focus
/// scope node must call [dispose] from its [State.dispose] method.
///
/// Once created, a [FocusNode] must be attached to the widget tree via a
/// [FocusAttachment] object. This attachment is created by calling [attach],
/// usually from the [State.initState] method. If the hosting widget is updated
/// to have a different focus node, then the updated node needs to be attached
/// in [State.didUpdateWidget], after calling [FocusAttachment.detach] on the
/// previous [FocusAttachment].
///
/// Because [FocusNode]s form a sparse representation of the widget tree, they
/// must be updated whenever the widget tree is rebuilt. This is done by calling
/// [FocusAttachment.reparent], usually from the [State.build] or
/// [State.didChangeDependencies] methods of the widget that represents the
/// focused region, so that the [BuildContext] assigned to the [FocusScopeNode]
/// can be tracked (the context is used to obtain the [RenderObject], from which
/// the geometry of focused regions can be determined).
///
/// Creating a [FocusNode] each time [State.build] is invoked will cause the
/// focus to be lost each time the widget is built, which is usually not desired
/// behavior (call [unfocus] if losing focus is desired).
///
/// If, as is common, the hosting [StatefulWidget] is also the owner of the
/// focus node, then it will also call [dispose] from its [State.dispose] (in
/// which case the [FocusAttachment.detach] may be skipped, since dispose will
/// automatically detach). If another object owns the focus node, then it must
/// call [dispose] when the node is done being used.
/// {@endtemplate}
///
/// {@template flutter.widgets.FocusNode.keyEvents}
/// ## Key Event Propagation
///
/// The [FocusManager] receives key events from [HardwareKeyboard] and will pass
/// them to the focused nodes. It starts with the node with the primary focus,
/// and will call the [onKeyEvent] callback for that node. If the callback
/// returns [KeyEventResult.ignored], indicating that it did not handle the
/// event, the [FocusManager] will move to the parent of that node and call its
/// [onKeyEvent]. If that [onKeyEvent] returns [KeyEventResult.handled], then it
/// will stop propagating the event. If it reaches the root [FocusScopeNode],
/// [FocusManager.rootScope], the event is discarded.
/// {@endtemplate}
///
/// ## Focus Traversal
///
/// The term _traversal_, sometimes called _tab traversal_, refers to moving the
/// focus from one widget to the next in a particular order (also sometimes
/// referred to as the _tab order_, since the TAB key is often bound to the
/// action to move to the next widget).
///
/// To give focus to the logical _next_ or _previous_ widget in the UI, call the
/// [nextFocus] or [previousFocus] methods. To give the focus to a widget in a
/// particular direction, call the [focusInDirection] method.
///
/// The policy for what the _next_ or _previous_ widget is, or the widget in a
/// particular direction, is determined by the [FocusTraversalPolicy] in force.
///
/// The ambient policy is determined by looking up the widget hierarchy for a
/// [FocusTraversalGroup] widget, and obtaining the focus traversal policy from
/// it. Different focus nodes can inherit difference policies, so part of the
/// app can go in a predefined order (using [OrderedTraversalPolicy]), and part
/// can go in reading order (using [ReadingOrderTraversalPolicy]), depending
/// upon the use case.
///
/// Predefined policies include [WidgetOrderTraversalPolicy],
/// [ReadingOrderTraversalPolicy], [OrderedTraversalPolicy], and
/// [DirectionalFocusTraversalPolicyMixin], but custom policies can be built
/// based upon these policies. See [FocusTraversalPolicy] for more information.
///
/// {@tool dartpad}
/// This example shows how a FocusNode should be managed if not using the
/// [Focus] or [FocusScope] widgets. See the [Focus] widget for a similar
/// example using [Focus] and [FocusScope] widgets.
///
/// ** See code in examples/api/lib/widgets/focus_manager/focus_node.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Focus], a widget that manages a [FocusNode] and provides access to focus
/// information and actions to its descendant widgets.
/// * [FocusTraversalGroup], a widget used to group together and configure the
/// focus traversal policy for a widget subtree.
/// * [FocusManager], a singleton that manages the primary focus and distributes
/// key events to focused nodes.
/// * [FocusTraversalPolicy], a class used to determine how to move the focus to
/// other nodes.
class FocusNode with DiagnosticableTreeMixin, ChangeNotifier {
/// Creates a focus node.
///
/// The [debugLabel] is ignored on release builds.
///
/// To receive key events that focuses on this node, pass a listener to
/// `onKeyEvent`.
FocusNode({
String? debugLabel,
@Deprecated(
'Use onKeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
this.onKey,
this.onKeyEvent,
bool skipTraversal = false,
bool canRequestFocus = true,
bool descendantsAreFocusable = true,
bool descendantsAreTraversable = true,
}) : _skipTraversal = skipTraversal,
_canRequestFocus = canRequestFocus,
_descendantsAreFocusable = descendantsAreFocusable,
_descendantsAreTraversable = descendantsAreTraversable {
// Set it via the setter so that it does nothing on release builds.
this.debugLabel = debugLabel;
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// If true, tells the focus traversal policy to skip over this node for
/// purposes of the traversal algorithm.
///
/// This may be used to place nodes in the focus tree that may be focused, but
/// not traversed, allowing them to receive key events as part of the focus
/// chain, but not be traversed to via focus traversal.
///
/// This is different from [canRequestFocus] because it only implies that the
/// node can't be reached via traversal, not that it can't be focused. It may
/// still be focused explicitly.
bool get skipTraversal {
if (_skipTraversal) {
return true;
}
for (final FocusNode ancestor in ancestors) {
if (!ancestor.descendantsAreTraversable) {
return true;
}
}
return false;
}
bool _skipTraversal;
set skipTraversal(bool value) {
if (value != _skipTraversal) {
_skipTraversal = value;
_manager?._markPropertiesChanged(this);
}
}
/// If true, this focus node may request the primary focus.
///
/// Defaults to true. Set to false if you want this node to do nothing when
/// [requestFocus] is called on it.
///
/// If set to false on a [FocusScopeNode], will cause all of the children of
/// the scope node to not be focusable.
///
/// If set to false on a [FocusNode], it will not affect the focusability of
/// children of the node.
///
/// The [hasFocus] member can still return true if this node is the ancestor
/// of a node with primary focus.
///
/// This is different than [skipTraversal] because [skipTraversal] still
/// allows the node to be focused, just not traversed to via the
/// [FocusTraversalPolicy].
///
/// Setting [canRequestFocus] to false implies that the node will also be
/// skipped for traversal purposes.
///
/// See also:
///
/// * [FocusTraversalGroup], a widget used to group together and configure the
/// focus traversal policy for a widget subtree.
/// * [FocusTraversalPolicy], a class that can be extended to describe a
/// traversal policy.
bool get canRequestFocus => _canRequestFocus && ancestors.every(_allowDescendantsToBeFocused);
static bool _allowDescendantsToBeFocused(FocusNode ancestor) => ancestor.descendantsAreFocusable;
bool _canRequestFocus;
@mustCallSuper
set canRequestFocus(bool value) {
if (value != _canRequestFocus) {
// Have to set this first before unfocusing, since it checks this to cull
// unfocusable, previously-focused children.
_canRequestFocus = value;
if (hasFocus && !value) {
unfocus(disposition: UnfocusDisposition.previouslyFocusedChild);
}
_manager?._markPropertiesChanged(this);
}
}
/// If false, will disable focus for all of this node's descendants.
///
/// Defaults to true. Does not affect focusability of this node: for that,
/// use [canRequestFocus].
///
/// If any descendants are focused when this is set to false, they will be
/// unfocused. When [descendantsAreFocusable] is set to true again, they will
/// not be refocused, although they will be able to accept focus again.
///
/// Does not affect the value of [canRequestFocus] on the descendants.
///
/// If a descendant node loses focus when this value is changed, the focus
/// will move to the scope enclosing this node.
///
/// See also:
///
/// * [ExcludeFocus], a widget that uses this property to conditionally
/// exclude focus for a subtree.
/// * [descendantsAreTraversable], which makes this widget's descendants
/// untraversable.
/// * [ExcludeFocusTraversal], a widget that conditionally excludes focus
/// traversal for a subtree.
/// * [Focus], a widget that exposes this setting as a parameter.
/// * [FocusTraversalGroup], a widget used to group together and configure
/// the focus traversal policy for a widget subtree that also has a
/// `descendantsAreFocusable` parameter that prevents its children from
/// being focused.
bool get descendantsAreFocusable => _descendantsAreFocusable;
bool _descendantsAreFocusable;
@mustCallSuper
set descendantsAreFocusable(bool value) {
if (value == _descendantsAreFocusable) {
return;
}
// Set _descendantsAreFocusable before unfocusing, so the scope won't try
// and focus any of the children here again if it is false.
_descendantsAreFocusable = value;
if (!value && hasFocus) {
unfocus(disposition: UnfocusDisposition.previouslyFocusedChild);
}
_manager?._markPropertiesChanged(this);
}
/// If false, tells the focus traversal policy to skip over for all of this
/// node's descendants for purposes of the traversal algorithm.
///
/// Defaults to true. Does not affect the focus traversal of this node: for
/// that, use [skipTraversal].
///
/// Does not affect the value of [FocusNode.skipTraversal] on the
/// descendants. Does not affect focusability of the descendants.
///
/// See also:
///
/// * [ExcludeFocusTraversal], a widget that uses this property to conditionally
/// exclude focus traversal for a subtree.
/// * [descendantsAreFocusable], which makes this widget's descendants
/// unfocusable.
/// * [ExcludeFocus], a widget that conditionally excludes focus for a subtree.
/// * [FocusTraversalGroup], a widget used to group together and configure
/// the focus traversal policy for a widget subtree that also has an
/// `descendantsAreFocusable` parameter that prevents its children from
/// being focused.
bool get descendantsAreTraversable => _descendantsAreTraversable;
bool _descendantsAreTraversable;
@mustCallSuper
set descendantsAreTraversable(bool value) {
if (value != _descendantsAreTraversable) {
_descendantsAreTraversable = value;
_manager?._markPropertiesChanged(this);
}
}
/// The context that was supplied to [attach].
///
/// This is typically the context for the widget that is being focused, as it
/// is used to determine the bounds of the widget.
BuildContext? get context => _context;
BuildContext? _context;
/// Called if this focus node receives a key event while focused (i.e. when
/// [hasFocus] returns true).
///
/// This property is deprecated and will be removed at a future date. Use
/// [onKeyEvent] instead.
///
/// This is a legacy API based on [RawKeyEvent] and will be deprecated in the
/// future. Prefer [onKeyEvent] instead.
///
/// {@macro flutter.widgets.FocusNode.keyEvents}
@Deprecated(
'Use onKeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
FocusOnKeyCallback? onKey;
/// Called if this focus node receives a key event while focused (i.e. when
/// [hasFocus] returns true).
///
/// {@macro flutter.widgets.FocusNode.keyEvents}
FocusOnKeyEventCallback? onKeyEvent;
FocusManager? _manager;
List<FocusNode>? _ancestors;
List<FocusNode>? _descendants;
bool _hasKeyboardToken = false;
/// Returns the parent node for this object.
///
/// All nodes except for the root [FocusScopeNode] ([FocusManager.rootScope])
/// will be given a parent when they are added to the focus tree, which is
/// done using [FocusAttachment.reparent].
FocusNode? get parent => _parent;
FocusNode? _parent;
/// An iterator over the children of this node.
Iterable<FocusNode> get children => _children;
final List<FocusNode> _children = <FocusNode>[];
/// An iterator over the children that are allowed to be traversed by the
/// [FocusTraversalPolicy].
///
/// Returns the list of focusable, traversable children of this node,
/// regardless of those settings on this focus node. Will return an empty
/// iterable if [descendantsAreFocusable] is false.
///
/// See also
///
/// * [traversalDescendants], which traverses all of the node's descendants,
/// not just the immediate children.
Iterable<FocusNode> get traversalChildren {
if (!descendantsAreFocusable) {
return const Iterable<FocusNode>.empty();
}
return children.where(
(FocusNode node) => !node.skipTraversal && node.canRequestFocus,
);
}
/// A debug label that is used for diagnostic output.
///
/// Will always return null in release builds.
String? get debugLabel => _debugLabel;
String? _debugLabel;
set debugLabel(String? value) {
assert(() {
// Only set the value in debug builds.
_debugLabel = value;
return true;
}());
}
FocusAttachment? _attachment;
/// An [Iterable] over the hierarchy of children below this one, in
/// depth-first order.
Iterable<FocusNode> get descendants {
if (_descendants == null) {
final List<FocusNode> result = <FocusNode>[];
for (final FocusNode child in _children) {
result.addAll(child.descendants);
result.add(child);
}
_descendants = result;
}
return _descendants!;
}
/// Returns all descendants which do not have the [skipTraversal] and do have
/// the [canRequestFocus] flag set.
Iterable<FocusNode> get traversalDescendants {
if (!descendantsAreFocusable) {
return const Iterable<FocusNode>.empty();
}
return descendants.where((FocusNode node) => !node.skipTraversal && node.canRequestFocus);
}
/// An [Iterable] over the ancestors of this node.
///
/// Iterates the ancestors of this node starting at the parent and iterating
/// over successively more remote ancestors of this node, ending at the root
/// [FocusScopeNode] ([FocusManager.rootScope]).
Iterable<FocusNode> get ancestors {
if (_ancestors == null) {
final List<FocusNode> result = <FocusNode>[];
FocusNode? parent = _parent;
while (parent != null) {
result.add(parent);
parent = parent._parent;
}
_ancestors = result;
}
return _ancestors!;
}
/// Whether this node has input focus.
///
/// A [FocusNode] has focus when it is an ancestor of a node that returns true
/// from [hasPrimaryFocus], or it has the primary focus itself.
///
/// The [hasFocus] accessor is different from [hasPrimaryFocus] in that
/// [hasFocus] is true if the node is anywhere in the focus chain, but for
/// [hasPrimaryFocus] the node must to be at the end of the chain to return
/// true.
///
/// A node that returns true for [hasFocus] will receive key events if none of
/// its focused descendants returned true from their [onKey] handler.
///
/// This object is a [ChangeNotifier], and notifies its [Listenable] listeners
/// (registered via [addListener]) whenever this value changes.
///
/// See also:
///
/// * [Focus.isAt], which is a static method that will return the focus
/// state of the nearest ancestor [Focus] widget's focus node.
bool get hasFocus => hasPrimaryFocus || (_manager?.primaryFocus?.ancestors.contains(this) ?? false);
/// Returns true if this node currently has the application-wide input focus.
///
/// A [FocusNode] has the primary focus when the node is focused in its
/// nearest ancestor [FocusScopeNode] and [hasFocus] is true for all its
/// ancestor nodes, but none of its descendants.
///
/// This is different from [hasFocus] in that [hasFocus] is true if the node
/// is anywhere in the focus chain, but here the node has to be at the end of
/// the chain to return true.
///
/// A node that returns true for [hasPrimaryFocus] will be the first node to
/// receive key events through its [onKey] handler.
///
/// This object notifies its listeners whenever this value changes.
bool get hasPrimaryFocus => _manager?.primaryFocus == this;
/// Returns the [FocusHighlightMode] that is currently in effect for this node.
FocusHighlightMode get highlightMode => FocusManager.instance.highlightMode;
/// Returns the nearest enclosing scope node above this node, including
/// this node, if it's a scope.
///
/// Returns null if no scope is found.
///
/// Use [enclosingScope] to look for scopes above this node.
FocusScopeNode? get nearestScope => enclosingScope;
FocusScopeNode? _enclosingScope;
void _clearEnclosingScopeCache() {
final FocusScopeNode? cachedScope = _enclosingScope;
if (cachedScope == null) {
return;
}
_enclosingScope = null;
if (children.isNotEmpty) {
for (final FocusNode child in children) {
if (identical(cachedScope, child._enclosingScope)) {
child._clearEnclosingScopeCache();
}
}
}
}
/// Returns the nearest enclosing scope node above this node, or null if the
/// node has not yet be added to the focus tree.
///
/// If this node is itself a scope, this will only return ancestors of this
/// scope.
///
/// Use [nearestScope] to start at this node instead of above it.
FocusScopeNode? get enclosingScope {
final FocusScopeNode? enclosingScope = _enclosingScope ??= parent?.nearestScope;
assert(enclosingScope == parent?.nearestScope, '$this has invalid scope cache: $_enclosingScope != ${parent?.nearestScope}');
return enclosingScope;
}
/// Returns the size of the attached widget's [RenderObject], in logical
/// units.
///
/// Size is the size of the transformed widget in global coordinates.
Size get size => rect.size;
/// Returns the global offset to the upper left corner of the attached
/// widget's [RenderObject], in logical units.
///
/// Offset is the offset of the transformed widget in global coordinates.
Offset get offset {
assert(
context != null,
"Tried to get the offset of a focus node that didn't have its context set yet.\n"
'The context needs to be set before trying to evaluate traversal policies. '
'Setting the context is typically done with the attach method.',
);
final RenderObject object = context!.findRenderObject()!;
return MatrixUtils.transformPoint(object.getTransformTo(null), object.semanticBounds.topLeft);
}
/// Returns the global rectangle of the attached widget's [RenderObject], in
/// logical units.
///
/// Rect is the rectangle of the transformed widget in global coordinates.
Rect get rect {
assert(
context != null,
"Tried to get the bounds of a focus node that didn't have its context set yet.\n"
'The context needs to be set before trying to evaluate traversal policies. '
'Setting the context is typically done with the attach method.',
);
final RenderObject object = context!.findRenderObject()!;
final Offset topLeft = MatrixUtils.transformPoint(object.getTransformTo(null), object.semanticBounds.topLeft);
final Offset bottomRight = MatrixUtils.transformPoint(object.getTransformTo(null), object.semanticBounds.bottomRight);
return Rect.fromLTRB(topLeft.dx, topLeft.dy, bottomRight.dx, bottomRight.dy);
}
/// Removes the focus on this node by moving the primary focus to another node.
///
/// This method removes focus from a node that has the primary focus, cancels
/// any outstanding requests to focus it, while setting the primary focus to
/// another node according to the `disposition`.
///
/// It is safe to call regardless of whether this node has ever requested
/// focus or not. If this node doesn't have focus or primary focus, nothing
/// happens.
///
/// The `disposition` argument determines which node will receive primary
/// focus after this one loses it.
///
/// If `disposition` is set to [UnfocusDisposition.scope] (the default), then
/// the previously focused node history of the enclosing scope will be
/// cleared, and the primary focus will be moved to the nearest enclosing
/// scope ancestor that is enabled for focus, ignoring the
/// [FocusScopeNode.focusedChild] for that scope.
///
/// If `disposition` is set to [UnfocusDisposition.previouslyFocusedChild],
/// then this node will be removed from the previously focused list in the
/// [enclosingScope], and the focus will be moved to the previously focused
/// node of the [enclosingScope], which (if it is a scope itself), will find
/// its focused child, etc., until a leaf focus node is found. If there is no
/// previously focused child, then the scope itself will receive focus, as if
/// [UnfocusDisposition.scope] were specified.
///
/// If you want this node to lose focus and the focus to move to the next or
/// previous node in the enclosing [FocusTraversalGroup], call [nextFocus] or
/// [previousFocus] instead of calling [unfocus].
///
/// {@tool dartpad}
/// This example shows the difference between the different [UnfocusDisposition]
/// values for [unfocus].
///
/// Try setting focus on the four text fields by selecting them, and then
/// select "UNFOCUS" to see what happens when the current
/// [FocusManager.primaryFocus] is unfocused.
///
/// Try pressing the TAB key after unfocusing to see what the next widget
/// chosen is.
///
/// ** See code in examples/api/lib/widgets/focus_manager/focus_node.unfocus.0.dart **
/// {@end-tool}
void unfocus({
UnfocusDisposition disposition = UnfocusDisposition.scope,
}) {
if (!hasFocus && (_manager == null || _manager!._markedForFocus != this)) {
return;
}
FocusScopeNode? scope = enclosingScope;
if (scope == null) {
// If the scope is null, then this is either the root node, or a node that
// is not yet in the tree, neither of which do anything when unfocused.
return;
}
switch (disposition) {
case UnfocusDisposition.scope:
// If it can't request focus, then don't modify its focused children.
if (scope.canRequestFocus) {
// Clearing the focused children here prevents re-focusing the node
// that we just unfocused if we immediately hit "next" after
// unfocusing, and also prevents choosing to refocus the next-to-last
// focused child if unfocus is called more than once.
scope._focusedChildren.clear();
}
while (!scope!.canRequestFocus) {
scope = scope.enclosingScope ?? _manager?.rootScope;
}
scope._doRequestFocus(findFirstFocus: false);
case UnfocusDisposition.previouslyFocusedChild:
// Select the most recent focused child from the nearest focusable scope
// and focus that. If there isn't one, focus the scope itself.
if (scope.canRequestFocus) {
scope._focusedChildren.remove(this);
}
while (!scope!.canRequestFocus) {
scope.enclosingScope?._focusedChildren.remove(scope);
scope = scope.enclosingScope ?? _manager?.rootScope;
}
scope._doRequestFocus(findFirstFocus: true);
}
assert(_focusDebug(() => 'Unfocused node:', () => <Object>['primary focus was $this', 'next focus will be ${_manager?._markedForFocus}']));
}
/// Removes the keyboard token from this focus node if it has one.
///
/// This mechanism helps distinguish between an input control gaining focus by
/// default and gaining focus as a result of an explicit user action.
///
/// When a focus node requests the focus (either via
/// [FocusScopeNode.requestFocus] or [FocusScopeNode.autofocus]), the focus
/// node receives a keyboard token if it does not already have one. Later,
/// when the focus node becomes focused, the widget that manages the
/// [TextInputConnection] should show the keyboard (i.e. call
/// [TextInputConnection.show]) only if it successfully consumes the keyboard
/// token from the focus node.
///
/// Returns true if this method successfully consumes the keyboard token.
bool consumeKeyboardToken() {
if (!_hasKeyboardToken) {
return false;
}
_hasKeyboardToken = false;
return true;
}
// Marks the node as being the next to be focused, meaning that it will become
// the primary focus and notify listeners of a focus change the next time
// focus is resolved by the manager. If something else calls _markNextFocus
// before then, then that node will become the next focus instead of the
// previous one.
void _markNextFocus(FocusNode newFocus) {
if (_manager != null) {
// If we have a manager, then let it handle the focus change.
_manager!._markNextFocus(this);
return;
}
// If we don't have a manager, then change the focus locally.
newFocus._setAsFocusedChildForScope();
newFocus._notify();
if (newFocus != this) {
_notify();
}
}
// Removes the given FocusNode and its children as a child of this node.
@mustCallSuper
void _removeChild(FocusNode node, {bool removeScopeFocus = true}) {
assert(_children.contains(node), "Tried to remove a node that wasn't a child.");
assert(node._parent == this);
assert(node._manager == _manager);
if (removeScopeFocus) {
final FocusScopeNode? nodeScope = node.enclosingScope;
if (nodeScope != null) {
nodeScope._focusedChildren.remove(node);
node.descendants.where((FocusNode descendant) {
return descendant.enclosingScope == nodeScope;
}).forEach(nodeScope._focusedChildren.remove);
}
}
node._parent = null;
node._clearEnclosingScopeCache();
_children.remove(node);
for (final FocusNode ancestor in ancestors) {
ancestor._descendants = null;
}
_descendants = null;
assert(_manager == null || !_manager!.rootScope.descendants.contains(node));
}
void _updateManager(FocusManager? manager) {
_manager = manager;
for (final FocusNode descendant in descendants) {
descendant._manager = manager;
descendant._ancestors = null;
}
}
// Used by FocusAttachment.reparent to perform the actual parenting operation.
@mustCallSuper
void _reparent(FocusNode child) {
assert(child != this, 'Tried to make a child into a parent of itself.');
if (child._parent == this) {
assert(_children.contains(child), "Found a node that says it's a child, but doesn't appear in the child list.");
// The child is already a child of this parent.
return;
}
assert(_manager == null || child != _manager!.rootScope, "Reparenting the root node isn't allowed.");
assert(!ancestors.contains(child), 'The supplied child is already an ancestor of this node. Loops are not allowed.');
final FocusScopeNode? oldScope = child.enclosingScope;
final bool hadFocus = child.hasFocus;
child._parent?._removeChild(child, removeScopeFocus: oldScope != nearestScope);
_children.add(child);
child._parent = this;
child._ancestors = null;
child._updateManager(_manager);
for (final FocusNode ancestor in child.ancestors) {
ancestor._descendants = null;
}
if (hadFocus) {
// Update the focus chain for the current focus without changing it.
_manager?.primaryFocus?._setAsFocusedChildForScope();
}
if (oldScope != null && child.context != null && child.enclosingScope != oldScope) {
FocusTraversalGroup.maybeOf(child.context!)?.changedScope(node: child, oldScope: oldScope);
}
if (child._requestFocusWhenReparented) {
child._doRequestFocus(findFirstFocus: true);
child._requestFocusWhenReparented = false;
}
}
/// Called by the _host_ [StatefulWidget] to attach a [FocusNode] to the
/// widget tree.
///
/// In order to attach a [FocusNode] to the widget tree, call [attach],
/// typically from the [StatefulWidget]'s [State.initState] method.
///
/// If the focus node in the host widget is swapped out, the new node will
/// need to be attached. [FocusAttachment.detach] should be called on the old
/// node, and then [attach] called on the new node. This typically happens in
/// the [State.didUpdateWidget] method.
///
/// To receive key events that focuses on this node, pass a listener to
/// `onKeyEvent`.
@mustCallSuper
FocusAttachment attach(
BuildContext? context, {
FocusOnKeyEventCallback? onKeyEvent,
@Deprecated(
'Use onKeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
FocusOnKeyCallback? onKey,
}) {
_context = context;
this.onKey = onKey ?? this.onKey;
this.onKeyEvent = onKeyEvent ?? this.onKeyEvent;
_attachment = FocusAttachment._(this);
return _attachment!;
}
@override
void dispose() {
// Detaching will also unfocus and clean up the manager's data structures.
_attachment?.detach();
super.dispose();
}
@mustCallSuper
void _notify() {
if (_parent == null) {
// no longer part of the tree, so don't notify.
return;
}
if (hasPrimaryFocus) {
_setAsFocusedChildForScope();
}
notifyListeners();
}
/// Requests the primary focus for this node, or for a supplied [node], which
/// will also give focus to its [ancestors].
///
/// If called without a node, request focus for this node. If the node hasn't
/// been added to the focus tree yet, then defer the focus request until it
/// is, allowing newly created widgets to request focus as soon as they are
/// added.
///
/// If the given [node] is not yet a part of the focus tree, then this method
/// will add the [node] as a child of this node before requesting focus.
///
/// If the given [node] is a [FocusScopeNode] and that focus scope node has a
/// non-null [FocusScopeNode.focusedChild], then request the focus for the
/// focused child. This process is recursive and continues until it encounters
/// either a focus scope node with a null focused child or an ordinary
/// (non-scope) [FocusNode] is found.
///
/// The node is notified that it has received the primary focus in a
/// microtask, so notification may lag the request by up to one frame.
void requestFocus([FocusNode? node]) {
if (node != null) {
if (node._parent == null) {
_reparent(node);
}
assert(node.ancestors.contains(this), 'Focus was requested for a node that is not a descendant of the scope from which it was requested.');
node._doRequestFocus(findFirstFocus: true);
return;
}
_doRequestFocus(findFirstFocus: true);
}
// This is overridden in FocusScopeNode.
void _doRequestFocus({required bool findFirstFocus}) {
if (!canRequestFocus) {
assert(_focusDebug(() => 'Node NOT requesting focus because canRequestFocus is false: $this'));
return;
}
// If the node isn't part of the tree, then we just defer the focus request
// until the next time it is reparented, so that it's possible to focus
// newly added widgets.
if (_parent == null) {
_requestFocusWhenReparented = true;
return;
}
_setAsFocusedChildForScope();
if (hasPrimaryFocus && (_manager!._markedForFocus == null || _manager!._markedForFocus == this)) {
return;
}
_hasKeyboardToken = true;
assert(_focusDebug(() => 'Node requesting focus: $this'));
_markNextFocus(this);
}
// If set to true, the node will request focus on this node the next time
// this node is reparented in the focus tree.
//
// Once requestFocus has been called at the next reparenting, this value
// will be reset to false.
//
// This will only force a call to requestFocus for the node once the next time
// the node is reparented. After that, _requestFocusWhenReparented would need
// to be set to true again to have it be focused again on the next
// reparenting.
//
// This is used when requestFocus is called and there is no parent yet.
bool _requestFocusWhenReparented = false;
/// Sets this node as the [FocusScopeNode.focusedChild] of the enclosing
/// scope.
///
/// Sets this node as the focused child for the enclosing scope, and that
/// scope as the focused child for the scope above it, etc., until it reaches
/// the root node. It doesn't change the primary focus, it just changes what
/// node would be focused if the enclosing scope receives focus, and keeps
/// track of previously focused children in that scope, so that if the focused
/// child in that scope is removed, the previous focus returns.
void _setAsFocusedChildForScope() {
FocusNode scopeFocus = this;
for (final FocusScopeNode ancestor in ancestors.whereType<FocusScopeNode>()) {
assert(scopeFocus != ancestor, 'Somehow made a loop by setting focusedChild to its scope.');
assert(_focusDebug(() => 'Setting $scopeFocus as focused child for scope:', () => <Object>[ancestor]));
// Remove it anywhere in the focused child history.
ancestor._focusedChildren.remove(scopeFocus);
// Add it to the end of the list, which is also the top of the queue: The
// end of the list represents the currently focused child.
ancestor._focusedChildren.add(scopeFocus);
scopeFocus = ancestor;
}
}
/// Request to move the focus to the next focus node, by calling the
/// [FocusTraversalPolicy.next] method.
///
/// Returns true if it successfully found a node and requested focus.
bool nextFocus() => FocusTraversalGroup.of(context!).next(this);
/// Request to move the focus to the previous focus node, by calling the
/// [FocusTraversalPolicy.previous] method.
///
/// Returns true if it successfully found a node and requested focus.
bool previousFocus() => FocusTraversalGroup.of(context!).previous(this);
/// Request to move the focus to the nearest focus node in the given
/// direction, by calling the [FocusTraversalPolicy.inDirection] method.
///
/// Returns true if it successfully found a node and requested focus.
bool focusInDirection(TraversalDirection direction) => FocusTraversalGroup.of(context!).inDirection(this, direction);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<BuildContext>('context', context, defaultValue: null));
properties.add(FlagProperty('descendantsAreFocusable', value: descendantsAreFocusable, ifFalse: 'DESCENDANTS UNFOCUSABLE', defaultValue: true));
properties.add(FlagProperty('descendantsAreTraversable', value: descendantsAreTraversable, ifFalse: 'DESCENDANTS UNTRAVERSABLE', defaultValue: true));
properties.add(FlagProperty('canRequestFocus', value: canRequestFocus, ifFalse: 'NOT FOCUSABLE', defaultValue: true));
properties.add(FlagProperty('hasFocus', value: hasFocus && !hasPrimaryFocus, ifTrue: 'IN FOCUS PATH', defaultValue: false));
properties.add(FlagProperty('hasPrimaryFocus', value: hasPrimaryFocus, ifTrue: 'PRIMARY FOCUS', defaultValue: false));
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
int count = 1;
return _children.map<DiagnosticsNode>((FocusNode child) {
return child.toDiagnosticsNode(name: 'Child ${count++}');
}).toList();
}
@override
String toStringShort() {
final bool hasDebugLabel = debugLabel != null && debugLabel!.isNotEmpty;
final String extraData = '${hasDebugLabel ? debugLabel : ''}'
'${hasFocus && hasDebugLabel ? ' ' : ''}'
'${hasFocus && !hasPrimaryFocus ? '[IN FOCUS PATH]' : ''}'
'${hasPrimaryFocus ? '[PRIMARY FOCUS]' : ''}';
return '${describeIdentity(this)}${extraData.isNotEmpty ? '($extraData)' : ''}';
}
}
/// A subclass of [FocusNode] that acts as a scope for its descendants,
/// maintaining information about which descendant is currently or was last
/// focused.
///
/// _Please see the [FocusScope] and [Focus] widgets, which are utility widgets
/// that manage their own [FocusScopeNode]s and [FocusNode]s, respectively. If
/// they aren't appropriate, [FocusScopeNode]s can be managed directly._
///
/// [FocusScopeNode] organizes [FocusNode]s into _scopes_. Scopes form sub-trees
/// of nodes that can be traversed as a group. Within a scope, the most recent
/// nodes to have focus are remembered, and if a node is focused and then
/// removed, the original node receives focus again.
///
/// From a [FocusScopeNode], calling [setFirstFocus], sets the given focus scope
/// as the [focusedChild] of this node, adopting if it isn't already part of the
/// focus tree.
///
/// {@macro flutter.widgets.FocusNode.lifecycle}
/// {@macro flutter.widgets.FocusNode.keyEvents}
///
/// See also:
///
/// * [Focus], a widget that manages a [FocusNode] and provides access to focus
/// information and actions to its descendant widgets.
/// * [FocusManager], a singleton that manages the primary focus and
/// distributes key events to focused nodes.
class FocusScopeNode extends FocusNode {
/// Creates a [FocusScopeNode].
///
/// All parameters are optional.
FocusScopeNode({
super.debugLabel,
super.onKeyEvent,
@Deprecated(
'Use onKeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
super.onKey,
super.skipTraversal,
super.canRequestFocus,
this.traversalEdgeBehavior = TraversalEdgeBehavior.closedLoop,
}) : super(descendantsAreFocusable: true);
@override
FocusScopeNode get nearestScope => this;
@override
bool get descendantsAreFocusable => _canRequestFocus && super.descendantsAreFocusable;
/// Controls the transfer of focus beyond the first and the last items of a
/// [FocusScopeNode].
///
/// Changing this field value has no immediate effect on the UI. Instead, next time
/// focus traversal takes place [FocusTraversalPolicy] will read this value
/// and apply the new behavior.
TraversalEdgeBehavior traversalEdgeBehavior;
/// Returns true if this scope is the focused child of its parent scope.
bool get isFirstFocus => enclosingScope!.focusedChild == this;
/// Returns the child of this node that should receive focus if this scope
/// node receives focus.
///
/// If [hasFocus] is true, then this points to the child of this node that is
/// currently focused.
///
/// Returns null if there is no currently focused child.
FocusNode? get focusedChild {
assert(_focusedChildren.isEmpty || _focusedChildren.last.enclosingScope == this, 'Focused child does not have the same idea of its enclosing scope as the scope does.');
return _focusedChildren.isNotEmpty ? _focusedChildren.last : null;
}
// A stack of the children that have been set as the focusedChild, most recent
// last (which is the top of the stack).
final List<FocusNode> _focusedChildren = <FocusNode>[];
/// An iterator over the children that are allowed to be traversed by the
/// [FocusTraversalPolicy].
///
/// Will return an empty iterable if this scope node is not focusable, or if
/// [descendantsAreFocusable] is false.
///
/// See also:
///
/// * [traversalDescendants], which traverses all of the node's descendants,
/// not just the immediate children.
@override
Iterable<FocusNode> get traversalChildren {
if (!canRequestFocus) {
return const Iterable<FocusNode>.empty();
}
return super.traversalChildren;
}
/// Returns all descendants which do not have the [skipTraversal] and do have
/// the [canRequestFocus] flag set.
///
/// Will return an empty iterable if this scope node is not focusable, or if
/// [descendantsAreFocusable] is false.
@override
Iterable<FocusNode> get traversalDescendants {
if (!canRequestFocus) {
return const Iterable<FocusNode>.empty();
}
return super.traversalDescendants;
}
/// Make the given [scope] the active child scope for this scope.
///
/// If the given [scope] is not yet a part of the focus tree, then add it to
/// the tree as a child of this scope. If it is already part of the focus
/// tree, the given scope must be a descendant of this scope.
void setFirstFocus(FocusScopeNode scope) {
assert(scope != this, 'Unexpected self-reference in setFirstFocus.');
assert(_focusDebug(() => 'Setting scope as first focus in $this to node:', () => <Object>[scope]));
if (scope._parent == null) {
_reparent(scope);
}
assert(scope.ancestors.contains(this), '$FocusScopeNode $scope must be a child of $this to set it as first focus.');
if (hasFocus) {
scope._doRequestFocus(findFirstFocus: true);
} else {
scope._setAsFocusedChildForScope();
}
}
/// If this scope lacks a focus, request that the given node become the focus.
///
/// If the given node is not yet part of the focus tree, then add it as a
/// child of this node.
///
/// Useful for widgets that wish to grab the focus if no other widget already
/// has the focus.
///
/// The node is notified that it has received the primary focus in a
/// microtask, so notification may lag the request by up to one frame.
void autofocus(FocusNode node) {
// Attach the node to the tree first, so in _applyFocusChange if the node
// is detached we don't add it back to the tree.
if (node._parent == null) {
_reparent(node);
}
assert(_manager != null);
assert(_focusDebug(() => 'Autofocus scheduled for $node: scope $this'));
_manager?._pendingAutofocuses.add(_Autofocus(scope: this, autofocusNode: node));
_manager?._markNeedsUpdate();
}
@override
void _doRequestFocus({required bool findFirstFocus}) {
// It is possible that a previously focused child is no longer focusable.
while (this.focusedChild != null && !this.focusedChild!.canRequestFocus) {
_focusedChildren.removeLast();
}
final FocusNode? focusedChild = this.focusedChild;
// If findFirstFocus is false, then the request is to make this scope the
// focus instead of looking for the ultimate first focus for this scope and
// its descendants.
if (!findFirstFocus || focusedChild == null) {
if (canRequestFocus) {
_setAsFocusedChildForScope();
_markNextFocus(this);
}
return;
}
focusedChild._doRequestFocus(findFirstFocus: true);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
if (_focusedChildren.isEmpty) {
return;
}
final List<String> childList = _focusedChildren.reversed.map<String>((FocusNode child) {
return child.toStringShort();
}).toList();
properties.add(IterableProperty<String>('focusedChildren', childList, defaultValue: const Iterable<String>.empty()));
properties.add(DiagnosticsProperty<TraversalEdgeBehavior>('traversalEdgeBehavior', traversalEdgeBehavior, defaultValue: TraversalEdgeBehavior.closedLoop));
}
}
/// An enum to describe which kind of focus highlight behavior to use when
/// displaying focus information.
enum FocusHighlightMode {
/// Touch interfaces will not show the focus highlight except for controls
/// which bring up the soft keyboard.
///
/// If a device that uses a traditional mouse and keyboard has a touch screen
/// attached, it can also enter `touch` mode if the user is using the touch
/// screen.
touch,
/// Traditional interfaces (keyboard and mouse) will show the currently
/// focused control via a focus highlight of some sort.
///
/// If a touch device (like a mobile phone) has a keyboard and/or mouse
/// attached, it also can enter `traditional` mode if the user is using these
/// input devices.
traditional,
}
/// An enum to describe how the current value of [FocusManager.highlightMode] is
/// determined. The strategy is set on [FocusManager.highlightStrategy].
enum FocusHighlightStrategy {
/// Automatic switches between the various highlight modes based on the last
/// kind of input that was received. This is the default.
automatic,
/// [FocusManager.highlightMode] always returns [FocusHighlightMode.touch].
alwaysTouch,
/// [FocusManager.highlightMode] always returns [FocusHighlightMode.traditional].
alwaysTraditional,
}
// By extending the WidgetsBindingObserver class,
// we can add a listener object to FocusManager as a private member.
class _AppLifecycleListener extends WidgetsBindingObserver {
_AppLifecycleListener(this.onLifecycleStateChanged);
final void Function(AppLifecycleState) onLifecycleStateChanged;
@override
void didChangeAppLifecycleState(AppLifecycleState state) => onLifecycleStateChanged(state);
}
/// Manages the focus tree.
///
/// The focus tree is a separate, sparser, tree from the widget tree that
/// maintains the hierarchical relationship between focusable widgets in the
/// widget tree.
///
/// The focus manager is responsible for tracking which [FocusNode] has the
/// primary input focus (the [primaryFocus]), holding the [FocusScopeNode] that
/// is the root of the focus tree (the [rootScope]), and what the current
/// [highlightMode] is. It also distributes [KeyEvent]s to the nodes in the
/// focus tree.
///
/// The singleton [FocusManager] instance is held by the [WidgetsBinding] as
/// [WidgetsBinding.focusManager], and can be conveniently accessed using the
/// [FocusManager.instance] static accessor.
///
/// To find the [FocusNode] for a given [BuildContext], use [Focus.of]. To find
/// the [FocusScopeNode] for a given [BuildContext], use [FocusScope.of].
///
/// If you would like notification whenever the [primaryFocus] changes, register
/// a listener with [addListener]. When you no longer want to receive these
/// events, as when your object is about to be disposed, you must unregister
/// with [removeListener] to avoid memory leaks. Removing listeners is typically
/// done in [State.dispose] on stateful widgets.
///
/// The [highlightMode] describes how focus highlights should be displayed on
/// components in the UI. The [highlightMode] changes are notified separately
/// via [addHighlightModeListener] and removed with
/// [removeHighlightModeListener]. The highlight mode changes when the user
/// switches from a mouse to a touch interface, or vice versa.
///
/// The widgets that are used to manage focus in the widget tree are:
///
/// * [Focus], a widget that manages a [FocusNode] in the focus tree so that
/// the focus tree reflects changes in the widget hierarchy.
/// * [FocusScope], a widget that manages a [FocusScopeNode] in the focus tree,
/// creating a new scope for restricting focus to a set of focus nodes.
/// * [FocusTraversalGroup], a widget that groups together nodes that should be
/// traversed using an order described by a given [FocusTraversalPolicy].
///
/// See also:
///
/// * [FocusNode], which is a node in the focus tree that can receive focus.
/// * [FocusScopeNode], which is a node in the focus tree used to collect
/// subtrees into groups and restrict focus to them.
/// * The [primaryFocus] global accessor, for convenient access from anywhere
/// to the current focus manager state.
class FocusManager with DiagnosticableTreeMixin, ChangeNotifier {
/// Creates an object that manages the focus tree.
///
/// This constructor is rarely called directly. To access the [FocusManager],
/// consider using the [FocusManager.instance] accessor instead (which gets it
/// from the [WidgetsBinding] singleton).
///
/// This newly constructed focus manager does not have the necessary event
/// handlers registered to allow it to manage focus. To register those event
/// handlers, callers must call [registerGlobalHandlers]. See the
/// documentation in that method for caveats to watch out for.
FocusManager() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
if (kIsWeb || defaultTargetPlatform != TargetPlatform.android) {
// It appears that some Android keyboard implementations can cause
// app lifecycle state changes: adding this listener would cause the
// text field to unfocus as the user is trying to type.
//
// Until this is resolved, we won't be adding the listener to Android apps.
// https://github.com/flutter/flutter/pull/142930#issuecomment-1981750069
_appLifecycleListener = _AppLifecycleListener(_appLifecycleChange);
WidgetsBinding.instance.addObserver(_appLifecycleListener!);
}
rootScope._manager = this;
}
/// Registers global input event handlers that are needed to manage focus.
///
/// This calls the [HardwareKeyboard.addHandler] on the shared instance of
/// [HardwareKeyboard] and adds a route to the global entry in the gesture
/// routing table. As such, only one [FocusManager] instance should register
/// its global handlers.
///
/// When this focus manager is no longer needed, calling [dispose] on it will
/// unregister these handlers.
void registerGlobalHandlers() => _highlightManager.registerGlobalHandlers();
@override
void dispose() {
if (_appLifecycleListener != null) {
WidgetsBinding.instance.removeObserver(_appLifecycleListener!);
}
_highlightManager.dispose();
rootScope.dispose();
super.dispose();
}
/// Provides convenient access to the current [FocusManager] singleton from
/// the [WidgetsBinding] instance.
static FocusManager get instance => WidgetsBinding.instance.focusManager;
final _HighlightModeManager _highlightManager = _HighlightModeManager();
/// Sets the strategy by which [highlightMode] is determined.
///
/// If set to [FocusHighlightStrategy.automatic], then the highlight mode will
/// change depending upon the interaction mode used last. For instance, if the
/// last interaction was a touch interaction, then [highlightMode] will return
/// [FocusHighlightMode.touch], and focus highlights will only appear on
/// widgets that bring up a soft keyboard. If the last interaction was a
/// non-touch interaction (hardware keyboard press, mouse click, etc.), then
/// [highlightMode] will return [FocusHighlightMode.traditional], and focus
/// highlights will appear on all widgets.
///
/// If set to [FocusHighlightStrategy.alwaysTouch] or
/// [FocusHighlightStrategy.alwaysTraditional], then [highlightMode] will
/// always return [FocusHighlightMode.touch] or
/// [FocusHighlightMode.traditional], respectively, regardless of the last UI
/// interaction type.
///
/// The initial value of [highlightMode] depends upon the value of
/// [defaultTargetPlatform] and [MouseTracker.mouseIsConnected] of
/// [RendererBinding.mouseTracker], making a guess about which interaction is
/// most appropriate for the initial interaction mode.
///
/// Defaults to [FocusHighlightStrategy.automatic].
FocusHighlightStrategy get highlightStrategy => _highlightManager.strategy;
set highlightStrategy(FocusHighlightStrategy value) {
if (_highlightManager.strategy == value) {
return;
}
_highlightManager.strategy = value;
}
/// Indicates the current interaction mode for focus highlights.
///
/// The value returned depends upon the [highlightStrategy] used, and possibly
/// (depending on the value of [highlightStrategy]) the most recent
/// interaction mode that they user used.
///
/// If [highlightMode] returns [FocusHighlightMode.touch], then widgets should
/// not draw their focus highlight unless they perform text entry.
///
/// If [highlightMode] returns [FocusHighlightMode.traditional], then widgets should
/// draw their focus highlight whenever they are focused.
// Don't want to set _highlightMode here, since it's possible for the target
// platform to change (especially in tests).
FocusHighlightMode get highlightMode => _highlightManager.highlightMode;
/// Register a closure to be called when the [FocusManager] notifies its listeners
/// that the value of [highlightMode] has changed.
void addHighlightModeListener(ValueChanged<FocusHighlightMode> listener) => _highlightManager.addListener(listener);
/// Remove a previously registered closure from the list of closures that the
/// [FocusManager] notifies.
void removeHighlightModeListener(ValueChanged<FocusHighlightMode> listener) => _highlightManager.removeListener(listener);
/// {@template flutter.widgets.focus_manager.FocusManager.addEarlyKeyEventHandler}
/// Adds a key event handler to a set of handlers that are called before any
/// key event handlers in the focus tree are called.
///
/// All of the handlers in the set will be called for every key event the
/// [FocusManager] receives. If any one of the handlers returns
/// [KeyEventResult.handled] or [KeyEventResult.skipRemainingHandlers], then
/// none of the handlers in the focus tree will be called.
///
/// If handlers are added while the existing callbacks are being invoked, they
/// will not be called until the next key event occurs.
///
/// See also:
///
/// * [removeEarlyKeyEventHandler], which removes handlers added by this
/// function.
/// * [addLateKeyEventHandler], which is a similar mechanism for adding
/// handlers that are invoked after the focus tree has had a chance to
/// handle an event.
/// {@endtemplate}
void addEarlyKeyEventHandler(OnKeyEventCallback handler) {
_highlightManager.addEarlyKeyEventHandler(handler);
}
/// {@template flutter.widgets.focus_manager.FocusManager.removeEarlyKeyEventHandler}
/// Removes a key handler added by calling [addEarlyKeyEventHandler].
///
/// If handlers are removed while the existing callbacks are being invoked,
/// they will continue to be called until the next key event is received.
///
/// See also:
///
/// * [addEarlyKeyEventHandler], which adds the handlers removed by this
/// function.
/// {@endtemplate}
void removeEarlyKeyEventHandler(OnKeyEventCallback handler) {
_highlightManager.removeEarlyKeyEventHandler(handler);
}
/// {@template flutter.widgets.focus_manager.FocusManager.addLateKeyEventHandler}
/// Adds a key event handler to a set of handlers that are called if none of
/// the key event handlers in the focus tree handle the event.
///
/// If the event reaches the root of the focus tree without being handled,
/// then all of the handlers in the set will be called. If any of them returns
/// [KeyEventResult.handled] or [KeyEventResult.skipRemainingHandlers], then
/// event propagation to the platform will be stopped.
///
/// If handlers are added while the existing callbacks are being invoked, they
/// will not be called until the next key event is not handled by the focus
/// tree.
///
/// See also:
///
/// * [removeLateKeyEventHandler], which removes handlers added by this
/// function.
/// * [addEarlyKeyEventHandler], which is a similar mechanism for adding
/// handlers that are invoked before the focus tree has had a chance to
/// handle an event.
/// {@endtemplate}
void addLateKeyEventHandler(OnKeyEventCallback handler) {
_highlightManager.addLateKeyEventHandler(handler);
}
/// {@template flutter.widgets.focus_manager.FocusManager.removeLateKeyEventHandler}
/// Removes a key handler added by calling [addLateKeyEventHandler].
///
/// If handlers are removed while the existing callbacks are being invoked,
/// they will continue to be called until the next key event is received.
///
/// See also:
///
/// * [addLateKeyEventHandler], which adds the handlers removed by this
/// function.
/// {@endtemplate}
void removeLateKeyEventHandler(OnKeyEventCallback handler) {
_highlightManager.removeLateKeyEventHandler(handler);
}
/// The root [FocusScopeNode] in the focus tree.
///
/// This field is rarely used directly. To find the nearest [FocusScopeNode]
/// for a given [FocusNode], call [FocusNode.nearestScope].
final FocusScopeNode rootScope = FocusScopeNode(debugLabel: 'Root Focus Scope');
/// The node that currently has the primary focus.
FocusNode? get primaryFocus => _primaryFocus;
FocusNode? _primaryFocus;
// The set of nodes that need to notify their listeners of changes at the next
// update.
final Set<FocusNode> _dirtyNodes = <FocusNode>{};
// Allows FocusManager to respond to app lifecycle state changes,
// temporarily suspending the primaryFocus when the app is inactive.
_AppLifecycleListener? _appLifecycleListener;
// Stores the node that was focused before the app lifecycle changed.
// Will be restored as the primary focus once app is resumed.
FocusNode? _suspendedNode;
void _appLifecycleChange(AppLifecycleState state) {
if (state == AppLifecycleState.resumed) {
if (_primaryFocus != rootScope) {
assert(_focusDebug(() => 'focus changed while app was paused, ignoring $_suspendedNode'));
_suspendedNode = null;
}
else if (_suspendedNode != null) {
assert(_focusDebug(() => 'requesting focus for $_suspendedNode'));
_suspendedNode!.requestFocus();
_suspendedNode = null;
}
} else if (_primaryFocus != rootScope) {
assert(_focusDebug(() => 'suspending $_primaryFocus'));
_markedForFocus = rootScope;
_suspendedNode = _primaryFocus;
applyFocusChangesIfNeeded();
}
}
// The node that has requested to have the primary focus, but hasn't been
// given it yet.
FocusNode? _markedForFocus;
void _markDetached(FocusNode node) {
// The node has been removed from the tree, so it no longer needs to be
// notified of changes.
assert(_focusDebug(() => 'Node was detached: $node'));
if (_primaryFocus == node) {
_primaryFocus = null;
}
if (_suspendedNode == node) {
_suspendedNode = null;
}
_dirtyNodes.remove(node);
}
void _markPropertiesChanged(FocusNode node) {
_markNeedsUpdate();
assert(_focusDebug(() => 'Properties changed for node $node.'));
_dirtyNodes.add(node);
}
void _markNextFocus(FocusNode node) {
if (_primaryFocus == node) {
// The caller asked for the current focus to be the next focus, so just
// pretend that didn't happen.
_markedForFocus = null;
} else {
_markedForFocus = node;
_markNeedsUpdate();
}
}
// The list of autofocus requests made since the last _applyFocusChange call.
final List<_Autofocus> _pendingAutofocuses = <_Autofocus>[];
// True indicates that there is an update pending.
bool _haveScheduledUpdate = false;
// Request that an update be scheduled, optionally requesting focus for the
// given newFocus node.
void _markNeedsUpdate() {
assert(_focusDebug(() => 'Scheduling update, current focus is $_primaryFocus, next focus will be $_markedForFocus'));
if (_haveScheduledUpdate) {
return;
}
_haveScheduledUpdate = true;
scheduleMicrotask(applyFocusChangesIfNeeded);
}
/// Applies any pending focus changes and notifies listeners that the focus
/// has changed.
///
/// Must not be called during the build phase. This method is meant to be
/// called in a post-frame callback or microtask when the pending focus
/// changes need to be resolved before something else occurs.
///
/// It can't be called during the build phase because not all listeners are
/// safe to be called with an update during a build.
///
/// Typically, this is called automatically by the [FocusManager], but
/// sometimes it is necessary to ensure that no focus changes are pending
/// before executing an action. For example, the [MenuAnchor] class uses this
/// to make sure that the previous focus has been restored before executing a
/// menu callback when a menu item is selected.
///
/// It is safe to call this if no focus changes are pending.
void applyFocusChangesIfNeeded() {
assert(
SchedulerBinding.instance.schedulerPhase != SchedulerPhase.persistentCallbacks,
'applyFocusChangesIfNeeded() should not be called during the build phase.'
);
_haveScheduledUpdate = false;
final FocusNode? previousFocus = _primaryFocus;
for (final _Autofocus autofocus in _pendingAutofocuses) {
autofocus.applyIfValid(this);
}
_pendingAutofocuses.clear();
if (_primaryFocus == null && _markedForFocus == null) {
// If we don't have any current focus, and nobody has asked to focus yet,
// then revert to the root scope.
_markedForFocus = rootScope;
}
assert(_focusDebug(() => 'Refreshing focus state. Next focus will be $_markedForFocus'));
// A node has requested to be the next focus, and isn't already the primary
// focus.
if (_markedForFocus != null && _markedForFocus != _primaryFocus) {
final Set<FocusNode> previousPath = previousFocus?.ancestors.toSet() ?? <FocusNode>{};
final Set<FocusNode> nextPath = _markedForFocus!.ancestors.toSet();
// Notify nodes that are newly focused.
_dirtyNodes.addAll(nextPath.difference(previousPath));
// Notify nodes that are no longer focused
_dirtyNodes.addAll(previousPath.difference(nextPath));
_primaryFocus = _markedForFocus;
_markedForFocus = null;
}
assert(_markedForFocus == null);
if (previousFocus != _primaryFocus) {
assert(_focusDebug(() => 'Updating focus from $previousFocus to $_primaryFocus'));
if (previousFocus != null) {
_dirtyNodes.add(previousFocus);
}
if (_primaryFocus != null) {
_dirtyNodes.add(_primaryFocus!);
}
}
for (final FocusNode node in _dirtyNodes) {
node._notify();
}
assert(_focusDebug(() => 'Notified ${_dirtyNodes.length} dirty nodes:', () => _dirtyNodes));
_dirtyNodes.clear();
if (previousFocus != _primaryFocus) {
notifyListeners();
}
assert(() {
if (debugFocusChanges) {
debugDumpFocusTree();
}
return true;
}());
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return <DiagnosticsNode>[
rootScope.toDiagnosticsNode(name: 'rootScope'),
];
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
properties.add(FlagProperty('haveScheduledUpdate', value: _haveScheduledUpdate, ifTrue: 'UPDATE SCHEDULED'));
properties.add(DiagnosticsProperty<FocusNode>('primaryFocus', primaryFocus, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('nextFocus', _markedForFocus, defaultValue: null));
final Element? element = primaryFocus?.context as Element?;
if (element != null) {
properties.add(DiagnosticsProperty<String>('primaryFocusCreator', element.debugGetCreatorChain(20)));
}
}
}
// A class to detect and manage the highlight mode transitions. An instance of
// this is owned by the FocusManager.
//
// This doesn't extend ChangeNotifier because the callback passes the updated
// value, and ChangeNotifier requires using VoidCallback.
class _HighlightModeManager {
_HighlightModeManager() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$_HighlightModeManager',
object: this,
);
}
}
// If set, indicates if the last interaction detected was touch or not. If
// null, no interactions have occurred yet.
bool? _lastInteractionWasTouch;
FocusHighlightMode get highlightMode => _highlightMode ?? _defaultModeForPlatform;
FocusHighlightMode? _highlightMode;
FocusHighlightStrategy get strategy => _strategy;
FocusHighlightStrategy _strategy = FocusHighlightStrategy.automatic;
set strategy(FocusHighlightStrategy value) {
if (_strategy == value) {
return;
}
_strategy = value;
updateMode();
}
/// {@macro flutter.widgets.focus_manager.FocusManager.addEarlyKeyEventHandler}
void addEarlyKeyEventHandler(OnKeyEventCallback callback) => _earlyKeyEventHandlers.add(callback);
/// {@macro flutter.widgets.focus_manager.FocusManager.removeEarlyKeyEventHandler}
void removeEarlyKeyEventHandler(OnKeyEventCallback callback) => _earlyKeyEventHandlers.remove(callback);
// The list of callbacks for early key handling.
final HashedObserverList<OnKeyEventCallback> _earlyKeyEventHandlers = HashedObserverList<OnKeyEventCallback>();
/// {@macro flutter.widgets.focus_manager.FocusManager.addLateKeyEventHandler}
void addLateKeyEventHandler(OnKeyEventCallback callback) => _lateKeyEventHandlers.add(callback);
/// {@macro flutter.widgets.focus_manager.FocusManager.removeLateKeyEventHandler}
void removeLateKeyEventHandler(OnKeyEventCallback callback) => _lateKeyEventHandlers.remove(callback);
// The list of callbacks for late key handling.
final HashedObserverList<OnKeyEventCallback> _lateKeyEventHandlers = HashedObserverList<OnKeyEventCallback>();
/// Register a closure to be called when the [FocusManager] notifies its
/// listeners that the value of [highlightMode] has changed.
void addListener(ValueChanged<FocusHighlightMode> listener) => _listeners.add(listener);
/// Remove a previously registered closure from the list of closures that the
/// [FocusManager] notifies.
void removeListener(ValueChanged<FocusHighlightMode> listener) => _listeners.remove(listener);
// The list of listeners for [highlightMode] state changes.
HashedObserverList<ValueChanged<FocusHighlightMode>> _listeners = HashedObserverList<ValueChanged<FocusHighlightMode>>();
void registerGlobalHandlers() {
assert(ServicesBinding.instance.keyEventManager.keyMessageHandler == null);
// TODO(gspencergoog): Remove this when the RawKeyEvent system is
// deprecated, and replace it with registering a handler on the
// HardwareKeyboard.
ServicesBinding.instance.keyEventManager.keyMessageHandler = handleKeyMessage;
GestureBinding.instance.pointerRouter.addGlobalRoute(handlePointerEvent);
}
@mustCallSuper
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
if (ServicesBinding.instance.keyEventManager.keyMessageHandler == handleKeyMessage) {
GestureBinding.instance.pointerRouter.removeGlobalRoute(handlePointerEvent);
ServicesBinding.instance.keyEventManager.keyMessageHandler = null;
}
_listeners = HashedObserverList<ValueChanged<FocusHighlightMode>>();
}
@pragma('vm:notify-debugger-on-exception')
void notifyListeners() {
if (_listeners.isEmpty) {
return;
}
final List<ValueChanged<FocusHighlightMode>> localListeners = List<ValueChanged<FocusHighlightMode>>.of(_listeners);
for (final ValueChanged<FocusHighlightMode> listener in localListeners) {
try {
if (_listeners.contains(listener)) {
listener(highlightMode);
}
} catch (exception, stack) {
InformationCollector? collector;
assert(() {
collector = () => <DiagnosticsNode>[
DiagnosticsProperty<_HighlightModeManager>(
'The $runtimeType sending notification was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
];
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets library',
context: ErrorDescription('while dispatching notifications for $runtimeType'),
informationCollector: collector,
));
}
}
}
void handlePointerEvent(PointerEvent event) {
final FocusHighlightMode expectedMode;
switch (event.kind) {
case PointerDeviceKind.touch:
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
_lastInteractionWasTouch = true;
expectedMode = FocusHighlightMode.touch;
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
case PointerDeviceKind.unknown:
_lastInteractionWasTouch = false;
expectedMode = FocusHighlightMode.traditional;
}
if (expectedMode != highlightMode) {
updateMode();
}
}
bool handleKeyMessage(KeyMessage message) {
// Update highlightMode first, since things responding to the keys might
// look at the highlight mode, and it should be accurate.
_lastInteractionWasTouch = false;
updateMode();
assert(_focusDebug(() => 'Received key event $message'));
if (FocusManager.instance.primaryFocus == null) {
assert(_focusDebug(() => 'No primary focus for key event, ignored: $message'));
return false;
}
bool handled = false;
// Check to see if any of the early handlers handle the key. If so, then
// return early.
if (_earlyKeyEventHandlers.isNotEmpty) {
final List<KeyEventResult> results = <KeyEventResult>[];
// Copy the list before iteration to prevent problems if the list gets
// modified during iteration.
final List<OnKeyEventCallback> iterationList = _earlyKeyEventHandlers.toList();
for (final OnKeyEventCallback callback in iterationList) {
for (final KeyEvent event in message.events) {
results.add(callback(event));
}
}
final KeyEventResult result = combineKeyEventResults(results);
switch (result) {
case KeyEventResult.ignored:
break;
case KeyEventResult.handled:
assert(_focusDebug(() => 'Key event $message handled by early key event callback.'));
handled = true;
case KeyEventResult.skipRemainingHandlers:
assert(_focusDebug(() => 'Key event $message propagation stopped by early key event callback.'));
handled = false;
}
}
if (handled) {
return true;
}
// Walk the current focus from the leaf to the root, calling each node's
// onKeyEvent on the way up, and if one responds that they handled it or
// want to stop propagation, stop.
for (final FocusNode node in <FocusNode>[
FocusManager.instance.primaryFocus!,
...FocusManager.instance.primaryFocus!.ancestors,
]) {
final List<KeyEventResult> results = <KeyEventResult>[];
if (node.onKeyEvent != null) {
for (final KeyEvent event in message.events) {
results.add(node.onKeyEvent!(node, event));
}
}
if (node.onKey != null && message.rawEvent != null) {
results.add(node.onKey!(node, message.rawEvent!));
}
final KeyEventResult result = combineKeyEventResults(results);
switch (result) {
case KeyEventResult.ignored:
continue;
case KeyEventResult.handled:
assert(_focusDebug(() => 'Node $node handled key event $message.'));
handled = true;
case KeyEventResult.skipRemainingHandlers:
assert(_focusDebug(() => 'Node $node stopped key event propagation: $message.'));
handled = false;
}
// Only KeyEventResult.ignored will continue the for loop. All other
// options will stop the event propagation.
assert(result != KeyEventResult.ignored);
break;
}
// Check to see if any late key event handlers want to handle the event.
if (!handled && _lateKeyEventHandlers.isNotEmpty) {
final List<KeyEventResult> results = <KeyEventResult>[];
// Copy the list before iteration to prevent problems if the list gets
// modified during iteration.
final List<OnKeyEventCallback> iterationList = _lateKeyEventHandlers.toList();
for (final OnKeyEventCallback callback in iterationList) {
for (final KeyEvent event in message.events) {
results.add(callback(event));
}
}
final KeyEventResult result = combineKeyEventResults(results);
switch (result) {
case KeyEventResult.ignored:
break;
case KeyEventResult.handled:
assert(_focusDebug(() => 'Key event $message handled by late key event callback.'));
handled = true;
case KeyEventResult.skipRemainingHandlers:
assert(_focusDebug(() => 'Key event $message propagation stopped by late key event callback.'));
handled = false;
}
}
if (!handled) {
assert(_focusDebug(() => 'Key event not handled by focus system: $message.'));
}
return handled;
}
// Update function to be called whenever the state relating to highlightMode
// changes.
void updateMode() {
final FocusHighlightMode newMode;
switch (strategy) {
case FocusHighlightStrategy.automatic:
if (_lastInteractionWasTouch == null) {
// If we don't have any information about the last interaction yet,
// then just rely on the default value for the platform, which will be
// determined based on the target platform if _highlightMode is not
// set.
return;
}
if (_lastInteractionWasTouch!) {
newMode = FocusHighlightMode.touch;
} else {
newMode = FocusHighlightMode.traditional;
}
case FocusHighlightStrategy.alwaysTouch:
newMode = FocusHighlightMode.touch;
case FocusHighlightStrategy.alwaysTraditional:
newMode = FocusHighlightMode.traditional;
}
// We can't just compare newMode with _highlightMode here, since
// _highlightMode could be null, so we want to compare with the return value
// for the getter, since that's what clients will be looking at.
final FocusHighlightMode oldMode = highlightMode;
_highlightMode = newMode;
if (highlightMode != oldMode) {
notifyListeners();
}
}
static FocusHighlightMode get _defaultModeForPlatform {
// Assume that if we're on one of the mobile platforms, and there's no mouse
// connected, that the initial interaction will be touch-based, and that
// it's traditional mouse and keyboard on all other platforms.
//
// This only affects the initial value: the ongoing value is updated to a
// known correct value as soon as any pointer/keyboard events are received.
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
if (WidgetsBinding.instance.mouseTracker.mouseIsConnected) {
return FocusHighlightMode.traditional;
}
return FocusHighlightMode.touch;
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return FocusHighlightMode.traditional;
}
}
}
/// Provides convenient access to the current [FocusManager.primaryFocus] from
/// the [WidgetsBinding] instance.
FocusNode? get primaryFocus => WidgetsBinding.instance.focusManager.primaryFocus;
/// Returns a text representation of the current focus tree, along with the
/// current attributes on each node.
///
/// Will return an empty string in release builds.
String debugDescribeFocusTree() {
String? result;
assert(() {
result = FocusManager.instance.toStringDeep();
return true;
}());
return result ?? '';
}
/// Prints a text representation of the current focus tree, along with the
/// current attributes on each node.
///
/// Will do nothing in release builds.
void debugDumpFocusTree() {
assert(() {
debugPrint(debugDescribeFocusTree());
return true;
}());
}
| flutter/packages/flutter/lib/src/widgets/focus_manager.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/focus_manager.dart",
"repo_id": "flutter",
"token_count": 27612
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'framework.dart';
/// An [InheritedWidget] that's intended to be used as the base class for models
/// whose dependents may only depend on one part or "aspect" of the overall
/// model.
///
/// An inherited widget's dependents are unconditionally rebuilt when the
/// inherited widget changes per [InheritedWidget.updateShouldNotify]. This
/// widget is similar except that dependents aren't rebuilt unconditionally.
///
/// Widgets that depend on an [InheritedModel] qualify their dependence with a
/// value that indicates what "aspect" of the model they depend on. When the
/// model is rebuilt, dependents will also be rebuilt, but only if there was a
/// change in the model that corresponds to the aspect they provided.
///
/// The type parameter `T` is the type of the model aspect objects.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ml5uefGgkaA}
///
/// Widgets create a dependency on an [InheritedModel] with a static method:
/// [InheritedModel.inheritFrom]. This method's `context` parameter defines the
/// subtree that will be rebuilt when the model changes. Typically the
/// `inheritFrom` method is called from a model-specific static `maybeOf` or
/// `of` methods, a convention that is present in many Flutter framework classes
/// which look things up. For example:
///
/// ```dart
/// class MyModel extends InheritedModel<String> {
/// const MyModel({super.key, required super.child});
///
/// // ...
/// static MyModel? maybeOf(BuildContext context, [String? aspect]) {
/// return InheritedModel.inheritFrom<MyModel>(context, aspect: aspect);
/// }
///
/// // ...
/// static MyModel of(BuildContext context, [String? aspect]) {
/// final MyModel? result = maybeOf(context, aspect);
/// assert(result != null, 'Unable to find an instance of MyModel...');
/// return result!;
/// }
/// }
/// ```
///
/// Calling `MyModel.of(context, 'foo')` or `MyModel.maybeOf(context,
/// 'foo')` means that `context` should only be rebuilt when the `foo` aspect of
/// `MyModel` changes. If the `aspect` is null, then the model supports all
/// aspects.
///
/// {@tool snippet}
/// When the inherited model is rebuilt the [updateShouldNotify] and
/// [updateShouldNotifyDependent] methods are used to decide what should be
/// rebuilt. If [updateShouldNotify] returns true, then the inherited model's
/// [updateShouldNotifyDependent] method is tested for each dependent and the
/// set of aspect objects it depends on. The [updateShouldNotifyDependent]
/// method must compare the set of aspect dependencies with the changes in the
/// model itself. For example:
///
/// ```dart
/// class ABModel extends InheritedModel<String> {
/// const ABModel({
/// super.key,
/// this.a,
/// this.b,
/// required super.child,
/// });
///
/// final int? a;
/// final int? b;
///
/// @override
/// bool updateShouldNotify(ABModel oldWidget) {
/// return a != oldWidget.a || b != oldWidget.b;
/// }
///
/// @override
/// bool updateShouldNotifyDependent(ABModel oldWidget, Set<String> dependencies) {
/// return (a != oldWidget.a && dependencies.contains('a'))
/// || (b != oldWidget.b && dependencies.contains('b'));
/// }
///
/// // ...
/// }
/// ```
/// {@end-tool}
///
/// In the previous example the dependencies checked by
/// [updateShouldNotifyDependent] are just the aspect strings passed to
/// `dependOnInheritedWidgetOfExactType`. They're represented as a [Set] because
/// one Widget can depend on more than one aspect of the model. If a widget
/// depends on the model but doesn't specify an aspect, then changes in the
/// model will cause the widget to be rebuilt unconditionally.
///
/// {@tool dartpad}
/// This example shows how to implement [InheritedModel] to rebuild a widget
/// based on a qualified dependence. When tapped on the "Resize Logo" button
/// only the logo widget is rebuilt while the background widget remains
/// unaffected.
///
/// ** See code in examples/api/lib/widgets/inherited_model/inherited_model.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [InheritedWidget], an inherited widget that only notifies dependents when
/// its value is different.
/// * [InheritedNotifier], an inherited widget whose value can be a
/// [Listenable], and which will notify dependents whenever the value sends
/// notifications.
abstract class InheritedModel<T> extends InheritedWidget {
/// Creates an inherited widget that supports dependencies qualified by
/// "aspects", i.e. a descendant widget can indicate that it should
/// only be rebuilt if a specific aspect of the model changes.
const InheritedModel({ super.key, required super.child });
@override
InheritedModelElement<T> createElement() => InheritedModelElement<T>(this);
/// Return true if the changes between this model and [oldWidget] match any
/// of the [dependencies].
@protected
bool updateShouldNotifyDependent(covariant InheritedModel<T> oldWidget, Set<T> dependencies);
/// Returns true if this model supports the given [aspect].
///
/// Returns true by default: this model supports all aspects.
///
/// Subclasses may override this method to indicate that they do not support
/// all model aspects. This is typically done when a model can be used
/// to "shadow" some aspects of an ancestor.
@protected
bool isSupportedAspect(Object aspect) => true;
// The [result] will be a list of all of context's type T ancestors concluding
// with the one that supports the specified model [aspect].
static void _findModels<T extends InheritedModel<Object>>(BuildContext context, Object aspect, List<InheritedElement> results) {
final InheritedElement? model = context.getElementForInheritedWidgetOfExactType<T>();
if (model == null) {
return;
}
results.add(model);
assert(model.widget is T);
final T modelWidget = model.widget as T;
if (modelWidget.isSupportedAspect(aspect)) {
return;
}
Element? modelParent;
model.visitAncestorElements((Element ancestor) {
modelParent = ancestor;
return false;
});
if (modelParent == null) {
return;
}
_findModels<T>(modelParent!, aspect, results);
}
/// Makes [context] dependent on the specified [aspect] of an [InheritedModel]
/// of type T.
///
/// When the given [aspect] of the model changes, the [context] will be
/// rebuilt. The [updateShouldNotifyDependent] method must determine if a
/// change in the model widget corresponds to an [aspect] value.
///
/// The dependencies created by this method target all [InheritedModel] ancestors
/// of type T up to and including the first one for which [isSupportedAspect]
/// returns true.
///
/// If [aspect] is null this method is the same as
/// `context.dependOnInheritedWidgetOfExactType<T>()`.
///
/// If no ancestor of type T exists, null is returned.
static T? inheritFrom<T extends InheritedModel<Object>>(BuildContext context, { Object? aspect }) {
if (aspect == null) {
return context.dependOnInheritedWidgetOfExactType<T>();
}
// Create a dependency on all of the type T ancestor models up until
// a model is found for which isSupportedAspect(aspect) is true.
final List<InheritedElement> models = <InheritedElement>[];
_findModels<T>(context, aspect, models);
if (models.isEmpty) {
return null;
}
final InheritedElement lastModel = models.last;
for (final InheritedElement model in models) {
final T value = context.dependOnInheritedElement(model, aspect: aspect) as T;
if (model == lastModel) {
return value;
}
}
assert(false);
return null;
}
}
/// An [Element] that uses a [InheritedModel] as its configuration.
class InheritedModelElement<T> extends InheritedElement {
/// Creates an element that uses the given widget as its configuration.
InheritedModelElement(InheritedModel<T> super.widget);
@override
void updateDependencies(Element dependent, Object? aspect) {
final Set<T>? dependencies = getDependencies(dependent) as Set<T>?;
if (dependencies != null && dependencies.isEmpty) {
return;
}
if (aspect == null) {
setDependencies(dependent, HashSet<T>());
} else {
assert(aspect is T);
setDependencies(dependent, (dependencies ?? HashSet<T>())..add(aspect as T));
}
}
@override
void notifyDependent(InheritedModel<T> oldWidget, Element dependent) {
final Set<T>? dependencies = getDependencies(dependent) as Set<T>?;
if (dependencies == null) {
return;
}
if (dependencies.isEmpty || (widget as InheritedModel<T>).updateShouldNotifyDependent(oldWidget, dependencies)) {
dependent.didChangeDependencies();
}
}
}
| flutter/packages/flutter/lib/src/widgets/inherited_model.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/inherited_model.dart",
"repo_id": "flutter",
"token_count": 2711
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'framework.dart';
/// Signature for [Notification] listeners.
///
/// Return true to cancel the notification bubbling. Return false to allow the
/// notification to continue to be dispatched to further ancestors.
///
/// [NotificationListener] is useful when listening scroll events
/// in [ListView],[NestedScrollView],[GridView] or any Scrolling widgets.
/// Used by [NotificationListener.onNotification].
typedef NotificationListenerCallback<T extends Notification> = bool Function(T notification);
/// A notification that can bubble up the widget tree.
///
/// You can determine the type of a notification using the `is` operator to
/// check the [runtimeType] of the notification.
///
/// To listen for notifications in a subtree, use a [NotificationListener].
///
/// To send a notification, call [dispatch] on the notification you wish to
/// send. The notification will be delivered to any [NotificationListener]
/// widgets with the appropriate type parameters that are ancestors of the given
/// [BuildContext].
///
/// {@tool dartpad}
/// This example shows a [NotificationListener] widget
/// that listens for [ScrollNotification] notifications. When a scroll
/// event occurs in the [NestedScrollView],
/// this widget is notified. The events could be either a
/// [ScrollStartNotification]or[ScrollEndNotification].
///
/// ** See code in examples/api/lib/widgets/notification_listener/notification.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ScrollNotification] which describes the notification lifecycle.
/// * [ScrollStartNotification] which returns the start position of scrolling.
/// * [ScrollEndNotification] which returns the end position of scrolling.
/// * [NestedScrollView] which creates a nested scroll view.
///
abstract class Notification {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const Notification();
/// Start bubbling this notification at the given build context.
///
/// The notification will be delivered to any [NotificationListener] widgets
/// with the appropriate type parameters that are ancestors of the given
/// [BuildContext]. If the [BuildContext] is null, the notification is not
/// dispatched.
void dispatch(BuildContext? target) {
target?.dispatchNotification(this);
}
@override
String toString() {
final List<String> description = <String>[];
debugFillDescription(description);
return '${objectRuntimeType(this, 'Notification')}(${description.join(", ")})';
}
/// Add additional information to the given description for use by [toString].
///
/// This method makes it easier for subclasses to coordinate to provide a
/// high-quality [toString] implementation. The [toString] implementation on
/// the [Notification] base class calls [debugFillDescription] to collect
/// useful information from subclasses to incorporate into its return value.
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.debugFillDescription(description)`.
@protected
@mustCallSuper
void debugFillDescription(List<String> description) { }
}
/// A widget that listens for [Notification]s bubbling up the tree.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=cAnFbFoGM50}
///
/// Notifications will trigger the [onNotification] callback only if their
/// [runtimeType] is a subtype of `T`.
///
/// To dispatch notifications, use the [Notification.dispatch] method.
class NotificationListener<T extends Notification> extends ProxyWidget {
/// Creates a widget that listens for notifications.
const NotificationListener({
super.key,
required super.child,
this.onNotification,
});
/// Called when a notification of the appropriate type arrives at this
/// location in the tree.
///
/// Return true to cancel the notification bubbling. Return false to
/// allow the notification to continue to be dispatched to further ancestors.
///
/// Notifications vary in terms of when they are dispatched. There are two
/// main possibilities: dispatch between frames, and dispatch during layout.
///
/// For notifications that dispatch during layout, such as those that inherit
/// from [LayoutChangedNotification], it is too late to call [State.setState]
/// in response to the notification (as layout is currently happening in a
/// descendant, by definition, since notifications bubble up the tree). For
/// widgets that depend on layout, consider a [LayoutBuilder] instead.
final NotificationListenerCallback<T>? onNotification;
@override
Element createElement() {
return _NotificationElement<T>(this);
}
}
/// An element used to host [NotificationListener] elements.
class _NotificationElement<T extends Notification> extends ProxyElement with NotifiableElementMixin {
_NotificationElement(NotificationListener<T> super.widget);
@override
bool onNotification(Notification notification) {
final NotificationListener<T> listener = widget as NotificationListener<T>;
if (listener.onNotification != null && notification is T) {
return listener.onNotification!(notification);
}
return false;
}
@override
void notifyClients(covariant ProxyWidget oldWidget) {
// Notification tree does not need to notify clients.
}
}
/// Indicates that the layout of one of the descendants of the object receiving
/// this notification has changed in some way, and that therefore any
/// assumptions about that layout are no longer valid.
///
/// Useful if, for instance, you're trying to align multiple descendants.
///
/// To listen for notifications in a subtree, use a
/// [NotificationListener<LayoutChangedNotification>].
///
/// To send a notification, call [dispatch] on the notification you wish to
/// send. The notification will be delivered to any [NotificationListener]
/// widgets with the appropriate type parameters that are ancestors of the given
/// [BuildContext].
///
/// In the widgets library, only the [SizeChangedLayoutNotifier] class and
/// [Scrollable] classes dispatch this notification (specifically, they dispatch
/// [SizeChangedLayoutNotification]s and [ScrollNotification]s respectively).
/// Transitions, in particular, do not. Changing one's layout in one's build
/// function does not cause this notification to be dispatched automatically. If
/// an ancestor expects to be notified for any layout change, make sure you
/// either only use widgets that never change layout, or that notify their
/// ancestors when appropriate, or alternatively, dispatch the notifications
/// yourself when appropriate.
///
/// Also, since this notification is sent when the layout is changed, it is only
/// useful for paint effects that depend on the layout. If you were to use this
/// notification to change the build, for instance, you would always be one
/// frame behind, which would look really ugly and laggy.
class LayoutChangedNotification extends Notification {
/// Create a new [LayoutChangedNotification].
const LayoutChangedNotification();
}
| flutter/packages/flutter/lib/src/widgets/notification_listener.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/notification_listener.dart",
"repo_id": "flutter",
"token_count": 1798
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
export 'package:flutter/services.dart' show RawKeyEvent;
/// A widget that calls a callback whenever the user presses or releases a key
/// on a keyboard.
///
/// The [RawKeyboardListener] is deprecated and will be removed. Use
/// [KeyboardListener] instead.
///
/// A [RawKeyboardListener] is useful for listening to raw key events and
/// hardware buttons that are represented as keys. Typically used by games and
/// other apps that use keyboards for purposes other than text entry.
///
/// For text entry, consider using a [EditableText], which integrates with
/// on-screen keyboards and input method editors (IMEs).
///
/// See also:
///
/// * [EditableText], which should be used instead of this widget for text
/// entry.
/// * [KeyboardListener], a similar widget based on the newer [HardwareKeyboard]
/// API.
@Deprecated(
'Use KeyboardListener instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyboardListener extends StatefulWidget {
/// Creates a widget that receives raw keyboard events.
///
/// For text entry, consider using a [EditableText], which integrates with
/// on-screen keyboards and input method editors (IMEs).
@Deprecated(
'Use KeyboardListener instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyboardListener({
super.key,
required this.focusNode,
this.autofocus = false,
this.includeSemantics = true,
this.onKey,
required this.child,
});
/// Controls whether this widget has keyboard focus.
final FocusNode focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.widgets.Focus.includeSemantics}
final bool includeSemantics;
/// Called whenever this widget receives a raw keyboard event.
final ValueChanged<RawKeyEvent>? onKey;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
State<RawKeyboardListener> createState() => _RawKeyboardListenerState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode));
}
}
class _RawKeyboardListenerState extends State<RawKeyboardListener> {
@override
void initState() {
super.initState();
widget.focusNode.addListener(_handleFocusChanged);
}
@override
void didUpdateWidget(RawKeyboardListener oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.focusNode != oldWidget.focusNode) {
oldWidget.focusNode.removeListener(_handleFocusChanged);
widget.focusNode.addListener(_handleFocusChanged);
}
}
@override
void dispose() {
widget.focusNode.removeListener(_handleFocusChanged);
_detachKeyboardIfAttached();
super.dispose();
}
void _handleFocusChanged() {
if (widget.focusNode.hasFocus) {
_attachKeyboardIfDetached();
} else {
_detachKeyboardIfAttached();
}
}
bool _listening = false;
void _attachKeyboardIfDetached() {
if (_listening) {
return;
}
RawKeyboard.instance.addListener(_handleRawKeyEvent);
_listening = true;
}
void _detachKeyboardIfAttached() {
if (!_listening) {
return;
}
RawKeyboard.instance.removeListener(_handleRawKeyEvent);
_listening = false;
}
void _handleRawKeyEvent(RawKeyEvent event) {
widget.onKey?.call(event);
}
@override
Widget build(BuildContext context) {
return Focus(
focusNode: widget.focusNode,
autofocus: widget.autofocus,
includeSemantics: widget.includeSemantics,
child: widget.child,
);
}
}
| flutter/packages/flutter/lib/src/widgets/raw_keyboard_listener.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/raw_keyboard_listener.dart",
"repo_id": "flutter",
"token_count": 1293
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/painting.dart' show AxisDirection;
import 'package:flutter/physics.dart';
import 'binding.dart' show WidgetsBinding;
import 'framework.dart';
import 'overscroll_indicator.dart';
import 'scroll_metrics.dart';
import 'scroll_simulation.dart';
import 'view.dart';
export 'package:flutter/physics.dart' show ScrollSpringSimulation, Simulation, Tolerance;
/// The rate at which scroll momentum will be decelerated.
enum ScrollDecelerationRate {
/// Standard deceleration, aligned with mobile software expectations.
normal,
/// Increased deceleration, aligned with desktop software expectations.
///
/// Appropriate for use with input devices more precise than touch screens,
/// such as trackpads or mouse wheels.
fast
}
// Examples can assume:
// class FooScrollPhysics extends ScrollPhysics {
// const FooScrollPhysics({ super.parent });
// @override
// FooScrollPhysics applyTo(ScrollPhysics? ancestor) {
// return FooScrollPhysics(parent: buildParent(ancestor));
// }
// }
// class BarScrollPhysics extends ScrollPhysics {
// const BarScrollPhysics({ super.parent });
// }
/// Determines the physics of a [Scrollable] widget.
///
/// For example, determines how the [Scrollable] will behave when the user
/// reaches the maximum scroll extent or when the user stops scrolling.
///
/// When starting a physics [Simulation], the current scroll position and
/// velocity are used as the initial conditions for the particle in the
/// simulation. The movement of the particle in the simulation is then used to
/// determine the scroll position for the widget.
///
/// Instead of creating your own subclasses, [parent] can be used to combine
/// [ScrollPhysics] objects of different types to get the desired scroll physics.
/// For example:
///
/// ```dart
/// const BouncingScrollPhysics(parent: AlwaysScrollableScrollPhysics())
/// ```
///
/// You can also use `applyTo`, which is useful when you already have
/// an instance of [ScrollPhysics]:
///
/// ```dart
/// ScrollPhysics physics = const BouncingScrollPhysics();
/// // ...
/// final ScrollPhysics mergedPhysics = physics.applyTo(const AlwaysScrollableScrollPhysics());
/// ```
///
/// When implementing a subclass, you must override [applyTo] so that it returns
/// an appropriate instance of your subclass. Otherwise, classes like
/// [Scrollable] that inform a [ScrollPosition] will combine them with
/// the default [ScrollPhysics] object instead of your custom subclass.
@immutable
class ScrollPhysics {
/// Creates an object with the default scroll physics.
const ScrollPhysics({ this.parent });
/// If non-null, determines the default behavior for each method.
///
/// If a subclass of [ScrollPhysics] does not override a method, that subclass
/// will inherit an implementation from this base class that defers to
/// [parent]. This mechanism lets you assemble novel combinations of
/// [ScrollPhysics] subclasses at runtime. For example:
///
/// ```dart
/// const BouncingScrollPhysics(parent: AlwaysScrollableScrollPhysics())
/// ```
///
/// will result in a [ScrollPhysics] that has the combined behavior
/// of [BouncingScrollPhysics] and [AlwaysScrollableScrollPhysics]:
/// behaviors that are not specified in [BouncingScrollPhysics]
/// (e.g. [shouldAcceptUserOffset]) will defer to [AlwaysScrollableScrollPhysics].
final ScrollPhysics? parent;
/// If [parent] is null then return ancestor, otherwise recursively build a
/// ScrollPhysics that has [ancestor] as its parent.
///
/// This method is typically used to define [applyTo] methods like:
///
/// ```dart
/// class MyScrollPhysics extends ScrollPhysics {
/// const MyScrollPhysics({ super.parent });
///
/// @override
/// MyScrollPhysics applyTo(ScrollPhysics? ancestor) {
/// return MyScrollPhysics(parent: buildParent(ancestor));
/// }
///
/// // ...
/// }
/// ```
@protected
ScrollPhysics? buildParent(ScrollPhysics? ancestor) => parent?.applyTo(ancestor) ?? ancestor;
/// Combines this [ScrollPhysics] instance with the given physics.
///
/// The returned object uses this instance's physics when it has an
/// opinion, and defers to the given `ancestor` object's physics
/// when it does not.
///
/// If [parent] is null then this returns a [ScrollPhysics] with the
/// same [runtimeType], but where the [parent] has been replaced
/// with the [ancestor].
///
/// If this scroll physics object already has a parent, then this
/// method is applied recursively and ancestor will appear at the
/// end of the existing chain of parents.
///
/// Calling this method with a null argument will copy the current
/// object. This is inefficient.
///
/// {@tool snippet}
///
/// In the following example, the [applyTo] method is used to combine the
/// scroll physics of two [ScrollPhysics] objects. The resulting [ScrollPhysics]
/// `x` has the same behavior as `y`.
///
/// ```dart
/// final FooScrollPhysics x = const FooScrollPhysics().applyTo(const BarScrollPhysics());
/// const FooScrollPhysics y = FooScrollPhysics(parent: BarScrollPhysics());
/// ```
/// {@end-tool}
///
/// ## Implementing [applyTo]
///
/// When creating a custom [ScrollPhysics] subclass, this method
/// must be implemented. If the physics class has no constructor
/// arguments, then implementing this method is merely a matter of
/// calling the constructor with a [parent] constructed using
/// [buildParent], as follows:
///
/// ```dart
/// class MyScrollPhysics extends ScrollPhysics {
/// const MyScrollPhysics({ super.parent });
///
/// @override
/// MyScrollPhysics applyTo(ScrollPhysics? ancestor) {
/// return MyScrollPhysics(parent: buildParent(ancestor));
/// }
///
/// // ...
/// }
/// ```
///
/// If the physics class has constructor arguments, they must be passed to
/// the constructor here as well, so as to create a clone.
///
/// See also:
///
/// * [buildParent], a utility method that's often used to define [applyTo]
/// methods for [ScrollPhysics] subclasses.
ScrollPhysics applyTo(ScrollPhysics? ancestor) {
return ScrollPhysics(parent: buildParent(ancestor));
}
/// Used by [DragScrollActivity] and other user-driven activities to convert
/// an offset in logical pixels as provided by the [DragUpdateDetails] into a
/// delta to apply (subtract from the current position) using
/// [ScrollActivityDelegate.setPixels].
///
/// This is used by some [ScrollPosition] subclasses to apply friction during
/// overscroll situations.
///
/// This method must not adjust parts of the offset that are entirely within
/// the bounds described by the given `position`.
///
/// The given `position` is only valid during this method call. Do not keep a
/// reference to it to use later, as the values may update, may not update, or
/// may update to reflect an entirely unrelated scrollable.
double applyPhysicsToUserOffset(ScrollMetrics position, double offset) {
return parent?.applyPhysicsToUserOffset(position, offset) ?? offset;
}
/// Whether the scrollable should let the user adjust the scroll offset, for
/// example by dragging. If [allowUserScrolling] is false, the scrollable
/// will never allow user input to change the scroll position.
///
/// By default, the user can manipulate the scroll offset if, and only if,
/// there is actually content outside the viewport to reveal.
///
/// The given `position` is only valid during this method call. Do not keep a
/// reference to it to use later, as the values may update, may not update, or
/// may update to reflect an entirely unrelated scrollable.
bool shouldAcceptUserOffset(ScrollMetrics position) {
if (!allowUserScrolling) {
return false;
}
if (parent == null) {
return position.pixels != 0.0 || position.minScrollExtent != position.maxScrollExtent;
}
return parent!.shouldAcceptUserOffset(position);
}
/// Provides a heuristic to determine if expensive frame-bound tasks should be
/// deferred.
///
/// The `velocity` parameter may be positive, negative, or zero.
///
/// The `context` parameter normally refers to the [BuildContext] of the widget
/// making the call, such as an [Image] widget in a [ListView].
///
/// This can be used to determine whether decoding or fetching complex data
/// for the currently visible part of the viewport should be delayed
/// to avoid doing work that will not have a chance to appear before a new
/// frame is rendered.
///
/// For example, a list of images could use this logic to delay decoding
/// images until scrolling is slow enough to actually render the decoded
/// image to the screen.
///
/// The default implementation is a heuristic that compares the current
/// scroll velocity in local logical pixels to the longest side of the window
/// in physical pixels. Implementers can change this heuristic by overriding
/// this method and providing their custom physics to the scrollable widget.
/// For example, an application that changes the local coordinate system with
/// a large perspective transform could provide a more or less aggressive
/// heuristic depending on whether the transform was increasing or decreasing
/// the overall scale between the global screen and local scrollable
/// coordinate systems.
///
/// The default implementation is stateless, and provides a point-in-time
/// decision about how fast the scrollable is scrolling. It would always
/// return true for a scrollable that is animating back and forth at high
/// velocity in a loop. It is assumed that callers will handle such
/// a case, or that a custom stateful implementation would be written that
/// tracks the sign of the velocity on successive calls.
///
/// Returning true from this method indicates that the current scroll velocity
/// is great enough that expensive operations impacting the UI should be
/// deferred.
bool recommendDeferredLoading(double velocity, ScrollMetrics metrics, BuildContext context) {
if (parent == null) {
final double maxPhysicalPixels = View.of(context).physicalSize.longestSide;
return velocity.abs() > maxPhysicalPixels;
}
return parent!.recommendDeferredLoading(velocity, metrics, context);
}
/// Determines the overscroll by applying the boundary conditions.
///
/// Called by [ScrollPosition.applyBoundaryConditions], which is called by
/// [ScrollPosition.setPixels] just before the [ScrollPosition.pixels] value
/// is updated, to determine how much of the offset is to be clamped off and
/// sent to [ScrollPosition.didOverscrollBy].
///
/// The `value` argument is guaranteed to not equal the [ScrollMetrics.pixels]
/// of the `position` argument when this is called.
///
/// It is possible for this method to be called when the `position` describes
/// an already-out-of-bounds position. In that case, the boundary conditions
/// should usually only prevent a further increase in the extent to which the
/// position is out of bounds, allowing a decrease to be applied successfully,
/// so that (for instance) an animation can smoothly snap an out of bounds
/// position to the bounds. See [BallisticScrollActivity].
///
/// This method must not clamp parts of the offset that are entirely within
/// the bounds described by the given `position`.
///
/// The given `position` is only valid during this method call. Do not keep a
/// reference to it to use later, as the values may update, may not update, or
/// may update to reflect an entirely unrelated scrollable.
///
/// ## Examples
///
/// [BouncingScrollPhysics] returns zero. In other words, it allows scrolling
/// past the boundary unhindered.
///
/// [ClampingScrollPhysics] returns the amount by which the value is beyond
/// the position or the boundary, whichever is furthest from the content. In
/// other words, it disallows scrolling past the boundary, but allows
/// scrolling back from being overscrolled, if for some reason the position
/// ends up overscrolled.
double applyBoundaryConditions(ScrollMetrics position, double value) {
return parent?.applyBoundaryConditions(position, value) ?? 0.0;
}
/// Describes what the scroll position should be given new viewport dimensions.
///
/// This is called by [ScrollPosition.correctForNewDimensions].
///
/// The arguments consist of the scroll metrics as they stood in the previous
/// frame and the scroll metrics as they now stand after the last layout,
/// including the position and minimum and maximum scroll extents; a flag
/// indicating if the current [ScrollActivity] considers that the user is
/// actively scrolling (see [ScrollActivity.isScrolling]); and the current
/// velocity of the scroll position, if it is being driven by the scroll
/// activity (this is 0.0 during a user gesture) (see
/// [ScrollActivity.velocity]).
///
/// The scroll metrics will be identical except for the
/// [ScrollMetrics.minScrollExtent] and [ScrollMetrics.maxScrollExtent]. They
/// are referred to as the `oldPosition` and `newPosition` (even though they
/// both technically have the same "position", in the form of
/// [ScrollMetrics.pixels]) because they are generated from the
/// [ScrollPosition] before and after updating the scroll extents.
///
/// If the returned value does not exactly match the scroll offset given by
/// the `newPosition` argument (see [ScrollMetrics.pixels]), then the
/// [ScrollPosition] will call [ScrollPosition.correctPixels] to update the
/// new scroll position to the returned value, and layout will be re-run. This
/// is expensive. The new value is subject to further manipulation by
/// [applyBoundaryConditions].
///
/// If the returned value _does_ match the `newPosition.pixels` scroll offset
/// exactly, then [ScrollPosition.applyNewDimensions] will be called next. In
/// that case, [applyBoundaryConditions] is not applied to the return value.
///
/// The given [ScrollMetrics] are only valid during this method call. Do not
/// keep references to them to use later, as the values may update, may not
/// update, or may update to reflect an entirely unrelated scrollable.
///
/// The default implementation returns the [ScrollMetrics.pixels] of the
/// `newPosition`, which indicates that the current scroll offset is
/// acceptable.
///
/// See also:
///
/// * [RangeMaintainingScrollPhysics], which is enabled by default, and
/// which prevents unexpected changes to the content dimensions from
/// causing the scroll position to get any further out of bounds.
double adjustPositionForNewDimensions({
required ScrollMetrics oldPosition,
required ScrollMetrics newPosition,
required bool isScrolling,
required double velocity,
}) {
if (parent == null) {
return newPosition.pixels;
}
return parent!.adjustPositionForNewDimensions(oldPosition: oldPosition, newPosition: newPosition, isScrolling: isScrolling, velocity: velocity);
}
/// Returns a simulation for ballistic scrolling starting from the given
/// position with the given velocity.
///
/// This is used by [ScrollPositionWithSingleContext] in the
/// [ScrollPositionWithSingleContext.goBallistic] method. If the result
/// is non-null, [ScrollPositionWithSingleContext] will begin a
/// [BallisticScrollActivity] with the returned value. Otherwise, it will
/// begin an idle activity instead.
///
/// The given `position` is only valid during this method call. Do not keep a
/// reference to it to use later, as the values may update, may not update, or
/// may update to reflect an entirely unrelated scrollable.
///
/// This method can potentially be called in every frame, even in the middle
/// of what the user perceives as a single ballistic scroll. For example, in
/// a [ListView] when previously off-screen items come into view and are laid
/// out, this method may be called with a new [ScrollMetrics.maxScrollExtent].
/// The method implementation should ensure that when the same ballistic
/// scroll motion is still intended, these calls have no side effects on the
/// physics beyond continuing that motion.
///
/// Generally this is ensured by having the [Simulation] conform to a physical
/// metaphor of a particle in ballistic flight, where the forces on the
/// particle depend only on its position, velocity, and environment, and not
/// on the current time or any internal state. This means that the
/// time-derivative of [Simulation.dx] should be possible to write
/// mathematically as a function purely of the values of [Simulation.x],
/// [Simulation.dx], and the parameters used to construct the [Simulation],
/// independent of the time.
// TODO(gnprice): Some scroll physics in the framework violate that invariant; fix them.
// An audit found three cases violating the invariant:
// https://github.com/flutter/flutter/issues/120338
// https://github.com/flutter/flutter/issues/120340
// https://github.com/flutter/flutter/issues/109675
Simulation? createBallisticSimulation(ScrollMetrics position, double velocity) {
return parent?.createBallisticSimulation(position, velocity);
}
static final SpringDescription _kDefaultSpring = SpringDescription.withDampingRatio(
mass: 0.5,
stiffness: 100.0,
ratio: 1.1,
);
/// The spring to use for ballistic simulations.
SpringDescription get spring => parent?.spring ?? _kDefaultSpring;
/// Deprecated. Call [toleranceFor] instead.
@Deprecated(
'Call toleranceFor instead. '
'This feature was deprecated after v3.7.0-13.0.pre.',
)
Tolerance get tolerance {
return toleranceFor(FixedScrollMetrics(
minScrollExtent: null,
maxScrollExtent: null,
pixels: null,
viewportDimension: null,
axisDirection: AxisDirection.down,
devicePixelRatio: WidgetsBinding.instance.window.devicePixelRatio,
));
}
/// The tolerance to use for ballistic simulations.
Tolerance toleranceFor(ScrollMetrics metrics) {
return parent?.toleranceFor(metrics) ?? Tolerance(
velocity: 1.0 / (0.050 * metrics.devicePixelRatio), // logical pixels per second
distance: 1.0 / metrics.devicePixelRatio, // logical pixels
);
}
/// The minimum distance an input pointer drag must have moved to be
/// considered a scroll fling gesture.
///
/// This value is typically compared with the distance traveled along the
/// scrolling axis.
///
/// See also:
///
/// * [VelocityTracker.getVelocityEstimate], which computes the velocity
/// of a press-drag-release gesture.
double get minFlingDistance => parent?.minFlingDistance ?? kTouchSlop;
/// The minimum velocity for an input pointer drag to be considered a
/// scroll fling.
///
/// This value is typically compared with the magnitude of fling gesture's
/// velocity along the scrolling axis.
///
/// See also:
///
/// * [VelocityTracker.getVelocityEstimate], which computes the velocity
/// of a press-drag-release gesture.
double get minFlingVelocity => parent?.minFlingVelocity ?? kMinFlingVelocity;
/// Scroll fling velocity magnitudes will be clamped to this value.
double get maxFlingVelocity => parent?.maxFlingVelocity ?? kMaxFlingVelocity;
/// Returns the velocity carried on repeated flings.
///
/// The function is applied to the existing scroll velocity when another
/// scroll drag is applied in the same direction.
///
/// By default, physics for platforms other than iOS doesn't carry momentum.
double carriedMomentum(double existingVelocity) {
return parent?.carriedMomentum(existingVelocity) ?? 0.0;
}
/// The minimum amount of pixel distance drags must move by to start motion
/// the first time or after each time the drag motion stopped.
///
/// If null, no minimum threshold is enforced.
double? get dragStartDistanceMotionThreshold => parent?.dragStartDistanceMotionThreshold;
/// Whether a viewport is allowed to change its scroll position implicitly in
/// response to a call to [RenderObject.showOnScreen].
///
/// [RenderObject.showOnScreen] is for example used to bring a text field
/// fully on screen after it has received focus. This property controls
/// whether the viewport associated with this object is allowed to change the
/// scroll position to fulfill such a request.
bool get allowImplicitScrolling => true;
/// Whether a viewport is allowed to change the scroll position as the result of user input.
bool get allowUserScrolling => true;
@override
String toString() {
if (parent == null) {
return objectRuntimeType(this, 'ScrollPhysics');
}
return '${objectRuntimeType(this, 'ScrollPhysics')} -> $parent';
}
}
/// Scroll physics that attempt to keep the scroll position in range when the
/// contents change dimensions suddenly.
///
/// This attempts to maintain the amount of overscroll or underscroll already present,
/// if the scroll position is already out of range _and_ the extents
/// have decreased, meaning that some content was removed. The reason for this
/// condition is that when new content is added, keeping the same overscroll
/// would mean that instead of showing it to the user, all of it is
/// being skipped by jumping right to the max extent.
///
/// If the scroll activity is animating the scroll position, sudden changes to
/// the scroll dimensions are allowed to happen (so as to prevent animations
/// from jumping back and forth between in-range and out-of-range values).
///
/// These physics should be combined with other scroll physics, e.g.
/// [BouncingScrollPhysics] or [ClampingScrollPhysics], to obtain a complete
/// description of typical scroll physics. See [applyTo].
///
/// ## Implementation details
///
/// Specifically, these physics perform two adjustments.
///
/// The first is to maintain overscroll when the position is out of range.
///
/// The second is to enforce the boundary when the position is in range.
///
/// If the current velocity is non-zero, neither adjustment is made. The
/// assumption is that there is an ongoing animation and therefore
/// further changing the scroll position would disrupt the experience.
///
/// If the extents haven't changed, then the overscroll adjustment is
/// not made. The assumption is that if the position is overscrolled,
/// it is intentional, otherwise the position could not have reached
/// that position. (Consider [ClampingScrollPhysics] vs
/// [BouncingScrollPhysics] for example.)
///
/// If the position itself changed since the last animation frame,
/// then the overscroll is not maintained. The assumption is similar
/// to the previous case: the position would not have been placed out
/// of range unless it was intentional.
///
/// In addition, if the position changed and the boundaries were and
/// still are finite, then the boundary isn't enforced either, for
/// the same reason. However, if any of the boundaries were or are
/// now infinite, the boundary _is_ enforced, on the assumption that
/// infinite boundaries indicate a lazy-loading scroll view, which
/// cannot enforce boundaries while the full list has not loaded.
///
/// If the range was out of range, then the boundary is not enforced
/// even if the range is not maintained. If the range is maintained,
/// then the distance between the old position and the old boundary is
/// applied to the new boundary to obtain the new position.
///
/// If the range was in range, and the boundary is to be enforced,
/// then the new position is obtained by deferring to the other physics,
/// if any, and then clamped to the new range.
class RangeMaintainingScrollPhysics extends ScrollPhysics {
/// Creates scroll physics that maintain the scroll position in range.
const RangeMaintainingScrollPhysics({ super.parent });
@override
RangeMaintainingScrollPhysics applyTo(ScrollPhysics? ancestor) {
return RangeMaintainingScrollPhysics(parent: buildParent(ancestor));
}
@override
double adjustPositionForNewDimensions({
required ScrollMetrics oldPosition,
required ScrollMetrics newPosition,
required bool isScrolling,
required double velocity,
}) {
bool maintainOverscroll = true;
bool enforceBoundary = true;
if (velocity != 0.0) {
// Don't try to adjust an animating position, the jumping around
// would be distracting.
maintainOverscroll = false;
enforceBoundary = false;
}
if ((oldPosition.minScrollExtent == newPosition.minScrollExtent) &&
(oldPosition.maxScrollExtent == newPosition.maxScrollExtent)) {
// If the extents haven't changed then ignore overscroll.
maintainOverscroll = false;
}
if (oldPosition.pixels != newPosition.pixels) {
// If the position has been changed already, then it might have
// been adjusted to expect new overscroll, so don't try to
// maintain the relative overscroll.
maintainOverscroll = false;
if (oldPosition.minScrollExtent.isFinite && oldPosition.maxScrollExtent.isFinite &&
newPosition.minScrollExtent.isFinite && newPosition.maxScrollExtent.isFinite) {
// In addition, if the position changed then we don't enforce the new
// boundary if both the new and previous boundaries are entirely finite.
// A common case where the position changes while one
// of the extents is infinite is a lazily-loaded list. (If the
// boundaries were finite, and the position changed, then we
// assume it was intentional.)
enforceBoundary = false;
}
}
if ((oldPosition.pixels < oldPosition.minScrollExtent) ||
(oldPosition.pixels > oldPosition.maxScrollExtent)) {
// If the old position was out of range, then we should
// not try to keep the new position in range.
enforceBoundary = false;
}
if (maintainOverscroll) {
// Force the new position to be no more out of range than it was before, if:
// * it was overscrolled, and
// * the extents have decreased, meaning that some content was removed. The
// reason for this condition is that when new content is added, keeping
// the same overscroll would mean that instead of showing it to the user,
// all of it is being skipped by jumping right to the max extent.
if (oldPosition.pixels < oldPosition.minScrollExtent &&
newPosition.minScrollExtent > oldPosition.minScrollExtent) {
final double oldDelta = oldPosition.minScrollExtent - oldPosition.pixels;
return newPosition.minScrollExtent - oldDelta;
}
if (oldPosition.pixels > oldPosition.maxScrollExtent &&
newPosition.maxScrollExtent < oldPosition.maxScrollExtent) {
final double oldDelta = oldPosition.pixels - oldPosition.maxScrollExtent;
return newPosition.maxScrollExtent + oldDelta;
}
}
// If we're not forcing the overscroll, defer to other physics.
double result = super.adjustPositionForNewDimensions(oldPosition: oldPosition, newPosition: newPosition, isScrolling: isScrolling, velocity: velocity);
if (enforceBoundary) {
// ...but if they put us out of range then reinforce the boundary.
result = clampDouble(result, newPosition.minScrollExtent, newPosition.maxScrollExtent);
}
return result;
}
}
/// Scroll physics for environments that allow the scroll offset to go beyond
/// the bounds of the content, but then bounce the content back to the edge of
/// those bounds.
///
/// This is the behavior typically seen on iOS.
///
/// [BouncingScrollPhysics] by itself will not create an overscroll effect if
/// the contents of the scroll view do not extend beyond the size of the
/// viewport. To create the overscroll and bounce effect regardless of the
/// length of your scroll view, combine with [AlwaysScrollableScrollPhysics].
///
/// {@tool snippet}
/// ```dart
/// const BouncingScrollPhysics(parent: AlwaysScrollableScrollPhysics())
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ScrollConfiguration], which uses this to provide the default
/// scroll behavior on iOS.
/// * [ClampingScrollPhysics], which is the analogous physics for Android's
/// clamping behavior.
/// * [ScrollPhysics], for more examples of combining [ScrollPhysics] objects
/// of different types to get the desired scroll physics.
class BouncingScrollPhysics extends ScrollPhysics {
/// Creates scroll physics that bounce back from the edge.
const BouncingScrollPhysics({
this.decelerationRate = ScrollDecelerationRate.normal,
super.parent,
});
/// Used to determine parameters for friction simulations.
final ScrollDecelerationRate decelerationRate;
@override
BouncingScrollPhysics applyTo(ScrollPhysics? ancestor) {
return BouncingScrollPhysics(
parent: buildParent(ancestor),
decelerationRate: decelerationRate
);
}
/// The multiple applied to overscroll to make it appear that scrolling past
/// the edge of the scrollable contents is harder than scrolling the list.
/// This is done by reducing the ratio of the scroll effect output vs the
/// scroll gesture input.
///
/// This factor starts at 0.52 and progressively becomes harder to overscroll
/// as more of the area past the edge is dragged in (represented by an increasing
/// `overscrollFraction` which starts at 0 when there is no overscroll).
double frictionFactor(double overscrollFraction) {
switch (decelerationRate) {
case ScrollDecelerationRate.fast:
return 0.26 * math.pow(1 - overscrollFraction, 2);
case ScrollDecelerationRate.normal:
return 0.52 * math.pow(1 - overscrollFraction, 2);
}
}
@override
double applyPhysicsToUserOffset(ScrollMetrics position, double offset) {
assert(offset != 0.0);
assert(position.minScrollExtent <= position.maxScrollExtent);
if (!position.outOfRange) {
return offset;
}
final double overscrollPastStart = math.max(position.minScrollExtent - position.pixels, 0.0);
final double overscrollPastEnd = math.max(position.pixels - position.maxScrollExtent, 0.0);
final double overscrollPast = math.max(overscrollPastStart, overscrollPastEnd);
final bool easing = (overscrollPastStart > 0.0 && offset < 0.0)
|| (overscrollPastEnd > 0.0 && offset > 0.0);
final double friction = easing
// Apply less resistance when easing the overscroll vs tensioning.
? frictionFactor((overscrollPast - offset.abs()) / position.viewportDimension)
: frictionFactor(overscrollPast / position.viewportDimension);
final double direction = offset.sign;
if (easing && decelerationRate == ScrollDecelerationRate.fast) {
return direction * offset.abs();
}
return direction * _applyFriction(overscrollPast, offset.abs(), friction);
}
static double _applyFriction(double extentOutside, double absDelta, double gamma) {
assert(absDelta > 0);
double total = 0.0;
if (extentOutside > 0) {
final double deltaToLimit = extentOutside / gamma;
if (absDelta < deltaToLimit) {
return absDelta * gamma;
}
total += extentOutside;
absDelta -= deltaToLimit;
}
return total + absDelta;
}
@override
double applyBoundaryConditions(ScrollMetrics position, double value) => 0.0;
@override
Simulation? createBallisticSimulation(ScrollMetrics position, double velocity) {
final Tolerance tolerance = toleranceFor(position);
if (velocity.abs() >= tolerance.velocity || position.outOfRange) {
return BouncingScrollSimulation(
spring: spring,
position: position.pixels,
velocity: velocity,
leadingExtent: position.minScrollExtent,
trailingExtent: position.maxScrollExtent,
tolerance: tolerance,
constantDeceleration: switch (decelerationRate) {
ScrollDecelerationRate.fast => 1400,
ScrollDecelerationRate.normal => 0,
},
);
}
return null;
}
// The ballistic simulation here decelerates more slowly than the one for
// ClampingScrollPhysics so we require a more deliberate input gesture
// to trigger a fling.
@override
double get minFlingVelocity => kMinFlingVelocity * 2.0;
// Methodology:
// 1- Use https://github.com/flutter/platform_tests/tree/master/scroll_overlay to test with
// Flutter and platform scroll views superimposed.
// 3- If the scrollables stopped overlapping at any moment, adjust the desired
// output value of this function at that input speed.
// 4- Feed new input/output set into a power curve fitter. Change function
// and repeat from 2.
// 5- Repeat from 2 with medium and slow flings.
/// Momentum build-up function that mimics iOS's scroll speed increase with repeated flings.
///
/// The velocity of the last fling is not an important factor. Existing speed
/// and (related) time since last fling are factors for the velocity transfer
/// calculations.
@override
double carriedMomentum(double existingVelocity) {
return existingVelocity.sign *
math.min(0.000816 * math.pow(existingVelocity.abs(), 1.967).toDouble(), 40000.0);
}
// Eyeballed from observation to counter the effect of an unintended scroll
// from the natural motion of lifting the finger after a scroll.
@override
double get dragStartDistanceMotionThreshold => 3.5;
@override
double get maxFlingVelocity {
return switch (decelerationRate) {
ScrollDecelerationRate.fast => kMaxFlingVelocity * 8.0,
ScrollDecelerationRate.normal => super.maxFlingVelocity,
};
}
@override
SpringDescription get spring {
switch (decelerationRate) {
case ScrollDecelerationRate.fast:
return SpringDescription.withDampingRatio(
mass: 0.3,
stiffness: 75.0,
ratio: 1.3,
);
case ScrollDecelerationRate.normal:
return super.spring;
}
}
}
/// Scroll physics for environments that prevent the scroll offset from reaching
/// beyond the bounds of the content.
///
/// This is the behavior typically seen on Android.
///
/// See also:
///
/// * [ScrollConfiguration], which uses this to provide the default
/// scroll behavior on Android.
/// * [BouncingScrollPhysics], which is the analogous physics for iOS' bouncing
/// behavior.
/// * [GlowingOverscrollIndicator], which is used by [ScrollConfiguration] to
/// provide the glowing effect that is usually found with this clamping effect
/// on Android. When using a [MaterialApp], the [GlowingOverscrollIndicator]'s
/// glow color is specified to use the overall theme's
/// [ColorScheme.secondary] color.
class ClampingScrollPhysics extends ScrollPhysics {
/// Creates scroll physics that prevent the scroll offset from exceeding the
/// bounds of the content.
const ClampingScrollPhysics({ super.parent });
@override
ClampingScrollPhysics applyTo(ScrollPhysics? ancestor) {
return ClampingScrollPhysics(parent: buildParent(ancestor));
}
@override
double applyBoundaryConditions(ScrollMetrics position, double value) {
assert(() {
if (value == position.pixels) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType.applyBoundaryConditions() was called redundantly.'),
ErrorDescription(
'The proposed new position, $value, is exactly equal to the current position of the '
'given ${position.runtimeType}, ${position.pixels}.\n'
'The applyBoundaryConditions method should only be called when the value is '
'going to actually change the pixels, otherwise it is redundant.',
),
DiagnosticsProperty<ScrollPhysics>('The physics object in question was', this, style: DiagnosticsTreeStyle.errorProperty),
DiagnosticsProperty<ScrollMetrics>('The position object in question was', position, style: DiagnosticsTreeStyle.errorProperty),
]);
}
return true;
}());
if (value < position.pixels && position.pixels <= position.minScrollExtent) {
// Underscroll.
return value - position.pixels;
}
if (position.maxScrollExtent <= position.pixels && position.pixels < value) {
// Overscroll.
return value - position.pixels;
}
if (value < position.minScrollExtent && position.minScrollExtent < position.pixels) {
// Hit top edge.
return value - position.minScrollExtent;
}
if (position.pixels < position.maxScrollExtent && position.maxScrollExtent < value) {
// Hit bottom edge.
return value - position.maxScrollExtent;
}
return 0.0;
}
@override
Simulation? createBallisticSimulation(ScrollMetrics position, double velocity) {
final Tolerance tolerance = toleranceFor(position);
if (position.outOfRange) {
double? end;
if (position.pixels > position.maxScrollExtent) {
end = position.maxScrollExtent;
}
if (position.pixels < position.minScrollExtent) {
end = position.minScrollExtent;
}
assert(end != null);
return ScrollSpringSimulation(
spring,
position.pixels,
end!,
math.min(0.0, velocity),
tolerance: tolerance,
);
}
if (velocity.abs() < tolerance.velocity) {
return null;
}
if (velocity > 0.0 && position.pixels >= position.maxScrollExtent) {
return null;
}
if (velocity < 0.0 && position.pixels <= position.minScrollExtent) {
return null;
}
return ClampingScrollSimulation(
position: position.pixels,
velocity: velocity,
tolerance: tolerance,
);
}
}
/// Scroll physics that always lets the user scroll.
///
/// This overrides the default behavior which is to disable scrolling
/// when there is no content to scroll. It does not override the
/// handling of overscrolling.
///
/// On Android, overscrolls will be clamped by default and result in an
/// overscroll glow. On iOS, overscrolls will load a spring that will return the
/// scroll view to its normal range when released.
///
/// See also:
///
/// * [ScrollPhysics], which can be used instead of this class when the default
/// behavior is desired instead.
/// * [BouncingScrollPhysics], which provides the bouncing overscroll behavior
/// found on iOS.
/// * [ClampingScrollPhysics], which provides the clamping overscroll behavior
/// found on Android.
class AlwaysScrollableScrollPhysics extends ScrollPhysics {
/// Creates scroll physics that always lets the user scroll.
const AlwaysScrollableScrollPhysics({ super.parent });
@override
AlwaysScrollableScrollPhysics applyTo(ScrollPhysics? ancestor) {
return AlwaysScrollableScrollPhysics(parent: buildParent(ancestor));
}
@override
bool shouldAcceptUserOffset(ScrollMetrics position) => true;
}
/// Scroll physics that does not allow the user to scroll.
///
/// See also:
///
/// * [ScrollPhysics], which can be used instead of this class when the default
/// behavior is desired instead.
/// * [BouncingScrollPhysics], which provides the bouncing overscroll behavior
/// found on iOS.
/// * [ClampingScrollPhysics], which provides the clamping overscroll behavior
/// found on Android.
class NeverScrollableScrollPhysics extends ScrollPhysics {
/// Creates scroll physics that does not let the user scroll.
const NeverScrollableScrollPhysics({ super.parent });
@override
NeverScrollableScrollPhysics applyTo(ScrollPhysics? ancestor) {
return NeverScrollableScrollPhysics(parent: buildParent(ancestor));
}
@override
bool get allowUserScrolling => false;
@override
bool get allowImplicitScrolling => false;
}
| flutter/packages/flutter/lib/src/widgets/scroll_physics.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_physics.dart",
"repo_id": "flutter",
"token_count": 11511
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection' show HashMap, SplayTreeMap;
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'automatic_keep_alive.dart';
import 'basic.dart';
import 'framework.dart';
import 'scroll_delegate.dart';
/// A base class for slivers that have [KeepAlive] children.
///
/// See also:
///
/// * [KeepAlive], which marks whether its child widget should be kept alive.
/// * [SliverChildBuilderDelegate] and [SliverChildListDelegate], slivers
/// which make use of the keep alive functionality through the
/// `addAutomaticKeepAlives` property.
/// * [SliverGrid] and [SliverList], two sliver widgets that are commonly
/// wrapped with [KeepAlive] widgets to preserve their sliver child subtrees.
abstract class SliverWithKeepAliveWidget extends RenderObjectWidget {
/// Initializes fields for subclasses.
const SliverWithKeepAliveWidget({
super.key,
});
@override
RenderSliverWithKeepAliveMixin createRenderObject(BuildContext context);
}
/// A base class for slivers that have multiple box children.
///
/// Helps subclasses build their children lazily using a [SliverChildDelegate].
///
/// The widgets returned by the [delegate] are cached and the delegate is only
/// consulted again if it changes and the new delegate's
/// [SliverChildDelegate.shouldRebuild] method returns true.
abstract class SliverMultiBoxAdaptorWidget extends SliverWithKeepAliveWidget {
/// Initializes fields for subclasses.
const SliverMultiBoxAdaptorWidget({
super.key,
required this.delegate,
});
/// {@template flutter.widgets.SliverMultiBoxAdaptorWidget.delegate}
/// The delegate that provides the children for this widget.
///
/// The children are constructed lazily using this delegate to avoid creating
/// more children than are visible through the [Viewport].
///
/// ## Using more than one delegate in a [Viewport]
///
/// If multiple delegates are used in a single scroll view, the first child of
/// each delegate will always be laid out, even if it extends beyond the
/// currently viewable area. This is because at least one child is required in
/// order to estimate the max scroll offset for the whole scroll view, as it
/// uses the currently built children to estimate the remaining children's
/// extent.
///
/// See also:
///
/// * [SliverChildBuilderDelegate] and [SliverChildListDelegate], which are
/// commonly used subclasses of [SliverChildDelegate] that use a builder
/// callback and an explicit child list, respectively.
/// {@endtemplate}
final SliverChildDelegate delegate;
@override
SliverMultiBoxAdaptorElement createElement() => SliverMultiBoxAdaptorElement(this);
@override
RenderSliverMultiBoxAdaptor createRenderObject(BuildContext context);
/// Returns an estimate of the max scroll extent for all the children.
///
/// Subclasses should override this function if they have additional
/// information about their max scroll extent.
///
/// This is used by [SliverMultiBoxAdaptorElement] to implement part of the
/// [RenderSliverBoxChildManager] API.
///
/// The default implementation defers to [delegate] via its
/// [SliverChildDelegate.estimateMaxScrollOffset] method.
double? estimateMaxScrollOffset(
SliverConstraints? constraints,
int firstIndex,
int lastIndex,
double leadingScrollOffset,
double trailingScrollOffset,
) {
assert(lastIndex >= firstIndex);
return delegate.estimateMaxScrollOffset(
firstIndex,
lastIndex,
leadingScrollOffset,
trailingScrollOffset,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverChildDelegate>('delegate', delegate));
}
}
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
///
/// Each child is forced to have the [SliverConstraints.crossAxisExtent] in the
/// cross axis but determines its own main axis extent.
///
/// [SliverList] determines its scroll offset by "dead reckoning" because
/// children outside the visible part of the sliver are not materialized, which
/// means [SliverList] cannot learn their main axis extent. Instead, newly
/// materialized children are placed adjacent to existing children.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ORiTTaVY6mM}
///
/// If the children have a fixed extent in the main axis, consider using
/// [SliverFixedExtentList] rather than [SliverList] because
/// [SliverFixedExtentList] does not need to perform layout on its children to
/// obtain their extent in the main axis and is therefore more efficient.
///
/// {@macro flutter.widgets.SliverChildDelegate.lifecycle}
///
/// See also:
///
/// * <https://flutter.dev/docs/development/ui/advanced/slivers>, a description
/// of what slivers are and how to use them.
/// * [SliverFixedExtentList], which is more efficient for children with
/// the same extent in the main axis.
/// * [SliverPrototypeExtentList], which is similar to [SliverFixedExtentList]
/// except that it uses a prototype list item instead of a pixel value to define
/// the main axis extent of each item.
/// * [SliverAnimatedList], which animates items added to or removed from a
/// list.
/// * [SliverGrid], which places multiple children in a two dimensional grid.
/// * [SliverAnimatedGrid], a sliver which animates items when they are
/// inserted into or removed from a grid.
class SliverList extends SliverMultiBoxAdaptorWidget {
/// Creates a sliver that places box children in a linear array.
const SliverList({
super.key,
required super.delegate,
});
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// This constructor is appropriate for sliver lists with a large (or
/// infinite) number of children because the builder is called only for those
/// children that are actually visible.
///
/// Providing a non-null `itemCount` improves the ability of the [SliverGrid]
/// to estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example, which would be provided in [CustomScrollView.slivers],
/// shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverList.builder(
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('list item $index'),
/// );
/// },
/// )
/// ```
/// {@end-tool}
SliverList.builder({
super.key,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// A sliver that places multiple box children, separated by box widgets, in a
/// linear array along the main axis.
///
/// This constructor is appropriate for sliver lists with a large (or
/// infinite) number of children because the builder is called only for those
/// children that are actually visible.
///
/// Providing a non-null `itemCount` improves the ability of the [SliverGrid]
/// to estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
///
/// The `separatorBuilder` is similar to `itemBuilder`, except it is the widget
/// that gets placed between itemBuilder(context, index) and itemBuilder(context, index + 1).
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example shows how to create a [SliverList] whose [Container] items
/// are separated by [Divider]s. The [SliverList] would be provided in
/// [CustomScrollView.slivers].
///
/// ```dart
/// SliverList.separated(
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('list item $index'),
/// );
/// },
/// separatorBuilder: (BuildContext context, int index) => const Divider(),
/// )
/// ```
/// {@end-tool}
SliverList.separated({
super.key,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
required NullableIndexedWidgetBuilder separatorBuilder,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
final int itemIndex = index ~/ 2;
final Widget? widget;
if (index.isEven) {
widget = itemBuilder(context, itemIndex);
} else {
widget = separatorBuilder(context, itemIndex);
assert(() {
if (widget == null) {
throw FlutterError('separatorBuilder cannot return null.');
}
return true;
}());
}
return widget;
},
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount == null ? null : math.max(0, itemCount * 2 - 1),
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
semanticIndexCallback: (Widget _, int index) {
return index.isEven ? index ~/ 2 : null;
},
));
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// This constructor uses a list of [Widget]s to build the sliver.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example, which would be provided in [CustomScrollView.slivers],
/// shows a list containing two [Text] widgets:
///
/// ```dart
/// SliverList.list(
/// children: const <Widget>[
/// Text('Hello'),
/// Text('World!'),
/// ],
/// );
/// ```
/// {@end-tool}
SliverList.list({
super.key,
required List<Widget> children,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
@override
SliverMultiBoxAdaptorElement createElement() => SliverMultiBoxAdaptorElement(this, replaceMovedChildren: true);
@override
RenderSliverList createRenderObject(BuildContext context) {
final SliverMultiBoxAdaptorElement element = context as SliverMultiBoxAdaptorElement;
return RenderSliverList(childManager: element);
}
}
/// A sliver that places multiple box children with the same main axis extent in
/// a linear array.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
///
/// [SliverFixedExtentList] places its children in a linear array along the main
/// axis starting at offset zero and without gaps. Each child is forced to have
/// the [itemExtent] in the main axis and the
/// [SliverConstraints.crossAxisExtent] in the cross axis.
///
/// [SliverFixedExtentList] is more efficient than [SliverList] because
/// [SliverFixedExtentList] does not need to perform layout on its children to
/// obtain their extent in the main axis.
///
/// {@tool snippet}
///
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverFixedExtentList(
/// itemExtent: 50.0,
/// delegate: SliverChildBuilderDelegate(
/// (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('list item $index'),
/// );
/// },
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@macro flutter.widgets.SliverChildDelegate.lifecycle}
///
/// See also:
///
/// * [SliverPrototypeExtentList], which is similar to [SliverFixedExtentList]
/// except that it uses a prototype list item instead of a pixel value to define
/// the main axis extent of each item.
/// * [SliverVariedExtentList], which supports children with varying (but known
/// upfront) extents.
/// * [SliverFillViewport], which determines the [itemExtent] based on
/// [SliverConstraints.viewportMainAxisExtent].
/// * [SliverList], which does not require its children to have the same
/// extent in the main axis.
class SliverFixedExtentList extends SliverMultiBoxAdaptorWidget {
/// Creates a sliver that places box children with the same main axis extent
/// in a linear array.
const SliverFixedExtentList({
super.key,
required super.delegate,
required this.itemExtent,
});
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// [SliverFixedExtentList] places its children in a linear array along the main
/// axis starting at offset zero and without gaps. Each child is forced to have
/// the [itemExtent] in the main axis and the
/// [SliverConstraints.crossAxisExtent] in the cross axis.
///
/// This constructor is appropriate for sliver lists with a large (or
/// infinite) number of children whose extent is already determined.
///
/// Providing a non-null `itemCount` improves the ability of the [SliverGrid]
/// to estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// The `itemExtent` argument is the extent of each item.
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
/// {@tool snippet}
///
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverFixedExtentList.builder(
/// itemExtent: 50.0,
/// itemBuilder: (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.lightBlue[100 * (index % 9)],
/// child: Text('list item $index'),
/// );
/// },
/// )
/// ```
/// {@end-tool}
SliverFixedExtentList.builder({
super.key,
required NullableIndexedWidgetBuilder itemBuilder,
required this.itemExtent,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// A sliver that places multiple box children in a linear array along the main
/// axis.
///
/// [SliverFixedExtentList] places its children in a linear array along the main
/// axis starting at offset zero and without gaps. Each child is forced to have
/// the [itemExtent] in the main axis and the
/// [SliverConstraints.crossAxisExtent] in the cross axis.
///
/// This constructor uses a list of [Widget]s to build the sliver.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
///
/// {@tool snippet}
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows an infinite number of items in varying shades of blue:
///
/// ```dart
/// SliverFixedExtentList.list(
/// itemExtent: 50.0,
/// children: const <Widget>[
/// Text('Hello'),
/// Text('World!'),
/// ],
/// );
/// ```
/// {@end-tool}
SliverFixedExtentList.list({
super.key,
required List<Widget> children,
required this.itemExtent,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildListDelegate(
children,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// The extent the children are forced to have in the main axis.
final double itemExtent;
@override
RenderSliverFixedExtentList createRenderObject(BuildContext context) {
final SliverMultiBoxAdaptorElement element = context as SliverMultiBoxAdaptorElement;
return RenderSliverFixedExtentList(childManager: element, itemExtent: itemExtent);
}
@override
void updateRenderObject(BuildContext context, RenderSliverFixedExtentList renderObject) {
renderObject.itemExtent = itemExtent;
}
}
/// A sliver that places multiple box children in a two dimensional arrangement.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
///
/// [SliverGrid] places its children in arbitrary positions determined by
/// [gridDelegate]. Each child is forced to have the size specified by the
/// [gridDelegate].
///
/// The main axis direction of a grid is the direction in which it scrolls; the
/// cross axis direction is the orthogonal direction.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ORiTTaVY6mM}
///
/// {@tool snippet}
///
/// This example, which would be inserted into a [CustomScrollView.slivers]
/// list, shows twenty boxes in a pretty teal grid:
///
/// ```dart
/// SliverGrid(
/// gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
/// maxCrossAxisExtent: 200.0,
/// mainAxisSpacing: 10.0,
/// crossAxisSpacing: 10.0,
/// childAspectRatio: 4.0,
/// ),
/// delegate: SliverChildBuilderDelegate(
/// (BuildContext context, int index) {
/// return Container(
/// alignment: Alignment.center,
/// color: Colors.teal[100 * (index % 9)],
/// child: Text('grid item $index'),
/// );
/// },
/// childCount: 20,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@macro flutter.widgets.SliverChildDelegate.lifecycle}
///
/// See also:
///
/// * [SliverList], which places its children in a linear array.
/// * [SliverFixedExtentList], which places its children in a linear
/// array with a fixed extent in the main axis.
/// * [SliverPrototypeExtentList], which is similar to [SliverFixedExtentList]
/// except that it uses a prototype list item instead of a pixel value to define
/// the main axis extent of each item.
class SliverGrid extends SliverMultiBoxAdaptorWidget {
/// Creates a sliver that places multiple box children in a two dimensional
/// arrangement.
const SliverGrid({
super.key,
required super.delegate,
required this.gridDelegate,
});
/// A sliver that creates a 2D array of widgets that are created on demand.
///
/// This constructor is appropriate for sliver grids with a large (or
/// infinite) number of children because the builder is called only for those
/// children that are actually visible.
///
/// Providing a non-null `itemCount` improves the ability of the [SliverGrid]
/// to estimate the maximum scroll extent.
///
/// `itemBuilder` will be called only with indices greater than or equal to
/// zero and less than `itemCount`.
///
/// {@macro flutter.widgets.ListView.builder.itemBuilder}
///
/// {@macro flutter.widgets.PageView.findChildIndexCallback}
///
/// The [gridDelegate] argument is required.
///
/// The `addAutomaticKeepAlives` argument corresponds to the
/// [SliverChildBuilderDelegate.addAutomaticKeepAlives] property. The
/// `addRepaintBoundaries` argument corresponds to the
/// [SliverChildBuilderDelegate.addRepaintBoundaries] property. The
/// `addSemanticIndexes` argument corresponds to the
/// [SliverChildBuilderDelegate.addSemanticIndexes] property.
SliverGrid.builder({
super.key,
required this.gridDelegate,
required NullableIndexedWidgetBuilder itemBuilder,
ChildIndexGetter? findChildIndexCallback,
int? itemCount,
bool addAutomaticKeepAlives = true,
bool addRepaintBoundaries = true,
bool addSemanticIndexes = true,
}) : super(delegate: SliverChildBuilderDelegate(
itemBuilder,
findChildIndexCallback: findChildIndexCallback,
childCount: itemCount,
addAutomaticKeepAlives: addAutomaticKeepAlives,
addRepaintBoundaries: addRepaintBoundaries,
addSemanticIndexes: addSemanticIndexes,
));
/// Creates a sliver that places multiple box children in a two dimensional
/// arrangement with a fixed number of tiles in the cross axis.
///
/// Uses a [SliverGridDelegateWithFixedCrossAxisCount] as the [gridDelegate],
/// and a [SliverChildListDelegate] as the [delegate].
///
/// See also:
///
/// * [GridView.count], the equivalent constructor for [GridView] widgets.
SliverGrid.count({
super.key,
required int crossAxisCount,
double mainAxisSpacing = 0.0,
double crossAxisSpacing = 0.0,
double childAspectRatio = 1.0,
List<Widget> children = const <Widget>[],
}) : gridDelegate = SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: crossAxisCount,
mainAxisSpacing: mainAxisSpacing,
crossAxisSpacing: crossAxisSpacing,
childAspectRatio: childAspectRatio,
),
super(delegate: SliverChildListDelegate(children));
/// Creates a sliver that places multiple box children in a two dimensional
/// arrangement with tiles that each have a maximum cross-axis extent.
///
/// Uses a [SliverGridDelegateWithMaxCrossAxisExtent] as the [gridDelegate],
/// and a [SliverChildListDelegate] as the [delegate].
///
/// See also:
///
/// * [GridView.extent], the equivalent constructor for [GridView] widgets.
SliverGrid.extent({
super.key,
required double maxCrossAxisExtent,
double mainAxisSpacing = 0.0,
double crossAxisSpacing = 0.0,
double childAspectRatio = 1.0,
List<Widget> children = const <Widget>[],
}) : gridDelegate = SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: maxCrossAxisExtent,
mainAxisSpacing: mainAxisSpacing,
crossAxisSpacing: crossAxisSpacing,
childAspectRatio: childAspectRatio,
),
super(delegate: SliverChildListDelegate(children));
/// The delegate that controls the size and position of the children.
final SliverGridDelegate gridDelegate;
@override
RenderSliverGrid createRenderObject(BuildContext context) {
final SliverMultiBoxAdaptorElement element = context as SliverMultiBoxAdaptorElement;
return RenderSliverGrid(childManager: element, gridDelegate: gridDelegate);
}
@override
void updateRenderObject(BuildContext context, RenderSliverGrid renderObject) {
renderObject.gridDelegate = gridDelegate;
}
@override
double estimateMaxScrollOffset(
SliverConstraints? constraints,
int firstIndex,
int lastIndex,
double leadingScrollOffset,
double trailingScrollOffset,
) {
return super.estimateMaxScrollOffset(
constraints,
firstIndex,
lastIndex,
leadingScrollOffset,
trailingScrollOffset,
) ?? gridDelegate.getLayout(constraints!).computeMaxScrollOffset(delegate.estimatedChildCount!);
}
}
/// An element that lazily builds children for a [SliverMultiBoxAdaptorWidget].
///
/// Implements [RenderSliverBoxChildManager], which lets this element manage
/// the children of subclasses of [RenderSliverMultiBoxAdaptor].
class SliverMultiBoxAdaptorElement extends RenderObjectElement implements RenderSliverBoxChildManager {
/// Creates an element that lazily builds children for the given widget.
///
/// If `replaceMovedChildren` is set to true, a new child is proactively
/// inflate for the index that was previously occupied by a child that moved
/// to a new index. The layout offset of the moved child is copied over to the
/// new child. RenderObjects, that depend on the layout offset of existing
/// children during [RenderObject.performLayout] should set this to true
/// (example: [RenderSliverList]). For RenderObjects that figure out the
/// layout offset of their children without looking at the layout offset of
/// existing children this should be set to false (example:
/// [RenderSliverFixedExtentList]) to avoid inflating unnecessary children.
SliverMultiBoxAdaptorElement(SliverMultiBoxAdaptorWidget super.widget, {bool replaceMovedChildren = false})
: _replaceMovedChildren = replaceMovedChildren;
final bool _replaceMovedChildren;
@override
RenderSliverMultiBoxAdaptor get renderObject => super.renderObject as RenderSliverMultiBoxAdaptor;
@override
void update(covariant SliverMultiBoxAdaptorWidget newWidget) {
final SliverMultiBoxAdaptorWidget oldWidget = widget as SliverMultiBoxAdaptorWidget;
super.update(newWidget);
final SliverChildDelegate newDelegate = newWidget.delegate;
final SliverChildDelegate oldDelegate = oldWidget.delegate;
if (newDelegate != oldDelegate &&
(newDelegate.runtimeType != oldDelegate.runtimeType || newDelegate.shouldRebuild(oldDelegate))) {
performRebuild();
}
}
final SplayTreeMap<int, Element?> _childElements = SplayTreeMap<int, Element?>();
RenderBox? _currentBeforeChild;
@override
void performRebuild() {
super.performRebuild();
_currentBeforeChild = null;
bool childrenUpdated = false;
assert(_currentlyUpdatingChildIndex == null);
try {
final SplayTreeMap<int, Element?> newChildren = SplayTreeMap<int, Element?>();
final Map<int, double> indexToLayoutOffset = HashMap<int, double>();
final SliverMultiBoxAdaptorWidget adaptorWidget = widget as SliverMultiBoxAdaptorWidget;
void processElement(int index) {
_currentlyUpdatingChildIndex = index;
if (_childElements[index] != null && _childElements[index] != newChildren[index]) {
// This index has an old child that isn't used anywhere and should be deactivated.
_childElements[index] = updateChild(_childElements[index], null, index);
childrenUpdated = true;
}
final Element? newChild = updateChild(newChildren[index], _build(index, adaptorWidget), index);
if (newChild != null) {
childrenUpdated = childrenUpdated || _childElements[index] != newChild;
_childElements[index] = newChild;
final SliverMultiBoxAdaptorParentData parentData = newChild.renderObject!.parentData! as SliverMultiBoxAdaptorParentData;
if (index == 0) {
parentData.layoutOffset = 0.0;
} else if (indexToLayoutOffset.containsKey(index)) {
parentData.layoutOffset = indexToLayoutOffset[index];
}
if (!parentData.keptAlive) {
_currentBeforeChild = newChild.renderObject as RenderBox?;
}
} else {
childrenUpdated = true;
_childElements.remove(index);
}
}
for (final int index in _childElements.keys.toList()) {
final Key? key = _childElements[index]!.widget.key;
final int? newIndex = key == null ? null : adaptorWidget.delegate.findIndexByKey(key);
final SliverMultiBoxAdaptorParentData? childParentData =
_childElements[index]!.renderObject?.parentData as SliverMultiBoxAdaptorParentData?;
if (childParentData != null && childParentData.layoutOffset != null) {
indexToLayoutOffset[index] = childParentData.layoutOffset!;
}
if (newIndex != null && newIndex != index) {
// The layout offset of the child being moved is no longer accurate.
if (childParentData != null) {
childParentData.layoutOffset = null;
}
newChildren[newIndex] = _childElements[index];
if (_replaceMovedChildren) {
// We need to make sure the original index gets processed.
newChildren.putIfAbsent(index, () => null);
}
// We do not want the remapped child to get deactivated during processElement.
_childElements.remove(index);
} else {
newChildren.putIfAbsent(index, () => _childElements[index]);
}
}
renderObject.debugChildIntegrityEnabled = false; // Moving children will temporary violate the integrity.
newChildren.keys.forEach(processElement);
// An element rebuild only updates existing children. The underflow check
// is here to make sure we look ahead one more child if we were at the end
// of the child list before the update. By doing so, we can update the max
// scroll offset during the layout phase. Otherwise, the layout phase may
// be skipped, and the scroll view may be stuck at the previous max
// scroll offset.
//
// This logic is not needed if any existing children has been updated,
// because we will not skip the layout phase if that happens.
if (!childrenUpdated && _didUnderflow) {
final int lastKey = _childElements.lastKey() ?? -1;
final int rightBoundary = lastKey + 1;
newChildren[rightBoundary] = _childElements[rightBoundary];
processElement(rightBoundary);
}
} finally {
_currentlyUpdatingChildIndex = null;
renderObject.debugChildIntegrityEnabled = true;
}
}
Widget? _build(int index, SliverMultiBoxAdaptorWidget widget) {
return widget.delegate.build(this, index);
}
@override
void createChild(int index, { required RenderBox? after }) {
assert(_currentlyUpdatingChildIndex == null);
owner!.buildScope(this, () {
final bool insertFirst = after == null;
assert(insertFirst || _childElements[index-1] != null);
_currentBeforeChild = insertFirst ? null : (_childElements[index-1]!.renderObject as RenderBox?);
Element? newChild;
try {
final SliverMultiBoxAdaptorWidget adaptorWidget = widget as SliverMultiBoxAdaptorWidget;
_currentlyUpdatingChildIndex = index;
newChild = updateChild(_childElements[index], _build(index, adaptorWidget), index);
} finally {
_currentlyUpdatingChildIndex = null;
}
if (newChild != null) {
_childElements[index] = newChild;
} else {
_childElements.remove(index);
}
});
}
@override
Element? updateChild(Element? child, Widget? newWidget, Object? newSlot) {
final SliverMultiBoxAdaptorParentData? oldParentData = child?.renderObject?.parentData as SliverMultiBoxAdaptorParentData?;
final Element? newChild = super.updateChild(child, newWidget, newSlot);
final SliverMultiBoxAdaptorParentData? newParentData = newChild?.renderObject?.parentData as SliverMultiBoxAdaptorParentData?;
// Preserve the old layoutOffset if the renderObject was swapped out.
if (oldParentData != newParentData && oldParentData != null && newParentData != null) {
newParentData.layoutOffset = oldParentData.layoutOffset;
}
return newChild;
}
@override
void forgetChild(Element child) {
assert(child.slot != null);
assert(_childElements.containsKey(child.slot));
_childElements.remove(child.slot);
super.forgetChild(child);
}
@override
void removeChild(RenderBox child) {
final int index = renderObject.indexOf(child);
assert(_currentlyUpdatingChildIndex == null);
assert(index >= 0);
owner!.buildScope(this, () {
assert(_childElements.containsKey(index));
try {
_currentlyUpdatingChildIndex = index;
final Element? result = updateChild(_childElements[index], null, index);
assert(result == null);
} finally {
_currentlyUpdatingChildIndex = null;
}
_childElements.remove(index);
assert(!_childElements.containsKey(index));
});
}
static double _extrapolateMaxScrollOffset(
int firstIndex,
int lastIndex,
double leadingScrollOffset,
double trailingScrollOffset,
int childCount,
) {
if (lastIndex == childCount - 1) {
return trailingScrollOffset;
}
final int reifiedCount = lastIndex - firstIndex + 1;
final double averageExtent = (trailingScrollOffset - leadingScrollOffset) / reifiedCount;
final int remainingCount = childCount - lastIndex - 1;
return trailingScrollOffset + averageExtent * remainingCount;
}
@override
double estimateMaxScrollOffset(
SliverConstraints? constraints, {
int? firstIndex,
int? lastIndex,
double? leadingScrollOffset,
double? trailingScrollOffset,
}) {
final int? childCount = estimatedChildCount;
if (childCount == null) {
return double.infinity;
}
return (widget as SliverMultiBoxAdaptorWidget).estimateMaxScrollOffset(
constraints,
firstIndex!,
lastIndex!,
leadingScrollOffset!,
trailingScrollOffset!,
) ?? _extrapolateMaxScrollOffset(
firstIndex,
lastIndex,
leadingScrollOffset,
trailingScrollOffset,
childCount,
);
}
@override
int? get estimatedChildCount => (widget as SliverMultiBoxAdaptorWidget).delegate.estimatedChildCount;
@override
int get childCount {
int? result = estimatedChildCount;
if (result == null) {
// Since childCount was called, we know that we reached the end of
// the list (as in, _build return null once), so we know that the
// list is finite.
// Let's do an open-ended binary search to find the end of the list
// manually.
int lo = 0;
int hi = 1;
final SliverMultiBoxAdaptorWidget adaptorWidget = widget as SliverMultiBoxAdaptorWidget;
const int max = kIsWeb
? 9007199254740992 // max safe integer on JS (from 0 to this number x != x+1)
: ((1 << 63) - 1);
while (_build(hi - 1, adaptorWidget) != null) {
lo = hi - 1;
if (hi < max ~/ 2) {
hi *= 2;
} else if (hi < max) {
hi = max;
} else {
throw FlutterError(
'Could not find the number of children in ${adaptorWidget.delegate}.\n'
"The childCount getter was called (implying that the delegate's builder returned null "
'for a positive index), but even building the child with index $hi (the maximum '
'possible integer) did not return null. Consider implementing childCount to avoid '
'the cost of searching for the final child.',
);
}
}
while (hi - lo > 1) {
final int mid = (hi - lo) ~/ 2 + lo;
if (_build(mid - 1, adaptorWidget) == null) {
hi = mid;
} else {
lo = mid;
}
}
result = lo;
}
return result;
}
@override
void didStartLayout() {
assert(debugAssertChildListLocked());
}
@override
void didFinishLayout() {
assert(debugAssertChildListLocked());
final int firstIndex = _childElements.firstKey() ?? 0;
final int lastIndex = _childElements.lastKey() ?? 0;
(widget as SliverMultiBoxAdaptorWidget).delegate.didFinishLayout(firstIndex, lastIndex);
}
int? _currentlyUpdatingChildIndex;
@override
bool debugAssertChildListLocked() {
assert(_currentlyUpdatingChildIndex == null);
return true;
}
@override
void didAdoptChild(RenderBox child) {
assert(_currentlyUpdatingChildIndex != null);
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
childParentData.index = _currentlyUpdatingChildIndex;
}
bool _didUnderflow = false;
@override
void setDidUnderflow(bool value) {
_didUnderflow = value;
}
@override
void insertRenderObjectChild(covariant RenderObject child, int slot) {
assert(_currentlyUpdatingChildIndex == slot);
assert(renderObject.debugValidateChild(child));
renderObject.insert(child as RenderBox, after: _currentBeforeChild);
assert(() {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
assert(slot == childParentData.index);
return true;
}());
}
@override
void moveRenderObjectChild(covariant RenderObject child, int oldSlot, int newSlot) {
assert(_currentlyUpdatingChildIndex == newSlot);
renderObject.move(child as RenderBox, after: _currentBeforeChild);
}
@override
void removeRenderObjectChild(covariant RenderObject child, int slot) {
assert(_currentlyUpdatingChildIndex != null);
renderObject.remove(child as RenderBox);
}
@override
void visitChildren(ElementVisitor visitor) {
// The toList() is to make a copy so that the underlying list can be modified by
// the visitor:
assert(!_childElements.values.any((Element? child) => child == null));
_childElements.values.cast<Element>().toList().forEach(visitor);
}
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
_childElements.values.cast<Element>().where((Element child) {
final SliverMultiBoxAdaptorParentData parentData = child.renderObject!.parentData! as SliverMultiBoxAdaptorParentData;
final double itemExtent = switch (renderObject.constraints.axis) {
Axis.horizontal => child.renderObject!.paintBounds.width,
Axis.vertical => child.renderObject!.paintBounds.height,
};
return parentData.layoutOffset != null &&
parentData.layoutOffset! < renderObject.constraints.scrollOffset + renderObject.constraints.remainingPaintExtent &&
parentData.layoutOffset! + itemExtent > renderObject.constraints.scrollOffset;
}).forEach(visitor);
}
}
/// A sliver widget that makes its sliver child partially transparent.
///
/// This class paints its sliver child into an intermediate buffer and then
/// blends the sliver back into the scene partially transparent.
///
/// For values of opacity other than 0.0 and 1.0, this class is relatively
/// expensive because it requires painting the sliver child into an intermediate
/// buffer. For the value 0.0, the sliver child is not painted at all.
/// For the value 1.0, the sliver child is painted immediately without an
/// intermediate buffer.
///
/// {@tool dartpad}
///
/// This example shows a [SliverList] when the `_visible` member field is true,
/// and hides it when it is false.
///
/// This is more efficient than adding and removing the sliver child widget from
/// the tree on demand, but it does not affect how much the list scrolls (the
/// [SliverList] is still present, merely invisible).
///
/// ** See code in examples/api/lib/widgets/sliver/sliver_opacity.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Opacity], which can apply a uniform alpha effect to its child using the
/// [RenderBox] layout protocol.
/// * [AnimatedOpacity], which uses an animation internally to efficiently
/// animate [Opacity].
/// * [SliverVisibility], which can hide a child more efficiently (albeit less
/// subtly, because it is either visible or hidden, rather than allowing
/// fractional opacity values). Specifically, the [SliverVisibility.maintain]
/// constructor is equivalent to using a sliver opacity widget with values of
/// `0.0` or `1.0`.
class SliverOpacity extends SingleChildRenderObjectWidget {
/// Creates a sliver that makes its sliver child partially transparent.
///
/// The [opacity] argument must be between zero and one, inclusive.
const SliverOpacity({
super.key,
required this.opacity,
this.alwaysIncludeSemantics = false,
Widget? sliver,
}) : assert(opacity >= 0.0 && opacity <= 1.0),
super(child: sliver);
/// The fraction to scale the sliver child's alpha value.
///
/// An opacity of 1.0 is fully opaque. An opacity of 0.0 is fully transparent
/// (i.e. invisible).
///
/// Values 1.0 and 0.0 are painted with a fast path. Other values
/// require painting the sliver child into an intermediate buffer, which is
/// expensive.
final double opacity;
/// Whether the semantic information of the sliver child is always included.
///
/// Defaults to false.
///
/// When true, regardless of the opacity settings, the sliver child semantic
/// information is exposed as if the widget were fully visible. This is
/// useful in cases where labels may be hidden during animations that
/// would otherwise contribute relevant semantics.
final bool alwaysIncludeSemantics;
@override
RenderSliverOpacity createRenderObject(BuildContext context) {
return RenderSliverOpacity(
opacity: opacity,
alwaysIncludeSemantics: alwaysIncludeSemantics,
);
}
@override
void updateRenderObject(BuildContext context, RenderSliverOpacity renderObject) {
renderObject
..opacity = opacity
..alwaysIncludeSemantics = alwaysIncludeSemantics;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<double>('opacity', opacity));
properties.add(FlagProperty(
'alwaysIncludeSemantics',
value: alwaysIncludeSemantics,
ifTrue: 'alwaysIncludeSemantics',
));
}
}
/// A sliver widget that is invisible during hit testing.
///
/// When [ignoring] is true, this widget (and its subtree) is invisible
/// to hit testing. It still consumes space during layout and paints its sliver
/// child as usual. It just cannot be the target of located events, because it
/// returns false from [RenderSliver.hitTest].
///
/// ## Semantics
///
/// Using this class may also affect how the semantics subtree underneath is
/// collected.
///
/// {@macro flutter.widgets.IgnorePointer.semantics}
///
/// {@macro flutter.widgets.IgnorePointer.ignoringSemantics}
///
/// See also:
///
/// * [IgnorePointer], the equivalent widget for boxes.
class SliverIgnorePointer extends SingleChildRenderObjectWidget {
/// Creates a sliver widget that is invisible to hit testing.
const SliverIgnorePointer({
super.key,
this.ignoring = true,
@Deprecated(
'Create a custom sliver ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
this.ignoringSemantics,
Widget? sliver,
}) : super(child: sliver);
/// Whether this sliver is ignored during hit testing.
///
/// Regardless of whether this sliver is ignored during hit testing, it will
/// still consume space during layout and be visible during painting.
///
/// {@macro flutter.widgets.IgnorePointer.semantics}
final bool ignoring;
/// Whether the semantics of this sliver is ignored when compiling the
/// semantics tree.
///
/// {@macro flutter.widgets.IgnorePointer.ignoringSemantics}
@Deprecated(
'Create a custom sliver ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
final bool? ignoringSemantics;
@override
RenderSliverIgnorePointer createRenderObject(BuildContext context) {
return RenderSliverIgnorePointer(
ignoring: ignoring,
ignoringSemantics: ignoringSemantics,
);
}
@override
void updateRenderObject(BuildContext context, RenderSliverIgnorePointer renderObject) {
renderObject
..ignoring = ignoring
..ignoringSemantics = ignoringSemantics;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('ignoring', ignoring));
properties.add(DiagnosticsProperty<bool>('ignoringSemantics', ignoringSemantics, defaultValue: null));
}
}
/// A sliver that lays its sliver child out as if it was in the tree, but
/// without painting anything, without making the sliver child available for hit
/// testing, and without taking any room in the parent.
///
/// Animations continue to run in offstage sliver children, and therefore use
/// battery and CPU time, regardless of whether the animations end up being
/// visible.
///
/// To hide a sliver widget from view while it is
/// not needed, prefer removing the widget from the tree entirely rather than
/// keeping it alive in an [Offstage] subtree.
///
/// See also:
///
/// * [Offstage], the equivalent widget for boxes.
class SliverOffstage extends SingleChildRenderObjectWidget {
/// Creates a sliver that visually hides its sliver child.
const SliverOffstage({
super.key,
this.offstage = true,
Widget? sliver,
}) : super(child: sliver);
/// Whether the sliver child is hidden from the rest of the tree.
///
/// If true, the sliver child is laid out as if it was in the tree, but
/// without painting anything, without making the child available for hit
/// testing, and without taking any room in the parent.
///
/// If false, the sliver child is included in the tree as normal.
final bool offstage;
@override
RenderSliverOffstage createRenderObject(BuildContext context) => RenderSliverOffstage(offstage: offstage);
@override
void updateRenderObject(BuildContext context, RenderSliverOffstage renderObject) {
renderObject.offstage = offstage;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('offstage', offstage));
}
@override
SingleChildRenderObjectElement createElement() => _SliverOffstageElement(this);
}
class _SliverOffstageElement extends SingleChildRenderObjectElement {
_SliverOffstageElement(SliverOffstage super.widget);
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
if (!(widget as SliverOffstage).offstage) {
super.debugVisitOnstageChildren(visitor);
}
}
}
/// Mark a child as needing to stay alive even when it's in a lazy list that
/// would otherwise remove it.
///
/// This widget is for use in a [RenderAbstractViewport]s, such as
/// [Viewport] or [TwoDimensionalViewport].
///
/// This widget is rarely used directly. The [SliverChildBuilderDelegate] and
/// [SliverChildListDelegate] delegates, used with [SliverList] and
/// [SliverGrid], as well as the scroll view counterparts [ListView] and
/// [GridView], have an `addAutomaticKeepAlives` feature, which is enabled by
/// default, and which causes [AutomaticKeepAlive] widgets to be inserted around
/// each child, causing [KeepAlive] widgets to be automatically added and
/// configured in response to [KeepAliveNotification]s.
///
/// The same `addAutomaticKeepAlives` feature is supported by the
/// [TwoDimensionalChildBuilderDelegate] and [TwoDimensionalChildListDelegate].
///
/// Therefore, to keep a widget alive, it is more common to use those
/// notifications than to directly deal with [KeepAlive] widgets.
///
/// In practice, the simplest way to deal with these notifications is to mix
/// [AutomaticKeepAliveClientMixin] into one's [State]. See the documentation
/// for that mixin class for details.
class KeepAlive extends ParentDataWidget<KeepAliveParentDataMixin> {
/// Marks a child as needing to remain alive.
const KeepAlive({
super.key,
required this.keepAlive,
required super.child,
});
/// Whether to keep the child alive.
///
/// If this is false, it is as if this widget was omitted.
final bool keepAlive;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is KeepAliveParentDataMixin);
final KeepAliveParentDataMixin parentData = renderObject.parentData! as KeepAliveParentDataMixin;
if (parentData.keepAlive != keepAlive) {
// No need to redo layout if it became true.
parentData.keepAlive = keepAlive;
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject && !keepAlive) {
targetParent.markNeedsLayout();
}
}
}
// We only return true if [keepAlive] is true, because turning _off_ keep
// alive requires a layout to do the garbage collection (but turning it on
// requires nothing, since by definition the widget is already alive and won't
// go away _unless_ we do a layout).
@override
bool debugCanApplyOutOfTurn() => keepAlive;
@override
Type get debugTypicalAncestorWidgetClass => throw FlutterError('Multiple Types are supported, use debugTypicalAncestorWidgetDescription.');
@override
String get debugTypicalAncestorWidgetDescription => 'SliverWithKeepAliveWidget or TwoDimensionalViewport';
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('keepAlive', keepAlive));
}
}
/// A sliver that constrains the cross axis extent of its sliver child.
///
/// The [SliverConstrainedCrossAxis] takes a [maxExtent] parameter and uses it as
/// the cross axis extent of the [SliverConstraints] passed to the sliver child.
/// The widget ensures that the [maxExtent] is a nonnegative value.
///
/// This is useful when you want to apply a custom cross-axis extent constraint
/// to a sliver child, as slivers typically consume the full cross axis extent.
///
/// This widget also sets its parent data's [SliverPhysicalParentData.crossAxisFlex]
/// to 0, so that it informs [SliverCrossAxisGroup] that it should not flex
/// in the cross axis direction.
///
/// {@tool dartpad}
/// In this sample the [SliverConstrainedCrossAxis] sizes its child so that the
/// cross axis extent takes up less space than the actual viewport.
///
/// ** See code in examples/api/lib/widgets/sliver/sliver_constrained_cross_axis.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverCrossAxisGroup], the widget which makes use of 0 flex factor set by
/// this widget.
class SliverConstrainedCrossAxis extends StatelessWidget {
/// Creates a sliver that constrains the cross axis extent of its sliver child.
///
/// The [maxExtent] parameter is required and must be nonnegative.
const SliverConstrainedCrossAxis({
super.key,
required this.maxExtent,
required this.sliver,
});
/// The cross axis extent to apply to the sliver child.
///
/// This value must be nonnegative.
final double maxExtent;
/// The widget below this widget in the tree.
///
/// Must be a sliver.
final Widget sliver;
@override
Widget build(BuildContext context) {
return _SliverZeroFlexParentDataWidget(
sliver: _SliverConstrainedCrossAxis(
maxExtent: maxExtent,
sliver: sliver,
)
);
}
}
class _SliverZeroFlexParentDataWidget extends ParentDataWidget<SliverPhysicalParentData> {
const _SliverZeroFlexParentDataWidget({
required Widget sliver,
}) : super(child: sliver);
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is SliverPhysicalParentData);
final SliverPhysicalParentData parentData = renderObject.parentData! as SliverPhysicalParentData;
bool needsLayout = false;
if (parentData.crossAxisFlex != 0) {
parentData.crossAxisFlex = 0;
needsLayout = true;
}
if (needsLayout) {
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => SliverCrossAxisGroup;
}
class _SliverConstrainedCrossAxis extends SingleChildRenderObjectWidget {
const _SliverConstrainedCrossAxis({
required this.maxExtent,
required Widget sliver,
}) : assert(maxExtent >= 0.0),
super(child: sliver);
/// The cross axis extent to apply to the sliver child.
///
/// This value must be nonnegative.
final double maxExtent;
@override
RenderSliverConstrainedCrossAxis createRenderObject(BuildContext context) {
return RenderSliverConstrainedCrossAxis(maxExtent: maxExtent);
}
@override
void updateRenderObject(BuildContext context, RenderSliverConstrainedCrossAxis renderObject) {
renderObject.maxExtent = maxExtent;
}
}
/// Set a flex factor for allocating space in the cross axis direction.
///
/// This is a [ParentDataWidget] to be used in [SliverCrossAxisGroup].
/// After all slivers with null or zero flex (e.g. [SliverConstrainedCrossAxis])
/// are laid out (which should determine their own [SliverGeometry.crossAxisExtent]),
/// the remaining space is laid out among the slivers with nonzero flex
/// proportionally to their flex value.
class SliverCrossAxisExpanded extends ParentDataWidget<SliverPhysicalContainerParentData> {
/// Creates an object that assigns a [flex] value to the child sliver.
///
/// The provided [flex] value must be greater than 0.
const SliverCrossAxisExpanded({
super.key,
required this.flex,
required Widget sliver,
}): assert(flex > 0 && flex < double.infinity),
super(child: sliver);
/// Flex value for allocating cross axis extent left after laying out the children with
/// constrained cross axis. The children with flex values will have the remaining extent
/// allocated proportionally to their flex value. This must an integer between
/// 0 and infinity, exclusive.
final int flex;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is SliverPhysicalContainerParentData);
assert(renderObject.parent is RenderSliverCrossAxisGroup);
final SliverPhysicalParentData parentData = renderObject.parentData! as SliverPhysicalParentData;
bool needsLayout = false;
if (parentData.crossAxisFlex != flex) {
parentData.crossAxisFlex = flex;
needsLayout = true;
}
if (needsLayout) {
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => SliverCrossAxisGroup;
}
/// A sliver that places multiple sliver children in a linear array along
/// the cross axis.
///
/// ## Layout algorithm
///
/// _This section describes how the framework causes [RenderSliverCrossAxisGroup]
/// to position its children._
///
/// Layout for a [RenderSliverCrossAxisGroup] has four steps:
///
/// 1. Layout each child with a null or zero flex factor with cross axis constraint
/// being whatever cross axis space is remaining after laying out any previous
/// sliver. Slivers with null or zero flex factor should determine their own
/// [SliverGeometry.crossAxisExtent]. For example, the [SliverConstrainedCrossAxis]
/// widget uses either [SliverConstrainedCrossAxis.maxExtent] or
/// [SliverConstraints.crossAxisExtent], deciding between whichever is smaller.
/// 2. Divide up the remaining cross axis space among the children with non-zero flex
/// factors according to their flex factor. For example, a child with a flex
/// factor of 2.0 will receive twice the amount of cross axis space as a child
/// with a flex factor 1.0.
/// 3. Layout each of the remaining children with the cross axis constraint
/// allocated in the previous step.
/// 4. Set the geometry to that of whichever child has the longest
/// [SliverGeometry.scrollExtent] with the [SliverGeometry.crossAxisExtent] adjusted
/// to [SliverConstraints.crossAxisExtent].
///
/// {@tool dartpad}
/// In this sample the [SliverCrossAxisGroup] sizes its three [children] so that
/// the first normal [SliverList] has a flex factor of 1, the second [SliverConstrainedCrossAxis]
/// has a flex factor of 0 and a maximum cross axis extent of 200.0, and the third
/// [SliverCrossAxisExpanded] has a flex factor of 2.
///
/// ** See code in examples/api/lib/widgets/sliver/sliver_cross_axis_group.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverCrossAxisExpanded], which is the [ParentDataWidget] for setting a flex
/// value to a widget.
/// * [SliverConstrainedCrossAxis], which is a [RenderObjectWidget] for setting
/// an extent to constrain the widget to.
/// * [SliverMainAxisGroup], which is the [RenderObjectWidget] for laying out
/// multiple slivers along the main axis.
class SliverCrossAxisGroup extends MultiChildRenderObjectWidget {
/// Creates a sliver that places sliver children in a linear array along
/// the cross axis.
const SliverCrossAxisGroup({
super.key,
required List<Widget> slivers,
}): super(children: slivers);
@override
RenderSliverCrossAxisGroup createRenderObject(BuildContext context) {
return RenderSliverCrossAxisGroup();
}
}
/// A sliver that places multiple sliver children in a linear array along
/// the main axis, one after another.
///
/// ## Layout algorithm
///
/// _This section describes how the framework causes [RenderSliverMainAxisGroup]
/// to position its children._
///
/// Layout for a [RenderSliverMainAxisGroup] has four steps:
///
/// 1. Keep track of an offset variable which is the total [SliverGeometry.scrollExtent]
/// of the slivers laid out so far.
/// 2. To determine the constraints for the next sliver child to layout, calculate the
/// amount of paint extent occupied from 0.0 to the offset variable and subtract this from
/// [SliverConstraints.remainingPaintExtent] minus to use as the child's
/// [SliverConstraints.remainingPaintExtent]. For the [SliverConstraints.scrollOffset],
/// take the provided constraint's value and subtract out the offset variable, using
/// 0.0 if negative.
/// 3. Once we finish laying out all the slivers, this offset variable represents
/// the total [SliverGeometry.scrollExtent] of the sliver group. Since it is possible
/// for specialized slivers to try to paint itself outside of the bounds of the
/// sliver group's scroll extent (see [SliverPersistentHeader]), we must do a
/// second pass to set a [SliverPhysicalParentData.paintOffset] to make sure it
/// is within the bounds of the sliver group.
/// 4. Finally, set the [RenderSliverMainAxisGroup.geometry] with the total
/// [SliverGeometry.scrollExtent], [SliverGeometry.paintExtent] calculated from
/// the constraints and [SliverGeometry.scrollExtent], and [SliverGeometry.maxPaintExtent].
///
/// {@tool dartpad}
/// In this sample the [CustomScrollView] renders a [SliverMainAxisGroup] and a
/// [SliverToBoxAdapter] with some content. The [SliverMainAxisGroup] renders a
/// [SliverAppBar], [SliverList], and [SliverToBoxAdapter]. Notice that when the
/// [SliverMainAxisGroup] goes out of view, so does the pinned [SliverAppBar].
///
/// ** See code in examples/api/lib/widgets/sliver/sliver_main_axis_group.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverPersistentHeader], which is a [RenderObjectWidget] which may require
/// adjustment to its [SliverPhysicalParentData.paintOffset] to make it fit
/// within the computed [SliverGeometry.scrollExtent] of the [SliverMainAxisGroup].
/// * [SliverCrossAxisGroup], which is the [RenderObjectWidget] for laying out
/// multiple slivers along the cross axis.
class SliverMainAxisGroup extends MultiChildRenderObjectWidget {
/// Creates a sliver that places sliver children in a linear array along
/// the main axis.
const SliverMainAxisGroup({
super.key,
required List<Widget> slivers,
}) : super(children: slivers);
@override
RenderSliverMainAxisGroup createRenderObject(BuildContext context) {
return RenderSliverMainAxisGroup();
}
}
| flutter/packages/flutter/lib/src/widgets/sliver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/sliver.dart",
"repo_id": "flutter",
"token_count": 19863
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
/// The position information for a text selection toolbar.
///
/// Typically, a menu will attempt to position itself at [primaryAnchor], and
/// if that's not possible, then it will use [secondaryAnchor] instead, if it
/// exists.
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar.anchors], which is of this type.
@immutable
class TextSelectionToolbarAnchors {
/// Creates an instance of [TextSelectionToolbarAnchors] directly from the
/// anchor points.
const TextSelectionToolbarAnchors({
required this.primaryAnchor,
this.secondaryAnchor,
});
/// Creates an instance of [TextSelectionToolbarAnchors] for some selection.
factory TextSelectionToolbarAnchors.fromSelection({
required RenderBox renderBox,
required double startGlyphHeight,
required double endGlyphHeight,
required List<TextSelectionPoint> selectionEndpoints,
}) {
final Rect editingRegion = Rect.fromPoints(
renderBox.localToGlobal(Offset.zero),
renderBox.localToGlobal(renderBox.size.bottomRight(Offset.zero)),
);
if (editingRegion.left.isNaN || editingRegion.top.isNaN
|| editingRegion.right.isNaN || editingRegion.bottom.isNaN) {
return const TextSelectionToolbarAnchors(primaryAnchor: Offset.zero);
}
final bool isMultiline = selectionEndpoints.last.point.dy - selectionEndpoints.first.point.dy >
endGlyphHeight / 2;
final Rect selectionRect = Rect.fromLTRB(
isMultiline
? editingRegion.left
: editingRegion.left + selectionEndpoints.first.point.dx,
editingRegion.top + selectionEndpoints.first.point.dy - startGlyphHeight,
isMultiline
? editingRegion.right
: editingRegion.left + selectionEndpoints.last.point.dx,
editingRegion.top + selectionEndpoints.last.point.dy,
);
return TextSelectionToolbarAnchors(
primaryAnchor: Offset(
selectionRect.left + selectionRect.width / 2,
clampDouble(selectionRect.top, editingRegion.top, editingRegion.bottom),
),
secondaryAnchor: Offset(
selectionRect.left + selectionRect.width / 2,
clampDouble(selectionRect.bottom, editingRegion.top, editingRegion.bottom),
),
);
}
/// The location that the toolbar should attempt to position itself at.
///
/// If the toolbar doesn't fit at this location, use [secondaryAnchor] if it
/// exists.
final Offset primaryAnchor;
/// The fallback position that should be used if [primaryAnchor] doesn't work.
final Offset? secondaryAnchor;
}
| flutter/packages/flutter/lib/src/widgets/text_selection_toolbar_anchors.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/text_selection_toolbar_anchors.dart",
"repo_id": "flutter",
"token_count": 938
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show ParagraphBuilder, PlaceholderAlignment;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
// Examples can assume:
// late WidgetSpan myWidgetSpan;
/// An immutable widget that is embedded inline within text.
///
/// The [child] property is the widget that will be embedded. Children are
/// constrained by the width of the paragraph.
///
/// The [child] property may contain its own [Widget] children (if applicable),
/// including [Text] and [RichText] widgets which may include additional
/// [WidgetSpan]s. Child [Text] and [RichText] widgets will be laid out
/// independently and occupy a rectangular space in the parent text layout.
///
/// [WidgetSpan]s will be ignored when passed into a [TextPainter] directly.
/// To properly layout and paint the [child] widget, [WidgetSpan] should be
/// passed into a [Text.rich] widget.
///
/// {@tool snippet}
///
/// A card with `Hello World!` embedded inline within a TextSpan tree.
///
/// ```dart
/// const Text.rich(
/// TextSpan(
/// children: <InlineSpan>[
/// TextSpan(text: 'Flutter is'),
/// WidgetSpan(
/// child: SizedBox(
/// width: 120,
/// height: 50,
/// child: Card(
/// child: Center(
/// child: Text('Hello World!')
/// )
/// ),
/// )
/// ),
/// TextSpan(text: 'the best!'),
/// ],
/// )
/// )
/// ```
/// {@end-tool}
///
/// [WidgetSpan] contributes the semantics of the [WidgetSpan.child] to the
/// semantics tree.
///
/// See also:
///
/// * [TextSpan], a node that represents text in an [InlineSpan] tree.
/// * [Text], a widget for showing uniformly-styled text.
/// * [RichText], a widget for finer control of text rendering.
/// * [TextPainter], a class for painting [InlineSpan] objects on a [Canvas].
@immutable
class WidgetSpan extends PlaceholderSpan {
/// Creates a [WidgetSpan] with the given values.
///
/// [WidgetSpan] is a leaf node in the [InlineSpan] tree. Child widgets are
/// constrained by the width of the paragraph they occupy. Child widget
/// heights are unconstrained, and may cause the text to overflow and be
/// ellipsized/truncated.
///
/// A [TextStyle] may be provided with the [style] property, but only the
/// decoration, foreground, background, and spacing options will be used.
const WidgetSpan({
required this.child,
super.alignment,
super.baseline,
super.style,
}) : assert(
baseline != null || !(
identical(alignment, ui.PlaceholderAlignment.aboveBaseline) ||
identical(alignment, ui.PlaceholderAlignment.belowBaseline) ||
identical(alignment, ui.PlaceholderAlignment.baseline)
),
);
/// Helper function for extracting [WidgetSpan]s in preorder, from the given
/// [InlineSpan] as a list of widgets.
///
/// The `textScaler` is the scaling strategy for scaling the content.
///
/// This function is used by [EditableText] and [RichText] so calling it
/// directly is rarely necessary.
static List<Widget> extractFromInlineSpan(InlineSpan span, TextScaler textScaler) {
final List<Widget> widgets = <Widget>[];
// _kEngineDefaultFontSize is the default font size to use when none of the
// ancestor spans specifies one.
final List<double> fontSizeStack = <double>[kDefaultFontSize];
int index = 0;
// This assumes an InlineSpan tree's logical order is equivalent to preorder.
bool visitSubtree(InlineSpan span) {
final double? fontSizeToPush = switch (span.style?.fontSize) {
final double size when size != fontSizeStack.last => size,
_ => null,
};
if (fontSizeToPush != null) {
fontSizeStack.add(fontSizeToPush);
}
if (span is WidgetSpan) {
final double fontSize = fontSizeStack.last;
final double textScaleFactor = fontSize == 0 ? 0 : textScaler.scale(fontSize) / fontSize;
widgets.add(
_WidgetSpanParentData(
span: span,
child: Semantics(
tagForChildren: PlaceholderSpanIndexSemanticsTag(index++),
child: _AutoScaleInlineWidget(span: span, textScaleFactor: textScaleFactor, child: span.child),
),
),
);
}
assert(
span is WidgetSpan || span is! PlaceholderSpan,
'$span is a PlaceholderSpan but not a WidgetSpan subclass. This is currently not supported.',
);
span.visitDirectChildren(visitSubtree);
if (fontSizeToPush != null) {
final double poppedFontSize = fontSizeStack.removeLast();
assert(fontSizeStack.isNotEmpty);
assert(poppedFontSize == fontSizeToPush);
}
return true;
}
visitSubtree(span);
return widgets;
}
/// The widget to embed inline within text.
final Widget child;
/// Adds a placeholder box to the paragraph builder if a size has been
/// calculated for the widget.
///
/// Sizes are provided through `dimensions`, which should contain a 1:1
/// in-order mapping of widget to laid-out dimensions. If no such dimension
/// is provided, the widget will be skipped.
///
/// The `textScaler` will be applied to the laid-out size of the widget.
@override
void build(ui.ParagraphBuilder builder, {
TextScaler textScaler = TextScaler.noScaling,
List<PlaceholderDimensions>? dimensions,
}) {
assert(debugAssertIsValid());
assert(dimensions != null);
final bool hasStyle = style != null;
if (hasStyle) {
builder.pushStyle(style!.getTextStyle(textScaler: textScaler));
}
assert(builder.placeholderCount < dimensions!.length);
final PlaceholderDimensions currentDimensions = dimensions![builder.placeholderCount];
builder.addPlaceholder(
currentDimensions.size.width,
currentDimensions.size.height,
alignment,
baseline: currentDimensions.baseline,
baselineOffset: currentDimensions.baselineOffset,
);
if (hasStyle) {
builder.pop();
}
}
/// Calls `visitor` on this [WidgetSpan]. There are no children spans to walk.
@override
bool visitChildren(InlineSpanVisitor visitor) => visitor(this);
@override
bool visitDirectChildren(InlineSpanVisitor visitor) => true;
@override
InlineSpan? getSpanForPositionVisitor(TextPosition position, Accumulator offset) {
if (position.offset == offset.value) {
return this;
}
offset.increment(1);
return null;
}
@override
int? codeUnitAtVisitor(int index, Accumulator offset) {
final int localOffset = index - offset.value;
assert(localOffset >= 0);
offset.increment(1);
return localOffset == 0 ? PlaceholderSpan.placeholderCodeUnit : null;
}
@override
RenderComparison compareTo(InlineSpan other) {
if (identical(this, other)) {
return RenderComparison.identical;
}
if (other.runtimeType != runtimeType) {
return RenderComparison.layout;
}
if ((style == null) != (other.style == null)) {
return RenderComparison.layout;
}
final WidgetSpan typedOther = other as WidgetSpan;
if (child != typedOther.child || alignment != typedOther.alignment) {
return RenderComparison.layout;
}
RenderComparison result = RenderComparison.identical;
if (style != null) {
final RenderComparison candidate = style!.compareTo(other.style!);
if (candidate.index > result.index) {
result = candidate;
}
if (result == RenderComparison.layout) {
return result;
}
}
return result;
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
if (super != other) {
return false;
}
return other is WidgetSpan
&& other.child == child
&& other.alignment == alignment
&& other.baseline == baseline;
}
@override
int get hashCode => Object.hash(super.hashCode, child, alignment, baseline);
/// Returns the text span that contains the given position in the text.
@override
InlineSpan? getSpanForPosition(TextPosition position) {
assert(debugAssertIsValid());
return null;
}
/// In debug mode, throws an exception if the object is not in a
/// valid configuration. Otherwise, returns true.
///
/// This is intended to be used as follows:
///
/// ```dart
/// assert(myWidgetSpan.debugAssertIsValid());
/// ```
@override
bool debugAssertIsValid() {
// WidgetSpans are always valid as asserts prevent invalid WidgetSpans
// from being constructed.
return true;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Widget>('widget', child));
}
}
// A ParentDataWidget that sets TextParentData.span.
class _WidgetSpanParentData extends ParentDataWidget<TextParentData> {
const _WidgetSpanParentData({ required this.span, required super.child });
final WidgetSpan span;
@override
void applyParentData(RenderObject renderObject) {
final TextParentData parentData = renderObject.parentData! as TextParentData;
parentData.span = span;
}
@override
Type get debugTypicalAncestorWidgetClass => RichText;
}
// A RenderObjectWidget that automatically applies text scaling on inline
// widgets.
//
// TODO(LongCatIsLooong): this shouldn't happen automatically, at least there
// should be a way to opt out: https://github.com/flutter/flutter/issues/126962
class _AutoScaleInlineWidget extends SingleChildRenderObjectWidget {
const _AutoScaleInlineWidget({ required this.span, required this.textScaleFactor, required super.child });
final WidgetSpan span;
final double textScaleFactor;
@override
_RenderScaledInlineWidget createRenderObject(BuildContext context) {
return _RenderScaledInlineWidget(span.alignment, span.baseline, textScaleFactor);
}
@override
void updateRenderObject(BuildContext context, _RenderScaledInlineWidget renderObject) {
renderObject
..alignment = span.alignment
..baseline = span.baseline
..scale = textScaleFactor;
}
}
class _RenderScaledInlineWidget extends RenderBox with RenderObjectWithChildMixin<RenderBox> {
_RenderScaledInlineWidget(this._alignment, this._baseline, this._scale);
double get scale => _scale;
double _scale;
set scale(double value) {
if (value == _scale) {
return;
}
assert(value > 0);
assert(value.isFinite);
_scale = value;
markNeedsLayout();
}
ui.PlaceholderAlignment get alignment => _alignment;
ui.PlaceholderAlignment _alignment;
set alignment(ui.PlaceholderAlignment value) {
if (_alignment == value) {
return;
}
_alignment = value;
markNeedsLayout();
}
TextBaseline? get baseline => _baseline;
TextBaseline? _baseline;
set baseline(TextBaseline? value) {
if (value == _baseline) {
return;
}
_baseline = value;
markNeedsLayout();
}
@override
double computeMaxIntrinsicHeight(double width) {
return (child?.computeMaxIntrinsicHeight(width / scale) ?? 0.0) * scale;
}
@override
double computeMaxIntrinsicWidth(double height) {
return (child?.computeMaxIntrinsicWidth(height / scale) ?? 0.0) * scale;
}
@override
double computeMinIntrinsicHeight(double width) {
return (child?.computeMinIntrinsicHeight(width / scale) ?? 0.0) * scale;
}
@override
double computeMinIntrinsicWidth(double height) {
return (child?.computeMinIntrinsicWidth(height / scale) ?? 0.0) * scale;
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
return switch (child?.getDistanceToActualBaseline(baseline)) {
null => super.computeDistanceToActualBaseline(baseline),
final double childBaseline => scale * childBaseline,
};
}
@override
Size computeDryLayout(BoxConstraints constraints) {
assert(!constraints.hasBoundedHeight);
final Size unscaledSize = child?.computeDryLayout(BoxConstraints(maxWidth: constraints.maxWidth / scale)) ?? Size.zero;
return constraints.constrain(unscaledSize * scale);
}
@override
void performLayout() {
final RenderBox? child = this.child;
if (child == null) {
return;
}
assert(!constraints.hasBoundedHeight);
// Only constrain the width to the maximum width of the paragraph.
// Leave height unconstrained, which will overflow if expanded past.
child.layout(BoxConstraints(maxWidth: constraints.maxWidth / scale), parentUsesSize: true);
size = constraints.constrain(child.size * scale);
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
transform.scale(scale, scale);
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderBox? child = this.child;
if (child == null) {
layer = null;
return;
}
if (scale == 1.0) {
context.paintChild(child, offset);
layer = null;
return;
}
layer = context.pushTransform(
needsCompositing,
offset,
Matrix4.diagonal3Values(scale, scale, 1.0),
(PaintingContext context, Offset offset) => context.paintChild(child, offset),
oldLayer: layer as TransformLayer?
);
}
@override
bool hitTestChildren(BoxHitTestResult result, {required Offset position}) {
final RenderBox? child = this.child;
if (child == null) {
return false;
}
return result.addWithPaintTransform(
transform: Matrix4.diagonal3Values(scale, scale, 1.0),
position: position,
hitTest: (BoxHitTestResult result, Offset transformedOffset) => child.hitTest(result, position: transformedOffset),
);
}
}
| flutter/packages/flutter/lib/src/widgets/widget_span.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/widget_span.dart",
"repo_id": "flutter",
"token_count": 4874
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/animation.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('awaiting animation controllers - using direct future', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
addTearDown(controller1.dispose);
final AnimationController controller2 = AnimationController(
duration: const Duration(milliseconds: 600),
vsync: const TestVSync(),
);
addTearDown(controller2.dispose);
final AnimationController controller3 = AnimationController(
duration: const Duration(milliseconds: 300),
vsync: const TestVSync(),
);
addTearDown(controller3.dispose);
final List<String> log = <String>[];
Future<void> runTest() async {
log.add('a'); // t=0
await controller1.forward(); // starts at t=0 again
log.add('b'); // wants to end at t=100 but missed frames until t=150
await controller2.forward(); // starts at t=150
log.add('c'); // wants to end at t=750 but missed frames until t=799
await controller3.forward(); // starts at t=799
log.add('d'); // wants to end at t=1099 but missed frames until t=1200
}
log.add('start');
runTest().then((void value) {
log.add('end');
});
await tester.pump(); // t=0
expect(log, <String>['start', 'a']);
await tester.pump(); // t=0 again
expect(log, <String>['start', 'a']);
await tester.pump(const Duration(milliseconds: 50)); // t=50
expect(log, <String>['start', 'a']);
await tester.pump(const Duration(milliseconds: 100)); // t=150
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 50)); // t=200
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 400)); // t=600
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 199)); // t=799
expect(log, <String>['start', 'a', 'b', 'c']);
await tester.pump(const Duration(milliseconds: 51)); // t=850
expect(log, <String>['start', 'a', 'b', 'c']);
await tester.pump(const Duration(milliseconds: 400)); // t=1200
expect(log, <String>['start', 'a', 'b', 'c', 'd', 'end']);
await tester.pump(const Duration(milliseconds: 400)); // t=1600
expect(log, <String>['start', 'a', 'b', 'c', 'd', 'end']);
});
testWidgets('awaiting animation controllers - using orCancel', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
addTearDown(controller1.dispose);
final AnimationController controller2 = AnimationController(
duration: const Duration(milliseconds: 600),
vsync: const TestVSync(),
);
addTearDown(controller2.dispose);
final AnimationController controller3 = AnimationController(
duration: const Duration(milliseconds: 300),
vsync: const TestVSync(),
);
addTearDown(controller3.dispose);
final List<String> log = <String>[];
Future<void> runTest() async {
log.add('a'); // t=0
await controller1.forward().orCancel; // starts at t=0 again
log.add('b'); // wants to end at t=100 but missed frames until t=150
await controller2.forward().orCancel; // starts at t=150
log.add('c'); // wants to end at t=750 but missed frames until t=799
await controller3.forward().orCancel; // starts at t=799
log.add('d'); // wants to end at t=1099 but missed frames until t=1200
}
log.add('start');
runTest().then((void value) {
log.add('end');
});
await tester.pump(); // t=0
expect(log, <String>['start', 'a']);
await tester.pump(); // t=0 again
expect(log, <String>['start', 'a']);
await tester.pump(const Duration(milliseconds: 50)); // t=50
expect(log, <String>['start', 'a']);
await tester.pump(const Duration(milliseconds: 100)); // t=150
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 50)); // t=200
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 400)); // t=600
expect(log, <String>['start', 'a', 'b']);
await tester.pump(const Duration(milliseconds: 199)); // t=799
expect(log, <String>['start', 'a', 'b', 'c']);
await tester.pump(const Duration(milliseconds: 51)); // t=850
expect(log, <String>['start', 'a', 'b', 'c']);
await tester.pump(const Duration(milliseconds: 400)); // t=1200
expect(log, <String>['start', 'a', 'b', 'c', 'd', 'end']);
await tester.pump(const Duration(milliseconds: 400)); // t=1600
expect(log, <String>['start', 'a', 'b', 'c', 'd', 'end']);
});
testWidgets('awaiting animation controllers and failing', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
final List<String> log = <String>[];
Future<void> runTest() async {
try {
log.add('start');
await controller1.forward().orCancel;
log.add('fail');
} on TickerCanceled {
log.add('caught');
}
}
runTest().then((void value) {
log.add('end');
});
await tester.pump(); // start ticker
expect(log, <String>['start']);
await tester.pump(const Duration(milliseconds: 50));
expect(log, <String>['start']);
controller1.dispose();
expect(log, <String>['start']);
await tester.idle();
expect(log, <String>['start', 'caught', 'end']);
});
testWidgets('creating orCancel future later', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
addTearDown(controller1.dispose);
final TickerFuture f = controller1.forward();
await tester.pump(); // start ticker
await tester.pump(const Duration(milliseconds: 200)); // end ticker
await f; // should be a no-op
await f.orCancel; // should create a resolved future
expect(true, isTrue); // should reach here
});
testWidgets('creating orCancel future later', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
addTearDown(controller1.dispose);
final TickerFuture f = controller1.forward();
await tester.pump(); // start ticker
controller1.stop(); // cancel ticker
bool ok = false;
try {
await f.orCancel; // should create a resolved future
} on TickerCanceled {
ok = true;
}
expect(ok, isTrue); // should reach here
});
testWidgets('TickerFuture is a Future', (WidgetTester tester) async {
final AnimationController controller1 = AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
addTearDown(controller1.dispose);
final TickerFuture f = controller1.forward();
await tester.pump(); // start ticker
await tester.pump(const Duration(milliseconds: 200)); // end ticker
expect(f.asStream().single, isA<Future<void>>());
await f.catchError((dynamic e) { throw 'do not reach'; });
expect(await f.then<bool>((_) => true), isTrue);
expect(f.whenComplete(() => false), isA<Future<void>>());
expect(f.timeout(const Duration(seconds: 5)), isA<Future<void>>());
});
}
| flutter/packages/flutter/test/animation/futures_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/futures_test.dart",
"repo_id": "flutter",
"token_count": 2907
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// reduced-test-set:
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../impeller_test_helpers.dart';
// TODO(yjbanov): on the web text rendered with perspective produces flaky goldens: https://github.com/flutter/flutter/issues/110785
const bool skipPerspectiveTextGoldens = isBrowser;
// A number of the hit tests below say "warnIfMissed: false". This is because
// the way the CupertinoPicker works, the hits don't actually reach the labels,
// the scroll view intercepts them.
// scrolling by this offset will move the picker to the next item
const Offset _kRowOffset = Offset(0.0, -50.0);
void main() {
group('Countdown timer picker', () {
testWidgets('initialTimerDuration falls within limit', (WidgetTester tester) async {
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(days: 1),
);
},
throwsAssertionError,
);
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(seconds: -1),
);
},
throwsAssertionError,
);
});
testWidgets('minuteInterval is positive and is a factor of 60', (WidgetTester tester) async {
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
minuteInterval: 0,
);
},
throwsAssertionError,
);
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
minuteInterval: -1,
);
},
throwsAssertionError,
);
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
minuteInterval: 7,
);
},
throwsAssertionError,
);
});
testWidgets('secondInterval is positive and is a factor of 60', (WidgetTester tester) async {
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
secondInterval: 0,
);
},
throwsAssertionError,
);
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
secondInterval: -1,
);
},
throwsAssertionError,
);
expect(
() {
CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
secondInterval: 7,
);
},
throwsAssertionError,
);
});
testWidgets('background color default value', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.backgroundColor != null), false);
});
testWidgets('background color can be null', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('specified background color is applied', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
backgroundColor: CupertinoColors.black,
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.backgroundColor != CupertinoColors.black), false);
});
testWidgets('specified item extent value is applied', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
itemExtent: 42,
onTimerDurationChanged: (_) { },
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.itemExtent != 42), false);
});
testWidgets('columns are ordered correctly when text direction is ltr', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(hours: 12, minutes: 30, seconds: 59),
),
),
);
Offset lastOffset = tester.getTopLeft(find.text('12'));
expect(tester.getTopLeft(find.text('hours')).dx > lastOffset.dx, true);
lastOffset = tester.getTopLeft(find.text('hours'));
expect(tester.getTopLeft(find.text('30')).dx > lastOffset.dx, true);
lastOffset = tester.getTopLeft(find.text('30'));
expect(tester.getTopLeft(find.text('min.')).dx > lastOffset.dx, true);
lastOffset = tester.getTopLeft(find.text('min.'));
expect(tester.getTopLeft(find.text('59')).dx > lastOffset.dx, true);
lastOffset = tester.getTopLeft(find.text('59'));
expect(tester.getTopLeft(find.text('sec.')).dx > lastOffset.dx, true);
});
testWidgets('columns are ordered correctly when text direction is rtl', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(hours: 12, minutes: 30, seconds: 59),
),
),
),
);
Offset lastOffset = tester.getTopLeft(find.text('12'));
expect(tester.getTopLeft(find.text('hours')).dx > lastOffset.dx, false);
lastOffset = tester.getTopLeft(find.text('hours'));
expect(tester.getTopLeft(find.text('30')).dx > lastOffset.dx, false);
lastOffset = tester.getTopLeft(find.text('30'));
expect(tester.getTopLeft(find.text('min.')).dx > lastOffset.dx, false);
lastOffset = tester.getTopLeft(find.text('min.'));
expect(tester.getTopLeft(find.text('59')).dx > lastOffset.dx, false);
lastOffset = tester.getTopLeft(find.text('59'));
expect(tester.getTopLeft(find.text('sec.')).dx > lastOffset.dx, false);
});
testWidgets('width of picker is consistent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(hours: 12, minutes: 30, seconds: 59),
),
),
),
);
// Distance between the first column and the last column.
final double distance = tester.getCenter(find.text('sec.')).dx - tester.getCenter(find.text('12')).dx;
await tester.pumpWidget(
CupertinoApp(
home: SizedBox(
height: 400.0,
width: 800.0,
child: CupertinoTimerPicker(
onTimerDurationChanged: (_) { },
initialTimerDuration: const Duration(hours: 12, minutes: 30, seconds: 59),
),
),
),
);
// Distance between the first and the last column should be the same.
expect(
tester.getCenter(find.text('sec.')).dx - tester.getCenter(find.text('12')).dx,
distance,
);
});
});
testWidgets('picker honors minuteInterval and secondInterval', (WidgetTester tester) async {
late Duration duration;
await tester.pumpWidget(
CupertinoApp(
home: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoTimerPicker(
minuteInterval: 10,
secondInterval: 12,
initialTimerDuration: const Duration(hours: 10, minutes: 40, seconds: 48),
onTimerDurationChanged: (Duration d) {
duration = d;
},
),
),
),
);
await tester.drag(find.text('40'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.drag(find.text('48'), -_kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(
duration,
const Duration(hours: 10, minutes: 50, seconds: 36),
);
});
group('Date picker', () {
testWidgets('initial date is set to default value', (WidgetTester tester) async {
final CupertinoDatePicker picker = CupertinoDatePicker(
onDateTimeChanged: (_) { },
);
expect(picker.initialDateTime, isNotNull);
});
testWidgets('background color default value', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
onDateTimeChanged: (_) { },
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.backgroundColor != null), false);
});
testWidgets('background color can be null', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
onDateTimeChanged: (_) { },
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('specified background color is applied', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
onDateTimeChanged: (_) { },
backgroundColor: CupertinoColors.black,
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.backgroundColor != CupertinoColors.black), false);
});
testWidgets('specified item extent value is applied', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoDatePicker(
itemExtent: 55,
onDateTimeChanged: (_) { },
),
),
);
final Iterable<CupertinoPicker> pickers = tester.allWidgets.whereType<CupertinoPicker>();
expect(pickers.any((CupertinoPicker picker) => picker.itemExtent != 55), false);
});
testWidgets('initial date honors minuteInterval', (WidgetTester tester) async {
late DateTime newDateTime;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
width: 400,
height: 400,
child: CupertinoDatePicker(
onDateTimeChanged: (DateTime d) => newDateTime = d,
initialDateTime: DateTime(2018, 10, 10, 10, 3),
minuteInterval: 3,
),
),
),
),
);
// Drag the minute picker to the next slot (03 -> 06).
// The `initialDateTime` and the `minuteInterval` values are specifically chosen
// so that `find.text` finds exactly one widget.
await tester.drag(find.text('03'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
expect(newDateTime.minute, 6);
});
test('initial date honors minimumDate & maximumDate', () {
expect(() {
CupertinoDatePicker(
onDateTimeChanged: (DateTime d) { },
initialDateTime: DateTime(2018, 10, 10),
minimumDate: DateTime(2018, 10, 11),
);
},
throwsAssertionError,
);
expect(() {
CupertinoDatePicker(
onDateTimeChanged: (DateTime d) { },
initialDateTime: DateTime(2018, 10, 10),
maximumDate: DateTime(2018, 10, 9),
);
},
throwsAssertionError,
);
});
testWidgets('changing initialDateTime after first build does not do anything', (WidgetTester tester) async {
late DateTime selectedDateTime;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
onDateTimeChanged: (DateTime dateTime) => selectedDateTime = dateTime,
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
await tester.drag(find.text('10'), const Offset(0.0, 32.0), pointer: 1, touchSlopY: 0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(selectedDateTime, DateTime(2018, 1, 1, 9, 30));
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
onDateTimeChanged: (DateTime dateTime) => selectedDateTime = dateTime,
// Change the initial date, but it shouldn't affect the present state.
initialDateTime: DateTime(2016, 4, 5, 15),
),
),
),
),
);
await tester.drag(find.text('9'), const Offset(0.0, 32.0), pointer: 1, touchSlopY: 0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Moving up an hour is still based on the original initial date time.
expect(selectedDateTime, DateTime(2018, 1, 1, 8, 30));
});
testWidgets('date picker has expected string', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 9, 15),
),
),
),
),
);
expect(find.text('September'), findsOneWidget);
expect(find.text('9'), findsOneWidget);
expect(find.text('2018'), findsOneWidget);
});
testWidgets('datetime picker has expected string', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 9, 15, 3, 14),
),
),
),
),
);
expect(find.text('Sat Sep 15'), findsOneWidget);
expect(find.text('3'), findsOneWidget);
expect(find.text('14'), findsOneWidget);
expect(find.text('AM'), findsOneWidget);
});
testWidgets('monthYear picker has expected string', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.monthYear,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 9),
),
),
),
),
);
expect(find.text('September'), findsOneWidget);
expect(find.text('2018'), findsOneWidget);
});
testWidgets('width of picker in date and time mode is consistent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: CupertinoDatePicker(
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
);
// Distance between the first column and the last column.
final double distance =
tester.getCenter(find.text('Mon Jan 1 ')).dx - tester.getCenter(find.text('AM')).dx;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 800.0,
child: CupertinoDatePicker(
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
// Distance between the first and the last column should be the same.
expect(
tester.getCenter(find.text('Mon Jan 1 ')).dx - tester.getCenter(find.text('AM')).dx,
distance,
);
});
testWidgets('width of picker in date mode is consistent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
// Distance between the first column and the last column.
final double distance =
tester.getCenter(find.text('January')).dx - tester.getCenter(find.text('2018')).dx;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 800.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
// Distance between the first and the last column should be the same.
expect(
tester.getCenter(find.text('January')).dx - tester.getCenter(find.text('2018')).dx,
distance,
);
});
testWidgets('width of picker in time mode is consistent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
// Distance between the first column and the last column.
final double distance =
tester.getCenter(find.text('10')).dx - tester.getCenter(find.text('AM')).dx;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 800.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
),
),
),
),
);
// Distance between the first and the last column should be the same.
expect(
tester.getCenter(find.text('10')).dx - tester.getCenter(find.text('AM')).dx,
moreOrLessEquals(distance),
);
});
testWidgets('width of picker in monthYear mode is consistent', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.monthYear,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018),
),
),
),
),
);
// Distance between the first column and the last column.
final double distance =
tester.getCenter(find.text('January')).dx - tester.getCenter(find.text('2018')).dx;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 800.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.monthYear,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018),
),
),
),
),
);
// Distance between the first and the last column should be the same.
expect(
tester.getCenter(find.text('January')).dx - tester.getCenter(find.text('2018')).dx,
distance,
);
});
testWidgets('wheel does not bend outwards', (WidgetTester tester) async {
final Widget dateWidget = CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 1, 1, 10, 30),
);
const String centerMonth = 'January';
const List<String> visibleMonthsExceptTheCenter = <String>[
'September',
'October',
'November',
'December',
'February',
'March',
'April',
'May',
];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: SizedBox(
height: 200.0,
width: 300.0,
child: dateWidget,
),
),
),
),
);
// The wheel does not bend outwards.
for (final String month in visibleMonthsExceptTheCenter) {
expect(
tester.getBottomLeft(find.text(centerMonth)).dx,
lessThan(tester.getBottomLeft(find.text(month)).dx),
);
}
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: SizedBox(
height: 200.0,
width: 3000.0,
child: dateWidget,
),
),
),
),
);
// The wheel does not bend outwards at large widths.
for (final String month in visibleMonthsExceptTheCenter) {
expect(
tester.getBottomLeft(find.text(centerMonth)).dx,
lessThan(tester.getBottomLeft(find.text(month)).dx),
);
}
});
testWidgets(
'non-selectable dates are greyed out, '
'when minimum date is unconstrained',
(WidgetTester tester) async {
final DateTime maximum = DateTime(2018, 6, 15);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
maximumDate: maximum,
onDateTimeChanged: (_) {},
initialDateTime: DateTime(2018, 6, 15),
),
),
),
),
);
// unconstrained bounds are not affected.
expect(
tester.widget<Text>(find.text('14')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
// the selected day is not affected.
expect(
tester.widget<Text>(find.text('15')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
// out of bounds and should be greyed out.
expect(
tester.widget<Text>(find.text('16')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
},
);
testWidgets(
'non-selectable dates are greyed out, '
'when maximum date is unconstrained',
(WidgetTester tester) async {
final DateTime minimum = DateTime(2018, 6, 15);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
minimumDate: minimum,
onDateTimeChanged: (_) {},
initialDateTime: DateTime(2018, 6, 15),
),
),
),
),
);
// out of bounds and should be greyed out.
expect(
tester.widget<Text>(find.text('14')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
// the selected day is not affected.
expect(
tester.widget<Text>(find.text('15')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
// unconstrained bounds are not affected.
expect(
tester.widget<Text>(find.text('16')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
},
);
testWidgets(
'non-selectable dates are greyed out, '
'months should be taken into account when greying out days',
(WidgetTester tester) async {
final DateTime minimum = DateTime(2018, 5, 15);
final DateTime maximum = DateTime(2018, 7, 15);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (_) {},
initialDateTime: DateTime(2018, 6, 15),
),
),
),
),
);
// days of a different min/max month are not affected.
expect(
tester.widget<Text>(find.text('14')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
expect(
tester.widget<Text>(find.text('16')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
},
);
testWidgets(
'non-selectable dates are greyed out, '
'years should be taken into account when greying out days',
(WidgetTester tester) async {
final DateTime minimum = DateTime(2017, 6, 15);
final DateTime maximum = DateTime(2019, 6, 15);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (_) {},
initialDateTime: DateTime(2018, 6, 15),
),
),
),
),
);
// days of a different min/max year are not affected.
expect(
tester.widget<Text>(find.text('14')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
expect(
tester.widget<Text>(find.text('16')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
},
);
testWidgets('picker automatically scrolls away from invalid date on month change', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2018, 3, 30),
),
),
),
),
);
await tester.drag(find.text('March'), const Offset(0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
// Momentarily, the 2018 and the incorrect 30 of February is aligned.
expect(
tester.getTopLeft(find.text('2018')).dy,
tester.getTopLeft(find.text('30')).dy,
);
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pump(); // Once to start the DrivenScrollActivity.
await tester.pump(const Duration(milliseconds: 500));
expect(
date,
DateTime(2018, 2, 28),
);
expect(
tester.getTopLeft(find.text('2018')).dy,
tester.getTopLeft(find.text('28')).dy,
);
});
testWidgets(
'date picker automatically scrolls away from invalid date, '
"and onDateTimeChanged doesn't report these dates",
(WidgetTester tester) async {
late DateTime date;
// 2016 is a leap year.
final DateTime minimum = DateTime(2016, 2, 29);
final DateTime maximum = DateTime(2018, 12, 31);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
// Callback doesn't transiently go into invalid dates.
expect(newDate.isAtSameMomentAs(minimum) || newDate.isAfter(minimum), isTrue);
expect(newDate.isAtSameMomentAs(maximum) || newDate.isBefore(maximum), isTrue);
},
initialDateTime: DateTime(2017, 2, 28),
),
),
),
),
);
// 2017 has 28 days in Feb so 29 is greyed out.
expect(
tester.widget<Text>(find.text('29')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
await tester.drag(find.text('2017'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pumpAndSettle(); // Now the autoscrolling should happen.
expect(
date,
DateTime(2016, 2, 29),
);
// 2016 has 29 days in Feb so 29 is not greyed out.
expect(
tester.widget<Text>(find.text('29')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
await tester.drag(find.text('2016'), const Offset(0.0, -32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pumpAndSettle();
expect(
date,
DateTime(2017, 2, 28),
);
expect(
tester.widget<Text>(find.text('29')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
},
);
testWidgets(
'dateTime picker automatically scrolls away from invalid date, '
"and onDateTimeChanged doesn't report these dates",
(WidgetTester tester) async {
late DateTime date;
final DateTime minimum = DateTime(2019, 11, 11, 3, 30);
final DateTime maximum = DateTime(2019, 11, 11, 14, 59, 59);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
// Callback doesn't transiently go into invalid dates.
expect(minimum.isAfter(newDate), isFalse);
expect(maximum.isBefore(newDate), isFalse);
},
initialDateTime: DateTime(2019, 11, 11, 4),
),
),
),
),
);
// 3:00 is valid but 2:00 should be invalid.
expect(
tester.widget<Text>(find.text('3')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
expect(
tester.widget<Text>(find.text('2')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
// 'PM' is greyed out.
expect(
tester.widget<Text>(find.text('PM')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
await tester.drag(find.text('AM'), const Offset(0.0, -32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pumpAndSettle(); // Now the autoscrolling should happen.
expect(
date,
DateTime(2019, 11, 11, 14, 59),
);
// 3'o clock and 'AM' are now greyed out.
expect(
tester.widget<Text>(find.text('AM')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
expect(
tester.widget<Text>(find.text('3')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
await tester.drag(find.text('PM'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pumpAndSettle();
// Returns to min date.
expect(
date,
DateTime(2019, 11, 11, 3, 30),
);
},
);
testWidgets(
'time picker automatically scrolls away from invalid date, '
"and onDateTimeChanged doesn't report these dates",
(WidgetTester tester) async {
late DateTime date;
final DateTime minimum = DateTime(2019, 11, 11, 3, 30);
final DateTime maximum = DateTime(2019, 11, 11, 14, 59, 59);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
// Callback doesn't transiently go into invalid dates.
expect(minimum.isAfter(newDate), isFalse);
expect(maximum.isBefore(newDate), isFalse);
},
initialDateTime: DateTime(2019, 11, 11, 4),
),
),
),
),
);
// 3:00 is valid but 2:00 should be invalid.
expect(
tester.widget<Text>(find.text('3')).style!.color,
isNot(isSameColorAs(CupertinoColors.inactiveGray.color)),
);
expect(
tester.widget<Text>(find.text('2')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
// 'PM' is greyed out.
expect(
tester.widget<Text>(find.text('PM')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
await tester.drag(find.text('AM'), const Offset(0.0, -32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pumpAndSettle(); // Now the autoscrolling should happen.
expect(
date,
DateTime(2019, 11, 11, 14, 59),
);
// 3'o clock and 'AM' are now greyed out.
expect(
tester.widget<Text>(find.text('AM')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
expect(
tester.widget<Text>(find.text('3')).style!.color,
isSameColorAs(CupertinoColors.inactiveGray.color),
);
await tester.drag(find.text('PM'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pumpAndSettle();
// Returns to min date.
expect(
date,
DateTime(2019, 11, 11, 3, 30),
);
},
);
testWidgets(
'monthYear picker automatically scrolls away from invalid date, '
"and onDateTimeChanged doesn't report these dates",
(WidgetTester tester) async {
late DateTime date;
final DateTime minimum = DateTime(2016, 2);
final DateTime maximum = DateTime(2018, 12);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.monthYear,
minimumDate: minimum,
maximumDate: maximum,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
// Callback doesn't transiently go into invalid dates.
expect(newDate.isAtSameMomentAs(minimum) || newDate.isAfter(minimum), isTrue);
expect(newDate.isAtSameMomentAs(maximum) || newDate.isBefore(maximum), isTrue);
},
initialDateTime: DateTime(2017, 2),
),
),
),
),
);
await tester.drag(find.text('2017'), const Offset(0.0, 100.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pumpAndSettle(); // Now the autoscrolling should happen.
expect(
date,
DateTime(2016, 2),
);
await tester.drag(find.text('2016'), const Offset(0.0, -100.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pumpAndSettle();
expect(
date,
DateTime(2018, 12),
);
await tester.drag(find.text('2016'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
await tester.pumpAndSettle();
expect(
date,
DateTime(2017, 12),
);
},
);
testWidgets('picker automatically scrolls away from invalid date on day change', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2018, 2, 27), // 2018 has 28 days in Feb.
),
),
),
),
);
await tester.drag(find.text('27'), const Offset(0.0, -32.0), pointer: 1, touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
expect(
date,
DateTime(2018, 2, 28),
);
await tester.drag(find.text('28'), const Offset(0.0, -32.0), pointer: 1, touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump(); // Once to trigger the post frame animate call.
// Callback doesn't transiently go into invalid dates.
expect(
date,
DateTime(2018, 2, 28),
);
// Momentarily, the invalid 29th of Feb is dragged into the middle.
expect(
tester.getTopLeft(find.text('2018')).dy,
tester.getTopLeft(find.text('29')).dy,
);
await tester.pump(); // Once to start the DrivenScrollActivity.
await tester.pump(const Duration(milliseconds: 500));
expect(
date,
DateTime(2018, 2, 28),
);
expect(
tester.getTopLeft(find.text('2018')).dy,
tester.getTopLeft(find.text('28')).dy,
);
});
testWidgets(
'date picker should only take into account the date part of minimumDate and maximumDate',
(WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/49606.
late DateTime date;
final DateTime minDate = DateTime(2020, 1, 1, 12);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
minimumDate: minDate,
onDateTimeChanged: (DateTime newDate) { date = newDate; },
initialDateTime: DateTime(2020, 1, 12),
),
),
),
),
);
// Scroll to 2019.
await tester.drag(find.text('2020'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pumpAndSettle();
expect(date.year, minDate.year);
expect(date.month, minDate.month);
expect(date.day, minDate.day);
},
);
testWidgets('date picker does not display previous day of minimumDate if it is set at midnight', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/72932
final DateTime minDate = DateTime(2019, 12, 31);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
minimumDate: minDate,
onDateTimeChanged: (DateTime newDate) { },
initialDateTime: minDate.add(const Duration(days: 1)),
),
),
),
),
);
expect(find.text('Mon Dec 30'), findsNothing);
});
group('Picker handles initial noon/midnight times', () {
testWidgets('midnight', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2019, 1, 1, 0, 15),
),
),
),
),
);
// 0:15 -> 0:16
await tester.drag(find.text('15'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 0, 16));
});
testWidgets('noon', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2019, 1, 1, 12, 15),
),
),
),
),
);
// 12:15 -> 12:16
await tester.drag(find.text('15'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 12, 16));
});
testWidgets('noon in 24 hour time', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
use24hFormat: true,
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2019, 1, 1, 12, 25),
),
),
),
),
);
// 12:25 -> 12:26
await tester.drag(find.text('25'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 12, 26));
});
});
testWidgets('picker persists am/pm value when scrolling hours', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2019, 1, 1, 3),
),
),
),
),
);
// 3:00 -> 15:00
await tester.drag(find.text('AM'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 15));
// 15:00 -> 16:00
await tester.drag(find.text('3'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 16));
// 16:00 -> 4:00
await tester.drag(find.text('PM'), -_kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 4));
// 4:00 -> 3:00
await tester.drag(find.text('4'), -_kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2019, 1, 1, 3));
});
testWidgets('picker automatically scrolls the am/pm column when the hour column changes enough', (WidgetTester tester) async {
late DateTime date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.time,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
initialDateTime: DateTime(2018, 1, 1, 11, 59),
),
),
),
),
);
const Offset deltaOffset = Offset(0.0, -18.0);
// 11:59 -> 12:59
await tester.drag(find.text('11'), _kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2018, 1, 1, 12, 59));
// 12:59 -> 11:59
await tester.drag(find.text('12'), -_kRowOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2018, 1, 1, 11, 59));
// 11:59 -> 9:59
await tester.drag(find.text('11'), -((_kRowOffset - deltaOffset) * 2 + deltaOffset), warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2018, 1, 1, 9, 59));
// 9:59 -> 15:59
await tester.drag(find.text('9'), (_kRowOffset - deltaOffset) * 6 + deltaOffset, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(date, DateTime(2018, 1, 1, 15, 59));
});
testWidgets('date picker given too narrow space horizontally shows message', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
// This is too small to draw the picker out fully.
width: 100,
child: CupertinoDatePicker(
initialDateTime: DateTime(2019, 1, 1, 4),
onDateTimeChanged: (_) {},
),
),
),
),
);
final dynamic exception = tester.takeException();
expect(exception, isFlutterError);
expect(
exception.toString(),
contains('Insufficient horizontal space to render the CupertinoDatePicker'),
);
});
testWidgets('DatePicker golden tests', (WidgetTester tester) async {
Widget buildApp(CupertinoDatePickerMode mode) {
return CupertinoApp(
home: Center(
child: SizedBox(
width: 500,
height: 400,
child: RepaintBoundary(
child: CupertinoDatePicker(
key: ValueKey<CupertinoDatePickerMode>(mode),
mode: mode,
initialDateTime: DateTime(2019, 1, 1, 4, 12, 30),
onDateTimeChanged: (_) {},
),
),
),
),
);
}
await tester.pumpWidget(buildApp(CupertinoDatePickerMode.time));
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoDatePicker),
matchesGoldenFile('date_picker_test.time.initial.png'),
);
}
await tester.pumpWidget(buildApp(CupertinoDatePickerMode.date));
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoDatePicker),
matchesGoldenFile('date_picker_test.date.initial.png'),
);
}
await tester.pumpWidget(buildApp(CupertinoDatePickerMode.monthYear));
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoDatePicker),
matchesGoldenFile('date_picker_test.monthyear.initial.png'),
);
}
await tester.pumpWidget(buildApp(CupertinoDatePickerMode.dateAndTime));
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoDatePicker),
matchesGoldenFile('date_picker_test.datetime.initial.png'),
);
}
// Slightly drag the hour component to make the current hour off-center.
await tester.drag(find.text('4'), Offset(0, _kRowOffset.dy / 2), warnIfMissed: false); // see top of file
await tester.pump();
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoDatePicker),
matchesGoldenFile('date_picker_test.datetime.drag.png'),
);
}
}, skip: impellerEnabled); // https://github.com/flutter/flutter/issues/143616
testWidgets('DatePicker displays the date in correct order', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
dateOrder: DatePickerDateOrder.ydm,
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime newDate) {},
initialDateTime: DateTime(2018, 1, 14, 10, 30),
),
),
),
),
);
expect(
tester.getTopLeft(find.text('2018')).dx,
lessThan(tester.getTopLeft(find.text('14')).dx),
);
expect(
tester.getTopLeft(find.text('14')).dx,
lessThan(tester.getTopLeft(find.text('January')).dx),
);
});
testWidgets('monthYear DatePicker displays the date in correct order', (WidgetTester tester) async {
Widget buildApp(DatePickerDateOrder order) {
return CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
key: ValueKey<DatePickerDateOrder>(order),
dateOrder: order,
mode: CupertinoDatePickerMode.monthYear,
onDateTimeChanged: (DateTime newDate) {},
initialDateTime: DateTime(2018, 1, 14, 10, 30),
),
),
),
);
}
await tester.pumpWidget(buildApp(DatePickerDateOrder.dmy));
expect(
tester.getTopLeft(find.text('January')).dx,
lessThan(tester.getTopLeft(find.text('2018')).dx),
);
await tester.pumpWidget(buildApp(DatePickerDateOrder.mdy));
expect(
tester.getTopLeft(find.text('January')).dx,
lessThan(tester.getTopLeft(find.text('2018')).dx),
);
await tester.pumpWidget(buildApp(DatePickerDateOrder.ydm));
expect(
tester.getTopLeft(find.text('2018')).dx,
lessThan(tester.getTopLeft(find.text('January')).dx),
);
await tester.pumpWidget(buildApp(DatePickerDateOrder.ymd));
expect(
tester.getTopLeft(find.text('2018')).dx,
lessThan(tester.getTopLeft(find.text('January')).dx),
);
});
testWidgets('DatePicker displays hours and minutes correctly in RTL', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: SizedBox(
width: 500,
height: 400,
child: CupertinoDatePicker(
initialDateTime: DateTime(2019, 1, 1, 4),
onDateTimeChanged: (_) {},
),
),
),
),
),
);
final double hourLeft = tester.getTopLeft(find.text('4')).dx;
final double minuteLeft = tester.getTopLeft(find.text('00')).dx;
expect(hourLeft, lessThan(minuteLeft));
});
});
testWidgets('TimerPicker golden tests', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
// Also check if the picker respects the theme.
theme: const CupertinoThemeData(
textTheme: CupertinoTextThemeData(
pickerTextStyle: TextStyle(
color: Color(0xFF663311),
fontSize: 21,
),
),
),
home: Center(
child: SizedBox(
width: 320,
height: 216,
child: RepaintBoundary(
child: CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hm,
initialTimerDuration: const Duration(hours: 23, minutes: 59),
onTimerDurationChanged: (_) {},
),
),
),
),
),
);
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoTimerPicker),
matchesGoldenFile('timer_picker_test.datetime.initial.png'),
);
}
// Slightly drag the minute component to make the current minute off-center.
await tester.drag(find.text('59'), Offset(0, _kRowOffset.dy / 2), warnIfMissed: false); // see top of file
await tester.pump();
if (!skipPerspectiveTextGoldens) {
await expectLater(
find.byType(CupertinoTimerPicker),
matchesGoldenFile('timer_picker_test.datetime.drag.png'),
);
}
}, skip: impellerEnabled); // https://github.com/flutter/flutter/issues/143616
testWidgets('TimerPicker only changes hour label after scrolling stops', (WidgetTester tester) async {
Duration? duration;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
width: 320,
height: 216,
child: CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hm,
initialTimerDuration: const Duration(hours: 2, minutes: 30),
onTimerDurationChanged: (Duration d) { duration = d; },
),
),
),
),
);
expect(duration, isNull);
expect(find.text('hour'), findsNothing);
expect(find.text('hours'), findsOneWidget);
await tester.drag(find.text('2'), Offset(0, -_kRowOffset.dy), warnIfMissed: false); // see top of file
// Duration should change but not the label.
expect(duration!.inHours, 1);
expect(find.text('hour'), findsNothing);
expect(find.text('hours'), findsOneWidget);
await tester.pumpAndSettle();
// Now the label should change.
expect(duration!.inHours, 1);
expect(find.text('hours'), findsNothing);
expect(find.text('hour'), findsOneWidget);
});
testWidgets('TimerPicker has intrinsic width and height', (WidgetTester tester) async {
const Key key = Key('key');
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
key: key,
mode: CupertinoTimerPickerMode.hm,
initialTimerDuration: const Duration(hours: 2, minutes: 30),
onTimerDurationChanged: (Duration d) {},
),
),
);
expect(tester.getSize(find.descendant(of: find.byKey(key), matching: find.byType(Row))), const Size(320, 216));
// Different modes shouldn't share state.
await tester.pumpWidget(const Placeholder());
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
key: key,
mode: CupertinoTimerPickerMode.ms,
initialTimerDuration: const Duration(minutes: 30, seconds: 3),
onTimerDurationChanged: (Duration d) {},
),
),
);
expect(tester.getSize(find.descendant(of: find.byKey(key), matching: find.byType(Row))), const Size(320, 216));
// Different modes shouldn't share state.
await tester.pumpWidget(const Placeholder());
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTimerPicker(
key: key,
initialTimerDuration: const Duration(hours: 5, minutes: 17, seconds: 19),
onTimerDurationChanged: (Duration d) {},
),
),
);
expect(tester.getSize(find.descendant(of: find.byKey(key), matching: find.byType(Row))), const Size(342, 216));
});
testWidgets('scrollController can be removed or added', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
late int lastSelectedItem;
void onSelectedItemChanged(int index) {
lastSelectedItem = index;
}
final FixedExtentScrollController scrollController1 = FixedExtentScrollController();
addTearDown(scrollController1.dispose);
await tester.pumpWidget(_buildPicker(
controller: scrollController1,
onSelectedItemChanged: onSelectedItemChanged,
));
tester.binding.pipelineOwner.semanticsOwner!.performAction(1, SemanticsAction.increase);
await tester.pumpAndSettle();
expect(lastSelectedItem, 1);
await tester.pumpWidget(_buildPicker(
onSelectedItemChanged: onSelectedItemChanged,
));
tester.binding.pipelineOwner.semanticsOwner!.performAction(1, SemanticsAction.increase);
await tester.pumpAndSettle();
expect(lastSelectedItem, 2);
final FixedExtentScrollController scrollController2 = FixedExtentScrollController();
addTearDown(scrollController2.dispose);
await tester.pumpWidget(_buildPicker(
controller: scrollController2,
onSelectedItemChanged: onSelectedItemChanged,
));
tester.binding.pipelineOwner.semanticsOwner!.performAction(1, SemanticsAction.increase);
await tester.pumpAndSettle();
expect(lastSelectedItem, 3);
handle.dispose();
});
testWidgets('CupertinoDataPicker does not provide invalid MediaQuery', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/47989.
Brightness brightness = Brightness.light;
late StateSetter setState;
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(
textTheme: CupertinoTextThemeData(
dateTimePickerTextStyle: TextStyle(
color: CupertinoDynamicColor.withBrightness(
color: Color(0xFFFFFFFF),
darkColor: Color(0xFF000000),
),
),
),
),
home: StatefulBuilder(builder: (BuildContext context, StateSetter stateSetter) {
setState = stateSetter;
return MediaQuery(
data: MediaQuery.of(context).copyWith(platformBrightness: brightness),
child: CupertinoDatePicker(
initialDateTime: DateTime(2019),
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime date) {},
),
);
}),
),
);
expect(
tester.widget<Text>(find.text('2019')).style!.color,
isSameColorAs(const Color(0xFFFFFFFF)),
);
setState(() { brightness = Brightness.dark; });
await tester.pump();
expect(
tester.widget<Text>(find.text('2019')).style!.color,
isSameColorAs(const Color(0xFF000000)),
);
});
testWidgets('picker exports semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
debugResetSemanticsIdCounter();
int? lastSelectedItem;
await tester.pumpWidget(_buildPicker(onSelectedItemChanged: (int index) {
lastSelectedItem = index;
}));
expect(tester.getSemantics(find.byType(CupertinoPicker)), matchesSemantics(
children: <Matcher>[
matchesSemantics(
hasIncreaseAction: true,
increasedValue: '1',
value: '0',
textDirection: TextDirection.ltr,
),
],
));
tester.binding.pipelineOwner.semanticsOwner!.performAction(1, SemanticsAction.increase);
await tester.pumpAndSettle();
expect(tester.getSemantics(find.byType(CupertinoPicker)), matchesSemantics(
children: <Matcher>[
matchesSemantics(
hasIncreaseAction: true,
hasDecreaseAction: true,
increasedValue: '2',
decreasedValue: '0',
value: '1',
textDirection: TextDirection.ltr,
),
],
));
expect(lastSelectedItem, 1);
handle.dispose();
});
// Regression test for https://github.com/flutter/flutter/issues/98567
testWidgets('picker semantics action test', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
debugResetSemanticsIdCounter();
final DateTime initialDate = DateTime(2018, 6, 8);
late DateTime? date;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
onDateTimeChanged: (DateTime newDate) => date = newDate,
initialDateTime: initialDate,
maximumDate: initialDate.add(const Duration(days: 2)),
minimumDate: initialDate.subtract(const Duration(days: 2)),
),
),
),
),
);
tester.binding.pipelineOwner.semanticsOwner!.performAction(4, SemanticsAction.decrease);
await tester.pumpAndSettle();
expect(date, DateTime(2018, 6, 7));
handle.dispose();
});
testWidgets('DatePicker adapts to MaterialApp dark mode', (WidgetTester tester) async {
Widget buildDatePicker(Brightness brightness) {
return MaterialApp(
theme: ThemeData(brightness: brightness),
home: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (DateTime neData) {},
initialDateTime: DateTime(2018, 10, 10),
),
);
}
// CupertinoDatePicker with light theme.
await tester.pumpWidget(buildDatePicker(Brightness.light));
RenderParagraph paragraph = tester.renderObject(find.text('October').first);
expect(paragraph.text.style!.color, CupertinoColors.label);
// Text style should not return unresolved color.
expect(paragraph.text.style!.color.toString().contains('UNRESOLVED'), isFalse);
// CupertinoDatePicker with dark theme.
await tester.pumpWidget(buildDatePicker(Brightness.dark));
paragraph = tester.renderObject(find.text('October').first);
expect(paragraph.text.style!.color, CupertinoColors.label);
// Text style should not return unresolved color.
expect(paragraph.text.style!.color.toString().contains('UNRESOLVED'), isFalse);
});
testWidgets('TimerPicker adapts to MaterialApp dark mode', (WidgetTester tester) async {
Widget buildTimerPicker(Brightness brightness) {
return MaterialApp(
theme: ThemeData(brightness: brightness),
home: CupertinoTimerPicker(
mode: CupertinoTimerPickerMode.hm,
onTimerDurationChanged: (Duration newDuration) {},
initialTimerDuration: const Duration(hours: 12, minutes: 30, seconds: 59),
),
);
}
// CupertinoTimerPicker with light theme.
await tester.pumpWidget(buildTimerPicker(Brightness.light));
RenderParagraph paragraph = tester.renderObject(find.text('hours'));
expect(paragraph.text.style!.color, CupertinoColors.label);
// Text style should not return unresolved color.
expect(paragraph.text.style!.color.toString().contains('UNRESOLVED'), isFalse);
// CupertinoTimerPicker with light theme.
await tester.pumpWidget(buildTimerPicker(Brightness.dark));
paragraph = tester.renderObject(find.text('hours'));
expect(paragraph.text.style!.color, CupertinoColors.label);
// Text style should not return unresolved color.
expect(paragraph.text.style!.color.toString().contains('UNRESOLVED'), isFalse);
});
testWidgets('TimerPicker minDate - maxDate with minuteInterval', (WidgetTester tester) async {
late DateTime date;
final DateTime minimum = DateTime(2022, 6, 14, 3, 31);
final DateTime initial = DateTime(2022, 6, 14, 3, 40);
final DateTime maximum = DateTime(2022, 6, 14, 3, 49);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
initialDateTime: initial,
minimumDate: minimum,
maximumDate: maximum,
minuteInterval: 5,
use24hFormat: true,
onDateTimeChanged: (DateTime newDate) {
date = newDate;
},
),
),
),
),
);
// Drag picker minutes to min date
await tester.drag(find.text('40'), const Offset(0.0, 32.0), touchSlopY: 0.0, warnIfMissed: false);
await tester.pumpAndSettle();
// Returns to min date.
expect(
date,
DateTime(2022, 6, 14, 3, 35),
);
// Drag picker minutes to max date
await tester.drag(find.text('50'), const Offset(0.0, -64.0), touchSlopY: 0.0, warnIfMissed: false);
await tester.pumpAndSettle();
// Returns to max date.
expect(
date,
DateTime(2022, 6, 14, 3, 45),
);
});
testWidgets('date picker has expected day of week', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: SizedBox(
height: 400.0,
width: 400.0,
child: CupertinoDatePicker(
mode: CupertinoDatePickerMode.date,
onDateTimeChanged: (_) { },
initialDateTime: DateTime(2018, 9, 15),
showDayOfWeek: true,
),
),
),
),
);
expect(find.text('September'), findsOneWidget);
expect(find.textContaining('Sat').last, findsOneWidget);
expect(find.textContaining('15').last, findsOneWidget);
expect(find.text('2018'), findsOneWidget);
});
}
Widget _buildPicker({
FixedExtentScrollController? controller,
required ValueChanged<int> onSelectedItemChanged,
}) {
return Directionality(
textDirection: TextDirection.ltr,
child: CupertinoPicker(
scrollController: controller,
itemExtent: 100.0,
onSelectedItemChanged: onSelectedItemChanged,
children: List<Widget>.generate(100, (int index) {
return Center(
child: SizedBox(
width: 400.0,
height: 100.0,
child: Text(index.toString()),
),
);
}),
),
);
}
| flutter/packages/flutter/test/cupertino/date_picker_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/date_picker_test.dart",
"repo_id": "flutter",
"token_count": 33401
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('test iOS page transition (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 150));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is moving to the left.
expect(widget1TransientTopLeft.dx, lessThan(widget1InitialTopLeft.dx));
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy, equals(widget1InitialTopLeft.dy));
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy, equals(widget2TopLeft.dy));
// Page 2 is coming in from the right.
expect(widget2TopLeft.dx, greaterThan(widget1InitialTopLeft.dx));
// Will need to be changed if the animation curve or duration changes.
expect(widget1TransientTopLeft.dx, moreOrLessEquals(158, epsilon: 1.0));
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is coming back from the left.
expect(widget1TransientTopLeft.dx, lessThan(widget1InitialTopLeft.dx));
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy, equals(widget1InitialTopLeft.dy));
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy, equals(widget2TopLeft.dy));
// Page 2 is leaving towards the right.
expect(widget2TopLeft.dx, greaterThan(widget1InitialTopLeft.dx));
// Will need to be changed if the animation curve or duration changes.
expect(widget1TransientTopLeft.dx, moreOrLessEquals(220, epsilon: 1.0));
await tester.pumpAndSettle();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft, equals(widget1TransientTopLeft));
});
testWidgets('test iOS page transition (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
localizationsDelegates: const <LocalizationsDelegate<dynamic>>[
RtlOverrideWidgetsDelegate(),
],
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
await tester.pump(); // to load the localization, since it doesn't use a synchronous future
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 150));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is moving to the right.
expect(widget1TransientTopLeft.dx, greaterThan(widget1InitialTopLeft.dx));
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy, equals(widget1InitialTopLeft.dy));
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy, equals(widget2TopLeft.dy));
// Page 2 is coming in from the left.
expect(widget2TopLeft.dx, lessThan(widget1InitialTopLeft.dx));
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is coming back from the right.
expect(widget1TransientTopLeft.dx, greaterThan(widget1InitialTopLeft.dx));
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy, equals(widget1InitialTopLeft.dy));
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy, equals(widget2TopLeft.dy));
// Page 2 is leaving towards the left.
expect(widget2TopLeft.dx, lessThan(widget1InitialTopLeft.dx));
await tester.pumpAndSettle();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft, equals(widget1TransientTopLeft));
});
testWidgets('test iOS fullscreen dialog transition', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(child: Text('Page 1')),
),
);
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).push(CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const Center(child: Text('Page 2'));
},
fullscreenDialog: true,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 doesn't move.
expect(widget1TransientTopLeft, equals(widget1InitialTopLeft));
// Fullscreen dialogs transitions vertically only.
expect(widget1InitialTopLeft.dx, equals(widget2TopLeft.dx));
// Page 2 is coming in from the bottom.
expect(widget2TopLeft.dy, greaterThan(widget1InitialTopLeft.dy));
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 doesn't move.
expect(widget1TransientTopLeft, equals(widget1InitialTopLeft));
// Fullscreen dialogs transitions vertically only.
expect(widget1InitialTopLeft.dx, equals(widget2TopLeft.dx));
// Page 2 is leaving towards the bottom.
expect(widget2TopLeft.dy, greaterThan(widget1InitialTopLeft.dy));
await tester.pumpAndSettle();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft, equals(widget1TransientTopLeft));
});
testWidgets('test only edge swipes work (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the middle to the right.
TestGesture gesture = await tester.startGesture(const Offset(200.0, 200.0));
await gesture.moveBy(const Offset(300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the right to the left.
gesture = await tester.startGesture(const Offset(795.0, 200.0));
await gesture.moveBy(const Offset(-300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the right to the further right.
gesture = await tester.startGesture(const Offset(795.0, 200.0));
await gesture.moveBy(const Offset(300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Now drag from the left edge.
gesture = await tester.startGesture(const Offset(5.0, 200.0));
await gesture.moveBy(const Offset(300.0, 0.0));
await tester.pump();
// Page 1 is now visible.
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), isOnstage);
});
testWidgets('test edge swipes work with media query padding (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? navigator) {
return MediaQuery(
data: const MediaQueryData(padding: EdgeInsets.only(left: 40)),
child: navigator!,
);
},
home: const Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) => const Center(child: Text('Page 1')),
),
);
await tester.pump();
await tester.pump(const Duration(seconds: 2));
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) => const Center(child: Text('Page 2')),
),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Now drag from the left edge.
final TestGesture gesture = await tester.startGesture(const Offset(35.0, 200.0));
await gesture.moveBy(const Offset(300.0, 0.0));
await tester.pump();
await tester.pumpAndSettle();
// Page 1 is now visible.
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), isOnstage);
});
testWidgets('test edge swipes work with media query padding (RLT)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? navigator) {
return Directionality(
textDirection: TextDirection.rtl,
child: MediaQuery(
data: const MediaQueryData(padding: EdgeInsets.only(right: 40)),
child: navigator!,
),
);
},
home: const Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) => const Center(child: Text('Page 1')),
),
);
await tester.pump();
await tester.pumpAndSettle();
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) => const Center(child: Text('Page 2')),
),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Now drag from the left edge.
final TestGesture gesture = await tester.startGesture(const Offset(765.0, 200.0));
await gesture.moveBy(const Offset(-300.0, 0.0));
await tester.pump();
await tester.pumpAndSettle();
// Page 1 is now visible.
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), isOnstage);
});
testWidgets('test only edge swipes work (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
localizationsDelegates: const <LocalizationsDelegate<dynamic>>[
RtlOverrideWidgetsDelegate(),
],
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
await tester.pump(); // to load the localization, since it doesn't use a synchronous future
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the middle to the left.
TestGesture gesture = await tester.startGesture(const Offset(200.0, 200.0));
await gesture.moveBy(const Offset(-300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the left to the right.
gesture = await tester.startGesture(const Offset(5.0, 200.0));
await gesture.moveBy(const Offset(300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from the left to the further left.
gesture = await tester.startGesture(const Offset(5.0, 200.0));
await gesture.moveBy(const Offset(-300.0, 0.0));
await tester.pump();
// Nothing should happen.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Now drag from the right edge.
gesture = await tester.startGesture(const Offset(795.0, 200.0));
await gesture.moveBy(const Offset(-300.0, 0.0));
await tester.pump();
// Page 1 is now visible.
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), isOnstage);
});
testWidgets('test edge swipe then drop back at starting point works', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
final TestGesture gesture = await tester.startGesture(const Offset(5, 200));
await gesture.moveBy(const Offset(300, 0));
await tester.pump();
// Bring it exactly back such that there's nothing to animate when releasing.
await gesture.moveBy(const Offset(-300, 0));
await gesture.up();
await tester.pump();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
});
testWidgets('CupertinoPage does not lose its state when transitioning out', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigator = GlobalKey<NavigatorState>();
await tester.pumpWidget(KeepsStateTestWidget(navigatorKey: navigator));
expect(find.text('subpage'), findsOneWidget);
expect(find.text('home'), findsNothing);
navigator.currentState!.pop();
await tester.pump();
expect(find.text('subpage'), findsOneWidget);
expect(find.text('home'), findsOneWidget);
});
testWidgets('CupertinoPage restores its state', (WidgetTester tester) async {
await tester.pumpWidget(
RootRestorationScope(
restorationId: 'root',
child: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Navigator(
onPopPage: (Route<dynamic> route, dynamic result) { return false; },
pages: const <Page<Object?>>[
CupertinoPage<void>(
restorationId: 'p1',
child: TestRestorableWidget(restorationId: 'p1'),
),
],
restorationScopeId: 'nav',
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return TestRestorableWidget(restorationId: settings.name!);
},
);
},
),
),
),
),
);
expect(find.text('p1'), findsOneWidget);
expect(find.text('count: 0'), findsOneWidget);
await tester.tap(find.text('increment'));
await tester.pump();
expect(find.text('count: 1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('p2');
await tester.pumpAndSettle();
expect(find.text('p1'), findsNothing);
expect(find.text('p2'), findsOneWidget);
await tester.tap(find.text('increment'));
await tester.pump();
await tester.tap(find.text('increment'));
await tester.pump();
expect(find.text('count: 2'), findsOneWidget);
await tester.restartAndRestore();
expect(find.text('p2'), findsOneWidget);
expect(find.text('count: 2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(find.text('p1'), findsOneWidget);
expect(find.text('count: 1'), findsOneWidget);
});
}
class RtlOverrideWidgetsDelegate extends LocalizationsDelegate<WidgetsLocalizations> {
const RtlOverrideWidgetsDelegate();
@override
bool isSupported(Locale locale) => true;
@override
Future<WidgetsLocalizations> load(Locale locale) async => const RtlOverrideWidgetsLocalization();
@override
bool shouldReload(LocalizationsDelegate<WidgetsLocalizations> oldDelegate) => false;
}
class RtlOverrideWidgetsLocalization extends DefaultWidgetsLocalizations {
const RtlOverrideWidgetsLocalization();
@override
TextDirection get textDirection => TextDirection.rtl;
}
class KeepsStateTestWidget extends StatefulWidget {
const KeepsStateTestWidget({super.key, this.navigatorKey});
final Key? navigatorKey;
@override
State<KeepsStateTestWidget> createState() => _KeepsStateTestWidgetState();
}
class _KeepsStateTestWidgetState extends State<KeepsStateTestWidget> {
String? _subpage = 'subpage';
@override
Widget build(BuildContext context) {
return CupertinoApp(
home: Navigator(
key: widget.navigatorKey,
pages: <Page<void>>[
const CupertinoPage<void>(child: Text('home')),
if (_subpage != null) CupertinoPage<void>(child: Text(_subpage!)),
],
onPopPage: (Route<dynamic> route, dynamic result) {
if (!route.didPop(result)) {
return false;
}
setState(() {
_subpage = null;
});
return true;
},
),
);
}
}
class TestRestorableWidget extends StatefulWidget {
const TestRestorableWidget({super.key, required this.restorationId});
final String restorationId;
@override
State<StatefulWidget> createState() => _TestRestorableWidgetState();
}
class _TestRestorableWidgetState extends State<TestRestorableWidget> with RestorationMixin {
@override
String? get restorationId => widget.restorationId;
final RestorableInt counter = RestorableInt(0);
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(counter, 'counter');
}
@override
void dispose() {
super.dispose();
counter.dispose();
}
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
Text(widget.restorationId),
Text('count: ${counter.value}'),
CupertinoButton(
onPressed: () {
setState(() {
counter.value++;
});
},
child: const Text('increment'),
),
],
);
}
}
| flutter/packages/flutter/test/cupertino/page_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/page_test.dart",
"repo_id": "flutter",
"token_count": 8275
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
const String text = 'Hello World! How are you? Life is good!';
const String alternativeText = 'Everything is awesome!!';
void main() {
testWidgets('CupertinoTextField restoration', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'app',
home: TestWidget(),
),
);
await restoreAndVerify(tester);
});
testWidgets('CupertinoTextField restoration with external controller', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'app',
home: TestWidget(
useExternal: true,
),
),
);
await restoreAndVerify(tester);
});
}
Future<void> restoreAndVerify(WidgetTester tester) async {
expect(find.text(text), findsNothing);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 0);
await tester.enterText(find.byType(CupertinoTextField), text);
await skipPastScrollingAnimation(tester);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 0);
await tester.drag(find.byType(Scrollable), const Offset(0, -80));
await skipPastScrollingAnimation(tester);
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
await tester.restartAndRestore();
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
final TestRestorationData data = await tester.getRestorationData();
await tester.enterText(find.byType(CupertinoTextField), alternativeText);
await skipPastScrollingAnimation(tester);
await tester.drag(find.byType(Scrollable), const Offset(0, 80));
await skipPastScrollingAnimation(tester);
expect(find.text(text), findsNothing);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, isNot(60));
await tester.restoreFrom(data);
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
}
class TestWidget extends StatefulWidget {
const TestWidget({super.key, this.useExternal = false});
final bool useExternal;
@override
TestWidgetState createState() => TestWidgetState();
}
class TestWidgetState extends State<TestWidget> with RestorationMixin {
final RestorableTextEditingController controller = RestorableTextEditingController();
@override
String get restorationId => 'widget';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(controller, 'controller');
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Material(
child: Align(
child: SizedBox(
width: 50,
child: CupertinoTextField(
restorationId: 'text',
maxLines: 3,
controller: widget.useExternal ? controller.value : null,
),
),
),
);
}
}
Future<void> skipPastScrollingAnimation(WidgetTester tester) async {
await tester.pump();
await tester.pump(const Duration(milliseconds: 200));
}
| flutter/packages/flutter/test/cupertino/text_field_restoration_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/text_field_restoration_test.dart",
"repo_id": "flutter",
"token_count": 1212
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('test Category constructor', () {
const List<String> sections = <String>[
'First section',
'Second section',
'Third section'
];
const Category category = Category(sections);
expect(category.sections, sections);
});
test('test DocumentationIcon constructor', () {
const DocumentationIcon docIcon = DocumentationIcon('Test String');
expect(docIcon.url, contains('Test String'));
});
test('test Summary constructor', () {
const Summary summary = Summary('Test String');
expect(summary.text, contains('Test String'));
});
}
| flutter/packages/flutter/test/foundation/annotations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/annotations_test.dart",
"repo_id": "flutter",
"token_count": 261
} | 711 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
const int primaryKey = 4;
class TestGestureArenaMember extends GestureArenaMember {
bool acceptRan = false;
@override
void acceptGesture(int key) {
expect(key, equals(primaryKey));
acceptRan = true;
}
bool rejectRan = false;
@override
void rejectGesture(int key) {
expect(key, equals(primaryKey));
rejectRan = true;
}
}
class GestureTester {
GestureArenaManager arena = GestureArenaManager();
TestGestureArenaMember first = TestGestureArenaMember();
TestGestureArenaMember second = TestGestureArenaMember();
late GestureArenaEntry firstEntry;
void addFirst() {
firstEntry = arena.add(primaryKey, first);
}
late GestureArenaEntry secondEntry;
void addSecond() {
secondEntry = arena.add(primaryKey, second);
}
void expectNothing() {
expect(first.acceptRan, isFalse);
expect(first.rejectRan, isFalse);
expect(second.acceptRan, isFalse);
expect(second.rejectRan, isFalse);
}
void expectFirstWin() {
expect(first.acceptRan, isTrue);
expect(first.rejectRan, isFalse);
expect(second.acceptRan, isFalse);
expect(second.rejectRan, isTrue);
}
void expectSecondWin() {
expect(first.acceptRan, isFalse);
expect(first.rejectRan, isTrue);
expect(second.acceptRan, isTrue);
expect(second.rejectRan, isFalse);
}
}
void main() {
test('Should win by accepting', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.expectFirstWin();
});
test('Should win by sweep', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.arena.sweep(primaryKey);
tester.expectFirstWin();
});
test('Should win on release after hold sweep release', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.arena.hold(primaryKey);
tester.expectNothing();
tester.arena.sweep(primaryKey);
tester.expectNothing();
tester.arena.release(primaryKey);
tester.expectFirstWin();
});
test('Should win on sweep after hold release sweep', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.arena.hold(primaryKey);
tester.expectNothing();
tester.arena.release(primaryKey);
tester.expectNothing();
tester.arena.sweep(primaryKey);
tester.expectFirstWin();
});
test('Only first winner should win', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.secondEntry.resolve(GestureDisposition.accepted);
tester.expectFirstWin();
});
test('Only first winner should win, regardless of order', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.arena.close(primaryKey);
tester.expectNothing();
tester.secondEntry.resolve(GestureDisposition.accepted);
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.expectSecondWin();
});
test('Win before close is delayed to close', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.expectNothing();
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.expectNothing();
tester.arena.close(primaryKey);
tester.expectFirstWin();
});
test('Win before close is delayed to close, and only first winner should win', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.expectNothing();
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.secondEntry.resolve(GestureDisposition.accepted);
tester.expectNothing();
tester.arena.close(primaryKey);
tester.expectFirstWin();
});
test('Win before close is delayed to close, and only first winner should win, regardless of order', () {
final GestureTester tester = GestureTester();
tester.addFirst();
tester.addSecond();
tester.expectNothing();
tester.secondEntry.resolve(GestureDisposition.accepted);
tester.firstEntry.resolve(GestureDisposition.accepted);
tester.expectNothing();
tester.arena.close(primaryKey);
tester.expectSecondWin();
});
}
| flutter/packages/flutter/test/gestures/arena_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/arena_test.dart",
"repo_id": "flutter",
"token_count": 1816
} | 712 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
import 'gesture_tester.dart';
class TestDrag extends Drag { }
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testGesture('MultiDrag: moving before delay rejects', (GestureTester tester) {
final DelayedMultiDragGestureRecognizer drag = DelayedMultiDragGestureRecognizer();
bool didStartDrag = false;
drag.onStart = (Offset position) {
didStartDrag = true;
return TestDrag();
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
tester.async.flushMicrotasks();
expect(didStartDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 60.0))); // move more than touch slop before delay expires
expect(didStartDrag, isFalse);
tester.async.elapse(kLongPressTimeout * 2); // expire delay
expect(didStartDrag, isFalse);
tester.route(pointer.move(const Offset(30.0, 120.0))); // move some more after delay expires
expect(didStartDrag, isFalse);
drag.dispose();
});
testGesture('MultiDrag: delay triggers', (GestureTester tester) {
final DelayedMultiDragGestureRecognizer drag = DelayedMultiDragGestureRecognizer();
bool didStartDrag = false;
drag.onStart = (Offset position) {
didStartDrag = true;
return TestDrag();
};
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
tester.async.flushMicrotasks();
expect(didStartDrag, isFalse);
tester.route(pointer.move(const Offset(20.0, 20.0))); // move less than touch slop before delay expires
expect(didStartDrag, isFalse);
tester.async.elapse(kLongPressTimeout * 2); // expire delay
expect(didStartDrag, isTrue);
tester.route(pointer.move(const Offset(30.0, 70.0))); // move more than touch slop after delay expires
expect(didStartDrag, isTrue);
drag.dispose();
});
testGesture('MultiDrag: can filter based on device kind', (GestureTester tester) {
final DelayedMultiDragGestureRecognizer drag = DelayedMultiDragGestureRecognizer(
supportedDevices: <PointerDeviceKind>{ PointerDeviceKind.touch },
);
bool didStartDrag = false;
drag.onStart = (Offset position) {
didStartDrag = true;
return TestDrag();
};
final TestPointer mousePointer = TestPointer(5, PointerDeviceKind.mouse);
final PointerDownEvent down = mousePointer.down(const Offset(10.0, 10.0));
drag.addPointer(down);
tester.closeArena(5);
expect(didStartDrag, isFalse);
tester.async.flushMicrotasks();
expect(didStartDrag, isFalse);
tester.route(mousePointer.move(const Offset(20.0, 20.0))); // move less than touch slop before delay expires
expect(didStartDrag, isFalse);
tester.async.elapse(kLongPressTimeout * 2); // expire delay
// Still false because it shouldn't recognize mouse events.
expect(didStartDrag, isFalse);
tester.route(mousePointer.move(const Offset(30.0, 70.0))); // move more than touch slop after delay expires
// And still false.
expect(didStartDrag, isFalse);
drag.dispose();
});
test('allowedButtonsFilter should work the same when null or not specified', () {
// Regression test for https://github.com/flutter/flutter/pull/122227
final ImmediateMultiDragGestureRecognizer recognizer1 = ImmediateMultiDragGestureRecognizer();
// ignore: avoid_redundant_argument_values
final ImmediateMultiDragGestureRecognizer recognizer2 = ImmediateMultiDragGestureRecognizer(allowedButtonsFilter: null);
// We want to test _allowedButtonsFilter, which is called in this method.
const PointerDownEvent allowedPointer = PointerDownEvent(timeStamp: Duration(days: 10));
// ignore: invalid_use_of_protected_member
expect(recognizer1.isPointerAllowed(allowedPointer), true);
// ignore: invalid_use_of_protected_member
expect(recognizer2.isPointerAllowed(allowedPointer), true);
const PointerDownEvent rejectedPointer = PointerDownEvent(timeStamp: Duration(days: 10), buttons: kMiddleMouseButton);
// ignore: invalid_use_of_protected_member
expect(recognizer1.isPointerAllowed(rejectedPointer), false);
// ignore: invalid_use_of_protected_member
expect(recognizer2.isPointerAllowed(rejectedPointer), false);
});
test('$MultiDragPointerState dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => _MultiDragPointerState(
Offset.zero,
PointerDeviceKind.touch,
null,
).dispose(),
_MultiDragPointerState,
),
areCreateAndDispose,
);
});
}
class _MultiDragPointerState extends MultiDragPointerState {
_MultiDragPointerState(
super.initialPosition,
super.kind,
super.gestureSettings,
);
@override
void accepted(GestureMultiDragStartCallback starter) {}
@override
void dispose() {
super.dispose();
}
}
| flutter/packages/flutter/test/gestures/multidrag_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/multidrag_test.dart",
"repo_id": "flutter",
"token_count": 1916
} | 713 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
const List<PointerEvent> velocityEventData = <PointerEvent>[
PointerDownEvent(
timeStamp: Duration(milliseconds: 216690896),
pointer: 1,
position: Offset(270.0, 538.2857055664062),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690906),
pointer: 1,
position: Offset(270.0, 538.2857055664062),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690951),
pointer: 1,
position: Offset(270.0, 530.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690959),
pointer: 1,
position: Offset(270.0, 526.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690967),
pointer: 1,
position: Offset(270.0, 521.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690975),
pointer: 1,
position: Offset(270.0, 515.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690983),
pointer: 1,
position: Offset(270.0, 506.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690991),
pointer: 1,
position: Offset(268.8571472167969, 496.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216690998),
pointer: 1,
position: Offset(267.4285583496094, 483.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691006),
pointer: 1,
position: Offset(266.28570556640625, 469.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691014),
pointer: 1,
position: Offset(265.4285583496094, 456.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691021),
pointer: 1,
position: Offset(264.28570556640625, 443.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691029),
pointer: 1,
position: Offset(264.0, 431.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691036),
pointer: 1,
position: Offset(263.4285583496094, 421.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691044),
pointer: 1,
position: Offset(263.4285583496094, 412.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691052),
pointer: 1,
position: Offset(263.4285583496094, 404.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691060),
pointer: 1,
position: Offset(263.4285583496094, 396.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691068),
pointer: 1,
position: Offset(264.5714416503906, 390.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691075),
pointer: 1,
position: Offset(265.1428527832031, 384.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691083),
pointer: 1,
position: Offset(266.0, 380.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691091),
pointer: 1,
position: Offset(266.5714416503906, 376.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691098),
pointer: 1,
position: Offset(267.1428527832031, 373.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691106),
pointer: 1,
position: Offset(267.71429443359375, 370.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691114),
pointer: 1,
position: Offset(268.28570556640625, 367.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691121),
pointer: 1,
position: Offset(268.5714416503906, 366.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691130),
pointer: 1,
position: Offset(268.8571472167969, 364.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691137),
pointer: 1,
position: Offset(269.1428527832031, 363.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691145),
pointer: 1,
position: Offset(269.1428527832031, 362.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691153),
pointer: 1,
position: Offset(269.4285583496094, 362.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691168),
pointer: 1,
position: Offset(268.5714416503906, 365.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691176),
pointer: 1,
position: Offset(267.1428527832031, 370.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691183),
pointer: 1,
position: Offset(265.4285583496094, 376.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691191),
pointer: 1,
position: Offset(263.1428527832031, 385.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691199),
pointer: 1,
position: Offset(261.4285583496094, 396.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691207),
pointer: 1,
position: Offset(259.71429443359375, 408.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691215),
pointer: 1,
position: Offset(258.28570556640625, 419.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691222),
pointer: 1,
position: Offset(257.4285583496094, 428.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691230),
pointer: 1,
position: Offset(256.28570556640625, 436.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691238),
pointer: 1,
position: Offset(255.7142791748047, 442.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691245),
pointer: 1,
position: Offset(255.14285278320312, 447.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691253),
pointer: 1,
position: Offset(254.85714721679688, 453.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691261),
pointer: 1,
position: Offset(254.57142639160156, 458.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691268),
pointer: 1,
position: Offset(254.2857208251953, 463.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691276),
pointer: 1,
position: Offset(254.2857208251953, 470.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691284),
pointer: 1,
position: Offset(254.2857208251953, 477.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691292),
pointer: 1,
position: Offset(255.7142791748047, 487.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691300),
pointer: 1,
position: Offset(256.8571472167969, 498.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691307),
pointer: 1,
position: Offset(258.28570556640625, 507.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691315),
pointer: 1,
position: Offset(259.4285583496094, 516.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691323),
pointer: 1,
position: Offset(260.28570556640625, 521.7142944335938),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216691338),
pointer: 1,
position: Offset(260.28570556640625, 521.7142944335938),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216691573),
pointer: 2,
position: Offset(266.0, 327.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691588),
pointer: 2,
position: Offset(266.0, 327.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691626),
pointer: 2,
position: Offset(261.1428527832031, 337.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691634),
pointer: 2,
position: Offset(258.28570556640625, 343.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691642),
pointer: 2,
position: Offset(254.57142639160156, 354.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691650),
pointer: 2,
position: Offset(250.2857208251953, 368.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691657),
pointer: 2,
position: Offset(247.42857360839844, 382.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691665),
pointer: 2,
position: Offset(245.14285278320312, 397.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691673),
pointer: 2,
position: Offset(243.14285278320312, 411.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691680),
pointer: 2,
position: Offset(242.2857208251953, 426.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691688),
pointer: 2,
position: Offset(241.7142791748047, 440.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691696),
pointer: 2,
position: Offset(241.7142791748047, 454.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691703),
pointer: 2,
position: Offset(242.57142639160156, 467.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691712),
pointer: 2,
position: Offset(243.42857360839844, 477.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691720),
pointer: 2,
position: Offset(244.85714721679688, 485.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691727),
pointer: 2,
position: Offset(246.2857208251953, 493.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216691735),
pointer: 2,
position: Offset(248.0, 499.71429443359375),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216691750),
pointer: 2,
position: Offset(248.0, 499.71429443359375),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216692255),
pointer: 3,
position: Offset(249.42857360839844, 351.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692270),
pointer: 3,
position: Offset(249.42857360839844, 351.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692309),
pointer: 3,
position: Offset(246.2857208251953, 361.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692317),
pointer: 3,
position: Offset(244.0, 368.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692325),
pointer: 3,
position: Offset(241.42857360839844, 377.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692333),
pointer: 3,
position: Offset(237.7142791748047, 391.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692340),
pointer: 3,
position: Offset(235.14285278320312, 406.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692348),
pointer: 3,
position: Offset(232.57142639160156, 421.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692356),
pointer: 3,
position: Offset(230.2857208251953, 436.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692363),
pointer: 3,
position: Offset(228.2857208251953, 451.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692371),
pointer: 3,
position: Offset(227.42857360839844, 466.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692378),
pointer: 3,
position: Offset(226.2857208251953, 479.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692387),
pointer: 3,
position: Offset(225.7142791748047, 491.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692395),
pointer: 3,
position: Offset(225.14285278320312, 501.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692402),
pointer: 3,
position: Offset(224.85714721679688, 509.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692410),
pointer: 3,
position: Offset(224.57142639160156, 514.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692418),
pointer: 3,
position: Offset(224.2857208251953, 519.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692425),
pointer: 3,
position: Offset(224.0, 523.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692433),
pointer: 3,
position: Offset(224.0, 527.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692441),
pointer: 3,
position: Offset(224.0, 530.5714111328125),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692448),
pointer: 3,
position: Offset(224.0, 533.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692456),
pointer: 3,
position: Offset(224.0, 535.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692464),
pointer: 3,
position: Offset(223.7142791748047, 536.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692472),
pointer: 3,
position: Offset(223.7142791748047, 538.2857055664062),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216692487),
pointer: 3,
position: Offset(223.7142791748047, 538.2857055664062),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216692678),
pointer: 4,
position: Offset(221.42857360839844, 526.2857055664062),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692701),
pointer: 4,
position: Offset(220.57142639160156, 514.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692708),
pointer: 4,
position: Offset(220.2857208251953, 508.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692716),
pointer: 4,
position: Offset(220.2857208251953, 498.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692724),
pointer: 4,
position: Offset(221.14285278320312, 484.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692732),
pointer: 4,
position: Offset(221.7142791748047, 469.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692740),
pointer: 4,
position: Offset(223.42857360839844, 453.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692748),
pointer: 4,
position: Offset(225.7142791748047, 436.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692755),
pointer: 4,
position: Offset(229.14285278320312, 418.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692763),
pointer: 4,
position: Offset(232.85714721679688, 400.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692770),
pointer: 4,
position: Offset(236.85714721679688, 382.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692778),
pointer: 4,
position: Offset(241.14285278320312, 366.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692786),
pointer: 4,
position: Offset(244.85714721679688, 350.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216692793),
pointer: 4,
position: Offset(249.14285278320312, 335.4285583496094),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216692809),
pointer: 4,
position: Offset(249.14285278320312, 335.4285583496094),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216693222),
pointer: 5,
position: Offset(224.0, 545.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693245),
pointer: 5,
position: Offset(224.0, 545.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693275),
pointer: 5,
position: Offset(222.85714721679688, 535.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693284),
pointer: 5,
position: Offset(222.85714721679688, 528.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693291),
pointer: 5,
position: Offset(222.2857208251953, 518.5714111328125),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693299),
pointer: 5,
position: Offset(222.0, 503.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693307),
pointer: 5,
position: Offset(222.0, 485.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693314),
pointer: 5,
position: Offset(221.7142791748047, 464.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216693322),
pointer: 5,
position: Offset(222.2857208251953, 440.28570556640625),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216693337),
pointer: 5,
position: Offset(222.2857208251953, 440.28570556640625),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216693985),
pointer: 6,
position: Offset(208.0, 544.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694047),
pointer: 6,
position: Offset(208.57142639160156, 532.2857055664062),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694054),
pointer: 6,
position: Offset(208.85714721679688, 525.7142944335938),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694062),
pointer: 6,
position: Offset(208.85714721679688, 515.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694070),
pointer: 6,
position: Offset(208.0, 501.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694077),
pointer: 6,
position: Offset(207.42857360839844, 487.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694085),
pointer: 6,
position: Offset(206.57142639160156, 472.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694092),
pointer: 6,
position: Offset(206.57142639160156, 458.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694100),
pointer: 6,
position: Offset(206.57142639160156, 446.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694108),
pointer: 6,
position: Offset(206.57142639160156, 434.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694116),
pointer: 6,
position: Offset(207.14285278320312, 423.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694124),
pointer: 6,
position: Offset(208.57142639160156, 412.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694131),
pointer: 6,
position: Offset(209.7142791748047, 402.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694139),
pointer: 6,
position: Offset(211.7142791748047, 393.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694147),
pointer: 6,
position: Offset(213.42857360839844, 385.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694154),
pointer: 6,
position: Offset(215.42857360839844, 378.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694162),
pointer: 6,
position: Offset(217.42857360839844, 371.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694169),
pointer: 6,
position: Offset(219.42857360839844, 366.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694177),
pointer: 6,
position: Offset(221.42857360839844, 360.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694185),
pointer: 6,
position: Offset(223.42857360839844, 356.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694193),
pointer: 6,
position: Offset(225.14285278320312, 352.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694201),
pointer: 6,
position: Offset(226.85714721679688, 348.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694209),
pointer: 6,
position: Offset(228.2857208251953, 346.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694216),
pointer: 6,
position: Offset(229.14285278320312, 343.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694224),
pointer: 6,
position: Offset(230.0, 342.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694232),
pointer: 6,
position: Offset(230.57142639160156, 340.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694239),
pointer: 6,
position: Offset(230.85714721679688, 339.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694247),
pointer: 6,
position: Offset(230.85714721679688, 339.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694262),
pointer: 6,
position: Offset(230.2857208251953, 342.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694270),
pointer: 6,
position: Offset(228.85714721679688, 346.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694278),
pointer: 6,
position: Offset(227.14285278320312, 352.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694286),
pointer: 6,
position: Offset(225.42857360839844, 359.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694294),
pointer: 6,
position: Offset(223.7142791748047, 367.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694301),
pointer: 6,
position: Offset(222.57142639160156, 376.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694309),
pointer: 6,
position: Offset(221.42857360839844, 384.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694317),
pointer: 6,
position: Offset(220.85714721679688, 392.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694324),
pointer: 6,
position: Offset(220.0, 400.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694332),
pointer: 6,
position: Offset(219.14285278320312, 409.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694339),
pointer: 6,
position: Offset(218.85714721679688, 419.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694348),
pointer: 6,
position: Offset(218.2857208251953, 428.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694356),
pointer: 6,
position: Offset(218.2857208251953, 438.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694363),
pointer: 6,
position: Offset(218.2857208251953, 447.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694371),
pointer: 6,
position: Offset(218.2857208251953, 455.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694379),
pointer: 6,
position: Offset(219.14285278320312, 462.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694386),
pointer: 6,
position: Offset(220.0, 469.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694394),
pointer: 6,
position: Offset(221.14285278320312, 475.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694401),
pointer: 6,
position: Offset(222.0, 480.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694409),
pointer: 6,
position: Offset(222.85714721679688, 485.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694417),
pointer: 6,
position: Offset(224.0, 489.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694425),
pointer: 6,
position: Offset(224.85714721679688, 492.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694433),
pointer: 6,
position: Offset(225.42857360839844, 495.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694440),
pointer: 6,
position: Offset(226.0, 497.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694448),
pointer: 6,
position: Offset(226.2857208251953, 498.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694456),
pointer: 6,
position: Offset(226.2857208251953, 498.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694471),
pointer: 6,
position: Offset(226.2857208251953, 498.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694479),
pointer: 6,
position: Offset(226.2857208251953, 496.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694486),
pointer: 6,
position: Offset(226.2857208251953, 493.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694494),
pointer: 6,
position: Offset(226.2857208251953, 490.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694502),
pointer: 6,
position: Offset(226.2857208251953, 486.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694510),
pointer: 6,
position: Offset(226.2857208251953, 480.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694518),
pointer: 6,
position: Offset(226.2857208251953, 475.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694525),
pointer: 6,
position: Offset(226.2857208251953, 468.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694533),
pointer: 6,
position: Offset(226.2857208251953, 461.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694541),
pointer: 6,
position: Offset(226.2857208251953, 452.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694548),
pointer: 6,
position: Offset(226.57142639160156, 442.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694556),
pointer: 6,
position: Offset(226.57142639160156, 432.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694564),
pointer: 6,
position: Offset(226.85714721679688, 423.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694571),
pointer: 6,
position: Offset(227.42857360839844, 416.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694580),
pointer: 6,
position: Offset(227.7142791748047, 410.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694587),
pointer: 6,
position: Offset(228.2857208251953, 404.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694595),
pointer: 6,
position: Offset(228.85714721679688, 399.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694603),
pointer: 6,
position: Offset(229.14285278320312, 395.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694610),
pointer: 6,
position: Offset(229.42857360839844, 392.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694618),
pointer: 6,
position: Offset(229.7142791748047, 390.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694625),
pointer: 6,
position: Offset(229.7142791748047, 388.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694633),
pointer: 6,
position: Offset(229.7142791748047, 386.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694641),
pointer: 6,
position: Offset(229.7142791748047, 386.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694648),
pointer: 6,
position: Offset(229.7142791748047, 386.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694657),
pointer: 6,
position: Offset(228.85714721679688, 386.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694665),
pointer: 6,
position: Offset(228.0, 388.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694672),
pointer: 6,
position: Offset(226.0, 392.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694680),
pointer: 6,
position: Offset(224.0, 397.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694688),
pointer: 6,
position: Offset(222.0, 404.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694695),
pointer: 6,
position: Offset(219.7142791748047, 411.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694703),
pointer: 6,
position: Offset(218.2857208251953, 418.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694710),
pointer: 6,
position: Offset(217.14285278320312, 425.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694718),
pointer: 6,
position: Offset(215.7142791748047, 433.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694726),
pointer: 6,
position: Offset(214.85714721679688, 442.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694734),
pointer: 6,
position: Offset(214.0, 454.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694742),
pointer: 6,
position: Offset(214.0, 469.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694749),
pointer: 6,
position: Offset(215.42857360839844, 485.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694757),
pointer: 6,
position: Offset(217.7142791748047, 502.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694765),
pointer: 6,
position: Offset(221.14285278320312, 521.4285888671875),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694772),
pointer: 6,
position: Offset(224.57142639160156, 541.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694780),
pointer: 6,
position: Offset(229.14285278320312, 561.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216694788),
pointer: 6,
position: Offset(233.42857360839844, 578.8571166992188),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216694802),
pointer: 6,
position: Offset(233.42857360839844, 578.8571166992188),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216695344),
pointer: 7,
position: Offset(253.42857360839844, 310.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695352),
pointer: 7,
position: Offset(253.42857360839844, 310.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695359),
pointer: 7,
position: Offset(252.85714721679688, 318.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695367),
pointer: 7,
position: Offset(251.14285278320312, 322.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695375),
pointer: 7,
position: Offset(248.85714721679688, 327.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695382),
pointer: 7,
position: Offset(246.0, 334.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695390),
pointer: 7,
position: Offset(242.57142639160156, 344.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695397),
pointer: 7,
position: Offset(238.85714721679688, 357.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695406),
pointer: 7,
position: Offset(235.7142791748047, 371.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695414),
pointer: 7,
position: Offset(232.2857208251953, 386.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695421),
pointer: 7,
position: Offset(229.42857360839844, 402.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695429),
pointer: 7,
position: Offset(227.42857360839844, 416.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695437),
pointer: 7,
position: Offset(226.2857208251953, 431.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695444),
pointer: 7,
position: Offset(226.2857208251953, 446.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695452),
pointer: 7,
position: Offset(227.7142791748047, 460.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695459),
pointer: 7,
position: Offset(230.0, 475.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695467),
pointer: 7,
position: Offset(232.2857208251953, 489.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695475),
pointer: 7,
position: Offset(235.7142791748047, 504.0),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216695490),
pointer: 7,
position: Offset(235.7142791748047, 504.0),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216695885),
pointer: 8,
position: Offset(238.85714721679688, 524.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695908),
pointer: 8,
position: Offset(236.2857208251953, 515.7142944335938),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695916),
pointer: 8,
position: Offset(234.85714721679688, 509.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695924),
pointer: 8,
position: Offset(232.57142639160156, 498.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695931),
pointer: 8,
position: Offset(230.57142639160156, 483.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695939),
pointer: 8,
position: Offset(229.14285278320312, 466.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695947),
pointer: 8,
position: Offset(229.14285278320312, 446.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695955),
pointer: 8,
position: Offset(230.57142639160156, 424.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695963),
pointer: 8,
position: Offset(232.57142639160156, 402.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695970),
pointer: 8,
position: Offset(235.14285278320312, 380.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216695978),
pointer: 8,
position: Offset(238.57142639160156, 359.4285583496094),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216695993),
pointer: 8,
position: Offset(238.57142639160156, 359.4285583496094),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216696429),
pointer: 9,
position: Offset(238.2857208251953, 568.5714111328125),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696459),
pointer: 9,
position: Offset(234.0, 560.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696467),
pointer: 9,
position: Offset(231.42857360839844, 553.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696475),
pointer: 9,
position: Offset(228.2857208251953, 543.1428833007812),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696483),
pointer: 9,
position: Offset(225.42857360839844, 528.8571166992188),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696491),
pointer: 9,
position: Offset(223.14285278320312, 512.2857055664062),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696498),
pointer: 9,
position: Offset(222.0, 495.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696506),
pointer: 9,
position: Offset(221.7142791748047, 477.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696514),
pointer: 9,
position: Offset(221.7142791748047, 458.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696521),
pointer: 9,
position: Offset(223.14285278320312, 438.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216696529),
pointer: 9,
position: Offset(224.2857208251953, 416.28570556640625),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216696544),
pointer: 9,
position: Offset(224.2857208251953, 416.28570556640625),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216696974),
pointer: 10,
position: Offset(218.57142639160156, 530.5714111328125),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697012),
pointer: 10,
position: Offset(220.2857208251953, 522.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697020),
pointer: 10,
position: Offset(221.14285278320312, 517.7142944335938),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697028),
pointer: 10,
position: Offset(222.2857208251953, 511.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697036),
pointer: 10,
position: Offset(224.0, 504.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697044),
pointer: 10,
position: Offset(227.14285278320312, 490.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697052),
pointer: 10,
position: Offset(229.42857360839844, 474.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697059),
pointer: 10,
position: Offset(231.42857360839844, 454.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697067),
pointer: 10,
position: Offset(233.7142791748047, 431.1428527832031),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216697082),
pointer: 10,
position: Offset(233.7142791748047, 431.1428527832031),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216697435),
pointer: 11,
position: Offset(257.1428527832031, 285.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697465),
pointer: 11,
position: Offset(251.7142791748047, 296.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697473),
pointer: 11,
position: Offset(248.2857208251953, 304.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697481),
pointer: 11,
position: Offset(244.57142639160156, 314.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697489),
pointer: 11,
position: Offset(240.2857208251953, 329.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697497),
pointer: 11,
position: Offset(236.85714721679688, 345.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697505),
pointer: 11,
position: Offset(233.7142791748047, 361.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697512),
pointer: 11,
position: Offset(231.14285278320312, 378.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697520),
pointer: 11,
position: Offset(229.42857360839844, 395.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697528),
pointer: 11,
position: Offset(229.42857360839844, 412.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697535),
pointer: 11,
position: Offset(230.85714721679688, 430.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697543),
pointer: 11,
position: Offset(233.42857360839844, 449.71429443359375),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216697558),
pointer: 11,
position: Offset(233.42857360839844, 449.71429443359375),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216697749),
pointer: 12,
position: Offset(246.0, 311.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697780),
pointer: 12,
position: Offset(244.57142639160156, 318.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697787),
pointer: 12,
position: Offset(243.14285278320312, 325.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697795),
pointer: 12,
position: Offset(241.42857360839844, 336.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697803),
pointer: 12,
position: Offset(239.7142791748047, 351.1428527832031),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697811),
pointer: 12,
position: Offset(238.2857208251953, 368.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697819),
pointer: 12,
position: Offset(238.0, 389.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697826),
pointer: 12,
position: Offset(239.14285278320312, 412.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697834),
pointer: 12,
position: Offset(242.2857208251953, 438.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697842),
pointer: 12,
position: Offset(247.42857360839844, 466.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216697849),
pointer: 12,
position: Offset(254.2857208251953, 497.71429443359375),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216697864),
pointer: 12,
position: Offset(254.2857208251953, 497.71429443359375),
),
PointerDownEvent(
timeStamp: Duration(milliseconds: 216698321),
pointer: 13,
position: Offset(250.0, 306.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698328),
pointer: 13,
position: Offset(250.0, 306.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698344),
pointer: 13,
position: Offset(249.14285278320312, 314.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698351),
pointer: 13,
position: Offset(247.42857360839844, 319.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698359),
pointer: 13,
position: Offset(245.14285278320312, 326.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698366),
pointer: 13,
position: Offset(241.7142791748047, 339.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698374),
pointer: 13,
position: Offset(238.57142639160156, 355.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698382),
pointer: 13,
position: Offset(236.2857208251953, 374.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698390),
pointer: 13,
position: Offset(235.14285278320312, 396.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698398),
pointer: 13,
position: Offset(236.57142639160156, 421.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698406),
pointer: 13,
position: Offset(241.14285278320312, 451.4285583496094),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216698421),
pointer: 13,
position: Offset(241.14285278320312, 451.4285583496094),
),
];
const List<PointerEvent> interruptedVelocityEventData = <PointerEvent>[
PointerDownEvent(
timeStamp: Duration(milliseconds: 216698321),
pointer: 13,
position: Offset(250.0, 306.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698328),
pointer: 13,
position: Offset(250.0, 306.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698344),
pointer: 13,
position: Offset(249.14285278320312, 314.0),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698351),
pointer: 13,
position: Offset(247.42857360839844, 319.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698359),
pointer: 13,
position: Offset(245.14285278320312, 326.8571472167969),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698366),
pointer: 13,
position: Offset(241.7142791748047, 339.4285583496094),
),
// The pointer "stops" here because we've introduced a 40+ms gap
// in the move event stream. See kAssumePointerMoveStoppedMilliseconds
// in velocity_tracker.dart.
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698374 + 40),
pointer: 13,
position: Offset(238.57142639160156, 355.71429443359375),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698382 + 40),
pointer: 13,
position: Offset(236.2857208251953, 374.28570556640625),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698390 + 40),
pointer: 13,
position: Offset(235.14285278320312, 396.5714416503906),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698398 + 40),
pointer: 13,
position: Offset(236.57142639160156, 421.4285583496094),
),
PointerMoveEvent(
timeStamp: Duration(milliseconds: 216698406 + 40),
pointer: 13,
position: Offset(241.14285278320312, 451.4285583496094),
),
PointerUpEvent(
timeStamp: Duration(milliseconds: 216698421 + 40),
pointer: 13,
position: Offset(241.14285278320312, 451.4285583496094),
),
];
| flutter/packages/flutter/test/gestures/velocity_tracker_data.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/velocity_tracker_data.dart",
"repo_id": "flutter",
"token_count": 20054
} | 714 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('MaterialPointArcTween control test', () {
final MaterialPointArcTween a = MaterialPointArcTween(
begin: Offset.zero,
end: const Offset(0.0, 10.0),
);
final MaterialPointArcTween b = MaterialPointArcTween(
begin: Offset.zero,
end: const Offset(0.0, 10.0),
);
expect(a, hasOneLineDescription);
expect(a.toString(), equals(b.toString()));
});
test('MaterialRectArcTween control test', () {
final MaterialRectArcTween a = MaterialRectArcTween(
begin: const Rect.fromLTWH(0.0, 0.0, 10.0, 10.0),
end: const Rect.fromLTWH(0.0, 10.0, 10.0, 10.0),
);
final MaterialRectArcTween b = MaterialRectArcTween(
begin: const Rect.fromLTWH(0.0, 0.0, 10.0, 10.0),
end: const Rect.fromLTWH(0.0, 10.0, 10.0, 10.0),
);
expect(a, hasOneLineDescription);
expect(a.toString(), equals(b.toString()));
});
test('on-axis MaterialPointArcTween', () {
MaterialPointArcTween tween = MaterialPointArcTween(
begin: Offset.zero,
end: const Offset(0.0, 10.0),
);
expect(tween.lerp(0.5), equals(const Offset(0.0, 5.0)));
expect(tween, hasOneLineDescription);
tween = MaterialPointArcTween(
begin: Offset.zero,
end: const Offset(10.0, 0.0),
);
expect(tween.lerp(0.5), equals(const Offset(5.0, 0.0)));
});
test('on-axis MaterialRectArcTween', () {
MaterialRectArcTween tween = MaterialRectArcTween(
begin: const Rect.fromLTWH(0.0, 0.0, 10.0, 10.0),
end: const Rect.fromLTWH(0.0, 10.0, 10.0, 10.0),
);
expect(tween.lerp(0.5), equals(const Rect.fromLTWH(0.0, 5.0, 10.0, 10.0)));
expect(tween, hasOneLineDescription);
tween = MaterialRectArcTween(
begin: const Rect.fromLTWH(0.0, 0.0, 10.0, 10.0),
end: const Rect.fromLTWH(10.0, 0.0, 10.0, 10.0),
);
expect(tween.lerp(0.5), equals(const Rect.fromLTWH(5.0, 0.0, 10.0, 10.0)));
});
test('MaterialPointArcTween', () {
const Offset begin = Offset(180.0, 110.0);
const Offset end = Offset(37.0, 250.0);
MaterialPointArcTween tween = MaterialPointArcTween(begin: begin, end: end);
expect(tween.lerp(0.0), begin);
expect(tween.lerp(0.25), within<Offset>(distance: 2.0, from: const Offset(126.0, 120.0)));
expect(tween.lerp(0.75), within<Offset>(distance: 2.0, from: const Offset(48.0, 196.0)));
expect(tween.lerp(1.0), end);
tween = MaterialPointArcTween(begin: end, end: begin);
expect(tween.lerp(0.0), end);
expect(tween.lerp(0.25), within<Offset>(distance: 2.0, from: const Offset(91.0, 239.0)));
expect(tween.lerp(0.75), within<Offset>(distance: 2.0, from: const Offset(168.3, 163.8)));
expect(tween.lerp(1.0), begin);
});
test('MaterialRectArcTween', () {
const Rect begin = Rect.fromLTRB(180.0, 100.0, 330.0, 200.0);
const Rect end = Rect.fromLTRB(32.0, 275.0, 132.0, 425.0);
bool sameRect(Rect a, Rect b) {
return (a.left - b.left).abs() < 2.0
&& (a.top - b.top).abs() < 2.0
&& (a.right - b.right).abs() < 2.0
&& (a.bottom - b.bottom).abs() < 2.0;
}
MaterialRectArcTween tween = MaterialRectArcTween(begin: begin, end: end);
expect(tween.lerp(0.0), begin);
expect(sameRect(tween.lerp(0.25), const Rect.fromLTRB(120.0, 113.0, 259.0, 237.0)), isTrue);
expect(sameRect(tween.lerp(0.75), const Rect.fromLTRB(42.3, 206.5, 153.5, 354.7)), isTrue);
expect(tween.lerp(1.0), end);
tween = MaterialRectArcTween(begin: end, end: begin);
expect(tween.lerp(0.0), end);
expect(sameRect(tween.lerp(0.25), const Rect.fromLTRB(92.0, 262.0, 203.0, 388.0)), isTrue);
expect(sameRect(tween.lerp(0.75), const Rect.fromLTRB(169.7, 168.5, 308.5, 270.3)), isTrue);
expect(tween.lerp(1.0), begin);
});
}
| flutter/packages/flutter/test/material/arc_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/arc_test.dart",
"repo_id": "flutter",
"token_count": 1777
} | 715 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('ButtonThemeData defaults', () {
const ButtonThemeData theme = ButtonThemeData();
expect(theme.textTheme, ButtonTextTheme.normal);
expect(theme.constraints, const BoxConstraints(minWidth: 88.0, minHeight: 36.0));
expect(theme.padding, const EdgeInsets.symmetric(horizontal: 16.0));
expect(theme.shape, const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(2.0)),
));
expect(theme.alignedDropdown, false);
expect(theme.layoutBehavior, ButtonBarLayoutBehavior.padded);
});
test('ButtonThemeData default overrides', () {
const ButtonThemeData theme = ButtonThemeData(
textTheme: ButtonTextTheme.primary,
minWidth: 100.0,
height: 200.0,
padding: EdgeInsets.zero,
shape: RoundedRectangleBorder(),
alignedDropdown: true,
);
expect(theme.textTheme, ButtonTextTheme.primary);
expect(theme.constraints, const BoxConstraints(minWidth: 100.0, minHeight: 200.0));
expect(theme.padding, EdgeInsets.zero);
expect(theme.shape, const RoundedRectangleBorder());
expect(theme.alignedDropdown, true);
});
test('ButtonThemeData.copyWith', () {
ButtonThemeData theme = const ButtonThemeData().copyWith();
expect(theme.textTheme, ButtonTextTheme.normal);
expect(theme.layoutBehavior, ButtonBarLayoutBehavior.padded);
expect(theme.constraints, const BoxConstraints(minWidth: 88.0, minHeight: 36.0));
expect(theme.padding, const EdgeInsets.symmetric(horizontal: 16.0));
expect(theme.shape, const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(2.0)),
));
expect(theme.alignedDropdown, false);
expect(theme.colorScheme, null);
theme = const ButtonThemeData().copyWith(
textTheme: ButtonTextTheme.primary,
layoutBehavior: ButtonBarLayoutBehavior.constrained,
minWidth: 100.0,
height: 200.0,
padding: EdgeInsets.zero,
shape: const StadiumBorder(),
alignedDropdown: true,
colorScheme: const ColorScheme.dark(),
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
);
expect(theme.textTheme, ButtonTextTheme.primary);
expect(theme.layoutBehavior, ButtonBarLayoutBehavior.constrained);
expect(theme.constraints, const BoxConstraints(minWidth: 100.0, minHeight: 200.0));
expect(theme.padding, EdgeInsets.zero);
expect(theme.shape, const StadiumBorder());
expect(theme.alignedDropdown, true);
expect(theme.colorScheme, const ColorScheme.dark());
});
testWidgets('ButtonTheme alignedDropdown', (WidgetTester tester) async {
final Key dropdownKey = UniqueKey();
Widget buildFrame({ required bool alignedDropdown, required TextDirection textDirection }) {
return MaterialApp(
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child!,
);
},
home: ButtonTheme(
alignedDropdown: alignedDropdown,
child: Material(
child: Builder(
builder: (BuildContext context) {
return Container(
alignment: Alignment.center,
child: DropdownButtonHideUnderline(
child: SizedBox(
width: 200.0,
child: DropdownButton<String>(
key: dropdownKey,
onChanged: (String? value) { },
value: 'foo',
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
value: 'foo',
child: Text('foo'),
),
DropdownMenuItem<String>(
value: 'bar',
child: Text('bar'),
),
],
),
),
),
);
},
),
),
),
);
}
final Finder button = find.byKey(dropdownKey);
final Finder menu = find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DropdownMenu<String>');
await tester.pumpWidget(
buildFrame(
alignedDropdown: false,
textDirection: TextDirection.ltr,
),
);
await tester.tap(button);
await tester.pumpAndSettle();
// 240 = 200.0 (button width) + _kUnalignedMenuMargin (20.0 left and right)
expect(tester.getSize(button).width, 200.0);
expect(tester.getSize(menu).width, 240.0);
// Dismiss the menu.
await tester.tapAt(Offset.zero);
await tester.pumpAndSettle();
expect(menu, findsNothing);
await tester.pumpWidget(
buildFrame(
alignedDropdown: true,
textDirection: TextDirection.ltr,
),
);
await tester.tap(button);
await tester.pumpAndSettle();
// Aligneddropdown: true means the button and menu widths match
expect(tester.getSize(button).width, 200.0);
expect(tester.getSize(menu).width, 200.0);
// There are two 'foo' widgets: the selected menu item's label and the drop
// down button's label. The should both appear at the same location.
final Finder fooText = find.text('foo');
expect(fooText, findsNWidgets(2));
expect(tester.getRect(fooText.at(0)), tester.getRect(fooText.at(1)));
// Dismiss the menu.
await tester.tapAt(Offset.zero);
await tester.pumpAndSettle();
expect(menu, findsNothing);
// Same test as above except RTL
await tester.pumpWidget(
buildFrame(
alignedDropdown: true,
textDirection: TextDirection.rtl,
),
);
await tester.tap(button);
await tester.pumpAndSettle();
expect(fooText, findsNWidgets(2));
expect(tester.getRect(fooText.at(0)), tester.getRect(fooText.at(1)));
});
}
| flutter/packages/flutter/test/material/button_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/button_theme_test.dart",
"repo_id": "flutter",
"token_count": 2646
} | 716 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/clipboard_utils.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
late DateTime firstDate;
late DateTime lastDate;
late DateTime? initialDate;
late DateTime today;
late SelectableDayPredicate? selectableDayPredicate;
late DatePickerEntryMode initialEntryMode;
late DatePickerMode initialCalendarMode;
late DatePickerEntryMode currentMode;
String? cancelText;
String? confirmText;
String? errorFormatText;
String? errorInvalidText;
String? fieldHintText;
String? fieldLabelText;
String? helpText;
TextInputType? keyboardType;
final Finder nextMonthIcon = find.byWidgetPredicate((Widget w) => w is IconButton && (w.tooltip?.startsWith('Next month') ?? false));
final Finder previousMonthIcon = find.byWidgetPredicate((Widget w) => w is IconButton && (w.tooltip?.startsWith('Previous month') ?? false));
final Finder switchToInputIcon = find.byIcon(Icons.edit);
final Finder switchToCalendarIcon = find.byIcon(Icons.calendar_today);
TextField textField(WidgetTester tester) {
return tester.widget<TextField>(find.byType(TextField));
}
setUp(() {
firstDate = DateTime(2001);
lastDate = DateTime(2031, DateTime.december, 31);
initialDate = DateTime(2016, DateTime.january, 15);
today = DateTime(2016, DateTime.january, 3);
selectableDayPredicate = null;
initialEntryMode = DatePickerEntryMode.calendar;
initialCalendarMode = DatePickerMode.day;
cancelText = null;
confirmText = null;
errorFormatText = null;
errorInvalidText = null;
fieldHintText = null;
fieldLabelText = null;
helpText = null;
keyboardType = null;
currentMode = initialEntryMode;
});
const Size wideWindowSize = Size(1920.0, 1080.0);
const Size narrowWindowSize = Size(1070.0, 1770.0);
Future<void> prepareDatePicker(
WidgetTester tester,
Future<void> Function(Future<DateTime?> date) callback, {
TextDirection textDirection = TextDirection.ltr,
bool useMaterial3 = false,
ThemeData? theme,
TextScaler textScaler = TextScaler.noScaling,
}) async {
late BuildContext buttonContext;
await tester.pumpWidget(MaterialApp(
theme: theme ?? ThemeData(useMaterial3: useMaterial3),
home: MediaQuery(
data: MediaQueryData(textScaler: textScaler),
child: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
buttonContext = context;
},
child: const Text('Go'),
);
},
),
),
),
));
await tester.tap(find.text('Go'));
expect(buttonContext, isNotNull);
final Future<DateTime?> date = showDatePicker(
context: buttonContext,
initialDate: initialDate,
firstDate: firstDate,
lastDate: lastDate,
currentDate: today,
selectableDayPredicate: selectableDayPredicate,
initialDatePickerMode: initialCalendarMode,
initialEntryMode: initialEntryMode,
cancelText: cancelText,
confirmText: confirmText,
errorFormatText: errorFormatText,
errorInvalidText: errorInvalidText,
fieldHintText: fieldHintText,
fieldLabelText: fieldLabelText,
helpText: helpText,
keyboardType: keyboardType,
onDatePickerModeChange: (DatePickerEntryMode value) {
currentMode = value;
},
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child ?? const SizedBox(),
);
},
);
await tester.pumpAndSettle(const Duration(seconds: 1));
await callback(date);
}
group('showDatePicker Dialog', () {
testWidgets('Default dialog size', (WidgetTester tester) async {
Future<void> showPicker(WidgetTester tester, Size size) async {
tester.view.physicalSize = size;
tester.view.devicePixelRatio = 1.0;
addTearDown(tester.view.reset);
await prepareDatePicker(tester, (Future<DateTime?> date) async {}, useMaterial3: true);
}
const Size calendarLandscapeDialogSize = Size(496.0, 346.0);
const Size calendarPortraitDialogSizeM3 = Size(328.0, 512.0);
// Test landscape layout.
await showPicker(tester, wideWindowSize);
Size dialogContainerSize = tester.getSize(find.byType(AnimatedContainer));
expect(dialogContainerSize, calendarLandscapeDialogSize);
// Close the dialog.
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// Test portrait layout.
await showPicker(tester, narrowWindowSize);
dialogContainerSize = tester.getSize(find.byType(AnimatedContainer));
expect(dialogContainerSize, calendarPortraitDialogSizeM3);
});
testWidgets('Default dialog properties', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surfaceContainerHigh);
expect(dialogMaterial.shadowColor, Colors.transparent);
expect(dialogMaterial.surfaceTintColor, Colors.transparent);
expect(dialogMaterial.elevation, 6.0);
expect(
dialogMaterial.shape,
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(28.0))),
);
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, const EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0));
}, useMaterial3: theme.useMaterial3);
});
testWidgets('Material3 uses sentence case labels', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.text('Select date'), findsOneWidget);
}, useMaterial3: true);
});
testWidgets('Cancel, confirm, and help text is used', (WidgetTester tester) async {
cancelText = 'nope';
confirmText = 'yep';
helpText = 'help';
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.text(cancelText!), findsOneWidget);
expect(find.text(confirmText!), findsOneWidget);
expect(find.text(helpText!), findsOneWidget);
});
});
testWidgets('Initial date is the default', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('OK'));
expect(await date, DateTime(2016, DateTime.january, 15));
});
});
testWidgets('Can cancel', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('CANCEL'));
expect(await date, isNull);
});
});
testWidgets('Can switch from calendar to input entry mode', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsNothing);
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsOneWidget);
});
});
testWidgets('Can switch from input to calendar entry mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.input;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsOneWidget);
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
});
testWidgets('Can not switch out of calendarOnly mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.calendarOnly;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
});
});
testWidgets('Can not switch out of inputOnly mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.inputOnly;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsOneWidget);
expect(find.byIcon(Icons.calendar_today), findsNothing);
});
});
testWidgets('Switching to input mode keeps selected date', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('12'));
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
await tester.tap(find.text('OK'));
expect(await date, DateTime(2016, DateTime.january, 12));
});
});
testWidgets('Input only mode should validate date', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.inputOnly;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Enter text input mode and type an invalid date to get error.
await tester.enterText(find.byType(TextField), '1234567');
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text('Invalid format.'), findsOneWidget);
});
});
testWidgets('Switching to input mode resets input error state', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Enter text input mode and type an invalid date to get error.
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
await tester.enterText(find.byType(TextField), '1234567');
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text('Invalid format.'), findsOneWidget);
// Toggle to calendar mode and then back to input mode
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
expect(find.text('Invalid format.'), findsNothing);
// Edit the text, the error should not be showing until ok is tapped
await tester.enterText(find.byType(TextField), '1234567');
await tester.pumpAndSettle();
expect(find.text('Invalid format.'), findsNothing);
});
});
testWidgets('builder parameter', (WidgetTester tester) async {
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child ?? const SizedBox(),
);
},
);
},
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
final double ltrOkRight = tester.getBottomRight(find.text('OK')).dx;
await tester.tap(find.text('OK')); // Dismiss the dialog.
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
// Verify that the time picker is being laid out RTL.
// We expect the left edge of the 'OK' button in the RTL
// layout to match the gap between right edge of the 'OK'
// button and the right edge of the 800 wide view.
expect(tester.getBottomLeft(find.text('OK')).dx, moreOrLessEquals(800 - ltrOkRight));
});
group('Barrier dismissible', () {
late _DatePickerObserver rootObserver;
setUp(() {
rootObserver = _DatePickerObserver();
});
testWidgets('Barrier is dismissible with default parameter', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context,
Widget? child) => const SizedBox(),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(rootObserver.datePickerCount, 1);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(rootObserver.datePickerCount, 0);
});
testWidgets('Barrier is not dismissible with barrierDismissible is false', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
barrierDismissible: false,
builder: (BuildContext context,
Widget? child) => const SizedBox(),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(rootObserver.datePickerCount, 1);
// Tap on the barrier, which shouldn't do anything this time.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(rootObserver.datePickerCount, 1);
});
});
testWidgets('Barrier color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context,
Widget? child) => const SizedBox(),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, Colors.black54);
// Dismiss the dialog.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showDatePicker(
context: context,
barrierColor: Colors.pink,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context,
Widget? child) => const SizedBox(),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, Colors.pink);
});
testWidgets('Barrier Label', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showDatePicker(
context: context,
barrierLabel: 'Custom Label',
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context,
Widget? child) => const SizedBox(),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).semanticsLabel, 'Custom Label');
});
testWidgets('uses nested navigator if useRootNavigator is false', (WidgetTester tester) async {
final _DatePickerObserver rootObserver = _DatePickerObserver();
final _DatePickerObserver nestedObserver = _DatePickerObserver();
await tester.pumpWidget(MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<dynamic>(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
showDatePicker(
context: context,
useRootNavigator: false,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
builder: (BuildContext context, Widget? child) => const SizedBox(),
);
},
child: const Text('Show Date Picker'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
expect(rootObserver.datePickerCount, 0);
expect(nestedObserver.datePickerCount, 1);
});
testWidgets('honors DialogTheme for shape and elevation', (WidgetTester tester) async {
// Test that the defaults work
const DialogTheme datePickerDefaultDialogTheme = DialogTheme(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(4.0)),
),
elevation: 24,
);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
);
},
);
},
),
),
),
);
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
final Material defaultDialogMaterial = tester.widget<Material>(find.descendant(
of: find.byType(Dialog), matching: find.byType(Material)).first);
expect(defaultDialogMaterial.shape, datePickerDefaultDialogTheme.shape);
expect(defaultDialogMaterial.elevation, datePickerDefaultDialogTheme.elevation);
// Test that it honors ThemeData.dialogTheme settings
const DialogTheme customDialogTheme = DialogTheme(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(40.0)),
),
elevation: 50,
);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.fallback(useMaterial3: false).copyWith(dialogTheme: customDialogTheme),
home: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
);
},
);
},
),
),
),
);
await tester.pump(); // start theme animation
await tester.pump(const Duration(seconds: 5)); // end theme animation
final Material themeDialogMaterial = tester.widget<Material>(find.descendant(of: find.byType(Dialog), matching: find.byType(Material)).first);
expect(themeDialogMaterial.shape, customDialogTheme.shape);
expect(themeDialogMaterial.elevation, customDialogTheme.elevation);
});
testWidgets('OK Cancel button layout', (WidgetTester tester) async {
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime(2016, DateTime.january, 15),
firstDate:DateTime(2001),
lastDate: DateTime(2031, DateTime.december, 31),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child ?? const SizedBox(),
);
},
);
},
);
},
),
),
),
);
}
// Default landscape layout.
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getBottomRight(find.text('OK')).dx, 622);
expect(tester.getBottomLeft(find.text('OK')).dx, 594);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 560);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getBottomRight(find.text('OK')).dx, 206);
expect(tester.getBottomLeft(find.text('OK')).dx, 178);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 324);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// Portrait layout.
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(900, 1200);
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getBottomRight(find.text('OK')).dx, 258);
expect(tester.getBottomLeft(find.text('OK')).dx, 230);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 196);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getBottomRight(find.text('OK')).dx, 70);
expect(tester.getBottomLeft(find.text('OK')).dx, 42);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 188);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
});
testWidgets('honors switchToInputEntryModeIcon', (WidgetTester tester) async {
Widget buildApp({bool? useMaterial3, Icon? switchToInputEntryModeIcon}) {
return MaterialApp(
theme: ThemeData(
useMaterial3: useMaterial3 ?? false,
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Click X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
switchToInputEntryModeIcon: switchToInputEntryModeIcon,
);
},
);
},
),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.edit), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(buildApp(useMaterial3: true));
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.edit_outlined), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(
buildApp(
switchToInputEntryModeIcon: const Icon(Icons.keyboard),
),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.keyboard), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
});
testWidgets('honors switchToCalendarEntryModeIcon', (WidgetTester tester) async {
Widget buildApp({bool? useMaterial3, Icon? switchToCalendarEntryModeIcon}) {
return MaterialApp(
theme: ThemeData(
useMaterial3: useMaterial3 ?? false,
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Click X'),
onPressed: () {
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
switchToCalendarEntryModeIcon: switchToCalendarEntryModeIcon,
initialEntryMode: DatePickerEntryMode.input,
);
},
);
},
),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.calendar_today), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(buildApp(useMaterial3: true));
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.calendar_today), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
await tester.pumpWidget(
buildApp(
switchToCalendarEntryModeIcon: const Icon(Icons.favorite),
),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.favorite), findsOneWidget);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
});
});
group('Calendar mode', () {
testWidgets('Default Calendar mode layout (Landscape)', (WidgetTester tester) async {
final Finder helpText = find.text('Select date');
final Finder headerText = find.text('Fri, Jan 15');
final Finder subHeaderText = find.text('January 2016');
final Finder cancelButtonText = find.text('Cancel');
final Finder okButtonText = find.text('OK');
const EdgeInsets insetPadding = EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0);
tester.view.physicalSize = wideWindowSize;
addTearDown(tester.view.reset);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: DatePickerDialog(
initialDate: initialDate,
firstDate: firstDate,
lastDate: lastDate,
),
),
));
expect(helpText, findsOneWidget);
expect(headerText, findsOneWidget);
expect(subHeaderText, findsOneWidget);
expect(cancelButtonText, findsOneWidget);
expect(okButtonText, findsOneWidget);
// Test help text position.
final Offset dialogTopLeft = tester.getTopLeft(find.byType(AnimatedContainer));
final Offset helpTextTopLeft = tester.getTopLeft(helpText);
expect(helpTextTopLeft.dx, dialogTopLeft.dx + (insetPadding.horizontal / 2));
expect(helpTextTopLeft.dy, dialogTopLeft.dy + 16.0);
// Test header text position.
final Offset headerTextTopLeft = tester.getTopLeft(headerText);
final Offset helpTextBottomLeft = tester.getBottomLeft(helpText);
expect(headerTextTopLeft.dx, dialogTopLeft.dx + (insetPadding.horizontal / 2));
expect(headerTextTopLeft.dy, helpTextBottomLeft.dy + 16.0);
// Test switch button position.
final Finder switchButtonM3 = find.widgetWithIcon(IconButton, Icons.edit_outlined);
final Offset switchButtonTopLeft = tester.getTopLeft(switchButtonM3);
final Offset headerTextBottomLeft = tester.getBottomLeft(headerText);
expect(switchButtonTopLeft.dx, dialogTopLeft.dx + 4.0);
expect(switchButtonTopLeft.dy, headerTextBottomLeft.dy);
// Test vertical divider position.
final Finder divider = find.byType(VerticalDivider);
final Offset dividerTopLeft = tester.getTopLeft(divider);
final Offset headerTextTopRight = tester.getTopRight(headerText);
expect(dividerTopLeft.dx, headerTextTopRight.dx + 16.0);
expect(dividerTopLeft.dy, dialogTopLeft.dy);
// Test sub header text position.
final Offset subHeaderTextTopLeft = tester.getTopLeft(subHeaderText);
final Offset dividerTopRight = tester.getTopRight(divider);
expect(subHeaderTextTopLeft.dx, dividerTopRight.dx + 24.0);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(subHeaderTextTopLeft.dy, dialogTopLeft.dy + 16.0);
}
// Test sub header icon position.
final Finder subHeaderIcon = find.byIcon(Icons.arrow_drop_down);
final Offset subHeaderIconTopLeft = tester.getTopLeft(subHeaderIcon);
final Offset subHeaderTextTopRight = tester.getTopRight(subHeaderText);
expect(subHeaderIconTopLeft.dx, subHeaderTextTopRight.dx);
expect(subHeaderIconTopLeft.dy, dialogTopLeft.dy + 14.0);
// Test calendar page view position.
final Finder calendarPageView = find.byType(PageView);
final Offset calendarPageViewTopLeft = tester.getTopLeft(calendarPageView);
final Offset subHeaderTextBottomLeft = tester.getBottomLeft(subHeaderText);
expect(calendarPageViewTopLeft.dx, dividerTopRight.dx);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(calendarPageViewTopLeft.dy, subHeaderTextBottomLeft.dy + 16.0);
}
// Test month navigation icons position.
final Finder previousMonthButton = find.widgetWithIcon(IconButton, Icons.chevron_left);
final Finder nextMonthButton = find.widgetWithIcon(IconButton, Icons.chevron_right);
final Offset previousMonthButtonTopRight = tester.getTopRight(previousMonthButton);
final Offset nextMonthButtonTopRight = tester.getTopRight(nextMonthButton);
final Offset dialogTopRight = tester.getTopRight(find.byType(AnimatedContainer));
expect(nextMonthButtonTopRight.dx, dialogTopRight.dx - 4.0);
expect(nextMonthButtonTopRight.dy, dialogTopRight.dy + 2.0);
expect(previousMonthButtonTopRight.dx, nextMonthButtonTopRight.dx - 48.0);
// Test action buttons position.
final Offset dialogBottomRight = tester.getBottomRight(find.byType(AnimatedContainer));
final Offset okButtonTopRight = tester.getTopRight(find.widgetWithText(TextButton, 'OK'));
final Offset cancelButtonTopRight = tester.getTopRight(find.widgetWithText(TextButton, 'Cancel'));
final Offset calendarPageViewBottomRight = tester.getBottomRight(calendarPageView);
expect(okButtonTopRight.dx, dialogBottomRight.dx - 8);
expect(okButtonTopRight.dy, calendarPageViewBottomRight.dy + 2);
final Offset okButtonTopLeft = tester.getTopLeft(find.widgetWithText(TextButton, 'OK'));
expect(cancelButtonTopRight.dx, okButtonTopLeft.dx - 8);
});
testWidgets('Default Calendar mode layout (Portrait)', (WidgetTester tester) async {
final Finder helpText = find.text('Select date');
final Finder headerText = find.text('Fri, Jan 15');
final Finder subHeaderText = find.text('January 2016');
final Finder cancelButtonText = find.text('Cancel');
final Finder okButtonText = find.text('OK');
tester.view.physicalSize = narrowWindowSize;
addTearDown(tester.view.reset);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: DatePickerDialog(
initialDate: initialDate,
firstDate: firstDate,
lastDate: lastDate,
),
),
));
expect(helpText, findsOneWidget);
expect(headerText, findsOneWidget);
expect(subHeaderText, findsOneWidget);
expect(cancelButtonText, findsOneWidget);
expect(okButtonText, findsOneWidget);
// Test help text position.
final Offset dialogTopLeft = tester.getTopLeft(find.byType(AnimatedContainer));
final Offset helpTextTopLeft = tester.getTopLeft(helpText);
expect(helpTextTopLeft.dx, dialogTopLeft.dx + 24.0);
expect(helpTextTopLeft.dy, dialogTopLeft.dy + 16.0);
// Test header text position
final Offset headerTextTextTopLeft = tester.getTopLeft(headerText);
final Offset helpTextBottomLeft = tester.getBottomLeft(helpText);
expect(headerTextTextTopLeft.dx, dialogTopLeft.dx + 24.0);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(headerTextTextTopLeft.dy, helpTextBottomLeft.dy + 28.0);
}
// Test switch button position.
final Finder switchButtonM3 = find.widgetWithIcon(IconButton, Icons.edit_outlined);
final Offset switchButtonTopRight = tester.getTopRight(switchButtonM3);
final Offset dialogTopRight = tester.getTopRight(find.byType(AnimatedContainer));
expect(switchButtonTopRight.dx, dialogTopRight.dx - 12.0);
expect(switchButtonTopRight.dy, headerTextTextTopLeft.dy - 4.0);
// Test horizontal divider position.
final Finder divider = find.byType(Divider);
final Offset dividerTopLeft = tester.getTopLeft(divider);
final Offset headerTextBottomLeft = tester.getBottomLeft(headerText);
expect(dividerTopLeft.dx, dialogTopLeft.dx);
expect(dividerTopLeft.dy, headerTextBottomLeft.dy + 16.0);
// Test subHeaderText position.
final Offset subHeaderTextTopLeft = tester.getTopLeft(subHeaderText);
final Offset dividerBottomLeft = tester.getBottomLeft(divider);
expect(subHeaderTextTopLeft.dx, dialogTopLeft.dx + 24.0);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(subHeaderTextTopLeft.dy, dividerBottomLeft.dy + 16.0);
}
// Test sub header icon position.
final Finder subHeaderIcon = find.byIcon(Icons.arrow_drop_down);
final Offset subHeaderIconTopLeft = tester.getTopLeft(subHeaderIcon);
final Offset subHeaderTextTopRight = tester.getTopRight(subHeaderText);
expect(subHeaderIconTopLeft.dx, subHeaderTextTopRight.dx);
expect(subHeaderIconTopLeft.dy, dividerBottomLeft.dy + 14.0);
// Test month navigation icons position.
final Finder previousMonthButton = find.widgetWithIcon(IconButton, Icons.chevron_left);
final Finder nextMonthButton = find.widgetWithIcon(IconButton, Icons.chevron_right);
final Offset previousMonthButtonTopRight = tester.getTopRight(previousMonthButton);
final Offset nextMonthButtonTopRight = tester.getTopRight(nextMonthButton);
expect(nextMonthButtonTopRight.dx, dialogTopRight.dx - 4.0);
expect(nextMonthButtonTopRight.dy, dividerBottomLeft.dy + 2.0);
expect(previousMonthButtonTopRight.dx, nextMonthButtonTopRight.dx - 48.0);
// Test calendar page view position.
final Finder calendarPageView = find.byType(PageView);
final Offset calendarPageViewTopLeft = tester.getTopLeft(calendarPageView);
final Offset subHeaderTextBottomLeft = tester.getBottomLeft(subHeaderText);
expect(calendarPageViewTopLeft.dx, dialogTopLeft.dx);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(calendarPageViewTopLeft.dy, subHeaderTextBottomLeft.dy + 16.0);
}
// Test action buttons position.
final Offset dialogBottomRight = tester.getBottomRight(find.byType(AnimatedContainer));
final Offset okButtonTopRight = tester.getTopRight(find.widgetWithText(TextButton, 'OK'));
final Offset cancelButtonTopRight = tester.getTopRight(find.widgetWithText(TextButton, 'Cancel'));
final Offset calendarPageViewBottomRight = tester.getBottomRight(calendarPageView);
final Offset okButtonTopLeft = tester.getTopLeft(find.widgetWithText(TextButton, 'OK'));
expect(okButtonTopRight.dx, dialogBottomRight.dx - 8);
expect(okButtonTopRight.dy, calendarPageViewBottomRight.dy + 2);
expect(cancelButtonTopRight.dx, okButtonTopLeft.dx - 8);
});
testWidgets('Can select a day', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('12'));
await tester.tap(find.text('OK'));
expect(await date, equals(DateTime(2016, DateTime.january, 12)));
});
});
testWidgets('Can select a month', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(previousMonthIcon);
await tester.pumpAndSettle(const Duration(seconds: 1));
await tester.tap(find.text('25'));
await tester.tap(find.text('OK'));
expect(await date, DateTime(2015, DateTime.december, 25));
});
});
testWidgets('Can select a year', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('January 2016')); // Switch to year mode.
await tester.pump();
await tester.tap(find.text('2018'));
await tester.pump();
expect(find.text('January 2018'), findsOneWidget);
});
});
testWidgets('Can select a day with no initial date', (WidgetTester tester) async {
initialDate = null;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('12'));
await tester.tap(find.text('OK'));
expect(await date, equals(DateTime(2016, DateTime.january, 12)));
});
});
testWidgets('Can select a month with no initial date', (WidgetTester tester) async {
initialDate = null;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(previousMonthIcon);
await tester.pumpAndSettle(const Duration(seconds: 1));
await tester.tap(find.text('25'));
await tester.tap(find.text('OK'));
expect(await date, DateTime(2015, DateTime.december, 25));
});
});
testWidgets('Can select a year with no initial date', (WidgetTester tester) async {
initialDate = null;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('January 2016')); // Switch to year mode.
await tester.pump();
await tester.tap(find.text('2018'));
await tester.pump();
expect(find.text('January 2018'), findsOneWidget);
});
});
testWidgets('Selecting date does not change displayed month', (WidgetTester tester) async {
initialDate = DateTime(2020, DateTime.march, 15);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(nextMonthIcon);
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text('April 2020'), findsOneWidget);
await tester.tap(find.text('25'));
await tester.pumpAndSettle();
expect(find.text('April 2020'), findsOneWidget);
// There isn't a 31 in April so there shouldn't be one if it is showing April
expect(find.text('31'), findsNothing);
});
});
testWidgets('Changing year does change selected date', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('January 2016'));
await tester.pump();
await tester.tap(find.text('2018'));
await tester.pump();
await tester.tap(find.text('OK'));
expect(await date, equals(DateTime(2018, DateTime.january, 15)));
});
});
testWidgets('Changing year does not change the month', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(nextMonthIcon);
await tester.pumpAndSettle();
await tester.tap(nextMonthIcon);
await tester.pumpAndSettle();
await tester.tap(find.text('March 2016'));
await tester.pumpAndSettle();
await tester.tap(find.text('2018'));
await tester.pumpAndSettle();
expect(find.text('March 2018'), findsOneWidget);
});
});
testWidgets('Can select a year and then a day', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('January 2016')); // Switch to year mode.
await tester.pump();
await tester.tap(find.text('2017'));
await tester.pump();
await tester.tap(find.text('19'));
await tester.tap(find.text('OK'));
expect(await date, DateTime(2017, DateTime.january, 19));
});
});
testWidgets('Current year is visible in year picker', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('January 2016')); // Switch to year mode.
await tester.pump();
expect(find.text('2016'), findsOneWidget);
});
});
testWidgets('Cannot select a day outside bounds', (WidgetTester tester) async {
initialDate = DateTime(2017, DateTime.january, 15);
firstDate = initialDate!;
lastDate = initialDate!;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Earlier than firstDate. Should be ignored.
await tester.tap(find.text('10'));
// Later than lastDate. Should be ignored.
await tester.tap(find.text('20'));
await tester.tap(find.text('OK'));
// We should still be on the initial date.
expect(await date, initialDate);
});
});
testWidgets('Cannot select a month past last date', (WidgetTester tester) async {
initialDate = DateTime(2017, DateTime.january, 15);
firstDate = initialDate!;
lastDate = DateTime(2017, DateTime.february, 20);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(nextMonthIcon);
await tester.pumpAndSettle(const Duration(seconds: 1));
// Shouldn't be possible to keep going into March.
expect(nextMonthIcon, findsNothing);
});
});
testWidgets('Cannot select a month before first date', (WidgetTester tester) async {
initialDate = DateTime(2017, DateTime.january, 15);
firstDate = DateTime(2016, DateTime.december, 10);
lastDate = initialDate!;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(previousMonthIcon);
await tester.pumpAndSettle(const Duration(seconds: 1));
// Shouldn't be possible to keep going into November.
expect(previousMonthIcon, findsNothing);
});
});
testWidgets('Cannot select disabled year', (WidgetTester tester) async {
initialDate = DateTime(2018, DateTime.july, 4);
firstDate = DateTime(2018, DateTime.june, 9);
lastDate = DateTime(2018, DateTime.december, 15);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('July 2018')); // Switch to year mode.
await tester.pumpAndSettle();
await tester.tap(find.text('2016')); // Disabled, doesn't change the year.
await tester.pumpAndSettle();
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(await date, DateTime(2018, DateTime.july, 4));
});
});
testWidgets('Selecting firstDate year respects firstDate', (WidgetTester tester) async {
initialDate = DateTime(2018, DateTime.may, 4);
firstDate = DateTime(2016, DateTime.june, 9);
lastDate = DateTime(2019, DateTime.january, 15);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('May 2018'));
await tester.pumpAndSettle();
await tester.tap(find.text('2016'));
await tester.pumpAndSettle();
// Month should be clamped to June as the range starts at June 2016
expect(find.text('June 2016'), findsOneWidget);
});
});
testWidgets('Selecting lastDate year respects lastDate', (WidgetTester tester) async {
initialDate = DateTime(2018, DateTime.may, 4);
firstDate = DateTime(2016, DateTime.june, 9);
lastDate = DateTime(2019, DateTime.january, 15);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('May 2018'));
await tester.pumpAndSettle();
await tester.tap(find.text('2019'));
await tester.pumpAndSettle();
// Month should be clamped to January as the range ends at January 2019
expect(find.text('January 2019'), findsOneWidget);
});
});
testWidgets('Only predicate days are selectable', (WidgetTester tester) async {
initialDate = DateTime(2017, DateTime.january, 16);
firstDate = DateTime(2017, DateTime.january, 10);
lastDate = DateTime(2017, DateTime.january, 20);
selectableDayPredicate = (DateTime day) => day.day.isEven;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('13')); // Odd, doesn't work.
await tester.tap(find.text('10')); // Even, works.
await tester.tap(find.text('17')); // Odd, doesn't work.
await tester.tap(find.text('OK'));
expect(await date, DateTime(2017, DateTime.january, 10));
});
});
testWidgets('Can select initial calendar picker mode', (WidgetTester tester) async {
initialDate = DateTime(2014, DateTime.january, 15);
initialCalendarMode = DatePickerMode.year;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.pump();
// 2018 wouldn't be available if the year picker wasn't showing.
// The initial current year is 2014.
await tester.tap(find.text('2018'));
await tester.pump();
expect(find.text('January 2018'), findsOneWidget);
});
});
testWidgets('currentDate is highlighted', (WidgetTester tester) async {
today = DateTime(2016, 1, 2);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.pump();
const Color todayColor = Color(0xff2196f3); // default primary color
expect(
Material.of(tester.element(find.text('2'))),
// The current day should be painted with a circle outline
paints..circle(color: todayColor, style: PaintingStyle.stroke, strokeWidth: 1.0),
);
});
});
testWidgets('Date picker dayOverlayColor resolves pressed state', (WidgetTester tester) async {
today = DateTime(2023, 5, 4);
final ThemeData theme = ThemeData();
final bool material3 = theme.useMaterial3;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.pump();
// Hovered.
final Offset center = tester.getCenter(find.text('30'));
final TestGesture gesture = await tester.createGesture(
kind: PointerDeviceKind.mouse,
);
await gesture.addPointer();
await gesture.moveTo(center);
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.text('30'))),
paints..circle(color: material3 ? theme.colorScheme.onSurfaceVariant.withOpacity(0.08) : theme.colorScheme.onSurfaceVariant.withOpacity(0.08)),
);
// Highlighted (pressed).
await gesture.down(center);
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.text('30'))),
paints..circle()..circle(color: material3 ? theme.colorScheme.onSurfaceVariant.withOpacity(0.1) : theme.colorScheme.onSurfaceVariant.withOpacity(0.12))
);
await gesture.up();
await tester.pumpAndSettle();
}, theme: theme);
});
testWidgets('Selecting date does not switch picker to year selection', (WidgetTester tester) async {
initialDate = DateTime(2020, DateTime.may, 10);
initialCalendarMode = DatePickerMode.year;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.pump();
await tester.tap(find.text('2017'));
await tester.pump();
expect(find.text('May 2017'), findsOneWidget);
await tester.tap(find.text('10'));
await tester.pump();
expect(find.text('May 2017'), findsOneWidget);
expect(find.text('2017'), findsNothing);
});
});
});
group('Input mode', () {
setUp(() {
firstDate = DateTime(2015);
lastDate = DateTime(2017, DateTime.december, 31);
initialDate = DateTime(2016, DateTime.january, 15);
initialEntryMode = DatePickerEntryMode.input;
});
testWidgets('Default InputDecoration', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final InputDecoration decoration = tester.widget<TextField>(
find.byType(TextField)).decoration!;
expect(decoration.border, const OutlineInputBorder());
expect(decoration.filled, false);
expect(decoration.hintText, 'mm/dd/yyyy');
expect(decoration.labelText, 'Enter Date');
expect(decoration.errorText, null);
}, useMaterial3: true);
});
testWidgets('Initial entry mode is used', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsOneWidget);
});
});
testWidgets('Hint, label, and help text is used', (WidgetTester tester) async {
cancelText = 'nope';
confirmText = 'yep';
fieldHintText = 'hint';
fieldLabelText = 'label';
helpText = 'help';
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.text(cancelText!), findsOneWidget);
expect(find.text(confirmText!), findsOneWidget);
expect(find.text(fieldHintText!), findsOneWidget);
expect(find.text(fieldLabelText!), findsOneWidget);
expect(find.text(helpText!), findsOneWidget);
});
});
testWidgets('KeyboardType is used', (WidgetTester tester) async {
keyboardType = TextInputType.text;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
expect(field.keyboardType, TextInputType.text);
});
});
testWidgets('Initial date is the default', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('OK'));
expect(await date, DateTime(2016, DateTime.january, 15));
});
});
testWidgets('Can toggle to calendar entry mode', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsOneWidget);
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
});
testWidgets('Toggle to calendar mode keeps selected date', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
await tester.enterText(find.byType(TextField), '12/25/2016');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
await tester.tap(find.text('OK'));
expect(await date, DateTime(2016, DateTime.december, 25));
});
});
testWidgets('Entered text returns date', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
await tester.enterText(find.byType(TextField), '12/25/2016');
await tester.tap(find.text('OK'));
expect(await date, DateTime(2016, DateTime.december, 25));
});
});
testWidgets('Too short entered text shows error', (WidgetTester tester) async {
errorFormatText = 'oops';
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
await tester.pumpAndSettle();
await tester.enterText(find.byType(TextField), '1225');
expect(find.text(errorFormatText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorFormatText!), findsOneWidget);
});
});
testWidgets('Bad format entered text shows error', (WidgetTester tester) async {
errorFormatText = 'oops';
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
await tester.pumpAndSettle();
await tester.enterText(find.byType(TextField), '20 days, 3 months, 2003');
expect(find.text('20 days, 3 months, 2003'), findsOneWidget);
expect(find.text(errorFormatText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorFormatText!), findsOneWidget);
});
});
testWidgets('Invalid entered text shows error', (WidgetTester tester) async {
errorInvalidText = 'oops';
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
await tester.pumpAndSettle();
await tester.enterText(find.byType(TextField), '08/10/1969');
expect(find.text(errorInvalidText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorInvalidText!), findsOneWidget);
});
});
testWidgets('Invalid entered text shows error on autovalidate', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/126397.
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final TextField field = textField(tester);
field.controller!.clear();
// Enter some text to trigger autovalidate.
await tester.enterText(find.byType(TextField), 'xyz');
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// Invalid format validation error should be shown.
expect(find.text('Invalid format.'), findsOneWidget);
// Clear the text.
field.controller!.clear();
// Enter an invalid date that is too long while autovalidate is still on.
await tester.enterText(find.byType(TextField), '10/05/2023666777889');
await tester.pump();
// Invalid format validation error should be shown.
expect(find.text('Invalid format.'), findsOneWidget);
// Should not throw an exception.
expect(tester.takeException(), null);
});
});
// This is a regression test for https://github.com/flutter/flutter/issues/131989.
testWidgets('Dialog contents do not overflow when resized during orientation change',
(WidgetTester tester) async {
addTearDown(tester.view.reset);
// Initial window size is wide for landscape mode.
tester.view.physicalSize = wideWindowSize;
tester.view.devicePixelRatio = 1.0;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Change window size to narrow for portrait mode.
tester.view.physicalSize = narrowWindowSize;
await tester.pump();
expect(tester.takeException(), null);
});
});
// This is a regression test for https://github.com/flutter/flutter/issues/139120.
testWidgets('Dialog contents are visible - textScaler 0.88, 1.0, 2.0',
(WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(400, 800);
tester.view.devicePixelRatio = 1.0;
final List<double> scales = <double>[0.88, 1.0, 2.0];
for (final double scale in scales) {
await tester.pumpWidget(
MaterialApp(
home: MediaQuery(
data: MediaQueryData(textScaler: TextScaler.linear(scale)),
child: Material(
child: DatePickerDialog(
firstDate: DateTime(2001),
lastDate: DateTime(2031, DateTime.december, 31),
initialDate: DateTime(2016, DateTime.january, 15),
initialEntryMode: DatePickerEntryMode.input,
),
),
),
),
);
await tester.pumpAndSettle();
await expectLater(find.byType(Dialog), matchesGoldenFile('date_picker.dialog.contents.visible.$scale.png'));
}
});
});
group('Semantics', () {
testWidgets('calendar mode', (WidgetTester tester) async {
final SemanticsHandle semantics = tester.ensureSemantics();
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Header
expect(tester.getSemantics(find.text('SELECT DATE')), matchesSemantics(
label: 'SELECT DATE\nFri, Jan 15',
));
expect(tester.getSemantics(find.text('3')), matchesSemantics(
label: '3, Sunday, January 3, 2016, Today',
isButton: true,
hasTapAction: true,
isFocusable: true,
));
// Input mode toggle button
expect(tester.getSemantics(switchToInputIcon), matchesSemantics(
tooltip: 'Switch to input',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
// The semantics of the CalendarDatePicker are tested in its tests.
// Ok/Cancel buttons
expect(tester.getSemantics(find.text('OK')), matchesSemantics(
label: 'OK',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
expect(tester.getSemantics(find.text('CANCEL')), matchesSemantics(
label: 'CANCEL',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
});
semantics.dispose();
});
testWidgets('input mode', (WidgetTester tester) async {
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
final MockClipboard mockClipboard = MockClipboard();
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, mockClipboard.handleMethodCall);
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
addTearDown(() => tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, null));
final SemanticsHandle semantics = tester.ensureSemantics();
initialEntryMode = DatePickerEntryMode.input;
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Header
expect(tester.getSemantics(find.text('SELECT DATE')), matchesSemantics(
label: 'SELECT DATE\nFri, Jan 15',
));
// Input mode toggle button
expect(tester.getSemantics(switchToCalendarIcon), matchesSemantics(
tooltip: 'Switch to calendar',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
expect(tester.getSemantics(find.byType(EditableText)), matchesSemantics(
label: 'Enter Date',
isEnabled: true,
hasEnabledState: true,
isTextField: true,
isFocused: true,
value: '01/15/2016',
hasTapAction: true,
hasSetTextAction: true,
hasSetSelectionAction: true,
hasCopyAction: true,
hasCutAction: true,
hasPasteAction: true,
hasMoveCursorBackwardByCharacterAction: true,
hasMoveCursorBackwardByWordAction: true,
));
// Ok/Cancel buttons
expect(tester.getSemantics(find.text('OK')), matchesSemantics(
label: 'OK',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
expect(tester.getSemantics(find.text('CANCEL')), matchesSemantics(
label: 'CANCEL',
isButton: true,
hasTapAction: true,
isEnabled: true,
hasEnabledState: true,
isFocusable: true,
));
});
semantics.dispose();
});
});
group('Keyboard navigation', () {
testWidgets('Can toggle to calendar entry mode', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.byType(TextField), findsNothing);
// Navigate to the entry toggle button and activate it
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should be in the input mode
expect(find.byType(TextField), findsOneWidget);
});
});
testWidgets('Can toggle to year mode', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.text('2016'), findsNothing);
// Navigate to the year selector and activate it
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// The years should be visible
expect(find.text('2016'), findsOneWidget);
});
});
testWidgets('Can navigate next/previous months', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
expect(find.text('January 2016'), findsOneWidget);
// Navigate to the previous month button and activate it twice
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should be showing Nov 2015
expect(find.text('November 2015'), findsOneWidget);
// Navigate to the next month button and activate it four times
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should be on Mar 2016
expect(find.text('March 2016'), findsOneWidget);
});
});
testWidgets('Can navigate date grid with arrow keys', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Navigate from Jan 15 to Jan 18 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Activate it
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 18
expect(await date, DateTime(2016, DateTime.january, 18));
});
});
testWidgets('Navigating with arrow keys scrolls months', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.pumpAndSettle();
// Navigate from Jan 15 to Dec 31 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Should have scrolled to Dec 2015
expect(find.text('December 2015'), findsOneWidget);
// Navigate from Dec 31 to Nov 26 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
// Should have scrolled to Nov 2015
expect(find.text('November 2015'), findsOneWidget);
// Activate it
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.pumpAndSettle();
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 18
expect(await date, DateTime(2015, DateTime.november, 26));
});
});
testWidgets('RTL text direction reverses the horizontal arrow key navigation', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.pumpAndSettle();
// Navigate from Jan 15 to 19 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Activate it
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.pumpAndSettle();
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 18
expect(await date, DateTime(2016, DateTime.january, 19));
}, textDirection: TextDirection.rtl);
});
});
group('Screen configurations', () {
// Test various combinations of screen sizes, orientations and text scales
// to ensure the layout doesn't overflow and cause an exception to be thrown.
// Regression tests for https://github.com/flutter/flutter/issues/21383
// Regression tests for https://github.com/flutter/flutter/issues/19744
// Regression tests for https://github.com/flutter/flutter/issues/17745
// Common screen size roughly based on a Pixel 1
const Size kCommonScreenSizePortrait = Size(1070, 1770);
const Size kCommonScreenSizeLandscape = Size(1770, 1070);
// Small screen size based on a LG K130
const Size kSmallScreenSizePortrait = Size(320, 521);
const Size kSmallScreenSizeLandscape = Size(521, 320);
Future<void> showPicker(WidgetTester tester, Size size, [double textScaleFactor = 1.0]) async {
tester.view.physicalSize = size;
tester.view.devicePixelRatio = 1.0;
addTearDown(tester.view.reset);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.text('OK'));
});
await tester.pumpAndSettle();
}
testWidgets('common screen size - portrait', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizePortrait);
expect(tester.takeException(), isNull);
});
testWidgets('common screen size - landscape', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizeLandscape);
expect(tester.takeException(), isNull);
});
testWidgets('common screen size - portrait - textScale 1.3', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizePortrait, 1.3);
expect(tester.takeException(), isNull);
});
testWidgets('common screen size - landscape - textScale 1.3', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizeLandscape, 1.3);
expect(tester.takeException(), isNull);
});
testWidgets('small screen size - portrait', (WidgetTester tester) async {
await showPicker(tester, kSmallScreenSizePortrait);
expect(tester.takeException(), isNull);
});
testWidgets('small screen size - landscape', (WidgetTester tester) async {
await showPicker(tester, kSmallScreenSizeLandscape);
expect(tester.takeException(), isNull);
});
testWidgets('small screen size - portrait -textScale 1.3', (WidgetTester tester) async {
await showPicker(tester, kSmallScreenSizePortrait, 1.3);
expect(tester.takeException(), isNull);
});
testWidgets('small screen size - landscape - textScale 1.3', (WidgetTester tester) async {
await showPicker(tester, kSmallScreenSizeLandscape, 1.3);
expect(tester.takeException(), isNull);
});
});
group('showDatePicker avoids overlapping display features', () {
testWidgets('positioning with anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(DatePickerDialog)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(DatePickerDialog)), const Offset(800.0, 600.0));
});
testWidgets('positioning with Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
);
await tester.pumpAndSettle();
// By default it should place the dialog on the right screen
expect(tester.getTopLeft(find.byType(DatePickerDialog)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(DatePickerDialog)), const Offset(800.0, 600.0));
});
testWidgets('positioning with defaults', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDatePicker(
context: context,
initialDate: DateTime.now(),
firstDate: DateTime(2018),
lastDate: DateTime(2030),
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(DatePickerDialog)), Offset.zero);
expect(tester.getBottomRight(find.byType(DatePickerDialog)), const Offset(390.0, 600.0));
});
});
testWidgets('DatePickerDialog is state restorable', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
restorationScopeId: 'app',
home: _RestorableDatePickerDialogTestWidget(),
),
);
// The date picker should be closed.
expect(find.byType(DatePickerDialog), findsNothing);
expect(find.text('25/7/2021'), findsOneWidget);
// Open the date picker.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(DatePickerDialog), findsOneWidget);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// The date picker should be open after restoring.
expect(find.byType(DatePickerDialog), findsOneWidget);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
// The date picker should be closed, the text value updated to the
// newly selected date.
expect(find.byType(DatePickerDialog), findsNothing);
expect(find.text('25/7/2021'), findsOneWidget);
// The date picker should be open after restoring.
await tester.restoreFrom(restorationData);
expect(find.byType(DatePickerDialog), findsOneWidget);
// Select a different date.
await tester.tap(find.text('30'));
await tester.pumpAndSettle();
// Restart after the new selection. It should remain selected.
await tester.restartAndRestore();
// Close the date picker.
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// The date picker should be closed, the text value updated to the
// newly selected date.
expect(find.byType(DatePickerDialog), findsNothing);
expect(find.text('30/7/2021'), findsOneWidget);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('DatePickerDialog state restoration - DatePickerEntryMode', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
restorationScopeId: 'app',
home: _RestorableDatePickerDialogTestWidget(
datePickerEntryMode: DatePickerEntryMode.calendarOnly,
),
),
);
// The date picker should be closed.
expect(find.byType(DatePickerDialog), findsNothing);
expect(find.text('25/7/2021'), findsOneWidget);
// Open the date picker.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(DatePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// The date picker should be open after restoring.
expect(find.byType(DatePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
// The date picker should be closed, the text value should be the same
// as before.
expect(find.byType(DatePickerDialog), findsNothing);
expect(find.text('25/7/2021'), findsOneWidget);
// The date picker should be open after restoring.
await tester.restoreFrom(restorationData);
expect(find.byType(DatePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('Test Callback on Toggle of DatePicker Mode', (WidgetTester tester) async {
prepareDatePicker(tester, (Future<DateTime?> date) async {
await tester.tap(find.byIcon(Icons.edit));
expect(currentMode, DatePickerEntryMode.input);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsOneWidget);
await tester.tap(find.byIcon(Icons.calendar_today));
expect(currentMode, DatePickerEntryMode.calendar);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
});
group('Landscape input-only date picker headers use headlineSmall', () {
// Regression test for https://github.com/flutter/flutter/issues/122056
// Common screen size roughly based on a Pixel 1
const Size kCommonScreenSizePortrait = Size(1070, 1770);
const Size kCommonScreenSizeLandscape = Size(1770, 1070);
Future<void> showPicker(WidgetTester tester, Size size) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = size;
tester.view.devicePixelRatio = 1.0;
initialEntryMode = DatePickerEntryMode.input;
await prepareDatePicker(tester, (Future<DateTime?> date) async { }, useMaterial3: true);
}
testWidgets('portrait', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizePortrait);
expect(tester.widget<Text>(find.text('Fri, Jan 15')).style?.fontSize, 32);
await tester.tap(find.text('Cancel'));
await tester.pumpAndSettle();
});
testWidgets('landscape', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizeLandscape);
expect(tester.widget<Text>(find.text('Fri, Jan 15')).style?.fontSize, 24);
await tester.tap(find.text('Cancel'));
await tester.pumpAndSettle();
});
});
group('Material 2', () {
// These tests are only relevant for Material 2. Once Material 2
// support is deprecated and the APIs are removed, these tests
// can be deleted.
group('showDatePicker Dialog', () {
testWidgets('Default dialog size', (WidgetTester tester) async {
Future<void> showPicker(WidgetTester tester, Size size) async {
tester.view.physicalSize = size;
tester.view.devicePixelRatio = 1.0;
addTearDown(tester.view.reset);
await prepareDatePicker(tester, (Future<DateTime?> date) async {});
}
const Size wideWindowSize = Size(1920.0, 1080.0);
const Size narrowWindowSize = Size(1070.0, 1770.0);
const Size calendarLandscapeDialogSize = Size(496.0, 346.0);
const Size calendarPortraitDialogSizeM2 = Size(330.0, 518.0);
// Test landscape layout.
await showPicker(tester, wideWindowSize);
Size dialogContainerSize = tester.getSize(find.byType(AnimatedContainer));
expect(dialogContainerSize, calendarLandscapeDialogSize);
// Close the dialog.
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
// Test portrait layout.
await showPicker(tester, narrowWindowSize);
dialogContainerSize = tester.getSize(find.byType(AnimatedContainer));
expect(dialogContainerSize, calendarPortraitDialogSizeM2);
});
testWidgets('Default dialog properties', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surface);
expect(dialogMaterial.shadowColor, theme.shadowColor);
expect(dialogMaterial.elevation, 24.0);
expect(
dialogMaterial.shape,
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))),
);
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, const EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0));
}, useMaterial3: theme.useMaterial3);
});
});
group('Input mode', () {
setUp(() {
firstDate = DateTime(2015);
lastDate = DateTime(2017, DateTime.december, 31);
initialDate = DateTime(2016, DateTime.january, 15);
initialEntryMode = DatePickerEntryMode.input;
});
testWidgets('Default InputDecoration', (WidgetTester tester) async {
await prepareDatePicker(tester, (Future<DateTime?> date) async {
final InputDecoration decoration = tester.widget<TextField>(
find.byType(TextField)).decoration!;
expect(decoration.border, const UnderlineInputBorder());
expect(decoration.filled, false);
expect(decoration.hintText, 'mm/dd/yyyy');
expect(decoration.labelText, 'Enter Date');
expect(decoration.errorText, null);
});
});
});
});
}
class _RestorableDatePickerDialogTestWidget extends StatefulWidget {
const _RestorableDatePickerDialogTestWidget({
this.datePickerEntryMode = DatePickerEntryMode.calendar,
});
final DatePickerEntryMode datePickerEntryMode;
@override
_RestorableDatePickerDialogTestWidgetState createState() => _RestorableDatePickerDialogTestWidgetState();
}
class _RestorableDatePickerDialogTestWidgetState extends State<_RestorableDatePickerDialogTestWidget> with RestorationMixin {
@override
String? get restorationId => 'scaffold_state';
final RestorableDateTime _selectedDate = RestorableDateTime(DateTime(2021, 7, 25));
late final RestorableRouteFuture<DateTime?> _restorableDatePickerRouteFuture = RestorableRouteFuture<DateTime?>(
onComplete: _selectDate,
onPresent: (NavigatorState navigator, Object? arguments) {
return navigator.restorablePush(
_datePickerRoute,
arguments: <String, dynamic>{
'selectedDate': _selectedDate.value.millisecondsSinceEpoch,
'datePickerEntryMode': widget.datePickerEntryMode.index,
},
);
},
);
@override
void dispose() {
_selectedDate.dispose();
_restorableDatePickerRouteFuture.dispose();
super.dispose();
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_selectedDate, 'selected_date');
registerForRestoration(_restorableDatePickerRouteFuture, 'date_picker_route_future');
}
void _selectDate(DateTime? newSelectedDate) {
if (newSelectedDate != null) {
setState(() { _selectedDate.value = newSelectedDate; });
}
}
@pragma('vm:entry-point')
static Route<DateTime> _datePickerRoute(
BuildContext context,
Object? arguments,
) {
return DialogRoute<DateTime>(
context: context,
builder: (BuildContext context) {
final Map<dynamic, dynamic> args = arguments! as Map<dynamic, dynamic>;
return DatePickerDialog(
restorationId: 'date_picker_dialog',
initialEntryMode: DatePickerEntryMode.values[args['datePickerEntryMode'] as int],
initialDate: DateTime.fromMillisecondsSinceEpoch(args['selectedDate'] as int),
firstDate: DateTime(2021),
lastDate: DateTime(2022),
);
},
);
}
@override
Widget build(BuildContext context) {
final DateTime selectedDateTime = _selectedDate.value;
// Example: "25/7/1994"
final String selectedDateTimeString = '${selectedDateTime.day}/${selectedDateTime.month}/${selectedDateTime.year}';
return Scaffold(
body: Center(
child: Column(
children: <Widget>[
OutlinedButton(
onPressed: () {
_restorableDatePickerRouteFuture.present();
},
child: const Text('X'),
),
Text(selectedDateTimeString),
],
),
),
);
}
}
class _DatePickerObserver extends NavigatorObserver {
int datePickerCount = 0;
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is DialogRoute) {
datePickerCount++;
}
super.didPush(route, previousRoute);
}
@override
void didPop(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is DialogRoute) {
datePickerCount--;
}
super.didPop(route, previousRoute);
}
}
| flutter/packages/flutter/test/material/date_picker_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/date_picker_test.dart",
"repo_id": "flutter",
"token_count": 39204
} | 717 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// no-shuffle:
// //TODO(gspencergoog): Remove this tag once this test's state leaks/test
// dependencies have been fixed.
// https://github.com/flutter/flutter/issues/85160
// Fails with "flutter test --test-randomize-ordering-seed=456"
// reduced-test-set:
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set', 'no-shuffle'])
library;
import 'dart:math' as math;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
import 'feedback_tester.dart';
const List<String> menuItems = <String>['one', 'two', 'three', 'four'];
void onChanged<T>(T _) { }
final Type dropdownButtonType = DropdownButton<String>(
onChanged: (_) { },
items: const <DropdownMenuItem<String>>[],
).runtimeType;
Finder _iconRichText(Key iconKey) {
return find.descendant(
of: find.byKey(iconKey),
matching: find.byType(RichText),
);
}
Widget buildDropdown({
required bool isFormField,
Key? buttonKey,
String? value = 'two',
ValueChanged<String?>? onChanged,
VoidCallback? onTap,
Widget? icon,
Color? iconDisabledColor,
Color? iconEnabledColor,
double iconSize = 24.0,
bool isDense = false,
bool isExpanded = false,
Widget? hint,
Widget? disabledHint,
Widget? underline,
List<String>? items = menuItems,
List<Widget> Function(BuildContext)? selectedItemBuilder,
double? itemHeight = kMinInteractiveDimension,
AlignmentDirectional alignment = AlignmentDirectional.centerStart,
TextDirection textDirection = TextDirection.ltr,
Size? mediaSize,
FocusNode? focusNode,
bool autofocus = false,
Color? focusColor,
Color? dropdownColor,
double? menuMaxHeight,
EdgeInsetsGeometry? padding,
}) {
final List<DropdownMenuItem<String>>? listItems = items?.map<DropdownMenuItem<String>>((String item) {
return DropdownMenuItem<String>(
key: ValueKey<String>(item),
value: item,
child: Text(item, key: ValueKey<String>('${item}Text')),
);
}).toList();
if (isFormField) {
return Form(
child: DropdownButtonFormField<String>(
key: buttonKey,
value: value,
hint: hint,
disabledHint: disabledHint,
onChanged: onChanged,
onTap: onTap,
icon: icon,
iconSize: iconSize,
iconDisabledColor: iconDisabledColor,
iconEnabledColor: iconEnabledColor,
isDense: isDense,
isExpanded: isExpanded,
// No underline attribute
focusNode: focusNode,
autofocus: autofocus,
focusColor: focusColor,
dropdownColor: dropdownColor,
items: listItems,
selectedItemBuilder: selectedItemBuilder,
itemHeight: itemHeight,
alignment: alignment,
menuMaxHeight: menuMaxHeight,
padding: padding,
),
);
}
return DropdownButton<String>(
key: buttonKey,
value: value,
hint: hint,
disabledHint: disabledHint,
onChanged: onChanged,
onTap: onTap,
icon: icon,
iconSize: iconSize,
iconDisabledColor: iconDisabledColor,
iconEnabledColor: iconEnabledColor,
isDense: isDense,
isExpanded: isExpanded,
underline: underline,
focusNode: focusNode,
autofocus: autofocus,
focusColor: focusColor,
dropdownColor: dropdownColor,
items: listItems,
selectedItemBuilder: selectedItemBuilder,
itemHeight: itemHeight,
alignment: alignment,
menuMaxHeight: menuMaxHeight,
padding: padding,
);
}
Widget buildFrame({
Key? buttonKey,
String? value = 'two',
ValueChanged<String?>? onChanged,
VoidCallback? onTap,
Widget? icon,
Color? iconDisabledColor,
Color? iconEnabledColor,
double iconSize = 24.0,
bool isDense = false,
bool isExpanded = false,
Widget? hint,
Widget? disabledHint,
Widget? underline,
List<String>? items = menuItems,
List<Widget> Function(BuildContext)? selectedItemBuilder,
double? itemHeight = kMinInteractiveDimension,
AlignmentDirectional alignment = AlignmentDirectional.centerStart,
TextDirection textDirection = TextDirection.ltr,
Size? mediaSize,
FocusNode? focusNode,
bool autofocus = false,
Color? focusColor,
Color? dropdownColor,
bool isFormField = false,
double? menuMaxHeight,
EdgeInsetsGeometry? padding,
Alignment dropdownAlignment = Alignment.center,
bool? useMaterial3,
}) {
return Theme(
data: ThemeData(useMaterial3: useMaterial3),
child: TestApp(
textDirection: textDirection,
mediaSize: mediaSize,
child: Material(
child: Align(
alignment: dropdownAlignment,
child: RepaintBoundary(
child: buildDropdown(
isFormField: isFormField,
buttonKey: buttonKey,
value: value,
hint: hint,
disabledHint: disabledHint,
onChanged: onChanged,
onTap: onTap,
icon: icon,
iconSize: iconSize,
iconDisabledColor: iconDisabledColor,
iconEnabledColor: iconEnabledColor,
isDense: isDense,
isExpanded: isExpanded,
underline: underline,
focusNode: focusNode,
autofocus: autofocus,
focusColor: focusColor,
dropdownColor: dropdownColor,
items: items,
selectedItemBuilder: selectedItemBuilder,
itemHeight: itemHeight,
alignment: alignment,
menuMaxHeight: menuMaxHeight,
padding: padding,
),
),
),
),
),
);
}
Widget buildDropdownWithHint({
required AlignmentDirectional alignment,
required bool isExpanded,
bool enableSelectedItemBuilder = false,
}){
return buildFrame(
useMaterial3: false,
mediaSize: const Size(800, 600),
itemHeight: 100.0,
alignment: alignment,
isExpanded: isExpanded,
selectedItemBuilder: enableSelectedItemBuilder
? (BuildContext context) {
return menuItems.map<Widget>((String item) {
return ColoredBox(
color: const Color(0xff00ff00),
child: Text(item),
);
}).toList();
}
: null,
hint: const Text('hint'),
);
}
class TestApp extends StatefulWidget {
const TestApp({
super.key,
required this.textDirection,
required this.child,
this.mediaSize,
});
final TextDirection textDirection;
final Widget child;
final Size? mediaSize;
@override
State<TestApp> createState() => _TestAppState();
}
class _TestAppState extends State<TestApp> {
@override
Widget build(BuildContext context) {
return Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
child: MediaQuery(
data: MediaQueryData.fromView(View.of(context)).copyWith(size: widget.mediaSize),
child: Directionality(
textDirection: widget.textDirection,
child: Navigator(
onGenerateRoute: (RouteSettings settings) {
assert(settings.name == '/');
return MaterialPageRoute<void>(
settings: settings,
builder: (BuildContext context) => widget.child,
);
},
),
),
),
);
}
}
// When the dropdown's menu is popped up, a RenderParagraph for the selected
// menu's text item will appear both in the dropdown button and in the menu.
// The RenderParagraphs should be aligned, i.e. they should have the same
// size and location.
void checkSelectedItemTextGeometry(WidgetTester tester, String value) {
final List<RenderBox> boxes = tester.renderObjectList<RenderBox>(find.byKey(ValueKey<String>('${value}Text'))).toList();
expect(boxes.length, equals(2));
final RenderBox box0 = boxes[0];
final RenderBox box1 = boxes[1];
expect(box0.localToGlobal(Offset.zero), equals(box1.localToGlobal(Offset.zero)));
expect(box0.size, equals(box1.size));
}
Future<void> checkDropdownColor(WidgetTester tester, {Color? color, bool isFormField = false }) async {
const String text = 'foo';
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: isFormField
? Form(
child: DropdownButtonFormField<String>(
dropdownColor: color,
value: text,
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
value: text,
child: Text(text),
),
],
onChanged: (_) {},
),
)
: DropdownButton<String>(
dropdownColor: color,
value: text,
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
value: text,
child: Text(text),
),
],
onChanged: (_) {},
),
),
),
);
await tester.tap(find.text(text));
await tester.pump();
expect(
find.ancestor(
of: find.text(text).last,
matching: find.byType(CustomPaint),
).at(2),
paints
..save()
..rrect()
..rrect()
..rrect()
..rrect(color: color ?? Colors.grey[50], hasMaskFilter: false),
);
}
void main() {
testWidgets('Default dropdown golden', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build() => buildFrame(buttonKey: buttonKey, onChanged: onChanged, useMaterial3: false);
await tester.pumpWidget(build());
final Finder buttonFinder = find.byKey(buttonKey);
assert(tester.renderObject(buttonFinder).attached);
await expectLater(
find.ancestor(of: buttonFinder, matching: find.byType(RepaintBoundary)).first,
matchesGoldenFile('dropdown_test.default.png'),
);
});
testWidgets('Expanded dropdown golden', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build() => buildFrame(buttonKey: buttonKey, isExpanded: true, onChanged: onChanged, useMaterial3: false);
await tester.pumpWidget(build());
final Finder buttonFinder = find.byKey(buttonKey);
assert(tester.renderObject(buttonFinder).attached);
await expectLater(
find.ancestor(of: buttonFinder, matching: find.byType(RepaintBoundary)).first,
matchesGoldenFile('dropdown_test.expanded.png'),
);
});
testWidgets('Dropdown button control test', (WidgetTester tester) async {
String? value = 'one';
void didChangeValue(String? newValue) {
value = newValue;
}
Widget build() => buildFrame(value: value, onChanged: didChangeValue);
await tester.pumpWidget(build());
await tester.tap(find.text('one'));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('one'));
await tester.tap(find.text('three').last);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('three'));
await tester.tap(find.text('three', skipOffstage: false), warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('three'));
await tester.pumpWidget(build());
await tester.tap(find.text('two').last);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('two'));
});
testWidgets('Dropdown button with no app', (WidgetTester tester) async {
String? value = 'one';
void didChangeValue(String? newValue) {
value = newValue;
}
Widget build() {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData.fromView(tester.view),
child: Navigator(
initialRoute: '/',
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return Material(
child: buildFrame(value: 'one', onChanged: didChangeValue),
);
},
);
},
),
),
);
}
await tester.pumpWidget(build());
await tester.tap(find.text('one'));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('one'));
await tester.tap(find.text('three').last);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('three'));
await tester.tap(find.text('three', skipOffstage: false), warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('three'));
await tester.pumpWidget(build());
await tester.tap(find.text('two').last);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('two'));
});
testWidgets('DropdownButton does not allow duplicate item values', (WidgetTester tester) async {
final List<DropdownMenuItem<String>> itemsWithDuplicateValues = <String>['a', 'b', 'c', 'c']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList();
await expectLater(
() => tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
value: 'c',
onChanged: (String? newValue) {},
items: itemsWithDuplicateValues,
),
),
),
),
throwsA(isAssertionError.having(
(AssertionError error) => error.toString(),
'.toString()',
contains("There should be exactly one item with [DropdownButton]'s value"),
)),
);
});
testWidgets('DropdownButton value should only appear in one menu item', (WidgetTester tester) async {
final List<DropdownMenuItem<String>> itemsWithDuplicateValues = <String>['a', 'b', 'c', 'd']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList();
await expectLater(
() => tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
value: 'e',
onChanged: (String? newValue) {},
items: itemsWithDuplicateValues,
),
),
),
),
throwsA(isAssertionError.having(
(AssertionError error) => error.toString(),
'.toString()',
contains("There should be exactly one item with [DropdownButton]'s value"),
)),
);
});
testWidgets('Dropdown form field uses form field state', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
String? value;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MaterialApp(
home: Material(
child: Form(
key: formKey,
child: DropdownButtonFormField<String>(
key: buttonKey,
value: value,
hint: const Text('Select Value'),
decoration: const InputDecoration(
prefixIcon: Icon(Icons.fastfood),
),
items: menuItems.map((String val) {
return DropdownMenuItem<String>(
value: val,
child: Text(val),
);
}).toList(),
validator: (String? v) => v == null ? 'Must select value' : null,
onChanged: (String? newValue) {},
onSaved: (String? v) {
setState(() {
value = v;
});
},
),
),
),
);
},
),
);
int getIndex() {
final IndexedStack stack = tester.element(find.byType(IndexedStack)).widget as IndexedStack;
return stack.index!;
}
// Initial value of null displays hint
expect(value, equals(null));
expect(getIndex(), 4);
await tester.tap(find.text('Select Value', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
await tester.tap(find.text('three').last);
await tester.pumpAndSettle();
expect(getIndex(), 2);
// Changes only made to FormField state until form saved
expect(value, equals(null));
final FormState form = formKey.currentState!;
form.save();
expect(value, equals('three'));
});
testWidgets('Dropdown in ListView', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/12053
// Positions a DropdownButton at the left and right edges of the screen,
// forcing it to be sized down to the viewport width
const String value = 'foo';
final UniqueKey itemKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Material(
child: ListView(
children: <Widget>[
DropdownButton<String>(
value: value,
items: <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
key: itemKey,
value: value,
child: const Text(value),
),
],
onChanged: (_) { },
),
],
),
),
),
);
await tester.tap(find.text(value));
await tester.pump();
final List<RenderBox> itemBoxes = tester.renderObjectList<RenderBox>(find.byKey(itemKey)).toList();
expect(itemBoxes[0].localToGlobal(Offset.zero).dx, equals(0.0));
expect(itemBoxes[1].localToGlobal(Offset.zero).dx, equals(16.0));
expect(itemBoxes[1].size.width, equals(800.0 - 16.0 * 2));
});
testWidgets('Dropdown menu can position correctly inside a nested navigator', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/66870
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
appBar: AppBar(),
body: Column(
children: <Widget>[
ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 500, maxHeight: 200),
child: Navigator(
onGenerateRoute: (RouteSettings s) {
return MaterialPageRoute<void>(builder: (BuildContext context) {
return Center(
child: DropdownButton<int>(
value: 1,
items: const <DropdownMenuItem<int>>[
DropdownMenuItem<int>(
value: 1,
child: Text('First Item'),
),
DropdownMenuItem<int>(
value: 2,
child: Text('Second Item'),
),
],
onChanged: (_) { },
),
);
});
},
),
),
],
),
),
),
);
await tester.tap(find.text('First Item'));
await tester.pump();
final RenderBox secondItem = tester.renderObjectList<RenderBox>(find.text('Second Item', skipOffstage: false)).toList()[1];
expect(secondItem.localToGlobal(Offset.zero).dx, equals(150.0));
expect(secondItem.localToGlobal(Offset.zero).dy, equals(176.0));
});
testWidgets('Dropdown screen edges', (WidgetTester tester) async {
int? value = 4;
final List<DropdownMenuItem<int>> items = <DropdownMenuItem<int>>[
for (int i = 0; i < 20; ++i) DropdownMenuItem<int>(value: i, child: Text('$i')),
];
void handleChanged(int? newValue) {
value = newValue;
}
final DropdownButton<int> button = DropdownButton<int>(
value: value,
onChanged: handleChanged,
items: items,
);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
alignment: Alignment.topCenter,
child: button,
),
),
),
);
await tester.tap(find.text('4'));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
// We should have two copies of item 5, one in the menu and one in the
// button itself.
expect(tester.elementList(find.text('5', skipOffstage: false)), hasLength(2));
expect(value, 4);
await tester.tap(find.byWidget(button, skipOffstage: false), warnIfMissed: false);
expect(value, 4);
// this waits for the route's completer to complete, which calls handleChanged
await tester.idle();
expect(value, 4);
});
for (final TextDirection textDirection in TextDirection.values) {
testWidgets('Dropdown button aligns selected menu item ($textDirection)', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build() => buildFrame(buttonKey: buttonKey, textDirection: textDirection, onChanged: onChanged, useMaterial3: false);
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
final Offset buttonOriginBeforeTap = buttonBox.localToGlobal(Offset.zero);
await tester.tap(find.text('two'));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
// Tapping the dropdown button should not cause it to move.
expect(buttonBox.localToGlobal(Offset.zero), equals(buttonOriginBeforeTap));
// The selected dropdown item is both in menu we just popped up, and in
// the IndexedStack contained by the dropdown button. Both of them should
// have the same origin and height as the dropdown button.
final List<RenderBox> itemBoxes = tester.renderObjectList<RenderBox>(find.byKey(const ValueKey<String>('two'))).toList();
expect(itemBoxes.length, equals(2));
for (final RenderBox itemBox in itemBoxes) {
assert(itemBox.attached);
switch (textDirection) {
case TextDirection.rtl:
expect(
buttonBox.localToGlobal(buttonBox.size.bottomRight(Offset.zero)),
equals(itemBox.localToGlobal(itemBox.size.bottomRight(Offset.zero))),
);
case TextDirection.ltr:
expect(buttonBox.localToGlobal(Offset.zero), equals(itemBox.localToGlobal(Offset.zero)));
}
expect(buttonBox.size.height, equals(itemBox.size.height));
}
// The two RenderParagraph objects, for the 'two' items' Text children,
// should have the same size and location.
checkSelectedItemTextGeometry(tester, 'two');
await tester.pumpWidget(Container()); // reset test
});
}
testWidgets('Arrow icon aligns with the edge of button when expanded', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build() => buildFrame(buttonKey: buttonKey, isExpanded: true, onChanged: onChanged);
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
final RenderBox arrowIcon = tester.renderObject<RenderBox>(find.byIcon(Icons.arrow_drop_down));
assert(arrowIcon.attached);
// Arrow icon should be aligned with far right of button when expanded
expect(
arrowIcon.localToGlobal(Offset.zero).dx,
buttonBox.size.centerRight(Offset(-arrowIcon.size.width, 0.0)).dx,
);
});
testWidgets('Dropdown button icon will accept widgets as icons', (WidgetTester tester) async {
final Widget customWidget = Container(
decoration: ShapeDecoration(
shape: CircleBorder(
side: BorderSide(
width: 5.0,
color: Colors.grey.shade700,
),
),
),
);
await tester.pumpWidget(buildFrame(
icon: customWidget,
onChanged: onChanged,
));
expect(find.byWidget(customWidget), findsOneWidget);
expect(find.byIcon(Icons.arrow_drop_down), findsNothing);
await tester.pumpWidget(buildFrame(
icon: const Icon(Icons.assessment),
onChanged: onChanged,
));
expect(find.byIcon(Icons.assessment), findsOneWidget);
expect(find.byIcon(Icons.arrow_drop_down), findsNothing);
});
testWidgets('Dropdown button icon should have default size and colors when not defined', (WidgetTester tester) async {
final Key iconKey = UniqueKey();
final Icon customIcon = Icon(Icons.assessment, key: iconKey);
await tester.pumpWidget(buildFrame(
icon: customIcon,
onChanged: onChanged,
));
// test for size
final RenderBox icon = tester.renderObject(find.byKey(iconKey));
expect(icon.size, const Size(24.0, 24.0));
// test for enabled color
final RichText enabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(enabledRichText.text.style!.color, Colors.grey.shade700);
// test for disabled color
await tester.pumpWidget(buildFrame(
icon: customIcon,
));
final RichText disabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(disabledRichText.text.style!.color, Colors.grey.shade400);
});
testWidgets('Dropdown button icon should have the passed in size and color instead of defaults', (WidgetTester tester) async {
final Key iconKey = UniqueKey();
final Icon customIcon = Icon(Icons.assessment, key: iconKey);
await tester.pumpWidget(buildFrame(
icon: customIcon,
iconSize: 30.0,
iconEnabledColor: Colors.pink,
iconDisabledColor: Colors.orange,
onChanged: onChanged,
));
// test for size
final RenderBox icon = tester.renderObject(find.byKey(iconKey));
expect(icon.size, const Size(30.0, 30.0));
// test for enabled color
final RichText enabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(enabledRichText.text.style!.color, Colors.pink);
// test for disabled color
await tester.pumpWidget(buildFrame(
icon: customIcon,
iconSize: 30.0,
iconEnabledColor: Colors.pink,
iconDisabledColor: Colors.orange,
));
final RichText disabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(disabledRichText.text.style!.color, Colors.orange);
});
testWidgets('Dropdown button should use its own size and color properties over those defined by the theme', (WidgetTester tester) async {
final Key iconKey = UniqueKey();
final Icon customIcon = Icon(
Icons.assessment,
key: iconKey,
size: 40.0,
color: Colors.yellow,
);
await tester.pumpWidget(buildFrame(
icon: customIcon,
iconSize: 30.0,
iconEnabledColor: Colors.pink,
iconDisabledColor: Colors.orange,
onChanged: onChanged,
));
// test for size
final RenderBox icon = tester.renderObject(find.byKey(iconKey));
expect(icon.size, const Size(40.0, 40.0));
// test for enabled color
final RichText enabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(enabledRichText.text.style!.color, Colors.yellow);
// test for disabled color
await tester.pumpWidget(buildFrame(
icon: customIcon,
iconSize: 30.0,
iconEnabledColor: Colors.pink,
iconDisabledColor: Colors.orange,
));
final RichText disabledRichText = tester.widget<RichText>(_iconRichText(iconKey));
expect(disabledRichText.text.style!.color, Colors.yellow);
});
testWidgets('Dropdown button with isDense:true aligns selected menu item', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build() => buildFrame(buttonKey: buttonKey, isDense: true, onChanged: onChanged);
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
await tester.tap(find.text('two'));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
// The selected dropdown item is both in menu we just popped up, and in
// the IndexedStack contained by the dropdown button. Both of them should
// have the same vertical center as the button.
final List<RenderBox> itemBoxes = tester.renderObjectList<RenderBox>(find.byKey(const ValueKey<String>('two'))).toList();
expect(itemBoxes.length, equals(2));
// When isDense is true, the button's height is reduced. The menu items'
// heights are not.
final double menuItemHeight = itemBoxes.map<double>((RenderBox box) => box.size.height).reduce(math.max);
expect(menuItemHeight, greaterThan(buttonBox.size.height));
for (final RenderBox itemBox in itemBoxes) {
assert(itemBox.attached);
final Offset buttonBoxCenter = buttonBox.size.center(buttonBox.localToGlobal(Offset.zero));
final Offset itemBoxCenter = itemBox.size.center(itemBox.localToGlobal(Offset.zero));
expect(buttonBoxCenter.dy, equals(itemBoxCenter.dy));
}
// The two RenderParagraph objects, for the 'two' items' Text children,
// should have the same size and location.
checkSelectedItemTextGeometry(tester, 'two');
});
testWidgets('Dropdown button can have a text style with no fontSize specified', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/33425
const String value = 'foo';
final UniqueKey itemKey = UniqueKey();
await tester.pumpWidget(TestApp(
textDirection: TextDirection.ltr,
child: Material(
child: DropdownButton<String>(
value: value,
items: <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
key: itemKey,
value: 'foo',
child: const Text(value),
),
],
isDense: true,
onChanged: (_) { },
style: const TextStyle(color: Colors.blue),
),
),
));
expect(tester.takeException(), isNull);
});
testWidgets('Dropdown menu scrolls to first item in long lists', (WidgetTester tester) async {
// Open the dropdown menu
final Key buttonKey = UniqueKey();
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
value: null, // nothing selected
items: List<String>.generate(/*length=*/ 100, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.byKey(buttonKey));
await tester.pump();
await tester.pumpAndSettle(); // finish the menu animation
// Find the first item in the scrollable dropdown list
final Finder menuItemFinder = find.byType(Scrollable);
final RenderBox menuItemContainer = tester.renderObject<RenderBox>(menuItemFinder);
final RenderBox firstItem = tester.renderObject<RenderBox>(
find.descendant(of: menuItemFinder, matching: find.byKey(const ValueKey<String>('0'))),
);
// List should be scrolled so that the first item is at the top. Menu items
// are offset 8.0 from the top edge of the scrollable menu.
const Offset selectedItemOffset = Offset(0.0, -8.0);
expect(
firstItem.size.topCenter(firstItem.localToGlobal(selectedItemOffset)).dy,
equals(menuItemContainer.size.topCenter(menuItemContainer.localToGlobal(Offset.zero)).dy),
);
});
testWidgets('Dropdown menu aligns selected item with button in long lists', (WidgetTester tester) async {
// Open the dropdown menu
final Key buttonKey = UniqueKey();
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
value: '50',
items: List<String>.generate(/*length=*/ 100, (int index) => index.toString()),
onChanged: onChanged,
));
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
await tester.tap(find.byKey(buttonKey));
await tester.pumpAndSettle(); // finish the menu animation
// Find the selected item in the scrollable dropdown list
final RenderBox selectedItem = tester.renderObject<RenderBox>(
find.descendant(of: find.byType(Scrollable), matching: find.byKey(const ValueKey<String>('50'))),
);
// List should be scrolled so that the selected item is in line with the button
expect(
selectedItem.size.center(selectedItem.localToGlobal(Offset.zero)).dy,
equals(buttonBox.size.center(buttonBox.localToGlobal(Offset.zero)).dy),
);
});
testWidgets('Dropdown menu scrolls to last item in long lists', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
value: '99',
items: List<String>.generate(/*length=*/ 100, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.byKey(buttonKey));
await tester.pump();
final ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
// Make sure there is no overscroll
expect(scrollController.offset, scrollController.position.maxScrollExtent);
// Find the selected item in the scrollable dropdown list
final Finder menuItemFinder = find.byType(Scrollable);
final RenderBox menuItemContainer = tester.renderObject<RenderBox>(menuItemFinder);
final RenderBox selectedItem = tester.renderObject<RenderBox>(
find.descendant(
of: menuItemFinder,
matching: find.byKey(const ValueKey<String>('99')),
),
);
// kMaterialListPadding.vertical is 8.
const Offset menuPaddingOffset = Offset(0.0, -8.0);
final Offset selectedItemOffset = selectedItem.localToGlobal(Offset.zero);
final Offset menuItemContainerOffset = menuItemContainer.localToGlobal(menuPaddingOffset);
// Selected item should be aligned to the bottom of the dropdown menu.
expect(
selectedItem.size.bottomCenter(selectedItemOffset).dy,
menuItemContainer.size.bottomCenter(menuItemContainerOffset).dy,
);
});
testWidgets('Size of DropdownButton with null value', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
String? value;
Widget build() => buildFrame(buttonKey: buttonKey, value: value, onChanged: onChanged);
await tester.pumpWidget(build());
final RenderBox buttonBoxNullValue = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxNullValue.attached);
value = 'three';
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
// A Dropdown button with a null value should be the same size as a
// one with a non-null value.
expect(buttonBox.localToGlobal(Offset.zero), equals(buttonBoxNullValue.localToGlobal(Offset.zero)));
expect(buttonBox.size, equals(buttonBoxNullValue.size));
});
testWidgets('Size of DropdownButton with no items', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/26419
final Key buttonKey = UniqueKey();
List<String>? items;
Widget build() => buildFrame(buttonKey: buttonKey, items: items, onChanged: onChanged);
await tester.pumpWidget(build());
final RenderBox buttonBoxNullItems = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxNullItems.attached);
items = <String>[];
await tester.pumpWidget(build());
final RenderBox buttonBoxEmptyItems = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxEmptyItems.attached);
items = <String>['one', 'two', 'three', 'four'];
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
// A Dropdown button with a null value should be the same size as a
// one with a non-null value.
expect(buttonBox.localToGlobal(Offset.zero), equals(buttonBoxNullItems.localToGlobal(Offset.zero)));
expect(buttonBox.size, equals(buttonBoxNullItems.size));
});
testWidgets('Layout of a DropdownButton with null value', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
String? value;
void onChanged(String? newValue) {
value = newValue;
}
Widget build() => buildFrame(buttonKey: buttonKey, value: value, onChanged: onChanged);
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
// Show the menu.
await tester.tap(find.byKey(buttonKey));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
// Tap on item 'one', which must appear over the button.
await tester.tap(find.byKey(buttonKey, skipOffstage: false), warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
await tester.pumpWidget(build());
expect(value, equals('one'));
});
testWidgets('Size of DropdownButton with null value and a hint', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
String? value;
// The hint will define the dropdown's width
Widget build() => buildFrame(buttonKey: buttonKey, value: value, hint: const Text('onetwothree'));
await tester.pumpWidget(build());
expect(find.text('onetwothree'), findsOneWidget);
final RenderBox buttonBoxHintValue = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxHintValue.attached);
value = 'three';
await tester.pumpWidget(build());
final RenderBox buttonBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBox.attached);
// A Dropdown button with a null value and a hint should be the same size as a
// one with a non-null value.
expect(buttonBox.localToGlobal(Offset.zero), equals(buttonBoxHintValue.localToGlobal(Offset.zero)));
expect(buttonBox.size, equals(buttonBoxHintValue.size));
});
testWidgets('Dropdown menus must fit within the screen', (WidgetTester tester) async {
// The dropdown menu isn't readily accessible. To find it we're assuming that it
// contains a ListView and that it's an instance of _DropdownMenu.
Rect getMenuRect() {
late Rect menuRect;
tester.element(find.byType(ListView)).visitAncestorElements((Element element) {
if (element.toString().startsWith('_DropdownMenu')) {
final RenderBox box = element.findRenderObject()! as RenderBox;
menuRect = box.localToGlobal(Offset.zero) & box.size;
return false;
}
return true;
});
return menuRect;
}
// In all of the tests that follow we're assuming that the dropdown menu
// is horizontally aligned with the center of the dropdown button and padded
// on the top, left, and right.
const EdgeInsets buttonPadding = EdgeInsets.only(top: 8.0, left: 16.0, right: 24.0);
Rect getExpandedButtonRect() {
final RenderBox box = tester.renderObject<RenderBox>(find.byType(dropdownButtonType));
final Rect buttonRect = box.localToGlobal(Offset.zero) & box.size;
return buttonPadding.inflateRect(buttonRect);
}
late Rect buttonRect;
late Rect menuRect;
Future<void> popUpAndDown(Widget frame) async {
await tester.pumpWidget(frame);
await tester.tap(find.byType(dropdownButtonType));
await tester.pumpAndSettle();
menuRect = getMenuRect();
buttonRect = getExpandedButtonRect();
await tester.tap(find.byType(dropdownButtonType, skipOffstage: false), warnIfMissed: false);
}
// Dropdown button is along the top of the app. The top of the menu is
// aligned with the top of the expanded button and shifted horizontally
// so that it fits within the frame.
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.topLeft, value: menuItems.last, onChanged: onChanged),
);
expect(menuRect.topLeft, Offset.zero);
expect(menuRect.topRight, Offset(menuRect.width, 0.0));
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.topCenter, value: menuItems.last, onChanged: onChanged),
);
expect(menuRect.topLeft, Offset(buttonRect.left, 0.0));
expect(menuRect.topRight, Offset(buttonRect.right, 0.0));
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.topRight, value: menuItems.last, onChanged: onChanged),
);
expect(menuRect.topLeft, Offset(800.0 - menuRect.width, 0.0));
expect(menuRect.topRight, const Offset(800.0, 0.0));
// Dropdown button is along the middle of the app. The top of the menu is
// aligned with the top of the expanded button (because the 1st item
// is selected) and shifted horizontally so that it fits within the frame.
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.centerLeft, value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.topLeft, Offset(0.0, buttonRect.top));
expect(menuRect.topRight, Offset(menuRect.width, buttonRect.top));
await popUpAndDown(
buildFrame(value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.topLeft, buttonRect.topLeft);
expect(menuRect.topRight, buttonRect.topRight);
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.centerRight, value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.topLeft, Offset(800.0 - menuRect.width, buttonRect.top));
expect(menuRect.topRight, Offset(800.0, buttonRect.top));
// Dropdown button is along the bottom of the app. The bottom of the menu is
// aligned with the bottom of the expanded button and shifted horizontally
// so that it fits within the frame.
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.bottomLeft, value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.bottomLeft, const Offset(0.0, 600.0));
expect(menuRect.bottomRight, Offset(menuRect.width, 600.0));
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.bottomCenter, value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.bottomLeft, Offset(buttonRect.left, 600.0));
expect(menuRect.bottomRight, Offset(buttonRect.right, 600.0));
await popUpAndDown(
buildFrame(dropdownAlignment: Alignment.bottomRight, value: menuItems.first, onChanged: onChanged),
);
expect(menuRect.bottomLeft, Offset(800.0 - menuRect.width, 600.0));
expect(menuRect.bottomRight, const Offset(800.0, 600.0));
});
testWidgets('Dropdown menus are dismissed on screen orientation changes, but not on keyboard hide', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(onChanged: onChanged, mediaSize: const Size(800, 600)));
await tester.tap(find.byType(dropdownButtonType));
await tester.pumpAndSettle();
expect(find.byType(ListView), findsOneWidget);
// Show a keyboard (simulate by shortening the height).
await tester.pumpWidget(buildFrame(onChanged: onChanged, mediaSize: const Size(800, 300)));
await tester.pump();
expect(find.byType(ListView, skipOffstage: false), findsOneWidget);
// Hide a keyboard again (simulate by increasing the height).
await tester.pumpWidget(buildFrame(onChanged: onChanged, mediaSize: const Size(800, 600)));
await tester.pump();
expect(find.byType(ListView, skipOffstage: false), findsOneWidget);
// Rotate the device (simulate by changing the aspect ratio).
await tester.pumpWidget(buildFrame(onChanged: onChanged, mediaSize: const Size(600, 800)));
await tester.pump();
expect(find.byType(ListView, skipOffstage: false), findsNothing);
});
testWidgets('Semantics Tree contains only selected element', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(buildFrame(onChanged: onChanged));
expect(semantics, isNot(includesNodeWith(label: menuItems[0])));
expect(semantics, includesNodeWith(label: menuItems[1]));
expect(semantics, isNot(includesNodeWith(label: menuItems[2])));
expect(semantics, isNot(includesNodeWith(label: menuItems[3])));
semantics.dispose();
});
testWidgets('Dropdown button includes semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
const Key key = Key('test');
await tester.pumpWidget(buildFrame(
buttonKey: key,
value: null,
onChanged: (String? _) { },
hint: const Text('test'),
));
// By default the hint contributes the label.
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
isButton: true,
label: 'test',
hasTapAction: true,
isFocusable: true,
));
await tester.pumpWidget(buildFrame(
buttonKey: key,
value: 'three',
onChanged: onChanged,
hint: const Text('test'),
));
// Displays label of select item and is no longer tappable.
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
isButton: true,
label: 'three',
hasTapAction: true,
isFocusable: true,
));
handle.dispose();
});
testWidgets('Dropdown menu includes semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
const Key key = Key('test');
await tester.pumpWidget(buildFrame(
buttonKey: key,
value: null,
onChanged: onChanged,
));
await tester.tap(find.byKey(key));
await tester.pumpAndSettle();
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Dismiss',
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
label: 'Popup menu',
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
children: <TestSemantics>[
TestSemantics(
label: 'one',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[
SemanticsFlag.isFocused,
SemanticsFlag.isFocusable,
],
tags: <SemanticsTag>[const SemanticsTag('RenderViewport.twoPane')],
actions: <SemanticsAction>[SemanticsAction.tap],
),
TestSemantics(
label: 'two',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[SemanticsFlag.isFocusable],
tags: <SemanticsTag>[const SemanticsTag('RenderViewport.twoPane')],
actions: <SemanticsAction>[SemanticsAction.tap],
),
TestSemantics(
label: 'three',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[SemanticsFlag.isFocusable],
tags: <SemanticsTag>[const SemanticsTag('RenderViewport.twoPane')],
actions: <SemanticsAction>[SemanticsAction.tap],
),
TestSemantics(
label: 'four',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[SemanticsFlag.isFocusable],
tags: <SemanticsTag>[const SemanticsTag('RenderViewport.twoPane')],
actions: <SemanticsAction>[SemanticsAction.tap],
),
],
),
],
),
],
),
],
),
],
), ignoreId: true, ignoreRect: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('disabledHint displays on empty items or onChanged', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build({ List<String>? items, ValueChanged<String?>? onChanged }) => buildFrame(
items: items,
onChanged: onChanged,
buttonKey: buttonKey,
value: null,
hint: const Text('enabled'),
disabledHint: const Text('disabled'),
);
// [disabledHint] should display when [items] is null
await tester.pumpWidget(build(onChanged: onChanged));
expect(find.text('enabled'), findsNothing);
expect(find.text('disabled'), findsOneWidget);
// [disabledHint] should display when [items] is an empty list.
await tester.pumpWidget(build(items: <String>[], onChanged: onChanged));
expect(find.text('enabled'), findsNothing);
expect(find.text('disabled'), findsOneWidget);
// [disabledHint] should display when [onChanged] is null
await tester.pumpWidget(build(items: menuItems));
expect(find.text('enabled'), findsNothing);
expect(find.text('disabled'), findsOneWidget);
final RenderBox disabledHintBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
// A Dropdown button with a disabled hint should be the same size as a
// one with a regular enabled hint.
await tester.pumpWidget(build(items: menuItems, onChanged: onChanged));
expect(find.text('disabled'), findsNothing);
expect(find.text('enabled'), findsOneWidget);
final RenderBox enabledHintBox = tester.renderObject<RenderBox>(find.byKey(buttonKey));
expect(enabledHintBox.localToGlobal(Offset.zero), equals(disabledHintBox.localToGlobal(Offset.zero)));
expect(enabledHintBox.size, equals(disabledHintBox.size));
});
// Regression test for https://github.com/flutter/flutter/issues/70177
testWidgets('disabledHint behavior test', (WidgetTester tester) async {
Widget build({ List<String>? items, ValueChanged<String?>? onChanged, String? value, Widget? hint, Widget? disabledHint }) => buildFrame(
items: items,
onChanged: onChanged,
value: value,
hint: hint,
disabledHint: disabledHint,
);
// The selected value should be displayed when the button is disabled.
await tester.pumpWidget(build(items: menuItems, value: 'two'));
// The dropdown icon and the selected menu item are vertically aligned.
expect(tester.getCenter(find.text('two')).dy, tester.getCenter(find.byType(Icon)).dy);
// If [value] is null, the button is enabled, hint is displayed.
await tester.pumpWidget(build(
items: menuItems,
onChanged: onChanged,
hint: const Text('hint'),
disabledHint: const Text('disabledHint'),
));
expect(tester.getCenter(find.text('hint')).dy, tester.getCenter(find.byType(Icon)).dy);
// If [value] is null, the button is disabled, [disabledHint] is displayed when [disabledHint] is non-null.
await tester.pumpWidget(build(
items: menuItems,
hint: const Text('hint'),
disabledHint: const Text('disabledHint'),
));
expect(tester.getCenter(find.text('disabledHint')).dy, tester.getCenter(find.byType(Icon)).dy);
// If [value] is null, the button is disabled, [hint] is displayed when [disabledHint] is null.
await tester.pumpWidget(build(
items: menuItems,
hint: const Text('hint'),
));
expect(tester.getCenter(find.text('hint')).dy, tester.getCenter(find.byType(Icon)).dy);
int? getIndex() {
final IndexedStack stack = tester.element(find.byType(IndexedStack)).widget as IndexedStack;
return stack.index;
}
// If [value], [hint] and [disabledHint] are null, the button is disabled, nothing displayed.
await tester.pumpWidget(build(
items: menuItems,
));
expect(getIndex(), null);
// If [value], [hint] and [disabledHint] are null, the button is enabled, nothing displayed.
await tester.pumpWidget(build(
items: menuItems,
onChanged: onChanged,
));
expect(getIndex(), null);
});
testWidgets('DropdownButton selected item color test', (WidgetTester tester) async {
Widget build({ ValueChanged<String?>? onChanged, String? value, Widget? hint, Widget? disabledHint }) {
return MaterialApp(
theme: ThemeData(
disabledColor: Colors.pink,
),
home: Scaffold(
body: Center(
child: Column(children: <Widget>[
DropdownButtonFormField<String>(
style: const TextStyle(
color: Colors.yellow,
),
disabledHint: disabledHint,
hint: hint,
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
value: 'one',
child: Text('one'),
),
DropdownMenuItem<String>(
value: 'two',
child: Text('two'),
),
],
value: value,
onChanged: onChanged,
),
]),
),
),
);
}
Color textColor(String text) {
return tester.renderObject<RenderParagraph>(find.text(text)).text.style!.color!;
}
// The selected value should be displayed when the button is enabled.
await tester.pumpWidget(build(onChanged: onChanged, value: 'two'));
// The dropdown icon and the selected menu item are vertically aligned.
expect(tester.getCenter(find.text('two')).dy, tester.getCenter(find.byType(Icon)).dy);
// Selected item has a normal color from [DropdownButtonFormField.style]
// when the button is enabled.
expect(textColor('two'), Colors.yellow);
// The selected value should be displayed when the button is disabled.
await tester.pumpWidget(build(value: 'two'));
expect(tester.getCenter(find.text('two')).dy, tester.getCenter(find.byType(Icon)).dy);
// Selected item has a disabled color from [theme.disabledColor]
// when the button is disable.
expect(textColor('two'), Colors.pink);
});
testWidgets(
'DropdownButton hint displays when the items list is empty, '
'items is null, and disabledHint is null',
(WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build({ List<String>? items }) {
return buildFrame(
items: items,
buttonKey: buttonKey,
value: null,
hint: const Text('hint used when disabled'),
);
}
// [hint] should display when [items] is null and [disabledHint] is not defined
await tester.pumpWidget(build());
expect(find.text('hint used when disabled'), findsOneWidget);
// [hint] should display when [items] is an empty list and [disabledHint] is not defined.
await tester.pumpWidget(build(items: <String>[]));
expect(find.text('hint used when disabled'), findsOneWidget);
},
);
testWidgets('DropdownButton disabledHint is null by default', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget build({ List<String>? items }) {
return buildFrame(
items: items,
buttonKey: buttonKey,
value: null,
hint: const Text('hint used when disabled'),
);
}
// [hint] should display when [items] is null and [disabledHint] is not defined
await tester.pumpWidget(build());
expect(find.text('hint used when disabled'), findsOneWidget);
// [hint] should display when [items] is an empty list and [disabledHint] is not defined.
await tester.pumpWidget(build(items: <String>[]));
expect(find.text('hint used when disabled'), findsOneWidget);
});
testWidgets('Size of largest widget is used DropdownButton when selectedItemBuilder is non-null', (WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
const String selectedItem = '25';
await tester.pumpWidget(buildFrame(
// To test the size constraints, the selected item should not be the
// largest item. This validates that the button sizes itself according
// to the largest item regardless of which one is selected.
value: selectedItem,
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
onChanged: (String? newValue) {},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, '25'),
);
// DropdownButton should be the height of the largest item
expect(dropdownButtonRenderBox.size.height, 100);
// DropdownButton should be width of largest item added to the icon size
expect(dropdownButtonRenderBox.size.width, 100 + 24.0);
});
testWidgets(
'Enabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null and hint is defined, but smaller than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
await tester.pumpWidget(buildFrame(
value: null,
// [hint] widget is smaller than largest selected item widget
hint: const SizedBox(
height: 50,
width: 50,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
onChanged: (String? newValue) {},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, 'hint'),
);
// DropdownButton should be the height of the largest item
expect(dropdownButtonRenderBox.size.height, 100);
// DropdownButton should be width of largest item added to the icon size
expect(dropdownButtonRenderBox.size.width, 100 + 24.0);
},
);
testWidgets(
'Enabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null and hint is defined, but larger than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
const String selectedItem = '25';
await tester.pumpWidget(buildFrame(
// To test the size constraints, the selected item should not be the
// largest item. This validates that the button sizes itself according
// to the largest item regardless of which one is selected.
value: selectedItem,
// [hint] widget is larger than largest selected item widget
hint: const SizedBox(
height: 125,
width: 125,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
onChanged: (String? newValue) {},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, '25'),
);
// DropdownButton should be the height of the largest item (hint inclusive)
expect(dropdownButtonRenderBox.size.height, 125);
// DropdownButton should be width of largest item (hint inclusive) added to the icon size
expect(dropdownButtonRenderBox.size.width, 125 + 24.0);
},
);
testWidgets(
'Disabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null, and hint is defined, but smaller than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
await tester.pumpWidget(buildFrame(
value: null,
// [hint] widget is smaller than largest selected item widget
hint: const SizedBox(
height: 50,
width: 50,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, 'hint'),
);
// DropdownButton should be the height of the largest item
expect(dropdownButtonRenderBox.size.height, 100);
// DropdownButton should be width of largest item added to the icon size
expect(dropdownButtonRenderBox.size.width, 100 + 24.0);
},
);
testWidgets(
'Disabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null and hint is defined, but larger than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
await tester.pumpWidget(buildFrame(
value: null,
// [hint] widget is larger than largest selected item widget
hint: const SizedBox(
height: 125,
width: 125,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, '25', skipOffstage: false),
);
// DropdownButton should be the height of the largest item (hint inclusive)
expect(dropdownButtonRenderBox.size.height, 125);
// DropdownButton should be width of largest item (hint inclusive) added to the icon size
expect(dropdownButtonRenderBox.size.width, 125 + 24.0);
},
);
testWidgets(
'Disabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null, and disabledHint is defined, but smaller than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
await tester.pumpWidget(buildFrame(
value: null,
// [hint] widget is smaller than largest selected item widget
disabledHint: const SizedBox(
height: 50,
width: 50,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, 'hint'),
);
// DropdownButton should be the height of the largest item
expect(dropdownButtonRenderBox.size.height, 100);
// DropdownButton should be width of largest item added to the icon size
expect(dropdownButtonRenderBox.size.width, 100 + 24.0);
},
);
testWidgets(
'Disabled button - Size of largest widget is used DropdownButton when selectedItemBuilder '
'is non-null and disabledHint is defined, but larger than largest selected item widget',
(WidgetTester tester) async {
final List<String> items = <String>['25', '50', '100'];
await tester.pumpWidget(buildFrame(
value: null,
// [hint] widget is larger than largest selected item widget
disabledHint: const SizedBox(
height: 125,
width: 125,
child: Text('hint'),
),
items: items,
itemHeight: null,
selectedItemBuilder: (BuildContext context) {
return items.map<Widget>((String item) {
return SizedBox(
height: double.parse(item),
width: double.parse(item),
child: Center(child: Text(item)),
);
}).toList();
},
));
final RenderBox dropdownButtonRenderBox = tester.renderObject<RenderBox>(
find.widgetWithText(Row, '25', skipOffstage: false),
);
// DropdownButton should be the height of the largest item (hint inclusive)
expect(dropdownButtonRenderBox.size.height, 125);
// DropdownButton should be width of largest item (hint inclusive) added to the icon size
expect(dropdownButtonRenderBox.size.width, 125 + 24.0);
},
);
testWidgets('Dropdown in middle showing middle item', (WidgetTester tester) async {
final List<DropdownMenuItem<int>> items = List<DropdownMenuItem<int>>.generate(
100,
(int i) => DropdownMenuItem<int>(value: i, child: Text('$i')),
);
final DropdownButton<int> button = DropdownButton<int>(
value: 50,
onChanged: (int? newValue) { },
items: items,
);
double getMenuScroll() {
double scrollPosition;
final ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
scrollPosition = scrollController.position.pixels;
return scrollPosition;
}
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
child: button,
),
),
),
);
await tester.tap(find.text('50'));
await tester.pumpAndSettle();
expect(getMenuScroll(), 2180.0);
});
testWidgets('Dropdown in top showing bottom item', (WidgetTester tester) async {
final List<DropdownMenuItem<int>> items = List<DropdownMenuItem<int>>.generate(
100,
(int i) => DropdownMenuItem<int>(value: i, child: Text('$i')),
);
final DropdownButton<int> button = DropdownButton<int>(
value: 99,
onChanged: (int? newValue) { },
items: items,
);
double getMenuScroll() {
double scrollPosition;
final ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
scrollPosition = scrollController.position.pixels;
return scrollPosition;
}
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
alignment: Alignment.topCenter,
child: button,
),
),
),
);
await tester.tap(find.text('99'));
await tester.pumpAndSettle();
expect(getMenuScroll(), 4312.0);
});
testWidgets('Dropdown in bottom showing top item', (WidgetTester tester) async {
final List<DropdownMenuItem<int>> items = List<DropdownMenuItem<int>>.generate(
100,
(int i) => DropdownMenuItem<int>(value: i, child: Text('$i')),
);
final DropdownButton<int> button = DropdownButton<int>(
value: 0,
onChanged: (int? newValue) { },
items: items,
);
double getMenuScroll() {
double scrollPosition;
final ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
scrollPosition = scrollController.position.pixels;
return scrollPosition;
}
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
alignment: Alignment.bottomCenter,
child: button,
),
),
),
);
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
expect(getMenuScroll(), 0.0);
});
testWidgets('Dropdown in center showing bottom item', (WidgetTester tester) async {
final List<DropdownMenuItem<int>> items = List<DropdownMenuItem<int>>.generate(
100,
(int i) => DropdownMenuItem<int>(value: i, child: Text('$i')),
);
final DropdownButton<int> button = DropdownButton<int>(
value: 99,
onChanged: (int? newValue) { },
items: items,
);
double getMenuScroll() {
double scrollPosition;
final ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
scrollPosition = scrollController.position.pixels;
return scrollPosition;
}
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
child: button,
),
),
),
);
await tester.tap(find.text('99'));
await tester.pumpAndSettle();
expect(getMenuScroll(), 4312.0);
});
testWidgets('Dropdown menu respects parent size limits', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/24417
int? selectedIndex;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
bottomNavigationBar: const SizedBox(height: 200),
body: Navigator(
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
builder: (BuildContext context) {
return SafeArea(
child: Container(
alignment: Alignment.topLeft,
// From material/dropdown.dart (menus are unaligned by default):
// _kUnalignedMenuMargin = EdgeInsetsDirectional.only(start: 16.0, end: 24.0)
// This padding ensures that the entire menu will be visible
padding: const EdgeInsetsDirectional.only(start: 16.0, end: 24.0),
child: DropdownButton<int>(
value: 12,
onChanged: (int? i) {
selectedIndex = i;
},
items: List<DropdownMenuItem<int>>.generate(100, (int i) {
return DropdownMenuItem<int>(value: i, child: Text('$i'));
}),
),
),
);
},
);
},
),
),
),
);
await tester.tap(find.text('12'));
await tester.pumpAndSettle();
expect(selectedIndex, null);
await tester.tap(find.text('13').last);
await tester.pumpAndSettle();
expect(selectedIndex, 13);
});
testWidgets('Dropdown button will accept widgets as its underline', (WidgetTester tester) async {
const BoxDecoration decoration = BoxDecoration(
border: Border(bottom: BorderSide(color: Color(0xFFCCBB00), width: 4.0)),
);
const BoxDecoration defaultDecoration = BoxDecoration(
border: Border(bottom: BorderSide(color: Color(0xFFBDBDBD), width: 0.0)),
);
final Widget customUnderline = Container(height: 4.0, decoration: decoration);
final Key buttonKey = UniqueKey();
final Finder decoratedBox = find.descendant(
of: find.byKey(buttonKey),
matching: find.byType(DecoratedBox),
);
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
underline: customUnderline,
onChanged: onChanged,
));
expect(tester.widgetList<DecoratedBox>(decoratedBox).last.decoration, decoration);
await tester.pumpWidget(buildFrame(buttonKey: buttonKey, onChanged: onChanged));
expect(tester.widgetList<DecoratedBox>(decoratedBox).last.decoration, defaultDecoration);
});
testWidgets('DropdownButton selectedItemBuilder builds custom buttons', (WidgetTester tester) async {
const List<String> items = <String>[
'One',
'Two',
'Three',
];
String? selectedItem = items[0];
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
value: selectedItem,
onChanged: (String? string) {
setState(() => selectedItem = string);
},
selectedItemBuilder: (BuildContext context) {
int index = 0;
return items.map((String string) {
index += 1;
return Text('$string as an Arabic numeral: $index');
}).toList();
},
items: items.map((String string) {
return DropdownMenuItem<String>(
value: string,
child: Text(string),
);
}).toList(),
),
),
);
},
),
);
expect(find.text('One as an Arabic numeral: 1'), findsOneWidget);
await tester.tap(find.text('One as an Arabic numeral: 1'));
await tester.pumpAndSettle();
await tester.tap(find.text('Two'));
await tester.pumpAndSettle();
expect(find.text('Two as an Arabic numeral: 2'), findsOneWidget);
});
testWidgets('DropdownButton uses default color when expanded', (WidgetTester tester) async {
await checkDropdownColor(tester);
});
testWidgets('DropdownButton uses dropdownColor when expanded', (WidgetTester tester) async {
await checkDropdownColor(tester, color: const Color.fromRGBO(120, 220, 70, 0.8));
});
testWidgets('DropdownButtonFormField uses dropdownColor when expanded', (WidgetTester tester) async {
await checkDropdownColor(tester, color: const Color.fromRGBO(120, 220, 70, 0.8), isFormField: true);
});
testWidgets('DropdownButton hint displays properly when selectedItemBuilder is defined', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/42340
final List<String> items = <String>['1', '2', '3'];
String? selectedItem;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
hint: const Text('Please select an item'),
value: selectedItem,
onChanged: (String? string) {
setState(() {
selectedItem = string;
});
},
selectedItemBuilder: (BuildContext context) {
return items.map((String item) {
return Text('You have selected: $item');
}).toList();
},
items: items.map((String item) {
return DropdownMenuItem<String>(
value: item,
child: Text(item),
);
}).toList(),
),
),
);
},
),
);
// Initially shows the hint text
expect(find.text('Please select an item'), findsOneWidget);
await tester.tap(find.text('Please select an item', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
await tester.tap(find.text('1'));
await tester.pumpAndSettle();
// Selecting an item should display its corresponding item builder
expect(find.text('You have selected: 1'), findsOneWidget);
});
testWidgets('Variable size and oversized menu items', (WidgetTester tester) async {
final List<double> itemHeights = <double>[30, 40, 50, 60];
double? dropdownValue = itemHeights[0];
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<double>(
onChanged: (double? value) {
setState(() { dropdownValue = value; });
},
value: dropdownValue,
itemHeight: null,
items: itemHeights.map<DropdownMenuItem<double>>((double value) {
return DropdownMenuItem<double>(
key: ValueKey<double>(value),
value: value,
child: Center(
child: Container(
width: 100,
height: value,
color: Colors.blue,
),
),
);
}).toList(),
);
},
),
),
),
);
}
final Finder dropdownIcon = find.byType(Icon);
final Finder item30 = find.byKey(const ValueKey<double>(30), skipOffstage: false);
final Finder item40 = find.byKey(const ValueKey<double>(40), skipOffstage: false);
final Finder item50 = find.byKey(const ValueKey<double>(50), skipOffstage: false);
final Finder item60 = find.byKey(const ValueKey<double>(60), skipOffstage: false);
// Only the DropdownButton is visible. It contains the selected item
// and a dropdown arrow icon.
await tester.pumpWidget(buildFrame());
expect(dropdownIcon, findsOneWidget);
expect(item30, findsOneWidget);
// All menu items have a minimum height of 48. The centers of the
// dropdown icon and the selected menu item are vertically aligned
// and horizontally adjacent.
expect(tester.getSize(item30), const Size(100, 48));
expect(tester.getCenter(item30).dy, tester.getCenter(dropdownIcon).dy);
expect(tester.getTopRight(item30).dx, tester.getTopLeft(dropdownIcon).dx);
// Show the popup menu.
await tester.tap(item30);
await tester.pumpAndSettle();
// Each item appears twice, once in the menu and once
// in the dropdown button's IndexedStack.
expect(item30.evaluate().length, 2);
expect(item40.evaluate().length, 2);
expect(item50.evaluate().length, 2);
expect(item60.evaluate().length, 2);
// Verify that the items have the expected sizes. The width of the items
// that appear in the menu is padded by 16 on the left and right.
expect(tester.getSize(item30.first), const Size(100, 48));
expect(tester.getSize(item40.first), const Size(100, 48));
expect(tester.getSize(item50.first), const Size(100, 50));
expect(tester.getSize(item60.first), const Size(100, 60));
expect(tester.getSize(item30.last), const Size(132, 48));
expect(tester.getSize(item40.last), const Size(132, 48));
expect(tester.getSize(item50.last), const Size(132, 50));
expect(tester.getSize(item60.last), const Size(132, 60));
// The vertical center of the selectedItem (item30) should
// line up with its button counterpart.
expect(tester.getCenter(item30.first).dy, tester.getCenter(item30.last).dy);
// The menu items should be arranged in a column.
expect(tester.getBottomLeft(item30.last), tester.getTopLeft(item40.last));
expect(tester.getBottomLeft(item40.last), tester.getTopLeft(item50.last));
expect(tester.getBottomLeft(item50.last), tester.getTopLeft(item60.last));
// Dismiss the menu by selecting item40 and then show the menu again.
await tester.tap(item40.last);
await tester.pumpAndSettle();
expect(dropdownValue, 40);
await tester.tap(item40.first);
await tester.pumpAndSettle();
// The vertical center of the selectedItem (item40) should
// line up with its button counterpart.
expect(tester.getCenter(item40.first).dy, tester.getCenter(item40.last).dy);
});
testWidgets('DropdownButton menu items do not resize when its route is popped', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/44877.
const List<String> items = <String>[
'one',
'two',
'three',
];
String? item = items[0];
late double textScale;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MaterialApp(
builder: (BuildContext context, Widget? child) {
textScale = MediaQuery.of(context).textScaler.scale(14) / 14;
return MediaQuery(
data: MediaQueryData(textScaler: TextScaler.linear(textScale)),
child: child!,
);
},
home: Scaffold(
body: DropdownButton<String>(
value: item,
items: items.map((String item) => DropdownMenuItem<String>(
value: item,
child: Text(item),
)).toList(),
onChanged: (String? newItem) {
setState(() {
item = newItem;
textScale += 0.1;
});
},
),
),
);
},
),
);
// Verify that the first item is showing.
expect(find.text('one'), findsOneWidget);
// Select a different item to trigger setState, which updates mediaQuery
// and forces a performLayout on the popped _DropdownRoute. This operation
// should not cause an exception.
await tester.tap(find.text('one'));
await tester.pumpAndSettle();
await tester.tap(find.text('two').last);
await tester.pumpAndSettle();
expect(find.text('two'), findsOneWidget);
});
testWidgets('DropdownButton hint is selected item', (WidgetTester tester) async {
const double hintPaddingOffset = 8;
const List<String> itemValues = <String>['item0', 'item1', 'item2', 'item3'];
String? selectedItem = 'item0';
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: ButtonTheme(
alignedDropdown: true,
child: DropdownButtonHideUnderline(
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
// The pretzel below is from an actual app. The price
// of limited configurability is keeping this working.
return DropdownButton<String>(
isExpanded: true,
elevation: 2,
hint: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
// Stack with a positioned widget is used to override the
// hard coded 16px margin in the dropdown code, so that
// this hint aligns "properly" with the menu.
return Stack(
clipBehavior: Clip.none,
alignment: Alignment.topCenter,
children: <Widget>[
PositionedDirectional(
width: constraints.maxWidth + hintPaddingOffset,
start: -hintPaddingOffset,
top: 4.0,
child: Text('-$selectedItem-'),
),
],
);
},
),
onChanged: (String? value) {
setState(() { selectedItem = value; });
},
icon: Container(),
items: itemValues.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
);
},
),
),
),
),
),
);
}
await tester.pumpWidget(buildFrame());
expect(tester.getTopLeft(find.text('-item0-')).dx, 8);
// Show the popup menu.
await tester.tap(find.text('-item0-', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.text('-item0-')).dx, 8);
});
testWidgets('DropdownButton can be focused, and has focusColor', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final UniqueKey buttonKey = UniqueKey();
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
await tester.pumpWidget(buildFrame(buttonKey: buttonKey, onChanged: onChanged, focusNode: focusNode, autofocus: true, useMaterial3: false));
await tester.pumpAndSettle(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
expect(find.byType(Material), paints..rect(rect: const Rect.fromLTRB(348.0, 276.0, 452.0, 324.0), color: const Color(0x1f000000)));
await tester.pumpWidget(buildFrame(buttonKey: buttonKey, onChanged: onChanged, focusNode: focusNode, focusColor: const Color(0xff00ff00), useMaterial3: false));
await tester.pumpAndSettle(); // Pump a frame for autofocus to take effect.
expect(find.byType(Material), paints..rect(rect: const Rect.fromLTRB(348.0, 276.0, 452.0, 324.0), color: const Color(0x1f00ff00)));
});
testWidgets('DropdownButtonFormField can be focused, and has focusColor', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final UniqueKey buttonKey = UniqueKey();
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButtonFormField');
addTearDown(focusNode.dispose);
await tester.pumpWidget(buildFrame(isFormField: true, buttonKey: buttonKey, onChanged: onChanged, focusNode: focusNode, autofocus: true));
await tester.pumpAndSettle(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
expect(find.byType(Material), paints ..rect(rect: const Rect.fromLTRB(0.0, 268.0, 800.0, 332.0), color: const Color(0x1f000000)));
await tester.pumpWidget(buildFrame(isFormField: true, buttonKey: buttonKey, onChanged: onChanged, focusNode: focusNode, focusColor: const Color(0xff00ff00)));
await tester.pumpAndSettle(); // Pump a frame for autofocus to take effect.
expect(find.byType(Material), paints ..rect(rect: const Rect.fromLTRB(0.0, 268.0, 800.0, 332.0), color: const Color(0x1f00ff00)));
});
testWidgets("DropdownButton won't be focused if not enabled", (WidgetTester tester) async {
final UniqueKey buttonKey = UniqueKey();
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
await tester.pumpWidget(buildFrame(buttonKey: buttonKey, focusNode: focusNode, autofocus: true, focusColor: const Color(0xff00ff00)));
await tester.pump(); // Pump a frame for autofocus to take effect (although it shouldn't).
expect(focusNode.hasPrimaryFocus, isFalse);
expect(find.byKey(buttonKey), isNot(paints ..rrect(rrect: const RRect.fromLTRBXY(0.0, 0.0, 104.0, 48.0, 4.0, 4.0), color: const Color(0xff00ff00))));
});
testWidgets('DropdownButton is activated with the enter key', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
String? value = 'one';
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<String>(
focusNode: focusNode,
autofocus: true,
onChanged: (String? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: menuItems.map<DropdownMenuItem<String>>((String item) {
return DropdownMenuItem<String>(
key: ValueKey<String>(item),
value: item,
child: Text(item, key: ValueKey<String>('${item}Text')),
);
}).toList(),
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('one'));
await tester.sendKeyEvent(LogicalKeyboardKey.tab); // Focus 'two'
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter); // Select 'two'.
await tester.pump();
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('two'));
});
// Regression test for https://github.com/flutter/flutter/issues/77655.
testWidgets('DropdownButton selecting a null valued item should be selected', (WidgetTester tester) async {
final List<MapEntry<String?, String>> items = <MapEntry<String?, String>>[
const MapEntry<String?, String>(null, 'None'),
const MapEntry<String?, String>('one', 'One'),
const MapEntry<String?, String>('two', 'Two'),
];
String? selectedItem = 'one';
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
value: selectedItem,
onChanged: (String? string) {
setState(() {
selectedItem = string;
});
},
items: items.map((MapEntry<String?, String> item) {
return DropdownMenuItem<String>(
value: item.key,
child: Text(item.value),
);
}).toList(),
),
),
);
},
),
);
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
await tester.tap(find.text('None').last);
await tester.pumpAndSettle();
expect(find.text('None'), findsOneWidget);
});
testWidgets('DropdownButton is activated with the space key', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
String? value = 'one';
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<String>(
focusNode: focusNode,
autofocus: true,
onChanged: (String? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: menuItems.map<DropdownMenuItem<String>>((String item) {
return DropdownMenuItem<String>(
key: ValueKey<String>(item),
value: item,
child: Text(item, key: ValueKey<String>('${item}Text')),
);
}).toList(),
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('one'));
await tester.sendKeyEvent(LogicalKeyboardKey.tab); // Focus 'two'
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.space); // Select 'two'.
await tester.pump();
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('two'));
});
testWidgets('Selected element is focused when dropdown is opened', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
String? value = 'one';
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<String>(
focusNode: focusNode,
autofocus: true,
onChanged: (String? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: menuItems.map<DropdownMenuItem<String>>((String item) {
return DropdownMenuItem<String>(
key: ValueKey<String>(item),
value: item,
child: Text(item, key: ValueKey<String>('Text $item')),
);
}).toList(),
);
},
),
),
),
));
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu open animation
expect(value, equals('one'));
expect(Focus.of(tester.element(find.byKey(const ValueKey<String>('one')).last)).hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.tab); // Focus 'two'
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter); // Select 'two' and close the dropdown.
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu close animation
expect(value, equals('two'));
// Now make sure that "two" is focused when we re-open the dropdown.
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu open animation
expect(value, equals('two'));
final Element element = tester.element(find.byKey(const ValueKey<String>('two')).last);
final FocusNode node = Focus.of(element);
expect(node.hasFocus, isTrue);
});
testWidgets('Selected element is correctly focused with dropdown that more items than fit on the screen', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
int? value = 1;
final List<int> hugeMenuItems = List<int>.generate(50, (int index) => index);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<int>(
focusNode: focusNode,
autofocus: true,
onChanged: (int? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: hugeMenuItems.map<DropdownMenuItem<int>>((int item) {
return DropdownMenuItem<int>(
key: ValueKey<int>(item),
value: item,
child: Text(item.toString(), key: ValueKey<String>('Text $item')),
);
}).toList(),
);
},
),
),
),
),
);
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu open animation
expect(value, equals(1));
expect(Focus.of(tester.element(find.byKey(const ValueKey<int>(1)).last)).hasPrimaryFocus, isTrue);
for (int i = 0; i < 41; ++i) {
await tester.sendKeyEvent(LogicalKeyboardKey.tab); // Move to the next one.
await tester.pumpAndSettle(const Duration(milliseconds: 200)); // Wait for it to animate the menu.
}
await tester.sendKeyEvent(LogicalKeyboardKey.enter); // Select '42' and close the dropdown.
await tester.pumpAndSettle(const Duration(seconds: 1)); // Finish the menu close animation
expect(value, equals(42));
// Now make sure that "42" is focused when we re-open the dropdown.
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu open animation
expect(value, equals(42));
final Element element = tester.element(find.byKey(const ValueKey<int>(42)).last);
final FocusNode node = Focus.of(element);
expect(node.hasFocus, isTrue);
});
testWidgets("Having a focused element doesn't interrupt scroll when flung by touch", (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
int? value = 1;
final List<int> hugeMenuItems = List<int>.generate(100, (int index) => index);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<int>(
focusNode: focusNode,
autofocus: true,
onChanged: (int? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: hugeMenuItems.map<DropdownMenuItem<int>>((int item) {
return DropdownMenuItem<int>(
key: ValueKey<int>(item),
value: item,
child: Text(item.toString(), key: ValueKey<String>('Text $item')),
);
}).toList(),
);
},
),
),
),
),
);
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pumpAndSettle();
expect(value, equals(1));
expect(Focus.of(tester.element(find.byKey(const ValueKey<int>(1)).last)).hasPrimaryFocus, isTrue);
// Move to an item very far down the menu.
for (int i = 0; i < 90; ++i) {
await tester.sendKeyEvent(LogicalKeyboardKey.tab); // Move to the next one.
await tester.pumpAndSettle(); // Wait for it to animate the menu.
}
expect(Focus.of(tester.element(find.byKey(const ValueKey<int>(91)).last)).hasPrimaryFocus, isTrue);
// Scroll back to the top using touch, and make sure we end up there.
final Finder menu = find.byWidgetPredicate((Widget widget) {
return widget.runtimeType.toString().startsWith('_DropdownMenu<');
});
final Rect menuRect = tester.getRect(menu).shift(tester.getTopLeft(menu));
for (int i = 0; i < 10; ++i) {
await tester.fling(menu, Offset(0.0, menuRect.height), 10.0);
}
await tester.pumpAndSettle();
// Make sure that we made it to the top and something didn't stop the
// scroll.
expect(find.byKey(const ValueKey<int>(1)), findsNWidgets(2));
expect(
tester.getRect(find.byKey(const ValueKey<int>(1)).last),
equals(const Rect.fromLTRB(372.0, 104.0, 436.0, 152.0)),
);
// Scrolling to the top again has removed the one the focus was on from the
// tree, causing it to lose focus.
expect(Focus.of(tester.element(find.byKey(const ValueKey<int>(91), skipOffstage: false).last)).hasPrimaryFocus, isFalse);
});
testWidgets('DropdownButton onTap callback can request focus', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton')..addListener(() { });
addTearDown(focusNode.dispose);
int? value = 1;
final List<int> hugeMenuItems = List<int>.generate(100, (int index) => index);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<int>(
focusNode: focusNode,
onChanged: (int? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: hugeMenuItems.map<DropdownMenuItem<int>>((int item) {
return DropdownMenuItem<int>(
key: ValueKey<int>(item),
value: item,
child: Text(item.toString()),
);
}).toList(),
);
},
),
),
),
),
);
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isFalse);
await tester.tap(find.text('1'));
await tester.pumpAndSettle();
// Close the dropdown menu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isTrue);
});
testWidgets('DropdownButton changes selected item with arrow keys', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'DropdownButton');
addTearDown(focusNode.dispose);
String? value = 'one';
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return DropdownButton<String>(
focusNode: focusNode,
autofocus: true,
onChanged: (String? newValue) {
setState(() {
value = newValue;
});
},
value: value,
itemHeight: null,
items: menuItems.map<DropdownMenuItem<String>>((String item) {
return DropdownMenuItem<String>(
key: ValueKey<String>(item),
value: item,
child: Text(item, key: ValueKey<String>('${item}Text')),
);
}).toList(),
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame());
await tester.pump(); // Pump a frame for autofocus to take effect.
expect(focusNode.hasPrimaryFocus, isTrue);
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('one'));
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown); // Focus 'two'.
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown); // Focus 'three'.
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp); // Back to 'two'.
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter); // Select 'two'.
await tester.pump();
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // finish the menu animation
expect(value, equals('two'));
});
testWidgets('DropdownButton onTap callback is called when defined', (WidgetTester tester) async {
int dropdownButtonTapCounter = 0;
String? value = 'one';
void onChanged(String? newValue) { value = newValue; }
void onTap() { dropdownButtonTapCounter += 1; }
Widget build() => buildFrame(
value: value,
onChanged: onChanged,
onTap: onTap,
);
await tester.pumpWidget(build());
expect(dropdownButtonTapCounter, 0);
// Tap dropdown button.
await tester.tap(find.text('one'));
await tester.pumpAndSettle();
expect(value, equals('one'));
expect(dropdownButtonTapCounter, 1); // Should update counter.
// Tap dropdown menu item.
await tester.tap(find.text('three').last);
await tester.pumpAndSettle();
expect(value, equals('three'));
expect(dropdownButtonTapCounter, 1); // Should not change.
// Tap dropdown button again.
await tester.tap(find.text('three', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
expect(value, equals('three'));
expect(dropdownButtonTapCounter, 2); // Should update counter.
// Tap dropdown menu item.
await tester.tap(find.text('two').last);
await tester.pumpAndSettle();
expect(value, equals('two'));
expect(dropdownButtonTapCounter, 2); // Should not change.
});
testWidgets('DropdownMenuItem onTap callback is called when defined', (WidgetTester tester) async {
String? value = 'one';
final List<int> menuItemTapCounters = <int>[0, 0, 0, 0];
void onChanged(String? newValue) { value = newValue; }
final List<VoidCallback> onTapCallbacks = <VoidCallback>[
() { menuItemTapCounters[0] += 1; },
() { menuItemTapCounters[1] += 1; },
() { menuItemTapCounters[2] += 1; },
() { menuItemTapCounters[3] += 1; },
];
int currentIndex = -1;
await tester.pumpWidget(
TestApp(
textDirection: TextDirection.ltr,
child: Material(
child: RepaintBoundary(
child: DropdownButton<String>(
value: value,
onChanged: onChanged,
items: menuItems.map<DropdownMenuItem<String>>((String item) {
currentIndex += 1;
return DropdownMenuItem<String>(
value: item,
onTap: onTapCallbacks[currentIndex],
child: Text(item),
);
}).toList(),
),
),
),
),
);
// Tap dropdown button.
await tester.tap(find.text('one'));
await tester.pumpAndSettle();
expect(value, equals('one'));
// Counters should still be zero.
expect(menuItemTapCounters, <int>[0, 0, 0, 0]);
// Tap dropdown menu item.
await tester.tap(find.text('three').last);
await tester.pumpAndSettle();
// Should update the counter for the third item (second index).
expect(value, equals('three'));
expect(menuItemTapCounters, <int>[0, 0, 1, 0]);
// Tap dropdown button again.
await tester.tap(find.text('three', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
// Should not change.
expect(value, equals('three'));
expect(menuItemTapCounters, <int>[0, 0, 1, 0]);
// Tap dropdown menu item.
await tester.tap(find.text('two').last);
await tester.pumpAndSettle();
// Should update the counter for the second item (first index).
expect(value, equals('two'));
expect(menuItemTapCounters, <int>[0, 1, 1, 0]);
// Tap dropdown button again.
await tester.tap(find.text('two', skipOffstage: false), warnIfMissed: false);
await tester.pumpAndSettle();
// Should not change.
expect(value, equals('two'));
expect(menuItemTapCounters, <int>[0, 1, 1, 0]);
// Tap the already selected menu item
await tester.tap(find.text('two').last);
await tester.pumpAndSettle();
// Should update the counter for the second item (first index), even
// though it was already selected.
expect(value, equals('two'));
expect(menuItemTapCounters, <int>[0, 2, 1, 0]);
});
testWidgets('Does not crash when option is selected without waiting for opening animation to complete', (WidgetTester tester) async {
// Regression test for b/171846624.
final List<String> options = <String>['first', 'second', 'third'];
String? value = options.first;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) => DropdownButton<String>(
value: value,
items: options.map((String s) => DropdownMenuItem<String>(
value: s,
child: Text(s),
)).toList(),
onChanged: (String? v) {
setState(() {
value = v;
});
},
),
),
),
),
);
expect(find.text('first').hitTestable(), findsOneWidget);
expect(find.text('second').hitTestable(), findsNothing);
expect(find.text('third').hitTestable(), findsNothing);
// Open dropdown.
await tester.tap(find.text('first').hitTestable());
await tester.pump();
expect(find.text('third').hitTestable(), findsOneWidget);
expect(find.text('first').hitTestable(), findsOneWidget);
expect(find.text('second').hitTestable(), findsOneWidget);
// Deliberately not waiting for opening animation to complete!
// Select an option in dropdown.
await tester.tap(find.text('third').hitTestable());
await tester.pump();
expect(find.text('third').hitTestable(), findsOneWidget);
expect(find.text('first').hitTestable(), findsNothing);
expect(find.text('second').hitTestable(), findsNothing);
});
testWidgets('Dropdown menu should persistently show a scrollbar if it is scrollable', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(
value: '0',
// menu is short enough to fit onto the screen.
items: List<String>.generate(/*length=*/10, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
ScrollController scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
// The scrollbar shouldn't show if the list fits into the screen.
expect(scrollController.position.maxScrollExtent, 0);
expect(find.byType(Scrollbar), isNot(paints..rect()));
await tester.tap(find.text('0').last);
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(
value: '0',
// menu is too long to fit onto the screen.
items: List<String>.generate(/*length=*/100, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
scrollController = PrimaryScrollController.of(tester.element(find.byType(ListView)));
// The scrollbar is shown when the list is longer than the height of the screen.
expect(scrollController.position.maxScrollExtent > 0, isTrue);
expect(find.byType(Scrollbar), paints..rect());
});
testWidgets("Dropdown menu's maximum height should be influenced by DropdownButton.menuMaxHeight.", (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(
value: '0',
items: List<String>.generate(/*length=*/64, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
final Element element = tester.element(find.byType(ListView));
double menuHeight = element.size!.height;
// The default maximum height should be one item height from the edge.
// https://material.io/design/components/menus.html#usage
final double mediaHeight = MediaQuery.of(element).size.height;
final double defaultMenuHeight = mediaHeight - (2 * kMinInteractiveDimension);
expect(menuHeight, defaultMenuHeight);
await tester.tap(find.text('0').last);
await tester.pumpAndSettle();
// Set menuMaxHeight which is less than defaultMenuHeight
await tester.pumpWidget(buildFrame(
value: '0',
items: List<String>.generate(/*length=*/64, (int index) => index.toString()),
onChanged: onChanged,
menuMaxHeight: 7 * kMinInteractiveDimension,
));
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
menuHeight = tester.element(find.byType(ListView)).size!.height;
expect(menuHeight == defaultMenuHeight, isFalse);
expect(menuHeight, kMinInteractiveDimension * 7);
await tester.tap(find.text('0').last);
await tester.pumpAndSettle();
// Set menuMaxHeight which is greater than defaultMenuHeight
await tester.pumpWidget(buildFrame(
value: '0',
items: List<String>.generate(/*length=*/64, (int index) => index.toString()),
onChanged: onChanged,
menuMaxHeight: mediaHeight,
));
await tester.tap(find.text('0'));
await tester.pumpAndSettle();
menuHeight = tester.element(find.byType(ListView)).size!.height;
expect(menuHeight, defaultMenuHeight);
});
// Regression test for https://github.com/flutter/flutter/issues/89029
testWidgets('menu position test with `menuMaxHeight`', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
value: '6',
items: List<String>.generate(/*length=*/64, (int index) => index.toString()),
onChanged: onChanged,
menuMaxHeight: 2 * kMinInteractiveDimension,
));
await tester.tap(find.text('6'));
await tester.pumpAndSettle();
final RenderBox menuBox = tester.renderObject(find.byType(ListView));
final RenderBox buttonBox = tester.renderObject(find.byKey(buttonKey));
// The menu's bottom should align with the drop-button's bottom.
expect(
menuBox.localToGlobal(menuBox.paintBounds.bottomCenter).dy,
buttonBox.localToGlobal(buttonBox.paintBounds.bottomCenter).dy,
);
});
// Regression test for https://github.com/flutter/flutter/issues/76614
testWidgets('Do not crash if used in very short screen', (WidgetTester tester) async {
// The default item height is 48.0 pixels and needs two items padding since
// the menu requires empty space surrounding the menu. Finally, the constraint height
// is 47.0 pixels for the menu rendering.
tester.view.physicalSize = const Size(800.0, 48.0 * 3 - 1.0);
tester.view.devicePixelRatio = 1;
addTearDown(tester.view.reset);
const String value = 'foo';
final UniqueKey itemKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Center(
child: DropdownButton<String>(
value: value,
items: <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
key: itemKey,
value: value,
child: const Text(value),
),
],
onChanged: (_) { },
),
),
),
),
);
await tester.tap(find.text(value));
await tester.pumpAndSettle();
final List<RenderBox> itemBoxes = tester.renderObjectList<RenderBox>(find.byKey(itemKey)).toList();
expect(itemBoxes[0].localToGlobal(Offset.zero).dx, 364.0);
expect(itemBoxes[0].localToGlobal(Offset.zero).dy, 47.5);
expect(itemBoxes[1].localToGlobal(Offset.zero).dx, 364.0);
expect(itemBoxes[1].localToGlobal(Offset.zero).dy, 47.5);
expect(
find.ancestor(
of: find.text(value).last,
matching: find.byType(CustomPaint),
).at(2),
paints
..save()
..rrect()
..rrect()
..rrect()
// The height of menu is 47.0.
..rrect(rrect: const RRect.fromLTRBXY(0.0, 0.0, 112.0, 47.0, 2.0, 2.0), color: Colors.grey[50], hasMaskFilter: false),
);
});
testWidgets('Tapping a disabled item should not close DropdownButton', (WidgetTester tester) async {
String? value = 'first';
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) => DropdownButton<String>(
value: value,
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
enabled: false,
child: Text('disabled'),
),
DropdownMenuItem<String>(
value: 'first',
child: Text('first'),
),
DropdownMenuItem<String>(
value: 'second',
child: Text('second'),
),
],
onChanged: (String? newValue) {
setState(() {
value = newValue;
});
},
),
),
),
),
);
// Open dropdown.
await tester.tap(find.text('first').hitTestable());
await tester.pumpAndSettle();
// Tap on a disabled item.
await tester.tap(find.text('disabled').hitTestable());
await tester.pumpAndSettle();
// The dropdown should still be open, i.e., there should be one widget with 'second' text.
expect(find.text('second').hitTestable(), findsOneWidget);
});
testWidgets('Disabled item should not be focusable', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DropdownButton<String>(
value: 'enabled',
onChanged: onChanged,
items: const <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
enabled: false,
child: Text('disabled'),
),
DropdownMenuItem<String>(
value: 'enabled',
child: Text('enabled'),
),
],
),
),
),
);
// Open dropdown.
await tester.tap(find.text('enabled').hitTestable());
await tester.pumpAndSettle();
// The `FocusNode` of [disabledItem] should be `null` as enabled is false.
final Element disabledItem = tester.element(find.text('disabled').hitTestable());
expect(Focus.maybeOf(disabledItem), null, reason: 'Disabled menu item should not be able to request focus');
});
testWidgets('alignment test', (WidgetTester tester) async {
final Key buttonKey = UniqueKey();
Widget buildFrame({AlignmentGeometry? buttonAlignment, AlignmentGeometry? menuAlignment}) {
return MaterialApp(
home: Scaffold(
body: Center(
child: DropdownButton<String>(
key: buttonKey,
alignment: buttonAlignment ?? AlignmentDirectional.centerStart,
value: 'enabled',
onChanged: onChanged,
items: <DropdownMenuItem<String>>[
DropdownMenuItem<String>(
alignment: buttonAlignment ?? AlignmentDirectional.centerStart,
enabled: false,
child: const Text('disabled'),
),
DropdownMenuItem<String>(
alignment: buttonAlignment ?? AlignmentDirectional.centerStart,
value: 'enabled',
child: const Text('enabled'),
),
],
),
),
),
);
}
await tester.pumpWidget(buildFrame());
final RenderBox buttonBox = tester.renderObject(find.byKey(buttonKey));
RenderBox selectedItemBox = tester.renderObject(find.text('enabled'));
// Default to center-start aligned.
expect(
buttonBox.localToGlobal(Offset(0.0, buttonBox.size.height / 2.0)),
selectedItemBox.localToGlobal(Offset(0.0, selectedItemBox.size.height / 2.0)),
);
await tester.pumpWidget(buildFrame(
buttonAlignment: AlignmentDirectional.center,
menuAlignment: AlignmentDirectional.center,
));
selectedItemBox = tester.renderObject(find.text('enabled'));
// Should be center-center aligned, the icon size is 24.0 pixels.
expect(
buttonBox.localToGlobal(Offset((buttonBox.size.width -24.0) / 2.0, buttonBox.size.height / 2.0)),
offsetMoreOrLessEquals(selectedItemBox.localToGlobal(Offset(selectedItemBox.size.width / 2.0, selectedItemBox.size.height / 2.0))),
);
// Open dropdown.
await tester.tap(find.text('enabled').hitTestable());
await tester.pumpAndSettle();
final RenderBox selectedItemBoxInMenu = tester.renderObjectList<RenderBox>(find.text('enabled')).toList()[1];
final Finder menu = find.byWidgetPredicate((Widget widget) {
return widget.runtimeType.toString().startsWith('_DropdownMenu<');
});
final Rect menuRect = tester.getRect(menu);
final Offset center = selectedItemBoxInMenu.localToGlobal(
Offset(selectedItemBoxInMenu.size.width / 2.0, selectedItemBoxInMenu.size.height / 2.0)
);
expect(center.dx, moreOrLessEquals(menuRect.topCenter.dx));
expect(
center.dy,
moreOrLessEquals(selectedItemBox.localToGlobal(Offset(selectedItemBox.size.width / 2.0, selectedItemBox.size.height / 2.0)).dy),
);
});
group('feedback', () {
late FeedbackTester feedback;
setUp(() {
feedback = FeedbackTester();
});
tearDown(() {
feedback.dispose();
});
Widget feedbackBoilerplate({bool? enableFeedback}) {
return MaterialApp(
home : Material(
child: DropdownButton<String>(
value: 'One',
enableFeedback: enableFeedback,
underline: Container(
height: 2,
color: Colors.deepPurpleAccent,
),
onChanged: (String? value) {},
items: <String>['One', 'Two'].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
),
);
}
testWidgets('Dropdown with enabled feedback', (WidgetTester tester) async {
const bool enableFeedback = true;
await tester.pumpWidget(feedbackBoilerplate(enableFeedback: enableFeedback));
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(InkWell, 'One').last);
await tester.pumpAndSettle();
expect(feedback.clickSoundCount, 1);
expect(feedback.hapticCount, 0);
});
testWidgets('Dropdown with disabled feedback', (WidgetTester tester) async {
const bool enableFeedback = false;
await tester.pumpWidget(feedbackBoilerplate(enableFeedback: enableFeedback));
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(InkWell, 'One').last);
await tester.pumpAndSettle();
expect(feedback.clickSoundCount, 0);
expect(feedback.hapticCount, 0);
});
testWidgets('Dropdown with enabled feedback by default', (WidgetTester tester) async {
await tester.pumpWidget(feedbackBoilerplate());
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(InkWell, 'Two').last);
await tester.pumpAndSettle();
expect(feedback.clickSoundCount, 1);
expect(feedback.hapticCount, 0);
});
});
testWidgets('DropdownButton changes mouse cursor when hovered', (WidgetTester tester) async {
const Key key = Key('testDropdownButton');
await tester.pumpWidget(
MaterialApp(
home: Material(
child: DropdownButton<String>(
key: key,
onChanged: (String? newValue) {},
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList()
),
),
),
);
final Finder dropdownButtonFinder = find.byKey(key);
final Offset onDropdownButton = tester.getCenter(dropdownButtonFinder);
final Offset offDropdownButton = tester.getBottomRight(dropdownButtonFinder) + const Offset(1, 1);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: onDropdownButton);
await tester.pump();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
await gesture.moveTo(offDropdownButton);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
// Test that mouse cursor doesn't change when button is disabled
await tester.pumpWidget(
MaterialApp(
home: Material(
child: DropdownButton<String>(
key: key,
onChanged: null,
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
),
),
);
await gesture.moveTo(onDropdownButton);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
await gesture.moveTo(offDropdownButton);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
});
testWidgets('Conflicting scrollbars are not applied by ScrollBehavior to Dropdown', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/83819
// Open the dropdown menu
final Key buttonKey = UniqueKey();
await tester.pumpWidget(buildFrame(
buttonKey: buttonKey,
value: null, // nothing selected
items: List<String>.generate(100, (int index) => index.toString()),
onChanged: onChanged,
));
await tester.tap(find.byKey(buttonKey));
await tester.pump();
await tester.pumpAndSettle(); // finish the menu animation
// The inherited ScrollBehavior should not apply Scrollbars since they are
// already built in to the widget. For iOS platform, ScrollBar directly returns
// CupertinoScrollbar
expect(find.byType(CupertinoScrollbar), debugDefaultTargetPlatformOverride == TargetPlatform.iOS ? findsOneWidget : findsNothing);
expect(find.byType(Scrollbar), findsOneWidget);
expect(find.byType(RawScrollbar), findsNothing);
}, variant: TargetPlatformVariant.all());
testWidgets('borderRadius property works properly', (WidgetTester tester) async {
const double radius = 20.0;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Center(
child: DropdownButton<String>(
borderRadius: BorderRadius.circular(radius),
value: 'One',
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (_) { },
),
),
),
),
);
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
expect(
find.ancestor(
of: find.text('One').last,
matching: find.byType(CustomPaint),
).at(2),
paints
..save()
..rrect()
..rrect()
..rrect()
..rrect(rrect: const RRect.fromLTRBXY(0.0, 0.0, 144.0, 208.0, radius, radius)),
);
});
// Regression test for https://github.com/flutter/flutter/issues/88574
testWidgets("specifying itemHeight affects popup menu items' height", (WidgetTester tester) async {
const String value = 'One';
const double itemHeight = 80;
final List<DropdownMenuItem<String>> menuItems = <String>[
value,
'Two',
'Free',
'Four',
].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: DropdownButton<String>(
value: value,
itemHeight: itemHeight,
onChanged: (_) {},
items: menuItems,
),
),
),
),
);
await tester.tap(find.text(value));
await tester.pumpAndSettle();
for (final DropdownMenuItem<String> item in menuItems) {
final Iterable<Element> elements = tester.elementList(find.byWidget(item));
for (final Element element in elements){
expect(element.size!.height, itemHeight);
}
}
});
// Regression test for https://github.com/flutter/flutter/issues/92438
testWidgets('Do not throw due to the double precision', (WidgetTester tester) async {
const String value = 'One';
const double itemHeight = 77.701;
final List<DropdownMenuItem<String>> menuItems = <String>[
value,
'Two',
'Free',
].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: DropdownButton<String>(
value: value,
itemHeight: itemHeight,
onChanged: (_) {},
items: menuItems,
),
),
),
),
);
await tester.tap(find.text(value));
await tester.pumpAndSettle();
expect(tester.takeException(), null);
});
testWidgets('BorderRadius property works properly for DropdownButtonFormField', (WidgetTester tester) async {
const double radius = 20.0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: DropdownButtonFormField<String>(
borderRadius: BorderRadius.circular(radius),
value: 'One',
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (_) { },
),
),
),
),
);
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
expect(
find.ancestor(
of: find.text('One').last,
matching: find.byType(CustomPaint),
).at(2),
paints
..save()
..rrect()
..rrect()
..rrect()
..rrect(rrect: const RRect.fromLTRBXY(0.0, 0.0, 800.0, 208.0, radius, radius)),
);
});
testWidgets('DropdownButton hint alignment', (WidgetTester tester) async {
const String hintText = 'hint';
// AlignmentDirectional.centerStart (default)
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerStart,
isExpanded: false,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.topStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topStart,
isExpanded: false,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.bottomStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomStart,
isExpanded: false,
));
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dy, 350.0);
// AlignmentDirectional.center
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.center,
isExpanded: false,
));
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dx, 388.0);
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dy, 300.0);
// AlignmentDirectional.topEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topEnd,
isExpanded: false,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.centerEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerEnd,
isExpanded: false,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.bottomEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomEnd,
isExpanded: false,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 334.0);
// DropdownButton with `isExpanded: true`
// AlignmentDirectional.centerStart (default)
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerStart,
isExpanded: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.topStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topStart,
isExpanded: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.bottomStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomStart,
isExpanded: true,
));
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dy, 350.0);
// AlignmentDirectional.center
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.center,
isExpanded: true,
));
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dx, 388.0);
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dy, 300.0);
// AlignmentDirectional.topEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topEnd,
isExpanded: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.centerEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerEnd,
isExpanded: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.bottomEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomEnd,
isExpanded: true,
));
expect(tester.getBottomRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getBottomRight(find.text(hintText,skipOffstage: false)).dy, 350.0);
});
testWidgets('DropdownButton hint alignment with selectedItemBuilder', (WidgetTester tester) async {
const String hintText = 'hint';
// AlignmentDirectional.centerStart (default)
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerStart,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.topStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topStart,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.bottomStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomStart,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dx, 348.0);
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dy, 350.0);
// AlignmentDirectional.center
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.center,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dx, 388.0);
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dy, 300.0);
// AlignmentDirectional.topEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topEnd,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.centerEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerEnd,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.bottomEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomEnd,
isExpanded: false,
enableSelectedItemBuilder: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 428.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 334.0);
// DropdownButton with `isExpanded: true`
// AlignmentDirectional.centerStart (default)
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerStart,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.topStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topStart,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getTopLeft(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.bottomStart
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomStart,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dx, 0.0);
expect(tester.getBottomLeft(find.text(hintText,skipOffstage: false)).dy, 350.0);
// AlignmentDirectional.center
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.center,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dx, 388.0);
expect(tester.getCenter(find.text(hintText,skipOffstage: false)).dy, 300.0);
// AlignmentDirectional.topEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.topEnd,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 250.0);
// AlignmentDirectional.centerEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.centerEnd,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getTopRight(find.text(hintText,skipOffstage: false)).dy, 292.0);
// AlignmentDirectional.bottomEnd
await tester.pumpWidget(buildDropdownWithHint(
alignment: AlignmentDirectional.bottomEnd,
isExpanded: true,
enableSelectedItemBuilder: true,
));
expect(tester.getBottomRight(find.text(hintText,skipOffstage: false)).dx, 776.0);
expect(tester.getBottomRight(find.text(hintText,skipOffstage: false)).dy, 350.0);
});
testWidgets('BorderRadius property clips dropdown button and dropdown menu', (WidgetTester tester) async {
const double radius = 20.0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: DropdownButtonFormField<String>(
borderRadius: BorderRadius.circular(radius),
value: 'One',
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
onChanged: (_) { },
),
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(DropdownButtonFormField<String>)));
await tester.pumpAndSettle();
final RenderObject inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rrect(rrect: RRect.fromLTRBR(0.0, 276.0, 800.0, 324.0, const Radius.circular(radius))));
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
final RenderClipRRect renderClip = tester.allRenderObjects.whereType<RenderClipRRect>().first;
expect(renderClip.borderRadius, BorderRadius.circular(radius));
});
testWidgets('Size of DropdownButton with padding', (WidgetTester tester) async {
const double padVertical = 5;
const double padHorizontal = 10;
final Key buttonKey = UniqueKey();
EdgeInsets? padding;
Widget build() => buildFrame(buttonKey: buttonKey, onChanged: onChanged, padding: padding);
await tester.pumpWidget(build());
final RenderBox buttonBoxNoPadding = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxNoPadding.attached);
final Size noPaddingSize = Size.copy(buttonBoxNoPadding.size);
padding = const EdgeInsets.symmetric(vertical: padVertical, horizontal: padHorizontal);
await tester.pumpWidget(build());
final RenderBox buttonBoxPadded = tester.renderObject<RenderBox>(find.byKey(buttonKey));
assert(buttonBoxPadded.attached);
final Size paddedSize = Size.copy(buttonBoxPadded.size);
// dropdowns with padding should be that much larger than with no padding
expect(noPaddingSize.height, equals(paddedSize.height - padVertical * 2));
expect(noPaddingSize.width, equals(paddedSize.width - padHorizontal * 2));
});
}
| flutter/packages/flutter/test/material/dropdown_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/dropdown_test.dart",
"repo_id": "flutter",
"token_count": 61403
} | 718 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('Basic floating action button locations', () {
testWidgets('still animates motion when the floating action button is null', (WidgetTester tester) async {
await tester.pumpWidget(_buildFrame(fab: null));
expect(find.byType(FloatingActionButton), findsNothing);
expect(tester.binding.transientCallbackCount, 0);
await tester.pumpWidget(_buildFrame(fab: null, location: FloatingActionButtonLocation.endFloat));
expect(find.byType(FloatingActionButton), findsNothing);
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpWidget(_buildFrame(fab: null, location: FloatingActionButtonLocation.centerFloat));
expect(find.byType(FloatingActionButton), findsNothing);
expect(tester.binding.transientCallbackCount, greaterThan(0));
});
testWidgets('moves fab from center to end and back', (WidgetTester tester) async {
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.endFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 356.0));
expect(tester.binding.transientCallbackCount, 0);
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.centerFloat));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(400.0, 356.0));
expect(tester.binding.transientCallbackCount, 0);
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.endFloat));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 356.0));
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('moves to and from custom-defined positions', (WidgetTester tester) async {
await tester.pumpWidget(_buildFrame(location: const _StartTopFloatingActionButtonLocation()));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(44.0, 56.0));
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.centerFloat));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(400.0, 356.0));
expect(tester.binding.transientCallbackCount, 0);
await tester.pumpWidget(_buildFrame(location: const _StartTopFloatingActionButtonLocation()));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(44.0, 56.0));
expect(tester.binding.transientCallbackCount, 0);
});
group('interrupts in-progress animations without jumps', () {
_GeometryListener? geometryListener;
ScaffoldGeometry? geometry;
_GeometryListenerState? listenerState;
Size? previousRect;
Iterable<double>? previousRotations;
// The maximum amounts we expect the fab width and height to change
// during one step of a transition.
const double maxDeltaWidth = 12.5;
const double maxDeltaHeight = 12.5;
// The maximum amounts we expect the fab icon to rotate during one step
// of a transition.
const double maxDeltaRotation = 0.09;
// We'll listen to the Scaffold's geometry for any 'jumps' to detect
// changes in the size and rotation of the fab.
void setupListener(WidgetTester tester) {
// Measure the delta in width and height of the fab, and check that it never grows
// by more than the expected maximum deltas.
void check() {
geometry = listenerState?.cache.value;
final Size? currentRect = geometry?.floatingActionButtonArea?.size;
// Measure the delta in width and height of the rect, and check that
// it never grows by more than a safe amount.
if (previousRect != null && currentRect != null) {
final double deltaWidth = currentRect.width - previousRect!.width;
final double deltaHeight = currentRect.height - previousRect!.height;
expect(
deltaWidth.abs(),
lessThanOrEqualTo(maxDeltaWidth),
reason: "The Floating Action Button's width should not change "
'faster than $maxDeltaWidth per animation step.\n'
'Previous rect: $previousRect, current rect: $currentRect',
);
expect(
deltaHeight.abs(),
lessThanOrEqualTo(maxDeltaHeight),
reason: "The Floating Action Button's width should not change "
'faster than $maxDeltaHeight per animation step.\n'
'Previous rect: $previousRect, current rect: $currentRect',
);
}
previousRect = currentRect;
// Measure the delta in rotation.
// Check that it never grows by more than a safe amount.
//
// There may be multiple transitions all active at
// the same time. We are concerned only with the closest one.
final Iterable<RotationTransition> rotationTransitions = tester.widgetList(
find.byType(RotationTransition),
);
final Iterable<double> currentRotations = rotationTransitions.map((RotationTransition t) => t.turns.value);
if (previousRotations != null && previousRotations!.isNotEmpty && currentRotations.isNotEmpty
&& previousRect != null && currentRect != null) {
final List<double> deltas = <double>[];
for (final double currentRotation in currentRotations) {
late double minDelta;
for (final double previousRotation in previousRotations!) {
final double delta = (previousRotation - currentRotation).abs();
minDelta = delta;
minDelta = min(delta, minDelta);
}
deltas.add(minDelta);
}
if (deltas.where((double delta) => delta < maxDeltaRotation).isEmpty) {
fail(
"The Floating Action Button's rotation should not change "
'faster than $maxDeltaRotation per animation step.\n'
'Detected deltas were: $deltas\n'
'Previous values: $previousRotations, current values: $currentRotations\n'
'Previous rect: $previousRect, current rect: $currentRect',
);
}
}
previousRotations = currentRotations;
}
listenerState = tester.state(find.byType(_GeometryListener));
listenerState!.geometryListenable!.addListener(check);
}
setUp(() {
// We create the geometry listener here, but it can only be set up
// after it is pumped into the widget tree and a tester is
// available.
geometryListener = const _GeometryListener();
geometry = null;
listenerState = null;
previousRect = null;
previousRotations = null;
});
testWidgets('moving the fab to centerFloat', (WidgetTester tester) async {
// Create a scaffold with the fab at endFloat
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.endFloat, listener: geometryListener));
setupListener(tester);
// Move the fab to centerFloat'
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.centerFloat, listener: geometryListener));
await tester.pumpAndSettle();
});
testWidgets('interrupting motion towards the StartTop location.', (WidgetTester tester) async {
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.centerFloat, listener: geometryListener));
setupListener(tester);
// Move the fab to the top start after creating the fab.
await tester.pumpWidget(_buildFrame(location: const _StartTopFloatingActionButtonLocation(), listener: geometryListener));
await tester.pump(kFloatingActionButtonSegue ~/ 2);
// Interrupt motion to move to the end float
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.endFloat, listener: geometryListener));
await tester.pumpAndSettle();
});
testWidgets('interrupting entrance to remove the fab.', (WidgetTester tester) async {
await tester.pumpWidget(_buildFrame(fab: null, location: FloatingActionButtonLocation.centerFloat, listener: geometryListener));
setupListener(tester);
// Animate the fab in.
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.endFloat, listener: geometryListener));
await tester.pump(kFloatingActionButtonSegue ~/ 2);
// Remove the fab.
await tester.pumpWidget(
_buildFrame(
fab: null,
location: FloatingActionButtonLocation.endFloat,
listener: geometryListener,
),
);
await tester.pumpAndSettle();
});
testWidgets('interrupting entrance of a new fab.', (WidgetTester tester) async {
await tester.pumpWidget(
_buildFrame(
fab: null,
location: FloatingActionButtonLocation.endFloat,
listener: geometryListener,
),
);
setupListener(tester);
// Bring in a new fab.
await tester.pumpWidget(_buildFrame(location: FloatingActionButtonLocation.centerFloat, listener: geometryListener));
await tester.pump(kFloatingActionButtonSegue ~/ 2);
// Interrupt motion to move the fab.
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endFloat,
listener: geometryListener,
),
);
await tester.pumpAndSettle();
});
});
});
testWidgets('Docked floating action button locations', (WidgetTester tester) async {
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endDocked,
bab: const SizedBox(height: 100.0),
viewInsets: EdgeInsets.zero,
),
);
// Scaffold 800x600, FAB is 56x56, BAB is 800x100, FAB's center is
// at the top of the BAB.
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 500.0));
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.centerDocked,
bab: const SizedBox(height: 100.0),
viewInsets: EdgeInsets.zero,
),
);
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(400.0, 500.0));
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endDocked,
bab: const SizedBox(height: 100.0),
viewInsets: EdgeInsets.zero,
),
);
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 500.0));
});
testWidgets('Docked floating action button locations: no BAB, small BAB', (WidgetTester tester) async {
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endDocked,
viewInsets: EdgeInsets.zero,
),
);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 572.0));
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endDocked,
bab: const SizedBox(height: 16.0),
viewInsets: EdgeInsets.zero,
),
);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 572.0));
});
testWidgets('Contained floating action button locations', (WidgetTester tester) async {
await tester.pumpWidget(
_buildFrame(
location: FloatingActionButtonLocation.endContained,
bab: const SizedBox(height: 100.0),
viewInsets: EdgeInsets.zero,
),
);
// Scaffold 800x600, FAB is 56x56, BAB is 800x100, FAB's center is
// at the top of the BAB.
// Formula: scaffold height - BAB height + FAB height / 2 + BAB top & bottom margins.
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(756.0, 550.0));
});
testWidgets('Mini-start-top floating action button location', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
floatingActionButton: FloatingActionButton(onPressed: () { }, mini: true),
floatingActionButtonLocation: FloatingActionButtonLocation.miniStartTop,
body: const Column(
children: <Widget>[
ListTile(
leading: CircleAvatar(),
),
],
),
),
),
);
expect(tester.getCenter(find.byType(FloatingActionButton)).dx, tester.getCenter(find.byType(CircleAvatar)).dx);
expect(tester.getCenter(find.byType(FloatingActionButton)).dy, kToolbarHeight);
});
testWidgets('Start-top floating action button location LTR', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
floatingActionButton: const FloatingActionButton(onPressed: null),
floatingActionButtonLocation: FloatingActionButtonLocation.startTop,
),
),
);
expect(tester.getRect(find.byType(FloatingActionButton)), rectMoreOrLessEquals(const Rect.fromLTWH(16.0, 28.0, 56.0, 56.0)));
});
testWidgets('End-top floating action button location RTL', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(),
floatingActionButton: const FloatingActionButton(onPressed: null),
floatingActionButtonLocation: FloatingActionButtonLocation.endTop,
),
),
),
);
expect(tester.getRect(find.byType(FloatingActionButton)), rectMoreOrLessEquals(const Rect.fromLTWH(16.0, 28.0, 56.0, 56.0)));
});
testWidgets('Start-top floating action button location RTL', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(),
floatingActionButton: const FloatingActionButton(onPressed: null),
floatingActionButtonLocation: FloatingActionButtonLocation.startTop,
),
),
),
);
expect(tester.getRect(find.byType(FloatingActionButton)), rectMoreOrLessEquals(const Rect.fromLTWH(800.0 - 56.0 - 16.0, 28.0, 56.0, 56.0)));
});
testWidgets('End-top floating action button location LTR', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
floatingActionButton: const FloatingActionButton(onPressed: null),
floatingActionButtonLocation: FloatingActionButtonLocation.endTop,
),
),
);
expect(tester.getRect(find.byType(FloatingActionButton)), rectMoreOrLessEquals(const Rect.fromLTWH(800.0 - 56.0 - 16.0, 28.0, 56.0, 56.0)));
});
group('New Floating Action Button Locations', () {
testWidgets('startTop', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _topOffsetY));
});
testWidgets('centerTop', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _topOffsetY));
});
testWidgets('endTop', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX, _topOffsetY));
});
testWidgets('startFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _floatOffsetY));
});
testWidgets('centerFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _floatOffsetY));
});
testWidgets('endFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX, _floatOffsetY));
});
testWidgets('startDocked', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _dockedOffsetY));
});
testWidgets('centerDocked', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _dockedOffsetY));
});
testWidgets('endDocked', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX, _dockedOffsetY));
});
testWidgets('endContained', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endContained));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX, _containedOffsetY));
});
testWidgets('miniStartTop', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniStartTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniLeftOffsetX, _topOffsetY));
});
testWidgets('miniEndTop', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniEndTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniRightOffsetX, _topOffsetY));
});
testWidgets('miniStartFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniStartFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniLeftOffsetX, _miniFloatOffsetY));
});
testWidgets('miniCenterFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniCenterFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _miniFloatOffsetY));
});
testWidgets('miniEndFloat', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniEndFloat));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniRightOffsetX, _miniFloatOffsetY));
});
testWidgets('miniStartDocked', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniStartDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniLeftOffsetX, _dockedOffsetY));
});
testWidgets('miniEndDocked', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniEndDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniRightOffsetX, _dockedOffsetY));
});
// Test a few RTL cases.
testWidgets('endTop, RTL', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endTop, textDirection: TextDirection.rtl));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _topOffsetY));
});
testWidgets('miniStartFloat, RTL', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.miniStartFloat, textDirection: TextDirection.rtl));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_miniRightOffsetX, _miniFloatOffsetY));
});
});
group('Custom Floating Action Button Locations', () {
testWidgets('Almost end float', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(_AlmostEndFloatFabLocation()));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX - 50, _floatOffsetY));
});
testWidgets('Almost end float, RTL', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(_AlmostEndFloatFabLocation(), textDirection: TextDirection.rtl));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX + 50, _floatOffsetY));
});
testWidgets('Quarter end top', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(_QuarterEndTopFabLocation()));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX * 0.75 + _leftOffsetX * 0.25, _topOffsetY));
});
testWidgets('Quarter end top, RTL', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(_QuarterEndTopFabLocation(), textDirection: TextDirection.rtl));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX * 0.75 + _rightOffsetX * 0.25, _topOffsetY));
});
});
group('Moves involving new locations', () {
testWidgets('Moves between new locations and new locations', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerTop));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _topOffsetY));
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startFloat));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _floatOffsetY));
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startDocked));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _dockedOffsetY));
});
testWidgets('Moves between new locations and old locations', (WidgetTester tester) async {
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.endDocked));
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_rightOffsetX, _dockedOffsetY));
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.startDocked));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_leftOffsetX, _dockedOffsetY));
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerFloat));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _floatOffsetY));
await tester.pumpWidget(_singleFabScaffold(FloatingActionButtonLocation.centerTop));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pumpAndSettle();
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)), const Offset(_centerOffsetX, _topOffsetY));
});
testWidgets('Moves between new locations and old locations with custom animator', (WidgetTester tester) async {
final FloatingActionButtonAnimator animator = _LinearMovementFabAnimator();
const Offset begin = Offset(_centerOffsetX, _topOffsetY);
const Offset end = Offset(_rightOffsetX - 50, _floatOffsetY);
final Duration animationDuration = kFloatingActionButtonSegue * 2;
await tester.pumpWidget(_singleFabScaffold(
FloatingActionButtonLocation.centerTop,
animator: animator,
));
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(tester.binding.transientCallbackCount, 0);
await tester.pumpWidget(_singleFabScaffold(
_AlmostEndFloatFabLocation(),
animator: animator,
));
expect(tester.binding.transientCallbackCount, greaterThan(0));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)), offsetMoreOrLessEquals(begin * 0.75 + end * 0.25));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)), offsetMoreOrLessEquals(begin * 0.5 + end * 0.5));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)), offsetMoreOrLessEquals(begin * 0.25 + end * 0.75));
await tester.pumpAndSettle();
expect(tester.getCenter(find.byType(FloatingActionButton)), end);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('Animator can be updated', (WidgetTester tester) async {
FloatingActionButtonAnimator fabAnimator = FloatingActionButtonAnimator.scaling;
FloatingActionButtonLocation fabLocation = FloatingActionButtonLocation.startFloat;
final Duration animationDuration = kFloatingActionButtonSegue * 2;
await tester.pumpWidget(_singleFabScaffold(
fabLocation,
animator: fabAnimator,
));
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(tester.binding.transientCallbackCount, 0);
expect(tester.getCenter(find.byType(FloatingActionButton)).dx, 44.0);
fabLocation = FloatingActionButtonLocation.endFloat;
await tester.pumpWidget(_singleFabScaffold(
fabLocation,
animator: fabAnimator,
));
expect(tester.getTopLeft(find.byType(FloatingActionButton)).dx, lessThan(16.0));
await tester.pump(animationDuration * 0.25);
expect(tester.getTopLeft(find.byType(FloatingActionButton)).dx, greaterThan(16));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)).dx, 756.0);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx, lessThan(800 - 16));
await tester.pump(animationDuration * 0.25);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx, lessThan(800 - 16));
await tester.pump(animationDuration * 0.25);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx, equals(800 - 16));
fabLocation = FloatingActionButtonLocation.startFloat;
fabAnimator = _NoScalingFabAnimator();
await tester.pumpWidget(_singleFabScaffold(
fabLocation,
animator: fabAnimator,
));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)).dx, 756.0);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx, equals(800 - 16));
await tester.pump(animationDuration * 0.25);
expect(tester.getCenter(find.byType(FloatingActionButton)).dx, 44.0);
expect(tester.getTopLeft(find.byType(FloatingActionButton)).dx, lessThan(16.0));
});
});
group('Locations account for safe interactive areas', () {
Widget buildTest(
FloatingActionButtonLocation location,
MediaQueryData data,
Key key, {
bool mini = false,
bool appBar = false,
bool bottomNavigationBar = false,
bool bottomSheet = false,
bool resizeToAvoidBottomInset = true,
}) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: MediaQuery(
data: data,
child: Scaffold(
resizeToAvoidBottomInset: resizeToAvoidBottomInset,
bottomSheet: bottomSheet ? const SizedBox(
height: 100,
child: Center(child: Text('BottomSheet')),
) : null,
appBar: appBar ? AppBar(title: const Text('Demo')) : null,
bottomNavigationBar: bottomNavigationBar ? BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.star),
label: '0',
),
BottomNavigationBarItem(
icon: Icon(Icons.star_border),
label: '1',
),
],
) : null,
floatingActionButtonLocation: location,
floatingActionButton: Builder(
builder: (BuildContext context) {
return FloatingActionButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('Snacky!')),
);
},
mini: mini,
key: key,
child: const Text('FAB'),
);
},
),
),
),
);
}
// Test float locations, for each (6), keyboard presented or not:
// - Default
// - with resizeToAvoidBottomInset: false
// - with BottomNavigationBar
// - with BottomNavigationBar and resizeToAvoidBottomInset: false
// - with BottomNavigationBar & BottomSheet
// - with BottomNavigationBar & BottomSheet, resizeToAvoidBottomInset: false
// - with BottomSheet
// - with BottomSheet and resizeToAvoidBottomInset: false
// - with SnackBar
Future<void> runFloatTests(
WidgetTester tester,
FloatingActionButtonLocation location, {
required Rect defaultRect,
required Rect bottomNavigationBarRect,
required Rect bottomSheetRect,
required Rect snackBarRect,
bool mini = false,
}) async {
const double keyboardHeight = 200.0;
const double viewPadding = 50.0;
final Key floatingActionButton = UniqueKey();
const double bottomNavHeight = 106.0;
// Default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(bottom: viewPadding)),
floatingActionButton,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect),
);
// Present keyboard and check position, should change
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect.translate(
0.0,
viewPadding - keyboardHeight,
)),
);
// With resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect),
);
// BottomNavigationBar default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
floatingActionButton,
bottomNavigationBar: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect),
);
// Present keyboard and check position, FAB position changes
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect.translate(
0.0,
-keyboardHeight + bottomNavHeight,
)),
);
// BottomNavigationBar with resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect),
);
// BottomNavigationBar + BottomSheet default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect.translate(
0.0,
-bottomNavHeight,
)),
);
// Present keyboard and check position, FAB position changes
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect.translate(
0.0,
-keyboardHeight,
)),
);
// BottomNavigationBar + BottomSheet with resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect.translate(
0.0,
-bottomNavHeight,
)),
);
// BottomSheet default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(bottom: viewPadding)),
floatingActionButton,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect),
);
// Present keyboard and check position, bottomSheet and FAB both resize
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect.translate(0.0, -keyboardHeight)),
);
// bottomSheet with resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default bottomSheet position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomSheet: true,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect),
);
// SnackBar default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(bottom: viewPadding)),
floatingActionButton,
mini: mini,
));
await tester.tap(find.byKey(floatingActionButton));
await tester.pumpAndSettle(); // Show SnackBar
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(snackBarRect),
);
// SnackBar when resized for presented keyboard
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
mini: mini,
));
await tester.tap(find.byKey(floatingActionButton));
await tester.pumpAndSettle(); // Show SnackBar
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(snackBarRect.translate(0.0, -keyboardHeight + kFloatingActionButtonMargin/2)),
);
}
testWidgets('startFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(16.0, 478.0, 72.0, 534.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(16.0, 422.0, 72.0, 478.0);
// Position relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(16.0, 472.0, 72.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(16.0, 478.0, 72.0, 534.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.startFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniStartFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(12.0, 490.0, 60.0, 538.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(12.0, 434.0, 60.0, 482.0);
// Positioned relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(12.0, 480.0, 60.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(12.0, 490.0, 60.0, 538.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.miniStartFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
testWidgets('centerFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(372.0, 478.0, 428.0, 534.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(372.0, 422.0, 428.0, 478.0);
// Positioned relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(372.0, 472.0, 428.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(372.0, 478.0, 428.0, 534.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.centerFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniCenterFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(376.0, 490.0, 424.0, 538.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(376.0, 434.0, 424.0, 482.0);
// Positioned relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(376.0, 480.0, 424.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(376.0, 490.0, 424.0, 538.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.miniCenterFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
testWidgets('endFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(728.0, 478.0, 784.0, 534.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(728.0, 422.0, 784.0, 478.0);
// Positioned relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(728.0, 472.0, 784.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(728.0, 478.0, 784.0, 534.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.endFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniEndFloat', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(740.0, 490.0, 788.0, 538.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(740.0, 434.0, 788.0, 482.0);
// Positioned relative to BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(740.0, 480.0, 788.0, 528.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(740.0, 490.0, 788.0, 538.0);
await runFloatTests(
tester,
FloatingActionButtonLocation.miniEndFloat,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
// Test docked locations, for each (6), keyboard presented or not.
// If keyboard is presented and resizeToAvoidBottomInset: true, test whether
// the FAB is away from the keyboard(and thus not clipped):
// - Default
// - Default with resizeToAvoidBottomInset: false
// - docked with BottomNavigationBar
// - docked with BottomNavigationBar and resizeToAvoidBottomInset: false
// - docked with BottomNavigationBar & BottomSheet
// - docked with BottomNavigationBar & BottomSheet, resizeToAvoidBottomInset: false
// - with SnackBar
Future<void> runDockedTests(
WidgetTester tester,
FloatingActionButtonLocation location, {
required Rect defaultRect,
required Rect bottomNavigationBarRect,
required Rect bottomSheetRect,
required Rect snackBarRect,
bool mini = false,
}) async {
const double keyboardHeight = 200.0;
const double viewPadding = 50.0;
const double bottomNavHeight = 106.0;
const double scaffoldHeight = 600.0;
final Key floatingActionButton = UniqueKey();
final double fabHeight = mini ? 48.0 : 56.0;
// Default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(bottom: viewPadding)),
floatingActionButton,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect),
);
// Present keyboard and check position, should change
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect.translate(
0.0,
viewPadding - keyboardHeight - kFloatingActionButtonMargin,
)),
);
// The FAB should be away from the keyboard
expect(
tester.getRect(find.byKey(floatingActionButton)).bottom,
lessThan(scaffoldHeight - keyboardHeight),
);
// With resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect),
);
// BottomNavigationBar default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
floatingActionButton,
bottomNavigationBar: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect),
);
// Present keyboard and check position, FAB position changes
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect.translate(
0.0,
bottomNavHeight + fabHeight / 2.0 - keyboardHeight - kFloatingActionButtonMargin - fabHeight,
)),
);
// The FAB should be away from the keyboard
expect(
tester.getRect(find.byKey(floatingActionButton)).bottom,
lessThan(scaffoldHeight - keyboardHeight),
);
// BottomNavigationBar with resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomNavigationBarRect),
);
// BottomNavigationBar + BottomSheet default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect),
);
// Present keyboard and check position, FAB position changes
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect.translate(
0.0,
-keyboardHeight + bottomNavHeight,
)),
);
// The FAB should be away from the keyboard
expect(
tester.getRect(find.byKey(floatingActionButton)).bottom,
lessThan(scaffoldHeight - keyboardHeight),
);
// BottomNavigationBar + BottomSheet with resizeToAvoidBottomInset: false
// With keyboard presented, should maintain default position
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
bottomNavigationBar: true,
bottomSheet: true,
resizeToAvoidBottomInset: false,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(bottomSheetRect),
);
// SnackBar default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(bottom: viewPadding)),
floatingActionButton,
mini: mini,
));
await tester.tap(find.byKey(floatingActionButton));
await tester.pumpAndSettle(); // Show SnackBar
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(snackBarRect),
);
// SnackBar with BottomNavigationBar
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
padding: EdgeInsets.only(bottom: viewPadding),
viewPadding: EdgeInsets.only(bottom: viewPadding),
),
floatingActionButton,
bottomNavigationBar: true,
mini: mini,
));
await tester.tap(find.byKey(floatingActionButton));
await tester.pumpAndSettle(); // Show SnackBar
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(snackBarRect.translate(0.0, -bottomNavHeight)),
);
// SnackBar when resized for presented keyboard
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: viewPadding),
viewInsets: EdgeInsets.only(bottom: keyboardHeight),
),
floatingActionButton,
mini: mini,
));
await tester.tap(find.byKey(floatingActionButton));
await tester.pumpAndSettle(); // Show SnackBar
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(snackBarRect.translate(0.0, -keyboardHeight)),
);
// The FAB should be away from the keyboard
expect(
tester.getRect(find.byKey(floatingActionButton)).bottom,
lessThan(scaffoldHeight - keyboardHeight),
);
}
testWidgets('startDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(16.0, 494.0, 72.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(16.0, 466.0, 72.0, 522.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(16.0, 366.0, 72.0, 422.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(16.0, 486.0, 72.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.startDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniStartDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(12.0, 502.0, 60.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(12.0, 470.0, 60.0, 518.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(12.0, 370.0, 60.0, 418.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(12.0, 494.0, 60.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.miniStartDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
testWidgets('centerDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(372.0, 494.0, 428.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(372.0, 466.0, 428.0, 522.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(372.0, 366.0, 428.0, 422.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(372.0, 486.0, 428.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.centerDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniCenterDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(376.0, 502.0, 424.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(376.0, 470.0, 424.0, 518.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(376.0, 370.0, 424.0, 418.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(376.0, 494.0, 424.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.miniCenterDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
testWidgets('endDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(728.0, 494.0, 784.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(728.0, 466.0, 784.0, 522.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(728.0, 366.0, 784.0, 422.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(728.0, 486.0, 784.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.endDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
);
});
testWidgets('miniEndDocked', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(740.0, 502.0, 788.0, 550.0);
// Positioned relative to BottomNavigationBar
const Rect bottomNavigationBarRect = Rect.fromLTRB(740.0, 470.0, 788.0, 518.0);
// Positioned relative to BottomNavigationBar & BottomSheet
const Rect bottomSheetRect = Rect.fromLTRB(740.0, 370.0, 788.0, 418.0);
// Positioned relative to SnackBar
const Rect snackBarRect = Rect.fromLTRB(740.0, 494.0, 788.0, 542.0);
await runDockedTests(
tester,
FloatingActionButtonLocation.miniEndDocked,
defaultRect: defaultRect,
bottomNavigationBarRect: bottomNavigationBarRect,
bottomSheetRect: bottomSheetRect,
snackBarRect: snackBarRect,
mini: true,
);
});
// Test top locations, for each (6):
// - Default
// - with an AppBar
Future<void> runTopTests(
WidgetTester tester,
FloatingActionButtonLocation location, {
required Rect defaultRect,
required Rect appBarRect,
bool mini = false,
}) async {
const double viewPadding = 50.0;
final Key floatingActionButton = UniqueKey();
// Default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(top: viewPadding)),
floatingActionButton,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(defaultRect),
);
// AppBar default
await tester.pumpWidget(buildTest(
location,
const MediaQueryData(viewPadding: EdgeInsets.only(top: viewPadding)),
floatingActionButton,
appBar: true,
mini: mini,
));
expect(
tester.getRect(find.byKey(floatingActionButton)),
rectMoreOrLessEquals(appBarRect),
);
}
testWidgets('startTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(16.0, 50.0, 72.0, 106.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(16.0, 28.0, 72.0, 84.0);
await runTopTests(
tester,
FloatingActionButtonLocation.startTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
);
});
testWidgets('miniStartTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(12.0, 50.0, 60.0, 98.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(12.0, 32.0, 60.0, 80.0);
await runTopTests(
tester,
FloatingActionButtonLocation.miniStartTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
mini: true,
);
});
testWidgets('centerTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(372.0, 50.0, 428.0, 106.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(372.0, 28.0, 428.0, 84.0);
await runTopTests(
tester,
FloatingActionButtonLocation.centerTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
);
});
testWidgets('miniCenterTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(376.0, 50.0, 424.0, 98.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(376.0, 32.0, 424.0, 80.0);
await runTopTests(
tester,
FloatingActionButtonLocation.miniCenterTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
mini: true,
);
});
testWidgets('endTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(728.0, 50.0, 784.0, 106.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(728.0, 28.0, 784.0, 84.0);
await runTopTests(
tester,
FloatingActionButtonLocation.endTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
);
});
testWidgets('miniEndTop', (WidgetTester tester) async {
const Rect defaultRect = Rect.fromLTRB(740.0, 50.0, 788.0, 98.0);
// Positioned relative to AppBar
const Rect appBarRect = Rect.fromLTRB(740.0, 32.0, 788.0, 80.0);
await runTopTests(
tester,
FloatingActionButtonLocation.miniEndTop,
defaultRect: defaultRect,
appBarRect: appBarRect,
mini: true,
);
});
});
}
class _GeometryListener extends StatefulWidget {
const _GeometryListener();
@override
State createState() => _GeometryListenerState();
}
class _GeometryListenerState extends State<_GeometryListener> {
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: cache,
);
}
int numNotifications = 0;
ValueListenable<ScaffoldGeometry>? geometryListenable;
late _GeometryCachePainter cache;
@override
void didChangeDependencies() {
super.didChangeDependencies();
final ValueListenable<ScaffoldGeometry> newListenable = Scaffold.geometryOf(context);
if (geometryListenable == newListenable) {
return;
}
if (geometryListenable != null) {
geometryListenable!.removeListener(onGeometryChanged);
}
geometryListenable = newListenable;
geometryListenable!.addListener(onGeometryChanged);
cache = _GeometryCachePainter(geometryListenable!);
}
void onGeometryChanged() {
numNotifications += 1;
}
}
const double _leftOffsetX = 44.0;
const double _centerOffsetX = 400.0;
const double _rightOffsetX = 756.0;
const double _miniLeftOffsetX = _leftOffsetX - kMiniButtonOffsetAdjustment;
const double _miniRightOffsetX = _rightOffsetX + kMiniButtonOffsetAdjustment;
const double _topOffsetY = 56.0;
const double _floatOffsetY = 500.0;
const double _dockedOffsetY = 544.0;
const double _containedOffsetY = 544.0 + 56.0 / 2;
const double _miniFloatOffsetY = _floatOffsetY + kMiniButtonOffsetAdjustment;
Widget _singleFabScaffold(FloatingActionButtonLocation location, {
bool useMaterial3 = false,
FloatingActionButtonAnimator? animator,
bool mini = false,
TextDirection textDirection = TextDirection.ltr,
}) {
return MaterialApp(
theme: ThemeData(useMaterial3: useMaterial3),
home: Directionality(
textDirection: textDirection,
child: Scaffold(
appBar: AppBar(
title: const Text('FloatingActionButtonLocation Test.'),
),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.school),
label: 'School',
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: () {},
mini: mini,
child: const Icon(Icons.beach_access),
),
floatingActionButtonLocation: location,
floatingActionButtonAnimator: animator,
),
),
);
}
// The Scaffold.geometryOf() value is only available at paint time.
// To fetch it for the tests we implement this CustomPainter that just
// caches the ScaffoldGeometry value in its paint method.
class _GeometryCachePainter extends CustomPainter {
_GeometryCachePainter(this.geometryListenable) : super(repaint: geometryListenable);
final ValueListenable<ScaffoldGeometry> geometryListenable;
late ScaffoldGeometry value;
@override
void paint(Canvas canvas, Size size) {
value = geometryListenable.value;
}
@override
bool shouldRepaint(_GeometryCachePainter oldDelegate) {
return true;
}
}
Widget _buildFrame({
FloatingActionButton? fab = const FloatingActionButton(
onPressed: null,
child: Text('1'),
),
FloatingActionButtonLocation? location,
_GeometryListener? listener,
TextDirection textDirection = TextDirection.ltr,
EdgeInsets viewInsets = const EdgeInsets.only(bottom: 200.0),
Widget? bab,
}) {
return Localizations(
locale: const Locale('en', 'us'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
child: Directionality(
textDirection: textDirection,
child: MediaQuery(
data: MediaQueryData(viewInsets: viewInsets),
child: Scaffold(
appBar: AppBar(title: const Text('FabLocation Test')),
floatingActionButtonLocation: location,
floatingActionButton: fab,
bottomNavigationBar: bab,
body: listener,
),
),
),
);
}
class _StartTopFloatingActionButtonLocation extends FloatingActionButtonLocation {
const _StartTopFloatingActionButtonLocation();
@override
Offset getOffset(ScaffoldPrelayoutGeometry scaffoldGeometry) {
double fabX;
switch (scaffoldGeometry.textDirection) {
case TextDirection.rtl:
final double startPadding = kFloatingActionButtonMargin + scaffoldGeometry.minInsets.right;
fabX = scaffoldGeometry.scaffoldSize.width - scaffoldGeometry.floatingActionButtonSize.width - startPadding;
case TextDirection.ltr:
final double startPadding = kFloatingActionButtonMargin + scaffoldGeometry.minInsets.left;
fabX = startPadding;
}
final double fabY = scaffoldGeometry.contentTop - (scaffoldGeometry.floatingActionButtonSize.height / 2.0);
return Offset(fabX, fabY);
}
}
class _AlmostEndFloatFabLocation extends StandardFabLocation
with FabEndOffsetX, FabFloatOffsetY {
@override
double getOffsetX (ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
final double directionalAdjustment =
scaffoldGeometry.textDirection == TextDirection.ltr ? -50.0 : 50.0;
return super.getOffsetX(scaffoldGeometry, adjustment) + directionalAdjustment;
}
}
class _QuarterEndTopFabLocation extends StandardFabLocation
with FabEndOffsetX, FabTopOffsetY {
@override
double getOffsetX (ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
return super.getOffsetX(scaffoldGeometry, adjustment) * 0.75
+ (FloatingActionButtonLocation.startFloat as StandardFabLocation)
.getOffsetX(scaffoldGeometry, adjustment) * 0.25;
}
}
class _LinearMovementFabAnimator extends FloatingActionButtonAnimator {
@override
Offset getOffset({required Offset begin, required Offset end, required double progress}) {
return Offset.lerp(begin, end, progress)!;
}
@override
Animation<double> getScaleAnimation({required Animation<double> parent}) {
return const AlwaysStoppedAnimation<double>(1.0);
}
@override
Animation<double> getRotationAnimation({required Animation<double> parent}) {
return const AlwaysStoppedAnimation<double>(1.0);
}
}
class _NoScalingFabAnimator extends FloatingActionButtonAnimator {
@override
Offset getOffset({required Offset begin, required Offset end, required double progress}) {
return progress < 0.5 ? begin : end;
}
@override
Animation<double> getScaleAnimation({required Animation<double> parent}) {
return const AlwaysStoppedAnimation<double>(1.0);
}
@override
Animation<double> getRotationAnimation({required Animation<double> parent}) {
return const AlwaysStoppedAnimation<double>(1.0);
}
}
| flutter/packages/flutter/test/material/floating_action_button_location_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/floating_action_button_location_test.dart",
"repo_id": "flutter",
"token_count": 27356
} | 719 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.