text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
// Any changes to this file should be reflected in the debugAssertAllGesturesVarsUnset()
// function below.
/// Whether to print the results of each hit test to the console.
///
/// When this is set, in debug mode, any time a hit test is triggered by the
/// [GestureBinding] the results are dumped to the console.
///
/// This has no effect in release builds.
bool debugPrintHitTestResults = false;
/// Whether to print the details of each mouse hover event to the console.
///
/// When this is set, in debug mode, any time a mouse hover event is triggered
/// by the [GestureBinding], the results are dumped to the console.
///
/// This has no effect in release builds, and only applies to mouse hover
/// events.
bool debugPrintMouseHoverEvents = false;
/// Prints information about gesture recognizers and gesture arenas.
///
/// This flag only has an effect in debug mode.
///
/// See also:
///
/// * [GestureArenaManager], the class that manages gesture arenas.
/// * [debugPrintRecognizerCallbacksTrace], for debugging issues with
/// gesture recognizers.
bool debugPrintGestureArenaDiagnostics = false;
/// Logs a message every time a gesture recognizer callback is invoked.
///
/// This flag only has an effect in debug mode.
///
/// This is specifically used by [GestureRecognizer.invokeCallback]. Gesture
/// recognizers that do not use this method to invoke callbacks may not honor
/// the [debugPrintRecognizerCallbacksTrace] flag.
///
/// See also:
///
/// * [debugPrintGestureArenaDiagnostics], for debugging issues with gesture
/// arenas.
bool debugPrintRecognizerCallbacksTrace = false;
/// Whether to print the resampling margin to the console.
///
/// When this is set, in debug mode, any time resampling is triggered by the
/// [GestureBinding] the resampling margin is dumped to the console. The
/// resampling margin is the delta between the time of the last received
/// touch event and the current sample time. Positive value indicates that
/// resampling is effective and the resampling offset can potentially be
/// reduced for improved latency. Negative value indicates that resampling
/// is failing and resampling offset needs to be increased for smooth
/// touch event processing.
///
/// This has no effect in release builds.
bool debugPrintResamplingMargin = false;
/// Returns true if none of the gestures library debug variables have been changed.
///
/// This function is used by the test framework to ensure that debug variables
/// haven't been inadvertently changed.
///
/// See [the gestures library](gestures/gestures-library.html) for a complete
/// list.
bool debugAssertAllGesturesVarsUnset(String reason) {
assert(() {
if (debugPrintHitTestResults ||
debugPrintGestureArenaDiagnostics ||
debugPrintRecognizerCallbacksTrace ||
debugPrintResamplingMargin) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
| flutter/packages/flutter/lib/src/gestures/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/debug.dart",
"repo_id": "flutter",
"token_count": 846
} | 671 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'events.dart';
export 'events.dart' show PointerEvent;
/// A callback used by [PointerEventResampler.sample] and
/// [PointerEventResampler.stop] to process a resampled `event`.
typedef HandleEventCallback = void Function(PointerEvent event);
/// Class for pointer event resampling.
///
/// An instance of this class can be used to resample one sequence
/// of pointer events. Multiple instances are expected to be used for
/// multi-touch support. The sampling frequency and the sampling
/// offset is determined by the caller.
///
/// This can be used to get smooth touch event processing at the cost
/// of adding some latency. Devices with low frequency sensors or when
/// the frequency is not a multiple of the display frequency
/// (e.g., 120Hz input and 90Hz display) benefit from this.
///
/// The following pointer event types are supported:
/// [PointerAddedEvent], [PointerHoverEvent], [PointerDownEvent],
/// [PointerMoveEvent], [PointerCancelEvent], [PointerUpEvent],
/// [PointerRemovedEvent].
///
/// Resampling is currently limited to event position and delta. All
/// pointer event types except [PointerAddedEvent] will be resampled.
/// [PointerHoverEvent] and [PointerMoveEvent] will only be generated
/// when the position has changed.
class PointerEventResampler {
// Events queued for processing.
final Queue<PointerEvent> _queuedEvents = Queue<PointerEvent>();
// Pointer state required for resampling.
PointerEvent? _last;
PointerEvent? _next;
Offset _position = Offset.zero;
bool _isTracked = false;
bool _isDown = false;
int _pointerIdentifier = 0;
int _hasButtons = 0;
PointerEvent _toHoverEvent(
PointerEvent event,
Offset position,
Offset delta,
Duration timeStamp,
int buttons,
) {
return PointerHoverEvent(
viewId: event.viewId,
timeStamp: timeStamp,
kind: event.kind,
device: event.device,
position: position,
delta: delta,
buttons: event.buttons,
obscured: event.obscured,
pressureMin: event.pressureMin,
pressureMax: event.pressureMax,
distance: event.distance,
distanceMax: event.distanceMax,
size: event.size,
radiusMajor: event.radiusMajor,
radiusMinor: event.radiusMinor,
radiusMin: event.radiusMin,
radiusMax: event.radiusMax,
orientation: event.orientation,
tilt: event.tilt,
synthesized: event.synthesized,
embedderId: event.embedderId,
);
}
PointerEvent _toMoveEvent(
PointerEvent event,
Offset position,
Offset delta,
int pointerIdentifier,
Duration timeStamp,
int buttons,
) {
return PointerMoveEvent(
viewId: event.viewId,
timeStamp: timeStamp,
pointer: pointerIdentifier,
kind: event.kind,
device: event.device,
position: position,
delta: delta,
buttons: buttons,
obscured: event.obscured,
pressure: event.pressure,
pressureMin: event.pressureMin,
pressureMax: event.pressureMax,
distanceMax: event.distanceMax,
size: event.size,
radiusMajor: event.radiusMajor,
radiusMinor: event.radiusMinor,
radiusMin: event.radiusMin,
radiusMax: event.radiusMax,
orientation: event.orientation,
tilt: event.tilt,
platformData: event.platformData,
synthesized: event.synthesized,
embedderId: event.embedderId,
);
}
PointerEvent _toMoveOrHoverEvent(
PointerEvent event,
Offset position,
Offset delta,
int pointerIdentifier,
Duration timeStamp,
bool isDown,
int buttons,
) {
return isDown
? _toMoveEvent(
event, position, delta, pointerIdentifier, timeStamp, buttons)
: _toHoverEvent(event, position, delta, timeStamp, buttons);
}
Offset _positionAt(Duration sampleTime) {
// Use `next` position by default.
double x = _next?.position.dx ?? 0.0;
double y = _next?.position.dy ?? 0.0;
final Duration nextTimeStamp = _next?.timeStamp ?? Duration.zero;
final Duration lastTimeStamp = _last?.timeStamp ?? Duration.zero;
// Resample if `next` time stamp is past `sampleTime`.
if (nextTimeStamp > sampleTime && nextTimeStamp > lastTimeStamp) {
final double interval = (nextTimeStamp - lastTimeStamp).inMicroseconds.toDouble();
final double scalar = (sampleTime - lastTimeStamp).inMicroseconds.toDouble() / interval;
final double lastX = _last?.position.dx ?? 0.0;
final double lastY = _last?.position.dy ?? 0.0;
x = lastX + (x - lastX) * scalar;
y = lastY + (y - lastY) * scalar;
}
return Offset(x, y);
}
void _processPointerEvents(Duration sampleTime) {
final Iterator<PointerEvent> it = _queuedEvents.iterator;
while (it.moveNext()) {
final PointerEvent event = it.current;
// Update both `last` and `next` pointer event if time stamp is older
// or equal to `sampleTime`.
if (event.timeStamp <= sampleTime || _last == null) {
_last = event;
_next = event;
continue;
}
// Update only `next` pointer event if time stamp is more recent than
// `sampleTime` and next event is not already more recent.
final Duration nextTimeStamp = _next?.timeStamp ?? Duration.zero;
if (nextTimeStamp < sampleTime) {
_next = event;
break;
}
}
}
void _dequeueAndSampleNonHoverOrMovePointerEventsUntil(
Duration sampleTime,
Duration nextSampleTime,
HandleEventCallback callback,
) {
Duration endTime = sampleTime;
// Scan queued events to determine end time.
final Iterator<PointerEvent> it = _queuedEvents.iterator;
while (it.moveNext()) {
final PointerEvent event = it.current;
// Potentially stop dispatching events if more recent than `sampleTime`.
if (event.timeStamp > sampleTime) {
// Definitely stop if more recent than `nextSampleTime`.
if (event.timeStamp >= nextSampleTime) {
break;
}
// Update `endTime` to allow early processing of up and removed
// events as this improves resampling of these events, which is
// important for fling animations.
if (event is PointerUpEvent || event is PointerRemovedEvent) {
endTime = event.timeStamp;
continue;
}
// Stop if event is not move or hover.
if (event is! PointerMoveEvent && event is! PointerHoverEvent) {
break;
}
}
}
while (_queuedEvents.isNotEmpty) {
final PointerEvent event = _queuedEvents.first;
// Stop dispatching events if more recent than `endTime`.
if (event.timeStamp > endTime) {
break;
}
final bool wasTracked = _isTracked;
final bool wasDown = _isDown;
final int hadButtons = _hasButtons;
// Update pointer state.
_isTracked = event is! PointerRemovedEvent;
_isDown = event.down;
_hasButtons = event.buttons;
// Position at `sampleTime`.
final Offset position = _positionAt(sampleTime);
// Initialize position if we are starting to track this pointer.
if (_isTracked && !wasTracked) {
_position = position;
}
// Current pointer identifier.
final int pointerIdentifier = event.pointer;
// Initialize pointer identifier for `move` events.
// Identifier is expected to be the same while `down`.
assert(!wasDown || _pointerIdentifier == pointerIdentifier);
_pointerIdentifier = pointerIdentifier;
// Skip `move` and `hover` events as they are automatically
// generated when the position has changed.
if (event is! PointerMoveEvent && event is! PointerHoverEvent) {
// Add synthetics `move` or `hover` event if position has changed.
//
// Devices without `hover` events are expected to always have
// `add` and `down` events with the same position and this logic will
// therefore never produce `hover` events.
if (position != _position) {
final Offset delta = position - _position;
callback(_toMoveOrHoverEvent(event, position, delta,
_pointerIdentifier, sampleTime, wasDown, hadButtons));
_position = position;
}
callback(event.copyWith(
position: position,
delta: Offset.zero,
pointer: pointerIdentifier,
timeStamp: sampleTime,
));
}
_queuedEvents.removeFirst();
}
}
void _samplePointerPosition(
Duration sampleTime,
HandleEventCallback callback,
) {
// Position at `sampleTime`.
final Offset position = _positionAt(sampleTime);
// Add `move` or `hover` events if position has changed.
final PointerEvent? next = _next;
if (position != _position && next != null) {
final Offset delta = position - _position;
callback(_toMoveOrHoverEvent(next, position, delta, _pointerIdentifier,
sampleTime, _isDown, _hasButtons));
_position = position;
}
}
/// Enqueue pointer `event` for resampling.
void addEvent(PointerEvent event) {
_queuedEvents.add(event);
}
/// Dispatch resampled pointer events for the specified `sampleTime`
/// by calling [callback].
///
/// This may dispatch multiple events if position is not the only
/// state that has changed since last sample.
///
/// Calling [callback] must not add or sample events.
///
/// Positive value for `nextSampleTime` allow early processing of
/// up and removed events. This improves resampling of these events,
/// which is important for fling animations.
void sample(
Duration sampleTime,
Duration nextSampleTime,
HandleEventCallback callback,
) {
_processPointerEvents(sampleTime);
// Dequeue and sample pointer events until `sampleTime`.
_dequeueAndSampleNonHoverOrMovePointerEventsUntil(sampleTime, nextSampleTime, callback);
// Dispatch resampled pointer location event if tracked.
if (_isTracked) {
_samplePointerPosition(sampleTime, callback);
}
}
/// Stop resampling.
///
/// This will dispatch pending events by calling [callback] and reset
/// internal state.
void stop(HandleEventCallback callback) {
while (_queuedEvents.isNotEmpty) {
callback(_queuedEvents.removeFirst());
}
_pointerIdentifier = 0;
_isDown = false;
_isTracked = false;
_position = Offset.zero;
_next = null;
_last = null;
}
/// Returns `true` if a call to [sample] can dispatch more events.
bool get hasPendingEvents => _queuedEvents.isNotEmpty;
/// Returns `true` if pointer is currently tracked.
bool get isTracked => _isTracked;
/// Returns `true` if pointer is currently down.
bool get isDown => _isDown;
}
| flutter/packages/flutter/lib/src/gestures/resampler.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/resampler.dart",
"repo_id": "flutter",
"token_count": 3918
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$close_menu = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(22.262051805115647, 21.908449654595582),
Offset(24.88919842700383, 17.94756272667581),
Offset(28.423231797685485, 16.307213722363326),
Offset(31.75801074104301, 16.114437816710378),
Offset(34.549940992662854, 16.70741310193702),
Offset(36.75456537179234, 17.655783514919893),
Offset(38.441715273516245, 18.694992104934485),
Offset(39.70097192738563, 19.67449366114299),
Offset(40.617793553920194, 20.51263931960471),
Offset(41.26084924113239, 21.170496215429807),
Offset(41.68247655138362, 21.634692364647904),
Offset(41.92023728365015, 21.90732809306482),
Offset(41.99983686928147, 21.999805299557877),
Offset(42.0, 22.0),
],
),
_PathCubicTo(
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(22.262051805115647, 21.908449654595582),
Offset(24.88919842700383, 17.94756272667581),
Offset(28.423231797685485, 16.307213722363326),
Offset(31.75801074104301, 16.114437816710378),
Offset(34.549940992662854, 16.70741310193702),
Offset(36.75456537179234, 17.655783514919893),
Offset(38.441715273516245, 18.694992104934485),
Offset(39.70097192738563, 19.67449366114299),
Offset(40.617793553920194, 20.51263931960471),
Offset(41.26084924113239, 21.170496215429807),
Offset(41.68247655138362, 21.634692364647904),
Offset(41.92023728365015, 21.90732809306482),
Offset(41.99983686928147, 21.999805299557877),
Offset(42.0, 22.0),
],
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(21.773286636989344, 25.561023502210293),
Offset(19.560850614934246, 28.209111964173573),
Offset(16.64772019146621, 28.96869418114919),
Offset(13.944774246803789, 28.61099302263078),
Offset(11.716530993310267, 27.687745622141946),
Offset(9.984477784090515, 26.54816750788367),
Offset(8.678832846158931, 25.4028848331679),
Offset(7.7182562555573995, 24.367892904410397),
Offset(7.027497048073013, 23.503119065863647),
Offset(6.547674641076508, 22.834089300900743),
Offset(6.235000119645385, 22.366158988430406),
Offset(6.059083538826545, 22.092725360840227),
Offset(6.000119863487498, 22.00019470067613),
Offset(5.9999999999999964, 22.000000000000007),
],
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(21.773286636989344, 25.561023502210293),
Offset(19.560850614934246, 28.209111964173573),
Offset(16.64772019146621, 28.96869418114919),
Offset(13.944774246803789, 28.61099302263078),
Offset(11.716530993310267, 27.687745622141946),
Offset(9.984477784090515, 26.54816750788367),
Offset(8.678832846158931, 25.4028848331679),
Offset(7.7182562555573995, 24.367892904410397),
Offset(7.027497048073013, 23.503119065863647),
Offset(6.547674641076508, 22.834089300900743),
Offset(6.235000119645385, 22.366158988430406),
Offset(6.059083538826545, 22.092725360840227),
Offset(6.000119863487498, 22.00019470067613),
Offset(5.9999999999999964, 22.000000000000007),
],
),
_PathCubicTo(
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(21.773286636989344, 25.561023502210293),
Offset(19.560850614934246, 28.209111964173573),
Offset(16.64772019146621, 28.96869418114919),
Offset(13.944774246803789, 28.61099302263078),
Offset(11.716530993310267, 27.687745622141946),
Offset(9.984477784090515, 26.54816750788367),
Offset(8.678832846158931, 25.4028848331679),
Offset(7.7182562555573995, 24.367892904410397),
Offset(7.027497048073013, 23.503119065863647),
Offset(6.547674641076508, 22.834089300900743),
Offset(6.235000119645385, 22.366158988430406),
Offset(6.059083538826545, 22.092725360840227),
Offset(6.000119863487498, 22.00019470067613),
Offset(5.9999999999999964, 22.000000000000007),
],
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(25.737948194884353, 26.091550345404418),
Offset(23.110801573005386, 30.05243727330644),
Offset(19.576768202341754, 31.692786277607382),
Offset(16.24198925903886, 31.88556218323219),
Offset(13.450059007427265, 31.292586898019646),
Offset(11.245434628207665, 30.344216485080107),
Offset(9.558284726386193, 29.305007895087492),
Offset(8.29902807271331, 28.325506338842494),
Offset(7.38220644607981, 27.487360680395284),
Offset(6.7391507588675985, 26.8295037845702),
Offset(6.3175234486163845, 26.36530763535209),
Offset(6.079762716249856, 26.0926719069357),
Offset(6.000163130618532, 26.000194700442123),
Offset(5.9999999999999964, 26.000000000000007),
],
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(25.737948194884353, 26.091550345404418),
Offset(23.110801573005386, 30.05243727330644),
Offset(19.576768202341754, 31.692786277607382),
Offset(16.24198925903886, 31.88556218323219),
Offset(13.450059007427265, 31.292586898019646),
Offset(11.245434628207665, 30.344216485080107),
Offset(9.558284726386193, 29.305007895087492),
Offset(8.29902807271331, 28.325506338842494),
Offset(7.38220644607981, 27.487360680395284),
Offset(6.7391507588675985, 26.8295037845702),
Offset(6.3175234486163845, 26.36530763535209),
Offset(6.079762716249856, 26.0926719069357),
Offset(6.000163130618532, 26.000194700442123),
Offset(5.9999999999999964, 26.000000000000007),
],
),
_PathCubicTo(
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(25.737948194884353, 26.091550345404418),
Offset(23.110801573005386, 30.05243727330644),
Offset(19.576768202341754, 31.692786277607382),
Offset(16.24198925903886, 31.88556218323219),
Offset(13.450059007427265, 31.292586898019646),
Offset(11.245434628207665, 30.344216485080107),
Offset(9.558284726386193, 29.305007895087492),
Offset(8.29902807271331, 28.325506338842494),
Offset(7.38220644607981, 27.487360680395284),
Offset(6.7391507588675985, 26.8295037845702),
Offset(6.3175234486163845, 26.36530763535209),
Offset(6.079762716249856, 26.0926719069357),
Offset(6.000163130618532, 26.000194700442123),
Offset(5.9999999999999964, 26.000000000000007),
],
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(26.226713363010656, 22.438976497789707),
Offset(28.439149385074973, 19.790888035808678),
Offset(31.352279808561033, 19.031305818821522),
Offset(34.05522575327808, 19.389006977311787),
Offset(36.28346900677985, 20.312254377814725),
Offset(38.015522215909485, 21.45183249211633),
Offset(39.32116715374351, 22.597115166854078),
Offset(40.281743744541544, 23.632107095575087),
Offset(40.972502951927, 24.496880934136346),
Offset(41.45232535892349, 25.165910699099264),
Offset(41.76499988035462, 25.633841011569586),
Offset(41.940916461073456, 25.907274639160292),
Offset(41.9998801364125, 25.99980529932387),
Offset(42.0, 26.0),
],
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(26.226713363010656, 22.438976497789707),
Offset(28.439149385074973, 19.790888035808678),
Offset(31.352279808561033, 19.031305818821522),
Offset(34.05522575327808, 19.389006977311787),
Offset(36.28346900677985, 20.312254377814725),
Offset(38.015522215909485, 21.45183249211633),
Offset(39.32116715374351, 22.597115166854078),
Offset(40.281743744541544, 23.632107095575087),
Offset(40.972502951927, 24.496880934136346),
Offset(41.45232535892349, 25.165910699099264),
Offset(41.76499988035462, 25.633841011569586),
Offset(41.940916461073456, 25.907274639160292),
Offset(41.9998801364125, 25.99980529932387),
Offset(42.0, 26.0),
],
),
_PathCubicTo(
<Offset>[
Offset(24.0, 22.0),
Offset(24.040185978508838, 22.000403768974525),
Offset(24.181019326543748, 22.00820884543142),
Offset(24.461936845635496, 22.054077506516684),
Offset(24.91719787006675, 22.22271328504796),
Offset(25.546020906725474, 22.73121343167271),
Offset(25.924910472907296, 23.45711910026985),
Offset(26.226713363010656, 22.438976497789707),
Offset(28.439149385074973, 19.790888035808678),
Offset(31.352279808561033, 19.031305818821522),
Offset(34.05522575327808, 19.389006977311787),
Offset(36.28346900677985, 20.312254377814725),
Offset(38.015522215909485, 21.45183249211633),
Offset(39.32116715374351, 22.597115166854078),
Offset(40.281743744541544, 23.632107095575087),
Offset(40.972502951927, 24.496880934136346),
Offset(41.45232535892349, 25.165910699099264),
Offset(41.76499988035462, 25.633841011569586),
Offset(41.940916461073456, 25.907274639160292),
Offset(41.9998801364125, 25.99980529932387),
Offset(42.0, 26.0),
],
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(22.262051805115647, 21.908449654595582),
Offset(24.88919842700383, 17.94756272667581),
Offset(28.423231797685485, 16.307213722363326),
Offset(31.75801074104301, 16.114437816710378),
Offset(34.549940992662854, 16.70741310193702),
Offset(36.75456537179234, 17.655783514919893),
Offset(38.441715273516245, 18.694992104934485),
Offset(39.70097192738563, 19.67449366114299),
Offset(40.617793553920194, 20.51263931960471),
Offset(41.26084924113239, 21.170496215429807),
Offset(41.68247655138362, 21.634692364647904),
Offset(41.92023728365015, 21.90732809306482),
Offset(41.99983686928147, 21.999805299557877),
Offset(42.0, 22.0),
],
<Offset>[
Offset(24.0, 26.0),
Offset(23.959814021491162, 25.999596231025475),
Offset(23.818980673456252, 25.99179115456858),
Offset(23.538063154364504, 25.945922493483316),
Offset(23.08280212993325, 25.77728671495204),
Offset(22.453979093274526, 25.26878656832729),
Offset(22.075089527092704, 24.54288089973015),
Offset(22.262051805115647, 21.908449654595582),
Offset(24.88919842700383, 17.94756272667581),
Offset(28.423231797685485, 16.307213722363326),
Offset(31.75801074104301, 16.114437816710378),
Offset(34.549940992662854, 16.70741310193702),
Offset(36.75456537179234, 17.655783514919893),
Offset(38.441715273516245, 18.694992104934485),
Offset(39.70097192738563, 19.67449366114299),
Offset(40.617793553920194, 20.51263931960471),
Offset(41.26084924113239, 21.170496215429807),
Offset(41.68247655138362, 21.634692364647904),
Offset(41.92023728365015, 21.90732809306482),
Offset(41.99983686928147, 21.999805299557877),
Offset(42.0, 22.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(9.857864376269049, 12.68629150101524),
Offset(10.0286848752889, 12.538401058257541),
Offset(10.632873267418944, 12.039156238673812),
Offset(11.869661541953974, 11.120999908012887),
Offset(14.01269232753313, 9.802147761233421),
Offset(17.63755687713399, 8.1644208393319),
Offset(21.40270587697591, 7.061028351517436),
Offset(25.372566440386116, 6.474033586994366),
Offset(29.043281052671396, 6.480169426635232),
Offset(32.12573791953512, 6.935455570385773),
Offset(34.627918413389764, 7.642591727654583),
Offset(36.617140423267614, 8.447772996169093),
Offset(38.17478812826869, 9.24831138340156),
Offset(39.37733102863164, 9.981782438812019),
Offset(40.28991005711473, 10.613640055506202),
Offset(40.96529784286365, 11.127522886831306),
Offset(41.445182774971684, 11.518668960278076),
Offset(41.761944370115955, 11.789503608737085),
Offset(41.94049711880572, 11.94681212872769),
Offset(41.99987560677617, 11.999888404963674),
Offset(42.0, 12.000000000000002),
],
),
_PathCubicTo(
<Offset>[
Offset(9.857864376269049, 12.68629150101524),
Offset(10.0286848752889, 12.538401058257541),
Offset(10.632873267418944, 12.039156238673812),
Offset(11.869661541953974, 11.120999908012887),
Offset(14.01269232753313, 9.802147761233421),
Offset(17.63755687713399, 8.1644208393319),
Offset(21.40270587697591, 7.061028351517436),
Offset(25.372566440386116, 6.474033586994366),
Offset(29.043281052671396, 6.480169426635232),
Offset(32.12573791953512, 6.935455570385773),
Offset(34.627918413389764, 7.642591727654583),
Offset(36.617140423267614, 8.447772996169093),
Offset(38.17478812826869, 9.24831138340156),
Offset(39.37733102863164, 9.981782438812019),
Offset(40.28991005711473, 10.613640055506202),
Offset(40.96529784286365, 11.127522886831306),
Offset(41.445182774971684, 11.518668960278076),
Offset(41.761944370115955, 11.789503608737085),
Offset(41.94049711880572, 11.94681212872769),
Offset(41.99987560677617, 11.999888404963674),
Offset(42.0, 12.000000000000002),
],
<Offset>[
Offset(35.31370849898477, 38.14213562373095),
Offset(35.09801389245515, 38.37497866886739),
Offset(34.30089322953512, 39.165247572901634),
Offset(32.51039870948586, 40.61608372177527),
Offset(28.957340520209982, 42.55359796419574),
Offset(22.04434118430577, 43.893684084885464),
Offset(14.726425304958642, 42.43654640742306),
Offset(8.351729390869856, 38.19612535493764),
Offset(4.454339035895707, 32.774349970923794),
Offset(2.747425156507667, 27.742058829873816),
Offset(2.3511131772704275, 23.586488483141284),
Offset(2.632378123985845, 20.323627974288197),
Offset(3.211174431823274, 17.82419135491087),
Offset(3.873722104158137, 15.939445851429909),
Offset(4.5046582506767905, 14.539930053688956),
Offset(5.04509760688121, 13.523188766482994),
Offset(5.468398892380936, 12.81134891500736),
Offset(5.766254411966681, 12.346553370240805),
Offset(5.940767729461317, 12.086396848425768),
Offset(5.999875607960821, 12.000180458137994),
Offset(5.9999999999999964, 12.000000000000005),
],
<Offset>[
Offset(35.31370849898477, 38.14213562373095),
Offset(35.09801389245515, 38.37497866886739),
Offset(34.30089322953512, 39.165247572901634),
Offset(32.51039870948586, 40.61608372177527),
Offset(28.957340520209982, 42.55359796419574),
Offset(22.04434118430577, 43.893684084885464),
Offset(14.726425304958642, 42.43654640742306),
Offset(8.351729390869856, 38.19612535493764),
Offset(4.454339035895707, 32.774349970923794),
Offset(2.747425156507667, 27.742058829873816),
Offset(2.3511131772704275, 23.586488483141284),
Offset(2.632378123985845, 20.323627974288197),
Offset(3.211174431823274, 17.82419135491087),
Offset(3.873722104158137, 15.939445851429909),
Offset(4.5046582506767905, 14.539930053688956),
Offset(5.04509760688121, 13.523188766482994),
Offset(5.468398892380936, 12.81134891500736),
Offset(5.766254411966681, 12.346553370240805),
Offset(5.940767729461317, 12.086396848425768),
Offset(5.999875607960821, 12.000180458137994),
Offset(5.9999999999999964, 12.000000000000005),
],
),
_PathCubicTo(
<Offset>[
Offset(35.31370849898477, 38.14213562373095),
Offset(35.09801389245515, 38.37497866886739),
Offset(34.30089322953512, 39.165247572901634),
Offset(32.51039870948586, 40.61608372177527),
Offset(28.957340520209982, 42.55359796419574),
Offset(22.04434118430577, 43.893684084885464),
Offset(14.726425304958642, 42.43654640742306),
Offset(8.351729390869856, 38.19612535493764),
Offset(4.454339035895707, 32.774349970923794),
Offset(2.747425156507667, 27.742058829873816),
Offset(2.3511131772704275, 23.586488483141284),
Offset(2.632378123985845, 20.323627974288197),
Offset(3.211174431823274, 17.82419135491087),
Offset(3.873722104158137, 15.939445851429909),
Offset(4.5046582506767905, 14.539930053688956),
Offset(5.04509760688121, 13.523188766482994),
Offset(5.468398892380936, 12.81134891500736),
Offset(5.766254411966681, 12.346553370240805),
Offset(5.940767729461317, 12.086396848425768),
Offset(5.999875607960821, 12.000180458137994),
Offset(5.9999999999999964, 12.000000000000005),
],
<Offset>[
Offset(38.14213562373095, 35.31370849898476),
Offset(37.96874473807846, 35.58949766696003),
Offset(37.314903377782656, 36.53546757711095),
Offset(35.78763024434835, 38.32266848093839),
Offset(32.59639054276135, 40.89308149834275),
Offset(26.01425932270061, 43.4040413840886),
Offset(18.657038422281488, 43.17835535986942),
Offset(11.876406253974665, 40.08732947155056),
Offset(7.375914651927772, 35.506454639454425),
Offset(5.059269963117448, 31.006315803543533),
Offset(4.122657261213394, 27.17280017604343),
Offset(3.9519175659990786, 24.099712674208394),
Offset(4.164049984213197, 21.709037321182585),
Offset(4.535684705560126, 19.884291287482522),
Offset(4.940912694919316, 18.516069143293173),
Offset(5.3112827046202895, 17.514322126036596),
Offset(5.612029998461967, 16.80876934640633),
Offset(5.828148829911537, 16.346074476701837),
Offset(5.9562771427611025, 16.086366780575148),
Offset(5.9999080583135225, 16.000180458006366),
Offset(5.9999999999999964, 16.000000000000007),
],
<Offset>[
Offset(38.14213562373095, 35.31370849898476),
Offset(37.96874473807846, 35.58949766696003),
Offset(37.314903377782656, 36.53546757711095),
Offset(35.78763024434835, 38.32266848093839),
Offset(32.59639054276135, 40.89308149834275),
Offset(26.01425932270061, 43.4040413840886),
Offset(18.657038422281488, 43.17835535986942),
Offset(11.876406253974665, 40.08732947155056),
Offset(7.375914651927772, 35.506454639454425),
Offset(5.059269963117448, 31.006315803543533),
Offset(4.122657261213394, 27.17280017604343),
Offset(3.9519175659990786, 24.099712674208394),
Offset(4.164049984213197, 21.709037321182585),
Offset(4.535684705560126, 19.884291287482522),
Offset(4.940912694919316, 18.516069143293173),
Offset(5.3112827046202895, 17.514322126036596),
Offset(5.612029998461967, 16.80876934640633),
Offset(5.828148829911537, 16.346074476701837),
Offset(5.9562771427611025, 16.086366780575148),
Offset(5.9999080583135225, 16.000180458006366),
Offset(5.9999999999999964, 16.000000000000007),
],
),
_PathCubicTo(
<Offset>[
Offset(38.14213562373095, 35.31370849898476),
Offset(37.96874473807846, 35.58949766696003),
Offset(37.314903377782656, 36.53546757711095),
Offset(35.78763024434835, 38.32266848093839),
Offset(32.59639054276135, 40.89308149834275),
Offset(26.01425932270061, 43.4040413840886),
Offset(18.657038422281488, 43.17835535986942),
Offset(11.876406253974665, 40.08732947155056),
Offset(7.375914651927772, 35.506454639454425),
Offset(5.059269963117448, 31.006315803543533),
Offset(4.122657261213394, 27.17280017604343),
Offset(3.9519175659990786, 24.099712674208394),
Offset(4.164049984213197, 21.709037321182585),
Offset(4.535684705560126, 19.884291287482522),
Offset(4.940912694919316, 18.516069143293173),
Offset(5.3112827046202895, 17.514322126036596),
Offset(5.612029998461967, 16.80876934640633),
Offset(5.828148829911537, 16.346074476701837),
Offset(5.9562771427611025, 16.086366780575148),
Offset(5.9999080583135225, 16.000180458006366),
Offset(5.9999999999999964, 16.000000000000007),
],
<Offset>[
Offset(12.68629150101524, 9.857864376269049),
Offset(12.899415720912216, 9.75292005635018),
Offset(13.64688341566648, 9.409376242883125),
Offset(15.146893076816461, 8.82758466717601),
Offset(17.651742350084497, 8.141631295380437),
Offset(21.607475015528827, 7.674778138535036),
Offset(25.333318994298757, 7.802837303963798),
Offset(28.897243303490924, 8.365237703607285),
Offset(31.96485666870346, 9.212274095165863),
Offset(34.437582726144896, 10.19971254405549),
Offset(36.399462497332735, 11.228903420556733),
Offset(37.93667986528085, 12.22385769608929),
Offset(39.127663680658614, 13.133157349673274),
Offset(40.039293630033626, 13.926627874864629),
Offset(40.72616450135726, 14.58977914511042),
Offset(41.231482940602724, 15.118656246384909),
Offset(41.588813881052715, 15.516089391677047),
Offset(41.82383878806081, 15.789024715198115),
Offset(41.956006532105505, 15.946782060877066),
Offset(41.99990805712887, 15.999888404832046),
Offset(42.0, 16.0),
],
<Offset>[
Offset(12.68629150101524, 9.857864376269049),
Offset(12.899415720912216, 9.75292005635018),
Offset(13.64688341566648, 9.409376242883125),
Offset(15.146893076816461, 8.82758466717601),
Offset(17.651742350084497, 8.141631295380437),
Offset(21.607475015528827, 7.674778138535036),
Offset(25.333318994298757, 7.802837303963798),
Offset(28.897243303490924, 8.365237703607285),
Offset(31.96485666870346, 9.212274095165863),
Offset(34.437582726144896, 10.19971254405549),
Offset(36.399462497332735, 11.228903420556733),
Offset(37.93667986528085, 12.22385769608929),
Offset(39.127663680658614, 13.133157349673274),
Offset(40.039293630033626, 13.926627874864629),
Offset(40.72616450135726, 14.58977914511042),
Offset(41.231482940602724, 15.118656246384909),
Offset(41.588813881052715, 15.516089391677047),
Offset(41.82383878806081, 15.789024715198115),
Offset(41.956006532105505, 15.946782060877066),
Offset(41.99990805712887, 15.999888404832046),
Offset(42.0, 16.0),
],
),
_PathCubicTo(
<Offset>[
Offset(12.68629150101524, 9.857864376269049),
Offset(12.899415720912216, 9.75292005635018),
Offset(13.64688341566648, 9.409376242883125),
Offset(15.146893076816461, 8.82758466717601),
Offset(17.651742350084497, 8.141631295380437),
Offset(21.607475015528827, 7.674778138535036),
Offset(25.333318994298757, 7.802837303963798),
Offset(28.897243303490924, 8.365237703607285),
Offset(31.96485666870346, 9.212274095165863),
Offset(34.437582726144896, 10.19971254405549),
Offset(36.399462497332735, 11.228903420556733),
Offset(37.93667986528085, 12.22385769608929),
Offset(39.127663680658614, 13.133157349673274),
Offset(40.039293630033626, 13.926627874864629),
Offset(40.72616450135726, 14.58977914511042),
Offset(41.231482940602724, 15.118656246384909),
Offset(41.588813881052715, 15.516089391677047),
Offset(41.82383878806081, 15.789024715198115),
Offset(41.956006532105505, 15.946782060877066),
Offset(41.99990805712887, 15.999888404832046),
Offset(42.0, 16.0),
],
<Offset>[
Offset(9.857864376269049, 12.68629150101524),
Offset(10.0286848752889, 12.538401058257541),
Offset(10.632873267418944, 12.039156238673812),
Offset(11.869661541953974, 11.120999908012887),
Offset(14.01269232753313, 9.802147761233421),
Offset(17.63755687713399, 8.1644208393319),
Offset(21.40270587697591, 7.061028351517436),
Offset(25.372566440386116, 6.474033586994366),
Offset(29.043281052671396, 6.480169426635232),
Offset(32.12573791953512, 6.935455570385773),
Offset(34.627918413389764, 7.642591727654583),
Offset(36.617140423267614, 8.447772996169093),
Offset(38.17478812826869, 9.24831138340156),
Offset(39.37733102863164, 9.981782438812019),
Offset(40.28991005711473, 10.613640055506202),
Offset(40.96529784286365, 11.127522886831306),
Offset(41.445182774971684, 11.518668960278076),
Offset(41.761944370115955, 11.789503608737085),
Offset(41.94049711880572, 11.94681212872769),
Offset(41.99987560677617, 11.999888404963674),
Offset(42.0, 12.000000000000002),
],
<Offset>[
Offset(9.857864376269049, 12.68629150101524),
Offset(10.0286848752889, 12.538401058257541),
Offset(10.632873267418944, 12.039156238673812),
Offset(11.869661541953974, 11.120999908012887),
Offset(14.01269232753313, 9.802147761233421),
Offset(17.63755687713399, 8.1644208393319),
Offset(21.40270587697591, 7.061028351517436),
Offset(25.372566440386116, 6.474033586994366),
Offset(29.043281052671396, 6.480169426635232),
Offset(32.12573791953512, 6.935455570385773),
Offset(34.627918413389764, 7.642591727654583),
Offset(36.617140423267614, 8.447772996169093),
Offset(38.17478812826869, 9.24831138340156),
Offset(39.37733102863164, 9.981782438812019),
Offset(40.28991005711473, 10.613640055506202),
Offset(40.96529784286365, 11.127522886831306),
Offset(41.445182774971684, 11.518668960278076),
Offset(41.761944370115955, 11.789503608737085),
Offset(41.94049711880572, 11.94681212872769),
Offset(41.99987560677617, 11.999888404963674),
Offset(42.0, 12.000000000000002),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(12.686291501015244, 38.14213562373095),
Offset(12.335961696184835, 37.78957826421869),
Offset(11.186164512970027, 36.485148967548454),
Offset(9.272264829328229, 33.57462824174341),
Offset(7.396433901397504, 28.01370476875244),
Offset(8.455221168610253, 18.46855392229577),
Offset(14.400164412415048, 10.886305797611346),
Offset(23.385743674953297, 7.627722418507858),
Offset(31.513897019057687, 8.97811161083639),
Offset(37.00821523813391, 12.590468749760188),
Offset(40.228807248234226, 16.687449599224042),
Offset(41.900283080823314, 20.466101465000598),
Offset(42.622994421193994, 23.65567978529433),
Offset(42.809759514234806, 26.219169470442615),
Offset(42.724595353131164, 28.207893098186),
Offset(42.529420070562, 29.69761906859474),
Offset(42.319508324086705, 30.763041306693708),
Offset(42.1473924208978, 31.468700863761335),
Offset(42.038312568419684, 31.86713222464318),
Offset(42.00008112385512, 31.999722191105658),
Offset(42.0, 32.0),
],
),
_PathCubicTo(
<Offset>[
Offset(12.686291501015244, 38.14213562373095),
Offset(12.335961696184835, 37.78957826421869),
Offset(11.186164512970027, 36.485148967548454),
Offset(9.272264829328229, 33.57462824174341),
Offset(7.396433901397504, 28.01370476875244),
Offset(8.455221168610253, 18.46855392229577),
Offset(14.400164412415048, 10.886305797611346),
Offset(23.385743674953297, 7.627722418507858),
Offset(31.513897019057687, 8.97811161083639),
Offset(37.00821523813391, 12.590468749760188),
Offset(40.228807248234226, 16.687449599224042),
Offset(41.900283080823314, 20.466101465000598),
Offset(42.622994421193994, 23.65567978529433),
Offset(42.809759514234806, 26.219169470442615),
Offset(42.724595353131164, 28.207893098186),
Offset(42.529420070562, 29.69761906859474),
Offset(42.319508324086705, 30.763041306693708),
Offset(42.1473924208978, 31.468700863761335),
Offset(42.038312568419684, 31.86713222464318),
Offset(42.00008112385512, 31.999722191105658),
Offset(42.0, 32.0),
],
<Offset>[
Offset(38.14213562373096, 12.686291501015244),
Offset(38.423106864699406, 12.98109469595424),
Offset(39.356600322670786, 14.070275639966862),
Offset(40.967250034331684, 16.50336839208347),
Offset(42.74943078741556, 21.21917673678452),
Offset(42.63823566995957, 29.761096576655866),
Offset(38.576286786907716, 37.560546310389874),
Offset(31.46737791244584, 42.70887388266861),
Offset(24.151451365764814, 44.21721480604141),
Offset(18.383677101408267, 43.39836675000767),
Offset(14.25135866363073, 41.61077532101089),
Offset(11.404888461060231, 39.59803568464825),
Offset(9.477230898414673, 37.70410889647938),
Offset(8.1829838828817, 36.06733728866804),
Offset(7.320063260869414, 34.72856080387442),
Offset(6.750940467797069, 33.68514929600791),
Offset(6.383985215921761, 32.91668448571468),
Offset(6.159364334665909, 32.39705126344063),
Offset(6.0390642630099585, 32.09977238767511),
Offset(6.000081127145819, 32.000208946289966),
Offset(5.9999999999999964, 32.00000000000001),
],
<Offset>[
Offset(38.14213562373096, 12.686291501015244),
Offset(38.423106864699406, 12.98109469595424),
Offset(39.356600322670786, 14.070275639966862),
Offset(40.967250034331684, 16.50336839208347),
Offset(42.74943078741556, 21.21917673678452),
Offset(42.63823566995957, 29.761096576655866),
Offset(38.576286786907716, 37.560546310389874),
Offset(31.46737791244584, 42.70887388266861),
Offset(24.151451365764814, 44.21721480604141),
Offset(18.383677101408267, 43.39836675000767),
Offset(14.25135866363073, 41.61077532101089),
Offset(11.404888461060231, 39.59803568464825),
Offset(9.477230898414673, 37.70410889647938),
Offset(8.1829838828817, 36.06733728866804),
Offset(7.320063260869414, 34.72856080387442),
Offset(6.750940467797069, 33.68514929600791),
Offset(6.383985215921761, 32.91668448571468),
Offset(6.159364334665909, 32.39705126344063),
Offset(6.0390642630099585, 32.09977238767511),
Offset(6.000081127145819, 32.000208946289966),
Offset(5.9999999999999964, 32.00000000000001),
],
),
_PathCubicTo(
<Offset>[
Offset(38.14213562373096, 12.686291501015244),
Offset(38.423106864699406, 12.98109469595424),
Offset(39.356600322670786, 14.070275639966862),
Offset(40.967250034331684, 16.50336839208347),
Offset(42.74943078741556, 21.21917673678452),
Offset(42.63823566995957, 29.761096576655866),
Offset(38.576286786907716, 37.560546310389874),
Offset(31.46737791244584, 42.70887388266861),
Offset(24.151451365764814, 44.21721480604141),
Offset(18.383677101408267, 43.39836675000767),
Offset(14.25135866363073, 41.61077532101089),
Offset(11.404888461060231, 39.59803568464825),
Offset(9.477230898414673, 37.70410889647938),
Offset(8.1829838828817, 36.06733728866804),
Offset(7.320063260869414, 34.72856080387442),
Offset(6.750940467797069, 33.68514929600791),
Offset(6.383985215921761, 32.91668448571468),
Offset(6.159364334665909, 32.39705126344063),
Offset(6.0390642630099585, 32.09977238767511),
Offset(6.000081127145819, 32.000208946289966),
Offset(5.9999999999999964, 32.00000000000001),
],
<Offset>[
Offset(35.31370849898477, 9.857864376269053),
Offset(35.6666086904478, 10.082523010563733),
Offset(36.86605884182839, 10.940227216666777),
Offset(39.07044338436947, 12.981703369305308),
Offset(41.99448322830801, 17.2910659716714),
Offset(43.892962631555136, 25.96298385428372),
Offset(41.540091288327545, 34.8743104910018),
Offset(35.36528363068592, 41.81091452294722),
Offset(28.066907276343148, 45.035264323073946),
Offset(21.806776879213544, 45.46775987631051),
Offset(17.0206170771626, 44.49715849707795),
Offset(13.530658929909972, 42.986412864621926),
Offset(11.038167466324122, 41.386971510121526),
Offset(9.277224751573414, 39.91475680326283),
Offset(8.044581894834794, 38.66239770301462),
Offset(7.193999381954086, 37.66053591853735),
Offset(6.6232789024796475, 36.90952038662189),
Offset(6.2625143790747195, 36.39572105079973),
Offset(6.064913170013508, 36.09968886605397),
Offset(6.000135211055188, 36.00020894592433),
Offset(5.9999999999999964, 36.00000000000001),
],
<Offset>[
Offset(35.31370849898477, 9.857864376269053),
Offset(35.6666086904478, 10.082523010563733),
Offset(36.86605884182839, 10.940227216666777),
Offset(39.07044338436947, 12.981703369305308),
Offset(41.99448322830801, 17.2910659716714),
Offset(43.892962631555136, 25.96298385428372),
Offset(41.540091288327545, 34.8743104910018),
Offset(35.36528363068592, 41.81091452294722),
Offset(28.066907276343148, 45.035264323073946),
Offset(21.806776879213544, 45.46775987631051),
Offset(17.0206170771626, 44.49715849707795),
Offset(13.530658929909972, 42.986412864621926),
Offset(11.038167466324122, 41.386971510121526),
Offset(9.277224751573414, 39.91475680326283),
Offset(8.044581894834794, 38.66239770301462),
Offset(7.193999381954086, 37.66053591853735),
Offset(6.6232789024796475, 36.90952038662189),
Offset(6.2625143790747195, 36.39572105079973),
Offset(6.064913170013508, 36.09968886605397),
Offset(6.000135211055188, 36.00020894592433),
Offset(5.9999999999999964, 36.00000000000001),
],
),
_PathCubicTo(
<Offset>[
Offset(35.31370849898477, 9.857864376269053),
Offset(35.6666086904478, 10.082523010563733),
Offset(36.86605884182839, 10.940227216666777),
Offset(39.07044338436947, 12.981703369305308),
Offset(41.99448322830801, 17.2910659716714),
Offset(43.892962631555136, 25.96298385428372),
Offset(41.540091288327545, 34.8743104910018),
Offset(35.36528363068592, 41.81091452294722),
Offset(28.066907276343148, 45.035264323073946),
Offset(21.806776879213544, 45.46775987631051),
Offset(17.0206170771626, 44.49715849707795),
Offset(13.530658929909972, 42.986412864621926),
Offset(11.038167466324122, 41.386971510121526),
Offset(9.277224751573414, 39.91475680326283),
Offset(8.044581894834794, 38.66239770301462),
Offset(7.193999381954086, 37.66053591853735),
Offset(6.6232789024796475, 36.90952038662189),
Offset(6.2625143790747195, 36.39572105079973),
Offset(6.064913170013508, 36.09968886605397),
Offset(6.000135211055188, 36.00020894592433),
Offset(5.9999999999999964, 36.00000000000001),
],
<Offset>[
Offset(9.857864376269053, 35.31370849898477),
Offset(9.579463521933231, 34.89100657882818),
Offset(8.695623032127628, 33.35510054424837),
Offset(7.375458179366014, 30.052963218965253),
Offset(6.641486342289959, 24.085594003639322),
Offset(9.70994813020582, 14.670441199923623),
Offset(17.36396891383488, 8.200069978223272),
Offset(27.283649393193382, 6.729763058786467),
Offset(35.429352929636025, 9.796161127868931),
Offset(40.431315015939184, 14.659861876063037),
Offset(42.99806566176609, 19.573832775291095),
Offset(44.02605354967305, 23.854478644974275),
Offset(44.183930989103445, 27.338542398936475),
Offset(43.90400038292652, 30.066588985037402),
Offset(43.44911398709654, 32.141729997326195),
Offset(42.97247898471902, 33.673005691124175),
Offset(42.55880201064459, 34.75587720760092),
Offset(42.25054246530661, 35.46737065112043),
Offset(42.064161475423234, 35.867048703022036),
Offset(42.00013520776449, 35.99972219074003),
Offset(42.0, 36.0),
],
<Offset>[
Offset(9.857864376269053, 35.31370849898477),
Offset(9.579463521933231, 34.89100657882818),
Offset(8.695623032127628, 33.35510054424837),
Offset(7.375458179366014, 30.052963218965253),
Offset(6.641486342289959, 24.085594003639322),
Offset(9.70994813020582, 14.670441199923623),
Offset(17.36396891383488, 8.200069978223272),
Offset(27.283649393193382, 6.729763058786467),
Offset(35.429352929636025, 9.796161127868931),
Offset(40.431315015939184, 14.659861876063037),
Offset(42.99806566176609, 19.573832775291095),
Offset(44.02605354967305, 23.854478644974275),
Offset(44.183930989103445, 27.338542398936475),
Offset(43.90400038292652, 30.066588985037402),
Offset(43.44911398709654, 32.141729997326195),
Offset(42.97247898471902, 33.673005691124175),
Offset(42.55880201064459, 34.75587720760092),
Offset(42.25054246530661, 35.46737065112043),
Offset(42.064161475423234, 35.867048703022036),
Offset(42.00013520776449, 35.99972219074003),
Offset(42.0, 36.0),
],
),
_PathCubicTo(
<Offset>[
Offset(9.857864376269053, 35.31370849898477),
Offset(9.579463521933231, 34.89100657882818),
Offset(8.695623032127628, 33.35510054424837),
Offset(7.375458179366014, 30.052963218965253),
Offset(6.641486342289959, 24.085594003639322),
Offset(9.70994813020582, 14.670441199923623),
Offset(17.36396891383488, 8.200069978223272),
Offset(27.283649393193382, 6.729763058786467),
Offset(35.429352929636025, 9.796161127868931),
Offset(40.431315015939184, 14.659861876063037),
Offset(42.99806566176609, 19.573832775291095),
Offset(44.02605354967305, 23.854478644974275),
Offset(44.183930989103445, 27.338542398936475),
Offset(43.90400038292652, 30.066588985037402),
Offset(43.44911398709654, 32.141729997326195),
Offset(42.97247898471902, 33.673005691124175),
Offset(42.55880201064459, 34.75587720760092),
Offset(42.25054246530661, 35.46737065112043),
Offset(42.064161475423234, 35.867048703022036),
Offset(42.00013520776449, 35.99972219074003),
Offset(42.0, 36.0),
],
<Offset>[
Offset(12.686291501015244, 38.14213562373095),
Offset(12.335961696184835, 37.78957826421869),
Offset(11.186164512970027, 36.485148967548454),
Offset(9.272264829328229, 33.57462824174341),
Offset(7.396433901397504, 28.01370476875244),
Offset(8.455221168610253, 18.46855392229577),
Offset(14.400164412415048, 10.886305797611346),
Offset(23.385743674953297, 7.627722418507858),
Offset(31.513897019057687, 8.97811161083639),
Offset(37.00821523813391, 12.590468749760188),
Offset(40.228807248234226, 16.687449599224042),
Offset(41.900283080823314, 20.466101465000598),
Offset(42.622994421193994, 23.65567978529433),
Offset(42.809759514234806, 26.219169470442615),
Offset(42.724595353131164, 28.207893098186),
Offset(42.529420070562, 29.69761906859474),
Offset(42.319508324086705, 30.763041306693708),
Offset(42.1473924208978, 31.468700863761335),
Offset(42.038312568419684, 31.86713222464318),
Offset(42.00008112385512, 31.999722191105658),
Offset(42.0, 32.0),
],
<Offset>[
Offset(12.686291501015244, 38.14213562373095),
Offset(12.335961696184835, 37.78957826421869),
Offset(11.186164512970027, 36.485148967548454),
Offset(9.272264829328229, 33.57462824174341),
Offset(7.396433901397504, 28.01370476875244),
Offset(8.455221168610253, 18.46855392229577),
Offset(14.400164412415048, 10.886305797611346),
Offset(23.385743674953297, 7.627722418507858),
Offset(31.513897019057687, 8.97811161083639),
Offset(37.00821523813391, 12.590468749760188),
Offset(40.228807248234226, 16.687449599224042),
Offset(41.900283080823314, 20.466101465000598),
Offset(42.622994421193994, 23.65567978529433),
Offset(42.809759514234806, 26.219169470442615),
Offset(42.724595353131164, 28.207893098186),
Offset(42.529420070562, 29.69761906859474),
Offset(42.319508324086705, 30.763041306693708),
Offset(42.1473924208978, 31.468700863761335),
Offset(42.038312568419684, 31.86713222464318),
Offset(42.00008112385512, 31.999722191105658),
Offset(42.0, 32.0),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/close_menu.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/close_menu.g.dart",
"repo_id": "flutter",
"token_count": 29556
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
import 'ink_well.dart';
import 'material.dart';
import 'text_form_field.dart';
import 'theme.dart';
/// {@macro flutter.widgets.RawAutocomplete.RawAutocomplete}
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=-Nny8kzW380}
///
/// {@tool dartpad}
/// This example shows how to create a very basic Autocomplete widget using the
/// default UI.
///
/// ** See code in examples/api/lib/material/autocomplete/autocomplete.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to create an Autocomplete widget with a custom type.
/// Try searching with text from the name or email field.
///
/// ** See code in examples/api/lib/material/autocomplete/autocomplete.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to create an Autocomplete widget whose options are
/// fetched over the network.
///
/// ** See code in examples/api/lib/material/autocomplete/autocomplete.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to create an Autocomplete widget whose options are
/// fetched over the network. It uses debouncing to wait to perform the network
/// request until after the user finishes typing.
///
/// ** See code in examples/api/lib/material/autocomplete/autocomplete.3.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to create an Autocomplete widget whose options are
/// fetched over the network. It includes both debouncing and error handling, so
/// that failed network requests show an error to the user and can be recovered
/// from. Try toggling the network Switch widget to simulate going offline.
///
/// ** See code in examples/api/lib/material/autocomplete/autocomplete.4.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RawAutocomplete], which is what Autocomplete is built upon, and which
/// contains more detailed examples.
class Autocomplete<T extends Object> extends StatelessWidget {
/// Creates an instance of [Autocomplete].
const Autocomplete({
super.key,
required this.optionsBuilder,
this.displayStringForOption = RawAutocomplete.defaultStringForOption,
this.fieldViewBuilder = _defaultFieldViewBuilder,
this.onSelected,
this.optionsMaxHeight = 200.0,
this.optionsViewBuilder,
this.optionsViewOpenDirection = OptionsViewOpenDirection.down,
this.initialValue,
});
/// {@macro flutter.widgets.RawAutocomplete.displayStringForOption}
final AutocompleteOptionToString<T> displayStringForOption;
/// {@macro flutter.widgets.RawAutocomplete.fieldViewBuilder}
///
/// If not provided, will build a standard Material-style text field by
/// default.
final AutocompleteFieldViewBuilder fieldViewBuilder;
/// {@macro flutter.widgets.RawAutocomplete.onSelected}
final AutocompleteOnSelected<T>? onSelected;
/// {@macro flutter.widgets.RawAutocomplete.optionsBuilder}
final AutocompleteOptionsBuilder<T> optionsBuilder;
/// {@macro flutter.widgets.RawAutocomplete.optionsViewBuilder}
///
/// If not provided, will build a standard Material-style list of results by
/// default.
final AutocompleteOptionsViewBuilder<T>? optionsViewBuilder;
/// {@macro flutter.widgets.RawAutocomplete.optionsViewOpenDirection}
final OptionsViewOpenDirection optionsViewOpenDirection;
/// The maximum height used for the default Material options list widget.
///
/// When [optionsViewBuilder] is `null`, this property sets the maximum height
/// that the options widget can occupy.
///
/// The default value is set to 200.
final double optionsMaxHeight;
/// {@macro flutter.widgets.RawAutocomplete.initialValue}
final TextEditingValue? initialValue;
static Widget _defaultFieldViewBuilder(BuildContext context, TextEditingController textEditingController, FocusNode focusNode, VoidCallback onFieldSubmitted) {
return _AutocompleteField(
focusNode: focusNode,
textEditingController: textEditingController,
onFieldSubmitted: onFieldSubmitted,
);
}
@override
Widget build(BuildContext context) {
return RawAutocomplete<T>(
displayStringForOption: displayStringForOption,
fieldViewBuilder: fieldViewBuilder,
initialValue: initialValue,
optionsBuilder: optionsBuilder,
optionsViewOpenDirection: optionsViewOpenDirection,
optionsViewBuilder: optionsViewBuilder ?? (BuildContext context, AutocompleteOnSelected<T> onSelected, Iterable<T> options) {
return _AutocompleteOptions<T>(
displayStringForOption: displayStringForOption,
onSelected: onSelected,
options: options,
openDirection: optionsViewOpenDirection,
maxOptionsHeight: optionsMaxHeight,
);
},
onSelected: onSelected,
);
}
}
// The default Material-style Autocomplete text field.
class _AutocompleteField extends StatelessWidget {
const _AutocompleteField({
required this.focusNode,
required this.textEditingController,
required this.onFieldSubmitted,
});
final FocusNode focusNode;
final VoidCallback onFieldSubmitted;
final TextEditingController textEditingController;
@override
Widget build(BuildContext context) {
return TextFormField(
controller: textEditingController,
focusNode: focusNode,
onFieldSubmitted: (String value) {
onFieldSubmitted();
},
);
}
}
// The default Material-style Autocomplete options.
class _AutocompleteOptions<T extends Object> extends StatelessWidget {
const _AutocompleteOptions({
super.key,
required this.displayStringForOption,
required this.onSelected,
required this.openDirection,
required this.options,
required this.maxOptionsHeight,
});
final AutocompleteOptionToString<T> displayStringForOption;
final AutocompleteOnSelected<T> onSelected;
final OptionsViewOpenDirection openDirection;
final Iterable<T> options;
final double maxOptionsHeight;
@override
Widget build(BuildContext context) {
final AlignmentDirectional optionsAlignment = switch (openDirection) {
OptionsViewOpenDirection.up => AlignmentDirectional.bottomStart,
OptionsViewOpenDirection.down => AlignmentDirectional.topStart,
};
return Align(
alignment: optionsAlignment,
child: Material(
elevation: 4.0,
child: ConstrainedBox(
constraints: BoxConstraints(maxHeight: maxOptionsHeight),
child: ListView.builder(
padding: EdgeInsets.zero,
shrinkWrap: true,
itemCount: options.length,
itemBuilder: (BuildContext context, int index) {
final T option = options.elementAt(index);
return InkWell(
onTap: () {
onSelected(option);
},
child: Builder(
builder: (BuildContext context) {
final bool highlight = AutocompleteHighlightedOption.of(context) == index;
if (highlight) {
SchedulerBinding.instance.addPostFrameCallback((Duration timeStamp) {
Scrollable.ensureVisible(context, alignment: 0.5);
}, debugLabel: 'AutocompleteOptions.ensureVisible');
}
return Container(
color: highlight ? Theme.of(context).focusColor : null,
padding: const EdgeInsets.all(16.0),
child: Text(displayStringForOption(option)),
);
}
),
);
},
),
),
),
);
}
}
| flutter/packages/flutter/lib/src/material/autocomplete.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/autocomplete.dart",
"repo_id": "flutter",
"token_count": 2785
} | 674 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'colors.dart';
import 'constants.dart';
import 'elevated_button.dart';
import 'filled_button.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_state.dart';
import 'outlined_button.dart';
import 'text_button.dart';
import 'theme_data.dart';
/// {@template flutter.material.ButtonStyleButton.iconAlignment}
/// Determines the alignment of the icon within the widgets such as:
/// - [ElevatedButton.icon],
/// - [FilledButton.icon],
/// - [FilledButton.tonalIcon].
/// - [OutlinedButton.icon],
/// - [TextButton.icon],
///
/// The effect of `iconAlignment` depends on [TextDirection]. If textDirection is
/// [TextDirection.ltr] then [IconAlignment.start] and [IconAlignment.end] align the
/// icon on the left or right respectively. If textDirection is [TextDirection.rtl] the
/// the alignments are reversed.
///
/// Defaults to [IconAlignment.start].
///
/// {@tool dartpad}
/// This sample demonstrates how to use `iconAlignment` to align the button icon to the start
/// or the end of the button.
///
/// ** See code in examples/api/lib/material/button_style_button/button_style_button.icon_alignment.0.dart **
/// {@end-tool}
///
/// {@endtemplate}
enum IconAlignment {
/// The icon is placed at the start of the button.
start,
/// The icon is placed at the end of the button.
end,
}
/// The base [StatefulWidget] class for buttons whose style is defined by a [ButtonStyle] object.
///
/// Concrete subclasses must override [defaultStyleOf] and [themeStyleOf].
///
/// See also:
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [FilledButton], a filled button that doesn't elevate when pressed.
/// * [FilledButton.tonal], a filled button variant that uses a secondary fill color.
/// * [OutlinedButton], a button with an outlined border and no fill color.
/// * [TextButton], a button with no outline or fill color.
/// * <https://m3.material.io/components/buttons/overview>, an overview of each of
/// the Material Design button types and how they should be used in designs.
abstract class ButtonStyleButton extends StatefulWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const ButtonStyleButton({
super.key,
required this.onPressed,
required this.onLongPress,
required this.onHover,
required this.onFocusChange,
required this.style,
required this.focusNode,
required this.autofocus,
required this.clipBehavior,
this.statesController,
this.isSemanticButton = true,
required this.child,
this.iconAlignment = IconAlignment.start,
});
/// Called when the button is tapped or otherwise activated.
///
/// If this callback and [onLongPress] are null, then the button will be disabled.
///
/// See also:
///
/// * [enabled], which is true if the button is enabled.
final VoidCallback? onPressed;
/// Called when the button is long-pressed.
///
/// If this callback and [onPressed] are null, then the button will be disabled.
///
/// See also:
///
/// * [enabled], which is true if the button is enabled.
final VoidCallback? onLongPress;
/// Called when a pointer enters or exits the button response area.
///
/// The value passed to the callback is true if a pointer has entered this
/// part of the material and false if a pointer has exited this part of the
/// material.
final ValueChanged<bool>? onHover;
/// Handler called when the focus changes.
///
/// Called with true if this widget's node gains focus, and false if it loses
/// focus.
final ValueChanged<bool>? onFocusChange;
/// Customizes this button's appearance.
///
/// Non-null properties of this style override the corresponding
/// properties in [themeStyleOf] and [defaultStyleOf]. [MaterialStateProperty]s
/// that resolve to non-null values will similarly override the corresponding
/// [MaterialStateProperty]s in [themeStyleOf] and [defaultStyleOf].
///
/// Null by default.
final ButtonStyle? style;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none] unless [ButtonStyle.backgroundBuilder] or
/// [ButtonStyle.foregroundBuilder] is specified. In those
/// cases the default is [Clip.antiAlias].
final Clip? clipBehavior;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.material.inkwell.statesController}
final MaterialStatesController? statesController;
/// Determine whether this subtree represents a button.
///
/// If this is null, the screen reader will not announce "button" when this
/// is focused. This is useful for [MenuItemButton] and [SubmenuButton] when we
/// traverse the menu system.
///
/// Defaults to true.
final bool? isSemanticButton;
/// Typically the button's label.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// {@macro flutter.material.ButtonStyleButton.iconAlignment}
final IconAlignment iconAlignment;
/// Returns a non-null [ButtonStyle] that's based primarily on the [Theme]'s
/// [ThemeData.textTheme] and [ThemeData.colorScheme].
///
/// The returned style can be overridden by the [style] parameter and
/// by the style returned by [themeStyleOf]. For example the default
/// style of the [TextButton] subclass can be overridden with its
/// [TextButton.style] constructor parameter, or with a
/// [TextButtonTheme].
///
/// Concrete button subclasses should return a ButtonStyle that
/// has no null properties, and where all of the [MaterialStateProperty]
/// properties resolve to non-null values.
///
/// See also:
///
/// * [themeStyleOf], Returns the ButtonStyle of this button's component theme.
@protected
ButtonStyle defaultStyleOf(BuildContext context);
/// Returns the ButtonStyle that belongs to the button's component theme.
///
/// The returned style can be overridden by the [style] parameter.
///
/// Concrete button subclasses should return the ButtonStyle for the
/// nearest subclass-specific inherited theme, and if no such theme
/// exists, then the same value from the overall [Theme].
///
/// See also:
///
/// * [defaultStyleOf], Returns the default [ButtonStyle] for this button.
@protected
ButtonStyle? themeStyleOf(BuildContext context);
/// Whether the button is enabled or disabled.
///
/// Buttons are disabled by default. To enable a button, set its [onPressed]
/// or [onLongPress] properties to a non-null value.
bool get enabled => onPressed != null || onLongPress != null;
@override
State<ButtonStyleButton> createState() => _ButtonStyleState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('enabled', value: enabled, ifFalse: 'disabled'));
properties.add(DiagnosticsProperty<ButtonStyle>('style', style, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode, defaultValue: null));
}
/// Returns null if [value] is null, otherwise `MaterialStatePropertyAll<T>(value)`.
///
/// A convenience method for subclasses.
static MaterialStateProperty<T>? allOrNull<T>(T? value) => value == null ? null : MaterialStatePropertyAll<T>(value);
/// A convenience method used by subclasses in the framework, that returns an
/// interpolated value based on the [fontSizeMultiplier] parameter:
///
/// * 0 - 1 [geometry1x]
/// * 1 - 2 lerp([geometry1x], [geometry2x], [fontSizeMultiplier] - 1)
/// * 2 - 3 lerp([geometry2x], [geometry3x], [fontSizeMultiplier] - 2)
/// * otherwise [geometry3x]
///
/// This method is used by the framework for estimating the default paddings to
/// use on a button with a text label, when the system text scaling setting
/// changes. It's usually supplied with empirical [geometry1x], [geometry2x],
/// [geometry3x] values adjusted for different system text scaling values, when
/// the unscaled font size is set to 14.0 (the default [TextTheme.labelLarge]
/// value).
///
/// The `fontSizeMultiplier` argument, for historical reasons, is the default
/// font size specified in the [ButtonStyle], scaled by the ambient font
/// scaler, then divided by 14.0 (the default font size used in buttons).
static EdgeInsetsGeometry scaledPadding(
EdgeInsetsGeometry geometry1x,
EdgeInsetsGeometry geometry2x,
EdgeInsetsGeometry geometry3x,
double fontSizeMultiplier,
) {
return switch (fontSizeMultiplier) {
<= 1 => geometry1x,
< 2 => EdgeInsetsGeometry.lerp(geometry1x, geometry2x, fontSizeMultiplier - 1)!,
< 3 => EdgeInsetsGeometry.lerp(geometry2x, geometry3x, fontSizeMultiplier - 2)!,
_ => geometry3x,
};
}
}
/// The base [State] class for buttons whose style is defined by a [ButtonStyle] object.
///
/// See also:
///
/// * [ButtonStyleButton], the [StatefulWidget] subclass for which this class is the [State].
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [FilledButton], a filled ButtonStyleButton that doesn't elevate when pressed.
/// * [OutlinedButton], similar to [TextButton], but with an outline.
/// * [TextButton], a simple button without a shadow.
class _ButtonStyleState extends State<ButtonStyleButton> with TickerProviderStateMixin {
AnimationController? controller;
double? elevation;
Color? backgroundColor;
MaterialStatesController? internalStatesController;
void handleStatesControllerChange() {
// Force a rebuild to resolve MaterialStateProperty properties
setState(() { });
}
MaterialStatesController get statesController => widget.statesController ?? internalStatesController!;
void initStatesController() {
if (widget.statesController == null) {
internalStatesController = MaterialStatesController();
}
statesController.update(MaterialState.disabled, !widget.enabled);
statesController.addListener(handleStatesControllerChange);
}
@override
void initState() {
super.initState();
initStatesController();
}
@override
void didUpdateWidget(ButtonStyleButton oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.statesController != oldWidget.statesController) {
oldWidget.statesController?.removeListener(handleStatesControllerChange);
if (widget.statesController != null) {
internalStatesController?.dispose();
internalStatesController = null;
}
initStatesController();
}
if (widget.enabled != oldWidget.enabled) {
statesController.update(MaterialState.disabled, !widget.enabled);
if (!widget.enabled) {
// The button may have been disabled while a press gesture is currently underway.
statesController.update(MaterialState.pressed, false);
}
}
}
@override
void dispose() {
statesController.removeListener(handleStatesControllerChange);
internalStatesController?.dispose();
controller?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final ButtonStyle? widgetStyle = widget.style;
final ButtonStyle? themeStyle = widget.themeStyleOf(context);
final ButtonStyle defaultStyle = widget.defaultStyleOf(context);
T? effectiveValue<T>(T? Function(ButtonStyle? style) getProperty) {
final T? widgetValue = getProperty(widgetStyle);
final T? themeValue = getProperty(themeStyle);
final T? defaultValue = getProperty(defaultStyle);
return widgetValue ?? themeValue ?? defaultValue;
}
T? resolve<T>(MaterialStateProperty<T>? Function(ButtonStyle? style) getProperty) {
return effectiveValue(
(ButtonStyle? style) {
return getProperty(style)?.resolve(statesController.value);
},
);
}
final double? resolvedElevation = resolve<double?>((ButtonStyle? style) => style?.elevation);
final TextStyle? resolvedTextStyle = resolve<TextStyle?>((ButtonStyle? style) => style?.textStyle);
Color? resolvedBackgroundColor = resolve<Color?>((ButtonStyle? style) => style?.backgroundColor);
final Color? resolvedForegroundColor = resolve<Color?>((ButtonStyle? style) => style?.foregroundColor);
final Color? resolvedShadowColor = resolve<Color?>((ButtonStyle? style) => style?.shadowColor);
final Color? resolvedSurfaceTintColor = resolve<Color?>((ButtonStyle? style) => style?.surfaceTintColor);
final EdgeInsetsGeometry? resolvedPadding = resolve<EdgeInsetsGeometry?>((ButtonStyle? style) => style?.padding);
final Size? resolvedMinimumSize = resolve<Size?>((ButtonStyle? style) => style?.minimumSize);
final Size? resolvedFixedSize = resolve<Size?>((ButtonStyle? style) => style?.fixedSize);
final Size? resolvedMaximumSize = resolve<Size?>((ButtonStyle? style) => style?.maximumSize);
final Color? resolvedIconColor = resolve<Color?>((ButtonStyle? style) => style?.iconColor);
final double? resolvedIconSize = resolve<double?>((ButtonStyle? style) => style?.iconSize);
final BorderSide? resolvedSide = resolve<BorderSide?>((ButtonStyle? style) => style?.side);
final OutlinedBorder? resolvedShape = resolve<OutlinedBorder?>((ButtonStyle? style) => style?.shape);
final MaterialStateMouseCursor mouseCursor = _MouseCursor(
(Set<MaterialState> states) => effectiveValue((ButtonStyle? style) => style?.mouseCursor?.resolve(states)),
);
final MaterialStateProperty<Color?> overlayColor = MaterialStateProperty.resolveWith<Color?>(
(Set<MaterialState> states) => effectiveValue((ButtonStyle? style) => style?.overlayColor?.resolve(states)),
);
final VisualDensity? resolvedVisualDensity = effectiveValue((ButtonStyle? style) => style?.visualDensity);
final MaterialTapTargetSize? resolvedTapTargetSize = effectiveValue((ButtonStyle? style) => style?.tapTargetSize);
final Duration? resolvedAnimationDuration = effectiveValue((ButtonStyle? style) => style?.animationDuration);
final bool? resolvedEnableFeedback = effectiveValue((ButtonStyle? style) => style?.enableFeedback);
final AlignmentGeometry? resolvedAlignment = effectiveValue((ButtonStyle? style) => style?.alignment);
final Offset densityAdjustment = resolvedVisualDensity!.baseSizeAdjustment;
final InteractiveInkFeatureFactory? resolvedSplashFactory = effectiveValue((ButtonStyle? style) => style?.splashFactory);
final ButtonLayerBuilder? resolvedBackgroundBuilder = effectiveValue((ButtonStyle? style) => style?.backgroundBuilder);
final ButtonLayerBuilder? resolvedForegroundBuilder = effectiveValue((ButtonStyle? style) => style?.foregroundBuilder);
final Clip effectiveClipBehavior = widget.clipBehavior
?? ((resolvedBackgroundBuilder ?? resolvedForegroundBuilder) != null ? Clip.antiAlias : Clip.none);
BoxConstraints effectiveConstraints = resolvedVisualDensity.effectiveConstraints(
BoxConstraints(
minWidth: resolvedMinimumSize!.width,
minHeight: resolvedMinimumSize.height,
maxWidth: resolvedMaximumSize!.width,
maxHeight: resolvedMaximumSize.height,
),
);
if (resolvedFixedSize != null) {
final Size size = effectiveConstraints.constrain(resolvedFixedSize);
if (size.width.isFinite) {
effectiveConstraints = effectiveConstraints.copyWith(
minWidth: size.width,
maxWidth: size.width,
);
}
if (size.height.isFinite) {
effectiveConstraints = effectiveConstraints.copyWith(
minHeight: size.height,
maxHeight: size.height,
);
}
}
// Per the Material Design team: don't allow the VisualDensity
// adjustment to reduce the width of the left/right padding. If we
// did, VisualDensity.compact, the default for desktop/web, would
// reduce the horizontal padding to zero.
final double dy = densityAdjustment.dy;
final double dx = math.max(0, densityAdjustment.dx);
final EdgeInsetsGeometry padding = resolvedPadding!
.add(EdgeInsets.fromLTRB(dx, dy, dx, dy))
.clamp(EdgeInsets.zero, EdgeInsetsGeometry.infinity);
// If an opaque button's background is becoming translucent while its
// elevation is changing, change the elevation first. Material implicitly
// animates its elevation but not its color. SKIA renders non-zero
// elevations as a shadow colored fill behind the Material's background.
if (resolvedAnimationDuration! > Duration.zero
&& elevation != null
&& backgroundColor != null
&& elevation != resolvedElevation
&& backgroundColor!.value != resolvedBackgroundColor!.value
&& backgroundColor!.opacity == 1
&& resolvedBackgroundColor.opacity < 1
&& resolvedElevation == 0) {
if (controller?.duration != resolvedAnimationDuration) {
controller?.dispose();
controller = AnimationController(
duration: resolvedAnimationDuration,
vsync: this,
)
..addStatusListener((AnimationStatus status) {
if (status == AnimationStatus.completed) {
setState(() { }); // Rebuild with the final background color.
}
});
}
resolvedBackgroundColor = backgroundColor; // Defer changing the background color.
controller!.value = 0;
controller!.forward();
}
elevation = resolvedElevation;
backgroundColor = resolvedBackgroundColor;
Widget effectiveChild = Padding(
padding: padding,
child: Align(
alignment: resolvedAlignment!,
widthFactor: 1.0,
heightFactor: 1.0,
child: resolvedForegroundBuilder != null
? resolvedForegroundBuilder(context, statesController.value, widget.child)
: widget.child,
),
);
if (resolvedBackgroundBuilder != null) {
effectiveChild = resolvedBackgroundBuilder(context, statesController.value, effectiveChild);
}
final Widget result = ConstrainedBox(
constraints: effectiveConstraints,
child: Material(
elevation: resolvedElevation!,
textStyle: resolvedTextStyle?.copyWith(color: resolvedForegroundColor),
shape: resolvedShape!.copyWith(side: resolvedSide),
color: resolvedBackgroundColor,
shadowColor: resolvedShadowColor,
surfaceTintColor: resolvedSurfaceTintColor,
type: resolvedBackgroundColor == null ? MaterialType.transparency : MaterialType.button,
animationDuration: resolvedAnimationDuration,
clipBehavior: effectiveClipBehavior,
child: InkWell(
onTap: widget.onPressed,
onLongPress: widget.onLongPress,
onHover: widget.onHover,
mouseCursor: mouseCursor,
enableFeedback: resolvedEnableFeedback,
focusNode: widget.focusNode,
canRequestFocus: widget.enabled,
onFocusChange: widget.onFocusChange,
autofocus: widget.autofocus,
splashFactory: resolvedSplashFactory,
overlayColor: overlayColor,
highlightColor: Colors.transparent,
customBorder: resolvedShape.copyWith(side: resolvedSide),
statesController: statesController,
child: IconTheme.merge(
data: IconThemeData(color: resolvedIconColor ?? resolvedForegroundColor, size: resolvedIconSize),
child: effectiveChild,
),
),
),
);
final Size minSize;
switch (resolvedTapTargetSize!) {
case MaterialTapTargetSize.padded:
minSize = Size(
kMinInteractiveDimension + densityAdjustment.dx,
kMinInteractiveDimension + densityAdjustment.dy,
);
assert(minSize.width >= 0.0);
assert(minSize.height >= 0.0);
case MaterialTapTargetSize.shrinkWrap:
minSize = Size.zero;
}
return Semantics(
container: true,
button: widget.isSemanticButton,
enabled: widget.enabled,
child: _InputPadding(
minSize: minSize,
child: result,
),
);
}
}
class _MouseCursor extends MaterialStateMouseCursor {
const _MouseCursor(this.resolveCallback);
final MaterialPropertyResolver<MouseCursor?> resolveCallback;
@override
MouseCursor resolve(Set<MaterialState> states) => resolveCallback(states)!;
@override
String get debugDescription => 'ButtonStyleButton_MouseCursor';
}
/// A widget to pad the area around a [ButtonStyleButton]'s inner [Material].
///
/// Redirect taps that occur in the padded area around the child to the center
/// of the child. This increases the size of the button and the button's
/// "tap target", but not its material or its ink splashes.
class _InputPadding extends SingleChildRenderObjectWidget {
const _InputPadding({
super.child,
required this.minSize,
});
final Size minSize;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderInputPadding(minSize);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderInputPadding renderObject) {
renderObject.minSize = minSize;
}
}
class _RenderInputPadding extends RenderShiftedBox {
_RenderInputPadding(this._minSize, [RenderBox? child]) : super(child);
Size get minSize => _minSize;
Size _minSize;
set minSize(Size value) {
if (_minSize == value) {
return;
}
_minSize = value;
markNeedsLayout();
}
@override
double computeMinIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMinIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMinIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMaxIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMaxIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
Size _computeSize({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
if (child != null) {
final Size childSize = layoutChild(child!, constraints);
final double height = math.max(childSize.width, minSize.width);
final double width = math.max(childSize.height, minSize.height);
return constraints.constrain(Size(height, width));
}
return Size.zero;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.dryLayoutChild,
);
}
@override
void performLayout() {
size = _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.layoutChild,
);
if (child != null) {
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Alignment.center.alongOffset(size - child!.size as Offset);
}
}
@override
bool hitTest(BoxHitTestResult result, { required Offset position }) {
if (super.hitTest(result, position: position)) {
return true;
}
final Offset center = child!.size.center(Offset.zero);
return result.addWithRawTransform(
transform: MatrixUtils.forceToPoint(center),
position: center,
hitTest: (BoxHitTestResult result, Offset position) {
assert(position == center);
return child!.hitTest(result, position: center);
},
);
}
}
| flutter/packages/flutter/lib/src/material/button_style_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/button_style_button.dart",
"repo_id": "flutter",
"token_count": 7758
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'checkbox.dart';
import 'constants.dart';
import 'data_table_theme.dart';
import 'debug.dart';
import 'divider.dart';
import 'dropdown.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_state.dart';
import 'theme.dart';
import 'tooltip.dart';
// Examples can assume:
// late BuildContext context;
// late List<DataColumn> _columns;
// late List<DataRow> _rows;
/// Signature for [DataColumn.onSort] callback.
typedef DataColumnSortCallback = void Function(int columnIndex, bool ascending);
/// Column configuration for a [DataTable].
///
/// One column configuration must be provided for each column to
/// display in the table. The list of [DataColumn] objects is passed
/// as the `columns` argument to the [DataTable.new] constructor.
@immutable
class DataColumn {
/// Creates the configuration for a column of a [DataTable].
const DataColumn({
required this.label,
this.tooltip,
this.numeric = false,
this.onSort,
this.mouseCursor,
});
/// The column heading.
///
/// Typically, this will be a [Text] widget. It could also be an
/// [Icon] (typically using size 18), or a [Row] with an icon and
/// some text.
///
/// The [label] is placed within a [Row] along with the
/// sort indicator (if applicable). By default, [label] only occupy minimal
/// space. It is recommended to place the label content in an [Expanded] or
/// [Flexible] as [label] to control how the content flexes. Otherwise,
/// an exception will occur when the available space is insufficient.
///
/// By default, [DefaultTextStyle.softWrap] of this subtree will be set to false.
/// Use [DefaultTextStyle.merge] to override it if needed.
///
/// The label should not include the sort indicator.
final Widget label;
/// The column heading's tooltip.
///
/// This is a longer description of the column heading, for cases
/// where the heading might have been abbreviated to keep the column
/// width to a reasonable size.
final String? tooltip;
/// Whether this column represents numeric data or not.
///
/// The contents of cells of columns containing numeric data are
/// right-aligned.
final bool numeric;
/// Called when the user asks to sort the table using this column.
///
/// If null, the column will not be considered sortable.
///
/// See [DataTable.sortColumnIndex] and [DataTable.sortAscending].
final DataColumnSortCallback? onSort;
bool get _debugInteractive => onSort != null;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// heading row.
///
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.disabled].
///
/// If this is null, then the value of [DataTableThemeData.headingCellCursor]
/// is used. If that's null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
/// * [MaterialStateMouseCursor], which can be used to create a [MouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
}
/// Row configuration and cell data for a [DataTable].
///
/// One row configuration must be provided for each row to
/// display in the table. The list of [DataRow] objects is passed
/// as the `rows` argument to the [DataTable.new] constructor.
///
/// The data for this row of the table is provided in the [cells]
/// property of the [DataRow] object.
@immutable
class DataRow {
/// Creates the configuration for a row of a [DataTable].
const DataRow({
this.key,
this.selected = false,
this.onSelectChanged,
this.onLongPress,
this.color,
this.mouseCursor,
required this.cells,
});
/// Creates the configuration for a row of a [DataTable], deriving
/// the key from a row index.
DataRow.byIndex({
int? index,
this.selected = false,
this.onSelectChanged,
this.onLongPress,
this.color,
this.mouseCursor,
required this.cells,
}) : key = ValueKey<int?>(index);
/// A [Key] that uniquely identifies this row. This is used to
/// ensure that if a row is added or removed, any stateful widgets
/// related to this row (e.g. an in-progress checkbox animation)
/// remain on the right row visually.
///
/// If the table never changes once created, no key is necessary.
final LocalKey? key;
/// Called when the user selects or unselects a selectable row.
///
/// If this is not null, then the row is selectable. The current
/// selection state of the row is given by [selected].
///
/// If any row is selectable, then the table's heading row will have
/// a checkbox that can be checked to select all selectable rows
/// (and which is checked if all the rows are selected), and each
/// subsequent row will have a checkbox to toggle just that row.
///
/// A row whose [onSelectChanged] callback is null is ignored for
/// the purposes of determining the state of the "all" checkbox,
/// and its checkbox is disabled.
///
/// If a [DataCell] in the row has its [DataCell.onTap] callback defined,
/// that callback behavior overrides the gesture behavior of the row for
/// that particular cell.
final ValueChanged<bool?>? onSelectChanged;
/// Called if the row is long-pressed.
///
/// If a [DataCell] in the row has its [DataCell.onTap], [DataCell.onDoubleTap],
/// [DataCell.onLongPress], [DataCell.onTapCancel] or [DataCell.onTapDown] callback defined,
/// that callback behavior overrides the gesture behavior of the row for
/// that particular cell.
final GestureLongPressCallback? onLongPress;
/// Whether the row is selected.
///
/// If [onSelectChanged] is non-null for any row in the table, then
/// a checkbox is shown at the start of each row. If the row is
/// selected (true), the checkbox will be checked and the row will
/// be highlighted.
///
/// Otherwise, the checkbox, if present, will not be checked.
final bool selected;
/// The data for this row.
///
/// There must be exactly as many cells as there are columns in the
/// table.
final List<DataCell> cells;
/// The color for the row.
///
/// By default, the color is transparent unless selected. Selected rows has
/// a grey translucent color.
///
/// The effective color can depend on the [MaterialState] state, if the
/// row is selected, pressed, hovered, focused, disabled or enabled. The
/// color is painted as an overlay to the row. To make sure that the row's
/// [InkWell] is visible (when pressed, hovered and focused), it is
/// recommended to use a translucent color.
///
/// If [onSelectChanged] or [onLongPress] is null, the row's [InkWell] will be disabled.
///
/// ```dart
/// DataRow(
/// color: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.selected)) {
/// return Theme.of(context).colorScheme.primary.withOpacity(0.08);
/// }
/// return null; // Use the default value.
/// }),
/// cells: const <DataCell>[
/// // ...
/// ],
/// )
/// ```
///
/// See also:
///
/// * The Material Design specification for overlay colors and how they
/// match a component's state:
/// <https://material.io/design/interaction/states.html#anatomy>.
final MaterialStateProperty<Color?>? color;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// data row.
///
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
///
/// If this is null, then the value of [DataTableThemeData.dataRowCursor]
/// is used. If that's null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
/// * [MaterialStateMouseCursor], which can be used to create a [MouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
bool get _debugInteractive => onSelectChanged != null || cells.any((DataCell cell) => cell._debugInteractive);
}
/// The data for a cell of a [DataTable].
///
/// One list of [DataCell] objects must be provided for each [DataRow]
/// in the [DataTable], in the new [DataRow] constructor's `cells`
/// argument.
@immutable
class DataCell {
/// Creates an object to hold the data for a cell in a [DataTable].
///
/// The first argument is the widget to show for the cell, typically
/// a [Text] or [DropdownButton] widget.
///
/// If the cell has no data, then a [Text] widget with placeholder
/// text should be provided instead, and then the [placeholder]
/// argument should be set to true.
const DataCell(
this.child, {
this.placeholder = false,
this.showEditIcon = false,
this.onTap,
this.onLongPress,
this.onTapDown,
this.onDoubleTap,
this.onTapCancel,
});
/// A cell that has no content and has zero width and height.
static const DataCell empty = DataCell(SizedBox.shrink());
/// The data for the row.
///
/// Typically a [Text] widget or a [DropdownButton] widget.
///
/// If the cell has no data, then a [Text] widget with placeholder
/// text should be provided instead, and [placeholder] should be set
/// to true.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Whether the [child] is actually a placeholder.
///
/// If this is true, the default text style for the cell is changed
/// to be appropriate for placeholder text.
final bool placeholder;
/// Whether to show an edit icon at the end of the cell.
///
/// This does not make the cell actually editable; the caller must
/// implement editing behavior if desired (initiated from the
/// [onTap] callback).
///
/// If this is set, [onTap] should also be set, otherwise tapping
/// the icon will have no effect.
final bool showEditIcon;
/// Called if the cell is tapped.
///
/// If non-null, tapping the cell will call this callback. If
/// null (including [onDoubleTap], [onLongPress], [onTapCancel] and [onTapDown]),
/// tapping the cell will attempt to select the row (if
/// [DataRow.onSelectChanged] is provided).
final GestureTapCallback? onTap;
/// Called when the cell is double tapped.
///
/// If non-null, tapping the cell will call this callback. If
/// null (including [onTap], [onLongPress], [onTapCancel] and [onTapDown]),
/// tapping the cell will attempt to select the row (if
/// [DataRow.onSelectChanged] is provided).
final GestureTapCallback? onDoubleTap;
/// Called if the cell is long-pressed.
///
/// If non-null, tapping the cell will invoke this callback. If
/// null (including [onDoubleTap], [onTap], [onTapCancel] and [onTapDown]),
/// tapping the cell will attempt to select the row (if
/// [DataRow.onSelectChanged] is provided).
final GestureLongPressCallback? onLongPress;
/// Called if the cell is tapped down.
///
/// If non-null, tapping the cell will call this callback. If
/// null (including [onTap] [onDoubleTap], [onLongPress] and [onTapCancel]),
/// tapping the cell will attempt to select the row (if
/// [DataRow.onSelectChanged] is provided).
final GestureTapDownCallback? onTapDown;
/// Called if the user cancels a tap was started on cell.
///
/// If non-null, canceling the tap gesture will invoke this callback.
/// If null (including [onTap], [onDoubleTap] and [onLongPress]),
/// tapping the cell will attempt to select the
/// row (if [DataRow.onSelectChanged] is provided).
final GestureTapCancelCallback? onTapCancel;
bool get _debugInteractive => onTap != null ||
onDoubleTap != null ||
onLongPress != null ||
onTapDown != null ||
onTapCancel != null;
}
/// A Material Design data table.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ktTajqbhIcY}
///
/// Displaying data in a table is expensive, because to lay out the
/// table all the data must be measured twice, once to negotiate the
/// dimensions to use for each column, and once to actually lay out
/// the table given the results of the negotiation.
///
/// For this reason, if you have a lot of data (say, more than a dozen
/// rows with a dozen columns, though the precise limits depend on the
/// target device), it is suggested that you use a
/// [PaginatedDataTable] which automatically splits the data into
/// multiple pages.
///
/// ## Performance considerations when wrapping [DataTable] with [SingleChildScrollView]
///
/// Wrapping a [DataTable] with [SingleChildScrollView] is expensive as [SingleChildScrollView]
/// mounts and paints the entire [DataTable] even when only some rows are visible. If scrolling in
/// one direction is necessary, then consider using a [CustomScrollView], otherwise use [PaginatedDataTable]
/// to split the data into smaller pages.
///
/// {@tool dartpad}
/// This sample shows how to display a [DataTable] with three columns: name, age, and
/// role. The columns are defined by three [DataColumn] objects. The table
/// contains three rows of data for three example users, the data for which
/// is defined by three [DataRow] objects.
///
/// 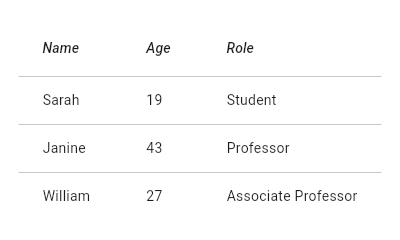
///
/// ** See code in examples/api/lib/material/data_table/data_table.0.dart **
/// {@end-tool}
///
///
/// {@tool dartpad}
/// This sample shows how to display a [DataTable] with alternate colors per
/// row, and a custom color for when the row is selected.
///
/// ** See code in examples/api/lib/material/data_table/data_table.1.dart **
/// {@end-tool}
///
/// [DataTable] can be sorted on the basis of any column in [columns] in
/// ascending or descending order. If [sortColumnIndex] is non-null, then the
/// table will be sorted by the values in the specified column. The boolean
/// [sortAscending] flag controls the sort order.
///
/// See also:
///
/// * [DataColumn], which describes a column in the data table.
/// * [DataRow], which contains the data for a row in the data table.
/// * [DataCell], which contains the data for a single cell in the data table.
/// * [PaginatedDataTable], which shows part of the data in a data table and
/// provides controls for paging through the remainder of the data.
/// * <https://material.io/design/components/data-tables.html>
class DataTable extends StatelessWidget {
/// Creates a widget describing a data table.
///
/// The [columns] argument must be a list of as many [DataColumn]
/// objects as the table is to have columns, ignoring the leading
/// checkbox column if any. The [columns] argument must have a
/// length greater than zero.
///
/// The [rows] argument must be a list of as many [DataRow] objects
/// as the table is to have rows, ignoring the leading heading row
/// that contains the column headings (derived from the [columns]
/// argument). There may be zero rows, but the rows argument must
/// not be null.
///
/// Each [DataRow] object in [rows] must have as many [DataCell]
/// objects in the [DataRow.cells] list as the table has columns.
///
/// If the table is sorted, the column that provides the current
/// primary key should be specified by index in [sortColumnIndex], 0
/// meaning the first column in [columns], 1 being the next one, and
/// so forth.
///
/// The actual sort order can be specified using [sortAscending]; if
/// the sort order is ascending, this should be true (the default),
/// otherwise it should be false.
DataTable({
super.key,
required this.columns,
this.sortColumnIndex,
this.sortAscending = true,
this.onSelectAll,
this.decoration,
this.dataRowColor,
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? dataRowHeight,
double? dataRowMinHeight,
double? dataRowMaxHeight,
this.dataTextStyle,
this.headingRowColor,
this.headingRowHeight,
this.headingTextStyle,
this.horizontalMargin,
this.columnSpacing,
this.showCheckboxColumn = true,
this.showBottomBorder = false,
this.dividerThickness,
required this.rows,
this.checkboxHorizontalMargin,
this.border,
this.clipBehavior = Clip.none,
}) : assert(columns.isNotEmpty),
assert(sortColumnIndex == null || (sortColumnIndex >= 0 && sortColumnIndex < columns.length)),
assert(!rows.any((DataRow row) => row.cells.length != columns.length), 'All rows must have the same number of cells as there are header cells (${columns.length})'),
assert(dividerThickness == null || dividerThickness >= 0),
assert(dataRowMinHeight == null || dataRowMaxHeight == null || dataRowMaxHeight >= dataRowMinHeight),
assert(dataRowHeight == null || (dataRowMinHeight == null && dataRowMaxHeight == null),
'dataRowHeight ($dataRowHeight) must not be set if dataRowMinHeight ($dataRowMinHeight) or dataRowMaxHeight ($dataRowMaxHeight) are set.'),
dataRowMinHeight = dataRowHeight ?? dataRowMinHeight,
dataRowMaxHeight = dataRowHeight ?? dataRowMaxHeight,
_onlyTextColumn = _initOnlyTextColumn(columns);
/// The configuration and labels for the columns in the table.
final List<DataColumn> columns;
/// The current primary sort key's column.
///
/// If non-null, indicates that the indicated column is the column
/// by which the data is sorted. The number must correspond to the
/// index of the relevant column in [columns].
///
/// Setting this will cause the relevant column to have a sort
/// indicator displayed.
///
/// When this is null, it implies that the table's sort order does
/// not correspond to any of the columns.
///
/// The direction of the sort is specified using [sortAscending].
final int? sortColumnIndex;
/// Whether the column mentioned in [sortColumnIndex], if any, is sorted
/// in ascending order.
///
/// If true, the order is ascending (meaning the rows with the
/// smallest values for the current sort column are first in the
/// table).
///
/// If false, the order is descending (meaning the rows with the
/// smallest values for the current sort column are last in the
/// table).
///
/// Ascending order is represented by an upwards-facing arrow.
final bool sortAscending;
/// Invoked when the user selects or unselects every row, using the
/// checkbox in the heading row.
///
/// If this is null, then the [DataRow.onSelectChanged] callback of
/// every row in the table is invoked appropriately instead.
///
/// To control whether a particular row is selectable or not, see
/// [DataRow.onSelectChanged]. This callback is only relevant if any
/// row is selectable.
final ValueSetter<bool?>? onSelectAll;
/// {@template flutter.material.dataTable.decoration}
/// The background and border decoration for the table.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.decoration] is used. By default there is no
/// decoration.
final Decoration? decoration;
/// {@template flutter.material.dataTable.dataRowColor}
/// The background color for the data rows.
///
/// The effective background color can be made to depend on the
/// [MaterialState] state, i.e. if the row is selected, pressed, hovered,
/// focused, disabled or enabled. The color is painted as an overlay to the
/// row. To make sure that the row's [InkWell] is visible (when pressed,
/// hovered and focused), it is recommended to use a translucent background
/// color.
///
/// If [DataRow.onSelectChanged] or [DataRow.onLongPress] is null, the row's
/// [InkWell] will be disabled.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dataRowColor] is used. By default, the
/// background color is transparent unless selected. Selected rows have a grey
/// translucent color. To set a different color for individual rows, see
/// [DataRow.color].
///
/// {@template flutter.material.DataTable.dataRowColor}
/// ```dart
/// DataTable(
/// dataRowColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.selected)) {
/// return Theme.of(context).colorScheme.primary.withOpacity(0.08);
/// }
/// return null; // Use the default value.
/// }),
/// columns: _columns,
/// rows: _rows,
/// )
/// ```
///
/// See also:
///
/// * The Material Design specification for overlay colors and how they
/// match a component's state:
/// <https://material.io/design/interaction/states.html#anatomy>.
/// {@endtemplate}
final MaterialStateProperty<Color?>? dataRowColor;
/// {@template flutter.material.dataTable.dataRowHeight}
/// The height of each row (excluding the row that contains column headings).
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dataRowHeight] is used. This value defaults
/// to [kMinInteractiveDimension] to adhere to the Material Design
/// specifications.
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? get dataRowHeight => dataRowMinHeight == dataRowMaxHeight ? dataRowMinHeight : null;
/// {@template flutter.material.dataTable.dataRowMinHeight}
/// The minimum height of each row (excluding the row that contains column headings).
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dataRowMinHeight] is used. This value defaults
/// to [kMinInteractiveDimension] to adhere to the Material Design
/// specifications.
final double? dataRowMinHeight;
/// {@template flutter.material.dataTable.dataRowMaxHeight}
/// The maximum height of each row (excluding the row that contains column headings).
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dataRowMaxHeight] is used. This value defaults
/// to [kMinInteractiveDimension] to adhere to the Material Design
/// specifications.
final double? dataRowMaxHeight;
/// {@template flutter.material.dataTable.dataTextStyle}
/// The text style for data rows.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dataTextStyle] is used. By default, the text
/// style is [TextTheme.bodyMedium].
final TextStyle? dataTextStyle;
/// {@template flutter.material.dataTable.headingRowColor}
/// The background color for the heading row.
///
/// The effective background color can be made to depend on the
/// [MaterialState] state, i.e. if the row is pressed, hovered, focused when
/// sorted. The color is painted as an overlay to the row. To make sure that
/// the row's [InkWell] is visible (when pressed, hovered and focused), it is
/// recommended to use a translucent color.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.headingRowColor] is used.
///
/// {@template flutter.material.DataTable.headingRowColor}
/// ```dart
/// DataTable(
/// columns: _columns,
/// rows: _rows,
/// headingRowColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.hovered)) {
/// return Theme.of(context).colorScheme.primary.withOpacity(0.08);
/// }
/// return null; // Use the default value.
/// }),
/// )
/// ```
///
/// See also:
///
/// * The Material Design specification for overlay colors and how they
/// match a component's state:
/// <https://material.io/design/interaction/states.html#anatomy>.
/// {@endtemplate}
final MaterialStateProperty<Color?>? headingRowColor;
/// {@template flutter.material.dataTable.headingRowHeight}
/// The height of the heading row.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.headingRowHeight] is used. This value
/// defaults to 56.0 to adhere to the Material Design specifications.
final double? headingRowHeight;
/// {@template flutter.material.dataTable.headingTextStyle}
/// The text style for the heading row.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.headingTextStyle] is used. By default, the
/// text style is [TextTheme.titleSmall].
final TextStyle? headingTextStyle;
/// {@template flutter.material.dataTable.horizontalMargin}
/// The horizontal margin between the edges of the table and the content
/// in the first and last cells of each row.
///
/// When a checkbox is displayed, it is also the margin between the checkbox
/// the content in the first data column.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.horizontalMargin] is used. This value
/// defaults to 24.0 to adhere to the Material Design specifications.
///
/// If [checkboxHorizontalMargin] is null, then [horizontalMargin] is also the
/// margin between the edge of the table and the checkbox, as well as the
/// margin between the checkbox and the content in the first data column.
final double? horizontalMargin;
/// {@template flutter.material.dataTable.columnSpacing}
/// The horizontal margin between the contents of each data column.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.columnSpacing] is used. This value defaults
/// to 56.0 to adhere to the Material Design specifications.
final double? columnSpacing;
/// {@template flutter.material.dataTable.showCheckboxColumn}
/// Whether the widget should display checkboxes for selectable rows.
///
/// If true, a [Checkbox] will be placed at the beginning of each row that is
/// selectable. However, if [DataRow.onSelectChanged] is not set for any row,
/// checkboxes will not be placed, even if this value is true.
///
/// If false, all rows will not display a [Checkbox].
/// {@endtemplate}
final bool showCheckboxColumn;
/// The data to show in each row (excluding the row that contains
/// the column headings).
///
/// The list may be empty.
final List<DataRow> rows;
/// {@template flutter.material.dataTable.dividerThickness}
/// The width of the divider that appears between [TableRow]s.
///
/// Must be greater than or equal to zero.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.dividerThickness] is used. This value
/// defaults to 1.0.
final double? dividerThickness;
/// Whether a border at the bottom of the table is displayed.
///
/// By default, a border is not shown at the bottom to allow for a border
/// around the table defined by [decoration].
final bool showBottomBorder;
/// {@template flutter.material.dataTable.checkboxHorizontalMargin}
/// Horizontal margin around the checkbox, if it is displayed.
/// {@endtemplate}
///
/// If null, [DataTableThemeData.checkboxHorizontalMargin] is used. If that is
/// also null, then [horizontalMargin] is used as the margin between the edge
/// of the table and the checkbox, as well as the margin between the checkbox
/// and the content in the first data column. This value defaults to 24.0.
final double? checkboxHorizontalMargin;
/// The style to use when painting the boundary and interior divisions of the table.
final TableBorder? border;
/// {@macro flutter.material.Material.clipBehavior}
///
/// This can be used to clip the content within the border of the [DataTable].
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
// Set by the constructor to the index of the only Column that is
// non-numeric, if there is exactly one, otherwise null.
final int? _onlyTextColumn;
static int? _initOnlyTextColumn(List<DataColumn> columns) {
int? result;
for (int index = 0; index < columns.length; index += 1) {
final DataColumn column = columns[index];
if (!column.numeric) {
if (result != null) {
return null;
}
result = index;
}
}
return result;
}
bool get _debugInteractive {
return columns.any((DataColumn column) => column._debugInteractive)
|| rows.any((DataRow row) => row._debugInteractive);
}
static final LocalKey _headingRowKey = UniqueKey();
void _handleSelectAll(bool? checked, bool someChecked) {
// If some checkboxes are checked, all checkboxes are selected. Otherwise,
// use the new checked value but default to false if it's null.
final bool effectiveChecked = someChecked || (checked ?? false);
if (onSelectAll != null) {
onSelectAll!(effectiveChecked);
} else {
for (final DataRow row in rows) {
if (row.onSelectChanged != null && row.selected != effectiveChecked) {
row.onSelectChanged!(effectiveChecked);
}
}
}
}
/// The default height of the heading row.
static const double _headingRowHeight = 56.0;
/// The default horizontal margin between the edges of the table and the content
/// in the first and last cells of each row.
static const double _horizontalMargin = 24.0;
/// The default horizontal margin between the contents of each data column.
static const double _columnSpacing = 56.0;
/// The default padding between the heading content and sort arrow.
static const double _sortArrowPadding = 2.0;
/// The default divider thickness.
static const double _dividerThickness = 1.0;
static const Duration _sortArrowAnimationDuration = Duration(milliseconds: 150);
Widget _buildCheckbox({
required BuildContext context,
required bool? checked,
required VoidCallback? onRowTap,
required ValueChanged<bool?>? onCheckboxChanged,
required MaterialStateProperty<Color?>? overlayColor,
required bool tristate,
MouseCursor? rowMouseCursor,
}) {
final ThemeData themeData = Theme.of(context);
final double effectiveHorizontalMargin = horizontalMargin
?? themeData.dataTableTheme.horizontalMargin
?? _horizontalMargin;
final double effectiveCheckboxHorizontalMarginStart = checkboxHorizontalMargin
?? themeData.dataTableTheme.checkboxHorizontalMargin
?? effectiveHorizontalMargin;
final double effectiveCheckboxHorizontalMarginEnd = checkboxHorizontalMargin
?? themeData.dataTableTheme.checkboxHorizontalMargin
?? effectiveHorizontalMargin / 2.0;
Widget contents = Semantics(
container: true,
child: Padding(
padding: EdgeInsetsDirectional.only(
start: effectiveCheckboxHorizontalMarginStart,
end: effectiveCheckboxHorizontalMarginEnd,
),
child: Center(
child: Checkbox(
value: checked,
onChanged: onCheckboxChanged,
tristate: tristate,
),
),
),
);
if (onRowTap != null) {
contents = TableRowInkWell(
onTap: onRowTap,
overlayColor: overlayColor,
mouseCursor: rowMouseCursor,
child: contents,
);
}
return TableCell(
verticalAlignment: TableCellVerticalAlignment.fill,
child: contents,
);
}
Widget _buildHeadingCell({
required BuildContext context,
required EdgeInsetsGeometry padding,
required Widget label,
required String? tooltip,
required bool numeric,
required VoidCallback? onSort,
required bool sorted,
required bool ascending,
required MaterialStateProperty<Color?>? overlayColor,
required MouseCursor? mouseCursor,
}) {
final ThemeData themeData = Theme.of(context);
final DataTableThemeData dataTableTheme = DataTableTheme.of(context);
label = Row(
textDirection: numeric ? TextDirection.rtl : null,
children: <Widget>[
label,
if (onSort != null)
...<Widget>[
_SortArrow(
visible: sorted,
up: sorted ? ascending : null,
duration: _sortArrowAnimationDuration,
),
const SizedBox(width: _sortArrowPadding),
],
],
);
final TextStyle effectiveHeadingTextStyle = headingTextStyle
?? dataTableTheme.headingTextStyle
?? themeData.dataTableTheme.headingTextStyle
?? themeData.textTheme.titleSmall!;
final double effectiveHeadingRowHeight = headingRowHeight
?? dataTableTheme.headingRowHeight
?? themeData.dataTableTheme.headingRowHeight
?? _headingRowHeight;
label = Container(
padding: padding,
height: effectiveHeadingRowHeight,
alignment: numeric ? Alignment.centerRight : AlignmentDirectional.centerStart,
child: AnimatedDefaultTextStyle(
style: DefaultTextStyle.of(context).style.merge(effectiveHeadingTextStyle),
softWrap: false,
duration: _sortArrowAnimationDuration,
child: label,
),
);
if (tooltip != null) {
label = Tooltip(
message: tooltip,
child: label,
);
}
// TODO(dkwingsmt): Only wrap Inkwell if onSort != null. Blocked by
// https://github.com/flutter/flutter/issues/51152
label = InkWell(
onTap: onSort,
overlayColor: overlayColor,
mouseCursor: mouseCursor,
child: label,
);
return label;
}
Widget _buildDataCell({
required BuildContext context,
required EdgeInsetsGeometry padding,
required Widget label,
required bool numeric,
required bool placeholder,
required bool showEditIcon,
required GestureTapCallback? onTap,
required VoidCallback? onSelectChanged,
required GestureTapCallback? onDoubleTap,
required GestureLongPressCallback? onLongPress,
required GestureTapDownCallback? onTapDown,
required GestureTapCancelCallback? onTapCancel,
required MaterialStateProperty<Color?>? overlayColor,
required GestureLongPressCallback? onRowLongPress,
required MouseCursor? mouseCursor,
}) {
final ThemeData themeData = Theme.of(context);
final DataTableThemeData dataTableTheme = DataTableTheme.of(context);
if (showEditIcon) {
const Widget icon = Icon(Icons.edit, size: 18.0);
label = Expanded(child: label);
label = Row(
textDirection: numeric ? TextDirection.rtl : null,
children: <Widget>[ label, icon ],
);
}
final TextStyle effectiveDataTextStyle = dataTextStyle
?? dataTableTheme.dataTextStyle
?? themeData.dataTableTheme.dataTextStyle
?? themeData.textTheme.bodyMedium!;
final double effectiveDataRowMinHeight = dataRowMinHeight
?? dataTableTheme.dataRowMinHeight
?? themeData.dataTableTheme.dataRowMinHeight
?? kMinInteractiveDimension;
final double effectiveDataRowMaxHeight = dataRowMaxHeight
?? dataTableTheme.dataRowMaxHeight
?? themeData.dataTableTheme.dataRowMaxHeight
?? kMinInteractiveDimension;
label = Container(
padding: padding,
constraints: BoxConstraints(minHeight: effectiveDataRowMinHeight, maxHeight: effectiveDataRowMaxHeight),
alignment: numeric ? Alignment.centerRight : AlignmentDirectional.centerStart,
child: DefaultTextStyle(
style: DefaultTextStyle.of(context).style
.merge(effectiveDataTextStyle)
.copyWith(color: placeholder ? effectiveDataTextStyle.color!.withOpacity(0.6) : null),
child: DropdownButtonHideUnderline(child: label),
),
);
if (onTap != null ||
onDoubleTap != null ||
onLongPress != null ||
onTapDown != null ||
onTapCancel != null) {
label = InkWell(
onTap: onTap,
onDoubleTap: onDoubleTap,
onLongPress: onLongPress,
onTapCancel: onTapCancel,
onTapDown: onTapDown,
overlayColor: overlayColor,
child: label,
);
} else if (onSelectChanged != null || onRowLongPress != null) {
label = TableRowInkWell(
onTap: onSelectChanged,
onLongPress: onRowLongPress,
overlayColor: overlayColor,
mouseCursor: mouseCursor,
child: label,
);
}
return label;
}
@override
Widget build(BuildContext context) {
assert(!_debugInteractive || debugCheckHasMaterial(context));
final ThemeData theme = Theme.of(context);
final DataTableThemeData dataTableTheme = DataTableTheme.of(context);
final MaterialStateProperty<Color?>? effectiveHeadingRowColor = headingRowColor
?? dataTableTheme.headingRowColor
?? theme.dataTableTheme.headingRowColor;
final MaterialStateProperty<Color?>? effectiveDataRowColor = dataRowColor
?? dataTableTheme.dataRowColor
?? theme.dataTableTheme.dataRowColor;
final MaterialStateProperty<Color?> defaultRowColor = MaterialStateProperty.resolveWith(
(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return theme.colorScheme.primary.withOpacity(0.08);
}
return null;
},
);
final bool anyRowSelectable = rows.any((DataRow row) => row.onSelectChanged != null);
final bool displayCheckboxColumn = showCheckboxColumn && anyRowSelectable;
final Iterable<DataRow> rowsWithCheckbox = displayCheckboxColumn ?
rows.where((DataRow row) => row.onSelectChanged != null) : <DataRow>[];
final Iterable<DataRow> rowsChecked = rowsWithCheckbox.where((DataRow row) => row.selected);
final bool allChecked = displayCheckboxColumn && rowsChecked.length == rowsWithCheckbox.length;
final bool anyChecked = displayCheckboxColumn && rowsChecked.isNotEmpty;
final bool someChecked = anyChecked && !allChecked;
final double effectiveHorizontalMargin = horizontalMargin
?? dataTableTheme.horizontalMargin
?? theme.dataTableTheme.horizontalMargin
?? _horizontalMargin;
final double effectiveCheckboxHorizontalMarginStart = checkboxHorizontalMargin
?? dataTableTheme.checkboxHorizontalMargin
?? theme.dataTableTheme.checkboxHorizontalMargin
?? effectiveHorizontalMargin;
final double effectiveCheckboxHorizontalMarginEnd = checkboxHorizontalMargin
?? dataTableTheme.checkboxHorizontalMargin
?? theme.dataTableTheme.checkboxHorizontalMargin
?? effectiveHorizontalMargin / 2.0;
final double effectiveColumnSpacing = columnSpacing
?? dataTableTheme.columnSpacing
?? theme.dataTableTheme.columnSpacing
?? _columnSpacing;
final List<TableColumnWidth> tableColumns = List<TableColumnWidth>.filled(columns.length + (displayCheckboxColumn ? 1 : 0), const _NullTableColumnWidth());
final List<TableRow> tableRows = List<TableRow>.generate(
rows.length + 1, // the +1 is for the header row
(int index) {
final bool isSelected = index > 0 && rows[index - 1].selected;
final bool isDisabled = index > 0 && anyRowSelectable && rows[index - 1].onSelectChanged == null;
final Set<MaterialState> states = <MaterialState>{
if (isSelected)
MaterialState.selected,
if (isDisabled)
MaterialState.disabled,
};
final Color? resolvedDataRowColor = index > 0 ? (rows[index - 1].color ?? effectiveDataRowColor)?.resolve(states) : null;
final Color? resolvedHeadingRowColor = effectiveHeadingRowColor?.resolve(<MaterialState>{});
final Color? rowColor = index > 0 ? resolvedDataRowColor : resolvedHeadingRowColor;
final BorderSide borderSide = Divider.createBorderSide(
context,
width: dividerThickness
?? dataTableTheme.dividerThickness
?? theme.dataTableTheme.dividerThickness
?? _dividerThickness,
);
final Border? border = showBottomBorder
? Border(bottom: borderSide)
: index == 0 ? null : Border(top: borderSide);
return TableRow(
key: index == 0 ? _headingRowKey : rows[index - 1].key,
decoration: BoxDecoration(
border: border,
color: rowColor ?? defaultRowColor.resolve(states),
),
children: List<Widget>.filled(tableColumns.length, const _NullWidget()),
);
},
);
int rowIndex;
int displayColumnIndex = 0;
if (displayCheckboxColumn) {
tableColumns[0] = FixedColumnWidth(effectiveCheckboxHorizontalMarginStart + Checkbox.width + effectiveCheckboxHorizontalMarginEnd);
tableRows[0].children[0] = _buildCheckbox(
context: context,
checked: someChecked ? null : allChecked,
onRowTap: null,
onCheckboxChanged: (bool? checked) => _handleSelectAll(checked, someChecked),
overlayColor: null,
tristate: true,
);
rowIndex = 1;
for (final DataRow row in rows) {
final Set<MaterialState> states = <MaterialState>{
if (row.selected)
MaterialState.selected,
};
tableRows[rowIndex].children[0] = _buildCheckbox(
context: context,
checked: row.selected,
onRowTap: row.onSelectChanged == null ? null : () => row.onSelectChanged?.call(!row.selected),
onCheckboxChanged: row.onSelectChanged,
overlayColor: row.color ?? effectiveDataRowColor,
rowMouseCursor: row.mouseCursor?.resolve(states) ?? dataTableTheme.dataRowCursor?.resolve(states),
tristate: false,
);
rowIndex += 1;
}
displayColumnIndex += 1;
}
for (int dataColumnIndex = 0; dataColumnIndex < columns.length; dataColumnIndex += 1) {
final DataColumn column = columns[dataColumnIndex];
final double paddingStart;
if (dataColumnIndex == 0 && displayCheckboxColumn && checkboxHorizontalMargin != null) {
paddingStart = effectiveHorizontalMargin;
} else if (dataColumnIndex == 0 && displayCheckboxColumn) {
paddingStart = effectiveHorizontalMargin / 2.0;
} else if (dataColumnIndex == 0 && !displayCheckboxColumn) {
paddingStart = effectiveHorizontalMargin;
} else {
paddingStart = effectiveColumnSpacing / 2.0;
}
final double paddingEnd;
if (dataColumnIndex == columns.length - 1) {
paddingEnd = effectiveHorizontalMargin;
} else {
paddingEnd = effectiveColumnSpacing / 2.0;
}
final EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(
start: paddingStart,
end: paddingEnd,
);
if (dataColumnIndex == _onlyTextColumn) {
tableColumns[displayColumnIndex] = const IntrinsicColumnWidth(flex: 1.0);
} else {
tableColumns[displayColumnIndex] = const IntrinsicColumnWidth();
}
final Set<MaterialState> headerStates = <MaterialState>{
if (column.onSort == null)
MaterialState.disabled,
};
tableRows[0].children[displayColumnIndex] = _buildHeadingCell(
context: context,
padding: padding,
label: column.label,
tooltip: column.tooltip,
numeric: column.numeric,
onSort: column.onSort != null ? () => column.onSort!(dataColumnIndex, sortColumnIndex != dataColumnIndex || !sortAscending) : null,
sorted: dataColumnIndex == sortColumnIndex,
ascending: sortAscending,
overlayColor: effectiveHeadingRowColor,
mouseCursor: column.mouseCursor?.resolve(headerStates) ?? dataTableTheme.headingCellCursor?.resolve(headerStates),
);
rowIndex = 1;
for (final DataRow row in rows) {
final Set<MaterialState> states = <MaterialState>{
if (row.selected)
MaterialState.selected,
};
final DataCell cell = row.cells[dataColumnIndex];
tableRows[rowIndex].children[displayColumnIndex] = _buildDataCell(
context: context,
padding: padding,
label: cell.child,
numeric: column.numeric,
placeholder: cell.placeholder,
showEditIcon: cell.showEditIcon,
onTap: cell.onTap,
onDoubleTap: cell.onDoubleTap,
onLongPress: cell.onLongPress,
onTapCancel: cell.onTapCancel,
onTapDown: cell.onTapDown,
onSelectChanged: row.onSelectChanged == null ? null : () => row.onSelectChanged?.call(!row.selected),
overlayColor: row.color ?? effectiveDataRowColor,
onRowLongPress: row.onLongPress,
mouseCursor: row.mouseCursor?.resolve(states) ?? dataTableTheme.dataRowCursor?.resolve(states),
);
rowIndex += 1;
}
displayColumnIndex += 1;
}
return Container(
decoration: decoration ?? dataTableTheme.decoration ?? theme.dataTableTheme.decoration,
child: Material(
type: MaterialType.transparency,
borderRadius: border?.borderRadius,
clipBehavior: clipBehavior,
child: Table(
columnWidths: tableColumns.asMap(),
defaultVerticalAlignment: TableCellVerticalAlignment.middle,
children: tableRows,
border: border,
),
),
);
}
}
/// A rectangular area of a Material that responds to touch but clips
/// its ink splashes to the current table row of the nearest table.
///
/// Must have an ancestor [Material] widget in which to cause ink
/// reactions and an ancestor [Table] widget to establish a row.
///
/// The [TableRowInkWell] must be in the same coordinate space (modulo
/// translations) as the [Table]. If it's rotated or scaled or
/// otherwise transformed, it will not be able to describe the
/// rectangle of the row in its own coordinate system as a [Rect], and
/// thus the splash will not occur. (In general, this is easy to
/// achieve: just put the [TableRowInkWell] as the direct child of the
/// [Table], and put the other contents of the cell inside it.)
///
/// See also:
///
/// * [DataTable], which makes use of [TableRowInkWell] when
/// [DataRow.onSelectChanged] is defined and [DataCell.onTap]
/// is not.
class TableRowInkWell extends InkResponse {
/// Creates an ink well for a table row.
const TableRowInkWell({
super.key,
super.child,
super.onTap,
super.onDoubleTap,
super.onLongPress,
super.onHighlightChanged,
super.onSecondaryTap,
super.onSecondaryTapDown,
super.overlayColor,
super.mouseCursor,
}) : super(
containedInkWell: true,
highlightShape: BoxShape.rectangle,
);
@override
RectCallback getRectCallback(RenderBox referenceBox) {
return () {
RenderObject cell = referenceBox;
RenderObject? table = cell.parent;
final Matrix4 transform = Matrix4.identity();
while (table is RenderObject && table is! RenderTable) {
table.applyPaintTransform(cell, transform);
assert(table == cell.parent);
cell = table;
table = table.parent;
}
if (table is RenderTable) {
final TableCellParentData cellParentData = cell.parentData! as TableCellParentData;
assert(cellParentData.y != null);
final Rect rect = table.getRowBox(cellParentData.y!);
// The rect is in the table's coordinate space. We need to change it to the
// TableRowInkWell's coordinate space.
table.applyPaintTransform(cell, transform);
final Offset? offset = MatrixUtils.getAsTranslation(transform);
if (offset != null) {
return rect.shift(-offset);
}
}
return Rect.zero;
};
}
@override
bool debugCheckContext(BuildContext context) {
assert(debugCheckHasTable(context));
return super.debugCheckContext(context);
}
}
class _SortArrow extends StatefulWidget {
const _SortArrow({
required this.visible,
required this.up,
required this.duration,
});
final bool visible;
final bool? up;
final Duration duration;
@override
_SortArrowState createState() => _SortArrowState();
}
class _SortArrowState extends State<_SortArrow> with TickerProviderStateMixin {
late AnimationController _opacityController;
late Animation<double> _opacityAnimation;
late AnimationController _orientationController;
late Animation<double> _orientationAnimation;
double _orientationOffset = 0.0;
bool? _up;
static final Animatable<double> _turnTween = Tween<double>(begin: 0.0, end: math.pi)
.chain(CurveTween(curve: Curves.easeIn));
@override
void initState() {
super.initState();
_up = widget.up;
_opacityAnimation = CurvedAnimation(
parent: _opacityController = AnimationController(
duration: widget.duration,
vsync: this,
),
curve: Curves.fastOutSlowIn,
)
..addListener(_rebuild);
_opacityController.value = widget.visible ? 1.0 : 0.0;
_orientationController = AnimationController(
duration: widget.duration,
vsync: this,
);
_orientationAnimation = _orientationController.drive(_turnTween)
..addListener(_rebuild)
..addStatusListener(_resetOrientationAnimation);
if (widget.visible) {
_orientationOffset = widget.up! ? 0.0 : math.pi;
}
}
void _rebuild() {
setState(() {
// The animations changed, so we need to rebuild.
});
}
void _resetOrientationAnimation(AnimationStatus status) {
if (status == AnimationStatus.completed) {
assert(_orientationAnimation.value == math.pi);
_orientationOffset += math.pi;
_orientationController.value = 0.0; // TODO(ianh): This triggers a pointless rebuild.
}
}
@override
void didUpdateWidget(_SortArrow oldWidget) {
super.didUpdateWidget(oldWidget);
bool skipArrow = false;
final bool? newUp = widget.up ?? _up;
if (oldWidget.visible != widget.visible) {
if (widget.visible && (_opacityController.status == AnimationStatus.dismissed)) {
_orientationController.stop();
_orientationController.value = 0.0;
_orientationOffset = newUp! ? 0.0 : math.pi;
skipArrow = true;
}
if (widget.visible) {
_opacityController.forward();
} else {
_opacityController.reverse();
}
}
if ((_up != newUp) && !skipArrow) {
if (_orientationController.status == AnimationStatus.dismissed) {
_orientationController.forward();
} else {
_orientationController.reverse();
}
}
_up = newUp;
}
@override
void dispose() {
_opacityController.dispose();
_orientationController.dispose();
super.dispose();
}
static const double _arrowIconBaselineOffset = -1.5;
static const double _arrowIconSize = 16.0;
@override
Widget build(BuildContext context) {
return FadeTransition(
opacity: _opacityAnimation,
child: Transform(
transform: Matrix4.rotationZ(_orientationOffset + _orientationAnimation.value)
..setTranslationRaw(0.0, _arrowIconBaselineOffset, 0.0),
alignment: Alignment.center,
child: const Icon(
Icons.arrow_upward,
size: _arrowIconSize,
),
),
);
}
}
class _NullTableColumnWidth extends TableColumnWidth {
const _NullTableColumnWidth();
@override
double maxIntrinsicWidth(Iterable<RenderBox> cells, double containerWidth) => throw UnimplementedError();
@override
double minIntrinsicWidth(Iterable<RenderBox> cells, double containerWidth) => throw UnimplementedError();
}
class _NullWidget extends Widget {
const _NullWidget();
@override
Element createElement() => throw UnimplementedError();
}
| flutter/packages/flutter/lib/src/material/data_table.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/data_table.dart",
"repo_id": "flutter",
"token_count": 17013
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [Drawer] widgets.
///
/// Descendant widgets obtain the current [DrawerThemeData] object
/// using `DrawerTheme.of(context)`. Instances of [DrawerThemeData] can be
/// customized with [DrawerThemeData.copyWith].
///
/// Typically a [DrawerThemeData] is specified as part of the
/// overall [Theme] with [ThemeData.drawerTheme].
///
/// All [DrawerThemeData] properties are `null` by default.
///
/// See also:
///
/// * [DrawerTheme], an [InheritedWidget] that propagates the theme down its
/// subtree.
/// * [ThemeData], which describes the overall theme information for the
/// application and can customize a drawer using [ThemeData.drawerTheme].
@immutable
class DrawerThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.drawerTheme] and
/// [DrawerTheme].
const DrawerThemeData({
this.backgroundColor,
this.scrimColor,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.shape,
this.endShape,
this.width,
});
/// Overrides the default value of [Drawer.backgroundColor].
final Color? backgroundColor;
/// Overrides the default value of [DrawerController.scrimColor].
final Color? scrimColor;
/// Overrides the default value of [Drawer.elevation].
final double? elevation;
/// Overrides the default value for [Drawer.shadowColor].
final Color? shadowColor;
/// Overrides the default value for [Drawer.surfaceTintColor].
final Color? surfaceTintColor;
/// Overrides the default value of [Drawer.shape].
final ShapeBorder? shape;
/// Overrides the default value of [Drawer.shape] for a end drawer.
final ShapeBorder? endShape;
/// Overrides the default value of [Drawer.width].
final double? width;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
DrawerThemeData copyWith({
Color? backgroundColor,
Color? scrimColor,
double? elevation,
Color? shadowColor,
Color? surfaceTintColor,
ShapeBorder? shape,
ShapeBorder? endShape,
double? width,
}) {
return DrawerThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
scrimColor: scrimColor ?? this.scrimColor,
elevation: elevation ?? this.elevation,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
shape: shape ?? this.shape,
endShape: endShape ?? this.endShape,
width: width ?? this.width,
);
}
/// Linearly interpolate between two drawer themes.
///
/// If both arguments are null then null is returned.
///
/// {@macro dart.ui.shadow.lerp}
static DrawerThemeData? lerp(DrawerThemeData? a, DrawerThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return DrawerThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
scrimColor: Color.lerp(a?.scrimColor, b?.scrimColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
shape: ShapeBorder.lerp(a?.shape, b?.shape, t),
endShape: ShapeBorder.lerp(a?.endShape, b?.endShape, t),
width: lerpDouble(a?.width, b?.width, t),
);
}
@override
int get hashCode => Object.hash(
backgroundColor,
scrimColor,
elevation,
shadowColor,
surfaceTintColor,
shape,
endShape,
width,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is DrawerThemeData
&& other.backgroundColor == backgroundColor
&& other.scrimColor == scrimColor
&& other.elevation == elevation
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.shape == shape
&& other.endShape == endShape
&& other.width == width;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('scrimColor', scrimColor, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('endShape', endShape, defaultValue: null));
properties.add(DoubleProperty('width', width, defaultValue: null));
}
}
/// An inherited widget that defines visual properties for [Drawer]s in this
/// widget's subtree.
///
/// Values specified here are used for [Drawer] properties that are not
/// given an explicit non-null value.
///
/// Using this would allow you to override the [ThemeData.drawerTheme].
class DrawerTheme extends InheritedTheme {
/// Creates a theme that defines the [DrawerThemeData] properties for a
/// [Drawer].
const DrawerTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the background color, scrim color, elevation, and shape for
/// descendant [Drawer] widgets.
final DrawerThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [DrawerTheme] widget, then
/// [ThemeData.drawerTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// DrawerThemeData theme = DrawerTheme.of(context);
/// ```
static DrawerThemeData of(BuildContext context) {
final DrawerTheme? drawerTheme = context.dependOnInheritedWidgetOfExactType<DrawerTheme>();
return drawerTheme?.data ?? Theme.of(context).drawerTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return DrawerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(DrawerTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/drawer_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/drawer_theme.dart",
"repo_id": "flutter",
"token_count": 2203
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button.dart';
import 'color_scheme.dart';
import 'floating_action_button_theme.dart';
import 'material_state.dart';
import 'scaffold.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'tooltip.dart';
class _DefaultHeroTag {
const _DefaultHeroTag();
@override
String toString() => '<default FloatingActionButton tag>';
}
enum _FloatingActionButtonType {
regular,
small,
large,
extended,
}
/// A Material Design floating action button.
///
/// A floating action button is a circular icon button that hovers over content
/// to promote a primary action in the application. Floating action buttons are
/// most commonly used in the [Scaffold.floatingActionButton] field.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=2uaoEDOgk_I}
///
/// Use at most a single floating action button per screen. Floating action
/// buttons should be used for positive actions such as "create", "share", or
/// "navigate". (If more than one floating action button is used within a
/// [Route], then make sure that each button has a unique [heroTag], otherwise
/// an exception will be thrown.)
///
/// If the [onPressed] callback is null, then the button will be disabled and
/// will not react to touch. It is highly discouraged to disable a floating
/// action button as there is no indication to the user that the button is
/// disabled. Consider changing the [backgroundColor] if disabling the floating
/// action button.
///
/// {@tool dartpad}
/// This example shows a [FloatingActionButton] in its usual position within a
/// [Scaffold]. Pressing the button cycles it through a few variations in its
/// [foregroundColor], [backgroundColor], and [shape]. The button automatically
/// animates its segue from one set of visual parameters to another.
///
/// ** See code in examples/api/lib/material/floating_action_button/floating_action_button.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows all the variants of [FloatingActionButton] widget as
/// described in: https://m3.material.io/components/floating-action-button/overview.
///
/// ** See code in examples/api/lib/material/floating_action_button/floating_action_button.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows [FloatingActionButton] with additional color mappings as
/// described in: https://m3.material.io/components/floating-action-button/overview.
///
/// ** See code in examples/api/lib/material/floating_action_button/floating_action_button.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Scaffold], in which floating action buttons typically live.
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * <https://material.io/design/components/buttons-floating-action-button.html>
/// * <https://m3.material.io/components/floating-action-button>
class FloatingActionButton extends StatelessWidget {
/// Creates a circular floating action button.
///
/// The [elevation], [highlightElevation], and [disabledElevation] parameters,
/// if specified, must be non-negative.
const FloatingActionButton({
super.key,
this.child,
this.tooltip,
this.foregroundColor,
this.backgroundColor,
this.focusColor,
this.hoverColor,
this.splashColor,
this.heroTag = const _DefaultHeroTag(),
this.elevation,
this.focusElevation,
this.hoverElevation,
this.highlightElevation,
this.disabledElevation,
required this.onPressed,
this.mouseCursor,
this.mini = false,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.materialTapTargetSize,
this.isExtended = false,
this.enableFeedback,
}) : assert(elevation == null || elevation >= 0.0),
assert(focusElevation == null || focusElevation >= 0.0),
assert(hoverElevation == null || hoverElevation >= 0.0),
assert(highlightElevation == null || highlightElevation >= 0.0),
assert(disabledElevation == null || disabledElevation >= 0.0),
_floatingActionButtonType = mini ? _FloatingActionButtonType.small : _FloatingActionButtonType.regular,
_extendedLabel = null,
extendedIconLabelSpacing = null,
extendedPadding = null,
extendedTextStyle = null;
/// Creates a small circular floating action button.
///
/// This constructor overrides the default size constraints of the floating
/// action button.
///
/// The [elevation], [focusElevation], [hoverElevation], [highlightElevation],
/// and [disabledElevation] parameters, if specified, must be non-negative.
const FloatingActionButton.small({
super.key,
this.child,
this.tooltip,
this.foregroundColor,
this.backgroundColor,
this.focusColor,
this.hoverColor,
this.splashColor,
this.heroTag = const _DefaultHeroTag(),
this.elevation,
this.focusElevation,
this.hoverElevation,
this.highlightElevation,
this.disabledElevation,
required this.onPressed,
this.mouseCursor,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.materialTapTargetSize,
this.enableFeedback,
}) : assert(elevation == null || elevation >= 0.0),
assert(focusElevation == null || focusElevation >= 0.0),
assert(hoverElevation == null || hoverElevation >= 0.0),
assert(highlightElevation == null || highlightElevation >= 0.0),
assert(disabledElevation == null || disabledElevation >= 0.0),
_floatingActionButtonType = _FloatingActionButtonType.small,
mini = true,
isExtended = false,
_extendedLabel = null,
extendedIconLabelSpacing = null,
extendedPadding = null,
extendedTextStyle = null;
/// Creates a large circular floating action button.
///
/// This constructor overrides the default size constraints of the floating
/// action button.
///
/// The [elevation], [focusElevation], [hoverElevation], [highlightElevation],
/// and [disabledElevation] parameters, if specified, must be non-negative.
const FloatingActionButton.large({
super.key,
this.child,
this.tooltip,
this.foregroundColor,
this.backgroundColor,
this.focusColor,
this.hoverColor,
this.splashColor,
this.heroTag = const _DefaultHeroTag(),
this.elevation,
this.focusElevation,
this.hoverElevation,
this.highlightElevation,
this.disabledElevation,
required this.onPressed,
this.mouseCursor,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.materialTapTargetSize,
this.enableFeedback,
}) : assert(elevation == null || elevation >= 0.0),
assert(focusElevation == null || focusElevation >= 0.0),
assert(hoverElevation == null || hoverElevation >= 0.0),
assert(highlightElevation == null || highlightElevation >= 0.0),
assert(disabledElevation == null || disabledElevation >= 0.0),
_floatingActionButtonType = _FloatingActionButtonType.large,
mini = false,
isExtended = false,
_extendedLabel = null,
extendedIconLabelSpacing = null,
extendedPadding = null,
extendedTextStyle = null;
/// Creates a wider [StadiumBorder]-shaped floating action button with
/// an optional [icon] and a [label].
///
/// The [elevation], [highlightElevation], and [disabledElevation] parameters,
/// if specified, must be non-negative.
///
/// See also:
/// * <https://m3.material.io/components/extended-fab>
const FloatingActionButton.extended({
super.key,
this.tooltip,
this.foregroundColor,
this.backgroundColor,
this.focusColor,
this.hoverColor,
this.heroTag = const _DefaultHeroTag(),
this.elevation,
this.focusElevation,
this.hoverElevation,
this.splashColor,
this.highlightElevation,
this.disabledElevation,
required this.onPressed,
this.mouseCursor = SystemMouseCursors.click,
this.shape,
this.isExtended = true,
this.materialTapTargetSize,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.extendedIconLabelSpacing,
this.extendedPadding,
this.extendedTextStyle,
Widget? icon,
required Widget label,
this.enableFeedback,
}) : assert(elevation == null || elevation >= 0.0),
assert(focusElevation == null || focusElevation >= 0.0),
assert(hoverElevation == null || hoverElevation >= 0.0),
assert(highlightElevation == null || highlightElevation >= 0.0),
assert(disabledElevation == null || disabledElevation >= 0.0),
mini = false,
_floatingActionButtonType = _FloatingActionButtonType.extended,
child = icon,
_extendedLabel = label;
/// The widget below this widget in the tree.
///
/// Typically an [Icon].
final Widget? child;
/// Text that describes the action that will occur when the button is pressed.
///
/// This text is displayed when the user long-presses on the button and is
/// used for accessibility.
final String? tooltip;
/// The default foreground color for icons and text within the button.
///
/// If this property is null, then the
/// [FloatingActionButtonThemeData.foregroundColor] of
/// [ThemeData.floatingActionButtonTheme] is used. If that property is also
/// null, then the [ColorScheme.onSecondary] color of [ThemeData.colorScheme]
/// is used.
final Color? foregroundColor;
/// The button's background color.
///
/// If this property is null, then the
/// [FloatingActionButtonThemeData.backgroundColor] of
/// [ThemeData.floatingActionButtonTheme] is used. If that property is also
/// null, then the [Theme]'s [ColorScheme.secondary] color is used.
final Color? backgroundColor;
/// The color to use for filling the button when the button has input focus.
///
/// In Material3, defaults to [ColorScheme.onPrimaryContainer] with opacity 0.1.
/// In Material 2, it defaults to [ThemeData.focusColor] for the current theme.
final Color? focusColor;
/// The color to use for filling the button when the button has a pointer
/// hovering over it.
///
/// Defaults to [ThemeData.hoverColor] for the current theme in Material 2. In
/// Material 3, defaults to [ColorScheme.onPrimaryContainer] with opacity 0.08.
final Color? hoverColor;
/// The splash color for this [FloatingActionButton]'s [InkWell].
///
/// If null, [FloatingActionButtonThemeData.splashColor] is used, if that is
/// null, [ThemeData.splashColor] is used in Material 2; [ColorScheme.onPrimaryContainer]
/// with opacity 0.1 is used in Material 3.
final Color? splashColor;
/// The tag to apply to the button's [Hero] widget.
///
/// Defaults to a tag that matches other floating action buttons.
///
/// Set this to null explicitly if you don't want the floating action button to
/// have a hero tag.
///
/// If this is not explicitly set, then there can only be one
/// [FloatingActionButton] per route (that is, per screen), since otherwise
/// there would be a tag conflict (multiple heroes on one route can't have the
/// same tag). The Material Design specification recommends only using one
/// floating action button per screen.
final Object? heroTag;
/// The callback that is called when the button is tapped or otherwise activated.
///
/// If this is set to null, the button will be disabled.
final VoidCallback? onPressed;
/// {@macro flutter.material.RawMaterialButton.mouseCursor}
///
/// If this property is null, [MaterialStateMouseCursor.clickable] will be used.
final MouseCursor? mouseCursor;
/// The z-coordinate at which to place this button relative to its parent.
///
/// This controls the size of the shadow below the floating action button.
///
/// Defaults to 6, the appropriate elevation for floating action buttons. The
/// value is always non-negative.
///
/// See also:
///
/// * [highlightElevation], the elevation when the button is pressed.
/// * [disabledElevation], the elevation when the button is disabled.
final double? elevation;
/// The z-coordinate at which to place this button relative to its parent when
/// the button has the input focus.
///
/// This controls the size of the shadow below the floating action button.
///
/// Defaults to 8, the appropriate elevation for floating action buttons
/// while they have focus. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [highlightElevation], the elevation when the button is pressed.
/// * [disabledElevation], the elevation when the button is disabled.
final double? focusElevation;
/// The z-coordinate at which to place this button relative to its parent when
/// the button is enabled and has a pointer hovering over it.
///
/// This controls the size of the shadow below the floating action button.
///
/// Defaults to 8, the appropriate elevation for floating action buttons while
/// they have a pointer hovering over them. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [highlightElevation], the elevation when the button is pressed.
/// * [disabledElevation], the elevation when the button is disabled.
final double? hoverElevation;
/// The z-coordinate at which to place this button relative to its parent when
/// the user is touching the button.
///
/// This controls the size of the shadow below the floating action button.
///
/// Defaults to 12, the appropriate elevation for floating action buttons
/// while they are being touched. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
final double? highlightElevation;
/// The z-coordinate at which to place this button when the button is disabled
/// ([onPressed] is null).
///
/// This controls the size of the shadow below the floating action button.
///
/// Defaults to the same value as [elevation]. Setting this to zero makes the
/// floating action button work similar to an [ElevatedButton] but the titular
/// "floating" effect is lost. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [highlightElevation], the elevation when the button is pressed.
final double? disabledElevation;
/// Controls the size of this button.
///
/// By default, floating action buttons are non-mini and have a height and
/// width of 56.0 logical pixels. Mini floating action buttons have a height
/// and width of 40.0 logical pixels with a layout width and height of 48.0
/// logical pixels. (The extra 4 pixels of padding on each side are added as a
/// result of the floating action button having [MaterialTapTargetSize.padded]
/// set on the underlying [RawMaterialButton.materialTapTargetSize].)
final bool mini;
/// The shape of the button's [Material].
///
/// The button's highlight and splash are clipped to this shape. If the
/// button has an elevation, then its drop shadow is defined by this
/// shape as well.
final ShapeBorder? shape;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// True if this is an "extended" floating action button.
///
/// Typically [extended] buttons have a [StadiumBorder] [shape]
/// and have been created with the [FloatingActionButton.extended]
/// constructor.
///
/// The [Scaffold] animates the appearance of ordinary floating
/// action buttons with scale and rotation transitions. Extended
/// floating action buttons are scaled and faded in.
final bool isExtended;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// Configures the minimum size of the tap target.
///
/// Defaults to [ThemeData.materialTapTargetSize].
///
/// See also:
///
/// * [MaterialTapTargetSize], for a description of how this affects tap targets.
final MaterialTapTargetSize? materialTapTargetSize;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// If null, [FloatingActionButtonThemeData.enableFeedback] is used.
/// If both are null, then default value is true.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// The spacing between the icon and the label for an extended
/// [FloatingActionButton].
///
/// If null, [FloatingActionButtonThemeData.extendedIconLabelSpacing] is used.
/// If that is also null, the default is 8.0.
final double? extendedIconLabelSpacing;
/// The padding for an extended [FloatingActionButton]'s content.
///
/// If null, [FloatingActionButtonThemeData.extendedPadding] is used. If that
/// is also null, the default is
/// `EdgeInsetsDirectional.only(start: 16.0, end: 20.0)` if an icon is
/// provided, and `EdgeInsetsDirectional.only(start: 20.0, end: 20.0)` if not.
final EdgeInsetsGeometry? extendedPadding;
/// The text style for an extended [FloatingActionButton]'s label.
///
/// If null, [FloatingActionButtonThemeData.extendedTextStyle] is used. If
/// that is also null, then [TextTheme.labelLarge] with a letter spacing of 1.2
/// is used.
final TextStyle? extendedTextStyle;
final _FloatingActionButtonType _floatingActionButtonType;
final Widget? _extendedLabel;
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final FloatingActionButtonThemeData floatingActionButtonTheme = theme.floatingActionButtonTheme;
final FloatingActionButtonThemeData defaults = theme.useMaterial3
? _FABDefaultsM3(context, _floatingActionButtonType, child != null)
: _FABDefaultsM2(context, _floatingActionButtonType, child != null);
final Color foregroundColor = this.foregroundColor
?? floatingActionButtonTheme.foregroundColor
?? defaults.foregroundColor!;
final Color backgroundColor = this.backgroundColor
?? floatingActionButtonTheme.backgroundColor
?? defaults.backgroundColor!;
final Color focusColor = this.focusColor
?? floatingActionButtonTheme.focusColor
?? defaults.focusColor!;
final Color hoverColor = this.hoverColor
?? floatingActionButtonTheme.hoverColor
?? defaults.hoverColor!;
final Color splashColor = this.splashColor
?? floatingActionButtonTheme.splashColor
?? defaults.splashColor!;
final double elevation = this.elevation
?? floatingActionButtonTheme.elevation
?? defaults.elevation!;
final double focusElevation = this.focusElevation
?? floatingActionButtonTheme.focusElevation
?? defaults.focusElevation!;
final double hoverElevation = this.hoverElevation
?? floatingActionButtonTheme.hoverElevation
?? defaults.hoverElevation!;
final double disabledElevation = this.disabledElevation
?? floatingActionButtonTheme.disabledElevation
?? defaults.disabledElevation
?? elevation;
final double highlightElevation = this.highlightElevation
?? floatingActionButtonTheme.highlightElevation
?? defaults.highlightElevation!;
final MaterialTapTargetSize materialTapTargetSize = this.materialTapTargetSize
?? theme.materialTapTargetSize;
final bool enableFeedback = this.enableFeedback
?? floatingActionButtonTheme.enableFeedback
?? defaults.enableFeedback!;
final double iconSize = floatingActionButtonTheme.iconSize
?? defaults.iconSize!;
final TextStyle extendedTextStyle = (this.extendedTextStyle
?? floatingActionButtonTheme.extendedTextStyle
?? defaults.extendedTextStyle!).copyWith(color: foregroundColor);
final ShapeBorder shape = this.shape
?? floatingActionButtonTheme.shape
?? defaults.shape!;
BoxConstraints sizeConstraints;
Widget? resolvedChild = child != null ? IconTheme.merge(
data: IconThemeData(size: iconSize),
child: child!,
) : child;
switch (_floatingActionButtonType) {
case _FloatingActionButtonType.regular:
sizeConstraints = floatingActionButtonTheme.sizeConstraints ?? defaults.sizeConstraints!;
case _FloatingActionButtonType.small:
sizeConstraints = floatingActionButtonTheme.smallSizeConstraints ?? defaults.smallSizeConstraints!;
case _FloatingActionButtonType.large:
sizeConstraints = floatingActionButtonTheme.largeSizeConstraints ?? defaults.largeSizeConstraints!;
case _FloatingActionButtonType.extended:
sizeConstraints = floatingActionButtonTheme.extendedSizeConstraints ?? defaults.extendedSizeConstraints!;
final double iconLabelSpacing = extendedIconLabelSpacing ?? floatingActionButtonTheme.extendedIconLabelSpacing ?? 8.0;
final EdgeInsetsGeometry padding = extendedPadding
?? floatingActionButtonTheme.extendedPadding
?? defaults.extendedPadding!;
resolvedChild = _ChildOverflowBox(
child: Padding(
padding: padding,
child: Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
if (child != null)
child!,
if (child != null && isExtended)
SizedBox(width: iconLabelSpacing),
if (isExtended)
_extendedLabel!,
],
),
),
);
}
Widget result = RawMaterialButton(
onPressed: onPressed,
mouseCursor: _EffectiveMouseCursor(mouseCursor, floatingActionButtonTheme.mouseCursor),
elevation: elevation,
focusElevation: focusElevation,
hoverElevation: hoverElevation,
highlightElevation: highlightElevation,
disabledElevation: disabledElevation,
constraints: sizeConstraints,
materialTapTargetSize: materialTapTargetSize,
fillColor: backgroundColor,
focusColor: focusColor,
hoverColor: hoverColor,
splashColor: splashColor,
textStyle: extendedTextStyle,
shape: shape,
clipBehavior: clipBehavior,
focusNode: focusNode,
autofocus: autofocus,
enableFeedback: enableFeedback,
child: resolvedChild,
);
if (tooltip != null) {
result = Tooltip(
message: tooltip,
child: result,
);
}
if (heroTag != null) {
result = Hero(
tag: heroTag!,
child: result,
);
}
return MergeSemantics(child: result);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ObjectFlagProperty<VoidCallback>('onPressed', onPressed, ifNull: 'disabled'));
properties.add(StringProperty('tooltip', tooltip, defaultValue: null));
properties.add(ColorProperty('foregroundColor', foregroundColor, defaultValue: null));
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: null));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: null));
properties.add(ObjectFlagProperty<Object>('heroTag', heroTag, ifPresent: 'hero'));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(DoubleProperty('focusElevation', focusElevation, defaultValue: null));
properties.add(DoubleProperty('hoverElevation', hoverElevation, defaultValue: null));
properties.add(DoubleProperty('highlightElevation', highlightElevation, defaultValue: null));
properties.add(DoubleProperty('disabledElevation', disabledElevation, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode, defaultValue: null));
properties.add(FlagProperty('isExtended', value: isExtended, ifTrue: 'extended'));
properties.add(DiagnosticsProperty<MaterialTapTargetSize>('materialTapTargetSize', materialTapTargetSize, defaultValue: null));
}
}
// This MaterialStateProperty is passed along to RawMaterialButton which
// resolves the property against MaterialState.pressed, MaterialState.hovered,
// MaterialState.focused, MaterialState.disabled.
class _EffectiveMouseCursor extends MaterialStateMouseCursor {
const _EffectiveMouseCursor(this.widgetCursor, this.themeCursor);
final MouseCursor? widgetCursor;
final MaterialStateProperty<MouseCursor?>? themeCursor;
@override
MouseCursor resolve(Set<MaterialState> states) {
return MaterialStateProperty.resolveAs<MouseCursor?>(widgetCursor, states)
?? themeCursor?.resolve(states)
?? MaterialStateMouseCursor.clickable.resolve(states);
}
@override
String get debugDescription => 'MaterialStateMouseCursor(FloatActionButton)';
}
// This widget's size matches its child's size unless its constraints
// force it to be larger or smaller. The child is centered.
//
// Used to encapsulate extended FABs whose size is fixed, using Row
// and MainAxisSize.min, to be as wide as their label and icon.
class _ChildOverflowBox extends SingleChildRenderObjectWidget {
const _ChildOverflowBox({
super.child,
});
@override
_RenderChildOverflowBox createRenderObject(BuildContext context) {
return _RenderChildOverflowBox(
textDirection: Directionality.of(context),
);
}
@override
void updateRenderObject(BuildContext context, _RenderChildOverflowBox renderObject) {
renderObject.textDirection = Directionality.of(context);
}
}
class _RenderChildOverflowBox extends RenderAligningShiftedBox {
_RenderChildOverflowBox({
super.textDirection,
}) : super(alignment: Alignment.center);
@override
double computeMinIntrinsicWidth(double height) => 0.0;
@override
double computeMinIntrinsicHeight(double width) => 0.0;
@override
Size computeDryLayout(BoxConstraints constraints) {
if (child != null) {
final Size childSize = child!.getDryLayout(const BoxConstraints());
return Size(
math.max(constraints.minWidth, math.min(constraints.maxWidth, childSize.width)),
math.max(constraints.minHeight, math.min(constraints.maxHeight, childSize.height)),
);
} else {
return constraints.biggest;
}
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
if (child != null) {
child!.layout(const BoxConstraints(), parentUsesSize: true);
size = Size(
math.max(constraints.minWidth, math.min(constraints.maxWidth, child!.size.width)),
math.max(constraints.minHeight, math.min(constraints.maxHeight, child!.size.height)),
);
alignChild();
} else {
size = constraints.biggest;
}
}
}
// Hand coded defaults based on Material Design 2.
class _FABDefaultsM2 extends FloatingActionButtonThemeData {
_FABDefaultsM2(BuildContext context, this.type, this.hasChild)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme,
super(
elevation: 6,
focusElevation: 6,
hoverElevation: 8,
highlightElevation: 12,
enableFeedback: true,
sizeConstraints: const BoxConstraints.tightFor(
width: 56.0,
height: 56.0,
),
smallSizeConstraints: const BoxConstraints.tightFor(
width: 40.0,
height: 40.0,
),
largeSizeConstraints: const BoxConstraints.tightFor(
width: 96.0,
height: 96.0,
),
extendedSizeConstraints: const BoxConstraints.tightFor(
height: 48.0,
),
extendedIconLabelSpacing: 8.0,
);
final _FloatingActionButtonType type;
final bool hasChild;
final ThemeData _theme;
final ColorScheme _colors;
bool get _isExtended => type == _FloatingActionButtonType.extended;
bool get _isLarge => type == _FloatingActionButtonType.large;
@override Color? get foregroundColor => _colors.onSecondary;
@override Color? get backgroundColor => _colors.secondary;
@override Color? get focusColor => _theme.focusColor;
@override Color? get hoverColor => _theme.hoverColor;
@override Color? get splashColor => _theme.splashColor;
@override ShapeBorder? get shape => _isExtended ? const StadiumBorder() : const CircleBorder();
@override double? get iconSize => _isLarge ? 36.0 : 24.0;
@override EdgeInsetsGeometry? get extendedPadding => EdgeInsetsDirectional.only(start: hasChild && _isExtended ? 16.0 : 20.0, end: 20.0);
@override TextStyle? get extendedTextStyle => _theme.textTheme.labelLarge!.copyWith(letterSpacing: 1.2);
}
// BEGIN GENERATED TOKEN PROPERTIES - FAB
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FABDefaultsM3 extends FloatingActionButtonThemeData {
_FABDefaultsM3(this.context, this.type, this.hasChild)
: super(
elevation: 6.0,
focusElevation: 6.0,
hoverElevation: 8.0,
highlightElevation: 6.0,
enableFeedback: true,
sizeConstraints: const BoxConstraints.tightFor(
width: 56.0,
height: 56.0,
),
smallSizeConstraints: const BoxConstraints.tightFor(
width: 40.0,
height: 40.0,
),
largeSizeConstraints: const BoxConstraints.tightFor(
width: 96.0,
height: 96.0,
),
extendedSizeConstraints: const BoxConstraints.tightFor(
height: 56.0,
),
extendedIconLabelSpacing: 8.0,
);
final BuildContext context;
final _FloatingActionButtonType type;
final bool hasChild;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
bool get _isExtended => type == _FloatingActionButtonType.extended;
@override Color? get foregroundColor => _colors.onPrimaryContainer;
@override Color? get backgroundColor => _colors.primaryContainer;
@override Color? get splashColor => _colors.onPrimaryContainer.withOpacity(0.1);
@override Color? get focusColor => _colors.onPrimaryContainer.withOpacity(0.1);
@override Color? get hoverColor => _colors.onPrimaryContainer.withOpacity(0.08);
@override
ShapeBorder? get shape {
return switch (type) {
_FloatingActionButtonType.regular => const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(16.0))),
_FloatingActionButtonType.small => const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(12.0))),
_FloatingActionButtonType.large => const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(28.0))),
_FloatingActionButtonType.extended => const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(16.0))),
};
}
@override
double? get iconSize {
return switch (type) {
_FloatingActionButtonType.regular => 24.0,
_FloatingActionButtonType.small => 24.0,
_FloatingActionButtonType.large => 36.0,
_FloatingActionButtonType.extended => 24.0,
};
}
@override EdgeInsetsGeometry? get extendedPadding => EdgeInsetsDirectional.only(start: hasChild && _isExtended ? 16.0 : 20.0, end: 20.0);
@override TextStyle? get extendedTextStyle => _textTheme.labelLarge;
}
// END GENERATED TOKEN PROPERTIES - FAB
| flutter/packages/flutter/lib/src/material/floating_action_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/floating_action_button.dart",
"repo_id": "flutter",
"token_count": 10703
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show clampDouble;
import 'package:flutter/widgets.dart';
import 'chip.dart';
import 'chip_theme.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'debug.dart';
import 'icons.dart';
import 'material_state.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
/// A Material Design input chip.
///
/// Input chips represent a complex piece of information, such as an entity
/// (person, place, or thing) or conversational text, in a compact form.
///
/// Input chips can be made selectable by setting [onSelected], deletable by
/// setting [onDeleted], and pressable like a button with [onPressed]. They have
/// a [label], and they can have a leading icon (see [avatar]) and a trailing
/// icon ([deleteIcon]). Colors and padding can be customized.
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// Input chips work together with other UI elements. They can appear:
///
/// * In a [Wrap] widget.
/// * In a horizontally scrollable list, for example configured such as a
/// [ListView] with [ListView.scrollDirection] set to [Axis.horizontal].
///
/// {@tool dartpad}
/// This example shows how to create [InputChip]s with [onSelected] and
/// [onDeleted] callbacks. When the user taps the chip, the chip will be selected.
/// When the user taps the delete icon, the chip will be deleted.
///
/// ** See code in examples/api/lib/material/input_chip/input_chip.0.dart **
/// {@end-tool}
///
///
/// {@tool dartpad}
/// The following example shows how to generate [InputChip]s from
/// user text input. When the user enters a pizza topping in the text field,
/// the user is presented with a list of suggestions. When selecting one of the
/// suggestions, an [InputChip] is generated in the text field.
///
/// ** See code in examples/api/lib/material/input_chip/input_chip.1.dart **
/// {@end-tool}
///
/// ## Material Design 3
///
/// [InputChip] can be used for Input chips from Material Design 3.
/// If [ThemeData.useMaterial3] is true, then [InputChip]
/// will be styled to match the Material Design 3 specification for Input
/// chips.
///
/// See also:
///
/// * [Chip], a chip that displays information and can be deleted.
/// * [ChoiceChip], allows a single selection from a set of options. Choice
/// chips contain related descriptive text or categories.
/// * [FilterChip], uses tags or descriptive words as a way to filter content.
/// * [ActionChip], represents an action related to primary content.
/// * [CircleAvatar], which shows images or initials of people.
/// * [Wrap], A widget that displays its children in multiple horizontal or
/// vertical runs.
/// * <https://material.io/design/components/chips.html>
class InputChip extends StatelessWidget
implements
ChipAttributes,
DeletableChipAttributes,
SelectableChipAttributes,
CheckmarkableChipAttributes,
DisabledChipAttributes,
TappableChipAttributes {
/// Creates an [InputChip].
///
/// The [onPressed] and [onSelected] callbacks must not both be specified at
/// the same time. When both [onPressed] and [onSelected] are null, the chip
/// will be disabled.
///
/// The [pressElevation] and [elevation] must be null or non-negative.
/// Typically, [pressElevation] is greater than [elevation].
const InputChip({
super.key,
this.avatar,
required this.label,
this.labelStyle,
this.labelPadding,
this.selected = false,
this.isEnabled = true,
this.onSelected,
this.deleteIcon,
this.onDeleted,
this.deleteIconColor,
this.deleteButtonTooltipMessage,
this.onPressed,
this.pressElevation,
this.disabledColor,
this.selectedColor,
this.tooltip,
this.side,
this.shape,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
this.color,
this.backgroundColor,
this.padding,
this.visualDensity,
this.materialTapTargetSize,
this.elevation,
this.shadowColor,
this.surfaceTintColor,
this.iconTheme,
this.selectedShadowColor,
this.showCheckmark,
this.checkmarkColor,
this.avatarBorder = const CircleBorder(),
this.avatarBoxConstraints,
this.deleteIconBoxConstraints,
}) : assert(pressElevation == null || pressElevation >= 0.0),
assert(elevation == null || elevation >= 0.0);
@override
final Widget? avatar;
@override
final Widget label;
@override
final TextStyle? labelStyle;
@override
final EdgeInsetsGeometry? labelPadding;
@override
final bool selected;
@override
final bool isEnabled;
@override
final ValueChanged<bool>? onSelected;
@override
final Widget? deleteIcon;
@override
final VoidCallback? onDeleted;
@override
final Color? deleteIconColor;
@override
final String? deleteButtonTooltipMessage;
@override
final VoidCallback? onPressed;
@override
final double? pressElevation;
@override
final Color? disabledColor;
@override
final Color? selectedColor;
@override
final String? tooltip;
@override
final BorderSide? side;
@override
final OutlinedBorder? shape;
@override
final Clip clipBehavior;
@override
final FocusNode? focusNode;
@override
final bool autofocus;
@override
final MaterialStateProperty<Color?>? color;
@override
final Color? backgroundColor;
@override
final EdgeInsetsGeometry? padding;
@override
final VisualDensity? visualDensity;
@override
final MaterialTapTargetSize? materialTapTargetSize;
@override
final double? elevation;
@override
final Color? shadowColor;
@override
final Color? surfaceTintColor;
@override
final Color? selectedShadowColor;
@override
final bool? showCheckmark;
@override
final Color? checkmarkColor;
@override
final ShapeBorder avatarBorder;
@override
final IconThemeData? iconTheme;
@override
final BoxConstraints? avatarBoxConstraints;
@override
final BoxConstraints? deleteIconBoxConstraints;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
final ChipThemeData? defaults = Theme.of(context).useMaterial3
? _InputChipDefaultsM3(context, isEnabled, selected)
: null;
final Widget? resolvedDeleteIcon = deleteIcon
?? (Theme.of(context).useMaterial3 ? const Icon(Icons.clear, size: 18) : null);
return RawChip(
defaultProperties: defaults,
avatar: avatar,
label: label,
labelStyle: labelStyle,
labelPadding: labelPadding,
deleteIcon: resolvedDeleteIcon,
onDeleted: onDeleted,
deleteIconColor: deleteIconColor,
deleteButtonTooltipMessage: deleteButtonTooltipMessage,
onSelected: onSelected,
onPressed: onPressed,
pressElevation: pressElevation,
selected: selected,
disabledColor: disabledColor,
selectedColor: selectedColor,
tooltip: tooltip,
side: side,
shape: shape,
clipBehavior: clipBehavior,
focusNode: focusNode,
autofocus: autofocus,
color: color,
backgroundColor: backgroundColor,
padding: padding,
visualDensity: visualDensity,
materialTapTargetSize: materialTapTargetSize,
elevation: elevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
selectedShadowColor: selectedShadowColor,
showCheckmark: showCheckmark,
checkmarkColor: checkmarkColor,
isEnabled: isEnabled && (onSelected != null || onDeleted != null || onPressed != null),
avatarBorder: avatarBorder,
iconTheme: iconTheme,
avatarBoxConstraints: avatarBoxConstraints,
deleteIconBoxConstraints: deleteIconBoxConstraints,
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - InputChip
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _InputChipDefaultsM3 extends ChipThemeData {
_InputChipDefaultsM3(this.context, this.isEnabled, this.isSelected)
: super(
elevation: 0.0,
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(8.0))),
showCheckmark: true,
);
final BuildContext context;
final bool isEnabled;
final bool isSelected;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
TextStyle? get labelStyle => _textTheme.labelLarge?.copyWith(
color: isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.onSurfaceVariant
: _colors.onSurface,
);
@override
MaterialStateProperty<Color?>? get color =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected) && states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
if (states.contains(MaterialState.disabled)) {
return null;
}
if (states.contains(MaterialState.selected)) {
return _colors.secondaryContainer;
}
return null;
});
@override
Color? get shadowColor => Colors.transparent;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
Color? get checkmarkColor => isEnabled
? isSelected
? _colors.primary
: _colors.onSurfaceVariant
: _colors.onSurface;
@override
Color? get deleteIconColor => isEnabled
? isSelected
? _colors.onSecondaryContainer
: _colors.onSurfaceVariant
: _colors.onSurface;
@override
BorderSide? get side => !isSelected
? isEnabled
? BorderSide(color: _colors.outline)
: BorderSide(color: _colors.onSurface.withOpacity(0.12))
: const BorderSide(color: Colors.transparent);
@override
IconThemeData? get iconTheme => IconThemeData(
color: isEnabled
? isSelected
? _colors.primary
: _colors.onSurfaceVariant
: _colors.onSurface,
size: 18.0,
);
@override
EdgeInsetsGeometry? get padding => const EdgeInsets.all(8.0);
/// The label padding of the chip scales with the font size specified in the
/// [labelStyle], and the system font size settings that scale font sizes
/// globally.
///
/// The chip at effective font size 14.0 starts with 8px on each side and as
/// the font size scales up to closer to 28.0, the label padding is linearly
/// interpolated from 8px to 4px. Once the label has a font size of 2 or
/// higher, label padding remains 4px.
@override
EdgeInsetsGeometry? get labelPadding {
final double fontSize = labelStyle?.fontSize ?? 14.0;
final double fontSizeRatio = MediaQuery.textScalerOf(context).scale(fontSize) / 14.0;
return EdgeInsets.lerp(
const EdgeInsets.symmetric(horizontal: 8.0),
const EdgeInsets.symmetric(horizontal: 4.0),
clampDouble(fontSizeRatio - 1.0, 0.0, 1.0),
)!;
}
}
// END GENERATED TOKEN PROPERTIES - InputChip
| flutter/packages/flutter/lib/src/material/input_chip.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/input_chip.dart",
"repo_id": "flutter",
"token_count": 3844
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'divider.dart';
import 'material.dart';
import 'theme.dart';
/// The base type for [MaterialSlice] and [MaterialGap].
///
/// All [MergeableMaterialItem] objects need a [LocalKey].
@immutable
abstract class MergeableMaterialItem {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const MergeableMaterialItem(this.key);
/// The key for this item of the list.
///
/// The key is used to match parts of the mergeable material from frame to
/// frame so that state is maintained appropriately even as slices are added
/// or removed.
final LocalKey key;
}
/// A class that can be used as a child to [MergeableMaterial]. It is a slice
/// of [Material] that animates merging with other slices.
///
/// All [MaterialSlice] objects need a [LocalKey].
class MaterialSlice extends MergeableMaterialItem {
/// Creates a slice of [Material] that's mergeable within a
/// [MergeableMaterial].
const MaterialSlice({
required LocalKey key,
required this.child,
this.color,
}) : super(key);
/// The contents of this slice.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Defines the color for the slice.
///
/// By default, the value of [color] is [ThemeData.cardColor].
final Color? color;
@override
String toString() {
return 'MergeableSlice(key: $key, child: $child, color: $color)';
}
}
/// A class that represents a gap within [MergeableMaterial].
///
/// All [MaterialGap] objects need a [LocalKey].
class MaterialGap extends MergeableMaterialItem {
/// Creates a Material gap with a given size.
const MaterialGap({
required LocalKey key,
this.size = 16.0,
}) : super(key);
/// The main axis extent of this gap. For example, if the [MergeableMaterial]
/// is vertical, then this is the height of the gap.
final double size;
@override
String toString() {
return 'MaterialGap(key: $key, child: $size)';
}
}
/// Displays a list of [MergeableMaterialItem] children. The list contains
/// [MaterialSlice] items whose boundaries are either "merged" with adjacent
/// items or separated by a [MaterialGap]. The [children] are distributed along
/// the given [mainAxis] in the same way as the children of a [ListBody]. When
/// the list of children changes, gaps are automatically animated open or closed
/// as needed.
///
/// To enable this widget to correlate its list of children with the previous
/// one, each child must specify a key.
///
/// When a new gap is added to the list of children the adjacent items are
/// animated apart. Similarly when a gap is removed the adjacent items are
/// brought back together.
///
/// When a new slice is added or removed, the app is responsible for animating
/// the transition of the slices, while the gaps will be animated automatically.
///
/// See also:
///
/// * [Card], a piece of material that does not support splitting and merging
/// but otherwise looks the same.
class MergeableMaterial extends StatefulWidget {
/// Creates a mergeable Material list of items.
const MergeableMaterial({
super.key,
this.mainAxis = Axis.vertical,
this.elevation = 2,
this.hasDividers = false,
this.children = const <MergeableMaterialItem>[],
this.dividerColor,
});
/// The children of the [MergeableMaterial].
final List<MergeableMaterialItem> children;
/// The main layout axis.
final Axis mainAxis;
/// The z-coordinate at which to place all the [Material] slices.
///
/// Defaults to 2, the appropriate elevation for cards.
final double elevation;
/// Whether connected pieces of [MaterialSlice] have dividers between them.
final bool hasDividers;
/// Defines color used for dividers if [hasDividers] is true.
///
/// If [dividerColor] is null, then [DividerThemeData.color] is used. If that
/// is null, then [ThemeData.dividerColor] is used.
final Color? dividerColor;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<Axis>('mainAxis', mainAxis));
properties.add(DoubleProperty('elevation', elevation));
}
@override
State<MergeableMaterial> createState() => _MergeableMaterialState();
}
class _AnimationTuple {
_AnimationTuple({
required this.controller,
required this.startAnimation,
required this.endAnimation,
required this.gapAnimation,
}) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/material.dart',
className: '$_AnimationTuple',
object: this,
);
}
}
final AnimationController controller;
final CurvedAnimation startAnimation;
final CurvedAnimation endAnimation;
final CurvedAnimation gapAnimation;
double gapStart = 0.0;
@mustCallSuper
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
controller.dispose();
startAnimation.dispose();
endAnimation.dispose();
gapAnimation.dispose();
}
}
class _MergeableMaterialState extends State<MergeableMaterial> with TickerProviderStateMixin {
late List<MergeableMaterialItem> _children;
final Map<LocalKey, _AnimationTuple?> _animationTuples = <LocalKey, _AnimationTuple?>{};
@override
void initState() {
super.initState();
_children = List<MergeableMaterialItem>.of(widget.children);
for (int i = 0; i < _children.length; i += 1) {
final MergeableMaterialItem child = _children[i];
if (child is MaterialGap) {
_initGap(child);
_animationTuples[child.key]!.controller.value = 1.0; // Gaps are initially full-sized.
}
}
assert(_debugGapsAreValid(_children));
}
void _initGap(MaterialGap gap) {
final AnimationController controller = AnimationController(
duration: kThemeAnimationDuration,
vsync: this,
);
final CurvedAnimation startAnimation = CurvedAnimation(
parent: controller,
curve: Curves.fastOutSlowIn,
);
final CurvedAnimation endAnimation = CurvedAnimation(
parent: controller,
curve: Curves.fastOutSlowIn,
);
final CurvedAnimation gapAnimation = CurvedAnimation(
parent: controller,
curve: Curves.fastOutSlowIn,
);
controller.addListener(_handleTick);
_animationTuples[gap.key] = _AnimationTuple(
controller: controller,
startAnimation: startAnimation,
endAnimation: endAnimation,
gapAnimation: gapAnimation,
);
}
@override
void dispose() {
for (final MergeableMaterialItem child in _children) {
if (child is MaterialGap) {
_animationTuples[child.key]!.dispose();
}
}
super.dispose();
}
void _handleTick() {
setState(() {
// The animation's state is our build state, and it changed already.
});
}
bool _debugHasConsecutiveGaps(List<MergeableMaterialItem> children) {
for (int i = 0; i < widget.children.length - 1; i += 1) {
if (widget.children[i] is MaterialGap &&
widget.children[i + 1] is MaterialGap) {
return true;
}
}
return false;
}
bool _debugGapsAreValid(List<MergeableMaterialItem> children) {
// Check for consecutive gaps.
if (_debugHasConsecutiveGaps(children)) {
return false;
}
// First and last children must not be gaps.
if (children.isNotEmpty) {
if (children.first is MaterialGap || children.last is MaterialGap) {
return false;
}
}
return true;
}
void _insertChild(int index, MergeableMaterialItem child) {
_children.insert(index, child);
if (child is MaterialGap) {
_initGap(child);
}
}
void _removeChild(int index) {
final MergeableMaterialItem child = _children.removeAt(index);
if (child is MaterialGap) {
_animationTuples[child.key]!.dispose();
_animationTuples[child.key] = null;
}
}
bool _isClosingGap(int index) {
if (index < _children.length - 1 && _children[index] is MaterialGap) {
return _animationTuples[_children[index].key]!.controller.status ==
AnimationStatus.reverse;
}
return false;
}
void _removeEmptyGaps() {
int j = 0;
while (j < _children.length) {
if (
_children[j] is MaterialGap &&
_animationTuples[_children[j].key]!.controller.status == AnimationStatus.dismissed
) {
_removeChild(j);
} else {
j += 1;
}
}
}
@override
void didUpdateWidget(MergeableMaterial oldWidget) {
super.didUpdateWidget(oldWidget);
final Set<LocalKey> oldKeys = oldWidget.children.map<LocalKey>(
(MergeableMaterialItem child) => child.key,
).toSet();
final Set<LocalKey> newKeys = widget.children.map<LocalKey>(
(MergeableMaterialItem child) => child.key,
).toSet();
final Set<LocalKey> newOnly = newKeys.difference(oldKeys);
final Set<LocalKey> oldOnly = oldKeys.difference(newKeys);
final List<MergeableMaterialItem> newChildren = widget.children;
int i = 0;
int j = 0;
assert(_debugGapsAreValid(newChildren));
_removeEmptyGaps();
while (i < newChildren.length && j < _children.length) {
if (newOnly.contains(newChildren[i].key) ||
oldOnly.contains(_children[j].key)) {
final int startNew = i;
final int startOld = j;
// Skip new keys.
while (newOnly.contains(newChildren[i].key)) {
i += 1;
}
// Skip old keys.
while (oldOnly.contains(_children[j].key) || _isClosingGap(j)) {
j += 1;
}
final int newLength = i - startNew;
final int oldLength = j - startOld;
if (newLength > 0) {
if (oldLength > 1 ||
oldLength == 1 && _children[startOld] is MaterialSlice) {
if (newLength == 1 && newChildren[startNew] is MaterialGap) {
// Shrink all gaps into the size of the new one.
double gapSizeSum = 0.0;
while (startOld < j) {
final MergeableMaterialItem child = _children[startOld];
if (child is MaterialGap) {
final MaterialGap gap = child;
gapSizeSum += gap.size;
}
_removeChild(startOld);
j -= 1;
}
_insertChild(startOld, newChildren[startNew]);
_animationTuples[newChildren[startNew].key]!
..gapStart = gapSizeSum
..controller.forward();
j += 1;
} else {
// No animation if replaced items are more than one.
for (int k = 0; k < oldLength; k += 1) {
_removeChild(startOld);
}
for (int k = 0; k < newLength; k += 1) {
_insertChild(startOld + k, newChildren[startNew + k]);
}
j += newLength - oldLength;
}
} else if (oldLength == 1) {
if (newLength == 1 && newChildren[startNew] is MaterialGap &&
_children[startOld].key == newChildren[startNew].key) {
/// Special case: gap added back.
_animationTuples[newChildren[startNew].key]!.controller.forward();
} else {
final double gapSize = _getGapSize(startOld);
_removeChild(startOld);
for (int k = 0; k < newLength; k += 1) {
_insertChild(startOld + k, newChildren[startNew + k]);
}
j += newLength - 1;
double gapSizeSum = 0.0;
for (int k = startNew; k < i; k += 1) {
final MergeableMaterialItem newChild = newChildren[k];
if (newChild is MaterialGap) {
gapSizeSum += newChild.size;
}
}
// All gaps get proportional sizes of the original gap and they will
// animate to their actual size.
for (int k = startNew; k < i; k += 1) {
final MergeableMaterialItem newChild = newChildren[k];
if (newChild is MaterialGap) {
_animationTuples[newChild.key]!.gapStart = gapSize * newChild.size / gapSizeSum;
_animationTuples[newChild.key]!.controller
..value = 0.0
..forward();
}
}
}
} else {
// Grow gaps.
for (int k = 0; k < newLength; k += 1) {
final MergeableMaterialItem newChild = newChildren[startNew + k];
_insertChild(startOld + k, newChild);
if (newChild is MaterialGap) {
_animationTuples[newChild.key]!.controller.forward();
}
}
j += newLength;
}
} else {
// If more than a gap disappeared, just remove slices and shrink gaps.
if (oldLength > 1 ||
oldLength == 1 && _children[startOld] is MaterialSlice) {
double gapSizeSum = 0.0;
while (startOld < j) {
final MergeableMaterialItem child = _children[startOld];
if (child is MaterialGap) {
gapSizeSum += child.size;
}
_removeChild(startOld);
j -= 1;
}
if (gapSizeSum != 0.0) {
final MaterialGap gap = MaterialGap(
key: UniqueKey(),
size: gapSizeSum,
);
_insertChild(startOld, gap);
_animationTuples[gap.key]!.gapStart = 0.0;
_animationTuples[gap.key]!.controller
..value = 1.0
..reverse();
j += 1;
}
} else if (oldLength == 1) {
// Shrink gap.
final MaterialGap gap = _children[startOld] as MaterialGap;
_animationTuples[gap.key]!.gapStart = 0.0;
_animationTuples[gap.key]!.controller.reverse();
}
}
} else {
// Check whether the items are the same type. If they are, it means that
// their places have been swapped.
if ((_children[j] is MaterialGap) == (newChildren[i] is MaterialGap)) {
_children[j] = newChildren[i];
i += 1;
j += 1;
} else {
// This is a closing gap which we need to skip.
assert(_children[j] is MaterialGap);
j += 1;
}
}
}
// Handle remaining items.
while (j < _children.length) {
_removeChild(j);
}
while (i < newChildren.length) {
final MergeableMaterialItem newChild = newChildren[i];
_insertChild(j, newChild);
if (newChild is MaterialGap) {
_animationTuples[newChild.key]!.controller.forward();
}
i += 1;
j += 1;
}
}
BorderRadius _borderRadius(int index, bool start, bool end) {
assert(kMaterialEdges[MaterialType.card]!.topLeft == kMaterialEdges[MaterialType.card]!.topRight);
assert(kMaterialEdges[MaterialType.card]!.topLeft == kMaterialEdges[MaterialType.card]!.bottomLeft);
assert(kMaterialEdges[MaterialType.card]!.topLeft == kMaterialEdges[MaterialType.card]!.bottomRight);
final Radius cardRadius = kMaterialEdges[MaterialType.card]!.topLeft;
Radius startRadius = Radius.zero;
Radius endRadius = Radius.zero;
if (index > 0 && _children[index - 1] is MaterialGap) {
startRadius = Radius.lerp(
Radius.zero,
cardRadius,
_animationTuples[_children[index - 1].key]!.startAnimation.value,
)!;
}
if (index < _children.length - 2 && _children[index + 1] is MaterialGap) {
endRadius = Radius.lerp(
Radius.zero,
cardRadius,
_animationTuples[_children[index + 1].key]!.endAnimation.value,
)!;
}
if (widget.mainAxis == Axis.vertical) {
return BorderRadius.vertical(
top: start ? cardRadius : startRadius,
bottom: end ? cardRadius : endRadius,
);
} else {
return BorderRadius.horizontal(
left: start ? cardRadius : startRadius,
right: end ? cardRadius : endRadius,
);
}
}
double _getGapSize(int index) {
final MaterialGap gap = _children[index] as MaterialGap;
return lerpDouble(
_animationTuples[gap.key]!.gapStart,
gap.size,
_animationTuples[gap.key]!.gapAnimation.value,
)!;
}
bool _willNeedDivider(int index) {
if (index < 0) {
return false;
}
if (index >= _children.length) {
return false;
}
return _children[index] is MaterialSlice || _isClosingGap(index);
}
@override
Widget build(BuildContext context) {
_removeEmptyGaps();
final List<Widget> widgets = <Widget>[];
List<Widget> slices = <Widget>[];
int i;
for (i = 0; i < _children.length; i += 1) {
if (_children[i] is MaterialGap) {
assert(slices.isNotEmpty);
widgets.add(
ListBody(
mainAxis: widget.mainAxis,
children: slices,
),
);
slices = <Widget>[];
widgets.add(
SizedBox(
width: widget.mainAxis == Axis.horizontal ? _getGapSize(i) : null,
height: widget.mainAxis == Axis.vertical ? _getGapSize(i) : null,
),
);
} else {
final MaterialSlice slice = _children[i] as MaterialSlice;
Widget child = slice.child;
if (widget.hasDividers) {
final bool hasTopDivider = _willNeedDivider(i - 1);
final bool hasBottomDivider = _willNeedDivider(i + 1);
final BorderSide divider = Divider.createBorderSide(
context,
width: 0.5, // TODO(ianh): This probably looks terrible when the dpr isn't a power of two.
color: widget.dividerColor,
);
final Border border;
if (i == 0) {
border = Border(
bottom: hasBottomDivider ? divider : BorderSide.none,
);
} else if (i == _children.length - 1) {
border = Border(
top: hasTopDivider ? divider : BorderSide.none,
);
} else {
border = Border(
top: hasTopDivider ? divider : BorderSide.none,
bottom: hasBottomDivider ? divider : BorderSide.none,
);
}
child = AnimatedContainer(
key: _MergeableMaterialSliceKey(_children[i].key),
decoration: BoxDecoration(border: border),
duration: kThemeAnimationDuration,
curve: Curves.fastOutSlowIn,
child: child,
);
}
slices.add(
Container(
decoration: BoxDecoration(
color: (_children[i] as MaterialSlice).color ?? Theme.of(context).cardColor,
borderRadius: _borderRadius(i, i == 0, i == _children.length - 1),
),
child: Material(
type: MaterialType.transparency,
child: child,
),
),
);
}
}
if (slices.isNotEmpty) {
widgets.add(
ListBody(
mainAxis: widget.mainAxis,
children: slices,
),
);
slices = <Widget>[];
}
return _MergeableMaterialListBody(
mainAxis: widget.mainAxis,
elevation: widget.elevation,
items: _children,
children: widgets,
);
}
}
// The parent hierarchy can change and lead to the slice being
// rebuilt. Using a global key solves the issue.
class _MergeableMaterialSliceKey extends GlobalKey {
const _MergeableMaterialSliceKey(this.value) : super.constructor();
final LocalKey value;
@override
bool operator ==(Object other) {
return other is _MergeableMaterialSliceKey
&& other.value == value;
}
@override
int get hashCode => value.hashCode;
@override
String toString() {
return '_MergeableMaterialSliceKey($value)';
}
}
class _MergeableMaterialListBody extends ListBody {
const _MergeableMaterialListBody({
required super.children,
super.mainAxis,
required this.items,
required this.elevation,
});
final List<MergeableMaterialItem> items;
final double elevation;
AxisDirection _getDirection(BuildContext context) {
return getAxisDirectionFromAxisReverseAndDirectionality(context, mainAxis, false);
}
@override
RenderListBody createRenderObject(BuildContext context) {
return _RenderMergeableMaterialListBody(
axisDirection: _getDirection(context),
elevation: elevation,
);
}
@override
void updateRenderObject(BuildContext context, RenderListBody renderObject) {
final _RenderMergeableMaterialListBody materialRenderListBody = renderObject as _RenderMergeableMaterialListBody;
materialRenderListBody
..axisDirection = _getDirection(context)
..elevation = elevation;
}
}
class _RenderMergeableMaterialListBody extends RenderListBody {
_RenderMergeableMaterialListBody({
super.axisDirection,
double elevation = 0.0,
}) : _elevation = elevation;
double get elevation => _elevation;
double _elevation;
set elevation(double value) {
if (value == _elevation) {
return;
}
_elevation = value;
markNeedsPaint();
}
void _paintShadows(Canvas canvas, Rect rect) {
// TODO(ianh): We should interpolate the border radii of the shadows the same way we do those of the visible Material slices.
if (elevation != 0) {
canvas.drawShadow(
Path()..addRRect(kMaterialEdges[MaterialType.card]!.toRRect(rect)),
Colors.black,
elevation,
true, // occluding object is not (necessarily) opaque
);
}
}
@override
void paint(PaintingContext context, Offset offset) {
RenderBox? child = firstChild;
int index = 0;
while (child != null) {
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
final Rect rect = (childParentData.offset + offset) & child.size;
if (index.isEven) {
_paintShadows(context.canvas, rect);
}
child = childParentData.nextSibling;
index += 1;
}
defaultPaint(context, offset);
}
}
| flutter/packages/flutter/lib/src/material/mergeable_material.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/mergeable_material.dart",
"repo_id": "flutter",
"token_count": 9377
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'color_scheme.dart';
import 'material.dart';
import 'progress_indicator_theme.dart';
import 'theme.dart';
const double _kMinCircularProgressIndicatorSize = 36.0;
const int _kIndeterminateLinearDuration = 1800;
const int _kIndeterminateCircularDuration = 1333 * 2222;
enum _ActivityIndicatorType { material, adaptive }
/// A base class for Material Design progress indicators.
///
/// This widget cannot be instantiated directly. For a linear progress
/// indicator, see [LinearProgressIndicator]. For a circular progress indicator,
/// see [CircularProgressIndicator].
///
/// See also:
///
/// * <https://material.io/components/progress-indicators>
abstract class ProgressIndicator extends StatefulWidget {
/// Creates a progress indicator.
///
/// {@template flutter.material.ProgressIndicator.ProgressIndicator}
/// The [value] argument can either be null for an indeterminate
/// progress indicator, or a non-null value between 0.0 and 1.0 for a
/// determinate progress indicator.
///
/// ## Accessibility
///
/// The [semanticsLabel] can be used to identify the purpose of this progress
/// bar for screen reading software. The [semanticsValue] property may be used
/// for determinate progress indicators to indicate how much progress has been made.
/// {@endtemplate}
const ProgressIndicator({
super.key,
this.value,
this.backgroundColor,
this.color,
this.valueColor,
this.semanticsLabel,
this.semanticsValue,
});
/// If non-null, the value of this progress indicator.
///
/// A value of 0.0 means no progress and 1.0 means that progress is complete.
/// The value will be clamped to be in the range 0.0-1.0.
///
/// If null, this progress indicator is indeterminate, which means the
/// indicator displays a predetermined animation that does not indicate how
/// much actual progress is being made.
final double? value;
/// The progress indicator's background color.
///
/// It is up to the subclass to implement this in whatever way makes sense
/// for the given use case. See the subclass documentation for details.
final Color? backgroundColor;
/// {@template flutter.progress_indicator.ProgressIndicator.color}
/// The progress indicator's color.
///
/// This is only used if [ProgressIndicator.valueColor] is null.
/// If [ProgressIndicator.color] is also null, then the ambient
/// [ProgressIndicatorThemeData.color] will be used. If that
/// is null then the current theme's [ColorScheme.primary] will
/// be used by default.
/// {@endtemplate}
final Color? color;
/// The progress indicator's color as an animated value.
///
/// If null, the progress indicator is rendered with [color]. If that is null,
/// then it will use the ambient [ProgressIndicatorThemeData.color]. If that
/// is also null then it defaults to the current theme's [ColorScheme.primary].
final Animation<Color?>? valueColor;
/// {@template flutter.progress_indicator.ProgressIndicator.semanticsLabel}
/// The [SemanticsProperties.label] for this progress indicator.
///
/// This value indicates the purpose of the progress bar, and will be
/// read out by screen readers to indicate the purpose of this progress
/// indicator.
/// {@endtemplate}
final String? semanticsLabel;
/// {@template flutter.progress_indicator.ProgressIndicator.semanticsValue}
/// The [SemanticsProperties.value] for this progress indicator.
///
/// This will be used in conjunction with the [semanticsLabel] by
/// screen reading software to identify the widget, and is primarily
/// intended for use with determinate progress indicators to announce
/// how far along they are.
///
/// For determinate progress indicators, this will be defaulted to
/// [ProgressIndicator.value] expressed as a percentage, i.e. `0.1` will
/// become '10%'.
/// {@endtemplate}
final String? semanticsValue;
Color _getValueColor(BuildContext context, {Color? defaultColor}) {
return valueColor?.value ??
color ??
ProgressIndicatorTheme.of(context).color ??
defaultColor ??
Theme.of(context).colorScheme.primary;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(PercentProperty('value', value, showName: false, ifNull: '<indeterminate>'));
}
Widget _buildSemanticsWrapper({
required BuildContext context,
required Widget child,
}) {
String? expandedSemanticsValue = semanticsValue;
if (value != null) {
expandedSemanticsValue ??= '${(value! * 100).round()}%';
}
return Semantics(
label: semanticsLabel,
value: expandedSemanticsValue,
child: child,
);
}
}
class _LinearProgressIndicatorPainter extends CustomPainter {
const _LinearProgressIndicatorPainter({
required this.backgroundColor,
required this.valueColor,
this.value,
required this.animationValue,
required this.textDirection,
required this.indicatorBorderRadius,
});
final Color backgroundColor;
final Color valueColor;
final double? value;
final double animationValue;
final TextDirection textDirection;
final BorderRadiusGeometry indicatorBorderRadius;
// The indeterminate progress animation displays two lines whose leading (head)
// and trailing (tail) endpoints are defined by the following four curves.
static const Curve line1Head = Interval(
0.0,
750.0 / _kIndeterminateLinearDuration,
curve: Cubic(0.2, 0.0, 0.8, 1.0),
);
static const Curve line1Tail = Interval(
333.0 / _kIndeterminateLinearDuration,
(333.0 + 750.0) / _kIndeterminateLinearDuration,
curve: Cubic(0.4, 0.0, 1.0, 1.0),
);
static const Curve line2Head = Interval(
1000.0 / _kIndeterminateLinearDuration,
(1000.0 + 567.0) / _kIndeterminateLinearDuration,
curve: Cubic(0.0, 0.0, 0.65, 1.0),
);
static const Curve line2Tail = Interval(
1267.0 / _kIndeterminateLinearDuration,
(1267.0 + 533.0) / _kIndeterminateLinearDuration,
curve: Cubic(0.10, 0.0, 0.45, 1.0),
);
@override
void paint(Canvas canvas, Size size) {
final Paint paint = Paint()
..color = backgroundColor
..style = PaintingStyle.fill;
paint.color = valueColor;
void drawBar(double x, double width) {
if (width <= 0.0) {
return;
}
final double left = switch (textDirection) {
TextDirection.rtl => size.width - width - x,
TextDirection.ltr => x,
};
final Rect rect = Offset(left, 0.0) & Size(width, size.height);
if (indicatorBorderRadius != BorderRadius.zero) {
final RRect rrect = indicatorBorderRadius.resolve(textDirection).toRRect(rect);
canvas.drawRRect(rrect, paint);
} else {
canvas.drawRect(rect, paint);
}
}
if (value != null) {
drawBar(0.0, clampDouble(value!, 0.0, 1.0) * size.width);
} else {
final double x1 = size.width * line1Tail.transform(animationValue);
final double width1 = size.width * line1Head.transform(animationValue) - x1;
final double x2 = size.width * line2Tail.transform(animationValue);
final double width2 = size.width * line2Head.transform(animationValue) - x2;
drawBar(x1, width1);
drawBar(x2, width2);
}
}
@override
bool shouldRepaint(_LinearProgressIndicatorPainter oldPainter) {
return oldPainter.backgroundColor != backgroundColor
|| oldPainter.valueColor != valueColor
|| oldPainter.value != value
|| oldPainter.animationValue != animationValue
|| oldPainter.textDirection != textDirection
|| oldPainter.indicatorBorderRadius != indicatorBorderRadius;
}
}
/// A Material Design linear progress indicator, also known as a progress bar.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=O-rhXZLtpv0}
///
/// A widget that shows progress along a line. There are two kinds of linear
/// progress indicators:
///
/// * _Determinate_. Determinate progress indicators have a specific value at
/// each point in time, and the value should increase monotonically from 0.0
/// to 1.0, at which time the indicator is complete. To create a determinate
/// progress indicator, use a non-null [value] between 0.0 and 1.0.
/// * _Indeterminate_. Indeterminate progress indicators do not have a specific
/// value at each point in time and instead indicate that progress is being
/// made without indicating how much progress remains. To create an
/// indeterminate progress indicator, use a null [value].
///
/// The indicator line is displayed with [valueColor], an animated value. To
/// specify a constant color value use: `AlwaysStoppedAnimation<Color>(color)`.
///
/// The minimum height of the indicator can be specified using [minHeight].
/// The indicator can be made taller by wrapping the widget with a [SizedBox].
///
/// {@tool dartpad}
/// This example shows a [LinearProgressIndicator] with a changing value.
///
/// ** See code in examples/api/lib/material/progress_indicator/linear_progress_indicator.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of a [LinearProgressIndicator] with a changing value.
/// When toggling the switch, [LinearProgressIndicator] uses a determinate value.
/// As described in: https://m3.material.io/components/progress-indicators/overview
///
/// ** See code in examples/api/lib/material/progress_indicator/linear_progress_indicator.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CircularProgressIndicator], which shows progress along a circular arc.
/// * [RefreshIndicator], which automatically displays a [CircularProgressIndicator]
/// when the underlying vertical scrollable is overscrolled.
/// * <https://material.io/design/components/progress-indicators.html#linear-progress-indicators>
class LinearProgressIndicator extends ProgressIndicator {
/// Creates a linear progress indicator.
///
/// {@macro flutter.material.ProgressIndicator.ProgressIndicator}
const LinearProgressIndicator({
super.key,
super.value,
super.backgroundColor,
super.color,
super.valueColor,
this.minHeight,
super.semanticsLabel,
super.semanticsValue,
this.borderRadius = BorderRadius.zero,
}) : assert(minHeight == null || minHeight > 0);
/// {@template flutter.material.LinearProgressIndicator.trackColor}
/// Color of the track being filled by the linear indicator.
///
/// If [LinearProgressIndicator.backgroundColor] is null then the
/// ambient [ProgressIndicatorThemeData.linearTrackColor] will be used.
/// If that is null, then the ambient theme's [ColorScheme.background]
/// will be used to draw the track.
/// {@endtemplate}
@override
Color? get backgroundColor => super.backgroundColor;
/// {@template flutter.material.LinearProgressIndicator.minHeight}
/// The minimum height of the line used to draw the linear indicator.
///
/// If [LinearProgressIndicator.minHeight] is null then it will use the
/// ambient [ProgressIndicatorThemeData.linearMinHeight]. If that is null
/// it will use 4dp.
/// {@endtemplate}
final double? minHeight;
/// The border radius of both the indicator and the track.
///
/// By default it is [BorderRadius.zero], which produces a rectangular shape
/// with a rectangular indicator.
final BorderRadiusGeometry borderRadius;
@override
State<LinearProgressIndicator> createState() => _LinearProgressIndicatorState();
}
class _LinearProgressIndicatorState extends State<LinearProgressIndicator> with SingleTickerProviderStateMixin {
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: _kIndeterminateLinearDuration),
vsync: this,
);
if (widget.value == null) {
_controller.repeat();
}
}
@override
void didUpdateWidget(LinearProgressIndicator oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.value == null && !_controller.isAnimating) {
_controller.repeat();
} else if (widget.value != null && _controller.isAnimating) {
_controller.stop();
}
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
Widget _buildIndicator(BuildContext context, double animationValue, TextDirection textDirection) {
final ProgressIndicatorThemeData defaults = Theme.of(context).useMaterial3
? _LinearProgressIndicatorDefaultsM3(context)
: _LinearProgressIndicatorDefaultsM2(context);
final ProgressIndicatorThemeData indicatorTheme = ProgressIndicatorTheme.of(context);
final Color trackColor = widget.backgroundColor ??
indicatorTheme.linearTrackColor ??
defaults.linearTrackColor!;
final double minHeight = widget.minHeight ??
indicatorTheme.linearMinHeight ??
defaults.linearMinHeight!;
return widget._buildSemanticsWrapper(
context: context,
child: Container(
// Clip is only needed with indeterminate progress indicators
clipBehavior: (widget.borderRadius != BorderRadius.zero && widget.value == null)
? Clip.antiAlias
: Clip.none,
decoration: ShapeDecoration(
color: trackColor,
shape: RoundedRectangleBorder(borderRadius: widget.borderRadius),
),
constraints: BoxConstraints(
minWidth: double.infinity,
minHeight: minHeight,
),
child: CustomPaint(
painter: _LinearProgressIndicatorPainter(
backgroundColor: trackColor,
valueColor: widget._getValueColor(context, defaultColor: defaults.color),
value: widget.value, // may be null
animationValue: animationValue, // ignored if widget.value is not null
textDirection: textDirection,
indicatorBorderRadius: widget.borderRadius,
),
),
),
);
}
@override
Widget build(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
if (widget.value != null) {
return _buildIndicator(context, _controller.value, textDirection);
}
return AnimatedBuilder(
animation: _controller.view,
builder: (BuildContext context, Widget? child) {
return _buildIndicator(context, _controller.value, textDirection);
},
);
}
}
class _CircularProgressIndicatorPainter extends CustomPainter {
_CircularProgressIndicatorPainter({
this.backgroundColor,
required this.valueColor,
required this.value,
required this.headValue,
required this.tailValue,
required this.offsetValue,
required this.rotationValue,
required this.strokeWidth,
required this.strokeAlign,
this.strokeCap,
}) : arcStart = value != null
? _startAngle
: _startAngle + tailValue * 3 / 2 * math.pi + rotationValue * math.pi * 2.0 + offsetValue * 0.5 * math.pi,
arcSweep = value != null
? clampDouble(value, 0.0, 1.0) * _sweep
: math.max(headValue * 3 / 2 * math.pi - tailValue * 3 / 2 * math.pi, _epsilon);
final Color? backgroundColor;
final Color valueColor;
final double? value;
final double headValue;
final double tailValue;
final double offsetValue;
final double rotationValue;
final double strokeWidth;
final double strokeAlign;
final double arcStart;
final double arcSweep;
final StrokeCap? strokeCap;
static const double _twoPi = math.pi * 2.0;
static const double _epsilon = .001;
// Canvas.drawArc(r, 0, 2*PI) doesn't draw anything, so just get close.
static const double _sweep = _twoPi - _epsilon;
static const double _startAngle = -math.pi / 2.0;
@override
void paint(Canvas canvas, Size size) {
final Paint paint = Paint()
..color = valueColor
..strokeWidth = strokeWidth
..style = PaintingStyle.stroke;
// Use the negative operator as intended to keep the exposed constant value
// as users are already familiar with.
final double strokeOffset = strokeWidth / 2 * -strokeAlign;
final Offset arcBaseOffset = Offset(strokeOffset, strokeOffset);
final Size arcActualSize = Size(
size.width - strokeOffset * 2,
size.height - strokeOffset * 2,
);
if (backgroundColor != null) {
final Paint backgroundPaint = Paint()
..color = backgroundColor!
..strokeWidth = strokeWidth
..style = PaintingStyle.stroke;
canvas.drawArc(
arcBaseOffset & arcActualSize,
0,
_sweep,
false,
backgroundPaint,
);
}
if (value == null && strokeCap == null) {
// Indeterminate
paint.strokeCap = StrokeCap.square;
} else {
// Butt when determinate (value != null) && strokeCap == null;
paint.strokeCap = strokeCap ?? StrokeCap.butt;
}
canvas.drawArc(
arcBaseOffset & arcActualSize,
arcStart,
arcSweep,
false,
paint,
);
}
@override
bool shouldRepaint(_CircularProgressIndicatorPainter oldPainter) {
return oldPainter.backgroundColor != backgroundColor
|| oldPainter.valueColor != valueColor
|| oldPainter.value != value
|| oldPainter.headValue != headValue
|| oldPainter.tailValue != tailValue
|| oldPainter.offsetValue != offsetValue
|| oldPainter.rotationValue != rotationValue
|| oldPainter.strokeWidth != strokeWidth
|| oldPainter.strokeAlign != strokeAlign
|| oldPainter.strokeCap != strokeCap;
}
}
/// A Material Design circular progress indicator, which spins to indicate that
/// the application is busy.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=O-rhXZLtpv0}
///
/// A widget that shows progress along a circle. There are two kinds of circular
/// progress indicators:
///
/// * _Determinate_. Determinate progress indicators have a specific value at
/// each point in time, and the value should increase monotonically from 0.0
/// to 1.0, at which time the indicator is complete. To create a determinate
/// progress indicator, use a non-null [value] between 0.0 and 1.0.
/// * _Indeterminate_. Indeterminate progress indicators do not have a specific
/// value at each point in time and instead indicate that progress is being
/// made without indicating how much progress remains. To create an
/// indeterminate progress indicator, use a null [value].
///
/// The indicator arc is displayed with [valueColor], an animated value. To
/// specify a constant color use: `AlwaysStoppedAnimation<Color>(color)`.
///
/// {@tool dartpad}
/// This example shows a [CircularProgressIndicator] with a changing value.
///
/// ** See code in examples/api/lib/material/progress_indicator/circular_progress_indicator.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of a [CircularProgressIndicator] with a changing value.
/// When toggling the switch, [CircularProgressIndicator] uses a determinate value.
/// As described in: https://m3.material.io/components/progress-indicators/overview
///
/// ** See code in examples/api/lib/material/progress_indicator/circular_progress_indicator.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [LinearProgressIndicator], which displays progress along a line.
/// * [RefreshIndicator], which automatically displays a [CircularProgressIndicator]
/// when the underlying vertical scrollable is overscrolled.
/// * <https://material.io/design/components/progress-indicators.html#circular-progress-indicators>
class CircularProgressIndicator extends ProgressIndicator {
/// Creates a circular progress indicator.
///
/// {@macro flutter.material.ProgressIndicator.ProgressIndicator}
const CircularProgressIndicator({
super.key,
super.value,
super.backgroundColor,
super.color,
super.valueColor,
this.strokeWidth = 4.0,
this.strokeAlign = strokeAlignCenter,
super.semanticsLabel,
super.semanticsValue,
this.strokeCap,
}) : _indicatorType = _ActivityIndicatorType.material;
/// Creates an adaptive progress indicator that is a
/// [CupertinoActivityIndicator] in iOS and [CircularProgressIndicator] in
/// material theme/non-iOS.
///
/// The [value], [valueColor], [strokeWidth], [semanticsLabel], and
/// [semanticsValue] will be ignored in iOS.
///
/// {@macro flutter.material.ProgressIndicator.ProgressIndicator}
const CircularProgressIndicator.adaptive({
super.key,
super.value,
super.backgroundColor,
super.valueColor,
this.strokeWidth = 4.0,
super.semanticsLabel,
super.semanticsValue,
this.strokeCap,
this.strokeAlign = strokeAlignCenter,
}) : _indicatorType = _ActivityIndicatorType.adaptive;
final _ActivityIndicatorType _indicatorType;
/// {@template flutter.material.CircularProgressIndicator.trackColor}
/// Color of the circular track being filled by the circular indicator.
///
/// If [CircularProgressIndicator.backgroundColor] is null then the
/// ambient [ProgressIndicatorThemeData.circularTrackColor] will be used.
/// If that is null, then the track will not be painted.
/// {@endtemplate}
@override
Color? get backgroundColor => super.backgroundColor;
/// The width of the line used to draw the circle.
final double strokeWidth;
/// The relative position of the stroke on a [CircularProgressIndicator].
///
/// Values typically range from -1.0 ([strokeAlignInside], inside stroke)
/// to 1.0 ([strokeAlignOutside], outside stroke),
/// without any bound constraints (e.g., a value of -2.0 is not typical, but allowed).
/// A value of 0 ([strokeAlignCenter], default) will center the border
/// on the edge of the widget.
final double strokeAlign;
/// The progress indicator's line ending.
///
/// This determines the shape of the stroke ends of the progress indicator.
/// By default, [strokeCap] is null.
/// When [value] is null (indeterminate), the stroke ends are set to
/// [StrokeCap.square]. When [value] is not null, the stroke
/// ends are set to [StrokeCap.butt].
///
/// Setting [strokeCap] to [StrokeCap.round] will result in a rounded end.
/// Setting [strokeCap] to [StrokeCap.butt] with [value] == null will result
/// in a slightly different indeterminate animation; the indicator completely
/// disappears and reappears on its minimum value.
/// Setting [strokeCap] to [StrokeCap.square] with [value] != null will
/// result in a different display of [value]. The indicator will start
/// drawing from slightly less than the start, and end slightly after
/// the end. This will produce an alternative result, as the
/// default behavior, for example, that a [value] of 0.5 starts at 90 degrees
/// and ends at 270 degrees. With [StrokeCap.square], it could start 85
/// degrees and end at 275 degrees.
final StrokeCap? strokeCap;
/// The indicator stroke is drawn fully inside of the indicator path.
///
/// This is a constant for use with [strokeAlign].
static const double strokeAlignInside = -1.0;
/// The indicator stroke is drawn on the center of the indicator path,
/// with half of the [strokeWidth] on the inside, and the other half
/// on the outside of the path.
///
/// This is a constant for use with [strokeAlign].
///
/// This is the default value for [strokeAlign].
static const double strokeAlignCenter = 0.0;
/// The indicator stroke is drawn on the outside of the indicator path.
///
/// This is a constant for use with [strokeAlign].
static const double strokeAlignOutside = 1.0;
@override
State<CircularProgressIndicator> createState() => _CircularProgressIndicatorState();
}
class _CircularProgressIndicatorState extends State<CircularProgressIndicator> with SingleTickerProviderStateMixin {
static const int _pathCount = _kIndeterminateCircularDuration ~/ 1333;
static const int _rotationCount = _kIndeterminateCircularDuration ~/ 2222;
static final Animatable<double> _strokeHeadTween = CurveTween(
curve: const Interval(0.0, 0.5, curve: Curves.fastOutSlowIn),
).chain(CurveTween(
curve: const SawTooth(_pathCount),
));
static final Animatable<double> _strokeTailTween = CurveTween(
curve: const Interval(0.5, 1.0, curve: Curves.fastOutSlowIn),
).chain(CurveTween(
curve: const SawTooth(_pathCount),
));
static final Animatable<double> _offsetTween = CurveTween(curve: const SawTooth(_pathCount));
static final Animatable<double> _rotationTween = CurveTween(curve: const SawTooth(_rotationCount));
late AnimationController _controller;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: _kIndeterminateCircularDuration),
vsync: this,
);
if (widget.value == null) {
_controller.repeat();
}
}
@override
void didUpdateWidget(CircularProgressIndicator oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.value == null && !_controller.isAnimating) {
_controller.repeat();
} else if (widget.value != null && _controller.isAnimating) {
_controller.stop();
}
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
Widget _buildCupertinoIndicator(BuildContext context) {
final Color? tickColor = widget.backgroundColor;
final double? value = widget.value;
if (value == null) {
return CupertinoActivityIndicator(
key: widget.key,
color: tickColor
);
}
return CupertinoActivityIndicator.partiallyRevealed(
key: widget.key,
color: tickColor,
progress: value
);
}
Widget _buildMaterialIndicator(BuildContext context, double headValue, double tailValue, double offsetValue, double rotationValue) {
final ProgressIndicatorThemeData defaults = Theme.of(context).useMaterial3
? _CircularProgressIndicatorDefaultsM3(context)
: _CircularProgressIndicatorDefaultsM2(context);
final Color? trackColor = widget.backgroundColor ?? ProgressIndicatorTheme.of(context).circularTrackColor;
return widget._buildSemanticsWrapper(
context: context,
child: Container(
constraints: const BoxConstraints(
minWidth: _kMinCircularProgressIndicatorSize,
minHeight: _kMinCircularProgressIndicatorSize,
),
child: CustomPaint(
painter: _CircularProgressIndicatorPainter(
backgroundColor: trackColor,
valueColor: widget._getValueColor(context, defaultColor: defaults.color),
value: widget.value, // may be null
headValue: headValue, // remaining arguments are ignored if widget.value is not null
tailValue: tailValue,
offsetValue: offsetValue,
rotationValue: rotationValue,
strokeWidth: widget.strokeWidth,
strokeAlign: widget.strokeAlign,
strokeCap: widget.strokeCap,
),
),
),
);
}
Widget _buildAnimation() {
return AnimatedBuilder(
animation: _controller,
builder: (BuildContext context, Widget? child) {
return _buildMaterialIndicator(
context,
_strokeHeadTween.evaluate(_controller),
_strokeTailTween.evaluate(_controller),
_offsetTween.evaluate(_controller),
_rotationTween.evaluate(_controller),
);
},
);
}
@override
Widget build(BuildContext context) {
switch (widget._indicatorType) {
case _ActivityIndicatorType.material:
if (widget.value != null) {
return _buildMaterialIndicator(context, 0.0, 0.0, 0, 0.0);
}
return _buildAnimation();
case _ActivityIndicatorType.adaptive:
final ThemeData theme = Theme.of(context);
switch (theme.platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return _buildCupertinoIndicator(context);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
if (widget.value != null) {
return _buildMaterialIndicator(context, 0.0, 0.0, 0, 0.0);
}
return _buildAnimation();
}
}
}
}
class _RefreshProgressIndicatorPainter extends _CircularProgressIndicatorPainter {
_RefreshProgressIndicatorPainter({
required super.valueColor,
required super.value,
required super.headValue,
required super.tailValue,
required super.offsetValue,
required super.rotationValue,
required super.strokeWidth,
required super.strokeAlign,
required this.arrowheadScale,
required super.strokeCap,
});
final double arrowheadScale;
void paintArrowhead(Canvas canvas, Size size) {
// ux, uy: a unit vector whose direction parallels the base of the arrowhead.
// (So ux, -uy points in the direction the arrowhead points.)
final double arcEnd = arcStart + arcSweep;
final double ux = math.cos(arcEnd);
final double uy = math.sin(arcEnd);
assert(size.width == size.height);
final double radius = size.width / 2.0;
final double arrowheadPointX = radius + ux * radius + -uy * strokeWidth * 2.0 * arrowheadScale;
final double arrowheadPointY = radius + uy * radius + ux * strokeWidth * 2.0 * arrowheadScale;
final double arrowheadRadius = strokeWidth * 2.0 * arrowheadScale;
final double innerRadius = radius - arrowheadRadius;
final double outerRadius = radius + arrowheadRadius;
final Path path = Path()
..moveTo(radius + ux * innerRadius, radius + uy * innerRadius)
..lineTo(radius + ux * outerRadius, radius + uy * outerRadius)
..lineTo(arrowheadPointX, arrowheadPointY)
..close();
final Paint paint = Paint()
..color = valueColor
..strokeWidth = strokeWidth
..style = PaintingStyle.fill;
canvas.drawPath(path, paint);
}
@override
void paint(Canvas canvas, Size size) {
super.paint(canvas, size);
if (arrowheadScale > 0.0) {
paintArrowhead(canvas, size);
}
}
}
/// An indicator for the progress of refreshing the contents of a widget.
///
/// Typically used for swipe-to-refresh interactions. See [RefreshIndicator] for
/// a complete implementation of swipe-to-refresh driven by a [Scrollable]
/// widget.
///
/// The indicator arc is displayed with [valueColor], an animated value. To
/// specify a constant color use: `AlwaysStoppedAnimation<Color>(color)`.
///
/// See also:
///
/// * [RefreshIndicator], which automatically displays a [CircularProgressIndicator]
/// when the underlying vertical scrollable is overscrolled.
class RefreshProgressIndicator extends CircularProgressIndicator {
/// Creates a refresh progress indicator.
///
/// Rather than creating a refresh progress indicator directly, consider using
/// a [RefreshIndicator] together with a [Scrollable] widget.
///
/// {@macro flutter.material.ProgressIndicator.ProgressIndicator}
const RefreshProgressIndicator({
super.key,
super.value,
super.backgroundColor,
super.color,
super.valueColor,
super.strokeWidth = defaultStrokeWidth, // Different default than CircularProgressIndicator.
super.strokeAlign,
super.semanticsLabel,
super.semanticsValue,
super.strokeCap,
this.elevation = 2.0,
this.indicatorMargin = const EdgeInsets.all(4.0),
this.indicatorPadding = const EdgeInsets.all(12.0),
});
/// {@macro flutter.material.material.elevation}
final double elevation;
/// The amount of space by which to inset the whole indicator.
/// It accommodates the [elevation] of the indicator.
final EdgeInsetsGeometry indicatorMargin;
/// The amount of space by which to inset the inner refresh indicator.
final EdgeInsetsGeometry indicatorPadding;
/// Default stroke width.
static const double defaultStrokeWidth = 2.5;
/// {@template flutter.material.RefreshProgressIndicator.backgroundColor}
/// Background color of that fills the circle under the refresh indicator.
///
/// If [RefreshIndicator.backgroundColor] is null then the
/// ambient [ProgressIndicatorThemeData.refreshBackgroundColor] will be used.
/// If that is null, then the ambient theme's [ThemeData.canvasColor]
/// will be used.
/// {@endtemplate}
@override
Color? get backgroundColor => super.backgroundColor;
@override
State<CircularProgressIndicator> createState() => _RefreshProgressIndicatorState();
}
class _RefreshProgressIndicatorState extends _CircularProgressIndicatorState {
static const double _indicatorSize = 41.0;
/// Interval for arrow head to fully grow.
static const double _strokeHeadInterval = 0.33;
late final Animatable<double> _convertTween = CurveTween(
curve: const Interval(0.1, _strokeHeadInterval),
);
late final Animatable<double> _additionalRotationTween = TweenSequence<double>(
<TweenSequenceItem<double>>[
// Makes arrow to expand a little bit earlier, to match the Android look.
TweenSequenceItem<double>(
tween: Tween<double>(begin: -0.1, end: -0.2),
weight: _strokeHeadInterval,
),
// Additional rotation after the arrow expanded
TweenSequenceItem<double>(
tween: Tween<double>(begin: -0.2, end: 1.35),
weight: 1 - _strokeHeadInterval,
),
],
);
// Last value received from the widget before null.
double? _lastValue;
/// Force casting the widget as [RefreshProgressIndicator].
@override
RefreshProgressIndicator get widget => super.widget as RefreshProgressIndicator;
// Always show the indeterminate version of the circular progress indicator.
//
// When value is non-null the sweep of the progress indicator arrow's arc
// varies from 0 to about 300 degrees.
//
// When value is null the arrow animation starting from wherever we left it.
@override
Widget build(BuildContext context) {
final double? value = widget.value;
if (value != null) {
_lastValue = value;
_controller.value = _convertTween.transform(value)
* (1333 / 2 / _kIndeterminateCircularDuration);
}
return _buildAnimation();
}
@override
Widget _buildAnimation() {
return AnimatedBuilder(
animation: _controller,
builder: (BuildContext context, Widget? child) {
return _buildMaterialIndicator(
context,
// Lengthen the arc a little
1.05 * _CircularProgressIndicatorState._strokeHeadTween.evaluate(_controller),
_CircularProgressIndicatorState._strokeTailTween.evaluate(_controller),
_CircularProgressIndicatorState._offsetTween.evaluate(_controller),
_CircularProgressIndicatorState._rotationTween.evaluate(_controller),
);
},
);
}
@override
Widget _buildMaterialIndicator(BuildContext context, double headValue, double tailValue, double offsetValue, double rotationValue) {
final double? value = widget.value;
final double arrowheadScale = value == null ? 0.0 : const Interval(0.1, _strokeHeadInterval).transform(value);
final double rotation;
if (value == null && _lastValue == null) {
rotation = 0.0;
} else {
rotation = math.pi * _additionalRotationTween.transform(value ?? _lastValue!);
}
Color valueColor = widget._getValueColor(context);
final double opacity = valueColor.opacity;
valueColor = valueColor.withOpacity(1.0);
final Color backgroundColor =
widget.backgroundColor ??
ProgressIndicatorTheme.of(context).refreshBackgroundColor ??
Theme.of(context).canvasColor;
return widget._buildSemanticsWrapper(
context: context,
child: Container(
width: _indicatorSize,
height: _indicatorSize,
margin: widget.indicatorMargin,
child: Material(
type: MaterialType.circle,
color: backgroundColor,
elevation: widget.elevation,
child: Padding(
padding: widget.indicatorPadding,
child: Opacity(
opacity: opacity,
child: Transform.rotate(
angle: rotation,
child: CustomPaint(
painter: _RefreshProgressIndicatorPainter(
valueColor: valueColor,
value: null, // Draw the indeterminate progress indicator.
headValue: headValue,
tailValue: tailValue,
offsetValue: offsetValue,
rotationValue: rotationValue,
strokeWidth: widget.strokeWidth,
strokeAlign: widget.strokeAlign,
arrowheadScale: arrowheadScale,
strokeCap: widget.strokeCap,
),
),
),
),
),
),
),
);
}
}
// Hand coded defaults based on Material Design 2.
class _CircularProgressIndicatorDefaultsM2 extends ProgressIndicatorThemeData {
_CircularProgressIndicatorDefaultsM2(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color get color => _colors.primary;
}
class _LinearProgressIndicatorDefaultsM2 extends ProgressIndicatorThemeData {
_LinearProgressIndicatorDefaultsM2(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color get color => _colors.primary;
@override
Color get linearTrackColor => _colors.background;
@override
double get linearMinHeight => 4.0;
}
// BEGIN GENERATED TOKEN PROPERTIES - ProgressIndicator
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _CircularProgressIndicatorDefaultsM3 extends ProgressIndicatorThemeData {
_CircularProgressIndicatorDefaultsM3(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color get color => _colors.primary;
}
class _LinearProgressIndicatorDefaultsM3 extends ProgressIndicatorThemeData {
_LinearProgressIndicatorDefaultsM3(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color get color => _colors.primary;
@override
Color get linearTrackColor => _colors.surfaceContainerHighest;
@override
double get linearMinHeight => 4.0;
}
// END GENERATED TOKEN PROPERTIES - ProgressIndicator
| flutter/packages/flutter/lib/src/material/progress_indicator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/progress_indicator.dart",
"repo_id": "flutter",
"token_count": 13017
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Overrides the default values of visual properties for descendant
/// [SegmentedButton] widgets.
///
/// Descendant widgets obtain the current [SegmentedButtonThemeData] object with
/// [SegmentedButtonTheme.of]. Instances of [SegmentedButtonTheme] can
/// be customized with [SegmentedButtonThemeData.copyWith].
///
/// Typically a [SegmentedButtonTheme] is specified as part of the overall
/// [Theme] with [ThemeData.segmentedButtonTheme].
///
/// All [SegmentedButtonThemeData] properties are null by default. When null,
/// the [SegmentedButton] computes its own default values, typically based on
/// the overall theme's [ThemeData.colorScheme], [ThemeData.textTheme], and
/// [ThemeData.iconTheme].
@immutable
class SegmentedButtonThemeData with Diagnosticable {
/// Creates a [SegmentedButtonThemeData] that can be used to override default properties
/// in a [SegmentedButtonTheme] widget.
const SegmentedButtonThemeData({
this.style,
this.selectedIcon,
});
/// Overrides the [SegmentedButton]'s default style.
///
/// Non-null properties or non-null resolved [MaterialStateProperty]
/// values override the default values used by [SegmentedButton].
///
/// If [style] is null, then this theme doesn't override anything.
final ButtonStyle? style;
/// Override for [SegmentedButton.selectedIcon] property.
///
/// If non-null, then [selectedIcon] will be used instead of default
/// value for [SegmentedButton.selectedIcon].
final Widget? selectedIcon;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
SegmentedButtonThemeData copyWith({
ButtonStyle? style,
Widget? selectedIcon,
}) {
return SegmentedButtonThemeData(
style: style ?? this.style,
selectedIcon: selectedIcon ?? this.selectedIcon,
);
}
/// Linearly interpolates between two segmented button themes.
static SegmentedButtonThemeData lerp(SegmentedButtonThemeData? a, SegmentedButtonThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return SegmentedButtonThemeData(
style: ButtonStyle.lerp(a?.style, b?.style, t),
selectedIcon: t < 0.5 ? a?.selectedIcon : b?.selectedIcon,
);
}
@override
int get hashCode => Object.hash(
style,
selectedIcon,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SegmentedButtonThemeData
&& other.style == style
&& other.selectedIcon == selectedIcon;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ButtonStyle>('style', style, defaultValue: null));
}
}
/// An inherited widget that defines the visual properties for
/// [SegmentedButton]s in this widget's subtree.
///
/// Values specified here are used for [SegmentedButton] properties that are not
/// given an explicit non-null value.
class SegmentedButtonTheme extends InheritedTheme {
/// Creates a [SegmentedButtonTheme] that controls visual parameters for
/// descendent [SegmentedButton]s.
const SegmentedButtonTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the visual properties used by descendant [SegmentedButton]
/// widgets.
final SegmentedButtonThemeData data;
/// The [data] from the closest instance of this class that encloses the given
/// context.
///
/// If there is no [SegmentedButtonTheme] in scope, this will return
/// [ThemeData.segmentedButtonTheme] from the ambient [Theme].
///
/// Typical usage is as follows:
///
/// ```dart
/// SegmentedButtonThemeData theme = SegmentedButtonTheme.of(context);
/// ```
///
/// See also:
///
/// * [maybeOf], which returns null if it doesn't find a
/// [SegmentedButtonTheme] ancestor.
static SegmentedButtonThemeData of(BuildContext context) {
return maybeOf(context) ?? Theme.of(context).segmentedButtonTheme;
}
/// The data from the closest instance of this class that encloses the given
/// context, if any.
///
/// Use this function if you want to allow situations where no
/// [SegmentedButtonTheme] is in scope. Prefer using [SegmentedButtonTheme.of]
/// in situations where a [SegmentedButtonThemeData] is expected to be
/// non-null.
///
/// If there is no [SegmentedButtonTheme] in scope, then this function will
/// return null.
///
/// Typical usage is as follows:
///
/// ```dart
/// SegmentedButtonThemeData? theme = SegmentedButtonTheme.maybeOf(context);
/// if (theme == null) {
/// // Do something else instead.
/// }
/// ```
///
/// See also:
///
/// * [of], which will return [ThemeData.segmentedButtonTheme] if it doesn't
/// find a [SegmentedButtonTheme] ancestor, instead of returning null.
static SegmentedButtonThemeData? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<SegmentedButtonTheme>()?.data;
}
@override
Widget wrap(BuildContext context, Widget child) {
return SegmentedButtonTheme(data: data, child: child);
}
@override
bool updateShouldNotify(SegmentedButtonTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/segmented_button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/segmented_button_theme.dart",
"repo_id": "flutter",
"token_count": 1725
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'constants.dart';
// Examples can assume:
// late BuildContext context;
/// Coordinates tab selection between a [TabBar] and a [TabBarView].
///
/// The [index] property is the index of the selected tab and the [animation]
/// represents the current scroll positions of the tab bar and the tab bar view.
/// The selected tab's index can be changed with [animateTo].
///
/// A stateful widget that builds a [TabBar] or a [TabBarView] can create
/// a [TabController] and share it directly.
///
/// When the [TabBar] and [TabBarView] don't have a convenient stateful
/// ancestor, a [TabController] can be shared by providing a
/// [DefaultTabController] inherited widget.
///
/// {@animation 700 540 https://flutter.github.io/assets-for-api-docs/assets/material/tabs.mp4}
///
/// {@tool snippet}
///
/// This widget introduces a [Scaffold] with an [AppBar] and a [TabBar].
///
/// ```dart
/// class MyTabbedPage extends StatefulWidget {
/// const MyTabbedPage({ super.key });
/// @override
/// State<MyTabbedPage> createState() => _MyTabbedPageState();
/// }
///
/// class _MyTabbedPageState extends State<MyTabbedPage> with SingleTickerProviderStateMixin {
/// static const List<Tab> myTabs = <Tab>[
/// Tab(text: 'LEFT'),
/// Tab(text: 'RIGHT'),
/// ];
///
/// late TabController _tabController;
///
/// @override
/// void initState() {
/// super.initState();
/// _tabController = TabController(vsync: this, length: myTabs.length);
/// }
///
/// @override
/// void dispose() {
/// _tabController.dispose();
/// super.dispose();
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// bottom: TabBar(
/// controller: _tabController,
/// tabs: myTabs,
/// ),
/// ),
/// body: TabBarView(
/// controller: _tabController,
/// children: myTabs.map((Tab tab) {
/// final String label = tab.text!.toLowerCase();
/// return Center(
/// child: Text(
/// 'This is the $label tab',
/// style: const TextStyle(fontSize: 36),
/// ),
/// );
/// }).toList(),
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to listen to page updates in [TabBar] and [TabBarView]
/// when using [DefaultTabController].
///
/// ** See code in examples/api/lib/material/tab_controller/tab_controller.1.dart **
/// {@end-tool}
///
class TabController extends ChangeNotifier {
/// Creates an object that manages the state required by [TabBar] and a
/// [TabBarView].
///
/// The [length] must not be negative. Typically it's a value greater than
/// one, i.e. typically there are two or more tabs. The [length] must match
/// [TabBar.tabs]'s and [TabBarView.children]'s length.
///
/// The `initialIndex` must be valid given [length]. If [length] is zero, then
/// `initialIndex` must be 0 (the default).
TabController({
int initialIndex = 0,
Duration? animationDuration,
required this.length,
required TickerProvider vsync,
}) : assert(length >= 0),
assert(initialIndex >= 0 && (length == 0 || initialIndex < length)),
_index = initialIndex,
_previousIndex = initialIndex,
_animationDuration = animationDuration ?? kTabScrollDuration,
_animationController = AnimationController.unbounded(
value: initialIndex.toDouble(),
vsync: vsync,
) {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
// Private constructor used by `_copyWith`. This allows a new TabController to
// be created without having to create a new animationController.
TabController._({
required int index,
required int previousIndex,
required AnimationController? animationController,
required Duration animationDuration,
required this.length,
}) : _index = index,
_previousIndex = previousIndex,
_animationController = animationController,
_animationDuration = animationDuration {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// Creates a new [TabController] with `index`, `previousIndex`, `length`, and
/// `animationDuration` if they are non-null.
///
/// This method is used by [DefaultTabController].
///
/// When [DefaultTabController.length] is updated, this method is called to
/// create a new [TabController] without creating a new [AnimationController].
TabController _copyWith({
required int? index,
required int? length,
required int? previousIndex,
required Duration? animationDuration,
}) {
if (index != null) {
_animationController!.value = index.toDouble();
}
return TabController._(
index: index ?? _index,
length: length ?? this.length,
animationController: _animationController,
previousIndex: previousIndex ?? _previousIndex,
animationDuration: animationDuration ?? _animationDuration,
);
}
/// An animation whose value represents the current position of the [TabBar]'s
/// selected tab indicator as well as the scrollOffsets of the [TabBar]
/// and [TabBarView].
///
/// The animation's value ranges from 0.0 to [length] - 1.0. After the
/// selected tab is changed, the animation's value equals [index]. The
/// animation's value can be [offset] by +/- 1.0 to reflect [TabBarView]
/// drag scrolling.
///
/// If this [TabController] was disposed, then return null.
Animation<double>? get animation => _animationController?.view;
AnimationController? _animationController;
/// Controls the duration of TabController and TabBarView animations.
///
/// Defaults to kTabScrollDuration.
Duration get animationDuration => _animationDuration;
final Duration _animationDuration;
/// The total number of tabs.
///
/// Typically greater than one. Must match [TabBar.tabs]'s and
/// [TabBarView.children]'s length.
final int length;
void _changeIndex(int value, { Duration? duration, Curve? curve }) {
assert(value >= 0 && (value < length || length == 0));
assert(duration != null || curve == null);
assert(_indexIsChangingCount >= 0);
if (value == _index || length < 2) {
return;
}
_previousIndex = index;
_index = value;
if (duration != null && duration > Duration.zero) {
_indexIsChangingCount += 1;
notifyListeners(); // Because the value of indexIsChanging may have changed.
_animationController!
.animateTo(_index.toDouble(), duration: duration, curve: curve!)
.whenCompleteOrCancel(() {
if (_animationController != null) { // don't notify if we've been disposed
_indexIsChangingCount -= 1;
notifyListeners();
}
});
} else {
_indexIsChangingCount += 1;
_animationController!.value = _index.toDouble();
_indexIsChangingCount -= 1;
notifyListeners();
}
}
/// The index of the currently selected tab.
///
/// Changing the index also updates [previousIndex], sets the [animation]'s
/// value to index, resets [indexIsChanging] to false, and notifies listeners.
///
/// To change the currently selected tab and play the [animation] use [animateTo].
///
/// The value of [index] must be valid given [length]. If [length] is zero,
/// then [index] will also be zero.
int get index => _index;
int _index;
set index(int value) {
_changeIndex(value);
}
/// The index of the previously selected tab.
///
/// Initially the same as [index].
int get previousIndex => _previousIndex;
int _previousIndex;
/// True while we're animating from [previousIndex] to [index] as a
/// consequence of calling [animateTo].
///
/// This value is true during the [animateTo] animation that's triggered when
/// the user taps a [TabBar] tab. It is false when [offset] is changing as a
/// consequence of the user dragging (and "flinging") the [TabBarView].
bool get indexIsChanging => _indexIsChangingCount != 0;
int _indexIsChangingCount = 0;
/// Immediately sets [index] and [previousIndex] and then plays the
/// [animation] from its current value to [index].
///
/// While the animation is running [indexIsChanging] is true. When the
/// animation completes [offset] will be 0.0.
void animateTo(int value, { Duration? duration, Curve curve = Curves.ease }) {
_changeIndex(value, duration: duration ?? _animationDuration, curve: curve);
}
/// The difference between the [animation]'s value and [index].
///
/// The offset value must be between -1.0 and 1.0.
///
/// This property is typically set by the [TabBarView] when the user
/// drags left or right. A value between -1.0 and 0.0 implies that the
/// TabBarView has been dragged to the left. Similarly a value between
/// 0.0 and 1.0 implies that the TabBarView has been dragged to the right.
double get offset => _animationController!.value - _index.toDouble();
set offset(double value) {
assert(value >= -1.0 && value <= 1.0);
assert(!indexIsChanging);
if (value == offset) {
return;
}
_animationController!.value = value + _index.toDouble();
}
@override
void dispose() {
_animationController?.dispose();
_animationController = null;
super.dispose();
}
}
class _TabControllerScope extends InheritedWidget {
const _TabControllerScope({
required this.controller,
required this.enabled,
required super.child,
});
final TabController controller;
final bool enabled;
@override
bool updateShouldNotify(_TabControllerScope old) {
return enabled != old.enabled || controller != old.controller;
}
}
/// The [TabController] for descendant widgets that don't specify one
/// explicitly.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=POtoEH-5l40}
///
/// [DefaultTabController] is an inherited widget that is used to share a
/// [TabController] with a [TabBar] or a [TabBarView]. It's used when sharing an
/// explicitly created [TabController] isn't convenient because the tab bar
/// widgets are created by a stateless parent widget or by different parent
/// widgets.
///
/// {@animation 700 540 https://flutter.github.io/assets-for-api-docs/assets/material/tabs.mp4}
///
/// ```dart
/// class MyDemo extends StatelessWidget {
/// const MyDemo({super.key});
///
/// static const List<Tab> myTabs = <Tab>[
/// Tab(text: 'LEFT'),
/// Tab(text: 'RIGHT'),
/// ];
///
/// @override
/// Widget build(BuildContext context) {
/// return DefaultTabController(
/// length: myTabs.length,
/// child: Scaffold(
/// appBar: AppBar(
/// bottom: const TabBar(
/// tabs: myTabs,
/// ),
/// ),
/// body: TabBarView(
/// children: myTabs.map((Tab tab) {
/// final String label = tab.text!.toLowerCase();
/// return Center(
/// child: Text(
/// 'This is the $label tab',
/// style: const TextStyle(fontSize: 36),
/// ),
/// );
/// }).toList(),
/// ),
/// ),
/// );
/// }
/// }
/// ```
class DefaultTabController extends StatefulWidget {
/// Creates a default tab controller for the given [child] widget.
///
/// The [length] argument is typically greater than one. The [length] must
/// match [TabBar.tabs]'s and [TabBarView.children]'s length.
const DefaultTabController({
super.key,
required this.length,
this.initialIndex = 0,
required this.child,
this.animationDuration,
}) : assert(length >= 0),
assert(length == 0 || (initialIndex >= 0 && initialIndex < length));
/// The total number of tabs.
///
/// Typically greater than one. Must match [TabBar.tabs]'s and
/// [TabBarView.children]'s length.
final int length;
/// The initial index of the selected tab.
///
/// Defaults to zero.
final int initialIndex;
/// Controls the duration of DefaultTabController and TabBarView animations.
///
/// Defaults to kTabScrollDuration.
final Duration? animationDuration;
/// The widget below this widget in the tree.
///
/// Typically a [Scaffold] whose [AppBar] includes a [TabBar].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The closest instance of [DefaultTabController] that encloses the given
/// context, or null if none is found.
///
/// {@tool snippet} Typical usage is as follows:
///
/// ```dart
/// TabController? controller = DefaultTabController.maybeOf(context);
/// ```
/// {@end-tool}
///
/// Calling this method will create a dependency on the closest
/// [DefaultTabController] in the [context], if there is one.
///
/// See also:
///
/// * [DefaultTabController.of], which is similar to this method, but asserts
/// if no [DefaultTabController] ancestor is found.
static TabController? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<_TabControllerScope>()?.controller;
}
/// The closest instance of [DefaultTabController] that encloses the given
/// context.
///
/// If no instance is found, this method will assert in debug mode and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest
/// [DefaultTabController] in the [context].
///
/// {@tool snippet} Typical usage is as follows:
///
/// ```dart
/// TabController controller = DefaultTabController.of(context);
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [DefaultTabController.maybeOf], which is similar to this method, but
/// returns null if no [DefaultTabController] ancestor is found.
static TabController of(BuildContext context) {
final TabController? controller = maybeOf(context);
assert(() {
if (controller == null) {
throw FlutterError(
'DefaultTabController.of() was called with a context that does not '
'contain a DefaultTabController widget.\n'
'No DefaultTabController widget ancestor could be found starting from '
'the context that was passed to DefaultTabController.of(). This can '
'happen because you are using a widget that looks for a DefaultTabController '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return controller!;
}
@override
State<DefaultTabController> createState() => _DefaultTabControllerState();
}
class _DefaultTabControllerState extends State<DefaultTabController> with SingleTickerProviderStateMixin {
late TabController _controller;
@override
void initState() {
super.initState();
_controller = TabController(
vsync: this,
length: widget.length,
initialIndex: widget.initialIndex,
animationDuration: widget.animationDuration,
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return _TabControllerScope(
controller: _controller,
enabled: TickerMode.of(context),
child: widget.child,
);
}
@override
void didUpdateWidget(DefaultTabController oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.length != widget.length) {
// If the length is shortened while the last tab is selected, we should
// automatically update the index of the controller to be the new last tab.
int? newIndex;
int previousIndex = _controller.previousIndex;
if (_controller.index >= widget.length) {
newIndex = math.max(0, widget.length - 1);
previousIndex = _controller.index;
}
_controller = _controller._copyWith(
length: widget.length,
animationDuration: widget.animationDuration,
index: newIndex,
previousIndex: previousIndex,
);
}
if (oldWidget.animationDuration != widget.animationDuration) {
_controller = _controller._copyWith(
length: widget.length,
animationDuration: widget.animationDuration,
index: _controller.index,
previousIndex: _controller.previousIndex,
);
}
}
}
| flutter/packages/flutter/lib/src/material/tab_controller.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/tab_controller.dart",
"repo_id": "flutter",
"token_count": 5619
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'colors.dart';
import 'input_decorator.dart';
import 'material_state.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the visual properties of the widget displayed with [showTimePicker].
///
/// Descendant widgets obtain the current [TimePickerThemeData] object using
/// `TimePickerTheme.of(context)`. Instances of [TimePickerThemeData]
/// can be customized with [TimePickerThemeData.copyWith].
///
/// Typically a [TimePickerThemeData] is specified as part of the overall
/// [Theme] with [ThemeData.timePickerTheme].
///
/// All [TimePickerThemeData] properties are `null` by default. When null,
/// [showTimePicker] will provide its own defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
/// * [TimePickerTheme], which describes the actual configuration of a time
/// picker theme.
@immutable
class TimePickerThemeData with Diagnosticable {
/// Creates a theme that can be used for [TimePickerTheme] or
/// [ThemeData.timePickerTheme].
const TimePickerThemeData({
this.backgroundColor,
this.cancelButtonStyle,
this.confirmButtonStyle,
this.dayPeriodBorderSide,
Color? dayPeriodColor,
this.dayPeriodShape,
this.dayPeriodTextColor,
this.dayPeriodTextStyle,
this.dialBackgroundColor,
this.dialHandColor,
this.dialTextColor,
this.dialTextStyle,
this.elevation,
this.entryModeIconColor,
this.helpTextStyle,
this.hourMinuteColor,
this.hourMinuteShape,
this.hourMinuteTextColor,
this.hourMinuteTextStyle,
this.inputDecorationTheme,
this.padding,
this.shape,
this.timeSelectorSeparatorColor,
this.timeSelectorSeparatorTextStyle,
}) : _dayPeriodColor = dayPeriodColor;
/// The background color of a time picker.
///
/// If this is null, the time picker defaults to the overall theme's
/// [ColorScheme.surfaceContainerHigh].
final Color? backgroundColor;
/// The style of the cancel button of a [TimePickerDialog].
final ButtonStyle? cancelButtonStyle;
/// The style of the confirm (OK) button of a [TimePickerDialog].
final ButtonStyle? confirmButtonStyle;
/// The color and weight of the day period's outline.
///
/// If this is null, the time picker defaults to:
///
/// ```dart
/// BorderSide(
/// color: Color.alphaBlend(
/// Theme.of(context).colorScheme.onSurface.withOpacity(0.38),
/// Theme.of(context).colorScheme.surface,
/// ),
/// ),
/// ```
final BorderSide? dayPeriodBorderSide;
/// The background color of the AM/PM toggle.
///
/// If [dayPeriodColor] is a [MaterialStateColor], then the effective
/// background color can depend on the [MaterialState.selected] state, i.e.
/// if the segment is selected or not.
///
/// By default, if the segment is selected, the overall theme's
/// `ColorScheme.primary.withOpacity(0.12)` is used when the overall theme's
/// brightness is [Brightness.light] and
/// `ColorScheme.primary.withOpacity(0.24)` is used when the overall theme's
/// brightness is [Brightness.dark].
/// If the segment is not selected, [Colors.transparent] is used to allow the
/// [Dialog]'s color to be used.
Color? get dayPeriodColor {
if (_dayPeriodColor == null || _dayPeriodColor is MaterialStateColor) {
return _dayPeriodColor;
}
return MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return _dayPeriodColor;
}
// The unselected day period should match the overall picker dialog color.
// Making it transparent enables that without being redundant and allows
// the optional elevation overlay for dark mode to be visible.
return Colors.transparent;
});
}
final Color? _dayPeriodColor;
/// The shape of the day period that the time picker uses.
///
/// If this is null, the time picker defaults to:
///
/// ```dart
/// const RoundedRectangleBorder(
/// borderRadius: BorderRadius.all(Radius.circular(4.0)),
/// side: BorderSide(),
/// )
/// ```
final OutlinedBorder? dayPeriodShape;
/// The color of the day period text that represents AM/PM.
///
/// If [dayPeriodTextColor] is a [MaterialStateColor], then the effective
/// text color can depend on the [MaterialState.selected] state, i.e. if the
/// text is selected or not.
///
/// By default the overall theme's [ColorScheme.primary] color is used when
/// the text is selected and `ColorScheme.onSurface.withOpacity(0.60)` when
/// it's not selected.
final Color? dayPeriodTextColor;
/// Used to configure the [TextStyle]s for the day period control.
///
/// If this is null, the time picker defaults to the overall theme's
/// [TextTheme.titleMedium].
final TextStyle? dayPeriodTextStyle;
/// The background color of the time picker dial when the entry mode is
/// [TimePickerEntryMode.dial] or [TimePickerEntryMode.dialOnly].
///
/// If this is null and [ThemeData.useMaterial3] is true, the time picker
/// dial background color defaults [ColorScheme.surfaceContainerHighest] color.
///
/// If this is null and [ThemeData.useMaterial3] is false, the time picker
/// dial background color defaults to [ColorScheme.onSurface] color with
/// an opacity of 0.08 when the overall theme's brightness is [Brightness.light]
/// and [ColorScheme.onSurface] color with an opacity of 0.12 when the overall
/// theme's brightness is [Brightness.dark].
final Color? dialBackgroundColor;
/// The color of the time picker dial's hand when the entry mode is
/// [TimePickerEntryMode.dial] or [TimePickerEntryMode.dialOnly].
///
/// If this is null, the time picker defaults to the overall theme's
/// [ColorScheme.primary].
final Color? dialHandColor;
/// The color of the dial text that represents specific hours and minutes.
///
/// If [dialTextColor] is a [MaterialStateColor], then the effective
/// text color can depend on the [MaterialState.selected] state, i.e. if the
/// text is selected or not.
///
/// If this color is null then the dial's text colors are based on the
/// theme's [ThemeData.colorScheme].
final Color? dialTextColor;
/// The [TextStyle] for the numbers on the time selection dial.
///
/// If [dialTextStyle]'s [TextStyle.color] is a [MaterialStateColor], then the
/// effective text color can depend on the [MaterialState.selected] state,
/// i.e. if the text is selected or not.
///
/// If this style is null then the dial's text style is based on the theme's
/// [ThemeData.textTheme].
final TextStyle? dialTextStyle;
/// The Material elevation for the time picker dialog.
final double? elevation;
/// The color of the entry mode [IconButton].
///
/// If this is null, the time picker defaults to:
///
///
/// ```dart
/// Theme.of(context).colorScheme.onSurface.withOpacity(
/// Theme.of(context).colorScheme.brightness == Brightness.dark ? 1.0 : 0.6,
/// )
/// ```
final Color? entryModeIconColor;
/// Used to configure the [TextStyle]s for the helper text in the header.
///
/// If this is null, the time picker defaults to the overall theme's
/// [TextTheme.labelSmall].
final TextStyle? helpTextStyle;
/// The background color of the hour and minute header segments.
///
/// If [hourMinuteColor] is a [MaterialStateColor], then the effective
/// background color can depend on the [MaterialState.selected] state, i.e.
/// if the segment is selected or not.
///
/// By default, if the segment is selected, the overall theme's
/// `ColorScheme.primary.withOpacity(0.12)` is used when the overall theme's
/// brightness is [Brightness.light] and
/// `ColorScheme.primary.withOpacity(0.24)` is used when the overall theme's
/// brightness is [Brightness.dark].
/// If the segment is not selected, the overall theme's
/// `ColorScheme.onSurface.withOpacity(0.12)` is used.
final Color? hourMinuteColor;
/// The shape of the hour and minute controls that the time picker uses.
///
/// If this is null, the time picker defaults to
/// `RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0)))`.
final ShapeBorder? hourMinuteShape;
/// The color of the header text that represents hours and minutes.
///
/// If [hourMinuteTextColor] is a [MaterialStateColor], then the effective
/// text color can depend on the [MaterialState.selected] state, i.e. if the
/// text is selected or not.
///
/// By default the overall theme's [ColorScheme.primary] color is used when
/// the text is selected and [ColorScheme.onSurface] when it's not selected.
final Color? hourMinuteTextColor;
/// Used to configure the [TextStyle]s for the hour/minute controls.
///
/// If this is null and entry mode is [TimePickerEntryMode.dial], the time
/// picker defaults to the overall theme's [TextTheme.displayLarge] with
/// the value of [hourMinuteTextColor].
///
/// If this is null and entry mode is [TimePickerEntryMode.input], the time
/// picker defaults to the overall theme's [TextTheme.displayMedium] with
/// the value of [hourMinuteTextColor].
///
/// If this is null and [ThemeData.useMaterial3] is false, the time picker
/// defaults to the overall theme's [TextTheme.displayMedium].
final TextStyle? hourMinuteTextStyle;
/// The input decoration theme for the [TextField]s in the time picker.
///
/// If this is null, the time picker provides its own defaults.
final InputDecorationTheme? inputDecorationTheme;
/// The padding around the time picker dialog when the entry mode is
/// [TimePickerEntryMode.dial] or [TimePickerEntryMode.dialOnly].
final EdgeInsetsGeometry? padding;
/// The shape of the [Dialog] that the time picker is presented in.
///
/// If this is null, the time picker defaults to
/// `RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0)))`.
final ShapeBorder? shape;
/// The color of the time selector separator between the hour and minute controls.
///
/// if this is null, the time picker defaults to the overall theme's
/// [ColorScheme.onSurface].
///
/// If this is null and [ThemeData.useMaterial3] is false, then defaults to the value of
/// [hourMinuteTextColor].
final MaterialStateProperty<Color?>? timeSelectorSeparatorColor;
/// Used to configure the text style for the time selector separator between the hour
/// and minute controls.
///
/// If this is null, the time picker defaults to the overall theme's
/// [TextTheme.displayLarge].
///
/// If this is null and [ThemeData.useMaterial3] is false, then defaults to the value of
/// [hourMinuteTextStyle].
final MaterialStateProperty<TextStyle?>? timeSelectorSeparatorTextStyle;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
TimePickerThemeData copyWith({
Color? backgroundColor,
ButtonStyle? cancelButtonStyle,
ButtonStyle? confirmButtonStyle,
ButtonStyle? dayPeriodButtonStyle,
BorderSide? dayPeriodBorderSide,
Color? dayPeriodColor,
OutlinedBorder? dayPeriodShape,
Color? dayPeriodTextColor,
TextStyle? dayPeriodTextStyle,
Color? dialBackgroundColor,
Color? dialHandColor,
Color? dialTextColor,
TextStyle? dialTextStyle,
double? elevation,
Color? entryModeIconColor,
TextStyle? helpTextStyle,
Color? hourMinuteColor,
ShapeBorder? hourMinuteShape,
Color? hourMinuteTextColor,
TextStyle? hourMinuteTextStyle,
InputDecorationTheme? inputDecorationTheme,
EdgeInsetsGeometry? padding,
ShapeBorder? shape,
MaterialStateProperty<Color?>? timeSelectorSeparatorColor,
MaterialStateProperty<TextStyle?>? timeSelectorSeparatorTextStyle,
}) {
return TimePickerThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
cancelButtonStyle: cancelButtonStyle ?? this.cancelButtonStyle,
confirmButtonStyle: confirmButtonStyle ?? this.confirmButtonStyle,
dayPeriodBorderSide: dayPeriodBorderSide ?? this.dayPeriodBorderSide,
dayPeriodColor: dayPeriodColor ?? this.dayPeriodColor,
dayPeriodShape: dayPeriodShape ?? this.dayPeriodShape,
dayPeriodTextColor: dayPeriodTextColor ?? this.dayPeriodTextColor,
dayPeriodTextStyle: dayPeriodTextStyle ?? this.dayPeriodTextStyle,
dialBackgroundColor: dialBackgroundColor ?? this.dialBackgroundColor,
dialHandColor: dialHandColor ?? this.dialHandColor,
dialTextColor: dialTextColor ?? this.dialTextColor,
dialTextStyle: dialTextStyle ?? this.dialTextStyle,
elevation: elevation ?? this.elevation,
entryModeIconColor: entryModeIconColor ?? this.entryModeIconColor,
helpTextStyle: helpTextStyle ?? this.helpTextStyle,
hourMinuteColor: hourMinuteColor ?? this.hourMinuteColor,
hourMinuteShape: hourMinuteShape ?? this.hourMinuteShape,
hourMinuteTextColor: hourMinuteTextColor ?? this.hourMinuteTextColor,
hourMinuteTextStyle: hourMinuteTextStyle ?? this.hourMinuteTextStyle,
inputDecorationTheme: inputDecorationTheme ?? this.inputDecorationTheme,
padding: padding ?? this.padding,
shape: shape ?? this.shape,
timeSelectorSeparatorColor: timeSelectorSeparatorColor ?? this.timeSelectorSeparatorColor,
timeSelectorSeparatorTextStyle: timeSelectorSeparatorTextStyle ?? this.timeSelectorSeparatorTextStyle,
);
}
/// Linearly interpolate between two time picker themes.
///
/// {@macro dart.ui.shadow.lerp}
static TimePickerThemeData lerp(TimePickerThemeData? a, TimePickerThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
// Workaround since BorderSide's lerp does not allow for null arguments.
BorderSide? lerpedBorderSide;
if (a?.dayPeriodBorderSide == null && b?.dayPeriodBorderSide == null) {
lerpedBorderSide = null;
} else if (a?.dayPeriodBorderSide == null) {
lerpedBorderSide = b?.dayPeriodBorderSide;
} else if (b?.dayPeriodBorderSide == null) {
lerpedBorderSide = a?.dayPeriodBorderSide;
} else {
lerpedBorderSide = BorderSide.lerp(a!.dayPeriodBorderSide!, b!.dayPeriodBorderSide!, t);
}
return TimePickerThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
cancelButtonStyle: ButtonStyle.lerp(a?.cancelButtonStyle, b?.cancelButtonStyle, t),
confirmButtonStyle: ButtonStyle.lerp(a?.confirmButtonStyle, b?.confirmButtonStyle, t),
dayPeriodBorderSide: lerpedBorderSide,
dayPeriodColor: Color.lerp(a?.dayPeriodColor, b?.dayPeriodColor, t),
dayPeriodShape: ShapeBorder.lerp(a?.dayPeriodShape, b?.dayPeriodShape, t) as OutlinedBorder?,
dayPeriodTextColor: Color.lerp(a?.dayPeriodTextColor, b?.dayPeriodTextColor, t),
dayPeriodTextStyle: TextStyle.lerp(a?.dayPeriodTextStyle, b?.dayPeriodTextStyle, t),
dialBackgroundColor: Color.lerp(a?.dialBackgroundColor, b?.dialBackgroundColor, t),
dialHandColor: Color.lerp(a?.dialHandColor, b?.dialHandColor, t),
dialTextColor: Color.lerp(a?.dialTextColor, b?.dialTextColor, t),
dialTextStyle: TextStyle.lerp(a?.dialTextStyle, b?.dialTextStyle, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
entryModeIconColor: Color.lerp(a?.entryModeIconColor, b?.entryModeIconColor, t),
helpTextStyle: TextStyle.lerp(a?.helpTextStyle, b?.helpTextStyle, t),
hourMinuteColor: Color.lerp(a?.hourMinuteColor, b?.hourMinuteColor, t),
hourMinuteShape: ShapeBorder.lerp(a?.hourMinuteShape, b?.hourMinuteShape, t),
hourMinuteTextColor: Color.lerp(a?.hourMinuteTextColor, b?.hourMinuteTextColor, t),
hourMinuteTextStyle: TextStyle.lerp(a?.hourMinuteTextStyle, b?.hourMinuteTextStyle, t),
inputDecorationTheme: t < 0.5 ? a?.inputDecorationTheme : b?.inputDecorationTheme,
padding: EdgeInsetsGeometry.lerp(a?.padding, b?.padding, t),
shape: ShapeBorder.lerp(a?.shape, b?.shape, t),
timeSelectorSeparatorColor: MaterialStateProperty.lerp<Color?>(a?.timeSelectorSeparatorColor, b?.timeSelectorSeparatorColor, t, Color.lerp),
timeSelectorSeparatorTextStyle: MaterialStateProperty.lerp<TextStyle?>(a?.timeSelectorSeparatorTextStyle, b?.timeSelectorSeparatorTextStyle, t, TextStyle.lerp),
);
}
@override
int get hashCode => Object.hashAll(<Object?>[
backgroundColor,
cancelButtonStyle,
confirmButtonStyle,
dayPeriodBorderSide,
dayPeriodColor,
dayPeriodShape,
dayPeriodTextColor,
dayPeriodTextStyle,
dialBackgroundColor,
dialHandColor,
dialTextColor,
dialTextStyle,
elevation,
entryModeIconColor,
helpTextStyle,
hourMinuteColor,
hourMinuteShape,
hourMinuteTextColor,
hourMinuteTextStyle,
inputDecorationTheme,
padding,
shape,
timeSelectorSeparatorColor,
timeSelectorSeparatorTextStyle,
]);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is TimePickerThemeData
&& other.backgroundColor == backgroundColor
&& other.cancelButtonStyle == cancelButtonStyle
&& other.confirmButtonStyle == confirmButtonStyle
&& other.dayPeriodBorderSide == dayPeriodBorderSide
&& other.dayPeriodColor == dayPeriodColor
&& other.dayPeriodShape == dayPeriodShape
&& other.dayPeriodTextColor == dayPeriodTextColor
&& other.dayPeriodTextStyle == dayPeriodTextStyle
&& other.dialBackgroundColor == dialBackgroundColor
&& other.dialHandColor == dialHandColor
&& other.dialTextColor == dialTextColor
&& other.dialTextStyle == dialTextStyle
&& other.elevation == elevation
&& other.entryModeIconColor == entryModeIconColor
&& other.helpTextStyle == helpTextStyle
&& other.hourMinuteColor == hourMinuteColor
&& other.hourMinuteShape == hourMinuteShape
&& other.hourMinuteTextColor == hourMinuteTextColor
&& other.hourMinuteTextStyle == hourMinuteTextStyle
&& other.inputDecorationTheme == inputDecorationTheme
&& other.padding == padding
&& other.shape == shape
&& other.timeSelectorSeparatorColor == timeSelectorSeparatorColor
&& other.timeSelectorSeparatorTextStyle == timeSelectorSeparatorTextStyle;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<ButtonStyle>('cancelButtonStyle', cancelButtonStyle, defaultValue: null));
properties.add(DiagnosticsProperty<ButtonStyle>('confirmButtonStyle', confirmButtonStyle, defaultValue: null));
properties.add(DiagnosticsProperty<BorderSide>('dayPeriodBorderSide', dayPeriodBorderSide, defaultValue: null));
properties.add(ColorProperty('dayPeriodColor', dayPeriodColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('dayPeriodShape', dayPeriodShape, defaultValue: null));
properties.add(ColorProperty('dayPeriodTextColor', dayPeriodTextColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('dayPeriodTextStyle', dayPeriodTextStyle, defaultValue: null));
properties.add(ColorProperty('dialBackgroundColor', dialBackgroundColor, defaultValue: null));
properties.add(ColorProperty('dialHandColor', dialHandColor, defaultValue: null));
properties.add(ColorProperty('dialTextColor', dialTextColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle?>('dialTextStyle', dialTextStyle, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(ColorProperty('entryModeIconColor', entryModeIconColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('helpTextStyle', helpTextStyle, defaultValue: null));
properties.add(ColorProperty('hourMinuteColor', hourMinuteColor, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('hourMinuteShape', hourMinuteShape, defaultValue: null));
properties.add(ColorProperty('hourMinuteTextColor', hourMinuteTextColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('hourMinuteTextStyle', hourMinuteTextStyle, defaultValue: null));
properties.add(DiagnosticsProperty<InputDecorationTheme>('inputDecorationTheme', inputDecorationTheme, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('timeSelectorSeparatorColor', timeSelectorSeparatorColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<TextStyle?>>('timeSelectorSeparatorTextStyle', timeSelectorSeparatorTextStyle, defaultValue: null));
}
}
/// An inherited widget that defines the configuration for time pickers
/// displayed using [showTimePicker] in this widget's subtree.
///
/// Values specified here are used for time picker properties that are not
/// given an explicit non-null value.
class TimePickerTheme extends InheritedTheme {
/// Creates a time picker theme that controls the configurations for
/// time pickers displayed in its widget subtree.
const TimePickerTheme({
super.key,
required this.data,
required super.child,
});
/// The properties for descendant time picker widgets.
final TimePickerThemeData data;
/// The [data] value of the closest [TimePickerTheme] ancestor.
///
/// If there is no ancestor, it returns [ThemeData.timePickerTheme].
/// Applications can assume that the returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// TimePickerThemeData theme = TimePickerTheme.of(context);
/// ```
static TimePickerThemeData of(BuildContext context) {
final TimePickerTheme? timePickerTheme = context.dependOnInheritedWidgetOfExactType<TimePickerTheme>();
return timePickerTheme?.data ?? Theme.of(context).timePickerTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return TimePickerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(TimePickerTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/time_picker_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/time_picker_theme.dart",
"repo_id": "flutter",
"token_count": 7306
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'edge_insets.dart';
/// The style of line to draw for a [BorderSide] in a [Border].
enum BorderStyle {
/// Skip the border.
none,
/// Draw the border as a solid line.
solid,
// if you add more, think about how they will lerp
}
/// A side of a border of a box.
///
/// A [Border] consists of four [BorderSide] objects: [Border.top],
/// [Border.left], [Border.right], and [Border.bottom].
///
/// Setting [BorderSide.width] to 0.0 will result in hairline rendering; see
/// [BorderSide.width] for a more involved explanation.
///
/// {@tool snippet}
/// This sample shows how [BorderSide] objects can be used in a [Container], via
/// a [BoxDecoration] and a [Border], to decorate some [Text]. In this example,
/// the text has a thick bar above it that is light blue, and a thick bar below
/// it that is a darker shade of blue.
///
/// ```dart
/// Container(
/// padding: const EdgeInsets.all(8.0),
/// decoration: BoxDecoration(
/// border: Border(
/// top: BorderSide(width: 16.0, color: Colors.lightBlue.shade50),
/// bottom: BorderSide(width: 16.0, color: Colors.lightBlue.shade900),
/// ),
/// ),
/// child: const Text('Flutter in the sky', textAlign: TextAlign.center),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Border], which uses [BorderSide] objects to represent its sides.
/// * [BoxDecoration], which optionally takes a [Border] object.
/// * [TableBorder], which is similar to [Border] but has two more sides
/// ([TableBorder.horizontalInside] and [TableBorder.verticalInside]), both
/// of which are also [BorderSide] objects.
@immutable
class BorderSide with Diagnosticable {
/// Creates the side of a border.
///
/// By default, the border is 1.0 logical pixels wide and solid black.
const BorderSide({
this.color = const Color(0xFF000000),
this.width = 1.0,
this.style = BorderStyle.solid,
this.strokeAlign = strokeAlignInside,
}) : assert(width >= 0.0);
/// Creates a [BorderSide] that represents the addition of the two given
/// [BorderSide]s.
///
/// It is only valid to call this if [canMerge] returns true for the two
/// sides.
///
/// If one of the sides is zero-width with [BorderStyle.none], then the other
/// side is return as-is. If both of the sides are zero-width with
/// [BorderStyle.none], then [BorderSide.none] is returned.
static BorderSide merge(BorderSide a, BorderSide b) {
assert(canMerge(a, b));
final bool aIsNone = a.style == BorderStyle.none && a.width == 0.0;
final bool bIsNone = b.style == BorderStyle.none && b.width == 0.0;
if (aIsNone && bIsNone) {
return BorderSide.none;
}
if (aIsNone) {
return b;
}
if (bIsNone) {
return a;
}
assert(a.color == b.color);
assert(a.style == b.style);
return BorderSide(
color: a.color, // == b.color
width: a.width + b.width,
strokeAlign: math.max(a.strokeAlign, b.strokeAlign),
style: a.style, // == b.style
);
}
/// The color of this side of the border.
final Color color;
/// The width of this side of the border, in logical pixels.
///
/// Setting width to 0.0 will result in a hairline border. This means that
/// the border will have the width of one physical pixel. Hairline
/// rendering takes shortcuts when the path overlaps a pixel more than once.
/// This means that it will render faster than otherwise, but it might
/// double-hit pixels, giving it a slightly darker/lighter result.
///
/// To omit the border entirely, set the [style] to [BorderStyle.none].
final double width;
/// The style of this side of the border.
///
/// To omit a side, set [style] to [BorderStyle.none]. This skips
/// painting the border, but the border still has a [width].
final BorderStyle style;
/// A hairline black border that is not rendered.
static const BorderSide none = BorderSide(width: 0.0, style: BorderStyle.none);
/// The relative position of the stroke on a [BorderSide] in an
/// [OutlinedBorder] or [Border].
///
/// Values typically range from -1.0 ([strokeAlignInside], inside border,
/// default) to 1.0 ([strokeAlignOutside], outside border), without any
/// bound constraints (e.g., a value of -2.0 is not typical, but allowed).
/// A value of 0 ([strokeAlignCenter]) will center the border on the edge
/// of the widget.
///
/// When set to [strokeAlignInside], the stroke is drawn completely inside
/// the widget. For [strokeAlignCenter] and [strokeAlignOutside], a property
/// such as [Container.clipBehavior] can be used in an outside widget to clip
/// it. If [Container.decoration] has a border, the container may incorporate
/// [width] as additional padding:
/// - [strokeAlignInside] provides padding with full [width].
/// - [strokeAlignCenter] provides padding with half [width].
/// - [strokeAlignOutside] provides zero padding, as stroke is drawn entirely outside.
///
/// This property is not honored by [toPaint] (because the [Paint] object
/// cannot represent it); it is intended that classes that use [BorderSide]
/// objects implement this property when painting borders by suitably
/// inflating or deflating their regions.
///
/// {@tool dartpad}
/// This example shows an animation of how [strokeAlign] affects the drawing
/// when applied to borders of various shapes.
///
/// ** See code in examples/api/lib/painting/borders/border_side.stroke_align.0.dart **
/// {@end-tool}
final double strokeAlign;
/// The border is drawn fully inside of the border path.
///
/// This is a constant for use with [strokeAlign].
///
/// This is the default value for [strokeAlign].
static const double strokeAlignInside = -1.0;
/// The border is drawn on the center of the border path, with half of the
/// [BorderSide.width] on the inside, and the other half on the outside of
/// the path.
///
/// This is a constant for use with [strokeAlign].
static const double strokeAlignCenter = 0.0;
/// The border is drawn on the outside of the border path.
///
/// This is a constant for use with [strokeAlign].
static const double strokeAlignOutside = 1.0;
/// Creates a copy of this border but with the given fields replaced with the new values.
BorderSide copyWith({
Color? color,
double? width,
BorderStyle? style,
double? strokeAlign,
}) {
return BorderSide(
color: color ?? this.color,
width: width ?? this.width,
style: style ?? this.style,
strokeAlign: strokeAlign ?? this.strokeAlign,
);
}
/// Creates a copy of this border side description but with the width scaled
/// by the factor `t`.
///
/// The `t` argument represents the multiplicand, or the position on the
/// timeline for an interpolation from nothing to `this`, with 0.0 meaning
/// that the object returned should be the nil variant of this object, 1.0
/// meaning that no change should be applied, returning `this` (or something
/// equivalent to `this`), and other values meaning that the object should be
/// multiplied by `t`. Negative values are treated like zero.
///
/// Since a zero width is normally painted as a hairline width rather than no
/// border at all, the zero factor is special-cased to instead change the
/// style to [BorderStyle.none].
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
BorderSide scale(double t) {
return BorderSide(
color: color,
width: math.max(0.0, width * t),
style: t <= 0.0 ? BorderStyle.none : style,
);
}
/// Create a [Paint] object that, if used to stroke a line, will draw the line
/// in this border's style.
///
/// The [strokeAlign] property is not reflected in the [Paint]; consumers must
/// implement that directly by inflating or deflating their region appropriately.
///
/// Not all borders use this method to paint their border sides. For example,
/// non-uniform rectangular [Border]s have beveled edges and so paint their
/// border sides as filled shapes rather than using a stroke.
Paint toPaint() {
switch (style) {
case BorderStyle.solid:
return Paint()
..color = color
..strokeWidth = width
..style = PaintingStyle.stroke;
case BorderStyle.none:
return Paint()
..color = const Color(0x00000000)
..strokeWidth = 0.0
..style = PaintingStyle.stroke;
}
}
/// Whether the two given [BorderSide]s can be merged using
/// [BorderSide.merge].
///
/// Two sides can be merged if one or both are zero-width with
/// [BorderStyle.none], or if they both have the same color and style.
static bool canMerge(BorderSide a, BorderSide b) {
if ((a.style == BorderStyle.none && a.width == 0.0) ||
(b.style == BorderStyle.none && b.width == 0.0)) {
return true;
}
return a.style == b.style
&& a.color == b.color;
}
/// Linearly interpolate between two border sides.
///
/// {@macro dart.ui.shadow.lerp}
static BorderSide lerp(BorderSide a, BorderSide b, double t) {
if (identical(a, b)) {
return a;
}
if (t == 0.0) {
return a;
}
if (t == 1.0) {
return b;
}
final double width = ui.lerpDouble(a.width, b.width, t)!;
if (width < 0.0) {
return BorderSide.none;
}
if (a.style == b.style && a.strokeAlign == b.strokeAlign) {
return BorderSide(
color: Color.lerp(a.color, b.color, t)!,
width: width,
style: a.style, // == b.style
strokeAlign: a.strokeAlign, // == b.strokeAlign
);
}
final Color colorA = switch (a.style) {
BorderStyle.solid => a.color,
BorderStyle.none => a.color.withAlpha(0x00),
};
final Color colorB = switch (b.style) {
BorderStyle.solid => b.color,
BorderStyle.none => b.color.withAlpha(0x00),
};
if (a.strokeAlign != b.strokeAlign) {
return BorderSide(
color: Color.lerp(colorA, colorB, t)!,
width: width,
strokeAlign: ui.lerpDouble(a.strokeAlign, b.strokeAlign, t)!,
);
}
return BorderSide(
color: Color.lerp(colorA, colorB, t)!,
width: width,
strokeAlign: a.strokeAlign, // == b.strokeAlign
);
}
/// Get the amount of the stroke width that lies inside of the [BorderSide].
///
/// For example, this will return the [width] for a [strokeAlign] of -1, half
/// the [width] for a [strokeAlign] of 0, and 0 for a [strokeAlign] of 1.
double get strokeInset => width * (1 - (1 + strokeAlign) / 2);
/// Get the amount of the stroke width that lies outside of the [BorderSide].
///
/// For example, this will return 0 for a [strokeAlign] of -1, half the
/// [width] for a [strokeAlign] of 0, and the [width] for a [strokeAlign]
/// of 1.
double get strokeOutset => width * (1 + strokeAlign) / 2;
/// The offset of the stroke, taking into account the stroke alignment.
///
/// For example, this will return the negative [width] of the stroke
/// for a [strokeAlign] of -1, 0 for a [strokeAlign] of 0, and the
/// [width] for a [strokeAlign] of -1.
double get strokeOffset => width * strokeAlign;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is BorderSide
&& other.color == color
&& other.width == width
&& other.style == style
&& other.strokeAlign == strokeAlign;
}
@override
int get hashCode => Object.hash(color, width, style, strokeAlign);
@override
String toStringShort() => 'BorderSide';
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Color>('color', color, defaultValue: const Color(0xFF000000)));
properties.add(DoubleProperty('width', width, defaultValue: 1.0));
properties.add(DoubleProperty('strokeAlign', strokeAlign, defaultValue: strokeAlignInside));
properties.add(EnumProperty<BorderStyle>('style', style, defaultValue: BorderStyle.solid));
}
}
/// Base class for shape outlines.
///
/// This class handles how to add multiple borders together. Subclasses define
/// various shapes, like circles ([CircleBorder]), rounded rectangles
/// ([RoundedRectangleBorder]), continuous rectangles
/// ([ContinuousRectangleBorder]), or beveled rectangles
/// ([BeveledRectangleBorder]).
///
/// See also:
///
/// * [ShapeDecoration], which can be used with [DecoratedBox] to show a shape.
/// * [Material] (and many other widgets in the Material library), which takes
/// a [ShapeBorder] to define its shape.
/// * [NotchedShape], which describes a shape with a hole in it.
@immutable
abstract class ShapeBorder {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const ShapeBorder();
/// The widths of the sides of this border represented as an [EdgeInsets].
///
/// Specifically, this is the amount by which a rectangle should be inset so
/// as to avoid painting over any important part of the border. It is the
/// amount by which additional borders will be inset before they are drawn.
///
/// This can be used, for example, with a [Padding] widget to inset a box by
/// the size of these borders.
///
/// Shapes that have a fixed ratio regardless of the area on which they are
/// painted, or that change their rendering based on the size they are given
/// when painting (for instance [CircleBorder]), will not return valid
/// [dimensions] information because they cannot know their eventual size when
/// computing their [dimensions].
EdgeInsetsGeometry get dimensions;
/// Attempts to create a new object that represents the amalgamation of `this`
/// border and the `other` border.
///
/// If the type of the other border isn't known, or the given instance cannot
/// be reasonably added to this instance, then this should return null.
///
/// This method is used by the [operator +] implementation.
///
/// The `reversed` argument is true if this object was the right operand of
/// the `+` operator, and false if it was the left operand.
@protected
ShapeBorder? add(ShapeBorder other, { bool reversed = false }) => null;
/// Creates a new border consisting of the two borders on either side of the
/// operator.
///
/// If the borders belong to classes that know how to add themselves, then
/// this results in a new border that represents the intelligent addition of
/// those two borders (see [add]). Otherwise, an object is returned that
/// merely paints the two borders sequentially, with the left hand operand on
/// the inside and the right hand operand on the outside.
ShapeBorder operator +(ShapeBorder other) {
return add(other) ?? other.add(this, reversed: true) ?? _CompoundBorder(<ShapeBorder>[other, this]);
}
/// Creates a copy of this border, scaled by the factor `t`.
///
/// Typically this means scaling the width of the border's side, but it can
/// also include scaling other artifacts of the border, e.g. the border radius
/// of a [RoundedRectangleBorder].
///
/// The `t` argument represents the multiplicand, or the position on the
/// timeline for an interpolation from nothing to `this`, with 0.0 meaning
/// that the object returned should be the nil variant of this object, 1.0
/// meaning that no change should be applied, returning `this` (or something
/// equivalent to `this`), and other values meaning that the object should be
/// multiplied by `t`. Negative values are allowed but may be meaningless
/// (they correspond to extrapolating the interpolation from this object to
/// nothing, and going beyond nothing)
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// See also:
///
/// * [BorderSide.scale], which most [ShapeBorder] subclasses defer to for
/// the actual computation.
ShapeBorder scale(double t);
/// Linearly interpolates from another [ShapeBorder] (possibly of another
/// class) to `this`.
///
/// When implementing this method in subclasses, return null if this class
/// cannot interpolate from `a`. In that case, [lerp] will try `a`'s [lerpTo]
/// method instead. If `a` is null, this must not return null.
///
/// The base class implementation handles the case of `a` being null by
/// deferring to [scale].
///
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `this` (or something equivalent to `this`), and values in
/// between meaning that the interpolation is at the relevant point on the
/// timeline between `a` and `this`. The interpolation can be extrapolated
/// beyond 0.0 and 1.0, so negative values and values greater than 1.0 are
/// valid (and can easily be generated by curves such as
/// [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// Instead of calling this directly, use [ShapeBorder.lerp].
@protected
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a == null) {
return scale(t);
}
return null;
}
/// Linearly interpolates from `this` to another [ShapeBorder] (possibly of
/// another class).
///
/// This is called if `b`'s [lerpTo] did not know how to handle this class.
///
/// When implementing this method in subclasses, return null if this class
/// cannot interpolate from `b`. In that case, [lerp] will apply a default
/// behavior instead. If `b` is null, this must not return null.
///
/// The base class implementation handles the case of `b` being null by
/// deferring to [scale].
///
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `this` (or something
/// equivalent to `this`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `this` and `b`. The interpolation can be extrapolated beyond 0.0
/// and 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// Instead of calling this directly, use [ShapeBorder.lerp].
@protected
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b == null) {
return scale(1.0 - t);
}
return null;
}
/// Linearly interpolates between two [ShapeBorder]s.
///
/// This defers to `b`'s [lerpTo] function if `b` is not null. If `b` is
/// null or if its [lerpTo] returns null, it uses `a`'s [lerpFrom]
/// function instead. If both return null, it returns `a` before `t=0.5`
/// and `b` after `t=0.5`.
///
/// {@macro dart.ui.shadow.lerp}
static ShapeBorder? lerp(ShapeBorder? a, ShapeBorder? b, double t) {
if (identical(a, b)) {
return a;
}
final ShapeBorder? result = b?.lerpFrom(a, t) ?? a?.lerpTo(b, t);
return result ?? (t < 0.5 ? a : b);
}
/// Create a [Path] that describes the outer edge of the border.
///
/// This path must not cross the path given by [getInnerPath] for the same
/// [Rect].
///
/// To obtain a [Path] that describes the area of the border itself, set the
/// [Path.fillType] of the returned object to [PathFillType.evenOdd], and add
/// to this object the path returned from [getInnerPath] (using
/// [Path.addPath]).
///
/// The `textDirection` argument must be provided non-null if the border
/// has a text direction dependency (for example if it is expressed in terms
/// of "start" and "end" instead of "left" and "right"). It may be null if
/// the border will not need the text direction to paint itself.
///
/// See also:
///
/// * [getInnerPath], which creates the path for the inner edge.
/// * [Path.contains], which can tell if an [Offset] is within a [Path].
Path getOuterPath(Rect rect, { TextDirection? textDirection });
/// Create a [Path] that describes the inner edge of the border.
///
/// This path must not cross the path given by [getOuterPath] for the same
/// [Rect].
///
/// To obtain a [Path] that describes the area of the border itself, set the
/// [Path.fillType] of the returned object to [PathFillType.evenOdd], and add
/// to this object the path returned from [getOuterPath] (using
/// [Path.addPath]).
///
/// The `textDirection` argument must be provided and non-null if the border
/// has a text direction dependency (for example if it is expressed in terms
/// of "start" and "end" instead of "left" and "right"). It may be null if
/// the border will not need the text direction to paint itself.
///
/// See also:
///
/// * [getOuterPath], which creates the path for the outer edge.
/// * [Path.contains], which can tell if an [Offset] is within a [Path].
Path getInnerPath(Rect rect, { TextDirection? textDirection });
/// Paint a canvas with the appropriate shape.
///
/// On [ShapeBorder] subclasses whose [preferPaintInterior] method returns
/// true, this should be faster than using [Canvas.drawPath] with the path
/// provided by [getOuterPath]. (If [preferPaintInterior] returns false,
/// then this method asserts in debug mode and does nothing in release mode.)
///
/// Subclasses are expected to implement this method when the [Canvas] API
/// has a dedicated method to draw the relevant shape. For example,
/// [CircleBorder] uses this to call [Canvas.drawCircle], and
/// [RoundedRectangleBorder] uses this to call [Canvas.drawRRect].
///
/// Subclasses that implement this must ensure that calling [paintInterior]
/// is semantically equivalent to (i.e. renders the same pixels as) calling
/// [Canvas.drawPath] with the same [Paint] and the [Path] returned from
/// [getOuterPath], and must also override [preferPaintInterior] to
/// return true.
///
/// For example, a shape that draws a rectangle might implement
/// [getOuterPath], [paintInterior], and [preferPaintInterior] as follows:
///
/// ```dart
/// class RectangleBorder extends OutlinedBorder {
/// // ...
///
/// @override
/// Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
/// return Path()
/// ..addRect(rect);
/// }
///
/// @override
/// void paintInterior(Canvas canvas, Rect rect, Paint paint, {TextDirection? textDirection}) {
/// canvas.drawRect(rect, paint);
/// }
///
/// @override
/// bool get preferPaintInterior => true;
///
/// // ...
/// }
/// ```
///
/// When a shape can only be drawn using path, [preferPaintInterior] must
/// return false. In that case, classes such as [ShapeDecoration] will cache
/// the path from [getOuterPath] and call [Canvas.drawPath] directly.
void paintInterior(Canvas canvas, Rect rect, Paint paint, {TextDirection? textDirection}) {
assert(!preferPaintInterior, '$runtimeType.preferPaintInterior returns true but $runtimeType.paintInterior is not implemented.');
assert(false, '$runtimeType.preferPaintInterior returns false, so it is an error to call its paintInterior method.');
}
/// Reports whether [paintInterior] is implemented.
///
/// Classes such as [ShapeDecoration] prefer to use [paintInterior] if this
/// getter returns true. This is intended to enable faster painting; instead
/// of computing a shape using [getOuterPath] and then drawing it using
/// [Canvas.drawPath], the path can be drawn directly to the [Canvas] using
/// dedicated methods such as [Canvas.drawRect] or [Canvas.drawCircle].
///
/// By default, this getter returns false.
///
/// Subclasses that implement [paintInterior] should override this to return
/// true. Subclasses should only override [paintInterior] if doing so enables
/// faster rendering than is possible with [Canvas.drawPath] (so, in
/// particular, subclasses should not call [Canvas.drawPath] in
/// [paintInterior]).
///
/// See also:
///
/// * [paintInterior], whose API documentation has an example implementation.
bool get preferPaintInterior => false;
/// Paints the border within the given [Rect] on the given [Canvas].
///
/// The `textDirection` argument must be provided and non-null if the border
/// has a text direction dependency (for example if it is expressed in terms
/// of "start" and "end" instead of "left" and "right"). It may be null if
/// the border will not need the text direction to paint itself.
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection });
@override
String toString() {
return '${objectRuntimeType(this, 'ShapeBorder')}()';
}
}
/// A ShapeBorder that draws an outline with the width and color specified
/// by [side].
@immutable
abstract class OutlinedBorder extends ShapeBorder {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const OutlinedBorder({ this.side = BorderSide.none });
@override
EdgeInsetsGeometry get dimensions => EdgeInsets.all(math.max(side.strokeInset, 0));
/// The border outline's color and weight.
///
/// If [side] is [BorderSide.none], which is the default, an outline is not drawn.
/// Otherwise the outline is centered over the shape's boundary.
final BorderSide side;
/// Returns a copy of this OutlinedBorder that draws its outline with the
/// specified [side], if [side] is non-null.
OutlinedBorder copyWith({ BorderSide? side });
@override
ShapeBorder scale(double t);
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a == null) {
return scale(t);
}
return null;
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b == null) {
return scale(1.0 - t);
}
return null;
}
/// Linearly interpolates between two [OutlinedBorder]s.
///
/// This defers to `b`'s [lerpTo] function if `b` is not null. If `b` is
/// null or if its [lerpTo] returns null, it uses `a`'s [lerpFrom]
/// function instead. If both return null, it returns `a` before `t=0.5`
/// and `b` after `t=0.5`.
///
/// {@macro dart.ui.shadow.lerp}
static OutlinedBorder? lerp(OutlinedBorder? a, OutlinedBorder? b, double t) {
if (identical(a, b)) {
return a;
}
final ShapeBorder? result = b?.lerpFrom(a, t) ?? a?.lerpTo(b, t);
return result as OutlinedBorder? ?? (t < 0.5 ? a : b);
}
}
/// Represents the addition of two otherwise-incompatible borders.
///
/// The borders are listed from the outside to the inside.
class _CompoundBorder extends ShapeBorder {
_CompoundBorder(this.borders)
: assert(borders.length >= 2),
assert(!borders.any((ShapeBorder border) => border is _CompoundBorder));
final List<ShapeBorder> borders;
@override
EdgeInsetsGeometry get dimensions {
return borders.fold<EdgeInsetsGeometry>(
EdgeInsets.zero,
(EdgeInsetsGeometry previousValue, ShapeBorder border) {
return previousValue.add(border.dimensions);
},
);
}
@override
ShapeBorder add(ShapeBorder other, { bool reversed = false }) {
// This wraps the list of borders with "other", or, if "reversed" is true,
// wraps "other" with the list of borders.
// If "reversed" is false, "other" should end up being at the start of the
// list, otherwise, if "reversed" is true, it should end up at the end.
// First, see if we can merge the new adjacent borders.
if (other is! _CompoundBorder) {
// Here, "ours" is the border at the side where we're adding the new
// border, and "merged" is the result of attempting to merge it with the
// new border. If it's null, it couldn't be merged.
final ShapeBorder ours = reversed ? borders.last : borders.first;
final ShapeBorder? merged = ours.add(other, reversed: reversed)
?? other.add(ours, reversed: !reversed);
if (merged != null) {
final List<ShapeBorder> result = <ShapeBorder>[...borders];
result[reversed ? result.length - 1 : 0] = merged;
return _CompoundBorder(result);
}
}
// We can't, so fall back to just adding the new border to the list.
final List<ShapeBorder> mergedBorders = <ShapeBorder>[
if (reversed) ...borders,
if (other is _CompoundBorder) ...other.borders
else other,
if (!reversed) ...borders,
];
return _CompoundBorder(mergedBorders);
}
@override
ShapeBorder scale(double t) {
return _CompoundBorder(
borders.map<ShapeBorder>((ShapeBorder border) => border.scale(t)).toList(),
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
return _CompoundBorder.lerp(a, this, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
return _CompoundBorder.lerp(this, b, t);
}
static _CompoundBorder lerp(ShapeBorder? a, ShapeBorder? b, double t) {
assert(a is _CompoundBorder || b is _CompoundBorder); // Not really necessary, but all call sites currently intend this.
final List<ShapeBorder?> aList = a is _CompoundBorder ? a.borders : <ShapeBorder?>[a];
final List<ShapeBorder?> bList = b is _CompoundBorder ? b.borders : <ShapeBorder?>[b];
final List<ShapeBorder> results = <ShapeBorder>[];
final int length = math.max(aList.length, bList.length);
for (int index = 0; index < length; index += 1) {
final ShapeBorder? localA = index < aList.length ? aList[index] : null;
final ShapeBorder? localB = index < bList.length ? bList[index] : null;
if (localA != null && localB != null) {
final ShapeBorder? localResult = localA.lerpTo(localB, t) ?? localB.lerpFrom(localA, t);
if (localResult != null) {
results.add(localResult);
continue;
}
}
// If we're changing from one shape to another, make sure the shape that is coming in
// is inserted before the shape that is going away, so that the outer path changes to
// the new border earlier rather than later. (This affects, among other things, where
// the ShapeDecoration class puts its background.)
if (localB != null) {
results.add(localB.scale(t));
}
if (localA != null) {
results.add(localA.scale(1.0 - t));
}
}
return _CompoundBorder(results);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
for (int index = 0; index < borders.length - 1; index += 1) {
rect = borders[index].dimensions.resolve(textDirection).deflateRect(rect);
}
return borders.last.getInnerPath(rect, textDirection: textDirection);
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return borders.first.getOuterPath(rect, textDirection: textDirection);
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
borders.first.paintInterior(canvas, rect, paint, textDirection: textDirection);
}
@override
bool get preferPaintInterior => borders.every((ShapeBorder border) => border.preferPaintInterior);
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
for (final ShapeBorder border in borders) {
border.paint(canvas, rect, textDirection: textDirection);
rect = border.dimensions.resolve(textDirection).deflateRect(rect);
}
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is _CompoundBorder
&& listEquals<ShapeBorder>(other.borders, borders);
}
@override
int get hashCode => Object.hashAll(borders);
@override
String toString() {
// We list them in reverse order because when adding two borders they end up
// in the list in the opposite order of what the source looks like: a + b =>
// [b, a]. We do this to make the painting code more optimal, and most of
// the rest of the code doesn't care, except toString() (for debugging).
return borders.reversed.map<String>((ShapeBorder border) => border.toString()).join(' + ');
}
}
/// Paints a border around the given rectangle on the canvas.
///
/// The four sides can be independently specified. They are painted in the order
/// top, right, bottom, left. This is only notable if the widths of the borders
/// and the size of the given rectangle are such that the border sides will
/// overlap each other. No effort is made to optimize the rendering of uniform
/// borders (where all the borders have the same configuration); to render a
/// uniform border, consider using [Canvas.drawRect] directly.
///
/// See also:
///
/// * [paintImage], which paints an image in a rectangle on a canvas.
/// * [Border], which uses this function to paint its border when the border is
/// not uniform.
/// * [BoxDecoration], which describes its border using the [Border] class.
void paintBorder(
Canvas canvas,
Rect rect, {
BorderSide top = BorderSide.none,
BorderSide right = BorderSide.none,
BorderSide bottom = BorderSide.none,
BorderSide left = BorderSide.none,
}) {
// We draw the borders as filled shapes, unless the borders are hairline
// borders, in which case we use PaintingStyle.stroke, with the stroke width
// specified here.
final Paint paint = Paint()
..strokeWidth = 0.0;
final Path path = Path();
switch (top.style) {
case BorderStyle.solid:
paint.color = top.color;
path.reset();
path.moveTo(rect.left, rect.top);
path.lineTo(rect.right, rect.top);
if (top.width == 0.0) {
paint.style = PaintingStyle.stroke;
} else {
paint.style = PaintingStyle.fill;
path.lineTo(rect.right - right.width, rect.top + top.width);
path.lineTo(rect.left + left.width, rect.top + top.width);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
switch (right.style) {
case BorderStyle.solid:
paint.color = right.color;
path.reset();
path.moveTo(rect.right, rect.top);
path.lineTo(rect.right, rect.bottom);
if (right.width == 0.0) {
paint.style = PaintingStyle.stroke;
} else {
paint.style = PaintingStyle.fill;
path.lineTo(rect.right - right.width, rect.bottom - bottom.width);
path.lineTo(rect.right - right.width, rect.top + top.width);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
switch (bottom.style) {
case BorderStyle.solid:
paint.color = bottom.color;
path.reset();
path.moveTo(rect.right, rect.bottom);
path.lineTo(rect.left, rect.bottom);
if (bottom.width == 0.0) {
paint.style = PaintingStyle.stroke;
} else {
paint.style = PaintingStyle.fill;
path.lineTo(rect.left + left.width, rect.bottom - bottom.width);
path.lineTo(rect.right - right.width, rect.bottom - bottom.width);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
switch (left.style) {
case BorderStyle.solid:
paint.color = left.color;
path.reset();
path.moveTo(rect.left, rect.bottom);
path.lineTo(rect.left, rect.top);
if (left.width == 0.0) {
paint.style = PaintingStyle.stroke;
} else {
paint.style = PaintingStyle.fill;
path.lineTo(rect.left + left.width, rect.top + top.width);
path.lineTo(rect.left + left.width, rect.bottom - bottom.width);
}
canvas.drawPath(path, paint);
case BorderStyle.none:
break;
}
}
| flutter/packages/flutter/lib/src/painting/borders.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/borders.dart",
"repo_id": "flutter",
"token_count": 11888
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:math' as math;
import 'dart:ui' as ui show Gradient, lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart';
import 'alignment.dart';
import 'basic_types.dart';
class _ColorsAndStops {
_ColorsAndStops(this.colors, this.stops);
final List<Color> colors;
final List<double> stops;
}
/// Calculate the color at position [t] of the gradient defined by [colors] and [stops].
Color _sample(List<Color> colors, List<double> stops, double t) {
assert(colors.isNotEmpty);
assert(stops.isNotEmpty);
if (t <= stops.first) {
return colors.first;
}
if (t >= stops.last) {
return colors.last;
}
final int index = stops.lastIndexWhere((double s) => s <= t);
assert(index != -1);
return Color.lerp(
colors[index], colors[index + 1],
(t - stops[index]) / (stops[index + 1] - stops[index]),
)!;
}
_ColorsAndStops _interpolateColorsAndStops(
List<Color> aColors,
List<double> aStops,
List<Color> bColors,
List<double> bStops,
double t,
) {
assert(aColors.length >= 2);
assert(bColors.length >= 2);
assert(aStops.length == aColors.length);
assert(bStops.length == bColors.length);
final SplayTreeSet<double> stops = SplayTreeSet<double>()
..addAll(aStops)
..addAll(bStops);
final List<double> interpolatedStops = stops.toList(growable: false);
final List<Color> interpolatedColors = interpolatedStops.map<Color>(
(double stop) => Color.lerp(_sample(aColors, aStops, stop), _sample(bColors, bStops, stop), t)!,
).toList(growable: false);
return _ColorsAndStops(interpolatedColors, interpolatedStops);
}
/// Base class for transforming gradient shaders without applying the same
/// transform to the entire canvas.
///
/// For example, a [SweepGradient] normally starts its gradation at 3 o'clock
/// and draws clockwise. To have the sweep appear to start at 6 o'clock, supply
/// a [GradientRotation] of `pi/4` radians (i.e. 45 degrees).
@immutable
abstract class GradientTransform {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const GradientTransform();
/// When a [Gradient] creates its [Shader], it will call this method to
/// determine what transform to apply to the shader for the given [Rect] and
/// [TextDirection].
///
/// Implementers may return null from this method, which achieves the same
/// final effect as returning [Matrix4.identity].
Matrix4? transform(Rect bounds, {TextDirection? textDirection});
}
/// A [GradientTransform] that rotates the gradient around the center-point of
/// its bounding box.
///
/// {@tool snippet}
///
/// This sample would rotate a sweep gradient by a quarter turn clockwise:
///
/// ```dart
/// const SweepGradient gradient = SweepGradient(
/// colors: <Color>[Color(0xFFFFFFFF), Color(0xFF009900)],
/// transform: GradientRotation(math.pi/4),
/// );
/// ```
/// {@end-tool}
@immutable
class GradientRotation extends GradientTransform {
/// Constructs a [GradientRotation] for the specified angle.
///
/// The angle is in radians in the clockwise direction.
const GradientRotation(this.radians);
/// The angle of rotation in radians in the clockwise direction.
final double radians;
@override
Matrix4 transform(Rect bounds, {TextDirection? textDirection}) {
final double sinRadians = math.sin(radians);
final double oneMinusCosRadians = 1 - math.cos(radians);
final Offset center = bounds.center;
final double originX = sinRadians * center.dy + oneMinusCosRadians * center.dx;
final double originY = -sinRadians * center.dx + oneMinusCosRadians * center.dy;
return Matrix4.identity()
..translate(originX, originY)
..rotateZ(radians);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is GradientRotation
&& other.radians == radians;
}
@override
int get hashCode => radians.hashCode;
@override
String toString() {
return '${objectRuntimeType(this, 'GradientRotation')}(radians: ${debugFormatDouble(radians)})';
}
}
/// A 2D gradient.
///
/// This is an interface that allows [LinearGradient], [RadialGradient], and
/// [SweepGradient] classes to be used interchangeably in [BoxDecoration]s.
///
/// See also:
///
/// * [Gradient](dart-ui/Gradient-class.html), the class in the [dart:ui] library.
///
@immutable
abstract class Gradient {
/// Initialize the gradient's colors and stops.
///
/// The [colors] argument must have at least two colors (the length is not
/// verified until the [createShader] method is called).
///
/// If specified, the [stops] argument must have the same number of entries as
/// [colors] (this is also not verified until the [createShader] method is
/// called).
///
/// The [transform] argument can be applied to transform _only_ the gradient,
/// without rotating the canvas itself or other geometry on the canvas. For
/// example, a `GradientRotation(math.pi/4)` will result in a [SweepGradient]
/// that starts from a position of 6 o'clock instead of 3 o'clock, assuming
/// no other rotation or perspective transformations have been applied to the
/// [Canvas]. If null, no transformation is applied.
const Gradient({
required this.colors,
this.stops,
this.transform,
});
/// The colors the gradient should obtain at each of the stops.
///
/// If [stops] is non-null, this list must have the same length as [stops].
///
/// This list must have at least two colors in it (otherwise, it's not a
/// gradient!).
final List<Color> colors;
/// A list of values from 0.0 to 1.0 that denote fractions along the gradient.
///
/// If non-null, this list must have the same length as [colors].
///
/// If the first value is not 0.0, then a stop with position 0.0 and a color
/// equal to the first color in [colors] is implied.
///
/// If the last value is not 1.0, then a stop with position 1.0 and a color
/// equal to the last color in [colors] is implied.
///
/// The values in the [stops] list must be in ascending order. If a value in
/// the [stops] list is less than an earlier value in the list, then its value
/// is assumed to equal the previous value.
///
/// If stops is null, then a set of uniformly distributed stops is implied,
/// with the first stop at 0.0 and the last stop at 1.0.
final List<double>? stops;
/// The transform, if any, to apply to the gradient.
///
/// This transform is in addition to any other transformations applied to the
/// canvas, but does not add any transformations to the canvas.
final GradientTransform? transform;
List<double> _impliedStops() {
if (stops != null) {
return stops!;
}
assert(colors.length >= 2, 'colors list must have at least two colors');
final double separation = 1.0 / (colors.length - 1);
return List<double>.generate(
colors.length,
(int index) => index * separation,
growable: false,
);
}
/// Creates a [Shader] for this gradient to fill the given rect.
///
/// If the gradient's configuration is text-direction-dependent, for example
/// it uses [AlignmentDirectional] objects instead of [Alignment]
/// objects, then the `textDirection` argument must not be null.
///
/// The shader's transform will be resolved from the [transform] of this
/// gradient.
@factory
Shader createShader(Rect rect, { TextDirection? textDirection });
/// Returns a new gradient with its properties scaled by the given factor.
///
/// A factor of 0.0 (or less) should result in a variant of the gradient that
/// is invisible; any two factors epsilon apart should be unnoticeably
/// different from each other at first glance. From this it follows that
/// scaling a gradient with values from 1.0 to 0.0 over time should cause the
/// gradient to smoothly disappear.
///
/// Typically this is the same as interpolating from null (with [lerp]).
Gradient scale(double factor);
/// Linearly interpolates from another [Gradient] to `this`.
///
/// When implementing this method in subclasses, return null if this class
/// cannot interpolate from `a`. In that case, [lerp] will try `a`'s [lerpTo]
/// method instead.
///
/// If `a` is null, this must not return null. The base class implements this
/// by deferring to [scale].
///
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `this` (or something equivalent to `this`), and values in
/// between meaning that the interpolation is at the relevant point on the
/// timeline between `a` and `this`. The interpolation can be extrapolated
/// beyond 0.0 and 1.0, so negative values and values greater than 1.0 are
/// valid (and can easily be generated by curves such as
/// [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// Instead of calling this directly, use [Gradient.lerp].
@protected
Gradient? lerpFrom(Gradient? a, double t) {
if (a == null) {
return scale(t);
}
return null;
}
/// Linearly interpolates from `this` to another [Gradient].
///
/// This is called if `b`'s [lerpTo] did not know how to handle this class.
///
/// When implementing this method in subclasses, return null if this class
/// cannot interpolate from `b`. In that case, [lerp] will apply a default
/// behavior instead.
///
/// If `b` is null, this must not return null. The base class implements this
/// by deferring to [scale].
///
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `this` (or something
/// equivalent to `this`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `this` and `b`. The interpolation can be extrapolated beyond 0.0
/// and 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
///
/// Instead of calling this directly, use [Gradient.lerp].
@protected
Gradient? lerpTo(Gradient? b, double t) {
if (b == null) {
return scale(1.0 - t);
}
return null;
}
/// Linearly interpolates between two [Gradient]s.
///
/// This defers to `b`'s [lerpTo] function if `b` is not null. If `b` is
/// null or if its [lerpTo] returns null, it uses `a`'s [lerpFrom]
/// function instead. If both return null, it returns `a` before `t == 0.5`
/// and `b` after `t == 0.5`.
///
/// {@macro dart.ui.shadow.lerp}
static Gradient? lerp(Gradient? a, Gradient? b, double t) {
if (identical(a, b)) {
return a;
}
Gradient? result;
if (b != null) {
result = b.lerpFrom(a, t); // if a is null, this must return non-null
}
if (result == null && a != null) {
result = a.lerpTo(b, t); // if b is null, this must return non-null
}
if (result != null) {
return result;
}
assert(a != null && b != null);
return t < 0.5 ? a!.scale(1.0 - (t * 2.0)) : b!.scale((t - 0.5) * 2.0);
}
Float64List? _resolveTransform(Rect bounds, TextDirection? textDirection) {
return transform?.transform(bounds, textDirection: textDirection)?.storage;
}
}
/// A 2D linear gradient.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=gYNTcgZVcWw}
///
/// This class is used by [BoxDecoration] to represent linear gradients. This
/// abstracts out the arguments to the [ui.Gradient.linear] constructor from
/// the `dart:ui` library.
///
/// A gradient has two anchor points, [begin] and [end]. The [begin] point
/// corresponds to 0.0, and the [end] point corresponds to 1.0. These points are
/// expressed in fractions, so that the same gradient can be reused with varying
/// sized boxes without changing the parameters. (This contrasts with [
/// ui.Gradient.linear], whose arguments are expressed in logical pixels.)
///
/// The [colors] are described by a list of [Color] objects. There must be at
/// least two colors. The [stops] list, if specified, must have the same length
/// as [colors]. It specifies fractions of the vector from start to end, between
/// 0.0 and 1.0, for each color. If it is null, a uniform distribution is
/// assumed.
///
/// The region of the canvas before [begin] and after [end] is colored according
/// to [tileMode].
///
/// Typically this class is used with [BoxDecoration], which does the painting.
/// To use a [LinearGradient] to paint on a canvas directly, see [createShader].
///
/// {@tool dartpad}
/// This sample draws a picture with a gradient sweeping through different
/// colors, by having a [Container] display a [BoxDecoration] with a
/// [LinearGradient].
///
/// ** See code in examples/api/lib/painting/gradient/linear_gradient.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RadialGradient], which displays a gradient in concentric circles, and
/// has an example which shows a different way to use [Gradient] objects.
/// * [SweepGradient], which displays a gradient in a sweeping arc around a
/// center point.
/// * [BoxDecoration], which can take a [LinearGradient] in its
/// [BoxDecoration.gradient] property.
class LinearGradient extends Gradient {
/// Creates a linear gradient.
///
/// If [stops] is non-null, it must have the same length as [colors].
const LinearGradient({
this.begin = Alignment.centerLeft,
this.end = Alignment.centerRight,
required super.colors,
super.stops,
this.tileMode = TileMode.clamp,
super.transform,
});
/// The offset at which stop 0.0 of the gradient is placed.
///
/// If this is an [Alignment], then it is expressed as a vector from
/// coordinate (0.0, 0.0), in a coordinate space that maps the center of the
/// paint box at (0.0, 0.0) and the bottom right at (1.0, 1.0).
///
/// For example, a begin offset of (-1.0, 0.0) is half way down the
/// left side of the box.
///
/// It can also be an [AlignmentDirectional], where the start is the
/// left in left-to-right contexts and the right in right-to-left contexts. If
/// a text-direction-dependent value is provided here, then the [createShader]
/// method will need to be given a [TextDirection].
final AlignmentGeometry begin;
/// The offset at which stop 1.0 of the gradient is placed.
///
/// If this is an [Alignment], then it is expressed as a vector from
/// coordinate (0.0, 0.0), in a coordinate space that maps the center of the
/// paint box at (0.0, 0.0) and the bottom right at (1.0, 1.0).
///
/// For example, a begin offset of (1.0, 0.0) is half way down the
/// right side of the box.
///
/// It can also be an [AlignmentDirectional], where the start is the left in
/// left-to-right contexts and the right in right-to-left contexts. If a
/// text-direction-dependent value is provided here, then the [createShader]
/// method will need to be given a [TextDirection].
final AlignmentGeometry end;
/// How this gradient should tile the plane beyond in the region before
/// [begin] and after [end].
///
/// For details, see [TileMode].
///
/// 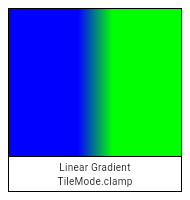
/// 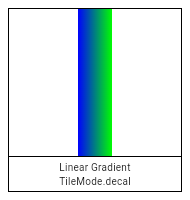
/// 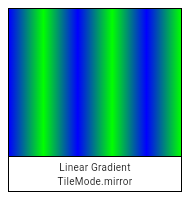
/// 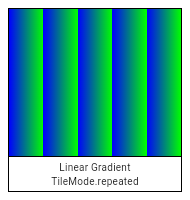
final TileMode tileMode;
@override
Shader createShader(Rect rect, { TextDirection? textDirection }) {
return ui.Gradient.linear(
begin.resolve(textDirection).withinRect(rect),
end.resolve(textDirection).withinRect(rect),
colors, _impliedStops(), tileMode, _resolveTransform(rect, textDirection),
);
}
/// Returns a new [LinearGradient] with its colors scaled by the given factor.
///
/// Since the alpha component of the Color is what is scaled, a factor
/// of 0.0 or less results in a gradient that is fully transparent.
@override
LinearGradient scale(double factor) {
return LinearGradient(
begin: begin,
end: end,
colors: colors.map<Color>((Color color) => Color.lerp(null, color, factor)!).toList(),
stops: stops,
tileMode: tileMode,
);
}
@override
Gradient? lerpFrom(Gradient? a, double t) {
if (a == null || (a is LinearGradient)) {
return LinearGradient.lerp(a as LinearGradient?, this, t);
}
return super.lerpFrom(a, t);
}
@override
Gradient? lerpTo(Gradient? b, double t) {
if (b == null || (b is LinearGradient)) {
return LinearGradient.lerp(this, b as LinearGradient?, t);
}
return super.lerpTo(b, t);
}
/// Linearly interpolate between two [LinearGradient]s.
///
/// If either gradient is null, this function linearly interpolates from
/// a gradient that matches the other gradient in [begin], [end], [stops] and
/// [tileMode] and with the same [colors] but transparent (using [scale]).
///
/// If neither gradient is null, they must have the same number of [colors].
///
/// The `t` argument represents a position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
/// 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
static LinearGradient? lerp(LinearGradient? a, LinearGradient? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
final _ColorsAndStops interpolated = _interpolateColorsAndStops(
a.colors,
a._impliedStops(),
b.colors,
b._impliedStops(),
t,
);
return LinearGradient(
begin: AlignmentGeometry.lerp(a.begin, b.begin, t)!,
end: AlignmentGeometry.lerp(a.end, b.end, t)!,
colors: interpolated.colors,
stops: interpolated.stops,
tileMode: t < 0.5 ? a.tileMode : b.tileMode, // TODO(ianh): interpolate tile mode
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is LinearGradient
&& other.begin == begin
&& other.end == end
&& other.tileMode == tileMode
&& other.transform == transform
&& listEquals<Color>(other.colors, colors)
&& listEquals<double>(other.stops, stops);
}
@override
int get hashCode => Object.hash(
begin,
end,
tileMode,
transform,
Object.hashAll(colors),
stops == null ? null : Object.hashAll(stops!),
);
@override
String toString() {
final List<String> description = <String>[
'begin: $begin',
'end: $end',
'colors: $colors',
if (stops != null) 'stops: $stops',
'tileMode: $tileMode',
if (transform != null) 'transform: $transform',
];
return '${objectRuntimeType(this, 'LinearGradient')}(${description.join(', ')})';
}
}
/// A 2D radial gradient.
///
/// This class is used by [BoxDecoration] to represent radial gradients. This
/// abstracts out the arguments to the [ui.Gradient.radial] constructor from
/// the `dart:ui` library.
///
/// A normal radial gradient has a [center] and a [radius]. The [center] point
/// corresponds to 0.0, and the ring at [radius] from the center corresponds
/// to 1.0. These lengths are expressed in fractions, so that the same gradient
/// can be reused with varying sized boxes without changing the parameters.
/// (This contrasts with [ui.Gradient.radial], whose arguments are expressed
/// in logical pixels.)
///
/// It is also possible to create a two-point (or focal pointed) radial gradient
/// (which is sometimes referred to as a two point conic gradient, but is not the
/// same as a CSS conic gradient which corresponds to a [SweepGradient]). A [focal]
/// point and [focalRadius] can be specified similarly to [center] and [radius],
/// which will make the rendered gradient appear to be pointed or directed in the
/// direction of the [focal] point. This is only important if [focal] and [center]
/// are not equal or [focalRadius] > 0.0 (as this case is visually identical to a
/// normal radial gradient). One important case to avoid is having [focal] and
/// [center] both resolve to [Offset.zero] when [focalRadius] > 0.0. In such a case,
/// a valid shader cannot be created by the framework.
///
/// The [colors] are described by a list of [Color] objects. There must be at
/// least two colors. The [stops] list, if specified, must have the same length
/// as [colors]. It specifies fractions of the radius between 0.0 and 1.0,
/// giving concentric rings for each color stop. If it is null, a uniform
/// distribution is assumed.
///
/// The region of the canvas beyond [radius] from the [center] is colored
/// according to [tileMode].
///
/// Typically this class is used with [BoxDecoration], which does the painting.
/// To use a [RadialGradient] to paint on a canvas directly, see [createShader].
///
/// {@tool snippet}
///
/// This function draws a gradient that looks like a sun in a blue sky.
///
/// ```dart
/// void paintSky(Canvas canvas, Rect rect) {
/// const RadialGradient gradient = RadialGradient(
/// center: Alignment(0.7, -0.6), // near the top right
/// radius: 0.2,
/// colors: <Color>[
/// Color(0xFFFFFF00), // yellow sun
/// Color(0xFF0099FF), // blue sky
/// ],
/// stops: <double>[0.4, 1.0],
/// );
/// // rect is the area we are painting over
/// final Paint paint = Paint()
/// ..shader = gradient.createShader(rect);
/// canvas.drawRect(rect, paint);
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [LinearGradient], which displays a gradient in parallel lines, and has an
/// example which shows a different way to use [Gradient] objects.
/// * [SweepGradient], which displays a gradient in a sweeping arc around a
/// center point.
/// * [BoxDecoration], which can take a [RadialGradient] in its
/// [BoxDecoration.gradient] property.
/// * [CustomPainter], which shows how to use the above sample code in a custom
/// painter.
class RadialGradient extends Gradient {
/// Creates a radial gradient.
///
/// If [stops] is non-null, it must have the same length as [colors].
const RadialGradient({
this.center = Alignment.center,
this.radius = 0.5,
required super.colors,
super.stops,
this.tileMode = TileMode.clamp,
this.focal,
this.focalRadius = 0.0,
super.transform,
});
/// The center of the gradient, as an offset into the (-1.0, -1.0) x (1.0, 1.0)
/// square describing the gradient which will be mapped onto the paint box.
///
/// For example, an alignment of (0.0, 0.0) will place the radial
/// gradient in the center of the box.
///
/// If this is an [Alignment], then it is expressed as a vector from
/// coordinate (0.0, 0.0), in a coordinate space that maps the center of the
/// paint box at (0.0, 0.0) and the bottom right at (1.0, 1.0).
///
/// It can also be an [AlignmentDirectional], where the start is the left in
/// left-to-right contexts and the right in right-to-left contexts. If a
/// text-direction-dependent value is provided here, then the [createShader]
/// method will need to be given a [TextDirection].
final AlignmentGeometry center;
/// The radius of the gradient, as a fraction of the shortest side
/// of the paint box.
///
/// For example, if a radial gradient is painted on a box that is
/// 100.0 pixels wide and 200.0 pixels tall, then a radius of 1.0
/// will place the 1.0 stop at 100.0 pixels from the [center].
final double radius;
/// How this gradient should tile the plane beyond the outer ring at [radius]
/// pixels from the [center].
///
/// For details, see [TileMode].
///
/// 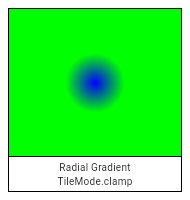
/// 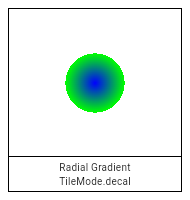
/// 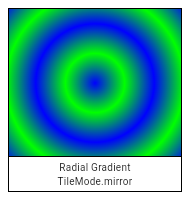
/// 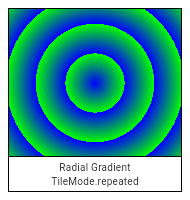
///
/// 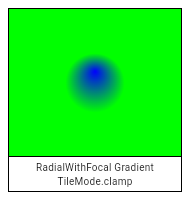
/// 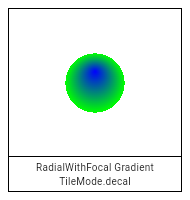
/// 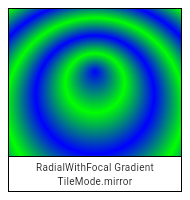
/// 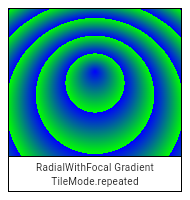
final TileMode tileMode;
/// The focal point of the gradient. If specified, the gradient will appear
/// to be focused along the vector from [center] to focal.
///
/// See [center] for a description of how the coordinates are mapped.
///
/// If this value is specified and [focalRadius] > 0.0, care should be taken
/// to ensure that either this value or [center] will not both resolve to
/// [Offset.zero], which would fail to create a valid gradient.
final AlignmentGeometry? focal;
/// The radius of the focal point of gradient, as a fraction of the shortest
/// side of the paint box.
///
/// For example, if a radial gradient is painted on a box that is
/// 100.0 pixels wide and 200.0 pixels tall, then a radius of 1.0
/// will place the 1.0 stop at 100.0 pixels from the [focal] point.
///
/// If this value is specified and is greater than 0.0, either [focal] or
/// [center] must not resolve to [Offset.zero], which would fail to create
/// a valid gradient.
final double focalRadius;
@override
Shader createShader(Rect rect, { TextDirection? textDirection }) {
return ui.Gradient.radial(
center.resolve(textDirection).withinRect(rect),
radius * rect.shortestSide,
colors, _impliedStops(), tileMode,
_resolveTransform(rect, textDirection),
focal?.resolve(textDirection).withinRect(rect),
focalRadius * rect.shortestSide,
);
}
/// Returns a new [RadialGradient] with its colors scaled by the given factor.
///
/// Since the alpha component of the Color is what is scaled, a factor
/// of 0.0 or less results in a gradient that is fully transparent.
@override
RadialGradient scale(double factor) {
return RadialGradient(
center: center,
radius: radius,
colors: colors.map<Color>((Color color) => Color.lerp(null, color, factor)!).toList(),
stops: stops,
tileMode: tileMode,
focal: focal,
focalRadius: focalRadius,
);
}
@override
Gradient? lerpFrom(Gradient? a, double t) {
if (a == null || (a is RadialGradient)) {
return RadialGradient.lerp(a as RadialGradient?, this, t);
}
return super.lerpFrom(a, t);
}
@override
Gradient? lerpTo(Gradient? b, double t) {
if (b == null || (b is RadialGradient)) {
return RadialGradient.lerp(this, b as RadialGradient?, t);
}
return super.lerpTo(b, t);
}
/// Linearly interpolate between two [RadialGradient]s.
///
/// If either gradient is null, this function linearly interpolates from
/// a gradient that matches the other gradient in [center], [radius], [stops] and
/// [tileMode] and with the same [colors] but transparent (using [scale]).
///
/// If neither gradient is null, they must have the same number of [colors].
///
/// The `t` argument represents a position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
/// 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
static RadialGradient? lerp(RadialGradient? a, RadialGradient? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
final _ColorsAndStops interpolated = _interpolateColorsAndStops(
a.colors,
a._impliedStops(),
b.colors,
b._impliedStops(),
t,
);
return RadialGradient(
center: AlignmentGeometry.lerp(a.center, b.center, t)!,
radius: math.max(0.0, ui.lerpDouble(a.radius, b.radius, t)!),
colors: interpolated.colors,
stops: interpolated.stops,
tileMode: t < 0.5 ? a.tileMode : b.tileMode, // TODO(ianh): interpolate tile mode
focal: AlignmentGeometry.lerp(a.focal, b.focal, t),
focalRadius: math.max(0.0, ui.lerpDouble(a.focalRadius, b.focalRadius, t)!),
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is RadialGradient
&& other.center == center
&& other.radius == radius
&& other.tileMode == tileMode
&& other.transform == transform
&& listEquals<Color>(other.colors, colors)
&& listEquals<double>(other.stops, stops)
&& other.focal == focal
&& other.focalRadius == focalRadius;
}
@override
int get hashCode => Object.hash(
center,
radius,
tileMode,
transform,
Object.hashAll(colors),
stops == null ? null : Object.hashAll(stops!),
focal,
focalRadius,
);
@override
String toString() {
final List<String> description = <String>[
'center: $center',
'radius: ${debugFormatDouble(radius)}',
'colors: $colors',
if (stops != null) 'stops: $stops',
'tileMode: $tileMode',
if (focal != null) 'focal: $focal',
'focalRadius: ${debugFormatDouble(focalRadius)}',
if (transform != null) 'transform: $transform',
];
return '${objectRuntimeType(this, 'RadialGradient')}(${description.join(', ')})';
}
}
/// A 2D sweep gradient.
///
/// This class is used by [BoxDecoration] to represent sweep gradients. This
/// abstracts out the arguments to the [ui.Gradient.sweep] constructor from
/// the `dart:ui` library.
///
/// A gradient has a [center], a [startAngle], and an [endAngle]. The [startAngle]
/// corresponds to 0.0, and the [endAngle] corresponds to 1.0. These angles are
/// expressed in radians.
///
/// The [colors] are described by a list of [Color] objects. There must be at
/// least two colors. The [stops] list, if specified, must have the same length
/// as [colors]. It specifies fractions of the vector from start to end, between
/// 0.0 and 1.0, for each color. If it is null, a uniform distribution is
/// assumed.
///
/// The region of the canvas before [startAngle] and after [endAngle] is colored
/// according to [tileMode].
///
/// Typically this class is used with [BoxDecoration], which does the painting.
/// To use a [SweepGradient] to paint on a canvas directly, see [createShader].
///
/// {@tool snippet}
///
/// This sample draws a different color in each quadrant.
///
/// ```dart
/// Container(
/// decoration: const BoxDecoration(
/// gradient: SweepGradient(
/// center: FractionalOffset.center,
/// colors: <Color>[
/// Color(0xFF4285F4), // blue
/// Color(0xFF34A853), // green
/// Color(0xFFFBBC05), // yellow
/// Color(0xFFEA4335), // red
/// Color(0xFF4285F4), // blue again to seamlessly transition to the start
/// ],
/// stops: <double>[0.0, 0.25, 0.5, 0.75, 1.0],
/// ),
/// )
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// This sample takes the above gradient and rotates it by `math.pi/4` radians,
/// i.e. 45 degrees.
///
/// ```dart
/// Container(
/// decoration: const BoxDecoration(
/// gradient: SweepGradient(
/// center: FractionalOffset.center,
/// colors: <Color>[
/// Color(0xFF4285F4), // blue
/// Color(0xFF34A853), // green
/// Color(0xFFFBBC05), // yellow
/// Color(0xFFEA4335), // red
/// Color(0xFF4285F4), // blue again to seamlessly transition to the start
/// ],
/// stops: <double>[0.0, 0.25, 0.5, 0.75, 1.0],
/// transform: GradientRotation(math.pi/4),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [LinearGradient], which displays a gradient in parallel lines, and has an
/// example which shows a different way to use [Gradient] objects.
/// * [RadialGradient], which displays a gradient in concentric circles, and
/// has an example which shows a different way to use [Gradient] objects.
/// * [BoxDecoration], which can take a [SweepGradient] in its
/// [BoxDecoration.gradient] property.
class SweepGradient extends Gradient {
/// Creates a sweep gradient.
///
/// If [stops] is non-null, it must have the same length as [colors].
const SweepGradient({
this.center = Alignment.center,
this.startAngle = 0.0,
this.endAngle = math.pi * 2,
required super.colors,
super.stops,
this.tileMode = TileMode.clamp,
super.transform,
});
/// The center of the gradient, as an offset into the (-1.0, -1.0) x (1.0, 1.0)
/// square describing the gradient which will be mapped onto the paint box.
///
/// For example, an alignment of (0.0, 0.0) will place the sweep
/// gradient in the center of the box.
///
/// If this is an [Alignment], then it is expressed as a vector from
/// coordinate (0.0, 0.0), in a coordinate space that maps the center of the
/// paint box at (0.0, 0.0) and the bottom right at (1.0, 1.0).
///
/// It can also be an [AlignmentDirectional], where the start is the left in
/// left-to-right contexts and the right in right-to-left contexts. If a
/// text-direction-dependent value is provided here, then the [createShader]
/// method will need to be given a [TextDirection].
final AlignmentGeometry center;
/// The angle in radians at which stop 0.0 of the gradient is placed.
///
/// Defaults to 0.0.
final double startAngle;
/// The angle in radians at which stop 1.0 of the gradient is placed.
///
/// Defaults to math.pi * 2.
final double endAngle;
/// How this gradient should tile the plane beyond in the region before
/// [startAngle] and after [endAngle].
///
/// For details, see [TileMode].
///
/// 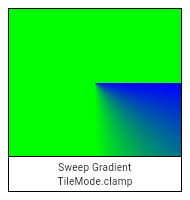
/// 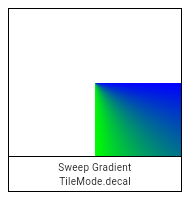
/// 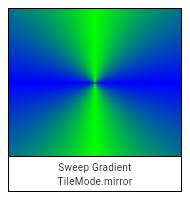
/// 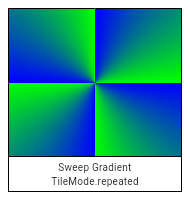
final TileMode tileMode;
@override
Shader createShader(Rect rect, { TextDirection? textDirection }) {
return ui.Gradient.sweep(
center.resolve(textDirection).withinRect(rect),
colors, _impliedStops(), tileMode,
startAngle,
endAngle,
_resolveTransform(rect, textDirection),
);
}
/// Returns a new [SweepGradient] with its colors scaled by the given factor.
///
/// Since the alpha component of the Color is what is scaled, a factor
/// of 0.0 or less results in a gradient that is fully transparent.
@override
SweepGradient scale(double factor) {
return SweepGradient(
center: center,
startAngle: startAngle,
endAngle: endAngle,
colors: colors.map<Color>((Color color) => Color.lerp(null, color, factor)!).toList(),
stops: stops,
tileMode: tileMode,
);
}
@override
Gradient? lerpFrom(Gradient? a, double t) {
if (a == null || (a is SweepGradient)) {
return SweepGradient.lerp(a as SweepGradient?, this, t);
}
return super.lerpFrom(a, t);
}
@override
Gradient? lerpTo(Gradient? b, double t) {
if (b == null || (b is SweepGradient)) {
return SweepGradient.lerp(this, b as SweepGradient?, t);
}
return super.lerpTo(b, t);
}
/// Linearly interpolate between two [SweepGradient]s.
///
/// If either gradient is null, then the non-null gradient is returned with
/// its color scaled in the same way as the [scale] function.
///
/// If neither gradient is null, they must have the same number of [colors].
///
/// The `t` argument represents a position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
/// 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
static SweepGradient? lerp(SweepGradient? a, SweepGradient? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b!.scale(t);
}
if (b == null) {
return a.scale(1.0 - t);
}
final _ColorsAndStops interpolated = _interpolateColorsAndStops(
a.colors,
a._impliedStops(),
b.colors,
b._impliedStops(),
t,
);
return SweepGradient(
center: AlignmentGeometry.lerp(a.center, b.center, t)!,
startAngle: math.max(0.0, ui.lerpDouble(a.startAngle, b.startAngle, t)!),
endAngle: math.max(0.0, ui.lerpDouble(a.endAngle, b.endAngle, t)!),
colors: interpolated.colors,
stops: interpolated.stops,
tileMode: t < 0.5 ? a.tileMode : b.tileMode, // TODO(ianh): interpolate tile mode
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SweepGradient
&& other.center == center
&& other.startAngle == startAngle
&& other.endAngle == endAngle
&& other.tileMode == tileMode
&& other.transform == transform
&& listEquals<Color>(other.colors, colors)
&& listEquals<double>(other.stops, stops);
}
@override
int get hashCode => Object.hash(
center,
startAngle,
endAngle,
tileMode,
transform,
Object.hashAll(colors),
stops == null ? null : Object.hashAll(stops!),
);
@override
String toString() {
final List<String> description = <String>[
'center: $center',
'startAngle: ${debugFormatDouble(startAngle)}',
'endAngle: ${debugFormatDouble(endAngle)}',
'colors: $colors',
if (stops != null) 'stops: $stops',
'tileMode: $tileMode',
if (transform != null) 'transform: $transform',
];
return '${objectRuntimeType(this, 'SweepGradient')}(${description.join(', ')})';
}
}
| flutter/packages/flutter/lib/src/painting/gradient.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/gradient.dart",
"repo_id": "flutter",
"token_count": 13892
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'border_radius.dart';
import 'borders.dart';
import 'circle_border.dart';
import 'rounded_rectangle_border.dart';
/// A border that fits a stadium-shaped border (a box with semicircles on the ends)
/// within the rectangle of the widget it is applied to.
///
/// Typically used with [ShapeDecoration] to draw a stadium border.
///
/// If the rectangle is taller than it is wide, then the semicircles will be on the
/// top and bottom, and on the left and right otherwise.
///
/// See also:
///
/// * [BorderSide], which is used to describe the border of the stadium.
class StadiumBorder extends OutlinedBorder {
/// Create a stadium border.
const StadiumBorder({ super.side });
@override
ShapeBorder scale(double t) => StadiumBorder(side: side.scale(t));
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is StadiumBorder) {
return StadiumBorder(side: BorderSide.lerp(a.side, side, t));
}
if (a is CircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
circularity: 1.0 - t,
eccentricity: a.eccentricity,
);
}
if (a is RoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: a.borderRadius,
rectilinearity: 1.0 - t,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is StadiumBorder) {
return StadiumBorder(side: BorderSide.lerp(side, b.side, t));
}
if (b is CircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
circularity: t,
eccentricity: b.eccentricity,
);
}
if (b is RoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: b.borderRadius,
rectilinearity: t,
);
}
return super.lerpTo(b, t);
}
@override
StadiumBorder copyWith({ BorderSide? side }) {
return StadiumBorder(side: side ?? this.side);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
final Radius radius = Radius.circular(rect.shortestSide / 2.0);
final RRect borderRect = RRect.fromRectAndRadius(rect, radius);
final RRect adjustedRect = borderRect.deflate(side.strokeInset);
return Path()
..addRRect(adjustedRect);
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
final Radius radius = Radius.circular(rect.shortestSide / 2.0);
return Path()
..addRRect(RRect.fromRectAndRadius(rect, radius));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
final Radius radius = Radius.circular(rect.shortestSide / 2.0);
canvas.drawRRect(RRect.fromRectAndRadius(rect, radius), paint);
}
@override
bool get preferPaintInterior => true;
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final Radius radius = Radius.circular(rect.shortestSide / 2);
final RRect borderRect = RRect.fromRectAndRadius(rect, radius);
canvas.drawRRect(borderRect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is StadiumBorder
&& other.side == side;
}
@override
int get hashCode => side.hashCode;
@override
String toString() {
return '${objectRuntimeType(this, 'StadiumBorder')}($side)';
}
}
// Class to help with transitioning to/from a CircleBorder.
class _StadiumToCircleBorder extends OutlinedBorder {
const _StadiumToCircleBorder({
super.side,
this.circularity = 0.0,
required this.eccentricity,
});
final double circularity;
final double eccentricity;
@override
ShapeBorder scale(double t) {
return _StadiumToCircleBorder(
side: side.scale(t),
circularity: t,
eccentricity: eccentricity,
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is StadiumBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
circularity: circularity * t,
eccentricity: eccentricity,
);
}
if (a is CircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
circularity: circularity + (1.0 - circularity) * (1.0 - t),
eccentricity: a.eccentricity,
);
}
if (a is _StadiumToCircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
circularity: ui.lerpDouble(a.circularity, circularity, t)!,
eccentricity: ui.lerpDouble(a.eccentricity, eccentricity, t)!,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is StadiumBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
circularity: circularity * (1.0 - t),
eccentricity: eccentricity,
);
}
if (b is CircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
circularity: circularity + (1.0 - circularity) * t,
eccentricity: b.eccentricity,
);
}
if (b is _StadiumToCircleBorder) {
return _StadiumToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
circularity: ui.lerpDouble(circularity, b.circularity, t)!,
eccentricity: ui.lerpDouble(eccentricity, b.eccentricity, t)!,
);
}
return super.lerpTo(b, t);
}
Rect _adjustRect(Rect rect) {
if (circularity == 0.0 || rect.width == rect.height) {
return rect;
}
if (rect.width < rect.height) {
final double partialDelta = (rect.height - rect.width) / 2;
final double delta = circularity * partialDelta * (1.0 - eccentricity);
return Rect.fromLTRB(
rect.left,
rect.top + delta,
rect.right,
rect.bottom - delta,
);
} else {
final double partialDelta = (rect.width - rect.height) / 2;
final double delta = circularity * partialDelta * (1.0 - eccentricity);
return Rect.fromLTRB(
rect.left + delta,
rect.top,
rect.right - delta,
rect.bottom,
);
}
}
BorderRadius _adjustBorderRadius(Rect rect) {
final BorderRadius circleRadius = BorderRadius.circular(rect.shortestSide / 2);
if (eccentricity != 0.0) {
if (rect.width < rect.height) {
return BorderRadius.lerp(
circleRadius,
BorderRadius.all(Radius.elliptical(rect.width / 2, (0.5 + eccentricity / 2) * rect.height / 2)),
circularity,
)!;
} else {
return BorderRadius.lerp(
circleRadius,
BorderRadius.all(Radius.elliptical((0.5 + eccentricity / 2) * rect.width / 2, rect.height / 2)),
circularity,
)!;
}
}
return circleRadius;
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
return Path()
..addRRect(_adjustBorderRadius(rect).toRRect(_adjustRect(rect)).deflate(side.strokeInset));
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return Path()
..addRRect(_adjustBorderRadius(rect).toRRect(_adjustRect(rect)));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
canvas.drawRRect(_adjustBorderRadius(rect).toRRect(_adjustRect(rect)), paint);
}
@override
bool get preferPaintInterior => true;
@override
_StadiumToCircleBorder copyWith({ BorderSide? side, double? circularity, double? eccentricity }) {
return _StadiumToCircleBorder(
side: side ?? this.side,
circularity: circularity ?? this.circularity,
eccentricity: eccentricity ?? this.eccentricity,
);
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final RRect borderRect = _adjustBorderRadius(rect).toRRect(_adjustRect(rect));
canvas.drawRRect(borderRect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _StadiumToCircleBorder
&& other.side == side
&& other.circularity == circularity;
}
@override
int get hashCode => Object.hash(side, circularity);
@override
String toString() {
if (eccentricity != 0.0) {
return 'StadiumBorder($side, ${(circularity * 100).toStringAsFixed(1)}% of the way to being a CircleBorder that is ${(eccentricity * 100).toStringAsFixed(1)}% oval)';
}
return 'StadiumBorder($side, ${(circularity * 100).toStringAsFixed(1)}% of the way to being a CircleBorder)';
}
}
// Class to help with transitioning to/from a RoundedRectBorder.
class _StadiumToRoundedRectangleBorder extends OutlinedBorder {
const _StadiumToRoundedRectangleBorder({
super.side,
this.borderRadius = BorderRadius.zero,
this.rectilinearity = 0.0,
});
final BorderRadiusGeometry borderRadius;
final double rectilinearity;
@override
ShapeBorder scale(double t) {
return _StadiumToRoundedRectangleBorder(
side: side.scale(t),
borderRadius: borderRadius * t,
rectilinearity: t,
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is StadiumBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: borderRadius,
rectilinearity: rectilinearity * t,
);
}
if (a is RoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: borderRadius,
rectilinearity: rectilinearity + (1.0 - rectilinearity) * (1.0 - t),
);
}
if (a is _StadiumToRoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, borderRadius, t)!,
rectilinearity: ui.lerpDouble(a.rectilinearity, rectilinearity, t)!,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is StadiumBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: borderRadius,
rectilinearity: rectilinearity * (1.0 - t),
);
}
if (b is RoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: borderRadius,
rectilinearity: rectilinearity + (1.0 - rectilinearity) * t,
);
}
if (b is _StadiumToRoundedRectangleBorder) {
return _StadiumToRoundedRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: BorderRadiusGeometry.lerp(borderRadius, b.borderRadius, t)!,
rectilinearity: ui.lerpDouble(rectilinearity, b.rectilinearity, t)!,
);
}
return super.lerpTo(b, t);
}
BorderRadiusGeometry _adjustBorderRadius(Rect rect) {
return BorderRadiusGeometry.lerp(
borderRadius,
BorderRadius.all(Radius.circular(rect.shortestSide / 2.0)),
1.0 - rectilinearity,
)!;
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
final RRect borderRect = _adjustBorderRadius(rect).resolve(textDirection).toRRect(rect);
final RRect adjustedRect = borderRect.deflate(ui.lerpDouble(side.width, 0, side.strokeAlign)!);
return Path()
..addRRect(adjustedRect);
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return Path()
..addRRect(_adjustBorderRadius(rect).resolve(textDirection).toRRect(rect));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
final BorderRadiusGeometry adjustedBorderRadius = _adjustBorderRadius(rect);
if (adjustedBorderRadius == BorderRadius.zero) {
canvas.drawRect(rect, paint);
} else {
canvas.drawRRect(adjustedBorderRadius.resolve(textDirection).toRRect(rect), paint);
}
}
@override
bool get preferPaintInterior => true;
@override
_StadiumToRoundedRectangleBorder copyWith({ BorderSide? side, BorderRadiusGeometry? borderRadius, double? rectilinearity }) {
return _StadiumToRoundedRectangleBorder(
side: side ?? this.side,
borderRadius: borderRadius ?? this.borderRadius,
rectilinearity: rectilinearity ?? this.rectilinearity,
);
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final BorderRadiusGeometry adjustedBorderRadius = _adjustBorderRadius(rect);
final RRect borderRect = adjustedBorderRadius.resolve(textDirection).toRRect(rect);
canvas.drawRRect(borderRect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _StadiumToRoundedRectangleBorder
&& other.side == side
&& other.borderRadius == borderRadius
&& other.rectilinearity == rectilinearity;
}
@override
int get hashCode => Object.hash(side, borderRadius, rectilinearity);
@override
String toString() {
return 'StadiumBorder($side, $borderRadius, '
'${(rectilinearity * 100).toStringAsFixed(1)}% of the way to being a '
'RoundedRectangleBorder)';
}
}
| flutter/packages/flutter/lib/src/painting/stadium_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/stadium_border.dart",
"repo_id": "flutter",
"token_count": 5738
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui show ViewConstraints, lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:vector_math/vector_math_64.dart';
import 'debug.dart';
import 'object.dart';
// Examples can assume:
// abstract class RenderBar extends RenderBox { }
// late RenderBox firstChild;
// void markNeedsLayout() { }
// This class should only be used in debug builds.
class _DebugSize extends Size {
_DebugSize(super.source, this._owner, this._canBeUsedByParent) : super.copy();
final RenderBox _owner;
final bool _canBeUsedByParent;
}
/// Immutable layout constraints for [RenderBox] layout.
///
/// A [Size] respects a [BoxConstraints] if, and only if, all of the following
/// relations hold:
///
/// * [minWidth] <= [Size.width] <= [maxWidth]
/// * [minHeight] <= [Size.height] <= [maxHeight]
///
/// The constraints themselves must satisfy these relations:
///
/// * 0.0 <= [minWidth] <= [maxWidth] <= [double.infinity]
/// * 0.0 <= [minHeight] <= [maxHeight] <= [double.infinity]
///
/// [double.infinity] is a legal value for each constraint.
///
/// ## The box layout model
///
/// Render objects in the Flutter framework are laid out by a one-pass layout
/// model which walks down the render tree passing constraints, then walks back
/// up the render tree passing concrete geometry.
///
/// For boxes, the constraints are [BoxConstraints], which, as described herein,
/// consist of four numbers: a minimum width [minWidth], a maximum width
/// [maxWidth], a minimum height [minHeight], and a maximum height [maxHeight].
///
/// The geometry for boxes consists of a [Size], which must satisfy the
/// constraints described above.
///
/// Each [RenderBox] (the objects that provide the layout models for box
/// widgets) receives [BoxConstraints] from its parent, then lays out each of
/// its children, then picks a [Size] that satisfies the [BoxConstraints].
///
/// Render objects position their children independently of laying them out.
/// Frequently, the parent will use the children's sizes to determine their
/// position. A child does not know its position and will not necessarily be
/// laid out again, or repainted, if its position changes.
///
/// ## Terminology
///
/// When the minimum constraints and the maximum constraint in an axis are the
/// same, that axis is _tightly_ constrained. See: [
/// BoxConstraints.tightFor], [BoxConstraints.tightForFinite], [tighten],
/// [hasTightWidth], [hasTightHeight], [isTight].
///
/// An axis with a minimum constraint of 0.0 is _loose_ (regardless of the
/// maximum constraint; if it is also 0.0, then the axis is simultaneously tight
/// and loose!). See: [BoxConstraints.loose], [loosen].
///
/// An axis whose maximum constraint is not infinite is _bounded_. See:
/// [hasBoundedWidth], [hasBoundedHeight].
///
/// An axis whose maximum constraint is infinite is _unbounded_. An axis is
/// _expanding_ if it is tightly infinite (its minimum and maximum constraints
/// are both infinite). See: [BoxConstraints.expand].
///
/// An axis whose _minimum_ constraint is infinite is just said to be _infinite_
/// (since by definition the maximum constraint must also be infinite in that
/// case). See: [hasInfiniteWidth], [hasInfiniteHeight].
///
/// A size is _constrained_ when it satisfies a [BoxConstraints] description.
/// See: [constrain], [constrainWidth], [constrainHeight],
/// [constrainDimensions], [constrainSizeAndAttemptToPreserveAspectRatio],
/// [isSatisfiedBy].
class BoxConstraints extends Constraints {
/// Creates box constraints with the given constraints.
const BoxConstraints({
this.minWidth = 0.0,
this.maxWidth = double.infinity,
this.minHeight = 0.0,
this.maxHeight = double.infinity,
});
/// Creates box constraints that is respected only by the given size.
BoxConstraints.tight(Size size)
: minWidth = size.width,
maxWidth = size.width,
minHeight = size.height,
maxHeight = size.height;
/// Creates box constraints that require the given width or height.
///
/// See also:
///
/// * [BoxConstraints.tightForFinite], which is similar but instead of
/// being tight if the value is non-null, is tight if the value is not
/// infinite.
const BoxConstraints.tightFor({
double? width,
double? height,
}) : minWidth = width ?? 0.0,
maxWidth = width ?? double.infinity,
minHeight = height ?? 0.0,
maxHeight = height ?? double.infinity;
/// Creates box constraints that require the given width or height, except if
/// they are infinite.
///
/// See also:
///
/// * [BoxConstraints.tightFor], which is similar but instead of being
/// tight if the value is not infinite, is tight if the value is non-null.
const BoxConstraints.tightForFinite({
double width = double.infinity,
double height = double.infinity,
}) : minWidth = width != double.infinity ? width : 0.0,
maxWidth = width != double.infinity ? width : double.infinity,
minHeight = height != double.infinity ? height : 0.0,
maxHeight = height != double.infinity ? height : double.infinity;
/// Creates box constraints that forbid sizes larger than the given size.
BoxConstraints.loose(Size size)
: minWidth = 0.0,
maxWidth = size.width,
minHeight = 0.0,
maxHeight = size.height;
/// Creates box constraints that expand to fill another box constraints.
///
/// If width or height is given, the constraints will require exactly the
/// given value in the given dimension.
const BoxConstraints.expand({
double? width,
double? height,
}) : minWidth = width ?? double.infinity,
maxWidth = width ?? double.infinity,
minHeight = height ?? double.infinity,
maxHeight = height ?? double.infinity;
/// Creates box constraints that match the given view constraints.
BoxConstraints.fromViewConstraints(ui.ViewConstraints constraints)
: minWidth = constraints.minWidth,
maxWidth = constraints.maxWidth,
minHeight = constraints.minHeight,
maxHeight = constraints.maxHeight;
/// The minimum width that satisfies the constraints.
final double minWidth;
/// The maximum width that satisfies the constraints.
///
/// Might be [double.infinity].
final double maxWidth;
/// The minimum height that satisfies the constraints.
final double minHeight;
/// The maximum height that satisfies the constraints.
///
/// Might be [double.infinity].
final double maxHeight;
/// Creates a copy of this box constraints but with the given fields replaced with the new values.
BoxConstraints copyWith({
double? minWidth,
double? maxWidth,
double? minHeight,
double? maxHeight,
}) {
return BoxConstraints(
minWidth: minWidth ?? this.minWidth,
maxWidth: maxWidth ?? this.maxWidth,
minHeight: minHeight ?? this.minHeight,
maxHeight: maxHeight ?? this.maxHeight,
);
}
/// Returns new box constraints that are smaller by the given edge dimensions.
BoxConstraints deflate(EdgeInsetsGeometry edges) {
assert(debugAssertIsValid());
final double horizontal = edges.horizontal;
final double vertical = edges.vertical;
final double deflatedMinWidth = math.max(0.0, minWidth - horizontal);
final double deflatedMinHeight = math.max(0.0, minHeight - vertical);
return BoxConstraints(
minWidth: deflatedMinWidth,
maxWidth: math.max(deflatedMinWidth, maxWidth - horizontal),
minHeight: deflatedMinHeight,
maxHeight: math.max(deflatedMinHeight, maxHeight - vertical),
);
}
/// Returns new box constraints that remove the minimum width and height requirements.
BoxConstraints loosen() {
assert(debugAssertIsValid());
return BoxConstraints(
maxWidth: maxWidth,
maxHeight: maxHeight,
);
}
/// Returns new box constraints that respect the given constraints while being
/// as close as possible to the original constraints.
BoxConstraints enforce(BoxConstraints constraints) {
return BoxConstraints(
minWidth: clampDouble(minWidth, constraints.minWidth, constraints.maxWidth),
maxWidth: clampDouble(maxWidth, constraints.minWidth, constraints.maxWidth),
minHeight: clampDouble(minHeight, constraints.minHeight, constraints.maxHeight),
maxHeight: clampDouble(maxHeight, constraints.minHeight, constraints.maxHeight),
);
}
/// Returns new box constraints with a tight width and/or height as close to
/// the given width and height as possible while still respecting the original
/// box constraints.
BoxConstraints tighten({ double? width, double? height }) {
return BoxConstraints(
minWidth: width == null ? minWidth : clampDouble(width, minWidth, maxWidth),
maxWidth: width == null ? maxWidth : clampDouble(width, minWidth, maxWidth),
minHeight: height == null ? minHeight : clampDouble(height, minHeight, maxHeight),
maxHeight: height == null ? maxHeight : clampDouble(height, minHeight, maxHeight),
);
}
/// A box constraints with the width and height constraints flipped.
BoxConstraints get flipped {
return BoxConstraints(
minWidth: minHeight,
maxWidth: maxHeight,
minHeight: minWidth,
maxHeight: maxWidth,
);
}
/// Returns box constraints with the same width constraints but with
/// unconstrained height.
BoxConstraints widthConstraints() => BoxConstraints(minWidth: minWidth, maxWidth: maxWidth);
/// Returns box constraints with the same height constraints but with
/// unconstrained width.
BoxConstraints heightConstraints() => BoxConstraints(minHeight: minHeight, maxHeight: maxHeight);
/// Returns the width that both satisfies the constraints and is as close as
/// possible to the given width.
double constrainWidth([ double width = double.infinity ]) {
assert(debugAssertIsValid());
return clampDouble(width, minWidth, maxWidth);
}
/// Returns the height that both satisfies the constraints and is as close as
/// possible to the given height.
double constrainHeight([ double height = double.infinity ]) {
assert(debugAssertIsValid());
return clampDouble(height, minHeight, maxHeight);
}
Size _debugPropagateDebugSize(Size size, Size result) {
assert(() {
if (size is _DebugSize) {
result = _DebugSize(result, size._owner, size._canBeUsedByParent);
}
return true;
}());
return result;
}
/// Returns the size that both satisfies the constraints and is as close as
/// possible to the given size.
///
/// See also:
///
/// * [constrainDimensions], which applies the same algorithm to
/// separately provided widths and heights.
Size constrain(Size size) {
Size result = Size(constrainWidth(size.width), constrainHeight(size.height));
assert(() {
result = _debugPropagateDebugSize(size, result);
return true;
}());
return result;
}
/// Returns the size that both satisfies the constraints and is as close as
/// possible to the given width and height.
///
/// When you already have a [Size], prefer [constrain], which applies the same
/// algorithm to a [Size] directly.
Size constrainDimensions(double width, double height) {
return Size(constrainWidth(width), constrainHeight(height));
}
/// Returns a size that attempts to meet the following conditions, in order:
///
/// * The size must satisfy these constraints.
/// * The aspect ratio of the returned size matches the aspect ratio of the
/// given size.
/// * The returned size is as big as possible while still being equal to or
/// smaller than the given size.
Size constrainSizeAndAttemptToPreserveAspectRatio(Size size) {
if (isTight) {
Size result = smallest;
assert(() {
result = _debugPropagateDebugSize(size, result);
return true;
}());
return result;
}
double width = size.width;
double height = size.height;
assert(width > 0.0);
assert(height > 0.0);
final double aspectRatio = width / height;
if (width > maxWidth) {
width = maxWidth;
height = width / aspectRatio;
}
if (height > maxHeight) {
height = maxHeight;
width = height * aspectRatio;
}
if (width < minWidth) {
width = minWidth;
height = width / aspectRatio;
}
if (height < minHeight) {
height = minHeight;
width = height * aspectRatio;
}
Size result = Size(constrainWidth(width), constrainHeight(height));
assert(() {
result = _debugPropagateDebugSize(size, result);
return true;
}());
return result;
}
/// The biggest size that satisfies the constraints.
Size get biggest => Size(constrainWidth(), constrainHeight());
/// The smallest size that satisfies the constraints.
Size get smallest => Size(constrainWidth(0.0), constrainHeight(0.0));
/// Whether there is exactly one width value that satisfies the constraints.
bool get hasTightWidth => minWidth >= maxWidth;
/// Whether there is exactly one height value that satisfies the constraints.
bool get hasTightHeight => minHeight >= maxHeight;
/// Whether there is exactly one size that satisfies the constraints.
@override
bool get isTight => hasTightWidth && hasTightHeight;
/// Whether there is an upper bound on the maximum width.
///
/// See also:
///
/// * [hasBoundedHeight], the equivalent for the vertical axis.
/// * [hasInfiniteWidth], which describes whether the minimum width
/// constraint is infinite.
bool get hasBoundedWidth => maxWidth < double.infinity;
/// Whether there is an upper bound on the maximum height.
///
/// See also:
///
/// * [hasBoundedWidth], the equivalent for the horizontal axis.
/// * [hasInfiniteHeight], which describes whether the minimum height
/// constraint is infinite.
bool get hasBoundedHeight => maxHeight < double.infinity;
/// Whether the width constraint is infinite.
///
/// Such a constraint is used to indicate that a box should grow as large as
/// some other constraint (in this case, horizontally). If constraints are
/// infinite, then they must have other (non-infinite) constraints [enforce]d
/// upon them, or must be [tighten]ed, before they can be used to derive a
/// [Size] for a [RenderBox.size].
///
/// See also:
///
/// * [hasInfiniteHeight], the equivalent for the vertical axis.
/// * [hasBoundedWidth], which describes whether the maximum width
/// constraint is finite.
bool get hasInfiniteWidth => minWidth >= double.infinity;
/// Whether the height constraint is infinite.
///
/// Such a constraint is used to indicate that a box should grow as large as
/// some other constraint (in this case, vertically). If constraints are
/// infinite, then they must have other (non-infinite) constraints [enforce]d
/// upon them, or must be [tighten]ed, before they can be used to derive a
/// [Size] for a [RenderBox.size].
///
/// See also:
///
/// * [hasInfiniteWidth], the equivalent for the horizontal axis.
/// * [hasBoundedHeight], which describes whether the maximum height
/// constraint is finite.
bool get hasInfiniteHeight => minHeight >= double.infinity;
/// Whether the given size satisfies the constraints.
bool isSatisfiedBy(Size size) {
assert(debugAssertIsValid());
return (minWidth <= size.width) && (size.width <= maxWidth) &&
(minHeight <= size.height) && (size.height <= maxHeight);
}
/// Scales each constraint parameter by the given factor.
BoxConstraints operator*(double factor) {
return BoxConstraints(
minWidth: minWidth * factor,
maxWidth: maxWidth * factor,
minHeight: minHeight * factor,
maxHeight: maxHeight * factor,
);
}
/// Scales each constraint parameter by the inverse of the given factor.
BoxConstraints operator/(double factor) {
return BoxConstraints(
minWidth: minWidth / factor,
maxWidth: maxWidth / factor,
minHeight: minHeight / factor,
maxHeight: maxHeight / factor,
);
}
/// Scales each constraint parameter by the inverse of the given factor, rounded to the nearest integer.
BoxConstraints operator~/(double factor) {
return BoxConstraints(
minWidth: (minWidth ~/ factor).toDouble(),
maxWidth: (maxWidth ~/ factor).toDouble(),
minHeight: (minHeight ~/ factor).toDouble(),
maxHeight: (maxHeight ~/ factor).toDouble(),
);
}
/// Computes the remainder of each constraint parameter by the given value.
BoxConstraints operator%(double value) {
return BoxConstraints(
minWidth: minWidth % value,
maxWidth: maxWidth % value,
minHeight: minHeight % value,
maxHeight: maxHeight % value,
);
}
/// Linearly interpolate between two BoxConstraints.
///
/// If either is null, this function interpolates from a [BoxConstraints]
/// object whose fields are all set to 0.0.
///
/// {@macro dart.ui.shadow.lerp}
static BoxConstraints? lerp(BoxConstraints? a, BoxConstraints? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b! * t;
}
if (b == null) {
return a * (1.0 - t);
}
assert(a.debugAssertIsValid());
assert(b.debugAssertIsValid());
assert((a.minWidth.isFinite && b.minWidth.isFinite) || (a.minWidth == double.infinity && b.minWidth == double.infinity), 'Cannot interpolate between finite constraints and unbounded constraints.');
assert((a.maxWidth.isFinite && b.maxWidth.isFinite) || (a.maxWidth == double.infinity && b.maxWidth == double.infinity), 'Cannot interpolate between finite constraints and unbounded constraints.');
assert((a.minHeight.isFinite && b.minHeight.isFinite) || (a.minHeight == double.infinity && b.minHeight == double.infinity), 'Cannot interpolate between finite constraints and unbounded constraints.');
assert((a.maxHeight.isFinite && b.maxHeight.isFinite) || (a.maxHeight == double.infinity && b.maxHeight == double.infinity), 'Cannot interpolate between finite constraints and unbounded constraints.');
return BoxConstraints(
minWidth: a.minWidth.isFinite ? ui.lerpDouble(a.minWidth, b.minWidth, t)! : double.infinity,
maxWidth: a.maxWidth.isFinite ? ui.lerpDouble(a.maxWidth, b.maxWidth, t)! : double.infinity,
minHeight: a.minHeight.isFinite ? ui.lerpDouble(a.minHeight, b.minHeight, t)! : double.infinity,
maxHeight: a.maxHeight.isFinite ? ui.lerpDouble(a.maxHeight, b.maxHeight, t)! : double.infinity,
);
}
/// Returns whether the object's constraints are normalized.
/// Constraints are normalized if the minimums are less than or
/// equal to the corresponding maximums.
///
/// For example, a BoxConstraints object with a minWidth of 100.0
/// and a maxWidth of 90.0 is not normalized.
///
/// Most of the APIs on BoxConstraints expect the constraints to be
/// normalized and have undefined behavior when they are not. In
/// debug mode, many of these APIs will assert if the constraints
/// are not normalized.
@override
bool get isNormalized {
return minWidth >= 0.0 &&
minWidth <= maxWidth &&
minHeight >= 0.0 &&
minHeight <= maxHeight;
}
@override
bool debugAssertIsValid({
bool isAppliedConstraint = false,
InformationCollector? informationCollector,
}) {
assert(() {
void throwError(DiagnosticsNode message) {
throw FlutterError.fromParts(<DiagnosticsNode>[
message,
if (informationCollector != null) ...informationCollector(),
DiagnosticsProperty<BoxConstraints>('The offending constraints were', this, style: DiagnosticsTreeStyle.errorProperty),
]);
}
if (minWidth.isNaN || maxWidth.isNaN || minHeight.isNaN || maxHeight.isNaN) {
final List<String> affectedFieldsList = <String>[
if (minWidth.isNaN) 'minWidth',
if (maxWidth.isNaN) 'maxWidth',
if (minHeight.isNaN) 'minHeight',
if (maxHeight.isNaN) 'maxHeight',
];
assert(affectedFieldsList.isNotEmpty);
if (affectedFieldsList.length > 1) {
affectedFieldsList.add('and ${affectedFieldsList.removeLast()}');
}
String whichFields = '';
if (affectedFieldsList.length > 2) {
whichFields = affectedFieldsList.join(', ');
} else if (affectedFieldsList.length == 2) {
whichFields = affectedFieldsList.join(' ');
} else {
whichFields = affectedFieldsList.single;
}
throwError(ErrorSummary('BoxConstraints has ${affectedFieldsList.length == 1 ? 'a NaN value' : 'NaN values' } in $whichFields.'));
}
if (minWidth < 0.0 && minHeight < 0.0) {
throwError(ErrorSummary('BoxConstraints has both a negative minimum width and a negative minimum height.'));
}
if (minWidth < 0.0) {
throwError(ErrorSummary('BoxConstraints has a negative minimum width.'));
}
if (minHeight < 0.0) {
throwError(ErrorSummary('BoxConstraints has a negative minimum height.'));
}
if (maxWidth < minWidth && maxHeight < minHeight) {
throwError(ErrorSummary('BoxConstraints has both width and height constraints non-normalized.'));
}
if (maxWidth < minWidth) {
throwError(ErrorSummary('BoxConstraints has non-normalized width constraints.'));
}
if (maxHeight < minHeight) {
throwError(ErrorSummary('BoxConstraints has non-normalized height constraints.'));
}
if (isAppliedConstraint) {
if (minWidth.isInfinite && minHeight.isInfinite) {
throwError(ErrorSummary('BoxConstraints forces an infinite width and infinite height.'));
}
if (minWidth.isInfinite) {
throwError(ErrorSummary('BoxConstraints forces an infinite width.'));
}
if (minHeight.isInfinite) {
throwError(ErrorSummary('BoxConstraints forces an infinite height.'));
}
}
assert(isNormalized);
return true;
}());
return isNormalized;
}
/// Returns a box constraints that [isNormalized].
///
/// The returned [maxWidth] is at least as large as the [minWidth]. Similarly,
/// the returned [maxHeight] is at least as large as the [minHeight].
BoxConstraints normalize() {
if (isNormalized) {
return this;
}
final double minWidth = this.minWidth >= 0.0 ? this.minWidth : 0.0;
final double minHeight = this.minHeight >= 0.0 ? this.minHeight : 0.0;
return BoxConstraints(
minWidth: minWidth,
maxWidth: minWidth > maxWidth ? minWidth : maxWidth,
minHeight: minHeight,
maxHeight: minHeight > maxHeight ? minHeight : maxHeight,
);
}
@override
bool operator ==(Object other) {
assert(debugAssertIsValid());
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
assert(other is BoxConstraints && other.debugAssertIsValid());
return other is BoxConstraints
&& other.minWidth == minWidth
&& other.maxWidth == maxWidth
&& other.minHeight == minHeight
&& other.maxHeight == maxHeight;
}
@override
int get hashCode {
assert(debugAssertIsValid());
return Object.hash(minWidth, maxWidth, minHeight, maxHeight);
}
@override
String toString() {
final String annotation = isNormalized ? '' : '; NOT NORMALIZED';
if (minWidth == double.infinity && minHeight == double.infinity) {
return 'BoxConstraints(biggest$annotation)';
}
if (minWidth == 0 && maxWidth == double.infinity &&
minHeight == 0 && maxHeight == double.infinity) {
return 'BoxConstraints(unconstrained$annotation)';
}
String describe(double min, double max, String dim) {
if (min == max) {
return '$dim=${min.toStringAsFixed(1)}';
}
return '${min.toStringAsFixed(1)}<=$dim<=${max.toStringAsFixed(1)}';
}
final String width = describe(minWidth, maxWidth, 'w');
final String height = describe(minHeight, maxHeight, 'h');
return 'BoxConstraints($width, $height$annotation)';
}
}
/// Method signature for hit testing a [RenderBox].
///
/// Used by [BoxHitTestResult.addWithPaintTransform] to hit test children
/// of a [RenderBox].
///
/// See also:
///
/// * [RenderBox.hitTest], which documents more details around hit testing
/// [RenderBox]es.
typedef BoxHitTest = bool Function(BoxHitTestResult result, Offset position);
/// Method signature for hit testing a [RenderBox] with a manually
/// managed position (one that is passed out-of-band).
///
/// Used by [RenderSliverSingleBoxAdapter.hitTestBoxChild] to hit test
/// [RenderBox] children of a [RenderSliver].
///
/// See also:
///
/// * [RenderBox.hitTest], which documents more details around hit testing
/// [RenderBox]es.
typedef BoxHitTestWithOutOfBandPosition = bool Function(BoxHitTestResult result);
/// The result of performing a hit test on [RenderBox]es.
///
/// An instance of this class is provided to [RenderBox.hitTest] to record the
/// result of the hit test.
class BoxHitTestResult extends HitTestResult {
/// Creates an empty hit test result for hit testing on [RenderBox].
BoxHitTestResult() : super();
/// Wraps `result` to create a [HitTestResult] that implements the
/// [BoxHitTestResult] protocol for hit testing on [RenderBox]es.
///
/// This method is used by [RenderObject]s that adapt between the
/// [RenderBox]-world and the non-[RenderBox]-world to convert a (subtype of)
/// [HitTestResult] to a [BoxHitTestResult] for hit testing on [RenderBox]es.
///
/// The [HitTestEntry] instances added to the returned [BoxHitTestResult] are
/// also added to the wrapped `result` (both share the same underlying data
/// structure to store [HitTestEntry] instances).
///
/// See also:
///
/// * [HitTestResult.wrap], which turns a [BoxHitTestResult] back into a
/// generic [HitTestResult].
/// * [SliverHitTestResult.wrap], which turns a [BoxHitTestResult] into a
/// [SliverHitTestResult] for hit testing on [RenderSliver] children.
BoxHitTestResult.wrap(super.result) : super.wrap();
/// Transforms `position` to the local coordinate system of a child for
/// hit-testing the child.
///
/// The actual hit testing of the child needs to be implemented in the
/// provided `hitTest` callback, which is invoked with the transformed
/// `position` as argument.
///
/// The provided paint `transform` (which describes the transform from the
/// child to the parent in 3D) is processed by
/// [PointerEvent.removePerspectiveTransform] to remove the
/// perspective component and inverted before it is used to transform
/// `position` from the coordinate system of the parent to the system of the
/// child.
///
/// If `transform` is null it will be treated as the identity transform and
/// `position` is provided to the `hitTest` callback as-is. If `transform`
/// cannot be inverted, the `hitTest` callback is not invoked and false is
/// returned. Otherwise, the return value of the `hitTest` callback is
/// returned.
///
/// The `position` argument may be null, which will be forwarded to the
/// `hitTest` callback as-is. Using null as the position can be useful if
/// the child speaks a different hit test protocol than the parent and the
/// position is not required to do the actual hit testing in that protocol.
///
/// The function returns the return value of the `hitTest` callback.
///
/// {@tool snippet}
/// This method is used in [RenderBox.hitTestChildren] when the child and
/// parent don't share the same origin.
///
/// ```dart
/// abstract class RenderFoo extends RenderBox {
/// final Matrix4 _effectiveTransform = Matrix4.rotationZ(50);
///
/// @override
/// void applyPaintTransform(RenderBox child, Matrix4 transform) {
/// transform.multiply(_effectiveTransform);
/// }
///
/// @override
/// bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
/// return result.addWithPaintTransform(
/// transform: _effectiveTransform,
/// position: position,
/// hitTest: (BoxHitTestResult result, Offset position) {
/// return super.hitTestChildren(result, position: position);
/// },
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [addWithPaintOffset], which can be used for `transform`s that are just
/// simple matrix translations by an [Offset].
/// * [addWithRawTransform], which takes a transform matrix that is directly
/// used to transform the position without any pre-processing.
bool addWithPaintTransform({
required Matrix4? transform,
required Offset position,
required BoxHitTest hitTest,
}) {
if (transform != null) {
transform = Matrix4.tryInvert(PointerEvent.removePerspectiveTransform(transform));
if (transform == null) {
// Objects are not visible on screen and cannot be hit-tested.
return false;
}
}
return addWithRawTransform(
transform: transform,
position: position,
hitTest: hitTest,
);
}
/// Convenience method for hit testing children, that are translated by
/// an [Offset].
///
/// The actual hit testing of the child needs to be implemented in the
/// provided `hitTest` callback, which is invoked with the transformed
/// `position` as argument.
///
/// This method can be used as a convenience over [addWithPaintTransform] if
/// a parent paints a child at an `offset`.
///
/// A null value for `offset` is treated as if [Offset.zero] was provided.
///
/// The function returns the return value of the `hitTest` callback.
///
/// See also:
///
/// * [addWithPaintTransform], which takes a generic paint transform matrix and
/// documents the intended usage of this API in more detail.
bool addWithPaintOffset({
required Offset? offset,
required Offset position,
required BoxHitTest hitTest,
}) {
final Offset transformedPosition = offset == null ? position : position - offset;
if (offset != null) {
pushOffset(-offset);
}
final bool isHit = hitTest(this, transformedPosition);
if (offset != null) {
popTransform();
}
return isHit;
}
/// Transforms `position` to the local coordinate system of a child for
/// hit-testing the child.
///
/// The actual hit testing of the child needs to be implemented in the
/// provided `hitTest` callback, which is invoked with the transformed
/// `position` as argument.
///
/// Unlike [addWithPaintTransform], the provided `transform` matrix is used
/// directly to transform `position` without any pre-processing.
///
/// If `transform` is null it will be treated as the identity transform ad
/// `position` is provided to the `hitTest` callback as-is.
///
/// The function returns the return value of the `hitTest` callback.
///
/// See also:
///
/// * [addWithPaintTransform], which accomplishes the same thing, but takes a
/// _paint_ transform matrix.
bool addWithRawTransform({
required Matrix4? transform,
required Offset position,
required BoxHitTest hitTest,
}) {
final Offset transformedPosition = transform == null ?
position : MatrixUtils.transformPoint(transform, position);
if (transform != null) {
pushTransform(transform);
}
final bool isHit = hitTest(this, transformedPosition);
if (transform != null) {
popTransform();
}
return isHit;
}
/// Pass-through method for adding a hit test while manually managing
/// the position transformation logic.
///
/// The actual hit testing of the child needs to be implemented in the
/// provided `hitTest` callback. The position needs to be handled by
/// the caller.
///
/// The function returns the return value of the `hitTest` callback.
///
/// A `paintOffset`, `paintTransform`, or `rawTransform` should be
/// passed to the method to update the hit test stack.
///
/// * `paintOffset` has the semantics of the `offset` passed to
/// [addWithPaintOffset].
///
/// * `paintTransform` has the semantics of the `transform` passed to
/// [addWithPaintTransform], except that it must be invertible; it
/// is the responsibility of the caller to ensure this.
///
/// * `rawTransform` has the semantics of the `transform` passed to
/// [addWithRawTransform].
///
/// Exactly one of these must be non-null.
///
/// See also:
///
/// * [addWithPaintTransform], which takes a generic paint transform matrix and
/// documents the intended usage of this API in more detail.
bool addWithOutOfBandPosition({
Offset? paintOffset,
Matrix4? paintTransform,
Matrix4? rawTransform,
required BoxHitTestWithOutOfBandPosition hitTest,
}) {
assert(
(paintOffset == null && paintTransform == null && rawTransform != null) ||
(paintOffset == null && paintTransform != null && rawTransform == null) ||
(paintOffset != null && paintTransform == null && rawTransform == null),
'Exactly one transform or offset argument must be provided.',
);
if (paintOffset != null) {
pushOffset(-paintOffset);
} else if (rawTransform != null) {
pushTransform(rawTransform);
} else {
assert(paintTransform != null);
paintTransform = Matrix4.tryInvert(PointerEvent.removePerspectiveTransform(paintTransform!));
assert(paintTransform != null, 'paintTransform must be invertible.');
pushTransform(paintTransform!);
}
final bool isHit = hitTest(this);
popTransform();
return isHit;
}
}
/// A hit test entry used by [RenderBox].
class BoxHitTestEntry extends HitTestEntry<RenderBox> {
/// Creates a box hit test entry.
BoxHitTestEntry(super.target, this.localPosition);
/// The position of the hit test in the local coordinates of [target].
final Offset localPosition;
@override
String toString() => '${describeIdentity(target)}@$localPosition';
}
/// Parent data used by [RenderBox] and its subclasses.
///
/// {@tool dartpad}
/// Parent data is used to communicate to a render object about its
/// children. In this example, there are two render objects that perform
/// text layout. They use parent data to identify the kind of child they
/// are laying out, and space the children accordingly.
///
/// ** See code in examples/api/lib/rendering/box/parent_data.0.dart **
/// {@end-tool}
class BoxParentData extends ParentData {
/// The offset at which to paint the child in the parent's coordinate system.
Offset offset = Offset.zero;
@override
String toString() => 'offset=$offset';
}
/// Abstract [ParentData] subclass for [RenderBox] subclasses that want the
/// [ContainerRenderObjectMixin].
///
/// This is a convenience class that mixes in the relevant classes with
/// the relevant type arguments.
abstract class ContainerBoxParentData<ChildType extends RenderObject> extends BoxParentData with ContainerParentDataMixin<ChildType> { }
/// A wrapper that represents the baseline location of a `RenderBox`.
extension type const BaselineOffset(double? offset) {
/// A value that indicates that the associated `RenderBox` does not have any
/// baselines.
///
/// [BaselineOffset.noBaseline] is an identity element in most binary
/// operations involving two [BaselineOffset]s (such as [minOf]), for render
/// objects with no baselines typically do not contribute to the baseline
/// offset of their parents.
static const BaselineOffset noBaseline = BaselineOffset(null);
/// Returns a new baseline location that is `offset` pixels further away from
/// the origin than `this`, or unchanged if `this` is [noBaseline].
BaselineOffset operator +(double offset) {
final double? value = this.offset;
return BaselineOffset(value == null ? null : value + offset);
}
/// Compares this [BaselineOffset] and `other`, and returns whichever is closer
/// to the origin.
///
/// When both `this` and `other` are [noBaseline], this method returns
/// [noBaseline]. When one of them is [noBaseline], this method returns the
/// other oprand that's not [noBaseline].
BaselineOffset minOf(BaselineOffset other) {
return switch ((this, other)) {
(final double lhs?, final double rhs?) => lhs >= rhs ? other : this,
(final double lhs?, null) => BaselineOffset(lhs),
(null, final BaselineOffset rhs) => rhs,
};
}
}
/// An interface that represents a memoized layout computation run by a [RenderBox].
///
/// Each subclass is inhabited by a single object. Each object represents the
/// signature of a memoized layout computation run by [RenderBox]. For instance,
/// the [dryLayout] object of the [_DryLayout] subclass represents the signature
/// of the [RenderBox.computeDryLayout] method: it takes a [BoxConstraints] (the
/// subclass's `Input` type parameter) and returns a [Size] (the subclass's
/// `Output` type parameter).
///
/// Subclasses do not own their own cache storage. Rather, their [memoize]
/// implementation takes a `cacheStorage`. If a prior computation with the same
/// input valus has already been memoized in `cacheStorage`, it returns the
/// memoized value without running `computer`. Otherwise the method runs the
/// `computer` to compute the return value, and caches the result to
/// `cacheStorage`.
///
/// The layout cache storage is typically cleared in `markNeedsLayout`, but is
/// usually kept across [RenderObject.layout] calls because the incoming
/// [BoxConstraints] is always an input of every layout computation.
abstract class _CachedLayoutCalculation<Input extends Object, Output> {
static const _DryLayout dryLayout = _DryLayout();
static const _Baseline baseline = _Baseline();
Output memoize(_LayoutCacheStorage cacheStorage, Input input, Output Function(Input) computer);
// Debug information that will be used to generate the Timeline event for this type of calculation.
Map<String, String> debugFillTimelineArguments(Map<String, String> timelineArguments, Input input);
String eventLabel(RenderBox renderBox);
}
final class _DryLayout implements _CachedLayoutCalculation<BoxConstraints, Size> {
const _DryLayout();
@override
Size memoize(_LayoutCacheStorage cacheStorage, BoxConstraints input, Size Function(BoxConstraints) computer) {
return (cacheStorage._cachedDryLayoutSizes ??= <BoxConstraints, Size>{}).putIfAbsent(input, () => computer(input));
}
@override
Map<String, String> debugFillTimelineArguments(Map<String, String> timelineArguments, BoxConstraints input) {
return timelineArguments..['getDryLayout constraints'] = '$input';
}
@override
String eventLabel(RenderBox renderBox) => '${renderBox.runtimeType}.getDryLayout';
}
final class _Baseline implements _CachedLayoutCalculation<(BoxConstraints, TextBaseline), BaselineOffset> {
const _Baseline();
@override
BaselineOffset memoize(_LayoutCacheStorage cacheStorage, (BoxConstraints, TextBaseline) input, BaselineOffset Function((BoxConstraints, TextBaseline)) computer) {
final Map<BoxConstraints, BaselineOffset> cache = switch (input.$2) {
TextBaseline.alphabetic => cacheStorage._cachedAlphabeticBaseline ??= <BoxConstraints, BaselineOffset>{},
TextBaseline.ideographic => cacheStorage._cachedIdeoBaseline ??= <BoxConstraints, BaselineOffset>{},
};
BaselineOffset ifAbsent() => computer(input);
return cache.putIfAbsent(input.$1, ifAbsent);
}
@override
Map<String, String> debugFillTimelineArguments(Map<String, String> timelineArguments, (BoxConstraints, TextBaseline) input) {
return timelineArguments
..['baseline type'] = '${input.$2}'
..['constraints'] = '${input.$1}';
}
@override
String eventLabel(RenderBox renderBox) => '${renderBox.runtimeType}.getDryBaseline';
}
// Intrinsic dimension calculation that computes the intrinsic width given the
// max height, or the intrinsic height given the max width.
enum _IntrinsicDimension implements _CachedLayoutCalculation<double, double> {
minWidth, maxWidth, minHeight, maxHeight;
@override
double memoize(_LayoutCacheStorage cacheStorage, double input, double Function(double) computer) {
return (cacheStorage._cachedIntrinsicDimensions ??= <(_IntrinsicDimension, double), double>{})
.putIfAbsent((this, input), () => computer(input));
}
@override
Map<String, String> debugFillTimelineArguments(Map<String, String> timelineArguments, double input) {
return timelineArguments
..['intrinsics dimension'] = name
..['intrinsics argument'] = '$input';
}
@override
String eventLabel(RenderBox renderBox) => '${renderBox.runtimeType} intrinsics';
}
final class _LayoutCacheStorage {
Map<(_IntrinsicDimension, double), double>? _cachedIntrinsicDimensions;
Map<BoxConstraints, Size>? _cachedDryLayoutSizes;
Map<BoxConstraints, BaselineOffset>? _cachedAlphabeticBaseline;
Map<BoxConstraints, BaselineOffset>? _cachedIdeoBaseline;
// Returns a boolean indicating whether the cache storage has cached
// intrinsics / dry layout data in it.
bool clear() {
final bool hasCache = (_cachedDryLayoutSizes?.isNotEmpty ?? false)
|| (_cachedIntrinsicDimensions?.isNotEmpty ?? false)
|| (_cachedAlphabeticBaseline?.isNotEmpty ?? false)
|| (_cachedIdeoBaseline?.isNotEmpty ?? false);
if (hasCache) {
_cachedDryLayoutSizes?.clear();
_cachedIntrinsicDimensions?.clear();
_cachedAlphabeticBaseline?.clear();
_cachedIdeoBaseline?.clear();
}
return hasCache;
}
}
/// A render object in a 2D Cartesian coordinate system.
///
/// The [size] of each box is expressed as a width and a height. Each box has
/// its own coordinate system in which its upper left corner is placed at (0,
/// 0). The lower right corner of the box is therefore at (width, height). The
/// box contains all the points including the upper left corner and extending
/// to, but not including, the lower right corner.
///
/// Box layout is performed by passing a [BoxConstraints] object down the tree.
/// The box constraints establish a min and max value for the child's width and
/// height. In determining its size, the child must respect the constraints
/// given to it by its parent.
///
/// This protocol is sufficient for expressing a number of common box layout
/// data flows. For example, to implement a width-in-height-out data flow, call
/// your child's [layout] function with a set of box constraints with a tight
/// width value (and pass true for parentUsesSize). After the child determines
/// its height, use the child's height to determine your size.
///
/// ## Writing a RenderBox subclass
///
/// One would implement a new [RenderBox] subclass to describe a new layout
/// model, new paint model, new hit-testing model, or new semantics model, while
/// remaining in the Cartesian space defined by the [RenderBox] protocol.
///
/// To create a new protocol, consider subclassing [RenderObject] instead.
///
/// ### Constructors and properties of a new RenderBox subclass
///
/// The constructor will typically take a named argument for each property of
/// the class. The value is then passed to a private field of the class and the
/// constructor asserts its correctness (e.g. if it should not be null, it
/// asserts it's not null).
///
/// Properties have the form of a getter/setter/field group like the following:
///
/// ```dart
/// AxisDirection get axis => _axis;
/// AxisDirection _axis = AxisDirection.down; // or initialized in constructor
/// set axis(AxisDirection value) {
/// if (value == _axis) {
/// return;
/// }
/// _axis = value;
/// markNeedsLayout();
/// }
/// ```
///
/// The setter will typically finish with either a call to [markNeedsLayout], if
/// the layout uses this property, or [markNeedsPaint], if only the painter
/// function does. (No need to call both, [markNeedsLayout] implies
/// [markNeedsPaint].)
///
/// Consider layout and paint to be expensive; be conservative about calling
/// [markNeedsLayout] or [markNeedsPaint]. They should only be called if the
/// layout (or paint, respectively) has actually changed.
///
/// ### Children
///
/// If a render object is a leaf, that is, it cannot have any children, then
/// ignore this section. (Examples of leaf render objects are [RenderImage] and
/// [RenderParagraph].)
///
/// For render objects with children, there are four possible scenarios:
///
/// * A single [RenderBox] child. In this scenario, consider inheriting from
/// [RenderProxyBox] (if the render object sizes itself to match the child) or
/// [RenderShiftedBox] (if the child will be smaller than the box and the box
/// will align the child inside itself).
///
/// * A single child, but it isn't a [RenderBox]. Use the
/// [RenderObjectWithChildMixin] mixin.
///
/// * A single list of children. Use the [ContainerRenderObjectMixin] mixin.
///
/// * A more complicated child model.
///
/// #### Using RenderProxyBox
///
/// By default, a [RenderProxyBox] render object sizes itself to fit its child, or
/// to be as small as possible if there is no child; it passes all hit testing
/// and painting on to the child, and intrinsic dimensions and baseline
/// measurements similarly are proxied to the child.
///
/// A subclass of [RenderProxyBox] just needs to override the parts of the
/// [RenderBox] protocol that matter. For example, [RenderOpacity] just
/// overrides the paint method (and [alwaysNeedsCompositing] to reflect what the
/// paint method does, and the [visitChildrenForSemantics] method so that the
/// child is hidden from accessibility tools when it's invisible), and adds an
/// [RenderOpacity.opacity] field.
///
/// [RenderProxyBox] assumes that the child is the size of the parent and
/// positioned at 0,0. If this is not true, then use [RenderShiftedBox] instead.
///
/// See
/// [proxy_box.dart](https://github.com/flutter/flutter/blob/master/packages/flutter/lib/src/rendering/proxy_box.dart)
/// for examples of inheriting from [RenderProxyBox].
///
/// #### Using RenderShiftedBox
///
/// By default, a [RenderShiftedBox] acts much like a [RenderProxyBox] but
/// without assuming that the child is positioned at 0,0 (the actual position
/// recorded in the child's [parentData] field is used), and without providing a
/// default layout algorithm.
///
/// See
/// [shifted_box.dart](https://github.com/flutter/flutter/blob/master/packages/flutter/lib/src/rendering/shifted_box.dart)
/// for examples of inheriting from [RenderShiftedBox].
///
/// #### Kinds of children and child-specific data
///
/// A [RenderBox] doesn't have to have [RenderBox] children. One can use another
/// subclass of [RenderObject] for a [RenderBox]'s children. See the discussion
/// at [RenderObject].
///
/// Children can have additional data owned by the parent but stored on the
/// child using the [parentData] field. The class used for that data must
/// inherit from [ParentData]. The [setupParentData] method is used to
/// initialize the [parentData] field of a child when the child is attached.
///
/// By convention, [RenderBox] objects that have [RenderBox] children use the
/// [BoxParentData] class, which has a [BoxParentData.offset] field to store the
/// position of the child relative to the parent. ([RenderProxyBox] does not
/// need this offset and therefore is an exception to this rule.)
///
/// #### Using RenderObjectWithChildMixin
///
/// If a render object has a single child but it isn't a [RenderBox], then the
/// [RenderObjectWithChildMixin] class, which is a mixin that will handle the
/// boilerplate of managing a child, will be useful.
///
/// It's a generic class with one type argument, the type of the child. For
/// example, if you are building a `RenderFoo` class which takes a single
/// `RenderBar` child, you would use the mixin as follows:
///
/// ```dart
/// class RenderFoo extends RenderBox
/// with RenderObjectWithChildMixin<RenderBar> {
/// // ...
/// }
/// ```
///
/// Since the `RenderFoo` class itself is still a [RenderBox] in this case, you
/// still have to implement the [RenderBox] layout algorithm, as well as
/// features like intrinsics and baselines, painting, and hit testing.
///
/// #### Using ContainerRenderObjectMixin
///
/// If a render box can have multiple children, then the
/// [ContainerRenderObjectMixin] mixin can be used to handle the boilerplate. It
/// uses a linked list to model the children in a manner that is easy to mutate
/// dynamically and that can be walked efficiently. Random access is not
/// efficient in this model; if you need random access to the children consider
/// the next section on more complicated child models.
///
/// The [ContainerRenderObjectMixin] class has two type arguments. The first is
/// the type of the child objects. The second is the type for their
/// [parentData]. The class used for [parentData] must itself have the
/// [ContainerParentDataMixin] class mixed into it; this is where
/// [ContainerRenderObjectMixin] stores the linked list. A [ParentData] class
/// can extend [ContainerBoxParentData]; this is essentially
/// [BoxParentData] mixed with [ContainerParentDataMixin]. For example, if a
/// `RenderFoo` class wanted to have a linked list of [RenderBox] children, one
/// might create a `FooParentData` class as follows:
///
/// ```dart
/// class FooParentData extends ContainerBoxParentData<RenderBox> {
/// // (any fields you might need for these children)
/// }
/// ```
///
/// When using [ContainerRenderObjectMixin] in a [RenderBox], consider mixing in
/// [RenderBoxContainerDefaultsMixin], which provides a collection of utility
/// methods that implement common parts of the [RenderBox] protocol (such as
/// painting the children).
///
/// The declaration of the `RenderFoo` class itself would thus look like this:
///
/// ```dart
/// // continuing from previous example...
/// class RenderFoo extends RenderBox with
/// ContainerRenderObjectMixin<RenderBox, FooParentData>,
/// RenderBoxContainerDefaultsMixin<RenderBox, FooParentData> {
/// // ...
/// }
/// ```
///
/// When walking the children (e.g. during layout), the following pattern is
/// commonly used (in this case assuming that the children are all [RenderBox]
/// objects and that this render object uses `FooParentData` objects for its
/// children's [parentData] fields):
///
/// ```dart
/// // continuing from previous example...
/// RenderBox? child = firstChild;
/// while (child != null) {
/// final FooParentData childParentData = child.parentData! as FooParentData;
/// // ...operate on child and childParentData...
/// assert(child.parentData == childParentData);
/// child = childParentData.nextSibling;
/// }
/// ```
///
/// #### More complicated child models
///
/// Render objects can have more complicated models, for example a map of
/// children keyed on an enum, or a 2D grid of efficiently randomly-accessible
/// children, or multiple lists of children, etc. If a render object has a model
/// that can't be handled by the mixins above, it must implement the
/// [RenderObject] child protocol, as follows:
///
/// * Any time a child is removed, call [dropChild] with the child.
///
/// * Any time a child is added, call [adoptChild] with the child.
///
/// * Implement the [attach] method such that it calls [attach] on each child.
///
/// * Implement the [detach] method such that it calls [detach] on each child.
///
/// * Implement the [redepthChildren] method such that it calls [redepthChild]
/// on each child.
///
/// * Implement the [visitChildren] method such that it calls its argument for
/// each child, typically in paint order (back-most to front-most).
///
/// * Implement [debugDescribeChildren] such that it outputs a [DiagnosticsNode]
/// for each child.
///
/// Implementing these seven bullet points is essentially all that the two
/// aforementioned mixins do.
///
/// ### Layout
///
/// [RenderBox] classes implement a layout algorithm. They have a set of
/// constraints provided to them, and they size themselves based on those
/// constraints and whatever other inputs they may have (for example, their
/// children or properties).
///
/// When implementing a [RenderBox] subclass, one must make a choice. Does it
/// size itself exclusively based on the constraints, or does it use any other
/// information in sizing itself? An example of sizing purely based on the
/// constraints would be growing to fit the parent.
///
/// Sizing purely based on the constraints allows the system to make some
/// significant optimizations. Classes that use this approach should override
/// [sizedByParent] to return true, and then override [computeDryLayout] to
/// compute the [Size] using nothing but the constraints, e.g.:
///
/// ```dart
/// @override
/// bool get sizedByParent => true;
///
/// @override
/// Size computeDryLayout(BoxConstraints constraints) {
/// return constraints.smallest;
/// }
/// ```
///
/// Otherwise, the size is set in the [performLayout] function.
///
/// The [performLayout] function is where render boxes decide, if they are not
/// [sizedByParent], what [size] they should be, and also where they decide
/// where their children should be.
///
/// #### Layout of RenderBox children
///
/// The [performLayout] function should call the [layout] function of each (box)
/// child, passing it a [BoxConstraints] object describing the constraints
/// within which the child can render. Passing tight constraints (see
/// [BoxConstraints.isTight]) to the child will allow the rendering library to
/// apply some optimizations, as it knows that if the constraints are tight, the
/// child's dimensions cannot change even if the layout of the child itself
/// changes.
///
/// If the [performLayout] function will use the child's size to affect other
/// aspects of the layout, for example if the render box sizes itself around the
/// child, or positions several children based on the size of those children,
/// then it must specify the `parentUsesSize` argument to the child's [layout]
/// function, setting it to true.
///
/// This flag turns off some optimizations; algorithms that do not rely on the
/// children's sizes will be more efficient. (In particular, relying on the
/// child's [size] means that if the child is marked dirty for layout, the
/// parent will probably also be marked dirty for layout, unless the
/// [constraints] given by the parent to the child were tight constraints.)
///
/// For [RenderBox] classes that do not inherit from [RenderProxyBox], once they
/// have laid out their children, they should also position them, by setting the
/// [BoxParentData.offset] field of each child's [parentData] object.
///
/// #### Layout of non-RenderBox children
///
/// The children of a [RenderBox] do not have to be [RenderBox]es themselves. If
/// they use another protocol (as discussed at [RenderObject]), then instead of
/// [BoxConstraints], the parent would pass in the appropriate [Constraints]
/// subclass, and instead of reading the child's size, the parent would read
/// whatever the output of [layout] is for that layout protocol. The
/// `parentUsesSize` flag is still used to indicate whether the parent is going
/// to read that output, and optimizations still kick in if the child has tight
/// constraints (as defined by [Constraints.isTight]).
///
/// ### Painting
///
/// To describe how a render box paints, implement the [paint] method. It is
/// given a [PaintingContext] object and an [Offset]. The painting context
/// provides methods to affect the layer tree as well as a
/// [PaintingContext.canvas] which can be used to add drawing commands. The
/// canvas object should not be cached across calls to the [PaintingContext]'s
/// methods; every time a method on [PaintingContext] is called, there is a
/// chance that the canvas will change identity. The offset specifies the
/// position of the top left corner of the box in the coordinate system of the
/// [PaintingContext.canvas].
///
/// To draw text on a canvas, use a [TextPainter].
///
/// To draw an image to a canvas, use the [paintImage] method.
///
/// A [RenderBox] that uses methods on [PaintingContext] that introduce new
/// layers should override the [alwaysNeedsCompositing] getter and set it to
/// true. If the object sometimes does and sometimes does not, it can have that
/// getter return true in some cases and false in others. In that case, whenever
/// the return value would change, call [markNeedsCompositingBitsUpdate]. (This
/// is done automatically when a child is added or removed, so you don't have to
/// call it explicitly if the [alwaysNeedsCompositing] getter only changes value
/// based on the presence or absence of children.)
///
/// Anytime anything changes on the object that would cause the [paint] method
/// to paint something different (but would not cause the layout to change),
/// the object should call [markNeedsPaint].
///
/// #### Painting children
///
/// The [paint] method's `context` argument has a [PaintingContext.paintChild]
/// method, which should be called for each child that is to be painted. It
/// should be given a reference to the child, and an [Offset] giving the
/// position of the child relative to the parent.
///
/// If the [paint] method applies a transform to the painting context before
/// painting children (or generally applies an additional offset beyond the
/// offset it was itself given as an argument), then the [applyPaintTransform]
/// method should also be overridden. That method must adjust the matrix that it
/// is given in the same manner as it transformed the painting context and
/// offset before painting the given child. This is used by the [globalToLocal]
/// and [localToGlobal] methods.
///
/// #### Hit Tests
///
/// Hit testing for render boxes is implemented by the [hitTest] method. The
/// default implementation of this method defers to [hitTestSelf] and
/// [hitTestChildren]. When implementing hit testing, you can either override
/// these latter two methods, or ignore them and just override [hitTest].
///
/// The [hitTest] method itself is given an [Offset], and must return true if the
/// object or one of its children has absorbed the hit (preventing objects below
/// this one from being hit), or false if the hit can continue to other objects
/// below this one.
///
/// For each child [RenderBox], the [hitTest] method on the child should be
/// called with the same [HitTestResult] argument and with the point transformed
/// into the child's coordinate space (in the same manner that the
/// [applyPaintTransform] method would). The default implementation defers to
/// [hitTestChildren] to call the children. [RenderBoxContainerDefaultsMixin]
/// provides a [RenderBoxContainerDefaultsMixin.defaultHitTestChildren] method
/// that does this assuming that the children are axis-aligned, not transformed,
/// and positioned according to the [BoxParentData.offset] field of the
/// [parentData]; more elaborate boxes can override [hitTestChildren]
/// accordingly.
///
/// If the object is hit, then it should also add itself to the [HitTestResult]
/// object that is given as an argument to the [hitTest] method, using
/// [HitTestResult.add]. The default implementation defers to [hitTestSelf] to
/// determine if the box is hit. If the object adds itself before the children
/// can add themselves, then it will be as if the object was above the children.
/// If it adds itself after the children, then it will be as if it was below the
/// children. Entries added to the [HitTestResult] object should use the
/// [BoxHitTestEntry] class. The entries are subsequently walked by the system
/// in the order they were added, and for each entry, the target's [handleEvent]
/// method is called, passing in the [HitTestEntry] object.
///
/// Hit testing cannot rely on painting having happened.
///
/// ### Semantics
///
/// For a render box to be accessible, implement the
/// [describeApproximatePaintClip], [visitChildrenForSemantics], and
/// [describeSemanticsConfiguration] methods. The default implementations are
/// sufficient for objects that only affect layout, but nodes that represent
/// interactive components or information (diagrams, text, images, etc) should
/// provide more complete implementations. For more information, see the
/// documentation for these members.
///
/// ### Intrinsics and Baselines
///
/// The layout, painting, hit testing, and semantics protocols are common to all
/// render objects. [RenderBox] objects must implement two additional protocols:
/// intrinsic sizing and baseline measurements.
///
/// There are four methods to implement for intrinsic sizing, to compute the
/// minimum and maximum intrinsic width and height of the box. The documentation
/// for these methods discusses the protocol in detail:
/// [computeMinIntrinsicWidth], [computeMaxIntrinsicWidth],
/// [computeMinIntrinsicHeight], [computeMaxIntrinsicHeight].
///
/// Be sure to set [debugCheckIntrinsicSizes] to true in your unit tests if you
/// do override any of these methods, which will add additional checks to
/// help validate your implementation.
///
/// In addition, if the box has any children, it must implement
/// [computeDistanceToActualBaseline]. [RenderProxyBox] provides a simple
/// implementation that forwards to the child; [RenderShiftedBox] provides an
/// implementation that offsets the child's baseline information by the position
/// of the child relative to the parent. If you do not inherited from either of
/// these classes, however, you must implement the algorithm yourself.
abstract class RenderBox extends RenderObject {
@override
void setupParentData(covariant RenderObject child) {
if (child.parentData is! BoxParentData) {
child.parentData = BoxParentData();
}
}
final _LayoutCacheStorage _layoutCacheStorage = _LayoutCacheStorage();
static int _debugIntrinsicsDepth = 0;
Output _computeIntrinsics<Input extends Object, Output>(
_CachedLayoutCalculation<Input, Output> type,
Input input,
Output Function(Input) computer,
) {
assert(RenderObject.debugCheckingIntrinsics || !debugDoingThisResize); // performResize should not depend on anything except the incoming constraints
bool shouldCache = true;
assert(() {
// we don't want the checked-mode intrinsic tests to affect
// who gets marked dirty, etc.
shouldCache = !RenderObject.debugCheckingIntrinsics;
return true;
}());
return shouldCache ? _computeWithTimeline(type, input, computer) : computer(input);
}
Output _computeWithTimeline<Input extends Object, Output>(
_CachedLayoutCalculation<Input, Output> type,
Input input,
Output Function(Input) computer,
) {
Map<String, String>? debugTimelineArguments;
assert(() {
final Map<String, String> arguments = debugEnhanceLayoutTimelineArguments
? toDiagnosticsNode().toTimelineArguments()!
: <String, String>{};
debugTimelineArguments = type.debugFillTimelineArguments(arguments, input);
return true;
}());
if (!kReleaseMode) {
if (debugProfileLayoutsEnabled || _debugIntrinsicsDepth == 0) {
FlutterTimeline.startSync(type.eventLabel(this), arguments: debugTimelineArguments);
}
_debugIntrinsicsDepth += 1;
}
final Output result = type.memoize(_layoutCacheStorage, input, computer);
if (!kReleaseMode) {
_debugIntrinsicsDepth -= 1;
if (debugProfileLayoutsEnabled || _debugIntrinsicsDepth == 0) {
FlutterTimeline.finishSync();
}
}
return result;
}
/// Returns the minimum width that this box could be without failing to
/// correctly paint its contents within itself, without clipping.
///
/// The height argument may give a specific height to assume. The given height
/// can be infinite, meaning that the intrinsic width in an unconstrained
/// environment is being requested. The given height should never be negative
/// or null.
///
/// This function should only be called on one's children. Calling this
/// function couples the child with the parent so that when the child's layout
/// changes, the parent is notified (via [markNeedsLayout]).
///
/// Calling this function is expensive as it can result in O(N^2) behavior.
///
/// Do not override this method. Instead, implement [computeMinIntrinsicWidth].
@mustCallSuper
double getMinIntrinsicWidth(double height) {
assert(() {
if (height < 0.0) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The height argument to getMinIntrinsicWidth was negative.'),
ErrorDescription('The argument to getMinIntrinsicWidth must not be negative or null.'),
ErrorHint(
'If you perform computations on another height before passing it to '
'getMinIntrinsicWidth, consider using math.max() or double.clamp() '
'to force the value into the valid range.',
),
]);
}
return true;
}());
return _computeIntrinsics(_IntrinsicDimension.minWidth, height, computeMinIntrinsicWidth);
}
/// Computes the value returned by [getMinIntrinsicWidth]. Do not call this
/// function directly, instead, call [getMinIntrinsicWidth].
///
/// Override in subclasses that implement [performLayout]. This method should
/// return the minimum width that this box could be without failing to
/// correctly paint its contents within itself, without clipping.
///
/// If the layout algorithm is independent of the context (e.g. it always
/// tries to be a particular size), or if the layout algorithm is
/// width-in-height-out, or if the layout algorithm uses both the incoming
/// width and height constraints (e.g. it always sizes itself to
/// [BoxConstraints.biggest]), then the `height` argument should be ignored.
///
/// If the layout algorithm is strictly height-in-width-out, or is
/// height-in-width-out when the width is unconstrained, then the height
/// argument is the height to use.
///
/// The `height` argument will never be negative or null. It may be infinite.
///
/// If this algorithm depends on the intrinsic dimensions of a child, the
/// intrinsic dimensions of that child should be obtained using the functions
/// whose names start with `get`, not `compute`.
///
/// This function should never return a negative or infinite value.
///
/// Be sure to set [debugCheckIntrinsicSizes] to true in your unit tests if
/// you do override this method, which will add additional checks to help
/// validate your implementation.
///
/// ## Examples
///
/// ### Text
///
/// English text is the canonical example of a width-in-height-out algorithm.
/// The `height` argument is therefore ignored.
///
/// Consider the string "Hello World". The _maximum_ intrinsic width (as
/// returned from [computeMaxIntrinsicWidth]) would be the width of the string
/// with no line breaks.
///
/// The minimum intrinsic width would be the width of the widest word, "Hello"
/// or "World". If the text is rendered in an even narrower width, however, it
/// might still not overflow. For example, maybe the rendering would put a
/// line-break half-way through the words, as in "Hel⁞lo⁞Wor⁞ld". However,
/// this wouldn't be a _correct_ rendering, and [computeMinIntrinsicWidth] is
/// defined as returning the minimum width that the box could be without
/// failing to _correctly_ paint the contents within itself.
///
/// The minimum intrinsic _height_ for a given width _smaller_ than the
/// minimum intrinsic width could therefore be greater than the minimum
/// intrinsic height for the minimum intrinsic width.
///
/// ### Viewports (e.g. scrolling lists)
///
/// Some render boxes are intended to clip their children. For example, the
/// render box for a scrolling list might always size itself to its parents'
/// size (or rather, to the maximum incoming constraints), regardless of the
/// children's sizes, and then clip the children and position them based on
/// the current scroll offset.
///
/// The intrinsic dimensions in these cases still depend on the children, even
/// though the layout algorithm sizes the box in a way independent of the
/// children. It is the size that is needed to paint the box's contents (in
/// this case, the children) _without clipping_ that matters.
///
/// ### When the intrinsic dimensions cannot be known
///
/// There are cases where render objects do not have an efficient way to
/// compute their intrinsic dimensions. For example, it may be prohibitively
/// expensive to reify and measure every child of a lazy viewport (viewports
/// generally only instantiate the actually visible children), or the
/// dimensions may be computed by a callback about which the render object
/// cannot reason.
///
/// In such cases, it may be impossible (or at least impractical) to actually
/// return a valid answer. In such cases, the intrinsic functions should throw
/// when [RenderObject.debugCheckingIntrinsics] is false and asserts are
/// enabled, and return 0.0 otherwise.
///
/// See the implementations of [LayoutBuilder] or [RenderViewportBase] for
/// examples (in particular,
/// [RenderViewportBase.debugThrowIfNotCheckingIntrinsics]).
///
/// ### Aspect-ratio-driven boxes
///
/// Some boxes always return a fixed size based on the constraints. For these
/// boxes, the intrinsic functions should return the appropriate size when the
/// incoming `height` or `width` argument is finite, treating that as a tight
/// constraint in the respective direction and treating the other direction's
/// constraints as unbounded. This is because the definitions of
/// [computeMinIntrinsicWidth] and [computeMinIntrinsicHeight] are in terms of
/// what the dimensions _could be_, and such boxes can only be one size in
/// such cases.
///
/// When the incoming argument is not finite, then they should return the
/// actual intrinsic dimensions based on the contents, as any other box would.
///
/// See also:
///
/// * [computeMaxIntrinsicWidth], which computes the smallest width beyond
/// which increasing the width never decreases the preferred height.
@protected
double computeMinIntrinsicWidth(double height) {
return 0.0;
}
/// Returns the smallest width beyond which increasing the width never
/// decreases the preferred height. The preferred height is the value that
/// would be returned by [getMinIntrinsicHeight] for that width.
///
/// The height argument may give a specific height to assume. The given height
/// can be infinite, meaning that the intrinsic width in an unconstrained
/// environment is being requested. The given height should never be negative
/// or null.
///
/// This function should only be called on one's children. Calling this
/// function couples the child with the parent so that when the child's layout
/// changes, the parent is notified (via [markNeedsLayout]).
///
/// Calling this function is expensive as it can result in O(N^2) behavior.
///
/// Do not override this method. Instead, implement
/// [computeMaxIntrinsicWidth].
@mustCallSuper
double getMaxIntrinsicWidth(double height) {
assert(() {
if (height < 0.0) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The height argument to getMaxIntrinsicWidth was negative.'),
ErrorDescription('The argument to getMaxIntrinsicWidth must not be negative or null.'),
ErrorHint(
'If you perform computations on another height before passing it to '
'getMaxIntrinsicWidth, consider using math.max() or double.clamp() '
'to force the value into the valid range.',
),
]);
}
return true;
}());
return _computeIntrinsics(_IntrinsicDimension.maxWidth, height, computeMaxIntrinsicWidth);
}
/// Computes the value returned by [getMaxIntrinsicWidth]. Do not call this
/// function directly, instead, call [getMaxIntrinsicWidth].
///
/// Override in subclasses that implement [performLayout]. This should return
/// the smallest width beyond which increasing the width never decreases the
/// preferred height. The preferred height is the value that would be returned
/// by [computeMinIntrinsicHeight] for that width.
///
/// If the layout algorithm is strictly height-in-width-out, or is
/// height-in-width-out when the width is unconstrained, then this should
/// return the same value as [computeMinIntrinsicWidth] for the same height.
///
/// Otherwise, the height argument should be ignored, and the returned value
/// should be equal to or bigger than the value returned by
/// [computeMinIntrinsicWidth].
///
/// The `height` argument will never be negative or null. It may be infinite.
///
/// The value returned by this method might not match the size that the object
/// would actually take. For example, a [RenderBox] subclass that always
/// exactly sizes itself using [BoxConstraints.biggest] might well size itself
/// bigger than its max intrinsic size.
///
/// If this algorithm depends on the intrinsic dimensions of a child, the
/// intrinsic dimensions of that child should be obtained using the functions
/// whose names start with `get`, not `compute`.
///
/// This function should never return a negative or infinite value.
///
/// Be sure to set [debugCheckIntrinsicSizes] to true in your unit tests if
/// you do override this method, which will add additional checks to help
/// validate your implementation.
///
/// See also:
///
/// * [computeMinIntrinsicWidth], which has usage examples.
@protected
double computeMaxIntrinsicWidth(double height) {
return 0.0;
}
/// Returns the minimum height that this box could be without failing to
/// correctly paint its contents within itself, without clipping.
///
/// The width argument may give a specific width to assume. The given width
/// can be infinite, meaning that the intrinsic height in an unconstrained
/// environment is being requested. The given width should never be negative
/// or null.
///
/// This function should only be called on one's children. Calling this
/// function couples the child with the parent so that when the child's layout
/// changes, the parent is notified (via [markNeedsLayout]).
///
/// Calling this function is expensive as it can result in O(N^2) behavior.
///
/// Do not override this method. Instead, implement
/// [computeMinIntrinsicHeight].
@mustCallSuper
double getMinIntrinsicHeight(double width) {
assert(() {
if (width < 0.0) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The width argument to getMinIntrinsicHeight was negative.'),
ErrorDescription('The argument to getMinIntrinsicHeight must not be negative or null.'),
ErrorHint(
'If you perform computations on another width before passing it to '
'getMinIntrinsicHeight, consider using math.max() or double.clamp() '
'to force the value into the valid range.',
),
]);
}
return true;
}());
return _computeIntrinsics(_IntrinsicDimension.minHeight, width, computeMinIntrinsicHeight);
}
/// Computes the value returned by [getMinIntrinsicHeight]. Do not call this
/// function directly, instead, call [getMinIntrinsicHeight].
///
/// Override in subclasses that implement [performLayout]. Should return the
/// minimum height that this box could be without failing to correctly paint
/// its contents within itself, without clipping.
///
/// If the layout algorithm is independent of the context (e.g. it always
/// tries to be a particular size), or if the layout algorithm is
/// height-in-width-out, or if the layout algorithm uses both the incoming
/// height and width constraints (e.g. it always sizes itself to
/// [BoxConstraints.biggest]), then the `width` argument should be ignored.
///
/// If the layout algorithm is strictly width-in-height-out, or is
/// width-in-height-out when the height is unconstrained, then the width
/// argument is the width to use.
///
/// The `width` argument will never be negative or null. It may be infinite.
///
/// If this algorithm depends on the intrinsic dimensions of a child, the
/// intrinsic dimensions of that child should be obtained using the functions
/// whose names start with `get`, not `compute`.
///
/// This function should never return a negative or infinite value.
///
/// Be sure to set [debugCheckIntrinsicSizes] to true in your unit tests if
/// you do override this method, which will add additional checks to help
/// validate your implementation.
///
/// See also:
///
/// * [computeMinIntrinsicWidth], which has usage examples.
/// * [computeMaxIntrinsicHeight], which computes the smallest height beyond
/// which increasing the height never decreases the preferred width.
@protected
double computeMinIntrinsicHeight(double width) {
return 0.0;
}
/// Returns the smallest height beyond which increasing the height never
/// decreases the preferred width. The preferred width is the value that
/// would be returned by [getMinIntrinsicWidth] for that height.
///
/// The width argument may give a specific width to assume. The given width
/// can be infinite, meaning that the intrinsic height in an unconstrained
/// environment is being requested. The given width should never be negative
/// or null.
///
/// This function should only be called on one's children. Calling this
/// function couples the child with the parent so that when the child's layout
/// changes, the parent is notified (via [markNeedsLayout]).
///
/// Calling this function is expensive as it can result in O(N^2) behavior.
///
/// Do not override this method. Instead, implement
/// [computeMaxIntrinsicHeight].
@mustCallSuper
double getMaxIntrinsicHeight(double width) {
assert(() {
if (width < 0.0) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The width argument to getMaxIntrinsicHeight was negative.'),
ErrorDescription('The argument to getMaxIntrinsicHeight must not be negative or null.'),
ErrorHint(
'If you perform computations on another width before passing it to '
'getMaxIntrinsicHeight, consider using math.max() or double.clamp() '
'to force the value into the valid range.',
),
]);
}
return true;
}());
return _computeIntrinsics(_IntrinsicDimension.maxHeight, width, computeMaxIntrinsicHeight);
}
/// Computes the value returned by [getMaxIntrinsicHeight]. Do not call this
/// function directly, instead, call [getMaxIntrinsicHeight].
///
/// Override in subclasses that implement [performLayout]. Should return the
/// smallest height beyond which increasing the height never decreases the
/// preferred width. The preferred width is the value that would be returned
/// by [computeMinIntrinsicWidth] for that height.
///
/// If the layout algorithm is strictly width-in-height-out, or is
/// width-in-height-out when the height is unconstrained, then this should
/// return the same value as [computeMinIntrinsicHeight] for the same width.
///
/// Otherwise, the width argument should be ignored, and the returned value
/// should be equal to or bigger than the value returned by
/// [computeMinIntrinsicHeight].
///
/// The `width` argument will never be negative or null. It may be infinite.
///
/// The value returned by this method might not match the size that the object
/// would actually take. For example, a [RenderBox] subclass that always
/// exactly sizes itself using [BoxConstraints.biggest] might well size itself
/// bigger than its max intrinsic size.
///
/// If this algorithm depends on the intrinsic dimensions of a child, the
/// intrinsic dimensions of that child should be obtained using the functions
/// whose names start with `get`, not `compute`.
///
/// This function should never return a negative or infinite value.
///
/// Be sure to set [debugCheckIntrinsicSizes] to true in your unit tests if
/// you do override this method, which will add additional checks to help
/// validate your implementation.
///
/// See also:
///
/// * [computeMinIntrinsicWidth], which has usage examples.
@protected
double computeMaxIntrinsicHeight(double width) {
return 0.0;
}
/// Returns the [Size] that this [RenderBox] would like to be given the
/// provided [BoxConstraints].
///
/// The size returned by this method is guaranteed to be the same size that
/// this [RenderBox] computes for itself during layout given the same
/// constraints.
///
/// This function should only be called on one's children. Calling this
/// function couples the child with the parent so that when the child's layout
/// changes, the parent is notified (via [markNeedsLayout]).
///
/// This layout is called "dry" layout as opposed to the regular "wet" layout
/// run performed by [performLayout] because it computes the desired size for
/// the given constraints without changing any internal state.
///
/// Calling this function is expensive as it can result in O(N^2) behavior.
///
/// Do not override this method. Instead, implement [computeDryLayout].
@mustCallSuper
Size getDryLayout(covariant BoxConstraints constraints) {
return _computeIntrinsics(_CachedLayoutCalculation.dryLayout, constraints, _computeDryLayout);
}
bool _computingThisDryLayout = false;
Size _computeDryLayout(BoxConstraints constraints) {
assert(() {
assert(!_computingThisDryLayout);
_computingThisDryLayout = true;
return true;
}());
final Size result = computeDryLayout(constraints);
assert(() {
assert(_computingThisDryLayout);
_computingThisDryLayout = false;
return true;
}());
return result;
}
/// Computes the value returned by [getDryLayout]. Do not call this
/// function directly, instead, call [getDryLayout].
///
/// Override in subclasses that implement [performLayout] or [performResize]
/// or when setting [sizedByParent] to true without overriding
/// [performResize]. This method should return the [Size] that this
/// [RenderBox] would like to be given the provided [BoxConstraints].
///
/// The size returned by this method must match the [size] that the
/// [RenderBox] will compute for itself in [performLayout] (or
/// [performResize], if [sizedByParent] is true).
///
/// If this algorithm depends on the size of a child, the size of that child
/// should be obtained using its [getDryLayout] method.
///
/// This layout is called "dry" layout as opposed to the regular "wet" layout
/// run performed by [performLayout] because it computes the desired size for
/// the given constraints without changing any internal state.
///
/// ### When the size cannot be known
///
/// There are cases where render objects do not have an efficient way to
/// compute their size. For example, the size may computed by a callback about
/// which the render object cannot reason.
///
/// In such cases, it may be impossible (or at least impractical) to actually
/// return a valid answer. In such cases, the function should call
/// [debugCannotComputeDryLayout] from within an assert and return a dummy
/// value of `const Size(0, 0)`.
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
assert(debugCannotComputeDryLayout(
error: FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The ${objectRuntimeType(this, 'RenderBox')} class does not implement "computeDryLayout".'),
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not\n'
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]),
));
return Size.zero;
}
/// Returns the distance from the top of the box to the first baseline of the
/// box's contents for the given `constraints`, or `null` if this [RenderBox]
/// does not have any baselines.
///
/// This method calls [computeDryBaseline] under the hood and caches the result.
/// [RenderBox] subclasses typically don't overridden [getDryBaseline]. Instead,
/// consider overriding [computeDryBaseline] such that it returns a baseline
/// location that is consistent with [getDistanceToActualBaseline]. See the
/// documentation for the [computeDryBaseline] method for more details.
///
/// This method is usually called by the [computeDryBaseline] or the
/// [computeDryLayout] implementation of a parent [RenderBox] to get the
/// baseline location of a [RenderBox] child. Unlike [getDistanceToBaseline],
/// this method takes a [BoxConstraints] as an argument and computes the
/// baseline location as if the [RenderBox] was laid out by the parent using
/// that [BoxConstraints].
///
/// The "dry" in the method name means this method, like [getDryLayout], has
/// no observable side effects when called, as opposed to "wet" layout methods
/// such as [performLayout] (which changes this [RenderBox]'s [size], and the
/// offsets of its children if any). Since this method does not depend on the
/// current layout, unlike [getDistanceToBaseline], it's ok to call this method
/// when this [RenderBox]'s layout is outdated.
///
/// Similar to the intrinsic width/height and [getDryLayout], calling this
/// function in [performLayout] is expensive, as it can result in O(N^2) layout
/// performance, where N is the number of render objects in the render subtree.
/// Typically this method should be only called by the parent [RenderBox]'s
/// [computeDryBaseline] or [computeDryLayout] implementation.
double? getDryBaseline(covariant BoxConstraints constraints, TextBaseline baseline) {
final double? baselineOffset = _computeIntrinsics(_CachedLayoutCalculation.baseline, (constraints, baseline), _computeDryBaseline).offset;
// This assert makes sure computeDryBaseline always gets called in debug mode,
// in case the computeDryBaseline implementation invokes debugCannotComputeDryLayout.
assert(baselineOffset == computeDryBaseline(constraints, baseline));
return baselineOffset;
}
bool _computingThisDryBaseline = false;
BaselineOffset _computeDryBaseline((BoxConstraints, TextBaseline) pair) {
assert(() {
assert(!_computingThisDryBaseline);
_computingThisDryBaseline = true;
return true;
}());
final BaselineOffset result = BaselineOffset(computeDryBaseline(pair.$1, pair.$2));
assert(() {
assert(_computingThisDryBaseline);
_computingThisDryBaseline = false;
return true;
}());
return result;
}
/// Computes the value returned by [getDryBaseline].
///
/// This method is for overriding only and shouldn't be called directly. To
/// get this [RenderBox]'s speculative baseline location for the given
/// `constraints`, call [getDryBaseline] instead.
///
/// The "dry" in the method name means the implementation must not produce
/// observable side effects when called. For example, it must not change the
/// [size] of the [RenderBox], or its children's paint offsets, otherwise that
/// would results in UI changes when [paint] is called, or hit-testing behavior
/// changes when [hitTest] is called. Moreover, accessing the current layout
/// of this [RenderBox] or child [RenderBox]es (including accessing [size], or
/// `child.size`) usually indicates a bug in the implementation, as the current
/// layout is typically calculated using a set of [BoxConstraints] that's
/// different from the `constraints` given as the first parameter. To get the
/// size of this [RenderBox] or a child [RenderBox] in this method's
/// implementation, use the [getDryLayout] method instead.
///
/// The implementation must return a value that represents the distance from
/// the top of the box to the first baseline of the box's contents, for the
/// given `constraints`, or `null` if the [RenderBox] has no baselines. It's
/// the same exact value [RenderBox.computeDistanceToActualBaseline] would
/// return, when this [RenderBox] was laid out at `constraints` in the same
/// exact state.
///
/// Not all [RenderBox]es support dry baseline computation. For example, to
/// compute the dry baseline of a [LayoutBuilder], its `builder` may have to
/// be called with different constraints, which may have side effects such as
/// updating the widget tree, violating the "dry" contract. In such cases the
/// [RenderBox] must call [debugCannotComputeDryLayout] in an assert, and
/// return a dummy baseline offset value (such as `null`).
@protected
double? computeDryBaseline(covariant BoxConstraints constraints, TextBaseline baseline) {
assert(debugCannotComputeDryLayout(
error: FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The ${objectRuntimeType(this, 'RenderBox')} class does not implement "computeDryBaseline".'),
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not\n'
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]),
));
return null;
}
static bool _debugDryLayoutCalculationValid = true;
/// Called from [computeDryLayout] or [computeDryBaseline] within an assert if
/// the given [RenderBox] subclass does not support calculating a dry layout.
///
/// When asserts are enabled and [debugCheckingIntrinsics] is not true, this
/// method will either throw the provided [FlutterError] or it will create and
/// throw a [FlutterError] with the provided `reason`. Otherwise, it will
/// return true.
///
/// One of the arguments has to be provided.
///
/// See also:
///
/// * [computeDryLayout], which lists some reasons why it may not be feasible
/// to compute the dry layout.
bool debugCannotComputeDryLayout({String? reason, FlutterError? error}) {
assert((reason == null) != (error == null));
assert(() {
if (!RenderObject.debugCheckingIntrinsics) {
if (reason != null) {
assert(error == null);
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The ${objectRuntimeType(this, 'RenderBox')} class does not support dry layout.'),
if (reason.isNotEmpty) ErrorDescription(reason),
]);
}
assert(error != null);
throw error!;
}
_debugDryLayoutCalculationValid = false;
return true;
}());
return true;
}
/// Whether this render object has undergone layout and has a [size].
bool get hasSize => _size != null;
/// The size of this render box computed during layout.
///
/// This value is stale whenever this object is marked as needing layout.
/// During [performLayout], do not read the size of a child unless you pass
/// true for parentUsesSize when calling the child's [layout] function.
///
/// The size of a box should be set only during the box's [performLayout] or
/// [performResize] functions. If you wish to change the size of a box outside
/// of those functions, call [markNeedsLayout] instead to schedule a layout of
/// the box.
Size get size {
assert(hasSize, 'RenderBox was not laid out: $this');
assert(() {
final Size? size = _size;
if (size is _DebugSize) {
assert(size._owner == this);
final RenderObject? parent = this.parent;
// Whether the size getter is accessed during layout (but not in a
// layout callback).
final bool doingRegularLayout = !(RenderObject.debugActiveLayout?.debugDoingThisLayoutWithCallback ?? true);
final bool sizeAccessAllowed = !doingRegularLayout
|| debugDoingThisResize
|| debugDoingThisLayout
|| _computingThisDryLayout
|| RenderObject.debugActiveLayout == parent && size._canBeUsedByParent;
assert(sizeAccessAllowed,
'RenderBox.size accessed beyond the scope of resize, layout, or '
'permitted parent access. RenderBox can always access its own size, '
'otherwise, the only object that is allowed to read RenderBox.size '
'is its parent, if they have said they will. It you hit this assert '
'trying to access a child\'s size, pass "parentUsesSize: true" to '
"that child's layout() in ${objectRuntimeType(this, 'RenderBox')}.performLayout.",
);
final RenderBox? renderBoxDoingDryBaseline = _computingThisDryBaseline
? this
: (parent is RenderBox && parent._computingThisDryBaseline ? parent : null);
assert(renderBoxDoingDryBaseline == null,
'RenderBox.size accessed in '
'${objectRuntimeType(renderBoxDoingDryBaseline, 'RenderBox')}.computeDryBaseline.'
'The computeDryBaseline method must not access '
'${renderBoxDoingDryBaseline == this ? "the RenderBox's own size" : "the size of its child"},'
"because it's established in peformLayout or peformResize using different BoxConstraints."
);
assert(size == _size);
}
return true;
}());
return _size ?? (throw StateError('RenderBox was not laid out: $runtimeType#${shortHash(this)}'));
}
Size? _size;
/// Setting the size, in debug mode, triggers some analysis of the render box,
/// as implemented by [debugAssertDoesMeetConstraints], including calling the intrinsic
/// sizing methods and checking that they meet certain invariants.
@protected
set size(Size value) {
assert(!(debugDoingThisResize && debugDoingThisLayout));
assert(sizedByParent || !debugDoingThisResize);
assert(() {
if ((sizedByParent && debugDoingThisResize) ||
(!sizedByParent && debugDoingThisLayout)) {
return true;
}
assert(!debugDoingThisResize);
final List<DiagnosticsNode> information = <DiagnosticsNode>[
ErrorSummary('RenderBox size setter called incorrectly.'),
];
if (debugDoingThisLayout) {
assert(sizedByParent);
information.add(ErrorDescription('It appears that the size setter was called from performLayout().'));
} else {
information.add(ErrorDescription(
'The size setter was called from outside layout (neither performResize() nor performLayout() were being run for this object).',
));
if (owner != null && owner!.debugDoingLayout) {
information.add(ErrorDescription('Only the object itself can set its size. It is a contract violation for other objects to set it.'));
}
}
if (sizedByParent) {
information.add(ErrorDescription('Because this RenderBox has sizedByParent set to true, it must set its size in performResize().'));
} else {
information.add(ErrorDescription('Because this RenderBox has sizedByParent set to false, it must set its size in performLayout().'));
}
throw FlutterError.fromParts(information);
}());
assert(() {
value = debugAdoptSize(value);
return true;
}());
_size = value;
assert(() {
debugAssertDoesMeetConstraints();
return true;
}());
}
/// Claims ownership of the given [Size].
///
/// In debug mode, the [RenderBox] class verifies that [Size] objects obtained
/// from other [RenderBox] objects are only used according to the semantics of
/// the [RenderBox] protocol, namely that a [Size] from a [RenderBox] can only
/// be used by its parent, and then only if `parentUsesSize` was set.
///
/// Sometimes, a [Size] that can validly be used ends up no longer being valid
/// over time. The common example is a [Size] taken from a child that is later
/// removed from the parent. In such cases, this method can be called to first
/// check whether the size can legitimately be used, and if so, to then create
/// a new [Size] that can be used going forward, regardless of what happens to
/// the original owner.
Size debugAdoptSize(Size value) {
Size result = value;
assert(() {
if (value is _DebugSize) {
if (value._owner != this) {
if (value._owner.parent != this) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The size property was assigned a size inappropriately.'),
describeForError('The following render object'),
value._owner.describeForError('...was assigned a size obtained from'),
ErrorDescription(
'However, this second render object is not, or is no longer, a '
'child of the first, and it is therefore a violation of the '
'RenderBox layout protocol to use that size in the layout of the '
'first render object.',
),
ErrorHint(
'If the size was obtained at a time where it was valid to read '
'the size (because the second render object above was a child '
'of the first at the time), then it should be adopted using '
'debugAdoptSize at that time.',
),
ErrorHint(
'If the size comes from a grandchild or a render object from an '
'entirely different part of the render tree, then there is no '
'way to be notified when the size changes and therefore attempts '
'to read that size are almost certainly a source of bugs. A different '
'approach should be used.',
),
]);
}
if (!value._canBeUsedByParent) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary("A child's size was used without setting parentUsesSize."),
describeForError('The following render object'),
value._owner.describeForError('...was assigned a size obtained from its child'),
ErrorDescription(
'However, when the child was laid out, the parentUsesSize argument '
'was not set or set to false. Subsequently this transpired to be '
'inaccurate: the size was nonetheless used by the parent.\n'
'It is important to tell the framework if the size will be used or not '
'as several important performance optimizations can be made if the '
'size will not be used by the parent.',
),
]);
}
}
}
result = _DebugSize(value, this, debugCanParentUseSize);
return true;
}());
return result;
}
@override
Rect get semanticBounds => Offset.zero & size;
@override
void debugResetSize() {
// updates the value of size._canBeUsedByParent if necessary
size = size; // ignore: no_self_assignments
}
static bool _debugDoingBaseline = false;
static bool _debugSetDoingBaseline(bool value) {
_debugDoingBaseline = value;
return true;
}
/// Returns the distance from the y-coordinate of the position of the box to
/// the y-coordinate of the first given baseline in the box's contents.
///
/// Used by certain layout models to align adjacent boxes on a common
/// baseline, regardless of padding, font size differences, etc. If there is
/// no baseline, this function returns the distance from the y-coordinate of
/// the position of the box to the y-coordinate of the bottom of the box
/// (i.e., the height of the box) unless the caller passes true
/// for `onlyReal`, in which case the function returns null.
///
/// Only call this function after calling [layout] on this box. You
/// are only allowed to call this from the parent of this box during
/// that parent's [performLayout] or [paint] functions.
///
/// When implementing a [RenderBox] subclass, to override the baseline
/// computation, override [computeDistanceToActualBaseline].
///
/// See also:
///
/// * [getDryBaseline], which returns the baseline location of this
/// [RenderBox] at a certain [BoxConstraints].
double? getDistanceToBaseline(TextBaseline baseline, { bool onlyReal = false }) {
assert(!_debugDoingBaseline, 'Please see the documentation for computeDistanceToActualBaseline for the required calling conventions of this method.');
assert(!debugNeedsLayout || RenderObject.debugCheckingIntrinsics);
assert(RenderObject.debugCheckingIntrinsics || switch (owner!) {
PipelineOwner(debugDoingLayout: true) => RenderObject.debugActiveLayout == parent && parent!.debugDoingThisLayout,
PipelineOwner(debugDoingPaint: true) => RenderObject.debugActivePaint == parent && parent!.debugDoingThisPaint || (RenderObject.debugActivePaint == this && debugDoingThisPaint),
PipelineOwner() => false,
});
assert(_debugSetDoingBaseline(true));
final double? result;
try {
result = getDistanceToActualBaseline(baseline);
} finally {
assert(_debugSetDoingBaseline(false));
}
if (result == null && !onlyReal) {
return size.height;
}
return result;
}
/// Calls [computeDistanceToActualBaseline] and caches the result.
///
/// This function must only be called from [getDistanceToBaseline] and
/// [computeDistanceToActualBaseline]. Do not call this function directly from
/// outside those two methods.
@protected
@mustCallSuper
double? getDistanceToActualBaseline(TextBaseline baseline) {
assert(_debugDoingBaseline, 'Please see the documentation for computeDistanceToActualBaseline for the required calling conventions of this method.');
return _computeIntrinsics(
_CachedLayoutCalculation.baseline,
(constraints, baseline),
((BoxConstraints, TextBaseline) pair) => BaselineOffset(computeDistanceToActualBaseline(pair.$2)),
).offset;
}
/// Returns the distance from the y-coordinate of the position of the box to
/// the y-coordinate of the first given baseline in the box's contents, if
/// any, or null otherwise.
///
/// Do not call this function directly. If you need to know the baseline of a
/// child from an invocation of [performLayout] or [paint], call
/// [getDistanceToBaseline].
///
/// Subclasses should override this method to supply the distances to their
/// baselines. When implementing this method, there are generally three
/// strategies:
///
/// * For classes that use the [ContainerRenderObjectMixin] child model,
/// consider mixing in the [RenderBoxContainerDefaultsMixin] class and
/// using
/// [RenderBoxContainerDefaultsMixin.defaultComputeDistanceToFirstActualBaseline].
///
/// * For classes that define a particular baseline themselves, return that
/// value directly.
///
/// * For classes that have a child to which they wish to defer the
/// computation, call [getDistanceToActualBaseline] on the child (not
/// [computeDistanceToActualBaseline], the internal implementation, and not
/// [getDistanceToBaseline], the public entry point for this API).
@protected
double? computeDistanceToActualBaseline(TextBaseline baseline) {
assert(_debugDoingBaseline, 'Please see the documentation for computeDistanceToActualBaseline for the required calling conventions of this method.');
return null;
}
/// The box constraints most recently received from the parent.
@override
BoxConstraints get constraints => super.constraints as BoxConstraints;
@override
void debugAssertDoesMeetConstraints() {
assert(() {
if (!hasSize) {
final DiagnosticsNode contract;
if (sizedByParent) {
contract = ErrorDescription('Because this RenderBox has sizedByParent set to true, it must set its size in performResize().');
} else {
contract = ErrorDescription('Because this RenderBox has sizedByParent set to false, it must set its size in performLayout().');
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('RenderBox did not set its size during layout.'),
contract,
ErrorDescription('It appears that this did not happen; layout completed, but the size property is still null.'),
DiagnosticsProperty<RenderBox>('The RenderBox in question is', this, style: DiagnosticsTreeStyle.errorProperty),
]);
}
// verify that the size is not infinite
if (!_size!.isFinite) {
final List<DiagnosticsNode> information = <DiagnosticsNode>[
ErrorSummary('$runtimeType object was given an infinite size during layout.'),
ErrorDescription(
'This probably means that it is a render object that tries to be '
'as big as possible, but it was put inside another render object '
'that allows its children to pick their own size.',
),
];
if (!constraints.hasBoundedWidth) {
RenderBox node = this;
while (!node.constraints.hasBoundedWidth && node.parent is RenderBox) {
node = node.parent! as RenderBox;
}
information.add(node.describeForError('The nearest ancestor providing an unbounded width constraint is'));
}
if (!constraints.hasBoundedHeight) {
RenderBox node = this;
while (!node.constraints.hasBoundedHeight && node.parent is RenderBox) {
node = node.parent! as RenderBox;
}
information.add(node.describeForError('The nearest ancestor providing an unbounded height constraint is'));
}
throw FlutterError.fromParts(<DiagnosticsNode>[
...information,
DiagnosticsProperty<BoxConstraints>('The constraints that applied to the $runtimeType were', constraints, style: DiagnosticsTreeStyle.errorProperty),
DiagnosticsProperty<Size>('The exact size it was given was', _size, style: DiagnosticsTreeStyle.errorProperty),
ErrorHint('See https://flutter.dev/docs/development/ui/layout/box-constraints for more information.'),
]);
}
// verify that the size is within the constraints
if (!constraints.isSatisfiedBy(_size!)) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType does not meet its constraints.'),
DiagnosticsProperty<BoxConstraints>('Constraints', constraints, style: DiagnosticsTreeStyle.errorProperty),
DiagnosticsProperty<Size>('Size', _size, style: DiagnosticsTreeStyle.errorProperty),
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not '
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]);
}
if (debugCheckIntrinsicSizes) {
// verify that the intrinsics are sane
assert(!RenderObject.debugCheckingIntrinsics);
RenderObject.debugCheckingIntrinsics = true;
final List<DiagnosticsNode> failures = <DiagnosticsNode>[];
double testIntrinsic(double Function(double extent) function, String name, double constraint) {
final double result = function(constraint);
if (result < 0) {
failures.add(ErrorDescription(' * $name($constraint) returned a negative value: $result'));
}
if (!result.isFinite) {
failures.add(ErrorDescription(' * $name($constraint) returned a non-finite value: $result'));
}
return result;
}
void testIntrinsicsForValues(double Function(double extent) getMin, double Function(double extent) getMax, String name, double constraint) {
final double min = testIntrinsic(getMin, 'getMinIntrinsic$name', constraint);
final double max = testIntrinsic(getMax, 'getMaxIntrinsic$name', constraint);
if (min > max) {
failures.add(ErrorDescription(' * getMinIntrinsic$name($constraint) returned a larger value ($min) than getMaxIntrinsic$name($constraint) ($max)'));
}
}
testIntrinsicsForValues(getMinIntrinsicWidth, getMaxIntrinsicWidth, 'Width', double.infinity);
testIntrinsicsForValues(getMinIntrinsicHeight, getMaxIntrinsicHeight, 'Height', double.infinity);
if (constraints.hasBoundedWidth) {
testIntrinsicsForValues(getMinIntrinsicWidth, getMaxIntrinsicWidth, 'Width', constraints.maxHeight);
}
if (constraints.hasBoundedHeight) {
testIntrinsicsForValues(getMinIntrinsicHeight, getMaxIntrinsicHeight, 'Height', constraints.maxWidth);
}
// TODO(ianh): Test that values are internally consistent in more ways than the above.
RenderObject.debugCheckingIntrinsics = false;
if (failures.isNotEmpty) {
// TODO(jacobr): consider nesting the failures object so it is collapsible.
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The intrinsic dimension methods of the $runtimeType class returned values that violate the intrinsic protocol contract.'),
ErrorDescription('The following ${failures.length > 1 ? "failures" : "failure"} was detected:'), // should this be tagged as an error or not?
...failures,
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not\n'
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]);
}
// Checking that getDryLayout computes the same size.
_debugDryLayoutCalculationValid = true;
RenderObject.debugCheckingIntrinsics = true;
final Size dryLayoutSize;
try {
dryLayoutSize = getDryLayout(constraints);
} finally {
RenderObject.debugCheckingIntrinsics = false;
}
if (_debugDryLayoutCalculationValid && dryLayoutSize != size) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The size given to the ${objectRuntimeType(this, 'RenderBox')} class differs from the size computed by computeDryLayout.'),
ErrorDescription(
'The size computed in ${sizedByParent ? 'performResize' : 'performLayout'} '
'is $size, which is different from $dryLayoutSize, which was computed by computeDryLayout.',
),
ErrorDescription(
'The constraints used were $constraints.',
),
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not\n'
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
),
]);
}
}
return true;
}());
}
void _debugVerifyDryBaselines() {
assert(() {
final List<DiagnosticsNode> messages = <DiagnosticsNode>[
ErrorDescription(
'The constraints used were $constraints.',
),
ErrorHint(
'If you are not writing your own RenderBox subclass, then this is not\n'
'your fault. Contact support: https://github.com/flutter/flutter/issues/new?template=2_bug.yml',
)
];
for (final TextBaseline baseline in TextBaseline.values) {
assert(!RenderObject.debugCheckingIntrinsics);
RenderObject.debugCheckingIntrinsics = true;
_debugDryLayoutCalculationValid = true;
final double? dryBaseline;
final double? realBaseline;
try {
dryBaseline = getDryBaseline(constraints, baseline);
realBaseline = getDistanceToBaseline(baseline, onlyReal: true);
} finally {
RenderObject.debugCheckingIntrinsics = false;
}
assert(!RenderObject.debugCheckingIntrinsics);
if (!_debugDryLayoutCalculationValid || dryBaseline == realBaseline) {
continue;
}
if ((dryBaseline == null) != (realBaseline == null)) {
final (String methodReturnedNull, String methodReturnedNonNull) = dryBaseline == null
? ('computeDryBaseline', 'computeDistanceToActualBaseline')
: ('computeDistanceToActualBaseline', 'computeDryBaseline');
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The $baseline location returned by ${objectRuntimeType(this, 'RenderBox')}.computeDistanceToActualBaseline '
'differs from the baseline location computed by computeDryBaseline.'
),
ErrorDescription(
'The $methodReturnedNull method returned null while the $methodReturnedNonNull returned a non-null $baseline of ${dryBaseline ?? realBaseline}. '
'Did you forget to implement $methodReturnedNull for ${objectRuntimeType(this, 'RenderBox')}?'
),
...messages,
]);
} else {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The $baseline location returned by ${objectRuntimeType(this, 'RenderBox')}.computeDistanceToActualBaseline '
'differs from the baseline location computed by computeDryBaseline.'
),
DiagnosticsProperty<RenderObject>(
'The RenderBox was',
this,
),
ErrorDescription(
'The computeDryBaseline method returned $dryBaseline,\n'
'while the computeDistanceToActualBaseline method returned $realBaseline.\n'
'Consider checking the implementations of the following methods on the ${objectRuntimeType(this, 'RenderBox')} class and make sure they are consistent:\n'
' * computeDistanceToActualBaseline\n'
' * computeDryBaseline\n'
' * performLayout\n'
),
...messages,
]);
}
}
return true;
}());
}
@override
void markNeedsLayout() {
// If `_layoutCacheStorage.clear` returns true, then this [RenderBox]'s layout
// is used by the parent's layout algorithm (it's possible that the parent
// only used the intrinsics for paint, but there's no good way to detect that
// so we conservatively assume it's a layout dependency).
//
// A render object's performLayout implementation may depend on the baseline
// location or the intrinsic dimensions of a descendant, even when there are
// relayout boundaries between them. The `_layoutCacheStorage` being non-empty
// indicates that the parent depended on this RenderBox's baseline location,
// or intrinsic sizes, and thus may need relayout, regardless of relayout
// boundaries.
//
// Some calculations may fail (dry baseline, for example). The layout
// dependency is still established, but only from the RenderBox that failed
// to compute the dry baseline to the ancestor that queried the dry baseline.
if (_layoutCacheStorage.clear() && parent != null) {
markParentNeedsLayout();
return;
}
super.markNeedsLayout();
}
/// {@macro flutter.rendering.RenderObject.performResize}
///
/// By default this method sets [size] to the result of [computeDryLayout]
/// called with the current [constraints]. Instead of overriding this method,
/// consider overriding [computeDryLayout].
@override
void performResize() {
// default behavior for subclasses that have sizedByParent = true
size = computeDryLayout(constraints);
assert(size.isFinite);
}
@override
void performLayout() {
assert(() {
if (!sizedByParent) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType did not implement performLayout().'),
ErrorHint(
'RenderBox subclasses need to either override performLayout() to '
'set a size and lay out any children, or, set sizedByParent to true '
'so that performResize() sizes the render object.',
),
]);
}
return true;
}());
}
/// Determines the set of render objects located at the given position.
///
/// Returns true, and adds any render objects that contain the point to the
/// given hit test result, if this render object or one of its descendants
/// absorbs the hit (preventing objects below this one from being hit).
/// Returns false if the hit can continue to other objects below this one.
///
/// The caller is responsible for transforming [position] from global
/// coordinates to its location relative to the origin of this [RenderBox].
/// This [RenderBox] is responsible for checking whether the given position is
/// within its bounds.
///
/// If transforming is necessary, [BoxHitTestResult.addWithPaintTransform],
/// [BoxHitTestResult.addWithPaintOffset], or
/// [BoxHitTestResult.addWithRawTransform] need to be invoked by the caller
/// to record the required transform operations in the [HitTestResult]. These
/// methods will also help with applying the transform to `position`.
///
/// Hit testing requires layout to be up-to-date but does not require painting
/// to be up-to-date. That means a render object can rely upon [performLayout]
/// having been called in [hitTest] but cannot rely upon [paint] having been
/// called. For example, a render object might be a child of a [RenderOpacity]
/// object, which calls [hitTest] on its children when its opacity is zero
/// even through it does not [paint] its children.
bool hitTest(BoxHitTestResult result, { required Offset position }) {
assert(() {
if (!hasSize) {
if (debugNeedsLayout) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot hit test a render box that has never been laid out.'),
describeForError('The hitTest() method was called on this RenderBox'),
ErrorDescription(
"Unfortunately, this object's geometry is not known at this time, "
'probably because it has never been laid out. '
'This means it cannot be accurately hit-tested.',
),
ErrorHint(
'If you are trying '
'to perform a hit test during the layout phase itself, make sure '
"you only hit test nodes that have completed layout (e.g. the node's "
'children, after their layout() method has been called).',
),
]);
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Cannot hit test a render box with no size.'),
describeForError('The hitTest() method was called on this RenderBox'),
ErrorDescription(
'Although this node is not marked as needing layout, '
'its size is not set.',
),
ErrorHint(
'A RenderBox object must have an '
'explicit size before it can be hit-tested. Make sure '
'that the RenderBox in question sets its size during layout.',
),
]);
}
return true;
}());
if (_size!.contains(position)) {
if (hitTestChildren(result, position: position) || hitTestSelf(position)) {
result.add(BoxHitTestEntry(this, position));
return true;
}
}
return false;
}
/// Override this method if this render object can be hit even if its
/// children were not hit.
///
/// Returns true if the specified `position` should be considered a hit
/// on this render object.
///
/// The caller is responsible for transforming [position] from global
/// coordinates to its location relative to the origin of this [RenderBox].
/// This [RenderBox] is responsible for checking whether the given position is
/// within its bounds.
///
/// Used by [hitTest]. If you override [hitTest] and do not call this
/// function, then you don't need to implement this function.
@protected
bool hitTestSelf(Offset position) => false;
/// Override this method to check whether any children are located at the
/// given position.
///
/// Subclasses should return true if at least one child reported a hit at the
/// specified position.
///
/// Typically children should be hit-tested in reverse paint order so that
/// hit tests at locations where children overlap hit the child that is
/// visually "on top" (i.e., paints later).
///
/// The caller is responsible for transforming [position] from global
/// coordinates to its location relative to the origin of this [RenderBox].
/// Likewise, this [RenderBox] is responsible for transforming the position
/// that it passes to its children when it calls [hitTest] on each child.
///
/// If transforming is necessary, [BoxHitTestResult.addWithPaintTransform],
/// [BoxHitTestResult.addWithPaintOffset], or
/// [BoxHitTestResult.addWithRawTransform] need to be invoked by subclasses to
/// record the required transform operations in the [BoxHitTestResult]. These
/// methods will also help with applying the transform to `position`.
///
/// Used by [hitTest]. If you override [hitTest] and do not call this
/// function, then you don't need to implement this function.
@protected
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) => false;
/// Multiply the transform from the parent's coordinate system to this box's
/// coordinate system into the given transform.
///
/// This function is used to convert coordinate systems between boxes.
/// Subclasses that apply transforms during painting should override this
/// function to factor those transforms into the calculation.
///
/// The [RenderBox] implementation takes care of adjusting the matrix for the
/// position of the given child as determined during layout and stored on the
/// child's [parentData] in the [BoxParentData.offset] field.
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
assert(child.parent == this);
assert(() {
if (child.parentData is! BoxParentData) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType does not implement applyPaintTransform.'),
describeForError('The following $runtimeType object'),
child.describeForError('...did not use a BoxParentData class for the parentData field of the following child'),
ErrorDescription('The $runtimeType class inherits from RenderBox.'),
ErrorHint(
'The default applyPaintTransform implementation provided by RenderBox assumes that the '
'children all use BoxParentData objects for their parentData field. '
'Since $runtimeType does not in fact use that ParentData class for its children, it must '
'provide an implementation of applyPaintTransform that supports the specific ParentData '
'subclass used by its children (which apparently is ${child.parentData.runtimeType}).',
),
]);
}
return true;
}());
final BoxParentData childParentData = child.parentData! as BoxParentData;
final Offset offset = childParentData.offset;
transform.translate(offset.dx, offset.dy);
}
/// Convert the given point from the global coordinate system in logical pixels
/// to the local coordinate system for this box.
///
/// This method will un-project the point from the screen onto the widget,
/// which makes it different from [MatrixUtils.transformPoint].
///
/// If the transform from global coordinates to local coordinates is
/// degenerate, this function returns [Offset.zero].
///
/// If `ancestor` is non-null, this function converts the given point from the
/// coordinate system of `ancestor` (which must be an ancestor of this render
/// object) instead of from the global coordinate system.
///
/// This method is implemented in terms of [getTransformTo].
Offset globalToLocal(Offset point, { RenderObject? ancestor }) {
// We want to find point (p) that corresponds to a given point on the
// screen (s), but that also physically resides on the local render plane,
// so that it is useful for visually accurate gesture processing in the
// local space. For that, we can't simply transform 2D screen point to
// the 3D local space since the screen space lacks the depth component |z|,
// and so there are many 3D points that correspond to the screen point.
// We must first unproject the screen point onto the render plane to find
// the true 3D point that corresponds to the screen point.
// We do orthogonal unprojection after undoing perspective, in local space.
// The render plane is specified by renderBox offset (o) and Z axis (n).
// Unprojection is done by finding the intersection of the view vector (d)
// with the local X-Y plane: (o-s).dot(n) == (p-s).dot(n), (p-s) == |z|*d.
final Matrix4 transform = getTransformTo(ancestor);
final double det = transform.invert();
if (det == 0.0) {
return Offset.zero;
}
final Vector3 n = Vector3(0.0, 0.0, 1.0);
final Vector3 i = transform.perspectiveTransform(Vector3(0.0, 0.0, 0.0));
final Vector3 d = transform.perspectiveTransform(Vector3(0.0, 0.0, 1.0)) - i;
final Vector3 s = transform.perspectiveTransform(Vector3(point.dx, point.dy, 0.0));
final Vector3 p = s - d * (n.dot(s) / n.dot(d));
return Offset(p.x, p.y);
}
/// Convert the given point from the local coordinate system for this box to
/// the global coordinate system in logical pixels.
///
/// If `ancestor` is non-null, this function converts the given point to the
/// coordinate system of `ancestor` (which must be an ancestor of this render
/// object) instead of to the global coordinate system.
///
/// This method is implemented in terms of [getTransformTo]. If the transform
/// matrix puts the given `point` on the line at infinity (for instance, when
/// the transform matrix is the zero matrix), this method returns (NaN, NaN).
Offset localToGlobal(Offset point, { RenderObject? ancestor }) {
return MatrixUtils.transformPoint(getTransformTo(ancestor), point);
}
/// Returns a rectangle that contains all the pixels painted by this box.
///
/// The paint bounds can be larger or smaller than [size], which is the amount
/// of space this box takes up during layout. For example, if this box casts a
/// shadow, that shadow might extend beyond the space allocated to this box
/// during layout.
///
/// The paint bounds are used to size the buffers into which this box paints.
/// If the box attempts to paints outside its paint bounds, there might not be
/// enough memory allocated to represent the box's visual appearance, which
/// can lead to undefined behavior.
///
/// The returned paint bounds are in the local coordinate system of this box.
@override
Rect get paintBounds => Offset.zero & size;
/// Override this method to handle pointer events that hit this render object.
///
/// For [RenderBox] objects, the `entry` argument is a [BoxHitTestEntry]. From this
/// object you can determine the [PointerDownEvent]'s position in local coordinates.
/// (This is useful because [PointerEvent.position] is in global coordinates.)
///
/// Implementations of this method should call [debugHandleEvent] as follows,
/// so that they support [debugPaintPointersEnabled]:
///
/// ```dart
/// class RenderFoo extends RenderBox {
/// // ...
///
/// @override
/// void handleEvent(PointerEvent event, HitTestEntry entry) {
/// assert(debugHandleEvent(event, entry));
/// // ... handle the event ...
/// }
///
/// // ...
/// }
/// ```
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
super.handleEvent(event, entry);
}
int _debugActivePointers = 0;
/// Implements the [debugPaintPointersEnabled] debugging feature.
///
/// [RenderBox] subclasses that implement [handleEvent] should call
/// [debugHandleEvent] from their [handleEvent] method, as follows:
///
/// ```dart
/// class RenderFoo extends RenderBox {
/// // ...
///
/// @override
/// void handleEvent(PointerEvent event, HitTestEntry entry) {
/// assert(debugHandleEvent(event, entry));
/// // ... handle the event ...
/// }
///
/// // ...
/// }
/// ```
///
/// If you call this for a [PointerDownEvent], make sure you also call it for
/// the corresponding [PointerUpEvent] or [PointerCancelEvent].
bool debugHandleEvent(PointerEvent event, HitTestEntry entry) {
assert(() {
if (debugPaintPointersEnabled) {
if (event is PointerDownEvent) {
_debugActivePointers += 1;
} else if (event is PointerUpEvent || event is PointerCancelEvent) {
_debugActivePointers -= 1;
}
markNeedsPaint();
}
return true;
}());
return true;
}
@override
void debugPaint(PaintingContext context, Offset offset) {
assert(() {
// Only perform the baseline checks after `PipelineOwner.flushLayout` completes.
// We can't run this check in the same places we run other intrinsics checks
// (in the `RenderBox.size` setter, or after `performResize`), because
// `getDistanceToBaseline` may depend on the layout of the child so it's
// the safest to only call `getDistanceToBaseline` after the entire tree
// finishes doing layout.
//
// Descendant `RenderObject`s typically call `debugPaint` before their
// parents do. This means the baseline implementations are checked from
// descendants to ancestors, allowing us to spot the `RenderBox` with an
// inconsistent implementation, instead of its ancestors that only reported
// inconsistent baseline values because one of its ancestors has an
// inconsistent implementation.
if (debugCheckIntrinsicSizes) {
_debugVerifyDryBaselines();
}
if (debugPaintSizeEnabled) {
debugPaintSize(context, offset);
}
if (debugPaintBaselinesEnabled) {
debugPaintBaselines(context, offset);
}
if (debugPaintPointersEnabled) {
debugPaintPointers(context, offset);
}
return true;
}());
}
/// In debug mode, paints a border around this render box.
///
/// Called for every [RenderBox] when [debugPaintSizeEnabled] is true.
@protected
@visibleForTesting
void debugPaintSize(PaintingContext context, Offset offset) {
assert(() {
final Paint paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1.0
..color = const Color(0xFF00FFFF);
context.canvas.drawRect((offset & size).deflate(0.5), paint);
return true;
}());
}
/// In debug mode, paints a line for each baseline.
///
/// Called for every [RenderBox] when [debugPaintBaselinesEnabled] is true.
@protected
void debugPaintBaselines(PaintingContext context, Offset offset) {
assert(() {
final Paint paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 0.25;
Path path;
// ideographic baseline
final double? baselineI = getDistanceToBaseline(TextBaseline.ideographic, onlyReal: true);
if (baselineI != null) {
paint.color = const Color(0xFFFFD000);
path = Path();
path.moveTo(offset.dx, offset.dy + baselineI);
path.lineTo(offset.dx + size.width, offset.dy + baselineI);
context.canvas.drawPath(path, paint);
}
// alphabetic baseline
final double? baselineA = getDistanceToBaseline(TextBaseline.alphabetic, onlyReal: true);
if (baselineA != null) {
paint.color = const Color(0xFF00FF00);
path = Path();
path.moveTo(offset.dx, offset.dy + baselineA);
path.lineTo(offset.dx + size.width, offset.dy + baselineA);
context.canvas.drawPath(path, paint);
}
return true;
}());
}
/// In debug mode, paints a rectangle if this render box has counted more
/// pointer downs than pointer up events.
///
/// Called for every [RenderBox] when [debugPaintPointersEnabled] is true.
///
/// By default, events are not counted. For details on how to ensure that
/// events are counted for your class, see [debugHandleEvent].
@protected
void debugPaintPointers(PaintingContext context, Offset offset) {
assert(() {
if (_debugActivePointers > 0) {
final Paint paint = Paint()
..color = Color(0x00BBBB | ((0x04000000 * depth) & 0xFF000000));
context.canvas.drawRect(offset & size, paint);
}
return true;
}());
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Size>('size', _size, missingIfNull: true));
}
}
/// A mixin that provides useful default behaviors for boxes with children
/// managed by the [ContainerRenderObjectMixin] mixin.
///
/// By convention, this class doesn't override any members of the superclass.
/// Instead, it provides helpful functions that subclasses can call as
/// appropriate.
mixin RenderBoxContainerDefaultsMixin<ChildType extends RenderBox, ParentDataType extends ContainerBoxParentData<ChildType>> implements ContainerRenderObjectMixin<ChildType, ParentDataType> {
/// Returns the baseline of the first child with a baseline.
///
/// Useful when the children are displayed vertically in the same order they
/// appear in the child list.
double? defaultComputeDistanceToFirstActualBaseline(TextBaseline baseline) {
assert(!debugNeedsLayout);
ChildType? child = firstChild;
while (child != null) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
final double? result = child.getDistanceToActualBaseline(baseline);
if (result != null) {
return result + childParentData.offset.dy;
}
child = childParentData.nextSibling;
}
return null;
}
/// Returns the minimum baseline value among every child.
///
/// Useful when the vertical position of the children isn't determined by the
/// order in the child list.
double? defaultComputeDistanceToHighestActualBaseline(TextBaseline baseline) {
assert(!debugNeedsLayout);
BaselineOffset minBaseline = BaselineOffset.noBaseline;
ChildType? child = firstChild;
while (child != null) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
final BaselineOffset candidate = BaselineOffset(child.getDistanceToActualBaseline(baseline)) + childParentData.offset.dy;
minBaseline = minBaseline.minOf(candidate);
child = childParentData.nextSibling;
}
return minBaseline.offset;
}
/// Performs a hit test on each child by walking the child list backwards.
///
/// Stops walking once after the first child reports that it contains the
/// given point. Returns whether any children contain the given point.
///
/// See also:
///
/// * [defaultPaint], which paints the children appropriate for this
/// hit-testing strategy.
bool defaultHitTestChildren(BoxHitTestResult result, { required Offset position }) {
ChildType? child = lastChild;
while (child != null) {
// The x, y parameters have the top left of the node's box as the origin.
final ParentDataType childParentData = child.parentData! as ParentDataType;
final bool isHit = result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child!.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
child = childParentData.previousSibling;
}
return false;
}
/// Paints each child by walking the child list forwards.
///
/// See also:
///
/// * [defaultHitTestChildren], which implements hit-testing of the children
/// in a manner appropriate for this painting strategy.
void defaultPaint(PaintingContext context, Offset offset) {
ChildType? child = firstChild;
while (child != null) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
context.paintChild(child, childParentData.offset + offset);
child = childParentData.nextSibling;
}
}
/// Returns a list containing the children of this render object.
///
/// This function is useful when you need random-access to the children of
/// this render object. If you're accessing the children in order, consider
/// walking the child list directly.
List<ChildType> getChildrenAsList() {
final List<ChildType> result = <ChildType>[];
RenderBox? child = firstChild;
while (child != null) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
result.add(child as ChildType);
child = childParentData.nextSibling;
}
return result;
}
}
| flutter/packages/flutter/lib/src/rendering/box.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/box.dart",
"repo_id": "flutter",
"token_count": 42776
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show PictureRecorder;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/painting.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/semantics.dart';
import 'debug.dart';
import 'layer.dart';
export 'package:flutter/foundation.dart' show
DiagnosticPropertiesBuilder,
DiagnosticsNode,
DiagnosticsProperty,
DoubleProperty,
EnumProperty,
ErrorDescription,
ErrorHint,
ErrorSummary,
FlagProperty,
FlutterError,
InformationCollector,
IntProperty,
StringProperty;
export 'package:flutter/gestures.dart' show HitTestEntry, HitTestResult;
export 'package:flutter/painting.dart';
/// Base class for data associated with a [RenderObject] by its parent.
///
/// Some render objects wish to store data on their children, such as the
/// children's input parameters to the parent's layout algorithm or the
/// children's position relative to other children.
///
/// See also:
///
/// * [RenderObject.setupParentData], which [RenderObject] subclasses may
/// override to attach specific types of parent data to children.
class ParentData {
/// Called when the RenderObject is removed from the tree.
@protected
@mustCallSuper
void detach() { }
@override
String toString() => '<none>';
}
/// Signature for painting into a [PaintingContext].
///
/// The `offset` argument is the offset from the origin of the coordinate system
/// of the [PaintingContext.canvas] to the coordinate system of the callee.
///
/// Used by many of the methods of [PaintingContext].
typedef PaintingContextCallback = void Function(PaintingContext context, Offset offset);
/// A place to paint.
///
/// Rather than holding a canvas directly, [RenderObject]s paint using a painting
/// context. The painting context has a [Canvas], which receives the
/// individual draw operations, and also has functions for painting child
/// render objects.
///
/// When painting a child render object, the canvas held by the painting context
/// can change because the draw operations issued before and after painting the
/// child might be recorded in separate compositing layers. For this reason, do
/// not hold a reference to the canvas across operations that might paint
/// child render objects.
///
/// New [PaintingContext] objects are created automatically when using
/// [PaintingContext.repaintCompositedChild] and [pushLayer].
class PaintingContext extends ClipContext {
/// Creates a painting context.
///
/// Typically only called by [PaintingContext.repaintCompositedChild]
/// and [pushLayer].
@protected
PaintingContext(this._containerLayer, this.estimatedBounds);
final ContainerLayer _containerLayer;
/// An estimate of the bounds within which the painting context's [canvas]
/// will record painting commands. This can be useful for debugging.
///
/// The canvas will allow painting outside these bounds.
///
/// The [estimatedBounds] rectangle is in the [canvas] coordinate system.
final Rect estimatedBounds;
/// Repaint the given render object.
///
/// The render object must be attached to a [PipelineOwner], must have a
/// composited layer, and must be in need of painting. The render object's
/// layer, if any, is re-used, along with any layers in the subtree that don't
/// need to be repainted.
///
/// See also:
///
/// * [RenderObject.isRepaintBoundary], which determines if a [RenderObject]
/// has a composited layer.
static void repaintCompositedChild(RenderObject child, { bool debugAlsoPaintedParent = false }) {
assert(child._needsPaint);
_repaintCompositedChild(
child,
debugAlsoPaintedParent: debugAlsoPaintedParent,
);
}
static void _repaintCompositedChild(
RenderObject child, {
bool debugAlsoPaintedParent = false,
PaintingContext? childContext,
}) {
assert(child.isRepaintBoundary);
assert(() {
// register the call for RepaintBoundary metrics
child.debugRegisterRepaintBoundaryPaint(
includedParent: debugAlsoPaintedParent,
includedChild: true,
);
return true;
}());
OffsetLayer? childLayer = child._layerHandle.layer as OffsetLayer?;
if (childLayer == null) {
assert(debugAlsoPaintedParent);
assert(child._layerHandle.layer == null);
// Not using the `layer` setter because the setter asserts that we not
// replace the layer for repaint boundaries. That assertion does not
// apply here because this is exactly the place designed to create a
// layer for repaint boundaries.
final OffsetLayer layer = child.updateCompositedLayer(oldLayer: null);
child._layerHandle.layer = childLayer = layer;
} else {
assert(debugAlsoPaintedParent || childLayer.attached);
Offset? debugOldOffset;
assert(() {
debugOldOffset = childLayer!.offset;
return true;
}());
childLayer.removeAllChildren();
final OffsetLayer updatedLayer = child.updateCompositedLayer(oldLayer: childLayer);
assert(identical(updatedLayer, childLayer),
'$child created a new layer instance $updatedLayer instead of reusing the '
'existing layer $childLayer. See the documentation of RenderObject.updateCompositedLayer '
'for more information on how to correctly implement this method.'
);
assert(debugOldOffset == updatedLayer.offset);
}
child._needsCompositedLayerUpdate = false;
assert(identical(childLayer, child._layerHandle.layer));
assert(child._layerHandle.layer is OffsetLayer);
assert(() {
childLayer!.debugCreator = child.debugCreator ?? child.runtimeType;
return true;
}());
childContext ??= PaintingContext(childLayer, child.paintBounds);
child._paintWithContext(childContext, Offset.zero);
// Double-check that the paint method did not replace the layer (the first
// check is done in the [layer] setter itself).
assert(identical(childLayer, child._layerHandle.layer));
childContext.stopRecordingIfNeeded();
}
/// Update the composited layer of [child] without repainting its children.
///
/// The render object must be attached to a [PipelineOwner], must have a
/// composited layer, and must be in need of a composited layer update but
/// not in need of painting. The render object's layer is re-used, and none
/// of its children are repaint or their layers updated.
///
/// See also:
///
/// * [RenderObject.isRepaintBoundary], which determines if a [RenderObject]
/// has a composited layer.
static void updateLayerProperties(RenderObject child) {
assert(child.isRepaintBoundary && child._wasRepaintBoundary);
assert(!child._needsPaint);
assert(child._layerHandle.layer != null);
final OffsetLayer childLayer = child._layerHandle.layer! as OffsetLayer;
Offset? debugOldOffset;
assert(() {
debugOldOffset = childLayer.offset;
return true;
}());
final OffsetLayer updatedLayer = child.updateCompositedLayer(oldLayer: childLayer);
assert(identical(updatedLayer, childLayer),
'$child created a new layer instance $updatedLayer instead of reusing the '
'existing layer $childLayer. See the documentation of RenderObject.updateCompositedLayer '
'for more information on how to correctly implement this method.'
);
assert(debugOldOffset == updatedLayer.offset);
child._needsCompositedLayerUpdate = false;
}
/// In debug mode, repaint the given render object using a custom painting
/// context that can record the results of the painting operation in addition
/// to performing the regular paint of the child.
///
/// See also:
///
/// * [repaintCompositedChild], for repainting a composited child without
/// instrumentation.
static void debugInstrumentRepaintCompositedChild(
RenderObject child, {
bool debugAlsoPaintedParent = false,
required PaintingContext customContext,
}) {
assert(() {
_repaintCompositedChild(
child,
debugAlsoPaintedParent: debugAlsoPaintedParent,
childContext: customContext,
);
return true;
}());
}
/// Paint a child [RenderObject].
///
/// If the child has its own composited layer, the child will be composited
/// into the layer subtree associated with this painting context. Otherwise,
/// the child will be painted into the current PictureLayer for this context.
void paintChild(RenderObject child, Offset offset) {
assert(() {
debugOnProfilePaint?.call(child);
return true;
}());
if (child.isRepaintBoundary) {
stopRecordingIfNeeded();
_compositeChild(child, offset);
// If a render object was a repaint boundary but no longer is one, this
// is where the framework managed layer is automatically disposed.
} else if (child._wasRepaintBoundary) {
assert(child._layerHandle.layer is OffsetLayer);
child._layerHandle.layer = null;
child._paintWithContext(this, offset);
} else {
child._paintWithContext(this, offset);
}
}
void _compositeChild(RenderObject child, Offset offset) {
assert(!_isRecording);
assert(child.isRepaintBoundary);
assert(_canvas == null || _canvas!.getSaveCount() == 1);
// Create a layer for our child, and paint the child into it.
if (child._needsPaint || !child._wasRepaintBoundary) {
repaintCompositedChild(child, debugAlsoPaintedParent: true);
} else {
if (child._needsCompositedLayerUpdate) {
updateLayerProperties(child);
}
assert(() {
// register the call for RepaintBoundary metrics
child.debugRegisterRepaintBoundaryPaint();
child._layerHandle.layer!.debugCreator = child.debugCreator ?? child;
return true;
}());
}
assert(child._layerHandle.layer is OffsetLayer);
final OffsetLayer childOffsetLayer = child._layerHandle.layer! as OffsetLayer;
childOffsetLayer.offset = offset;
appendLayer(childOffsetLayer);
}
/// Adds a layer to the recording requiring that the recording is already
/// stopped.
///
/// Do not call this function directly: call [addLayer] or [pushLayer]
/// instead. This function is called internally when all layers not
/// generated from the [canvas] are added.
///
/// Subclasses that need to customize how layers are added should override
/// this method.
@protected
void appendLayer(Layer layer) {
assert(!_isRecording);
layer.remove();
_containerLayer.append(layer);
}
bool get _isRecording {
final bool hasCanvas = _canvas != null;
assert(() {
if (hasCanvas) {
assert(_currentLayer != null);
assert(_recorder != null);
assert(_canvas != null);
} else {
assert(_currentLayer == null);
assert(_recorder == null);
assert(_canvas == null);
}
return true;
}());
return hasCanvas;
}
// Recording state
PictureLayer? _currentLayer;
ui.PictureRecorder? _recorder;
Canvas? _canvas;
/// The canvas on which to paint.
///
/// The current canvas can change whenever you paint a child using this
/// context, which means it's fragile to hold a reference to the canvas
/// returned by this getter.
@override
Canvas get canvas {
if (_canvas == null) {
_startRecording();
}
assert(_currentLayer != null);
return _canvas!;
}
void _startRecording() {
assert(!_isRecording);
_currentLayer = PictureLayer(estimatedBounds);
_recorder = ui.PictureRecorder();
_canvas = Canvas(_recorder!);
_containerLayer.append(_currentLayer!);
}
/// Adds a [CompositionCallback] for the current [ContainerLayer] used by this
/// context.
///
/// Composition callbacks are called whenever the layer tree containing the
/// current layer of this painting context gets composited, or when it gets
/// detached and will not be rendered again. This happens regardless of
/// whether the layer is added via retained rendering or not.
///
/// {@macro flutter.rendering.Layer.compositionCallbacks}
///
/// See also:
/// * [Layer.addCompositionCallback].
VoidCallback addCompositionCallback(CompositionCallback callback) {
return _containerLayer.addCompositionCallback(callback);
}
/// Stop recording to a canvas if recording has started.
///
/// Do not call this function directly: functions in this class will call
/// this method as needed. This function is called internally to ensure that
/// recording is stopped before adding layers or finalizing the results of a
/// paint.
///
/// Subclasses that need to customize how recording to a canvas is performed
/// should override this method to save the results of the custom canvas
/// recordings.
@protected
@mustCallSuper
void stopRecordingIfNeeded() {
if (!_isRecording) {
return;
}
assert(() {
if (debugRepaintRainbowEnabled) {
final Paint paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 6.0
..color = debugCurrentRepaintColor.toColor();
canvas.drawRect(estimatedBounds.deflate(3.0), paint);
}
if (debugPaintLayerBordersEnabled) {
final Paint paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1.0
..color = const Color(0xFFFF9800);
canvas.drawRect(estimatedBounds, paint);
}
return true;
}());
_currentLayer!.picture = _recorder!.endRecording();
_currentLayer = null;
_recorder = null;
_canvas = null;
}
/// Hints that the painting in the current layer is complex and would benefit
/// from caching.
///
/// If this hint is not set, the compositor will apply its own heuristics to
/// decide whether the current layer is complex enough to benefit from
/// caching.
///
/// Calling this ensures a [Canvas] is available. Only draw calls on the
/// current canvas will be hinted; the hint is not propagated to new canvases
/// created after a new layer is added to the painting context (e.g. with
/// [addLayer] or [pushLayer]).
void setIsComplexHint() {
if (_currentLayer == null) {
_startRecording();
}
_currentLayer!.isComplexHint = true;
}
/// Hints that the painting in the current layer is likely to change next frame.
///
/// This hint tells the compositor not to cache the current layer because the
/// cache will not be used in the future. If this hint is not set, the
/// compositor will apply its own heuristics to decide whether the current
/// layer is likely to be reused in the future.
///
/// Calling this ensures a [Canvas] is available. Only draw calls on the
/// current canvas will be hinted; the hint is not propagated to new canvases
/// created after a new layer is added to the painting context (e.g. with
/// [addLayer] or [pushLayer]).
void setWillChangeHint() {
if (_currentLayer == null) {
_startRecording();
}
_currentLayer!.willChangeHint = true;
}
/// Adds a composited leaf layer to the recording.
///
/// After calling this function, the [canvas] property will change to refer to
/// a new [Canvas] that draws on top of the given layer.
///
/// A [RenderObject] that uses this function is very likely to require its
/// [RenderObject.alwaysNeedsCompositing] property to return true. That informs
/// ancestor render objects that this render object will include a composited
/// layer, which, for example, causes them to use composited clips.
///
/// See also:
///
/// * [pushLayer], for adding a layer and painting further contents within
/// it.
void addLayer(Layer layer) {
stopRecordingIfNeeded();
appendLayer(layer);
}
/// Appends the given layer to the recording, and calls the `painter` callback
/// with that layer, providing the `childPaintBounds` as the estimated paint
/// bounds of the child. The `childPaintBounds` can be used for debugging but
/// have no effect on painting.
///
/// The given layer must be an unattached orphan. (Providing a newly created
/// object, rather than reusing an existing layer, satisfies that
/// requirement.)
///
/// {@template flutter.rendering.PaintingContext.pushLayer.offset}
/// The `offset` is the offset to pass to the `painter`. In particular, it is
/// not an offset applied to the layer itself. Layers conceptually by default
/// have no position or size, though they can transform their contents. For
/// example, an [OffsetLayer] applies an offset to its children.
/// {@endtemplate}
///
/// If the `childPaintBounds` are not specified then the current layer's paint
/// bounds are used. This is appropriate if the child layer does not apply any
/// transformation or clipping to its contents. The `childPaintBounds`, if
/// specified, must be in the coordinate system of the new layer (i.e. as seen
/// by its children after it applies whatever transform to its contents), and
/// should not go outside the current layer's paint bounds.
///
/// See also:
///
/// * [addLayer], for pushing a layer without painting further contents
/// within it.
void pushLayer(ContainerLayer childLayer, PaintingContextCallback painter, Offset offset, { Rect? childPaintBounds }) {
// If a layer is being reused, it may already contain children. We remove
// them so that `painter` can add children that are relevant for this frame.
if (childLayer.hasChildren) {
childLayer.removeAllChildren();
}
stopRecordingIfNeeded();
appendLayer(childLayer);
final PaintingContext childContext = createChildContext(childLayer, childPaintBounds ?? estimatedBounds);
painter(childContext, offset);
childContext.stopRecordingIfNeeded();
}
/// Creates a painting context configured to paint into [childLayer].
///
/// The `bounds` are estimated paint bounds for debugging purposes.
@protected
PaintingContext createChildContext(ContainerLayer childLayer, Rect bounds) {
return PaintingContext(childLayer, bounds);
}
/// Clip further painting using a rectangle.
///
/// {@template flutter.rendering.PaintingContext.pushClipRect.needsCompositing}
/// The `needsCompositing` argument specifies whether the child needs
/// compositing. Typically this matches the value of
/// [RenderObject.needsCompositing] for the caller. If false, this method
/// returns null, indicating that a layer is no longer necessary. If a render
/// object calling this method stores the `oldLayer` in its
/// [RenderObject.layer] field, it should set that field to null.
///
/// When `needsCompositing` is false, this method will use a more efficient
/// way to apply the layer effect than actually creating a layer.
/// {@endtemplate}
///
/// {@template flutter.rendering.PaintingContext.pushClipRect.offset}
/// The `offset` argument is the offset from the origin of the canvas'
/// coordinate system to the origin of the caller's coordinate system.
/// {@endtemplate}
///
/// The `clipRect` is the rectangle (in the caller's coordinate system) to use
/// to clip the painting done by [painter]. It should not include the
/// `offset`.
///
/// The `painter` callback will be called while the `clipRect` is applied. It
/// is called synchronously during the call to [pushClipRect].
///
/// The `clipBehavior` argument controls how the rectangle is clipped.
///
/// {@template flutter.rendering.PaintingContext.pushClipRect.oldLayer}
/// For the `oldLayer` argument, specify the layer created in the previous
/// frame. This gives the engine more information for performance
/// optimizations. Typically this is the value of [RenderObject.layer] that a
/// render object creates once, then reuses for all subsequent frames until a
/// layer is no longer needed (e.g. the render object no longer needs
/// compositing) or until the render object changes the type of the layer
/// (e.g. from opacity layer to a clip rect layer).
/// {@endtemplate}
ClipRectLayer? pushClipRect(bool needsCompositing, Offset offset, Rect clipRect, PaintingContextCallback painter, { Clip clipBehavior = Clip.hardEdge, ClipRectLayer? oldLayer }) {
if (clipBehavior == Clip.none) {
painter(this, offset);
return null;
}
final Rect offsetClipRect = clipRect.shift(offset);
if (needsCompositing) {
final ClipRectLayer layer = oldLayer ?? ClipRectLayer();
layer
..clipRect = offsetClipRect
..clipBehavior = clipBehavior;
pushLayer(layer, painter, offset, childPaintBounds: offsetClipRect);
return layer;
} else {
clipRectAndPaint(offsetClipRect, clipBehavior, offsetClipRect, () => painter(this, offset));
return null;
}
}
/// Clip further painting using a rounded rectangle.
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.needsCompositing}
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.offset}
///
/// The `bounds` argument is used to specify the region of the canvas (in the
/// caller's coordinate system) into which `painter` will paint.
///
/// The `clipRRect` argument specifies the rounded-rectangle (in the caller's
/// coordinate system) to use to clip the painting done by `painter`. It
/// should not include the `offset`.
///
/// The `painter` callback will be called while the `clipRRect` is applied. It
/// is called synchronously during the call to [pushClipRRect].
///
/// The `clipBehavior` argument controls how the rounded rectangle is clipped.
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.oldLayer}
ClipRRectLayer? pushClipRRect(bool needsCompositing, Offset offset, Rect bounds, RRect clipRRect, PaintingContextCallback painter, { Clip clipBehavior = Clip.antiAlias, ClipRRectLayer? oldLayer }) {
if (clipBehavior == Clip.none) {
painter(this, offset);
return null;
}
final Rect offsetBounds = bounds.shift(offset);
final RRect offsetClipRRect = clipRRect.shift(offset);
if (needsCompositing) {
final ClipRRectLayer layer = oldLayer ?? ClipRRectLayer();
layer
..clipRRect = offsetClipRRect
..clipBehavior = clipBehavior;
pushLayer(layer, painter, offset, childPaintBounds: offsetBounds);
return layer;
} else {
clipRRectAndPaint(offsetClipRRect, clipBehavior, offsetBounds, () => painter(this, offset));
return null;
}
}
/// Clip further painting using a path.
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.needsCompositing}
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.offset}
///
/// The `bounds` argument is used to specify the region of the canvas (in the
/// caller's coordinate system) into which `painter` will paint.
///
/// The `clipPath` argument specifies the [Path] (in the caller's coordinate
/// system) to use to clip the painting done by `painter`. It should not
/// include the `offset`.
///
/// The `painter` callback will be called while the `clipPath` is applied. It
/// is called synchronously during the call to [pushClipPath].
///
/// The `clipBehavior` argument controls how the path is clipped.
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.oldLayer}
ClipPathLayer? pushClipPath(bool needsCompositing, Offset offset, Rect bounds, Path clipPath, PaintingContextCallback painter, { Clip clipBehavior = Clip.antiAlias, ClipPathLayer? oldLayer }) {
if (clipBehavior == Clip.none) {
painter(this, offset);
return null;
}
final Rect offsetBounds = bounds.shift(offset);
final Path offsetClipPath = clipPath.shift(offset);
if (needsCompositing) {
final ClipPathLayer layer = oldLayer ?? ClipPathLayer();
layer
..clipPath = offsetClipPath
..clipBehavior = clipBehavior;
pushLayer(layer, painter, offset, childPaintBounds: offsetBounds);
return layer;
} else {
clipPathAndPaint(offsetClipPath, clipBehavior, offsetBounds, () => painter(this, offset));
return null;
}
}
/// Blend further painting with a color filter.
///
/// {@macro flutter.rendering.PaintingContext.pushLayer.offset}
///
/// The `colorFilter` argument is the [ColorFilter] value to use when blending
/// the painting done by `painter`.
///
/// The `painter` callback will be called while the `colorFilter` is applied.
/// It is called synchronously during the call to [pushColorFilter].
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.oldLayer}
///
/// A [RenderObject] that uses this function is very likely to require its
/// [RenderObject.alwaysNeedsCompositing] property to return true. That informs
/// ancestor render objects that this render object will include a composited
/// layer, which, for example, causes them to use composited clips.
ColorFilterLayer pushColorFilter(Offset offset, ColorFilter colorFilter, PaintingContextCallback painter, { ColorFilterLayer? oldLayer }) {
final ColorFilterLayer layer = oldLayer ?? ColorFilterLayer();
layer.colorFilter = colorFilter;
pushLayer(layer, painter, offset);
return layer;
}
/// Transform further painting using a matrix.
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.needsCompositing}
///
/// The `offset` argument is the offset to pass to `painter` and the offset to
/// the origin used by `transform`.
///
/// The `transform` argument is the [Matrix4] with which to transform the
/// coordinate system while calling `painter`. It should not include `offset`.
/// It is applied effectively after applying `offset`.
///
/// The `painter` callback will be called while the `transform` is applied. It
/// is called synchronously during the call to [pushTransform].
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.oldLayer}
TransformLayer? pushTransform(bool needsCompositing, Offset offset, Matrix4 transform, PaintingContextCallback painter, { TransformLayer? oldLayer }) {
final Matrix4 effectiveTransform = Matrix4.translationValues(offset.dx, offset.dy, 0.0)
..multiply(transform)..translate(-offset.dx, -offset.dy);
if (needsCompositing) {
final TransformLayer layer = oldLayer ?? TransformLayer();
layer.transform = effectiveTransform;
pushLayer(
layer,
painter,
offset,
childPaintBounds: MatrixUtils.inverseTransformRect(effectiveTransform, estimatedBounds),
);
return layer;
} else {
canvas
..save()
..transform(effectiveTransform.storage);
painter(this, offset);
canvas.restore();
return null;
}
}
/// Blend further painting with an alpha value.
///
/// The `offset` argument indicates an offset to apply to all the children
/// (the rendering created by `painter`).
///
/// The `alpha` argument is the alpha value to use when blending the painting
/// done by `painter`. An alpha value of 0 means the painting is fully
/// transparent and an alpha value of 255 means the painting is fully opaque.
///
/// The `painter` callback will be called while the `alpha` is applied. It
/// is called synchronously during the call to [pushOpacity].
///
/// {@macro flutter.rendering.PaintingContext.pushClipRect.oldLayer}
///
/// A [RenderObject] that uses this function is very likely to require its
/// [RenderObject.alwaysNeedsCompositing] property to return true. That informs
/// ancestor render objects that this render object will include a composited
/// layer, which, for example, causes them to use composited clips.
OpacityLayer pushOpacity(Offset offset, int alpha, PaintingContextCallback painter, { OpacityLayer? oldLayer }) {
final OpacityLayer layer = oldLayer ?? OpacityLayer();
layer
..alpha = alpha
..offset = offset;
pushLayer(layer, painter, Offset.zero);
return layer;
}
@override
String toString() => '${objectRuntimeType(this, 'PaintingContext')}#$hashCode(layer: $_containerLayer, canvas bounds: $estimatedBounds)';
}
/// An abstract set of layout constraints.
///
/// Concrete layout models (such as box) will create concrete subclasses to
/// communicate layout constraints between parents and children.
///
/// ## Writing a Constraints subclass
///
/// When creating a new [RenderObject] subclass with a new layout protocol, one
/// will usually need to create a new [Constraints] subclass to express the
/// input to the layout algorithms.
///
/// A [Constraints] subclass should be immutable (all fields final). There are
/// several members to implement, in addition to whatever fields, constructors,
/// and helper methods one may find useful for a particular layout protocol:
///
/// * The [isTight] getter, which should return true if the object represents a
/// case where the [RenderObject] class has no choice for how to lay itself
/// out. For example, [BoxConstraints] returns true for [isTight] when both
/// the minimum and maximum widths and the minimum and maximum heights are
/// equal.
///
/// * The [isNormalized] getter, which should return true if the object
/// represents its data in its canonical form. Sometimes, it is possible for
/// fields to be redundant with each other, such that several different
/// representations have the same implications. For example, a
/// [BoxConstraints] instance with its minimum width greater than its maximum
/// width is equivalent to one where the maximum width is set to that minimum
/// width (`2<w<1` is equivalent to `2<w<2`, since minimum constraints have
/// priority). This getter is used by the default implementation of
/// [debugAssertIsValid].
///
/// * The [debugAssertIsValid] method, which should assert if there's anything
/// wrong with the constraints object. (We use this approach rather than
/// asserting in constructors so that our constructors can be `const` and so
/// that it is possible to create invalid constraints temporarily while
/// building valid ones.) See the implementation of
/// [BoxConstraints.debugAssertIsValid] for an example of the detailed checks
/// that can be made.
///
/// * The [==] operator and the [hashCode] getter, so that constraints can be
/// compared for equality. If a render object is given constraints that are
/// equal, then the rendering library will avoid laying the object out again
/// if it is not dirty.
///
/// * The [toString] method, which should describe the constraints so that they
/// appear in a usefully readable form in the output of [debugDumpRenderTree].
@immutable
abstract class Constraints {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const Constraints();
/// Whether there is exactly one size possible given these constraints.
bool get isTight;
/// Whether the constraint is expressed in a consistent manner.
bool get isNormalized;
/// Asserts that the constraints are valid.
///
/// This might involve checks more detailed than [isNormalized].
///
/// For example, the [BoxConstraints] subclass verifies that the constraints
/// are not [double.nan].
///
/// If the `isAppliedConstraint` argument is true, then even stricter rules
/// are enforced. This argument is set to true when checking constraints that
/// are about to be applied to a [RenderObject] during layout, as opposed to
/// constraints that may be further affected by other constraints. For
/// example, the asserts for verifying the validity of
/// [RenderConstrainedBox.additionalConstraints] do not set this argument, but
/// the asserts for verifying the argument passed to the [RenderObject.layout]
/// method do.
///
/// The `informationCollector` argument takes an optional callback which is
/// called when an exception is to be thrown. The collected information is
/// then included in the message after the error line.
///
/// Returns the same as [isNormalized] if asserts are disabled.
bool debugAssertIsValid({
bool isAppliedConstraint = false,
InformationCollector? informationCollector,
}) {
assert(isNormalized);
return isNormalized;
}
}
/// Signature for a function that is called for each [RenderObject].
///
/// Used by [RenderObject.visitChildren] and [RenderObject.visitChildrenForSemantics].
typedef RenderObjectVisitor = void Function(RenderObject child);
/// Signature for a function that is called during layout.
///
/// Used by [RenderObject.invokeLayoutCallback].
typedef LayoutCallback<T extends Constraints> = void Function(T constraints);
class _LocalSemanticsHandle implements SemanticsHandle {
_LocalSemanticsHandle._(PipelineOwner owner, this.listener)
: _owner = owner {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/rendering.dart',
className: '$_LocalSemanticsHandle',
object: this,
);
}
if (listener != null) {
_owner.semanticsOwner!.addListener(listener!);
}
}
final PipelineOwner _owner;
/// The callback that will be notified when the semantics tree updates.
final VoidCallback? listener;
@override
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
if (listener != null) {
_owner.semanticsOwner!.removeListener(listener!);
}
_owner._didDisposeSemanticsHandle();
}
}
/// The pipeline owner manages the rendering pipeline.
///
/// The pipeline owner provides an interface for driving the rendering pipeline
/// and stores the state about which render objects have requested to be visited
/// in each stage of the pipeline. To flush the pipeline, call the following
/// functions in order:
///
/// 1. [flushLayout] updates any render objects that need to compute their
/// layout. During this phase, the size and position of each render
/// object is calculated. Render objects might dirty their painting or
/// compositing state during this phase.
/// 2. [flushCompositingBits] updates any render objects that have dirty
/// compositing bits. During this phase, each render object learns whether
/// any of its children require compositing. This information is used during
/// the painting phase when selecting how to implement visual effects such as
/// clipping. If a render object has a composited child, it needs to use a
/// [Layer] to create the clip in order for the clip to apply to the
/// composited child (which will be painted into its own [Layer]).
/// 3. [flushPaint] visits any render objects that need to paint. During this
/// phase, render objects get a chance to record painting commands into
/// [PictureLayer]s and construct other composited [Layer]s.
/// 4. Finally, if semantics are enabled, [flushSemantics] will compile the
/// semantics for the render objects. This semantic information is used by
/// assistive technology to improve the accessibility of the render tree.
///
/// The [RendererBinding] holds the pipeline owner for the render objects that
/// are visible on screen. You can create other pipeline owners to manage
/// off-screen objects, which can flush their pipelines independently of the
/// on-screen render objects.
///
/// [PipelineOwner]s can be organized in a tree to manage multiple render trees,
/// where each [PipelineOwner] is responsible for one of the render trees. To
/// build or modify the tree, call [adoptChild] or [dropChild]. During each of
/// the different flush phases described above, a [PipelineOwner] will first
/// perform the phase on the nodes it manages in its own render tree before
/// calling the same flush method on its children. No assumption must be made
/// about the order in which child [PipelineOwner]s are flushed.
///
/// A [PipelineOwner] may also be [attach]ed to a [PipelineManifold], which
/// gives it access to platform functionality usually exposed by the bindings
/// without tying it to a specific binding implementation. All [PipelineOwner]s
/// in a given tree must be attached to the same [PipelineManifold]. This
/// happens automatically during [adoptChild].
class PipelineOwner with DiagnosticableTreeMixin {
/// Creates a pipeline owner.
///
/// Typically created by the binding (e.g., [RendererBinding]), but can be
/// created separately from the binding to drive off-screen render objects
/// through the rendering pipeline.
PipelineOwner({
this.onNeedVisualUpdate,
this.onSemanticsOwnerCreated,
this.onSemanticsUpdate,
this.onSemanticsOwnerDisposed,
}){
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/rendering.dart',
className: '$PipelineOwner',
object: this,
);
}
}
/// Called when a render object associated with this pipeline owner wishes to
/// update its visual appearance.
///
/// Typical implementations of this function will schedule a task to flush the
/// various stages of the pipeline. This function might be called multiple
/// times in quick succession. Implementations should take care to discard
/// duplicate calls quickly.
///
/// When the [PipelineOwner] is attached to a [PipelineManifold] and
/// [onNeedVisualUpdate] is provided, the [onNeedVisualUpdate] callback is
/// invoked instead of calling [PipelineManifold.requestVisualUpdate].
final VoidCallback? onNeedVisualUpdate;
/// Called whenever this pipeline owner creates a semantics object.
///
/// Typical implementations will schedule the creation of the initial
/// semantics tree.
final VoidCallback? onSemanticsOwnerCreated;
/// Called whenever this pipeline owner's semantics owner emits a [SemanticsUpdate].
///
/// Typical implementations will delegate the [SemanticsUpdate] to a [FlutterView]
/// that can handle the [SemanticsUpdate].
final SemanticsUpdateCallback? onSemanticsUpdate;
/// Called whenever this pipeline owner disposes its semantics owner.
///
/// Typical implementations will tear down the semantics tree.
final VoidCallback? onSemanticsOwnerDisposed;
/// Calls [onNeedVisualUpdate] if [onNeedVisualUpdate] is not null.
///
/// Used to notify the pipeline owner that an associated render object wishes
/// to update its visual appearance.
void requestVisualUpdate() {
if (onNeedVisualUpdate != null) {
onNeedVisualUpdate!();
} else {
_manifold?.requestVisualUpdate();
}
}
/// The unique object managed by this pipeline that has no parent.
RenderObject? get rootNode => _rootNode;
RenderObject? _rootNode;
set rootNode(RenderObject? value) {
if (_rootNode == value) {
return;
}
_rootNode?.detach();
_rootNode = value;
_rootNode?.attach(this);
}
// Whether the current [flushLayout] call should pause to incorporate the
// [RenderObject]s in `_nodesNeedingLayout` into the current dirty list,
// before continuing to process dirty relayout boundaries.
//
// This flag is set to true when a [RenderObject.invokeLayoutCallback]
// returns, to avoid laying out dirty relayout boundaries in an incorrect
// order and causing them to be laid out more than once per frame. See
// layout_builder_mutations_test.dart for an example.
//
// The new dirty nodes are not immediately merged after a
// [RenderObject.invokeLayoutCallback] call because we may encounter multiple
// such calls while processing a single relayout boundary in [flushLayout].
// Batching new dirty nodes can reduce the number of merges [flushLayout]
// has to perform.
bool _shouldMergeDirtyNodes = false;
List<RenderObject> _nodesNeedingLayout = <RenderObject>[];
/// Whether this pipeline is currently in the layout phase.
///
/// Specifically, whether [flushLayout] is currently running.
///
/// Only valid when asserts are enabled; in release builds, this
/// always returns false.
bool get debugDoingLayout => _debugDoingLayout;
bool _debugDoingLayout = false;
bool _debugDoingChildLayout = false;
/// Update the layout information for all dirty render objects.
///
/// This function is one of the core stages of the rendering pipeline. Layout
/// information is cleaned prior to painting so that render objects will
/// appear on screen in their up-to-date locations.
///
/// See [RendererBinding] for an example of how this function is used.
void flushLayout() {
if (!kReleaseMode) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (debugEnhanceLayoutTimelineArguments) {
debugTimelineArguments = <String, String>{
'dirty count': '${_nodesNeedingLayout.length}',
'dirty list': '$_nodesNeedingLayout',
};
}
return true;
}());
FlutterTimeline.startSync(
'LAYOUT$_debugRootSuffixForTimelineEventNames',
arguments: debugTimelineArguments,
);
}
assert(() {
_debugDoingLayout = true;
return true;
}());
try {
while (_nodesNeedingLayout.isNotEmpty) {
assert(!_shouldMergeDirtyNodes);
final List<RenderObject> dirtyNodes = _nodesNeedingLayout;
_nodesNeedingLayout = <RenderObject>[];
dirtyNodes.sort((RenderObject a, RenderObject b) => a.depth - b.depth);
for (int i = 0; i < dirtyNodes.length; i++) {
if (_shouldMergeDirtyNodes) {
_shouldMergeDirtyNodes = false;
if (_nodesNeedingLayout.isNotEmpty) {
_nodesNeedingLayout.addAll(dirtyNodes.getRange(i, dirtyNodes.length));
break;
}
}
final RenderObject node = dirtyNodes[i];
if (node._needsLayout && node.owner == this) {
node._layoutWithoutResize();
}
}
// No need to merge dirty nodes generated from processing the last
// relayout boundary back.
_shouldMergeDirtyNodes = false;
}
assert(() {
_debugDoingChildLayout = true;
return true;
}());
for (final PipelineOwner child in _children) {
child.flushLayout();
}
assert(_nodesNeedingLayout.isEmpty, 'Child PipelineOwners must not dirty nodes in their parent.');
} finally {
_shouldMergeDirtyNodes = false;
assert(() {
_debugDoingLayout = false;
_debugDoingChildLayout = false;
return true;
}());
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
}
// This flag is used to allow the kinds of mutations performed by GlobalKey
// reparenting while a LayoutBuilder is being rebuilt and in so doing tries to
// move a node from another LayoutBuilder subtree that hasn't been updated
// yet. To set this, call [_enableMutationsToDirtySubtrees], which is called
// by [RenderObject.invokeLayoutCallback].
bool _debugAllowMutationsToDirtySubtrees = false;
// See [RenderObject.invokeLayoutCallback].
void _enableMutationsToDirtySubtrees(VoidCallback callback) {
assert(_debugDoingLayout);
bool? oldState;
assert(() {
oldState = _debugAllowMutationsToDirtySubtrees;
_debugAllowMutationsToDirtySubtrees = true;
return true;
}());
try {
callback();
} finally {
_shouldMergeDirtyNodes = true;
assert(() {
_debugAllowMutationsToDirtySubtrees = oldState!;
return true;
}());
}
}
final List<RenderObject> _nodesNeedingCompositingBitsUpdate = <RenderObject>[];
/// Updates the [RenderObject.needsCompositing] bits.
///
/// Called as part of the rendering pipeline after [flushLayout] and before
/// [flushPaint].
void flushCompositingBits() {
if (!kReleaseMode) {
FlutterTimeline.startSync('UPDATING COMPOSITING BITS$_debugRootSuffixForTimelineEventNames');
}
_nodesNeedingCompositingBitsUpdate.sort((RenderObject a, RenderObject b) => a.depth - b.depth);
for (final RenderObject node in _nodesNeedingCompositingBitsUpdate) {
if (node._needsCompositingBitsUpdate && node.owner == this) {
node._updateCompositingBits();
}
}
_nodesNeedingCompositingBitsUpdate.clear();
for (final PipelineOwner child in _children) {
child.flushCompositingBits();
}
assert(_nodesNeedingCompositingBitsUpdate.isEmpty, 'Child PipelineOwners must not dirty nodes in their parent.');
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
List<RenderObject> _nodesNeedingPaint = <RenderObject>[];
/// Whether this pipeline is currently in the paint phase.
///
/// Specifically, whether [flushPaint] is currently running.
///
/// Only valid when asserts are enabled. In release builds,
/// this always returns false.
bool get debugDoingPaint => _debugDoingPaint;
bool _debugDoingPaint = false;
/// Update the display lists for all render objects.
///
/// This function is one of the core stages of the rendering pipeline.
/// Painting occurs after layout and before the scene is recomposited so that
/// scene is composited with up-to-date display lists for every render object.
///
/// See [RendererBinding] for an example of how this function is used.
void flushPaint() {
if (!kReleaseMode) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (debugEnhancePaintTimelineArguments) {
debugTimelineArguments = <String, String>{
'dirty count': '${_nodesNeedingPaint.length}',
'dirty list': '$_nodesNeedingPaint',
};
}
return true;
}());
FlutterTimeline.startSync(
'PAINT$_debugRootSuffixForTimelineEventNames',
arguments: debugTimelineArguments,
);
}
try {
assert(() {
_debugDoingPaint = true;
return true;
}());
final List<RenderObject> dirtyNodes = _nodesNeedingPaint;
_nodesNeedingPaint = <RenderObject>[];
// Sort the dirty nodes in reverse order (deepest first).
for (final RenderObject node in dirtyNodes..sort((RenderObject a, RenderObject b) => b.depth - a.depth)) {
assert(node._layerHandle.layer != null);
if ((node._needsPaint || node._needsCompositedLayerUpdate) && node.owner == this) {
if (node._layerHandle.layer!.attached) {
assert(node.isRepaintBoundary);
if (node._needsPaint) {
PaintingContext.repaintCompositedChild(node);
} else {
PaintingContext.updateLayerProperties(node);
}
} else {
node._skippedPaintingOnLayer();
}
}
}
for (final PipelineOwner child in _children) {
child.flushPaint();
}
assert(_nodesNeedingPaint.isEmpty, 'Child PipelineOwners must not dirty nodes in their parent.');
} finally {
assert(() {
_debugDoingPaint = false;
return true;
}());
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
}
/// The object that is managing semantics for this pipeline owner, if any.
///
/// An owner is created by [ensureSemantics] or when the [PipelineManifold] to
/// which this owner is connected has [PipelineManifold.semanticsEnabled] set
/// to true. The owner is valid for as long as
/// [PipelineManifold.semanticsEnabled] remains true or while there are
/// outstanding [SemanticsHandle]s from calls to [ensureSemantics]. The
/// [semanticsOwner] field will revert to null once both conditions are no
/// longer met.
///
/// When [semanticsOwner] is null, the [PipelineOwner] skips all steps
/// relating to semantics.
SemanticsOwner? get semanticsOwner => _semanticsOwner;
SemanticsOwner? _semanticsOwner;
/// The number of clients registered to listen for semantics.
///
/// The number is increased whenever [ensureSemantics] is called and decreased
/// when [SemanticsHandle.dispose] is called.
int get debugOutstandingSemanticsHandles => _outstandingSemanticsHandles;
int _outstandingSemanticsHandles = 0;
/// Opens a [SemanticsHandle] and calls [listener] whenever the semantics tree
/// generated from the render tree owned by this [PipelineOwner] updates.
///
/// Calling this method only ensures that this particular [PipelineOwner] will
/// generate a semantics tree. Consider calling
/// [SemanticsBinding.ensureSemantics] instead to turn on semantics globally
/// for the entire app.
///
/// The [PipelineOwner] updates the semantics tree only when there are clients
/// that wish to use the semantics tree. These clients express their interest
/// by holding [SemanticsHandle] objects that notify them whenever the
/// semantics tree updates.
///
/// Clients can close their [SemanticsHandle] by calling
/// [SemanticsHandle.dispose]. Once all the outstanding [SemanticsHandle]
/// objects for a given [PipelineOwner] are closed, the [PipelineOwner] stops
/// maintaining the semantics tree.
SemanticsHandle ensureSemantics({ VoidCallback? listener }) {
_outstandingSemanticsHandles += 1;
_updateSemanticsOwner();
return _LocalSemanticsHandle._(this, listener);
}
void _updateSemanticsOwner() {
if ((_manifold?.semanticsEnabled ?? false) || _outstandingSemanticsHandles > 0) {
if (_semanticsOwner == null) {
assert(onSemanticsUpdate != null, 'Attempted to enable semantics without configuring an onSemanticsUpdate callback.');
_semanticsOwner = SemanticsOwner(onSemanticsUpdate: onSemanticsUpdate!);
onSemanticsOwnerCreated?.call();
}
} else if (_semanticsOwner != null) {
_semanticsOwner?.dispose();
_semanticsOwner = null;
onSemanticsOwnerDisposed?.call();
}
}
void _didDisposeSemanticsHandle() {
assert(_semanticsOwner != null);
_outstandingSemanticsHandles -= 1;
_updateSemanticsOwner();
}
bool _debugDoingSemantics = false;
final Set<RenderObject> _nodesNeedingSemantics = <RenderObject>{};
/// Update the semantics for render objects marked as needing a semantics
/// update.
///
/// Initially, only the root node, as scheduled by
/// [RenderObject.scheduleInitialSemantics], needs a semantics update.
///
/// This function is one of the core stages of the rendering pipeline. The
/// semantics are compiled after painting and only after
/// [RenderObject.scheduleInitialSemantics] has been called.
///
/// See [RendererBinding] for an example of how this function is used.
void flushSemantics() {
if (_semanticsOwner == null) {
return;
}
if (!kReleaseMode) {
FlutterTimeline.startSync('SEMANTICS$_debugRootSuffixForTimelineEventNames');
}
assert(_semanticsOwner != null);
assert(() {
_debugDoingSemantics = true;
return true;
}());
try {
final List<RenderObject> nodesToProcess = _nodesNeedingSemantics.toList()
..sort((RenderObject a, RenderObject b) => a.depth - b.depth);
_nodesNeedingSemantics.clear();
for (final RenderObject node in nodesToProcess) {
if (node._needsSemanticsUpdate && node.owner == this) {
node._updateSemantics();
}
}
_semanticsOwner!.sendSemanticsUpdate();
for (final PipelineOwner child in _children) {
child.flushSemantics();
}
assert(_nodesNeedingSemantics.isEmpty, 'Child PipelineOwners must not dirty nodes in their parent.');
} finally {
assert(() {
_debugDoingSemantics = false;
return true;
}());
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return <DiagnosticsNode>[
for (final PipelineOwner child in _children)
child.toDiagnosticsNode(),
];
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<RenderObject>('rootNode', rootNode, defaultValue: null));
}
// TREE MANAGEMENT
final Set<PipelineOwner> _children = <PipelineOwner>{};
PipelineManifold? _manifold;
PipelineOwner? _debugParent;
bool _debugSetParent(PipelineOwner child, PipelineOwner? parent) {
child._debugParent = parent;
return true;
}
String get _debugRootSuffixForTimelineEventNames => _debugParent == null ? ' (root)' : '';
/// Mark this [PipelineOwner] as attached to the given [PipelineManifold].
///
/// Typically, this is only called directly on the root [PipelineOwner].
/// Children are automatically attached to their parent's [PipelineManifold]
/// when [adoptChild] is called.
void attach(PipelineManifold manifold) {
assert(_manifold == null);
_manifold = manifold;
_manifold!.addListener(_updateSemanticsOwner);
_updateSemanticsOwner();
for (final PipelineOwner child in _children) {
child.attach(manifold);
}
}
/// Mark this [PipelineOwner] as detached.
///
/// Typically, this is only called directly on the root [PipelineOwner].
/// Children are automatically detached from their parent's [PipelineManifold]
/// when [dropChild] is called.
void detach() {
assert(_manifold != null);
_manifold!.removeListener(_updateSemanticsOwner);
_manifold = null;
// Not updating the semantics owner here to not disrupt any of its clients
// in case we get re-attached. If necessary, semantics owner will be updated
// in "attach", or disposed in "dispose", if not reattached.
for (final PipelineOwner child in _children) {
child.detach();
}
}
// In theory, child list modifications are also disallowed between
// _debugDoingChildrenLayout and _debugDoingPaint as well as between
// _debugDoingPaint and _debugDoingSemantics. However, since the associated
// flush methods are usually called back to back, this gets us close enough.
bool get _debugAllowChildListModifications => !_debugDoingChildLayout && !_debugDoingPaint && !_debugDoingSemantics;
/// Adds `child` to this [PipelineOwner].
///
/// During the phases of frame production (see [RendererBinding.drawFrame]),
/// the parent [PipelineOwner] will complete a phase for the nodes it owns
/// directly before invoking the flush method corresponding to the current
/// phase on its child [PipelineOwner]s. For example, during layout, the
/// parent [PipelineOwner] will first lay out its own nodes before calling
/// [flushLayout] on its children. During paint, it will first paint its own
/// nodes before calling [flushPaint] on its children. This order also applies
/// for all the other phases.
///
/// No assumptions must be made about the order in which child
/// [PipelineOwner]s are flushed.
///
/// No new children may be added after the [PipelineOwner] has started calling
/// [flushLayout] on any of its children until the end of the current frame.
///
/// To remove a child, call [dropChild].
void adoptChild(PipelineOwner child) {
assert(child._debugParent == null);
assert(!_children.contains(child));
assert(_debugAllowChildListModifications, 'Cannot modify child list after layout.');
_children.add(child);
if (!kReleaseMode) {
_debugSetParent(child, this);
}
if (_manifold != null) {
child.attach(_manifold!);
}
}
/// Removes a child [PipelineOwner] previously added via [adoptChild].
///
/// This node will cease to call the flush methods on the `child` during frame
/// production.
///
/// No children may be removed after the [PipelineOwner] has started calling
/// [flushLayout] on any of its children until the end of the current frame.
void dropChild(PipelineOwner child) {
assert(child._debugParent == this);
assert(_children.contains(child));
assert(_debugAllowChildListModifications, 'Cannot modify child list after layout.');
_children.remove(child);
if (!kReleaseMode) {
_debugSetParent(child, null);
}
if (_manifold != null) {
child.detach();
}
}
/// Calls `visitor` for each immediate child of this [PipelineOwner].
///
/// See also:
///
/// * [adoptChild] to add a child.
/// * [dropChild] to remove a child.
void visitChildren(PipelineOwnerVisitor visitor) {
_children.forEach(visitor);
}
/// Release any resources held by this pipeline owner.
///
/// Prior to calling this method the pipeline owner must be removed from the
/// pipeline owner tree, i.e. it must have neither a parent nor any children
/// (see [dropChild]). It also must be [detach]ed from any [PipelineManifold].
///
/// The object is no longer usable after calling dispose.
void dispose() {
assert(_children.isEmpty);
assert(rootNode == null);
assert(_manifold == null);
assert(_debugParent == null);
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_semanticsOwner?.dispose();
_semanticsOwner = null;
_nodesNeedingLayout.clear();
_nodesNeedingCompositingBitsUpdate.clear();
_nodesNeedingPaint.clear();
_nodesNeedingSemantics.clear();
}
}
/// Signature for the callback to [PipelineOwner.visitChildren].
///
/// The argument is the child being visited.
typedef PipelineOwnerVisitor = void Function(PipelineOwner child);
/// Manages a tree of [PipelineOwner]s.
///
/// All [PipelineOwner]s within a tree are attached to the same
/// [PipelineManifold], which gives them access to shared functionality such
/// as requesting a visual update (by calling [requestVisualUpdate]). As such,
/// the [PipelineManifold] gives the [PipelineOwner]s access to functionality
/// usually provided by the bindings without tying the [PipelineOwner]s to a
/// particular binding implementation.
///
/// The root of the [PipelineOwner] tree is attached to a [PipelineManifold] by
/// passing the manifold to [PipelineOwner.attach]. Children are attached to the
/// same [PipelineManifold] as their parent when they are adopted via
/// [PipelineOwner.adoptChild].
///
/// [PipelineOwner]s can register listeners with the [PipelineManifold] to be
/// informed when certain values provided by the [PipelineManifold] change.
abstract class PipelineManifold implements Listenable {
/// Whether [PipelineOwner]s connected to this [PipelineManifold] should
/// collect semantics information and produce a semantics tree.
///
/// The [PipelineManifold] notifies its listeners (managed with [addListener]
/// and [removeListener]) when this property changes its value.
///
/// See also:
///
/// * [SemanticsBinding.semanticsEnabled], which [PipelineManifold]
/// implementations typically use to back this property.
bool get semanticsEnabled;
/// Called by a [PipelineOwner] connected to this [PipelineManifold] when a
/// [RenderObject] associated with that pipeline owner wishes to update its
/// visual appearance.
///
/// Typical implementations of this function will schedule a task to flush the
/// various stages of the pipeline. This function might be called multiple
/// times in quick succession. Implementations should take care to discard
/// duplicate calls quickly.
///
/// A [PipelineOwner] connected to this [PipelineManifold] will call
/// [PipelineOwner.onNeedVisualUpdate] instead of this method if it has been
/// configured with a non-null [PipelineOwner.onNeedVisualUpdate] callback.
///
/// See also:
///
/// * [SchedulerBinding.ensureVisualUpdate], which [PipelineManifold]
/// implementations typically call to implement this method.
void requestVisualUpdate();
}
const String _flutterRenderingLibrary = 'package:flutter/rendering.dart';
/// An object in the render tree.
///
/// The [RenderObject] class hierarchy is the core of the rendering
/// library's reason for being.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=zmbmrw07qBc}
///
/// [RenderObject]s have a [parent], and have a slot called [parentData] in
/// which the parent [RenderObject] can store child-specific data, for example,
/// the child position. The [RenderObject] class also implements the basic
/// layout and paint protocols.
///
/// The [RenderObject] class, however, does not define a child model (e.g.
/// whether a node has zero, one, or more children). It also doesn't define a
/// coordinate system (e.g. whether children are positioned in Cartesian
/// coordinates, in polar coordinates, etc) or a specific layout protocol (e.g.
/// whether the layout is width-in-height-out, or constraint-in-size-out, or
/// whether the parent sets the size and position of the child before or after
/// the child lays out, etc; or indeed whether the children are allowed to read
/// their parent's [parentData] slot).
///
/// The [RenderBox] subclass introduces the opinion that the layout
/// system uses Cartesian coordinates.
///
/// ## Lifecycle
///
/// A [RenderObject] must [dispose] when it is no longer needed. The creator
/// of the object is responsible for disposing of it. Typically, the creator is
/// a [RenderObjectElement], and that element will dispose the object it creates
/// when it is unmounted.
///
/// [RenderObject]s are responsible for cleaning up any expensive resources
/// they hold when [dispose] is called, such as [Picture] or [Image] objects.
/// This includes any [Layer]s that the render object has directly created. The
/// base implementation of dispose will nullify the [layer] property. Subclasses
/// must also nullify any other layer(s) it directly creates.
///
/// ## Writing a RenderObject subclass
///
/// In most cases, subclassing [RenderObject] itself is overkill, and
/// [RenderBox] would be a better starting point. However, if a render object
/// doesn't want to use a Cartesian coordinate system, then it should indeed
/// inherit from [RenderObject] directly. This allows it to define its own
/// layout protocol by using a new subclass of [Constraints] rather than using
/// [BoxConstraints], and by potentially using an entirely new set of objects
/// and values to represent the result of the output rather than just a [Size].
/// This increased flexibility comes at the cost of not being able to rely on
/// the features of [RenderBox]. For example, [RenderBox] implements an
/// intrinsic sizing protocol that allows you to measure a child without fully
/// laying it out, in such a way that if that child changes size, the parent
/// will be laid out again (to take into account the new dimensions of the
/// child). This is a subtle and bug-prone feature to get right.
///
/// Most aspects of writing a [RenderBox] apply to writing a [RenderObject] as
/// well, and therefore the discussion at [RenderBox] is recommended background
/// reading. The main differences are around layout and hit testing, since those
/// are the aspects that [RenderBox] primarily specializes.
///
/// ### Layout
///
/// A layout protocol begins with a subclass of [Constraints]. See the
/// discussion at [Constraints] for more information on how to write a
/// [Constraints] subclass.
///
/// The [performLayout] method should take the [constraints], and apply them.
/// The output of the layout algorithm is fields set on the object that describe
/// the geometry of the object for the purposes of the parent's layout. For
/// example, with [RenderBox] the output is the [RenderBox.size] field. This
/// output should only be read by the parent if the parent specified
/// `parentUsesSize` as true when calling [layout] on the child.
///
/// Anytime anything changes on a render object that would affect the layout of
/// that object, it should call [markNeedsLayout].
///
/// ### Hit Testing
///
/// Hit testing is even more open-ended than layout. There is no method to
/// override, you are expected to provide one.
///
/// The general behavior of your hit-testing method should be similar to the
/// behavior described for [RenderBox]. The main difference is that the input
/// need not be an [Offset]. You are also allowed to use a different subclass of
/// [HitTestEntry] when adding entries to the [HitTestResult]. When the
/// [handleEvent] method is called, the same object that was added to the
/// [HitTestResult] will be passed in, so it can be used to track information
/// like the precise coordinate of the hit, in whatever coordinate system is
/// used by the new layout protocol.
///
/// ### Adapting from one protocol to another
///
/// In general, the root of a Flutter render object tree is a [RenderView]. This
/// object has a single child, which must be a [RenderBox]. Thus, if you want to
/// have a custom [RenderObject] subclass in the render tree, you have two
/// choices: you either need to replace the [RenderView] itself, or you need to
/// have a [RenderBox] that has your class as its child. (The latter is the much
/// more common case.)
///
/// This [RenderBox] subclass converts from the box protocol to the protocol of
/// your class.
///
/// In particular, this means that for hit testing it overrides
/// [RenderBox.hitTest], and calls whatever method you have in your class for
/// hit testing.
///
/// Similarly, it overrides [performLayout] to create a [Constraints] object
/// appropriate for your class and passes that to the child's [layout] method.
///
/// ### Layout interactions between render objects
///
/// In general, the layout of a render object should only depend on the output of
/// its child's layout, and then only if `parentUsesSize` is set to true in the
/// [layout] call. Furthermore, if it is set to true, the parent must call the
/// child's [layout] if the child is to be rendered, because otherwise the
/// parent will not be notified when the child changes its layout outputs.
///
/// It is possible to set up render object protocols that transfer additional
/// information. For example, in the [RenderBox] protocol you can query your
/// children's intrinsic dimensions and baseline geometry. However, if this is
/// done then it is imperative that the child call [markNeedsLayout] on the
/// parent any time that additional information changes, if the parent used it
/// in the last layout phase. For an example of how to implement this, see the
/// [RenderBox.markNeedsLayout] method. It overrides
/// [RenderObject.markNeedsLayout] so that if a parent has queried the intrinsic
/// or baseline information, it gets marked dirty whenever the child's geometry
/// changes.
abstract class RenderObject with DiagnosticableTreeMixin implements HitTestTarget {
/// Initializes internal fields for subclasses.
RenderObject() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterRenderingLibrary,
className: '$RenderObject',
object: this,
);
}
_needsCompositing = isRepaintBoundary || alwaysNeedsCompositing;
_wasRepaintBoundary = isRepaintBoundary;
}
/// Cause the entire subtree rooted at the given [RenderObject] to be marked
/// dirty for layout, paint, etc, so that the effects of a hot reload can be
/// seen, or so that the effect of changing a global debug flag (such as
/// [debugPaintSizeEnabled]) can be applied.
///
/// This is called by the [RendererBinding] in response to the
/// `ext.flutter.reassemble` hook, which is used by development tools when the
/// application code has changed, to cause the widget tree to pick up any
/// changed implementations.
///
/// This is expensive and should not be called except during development.
///
/// See also:
///
/// * [BindingBase.reassembleApplication]
void reassemble() {
markNeedsLayout();
markNeedsCompositingBitsUpdate();
markNeedsPaint();
markNeedsSemanticsUpdate();
visitChildren((RenderObject child) {
child.reassemble();
});
}
/// Whether this has been disposed.
///
/// If asserts are disabled, this property is always null.
bool? get debugDisposed {
bool? disposed;
assert(() {
disposed = _debugDisposed;
return true;
}());
return disposed;
}
bool _debugDisposed = false;
/// Release any resources held by this render object.
///
/// The object that creates a RenderObject is in charge of disposing it.
/// If this render object has created any children directly, it must dispose
/// of those children in this method as well. It must not dispose of any
/// children that were created by some other object, such as
/// a [RenderObjectElement]. Those children will be disposed when that
/// element unmounts, which may be delayed if the element is moved to another
/// part of the tree.
///
/// Implementations of this method must end with a call to the inherited
/// method, as in `super.dispose()`.
///
/// The object is no longer usable after calling dispose.
@mustCallSuper
void dispose() {
assert(!_debugDisposed);
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_layerHandle.layer = null;
assert(() {
// TODO(dnfield): Enable this assert once clients have had a chance to
// migrate.
// visitChildren((RenderObject child) {
// assert(
// child.debugDisposed!,
// '${child.runtimeType} (child of $runtimeType) must be disposed before calling super.dispose().',
// );
// });
_debugDisposed = true;
return true;
}());
}
// LAYOUT
/// Data for use by the parent render object.
///
/// The parent data is used by the render object that lays out this object
/// (typically this object's parent in the render tree) to store information
/// relevant to itself and to any other nodes who happen to know exactly what
/// the data means. The parent data is opaque to the child.
///
/// * The parent data field must not be directly set, except by calling
/// [setupParentData] on the parent node.
/// * The parent data can be set before the child is added to the parent, by
/// calling [setupParentData] on the future parent node.
/// * The conventions for using the parent data depend on the layout protocol
/// used between the parent and child. For example, in box layout, the
/// parent data is completely opaque but in sector layout the child is
/// permitted to read some fields of the parent data.
ParentData? parentData;
/// Override to setup parent data correctly for your children.
///
/// You can call this function to set up the parent data for child before the
/// child is added to the parent's child list.
void setupParentData(covariant RenderObject child) {
assert(_debugCanPerformMutations);
if (child.parentData is! ParentData) {
child.parentData = ParentData();
}
}
/// The depth of this render object in the render tree.
///
/// The depth of nodes in a tree monotonically increases as you traverse down
/// the tree: a node always has a [depth] greater than its ancestors.
/// There's no guarantee regarding depth between siblings.
///
/// The [depth] of a child can be more than one greater than the [depth] of
/// the parent, because the [depth] values are never decreased: all that
/// matters is that it's greater than the parent. Consider a tree with a root
/// node A, a child B, and a grandchild C. Initially, A will have [depth] 0,
/// B [depth] 1, and C [depth] 2. If C is moved to be a child of A,
/// sibling of B, then the numbers won't change. C's [depth] will still be 2.
///
/// The depth of a node is used to ensure that nodes are processed in
/// depth order. The [depth] is automatically maintained by the [adoptChild]
/// and [dropChild] methods.
int get depth => _depth;
int _depth = 0;
/// Adjust the [depth] of the given [child] to be greater than this node's own
/// [depth].
///
/// Only call this method from overrides of [redepthChildren].
@protected
void redepthChild(RenderObject child) {
assert(child.owner == owner);
if (child._depth <= _depth) {
child._depth = _depth + 1;
child.redepthChildren();
}
}
/// Adjust the [depth] of this node's children, if any.
///
/// Override this method in subclasses with child nodes to call [redepthChild]
/// for each child. Do not call this method directly.
@protected
void redepthChildren() { }
/// The parent of this render object in the render tree.
///
/// The [parent] of the root node in the render tree is null.
RenderObject? get parent => _parent;
RenderObject? _parent;
/// Called by subclasses when they decide a render object is a child.
///
/// Only for use by subclasses when changing their child lists. Calling this
/// in other cases will lead to an inconsistent tree and probably cause crashes.
@mustCallSuper
@protected
void adoptChild(RenderObject child) {
assert(child._parent == null);
assert(() {
RenderObject node = this;
while (node.parent != null) {
node = node.parent!;
}
assert(node != child); // indicates we are about to create a cycle
return true;
}());
setupParentData(child);
markNeedsLayout();
markNeedsCompositingBitsUpdate();
markNeedsSemanticsUpdate();
child._parent = this;
if (attached) {
child.attach(_owner!);
}
redepthChild(child);
}
/// Called by subclasses when they decide a render object is no longer a child.
///
/// Only for use by subclasses when changing their child lists. Calling this
/// in other cases will lead to an inconsistent tree and probably cause crashes.
@mustCallSuper
@protected
void dropChild(RenderObject child) {
assert(child._parent == this);
assert(child.attached == attached);
assert(child.parentData != null);
child._cleanRelayoutBoundary();
child.parentData!.detach();
child.parentData = null;
child._parent = null;
if (attached) {
child.detach();
}
markNeedsLayout();
markNeedsCompositingBitsUpdate();
markNeedsSemanticsUpdate();
}
/// Calls visitor for each immediate child of this render object.
///
/// Override in subclasses with children and call the visitor for each child.
void visitChildren(RenderObjectVisitor visitor) { }
/// The object responsible for creating this render object.
///
/// Used in debug messages.
///
/// See also:
///
/// * [DebugCreator], which the [widgets] library uses as values for this field.
Object? debugCreator;
void _reportException(String method, Object exception, StackTrace stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'rendering library',
context: ErrorDescription('during $method()'),
informationCollector: () => <DiagnosticsNode>[
// debugCreator should always be null outside of debugMode, but we want
// the tree shaker to notice this.
if (kDebugMode && debugCreator != null)
DiagnosticsDebugCreator(debugCreator!),
describeForError('The following RenderObject was being processed when the exception was fired'),
// TODO(jacobr): this error message has a code smell. Consider whether
// displaying the truncated children is really useful for command line
// users. Inspector users can see the full tree by clicking on the
// render object so this may not be that useful.
describeForError('RenderObject', style: DiagnosticsTreeStyle.truncateChildren),
],
));
}
/// Whether [performResize] for this render object is currently running.
///
/// Only valid when asserts are enabled. In release builds, always returns
/// false.
bool get debugDoingThisResize => _debugDoingThisResize;
bool _debugDoingThisResize = false;
/// Whether [performLayout] for this render object is currently running.
///
/// Only valid when asserts are enabled. In release builds, always returns
/// false.
bool get debugDoingThisLayout => _debugDoingThisLayout;
bool _debugDoingThisLayout = false;
/// The render object that is actively computing layout.
///
/// Only valid when asserts are enabled. In release builds, always returns
/// null.
static RenderObject? get debugActiveLayout => _debugActiveLayout;
static RenderObject? _debugActiveLayout;
/// Set [debugActiveLayout] to null when [inner] callback is called.
/// This is useful when you have to temporarily clear that variable to
/// disable some false-positive checks, such as when computing toStringDeep
/// or using custom trees.
@pragma('dart2js:tryInline')
@pragma('vm:prefer-inline')
@pragma('wasm:prefer-inline')
static T _withDebugActiveLayoutCleared<T>(T Function() inner) {
RenderObject? debugPreviousActiveLayout;
assert(() {
debugPreviousActiveLayout = _debugActiveLayout;
_debugActiveLayout = null;
return true;
}());
final T result = inner();
assert(() {
_debugActiveLayout = debugPreviousActiveLayout;
return true;
}());
return result;
}
/// Whether the parent render object is permitted to use this render object's
/// size.
///
/// Determined by the `parentUsesSize` parameter to [layout].
///
/// Only valid when asserts are enabled. In release builds, throws.
bool get debugCanParentUseSize => _debugCanParentUseSize!;
bool? _debugCanParentUseSize;
bool _debugMutationsLocked = false;
/// Whether tree mutations are currently permitted.
///
/// This is only useful during layout. One should also not mutate the tree at
/// other times (e.g. during paint or while assembling the semantic tree) but
/// this function does not currently enforce those conventions.
///
/// Only valid when asserts are enabled. This will throw in release builds.
bool get _debugCanPerformMutations {
late bool result;
assert(() {
if (_debugDisposed) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A disposed RenderObject was mutated.'),
DiagnosticsProperty<RenderObject>(
'The disposed RenderObject was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
final PipelineOwner? owner = this.owner;
// Detached nodes are allowed to mutate and the "can perform mutations"
// check will be performed when they re-attach. This assert is only useful
// during layout.
if (owner == null || !owner.debugDoingLayout) {
result = true;
return true;
}
RenderObject? activeLayoutRoot = this;
while (activeLayoutRoot != null) {
final bool mutationsToDirtySubtreesAllowed = activeLayoutRoot.owner?._debugAllowMutationsToDirtySubtrees ?? false;
final bool doingLayoutWithCallback = activeLayoutRoot._doingThisLayoutWithCallback;
// Mutations on this subtree is allowed when:
// - the "activeLayoutRoot" subtree is being mutated in a layout callback.
// - a different part of the render tree is doing a layout callback,
// and this subtree is being reparented to that subtree, as a result
// of global key reparenting.
if (doingLayoutWithCallback || mutationsToDirtySubtreesAllowed && activeLayoutRoot._needsLayout) {
result = true;
return true;
}
if (!activeLayoutRoot._debugMutationsLocked) {
final RenderObject? p = activeLayoutRoot.debugLayoutParent;
activeLayoutRoot = p is RenderObject ? p : null;
} else {
// activeLayoutRoot found.
break;
}
}
final RenderObject debugActiveLayout = RenderObject.debugActiveLayout!;
final String culpritMethodName = debugActiveLayout.debugDoingThisLayout ? 'performLayout' : 'performResize';
final String culpritFullMethodName = '${debugActiveLayout.runtimeType}.$culpritMethodName';
result = false;
if (activeLayoutRoot == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A $runtimeType was mutated in $culpritFullMethodName.'),
ErrorDescription(
'The RenderObject was mutated when none of its ancestors is actively performing layout.',
),
DiagnosticsProperty<RenderObject>(
'The RenderObject being mutated was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
DiagnosticsProperty<RenderObject>(
'The RenderObject that was mutating the said $runtimeType was',
debugActiveLayout,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
if (activeLayoutRoot == this) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A $runtimeType was mutated in its own $culpritMethodName implementation.'),
ErrorDescription('A RenderObject must not re-dirty itself while still being laid out.'),
DiagnosticsProperty<RenderObject>(
'The RenderObject being mutated was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
ErrorHint('Consider using the LayoutBuilder widget to dynamically change a subtree during layout.'),
]);
}
final ErrorSummary summary = ErrorSummary('A $runtimeType was mutated in $culpritFullMethodName.');
final bool isMutatedByAncestor = activeLayoutRoot == debugActiveLayout;
final String description = isMutatedByAncestor
? 'A RenderObject must not mutate its descendants in its $culpritMethodName method.'
: 'A RenderObject must not mutate another RenderObject from a different render subtree '
'in its $culpritMethodName method.';
throw FlutterError.fromParts(<DiagnosticsNode>[
summary,
ErrorDescription(description),
DiagnosticsProperty<RenderObject>(
'The RenderObject being mutated was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
DiagnosticsProperty<RenderObject>(
'The ${isMutatedByAncestor ? 'ancestor ' : ''}RenderObject that was mutating the said $runtimeType was',
debugActiveLayout,
style: DiagnosticsTreeStyle.errorProperty,
),
if (!isMutatedByAncestor) DiagnosticsProperty<RenderObject>(
'Their common ancestor was',
activeLayoutRoot,
style: DiagnosticsTreeStyle.errorProperty,
),
ErrorHint(
'Mutating the layout of another RenderObject may cause some RenderObjects in its subtree to be laid out more than once. '
'Consider using the LayoutBuilder widget to dynamically mutate a subtree during layout.'
),
]);
}());
return result;
}
/// The [RenderObject] that's expected to call [layout] on this [RenderObject]
/// in its [performLayout] implementation.
///
/// This method is used to implement an assert that ensures the render subtree
/// actively performing layout can not get accidentally mutated. It's only
/// implemented in debug mode and always returns null in release mode.
///
/// The default implementation returns [parent] and overriding is rarely
/// needed. A [RenderObject] subclass that expects its
/// [RenderObject.performLayout] to be called from a different [RenderObject]
/// that's not its [parent] should override this property to return the actual
/// layout parent.
@protected
RenderObject? get debugLayoutParent {
RenderObject? layoutParent;
assert(() {
layoutParent = parent;
return true;
}());
return layoutParent;
}
/// The owner for this render object (null if unattached).
///
/// The entire render tree that this render object belongs to
/// will have the same owner.
PipelineOwner? get owner => _owner;
PipelineOwner? _owner;
/// Whether the render tree this render object belongs to is attached to a [PipelineOwner].
///
/// This becomes true during the call to [attach].
///
/// This becomes false during the call to [detach].
bool get attached => _owner != null;
/// Mark this render object as attached to the given owner.
///
/// Typically called only from the [parent]'s [attach] method, and by the
/// [owner] to mark the root of a tree as attached.
///
/// Subclasses with children should override this method to
/// [attach] all their children to the same [owner]
/// after calling the inherited method, as in `super.attach(owner)`.
@mustCallSuper
void attach(PipelineOwner owner) {
assert(!_debugDisposed);
assert(_owner == null);
_owner = owner;
// If the node was dirtied in some way while unattached, make sure to add
// it to the appropriate dirty list now that an owner is available
if (_needsLayout && _relayoutBoundary != null) {
// Don't enter this block if we've never laid out at all;
// scheduleInitialLayout() will handle it
_needsLayout = false;
markNeedsLayout();
}
if (_needsCompositingBitsUpdate) {
_needsCompositingBitsUpdate = false;
markNeedsCompositingBitsUpdate();
}
if (_needsPaint && _layerHandle.layer != null) {
// Don't enter this block if we've never painted at all;
// scheduleInitialPaint() will handle it
_needsPaint = false;
markNeedsPaint();
}
if (_needsSemanticsUpdate && _semanticsConfiguration.isSemanticBoundary) {
// Don't enter this block if we've never updated semantics at all;
// scheduleInitialSemantics() will handle it
_needsSemanticsUpdate = false;
markNeedsSemanticsUpdate();
}
}
/// Mark this render object as detached from its [PipelineOwner].
///
/// Typically called only from the [parent]'s [detach], and by the [owner] to
/// mark the root of a tree as detached.
///
/// Subclasses with children should override this method to
/// [detach] all their children after calling the inherited method,
/// as in `super.detach()`.
@mustCallSuper
void detach() {
assert(_owner != null);
_owner = null;
assert(parent == null || attached == parent!.attached);
}
/// Whether this render object's layout information is dirty.
///
/// This is only set in debug mode. In general, render objects should not need
/// to condition their runtime behavior on whether they are dirty or not,
/// since they should only be marked dirty immediately prior to being laid
/// out and painted. In release builds, this throws.
///
/// It is intended to be used by tests and asserts.
bool get debugNeedsLayout {
late bool result;
assert(() {
result = _needsLayout;
return true;
}());
return result;
}
bool _needsLayout = true;
RenderObject? _relayoutBoundary;
/// Whether [invokeLayoutCallback] for this render object is currently running.
bool get debugDoingThisLayoutWithCallback => _doingThisLayoutWithCallback;
bool _doingThisLayoutWithCallback = false;
/// The layout constraints most recently supplied by the parent.
///
/// If layout has not yet happened, accessing this getter will
/// throw a [StateError] exception.
@protected
Constraints get constraints {
if (_constraints == null) {
throw StateError('A RenderObject does not have any constraints before it has been laid out.');
}
return _constraints!;
}
Constraints? _constraints;
/// Verify that the object's constraints are being met. Override
/// this function in a subclass to verify that your state matches
/// the constraints object. This function is only called in checked
/// mode and only when needsLayout is false. If the constraints are
/// not met, it should assert or throw an exception.
@protected
void debugAssertDoesMeetConstraints();
/// When true, a debug method ([debugAssertDoesMeetConstraints], for instance)
/// is currently executing asserts for verifying the consistent behavior of
/// intrinsic dimensions methods.
///
/// This is typically set by framework debug methods. It is read by tests to
/// selectively ignore custom layout callbacks. It should not be set outside of
/// intrinsic-checking debug methods, and should not be checked in release mode
/// (where it will always be false).
static bool debugCheckingIntrinsics = false;
bool _debugSubtreeRelayoutRootAlreadyMarkedNeedsLayout() {
if (_relayoutBoundary == null) {
// We don't know where our relayout boundary is yet.
return true;
}
RenderObject node = this;
while (node != _relayoutBoundary) {
assert(node._relayoutBoundary == _relayoutBoundary);
assert(node.parent != null);
node = node.parent!;
if ((!node._needsLayout) && (!node._debugDoingThisLayout)) {
return false;
}
}
assert(node._relayoutBoundary == node);
return true;
}
/// Mark this render object's layout information as dirty, and either register
/// this object with its [PipelineOwner], or defer to the parent, depending on
/// whether this object is a relayout boundary or not respectively.
///
/// ## Background
///
/// Rather than eagerly updating layout information in response to writes into
/// a render object, we instead mark the layout information as dirty, which
/// schedules a visual update. As part of the visual update, the rendering
/// pipeline updates the render object's layout information.
///
/// This mechanism batches the layout work so that multiple sequential writes
/// are coalesced, removing redundant computation.
///
/// If a render object's parent indicates that it uses the size of one of its
/// render object children when computing its layout information, this
/// function, when called for the child, will also mark the parent as needing
/// layout. In that case, since both the parent and the child need to have
/// their layout recomputed, the pipeline owner is only notified about the
/// parent; when the parent is laid out, it will call the child's [layout]
/// method and thus the child will be laid out as well.
///
/// Once [markNeedsLayout] has been called on a render object,
/// [debugNeedsLayout] returns true for that render object until just after
/// the pipeline owner has called [layout] on the render object.
///
/// ## Special cases
///
/// Some subclasses of [RenderObject], notably [RenderBox], have other
/// situations in which the parent needs to be notified if the child is
/// dirtied (e.g., if the child's intrinsic dimensions or baseline changes).
/// Such subclasses override markNeedsLayout and either call
/// `super.markNeedsLayout()`, in the normal case, or call
/// [markParentNeedsLayout], in the case where the parent needs to be laid out
/// as well as the child.
///
/// If [sizedByParent] has changed, calls
/// [markNeedsLayoutForSizedByParentChange] instead of [markNeedsLayout].
void markNeedsLayout() {
assert(_debugCanPerformMutations);
if (_needsLayout) {
assert(_debugSubtreeRelayoutRootAlreadyMarkedNeedsLayout());
return;
}
if (_relayoutBoundary == null) {
_needsLayout = true;
if (parent != null) {
// _relayoutBoundary is cleaned by an ancestor in RenderObject.layout.
// Conservatively mark everything dirty until it reaches the closest
// known relayout boundary.
markParentNeedsLayout();
}
return;
}
if (_relayoutBoundary != this) {
markParentNeedsLayout();
} else {
_needsLayout = true;
if (owner != null) {
assert(() {
if (debugPrintMarkNeedsLayoutStacks) {
debugPrintStack(label: 'markNeedsLayout() called for $this');
}
return true;
}());
owner!._nodesNeedingLayout.add(this);
owner!.requestVisualUpdate();
}
}
}
/// Mark this render object's layout information as dirty, and then defer to
/// the parent.
///
/// This function should only be called from [markNeedsLayout] or
/// [markNeedsLayoutForSizedByParentChange] implementations of subclasses that
/// introduce more reasons for deferring the handling of dirty layout to the
/// parent. See [markNeedsLayout] for details.
///
/// Only call this if [parent] is not null.
@protected
void markParentNeedsLayout() {
assert(_debugCanPerformMutations);
_needsLayout = true;
assert(this.parent != null);
final RenderObject parent = this.parent!;
if (!_doingThisLayoutWithCallback) {
parent.markNeedsLayout();
} else {
assert(parent._debugDoingThisLayout);
}
assert(parent == this.parent);
}
/// Mark this render object's layout information as dirty (like
/// [markNeedsLayout]), and additionally also handle any necessary work to
/// handle the case where [sizedByParent] has changed value.
///
/// This should be called whenever [sizedByParent] might have changed.
///
/// Only call this if [parent] is not null.
void markNeedsLayoutForSizedByParentChange() {
markNeedsLayout();
markParentNeedsLayout();
}
void _cleanRelayoutBoundary() {
if (_relayoutBoundary != this) {
_relayoutBoundary = null;
visitChildren(_cleanChildRelayoutBoundary);
}
}
void _propagateRelayoutBoundary() {
if (_relayoutBoundary == this) {
return;
}
final RenderObject? parentRelayoutBoundary = parent?._relayoutBoundary;
assert(parentRelayoutBoundary != null);
if (parentRelayoutBoundary != _relayoutBoundary) {
_relayoutBoundary = parentRelayoutBoundary;
visitChildren(_propagateRelayoutBoundaryToChild);
}
}
// Reduces closure allocation for visitChildren use cases.
static void _cleanChildRelayoutBoundary(RenderObject child) {
child._cleanRelayoutBoundary();
}
static void _propagateRelayoutBoundaryToChild(RenderObject child) {
child._propagateRelayoutBoundary();
}
/// Bootstrap the rendering pipeline by scheduling the very first layout.
///
/// Requires this render object to be attached and that this render object
/// is the root of the render tree.
///
/// See [RenderView] for an example of how this function is used.
void scheduleInitialLayout() {
assert(!_debugDisposed);
assert(attached);
assert(parent is! RenderObject);
assert(!owner!._debugDoingLayout);
assert(_relayoutBoundary == null);
_relayoutBoundary = this;
assert(() {
_debugCanParentUseSize = false;
return true;
}());
owner!._nodesNeedingLayout.add(this);
}
@pragma('vm:notify-debugger-on-exception')
void _layoutWithoutResize() {
assert(_relayoutBoundary == this);
RenderObject? debugPreviousActiveLayout;
assert(!_debugMutationsLocked);
assert(!_doingThisLayoutWithCallback);
assert(_debugCanParentUseSize != null);
assert(() {
_debugMutationsLocked = true;
_debugDoingThisLayout = true;
debugPreviousActiveLayout = _debugActiveLayout;
_debugActiveLayout = this;
if (debugPrintLayouts) {
debugPrint('Laying out (without resize) $this');
}
return true;
}());
try {
performLayout();
markNeedsSemanticsUpdate();
} catch (e, stack) {
_reportException('performLayout', e, stack);
}
assert(() {
_debugActiveLayout = debugPreviousActiveLayout;
_debugDoingThisLayout = false;
_debugMutationsLocked = false;
return true;
}());
_needsLayout = false;
markNeedsPaint();
}
/// Compute the layout for this render object.
///
/// This method is the main entry point for parents to ask their children to
/// update their layout information. The parent passes a constraints object,
/// which informs the child as to which layouts are permissible. The child is
/// required to obey the given constraints.
///
/// If the parent reads information computed during the child's layout, the
/// parent must pass true for `parentUsesSize`. In that case, the parent will
/// be marked as needing layout whenever the child is marked as needing layout
/// because the parent's layout information depends on the child's layout
/// information. If the parent uses the default value (false) for
/// `parentUsesSize`, the child can change its layout information (subject to
/// the given constraints) without informing the parent.
///
/// Subclasses should not override [layout] directly. Instead, they should
/// override [performResize] and/or [performLayout]. The [layout] method
/// delegates the actual work to [performResize] and [performLayout].
///
/// The parent's [performLayout] method should call the [layout] of all its
/// children unconditionally. It is the [layout] method's responsibility (as
/// implemented here) to return early if the child does not need to do any
/// work to update its layout information.
@pragma('vm:notify-debugger-on-exception')
void layout(Constraints constraints, { bool parentUsesSize = false }) {
assert(!_debugDisposed);
if (!kReleaseMode && debugProfileLayoutsEnabled) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (debugEnhanceLayoutTimelineArguments) {
debugTimelineArguments = toDiagnosticsNode().toTimelineArguments();
}
return true;
}());
FlutterTimeline.startSync(
'$runtimeType',
arguments: debugTimelineArguments,
);
}
assert(constraints.debugAssertIsValid(
isAppliedConstraint: true,
informationCollector: () {
final List<String> stack = StackTrace.current.toString().split('\n');
int? targetFrame;
final Pattern layoutFramePattern = RegExp(r'^#[0-9]+ +Render(?:Object|Box).layout \(');
for (int i = 0; i < stack.length; i += 1) {
if (layoutFramePattern.matchAsPrefix(stack[i]) != null) {
targetFrame = i + 1;
} else if (targetFrame != null) {
break;
}
}
if (targetFrame != null && targetFrame < stack.length) {
final Pattern targetFramePattern = RegExp(r'^#[0-9]+ +(.+)$');
final Match? targetFrameMatch = targetFramePattern.matchAsPrefix(stack[targetFrame]);
final String? problemFunction = (targetFrameMatch != null && targetFrameMatch.groupCount > 0) ? targetFrameMatch.group(1) : stack[targetFrame].trim();
return <DiagnosticsNode>[
ErrorDescription(
"These invalid constraints were provided to $runtimeType's layout() "
'function by the following function, which probably computed the '
'invalid constraints in question:\n'
' $problemFunction',
),
];
}
return <DiagnosticsNode>[];
},
));
assert(!_debugDoingThisResize);
assert(!_debugDoingThisLayout);
final bool isRelayoutBoundary = !parentUsesSize || sizedByParent || constraints.isTight || parent is! RenderObject;
final RenderObject relayoutBoundary = isRelayoutBoundary ? this : parent!._relayoutBoundary!;
assert(() {
_debugCanParentUseSize = parentUsesSize;
return true;
}());
if (!_needsLayout && constraints == _constraints) {
assert(() {
// in case parentUsesSize changed since the last invocation, set size
// to itself, so it has the right internal debug values.
_debugDoingThisResize = sizedByParent;
_debugDoingThisLayout = !sizedByParent;
final RenderObject? debugPreviousActiveLayout = _debugActiveLayout;
_debugActiveLayout = this;
debugResetSize();
_debugActiveLayout = debugPreviousActiveLayout;
_debugDoingThisLayout = false;
_debugDoingThisResize = false;
return true;
}());
if (relayoutBoundary != _relayoutBoundary) {
_relayoutBoundary = relayoutBoundary;
visitChildren(_propagateRelayoutBoundaryToChild);
}
if (!kReleaseMode && debugProfileLayoutsEnabled) {
FlutterTimeline.finishSync();
}
return;
}
_constraints = constraints;
if (_relayoutBoundary != null && relayoutBoundary != _relayoutBoundary) {
// The local relayout boundary has changed, must notify children in case
// they also need updating. Otherwise, they will be confused about what
// their actual relayout boundary is later.
visitChildren(_cleanChildRelayoutBoundary);
}
_relayoutBoundary = relayoutBoundary;
assert(!_debugMutationsLocked);
assert(!_doingThisLayoutWithCallback);
assert(() {
_debugMutationsLocked = true;
if (debugPrintLayouts) {
debugPrint('Laying out (${sizedByParent ? "with separate resize" : "with resize allowed"}) $this');
}
return true;
}());
if (sizedByParent) {
assert(() {
_debugDoingThisResize = true;
return true;
}());
try {
performResize();
assert(() {
debugAssertDoesMeetConstraints();
return true;
}());
} catch (e, stack) {
_reportException('performResize', e, stack);
}
assert(() {
_debugDoingThisResize = false;
return true;
}());
}
RenderObject? debugPreviousActiveLayout;
assert(() {
_debugDoingThisLayout = true;
debugPreviousActiveLayout = _debugActiveLayout;
_debugActiveLayout = this;
return true;
}());
try {
performLayout();
markNeedsSemanticsUpdate();
assert(() {
debugAssertDoesMeetConstraints();
return true;
}());
} catch (e, stack) {
_reportException('performLayout', e, stack);
}
assert(() {
_debugActiveLayout = debugPreviousActiveLayout;
_debugDoingThisLayout = false;
_debugMutationsLocked = false;
return true;
}());
_needsLayout = false;
markNeedsPaint();
if (!kReleaseMode && debugProfileLayoutsEnabled) {
FlutterTimeline.finishSync();
}
}
/// If a subclass has a "size" (the state controlled by `parentUsesSize`,
/// whatever it is in the subclass, e.g. the actual `size` property of
/// [RenderBox]), and the subclass verifies that in debug mode this "size"
/// property isn't used when [debugCanParentUseSize] isn't set, then that
/// subclass should override [debugResetSize] to reapply the current values of
/// [debugCanParentUseSize] to that state.
@protected
void debugResetSize() { }
/// Whether the constraints are the only input to the sizing algorithm (in
/// particular, child nodes have no impact).
///
/// Returning false is always correct, but returning true can be more
/// efficient when computing the size of this render object because we don't
/// need to recompute the size if the constraints don't change.
///
/// Typically, subclasses will always return the same value. If the value can
/// change, then, when it does change, the subclass should make sure to call
/// [markNeedsLayoutForSizedByParentChange].
///
/// Subclasses that return true must not change the dimensions of this render
/// object in [performLayout]. Instead, that work should be done by
/// [performResize] or - for subclasses of [RenderBox] - in
/// [RenderBox.computeDryLayout].
@protected
bool get sizedByParent => false;
/// {@template flutter.rendering.RenderObject.performResize}
/// Updates the render objects size using only the constraints.
///
/// Do not call this function directly: call [layout] instead. This function
/// is called by [layout] when there is actually work to be done by this
/// render object during layout. The layout constraints provided by your
/// parent are available via the [constraints] getter.
///
/// This function is called only if [sizedByParent] is true.
/// {@endtemplate}
///
/// Subclasses that set [sizedByParent] to true should override this method to
/// compute their size. Subclasses of [RenderBox] should consider overriding
/// [RenderBox.computeDryLayout] instead.
@protected
void performResize();
/// Do the work of computing the layout for this render object.
///
/// Do not call this function directly: call [layout] instead. This function
/// is called by [layout] when there is actually work to be done by this
/// render object during layout. The layout constraints provided by your
/// parent are available via the [constraints] getter.
///
/// If [sizedByParent] is true, then this function should not actually change
/// the dimensions of this render object. Instead, that work should be done by
/// [performResize]. If [sizedByParent] is false, then this function should
/// both change the dimensions of this render object and instruct its children
/// to layout.
///
/// In implementing this function, you must call [layout] on each of your
/// children, passing true for parentUsesSize if your layout information is
/// dependent on your child's layout information. Passing true for
/// parentUsesSize ensures that this render object will undergo layout if the
/// child undergoes layout. Otherwise, the child can change its layout
/// information without informing this render object.
@protected
void performLayout();
/// Allows mutations to be made to this object's child list (and any
/// descendants) as well as to any other dirty nodes in the render tree owned
/// by the same [PipelineOwner] as this object. The `callback` argument is
/// invoked synchronously, and the mutations are allowed only during that
/// callback's execution.
///
/// This exists to allow child lists to be built on-demand during layout (e.g.
/// based on the object's size), and to enable nodes to be moved around the
/// tree as this happens (e.g. to handle [GlobalKey] reparenting), while still
/// ensuring that any particular node is only laid out once per frame.
///
/// Calling this function disables a number of assertions that are intended to
/// catch likely bugs. As such, using this function is generally discouraged.
///
/// This function can only be called during layout.
@protected
void invokeLayoutCallback<T extends Constraints>(LayoutCallback<T> callback) {
assert(_debugMutationsLocked);
assert(_debugDoingThisLayout);
assert(!_doingThisLayoutWithCallback);
_doingThisLayoutWithCallback = true;
try {
owner!._enableMutationsToDirtySubtrees(() { callback(constraints as T); });
} finally {
_doingThisLayoutWithCallback = false;
}
}
// PAINTING
/// Whether [paint] for this render object is currently running.
///
/// Only valid when asserts are enabled. In release builds, always returns
/// false.
bool get debugDoingThisPaint => _debugDoingThisPaint;
bool _debugDoingThisPaint = false;
/// The render object that is actively painting.
///
/// Only valid when asserts are enabled. In release builds, always returns
/// null.
static RenderObject? get debugActivePaint => _debugActivePaint;
static RenderObject? _debugActivePaint;
/// Whether this render object repaints separately from its parent.
///
/// Override this in subclasses to indicate that instances of your class ought
/// to repaint independently. For example, render objects that repaint
/// frequently might want to repaint themselves without requiring their parent
/// to repaint.
///
/// If this getter returns true, the [paintBounds] are applied to this object
/// and all descendants. The framework invokes [RenderObject.updateCompositedLayer]
/// to create an [OffsetLayer] and assigns it to the [layer] field.
/// Render objects that declare themselves as repaint boundaries must not replace
/// the layer created by the framework.
///
/// If the value of this getter changes, [markNeedsCompositingBitsUpdate] must
/// be called.
///
/// See [RepaintBoundary] for more information about how repaint boundaries function.
bool get isRepaintBoundary => false;
/// Called, in debug mode, if [isRepaintBoundary] is true, when either the
/// this render object or its parent attempt to paint.
///
/// This can be used to record metrics about whether the node should actually
/// be a repaint boundary.
void debugRegisterRepaintBoundaryPaint({ bool includedParent = true, bool includedChild = false }) { }
/// Whether this render object always needs compositing.
///
/// Override this in subclasses to indicate that your paint function always
/// creates at least one composited layer. For example, videos should return
/// true if they use hardware decoders.
///
/// You must call [markNeedsCompositingBitsUpdate] if the value of this getter
/// changes. (This is implied when [adoptChild] or [dropChild] are called.)
@protected
bool get alwaysNeedsCompositing => false;
late bool _wasRepaintBoundary;
/// Update the composited layer owned by this render object.
///
/// This method is called by the framework when [isRepaintBoundary] is true.
///
/// If [oldLayer] is `null`, this method must return a new [OffsetLayer]
/// (or subtype thereof). If [oldLayer] is not `null`, then this method must
/// reuse the layer instance that is provided - it is an error to create a new
/// layer in this instance. The layer will be disposed by the framework when
/// either the render object is disposed or if it is no longer a repaint
/// boundary.
///
/// The [OffsetLayer.offset] property will be managed by the framework and
/// must not be updated by this method.
///
/// If a property of the composited layer needs to be updated, the render object
/// must call [markNeedsCompositedLayerUpdate] which will schedule this method
/// to be called without repainting children. If this widget was marked as
/// needing to paint and needing a composited layer update, this method is only
/// called once.
// TODO(jonahwilliams): https://github.com/flutter/flutter/issues/102102 revisit the
// constraint that the instance/type of layer cannot be changed at runtime.
OffsetLayer updateCompositedLayer({required covariant OffsetLayer? oldLayer}) {
assert(isRepaintBoundary);
return oldLayer ?? OffsetLayer();
}
/// The compositing layer that this render object uses to repaint.
///
/// If this render object is not a repaint boundary, it is the responsibility
/// of the [paint] method to populate this field. If [needsCompositing] is
/// true, this field may be populated with the root-most layer used by the
/// render object implementation. When repainting, instead of creating a new
/// layer the render object may update the layer stored in this field for better
/// performance. It is also OK to leave this field as null and create a new
/// layer on every repaint, but without the performance benefit. If
/// [needsCompositing] is false, this field must be set to null either by
/// never populating this field, or by setting it to null when the value of
/// [needsCompositing] changes from true to false.
///
/// If a new layer is created and stored in some other field on the render
/// object, the render object must use a [LayerHandle] to store it. A layer
/// handle will prevent the layer from being disposed before the render
/// object is finished with it, and it will also make sure that the layer
/// gets appropriately disposed when the render object creates a replacement
/// or nulls it out. The render object must null out the [LayerHandle.layer]
/// in its [dispose] method.
///
/// If this render object is a repaint boundary, the framework automatically
/// creates an [OffsetLayer] and populates this field prior to calling the
/// [paint] method. The [paint] method must not replace the value of this
/// field.
@protected
ContainerLayer? get layer {
assert(!isRepaintBoundary || _layerHandle.layer == null || _layerHandle.layer is OffsetLayer);
return _layerHandle.layer;
}
@protected
set layer(ContainerLayer? newLayer) {
assert(
!isRepaintBoundary,
'Attempted to set a layer to a repaint boundary render object.\n'
'The framework creates and assigns an OffsetLayer to a repaint '
'boundary automatically.',
);
_layerHandle.layer = newLayer;
}
final LayerHandle<ContainerLayer> _layerHandle = LayerHandle<ContainerLayer>();
/// In debug mode, the compositing layer that this render object uses to repaint.
///
/// This getter is intended for debugging purposes only. In release builds, it
/// always returns null. In debug builds, it returns the layer even if the layer
/// is dirty.
///
/// For production code, consider [layer].
ContainerLayer? get debugLayer {
ContainerLayer? result;
assert(() {
result = _layerHandle.layer;
return true;
}());
return result;
}
bool _needsCompositingBitsUpdate = false; // set to true when a child is added
/// Mark the compositing state for this render object as dirty.
///
/// This is called to indicate that the value for [needsCompositing] needs to
/// be recomputed during the next [PipelineOwner.flushCompositingBits] engine
/// phase.
///
/// When the subtree is mutated, we need to recompute our
/// [needsCompositing] bit, and some of our ancestors need to do the
/// same (in case ours changed in a way that will change theirs). To
/// this end, [adoptChild] and [dropChild] call this method, and, as
/// necessary, this method calls the parent's, etc, walking up the
/// tree to mark all the nodes that need updating.
///
/// This method does not schedule a rendering frame, because since
/// it cannot be the case that _only_ the compositing bits changed,
/// something else will have scheduled a frame for us.
void markNeedsCompositingBitsUpdate() {
assert(!_debugDisposed);
if (_needsCompositingBitsUpdate) {
return;
}
_needsCompositingBitsUpdate = true;
if (parent is RenderObject) {
final RenderObject parent = this.parent!;
if (parent._needsCompositingBitsUpdate) {
return;
}
if ((!_wasRepaintBoundary || !isRepaintBoundary) && !parent.isRepaintBoundary) {
parent.markNeedsCompositingBitsUpdate();
return;
}
}
// parent is fine (or there isn't one), but we are dirty
if (owner != null) {
owner!._nodesNeedingCompositingBitsUpdate.add(this);
}
}
late bool _needsCompositing; // initialized in the constructor
/// Whether we or one of our descendants has a compositing layer.
///
/// If this node needs compositing as indicated by this bit, then all ancestor
/// nodes will also need compositing.
///
/// Only legal to call after [PipelineOwner.flushLayout] and
/// [PipelineOwner.flushCompositingBits] have been called.
bool get needsCompositing {
assert(!_needsCompositingBitsUpdate); // make sure we don't use this bit when it is dirty
return _needsCompositing;
}
void _updateCompositingBits() {
if (!_needsCompositingBitsUpdate) {
return;
}
final bool oldNeedsCompositing = _needsCompositing;
_needsCompositing = false;
visitChildren((RenderObject child) {
child._updateCompositingBits();
if (child.needsCompositing) {
_needsCompositing = true;
}
});
if (isRepaintBoundary || alwaysNeedsCompositing) {
_needsCompositing = true;
}
// If a node was previously a repaint boundary, but no longer is one, then
// regardless of its compositing state we need to find a new parent to
// paint from. To do this, we mark it clean again so that the traversal
// in markNeedsPaint is not short-circuited. It is removed from _nodesNeedingPaint
// so that we do not attempt to paint from it after locating a parent.
if (!isRepaintBoundary && _wasRepaintBoundary) {
_needsPaint = false;
_needsCompositedLayerUpdate = false;
owner?._nodesNeedingPaint.remove(this);
_needsCompositingBitsUpdate = false;
markNeedsPaint();
} else if (oldNeedsCompositing != _needsCompositing) {
_needsCompositingBitsUpdate = false;
markNeedsPaint();
} else {
_needsCompositingBitsUpdate = false;
}
}
/// Whether this render object's paint information is dirty.
///
/// This is only set in debug mode. In general, render objects should not need
/// to condition their runtime behavior on whether they are dirty or not,
/// since they should only be marked dirty immediately prior to being laid
/// out and painted. (In release builds, this throws.)
///
/// It is intended to be used by tests and asserts.
///
/// It is possible (and indeed, quite common) for [debugNeedsPaint] to be
/// false and [debugNeedsLayout] to be true. The render object will still be
/// repainted in the next frame when this is the case, because the
/// [markNeedsPaint] method is implicitly called by the framework after a
/// render object is laid out, prior to the paint phase.
bool get debugNeedsPaint {
late bool result;
assert(() {
result = _needsPaint;
return true;
}());
return result;
}
bool _needsPaint = true;
/// Whether this render object's layer information is dirty.
///
/// This is only set in debug mode. In general, render objects should not need
/// to condition their runtime behavior on whether they are dirty or not,
/// since they should only be marked dirty immediately prior to being laid
/// out and painted. (In release builds, this throws.)
///
/// It is intended to be used by tests and asserts.
bool get debugNeedsCompositedLayerUpdate {
late bool result;
assert(() {
result = _needsCompositedLayerUpdate;
return true;
}());
return result;
}
bool _needsCompositedLayerUpdate = false;
/// Mark this render object as having changed its visual appearance.
///
/// Rather than eagerly updating this render object's display list
/// in response to writes, we instead mark the render object as needing to
/// paint, which schedules a visual update. As part of the visual update, the
/// rendering pipeline will give this render object an opportunity to update
/// its display list.
///
/// This mechanism batches the painting work so that multiple sequential
/// writes are coalesced, removing redundant computation.
///
/// Once [markNeedsPaint] has been called on a render object,
/// [debugNeedsPaint] returns true for that render object until just after
/// the pipeline owner has called [paint] on the render object.
///
/// See also:
///
/// * [RepaintBoundary], to scope a subtree of render objects to their own
/// layer, thus limiting the number of nodes that [markNeedsPaint] must mark
/// dirty.
void markNeedsPaint() {
assert(!_debugDisposed);
assert(owner == null || !owner!.debugDoingPaint);
if (_needsPaint) {
return;
}
_needsPaint = true;
// If this was not previously a repaint boundary it will not have
// a layer we can paint from.
if (isRepaintBoundary && _wasRepaintBoundary) {
assert(() {
if (debugPrintMarkNeedsPaintStacks) {
debugPrintStack(label: 'markNeedsPaint() called for $this');
}
return true;
}());
// If we always have our own layer, then we can just repaint
// ourselves without involving any other nodes.
assert(_layerHandle.layer is OffsetLayer);
if (owner != null) {
owner!._nodesNeedingPaint.add(this);
owner!.requestVisualUpdate();
}
} else if (parent is RenderObject) {
parent!.markNeedsPaint();
} else {
assert(() {
if (debugPrintMarkNeedsPaintStacks) {
debugPrintStack(label: 'markNeedsPaint() called for $this (root of render tree)');
}
return true;
}());
// If we are the root of the render tree and not a repaint boundary
// then we have to paint ourselves, since nobody else can paint us.
// We don't add ourselves to _nodesNeedingPaint in this case,
// because the root is always told to paint regardless.
//
// Trees rooted at a RenderView do not go through this
// code path because RenderViews are repaint boundaries.
if (owner != null) {
owner!.requestVisualUpdate();
}
}
}
/// Mark this render object as having changed a property on its composited
/// layer.
///
/// Render objects that have a composited layer have [isRepaintBoundary] equal
/// to true may update the properties of that composited layer without repainting
/// their children. If this render object is a repaint boundary but does
/// not yet have a composited layer created for it, this method will instead
/// mark the nearest repaint boundary parent as needing to be painted.
///
/// If this method is called on a render object that is not a repaint boundary
/// or is a repaint boundary but hasn't been composited yet, it is equivalent
/// to calling [markNeedsPaint].
///
/// See also:
///
/// * [RenderOpacity], which uses this method when its opacity is updated to
/// update the layer opacity without repainting children.
void markNeedsCompositedLayerUpdate() {
assert(!_debugDisposed);
assert(owner == null || !owner!.debugDoingPaint);
if (_needsCompositedLayerUpdate || _needsPaint) {
return;
}
_needsCompositedLayerUpdate = true;
// If this was not previously a repaint boundary it will not have
// a layer we can paint from.
if (isRepaintBoundary && _wasRepaintBoundary) {
// If we always have our own layer, then we can just repaint
// ourselves without involving any other nodes.
assert(_layerHandle.layer != null);
if (owner != null) {
owner!._nodesNeedingPaint.add(this);
owner!.requestVisualUpdate();
}
} else {
markNeedsPaint();
}
}
// Called when flushPaint() tries to make us paint but our layer is detached.
// To make sure that our subtree is repainted when it's finally reattached,
// even in the case where some ancestor layer is itself never marked dirty, we
// have to mark our entire detached subtree as dirty and needing to be
// repainted. That way, we'll eventually be repainted.
void _skippedPaintingOnLayer() {
assert(attached);
assert(isRepaintBoundary);
assert(_needsPaint || _needsCompositedLayerUpdate);
assert(_layerHandle.layer != null);
assert(!_layerHandle.layer!.attached);
RenderObject? node = parent;
while (node is RenderObject) {
if (node.isRepaintBoundary) {
if (node._layerHandle.layer == null) {
// Looks like the subtree here has never been painted. Let it handle itself.
break;
}
if (node._layerHandle.layer!.attached) {
// It's the one that detached us, so it's the one that will decide to repaint us.
break;
}
node._needsPaint = true;
}
node = node.parent;
}
}
/// Bootstrap the rendering pipeline by scheduling the very first paint.
///
/// Requires that this render object is attached, is the root of the render
/// tree, and has a composited layer.
///
/// See [RenderView] for an example of how this function is used.
void scheduleInitialPaint(ContainerLayer rootLayer) {
assert(rootLayer.attached);
assert(attached);
assert(parent is! RenderObject);
assert(!owner!._debugDoingPaint);
assert(isRepaintBoundary);
assert(_layerHandle.layer == null);
_layerHandle.layer = rootLayer;
assert(_needsPaint);
owner!._nodesNeedingPaint.add(this);
}
/// Replace the layer. This is only valid for the root of a render
/// object subtree (whatever object [scheduleInitialPaint] was
/// called on).
///
/// This might be called if, e.g., the device pixel ratio changed.
void replaceRootLayer(OffsetLayer rootLayer) {
assert(!_debugDisposed);
assert(rootLayer.attached);
assert(attached);
assert(parent is! RenderObject);
assert(!owner!._debugDoingPaint);
assert(isRepaintBoundary);
assert(_layerHandle.layer != null); // use scheduleInitialPaint the first time
_layerHandle.layer!.detach();
_layerHandle.layer = rootLayer;
markNeedsPaint();
}
void _paintWithContext(PaintingContext context, Offset offset) {
assert(!_debugDisposed);
assert(() {
if (_debugDoingThisPaint) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Tried to paint a RenderObject reentrantly.'),
describeForError(
'The following RenderObject was already being painted when it was '
'painted again',
),
ErrorDescription(
'Since this typically indicates an infinite recursion, it is '
'disallowed.',
),
]);
}
return true;
}());
// If we still need layout, then that means that we were skipped in the
// layout phase and therefore don't need painting. We might not know that
// yet (that is, our layer might not have been detached yet), because the
// same node that skipped us in layout is above us in the tree (obviously)
// and therefore may not have had a chance to paint yet (since the tree
// paints in reverse order). In particular this will happen if they have
// a different layer, because there's a repaint boundary between us.
if (_needsLayout) {
return;
}
if (!kReleaseMode && debugProfilePaintsEnabled) {
Map<String, String>? debugTimelineArguments;
assert(() {
if (debugEnhancePaintTimelineArguments) {
debugTimelineArguments = toDiagnosticsNode().toTimelineArguments();
}
return true;
}());
FlutterTimeline.startSync(
'$runtimeType',
arguments: debugTimelineArguments,
);
}
assert(() {
if (_needsCompositingBitsUpdate) {
if (parent is RenderObject) {
final RenderObject parent = this.parent!;
bool visitedByParent = false;
parent.visitChildren((RenderObject child) {
if (child == this) {
visitedByParent = true;
}
});
if (!visitedByParent) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
"A RenderObject was not visited by the parent's visitChildren "
'during paint.',
),
parent.describeForError(
'The parent was',
),
describeForError(
'The child that was not visited was',
),
ErrorDescription(
'A RenderObject with children must implement visitChildren and '
'call the visitor exactly once for each child; it also should not '
'paint children that were removed with dropChild.',
),
ErrorHint(
'This usually indicates an error in the Flutter framework itself.',
),
]);
}
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'Tried to paint a RenderObject before its compositing bits were '
'updated.',
),
describeForError(
'The following RenderObject was marked as having dirty compositing '
'bits at the time that it was painted',
),
ErrorDescription(
'A RenderObject that still has dirty compositing bits cannot be '
'painted because this indicates that the tree has not yet been '
'properly configured for creating the layer tree.',
),
ErrorHint(
'This usually indicates an error in the Flutter framework itself.',
),
]);
}
return true;
}());
RenderObject? debugLastActivePaint;
assert(() {
_debugDoingThisPaint = true;
debugLastActivePaint = _debugActivePaint;
_debugActivePaint = this;
assert(!isRepaintBoundary || _layerHandle.layer != null);
return true;
}());
_needsPaint = false;
_needsCompositedLayerUpdate = false;
_wasRepaintBoundary = isRepaintBoundary;
try {
paint(context, offset);
assert(!_needsLayout); // check that the paint() method didn't mark us dirty again
assert(!_needsPaint); // check that the paint() method didn't mark us dirty again
} catch (e, stack) {
_reportException('paint', e, stack);
}
assert(() {
debugPaint(context, offset);
_debugActivePaint = debugLastActivePaint;
_debugDoingThisPaint = false;
return true;
}());
if (!kReleaseMode && debugProfilePaintsEnabled) {
FlutterTimeline.finishSync();
}
}
/// An estimate of the bounds within which this render object will paint.
/// Useful for debugging flags such as [debugPaintLayerBordersEnabled].
///
/// These are also the bounds used by [showOnScreen] to make a [RenderObject]
/// visible on screen.
Rect get paintBounds;
/// Override this method to paint debugging information.
void debugPaint(PaintingContext context, Offset offset) { }
/// Paint this render object into the given context at the given offset.
///
/// Subclasses should override this method to provide a visual appearance
/// for themselves. The render object's local coordinate system is
/// axis-aligned with the coordinate system of the context's canvas and the
/// render object's local origin (i.e, x=0 and y=0) is placed at the given
/// offset in the context's canvas.
///
/// Do not call this function directly. If you wish to paint yourself, call
/// [markNeedsPaint] instead to schedule a call to this function. If you wish
/// to paint one of your children, call [PaintingContext.paintChild] on the
/// given `context`.
///
/// When painting one of your children (via a paint child function on the
/// given context), the current canvas held by the context might change
/// because draw operations before and after painting children might need to
/// be recorded on separate compositing layers.
void paint(PaintingContext context, Offset offset) { }
/// Applies the transform that would be applied when painting the given child
/// to the given matrix.
///
/// Used by coordinate conversion functions to translate coordinates local to
/// one render object into coordinates local to another render object.
///
/// Some RenderObjects will provide a zeroed out matrix in this method,
/// indicating that the child should not paint anything or respond to hit
/// tests currently. A parent may supply a non-zero matrix even though it
/// does not paint its child currently, for example if the parent is a
/// [RenderOffstage] with `offstage` set to true. In both of these cases,
/// the parent must return `false` from [paintsChild].
void applyPaintTransform(covariant RenderObject child, Matrix4 transform) {
assert(child.parent == this);
}
/// Whether the given child would be painted if [paint] were called.
///
/// Some RenderObjects skip painting their children if they are configured to
/// not produce any visible effects. For example, a [RenderOffstage] with
/// its `offstage` property set to true, or a [RenderOpacity] with its opacity
/// value set to zero.
///
/// In these cases, the parent may still supply a non-zero matrix in
/// [applyPaintTransform] to inform callers about where it would paint the
/// child if the child were painted at all. Alternatively, the parent may
/// supply a zeroed out matrix if it would not otherwise be able to determine
/// a valid matrix for the child and thus cannot meaningfully determine where
/// the child would paint.
bool paintsChild(covariant RenderObject child) {
assert(child.parent == this);
return true;
}
/// {@template flutter.rendering.RenderObject.getTransformTo}
/// Applies the paint transform up the tree to `ancestor`.
///
/// Returns a matrix that maps the local paint coordinate system to the
/// coordinate system of `ancestor`.
///
/// If `ancestor` is null, this method returns a matrix that maps from the
/// local paint coordinate system to the coordinate system of the
/// [PipelineOwner.rootNode].
/// {@endtemplate}
///
/// For the render tree owned by the [RendererBinding] (i.e. for the main
/// render tree displayed on the device) this means that this method maps to
/// the global coordinate system in logical pixels. To get physical pixels,
/// use [applyPaintTransform] from the [RenderView] to further transform the
/// coordinate.
Matrix4 getTransformTo(RenderObject? ancestor) {
final bool ancestorSpecified = ancestor != null;
assert(attached);
if (ancestor == null) {
final RenderObject? rootNode = owner!.rootNode;
if (rootNode is RenderObject) {
ancestor = rootNode;
}
}
final List<RenderObject> renderers = <RenderObject>[];
for (RenderObject renderer = this; renderer != ancestor; renderer = renderer.parent!) {
renderers.add(renderer);
assert(renderer.parent != null); // Failed to find ancestor in parent chain.
}
if (ancestorSpecified) {
renderers.add(ancestor!);
}
final Matrix4 transform = Matrix4.identity();
for (int index = renderers.length - 1; index > 0; index -= 1) {
renderers[index].applyPaintTransform(renderers[index - 1], transform);
}
return transform;
}
/// Returns a rect in this object's coordinate system that describes
/// the approximate bounding box of the clip rect that would be
/// applied to the given child during the paint phase, if any.
///
/// Returns null if the child would not be clipped.
///
/// This is used in the semantics phase to avoid including children
/// that are not physically visible.
///
/// RenderObjects that respect a [Clip] behavior when painting _must_ respect
/// that same behavior when describing this value. For example, if passing
/// [Clip.none] to [PaintingContext.pushClipRect] as the `clipBehavior`, then
/// the implementation of this method must return null.
Rect? describeApproximatePaintClip(covariant RenderObject child) => null;
/// Returns a rect in this object's coordinate system that describes
/// which [SemanticsNode]s produced by the `child` should be included in the
/// semantics tree. [SemanticsNode]s from the `child` that are positioned
/// outside of this rect will be dropped. Child [SemanticsNode]s that are
/// positioned inside this rect, but outside of [describeApproximatePaintClip]
/// will be included in the tree marked as hidden. Child [SemanticsNode]s
/// that are inside of both rect will be included in the tree as regular
/// nodes.
///
/// This method only returns a non-null value if the semantics clip rect
/// is different from the rect returned by [describeApproximatePaintClip].
/// If the semantics clip rect and the paint clip rect are the same, this
/// method returns null.
///
/// A viewport would typically implement this method to include semantic nodes
/// in the semantics tree that are currently hidden just before the leading
/// or just after the trailing edge. These nodes have to be included in the
/// semantics tree to implement implicit accessibility scrolling on iOS where
/// the viewport scrolls implicitly when moving the accessibility focus from
/// the last visible node in the viewport to the first hidden one.
///
/// See also:
///
/// * [RenderViewportBase.cacheExtent], used by viewports to extend their
/// semantics clip beyond their approximate paint clip.
Rect? describeSemanticsClip(covariant RenderObject? child) => null;
// SEMANTICS
/// Bootstrap the semantics reporting mechanism by marking this node
/// as needing a semantics update.
///
/// Requires that this render object is attached, and is the root of
/// the render tree.
///
/// See [RendererBinding] for an example of how this function is used.
void scheduleInitialSemantics() {
assert(!_debugDisposed);
assert(attached);
assert(parent is! RenderObject);
assert(!owner!._debugDoingSemantics);
assert(_semantics == null);
assert(_needsSemanticsUpdate);
assert(owner!._semanticsOwner != null);
owner!._nodesNeedingSemantics.add(this);
owner!.requestVisualUpdate();
}
/// Report the semantics of this node, for example for accessibility purposes.
///
/// This method should be overridden by subclasses that have interesting
/// semantic information.
///
/// The given [SemanticsConfiguration] object is mutable and should be
/// annotated in a manner that describes the current state. No reference
/// should be kept to that object; mutating it outside of the context of the
/// [describeSemanticsConfiguration] call (for example as a result of
/// asynchronous computation) will at best have no useful effect and at worse
/// will cause crashes as the data will be in an inconsistent state.
///
/// {@tool snippet}
///
/// The following snippet will describe the node as a button that responds to
/// tap actions.
///
/// ```dart
/// abstract class SemanticButtonRenderObject extends RenderObject {
/// @override
/// void describeSemanticsConfiguration(SemanticsConfiguration config) {
/// super.describeSemanticsConfiguration(config);
/// config
/// ..onTap = _handleTap
/// ..label = 'I am a button'
/// ..isButton = true;
/// }
///
/// void _handleTap() {
/// // Do something.
/// }
/// }
/// ```
/// {@end-tool}
@protected
void describeSemanticsConfiguration(SemanticsConfiguration config) {
// Nothing to do by default.
}
/// Sends a [SemanticsEvent] associated with this render object's [SemanticsNode].
///
/// If this render object has no semantics information, the first parent
/// render object with a non-null semantic node is used.
///
/// If semantics are disabled, no events are dispatched.
///
/// See [SemanticsNode.sendEvent] for a full description of the behavior.
void sendSemanticsEvent(SemanticsEvent semanticsEvent) {
if (owner!.semanticsOwner == null) {
return;
}
if (_semantics != null && !_semantics!.isMergedIntoParent) {
_semantics!.sendEvent(semanticsEvent);
} else if (parent != null) {
parent!.sendSemanticsEvent(semanticsEvent);
}
}
// Use [_semanticsConfiguration] to access.
SemanticsConfiguration? _cachedSemanticsConfiguration;
SemanticsConfiguration get _semanticsConfiguration {
if (_cachedSemanticsConfiguration == null) {
_cachedSemanticsConfiguration = SemanticsConfiguration();
describeSemanticsConfiguration(_cachedSemanticsConfiguration!);
assert(
!_cachedSemanticsConfiguration!.explicitChildNodes || _cachedSemanticsConfiguration!.childConfigurationsDelegate == null,
'A SemanticsConfiguration with explicitChildNode set to true cannot have a non-null childConfigsDelegate.',
);
}
return _cachedSemanticsConfiguration!;
}
/// The bounding box, in the local coordinate system, of this
/// object, for accessibility purposes.
Rect get semanticBounds;
bool _needsSemanticsUpdate = true;
SemanticsNode? _semantics;
/// The semantics of this render object.
///
/// Exposed only for testing and debugging. To learn about the semantics of
/// render objects in production, obtain a [SemanticsHandle] from
/// [PipelineOwner.ensureSemantics].
///
/// Only valid in debug and profile mode. In release builds, always returns
/// null.
SemanticsNode? get debugSemantics {
if (!kReleaseMode) {
return _semantics;
}
return null;
}
/// Removes all semantics from this render object and its descendants.
///
/// Should only be called on objects whose [parent] is not a [RenderObject].
///
/// Override this method if you instantiate new [SemanticsNode]s in an
/// overridden [assembleSemanticsNode] method, to dispose of those nodes.
@mustCallSuper
void clearSemantics() {
_needsSemanticsUpdate = true;
_semantics = null;
visitChildren((RenderObject child) {
child.clearSemantics();
});
}
/// Mark this node as needing an update to its semantics description.
///
/// This must be called whenever the semantics configuration of this
/// [RenderObject] as annotated by [describeSemanticsConfiguration] changes in
/// any way to update the semantics tree.
void markNeedsSemanticsUpdate() {
assert(!_debugDisposed);
assert(!attached || !owner!._debugDoingSemantics);
if (!attached || owner!._semanticsOwner == null) {
_cachedSemanticsConfiguration = null;
return;
}
// Dirty the semantics tree starting at `this` until we have reached a
// RenderObject that is a semantics boundary. All semantics past this
// RenderObject are still up-to date. Therefore, we will later only rebuild
// the semantics subtree starting at the identified semantics boundary.
final bool wasSemanticsBoundary = _semantics != null && (_cachedSemanticsConfiguration?.isSemanticBoundary ?? false);
bool mayProduceSiblingNodes =
_cachedSemanticsConfiguration?.childConfigurationsDelegate != null ||
_semanticsConfiguration.childConfigurationsDelegate != null;
_cachedSemanticsConfiguration = null;
bool isEffectiveSemanticsBoundary = _semanticsConfiguration.isSemanticBoundary && wasSemanticsBoundary;
RenderObject node = this;
// The sibling nodes will be attached to the parent of immediate semantics
// node, thus marking this semantics boundary dirty is not enough, it needs
// to find the first parent semantics boundary that does not have any
// possible sibling node.
while (node.parent != null && (mayProduceSiblingNodes || !isEffectiveSemanticsBoundary)) {
if (node != this && node._needsSemanticsUpdate) {
break;
}
node._needsSemanticsUpdate = true;
// Since this node is a semantics boundary, the produced sibling nodes will
// be attached to the parent semantics boundary. Thus, these sibling nodes
// will not be carried to the next loop.
if (isEffectiveSemanticsBoundary) {
mayProduceSiblingNodes = false;
}
node = node.parent!;
isEffectiveSemanticsBoundary = node._semanticsConfiguration.isSemanticBoundary;
if (isEffectiveSemanticsBoundary && node._semantics == null) {
// We have reached a semantics boundary that doesn't own a semantics node.
// That means the semantics of this branch are currently blocked and will
// not appear in the semantics tree. We can abort the walk here.
return;
}
}
if (node != this && _semantics != null && _needsSemanticsUpdate) {
// If `this` node has already been added to [owner._nodesNeedingSemantics]
// remove it as it is no longer guaranteed that its semantics
// node will continue to be in the tree. If it still is in the tree, the
// ancestor `node` added to [owner._nodesNeedingSemantics] at the end of
// this block will ensure that the semantics of `this` node actually gets
// updated.
// (See semantics_10_test.dart for an example why this is required).
owner!._nodesNeedingSemantics.remove(this);
}
if (!node._needsSemanticsUpdate) {
node._needsSemanticsUpdate = true;
if (owner != null) {
assert(node._semanticsConfiguration.isSemanticBoundary || node.parent == null);
owner!._nodesNeedingSemantics.add(node);
owner!.requestVisualUpdate();
}
}
}
/// Updates the semantic information of the render object.
void _updateSemantics() {
assert(_semanticsConfiguration.isSemanticBoundary || parent == null);
if (_needsLayout) {
// There's not enough information in this subtree to compute semantics.
// The subtree is probably being kept alive by a viewport but not laid out.
return;
}
if (!kReleaseMode) {
FlutterTimeline.startSync('Semantics.GetFragment');
}
final _SemanticsFragment fragment = _getSemanticsForParent(
mergeIntoParent: _semantics?.parent?.isPartOfNodeMerging ?? false,
blockUserActions: _semantics?.areUserActionsBlocked ?? false,
);
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
assert(fragment is _InterestingSemanticsFragment);
final _InterestingSemanticsFragment interestingFragment = fragment as _InterestingSemanticsFragment;
final List<SemanticsNode> result = <SemanticsNode>[];
final List<SemanticsNode> siblingNodes = <SemanticsNode>[];
if (!kReleaseMode) {
FlutterTimeline.startSync('Semantics.compileChildren');
}
interestingFragment.compileChildren(
parentSemanticsClipRect: _semantics?.parentSemanticsClipRect,
parentPaintClipRect: _semantics?.parentPaintClipRect,
elevationAdjustment: _semantics?.elevationAdjustment ?? 0.0,
result: result,
siblingNodes: siblingNodes,
);
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
// Result may contain sibling nodes that are irrelevant for this update.
assert(interestingFragment.config == null && result.any((SemanticsNode node) => node == _semantics));
}
/// Returns the semantics that this node would like to add to its parent.
_SemanticsFragment _getSemanticsForParent({
required bool mergeIntoParent,
required bool blockUserActions,
}) {
assert(!_needsLayout, 'Updated layout information required for $this to calculate semantics.');
final SemanticsConfiguration config = _semanticsConfiguration;
bool dropSemanticsOfPreviousSiblings = config.isBlockingSemanticsOfPreviouslyPaintedNodes;
bool producesForkingFragment = !config.hasBeenAnnotated && !config.isSemanticBoundary;
final bool blockChildInteractions = blockUserActions || config.isBlockingUserActions;
final bool childrenMergeIntoParent = mergeIntoParent || config.isMergingSemanticsOfDescendants;
final List<SemanticsConfiguration> childConfigurations = <SemanticsConfiguration>[];
final bool explicitChildNode = config.explicitChildNodes || parent == null;
final ChildSemanticsConfigurationsDelegate? childConfigurationsDelegate = config.childConfigurationsDelegate;
final Map<SemanticsConfiguration, _InterestingSemanticsFragment> configToFragment = <SemanticsConfiguration, _InterestingSemanticsFragment>{};
final List<_InterestingSemanticsFragment> mergeUpFragments = <_InterestingSemanticsFragment>[];
final List<List<_InterestingSemanticsFragment>> siblingMergeFragmentGroups = <List<_InterestingSemanticsFragment>>[];
final bool hasTags = config.tagsForChildren?.isNotEmpty ?? false;
visitChildrenForSemantics((RenderObject renderChild) {
assert(!_needsLayout);
final _SemanticsFragment parentFragment = renderChild._getSemanticsForParent(
mergeIntoParent: childrenMergeIntoParent,
blockUserActions: blockChildInteractions,
);
if (parentFragment.dropsSemanticsOfPreviousSiblings) {
childConfigurations.clear();
mergeUpFragments.clear();
siblingMergeFragmentGroups.clear();
if (!config.isSemanticBoundary) {
dropSemanticsOfPreviousSiblings = true;
}
}
for (final _InterestingSemanticsFragment fragment in parentFragment.mergeUpFragments) {
fragment.addAncestor(this);
if (hasTags) {
fragment.addTags(config.tagsForChildren!);
}
if (childConfigurationsDelegate != null && fragment.config != null) {
// This fragment need to go through delegate to determine whether it
// merge up or not.
childConfigurations.add(fragment.config!);
configToFragment[fragment.config!] = fragment;
} else {
mergeUpFragments.add(fragment);
}
}
if (parentFragment is _ContainerSemanticsFragment) {
// Container fragments needs to propagate sibling merge group to be
// compiled by _SwitchableSemanticsFragment.
for (final List<_InterestingSemanticsFragment> siblingMergeGroup in parentFragment.siblingMergeGroups) {
for (final _InterestingSemanticsFragment siblingMergingFragment in siblingMergeGroup) {
siblingMergingFragment.addAncestor(this);
if (hasTags) {
siblingMergingFragment.addTags(config.tagsForChildren!);
}
}
siblingMergeFragmentGroups.add(siblingMergeGroup);
}
}
});
assert(childConfigurationsDelegate != null || configToFragment.isEmpty);
if (explicitChildNode) {
for (final _InterestingSemanticsFragment fragment in mergeUpFragments) {
fragment.markAsExplicit();
}
} else if (childConfigurationsDelegate != null) {
final ChildSemanticsConfigurationsResult result = childConfigurationsDelegate(childConfigurations);
mergeUpFragments.addAll(
result.mergeUp.map<_InterestingSemanticsFragment>((SemanticsConfiguration config) {
final _InterestingSemanticsFragment? fragment = configToFragment[config];
if (fragment == null) {
// Parent fragment of Incomplete fragments can't be a forking
// fragment since they need to be merged.
producesForkingFragment = false;
return _IncompleteSemanticsFragment(config: config, owner: this);
}
return fragment;
}),
);
for (final Iterable<SemanticsConfiguration> group in result.siblingMergeGroups) {
siblingMergeFragmentGroups.add(
group.map<_InterestingSemanticsFragment>((SemanticsConfiguration config) {
return configToFragment[config] ?? _IncompleteSemanticsFragment(config: config, owner: this);
}).toList(),
);
}
}
_needsSemanticsUpdate = false;
final _SemanticsFragment result;
if (parent == null) {
assert(!config.hasBeenAnnotated);
assert(!mergeIntoParent);
assert(siblingMergeFragmentGroups.isEmpty);
_marksExplicitInMergeGroup(mergeUpFragments, isMergeUp: true);
siblingMergeFragmentGroups.forEach(_marksExplicitInMergeGroup);
result = _RootSemanticsFragment(
owner: this,
dropsSemanticsOfPreviousSiblings: dropSemanticsOfPreviousSiblings,
);
} else if (producesForkingFragment) {
result = _ContainerSemanticsFragment(
siblingMergeGroups: siblingMergeFragmentGroups,
dropsSemanticsOfPreviousSiblings: dropSemanticsOfPreviousSiblings,
);
} else {
_marksExplicitInMergeGroup(mergeUpFragments, isMergeUp: true);
siblingMergeFragmentGroups.forEach(_marksExplicitInMergeGroup);
result = _SwitchableSemanticsFragment(
config: config,
blockUserActions: blockUserActions,
mergeIntoParent: mergeIntoParent,
siblingMergeGroups: siblingMergeFragmentGroups,
owner: this,
dropsSemanticsOfPreviousSiblings: dropSemanticsOfPreviousSiblings,
);
if (config.isSemanticBoundary) {
final _SwitchableSemanticsFragment fragment = result as _SwitchableSemanticsFragment;
fragment.markAsExplicit();
}
}
result.addAll(mergeUpFragments);
return result;
}
void _marksExplicitInMergeGroup(List<_InterestingSemanticsFragment> mergeGroup, {bool isMergeUp = false}) {
final Set<_InterestingSemanticsFragment> toBeExplicit = <_InterestingSemanticsFragment>{};
for (int i = 0; i < mergeGroup.length; i += 1) {
final _InterestingSemanticsFragment fragment = mergeGroup[i];
if (!fragment.hasConfigForParent) {
continue;
}
if (isMergeUp && !_semanticsConfiguration.isCompatibleWith(fragment.config)) {
toBeExplicit.add(fragment);
}
final int siblingLength = i;
for (int j = 0; j < siblingLength; j += 1) {
final _InterestingSemanticsFragment siblingFragment = mergeGroup[j];
if (!fragment.config!.isCompatibleWith(siblingFragment.config)) {
toBeExplicit.add(fragment);
toBeExplicit.add(siblingFragment);
}
}
}
for (final _InterestingSemanticsFragment fragment in toBeExplicit) {
fragment.markAsExplicit();
}
}
/// Called when collecting the semantics of this node.
///
/// The implementation has to return the children in paint order skipping all
/// children that are not semantically relevant (e.g. because they are
/// invisible).
///
/// The default implementation mirrors the behavior of
/// [visitChildren] (which is supposed to walk all the children).
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
visitChildren(visitor);
}
/// Assemble the [SemanticsNode] for this [RenderObject].
///
/// If [describeSemanticsConfiguration] sets
/// [SemanticsConfiguration.isSemanticBoundary] to true, this method is called
/// with the `node` created for this [RenderObject], the `config` to be
/// applied to that node and the `children` [SemanticsNode]s that descendants
/// of this RenderObject have generated.
///
/// By default, the method will annotate `node` with `config` and add the
/// `children` to it.
///
/// Subclasses can override this method to add additional [SemanticsNode]s
/// to the tree. If new [SemanticsNode]s are instantiated in this method
/// they must be disposed in [clearSemantics].
void assembleSemanticsNode(
SemanticsNode node,
SemanticsConfiguration config,
Iterable<SemanticsNode> children,
) {
assert(node == _semantics);
// TODO(a14n): remove the following cast by updating type of parameter in either updateWith or assembleSemanticsNode
node.updateWith(config: config, childrenInInversePaintOrder: children as List<SemanticsNode>);
}
// EVENTS
/// Override this method to handle pointer events that hit this render object.
@override
void handleEvent(PointerEvent event, covariant HitTestEntry entry) { }
// HIT TESTING
// RenderObject subclasses are expected to have a method like the following
// (with the signature being whatever passes for coordinates for this
// particular class):
//
// bool hitTest(HitTestResult result, { Offset position }) {
// // If the given position is not inside this node, then return false.
// // Otherwise:
// // For each child that intersects the position, in z-order starting from
// // the top, call hitTest() for that child, passing it /result/, and the
// // coordinates converted to the child's coordinate origin, and stop at
// // the first child that returns true.
// // Then, add yourself to /result/, and return true.
// }
//
// If you add yourself to /result/ and still return false, then that means you
// will see events but so will objects below you.
/// Returns a human understandable name.
@override
String toStringShort() {
String header = describeIdentity(this);
if (!kReleaseMode) {
if (_debugDisposed) {
header += ' DISPOSED';
return header;
}
if (_relayoutBoundary != null && _relayoutBoundary != this) {
int count = 1;
RenderObject? target = parent;
while (target != null && target != _relayoutBoundary) {
target = target.parent;
count += 1;
}
header += ' relayoutBoundary=up$count';
}
if (_needsLayout) {
header += ' NEEDS-LAYOUT';
}
if (_needsPaint) {
header += ' NEEDS-PAINT';
}
if (_needsCompositingBitsUpdate) {
header += ' NEEDS-COMPOSITING-BITS-UPDATE';
}
if (!attached) {
header += ' DETACHED';
}
}
return header;
}
@override
String toString({ DiagnosticLevel minLevel = DiagnosticLevel.info }) => toStringShort();
/// Returns a description of the tree rooted at this node.
/// If the prefix argument is provided, then every line in the output
/// will be prefixed by that string.
@override
String toStringDeep({
String prefixLineOne = '',
String? prefixOtherLines = '',
DiagnosticLevel minLevel = DiagnosticLevel.debug,
}) {
return _withDebugActiveLayoutCleared(() => super.toStringDeep(
prefixLineOne: prefixLineOne,
prefixOtherLines: prefixOtherLines,
minLevel: minLevel,
));
}
/// Returns a one-line detailed description of the render object.
/// This description is often somewhat long.
///
/// This includes the same information for this RenderObject as given by
/// [toStringDeep], but does not recurse to any children.
@override
String toStringShallow({
String joiner = ', ',
DiagnosticLevel minLevel = DiagnosticLevel.debug,
}) {
return _withDebugActiveLayoutCleared(() => super.toStringShallow(joiner: joiner, minLevel: minLevel));
}
@protected
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('needsCompositing', value: _needsCompositing, ifTrue: 'needs compositing'));
properties.add(DiagnosticsProperty<Object?>('creator', debugCreator, defaultValue: null, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ParentData>('parentData', parentData, tooltip: (_debugCanParentUseSize ?? false) ? 'can use size' : null, missingIfNull: true));
properties.add(DiagnosticsProperty<Constraints>('constraints', _constraints, missingIfNull: true));
// don't access it via the "layer" getter since that's only valid when we don't need paint
properties.add(DiagnosticsProperty<ContainerLayer>('layer', _layerHandle.layer, defaultValue: null));
properties.add(DiagnosticsProperty<SemanticsNode>('semantics node', _semantics, defaultValue: null));
properties.add(FlagProperty(
'isBlockingSemanticsOfPreviouslyPaintedNodes',
value: _semanticsConfiguration.isBlockingSemanticsOfPreviouslyPaintedNodes,
ifTrue: 'blocks semantics of earlier render objects below the common boundary',
));
properties.add(FlagProperty('isSemanticBoundary', value: _semanticsConfiguration.isSemanticBoundary, ifTrue: 'semantic boundary'));
}
@override
List<DiagnosticsNode> debugDescribeChildren() => <DiagnosticsNode>[];
/// Attempt to make (a portion of) this or a descendant [RenderObject] visible
/// on screen.
///
/// If `descendant` is provided, that [RenderObject] is made visible. If
/// `descendant` is omitted, this [RenderObject] is made visible.
///
/// The optional `rect` parameter describes which area of that [RenderObject]
/// should be shown on screen. If `rect` is null, the entire
/// [RenderObject] (as defined by its [paintBounds]) will be revealed. The
/// `rect` parameter is interpreted relative to the coordinate system of
/// `descendant` if that argument is provided and relative to this
/// [RenderObject] otherwise.
///
/// The `duration` parameter can be set to a non-zero value to bring the
/// target object on screen in an animation defined by `curve`.
///
/// See also:
///
/// * [RenderViewportBase.showInViewport], which [RenderViewportBase] and
/// [SingleChildScrollView] delegate this method to.
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
if (parent is RenderObject) {
parent!.showOnScreen(
descendant: descendant ?? this,
rect: rect,
duration: duration,
curve: curve,
);
}
}
/// Adds a debug representation of a [RenderObject] optimized for including in
/// error messages.
///
/// The default [style] of [DiagnosticsTreeStyle.shallow] ensures that all of
/// the properties of the render object are included in the error output but
/// none of the children of the object are.
///
/// You should always include a RenderObject in an error message if it is the
/// [RenderObject] causing the failure or contract violation of the error.
DiagnosticsNode describeForError(String name, { DiagnosticsTreeStyle style = DiagnosticsTreeStyle.shallow }) {
return toDiagnosticsNode(name: name, style: style);
}
}
/// Generic mixin for render objects with one child.
///
/// Provides a child model for a render object subclass that has
/// a unique child, which is accessible via the [child] getter.
///
/// This mixin is typically used to implement render objects created
/// in a [SingleChildRenderObjectWidget].
mixin RenderObjectWithChildMixin<ChildType extends RenderObject> on RenderObject {
/// Checks whether the given render object has the correct [runtimeType] to be
/// a child of this render object.
///
/// Does nothing if assertions are disabled.
///
/// Always returns true.
bool debugValidateChild(RenderObject child) {
assert(() {
if (child is! ChildType) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'A $runtimeType expected a child of type $ChildType but received a '
'child of type ${child.runtimeType}.',
),
ErrorDescription(
'RenderObjects expect specific types of children because they '
'coordinate with their children during layout and paint. For '
'example, a RenderSliver cannot be the child of a RenderBox because '
'a RenderSliver does not understand the RenderBox layout protocol.',
),
ErrorSpacer(),
DiagnosticsProperty<Object?>(
'The $runtimeType that expected a $ChildType child was created by',
debugCreator,
style: DiagnosticsTreeStyle.errorProperty,
),
ErrorSpacer(),
DiagnosticsProperty<Object?>(
'The ${child.runtimeType} that did not match the expected child type '
'was created by',
child.debugCreator,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
return true;
}());
return true;
}
ChildType? _child;
/// The render object's unique child.
ChildType? get child => _child;
set child(ChildType? value) {
if (_child != null) {
dropChild(_child!);
}
_child = value;
if (_child != null) {
adoptChild(_child!);
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_child?.attach(owner);
}
@override
void detach() {
super.detach();
_child?.detach();
}
@override
void redepthChildren() {
if (_child != null) {
redepthChild(_child!);
}
}
@override
void visitChildren(RenderObjectVisitor visitor) {
if (_child != null) {
visitor(_child!);
}
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return child != null ? <DiagnosticsNode>[child!.toDiagnosticsNode(name: 'child')] : <DiagnosticsNode>[];
}
}
/// Parent data to support a doubly-linked list of children.
///
/// The children can be traversed using [nextSibling] or [previousSibling],
/// which can be called on the parent data of the render objects
/// obtained via [ContainerRenderObjectMixin.firstChild] or
/// [ContainerRenderObjectMixin.lastChild].
mixin ContainerParentDataMixin<ChildType extends RenderObject> on ParentData {
/// The previous sibling in the parent's child list.
ChildType? previousSibling;
/// The next sibling in the parent's child list.
ChildType? nextSibling;
/// Clear the sibling pointers.
@override
void detach() {
assert(previousSibling == null, 'Pointers to siblings must be nulled before detaching ParentData.');
assert(nextSibling == null, 'Pointers to siblings must be nulled before detaching ParentData.');
super.detach();
}
}
/// Generic mixin for render objects with a list of children.
///
/// Provides a child model for a render object subclass that has a doubly-linked
/// list of children.
///
/// The [ChildType] specifies the type of the children (extending [RenderObject]),
/// e.g. [RenderBox].
///
/// [ParentDataType] stores parent container data on its child render objects.
/// It must extend [ContainerParentDataMixin], which provides the interface
/// for visiting children. This data is populated by
/// [RenderObject.setupParentData] implemented by the class using this mixin.
///
/// When using [RenderBox] as the child type, you will usually want to make use of
/// [RenderBoxContainerDefaultsMixin] and extend [ContainerBoxParentData] for the
/// parent data.
///
/// Moreover, this is a required mixin for render objects returned to [MultiChildRenderObjectWidget].
///
/// See also:
///
/// * [SlottedContainerRenderObjectMixin], which organizes its children
/// in different named slots.
mixin ContainerRenderObjectMixin<ChildType extends RenderObject, ParentDataType extends ContainerParentDataMixin<ChildType>> on RenderObject {
bool _debugUltimatePreviousSiblingOf(ChildType child, { ChildType? equals }) {
ParentDataType childParentData = child.parentData! as ParentDataType;
while (childParentData.previousSibling != null) {
assert(childParentData.previousSibling != child);
child = childParentData.previousSibling!;
childParentData = child.parentData! as ParentDataType;
}
return child == equals;
}
bool _debugUltimateNextSiblingOf(ChildType child, { ChildType? equals }) {
ParentDataType childParentData = child.parentData! as ParentDataType;
while (childParentData.nextSibling != null) {
assert(childParentData.nextSibling != child);
child = childParentData.nextSibling!;
childParentData = child.parentData! as ParentDataType;
}
return child == equals;
}
int _childCount = 0;
/// The number of children.
int get childCount => _childCount;
/// Checks whether the given render object has the correct [runtimeType] to be
/// a child of this render object.
///
/// Does nothing if assertions are disabled.
///
/// Always returns true.
bool debugValidateChild(RenderObject child) {
assert(() {
if (child is! ChildType) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'A $runtimeType expected a child of type $ChildType but received a '
'child of type ${child.runtimeType}.',
),
ErrorDescription(
'RenderObjects expect specific types of children because they '
'coordinate with their children during layout and paint. For '
'example, a RenderSliver cannot be the child of a RenderBox because '
'a RenderSliver does not understand the RenderBox layout protocol.',
),
ErrorSpacer(),
DiagnosticsProperty<Object?>(
'The $runtimeType that expected a $ChildType child was created by',
debugCreator,
style: DiagnosticsTreeStyle.errorProperty,
),
ErrorSpacer(),
DiagnosticsProperty<Object?>(
'The ${child.runtimeType} that did not match the expected child type '
'was created by',
child.debugCreator,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
return true;
}());
return true;
}
ChildType? _firstChild;
ChildType? _lastChild;
void _insertIntoChildList(ChildType child, { ChildType? after }) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
assert(childParentData.nextSibling == null);
assert(childParentData.previousSibling == null);
_childCount += 1;
assert(_childCount > 0);
if (after == null) {
// insert at the start (_firstChild)
childParentData.nextSibling = _firstChild;
if (_firstChild != null) {
final ParentDataType firstChildParentData = _firstChild!.parentData! as ParentDataType;
firstChildParentData.previousSibling = child;
}
_firstChild = child;
_lastChild ??= child;
} else {
assert(_firstChild != null);
assert(_lastChild != null);
assert(_debugUltimatePreviousSiblingOf(after, equals: _firstChild));
assert(_debugUltimateNextSiblingOf(after, equals: _lastChild));
final ParentDataType afterParentData = after.parentData! as ParentDataType;
if (afterParentData.nextSibling == null) {
// insert at the end (_lastChild); we'll end up with two or more children
assert(after == _lastChild);
childParentData.previousSibling = after;
afterParentData.nextSibling = child;
_lastChild = child;
} else {
// insert in the middle; we'll end up with three or more children
// set up links from child to siblings
childParentData.nextSibling = afterParentData.nextSibling;
childParentData.previousSibling = after;
// set up links from siblings to child
final ParentDataType childPreviousSiblingParentData = childParentData.previousSibling!.parentData! as ParentDataType;
final ParentDataType childNextSiblingParentData = childParentData.nextSibling!.parentData! as ParentDataType;
childPreviousSiblingParentData.nextSibling = child;
childNextSiblingParentData.previousSibling = child;
assert(afterParentData.nextSibling == child);
}
}
}
/// Insert child into this render object's child list after the given child.
///
/// If `after` is null, then this inserts the child at the start of the list,
/// and the child becomes the new [firstChild].
void insert(ChildType child, { ChildType? after }) {
assert(child != this, 'A RenderObject cannot be inserted into itself.');
assert(after != this, 'A RenderObject cannot simultaneously be both the parent and the sibling of another RenderObject.');
assert(child != after, 'A RenderObject cannot be inserted after itself.');
assert(child != _firstChild);
assert(child != _lastChild);
adoptChild(child);
_insertIntoChildList(child, after: after);
}
/// Append child to the end of this render object's child list.
void add(ChildType child) {
insert(child, after: _lastChild);
}
/// Add all the children to the end of this render object's child list.
void addAll(List<ChildType>? children) {
children?.forEach(add);
}
void _removeFromChildList(ChildType child) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
assert(_debugUltimatePreviousSiblingOf(child, equals: _firstChild));
assert(_debugUltimateNextSiblingOf(child, equals: _lastChild));
assert(_childCount >= 0);
if (childParentData.previousSibling == null) {
assert(_firstChild == child);
_firstChild = childParentData.nextSibling;
} else {
final ParentDataType childPreviousSiblingParentData = childParentData.previousSibling!.parentData! as ParentDataType;
childPreviousSiblingParentData.nextSibling = childParentData.nextSibling;
}
if (childParentData.nextSibling == null) {
assert(_lastChild == child);
_lastChild = childParentData.previousSibling;
} else {
final ParentDataType childNextSiblingParentData = childParentData.nextSibling!.parentData! as ParentDataType;
childNextSiblingParentData.previousSibling = childParentData.previousSibling;
}
childParentData.previousSibling = null;
childParentData.nextSibling = null;
_childCount -= 1;
}
/// Remove this child from the child list.
///
/// Requires the child to be present in the child list.
void remove(ChildType child) {
_removeFromChildList(child);
dropChild(child);
}
/// Remove all their children from this render object's child list.
///
/// More efficient than removing them individually.
void removeAll() {
ChildType? child = _firstChild;
while (child != null) {
final ParentDataType childParentData = child.parentData! as ParentDataType;
final ChildType? next = childParentData.nextSibling;
childParentData.previousSibling = null;
childParentData.nextSibling = null;
dropChild(child);
child = next;
}
_firstChild = null;
_lastChild = null;
_childCount = 0;
}
/// Move the given `child` in the child list to be after another child.
///
/// More efficient than removing and re-adding the child. Requires the child
/// to already be in the child list at some position. Pass null for `after` to
/// move the child to the start of the child list.
void move(ChildType child, { ChildType? after }) {
assert(child != this);
assert(after != this);
assert(child != after);
assert(child.parent == this);
final ParentDataType childParentData = child.parentData! as ParentDataType;
if (childParentData.previousSibling == after) {
return;
}
_removeFromChildList(child);
_insertIntoChildList(child, after: after);
markNeedsLayout();
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
ChildType? child = _firstChild;
while (child != null) {
child.attach(owner);
final ParentDataType childParentData = child.parentData! as ParentDataType;
child = childParentData.nextSibling;
}
}
@override
void detach() {
super.detach();
ChildType? child = _firstChild;
while (child != null) {
child.detach();
final ParentDataType childParentData = child.parentData! as ParentDataType;
child = childParentData.nextSibling;
}
}
@override
void redepthChildren() {
ChildType? child = _firstChild;
while (child != null) {
redepthChild(child);
final ParentDataType childParentData = child.parentData! as ParentDataType;
child = childParentData.nextSibling;
}
}
@override
void visitChildren(RenderObjectVisitor visitor) {
ChildType? child = _firstChild;
while (child != null) {
visitor(child);
final ParentDataType childParentData = child.parentData! as ParentDataType;
child = childParentData.nextSibling;
}
}
/// The first child in the child list.
ChildType? get firstChild => _firstChild;
/// The last child in the child list.
ChildType? get lastChild => _lastChild;
/// The previous child before the given child in the child list.
ChildType? childBefore(ChildType child) {
assert(child.parent == this);
final ParentDataType childParentData = child.parentData! as ParentDataType;
return childParentData.previousSibling;
}
/// The next child after the given child in the child list.
ChildType? childAfter(ChildType child) {
assert(child.parent == this);
final ParentDataType childParentData = child.parentData! as ParentDataType;
return childParentData.nextSibling;
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> children = <DiagnosticsNode>[];
if (firstChild != null) {
ChildType child = firstChild!;
int count = 1;
while (true) {
children.add(child.toDiagnosticsNode(name: 'child $count'));
if (child == lastChild) {
break;
}
count += 1;
final ParentDataType childParentData = child.parentData! as ParentDataType;
child = childParentData.nextSibling!;
}
}
return children;
}
}
/// Mixin for [RenderObject] that will call [systemFontsDidChange] whenever the
/// system fonts change.
///
/// System fonts can change when the OS installs or removes a font. Use this
/// mixin if the [RenderObject] uses [TextPainter] or [Paragraph] to correctly
/// update the text when it happens.
mixin RelayoutWhenSystemFontsChangeMixin on RenderObject {
/// A callback that is called when system fonts have changed.
///
/// The framework defers the invocation of the callback to the
/// [SchedulerPhase.transientCallbacks] phase to ensure that the
/// [RenderObject]'s text layout is still valid when user interactions are in
/// progress (which usually take place during the [SchedulerPhase.idle] phase).
///
/// By default, [markNeedsLayout] is called on the [RenderObject]
/// implementing this mixin.
///
/// Subclass should override this method to clear any extra cache that depend
/// on font-related metrics.
@protected
@mustCallSuper
void systemFontsDidChange() {
markNeedsLayout();
}
bool _hasPendingSystemFontsDidChangeCallBack = false;
void _scheduleSystemFontsUpdate() {
assert(
SchedulerBinding.instance.schedulerPhase == SchedulerPhase.idle,
'${objectRuntimeType(this, "RelayoutWhenSystemFontsChangeMixin")}._scheduleSystemFontsUpdate() '
'called during ${SchedulerBinding.instance.schedulerPhase}.',
);
if (_hasPendingSystemFontsDidChangeCallBack) {
return;
}
_hasPendingSystemFontsDidChangeCallBack = true;
SchedulerBinding.instance.scheduleFrameCallback((Duration timeStamp) {
assert(_hasPendingSystemFontsDidChangeCallBack);
_hasPendingSystemFontsDidChangeCallBack = false;
assert(
attached || (debugDisposed ?? true),
'$this is detached during ${SchedulerBinding.instance.schedulerPhase} but is not disposed.',
);
if (attached) {
systemFontsDidChange();
}
});
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
// If there's a pending callback that would imply this node was detached
// between the idle phase and the next transientCallbacks phase. The tree
// can not be mutated between those two phases so that should never happen.
assert(!_hasPendingSystemFontsDidChangeCallBack);
PaintingBinding.instance.systemFonts.addListener(_scheduleSystemFontsUpdate);
}
@override
void detach() {
assert(!_hasPendingSystemFontsDidChangeCallBack);
PaintingBinding.instance.systemFonts.removeListener(_scheduleSystemFontsUpdate);
super.detach();
}
}
/// Describes the semantics information a [RenderObject] wants to add to its
/// parent.
///
/// It has two notable subclasses:
/// * [_InterestingSemanticsFragment] describing actual semantic information to
/// be added to the parent.
/// * [_ContainerSemanticsFragment]: a container class to transport the semantic
/// information of multiple [_InterestingSemanticsFragment] to a parent.
abstract class _SemanticsFragment {
_SemanticsFragment({
required this.dropsSemanticsOfPreviousSiblings,
});
/// Incorporate the fragments of children into this fragment.
void addAll(Iterable<_InterestingSemanticsFragment> fragments);
/// Whether this fragment wants to make the semantics information of
/// previously painted [RenderObject]s unreachable for accessibility purposes.
///
/// See also:
///
/// * [SemanticsConfiguration.isBlockingSemanticsOfPreviouslyPaintedNodes]
/// describes what semantics are dropped in more detail.
final bool dropsSemanticsOfPreviousSiblings;
/// Returns [_InterestingSemanticsFragment] describing the actual semantic
/// information that this fragment wants to add to the parent.
List<_InterestingSemanticsFragment> get mergeUpFragments;
}
/// A container used when a [RenderObject] wants to add multiple independent
/// [_InterestingSemanticsFragment] to its parent.
///
/// The [_InterestingSemanticsFragment] to be added to the parent can be
/// obtained via [mergeUpFragments].
class _ContainerSemanticsFragment extends _SemanticsFragment {
_ContainerSemanticsFragment({
required super.dropsSemanticsOfPreviousSiblings,
required this.siblingMergeGroups,
});
final List<List<_InterestingSemanticsFragment>> siblingMergeGroups;
@override
void addAll(Iterable<_InterestingSemanticsFragment> fragments) {
mergeUpFragments.addAll(fragments);
}
@override
final List<_InterestingSemanticsFragment> mergeUpFragments = <_InterestingSemanticsFragment>[];
}
/// A [_SemanticsFragment] that describes which concrete semantic information
/// a [RenderObject] wants to add to the [SemanticsNode] of its parent.
///
/// Specifically, it describes which children (as returned by [compileChildren])
/// should be added to the parent's [SemanticsNode] and which [config] should be
/// merged into the parent's [SemanticsNode].
abstract class _InterestingSemanticsFragment extends _SemanticsFragment {
_InterestingSemanticsFragment({
required RenderObject owner,
required super.dropsSemanticsOfPreviousSiblings,
}) : _ancestorChain = <RenderObject>[owner];
/// The [RenderObject] that owns this fragment (and any new [SemanticsNode]
/// introduced by it).
RenderObject get owner => _ancestorChain.first;
final List<RenderObject> _ancestorChain;
/// The children to be added to the parent.
///
/// See also:
///
/// * [SemanticsNode.parentSemanticsClipRect] for the source and definition
/// of the `parentSemanticsClipRect` argument.
/// * [SemanticsNode.parentPaintClipRect] for the source and definition
/// of the `parentPaintClipRect` argument.
/// * [SemanticsNode.elevationAdjustment] for the source and definition
/// of the `elevationAdjustment` argument.
void compileChildren({
required Rect? parentSemanticsClipRect,
required Rect? parentPaintClipRect,
required double elevationAdjustment,
required List<SemanticsNode> result,
required List<SemanticsNode> siblingNodes,
});
/// The [SemanticsConfiguration] the child wants to merge into the parent's
/// [SemanticsNode] or null if it doesn't want to merge anything.
SemanticsConfiguration? get config;
/// Disallows this fragment to merge any configuration into its parent's
/// [SemanticsNode].
///
/// After calling this, the fragment will only produce children to be added
/// to the parent and it will return null for [config].
void markAsExplicit();
/// Consume the fragments of children.
///
/// For each provided fragment it will add that fragment's children to
/// this fragment's children (as returned by [compileChildren]) and merge that
/// fragment's [config] into this fragment's [config].
///
/// If a provided fragment should not merge anything into [config] call
/// [markAsExplicit] before passing the fragment to this method.
@override
void addAll(Iterable<_InterestingSemanticsFragment> fragments);
/// Whether this fragment wants to add any semantic information to the parent
/// [SemanticsNode].
bool get hasConfigForParent => config != null;
@override
List<_InterestingSemanticsFragment> get mergeUpFragments => <_InterestingSemanticsFragment>[this];
Set<SemanticsTag>? _tagsForChildren;
/// Tag all children produced by [compileChildren] with `tags`.
///
/// `tags` must not be empty.
void addTags(Iterable<SemanticsTag> tags) {
assert(tags.isNotEmpty);
_tagsForChildren ??= <SemanticsTag>{};
_tagsForChildren!.addAll(tags);
}
/// Adds the geometric information of `ancestor` to this object.
///
/// Those information are required to properly compute the value for
/// [SemanticsNode.transform], [SemanticsNode.clipRect], and
/// [SemanticsNode.rect].
///
/// Ancestors have to be added in order from [owner] up until the next
/// [RenderObject] that owns a [SemanticsNode] is reached.
void addAncestor(RenderObject ancestor) {
_ancestorChain.add(ancestor);
}
}
/// An [_InterestingSemanticsFragment] that produces the root [SemanticsNode] of
/// the semantics tree.
///
/// The root node is available as the only element in the Iterable returned by
/// [children].
class _RootSemanticsFragment extends _InterestingSemanticsFragment {
_RootSemanticsFragment({
required super.owner,
required super.dropsSemanticsOfPreviousSiblings,
});
@override
void compileChildren({
Rect? parentSemanticsClipRect,
Rect? parentPaintClipRect,
required double elevationAdjustment,
required List<SemanticsNode> result,
required List<SemanticsNode> siblingNodes,
}) {
assert(_tagsForChildren == null || _tagsForChildren!.isEmpty);
assert(parentSemanticsClipRect == null);
assert(parentPaintClipRect == null);
assert(_ancestorChain.length == 1);
assert(elevationAdjustment == 0.0);
owner._semantics ??= SemanticsNode.root(
showOnScreen: owner.showOnScreen,
owner: owner.owner!.semanticsOwner!,
);
final SemanticsNode node = owner._semantics!;
assert(MatrixUtils.matrixEquals(node.transform, Matrix4.identity()));
assert(node.parentSemanticsClipRect == null);
assert(node.parentPaintClipRect == null);
node.rect = owner.semanticBounds;
final List<SemanticsNode> children = <SemanticsNode>[];
for (final _InterestingSemanticsFragment fragment in _children) {
assert(fragment.config == null);
fragment.compileChildren(
parentSemanticsClipRect: parentSemanticsClipRect,
parentPaintClipRect: parentPaintClipRect,
elevationAdjustment: 0.0,
result: children,
siblingNodes: siblingNodes,
);
}
// Root node does not have a parent and thus can't attach sibling nodes.
assert(siblingNodes.isEmpty);
node.updateWith(config: null, childrenInInversePaintOrder: children);
// The root node is the only semantics node allowed to be invisible. This
// can happen when the canvas the app is drawn on has a size of 0 by 0
// pixel. If this happens, the root node must not have any children (because
// these would be invisible as well and are therefore excluded from the
// tree).
assert(!node.isInvisible || children.isEmpty);
result.add(node);
}
@override
SemanticsConfiguration? get config => null;
final List<_InterestingSemanticsFragment> _children = <_InterestingSemanticsFragment>[];
@override
void markAsExplicit() {
// nothing to do, we are always explicit.
}
@override
void addAll(Iterable<_InterestingSemanticsFragment> fragments) {
_children.addAll(fragments);
}
}
/// A fragment with partial information that must not form an explicit
/// semantics node without merging into another _SwitchableSemanticsFragment.
///
/// This fragment is generated from synthetic SemanticsConfiguration returned from
/// [SemanticsConfiguration.childConfigurationsDelegate].
class _IncompleteSemanticsFragment extends _InterestingSemanticsFragment {
_IncompleteSemanticsFragment({
required this.config,
required super.owner,
}) : super(dropsSemanticsOfPreviousSiblings: false);
@override
void addAll(Iterable<_InterestingSemanticsFragment> fragments) {
assert(false, 'This fragment must be a leaf node');
}
@override
void compileChildren({
required Rect? parentSemanticsClipRect,
required Rect? parentPaintClipRect,
required double elevationAdjustment,
required List<SemanticsNode> result,
required List<SemanticsNode> siblingNodes,
}) {
// There is nothing to do because this fragment must be a leaf node and
// must not be explicit.
}
@override
final SemanticsConfiguration config;
@override
void markAsExplicit() {
assert(
false,
'SemanticsConfiguration created in '
'SemanticsConfiguration.childConfigurationsDelegate must not produce '
'its own semantics node'
);
}
}
/// An [_InterestingSemanticsFragment] that can be told to only add explicit
/// [SemanticsNode]s to the parent.
///
/// If [markAsExplicit] was not called before this fragment is added to
/// another fragment it will merge [config] into the parent's [SemanticsNode]
/// and add its [children] to it.
///
/// If [markAsExplicit] was called before adding this fragment to another
/// fragment it will create a new [SemanticsNode]. The newly created node will
/// be annotated with the [SemanticsConfiguration] that - without the call to
/// [markAsExplicit] - would have been merged into the parent's [SemanticsNode].
/// Similarly, the new node will also take over the children that otherwise
/// would have been added to the parent's [SemanticsNode].
///
/// After a call to [markAsExplicit] the only element returned by [children]
/// is the newly created node and [config] will return null as the fragment
/// no longer wants to merge any semantic information into the parent's
/// [SemanticsNode].
class _SwitchableSemanticsFragment extends _InterestingSemanticsFragment {
_SwitchableSemanticsFragment({
required bool mergeIntoParent,
required bool blockUserActions,
required SemanticsConfiguration config,
required List<List<_InterestingSemanticsFragment>> siblingMergeGroups,
required super.owner,
required super.dropsSemanticsOfPreviousSiblings,
}) : _siblingMergeGroups = siblingMergeGroups,
_mergeIntoParent = mergeIntoParent,
_config = config {
if (blockUserActions && !_config.isBlockingUserActions) {
_ensureConfigIsWritable();
_config.isBlockingUserActions = true;
}
}
final bool _mergeIntoParent;
SemanticsConfiguration _config;
bool _isConfigWritable = false;
bool _mergesToSibling = false;
final List<List<_InterestingSemanticsFragment>> _siblingMergeGroups;
void _mergeSiblingGroup(Rect? parentSemanticsClipRect, Rect? parentPaintClipRect, List<SemanticsNode> result, Set<int> usedSemanticsIds) {
for (final List<_InterestingSemanticsFragment> group in _siblingMergeGroups) {
Rect? rect;
Rect? semanticsClipRect;
Rect? paintClipRect;
SemanticsConfiguration? configuration;
// Use empty set because the _tagsForChildren may not contains all of the
// tags if this fragment is not explicit. The _tagsForChildren are added
// to sibling nodes at the end of compileChildren if this fragment is
// explicit.
final Set<SemanticsTag> tags = <SemanticsTag>{};
SemanticsNode? node;
for (final _InterestingSemanticsFragment fragment in group) {
if (fragment.config != null) {
final _SwitchableSemanticsFragment switchableFragment = fragment as _SwitchableSemanticsFragment;
switchableFragment._mergesToSibling = true;
node ??= fragment.owner._semantics;
configuration ??= SemanticsConfiguration();
configuration.absorb(switchableFragment.config!);
// It is a child fragment of a _SwitchableFragment, it must have a
// geometry.
final _SemanticsGeometry geometry = switchableFragment._computeSemanticsGeometry(
parentSemanticsClipRect: parentSemanticsClipRect,
parentPaintClipRect: parentPaintClipRect,
)!;
final Rect fragmentRect = MatrixUtils.transformRect(geometry.transform, geometry.rect);
rect = rect?.expandToInclude(fragmentRect) ?? fragmentRect;
if (geometry.semanticsClipRect != null) {
final Rect rect = MatrixUtils.transformRect(geometry.transform, geometry.semanticsClipRect!);
semanticsClipRect = semanticsClipRect?.intersect(rect) ?? rect;
}
if (geometry.paintClipRect != null) {
final Rect rect = MatrixUtils.transformRect(geometry.transform, geometry.paintClipRect!);
paintClipRect = paintClipRect?.intersect(rect) ?? rect;
}
if (switchableFragment._tagsForChildren != null) {
tags.addAll(switchableFragment._tagsForChildren!);
}
}
}
// Can be null if all fragments in group are marked as explicit.
if (configuration != null && !rect!.isEmpty) {
if (node == null || usedSemanticsIds.contains(node.id)) {
node = SemanticsNode(showOnScreen: owner.showOnScreen);
}
usedSemanticsIds.add(node.id);
node
..tags = tags
..rect = rect
..transform = null // Will be set when compiling immediate parent node.
..parentSemanticsClipRect = semanticsClipRect
..parentPaintClipRect = paintClipRect;
for (final _InterestingSemanticsFragment fragment in group) {
if (fragment.config != null) {
fragment.owner._semantics = node;
}
}
node.updateWith(config: configuration);
result.add(node);
}
}
}
final List<_InterestingSemanticsFragment> _children = <_InterestingSemanticsFragment>[];
@override
void compileChildren({
Rect? parentSemanticsClipRect,
Rect? parentPaintClipRect,
required double elevationAdjustment,
required List<SemanticsNode> result,
required List<SemanticsNode> siblingNodes,
}) {
final Set<int> usedSemanticsIds = <int>{};
Iterable<_InterestingSemanticsFragment> compilingFragments = _children;
for (final List<_InterestingSemanticsFragment> siblingGroup in _siblingMergeGroups) {
compilingFragments = compilingFragments.followedBy(siblingGroup);
}
if (!_isExplicit) {
if (!_mergesToSibling) {
owner._semantics = null;
}
_mergeSiblingGroup(
parentSemanticsClipRect,
parentPaintClipRect,
siblingNodes,
usedSemanticsIds,
);
for (final _InterestingSemanticsFragment fragment in compilingFragments) {
assert(_ancestorChain.first == fragment._ancestorChain.last);
if (fragment is _SwitchableSemanticsFragment) {
// Cached semantics node may be part of sibling merging group prior
// to this update. In this case, the semantics node may continue to
// be reused in that sibling merging group.
if (fragment._isExplicit &&
fragment.owner._semantics != null &&
usedSemanticsIds.contains(fragment.owner._semantics!.id)) {
fragment.owner._semantics = null;
}
}
fragment._ancestorChain.addAll(_ancestorChain.skip(1));
fragment.compileChildren(
parentSemanticsClipRect: parentSemanticsClipRect,
parentPaintClipRect: parentPaintClipRect,
// The fragment is not explicit, its elevation has been absorbed by
// the parent config (as thickness). We still need to make sure that
// its children are placed at the elevation dictated by this config.
elevationAdjustment: elevationAdjustment + _config.elevation,
result: result,
siblingNodes: siblingNodes,
);
}
return;
}
final _SemanticsGeometry? geometry = _computeSemanticsGeometry(
parentSemanticsClipRect: parentSemanticsClipRect,
parentPaintClipRect: parentPaintClipRect,
);
if (!_mergeIntoParent && (geometry?.dropFromTree ?? false)) {
return; // Drop the node, it's not going to be visible.
}
final SemanticsNode node = (owner._semantics ??= SemanticsNode(showOnScreen: owner.showOnScreen))
..tags = _tagsForChildren;
node.elevationAdjustment = elevationAdjustment;
if (elevationAdjustment != 0.0) {
_ensureConfigIsWritable();
_config.elevation += elevationAdjustment;
}
if (geometry != null) {
assert(_needsGeometryUpdate);
node
..rect = geometry.rect
..transform = geometry.transform
..parentSemanticsClipRect = geometry.semanticsClipRect
..parentPaintClipRect = geometry.paintClipRect;
if (!_mergeIntoParent && geometry.markAsHidden) {
_ensureConfigIsWritable();
_config.isHidden = true;
}
}
final List<SemanticsNode> children = <SemanticsNode>[];
_mergeSiblingGroup(
node.parentSemanticsClipRect,
node.parentPaintClipRect,
siblingNodes,
usedSemanticsIds,
);
for (final _InterestingSemanticsFragment fragment in compilingFragments) {
if (fragment is _SwitchableSemanticsFragment) {
// Cached semantics node may be part of sibling merging group prior
// to this update. In this case, the semantics node may continue to
// be reused in that sibling merging group.
if (fragment._isExplicit &&
fragment.owner._semantics != null &&
usedSemanticsIds.contains(fragment.owner._semantics!.id)) {
fragment.owner._semantics = null;
}
}
final List<SemanticsNode> childSiblingNodes = <SemanticsNode>[];
fragment.compileChildren(
parentSemanticsClipRect: node.parentSemanticsClipRect,
parentPaintClipRect: node.parentPaintClipRect,
elevationAdjustment: 0.0,
result: children,
siblingNodes: childSiblingNodes,
);
siblingNodes.addAll(childSiblingNodes);
}
if (_config.isSemanticBoundary) {
owner.assembleSemanticsNode(node, _config, children);
} else {
node.updateWith(config: _config, childrenInInversePaintOrder: children);
}
result.add(node);
// Sibling node needs to attach to the parent of an explicit node.
for (final SemanticsNode siblingNode in siblingNodes) {
// sibling nodes are in the same coordinate of the immediate explicit node.
// They need to share the same transform if they are going to attach to the
// parent of the immediate explicit node.
assert(siblingNode.transform == null);
siblingNode.transform = node.transform;
if (_tagsForChildren != null) {
siblingNode.tags ??= <SemanticsTag>{};
siblingNode.tags!.addAll(_tagsForChildren!);
}
}
result.addAll(siblingNodes);
siblingNodes.clear();
}
_SemanticsGeometry? _computeSemanticsGeometry({
required Rect? parentSemanticsClipRect,
required Rect? parentPaintClipRect,
}) {
return _needsGeometryUpdate
? _SemanticsGeometry(parentSemanticsClipRect: parentSemanticsClipRect, parentPaintClipRect: parentPaintClipRect, ancestors: _ancestorChain)
: null;
}
@override
SemanticsConfiguration? get config {
return _isExplicit ? null : _config;
}
@override
void addAll(Iterable<_InterestingSemanticsFragment> fragments) {
for (final _InterestingSemanticsFragment fragment in fragments) {
_children.add(fragment);
if (fragment.config == null) {
continue;
}
_ensureConfigIsWritable();
_config.absorb(fragment.config!);
}
}
@override
void addTags(Iterable<SemanticsTag> tags) {
super.addTags(tags);
// _ContainerSemanticsFragments add their tags to child fragments through
// this method. This fragment must make sure its _config is in sync.
if (tags.isNotEmpty) {
_ensureConfigIsWritable();
tags.forEach(_config.addTagForChildren);
}
}
void _ensureConfigIsWritable() {
if (!_isConfigWritable) {
_config = _config.copy();
_isConfigWritable = true;
}
}
bool _isExplicit = false;
@override
void markAsExplicit() {
_isExplicit = true;
}
bool get _needsGeometryUpdate => _ancestorChain.length > 1;
}
/// Helper class that keeps track of the geometry of a [SemanticsNode].
///
/// It is used to annotate a [SemanticsNode] with the current information for
/// [SemanticsNode.rect] and [SemanticsNode.transform].
class _SemanticsGeometry {
/// The `parentClippingRect` may be null if no clip is to be applied.
///
/// The `ancestors` list has to include all [RenderObject] in order that are
/// located between the [SemanticsNode] whose geometry is represented here
/// (first [RenderObject] in the list) and its closest ancestor [RenderObject]
/// that also owns its own [SemanticsNode] (last [RenderObject] in the list).
_SemanticsGeometry({
required Rect? parentSemanticsClipRect,
required Rect? parentPaintClipRect,
required List<RenderObject> ancestors,
}) {
_computeValues(parentSemanticsClipRect, parentPaintClipRect, ancestors);
}
Rect? _paintClipRect;
Rect? _semanticsClipRect;
late Matrix4 _transform;
late Rect _rect;
/// Value for [SemanticsNode.transform].
Matrix4 get transform => _transform;
/// Value for [SemanticsNode.parentSemanticsClipRect].
Rect? get semanticsClipRect => _semanticsClipRect;
/// Value for [SemanticsNode.parentPaintClipRect].
Rect? get paintClipRect => _paintClipRect;
/// Value for [SemanticsNode.rect].
Rect get rect => _rect;
/// Computes values, ensuring `rect` is properly bounded by ancestor clipping rects.
///
/// See also:
///
/// * [RenderObject.describeSemanticsClip], typically used to determine `parentSemanticsClipRect`.
/// * [RenderObject.describeApproximatePaintClip], typically used to determine `parentPaintClipRect`.
void _computeValues(Rect? parentSemanticsClipRect, Rect? parentPaintClipRect, List<RenderObject> ancestors) {
assert(ancestors.length > 1);
_transform = Matrix4.identity();
_semanticsClipRect = parentSemanticsClipRect;
_paintClipRect = parentPaintClipRect;
for (int index = ancestors.length-1; index > 0; index -= 1) {
final RenderObject semanticsParent = ancestors[index];
final RenderObject semanticsChild = ancestors[index-1];
_applyIntermediatePaintTransforms(semanticsParent, semanticsChild, _transform);
if (identical(semanticsParent, semanticsChild.parent)) {
// The easier and more common case: semanticsParent is directly
// responsible for painting (and potentially clipping) the semanticsChild
// RenderObject.
_computeClipRect(semanticsParent, semanticsChild, _semanticsClipRect, _paintClipRect);
} else {
// Otherwise we have to find the closest ancestor RenderObject that
// has up-to-date semantics geometry and compute the clip rects from there.
//
// Currently it can only happen when the subtree contains an OverlayPortal.
final List<RenderObject> clipPath = <RenderObject>[semanticsChild];
RenderObject? ancestor = semanticsChild.parent;
while (ancestor != null && ancestor._semantics == null) {
clipPath.add(ancestor);
ancestor = ancestor.parent;
}
_paintClipRect = ancestor?._semantics?.parentPaintClipRect;
_semanticsClipRect = ancestor?._semantics?.parentSemanticsClipRect;
if (ancestor != null) {
assert(ancestor._semantics != null);
assert(!ancestor._needsSemanticsUpdate);
RenderObject parent = ancestor;
for (int i = clipPath.length - 1; i >= 0; i -= 1) {
_computeClipRect(parent, clipPath[i], _semanticsClipRect, _paintClipRect);
parent = clipPath[i];
}
}
}
}
final RenderObject owner = ancestors.first;
_rect = _semanticsClipRect?.intersect(owner.semanticBounds) ?? owner.semanticBounds;
if (_paintClipRect != null) {
final Rect paintRect = _paintClipRect!.intersect(_rect);
_markAsHidden = paintRect.isEmpty && !_rect.isEmpty;
if (!_markAsHidden) {
_rect = paintRect;
}
}
}
/// From parent to child coordinate system.
static Rect? _transformRect(Rect? rect, Matrix4 transform) {
if (rect == null) {
return null;
}
if (rect.isEmpty || transform.isZero()) {
return Rect.zero;
}
return MatrixUtils.inverseTransformRect(transform, rect);
}
// Computes the paint transform from `childFragmentOwner` to
// `parentFragmentOwner` and applies the paint transform to `transform` in
// place.
//
// The `parentFragmentOwner` and `childFragmentOwner` [RenderObject]s must be
// in the same render tree (so they have a common ancestor).
static void _applyIntermediatePaintTransforms(
RenderObject parentFragmentOwner,
RenderObject childFragmentOwner,
Matrix4 transform,
) {
Matrix4? parentToCommonAncestorTransform;
RenderObject from = childFragmentOwner;
RenderObject to = parentFragmentOwner;
while (!identical(from, to)) {
final int fromDepth = from.depth;
final int toDepth = to.depth;
if (fromDepth >= toDepth) {
assert(from.parent != null, '$parentFragmentOwner and $childFragmentOwner are not in the same render tree.');
final RenderObject fromParent = from.parent!;
fromParent.applyPaintTransform(from, transform);
from = fromParent;
}
if (fromDepth <= toDepth) {
assert(to.parent != null, '$parentFragmentOwner and $childFragmentOwner are not in the same render tree.');
final RenderObject toParent = to.parent!;
toParent.applyPaintTransform(to, parentToCommonAncestorTransform ??= Matrix4.identity());
to = toParent;
}
}
if (parentToCommonAncestorTransform != null) {
if (parentToCommonAncestorTransform.invert() != 0) {
transform.multiply(parentToCommonAncestorTransform);
} else {
transform.setZero();
}
}
}
// A matrix used to store transient transform data.
//
// Reusing this matrix avoids allocating a new matrix every time a temporary
// matrix is needed.
//
// This instance should never be returned to the caller. Otherwise, the data
// stored in it will be overwritten unpredictably by subsequent reuses.
static final Matrix4 _temporaryTransformHolder = Matrix4.zero();
// Computes the semantics and painting clip rects for the given child and
// assigns the rects to _semanticsClipRect and _paintClipRect respectively.
//
// The caller must guarantee that child.parent == parent. The resulting rects
// are in `child`'s coordinate system.
void _computeClipRect(RenderObject parent, RenderObject child, Rect? parentSemanticsClipRect, Rect? parentPaintClipRect) {
assert(identical(child.parent, parent));
// Computes the paint transform from child to parent. The _transformRect
// method will compute the inverse.
_temporaryTransformHolder.setIdentity(); // clears data from previous call(s)
parent.applyPaintTransform(child, _temporaryTransformHolder);
final Rect? additionalPaintClip = parent.describeApproximatePaintClip(child);
_paintClipRect = _transformRect(
_intersectRects(additionalPaintClip, parentPaintClipRect),
_temporaryTransformHolder,
);
if (_paintClipRect == null) {
_semanticsClipRect = null;
} else {
final Rect? semanticsClip = parent.describeSemanticsClip(child) ?? _intersectRects(parentSemanticsClipRect, additionalPaintClip);
_semanticsClipRect = _transformRect(semanticsClip, _temporaryTransformHolder);
}
}
static Rect? _intersectRects(Rect? a, Rect? b) {
if (b == null) {
return a;
}
return a?.intersect(b) ?? b;
}
/// Whether the [SemanticsNode] annotated with the geometric information tracked
/// by this object can be dropped from the semantics tree without losing
/// semantics information.
bool get dropFromTree {
return _rect.isEmpty || _transform.isZero();
}
/// Whether the [SemanticsNode] annotated with the geometric information
/// tracked by this object should be marked as hidden because it is not
/// visible on screen.
///
/// Hidden elements should still be included in the tree to work around
/// platform limitations (e.g. accessibility scrolling on iOS).
///
/// See also:
///
/// * [SemanticsFlag.isHidden] for the purpose of marking a node as hidden.
bool get markAsHidden => _markAsHidden;
bool _markAsHidden = false;
}
/// A class that creates [DiagnosticsNode] by wrapping [RenderObject.debugCreator].
///
/// Attach a [DiagnosticsDebugCreator] into [FlutterErrorDetails.informationCollector]
/// when a [RenderObject.debugCreator] is available. This will lead to improved
/// error message.
class DiagnosticsDebugCreator extends DiagnosticsProperty<Object> {
/// Create a [DiagnosticsProperty] with its [value] initialized to input
/// [RenderObject.debugCreator].
DiagnosticsDebugCreator(Object value)
: super(
'debugCreator',
value,
level: DiagnosticLevel.hidden,
);
}
| flutter/packages/flutter/lib/src/rendering/object.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/object.dart",
"repo_id": "flutter",
"token_count": 66439
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart';
import 'box.dart';
import 'object.dart';
import 'sliver.dart';
/// A delegate used by [RenderSliverMultiBoxAdaptor] to manage its children.
///
/// [RenderSliverMultiBoxAdaptor] objects reify their children lazily to avoid
/// spending resources on children that are not visible in the viewport. This
/// delegate lets these objects create and remove children as well as estimate
/// the total scroll offset extent occupied by the full child list.
abstract class RenderSliverBoxChildManager {
/// Called during layout when a new child is needed. The child should be
/// inserted into the child list in the appropriate position, after the
/// `after` child (at the start of the list if `after` is null). Its index and
/// scroll offsets will automatically be set appropriately.
///
/// The `index` argument gives the index of the child to show. It is possible
/// for negative indices to be requested. For example: if the user scrolls
/// from child 0 to child 10, and then those children get much smaller, and
/// then the user scrolls back up again, this method will eventually be asked
/// to produce a child for index -1.
///
/// If no child corresponds to `index`, then do nothing.
///
/// Which child is indicated by index zero depends on the [GrowthDirection]
/// specified in the `constraints` of the [RenderSliverMultiBoxAdaptor]. For
/// example if the children are the alphabet, then if
/// [SliverConstraints.growthDirection] is [GrowthDirection.forward] then
/// index zero is A, and index 25 is Z. On the other hand if
/// [SliverConstraints.growthDirection] is [GrowthDirection.reverse] then
/// index zero is Z, and index 25 is A.
///
/// During a call to [createChild] it is valid to remove other children from
/// the [RenderSliverMultiBoxAdaptor] object if they were not created during
/// this frame and have not yet been updated during this frame. It is not
/// valid to add any other children to this render object.
void createChild(int index, { required RenderBox? after });
/// Remove the given child from the child list.
///
/// Called by [RenderSliverMultiBoxAdaptor.collectGarbage], which itself is
/// called from [RenderSliverMultiBoxAdaptor]'s `performLayout`.
///
/// The index of the given child can be obtained using the
/// [RenderSliverMultiBoxAdaptor.indexOf] method, which reads it from the
/// [SliverMultiBoxAdaptorParentData.index] field of the child's
/// [RenderObject.parentData].
void removeChild(RenderBox child);
/// Called to estimate the total scrollable extents of this object.
///
/// Must return the total distance from the start of the child with the
/// earliest possible index to the end of the child with the last possible
/// index.
double estimateMaxScrollOffset(
SliverConstraints constraints, {
int? firstIndex,
int? lastIndex,
double? leadingScrollOffset,
double? trailingScrollOffset,
});
/// Called to obtain a precise measure of the total number of children.
///
/// Must return the number that is one greater than the greatest `index` for
/// which `createChild` will actually create a child.
///
/// This is used when [createChild] cannot add a child for a positive `index`,
/// to determine the precise dimensions of the sliver. It must return an
/// accurate and precise non-null value. It will not be called if
/// [createChild] is always able to create a child (e.g. for an infinite
/// list).
int get childCount;
/// The best available estimate of [childCount], or null if no estimate is available.
///
/// This differs from [childCount] in that [childCount] never returns null (and must
/// not be accessed if the child count is not yet available, meaning the [createChild]
/// method has not been provided an index that does not create a child).
///
/// See also:
///
/// * [SliverChildDelegate.estimatedChildCount], to which this getter defers.
int? get estimatedChildCount => null;
/// Called during [RenderSliverMultiBoxAdaptor.adoptChild] or
/// [RenderSliverMultiBoxAdaptor.move].
///
/// Subclasses must ensure that the [SliverMultiBoxAdaptorParentData.index]
/// field of the child's [RenderObject.parentData] accurately reflects the
/// child's index in the child list after this function returns.
void didAdoptChild(RenderBox child);
/// Called during layout to indicate whether this object provided insufficient
/// children for the [RenderSliverMultiBoxAdaptor] to fill the
/// [SliverConstraints.remainingPaintExtent].
///
/// Typically called unconditionally at the start of layout with false and
/// then later called with true when the [RenderSliverMultiBoxAdaptor]
/// fails to create a child required to fill the
/// [SliverConstraints.remainingPaintExtent].
///
/// Useful for subclasses to determine whether newly added children could
/// affect the visible contents of the [RenderSliverMultiBoxAdaptor].
void setDidUnderflow(bool value);
/// Called at the beginning of layout to indicate that layout is about to
/// occur.
void didStartLayout() { }
/// Called at the end of layout to indicate that layout is now complete.
void didFinishLayout() { }
/// In debug mode, asserts that this manager is not expecting any
/// modifications to the [RenderSliverMultiBoxAdaptor]'s child list.
///
/// This function always returns true.
///
/// The manager is not required to track whether it is expecting modifications
/// to the [RenderSliverMultiBoxAdaptor]'s child list and can return
/// true without making any assertions.
bool debugAssertChildListLocked() => true;
}
/// Parent data structure used by [RenderSliverWithKeepAliveMixin].
mixin KeepAliveParentDataMixin implements ParentData {
/// Whether to keep the child alive even when it is no longer visible.
bool keepAlive = false;
/// Whether the widget is currently being kept alive, i.e. has [keepAlive] set
/// to true and is offscreen.
bool get keptAlive;
}
/// This class exists to dissociate [KeepAlive] from [RenderSliverMultiBoxAdaptor].
///
/// [RenderSliverWithKeepAliveMixin.setupParentData] must be implemented to use
/// a parentData class that uses the right mixin or whatever is appropriate.
mixin RenderSliverWithKeepAliveMixin implements RenderSliver {
/// Alerts the developer that the child's parentData needs to be of type
/// [KeepAliveParentDataMixin].
@override
void setupParentData(RenderObject child) {
assert(child.parentData is KeepAliveParentDataMixin);
}
}
/// Parent data structure used by [RenderSliverMultiBoxAdaptor].
class SliverMultiBoxAdaptorParentData extends SliverLogicalParentData with ContainerParentDataMixin<RenderBox>, KeepAliveParentDataMixin {
/// The index of this child according to the [RenderSliverBoxChildManager].
int? index;
@override
bool get keptAlive => _keptAlive;
bool _keptAlive = false;
@override
String toString() => 'index=$index; ${keepAlive ? "keepAlive; " : ""}${super.toString()}';
}
/// A sliver with multiple box children.
///
/// [RenderSliverMultiBoxAdaptor] is a base class for slivers that have multiple
/// box children. The children are managed by a [RenderSliverBoxChildManager],
/// which lets subclasses create children lazily during layout. Typically
/// subclasses will create only those children that are actually needed to fill
/// the [SliverConstraints.remainingPaintExtent].
///
/// The contract for adding and removing children from this render object is
/// more strict than for normal render objects:
///
/// * Children can be removed except during a layout pass if they have already
/// been laid out during that layout pass.
/// * Children cannot be added except during a call to [childManager], and
/// then only if there is no child corresponding to that index (or the child
/// corresponding to that index was first removed).
///
/// See also:
///
/// * [RenderSliverToBoxAdapter], which has a single box child.
/// * [RenderSliverList], which places its children in a linear
/// array.
/// * [RenderSliverFixedExtentList], which places its children in a linear
/// array with a fixed extent in the main axis.
/// * [RenderSliverGrid], which places its children in arbitrary positions.
abstract class RenderSliverMultiBoxAdaptor extends RenderSliver
with ContainerRenderObjectMixin<RenderBox, SliverMultiBoxAdaptorParentData>,
RenderSliverHelpers, RenderSliverWithKeepAliveMixin {
/// Creates a sliver with multiple box children.
RenderSliverMultiBoxAdaptor({
required RenderSliverBoxChildManager childManager,
}) : _childManager = childManager {
assert(() {
_debugDanglingKeepAlives = <RenderBox>[];
return true;
}());
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverMultiBoxAdaptorParentData) {
child.parentData = SliverMultiBoxAdaptorParentData();
}
}
/// The delegate that manages the children of this object.
///
/// Rather than having a concrete list of children, a
/// [RenderSliverMultiBoxAdaptor] uses a [RenderSliverBoxChildManager] to
/// create children during layout in order to fill the
/// [SliverConstraints.remainingPaintExtent].
@protected
RenderSliverBoxChildManager get childManager => _childManager;
final RenderSliverBoxChildManager _childManager;
/// The nodes being kept alive despite not being visible.
final Map<int, RenderBox> _keepAliveBucket = <int, RenderBox>{};
late List<RenderBox> _debugDanglingKeepAlives;
/// Indicates whether integrity check is enabled.
///
/// Setting this property to true will immediately perform an integrity check.
///
/// The integrity check consists of:
///
/// 1. Verify that the children index in childList is in ascending order.
/// 2. Verify that there is no dangling keepalive child as the result of [move].
bool get debugChildIntegrityEnabled => _debugChildIntegrityEnabled;
bool _debugChildIntegrityEnabled = true;
set debugChildIntegrityEnabled(bool enabled) {
assert(() {
_debugChildIntegrityEnabled = enabled;
return _debugVerifyChildOrder() &&
(!_debugChildIntegrityEnabled || _debugDanglingKeepAlives.isEmpty);
}());
}
@override
void adoptChild(RenderObject child) {
super.adoptChild(child);
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
if (!childParentData._keptAlive) {
childManager.didAdoptChild(child as RenderBox);
}
}
bool _debugAssertChildListLocked() => childManager.debugAssertChildListLocked();
/// Verify that the child list index is in strictly increasing order.
///
/// This has no effect in release builds.
bool _debugVerifyChildOrder() {
if (_debugChildIntegrityEnabled) {
RenderBox? child = firstChild;
int index;
while (child != null) {
index = indexOf(child);
child = childAfter(child);
assert(child == null || indexOf(child) > index);
}
}
return true;
}
@override
void insert(RenderBox child, { RenderBox? after }) {
assert(!_keepAliveBucket.containsValue(child));
super.insert(child, after: after);
assert(firstChild != null);
assert(_debugVerifyChildOrder());
}
@override
void move(RenderBox child, { RenderBox? after }) {
// There are two scenarios:
//
// 1. The child is not keptAlive.
// The child is in the childList maintained by ContainerRenderObjectMixin.
// We can call super.move and update parentData with the new slot.
//
// 2. The child is keptAlive.
// In this case, the child is no longer in the childList but might be stored in
// [_keepAliveBucket]. We need to update the location of the child in the bucket.
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
if (!childParentData.keptAlive) {
super.move(child, after: after);
childManager.didAdoptChild(child); // updates the slot in the parentData
// Its slot may change even if super.move does not change the position.
// In this case, we still want to mark as needs layout.
markNeedsLayout();
} else {
// If the child in the bucket is not current child, that means someone has
// already moved and replaced current child, and we cannot remove this child.
if (_keepAliveBucket[childParentData.index] == child) {
_keepAliveBucket.remove(childParentData.index);
}
assert(() {
_debugDanglingKeepAlives.remove(child);
return true;
}());
// Update the slot and reinsert back to _keepAliveBucket in the new slot.
childManager.didAdoptChild(child);
// If there is an existing child in the new slot, that mean that child will
// be moved to other index. In other cases, the existing child should have been
// removed by updateChild. Thus, it is ok to overwrite it.
assert(() {
if (_keepAliveBucket.containsKey(childParentData.index)) {
_debugDanglingKeepAlives.add(_keepAliveBucket[childParentData.index]!);
}
return true;
}());
_keepAliveBucket[childParentData.index!] = child;
}
}
@override
void remove(RenderBox child) {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
if (!childParentData._keptAlive) {
super.remove(child);
return;
}
assert(_keepAliveBucket[childParentData.index] == child);
assert(() {
_debugDanglingKeepAlives.remove(child);
return true;
}());
_keepAliveBucket.remove(childParentData.index);
dropChild(child);
}
@override
void removeAll() {
super.removeAll();
_keepAliveBucket.values.forEach(dropChild);
_keepAliveBucket.clear();
}
void _createOrObtainChild(int index, { required RenderBox? after }) {
invokeLayoutCallback<SliverConstraints>((SliverConstraints constraints) {
assert(constraints == this.constraints);
if (_keepAliveBucket.containsKey(index)) {
final RenderBox child = _keepAliveBucket.remove(index)!;
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
assert(childParentData._keptAlive);
dropChild(child);
child.parentData = childParentData;
insert(child, after: after);
childParentData._keptAlive = false;
} else {
_childManager.createChild(index, after: after);
}
});
}
void _destroyOrCacheChild(RenderBox child) {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
if (childParentData.keepAlive) {
assert(!childParentData._keptAlive);
remove(child);
_keepAliveBucket[childParentData.index!] = child;
child.parentData = childParentData;
super.adoptChild(child);
childParentData._keptAlive = true;
} else {
assert(child.parent == this);
_childManager.removeChild(child);
assert(child.parent == null);
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
for (final RenderBox child in _keepAliveBucket.values) {
child.attach(owner);
}
}
@override
void detach() {
super.detach();
for (final RenderBox child in _keepAliveBucket.values) {
child.detach();
}
}
@override
void redepthChildren() {
super.redepthChildren();
_keepAliveBucket.values.forEach(redepthChild);
}
@override
void visitChildren(RenderObjectVisitor visitor) {
super.visitChildren(visitor);
_keepAliveBucket.values.forEach(visitor);
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
super.visitChildren(visitor);
// Do not visit children in [_keepAliveBucket].
}
/// Called during layout to create and add the child with the given index and
/// scroll offset.
///
/// Calls [RenderSliverBoxChildManager.createChild] to actually create and add
/// the child if necessary. The child may instead be obtained from a cache;
/// see [SliverMultiBoxAdaptorParentData.keepAlive].
///
/// Returns false if there was no cached child and `createChild` did not add
/// any child, otherwise returns true.
///
/// Does not layout the new child.
///
/// When this is called, there are no visible children, so no children can be
/// removed during the call to `createChild`. No child should be added during
/// that call either, except for the one that is created and returned by
/// `createChild`.
@protected
bool addInitialChild({ int index = 0, double layoutOffset = 0.0 }) {
assert(_debugAssertChildListLocked());
assert(firstChild == null);
_createOrObtainChild(index, after: null);
if (firstChild != null) {
assert(firstChild == lastChild);
assert(indexOf(firstChild!) == index);
final SliverMultiBoxAdaptorParentData firstChildParentData = firstChild!.parentData! as SliverMultiBoxAdaptorParentData;
firstChildParentData.layoutOffset = layoutOffset;
return true;
}
childManager.setDidUnderflow(true);
return false;
}
/// Called during layout to create, add, and layout the child before
/// [firstChild].
///
/// Calls [RenderSliverBoxChildManager.createChild] to actually create and add
/// the child if necessary. The child may instead be obtained from a cache;
/// see [SliverMultiBoxAdaptorParentData.keepAlive].
///
/// Returns the new child or null if no child was obtained.
///
/// The child that was previously the first child, as well as any subsequent
/// children, may be removed by this call if they have not yet been laid out
/// during this layout pass. No child should be added during that call except
/// for the one that is created and returned by `createChild`.
@protected
RenderBox? insertAndLayoutLeadingChild(
BoxConstraints childConstraints, {
bool parentUsesSize = false,
}) {
assert(_debugAssertChildListLocked());
final int index = indexOf(firstChild!) - 1;
_createOrObtainChild(index, after: null);
if (indexOf(firstChild!) == index) {
firstChild!.layout(childConstraints, parentUsesSize: parentUsesSize);
return firstChild;
}
childManager.setDidUnderflow(true);
return null;
}
/// Called during layout to create, add, and layout the child after
/// the given child.
///
/// Calls [RenderSliverBoxChildManager.createChild] to actually create and add
/// the child if necessary. The child may instead be obtained from a cache;
/// see [SliverMultiBoxAdaptorParentData.keepAlive].
///
/// Returns the new child. It is the responsibility of the caller to configure
/// the child's scroll offset.
///
/// Children after the `after` child may be removed in the process. Only the
/// new child may be added.
@protected
RenderBox? insertAndLayoutChild(
BoxConstraints childConstraints, {
required RenderBox? after,
bool parentUsesSize = false,
}) {
assert(_debugAssertChildListLocked());
assert(after != null);
final int index = indexOf(after!) + 1;
_createOrObtainChild(index, after: after);
final RenderBox? child = childAfter(after);
if (child != null && indexOf(child) == index) {
child.layout(childConstraints, parentUsesSize: parentUsesSize);
return child;
}
childManager.setDidUnderflow(true);
return null;
}
/// Returns the number of children preceding the `firstIndex` that need to be
/// garbage collected.
///
/// See also:
///
/// * [collectGarbage], which takes the leading and trailing number of
/// children to be garbage collected.
/// * [calculateTrailingGarbage], which similarly returns the number of
/// trailing children to be garbage collected.
@visibleForTesting
@protected
int calculateLeadingGarbage({required int firstIndex}) {
RenderBox? walker = firstChild;
int leadingGarbage = 0;
while (walker != null && indexOf(walker) < firstIndex) {
leadingGarbage += 1;
walker = childAfter(walker);
}
return leadingGarbage;
}
/// Returns the number of children following the `lastIndex` that need to be
/// garbage collected.
///
/// See also:
///
/// * [collectGarbage], which takes the leading and trailing number of
/// children to be garbage collected.
/// * [calculateLeadingGarbage], which similarly returns the number of
/// leading children to be garbage collected.
@visibleForTesting
@protected
int calculateTrailingGarbage({required int lastIndex}) {
RenderBox? walker = lastChild;
int trailingGarbage = 0;
while (walker != null && indexOf(walker) > lastIndex) {
trailingGarbage += 1;
walker = childBefore(walker);
}
return trailingGarbage;
}
/// Called after layout with the number of children that can be garbage
/// collected at the head and tail of the child list.
///
/// Children whose [SliverMultiBoxAdaptorParentData.keepAlive] property is
/// set to true will be removed to a cache instead of being dropped.
///
/// This method also collects any children that were previously kept alive but
/// are now no longer necessary. As such, it should be called every time
/// [performLayout] is run, even if the arguments are both zero.
///
/// See also:
///
/// * [calculateLeadingGarbage], which can be used to determine
/// `leadingGarbage` here.
/// * [calculateTrailingGarbage], which can be used to determine
/// `trailingGarbage` here.
@protected
void collectGarbage(int leadingGarbage, int trailingGarbage) {
assert(_debugAssertChildListLocked());
assert(childCount >= leadingGarbage + trailingGarbage);
invokeLayoutCallback<SliverConstraints>((SliverConstraints constraints) {
while (leadingGarbage > 0) {
_destroyOrCacheChild(firstChild!);
leadingGarbage -= 1;
}
while (trailingGarbage > 0) {
_destroyOrCacheChild(lastChild!);
trailingGarbage -= 1;
}
// Ask the child manager to remove the children that are no longer being
// kept alive. (This should cause _keepAliveBucket to change, so we have
// to prepare our list ahead of time.)
_keepAliveBucket.values.where((RenderBox child) {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
return !childParentData.keepAlive;
}).toList().forEach(_childManager.removeChild);
assert(_keepAliveBucket.values.where((RenderBox child) {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
return !childParentData.keepAlive;
}).isEmpty);
});
}
/// Returns the index of the given child, as given by the
/// [SliverMultiBoxAdaptorParentData.index] field of the child's [parentData].
int indexOf(RenderBox child) {
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
assert(childParentData.index != null);
return childParentData.index!;
}
/// Returns the dimension of the given child in the main axis, as given by the
/// child's [RenderBox.size] property. This is only valid after layout.
@protected
double paintExtentOf(RenderBox child) {
assert(child.hasSize);
return switch (constraints.axis) {
Axis.horizontal => child.size.width,
Axis.vertical => child.size.height,
};
}
@override
bool hitTestChildren(SliverHitTestResult result, { required double mainAxisPosition, required double crossAxisPosition }) {
RenderBox? child = lastChild;
final BoxHitTestResult boxResult = BoxHitTestResult.wrap(result);
while (child != null) {
if (hitTestBoxChild(boxResult, child, mainAxisPosition: mainAxisPosition, crossAxisPosition: crossAxisPosition)) {
return true;
}
child = childBefore(child);
}
return false;
}
@override
double childMainAxisPosition(RenderBox child) {
return childScrollOffset(child)! - constraints.scrollOffset;
}
@override
double? childScrollOffset(RenderObject child) {
assert(child.parent == this);
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
return childParentData.layoutOffset;
}
@override
bool paintsChild(RenderBox child) {
final SliverMultiBoxAdaptorParentData? childParentData = child.parentData as SliverMultiBoxAdaptorParentData?;
return childParentData?.index != null &&
!_keepAliveBucket.containsKey(childParentData!.index);
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
if (!paintsChild(child)) {
// This can happen if some child asks for the global transform even though
// they are not getting painted. In that case, the transform sets set to
// zero since [applyPaintTransformForBoxChild] would end up throwing due
// to the child not being configured correctly for applying a transform.
// There's no assert here because asking for the paint transform is a
// valid thing to do even if a child would not be painted, but there is no
// meaningful non-zero matrix to use in this case.
transform.setZero();
} else {
applyPaintTransformForBoxChild(child, transform);
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (firstChild == null) {
return;
}
// offset is to the top-left corner, regardless of our axis direction.
// originOffset gives us the delta from the real origin to the origin in the axis direction.
final Offset mainAxisUnit, crossAxisUnit, originOffset;
final bool addExtent;
switch (applyGrowthDirectionToAxisDirection(constraints.axisDirection, constraints.growthDirection)) {
case AxisDirection.up:
mainAxisUnit = const Offset(0.0, -1.0);
crossAxisUnit = const Offset(1.0, 0.0);
originOffset = offset + Offset(0.0, geometry!.paintExtent);
addExtent = true;
case AxisDirection.right:
mainAxisUnit = const Offset(1.0, 0.0);
crossAxisUnit = const Offset(0.0, 1.0);
originOffset = offset;
addExtent = false;
case AxisDirection.down:
mainAxisUnit = const Offset(0.0, 1.0);
crossAxisUnit = const Offset(1.0, 0.0);
originOffset = offset;
addExtent = false;
case AxisDirection.left:
mainAxisUnit = const Offset(-1.0, 0.0);
crossAxisUnit = const Offset(0.0, 1.0);
originOffset = offset + Offset(geometry!.paintExtent, 0.0);
addExtent = true;
}
RenderBox? child = firstChild;
while (child != null) {
final double mainAxisDelta = childMainAxisPosition(child);
final double crossAxisDelta = childCrossAxisPosition(child);
Offset childOffset = Offset(
originOffset.dx + mainAxisUnit.dx * mainAxisDelta + crossAxisUnit.dx * crossAxisDelta,
originOffset.dy + mainAxisUnit.dy * mainAxisDelta + crossAxisUnit.dy * crossAxisDelta,
);
if (addExtent) {
childOffset += mainAxisUnit * paintExtentOf(child);
}
// If the child's visible interval (mainAxisDelta, mainAxisDelta + paintExtentOf(child))
// does not intersect the paint extent interval (0, constraints.remainingPaintExtent), it's hidden.
if (mainAxisDelta < constraints.remainingPaintExtent && mainAxisDelta + paintExtentOf(child) > 0) {
context.paintChild(child, childOffset);
}
child = childAfter(child);
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsNode.message(firstChild != null ? 'currently live children: ${indexOf(firstChild!)} to ${indexOf(lastChild!)}' : 'no children current live'));
}
/// Asserts that the reified child list is not empty and has a contiguous
/// sequence of indices.
///
/// Always returns true.
bool debugAssertChildListIsNonEmptyAndContiguous() {
assert(() {
assert(firstChild != null);
int index = indexOf(firstChild!);
RenderBox? child = childAfter(firstChild!);
while (child != null) {
index += 1;
assert(indexOf(child) == index);
child = childAfter(child);
}
return true;
}());
return true;
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> children = <DiagnosticsNode>[];
if (firstChild != null) {
RenderBox? child = firstChild;
while (true) {
final SliverMultiBoxAdaptorParentData childParentData = child!.parentData! as SliverMultiBoxAdaptorParentData;
children.add(child.toDiagnosticsNode(name: 'child with index ${childParentData.index}'));
if (child == lastChild) {
break;
}
child = childParentData.nextSibling;
}
}
if (_keepAliveBucket.isNotEmpty) {
final List<int> indices = _keepAliveBucket.keys.toList()..sort();
for (final int index in indices) {
children.add(_keepAliveBucket[index]!.toDiagnosticsNode(
name: 'child with index $index (kept alive but not laid out)',
style: DiagnosticsTreeStyle.offstage,
));
}
}
return children;
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_multi_box_adaptor.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_multi_box_adaptor.dart",
"repo_id": "flutter",
"token_count": 9477
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'binding.dart';
export 'dart:ui' show VoidCallback;
export 'package:flutter/foundation.dart' show DiagnosticsNode;
/// Signature for the callback passed to the [Ticker] class's constructor.
///
/// The argument is the time that the object had spent enabled so far
/// at the time of the callback being called.
typedef TickerCallback = void Function(Duration elapsed);
/// An interface implemented by classes that can vend [Ticker] objects.
///
/// Tickers can be used by any object that wants to be notified whenever a frame
/// triggers, but are most commonly used indirectly via an
/// [AnimationController]. [AnimationController]s need a [TickerProvider] to
/// obtain their [Ticker]. If you are creating an [AnimationController] from a
/// [State], then you can use the [TickerProviderStateMixin] and
/// [SingleTickerProviderStateMixin] classes to obtain a suitable
/// [TickerProvider]. The widget test framework [WidgetTester] object can be
/// used as a ticker provider in the context of tests. In other contexts, you
/// will have to either pass a [TickerProvider] from a higher level (e.g.
/// indirectly from a [State] that mixes in [TickerProviderStateMixin]), or
/// create a custom [TickerProvider] subclass.
abstract class TickerProvider {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const TickerProvider();
/// Creates a ticker with the given callback.
///
/// The kind of ticker provided depends on the kind of ticker provider.
@factory
Ticker createTicker(TickerCallback onTick);
}
// TODO(jacobr): make Ticker use Diagnosticable to simplify reporting errors
// related to a ticker.
/// Calls its callback once per animation frame.
///
/// When created, a ticker is initially disabled. Call [start] to
/// enable the ticker.
///
/// A [Ticker] can be silenced by setting [muted] to true. While silenced, time
/// still elapses, and [start] and [stop] can still be called, but no callbacks
/// are called.
///
/// By convention, the [start] and [stop] methods are used by the ticker's
/// consumer, and the [muted] property is controlled by the [TickerProvider]
/// that created the ticker.
///
/// Tickers are driven by the [SchedulerBinding]. See
/// [SchedulerBinding.scheduleFrameCallback].
class Ticker {
/// Creates a ticker that will call the provided callback once per frame while
/// running.
///
/// An optional label can be provided for debugging purposes. That label
/// will appear in the [toString] output in debug builds.
Ticker(this._onTick, { this.debugLabel }) {
assert(() {
_debugCreationStack = StackTrace.current;
return true;
}());
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/scheduler.dart',
className: '$Ticker',
object: this,
);
}
}
TickerFuture? _future;
/// Whether this ticker has been silenced.
///
/// While silenced, a ticker's clock can still run, but the callback will not
/// be called.
bool get muted => _muted;
bool _muted = false;
/// When set to true, silences the ticker, so that it is no longer ticking. If
/// a tick is already scheduled, it will unschedule it. This will not
/// unschedule the next frame, though.
///
/// When set to false, unsilences the ticker, potentially scheduling a frame
/// to handle the next tick.
///
/// By convention, the [muted] property is controlled by the object that
/// created the [Ticker] (typically a [TickerProvider]), not the object that
/// listens to the ticker's ticks.
set muted(bool value) {
if (value == muted) {
return;
}
_muted = value;
if (value) {
unscheduleTick();
} else if (shouldScheduleTick) {
scheduleTick();
}
}
/// Whether this [Ticker] has scheduled a call to call its callback
/// on the next frame.
///
/// A ticker that is [muted] can be active (see [isActive]) yet not be
/// ticking. In that case, the ticker will not call its callback, and
/// [isTicking] will be false, but time will still be progressing.
///
/// This will return false if the [SchedulerBinding.lifecycleState] is one
/// that indicates the application is not currently visible (e.g. if the
/// device's screen is turned off).
bool get isTicking {
if (_future == null) {
return false;
}
if (muted) {
return false;
}
if (SchedulerBinding.instance.framesEnabled) {
return true;
}
if (SchedulerBinding.instance.schedulerPhase != SchedulerPhase.idle) {
return true;
} // for example, we might be in a warm-up frame or forced frame
return false;
}
/// Whether time is elapsing for this [Ticker]. Becomes true when [start] is
/// called and false when [stop] is called.
///
/// A ticker can be active yet not be actually ticking (i.e. not be calling
/// the callback). To determine if a ticker is actually ticking, use
/// [isTicking].
bool get isActive => _future != null;
Duration? _startTime;
/// Starts the clock for this [Ticker]. If the ticker is not [muted], then this
/// also starts calling the ticker's callback once per animation frame.
///
/// The returned future resolves once the ticker [stop]s ticking. If the
/// ticker is disposed, the future does not resolve. A derivative future is
/// available from the returned [TickerFuture] object that resolves with an
/// error in that case, via [TickerFuture.orCancel].
///
/// Calling this sets [isActive] to true.
///
/// This method cannot be called while the ticker is active. To restart the
/// ticker, first [stop] it.
///
/// By convention, this method is used by the object that receives the ticks
/// (as opposed to the [TickerProvider] which created the ticker).
TickerFuture start() {
assert(() {
if (isActive) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A ticker was started twice.'),
ErrorDescription('A ticker that is already active cannot be started again without first stopping it.'),
describeForError('The affected ticker was'),
]);
}
return true;
}());
assert(_startTime == null);
_future = TickerFuture._();
if (shouldScheduleTick) {
scheduleTick();
}
if (SchedulerBinding.instance.schedulerPhase.index > SchedulerPhase.idle.index &&
SchedulerBinding.instance.schedulerPhase.index < SchedulerPhase.postFrameCallbacks.index) {
_startTime = SchedulerBinding.instance.currentFrameTimeStamp;
}
return _future!;
}
/// Adds a debug representation of a [Ticker] optimized for including in error
/// messages.
DiagnosticsNode describeForError(String name) {
// TODO(jacobr): make this more structured.
return DiagnosticsProperty<Ticker>(name, this, description: toString(debugIncludeStack: true));
}
/// Stops calling this [Ticker]'s callback.
///
/// If called with the `canceled` argument set to false (the default), causes
/// the future returned by [start] to resolve. If called with the `canceled`
/// argument set to true, the future does not resolve, and the future obtained
/// from [TickerFuture.orCancel], if any, resolves with a [TickerCanceled]
/// error.
///
/// Calling this sets [isActive] to false.
///
/// This method does nothing if called when the ticker is inactive.
///
/// By convention, this method is used by the object that receives the ticks
/// (as opposed to the [TickerProvider] which created the ticker).
void stop({ bool canceled = false }) {
if (!isActive) {
return;
}
// We take the _future into a local variable so that isTicking is false
// when we actually complete the future (isTicking uses _future to
// determine its state).
final TickerFuture localFuture = _future!;
_future = null;
_startTime = null;
assert(!isActive);
unscheduleTick();
if (canceled) {
localFuture._cancel(this);
} else {
localFuture._complete();
}
}
final TickerCallback _onTick;
int? _animationId;
/// Whether this [Ticker] has already scheduled a frame callback.
@protected
bool get scheduled => _animationId != null;
/// Whether a tick should be scheduled.
///
/// If this is true, then calling [scheduleTick] should succeed.
///
/// Reasons why a tick should not be scheduled include:
///
/// * A tick has already been scheduled for the coming frame.
/// * The ticker is not active ([start] has not been called).
/// * The ticker is not ticking, e.g. because it is [muted] (see [isTicking]).
@protected
bool get shouldScheduleTick => !muted && isActive && !scheduled;
void _tick(Duration timeStamp) {
assert(isTicking);
assert(scheduled);
_animationId = null;
_startTime ??= timeStamp;
_onTick(timeStamp - _startTime!);
// The onTick callback may have scheduled another tick already, for
// example by calling stop then start again.
if (shouldScheduleTick) {
scheduleTick(rescheduling: true);
}
}
/// Schedules a tick for the next frame.
///
/// This should only be called if [shouldScheduleTick] is true.
@protected
void scheduleTick({ bool rescheduling = false }) {
assert(!scheduled);
assert(shouldScheduleTick);
_animationId = SchedulerBinding.instance.scheduleFrameCallback(_tick, rescheduling: rescheduling);
}
/// Cancels the frame callback that was requested by [scheduleTick], if any.
///
/// Calling this method when no tick is [scheduled] is harmless.
///
/// This method should not be called when [shouldScheduleTick] would return
/// true if no tick was scheduled.
@protected
void unscheduleTick() {
if (scheduled) {
SchedulerBinding.instance.cancelFrameCallbackWithId(_animationId!);
_animationId = null;
}
assert(!shouldScheduleTick);
}
/// Makes this [Ticker] take the state of another ticker, and disposes the
/// other ticker.
///
/// This is useful if an object with a [Ticker] is given a new
/// [TickerProvider] but needs to maintain continuity. In particular, this
/// maintains the identity of the [TickerFuture] returned by the [start]
/// function of the original [Ticker] if the original ticker is active.
///
/// This ticker must not be active when this method is called.
void absorbTicker(Ticker originalTicker) {
assert(!isActive);
assert(_future == null);
assert(_startTime == null);
assert(_animationId == null);
assert((originalTicker._future == null) == (originalTicker._startTime == null), 'Cannot absorb Ticker after it has been disposed.');
if (originalTicker._future != null) {
_future = originalTicker._future;
_startTime = originalTicker._startTime;
if (shouldScheduleTick) {
scheduleTick();
}
originalTicker._future = null; // so that it doesn't get disposed when we dispose of originalTicker
originalTicker.unscheduleTick();
}
originalTicker.dispose();
}
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// It is legal to call this method while [isActive] is true, in which case:
///
/// * The frame callback that was requested by [scheduleTick], if any, is
/// canceled.
/// * The future that was returned by [start] does not resolve.
/// * The future obtained from [TickerFuture.orCancel], if any, resolves
/// with a [TickerCanceled] error.
@mustCallSuper
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
if (_future != null) {
final TickerFuture localFuture = _future!;
_future = null;
assert(!isActive);
unscheduleTick();
localFuture._cancel(this);
}
assert(() {
// We intentionally don't null out _startTime. This means that if start()
// was ever called, the object is now in a bogus state. This weakly helps
// catch cases of use-after-dispose.
_startTime = Duration.zero;
return true;
}());
}
/// An optional label can be provided for debugging purposes.
///
/// This label will appear in the [toString] output in debug builds.
final String? debugLabel;
late StackTrace _debugCreationStack;
@override
String toString({ bool debugIncludeStack = false }) {
final StringBuffer buffer = StringBuffer();
buffer.write('${objectRuntimeType(this, 'Ticker')}(');
assert(() {
buffer.write(debugLabel ?? '');
return true;
}());
buffer.write(')');
assert(() {
if (debugIncludeStack) {
buffer.writeln();
buffer.writeln('The stack trace when the $runtimeType was actually created was:');
FlutterError.defaultStackFilter(_debugCreationStack.toString().trimRight().split('\n')).forEach(buffer.writeln);
}
return true;
}());
return buffer.toString();
}
}
/// An object representing an ongoing [Ticker] sequence.
///
/// The [Ticker.start] method returns a [TickerFuture]. The [TickerFuture] will
/// complete successfully if the [Ticker] is stopped using [Ticker.stop] with
/// the `canceled` argument set to false (the default).
///
/// If the [Ticker] is disposed without being stopped, or if it is stopped with
/// `canceled` set to true, then this Future will never complete.
///
/// This class works like a normal [Future], but has an additional property,
/// [orCancel], which returns a derivative [Future] that completes with an error
/// if the [Ticker] that returned the [TickerFuture] was stopped with `canceled`
/// set to true, or if it was disposed without being stopped.
///
/// To run a callback when either this future resolves or when the ticker is
/// canceled, use [whenCompleteOrCancel].
class TickerFuture implements Future<void> {
TickerFuture._();
/// Creates a [TickerFuture] instance that represents an already-complete
/// [Ticker] sequence.
///
/// This is useful for implementing objects that normally defer to a [Ticker]
/// but sometimes can skip the ticker because the animation is of zero
/// duration, but which still need to represent the completed animation in the
/// form of a [TickerFuture].
TickerFuture.complete() {
_complete();
}
final Completer<void> _primaryCompleter = Completer<void>();
Completer<void>? _secondaryCompleter;
bool? _completed; // null means unresolved, true means complete, false means canceled
void _complete() {
assert(_completed == null);
_completed = true;
_primaryCompleter.complete();
_secondaryCompleter?.complete();
}
void _cancel(Ticker ticker) {
assert(_completed == null);
_completed = false;
_secondaryCompleter?.completeError(TickerCanceled(ticker));
}
/// Calls `callback` either when this future resolves or when the ticker is
/// canceled.
///
/// Calling this method registers an exception handler for the [orCancel]
/// future, so even if the [orCancel] property is accessed, canceling the
/// ticker will not cause an uncaught exception in the current zone.
void whenCompleteOrCancel(VoidCallback callback) {
void thunk(dynamic value) {
callback();
}
orCancel.then<void>(thunk, onError: thunk);
}
/// A future that resolves when this future resolves or throws when the ticker
/// is canceled.
///
/// If this property is never accessed, then canceling the ticker does not
/// throw any exceptions. Once this property is accessed, though, if the
/// corresponding ticker is canceled, then the [Future] returned by this
/// getter will complete with an error, and if that error is not caught, there
/// will be an uncaught exception in the current zone.
Future<void> get orCancel {
if (_secondaryCompleter == null) {
_secondaryCompleter = Completer<void>();
if (_completed != null) {
if (_completed!) {
_secondaryCompleter!.complete();
} else {
_secondaryCompleter!.completeError(const TickerCanceled());
}
}
}
return _secondaryCompleter!.future;
}
@override
Stream<void> asStream() {
return _primaryCompleter.future.asStream();
}
@override
Future<void> catchError(Function onError, { bool Function(Object)? test }) {
return _primaryCompleter.future.catchError(onError, test: test);
}
@override
Future<R> then<R>(FutureOr<R> Function(void value) onValue, { Function? onError }) {
return _primaryCompleter.future.then<R>(onValue, onError: onError);
}
@override
Future<void> timeout(Duration timeLimit, { FutureOr<void> Function()? onTimeout }) {
return _primaryCompleter.future.timeout(timeLimit, onTimeout: onTimeout);
}
@override
Future<void> whenComplete(dynamic Function() action) {
return _primaryCompleter.future.whenComplete(action);
}
@override
String toString() => '${describeIdentity(this)}(${ _completed == null ? "active" : _completed! ? "complete" : "canceled" })';
}
/// Exception thrown by [Ticker] objects on the [TickerFuture.orCancel] future
/// when the ticker is canceled.
class TickerCanceled implements Exception {
/// Creates a canceled-ticker exception.
const TickerCanceled([this.ticker]);
/// Reference to the [Ticker] object that was canceled.
///
/// This may be null in the case that the [Future] created for
/// [TickerFuture.orCancel] was created after the ticker was canceled.
final Ticker? ticker;
@override
String toString() {
if (ticker != null) {
return 'This ticker was canceled: $ticker';
}
return 'The ticker was canceled before the "orCancel" property was first used.';
}
}
| flutter/packages/flutter/lib/src/scheduler/ticker.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/scheduler/ticker.dart",
"repo_id": "flutter",
"token_count": 5766
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'system_channels.dart';
// Examples can assume:
// // so that we can import the fake "split_component.dart" in an example below:
// // ignore_for_file: uri_does_not_exist
/// Manages the installation and loading of deferred components.
///
/// Deferred components allow Flutter applications to download precompiled AOT
/// dart code and assets at runtime, reducing the install size of apps and
/// avoiding installing unnecessary code/assets on end user devices. Common
/// use cases include deferring installation of advanced or infrequently
/// used features and limiting locale specific features to users of matching
/// locales. Deferred components can only deliver split off parts of the same
/// app that was built and installed on the device. It cannot load new code
/// written after the app is distributed.
///
/// Deferred components are currently an Android-only feature. The methods in
/// this class are a no-op and all assets and dart code are already available
/// without installation if called on other platforms.
abstract final class DeferredComponent {
// TODO(garyq): We should eventually expand this to install components by loadingUnitId
// as well as componentName, but currently, loadingUnitId is opaque to the dart code
// so this is not possible. The API has been left flexible to allow adding
// loadingUnitId as a parameter.
/// Requests that an assets-only deferred component identified by the [componentName]
/// be downloaded and installed.
///
/// This method returns a Future<void> that will complete when the feature is
/// installed and any assets are ready to be used. When an error occurs, the
/// future will complete with an error.
///
/// This method should be used for asset-only deferred components or loading just
/// the assets from a component with both dart code and assets. Deferred components
/// containing dart code should call `loadLibrary()` on a deferred imported
/// library's prefix to ensure that the dart code is properly loaded as
/// `loadLibrary()` will provide the loading unit ID needed for the Dart
/// library loading process. For example:
///
/// ```dart
/// import 'split_component.dart' deferred as split_component;
/// // ...
/// void _showSplit() {
/// // ...
/// split_component.loadLibrary();
/// // ...
/// }
/// ```
///
/// This method will not load associated dart libraries contained in the component,
/// though it will download the files necessary and subsequent calls to `loadLibrary()`
/// to load will complete faster.
///
/// Assets installed by this method may be accessed in the same way as any other
/// local asset by providing a string path to the asset.
///
/// See also:
///
/// * [uninstallDeferredComponent], a method to request the uninstall of a component.
/// * [loadLibrary](https://dart.dev/guides/language/language-tour#lazily-loading-a-library),
/// the Dart method to trigger the installation of the corresponding deferred component that
/// contains the Dart library.
static Future<void> installDeferredComponent({required String componentName}) async {
await SystemChannels.deferredComponent.invokeMethod<void>(
'installDeferredComponent',
<String, dynamic>{ 'loadingUnitId': -1, 'componentName': componentName },
);
}
/// Requests that a deferred component identified by the [componentName] be
/// uninstalled.
///
/// Since uninstallation typically requires significant disk i/o, this method only
/// signals the intent to uninstall. Completion of the returned future indicates
/// that the request to uninstall has been registered. Actual uninstallation (e.g.,
/// removal of assets and files) may occur at a later time. However, once uninstallation
/// is requested, the deferred component should not be used anymore until
/// [installDeferredComponent] or `loadLibrary()` is called again.
///
/// It is safe to request an uninstall when dart code from the component is in use,
/// but assets from the component should not be used once the component uninstall is
/// requested. The dart code will remain usable in the app's current session but
/// is not guaranteed to work in future sessions.
///
/// See also:
///
/// * [installDeferredComponent], a method to install asset-only components.
/// * [loadLibrary](https://api.dart.dev/dart-mirrors/LibraryDependencyMirror/loadLibrary.html),
/// the dart method to trigger the installation of the corresponding deferred component that
/// contains the dart library.
static Future<void> uninstallDeferredComponent({required String componentName}) async {
await SystemChannels.deferredComponent.invokeMethod<void>(
'uninstallDeferredComponent',
<String, dynamic>{ 'loadingUnitId': -1, 'componentName': componentName },
);
}
}
| flutter/packages/flutter/lib/src/services/deferred_component.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/deferred_component.dart",
"repo_id": "flutter",
"token_count": 1273
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter/foundation.dart';
import 'binding.dart';
import 'hardware_keyboard.dart';
import 'raw_keyboard_android.dart';
import 'raw_keyboard_fuchsia.dart';
import 'raw_keyboard_ios.dart';
import 'raw_keyboard_linux.dart';
import 'raw_keyboard_macos.dart';
import 'raw_keyboard_web.dart';
import 'raw_keyboard_windows.dart';
import 'system_channels.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder, ValueChanged;
export 'keyboard_key.g.dart' show LogicalKeyboardKey, PhysicalKeyboardKey;
/// An enum describing the side of the keyboard that a key is on, to allow
/// discrimination between which key is pressed (e.g. the left or right SHIFT
/// key).
///
/// This enum is deprecated and will be removed. There is no direct substitute
/// planned, since this enum will no longer be necessary once [RawKeyEvent] and
/// associated APIs are removed.
///
/// See also:
///
/// * [RawKeyEventData.isModifierPressed], which accepts this enum as an
/// argument.
@Deprecated(
'No longer supported. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
enum KeyboardSide {
/// Matches if either the left, right or both versions of the key are pressed.
any,
/// Matches the left version of the key.
left,
/// Matches the right version of the key.
right,
/// Matches the left and right version of the key pressed simultaneously.
all,
}
/// An enum describing the type of modifier key that is being pressed.
///
/// This enum is deprecated and will be removed. There is no direct substitute
/// planned, since this enum will no longer be necessary once [RawKeyEvent] and
/// associated APIs are removed.
///
/// See also:
///
/// * [RawKeyEventData.isModifierPressed], which accepts this enum as an
/// argument.
@Deprecated(
'No longer supported. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
enum ModifierKey {
/// The CTRL modifier key.
///
/// Typically, there are two of these.
controlModifier,
/// The SHIFT modifier key.
///
/// Typically, there are two of these.
shiftModifier,
/// The ALT modifier key.
///
/// Typically, there are two of these.
altModifier,
/// The META modifier key.
///
/// Typically, there are two of these. This is, for example, the Windows key
/// on Windows (⊞), the Command (⌘) key on macOS and iOS, and the Search (🔍)
/// key on Android.
metaModifier,
/// The CAPS LOCK modifier key.
///
/// Typically, there is one of these. Only shown as "pressed" when the caps
/// lock is on, so on a key up when the mode is turned on, on each key press
/// when it's enabled, and on a key down when it is turned off.
capsLockModifier,
/// The NUM LOCK modifier key.
///
/// Typically, there is one of these. Only shown as "pressed" when the num
/// lock is on, so on a key up when the mode is turned on, on each key press
/// when it's enabled, and on a key down when it is turned off.
numLockModifier,
/// The SCROLL LOCK modifier key.
///
/// Typically, there is one of these. Only shown as "pressed" when the scroll
/// lock is on, so on a key up when the mode is turned on, on each key press
/// when it's enabled, and on a key down when it is turned off.
scrollLockModifier,
/// The FUNCTION (Fn) modifier key.
///
/// Typically, there is one of these.
functionModifier,
/// The SYMBOL modifier key.
///
/// Typically, there is one of these.
symbolModifier,
}
/// Base class for platform-specific key event data.
///
/// This class is deprecated, and will be removed. Platform specific key event
/// data will no be longer available. See [KeyEvent] for what is available.
///
/// This base class exists to have a common type to use for each of the
/// target platform's key event data structures.
///
/// See also:
///
/// * [RawKeyEventDataAndroid], a specialization for Android.
/// * [RawKeyEventDataFuchsia], a specialization for Fuchsia.
/// * [RawKeyDownEvent] and [RawKeyUpEvent], the classes that hold the
/// reference to [RawKeyEventData] subclasses.
/// * [RawKeyboard], which uses these interfaces to expose key data.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
@immutable
abstract class RawKeyEventData with Diagnosticable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEventData();
/// Returns true if the given [ModifierKey] was pressed at the time of this
/// event.
///
/// This method is deprecated and will be removed. For equivalent information,
/// inspect [HardwareKeyboard.logicalKeysPressed] instead.
///
/// If [side] is specified, then this restricts its check to the specified
/// side of the keyboard. Defaults to checking for the key being down on
/// either side of the keyboard. If there is only one instance of the key on
/// the keyboard, then [side] is ignored.
@Deprecated(
'No longer available. Inspect HardwareKeyboard.instance.logicalKeysPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool isModifierPressed(ModifierKey key, { KeyboardSide side = KeyboardSide.any });
/// Returns a [KeyboardSide] enum value that describes which side or sides of
/// the given keyboard modifier key were pressed at the time of this event.
///
/// This method is deprecated and will be removed.
///
/// If the modifier key wasn't pressed at the time of this event, returns
/// null. If the given key only appears in one place on the keyboard, returns
/// [KeyboardSide.all] if pressed. If the given platform does not specify
/// the side, return [KeyboardSide.any].
@Deprecated(
'No longer available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
KeyboardSide? getModifierSide(ModifierKey key);
/// Returns true if a CTRL modifier key was pressed at the time of this event,
/// regardless of which side of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isControlPressed] instead.
///
/// Use [isModifierPressed] if you need to know which control key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isControlPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isControlPressed => isModifierPressed(ModifierKey.controlModifier);
/// Returns true if a SHIFT modifier key was pressed at the time of this
/// event, regardless of which side of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isShiftPressed] instead.
///
/// Use [isModifierPressed] if you need to know which shift key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isShiftPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isShiftPressed => isModifierPressed(ModifierKey.shiftModifier);
/// Returns true if a ALT modifier key was pressed at the time of this event,
/// regardless of which side of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isAltPressed] instead.
///
/// Use [isModifierPressed] if you need to know which alt key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isAltPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isAltPressed => isModifierPressed(ModifierKey.altModifier);
/// Returns true if a META modifier key was pressed at the time of this event,
/// regardless of which side of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isMetaPressed] instead.
///
/// Use [isModifierPressed] if you need to know which meta key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isMetaPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isMetaPressed => isModifierPressed(ModifierKey.metaModifier);
/// Returns a map of modifier keys that were pressed at the time of this
/// event, and the keyboard side or sides that the key was on.
///
/// This method is deprecated and will be removed. For equivalent information,
/// inspect [HardwareKeyboard.logicalKeysPressed] instead.
@Deprecated(
'No longer available. Inspect HardwareKeyboard.instance.logicalKeysPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
Map<ModifierKey, KeyboardSide> get modifiersPressed {
final Map<ModifierKey, KeyboardSide> result = <ModifierKey, KeyboardSide>{};
for (final ModifierKey key in ModifierKey.values) {
if (isModifierPressed(key)) {
final KeyboardSide? side = getModifierSide(key);
if (side != null) {
result[key] = side;
}
assert(() {
if (side == null) {
debugPrint(
'Raw key data is returning inconsistent information for '
'pressed modifiers. isModifierPressed returns true for $key '
'being pressed, but when getModifierSide is called, it says '
'that no modifiers are pressed.',
);
if (this is RawKeyEventDataAndroid) {
debugPrint('Android raw key metaState: ${(this as RawKeyEventDataAndroid).metaState}');
}
}
return true;
}());
}
}
return result;
}
/// Returns an object representing the physical location of this key on a
/// QWERTY keyboard.
///
/// {@macro flutter.services.RawKeyEvent.physicalKey}
///
/// See also:
///
/// * [logicalKey] for the non-location-specific key generated by this event.
/// * [RawKeyEvent.physicalKey], where this value is available on the event.
PhysicalKeyboardKey get physicalKey;
/// Returns an object representing the logical key that was pressed.
///
/// {@macro flutter.services.RawKeyEvent.logicalKey}
///
/// See also:
///
/// * [physicalKey] for the location-specific key generated by this event.
/// * [RawKeyEvent.logicalKey], where this value is available on the event.
LogicalKeyboardKey get logicalKey;
/// Returns the Unicode string representing the label on this key.
///
/// This value is an empty string if there's no key label data for a key.
///
/// {@template flutter.services.RawKeyEventData.keyLabel}
/// Do not use the [keyLabel] to compose a text string: it will be missing
/// special processing for Unicode strings for combining characters and other
/// special characters, and the effects of modifiers.
///
/// If you are looking for the character produced by a key event, use
/// [RawKeyEvent.character] instead.
///
/// If you are composing text strings, use the [TextField] or
/// [CupertinoTextField] widgets, since those automatically handle many of the
/// complexities of managing keyboard input, like showing a soft keyboard or
/// interacting with an input method editor (IME).
/// {@endtemplate}
String get keyLabel;
/// Whether a key down event, and likewise its accompanying key up event,
/// should be dispatched.
///
/// Certain events on some platforms should not be dispatched to listeners
/// according to Flutter's event model. For example, on macOS, Fn keys are
/// skipped to be consistent with other platform. On Win32, events dispatched
/// for IME (`VK_PROCESSKEY`) are also skipped.
///
/// This method will be called upon every down events. By default, this method
/// always return true. Subclasses should override this method to define the
/// filtering rule for the platform. If this method returns false for an event
/// message, the event will not be dispatched to listeners, but respond with
/// "handled: true" immediately. Moreover, the following up event with the
/// same physical key will also be skipped.
bool shouldDispatchEvent() {
return true;
}
}
/// Defines the interface for raw key events.
///
/// This type of event has been deprecated, and will be removed at a future
/// date. Instead of using this, use the [KeyEvent] class instead, which means
/// you will use [Focus.onKeyEvent], [HardwareKeyboard] and [KeyboardListener]
/// to get these events instead of the raw key event equivalents.
///
/// Raw key events pass through as much information as possible from the
/// underlying platform's key events, which allows them to provide a high level
/// of fidelity but a low level of portability.
///
/// The event also provides an abstraction for the [physicalKey] and the
/// [logicalKey], describing the physical location of the key, and the logical
/// meaning of the key, respectively. These are more portable representations of
/// the key events, and should produce the same results regardless of platform.
///
/// See also:
///
/// * [LogicalKeyboardKey], an object that describes the logical meaning of a
/// key.
/// * [PhysicalKeyboardKey], an object that describes the physical location of a
/// key.
/// * [RawKeyDownEvent], a specialization for events representing the user
/// pressing a key.
/// * [RawKeyUpEvent], a specialization for events representing the user
/// releasing a key.
/// * [RawKeyboard], which uses this interface to expose key data.
/// * [RawKeyboardListener], a widget that listens for raw key events.
@Deprecated(
'Use KeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
@immutable
abstract class RawKeyEvent with Diagnosticable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
@Deprecated(
'Use KeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEvent({
required this.data,
this.character,
this.repeat = false,
});
/// Creates a concrete [RawKeyEvent] class from a message in the form received
/// on the [SystemChannels.keyEvent] channel.
///
/// [RawKeyEvent.repeat] will be derived from the current keyboard state,
/// instead of using the message information.
@Deprecated(
'No longer supported. Use KeyEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
factory RawKeyEvent.fromMessage(Map<String, Object?> message) {
String? character;
RawKeyEventData dataFromWeb() {
final String? key = message['key'] as String?;
if (key != null && key.isNotEmpty && key.length == 1) {
character = key;
}
return RawKeyEventDataWeb(
code: message['code'] as String? ?? '',
key: key ?? '',
location: message['location'] as int? ?? 0,
metaState: message['metaState'] as int? ?? 0,
keyCode: message['keyCode'] as int? ?? 0,
);
}
final RawKeyEventData data;
if (kIsWeb) {
data = dataFromWeb();
} else {
final String keymap = message['keymap']! as String;
switch (keymap) {
case 'android':
data = RawKeyEventDataAndroid(
flags: message['flags'] as int? ?? 0,
codePoint: message['codePoint'] as int? ?? 0,
keyCode: message['keyCode'] as int? ?? 0,
plainCodePoint: message['plainCodePoint'] as int? ?? 0,
scanCode: message['scanCode'] as int? ?? 0,
metaState: message['metaState'] as int? ?? 0,
eventSource: message['source'] as int? ?? 0,
vendorId: message['vendorId'] as int? ?? 0,
productId: message['productId'] as int? ?? 0,
deviceId: message['deviceId'] as int? ?? 0,
repeatCount: message['repeatCount'] as int? ?? 0,
);
if (message.containsKey('character')) {
character = message['character'] as String?;
}
case 'fuchsia':
final int codePoint = message['codePoint'] as int? ?? 0;
data = RawKeyEventDataFuchsia(
hidUsage: message['hidUsage'] as int? ?? 0,
codePoint: codePoint,
modifiers: message['modifiers'] as int? ?? 0,
);
if (codePoint != 0) {
character = String.fromCharCode(codePoint);
}
case 'macos':
data = RawKeyEventDataMacOs(
characters: message['characters'] as String? ?? '',
charactersIgnoringModifiers: message['charactersIgnoringModifiers'] as String? ?? '',
keyCode: message['keyCode'] as int? ?? 0,
modifiers: message['modifiers'] as int? ?? 0,
specifiedLogicalKey: message['specifiedLogicalKey'] as int?,
);
character = message['characters'] as String?;
case 'ios':
data = RawKeyEventDataIos(
characters: message['characters'] as String? ?? '',
charactersIgnoringModifiers: message['charactersIgnoringModifiers'] as String? ?? '',
keyCode: message['keyCode'] as int? ?? 0,
modifiers: message['modifiers'] as int? ?? 0,
);
final Object? characters = message['characters'];
if (characters is String && characters.isNotEmpty) {
character = characters;
}
case 'linux':
final int unicodeScalarValues = message['unicodeScalarValues'] as int? ?? 0;
data = RawKeyEventDataLinux(
keyHelper: KeyHelper(message['toolkit'] as String? ?? ''),
unicodeScalarValues: unicodeScalarValues,
keyCode: message['keyCode'] as int? ?? 0,
scanCode: message['scanCode'] as int? ?? 0,
modifiers: message['modifiers'] as int? ?? 0,
isDown: message['type'] == 'keydown',
specifiedLogicalKey: message['specifiedLogicalKey'] as int?,
);
if (unicodeScalarValues != 0) {
character = String.fromCharCode(unicodeScalarValues);
}
case 'windows':
final int characterCodePoint = message['characterCodePoint'] as int? ?? 0;
data = RawKeyEventDataWindows(
keyCode: message['keyCode'] as int? ?? 0,
scanCode: message['scanCode'] as int? ?? 0,
characterCodePoint: characterCodePoint,
modifiers: message['modifiers'] as int? ?? 0,
);
if (characterCodePoint != 0) {
character = String.fromCharCode(characterCodePoint);
}
case 'web':
data = dataFromWeb();
default:
/// This exception would only be hit on platforms that haven't yet
/// implemented raw key events, but will only be triggered if the
/// engine for those platforms sends raw key event messages in the
/// first place.
throw FlutterError('Unknown keymap for key events: $keymap');
}
}
final bool repeat = RawKeyboard.instance.physicalKeysPressed.contains(data.physicalKey);
final String type = message['type']! as String;
return switch (type) {
'keydown' => RawKeyDownEvent(data: data, character: character, repeat: repeat),
'keyup' => RawKeyUpEvent(data: data),
_ => throw FlutterError('Unknown key event type: $type'),
};
}
/// Returns true if the given [LogicalKeyboardKey] is pressed.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isLogicalKeyPressed] instead.
@Deprecated(
'Use HardwareKeyboard.instance.isLogicalKeyPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool isKeyPressed(LogicalKeyboardKey key) => RawKeyboard.instance.keysPressed.contains(key);
/// Returns true if a CTRL modifier key is pressed, regardless of which side
/// of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isControlPressed] instead.
///
/// Use [isKeyPressed] if you need to know which control key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isControlPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isControlPressed {
return isKeyPressed(LogicalKeyboardKey.controlLeft) || isKeyPressed(LogicalKeyboardKey.controlRight);
}
/// Returns true if a SHIFT modifier key is pressed, regardless of which side
/// of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isShiftPressed] instead.
///
/// Use [isKeyPressed] if you need to know which shift key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isShiftPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isShiftPressed {
return isKeyPressed(LogicalKeyboardKey.shiftLeft) || isKeyPressed(LogicalKeyboardKey.shiftRight);
}
/// Returns true if a ALT modifier key is pressed, regardless of which side
/// of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isAltPressed] instead.
///
/// The `AltGr` key that appears on some keyboards is considered to be
/// the same as [LogicalKeyboardKey.altRight] on some platforms (notably
/// Android). On platforms that can distinguish between `altRight` and
/// `altGr`, a press of `AltGr` will not return true here, and will need to be
/// tested for separately.
///
/// Use [isKeyPressed] if you need to know which alt key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isAltPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isAltPressed {
return isKeyPressed(LogicalKeyboardKey.altLeft) || isKeyPressed(LogicalKeyboardKey.altRight);
}
/// Returns true if a META modifier key is pressed, regardless of which side
/// of the keyboard it is on.
///
/// This method is deprecated and will be removed. Use
/// [HardwareKeyboard.isMetaPressed] instead.
///
/// Use [isKeyPressed] if you need to know which meta key was pressed.
@Deprecated(
'Use HardwareKeyboard.instance.isMetaPressed instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
bool get isMetaPressed {
return isKeyPressed(LogicalKeyboardKey.metaLeft) || isKeyPressed(LogicalKeyboardKey.metaRight);
}
/// Returns an object representing the physical location of this key.
///
/// {@template flutter.services.RawKeyEvent.physicalKey}
/// The [PhysicalKeyboardKey] ignores the key map, modifier keys (like SHIFT),
/// and the label on the key. It describes the location of the key as if it
/// were on a QWERTY keyboard regardless of the keyboard mapping in effect.
///
/// [PhysicalKeyboardKey]s are used to describe and test for keys in a
/// particular location.
///
/// For instance, if you wanted to make a game where the key to the right of
/// the CAPS LOCK key made the player move left, you would be comparing the
/// result of this [physicalKey] with [PhysicalKeyboardKey.keyA], since that
/// is the key next to the CAPS LOCK key on a QWERTY keyboard. This would
/// return the same thing even on a French keyboard where the key next to the
/// CAPS LOCK produces a "Q" when pressed.
///
/// If you want to make your app respond to a key with a particular character
/// on it regardless of location of the key, use [RawKeyEvent.logicalKey] instead.
/// {@endtemplate}
///
/// See also:
///
/// * [logicalKey] for the non-location specific key generated by this event.
/// * [character] for the character generated by this keypress (if any).
PhysicalKeyboardKey get physicalKey => data.physicalKey;
/// Returns an object representing the logical key that was pressed.
///
/// {@template flutter.services.RawKeyEvent.logicalKey}
/// This method takes into account the key map and modifier keys (like SHIFT)
/// to determine which logical key to return.
///
/// If you are looking for the character produced by a key event, use
/// [RawKeyEvent.character] instead.
///
/// If you are collecting text strings, use the [TextField] or
/// [CupertinoTextField] widgets, since those automatically handle many of the
/// complexities of managing keyboard input, like showing a soft keyboard or
/// interacting with an input method editor (IME).
/// {@endtemplate}
LogicalKeyboardKey get logicalKey => data.logicalKey;
/// Returns the Unicode character (grapheme cluster) completed by this
/// keystroke, if any.
///
/// This will only return a character if this keystroke, combined with any
/// preceding keystroke(s), generated a character, and only on a "key down"
/// event. It will return null if no character has been generated by the
/// keystroke (e.g. a "dead" or "combining" key), or if the corresponding key
/// is a key without a visual representation, such as a modifier key or a
/// control key.
///
/// This can return multiple Unicode code points, since some characters (more
/// accurately referred to as grapheme clusters) are made up of more than one
/// code point.
///
/// The [character] doesn't take into account edits by an input method editor
/// (IME), or manage the visibility of the soft keyboard on touch devices. For
/// composing text, use the [TextField] or [CupertinoTextField] widgets, since
/// those automatically handle many of the complexities of managing keyboard
/// input.
final String? character;
/// Whether this is a repeated down event.
///
/// When a key is held down, the systems usually fire a down event and then
/// a series of repeated down events. The [repeat] is false for the
/// first event and true for the following events.
///
/// The [repeat] attribute is always false for [RawKeyUpEvent]s.
final bool repeat;
/// Platform-specific information about the key event.
final RawKeyEventData data;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<LogicalKeyboardKey>('logicalKey', logicalKey));
properties.add(DiagnosticsProperty<PhysicalKeyboardKey>('physicalKey', physicalKey));
if (this is RawKeyDownEvent) {
properties.add(DiagnosticsProperty<bool>('repeat', repeat));
}
}
}
/// The user has pressed a key on the keyboard.
///
/// This class has been deprecated and will be removed. Use [KeyDownEvent]
/// instead.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Use KeyDownEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyDownEvent extends RawKeyEvent {
/// Creates a key event that represents the user pressing a key.
@Deprecated(
'Use KeyDownEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyDownEvent({
required super.data,
super.character,
super.repeat,
});
}
/// The user has released a key on the keyboard.
///
/// This class has been deprecated and will be removed. Use [KeyUpEvent]
/// instead.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Use KeyUpEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyUpEvent extends RawKeyEvent {
/// Creates a key event that represents the user releasing a key.
@Deprecated(
'Use KeyUpEvent instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyUpEvent({
required super.data,
super.character,
}) : super(repeat: false);
}
/// A callback type used by [RawKeyboard.keyEventHandler] to send key events to
/// a handler that can determine if the key has been handled or not.
///
/// This typedef has been deprecated and will be removed. Use [KeyEventCallback]
/// instead.
///
/// The handler should return true if the key has been handled, and false if the
/// key was not handled. It must not return null.
@Deprecated(
'Use KeyEventCallback instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
typedef RawKeyEventHandler = bool Function(RawKeyEvent event);
/// An interface for listening to raw key events.
///
/// This class is deprecated and will be removed at a future date. The
/// [HardwareKeyboard] class is its replacement.
///
/// Raw key events pass through as much information as possible from the
/// underlying platform's key events, which makes them provide a high level of
/// fidelity but a low level of portability.
///
/// A [RawKeyboard] is useful for listening to raw key events and hardware
/// buttons that are represented as keys. Typically used by games and other apps
/// that use keyboards for purposes other than text entry.
///
/// These key events are typically only key events generated by a hardware
/// keyboard, and not those from software keyboards or input method editors.
///
/// ## Compared to [HardwareKeyboard]
///
/// Behavior-wise, [RawKeyboard] provides a less unified, less regular event
/// model than [HardwareKeyboard]. For example:
///
/// * Down events might not be matched with an up event, and vice versa (the set
/// of pressed keys is silently updated).
/// * The logical key of the down event might not be the same as that of the up
/// event.
/// * Down events and repeat events are not easily distinguishable (must be
/// tracked manually).
/// * Lock modes (such as CapsLock) only have their "enabled" state recorded.
/// There's no way to acquire their pressing state.
///
/// See also:
///
/// * [RawKeyDownEvent] and [RawKeyUpEvent], the classes used to describe
/// specific raw key events.
/// * [RawKeyboardListener], a widget that listens for raw key events.
/// * [SystemChannels.keyEvent], the low-level channel used for receiving events
/// from the system.
/// * [HardwareKeyboard], the recommended replacement.
@Deprecated(
'Use HardwareKeyboard instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
class RawKeyboard {
@Deprecated(
'Use HardwareKeyboard instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
RawKeyboard._();
/// The shared instance of [RawKeyboard].
@Deprecated(
'Use HardwareKeyboard.instance instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
static final RawKeyboard instance = RawKeyboard._();
final List<ValueChanged<RawKeyEvent>> _listeners = <ValueChanged<RawKeyEvent>>[];
/// Register a listener that is called every time the user presses or releases
/// a hardware keyboard key.
///
/// Since the listeners have no way to indicate what they did with the event,
/// listeners are assumed to not handle the key event. These events will also
/// be distributed to other listeners, and to the [keyEventHandler].
///
/// Most applications prefer to use the focus system (see [Focus] and
/// [FocusManager]) to receive key events to the focused control instead of
/// this kind of passive listener.
///
/// Listeners can be removed with [removeListener].
void addListener(ValueChanged<RawKeyEvent> listener) {
_listeners.add(listener);
}
/// Stop calling the given listener every time the user presses or releases a
/// hardware keyboard key.
///
/// Listeners can be added with [addListener].
void removeListener(ValueChanged<RawKeyEvent> listener) {
_listeners.remove(listener);
}
/// A handler for raw hardware keyboard events that will stop propagation if
/// the handler returns true.
///
/// This property is only a wrapper over [KeyEventManager.keyMessageHandler],
/// and is kept only for backward compatibility. New code should use
/// [KeyEventManager.keyMessageHandler] to set custom global key event
/// handler. Setting [keyEventHandler] will cause
/// [KeyEventManager.keyMessageHandler] to be set with a converted handler.
/// If [KeyEventManager.keyMessageHandler] is set by [FocusManager] (the most
/// common situation), then the exact value of [keyEventHandler] is a dummy
/// callback and must not be invoked.
RawKeyEventHandler? get keyEventHandler {
if (ServicesBinding.instance.keyEventManager.keyMessageHandler != _cachedKeyMessageHandler) {
_cachedKeyMessageHandler = ServicesBinding.instance.keyEventManager.keyMessageHandler;
_cachedKeyEventHandler = _cachedKeyMessageHandler == null ?
null :
(RawKeyEvent event) {
assert(false,
'The RawKeyboard.instance.keyEventHandler assigned by Flutter is a dummy '
'callback kept for compatibility and should not be directly called. Use '
'ServicesBinding.instance!.keyMessageHandler instead.');
return true;
};
}
return _cachedKeyEventHandler;
}
RawKeyEventHandler? _cachedKeyEventHandler;
KeyMessageHandler? _cachedKeyMessageHandler;
set keyEventHandler(RawKeyEventHandler? handler) {
_cachedKeyEventHandler = handler;
_cachedKeyMessageHandler = handler == null ?
null :
(KeyMessage message) {
if (message.rawEvent != null) {
return handler(message.rawEvent!);
}
return false;
};
ServicesBinding.instance.keyEventManager.keyMessageHandler = _cachedKeyMessageHandler;
}
/// Process a new [RawKeyEvent] by recording the state changes and
/// dispatching to listeners.
bool handleRawKeyEvent(RawKeyEvent event) {
if (event is RawKeyDownEvent) {
_keysPressed[event.physicalKey] = event.logicalKey;
} else if (event is RawKeyUpEvent) {
// Use the physical key in the key up event to find the physical key from
// the corresponding key down event and remove it, even if the logical
// keys don't match.
_keysPressed.remove(event.physicalKey);
}
// Make sure that the modifiers reflect reality, in case a modifier key was
// pressed/released while the app didn't have focus.
_synchronizeModifiers(event);
assert(
event is! RawKeyDownEvent || _keysPressed.isNotEmpty,
'Attempted to send a key down event when no keys are in keysPressed. '
"This state can occur if the key event being sent doesn't properly "
'set its modifier flags. This was the event: $event and its data: '
'${event.data}',
);
// Send the event to passive listeners.
for (final ValueChanged<RawKeyEvent> listener in List<ValueChanged<RawKeyEvent>>.of(_listeners)) {
try {
if (_listeners.contains(listener)) {
listener(event);
}
} catch (exception, stack) {
InformationCollector? collector;
assert(() {
collector = () => <DiagnosticsNode>[
DiagnosticsProperty<RawKeyEvent>('Event', event),
];
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context: ErrorDescription('while processing a raw key listener'),
informationCollector: collector,
));
}
}
return false;
}
static final Map<_ModifierSidePair, Set<PhysicalKeyboardKey>> _modifierKeyMap = <_ModifierSidePair, Set<PhysicalKeyboardKey>>{
const _ModifierSidePair(ModifierKey.altModifier, KeyboardSide.left): <PhysicalKeyboardKey>{PhysicalKeyboardKey.altLeft},
const _ModifierSidePair(ModifierKey.altModifier, KeyboardSide.right): <PhysicalKeyboardKey>{PhysicalKeyboardKey.altRight},
const _ModifierSidePair(ModifierKey.altModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.altLeft, PhysicalKeyboardKey.altRight},
const _ModifierSidePair(ModifierKey.altModifier, KeyboardSide.any): <PhysicalKeyboardKey>{PhysicalKeyboardKey.altLeft},
const _ModifierSidePair(ModifierKey.shiftModifier, KeyboardSide.left): <PhysicalKeyboardKey>{PhysicalKeyboardKey.shiftLeft},
const _ModifierSidePair(ModifierKey.shiftModifier, KeyboardSide.right): <PhysicalKeyboardKey>{PhysicalKeyboardKey.shiftRight},
const _ModifierSidePair(ModifierKey.shiftModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.shiftLeft, PhysicalKeyboardKey.shiftRight},
const _ModifierSidePair(ModifierKey.shiftModifier, KeyboardSide.any): <PhysicalKeyboardKey>{PhysicalKeyboardKey.shiftLeft},
const _ModifierSidePair(ModifierKey.controlModifier, KeyboardSide.left): <PhysicalKeyboardKey>{PhysicalKeyboardKey.controlLeft},
const _ModifierSidePair(ModifierKey.controlModifier, KeyboardSide.right): <PhysicalKeyboardKey>{PhysicalKeyboardKey.controlRight},
const _ModifierSidePair(ModifierKey.controlModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.controlLeft, PhysicalKeyboardKey.controlRight},
const _ModifierSidePair(ModifierKey.controlModifier, KeyboardSide.any): <PhysicalKeyboardKey>{PhysicalKeyboardKey.controlLeft},
const _ModifierSidePair(ModifierKey.metaModifier, KeyboardSide.left): <PhysicalKeyboardKey>{PhysicalKeyboardKey.metaLeft},
const _ModifierSidePair(ModifierKey.metaModifier, KeyboardSide.right): <PhysicalKeyboardKey>{PhysicalKeyboardKey.metaRight},
const _ModifierSidePair(ModifierKey.metaModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.metaLeft, PhysicalKeyboardKey.metaRight},
const _ModifierSidePair(ModifierKey.metaModifier, KeyboardSide.any): <PhysicalKeyboardKey>{PhysicalKeyboardKey.metaLeft},
const _ModifierSidePair(ModifierKey.capsLockModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.capsLock},
const _ModifierSidePair(ModifierKey.numLockModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.numLock},
const _ModifierSidePair(ModifierKey.scrollLockModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.scrollLock},
const _ModifierSidePair(ModifierKey.functionModifier, KeyboardSide.all): <PhysicalKeyboardKey>{PhysicalKeyboardKey.fn},
// The symbolModifier doesn't have a key representation on any of the
// platforms, so don't map it here.
};
// The map of all modifier keys except Fn, since that is treated differently
// on some platforms.
static final Map<PhysicalKeyboardKey, LogicalKeyboardKey> _allModifiersExceptFn = <PhysicalKeyboardKey, LogicalKeyboardKey>{
PhysicalKeyboardKey.altLeft: LogicalKeyboardKey.altLeft,
PhysicalKeyboardKey.altRight: LogicalKeyboardKey.altRight,
PhysicalKeyboardKey.shiftLeft: LogicalKeyboardKey.shiftLeft,
PhysicalKeyboardKey.shiftRight: LogicalKeyboardKey.shiftRight,
PhysicalKeyboardKey.controlLeft: LogicalKeyboardKey.controlLeft,
PhysicalKeyboardKey.controlRight: LogicalKeyboardKey.controlRight,
PhysicalKeyboardKey.metaLeft: LogicalKeyboardKey.metaLeft,
PhysicalKeyboardKey.metaRight: LogicalKeyboardKey.metaRight,
PhysicalKeyboardKey.capsLock: LogicalKeyboardKey.capsLock,
PhysicalKeyboardKey.numLock: LogicalKeyboardKey.numLock,
PhysicalKeyboardKey.scrollLock: LogicalKeyboardKey.scrollLock,
};
// The map of all modifier keys that are represented in modifier key bit
// masks on all platforms, so that they can be cleared out of pressedKeys when
// synchronizing.
static final Map<PhysicalKeyboardKey, LogicalKeyboardKey> _allModifiers = <PhysicalKeyboardKey, LogicalKeyboardKey>{
PhysicalKeyboardKey.fn: LogicalKeyboardKey.fn,
..._allModifiersExceptFn,
};
void _synchronizeModifiers(RawKeyEvent event) {
// Compare modifier states to the ground truth as specified by
// [RawKeyEvent.data.modifiersPressed] and update unsynchronized ones.
//
// This function will update the state of modifier keys in `_keysPressed` so
// that they match the ones given by [RawKeyEvent.data.modifiersPressed].
// For a `modifiersPressed` result of anything but [KeyboardSide.any], the
// states in `_keysPressed` will be updated to exactly match the result,
// i.e. exactly one of "no down", "left down", "right down" or "both down".
//
// If `modifiersPressed` returns [KeyboardSide.any], the states in
// `_keysPressed` will be updated to a rough match, i.e. "either side down"
// or "no down". If `_keysPressed` has no modifier down, a
// [KeyboardSide.any] will synchronize by forcing the left modifier down. If
// `_keysPressed` has any modifier down, a [KeyboardSide.any] will not cause
// a state change.
final Map<ModifierKey, KeyboardSide?> modifiersPressed = event.data.modifiersPressed;
final Map<PhysicalKeyboardKey, LogicalKeyboardKey> modifierKeys = <PhysicalKeyboardKey, LogicalKeyboardKey>{};
// Physical keys that whose modifiers are pressed at any side.
final Set<PhysicalKeyboardKey> anySideKeys = <PhysicalKeyboardKey>{};
final Set<PhysicalKeyboardKey> keysPressedAfterEvent = <PhysicalKeyboardKey>{
..._keysPressed.keys,
if (event is RawKeyDownEvent) event.physicalKey,
};
ModifierKey? thisKeyModifier;
for (final ModifierKey key in ModifierKey.values) {
final Set<PhysicalKeyboardKey>? thisModifierKeys = _modifierKeyMap[_ModifierSidePair(key, KeyboardSide.all)];
if (thisModifierKeys == null) {
continue;
}
if (thisModifierKeys.contains(event.physicalKey)) {
thisKeyModifier = key;
}
if (modifiersPressed[key] == KeyboardSide.any) {
anySideKeys.addAll(thisModifierKeys);
if (thisModifierKeys.any(keysPressedAfterEvent.contains)) {
continue;
}
}
final Set<PhysicalKeyboardKey>? mappedKeys = modifiersPressed[key] == null ?
<PhysicalKeyboardKey>{} : _modifierKeyMap[_ModifierSidePair(key, modifiersPressed[key])];
assert(() {
if (mappedKeys == null) {
debugPrint(
'Platform key support for ${Platform.operatingSystem} is '
'producing unsupported modifier combinations for '
'modifier $key on side ${modifiersPressed[key]}.',
);
if (event.data is RawKeyEventDataAndroid) {
debugPrint('Android raw key metaState: ${(event.data as RawKeyEventDataAndroid).metaState}');
}
}
return true;
}());
if (mappedKeys == null) {
continue;
}
for (final PhysicalKeyboardKey physicalModifier in mappedKeys) {
modifierKeys[physicalModifier] = _allModifiers[physicalModifier]!;
}
}
// On Linux, CapsLock key can be mapped to a non-modifier logical key:
// https://github.com/flutter/flutter/issues/114591.
// This is also affecting Flutter Web on Linux.
final bool nonModifierCapsLock = (event.data is RawKeyEventDataLinux || event.data is RawKeyEventDataWeb)
&& _keysPressed[PhysicalKeyboardKey.capsLock] != null
&& _keysPressed[PhysicalKeyboardKey.capsLock] != LogicalKeyboardKey.capsLock;
for (final PhysicalKeyboardKey physicalKey in _allModifiersExceptFn.keys) {
final bool skipReleasingKey = nonModifierCapsLock && physicalKey == PhysicalKeyboardKey.capsLock;
if (!anySideKeys.contains(physicalKey) && !skipReleasingKey) {
_keysPressed.remove(physicalKey);
}
}
if (event.data is! RawKeyEventDataFuchsia && event.data is! RawKeyEventDataMacOs) {
// On Fuchsia and macOS, the Fn key is not considered a modifier key.
_keysPressed.remove(PhysicalKeyboardKey.fn);
}
_keysPressed.addAll(modifierKeys);
// In rare cases, the event presses a modifier key but the key does not
// exist in the modifier list. Enforce the pressing state.
if (event is RawKeyDownEvent && thisKeyModifier != null
&& !_keysPressed.containsKey(event.physicalKey)) {
// This inconsistency is found on Linux GTK for AltRight:
// https://github.com/flutter/flutter/issues/93278
// And also on Android and iOS:
// https://github.com/flutter/flutter/issues/101090
if ((event.data is RawKeyEventDataLinux && event.physicalKey == PhysicalKeyboardKey.altRight)
|| event.data is RawKeyEventDataIos
|| event.data is RawKeyEventDataAndroid) {
final LogicalKeyboardKey? logicalKey = _allModifiersExceptFn[event.physicalKey];
if (logicalKey != null) {
_keysPressed[event.physicalKey] = logicalKey;
}
}
// On Web, PhysicalKeyboardKey.altRight can be map to LogicalKeyboardKey.altGraph or
// LogicalKeyboardKey.altRight:
// https://github.com/flutter/flutter/issues/113836
if (event.data is RawKeyEventDataWeb && event.physicalKey == PhysicalKeyboardKey.altRight) {
_keysPressed[event.physicalKey] = event.logicalKey;
}
}
}
final Map<PhysicalKeyboardKey, LogicalKeyboardKey> _keysPressed = <PhysicalKeyboardKey, LogicalKeyboardKey>{};
/// Returns the set of keys currently pressed.
Set<LogicalKeyboardKey> get keysPressed => _keysPressed.values.toSet();
/// Returns the set of physical keys currently pressed.
Set<PhysicalKeyboardKey> get physicalKeysPressed => _keysPressed.keys.toSet();
/// Returns the logical key that corresponds to the given pressed physical key.
///
/// Returns null if the physical key is not currently pressed.
LogicalKeyboardKey? lookUpLayout(PhysicalKeyboardKey physicalKey) => _keysPressed[physicalKey];
/// Clears the list of keys returned from [keysPressed].
///
/// This is used by the testing framework to make sure tests are hermetic.
@visibleForTesting
void clearKeysPressed() => _keysPressed.clear();
}
@immutable
class _ModifierSidePair {
const _ModifierSidePair(this.modifier, this.side);
final ModifierKey modifier;
final KeyboardSide? side;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _ModifierSidePair
&& other.modifier == modifier
&& other.side == side;
}
@override
int get hashCode => Object.hash(modifier, side);
}
| flutter/packages/flutter/lib/src/services/raw_keyboard.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/raw_keyboard.dart",
"repo_id": "flutter",
"token_count": 14844
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show TextAffinity, TextPosition, TextRange;
import 'package:flutter/foundation.dart';
export 'dart:ui' show TextAffinity, TextPosition;
/// A range of text that represents a selection.
@immutable
class TextSelection extends TextRange {
/// Creates a text selection.
const TextSelection({
required this.baseOffset,
required this.extentOffset,
this.affinity = TextAffinity.downstream,
this.isDirectional = false,
}) : super(
start: baseOffset < extentOffset ? baseOffset : extentOffset,
end: baseOffset < extentOffset ? extentOffset : baseOffset,
);
/// Creates a collapsed selection at the given offset.
///
/// A collapsed selection starts and ends at the same offset, which means it
/// contains zero characters but instead serves as an insertion point in the
/// text.
const TextSelection.collapsed({
required int offset,
this.affinity = TextAffinity.downstream,
}) : baseOffset = offset,
extentOffset = offset,
isDirectional = false,
super.collapsed(offset);
/// Creates a collapsed selection at the given text position.
///
/// A collapsed selection starts and ends at the same offset, which means it
/// contains zero characters but instead serves as an insertion point in the
/// text.
TextSelection.fromPosition(TextPosition position)
: baseOffset = position.offset,
extentOffset = position.offset,
affinity = position.affinity,
isDirectional = false,
super.collapsed(position.offset);
/// The offset at which the selection originates.
///
/// Might be larger than, smaller than, or equal to extent.
final int baseOffset;
/// The offset at which the selection terminates.
///
/// When the user uses the arrow keys to adjust the selection, this is the
/// value that changes. Similarly, if the current theme paints a caret on one
/// side of the selection, this is the location at which to paint the caret.
///
/// Might be larger than, smaller than, or equal to base.
final int extentOffset;
/// If the text range is collapsed and has more than one visual location
/// (e.g., occurs at a line break), which of the two locations to use when
/// painting the caret.
final TextAffinity affinity;
/// Whether this selection has disambiguated its base and extent.
///
/// On some platforms, the base and extent are not disambiguated until the
/// first time the user adjusts the selection. At that point, either the start
/// or the end of the selection becomes the base and the other one becomes the
/// extent and is adjusted.
final bool isDirectional;
/// The position at which the selection originates.
///
/// {@template flutter.services.TextSelection.TextAffinity}
/// The [TextAffinity] of the resulting [TextPosition] is based on the
/// relative logical position in the text to the other selection endpoint:
/// * if [baseOffset] < [extentOffset], [base] will have
/// [TextAffinity.downstream] and [extent] will have
/// [TextAffinity.upstream].
/// * if [baseOffset] > [extentOffset], [base] will have
/// [TextAffinity.upstream] and [extent] will have
/// [TextAffinity.downstream].
/// * if [baseOffset] == [extentOffset], [base] and [extent] will both have
/// the collapsed selection's [affinity].
/// {@endtemplate}
///
/// Might be larger than, smaller than, or equal to extent.
TextPosition get base {
final TextAffinity affinity;
if (!isValid || baseOffset == extentOffset) {
affinity = this.affinity;
} else if (baseOffset < extentOffset) {
affinity = TextAffinity.downstream;
} else {
affinity = TextAffinity.upstream;
}
return TextPosition(offset: baseOffset, affinity: affinity);
}
/// The position at which the selection terminates.
///
/// When the user uses the arrow keys to adjust the selection, this is the
/// value that changes. Similarly, if the current theme paints a caret on one
/// side of the selection, this is the location at which to paint the caret.
///
/// {@macro flutter.services.TextSelection.TextAffinity}
///
/// Might be larger than, smaller than, or equal to base.
TextPosition get extent {
final TextAffinity affinity;
if (!isValid || baseOffset == extentOffset) {
affinity = this.affinity;
} else if (baseOffset < extentOffset) {
affinity = TextAffinity.upstream;
} else {
affinity = TextAffinity.downstream;
}
return TextPosition(offset: extentOffset, affinity: affinity);
}
@override
String toString() {
final String typeName = objectRuntimeType(this, 'TextSelection');
if (!isValid) {
return '$typeName.invalid';
}
return isCollapsed
? '$typeName.collapsed(offset: $baseOffset, affinity: $affinity, isDirectional: $isDirectional)'
: '$typeName(baseOffset: $baseOffset, extentOffset: $extentOffset, isDirectional: $isDirectional)';
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other is! TextSelection) {
return false;
}
if (!isValid) {
return !other.isValid;
}
return other.baseOffset == baseOffset
&& other.extentOffset == extentOffset
&& (!isCollapsed || other.affinity == affinity)
&& other.isDirectional == isDirectional;
}
@override
int get hashCode {
if (!isValid) {
return Object.hash(-1.hashCode, -1.hashCode, TextAffinity.downstream.hashCode);
}
final int affinityHash = isCollapsed ? affinity.hashCode : TextAffinity.downstream.hashCode;
return Object.hash(baseOffset.hashCode, extentOffset.hashCode, affinityHash, isDirectional.hashCode);
}
/// Creates a new [TextSelection] based on the current selection, with the
/// provided parameters overridden.
TextSelection copyWith({
int? baseOffset,
int? extentOffset,
TextAffinity? affinity,
bool? isDirectional,
}) {
return TextSelection(
baseOffset: baseOffset ?? this.baseOffset,
extentOffset: extentOffset ?? this.extentOffset,
affinity: affinity ?? this.affinity,
isDirectional: isDirectional ?? this.isDirectional,
);
}
/// Returns the smallest [TextSelection] that this could expand to in order to
/// include the given [TextPosition].
///
/// If the given [TextPosition] is already inside of the selection, then
/// returns `this` without change.
///
/// The returned selection will always be a strict superset of the current
/// selection. In other words, the selection grows to include the given
/// [TextPosition].
///
/// If extentAtIndex is true, then the [TextSelection.extentOffset] will be
/// placed at the given index regardless of the original order of it and
/// [TextSelection.baseOffset]. Otherwise, their order will be preserved.
///
/// ## Difference with [extendTo]
/// In contrast with this method, [extendTo] is a pivot; it holds
/// [TextSelection.baseOffset] fixed while moving [TextSelection.extentOffset]
/// to the given [TextPosition]. It doesn't strictly grow the selection and
/// may collapse it or flip its order.
TextSelection expandTo(TextPosition position, [bool extentAtIndex = false]) {
// If position is already within in the selection, there's nothing to do.
if (position.offset >= start && position.offset <= end) {
return this;
}
final bool normalized = baseOffset <= extentOffset;
if (position.offset <= start) {
// Here the position is somewhere before the selection: ..|..[...]....
if (extentAtIndex) {
return copyWith(
baseOffset: end,
extentOffset: position.offset,
affinity: position.affinity,
);
}
return copyWith(
baseOffset: normalized ? position.offset : baseOffset,
extentOffset: normalized ? extentOffset : position.offset,
);
}
// Here the position is somewhere after the selection: ....[...]..|..
if (extentAtIndex) {
return copyWith(
baseOffset: start,
extentOffset: position.offset,
affinity: position.affinity,
);
}
return copyWith(
baseOffset: normalized ? baseOffset : position.offset,
extentOffset: normalized ? position.offset : extentOffset,
);
}
/// Keeping the selection's [TextSelection.baseOffset] fixed, pivot the
/// [TextSelection.extentOffset] to the given [TextPosition].
///
/// In some cases, the [TextSelection.baseOffset] and
/// [TextSelection.extentOffset] may flip during this operation, and/or the
/// size of the selection may shrink.
///
/// ## Difference with [expandTo]
/// In contrast with this method, [expandTo] is strictly growth; the
/// selection is grown to include the given [TextPosition] and will never
/// shrink.
TextSelection extendTo(TextPosition position) {
// If the selection's extent is at the position already, then nothing
// happens.
if (extent == position) {
return this;
}
return copyWith(
extentOffset: position.offset,
affinity: position.affinity,
);
}
}
| flutter/packages/flutter/lib/src/services/text_editing.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/text_editing.dart",
"repo_id": "flutter",
"token_count": 2944
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic.dart';
import 'framework.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
// Internal representation of a child that, now or in the past, was set on the
// AnimatedSwitcher.child field, but is now in the process of
// transitioning. The internal representation includes fields that we don't want
// to expose to the public API (like the controller).
class _ChildEntry {
_ChildEntry({
required this.controller,
required this.animation,
required this.transition,
required this.widgetChild,
});
// The animation controller for the child's transition.
final AnimationController controller;
// The (curved) animation being used to drive the transition.
final CurvedAnimation animation;
// The currently built transition for this child.
Widget transition;
// The widget's child at the time this entry was created or updated.
// Used to rebuild the transition if necessary.
Widget widgetChild;
@override
String toString() => 'Entry#${shortHash(this)}($widgetChild)';
}
/// Signature for builders used to generate custom transitions for
/// [AnimatedSwitcher].
///
/// The `child` should be transitioning in when the `animation` is running in
/// the forward direction.
///
/// The function should return a widget which wraps the given `child`. It may
/// also use the `animation` to inform its transition. It must not return null.
typedef AnimatedSwitcherTransitionBuilder = Widget Function(Widget child, Animation<double> animation);
/// Signature for builders used to generate custom layouts for
/// [AnimatedSwitcher].
///
/// The builder should return a widget which contains the given children, laid
/// out as desired. It must not return null. The builder should be able to
/// handle an empty list of `previousChildren`, or a null `currentChild`.
///
/// The `previousChildren` list is an unmodifiable list, sorted with the oldest
/// at the beginning and the newest at the end. It does not include the
/// `currentChild`.
typedef AnimatedSwitcherLayoutBuilder = Widget Function(Widget? currentChild, List<Widget> previousChildren);
/// A widget that by default does a cross-fade between a new widget and the
/// widget previously set on the [AnimatedSwitcher] as a child.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=2W7POjFb88g}
///
/// If they are swapped fast enough (i.e. before [duration] elapses), more than
/// one previous child can exist and be transitioning out while the newest one
/// is transitioning in.
///
/// If the "new" child is the same widget type and key as the "old" child, but
/// with different parameters, then [AnimatedSwitcher] will *not* do a
/// transition between them, since as far as the framework is concerned, they
/// are the same widget and the existing widget can be updated with the new
/// parameters. To force the transition to occur, set a [Key] on each child
/// widget that you wish to be considered unique (typically a [ValueKey] on the
/// widget data that distinguishes this child from the others).
///
/// The same key can be used for a new child as was used for an already-outgoing
/// child; the two will not be considered related. (For example, if a progress
/// indicator with key A is first shown, then an image with key B, then another
/// progress indicator with key A again, all in rapid succession, then the old
/// progress indicator and the image will be fading out while a new progress
/// indicator is fading in.)
///
/// The type of transition can be changed from a cross-fade to a custom
/// transition by setting the [transitionBuilder].
///
/// {@tool dartpad}
/// This sample shows a counter that animates the scale of a text widget
/// whenever the value changes.
///
/// ** See code in examples/api/lib/widgets/animated_switcher/animated_switcher.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedCrossFade], which only fades between two children, but also
/// interpolates their sizes, and is reversible.
/// * [AnimatedOpacity], which can be used to switch between nothingness and
/// a given child by fading the child in and out.
/// * [FadeTransition], which [AnimatedSwitcher] uses to perform the transition.
class AnimatedSwitcher extends StatefulWidget {
/// Creates an [AnimatedSwitcher].
const AnimatedSwitcher({
super.key,
this.child,
required this.duration,
this.reverseDuration,
this.switchInCurve = Curves.linear,
this.switchOutCurve = Curves.linear,
this.transitionBuilder = AnimatedSwitcher.defaultTransitionBuilder,
this.layoutBuilder = AnimatedSwitcher.defaultLayoutBuilder,
});
/// The current child widget to display. If there was a previous child, then
/// that child will be faded out using the [switchOutCurve], while the new
/// child is faded in with the [switchInCurve], over the [duration].
///
/// If there was no previous child, then this child will fade in using the
/// [switchInCurve] over the [duration].
///
/// The child is considered to be "new" if it has a different type or [Key]
/// (see [Widget.canUpdate]).
///
/// To change the kind of transition used, see [transitionBuilder].
final Widget? child;
/// The duration of the transition from the old [child] value to the new one.
///
/// This duration is applied to the given [child] when that property is set to
/// a new child. The same duration is used when fading out, unless
/// [reverseDuration] is set. Changing [duration] will not affect the
/// durations of transitions already in progress.
final Duration duration;
/// The duration of the transition from the new [child] value to the old one.
///
/// This duration is applied to the given [child] when that property is set to
/// a new child. Changing [reverseDuration] will not affect the durations of
/// transitions already in progress.
///
/// If not set, then the value of [duration] is used by default.
final Duration? reverseDuration;
/// The animation curve to use when transitioning in a new [child].
///
/// This curve is applied to the given [child] when that property is set to a
/// new child. Changing [switchInCurve] will not affect the curve of a
/// transition already in progress.
///
/// The [switchOutCurve] is used when fading out, except that if [child] is
/// changed while the current child is in the middle of fading in,
/// [switchInCurve] will be run in reverse from that point instead of jumping
/// to the corresponding point on [switchOutCurve].
final Curve switchInCurve;
/// The animation curve to use when transitioning a previous [child] out.
///
/// This curve is applied to the [child] when the child is faded in (or when
/// the widget is created, for the first child). Changing [switchOutCurve]
/// will not affect the curves of already-visible widgets, it only affects the
/// curves of future children.
///
/// If [child] is changed while the current child is in the middle of fading
/// in, [switchInCurve] will be run in reverse from that point instead of
/// jumping to the corresponding point on [switchOutCurve].
final Curve switchOutCurve;
/// A function that wraps a new [child] with an animation that transitions
/// the [child] in when the animation runs in the forward direction and out
/// when the animation runs in the reverse direction. This is only called
/// when a new [child] is set (not for each build), or when a new
/// [transitionBuilder] is set. If a new [transitionBuilder] is set, then
/// the transition is rebuilt for the current child and all previous children
/// using the new [transitionBuilder]. The function must not return null.
///
/// The default is [AnimatedSwitcher.defaultTransitionBuilder].
///
/// The animation provided to the builder has the [duration] and
/// [switchInCurve] or [switchOutCurve] applied as provided when the
/// corresponding [child] was first provided.
///
/// See also:
///
/// * [AnimatedSwitcherTransitionBuilder] for more information about
/// how a transition builder should function.
final AnimatedSwitcherTransitionBuilder transitionBuilder;
/// A function that wraps all of the children that are transitioning out, and
/// the [child] that's transitioning in, with a widget that lays all of them
/// out. This is called every time this widget is built. The function must not
/// return null.
///
/// The default is [AnimatedSwitcher.defaultLayoutBuilder].
///
/// See also:
///
/// * [AnimatedSwitcherLayoutBuilder] for more information about
/// how a layout builder should function.
final AnimatedSwitcherLayoutBuilder layoutBuilder;
@override
State<AnimatedSwitcher> createState() => _AnimatedSwitcherState();
/// The transition builder used as the default value of [transitionBuilder].
///
/// The new child is given a [FadeTransition] which increases opacity as
/// the animation goes from 0.0 to 1.0, and decreases when the animation is
/// reversed.
///
/// This is an [AnimatedSwitcherTransitionBuilder] function.
static Widget defaultTransitionBuilder(Widget child, Animation<double> animation) {
return FadeTransition(
key: ValueKey<Key?>(child.key),
opacity: animation,
child: child,
);
}
/// The layout builder used as the default value of [layoutBuilder].
///
/// The new child is placed in a [Stack] that sizes itself to match the
/// largest of the child or a previous child. The children are centered on
/// each other.
///
/// This is an [AnimatedSwitcherLayoutBuilder] function.
static Widget defaultLayoutBuilder(Widget? currentChild, List<Widget> previousChildren) {
return Stack(
alignment: Alignment.center,
children: <Widget>[
...previousChildren,
if (currentChild != null) currentChild,
],
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('duration', duration.inMilliseconds, unit: 'ms'));
properties.add(IntProperty('reverseDuration', reverseDuration?.inMilliseconds, unit: 'ms', defaultValue: null));
}
}
class _AnimatedSwitcherState extends State<AnimatedSwitcher> with TickerProviderStateMixin {
_ChildEntry? _currentEntry;
final Set<_ChildEntry> _outgoingEntries = <_ChildEntry>{};
List<Widget>? _outgoingWidgets = const <Widget>[];
int _childNumber = 0;
@override
void initState() {
super.initState();
_addEntryForNewChild(animate: false);
}
@override
void didUpdateWidget(AnimatedSwitcher oldWidget) {
super.didUpdateWidget(oldWidget);
// If the transition builder changed, then update all of the previous
// transitions.
if (widget.transitionBuilder != oldWidget.transitionBuilder) {
_outgoingEntries.forEach(_updateTransitionForEntry);
if (_currentEntry != null) {
_updateTransitionForEntry(_currentEntry!);
}
_markChildWidgetCacheAsDirty();
}
final bool hasNewChild = widget.child != null;
final bool hasOldChild = _currentEntry != null;
if (hasNewChild != hasOldChild ||
hasNewChild && !Widget.canUpdate(widget.child!, _currentEntry!.widgetChild)) {
// Child has changed, fade current entry out and add new entry.
_childNumber += 1;
_addEntryForNewChild(animate: true);
} else if (_currentEntry != null) {
assert(hasOldChild && hasNewChild);
assert(Widget.canUpdate(widget.child!, _currentEntry!.widgetChild));
// Child has been updated. Make sure we update the child widget and
// transition in _currentEntry even though we're not going to start a new
// animation, but keep the key from the previous transition so that we
// update the transition instead of replacing it.
_currentEntry!.widgetChild = widget.child!;
_updateTransitionForEntry(_currentEntry!); // uses entry.widgetChild
_markChildWidgetCacheAsDirty();
}
}
void _addEntryForNewChild({ required bool animate }) {
assert(animate || _currentEntry == null);
if (_currentEntry != null) {
assert(animate);
assert(!_outgoingEntries.contains(_currentEntry));
_outgoingEntries.add(_currentEntry!);
_currentEntry!.controller.reverse();
_markChildWidgetCacheAsDirty();
_currentEntry = null;
}
if (widget.child == null) {
return;
}
final AnimationController controller = AnimationController(
duration: widget.duration,
reverseDuration: widget.reverseDuration,
vsync: this,
);
final CurvedAnimation animation = CurvedAnimation(
parent: controller,
curve: widget.switchInCurve,
reverseCurve: widget.switchOutCurve,
);
_currentEntry = _newEntry(
child: widget.child!,
controller: controller,
animation: animation,
builder: widget.transitionBuilder,
);
if (animate) {
controller.forward();
} else {
assert(_outgoingEntries.isEmpty);
controller.value = 1.0;
}
}
_ChildEntry _newEntry({
required Widget child,
required AnimatedSwitcherTransitionBuilder builder,
required AnimationController controller,
required CurvedAnimation animation,
}) {
final _ChildEntry entry = _ChildEntry(
widgetChild: child,
transition: KeyedSubtree.wrap(builder(child, animation), _childNumber),
animation: animation,
controller: controller,
);
animation.addStatusListener((AnimationStatus status) {
if (status == AnimationStatus.dismissed) {
setState(() {
assert(mounted);
assert(_outgoingEntries.contains(entry));
_outgoingEntries.remove(entry);
_markChildWidgetCacheAsDirty();
});
controller.dispose();
animation.dispose();
}
});
return entry;
}
void _markChildWidgetCacheAsDirty() {
_outgoingWidgets = null;
}
void _updateTransitionForEntry(_ChildEntry entry) {
entry.transition = KeyedSubtree(
key: entry.transition.key,
child: widget.transitionBuilder(entry.widgetChild, entry.animation),
);
}
void _rebuildOutgoingWidgetsIfNeeded() {
_outgoingWidgets ??= List<Widget>.unmodifiable(
_outgoingEntries.map<Widget>((_ChildEntry entry) => entry.transition),
);
assert(_outgoingEntries.length == _outgoingWidgets!.length);
assert(_outgoingEntries.isEmpty || _outgoingEntries.last.transition == _outgoingWidgets!.last);
}
@override
void dispose() {
if (_currentEntry != null) {
_currentEntry!.controller.dispose();
_currentEntry!.animation.dispose();
}
for (final _ChildEntry entry in _outgoingEntries) {
entry.controller.dispose();
entry.animation.dispose();
}
super.dispose();
}
@override
Widget build(BuildContext context) {
_rebuildOutgoingWidgetsIfNeeded();
return widget.layoutBuilder(_currentEntry?.transition, _outgoingWidgets!.where((Widget outgoing) => outgoing.key != _currentEntry?.transition.key).toSet().toList());
}
}
| flutter/packages/flutter/lib/src/widgets/animated_switcher.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/animated_switcher.dart",
"repo_id": "flutter",
"token_count": 4532
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'framework.dart';
import 'inherited_theme.dart';
import 'navigator.dart';
import 'overlay.dart';
/// Builds and manages a context menu at a given location.
///
/// There can only ever be one context menu shown at a given time in the entire
/// app. Calling [show] on one instance of this class will hide any other
/// shown instances.
///
/// {@tool dartpad}
/// This example shows how to use a GestureDetector to show a context menu
/// anywhere in a widget subtree that receives a right click or long press.
///
/// ** See code in examples/api/lib/material/context_menu/context_menu_controller.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [BrowserContextMenu], which allows the browser's context menu on web to
/// be disabled and Flutter-rendered context menus to appear.
class ContextMenuController {
/// Creates a context menu that can be shown with [show].
ContextMenuController({
this.onRemove,
});
/// Called when this menu is removed.
final VoidCallback? onRemove;
/// The currently shown instance, if any.
static ContextMenuController? _shownInstance;
// The OverlayEntry is static because only one context menu can be displayed
// at one time.
static OverlayEntry? _menuOverlayEntry;
/// Shows the given context menu.
///
/// Since there can only be one shown context menu at a time, calling this
/// will also remove any other context menu that is visible.
void show({
required BuildContext context,
required WidgetBuilder contextMenuBuilder,
Widget? debugRequiredFor,
}) {
removeAny();
final OverlayState overlayState = Overlay.of(
context,
rootOverlay: true,
debugRequiredFor: debugRequiredFor,
);
final CapturedThemes capturedThemes = InheritedTheme.capture(
from: context,
to: Navigator.maybeOf(context)?.context,
);
_menuOverlayEntry = OverlayEntry(
builder: (BuildContext context) {
return capturedThemes.wrap(contextMenuBuilder(context));
},
);
overlayState.insert(_menuOverlayEntry!);
_shownInstance = this;
}
/// Remove the currently shown context menu from the UI.
///
/// Does nothing if no context menu is currently shown.
///
/// If a menu is removed, and that menu provided an [onRemove] callback when
/// it was created, then that callback will be called.
///
/// See also:
///
/// * [remove], which removes only the current instance.
static void removeAny() {
_menuOverlayEntry?.remove();
_menuOverlayEntry?.dispose();
_menuOverlayEntry = null;
if (_shownInstance != null) {
_shownInstance!.onRemove?.call();
_shownInstance = null;
}
}
/// True if and only if this menu is currently being shown.
bool get isShown => _shownInstance == this;
/// Cause the underlying [OverlayEntry] to rebuild during the next pipeline
/// flush.
///
/// It's necessary to call this function if the output of [contextMenuBuilder]
/// has changed.
///
/// Errors if the context menu is not currently shown.
///
/// See also:
///
/// * [OverlayEntry.markNeedsBuild]
void markNeedsBuild() {
assert(isShown);
_menuOverlayEntry?.markNeedsBuild();
}
/// Remove this menu from the UI.
///
/// Does nothing if this instance is not currently shown. In other words, if
/// another context menu is currently shown, that menu will not be removed.
///
/// This method should only be called once. The instance cannot be shown again
/// after removing. Create a new instance.
///
/// If an [onRemove] method was given to this instance, it will be called.
///
/// See also:
///
/// * [removeAny], which removes any shown instance of the context menu.
void remove() {
if (!isShown) {
return;
}
removeAny();
}
}
| flutter/packages/flutter/lib/src/widgets/context_menu_controller.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/context_menu_controller.dart",
"repo_id": "flutter",
"token_count": 1201
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'actions.dart';
import 'basic.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'scroll_position.dart';
import 'scrollable.dart';
// Examples can assume:
// late BuildContext context;
// FocusNode focusNode = FocusNode();
// BuildContext/Element doesn't have a parent accessor, but it can be simulated
// with visitAncestorElements. _getAncestor is needed because
// context.getElementForInheritedWidgetOfExactType will return itself if it
// happens to be of the correct type. _getAncestor should be O(count), since we
// always return false at a specific ancestor. By default it returns the parent,
// which is O(1).
BuildContext? _getAncestor(BuildContext context, {int count = 1}) {
BuildContext? target;
context.visitAncestorElements((Element ancestor) {
count--;
if (count == 0) {
target = ancestor;
return false;
}
return true;
});
return target;
}
/// Signature for the callback that's called when a traversal policy
/// requests focus.
typedef TraversalRequestFocusCallback = void Function(
FocusNode node, {
ScrollPositionAlignmentPolicy? alignmentPolicy,
double? alignment,
Duration? duration,
Curve? curve,
});
// A class to temporarily hold information about FocusTraversalGroups when
// sorting their contents.
class _FocusTraversalGroupInfo {
_FocusTraversalGroupInfo(
_FocusTraversalGroupNode? group, {
FocusTraversalPolicy? defaultPolicy,
List<FocusNode>? members,
}) : groupNode = group,
policy = group?.policy ?? defaultPolicy ?? ReadingOrderTraversalPolicy(),
members = members ?? <FocusNode>[];
final FocusNode? groupNode;
final FocusTraversalPolicy policy;
final List<FocusNode> members;
}
/// A direction along either the horizontal or vertical axes.
///
/// This is used by the [DirectionalFocusTraversalPolicyMixin], and
/// [FocusNode.focusInDirection] to indicate which direction to look in for the
/// next focus.
enum TraversalDirection {
/// Indicates a direction above the currently focused widget.
up,
/// Indicates a direction to the right of the currently focused widget.
///
/// This direction is unaffected by the [Directionality] of the current
/// context.
right,
/// Indicates a direction below the currently focused widget.
down,
/// Indicates a direction to the left of the currently focused widget.
///
/// This direction is unaffected by the [Directionality] of the current
/// context.
left,
}
/// Controls the transfer of focus beyond the first and the last items of a
/// [FocusScopeNode].
///
/// This enumeration only controls the traversal behavior performed by
/// [FocusTraversalPolicy]. Other methods of focus transfer, such as direct
/// calls to [FocusNode.requestFocus] and [FocusNode.unfocus], are not affected
/// by this enumeration.
///
/// See also:
///
/// * [FocusTraversalPolicy], which implements the logic behind this enum.
/// * [FocusScopeNode], which is configured by this enum.
enum TraversalEdgeBehavior {
/// Keeps the focus among the items of the focus scope.
///
/// Requesting the next focus after the last focusable item will transfer the
/// focus to the first item, and requesting focus previous to the first item
/// will transfer the focus to the last item, thus forming a closed loop of
/// focusable items.
closedLoop,
/// Allows the focus to leave the [FlutterView].
///
/// Requesting next focus after the last focusable item or previous to the
/// first item will unfocus any focused nodes. If the focus traversal action
/// was initiated by the embedder (e.g. the Flutter Engine) the embedder
/// receives a result indicating that the focus is no longer within the
/// current [FlutterView]. For example, [NextFocusAction] invoked via keyboard
/// (typically the TAB key) would receive [KeyEventResult.skipRemainingHandlers]
/// allowing the embedder handle the shortcut. On the web, typically the
/// control is transferred to the browser, allowing the user to reach the
/// address bar, escape an `iframe`, or focus on HTML elements other than
/// those managed by Flutter.
leaveFlutterView,
/// Allows focus to traverse up to parent scope.
///
/// When reaching the edge of the current scope, requesting the next focus
/// will look up to the parent scope of the current scope and focus the focus
/// node next to the current scope.
///
/// If there is no parent scope above the current scope, fallback to
/// [closedLoop] behavior.
parentScope,
}
/// Determines how focusable widgets are traversed within a [FocusTraversalGroup].
///
/// The focus traversal policy is what determines which widget is "next",
/// "previous", or in a direction from the widget associated with the currently
/// focused [FocusNode] (usually a [Focus] widget).
///
/// One of the pre-defined subclasses may be used, or define a custom policy to
/// create a unique focus order.
///
/// When defining your own, your subclass should implement [sortDescendants] to
/// provide the order in which you would like the descendants to be traversed.
///
/// See also:
///
/// * [FocusNode], for a description of the focus system.
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [FocusNode], which is affected by the traversal policy.
/// * [WidgetOrderTraversalPolicy], a policy that relies on the widget
/// creation order to describe the order of traversal.
/// * [ReadingOrderTraversalPolicy], a policy that describes the order as the
/// natural "reading order" for the current [Directionality].
/// * [OrderedTraversalPolicy], a policy that describes the order
/// explicitly using [FocusTraversalOrder] widgets.
/// * [DirectionalFocusTraversalPolicyMixin] a mixin class that implements
/// focus traversal in a direction.
@immutable
abstract class FocusTraversalPolicy with Diagnosticable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
///
/// {@template flutter.widgets.FocusTraversalPolicy.requestFocusCallback}
/// The `requestFocusCallback` can be used to override the default behavior
/// of the focus requests. If `requestFocusCallback`
/// is null, it defaults to [FocusTraversalPolicy.defaultTraversalRequestFocusCallback].
/// {@endtemplate}
const FocusTraversalPolicy({
TraversalRequestFocusCallback? requestFocusCallback
}) : requestFocusCallback = requestFocusCallback ?? defaultTraversalRequestFocusCallback;
/// The callback used to move the focus from one focus node to another when
/// traversing them using a keyboard. By default it requests focus on the next
/// node and ensures the node is visible if it's in a scrollable.
final TraversalRequestFocusCallback requestFocusCallback;
/// The default value for [requestFocusCallback].
/// Requests focus from `node` and ensures the node is visible
/// by calling [Scrollable.ensureVisible].
static void defaultTraversalRequestFocusCallback(
FocusNode node, {
ScrollPositionAlignmentPolicy? alignmentPolicy,
double? alignment,
Duration? duration,
Curve? curve,
}) {
node.requestFocus();
Scrollable.ensureVisible(
node.context!,
alignment: alignment ?? 1,
alignmentPolicy: alignmentPolicy ?? ScrollPositionAlignmentPolicy.explicit,
duration: duration ?? Duration.zero,
curve: curve ?? Curves.ease,
);
}
/// Request focus on a focus node as a result of a tab traversal.
///
/// If the `node` is a [FocusScopeNode], this method will recursively find
/// the next focus from its descendants until it find a regular [FocusNode].
///
/// Returns true if this method focused a new focus node.
bool _requestTabTraversalFocus(
FocusNode node, {
ScrollPositionAlignmentPolicy? alignmentPolicy,
double? alignment,
Duration? duration,
Curve? curve,
required bool forward,
}) {
if (node is FocusScopeNode) {
if (node.focusedChild != null) {
// Can't stop here as the `focusedChild` may be a focus scope node
// without a first focus. The first focus will be picked in the
// next iteration.
return _requestTabTraversalFocus(
node.focusedChild!,
alignmentPolicy: alignmentPolicy,
alignment: alignment,
duration: duration,
curve: curve,
forward: forward,
);
}
final List<FocusNode> sortedChildren = _sortAllDescendants(node, node);
if (sortedChildren.isNotEmpty) {
_requestTabTraversalFocus(
forward ? sortedChildren.first : sortedChildren.last,
alignmentPolicy: alignmentPolicy,
alignment: alignment,
duration: duration,
curve: curve,
forward: forward,
);
// Regardless if _requestTabTraversalFocus return true or false, a first
// focus has been picked.
return true;
}
}
final bool nodeHadPrimaryFocus = node.hasPrimaryFocus;
requestFocusCallback(
node,
alignmentPolicy: alignmentPolicy,
alignment: alignment,
duration: duration,
curve: curve,
);
return !nodeHadPrimaryFocus;
}
/// Returns the node that should receive focus if focus is traversing
/// forwards, and there is no current focus.
///
/// The node returned is the node that should receive focus if focus is
/// traversing forwards (i.e. with [next]), and there is no current focus in
/// the nearest [FocusScopeNode] that `currentNode` belongs to.
///
/// If `ignoreCurrentFocus` is false or not given, this function returns the
/// [FocusScopeNode.focusedChild], if set, on the nearest scope of the
/// `currentNode`, otherwise, returns the first node from [sortDescendants],
/// or the given `currentNode` if there are no descendants.
///
/// If `ignoreCurrentFocus` is true, then the algorithm returns the first node
/// from [sortDescendants], or the given `currentNode` if there are no
/// descendants.
///
/// See also:
///
/// * [next], the function that is called to move the focus to the next node.
/// * [DirectionalFocusTraversalPolicyMixin.findFirstFocusInDirection], a
/// function that finds the first focusable widget in a particular
/// direction.
FocusNode? findFirstFocus(FocusNode currentNode, {bool ignoreCurrentFocus = false}) {
return _findInitialFocus(currentNode, ignoreCurrentFocus: ignoreCurrentFocus);
}
/// Returns the node that should receive focus if focus is traversing
/// backwards, and there is no current focus.
///
/// The node returned is the one that should receive focus if focus is
/// traversing backwards (i.e. with [previous]), and there is no current focus
/// in the nearest [FocusScopeNode] that `currentNode` belongs to.
///
/// If `ignoreCurrentFocus` is false or not given, this function returns the
/// [FocusScopeNode.focusedChild], if set, on the nearest scope of the
/// `currentNode`, otherwise, returns the last node from [sortDescendants],
/// or the given `currentNode` if there are no descendants.
///
/// If `ignoreCurrentFocus` is true, then the algorithm returns the last node
/// from [sortDescendants], or the given `currentNode` if there are no
/// descendants.
///
/// See also:
///
/// * [previous], the function that is called to move the focus to the previous node.
/// * [DirectionalFocusTraversalPolicyMixin.findFirstFocusInDirection], a
/// function that finds the first focusable widget in a particular direction.
FocusNode findLastFocus(FocusNode currentNode, {bool ignoreCurrentFocus = false}) {
return _findInitialFocus(currentNode, fromEnd: true, ignoreCurrentFocus: ignoreCurrentFocus);
}
FocusNode _findInitialFocus(FocusNode currentNode, {bool fromEnd = false, bool ignoreCurrentFocus = false}) {
final FocusScopeNode scope = currentNode.nearestScope!;
FocusNode? candidate = scope.focusedChild;
if (ignoreCurrentFocus || candidate == null && scope.descendants.isNotEmpty) {
final Iterable<FocusNode> sorted = _sortAllDescendants(scope, currentNode).where((FocusNode node) => _canRequestTraversalFocus(node));
if (sorted.isEmpty) {
candidate = null;
} else {
candidate = fromEnd ? sorted.last : sorted.first;
}
}
// If we still didn't find any candidate, use the current node as a
// fallback.
candidate ??= currentNode;
return candidate;
}
/// Returns the first node in the given `direction` that should receive focus
/// if there is no current focus in the scope to which the `currentNode`
/// belongs.
///
/// This is typically used by [inDirection] to determine which node to focus
/// if it is called when no node is currently focused.
FocusNode? findFirstFocusInDirection(FocusNode currentNode, TraversalDirection direction);
/// Clears the data associated with the given [FocusScopeNode] for this object.
///
/// This is used to indicate that the focus policy has changed its mode, and
/// so any cached policy data should be invalidated. For example, changing the
/// direction in which focus is moving, or changing from directional to
/// next/previous navigation modes.
///
/// The default implementation does nothing.
@mustCallSuper
void invalidateScopeData(FocusScopeNode node) {}
/// This is called whenever the given [node] is re-parented into a new scope,
/// so that the policy has a chance to update or invalidate any cached data
/// that it maintains per scope about the node.
///
/// The [oldScope] is the previous scope that this node belonged to, if any.
///
/// The default implementation does nothing.
@mustCallSuper
void changedScope({FocusNode? node, FocusScopeNode? oldScope}) {}
/// Focuses the next widget in the focus scope that contains the given
/// [currentNode].
///
/// This should determine what the next node to receive focus should be by
/// inspecting the node tree, and then calling [FocusNode.requestFocus] on
/// the node that has been selected.
///
/// Returns true if it successfully found a node and requested focus.
bool next(FocusNode currentNode) => _moveFocus(currentNode, forward: true);
/// Focuses the previous widget in the focus scope that contains the given
/// [currentNode].
///
/// This should determine what the previous node to receive focus should be by
/// inspecting the node tree, and then calling [FocusNode.requestFocus] on
/// the node that has been selected.
///
/// Returns true if it successfully found a node and requested focus.
bool previous(FocusNode currentNode) => _moveFocus(currentNode, forward: false);
/// Focuses the next widget in the given [direction] in the focus scope that
/// contains the given [currentNode].
///
/// This should determine what the next node to receive focus in the given
/// [direction] should be by inspecting the node tree, and then calling
/// [FocusNode.requestFocus] on the node that has been selected.
///
/// Returns true if it successfully found a node and requested focus.
bool inDirection(FocusNode currentNode, TraversalDirection direction);
/// Sorts the given `descendants` into focus order.
///
/// Subclasses should override this to implement a different sort for [next]
/// and [previous] to use in their ordering. If the returned iterable omits a
/// node that is a descendant of the given scope, then the user will be unable
/// to use next/previous keyboard traversal to reach that node.
///
/// The node used to initiate the traversal (the one passed to [next] or
/// [previous]) is passed as `currentNode`.
///
/// Having the current node in the list is what allows the algorithm to
/// determine which nodes are adjacent to the current node. If the
/// `currentNode` is removed from the list, then the focus will be unchanged
/// when [next] or [previous] are called, and they will return false.
///
/// This is not used for directional focus ([inDirection]), only for
/// determining the focus order for [next] and [previous].
///
/// When implementing an override for this function, be sure to use
/// [mergeSort] instead of Dart's default list sorting algorithm when sorting
/// items, since the default algorithm is not stable (items deemed to be equal
/// can appear in arbitrary order, and change positions between sorts), whereas
/// [mergeSort] is stable.
@protected
Iterable<FocusNode> sortDescendants(Iterable<FocusNode> descendants, FocusNode currentNode);
static bool _canRequestTraversalFocus(FocusNode node) {
return node.canRequestFocus && !node.skipTraversal;
}
static Iterable<FocusNode> _getDescendantsWithoutExpandingScope(FocusNode node) {
final List<FocusNode> result = <FocusNode>[];
for (final FocusNode child in node.children) {
result.add(child);
if (child is! FocusScopeNode) {
result.addAll(_getDescendantsWithoutExpandingScope(child));
}
}
return result;
}
static Map<FocusNode?, _FocusTraversalGroupInfo> _findGroups(FocusScopeNode scope, _FocusTraversalGroupNode? scopeGroupNode, FocusNode currentNode) {
final FocusTraversalPolicy defaultPolicy = scopeGroupNode?.policy ?? ReadingOrderTraversalPolicy();
final Map<FocusNode?, _FocusTraversalGroupInfo> groups = <FocusNode?, _FocusTraversalGroupInfo>{};
for (final FocusNode node in _getDescendantsWithoutExpandingScope(scope)) {
final _FocusTraversalGroupNode? groupNode = FocusTraversalGroup._getGroupNode(node);
// Group nodes need to be added to their parent's node, or to the "null"
// node if no parent is found. This creates the hierarchy of group nodes
// and makes it so the entire group is sorted along with the other members
// of the parent group.
if (node == groupNode) {
// To find the parent of the group node, we need to skip over the parent
// of the Focus node added in _FocusTraversalGroupState.build, and start
// looking with that node's parent, since _getGroupNode will return the
// node it was called on if it matches the type.
final _FocusTraversalGroupNode? parentGroup = FocusTraversalGroup._getGroupNode(groupNode!.parent!);
groups[parentGroup] ??= _FocusTraversalGroupInfo(parentGroup, members: <FocusNode>[], defaultPolicy: defaultPolicy);
assert(!groups[parentGroup]!.members.contains(node));
groups[parentGroup]!.members.add(groupNode);
continue;
}
// Skip non-focusable and non-traversable nodes in the same way that
// FocusScopeNode.traversalDescendants would.
//
// Current focused node needs to be in the group so that the caller can
// find the next traversable node from the current focused node.
if (node == currentNode || (node.canRequestFocus && !node.skipTraversal)) {
groups[groupNode] ??= _FocusTraversalGroupInfo(groupNode, members: <FocusNode>[], defaultPolicy: defaultPolicy);
assert(!groups[groupNode]!.members.contains(node));
groups[groupNode]!.members.add(node);
}
}
return groups;
}
// Sort all descendants, taking into account the FocusTraversalGroup
// that they are each in, and filtering out non-traversable/focusable nodes.
static List<FocusNode> _sortAllDescendants(FocusScopeNode scope, FocusNode currentNode) {
final _FocusTraversalGroupNode? scopeGroupNode = FocusTraversalGroup._getGroupNode(scope);
// Build the sorting data structure, separating descendants into groups.
final Map<FocusNode?, _FocusTraversalGroupInfo> groups = _findGroups(scope, scopeGroupNode, currentNode);
// Sort the member lists using the individual policy sorts.
for (final FocusNode? key in groups.keys) {
final List<FocusNode> sortedMembers = groups[key]!.policy.sortDescendants(groups[key]!.members, currentNode).toList();
groups[key]!.members.clear();
groups[key]!.members.addAll(sortedMembers);
}
// Traverse the group tree, adding the children of members in the order they
// appear in the member lists.
final List<FocusNode> sortedDescendants = <FocusNode>[];
void visitGroups(_FocusTraversalGroupInfo info) {
for (final FocusNode node in info.members) {
if (groups.containsKey(node)) {
// This is a policy group focus node. Replace it with the members of
// the corresponding policy group.
visitGroups(groups[node]!);
} else {
sortedDescendants.add(node);
}
}
}
// Visit the children of the scope, if any.
if (groups.isNotEmpty && groups.containsKey(scopeGroupNode)) {
visitGroups(groups[scopeGroupNode]!);
}
// Remove the FocusTraversalGroup nodes themselves, which aren't focusable.
// They were left in above because they were needed to find their members
// during sorting.
sortedDescendants.removeWhere((FocusNode node) {
return node != currentNode && !_canRequestTraversalFocus(node);
});
// Sanity check to make sure that the algorithm above doesn't diverge from
// the one in FocusScopeNode.traversalDescendants in terms of which nodes it
// finds.
assert((){
final Set<FocusNode> difference = sortedDescendants.toSet().difference(scope.traversalDescendants.toSet());
if (!_canRequestTraversalFocus(currentNode)) {
// The scope.traversalDescendants will not contain currentNode if it
// skips traversal or not focusable.
assert(
difference.isEmpty || (difference.length == 1 && difference.contains(currentNode)),
'Difference between sorted descendants and FocusScopeNode.traversalDescendants contains '
'something other than the current skipped node. This is the difference: $difference',
);
return true;
}
assert(
difference.isEmpty,
'Sorted descendants contains different nodes than FocusScopeNode.traversalDescendants would. '
'These are the different nodes: $difference',
);
return true;
}());
return sortedDescendants;
}
/// Moves the focus to the next node in the FocusScopeNode nearest to the
/// currentNode argument, either in a forward or reverse direction, depending
/// on the value of the forward argument.
///
/// This function is called by the next and previous members to move to the
/// next or previous node, respectively.
///
/// Uses [findFirstFocus]/[findLastFocus] to find the first/last node if there is
/// no [FocusScopeNode.focusedChild] set. If there is a focused child for the
/// scope, then it calls sortDescendants to get a sorted list of descendants,
/// and then finds the node after the current first focus of the scope if
/// forward is true, and the node before it if forward is false.
///
/// Returns true if a node requested focus.
@protected
bool _moveFocus(FocusNode currentNode, {required bool forward}) {
final FocusScopeNode nearestScope = currentNode.nearestScope!;
invalidateScopeData(nearestScope);
FocusNode? focusedChild = nearestScope.focusedChild;
if (focusedChild == null) {
final FocusNode? firstFocus = forward ? findFirstFocus(currentNode) : findLastFocus(currentNode);
if (firstFocus != null) {
return _requestTabTraversalFocus(
firstFocus,
alignmentPolicy: forward ? ScrollPositionAlignmentPolicy.keepVisibleAtEnd : ScrollPositionAlignmentPolicy.keepVisibleAtStart,
forward: forward,
);
}
}
focusedChild ??= nearestScope;
final List<FocusNode> sortedNodes = _sortAllDescendants(nearestScope, focusedChild);
assert(sortedNodes.contains(focusedChild));
if (forward && focusedChild == sortedNodes.last) {
switch (nearestScope.traversalEdgeBehavior) {
case TraversalEdgeBehavior.leaveFlutterView:
focusedChild.unfocus();
return false;
case TraversalEdgeBehavior.parentScope:
final FocusScopeNode? parentScope = nearestScope.enclosingScope;
if (parentScope != null && parentScope != FocusManager.instance.rootScope) {
focusedChild.unfocus();
parentScope.nextFocus();
// Verify the focus really has changed.
return focusedChild.enclosingScope?.focusedChild != focusedChild;
}
// No valid parent scope. Fallback to closed loop behavior.
return _requestTabTraversalFocus(
sortedNodes.first,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
forward: forward,
);
case TraversalEdgeBehavior.closedLoop:
return _requestTabTraversalFocus(
sortedNodes.first,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
forward: forward,
);
}
}
if (!forward && focusedChild == sortedNodes.first) {
switch (nearestScope.traversalEdgeBehavior) {
case TraversalEdgeBehavior.leaveFlutterView:
focusedChild.unfocus();
return false;
case TraversalEdgeBehavior.parentScope:
final FocusScopeNode? parentScope = nearestScope.enclosingScope;
if (parentScope != null && parentScope != FocusManager.instance.rootScope) {
focusedChild.unfocus();
parentScope.previousFocus();
// Verify the focus really has changed.
return focusedChild.enclosingScope?.focusedChild != focusedChild;
}
// No valid parent scope. Fallback to closed loop behavior.
return _requestTabTraversalFocus(
sortedNodes.last,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
forward: forward,
);
case TraversalEdgeBehavior.closedLoop:
return _requestTabTraversalFocus(
sortedNodes.last,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
forward: forward,
);
}
}
final Iterable<FocusNode> maybeFlipped = forward ? sortedNodes : sortedNodes.reversed;
FocusNode? previousNode;
for (final FocusNode node in maybeFlipped) {
if (previousNode == focusedChild) {
return _requestTabTraversalFocus(
node,
alignmentPolicy: forward ? ScrollPositionAlignmentPolicy.keepVisibleAtEnd : ScrollPositionAlignmentPolicy.keepVisibleAtStart,
forward: forward,
);
}
previousNode = node;
}
return false;
}
}
// A policy data object for use by the DirectionalFocusTraversalPolicyMixin so
// it can keep track of the traversal history.
class _DirectionalPolicyDataEntry {
const _DirectionalPolicyDataEntry({required this.direction, required this.node});
final TraversalDirection direction;
final FocusNode node;
}
class _DirectionalPolicyData {
const _DirectionalPolicyData({required this.history});
/// A queue of entries that describe the path taken to the current node.
final List<_DirectionalPolicyDataEntry> history;
}
/// A mixin class that provides an implementation for finding a node in a
/// particular direction.
///
/// This can be mixed in to other [FocusTraversalPolicy] implementations that
/// only want to implement new next/previous policies.
///
/// Since hysteresis in the navigation order is undesirable, this implementation
/// maintains a stack of previous locations that have been visited on the policy
/// data for the affected [FocusScopeNode]. If the previous direction was the
/// opposite of the current direction, then the this policy will request focus
/// on the previously focused node. Change to another direction other than the
/// current one or its opposite will clear the stack.
///
/// For instance, if the focus moves down, down, down, and then up, up, up, it
/// will follow the same path through the widgets in both directions. However,
/// if it moves down, down, down, left, right, and then up, up, up, it may not
/// follow the same path on the way up as it did on the way down, since changing
/// the axis of motion resets the history.
///
/// This class implements an algorithm that considers an infinite band extending
/// along the direction of movement, the width or height (depending on
/// direction) of the currently focused widget, and finds the closest widget in
/// that band along the direction of movement. If nothing is found in that band,
/// then it picks the widget with an edge closest to the band in the
/// perpendicular direction. If two out-of-band widgets are the same distance
/// from the band, then it picks the one closest along the direction of
/// movement.
///
/// The goal of this algorithm is to pick a widget that (to the user) doesn't
/// appear to traverse along the wrong axis, as it might if it only sorted
/// widgets by distance along one axis, but also jumps to the next logical
/// widget in a direction without skipping over widgets.
///
/// See also:
///
/// * [FocusNode], for a description of the focus system.
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [WidgetOrderTraversalPolicy], a policy that relies on the widget creation
/// order to describe the order of traversal.
/// * [ReadingOrderTraversalPolicy], a policy that describes the order as the
/// natural "reading order" for the current [Directionality].
/// * [OrderedTraversalPolicy], a policy that describes the order explicitly
/// using [FocusTraversalOrder] widgets.
mixin DirectionalFocusTraversalPolicyMixin on FocusTraversalPolicy {
final Map<FocusScopeNode, _DirectionalPolicyData> _policyData = <FocusScopeNode, _DirectionalPolicyData>{};
@override
void invalidateScopeData(FocusScopeNode node) {
super.invalidateScopeData(node);
_policyData.remove(node);
}
@override
void changedScope({FocusNode? node, FocusScopeNode? oldScope}) {
super.changedScope(node: node, oldScope: oldScope);
if (oldScope != null) {
_policyData[oldScope]?.history.removeWhere((_DirectionalPolicyDataEntry entry) {
return entry.node == node;
});
}
}
@override
FocusNode? findFirstFocusInDirection(FocusNode currentNode, TraversalDirection direction) {
switch (direction) {
case TraversalDirection.up:
// Find the bottom-most node so we can go up from there.
return _sortAndFindInitial(currentNode, vertical: true, first: false);
case TraversalDirection.down:
// Find the top-most node so we can go down from there.
return _sortAndFindInitial(currentNode, vertical: true, first: true);
case TraversalDirection.left:
// Find the right-most node so we can go left from there.
return _sortAndFindInitial(currentNode, vertical: false, first: false);
case TraversalDirection.right:
// Find the left-most node so we can go right from there.
return _sortAndFindInitial(currentNode, vertical: false, first: true);
}
}
FocusNode? _sortAndFindInitial(FocusNode currentNode, {required bool vertical, required bool first}) {
final Iterable<FocusNode> nodes = currentNode.nearestScope!.traversalDescendants;
final List<FocusNode> sorted = nodes.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode a, FocusNode b) {
if (vertical) {
if (first) {
return a.rect.top.compareTo(b.rect.top);
} else {
return b.rect.bottom.compareTo(a.rect.bottom);
}
} else {
if (first) {
return a.rect.left.compareTo(b.rect.left);
} else {
return b.rect.right.compareTo(a.rect.right);
}
}
});
if (sorted.isNotEmpty) {
return sorted.first;
}
return null;
}
static int _verticalCompare(Offset target, Offset a, Offset b) {
return (a.dy - target.dy).abs().compareTo((b.dy - target.dy).abs());
}
static int _horizontalCompare(Offset target, Offset a, Offset b) {
return (a.dx - target.dx).abs().compareTo((b.dx - target.dx).abs());
}
// Sort the ones that are closest to target vertically first, and if two are
// the same vertical distance, pick the one that is closest horizontally.
static Iterable<FocusNode> _sortByDistancePreferVertical(Offset target, Iterable<FocusNode> nodes) {
final List<FocusNode> sorted = nodes.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode nodeA, FocusNode nodeB) {
final Offset a = nodeA.rect.center;
final Offset b = nodeB.rect.center;
final int vertical = _verticalCompare(target, a, b);
if (vertical == 0) {
return _horizontalCompare(target, a, b);
}
return vertical;
});
return sorted;
}
// Sort the ones that are closest horizontally first, and if two are the same
// horizontal distance, pick the one that is closest vertically.
static Iterable<FocusNode> _sortByDistancePreferHorizontal(Offset target, Iterable<FocusNode> nodes) {
final List<FocusNode> sorted = nodes.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode nodeA, FocusNode nodeB) {
final Offset a = nodeA.rect.center;
final Offset b = nodeB.rect.center;
final int horizontal = _horizontalCompare(target, a, b);
if (horizontal == 0) {
return _verticalCompare(target, a, b);
}
return horizontal;
});
return sorted;
}
static int _verticalCompareClosestEdge(Offset target, Rect a, Rect b) {
// Find which edge is closest to the target for each.
final double aCoord = (a.top - target.dy).abs() < (a.bottom - target.dy).abs() ? a.top : a.bottom;
final double bCoord = (b.top - target.dy).abs() < (b.bottom - target.dy).abs() ? b.top : b.bottom;
return (aCoord - target.dy).abs().compareTo((bCoord - target.dy).abs());
}
static int _horizontalCompareClosestEdge(Offset target, Rect a, Rect b) {
// Find which edge is closest to the target for each.
final double aCoord = (a.left - target.dx).abs() < (a.right - target.dx).abs() ? a.left : a.right;
final double bCoord = (b.left - target.dx).abs() < (b.right - target.dx).abs() ? b.left : b.right;
return (aCoord - target.dx).abs().compareTo((bCoord - target.dx).abs());
}
// Sort the ones that have edges that are closest horizontally first, and if
// two are the same horizontal distance, pick the one that is closest
// vertically.
static Iterable<FocusNode> _sortClosestEdgesByDistancePreferHorizontal(Offset target, Iterable<FocusNode> nodes) {
final List<FocusNode> sorted = nodes.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode nodeA, FocusNode nodeB) {
final int horizontal = _horizontalCompareClosestEdge(target, nodeA.rect, nodeB.rect);
if (horizontal == 0) {
// If they're the same distance horizontally, pick the closest one
// vertically.
return _verticalCompare(target, nodeA.rect.center, nodeB.rect.center);
}
return horizontal;
});
return sorted;
}
// Sort the ones that have edges that are closest vertically first, and if
// two are the same vertical distance, pick the one that is closest
// horizontally.
static Iterable<FocusNode> _sortClosestEdgesByDistancePreferVertical(Offset target, Iterable<FocusNode> nodes) {
final List<FocusNode> sorted = nodes.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode nodeA, FocusNode nodeB) {
final int vertical = _verticalCompareClosestEdge(target, nodeA.rect, nodeB.rect);
if (vertical == 0) {
// If they're the same distance vertically, pick the closest one
// horizontally.
return _horizontalCompare(target, nodeA.rect.center, nodeB.rect.center);
}
return vertical;
});
return sorted;
}
// Sorts nodes from left to right horizontally, and removes nodes that are
// either to the right of the left side of the target node if we're going
// left, or to the left of the right side of the target node if we're going
// right.
//
// This doesn't need to take into account directionality because it is
// typically intending to actually go left or right, not in a reading
// direction.
Iterable<FocusNode> _sortAndFilterHorizontally(
TraversalDirection direction,
Rect target,
Iterable<FocusNode> nodes,
) {
assert(direction == TraversalDirection.left || direction == TraversalDirection.right);
final Iterable<FocusNode> filtered;
switch (direction) {
case TraversalDirection.left:
filtered = nodes.where((FocusNode node) => node.rect != target && node.rect.center.dx <= target.left);
case TraversalDirection.right:
filtered = nodes.where((FocusNode node) => node.rect != target && node.rect.center.dx >= target.right);
case TraversalDirection.up:
case TraversalDirection.down:
throw ArgumentError('Invalid direction $direction');
}
final List<FocusNode> sorted = filtered.toList();
// Sort all nodes from left to right.
mergeSort<FocusNode>(sorted, compare: (FocusNode a, FocusNode b) => a.rect.center.dx.compareTo(b.rect.center.dx));
return sorted;
}
// Sorts nodes from top to bottom vertically, and removes nodes that are
// either below the top of the target node if we're going up, or above the
// bottom of the target node if we're going down.
Iterable<FocusNode> _sortAndFilterVertically(
TraversalDirection direction,
Rect target,
Iterable<FocusNode> nodes,
) {
assert(direction == TraversalDirection.up || direction == TraversalDirection.down);
final Iterable<FocusNode> filtered;
switch (direction) {
case TraversalDirection.up:
filtered = nodes.where((FocusNode node) => node.rect != target && node.rect.center.dy <= target.top);
case TraversalDirection.down:
filtered = nodes.where((FocusNode node) => node.rect != target && node.rect.center.dy >= target.bottom);
case TraversalDirection.left:
case TraversalDirection.right:
throw ArgumentError('Invalid direction $direction');
}
final List<FocusNode> sorted = filtered.toList();
mergeSort<FocusNode>(sorted, compare: (FocusNode a, FocusNode b) => a.rect.center.dy.compareTo(b.rect.center.dy));
return sorted;
}
// Updates the policy data to keep the previously visited node so that we can
// avoid hysteresis when we change directions in navigation.
//
// Returns true if focus was requested on a previous node.
bool _popPolicyDataIfNeeded(TraversalDirection direction, FocusScopeNode nearestScope, FocusNode focusedChild) {
final _DirectionalPolicyData? policyData = _policyData[nearestScope];
if (policyData != null && policyData.history.isNotEmpty && policyData.history.first.direction != direction) {
if (policyData.history.last.node.parent == null) {
// If a node has been removed from the tree, then we should stop
// referencing it and reset the scope data so that we don't try and
// request focus on it. This can happen in slivers where the rendered
// node has been unmounted. This has the side effect that hysteresis
// might not be avoided when items that go off screen get unmounted.
invalidateScopeData(nearestScope);
return false;
}
// Returns true if successfully popped the history.
bool popOrInvalidate(TraversalDirection direction) {
final FocusNode lastNode = policyData.history.removeLast().node;
if (Scrollable.maybeOf(lastNode.context!) != Scrollable.maybeOf(primaryFocus!.context!)) {
invalidateScopeData(nearestScope);
return false;
}
final ScrollPositionAlignmentPolicy alignmentPolicy;
switch (direction) {
case TraversalDirection.up:
case TraversalDirection.left:
alignmentPolicy = ScrollPositionAlignmentPolicy.keepVisibleAtStart;
case TraversalDirection.right:
case TraversalDirection.down:
alignmentPolicy = ScrollPositionAlignmentPolicy.keepVisibleAtEnd;
}
requestFocusCallback(
lastNode,
alignmentPolicy: alignmentPolicy,
);
return true;
}
switch (direction) {
case TraversalDirection.down:
case TraversalDirection.up:
switch (policyData.history.first.direction) {
case TraversalDirection.left:
case TraversalDirection.right:
// Reset the policy data if we change directions.
invalidateScopeData(nearestScope);
case TraversalDirection.up:
case TraversalDirection.down:
if (popOrInvalidate(direction)) {
return true;
}
}
case TraversalDirection.left:
case TraversalDirection.right:
switch (policyData.history.first.direction) {
case TraversalDirection.left:
case TraversalDirection.right:
if (popOrInvalidate(direction)) {
return true;
}
case TraversalDirection.up:
case TraversalDirection.down:
// Reset the policy data if we change directions.
invalidateScopeData(nearestScope);
}
}
}
if (policyData != null && policyData.history.isEmpty) {
invalidateScopeData(nearestScope);
}
return false;
}
void _pushPolicyData(TraversalDirection direction, FocusScopeNode nearestScope, FocusNode focusedChild) {
final _DirectionalPolicyData? policyData = _policyData[nearestScope];
final _DirectionalPolicyDataEntry newEntry = _DirectionalPolicyDataEntry(node: focusedChild, direction: direction);
if (policyData != null) {
policyData.history.add(newEntry);
} else {
_policyData[nearestScope] = _DirectionalPolicyData(history: <_DirectionalPolicyDataEntry>[newEntry]);
}
}
/// Focuses the next widget in the given [direction] in the [FocusScope] that
/// contains the [currentNode].
///
/// This determines what the next node to receive focus in the given
/// [direction] will be by inspecting the node tree, and then calling
/// [FocusNode.requestFocus] on it.
///
/// Returns true if it successfully found a node and requested focus.
///
/// Maintains a stack of previous locations that have been visited on the
/// policy data for the affected [FocusScopeNode]. If the previous direction
/// was the opposite of the current direction, then the this policy will
/// request focus on the previously focused node. Change to another direction
/// other than the current one or its opposite will clear the stack.
///
/// If this function returns true when called by a subclass, then the subclass
/// should return true and not request focus from any node.
@mustCallSuper
@override
bool inDirection(FocusNode currentNode, TraversalDirection direction) {
final FocusScopeNode nearestScope = currentNode.nearestScope!;
final FocusNode? focusedChild = nearestScope.focusedChild;
if (focusedChild == null) {
final FocusNode firstFocus = findFirstFocusInDirection(currentNode, direction) ?? currentNode;
switch (direction) {
case TraversalDirection.up:
case TraversalDirection.left:
requestFocusCallback(
firstFocus,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
case TraversalDirection.right:
case TraversalDirection.down:
requestFocusCallback(
firstFocus,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
);
}
return true;
}
if (_popPolicyDataIfNeeded(direction, nearestScope, focusedChild)) {
return true;
}
FocusNode? found;
final ScrollableState? focusedScrollable = Scrollable.maybeOf(focusedChild.context!);
switch (direction) {
case TraversalDirection.down:
case TraversalDirection.up:
Iterable<FocusNode> eligibleNodes = _sortAndFilterVertically(direction, focusedChild.rect, nearestScope.traversalDescendants);
if (eligibleNodes.isEmpty) {
break;
}
if (focusedScrollable != null && !focusedScrollable.position.atEdge) {
final Iterable<FocusNode> filteredEligibleNodes = eligibleNodes.where((FocusNode node) => Scrollable.maybeOf(node.context!) == focusedScrollable);
if (filteredEligibleNodes.isNotEmpty) {
eligibleNodes = filteredEligibleNodes;
}
}
if (direction == TraversalDirection.up) {
eligibleNodes = eligibleNodes.toList().reversed;
}
// Find any nodes that intersect the band of the focused child.
final Rect band = Rect.fromLTRB(focusedChild.rect.left, -double.infinity, focusedChild.rect.right, double.infinity);
final Iterable<FocusNode> inBand = eligibleNodes.where((FocusNode node) => !node.rect.intersect(band).isEmpty);
if (inBand.isNotEmpty) {
found = _sortByDistancePreferVertical(focusedChild.rect.center, inBand).first;
break;
}
// Only out-of-band targets are eligible, so pick the one that is
// closest to the center line horizontally, and if any are the same
// distance horizontally, pick the closest one of those vertically.
found = _sortClosestEdgesByDistancePreferHorizontal(focusedChild.rect.center, eligibleNodes).first;
case TraversalDirection.right:
case TraversalDirection.left:
Iterable<FocusNode> eligibleNodes = _sortAndFilterHorizontally(direction, focusedChild.rect, nearestScope.traversalDescendants);
if (eligibleNodes.isEmpty) {
break;
}
if (focusedScrollable != null && !focusedScrollable.position.atEdge) {
final Iterable<FocusNode> filteredEligibleNodes = eligibleNodes.where((FocusNode node) => Scrollable.maybeOf(node.context!) == focusedScrollable);
if (filteredEligibleNodes.isNotEmpty) {
eligibleNodes = filteredEligibleNodes;
}
}
if (direction == TraversalDirection.left) {
eligibleNodes = eligibleNodes.toList().reversed;
}
// Find any nodes that intersect the band of the focused child.
final Rect band = Rect.fromLTRB(-double.infinity, focusedChild.rect.top, double.infinity, focusedChild.rect.bottom);
final Iterable<FocusNode> inBand = eligibleNodes.where((FocusNode node) => !node.rect.intersect(band).isEmpty);
if (inBand.isNotEmpty) {
found = _sortByDistancePreferHorizontal(focusedChild.rect.center, inBand).first;
break;
}
// Only out-of-band targets are eligible, so pick the one that is
// closest to the center line vertically, and if any are the same
// distance vertically, pick the closest one of those horizontally.
found = _sortClosestEdgesByDistancePreferVertical(focusedChild.rect.center, eligibleNodes).first;
}
if (found != null) {
_pushPolicyData(direction, nearestScope, focusedChild);
switch (direction) {
case TraversalDirection.up:
case TraversalDirection.left:
requestFocusCallback(
found,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtStart,
);
case TraversalDirection.down:
case TraversalDirection.right:
requestFocusCallback(
found,
alignmentPolicy: ScrollPositionAlignmentPolicy.keepVisibleAtEnd,
);
}
return true;
}
return false;
}
}
/// A [FocusTraversalPolicy] that traverses the focus order in widget hierarchy
/// order.
///
/// This policy is used when the order desired is the order in which widgets are
/// created in the widget hierarchy.
///
/// See also:
///
/// * [FocusNode], for a description of the focus system.
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [ReadingOrderTraversalPolicy], a policy that describes the order as the
/// natural "reading order" for the current [Directionality].
/// * [DirectionalFocusTraversalPolicyMixin] a mixin class that implements
/// focus traversal in a direction.
/// * [OrderedTraversalPolicy], a policy that describes the order
/// explicitly using [FocusTraversalOrder] widgets.
class WidgetOrderTraversalPolicy extends FocusTraversalPolicy with DirectionalFocusTraversalPolicyMixin {
/// Constructs a traversal policy that orders widgets for keyboard traversal
/// based on the widget hierarchy order.
///
/// {@macro flutter.widgets.FocusTraversalPolicy.requestFocusCallback}
WidgetOrderTraversalPolicy({super.requestFocusCallback});
@override
Iterable<FocusNode> sortDescendants(Iterable<FocusNode> descendants, FocusNode currentNode) => descendants;
}
// This class exists mainly for efficiency reasons: the rect is copied out of
// the node, because it will be accessed many times in the reading order
// algorithm, and the FocusNode.rect accessor does coordinate transformation. If
// not for this optimization, it could just be removed, and the node used
// directly.
//
// It's also a convenient place to put some utility functions having to do with
// the sort data.
class _ReadingOrderSortData with Diagnosticable {
_ReadingOrderSortData(this.node)
: rect = node.rect,
directionality = _findDirectionality(node.context!);
final TextDirection? directionality;
final Rect rect;
final FocusNode node;
// Find the directionality in force for a build context without creating a
// dependency.
static TextDirection? _findDirectionality(BuildContext context) {
return context.getInheritedWidgetOfExactType<Directionality>()?.textDirection;
}
/// Finds the common Directional ancestor of an entire list of groups.
static TextDirection? commonDirectionalityOf(List<_ReadingOrderSortData> list) {
final Iterable<Set<Directionality>> allAncestors = list.map<Set<Directionality>>((_ReadingOrderSortData member) => member.directionalAncestors.toSet());
Set<Directionality>? common;
for (final Set<Directionality> ancestorSet in allAncestors) {
common ??= ancestorSet;
common = common.intersection(ancestorSet);
}
if (common!.isEmpty) {
// If there is no common ancestor, then arbitrarily pick the
// directionality of the first group, which is the equivalent of the
// "first strongly typed" item in a bidirectional algorithm.
return list.first.directionality;
}
// Find the closest common ancestor. The memberAncestors list contains the
// ancestors for all members, but the first member's ancestry was
// added in order from nearest to furthest, so we can still use that
// to determine the closest one.
return list.first.directionalAncestors.firstWhere(common.contains).textDirection;
}
static void sortWithDirectionality(List<_ReadingOrderSortData> list, TextDirection directionality) {
mergeSort<_ReadingOrderSortData>(
list,
compare: (_ReadingOrderSortData a, _ReadingOrderSortData b) => switch (directionality) {
TextDirection.ltr => a.rect.left.compareTo(b.rect.left),
TextDirection.rtl => b.rect.right.compareTo(a.rect.right),
},
);
}
/// Returns the list of Directionality ancestors, in order from nearest to
/// furthest.
Iterable<Directionality> get directionalAncestors {
List<Directionality> getDirectionalityAncestors(BuildContext context) {
final List<Directionality> result = <Directionality>[];
InheritedElement? directionalityElement = context.getElementForInheritedWidgetOfExactType<Directionality>();
while (directionalityElement != null) {
result.add(directionalityElement.widget as Directionality);
directionalityElement = _getAncestor(directionalityElement)?.getElementForInheritedWidgetOfExactType<Directionality>();
}
return result;
}
_directionalAncestors ??= getDirectionalityAncestors(node.context!);
return _directionalAncestors!;
}
List<Directionality>? _directionalAncestors;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<TextDirection>('directionality', directionality));
properties.add(StringProperty('name', node.debugLabel, defaultValue: null));
properties.add(DiagnosticsProperty<Rect>('rect', rect));
}
}
// A class for containing group data while sorting in reading order while taking
// into account the ambient directionality.
class _ReadingOrderDirectionalGroupData with Diagnosticable {
_ReadingOrderDirectionalGroupData(this.members);
final List<_ReadingOrderSortData> members;
TextDirection? get directionality => members.first.directionality;
Rect? _rect;
Rect get rect {
if (_rect == null) {
for (final Rect rect in members.map<Rect>((_ReadingOrderSortData data) => data.rect)) {
_rect ??= rect;
_rect = _rect!.expandToInclude(rect);
}
}
return _rect!;
}
List<Directionality> get memberAncestors {
if (_memberAncestors == null) {
_memberAncestors = <Directionality>[];
for (final _ReadingOrderSortData member in members) {
_memberAncestors!.addAll(member.directionalAncestors);
}
}
return _memberAncestors!;
}
List<Directionality>? _memberAncestors;
static void sortWithDirectionality(List<_ReadingOrderDirectionalGroupData> list, TextDirection directionality) {
mergeSort<_ReadingOrderDirectionalGroupData>(
list,
compare: (_ReadingOrderDirectionalGroupData a, _ReadingOrderDirectionalGroupData b) => switch (directionality) {
TextDirection.ltr => a.rect.left.compareTo(b.rect.left),
TextDirection.rtl => b.rect.right.compareTo(a.rect.right),
},
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<TextDirection>('directionality', directionality));
properties.add(DiagnosticsProperty<Rect>('rect', rect));
properties.add(IterableProperty<String>('members', members.map<String>((_ReadingOrderSortData member) {
return '"${member.node.debugLabel}"(${member.rect})';
})));
}
}
/// Traverses the focus order in "reading order".
///
/// By default, reading order traversal goes in the reading direction, and then
/// down, using this algorithm:
///
/// 1. Find the node rectangle that has the highest `top` on the screen.
/// 2. Find any other nodes that intersect the infinite horizontal band defined
/// by the highest rectangle's top and bottom edges.
/// 3. Pick the closest to the beginning of the reading order from among the
/// nodes discovered above.
///
/// It uses the ambient [Directionality] in the context for the enclosing
/// [FocusTraversalGroup] to determine which direction is "reading order".
///
/// See also:
///
/// * [FocusNode], for a description of the focus system.
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [WidgetOrderTraversalPolicy], a policy that relies on the widget
/// creation order to describe the order of traversal.
/// * [DirectionalFocusTraversalPolicyMixin] a mixin class that implements
/// focus traversal in a direction.
/// * [OrderedTraversalPolicy], a policy that describes the order
/// explicitly using [FocusTraversalOrder] widgets.
class ReadingOrderTraversalPolicy extends FocusTraversalPolicy with DirectionalFocusTraversalPolicyMixin {
/// Constructs a traversal policy that orders the widgets in "reading order".
///
/// {@macro flutter.widgets.FocusTraversalPolicy.requestFocusCallback}
ReadingOrderTraversalPolicy({super.requestFocusCallback});
// Collects the given candidates into groups by directionality. The candidates
// have already been sorted as if they all had the directionality of the
// nearest Directionality ancestor.
List<_ReadingOrderDirectionalGroupData> _collectDirectionalityGroups(Iterable<_ReadingOrderSortData> candidates) {
TextDirection? currentDirection = candidates.first.directionality;
List<_ReadingOrderSortData> currentGroup = <_ReadingOrderSortData>[];
final List<_ReadingOrderDirectionalGroupData> result = <_ReadingOrderDirectionalGroupData>[];
// Split candidates into runs of the same directionality.
for (final _ReadingOrderSortData candidate in candidates) {
if (candidate.directionality == currentDirection) {
currentGroup.add(candidate);
continue;
}
currentDirection = candidate.directionality;
result.add(_ReadingOrderDirectionalGroupData(currentGroup));
currentGroup = <_ReadingOrderSortData>[candidate];
}
if (currentGroup.isNotEmpty) {
result.add(_ReadingOrderDirectionalGroupData(currentGroup));
}
// Sort each group separately. Each group has the same directionality.
for (final _ReadingOrderDirectionalGroupData bandGroup in result) {
if (bandGroup.members.length == 1) {
continue; // No need to sort one node.
}
_ReadingOrderSortData.sortWithDirectionality(bandGroup.members, bandGroup.directionality!);
}
return result;
}
_ReadingOrderSortData _pickNext(List<_ReadingOrderSortData> candidates) {
// Find the topmost node by sorting on the top of the rectangles.
mergeSort<_ReadingOrderSortData>(candidates, compare: (_ReadingOrderSortData a, _ReadingOrderSortData b) => a.rect.top.compareTo(b.rect.top));
final _ReadingOrderSortData topmost = candidates.first;
// Find the candidates that are in the same horizontal band as the current one.
List<_ReadingOrderSortData> inBand(_ReadingOrderSortData current, Iterable<_ReadingOrderSortData> candidates) {
final Rect band = Rect.fromLTRB(double.negativeInfinity, current.rect.top, double.infinity, current.rect.bottom);
return candidates.where((_ReadingOrderSortData item) {
return !item.rect.intersect(band).isEmpty;
}).toList();
}
final List<_ReadingOrderSortData> inBandOfTop = inBand(topmost, candidates);
// It has to have at least topmost in it if the topmost is not degenerate.
assert(topmost.rect.isEmpty || inBandOfTop.isNotEmpty);
// The topmost rect is in a band by itself, so just return that one.
if (inBandOfTop.length <= 1) {
return topmost;
}
// Now that we know there are others in the same band as the topmost, then pick
// the one at the beginning, depending on the text direction in force.
// Find out the directionality of the nearest common Directionality
// ancestor for all nodes. This provides a base directionality to use for
// the ordering of the groups.
final TextDirection? nearestCommonDirectionality = _ReadingOrderSortData.commonDirectionalityOf(inBandOfTop);
// Do an initial common-directionality-based sort to get consistent geometric
// ordering for grouping into directionality groups. It has to use the
// common directionality to be able to group into sane groups for the
// given directionality, since rectangles can overlap and give different
// results for different directionalities.
_ReadingOrderSortData.sortWithDirectionality(inBandOfTop, nearestCommonDirectionality!);
// Collect the top band into internally sorted groups with shared directionality.
final List<_ReadingOrderDirectionalGroupData> bandGroups = _collectDirectionalityGroups(inBandOfTop);
if (bandGroups.length == 1) {
// There's only one directionality group, so just send back the first
// one in that group, since it's already sorted.
return bandGroups.first.members.first;
}
// Sort the groups based on the common directionality and bounding boxes.
_ReadingOrderDirectionalGroupData.sortWithDirectionality(bandGroups, nearestCommonDirectionality);
return bandGroups.first.members.first;
}
// Sorts the list of nodes based on their geometry into the desired reading
// order based on the directionality of the context for each node.
@override
Iterable<FocusNode> sortDescendants(Iterable<FocusNode> descendants, FocusNode currentNode) {
if (descendants.length <= 1) {
return descendants;
}
final List<_ReadingOrderSortData> data = <_ReadingOrderSortData>[
for (final FocusNode node in descendants) _ReadingOrderSortData(node),
];
final List<FocusNode> sortedList = <FocusNode>[];
final List<_ReadingOrderSortData> unplaced = data;
// Pick the initial widget as the one that is at the beginning of the band
// of the topmost, or the topmost, if there are no others in its band.
_ReadingOrderSortData current = _pickNext(unplaced);
sortedList.add(current.node);
unplaced.remove(current);
// Go through each node, picking the next one after eliminating the previous
// one, since removing the previously picked node will expose a new band in
// which to choose candidates.
while (unplaced.isNotEmpty) {
final _ReadingOrderSortData next = _pickNext(unplaced);
current = next;
sortedList.add(current.node);
unplaced.remove(current);
}
return sortedList;
}
}
/// Base class for all sort orders for [OrderedTraversalPolicy] traversal.
///
/// {@template flutter.widgets.FocusOrder.comparable}
/// Only orders of the same type are comparable. If a set of widgets in the same
/// [FocusTraversalGroup] contains orders that are not comparable with each
/// other, it will assert, since the ordering between such keys is undefined. To
/// avoid collisions, use a [FocusTraversalGroup] to group similarly ordered
/// widgets together.
///
/// When overriding, [FocusOrder.doCompare] must be overridden instead of
/// [FocusOrder.compareTo], which calls [FocusOrder.doCompare] to do the actual
/// comparison.
/// {@endtemplate}
///
/// See also:
///
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [FocusTraversalOrder], a widget that assigns an order to a widget subtree
/// for the [OrderedTraversalPolicy] to use.
/// * [NumericFocusOrder], for a focus order that describes its order with a
/// `double`.
/// * [LexicalFocusOrder], a focus order that assigns a string-based lexical
/// traversal order to a [FocusTraversalOrder] widget.
@immutable
abstract class FocusOrder with Diagnosticable implements Comparable<FocusOrder> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const FocusOrder();
/// Compares this object to another [Comparable].
///
/// When overriding [FocusOrder], implement [doCompare] instead of this
/// function to do the actual comparison.
///
/// Returns a value like a [Comparator] when comparing `this` to [other].
/// That is, it returns a negative integer if `this` is ordered before [other],
/// a positive integer if `this` is ordered after [other],
/// and zero if `this` and [other] are ordered together.
///
/// The [other] argument must be a value that is comparable to this object.
@override
@nonVirtual
int compareTo(FocusOrder other) {
assert(
runtimeType == other.runtimeType,
"The sorting algorithm must not compare incomparable keys, since they don't "
'know how to order themselves relative to each other. Comparing $this with $other',
);
return doCompare(other);
}
/// The subclass implementation called by [compareTo] to compare orders.
///
/// The argument is guaranteed to be of the same [runtimeType] as this object.
///
/// The method should return a negative number if this object comes earlier in
/// the sort order than the `other` argument; and a positive number if it
/// comes later in the sort order than `other`. Returning zero causes the
/// system to fall back to the secondary sort order defined by
/// [OrderedTraversalPolicy.secondary]
@protected
int doCompare(covariant FocusOrder other);
}
/// Can be given to a [FocusTraversalOrder] widget to assign a numerical order
/// to a widget subtree that is using a [OrderedTraversalPolicy] to define the
/// order in which widgets should be traversed with the keyboard.
///
/// {@macro flutter.widgets.FocusOrder.comparable}
///
/// See also:
///
/// * [FocusTraversalOrder], a widget that assigns an order to a widget subtree
/// for the [OrderedTraversalPolicy] to use.
class NumericFocusOrder extends FocusOrder {
/// Creates an object that describes a focus traversal order numerically.
const NumericFocusOrder(this.order);
/// The numerical order to assign to the widget subtree using
/// [FocusTraversalOrder].
///
/// Determines the placement of this widget in a sequence of widgets that defines
/// the order in which this node is traversed by the focus policy.
///
/// Lower values will be traversed first.
final double order;
@override
int doCompare(NumericFocusOrder other) => order.compareTo(other.order);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('order', order));
}
}
/// Can be given to a [FocusTraversalOrder] widget to use a String to assign a
/// lexical order to a widget subtree that is using a
/// [OrderedTraversalPolicy] to define the order in which widgets should be
/// traversed with the keyboard.
///
/// This sorts strings using Dart's default string comparison, which is not
/// locale-specific.
///
/// {@macro flutter.widgets.FocusOrder.comparable}
///
/// See also:
///
/// * [FocusTraversalOrder], a widget that assigns an order to a widget subtree
/// for the [OrderedTraversalPolicy] to use.
class LexicalFocusOrder extends FocusOrder {
/// Creates an object that describes a focus traversal order lexically.
const LexicalFocusOrder(this.order);
/// The String that defines the lexical order to assign to the widget subtree
/// using [FocusTraversalOrder].
///
/// Determines the placement of this widget in a sequence of widgets that defines
/// the order in which this node is traversed by the focus policy.
///
/// Lower lexical values will be traversed first (e.g. 'a' comes before 'z').
final String order;
@override
int doCompare(LexicalFocusOrder other) => order.compareTo(other.order);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('order', order));
}
}
// Used to help sort the focus nodes in an OrderedFocusTraversalPolicy.
class _OrderedFocusInfo {
const _OrderedFocusInfo({required this.node, required this.order});
final FocusNode node;
final FocusOrder order;
}
/// A [FocusTraversalPolicy] that orders nodes by an explicit order that resides
/// in the nearest [FocusTraversalOrder] widget ancestor.
///
/// {@macro flutter.widgets.FocusOrder.comparable}
///
/// {@tool dartpad}
/// This sample shows how to assign a traversal order to a widget. In the
/// example, the focus order goes from bottom right (the "One" button) to top
/// left (the "Six" button).
///
/// ** See code in examples/api/lib/widgets/focus_traversal/ordered_traversal_policy.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [FocusTraversalGroup], a widget that groups together and imposes a
/// traversal policy on the [Focus] nodes below it in the widget hierarchy.
/// * [WidgetOrderTraversalPolicy], a policy that relies on the widget
/// creation order to describe the order of traversal.
/// * [ReadingOrderTraversalPolicy], a policy that describes the order as the
/// natural "reading order" for the current [Directionality].
/// * [NumericFocusOrder], a focus order that assigns a numeric traversal order
/// to a [FocusTraversalOrder] widget.
/// * [LexicalFocusOrder], a focus order that assigns a string-based lexical
/// traversal order to a [FocusTraversalOrder] widget.
/// * [FocusOrder], an abstract base class for all types of focus traversal
/// orderings.
class OrderedTraversalPolicy extends FocusTraversalPolicy with DirectionalFocusTraversalPolicyMixin {
/// Constructs a traversal policy that orders widgets for keyboard traversal
/// based on an explicit order.
///
/// If [secondary] is null, it will default to [ReadingOrderTraversalPolicy].
OrderedTraversalPolicy({this.secondary, super.requestFocusCallback});
/// This is the policy that is used when a node doesn't have an order
/// assigned, or when multiple nodes have orders which are identical.
///
/// If not set, this defaults to [ReadingOrderTraversalPolicy].
///
/// This policy determines the secondary sorting order of nodes which evaluate
/// as having an identical order (including those with no order specified).
///
/// Nodes with no order specified will be sorted after nodes with an explicit
/// order.
final FocusTraversalPolicy? secondary;
@override
Iterable<FocusNode> sortDescendants(Iterable<FocusNode> descendants, FocusNode currentNode) {
final FocusTraversalPolicy secondaryPolicy = secondary ?? ReadingOrderTraversalPolicy();
final Iterable<FocusNode> sortedDescendants = secondaryPolicy.sortDescendants(descendants, currentNode);
final List<FocusNode> unordered = <FocusNode>[];
final List<_OrderedFocusInfo> ordered = <_OrderedFocusInfo>[];
for (final FocusNode node in sortedDescendants) {
final FocusOrder? order = FocusTraversalOrder.maybeOf(node.context!);
if (order != null) {
ordered.add(_OrderedFocusInfo(node: node, order: order));
} else {
unordered.add(node);
}
}
mergeSort<_OrderedFocusInfo>(ordered, compare: (_OrderedFocusInfo a, _OrderedFocusInfo b) {
assert(
a.order.runtimeType == b.order.runtimeType,
'When sorting nodes for determining focus order, the order (${a.order}) of '
"node ${a.node}, isn't the same type as the order (${b.order}) of ${b.node}. "
"Incompatible order types can't be compared. Use a FocusTraversalGroup to group "
'similar orders together.',
);
return a.order.compareTo(b.order);
});
return ordered.map<FocusNode>((_OrderedFocusInfo info) => info.node).followedBy(unordered);
}
}
/// An inherited widget that describes the order in which its child subtree
/// should be traversed.
///
/// {@macro flutter.widgets.FocusOrder.comparable}
///
/// The order for a widget is determined by the [FocusOrder] returned by
/// [FocusTraversalOrder.of] for a particular context.
class FocusTraversalOrder extends InheritedWidget {
/// Creates an inherited widget used to describe the focus order of
/// the [child] subtree.
const FocusTraversalOrder({super.key, required this.order, required super.child});
/// The order for the widget descendants of this [FocusTraversalOrder].
final FocusOrder order;
/// Finds the [FocusOrder] in the nearest ancestor [FocusTraversalOrder] widget.
///
/// It does not create a rebuild dependency because changing the traversal
/// order doesn't change the widget tree, so nothing needs to be rebuilt as a
/// result of an order change.
///
/// If no [FocusTraversalOrder] ancestor exists, or the order is null, this
/// will assert in debug mode, and throw an exception in release mode.
static FocusOrder of(BuildContext context) {
final FocusTraversalOrder? marker = context.getInheritedWidgetOfExactType<FocusTraversalOrder>();
assert(() {
if (marker == null) {
throw FlutterError(
'FocusTraversalOrder.of() was called with a context that '
'does not contain a FocusTraversalOrder widget. No TraversalOrder widget '
'ancestor could be found starting from the context that was passed to '
'FocusTraversalOrder.of().\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return marker!.order;
}
/// Finds the [FocusOrder] in the nearest ancestor [FocusTraversalOrder] widget.
///
/// It does not create a rebuild dependency because changing the traversal
/// order doesn't change the widget tree, so nothing needs to be rebuilt as a
/// result of an order change.
///
/// If no [FocusTraversalOrder] ancestor exists, or the order is null, returns null.
static FocusOrder? maybeOf(BuildContext context) {
final FocusTraversalOrder? marker = context.getInheritedWidgetOfExactType<FocusTraversalOrder>();
return marker?.order;
}
// Since the order of traversal doesn't affect display of anything, we don't
// need to force a rebuild of anything that depends upon it.
@override
bool updateShouldNotify(InheritedWidget oldWidget) => false;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<FocusOrder>('order', order));
}
}
/// A widget that describes the inherited focus policy for focus traversal for
/// its descendants, grouping them into a separate traversal group.
///
/// A traversal group is treated as one entity when sorted by the traversal
/// algorithm, so it can be used to segregate different parts of the widget tree
/// that need to be sorted using different algorithms and/or sort orders when
/// using an [OrderedTraversalPolicy].
///
/// Within the group, it will use the given [policy] to order the elements. The
/// group itself will be ordered using the parent group's policy.
///
/// By default, traverses in reading order using [ReadingOrderTraversalPolicy].
///
/// To prevent the members of the group from being focused, set the
/// [descendantsAreFocusable] attribute to false.
///
/// {@tool dartpad}
/// This sample shows three rows of buttons, each grouped by a
/// [FocusTraversalGroup], each with different traversal order policies. Use tab
/// traversal to see the order they are traversed in. The first row follows a
/// numerical order, the second follows a lexical order (ordered to traverse
/// right to left), and the third ignores the numerical order assigned to it and
/// traverses in widget order.
///
/// ** See code in examples/api/lib/widgets/focus_traversal/focus_traversal_group.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [FocusNode], for a description of the focus system.
/// * [WidgetOrderTraversalPolicy], a policy that relies on the widget
/// creation order to describe the order of traversal.
/// * [ReadingOrderTraversalPolicy], a policy that describes the order as the
/// natural "reading order" for the current [Directionality].
/// * [DirectionalFocusTraversalPolicyMixin] a mixin class that implements
/// focus traversal in a direction.
class FocusTraversalGroup extends StatefulWidget {
/// Creates a [FocusTraversalGroup] object.
FocusTraversalGroup({
super.key,
FocusTraversalPolicy? policy,
this.descendantsAreFocusable = true,
this.descendantsAreTraversable = true,
required this.child,
}) : policy = policy ?? ReadingOrderTraversalPolicy();
/// The policy used to move the focus from one focus node to another when
/// traversing them using a keyboard.
///
/// If not specified, traverses in reading order using
/// [ReadingOrderTraversalPolicy].
///
/// See also:
///
/// * [FocusTraversalPolicy] for the API used to impose traversal order
/// policy.
/// * [WidgetOrderTraversalPolicy] for a traversal policy that traverses
/// nodes in the order they are added to the widget tree.
/// * [ReadingOrderTraversalPolicy] for a traversal policy that traverses
/// nodes in the reading order defined in the widget tree, and then top to
/// bottom.
final FocusTraversalPolicy policy;
/// {@macro flutter.widgets.Focus.descendantsAreFocusable}
final bool descendantsAreFocusable;
/// {@macro flutter.widgets.Focus.descendantsAreTraversable}
final bool descendantsAreTraversable;
/// The child widget of this [FocusTraversalGroup].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Returns the [FocusTraversalPolicy] that applies to the nearest ancestor of
/// the given [FocusNode].
///
/// Will return null if no [FocusTraversalPolicy] ancestor applies to the
/// given [FocusNode].
///
/// The [FocusTraversalPolicy] is set by introducing a [FocusTraversalGroup]
/// into the widget tree, which will associate a policy with the focus tree
/// under the nearest ancestor [Focus] widget.
///
/// This function differs from [maybeOf] in that it takes a [FocusNode] and
/// only traverses the focus tree to determine the policy in effect. Unlike
/// this function, the [maybeOf] function takes a [BuildContext] and first
/// walks up the widget tree to find the nearest ancestor [Focus] or
/// [FocusScope] widget, and then calls this function with the focus node
/// associated with that widget to determine the policy in effect.
static FocusTraversalPolicy? maybeOfNode(FocusNode node) {
return _getGroupNode(node)?.policy;
}
static _FocusTraversalGroupNode? _getGroupNode(FocusNode node) {
while (node.parent != null) {
if (node.context == null) {
return null;
}
if (node is _FocusTraversalGroupNode) {
return node;
}
node = node.parent!;
}
return null;
}
/// Returns the [FocusTraversalPolicy] that applies to the [FocusNode] of the
/// nearest ancestor [Focus] widget, given a [BuildContext].
///
/// Will throw a [FlutterError] in debug mode, and throw a null check
/// exception in release mode, if no [Focus] ancestor is found, or if no
/// [FocusTraversalPolicy] applies to the associated [FocusNode].
///
/// {@template flutter.widgets.focus_traversal.FocusTraversalGroup.of}
/// This function looks up the nearest ancestor [Focus] (or [FocusScope])
/// widget, and uses its [FocusNode] (or [FocusScopeNode]) to walk up the
/// focus tree to find the applicable [FocusTraversalPolicy] for that node.
///
/// Calling this function does not create a rebuild dependency because
/// changing the traversal order doesn't change the widget tree, so nothing
/// needs to be rebuilt as a result of an order change.
///
/// The [FocusTraversalPolicy] is set by introducing a [FocusTraversalGroup]
/// into the widget tree, which will associate a policy with the focus tree
/// under the nearest ancestor [Focus] widget.
/// {@endtemplate}
///
/// See also:
///
/// * [maybeOf] for a similar function that will return null if no
/// [FocusTraversalGroup] ancestor is found.
/// * [maybeOfNode] for a function that will look for a policy using a given
/// [FocusNode], and return null if no policy applies.
static FocusTraversalPolicy of(BuildContext context) {
final FocusTraversalPolicy? policy = maybeOf(context);
assert(() {
if (policy == null) {
throw FlutterError(
'Unable to find a Focus or FocusScope widget in the given context, or the FocusNode '
'from with the widget that was found is not associated with a FocusTraversalPolicy.\n'
'FocusTraversalGroup.of() was called with a context that does not contain a '
'Focus or FocusScope widget, or there was no FocusTraversalPolicy in effect.\n'
'This can happen if there is not a FocusTraversalGroup that defines the policy, '
'or if the context comes from a widget that is above the WidgetsApp, MaterialApp, '
'or CupertinoApp widget (those widgets introduce an implicit default policy) \n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return policy!;
}
/// Returns the [FocusTraversalPolicy] that applies to the [FocusNode] of the
/// nearest ancestor [Focus] widget, or null, given a [BuildContext].
///
/// Will return null if it doesn't find an ancestor [Focus] or [FocusScope]
/// widget, or doesn't find a [FocusTraversalPolicy] that applies to the node.
///
/// {@macro flutter.widgets.focus_traversal.FocusTraversalGroup.of}
///
/// See also:
///
/// * [maybeOfNode] for a similar function that will look for a policy using a
/// given [FocusNode].
/// * [of] for a similar function that will throw if no [FocusTraversalPolicy]
/// applies.
static FocusTraversalPolicy? maybeOf(BuildContext context) {
final FocusNode? node = Focus.maybeOf(context, scopeOk: true, createDependency: false);
if (node == null) {
return null;
}
return FocusTraversalGroup.maybeOfNode(node);
}
@override
State<FocusTraversalGroup> createState() => _FocusTraversalGroupState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<FocusTraversalPolicy>('policy', policy));
}
}
// A special focus node subclass that only FocusTraversalGroup uses so that it
// can be used to cache the policy in the focus tree, and so that the traversal
// code can find groups in the focus tree.
class _FocusTraversalGroupNode extends FocusNode {
_FocusTraversalGroupNode({
super.debugLabel,
required this.policy,
}) {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
FocusTraversalPolicy policy;
}
class _FocusTraversalGroupState extends State<FocusTraversalGroup> {
// The internal focus node used to collect the children of this node into a
// group, and to provide a context for the traversal algorithm to sort the
// group with. It's a special subclass of FocusNode just so that it can be
// identified when walking the focus tree during traversal, and hold the
// current policy.
late final _FocusTraversalGroupNode focusNode = _FocusTraversalGroupNode(
debugLabel: 'FocusTraversalGroup',
policy: widget.policy,
);
@override
void dispose() {
focusNode.dispose();
super.dispose();
}
@override
void didUpdateWidget (FocusTraversalGroup oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.policy != widget.policy) {
focusNode.policy = widget.policy;
}
}
@override
Widget build(BuildContext context) {
return Focus(
focusNode: focusNode,
canRequestFocus: false,
skipTraversal: true,
includeSemantics: false,
descendantsAreFocusable: widget.descendantsAreFocusable,
descendantsAreTraversable: widget.descendantsAreTraversable,
child: widget.child,
);
}
}
/// An intent for use with the [RequestFocusAction], which supplies the
/// [FocusNode] that should be focused.
class RequestFocusIntent extends Intent {
/// Creates an intent used with [RequestFocusAction].
///
/// {@macro flutter.widgets.FocusTraversalPolicy.requestFocusCallback}
const RequestFocusIntent(this.focusNode, {
TraversalRequestFocusCallback? requestFocusCallback
}) : requestFocusCallback = requestFocusCallback ?? FocusTraversalPolicy.defaultTraversalRequestFocusCallback;
/// The callback used to move the focus to the node [focusNode].
/// By default it requests focus on the node and ensures the node is visible
/// if it's in a scrollable.
final TraversalRequestFocusCallback requestFocusCallback;
/// The [FocusNode] that is to be focused.
final FocusNode focusNode;
}
/// An [Action] that requests the focus on the node it is given in its
/// [RequestFocusIntent].
///
/// This action can be used to request focus for a particular node, by calling
/// [Action.invoke] like so:
///
/// ```dart
/// Actions.invoke(context, RequestFocusIntent(focusNode));
/// ```
///
/// Where the `focusNode` is the node for which the focus will be requested.
///
/// The difference between requesting focus in this way versus calling
/// [FocusNode.requestFocus] directly is that it will use the [Action]
/// registered in the nearest [Actions] widget associated with
/// [RequestFocusIntent] to make the request, rather than just requesting focus
/// directly. This allows the action to have additional side effects, like
/// logging, or undo and redo functionality.
///
/// This [RequestFocusAction] class is the default action associated with the
/// [RequestFocusIntent] in the [WidgetsApp]. It requests focus. You
/// can redefine the associated action with your own [Actions] widget.
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class RequestFocusAction extends Action<RequestFocusIntent> {
@override
void invoke(RequestFocusIntent intent) {
intent.requestFocusCallback(intent.focusNode);
}
}
/// An [Intent] bound to [NextFocusAction], which moves the focus to the next
/// focusable node in the focus traversal order.
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class NextFocusIntent extends Intent {
/// Creates an intent that is used with [NextFocusAction].
const NextFocusIntent();
}
/// An [Action] that moves the focus to the next focusable node in the focus
/// order.
///
/// This action is the default action registered for the [NextFocusIntent], and
/// by default is bound to the [LogicalKeyboardKey.tab] key in the [WidgetsApp].
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class NextFocusAction extends Action<NextFocusIntent> {
/// Attempts to pass the focus to the next widget.
///
/// Returns true if a widget was focused as a result of invoking this action.
///
/// Returns false when the traversal reached the end and the engine must pass
/// focus to platform UI.
@override
bool invoke(NextFocusIntent intent) {
return primaryFocus!.nextFocus();
}
@override
KeyEventResult toKeyEventResult(NextFocusIntent intent, bool invokeResult) {
return invokeResult ? KeyEventResult.handled : KeyEventResult.skipRemainingHandlers;
}
}
/// An [Intent] bound to [PreviousFocusAction], which moves the focus to the
/// previous focusable node in the focus traversal order.
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class PreviousFocusIntent extends Intent {
/// Creates an intent that is used with [PreviousFocusAction].
const PreviousFocusIntent();
}
/// An [Action] that moves the focus to the previous focusable node in the focus
/// order.
///
/// This action is the default action registered for the [PreviousFocusIntent],
/// and by default is bound to a combination of the [LogicalKeyboardKey.tab] key
/// and the [LogicalKeyboardKey.shift] key in the [WidgetsApp].
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class PreviousFocusAction extends Action<PreviousFocusIntent> {
/// Attempts to pass the focus to the previous widget.
///
/// Returns true if a widget was focused as a result of invoking this action.
///
/// Returns false when the traversal reached the beginning and the engine must
/// pass focus to platform UI.
@override
bool invoke(PreviousFocusIntent intent) {
return primaryFocus!.previousFocus();
}
@override
KeyEventResult toKeyEventResult(PreviousFocusIntent intent, bool invokeResult) {
return invokeResult ? KeyEventResult.handled : KeyEventResult.skipRemainingHandlers;
}
}
/// An [Intent] that represents moving to the next focusable node in the given
/// [direction].
///
/// This is the [Intent] bound by default to the [LogicalKeyboardKey.arrowUp],
/// [LogicalKeyboardKey.arrowDown], [LogicalKeyboardKey.arrowLeft], and
/// [LogicalKeyboardKey.arrowRight] keys in the [WidgetsApp], with the
/// appropriate associated directions.
///
/// See [FocusTraversalPolicy] for more information about focus traversal.
class DirectionalFocusIntent extends Intent {
/// Creates an intent used to move the focus in the given [direction].
const DirectionalFocusIntent(this.direction, {this.ignoreTextFields = true});
/// The direction in which to look for the next focusable node when the
/// associated [DirectionalFocusAction] is invoked.
final TraversalDirection direction;
/// If true, then directional focus actions that occur within a text field
/// will not happen when the focus node which received the key is a text
/// field.
///
/// Defaults to true.
final bool ignoreTextFields;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<TraversalDirection>('direction', direction));
}
}
/// An [Action] that moves the focus to the focusable node in the direction
/// configured by the associated [DirectionalFocusIntent.direction].
///
/// This is the [Action] associated with [DirectionalFocusIntent] and bound by
/// default to the [LogicalKeyboardKey.arrowUp], [LogicalKeyboardKey.arrowDown],
/// [LogicalKeyboardKey.arrowLeft], and [LogicalKeyboardKey.arrowRight] keys in
/// the [WidgetsApp], with the appropriate associated directions.
class DirectionalFocusAction extends Action<DirectionalFocusIntent> {
/// Creates a [DirectionalFocusAction].
DirectionalFocusAction() : _isForTextField = false;
/// Creates a [DirectionalFocusAction] that ignores [DirectionalFocusIntent]s
/// whose `ignoreTextFields` field is true.
DirectionalFocusAction.forTextField() : _isForTextField = true;
// Whether this action is defined in a text field.
final bool _isForTextField;
@override
void invoke(DirectionalFocusIntent intent) {
if (!intent.ignoreTextFields || !_isForTextField) {
primaryFocus!.focusInDirection(intent.direction);
}
}
}
/// A widget that controls whether or not the descendants of this widget are
/// traversable.
///
/// Does not affect the value of [FocusNode.skipTraversal] of the descendants.
///
/// See also:
///
/// * [Focus], a widget for adding and managing a [FocusNode] in the widget tree.
/// * [ExcludeFocus], a widget that excludes its descendants from focusability.
/// * [FocusTraversalGroup], a widget that groups widgets for focus traversal,
/// and can also be used in the same way as this widget by setting its
/// `descendantsAreFocusable` attribute.
class ExcludeFocusTraversal extends StatelessWidget {
/// Const constructor for [ExcludeFocusTraversal] widget.
const ExcludeFocusTraversal({
super.key,
this.excluding = true,
required this.child,
});
/// If true, will make this widget's descendants untraversable.
///
/// Defaults to true.
///
/// Does not affect the value of [FocusNode.skipTraversal] on the descendants.
///
/// See also:
///
/// * [Focus.descendantsAreTraversable], the attribute of a [Focus] widget that
/// controls this same property for focus widgets.
/// * [FocusTraversalGroup], a widget used to group together and configure the
/// focus traversal policy for a widget subtree that has a
/// `descendantsAreFocusable` parameter to conditionally block focus for a
/// subtree.
final bool excluding;
/// The child widget of this [ExcludeFocusTraversal].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
Widget build(BuildContext context) {
return Focus(
canRequestFocus: false,
skipTraversal: true,
includeSemantics: false,
descendantsAreTraversable: !excluding,
child: child,
);
}
}
| flutter/packages/flutter/lib/src/widgets/focus_traversal.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/focus_traversal.dart",
"repo_id": "flutter",
"token_count": 28533
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'framework.dart';
// Examples can assume:
// TooltipThemeData data = const TooltipThemeData();
/// An [InheritedWidget] that defines visual properties like colors
/// and text styles, which the [child]'s subtree depends on.
///
/// The [wrap] method is used by [captureAll] and [CapturedThemes.wrap] to
/// construct a widget that will wrap a child in all of the inherited themes
/// which are present in a specified part of the widget tree.
///
/// A widget that's shown in a different context from the one it's built in,
/// like the contents of a new route or an overlay, will be able to see the
/// ancestor inherited themes of the context it was built in.
///
/// {@tool dartpad}
/// This example demonstrates how `InheritedTheme.capture()` can be used
/// to wrap the contents of a new route with the inherited themes that
/// are present when the route was built - but are not present when route
/// is actually shown.
///
/// If the same code is run without `InheritedTheme.capture(), the
/// new route's Text widget will inherit the "something must be wrong"
/// fallback text style, rather than the default text style defined in MyApp.
///
/// ** See code in examples/api/lib/widgets/inherited_theme/inherited_theme.0.dart **
/// {@end-tool}
abstract class InheritedTheme extends InheritedWidget {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const InheritedTheme({
super.key,
required super.child,
});
/// Return a copy of this inherited theme with the specified [child].
///
/// This implementation for [TooltipTheme] is typical:
///
/// ```dart
/// Widget wrap(BuildContext context, Widget child) {
/// return TooltipTheme(data: data, child: child);
/// }
/// ```
Widget wrap(BuildContext context, Widget child);
/// Returns a widget that will [wrap] `child` in all of the inherited themes
/// which are present between `context` and the specified `to`
/// [BuildContext].
///
/// The `to` context must be an ancestor of `context`. If `to` is not
/// specified, all inherited themes up to the root of the widget tree are
/// captured.
///
/// After calling this method, the themes present between `context` and `to`
/// are frozen for the provided `child`. If the themes (or their theme data)
/// change in the original subtree, those changes will not be visible to
/// the wrapped `child` - unless this method is called again to re-wrap the
/// child.
static Widget captureAll(BuildContext context, Widget child, {BuildContext? to}) {
return capture(from: context, to: to).wrap(child);
}
/// Returns a [CapturedThemes] object that includes all the [InheritedTheme]s
/// between the given `from` and `to` [BuildContext]s.
///
/// The `to` context must be an ancestor of the `from` context. If `to` is
/// null, all ancestor inherited themes of `from` up to the root of the
/// widget tree are captured.
///
/// After calling this method, the themes present between `from` and `to` are
/// frozen in the returned [CapturedThemes] object. If the themes (or their
/// theme data) change in the original subtree, those changes will not be
/// applied to the themes captured in the [CapturedThemes] object - unless
/// this method is called again to re-capture the updated themes.
///
/// To wrap a [Widget] in the captured themes, call [CapturedThemes.wrap].
///
/// This method can be expensive if there are many widgets between `from` and
/// `to` (it walks the element tree between those nodes).
static CapturedThemes capture({ required BuildContext from, required BuildContext? to }) {
if (from == to) {
// Nothing to capture.
return CapturedThemes._(const <InheritedTheme>[]);
}
final List<InheritedTheme> themes = <InheritedTheme>[];
final Set<Type> themeTypes = <Type>{};
late bool debugDidFindAncestor;
assert(() {
debugDidFindAncestor = to == null;
return true;
}());
from.visitAncestorElements((Element ancestor) {
if (ancestor == to) {
assert(() {
debugDidFindAncestor = true;
return true;
}());
return false;
}
if (ancestor is InheritedElement && ancestor.widget is InheritedTheme) {
final InheritedTheme theme = ancestor.widget as InheritedTheme;
final Type themeType = theme.runtimeType;
// Only remember the first theme of any type. This assumes
// that inherited themes completely shadow ancestors of the
// same type.
if (!themeTypes.contains(themeType)) {
themeTypes.add(themeType);
themes.add(theme);
}
}
return true;
});
assert(debugDidFindAncestor, 'The provided `to` context must be an ancestor of the `from` context.');
return CapturedThemes._(themes);
}
}
/// Stores a list of captured [InheritedTheme]s that can be wrapped around a
/// child [Widget].
///
/// Used as return type by [InheritedTheme.capture].
class CapturedThemes {
CapturedThemes._(this._themes);
final List<InheritedTheme> _themes;
/// Wraps a `child` [Widget] in the [InheritedTheme]s captured in this object.
Widget wrap(Widget child) {
return _CaptureAll(themes: _themes, child: child);
}
}
class _CaptureAll extends StatelessWidget {
const _CaptureAll({
required this.themes,
required this.child,
});
final List<InheritedTheme> themes;
final Widget child;
@override
Widget build(BuildContext context) {
Widget wrappedChild = child;
for (final InheritedTheme theme in themes) {
wrappedChild = theme.wrap(context, wrappedChild);
}
return wrappedChild;
}
}
| flutter/packages/flutter/lib/src/widgets/inherited_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/inherited_theme.dart",
"repo_id": "flutter",
"token_count": 1814
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
/// Defines the horizontal alignment of [OverflowBar] children
/// when they're laid out in an overflow column.
///
/// This value must be interpreted relative to the ambient
/// [TextDirection].
enum OverflowBarAlignment {
/// Each child is left-aligned for [TextDirection.ltr],
/// right-aligned for [TextDirection.rtl].
start,
/// Each child is right-aligned for [TextDirection.ltr],
/// left-aligned for [TextDirection.rtl].
end,
/// Each child is horizontally centered.
center,
}
/// A widget that lays out its [children] in a row unless they
/// "overflow" the available horizontal space, in which case it lays
/// them out in a column instead.
///
/// This widget's width will expand to contain its children and the
/// specified [spacing] until it overflows. The overflow column will
/// consume all of the available width. The [overflowAlignment]
/// defines how each child will be aligned within the overflow column
/// and the [overflowSpacing] defines the gap between each child.
///
/// The order that the children appear in the horizontal layout
/// is defined by the [textDirection], just like the [Row] widget.
/// If the layout overflows, then children's order within their
/// column is specified by [overflowDirection] instead.
///
/// {@tool dartpad}
/// This example defines a simple approximation of a dialog
/// layout, where the layout of the dialog's action buttons are
/// defined by an [OverflowBar]. The content is wrapped in a
/// [SingleChildScrollView], so that if overflow occurs, the
/// action buttons will still be accessible by scrolling,
/// no matter how much vertical space is available.
///
/// ** See code in examples/api/lib/widgets/overflow_bar/overflow_bar.0.dart **
/// {@end-tool}
class OverflowBar extends MultiChildRenderObjectWidget {
/// Constructs an OverflowBar.
const OverflowBar({
super.key,
this.spacing = 0.0,
this.alignment,
this.overflowSpacing = 0.0,
this.overflowAlignment = OverflowBarAlignment.start,
this.overflowDirection = VerticalDirection.down,
this.textDirection,
super.children,
});
/// The width of the gap between [children] for the default
/// horizontal layout.
///
/// If the horizontal layout overflows, then [overflowSpacing] is
/// used instead.
///
/// Defaults to 0.0.
final double spacing;
/// Defines the [children]'s horizontal layout according to the same
/// rules as for [Row.mainAxisAlignment].
///
/// If this property is non-null, and the [children], separated by
/// [spacing], fit within the available width, then the overflow
/// bar will be as wide as possible. If the children do not fit
/// within the available width, then this property is ignored and
/// [overflowAlignment] applies instead.
///
/// If this property is null (the default) then the overflow bar
/// will be no wider than needed to layout the [children] separated
/// by [spacing], modulo the incoming constraints.
///
/// If [alignment] is one of [MainAxisAlignment.spaceAround],
/// [MainAxisAlignment.spaceBetween], or
/// [MainAxisAlignment.spaceEvenly], then the [spacing] parameter is
/// only used to see if the horizontal layout will overflow.
///
/// Defaults to null.
///
/// See also:
///
/// * [overflowAlignment], the horizontal alignment of the [children] within
/// the vertical "overflow" layout.
///
final MainAxisAlignment? alignment;
/// The height of the gap between [children] in the vertical
/// "overflow" layout.
///
/// This parameter is only used if the horizontal layout overflows, i.e.
/// if there isn't enough horizontal room for the [children] and [spacing].
///
/// Defaults to 0.0.
///
/// See also:
///
/// * [spacing], The width of the gap between each pair of children
/// for the default horizontal layout.
final double overflowSpacing;
/// The horizontal alignment of the [children] within the vertical
/// "overflow" layout.
///
/// This parameter is only used if the horizontal layout overflows, i.e.
/// if there isn't enough horizontal room for the [children] and [spacing].
/// In that case the overflow bar will expand to fill the available
/// width and it will layout its [children] in a column. The
/// horizontal alignment of each child within that column is
/// defined by this parameter and the [textDirection]. If the
/// [textDirection] is [TextDirection.ltr] then each child will be
/// aligned with the left edge of the available space for
/// [OverflowBarAlignment.start], with the right edge of the
/// available space for [OverflowBarAlignment.end]. Similarly, if the
/// [textDirection] is [TextDirection.rtl] then each child will
/// be aligned with the right edge of the available space for
/// [OverflowBarAlignment.start], and with the left edge of the
/// available space for [OverflowBarAlignment.end]. For
/// [OverflowBarAlignment.center] each child is horizontally
/// centered within the available space.
///
/// Defaults to [OverflowBarAlignment.start].
///
/// See also:
///
/// * [alignment], which defines the [children]'s horizontal layout
/// (according to the same rules as for [Row.mainAxisAlignment]) when
/// the children, separated by [spacing], fit within the available space.
/// * [overflowDirection], which defines the order that the
/// [OverflowBar]'s children appear in, if the horizontal layout
/// overflows.
final OverflowBarAlignment overflowAlignment;
/// Defines the order that the [children] appear in, if
/// the horizontal layout overflows.
///
/// This parameter is only used if the horizontal layout overflows, i.e.
/// if there isn't enough horizontal room for the [children] and [spacing].
///
/// If the children do not fit into a single row, then they
/// are arranged in a column. The first child is at the top of the
/// column if this property is set to [VerticalDirection.down], since it
/// "starts" at the top and "ends" at the bottom. On the other hand,
/// the first child will be at the bottom of the column if this
/// property is set to [VerticalDirection.up], since it "starts" at the
/// bottom and "ends" at the top.
///
/// Defaults to [VerticalDirection.down].
///
/// See also:
///
/// * [overflowAlignment], which defines the horizontal alignment
/// of the children within the vertical "overflow" layout.
final VerticalDirection overflowDirection;
/// Determines the order that the [children] appear in for the default
/// horizontal layout, and the interpretation of
/// [OverflowBarAlignment.start] and [OverflowBarAlignment.end] for
/// the vertical overflow layout.
///
/// For the default horizontal layout, if [textDirection] is
/// [TextDirection.rtl] then the last child is laid out first. If
/// [textDirection] is [TextDirection.ltr] then the first child is
/// laid out first.
///
/// If this parameter is null, then the value of
/// `Directionality.of(context)` is used.
///
/// See also:
///
/// * [overflowDirection], which defines the order that the
/// [OverflowBar]'s children appear in, if the horizontal layout
/// overflows.
/// * [Directionality], which defines the ambient directionality of
/// text and text-direction-sensitive render objects.
final TextDirection? textDirection;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderOverflowBar(
spacing: spacing,
alignment: alignment,
overflowSpacing: overflowSpacing,
overflowAlignment: overflowAlignment,
overflowDirection: overflowDirection,
textDirection: textDirection ?? Directionality.of(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderObject renderObject) {
(renderObject as _RenderOverflowBar)
..spacing = spacing
..alignment = alignment
..overflowSpacing = overflowSpacing
..overflowAlignment = overflowAlignment
..overflowDirection = overflowDirection
..textDirection = textDirection ?? Directionality.of(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('spacing', spacing, defaultValue: 0));
properties.add(EnumProperty<MainAxisAlignment>('alignment', alignment, defaultValue: null));
properties.add(DoubleProperty('overflowSpacing', overflowSpacing, defaultValue: 0));
properties.add(EnumProperty<OverflowBarAlignment>('overflowAlignment', overflowAlignment, defaultValue: OverflowBarAlignment.start));
properties.add(EnumProperty<VerticalDirection>('overflowDirection', overflowDirection, defaultValue: VerticalDirection.down));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
class _OverflowBarParentData extends ContainerBoxParentData<RenderBox> { }
class _RenderOverflowBar extends RenderBox
with ContainerRenderObjectMixin<RenderBox, _OverflowBarParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, _OverflowBarParentData> {
_RenderOverflowBar({
List<RenderBox>? children,
double spacing = 0.0,
MainAxisAlignment? alignment,
double overflowSpacing = 0.0,
OverflowBarAlignment overflowAlignment = OverflowBarAlignment.start,
VerticalDirection overflowDirection = VerticalDirection.down,
required TextDirection textDirection,
}) : _spacing = spacing,
_alignment = alignment,
_overflowSpacing = overflowSpacing,
_overflowAlignment = overflowAlignment,
_overflowDirection = overflowDirection,
_textDirection = textDirection {
addAll(children);
}
double get spacing => _spacing;
double _spacing;
set spacing (double value) {
if (_spacing == value) {
return;
}
_spacing = value;
markNeedsLayout();
}
MainAxisAlignment? get alignment => _alignment;
MainAxisAlignment? _alignment;
set alignment (MainAxisAlignment? value) {
if (_alignment == value) {
return;
}
_alignment = value;
markNeedsLayout();
}
double get overflowSpacing => _overflowSpacing;
double _overflowSpacing;
set overflowSpacing (double value) {
if (_overflowSpacing == value) {
return;
}
_overflowSpacing = value;
markNeedsLayout();
}
OverflowBarAlignment get overflowAlignment => _overflowAlignment;
OverflowBarAlignment _overflowAlignment;
set overflowAlignment (OverflowBarAlignment value) {
if (_overflowAlignment == value) {
return;
}
_overflowAlignment = value;
markNeedsLayout();
}
VerticalDirection get overflowDirection => _overflowDirection;
VerticalDirection _overflowDirection;
set overflowDirection (VerticalDirection value) {
if (_overflowDirection == value) {
return;
}
_overflowDirection = value;
markNeedsLayout();
}
TextDirection get textDirection => _textDirection;
TextDirection _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
markNeedsLayout();
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! _OverflowBarParentData) {
child.parentData = _OverflowBarParentData();
}
}
@override
double computeMinIntrinsicHeight(double width) {
RenderBox? child = firstChild;
if (child == null) {
return 0;
}
double barWidth = 0.0;
while (child != null) {
barWidth += child.getMinIntrinsicWidth(double.infinity);
child = childAfter(child);
}
barWidth += spacing * (childCount - 1);
double height = 0.0;
if (barWidth > width) {
child = firstChild;
while (child != null) {
height += child.getMinIntrinsicHeight(width);
child = childAfter(child);
}
return height + overflowSpacing * (childCount - 1);
} else {
child = firstChild;
while (child != null) {
height = math.max(height, child.getMinIntrinsicHeight(width));
child = childAfter(child);
}
return height;
}
}
@override
double computeMaxIntrinsicHeight(double width) {
RenderBox? child = firstChild;
if (child == null) {
return 0;
}
double barWidth = 0.0;
while (child != null) {
barWidth += child.getMinIntrinsicWidth(double.infinity);
child = childAfter(child);
}
barWidth += spacing * (childCount - 1);
double height = 0.0;
if (barWidth > width) {
child = firstChild;
while (child != null) {
height += child.getMaxIntrinsicHeight(width);
child = childAfter(child);
}
return height + overflowSpacing * (childCount - 1);
} else {
child = firstChild;
while (child != null) {
height = math.max(height, child.getMaxIntrinsicHeight(width));
child = childAfter(child);
}
return height;
}
}
@override
double computeMinIntrinsicWidth(double height) {
RenderBox? child = firstChild;
if (child == null) {
return 0;
}
double width = 0.0;
while (child != null) {
width += child.getMinIntrinsicWidth(double.infinity);
child = childAfter(child);
}
return width + spacing * (childCount - 1);
}
@override
double computeMaxIntrinsicWidth(double height) {
RenderBox? child = firstChild;
if (child == null) {
return 0;
}
double width = 0.0;
while (child != null) {
width += child.getMaxIntrinsicWidth(double.infinity);
child = childAfter(child);
}
return width + spacing * (childCount - 1);
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
return defaultComputeDistanceToHighestActualBaseline(baseline);
}
@override
Size computeDryLayout(BoxConstraints constraints) {
RenderBox? child = firstChild;
if (child == null) {
return constraints.smallest;
}
final BoxConstraints childConstraints = constraints.loosen();
double childrenWidth = 0.0;
double maxChildHeight = 0.0;
double y = 0.0;
while (child != null) {
final Size childSize = child.getDryLayout(childConstraints);
childrenWidth += childSize.width;
maxChildHeight = math.max(maxChildHeight, childSize.height);
y += childSize.height + overflowSpacing;
child = childAfter(child);
}
final double actualWidth = childrenWidth + spacing * (childCount - 1);
if (actualWidth > constraints.maxWidth) {
return constraints.constrain(Size(constraints.maxWidth, y - overflowSpacing));
} else {
final double overallWidth = alignment == null ? actualWidth : constraints.maxWidth;
return constraints.constrain(Size(overallWidth, maxChildHeight));
}
}
@override
void performLayout() {
RenderBox? child = firstChild;
if (child == null) {
size = constraints.smallest;
return;
}
final BoxConstraints childConstraints = constraints.loosen();
double childrenWidth = 0;
double maxChildHeight = 0;
double maxChildWidth = 0;
while (child != null) {
child.layout(childConstraints, parentUsesSize: true);
childrenWidth += child.size.width;
maxChildHeight = math.max(maxChildHeight, child.size.height);
maxChildWidth = math.max(maxChildWidth, child.size.width);
child = childAfter(child);
}
final bool rtl = textDirection == TextDirection.rtl;
final double actualWidth = childrenWidth + spacing * (childCount - 1);
if (actualWidth > constraints.maxWidth) {
// Overflow vertical layout
child = overflowDirection == VerticalDirection.down ? firstChild : lastChild;
RenderBox? nextChild() => overflowDirection == VerticalDirection.down ? childAfter(child!) : childBefore(child!);
double y = 0;
while (child != null) {
final _OverflowBarParentData childParentData = child.parentData! as _OverflowBarParentData;
final double x = switch (overflowAlignment) {
OverflowBarAlignment.center => (constraints.maxWidth - child.size.width) / 2,
OverflowBarAlignment.start => rtl ? constraints.maxWidth - child.size.width : 0,
OverflowBarAlignment.end => rtl ? 0 : constraints.maxWidth - child.size.width,
};
childParentData.offset = Offset(x, y);
y += child.size.height + overflowSpacing;
child = nextChild();
}
size = constraints.constrain(Size(constraints.maxWidth, y - overflowSpacing));
} else {
// Default horizontal layout
child = firstChild;
final double firstChildWidth = child!.size.width;
final double overallWidth = alignment == null ? actualWidth : constraints.maxWidth;
size = constraints.constrain(Size(overallWidth, maxChildHeight));
late double x; // initial value: origin of the first child
double layoutSpacing = spacing; // space between children
switch (alignment) {
case null:
x = rtl ? size.width - firstChildWidth : 0;
case MainAxisAlignment.start:
x = rtl ? size.width - firstChildWidth : 0;
case MainAxisAlignment.center:
final double halfRemainingWidth = (size.width - actualWidth) / 2;
x = rtl ? size.width - halfRemainingWidth - firstChildWidth : halfRemainingWidth;
case MainAxisAlignment.end:
x = rtl ? actualWidth - firstChildWidth : size.width - actualWidth;
case MainAxisAlignment.spaceBetween:
layoutSpacing = (size.width - childrenWidth) / (childCount - 1);
x = rtl ? size.width - firstChildWidth : 0;
case MainAxisAlignment.spaceAround:
layoutSpacing = childCount > 0 ? (size.width - childrenWidth) / childCount : 0;
x = rtl ? size.width - layoutSpacing / 2 - firstChildWidth : layoutSpacing / 2;
case MainAxisAlignment.spaceEvenly:
layoutSpacing = (size.width - childrenWidth) / (childCount + 1);
x = rtl ? size.width - layoutSpacing - firstChildWidth : layoutSpacing;
}
while (child != null) {
final _OverflowBarParentData childParentData = child.parentData! as _OverflowBarParentData;
childParentData.offset = Offset(x, (maxChildHeight - child.size.height) / 2);
// x is the horizontal origin of child. To advance x to the next child's
// origin for LTR: add the width of the current child. To advance x to
// the origin of the next child for RTL: subtract the width of the next
// child (if there is one).
if (!rtl) {
x += child.size.width + layoutSpacing;
}
child = childAfter(child);
if (rtl && child != null) {
x -= child.size.width + layoutSpacing;
}
}
}
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
return defaultHitTestChildren(result, position: position);
}
@override
void paint(PaintingContext context, Offset offset) {
defaultPaint(context, offset);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('spacing', spacing, defaultValue: 0));
properties.add(DoubleProperty('overflowSpacing', overflowSpacing, defaultValue: 0));
properties.add(EnumProperty<OverflowBarAlignment>('overflowAlignment', overflowAlignment, defaultValue: OverflowBarAlignment.start));
properties.add(EnumProperty<VerticalDirection>('overflowDirection', overflowDirection, defaultValue: VerticalDirection.down));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/overflow_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/overflow_bar.dart",
"repo_id": "flutter",
"token_count": 6629
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'framework.dart';
export 'package:flutter/services.dart' show RestorationBucket;
/// Creates a new scope for restoration IDs used by descendant widgets to claim
/// [RestorationBucket]s.
///
/// {@template flutter.widgets.RestorationScope}
/// A restoration scope inserts a [RestorationBucket] into the widget tree,
/// which descendant widgets can access via [RestorationScope.of]. It is
/// uncommon for descendants to directly store data in this bucket. Instead,
/// descendant widgets should consider storing their own restoration data in a
/// child bucket claimed with [RestorationBucket.claimChild] from the bucket
/// provided by this scope.
/// {@endtemplate}
///
/// The bucket inserted into the widget tree by this scope has been claimed from
/// the surrounding [RestorationScope] using the provided [restorationId]. If
/// the [RestorationScope] is moved to a different part of the widget tree under
/// a different [RestorationScope], the bucket owned by this scope with all its
/// children and the data contained in them is moved to the new scope as well.
///
/// This widget will not make a [RestorationBucket] available to descendants if
/// [restorationId] is null or when there is no surrounding restoration scope to
/// claim a bucket from. In this case, descendant widgets invoking
/// [RestorationScope.of] will receive null as a return value indicating that no
/// bucket is available for storing restoration data. This will turn off state
/// restoration for the widget subtree.
///
/// See also:
///
/// * [RootRestorationScope], which inserts the root bucket provided by
/// the [RestorationManager] into the widget tree and makes it accessible
/// for descendants via [RestorationScope.of].
/// * [UnmanagedRestorationScope], which inserts a provided [RestorationBucket]
/// into the widget tree and makes it accessible for descendants via
/// [RestorationScope.of].
/// * [RestorationMixin], which may be used in [State] objects to manage the
/// restoration data of a [StatefulWidget] instead of manually interacting
/// with [RestorationScope]s and [RestorationBucket]s.
/// * [RestorationManager], which describes the basic concepts of state
/// restoration in Flutter.
class RestorationScope extends StatefulWidget {
/// Creates a [RestorationScope].
///
/// Providing null as the [restorationId] turns off state restoration for
/// the [child] and its descendants.
const RestorationScope({
super.key,
required this.restorationId,
required this.child,
});
/// Returns the [RestorationBucket] inserted into the widget tree by the
/// closest ancestor [RestorationScope] of `context`.
///
/// {@template flutter.widgets.restoration.RestorationScope.bucket_warning}
/// To avoid accidentally overwriting data already stored in the bucket by its
/// owner, data should not be stored directly in the bucket returned by this
/// method. Instead, consider claiming a child bucket from the returned bucket
/// (via [RestorationBucket.claimChild]) and store the restoration data in
/// that child.
/// {@endtemplate}
///
/// This method returns null if state restoration is turned off for this
/// subtree.
///
/// Calling this method will create a dependency on the closest
/// [RestorationScope] in the [context], if there is one.
///
/// See also:
///
/// * [RestorationScope.maybeOf], which is similar to this method, but asserts
/// if no [RestorationScope] ancestor is found.
static RestorationBucket? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<UnmanagedRestorationScope>()?.bucket;
}
/// Returns the [RestorationBucket] inserted into the widget tree by the
/// closest ancestor [RestorationScope] of `context`.
///
/// {@macro flutter.widgets.restoration.RestorationScope.bucket_warning}
///
/// This method will assert in debug mode and throw an exception in release
/// mode if state restoration is turned off for this subtree.
///
/// Calling this method will create a dependency on the closest
/// [RestorationScope] in the [context].
///
/// See also:
///
/// * [RestorationScope.maybeOf], which is similar to this method, but returns
/// null if no [RestorationScope] ancestor is found.
static RestorationBucket of(BuildContext context) {
final RestorationBucket? bucket = maybeOf(context);
assert(() {
if (bucket == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'RestorationScope.of() was called with a context that does not '
'contain a RestorationScope widget. '
),
ErrorDescription(
'No RestorationScope widget ancestor could be found starting from '
'the context that was passed to RestorationScope.of(). This can '
'happen because you are using a widget that looks for a '
'RestorationScope ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context'
),
ErrorHint(
'State restoration must be enabled for a RestorationScope to exist. '
'This can be done by passing a restorationScopeId to MaterialApp, '
'CupertinoApp, or WidgetsApp at the root of the widget tree or by '
'wrapping the widget tree in a RootRestorationScope.'
),
],
);
}
return true;
}());
return bucket!;
}
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The restoration ID used by this widget to obtain a child bucket from the
/// surrounding [RestorationScope].
///
/// The child bucket obtained from the surrounding scope is made available to
/// descendant widgets via [RestorationScope.of].
///
/// If this is null, [RestorationScope.of] invoked by descendants will return
/// null which effectively turns off state restoration for this subtree.
final String? restorationId;
@override
State<RestorationScope> createState() => _RestorationScopeState();
}
class _RestorationScopeState extends State<RestorationScope> with RestorationMixin {
@override
String? get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
// Nothing to do.
// The bucket gets injected into the widget tree in the build method.
}
@override
Widget build(BuildContext context) {
return UnmanagedRestorationScope(
bucket: bucket, // `bucket` is provided by the RestorationMixin.
child: widget.child,
);
}
}
/// Inserts a provided [RestorationBucket] into the widget tree and makes it
/// available to descendants via [RestorationScope.of].
///
/// {@macro flutter.widgets.RestorationScope}
///
/// If [bucket] is null, no restoration bucket is made available to descendant
/// widgets ([RestorationScope.of] invoked from a descendant will return null).
/// This effectively turns off state restoration for the subtree because no
/// bucket for storing restoration data is made available.
///
/// See also:
///
/// * [RestorationScope], which inserts a bucket obtained from a surrounding
/// restoration scope into the widget tree and makes it accessible
/// for descendants via [RestorationScope.of].
/// * [RootRestorationScope], which inserts the root bucket provided by
/// the [RestorationManager] into the widget tree and makes it accessible
/// for descendants via [RestorationScope.of].
/// * [RestorationMixin], which may be used in [State] objects to manage the
/// restoration data of a [StatefulWidget] instead of manually interacting
/// with [RestorationScope]s and [RestorationBucket]s.
/// * [RestorationManager], which describes the basic concepts of state
/// restoration in Flutter.
class UnmanagedRestorationScope extends InheritedWidget {
/// Creates an [UnmanagedRestorationScope].
///
/// When [bucket] is null state restoration is turned off for the [child] and
/// its descendants.
const UnmanagedRestorationScope({
super.key,
this.bucket,
required super.child,
});
/// The [RestorationBucket] that this widget will insert into the widget tree.
///
/// Descendant widgets may obtain this bucket via [RestorationScope.of].
final RestorationBucket? bucket;
@override
bool updateShouldNotify(UnmanagedRestorationScope oldWidget) {
return oldWidget.bucket != bucket;
}
}
/// Inserts a child bucket of [RestorationManager.rootBucket] into the widget
/// tree and makes it available to descendants via [RestorationScope.of].
///
/// This widget is usually used near the root of the widget tree to enable the
/// state restoration functionality for the application. For all other use
/// cases, consider using a regular [RestorationScope] instead.
///
/// The root restoration bucket can only be retrieved asynchronously from the
/// [RestorationManager]. To ensure that the provided [child] has its
/// restoration data available the first time it builds, the
/// [RootRestorationScope] will build an empty [Container] instead of the actual
/// [child] until the root bucket is available. To hide the empty container from
/// the eyes of users, the [RootRestorationScope] also delays rendering the
/// first frame while the container is shown. On platforms that show a splash
/// screen on app launch the splash screen is kept up (hiding the empty
/// container) until the bucket is available and the [child] is ready to be
/// build.
///
/// The exact behavior of this widget depends on its ancestors: When the
/// [RootRestorationScope] does not find an ancestor restoration bucket via
/// [RestorationScope.of] it will claim a child bucket from the root restoration
/// bucket ([RestorationManager.rootBucket]) using the provided [restorationId]
/// and inserts that bucket into the widget tree where descendants may access it
/// via [RestorationScope.of]. If the [RootRestorationScope] finds a non-null
/// ancestor restoration bucket via [RestorationScope.of] it will behave like a
/// regular [RestorationScope] instead: It will claim a child bucket from that
/// ancestor and insert that child into the widget tree.
///
/// Unlike the [RestorationScope] widget, the [RootRestorationScope] will
/// guarantee that descendants have a bucket available for storing restoration
/// data as long as [restorationId] is not null and [RestorationManager] is
/// able to provide a root bucket. In other words, it will force-enable
/// state restoration for the subtree if [restorationId] is not null.
///
/// If [restorationId] is null, no bucket is made available to descendants,
/// which effectively turns off state restoration for this subtree.
///
/// See also:
///
/// * [RestorationScope], which inserts a bucket obtained from a surrounding
/// restoration scope into the widget tree and makes it accessible
/// for descendants via [RestorationScope.of].
/// * [UnmanagedRestorationScope], which inserts a provided [RestorationBucket]
/// into the widget tree and makes it accessible for descendants via
/// [RestorationScope.of].
/// * [RestorationMixin], which may be used in [State] objects to manage the
/// restoration data of a [StatefulWidget] instead of manually interacting
/// with [RestorationScope]s and [RestorationBucket]s.
/// * [RestorationManager], which describes the basic concepts of state
/// restoration in Flutter.
class RootRestorationScope extends StatefulWidget {
/// Creates a [RootRestorationScope].
///
/// Providing null as the [restorationId] turns off state restoration for
/// the [child] and its descendants.
const RootRestorationScope({
super.key,
required this.restorationId,
required this.child,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The restoration ID used to identify the child bucket that this widget
/// will insert into the tree.
///
/// If this is null, no bucket is made available to descendants and state
/// restoration for the subtree is essentially turned off.
final String? restorationId;
@override
State<RootRestorationScope> createState() => _RootRestorationScopeState();
}
class _RootRestorationScopeState extends State<RootRestorationScope> {
bool? _okToRenderBlankContainer;
bool _rootBucketValid = false;
RestorationBucket? _rootBucket;
RestorationBucket? _ancestorBucket;
@override
void didChangeDependencies() {
super.didChangeDependencies();
_ancestorBucket = RestorationScope.maybeOf(context);
_loadRootBucketIfNecessary();
_okToRenderBlankContainer ??= widget.restorationId != null && _needsRootBucketInserted;
}
@override
void didUpdateWidget(RootRestorationScope oldWidget) {
super.didUpdateWidget(oldWidget);
_loadRootBucketIfNecessary();
}
bool get _needsRootBucketInserted => _ancestorBucket == null;
bool get _isWaitingForRootBucket {
return widget.restorationId != null && _needsRootBucketInserted && !_rootBucketValid;
}
bool _isLoadingRootBucket = false;
void _loadRootBucketIfNecessary() {
if (_isWaitingForRootBucket && !_isLoadingRootBucket) {
_isLoadingRootBucket = true;
RendererBinding.instance.deferFirstFrame();
ServicesBinding.instance.restorationManager.rootBucket.then((RestorationBucket? bucket) {
_isLoadingRootBucket = false;
if (mounted) {
ServicesBinding.instance.restorationManager.addListener(_replaceRootBucket);
setState(() {
_rootBucket = bucket;
_rootBucketValid = true;
_okToRenderBlankContainer = false;
});
}
RendererBinding.instance.allowFirstFrame();
});
}
}
void _replaceRootBucket() {
_rootBucketValid = false;
_rootBucket = null;
ServicesBinding.instance.restorationManager.removeListener(_replaceRootBucket);
_loadRootBucketIfNecessary();
assert(!_isWaitingForRootBucket); // Ensure that load finished synchronously.
}
@override
void dispose() {
if (_rootBucketValid) {
ServicesBinding.instance.restorationManager.removeListener(_replaceRootBucket);
}
super.dispose();
}
@override
Widget build(BuildContext context) {
if (_okToRenderBlankContainer! && _isWaitingForRootBucket) {
return const SizedBox.shrink();
}
return UnmanagedRestorationScope(
bucket: _ancestorBucket ?? _rootBucket,
child: RestorationScope(
restorationId: widget.restorationId,
child: widget.child,
),
);
}
}
/// Manages an object of type `T`, whose value a [State] object wants to have
/// restored during state restoration.
///
/// The property wraps an object of type `T`. It knows how to store its value in
/// the restoration data and it knows how to re-instantiate that object from the
/// information it previously stored in the restoration data.
///
/// The knowledge of how to store the wrapped object in the restoration data is
/// encoded in the [toPrimitives] method and the knowledge of how to
/// re-instantiate the object from that data is encoded in the [fromPrimitives]
/// method. A call to [toPrimitives] must return a representation of the wrapped
/// object that can be serialized with the [StandardMessageCodec]. If any
/// collections (e.g. [List]s, [Map]s, etc.) are returned, they must not be
/// modified after they have been returned from [toPrimitives]. At a later point
/// in time (which may be after the application restarted), the data obtained
/// from [toPrimitives] may be handed back to the property's [fromPrimitives]
/// method to restore it to the previous state described by that data.
///
/// A [RestorableProperty] needs to be registered to a [RestorationMixin] using
/// a restoration ID that is unique within the mixin. The [RestorationMixin]
/// provides and manages the [RestorationBucket], in which the data returned by
/// [toPrimitives] is stored.
///
/// Whenever the value returned by [toPrimitives] (or the [enabled] getter)
/// changes, the [RestorableProperty] must call [notifyListeners]. This will
/// trigger the [RestorationMixin] to update the data it has stored for the
/// property in its [RestorationBucket] to the latest information returned by
/// [toPrimitives].
///
/// When the property is registered with the [RestorationMixin], the mixin
/// checks whether there is any restoration data available for the property. If
/// data is available, the mixin calls [fromPrimitives] on the property, which
/// must return an object that matches the object the property wrapped when the
/// provided restoration data was obtained from [toPrimitives]. If no
/// restoration data is available to restore the property's wrapped object from,
/// the mixin calls [createDefaultValue]. The value returned by either of those
/// methods is then handed to the property's [initWithValue] method.
///
/// Usually, subclasses of [RestorableProperty] hold on to the value provided to
/// them in [initWithValue] and make it accessible to the [State] object that
/// owns the property. This [RestorableProperty] base class, however, has no
/// opinion about what to do with the value provided to [initWithValue].
///
/// The [RestorationMixin] may call [fromPrimitives]/[createDefaultValue]
/// followed by [initWithValue] multiple times throughout the life of a
/// [RestorableProperty]: Whenever new restoration data is made available to the
/// [RestorationMixin] the property is registered with, the cycle of calling
/// [fromPrimitives] (if the new restoration data contains information to
/// restore the property from) or [createDefaultValue] (if no information for
/// the property is available in the new restoration data) followed by a call to
/// [initWithValue] repeats. Whenever [initWithValue] is called, the property
/// should forget the old value it was wrapping and re-initialize itself with
/// the newly provided value.
///
/// In a typical use case, a subclass of [RestorableProperty] is instantiated
/// either to initialize a member variable of a [State] object or within
/// [State.initState]. It is then registered to a [RestorationMixin] in
/// [RestorationMixin.restoreState] and later [dispose]ed in [State.dispose].
/// For less common use cases (e.g. if the value stored in a
/// [RestorableProperty] is only needed while the [State] object is in a certain
/// state), a [RestorableProperty] may be registered with a [RestorationMixin]
/// any time after [RestorationMixin.restoreState] has been called for the first
/// time. A [RestorableProperty] may also be unregistered from a
/// [RestorationMixin] before the owning [State] object is disposed by calling
/// [RestorationMixin.unregisterFromRestoration]. This is uncommon, though, and
/// will delete the information that the property contributed from the
/// restoration data (meaning the value of the property will no longer be
/// restored during a future state restoration).
///
/// See also:
///
/// * [RestorableValue], which is a [RestorableProperty] that makes the wrapped
/// value accessible to the owning [State] object via a `value`
/// getter and setter.
/// * [RestorationMixin], to which a [RestorableProperty] must be registered.
/// * [RestorationManager], which describes how state restoration works in
/// Flutter.
abstract class RestorableProperty<T> extends ChangeNotifier {
/// Creates a [RestorableProperty].
RestorableProperty(){
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// Called by the [RestorationMixin] if no restoration data is available to
/// restore the value of the property from to obtain the default value for the
/// property.
///
/// The method returns the default value that the property should wrap if no
/// restoration data is available. After this is called, [initWithValue] will
/// be called with this method's return value.
///
/// The method may be called multiple times throughout the life of the
/// [RestorableProperty]. Whenever new restoration data has been provided to
/// the [RestorationMixin] the property is registered to, either this method
/// or [fromPrimitives] is called before [initWithValue] is invoked.
T createDefaultValue();
/// Called by the [RestorationMixin] to convert the `data` previously
/// retrieved from [toPrimitives] back into an object of type `T` that this
/// property should wrap.
///
/// The object returned by this method is passed to [initWithValue] to restore
/// the value that this property is wrapping to the value described by the
/// provided `data`.
///
/// The method may be called multiple times throughout the life of the
/// [RestorableProperty]. Whenever new restoration data has been provided to
/// the [RestorationMixin] the property is registered to, either this method
/// or [createDefaultValue] is called before [initWithValue] is invoked.
T fromPrimitives(Object? data);
/// Called by the [RestorationMixin] with the `value` returned by either
/// [createDefaultValue] or [fromPrimitives] to set the value that this
/// property currently wraps.
///
/// The [initWithValue] method may be called multiple times throughout the
/// life of the [RestorableProperty] whenever new restoration data has been
/// provided to the [RestorationMixin] the property is registered to. When
/// [initWithValue] is called, the property should forget its previous value
/// and re-initialize itself to the newly provided `value`.
void initWithValue(T value);
/// Called by the [RestorationMixin] to retrieve the information that this
/// property wants to store in the restoration data.
///
/// The returned object must be serializable with the [StandardMessageCodec]
/// and if it includes any collections, those should not be modified after
/// they have been returned by this method.
///
/// The information returned by this method may be handed back to the property
/// in a call to [fromPrimitives] at a later point in time (possibly after the
/// application restarted) to restore the value that the property is currently
/// wrapping.
///
/// When the value returned by this method changes, the property must call
/// [notifyListeners]. The [RestorationMixin] will invoke this method whenever
/// the property's listeners are notified.
Object? toPrimitives();
/// Whether the object currently returned by [toPrimitives] should be included
/// in the restoration state.
///
/// When this returns false, no information is included in the restoration
/// data for this property and the property will be initialized to its default
/// value (obtained from [createDefaultValue]) the next time that restoration
/// data is used for state restoration.
///
/// Whenever the value returned by this getter changes, [notifyListeners] must
/// be called. When the value changes from true to false, the information last
/// retrieved from [toPrimitives] is removed from the restoration data. When
/// it changes from false to true, [toPrimitives] is invoked to add the latest
/// restoration information provided by this property to the restoration data.
bool get enabled => true;
bool _disposed = false;
@override
void dispose() {
assert(ChangeNotifier.debugAssertNotDisposed(this)); // FYI, This uses ChangeNotifier's _debugDisposed, not _disposed.
_owner?._unregister(this);
super.dispose();
_disposed = true;
}
// ID under which the property has been registered with the RestorationMixin.
String? _restorationId;
RestorationMixin? _owner;
void _register(String restorationId, RestorationMixin owner) {
assert(ChangeNotifier.debugAssertNotDisposed(this));
_restorationId = restorationId;
_owner = owner;
}
void _unregister() {
assert(ChangeNotifier.debugAssertNotDisposed(this));
assert(_restorationId != null);
assert(_owner != null);
_restorationId = null;
_owner = null;
}
/// The [State] object that this property is registered with.
///
/// Must only be called when [isRegistered] is true.
@protected
State get state {
assert(isRegistered);
assert(ChangeNotifier.debugAssertNotDisposed(this));
return _owner!;
}
/// Whether this property is currently registered with a [RestorationMixin].
@protected
bool get isRegistered {
assert(ChangeNotifier.debugAssertNotDisposed(this));
return _restorationId != null;
}
}
/// Manages the restoration data for a [State] object of a [StatefulWidget].
///
/// Restoration data can be serialized out and, at a later point in time, be
/// used to restore the stateful members in the [State] object to the same
/// values they had when the data was generated.
///
/// This mixin organizes the restoration data of a [State] object in
/// [RestorableProperty]. All the information that the [State] object wants to
/// get restored during state restoration need to be saved in a subclass of
/// [RestorableProperty]. For example, to restore the count value in a counter
/// app, that value should be stored in a member variable of type
/// [RestorableInt] instead of a plain member variable of type [int].
///
/// The mixin ensures that the current values of the [RestorableProperty]s are
/// serialized as part of the restoration state. It is up to the [State] to
/// ensure that the data stored in the properties is always up to date. When the
/// widget is restored from previously generated restoration data, the values of
/// the [RestorableProperty]s are automatically restored to the values that had
/// when the restoration data was serialized out.
///
/// Within a [State] that uses this mixin, [RestorableProperty]s are usually
/// instantiated to initialize member variables. Users of the mixin must
/// override [restoreState] and register their previously instantiated
/// [RestorableProperty]s in this method by calling [registerForRestoration].
/// The mixin calls this method for the first time right after
/// [State.initState]. After registration, the values stored in the property
/// have either been restored to their previous value or - if no restoration
/// data for restoring is available - they are initialized with a
/// property-specific default value. At the end of a [State] object's life
/// cycle, all restorable properties must be disposed in [State.dispose].
///
/// In addition to being invoked right after [State.initState], [restoreState]
/// is invoked again when new restoration data has been provided to the mixin.
/// When this happens, the [State] object must re-register all properties with
/// [registerForRestoration] again to restore them to their previous values as
/// described by the new restoration data. All initialization logic that depends
/// on the current value of a restorable property should be included in the
/// [restoreState] method to ensure it re-executes when the properties are
/// restored to a different value during the life time of the [State] object.
///
/// Internally, the mixin stores the restoration data from all registered
/// properties in a [RestorationBucket] claimed from the surrounding
/// [RestorationScope] using the [State]-provided [restorationId]. The
/// [restorationId] must be unique in the surrounding [RestorationScope]. State
/// restoration is disabled for the [State] object using this mixin if
/// [restorationId] is null or when there is no surrounding [RestorationScope].
/// In that case, the values of the registered properties will not be restored
/// during state restoration.
///
/// The [RestorationBucket] used to store the registered properties is available
/// via the [bucket] getter. Interacting directly with the bucket is uncommon,
/// but the [State] object may make this bucket available for its descendants to
/// claim child buckets from. For that, the [bucket] is injected into the widget
/// tree in [State.build] with the help of an [UnmanagedRestorationScope].
///
/// The [bucket] getter returns null if state restoration is turned off. If
/// state restoration is turned on or off during the lifetime of the widget
/// (e.g. because [restorationId] changes from null to non-null) the value
/// returned by the getter will also change from null to non-null or vice versa.
/// The mixin calls [didToggleBucket] on itself to notify the [State] object
/// about this change. Overriding this method is not necessary as long as the
/// [State] object does not directly interact with the [bucket].
///
/// Whenever the value returned by [restorationId] changes,
/// [didUpdateRestorationId] must be called (unless the change already triggers
/// a call to [didUpdateWidget]).
///
/// {@tool dartpad}
/// This example demonstrates how to make a simple counter app restorable by
/// using the [RestorationMixin] and a [RestorableInt].
///
/// ** See code in examples/api/lib/widgets/restoration/restoration_mixin.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RestorableProperty], which is the base class for all restoration
/// properties managed by this mixin.
/// * [RestorationManager], which describes how state restoration in Flutter
/// works.
/// * [RestorationScope], which creates a new namespace for restoration IDs
/// in the widget tree.
@optionalTypeArgs
mixin RestorationMixin<S extends StatefulWidget> on State<S> {
/// The restoration ID used for the [RestorationBucket] in which the mixin
/// will store the restoration data of all registered properties.
///
/// The restoration ID is used to claim a child [RestorationScope] from the
/// surrounding [RestorationScope] (accessed via [RestorationScope.of]) and
/// the ID must be unique in that scope (otherwise an exception is triggered
/// in debug mode).
///
/// State restoration for this mixin is turned off when this getter returns
/// null or when there is no surrounding [RestorationScope] available. When
/// state restoration is turned off, the values of the registered properties
/// cannot be restored.
///
/// Whenever the value returned by this getter changes,
/// [didUpdateRestorationId] must be called unless the (unless the change
/// already triggered a call to [didUpdateWidget]).
///
/// The restoration ID returned by this getter is often provided in the
/// constructor of the [StatefulWidget] that this [State] object is associated
/// with.
@protected
String? get restorationId;
/// The [RestorationBucket] used for the restoration data of the
/// [RestorableProperty]s registered to this mixin.
///
/// The bucket has been claimed from the surrounding [RestorationScope] using
/// [restorationId].
///
/// The getter returns null if state restoration is turned off. When state
/// restoration is turned on or off during the lifetime of this mixin (and
/// hence the return value of this getter switches between null and non-null)
/// [didToggleBucket] is called.
///
/// Interacting directly with this bucket is uncommon. However, the bucket may
/// be injected into the widget tree in the [State]'s `build` method using an
/// [UnmanagedRestorationScope]. That allows descendants to claim child
/// buckets from this bucket for their own restoration needs.
RestorationBucket? get bucket => _bucket;
RestorationBucket? _bucket;
/// Called to initialize or restore the [RestorableProperty]s used by the
/// [State] object.
///
/// This method is always invoked at least once right after [State.initState]
/// to register the [RestorableProperty]s with the mixin even when state
/// restoration is turned off or no restoration data is available for this
/// [State] object.
///
/// Typically, [registerForRestoration] is called from this method to register
/// all [RestorableProperty]s used by the [State] object with the mixin. The
/// registration will either restore the property's value to the value
/// described by the restoration data, if available, or, if no restoration
/// data is available - initialize it to a property-specific default value.
///
/// The method is called again whenever new restoration data (in the form of a
/// new [bucket]) has been provided to the mixin. When that happens, the
/// [State] object must re-register all previously registered properties,
/// which will restore their values to the value described by the new
/// restoration data.
///
/// Since the method may change the value of the registered properties when
/// new restoration state is provided, all initialization logic that depends
/// on a specific value of a [RestorableProperty] should be included in this
/// method. That way, that logic re-executes when the [RestorableProperty]s
/// have their values restored from newly provided restoration data.
///
/// The first time the method is invoked, the provided `oldBucket` argument is
/// always null. In subsequent calls triggered by new restoration data in the
/// form of a new bucket, the argument given is the previous value of
/// [bucket].
@mustCallSuper
@protected
void restoreState(RestorationBucket? oldBucket, bool initialRestore);
/// Called when [bucket] switches between null and non-null values.
///
/// [State] objects that wish to directly interact with the bucket may
/// override this method to store additional values in the bucket when one
/// becomes available or to save values stored in a bucket elsewhere when the
/// bucket goes away. This is uncommon and storing those values in
/// [RestorableProperty]s should be considered instead.
///
/// The `oldBucket` is provided to the method when the [bucket] getter changes
/// from non-null to null. The `oldBucket` argument is null when the [bucket]
/// changes from null to non-null.
///
/// See also:
///
/// * [restoreState], which is called when the [bucket] changes from one
/// non-null value to another non-null value.
@mustCallSuper
@protected
void didToggleBucket(RestorationBucket? oldBucket) {
// When a bucket is replaced, must `restoreState` is called instead.
assert(_bucket?.isReplacing != true);
}
// Maps properties to their listeners.
final Map<RestorableProperty<Object?>, VoidCallback> _properties = <RestorableProperty<Object?>, VoidCallback>{};
/// Registers a [RestorableProperty] for state restoration.
///
/// The registration associates the provided `property` with the provided
/// `restorationId`. If restoration data is available for the provided
/// `restorationId`, the property's value is restored to the value described
/// by the restoration data. If no restoration data is available, the property
/// will be initialized to a property-specific default value.
///
/// Each property within a [State] object must be registered under a unique
/// ID. Only registered properties will have their values restored during
/// state restoration.
///
/// Typically, this method is called from within [restoreState] to register
/// all restorable properties of the owning [State] object. However, if a
/// given [RestorableProperty] is only needed when certain conditions are met
/// within the [State], [registerForRestoration] may also be called at any
/// time after [restoreState] has been invoked for the first time.
///
/// A property that has been registered outside of [restoreState] must be
/// re-registered within [restoreState] the next time that method is called
/// unless it has been unregistered with [unregisterFromRestoration].
@protected
void registerForRestoration(RestorableProperty<Object?> property, String restorationId) {
assert(property._restorationId == null || (_debugDoingRestore && property._restorationId == restorationId),
'Property is already registered under ${property._restorationId}.',
);
assert(_debugDoingRestore || !_properties.keys.map((RestorableProperty<Object?> r) => r._restorationId).contains(restorationId),
'"$restorationId" is already registered to another property.',
);
final bool hasSerializedValue = bucket?.contains(restorationId) ?? false;
final Object? initialValue = hasSerializedValue
? property.fromPrimitives(bucket!.read<Object>(restorationId))
: property.createDefaultValue();
if (!property.isRegistered) {
property._register(restorationId, this);
void listener() {
if (bucket == null) {
return;
}
_updateProperty(property);
}
property.addListener(listener);
_properties[property] = listener;
}
assert(
property._restorationId == restorationId &&
property._owner == this &&
_properties.containsKey(property),
);
property.initWithValue(initialValue);
if (!hasSerializedValue && property.enabled && bucket != null) {
_updateProperty(property);
}
assert(() {
_debugPropertiesWaitingForReregistration?.remove(property);
return true;
}());
}
/// Unregisters a [RestorableProperty] from state restoration.
///
/// The value of the `property` is removed from the restoration data and it
/// will not be restored if that data is used in a future state restoration.
///
/// Calling this method is uncommon, but may be necessary if the data of a
/// [RestorableProperty] is only relevant when the [State] object is in a
/// certain state. When the data of a property is no longer necessary to
/// restore the internal state of a [State] object, it may be removed from the
/// restoration data by calling this method.
@protected
void unregisterFromRestoration(RestorableProperty<Object?> property) {
assert(property._owner == this);
_bucket?.remove<Object?>(property._restorationId!);
_unregister(property);
}
/// Must be called when the value returned by [restorationId] changes.
///
/// This method is automatically called from [didUpdateWidget]. Therefore,
/// manually invoking this method may be omitted when the change in
/// [restorationId] was caused by an updated widget.
@protected
void didUpdateRestorationId() {
// There's nothing to do if:
// - We don't have a parent to claim a bucket from.
// - Our current bucket already uses the provided restoration ID.
// - There's a restore pending, which means that didChangeDependencies
// will be called and we handle the rename there.
if (_currentParent == null || _bucket?.restorationId == restorationId || restorePending) {
return;
}
final RestorationBucket? oldBucket = _bucket;
assert(!restorePending);
final bool didReplaceBucket = _updateBucketIfNecessary(parent: _currentParent, restorePending: false);
if (didReplaceBucket) {
assert(oldBucket != _bucket);
assert(_bucket == null || oldBucket == null);
oldBucket?.dispose();
}
}
@override
void didUpdateWidget(S oldWidget) {
super.didUpdateWidget(oldWidget);
didUpdateRestorationId();
}
/// Whether [restoreState] will be called at the beginning of the next build
/// phase.
///
/// Returns true when new restoration data has been provided to the mixin, but
/// the registered [RestorableProperty]s have not been restored to their new
/// values (as described by the new restoration data) yet. The properties will
/// get the values restored when [restoreState] is invoked at the beginning of
/// the next build cycle.
///
/// While this is true, [bucket] will also still return the old bucket with
/// the old restoration data. It will update to the new bucket with the new
/// data just before [restoreState] is invoked.
bool get restorePending {
if (_firstRestorePending) {
return true;
}
if (restorationId == null) {
return false;
}
final RestorationBucket? potentialNewParent = RestorationScope.maybeOf(context);
return potentialNewParent != _currentParent && (potentialNewParent?.isReplacing ?? false);
}
List<RestorableProperty<Object?>>? _debugPropertiesWaitingForReregistration;
bool get _debugDoingRestore => _debugPropertiesWaitingForReregistration != null;
bool _firstRestorePending = true;
RestorationBucket? _currentParent;
@override
void didChangeDependencies() {
super.didChangeDependencies();
final RestorationBucket? oldBucket = _bucket;
final bool needsRestore = restorePending;
_currentParent = RestorationScope.maybeOf(context);
final bool didReplaceBucket = _updateBucketIfNecessary(parent: _currentParent, restorePending: needsRestore);
if (needsRestore) {
_doRestore(oldBucket);
}
if (didReplaceBucket) {
assert(oldBucket != _bucket);
oldBucket?.dispose();
}
}
void _doRestore(RestorationBucket? oldBucket) {
assert(() {
_debugPropertiesWaitingForReregistration = _properties.keys.toList();
return true;
}());
restoreState(oldBucket, _firstRestorePending);
_firstRestorePending = false;
assert(() {
if (_debugPropertiesWaitingForReregistration!.isNotEmpty) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'Previously registered RestorableProperties must be re-registered in "restoreState".',
),
ErrorDescription(
'The RestorableProperties with the following IDs were not re-registered to $this when '
'"restoreState" was called:',
),
..._debugPropertiesWaitingForReregistration!.map((RestorableProperty<Object?> property) => ErrorDescription(
' * ${property._restorationId}',
)),
]);
}
_debugPropertiesWaitingForReregistration = null;
return true;
}());
}
// Returns true if `bucket` has been replaced with a new bucket. It's the
// responsibility of the caller to dispose the old bucket when this returns true.
bool _updateBucketIfNecessary({
required RestorationBucket? parent,
required bool restorePending,
}) {
if (restorationId == null || parent == null) {
final bool didReplace = _setNewBucketIfNecessary(newBucket: null, restorePending: restorePending);
assert(_bucket == null);
return didReplace;
}
assert(restorationId != null);
if (restorePending || _bucket == null) {
final RestorationBucket newBucket = parent.claimChild(restorationId!, debugOwner: this);
final bool didReplace = _setNewBucketIfNecessary(newBucket: newBucket, restorePending: restorePending);
assert(_bucket == newBucket);
return didReplace;
}
// We have an existing bucket, make sure it has the right parent and id.
assert(_bucket != null);
assert(!restorePending);
_bucket!.rename(restorationId!);
parent.adoptChild(_bucket!);
return false;
}
// Returns true if `bucket` has been replaced with a new bucket. It's the
// responsibility of the caller to dispose the old bucket when this returns true.
bool _setNewBucketIfNecessary({required RestorationBucket? newBucket, required bool restorePending}) {
if (newBucket == _bucket) {
return false;
}
final RestorationBucket? oldBucket = _bucket;
_bucket = newBucket;
if (!restorePending) {
// Write the current property values into the new bucket to persist them.
if (_bucket != null) {
_properties.keys.forEach(_updateProperty);
}
didToggleBucket(oldBucket);
}
return true;
}
void _updateProperty(RestorableProperty<Object?> property) {
if (property.enabled) {
_bucket?.write(property._restorationId!, property.toPrimitives());
} else {
_bucket?.remove<Object>(property._restorationId!);
}
}
void _unregister(RestorableProperty<Object?> property) {
final VoidCallback listener = _properties.remove(property)!;
assert(() {
_debugPropertiesWaitingForReregistration?.remove(property);
return true;
}());
property.removeListener(listener);
property._unregister();
}
@override
void dispose() {
_properties.forEach((RestorableProperty<Object?> property, VoidCallback listener) {
if (!property._disposed) {
property.removeListener(listener);
}
});
_bucket?.dispose();
_bucket = null;
super.dispose();
}
}
| flutter/packages/flutter/lib/src/widgets/restoration.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/restoration.dart",
"repo_id": "flutter",
"token_count": 12580
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/gestures.dart';
import 'package:flutter/physics.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'scroll_activity.dart';
import 'scroll_context.dart';
import 'scroll_notification.dart';
import 'scroll_physics.dart';
import 'scroll_position.dart';
/// A scroll position that manages scroll activities for a single
/// [ScrollContext].
///
/// This class is a concrete subclass of [ScrollPosition] logic that handles a
/// single [ScrollContext], such as a [Scrollable]. An instance of this class
/// manages [ScrollActivity] instances, which change what content is visible in
/// the [Scrollable]'s [Viewport].
///
/// {@macro flutter.widgets.scrollPosition.listening}
///
/// See also:
///
/// * [ScrollPosition], which defines the underlying model for a position
/// within a [Scrollable] but is agnostic as to how that position is
/// changed.
/// * [ScrollView] and its subclasses such as [ListView], which use
/// [ScrollPositionWithSingleContext] to manage their scroll position.
/// * [ScrollController], which can manipulate one or more [ScrollPosition]s,
/// and which uses [ScrollPositionWithSingleContext] as its default class for
/// scroll positions.
class ScrollPositionWithSingleContext extends ScrollPosition implements ScrollActivityDelegate {
/// Create a [ScrollPosition] object that manages its behavior using
/// [ScrollActivity] objects.
///
/// The `initialPixels` argument can be null, but in that case it is
/// imperative that the value be set, using [correctPixels], as soon as
/// [applyNewDimensions] is invoked, before calling the inherited
/// implementation of that method.
///
/// If [keepScrollOffset] is true (the default), the current scroll offset is
/// saved with [PageStorage] and restored it if this scroll position's scrollable
/// is recreated.
ScrollPositionWithSingleContext({
required super.physics,
required super.context,
double? initialPixels = 0.0,
super.keepScrollOffset,
super.oldPosition,
super.debugLabel,
}) {
// If oldPosition is not null, the superclass will first call absorb(),
// which may set _pixels and _activity.
if (!hasPixels && initialPixels != null) {
correctPixels(initialPixels);
}
if (activity == null) {
goIdle();
}
assert(activity != null);
}
/// Velocity from a previous activity temporarily held by [hold] to potentially
/// transfer to a next activity.
double _heldPreviousVelocity = 0.0;
@override
AxisDirection get axisDirection => context.axisDirection;
@override
double setPixels(double newPixels) {
assert(activity!.isScrolling);
return super.setPixels(newPixels);
}
@override
void absorb(ScrollPosition other) {
super.absorb(other);
if (other is! ScrollPositionWithSingleContext) {
goIdle();
return;
}
activity!.updateDelegate(this);
_userScrollDirection = other._userScrollDirection;
assert(_currentDrag == null);
if (other._currentDrag != null) {
_currentDrag = other._currentDrag;
_currentDrag!.updateDelegate(this);
other._currentDrag = null;
}
}
@override
void applyNewDimensions() {
super.applyNewDimensions();
context.setCanDrag(physics.shouldAcceptUserOffset(this));
}
@override
void beginActivity(ScrollActivity? newActivity) {
_heldPreviousVelocity = 0.0;
if (newActivity == null) {
return;
}
assert(newActivity.delegate == this);
super.beginActivity(newActivity);
_currentDrag?.dispose();
_currentDrag = null;
if (!activity!.isScrolling) {
updateUserScrollDirection(ScrollDirection.idle);
}
}
@override
void applyUserOffset(double delta) {
updateUserScrollDirection(delta > 0.0 ? ScrollDirection.forward : ScrollDirection.reverse);
setPixels(pixels - physics.applyPhysicsToUserOffset(this, delta));
}
@override
void goIdle() {
beginActivity(IdleScrollActivity(this));
}
/// Start a physics-driven simulation that settles the [pixels] position,
/// starting at a particular velocity.
///
/// This method defers to [ScrollPhysics.createBallisticSimulation], which
/// typically provides a bounce simulation when the current position is out of
/// bounds and a friction simulation when the position is in bounds but has a
/// non-zero velocity.
///
/// The velocity should be in logical pixels per second.
@override
void goBallistic(double velocity) {
assert(hasPixels);
final Simulation? simulation = physics.createBallisticSimulation(this, velocity);
if (simulation != null) {
beginActivity(BallisticScrollActivity(
this,
simulation,
context.vsync,
activity?.shouldIgnorePointer ?? true,
));
} else {
goIdle();
}
}
@override
ScrollDirection get userScrollDirection => _userScrollDirection;
ScrollDirection _userScrollDirection = ScrollDirection.idle;
/// Set [userScrollDirection] to the given value.
///
/// If this changes the value, then a [UserScrollNotification] is dispatched.
@protected
@visibleForTesting
void updateUserScrollDirection(ScrollDirection value) {
if (userScrollDirection == value) {
return;
}
_userScrollDirection = value;
didUpdateScrollDirection(value);
}
@override
Future<void> animateTo(
double to, {
required Duration duration,
required Curve curve,
}) {
if (nearEqual(to, pixels, physics.toleranceFor(this).distance)) {
// Skip the animation, go straight to the position as we are already close.
jumpTo(to);
return Future<void>.value();
}
final DrivenScrollActivity activity = DrivenScrollActivity(
this,
from: pixels,
to: to,
duration: duration,
curve: curve,
vsync: context.vsync,
);
beginActivity(activity);
return activity.done;
}
@override
void jumpTo(double value) {
goIdle();
if (pixels != value) {
final double oldPixels = pixels;
forcePixels(value);
didStartScroll();
didUpdateScrollPositionBy(pixels - oldPixels);
didEndScroll();
}
goBallistic(0.0);
}
@override
void pointerScroll(double delta) {
// If an update is made to pointer scrolling here, consider if the same
// (or similar) change should be made in
// _NestedScrollCoordinator.pointerScroll.
if (delta == 0.0) {
goBallistic(0.0);
return;
}
final double targetPixels =
math.min(math.max(pixels + delta, minScrollExtent), maxScrollExtent);
if (targetPixels != pixels) {
goIdle();
updateUserScrollDirection(
-delta > 0.0 ? ScrollDirection.forward : ScrollDirection.reverse,
);
final double oldPixels = pixels;
// Set the notifier before calling force pixels.
// This is set to false again after going ballistic below.
isScrollingNotifier.value = true;
forcePixels(targetPixels);
didStartScroll();
didUpdateScrollPositionBy(pixels - oldPixels);
didEndScroll();
goBallistic(0.0);
}
}
@Deprecated('This will lead to bugs.') // flutter_ignore: deprecation_syntax, https://github.com/flutter/flutter/issues/44609
@override
void jumpToWithoutSettling(double value) {
goIdle();
if (pixels != value) {
final double oldPixels = pixels;
forcePixels(value);
didStartScroll();
didUpdateScrollPositionBy(pixels - oldPixels);
didEndScroll();
}
}
@override
ScrollHoldController hold(VoidCallback holdCancelCallback) {
final double previousVelocity = activity!.velocity;
final HoldScrollActivity holdActivity = HoldScrollActivity(
delegate: this,
onHoldCanceled: holdCancelCallback,
);
beginActivity(holdActivity);
_heldPreviousVelocity = previousVelocity;
return holdActivity;
}
ScrollDragController? _currentDrag;
@override
Drag drag(DragStartDetails details, VoidCallback dragCancelCallback) {
final ScrollDragController drag = ScrollDragController(
delegate: this,
details: details,
onDragCanceled: dragCancelCallback,
carriedVelocity: physics.carriedMomentum(_heldPreviousVelocity),
motionStartDistanceThreshold: physics.dragStartDistanceMotionThreshold,
);
beginActivity(DragScrollActivity(this, drag));
assert(_currentDrag == null);
_currentDrag = drag;
return drag;
}
@override
void dispose() {
_currentDrag?.dispose();
_currentDrag = null;
super.dispose();
}
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('${context.runtimeType}');
description.add('$physics');
description.add('$activity');
description.add('$userScrollDirection');
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_position_with_single_context.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_position_with_single_context.dart",
"repo_id": "flutter",
"token_count": 3025
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
import 'layout_builder.dart';
/// The signature of the [SliverLayoutBuilder] builder function.
typedef SliverLayoutWidgetBuilder = Widget Function(BuildContext context, SliverConstraints constraints);
/// Builds a sliver widget tree that can depend on its own [SliverConstraints].
///
/// Similar to the [LayoutBuilder] widget except its builder should return a sliver
/// widget, and [SliverLayoutBuilder] is itself a sliver. The framework calls the
/// [builder] function at layout time and provides the current [SliverConstraints].
/// The [SliverLayoutBuilder]'s final [SliverGeometry] will match the [SliverGeometry]
/// of its child.
///
/// {@macro flutter.widgets.ConstrainedLayoutBuilder}
///
/// See also:
///
/// * [LayoutBuilder], the non-sliver version of this widget.
class SliverLayoutBuilder extends ConstrainedLayoutBuilder<SliverConstraints> {
/// Creates a sliver widget that defers its building until layout.
const SliverLayoutBuilder({
super.key,
required super.builder,
});
@override
RenderObject createRenderObject(BuildContext context) => _RenderSliverLayoutBuilder();
}
class _RenderSliverLayoutBuilder extends RenderSliver with RenderObjectWithChildMixin<RenderSliver>, RenderConstrainedLayoutBuilder<SliverConstraints, RenderSliver> {
@override
double childMainAxisPosition(RenderObject child) {
assert(child == this.child);
return 0;
}
@override
void performLayout() {
rebuildIfNecessary();
child?.layout(constraints, parentUsesSize: true);
geometry = child?.geometry ?? SliverGeometry.zero;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
assert(child == this.child);
// child's offset is always (0, 0), transform.translate(0, 0) does not mutate the transform.
}
@override
void paint(PaintingContext context, Offset offset) {
// This renderObject does not introduce additional offset to child's position.
if (child?.geometry?.visible ?? false) {
context.paintChild(child!, offset);
}
}
@override
bool hitTestChildren(SliverHitTestResult result, {required double mainAxisPosition, required double crossAxisPosition}) {
return child != null
&& child!.geometry!.hitTestExtent > 0
&& child!.hitTest(result, mainAxisPosition: mainAxisPosition, crossAxisPosition: crossAxisPosition);
}
}
| flutter/packages/flutter/lib/src/widgets/sliver_layout_builder.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/sliver_layout_builder.dart",
"repo_id": "flutter",
"token_count": 772
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
/// A rectangle upon which a backend texture is mapped.
///
/// Backend textures are images that can be applied (mapped) to an area of the
/// Flutter view. They are created, managed, and updated using a
/// platform-specific texture registry. This is typically done by a plugin
/// that integrates with host platform video player, camera, or OpenGL APIs,
/// or similar image sources.
///
/// A texture widget refers to its backend texture using an integer ID. Texture
/// IDs are obtained from the texture registry and are scoped to the Flutter
/// view. Texture IDs may be reused after deregistration, at the discretion
/// of the registry. The use of texture IDs currently unknown to the registry
/// will silently result in a blank rectangle.
///
/// Texture widgets are repainted autonomously as dictated by the backend (e.g.
/// on arrival of a video frame). Such repainting generally does not involve
/// executing Dart code.
///
/// The size of the rectangle is determined by its parent widget, and the
/// texture is automatically scaled to fit.
///
/// See also:
///
/// * [TextureRegistry](/javadoc/io/flutter/view/TextureRegistry.html)
/// for how to create and manage backend textures on Android.
/// * [TextureRegistry Protocol](/ios-embedder/protocol_flutter_texture_registry-p.html)
/// for how to create and manage backend textures on iOS.
class Texture extends LeafRenderObjectWidget {
/// Creates a widget backed by the texture identified by [textureId], and use
/// [filterQuality] to set texture's [FilterQuality].
const Texture({
super.key,
required this.textureId,
this.freeze = false,
this.filterQuality = FilterQuality.low,
});
/// The identity of the backend texture.
final int textureId;
/// When true the texture will not be updated with new frames.
final bool freeze;
/// {@template flutter.widgets.Texture.filterQuality}
/// The quality of sampling the texture and rendering it on screen.
///
/// When the texture is scaled, a default [FilterQuality.low] is used for a higher quality but slower
/// interpolation (typically bilinear). It can be changed to [FilterQuality.none] for a lower quality but
/// faster interpolation (typically nearest-neighbor). See also [FilterQuality.medium] and
/// [FilterQuality.high] for more options.
/// {@endtemplate}
final FilterQuality filterQuality;
@override
TextureBox createRenderObject(BuildContext context) => TextureBox(textureId: textureId, freeze: freeze, filterQuality: filterQuality);
@override
void updateRenderObject(BuildContext context, TextureBox renderObject) {
renderObject.textureId = textureId;
renderObject.freeze = freeze;
renderObject.filterQuality = filterQuality;
}
}
| flutter/packages/flutter/lib/src/widgets/texture.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/texture.dart",
"repo_id": "flutter",
"token_count": 775
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'framework.dart';
import 'navigator.dart';
import 'routes.dart';
/// Registers a callback to veto attempts by the user to dismiss the enclosing
/// [ModalRoute].
///
/// See also:
///
/// * [ModalRoute.addScopedWillPopCallback] and [ModalRoute.removeScopedWillPopCallback],
/// which this widget uses to register and unregister [onWillPop].
/// * [Form], which provides an `onWillPop` callback that enables the form
/// to veto a `pop` initiated by the app's back button.
@Deprecated(
'Use PopScope instead. '
'This feature was deprecated after v3.12.0-1.0.pre.',
)
class WillPopScope extends StatefulWidget {
/// Creates a widget that registers a callback to veto attempts by the user to
/// dismiss the enclosing [ModalRoute].
@Deprecated(
'Use PopScope instead. '
'This feature was deprecated after v3.12.0-1.0.pre.',
)
const WillPopScope({
super.key,
required this.child,
required this.onWillPop,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Called to veto attempts by the user to dismiss the enclosing [ModalRoute].
///
/// If the callback returns a Future that resolves to false, the enclosing
/// route will not be popped.
final WillPopCallback? onWillPop;
@override
State<WillPopScope> createState() => _WillPopScopeState();
}
class _WillPopScopeState extends State<WillPopScope> {
ModalRoute<dynamic>? _route;
@override
void didChangeDependencies() {
super.didChangeDependencies();
if (widget.onWillPop != null) {
_route?.removeScopedWillPopCallback(widget.onWillPop!);
}
_route = ModalRoute.of(context);
if (widget.onWillPop != null) {
_route?.addScopedWillPopCallback(widget.onWillPop!);
}
}
@override
void didUpdateWidget(WillPopScope oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.onWillPop != oldWidget.onWillPop && _route != null) {
if (oldWidget.onWillPop != null) {
_route!.removeScopedWillPopCallback(oldWidget.onWillPop!);
}
if (widget.onWillPop != null) {
_route!.addScopedWillPopCallback(widget.onWillPop!);
}
}
}
@override
void dispose() {
if (widget.onWillPop != null) {
_route?.removeScopedWillPopCallback(widget.onWillPop!);
}
super.dispose();
}
@override
Widget build(BuildContext context) => widget.child;
}
| flutter/packages/flutter/lib/src/widgets/will_pop_scope.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/will_pop_scope.dart",
"repo_id": "flutter",
"token_count": 891
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('AnimationLocalStatusListenersMixin with AnimationLazyListenerMixin - removing unregistered listener is no-op', () {
final _TestAnimationLocalStatusListeners uut = _TestAnimationLocalStatusListeners();
void fakeListener(AnimationStatus status) { }
uut.removeStatusListener(fakeListener);
expect(uut.callsToStart, 0);
expect(uut.callsToStop, 0);
});
test('AnimationLocalListenersMixin with AnimationLazyListenerMixin - removing unregistered listener is no-op', () {
final _TestAnimationLocalListeners uut = _TestAnimationLocalListeners();
void fakeListener() { }
uut.removeListener(fakeListener);
expect(uut.callsToStart, 0);
expect(uut.callsToStop, 0);
});
}
class _TestAnimationLocalStatusListeners with AnimationLocalStatusListenersMixin, AnimationLazyListenerMixin {
int callsToStart = 0;
int callsToStop = 0;
@override
void didStartListening() {
callsToStart += 1;
}
@override
void didStopListening() {
callsToStop += 1;
}
}
class _TestAnimationLocalListeners with AnimationLocalListenersMixin, AnimationLazyListenerMixin {
int callsToStart = 0;
int callsToStop = 0;
@override
void didStartListening() {
callsToStart += 1;
}
@override
void didStopListening() {
callsToStop += 1;
}
}
| flutter/packages/flutter/test/animation/listener_helpers_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/listener_helpers_test.dart",
"repo_id": "flutter",
"token_count": 504
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
testWidgets('can press', (WidgetTester tester) async {
bool pressed = false;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbarButton(
onPressed: () {
pressed = true;
},
child: const Text('Tap me'),
),
),
),
);
expect(pressed, false);
await tester.tap(find.byType(CupertinoDesktopTextSelectionToolbarButton));
expect(pressed, true);
});
testWidgets('keeps contrast with background on hover',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbarButton.text(
text: 'Tap me',
onPressed: () {},
),
),
),
);
final BuildContext context =
tester.element(find.byType(CupertinoDesktopTextSelectionToolbarButton));
// The Text color is a CupertinoDynamicColor so we have to compare the color
// values instead of just comparing the colors themselves.
expect(
(tester.firstWidget(find.text('Tap me')) as Text).style!.color!.value,
CupertinoColors.black.value,
);
// Hover gesture
final TestGesture gesture =
await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer(location: Offset.zero);
addTearDown(gesture.removePointer);
await tester.pump();
await gesture.moveTo(tester
.getCenter(find.byType(CupertinoDesktopTextSelectionToolbarButton)));
await tester.pumpAndSettle();
// The color here should be a standard Color, there's no need to use value.
expect(
(tester.firstWidget(find.text('Tap me')) as Text).style!.color,
CupertinoTheme.of(context).primaryContrastingColor,
);
});
testWidgets('pressedOpacity defaults to 0.1', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbarButton(
onPressed: () { },
child: const Text('Tap me'),
),
),
),
);
// Original at full opacity.
FadeTransition opacity = tester.widget(find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbarButton),
matching: find.byType(FadeTransition),
));
expect(opacity.opacity.value, 1.0);
// Make a "down" gesture on the button.
final Offset center = tester
.getCenter(find.byType(CupertinoDesktopTextSelectionToolbarButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
// Opacity reduces during the down gesture.
opacity = tester.widget(find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbarButton),
matching: find.byType(FadeTransition),
));
expect(opacity.opacity.value, 0.7);
// Release the down gesture.
await gesture.up();
await tester.pumpAndSettle();
// Opacity is back to normal.
opacity = tester.widget(find.descendant(
of: find.byType(CupertinoDesktopTextSelectionToolbarButton),
matching: find.byType(FadeTransition),
));
expect(opacity.opacity.value, 1.0);
});
testWidgets('passing null to onPressed disables the button', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoDesktopTextSelectionToolbarButton(
onPressed: null,
child: Text('Tap me'),
),
),
),
);
expect(find.byType(CupertinoButton), findsOneWidget);
final CupertinoButton button = tester.widget(find.byType(CupertinoButton));
expect(button.enabled, isFalse);
});
}
| flutter/packages/flutter/test/cupertino/desktop_text_selection_toolbar_button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/desktop_text_selection_toolbar_button_test.dart",
"repo_id": "flutter",
"token_count": 1647
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('Radio control test', (WidgetTester tester) async {
final Key key = UniqueKey();
final List<int?> log = <int?>[];
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: 2,
onChanged: log.add,
),
),
));
await tester.tap(find.byKey(key));
expect(log, equals(<int>[1]));
log.clear();
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: 1,
onChanged: log.add,
activeColor: CupertinoColors.systemGreen,
),
),
));
await tester.tap(find.byKey(key));
expect(log, isEmpty);
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: 2,
onChanged: null,
),
),
));
await tester.tap(find.byKey(key));
expect(log, isEmpty);
});
testWidgets('Radio can be toggled when toggleable is set', (WidgetTester tester) async {
final Key key = UniqueKey();
final List<int?> log = <int?>[];
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: 2,
onChanged: log.add,
toggleable: true,
),
),
));
await tester.tap(find.byKey(key));
expect(log, equals(<int>[1]));
log.clear();
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: 1,
onChanged: log.add,
toggleable: true,
),
),
));
await tester.tap(find.byKey(key));
expect(log, equals(<int?>[null]));
log.clear();
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: null,
onChanged: log.add,
toggleable: true,
),
),
));
await tester.tap(find.byKey(key));
expect(log, equals(<int>[1]));
});
testWidgets('Radio selected semantics - platform adaptive', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 1,
onChanged: (int? i) { },
),
),
));
final bool isApple = defaultTargetPlatform == TargetPlatform.iOS ||
defaultTargetPlatform == TargetPlatform.macOS;
expect(
semantics,
includesNodeWith(
flags: <SemanticsFlag>[
SemanticsFlag.isInMutuallyExclusiveGroup,
SemanticsFlag.hasCheckedState,
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
SemanticsFlag.isChecked,
if (isApple) SemanticsFlag.isSelected,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
),
);
semantics.dispose();
}, variant: TargetPlatformVariant.all());
testWidgets('Radio semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 2,
onChanged: (int? i) { },
),
),
));
expect(tester.getSemantics(find.byType(Focus).last), matchesSemantics(
hasCheckedState: true,
hasEnabledState: true,
isEnabled: true,
hasTapAction: true,
isFocusable: true,
isInMutuallyExclusiveGroup: true,
));
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 2,
groupValue: 2,
onChanged: (int? i) { },
),
),
));
expect(tester.getSemantics(find.byType(Focus).last), matchesSemantics(
hasCheckedState: true,
hasEnabledState: true,
isEnabled: true,
hasTapAction: true,
isFocusable: true,
isInMutuallyExclusiveGroup: true,
isChecked: true,
));
await tester.pumpWidget(const CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 2,
onChanged: null,
),
),
));
expect(tester.getSemantics(find.byType(Focus).last), matchesSemantics(
hasCheckedState: true,
hasEnabledState: true,
isFocusable: true,
isInMutuallyExclusiveGroup: true,
));
await tester.pump();
// Now the isFocusable should be gone.
expect(tester.getSemantics(find.byType(Focus).last), matchesSemantics(
hasCheckedState: true,
hasEnabledState: true,
isInMutuallyExclusiveGroup: true,
));
await tester.pumpWidget(const CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 2,
groupValue: 2,
onChanged: null,
),
),
));
expect(tester.getSemantics(find.byType(Focus).last), matchesSemantics(
hasCheckedState: true,
hasEnabledState: true,
isChecked: true,
isInMutuallyExclusiveGroup: true,
));
semantics.dispose();
});
testWidgets('has semantic events', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final Key key = UniqueKey();
dynamic semanticEvent;
int? radioValue = 2;
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, (dynamic message) async {
semanticEvent = message;
});
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
key: key,
value: 1,
groupValue: radioValue,
onChanged: (int? i) {
radioValue = i;
},
),
),
));
await tester.tap(find.byKey(key));
final RenderObject object = tester.firstRenderObject(find.byKey(key));
expect(radioValue, 1);
expect(semanticEvent, <String, dynamic>{
'type': 'tap',
'nodeId': object.debugSemantics!.id,
'data': <String, dynamic>{},
});
expect(object.debugSemantics!.getSemanticsData().hasAction(SemanticsAction.tap), true);
semantics.dispose();
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, null);
});
testWidgets('Radio can be controlled by keyboard shortcuts', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
int? groupValue = 1;
const Key radioKey0 = Key('radio0');
const Key radioKey1 = Key('radio1');
const Key radioKey2 = Key('radio2');
final FocusNode focusNode2 = FocusNode(debugLabel: 'radio2');
addTearDown(focusNode2.dispose);
Widget buildApp({bool enabled = true}) {
return CupertinoApp(
home: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return SizedBox(
width: 200,
height: 100,
child: Row(
children: <Widget>[
CupertinoRadio<int>(
key: radioKey0,
value: 0,
onChanged: enabled ? (int? newValue) {
setState(() {
groupValue = newValue;
});
} : null,
groupValue: groupValue,
autofocus: true,
),
CupertinoRadio<int>(
key: radioKey1,
value: 1,
onChanged: enabled ? (int? newValue) {
setState(() {
groupValue = newValue;
});
} : null,
groupValue: groupValue,
),
CupertinoRadio<int>(
key: radioKey2,
value: 2,
onChanged: enabled ? (int? newValue) {
setState(() {
groupValue = newValue;
});
} : null,
groupValue: groupValue,
focusNode: focusNode2,
),
],
),
);
}),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pumpAndSettle();
// On web, radios don't respond to the enter key.
expect(groupValue, kIsWeb ? equals(1) : equals(0));
focusNode2.requestFocus();
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
expect(groupValue, equals(2));
});
testWidgets('Show a checkmark when useCheckmarkStyle is true', (WidgetTester tester) async {
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 1,
onChanged: (int? i) { },
),
),
));
await tester.pumpAndSettle();
// Has no checkmark when useCheckmarkStyle is false
expect(
tester.firstRenderObject<RenderBox>(find.byType(CupertinoRadio<int>)),
isNot(paints..path())
);
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 2,
useCheckmarkStyle: true,
onChanged: (int? i) { },
),
),
));
await tester.pumpAndSettle();
// Has no checkmark when group value doesn't match the value
expect(
tester.firstRenderObject<RenderBox>(find.byType(CupertinoRadio<int>)),
isNot(paints..path())
);
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoRadio<int>(
value: 1,
groupValue: 1,
useCheckmarkStyle: true,
onChanged: (int? i) { },
),
),
));
await tester.pumpAndSettle();
// Draws a path to show the checkmark when toggled on
expect(
tester.firstRenderObject<RenderBox>(find.byType(CupertinoRadio<int>)),
paints..path()
);
});
testWidgets('Do not crash when widget disappears while pointer is down', (WidgetTester tester) async {
final Key key = UniqueKey();
Widget buildRadio(bool show) {
return CupertinoApp(
home: Center(
child: show ? CupertinoRadio<bool>(key: key, value: true, groupValue: false, onChanged: (_) { }) : Container(),
),
);
}
await tester.pumpWidget(buildRadio(true));
final Offset center = tester.getCenter(find.byKey(key));
// Put a pointer down on the screen.
final TestGesture gesture = await tester.startGesture(center);
await tester.pump();
// While the pointer is down, the widget disappears.
await tester.pumpWidget(buildRadio(false));
expect(find.byKey(key), findsNothing);
// Release pointer after widget disappeared.
await gesture.up();
});
}
| flutter/packages/flutter/test/cupertino/radio_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/radio_test.dart",
"repo_id": "flutter",
"token_count": 5522
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
const String text = 'Hello World! How are you? Life is good!';
const String alternativeText = 'Everything is awesome!!';
void main() {
testWidgets('CupertinoTextFormFieldRow restoration', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'app',
home: RestorableTestWidget(),
),
);
await restoreAndVerify(tester);
});
testWidgets('CupertinoTextFormFieldRow restoration with external controller', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
restorationScopeId: 'root',
home: RestorableTestWidget(
useExternalController: true,
),
),
);
await restoreAndVerify(tester);
});
testWidgets('State restoration (No Form ancestor) - onUserInteraction error text validation', (WidgetTester tester) async {
String? errorText(String? value) => '$value/error';
late GlobalKey<FormFieldState<String>> formState;
Widget builder() {
return CupertinoApp(
restorationScopeId: 'app',
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter state) {
formState = GlobalKey<FormFieldState<String>>();
return Material(
child: CupertinoTextFormFieldRow(
key: formState,
autovalidateMode: AutovalidateMode.onUserInteraction,
restorationId: 'text_form_field',
initialValue: 'foo',
validator: errorText,
),
);
},
),
),
),
),
);
}
await tester.pumpWidget(builder());
// No error text is visible yet.
expect(find.text(errorText('foo')!), findsNothing);
await tester.enterText(find.byType(CupertinoTextFormFieldRow), 'bar');
await tester.pumpAndSettle();
expect(find.text(errorText('bar')!), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.restartAndRestore();
// Error text should be present after restart and restore.
expect(find.text(errorText('bar')!), findsOneWidget);
// Resetting the form state should remove the error text.
formState.currentState!.reset();
await tester.pumpAndSettle();
expect(find.text(errorText('bar')!), findsNothing);
await tester.restartAndRestore();
// Error text should still be removed after restart and restore.
expect(find.text(errorText('bar')!), findsNothing);
await tester.restoreFrom(data);
expect(find.text(errorText('bar')!), findsOneWidget);
});
testWidgets('State Restoration (No Form ancestor) - validator sets the error text only when validate is called', (WidgetTester tester) async {
String? errorText(String? value) => '$value/error';
late GlobalKey<FormFieldState<String>> formState;
Widget builder(AutovalidateMode mode) {
return CupertinoApp(
restorationScopeId: 'app',
home: MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter state) {
formState = GlobalKey<FormFieldState<String>>();
return Material(
child: CupertinoTextFormFieldRow(
key: formState,
restorationId: 'form_field',
autovalidateMode: mode,
initialValue: 'foo',
validator: errorText,
),
);
},
),
),
),
),
);
}
// Start off not autovalidating.
await tester.pumpWidget(builder(AutovalidateMode.disabled));
Future<void> checkErrorText(String testValue) async {
formState.currentState!.reset();
await tester.pumpWidget(builder(AutovalidateMode.disabled));
await tester.enterText(find.byType(CupertinoTextFormFieldRow), testValue);
await tester.pump();
// We have to manually validate if we're not autovalidating.
expect(find.text(errorText(testValue)!), findsNothing);
formState.currentState!.validate();
await tester.pump();
expect(find.text(errorText(testValue)!), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.restartAndRestore();
// Error text should be present after restart and restore.
expect(find.text(errorText(testValue)!), findsOneWidget);
formState.currentState!.reset();
await tester.pumpAndSettle();
expect(find.text(errorText(testValue)!), findsNothing);
await tester.restoreFrom(data);
expect(find.text(errorText(testValue)!), findsOneWidget);
// Try again with autovalidation. Should validate immediately.
formState.currentState!.reset();
await tester.pumpWidget(builder(AutovalidateMode.always));
await tester.enterText(find.byType(CupertinoTextFormFieldRow), testValue);
await tester.pump();
expect(find.text(errorText(testValue)!), findsOneWidget);
await tester.restartAndRestore();
// Error text should be present after restart and restore.
expect(find.text(errorText(testValue)!), findsOneWidget);
}
await checkErrorText('Test');
await checkErrorText('');
});
}
Future<void> restoreAndVerify(WidgetTester tester) async {
expect(find.text(text), findsNothing);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 0);
await tester.enterText(find.byType(CupertinoTextFormFieldRow), text);
await skipPastScrollingAnimation(tester);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 0);
await tester.drag(find.byType(Scrollable), const Offset(0, -80));
await skipPastScrollingAnimation(tester);
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
await tester.restartAndRestore();
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
final TestRestorationData data = await tester.getRestorationData();
await tester.enterText(find.byType(CupertinoTextFormFieldRow), alternativeText);
await skipPastScrollingAnimation(tester);
await tester.drag(find.byType(Scrollable), const Offset(0, 80));
await skipPastScrollingAnimation(tester);
expect(find.text(text), findsNothing);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, isNot(60));
await tester.restoreFrom(data);
expect(find.text(text), findsOneWidget);
expect(tester.state<ScrollableState>(find.byType(Scrollable)).position.pixels, 60);
}
class RestorableTestWidget extends StatefulWidget {
const RestorableTestWidget({super.key, this.useExternalController = false});
final bool useExternalController;
@override
RestorableTestWidgetState createState() => RestorableTestWidgetState();
}
class RestorableTestWidgetState extends State<RestorableTestWidget> with RestorationMixin {
final RestorableTextEditingController controller = RestorableTextEditingController();
@override
String get restorationId => 'widget';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(controller, 'controller');
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Material(
child: Align(
child: SizedBox(
width: 50,
child: CupertinoTextFormFieldRow(
restorationId: 'text',
maxLines: 3,
controller: widget.useExternalController ? controller.value : null,
),
),
),
);
}
}
Future<void> skipPastScrollingAnimation(WidgetTester tester) async {
await tester.pump();
await tester.pump(const Duration(milliseconds: 200));
}
| flutter/packages/flutter/test/cupertino/text_form_field_row_restoration_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/text_form_field_row_restoration_test.dart",
"repo_id": "flutter",
"token_count": 3342
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/src/foundation/basic_types.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('lerp Duration', () {
test('linearly interpolates between positive Durations', () {
expect(
lerpDuration(const Duration(seconds: 1), const Duration(seconds: 2), 0.5),
const Duration(milliseconds: 1500),
);
});
test('linearly interpolates between negative Durations', () {
expect(
lerpDuration(const Duration(seconds: -1), const Duration(seconds: -2), 0.5),
const Duration(milliseconds: -1500),
);
});
test('linearly interpolates between positive and negative Durations', () {
expect(
lerpDuration(const Duration(seconds: -1), const Duration(seconds:2), 0.5),
const Duration(milliseconds: 500),
);
});
test('starts at first Duration', () {
expect(
lerpDuration(const Duration(seconds: 1), const Duration(seconds: 2), 0),
const Duration(seconds: 1),
);
});
test('ends at second Duration', () {
expect(
lerpDuration(const Duration(seconds: 1), const Duration(seconds: 2), 1),
const Duration(seconds: 2),
);
});
test('time values beyond 1.0 have a multiplier effect', () {
expect(
lerpDuration(const Duration(seconds: 1), const Duration(seconds: 2), 5),
const Duration(seconds: 6),
);
expect(
lerpDuration(const Duration(seconds: -1), const Duration(seconds: -2), 5),
const Duration(seconds: -6),
);
});
});
}
| flutter/packages/flutter/test/foundation/basic_types_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/basic_types_test.dart",
"repo_id": "flutter",
"token_count": 661
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'dart:isolate';
import 'package:file/file.dart';
import 'package:file/local.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:platform/platform.dart';
final Matcher throwsRemoteError = throwsA(isA<RemoteError>());
int test1(int value) {
return value + 1;
}
int test2(int value) {
throw 2;
}
int test3(int value) {
Isolate.exit();
}
int test4(int value) {
Isolate.current.kill();
return value + 1;
}
int test5(int value) {
Isolate.current.kill(priority: Isolate.immediate);
return value + 1;
}
Future<int> test1Async(int value) async {
return value + 1;
}
Future<int> test2Async(int value) async {
throw 2;
}
Future<int> test3Async(int value) async {
Isolate.exit();
}
Future<int> test4Async(int value) async {
Isolate.current.kill();
return value + 1;
}
Future<int> test5Async(int value) async {
Isolate.current.kill(priority: Isolate.immediate);
return value + 1;
}
Future<int> test1CallCompute(int value) {
return compute(test1, value);
}
Future<int> test2CallCompute(int value) {
return compute(test2, value);
}
Future<int> test3CallCompute(int value) {
return compute(test3, value);
}
Future<int> test4CallCompute(int value) {
return compute(test4, value);
}
Future<int> test5CallCompute(int value) {
return compute(test5, value);
}
Future<void> expectFileSuccessfullyCompletes(String filename) async {
// Run a Dart script that calls compute().
// The Dart process will terminate only if the script exits cleanly with
// all isolate ports closed.
const FileSystem fs = LocalFileSystem();
const Platform platform = LocalPlatform();
final String flutterRoot = platform.environment['FLUTTER_ROOT']!;
final String dartPath =
fs.path.join(flutterRoot, 'bin', 'cache', 'dart-sdk', 'bin', 'dart');
final String scriptPath =
fs.path.join(flutterRoot, 'packages', 'flutter', 'test', 'foundation', filename);
// Enable asserts to also catch potentially invalid assertions.
final ProcessResult result = await Process.run(
dartPath, <String>['run', '--enable-asserts', scriptPath]);
expect(result.exitCode, 0);
}
class ComputeTestSubject {
ComputeTestSubject(this.base, [this.additional]);
final int base;
final dynamic additional;
int method(int x) {
return base * x;
}
static int staticMethod(int square) {
return square * square;
}
}
Future<int> computeStaticMethod(int square) {
return compute(ComputeTestSubject.staticMethod, square);
}
Future<int> computeClosure(int square) {
return compute((_) => square * square, null);
}
Future<int> computeInvalidClosure(int square) {
final ReceivePort r = ReceivePort();
return compute((_) {
r.sendPort.send('Computing!');
return square * square;
}, null);
}
Future<int> computeInstanceMethod(int square) {
final ComputeTestSubject subject = ComputeTestSubject(square);
return compute(subject.method, square);
}
Future<int> computeInvalidInstanceMethod(int square) {
final ComputeTestSubject subject = ComputeTestSubject(square, ReceivePort());
expect(subject.additional, isA<ReceivePort>());
return compute(subject.method, square);
}
dynamic testInvalidResponse(int square) {
final ReceivePort r = ReceivePort();
try {
return r;
} finally {
r.close();
}
}
dynamic testInvalidError(int square) {
final ReceivePort r = ReceivePort();
try {
throw r;
} finally {
r.close();
}
}
String? testDebugName(_) {
return Isolate.current.debugName;
}
int? testReturnNull(_) {
return null;
}
void main() {
test('compute()', () async {
expect(await compute(test1, 0), 1);
expect(compute(test2, 0), throwsA(2));
expect(compute(test3, 0), throwsRemoteError);
expect(await compute(test4, 0), 1);
expect(compute(test5, 0), throwsRemoteError);
expect(await compute(test1Async, 0), 1);
expect(compute(test2Async, 0), throwsA(2));
expect(compute(test3Async, 0), throwsRemoteError);
expect(await compute(test4Async, 0), 1);
expect(compute(test5Async, 0), throwsRemoteError);
expect(await compute(test1CallCompute, 0), 1);
expect(compute(test2CallCompute, 0), throwsA(2));
expect(compute(test3CallCompute, 0), throwsRemoteError);
expect(await compute(test4CallCompute, 0), 1);
expect(compute(test5CallCompute, 0), throwsRemoteError);
expect(compute(testInvalidResponse, 0), throwsRemoteError);
expect(compute(testInvalidError, 0), throwsRemoteError);
expect(await computeStaticMethod(10), 100);
expect(await computeClosure(10), 100);
expect(computeInvalidClosure(10), throwsArgumentError);
expect(await computeInstanceMethod(10), 100);
expect(computeInvalidInstanceMethod(10), throwsArgumentError);
expect(await compute(testDebugName, null, debugLabel: 'debug_name'),
'debug_name');
expect(await compute(testReturnNull, null), null);
}, skip: kIsWeb); // [intended] isn't supported on the web.
group('compute() closes all ports', () {
test('with valid message', () async {
await expectFileSuccessfullyCompletes('_compute_caller.dart');
});
test('with invalid message', () async {
await expectFileSuccessfullyCompletes(
'_compute_caller_invalid_message.dart');
});
test('with valid error', () async {
await expectFileSuccessfullyCompletes('_compute_caller.dart');
});
test('with invalid error', () async {
await expectFileSuccessfullyCompletes(
'_compute_caller_invalid_message.dart');
});
}, skip: kIsWeb); // [intended] isn't supported on the web.
}
| flutter/packages/flutter/test/foundation/isolates_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/isolates_test.dart",
"repo_id": "flutter",
"token_count": 1990
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:fake_async/fake_async.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import 'gesture_tester.dart';
class TestGestureArenaMember extends GestureArenaMember {
@override
void acceptGesture(int key) {
accepted = true;
}
@override
void rejectGesture(int key) {
rejected = true;
}
bool accepted = false;
bool rejected = false;
}
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
late DoubleTapGestureRecognizer tap;
bool doubleTapRecognized = false;
TapDownDetails? doubleTapDownDetails;
bool doubleTapCanceled = false;
setUp(() {
tap = DoubleTapGestureRecognizer();
addTearDown(tap.dispose);
doubleTapRecognized = false;
tap.onDoubleTap = () {
expect(doubleTapRecognized, isFalse);
doubleTapRecognized = true;
};
doubleTapDownDetails = null;
tap.onDoubleTapDown = (TapDownDetails details) {
expect(doubleTapDownDetails, isNull);
doubleTapDownDetails = details;
};
doubleTapCanceled = false;
tap.onDoubleTapCancel = () {
expect(doubleTapCanceled, isFalse);
doubleTapCanceled = true;
};
});
tearDown(() {
tap.dispose();
});
// Down/up pair 1: normal tap sequence
const PointerDownEvent down1 = PointerDownEvent(
pointer: 1,
position: Offset(10.0, 10.0),
);
const PointerUpEvent up1 = PointerUpEvent(
pointer: 1,
position: Offset(11.0, 9.0),
);
// Down/up pair 2: normal tap sequence close to pair 1
const PointerDownEvent down2 = PointerDownEvent(
pointer: 2,
position: Offset(12.0, 12.0),
);
const PointerUpEvent up2 = PointerUpEvent(
pointer: 2,
position: Offset(13.0, 11.0),
);
// Down/up pair 3: normal tap sequence far away from pair 1
const PointerDownEvent down3 = PointerDownEvent(
pointer: 3,
position: Offset(130.0, 130.0),
);
const PointerUpEvent up3 = PointerUpEvent(
pointer: 3,
position: Offset(131.0, 129.0),
);
// Down/move/up sequence 4: intervening motion
const PointerDownEvent down4 = PointerDownEvent(
pointer: 4,
position: Offset(10.0, 10.0),
);
const PointerMoveEvent move4 = PointerMoveEvent(
pointer: 4,
position: Offset(25.0, 25.0),
);
const PointerUpEvent up4 = PointerUpEvent(
pointer: 4,
position: Offset(25.0, 25.0),
);
// Down/up pair 5: normal tap sequence identical to pair 1
const PointerDownEvent down5 = PointerDownEvent(
pointer: 5,
position: Offset(10.0, 10.0),
);
const PointerUpEvent up5 = PointerUpEvent(
pointer: 5,
position: Offset(11.0, 9.0),
);
// Down/up pair 6: normal tap sequence close to pair 1 but on secondary button
const PointerDownEvent down6 = PointerDownEvent(
pointer: 6,
position: Offset(10.0, 10.0),
buttons: kSecondaryMouseButton,
);
const PointerUpEvent up6 = PointerUpEvent(
pointer: 6,
position: Offset(11.0, 9.0),
);
testGesture('Should recognize double tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down2);
tester.closeArena(2);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down2.position);
expect(doubleTapDownDetails!.localPosition, down2.localPosition);
tester.route(down2);
expect(doubleTapRecognized, isFalse);
tester.route(up2);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should recognize double tap with secondaryButton', (GestureTester tester) {
final DoubleTapGestureRecognizer tapSecondary = DoubleTapGestureRecognizer(
allowedButtonsFilter: (int buttons) => buttons == kSecondaryButton,
);
addTearDown(tapSecondary.dispose);
tapSecondary.onDoubleTap = () {
doubleTapRecognized = true;
};
tapSecondary.onDoubleTapDown = (TapDownDetails details) {
doubleTapDownDetails = details;
};
tapSecondary.onDoubleTapCancel = () {
doubleTapCanceled = true;
};
// Down/up pair 7: normal tap sequence close to pair 6
const PointerDownEvent down7 = PointerDownEvent(
pointer: 7,
position: Offset(10.0, 10.0),
buttons: kSecondaryMouseButton,
);
const PointerUpEvent up7 = PointerUpEvent(
pointer: 7,
position: Offset(11.0, 9.0),
);
tapSecondary.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tapSecondary.addPointer(down7);
tester.closeArena(7);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down7.position);
expect(doubleTapDownDetails!.localPosition, down7.localPosition);
tester.route(down7);
expect(doubleTapRecognized, isFalse);
tester.route(up7);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapCanceled, isFalse);
});
testGesture('Inter-tap distance cancels double tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tap.addPointer(down3);
tester.closeArena(3);
tester.route(down3);
tester.route(up3);
GestureBinding.instance.gestureArena.sweep(3);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Intra-tap distance cancels double tap', (GestureTester tester) {
tap.addPointer(down4);
tester.closeArena(4);
tester.route(down4);
tester.route(move4);
tester.route(up4);
GestureBinding.instance.gestureArena.sweep(4);
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down2);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Inter-tap delay cancels double tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(const Duration(milliseconds: 5000));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Inter-tap delay resets double tap, allowing third tap to be a double-tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(const Duration(milliseconds: 5000));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down5.position);
expect(doubleTapDownDetails!.localPosition, down5.localPosition);
tester.route(up5);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(5);
expect(doubleTapCanceled, isFalse);
});
testGesture('Intra-tap delay does not cancel double tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.async.elapse(const Duration(milliseconds: 1000));
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapDownDetails, isNull);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down2.position);
expect(doubleTapDownDetails!.localPosition, down2.localPosition);
tester.route(up2);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should not recognize two overlapping taps', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should recognize one tap of group followed by second tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down1.position);
expect(doubleTapDownDetails!.localPosition, down1.localPosition);
tester.route(up1);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should cancel on arena reject during first tap', (GestureTester tester) {
tap.addPointer(down1);
final TestGestureArenaMember member = TestGestureArenaMember();
final GestureArenaEntry entry = GestureBinding.instance.gestureArena.add(1, member);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
entry.resolve(GestureDisposition.accepted);
expect(member.accepted, isTrue);
GestureBinding.instance.gestureArena.sweep(1);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should cancel on arena reject between taps', (GestureTester tester) {
tap.addPointer(down1);
final TestGestureArenaMember member = TestGestureArenaMember();
final GestureArenaEntry entry = GestureBinding.instance.gestureArena.add(1, member);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
entry.resolve(GestureDisposition.accepted);
expect(member.accepted, isTrue);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Should cancel on arena reject during last tap', (GestureTester tester) {
tap.addPointer(down1);
final TestGestureArenaMember member = TestGestureArenaMember();
final GestureArenaEntry entry = GestureBinding.instance.gestureArena.add(1, member);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down2.position);
expect(doubleTapDownDetails!.localPosition, down2.localPosition);
expect(doubleTapCanceled, isFalse);
entry.resolve(GestureDisposition.accepted);
expect(member.accepted, isTrue);
expect(doubleTapCanceled, isTrue);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
});
testGesture('Passive gesture should trigger on double tap cancel', (GestureTester tester) {
FakeAsync().run((FakeAsync async) {
tap.addPointer(down1);
final TestGestureArenaMember member = TestGestureArenaMember();
GestureBinding.instance.gestureArena.add(1, member);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(member.accepted, isFalse);
async.elapse(const Duration(milliseconds: 5000));
expect(member.accepted, isTrue);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
});
testGesture('Should not recognize two over-rapid taps', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(const Duration(milliseconds: 10));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Over-rapid taps resets double tap, allowing third tap to be a double-tap', (GestureTester tester) {
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(const Duration(milliseconds: 10));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down5.position);
expect(doubleTapDownDetails!.localPosition, down5.localPosition);
tester.route(up5);
expect(doubleTapRecognized, isTrue);
GestureBinding.instance.gestureArena.sweep(5);
expect(doubleTapCanceled, isFalse);
});
group('Enforce consistent-button restriction:', () {
testGesture('Button change should interrupt existing sequence', (GestureTester tester) {
// Down1 -> down6 (different button from 1) -> down2 (same button as 1)
// Down1 and down2 could've been a double tap, but is interrupted by down 6.
const Duration interval = Duration(milliseconds: 100);
assert(interval * 2 < kDoubleTapTimeout);
assert(interval > kDoubleTapMinTime);
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(interval);
tap.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
tester.async.elapse(interval);
expect(doubleTapRecognized, isFalse);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Button change with allowedButtonsFilter should interrupt existing sequence', (GestureTester tester) {
final DoubleTapGestureRecognizer tapPrimary = DoubleTapGestureRecognizer(
allowedButtonsFilter: (int buttons) => buttons == kPrimaryButton,
);
addTearDown(tapPrimary.dispose);
tapPrimary.onDoubleTap = () {
doubleTapRecognized = true;
};
tapPrimary.onDoubleTapDown = (TapDownDetails details) {
doubleTapDownDetails = details;
};
tapPrimary.onDoubleTapCancel = () {
doubleTapCanceled = true;
};
// Down1 -> down6 (different button from 1) -> down2 (same button as 1)
// Down1 and down2 could've been a double tap, but is interrupted by down 6.
// Down6 gets ignored because it's not a primary button. Regardless, the state
// is reset.
const Duration interval = Duration(milliseconds: 100);
assert(interval * 2 < kDoubleTapTimeout);
assert(interval > kDoubleTapMinTime);
tapPrimary.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.async.elapse(interval);
tapPrimary.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
tester.async.elapse(interval);
expect(doubleTapRecognized, isFalse);
tapPrimary.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
expect(doubleTapCanceled, isFalse);
});
testGesture('Button change should start a valid sequence', (GestureTester tester) {
// Down6 -> down1 (different button from 6) -> down2 (same button as 1)
const Duration interval = Duration(milliseconds: 100);
assert(interval * 2 < kDoubleTapTimeout);
assert(interval > kDoubleTapMinTime);
tap.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
tester.async.elapse(interval);
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(doubleTapRecognized, isFalse);
expect(doubleTapDownDetails, isNull);
tester.async.elapse(interval);
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
expect(doubleTapDownDetails, isNotNull);
expect(doubleTapDownDetails!.globalPosition, down2.position);
expect(doubleTapDownDetails!.localPosition, down2.localPosition);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(doubleTapRecognized, isTrue);
expect(doubleTapCanceled, isFalse);
});
});
group('Recognizers listening on different buttons do not form competition:', () {
// This test is assisted by tap recognizers. If a tap gesture has
// no competing recognizers, a pointer down event triggers its onTapDown
// immediately; if there are competitors, onTapDown is triggered after a
// timeout.
// The following tests make sure that double tap recognizers do not form
// competition with a tap gesture recognizer listening on a different button.
final List<String> recognized = <String>[];
late TapGestureRecognizer tapPrimary;
late TapGestureRecognizer tapSecondary;
late DoubleTapGestureRecognizer doubleTap;
setUp(() {
tapPrimary = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
recognized.add('tapPrimary');
};
addTearDown(tapPrimary.dispose);
tapSecondary = TapGestureRecognizer()
..onSecondaryTapDown = (TapDownDetails details) {
recognized.add('tapSecondary');
};
addTearDown(tapSecondary.dispose);
doubleTap = DoubleTapGestureRecognizer()
..onDoubleTap = () {
recognized.add('doubleTap');
};
addTearDown(doubleTap.dispose);
});
tearDown(() {
recognized.clear();
tapPrimary.dispose();
tapSecondary.dispose();
doubleTap.dispose();
});
testGesture('A primary double tap recognizer does not form competition with a secondary tap recognizer', (GestureTester tester) {
doubleTap.addPointer(down6);
tapSecondary.addPointer(down6);
tester.closeArena(down6.pointer);
tester.route(down6);
expect(recognized, <String>['tapSecondary']);
});
testGesture('A primary double tap recognizer forms competition with a primary tap recognizer', (GestureTester tester) {
doubleTap.addPointer(down1);
tapPrimary.addPointer(down1);
tester.closeArena(down1.pointer);
tester.route(down1);
expect(recognized, <String>[]);
tester.async.elapse(const Duration(milliseconds: 300));
expect(recognized, <String>['tapPrimary']);
});
});
testGesture('A secondary double tap should not trigger primary', (GestureTester tester) {
final List<String> recognized = <String>[];
final DoubleTapGestureRecognizer doubleTap = DoubleTapGestureRecognizer()
..onDoubleTap = () {
recognized.add('primary');
};
addTearDown(doubleTap.dispose);
// Down/up pair 7: normal tap sequence close to pair 6
const PointerDownEvent down7 = PointerDownEvent(
pointer: 7,
position: Offset(10.0, 10.0),
buttons: kSecondaryMouseButton,
);
const PointerUpEvent up7 = PointerUpEvent(
pointer: 7,
position: Offset(11.0, 9.0),
);
doubleTap.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
tester.async.elapse(const Duration(milliseconds: 100));
doubleTap.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
tester.route(up7);
expect(recognized, <String>[]);
recognized.clear();
doubleTap.dispose();
});
testGesture('Buttons filter should cancel invalid taps', (GestureTester tester) {
final List<String> recognized = <String>[];
final DoubleTapGestureRecognizer doubleTap = DoubleTapGestureRecognizer(
allowedButtonsFilter: (int buttons) => false,
)
..onDoubleTap = () {
recognized.add('primary');
};
addTearDown(doubleTap.dispose);
// Down/up pair 7: normal tap sequence close to pair 6
const PointerDownEvent down7 = PointerDownEvent(
pointer: 7,
position: Offset(10.0, 10.0),
);
const PointerUpEvent up7 = PointerUpEvent(
pointer: 7,
position: Offset(11.0, 9.0),
);
doubleTap.addPointer(down7);
tester.closeArena(7);
tester.route(down7);
tester.route(up7);
GestureBinding.instance.gestureArena.sweep(7);
tester.async.elapse(const Duration(milliseconds: 100));
doubleTap.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(up6);
expect(recognized, <String>[]);
recognized.clear();
doubleTap.dispose();
});
// Regression test for https://github.com/flutter/flutter/issues/73667
testGesture('Unfinished DoubleTap does not prevent competing Tap', (GestureTester tester) {
int tapCount = 0;
final DoubleTapGestureRecognizer doubleTap = DoubleTapGestureRecognizer()
..onDoubleTap = () {};
addTearDown(doubleTap.dispose);
final TapGestureRecognizer tap = TapGestureRecognizer()
..onTap = () => tapCount++;
addTearDown(tap.dispose);
// Open a arena with 2 members and holding.
doubleTap.addPointer(down1);
tap.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
// Open a new arena with only one TapGestureRecognizer.
tester.async.elapse(const Duration(milliseconds: 100));
tap.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
final PointerMoveEvent move2 = PointerMoveEvent(pointer: 2, position: down2.position);
tester.route(move2);
tester.route(up2);
expect(tapCount, 1); // The second tap will win immediately.
GestureBinding.instance.gestureArena.sweep(2);
// Finish the previous gesture arena.
tester.async.elapse(const Duration(milliseconds: 300));
expect(tapCount, 1); // The first tap should not trigger onTap callback though it wins the arena.
tap.dispose();
doubleTap.dispose();
});
}
| flutter/packages/flutter/test/gestures/double_tap_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/double_tap_test.dart",
"repo_id": "flutter",
"token_count": 9906
} | 711 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('Should route pointers', () {
bool callbackRan = false;
void callback(PointerEvent event) {
callbackRan = true;
}
final TestPointer pointer2 = TestPointer(2);
final TestPointer pointer3 = TestPointer(3);
final PointerRouter router = PointerRouter();
router.addRoute(3, callback);
router.route(pointer2.down(Offset.zero));
expect(callbackRan, isFalse);
router.route(pointer3.down(Offset.zero));
expect(callbackRan, isTrue);
callbackRan = false;
router.removeRoute(3, callback);
router.route(pointer3.up());
expect(callbackRan, isFalse);
});
test('Supports re-entrant cancellation', () {
bool callbackRan = false;
void callback(PointerEvent event) {
callbackRan = true;
}
final PointerRouter router = PointerRouter();
router.addRoute(2, (PointerEvent event) {
router.removeRoute(2, callback);
});
router.addRoute(2, callback);
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(callbackRan, isFalse);
});
test('Supports global callbacks', () {
bool secondCallbackRan = false;
void secondCallback(PointerEvent event) {
secondCallbackRan = true;
}
bool firstCallbackRan = false;
final PointerRouter router = PointerRouter();
router.addGlobalRoute((PointerEvent event) {
firstCallbackRan = true;
router.addGlobalRoute(secondCallback);
});
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(firstCallbackRan, isTrue);
expect(secondCallbackRan, isFalse);
});
test('Supports re-entrant global cancellation', () {
bool callbackRan = false;
void callback(PointerEvent event) {
callbackRan = true;
}
final PointerRouter router = PointerRouter();
router.addGlobalRoute((PointerEvent event) {
router.removeGlobalRoute(callback);
});
router.addGlobalRoute(callback);
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(callbackRan, isFalse);
});
test('Per-pointer callbacks cannot re-entrantly add global routes', () {
bool callbackRan = false;
void callback(PointerEvent event) {
callbackRan = true;
}
final PointerRouter router = PointerRouter();
bool perPointerCallbackRan = false;
router.addRoute(2, (PointerEvent event) {
perPointerCallbackRan = true;
router.addGlobalRoute(callback);
});
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(perPointerCallbackRan, isTrue);
expect(callbackRan, isFalse);
});
test('Per-pointer callbacks happen before global callbacks', () {
final List<String> log = <String>[];
final PointerRouter router = PointerRouter();
router.addGlobalRoute((PointerEvent event) {
log.add('global 1');
});
router.addRoute(2, (PointerEvent event) {
log.add('per-pointer 1');
});
router.addGlobalRoute((PointerEvent event) {
log.add('global 2');
});
router.addRoute(2, (PointerEvent event) {
log.add('per-pointer 2');
});
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(log, equals(<String>[
'per-pointer 1',
'per-pointer 2',
'global 1',
'global 2',
]));
});
test('Exceptions do not stop pointer routing', () {
final List<String> log = <String>[];
final PointerRouter router = PointerRouter();
router.addRoute(2, (PointerEvent event) {
log.add('per-pointer 1');
});
router.addRoute(2, (PointerEvent event) {
log.add('per-pointer 2');
throw 'Having a bad day!';
});
router.addRoute(2, (PointerEvent event) {
log.add('per-pointer 3');
});
final FlutterExceptionHandler? previousErrorHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails details) {
log.add('error report');
};
final TestPointer pointer2 = TestPointer(2);
router.route(pointer2.down(Offset.zero));
expect(log, equals(<String>[
'per-pointer 1',
'per-pointer 2',
'error report',
'per-pointer 3',
]));
FlutterError.onError = previousErrorHandler;
});
test('Exceptions include router, route & event', () {
try {
final PointerRouter router = PointerRouter();
router.addRoute(2, (PointerEvent event) => throw 'Pointer exception');
} catch (e) {
expect(e, contains("router: Instance of 'PointerRouter'"));
expect(e, contains('route: Closure: (PointerEvent) => Null'));
expect(e, contains('event: PointerDownEvent#[a-zA-Z0-9]{5}(position: Offset(0.0, 0.0))'));
}
});
test('Should transform events', () {
final List<PointerEvent> events = <PointerEvent>[];
final List<PointerEvent> globalEvents = <PointerEvent>[];
final PointerRouter router = PointerRouter();
final Matrix4 transform = (Matrix4.identity()..scale(1 / 2.0, 1 / 2.0, 1.0)).multiplied(Matrix4.translationValues(-10, -30, 0));
router.addRoute(1, (PointerEvent event) {
events.add(event);
}, transform);
router.addGlobalRoute((PointerEvent event) {
globalEvents.add(event);
}, transform);
final TestPointer pointer1 = TestPointer();
const Offset firstPosition = Offset(16, 36);
router.route(pointer1.down(firstPosition));
expect(events.single.transform, transform);
expect(events.single.position, firstPosition);
expect(events.single.delta, Offset.zero);
expect(events.single.localPosition, const Offset(3, 3));
expect(events.single.localDelta, Offset.zero);
expect(globalEvents.single.transform, transform);
expect(globalEvents.single.position, firstPosition);
expect(globalEvents.single.delta, Offset.zero);
expect(globalEvents.single.localPosition, const Offset(3, 3));
expect(globalEvents.single.localDelta, Offset.zero);
events.clear();
globalEvents.clear();
const Offset newPosition = Offset(20, 40);
router.route(pointer1.move(newPosition));
expect(events.single.transform, transform);
expect(events.single.position, newPosition);
expect(events.single.delta, newPosition - firstPosition);
expect(events.single.localPosition, const Offset(5, 5));
expect(events.single.localDelta, const Offset(2, 2));
expect(globalEvents.single.transform, transform);
expect(globalEvents.single.position, newPosition);
expect(globalEvents.single.delta, newPosition - firstPosition);
expect(globalEvents.single.localPosition, const Offset(5, 5));
expect(globalEvents.single.localDelta, const Offset(2, 2));
});
}
| flutter/packages/flutter/test/gestures/pointer_router_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/pointer_router_test.dart",
"repo_id": "flutter",
"token_count": 2546
} | 712 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('BackButton control test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Home')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(
child: Center(
child: BackButton(),
),
);
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
await tester.tap(find.byType(BackButton));
await tester.pumpAndSettle();
expect(find.text('Home'), findsOneWidget);
});
testWidgets('BackButton onPressed overrides default pop behavior', (WidgetTester tester) async {
bool customCallbackWasCalled = false;
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Home')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return Material(
child: Center(
child: BackButton(onPressed: () => customCallbackWasCalled = true),
),
);
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
expect(find.text('Home'), findsNothing); // Start off on the second page.
expect(customCallbackWasCalled, false); // customCallbackWasCalled should still be false.
await tester.tap(find.byType(BackButton));
await tester.pumpAndSettle();
// We're still on the second page.
expect(find.text('Home'), findsNothing);
// But the custom callback is called.
expect(customCallbackWasCalled, true);
});
testWidgets('BackButton icon', (WidgetTester tester) async {
final Key androidKey = UniqueKey();
final Key iOSKey = UniqueKey();
final Key linuxKey = UniqueKey();
final Key macOSKey = UniqueKey();
final Key windowsKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Column(
children: <Widget>[
Theme(
data: ThemeData(platform: TargetPlatform.android),
child: BackButtonIcon(key: androidKey),
),
Theme(
data: ThemeData(platform: TargetPlatform.iOS),
child: BackButtonIcon(key: iOSKey),
),
Theme(
data: ThemeData(platform: TargetPlatform.linux),
child: BackButtonIcon(key: linuxKey),
),
Theme(
data: ThemeData(platform: TargetPlatform.macOS),
child: BackButtonIcon(key: macOSKey),
),
Theme(
data: ThemeData(platform: TargetPlatform.windows),
child: BackButtonIcon(key: windowsKey),
),
],
),
),
);
final Icon androidIcon = tester.widget(find.descendant(of: find.byKey(androidKey), matching: find.byType(Icon)));
final Icon iOSIcon = tester.widget(find.descendant(of: find.byKey(iOSKey), matching: find.byType(Icon)));
final Icon linuxIcon = tester.widget(find.descendant(of: find.byKey(linuxKey), matching: find.byType(Icon)));
final Icon macOSIcon = tester.widget(find.descendant(of: find.byKey(macOSKey), matching: find.byType(Icon)));
final Icon windowsIcon = tester.widget(find.descendant(of: find.byKey(windowsKey), matching: find.byType(Icon)));
expect(iOSIcon.icon == androidIcon.icon, kIsWeb ? isTrue : isFalse);
expect(linuxIcon.icon == androidIcon.icon, isTrue);
expect(macOSIcon.icon == androidIcon.icon, kIsWeb ? isTrue : isFalse);
expect(macOSIcon.icon == iOSIcon.icon, isTrue);
expect(windowsIcon.icon == androidIcon.icon, isTrue);
});
testWidgets('BackButton color', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: BackButton(
color: Colors.red,
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(BackButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('BackButton color with ButtonStyle', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Material(
child: BackButton(
style: ButtonStyle(
iconColor: MaterialStatePropertyAll<Color>(Colors.red),
),
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(BackButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('BackButton.style.iconColor parameter overrides BackButton.color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Material(
child: BackButton(
color: Colors.green,
style: ButtonStyle(
iconColor: MaterialStatePropertyAll<Color>(Colors.red),
),
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(BackButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('BackButton semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Home')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(
child: Center(
child: BackButton(),
),
);
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
final String? expectedLabel;
switch (defaultTargetPlatform) {
case TargetPlatform.android:
expectedLabel = 'Back';
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
expectedLabel = null;
}
expect(tester.getSemantics(find.byType(BackButton)), matchesSemantics(
tooltip: 'Back',
label: expectedLabel,
isButton: true,
hasEnabledState: true,
isEnabled: true,
hasTapAction: true,
isFocusable: true,
));
handle.dispose();
}, variant: TargetPlatformVariant.all());
testWidgets('CloseButton semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Home')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(
child: Center(
child: CloseButton(),
),
);
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
final String? expectedLabel;
switch (defaultTargetPlatform) {
case TargetPlatform.android:
expectedLabel = 'Close';
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
expectedLabel = null;
}
expect(tester.getSemantics(find.byType(CloseButton)), matchesSemantics(
tooltip: 'Close',
label: expectedLabel,
isButton: true,
hasEnabledState: true,
isEnabled: true,
hasTapAction: true,
isFocusable: true,
));
handle.dispose();
}, variant: TargetPlatformVariant.all());
testWidgets('CloseButton color', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: CloseButton(
color: Colors.red,
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(CloseButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('CloseButton color with ButtonStyle', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Material(
child: CloseButton(
style: ButtonStyle(
iconColor: MaterialStatePropertyAll<Color>(Colors.red),
),
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(CloseButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('CloseButton.style.iconColor parameter overrides CloseButton.color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Material(
child: CloseButton(
color: Colors.green,
style: ButtonStyle(
iconColor: MaterialStatePropertyAll<Color>(Colors.red),
),
),
),
),
);
final RichText iconText = tester.firstWidget(find.descendant(
of: find.byType(CloseButton),
matching: find.byType(RichText),
));
expect(iconText.text.style!.color, Colors.red);
});
testWidgets('CloseButton onPressed overrides default pop behavior', (WidgetTester tester) async {
bool customCallbackWasCalled = false;
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Home')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return Material(
child: Center(
child: CloseButton(onPressed: () => customCallbackWasCalled = true),
),
);
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
expect(find.text('Home'), findsNothing); // Start off on the second page.
expect(customCallbackWasCalled, false); // customCallbackWasCalled should still be false.
await tester.tap(find.byType(CloseButton));
await tester.pumpAndSettle();
// We're still on the second page.
expect(find.text('Home'), findsNothing);
// The custom callback is called, setting customCallbackWasCalled to true.
expect(customCallbackWasCalled, true);
});
}
| flutter/packages/flutter/test/material/back_button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/back_button_test.dart",
"repo_id": "flutter",
"token_count": 4680
} | 713 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('Material3 - Card defaults (Elevated card)', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final ColorScheme colors = theme.colorScheme;
await tester.pumpWidget(MaterialApp(
theme: theme,
home: const Scaffold(
body: Card(),
),
));
final Container container = _getCardContainer(tester);
final Material material = _getCardMaterial(tester);
expect(material.clipBehavior, Clip.none);
expect(material.elevation, 1.0);
expect(container.margin, const EdgeInsets.all(4.0));
expect(material.color, colors.surfaceContainerLow);
expect(material.shadowColor, colors.shadow);
expect(material.surfaceTintColor, Colors.transparent); // Don't use surface tint. Toned surface container is used instead.
expect(material.shape, const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12.0)),
));
});
testWidgets('Material3 - Card.filled defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final ColorScheme colors = theme.colorScheme;
await tester.pumpWidget(MaterialApp(
theme: theme,
home: const Scaffold(
body: Card.filled(),
),
));
final Container container = _getCardContainer(tester);
final Material material = _getCardMaterial(tester);
expect(material.clipBehavior, Clip.none);
expect(material.elevation, 0.0);
expect(container.margin, const EdgeInsets.all(4.0));
expect(material.color, colors.surfaceContainerHighest);
expect(material.shadowColor, colors.shadow);
expect(material.surfaceTintColor, Colors.transparent);
expect(material.shape, const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12.0)),
));
});
testWidgets('Material3 - Card.outlined defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final ColorScheme colors = theme.colorScheme;
await tester.pumpWidget(MaterialApp(
theme: theme,
home: const Scaffold(
body: Card.outlined(),
),
));
final Container container = _getCardContainer(tester);
final Material material = _getCardMaterial(tester);
expect(material.clipBehavior, Clip.none);
expect(material.elevation, 0.0);
expect(container.margin, const EdgeInsets.all(4.0));
expect(material.color, colors.surface);
expect(material.shadowColor, colors.shadow);
expect(material.surfaceTintColor, Colors.transparent);
expect(material.shape, RoundedRectangleBorder(
side: BorderSide(color: colors.outlineVariant),
borderRadius: const BorderRadius.all(Radius.circular(12.0)),
));
});
testWidgets('Card can take semantic text from multiple children', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Card(
semanticContainer: false,
child: Column(
children: <Widget>[
const Text('I am text!'),
const Text('Moar text!!1'),
ElevatedButton(
onPressed: () { },
child: const Text('Button'),
),
],
),
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
elevation: 1.0,
thickness: 0.0,
children: <TestSemantics>[
TestSemantics(
id: 2,
label: 'I am text!',
textDirection: TextDirection.ltr,
),
TestSemantics(
id: 3,
label: 'Moar text!!1',
textDirection: TextDirection.ltr,
),
TestSemantics(
id: 4,
label: 'Button',
textDirection: TextDirection.ltr,
actions: <SemanticsAction>[
SemanticsAction.tap,
],
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isButton,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
],
),
],
),
],
),
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Card merges children when it is a semanticContainer', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
debugResetSemanticsIdCounter();
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Card(
child: Column(
children: <Widget>[
Text('First child'),
Text('Second child'),
],
),
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
label: 'First child\nSecond child',
textDirection: TextDirection.ltr,
),
],
),
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Card margin', (WidgetTester tester) async {
const Key contentsKey = ValueKey<String>('contents');
await tester.pumpWidget(
Container(
alignment: Alignment.topLeft,
child: Card(
child: Container(
key: contentsKey,
color: const Color(0xFF00FF00),
width: 100.0,
height: 100.0,
),
),
),
);
// Default margin is 4
expect(tester.getTopLeft(find.byType(Card)), Offset.zero);
expect(tester.getSize(find.byType(Card)), const Size(108.0, 108.0));
expect(tester.getTopLeft(find.byKey(contentsKey)), const Offset(4.0, 4.0));
expect(tester.getSize(find.byKey(contentsKey)), const Size(100.0, 100.0));
await tester.pumpWidget(
Container(
alignment: Alignment.topLeft,
child: Card(
margin: EdgeInsets.zero,
child: Container(
key: contentsKey,
color: const Color(0xFF00FF00),
width: 100.0,
height: 100.0,
),
),
),
);
// Specified margin is zero
expect(tester.getTopLeft(find.byType(Card)), Offset.zero);
expect(tester.getSize(find.byType(Card)), const Size(100.0, 100.0));
expect(tester.getTopLeft(find.byKey(contentsKey)), Offset.zero);
expect(tester.getSize(find.byKey(contentsKey)), const Size(100.0, 100.0));
});
testWidgets('Card clipBehavior property passes through to the Material', (WidgetTester tester) async {
await tester.pumpWidget(const Card());
expect(tester.widget<Material>(find.byType(Material)).clipBehavior, Clip.none);
await tester.pumpWidget(const Card(clipBehavior: Clip.antiAlias));
expect(tester.widget<Material>(find.byType(Material)).clipBehavior, Clip.antiAlias);
});
testWidgets('Card clipBehavior property defers to theme when null', (WidgetTester tester) async {
await tester.pumpWidget(Builder(builder: (BuildContext context) {
final ThemeData themeData = Theme.of(context);
return Theme(
data: themeData.copyWith(
cardTheme: themeData.cardTheme.copyWith(
clipBehavior: Clip.antiAliasWithSaveLayer,
),
),
child: const Card(),
);
}));
expect(tester.widget<Material>(find.byType(Material)).clipBehavior, Clip.antiAliasWithSaveLayer);
});
testWidgets('Card shadowColor', (WidgetTester tester) async {
Material getCardMaterial(WidgetTester tester) {
return tester.widget<Material>(
find.descendant(
of: find.byType(Card),
matching: find.byType(Material),
),
);
}
Card getCard(WidgetTester tester) {
return tester.widget<Card>(
find.byType(Card),
);
}
await tester.pumpWidget(
const Card(),
);
expect(getCard(tester).shadowColor, null);
expect(getCardMaterial(tester).shadowColor, const Color(0xFF000000));
await tester.pumpWidget(
const Card(
shadowColor: Colors.red,
),
);
expect(getCardMaterial(tester).shadowColor, getCard(tester).shadowColor);
expect(getCardMaterial(tester).shadowColor, Colors.red);
});
}
Material _getCardMaterial(WidgetTester tester) {
return tester.widget<Material>(
find.descendant(
of: find.byType(Card),
matching: find.byType(Material),
),
);
}
Container _getCardContainer(WidgetTester tester) {
return tester.widget<Container>(
find.descendant(
of: find.byType(Card),
matching: find.byType(Container),
),
);
}
| flutter/packages/flutter/test/material/card_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/card_test.dart",
"repo_id": "flutter",
"token_count": 4154
} | 714 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'feedback_tester.dart';
void main() {
late DateTime firstDate;
late DateTime lastDate;
late DateTime? currentDate;
late DateTimeRange? initialDateRange;
late DatePickerEntryMode initialEntryMode = DatePickerEntryMode.calendar;
String? cancelText;
String? confirmText;
String? errorInvalidRangeText;
String? errorFormatText;
String? errorInvalidText;
String? fieldStartHintText;
String? fieldEndHintText;
String? fieldStartLabelText;
String? fieldEndLabelText;
String? helpText;
String? saveText;
setUp(() {
firstDate = DateTime(2015);
lastDate = DateTime(2016, DateTime.december, 31);
currentDate = null;
initialDateRange = DateTimeRange(
start: DateTime(2016, DateTime.january, 15),
end: DateTime(2016, DateTime.january, 25),
);
initialEntryMode = DatePickerEntryMode.calendar;
cancelText = null;
confirmText = null;
errorInvalidRangeText = null;
errorFormatText = null;
errorInvalidText = null;
fieldStartHintText = null;
fieldEndHintText = null;
fieldStartLabelText = null;
fieldEndLabelText = null;
helpText = null;
saveText = null;
});
const Size wideWindowSize = Size(1920.0, 1080.0);
const Size narrowWindowSize = Size(1070.0, 1770.0);
Future<void> preparePicker(
WidgetTester tester,
Future<void> Function(Future<DateTimeRange?> date) callback, {
TextDirection textDirection = TextDirection.ltr,
bool useMaterial3 = false,
}) async {
late BuildContext buttonContext;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: useMaterial3),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
buttonContext = context;
},
child: const Text('Go'),
);
},
),
),
));
await tester.tap(find.text('Go'));
expect(buttonContext, isNotNull);
final Future<DateTimeRange?> range = showDateRangePicker(
context: buttonContext,
initialDateRange: initialDateRange,
firstDate: firstDate,
lastDate: lastDate,
currentDate: currentDate,
initialEntryMode: initialEntryMode,
cancelText: cancelText,
confirmText: confirmText,
errorInvalidRangeText: errorInvalidRangeText,
errorFormatText: errorFormatText,
errorInvalidText: errorInvalidText,
fieldStartHintText: fieldStartHintText,
fieldEndHintText: fieldEndHintText,
fieldStartLabelText: fieldStartLabelText,
fieldEndLabelText: fieldEndLabelText,
helpText: helpText,
saveText: saveText,
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child ?? const SizedBox(),
);
},
);
await tester.pumpAndSettle(const Duration(seconds: 1));
await callback(range);
}
testWidgets('Default layout (calendar mode)', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Finder helpText = find.text('Select range');
final Finder firstDateHeaderText = find.text('Jan 15');
final Finder lastDateHeaderText = find.text('Jan 25, 2016');
final Finder saveText = find.text('Save');
expect(helpText, findsOneWidget);
expect(firstDateHeaderText, findsOneWidget);
expect(lastDateHeaderText, findsOneWidget);
expect(saveText, findsOneWidget);
// Test the close button position.
final Offset closeButtonBottomRight = tester.getBottomRight(find.byType(CloseButton));
final Offset helpTextTopLeft = tester.getTopLeft(helpText);
expect(closeButtonBottomRight.dx, 56.0);
expect(closeButtonBottomRight.dy, helpTextTopLeft.dy);
// Test the save and entry buttons position.
final Offset saveButtonBottomLeft = tester.getBottomLeft(find.byType(TextButton));
final Offset entryButtonBottomLeft = tester.getBottomLeft(
find.widgetWithIcon(IconButton, Icons.edit_outlined),
);
expect(saveButtonBottomLeft.dx, moreOrLessEquals(711.6, epsilon: 1e-5));
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(saveButtonBottomLeft.dy, helpTextTopLeft.dy);
}
expect(entryButtonBottomLeft.dx, saveButtonBottomLeft.dx - 48.0);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(entryButtonBottomLeft.dy, helpTextTopLeft.dy);
}
// Test help text position.
final Offset helpTextBottomLeft = tester.getBottomLeft(helpText);
expect(helpTextBottomLeft.dx, 72.0);
if (!kIsWeb || isCanvasKit) { // https://github.com/flutter/flutter/issues/99933
expect(helpTextBottomLeft.dy, closeButtonBottomRight.dy + 20.0);
}
// Test the header position.
final Offset firstDateHeaderTopLeft = tester.getTopLeft(firstDateHeaderText);
final Offset lastDateHeaderTopLeft = tester.getTopLeft(lastDateHeaderText);
expect(firstDateHeaderTopLeft.dx, 72.0);
expect(firstDateHeaderTopLeft.dy, helpTextBottomLeft.dy + 8.0);
final Offset firstDateHeaderTopRight = tester.getTopRight(firstDateHeaderText);
expect(lastDateHeaderTopLeft.dx, firstDateHeaderTopRight.dx + 66.0);
expect(lastDateHeaderTopLeft.dy, helpTextBottomLeft.dy + 8.0);
// Test the day headers position.
final Offset dayHeadersGridTopLeft = tester.getTopLeft(find.byType(GridView).first);
final Offset firstDateHeaderBottomLeft = tester.getBottomLeft(firstDateHeaderText);
expect(dayHeadersGridTopLeft.dx, (800 - 384) / 2);
expect(dayHeadersGridTopLeft.dy, firstDateHeaderBottomLeft.dy + 16.0);
// Test the calendar custom scroll view position.
final Offset calendarScrollViewTopLeft = tester.getTopLeft(find.byType(CustomScrollView));
final Offset dayHeadersGridBottomLeft = tester.getBottomLeft(find.byType(GridView).first);
expect(calendarScrollViewTopLeft.dx, 0.0);
expect(calendarScrollViewTopLeft.dy, dayHeadersGridBottomLeft.dy);
}, useMaterial3: true);
});
testWidgets('Default Dialog properties (calendar mode)', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surfaceContainerHigh);
expect(dialogMaterial.shadowColor, Colors.transparent);
expect(dialogMaterial.surfaceTintColor, Colors.transparent);
expect(dialogMaterial.elevation, 0.0);
expect(dialogMaterial.shape, const RoundedRectangleBorder());
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, EdgeInsets.zero);
}, useMaterial3: theme.useMaterial3);
});
testWidgets('Default Dialog properties (input mode)', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surfaceContainerHigh);
expect(dialogMaterial.shadowColor, Colors.transparent);
expect(dialogMaterial.surfaceTintColor, Colors.transparent);
expect(dialogMaterial.elevation, 0.0);
expect(dialogMaterial.shape, const RoundedRectangleBorder());
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, EdgeInsets.zero);
}, useMaterial3: theme.useMaterial3);
});
testWidgets('Scaffold and AppBar defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Scaffold scaffold = tester.widget<Scaffold>(find.byType(Scaffold));
expect(scaffold.backgroundColor, null);
final AppBar appBar = tester.widget<AppBar>(find.byType(AppBar));
final IconThemeData iconTheme = IconThemeData(color: theme.colorScheme.onSurfaceVariant);
expect(appBar.iconTheme, iconTheme);
expect(appBar.actionsIconTheme, iconTheme);
expect(appBar.elevation, 0);
expect(appBar.scrolledUnderElevation, 0);
expect(appBar.backgroundColor, Colors.transparent);
}, useMaterial3: theme.useMaterial3);
});
group('Landscape input-only date picker headers use headlineSmall', () {
// Regression test for https://github.com/flutter/flutter/issues/122056
// Common screen size roughly based on a Pixel 1
const Size kCommonScreenSizePortrait = Size(1070, 1770);
const Size kCommonScreenSizeLandscape = Size(1770, 1070);
Future<void> showPicker(WidgetTester tester, Size size) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = size;
tester.view.devicePixelRatio = 1.0;
initialEntryMode = DatePickerEntryMode.input;
await preparePicker(tester, (Future<DateTimeRange?> range) async { }, useMaterial3: true);
}
testWidgets('portrait', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizePortrait);
expect(tester.widget<Text>(find.text('Jan 15 – Jan 25, 2016')).style?.fontSize, 32);
await tester.tap(find.text('Cancel'));
await tester.pumpAndSettle();
});
testWidgets('landscape', (WidgetTester tester) async {
await showPicker(tester, kCommonScreenSizeLandscape);
expect(tester.widget<Text>(find.text('Jan 15 – Jan 25, 2016')).style?.fontSize, 24);
await tester.tap(find.text('Cancel'));
await tester.pumpAndSettle();
});
});
testWidgets('Save and help text is used', (WidgetTester tester) async {
helpText = 'help';
saveText = 'make it so';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.text(helpText!), findsOneWidget);
expect(find.text(saveText!), findsOneWidget);
});
});
testWidgets('Material3 has sentence case labels', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.text('Save'), findsOneWidget);
expect(find.text('Select range'), findsOneWidget);
}, useMaterial3: true);
});
testWidgets('Initial date is the default', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('SAVE'));
expect(
await range,
DateTimeRange(
start: DateTime(2016, DateTime.january, 15),
end: DateTime(2016, DateTime.january, 25),
),
);
});
});
testWidgets('Last month header should be visible if last date is selected', (WidgetTester tester) async {
firstDate = DateTime(2015);
lastDate = DateTime(2016, DateTime.december, 31);
initialDateRange = DateTimeRange(
start: lastDate,
end: lastDate,
);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// December header should be showing, but no November
expect(find.text('December 2016'), findsOneWidget);
expect(find.text('November 2016'), findsNothing);
});
});
testWidgets('First month header should be visible if first date is selected', (WidgetTester tester) async {
firstDate = DateTime(2015);
lastDate = DateTime(2016, DateTime.december, 31);
initialDateRange = DateTimeRange(
start: firstDate,
end: firstDate,
);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// January and February headers should be showing, but no March
expect(find.text('January 2015'), findsOneWidget);
expect(find.text('February 2015'), findsOneWidget);
expect(find.text('March 2015'), findsNothing);
});
});
testWidgets('Current month header should be visible if no date is selected', (WidgetTester tester) async {
firstDate = DateTime(2015);
lastDate = DateTime(2016, DateTime.december, 31);
currentDate = DateTime(2016, DateTime.september);
initialDateRange = null;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// September and October headers should be showing, but no August
expect(find.text('September 2016'), findsOneWidget);
expect(find.text('October 2016'), findsOneWidget);
expect(find.text('August 2016'), findsNothing);
});
});
testWidgets('Can cancel', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.byIcon(Icons.close));
expect(await range, isNull);
});
});
testWidgets('Can select a range', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('12').first);
await tester.tap(find.text('14').first);
await tester.tap(find.text('SAVE'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 12),
end: DateTime(2016, DateTime.january, 14),
));
});
});
testWidgets('Tapping earlier date resets selected range', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('12').first);
await tester.tap(find.text('11').first);
await tester.tap(find.text('15').first);
await tester.tap(find.text('SAVE'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 11),
end: DateTime(2016, DateTime.january, 15),
));
});
});
testWidgets('Can select single day range', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('12').first);
await tester.tap(find.text('12').first);
await tester.tap(find.text('SAVE'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 12),
end: DateTime(2016, DateTime.january, 12),
));
});
});
testWidgets('Cannot select a day outside bounds', (WidgetTester tester) async {
initialDateRange = DateTimeRange(
start: DateTime(2017, DateTime.january, 13),
end: DateTime(2017, DateTime.january, 15),
);
firstDate = DateTime(2017, DateTime.january, 12);
lastDate = DateTime(2017, DateTime.january, 16);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// Earlier than firstDate. Should be ignored.
await tester.tap(find.text('10'));
// Later than lastDate. Should be ignored.
await tester.tap(find.text('20'));
await tester.tap(find.text('SAVE'));
// We should still be on the initial date.
expect(await range, initialDateRange);
});
});
testWidgets('Can switch from calendar to input entry mode', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNothing);
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNWidgets(2));
});
});
testWidgets('Can switch from input to calendar entry mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.input;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNWidgets(2));
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
});
testWidgets('Can not switch out of calendarOnly mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.calendarOnly;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
});
});
testWidgets('Can not switch out of inputOnly mode', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.inputOnly;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNWidgets(2));
expect(find.byIcon(Icons.calendar_today), findsNothing);
});
});
testWidgets('Input only mode should validate date', (WidgetTester tester) async {
initialEntryMode = DatePickerEntryMode.inputOnly;
errorInvalidText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '08/08/2014');
await tester.enterText(find.byType(TextField).at(1), '08/08/2014');
expect(find.text(errorInvalidText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorInvalidText!), findsNWidgets(2));
});
});
testWidgets('Switching to input mode keeps selected date', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('12').first);
await tester.tap(find.text('14').first);
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
await tester.tap(find.text('OK'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 12),
end: DateTime(2016, DateTime.january, 14),
));
});
});
group('Toggle from input entry mode validates dates', () {
setUp(() {
initialEntryMode = DatePickerEntryMode.input;
});
testWidgets('Invalid start date', (WidgetTester tester) async {
// Invalid start date should have neither a start nor end date selected in
// calendar mode
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/27/1918');
await tester.enterText(find.byType(TextField).at(1), '12/25/2016');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.text('Start Date'), findsOneWidget);
expect(find.text('End Date'), findsOneWidget);
});
});
testWidgets('Invalid end date', (WidgetTester tester) async {
// Invalid end date should only have a start date selected
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/24/2016');
await tester.enterText(find.byType(TextField).at(1), '12/25/2050');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.text('Dec 24'), findsOneWidget);
expect(find.text('End Date'), findsOneWidget);
});
});
testWidgets('Invalid range', (WidgetTester tester) async {
// Start date after end date should just use the start date
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/25/2016');
await tester.enterText(find.byType(TextField).at(1), '12/24/2016');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.text('Dec 25'), findsOneWidget);
expect(find.text('End Date'), findsOneWidget);
});
});
});
testWidgets('OK Cancel button layout', (WidgetTester tester) async {
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showDateRangePicker(
context: context,
firstDate:DateTime(2001),
lastDate: DateTime(2031, DateTime.december, 31),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child ?? const SizedBox(),
);
},
);
},
);
},
),
),
),
);
}
Future<void> showOkCancelDialog(TextDirection textDirection) async {
await tester.pumpWidget(buildFrame(textDirection));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
await tester.tap(find.byIcon(Icons.edit));
await tester.pumpAndSettle();
}
Future<void> dismissOkCancelDialog() async {
await tester.tap(find.text('CANCEL'));
await tester.pumpAndSettle();
}
await showOkCancelDialog(TextDirection.ltr);
expect(tester.getBottomRight(find.text('OK')).dx, 622);
expect(tester.getBottomLeft(find.text('OK')).dx, 594);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 560);
await dismissOkCancelDialog();
await showOkCancelDialog(TextDirection.rtl);
expect(tester.getBottomRight(find.text('OK')).dx, 206);
expect(tester.getBottomLeft(find.text('OK')).dx, 178);
expect(tester.getBottomRight(find.text('CANCEL')).dx, 324);
await dismissOkCancelDialog();
});
group('Haptic feedback', () {
const Duration hapticFeedbackInterval = Duration(milliseconds: 10);
late FeedbackTester feedback;
setUp(() {
feedback = FeedbackTester();
initialDateRange = DateTimeRange(
start: DateTime(2017, DateTime.january, 15),
end: DateTime(2017, DateTime.january, 17),
);
firstDate = DateTime(2017, DateTime.january, 10);
lastDate = DateTime(2018, DateTime.january, 20);
});
tearDown(() {
feedback.dispose();
});
testWidgets('Selecting dates vibrates', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('10').first);
await tester.pump(hapticFeedbackInterval);
expect(feedback.hapticCount, 1);
await tester.tap(find.text('12').first);
await tester.pump(hapticFeedbackInterval);
expect(feedback.hapticCount, 2);
await tester.tap(find.text('14').first);
await tester.pump(hapticFeedbackInterval);
expect(feedback.hapticCount, 3);
});
});
testWidgets('Tapping unselectable date does not vibrate', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('8').first);
await tester.pump(hapticFeedbackInterval);
expect(feedback.hapticCount, 0);
});
});
});
group('Keyboard navigation', () {
testWidgets('Can toggle to calendar entry mode', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNothing);
// Navigate to the entry toggle button and activate it
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should be in the input mode
expect(find.byType(TextField), findsNWidgets(2));
});
});
testWidgets('Can navigate date grid with arrow keys', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.pumpAndSettle();
// Navigate from Jan 15 to Jan 18 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Activate it to select the beginning of the range
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate to Jan 29
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
// Activate it to select the end of the range
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 18 - Jan 29
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 18),
end: DateTime(2016, DateTime.january, 29),
));
});
});
testWidgets('Navigating with arrow keys scrolls as needed', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// Jan and Feb headers should be showing, but no March
expect(find.text('January 2016'), findsOneWidget);
expect(find.text('February 2016'), findsOneWidget);
expect(find.text('March 2016'), findsNothing);
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Navigate from Jan 15 to Jan 18 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
// Activate it to select the beginning of the range
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate to Mar 17
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
// Jan should have scrolled off, Mar should be visible
expect(find.text('January 2016'), findsNothing);
expect(find.text('February 2016'), findsOneWidget);
expect(find.text('March 2016'), findsOneWidget);
// Activate it to select the end of the range
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 18 - Mar 17
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 18),
end: DateTime(2016, DateTime.march, 17),
));
});
});
testWidgets('RTL text direction reverses the horizontal arrow key navigation', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// Navigate to the grid
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Navigate from Jan 15 to 19 with arrow keys
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Activate it
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate to Jan 21
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
// Activate it
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Navigate out of the grid and to the OK button
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
await tester.sendKeyEvent(LogicalKeyboardKey.tab);
// Activate OK
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Should have selected Jan 19 - Mar 21
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.january, 19),
end: DateTime(2016, DateTime.january, 21),
));
}, textDirection: TextDirection.rtl);
});
});
group('Input mode', () {
setUp(() {
firstDate = DateTime(2015);
lastDate = DateTime(2017, DateTime.december, 31);
initialDateRange = DateTimeRange(
start: DateTime(2017, DateTime.january, 15),
end: DateTime(2017, DateTime.january, 17),
);
initialEntryMode = DatePickerEntryMode.input;
});
testWidgets('Default Dialog properties (input mode)', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surfaceContainerHigh);
expect(dialogMaterial.shadowColor, Colors.transparent);
expect(dialogMaterial.surfaceTintColor, Colors.transparent);
expect(dialogMaterial.elevation, 6.0);
expect(
dialogMaterial.shape,
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(28.0))),
);
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, const EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0));
}, useMaterial3: theme.useMaterial3);
});
testWidgets('Default InputDecoration', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final InputDecoration startDateDecoration = tester.widget<TextField>(
find.byType(TextField).first).decoration!;
expect(startDateDecoration.border, const OutlineInputBorder());
expect(startDateDecoration.filled, false);
expect(startDateDecoration.hintText, 'mm/dd/yyyy');
expect(startDateDecoration.labelText, 'Start Date');
expect(startDateDecoration.errorText, null);
final InputDecoration endDateDecoration = tester.widget<TextField>(
find.byType(TextField).last).decoration!;
expect(endDateDecoration.border, const OutlineInputBorder());
expect(endDateDecoration.filled, false);
expect(endDateDecoration.hintText, 'mm/dd/yyyy');
expect(endDateDecoration.labelText, 'End Date');
expect(endDateDecoration.errorText, null);
}, useMaterial3: true);
});
testWidgets('Initial entry mode is used', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNWidgets(2));
});
});
testWidgets('All custom strings are used', (WidgetTester tester) async {
initialDateRange = null;
cancelText = 'nope';
confirmText = 'yep';
fieldStartHintText = 'hint1';
fieldEndHintText = 'hint2';
fieldStartLabelText = 'label1';
fieldEndLabelText = 'label2';
helpText = 'help';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.text(cancelText!), findsOneWidget);
expect(find.text(confirmText!), findsOneWidget);
expect(find.text(fieldStartHintText!), findsOneWidget);
expect(find.text(fieldEndHintText!), findsOneWidget);
expect(find.text(fieldStartLabelText!), findsOneWidget);
expect(find.text(fieldEndLabelText!), findsOneWidget);
expect(find.text(helpText!), findsOneWidget);
});
});
testWidgets('Initial date is the default', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.tap(find.text('OK'));
expect(await range, DateTimeRange(
start: DateTime(2017, DateTime.january, 15),
end: DateTime(2017, DateTime.january, 17),
));
});
});
testWidgets('Can toggle to calendar entry mode', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(find.byType(TextField), findsNWidgets(2));
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
});
testWidgets('Toggle to calendar mode keeps selected date', (WidgetTester tester) async {
initialDateRange = null;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/25/2016');
await tester.enterText(find.byType(TextField).at(1), '12/27/2016');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
await tester.tap(find.text('SAVE'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.december, 25),
end: DateTime(2016, DateTime.december, 27),
));
});
});
testWidgets('Entered text returns range', (WidgetTester tester) async {
initialDateRange = null;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/25/2016');
await tester.enterText(find.byType(TextField).at(1), '12/27/2016');
await tester.tap(find.text('OK'));
expect(await range, DateTimeRange(
start: DateTime(2016, DateTime.december, 25),
end: DateTime(2016, DateTime.december, 27),
));
});
});
testWidgets('Too short entered text shows error', (WidgetTester tester) async {
initialDateRange = null;
errorFormatText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/25');
await tester.enterText(find.byType(TextField).at(1), '12/25');
expect(find.text(errorFormatText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorFormatText!), findsNWidgets(2));
});
});
testWidgets('Bad format entered text shows error', (WidgetTester tester) async {
initialDateRange = null;
errorFormatText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '20202014');
await tester.enterText(find.byType(TextField).at(1), '20212014');
expect(find.text(errorFormatText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorFormatText!), findsNWidgets(2));
});
});
testWidgets('Invalid entered text shows error', (WidgetTester tester) async {
initialDateRange = null;
errorInvalidText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '08/08/2014');
await tester.enterText(find.byType(TextField).at(1), '08/08/2014');
expect(find.text(errorInvalidText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorInvalidText!), findsNWidgets(2));
});
});
testWidgets('End before start date shows error', (WidgetTester tester) async {
initialDateRange = null;
errorInvalidRangeText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/27/2016');
await tester.enterText(find.byType(TextField).at(1), '12/25/2016');
expect(find.text(errorInvalidRangeText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorInvalidRangeText!), findsOneWidget);
});
});
testWidgets('Error text only displayed for invalid date', (WidgetTester tester) async {
initialDateRange = null;
errorInvalidText = 'oops';
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/27/2016');
await tester.enterText(find.byType(TextField).at(1), '01/01/2018');
expect(find.text(errorInvalidText!), findsNothing);
await tester.tap(find.text('OK'));
await tester.pumpAndSettle();
expect(find.text(errorInvalidText!), findsOneWidget);
});
});
testWidgets('End before start date does not get passed to calendar mode', (WidgetTester tester) async {
initialDateRange = null;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
await tester.enterText(find.byType(TextField).at(0), '12/27/2016');
await tester.enterText(find.byType(TextField).at(1), '12/25/2016');
await tester.tap(find.byIcon(Icons.calendar_today));
await tester.pumpAndSettle();
await tester.tap(find.text('SAVE'));
await tester.pumpAndSettle();
// Save button should be disabled, so dialog should still be up
// with the first date selected, but no end date
expect(find.text('Dec 27'), findsOneWidget);
expect(find.text('End Date'), findsOneWidget);
});
});
testWidgets('InputDecorationTheme is honored', (WidgetTester tester) async {
// Given a custom paint for an input decoration, extract the border and
// fill color and test them against the expected values.
void testInputDecorator(CustomPaint decoratorPaint, InputBorder expectedBorder, Color expectedContainerColor) {
final dynamic/*_InputBorderPainter*/ inputBorderPainter = decoratorPaint.foregroundPainter;
// ignore: avoid_dynamic_calls
final dynamic/*_InputBorderTween*/ inputBorderTween = inputBorderPainter.border;
// ignore: avoid_dynamic_calls
final Animation<double> animation = inputBorderPainter.borderAnimation as Animation<double>;
// ignore: avoid_dynamic_calls
final InputBorder actualBorder = inputBorderTween.evaluate(animation) as InputBorder;
// ignore: avoid_dynamic_calls
final Color containerColor = inputBorderPainter.blendedColor as Color;
expect(actualBorder, equals(expectedBorder));
expect(containerColor, equals(expectedContainerColor));
}
late BuildContext buttonContext;
const InputBorder border = InputBorder.none;
await tester.pumpWidget(MaterialApp(
theme: ThemeData.light().copyWith(
inputDecorationTheme: const InputDecorationTheme(
border: border,
),
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
buttonContext = context;
},
child: const Text('Go'),
);
},
),
),
));
await tester.tap(find.text('Go'));
expect(buttonContext, isNotNull);
showDateRangePicker(
context: buttonContext,
initialDateRange: initialDateRange,
firstDate: firstDate,
lastDate: lastDate,
initialEntryMode: DatePickerEntryMode.input,
);
await tester.pumpAndSettle();
final Finder borderContainers = find.descendant(
of: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_BorderContainer'),
matching: find.byWidgetPredicate((Widget w) => w is CustomPaint),
);
// Test the start date text field
testInputDecorator(tester.widget(borderContainers.first), border, Colors.transparent);
// Test the end date text field
testInputDecorator(tester.widget(borderContainers.last), border, Colors.transparent);
});
// This is a regression test for https://github.com/flutter/flutter/issues/131989.
testWidgets('Dialog contents do not overflow when resized from landscape to portrait',
(WidgetTester tester) async {
addTearDown(tester.view.reset);
// Initial window size is wide for landscape mode.
tester.view.physicalSize = wideWindowSize;
tester.view.devicePixelRatio = 1.0;
await preparePicker(tester, (Future<DateTimeRange?> range) async {
// Change window size to narrow for portrait mode.
tester.view.physicalSize = narrowWindowSize;
await tester.pump();
expect(tester.takeException(), null);
});
});
});
testWidgets('DatePickerDialog is state restorable', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
restorationScopeId: 'app',
home: const _RestorableDateRangePickerDialogTestWidget(),
),
);
// The date range picker should be closed.
expect(find.byType(DateRangePickerDialog), findsNothing);
expect(find.text('1/1/2021 to 5/1/2021'), findsOneWidget);
// Open the date range picker.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(DateRangePickerDialog), findsOneWidget);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// The date range picker should be open after restoring.
expect(find.byType(DateRangePickerDialog), findsOneWidget);
// Close the date range picker.
await tester.tap(find.byIcon(Icons.close));
await tester.pumpAndSettle();
// The date range picker should be closed, the text value updated to the
// newly selected date.
expect(find.byType(DateRangePickerDialog), findsNothing);
expect(find.text('1/1/2021 to 5/1/2021'), findsOneWidget);
// The date range picker should be open after restoring.
await tester.restoreFrom(restorationData);
expect(find.byType(DateRangePickerDialog), findsOneWidget);
// // Select a different date and close the date range picker.
await tester.tap(find.text('12').first);
await tester.pumpAndSettle();
await tester.tap(find.text('14').first);
await tester.pumpAndSettle();
// Restart after the new selection. It should remain selected.
await tester.restartAndRestore();
// Close the date range picker.
await tester.tap(find.text('SAVE'));
await tester.pumpAndSettle();
// The date range picker should be closed, the text value updated to the
// newly selected date.
expect(find.byType(DateRangePickerDialog), findsNothing);
expect(find.text('12/1/2021 to 14/1/2021'), findsOneWidget);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
testWidgets('DateRangePickerDialog state restoration - DatePickerEntryMode', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
restorationScopeId: 'app',
home: _RestorableDateRangePickerDialogTestWidget(
datePickerEntryMode: DatePickerEntryMode.calendarOnly,
),
),
);
// The date range picker should be closed.
expect(find.byType(DateRangePickerDialog), findsNothing);
expect(find.text('1/1/2021 to 5/1/2021'), findsOneWidget);
// Open the date range picker.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(find.byType(DateRangePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// The date range picker should be open after restoring.
expect(find.byType(DateRangePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
// Tap on the barrier.
await tester.tap(find.byIcon(Icons.close));
await tester.pumpAndSettle();
// The date range picker should be closed, the text value should be the same
// as before.
expect(find.byType(DateRangePickerDialog), findsNothing);
expect(find.text('1/1/2021 to 5/1/2021'), findsOneWidget);
// The date range picker should be open after restoring.
await tester.restoreFrom(restorationData);
expect(find.byType(DateRangePickerDialog), findsOneWidget);
// Only in calendar mode and cannot switch out.
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.edit), findsNothing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/33615
group('showDateRangePicker avoids overlapping display features', () {
testWidgets('positioning with anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDateRangePicker(
context: context,
firstDate: DateTime(2018),
lastDate: DateTime(2030),
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(DateRangePickerDialog)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(DateRangePickerDialog)), const Offset(800.0, 600.0));
});
testWidgets('positioning with Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDateRangePicker(
context: context,
firstDate: DateTime(2018),
lastDate: DateTime(2030),
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// By default it should place the dialog on the right screen
expect(tester.getTopLeft(find.byType(DateRangePickerDialog)), const Offset(410.0, 0.0));
expect(tester.getBottomRight(find.byType(DateRangePickerDialog)), const Offset(800.0, 600.0));
});
testWidgets('positioning with defaults', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showDateRangePicker(
context: context,
firstDate: DateTime(2018),
lastDate: DateTime(2030),
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(DateRangePickerDialog)), Offset.zero);
expect(tester.getBottomRight(find.byType(DateRangePickerDialog)), const Offset(390.0, 600.0));
});
});
group('Semantics', () {
testWidgets('calendar mode', (WidgetTester tester) async {
final SemanticsHandle semantics = tester.ensureSemantics();
currentDate = DateTime(2016, DateTime.january, 30);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
expect(
tester.getSemantics(find.text('30')),
matchesSemantics(
label: '30, Saturday, January 30, 2016, Today',
hasTapAction: true,
isFocusable: true,
),
);
});
semantics.dispose();
});
});
for (final TextInputType? keyboardType in <TextInputType?>[null, TextInputType.emailAddress]) {
testWidgets('DateRangePicker takes keyboardType $keyboardType', (WidgetTester tester) async {
late BuildContext buttonContext;
const InputBorder border = InputBorder.none;
await tester.pumpWidget(MaterialApp(
theme: ThemeData.light().copyWith(
inputDecorationTheme: const InputDecorationTheme(
border: border,
),
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
buttonContext = context;
},
child: const Text('Go'),
);
},
),
),
));
await tester.tap(find.text('Go'));
expect(buttonContext, isNotNull);
if (keyboardType == null) {
// If no keyboardType, expect the default.
showDateRangePicker(
context: buttonContext,
initialDateRange: initialDateRange,
firstDate: firstDate,
lastDate: lastDate,
initialEntryMode: DatePickerEntryMode.input,
);
} else {
// If there is a keyboardType, expect it to be passed through.
showDateRangePicker(
context: buttonContext,
initialDateRange: initialDateRange,
firstDate: firstDate,
lastDate: lastDate,
initialEntryMode: DatePickerEntryMode.input,
keyboardType: keyboardType,
);
}
await tester.pumpAndSettle();
final DateRangePickerDialog picker = tester.widget(find.byType(DateRangePickerDialog));
expect(picker.keyboardType, keyboardType ?? TextInputType.datetime);
});
}
testWidgets('honors switchToInputEntryModeIcon', (WidgetTester tester) async {
Widget buildApp({bool? useMaterial3, Icon? switchToInputEntryModeIcon}) {
return MaterialApp(
theme: ThemeData(
useMaterial3: useMaterial3 ?? false,
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Click X'),
onPressed: () {
showDateRangePicker(
context: context,
firstDate: DateTime(2020),
lastDate: DateTime(2030),
switchToInputEntryModeIcon: switchToInputEntryModeIcon,
);
},
);
},
),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.edit), findsOneWidget);
await tester.tap(find.byIcon(Icons.close));
await tester.pumpAndSettle();
await tester.pumpWidget(buildApp(useMaterial3: true));
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.edit_outlined), findsOneWidget);
await tester.tap(find.byIcon(Icons.close));
await tester.pumpAndSettle();
await tester.pumpWidget(
buildApp(
switchToInputEntryModeIcon: const Icon(Icons.keyboard),
),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.keyboard), findsOneWidget);
await tester.tap(find.byIcon(Icons.close));
await tester.pumpAndSettle();
});
testWidgets('honors switchToCalendarEntryModeIcon', (WidgetTester tester) async {
Widget buildApp({bool? useMaterial3, Icon? switchToCalendarEntryModeIcon}) {
return MaterialApp(
theme: ThemeData(
useMaterial3: useMaterial3 ?? false,
),
home: Material(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Click X'),
onPressed: () {
showDateRangePicker(
context: context,
firstDate: DateTime(2020),
lastDate: DateTime(2030),
switchToCalendarEntryModeIcon: switchToCalendarEntryModeIcon,
initialEntryMode: DatePickerEntryMode.input,
cancelText: 'CANCEL',
);
},
);
},
),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.calendar_today), findsOneWidget);
await tester.tap(find.text('CANCEL'));
await tester.pumpAndSettle();
await tester.pumpWidget(buildApp(useMaterial3: true));
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.calendar_today), findsOneWidget);
await tester.tap(find.text('CANCEL'));
await tester.pumpAndSettle();
await tester.pumpWidget(
buildApp(
switchToCalendarEntryModeIcon: const Icon(Icons.favorite),
),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.favorite), findsOneWidget);
await tester.tap(find.text('CANCEL'));
await tester.pumpAndSettle();
});
group('Material 2', () {
// These tests are only relevant for Material 2. Once Material 2
// support is deprecated and the APIs are removed, these tests
// can be deleted.
testWidgets('Default layout (calendar mode)', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Finder helpText = find.text('SELECT RANGE');
final Finder firstDateHeaderText = find.text('Jan 15');
final Finder lastDateHeaderText = find.text('Jan 25, 2016');
final Finder saveText = find.text('SAVE');
expect(helpText, findsOneWidget);
expect(firstDateHeaderText, findsOneWidget);
expect(lastDateHeaderText, findsOneWidget);
expect(saveText, findsOneWidget);
// Test the close button position.
final Offset closeButtonBottomRight = tester.getBottomRight(find.byType(CloseButton));
final Offset helpTextTopLeft = tester.getTopLeft(helpText);
expect(closeButtonBottomRight.dx, 56.0);
expect(closeButtonBottomRight.dy, helpTextTopLeft.dy - 6.0);
// Test the save and entry buttons position.
final Offset saveButtonBottomLeft = tester.getBottomLeft(find.byType(TextButton));
final Offset entryButtonBottomLeft = tester.getBottomLeft(
find.widgetWithIcon(IconButton, Icons.edit),
);
expect(saveButtonBottomLeft.dx, 800 - 80.0);
expect(saveButtonBottomLeft.dy, helpTextTopLeft.dy - 6.0);
expect(entryButtonBottomLeft.dx, saveButtonBottomLeft.dx - 48.0);
expect(entryButtonBottomLeft.dy, helpTextTopLeft.dy - 6.0);
// Test help text position.
final Offset helpTextBottomLeft = tester.getBottomLeft(helpText);
expect(helpTextBottomLeft.dx, 72.0);
expect(helpTextBottomLeft.dy, closeButtonBottomRight.dy + 16.0);
// Test the header position.
final Offset firstDateHeaderTopLeft = tester.getTopLeft(firstDateHeaderText);
final Offset lastDateHeaderTopLeft = tester.getTopLeft(lastDateHeaderText);
expect(firstDateHeaderTopLeft.dx, 72.0);
expect(firstDateHeaderTopLeft.dy, helpTextBottomLeft.dy + 8.0);
final Offset firstDateHeaderTopRight = tester.getTopRight(firstDateHeaderText);
expect(lastDateHeaderTopLeft.dx, firstDateHeaderTopRight.dx + 72.0);
expect(lastDateHeaderTopLeft.dy, helpTextBottomLeft.dy + 8.0);
// Test the day headers position.
final Offset dayHeadersGridTopLeft = tester.getTopLeft(find.byType(GridView).first);
final Offset firstDateHeaderBottomLeft = tester.getBottomLeft(firstDateHeaderText);
expect(dayHeadersGridTopLeft.dx, (800 - 384) / 2);
expect(dayHeadersGridTopLeft.dy, firstDateHeaderBottomLeft.dy + 16.0);
// Test the calendar custom scroll view position.
final Offset calendarScrollViewTopLeft = tester.getTopLeft(find.byType(CustomScrollView));
final Offset dayHeadersGridBottomLeft = tester.getBottomLeft(find.byType(GridView).first);
expect(calendarScrollViewTopLeft.dx, 0.0);
expect(calendarScrollViewTopLeft.dy, dayHeadersGridBottomLeft.dy);
});
});
testWidgets('Default Dialog properties (calendar mode)', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surface);
expect(dialogMaterial.shadowColor, Colors.transparent);
expect(dialogMaterial.surfaceTintColor, Colors.transparent);
expect(dialogMaterial.elevation, 0.0);
expect(dialogMaterial.shape, const RoundedRectangleBorder());
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, EdgeInsets.zero);
});
});
testWidgets('Scaffold and AppBar defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Scaffold scaffold = tester.widget<Scaffold>(find.byType(Scaffold));
expect(scaffold.backgroundColor, theme.colorScheme.surface);
final AppBar appBar = tester.widget<AppBar>(find.byType(AppBar));
final IconThemeData iconTheme = IconThemeData(color: theme.colorScheme.onPrimary);
expect(appBar.iconTheme, iconTheme);
expect(appBar.actionsIconTheme, iconTheme);
expect(appBar.elevation, null);
expect(appBar.scrolledUnderElevation, null);
expect(appBar.backgroundColor, null);
});
});
group('Input mode', () {
setUp(() {
firstDate = DateTime(2015);
lastDate = DateTime(2017, DateTime.december, 31);
initialDateRange = DateTimeRange(
start: DateTime(2017, DateTime.january, 15),
end: DateTime(2017, DateTime.january, 17),
);
initialEntryMode = DatePickerEntryMode.input;
});
testWidgets('Default Dialog properties (input mode)', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final Material dialogMaterial = tester.widget<Material>(
find.descendant(of: find.byType(Dialog),
matching: find.byType(Material),
).first);
expect(dialogMaterial.color, theme.colorScheme.surface);
expect(dialogMaterial.shadowColor, theme.shadowColor);
expect(dialogMaterial.surfaceTintColor, null);
expect(dialogMaterial.elevation, 24.0);
expect(
dialogMaterial.shape,
const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))),
);
expect(dialogMaterial.clipBehavior, Clip.antiAlias);
final Dialog dialog = tester.widget<Dialog>(find.byType(Dialog));
expect(dialog.insetPadding, const EdgeInsets.symmetric(horizontal: 16.0, vertical: 24.0));
});
});
testWidgets('Default InputDecoration', (WidgetTester tester) async {
await preparePicker(tester, (Future<DateTimeRange?> range) async {
final InputDecoration startDateDecoration = tester.widget<TextField>(
find.byType(TextField).first).decoration!;
expect(startDateDecoration.border, const UnderlineInputBorder());
expect(startDateDecoration.filled, false);
expect(startDateDecoration.hintText, 'mm/dd/yyyy');
expect(startDateDecoration.labelText, 'Start Date');
expect(startDateDecoration.errorText, null);
final InputDecoration endDateDecoration = tester.widget<TextField>(
find.byType(TextField).last).decoration!;
expect(endDateDecoration.border, const UnderlineInputBorder());
expect(endDateDecoration.filled, false);
expect(endDateDecoration.hintText, 'mm/dd/yyyy');
expect(endDateDecoration.labelText, 'End Date');
expect(endDateDecoration.errorText, null);
});
});
});
});
}
class _RestorableDateRangePickerDialogTestWidget extends StatefulWidget {
const _RestorableDateRangePickerDialogTestWidget({
this.datePickerEntryMode = DatePickerEntryMode.calendar,
});
final DatePickerEntryMode datePickerEntryMode;
@override
_RestorableDateRangePickerDialogTestWidgetState createState() => _RestorableDateRangePickerDialogTestWidgetState();
}
class _RestorableDateRangePickerDialogTestWidgetState extends State<_RestorableDateRangePickerDialogTestWidget> with RestorationMixin {
@override
String? get restorationId => 'scaffold_state';
final RestorableDateTimeN _startDate = RestorableDateTimeN(DateTime(2021));
final RestorableDateTimeN _endDate = RestorableDateTimeN(DateTime(2021, 1, 5));
late final RestorableRouteFuture<DateTimeRange?> _restorableDateRangePickerRouteFuture = RestorableRouteFuture<DateTimeRange?>(
onComplete: _selectDateRange,
onPresent: (NavigatorState navigator, Object? arguments) {
return navigator.restorablePush(
_dateRangePickerRoute,
arguments: <String, dynamic>{
'datePickerEntryMode': widget.datePickerEntryMode.index,
},
);
},
);
@override
void dispose() {
_startDate.dispose();
_endDate.dispose();
_restorableDateRangePickerRouteFuture.dispose();
super.dispose();
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_startDate, 'start_date');
registerForRestoration(_endDate, 'end_date');
registerForRestoration(_restorableDateRangePickerRouteFuture, 'date_picker_route_future');
}
void _selectDateRange(DateTimeRange? newSelectedDate) {
if (newSelectedDate != null) {
setState(() {
_startDate.value = newSelectedDate.start;
_endDate.value = newSelectedDate.end;
});
}
}
@pragma('vm:entry-point')
static Route<DateTimeRange?> _dateRangePickerRoute(
BuildContext context,
Object? arguments,
) {
return DialogRoute<DateTimeRange?>(
context: context,
builder: (BuildContext context) {
final Map<dynamic, dynamic> args = arguments! as Map<dynamic, dynamic>;
return DateRangePickerDialog(
restorationId: 'date_picker_dialog',
initialEntryMode: DatePickerEntryMode.values[args['datePickerEntryMode'] as int],
firstDate: DateTime(2021),
currentDate: DateTime(2021, 1, 25),
lastDate: DateTime(2022),
);
},
);
}
@override
Widget build(BuildContext context) {
final DateTime? startDateTime = _startDate.value;
final DateTime? endDateTime = _endDate.value;
// Example: "25/7/1994"
final String startDateTimeString = '${startDateTime?.day}/${startDateTime?.month}/${startDateTime?.year}';
final String endDateTimeString = '${endDateTime?.day}/${endDateTime?.month}/${endDateTime?.year}';
return Scaffold(
body: Center(
child: Column(
children: <Widget>[
OutlinedButton(
onPressed: () {
_restorableDateRangePickerRouteFuture.present();
},
child: const Text('X'),
),
Text('$startDateTimeString to $endDateTimeString'),
],
),
),
);
}
}
| flutter/packages/flutter/test/material/date_range_picker_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/date_range_picker_test.dart",
"repo_id": "flutter",
"token_count": 27625
} | 715 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('ElevatedButtonThemeData lerp special cases', () {
expect(ElevatedButtonThemeData.lerp(null, null, 0), null);
const ElevatedButtonThemeData data = ElevatedButtonThemeData();
expect(identical(ElevatedButtonThemeData.lerp(data, data, 0.5), data), true);
});
testWidgets('Material3: Passing no ElevatedButtonTheme returns defaults', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(colorScheme: colorScheme, useMaterial3: true),
home: Scaffold(
body: Center(
child: ElevatedButton(
onPressed: () { },
child: const Text('button'),
),
),
),
),
);
final Finder buttonMaterial = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderRadius, null);
expect(material.color, colorScheme.surface);
expect(material.elevation, 1);
expect(material.shadowColor, colorScheme.shadow);
expect(material.shape, const StadiumBorder());
expect(material.textStyle!.color, colorScheme.primary);
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center);
});
testWidgets('Material2: Passing no ElevatedButtonTheme returns defaults', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(colorScheme: colorScheme, useMaterial3: false),
home: Scaffold(
body: Center(
child: ElevatedButton(
onPressed: () { },
child: const Text('button'),
),
),
),
),
);
final Finder buttonMaterial = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderRadius, null);
expect(material.color, colorScheme.primary);
expect(material.elevation, 2);
expect(material.shadowColor, const Color(0xff000000));
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))));
expect(material.textStyle!.color, colorScheme.onPrimary);
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center);
});
group('[Theme, TextTheme, ElevatedButton style overrides]', () {
const Color foregroundColor = Color(0xff000001);
const Color backgroundColor = Color(0xff000002);
const Color disabledColor = Color(0xff000003);
const Color shadowColor = Color(0xff000004);
const double elevation = 1;
const TextStyle textStyle = TextStyle(fontSize: 12.0);
const EdgeInsets padding = EdgeInsets.all(3);
const Size minimumSize = Size(200, 200);
const BorderSide side = BorderSide(color: Colors.green, width: 2);
const OutlinedBorder shape = RoundedRectangleBorder(side: side, borderRadius: BorderRadius.all(Radius.circular(2)));
const MouseCursor enabledMouseCursor = SystemMouseCursors.text;
const MouseCursor disabledMouseCursor = SystemMouseCursors.grab;
const MaterialTapTargetSize tapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Duration animationDuration = Duration(milliseconds: 25);
const bool enableFeedback = false;
const AlignmentGeometry alignment = Alignment.centerLeft;
final ButtonStyle style = ElevatedButton.styleFrom(
foregroundColor: foregroundColor,
disabledForegroundColor: disabledColor,
backgroundColor: backgroundColor,
disabledBackgroundColor: disabledColor,
shadowColor: shadowColor,
elevation: elevation,
textStyle: textStyle,
padding: padding,
minimumSize: minimumSize,
side: side,
shape: shape,
enabledMouseCursor: enabledMouseCursor,
disabledMouseCursor: disabledMouseCursor,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
);
Widget buildFrame({ ButtonStyle? buttonStyle, ButtonStyle? themeStyle, ButtonStyle? overallStyle }) {
final Widget child = Builder(
builder: (BuildContext context) {
return ElevatedButton(
style: buttonStyle,
onPressed: () { },
child: const Text('button'),
);
},
);
return MaterialApp(
theme: ThemeData.from(useMaterial3: false, colorScheme: const ColorScheme.light()).copyWith(
elevatedButtonTheme: ElevatedButtonThemeData(style: overallStyle),
),
home: Scaffold(
body: Center(
// If the ElevatedButtonTheme widget is present, it's used
// instead of the Theme's ThemeData.ElevatedButtonTheme.
child: themeStyle == null ? child : ElevatedButtonTheme(
data: ElevatedButtonThemeData(style: themeStyle),
child: child,
),
),
),
);
}
final Finder findMaterial = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(Material),
);
final Finder findInkWell = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(InkWell),
);
const Set<MaterialState> enabled = <MaterialState>{};
const Set<MaterialState> disabled = <MaterialState>{ MaterialState.disabled };
const Set<MaterialState> hovered = <MaterialState>{ MaterialState.hovered };
const Set<MaterialState> focused = <MaterialState>{ MaterialState.focused };
const Set<MaterialState> pressed = <MaterialState>{ MaterialState.pressed };
void checkButton(WidgetTester tester) {
final Material material = tester.widget<Material>(findMaterial);
final InkWell inkWell = tester.widget<InkWell>(findInkWell);
expect(material.textStyle!.color, foregroundColor);
expect(material.textStyle!.fontSize, 12);
expect(material.color, backgroundColor);
expect(material.shadowColor, shadowColor);
expect(material.elevation, elevation);
expect(MaterialStateProperty.resolveAs<MouseCursor>(inkWell.mouseCursor!, enabled), enabledMouseCursor);
expect(MaterialStateProperty.resolveAs<MouseCursor>(inkWell.mouseCursor!, disabled), disabledMouseCursor);
expect(inkWell.overlayColor!.resolve(hovered), foregroundColor.withOpacity(0.08));
expect(inkWell.overlayColor!.resolve(focused), foregroundColor.withOpacity(0.1));
expect(inkWell.overlayColor!.resolve(pressed), foregroundColor.withOpacity(0.1));
expect(inkWell.enableFeedback, enableFeedback);
expect(material.borderRadius, null);
expect(material.shape, shape);
expect(material.animationDuration, animationDuration);
expect(tester.getSize(find.byType(ElevatedButton)), const Size(200, 200));
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, alignment);
}
testWidgets('Button style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(themeStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(overallStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
// Same as the previous tests with empty ButtonStyle's instead of null.
testWidgets('Button style overrides defaults, empty theme and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style, themeStyle: const ButtonStyle(), overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults, empty button and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), themeStyle: style, overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults, null theme and empty overall style', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), overallStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
});
testWidgets('Material 3: Theme shadowColor', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Color shadowColor = Color(0xff000001);
const Color overriddenColor = Color(0xff000002);
Widget buildFrame({ Color? overallShadowColor, Color? themeShadowColor, Color? shadowColor }) {
return MaterialApp(
theme: ThemeData.from(
useMaterial3: true,
colorScheme: colorScheme.copyWith(shadow: overallShadowColor),
),
home: Scaffold(
body: Center(
child: ElevatedButtonTheme(
data: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
shadowColor: themeShadowColor,
),
),
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
style: ElevatedButton.styleFrom(
shadowColor: shadowColor,
),
onPressed: () { },
child: const Text('button'),
);
},
),
),
),
),
);
}
final Finder buttonMaterialFinder = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(Material),
);
await tester.pumpWidget(buildFrame());
Material material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.black); //default
await tester.pumpWidget(buildFrame(overallShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(overallShadowColor: overriddenColor, themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: overriddenColor, shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
});
testWidgets('Material 2: Theme shadowColor', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Color shadowColor = Color(0xff000001);
const Color overriddenColor = Color(0xff000002);
Widget buildFrame({ Color? overallShadowColor, Color? themeShadowColor, Color? shadowColor }) {
return MaterialApp(
theme: ThemeData.from(useMaterial3: false, colorScheme: colorScheme).copyWith(
shadowColor: overallShadowColor,
),
home: Scaffold(
body: Center(
child: ElevatedButtonTheme(
data: ElevatedButtonThemeData(
style: ElevatedButton.styleFrom(
shadowColor: themeShadowColor,
),
),
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
style: ElevatedButton.styleFrom(
shadowColor: shadowColor,
),
onPressed: () { },
child: const Text('button'),
);
},
),
),
),
),
);
}
final Finder buttonMaterialFinder = find.descendant(
of: find.byType(ElevatedButton),
matching: find.byType(Material),
);
await tester.pumpWidget(buildFrame());
Material material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.black); //default
await tester.pumpWidget(buildFrame(overallShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(overallShadowColor: overriddenColor, themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: overriddenColor, shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
});
}
| flutter/packages/flutter/test/material/elevated_button_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/elevated_button_theme_test.dart",
"repo_id": "flutter",
"token_count": 5703
} | 716 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('FloatingActionButtonThemeData copyWith, ==, hashCode basics', () {
expect(const FloatingActionButtonThemeData(), const FloatingActionButtonThemeData().copyWith());
expect(const FloatingActionButtonThemeData().hashCode, const FloatingActionButtonThemeData().copyWith().hashCode);
});
test('FloatingActionButtonThemeData lerp special cases', () {
expect(FloatingActionButtonThemeData.lerp(null, null, 0), null);
const FloatingActionButtonThemeData data = FloatingActionButtonThemeData();
expect(identical(FloatingActionButtonThemeData.lerp(data, data, 0.5), data), true);
});
testWidgets('Material3: Default values are used when no FloatingActionButton or FloatingActionButtonThemeData properties are specified', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(MaterialApp(
theme: ThemeData.from(useMaterial3: true, colorScheme: colorScheme),
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).fillColor, colorScheme.primaryContainer);
expect(_getRichText(tester).text.style!.color, colorScheme.onPrimaryContainer);
// These defaults come directly from the [FloatingActionButton].
expect(_getRawMaterialButton(tester).elevation, 6);
expect(_getRawMaterialButton(tester).highlightElevation, 6);
expect(_getRawMaterialButton(tester).shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(16.0))));
expect(_getRawMaterialButton(tester).splashColor, colorScheme.onPrimaryContainer.withOpacity(0.1));
expect(_getRawMaterialButton(tester).constraints, const BoxConstraints.tightFor(width: 56.0, height: 56.0));
expect(_getIconSize(tester).width, 24.0);
expect(_getIconSize(tester).height, 24.0);
});
testWidgets('Material2: Default values are used when no FloatingActionButton or FloatingActionButtonThemeData properties are specified', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(MaterialApp(
theme: ThemeData.from(useMaterial3: false, colorScheme: colorScheme),
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).fillColor, colorScheme.secondary);
expect(_getRichText(tester).text.style!.color, colorScheme.onSecondary);
// These defaults come directly from the [FloatingActionButton].
expect(_getRawMaterialButton(tester).elevation, 6);
expect(_getRawMaterialButton(tester).highlightElevation, 12);
expect(_getRawMaterialButton(tester).shape, const CircleBorder());
expect(_getRawMaterialButton(tester).splashColor, ThemeData().splashColor);
expect(_getRawMaterialButton(tester).constraints, const BoxConstraints.tightFor(width: 56.0, height: 56.0));
expect(_getIconSize(tester).width, 24.0);
expect(_getIconSize(tester).height, 24.0);
});
testWidgets('FloatingActionButtonThemeData values are used when no FloatingActionButton properties are specified', (WidgetTester tester) async {
const Color backgroundColor = Color(0xBEEFBEEF);
const Color foregroundColor = Color(0xFACEFACE);
const Color splashColor = Color(0xCAFEFEED);
const double elevation = 7;
const double disabledElevation = 1;
const double highlightElevation = 13;
const ShapeBorder shape = StadiumBorder();
const BoxConstraints constraints = BoxConstraints.tightFor(width: 100.0, height: 100.0);
await tester.pumpWidget(MaterialApp(
theme: ThemeData().copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
backgroundColor: backgroundColor,
foregroundColor: foregroundColor,
splashColor: splashColor,
elevation: elevation,
disabledElevation: disabledElevation,
highlightElevation: highlightElevation,
shape: shape,
sizeConstraints: constraints,
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).fillColor, backgroundColor);
expect(_getRichText(tester).text.style!.color, foregroundColor);
expect(_getRawMaterialButton(tester).elevation, elevation);
expect(_getRawMaterialButton(tester).disabledElevation, disabledElevation);
expect(_getRawMaterialButton(tester).highlightElevation, highlightElevation);
expect(_getRawMaterialButton(tester).shape, shape);
expect(_getRawMaterialButton(tester).splashColor, splashColor);
expect(_getRawMaterialButton(tester).constraints, constraints);
});
testWidgets('FloatingActionButton values take priority over FloatingActionButtonThemeData values when both properties are specified', (WidgetTester tester) async {
const Color backgroundColor = Color(0x00000001);
const Color foregroundColor = Color(0x00000002);
const Color splashColor = Color(0x00000003);
const double elevation = 7;
const double disabledElevation = 1;
const double highlightElevation = 13;
const ShapeBorder shape = StadiumBorder();
await tester.pumpWidget(MaterialApp(
theme: ThemeData().copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
backgroundColor: Color(0x00000004),
foregroundColor: Color(0x00000005),
splashColor: Color(0x00000006),
elevation: 23,
disabledElevation: 11,
highlightElevation: 43,
shape: BeveledRectangleBorder(),
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
backgroundColor: backgroundColor,
foregroundColor: foregroundColor,
splashColor: splashColor,
elevation: elevation,
disabledElevation: disabledElevation,
highlightElevation: highlightElevation,
shape: shape,
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).fillColor, backgroundColor);
expect(_getRichText(tester).text.style!.color, foregroundColor);
expect(_getRawMaterialButton(tester).elevation, elevation);
expect(_getRawMaterialButton(tester).disabledElevation, disabledElevation);
expect(_getRawMaterialButton(tester).highlightElevation, highlightElevation);
expect(_getRawMaterialButton(tester).shape, shape);
expect(_getRawMaterialButton(tester).splashColor, splashColor);
});
testWidgets('FloatingActionButton uses a custom shape when specified in the theme', (WidgetTester tester) async {
const ShapeBorder customShape = BeveledRectangleBorder();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
shape: customShape,
),
),
));
expect(_getRawMaterialButton(tester).shape, customShape);
});
testWidgets('FloatingActionButton.small uses custom constraints when specified in the theme', (WidgetTester tester) async {
const BoxConstraints constraints = BoxConstraints.tightFor(width: 100.0, height: 100.0);
const double iconSize = 24.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData().copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
smallSizeConstraints: constraints,
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.small(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).constraints, constraints);
expect(_getIconSize(tester).width, iconSize);
expect(_getIconSize(tester).height, iconSize);
});
testWidgets('FloatingActionButton.large uses custom constraints when specified in the theme', (WidgetTester tester) async {
const BoxConstraints constraints = BoxConstraints.tightFor(width: 100.0, height: 100.0);
const double iconSize = 36.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData().copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
largeSizeConstraints: constraints,
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.large(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
expect(_getRawMaterialButton(tester).constraints, constraints);
expect(_getIconSize(tester).width, iconSize);
expect(_getIconSize(tester).height, iconSize);
});
testWidgets('Material3: FloatingActionButton.extended uses custom properties when specified in the theme', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Key iconKey = Key('icon');
const Key labelKey = Key('label');
const BoxConstraints constraints = BoxConstraints.tightFor(height: 100.0);
const double iconLabelSpacing = 33.0;
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(start: 5.0, end: 6.0);
const TextStyle textStyle = TextStyle(letterSpacing: 2.0);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
colorScheme: colorScheme,
).copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
extendedSizeConstraints: constraints,
extendedIconLabelSpacing: iconLabelSpacing,
extendedPadding: padding,
extendedTextStyle: textStyle,
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.extended(
onPressed: () { },
label: const Text('Extended', key: labelKey),
icon: const Icon(Icons.add, key: iconKey),
),
),
));
expect(_getRawMaterialButton(tester).constraints, constraints);
expect(tester.getTopLeft(find.byKey(labelKey)).dx - tester.getTopRight(find.byKey(iconKey)).dx, iconLabelSpacing);
expect(tester.getTopLeft(find.byKey(iconKey)).dx - tester.getTopLeft(find.byType(FloatingActionButton)).dx, padding.start);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx - tester.getTopRight(find.byKey(labelKey)).dx, padding.end);
expect(_getRawMaterialButton(tester).textStyle, textStyle.copyWith(color: colorScheme.onPrimaryContainer));
});
testWidgets('Material2: FloatingActionButton.extended uses custom properties when specified in the theme', (WidgetTester tester) async {
const Key iconKey = Key('icon');
const Key labelKey = Key('label');
const BoxConstraints constraints = BoxConstraints.tightFor(height: 100.0);
const double iconLabelSpacing = 33.0;
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(start: 5.0, end: 6.0);
const TextStyle textStyle = TextStyle(letterSpacing: 2.0);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false).copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
extendedSizeConstraints: constraints,
extendedIconLabelSpacing: iconLabelSpacing,
extendedPadding: padding,
extendedTextStyle: textStyle,
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.extended(
onPressed: () { },
label: const Text('Extended', key: labelKey),
icon: const Icon(Icons.add, key: iconKey),
),
),
));
expect(_getRawMaterialButton(tester).constraints, constraints);
expect(tester.getTopLeft(find.byKey(labelKey)).dx - tester.getTopRight(find.byKey(iconKey)).dx, iconLabelSpacing);
expect(tester.getTopLeft(find.byKey(iconKey)).dx - tester.getTopLeft(find.byType(FloatingActionButton)).dx, padding.start);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx - tester.getTopRight(find.byKey(labelKey)).dx, padding.end);
// The color comes from the default color scheme's onSecondary value.
expect(_getRawMaterialButton(tester).textStyle, textStyle.copyWith(color: const Color(0xffffffff)));
});
testWidgets('Material3: FloatingActionButton.extended custom properties takes priority over FloatingActionButtonThemeData spacing', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Key iconKey = Key('icon');
const Key labelKey = Key('label');
const double iconLabelSpacing = 33.0;
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(start: 5.0, end: 6.0);
const TextStyle textStyle = TextStyle(letterSpacing: 2.0);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
colorScheme: colorScheme,
).copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
extendedIconLabelSpacing: 25.0,
extendedPadding: EdgeInsetsDirectional.only(start: 7.0, end: 8.0),
extendedTextStyle: TextStyle(letterSpacing: 3.0),
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.extended(
onPressed: () { },
label: const Text('Extended', key: labelKey),
icon: const Icon(Icons.add, key: iconKey),
extendedIconLabelSpacing: iconLabelSpacing,
extendedPadding: padding,
extendedTextStyle: textStyle,
),
),
));
expect(tester.getTopLeft(find.byKey(labelKey)).dx - tester.getTopRight(find.byKey(iconKey)).dx, iconLabelSpacing);
expect(tester.getTopLeft(find.byKey(iconKey)).dx - tester.getTopLeft(find.byType(FloatingActionButton)).dx, padding.start);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx - tester.getTopRight(find.byKey(labelKey)).dx, padding.end);
expect(_getRawMaterialButton(tester).textStyle, textStyle.copyWith(color: colorScheme.onPrimaryContainer));
});
testWidgets('Material2: FloatingActionButton.extended custom properties takes priority over FloatingActionButtonThemeData spacing', (WidgetTester tester) async {
const Key iconKey = Key('icon');
const Key labelKey = Key('label');
const double iconLabelSpacing = 33.0;
const EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(start: 5.0, end: 6.0);
const TextStyle textStyle = TextStyle(letterSpacing: 2.0);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false).copyWith(
floatingActionButtonTheme: const FloatingActionButtonThemeData(
extendedIconLabelSpacing: 25.0,
extendedPadding: EdgeInsetsDirectional.only(start: 7.0, end: 8.0),
extendedTextStyle: TextStyle(letterSpacing: 3.0),
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton.extended(
onPressed: () { },
label: const Text('Extended', key: labelKey),
icon: const Icon(Icons.add, key: iconKey),
extendedIconLabelSpacing: iconLabelSpacing,
extendedPadding: padding,
extendedTextStyle: textStyle,
),
),
));
expect(tester.getTopLeft(find.byKey(labelKey)).dx - tester.getTopRight(find.byKey(iconKey)).dx, iconLabelSpacing);
expect(tester.getTopLeft(find.byKey(iconKey)).dx - tester.getTopLeft(find.byType(FloatingActionButton)).dx, padding.start);
expect(tester.getTopRight(find.byType(FloatingActionButton)).dx - tester.getTopRight(find.byKey(labelKey)).dx, padding.end);
// The color comes from the default color scheme's onSecondary value.
expect(_getRawMaterialButton(tester).textStyle, textStyle.copyWith(color: const Color(0xffffffff)));
});
testWidgets('default FloatingActionButton debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const FloatingActionButtonThemeData ().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('Material implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const FloatingActionButtonThemeData(
foregroundColor: Color(0xFEEDFEED),
backgroundColor: Color(0xCAFECAFE),
focusColor: Color(0xFEEDFEE1),
hoverColor: Color(0xFEEDFEE2),
splashColor: Color(0xFEEDFEE3),
elevation: 23,
focusElevation: 9,
hoverElevation: 10,
disabledElevation: 11,
highlightElevation: 43,
shape: BeveledRectangleBorder(),
enableFeedback: true,
iconSize: 42,
sizeConstraints: BoxConstraints.tightFor(width: 100.0, height: 100.0),
smallSizeConstraints: BoxConstraints.tightFor(width: 101.0, height: 101.0),
largeSizeConstraints: BoxConstraints.tightFor(width: 102.0, height: 102.0),
extendedSizeConstraints: BoxConstraints(minHeight: 103.0, maxHeight: 103.0),
extendedIconLabelSpacing: 12,
extendedPadding: EdgeInsetsDirectional.only(start: 7.0, end: 8.0),
extendedTextStyle: TextStyle(letterSpacing: 2.0),
mouseCursor: MaterialStateMouseCursor.clickable,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'foregroundColor: Color(0xfeedfeed)',
'backgroundColor: Color(0xcafecafe)',
'focusColor: Color(0xfeedfee1)',
'hoverColor: Color(0xfeedfee2)',
'splashColor: Color(0xfeedfee3)',
'elevation: 23.0',
'focusElevation: 9.0',
'hoverElevation: 10.0',
'disabledElevation: 11.0',
'highlightElevation: 43.0',
'shape: BeveledRectangleBorder(BorderSide(width: 0.0, style: none), BorderRadius.zero)',
'enableFeedback: true',
'iconSize: 42.0',
'sizeConstraints: BoxConstraints(w=100.0, h=100.0)',
'smallSizeConstraints: BoxConstraints(w=101.0, h=101.0)',
'largeSizeConstraints: BoxConstraints(w=102.0, h=102.0)',
'extendedSizeConstraints: BoxConstraints(0.0<=w<=Infinity, h=103.0)',
'extendedIconLabelSpacing: 12.0',
'extendedPadding: EdgeInsetsDirectional(7.0, 0.0, 8.0, 0.0)',
'extendedTextStyle: TextStyle(inherit: true, letterSpacing: 2.0)',
'mouseCursor: WidgetStateMouseCursor(clickable)',
]);
});
testWidgets('FloatingActionButton.mouseCursor uses FloatingActionButtonThemeData.mouseCursor when specified.', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData().copyWith(
floatingActionButtonTheme: FloatingActionButtonThemeData(
mouseCursor: MaterialStateProperty.all(SystemMouseCursors.text),
),
),
home: Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: () { },
child: const Icon(Icons.add),
),
),
));
await tester.pumpAndSettle();
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
addTearDown(gesture.removePointer);
await gesture.moveTo(tester.getCenter(find.byType(FloatingActionButton)));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
});
}
RawMaterialButton _getRawMaterialButton(WidgetTester tester) {
return tester.widget<RawMaterialButton>(
find.descendant(
of: find.byType(FloatingActionButton),
matching: find.byType(RawMaterialButton),
),
);
}
RichText _getRichText(WidgetTester tester) {
return tester.widget<RichText>(
find.descendant(
of: find.byType(FloatingActionButton),
matching: find.byType(RichText),
),
);
}
SizedBox _getIconSize(WidgetTester tester) {
return tester.widget<SizedBox>(
find.descendant(
of: find.descendant(
of: find.byType(FloatingActionButton),
matching: find.byType(Icon),
),
matching: find.byType(SizedBox),
),
);
}
| flutter/packages/flutter/test/material/floating_action_button_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/floating_action_button_theme_test.dart",
"repo_id": "flutter",
"token_count": 7675
} | 717 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('English translations exist for all MaterialLocalizations properties', (WidgetTester tester) async {
const MaterialLocalizations localizations = DefaultMaterialLocalizations();
expect(localizations.openAppDrawerTooltip, isNotNull);
expect(localizations.backButtonTooltip, isNotNull);
expect(localizations.clearButtonTooltip, isNotNull);
expect(localizations.closeButtonTooltip, isNotNull);
expect(localizations.deleteButtonTooltip, isNotNull);
expect(localizations.moreButtonTooltip, isNotNull);
expect(localizations.nextMonthTooltip, isNotNull);
expect(localizations.previousMonthTooltip, isNotNull);
expect(localizations.nextPageTooltip, isNotNull);
expect(localizations.previousPageTooltip, isNotNull);
expect(localizations.firstPageTooltip, isNotNull);
expect(localizations.lastPageTooltip, isNotNull);
expect(localizations.showMenuTooltip, isNotNull);
expect(localizations.licensesPageTitle, isNotNull);
expect(localizations.rowsPerPageTitle, isNotNull);
expect(localizations.cancelButtonLabel, isNotNull);
expect(localizations.closeButtonLabel, isNotNull);
expect(localizations.continueButtonLabel, isNotNull);
expect(localizations.copyButtonLabel, isNotNull);
expect(localizations.cutButtonLabel, isNotNull);
expect(localizations.scanTextButtonLabel, isNotNull);
expect(localizations.lookUpButtonLabel, isNotNull);
expect(localizations.searchWebButtonLabel, isNotNull);
expect(localizations.shareButtonLabel, isNotNull);
expect(localizations.okButtonLabel, isNotNull);
expect(localizations.pasteButtonLabel, isNotNull);
expect(localizations.selectAllButtonLabel, isNotNull);
expect(localizations.viewLicensesButtonLabel, isNotNull);
expect(localizations.anteMeridiemAbbreviation, isNotNull);
expect(localizations.postMeridiemAbbreviation, isNotNull);
expect(localizations.timePickerHourModeAnnouncement, isNotNull);
expect(localizations.timePickerMinuteModeAnnouncement, isNotNull);
expect(localizations.modalBarrierDismissLabel, isNotNull);
expect(localizations.menuDismissLabel, isNotNull);
expect(localizations.drawerLabel, isNotNull);
expect(localizations.menuBarMenuLabel, isNotNull);
expect(localizations.popupMenuLabel, isNotNull);
expect(localizations.dialogLabel, isNotNull);
expect(localizations.alertDialogLabel, isNotNull);
expect(localizations.searchFieldLabel, isNotNull);
expect(localizations.dateSeparator, isNotNull);
expect(localizations.dateHelpText, isNotNull);
expect(localizations.selectYearSemanticsLabel, isNotNull);
expect(localizations.unspecifiedDate, isNotNull);
expect(localizations.unspecifiedDateRange, isNotNull);
expect(localizations.dateInputLabel, isNotNull);
expect(localizations.dateRangeStartLabel, isNotNull);
expect(localizations.dateRangeEndLabel, isNotNull);
expect(localizations.invalidDateFormatLabel, isNotNull);
expect(localizations.invalidDateRangeLabel, isNotNull);
expect(localizations.dateOutOfRangeLabel, isNotNull);
expect(localizations.saveButtonLabel, isNotNull);
expect(localizations.datePickerHelpText, isNotNull);
expect(localizations.dateRangePickerHelpText, isNotNull);
expect(localizations.calendarModeButtonLabel, isNotNull);
expect(localizations.inputDateModeButtonLabel, isNotNull);
expect(localizations.timePickerDialHelpText, isNotNull);
expect(localizations.timePickerInputHelpText, isNotNull);
expect(localizations.timePickerHourLabel, isNotNull);
expect(localizations.timePickerMinuteLabel, isNotNull);
expect(localizations.invalidTimeLabel, isNotNull);
expect(localizations.dialModeButtonLabel, isNotNull);
expect(localizations.inputTimeModeButtonLabel, isNotNull);
expect(localizations.signedInLabel, isNotNull);
expect(localizations.hideAccountsLabel, isNotNull);
expect(localizations.showAccountsLabel, isNotNull);
expect(localizations.reorderItemToStart, isNotNull);
expect(localizations.reorderItemToEnd, isNotNull);
expect(localizations.reorderItemUp, isNotNull);
expect(localizations.reorderItemDown, isNotNull);
expect(localizations.reorderItemLeft, isNotNull);
expect(localizations.reorderItemRight, isNotNull);
expect(localizations.expandedIconTapHint, isNotNull);
expect(localizations.collapsedIconTapHint, isNotNull);
expect(localizations.expansionTileExpandedHint, isNotNull);
expect(localizations.expansionTileCollapsedHint, isNotNull);
expect(localizations.expandedHint, isNotNull);
expect(localizations.collapsedHint, isNotNull);
expect(localizations.keyboardKeyAlt, isNotNull);
expect(localizations.keyboardKeyAltGraph, isNotNull);
expect(localizations.keyboardKeyBackspace, isNotNull);
expect(localizations.keyboardKeyCapsLock, isNotNull);
expect(localizations.keyboardKeyChannelDown, isNotNull);
expect(localizations.keyboardKeyChannelUp, isNotNull);
expect(localizations.keyboardKeyControl, isNotNull);
expect(localizations.keyboardKeyDelete, isNotNull);
expect(localizations.keyboardKeyEject, isNotNull);
expect(localizations.keyboardKeyEnd, isNotNull);
expect(localizations.keyboardKeyEscape, isNotNull);
expect(localizations.keyboardKeyFn, isNotNull);
expect(localizations.keyboardKeyHome, isNotNull);
expect(localizations.keyboardKeyInsert, isNotNull);
expect(localizations.keyboardKeyMeta, isNotNull);
expect(localizations.keyboardKeyMetaMacOs, isNotNull);
expect(localizations.keyboardKeyMetaWindows, isNotNull);
expect(localizations.keyboardKeyNumLock, isNotNull);
expect(localizations.keyboardKeyNumpad1, isNotNull);
expect(localizations.keyboardKeyNumpad2, isNotNull);
expect(localizations.keyboardKeyNumpad3, isNotNull);
expect(localizations.keyboardKeyNumpad4, isNotNull);
expect(localizations.keyboardKeyNumpad5, isNotNull);
expect(localizations.keyboardKeyNumpad6, isNotNull);
expect(localizations.keyboardKeyNumpad7, isNotNull);
expect(localizations.keyboardKeyNumpad8, isNotNull);
expect(localizations.keyboardKeyNumpad9, isNotNull);
expect(localizations.keyboardKeyNumpad0, isNotNull);
expect(localizations.keyboardKeyNumpadAdd, isNotNull);
expect(localizations.keyboardKeyNumpadComma, isNotNull);
expect(localizations.keyboardKeyNumpadDecimal, isNotNull);
expect(localizations.keyboardKeyNumpadDivide, isNotNull);
expect(localizations.keyboardKeyNumpadEnter, isNotNull);
expect(localizations.keyboardKeyNumpadEqual, isNotNull);
expect(localizations.keyboardKeyNumpadMultiply, isNotNull);
expect(localizations.keyboardKeyNumpadParenLeft, isNotNull);
expect(localizations.keyboardKeyNumpadParenRight, isNotNull);
expect(localizations.keyboardKeyNumpadSubtract, isNotNull);
expect(localizations.keyboardKeyPageDown, isNotNull);
expect(localizations.keyboardKeyPageUp, isNotNull);
expect(localizations.keyboardKeyPower, isNotNull);
expect(localizations.keyboardKeyPowerOff, isNotNull);
expect(localizations.keyboardKeyPrintScreen, isNotNull);
expect(localizations.keyboardKeyScrollLock, isNotNull);
expect(localizations.keyboardKeySelect, isNotNull);
expect(localizations.keyboardKeyShift, isNotNull);
expect(localizations.keyboardKeySpace, isNotNull);
expect(localizations.currentDateLabel, isNotNull);
expect(localizations.scrimLabel, isNotNull);
expect(localizations.bottomSheetLabel, isNotNull);
expect(localizations.selectedDateLabel, isNotNull);
expect(localizations.scrimOnTapHint('FOO'), contains('FOO'));
expect(localizations.aboutListTileTitle('FOO'), isNotNull);
expect(localizations.aboutListTileTitle('FOO'), contains('FOO'));
expect(localizations.selectedRowCountTitle(0), isNotNull);
expect(localizations.selectedRowCountTitle(1), isNotNull);
expect(localizations.selectedRowCountTitle(2), isNotNull);
expect(localizations.selectedRowCountTitle(100), isNotNull);
expect(localizations.selectedRowCountTitle(0).contains(r'$selectedRowCount'), isFalse);
expect(localizations.selectedRowCountTitle(1).contains(r'$selectedRowCount'), isFalse);
expect(localizations.selectedRowCountTitle(2).contains(r'$selectedRowCount'), isFalse);
expect(localizations.selectedRowCountTitle(100).contains(r'$selectedRowCount'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, true), isNotNull);
expect(localizations.pageRowsInfoTitle(1, 10, 100, false), isNotNull);
expect(localizations.pageRowsInfoTitle(1, 10, 100, true).contains(r'$firstRow'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, true).contains(r'$lastRow'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, true).contains(r'$rowCount'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, false).contains(r'$firstRow'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, false).contains(r'$lastRow'), isFalse);
expect(localizations.pageRowsInfoTitle(1, 10, 100, false).contains(r'$rowCount'), isFalse);
expect(localizations.licensesPackageDetailText(0), isNotNull);
expect(localizations.licensesPackageDetailText(1), isNotNull);
expect(localizations.licensesPackageDetailText(2), isNotNull);
expect(localizations.licensesPackageDetailText(100), isNotNull);
expect(localizations.licensesPackageDetailText(1).contains(r'$licensesCount'), isFalse);
expect(localizations.licensesPackageDetailText(2).contains(r'$licensesCount'), isFalse);
expect(localizations.licensesPackageDetailText(100).contains(r'$licensesCount'), isFalse);
});
testWidgets('MaterialLocalizations.of throws', (WidgetTester tester) async {
final GlobalKey noLocalizationsAvailable = GlobalKey();
final GlobalKey localizationsAvailable = GlobalKey();
await tester.pumpWidget(
Container(
key: noLocalizationsAvailable,
child: MaterialApp(
home: Container(
key: localizationsAvailable,
),
),
),
);
expect(() => MaterialLocalizations.of(noLocalizationsAvailable.currentContext!), throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
contains('No MaterialLocalizations found'),
)));
expect(MaterialLocalizations.of(localizationsAvailable.currentContext!), isA<MaterialLocalizations>());
});
testWidgets("parseCompactDate doesn't throw an exception on invalid text", (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/126397.
final GlobalKey localizations = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Material(
key: localizations,
child: const SizedBox.expand(),
),
),
);
final MaterialLocalizations materialLocalizations = MaterialLocalizations.of(localizations.currentContext!);
expect(materialLocalizations.parseCompactDate('10/05/2023'), isNotNull);
expect(tester.takeException(), null);
expect(materialLocalizations.parseCompactDate('10/05/2023666777889'), null);
expect(tester.takeException(), null);
});
}
| flutter/packages/flutter/test/material/localizations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/localizations_test.dart",
"repo_id": "flutter",
"token_count": 3826
} | 718 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('NavigationDrawerThemeData copyWith, ==, hashCode, basics', () {
expect(const NavigationDrawerThemeData(), const NavigationDrawerThemeData().copyWith());
expect(const NavigationDrawerThemeData().hashCode, const NavigationDrawerThemeData().copyWith().hashCode);
});
test('NavigationDrawerThemeData lerp special cases', () {
expect(NavigationDrawerThemeData.lerp(null, null, 0), null);
const NavigationDrawerThemeData data = NavigationDrawerThemeData();
expect(identical(NavigationDrawerThemeData.lerp(data, data, 0.5), data), true);
});
testWidgets('Default debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const NavigationDrawerThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('NavigationDrawerThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const NavigationDrawerThemeData(
tileHeight: 50,
backgroundColor: Color(0x00000099),
elevation: 5.0,
shadowColor: Color(0x00000098),
surfaceTintColor: Color(0x00000097),
indicatorColor: Color(0x00000096),
indicatorShape: RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2.0))),
indicatorSize: Size(10, 10),
labelTextStyle: MaterialStatePropertyAll<TextStyle>(TextStyle(fontSize: 7.0)),
iconTheme: MaterialStatePropertyAll<IconThemeData>(IconThemeData(color: Color(0x00000095))),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, equalsIgnoringHashCodes(
<String>[
'tileHeight: 50.0',
'backgroundColor: Color(0x00000099)',
'elevation: 5.0',
'shadowColor: Color(0x00000098)',
'surfaceTintColor: Color(0x00000097)',
'indicatorColor: Color(0x00000096)',
'indicatorShape: RoundedRectangleBorder(BorderSide(width: 0.0, style: none), BorderRadius.circular(2.0))',
'indicatorSize: Size(10.0, 10.0)',
'labelTextStyle: WidgetStatePropertyAll(TextStyle(inherit: true, size: 7.0))',
'iconTheme: WidgetStatePropertyAll(IconThemeData#00000(color: Color(0x00000095)))'
],
));
});
testWidgets(
'NavigationDrawerThemeData values are used when no NavigationDrawer properties are specified',
(WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const NavigationDrawerThemeData navigationDrawerTheme = NavigationDrawerThemeData(
backgroundColor: Color(0x00000001),
elevation: 7.0,
shadowColor: Color(0x00000002),
surfaceTintColor: Color(0x00000003),
indicatorColor: Color(0x00000004),
indicatorShape: RoundedRectangleBorder(borderRadius: BorderRadius.only(topRight: Radius.circular(16.0))),
labelTextStyle:MaterialStatePropertyAll<TextStyle>(TextStyle(fontSize: 7.0)),
iconTheme: MaterialStatePropertyAll<IconThemeData>(IconThemeData(color: Color(0x00000005))),
);
await tester.pumpWidget(
_buildWidget(
scaffoldKey,
NavigationDrawer(
children: const <Widget>[
Text('Headline'),
NavigationDrawerDestination(
icon: Icon(Icons.ac_unit),
label: Text('AC'),
),
NavigationDrawerDestination(
icon: Icon(Icons.access_alarm),
label: Text('Alarm'),
),
],
onDestinationSelected: (int i) {},
),
theme: ThemeData(
navigationDrawerTheme: navigationDrawerTheme,
),
),
);
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(seconds: 1));
// Test drawer Material.
expect(_getMaterial(tester).color, navigationDrawerTheme.backgroundColor);
expect(_getMaterial(tester).surfaceTintColor, navigationDrawerTheme.surfaceTintColor);
expect(_getMaterial(tester).shadowColor, navigationDrawerTheme.shadowColor);
expect(_getMaterial(tester).elevation, 7);
// Test indicator decoration.
expect(_getIndicatorDecoration(tester)?.color, navigationDrawerTheme.indicatorColor);
expect(_getIndicatorDecoration(tester)?.shape, navigationDrawerTheme.indicatorShape);
// Test icon.
expect(
_iconStyle(tester, Icons.ac_unit)?.color,
navigationDrawerTheme.iconTheme?.resolve(<MaterialState>{})?.color,
);
expect(
_iconStyle(tester, Icons.access_alarm)?.color,
navigationDrawerTheme.iconTheme?.resolve(<MaterialState>{})?.color,
);
// Test label.
expect(
_labelStyle(tester, 'AC'),
navigationDrawerTheme.labelTextStyle?.resolve(<MaterialState>{})
);
expect(
_labelStyle(tester, 'Alarm'),
navigationDrawerTheme.labelTextStyle?.resolve(<MaterialState>{})
);
});
testWidgets(
'NavigationDrawer values take priority over NavigationDrawerThemeData values when both properties are specified',
(WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const NavigationDrawerThemeData navigationDrawerTheme = NavigationDrawerThemeData(
backgroundColor: Color(0x00000001),
elevation: 7.0,
shadowColor: Color(0x00000002),
surfaceTintColor: Color(0x00000003),
indicatorColor: Color(0x00000004),
indicatorShape: RoundedRectangleBorder(borderRadius: BorderRadius.only(topRight: Radius.circular(16.0))),
labelTextStyle:MaterialStatePropertyAll<TextStyle>(TextStyle(fontSize: 7.0)),
iconTheme: MaterialStatePropertyAll<IconThemeData>(IconThemeData(color: Color(0x00000005))),
);
const Color backgroundColor = Color(0x00000009);
const double elevation = 14.0;
const Color shadowColor = Color(0x00000008);
const Color surfaceTintColor = Color(0x00000007);
const RoundedRectangleBorder indicatorShape = RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(32.0)));
const Color indicatorColor = Color(0x00000006);
await tester.pumpWidget(
_buildWidget(
scaffoldKey,
NavigationDrawer(
backgroundColor: backgroundColor,
elevation: elevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
indicatorShape: indicatorShape,
indicatorColor: indicatorColor,
children: const <Widget>[
Text('Headline'),
NavigationDrawerDestination(
icon: Icon(Icons.ac_unit),
label: Text('AC'),
),
NavigationDrawerDestination(
icon: Icon(Icons.access_alarm),
label: Text('Alarm'),
),
],
onDestinationSelected: (int i) {},
),
theme: ThemeData(
navigationDrawerTheme: navigationDrawerTheme,
),
),
);
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(seconds: 1));
// Test drawer Material.
expect(_getMaterial(tester).color, backgroundColor);
expect(_getMaterial(tester).surfaceTintColor, surfaceTintColor);
expect(_getMaterial(tester).shadowColor, shadowColor);
expect(_getMaterial(tester).elevation, elevation);
// Test indicator decoration.
expect(_getIndicatorDecoration(tester)?.color, indicatorColor);
expect(_getIndicatorDecoration(tester)?.shape, indicatorShape);
});
testWidgets('Local NavigationDrawerTheme takes priority over ThemeData.navigationDrawerTheme', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const Color backgroundColor = Color(0x00000009);
const double elevation = 7.0;
const Color shadowColor = Color(0x00000008);
const Color surfaceTintColor = Color(0x00000007);
const Color iconColor = Color(0x00000006);
const TextStyle labelStyle = TextStyle(fontSize: 7.0);
const ShapeBorder indicatorShape = CircleBorder();
const Color indicatorColor = Color(0x00000005);
await tester.pumpWidget(
_buildWidget(
scaffoldKey,
NavigationDrawerTheme(
data: const NavigationDrawerThemeData(
backgroundColor: backgroundColor,
elevation: elevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
indicatorShape: indicatorShape,
indicatorColor: indicatorColor,
labelTextStyle:MaterialStatePropertyAll<TextStyle>(TextStyle(fontSize: 7.0)),
iconTheme: MaterialStatePropertyAll<IconThemeData>(IconThemeData(color: iconColor)),
),
child: NavigationDrawer(
children: const <Widget>[
Text('Headline'),
NavigationDrawerDestination(
icon: Icon(Icons.ac_unit),
label: Text('AC'),
),
NavigationDrawerDestination(
icon: Icon(Icons.access_alarm),
label: Text('Alarm'),
),
],
onDestinationSelected: (int i) {},
),
),
theme: ThemeData(
navigationDrawerTheme: const NavigationDrawerThemeData(
backgroundColor: Color(0x00000001),
elevation: 7.0,
shadowColor: Color(0x00000002),
surfaceTintColor: Color(0x00000003),
indicatorColor: Color(0x00000004),
indicatorShape: RoundedRectangleBorder(borderRadius: BorderRadius.only(topRight: Radius.circular(16.0))),
labelTextStyle:MaterialStatePropertyAll<TextStyle>(TextStyle(fontSize: 7.0)),
iconTheme: MaterialStatePropertyAll<IconThemeData>(IconThemeData(color: Color(0x00000005))),
),
),
),
);
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(seconds: 1));
// Test drawer Material.
expect(_getMaterial(tester).color, backgroundColor);
expect(_getMaterial(tester).surfaceTintColor, surfaceTintColor);
expect(_getMaterial(tester).shadowColor, shadowColor);
expect(_getMaterial(tester).elevation, elevation);
// Test indicator decoration.
expect(_getIndicatorDecoration(tester)?.color, indicatorColor);
expect(_getIndicatorDecoration(tester)?.shape, indicatorShape);
// Test icon.
expect(_iconStyle(tester, Icons.ac_unit)?.color, iconColor);
expect(_iconStyle(tester, Icons.access_alarm)?.color, iconColor);
// Test label.
expect(_labelStyle(tester, 'AC'), labelStyle);
expect(_labelStyle(tester, 'Alarm'), labelStyle);
});
}
Widget _buildWidget(GlobalKey<ScaffoldState> scaffoldKey, Widget child, { ThemeData? theme }) {
return MaterialApp(
theme: theme,
home: Scaffold(
key: scaffoldKey,
drawer: child,
body: Container(),
),
);
}
Material _getMaterial(WidgetTester tester) {
return tester.firstWidget<Material>(find.descendant(
of: find.byType(NavigationDrawer),
matching: find.byType(Material),
));
}
ShapeDecoration? _getIndicatorDecoration(WidgetTester tester) {
return tester.firstWidget<Container>(find.descendant(
of: find.byType(FadeTransition),
matching: find.byType(Container),
)).decoration as ShapeDecoration?;
}
TextStyle? _iconStyle(WidgetTester tester, IconData icon) {
return tester.widget<RichText>(
find.descendant(of: find.byIcon(icon),
matching: find.byType(RichText)),
).text.style;
}
TextStyle? _labelStyle(WidgetTester tester, String label) {
return tester.widget<RichText>(find.descendant(
of: find.text(label),
matching: find.byType(RichText),
)).text.style;
}
| flutter/packages/flutter/test/material/navigation_drawer_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/navigation_drawer_theme_test.dart",
"repo_id": "flutter",
"token_count": 4996
} | 719 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('RadioThemeData copyWith, ==, hashCode basics', () {
expect(const RadioThemeData(), const RadioThemeData().copyWith());
expect(const RadioThemeData().hashCode, const RadioThemeData().copyWith().hashCode);
});
test('RadioThemeData lerp special cases', () {
expect(RadioThemeData.lerp(null, null, 0), const RadioThemeData());
const RadioThemeData data = RadioThemeData();
expect(identical(RadioThemeData.lerp(data, data, 0.5), data), true);
});
test('RadioThemeData defaults', () {
const RadioThemeData themeData = RadioThemeData();
expect(themeData.mouseCursor, null);
expect(themeData.fillColor, null);
expect(themeData.overlayColor, null);
expect(themeData.splashRadius, null);
expect(themeData.materialTapTargetSize, null);
expect(themeData.visualDensity, null);
const RadioTheme theme = RadioTheme(data: RadioThemeData(), child: SizedBox());
expect(theme.data.mouseCursor, null);
expect(theme.data.fillColor, null);
expect(theme.data.overlayColor, null);
expect(theme.data.splashRadius, null);
expect(theme.data.materialTapTargetSize, null);
expect(theme.data.visualDensity, null);
});
testWidgets('Default RadioThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const RadioThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('RadioThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const RadioThemeData(
mouseCursor: MaterialStatePropertyAll<MouseCursor>(SystemMouseCursors.click),
fillColor: MaterialStatePropertyAll<Color>(Color(0xfffffff0)),
overlayColor: MaterialStatePropertyAll<Color>(Color(0xfffffff1)),
splashRadius: 1.0,
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
visualDensity: VisualDensity.standard,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(
description,
equalsIgnoringHashCodes(<String>[
'mouseCursor: WidgetStatePropertyAll(SystemMouseCursor(click))',
'fillColor: WidgetStatePropertyAll(Color(0xfffffff0))',
'overlayColor: WidgetStatePropertyAll(Color(0xfffffff1))',
'splashRadius: 1.0',
'materialTapTargetSize: MaterialTapTargetSize.shrinkWrap',
'visualDensity: VisualDensity#00000(h: 0.0, v: 0.0)',
]),
);
});
testWidgets('Radio is themeable', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const Color defaultFillColor = Color(0xfffffff0);
const Color selectedFillColor = Color(0xfffffff1);
const Color focusOverlayColor = Color(0xfffffff2);
const Color hoverOverlayColor = Color(0xfffffff3);
const double splashRadius = 1.0;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const VisualDensity visualDensity = VisualDensity(horizontal: 1, vertical: 1);
Widget buildRadio({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: ThemeData(
radioTheme: RadioThemeData(
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(mouseCursor),
fillColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedFillColor;
}
return defaultFillColor;
}),
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return focusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverOverlayColor;
}
return null;
}),
splashRadius: splashRadius,
materialTapTargetSize: materialTapTargetSize,
visualDensity: visualDensity,
),
),
home: Scaffold(
body: Radio<int>(
onChanged: (int? int) {},
value: selected ? 1 : 0,
groupValue: 1,
autofocus: autofocus,
),
),
);
}
// Radio.
await tester.pumpWidget(buildRadio());
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: defaultFillColor));
// Size from MaterialTapTargetSize.shrinkWrap with added VisualDensity.
expect(tester.getSize(_findRadio()), const Size(40.0, 40.0) + visualDensity.baseSizeAdjustment);
// Selected radio.
await tester.pumpWidget(buildRadio(selected: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: selectedFillColor));
// Radio with hover.
await tester.pumpWidget(buildRadio());
await _pointGestureToRadio(tester);
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: hoverOverlayColor));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Radio with focus.
await tester.pumpWidget(buildRadio(autofocus: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: focusOverlayColor, radius: splashRadius));
});
testWidgets('Radio properties are taken over the theme values', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const MouseCursor themeMouseCursor = SystemMouseCursors.click;
const Color themeDefaultFillColor = Color(0xfffffff0);
const Color themeSelectedFillColor = Color(0xfffffff1);
const Color themeFocusOverlayColor = Color(0xfffffff2);
const Color themeHoverOverlayColor = Color(0xfffffff3);
const double themeSplashRadius = 1.0;
const MaterialTapTargetSize themeMaterialTapTargetSize = MaterialTapTargetSize.padded;
const VisualDensity themeVisualDensity = VisualDensity.standard;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const Color defaultFillColor = Color(0xfffffff0);
const Color selectedFillColor = Color(0xfffffff1);
const Color focusColor = Color(0xfffffff2);
const Color hoverColor = Color(0xfffffff3);
const double splashRadius = 2.0;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const VisualDensity visualDensity = VisualDensity(horizontal: 1, vertical: 1);
Widget buildRadio({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: ThemeData(
radioTheme: RadioThemeData(
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(themeMouseCursor),
fillColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedFillColor;
}
return themeDefaultFillColor;
}),
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return themeFocusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return themeHoverOverlayColor;
}
return null;
}),
splashRadius: themeSplashRadius,
materialTapTargetSize: themeMaterialTapTargetSize,
visualDensity: themeVisualDensity,
),
),
home: Scaffold(
body: Radio<int>(
onChanged: (int? int) {},
value: selected ? 0 : 1,
groupValue: 0,
autofocus: autofocus,
mouseCursor: mouseCursor,
fillColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedFillColor;
}
return defaultFillColor;
}),
focusColor: focusColor,
hoverColor: hoverColor,
splashRadius: splashRadius,
materialTapTargetSize: materialTapTargetSize,
visualDensity: visualDensity,
),
),
);
}
// Radio.
await tester.pumpWidget(buildRadio());
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: defaultFillColor));
// Size from MaterialTapTargetSize.shrinkWrap with added VisualDensity.
expect(tester.getSize(_findRadio()), const Size(40.0, 40.0) + visualDensity.baseSizeAdjustment);
// Selected radio.
await tester.pumpWidget(buildRadio(selected: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: selectedFillColor));
// Radio with hover.
await tester.pumpWidget(buildRadio());
await _pointGestureToRadio(tester);
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: hoverColor));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Radio with focus.
await tester.pumpWidget(buildRadio(autofocus: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: focusColor, radius: splashRadius));
});
testWidgets('Radio activeColor property is taken over the theme', (WidgetTester tester) async {
const Color themeDefaultFillColor = Color(0xfffffff0);
const Color themeSelectedFillColor = Color(0xfffffff1);
const Color selectedFillColor = Color(0xfffffff1);
Widget buildRadio({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: ThemeData(
radioTheme: RadioThemeData(
fillColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedFillColor;
}
return themeDefaultFillColor;
}),
),
),
home: Scaffold(
body: Radio<int>(
onChanged: (int? int) {},
value: selected ? 0 : 1,
groupValue: 0,
autofocus: autofocus,
activeColor: selectedFillColor,
),
),
);
}
// Radio.
await tester.pumpWidget(buildRadio());
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: themeDefaultFillColor));
// Selected radio.
await tester.pumpWidget(buildRadio(selected: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: selectedFillColor));
});
testWidgets('Radio theme overlay color resolves in active/pressed states', (WidgetTester tester) async {
const Color activePressedOverlayColor = Color(0xFF000001);
const Color inactivePressedOverlayColor = Color(0xFF000002);
Color? getOverlayColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
if (states.contains(MaterialState.selected)) {
return activePressedOverlayColor;
}
return inactivePressedOverlayColor;
}
return null;
}
const double splashRadius = 24.0;
Widget buildRadio({required bool active}) {
return MaterialApp(
theme: ThemeData(
radioTheme: RadioThemeData(
overlayColor: MaterialStateProperty.resolveWith(getOverlayColor),
splashRadius: splashRadius,
),
),
home: Scaffold(
body: Radio<int>(
value: active ? 1 : 0,
groupValue: 1,
onChanged: (_) { },
),
),
);
}
await tester.pumpWidget(buildRadio(active: false));
await tester.press(_findRadio());
await tester.pumpAndSettle();
expect(
_getRadioMaterial(tester),
paints
..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
),
reason: 'Inactive pressed Radio should have overlay color: $inactivePressedOverlayColor',
);
await tester.pumpWidget(buildRadio(active: true));
await tester.press(_findRadio());
await tester.pumpAndSettle();
expect(
_getRadioMaterial(tester),
paints
..circle(
color: activePressedOverlayColor,
radius: splashRadius,
),
reason: 'Active pressed Radio should have overlay color: $activePressedOverlayColor',
);
});
testWidgets('Local RadioTheme can override global RadioTheme', (WidgetTester tester) async {
const Color globalThemeFillColor = Color(0xfffffff1);
const Color localThemeFillColor = Color(0xffff0000);
Widget buildRadio({required bool active}) {
return MaterialApp(
theme: ThemeData(
radioTheme: const RadioThemeData(
fillColor: MaterialStatePropertyAll<Color>(globalThemeFillColor),
),
),
home: Scaffold(
body: RadioTheme(
data: const RadioThemeData(
fillColor: MaterialStatePropertyAll<Color>(localThemeFillColor),
),
child: Radio<int>(
value: active ? 1 : 0,
groupValue: 1,
onChanged: (_) { },
),
),
),
);
}
await tester.pumpWidget(buildRadio(active: true));
await tester.pumpAndSettle();
expect(_getRadioMaterial(tester), paints..circle(color: localThemeFillColor));
});
}
Finder _findRadio() {
return find.byWidgetPredicate((Widget widget) => widget is Radio<int>);
}
Future<void> _pointGestureToRadio(WidgetTester tester) async {
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
addTearDown(gesture.removePointer);
await gesture.moveTo(tester.getCenter(_findRadio()));
}
MaterialInkController? _getRadioMaterial(WidgetTester tester) {
return Material.of(tester.element(_findRadio()));
}
| flutter/packages/flutter/test/material/radio_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/radio_theme_test.dart",
"repo_id": "flutter",
"token_count": 5890
} | 720 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'package:flutter/src/physics/utils.dart' show nearEqual;
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
// A thumb shape that also logs its repaint center.
class LoggingThumbShape extends SliderComponentShape {
LoggingThumbShape(this.log);
final List<Offset> log;
@override
Size getPreferredSize(bool isEnabled, bool isDiscrete) {
return const Size(10.0, 10.0);
}
@override
void paint(
PaintingContext context,
Offset thumbCenter, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
log.add(thumbCenter);
final Paint thumbPaint = Paint()..color = Colors.red;
context.canvas.drawCircle(thumbCenter, 5.0, thumbPaint);
}
}
class TallSliderTickMarkShape extends SliderTickMarkShape {
@override
Size getPreferredSize({required SliderThemeData sliderTheme, required bool isEnabled}) {
return const Size(10.0, 200.0);
}
@override
void paint(
PaintingContext context,
Offset offset, {
required Offset thumbCenter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required bool isEnabled,
required TextDirection textDirection,
}) {
final Paint paint = Paint()..color = Colors.red;
context.canvas.drawRect(Rect.fromLTWH(offset.dx, offset.dy, 10.0, 20.0), paint);
}
}
class _StateDependentMouseCursor extends MaterialStateMouseCursor {
const _StateDependentMouseCursor({
this.disabled = SystemMouseCursors.none,
this.dragged = SystemMouseCursors.none,
this.hovered = SystemMouseCursors.none,
});
final MouseCursor disabled;
final MouseCursor hovered;
final MouseCursor dragged;
@override
MouseCursor resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabled;
}
if (states.contains(MaterialState.dragged)) {
return dragged;
}
if (states.contains(MaterialState.hovered)) {
return hovered;
}
return SystemMouseCursors.none;
}
@override
String get debugDescription => '_StateDependentMouseCursor';
}
void main() {
testWidgets('The initial value should respect the discrete value', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.20;
final List<Offset> log = <Offset>[];
final LoggingThumbShape loggingThumb = LoggingThumbShape(log);
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
final SliderThemeData sliderTheme = SliderTheme.of(context).copyWith(thumbShape: loggingThumb);
return Material(
child: Center(
child: SliderTheme(
data: sliderTheme,
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
);
},
),
),
),
);
expect(value, equals(0.20));
expect(log.length, 1);
expect(log[0], const Offset(212.0, 300.0));
});
testWidgets('Slider can move when tapped (LTR)', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
double? startValue;
double? endValue;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
onChangeStart: (double value) {
startValue = value;
},
onChangeEnd: (double value) {
endValue = value;
},
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
expect(startValue, equals(0.0));
expect(endValue, equals(0.5));
startValue = null;
endValue = null;
await tester.pump(); // No animation should start.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
final Offset topLeft = tester.getTopLeft(find.byKey(sliderKey));
final Offset bottomRight = tester.getBottomRight(find.byKey(sliderKey));
final Offset target = topLeft + (bottomRight - topLeft) / 4.0;
await tester.tapAt(target);
expect(value, moreOrLessEquals(0.25, epsilon: 0.05));
expect(startValue, equals(0.5));
expect(endValue, moreOrLessEquals(0.25, epsilon: 0.05));
await tester.pump(); // No animation should start.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
});
testWidgets('Slider can move when tapped (RTL)', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
await tester.pump(); // No animation should start.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
final Offset topLeft = tester.getTopLeft(find.byKey(sliderKey));
final Offset bottomRight = tester.getBottomRight(find.byKey(sliderKey));
final Offset target = topLeft + (bottomRight - topLeft) / 4.0;
await tester.tapAt(target);
expect(value, moreOrLessEquals(0.75, epsilon: 0.05));
await tester.pump(); // No animation should start.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
});
testWidgets("Slider doesn't send duplicate change events if tapped on the same value", (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
late double startValue;
late double endValue;
int updates = 0;
int startValueUpdates = 0;
int endValueUpdates = 0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
onChanged: (double newValue) {
setState(() {
updates++;
value = newValue;
});
},
onChangeStart: (double value) {
startValueUpdates++;
startValue = value;
},
onChangeEnd: (double value) {
endValueUpdates++;
endValue = value;
},
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
expect(startValue, equals(0.0));
expect(endValue, equals(0.5));
await tester.pump();
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
await tester.pump();
expect(updates, equals(1));
expect(startValueUpdates, equals(2));
expect(endValueUpdates, equals(2));
});
testWidgets('Value indicator shows for a bit after being tapped', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
await tester.pump(const Duration(milliseconds: 100));
// Starts with the position animation and value indicator
expect(SchedulerBinding.instance.transientCallbackCount, equals(2));
await tester.pump(const Duration(milliseconds: 100));
// Value indicator is longer than position.
expect(SchedulerBinding.instance.transientCallbackCount, equals(1));
await tester.pump(const Duration(milliseconds: 100));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.pump(const Duration(milliseconds: 100));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.pump(const Duration(milliseconds: 100));
// Shown for long enough, value indicator is animated closed.
expect(SchedulerBinding.instance.transientCallbackCount, equals(1));
await tester.pump(const Duration(milliseconds: 101));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
});
testWidgets('Discrete Slider repaints and animates when dragged', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
final List<Offset> log = <Offset>[];
final LoggingThumbShape loggingThumb = LoggingThumbShape(log);
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
final SliderThemeData sliderTheme = SliderTheme.of(context).copyWith(thumbShape: loggingThumb);
return Material(
child: Center(
child: SliderTheme(
data: sliderTheme,
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
);
},
),
),
),
);
final List<Offset> expectedLog = <Offset>[
const Offset(24.0, 300.0),
const Offset(24.0, 300.0),
const Offset(400.0, 300.0),
];
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(value, equals(0.5));
expect(log.length, 3);
expect(log, orderedEquals(expectedLog));
await gesture.moveBy(const Offset(-500.0, 0.0));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(value, equals(0.0));
expect(log.length, 5);
expect(log.last.dx, moreOrLessEquals(386.6, epsilon: 0.1));
// With no more gesture or value changes, the thumb position should still
// be redrawn in the animated position.
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(value, equals(0.0));
expect(log.length, 7);
expect(log.last.dx, moreOrLessEquals(344.5, epsilon: 0.1));
// Final position.
await tester.pump(const Duration(milliseconds: 80));
expectedLog.add(const Offset(24.0, 300.0));
expect(value, equals(0.0));
expect(log.length, 8);
expect(log.last.dx, moreOrLessEquals(24.0, epsilon: 0.1));
await gesture.up();
});
testWidgets("Slider doesn't send duplicate change events if tapped on the same value", (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
int updates = 0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
onChanged: (double newValue) {
setState(() {
updates++;
value = newValue;
});
},
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
await tester.pump();
await tester.tap(find.byKey(sliderKey));
expect(value, equals(0.5));
await tester.pump();
expect(updates, equals(1));
});
testWidgets('discrete Slider repaints when dragged', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
final List<Offset> log = <Offset>[];
final LoggingThumbShape loggingThumb = LoggingThumbShape(log);
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
final SliderThemeData sliderTheme = SliderTheme.of(context).copyWith(thumbShape: loggingThumb);
return Material(
child: Center(
child: SliderTheme(
data: sliderTheme,
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
);
},
),
),
),
);
final List<Offset> expectedLog = <Offset>[
const Offset(24.0, 300.0),
const Offset(24.0, 300.0),
const Offset(400.0, 300.0),
];
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(value, equals(0.5));
expect(log.length, 3);
expect(log, orderedEquals(expectedLog));
await gesture.moveBy(const Offset(-500.0, 0.0));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(value, equals(0.0));
expect(log.length, 5);
expect(log.last.dx, moreOrLessEquals(386.6, epsilon: 0.1));
// With no more gesture or value changes, the thumb position should still
// be redrawn in the animated position.
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(value, equals(0.0));
expect(log.length, 7);
expect(log.last.dx, moreOrLessEquals(344.5, epsilon: 0.1));
// Final position.
await tester.pump(const Duration(milliseconds: 80));
expectedLog.add(const Offset(24.0, 300.0));
expect(value, equals(0.0));
expect(log.length, 8);
expect(log.last.dx, moreOrLessEquals(24.0, epsilon: 0.1));
await gesture.up();
});
testWidgets('Slider take on discrete values', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: SizedBox(
width: 144.0 + 2 * 16.0, // _kPreferredTotalWidth
child: Slider(
key: sliderKey,
max: 100.0,
divisions: 10,
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
);
},
),
),
),
);
expect(value, equals(0.0));
await tester.tap(find.byKey(sliderKey));
expect(value, equals(50.0));
await tester.drag(find.byKey(sliderKey), const Offset(5.0, 0.0));
expect(value, equals(50.0));
await tester.drag(find.byKey(sliderKey), const Offset(40.0, 0.0));
expect(value, equals(80.0));
await tester.pump(); // Starts animation.
expect(SchedulerBinding.instance.transientCallbackCount, greaterThan(0));
await tester.pump(const Duration(milliseconds: 200));
await tester.pump(const Duration(milliseconds: 200));
await tester.pump(const Duration(milliseconds: 200));
await tester.pump(const Duration(milliseconds: 200));
// Animation complete.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
});
testWidgets('Slider can be given zero values', (WidgetTester tester) async {
final List<double> log = <double>[];
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.0,
onChanged: (double newValue) {
log.add(newValue);
},
),
),
),
),
);
await tester.tap(find.byType(Slider));
expect(log, <double>[0.5]);
log.clear();
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.0,
max: 0.0,
onChanged: (double newValue) {
log.add(newValue);
},
),
),
),
),
);
await tester.tap(find.byType(Slider));
expect(log, <double>[]);
log.clear();
});
testWidgets('Slider can tap in vertical scroller', (WidgetTester tester) async {
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: ListView(
children: <Widget>[
Slider(
value: value,
onChanged: (double newValue) {
value = newValue;
},
),
Container(
height: 2000.0,
),
],
),
),
),
),
);
await tester.tap(find.byType(Slider));
expect(value, equals(0.5));
});
testWidgets('Slider drags immediately (LTR)', (WidgetTester tester) async {
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Slider(
value: value,
onChanged: (double newValue) {
value = newValue;
},
),
),
),
),
),
);
final Offset center = tester.getCenter(find.byType(Slider));
final TestGesture gesture = await tester.startGesture(center);
expect(value, equals(0.5));
await gesture.moveBy(const Offset(1.0, 0.0));
expect(value, greaterThan(0.5));
await gesture.up();
});
testWidgets('Slider drags immediately (RTL)', (WidgetTester tester) async {
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Material(
child: Center(
child: Slider(
value: value,
onChanged: (double newValue) {
value = newValue;
},
),
),
),
),
),
);
final Offset center = tester.getCenter(find.byType(Slider));
final TestGesture gesture = await tester.startGesture(center);
expect(value, equals(0.5));
await gesture.moveBy(const Offset(1.0, 0.0));
expect(value, lessThan(0.5));
await gesture.up();
});
testWidgets('Slider onChangeStart and onChangeEnd fire once', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/28115
int startFired = 0;
int endFired = 0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: GestureDetector(
onHorizontalDragUpdate: (_) { },
child: Slider(
value: 0.0,
onChanged: (double newValue) { },
onChangeStart: (double value) {
startFired += 1;
},
onChangeEnd: (double value) {
endFired += 1;
},
),
),
),
),
),
),
);
await tester.timedDrag(
find.byType(Slider),
const Offset(20.0, 0.0),
const Duration(milliseconds: 100),
);
expect(startFired, equals(1));
expect(endFired, equals(1));
});
testWidgets('Slider sizing', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byType(Slider)).size, const Size(800.0, 600.0));
await tester.pumpWidget(
const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: IntrinsicWidth(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byType(Slider)).size, const Size(144.0 + 2.0 * 24.0, 600.0));
await tester.pumpWidget(
const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: OverflowBox(
maxWidth: double.infinity,
maxHeight: double.infinity,
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byType(Slider)).size, const Size(144.0 + 2.0 * 24.0, 48.0));
});
testWidgets('Slider respects textScaleFactor', (WidgetTester tester) async {
debugDisableShadows = false;
try {
final Key sliderKey = UniqueKey();
double value = 0.0;
Widget buildSlider({
required double textScaleFactor,
bool isDiscrete = true,
ShowValueIndicator show = ShowValueIndicator.onlyForDiscrete,
}) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return MediaQuery(
data: MediaQueryData(textScaler: TextScaler.linear(textScaleFactor)),
child: Material(
child: Theme(
data: Theme.of(context).copyWith(
sliderTheme: Theme.of(context).sliderTheme.copyWith(showValueIndicator: show),
),
child: Center(
child: OverflowBox(
maxWidth: double.infinity,
maxHeight: double.infinity,
child: Slider(
key: sliderKey,
max: 100.0,
divisions: isDiscrete ? 10 : null,
label: '${value.round()}',
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
),
),
);
},
),
),
);
}
await tester.pumpWidget(buildSlider(textScaleFactor: 1.0));
Offset center = tester.getCenter(find.byType(Slider));
TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
expect(
tester.renderObject(find.byType(Overlay)),
paints
..path(
includes: const <Offset>[
Offset.zero,
Offset(0.0, -8.0),
Offset(-276.0, -16.0),
Offset(-216.0, -16.0),
],
color: const Color(0xf55f5f5f),
)
..paragraph(),
);
await gesture.up();
await tester.pumpAndSettle();
await tester.pumpWidget(buildSlider(textScaleFactor: 2.0));
center = tester.getCenter(find.byType(Slider));
gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
expect(
tester.renderObject(find.byType(Overlay)),
paints
..path(
includes: const <Offset>[
Offset.zero,
Offset(0.0, -8.0),
Offset(-304.0, -16.0),
Offset(-216.0, -16.0),
],
color: const Color(0xf55f5f5f),
)
..paragraph(),
);
await gesture.up();
await tester.pumpAndSettle();
// Check continuous
await tester.pumpWidget(buildSlider(
textScaleFactor: 1.0,
isDiscrete: false,
show: ShowValueIndicator.onlyForContinuous,
));
center = tester.getCenter(find.byType(Slider));
gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
expect(tester.renderObject(find.byType(Overlay)),
paints
..path(
includes: const <Offset>[
Offset.zero,
Offset(0.0, -8.0),
Offset(-276.0, -16.0),
Offset(-216.0, -16.0),
],
color: const Color(0xf55f5f5f),
)
..paragraph(),
);
await gesture.up();
await tester.pumpAndSettle();
await tester.pumpWidget(buildSlider(
textScaleFactor: 2.0,
isDiscrete: false,
show: ShowValueIndicator.onlyForContinuous,
));
center = tester.getCenter(find.byType(Slider));
gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
expect(
tester.renderObject(find.byType(Overlay)),
paints
..path(
includes: const <Offset>[
Offset.zero,
Offset(0.0, -8.0),
Offset(-276.0, -16.0),
Offset(-216.0, -16.0),
],
color: const Color(0xf55f5f5f),
)
..paragraph(),
);
await gesture.up();
await tester.pumpAndSettle();
} finally {
debugDisableShadows = true;
}
});
testWidgets('Tick marks are skipped when they are too dense', (WidgetTester tester) async {
Widget buildSlider({
required int divisions,
}) {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Slider(
max: 100.0,
divisions: divisions,
value: 0.25,
onChanged: (double newValue) { },
),
),
),
),
);
}
// Pump a slider with a reasonable amount of divisions to verify that the
// tick marks are drawn when the number of tick marks is not too dense.
await tester.pumpWidget(
buildSlider(
divisions: 4,
),
);
final MaterialInkController material = Material.of(tester.element(find.byType(Slider)));
// 5 tick marks and a thumb.
expect(material, paintsExactlyCountTimes(#drawCircle, 6));
// 200 divisions will produce a tick interval off less than 6,
// which would be too dense to draw.
await tester.pumpWidget(
buildSlider(
divisions: 200,
),
);
// No tick marks are drawn because they are too dense, but the thumb is
// still drawn.
expect(material, paintsExactlyCountTimes(#drawCircle, 1));
});
testWidgets('Slider has correct animations when reparented', (WidgetTester tester) async {
final Key sliderKey = GlobalKey(debugLabel: 'A');
double value = 0.0;
Widget buildSlider(int parents) {
Widget createParents(int parents, StateSetter setState) {
Widget slider = Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
);
for (int i = 0; i < parents; ++i) {
slider = Column(children: <Widget>[slider]);
}
return slider;
}
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: createParents(parents, setState),
);
},
),
),
);
}
Future<void> testReparenting(bool reparent) async {
final MaterialInkController material = Material.of(tester.element(find.byType(Slider)));
final Offset center = tester.getCenter(find.byType(Slider));
// Move to 0.0.
TestGesture gesture = await tester.startGesture(Offset.zero);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
expect(
material,
paints
..circle(x: 26.0, y: 24.0, radius: 1.0)
..circle(x: 213.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 1.0)
..circle(x: 587.0, y: 24.0, radius: 1.0)
..circle(x: 774.0, y: 24.0, radius: 1.0)
..circle(x: 24.0, y: 24.0, radius: 10.0),
);
gesture = await tester.startGesture(center);
await tester.pump();
// Wait for animations to start.
await tester.pump(const Duration(milliseconds: 25));
expect(SchedulerBinding.instance.transientCallbackCount, equals(2));
expect(
material,
paints
..circle(x: 111.20703125, y: 24.0, radius: 5.687664985656738)
..circle(x: 26.0, y: 24.0, radius: 1.0)
..circle(x: 213.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 1.0)
..circle(x: 587.0, y: 24.0, radius: 1.0)
..circle(x: 774.0, y: 24.0, radius: 1.0)
..circle(x: 111.20703125, y: 24.0, radius: 10.0),
);
// Reparenting in the middle of an animation should do nothing.
if (reparent) {
await tester.pumpWidget(buildSlider(2));
}
// Move a little further in the animations.
await tester.pump(const Duration(milliseconds: 10));
expect(SchedulerBinding.instance.transientCallbackCount, equals(2));
expect(
material,
paints
..circle(x: 190.0135726928711, y: 24.0, radius: 12.0)
..circle(x: 26.0, y: 24.0, radius: 1.0)
..circle(x: 213.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 1.0)
..circle(x: 587.0, y: 24.0, radius: 1.0)
..circle(x: 774.0, y: 24.0, radius: 1.0)
..circle(x: 190.0135726928711, y: 24.0, radius: 10.0),
);
// Wait for animations to finish.
await tester.pumpAndSettle();
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
expect(
material,
paints
..circle(x: 400.0, y: 24.0, radius: 24.0)
..circle(x: 26.0, y: 24.0, radius: 1.0)
..circle(x: 213.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 1.0)
..circle(x: 587.0, y: 24.0, radius: 1.0)
..circle(x: 774.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 10.0),
);
await gesture.up();
await tester.pumpAndSettle();
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
expect(
material,
paints
..circle(x: 26.0, y: 24.0, radius: 1.0)
..circle(x: 213.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 1.0)
..circle(x: 587.0, y: 24.0, radius: 1.0)
..circle(x: 774.0, y: 24.0, radius: 1.0)
..circle(x: 400.0, y: 24.0, radius: 10.0),
);
}
await tester.pumpWidget(buildSlider(1));
// Do it once without reparenting in the middle of an animation
await testReparenting(false);
// Now do it again with reparenting in the middle of an animation.
await testReparenting(true);
});
testWidgets('Slider Semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.5,
onChanged: (double v) { },
),
),
),
));
await tester.pumpAndSettle();
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
SemanticsFlag.isSlider,
],
actions: <SemanticsAction>[
SemanticsAction.increase,
SemanticsAction.decrease,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
// Disable slider
await tester.pumpWidget(const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
// isFocusable is delayed by 1 frame.
SemanticsFlag.isFocusable,
SemanticsFlag.isSlider,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
await tester.pump();
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isSlider,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.android, TargetPlatform.fuchsia, TargetPlatform.linux }));
testWidgets('Slider Semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
home: Theme(
data: ThemeData.light(),
child: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 100.0,
max: 200.0,
onChanged: (double v) { },
),
),
),
),
),
);
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[SemanticsFlag.hasEnabledState, SemanticsFlag.isEnabled, SemanticsFlag.isFocusable, SemanticsFlag.isSlider],
actions: <SemanticsAction>[SemanticsAction.increase, SemanticsAction.decrease],
value: '50%',
increasedValue: '60%',
decreasedValue: '40%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
// Disable slider
await tester.pumpWidget(const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 5,
flags: <SemanticsFlag>[SemanticsFlag.hasEnabledState, SemanticsFlag.isSlider],
value: '50%',
increasedValue: '60%',
decreasedValue: '40%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Slider Semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.5,
onChanged: (double v) { },
),
),
),
));
await tester.pumpAndSettle();
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
SemanticsFlag.isSlider,
],
actions: <SemanticsAction>[
SemanticsAction.increase,
SemanticsAction.decrease,
SemanticsAction.didGainAccessibilityFocus,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
// Disable slider
await tester.pumpWidget(const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
// isFocusable is delayed by 1 frame.
SemanticsFlag.isFocusable,
SemanticsFlag.isSlider,
],
actions: <SemanticsAction>[
SemanticsAction.didGainAccessibilityFocus,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
await tester.pump();
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isSlider,
],
actions: <SemanticsAction>[
SemanticsAction.didGainAccessibilityFocus,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.windows }));
testWidgets('Slider semantics with custom formatter', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 40.0,
max: 200.0,
divisions: 10,
semanticFormatterCallback: (double value) => value.round().toString(),
onChanged: (double v) { },
),
),
),
));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[SemanticsFlag.hasEnabledState, SemanticsFlag.isEnabled, SemanticsFlag.isFocusable, SemanticsFlag.isSlider],
actions: <SemanticsAction>[SemanticsAction.increase, SemanticsAction.decrease],
value: '40',
increasedValue: '60',
decreasedValue: '20',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
});
// Regression test for https://github.com/flutter/flutter/issues/101868
testWidgets('Slider.label info should not write to semantic node', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Slider(
value: 40.0,
max: 200.0,
divisions: 10,
semanticFormatterCallback: (double value) => value.round().toString(),
onChanged: (double v) { },
label: 'Bingo',
),
),
),
));
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[SemanticsFlag.hasEnabledState, SemanticsFlag.isEnabled, SemanticsFlag.isFocusable, SemanticsFlag.isSlider],
actions: <SemanticsAction>[SemanticsAction.increase, SemanticsAction.decrease],
value: '40',
increasedValue: '60',
decreasedValue: '20',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
});
testWidgets('Material3 - Slider is focusable and has correct focus color', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Slider');
addTearDown(focusNode.dispose);
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final ThemeData theme = ThemeData();
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
autofocus: true,
focusNode: focusNode,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Check that the overlay shows when focused.
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isTrue);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.1)),
);
// Check that the overlay does not show when unfocused and disabled.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isFalse);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.1))),
);
});
testWidgets('Slider has correct focus color from overlayColor property', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Slider');
addTearDown(focusNode.dispose);
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
overlayColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return Colors.purple[500]!;
}
return Colors.transparent;
}),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
autofocus: true,
focusNode: focusNode,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Check that the overlay shows when focused.
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isTrue);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: Colors.purple[500]),
);
// Check that the overlay does not show when focused and disabled.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isFalse);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.purple[500])),
);
});
testWidgets('Slider can be hovered and has correct hover color', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final ThemeData theme = ThemeData(useMaterial3: true);
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not hovered.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.orange[500])),
);
// Start hovering.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Slider)));
// Slider has overlay when enabled and hovered.
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.08)),
);
// Slider still shows correct hovered color after pressing/dragging
await gesture.down(tester.getCenter(find.byType(Slider)));
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
await gesture.moveTo(const Offset(0.0, 100.0));
await tester.pumpAndSettle();
await gesture.moveTo(tester.getCenter(find.byType(Slider)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.08)),
);
// Slider does not have an overlay when disabled and hovered.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.orange[500])),
);
});
testWidgets('Slider has correct hovered color from overlayColor property', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
overlayColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.hovered)) {
return Colors.cyan[500]!;
}
return Colors.transparent;
}),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not hovered.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.cyan[500])),
);
// Start hovering.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Slider)));
// Slider has overlay when enabled and hovered.
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: Colors.cyan[500]),
);
// Slider does not have an overlay when disabled and hovered.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.cyan[500])),
);
});
testWidgets('Material3 - Slider is draggable and has correct dragged color', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
final ThemeData theme = ThemeData();
final Key sliderKey = UniqueKey();
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
key: sliderKey,
value: value,
focusNode: focusNode,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not dragged.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.1))),
);
// Start dragging.
final TestGesture drag = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump(kPressTimeout);
// Less than configured touch slop, more than default touch slop
await drag.moveBy(const Offset(19.0, 0));
await tester.pump();
// Slider has overlay when enabled and dragged.
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.1)),
);
await drag.up();
await tester.pumpAndSettle();
// Slider without focus doesn't have overlay when enabled and dragged.
expect(focusNode.hasFocus, false);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.1))),
);
// Slider has overlay when enabled, dragged and focused.
focusNode.requestFocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, true);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.1)),
);
});
testWidgets('Slider has correct dragged color from overlayColor property', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
final Key sliderKey = UniqueKey();
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
key: sliderKey,
value: value,
focusNode: focusNode,
overlayColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.dragged)) {
return Colors.lime[500]!;
}
return Colors.transparent;
}),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not dragged.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.lime[500])),
);
// Start dragging.
final TestGesture drag = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump(kPressTimeout);
// Less than configured touch slop, more than default touch slop
await drag.moveBy(const Offset(19.0, 0));
await tester.pump();
// Slider has overlay when enabled and dragged.
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: Colors.lime[500]),
);
await drag.up();
await tester.pumpAndSettle();
// Slider without focus doesn't have overlay when enabled and dragged.
expect(focusNode.hasFocus, false);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: Colors.lime[500])),
);
});
testWidgets('OverlayColor property is correctly applied when activeColor is also provided', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Slider');
addTearDown(focusNode.dispose);
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
const Color activeColor = Color(0xffff0000);
const Color overlayColor = Color(0xff0000ff);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
activeColor: activeColor,
overlayColor: const MaterialStatePropertyAll<Color?>(overlayColor),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
focusNode: focusNode,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
final MaterialInkController material = Material.of(tester.element(find.byType(Slider)));
// Check that thumb color is using active color.
expect(material, paints..circle(color: activeColor));
focusNode.requestFocus();
await tester.pumpAndSettle();
// Check that the overlay shows when focused.
expect(focusNode.hasPrimaryFocus, isTrue);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: overlayColor),
);
// Check that the overlay does not show when focused and disabled.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isFalse);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
});
testWidgets('Slider can be incremented and decremented by keyboard shortcuts - LTR', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double startValue = 0.0;
double currentValue = 0.5;
double endValue = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: currentValue,
onChangeStart: (double newValue) {
setState(() {
startValue = newValue;
});
},
onChanged: (double newValue) {
setState(() {
currentValue = newValue;
});
},
onChangeEnd: (double newValue) {
setState(() {
endValue = newValue;
});
},
autofocus: true,
);
}),
),
),
),
);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.55);
expect(endValue, 0.55);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(startValue, 0.55);
expect(currentValue, 0.5);
expect(endValue, 0.5);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.55);
expect(endValue, 0.55);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
expect(startValue, 0.55);
expect(currentValue, 0.5);
expect(endValue, 0.5);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.android, TargetPlatform.fuchsia, TargetPlatform.linux, TargetPlatform.windows }));
testWidgets('Slider can be incremented and decremented by keyboard shortcuts - LTR', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double startValue = 0.0;
double currentValue = 0.5;
double endValue = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: currentValue,
onChangeStart: (double newValue) {
setState(() {
startValue = newValue;
});
},
onChanged: (double newValue) {
setState(() {
currentValue = newValue;
});
},
onChangeEnd: (double newValue) {
setState(() {
endValue = newValue;
});
},
autofocus: true,
);
}),
),
),
),
);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.6);
expect(endValue, 0.6);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(startValue, 0.6);
expect(currentValue, 0.5);
expect(endValue, 0.5);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.6);
expect(endValue, 0.6);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
expect(startValue, 0.6);
expect(currentValue, 0.5);
expect(endValue, 0.5);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Slider can be incremented and decremented by keyboard shortcuts - RTL', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double startValue = 0.0;
double currentValue = 0.5;
double endValue = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.rtl,
child: Slider(
value: currentValue,
onChangeStart: (double newValue) {
setState(() {
startValue = newValue;
});
},
onChanged: (double newValue) {
setState(() {
currentValue = newValue;
});
},
onChangeEnd: (double newValue) {
setState(() {
endValue = newValue;
});
},
autofocus: true,
),
);
}),
),
),
),
);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.45);
expect(endValue, 0.45);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(startValue, 0.45);
expect(currentValue, 0.5);
expect(endValue, 0.5);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.55);
expect(endValue, 0.55);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
expect(startValue, 0.55);
expect(currentValue, 0.5);
expect(endValue, 0.5);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.android, TargetPlatform.fuchsia, TargetPlatform.linux, TargetPlatform.windows }));
testWidgets('Slider can be incremented and decremented by keyboard shortcuts - RTL', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double startValue = 0.0;
double currentValue = 0.5;
double endValue = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Directionality(
textDirection: TextDirection.rtl,
child: Slider(
value: currentValue,
onChangeStart: (double newValue) {
setState(() {
startValue = newValue;
});
},
onChanged: (double newValue) {
setState(() {
currentValue = newValue;
});
},
onChangeEnd: (double newValue) {
setState(() {
endValue = newValue;
});
},
autofocus: true,
),
);
}),
),
),
),
);
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.4);
expect(endValue, 0.4);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(startValue, 0.4);
expect(currentValue, 0.5);
expect(endValue, 0.5);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(startValue, 0.5);
expect(currentValue, 0.6);
expect(endValue, 0.6);
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
expect(startValue, 0.6);
expect(currentValue, 0.5);
expect(endValue, 0.5);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('In directional nav, Slider can be navigated out of by using up and down arrows', (WidgetTester tester) async {
const Map<ShortcutActivator, Intent> shortcuts = <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.arrowLeft): DirectionalFocusIntent(TraversalDirection.left),
SingleActivator(LogicalKeyboardKey.arrowRight): DirectionalFocusIntent(TraversalDirection.right),
SingleActivator(LogicalKeyboardKey.arrowDown): DirectionalFocusIntent(TraversalDirection.down),
SingleActivator(LogicalKeyboardKey.arrowUp): DirectionalFocusIntent(TraversalDirection.up),
};
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double topSliderValue = 0.5;
double bottomSliderValue = 0.5;
await tester.pumpWidget(
MaterialApp(
home: Shortcuts(
shortcuts: shortcuts,
child: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return MediaQuery(
data: const MediaQueryData(navigationMode: NavigationMode.directional),
child: Column(
children: <Widget>[
Slider(
value: topSliderValue,
onChanged: (double newValue) {
setState(() {
topSliderValue = newValue;
});
},
autofocus: true,
),
Slider(
value: bottomSliderValue,
onChanged: (double newValue) {
setState(() {
bottomSliderValue = newValue;
});
},
),
]
),
);
}),
),
),
),
),
);
await tester.pumpAndSettle();
// The top slider is auto-focused and can be adjusted with left and right arrow keys.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(topSliderValue, 0.55, reason: 'focused top Slider increased after first arrowRight');
expect(bottomSliderValue, 0.5, reason: 'unfocused bottom Slider unaffected by first arrowRight');
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'focused top Slider decreased after first arrowLeft');
expect(bottomSliderValue, 0.5, reason: 'unfocused bottom Slider unaffected by first arrowLeft');
// Pressing the down-arrow key moves focus down to the bottom slider
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'arrowDown unfocuses top Slider, does not alter its value');
expect(bottomSliderValue, 0.5, reason: 'arrowDown focuses bottom Slider, does not alter its value');
// The bottom slider is now focused and can be adjusted with left and right arrow keys.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'unfocused top Slider unaffected by second arrowRight');
expect(bottomSliderValue, 0.55, reason: 'focused bottom Slider increased by second arrowRight');
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'unfocused top Slider unaffected by second arrowLeft');
expect(bottomSliderValue, 0.5, reason: 'focused bottom Slider decreased by second arrowLeft');
// Pressing the up-arrow key moves focus back up to the top slider
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'arrowUp focuses top Slider, does not alter its value');
expect(bottomSliderValue, 0.5, reason: 'arrowUp unfocuses bottom Slider, does not alter its value');
// The top slider is now focused again and can be adjusted with left and right arrow keys.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowRight);
await tester.pumpAndSettle();
expect(topSliderValue, 0.55, reason: 'focused top Slider increased after third arrowRight');
expect(bottomSliderValue, 0.5, reason: 'unfocused bottom Slider unaffected by third arrowRight');
await tester.sendKeyEvent(LogicalKeyboardKey.arrowLeft);
await tester.pumpAndSettle();
expect(topSliderValue, 0.5, reason: 'focused top Slider decreased after third arrowRight');
expect(bottomSliderValue, 0.5, reason: 'unfocused bottom Slider unaffected by third arrowRight');
});
testWidgets('Slider gains keyboard focus when it gains semantics focus on Windows', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final SemanticsOwner semanticsOwner = tester.binding.pipelineOwner.semanticsOwner!;
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Slider(
value: 0.5,
onChanged: (double _) {},
focusNode: focusNode,
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
id: 1,
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
id: 2,
children: <TestSemantics>[
TestSemantics(
id: 3,
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
id: 4,
flags: <SemanticsFlag>[
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isFocusable,
SemanticsFlag.isSlider,
],
actions: <SemanticsAction>[
SemanticsAction.increase,
SemanticsAction.decrease,
SemanticsAction.didGainAccessibilityFocus,
],
value: '50%',
increasedValue: '55%',
decreasedValue: '45%',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
));
expect(focusNode.hasFocus, isFalse);
semanticsOwner.performAction(4, SemanticsAction.didGainAccessibilityFocus);
await tester.pumpAndSettle();
expect(focusNode.hasFocus, isTrue);
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.windows }));
testWidgets('Value indicator appears when it should', (WidgetTester tester) async {
final ThemeData baseTheme = ThemeData(
platform: TargetPlatform.android,
primarySwatch: Colors.blue,
);
SliderThemeData theme = baseTheme.sliderTheme;
double value = 0.45;
Widget buildApp({ required SliderThemeData sliderTheme, int? divisions, bool enabled = true }) {
final ValueChanged<double>? onChanged = enabled ? (double d) => value = d : null;
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Theme(
data: baseTheme,
child: SliderTheme(
data: sliderTheme,
child: Slider(
value: value,
label: '$value',
divisions: divisions,
onChanged: onChanged,
),
),
),
),
),
),
);
}
Future<void> expectValueIndicator({
required bool isVisible,
required SliderThemeData theme,
int? divisions,
bool enabled = true,
}) async {
// Discrete enabled widget.
await tester.pumpWidget(buildApp(sliderTheme: theme, divisions: divisions, enabled: enabled));
final Offset center = tester.getCenter(find.byType(Slider));
final TestGesture gesture = await tester.startGesture(center);
// Wait for value indicator animation to finish.
await tester.pumpAndSettle();
final RenderBox valueIndicatorBox = tester.renderObject(find.byType(Overlay));
expect(
valueIndicatorBox,
isVisible
? (paints..path(color: theme.valueIndicatorColor)..paragraph())
: isNot(paints..path(color: theme.valueIndicatorColor)..paragraph()),
);
await gesture.up();
}
// Default (showValueIndicator set to onlyForDiscrete).
await expectValueIndicator(isVisible: true, theme: theme, divisions: 3);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3, enabled: false);
await expectValueIndicator(isVisible: false, theme: theme);
await expectValueIndicator(isVisible: false, theme: theme, enabled: false);
// With showValueIndicator set to onlyForContinuous.
theme = theme.copyWith(showValueIndicator: ShowValueIndicator.onlyForContinuous);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3, enabled: false);
await expectValueIndicator(isVisible: true, theme: theme);
await expectValueIndicator(isVisible: false, theme: theme, enabled: false);
// discrete enabled widget with showValueIndicator set to always.
theme = theme.copyWith(showValueIndicator: ShowValueIndicator.always);
await expectValueIndicator(isVisible: true, theme: theme, divisions: 3);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3, enabled: false);
await expectValueIndicator(isVisible: true, theme: theme);
await expectValueIndicator(isVisible: false, theme: theme, enabled: false);
// discrete enabled widget with showValueIndicator set to never.
theme = theme.copyWith(showValueIndicator: ShowValueIndicator.never);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3);
await expectValueIndicator(isVisible: false, theme: theme, divisions: 3, enabled: false);
await expectValueIndicator(isVisible: false, theme: theme);
await expectValueIndicator(isVisible: false, theme: theme, enabled: false);
});
testWidgets("Slider doesn't start any animations after dispose", (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
);
},
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pumpAndSettle();
expect(value, equals(0.5));
await gesture.moveBy(const Offset(-500.0, 0.0));
await tester.pumpAndSettle();
// Change the tree to dispose the original widget.
await tester.pumpWidget(Container());
expect(await tester.pumpAndSettle(), equals(1));
await gesture.up();
});
testWidgets('Slider removes value indicator from overlay if Slider gets disposed without value indicator animation completing.', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
const Color fillColor = Color(0xf55f5f5f);
double value = 0.0;
Widget buildApp({
int? divisions,
bool enabled = true,
}) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Builder(
// The builder is used to pass the context from the MaterialApp widget
// to the [Navigator]. This context is required in order for the
// Navigator to work.
builder: (BuildContext context) {
return Column(
children: <Widget>[
Slider(
key: sliderKey,
max: 100.0,
divisions: divisions,
label: '${value.round()}',
value: value,
onChanged: (double newValue) {
value = newValue;
},
),
ElevatedButton(
child: const Text('Next'),
onPressed: () {
Navigator.of(context).pushReplacement(
MaterialPageRoute<void>(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('Inner page'),
onPressed: () { Navigator.of(context).pop(); },
);
},
),
);
},
),
],
);
},
),
),
);
}
await tester.pumpWidget(buildApp(divisions: 3));
final RenderObject valueIndicatorBox = tester.renderObject(find.byType(Overlay));
final Offset topRight = tester.getTopRight(find.byType(Slider)).translate(-24, 0);
final TestGesture gesture = await tester.startGesture(topRight);
// Wait for value indicator animation to finish.
await tester.pumpAndSettle();
expect(find.byType(Slider), isNotNull);
expect(
valueIndicatorBox,
paints
// Represents the raised button with text, next.
..path(color: Colors.black)
..paragraph()
// Represents the Slider.
..path(color: fillColor)
..paragraph(),
);
expect(valueIndicatorBox, paintsExactlyCountTimes(#drawPath, 4));
expect(valueIndicatorBox, paintsExactlyCountTimes(#drawParagraph, 2));
await tester.tap(find.text('Next'));
await tester.pumpAndSettle();
expect(find.byType(Slider), findsNothing);
expect(
valueIndicatorBox,
isNot(
paints
..path(color: fillColor)
..paragraph(),
),
);
// Represents the ElevatedButton with inner Text, inner page.
expect(valueIndicatorBox, paintsExactlyCountTimes(#drawPath, 2));
expect(valueIndicatorBox, paintsExactlyCountTimes(#drawParagraph, 1));
// Don't stop holding the value indicator.
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Slider.adaptive', (WidgetTester tester) async {
double value = 0.5;
Widget buildFrame(TargetPlatform platform) {
return MaterialApp(
theme: ThemeData(platform: platform),
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: Slider.adaptive(
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
);
},
),
);
}
for (final TargetPlatform platform in <TargetPlatform>[TargetPlatform.iOS, TargetPlatform.macOS]) {
value = 0.5;
await tester.pumpWidget(buildFrame(platform));
expect(find.byType(Slider), findsOneWidget);
expect(find.byType(CupertinoSlider), findsOneWidget);
expect(value, 0.5, reason: 'on ${platform.name}');
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CupertinoSlider)));
// Drag to the right end of the track.
await gesture.moveBy(const Offset(600.0, 0.0));
expect(value, 1.0, reason: 'on ${platform.name}');
await gesture.up();
}
for (final TargetPlatform platform in <TargetPlatform>[TargetPlatform.android, TargetPlatform.fuchsia, TargetPlatform.linux, TargetPlatform.windows]) {
value = 0.5;
await tester.pumpWidget(buildFrame(platform));
await tester.pumpAndSettle(); // Finish the theme change animation.
expect(find.byType(Slider), findsOneWidget);
expect(find.byType(CupertinoSlider), findsNothing);
expect(value, 0.5, reason: 'on ${platform.name}');
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(Slider)));
// Drag to the right end of the track.
await gesture.moveBy(const Offset(600.0, 0.0));
expect(value, 1.0, reason: 'on ${platform.name}');
await gesture.up();
}
});
testWidgets('Slider respects height from theme', (WidgetTester tester) async {
final Key sliderKey = UniqueKey();
double value = 0.0;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
final SliderThemeData sliderTheme = SliderTheme.of(context).copyWith(tickMarkShape: TallSliderTickMarkShape());
return Material(
child: Center(
child: IntrinsicHeight(
child: SliderTheme(
data: sliderTheme,
child: Slider(
key: sliderKey,
value: value,
divisions: 4,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
),
);
},
),
),
),
);
final RenderBox renderObject = tester.renderObject<RenderBox>(find.byType(Slider));
expect(renderObject.size.height, 200);
});
testWidgets('Slider changes mouse cursor when hovered', (WidgetTester tester) async {
// Test Slider() constructor
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: Slider(
mouseCursor: SystemMouseCursors.text,
value: 0.5,
onChanged: (double newValue) { },
),
),
),
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: tester.getCenter(find.byType(Slider)));
await tester.pump();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Test Slider.adaptive() constructor
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: Slider.adaptive(
mouseCursor: SystemMouseCursors.text,
value: 0.5,
onChanged: (double newValue) { },
),
),
),
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
// Test default cursor
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: Slider(
value: 0.5,
onChanged: (double newValue) { },
),
),
),
),
),
),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
});
testWidgets('Slider MaterialStateMouseCursor resolves correctly', (WidgetTester tester) async {
const MouseCursor disabledCursor = SystemMouseCursors.basic;
const MouseCursor hoveredCursor = SystemMouseCursors.grab;
const MouseCursor draggedCursor = SystemMouseCursors.move;
Widget buildFrame({ required bool enabled }) {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: MouseRegion(
cursor: SystemMouseCursors.forbidden,
child: Slider(
mouseCursor: const _StateDependentMouseCursor(
disabled: disabledCursor,
hovered: hoveredCursor,
dragged: draggedCursor,
),
value: 0.5,
onChanged: enabled ? (double newValue) { } : null,
),
),
),
),
),
);
}
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: Offset.zero);
await tester.pumpWidget(buildFrame(enabled: false));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), disabledCursor);
await tester.pumpWidget(buildFrame(enabled: true));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.none);
await gesture.moveTo(tester.getCenter(find.byType(Slider))); // start hover
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), hoveredCursor);
await tester.timedDrag(
find.byType(Slider),
const Offset(20.0, 0.0),
const Duration(milliseconds: 100),
);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.move);
});
testWidgets('Slider implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const Slider(
activeColor: Colors.blue,
divisions: 10,
inactiveColor: Colors.grey,
secondaryActiveColor: Colors.blueGrey,
label: 'Set a value',
max: 100.0,
onChanged: null,
value: 50.0,
secondaryTrackValue: 75.0,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString()).toList();
expect(description, <String>[
'value: 50.0',
'secondaryTrackValue: 75.0',
'disabled',
'min: 0.0',
'max: 100.0',
'divisions: 10',
'label: "Set a value"',
'activeColor: MaterialColor(primary value: Color(0xff2196f3))',
'inactiveColor: MaterialColor(primary value: Color(0xff9e9e9e))',
'secondaryActiveColor: MaterialColor(primary value: Color(0xff607d8b))',
]);
});
testWidgets('Slider track paints correctly when the shape is rectangular', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
sliderTheme: const SliderThemeData(
trackShape: RectangularSliderTrackShape(),
),
),
home: const Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
),
),
);
// _RenderSlider is the last render object in the tree.
final RenderObject renderObject = tester.allRenderObjects.last;
// The active track rect should start at 24.0 pixels,
// and there should not have a gap between active and inactive track.
expect(
renderObject,
paints
..rect(rect: const Rect.fromLTRB(24.0, 298.0, 400.0, 302.0)) // active track Rect.
..rect(rect: const Rect.fromLTRB(400.0, 298.0, 776.0, 302.0)), // inactive track Rect.
);
});
testWidgets('SliderTheme change should trigger re-layout', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/118955
double sliderValue = 0.0;
Widget buildFrame(ThemeMode themeMode) {
return MaterialApp(
themeMode: themeMode,
theme: ThemeData(brightness: Brightness.light, useMaterial3: true),
darkTheme: ThemeData(brightness: Brightness.dark, useMaterial3: true),
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: SizedBox(
height: 10.0,
width: 10.0,
child: Slider(
value: sliderValue,
label: 'label',
onChanged: (double value) => sliderValue = value,
),
),
),
),
),
);
}
await tester.pumpWidget(buildFrame(ThemeMode.light));
// _RenderSlider is the last render object in the tree.
final RenderObject renderObject = tester.allRenderObjects.last;
expect(renderObject.debugNeedsLayout, false);
await tester.pumpWidget(buildFrame(ThemeMode.dark));
await tester.pump(
const Duration(milliseconds: 100), // to let the theme animate
EnginePhase.build,
);
expect(renderObject.debugNeedsLayout, true);
// Pump the rest of the frames to complete the test.
await tester.pumpAndSettle();
});
testWidgets('Slider can be painted in a narrower constraint', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: SizedBox(
height: 10.0,
width: 10.0,
child: Slider(
value: 0.5,
onChanged: null,
),
),
),
),
),
),
);
// _RenderSlider is the last render object in the tree.
final RenderObject renderObject = tester.allRenderObjects.last;
expect(
renderObject,
paints
// active track RRect
..rrect(rrect: RRect.fromLTRBAndCorners(-14.0, 2.0, 5.0, 8.0, topLeft: const Radius.circular(3.0), bottomLeft: const Radius.circular(3.0)))
// inactive track RRect
..rrect(rrect: RRect.fromLTRBAndCorners(5.0, 3.0, 24.0, 7.0, topRight: const Radius.circular(2.0), bottomRight: const Radius.circular(2.0)))
// thumb
..circle(x: 5.0, y: 5.0, radius: 10.0, ),
);
});
testWidgets('Update the divisions and value at the same time for Slider', (WidgetTester tester) async {
// Regress test for https://github.com/flutter/flutter/issues/65943
Widget buildFrame(double maxValue) {
return MaterialApp(
home: Material(
child: Center(
child: Slider.adaptive(
value: 5,
max: maxValue,
divisions: maxValue.toInt(),
onChanged: (double newValue) {},
),
),
),
);
}
await tester.pumpWidget(buildFrame(10));
// _RenderSlider is the last render object in the tree.
final RenderObject renderObject = tester.allRenderObjects.last;
// Update the divisions from 10 to 15, the thumb should be paint at the correct position.
await tester.pumpWidget(buildFrame(15));
await tester.pumpAndSettle(); // Finish the animation.
late RRect activeTrackRRect;
expect(renderObject, paints..something((Symbol method, List<dynamic> arguments) {
if (method != #drawRRect) {
return false;
}
activeTrackRRect = arguments[0] as RRect;
return true;
}));
// The thumb should at one-third(5 / 15) of the Slider.
// The right of the active track shape is the position of the thumb.
// 24.0 is the default margin, (800.0 - 24.0 - 24.0) is the slider's width.
expect(nearEqual(activeTrackRRect.right, (800.0 - 24.0 - 24.0) * (5 / 15) + 24.0, 0.01), true);
});
testWidgets('Slider paints thumbColor', (WidgetTester tester) async {
const Color color = Color(0xffffc107);
final Widget sliderAdaptive = MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
home: Material(
child: Slider(
value: 0,
onChanged: (double newValue) {},
thumbColor: color,
),
),
);
await tester.pumpWidget(sliderAdaptive);
await tester.pumpAndSettle();
final MaterialInkController material =
Material.of(tester.element(find.byType(Slider)));
expect(material, paints..circle(color: color));
});
testWidgets('Slider.adaptive paints thumbColor on Android',
(WidgetTester tester) async {
const Color color = Color(0xffffc107);
final Widget sliderAdaptive = MaterialApp(
theme: ThemeData(platform: TargetPlatform.android),
home: Material(
child: Slider.adaptive(
value: 0,
onChanged: (double newValue) {},
thumbColor: color,
),
),
);
await tester.pumpWidget(sliderAdaptive);
await tester.pumpAndSettle();
final MaterialInkController material =
Material.of(tester.element(find.byType(Slider)));
expect(material, paints..circle(color: color));
});
testWidgets('If thumbColor is null, it defaults to CupertinoColors.white',
(WidgetTester tester) async {
final Widget sliderAdaptive = MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
home: Material(
child: Slider.adaptive(
value: 0,
onChanged: (double newValue) {},
),
),
);
await tester.pumpWidget(sliderAdaptive);
await tester.pumpAndSettle();
final MaterialInkController material =
Material.of(tester.element(find.byType(CupertinoSlider)));
expect(
material,
paints
..rrect()
..rrect()
..rrect()
..rrect()
..rrect()
..rrect(color: CupertinoColors.white),
);
});
testWidgets('Slider.adaptive passes thumbColor to CupertinoSlider',
(WidgetTester tester) async {
const Color color = Color(0xffffc107);
final Widget sliderAdaptive = MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
home: Material(
child: Slider.adaptive(
value: 0,
onChanged: (double newValue) {},
thumbColor: color,
),
),
);
await tester.pumpWidget(sliderAdaptive);
await tester.pumpAndSettle();
final MaterialInkController material =
Material.of(tester.element(find.byType(CupertinoSlider)));
expect(
material,
paints..rrect()..rrect()..rrect()..rrect()..rrect()..rrect(color: color),
);
});
// Regression test for https://github.com/flutter/flutter/issues/103566
testWidgets('Drag gesture uses provided gesture settings', (WidgetTester tester) async {
double value = 0.5;
bool dragStarted = false;
final Key sliderKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: GestureDetector(
behavior: HitTestBehavior.deferToChild,
onHorizontalDragStart: (DragStartDetails details) {
dragStarted = true;
},
child: MediaQuery(
data: MediaQuery.of(context).copyWith(gestureSettings: const DeviceGestureSettings(touchSlop: 20)),
child: Slider(
value: value,
key: sliderKey,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
),
),
),
),
);
},
),
),
),
);
TestGesture drag = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump(kPressTimeout);
// Less than configured touch slop, more than default touch slop
await drag.moveBy(const Offset(19.0, 0));
await tester.pump();
expect(value, 0.5);
expect(dragStarted, true);
dragStarted = false;
await drag.up();
await tester.pumpAndSettle();
drag = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump(kPressTimeout);
bool sliderEnd = false;
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: GestureDetector(
behavior: HitTestBehavior.deferToChild,
onHorizontalDragStart: (DragStartDetails details) {
dragStarted = true;
},
child: MediaQuery(
data: MediaQuery.of(context).copyWith(gestureSettings: const DeviceGestureSettings(touchSlop: 10)),
child: Slider(
value: value,
key: sliderKey,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
onChangeEnd: (double endValue) {
sliderEnd = true;
},
),
),
),
),
);
},
),
),
),
);
// More than touch slop.
await drag.moveBy(const Offset(12.0, 0));
await drag.up();
await tester.pumpAndSettle();
expect(sliderEnd, true);
expect(dragStarted, false);
});
// Regression test for https://github.com/flutter/flutter/issues/139281
testWidgets('Slider does not request focus when the value is changed', (WidgetTester tester) async {
double value = 0.5;
await tester.pumpWidget(MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
}
);
}),
),
),
));
// Initially, the slider does not have focus whe enabled and not tapped.
await tester.pumpAndSettle();
expect(value, equals(0.5));
// Get FocusNode from the state of the slider to include auto-generated FocusNode.
// ignore: invalid_assignment
final FocusNode focusNode = (tester.firstState(find.byType(Slider)) as dynamic).focusNode;
// The slider does not have focus.
expect(focusNode.hasFocus, false);
final Offset sliderCenter = tester.getCenter(find.byType(Slider));
final Offset tapLocation = Offset(sliderCenter.dx + 50, sliderCenter.dy);
// Tap on the slider to change the value.
final TestGesture gesture = await tester.createGesture();
await gesture.addPointer();
await gesture.down(tapLocation);
await gesture.up();
await tester.pumpAndSettle();
expect(value, isNot(equals(0.5)));
// The slider does not have focus after the value is changed.
expect(focusNode.hasFocus, false);
});
// Regression test for https://github.com/flutter/flutter/issues/139281
testWidgets('Overlay remains when Slider thumb is interacted', (WidgetTester tester) async {
double value = 0.5;
const Color overlayColor = Color(0xffff0000);
await tester.pumpWidget(MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
overlayColor: const MaterialStatePropertyAll<Color?>(overlayColor),
onChanged: (double newValue) {
setState(() {
value = newValue;
});
}
);
}),
),
),
));
// Slider does not have overlay when enabled and not tapped.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
final Offset sliderCenter = tester.getCenter(find.byType(Slider));
// Tap and hold down on the thumb to keep it active.
final TestGesture gesture = await tester.createGesture();
await gesture.addPointer();
await gesture.down(sliderCenter);
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: overlayColor),
);
// Hover on the slider but outside the thumb.
await gesture.moveTo(tester.getTopLeft(find.byType(Slider)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: overlayColor),
);
// Tap up on the slider.
await gesture.up();
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
});
testWidgets('Overlay appear only when hovered on the thumb on desktop', (WidgetTester tester) async {
double value = 0.5;
const Color overlayColor = Color(0xffff0000);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
overlayColor: const MaterialStatePropertyAll<Color?>(overlayColor),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not hovered.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
// Hover on the slider but outside the thumb.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getTopLeft(find.byType(Slider)));
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
// Hover on the thumb.
await gesture.moveTo(tester.getCenter(find.byType(Slider)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: overlayColor),
);
// Hover on the slider but outside the thumb.
await gesture.moveTo(tester.getBottomRight(find.byType(Slider)));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
}, variant: TargetPlatformVariant.desktop());
testWidgets('Overlay remains when Slider is in focus on desktop', (WidgetTester tester) async {
double value = 0.5;
const Color overlayColor = Color(0xffff0000);
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
focusNode: focusNode,
overlayColor: const MaterialStatePropertyAll<Color?>(overlayColor),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not tapped.
await tester.pumpAndSettle();
expect(focusNode.hasFocus, false);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
final Offset sliderCenter = tester.getCenter(find.byType(Slider));
Offset tapLocation = Offset(sliderCenter.dx + 50, sliderCenter.dy);
// Tap somewhere to bring overlay.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.down(tapLocation);
await gesture.up();
focusNode.requestFocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, true);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: overlayColor),
);
tapLocation = Offset(sliderCenter.dx - 50, sliderCenter.dy);
await gesture.down(tapLocation);
await gesture.up();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, true);
// Overlay is removed when adjusted with a tap.
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: overlayColor)),
);
}, variant: TargetPlatformVariant.desktop());
// Regression test for https://github.com/flutter/flutter/issues/123313, which only occurs on desktop platforms.
testWidgets('Value indicator disappears after adjusting the slider on desktop', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
const double currentValue = 0.5;
await tester.pumpWidget(MaterialApp(
theme: theme,
home: Material(
child: Center(
child: Slider(
value: currentValue,
divisions: 5,
label: currentValue.toStringAsFixed(1),
onChanged: (_) {},
),
),
),
));
// Slider does not show value indicator initially.
await tester.pumpAndSettle();
RenderBox valueIndicatorBox = tester.renderObject(find.byType(Overlay));
expect(
valueIndicatorBox,
isNot(paints..scale()..path(color: theme.colorScheme.primary)),
);
final Offset sliderCenter = tester.getCenter(find.byType(Slider));
final Offset tapLocation = Offset(sliderCenter.dx + 50, sliderCenter.dy);
// Tap the slider by mouse to bring up the value indicator.
await tester.tapAt(tapLocation, kind: PointerDeviceKind.mouse);
await tester.pumpAndSettle();
// Value indicator is visible.
valueIndicatorBox = tester.renderObject(find.byType(Overlay));
expect(
valueIndicatorBox,
paints..scale()..path(color: theme.colorScheme.primary),
);
// Wait for the value indicator to disappear.
await tester.pumpAndSettle(const Duration(seconds: 2));
// Value indicator is no longer visible.
expect(
valueIndicatorBox,
isNot(paints..scale()..path(color: theme.colorScheme.primary)),
);
}, variant: TargetPlatformVariant.desktop());
testWidgets('Value indicator remains when Slider is in focus on desktop', (WidgetTester tester) async {
double value = 0.5;
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: ThemeData(
sliderTheme: const SliderThemeData(
showValueIndicator: ShowValueIndicator.always,
),
),
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
focusNode: focusNode,
divisions: 5,
label: value.toStringAsFixed(1),
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not show value indicator without focus.
await tester.pumpAndSettle();
expect(focusNode.hasFocus, false);
RenderBox valueIndicatorBox = tester.renderObject(find.byType(Overlay));
expect(
valueIndicatorBox,
isNot(paints..path(color: const Color(0xff000000))..paragraph()),
);
final Offset sliderCenter = tester.getCenter(find.byType(Slider));
final Offset tapLocation = Offset(sliderCenter.dx + 50, sliderCenter.dy);
// Tap somewhere to bring value indicator.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.down(tapLocation);
await gesture.up();
focusNode.requestFocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, true);
valueIndicatorBox = tester.renderObject(find.byType(Overlay));
expect(
valueIndicatorBox,
paints..path(color: const Color(0xff000000))..paragraph(),
);
focusNode.unfocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, false);
expect(
valueIndicatorBox,
isNot(paints..path(color: const Color(0xff000000))..paragraph()),
);
}, variant: TargetPlatformVariant.desktop());
testWidgets('Event on Slider should perform no-op if already unmounted', (WidgetTester tester) async {
// Test covering crashing found in Google internal issue b/192329942.
double value = 0.0;
final ValueNotifier<bool> shouldShowSliderListenable =
ValueNotifier<bool>(true);
addTearDown(shouldShowSliderListenable.dispose);
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Center(
child: ValueListenableBuilder<bool>(
valueListenable: shouldShowSliderListenable,
builder: (BuildContext context, bool shouldShowSlider, _) {
return GestureDetector(
behavior: HitTestBehavior.translucent,
// Note: it is important that `onTap` is non-null so
// [GestureDetector] will register tap events.
onTap: () {},
child: shouldShowSlider
? Slider(
value: value,
onChanged: (double newValue) {
setState(() {
value = newValue;
});
},
)
: const SizedBox.expand(),
);
},
),
),
);
},
),
),
),
);
// Move Slider.
final TestGesture gesture = await tester
.startGesture(tester.getRect(find.byType(Slider)).center);
await gesture.moveBy(const Offset(1.0, 0.0));
await tester.pumpAndSettle();
// Hide Slider. Slider will dispose and unmount.
shouldShowSliderListenable.value = false;
await tester.pumpAndSettle();
// Move Slider after unmounted.
await gesture.moveBy(const Offset(1.0, 0.0));
await tester.pumpAndSettle();
expect(tester.takeException(), null);
});
group('Material 2', () {
// These tests are only relevant for Material 2. Once Material 2
// support is deprecated and the APIs are removed, these tests
// can be deleted.
testWidgets('Slider can be hovered and has correct hover color', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final ThemeData theme = ThemeData(useMaterial3: false);
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not hovered.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.12))),
);
// Start hovering.
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Slider)));
// Slider has overlay when enabled and hovered.
await tester.pumpWidget(buildApp());
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.12)),
);
// Slider does not have an overlay when disabled and hovered.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.12))),
);
});
testWidgets('Material2 - Slider is focusable and has correct focus color', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Slider');
addTearDown(focusNode.dispose);
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
final ThemeData theme = ThemeData(useMaterial3: false);
double value = 0.5;
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
value: value,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
autofocus: true,
focusNode: focusNode,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Check that the overlay shows when focused.
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isTrue);
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.12)),
);
// Check that the overlay does not show when unfocused and disabled.
await tester.pumpWidget(buildApp(enabled: false));
await tester.pumpAndSettle();
expect(focusNode.hasPrimaryFocus, isFalse);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.12))),
);
});
testWidgets('Material2 - Slider is draggable and has correct dragged color', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
double value = 0.5;
final ThemeData theme = ThemeData(useMaterial3: false);
final Key sliderKey = UniqueKey();
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
Widget buildApp({bool enabled = true}) {
return MaterialApp(
theme: theme,
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Slider(
key: sliderKey,
value: value,
focusNode: focusNode,
onChanged: enabled
? (double newValue) {
setState(() {
value = newValue;
});
}
: null,
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
// Slider does not have overlay when enabled and not dragged.
await tester.pumpAndSettle();
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.12))),
);
// Start dragging.
final TestGesture drag = await tester.startGesture(tester.getCenter(find.byKey(sliderKey)));
await tester.pump(kPressTimeout);
// Less than configured touch slop, more than default touch slop
await drag.moveBy(const Offset(19.0, 0));
await tester.pump();
// Slider has overlay when enabled and dragged.
expect(
Material.of(tester.element(find.byType(Slider))),
paints..circle(color: theme.colorScheme.primary.withOpacity(0.12)),
);
await drag.up();
await tester.pumpAndSettle();
// Slider without focus doesn't have overlay when enabled and dragged.
expect(focusNode.hasFocus, false);
expect(
Material.of(tester.element(find.byType(Slider))),
isNot(paints..circle(color: theme.colorScheme.primary.withOpacity(0.12))),
);
});
});
group('Slider.allowedInteraction', () {
testWidgets('SliderInteraction.tapOnly', (WidgetTester tester) async {
double value = 1.0;
final Key sliderKey = UniqueKey();
// (slider's left padding (overlayRadius), windowHeight / 2)
const Offset startOfTheSliderTrack = Offset(24, 300);
const Offset centerOfTheSlideTrack = Offset(400, 300);
final List<String> logs = <String>[];
Widget buildWidget() => MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext _, StateSetter setState) {
return Slider(
value: value,
key: sliderKey,
allowedInteraction: SliderInteraction.tapOnly,
onChangeStart: (double newValue) {
logs.add('onChangeStart');
},
onChanged: (double newValue) {
logs.add('onChanged');
setState(() {
value = newValue;
});
},
onChangeEnd: (double newValue) {
logs.add('onChangeEnd');
},
);
}),
),
),
);
// allow tap only
await tester.pumpWidget(buildWidget());
expect(logs, isEmpty);
// test tap
final TestGesture gesture = await tester.startGesture(centerOfTheSlideTrack);
await tester.pump();
// changes from 1.0 -> 0.5
expect(value, 0.5);
expect(logs, <String>['onChangeStart', 'onChanged']);
// test slide
await gesture.moveTo(startOfTheSliderTrack);
await tester.pump();
// has no effect, remains 0.5
expect(value, 0.5);
expect(logs, <String>['onChangeStart', 'onChanged']);
await gesture.up();
await tester.pump();
expect(logs, <String>['onChangeStart', 'onChanged', 'onChangeEnd']);
});
testWidgets('SliderInteraction.tapAndSlide (default)', (WidgetTester tester) async {
double value = 1.0;
final Key sliderKey = UniqueKey();
// (slider's left padding (overlayRadius), windowHeight / 2)
const Offset startOfTheSliderTrack = Offset(24, 300);
const Offset centerOfTheSlideTrack = Offset(400, 300);
const Offset endOfTheSliderTrack = Offset(800 - 24, 300);
final List<String> logs = <String>[];
Widget buildWidget() => MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext _, StateSetter setState) {
return Slider(
value: value,
key: sliderKey,
onChangeStart: (double newValue) {
logs.add('onChangeStart');
},
onChanged: (double newValue) {
logs.add('onChanged');
setState(() {
value = newValue;
});
},
onChangeEnd: (double newValue) {
logs.add('onChangeEnd');
},
);
}),
),
),
);
await tester.pumpWidget(buildWidget());
expect(logs, isEmpty);
// Test tap.
final TestGesture gesture = await tester.startGesture(centerOfTheSlideTrack);
await tester.pump();
// changes from 1.0 -> 0.5
expect(value, 0.5);
expect(logs, <String>['onChangeStart', 'onChanged']);
// test slide
await gesture.moveTo(startOfTheSliderTrack);
await tester.pump();
// changes from 0.5 -> 0.0
expect(value, 0.0);
await gesture.moveTo(endOfTheSliderTrack);
await tester.pump();
// changes from 0.0 -> 1.0
expect(value, 1.0);
expect(logs, <String>['onChangeStart', 'onChanged', 'onChanged', 'onChanged']);
await gesture.up();
await tester.pump();
expect(logs, <String>['onChangeStart', 'onChanged', 'onChanged', 'onChanged', 'onChangeEnd']);
});
testWidgets('SliderInteraction.slideOnly', (WidgetTester tester) async {
double value = 1.0;
final Key sliderKey = UniqueKey();
// (slider's left padding (overlayRadius), windowHeight / 2)
const Offset startOfTheSliderTrack = Offset(24, 300);
const Offset centerOfTheSlideTrack = Offset(400, 300);
const Offset endOfTheSliderTrack = Offset(800 - 24, 300);
final List<String> logs = <String>[];
Widget buildApp() {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext _, StateSetter setState) {
return Slider(
value: value,
key: sliderKey,
allowedInteraction: SliderInteraction.slideOnly,
onChangeStart: (double newValue) {
logs.add('onChangeStart');
},
onChanged: (double newValue) {
logs.add('onChanged');
setState(() {
value = newValue;
});
},
onChangeEnd: (double newValue) {
logs.add('onChangeEnd');
},
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
expect(logs, isEmpty);
// test tap
final TestGesture gesture = await tester.startGesture(centerOfTheSlideTrack);
await tester.pump();
// has no effect as tap is disabled, remains 1.0
expect(value, 1.0);
expect(logs, <String>['onChangeStart']);
// test slide
await gesture.moveTo(startOfTheSliderTrack);
await tester.pump();
// changes from 1.0 -> 0.5
expect(value, 0.5);
await gesture.moveTo(endOfTheSliderTrack);
await tester.pump();
// changes from 0.0 -> 1.0
expect(value, 1.0);
expect(logs, <String>['onChangeStart', 'onChanged', 'onChanged']);
await gesture.up();
await tester.pump();
expect(logs, <String>['onChangeStart', 'onChanged', 'onChanged', 'onChangeEnd']);
});
testWidgets('SliderInteraction.slideThumb', (WidgetTester tester) async {
double value = 1.0;
final Key sliderKey = UniqueKey();
// (slider's left padding (overlayRadius), windowHeight / 2)
const Offset startOfTheSliderTrack = Offset(24, 300);
const Offset centerOfTheSliderTrack = Offset(400, 300);
const Offset endOfTheSliderTrack = Offset(800 - 24, 300);
final List<String> logs = <String>[];
Widget buildApp() {
return MaterialApp(
home: Material(
child: Center(
child: StatefulBuilder(builder: (BuildContext _, StateSetter setState) {
return Slider(
value: value,
key: sliderKey,
allowedInteraction: SliderInteraction.slideThumb,
onChangeStart: (double newValue) {
logs.add('onChangeStart');
},
onChanged: (double newValue) {
logs.add('onChanged');
setState(() {
value = newValue;
});
},
onChangeEnd: (double newValue) {
logs.add('onChangeEnd');
},
);
}),
),
),
);
}
await tester.pumpWidget(buildApp());
expect(logs, isEmpty);
// test tap
final TestGesture gesture = await tester.startGesture(centerOfTheSliderTrack);
await tester.pump();
// has no effect, remains 1.0
expect(value, 1.0);
expect(logs, isEmpty);
// test slide
await gesture.moveTo(startOfTheSliderTrack);
await tester.pump();
// has no effect, remains 1.0
expect(value, 1.0);
expect(logs, isEmpty);
// test slide thumb
await gesture.up();
await gesture.down(endOfTheSliderTrack); // where the thumb is
await tester.pump();
// has no effect, remains 1.0
expect(value, 1.0);
expect(logs, <String>['onChangeStart']);
await gesture.moveTo(centerOfTheSliderTrack);
await tester.pump();
// changes from 1.0 -> 0.5
expect(value, 0.5);
expect(logs, <String>['onChangeStart', 'onChanged']);
// test tap inside overlay but not on thumb, then slide
await gesture.up();
// default overlay radius is 12, so 10 is inside the overlay
await gesture.down(centerOfTheSliderTrack.translate(-10, 0));
await tester.pump();
// changes from 1.0 -> 0.5
expect(value, 0.5);
expect(logs, <String>['onChangeStart', 'onChanged', 'onChangeEnd', 'onChangeStart']);
await gesture.moveTo(endOfTheSliderTrack.translate(-10, 0));
await tester.pump();
// changes from 0.5 -> 1.0
expect(value, 1.0);
expect(logs, <String>['onChangeStart', 'onChanged', 'onChangeEnd', 'onChangeStart', 'onChanged']);
await gesture.up();
await tester.pump();
expect(
logs,
<String>['onChangeStart', 'onChanged', 'onChangeEnd', 'onChangeStart', 'onChanged', 'onChangeEnd'],
);
});
});
// This is a regression test for https://github.com/flutter/flutter/issues/143524.
testWidgets('Discrete Slider.onChanged is called only once', (WidgetTester tester) async {
int onChangeCallbackCount = 0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: Slider(
max: 5,
divisions: 5,
value: 0,
onChanged: (double newValue) {
onChangeCallbackCount++;
},
),
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getTopLeft(find.byType(Slider)));
await tester.pump(kLongPressTimeout);
await gesture.moveBy(const Offset(160.0, 0.0));
await gesture.moveBy(const Offset(1.0, 0.0));
await gesture.moveBy(const Offset(1.0, 0.0));
expect(onChangeCallbackCount, 1);
});
}
| flutter/packages/flutter/test/material/slider_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/slider_test.dart",
"repo_id": "flutter",
"token_count": 69999
} | 721 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('TextButtonTheme lerp special cases', () {
expect(TextButtonThemeData.lerp(null, null, 0), null);
const TextButtonThemeData data = TextButtonThemeData();
expect(identical(TextButtonThemeData.lerp(data, data, 0.5), data), true);
});
testWidgets('Material3: Passing no TextButtonTheme returns defaults', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(useMaterial3: true, colorScheme: colorScheme),
home: Scaffold(
body: Center(
child: TextButton(
onPressed: () { },
child: const Text('button'),
),
),
),
),
);
final Finder buttonMaterial = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderRadius, null);
expect(material.color, Colors.transparent);
expect(material.elevation, 0.0);
expect(material.shadowColor, Colors.transparent);
expect(material.shape, const StadiumBorder());
expect(material.textStyle!.color, colorScheme.primary);
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center);
});
testWidgets('Material2: Passing no TextButtonTheme returns defaults', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(useMaterial3: false, colorScheme: colorScheme),
home: Scaffold(
body: Center(
child: TextButton(
onPressed: () { },
child: const Text('button'),
),
),
),
),
);
final Finder buttonMaterial = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderRadius, null);
expect(material.color, Colors.transparent);
expect(material.elevation, 0.0);
expect(material.shadowColor, const Color(0xff000000));
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))));
expect(material.textStyle!.color, colorScheme.primary);
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center);
});
group('[Theme, TextTheme, TextButton style overrides]', () {
const Color foregroundColor = Color(0xff000001);
const Color backgroundColor = Color(0xff000002);
const Color disabledColor = Color(0xff000003);
const Color shadowColor = Color(0xff000004);
const double elevation = 3;
const TextStyle textStyle = TextStyle(fontSize: 12.0);
const EdgeInsets padding = EdgeInsets.all(3);
const Size minimumSize = Size(200, 200);
const BorderSide side = BorderSide(color: Colors.green, width: 2);
const OutlinedBorder shape = RoundedRectangleBorder(side: side, borderRadius: BorderRadius.all(Radius.circular(2)));
const MouseCursor enabledMouseCursor = SystemMouseCursors.text;
const MouseCursor disabledMouseCursor = SystemMouseCursors.grab;
const MaterialTapTargetSize tapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Duration animationDuration = Duration(milliseconds: 25);
const bool enableFeedback = false;
const AlignmentGeometry alignment = Alignment.centerLeft;
final Key backgroundKey = UniqueKey();
final Key foregroundKey = UniqueKey();
Widget backgroundBuilder(BuildContext context, Set<MaterialState> states, Widget? child) {
return KeyedSubtree(key: backgroundKey, child: child!);
}
Widget foregroundBuilder(BuildContext context, Set<MaterialState> states, Widget? child) {
return KeyedSubtree(key: foregroundKey, child: child!);
}
final ButtonStyle style = TextButton.styleFrom(
foregroundColor: foregroundColor,
disabledForegroundColor: disabledColor,
backgroundColor: backgroundColor,
disabledBackgroundColor: disabledColor,
shadowColor: shadowColor,
elevation: elevation,
textStyle: textStyle,
padding: padding,
minimumSize: minimumSize,
side: side,
shape: shape,
enabledMouseCursor: enabledMouseCursor,
disabledMouseCursor: disabledMouseCursor,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
backgroundBuilder: backgroundBuilder,
foregroundBuilder: foregroundBuilder,
);
Widget buildFrame({ ButtonStyle? buttonStyle, ButtonStyle? themeStyle, ButtonStyle? overallStyle }) {
final Widget child = Builder(
builder: (BuildContext context) {
return TextButton(
style: buttonStyle,
onPressed: () { },
child: const Text('button'),
);
},
);
return MaterialApp(
theme: ThemeData.from(colorScheme: const ColorScheme.light()).copyWith(
textButtonTheme: TextButtonThemeData(style: overallStyle),
),
home: Scaffold(
body: Center(
// If the TextButtonTheme widget is present, it's used
// instead of the Theme's ThemeData.textButtonTheme.
child: themeStyle == null ? child : TextButtonTheme(
data: TextButtonThemeData(style: themeStyle),
child: child,
),
),
),
);
}
final Finder findMaterial = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
);
final Finder findInkWell = find.descendant(
of: find.byType(TextButton),
matching: find.byType(InkWell),
);
const Set<MaterialState> enabled = <MaterialState>{};
const Set<MaterialState> disabled = <MaterialState>{ MaterialState.disabled };
const Set<MaterialState> hovered = <MaterialState>{ MaterialState.hovered };
const Set<MaterialState> focused = <MaterialState>{ MaterialState.focused };
void checkButton(WidgetTester tester) {
final Material material = tester.widget<Material>(findMaterial);
final InkWell inkWell = tester.widget<InkWell>(findInkWell);
expect(material.textStyle!.color, foregroundColor);
expect(material.textStyle!.fontSize, 12);
expect(material.color, backgroundColor);
expect(material.shadowColor, shadowColor);
expect(material.elevation, elevation);
expect(MaterialStateProperty.resolveAs<MouseCursor?>(inkWell.mouseCursor, enabled), enabledMouseCursor);
expect(MaterialStateProperty.resolveAs<MouseCursor?>(inkWell.mouseCursor, disabled), disabledMouseCursor);
expect(inkWell.overlayColor!.resolve(hovered), foregroundColor.withOpacity(0.08));
expect(inkWell.overlayColor!.resolve(focused), foregroundColor.withOpacity(0.1));
expect(inkWell.enableFeedback, enableFeedback);
expect(material.borderRadius, null);
expect(material.shape, shape);
expect(material.animationDuration, animationDuration);
expect(tester.getSize(find.byType(TextButton)), const Size(200, 200));
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, alignment);
expect(find.descendant(of: findMaterial, matching: find.byKey(backgroundKey)), findsOneWidget);
expect(find.descendant(of: findInkWell, matching: find.byKey(foregroundKey)), findsOneWidget);
}
testWidgets('Button style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(themeStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(overallStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
// Same as the previous tests with empty ButtonStyle's instead of null.
testWidgets('Button style overrides defaults, empty theme and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style, themeStyle: const ButtonStyle(), overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults, empty button and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), themeStyle: style, overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults, null theme and empty overall style', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), overallStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
});
testWidgets('Material3: Theme shadowColor', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Color shadowColor = Color(0xff000001);
const Color overriddenColor = Color(0xff000002);
Widget buildFrame({ Color? overallShadowColor, Color? themeShadowColor, Color? shadowColor }) {
return MaterialApp(
theme: ThemeData.from(
useMaterial3: true,
colorScheme: colorScheme.copyWith(shadow: overallShadowColor),
),
home: Scaffold(
body: Center(
child: TextButtonTheme(
data: TextButtonThemeData(
style: TextButton.styleFrom(
shadowColor: themeShadowColor,
),
),
child: Builder(
builder: (BuildContext context) {
return TextButton(
style: TextButton.styleFrom(
shadowColor: shadowColor,
),
onPressed: () { },
child: const Text('button'),
);
},
),
),
),
),
);
}
final Finder buttonMaterialFinder = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
);
await tester.pumpWidget(buildFrame());
Material material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.transparent);
await tester.pumpWidget(buildFrame(overallShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.transparent);
await tester.pumpWidget(buildFrame(themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(overallShadowColor: overriddenColor, themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: overriddenColor, shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
});
testWidgets('Material2: Theme shadowColor', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Color shadowColor = Color(0xff000001);
const Color overriddenColor = Color(0xff000002);
Widget buildFrame({ Color? overallShadowColor, Color? themeShadowColor, Color? shadowColor }) {
return MaterialApp(
theme: ThemeData.from(useMaterial3: false, colorScheme: colorScheme).copyWith(
shadowColor: overallShadowColor,
),
home: Scaffold(
body: Center(
child: TextButtonTheme(
data: TextButtonThemeData(
style: TextButton.styleFrom(
shadowColor: themeShadowColor,
),
),
child: Builder(
builder: (BuildContext context) {
return TextButton(
style: TextButton.styleFrom(
shadowColor: shadowColor,
),
onPressed: () { },
child: const Text('button'),
);
},
),
),
),
),
);
}
final Finder buttonMaterialFinder = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
);
await tester.pumpWidget(buildFrame());
Material material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.black); //default
await tester.pumpWidget(buildFrame(overallShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(overallShadowColor: overriddenColor, themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: overriddenColor, shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
});
}
| flutter/packages/flutter/test/material/text_button_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/text_button_theme_test.dart",
"repo_id": "flutter",
"token_count": 5822
} | 722 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('!chrome')
library;
import 'dart:math' as math;
import 'dart:ui';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
import 'feedback_tester.dart';
void main() {
const String okString = 'OK';
const String amString = 'AM';
const String pmString = 'PM';
Material getMaterialFromDialog(WidgetTester tester) {
return tester.widget<Material>(find.descendant(of: find.byType(Dialog), matching: find.byType(Material)).first);
}
testWidgets('Material2 - Dialog size - dial mode', (WidgetTester tester) async {
addTearDown(tester.view.reset);
const Size timePickerPortraitSize = Size(310, 468);
const Size timePickerLandscapeSize = Size(524, 342);
const Size timePickerLandscapeSizeM2 = Size(508, 300);
const EdgeInsets padding = EdgeInsets.fromLTRB(8, 18, 8, 8);
double width;
double height;
// portrait
tester.view.physicalSize = const Size(800, 800.5);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(tester, materialType: MaterialType.material2);
width = timePickerPortraitSize.width + padding.horizontal;
height = timePickerPortraitSize.height + padding.vertical;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
// landscape
tester.view.physicalSize = const Size(800.5, 800);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
materialType: MaterialType.material2,
);
width = timePickerLandscapeSize.width + padding.horizontal;
height = timePickerLandscapeSizeM2.height + padding.vertical;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
});
testWidgets('Material2 - Dialog size - input mode', (WidgetTester tester) async {
const TimePickerEntryMode entryMode = TimePickerEntryMode.input;
const Size timePickerInputSize = Size(312, 216);
const Size dayPeriodPortraitSize = Size(52, 80);
const EdgeInsets padding = EdgeInsets.fromLTRB(8, 18, 8, 8);
final double height = timePickerInputSize.height + padding.vertical;
double width;
await mediaQueryBoilerplate(
tester,
entryMode: entryMode,
materialType: MaterialType.material2,
);
width = timePickerInputSize.width + padding.horizontal;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: entryMode,
materialType: MaterialType.material2,
);
width = timePickerInputSize.width - dayPeriodPortraitSize.width - 12 + padding.horizontal + 16;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
});
testWidgets('Material2 - respects MediaQueryData.alwaysUse24HourFormat == true', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, materialType: MaterialType.material2);
final List<String> labels00To22 = List<String>.generate(12, (int index) {
return (index * 2).toString().padLeft(2, '0');
});
final CustomPaint dialPaint = tester.widget(findDialPaint);
final dynamic dialPainter = dialPaint.painter;
// ignore: avoid_dynamic_calls
final List<dynamic> primaryLabels = dialPainter.primaryLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(primaryLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels00To22);
// ignore: avoid_dynamic_calls
final List<dynamic> selectedLabels = dialPainter.selectedLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(selectedLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels00To22);
});
testWidgets('Material3 - Dialog size - dial mode', (WidgetTester tester) async {
addTearDown(tester.view.reset);
const Size timePickerPortraitSize = Size(310, 468);
const Size timePickerLandscapeSize = Size(524, 342);
const EdgeInsets padding = EdgeInsets.all(24.0);
double width;
double height;
// portrait
tester.view.physicalSize = const Size(800, 800.5);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(tester, materialType: MaterialType.material3);
width = timePickerPortraitSize.width + padding.horizontal;
height = timePickerPortraitSize.height + padding.vertical;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
// landscape
tester.view.physicalSize = const Size(800.5, 800);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
materialType: MaterialType.material3,
);
width = timePickerLandscapeSize.width + padding.horizontal;
height = timePickerLandscapeSize.height + padding.vertical;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
});
testWidgets('Material3 - Dialog size - input mode', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
const TimePickerEntryMode entryMode = TimePickerEntryMode.input;
const double textScaleFactor = 1.0;
const Size timePickerMinInputSize = Size(312, 216);
const Size dayPeriodPortraitSize = Size(52, 80);
const EdgeInsets padding = EdgeInsets.all(24.0);
final double height = timePickerMinInputSize.height * textScaleFactor + padding.vertical;
double width;
await mediaQueryBoilerplate(
tester,
entryMode: entryMode,
materialType: MaterialType.material3,
);
width = timePickerMinInputSize.width - (theme.useMaterial3 ? 32 : 0) + padding.horizontal;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: entryMode,
materialType: MaterialType.material3,
);
width = timePickerMinInputSize.width - dayPeriodPortraitSize.width - 12 + padding.horizontal;
expect(
tester.getSize(find.byWidget(getMaterialFromDialog(tester))),
Size(width, height),
);
});
testWidgets('Material3 - respects MediaQueryData.alwaysUse24HourFormat == true', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, materialType: MaterialType.material3);
final List<String> labels00To23 = List<String>.generate(24, (int index) {
return index == 0 ? '00' : index.toString();
});
final List<bool> inner0To23 = List<bool>.generate(24, (int index) => index >= 12);
final CustomPaint dialPaint = tester.widget(findDialPaint);
final dynamic dialPainter = dialPaint.painter;
// ignore: avoid_dynamic_calls
final List<dynamic> primaryLabels = dialPainter.primaryLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(primaryLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels00To23);
// ignore: avoid_dynamic_calls
expect(primaryLabels.map<bool>((dynamic tp) => tp.inner as bool), inner0To23);
// ignore: avoid_dynamic_calls
final List<dynamic> selectedLabels = dialPainter.selectedLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(selectedLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels00To23);
// ignore: avoid_dynamic_calls
expect(selectedLabels.map<bool>((dynamic tp) => tp.inner as bool), inner0To23);
});
testWidgets('Material3 - Dial background uses correct default color', (WidgetTester tester) async {
ThemeData theme = ThemeData(useMaterial3: true);
Widget buildTimePicker(ThemeData themeData) {
return MaterialApp(
theme: themeData,
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
);
},
);
},
),
),
),
);
}
await tester.pumpWidget(buildTimePicker(theme));
// Open the time picker dialog.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
// Test default dial background color.
RenderBox dial = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(
dial,
paints
..circle(color: theme.colorScheme.surfaceContainerHighest) // Dial background color.
..circle(color: Color(theme.colorScheme.primary.value)), // Dial hand color.
);
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
// Test dial background color when theme color scheme is changed.
theme = theme.copyWith(
colorScheme: theme.colorScheme.copyWith(
surfaceVariant: const Color(0xffff0000),
),
);
await tester.pumpWidget(buildTimePicker(theme));
// Open the time picker dialog.
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
dial = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(
dial,
paints
..circle(color: theme.colorScheme.surfaceContainerHighest) // Dial background color.
..circle(color: Color(theme.colorScheme.primary.value)), // Dial hand color.
);
});
for (final MaterialType materialType in MaterialType.values) {
group('Dial (${materialType.name})', () {
testWidgets('tap-select an hour', (WidgetTester tester) async {
TimeOfDay? result;
Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time;
}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy - 50)); // 12:00 AM
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 0, minute: 0)));
center = (await startPicker(tester, (TimeOfDay? time) {
result = time;
}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx + 50, center.dy));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 3, minute: 0)));
center = (await startPicker(tester, (TimeOfDay? time) {
result = time;
}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy + 50));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 0)));
center = (await startPicker(tester, (TimeOfDay? time) {
result = time;
}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy + 50));
await tester.tapAt(Offset(center.dx - 50, center.dy));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 9, minute: 0)));
});
testWidgets('drag-select an hour', (WidgetTester tester) async {
late TimeOfDay result;
final Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType))!;
final Offset hour0 = Offset(center.dx, center.dy - 50); // 12:00 AM
final Offset hour3 = Offset(center.dx + 50, center.dy);
final Offset hour6 = Offset(center.dx, center.dy + 50);
final Offset hour9 = Offset(center.dx - 50, center.dy);
TestGesture gesture;
gesture = await tester.startGesture(hour3);
await gesture.moveBy(hour0 - hour3);
await gesture.up();
await finishPicker(tester);
expect(result.hour, 0);
expect(
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType),
equals(center),
);
gesture = await tester.startGesture(hour0);
await gesture.moveBy(hour3 - hour0);
await gesture.up();
await finishPicker(tester);
expect(result.hour, 3);
expect(
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType),
equals(center),
);
gesture = await tester.startGesture(hour3);
await gesture.moveBy(hour6 - hour3);
await gesture.up();
await finishPicker(tester);
expect(result.hour, equals(6));
expect(
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType),
equals(center),
);
gesture = await tester.startGesture(hour6);
await gesture.moveBy(hour9 - hour6);
await gesture.up();
await finishPicker(tester);
expect(result.hour, equals(9));
});
testWidgets('tap-select switches from hour to minute', (WidgetTester tester) async {
late TimeOfDay result;
final Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType))!;
final Offset hour6 = Offset(center.dx, center.dy + 50); // 6:00
final Offset min45 = Offset(center.dx - 50, center.dy); // 45 mins (or 9:00 hours)
await tester.tapAt(hour6);
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(min45);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 45)));
});
testWidgets('drag-select switches from hour to minute', (WidgetTester tester) async {
late TimeOfDay result;
final Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType))!;
final Offset hour3 = Offset(center.dx + 50, center.dy);
final Offset hour6 = Offset(center.dx, center.dy + 50);
final Offset hour9 = Offset(center.dx - 50, center.dy);
TestGesture gesture = await tester.startGesture(hour6);
await gesture.moveBy(hour9 - hour6);
await gesture.up();
await tester.pump(const Duration(milliseconds: 50));
gesture = await tester.startGesture(hour6);
await gesture.moveBy(hour3 - hour6);
await gesture.up();
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 9, minute: 15)));
});
testWidgets('tap-select rounds down to nearest 5 minute increment', (WidgetTester tester) async {
late TimeOfDay result;
final Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType))!;
final Offset hour6 = Offset(center.dx, center.dy + 50); // 6:00
final Offset min46 = Offset(center.dx - 50, center.dy - 5); // 46 mins
await tester.tapAt(hour6);
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(min46);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 45)));
});
testWidgets('tap-select rounds up to nearest 5 minute increment', (WidgetTester tester) async {
late TimeOfDay result;
final Offset center = (await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, materialType: materialType))!;
final Offset hour6 = Offset(center.dx, center.dy + 50); // 6:00
final Offset min48 = Offset(center.dx - 50, center.dy - 15); // 48 mins
await tester.tapAt(hour6);
await tester.pump(const Duration(milliseconds: 50));
await tester.tapAt(min48);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 50)));
});
});
group('Dial Haptic Feedback (${materialType.name})', () {
const Duration kFastFeedbackInterval = Duration(milliseconds: 10);
const Duration kSlowFeedbackInterval = Duration(milliseconds: 200);
late FeedbackTester feedback;
setUp(() {
feedback = FeedbackTester();
});
tearDown(() {
feedback.dispose();
});
testWidgets('tap-select vibrates once', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy - 50));
await finishPicker(tester);
expect(feedback.hapticCount, 1);
});
testWidgets('quick successive tap-selects vibrate once', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy - 50));
await tester.pump(kFastFeedbackInterval);
await tester.tapAt(Offset(center.dx, center.dy + 50));
await finishPicker(tester);
expect(feedback.hapticCount, 1);
});
testWidgets('slow successive tap-selects vibrate once per tap', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
await tester.tapAt(Offset(center.dx, center.dy - 50));
await tester.pump(kSlowFeedbackInterval);
await tester.tapAt(Offset(center.dx, center.dy + 50));
await tester.pump(kSlowFeedbackInterval);
await tester.tapAt(Offset(center.dx, center.dy - 50));
await finishPicker(tester);
expect(feedback.hapticCount, 3);
});
testWidgets('drag-select vibrates once', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
final Offset hour0 = Offset(center.dx, center.dy - 50);
final Offset hour3 = Offset(center.dx + 50, center.dy);
final TestGesture gesture = await tester.startGesture(hour3);
await gesture.moveBy(hour0 - hour3);
await gesture.up();
await finishPicker(tester);
expect(feedback.hapticCount, 1);
});
testWidgets('quick drag-select vibrates once', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
final Offset hour0 = Offset(center.dx, center.dy - 50);
final Offset hour3 = Offset(center.dx + 50, center.dy);
final TestGesture gesture = await tester.startGesture(hour3);
await gesture.moveBy(hour0 - hour3);
await tester.pump(kFastFeedbackInterval);
await gesture.moveBy(hour3 - hour0);
await tester.pump(kFastFeedbackInterval);
await gesture.moveBy(hour0 - hour3);
await gesture.up();
await finishPicker(tester);
expect(feedback.hapticCount, 1);
});
testWidgets('slow drag-select vibrates once', (WidgetTester tester) async {
final Offset center = (await startPicker(tester, (TimeOfDay? time) {}, materialType: materialType))!;
final Offset hour0 = Offset(center.dx, center.dy - 50);
final Offset hour3 = Offset(center.dx + 50, center.dy);
final TestGesture gesture = await tester.startGesture(hour3);
await gesture.moveBy(hour0 - hour3);
await tester.pump(kSlowFeedbackInterval);
await gesture.moveBy(hour3 - hour0);
await tester.pump(kSlowFeedbackInterval);
await gesture.moveBy(hour0 - hour3);
await gesture.up();
await finishPicker(tester);
expect(feedback.hapticCount, 3);
});
});
group('Dialog (${materialType.name})', () {
testWidgets('Material2 - Widgets have correct label capitalization', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {}, materialType: MaterialType.material2);
expect(find.text('SELECT TIME'), findsOneWidget);
expect(find.text('CANCEL'), findsOneWidget);
});
testWidgets('Material3 - Widgets have correct label capitalization', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {}, materialType: MaterialType.material3);
expect(find.text('Select time'), findsOneWidget);
expect(find.text('Cancel'), findsOneWidget);
});
testWidgets('Material2 - Widgets have correct label capitalization in input mode', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {},
entryMode: TimePickerEntryMode.input, materialType: MaterialType.material2
);
expect(find.text('ENTER TIME'), findsOneWidget);
expect(find.text('CANCEL'), findsOneWidget);
});
testWidgets('Material3 - Widgets have correct label capitalization in input mode', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {},
entryMode: TimePickerEntryMode.input, materialType: MaterialType.material3
);
expect(find.text('Enter time'), findsOneWidget);
expect(find.text('Cancel'), findsOneWidget);
});
testWidgets('respects MediaQueryData.alwaysUse24HourFormat == false', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, materialType: materialType);
const List<String> labels12To11 = <String>['12', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10', '11'];
final CustomPaint dialPaint = tester.widget(findDialPaint);
final dynamic dialPainter = dialPaint.painter;
// ignore: avoid_dynamic_calls
final List<dynamic> primaryLabels = dialPainter.primaryLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(primaryLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels12To11);
// ignore: avoid_dynamic_calls
final List<dynamic> selectedLabels = dialPainter.selectedLabels as List<dynamic>;
// ignore: avoid_dynamic_calls
expect(selectedLabels.map<String>((dynamic tp) => tp.painter.text.text as String), labels12To11);
});
testWidgets('when change orientation, should reflect in render objects', (WidgetTester tester) async {
addTearDown(tester.view.reset);
// portrait
tester.view.physicalSize = const Size(800, 800.5);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(tester, materialType: materialType);
RenderObject render = tester.renderObject(
find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DayPeriodInputPadding'),
);
expect((render as dynamic).orientation, Orientation.portrait); // ignore: avoid_dynamic_calls
// landscape
tester.view.physicalSize = const Size(800.5, 800);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(tester, tapButton: false, materialType: materialType);
render = tester.renderObject(
find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DayPeriodInputPadding'),
);
expect((render as dynamic).orientation, Orientation.landscape);
});
testWidgets('setting orientation should override MediaQuery orientation', (WidgetTester tester) async {
addTearDown(tester.view.reset);
// portrait media query
tester.view.physicalSize = const Size(800, 800.5);
tester.view.devicePixelRatio = 1;
await mediaQueryBoilerplate(tester, orientation: Orientation.landscape, materialType: materialType);
final RenderObject render = tester.renderObject(
find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DayPeriodInputPadding'),
);
expect((render as dynamic).orientation, Orientation.landscape);
});
testWidgets('builder parameter', (WidgetTester tester) async {
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child!,
);
},
);
},
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
final double ltrOkRight = tester.getBottomRight(find.text(okString)).dx;
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
// Verify that the time picker is being laid out RTL.
// We expect the left edge of the 'OK' button in the RTL
// layout to match the gap between right edge of the 'OK'
// button and the right edge of the 800 wide view.
expect(tester.getBottomLeft(find.text(okString)).dx, 800 - ltrOkRight);
});
group('Barrier dismissible', () {
late PickerObserver rootObserver;
setUp(() {
rootObserver = PickerObserver();
});
testWidgets('Barrier is dismissible with default parameter', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(rootObserver.pickerCount, 1);
// Tap on the barrier.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(rootObserver.pickerCount, 0);
});
testWidgets('Barrier is not dismissible with barrierDismissible is false', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () =>
showTimePicker(
context: context,
barrierDismissible: false,
initialTime: const TimeOfDay(hour: 7, minute: 0),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(rootObserver.pickerCount, 1);
// Tap on the barrier, which shouldn't do anything this time.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpAndSettle();
expect(rootObserver.pickerCount, 1);
});
});
testWidgets('Barrier color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () => showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, Colors.black54);
// Dismiss the dialog.
await tester.tapAt(const Offset(10.0, 10.0));
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () => showTimePicker(
context: context,
barrierColor: Colors.pink,
initialTime: const TimeOfDay(hour: 7, minute: 0),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).color, Colors.pink);
});
testWidgets('Barrier Label', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () => showTimePicker(
context: context,
barrierLabel: 'Custom Label',
initialTime: const TimeOfDay(hour: 7, minute: 0),
),
);
},
),
),
),
),
);
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(tester.widget<ModalBarrier>(find.byType(ModalBarrier).last).semanticsLabel, 'Custom Label');
});
testWidgets('uses root navigator by default', (WidgetTester tester) async {
final PickerObserver rootObserver = PickerObserver();
final PickerObserver nestedObserver = PickerObserver();
await tester.pumpWidget(MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<dynamic>(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
);
},
child: const Text('Show Picker'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
expect(rootObserver.pickerCount, 1);
expect(nestedObserver.pickerCount, 0);
});
testWidgets('uses nested navigator if useRootNavigator is false', (WidgetTester tester) async {
final PickerObserver rootObserver = PickerObserver();
final PickerObserver nestedObserver = PickerObserver();
await tester.pumpWidget(MaterialApp(
navigatorObservers: <NavigatorObserver>[rootObserver],
home: Navigator(
observers: <NavigatorObserver>[nestedObserver],
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<dynamic>(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () {
showTimePicker(
context: context,
useRootNavigator: false,
initialTime: const TimeOfDay(hour: 7, minute: 0),
);
},
child: const Text('Show Picker'),
);
},
);
},
),
));
// Open the dialog.
await tester.tap(find.byType(ElevatedButton));
expect(rootObserver.pickerCount, 0);
expect(nestedObserver.pickerCount, 1);
});
testWidgets('optional text parameters are utilized', (WidgetTester tester) async {
const String cancelText = 'Custom Cancel';
const String confirmText = 'Custom OK';
const String helperText = 'Custom Help';
await tester.pumpWidget(MaterialApp(
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () async {
await showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
cancelText: cancelText,
confirmText: confirmText,
helpText: helperText,
);
},
);
},
),
),
),
));
// Open the picker.
await tester.tap(find.text('X'));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text(cancelText), findsOneWidget);
expect(find.text(confirmText), findsOneWidget);
expect(find.text(helperText), findsOneWidget);
});
testWidgets('Material2 - OK Cancel button and helpText layout', (WidgetTester tester) async {
const String selectTimeString = 'SELECT TIME';
const String cancelString = 'CANCEL';
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child!,
);
},
);
},
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.text(selectTimeString)), equals(const Offset(154, 155)));
expect(tester.getBottomRight(find.text(selectTimeString)), equals(
const Offset(280.5, 165),
));
expect(tester.getBottomRight(find.text(okString)).dx, 644);
expect(tester.getBottomLeft(find.text(okString)).dx, 616);
expect(tester.getBottomRight(find.text(cancelString)).dx, 582);
await tester.tap(find.text(okString));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.text(selectTimeString)), equals(
const Offset(519.5, 155),
));
expect(tester.getBottomRight(find.text(selectTimeString)), equals(const Offset(646, 165)));
expect(tester.getBottomLeft(find.text(okString)).dx, 156);
expect(tester.getBottomRight(find.text(okString)).dx, 184);
expect(tester.getBottomLeft(find.text(cancelString)).dx, 218);
await tester.tap(find.text(okString));
await tester.pumpAndSettle();
});
testWidgets('Material3 - OK Cancel button and helpText layout', (WidgetTester tester) async {
const String selectTimeString = 'Select time';
const String cancelString = 'Cancel';
Widget buildFrame(TextDirection textDirection) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () {
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: textDirection,
child: child!,
);
},
);
},
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame(TextDirection.ltr));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.text(selectTimeString)), equals(const Offset(138, 129)));
expect(
tester.getBottomRight(find.text(selectTimeString)),
const Offset(294.75, 149.0),
);
expect(
tester.getBottomLeft(find.text(okString)).dx,
moreOrLessEquals(615.9, epsilon: 0.001),
);
expect(tester.getBottomRight(find.text(cancelString)).dx, 578);
await tester.tap(find.text(okString));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(TextDirection.rtl));
await tester.tap(find.text('X'));
await tester.pumpAndSettle();
expect(
tester.getTopLeft(find.text(selectTimeString)),
equals(const Offset(505.25, 129.0)),
);
expect(tester.getBottomRight(find.text(selectTimeString)), equals(const Offset(662, 149)));
expect(
tester.getBottomLeft(find.text(okString)).dx,
moreOrLessEquals(155.9, epsilon: 0.001),
);
expect(
tester.getBottomRight(find.text(okString)).dx,
moreOrLessEquals(184.1, epsilon: 0.001),
);
expect(tester.getBottomLeft(find.text(cancelString)).dx, 222);
await tester.tap(find.text(okString));
await tester.pumpAndSettle();
});
testWidgets('text scale affects certain elements and not others', (WidgetTester tester) async {
await mediaQueryBoilerplate(
tester,
initialTime: const TimeOfDay(hour: 7, minute: 41),
materialType: materialType,
);
final double minutesDisplayHeight = tester.getSize(find.text('41')).height;
final double amHeight = tester.getSize(find.text(amString)).height;
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
// Verify that the time display is not affected by text scale.
await mediaQueryBoilerplate(
tester,
textScaler: const TextScaler.linear(2),
initialTime: const TimeOfDay(hour: 7, minute: 41),
materialType: materialType,
);
final double amHeight2x = tester.getSize(find.text(amString)).height;
expect(tester.getSize(find.text('41')).height, equals(minutesDisplayHeight));
expect(amHeight2x, math.min(38.0, amHeight * 2));
await tester.tap(find.text(okString)); // dismiss the dialog
await tester.pumpAndSettle();
// Verify that text scale for AM/PM is at most 2x.
await mediaQueryBoilerplate(
tester,
textScaler: const TextScaler.linear(3),
initialTime: const TimeOfDay(hour: 7, minute: 41),
materialType: materialType,
);
expect(tester.getSize(find.text('41')).height, equals(minutesDisplayHeight));
expect(tester.getSize(find.text(amString)).height, math.min(38.0, amHeight * 2));
});
group('showTimePicker avoids overlapping display features', () {
testWidgets('positioning with anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(TimePickerDialog)), const Offset(410, 0));
expect(tester.getBottomRight(find.byType(TimePickerDialog)), const Offset(800, 600));
});
testWidgets('positioning with Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
// By default it should place the dialog on the right screen
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
);
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.byType(TimePickerDialog)), const Offset(410, 0));
expect(tester.getBottomRight(find.byType(TimePickerDialog)), const Offset(800, 600));
});
testWidgets('positioning with defaults', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
// By default it should place the dialog on the left screen
showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
);
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.byType(TimePickerDialog)), Offset.zero);
expect(tester.getBottomRight(find.byType(TimePickerDialog)), const Offset(390, 600));
});
});
group('Works for various view sizes', () {
for (final Size size in const <Size>[Size(100, 100), Size(300, 300), Size(800, 600)]) {
testWidgets('Draws dial without overflows at $size', (WidgetTester tester) async {
tester.view.physicalSize = size;
addTearDown(tester.view.reset);
await mediaQueryBoilerplate(tester, entryMode: TimePickerEntryMode.input, materialType: materialType);
await tester.pumpAndSettle();
expect(tester.takeException(), isNot(throwsAssertionError));
});
testWidgets('Draws input without overflows at $size', (WidgetTester tester) async {
tester.view.physicalSize = size;
addTearDown(tester.view.reset);
await mediaQueryBoilerplate(tester, materialType: materialType);
await tester.pumpAndSettle();
expect(tester.takeException(), isNot(throwsAssertionError));
});
}
});
});
group('Time picker - A11y and Semantics (${materialType.name})', () {
testWidgets('provides semantics information for AM/PM indicator', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await mediaQueryBoilerplate(tester, materialType: materialType);
expect(
semantics,
includesNodeWith(
label: amString,
actions: <SemanticsAction>[SemanticsAction.tap],
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isChecked,
SemanticsFlag.isInMutuallyExclusiveGroup,
SemanticsFlag.hasCheckedState,
SemanticsFlag.isFocusable,
],
),
);
expect(
semantics,
includesNodeWith(
label: pmString,
actions: <SemanticsAction>[SemanticsAction.tap],
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isInMutuallyExclusiveGroup,
SemanticsFlag.hasCheckedState,
SemanticsFlag.isFocusable,
],
),
);
semantics.dispose();
});
testWidgets('Material2 - provides semantics information for header and footer', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, materialType: MaterialType.material2);
expect(semantics, isNot(includesNodeWith(label: ':')));
expect(
semantics.nodesWith(value: 'Select minutes 00'),
hasLength(1),
reason: '00 appears once in the header',
);
expect(
semantics.nodesWith(value: 'Select hours 07'),
hasLength(1),
reason: '07 appears once in the header',
);
expect(semantics, includesNodeWith(label: 'CANCEL'));
expect(semantics, includesNodeWith(label: okString));
// In 24-hour mode we don't have AM/PM control.
expect(semantics, isNot(includesNodeWith(label: amString)));
expect(semantics, isNot(includesNodeWith(label: pmString)));
semantics.dispose();
});
testWidgets('Material3 - provides semantics information for header and footer', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, materialType: MaterialType.material3);
expect(semantics, isNot(includesNodeWith(label: ':')));
expect(
semantics.nodesWith(value: 'Select minutes 00'),
hasLength(1),
reason: '00 appears once in the header',
);
expect(
semantics.nodesWith(value: 'Select hours 07'),
hasLength(1),
reason: '07 appears once in the header',
);
expect(semantics, includesNodeWith(label: 'Cancel'));
expect(semantics, includesNodeWith(label: okString));
// In 24-hour mode we don't have AM/PM control.
expect(semantics, isNot(includesNodeWith(label: amString)));
expect(semantics, isNot(includesNodeWith(label: pmString)));
semantics.dispose();
});
testWidgets('provides semantics information for text fields', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
accessibleNavigation: true,
materialType: materialType,
);
expect(
semantics,
includesNodeWith(
label: 'Hour',
value: '07',
actions: <SemanticsAction>[SemanticsAction.tap],
flags: <SemanticsFlag>[
SemanticsFlag.isTextField,
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isMultiline,
],
),
);
expect(
semantics,
includesNodeWith(
label: 'Minute',
value: '00',
actions: <SemanticsAction>[SemanticsAction.tap],
flags: <SemanticsFlag>[
SemanticsFlag.isTextField,
SemanticsFlag.hasEnabledState,
SemanticsFlag.isEnabled,
SemanticsFlag.isMultiline,
],
),
);
semantics.dispose();
});
testWidgets('can increment and decrement hours', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
Future<void> actAndExpect({
required String initialValue,
required SemanticsAction action,
required String finalValue,
}) async {
final SemanticsNode elevenHours = semantics
.nodesWith(
value: 'Select hours $initialValue',
ancestor: tester.renderObject(_hourControl).debugSemantics,
)
.single;
tester.binding.pipelineOwner.semanticsOwner!.performAction(elevenHours.id, action);
await tester.pumpAndSettle();
expect(
find.descendant(of: _hourControl, matching: find.text(finalValue)),
findsOneWidget,
);
}
// 12-hour format
await mediaQueryBoilerplate(
tester,
initialTime: const TimeOfDay(hour: 11, minute: 0),
materialType: materialType,
);
await actAndExpect(
initialValue: '11',
action: SemanticsAction.increase,
finalValue: '12',
);
await actAndExpect(
initialValue: '12',
action: SemanticsAction.increase,
finalValue: '1',
);
// Ensure we preserve day period as we roll over.
final dynamic pickerState = tester.state(_timePicker);
// ignore: avoid_dynamic_calls
expect(pickerState.selectedTime.value, const TimeOfDay(hour: 1, minute: 0));
await actAndExpect(
initialValue: '1',
action: SemanticsAction.decrease,
finalValue: '12',
);
await tester.pumpWidget(Container()); // clear old boilerplate
// 24-hour format
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
initialTime: const TimeOfDay(hour: 23, minute: 0),
materialType: materialType,
);
await actAndExpect(
initialValue: '23',
action: SemanticsAction.increase,
finalValue: '00',
);
await actAndExpect(
initialValue: '00',
action: SemanticsAction.increase,
finalValue: '01',
);
await actAndExpect(
initialValue: '01',
action: SemanticsAction.decrease,
finalValue: '00',
);
await actAndExpect(
initialValue: '00',
action: SemanticsAction.decrease,
finalValue: '23',
);
semantics.dispose();
});
testWidgets('can increment and decrement minutes', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
Future<void> actAndExpect({
required String initialValue,
required SemanticsAction action,
required String finalValue,
}) async {
final SemanticsNode elevenHours = semantics
.nodesWith(
value: 'Select minutes $initialValue',
ancestor: tester.renderObject(_minuteControl).debugSemantics,
)
.single;
tester.binding.pipelineOwner.semanticsOwner!.performAction(elevenHours.id, action);
await tester.pumpAndSettle();
expect(
find.descendant(of: _minuteControl, matching: find.text(finalValue)),
findsOneWidget,
);
}
await mediaQueryBoilerplate(
tester,
initialTime: const TimeOfDay(hour: 11, minute: 58),
materialType: materialType,
);
await actAndExpect(
initialValue: '58',
action: SemanticsAction.increase,
finalValue: '59',
);
await actAndExpect(
initialValue: '59',
action: SemanticsAction.increase,
finalValue: '00',
);
// Ensure we preserve hour period as we roll over.
final dynamic pickerState = tester.state(_timePicker);
// ignore: avoid_dynamic_calls
expect(pickerState.selectedTime.value, const TimeOfDay(hour: 11, minute: 0));
await actAndExpect(
initialValue: '00',
action: SemanticsAction.decrease,
finalValue: '59',
);
await actAndExpect(
initialValue: '59',
action: SemanticsAction.decrease,
finalValue: '58',
);
semantics.dispose();
});
testWidgets('header touch regions are large enough', (WidgetTester tester) async {
// Ensure picker is displayed in portrait mode.
tester.view.physicalSize = const Size(400, 800);
tester.view.devicePixelRatio = 1;
addTearDown(tester.view.reset);
await mediaQueryBoilerplate(tester, materialType: materialType);
final Size dayPeriodControlSize = tester.getSize(
find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DayPeriodControl'),
);
expect(dayPeriodControlSize.width, greaterThanOrEqualTo(48));
expect(dayPeriodControlSize.height, greaterThanOrEqualTo(80));
final Size hourSize = tester.getSize(find.ancestor(
of: find.text('7'),
matching: find.byType(InkWell),
));
expect(hourSize.width, greaterThanOrEqualTo(48));
expect(hourSize.height, greaterThanOrEqualTo(48));
final Size minuteSize = tester.getSize(find.ancestor(
of: find.text('00'),
matching: find.byType(InkWell),
));
expect(minuteSize.width, greaterThanOrEqualTo(48));
expect(minuteSize.height, greaterThanOrEqualTo(48));
});
});
group('Time picker - Input (${materialType.name})', () {
testWidgets('Initial entry mode is used', (WidgetTester tester) async {
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
materialType: materialType,
);
expect(find.byType(TextField), findsNWidgets(2));
});
testWidgets('Initial time is the default', (WidgetTester tester) async {
late TimeOfDay result;
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, entryMode: TimePickerEntryMode.input, materialType: materialType);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 7, minute: 0)));
});
testWidgets('Help text is used - Input', (WidgetTester tester) async {
const String helpText = 'help';
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
helpText: helpText,
materialType: materialType,
);
expect(find.text(helpText), findsOneWidget);
});
testWidgets('Help text is used in Material3 - Input', (WidgetTester tester) async {
const String helpText = 'help';
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
helpText: helpText,
materialType: materialType,
);
expect(find.text(helpText), findsOneWidget);
});
testWidgets('Hour label text is used - Input', (WidgetTester tester) async {
const String hourLabelText = 'Custom hour label';
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
hourLabelText: hourLabelText,
materialType: materialType,
);
expect(find.text(hourLabelText), findsOneWidget);
});
testWidgets('Minute label text is used - Input', (WidgetTester tester) async {
const String minuteLabelText = 'Custom minute label';
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
minuteLabelText: minuteLabelText,
materialType: materialType,
);
expect(find.text(minuteLabelText), findsOneWidget);
});
testWidgets('Invalid error text is used - Input', (WidgetTester tester) async {
const String errorInvalidText = 'Custom validation error';
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
errorInvalidText: errorInvalidText,
materialType: materialType,
);
// Input invalid time (hour) to force validation error
await tester.enterText(find.byType(TextField).first, '88');
final MaterialLocalizations materialLocalizations = MaterialLocalizations.of(
tester.element(find.byType(TextButton).first),
);
// Tap the ok button to trigger the validation error with custom translation
await tester.tap(find.text(materialLocalizations.okButtonLabel));
await tester.pumpAndSettle(const Duration(seconds: 1));
expect(find.text(errorInvalidText), findsOneWidget);
});
testWidgets('Can switch from input to dial entry mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
materialType: materialType,
);
await tester.tap(find.byIcon(Icons.access_time));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
testWidgets('Can switch from dial to input entry mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, materialType: materialType);
await tester.tap(find.byIcon(Icons.keyboard_outlined));
await tester.pumpAndSettle();
expect(find.byType(TextField), findsWidgets);
});
testWidgets('Can not switch out of inputOnly mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.inputOnly,
materialType: materialType,
);
expect(find.byType(TextField), findsWidgets);
expect(find.byIcon(Icons.access_time), findsNothing);
});
testWidgets('Can not switch out of dialOnly mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.dialOnly,
materialType: materialType,
);
expect(find.byType(TextField), findsNothing);
expect(find.byIcon(Icons.keyboard_outlined), findsNothing);
});
testWidgets('Switching to dial entry mode triggers entry callback', (WidgetTester tester) async {
bool triggeredCallback = false;
await mediaQueryBoilerplate(
tester,
alwaysUse24HourFormat: true,
entryMode: TimePickerEntryMode.input,
onEntryModeChange: (TimePickerEntryMode mode) {
if (mode == TimePickerEntryMode.dial) {
triggeredCallback = true;
}
},
materialType: materialType,
);
await tester.tap(find.byIcon(Icons.access_time));
await tester.pumpAndSettle();
expect(triggeredCallback, true);
});
testWidgets('Switching to input entry mode triggers entry callback', (WidgetTester tester) async {
bool triggeredCallback = false;
await mediaQueryBoilerplate(tester, alwaysUse24HourFormat: true, onEntryModeChange: (TimePickerEntryMode mode) {
if (mode == TimePickerEntryMode.input) {
triggeredCallback = true;
}
}, materialType: materialType);
await tester.tap(find.byIcon(Icons.keyboard_outlined));
await tester.pumpAndSettle();
expect(triggeredCallback, true);
});
testWidgets('Can double tap hours (when selected) to enter input mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, materialType: materialType);
final Finder hourFinder = find.ancestor(
of: find.text('7'),
matching: find.byType(InkWell),
);
expect(find.byType(TextField), findsNothing);
// Double tap the hour.
await tester.tap(hourFinder);
await tester.pump(const Duration(milliseconds: 100));
await tester.tap(hourFinder);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsWidgets);
});
testWidgets('Can not double tap hours (when not selected) to enter input mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, materialType: materialType);
final Finder hourFinder = find.ancestor(
of: find.text('7'),
matching: find.byType(InkWell),
);
final Finder minuteFinder = find.ancestor(
of: find.text('00'),
matching: find.byType(InkWell),
);
expect(find.byType(TextField), findsNothing);
// Switch to minutes mode.
await tester.tap(minuteFinder);
await tester.pumpAndSettle();
// Double tap the hour.
await tester.tap(hourFinder);
await tester.pump(const Duration(milliseconds: 100));
await tester.tap(hourFinder);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
testWidgets('Can double tap minutes (when selected) to enter input mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, materialType: materialType);
final Finder minuteFinder = find.ancestor(
of: find.text('00'),
matching: find.byType(InkWell),
);
expect(find.byType(TextField), findsNothing);
// Switch to minutes mode.
await tester.tap(minuteFinder);
await tester.pumpAndSettle();
// Double tap the minutes.
await tester.tap(minuteFinder);
await tester.pump(const Duration(milliseconds: 100));
await tester.tap(minuteFinder);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsWidgets);
});
testWidgets('Can not double tap minutes (when not selected) to enter input mode', (WidgetTester tester) async {
await mediaQueryBoilerplate(tester, materialType: materialType);
final Finder minuteFinder = find.ancestor(
of: find.text('00'),
matching: find.byType(InkWell),
);
expect(find.byType(TextField), findsNothing);
// Double tap the minutes.
await tester.tap(minuteFinder);
await tester.pump(const Duration(milliseconds: 100));
await tester.tap(minuteFinder);
await tester.pumpAndSettle();
expect(find.byType(TextField), findsNothing);
});
testWidgets('Entered text returns time', (WidgetTester tester) async {
late TimeOfDay result;
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, entryMode: TimePickerEntryMode.input, materialType: materialType);
await tester.enterText(find.byType(TextField).first, '9');
await tester.enterText(find.byType(TextField).last, '12');
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 9, minute: 12)));
});
testWidgets('Toggle to dial mode keeps selected time', (WidgetTester tester) async {
late TimeOfDay result;
await startPicker(tester, (TimeOfDay? time) {
result = time!;
}, entryMode: TimePickerEntryMode.input, materialType: materialType);
await tester.enterText(find.byType(TextField).first, '8');
await tester.enterText(find.byType(TextField).last, '15');
await tester.tap(find.byIcon(Icons.access_time));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 8, minute: 15)));
});
testWidgets('Invalid text prevents dismissing', (WidgetTester tester) async {
TimeOfDay? result;
await startPicker(tester, (TimeOfDay? time) {
result = time;
}, entryMode: TimePickerEntryMode.input, materialType: materialType);
// Invalid hour.
await tester.enterText(find.byType(TextField).first, '88');
await tester.enterText(find.byType(TextField).last, '15');
await finishPicker(tester);
expect(result, null);
// Invalid minute.
await tester.enterText(find.byType(TextField).first, '8');
await tester.enterText(find.byType(TextField).last, '95');
await finishPicker(tester);
expect(result, null);
await tester.enterText(find.byType(TextField).first, '8');
await tester.enterText(find.byType(TextField).last, '15');
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 8, minute: 15)));
});
// Fixes regression that was reverted in https://github.com/flutter/flutter/pull/64094#pullrequestreview-469836378.
testWidgets('Ensure hour/minute fields are top-aligned with the separator', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {},
entryMode: TimePickerEntryMode.input, materialType: materialType);
final double hourFieldTop =
tester.getTopLeft(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_HourTextField')).dy;
final double minuteFieldTop =
tester.getTopLeft(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_MinuteTextField')).dy;
final double separatorTop =
tester.getTopLeft(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_TimeSelectorSeparator')).dy;
expect(hourFieldTop, separatorTop);
expect(minuteFieldTop, separatorTop);
});
testWidgets('Can switch between hour/minute fields using keyboard input action', (WidgetTester tester) async {
await startPicker(tester, (TimeOfDay? time) {},
entryMode: TimePickerEntryMode.input, materialType: materialType);
final Finder hourFinder = find.byType(TextField).first;
final TextField hourField = tester.widget(hourFinder);
await tester.tap(hourFinder);
expect(hourField.focusNode!.hasFocus, isTrue);
await tester.enterText(find.byType(TextField).first, '08');
final Finder minuteFinder = find.byType(TextField).last;
final TextField minuteField = tester.widget(minuteFinder);
expect(hourField.focusNode!.hasFocus, isFalse);
expect(minuteField.focusNode!.hasFocus, isTrue);
expect(tester.testTextInput.setClientArgs!['inputAction'], equals('TextInputAction.done'));
await tester.testTextInput.receiveAction(TextInputAction.done);
expect(hourField.focusNode!.hasFocus, isFalse);
expect(minuteField.focusNode!.hasFocus, isFalse);
});
});
group('Time picker - Restoration (${materialType.name})', () {
testWidgets('Time Picker state restoration test - dial mode', (WidgetTester tester) async {
TimeOfDay? result;
final Offset center = (await startPicker(
tester,
(TimeOfDay? time) {
result = time;
},
restorationId: 'restorable_time_picker',
materialType: materialType,
))!;
final Offset hour6 = Offset(center.dx, center.dy + 50); // 6:00
final Offset min45 = Offset(center.dx - 50, center.dy); // 45 mins (or 9:00 hours)
await tester.tapAt(hour6);
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
await tester.tapAt(min45);
await tester.pump(const Duration(milliseconds: 50));
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// Setting to PM adds 12 hours (18:45)
await tester.tap(find.text(pmString));
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 18, minute: 45)));
// Test restoring from before PM was selected (6:45)
await tester.restoreFrom(restorationData);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 45)));
});
testWidgets('Time Picker state restoration test - input mode', (WidgetTester tester) async {
TimeOfDay? result;
await startPicker(
tester,
(TimeOfDay? time) {
result = time;
},
entryMode: TimePickerEntryMode.input,
restorationId: 'restorable_time_picker',
materialType: materialType,
);
await tester.enterText(find.byType(TextField).first, '9');
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
await tester.enterText(find.byType(TextField).last, '12');
await tester.pump(const Duration(milliseconds: 50));
final TestRestorationData restorationData = await tester.getRestorationData();
await tester.restartAndRestore();
// Setting to PM adds 12 hours (21:12)
await tester.tap(find.text(pmString));
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 21, minute: 12)));
// Restoring from before PM was set (9:12)
await tester.restoreFrom(restorationData);
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 9, minute: 12)));
});
testWidgets('Time Picker state restoration test - switching modes', (WidgetTester tester) async {
TimeOfDay? result;
final Offset center = (await startPicker(
tester,
(TimeOfDay? time) {
result = time;
},
restorationId: 'restorable_time_picker',
materialType: materialType,
))!;
final TestRestorationData restorationData = await tester.getRestorationData();
// Switch to input mode from dial mode.
await tester.tap(find.byIcon(Icons.keyboard_outlined));
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
// Select time using input mode controls.
await tester.enterText(find.byType(TextField).first, '9');
await tester.enterText(find.byType(TextField).last, '12');
await tester.pump(const Duration(milliseconds: 50));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 9, minute: 12)));
// Restoring from dial mode.
await tester.restoreFrom(restorationData);
final Offset hour6 = Offset(center.dx, center.dy + 50); // 6:00
final Offset min45 = Offset(center.dx - 50, center.dy); // 45 mins (or 9:00 hours)
await tester.tapAt(hour6);
await tester.pump(const Duration(milliseconds: 50));
await tester.restartAndRestore();
await tester.tapAt(min45);
await tester.pump(const Duration(milliseconds: 50));
await finishPicker(tester);
expect(result, equals(const TimeOfDay(hour: 6, minute: 45)));
});
});
}
testWidgets('Material3 - Time selector separator default text style', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
await startPicker(
tester,
(TimeOfDay? value) { },
theme: theme,
);
final RenderParagraph paragraph = tester.renderObject(find.text(':'));
expect(paragraph.text.style!.color, theme.colorScheme.onSurface);
expect(paragraph.text.style!.fontSize, 57.0);
});
testWidgets('Material2 - Time selector separator default text style', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
await startPicker(
tester,
(TimeOfDay? value) { },
theme: theme,
);
final RenderParagraph paragraph = tester.renderObject(find.text(':'));
expect(paragraph.text.style!.color, theme.colorScheme.onSurface);
expect(paragraph.text.style!.fontSize, 56.0);
});
}
final Finder findDialPaint = find.descendant(
of: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_Dial'),
matching: find.byWidgetPredicate((Widget w) => w is CustomPaint),
);
class PickerObserver extends NavigatorObserver {
int pickerCount = 0;
@override
void didPush(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is DialogRoute) {
pickerCount++;
}
super.didPush(route, previousRoute);
}
@override
void didPop(Route<dynamic> route, Route<dynamic>? previousRoute) {
if (route is DialogRoute) {
pickerCount--;
}
super.didPop(route, previousRoute);
}
}
Future<void> mediaQueryBoilerplate(
WidgetTester tester, {
bool alwaysUse24HourFormat = false,
TimeOfDay initialTime = const TimeOfDay(hour: 7, minute: 0),
TextScaler textScaler = TextScaler.noScaling,
TimePickerEntryMode entryMode = TimePickerEntryMode.dial,
String? helpText,
String? hourLabelText,
String? minuteLabelText,
String? errorInvalidText,
bool accessibleNavigation = false,
EntryModeChangeCallback? onEntryModeChange,
bool tapButton = true,
required MaterialType materialType,
Orientation? orientation,
}) async {
await tester.pumpWidget(
Theme(
data: ThemeData(useMaterial3: materialType == MaterialType.material3),
child: Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: MediaQuery(
data: MediaQueryData(
alwaysUse24HourFormat: alwaysUse24HourFormat,
textScaler: textScaler,
accessibleNavigation: accessibleNavigation,
size: tester.view.physicalSize / tester.view.devicePixelRatio,
),
child: Material(
child: Center(
child: Directionality(
textDirection: TextDirection.ltr,
child: Navigator(
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(builder: (BuildContext context) {
return TextButton(
onPressed: () {
showTimePicker(
context: context,
initialTime: initialTime,
initialEntryMode: entryMode,
helpText: helpText,
hourLabelText: hourLabelText,
minuteLabelText: minuteLabelText,
errorInvalidText: errorInvalidText,
onEntryModeChanged: onEntryModeChange,
orientation: orientation,
);
},
child: const Text('X'),
);
});
},
),
),
),
),
),
),
),
);
if (tapButton) {
await tester.tap(find.text('X'));
}
await tester.pumpAndSettle();
}
final Finder _hourControl = find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_HourControl');
final Finder _minuteControl = find.byWidgetPredicate((Widget widget) => '${widget.runtimeType}' == '_MinuteControl');
final Finder _timePicker = find.byWidgetPredicate((Widget widget) => '${widget.runtimeType}' == '_TimePicker');
class _TimePickerLauncher extends StatefulWidget {
const _TimePickerLauncher({
required this.onChanged,
this.entryMode = TimePickerEntryMode.dial,
this.restorationId,
});
final ValueChanged<TimeOfDay?> onChanged;
final TimePickerEntryMode entryMode;
final String? restorationId;
@override
_TimePickerLauncherState createState() => _TimePickerLauncherState();
}
class _TimePickerLauncherState extends State<_TimePickerLauncher> with RestorationMixin {
@override
String? get restorationId => widget.restorationId;
late final RestorableRouteFuture<TimeOfDay?> _restorableTimePickerRouteFuture = RestorableRouteFuture<TimeOfDay?>(
onComplete: _selectTime,
onPresent: (NavigatorState navigator, Object? arguments) {
return navigator.restorablePush(
_timePickerRoute,
arguments: <String, String>{
'entry_mode': widget.entryMode.name,
},
);
},
);
@override
void dispose() {
_restorableTimePickerRouteFuture.dispose();
super.dispose();
}
@pragma('vm:entry-point')
static Route<TimeOfDay> _timePickerRoute(
BuildContext context,
Object? arguments,
) {
final Map<dynamic, dynamic> args = arguments! as Map<dynamic, dynamic>;
final TimePickerEntryMode entryMode = TimePickerEntryMode.values.firstWhere(
(TimePickerEntryMode element) => element.name == args['entry_mode'],
);
return DialogRoute<TimeOfDay>(
context: context,
builder: (BuildContext context) {
return TimePickerDialog(
restorationId: 'time_picker_dialog',
initialTime: const TimeOfDay(hour: 7, minute: 0),
initialEntryMode: entryMode,
);
},
);
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_restorableTimePickerRouteFuture, 'time_picker_route_future');
}
void _selectTime(TimeOfDay? newSelectedTime) {
widget.onChanged(newSelectedTime);
}
@override
Widget build(BuildContext context) {
return Material(
child: Center(
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('X'),
onPressed: () async {
if (widget.restorationId == null) {
widget.onChanged(await showTimePicker(
context: context,
initialTime: const TimeOfDay(hour: 7, minute: 0),
initialEntryMode: widget.entryMode,
));
} else {
_restorableTimePickerRouteFuture.present();
}
},
);
},
),
),
);
}
}
// The version of material design layout, etc. to test. Corresponds to
// useMaterial3 true/false in the ThemeData, but used an enum here so that it
// wasn't just a boolean, for easier identification of the name of the mode in
// tests.
enum MaterialType {
material2,
material3,
}
Future<Offset?> startPicker(
WidgetTester tester,
ValueChanged<TimeOfDay?> onChanged, {
TimePickerEntryMode entryMode = TimePickerEntryMode.dial,
String? restorationId,
ThemeData? theme,
MaterialType? materialType,
}) async {
await tester.pumpWidget(MaterialApp(
theme: theme ?? ThemeData(useMaterial3: materialType == MaterialType.material3),
restorationScopeId: 'app',
locale: const Locale('en', 'US'),
home: _TimePickerLauncher(
onChanged: onChanged,
entryMode: entryMode,
restorationId: restorationId,
),
));
await tester.tap(find.text('X'));
await tester.pumpAndSettle(const Duration(seconds: 1));
return entryMode == TimePickerEntryMode.dial
? tester.getCenter(find.byKey(const ValueKey<String>('time-picker-dial')))
: null;
}
Future<void> finishPicker(WidgetTester tester) async {
final MaterialLocalizations materialLocalizations = MaterialLocalizations.of(
tester.element(find.byType(ElevatedButton)),
);
await tester.tap(find.text(materialLocalizations.okButtonLabel));
await tester.pumpAndSettle(const Duration(seconds: 1));
}
| flutter/packages/flutter/test/material/time_picker_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/time_picker_test.dart",
"repo_id": "flutter",
"token_count": 37649
} | 723 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('BeveledRectangleBorder defaults', () {
const BeveledRectangleBorder border = BeveledRectangleBorder();
expect(border.side, BorderSide.none);
expect(border.borderRadius, BorderRadius.zero);
});
test('BeveledRectangleBorder copyWith, ==, hashCode', () {
expect(const BeveledRectangleBorder(), const BeveledRectangleBorder().copyWith());
expect(const BeveledRectangleBorder().hashCode, const BeveledRectangleBorder().copyWith().hashCode);
const BorderSide side = BorderSide(width: 10.0, color: Color(0xff123456));
const BorderRadius radius = BorderRadius.all(Radius.circular(16.0));
const BorderRadiusDirectional directionalRadius = BorderRadiusDirectional.all(Radius.circular(16.0));
expect(
const BeveledRectangleBorder().copyWith(side: side, borderRadius: radius),
const BeveledRectangleBorder(side: side, borderRadius: radius),
);
expect(
const BeveledRectangleBorder().copyWith(side: side, borderRadius: directionalRadius),
const BeveledRectangleBorder(side: side, borderRadius: directionalRadius),
);
});
test('BeveledRectangleBorder scale and lerp', () {
const BeveledRectangleBorder c10 = BeveledRectangleBorder(side: BorderSide(width: 10.0), borderRadius: BorderRadius.all(Radius.circular(100.0)));
const BeveledRectangleBorder c15 = BeveledRectangleBorder(side: BorderSide(width: 15.0), borderRadius: BorderRadius.all(Radius.circular(150.0)));
const BeveledRectangleBorder c20 = BeveledRectangleBorder(side: BorderSide(width: 20.0), borderRadius: BorderRadius.all(Radius.circular(200.0)));
expect(c10.dimensions, const EdgeInsets.all(10.0));
expect(c10.scale(2.0), c20);
expect(c20.scale(0.5), c10);
expect(ShapeBorder.lerp(c10, c20, 0.0), c10);
expect(ShapeBorder.lerp(c10, c20, 0.5), c15);
expect(ShapeBorder.lerp(c10, c20, 1.0), c20);
});
test('BeveledRectangleBorder BorderRadius.zero', () {
const Rect rect1 = Rect.fromLTRB(10.0, 20.0, 30.0, 40.0);
final Matcher looksLikeRect1 = isPathThat(
includes: const <Offset>[ Offset(10.0, 20.0), Offset(20.0, 30.0) ],
excludes: const <Offset>[ Offset(9.0, 19.0), Offset(31.0, 41.0) ],
);
// Default border radius and border side are zero, i.e. just a rectangle.
expect(const BeveledRectangleBorder().getOuterPath(rect1), looksLikeRect1);
expect(const BeveledRectangleBorder().getInnerPath(rect1), looksLikeRect1);
// Represents the inner path when borderSide.width = 4, which is just rect1
// inset by 4 on all sides.
final Matcher looksLikeInnerPath = isPathThat(
includes: const <Offset>[ Offset(14.0, 24.0), Offset(16.0, 26.0) ],
excludes: const <Offset>[ Offset(9.0, 23.0), Offset(27.0, 37.0) ],
);
const BorderSide side = BorderSide(width: 4.0);
expect(const BeveledRectangleBorder(side: side).getOuterPath(rect1), looksLikeRect1);
expect(const BeveledRectangleBorder(side: side).getInnerPath(rect1), looksLikeInnerPath);
});
test('BeveledRectangleBorder non-zero BorderRadius', () {
const Rect rect = Rect.fromLTRB(10.0, 20.0, 30.0, 40.0);
final Matcher looksLikeRect = isPathThat(
includes: const <Offset>[ Offset(15.0, 25.0), Offset(20.0, 30.0) ],
excludes: const <Offset>[ Offset(10.0, 20.0), Offset(30.0, 40.0) ],
);
const BeveledRectangleBorder border = BeveledRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(5.0)),
);
expect(border.getOuterPath(rect), looksLikeRect);
expect(border.getInnerPath(rect), looksLikeRect);
});
test('BeveledRectangleBorder non-zero BorderRadiusDirectional', () {
const Rect rect = Rect.fromLTRB(10.0, 20.0, 30.0, 40.0);
final Matcher looksLikeRectLtr = isPathThat(
includes: const <Offset>[Offset(15.0, 25.0), Offset(20.0, 30.0)],
excludes: const <Offset>[Offset(10.0, 20.0), Offset(10.0, 40.0)],
);
const BeveledRectangleBorder border = BeveledRectangleBorder(
borderRadius: BorderRadiusDirectional.only(
topStart: Radius.circular(5.0),
bottomStart: Radius.circular(5.0),
),
);
// Test ltr situation
expect(border.getOuterPath(rect,textDirection: TextDirection.ltr), looksLikeRectLtr);
expect(border.getInnerPath(rect,textDirection: TextDirection.ltr), looksLikeRectLtr);
final Matcher looksLikeRectRtl = isPathThat(
includes: const <Offset>[Offset(25.0, 35.0), Offset(25.0, 25.0)],
excludes: const <Offset>[Offset(30.0, 20.0), Offset(30.0, 40.0)],
);
// Test Rtl situation
expect(border.getOuterPath(rect,textDirection: TextDirection.rtl), looksLikeRectRtl);
expect(border.getInnerPath(rect,textDirection: TextDirection.rtl), looksLikeRectRtl);
});
test('BeveledRectangleBorder with StrokeAlign', () {
const BorderRadius borderRadius = BorderRadius.all(Radius.circular(10));
const BeveledRectangleBorder inside = BeveledRectangleBorder(side: BorderSide(width: 10.0), borderRadius: borderRadius);
const BeveledRectangleBorder center = BeveledRectangleBorder(side: BorderSide(width: 10.0, strokeAlign: BorderSide.strokeAlignCenter), borderRadius: borderRadius);
const BeveledRectangleBorder outside = BeveledRectangleBorder(side: BorderSide(width: 10.0, strokeAlign: BorderSide.strokeAlignOutside), borderRadius: borderRadius);
expect(inside.dimensions, const EdgeInsets.all(10.0));
expect(center.dimensions, const EdgeInsets.all(5.0));
expect(outside.dimensions, EdgeInsets.zero);
const Rect rect = Rect.fromLTWH(0.0, 0.0, 120.0, 40.0);
expect(inside.getInnerPath(rect), isPathThat(
includes: const <Offset>[ Offset(10, 20), Offset(100, 10), Offset(50, 30), Offset(50, 20) ],
excludes: const <Offset>[ Offset(9, 9), Offset(100, 0), Offset(110, 31), Offset(9, 31) ],
));
expect(center.getInnerPath(rect), isPathThat(
includes: const <Offset>[ Offset(9, 9), Offset(100, 10), Offset(110, 31), Offset(9, 31) ],
excludes: const <Offset>[ Offset(4, 4), Offset(100, 0), Offset(116, 31), Offset(4, 31) ],
));
expect(outside.getInnerPath(rect), isPathThat(
includes: const <Offset>[ Offset(5, 5), Offset(110, 0), Offset(116, 31), Offset(4, 31) ],
excludes: const <Offset>[ Offset.zero, Offset(120, 0), Offset(120, 31), Offset(0, 31) ],
));
});
}
| flutter/packages/flutter/test/painting/beveled_rectangle_border_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/beveled_rectangle_border_test.dart",
"repo_id": "flutter",
"token_count": 2490
} | 724 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show Codec, FrameInfo, instantiateImageCodec;
import 'package:flutter/foundation.dart';
/// A [ui.Codec] implementation for testing that pre-fetches all the image
/// frames, and provides synchronous [getNextFrame] implementation.
///
/// This is useful for running in the test Zone, where it is tricky to receive
/// callbacks originating from the IO thread.
class FakeCodec implements ui.Codec {
FakeCodec._(this._frameCount, this._repetitionCount, this._frameInfos);
final int _frameCount;
final int _repetitionCount;
final List<ui.FrameInfo> _frameInfos;
int _nextFrame = 0;
int _numFramesAsked = 0;
/// Creates a FakeCodec from encoded image data.
///
/// Only call this method outside of the test zone.
static Future<FakeCodec> fromData(Uint8List data) async {
final ui.Codec codec = await ui.instantiateImageCodec(data);
final int frameCount = codec.frameCount;
final List<ui.FrameInfo> frameInfos = <ui.FrameInfo>[];
for (int i = 0; i < frameCount; i += 1) {
frameInfos.add(await codec.getNextFrame());
}
return FakeCodec._(frameCount, codec.repetitionCount, frameInfos);
}
@override
int get frameCount => _frameCount;
@override
int get repetitionCount => _repetitionCount;
int get numFramesAsked => _numFramesAsked;
@override
Future<ui.FrameInfo> getNextFrame() {
_numFramesAsked += 1;
final SynchronousFuture<ui.FrameInfo> result =
SynchronousFuture<ui.FrameInfo>(_frameInfos[_nextFrame]);
_nextFrame = (_nextFrame + 1) % _frameCount;
return result;
}
@override
void dispose() { }
}
| flutter/packages/flutter/test/painting/fake_codec.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/fake_codec.dart",
"repo_id": "flutter",
"token_count": 580
} | 725 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
class TestAssetBundle extends CachingAssetBundle {
TestAssetBundle(this._assetBundleMap);
final Map<String, List<Map<Object?, Object?>>> _assetBundleMap;
Map<String, int> loadCallCount = <String, int>{};
@override
Future<ByteData> load(String key) async {
if (key == 'AssetManifest.bin') {
return const StandardMessageCodec().encodeMessage(_assetBundleMap)!;
}
if (key == 'AssetManifest.bin.json') {
// Encode the manifest data that will be used by the app
final ByteData data = const StandardMessageCodec().encodeMessage(_assetBundleMap)!;
// Simulate the behavior of NetworkAssetBundle.load here, for web tests
return ByteData.sublistView(
utf8.encode(
json.encode(
base64.encode(
// Encode only the actual bytes of the buffer, and no more...
data.buffer.asUint8List(data.offsetInBytes, data.lengthInBytes)
)
)
)
);
}
loadCallCount[key] = loadCallCount[key] ?? 0 + 1;
if (key == 'one') {
return ByteData(1)
..setInt8(0, 49);
}
throw FlutterError('key not found');
}
@override
Future<ui.ImmutableBuffer> loadBuffer(String key) async {
final ByteData data = await load(key);
return ui.ImmutableBuffer.fromUint8List(data.buffer.asUint8List());
}
}
void main() {
group('1.0 scale device tests', () {
void buildAndTestWithOneAsset(String mainAssetPath) {
final Map<String, List<Map<Object?, Object?>>> assetBundleMap =
<String, List<Map<Object?, Object?>>>{};
final AssetImage assetImage = AssetImage(
mainAssetPath,
bundle: TestAssetBundle(assetBundleMap),
);
const ImageConfiguration configuration = ImageConfiguration.empty;
assetImage.obtainKey(configuration)
.then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, mainAssetPath);
expect(bundleKey.scale, 1.0);
}));
}
test('When asset is main variant check scale is 1.0', () {
buildAndTestWithOneAsset('assets/normalFolder/normalFile.png');
});
test('When asset path and key are the same string even though it could be took as a 3.0x variant', () async {
buildAndTestWithOneAsset('assets/parentFolder/3.0x/normalFile.png');
});
test('When asset path contains variant identifier as part of parent folder name scale is 1.0', () {
buildAndTestWithOneAsset('assets/parentFolder/__3.0x__/leafFolder/normalFile.png');
});
test('When asset path contains variant identifier as part of leaf folder name scale is 1.0', () {
buildAndTestWithOneAsset('assets/parentFolder/__3.0x_leaf_folder_/normalFile.png');
});
test('When asset path contains variant identifier as part of parent folder name scale is 1.0', () {
buildAndTestWithOneAsset('assets/parentFolder/__3.0x__/leafFolder/normalFile.png');
});
test('When asset path contains variant identifier in parent folder scale is 1.0', () {
buildAndTestWithOneAsset('assets/parentFolder/3.0x/leafFolder/normalFile.png');
});
});
group('High-res device behavior tests', () {
test('When asset is not main variant check scale is not 1.0', () {
const String mainAssetPath = 'assets/normalFolder/normalFile.png';
const String variantPath = 'assets/normalFolder/3.0x/normalFile.png';
final Map<String, List<Map<Object?, Object?>>> assetBundleMap =
<String, List<Map<Object?, Object?>>>{};
final Map<Object?, Object?> mainAssetVariantManifestEntry = <Object?, Object?>{};
mainAssetVariantManifestEntry['asset'] = variantPath;
mainAssetVariantManifestEntry['dpr'] = 3.0;
assetBundleMap[mainAssetPath] = <Map<Object?, Object?>>[mainAssetVariantManifestEntry];
final TestAssetBundle testAssetBundle = TestAssetBundle(assetBundleMap);
final AssetImage assetImage = AssetImage(
mainAssetPath,
bundle: testAssetBundle,
);
assetImage.obtainKey(ImageConfiguration.empty)
.then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, mainAssetPath);
expect(bundleKey.scale, 1.0);
}));
assetImage.obtainKey(ImageConfiguration(
bundle: testAssetBundle,
devicePixelRatio: 3.0,
)).then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, variantPath);
expect(bundleKey.scale, 3.0);
}));
});
test('When high-res device and high-res asset not present in bundle then return main variant', () {
const String mainAssetPath = 'assets/normalFolder/normalFile.png';
final Map<String, List<Map<Object?, Object?>>> assetBundleMap =
<String, List<Map<Object?, Object?>>>{};
assetBundleMap[mainAssetPath] = <Map<Object?, Object?>>[];
final TestAssetBundle testAssetBundle = TestAssetBundle(assetBundleMap);
final AssetImage assetImage = AssetImage(
mainAssetPath,
bundle: TestAssetBundle(assetBundleMap),
);
assetImage.obtainKey(ImageConfiguration.empty)
.then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, mainAssetPath);
expect(bundleKey.scale, 1.0);
}));
assetImage.obtainKey(ImageConfiguration(
bundle: testAssetBundle,
devicePixelRatio: 3.0,
)).then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, mainAssetPath);
expect(bundleKey.scale, 1.0);
}));
});
});
group('Regression - When assets available are 1.0 and 3.0 check devices with a range of scales', () {
const String mainAssetPath = 'assets/normalFolder/normalFile.png';
const String variantPath = 'assets/normalFolder/3.0x/normalFile.png';
void buildBundleAndTestVariantLogic(
double deviceRatio,
double chosenAssetRatio,
String expectedAssetPath,
) {
const Map<String, List<Map<Object?, Object?>>> assetManifest =
<String, List<Map<Object?, Object?>>>{
'assets/normalFolder/normalFile.png': <Map<Object?, Object?>>[
<Object?, Object?>{'asset': 'assets/normalFolder/normalFile.png'},
<Object?, Object?>{
'asset': 'assets/normalFolder/3.0x/normalFile.png',
'dpr': 3.0
},
]
};
final TestAssetBundle testAssetBundle = TestAssetBundle(assetManifest);
final AssetImage assetImage = AssetImage(
mainAssetPath,
bundle: testAssetBundle,
);
// we have 1.0 and 3.0, asking for 1.5 should give
assetImage.obtainKey(ImageConfiguration(
bundle: testAssetBundle,
devicePixelRatio: deviceRatio,
)).then(expectAsync1((AssetBundleImageKey bundleKey) {
expect(bundleKey.name, expectedAssetPath);
expect(bundleKey.scale, chosenAssetRatio);
}));
}
test('Obvious case 1.0 - we have exact asset', () {
buildBundleAndTestVariantLogic(1.0, 1.0, mainAssetPath);
});
test('Obvious case 3.0 - we have exact asset', () {
buildBundleAndTestVariantLogic(3.0, 3.0, variantPath);
});
test('Typical case 2.0', () {
buildBundleAndTestVariantLogic(2.0, 1.0, mainAssetPath);
});
test('Borderline case 2.01', () {
buildBundleAndTestVariantLogic(2.01, 3.0, variantPath);
});
test('Borderline case 2.9', () {
buildBundleAndTestVariantLogic(2.9, 3.0, variantPath);
});
test('Typical case 4.0', () {
buildBundleAndTestVariantLogic(4.0, 3.0, variantPath);
});
});
}
| flutter/packages/flutter/test/painting/image_resolution_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/image_resolution_test.dart",
"repo_id": "flutter",
"token_count": 3119
} | 726 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
Future<void> testBorder(WidgetTester tester, String name, StarBorder border,
{ShapeBorder? lerpTo, ShapeBorder? lerpFrom, double lerpAmount = 0}) async {
assert(lerpTo == null || lerpFrom == null); // They can't both be set.
ShapeBorder shape;
if (lerpTo != null) {
shape = border.lerpTo(lerpTo, lerpAmount)!;
} else if (lerpFrom != null) {
shape = border.lerpFrom(lerpFrom, lerpAmount)!;
} else {
shape = border;
}
await tester.pumpWidget(
Container(
alignment: Alignment.center,
width: 200,
height: 100,
decoration: ShapeDecoration(
color: const Color(0xff000000),
shape: shape,
),
),
);
await expectLater(
find.byType(Container),
matchesGoldenFile('painting.star_border.$name.png'),
);
}
test('StarBorder defaults', () {
const StarBorder star = StarBorder();
expect(star.side, BorderSide.none);
expect(star.points, 5);
expect(star.innerRadiusRatio, 0.4);
expect(star.rotation, 0);
expect(star.pointRounding, 0);
expect(star.valleyRounding, 0);
expect(star.squash, 0);
const StarBorder polygon = StarBorder.polygon();
expect(polygon.points, 5);
expect(polygon.pointRounding, 0);
expect(polygon.rotation, 0);
expect(polygon.squash, 0);
});
test('StarBorder copyWith, ==, hashCode', () {
const BorderSide side = BorderSide(width: 10.0, color: Color(0xff123456));
final StarBorder copy = const StarBorder().copyWith(
side: side,
points: 3,
innerRadiusRatio: 0.1,
pointRounding: 0.2,
valleyRounding: 0.3,
rotation: 180,
squash: 0.4,
);
const StarBorder expected = StarBorder(
side: side,
points: 3,
innerRadiusRatio: 0.1,
pointRounding: 0.2,
valleyRounding: 0.3,
rotation: 180,
squash: 0.4,
);
expect(const StarBorder(), equals(const StarBorder().copyWith()));
expect(copy, equals(expected));
expect(copy.hashCode, equals(expected.hashCode));
// Test that all properties are checked in operator==
expect(const StarBorder(), isNot(equals(const StarBorderSubclass())));
expect(copy, isNot(equals('Not a StarBorder')));
// Test that two StarBorders where the only difference is polygon vs star
// constructor compare as different (which they are, because
// _innerRadiusRatio is null on the polygon).
expect(
const StarBorder(
points: 3,
innerRadiusRatio: 1,
pointRounding: 0.2,
rotation: 180,
squash: 0.4,
),
isNot(equals(
const StarBorder.polygon(
sides: 3,
pointRounding: 0.2,
rotation: 180,
squash: 0.4,
),
)),
);
// Test that copies are unequal whenever any one of the properties changes.
expect(copy, equals(copy));
expect(copy, isNot(equals(copy.copyWith(side: const BorderSide()))));
expect(copy, isNot(equals(copy.copyWith(points: 10))));
expect(copy, isNot(equals(copy.copyWith(innerRadiusRatio: 0.5))));
expect(copy, isNot(equals(copy.copyWith(pointRounding: 0.5))));
expect(copy, isNot(equals(copy.copyWith(valleyRounding: 0.5))));
expect(copy, isNot(equals(copy.copyWith(rotation: 10))));
expect(copy, isNot(equals(copy.copyWith(squash: 0.0))));
});
testWidgets('StarBorder basic geometry', (WidgetTester tester) async {
await testBorder(tester, 'basic_star', const StarBorder());
await testBorder(tester, 'basic_polygon', const StarBorder.polygon());
});
testWidgets('StarBorder parameters', (WidgetTester tester) async {
await testBorder(tester, 'points_6', const StarBorder(points: 6));
await testBorder(tester, 'points_2', const StarBorder(points: 2));
await testBorder(tester, 'inner_radius_0', const StarBorder(innerRadiusRatio: 0.0));
await testBorder(tester, 'inner_radius_20', const StarBorder(innerRadiusRatio: 0.2));
await testBorder(tester, 'inner_radius_70', const StarBorder(innerRadiusRatio: 0.7));
await testBorder(tester, 'point_rounding_20', const StarBorder(pointRounding: 0.2));
await testBorder(tester, 'point_rounding_70', const StarBorder(pointRounding: 0.7));
await testBorder(tester, 'point_rounding_100', const StarBorder(pointRounding: 1.0));
await testBorder(tester, 'valley_rounding_20', const StarBorder(valleyRounding: 0.2));
await testBorder(tester, 'valley_rounding_70', const StarBorder(valleyRounding: 0.7));
await testBorder(tester, 'valley_rounding_100', const StarBorder(valleyRounding: 1.0));
await testBorder(tester, 'squash_2', const StarBorder(squash: 0.2));
await testBorder(tester, 'squash_7', const StarBorder(squash: 0.7));
await testBorder(tester, 'squash_10', const StarBorder(squash: 1.0));
await testBorder(tester, 'rotate_27', const StarBorder(rotation: 27));
await testBorder(tester, 'rotate_270', const StarBorder(rotation: 270));
await testBorder(tester, 'rotate_360', const StarBorder(rotation: 360));
await testBorder(tester, 'side_none', const StarBorder(side: BorderSide(style: BorderStyle.none)));
await testBorder(tester, 'side_1', const StarBorder(side: BorderSide(color: Color(0xffff0000))));
await testBorder(tester, 'side_10', const StarBorder(side: BorderSide(color: Color(0xffff0000), width: 10)));
await testBorder(tester, 'side_align_center', const StarBorder(side: BorderSide(color: Color(0xffff0000), strokeAlign: BorderSide.strokeAlignCenter)));
await testBorder(tester, 'side_align_outside', const StarBorder(side: BorderSide(color: Color(0xffff0000), strokeAlign: BorderSide.strokeAlignOutside)));
});
testWidgets('StarBorder.polygon parameters', (WidgetTester tester) async {
await testBorder(tester, 'poly_sides_6', const StarBorder.polygon(sides: 6));
await testBorder(tester, 'poly_sides_2', const StarBorder.polygon(sides: 2));
await testBorder(tester, 'poly_point_rounding_20', const StarBorder.polygon(pointRounding: 0.2));
await testBorder(tester, 'poly_point_rounding_70', const StarBorder.polygon(pointRounding: 0.7));
await testBorder(tester, 'poly_point_rounding_100', const StarBorder.polygon(pointRounding: 1.0));
await testBorder(tester, 'poly_squash_20', const StarBorder.polygon(squash: 0.2));
await testBorder(tester, 'poly_squash_70', const StarBorder.polygon(squash: 0.7));
await testBorder(tester, 'poly_squash_100', const StarBorder.polygon(squash: 1.0));
await testBorder(tester, 'poly_rotate_27', const StarBorder.polygon(rotation: 27));
await testBorder(tester, 'poly_rotate_270', const StarBorder.polygon(rotation: 270));
await testBorder(tester, 'poly_rotate_360', const StarBorder.polygon(rotation: 360));
await testBorder(tester, 'poly_side_none', const StarBorder.polygon(side: BorderSide(style: BorderStyle.none)));
await testBorder(tester, 'poly_side_1', const StarBorder.polygon(side: BorderSide(color: Color(0xffff0000))));
await testBorder(tester, 'poly_side_10', const StarBorder.polygon(side: BorderSide(color: Color(0xffff0000), width: 10)));
await testBorder(tester, 'poly_side_align_center', const StarBorder.polygon(side: BorderSide(color: Color(0xffff0000), strokeAlign: BorderSide.strokeAlignCenter)));
await testBorder(tester, 'poly_side_align_outside', const StarBorder.polygon(side: BorderSide(color: Color(0xffff0000), strokeAlign: BorderSide.strokeAlignOutside)));
});
testWidgets("StarBorder doesn't try to scale an infinite scale matrix", (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100,
height: 100,
child: Stack(
children: <Widget>[
Positioned.fromRelativeRect(
rect: const RelativeRect.fromLTRB(100, 100, 100, 100),
child: Container(
decoration: const ShapeDecoration(
color: Colors.green,
shape: StarBorder(),
),
),
),
],
),
),
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('StarBorder lerped with StarBorder', (WidgetTester tester) async {
const StarBorder from = StarBorder();
const ShapeBorder otherBorder = StarBorder(
points: 6,
pointRounding: 0.5,
valleyRounding: 0.5,
innerRadiusRatio: 0.5,
rotation: 90,
);
await testBorder(tester, 'to_star_border_20', from, lerpTo: otherBorder, lerpAmount: 0.2);
await testBorder(tester, 'to_star_border_70', from, lerpTo: otherBorder, lerpAmount: 0.7);
await testBorder(tester, 'to_star_border_100', from, lerpTo: otherBorder, lerpAmount: 1.0);
await testBorder(tester, 'from_star_border_20', from, lerpFrom: otherBorder, lerpAmount: 0.2);
await testBorder(tester, 'from_star_border_70', from, lerpFrom: otherBorder, lerpAmount: 0.7);
await testBorder(tester, 'from_star_border_100', from, lerpFrom: otherBorder, lerpAmount: 1.0);
});
testWidgets('StarBorder lerped with CircleBorder', (WidgetTester tester) async {
const StarBorder from = StarBorder();
const ShapeBorder otherBorder = CircleBorder();
const ShapeBorder eccentricCircle = CircleBorder(eccentricity: 0.6);
await testBorder(tester, 'to_circle_border_20', from, lerpTo: otherBorder, lerpAmount: 0.2);
await testBorder(tester, 'to_circle_border_70', from, lerpTo: otherBorder, lerpAmount: 0.7);
await testBorder(tester, 'to_circle_border_100', from, lerpTo: otherBorder, lerpAmount: 1.0);
await testBorder(tester, 'from_circle_border_20', from, lerpFrom: otherBorder, lerpAmount: 0.2);
await testBorder(tester, 'from_circle_border_70', from, lerpFrom: otherBorder, lerpAmount: 0.7);
await testBorder(tester, 'from_circle_border_100', from, lerpFrom: otherBorder, lerpAmount: 1.0);
await testBorder(tester, 'to_eccentric_circle_border_20', from, lerpTo: eccentricCircle, lerpAmount: 0.2);
await testBorder(tester, 'to_eccentric_circle_border_70', from, lerpTo: eccentricCircle, lerpAmount: 0.7);
await testBorder(tester, 'to_eccentric_circle_border_100', from, lerpTo: eccentricCircle, lerpAmount: 1.0);
await testBorder(tester, 'from_eccentric_circle_border_20', from, lerpFrom: eccentricCircle, lerpAmount: 0.2);
await testBorder(tester, 'from_eccentric_circle_border_70', from, lerpFrom: eccentricCircle, lerpAmount: 0.7);
await testBorder(tester, 'from_eccentric_circle_border_100', from, lerpFrom: eccentricCircle, lerpAmount: 1.0);
});
testWidgets('StarBorder lerped with RoundedRectangleBorder', (WidgetTester tester) async {
const StarBorder from = StarBorder();
const RoundedRectangleBorder rectangleBorder = RoundedRectangleBorder();
await testBorder(tester, 'to_rect_border_20', from, lerpTo: rectangleBorder, lerpAmount: 0.2);
await testBorder(tester, 'to_rect_border_70', from, lerpTo: rectangleBorder, lerpAmount: 0.7);
await testBorder(tester, 'to_rect_border_100', from, lerpTo: rectangleBorder, lerpAmount: 1.0);
await testBorder(tester, 'from_rect_border_20', from, lerpFrom: rectangleBorder, lerpAmount: 0.2);
await testBorder(tester, 'from_rect_border_70', from, lerpFrom: rectangleBorder, lerpAmount: 0.7);
await testBorder(tester, 'from_rect_border_100', from, lerpFrom: rectangleBorder, lerpAmount: 1.0);
const RoundedRectangleBorder roundedRectBorder = RoundedRectangleBorder(
borderRadius: BorderRadius.only(
bottomLeft: Radius.circular(10.0),
bottomRight: Radius.circular(10.0),
),
);
await testBorder(tester, 'to_rrect_border_20', from, lerpTo: roundedRectBorder, lerpAmount: 0.2);
await testBorder(tester, 'to_rrect_border_70', from, lerpTo: roundedRectBorder, lerpAmount: 0.7);
await testBorder(tester, 'to_rrect_border_100', from, lerpTo: roundedRectBorder, lerpAmount: 1.0);
await testBorder(tester, 'from_rrect_border_20', from, lerpFrom: roundedRectBorder, lerpAmount: 0.2);
await testBorder(tester, 'from_rrect_border_70', from, lerpFrom: roundedRectBorder, lerpAmount: 0.7);
await testBorder(tester, 'from_rrect_border_100', from, lerpFrom: roundedRectBorder, lerpAmount: 1.0);
});
testWidgets('StarBorder lerped with StadiumBorder', (WidgetTester tester) async {
const StarBorder from = StarBorder();
const StadiumBorder stadiumBorder = StadiumBorder();
await testBorder(tester, 'to_stadium_border_20', from, lerpTo: stadiumBorder, lerpAmount: 0.2);
await testBorder(tester, 'to_stadium_border_70', from, lerpTo: stadiumBorder, lerpAmount: 0.7);
await testBorder(tester, 'to_stadium_border_100', from, lerpTo: stadiumBorder, lerpAmount: 1.0);
await testBorder(tester, 'from_stadium_border_20', from, lerpFrom: stadiumBorder, lerpAmount: 0.2);
await testBorder(tester, 'from_stadium_border_70', from, lerpFrom: stadiumBorder, lerpAmount: 0.7);
await testBorder(tester, 'from_stadium_border_100', from, lerpFrom: stadiumBorder, lerpAmount: 1.0);
});
}
class StarBorderSubclass extends StarBorder {
const StarBorderSubclass();
}
| flutter/packages/flutter/test/painting/star_border_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/star_border_test.dart",
"repo_id": "flutter",
"token_count": 5254
} | 727 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('$AnnotatedRegion find', () {
test('finds the first value in a OffsetLayer when sized', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<OffsetLayer> layers = <OffsetLayer>[
OffsetLayer(),
OffsetLayer(offset: const Offset(0.0, 100.0)),
OffsetLayer(offset: const Offset(0.0, 200.0)),
];
int i = 0;
for (final OffsetLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i, size: const Size(200.0, 100.0)));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.find<int>(const Offset(0.0, 1.0)), 0);
expect(containerLayer.find<int>(const Offset(0.0, 101.0)), 1);
expect(containerLayer.find<int>(const Offset(0.0, 201.0)), 2);
});
test('finds a value within the clip in a ClipRectLayer', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<ClipRectLayer> layers = <ClipRectLayer>[
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 0.0, 100.0, 100.0)),
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 100.0, 100.0, 200.0)),
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 200.0, 100.0, 300.0)),
];
int i = 0;
for (final ClipRectLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.find<int>(const Offset(0.0, 1.0)), 0);
expect(containerLayer.find<int>(const Offset(0.0, 101.0)), 1);
expect(containerLayer.find<int>(const Offset(0.0, 201.0)), 2);
});
test('finds a value within the clip in a ClipRRectLayer', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<ClipRRectLayer> layers = <ClipRRectLayer>[
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 0.0, 100.0, 100.0, const Radius.circular(4.0))),
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 100.0, 100.0, 200.0, const Radius.circular(4.0))),
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 200.0, 100.0, 300.0, const Radius.circular(4.0))),
];
int i = 0;
for (final ClipRRectLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.find<int>(const Offset(5.0, 5.0)), 0);
expect(containerLayer.find<int>(const Offset(5.0, 105.0)), 1);
expect(containerLayer.find<int>(const Offset(5.0, 205.0)), 2);
});
test('finds a value under a TransformLayer', () {
final Matrix4 transform = Matrix4(
2.625, 0.0, 0.0, 0.0,
0.0, 2.625, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
);
final TransformLayer transformLayer = TransformLayer(transform: transform);
final List<OffsetLayer> layers = <OffsetLayer>[
OffsetLayer(),
OffsetLayer(offset: const Offset(0.0, 100.0)),
OffsetLayer(offset: const Offset(0.0, 200.0)),
];
int i = 0;
for (final OffsetLayer layer in layers) {
final AnnotatedRegionLayer<int> annotatedRegionLayer = AnnotatedRegionLayer<int>(i, size: const Size(100.0, 100.0));
layer.append(annotatedRegionLayer);
transformLayer.append(layer);
i += 1;
}
expect(transformLayer.find<int>(const Offset(0.0, 100.0)), 0);
expect(transformLayer.find<int>(const Offset(0.0, 200.0)), 0);
expect(transformLayer.find<int>(const Offset(0.0, 270.0)), 1);
expect(transformLayer.find<int>(const Offset(0.0, 400.0)), 1);
expect(transformLayer.find<int>(const Offset(0.0, 530.0)), 2);
});
test('looks for child AnnotatedRegions before parents', () {
final AnnotatedRegionLayer<int> parent = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<int> child = AnnotatedRegionLayer<int>(2);
final ContainerLayer layer = ContainerLayer();
parent.append(child);
layer.append(parent);
expect(parent.find<int>(Offset.zero), 2);
});
test('looks for correct type', () {
final AnnotatedRegionLayer<int> child1 = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<String> child2 = AnnotatedRegionLayer<String>('hello');
final ContainerLayer layer = ContainerLayer();
layer.append(child2);
layer.append(child1);
expect(layer.find<String>(Offset.zero), 'hello');
});
test('does not clip Layer.find on an AnnotatedRegion with an unrelated type', () {
final AnnotatedRegionLayer<int> child = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<String> parent = AnnotatedRegionLayer<String>('hello', size: const Size(10.0, 10.0));
final ContainerLayer layer = ContainerLayer();
parent.append(child);
layer.append(parent);
expect(layer.find<int>(const Offset(100.0, 100.0)), 1);
});
test('handles non-invertible transforms', () {
final AnnotatedRegionLayer<int> child = AnnotatedRegionLayer<int>(1);
final TransformLayer parent = TransformLayer(transform: Matrix4.diagonal3Values(0.0, 1.0, 1.0));
parent.append(child);
expect(parent.find<int>(Offset.zero), null);
parent.transform = Matrix4.diagonal3Values(1.0, 1.0, 1.0);
expect(parent.find<int>(Offset.zero), 1);
});
});
group('$AnnotatedRegion findAllAnnotations', () {
test('finds the first value in a OffsetLayer when sized', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<OffsetLayer> layers = <OffsetLayer>[
OffsetLayer(),
OffsetLayer(offset: const Offset(0.0, 100.0)),
OffsetLayer(offset: const Offset(0.0, 200.0)),
];
int i = 0;
for (final OffsetLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i, size: const Size(200.0, 100.0)));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 1.0)).annotations.toList(), equals(<int>[0]));
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 101.0)).annotations.toList(), equals(<int>[1]));
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 201.0)).annotations.toList(), equals(<int>[2]));
});
test('finds a value within the clip in a ClipRectLayer', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<ClipRectLayer> layers = <ClipRectLayer>[
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 0.0, 100.0, 100.0)),
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 100.0, 100.0, 200.0)),
ClipRectLayer(clipRect: const Rect.fromLTRB(0.0, 200.0, 100.0, 300.0)),
];
int i = 0;
for (final ClipRectLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 1.0)).annotations.toList(), equals(<int>[0]));
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 101.0)).annotations.toList(), equals(<int>[1]));
expect(containerLayer.findAllAnnotations<int>(const Offset(0.0, 201.0)).annotations.toList(), equals(<int>[2]));
});
test('finds a value within the clip in a ClipRRectLayer', () {
final ContainerLayer containerLayer = ContainerLayer();
final List<ClipRRectLayer> layers = <ClipRRectLayer>[
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 0.0, 100.0, 100.0, const Radius.circular(4.0))),
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 100.0, 100.0, 200.0, const Radius.circular(4.0))),
ClipRRectLayer(clipRRect: RRect.fromLTRBR(0.0, 200.0, 100.0, 300.0, const Radius.circular(4.0))),
];
int i = 0;
for (final ClipRRectLayer layer in layers) {
layer.append(AnnotatedRegionLayer<int>(i));
containerLayer.append(layer);
i += 1;
}
expect(containerLayer.findAllAnnotations<int>(const Offset(5.0, 5.0)).annotations.toList(), equals(<int>[0]));
expect(containerLayer.findAllAnnotations<int>(const Offset(5.0, 105.0)).annotations.toList(), equals(<int>[1]));
expect(containerLayer.findAllAnnotations<int>(const Offset(5.0, 205.0)).annotations.toList(), equals(<int>[2]));
});
test('finds a value under a TransformLayer', () {
final Matrix4 transform = Matrix4(
2.625, 0.0, 0.0, 0.0,
0.0, 2.625, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
);
final TransformLayer transformLayer = TransformLayer(transform: transform);
final List<OffsetLayer> layers = <OffsetLayer>[
OffsetLayer(),
OffsetLayer(offset: const Offset(0.0, 100.0)),
OffsetLayer(offset: const Offset(0.0, 200.0)),
];
int i = 0;
for (final OffsetLayer layer in layers) {
final AnnotatedRegionLayer<int> annotatedRegionLayer = AnnotatedRegionLayer<int>(i, size: const Size(100.0, 100.0));
layer.append(annotatedRegionLayer);
transformLayer.append(layer);
i += 1;
}
expect(transformLayer.findAllAnnotations<int>(const Offset(0.0, 100.0)).annotations.toList(), equals(<int>[0]));
expect(transformLayer.findAllAnnotations<int>(const Offset(0.0, 200.0)).annotations.toList(), equals(<int>[0]));
expect(transformLayer.findAllAnnotations<int>(const Offset(0.0, 270.0)).annotations.toList(), equals(<int>[1]));
expect(transformLayer.findAllAnnotations<int>(const Offset(0.0, 400.0)).annotations.toList(), equals(<int>[1]));
expect(transformLayer.findAllAnnotations<int>(const Offset(0.0, 530.0)).annotations.toList(), equals(<int>[2]));
});
test('finds multiple nested, overlapping regions', () {
final ContainerLayer parent = ContainerLayer();
int index = 0;
final List<AnnotatedRegionLayer<int>> layers = <AnnotatedRegionLayer<int>>[
AnnotatedRegionLayer<int>(index++, size: const Size(100.0, 100.0)),
AnnotatedRegionLayer<int>(index++, size: const Size(100.0, 100.0)),
];
for (final ContainerLayer layer in layers) {
final AnnotatedRegionLayer<int> annotatedRegionLayer = AnnotatedRegionLayer<int>(index++, size: const Size(100.0, 100.0));
layer.append(annotatedRegionLayer);
parent.append(layer);
}
expect(parent.findAllAnnotations<int>(Offset.zero).annotations.toList(), equals(<int>[3, 1, 2, 0,]));
});
test('looks for child AnnotatedRegions before parents', () {
final AnnotatedRegionLayer<int> parent = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<int> child1 = AnnotatedRegionLayer<int>(2);
final AnnotatedRegionLayer<int> child2 = AnnotatedRegionLayer<int>(3);
final AnnotatedRegionLayer<int> child3 = AnnotatedRegionLayer<int>(4);
final ContainerLayer layer = ContainerLayer();
parent.append(child1);
parent.append(child2);
parent.append(child3);
layer.append(parent);
expect(parent.findAllAnnotations<int>(Offset.zero).annotations.toList(), equals(<int>[4, 3, 2, 1]));
});
test('looks for correct type', () {
final AnnotatedRegionLayer<int> child1 = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<String> child2 = AnnotatedRegionLayer<String>('hello');
final ContainerLayer layer = ContainerLayer();
layer.append(child2);
layer.append(child1);
expect(layer.findAllAnnotations<String>(Offset.zero).annotations.toList(), equals(<String>['hello']));
});
test('does not clip Layer.find on an AnnotatedRegion with an unrelated type', () {
final AnnotatedRegionLayer<int> child = AnnotatedRegionLayer<int>(1);
final AnnotatedRegionLayer<String> parent = AnnotatedRegionLayer<String>('hello', size: const Size(10.0, 10.0));
final ContainerLayer layer = ContainerLayer();
parent.append(child);
layer.append(parent);
expect(layer.findAllAnnotations<int>(const Offset(100.0, 100.0)).annotations.toList(), equals(<int>[1]));
});
test('handles non-invertible transforms', () {
final AnnotatedRegionLayer<int> child = AnnotatedRegionLayer<int>(1);
final TransformLayer parent = TransformLayer(transform: Matrix4.diagonal3Values(0.0, 1.0, 1.0));
parent.append(child);
expect(parent.findAllAnnotations<int>(Offset.zero).annotations.toList(), equals(<int>[]));
parent.transform = Matrix4.diagonal3Values(1.0, 1.0, 1.0);
expect(parent.findAllAnnotations<int>(Offset.zero).annotations.toList(), equals(<int>[1]));
});
});
}
| flutter/packages/flutter/test/rendering/annotated_region_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/annotated_region_test.dart",
"repo_id": "flutter",
"token_count": 5186
} | 728 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
/// Unit tests error.dart's usage via ErrorWidget.
void main() {
const String errorMessage = 'Some error message';
testWidgets('test draw error paragraph', (WidgetTester tester) async {
await tester.pumpWidget(ErrorWidget(Exception(errorMessage)));
expect(
find.byType(ErrorWidget),
paints
..rect(rect: const Rect.fromLTWH(0.0, 0.0, 800.0, 600.0))
..paragraph(offset: const Offset(64.0, 96.0)),
);
final Widget error = Builder(builder: (BuildContext context) => throw 'pillow');
await tester.pumpWidget(Center(child: SizedBox(width: 100.0, child: error)));
expect(tester.takeException(), 'pillow');
expect(
find.byType(ErrorWidget),
paints
..rect(rect: const Rect.fromLTWH(0.0, 0.0, 100.0, 600.0))
..paragraph(offset: const Offset(0.0, 96.0)),
);
await tester.pumpWidget(Center(child: SizedBox(height: 100.0, child: error)));
expect(tester.takeException(), null);
await tester.pumpWidget(Center(child: SizedBox(key: UniqueKey(), height: 100.0, child: error)));
expect(tester.takeException(), 'pillow');
expect(
find.byType(ErrorWidget),
paints
..rect(rect: const Rect.fromLTWH(0.0, 0.0, 800.0, 100.0))
..paragraph(offset: const Offset(64.0, 0.0)),
);
RenderErrorBox.minimumWidth = 800.0;
await tester.pumpWidget(Center(child: error));
expect(tester.takeException(), 'pillow');
expect(
find.byType(ErrorWidget),
paints
..rect(rect: const Rect.fromLTWH(0.0, 0.0, 800.0, 600.0))
..paragraph(offset: const Offset(0.0, 96.0)),
);
await tester.pumpWidget(Center(child: error));
expect(tester.takeException(), null);
expect(
find.byType(ErrorWidget),
paints
..rect(color: const Color(0xF0900000))
..paragraph(),
);
RenderErrorBox.backgroundColor = const Color(0xFF112233);
await tester.pumpWidget(Center(child: error));
expect(tester.takeException(), null);
expect(
find.byType(ErrorWidget),
paints
..rect(color: const Color(0xFF112233))
..paragraph(),
);
});
}
| flutter/packages/flutter/test/rendering/error_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/error_test.dart",
"repo_id": "flutter",
"token_count": 970
} | 729 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
class RenderLayoutTestBox extends RenderProxyBox {
RenderLayoutTestBox(this.onLayout, {
this.onPerformLayout,
});
final VoidCallback onLayout;
final VoidCallback? onPerformLayout;
@override
void layout(Constraints constraints, { bool parentUsesSize = false }) {
// Doing this in tests is ok, but if you're writing your own
// render object, you want to override performLayout(), not
// layout(). Overriding layout() would remove many critical
// performance optimizations of the rendering system, as well as
// many bypassing many checked-mode integrity checks.
super.layout(constraints, parentUsesSize: parentUsesSize);
onLayout();
}
@override
bool get sizedByParent => true;
@override
void performLayout() {
child?.layout(constraints, parentUsesSize: true);
onPerformLayout?.call();
}
}
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('moving children', () {
RenderBox child1, child2;
bool movedChild1 = false;
bool movedChild2 = false;
final RenderFlex block = RenderFlex(textDirection: TextDirection.ltr);
block.add(child1 = RenderLayoutTestBox(() { movedChild1 = true; }));
block.add(child2 = RenderLayoutTestBox(() { movedChild2 = true; }));
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
layout(block);
expect(movedChild1, isTrue);
expect(movedChild2, isTrue);
movedChild1 = false;
movedChild2 = false;
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
pumpFrame();
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
block.move(child1, after: child2);
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
pumpFrame();
expect(movedChild1, isTrue);
expect(movedChild2, isTrue);
movedChild1 = false;
movedChild2 = false;
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
pumpFrame();
expect(movedChild1, isFalse);
expect(movedChild2, isFalse);
});
group('Throws when illegal mutations are attempted: ', () {
FlutterError catchLayoutError(RenderBox box) {
Object? error;
layout(box, onErrors: () {
error = TestRenderingFlutterBinding.instance.takeFlutterErrorDetails()!.exception;
});
expect(error, isFlutterError);
return error! as FlutterError;
}
test('on disposed render objects', () {
final RenderBox box = RenderLayoutTestBox(() {});
box.dispose();
Object? error;
try {
box.markNeedsLayout();
} catch (e) {
error = e;
}
expect(error, isFlutterError);
expect(
(error! as FlutterError).message,
equalsIgnoringWhitespace(
'A disposed RenderObject was mutated.\n'
'The disposed RenderObject was:\n'
'${box.toStringShort()}'
)
);
});
test('marking itself dirty in performLayout', () {
late RenderBox child1;
final RenderFlex block = RenderFlex(textDirection: TextDirection.ltr);
block.add(child1 = RenderLayoutTestBox(() {}, onPerformLayout: () { child1.markNeedsLayout(); }));
expect(
catchLayoutError(block).message,
equalsIgnoringWhitespace(
'A RenderLayoutTestBox was mutated in its own performLayout implementation.\n'
'A RenderObject must not re-dirty itself while still being laid out.\n'
'The RenderObject being mutated was:\n'
'${child1.toStringShort()}\n'
'Consider using the LayoutBuilder widget to dynamically change a subtree during layout.'
)
);
});
test('marking a sibling dirty in performLayout', () {
late RenderBox child1, child2;
final RenderFlex block = RenderFlex(textDirection: TextDirection.ltr);
block.add(child1 = RenderLayoutTestBox(() {}));
block.add(child2 = RenderLayoutTestBox(() {}, onPerformLayout: () { child1.markNeedsLayout(); }));
expect(
catchLayoutError(block).message,
equalsIgnoringWhitespace(
'A RenderLayoutTestBox was mutated in RenderLayoutTestBox.performLayout.\n'
'A RenderObject must not mutate another RenderObject from a different render subtree in its performLayout method.\n'
'The RenderObject being mutated was:\n'
'${child1.toStringShort()}\n'
'The RenderObject that was mutating the said RenderLayoutTestBox was:\n'
'${child2.toStringShort()}\n'
'Their common ancestor was:\n'
'${block.toStringShort()}\n'
'Mutating the layout of another RenderObject may cause some RenderObjects in its subtree to be laid out more than once. Consider using the LayoutBuilder widget to dynamically mutate a subtree during layout.'
)
);
});
test('marking a descendant dirty in performLayout', () {
late RenderBox child1;
final RenderFlex block = RenderFlex(textDirection: TextDirection.ltr);
block.add(child1 = RenderLayoutTestBox(() {}));
block.add(RenderLayoutTestBox(child1.markNeedsLayout));
expect(
catchLayoutError(block).message,
equalsIgnoringWhitespace(
'A RenderLayoutTestBox was mutated in RenderFlex.performLayout.\n'
'A RenderObject must not mutate its descendants in its performLayout method.\n'
'The RenderObject being mutated was:\n'
'${child1.toStringShort()}\n'
'The ancestor RenderObject that was mutating the said RenderLayoutTestBox was:\n'
'${block.toStringShort()}\n'
'Mutating the layout of another RenderObject may cause some RenderObjects in its subtree to be laid out more than once. Consider using the LayoutBuilder widget to dynamically mutate a subtree during layout.'
),
);
});
test('marking an out-of-band mutation in performLayout', () {
late RenderProxyBox child1, child11, child2, child21;
final RenderFlex block = RenderFlex(textDirection: TextDirection.ltr);
block.add(child1 = RenderLayoutTestBox(() {}));
block.add(child2 = RenderLayoutTestBox(() {}));
child1.child = child11 = RenderLayoutTestBox(() {});
layout(block);
expect(block.debugNeedsLayout, false);
expect(child1.debugNeedsLayout, false);
expect(child11.debugNeedsLayout, false);
expect(child2.debugNeedsLayout, false);
// Add a new child to child2 which is a relayout boundary.
child2.child = child21 = RenderLayoutTestBox(() {}, onPerformLayout: child11.markNeedsLayout);
FlutterError? error;
pumpFrame(onErrors: () {
error = TestRenderingFlutterBinding.instance.takeFlutterErrorDetails()!.exception as FlutterError;
});
expect(
error?.message,
equalsIgnoringWhitespace(
'A RenderLayoutTestBox was mutated in RenderLayoutTestBox.performLayout.\n'
'The RenderObject was mutated when none of its ancestors is actively performing layout.\n'
'The RenderObject being mutated was:\n'
'${child11.toStringShort()}\n'
'The RenderObject that was mutating the said RenderLayoutTestBox was:\n'
'${child21.toStringShort()}'
),
);
});
});
}
| flutter/packages/flutter/test/rendering/mutations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/mutations_test.dart",
"repo_id": "flutter",
"token_count": 2797
} | 730 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
class TestTree {
TestTree() {
// incoming constraints are tight 800x600
root = RenderPositionedBox(
child: RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(width: 800.0),
// Place the child to be evaluated within both a repaint boundary and a
// layout-root element (in this case a tightly constrained box). Otherwise
// the act of transplanting the root into a new container will cause the
// relayout/repaint of the new parent node to satisfy the test.
child: RenderRepaintBoundary(
child: RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(height: 20.0, width: 20.0),
child: RenderRepaintBoundary(
child: RenderCustomPaint(
painter: TestCallbackPainter(
onPaint: () { painted = true; },
),
child: RenderPositionedBox(
child: child = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(height: 20.0, width: 20.0),
child: RenderSemanticsAnnotations(
textDirection: TextDirection.ltr,
properties: const SemanticsProperties(label: 'Hello there foo'),
),
),
),
),
),
),
),
),
);
}
late RenderBox root;
late RenderConstrainedBox child;
bool painted = false;
}
class MutableCompositor extends RenderProxyBox {
MutableCompositor({ required RenderBox child }) : super(child);
bool _alwaysComposite = false;
@override
bool get alwaysNeedsCompositing => _alwaysComposite;
}
class TestCompositingBitsTree {
TestCompositingBitsTree() {
// incoming constraints are tight 800x600
root = RenderPositionedBox(
child: RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(width: 800.0),
// Place the child to be evaluated within a repaint boundary. Otherwise
// the act of transplanting the root into a new container will cause the
// repaint of the new parent node to satisfy the test.
child: RenderRepaintBoundary(
child: compositor = MutableCompositor(
child: RenderCustomPaint(
painter: TestCallbackPainter(
onPaint: () { painted = true; },
),
child: child = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(height: 20.0, width: 20.0),
),
),
),
),
),
);
}
late RenderBox root;
late MutableCompositor compositor;
late RenderConstrainedBox child;
bool painted = false;
}
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('objects can be detached and re-attached: layout', () {
final TestTree testTree = TestTree();
// Lay out
layout(testTree.root);
expect(testTree.child.size, equals(const Size(20.0, 20.0)));
// Remove testTree from the custom render view
TestRenderingFlutterBinding.instance.renderView.child = null;
expect(testTree.child.owner, isNull);
// Dirty one of the elements
testTree.child.additionalConstraints =
const BoxConstraints.tightFor(height: 5.0, width: 5.0);
// Lay out again
layout(testTree.root);
expect(testTree.child.size, equals(const Size(5.0, 5.0)));
});
test('objects can be detached and re-attached: compositingBits', () {
final TestCompositingBitsTree testTree = TestCompositingBitsTree();
// Lay out, composite, and paint
layout(testTree.root, phase: EnginePhase.paint);
expect(testTree.painted, isTrue);
// Remove testTree from the custom render view
TestRenderingFlutterBinding.instance.renderView.child = null;
expect(testTree.child.owner, isNull);
// Dirty one of the elements
testTree.compositor._alwaysComposite = true;
testTree.child.markNeedsCompositingBitsUpdate();
testTree.painted = false;
// Lay out, composite, and paint again
layout(testTree.root, phase: EnginePhase.paint);
expect(testTree.painted, isTrue);
});
test('objects can be detached and re-attached: paint', () {
final TestTree testTree = TestTree();
// Lay out, composite, and paint
layout(testTree.root, phase: EnginePhase.paint);
expect(testTree.painted, isTrue);
// Remove testTree from the custom render view
TestRenderingFlutterBinding.instance.renderView.child = null;
expect(testTree.child.owner, isNull);
// Dirty one of the elements
testTree.child.markNeedsPaint();
testTree.painted = false;
// Lay out, composite, and paint again
layout(testTree.root, phase: EnginePhase.paint);
expect(testTree.painted, isTrue);
});
test('objects can be detached and re-attached: semantics (no change)', () {
final TestTree testTree = TestTree();
int semanticsUpdateCount = 0;
final SemanticsHandle semanticsHandle = TestRenderingFlutterBinding.instance.pipelineOwner.ensureSemantics(
listener: () {
++semanticsUpdateCount;
},
);
// Lay out, composite, paint, and update semantics
layout(testTree.root, phase: EnginePhase.flushSemantics);
expect(semanticsUpdateCount, 1);
// Remove testTree from the custom render view
TestRenderingFlutterBinding.instance.renderView.child = null;
expect(testTree.child.owner, isNull);
// Dirty one of the elements
semanticsUpdateCount = 0;
testTree.child.markNeedsSemanticsUpdate();
expect(semanticsUpdateCount, 0);
// Lay out, composite, paint, and update semantics again
layout(testTree.root, phase: EnginePhase.flushSemantics);
expect(semanticsUpdateCount, 0); // no semantics have changed.
semanticsHandle.dispose();
});
test('objects can be detached and re-attached: semantics (with change)', () {
final TestTree testTree = TestTree();
int semanticsUpdateCount = 0;
final SemanticsHandle semanticsHandle = TestRenderingFlutterBinding.instance.pipelineOwner.ensureSemantics(
listener: () {
++semanticsUpdateCount;
},
);
// Lay out, composite, paint, and update semantics
layout(testTree.root, phase: EnginePhase.flushSemantics);
expect(semanticsUpdateCount, 1);
// Remove testTree from the custom render view
TestRenderingFlutterBinding.instance.renderView.child = null;
expect(testTree.child.owner, isNull);
// Dirty one of the elements
semanticsUpdateCount = 0;
testTree.child.additionalConstraints = const BoxConstraints.tightFor(height: 20.0, width: 30.0);
testTree.child.markNeedsSemanticsUpdate();
expect(semanticsUpdateCount, 0);
// Lay out, composite, paint, and update semantics again
layout(testTree.root, phase: EnginePhase.flushSemantics);
expect(semanticsUpdateCount, 1); // semantics have changed.
semanticsHandle.dispose();
});
}
| flutter/packages/flutter/test/rendering/reattach_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/reattach_test.dart",
"repo_id": "flutter",
"token_count": 2689
} | 731 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
int layouts = 0;
class RenderLayoutWatcher extends RenderProxyBox {
RenderLayoutWatcher(RenderBox super.child);
@override
void performLayout() {
layouts += 1;
super.performLayout();
}
}
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('RenderViewport basic test - impact of layout', () {
RenderSliverToBoxAdapter sliver;
RenderViewport viewport;
RenderBox box;
final RenderLayoutWatcher root = RenderLayoutWatcher(
viewport = RenderViewport(
crossAxisDirection: AxisDirection.right,
offset: ViewportOffset.zero(),
children: <RenderSliver>[
sliver = RenderSliverToBoxAdapter(child: box = RenderSizedBox(const Size(100.0, 400.0))),
],
),
);
expect(layouts, 0);
layout(root);
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 200.0));
sliver.child = box = RenderSizedBox(const Size(100.0, 300.0));
expect(layouts, 1);
pumpFrame();
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 150.0));
viewport.offset = ViewportOffset.fixed(20.0);
expect(layouts, 1);
pumpFrame();
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 130.0));
viewport.offset = ViewportOffset.fixed(-20.0);
expect(layouts, 1);
pumpFrame();
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 170.0));
viewport.anchor = 20.0 / 600.0;
expect(layouts, 1);
pumpFrame();
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 190.0));
viewport.axisDirection = AxisDirection.up;
expect(layouts, 1);
pumpFrame();
expect(layouts, 1);
expect(box.localToGlobal(box.size.center(Offset.zero)), const Offset(400.0, 600.0 - 190.0));
});
}
| flutter/packages/flutter/test/rendering/slivers_layout_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/slivers_layout_test.dart",
"repo_id": "flutter",
"token_count": 853
} | 732 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
void main() {
late SchedulerBinding binding;
setUpAll(() {
WidgetsFlutterBinding.ensureInitialized();
binding = SchedulerBinding.instance;
});
test('PerformanceModeHandler make one request', () async {
final PerformanceModeRequestHandle? requestHandle = binding.requestPerformanceMode(DartPerformanceMode.latency);
expect(requestHandle, isNotNull);
expect(binding.debugGetRequestedPerformanceMode(), equals(DartPerformanceMode.latency));
requestHandle?.dispose();
expect(binding.debugGetRequestedPerformanceMode(), isNull);
});
test('PerformanceModeHandler make conflicting requests', () async {
final PerformanceModeRequestHandle? requestHandle1 = binding.requestPerformanceMode(DartPerformanceMode.latency);
expect(requestHandle1, isNotNull);
final PerformanceModeRequestHandle? requestHandle2 = binding.requestPerformanceMode(DartPerformanceMode.throughput);
expect(requestHandle2, isNull);
expect(binding.debugGetRequestedPerformanceMode(), equals(DartPerformanceMode.latency));
requestHandle1?.dispose();
expect(binding.debugGetRequestedPerformanceMode(), isNull);
});
test('PerformanceModeHandler revert only after last requestor disposed',
() async {
final PerformanceModeRequestHandle? requestHandle1 = binding.requestPerformanceMode(DartPerformanceMode.latency);
expect(requestHandle1, isNotNull);
expect(binding.debugGetRequestedPerformanceMode(), equals(DartPerformanceMode.latency));
final PerformanceModeRequestHandle? requestHandle2 = binding.requestPerformanceMode(DartPerformanceMode.latency);
expect(requestHandle2, isNotNull);
expect(binding.debugGetRequestedPerformanceMode(), equals(DartPerformanceMode.latency));
requestHandle1?.dispose();
expect(binding.debugGetRequestedPerformanceMode(), equals(DartPerformanceMode.latency));
requestHandle2?.dispose();
expect(binding.debugGetRequestedPerformanceMode(), isNull);
});
test('PerformanceModeRequestHandle dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => binding.requestPerformanceMode(DartPerformanceMode.latency)!.dispose(),
PerformanceModeRequestHandle,
),
areCreateAndDispose,
);
});
}
| flutter/packages/flutter/test/scheduler/performance_mode_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/scheduler/performance_mode_test.dart",
"repo_id": "flutter",
"token_count": 780
} | 733 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'text_input_utils.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('AutofillClient', () {
late FakeTextChannel fakeTextChannel;
final FakeAutofillScope scope = FakeAutofillScope();
setUp(() {
fakeTextChannel = FakeTextChannel((MethodCall call) async {});
TextInput.setChannel(fakeTextChannel);
scope.clients.clear();
});
tearDown(() {
TextInputConnection.debugResetId();
TextInput.setChannel(SystemChannels.textInput);
});
test('Does not throw if the hint list is empty', () async {
Object? exception;
try {
const AutofillConfiguration(
uniqueIdentifier: 'id',
autofillHints: <String>[],
currentEditingValue: TextEditingValue.empty,
);
} catch (e) {
exception = e;
}
expect(exception, isNull);
});
test(
'AutofillClients send the correct configuration to the platform and responds to updateEditingStateWithTag method correctly',
() async {
final FakeAutofillClient client1 = FakeAutofillClient(const TextEditingValue(text: 'test1'));
final FakeAutofillClient client2 = FakeAutofillClient(const TextEditingValue(text: 'test2'));
client1.textInputConfiguration = TextInputConfiguration(
autofillConfiguration: AutofillConfiguration(
uniqueIdentifier: client1.autofillId,
autofillHints: const <String>['client1'],
currentEditingValue: client1.currentTextEditingValue,
),
);
client2.textInputConfiguration = TextInputConfiguration(
autofillConfiguration: AutofillConfiguration(
uniqueIdentifier: client2.autofillId,
autofillHints: const <String>['client2'],
currentEditingValue: client2.currentTextEditingValue,
),
);
scope.register(client1);
scope.register(client2);
client1.currentAutofillScope = scope;
client2.currentAutofillScope = scope;
scope.attach(client1, client1.textInputConfiguration);
final Map<String, dynamic> expectedConfiguration = client1.textInputConfiguration.toJson();
expectedConfiguration['fields'] = <Map<String, dynamic>>[
client1.textInputConfiguration.toJson(),
client2.textInputConfiguration.toJson(),
];
fakeTextChannel.validateOutgoingMethodCalls(<MethodCall>[
MethodCall('TextInput.setClient', <dynamic>[1, expectedConfiguration]),
]);
const TextEditingValue text2 = TextEditingValue(text: 'Text 2');
fakeTextChannel.incoming?.call(MethodCall(
'TextInputClient.updateEditingStateWithTag',
<dynamic>[0, <String, dynamic>{ client2.autofillId : text2.toJSON() }],
));
expect(client2.currentTextEditingValue, text2);
},
);
});
}
class FakeAutofillClient implements TextInputClient, AutofillClient {
FakeAutofillClient(this.currentTextEditingValue);
@override
String get autofillId => hashCode.toString();
@override
late TextInputConfiguration textInputConfiguration;
@override
void updateEditingValue(TextEditingValue newEditingValue) {
currentTextEditingValue = newEditingValue;
latestMethodCall = 'updateEditingValue';
}
@override
AutofillScope? currentAutofillScope;
String latestMethodCall = '';
@override
TextEditingValue currentTextEditingValue;
@override
void performAction(TextInputAction action) {
latestMethodCall = 'performAction';
}
@override
void performPrivateCommand(String action, Map<String, dynamic> data) {
latestMethodCall = 'performPrivateCommand';
}
@override
void insertContent(KeyboardInsertedContent content) {
latestMethodCall = 'commitContent';
}
@override
void updateFloatingCursor(RawFloatingCursorPoint point) {
latestMethodCall = 'updateFloatingCursor';
}
@override
void connectionClosed() {
latestMethodCall = 'connectionClosed';
}
@override
void showAutocorrectionPromptRect(int start, int end) {
latestMethodCall = 'showAutocorrectionPromptRect';
}
@override
void didChangeInputControl(TextInputControl? oldControl, TextInputControl? newControl) {
latestMethodCall = 'didChangeInputControl';
}
@override
void autofill(TextEditingValue newEditingValue) => updateEditingValue(newEditingValue);
@override
void showToolbar() {
latestMethodCall = 'showToolbar';
}
@override
void insertTextPlaceholder(Size size) {
latestMethodCall = 'insertTextPlaceholder';
}
@override
void removeTextPlaceholder() {
latestMethodCall = 'removeTextPlaceholder';
}
@override
void performSelector(String selectorName) {
latestMethodCall = 'performSelector';
}
}
class FakeAutofillScope with AutofillScopeMixin implements AutofillScope {
final Map<String, AutofillClient> clients = <String, AutofillClient>{};
@override
Iterable<AutofillClient> get autofillClients => clients.values;
@override
AutofillClient getAutofillClient(String autofillId) => clients[autofillId]!;
void register(AutofillClient client) {
clients.putIfAbsent(client.autofillId, () => client);
}
}
| flutter/packages/flutter/test/services/autofill_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/autofill_test.dart",
"repo_id": "flutter",
"token_count": 1970
} | 734 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This files contains message codec tests that are supported both on the Web
// and in the VM. For VM-only tests see message_codecs_vm_test.dart.
import 'dart:convert';
import 'dart:typed_data';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'message_codecs_testing.dart';
void main() {
group('Binary codec', () {
const MessageCodec<ByteData?> binary = BinaryCodec();
test('should encode and decode simple messages', () {
checkEncodeDecode<ByteData?>(binary, null);
checkEncodeDecode<ByteData?>(binary, ByteData(0));
checkEncodeDecode<ByteData?>(binary, ByteData(4)..setInt32(0, -7));
});
});
group('String codec', () {
const MessageCodec<String?> string = StringCodec();
test('should encode and decode simple messages', () {
checkEncodeDecode<String?>(string, null);
checkEncodeDecode<String?>(string, '');
checkEncodeDecode<String?>(string, 'hello');
checkEncodeDecode<String?>(string, 'special chars >\u263A\u{1F602}<');
});
test('ByteData with offset', () {
const MessageCodec<String?> string = StringCodec();
final ByteData helloWorldByteData = string.encodeMessage('hello world')!;
final ByteData helloByteData = string.encodeMessage('hello')!;
final ByteData offsetByteData = ByteData.view(
helloWorldByteData.buffer,
helloByteData.lengthInBytes,
helloWorldByteData.lengthInBytes - helloByteData.lengthInBytes,
);
expect(string.decodeMessage(offsetByteData), ' world');
});
});
group('Standard method codec', () {
const MethodCodec method = StandardMethodCodec();
const StandardMessageCodec messageCodec = StandardMessageCodec();
test('Should encode and decode objects produced from codec', () {
final ByteData? data = messageCodec.encodeMessage(<Object, Object>{
'foo': true,
3: 'fizz',
});
expect(messageCodec.decodeMessage(data), <Object?, Object?>{
'foo': true,
3: 'fizz',
});
});
test('should decode error envelope without native stacktrace', () {
final ByteData errorData = method.encodeErrorEnvelope(
code: 'errorCode',
message: 'errorMessage',
details: 'errorDetails',
);
expect(
() => method.decodeEnvelope(errorData),
throwsA(predicate(
(PlatformException e) =>
e.code == 'errorCode' &&
e.message == 'errorMessage' &&
e.details == 'errorDetails',
)),
);
});
test('should decode error envelope with native stacktrace.', () {
final WriteBuffer buffer = WriteBuffer();
buffer.putUint8(1);
messageCodec.writeValue(buffer, 'errorCode');
messageCodec.writeValue(buffer, 'errorMessage');
messageCodec.writeValue(buffer, 'errorDetails');
messageCodec.writeValue(buffer, 'errorStacktrace');
final ByteData errorData = buffer.done();
expect(
() => method.decodeEnvelope(errorData),
throwsA(predicate((PlatformException e) => e.stacktrace == 'errorStacktrace')),
);
});
test('should allow null error message,', () {
final ByteData errorData = method.encodeErrorEnvelope(
code: 'errorCode',
details: 'errorDetails',
);
expect(
() => method.decodeEnvelope(errorData),
throwsA(
predicate((PlatformException e) {
return e.code == 'errorCode' &&
e.message == null &&
e.details == 'errorDetails';
}),
),
);
});
});
group('Json method codec', () {
const JsonCodec json = JsonCodec();
const StringCodec stringCodec = StringCodec();
const JSONMethodCodec jsonMethodCodec = JSONMethodCodec();
test('should decode error envelope without native stacktrace', () {
final ByteData errorData = jsonMethodCodec.encodeErrorEnvelope(
code: 'errorCode',
message: 'errorMessage',
details: 'errorDetails',
);
expect(
() => jsonMethodCodec.decodeEnvelope(errorData),
throwsA(predicate(
(PlatformException e) =>
e.code == 'errorCode' &&
e.message == 'errorMessage' &&
e.details == 'errorDetails',
)),
);
});
test('should decode error envelope with native stacktrace.', () {
final ByteData? errorData = stringCodec.encodeMessage(json.encode(<dynamic>[
'errorCode',
'errorMessage',
'errorDetails',
'errorStacktrace',
]));
expect(
() => jsonMethodCodec.decodeEnvelope(errorData!),
throwsA(predicate((PlatformException e) => e.stacktrace == 'errorStacktrace')),
);
});
});
group('JSON message codec', () {
const MessageCodec<dynamic> json = JSONMessageCodec();
test('should encode and decode simple messages', () {
checkEncodeDecode<dynamic>(json, null);
checkEncodeDecode<dynamic>(json, true);
checkEncodeDecode<dynamic>(json, false);
checkEncodeDecode<dynamic>(json, 7);
checkEncodeDecode<dynamic>(json, -7);
checkEncodeDecode<dynamic>(json, 98742923489);
checkEncodeDecode<dynamic>(json, -98742923489);
checkEncodeDecode<dynamic>(json, 3.14);
checkEncodeDecode<dynamic>(json, '');
checkEncodeDecode<dynamic>(json, 'hello');
checkEncodeDecode<dynamic>(json, 'special chars >\u263A\u{1F602}<');
});
test('should encode and decode composite message', () {
final List<dynamic> message = <dynamic>[
null,
true,
false,
-707,
-7000000007,
-3.14,
'',
'hello',
<dynamic>['nested', <dynamic>[]],
<dynamic, dynamic>{'a': 'nested', 'b': <dynamic, dynamic>{}},
'world',
];
checkEncodeDecode<dynamic>(json, message);
});
});
group('Standard message codec', () {
const MessageCodec<dynamic> standard = StandardMessageCodec();
test('should encode sizes correctly at boundary cases', () {
checkEncoding<dynamic>(
standard,
Uint8List(253),
<int>[8, 253, ...List<int>.filled(253, 0)],
);
checkEncoding<dynamic>(
standard,
Uint8List(254),
<int>[8, 254, 254, 0, ...List<int>.filled(254, 0)],
);
checkEncoding<dynamic>(
standard,
Uint8List(0xffff),
<int>[8, 254, 0xff, 0xff, ...List<int>.filled(0xffff, 0)],
);
checkEncoding<dynamic>(
standard,
Uint8List(0xffff + 1),
<int>[8, 255, 0, 0, 1, 0, ...List<int>.filled(0xffff + 1, 0)],
);
});
test('should encode and decode simple messages', () {
checkEncodeDecode<dynamic>(standard, null);
checkEncodeDecode<dynamic>(standard, true);
checkEncodeDecode<dynamic>(standard, false);
checkEncodeDecode<dynamic>(standard, 7);
checkEncodeDecode<dynamic>(standard, -7);
checkEncodeDecode<dynamic>(standard, 98742923489);
checkEncodeDecode<dynamic>(standard, -98742923489);
checkEncodeDecode<dynamic>(standard, 3.14);
checkEncodeDecode<dynamic>(standard, double.infinity);
checkEncodeDecode<dynamic>(standard, double.nan);
checkEncodeDecode<dynamic>(standard, '');
checkEncodeDecode<dynamic>(standard, 'hello');
checkEncodeDecode<dynamic>(standard, 'special chars >\u263A\u{1F602}<');
});
test('should encode and decode composite message', () {
final List<dynamic> message = <dynamic>[
null,
true,
false,
-707,
-7000000007,
-3.14,
'',
'hello',
Uint8List.fromList(<int>[0xBA, 0x5E, 0xBA, 0x11]),
Int32List.fromList(<int>[-0x7fffffff - 1, 0, 0x7fffffff]),
null, // ensures the offset of the following list is unaligned.
null, // ensures the offset of the following list is unaligned.
Float64List.fromList(<double>[
double.negativeInfinity,
-double.maxFinite,
-double.minPositive,
-0.0,
0.0,
double.minPositive,
double.maxFinite,
double.infinity,
double.nan,
]),
Float32List.fromList(<double>[
double.negativeInfinity,
-double.maxFinite,
-double.minPositive,
-0.0,
0.0,
double.minPositive,
double.maxFinite,
double.infinity,
double.nan,
]),
<dynamic>['nested', <dynamic>[]],
<dynamic, dynamic>{'a': 'nested', null: <dynamic, dynamic>{}},
'world',
];
checkEncodeDecode<dynamic>(standard, message);
});
test('should align doubles to 8 bytes', () {
checkEncoding<dynamic>(
standard,
1.0,
<int>[
6,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0xf0,
0x3f,
],
);
});
});
test('toString works as intended', () async {
const MethodCall methodCall = MethodCall('sample method');
final PlatformException platformException = PlatformException(code: '100');
final MissingPluginException missingPluginException = MissingPluginException();
expect(methodCall.toString(), 'MethodCall(sample method, null)');
expect(platformException.toString(), 'PlatformException(100, null, null, null)');
expect(missingPluginException.toString(), 'MissingPluginException(null)');
});
}
| flutter/packages/flutter/test/services/message_codecs_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/message_codecs_test.dart",
"repo_id": "flutter",
"token_count": 4217
} | 735 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
class _ConsistentTextRangeImplementationMatcher extends Matcher {
_ConsistentTextRangeImplementationMatcher(int length)
: range = TextRange(start: -1, end: length + 1),
assert(length >= 0);
final TextRange range;
@override
Description describe(Description description) {
return description.add('The implementation of TextBoundary.getTextBoundaryAt is consistent with its other methods.');
}
@override
Description describeMismatch(dynamic item, Description mismatchDescription, Map<dynamic, dynamic> matchState, bool verbose) {
final TextBoundary boundary = matchState['textBoundary'] as TextBoundary;
final int position = matchState['position'] as int;
final int leading = boundary.getLeadingTextBoundaryAt(position) ?? -1;
final int trailing = boundary.getTrailingTextBoundaryAt(position) ?? -1;
return mismatchDescription.add(
'at position $position, expected ${TextRange(start: leading, end: trailing)} but got ${boundary.getTextBoundaryAt(position)}',
);
}
@override
bool matches(dynamic item, Map<dynamic, dynamic> matchState) {
for (int i = range.start; i <= range.end; i++) {
final int? leading = (item as TextBoundary).getLeadingTextBoundaryAt(i);
final int? trailing = item.getTrailingTextBoundaryAt(i);
final TextRange boundary = item.getTextBoundaryAt(i);
final bool consistent = boundary.start == (leading ?? -1) && boundary.end == (trailing ?? -1);
if (!consistent) {
matchState['textBoundary'] = item;
matchState['position'] = i;
return false;
}
}
return true;
}
}
Matcher _hasConsistentTextRangeImplementationWithinRange(int length) => _ConsistentTextRangeImplementationMatcher(length);
void main() {
test('Character boundary works', () {
const CharacterBoundary boundary = CharacterBoundary('abc');
expect(boundary, _hasConsistentTextRangeImplementationWithinRange(3));
expect(boundary.getLeadingTextBoundaryAt(-1), null);
expect(boundary.getTrailingTextBoundaryAt(-1), 0);
expect(boundary.getLeadingTextBoundaryAt(0), 0);
expect(boundary.getTrailingTextBoundaryAt(0), 1);
expect(boundary.getLeadingTextBoundaryAt(1), 1);
expect(boundary.getTrailingTextBoundaryAt(1), 2);
expect(boundary.getLeadingTextBoundaryAt(2), 2);
expect(boundary.getTrailingTextBoundaryAt(2), 3);
expect(boundary.getLeadingTextBoundaryAt(3), 3);
expect(boundary.getTrailingTextBoundaryAt(3), null);
expect(boundary.getLeadingTextBoundaryAt(4), 3);
expect(boundary.getTrailingTextBoundaryAt(4), null);
});
test('Character boundary works with grapheme', () {
const String text = 'a❄︎c';
const CharacterBoundary boundary = CharacterBoundary(text);
expect(boundary, _hasConsistentTextRangeImplementationWithinRange(text.length));
expect(boundary.getLeadingTextBoundaryAt(-1), null);
expect(boundary.getTrailingTextBoundaryAt(-1), 0);
expect(boundary.getLeadingTextBoundaryAt(0), 0);
expect(boundary.getTrailingTextBoundaryAt(0), 1);
// The `❄` takes two character length.
expect(boundary.getLeadingTextBoundaryAt(1), 1);
expect(boundary.getTrailingTextBoundaryAt(1), 3);
expect(boundary.getLeadingTextBoundaryAt(2), 1);
expect(boundary.getTrailingTextBoundaryAt(2), 3);
expect(boundary.getLeadingTextBoundaryAt(3), 3);
expect(boundary.getTrailingTextBoundaryAt(3), 4);
expect(boundary.getLeadingTextBoundaryAt(text.length), text.length);
expect(boundary.getTrailingTextBoundaryAt(text.length), null);
});
test('wordBoundary.moveByWordBoundary', () {
const String text = 'ABC ABC\n' // [0, 10)
'AÁ Á\n' // [10, 20)
' \n' // [20, 30)
'ABC!!!ABC\n' // [30, 40)
' !ABC !!\n' // [40, 50)
'A 𑗋𑗋 A\n'; // [50, 60)
final TextPainter textPainter = TextPainter()
..textDirection = TextDirection.ltr
..text = const TextSpan(text: text)
..layout();
final TextBoundary boundary = textPainter.wordBoundaries.moveByWordBoundary;
// 4 points to the 2nd whitespace in the first line.
// Don't break between horizontal spaces and letters/numbers.
expect(boundary.getLeadingTextBoundaryAt(4), 0);
expect(boundary.getTrailingTextBoundaryAt(4), 9);
// Works when words are starting/ending with a combining diacritical mark.
expect(boundary.getLeadingTextBoundaryAt(14), 10);
expect(boundary.getTrailingTextBoundaryAt(14), 19);
// Do break before and after newlines.
expect(boundary.getLeadingTextBoundaryAt(24), 20);
expect(boundary.getTrailingTextBoundaryAt(24), 29);
// Do not break on punctuations.
expect(boundary.getLeadingTextBoundaryAt(34), 30);
expect(boundary.getTrailingTextBoundaryAt(34), 39);
// Ok to break if next to punctuations or separating spaces.
expect(boundary.getLeadingTextBoundaryAt(44), 43);
expect(boundary.getTrailingTextBoundaryAt(44), 46);
// 44 points to a low surrogate of a punctuation.
expect(boundary.getLeadingTextBoundaryAt(54), 50);
expect(boundary.getTrailingTextBoundaryAt(54), 59);
});
test('line boundary works', () {
final LineBoundary boundary = LineBoundary(TestTextLayoutMetrics());
expect(boundary.getLeadingTextBoundaryAt(3), TestTextLayoutMetrics.lineAt3.start);
expect(boundary.getTrailingTextBoundaryAt(3), TestTextLayoutMetrics.lineAt3.end);
expect(boundary.getTextBoundaryAt(3), TestTextLayoutMetrics.lineAt3);
});
group('paragraph boundary', () {
test('works for simple cases', () {
const String textA= 'abcd efg hi\njklmno\npqrstuv';
const ParagraphBoundary boundaryA = ParagraphBoundary(textA);
// Position enclosed inside of paragraph, 'abcd efg h|i\n'.
const int position = 10;
// The range includes the line terminator.
expect(boundaryA.getLeadingTextBoundaryAt(position), 0);
expect(boundaryA.getTrailingTextBoundaryAt(position), 12);
// This text includes a carriage return followed by a line feed.
const String textB = 'abcd efg hi\r\njklmno\npqrstuv';
const ParagraphBoundary boundaryB = ParagraphBoundary(textB);
expect(boundaryB.getLeadingTextBoundaryAt(position), 0);
expect(boundaryB.getTrailingTextBoundaryAt(position), 13);
const String textF = 'Now is the time for\n' // 20
'all good people\n' // 20 + 16 => 36
'to come to the aid\n' // 36 + 19 => 55
'of their country.'; // 55 + 17 => 72
const ParagraphBoundary boundaryF = ParagraphBoundary(textF);
const int positionF = 11;
expect(boundaryF.getLeadingTextBoundaryAt(positionF), 0);
expect(boundaryF.getTrailingTextBoundaryAt(positionF), 20);
});
test('works for consecutive line terminators involving CRLF', () {
const String textI = 'Now is the time for\n' // 20
'all good people\n\r\n' // 20 + 16 => 38
'to come to the aid\n' // 38 + 19 => 57
'of their country.'; // 57 + 17 => 74
const ParagraphBoundary boundaryI = ParagraphBoundary(textI);
const int positionI = 56;// \n at the end of the third line.
const int positionJ = 38;// t at beginning of third line.
const int positionK = 37;// \n at end of second line.
expect(boundaryI.getLeadingTextBoundaryAt(positionI), 38);
expect(boundaryI.getTrailingTextBoundaryAt(positionI), 57);
expect(boundaryI.getLeadingTextBoundaryAt(positionJ), 38);
expect(boundaryI.getTrailingTextBoundaryAt(positionJ), 57);
expect(boundaryI.getLeadingTextBoundaryAt(positionK), 36);
expect(boundaryI.getTrailingTextBoundaryAt(positionK), 38);
});
test('works for consecutive line terminators', () {
const String textI = 'Now is the time for\n' // 20
'all good people\n\n' // 20 + 16 => 37
'to come to the aid\n' // 37 + 19 => 56
'of their country.'; // 56 + 17 => 73
const ParagraphBoundary boundaryI = ParagraphBoundary(textI);
const int positionI = 55;// \n at the end of the third line.
const int positionJ = 37;// t at beginning of third line.
const int positionK = 36;// \n at end of second line.
expect(boundaryI.getLeadingTextBoundaryAt(positionI), 37);
expect(boundaryI.getTrailingTextBoundaryAt(positionI), 56);
expect(boundaryI.getLeadingTextBoundaryAt(positionJ), 37);
expect(boundaryI.getTrailingTextBoundaryAt(positionJ), 56);
expect(boundaryI.getLeadingTextBoundaryAt(positionK), 36);
expect(boundaryI.getTrailingTextBoundaryAt(positionK), 37);
});
test('leading boundary works for consecutive CRLF', () {
// This text includes multiple consecutive carriage returns followed by line feeds (CRLF).
const String textH = 'abcd efg hi\r\n\r\n\r\n\r\n\r\n\r\n\r\n\n\n\n\n\njklmno\npqrstuv';
const ParagraphBoundary boundaryH = ParagraphBoundary(textH);
const int positionH = 18;
expect(boundaryH.getLeadingTextBoundaryAt(positionH), 17);
expect(boundaryH.getTrailingTextBoundaryAt(positionH), 19);
});
test('trailing boundary works for consecutive CRLF', () {
// This text includes multiple consecutive carriage returns followed by line feeds (CRLF).
const String textG = 'abcd efg hi\r\n\n\n\n\n\n\r\n\r\n\r\n\r\n\n\n\n\n\njklmno\npqrstuv';
const ParagraphBoundary boundaryG = ParagraphBoundary(textG);
const int positionG = 18;
expect(boundaryG.getLeadingTextBoundaryAt(positionG), 18);
expect(boundaryG.getTrailingTextBoundaryAt(positionG), 20);
});
test('works when position is between two CRLF', () {
const String textE = 'abcd efg hi\r\nhello\r\n\n';
const ParagraphBoundary boundaryE = ParagraphBoundary(textE);
// Position enclosed inside of paragraph, 'abcd efg hi\r\nhello\r\n\n'.
const int positionE = 16;
expect(boundaryE.getLeadingTextBoundaryAt(positionE), 13);
expect(boundaryE.getTrailingTextBoundaryAt(positionE), 20);
});
test('works for multiple consecutive line terminators', () {
// This text includes multiple consecutive line terminators.
const String textC = 'abcd efg hi\r\n\n\n\n\n\n\n\n\n\n\n\njklmno\npqrstuv';
const ParagraphBoundary boundaryC = ParagraphBoundary(textC);
// Position enclosed inside of paragraph, 'abcd efg hi\r\n\n\n\n\n\n|\n\n\n\n\n\njklmno\npqrstuv'.
const int positionC = 18;
expect(boundaryC.getLeadingTextBoundaryAt(positionC), 18);
expect(boundaryC.getTrailingTextBoundaryAt(positionC), 19);
const String textD = 'abcd efg hi\r\n\n\n\n';
const ParagraphBoundary boundaryD = ParagraphBoundary(textD);
// Position enclosed inside of paragraph, 'abcd efg hi\r\n\n|\n\n'.
const int positionD = 14;
expect(boundaryD.getLeadingTextBoundaryAt(positionD), 14);
expect(boundaryD.getTrailingTextBoundaryAt(positionD), 15);
});
});
test('document boundary works', () {
const String text = 'abcd efg hi\njklmno\npqrstuv';
const DocumentBoundary boundary = DocumentBoundary(text);
expect(boundary, _hasConsistentTextRangeImplementationWithinRange(text.length));
expect(boundary.getLeadingTextBoundaryAt(-1), null);
expect(boundary.getTrailingTextBoundaryAt(-1), text.length);
expect(boundary.getLeadingTextBoundaryAt(0), 0);
expect(boundary.getTrailingTextBoundaryAt(0), text.length);
expect(boundary.getLeadingTextBoundaryAt(10), 0);
expect(boundary.getTrailingTextBoundaryAt(10), text.length);
expect(boundary.getLeadingTextBoundaryAt(text.length), 0);
expect(boundary.getTrailingTextBoundaryAt(text.length), null);
expect(boundary.getLeadingTextBoundaryAt(text.length + 1), 0);
expect(boundary.getTrailingTextBoundaryAt(text.length + 1), null);
});
}
class TestTextLayoutMetrics extends TextLayoutMetrics {
static const TextSelection lineAt3 = TextSelection(baseOffset: 0, extentOffset: 10);
static const TextRange wordBoundaryAt3 = TextRange(start: 4, end: 7);
@override
TextSelection getLineAtOffset(TextPosition position) {
if (position.offset == 3) {
return lineAt3;
}
throw UnimplementedError();
}
@override
TextPosition getTextPositionAbove(TextPosition position) {
throw UnimplementedError();
}
@override
TextPosition getTextPositionBelow(TextPosition position) {
throw UnimplementedError();
}
@override
TextRange getWordBoundary(TextPosition position) {
if (position.offset == 3) {
return wordBoundaryAt3;
}
throw UnimplementedError();
}
}
| flutter/packages/flutter/test/services/text_boundary_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/text_boundary_test.dart",
"repo_id": "flutter",
"token_count": 5058
} | 736 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('RenderAnimatedOpacityMixin does not drop layer when animating to 1', (WidgetTester tester) async {
RenderTestObject.paintCount = 0;
final AnimationController controller = AnimationController(vsync: const TestVSync(), duration: const Duration(seconds: 1));
addTearDown(controller.dispose);
final Tween<double> opacityTween = Tween<double>(begin: 0, end: 1);
await tester.pumpWidget(
ColoredBox(
color: Colors.red,
child: FadeTransition(
opacity: controller.drive(opacityTween),
child: const TestWidget(),
),
)
);
expect(RenderTestObject.paintCount, 0);
controller.forward();
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(RenderTestObject.paintCount, 1);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(RenderTestObject.paintCount, 1);
controller.stop();
await tester.pump();
expect(RenderTestObject.paintCount, 1);
});
testWidgets('RenderAnimatedOpacityMixin avoids repainting child as it animates', (WidgetTester tester) async {
RenderTestObject.paintCount = 0;
final AnimationController controller = AnimationController(vsync: const TestVSync(), duration: const Duration(seconds: 1));
addTearDown(controller.dispose);
final Tween<double> opacityTween = Tween<double>(begin: 0, end: 0.99); // Layer is dropped at 1
await tester.pumpWidget(
ColoredBox(
color: Colors.red,
child: FadeTransition(
opacity: controller.drive(opacityTween),
child: const TestWidget(),
),
)
);
expect(RenderTestObject.paintCount, 0);
controller.forward();
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(RenderTestObject.paintCount, 1);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(RenderTestObject.paintCount, 1);
controller.stop();
await tester.pump();
expect(RenderTestObject.paintCount, 1);
});
testWidgets('RenderAnimatedOpacityMixin allows opacity layer to be disposed when animating to 0 opacity', (WidgetTester tester) async {
RenderTestObject.paintCount = 0;
final AnimationController controller = AnimationController(vsync: const TestVSync(), duration: const Duration(seconds: 1));
addTearDown(controller.dispose);
final Tween<double> opacityTween = Tween<double>(begin: 0.99, end: 0);
await tester.pumpWidget(
ColoredBox(
color: Colors.red,
child: FadeTransition(
opacity: controller.drive(opacityTween),
child: const TestWidget(),
),
)
);
expect(RenderTestObject.paintCount, 1);
expect(tester.layers, contains(isA<OpacityLayer>()));
controller.forward();
await tester.pump();
await tester.pump(const Duration(seconds: 2));
expect(RenderTestObject.paintCount, 1);
controller.stop();
await tester.pump();
expect(tester.layers, isNot(contains(isA<OpacityLayer>())));
});
}
class TestWidget extends SingleChildRenderObjectWidget {
const TestWidget({super.key, super.child});
@override
RenderObject createRenderObject(BuildContext context) {
return RenderTestObject();
}
}
class RenderTestObject extends RenderProxyBox {
static int paintCount = 0;
@override
void paint(PaintingContext context, Offset offset) {
paintCount += 1;
super.paint(context, offset);
}
}
| flutter/packages/flutter/test/widgets/animated_opacity_repaint_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/animated_opacity_repaint_test.dart",
"repo_id": "flutter",
"token_count": 1365
} | 737 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
class Leaf extends StatefulWidget {
const Leaf({ required Key key, required this.child }) : super(key: key);
final Widget child;
@override
State<Leaf> createState() => _LeafState();
}
class _LeafState extends State<Leaf> {
bool _keepAlive = false;
KeepAliveHandle? _handle;
@override
void deactivate() {
_handle?.dispose();
_handle = null;
super.deactivate();
}
void setKeepAlive(bool value) {
_keepAlive = value;
if (_keepAlive) {
if (_handle == null) {
_handle = KeepAliveHandle();
KeepAliveNotification(_handle!).dispatch(context);
}
} else {
_handle?.dispose();
_handle = null;
}
}
@override
Widget build(BuildContext context) {
if (_keepAlive && _handle == null) {
_handle = KeepAliveHandle();
KeepAliveNotification(_handle!).dispatch(context);
}
return widget.child;
}
}
List<Widget> generateList(Widget child, { required bool impliedMode }) {
return List<Widget>.generate(
100,
(int index) {
final Widget result = Leaf(
key: GlobalObjectKey<_LeafState>(index),
child: child,
);
if (impliedMode) {
return result;
}
return AutomaticKeepAlive(child: result);
},
growable: false,
);
}
void tests({ required bool impliedMode }) {
testWidgets('AutomaticKeepAlive with ListView with itemExtent', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: impliedMode,
addRepaintBoundaries: impliedMode,
addSemanticIndexes: false,
itemExtent: 12.3, // about 50 widgets visible
cacheExtent: 0.0,
children: generateList(const Placeholder(), impliedMode: impliedMode),
),
),
);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
await tester.drag(find.byType(ListView), const Offset(0.0, -300.0)); // about 25 widgets' worth
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(true);
await tester.drag(find.byType(ListView), const Offset(0.0, 300.0)); // back to top
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
});
testWidgets('AutomaticKeepAlive with ListView without itemExtent', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: impliedMode,
addRepaintBoundaries: impliedMode,
addSemanticIndexes: false,
cacheExtent: 0.0,
children: generateList(
const SizedBox(height: 12.3, child: Placeholder()), // about 50 widgets visible
impliedMode: impliedMode,
),
),
),
);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
await tester.drag(find.byType(ListView), const Offset(0.0, -300.0)); // about 25 widgets' worth
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(true);
await tester.drag(find.byType(ListView), const Offset(0.0, 300.0)); // back to top
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
});
testWidgets('AutomaticKeepAlive with GridView', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: GridView.count(
addAutomaticKeepAlives: impliedMode,
addRepaintBoundaries: impliedMode,
addSemanticIndexes: false,
crossAxisCount: 2,
childAspectRatio: 400.0 / 24.6, // about 50 widgets visible
cacheExtent: 0.0,
children: generateList(
const Placeholder(),
impliedMode: impliedMode,
),
),
),
);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
await tester.drag(find.byType(GridView), const Offset(0.0, -300.0)); // about 25 widgets' worth
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(true);
await tester.drag(find.byType(GridView), const Offset(0.0, 300.0)); // back to top
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(60).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(30)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(59), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(60), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(61), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(90), skipOffstage: false), findsNothing);
});
}
void main() {
group('Explicit automatic keep-alive', () { tests(impliedMode: false); });
group('Implied automatic keep-alive', () { tests(impliedMode: true); });
testWidgets('AutomaticKeepAlive double', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: false,
addRepaintBoundaries: false,
addSemanticIndexes: false,
cacheExtent: 0.0,
children: const <Widget>[
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(0), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(1), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
key: GlobalObjectKey<_LeafState>(2),
height: 400.0,
),
),
AutomaticKeepAlive(
child: SizedBox(
key: GlobalObjectKey<_LeafState>(3),
height: 400.0,
),
),
],
),
),
);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
await tester.drag(find.byType(ListView), const Offset(0.0, -1000.0)); // move to bottom
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
await tester.drag(find.byType(ListView), const Offset(0.0, 1000.0)); // move to top
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(0).currentState!.setKeepAlive(true);
await tester.drag(find.byType(ListView), const Offset(0.0, -1000.0)); // move to bottom
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
const GlobalObjectKey<_LeafState>(1).currentState!.setKeepAlive(true);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
const GlobalObjectKey<_LeafState>(0).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
const GlobalObjectKey<_LeafState>(1).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
});
testWidgets('AutomaticKeepAlive double 2', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: false,
addRepaintBoundaries: false,
addSemanticIndexes: false,
cacheExtent: 0.0,
children: const <Widget>[
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(0), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(1), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(2), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(3), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(4), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(5), child: Placeholder()),
]),
),
),
],
),
),
);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5), skipOffstage: false), findsNothing);
const GlobalObjectKey<_LeafState>(0).currentState!.setKeepAlive(true);
await tester.drag(find.byType(ListView), const Offset(0.0, -1000.0)); // move to bottom
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5)), findsOneWidget);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: false,
addRepaintBoundaries: false,
addSemanticIndexes: false,
cacheExtent: 0.0,
children: const <Widget>[
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(1), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(2), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(3), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(4), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(5), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(0), child: Placeholder()),
]),
),
),
],
),
));
await tester.pump(); // Sometimes AutomaticKeepAlive needs an extra pump to clean things up.
expect(find.byKey(const GlobalObjectKey<_LeafState>(1), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsOneWidget);
await tester.drag(find.byType(ListView), const Offset(0.0, 1000.0)); // move to top
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5)), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0)), findsNothing);
const GlobalObjectKey<_LeafState>(0).currentState!.setKeepAlive(false);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsNothing);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ListView(
addAutomaticKeepAlives: false,
addRepaintBoundaries: false,
addSemanticIndexes: false,
cacheExtent: 0.0,
children: const <Widget>[
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(1), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(2), child: Placeholder()),
]),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(),
),
),
AutomaticKeepAlive(
child: SizedBox(
height: 400.0,
child: Stack(children: <Widget>[
Leaf(key: GlobalObjectKey<_LeafState>(3), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(4), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(5), child: Placeholder()),
Leaf(key: GlobalObjectKey<_LeafState>(0), child: Placeholder()),
]),
),
),
],
),
));
await tester.pump(); // Sometimes AutomaticKeepAlive needs an extra pump to clean things up.
expect(find.byKey(const GlobalObjectKey<_LeafState>(1)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(2)), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_LeafState>(3), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(4), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(5), skipOffstage: false), findsNothing);
expect(find.byKey(const GlobalObjectKey<_LeafState>(0), skipOffstage: false), findsNothing);
});
testWidgets('AutomaticKeepAlive with keepAlive set to true before initState', (WidgetTester tester) async {
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ListView.builder(
dragStartBehavior: DragStartBehavior.down,
addSemanticIndexes: false,
itemCount: 50,
itemBuilder: (BuildContext context, int index) {
if (index == 0) {
return const _AlwaysKeepAlive(
key: GlobalObjectKey<_AlwaysKeepAliveState>(0),
);
}
return SizedBox(
height: 44.0,
child: Text('FooBar $index'),
);
},
),
));
expect(find.text('keep me alive'), findsOneWidget);
expect(find.text('FooBar 1'), findsOneWidget);
expect(find.text('FooBar 2'), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_AlwaysKeepAliveState>(0)), findsOneWidget);
await tester.drag(find.byType(ListView), const Offset(0.0, -1000.0)); // move to bottom
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_AlwaysKeepAliveState>(0), skipOffstage: false), findsOneWidget);
expect(find.text('keep me alive', skipOffstage: false), findsOneWidget);
expect(find.text('FooBar 1'), findsNothing);
expect(find.text('FooBar 2'), findsNothing);
});
testWidgets('AutomaticKeepAlive with keepAlive set to true before initState and widget goes out of scope', (WidgetTester tester) async {
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ListView.builder(
addSemanticIndexes: false,
itemCount: 250,
itemBuilder: (BuildContext context, int index) {
if (index.isEven) {
return _AlwaysKeepAlive(
key: GlobalObjectKey<_AlwaysKeepAliveState>(index),
);
}
return SizedBox(
height: 44.0,
child: Text('FooBar $index'),
);
},
),
));
expect(find.text('keep me alive'), findsNWidgets(7));
expect(find.text('FooBar 1'), findsOneWidget);
expect(find.text('FooBar 3'), findsOneWidget);
expect(find.byKey(const GlobalObjectKey<_AlwaysKeepAliveState>(0)), findsOneWidget);
final ScrollableState state = tester.state(find.byType(Scrollable));
final ScrollPosition position = state.position;
position.jumpTo(3025.0);
await tester.pump();
expect(find.byKey(const GlobalObjectKey<_AlwaysKeepAliveState>(0), skipOffstage: false), findsOneWidget);
expect(find.text('keep me alive', skipOffstage: false), findsNWidgets(23));
expect(find.text('FooBar 1'), findsNothing);
expect(find.text('FooBar 3'), findsNothing);
expect(find.text('FooBar 73'), findsOneWidget);
});
testWidgets('AutomaticKeepAlive with SliverKeepAliveWidget', (WidgetTester tester) async {
// We're just doing a basic test here to make sure that the functionality of
// RenderSliverWithKeepAliveMixin doesn't get regressed or deleted. As testing
// the full functionality would be cumbersome.
final RenderSliverMultiBoxAdaptorAlt alternate = RenderSliverMultiBoxAdaptorAlt();
addTearDown(alternate.dispose);
final RenderBox child = RenderBoxKeepAlive();
addTearDown(child.dispose);
alternate.insert(child);
expect(alternate.children.length, 1);
});
testWidgets('Keep alive Listenable has its listener removed once called', (WidgetTester tester) async {
final LeakCheckerHandle handle = LeakCheckerHandle();
addTearDown(handle.dispose);
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: ListView.builder(
itemCount: 1,
itemBuilder: (BuildContext context, int index) {
return const KeepAliveListenableLeakChecker(key: GlobalObjectKey<_KeepAliveListenableLeakCheckerState>(0));
},
),
));
final _KeepAliveListenableLeakCheckerState state = const GlobalObjectKey<_KeepAliveListenableLeakCheckerState>(0).currentState!;
expect(handle.hasListeners, false);
state.dispatch(handle);
expect(handle.hasListeners, true);
handle.notifyListeners();
expect(handle.hasListeners, false);
});
}
class _AlwaysKeepAlive extends StatefulWidget {
const _AlwaysKeepAlive({ required Key key }) : super(key: key);
@override
State<StatefulWidget> createState() => _AlwaysKeepAliveState();
}
class _AlwaysKeepAliveState extends State<_AlwaysKeepAlive> with AutomaticKeepAliveClientMixin<_AlwaysKeepAlive> {
@override
bool get wantKeepAlive => true;
@override
Widget build(BuildContext context) {
super.build(context);
return const SizedBox(
height: 48.0,
child: Text('keep me alive'),
);
}
}
class RenderBoxKeepAlive extends RenderBox { }
mixin KeepAliveParentDataMixinAlt implements KeepAliveParentDataMixin {
@override
bool keptAlive = false;
@override
bool keepAlive = false;
}
class RenderSliverMultiBoxAdaptorAlt extends RenderSliver with
KeepAliveParentDataMixinAlt,
RenderSliverHelpers,
RenderSliverWithKeepAliveMixin {
final List<RenderBox> children = <RenderBox>[];
void insert(RenderBox child, { RenderBox? after }) {
children.add(child);
}
@override
void visitChildren(RenderObjectVisitor visitor) {
children.forEach(visitor);
}
@override
void performLayout() { }
}
class LeakCheckerHandle with ChangeNotifier {
LeakCheckerHandle() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
@override
bool get hasListeners => super.hasListeners;
}
class KeepAliveListenableLeakChecker extends StatefulWidget {
const KeepAliveListenableLeakChecker({super.key});
@override
State<KeepAliveListenableLeakChecker> createState() => _KeepAliveListenableLeakCheckerState();
}
class _KeepAliveListenableLeakCheckerState extends State<KeepAliveListenableLeakChecker> {
void dispatch(Listenable handle) {
KeepAliveNotification(handle).dispatch(context);
}
@override
Widget build(BuildContext context) {
return const Placeholder();
}
}
| flutter/packages/flutter/test/widgets/automatic_keep_alive_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/automatic_keep_alive_test.dart",
"repo_id": "flutter",
"token_count": 11958
} | 738 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
Future<void> main() async {
testWidgets('Build method that returns context.widget throws FlutterError', (WidgetTester tester) async {
// Regression test for: https://github.com/flutter/flutter/issues/25041
await tester.pumpWidget(
Builder(builder: (BuildContext context) => context.widget),
);
expect(tester.takeException(), isFlutterError);
});
}
| flutter/packages/flutter/test/widgets/build_fail_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/build_fail_test.dart",
"repo_id": "flutter",
"token_count": 197
} | 739 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
// Regression test for https://github.com/flutter/flutter/issues/96024
testWidgets('CustomScrollView.center update test 1', (WidgetTester tester) async {
final Key centerKey = UniqueKey();
late StateSetter setState;
bool hasKey = false;
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
center: centerKey,
slivers: <Widget>[
const SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
StatefulBuilder(
key: centerKey,
builder: (BuildContext context, StateSetter setter) {
setState = setter;
if (hasKey) {
return const SliverToBoxAdapter(
key: Key('b'),
child: SizedBox(height: 100.0),
);
} else {
return const SliverToBoxAdapter(
child: SizedBox(height: 100.0),
);
}
},
),
],
),
));
await tester.pumpAndSettle();
// Change the center key will trigger the old RenderObject remove and a new
// RenderObject insert.
setState(() {
hasKey = true;
});
await tester.pumpAndSettle();
// Pass without throw.
});
testWidgets('CustomScrollView.center update test 2', (WidgetTester tester) async {
const List<Widget> slivers1 = <Widget>[
SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('b'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('c'), child: SizedBox(height: 100.0)),
];
const List<Widget> slivers2 = <Widget>[
SliverToBoxAdapter(key: Key('c'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('d'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
];
Widget buildFrame(List<Widget> slivers, Key center) {
return Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
center: center,
slivers: slivers,
),
);
}
await tester.pumpWidget(buildFrame(slivers1, const Key('b')));
await tester.pumpAndSettle();
await tester.pumpWidget(buildFrame(slivers2, const Key('d')));
await tester.pumpAndSettle();
// Pass without throw.
});
testWidgets('CustomScrollView.center', (WidgetTester tester) async {
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('b'), child: SizedBox(height: 100.0)),
],
center: Key('a'),
),
));
await tester.pumpAndSettle();
expect(
tester.getRect(find.descendant(of: find.byKey(const Key('a')), matching: find.byType(SizedBox))),
const Rect.fromLTRB(0.0, 0.0, 800.0, 100.0),
);
expect(
tester.getRect(find.descendant(of: find.byKey(const Key('b')), matching: find.byType(SizedBox))),
const Rect.fromLTRB(0.0, 100.0, 800.0, 200.0),
);
});
testWidgets('CustomScrollView.center', (WidgetTester tester) async {
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('b'), child: SizedBox(height: 100.0)),
],
center: Key('b'),
),
));
await tester.pumpAndSettle();
expect(
tester.getRect(
find.descendant(
of: find.byKey(const Key('a'), skipOffstage: false),
matching: find.byType(SizedBox, skipOffstage: false),
),
),
const Rect.fromLTRB(0.0, -100.0, 800.0, 0.0),
);
expect(
tester.getRect(
find.descendant(
of: find.byKey(const Key('b')),
matching: find.byType(SizedBox),
),
),
const Rect.fromLTRB(0.0, 0.0, 800.0, 100.0),
);
});
testWidgets('CustomScrollView.anchor', (WidgetTester tester) async {
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverToBoxAdapter(key: Key('a'), child: SizedBox(height: 100.0)),
SliverToBoxAdapter(key: Key('b'), child: SizedBox(height: 100.0)),
],
center: Key('b'),
anchor: 1.0,
),
));
await tester.pumpAndSettle();
expect(
tester.getRect(
find.descendant(
of: find.byKey(const Key('a')),
matching: find.byType(SizedBox),
),
),
const Rect.fromLTRB(0.0, 500.0, 800.0, 600.0),
);
expect(
tester.getRect(
find.descendant(
of: find.byKey(const Key('b'), skipOffstage: false),
matching: find.byType(SizedBox, skipOffstage: false),
),
),
const Rect.fromLTRB(0.0, 600.0, 800.0, 700.0),
);
});
}
| flutter/packages/flutter/test/widgets/custom_scroll_view_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/custom_scroll_view_test.dart",
"repo_id": "flutter",
"token_count": 2467
} | 740 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'semantics_tester.dart';
void main() {
testWidgets('Drawer control test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
late BuildContext savedContext;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Scaffold(
key: scaffoldKey,
drawer: const Text('drawer'),
body: Container(),
);
},
),
),
);
await tester.pump(); // no effect
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pump(); // drawer should be starting to animate in
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsOneWidget);
Navigator.pop(savedContext);
await tester.pump(); // drawer should be starting to animate away
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsNothing);
});
testWidgets('Drawer tap test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
drawer: const Text('drawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pump(); // drawer should be starting to animate in
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsOneWidget);
await tester.tap(find.text('drawer'));
await tester.pump(); // nothing should have happened
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // ditto
expect(find.text('drawer'), findsOneWidget);
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
// drawer should be starting to animate away
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsNothing);
});
testWidgets('Drawer hover test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
final List<String> logs = <String>[];
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
// Start out of hoverTarget
await gesture.addPointer(location: const Offset(100, 100));
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
drawer: const Text('drawer'),
body: Align(
alignment: Alignment.topLeft,
child: MouseRegion(
onEnter: (_) { logs.add('enter'); },
onHover: (_) { logs.add('hover'); },
onExit: (_) { logs.add('exit'); },
child: const SizedBox(width: 10, height: 10),
),
),
),
),
);
expect(logs, isEmpty);
expect(find.text('drawer'), findsNothing);
// When drawer is closed, hover is interactable
await gesture.moveTo(const Offset(5, 5));
await tester.pump(); // no effect
expect(logs, <String>['enter', 'hover']);
logs.clear();
await gesture.moveTo(const Offset(20, 20));
await tester.pump(); // no effect
expect(logs, <String>['exit']);
logs.clear();
// When drawer is open, hover is uninteractable
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsOneWidget);
await gesture.moveTo(const Offset(5, 5));
await tester.pump(); // no effect
expect(logs, isEmpty);
logs.clear();
await gesture.moveTo(const Offset(20, 20));
await tester.pump(); // no effect
expect(logs, isEmpty);
logs.clear();
// Close drawer, hover is interactable again
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsNothing);
await gesture.moveTo(const Offset(5, 5));
await tester.pump(); // no effect
expect(logs, <String>['enter', 'hover']);
logs.clear();
await gesture.moveTo(const Offset(20, 20));
await tester.pump(); // no effect
expect(logs, <String>['exit']);
logs.clear();
});
testWidgets('Drawer drag cancel resume (LTR)', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
drawerDragStartBehavior: DragStartBehavior.down,
key: scaffoldKey,
drawer: Drawer(
child: ListView(
children: <Widget>[
const Text('drawer'),
Container(
height: 1000.0,
color: Colors.blue[500],
),
],
),
),
body: Container(),
),
),
);
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pump(); // drawer should be starting to animate in
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsOneWidget);
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
// drawer should be starting to animate away
final double textLeft = tester.getTopLeft(find.text('drawer')).dx;
expect(textLeft, lessThan(0.0));
final TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
// drawer should be stopped.
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(tester.getTopLeft(find.text('drawer')).dx, equals(textLeft));
await gesture.moveBy(const Offset(50.0, 0.0));
// drawer should be returning to visible
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(tester.getTopLeft(find.text('drawer')).dx, equals(0.0));
await gesture.up();
});
testWidgets('Drawer drag cancel resume (RTL)', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
drawerDragStartBehavior: DragStartBehavior.down,
key: scaffoldKey,
drawer: Drawer(
child: ListView(
children: <Widget>[
const Text('drawer'),
Container(
height: 1000.0,
color: Colors.blue[500],
),
],
),
),
body: Container(),
),
),
),
);
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pump(); // drawer should be starting to animate in
expect(find.text('drawer'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('drawer'), findsOneWidget);
await tester.tapAt(const Offset(50.0, 100.0)); // on the mask
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
// drawer should be starting to animate away
final double textRight = tester.getTopRight(find.text('drawer')).dx;
expect(textRight, greaterThan(800.0));
final TestGesture gesture = await tester.startGesture(const Offset(700.0, 100.0));
// drawer should be stopped.
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(tester.getTopRight(find.text('drawer')).dx, equals(textRight));
await gesture.moveBy(const Offset(-50.0, 0.0));
// drawer should be returning to visible
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(tester.getTopRight(find.text('drawer')).dx, equals(800.0));
await gesture.up();
});
testWidgets('Drawer navigator back button', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
bool buttonPressed = false;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
drawer: Drawer(
child: ListView(
children: <Widget>[
const Text('drawer'),
TextButton(
child: const Text('close'),
onPressed: () => Navigator.pop(context),
),
],
),
),
body: TextButton(
child: const Text('button'),
onPressed: () { buttonPressed = true; },
),
);
},
),
),
);
// Open the drawer.
scaffoldKey.currentState!.openDrawer();
await tester.pump(); // drawer should be starting to animate in
expect(find.text('drawer'), findsOneWidget);
// Tap the close button to pop the drawer route.
await tester.pump(const Duration(milliseconds: 100));
await tester.tap(find.text('close'));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('drawer'), findsNothing);
// Confirm that a button in the scaffold body is still clickable.
await tester.tap(find.text('button'));
expect(buttonPressed, equals(true));
});
testWidgets('Dismissible ModalBarrier includes button in semantic tree', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
drawer: const Drawer(),
);
},
),
),
);
// Open the drawer.
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(milliseconds: 100));
expect(semantics, includesNodeWith(actions: <SemanticsAction>[SemanticsAction.tap]));
expect(semantics, includesNodeWith(label: 'Dismiss'));
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Dismissible ModalBarrier is hidden on Android (back button is used to dismiss)', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
drawer: const Drawer(),
body: Container(),
);
},
),
),
);
// Open the drawer.
scaffoldKey.currentState!.openDrawer();
await tester.pump(const Duration(milliseconds: 100));
expect(semantics, isNot(includesNodeWith(actions: <SemanticsAction>[SemanticsAction.tap])));
expect(semantics, isNot(includesNodeWith(label: 'Dismiss')));
semantics.dispose();
}, variant: TargetPlatformVariant.only(TargetPlatform.android));
testWidgets('Drawer contains route semantics flags', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
return Scaffold(
key: scaffoldKey,
drawer: const Drawer(),
body: Container(),
);
},
),
),
);
// Open the drawer.
scaffoldKey.currentState!.openDrawer();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(semantics, includesNodeWith(
label: 'Navigation menu',
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
));
semantics.dispose();
});
}
| flutter/packages/flutter/test/widgets/drawer_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/drawer_test.dart",
"repo_id": "flutter",
"token_count": 5511
} | 741 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/semantics.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'semantics_tester.dart';
void main() {
group('FocusScope', () {
testWidgets('Can focus', (WidgetTester tester) async {
final GlobalKey<TestFocusState> key = GlobalKey();
await tester.pumpWidget(
TestFocus(key: key),
);
expect(key.currentState!.focusNode.hasFocus, isFalse);
FocusScope.of(key.currentContext!).requestFocus(key.currentState!.focusNode);
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
});
testWidgets('Can unfocus', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
TestFocus(key: keyA),
TestFocus(key: keyB, name: 'b'),
],
),
);
expect(keyA.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
// Set focus to the "B" node to unfocus the "A" node.
FocusScope.of(keyB.currentContext!).requestFocus(keyB.currentState!.focusNode);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
});
testWidgets('Autofocus works', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
TestFocus(key: keyA),
TestFocus(key: keyB, name: 'b', autofocus: true),
],
),
);
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
});
testWidgets('Can have multiple focused children and they update accordingly', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
TestFocus(
key: keyA,
autofocus: true,
),
TestFocus(
key: keyB,
name: 'b',
),
],
),
);
// Autofocus is delayed one frame.
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.tap(find.text('A FOCUSED'));
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.tap(find.text('b'));
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
await tester.tap(find.text('a'));
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
});
// This moves a focus node first into a focus scope that is added to its
// parent, and then out of that focus scope again.
testWidgets('Can move focus in and out of FocusScope', (WidgetTester tester) async {
final FocusScopeNode parentFocusScope = FocusScopeNode(debugLabel: 'Parent Scope Node');
addTearDown(parentFocusScope.dispose);
final FocusScopeNode childFocusScope = FocusScopeNode(debugLabel: 'Child Scope Node');
addTearDown(childFocusScope.dispose);
final GlobalKey<TestFocusState> key = GlobalKey();
// Initially create the focus inside of the parent FocusScope.
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
autofocus: true,
child: Column(
children: <Widget>[
TestFocus(
key: key,
debugLabel: 'Child',
),
],
),
),
);
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
FocusScope.of(key.currentContext!).requestFocus(key.currentState!.focusNode);
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(parentFocusScope, hasAGoodToStringDeep);
expect(
parentFocusScope.toStringDeep(),
equalsIgnoringHashCodes(
'FocusScopeNode#00000(Parent Scope Node [IN FOCUS PATH])\n'
' │ context: FocusScope\n'
' │ IN FOCUS PATH\n'
' │ focusedChildren: FocusNode#00000(Child [PRIMARY FOCUS])\n'
' │\n'
' └─Child 1: FocusNode#00000(Child [PRIMARY FOCUS])\n'
' context: Focus\n'
' PRIMARY FOCUS\n',
),
);
expect(FocusManager.instance.rootScope, hasAGoodToStringDeep);
expect(
FocusManager.instance.rootScope.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'FocusScopeNode#00000(Root Focus Scope [IN FOCUS PATH])\n'
' │ IN FOCUS PATH\n'
' │ focusedChildren: FocusScopeNode#00000(Parent Scope Node [IN FOCUS\n'
' │ PATH])\n'
' │\n'
' └─Child 1: FocusScopeNode#00000(Parent Scope Node [IN FOCUS PATH])\n'
' │ context: FocusScope\n'
' │ IN FOCUS PATH\n'
' │ focusedChildren: FocusNode#00000(Child [PRIMARY FOCUS])\n'
' │\n'
' └─Child 1: FocusNode#00000(Child [PRIMARY FOCUS])\n'
' context: Focus\n'
' PRIMARY FOCUS\n',
),
);
// Add the child focus scope to the focus tree.
final FocusAttachment childAttachment = childFocusScope.attach(key.currentContext);
parentFocusScope.setFirstFocus(childFocusScope);
await tester.pumpAndSettle();
expect(childFocusScope.isFirstFocus, isTrue);
// Now add the child focus scope with no child focusable in it to the tree.
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
child: Column(
children: <Widget>[
TestFocus(
key: key,
debugLabel: 'Child',
),
FocusScope(
debugLabel: 'Child Scope',
node: childFocusScope,
child: Container(),
),
],
),
),
);
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
// Now move the existing focus node into the child focus scope.
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
child: Column(
children: <Widget>[
FocusScope(
debugLabel: 'Child Scope',
node: childFocusScope,
child: TestFocus(
key: key,
debugLabel: 'Child',
),
),
],
),
),
);
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
// Now remove the child focus scope.
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
child: Column(
children: <Widget>[
TestFocus(
key: key,
debugLabel: 'Child',
),
],
),
),
);
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
// Must detach the child because we had to attach it in order to call
// setFirstFocus before adding to the widget.
childAttachment.detach();
});
testWidgets('Setting first focus requests focus for the scope properly.', (WidgetTester tester) async {
final FocusScopeNode parentFocusScope = FocusScopeNode(debugLabel: 'Parent Scope Node');
addTearDown(parentFocusScope.dispose);
final FocusScopeNode childFocusScope1 = FocusScopeNode(debugLabel: 'Child Scope Node 1');
addTearDown(childFocusScope1.dispose);
final FocusScopeNode childFocusScope2 = FocusScopeNode(debugLabel: 'Child Scope Node 2');
addTearDown(childFocusScope2.dispose);
final GlobalKey<TestFocusState> keyA = GlobalKey(debugLabel: 'Key A');
final GlobalKey<TestFocusState> keyB = GlobalKey(debugLabel: 'Key B');
final GlobalKey<TestFocusState> keyC = GlobalKey(debugLabel: 'Key C');
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
child: Column(
children: <Widget>[
FocusScope(
debugLabel: 'Child Scope 1',
node: childFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
key: keyA,
autofocus: true,
debugLabel: 'Child A',
),
TestFocus(
key: keyB,
name: 'b',
debugLabel: 'Child B',
),
],
),
),
FocusScope(
debugLabel: 'Child Scope 2',
node: childFocusScope2,
child: TestFocus(
key: keyC,
name: 'c',
debugLabel: 'Child C',
),
),
],
),
),
);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
parentFocusScope.setFirstFocus(childFocusScope2);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('a'), findsOneWidget);
parentFocusScope.setFirstFocus(childFocusScope1);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
keyB.currentState!.focusNode.requestFocus();
await tester.pumpAndSettle();
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
expect(parentFocusScope.isFirstFocus, isTrue);
expect(childFocusScope1.isFirstFocus, isTrue);
parentFocusScope.setFirstFocus(childFocusScope2);
await tester.pumpAndSettle();
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
expect(parentFocusScope.isFirstFocus, isTrue);
expect(childFocusScope1.isFirstFocus, isFalse);
expect(childFocusScope2.isFirstFocus, isTrue);
keyC.currentState!.focusNode.requestFocus();
await tester.pumpAndSettle();
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
expect(keyC.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('C FOCUSED'), findsOneWidget);
expect(parentFocusScope.isFirstFocus, isTrue);
expect(childFocusScope1.isFirstFocus, isFalse);
expect(childFocusScope2.isFirstFocus, isTrue);
childFocusScope1.requestFocus();
await tester.pumpAndSettle();
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
expect(keyC.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('c'), findsOneWidget);
expect(parentFocusScope.isFirstFocus, isTrue);
expect(childFocusScope1.isFirstFocus, isTrue);
expect(childFocusScope2.isFirstFocus, isFalse);
});
testWidgets('Removing focused widget moves focus to next widget', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
TestFocus(
key: keyA,
),
TestFocus(
key: keyB,
name: 'b',
),
],
),
);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(
Column(
children: <Widget>[
TestFocus(
key: keyB,
name: 'b',
),
],
),
);
await tester.pump();
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
});
testWidgets('Adding a new FocusScope attaches the child to its parent.', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final FocusScopeNode parentFocusScope = FocusScopeNode(debugLabel: 'Parent Scope Node');
addTearDown(parentFocusScope.dispose);
final FocusScopeNode childFocusScope = FocusScopeNode(debugLabel: 'Child Scope Node');
addTearDown(childFocusScope.dispose);
await tester.pumpWidget(
FocusScope(
node: childFocusScope,
child: TestFocus(
debugLabel: 'Child',
key: keyA,
),
),
);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
expect(FocusScope.of(keyA.currentContext!), equals(childFocusScope));
expect(Focus.of(keyA.currentContext!, scopeOk: true), equals(childFocusScope));
FocusManager.instance.rootScope.setFirstFocus(FocusScope.of(keyA.currentContext!));
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(childFocusScope.isFirstFocus, isTrue);
await tester.pumpWidget(
FocusScope(
node: parentFocusScope,
child: FocusScope(
node: childFocusScope,
child: TestFocus(
debugLabel: 'Child',
key: keyA,
),
),
),
);
await tester.pump();
expect(childFocusScope.isFirstFocus, isTrue);
// Node keeps it's focus when moved to the new scope.
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
});
testWidgets('Setting parentNode determines focus tree hierarchy.', (WidgetTester tester) async {
final FocusNode topNode = FocusNode(debugLabel: 'Top');
addTearDown(topNode.dispose);
final FocusNode parentNode = FocusNode(debugLabel: 'Parent');
addTearDown(parentNode.dispose);
final FocusNode childNode = FocusNode(debugLabel: 'Child');
addTearDown(childNode.dispose);
final FocusNode insertedNode = FocusNode(debugLabel: 'Inserted');
addTearDown(insertedNode.dispose);
await tester.pumpWidget(
FocusScope(
child: Focus.withExternalFocusNode(
focusNode: topNode,
child: Column(
children: <Widget>[
Focus.withExternalFocusNode(
focusNode: parentNode,
child: const SizedBox(),
),
Focus.withExternalFocusNode(
focusNode: childNode,
parentNode: parentNode,
autofocus: true,
child: const SizedBox(),
)
],
),
),
),
);
await tester.pump();
expect(childNode.hasPrimaryFocus, isTrue);
expect(parentNode.hasFocus, isTrue);
expect(topNode.hasFocus, isTrue);
// Check that inserting a Focus in between doesn't reparent the child.
await tester.pumpWidget(
FocusScope(
child: Focus.withExternalFocusNode(
focusNode: topNode,
child: Column(
children: <Widget>[
Focus.withExternalFocusNode(
focusNode: parentNode,
child: const SizedBox(),
),
Focus.withExternalFocusNode(
focusNode: insertedNode,
child: Focus.withExternalFocusNode(
focusNode: childNode,
parentNode: parentNode,
autofocus: true,
child: const SizedBox(),
),
)
],
),
),
),
);
await tester.pump();
expect(childNode.hasPrimaryFocus, isTrue);
expect(parentNode.hasFocus, isTrue);
expect(topNode.hasFocus, isTrue);
expect(insertedNode.hasFocus, isFalse);
});
testWidgets('Setting parentNode determines focus scope tree hierarchy.', (WidgetTester tester) async {
final FocusScopeNode topNode = FocusScopeNode(debugLabel: 'Top');
addTearDown(topNode.dispose);
final FocusScopeNode parentNode = FocusScopeNode(debugLabel: 'Parent');
addTearDown(parentNode.dispose);
final FocusScopeNode childNode = FocusScopeNode(debugLabel: 'Child');
addTearDown(childNode.dispose);
final FocusScopeNode insertedNode = FocusScopeNode(debugLabel: 'Inserted');
addTearDown(insertedNode.dispose);
await tester.pumpWidget(
FocusScope.withExternalFocusNode(
focusScopeNode: topNode,
child: Column(
children: <Widget>[
FocusScope.withExternalFocusNode(
focusScopeNode: parentNode,
child: const SizedBox(),
),
FocusScope.withExternalFocusNode(
focusScopeNode: childNode,
parentNode: parentNode,
child: const Focus(
autofocus: true,
child: SizedBox(),
),
)
],
),
),
);
await tester.pump();
expect(childNode.hasFocus, isTrue);
expect(parentNode.hasFocus, isTrue);
expect(topNode.hasFocus, isTrue);
// Check that inserting a Focus in between doesn't reparent the child.
await tester.pumpWidget(
FocusScope.withExternalFocusNode(
focusScopeNode: topNode,
child: Column(
children: <Widget>[
FocusScope.withExternalFocusNode(
focusScopeNode: parentNode,
child: const SizedBox(),
),
FocusScope.withExternalFocusNode(
focusScopeNode: insertedNode,
child: FocusScope.withExternalFocusNode(
focusScopeNode: childNode,
parentNode: parentNode,
child: const Focus(
autofocus: true,
child: SizedBox(),
),
),
)
],
),
),
);
await tester.pump();
expect(childNode.hasFocus, isTrue);
expect(parentNode.hasFocus, isTrue);
expect(topNode.hasFocus, isTrue);
expect(insertedNode.hasFocus, isFalse);
});
// Arguably, this isn't correct behavior, but it is what happens now.
testWidgets("Removing focused widget doesn't move focus to next widget within FocusScope", (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
final FocusScopeNode parentFocusScope = FocusScopeNode(debugLabel: 'Parent Scope');
addTearDown(parentFocusScope.dispose);
await tester.pumpWidget(
FocusScope(
debugLabel: 'Parent Scope',
node: parentFocusScope,
autofocus: true,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Widget A',
key: keyA,
),
TestFocus(
debugLabel: 'Widget B',
key: keyB,
name: 'b',
),
],
),
),
);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode scope = FocusScope.of(keyA.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(scope);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(
FocusScope(
node: parentFocusScope,
child: Column(
children: <Widget>[
TestFocus(
key: keyB,
name: 'b',
),
],
),
),
);
await tester.pump();
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
});
testWidgets('Removing a FocusScope removes its node from the tree', (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
final GlobalKey<TestFocusState> scopeKeyA = GlobalKey();
final GlobalKey<TestFocusState> scopeKeyB = GlobalKey();
final FocusScopeNode parentFocusScope = FocusScopeNode(debugLabel: 'Parent Scope');
addTearDown(parentFocusScope.dispose);
// This checks both FocusScopes that have their own nodes, as well as those
// that use external nodes.
await tester.pumpWidget(
FocusTraversalGroup(
child: Column(
children: <Widget>[
FocusScope(
key: scopeKeyA,
node: parentFocusScope,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child A',
key: keyA,
),
],
),
),
FocusScope(
key: scopeKeyB,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
),
],
),
),
],
),
),
);
FocusScope.of(keyB.currentContext!).requestFocus(keyB.currentState!.focusNode);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode aScope = FocusScope.of(keyA.currentContext!);
final FocusScopeNode bScope = FocusScope.of(keyB.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(bScope);
FocusManager.instance.rootScope.setFirstFocus(aScope);
await tester.pumpAndSettle();
expect(FocusScope.of(keyA.currentContext!).isFirstFocus, isTrue);
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(Container());
expect(FocusManager.instance.rootScope.children, isEmpty);
});
// By "pinned", it means kept in the tree by a GlobalKey.
testWidgets("Removing pinned focused scope doesn't move focus to focused widget within next FocusScope", (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
final GlobalKey<TestFocusState> scopeKeyA = GlobalKey();
final GlobalKey<TestFocusState> scopeKeyB = GlobalKey();
final FocusScopeNode parentFocusScope1 = FocusScopeNode(debugLabel: 'Parent Scope 1');
addTearDown(parentFocusScope1.dispose);
final FocusScopeNode parentFocusScope2 = FocusScopeNode(debugLabel: 'Parent Scope 2');
addTearDown(parentFocusScope2.dispose);
await tester.pumpWidget(
FocusTraversalGroup(
child: Column(
children: <Widget>[
FocusScope(
key: scopeKeyA,
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child A',
key: keyA,
),
],
),
),
FocusScope(
key: scopeKeyB,
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
),
],
),
),
],
),
),
);
FocusScope.of(keyB.currentContext!).requestFocus(keyB.currentState!.focusNode);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode bScope = FocusScope.of(keyB.currentContext!);
final FocusScopeNode aScope = FocusScope.of(keyA.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(bScope);
FocusManager.instance.rootScope.setFirstFocus(aScope);
await tester.pumpAndSettle();
expect(FocusScope.of(keyA.currentContext!).isFirstFocus, isTrue);
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(
FocusTraversalGroup(
child: Column(
children: <Widget>[
FocusScope(
key: scopeKeyB,
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
autofocus: true,
),
],
),
),
],
),
),
);
await tester.pump();
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
});
testWidgets("Removing unpinned focused scope doesn't move focus to focused widget within next FocusScope", (WidgetTester tester) async {
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
final FocusScopeNode parentFocusScope1 = FocusScopeNode(debugLabel: 'Parent Scope 1');
addTearDown(parentFocusScope1.dispose);
final FocusScopeNode parentFocusScope2 = FocusScopeNode(debugLabel: 'Parent Scope 2');
addTearDown(parentFocusScope2.dispose);
await tester.pumpWidget(
FocusTraversalGroup(
child: Column(
children: <Widget>[
FocusScope(
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child A',
key: keyA,
),
],
),
),
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
),
],
),
),
],
),
),
);
FocusScope.of(keyB.currentContext!).requestFocus(keyB.currentState!.focusNode);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode bScope = FocusScope.of(keyB.currentContext!);
final FocusScopeNode aScope = FocusScope.of(keyA.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(bScope);
FocusManager.instance.rootScope.setFirstFocus(aScope);
await tester.pumpAndSettle();
expect(FocusScope.of(keyA.currentContext!).isFirstFocus, isTrue);
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(
FocusTraversalGroup(
child: Column(
children: <Widget>[
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
autofocus: true,
),
],
),
),
],
),
),
);
await tester.pump();
expect(keyB.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('B FOCUSED'), findsOneWidget);
});
testWidgets('Moving widget from one scope to another retains focus', (WidgetTester tester) async {
final FocusScopeNode parentFocusScope1 = FocusScopeNode();
addTearDown(parentFocusScope1.dispose);
final FocusScopeNode parentFocusScope2 = FocusScopeNode();
addTearDown(parentFocusScope2.dispose);
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
FocusScope(
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
key: keyA,
),
],
),
),
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
key: keyB,
name: 'b',
),
],
),
),
],
),
);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode aScope = FocusScope.of(keyA.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(aScope);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
await tester.pumpWidget(
Column(
children: <Widget>[
FocusScope(
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
key: keyB,
name: 'b',
),
],
),
),
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
key: keyA,
),
],
),
),
],
),
);
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
});
testWidgets('Moving FocusScopeNodes retains focus', (WidgetTester tester) async {
final FocusScopeNode parentFocusScope1 = FocusScopeNode(debugLabel: 'Scope 1');
addTearDown(parentFocusScope1.dispose);
final FocusScopeNode parentFocusScope2 = FocusScopeNode(debugLabel: 'Scope 2');
addTearDown(parentFocusScope2.dispose);
final GlobalKey<TestFocusState> keyA = GlobalKey();
final GlobalKey<TestFocusState> keyB = GlobalKey();
await tester.pumpWidget(
Column(
children: <Widget>[
FocusScope(
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child A',
key: keyA,
),
],
),
),
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
),
],
),
),
],
),
);
FocusScope.of(keyA.currentContext!).requestFocus(keyA.currentState!.focusNode);
final FocusScopeNode aScope = FocusScope.of(keyA.currentContext!);
FocusManager.instance.rootScope.setFirstFocus(aScope);
await tester.pumpAndSettle();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
// This just swaps the FocusScopeNodes that the FocusScopes have in them.
await tester.pumpWidget(
Column(
children: <Widget>[
FocusScope(
node: parentFocusScope2,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child A',
key: keyA,
),
],
),
),
FocusScope(
node: parentFocusScope1,
child: Column(
children: <Widget>[
TestFocus(
debugLabel: 'Child B',
key: keyB,
name: 'b',
),
],
),
),
],
),
);
await tester.pump();
expect(keyA.currentState!.focusNode.hasFocus, isTrue);
expect(find.text('A FOCUSED'), findsOneWidget);
expect(keyB.currentState!.focusNode.hasFocus, isFalse);
expect(find.text('b'), findsOneWidget);
});
testWidgets('Can focus root node.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
await tester.pumpWidget(
Focus(
key: key1,
child: Container(),
),
);
final Element firstElement = tester.element(find.byKey(key1));
final FocusScopeNode rootNode = FocusScope.of(firstElement);
rootNode.requestFocus();
await tester.pump();
expect(rootNode.hasFocus, isTrue);
expect(rootNode, equals(firstElement.owner!.focusManager.rootScope));
});
testWidgets('Can autofocus a node.', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode(debugLabel: 'Test Node');
addTearDown(focusNode.dispose);
await tester.pumpWidget(
Focus(
focusNode: focusNode,
child: Container(),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isFalse);
await tester.pumpWidget(
Focus(
autofocus: true,
focusNode: focusNode,
child: Container(),
),
);
await tester.pump();
expect(focusNode.hasPrimaryFocus, isTrue);
});
testWidgets("Won't autofocus a node if one is already focused.", (WidgetTester tester) async {
final FocusNode focusNodeA = FocusNode(debugLabel: 'Test Node A');
addTearDown(focusNodeA.dispose);
final FocusNode focusNodeB = FocusNode(debugLabel: 'Test Node B');
addTearDown(focusNodeB.dispose);
await tester.pumpWidget(
Column(
children: <Widget>[
Focus(
focusNode: focusNodeA,
autofocus: true,
child: Container(),
),
],
),
);
await tester.pump();
expect(focusNodeA.hasPrimaryFocus, isTrue);
await tester.pumpWidget(
Column(
children: <Widget>[
Focus(
focusNode: focusNodeA,
child: Container(),
),
Focus(
focusNode: focusNodeB,
autofocus: true,
child: Container(),
),
],
),
);
await tester.pump();
expect(focusNodeB.hasPrimaryFocus, isFalse);
expect(focusNodeA.hasPrimaryFocus, isTrue);
});
testWidgets("FocusScope doesn't update the focusNode attributes when the widget updates if withExternalFocusNode is used", (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final FocusScopeNode focusScopeNode = FocusScopeNode();
addTearDown(focusScopeNode.dispose);
bool? keyEventHandled;
KeyEventResult handleCallback(FocusNode node, RawKeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult handleEventCallback(FocusNode node, KeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult ignoreCallback(FocusNode node, RawKeyEvent event) => KeyEventResult.ignored;
KeyEventResult ignoreEventCallback(FocusNode node, KeyEvent event) => KeyEventResult.ignored;
focusScopeNode.onKey = ignoreCallback;
focusScopeNode.onKeyEvent = ignoreEventCallback;
focusScopeNode.descendantsAreFocusable = false;
focusScopeNode.descendantsAreTraversable = false;
focusScopeNode.skipTraversal = false;
focusScopeNode.canRequestFocus = true;
FocusScope focusScopeWidget = FocusScope.withExternalFocusNode(
focusScopeNode: focusScopeNode,
child: Container(key: key1),
);
await tester.pumpWidget(focusScopeWidget);
expect(focusScopeNode.onKey, equals(ignoreCallback));
expect(focusScopeNode.onKeyEvent, equals(ignoreEventCallback));
expect(focusScopeNode.descendantsAreFocusable, isFalse);
expect(focusScopeNode.descendantsAreTraversable, isFalse);
expect(focusScopeNode.skipTraversal, isFalse);
expect(focusScopeNode.canRequestFocus, isTrue);
expect(focusScopeWidget.onKey, equals(focusScopeNode.onKey));
expect(focusScopeWidget.onKeyEvent, equals(focusScopeNode.onKeyEvent));
expect(focusScopeWidget.descendantsAreFocusable, equals(focusScopeNode.descendantsAreFocusable));
expect(focusScopeWidget.descendantsAreTraversable, equals(focusScopeNode.descendantsAreTraversable));
expect(focusScopeWidget.skipTraversal, equals(focusScopeNode.skipTraversal));
expect(focusScopeWidget.canRequestFocus, equals(focusScopeNode.canRequestFocus));
FocusScope.of(key1.currentContext!).requestFocus();
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isNull);
focusScopeNode.onKey = handleCallback;
focusScopeNode.onKeyEvent = handleEventCallback;
focusScopeNode.descendantsAreFocusable = true;
focusScopeNode.descendantsAreTraversable = true;
focusScopeWidget = FocusScope.withExternalFocusNode(
focusScopeNode: focusScopeNode,
child: Container(key: key1),
);
await tester.pumpWidget(focusScopeWidget);
expect(focusScopeNode.onKey, equals(handleCallback));
expect(focusScopeNode.onKeyEvent, equals(handleEventCallback));
expect(focusScopeNode.descendantsAreFocusable, isTrue);
expect(focusScopeNode.descendantsAreTraversable, isTrue);
expect(focusScopeNode.skipTraversal, isFalse);
expect(focusScopeNode.canRequestFocus, isTrue);
expect(focusScopeWidget.onKey, equals(focusScopeNode.onKey));
expect(focusScopeWidget.onKeyEvent, equals(focusScopeNode.onKeyEvent));
expect(focusScopeWidget.descendantsAreFocusable, equals(focusScopeNode.descendantsAreFocusable));
expect(focusScopeWidget.descendantsAreTraversable, equals(focusScopeNode.descendantsAreTraversable));
expect(focusScopeWidget.skipTraversal, equals(focusScopeNode.skipTraversal));
expect(focusScopeWidget.canRequestFocus, equals(focusScopeNode.canRequestFocus));
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isTrue);
});
});
group('Focus', () {
testWidgets('Focus.of stops at the nearest Focus widget.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final GlobalKey key2 = GlobalKey(debugLabel: '2');
final GlobalKey key3 = GlobalKey(debugLabel: '3');
final GlobalKey key4 = GlobalKey(debugLabel: '4');
final GlobalKey key5 = GlobalKey(debugLabel: '5');
final GlobalKey key6 = GlobalKey(debugLabel: '6');
final FocusScopeNode scopeNode = FocusScopeNode();
addTearDown(scopeNode.dispose);
await tester.pumpWidget(
FocusScope(
key: key1,
node: scopeNode,
debugLabel: 'Key 1',
child: Container(
key: key2,
child: Focus(
debugLabel: 'Key 3',
key: key3,
child: Container(
key: key4,
child: Focus(
debugLabel: 'Key 5',
key: key5,
child: Container(
key: key6,
),
),
),
),
),
),
);
final Element element1 = tester.element(find.byKey(key1));
final Element element2 = tester.element(find.byKey(key2));
final Element element3 = tester.element(find.byKey(key3));
final Element element4 = tester.element(find.byKey(key4));
final Element element5 = tester.element(find.byKey(key5));
final Element element6 = tester.element(find.byKey(key6));
final FocusNode root = element1.owner!.focusManager.rootScope;
expect(Focus.maybeOf(element1), isNull);
expect(Focus.maybeOf(element2), isNull);
expect(Focus.maybeOf(element3), isNull);
expect(Focus.of(element4).parent!.parent, equals(root));
expect(Focus.of(element5).parent!.parent, equals(root));
expect(Focus.of(element6).parent!.parent!.parent, equals(root));
});
testWidgets('Can traverse Focus children.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final GlobalKey key2 = GlobalKey(debugLabel: '2');
final GlobalKey key3 = GlobalKey(debugLabel: '3');
final GlobalKey key4 = GlobalKey(debugLabel: '4');
final GlobalKey key5 = GlobalKey(debugLabel: '5');
final GlobalKey key6 = GlobalKey(debugLabel: '6');
final GlobalKey key7 = GlobalKey(debugLabel: '7');
final GlobalKey key8 = GlobalKey(debugLabel: '8');
await tester.pumpWidget(
Focus(
child: Column(
key: key1,
children: <Widget>[
Focus(
key: key2,
child: Focus(
key: key3,
child: Container(),
),
),
Focus(
key: key4,
child: Focus(
key: key5,
child: Container(),
),
),
Focus(
key: key6,
child: Column(
children: <Widget>[
Focus(
key: key7,
child: Container(),
),
Focus(
key: key8,
child: Container(),
),
],
),
),
],
),
),
);
final Element firstScope = tester.element(find.byKey(key1));
final List<FocusNode> nodes = <FocusNode>[];
final List<Key> keys = <Key>[];
bool visitor(FocusNode node) {
nodes.add(node);
keys.add(node.context!.widget.key!);
return true;
}
await tester.pump();
Focus.of(firstScope).descendants.forEach(visitor);
expect(nodes.length, equals(7));
expect(keys.length, equals(7));
// Depth first.
expect(keys, equals(<Key>[key3, key2, key5, key4, key7, key8, key6]));
// Just traverses a sub-tree.
final Element secondScope = tester.element(find.byKey(key7));
nodes.clear();
keys.clear();
Focus.of(secondScope).descendants.forEach(visitor);
expect(nodes.length, equals(2));
expect(keys, equals(<Key>[key7, key8]));
});
testWidgets('Can set focus.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
late bool gotFocus;
await tester.pumpWidget(
Focus(
onFocusChange: (bool focused) => gotFocus = focused,
child: Container(key: key1),
),
);
final Element firstNode = tester.element(find.byKey(key1));
final FocusNode node = Focus.of(firstNode);
node.requestFocus();
await tester.pump();
expect(gotFocus, isTrue);
expect(node.hasFocus, isTrue);
});
testWidgets('Focus is ignored when set to not focusable.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
bool? gotFocus;
await tester.pumpWidget(
Focus(
canRequestFocus: false,
onFocusChange: (bool focused) => gotFocus = focused,
child: Container(key: key1),
),
);
final Element firstNode = tester.element(find.byKey(key1));
final FocusNode node = Focus.of(firstNode);
node.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(node.hasFocus, isFalse);
});
testWidgets('Focus is lost when set to not focusable.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
bool? gotFocus;
await tester.pumpWidget(
Focus(
autofocus: true,
canRequestFocus: true,
onFocusChange: (bool focused) => gotFocus = focused,
child: Container(key: key1),
),
);
Element firstNode = tester.element(find.byKey(key1));
FocusNode node = Focus.of(firstNode);
node.requestFocus();
await tester.pump();
expect(gotFocus, isTrue);
expect(node.hasFocus, isTrue);
gotFocus = null;
await tester.pumpWidget(
Focus(
canRequestFocus: false,
onFocusChange: (bool focused) => gotFocus = focused,
child: Container(key: key1),
),
);
firstNode = tester.element(find.byKey(key1));
node = Focus.of(firstNode);
node.requestFocus();
await tester.pump();
expect(gotFocus, false);
expect(node.hasFocus, isFalse);
});
testWidgets('Child of unfocusable Focus can get focus.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final GlobalKey key2 = GlobalKey(debugLabel: '2');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? gotFocus;
await tester.pumpWidget(
Focus(
canRequestFocus: false,
onFocusChange: (bool focused) => gotFocus = focused,
child: Focus(key: key1, focusNode: focusNode, child: Container(key: key2)),
),
);
final Element childWidget = tester.element(find.byKey(key1));
final FocusNode unfocusableNode = Focus.of(childWidget);
unfocusableNode.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(unfocusableNode.hasFocus, isFalse);
final Element containerWidget = tester.element(find.byKey(key2));
final FocusNode focusableNode = Focus.of(containerWidget);
focusableNode.requestFocus();
await tester.pump();
expect(gotFocus, isTrue);
expect(unfocusableNode.hasFocus, isTrue);
});
testWidgets('Nodes are removed when all Focuses are removed.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
late bool gotFocus;
await tester.pumpWidget(
FocusScope(
child: Focus(
onFocusChange: (bool focused) => gotFocus = focused,
child: Container(key: key1),
),
),
);
final Element firstNode = tester.element(find.byKey(key1));
final FocusNode node = Focus.of(firstNode);
node.requestFocus();
await tester.pump();
expect(gotFocus, isTrue);
expect(node.hasFocus, isTrue);
await tester.pumpWidget(Container());
expect(FocusManager.instance.rootScope.descendants, isEmpty);
});
testWidgets('Focus widgets set Semantics information about focus', (WidgetTester tester) async {
final GlobalKey<TestFocusState> key = GlobalKey();
await tester.pumpWidget(
TestFocus(key: key),
);
final SemanticsNode semantics = tester.getSemantics(find.byKey(key));
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(semantics.hasFlag(SemanticsFlag.isFocused), isFalse);
expect(semantics.hasFlag(SemanticsFlag.isFocusable), isTrue);
FocusScope.of(key.currentContext!).requestFocus(key.currentState!.focusNode);
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isTrue);
expect(semantics.hasFlag(SemanticsFlag.isFocused), isTrue);
expect(semantics.hasFlag(SemanticsFlag.isFocusable), isTrue);
key.currentState!.focusNode.canRequestFocus = false;
await tester.pumpAndSettle();
expect(key.currentState!.focusNode.hasFocus, isFalse);
expect(key.currentState!.focusNode.canRequestFocus, isFalse);
expect(semantics.hasFlag(SemanticsFlag.isFocused), isFalse);
expect(semantics.hasFlag(SemanticsFlag.isFocusable), isFalse);
});
testWidgets('Setting canRequestFocus on focus node causes update.', (WidgetTester tester) async {
final GlobalKey<TestFocusState> key = GlobalKey();
final TestFocus testFocus = TestFocus(key: key);
await tester.pumpWidget(
testFocus,
);
await tester.pumpAndSettle();
key.currentState!.built = false;
key.currentState!.focusNode.canRequestFocus = false;
await tester.pumpAndSettle();
key.currentState!.built = true;
expect(key.currentState!.focusNode.canRequestFocus, isFalse);
});
testWidgets('canRequestFocus causes descendants of scope to be skipped.', (WidgetTester tester) async {
final GlobalKey scope1 = GlobalKey(debugLabel: 'scope1');
final GlobalKey scope2 = GlobalKey(debugLabel: 'scope2');
final GlobalKey focus1 = GlobalKey(debugLabel: 'focus1');
final GlobalKey focus2 = GlobalKey(debugLabel: 'focus2');
final GlobalKey container1 = GlobalKey(debugLabel: 'container');
Future<void> pumpTest({
bool allowScope1 = true,
bool allowScope2 = true,
bool allowFocus1 = true,
bool allowFocus2 = true,
}) async {
await tester.pumpWidget(
FocusScope(
key: scope1,
canRequestFocus: allowScope1,
child: FocusScope(
key: scope2,
canRequestFocus: allowScope2,
child: Focus(
key: focus1,
canRequestFocus: allowFocus1,
child: Focus(
key: focus2,
canRequestFocus: allowFocus2,
child: Container(
key: container1,
),
),
),
),
),
);
await tester.pump();
}
// Check childless node (focus2).
await pumpTest();
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
await pumpTest(allowFocus2: false);
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
await pumpTest();
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
// Check FocusNode with child (focus1). Shouldn't affect children.
await pumpTest(allowFocus1: false);
expect(Focus.of(container1.currentContext!).hasFocus, isTrue); // focus2 has focus.
Focus.of(focus2.currentContext!).requestFocus(); // Try to focus focus1
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue); // focus2 still has focus.
Focus.of(container1.currentContext!).requestFocus(); // Now try to focus focus2
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
await pumpTest();
// Try again, now that we've set focus1's canRequestFocus to true again.
Focus.of(container1.currentContext!).unfocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
// Check FocusScopeNode with only FocusNode children (scope2). Should affect children.
await pumpTest(allowScope2: false);
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
FocusScope.of(focus1.currentContext!).requestFocus(); // Try to focus scope2
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(focus2.currentContext!).requestFocus(); // Try to focus focus1
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(container1.currentContext!).requestFocus(); // Try to focus focus2
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
await pumpTest();
// Try again, now that we've set scope2's canRequestFocus to true again.
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
// Check FocusScopeNode with both FocusNode children and FocusScope children (scope1). Should affect children.
await pumpTest(allowScope1: false);
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
FocusScope.of(scope2.currentContext!).requestFocus(); // Try to focus scope1
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
FocusScope.of(focus1.currentContext!).requestFocus(); // Try to focus scope2
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(focus2.currentContext!).requestFocus(); // Try to focus focus1
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
Focus.of(container1.currentContext!).requestFocus(); // Try to focus focus2
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isFalse);
await pumpTest();
// Try again, now that we've set scope1's canRequestFocus to true again.
Focus.of(container1.currentContext!).requestFocus();
await tester.pump();
expect(Focus.of(container1.currentContext!).hasFocus, isTrue);
});
testWidgets('skipTraversal works as expected.', (WidgetTester tester) async {
final FocusScopeNode scope1 = FocusScopeNode(debugLabel: 'scope1');
addTearDown(scope1.dispose);
final FocusScopeNode scope2 = FocusScopeNode(debugLabel: 'scope2');
addTearDown(scope2.dispose);
final FocusNode focus1 = FocusNode(debugLabel: 'focus1');
addTearDown(focus1.dispose);
final FocusNode focus2 = FocusNode(debugLabel: 'focus2');
addTearDown(focus2.dispose);
Future<void> pumpTest({
bool traverseScope1 = false,
bool traverseScope2 = false,
bool traverseFocus1 = false,
bool traverseFocus2 = false,
}) async {
await tester.pumpWidget(
FocusScope(
node: scope1,
skipTraversal: traverseScope1,
child: FocusScope(
node: scope2,
skipTraversal: traverseScope2,
child: Focus(
focusNode: focus1,
skipTraversal: traverseFocus1,
child: Focus(
focusNode: focus2,
skipTraversal: traverseFocus2,
child: Container(),
),
),
),
),
);
await tester.pump();
}
await pumpTest();
expect(scope1.traversalDescendants, equals(<FocusNode>[focus2, focus1, scope2]));
// Check childless node (focus2).
await pumpTest(traverseFocus2: true);
expect(scope1.traversalDescendants, equals(<FocusNode>[focus1, scope2]));
// Check FocusNode with child (focus1). Shouldn't affect children.
await pumpTest(traverseFocus1: true);
expect(scope1.traversalDescendants, equals(<FocusNode>[focus2, scope2]));
// Check FocusScopeNode with only FocusNode children (scope2). Should affect children.
await pumpTest(traverseScope2: true);
expect(scope1.traversalDescendants, equals(<FocusNode>[focus2, focus1]));
// Check FocusScopeNode with both FocusNode children and FocusScope children (scope1). Should affect children.
await pumpTest(traverseScope1: true);
expect(scope1.traversalDescendants, equals(<FocusNode>[focus2, focus1, scope2]));
});
testWidgets('descendantsAreFocusable works as expected.', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final GlobalKey key2 = GlobalKey(debugLabel: '2');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? gotFocus;
await tester.pumpWidget(
Focus(
descendantsAreFocusable: false,
child: Focus(
onFocusChange: (bool focused) => gotFocus = focused,
child: Focus(
key: key1,
focusNode: focusNode,
child: Container(key: key2),
),
),
),
);
final Element childWidget = tester.element(find.byKey(key1));
final FocusNode unfocusableNode = Focus.of(childWidget);
final Element containerWidget = tester.element(find.byKey(key2));
final FocusNode containerNode = Focus.of(containerWidget);
unfocusableNode.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(containerNode.hasFocus, isFalse);
expect(unfocusableNode.hasFocus, isFalse);
containerNode.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(containerNode.hasFocus, isFalse);
expect(unfocusableNode.hasFocus, isFalse);
});
testWidgets('descendantsAreTraversable works as expected.', (WidgetTester tester) async {
final FocusScopeNode scopeNode = FocusScopeNode(debugLabel: 'scope');
addTearDown(scopeNode.dispose);
final FocusNode node1 = FocusNode(debugLabel: 'node 1');
addTearDown(node1.dispose);
final FocusNode node2 = FocusNode(debugLabel: 'node 2');
addTearDown(node2.dispose);
final FocusNode node3 = FocusNode(debugLabel: 'node 3');
addTearDown(node3.dispose);
await tester.pumpWidget(
FocusScope(
node: scopeNode,
child: Column(
children: <Widget>[
Focus(
focusNode: node1,
child: Container(),
),
Focus(
focusNode: node2,
descendantsAreTraversable: false,
child: Focus(
focusNode: node3,
child: Container(),
)
),
],
),
),
);
await tester.pump();
expect(scopeNode.traversalDescendants, equals(<FocusNode>[node1, node2]));
expect(node2.traversalDescendants, equals(<FocusNode>[]));
});
testWidgets("Focus doesn't introduce a Semantics node when includeSemantics is false", (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(Focus(includeSemantics: false, child: Container()));
final TestSemantics expectedSemantics = TestSemantics.root();
expect(semantics, hasSemantics(expectedSemantics));
semantics.dispose();
});
testWidgets('Focus updates the onKey handler when the widget updates', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? keyEventHandled;
KeyEventResult handleCallback(FocusNode node, RawKeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult ignoreCallback(FocusNode node, RawKeyEvent event) => KeyEventResult.ignored;
Focus focusWidget = Focus(
onKey: ignoreCallback, // This one does nothing.
focusNode: focusNode,
skipTraversal: true,
canRequestFocus: true,
child: Container(key: key1),
);
focusNode.onKeyEvent = null;
await tester.pumpWidget(focusWidget);
expect(focusNode.onKey, equals(ignoreCallback));
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
Focus.of(key1.currentContext!).requestFocus();
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isNull);
focusWidget = Focus(
onKey: handleCallback,
focusNode: focusNode,
skipTraversal: true,
canRequestFocus: true,
child: Container(key: key1),
);
await tester.pumpWidget(focusWidget);
expect(focusNode.onKey, equals(handleCallback));
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isTrue);
});
testWidgets('Focus updates the onKeyEvent handler when the widget updates', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? keyEventHandled;
KeyEventResult handleEventCallback(FocusNode node, KeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult ignoreEventCallback(FocusNode node, KeyEvent event) => KeyEventResult.ignored;
Focus focusWidget = Focus(
onKeyEvent: ignoreEventCallback, // This one does nothing.
focusNode: focusNode,
skipTraversal: true,
canRequestFocus: true,
child: Container(key: key1),
);
focusNode.onKeyEvent = null;
await tester.pumpWidget(focusWidget);
expect(focusNode.onKeyEvent, equals(ignoreEventCallback));
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
Focus.of(key1.currentContext!).requestFocus();
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isNull);
focusWidget = Focus(
onKeyEvent: handleEventCallback,
focusNode: focusNode,
skipTraversal: true,
canRequestFocus: true,
child: Container(key: key1),
);
await tester.pumpWidget(focusWidget);
expect(focusNode.onKeyEvent, equals(handleEventCallback));
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isTrue);
});
testWidgets("Focus doesn't update the focusNode attributes when the widget updates if withExternalFocusNode is used", (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? keyEventHandled;
KeyEventResult handleCallback(FocusNode node, RawKeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult handleEventCallback(FocusNode node, KeyEvent event) {
keyEventHandled = true;
return KeyEventResult.handled;
}
KeyEventResult ignoreCallback(FocusNode node, RawKeyEvent event) => KeyEventResult.ignored;
KeyEventResult ignoreEventCallback(FocusNode node, KeyEvent event) => KeyEventResult.ignored;
focusNode.onKey = ignoreCallback;
focusNode.onKeyEvent = ignoreEventCallback;
focusNode.descendantsAreFocusable = false;
focusNode.descendantsAreTraversable = false;
focusNode.skipTraversal = false;
focusNode.canRequestFocus = true;
Focus focusWidget = Focus.withExternalFocusNode(
focusNode: focusNode,
child: Container(key: key1),
);
await tester.pumpWidget(focusWidget);
expect(focusNode.onKey, equals(ignoreCallback));
expect(focusNode.onKeyEvent, equals(ignoreEventCallback));
expect(focusNode.descendantsAreFocusable, isFalse);
expect(focusNode.descendantsAreTraversable, isFalse);
expect(focusNode.skipTraversal, isFalse);
expect(focusNode.canRequestFocus, isTrue);
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.descendantsAreTraversable, equals(focusNode.descendantsAreTraversable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
Focus.of(key1.currentContext!).requestFocus();
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isNull);
focusNode.onKey = handleCallback;
focusNode.onKeyEvent = handleEventCallback;
focusNode.descendantsAreFocusable = true;
focusNode.descendantsAreTraversable = true;
focusWidget = Focus.withExternalFocusNode(
focusNode: focusNode,
child: Container(key: key1),
);
await tester.pumpWidget(focusWidget);
expect(focusNode.onKey, equals(handleCallback));
expect(focusNode.onKeyEvent, equals(handleEventCallback));
expect(focusNode.descendantsAreFocusable, isTrue);
expect(focusNode.descendantsAreTraversable, isTrue);
expect(focusNode.skipTraversal, isFalse);
expect(focusNode.canRequestFocus, isTrue);
expect(focusWidget.onKey, equals(focusNode.onKey));
expect(focusWidget.onKeyEvent, equals(focusNode.onKeyEvent));
expect(focusWidget.descendantsAreFocusable, equals(focusNode.descendantsAreFocusable));
expect(focusWidget.descendantsAreTraversable, equals(focusNode.descendantsAreTraversable));
expect(focusWidget.skipTraversal, equals(focusNode.skipTraversal));
expect(focusWidget.canRequestFocus, equals(focusNode.canRequestFocus));
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
expect(keyEventHandled, isTrue);
});
testWidgets('Focus does not update the focusNode attributes when the widget updates if withExternalFocusNode is used 2', (WidgetTester tester) async {
final TestExternalFocusNode focusNode = TestExternalFocusNode();
assert(!focusNode.isModified);
addTearDown(focusNode.dispose);
final Focus focusWidget = Focus.withExternalFocusNode(
focusNode: focusNode,
child: Container(),
);
await tester.pumpWidget(focusWidget);
expect(focusNode.isModified, isFalse);
await tester.pumpWidget(const SizedBox());
});
testWidgets('Focus passes changes in attribute values to its focus node', (WidgetTester tester) async {
await tester.pumpWidget(
Focus(
child: Container(),
),
);
});
});
group('ExcludeFocus', () {
testWidgets("Descendants of ExcludeFocus aren't focusable.", (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey(debugLabel: '1');
final GlobalKey key2 = GlobalKey(debugLabel: '2');
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
bool? gotFocus;
await tester.pumpWidget(
ExcludeFocus(
child: Focus(
onFocusChange: (bool focused) => gotFocus = focused,
child: Focus(
key: key1,
focusNode: focusNode,
child: Container(key: key2),
),
),
),
);
final Element childWidget = tester.element(find.byKey(key1));
final FocusNode unfocusableNode = Focus.of(childWidget);
final Element containerWidget = tester.element(find.byKey(key2));
final FocusNode containerNode = Focus.of(containerWidget);
unfocusableNode.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(containerNode.hasFocus, isFalse);
expect(unfocusableNode.hasFocus, isFalse);
containerNode.requestFocus();
await tester.pump();
expect(gotFocus, isNull);
expect(containerNode.hasFocus, isFalse);
expect(unfocusableNode.hasFocus, isFalse);
});
// Regression test for https://github.com/flutter/flutter/issues/61700
testWidgets("ExcludeFocus doesn't transfer focus to another descendant.", (WidgetTester tester) async {
final FocusNode parentFocusNode = FocusNode(debugLabel: 'group');
addTearDown(parentFocusNode.dispose);
final FocusNode focusNode1 = FocusNode(debugLabel: 'node 1');
addTearDown(focusNode1.dispose);
final FocusNode focusNode2 = FocusNode(debugLabel: 'node 2');
addTearDown(focusNode2.dispose);
await tester.pumpWidget(
ExcludeFocus(
excluding: false,
child: Focus(
focusNode: parentFocusNode,
child: Column(
children: <Widget>[
Focus(
autofocus: true,
focusNode: focusNode1,
child: Container(),
),
Focus(
focusNode: focusNode2,
child: Container(),
),
],
),
),
),
);
await tester.pump();
expect(parentFocusNode.hasFocus, isTrue);
expect(focusNode1.hasPrimaryFocus, isTrue);
expect(focusNode2.hasFocus, isFalse);
// Move focus to the second node to create some focus history for the scope.
focusNode2.requestFocus();
await tester.pump();
expect(parentFocusNode.hasFocus, isTrue);
expect(focusNode1.hasFocus, isFalse);
expect(focusNode2.hasPrimaryFocus, isTrue);
// Now turn off the focus for the subtree.
await tester.pumpWidget(
ExcludeFocus(
child: Focus(
focusNode: parentFocusNode,
child: Column(
children: <Widget>[
Focus(
autofocus: true,
focusNode: focusNode1,
child: Container(),
),
Focus(
focusNode: focusNode2,
child: Container(),
),
],
),
),
),
);
await tester.pump();
expect(focusNode1.hasFocus, isFalse);
expect(focusNode2.hasFocus, isFalse);
expect(parentFocusNode.hasFocus, isFalse);
expect(parentFocusNode.enclosingScope!.hasPrimaryFocus, isTrue);
});
testWidgets("ExcludeFocus doesn't introduce a Semantics node", (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(ExcludeFocus(child: Container()));
final TestSemantics expectedSemantics = TestSemantics.root();
expect(semantics, hasSemantics(expectedSemantics));
semantics.dispose();
});
// Regression test for https://github.com/flutter/flutter/issues/92693
testWidgets('Setting parent FocusScope.canRequestFocus to false, does not set descendant Focus._internalNode._canRequestFocus to false', (WidgetTester tester) async {
final FocusNode childFocusNode = FocusNode(debugLabel: 'node 1');
addTearDown(childFocusNode.dispose);
Widget buildFocusTree({required bool parentCanRequestFocus}) {
return FocusScope(
canRequestFocus: parentCanRequestFocus,
child: Column(
children: <Widget>[
Focus(
focusNode: childFocusNode,
child: Container(),
),
],
),
);
}
// childFocusNode.canRequestFocus is true when parent canRequestFocus is true
await tester.pumpWidget(buildFocusTree(parentCanRequestFocus: true));
expect(childFocusNode.canRequestFocus, isTrue);
// childFocusNode.canRequestFocus is false when parent canRequestFocus is false
await tester.pumpWidget(buildFocusTree(parentCanRequestFocus: false));
expect(childFocusNode.canRequestFocus, isFalse);
// childFocusNode.canRequestFocus is true again when parent canRequestFocus is changed back to true
await tester.pumpWidget(buildFocusTree(parentCanRequestFocus: true));
expect(childFocusNode.canRequestFocus, isTrue);
});
});
}
class TestFocus extends StatefulWidget {
const TestFocus({
super.key,
this.debugLabel,
this.name = 'a',
this.autofocus = false,
this.parentNode,
});
final String? debugLabel;
final String name;
final bool autofocus;
final FocusNode? parentNode;
@override
TestFocusState createState() => TestFocusState();
}
class TestFocusState extends State<TestFocus> {
late FocusNode focusNode;
late String _label;
bool built = false;
@override
void dispose() {
focusNode.removeListener(_updateLabel);
focusNode.dispose();
super.dispose();
}
String get label => focusNode.hasFocus ? '${widget.name.toUpperCase()} FOCUSED' : widget.name.toLowerCase();
@override
void initState() {
super.initState();
focusNode = FocusNode(debugLabel: widget.debugLabel);
_label = label;
focusNode.addListener(_updateLabel);
}
void _updateLabel() {
setState(() {
_label = label;
});
}
@override
Widget build(BuildContext context) {
built = true;
return GestureDetector(
onTap: () {
FocusScope.of(context).requestFocus(focusNode);
},
child: Focus(
autofocus: widget.autofocus,
focusNode: focusNode,
parentNode: widget.parentNode,
debugLabel: widget.debugLabel,
child: Text(
_label,
textDirection: TextDirection.ltr,
),
),
);
}
}
class TestExternalFocusNode extends FocusNode {
TestExternalFocusNode();
bool isModified = false;
@override
FocusOnKeyEventCallback? get onKeyEvent => _onKeyEvent;
FocusOnKeyEventCallback? _onKeyEvent;
@override
set onKeyEvent(FocusOnKeyEventCallback? newValue) {
if (newValue != _onKeyEvent) {
_onKeyEvent = newValue;
isModified = true;
}
}
@override
set descendantsAreFocusable(bool newValue) {
super.descendantsAreFocusable = newValue;
isModified = true;
}
@override
set descendantsAreTraversable(bool newValue) {
super.descendantsAreTraversable = newValue;
isModified = true;
}
@override
set skipTraversal(bool newValue) {
super.skipTraversal = newValue;
isModified = true;
}
@override
set canRequestFocus(bool newValue) {
super.canRequestFocus = newValue;
isModified = true;
}
}
| flutter/packages/flutter/test/widgets/focus_scope_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/focus_scope_test.dart",
"repo_id": "flutter",
"token_count": 35276
} | 742 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('chrome')
library;
import 'dart:async';
import 'dart:ui_web' as ui_web;
import 'package:collection/collection.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/src/widgets/_html_element_view_web.dart'
show debugOverridePlatformViewRegistry;
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:web/web.dart' as web;
final Object _mockHtmlElement = Object();
Object _mockViewFactory(int id, {Object? params}) {
return _mockHtmlElement;
}
void main() {
late FakePlatformViewRegistry fakePlatformViewRegistry;
setUp(() {
fakePlatformViewRegistry = FakePlatformViewRegistry();
// Simulate the engine registering default factories.
fakePlatformViewRegistry.registerViewFactory(ui_web.PlatformViewRegistry.defaultVisibleViewType, (int viewId, {Object? params}) {
params!;
params as Map<Object?, Object?>;
return web.document.createElement(params['tagName']! as String);
});
fakePlatformViewRegistry.registerViewFactory(ui_web.PlatformViewRegistry.defaultInvisibleViewType, (int viewId, {Object? params}) {
params!;
params as Map<Object?, Object?>;
return web.document.createElement(params['tagName']! as String);
});
});
group('HtmlElementView', () {
testWidgets('Create HTML view', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview'),
),
),
);
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 1, viewType: 'webview', params: null, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('Create HTML view with PlatformViewCreatedCallback', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
bool hasPlatformViewCreated = false;
void onPlatformViewCreatedCallBack(int id) {
hasPlatformViewCreated = true;
}
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(
viewType: 'webview',
onPlatformViewCreated: onPlatformViewCreatedCallBack,
),
),
),
);
// Check the onPlatformViewCreatedCallBack has been called.
expect(hasPlatformViewCreated, true);
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 1, viewType: 'webview', params: null, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('Create HTML view with creation params', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
await tester.pumpWidget(
const Column(
children: <Widget>[
SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(
viewType: 'webview',
creationParams: 'foobar',
),
),
SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(
viewType: 'webview',
creationParams: 123,
),
),
],
),
);
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 1, viewType: 'webview', params: 'foobar', htmlElement: _mockHtmlElement),
(id: currentViewId + 2, viewType: 'webview', params: 123, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('Resize HTML view', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview'),
),
),
);
final Completer<void> resizeCompleter = Completer<void>();
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 100.0,
height: 50.0,
child: HtmlElementView(viewType: 'webview'),
),
),
);
resizeCompleter.complete();
await tester.pump();
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 1, viewType: 'webview', params: null, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('Change HTML view type', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
fakePlatformViewRegistry.registerViewFactory('maps', _mockViewFactory);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview'),
),
),
);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'maps'),
),
),
);
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 2, viewType: 'maps', params: null, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('Dispose HTML view', (WidgetTester tester) async {
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview'),
),
),
);
await tester.pumpWidget(
const Center(
child: SizedBox(
width: 200.0,
height: 100.0,
),
),
);
expect(
fakePlatformViewRegistry.views,
isEmpty,
);
});
testWidgets('HTML view survives widget tree change', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview', key: key),
),
),
);
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(viewType: 'webview', key: key),
),
),
);
expect(
fakePlatformViewRegistry.views,
unorderedEquals(<FakePlatformView>[
(id: currentViewId + 1, viewType: 'webview', params: null, htmlElement: _mockHtmlElement),
]),
);
});
testWidgets('HtmlElementView has correct semantics', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
expect(currentViewId, greaterThanOrEqualTo(0));
fakePlatformViewRegistry.registerViewFactory('webview', _mockViewFactory);
await tester.pumpWidget(
Semantics(
container: true,
child: const Align(
alignment: Alignment.bottomRight,
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView(
viewType: 'webview',
),
),
),
),
);
// First frame is before the platform view was created so the render object
// is not yet in the tree.
await tester.pump();
// The platform view ID is set on the child of the HtmlElementView render object.
final SemanticsNode semantics = tester.getSemantics(find.byType(PlatformViewSurface));
expect(semantics.platformViewId, currentViewId + 1);
expect(semantics.rect, const Rect.fromLTWH(0, 0, 200, 100));
// A 200x100 rect positioned at bottom right of a 800x600 box.
expect(semantics.transform, Matrix4.translationValues(600, 500, 0));
expect(semantics.childrenCount, 0);
handle.dispose();
});
});
group('HtmlElementView.fromTagName', () {
setUp(() {
debugOverridePlatformViewRegistry = fakePlatformViewRegistry;
});
tearDown(() {
debugOverridePlatformViewRegistry = null;
});
testWidgets('Create platform view from tagName', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView.fromTagName(tagName: 'div'),
),
),
);
await tester.pumpAndSettle();
expect(fakePlatformViewRegistry.views, hasLength(1));
final FakePlatformView fakePlatformView = fakePlatformViewRegistry.views.single;
expect(fakePlatformView.id, currentViewId + 1);
expect(fakePlatformView.viewType, ui_web.PlatformViewRegistry.defaultVisibleViewType);
expect(fakePlatformView.params, <dynamic, dynamic>{'tagName': 'div'});
// The HTML element should be a div.
final web.HTMLElement htmlElement = fakePlatformView.htmlElement as web.HTMLElement;
expect(htmlElement.tagName, equalsIgnoringCase('div'));
});
testWidgets('Create invisible platform view', (WidgetTester tester) async {
final int currentViewId = platformViewsRegistry.getNextPlatformViewId();
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView.fromTagName(tagName: 'script', isVisible: false),
),
),
);
await tester.pumpAndSettle();
expect(fakePlatformViewRegistry.views, hasLength(1));
final FakePlatformView fakePlatformView = fakePlatformViewRegistry.views.single;
expect(fakePlatformView.id, currentViewId + 1);
// The view should be invisible.
expect(fakePlatformView.viewType, ui_web.PlatformViewRegistry.defaultInvisibleViewType);
expect(fakePlatformView.params, <dynamic, dynamic>{'tagName': 'script'});
// The HTML element should be a script.
final web.HTMLElement htmlElement = fakePlatformView.htmlElement as web.HTMLElement;
expect(htmlElement.tagName, equalsIgnoringCase('script'));
});
testWidgets('onElementCreated', (WidgetTester tester) async {
final List<Object> createdElements = <Object>[];
void onElementCreated(Object element) {
createdElements.add(element);
}
await tester.pumpWidget(
Center(
child: SizedBox(
width: 200.0,
height: 100.0,
child: HtmlElementView.fromTagName(
tagName: 'table',
onElementCreated: onElementCreated,
),
),
),
);
await tester.pumpAndSettle();
expect(fakePlatformViewRegistry.views, hasLength(1));
final FakePlatformView fakePlatformView = fakePlatformViewRegistry.views.single;
expect(createdElements, hasLength(1));
final Object createdElement = createdElements.single;
expect(createdElement, fakePlatformView.htmlElement);
});
});
}
typedef FakeViewFactory = ({
String viewType,
bool isVisible,
Function viewFactory,
});
typedef FakePlatformView = ({
int id,
String viewType,
Object? params,
Object htmlElement,
});
class FakePlatformViewRegistry implements ui_web.PlatformViewRegistry {
FakePlatformViewRegistry() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform_views, _onMethodCall);
}
Set<FakePlatformView> get views => Set<FakePlatformView>.unmodifiable(_views);
final Set<FakePlatformView> _views = <FakePlatformView>{};
final Set<FakeViewFactory> _registeredViewTypes = <FakeViewFactory>{};
@override
bool registerViewFactory(String viewType, Function viewFactory, {bool isVisible = true}) {
if (_findRegisteredViewFactory(viewType) != null) {
return false;
}
_registeredViewTypes.add((
viewType: viewType,
isVisible: isVisible,
viewFactory: viewFactory,
));
return true;
}
@override
Object getViewById(int viewId) {
return _findViewById(viewId)!.htmlElement;
}
FakeViewFactory? _findRegisteredViewFactory(String viewType) {
return _registeredViewTypes.singleWhereOrNull(
(FakeViewFactory registered) => registered.viewType == viewType,
);
}
FakePlatformView? _findViewById(int viewId) {
return _views.singleWhereOrNull(
(FakePlatformView view) => view.id == viewId,
);
}
Future<dynamic> _onMethodCall(MethodCall call) {
return switch (call.method) {
'create' => _create(call),
'dispose' => _dispose(call),
_ => Future<dynamic>.sync(() => null),
};
}
Future<dynamic> _create(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final String viewType = args['viewType'] as String;
final Object? params = args['params'];
if (_findViewById(id) != null) {
throw PlatformException(
code: 'error',
message: 'Trying to create an already created platform view, view id: $id',
);
}
final FakeViewFactory? registered = _findRegisteredViewFactory(viewType);
if (registered == null) {
throw PlatformException(
code: 'error',
message: 'Trying to create a platform view of unregistered type: $viewType',
);
}
final ui_web.ParameterizedPlatformViewFactory viewFactory =
registered.viewFactory as ui_web.ParameterizedPlatformViewFactory;
_views.add((
id: id,
viewType: viewType,
params: params,
htmlElement: viewFactory(id, params: params),
));
return null;
}
Future<dynamic> _dispose(MethodCall call) async {
final int id = call.arguments as int;
final FakePlatformView? view = _findViewById(id);
if (view == null) {
throw PlatformException(
code: 'error',
message: 'Trying to dispose a platform view with unknown id: $id',
);
}
_views.remove(view);
return null;
}
}
| flutter/packages/flutter/test/widgets/html_element_view_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/html_element_view_test.dart",
"repo_id": "flutter",
"token_count": 6512
} | 743 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/src/widgets/basic.dart';
import 'package:flutter/src/widgets/framework.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('InheritedWidget dependencies show up in diagnostic properties', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(Directionality(
key: key,
textDirection: TextDirection.ltr,
child: Builder(builder: (BuildContext context) {
Directionality.of(context);
return const SizedBox();
}),
));
final InheritedElement element = key.currentContext! as InheritedElement;
expect(
element.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'Directionality-[GlobalKey#00000](textDirection: ltr)\n'
'└Builder(dependencies: [Directionality-[GlobalKey#00000]])\n'
' └SizedBox(renderObject: RenderConstrainedBox#00000)\n',
),
);
await tester.pumpWidget(Directionality(
key: key,
textDirection: TextDirection.rtl,
child: Builder(builder: (BuildContext context) {
Directionality.of(context);
return const SizedBox();
}),
));
expect(
element.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'Directionality-[GlobalKey#00000](textDirection: rtl)\n'
'└Builder(dependencies: [Directionality-[GlobalKey#00000]])\n'
' └SizedBox(renderObject: RenderConstrainedBox#00000)\n',
),
);
});
}
| flutter/packages/flutter/test/widgets/inherited_dependencies_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/inherited_dependencies_test.dart",
"repo_id": "flutter",
"token_count": 644
} | 744 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('LayoutBuilder parent size', (WidgetTester tester) async {
late Size layoutBuilderSize;
final Key childKey = UniqueKey();
final Key parentKey = UniqueKey();
await tester.pumpWidget(
Center(
child: ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 100.0, maxHeight: 200.0),
child: LayoutBuilder(
key: parentKey,
builder: (BuildContext context, BoxConstraints constraints) {
layoutBuilderSize = constraints.biggest;
return SizedBox(
key: childKey,
width: layoutBuilderSize.width / 2.0,
height: layoutBuilderSize.height / 2.0,
);
},
),
),
),
);
expect(layoutBuilderSize, const Size(100.0, 200.0));
final RenderBox parentBox = tester.renderObject(find.byKey(parentKey));
expect(parentBox.size, equals(const Size(50.0, 100.0)));
final RenderBox childBox = tester.renderObject(find.byKey(childKey));
expect(childBox.size, equals(const Size(50.0, 100.0)));
});
testWidgets('SliverLayoutBuilder parent geometry', (WidgetTester tester) async {
late SliverConstraints parentConstraints1;
late SliverConstraints parentConstraints2;
final Key childKey1 = UniqueKey();
final Key parentKey1 = UniqueKey();
final Key childKey2 = UniqueKey();
final Key parentKey2 = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverLayoutBuilder(
key: parentKey1,
builder: (BuildContext context, SliverConstraints constraint) {
parentConstraints1 = constraint;
return SliverPadding(key: childKey1, padding: const EdgeInsets.fromLTRB(1, 2, 3, 4));
},
),
SliverLayoutBuilder(
key: parentKey2,
builder: (BuildContext context, SliverConstraints constraint) {
parentConstraints2 = constraint;
return SliverPadding(key: childKey2, padding: const EdgeInsets.fromLTRB(5, 7, 11, 13));
},
),
],
),
),
);
expect(parentConstraints1.crossAxisExtent, 800);
expect(parentConstraints1.remainingPaintExtent, 600);
expect(parentConstraints2.crossAxisExtent, 800);
expect(parentConstraints2.remainingPaintExtent, 600 - 2 - 4);
final RenderSliver parentSliver1 = tester.renderObject(find.byKey(parentKey1));
final RenderSliver parentSliver2 = tester.renderObject(find.byKey(parentKey2));
// scrollExtent == top + bottom.
expect(parentSliver1.geometry!.scrollExtent, 2 + 4);
expect(parentSliver2.geometry!.scrollExtent, 7 + 13);
final RenderSliver childSliver1 = tester.renderObject(find.byKey(childKey1));
final RenderSliver childSliver2 = tester.renderObject(find.byKey(childKey2));
expect(childSliver1.geometry, parentSliver1.geometry);
expect(childSliver2.geometry, parentSliver2.geometry);
});
testWidgets('LayoutBuilder stateful child', (WidgetTester tester) async {
late Size layoutBuilderSize;
late StateSetter setState;
final Key childKey = UniqueKey();
final Key parentKey = UniqueKey();
double childWidth = 10.0;
double childHeight = 20.0;
await tester.pumpWidget(
Center(
child: LayoutBuilder(
key: parentKey,
builder: (BuildContext context, BoxConstraints constraints) {
layoutBuilderSize = constraints.biggest;
return StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return SizedBox(
key: childKey,
width: childWidth,
height: childHeight,
);
},
);
},
),
),
);
expect(layoutBuilderSize, equals(const Size(800.0, 600.0)));
RenderBox parentBox = tester.renderObject(find.byKey(parentKey));
expect(parentBox.size, equals(const Size(10.0, 20.0)));
RenderBox childBox = tester.renderObject(find.byKey(childKey));
expect(childBox.size, equals(const Size(10.0, 20.0)));
setState(() {
childWidth = 100.0;
childHeight = 200.0;
});
await tester.pump();
parentBox = tester.renderObject(find.byKey(parentKey));
expect(parentBox.size, equals(const Size(100.0, 200.0)));
childBox = tester.renderObject(find.byKey(childKey));
expect(childBox.size, equals(const Size(100.0, 200.0)));
});
testWidgets('SliverLayoutBuilder stateful descendants', (WidgetTester tester) async {
late StateSetter setState;
double childWidth = 10.0;
double childHeight = 20.0;
final Key parentKey = UniqueKey();
final Key childKey = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverLayoutBuilder(
key: parentKey,
builder: (BuildContext context, SliverConstraints constraint) {
return SliverToBoxAdapter(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return SizedBox(
key: childKey,
width: childWidth,
height: childHeight,
);
},
),
);
},
),
],
),
),
);
RenderBox childBox = tester.renderObject(find.byKey(childKey));
RenderSliver parentSliver = tester.renderObject(find.byKey(parentKey));
expect(childBox.size.width, 800);
expect(childBox.size.height, childHeight);
expect(parentSliver.geometry!.scrollExtent, childHeight);
expect(parentSliver.geometry!.paintExtent, childHeight);
setState(() {
childWidth = 100.0;
childHeight = 200.0;
});
await tester.pump();
childBox = tester.renderObject(find.byKey(childKey));
parentSliver = tester.renderObject(find.byKey(parentKey));
expect(childBox.size.width, 800);
expect(childBox.size.height, childHeight);
expect(parentSliver.geometry!.scrollExtent, childHeight);
expect(parentSliver.geometry!.paintExtent, childHeight);
// Make child wider and higher than the viewport.
setState(() {
childWidth = 900.0;
childHeight = 900.0;
});
await tester.pump();
childBox = tester.renderObject(find.byKey(childKey));
parentSliver = tester.renderObject(find.byKey(parentKey));
expect(childBox.size.width, 800);
expect(childBox.size.height, childHeight);
expect(parentSliver.geometry!.scrollExtent, childHeight);
expect(parentSliver.geometry!.paintExtent, 600);
});
testWidgets('LayoutBuilder stateful parent', (WidgetTester tester) async {
late Size layoutBuilderSize;
late StateSetter setState;
final Key childKey = UniqueKey();
double childWidth = 10.0;
double childHeight = 20.0;
await tester.pumpWidget(
Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return SizedBox(
width: childWidth,
height: childHeight,
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
layoutBuilderSize = constraints.biggest;
return SizedBox(
key: childKey,
width: layoutBuilderSize.width,
height: layoutBuilderSize.height,
);
},
),
);
},
),
),
);
expect(layoutBuilderSize, equals(const Size(10.0, 20.0)));
RenderBox box = tester.renderObject(find.byKey(childKey));
expect(box.size, equals(const Size(10.0, 20.0)));
setState(() {
childWidth = 100.0;
childHeight = 200.0;
});
await tester.pump();
box = tester.renderObject(find.byKey(childKey));
expect(box.size, equals(const Size(100.0, 200.0)));
});
testWidgets('LayoutBuilder and Inherited -- do not rebuild when not using inherited', (WidgetTester tester) async {
int built = 0;
final Widget target = LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
built += 1;
return Container();
},
);
expect(built, 0);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(400.0, 300.0)),
child: target,
));
expect(built, 1);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(300.0, 400.0)),
child: target,
));
expect(built, 1);
});
testWidgets('LayoutBuilder and Inherited -- do rebuild when using inherited', (WidgetTester tester) async {
int built = 0;
final Widget target = LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
built += 1;
MediaQuery.of(context);
return Container();
},
);
expect(built, 0);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(400.0, 300.0)),
child: target,
));
expect(built, 1);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(300.0, 400.0)),
child: target,
));
expect(built, 2);
});
testWidgets('SliverLayoutBuilder and Inherited -- do not rebuild when not using inherited', (WidgetTester tester) async {
int built = 0;
final Widget target = Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverLayoutBuilder(
builder: (BuildContext context, SliverConstraints constraint) {
built++;
return SliverToBoxAdapter(child: Container());
},
),
],
),
);
expect(built, 0);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(400.0, 300.0)),
child: target,
));
expect(built, 1);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(300.0, 400.0)),
child: target,
));
expect(built, 1);
});
testWidgets(
'SliverLayoutBuilder and Inherited -- do rebuild when not using inherited',
(WidgetTester tester) async {
int built = 0;
final Widget target = Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverLayoutBuilder(
builder: (BuildContext context, SliverConstraints constraint) {
built++;
MediaQuery.of(context);
return SliverToBoxAdapter(child: Container());
},
),
],
),
);
expect(built, 0);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(400.0, 300.0)),
child: target,
));
expect(built, 1);
await tester.pumpWidget(MediaQuery(
data: const MediaQueryData(size: Size(300.0, 400.0)),
child: target,
));
expect(built, 2);
},
);
testWidgets('nested SliverLayoutBuilder', (WidgetTester tester) async {
late SliverConstraints parentConstraints1;
late SliverConstraints parentConstraints2;
final Key childKey = UniqueKey();
final Key parentKey1 = UniqueKey();
final Key parentKey2 = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
slivers: <Widget>[
SliverLayoutBuilder(
key: parentKey1,
builder: (BuildContext context, SliverConstraints constraint) {
parentConstraints1 = constraint;
return SliverLayoutBuilder(
key: parentKey2,
builder: (BuildContext context, SliverConstraints constraint) {
parentConstraints2 = constraint;
return SliverPadding(key: childKey, padding: const EdgeInsets.fromLTRB(1, 2, 3, 4));
},
);
},
),
],
),
),
);
expect(parentConstraints1, parentConstraints2);
expect(parentConstraints1.crossAxisExtent, 800);
expect(parentConstraints1.remainingPaintExtent, 600);
final RenderSliver parentSliver1 = tester.renderObject(find.byKey(parentKey1));
final RenderSliver parentSliver2 = tester.renderObject(find.byKey(parentKey2));
// scrollExtent == top + bottom.
expect(parentSliver1.geometry!.scrollExtent, 2 + 4);
final RenderSliver childSliver = tester.renderObject(find.byKey(childKey));
expect(childSliver.geometry, parentSliver1.geometry);
expect(parentSliver1.geometry, parentSliver2.geometry);
});
testWidgets('localToGlobal works with SliverLayoutBuilder', (WidgetTester tester) async {
final Key childKey1 = UniqueKey();
final Key childKey2 = UniqueKey();
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
const SliverToBoxAdapter(
child: SizedBox(height: 300),
),
SliverLayoutBuilder(
builder: (BuildContext context, SliverConstraints constraint) => SliverToBoxAdapter(
child: SizedBox(key: childKey1, height: 200),
),
),
SliverToBoxAdapter(
child: SizedBox(key: childKey2, height: 100),
),
],
),
),
);
final RenderBox renderChild1 = tester.renderObject(find.byKey(childKey1));
final RenderBox renderChild2 = tester.renderObject(find.byKey(childKey2));
// Test with scrollController.scrollOffset = 0.
expect(
renderChild1.localToGlobal(const Offset(100, 100)),
const Offset(100, 300.0 + 100),
);
expect(
renderChild2.localToGlobal(const Offset(100, 100)),
const Offset(100, 300.0 + 200 + 100),
);
scrollController.jumpTo(100);
await tester.pump();
expect(
renderChild1.localToGlobal(const Offset(100, 100)),
// -100 because the scroll offset is now 100.
const Offset(100, 300.0 + 100 - 100),
);
expect(
renderChild2.localToGlobal(const Offset(100, 100)),
// -100 because the scroll offset is now 100.
const Offset(100, 300.0 + 100 + 200 - 100),
);
});
testWidgets('hitTest works within SliverLayoutBuilder', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
List<int> hitCounts = <int> [0, 0, 0];
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Padding(
padding: const EdgeInsets.all(50),
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
SliverToBoxAdapter(
child: SizedBox(
height: 200,
child: GestureDetector(onTap: () => hitCounts[0]++),
),
),
SliverLayoutBuilder(
builder: (BuildContext context, SliverConstraints constraint) => SliverToBoxAdapter(
child: SizedBox(
height: 200,
child: GestureDetector(onTap: () => hitCounts[1]++),
),
),
),
SliverToBoxAdapter(
child: SizedBox(
height: 200,
child: GestureDetector(onTap: () => hitCounts[2]++),
),
),
],
),
),
),
);
// Tap item 1.
await tester.tapAt(const Offset(300, 50.0 + 100));
await tester.pump();
expect(hitCounts, const <int> [1, 0, 0]);
// Tap item 2.
await tester.tapAt(const Offset(300, 50.0 + 100 + 200));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 0]);
// Tap item 3. Shift the touch point up to ensure the touch lands within the viewport.
await tester.tapAt(const Offset(300, 50.0 + 200 + 200 + 10));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
// Scrolling doesn't break it.
hitCounts = <int> [0, 0, 0];
scrollController.jumpTo(100);
await tester.pump();
// Tap item 1.
await tester.tapAt(const Offset(300, 50.0 + 100 - 100));
await tester.pump();
expect(hitCounts, const <int> [1, 0, 0]);
// Tap item 2.
await tester.tapAt(const Offset(300, 50.0 + 100 + 200 - 100));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 0]);
// Tap item 3.
await tester.tapAt(const Offset(300, 50.0 + 100 + 200 + 200 - 100));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
// Tapping outside of the viewport shouldn't do anything.
await tester.tapAt(const Offset(300, 1));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
await tester.tapAt(const Offset(300, 599));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
await tester.tapAt(const Offset(1, 100));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
await tester.tapAt(const Offset(799, 100));
await tester.pump();
expect(hitCounts, const <int> [1, 1, 1]);
// Tap the no-content area in the viewport shouldn't do anything
hitCounts = <int> [0, 0, 0];
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
SliverToBoxAdapter(
child: SizedBox(
height: 100,
child: GestureDetector(onTap: () => hitCounts[0]++),
),
),
SliverLayoutBuilder(
builder: (BuildContext context, SliverConstraints constraint) => SliverToBoxAdapter(
child: SizedBox(
height: 100,
child: GestureDetector(onTap: () => hitCounts[1]++),
),
),
),
SliverToBoxAdapter(
child: SizedBox(
height: 100,
child: GestureDetector(onTap: () => hitCounts[2]++),
),
),
],
),
),
);
await tester.tapAt(const Offset(300, 301));
await tester.pump();
expect(hitCounts, const <int> [0, 0, 0]);
});
testWidgets('LayoutBuilder does not call builder when layout happens but layout constraints do not change', (WidgetTester tester) async {
int builderInvocationCount = 0;
Future<void> pumpTestWidget(Size size) async {
await tester.pumpWidget(
// Center is used to give the SizedBox the power to determine constraints for LayoutBuilder
Center(
child: SizedBox.fromSize(
size: size,
child: LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
builderInvocationCount += 1;
return const _LayoutSpy();
}),
),
),
);
}
await pumpTestWidget(const Size(10, 10));
final _RenderLayoutSpy spy = tester.renderObject(find.byType(_LayoutSpy));
// The child is laid out once the first time.
expect(spy.performLayoutCount, 1);
expect(spy.performResizeCount, 1);
// The initial `pumpWidget` will trigger `performRebuild`, asking for
// builder invocation.
expect(builderInvocationCount, 1);
// Invalidate the layout without changing the constraints.
tester.renderObject(find.byType(LayoutBuilder)).markNeedsLayout();
// The second pump will not go through the `performRebuild` or `update`, and
// only judge the need for builder invocation based on constraints, which
// didn't change, so we don't expect any counters to go up.
await tester.pump();
expect(builderInvocationCount, 1);
expect(spy.performLayoutCount, 1);
expect(spy.performResizeCount, 1);
// Cause the `update` to be called (but not `performRebuild`), triggering
// builder invocation.
await pumpTestWidget(const Size(10, 10));
expect(builderInvocationCount, 2);
// The spy does not invalidate its layout on widget update, so no
// layout-related methods should be called.
expect(spy.performLayoutCount, 1);
expect(spy.performResizeCount, 1);
// Have the child request layout and verify that the child gets laid out
// despite layout constraints remaining constant.
spy.markNeedsLayout();
await tester.pump();
// Builder is not invoked. This was a layout-only pump with the same parent
// constraints.
expect(builderInvocationCount, 2);
// Expect performLayout to be called.
expect(spy.performLayoutCount, 2);
// performResize should not be called because the spy sets sizedByParent,
// and the constraints did not change.
expect(spy.performResizeCount, 1);
// Change the parent size, triggering constraint change.
await pumpTestWidget(const Size(20, 20));
// We should see everything invoked once.
expect(builderInvocationCount, 3);
expect(spy.performLayoutCount, 3);
expect(spy.performResizeCount, 2);
});
testWidgets('LayoutBuilder descendant widget can access [RenderBox.size] when rebuilding during layout', (WidgetTester tester) async {
Size? childSize;
int buildCount = 0;
Future<void> pumpTestWidget(Size size) async {
await tester.pumpWidget(
// Center is used to give the SizedBox the power to determine constraints for LayoutBuilder
Center(
child: SizedBox.fromSize(
size: size,
child: LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
buildCount++;
if (buildCount > 1) {
final _RenderLayoutSpy spy = tester.renderObject(find.byType(_LayoutSpy));
childSize = spy.size;
}
return const ColoredBox(
color: Color(0xffffffff),
child: _LayoutSpy(),
);
}),
),
),
);
}
await pumpTestWidget(const Size(10.0, 10.0));
expect(childSize, isNull);
await pumpTestWidget(const Size(10.0, 10.0));
expect(childSize, const Size(10.0, 10.0));
});
testWidgets('LayoutBuilder will only invoke builder if updateShouldRebuild returns true', (WidgetTester tester) async {
int buildCount = 0;
int paintCount = 0;
Offset? mostRecentOffset;
void handleChildWasPainted(Offset extraOffset) {
paintCount++;
mostRecentOffset = extraOffset;
}
Future<void> pumpWidget(String text, double offsetPercentage) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100,
height: 100,
child: _SmartLayoutBuilder(
text: text,
offsetPercentage: offsetPercentage,
onChildWasPainted: handleChildWasPainted,
builder: (BuildContext context, BoxConstraints constraints) {
buildCount++;
return Text(text);
},
),
),
),
),
);
}
await pumpWidget('aaa', 0.2);
expect(find.text('aaa'), findsOneWidget);
expect(buildCount, 1);
expect(paintCount, 1);
expect(mostRecentOffset, const Offset(20, 20));
await pumpWidget('aaa', 0.4);
expect(find.text('aaa'), findsOneWidget);
expect(buildCount, 1);
expect(paintCount, 2);
expect(mostRecentOffset, const Offset(40, 40));
await pumpWidget('bbb', 0.6);
expect(find.text('aaa'), findsNothing);
expect(find.text('bbb'), findsOneWidget);
expect(buildCount, 2);
expect(paintCount, 3);
expect(mostRecentOffset, const Offset(60, 60));
});
}
class _SmartLayoutBuilder extends ConstrainedLayoutBuilder<BoxConstraints> {
const _SmartLayoutBuilder({
required this.text,
required this.offsetPercentage,
required this.onChildWasPainted,
required super.builder,
});
final String text;
final double offsetPercentage;
final _OnChildWasPaintedCallback onChildWasPainted;
@override
bool updateShouldRebuild(_SmartLayoutBuilder oldWidget) {
// Because this is a private widget and thus local to this file, we know
// that only the [text] property affects the builder; the other properties
// only affect painting.
return text != oldWidget.text;
}
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderSmartLayoutBuilder(
offsetPercentage: offsetPercentage,
onChildWasPainted: onChildWasPainted,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSmartLayoutBuilder renderObject) {
renderObject
..offsetPercentage = offsetPercentage
..onChildWasPainted = onChildWasPainted;
}
}
typedef _OnChildWasPaintedCallback = void Function(Offset extraOffset);
class _RenderSmartLayoutBuilder extends RenderProxyBox
with RenderConstrainedLayoutBuilder<BoxConstraints, RenderBox> {
_RenderSmartLayoutBuilder({
required double offsetPercentage,
required this.onChildWasPainted,
}) : _offsetPercentage = offsetPercentage;
double _offsetPercentage;
double get offsetPercentage => _offsetPercentage;
set offsetPercentage(double value) {
if (value != _offsetPercentage) {
_offsetPercentage = value;
markNeedsPaint();
}
}
_OnChildWasPaintedCallback onChildWasPainted;
@override
bool get sizedByParent => true;
@override
Size computeDryLayout(BoxConstraints constraints) {
return constraints.biggest;
}
@override
void performLayout() {
rebuildIfNecessary();
child?.layout(constraints);
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null) {
final Offset extraOffset = Offset(
size.width * offsetPercentage,
size.height * offsetPercentage,
);
context.paintChild(child!, offset + extraOffset);
onChildWasPainted(extraOffset);
}
}
}
class _LayoutSpy extends LeafRenderObjectWidget {
const _LayoutSpy();
@override
LeafRenderObjectElement createElement() => _LayoutSpyElement(this);
@override
RenderObject createRenderObject(BuildContext context) => _RenderLayoutSpy();
}
class _LayoutSpyElement extends LeafRenderObjectElement {
_LayoutSpyElement(super.widget);
}
class _RenderLayoutSpy extends RenderBox {
int performLayoutCount = 0;
int performResizeCount = 0;
@override
bool get sizedByParent => true;
@override
void performResize() {
performResizeCount += 1;
size = constraints.biggest;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return constraints.biggest;
}
@override
void performLayout() {
performLayoutCount += 1;
}
}
| flutter/packages/flutter/test/widgets/layout_builder_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/layout_builder_test.dart",
"repo_id": "flutter",
"token_count": 11818
} | 745 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ListView.builder() fixed itemExtent, scroll to end, append, scroll', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/9506
Widget buildFrame(int itemCount) {
return Directionality(
textDirection: TextDirection.ltr,
child: ListView.builder(
dragStartBehavior: DragStartBehavior.down,
itemExtent: 200.0,
itemCount: itemCount,
itemBuilder: (BuildContext context, int index) => Text('item $index'),
),
);
}
await tester.pumpWidget(buildFrame(3));
expect(find.text('item 0'), findsOneWidget);
expect(find.text('item 1'), findsOneWidget);
expect(find.text('item 2'), findsOneWidget);
await tester.pumpWidget(buildFrame(4));
expect(find.text('item 3'), findsNothing);
final TestGesture gesture = await tester.startGesture(const Offset(0.0, 300.0));
await gesture.moveBy(const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(find.text('item 3'), findsOneWidget);
});
testWidgets('ListView.builder() fixed itemExtent, scroll to end, append, scroll', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/9506
Widget buildFrame(int itemCount) {
return Directionality(
textDirection: TextDirection.ltr,
child: ListView.builder(
dragStartBehavior: DragStartBehavior.down,
itemCount: itemCount,
itemBuilder: (BuildContext context, int index) {
return SizedBox(
height: 200.0,
child: Text('item $index'),
);
},
),
);
}
await tester.pumpWidget(buildFrame(3));
expect(find.text('item 0'), findsOneWidget);
expect(find.text('item 1'), findsOneWidget);
expect(find.text('item 2'), findsOneWidget);
await tester.pumpWidget(buildFrame(4));
final TestGesture gesture = await tester.startGesture(const Offset(0.0, 300.0));
await gesture.moveBy(const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(find.text('item 3'), findsOneWidget);
});
}
| flutter/packages/flutter/test/widgets/listview_end_append_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/listview_end_append_test.dart",
"repo_id": "flutter",
"token_count": 969
} | 746 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'test_widgets.dart';
class TestCustomPainter extends CustomPainter {
TestCustomPainter({ required this.log, required this.name });
final List<String> log;
final String name;
@override
void paint(Canvas canvas, Size size) {
log.add(name);
}
@override
bool shouldRepaint(TestCustomPainter oldPainter) {
return name != oldPainter.name
|| log != oldPainter.log;
}
}
void main() {
testWidgets('Do we paint when coming back from a navigation', (WidgetTester tester) async {
final List<String> log = <String>[];
log.add('0');
await tester.pumpWidget(
MaterialApp(
routes: <String, WidgetBuilder>{
'/': (BuildContext context) => RepaintBoundary(
child: RepaintBoundary(
child: FlipWidget(
left: CustomPaint(
painter: TestCustomPainter(
log: log,
name: 'left',
),
),
right: CustomPaint(
painter: TestCustomPainter(
log: log,
name: 'right',
),
),
),
),
),
'/second': (BuildContext context) => Container(),
},
),
);
log.add('1');
final NavigatorState navigator = tester.state<NavigatorState>(find.byType(Navigator));
navigator.pushNamed('/second');
log.add('2');
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 2);
log.add('3');
flipStatefulWidget(tester, skipOffstage: false);
log.add('4');
navigator.pop();
log.add('5');
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 2);
log.add('6');
flipStatefulWidget(tester);
expect(await tester.pumpAndSettle(), 1);
log.add('7');
expect(log, <String>[
'0',
'left',
'1',
'2',
'3',
'4',
'5',
'right',
'6',
'left',
'7',
]);
});
}
| flutter/packages/flutter/test/widgets/navigator_and_layers_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/navigator_and_layers_test.dart",
"repo_id": "flutter",
"token_count": 1062
} | 747 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
Widget buildTest(
GlobalKey box1Key,
GlobalKey box2Key,
GlobalKey box3Key,
ScrollController controller, {
Axis axis = Axis.vertical,
bool reverse = false,
TextDirection textDirection = TextDirection.ltr,
double boxHeight = 250.0,
double boxWidth = 300.0,
ScrollPhysics? physics,
}) {
final AxisDirection axisDirection;
switch (axis) {
case Axis.horizontal:
if (textDirection == TextDirection.rtl) {
axisDirection = reverse ? AxisDirection.right : AxisDirection.left;
} else {
axisDirection = reverse ? AxisDirection.left : AxisDirection.right;
}
case Axis.vertical:
axisDirection = reverse ? AxisDirection.up : AxisDirection.down;
}
return Directionality(
textDirection: textDirection,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: StretchingOverscrollIndicator(
axisDirection: axisDirection,
child: CustomScrollView(
physics: physics,
reverse: reverse,
scrollDirection: axis,
controller: controller,
slivers: <Widget>[
SliverToBoxAdapter(child: Container(
color: const Color(0xD0FF0000),
key: box1Key,
height: boxHeight,
width: boxWidth,
)),
SliverToBoxAdapter(child: Container(
color: const Color(0xFFFFFF00),
key: box2Key,
height: boxHeight,
width: boxWidth,
)),
SliverToBoxAdapter(child: Container(
color: const Color(0xFF6200EA),
key: box3Key,
height: boxHeight,
width: boxWidth,
)),
],
),
),
),
),
);
}
testWidgets('Stretch overscroll will do nothing when axes do not match', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: StretchingOverscrollIndicator(
axisDirection: AxisDirection.right,
child: CustomScrollView(
controller: controller,
slivers: <Widget>[
SliverToBoxAdapter(child: Container(
color: const Color(0xD0FF0000),
key: box1Key,
height: 250.0,
)),
SliverToBoxAdapter(child: Container(
color: const Color(0xFFFFFF00),
key: box2Key,
height: 250.0,
width: 300.0,
)),
],
),
),
),
),
)
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start, no stretching occurs.
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dy, 250.0);
await gesture.up();
await tester.pumpAndSettle();
// Overscroll released
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
});
testWidgets('Stretch overscroll vertically', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.vertical.start.png'),
);
TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dy, greaterThan(255.0));
expect(box3.localToGlobal(Offset.zero).dy, greaterThan(510.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.vertical.start.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back to the start
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
// Jump to end of the list
controller.jumpTo(controller.position.maxScrollExtent);
await tester.pumpAndSettle();
expect(controller.offset, 150.0);
expect(box1.localToGlobal(Offset.zero).dy, -150.0);
expect(box2.localToGlobal(Offset.zero).dy, 100.0);
expect(box3.localToGlobal(Offset.zero).dy, 350.0);
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.vertical.end.png'),
);
gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the end
await gesture.moveBy(const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-165));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(90.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(350.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.vertical.end.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back
expect(box1.localToGlobal(Offset.zero).dy, -150.0);
expect(box2.localToGlobal(Offset.zero).dy, 100.0);
expect(box3.localToGlobal(Offset.zero).dy, 350.0);
});
testWidgets('Stretch overscroll works in reverse - vertical', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller, reverse: true),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), const Offset(0.0, 350.0));
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 100.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, -150.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll
await gesture.moveBy(const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dy, lessThan(350.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(100.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(-150.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.vertical.reverse.png'),
);
});
testWidgets('Stretch overscroll works in reverse - horizontal', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
axis: Axis.horizontal,
reverse: true,
),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), const Offset(500.0, 0.0));
expect(box2.localToGlobal(Offset.zero), const Offset(200.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(-100.0, 0.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll
await gesture.moveBy(const Offset(-200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dx, lessThan(500.0));
expect(box2.localToGlobal(Offset.zero).dx, lessThan(200.0));
expect(box3.localToGlobal(Offset.zero).dx, lessThan(-100.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.reverse.png'),
);
});
testWidgets('Stretch overscroll works in reverse - horizontal - RTL', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
axis: Axis.horizontal,
reverse: true,
textDirection: TextDirection.rtl,
)
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(300.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(600.0, 0.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.reverse.rtl.start.png'),
);
TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start
await gesture.moveBy(const Offset(200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dx, greaterThan(305.0));
expect(box3.localToGlobal(Offset.zero).dx, greaterThan(610.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.reverse.rtl.start.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back to the start
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(300.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(600.0, 0.0));
// Jump to end of the list
controller.jumpTo(controller.position.maxScrollExtent);
await tester.pumpAndSettle();
expect(controller.offset, 100.0);
expect(box1.localToGlobal(Offset.zero).dx, -100.0);
expect(box2.localToGlobal(Offset.zero).dx, 200.0);
expect(box3.localToGlobal(Offset.zero).dx, 500.0);
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.reverse.rtl.end.png'),
);
gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the end
await gesture.moveBy(const Offset(-200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dx, lessThan(-116.0));
expect(box2.localToGlobal(Offset.zero).dx, lessThan(190.0));
expect(box3.localToGlobal(Offset.zero).dx, lessThan(500.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.reverse.rtl.end.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back
expect(box1.localToGlobal(Offset.zero).dx, -100.0);
expect(box2.localToGlobal(Offset.zero).dx, 200.0);
expect(box3.localToGlobal(Offset.zero).dx, 500.0);
});
testWidgets('Stretch overscroll horizontally', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller, axis: Axis.horizontal)
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(300.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(600.0, 0.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.start.png'),
);
TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start
await gesture.moveBy(const Offset(200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dx, greaterThan(305.0));
expect(box3.localToGlobal(Offset.zero).dx, greaterThan(610.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.start.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back to the start
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(300.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(600.0, 0.0));
// Jump to end of the list
controller.jumpTo(controller.position.maxScrollExtent);
await tester.pumpAndSettle();
expect(controller.offset, 100.0);
expect(box1.localToGlobal(Offset.zero).dx, -100.0);
expect(box2.localToGlobal(Offset.zero).dx, 200.0);
expect(box3.localToGlobal(Offset.zero).dx, 500.0);
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.end.png'),
);
gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the end
await gesture.moveBy(const Offset(-200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dx, lessThan(-116.0));
expect(box2.localToGlobal(Offset.zero).dx, lessThan(190.0));
expect(box3.localToGlobal(Offset.zero).dx, lessThan(500.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.end.stretched.png'),
);
await gesture.up();
await tester.pumpAndSettle();
// Stretch released back
expect(box1.localToGlobal(Offset.zero).dx, -100.0);
expect(box2.localToGlobal(Offset.zero).dx, 200.0);
expect(box3.localToGlobal(Offset.zero).dx, 500.0);
});
testWidgets('Stretch overscroll horizontally RTL', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
axis: Axis.horizontal,
textDirection: TextDirection.rtl,
)
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), const Offset(500.0, 0.0));
expect(box2.localToGlobal(Offset.zero), const Offset(200.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(-100.0, 0.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll
await gesture.moveBy(const Offset(-200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dx, lessThan(500.0));
expect(box2.localToGlobal(Offset.zero).dx, lessThan(200.0));
expect(box3.localToGlobal(Offset.zero).dx, lessThan(-100.0));
await expectLater(
find.byType(CustomScrollView),
matchesGoldenFile('overscroll_stretch.horizontal.rtl.png'),
);
});
testWidgets('Disallow stretching overscroll', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
double indicatorNotification = 0;
await tester.pumpWidget(
NotificationListener<OverscrollIndicatorNotification>(
onNotification: (OverscrollIndicatorNotification notification) {
notification.disallowIndicator();
indicatorNotification += 1;
return false;
},
child: buildTest(box1Key, box2Key, box3Key, controller),
)
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(indicatorNotification, 0.0);
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start, should not stretch
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(indicatorNotification, 1.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Stretch does not overflow bounds of container', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/90197
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: Column(
children: <Widget>[
StretchingOverscrollIndicator(
axisDirection: AxisDirection.down,
child: SizedBox(
height: 300,
child: ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index){
return Padding(
padding: const EdgeInsets.all(10.0),
child: Text('Index $index'),
);
},
),
),
),
Opacity(
opacity: 0.5,
child: Container(
color: const Color(0xD0FF0000),
height: 100,
),
),
],
),
),
),
));
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, 51.0);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll the start.
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, greaterThan(0));
// Image should not show the text overlapping the red area below the list.
await expectLater(
find.byType(Column),
matchesGoldenFile('overscroll_stretch.no_overflow.png'),
);
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Clip behavior is updated as needed', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/97867
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: Column(
children: <Widget>[
StretchingOverscrollIndicator(
axisDirection: AxisDirection.down,
child: SizedBox(
height: 300,
child: ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index){
return Padding(
padding: const EdgeInsets.all(10.0),
child: Text('Index $index'),
);
},
),
),
),
Opacity(
opacity: 0.5,
child: Container(
color: const Color(0xD0FF0000),
height: 100,
),
),
],
),
),
),
),
);
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, 51.0);
RenderClipRect renderClip = tester.allRenderObjects.whereType<RenderClipRect>().first;
// Currently not clipping
expect(renderClip.clipBehavior, equals(Clip.none));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll the start.
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, greaterThan(0));
renderClip = tester.allRenderObjects.whereType<RenderClipRect>().first;
// Now clipping
expect(renderClip.clipBehavior, equals(Clip.hardEdge));
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('clipBehavior parameter updates overscroll clipping behavior', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/103491
Widget buildFrame(Clip clipBehavior) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(size: Size(800.0, 600.0)),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: Column(
children: <Widget>[
StretchingOverscrollIndicator(
axisDirection: AxisDirection.down,
clipBehavior: clipBehavior,
child: SizedBox(
height: 300,
child: ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index){
return Padding(
padding: const EdgeInsets.all(10.0),
child: Text('Index $index'),
);
},
),
),
),
Opacity(
opacity: 0.5,
child: Container(
color: const Color(0xD0FF0000),
height: 100,
),
),
],
),
),
),
);
}
await tester.pumpWidget(buildFrame(Clip.none));
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, 51.0);
RenderClipRect renderClip = tester.allRenderObjects.whereType<RenderClipRect>().first;
// Currently not clipping
expect(renderClip.clipBehavior, equals(Clip.none));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll the start.
await gesture.moveBy(const Offset(0.0, 200.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, greaterThan(0));
renderClip = tester.allRenderObjects.whereType<RenderClipRect>().first;
// Now clipping
expect(renderClip.clipBehavior, equals(Clip.none));
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Stretch limit', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/99264
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: StretchingOverscrollIndicator(
axisDirection: AxisDirection.down,
child: SizedBox(
height: 300,
child: ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index){
return Padding(
padding: const EdgeInsets.all(10.0),
child: Text('Index $index'),
);
},
),
),
),
),
)
)
);
const double maxStretchLocation = 52.63178407049861;
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, 51.0);
TestGesture pointer = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll beyond the limit (the viewport is 600.0).
await pointer.moveBy(const Offset(0.0, 610.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, maxStretchLocation);
pointer = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll way way beyond the limit
await pointer.moveBy(const Offset(0.0, 1000.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, maxStretchLocation);
await pointer.up();
await tester.pumpAndSettle();
});
testWidgets('Multiple pointers will not exceed stretch limit', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/99264
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: ScrollConfiguration(
behavior: const ScrollBehavior().copyWith(overscroll: false),
child: StretchingOverscrollIndicator(
axisDirection: AxisDirection.down,
child: SizedBox(
height: 300,
child: ListView.builder(
itemCount: 20,
itemBuilder: (BuildContext context, int index){
return Padding(
padding: const EdgeInsets.all(10.0),
child: Text('Index $index'),
);
},
),
),
),
),
)
)
);
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, 51.0);
final TestGesture pointer1 = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Overscroll the start.
await pointer1.moveBy(const Offset(0.0, 210.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
double lastStretchedLocation = tester.getCenter(find.text('Index 1')).dy;
expect(lastStretchedLocation, greaterThan(51.0));
final TestGesture pointer2 = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Add overscroll from an additional pointer
await pointer2.moveBy(const Offset(0.0, 210.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, greaterThan(lastStretchedLocation));
lastStretchedLocation = tester.getCenter(find.text('Index 1')).dy;
final TestGesture pointer3 = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Add overscroll from an additional pointer, exceeding the max stretch (600)
await pointer3.moveBy(const Offset(0.0, 210.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, greaterThan(lastStretchedLocation));
lastStretchedLocation = tester.getCenter(find.text('Index 1')).dy;
final TestGesture pointer4 = await tester.startGesture(tester.getCenter(find.text('Index 1')));
// Since we have maxed out the overscroll, it should not have stretched
// further, regardless of the number of pointers.
await pointer4.moveBy(const Offset(0.0, 210.0));
await tester.pumpAndSettle();
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getCenter(find.text('Index 1')).dy, lastStretchedLocation);
await pointer1.up();
await pointer2.up();
await pointer3.up();
await pointer4.up();
await tester.pumpAndSettle();
});
testWidgets('Stretch overscroll vertically, change direction mid scroll', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
// Setting the `boxHeight` to 100.0 will make the boxes fit in the
// scrollable viewport.
boxHeight: 100,
// To make the scroll view in the test still scrollable, we need to add
// the `AlwaysScrollableScrollPhysics`.
physics: const AlwaysScrollableScrollPhysics(),
),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 100.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 200.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start
await gesture.moveBy(const Offset(0.0, 600.0));
await tester.pumpAndSettle();
// The boxes should now be at different locations because of the scaling.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dy, greaterThan(103.0));
expect(box3.localToGlobal(Offset.zero).dy, greaterThan(206.0));
// Move the pointer up a miniscule amount to trigger a directional change.
await gesture.moveBy(const Offset(0.0, -20.0));
await tester.pumpAndSettle();
// The boxes should remain roughly at the same locations, since the pointer
// didn't move far.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dy, greaterThan(103.0));
expect(box3.localToGlobal(Offset.zero).dy, greaterThan(206.0));
// Now make the pointer overscroll to the end
await gesture.moveBy(const Offset(0.0, -1200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-19.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(85.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(188.0));
// Release the pointer
await gesture.up();
await tester.pumpAndSettle();
// Now the boxes should be back to their original locations.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 100.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 200.0));
});
testWidgets('Stretch overscroll horizontally, change direction mid scroll', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
// Setting the `boxWidth` to 100.0 will make the boxes fit in the
// scrollable viewport.
boxWidth: 100,
// To make the scroll view in the test still scrollable, we need to add
// the `AlwaysScrollableScrollPhysics`.
physics: const AlwaysScrollableScrollPhysics(),
axis: Axis.horizontal,
),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(100.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(200.0, 0.0));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll the start
await gesture.moveBy(const Offset(600.0, 0.0));
await tester.pumpAndSettle();
// The boxes should now be at different locations because of the scaling.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dx, greaterThan(102.0));
expect(box3.localToGlobal(Offset.zero).dx, greaterThan(205.0));
// Move the pointer up a miniscule amount to trigger a directional change.
await gesture.moveBy(const Offset(-20.0, 0.0));
await tester.pumpAndSettle();
// The boxes should remain roughly at the same locations, since the pointer
// didn't move far.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero).dx, greaterThan(102.0));
expect(box3.localToGlobal(Offset.zero).dx, greaterThan(205.0));
// Now make the pointer overscroll to the end
await gesture.moveBy(const Offset(-1200.0, 0.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dx, lessThan(-19.0));
expect(box2.localToGlobal(Offset.zero).dx, lessThan(85.0));
expect(box3.localToGlobal(Offset.zero).dx, lessThan(188.0));
// Release the pointer
await gesture.up();
await tester.pumpAndSettle();
// Now the boxes should be back to their original locations.
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(100.0, 0.0));
expect(box3.localToGlobal(Offset.zero), const Offset(200.0, 0.0));
});
testWidgets('Fling toward the trailing edge causes stretch toward the leading edge', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
await tester.fling(find.byType(CustomScrollView), const Offset(0.0, -50.0), 10000.0);
await tester.pump(const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 100));
// The boxes should now be at different locations because of the scaling.
expect(controller.offset, 150.0);
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-160.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(93.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(347.0));
await tester.pumpAndSettle();
// The boxes should now be at their final position.
expect(controller.offset, 150.0);
expect(box1.localToGlobal(Offset.zero).dy, -150.0);
expect(box2.localToGlobal(Offset.zero).dy, 100.0);
expect(box3.localToGlobal(Offset.zero).dy, 350.0);
});
testWidgets('Fling toward the leading edge causes stretch toward the trailing edge', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero), Offset.zero);
expect(box2.localToGlobal(Offset.zero), const Offset(0.0, 250.0));
expect(box3.localToGlobal(Offset.zero), const Offset(0.0, 500.0));
// We fling to the trailing edge and let it settle.
await tester.fling(find.byType(CustomScrollView), const Offset(0.0, -50.0), 10000.0);
await tester.pumpAndSettle();
// We are now at the trailing edge
expect(controller.offset, 150.0);
expect(box1.localToGlobal(Offset.zero).dy, -150.0);
expect(box2.localToGlobal(Offset.zero).dy, 100.0);
expect(box3.localToGlobal(Offset.zero).dy, 350.0);
// Now fling to the leading edge
await tester.fling(find.byType(CustomScrollView), const Offset(0.0, 50.0), 10000.0);
await tester.pump(const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 100));
await tester.pump(const Duration(milliseconds: 100));
// The boxes should now be at different locations because of the scaling.
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero).dy, 0.0);
expect(box2.localToGlobal(Offset.zero).dy, greaterThan(254.0));
expect(box3.localToGlobal(Offset.zero).dy, greaterThan(508.0));
await tester.pumpAndSettle();
// The boxes should now be at their final position.
expect(controller.offset, 0.0);
expect(box1.localToGlobal(Offset.zero).dy, 0.0);
expect(box2.localToGlobal(Offset.zero).dy, 250.0);
expect(box3.localToGlobal(Offset.zero).dy, 500.0);
});
testWidgets('changing scroll direction during recede animation will not change the stretch direction', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(box1Key, box2Key, box3Key, controller, boxHeight: 205.0),
);
expect(find.byType(StretchingOverscrollIndicator), findsOneWidget);
expect(find.byType(GlowingOverscrollIndicator), findsNothing);
final RenderBox box1 = tester.renderObject(find.byKey(box1Key));
final RenderBox box2 = tester.renderObject(find.byKey(box2Key));
final RenderBox box3 = tester.renderObject(find.byKey(box3Key));
// Fling to the trailing edge
await tester.fling(find.byType(CustomScrollView), const Offset(0.0, -50.0), 10000.0);
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dy, -15.0);
expect(box2.localToGlobal(Offset.zero).dy, 190.0);
expect(box3.localToGlobal(Offset.zero).dy, 395.0);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll to the trailing edge
await gesture.moveBy(const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-25.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(185.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(392.0));
// This will trigger the recede animation
// The y offset of the boxes should be increasing, since the boxes were stretched
// toward the leading edge.
await gesture.moveBy(const Offset(0.0, 150.0));
await tester.pump(const Duration(milliseconds: 100));
// Explicitly check that the box1 offset is not 0.0, since this would probably mean that
// the stretch direction is wrong.
expect(box1.localToGlobal(Offset.zero).dy, isNot(0.0));
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-12.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(197.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(407.0));
await tester.pump(const Duration(milliseconds: 100));
expect(box1.localToGlobal(Offset.zero).dy, lessThan(-6.0));
expect(box2.localToGlobal(Offset.zero).dy, lessThan(201.0));
expect(box3.localToGlobal(Offset.zero).dy, lessThan(408.0));
await tester.pumpAndSettle();
// The recede animation is done now, we should now be at the leading edge.
expect(box1.localToGlobal(Offset.zero).dy, 0.0);
expect(box2.localToGlobal(Offset.zero).dy, 205.0);
expect(box3.localToGlobal(Offset.zero).dy, 410.0);
await gesture.up();
});
testWidgets('Stretch overscroll only uses image filter during stretch effect', (WidgetTester tester) async {
final GlobalKey box1Key = GlobalKey();
final GlobalKey box2Key = GlobalKey();
final GlobalKey box3Key = GlobalKey();
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(
buildTest(
box1Key,
box2Key,
box3Key,
controller,
axis: Axis.horizontal,
)
);
expect(tester.layers, isNot(contains(isA<ImageFilterLayer>())));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
// Overscroll
await gesture.moveBy(const Offset(200.0, 0.0));
await tester.pumpAndSettle();
expect(tester.layers, contains(isA<ImageFilterLayer>()));
});
}
| flutter/packages/flutter/test/widgets/overscroll_stretch_indicator_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/overscroll_stretch_indicator_test.dart",
"repo_id": "flutter",
"token_count": 19142
} | 748 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
class ExpandingBox extends StatefulWidget {
const ExpandingBox({ super.key, required this.collapsedSize, required this.expandedSize });
final double collapsedSize;
final double expandedSize;
@override
State<ExpandingBox> createState() => _ExpandingBoxState();
}
class _ExpandingBoxState extends State<ExpandingBox> with AutomaticKeepAliveClientMixin<ExpandingBox> {
late double _height;
@override
void initState() {
super.initState();
_height = widget.collapsedSize;
}
void toggleSize() {
setState(() {
_height = _height == widget.collapsedSize ? widget.expandedSize : widget.collapsedSize;
});
}
@override
Widget build(BuildContext context) {
super.build(context);
return Container(
height: _height,
color: Colors.green,
child: Align(
alignment: Alignment.bottomCenter,
child: TextButton(
onPressed: toggleSize,
child: const Text('Collapse'),
),
),
);
}
@override
bool get wantKeepAlive => true;
}
void main() {
testWidgets('shrink listview', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: ListView.builder(
itemBuilder: (BuildContext context, int index) => index == 0
? const ExpandingBox(collapsedSize: 400, expandedSize: 1200)
: Container(height: 300, color: Colors.red),
itemCount: 2,
),
));
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
expect(position.activity, isInstanceOf<IdleScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 0.0);
await tester.tap(find.byType(TextButton));
await tester.pump();
final TestGesture drag1 = await tester.startGesture(const Offset(10.0, 500.0));
await tester.pump();
await drag1.moveTo(const Offset(10.0, 0.0));
await tester.pump();
await drag1.up();
await tester.pump();
expect(position.pixels, moreOrLessEquals(500.0));
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 900.0);
final TestGesture drag2 = await tester.startGesture(const Offset(10.0, 500.0));
await tester.pump();
await drag2.moveTo(const Offset(10.0, 100.0));
await tester.pump();
await drag2.up();
await tester.pump();
expect(position.maxScrollExtent, 900.0);
expect(position.pixels, moreOrLessEquals(900.0));
await tester.pump();
await tester.tap(find.byType(TextButton));
await tester.pump();
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 100.0);
});
testWidgets('shrink listview while dragging', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: ListView.builder(
itemBuilder: (BuildContext context, int index) => index == 0
? const ExpandingBox(collapsedSize: 400, expandedSize: 1200)
: Container(height: 300, color: Colors.red),
itemCount: 2,
),
));
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
expect(position.activity, isInstanceOf<IdleScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 0.0);
await tester.tap(find.byType(TextButton));
await tester.pump(); // start button animation
await tester.pump(const Duration(seconds: 1)); // finish button animation
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 1800.0);
expect(position.pixels, 0.0);
final TestGesture drag1 = await tester.startGesture(const Offset(10.0, 500.0));
expect(await tester.pumpAndSettle(), 1); // Nothing to animate
await drag1.moveTo(const Offset(10.0, 0.0));
expect(await tester.pumpAndSettle(), 2); // Nothing to animate, only one semantics update
await drag1.up();
expect(await tester.pumpAndSettle(), 1); // Nothing to animate
expect(position.pixels, moreOrLessEquals(500.0));
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 900.0);
final TestGesture drag2 = await tester.startGesture(const Offset(10.0, 500.0));
expect(await tester.pumpAndSettle(), 1); // Nothing to animate
await drag2.moveTo(const Offset(10.0, 100.0));
expect(await tester.pumpAndSettle(), 2); // Nothing to animate, only one semantics update
expect(position.maxScrollExtent, 900.0);
expect(position.pixels, lessThanOrEqualTo(900.0));
expect(position.activity, isInstanceOf<DragScrollActivity>());
final _ExpandingBoxState expandingBoxState = tester.state<_ExpandingBoxState>(find.byType(ExpandingBox));
expandingBoxState.toggleSize();
expect(await tester.pumpAndSettle(), 2); // Nothing to animate, only one semantics update
expect(position.activity, isInstanceOf<DragScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 100.0);
await drag2.moveTo(const Offset(10.0, 150.0));
await drag2.up();
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 50.0);
expect(await tester.pumpAndSettle(), 2); // Nothing to animate, only one semantics update
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 50.0);
});
testWidgets('shrink listview while ballistic', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: GestureDetector(
onTap: () { assert(false); },
child: ListView.builder(
physics: const RangeMaintainingScrollPhysics(parent: BouncingScrollPhysics()),
itemBuilder: (BuildContext context, int index) => index == 0
? const ExpandingBox(collapsedSize: 400, expandedSize: 1200)
: Container(height: 300, color: Colors.red),
itemCount: 2,
),
),
));
final _ExpandingBoxState expandingBoxState = tester.state<_ExpandingBoxState>(find.byType(ExpandingBox));
expandingBoxState.toggleSize();
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
expect(position.activity, isInstanceOf<IdleScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 0.0);
await tester.pump();
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 1800.0);
expect(position.pixels, 0.0);
final TestGesture drag1 = await tester.startGesture(const Offset(10.0, 10.0));
await tester.pump();
expect(position.activity, isInstanceOf<HoldScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 1800.0);
expect(position.pixels, 0.0);
await drag1.moveTo(const Offset(10.0, 50.0)); // to get past the slop and trigger the drag
await drag1.moveTo(const Offset(10.0, 550.0));
expect(position.pixels, -500.0);
await tester.pump();
expect(position.activity, isInstanceOf<DragScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 1800.0);
expect(position.pixels, -500.0);
await drag1.up();
await tester.pump();
expect(position.activity, isInstanceOf<BallisticScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 1800.0);
expect(position.pixels, -500.0);
expandingBoxState.toggleSize();
await tester.pump(); // apply physics without moving clock forward
expect(position.activity, isInstanceOf<BallisticScrollActivity>());
// TODO(ianh): Determine why the maxScrollOffset is 200.0 here instead of 100.0 or double.infinity.
// expect(position.minScrollExtent, 0.0);
// expect(position.maxScrollExtent, 100.0);
expect(position.pixels, -500.0);
await tester.pumpAndSettle(); // ignoring the exact effects of the animation
expect(position.activity, isInstanceOf<IdleScrollActivity>());
expect(position.minScrollExtent, 0.0);
expect(position.maxScrollExtent, 100.0);
expect(position.pixels, 0.0);
});
testWidgets('expanding page views', (WidgetTester tester) async {
await tester.pumpWidget(const Padding(padding: EdgeInsets.only(right: 200.0), child: TabBarDemo()));
await tester.tap(find.text('bike'));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
final Rect bike1 = tester.getRect(find.byIcon(Icons.directions_bike));
await tester.pumpWidget(const Padding(padding: EdgeInsets.zero, child: TabBarDemo()));
final Rect bike2 = tester.getRect(find.byIcon(Icons.directions_bike));
expect(bike2.center, bike1.shift(const Offset(100.0, 0.0)).center);
});
testWidgets('changing the size of the viewport when overscrolled', (WidgetTester tester) async {
Widget build(double height) {
return Directionality(
textDirection: TextDirection.rtl,
child: ScrollConfiguration(
behavior: const RangeMaintainingTestScrollBehavior(),
child: Align(
alignment: Alignment.topLeft,
child: SizedBox(
height: height,
width: 100.0,
child: ListView(
children: const <Widget>[SizedBox(height: 100.0, child: Placeholder())],
),
),
),
),
);
}
await tester.pumpWidget(build(200.0));
// to verify that changing the size of the viewport while you are overdragged does not change the
// scroll position, we must ensure that:
// - velocity is zero
// - scroll extents have changed
// - position does not change at the same time
// - old position is out of old range AND new range
await tester.drag(find.byType(Placeholder), const Offset(0.0, 100.0), touchSlopY: 0.0, warnIfMissed: false); // it'll hit the scrollable
await tester.pump();
final Rect oldPosition = tester.getRect(find.byType(Placeholder));
await tester.pumpWidget(build(220.0));
final Rect newPosition = tester.getRect(find.byType(Placeholder));
expect(oldPosition, newPosition);
});
testWidgets('inserting and removing an item when overscrolled', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/62890
const double itemExtent = 100.0;
final UniqueKey key = UniqueKey();
final Finder finder = find.byKey(key);
Widget build({required bool twoItems}) {
return Directionality(
textDirection: TextDirection.rtl,
child: ScrollConfiguration(
behavior: const RangeMaintainingTestScrollBehavior(),
child: Align(
child: SizedBox(
width: 100.0,
height: 100.0,
child: ListView(
children: <Widget>[
SizedBox(height: itemExtent, child: Placeholder(key: key)),
if (twoItems)
const SizedBox(height: itemExtent, child: Placeholder()),
],
),
),
),
),
);
}
await tester.pumpWidget(build(twoItems: false));
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
// overscroll bottom
final TestGesture drag1 = await tester.startGesture(tester.getCenter(finder));
await tester.pump();
await drag1.moveBy(const Offset(0.0, -50.0));
await tester.pump();
final double oldOverscroll1 = position.pixels - position.maxScrollExtent;
final Rect oldPosition1 = tester.getRect(finder);
await tester.pumpWidget(build(twoItems: true));
// verify inserting new item didn't change the position of the first one
expect(oldPosition1, tester.getRect(finder));
// verify the overscroll changed by the size of the added item
final double newOverscroll1 = position.pixels - position.maxScrollExtent;
expect(oldOverscroll1, isPositive);
expect(newOverscroll1, isNegative);
expect(newOverscroll1, oldOverscroll1 - itemExtent);
await drag1.up();
// verify there's no ballistic animation, because we weren't overscrolled
expect(await tester.pumpAndSettle(), 1);
// overscroll bottom
final TestGesture drag2 = await tester.startGesture(tester.getCenter(finder));
await tester.pump();
await drag2.moveBy(const Offset(0.0, -100.0));
await tester.pump();
final double oldOverscroll2 = position.pixels - position.maxScrollExtent;
// should find nothing because item is not visible
expect(finder, findsNothing);
await tester.pumpWidget(build(twoItems: false));
// verify removing an item changed the position of the first one, because prior it was not visible
expect(oldPosition1, tester.getRect(finder));
// verify the overscroll was maintained
final double newOverscroll2 = position.pixels - position.maxScrollExtent;
expect(oldOverscroll2, isPositive);
expect(oldOverscroll2, newOverscroll2);
await drag2.up();
// verify there's a ballistic animation from overscroll
expect(await tester.pumpAndSettle(), 9);
});
}
class TabBarDemo extends StatelessWidget {
const TabBarDemo({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: DefaultTabController(
length: 3,
child: Scaffold(
appBar: AppBar(
bottom: const TabBar(
tabs: <Widget>[
Tab(text: 'car'),
Tab(text: 'transit'),
Tab(text: 'bike'),
],
),
title: const Text('Tabs Demo'),
),
body: const TabBarView(
children: <Widget>[
Icon(Icons.directions_car),
Icon(Icons.directions_transit),
Icon(Icons.directions_bike),
],
),
),
),
);
}
}
class RangeMaintainingTestScrollBehavior extends ScrollBehavior {
const RangeMaintainingTestScrollBehavior();
@override
TargetPlatform getPlatform(BuildContext context) => defaultTargetPlatform;
@override
Widget buildOverscrollIndicator(BuildContext context, Widget child, ScrollableDetails details) {
return child;
}
@override
Widget buildScrollbar(BuildContext context, Widget child, ScrollableDetails details) {
return child;
}
@override
GestureVelocityTrackerBuilder velocityTrackerBuilder(BuildContext context) {
return (PointerEvent event) => VelocityTracker.withKind(event.kind);
}
@override
ScrollPhysics getScrollPhysics(BuildContext context) {
return const BouncingScrollPhysics(parent: RangeMaintainingScrollPhysics());
}
}
| flutter/packages/flutter/test/widgets/range_maintaining_scroll_physics_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/range_maintaining_scroll_physics_test.dart",
"repo_id": "flutter",
"token_count": 5774
} | 749 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Rotated box control test', (WidgetTester tester) async {
final List<String> log = <String>[];
final Key rotatedBoxKey = UniqueKey();
await tester.pumpWidget(
Center(
child: RotatedBox(
key: rotatedBoxKey,
quarterTurns: 1,
child: Row(
textDirection: TextDirection.ltr,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
GestureDetector(
onTap: () { log.add('left'); },
child: Container(
width: 100.0,
height: 40.0,
color: Colors.blue[500],
),
),
GestureDetector(
onTap: () { log.add('right'); },
child: Container(
width: 75.0,
height: 65.0,
color: Colors.blue[500],
),
),
],
),
),
),
);
final RenderBox box = tester.renderObject(find.byKey(rotatedBoxKey));
expect(box.size.width, equals(65.0));
expect(box.size.height, equals(175.0));
await tester.tapAt(const Offset(420.0, 280.0));
expect(log, equals(<String>['left']));
log.clear();
await tester.tapAt(const Offset(380.0, 320.0));
expect(log, equals(<String>['right']));
log.clear();
});
}
| flutter/packages/flutter/test/widgets/rotated_box_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/rotated_box_test.dart",
"repo_id": "flutter",
"token_count": 828
} | 750 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ScrollMetricsNotification test', (WidgetTester tester) async {
final List<Notification> events = <Notification>[];
Widget buildFrame(double height) {
return NotificationListener<Notification>(
onNotification: (Notification value) {
events.add(value);
return false;
},
child: SingleChildScrollView(
child: SizedBox(height: height),
),
);
}
await tester.pumpWidget(buildFrame(1200.0));
expect(events.length, 1);
ScrollMetricsNotification event = events[0] as ScrollMetricsNotification;
expect(event.metrics.extentBefore, 0.0);
expect(event.metrics.extentInside, 600.0);
expect(event.metrics.extentAfter, 600.0);
expect(event.metrics.extentTotal, 1200.0);
events.clear();
await tester.pumpWidget(buildFrame(1000.0));
// Change the content dimensions will trigger a new event.
expect(events.length, 1);
event = events[0] as ScrollMetricsNotification;
expect(event.metrics.extentBefore, 0.0);
expect(event.metrics.extentInside, 600.0);
expect(event.metrics.extentAfter, 400.0);
expect(event.metrics.extentTotal, 1000.0);
events.clear();
final TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pump(const Duration(seconds: 1));
await gesture.moveBy(const Offset(-10.0, -10.0));
await tester.pump(const Duration(seconds: 1));
await gesture.up();
await tester.pump(const Duration(seconds: 1));
expect(events.length, 5);
// user scroll do not trigger the ScrollMetricsNotification.
expect(events[0] is ScrollStartNotification, true);
expect(events[1] is UserScrollNotification, true);
expect(events[2] is ScrollUpdateNotification, true);
expect(events[3] is ScrollEndNotification, true);
expect(events[4] is UserScrollNotification, true);
events.clear();
// Change the content dimensions again.
await tester.pumpWidget(buildFrame(500.0));
expect(events.length, 1);
event = events[0] as ScrollMetricsNotification;
expect(event.metrics.extentBefore, 0.0);
expect(event.metrics.extentInside, 600.0);
expect(event.metrics.extentAfter, 0.0);
expect(event.metrics.extentTotal, 600.0);
events.clear();
// The content dimensions does not change.
await tester.pumpWidget(buildFrame(500.0));
expect(events.length, 0);
});
testWidgets('Scroll notification basics', (WidgetTester tester) async {
late ScrollNotification notification;
await tester.pumpWidget(NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification value) {
if (value is ScrollStartNotification || value is ScrollUpdateNotification || value is ScrollEndNotification) {
notification = value;
}
return false;
},
child: const SingleChildScrollView(
child: SizedBox(height: 1200.0),
),
));
final TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pump(const Duration(seconds: 1));
expect(notification, isA<ScrollStartNotification>());
expect(notification.depth, equals(0));
final ScrollStartNotification start = notification as ScrollStartNotification;
expect(start.dragDetails, isNotNull);
expect(start.dragDetails!.globalPosition, equals(const Offset(100.0, 100.0)));
await gesture.moveBy(const Offset(-10.0, -10.0));
await tester.pump(const Duration(seconds: 1));
expect(notification, isA<ScrollUpdateNotification>());
expect(notification.depth, equals(0));
final ScrollUpdateNotification update = notification as ScrollUpdateNotification;
expect(update.dragDetails, isNotNull);
expect(update.dragDetails!.globalPosition, equals(const Offset(90.0, 90.0)));
expect(update.dragDetails!.delta, equals(const Offset(0.0, -10.0)));
await gesture.up();
await tester.pump(const Duration(seconds: 1));
expect(notification, isA<ScrollEndNotification>());
expect(notification.depth, equals(0));
final ScrollEndNotification end = notification as ScrollEndNotification;
expect(end.dragDetails, isNotNull);
expect(end.dragDetails!.velocity, equals(Velocity.zero));
});
testWidgets('Scroll notification depth', (WidgetTester tester) async {
final List<Type> depth0Types = <Type>[];
final List<Type> depth1Types = <Type>[];
final List<int> depth0Values = <int>[];
final List<int> depth1Values = <int>[];
await tester.pumpWidget(NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification value) {
depth1Types.add(value.runtimeType);
depth1Values.add(value.depth);
return false;
},
child: SingleChildScrollView(
dragStartBehavior: DragStartBehavior.down,
child: SizedBox(
height: 1200.0,
child: NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification value) {
depth0Types.add(value.runtimeType);
depth0Values.add(value.depth);
return false;
},
child: Container(
padding: const EdgeInsets.all(50.0),
child: const SingleChildScrollView(
dragStartBehavior: DragStartBehavior.down,
child: SizedBox(height: 1200.0),
),
),
),
),
),
));
final TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pump(const Duration(seconds: 1));
await gesture.moveBy(const Offset(-10.0, -40.0));
await tester.pump(const Duration(seconds: 1));
await gesture.up();
await tester.pump(const Duration(seconds: 1));
final List<Type> types = <Type>[
ScrollStartNotification,
UserScrollNotification,
ScrollUpdateNotification,
ScrollEndNotification,
UserScrollNotification,
];
expect(depth0Types, equals(types));
expect(depth1Types, equals(types));
expect(depth0Values, equals(<int>[0, 0, 0, 0, 0]));
expect(depth1Values, equals(<int>[1, 1, 1, 1, 1]));
});
testWidgets('ScrollNotifications bubble past Scaffold Material', (WidgetTester tester) async {
final List<Type> notificationTypes = <Type>[];
await tester.pumpWidget(
MaterialApp(
home: NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification value) {
notificationTypes.add(value.runtimeType);
return false;
},
child: Scaffold(
body: SizedBox.expand(
child: SingleChildScrollView(
dragStartBehavior: DragStartBehavior.down,
child: SizedBox(
height: 1200.0,
child: Container(
padding: const EdgeInsets.all(50.0),
child: const SingleChildScrollView(
dragStartBehavior: DragStartBehavior.down,
child: SizedBox(height: 1200.0),
),
),
),
),
),
),
),
),
);
final TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pump(const Duration(seconds: 1));
await gesture.moveBy(const Offset(-10.0, -40.0));
await tester.pump(const Duration(seconds: 1));
await gesture.up();
await tester.pump(const Duration(seconds: 1));
final List<Type> types = <Type>[
ScrollStartNotification,
UserScrollNotification,
ScrollUpdateNotification,
ScrollEndNotification,
UserScrollNotification,
];
expect(notificationTypes, equals(types));
});
testWidgets('ScrollNotificationObserver', (WidgetTester tester) async {
late ScrollNotificationObserverState observer;
ScrollNotification? notification;
void handleNotification(ScrollNotification value) {
if (value is ScrollStartNotification || value is ScrollUpdateNotification || value is ScrollEndNotification) {
notification = value;
}
}
await tester.pumpWidget(
ScrollNotificationObserver(
child: Builder(
builder: (BuildContext context) {
observer = ScrollNotificationObserver.of(context);
return const SingleChildScrollView(
child: SizedBox(height: 1200.0),
);
},
),
),
);
observer.addListener(handleNotification);
TestGesture gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pumpAndSettle();
expect(notification, isA<ScrollStartNotification>());
expect(notification!.depth, equals(0));
final ScrollStartNotification start = notification! as ScrollStartNotification;
expect(start.dragDetails, isNotNull);
expect(start.dragDetails!.globalPosition, equals(const Offset(100.0, 100.0)));
await gesture.moveBy(const Offset(-10.0, -10.0));
await tester.pumpAndSettle();
expect(notification, isA<ScrollUpdateNotification>());
expect(notification!.depth, equals(0));
final ScrollUpdateNotification update = notification! as ScrollUpdateNotification;
expect(update.dragDetails, isNotNull);
expect(update.dragDetails!.globalPosition, equals(const Offset(90.0, 90.0)));
expect(update.dragDetails!.delta, equals(const Offset(0.0, -10.0)));
await gesture.up();
await tester.pumpAndSettle();
expect(notification, isA<ScrollEndNotification>());
expect(notification!.depth, equals(0));
final ScrollEndNotification end = notification! as ScrollEndNotification;
expect(end.dragDetails, isNotNull);
expect(end.dragDetails!.velocity, equals(Velocity.zero));
observer.removeListener(handleNotification);
notification = null;
gesture = await tester.startGesture(const Offset(100.0, 100.0));
await tester.pumpAndSettle();
expect(notification, isNull);
await gesture.moveBy(const Offset(-10.0, -10.0));
await tester.pumpAndSettle();
expect(notification, isNull);
await gesture.up();
await tester.pumpAndSettle();
expect(notification, isNull);
});
}
| flutter/packages/flutter/test/widgets/scroll_notification_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/scroll_notification_test.dart",
"repo_id": "flutter",
"token_count": 4068
} | 751 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'semantics_tester.dart';
void main() {
testWidgets('Traversal Order of SliverList', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final ScrollController controller = ScrollController(initialScrollOffset: 3000.0);
addTearDown(controller.dispose);
final List<Widget> listChildren = List<Widget>.generate(30, (int i) {
return SizedBox(
height: 200.0,
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Semantics(
container: true,
child: Text('Item ${i}a'),
),
Semantics(
container: true,
child: Text('item ${i}b'),
),
],
),
);
});
await tester.pumpWidget(
Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
controller: controller,
semanticChildCount: 30,
slivers: <Widget>[
SliverList(
delegate: SliverChildListDelegate(listChildren),
),
],
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
scrollIndex: 15,
scrollChildren: 30,
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 13a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 13b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 14a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 14b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Item 15a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 15b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Item 16a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 16b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Item 17a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 17b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 18a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 18b',
textDirection: TextDirection.ltr,
),
],
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 19a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 19b',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
],
),
ignoreId: true,
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Traversal Order of SliverFixedExtentList', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final ScrollController controller = ScrollController(initialScrollOffset: 3000.0);
addTearDown(controller.dispose);
final List<Widget> listChildren = List<Widget>.generate(30, (int i) {
return SizedBox(
height: 200.0,
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Semantics(
container: true,
child: Text('Item ${i}a'),
),
Semantics(
container: true,
child: Text('item ${i}b'),
),
],
),
);
});
await tester.pumpWidget(
Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
controller: controller,
slivers: <Widget>[
SliverFixedExtentList(
itemExtent: 200.0,
delegate: SliverChildListDelegate(listChildren, addSemanticIndexes: false),
),
],
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 13a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 13b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 14a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 14b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 15a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 15b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 16a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 16b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 17a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 17b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 18a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 18b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 19a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 19b',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreId: true,
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Traversal Order of SliverGrid', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final ScrollController controller = ScrollController(initialScrollOffset: 1600.0);
addTearDown(controller.dispose);
final List<Widget> listChildren = List<Widget>.generate(30, (int i) {
return SizedBox(
height: 200.0,
child: Text('Item $i'),
);
});
await tester.pumpWidget(
Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
controller: controller,
slivers: <Widget>[
SliverGrid.count(
crossAxisCount: 2,
crossAxisSpacing: 400.0,
children: listChildren,
),
],
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 12',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 13',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 14',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 15',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 16',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 17',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 18',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 19',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 20',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 21',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 22',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 23',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 24',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 25',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreId: true,
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Traversal Order of List of individual slivers', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final ScrollController controller = ScrollController(initialScrollOffset: 3000.0);
addTearDown(controller.dispose);
final List<Widget> listChildren = List<Widget>.generate(30, (int i) {
return SliverToBoxAdapter(
child: SizedBox(
height: 200.0,
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Semantics(
container: true,
child: Text('Item ${i}a'),
),
Semantics(
container: true,
child: Text('item ${i}b'),
),
],
),
),
);
});
await tester.pumpWidget(
Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CustomScrollView(
controller: controller,
slivers: listChildren,
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 13a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 13b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 14a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 14b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 15a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 15b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 16a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 16b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Item 17a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'item 17b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 18a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 18b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'Item 19a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: 'item 19b',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreId: true,
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Traversal Order of in a SingleChildScrollView', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final ScrollController controller = ScrollController(initialScrollOffset: 3000.0);
addTearDown(controller.dispose);
final List<Widget> listChildren = List<Widget>.generate(30, (int i) {
return SizedBox(
height: 200.0,
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Semantics(
container: true,
child: Text('Item ${i}a'),
),
Semantics(
container: true,
child: Text('item ${i}b'),
),
],
),
);
});
await tester.pumpWidget(
Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: SingleChildScrollView(
controller: controller,
child: Column(
children: listChildren,
),
),
),
),
),
);
final List<TestSemantics> children = <TestSemantics>[];
for (int index = 0; index < 30; index += 1) {
final bool isHidden = index < 15 || index > 17;
children.add(
TestSemantics(
flags: isHidden ? <SemanticsFlag>[SemanticsFlag.isHidden] : 0,
label: 'Item ${index}a',
textDirection: TextDirection.ltr,
),
);
children.add(
TestSemantics(
flags: isHidden ? <SemanticsFlag>[SemanticsFlag.isHidden] : 0,
label: 'item ${index}b',
textDirection: TextDirection.ltr,
),
);
}
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: children,
),
],
),
],
),
ignoreId: true,
ignoreTransform: true,
ignoreRect: true,
));
semantics.dispose();
});
testWidgets('Traversal Order with center child', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(Semantics(
textDirection: TextDirection.ltr,
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: Scrollable(
viewportBuilder: (BuildContext context, ViewportOffset offset) {
return Viewport(
offset: offset,
center: const ValueKey<int>(0),
slivers: List<Widget>.generate(30, (int i) {
final int item = i - 15;
return SliverToBoxAdapter(
key: ValueKey<int>(item),
child: SizedBox(
height: 200.0,
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Semantics(
container: true,
child: Text('${item}a'),
),
Semantics(
container: true,
child: Text('${item}b'),
),
],
),
),
);
}),
);
},
),
),
),
));
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[
SemanticsFlag.hasImplicitScrolling,
],
actions: <SemanticsAction>[
SemanticsAction.scrollUp,
SemanticsAction.scrollDown,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '-2a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '-2b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '-1a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '-1b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '0a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '0b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '1a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '1b',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '2a',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: '2b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '3a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '3b',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '4a',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isHidden],
label: '4b',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
ignoreId: true,
));
semantics.dispose();
});
}
| flutter/packages/flutter/test/widgets/scrollable_semantics_traversal_order_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/scrollable_semantics_traversal_order_test.dart",
"repo_id": "flutter",
"token_count": 18174
} | 752 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'semantics_tester.dart';
void main() {
testWidgets('Semantics 8 - Merging with reset', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MergeSemantics(
child: Semantics(
container: true,
child: Semantics(
container: true,
child: Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
Semantics(
checked: true,
),
Semantics(
label: 'label',
textDirection: TextDirection.ltr,
),
],
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
id: 1,
flags: SemanticsFlag.hasCheckedState.index | SemanticsFlag.isChecked.index,
label: 'label',
textDirection: TextDirection.ltr,
rect: TestSemantics.fullScreen,
),
],
),
));
// switch the order of the inner Semantics node to trigger a reset
await tester.pumpWidget(
MergeSemantics(
child: Semantics(
container: true,
child: Semantics(
container: true,
child: Stack(
textDirection: TextDirection.ltr,
children: <Widget>[
Semantics(
label: 'label',
textDirection: TextDirection.ltr,
),
Semantics(
checked: true,
),
],
),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
id: 1,
flags: SemanticsFlag.hasCheckedState.index | SemanticsFlag.isChecked.index,
label: 'label',
textDirection: TextDirection.ltr,
rect: TestSemantics.fullScreen,
),
],
),
));
semantics.dispose();
});
}
| flutter/packages/flutter/test/widgets/semantics_8_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/semantics_8_test.dart",
"repo_id": "flutter",
"token_count": 1276
} | 753 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
late ChangerState changer;
class Changer extends StatefulWidget {
const Changer(this.child, { super.key });
final Widget child;
@override
ChangerState createState() => ChangerState();
}
class ChangerState extends State<Changer> {
bool _state = false;
@override
void initState() {
super.initState();
changer = this;
}
void test() { setState(() { _state = true; }); }
@override
Widget build(BuildContext context) => _state ? Wrapper(widget.child) : widget.child;
}
class Wrapper extends StatelessWidget {
const Wrapper(this.child, { super.key });
final Widget child;
@override
Widget build(BuildContext context) => child;
}
class Leaf extends StatefulWidget {
const Leaf({ super.key });
@override
LeafState createState() => LeafState();
}
class LeafState extends State<Leaf> {
@override
Widget build(BuildContext context) => const Text('leaf', textDirection: TextDirection.ltr);
}
void main() {
testWidgets('three-way setState() smoke test', (WidgetTester tester) async {
await tester.pumpWidget(const Changer(Wrapper(Leaf())));
await tester.pumpWidget(const Changer(Wrapper(Leaf())));
changer.test();
await tester.pump();
});
}
| flutter/packages/flutter/test/widgets/set_state_3_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/set_state_3_test.dart",
"repo_id": "flutter",
"token_count": 478
} | 754 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../rendering/sliver_utils.dart';
const double VIEWPORT_HEIGHT = 600;
const double VIEWPORT_WIDTH = 300;
void main() {
testWidgets('SliverCrossAxisGroup is laid out properly', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
_buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item')),
_buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item')),
]),
);
await tester.pumpAndSettle();
expect(controller.offset, 0);
expect(find.text('Group 0 Tile 0'), findsOneWidget);
expect(find.text('Group 0 Tile 1'), findsOneWidget);
expect(find.text('Group 0 Tile 2'), findsNothing);
expect(find.text('Group 1 Tile 0'), findsOneWidget);
expect(find.text('Group 1 Tile 2'), findsOneWidget);
expect(find.text('Group 1 Tile 3'), findsNothing);
const double scrollOffset = 18 * 300.0;
controller.jumpTo(scrollOffset);
await tester.pumpAndSettle();
expect(controller.offset, scrollOffset);
expect(find.text('Group 0 Tile 17'), findsNothing);
expect(find.text('Group 0 Tile 18'), findsOneWidget);
expect(find.text('Group 0 Tile 19'), findsOneWidget);
expect(find.text('Group 1 Tile 19'), findsNothing);
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
expect(first.constraints.crossAxisExtent, equals(VIEWPORT_WIDTH / 2));
expect(second.constraints.crossAxisExtent, equals(VIEWPORT_WIDTH / 2));
expect((first.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
expect((second.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(VIEWPORT_WIDTH / 2));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('SliverExpanded is laid out properly', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverCrossAxisExpanded(
flex: 3,
sliver: _buildSliverList(
itemMainAxisExtent: 300,
items: items,
label: (int item) => Text('Group 0 Tile $item')
),
),
SliverCrossAxisExpanded(
flex: 2,
sliver: _buildSliverList(
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 1 Tile $item')
),
),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
expect(first.constraints.crossAxisExtent, equals(3 * VIEWPORT_WIDTH / 5));
expect(second.constraints.crossAxisExtent, equals(2 * VIEWPORT_WIDTH / 5));
expect((first.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
expect((second.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(3 * VIEWPORT_WIDTH / 5));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('SliverConstrainedCrossAxis is laid out properly', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverConstrainedCrossAxis(maxExtent: 60, sliver: _buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item'))),
SliverConstrainedCrossAxis(maxExtent: 120, sliver: _buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item'))),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
expect(first.constraints.crossAxisExtent, equals(60));
expect(second.constraints.crossAxisExtent, equals(120));
// Check that their parent SliverConstrainedCrossAxis have the correct paintOffsets.
final List<RenderSliverConstrainedCrossAxis> renderSliversConstrained = tester.renderObjectList<RenderSliverConstrainedCrossAxis>(find.byType(SliverConstrainedCrossAxis)).toList();
final RenderSliverConstrainedCrossAxis firstConstrained = renderSliversConstrained[0];
final RenderSliverConstrainedCrossAxis secondConstrained = renderSliversConstrained[1];
expect((firstConstrained.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
expect((secondConstrained.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(60));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Mix of slivers is laid out properly', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverConstrainedCrossAxis(maxExtent: 30, sliver: _buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item'))),
SliverCrossAxisExpanded(flex: 2, sliver: _buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item'))),
_buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 2 Tile $item')),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
final RenderSliverList third = renderSlivers[2];
expect(first.constraints.crossAxisExtent, equals(30));
expect(second.constraints.crossAxisExtent, equals(180));
expect(third.constraints.crossAxisExtent, equals(90));
// Check that paint offset for sliver children are correct as well.
final RenderSliverCrossAxisGroup sliverCrossAxisRenderObject = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
RenderSliver child = sliverCrossAxisRenderObject.firstChild!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(30));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(210));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Mix of slivers is laid out properly when horizontal', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
scrollDirection: Axis.horizontal,
slivers: <Widget>[
SliverConstrainedCrossAxis(
maxExtent: 30,
sliver: _buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 300,
items: items,
label: (int item) => Text('Group 0 Tile $item')
)
),
SliverCrossAxisExpanded(
flex: 2,
sliver: _buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 1 Tile $item')
)
),
_buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 2 Tile $item')
),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
final RenderSliverList third = renderSlivers[2];
expect(first.constraints.crossAxisExtent, equals(30));
expect(second.constraints.crossAxisExtent, equals(380));
expect(third.constraints.crossAxisExtent, equals(190));
// Check that paint offset for sliver children are correct as well.
final RenderSliverCrossAxisGroup sliverCrossAxisRenderObject = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
RenderSliver child = sliverCrossAxisRenderObject.firstChild!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(30));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(410));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Mix of slivers is laid out properly when reversed horizontal', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
scrollDirection: Axis.horizontal,
reverse: true,
slivers: <Widget>[
SliverConstrainedCrossAxis(
maxExtent: 30,
sliver: _buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 300,
items: items,
label: (int item) => Text('Group 0 Tile $item')
)
),
SliverCrossAxisExpanded(
flex: 2,
sliver: _buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 1 Tile $item')
)
),
_buildSliverList(
scrollDirection: Axis.horizontal,
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 2 Tile $item')
),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
final RenderSliverList third = renderSlivers[2];
expect(first.constraints.crossAxisExtent, equals(30));
expect(second.constraints.crossAxisExtent, equals(380));
expect(third.constraints.crossAxisExtent, equals(190));
// Check that paint offset for sliver children are correct as well.
final RenderSliverCrossAxisGroup sliverCrossAxisRenderObject = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
RenderSliver child = sliverCrossAxisRenderObject.firstChild!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(30));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(410));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Mix of slivers is laid out properly when reversed vertical', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
reverse: true,
slivers: <Widget>[
SliverConstrainedCrossAxis(
maxExtent: 30,
sliver: _buildSliverList(
itemMainAxisExtent: 300,
items: items,
label: (int item) => Text('Group 0 Tile $item')
)
),
SliverCrossAxisExpanded(
flex: 2,
sliver: _buildSliverList(
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 1 Tile $item')
)
),
_buildSliverList(
itemMainAxisExtent: 200,
items: items,
label: (int item) => Text('Group 2 Tile $item')
),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
final RenderSliverList third = renderSlivers[2];
expect(first.constraints.crossAxisExtent, equals(30));
expect(second.constraints.crossAxisExtent, equals(180));
expect(third.constraints.crossAxisExtent, equals(90));
// Check that paint offset for sliver children are correct as well.
final RenderSliverCrossAxisGroup sliverCrossAxisRenderObject = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
RenderSliver child = sliverCrossAxisRenderObject.firstChild!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(30));
child = sliverCrossAxisRenderObject.childAfter(child)!;
expect((child.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(210));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Assertion error when SliverExpanded is used outside of SliverCrossAxisGroup', (WidgetTester tester) async {
final List<FlutterErrorDetails> errors = <FlutterErrorDetails>[];
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails error) => errors.add(error);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: CustomScrollView(
slivers: <Widget>[
SliverCrossAxisExpanded(
flex: 2,
sliver: SliverToBoxAdapter(
child: Text('Hello World'),
),
),
],
),
),
),
);
FlutterError.onError = oldHandler;
expect(errors, isNotEmpty);
final AssertionError error = errors.first.exception as AssertionError;
expect(
error.toString(),
contains('renderObject.parent is RenderSliverCrossAxisGroup'),
);
});
testWidgets('Hit test works properly on various parts of SliverCrossAxisGroup', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
String? clickedTile;
int group = 0;
int tile = 0;
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
_buildSliverList(
itemMainAxisExtent: 300,
items: items,
label: (int item) => tile == item && group == 0
? TextButton(
onPressed: () => clickedTile = 'Group 0 Tile $item',
child: Text('Group 0 Tile $item'),
)
: Text('Group 0 Tile $item'),
),
_buildSliverList(
items: items,
label: (int item) => tile == item && group == 1
? TextButton(
onPressed: () => clickedTile = 'Group 1 Tile $item',
child: Text('Group 1 Tile $item'),
)
: Text('Group 1 Tile $item'),
),
]),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(TextButton));
await tester.pumpAndSettle();
expect(clickedTile, equals('Group 0 Tile 0'));
clickedTile = null;
group = 1;
tile = 2;
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
_buildSliverList(
itemMainAxisExtent: 300,
items: items,
label: (int item) => tile == item && group == 0
? TextButton(
onPressed: () => clickedTile = 'Group 0 Tile $item',
child: Text('Group 0 Tile $item'),
)
: Text('Group 0 Tile $item'),
),
_buildSliverList(
items: items,
label: (int item) => tile == item && group == 1
? TextButton(
onPressed: () => clickedTile = 'Group 1 Tile $item',
child: Text('Group 1 Tile $item'),
)
: Text('Group 1 Tile $item'),
),
]),
);
await tester.pumpAndSettle();
await tester.tap(find.byType(TextButton));
await tester.pumpAndSettle();
expect(clickedTile, equals('Group 1 Tile 2'));
});
testWidgets('Constrained sliver takes up remaining space', (WidgetTester tester) async {
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverConstrainedCrossAxis(maxExtent: 200, sliver: _buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item'))),
SliverConstrainedCrossAxis(maxExtent: 200, sliver: _buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item'))),
]),
);
await tester.pumpAndSettle();
final List<RenderSliverList> renderSlivers = tester.renderObjectList<RenderSliverList>(find.byType(SliverList)).toList();
final RenderSliverList first = renderSlivers[0];
final RenderSliverList second = renderSlivers[1];
expect(first.constraints.crossAxisExtent, equals(200));
expect(second.constraints.crossAxisExtent, equals(100));
// Check that their parent SliverConstrainedCrossAxis have the correct paintOffsets.
final List<RenderSliverConstrainedCrossAxis> renderSliversConstrained = tester.renderObjectList<RenderSliverConstrainedCrossAxis>(find.byType(SliverConstrainedCrossAxis)).toList();
final RenderSliverConstrainedCrossAxis firstConstrained = renderSliversConstrained[0];
final RenderSliverConstrainedCrossAxis secondConstrained = renderSliversConstrained[1];
expect((firstConstrained.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(0));
expect((secondConstrained.parentData! as SliverPhysicalParentData).paintOffset.dx, equals(200));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject<RenderSliverCrossAxisGroup>(find.byType(SliverCrossAxisGroup));
expect(renderGroup.geometry!.scrollExtent, equals(300 * 20));
});
testWidgets('Assertion error when constrained widget runs out of cross axis extent', (WidgetTester tester) async {
final List<FlutterErrorDetails> errors = <FlutterErrorDetails>[];
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails error) => errors.add(error);
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverConstrainedCrossAxis(maxExtent: 400, sliver: _buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item'))),
SliverConstrainedCrossAxis(maxExtent: 200, sliver: _buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item'))),
]),
);
await tester.pumpAndSettle();
FlutterError.onError = oldHandler;
expect(errors, isNotEmpty);
final AssertionError error = errors.first.exception as AssertionError;
expect(
error.toString(),
contains('SliverCrossAxisGroup ran out of extent before child could be laid out.'),
);
});
testWidgets('Assertion error when expanded widget runs out of cross axis extent', (WidgetTester tester) async {
final List<FlutterErrorDetails> errors = <FlutterErrorDetails>[];
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails error) => errors.add(error);
final List<int> items = List<int>.generate(20, (int i) => i);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
SliverConstrainedCrossAxis(maxExtent: 200, sliver: _buildSliverList(itemMainAxisExtent: 300, items: items, label: (int item) => Text('Group 0 Tile $item'))),
SliverConstrainedCrossAxis(maxExtent: 100, sliver: _buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 1 Tile $item'))),
_buildSliverList(itemMainAxisExtent: 200, items: items, label: (int item) => Text('Group 2 Tile $item')),
]),
);
await tester.pumpAndSettle();
FlutterError.onError = oldHandler;
expect(errors, isNotEmpty);
final AssertionError error = errors.first.exception as AssertionError;
expect(
error.toString(),
contains('SliverCrossAxisGroup ran out of extent before child could be laid out.'),
);
});
testWidgets('applyPaintTransform is implemented properly', (WidgetTester tester) async {
await tester.pumpWidget(_buildSliverCrossAxisGroup(
slivers: <Widget>[
const SliverToBoxAdapter(child: Text('first box')),
const SliverToBoxAdapter(child: Text('second box')),
]),
);
await tester.pumpAndSettle();
// localToGlobal calculates offset via applyPaintTransform
final RenderBox first = tester.renderObject(find.text('first box'));
final RenderBox second = tester.renderObject(find.text('second box'));
expect(first.localToGlobal(Offset.zero), Offset.zero);
expect(second.localToGlobal(Offset.zero), const Offset(VIEWPORT_WIDTH / 2, 0));
});
testWidgets('SliverPinnedPersistentHeader is painted within bounds of SliverCrossAxisGroup', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(),
pinned: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(560);
await tester.pumpAndSettle();
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
// Paint extent after header's layout is 60.0, so we must offset by -20.0 to fit within the 40.0 remaining extent.
expect(renderHeader.geometry!.paintExtent, equals(60.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-20.0));
});
testWidgets('SliverFloatingPersistentHeader is painted within bounds of SliverCrossAxisGroup', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(),
floating: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600.0);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(const Offset(150.0, 300.0));
await gesture.moveBy(const Offset(0.0, 40));
await tester.pump();
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
// Paint extent after header's layout is 40.0, so no need to correct the paintOffset.
expect(renderHeader.geometry!.paintExtent, equals(40.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
});
testWidgets('SliverPinnedPersistentHeader is painted within bounds of SliverCrossAxisGroup with different minExtent/maxExtent', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(minExtent: 40.0),
pinned: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(570);
await tester.pumpAndSettle();
// Paint extent of the header is 40.0, so we must provide an offset of -10.0 to make it fit in the 30.0 remaining paint extent of the group.
expect(renderHeader.geometry!.paintExtent, equals(40.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-10.0));
// Pinned headers should not expand to the maximum extent unless the scroll offset is at the top of the sliver group.
controller.jumpTo(550);
await tester.pumpAndSettle();
expect(renderHeader.geometry!.paintExtent, equals(40.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
});
testWidgets('SliverFloatingPersistentHeader is painted within bounds of SliverCrossAxisGroup with different minExtent/maxExtent', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(minExtent: 40.0),
floating: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(const Offset(150.0, 300.0));
await gesture.moveBy(const Offset(0.0, 30.0));
await tester.pump();
// Paint extent after header's layout is 30.0, so no need to correct the paintOffset.
expect(renderHeader.geometry!.paintExtent, equals(30.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
// Floating headers should expand to maximum extent as we continue scrolling.
await gesture.moveBy(const Offset(0.0, 20.0));
await tester.pump();
expect(renderHeader.geometry!.paintExtent, equals(50.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
});
testWidgets('SliverPinnedFloatingPersistentHeader is painted within bounds of SliverCrossAxisGroup with different minExtent/maxExtent', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(minExtent: 40.0),
pinned: true,
floating: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(const Offset(150.0, 300.0));
await gesture.moveBy(const Offset(0.0, 30.0));
await tester.pump();
// Paint extent after header's layout is 40.0, so we need to adjust by -10.0.
expect(renderHeader.geometry!.paintExtent, equals(40.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-10.0));
// Pinned floating headers should expand to maximum extent as we continue scrolling.
await gesture.moveBy(const Offset(0.0, 20.0));
await tester.pump();
expect(renderHeader.geometry!.paintExtent, equals(50.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
});
testWidgets('SliverAppBar with floating: false, pinned: false, snap: false is painted within bounds of SliverCrossAxisGroup', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
const SliverAppBar(
toolbarHeight: 30,
expandedHeight: 60,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
controller.jumpTo(570);
await tester.pumpAndSettle();
// At a scroll offset of 570, a normal scrolling header should be out of view.
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderHeader.geometry!.paintExtent, equals(0.0));
});
testWidgets('SliverAppBar with floating: true, pinned: false, snap: true is painted within bounds of SliverCrossAxisGroup', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
const SliverAppBar(
toolbarHeight: 30,
expandedHeight: 60,
floating: true,
snap: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(const Offset(150.0, 300.0));
await gesture.moveBy(const Offset(0.0, 10));
await tester.pump();
// The snap animation does not go through until the gesture is released.
expect(renderHeader.geometry!.paintExtent, equals(10));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
// Once it is released, the header's paint extent becomes the maximum and the group sets an offset of -50.0.
await gesture.up();
await tester.pumpAndSettle();
expect(renderHeader.geometry!.paintExtent, equals(60));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-50.0));
});
testWidgets('SliverAppBar with floating: true, pinned: true, snap: true is painted within bounds of SliverCrossAxisGroup', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
const SliverAppBar(
toolbarHeight: 30,
expandedHeight: 60,
floating: true,
pinned: true,
snap: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(const Offset(150.0, 300.0));
await gesture.moveBy(const Offset(0.0, 10));
await tester.pump();
expect(renderHeader.geometry!.paintExtent, equals(30.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-20.0));
// Once we lift the gesture up, the animation should finish.
await gesture.up();
await tester.pumpAndSettle();
expect(renderHeader.geometry!.paintExtent, equals(60.0));
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(-50.0));
});
testWidgets('SliverFloatingPersistentHeader scroll direction is not affected by controller.jumpTo', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
await tester.pumpWidget(_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 600)),
SliverPersistentHeader(
delegate: TestDelegate(),
floating: true,
),
],
otherSlivers: <Widget>[
const SliverToBoxAdapter(child: SizedBox(height: 2400)),
],
));
await tester.pumpAndSettle();
final RenderSliverCrossAxisGroup renderGroup = tester.renderObject(find.byType(SliverCrossAxisGroup)) as RenderSliverCrossAxisGroup;
final RenderSliverPersistentHeader renderHeader = tester.renderObject(find.byType(SliverPersistentHeader)) as RenderSliverPersistentHeader;
expect(renderGroup.geometry!.scrollExtent, equals(600));
controller.jumpTo(600);
await tester.pumpAndSettle();
controller.jumpTo(570);
await tester.pumpAndSettle();
// If renderHeader._lastStartedScrollDirection is not ScrollDirection.forward, then we shouldn't see the header at all.
expect((renderHeader.parentData! as SliverPhysicalParentData).paintOffset.dy, equals(0.0));
});
testWidgets('SliverCrossAxisGroup skips painting invisible children', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(controller.dispose);
int counter = 0;
void incrementCounter() {
counter += 1;
}
await tester.pumpWidget(
_buildSliverCrossAxisGroup(
controller: controller,
slivers: <Widget>[
MockSliverToBoxAdapter(
incrementCounter: incrementCounter,
child: Container(
height: 1000,
decoration: const BoxDecoration(color: Colors.amber),
),
),
MockSliverToBoxAdapter(
incrementCounter: incrementCounter,
child: Container(
height: 400,
decoration: const BoxDecoration(color: Colors.amber)
),
),
MockSliverToBoxAdapter(
incrementCounter: incrementCounter,
child: Container(
height: 500,
decoration: const BoxDecoration(color: Colors.amber)
),
),
MockSliverToBoxAdapter(
incrementCounter: incrementCounter,
child: Container(
height: 300,
decoration: const BoxDecoration(color: Colors.amber)
),
),
],
),
);
expect(counter, equals(4));
// Reset paint counter.
counter = 0;
controller.jumpTo(400);
await tester.pumpAndSettle();
expect(controller.offset, 400);
expect(counter, equals(2));
});
}
Widget _buildSliverList({
double itemMainAxisExtent = 100,
List<int> items = const <int>[],
required Widget Function(int) label,
Axis scrollDirection = Axis.vertical,
}) {
return SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int i) {
return scrollDirection == Axis.vertical
? SizedBox(
key: ValueKey<int>(items[i]),
height: itemMainAxisExtent,
child: label(items[i]),
)
: SizedBox(
key: ValueKey<int>(items[i]),
width: itemMainAxisExtent,
child: label(items[i]));
},
findChildIndexCallback: (Key key) {
final ValueKey<int> valueKey = key as ValueKey<int>;
final int index = items.indexOf(valueKey.value);
return index == -1 ? null : index;
},
childCount: items.length,
),
);
}
Widget _buildSliverCrossAxisGroup({
required List<Widget> slivers,
ScrollController? controller,
double viewportHeight = VIEWPORT_HEIGHT,
double viewportWidth = VIEWPORT_WIDTH,
Axis scrollDirection = Axis.vertical,
bool reverse = false,
List<Widget> otherSlivers = const <Widget>[],
}) {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: SizedBox(
height: viewportHeight,
width: viewportWidth,
child: CustomScrollView(
scrollDirection: scrollDirection,
reverse: reverse,
controller: controller,
slivers: <Widget>[SliverCrossAxisGroup(slivers: slivers), ...otherSlivers],
),
),
),
)
);
}
class TestDelegate extends SliverPersistentHeaderDelegate {
TestDelegate({ this.maxExtent = 60.0, this.minExtent = 60.0 });
@override
final double maxExtent;
@override
final double minExtent;
@override
Widget build(BuildContext context, double shrinkOffset, bool overlapsContent) {
return Container(height: maxExtent);
}
@override
bool shouldRebuild(TestDelegate oldDelegate) => true;
}
| flutter/packages/flutter/test/widgets/sliver_cross_axis_group_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/sliver_cross_axis_group_test.dart",
"repo_id": "flutter",
"token_count": 15691
} | 755 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
class TestSliverPersistentHeaderDelegate extends SliverPersistentHeaderDelegate {
TestSliverPersistentHeaderDelegate(this._maxExtent);
final double _maxExtent;
@override
double get maxExtent => _maxExtent;
@override
double get minExtent => 16.0;
@override
Widget build(BuildContext context, double shrinkOffset, bool overlapsContent) {
return Column(
children: <Widget>[
Container(height: minExtent),
Expanded(child: Container()),
],
);
}
@override
bool shouldRebuild(TestSliverPersistentHeaderDelegate oldDelegate) => false;
}
class TestBehavior extends ScrollBehavior {
const TestBehavior();
@override
Widget buildOverscrollIndicator(BuildContext context, Widget child, ScrollableDetails details) {
return GlowingOverscrollIndicator(
axisDirection: details.direction,
color: const Color(0xFFFFFFFF),
child: child,
);
}
}
class TestScrollPhysics extends ClampingScrollPhysics {
const TestScrollPhysics({ super.parent });
@override
TestScrollPhysics applyTo(ScrollPhysics? ancestor) {
return TestScrollPhysics(parent: parent?.applyTo(ancestor) ?? ancestor);
}
@override
Tolerance toleranceFor(ScrollMetrics metrics) => const Tolerance(velocity: 20.0, distance: 1.0);
}
void main() {
testWidgets('Evil test of sliver features - 1', (WidgetTester tester) async {
final GlobalKey centerKey = GlobalKey();
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: Directionality(
textDirection: TextDirection.ltr,
child: ScrollConfiguration(
behavior: const TestBehavior(),
child: Scrollbar(
child: Scrollable(
physics: const TestScrollPhysics(),
viewportBuilder: (BuildContext context, ViewportOffset offset) {
return Viewport(
anchor: 0.25,
offset: offset,
center: centerKey,
slivers: <Widget>[
SliverToBoxAdapter(child: Container(height: 5.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(150.0), pinned: true),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPadding(
padding: const EdgeInsets.all(50.0),
sliver: SliverToBoxAdapter(child: Container(height: 520.0)),
),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(150.0), floating: true),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(key: centerKey, child: Container(height: 520.0)), // ------------------------ CENTER ------------------------
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(150.0), pinned: true),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPadding(
padding: const EdgeInsets.all(50.0),
sliver: SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
SliverToBoxAdapter(child: Container(height: 5.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
SliverToBoxAdapter(child: Container(height: 5.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
SliverToBoxAdapter(child: Container(height: 5.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0), pinned: true),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(150.0), floating: true),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(150.0), floating: true),
SliverToBoxAdapter(child: Container(height: 5.0)),
SliverList(
delegate: SliverChildListDelegate(<Widget>[
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
Container(height: 50.0),
]),
),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0)),
SliverPersistentHeader(delegate: TestSliverPersistentHeaderDelegate(250.0)),
SliverPadding(
padding: const EdgeInsets.symmetric(horizontal: 50.0),
sliver: SliverToBoxAdapter(child: Container(height: 520.0)),
),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 520.0)),
SliverToBoxAdapter(child: Container(height: 5.0)),
],
);
},
),
),
),
),
),
);
final ScrollPosition position = tester.state<ScrollableState>(find.byType(Scrollable)).position;
position.animateTo(10000.0, curve: Curves.linear, duration: const Duration(minutes: 1));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 50));
await tester.pumpAndSettle(const Duration(milliseconds: 122));
position.animateTo(-10000.0, curve: Curves.linear, duration: const Duration(minutes: 1));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 50));
await tester.pumpAndSettle(const Duration(milliseconds: 122));
position.animateTo(10000.0, curve: Curves.linear, duration: const Duration(minutes: 1));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 50));
await tester.pumpAndSettle(const Duration(milliseconds: 122));
position.animateTo(-10000.0, curve: Curves.linear, duration: const Duration(seconds: 1));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 50));
await tester.pumpAndSettle(const Duration(milliseconds: 122));
position.animateTo(10000.0, curve: Curves.linear, duration: const Duration(seconds: 1));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 10));
await tester.pump(const Duration(milliseconds: 50));
await tester.pumpAndSettle(const Duration(milliseconds: 122));
});
testWidgets('Removing offscreen items above and rescrolling does not crash', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: CustomScrollView(
cacheExtent: 0.0,
slivers: <Widget>[
SliverFixedExtentList(
itemExtent: 100.0,
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return ColoredBox(
color: Colors.blue,
child: Text(index.toString()),
);
},
childCount: 30,
),
),
],
),
));
await tester.drag(find.text('5'), const Offset(0.0, -500.0));
await tester.pump();
// Screen is 600px high. Moved bottom item 500px up. It's now at the top.
expect(tester.getTopLeft(find.widgetWithText(ColoredBox, '5')).dy, 0.0);
expect(tester.getBottomLeft(find.widgetWithText(ColoredBox, '10')).dy, 600.0);
// Stop returning the first 3 items.
await tester.pumpWidget(MaterialApp(
home: CustomScrollView(
cacheExtent: 0.0,
slivers: <Widget>[
SliverFixedExtentList(
itemExtent: 100.0,
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
if (index > 3) {
return ColoredBox(
color: Colors.blue,
child: Text(index.toString()),
);
}
return null;
},
childCount: 30,
),
),
],
),
));
await tester.drag(find.text('5'), const Offset(0.0, 400.0));
await tester.pump();
// Move up by 4 items, meaning item 1 would have been at the top but
// 0 through 3 no longer exist, so item 4, 3 items down, is the first one.
// Item 4 is also shifted to the top.
expect(tester.getTopLeft(find.widgetWithText(ColoredBox, '4')).dy, 0.0);
// Because the screen is still 600px, item 9 is now visible at the bottom instead
// of what's supposed to be item 6 had we not re-shifted.
expect(tester.getBottomLeft(find.widgetWithText(ColoredBox, '9')).dy, 600.0);
});
}
| flutter/packages/flutter/test/widgets/slivers_evil_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/slivers_evil_test.dart",
"repo_id": "flutter",
"token_count": 5146
} | 756 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
class TestStatefulWidget extends StatefulWidget {
const TestStatefulWidget({ super.key });
@override
TestStatefulWidgetState createState() => TestStatefulWidgetState();
}
class TestStatefulWidgetState extends State<TestStatefulWidget> {
@override
Widget build(BuildContext context) => Container();
}
class TestChildWidget extends StatefulWidget {
const TestChildWidget({ super.key });
@override
TestChildState createState() => TestChildState();
}
class TestChildState extends State<TestChildWidget> {
bool toggle = true;
void toggleMe() {
setState(() { toggle = !toggle; });
}
@override
Widget build(BuildContext context) => toggle ? const SizedBox() : const Text('CRASHHH');
}
void main() {
testWidgets('Table widget - empty', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(),
),
);
});
testWidgets('Table widget - control test', (WidgetTester tester) async {
Future<void> run(TextDirection textDirection) async {
await tester.pumpWidget(
Directionality(
textDirection: textDirection,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAAAAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
final RenderBox boxA = tester.renderObject(find.text('AAAAAA'));
final RenderBox boxD = tester.renderObject(find.text('D'));
final RenderBox boxG = tester.renderObject(find.text('G'));
final RenderBox boxB = tester.renderObject(find.text('B'));
expect(boxA.size, equals(boxD.size));
expect(boxA.size, equals(boxG.size));
expect(boxA.size, equals(boxB.size));
}
await run(TextDirection.ltr);
await tester.pumpWidget(Container());
await run(TextDirection.rtl);
});
testWidgets('Table widget can be detached and re-attached', (WidgetTester tester) async {
final Widget table = Table(
key: GlobalKey(),
children: const <TableRow>[
TableRow(
decoration: BoxDecoration(
color: Colors.yellow,
),
children: <Widget>[Placeholder()],
),
],
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: table,
),
),
);
// Move table to a different location to simulate detaching and re-attaching effect.
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Center(
child: table,
),
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('Table widget - column offset (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Table(
columnWidths: const <int, TableColumnWidth>{
0: FixedColumnWidth(100.0),
1: FixedColumnWidth(110.0),
2: FixedColumnWidth(125.0),
},
defaultColumnWidth: const FixedColumnWidth(333.0),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A1'), Text('B1'), Text('C1'),
],
),
TableRow(
children: <Widget>[
Text('A2'), Text('B2'), Text('C2'),
],
),
TableRow(
children: <Widget>[
Text('A3'), Text('B3'), Text('C3'),
],
),
],
),
),
),
);
final Rect table = tester.getRect(find.byType(Table));
final Rect a1 = tester.getRect(find.text('A1'));
final Rect a2 = tester.getRect(find.text('A2'));
final Rect a3 = tester.getRect(find.text('A3'));
final Rect b1 = tester.getRect(find.text('B1'));
final Rect b2 = tester.getRect(find.text('B2'));
final Rect b3 = tester.getRect(find.text('B3'));
final Rect c1 = tester.getRect(find.text('C1'));
final Rect c2 = tester.getRect(find.text('C2'));
final Rect c3 = tester.getRect(find.text('C3'));
expect(a1.width, equals(100.0));
expect(a2.width, equals(100.0));
expect(a3.width, equals(100.0));
expect(b1.width, equals(110.0));
expect(b2.width, equals(110.0));
expect(b3.width, equals(110.0));
expect(c1.width, equals(125.0));
expect(c2.width, equals(125.0));
expect(c3.width, equals(125.0));
expect(table.width, equals(335.0));
expect(a1.left, equals(table.left));
expect(a2.left, equals(a1.left));
expect(a3.left, equals(a1.left));
expect(b1.left, equals(table.left + a1.width));
expect(b2.left, equals(b1.left));
expect(b3.left, equals(b1.left));
expect(c1.left, equals(table.left + a1.width + b1.width));
expect(c2.left, equals(c1.left));
expect(c3.left, equals(c1.left));
});
testWidgets('Table widget - column offset (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: Table(
columnWidths: const <int, TableColumnWidth>{
0: FixedColumnWidth(100.0),
1: FixedColumnWidth(110.0),
2: FixedColumnWidth(125.0),
},
defaultColumnWidth: const FixedColumnWidth(333.0),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A1'), Text('B1'), Text('C1'),
],
),
TableRow(
children: <Widget>[
Text('A2'), Text('B2'), Text('C2'),
],
),
TableRow(
children: <Widget>[
Text('A3'), Text('B3'), Text('C3'),
],
),
],
),
),
),
);
final Rect table = tester.getRect(find.byType(Table));
final Rect a1 = tester.getRect(find.text('A1'));
final Rect a2 = tester.getRect(find.text('A2'));
final Rect a3 = tester.getRect(find.text('A3'));
final Rect b1 = tester.getRect(find.text('B1'));
final Rect b2 = tester.getRect(find.text('B2'));
final Rect b3 = tester.getRect(find.text('B3'));
final Rect c1 = tester.getRect(find.text('C1'));
final Rect c2 = tester.getRect(find.text('C2'));
final Rect c3 = tester.getRect(find.text('C3'));
expect(a1.width, equals(100.0));
expect(a2.width, equals(100.0));
expect(a3.width, equals(100.0));
expect(b1.width, equals(110.0));
expect(b2.width, equals(110.0));
expect(b3.width, equals(110.0));
expect(c1.width, equals(125.0));
expect(c2.width, equals(125.0));
expect(c3.width, equals(125.0));
expect(table.width, equals(335.0));
expect(a1.right, equals(table.right));
expect(a2.right, equals(a1.right));
expect(a3.right, equals(a1.right));
expect(b1.right, equals(table.right - a1.width));
expect(b2.right, equals(b1.right));
expect(b3.right, equals(b1.right));
expect(c1.right, equals(table.right - a1.width - b1.width));
expect(c2.right, equals(c1.right));
expect(c3.right, equals(c1.right));
});
testWidgets('Table border - smoke test', (WidgetTester tester) async {
Future<void> run(TextDirection textDirection) async {
await tester.pumpWidget(
Directionality(
textDirection: textDirection,
child: Table(
border: TableBorder.all(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAAAAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
}
await run(TextDirection.ltr);
await tester.pumpWidget(Container());
await run(TextDirection.rtl);
});
testWidgets('Table widget - changing table dimensions', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('E'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('I'),
],
),
],
),
),
);
final RenderBox boxA1 = tester.renderObject(find.text('A'));
final RenderBox boxG1 = tester.renderObject(find.text('G'));
expect(boxA1, isNotNull);
expect(boxG1, isNotNull);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('a'), Text('b'), Text('c'), Text('d'),
],
),
TableRow(
children: <Widget>[
Text('e'), Text('f'), Text('g'), Text('h'),
],
),
],
),
),
);
final RenderBox boxA2 = tester.renderObject(find.text('a'));
final RenderBox boxG2 = tester.renderObject(find.text('g'));
expect(boxA2, isNotNull);
expect(boxG2, isNotNull);
expect(boxA1, equals(boxA2));
expect(boxG1, isNot(equals(boxG2)));
});
testWidgets('Really small deficit double precision error', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/27083
const SizedBox cell = SizedBox(width: 16, height: 16);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
cell, cell, cell, cell, cell, cell,
],
),
TableRow(
children: <Widget>[
cell, cell, cell, cell, cell, cell,
],
),
],
),
),
);
// If the above bug is present this test will never terminate.
});
testWidgets('Calculating flex columns with small width deficit', (WidgetTester tester) async {
const SizedBox cell = SizedBox(width: 1, height: 1);
// If the error is present, pumpWidget() will fail due to an unsatisfied
// assertion during the layout phase.
await tester.pumpWidget(
ConstrainedBox(
constraints: BoxConstraints.tight(const Size(600, 800)),
child: Directionality(
textDirection: TextDirection.ltr,
child: Table(
columnWidths: const <int, TableColumnWidth>{
0: FlexColumnWidth(),
1: FlexColumnWidth(0.123),
2: FlexColumnWidth(0.123),
3: FlexColumnWidth(0.123),
4: FlexColumnWidth(0.123),
5: FlexColumnWidth(0.123),
6: FlexColumnWidth(0.123),
},
children: <TableRow>[
TableRow(children: List<Widget>.filled(7, cell)),
TableRow(children: List<Widget>.filled(7, cell)),
],
),
),
),
);
expect(tester.takeException(), null);
});
testWidgets('Table widget - repump test', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAAAAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('E'), Text('FFFFFF'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
final RenderBox boxA = tester.renderObject(find.text('AAA'));
final RenderBox boxD = tester.renderObject(find.text('D'));
final RenderBox boxG = tester.renderObject(find.text('G'));
final RenderBox boxB = tester.renderObject(find.text('B'));
expect(boxA.size, equals(boxD.size));
expect(boxA.size, equals(boxG.size));
expect(boxA.size, equals(boxB.size));
});
testWidgets('Table widget - intrinsic sizing test', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
defaultColumnWidth: const IntrinsicColumnWidth(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('E'), Text('FFFFFF'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
final RenderBox boxA = tester.renderObject(find.text('AAA'));
final RenderBox boxD = tester.renderObject(find.text('D'));
final RenderBox boxG = tester.renderObject(find.text('G'));
final RenderBox boxB = tester.renderObject(find.text('B'));
expect(boxA.size, equals(boxD.size));
expect(boxA.size, equals(boxG.size));
expect(boxA.size.width, greaterThan(boxB.size.width));
expect(boxA.size.height, equals(boxB.size.height));
});
testWidgets('Table widget - intrinsic sizing test, resizing', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
defaultColumnWidth: const IntrinsicColumnWidth(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAAAAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
defaultColumnWidth: const IntrinsicColumnWidth(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
final RenderBox boxA = tester.renderObject(find.text('A'));
final RenderBox boxD = tester.renderObject(find.text('D'));
final RenderBox boxG = tester.renderObject(find.text('G'));
final RenderBox boxB = tester.renderObject(find.text('B'));
expect(boxA.size, equals(boxD.size));
expect(boxA.size, equals(boxG.size));
expect(boxA.size.width, lessThan(boxB.size.width));
expect(boxA.size.height, equals(boxB.size.height));
});
testWidgets('Table widget - intrinsic sizing test, changing column widths', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('E'), Text('FFFFFF'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
defaultColumnWidth: const IntrinsicColumnWidth(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('AAA'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('E'), Text('FFFFFF'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
),
);
final RenderBox boxA = tester.renderObject(find.text('AAA'));
final RenderBox boxD = tester.renderObject(find.text('D'));
final RenderBox boxG = tester.renderObject(find.text('G'));
final RenderBox boxB = tester.renderObject(find.text('B'));
expect(boxA.size, equals(boxD.size));
expect(boxA.size, equals(boxG.size));
expect(boxA.size.width, greaterThan(boxB.size.width));
expect(boxA.size.height, equals(boxB.size.height));
});
testWidgets('Table widget - moving test', (WidgetTester tester) async {
final List<BuildContext> contexts = <BuildContext>[];
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: <TableRow>[
TableRow(
key: const ValueKey<int>(1),
children: <Widget>[
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
contexts.add(context);
return const Text('A');
},
),
],
),
const TableRow(
children: <Widget>[
Text('b'),
],
),
],
),
),
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: <TableRow>[
const TableRow(
children: <Widget>[
Text('b'),
],
),
TableRow(
key: const ValueKey<int>(1),
children: <Widget>[
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
contexts.add(context);
return const Text('A');
},
),
],
),
],
),
),
);
expect(contexts.length, equals(2));
expect(contexts[0], equals(contexts[1]));
});
testWidgets('Table widget - keyed rows', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
key: ValueKey<int>(1),
children: <Widget>[
TestStatefulWidget(key: ValueKey<int>(11)),
TestStatefulWidget(key: ValueKey<int>(12)),
],
),
TableRow(
key: ValueKey<int>(2),
children: <Widget>[
TestStatefulWidget(key: ValueKey<int>(21)),
TestStatefulWidget(key: ValueKey<int>(22)),
],
),
],
),
),
);
final TestStatefulWidgetState state11 = tester.state(find.byKey(const ValueKey<int>(11)));
final TestStatefulWidgetState state12 = tester.state(find.byKey(const ValueKey<int>(12)));
final TestStatefulWidgetState state21 = tester.state(find.byKey(const ValueKey<int>(21)));
final TestStatefulWidgetState state22 = tester.state(find.byKey(const ValueKey<int>(22)));
expect(state11.mounted, isTrue);
expect(state12.mounted, isTrue);
expect(state21.mounted, isTrue);
expect(state22.mounted, isTrue);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
key: ValueKey<int>(2),
children: <Widget>[
TestStatefulWidget(key: ValueKey<int>(21)),
TestStatefulWidget(key: ValueKey<int>(22)),
],
),
],
),
),
);
expect(state11.mounted, isFalse);
expect(state12.mounted, isFalse);
expect(state21.mounted, isTrue);
expect(state22.mounted, isTrue);
});
testWidgets('Table widget - global key reparenting', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
final Key tableKey = UniqueKey();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Column(
children: <Widget> [
Expanded(
key: tableKey,
child: Table(
children: <TableRow>[
TableRow(
children: <Widget>[
Container(key: const ValueKey<int>(1)),
TestStatefulWidget(key: key),
Container(key: const ValueKey<int>(2)),
],
),
],
),
),
],
),
),
);
final RenderTable table = tester.renderObject(find.byType(Table));
expect(table.row(0).length, 3);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Column(
children: <Widget> [
Expanded(child: TestStatefulWidget(key: key)),
Expanded(
key: tableKey,
child: Table(
children: <TableRow>[
TableRow(
children: <Widget>[
Container(key: const ValueKey<int>(1)),
Container(key: const ValueKey<int>(2)),
],
),
],
),
),
],
),
),
);
expect(tester.renderObject(find.byType(Table)), equals(table));
expect(table.row(0).length, 2);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Column(
children: <Widget> [
Expanded(
key: tableKey,
child: Table(
children: <TableRow>[
TableRow(
children: <Widget>[
Container(key: const ValueKey<int>(1)),
TestStatefulWidget(key: key),
Container(key: const ValueKey<int>(2)),
],
),
],
),
),
],
),
),
);
expect(tester.renderObject(find.byType(Table)), equals(table));
expect(table.row(0).length, 3);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Column(
children: <Widget> [
Expanded(
key: tableKey,
child: Table(
children: <TableRow>[
TableRow(
children: <Widget>[
Container(key: const ValueKey<int>(1)),
Container(key: const ValueKey<int>(2)),
],
),
],
),
),
Expanded(child: TestStatefulWidget(key: key)),
],
),
),
);
expect(tester.renderObject(find.byType(Table)), equals(table));
expect(table.row(0).length, 2);
});
testWidgets('Table widget diagnostics', (WidgetTester tester) async {
GlobalKey key0;
final Widget table = Directionality(
textDirection: TextDirection.ltr,
child: Table(
key: key0 = GlobalKey(),
defaultColumnWidth: const IntrinsicColumnWidth(),
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A'), Text('B'), Text('C'),
],
),
TableRow(
children: <Widget>[
Text('D'), Text('EEE'), Text('F'),
],
),
TableRow(
children: <Widget>[
Text('G'), Text('H'), Text('III'),
],
),
],
),
);
await tester.pumpWidget(table);
final RenderObjectElement element = key0.currentContext! as RenderObjectElement;
expect(element, hasAGoodToStringDeep);
expect(
element.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'Table-[GlobalKey#00000](dependencies: [Directionality, MediaQuery], renderObject: RenderTable#00000)\n'
'├Text("A", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "A", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("B", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "B", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("C", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "C", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("D", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "D", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("EEE", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "EEE", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("F", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "F", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("G", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "G", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'├Text("H", dependencies: [MediaQuery])\n'
'│└RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "H", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n'
'└Text("III", dependencies: [MediaQuery])\n'
' └RichText(softWrap: wrapping at box width, maxLines: unlimited, text: "III", dependencies: [Directionality], renderObject: RenderParagraph#00000 relayoutBoundary=up1)\n',
),
);
});
// Regression test for https://github.com/flutter/flutter/issues/31473.
testWidgets(
'Does not crash if a child RenderObject is replaced by another RenderObject of a different type',
(WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(children: const <TableRow>[TableRow(children: <Widget>[TestChildWidget()])]),
),
);
expect(find.text('CRASHHH'), findsNothing);
final TestChildState state = tester.state(find.byType(TestChildWidget));
state.toggleMe();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(children: const <TableRow>[TableRow(children: <Widget>[TestChildWidget()])]),
),
);
// Should not crash.
expect(find.text('CRASHHH'), findsOneWidget);
},
);
testWidgets('Table widget - Default textBaseline is null', (WidgetTester tester) async {
expect(
() => Table(defaultVerticalAlignment: TableCellVerticalAlignment.baseline),
throwsA(
isAssertionError
.having((AssertionError error) => error.message, 'exception message', contains('baseline')),
),
);
});
testWidgets(
'Table widget requires all TableRows to have same number of children',
(WidgetTester tester) async {
FlutterError? error;
try {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(children: <Widget>[Text('Some Text')]),
TableRow(),
],
),
),
);
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error!.toStringDeep(), contains('Table contains irregular row lengths.'));
}
},
);
testWidgets('Can replace child with a different RenderObject type', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/69395.
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Table(children: const <TableRow>[
TableRow(children: <Widget>[
TestChildWidget(),
TestChildWidget(),
TestChildWidget(),
]),
TableRow(children: <Widget>[
TestChildWidget(),
TestChildWidget(),
TestChildWidget(),
]),
]),
),
);
final RenderTable table = tester.renderObject(find.byType(Table));
expect(find.text('CRASHHH'), findsNothing);
expect(find.byType(SizedBox), findsNWidgets(3 * 2));
final Type toBeReplaced = table.column(2).last.runtimeType;
final TestChildState state = tester.state(find.byType(TestChildWidget).last);
state.toggleMe();
await tester.pump();
expect(find.byType(SizedBox), findsNWidgets(5));
expect(find.text('CRASHHH'), findsOneWidget);
// The RenderObject got replaced by a different type.
expect(table.column(2).last.runtimeType, isNot(toBeReplaced));
});
testWidgets('Do not crash if a child that has not been laid out in a previous build is removed', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/60488.
Widget buildTable(Key key) {
return Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: <TableRow>[
TableRow(
children: <Widget>[
KeyedSubtree(
key: key,
child: const Text('Hello'),
),
],
),
],
),
);
}
await tester.pumpWidget(
buildTable(const ValueKey<int>(1)),
phase: EnginePhase.build, // Children are not laid out!
);
await tester.pumpWidget(
buildTable(const ValueKey<int>(2)),
);
expect(tester.takeException(), isNull);
expect(find.text('Hello'), findsOneWidget);
});
testWidgets('TableRow with no children throws an error message', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/119541.
String result = 'no exception';
// Test TableRow with children.
try {
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(
children: <Widget>[
Text('A'),
],
),
],
),
));
} on FlutterError catch (e) {
result = e.toString();
}
expect(result, 'no exception');
// Test TableRow with no children.
try {
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: Table(
children: const <TableRow>[
TableRow(),
],
),
));
} on FlutterError catch (e) {
result = e.toString();
}
expect(
result,
'One or more TableRow have no children.\n'
'Every TableRow in a Table must have at least one child, so there is no empty row.',
);
});
testWidgets('Set defaultVerticalAlignment to intrinsic height and check their heights', (WidgetTester tester) async {
final Widget table = Directionality(
textDirection: TextDirection.ltr,
child: Table(
defaultVerticalAlignment: TableCellVerticalAlignment.intrinsicHeight,
children: const <TableRow>[
TableRow(
children: <Widget>[
SizedBox(height: 100, child: Text('A')),
SizedBox(height: 200, child: Text('B')),
],
),
TableRow(
children: <Widget>[
SizedBox(height: 200, child: Text('C')),
SizedBox(height: 300, child: Text('D')),
],
),
],
),
);
// load and check if render object was created.
await tester.pumpWidget(table);
expect(find.byWidget(table), findsOneWidget);
final RenderBox boxA = tester.renderObject(find.text('A'));
final RenderBox boxB = tester.renderObject(find.text('B'));
// boxA and boxB must be the same height, even though boxB is higher than boxA initially.
expect(boxA.size.height, equals(boxB.size.height));
final RenderBox boxC = tester.renderObject(find.text('C'));
final RenderBox boxD = tester.renderObject(find.text('D'));
// boxC and boxD must be the same height, even though boxD is higher than boxC initially.
expect(boxC.size.height, equals(boxD.size.height));
// boxD (300.0h) should be higher than boxA (200.0h) which has the same height of boxB.
expect(boxD.size.height, greaterThan(boxA.size.height));
});
// TODO(ianh): Test handling of TableCell object
}
| flutter/packages/flutter/test/widgets/table_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/table_test.dart",
"repo_id": "flutter",
"token_count": 17416
} | 757 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:math' as math;
import 'dart:ui' as ui;
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:vector_math/vector_math_64.dart';
void main() {
testWidgets('Transform origin', (WidgetTester tester) async {
bool didReceiveTap = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
Positioned(
top: 100.0,
left: 100.0,
child: Container(
width: 100.0,
height: 100.0,
color: const Color(0xFF0000FF),
),
),
Positioned(
top: 100.0,
left: 100.0,
child: SizedBox(
width: 100.0,
height: 100.0,
child: Transform(
transform: Matrix4.diagonal3Values(0.5, 0.5, 1.0),
origin: const Offset(100.0, 50.0),
child: GestureDetector(
onTap: () {
didReceiveTap = true;
},
child: Container(
color: const Color(0xFF00FFFF),
),
),
),
),
),
],
),
),
);
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(110.0, 110.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isTrue);
});
testWidgets('Transform alignment', (WidgetTester tester) async {
bool didReceiveTap = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
Positioned(
top: 100.0,
left: 100.0,
child: Container(
width: 100.0,
height: 100.0,
color: const Color(0xFF0000FF),
),
),
Positioned(
top: 100.0,
left: 100.0,
child: SizedBox(
width: 100.0,
height: 100.0,
child: Transform(
transform: Matrix4.diagonal3Values(0.5, 0.5, 1.0),
alignment: Alignment.centerRight,
child: GestureDetector(
onTap: () {
didReceiveTap = true;
},
child: Container(
color: const Color(0xFF00FFFF),
),
),
),
),
),
],
),
),
);
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(110.0, 110.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isTrue);
});
testWidgets('Transform AlignmentDirectional alignment', (WidgetTester tester) async {
bool didReceiveTap = false;
Widget buildFrame(TextDirection textDirection, AlignmentGeometry alignment) {
return Directionality(
textDirection: textDirection,
child: Stack(
children: <Widget>[
Positioned(
top: 100.0,
left: 100.0,
child: Container(
width: 100.0,
height: 100.0,
color: const Color(0xFF0000FF),
),
),
Positioned(
top: 100.0,
left: 100.0,
child: SizedBox(
width: 100.0,
height: 100.0,
child: Transform(
transform: Matrix4.diagonal3Values(0.5, 0.5, 1.0),
alignment: alignment,
child: GestureDetector(
onTap: () {
didReceiveTap = true;
},
child: Container(
color: const Color(0xFF00FFFF),
),
),
),
),
),
],
),
);
}
await tester.pumpWidget(buildFrame(TextDirection.ltr, AlignmentDirectional.centerEnd));
didReceiveTap = false;
await tester.tapAt(const Offset(110.0, 110.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isTrue);
await tester.pumpWidget(buildFrame(TextDirection.rtl, AlignmentDirectional.centerStart));
didReceiveTap = false;
await tester.tapAt(const Offset(110.0, 110.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isTrue);
await tester.pumpWidget(buildFrame(TextDirection.ltr, AlignmentDirectional.centerStart));
didReceiveTap = false;
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(110.0, 150.0));
expect(didReceiveTap, isTrue);
await tester.pumpWidget(buildFrame(TextDirection.rtl, AlignmentDirectional.centerEnd));
didReceiveTap = false;
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(110.0, 150.0));
expect(didReceiveTap, isTrue);
});
testWidgets('Transform offset + alignment', (WidgetTester tester) async {
bool didReceiveTap = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Stack(
children: <Widget>[
Positioned(
top: 100.0,
left: 100.0,
child: Container(
width: 100.0,
height: 100.0,
color: const Color(0xFF0000FF),
),
),
Positioned(
top: 100.0,
left: 100.0,
child: SizedBox(
width: 100.0,
height: 100.0,
child: Transform(
transform: Matrix4.diagonal3Values(0.5, 0.5, 1.0),
origin: const Offset(100.0, 0.0),
alignment: Alignment.centerLeft,
child: GestureDetector(
onTap: () {
didReceiveTap = true;
},
child: Container(
color: const Color(0xFF00FFFF),
),
),
),
),
),
],
),
),
);
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(110.0, 110.0));
expect(didReceiveTap, isFalse);
await tester.tapAt(const Offset(190.0, 150.0));
expect(didReceiveTap, isTrue);
});
testWidgets('Composited transform offset', (WidgetTester tester) async {
await tester.pumpWidget(
Center(
child: SizedBox(
width: 400.0,
height: 300.0,
child: ClipRect(
child: Transform(
transform: Matrix4.diagonal3Values(0.5, 0.5, 1.0),
child: RepaintBoundary(
child: Container(
color: const Color(0xFF00FF00),
),
),
),
),
),
),
);
final List<Layer> layers = tester.layers
..retainWhere((Layer layer) => layer is TransformLayer);
expect(layers.length, 2);
// The first transform is from the render view.
final TransformLayer layer = layers[1] as TransformLayer;
final Matrix4 transform = layer.transform!;
expect(transform.getTranslation(), equals(Vector3(100.0, 75.0, 0.0)));
});
testWidgets('Transform.rotate', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 2.0,
child: RepaintBoundary(child: Container()),
),
);
final List<Layer> layers = tester.layers
..retainWhere((Layer layer) => layer is TransformLayer);
expect(layers.length, 2);
// The first transform is from the render view.
final TransformLayer layer = layers[1] as TransformLayer;
final Matrix4 transform = layer.transform!;
expect(transform.storage, <dynamic>[
moreOrLessEquals(0.0), 1.0, 0.0, 0.0,
-1.0, moreOrLessEquals(0.0), 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
700.0, -100.0, 0.0, 1.0,
]);
});
testWidgets('applyPaintTransform of Transform in Padding', (WidgetTester tester) async {
await tester.pumpWidget(
Padding(
padding: const EdgeInsets.only(
left: 30.0,
top: 20.0,
right: 50.0,
bottom: 70.0,
),
child: Transform(
transform: Matrix4.diagonal3Values(2.0, 2.0, 2.0),
child: const Placeholder(),
),
),
);
expect(tester.getTopLeft(find.byType(Placeholder)), const Offset(30.0, 20.0));
});
testWidgets('Transform.translate', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.translate(
offset: const Offset(100.0, 50.0),
child: RepaintBoundary(child: Container()),
),
);
// This should not cause a transform layer to be inserted.
final List<Layer> layers = tester.layers
..retainWhere((Layer layer) => layer is TransformLayer);
expect(layers.length, 1); // only the render view
expect(tester.getTopLeft(find.byType(Container)), const Offset(100.0, 50.0));
});
testWidgets('Transform.scale', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.scale(
scale: 2.0,
child: RepaintBoundary(child: Container()),
),
);
final List<Layer> layers = tester.layers
..retainWhere((Layer layer) => layer is TransformLayer);
expect(layers.length, 2);
// The first transform is from the render view.
final TransformLayer layer = layers[1] as TransformLayer;
final Matrix4 transform = layer.transform!;
expect(transform.storage, <dynamic>[
// These are column-major, not row-major.
2.0, 0.0, 0.0, 0.0,
0.0, 2.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
-400.0, -300.0, 0.0, 1.0, // it's 1600x1200, centered in an 800x600 square
]);
});
testWidgets('Transform with nan value short-circuits rendering', (WidgetTester tester) async {
await tester.pumpWidget(
Transform(
transform: Matrix4.identity()
..storage[0] = double.nan,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1));
});
testWidgets('Transform with inf value short-circuits rendering', (WidgetTester tester) async {
await tester.pumpWidget(
Transform(
transform: Matrix4.identity()
..storage[0] = double.infinity,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1));
});
testWidgets('Transform with -inf value short-circuits rendering', (WidgetTester tester) async {
await tester.pumpWidget(
Transform(
transform: Matrix4.identity()
..storage[0] = double.negativeInfinity,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1));
});
testWidgets('Transform.rotate does not remove layers due to singular short-circuit', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 2,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(3));
});
testWidgets('Transform.rotate creates nice rotation matrices for 0, 90, 180, 270 degrees', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 2,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers[1], isA<TransformLayer>()
.having((TransformLayer layer) => layer.transform, 'transform', equals(Matrix4.fromList(<double>[
0.0, -1.0, 0.0, 700.0,
1.0, 0.0, 0.0, -100.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
])..transpose()))
);
await tester.pumpWidget(
Transform.rotate(
angle: math.pi,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers[1], isA<TransformLayer>()
.having((TransformLayer layer) => layer.transform, 'transform', equals(Matrix4.fromList(<double>[
-1.0, 0.0, 0.0, 800.0,
0.0, -1.0, 0.0, 600.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
])..transpose()))
);
await tester.pumpWidget(
Transform.rotate(
angle: 3 * math.pi / 2,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers[1], isA<TransformLayer>()
.having((TransformLayer layer) => layer.transform, 'transform', equals(Matrix4.fromList(<double>[
0.0, 1.0, 0.0, 100.0,
-1.0, 0.0, 0.0, 700.0,
0.0, 0.0, 1.0, 0.0,
0.0, 0.0, 0.0, 1.0,
])..transpose()))
);
await tester.pumpWidget(
Transform.rotate(
angle: 0,
child: RepaintBoundary(child: Container()),
),
);
// No transform layer created
expect(tester.layers[1], isA<OffsetLayer>());
expect(tester.layers, hasLength(2));
});
testWidgets('Transform.scale with 0.0 does not paint child layers', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.scale(
scale: 0.0,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1)); // root transform layer
await tester.pumpWidget(
Transform.scale(
scaleX: 0.0,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1));
await tester.pumpWidget(
Transform.scale(
scaleY: 0.0,
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(1));
await tester.pumpWidget(
Transform.scale(
scale: 0.01, // small but non-zero
child: RepaintBoundary(child: Container()),
),
);
expect(tester.layers, hasLength(3));
});
testWidgets('Translated child into translated box - hit test', (WidgetTester tester) async {
final GlobalKey key1 = GlobalKey();
bool pointerDown = false;
await tester.pumpWidget(
Transform.translate(
offset: const Offset(100.0, 50.0),
child: Transform.translate(
offset: const Offset(1000.0, 1000.0),
child: Listener(
onPointerDown: (PointerDownEvent event) {
pointerDown = true;
},
child: Container(
key: key1,
color: const Color(0xFF000000),
),
),
),
),
);
expect(pointerDown, isFalse);
await tester.tap(find.byKey(key1));
expect(pointerDown, isTrue);
});
Widget generateTransform(bool needsCompositing, double angle) {
final Widget customPaint = CustomPaint(painter: TestRectPainter());
return Transform(
transform: MatrixUtils.createCylindricalProjectionTransform(
radius: 100,
angle: angle,
perspective: 0.003,
),
// A RepaintBoundary child forces the Transform to needsCompositing
child: needsCompositing ? RepaintBoundary(child: customPaint) : customPaint,
);
}
testWidgets(
'3D transform renders the same with or without needsCompositing',
(WidgetTester tester) async {
for (double angle = 0; angle <= math.pi/4; angle += 0.01) {
await tester.pumpWidget(RepaintBoundary(child: generateTransform(true, angle)));
final RenderBox renderBox = tester.binding.renderView.child!;
final OffsetLayer layer = renderBox.debugLayer! as OffsetLayer;
final ui.Image imageWithCompositing = await layer.toImage(renderBox.paintBounds);
addTearDown(imageWithCompositing.dispose);
await tester.pumpWidget(RepaintBoundary(child: generateTransform(false, angle)));
await expectLater(find.byType(RepaintBoundary).first, matchesReferenceImage(imageWithCompositing));
}
},
skip: isBrowser, // due to https://github.com/flutter/flutter/issues/49857
);
List<double> extractMatrix(ui.ImageFilter? filter) {
final List<String> numbers = filter.toString().split('[').last.split(']').first.split(',');
return numbers.map<double>((String str) => double.parse(str.trim())).toList();
}
testWidgets('Transform.translate with FilterQuality produces filter layer', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.translate(
offset: const Offset(25.0, 25.0),
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>().length, 1);
final ImageFilterLayer layer = tester.layers.whereType<ImageFilterLayer>().first;
expect(extractMatrix(layer.imageFilter), <double>[
1.0, 0.0, 0.0, 0.0,
0.0, 1.0, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
25.0, 25.0, 0.0, 1.0,
]);
});
testWidgets('Transform.scale with FilterQuality produces filter layer', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.scale(
scale: 3.14159,
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>().length, 1);
final ImageFilterLayer layer = tester.layers.whereType<ImageFilterLayer>().first;
expect(extractMatrix(layer.imageFilter), <double>[
3.14159, 0.0, 0.0, 0.0,
0.0, 3.14159, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
-856.636, -642.477, 0.0, 1.0,
]);
});
testWidgets('Transform.rotate with FilterQuality produces filter layer', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 4,
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>().length, 1);
final ImageFilterLayer layer = tester.layers.whereType<ImageFilterLayer>().first;
expect(extractMatrix(layer.imageFilter), <dynamic>[
moreOrLessEquals(0.7071067811865476), moreOrLessEquals(0.7071067811865475), 0.0, 0.0,
moreOrLessEquals(-0.7071067811865475), moreOrLessEquals(0.7071067811865476), 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
moreOrLessEquals(329.28932188134524), moreOrLessEquals(-194.97474683058329), 0.0, 1.0,
]);
});
testWidgets('Offset Transform.rotate with FilterQuality produces filter layer', (WidgetTester tester) async {
await tester.pumpWidget(
SizedBox(width: 400, height: 400,
child: Center(
child: Transform.rotate(
angle: math.pi / 4,
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
),
),
);
expect(tester.layers.whereType<ImageFilterLayer>().length, 1);
final ImageFilterLayer layer = tester.layers.whereType<ImageFilterLayer>().first;
expect(extractMatrix(layer.imageFilter), <dynamic>[
moreOrLessEquals(0.7071067811865476), moreOrLessEquals(0.7071067811865475), 0.0, 0.0,
moreOrLessEquals(-0.7071067811865475), moreOrLessEquals(0.7071067811865476), 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
moreOrLessEquals(329.28932188134524), moreOrLessEquals(-194.97474683058329), 0.0, 1.0,
]);
});
testWidgets('Transform layers update to match child and filterQuality', (WidgetTester tester) async {
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 4,
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>(), hasLength(1));
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 4,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>(), isEmpty);
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 4,
filterQuality: FilterQuality.low,
),
);
expect(tester.layers.whereType<ImageFilterLayer>(), isEmpty);
await tester.pumpWidget(
Transform.rotate(
angle: math.pi / 4,
filterQuality: FilterQuality.low,
child: const SizedBox(width: 100, height: 100),
),
);
expect(tester.layers.whereType<ImageFilterLayer>(), hasLength(1));
});
testWidgets('Transform layers with filterQuality golden', (WidgetTester tester) async {
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: GridView.count(
crossAxisCount: 3,
children: <Widget>[
Transform.rotate(
angle: math.pi / 6,
child: Center(child: Container(width: 100, height: 20, color: const Color(0xffffff00))),
),
Transform.scale(
scale: 1.5,
child: Center(child: Container(width: 100, height: 20, color: const Color(0xffffff00))),
),
Transform.translate(
offset: const Offset(20.0, 60.0),
child: Center(child: Container(width: 100, height: 20, color: const Color(0xffffff00))),
),
Transform.rotate(
angle: math.pi / 6,
filterQuality: FilterQuality.low,
child: Center(child: Container(width: 100, height: 20, color: const Color(0xff00ff00))),
),
Transform.scale(
scale: 1.5,
filterQuality: FilterQuality.low,
child: Center(child: Container(width: 100, height: 20, color: const Color(0xff00ff00))),
),
Transform.translate(
offset: const Offset(20.0, 60.0),
filterQuality: FilterQuality.low,
child: Center(child: Container(width: 100, height: 20, color: const Color(0xff00ff00))),
),
],
),
),
);
await expectLater(
find.byType(GridView),
matchesGoldenFile('transform_golden.BitmapRotate.png'),
);
});
testWidgets("Transform.scale() does not accept all three 'scale', 'scaleX' and 'scaleY' parameters to be non-null", (WidgetTester tester) async {
await expectLater(() {
tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Transform.scale(
scale: 1.0,
scaleX: 1.0,
scaleY: 1.0,
child: const SizedBox(
height: 100,
width: 100,
),
),
)));
}, throwsAssertionError);
});
testWidgets("Transform.scale() needs at least one of 'scale', 'scaleX' and 'scaleY' to be non-null, otherwise throws AssertionError", (WidgetTester tester) async {
await expectLater(() {
tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Transform.scale(
child: const SizedBox(
height: 100,
width: 100,
),
),
)));
}, throwsAssertionError);
});
testWidgets("Transform.scale() scales widget uniformly with 'scale' parameter", (WidgetTester tester) async {
const double scale = 1.5;
const double height = 100;
const double width = 150;
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: SizedBox(
height: 400,
width: 400,
child: Center(
child: Transform.scale(
scale: scale,
child: Container(
height: height,
width: width,
decoration: const BoxDecoration(),
),
),
),
)));
const Size target = Size(width * scale, height * scale);
expect(tester.getBottomRight(find.byType(Container)), target.bottomRight(tester.getTopLeft(find.byType(Container))));
});
testWidgets("Transform.scale() scales widget according to 'scaleX' and 'scaleY'", (WidgetTester tester) async {
const double scaleX = 1.5;
const double scaleY = 1.2;
const double height = 100;
const double width = 150;
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: SizedBox(
height: 400,
width: 400,
child: Center(
child: Transform.scale(
scaleX: scaleX,
scaleY: scaleY,
child: Container(
height: height,
width: width,
decoration: const BoxDecoration(),
),
),
),
)));
const Size target = Size(width * scaleX, height * scaleY);
expect(tester.getBottomRight(find.byType(Container)), target.bottomRight(tester.getTopLeft(find.byType(Container))));
});
testWidgets(
'Transform.flip does flip child correctly',
(WidgetTester tester) async {
const Offset topRight = Offset(60, 20);
const Offset bottomLeft = Offset(20, 60);
const Offset bottomRight = Offset(60, 60);
bool tappedRed = false;
const Widget square = SizedBox.square(dimension: 40);
final Widget child = Column(
mainAxisSize: MainAxisSize.min,
children: <Widget> [
Row(mainAxisSize: MainAxisSize.min, children: <Widget>[
GestureDetector(
onTap: () => tappedRed = true,
child: const ColoredBox(color: Color(0xffff0000), child: square),
),
const ColoredBox(color: Color(0xff00ff00), child: square),
]),
const Row(mainAxisSize: MainAxisSize.min, children: <Widget>[
ColoredBox(color: Color(0xff0000ff), child: square),
ColoredBox(color: Color(0xffeeff00), child: square),
]),
],
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: Transform.flip(
flipX: true,
child: child,
),
),
),
);
await tester.pumpAndSettle();
await tester.tapAt(topRight);
expect(tappedRed, isTrue, reason: 'Transform.flip cannot flipX');
tappedRed = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: Transform.flip(
flipY: true,
child: child,
),
),
),
);
await tester.pumpAndSettle();
await tester.tapAt(bottomLeft);
expect(tappedRed, isTrue, reason: 'Transform.flip cannot flipY');
tappedRed = false;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: Transform.flip(
flipX: true,
flipY: true,
child: child,
),
),
),
);
await tester.pumpAndSettle();
await tester.tapAt(bottomRight);
expect(
tappedRed,
isTrue,
reason: 'Transform.flip cannot flipX and flipY together',
);
},
);
}
class TestRectPainter extends CustomPainter {
@override
void paint(ui.Canvas canvas, ui.Size size) {
canvas.drawRect(
const Offset(200, 200) & const Size(10, 10),
Paint()..color = const Color(0xFFFF0000),
);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
| flutter/packages/flutter/test/widgets/transform_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/transform_test.dart",
"repo_id": "flutter",
"token_count": 13498
} | 758 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('WidgetSpan codeUnitAt', () {
const InlineSpan span = WidgetSpan(child: SizedBox());
expect(span.codeUnitAt(-1), isNull);
expect(span.codeUnitAt(0), PlaceholderSpan.placeholderCodeUnit);
expect(span.codeUnitAt(1), isNull);
expect(span.codeUnitAt(2), isNull);
const InlineSpan nestedSpan = TextSpan(
text: 'AAA',
children: <InlineSpan>[span, span],
);
expect(nestedSpan.codeUnitAt(-1), isNull);
expect(nestedSpan.codeUnitAt(0), 65);
expect(nestedSpan.codeUnitAt(1), 65);
expect(nestedSpan.codeUnitAt(2), 65);
expect(nestedSpan.codeUnitAt(3), PlaceholderSpan.placeholderCodeUnit);
expect(nestedSpan.codeUnitAt(4), PlaceholderSpan.placeholderCodeUnit);
expect(nestedSpan.codeUnitAt(5), isNull);
});
test('WidgetSpan.extractFromInlineSpan applies the correct scaling factor', () {
const WidgetSpan a = WidgetSpan(child: SizedBox(), style: TextStyle(fontSize: 0));
const WidgetSpan b = WidgetSpan(child: SizedBox(), style: TextStyle(fontSize: 10));
const WidgetSpan c = WidgetSpan(child: SizedBox());
const WidgetSpan d = WidgetSpan(child: SizedBox(), style: TextStyle(letterSpacing: 999));
const TextSpan span = TextSpan(
children: <InlineSpan>[
a, // fontSize = 0.
TextSpan(
children: <InlineSpan>[
b, // fontSize = 10.
c, // fontSize = 20.
],
style: TextStyle(fontSize: 20),
),
d, // fontSize = 14.
]
);
double effectiveTextScaleFactorFromWidget(Widget widget) {
final Semantics child = (widget as ProxyWidget).child as Semantics;
final dynamic grandChild = child.child;
final double textScaleFactor = grandChild.textScaleFactor as double; // ignore: avoid_dynamic_calls
return textScaleFactor;
}
final List<double> textScaleFactors = WidgetSpan.extractFromInlineSpan(span, const _QuadraticScaler())
.map(effectiveTextScaleFactorFromWidget).toList();
expect(textScaleFactors, <double>[
0, // a
10, // b
20, // c
14, // d
]);
});
}
class _QuadraticScaler extends TextScaler {
const _QuadraticScaler();
@override
double scale(double fontSize) => fontSize * fontSize;
@override
double get textScaleFactor => throw UnimplementedError();
}
| flutter/packages/flutter/test/widgets/widget_span_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/widget_span_test.dart",
"repo_id": "flutter",
"token_count": 1020
} | 759 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
// Changes made in https://github.com/flutter/flutter/pull/86198
AppBarTheme appBarTheme = AppBarTheme();
appBarTheme = AppBarTheme(systemOverlayStyle: SystemUiOverlayStyle.dark);
appBarTheme = AppBarTheme(systemOverlayStyle: SystemUiOverlayStyle.light);
appBarTheme = AppBarTheme(error: '');
appBarTheme = appBarTheme.copyWith(error: '');
appBarTheme = appBarTheme.copyWith(systemOverlayStyle: SystemUiOverlayStyle.dark);
appBarTheme = appBarTheme.copyWith(systemOverlayStyle: SystemUiOverlayStyle.light);
appBarTheme.systemOverlayStyle;
TextTheme myTextTheme = TextTheme();
AppBarTheme appBarTheme = AppBarTheme();
appBarTheme = AppBarTheme(toolbarTextStyle: myTextTheme.bodyMedium, titleTextStyle: myTextTheme.titleLarge);
appBarTheme = AppBarTheme(toolbarTextStyle: myTextTheme.bodyMedium, titleTextStyle: myTextTheme.titleLarge);
appBarTheme = appBarTheme.copyWith(toolbarTextStyle: myTextTheme.bodyMedium, titleTextStyle: myTextTheme.titleLarge);
appBarTheme = appBarTheme.copyWith(toolbarTextStyle: myTextTheme.bodyMedium, titleTextStyle: myTextTheme.titleLarge);
AppBarTheme appBarTheme = AppBarTheme();
appBarTheme = AppBarTheme();
appBarTheme = AppBarTheme();
appBarTheme = appBarTheme.copyWith();
appBarTheme = appBarTheme.copyWith();
appBarTheme.backwardsCompatibility; // Removing field reference not supported.
AppBarTheme appBarTheme = AppBarTheme();
appBarTheme.backgroundColor;
}
| flutter/packages/flutter/test_fixes/material/app_bar_theme.dart.expect/0 | {
"file_path": "flutter/packages/flutter/test_fixes/material/app_bar_theme.dart.expect",
"repo_id": "flutter",
"token_count": 517
} | 760 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
void main() {
// Change made in https://github.com/flutter/flutter/pull/121152
final EdgeInsets insets = EdgeInsets.fromViewPadding(ViewPadding.zero, 3.0);
// Change made in https://github.com/flutter/flutter/pull/128522
const TextStyle textStyle = TextStyle()
..getTextStyle(textScaler: TextScaler.linear(math.min(_kTextScaleFactor, 1.0)))
..getTextStyle();
TextPainter(text: inlineSpan);
TextPainter(textScaler: TextScaler.linear(someValue));
TextPainter.computeWidth(textScaler: TextScaler.linear(textScaleFactor));
TextPainter.computeMaxIntrinsicWidth(textScaler: TextScaler.linear(textScaleFactor));
}
| flutter/packages/flutter/test_fixes/painting/painting.dart.expect/0 | {
"file_path": "flutter/packages/flutter/test_fixes/painting/painting.dart.expect",
"repo_id": "flutter",
"token_count": 274
} | 761 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
void main() {
// Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
ListWheelScrollView listWheelScrollView = ListWheelScrollView();
listWheelScrollView = ListWheelScrollView(clipBehavior: Clip.hardEdge);
listWheelScrollView = ListWheelScrollView(clipBehavior: Clip.none);
listWheelScrollView = ListWheelScrollView(error: '');
listWheelScrollView = ListWheelScrollView.useDelegate();
listWheelScrollView = ListWheelScrollView.useDelegate(clipBehavior: Clip.hardEdge);
listWheelScrollView = ListWheelScrollView.useDelegate(clipBehavior: Clip.none);
listWheelScrollView = ListWheelScrollView.useDelegate(error: '');
listWheelScrollView.clipBehavior;
}
| flutter/packages/flutter/test_fixes/widgets/list_wheel_scroll_view.dart.expect/0 | {
"file_path": "flutter/packages/flutter/test_fixes/widgets/list_wheel_scroll_view.dart.expect",
"repo_id": "flutter",
"token_count": 257
} | 762 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' show FileSystemException, stderr;
/// Standard error thrown by Flutter Driver API.
class DriverError extends Error {
/// Create an error with a [message] and (optionally) the [originalError] and
/// [originalStackTrace] that caused it.
DriverError(this.message, [this.originalError, this.originalStackTrace]);
/// Human-readable error message.
final String message;
/// The error object that was caught and wrapped by this error object, if any.
final Object? originalError;
/// The stack trace that was caught and wrapped by this error object, if any.
final Object? originalStackTrace;
@override
String toString() {
if (originalError == null) {
return 'DriverError: $message\n';
}
return '''
DriverError: $message
Original error: $originalError
Original stack trace:
$originalStackTrace
''';
}
}
/// Signature for [driverLog].
///
/// The first argument is a string representing the source of the message,
/// typically the class name or library name calling the method.
///
/// The second argument is the message being logged.
typedef DriverLogCallback = void Function(String source, String message);
/// Print the given message to the console.
///
/// The first argument is a string representing the source of the message.
///
/// The second argument is the message being logged.
///
/// This can be set to a different callback to override the handling of log
/// messages from the driver subsystem.
///
/// The default implementation prints `"$source: $message"` to stderr.
DriverLogCallback driverLog = _defaultDriverLogger;
void _defaultDriverLogger(String source, String message) {
try {
stderr.writeln('$source: $message');
} on FileSystemException {
// May encounter IO error: https://github.com/flutter/flutter/issues/69314
}
}
| flutter/packages/flutter_driver/lib/src/common/error.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/common/error.dart",
"repo_id": "flutter",
"token_count": 537
} | 763 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' show Platform;
import 'package:file/file.dart';
import 'package:file/local.dart';
import 'package:file/memory.dart';
/// The file system implementation used by this library.
///
/// See [useMemoryFileSystemForTesting] and [restoreFileSystem].
FileSystem fs = const LocalFileSystem();
/// Overrides the file system so it can be tested without hitting the hard
/// drive.
void useMemoryFileSystemForTesting() {
fs = MemoryFileSystem();
}
/// Restores the file system to the default local file system implementation.
void restoreFileSystem() {
fs = const LocalFileSystem();
}
/// Flutter Driver test output directory.
///
/// Tests should write any output files to this directory. Defaults to the path
/// set in the FLUTTER_TEST_OUTPUTS_DIR environment variable, or `build` if
/// unset.
String get testOutputsDirectory => Platform.environment['FLUTTER_TEST_OUTPUTS_DIR'] ?? 'build';
| flutter/packages/flutter_driver/lib/src/driver/common.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/driver/common.dart",
"repo_id": "flutter",
"token_count": 298
} | 764 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The dart:io implementation of [registerWebServiceExtension].
///
/// See also:
///
/// * [_extension_web.dart], which has the dart:html implementation
void registerWebServiceExtension(Future<Map<String, dynamic>> Function(Map<String, String>) call) {
throw UnsupportedError('Use registerServiceExtension instead');
}
| flutter/packages/flutter_driver/lib/src/extension/_extension_io.dart/0 | {
"file_path": "flutter/packages/flutter_driver/lib/src/extension/_extension_io.dart",
"repo_id": "flutter",
"token_count": 130
} | 765 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_driver/driver_extension.dart';
import 'package:flutter_driver/src/common/find.dart';
import 'package:flutter_test/flutter_test.dart';
import 'stub_finder.dart';
class StubFinderExtension extends FinderExtension {
@override
Finder createFinder(
SerializableFinder finder,
CreateFinderFactory finderFactory,
) {
return find.byWidgetPredicate((Widget widget) {
final Key? key = widget.key;
if (key is! ValueKey<String>) {
return false;
}
return key.value == (finder as StubFinder).keyString;
});
}
@override
SerializableFinder deserialize(
Map<String, String> params,
DeserializeFinderFactory finderFactory,
) {
return StubFinder(params['keyString']!);
}
@override
String get finderType => 'Stub';
}
| flutter/packages/flutter_driver/test/src/real_tests/stubs/stub_finder_extension.dart/0 | {
"file_path": "flutter/packages/flutter_driver/test/src/real_tests/stubs/stub_finder_extension.dart",
"repo_id": "flutter",
"token_count": 353
} | 766 |
{
"datePickerHourSemanticsLabelFew": "$hour гадзіны нуль хвілін",
"datePickerHourSemanticsLabelMany": "$hour гадзін нуль хвілін",
"datePickerMinuteSemanticsLabelFew": "$minute хвіліны",
"datePickerMinuteSemanticsLabelMany": "$minute хвілін",
"timerPickerHourLabelFew": "гадзіны",
"timerPickerHourLabelMany": "гадзін",
"timerPickerMinuteLabelFew": "хв",
"timerPickerMinuteLabelMany": "хв",
"timerPickerSecondLabelFew": "с",
"timerPickerSecondLabelMany": "с",
"datePickerHourSemanticsLabelOne": "$hour гадзіна нуль хвілін",
"datePickerHourSemanticsLabelOther": "$hour гадзіны нуль хвілін",
"datePickerMinuteSemanticsLabelOne": "1 хвіліна",
"datePickerMinuteSemanticsLabelOther": "$minute хвіліны",
"datePickerDateOrder": "mdy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "раніцы",
"postMeridiemAbbreviation": "вечара",
"todayLabel": "Сёння",
"alertDialogLabel": "Абвестка",
"timerPickerHourLabelOne": "гадзіна",
"timerPickerHourLabelOther": "гадзіны",
"timerPickerMinuteLabelOne": "хв",
"timerPickerMinuteLabelOther": "хв",
"timerPickerSecondLabelOne": "с",
"timerPickerSecondLabelOther": "с",
"cutButtonLabel": "Выразаць",
"copyButtonLabel": "Капіраваць",
"pasteButtonLabel": "Уставіць",
"clearButtonLabel": "Clear",
"selectAllButtonLabel": "Выбраць усе",
"tabSemanticsLabel": "Укладка $tabIndex з $tabCount",
"modalBarrierDismissLabel": "Адхіліць",
"searchTextFieldPlaceholderLabel": "Пошук",
"noSpellCheckReplacementsLabel": "Замен не знойдзена",
"menuDismissLabel": "Закрыць меню",
"lookUpButtonLabel": "Знайсці",
"searchWebButtonLabel": "Пошук у сетцы",
"shareButtonLabel": "Абагуліць..."
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_be.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_be.arb",
"repo_id": "flutter",
"token_count": 881
} | 767 |
{
"datePickerHourSemanticsLabelOne": "$hour en punto",
"datePickerHourSemanticsLabelOther": "$hour en punto",
"datePickerMinuteSemanticsLabelOne": "1 minuto",
"datePickerMinuteSemanticsLabelOther": "$minute minutos",
"datePickerDateOrder": "dmy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "a.m.",
"postMeridiemAbbreviation": "p.m.",
"todayLabel": "Hoxe",
"alertDialogLabel": "Alerta",
"timerPickerHourLabelOne": "hora",
"timerPickerHourLabelOther": "horas",
"timerPickerMinuteLabelOne": "min",
"timerPickerMinuteLabelOther": "min",
"timerPickerSecondLabelOne": "s",
"timerPickerSecondLabelOther": "s",
"cutButtonLabel": "Cortar",
"copyButtonLabel": "Copiar",
"pasteButtonLabel": "Pegar",
"selectAllButtonLabel": "Seleccionar todo",
"tabSemanticsLabel": "Pestana $tabIndex de $tabCount",
"modalBarrierDismissLabel": "Ignorar",
"searchTextFieldPlaceholderLabel": "Fai unha busca",
"noSpellCheckReplacementsLabel": "Non se encontrou ningunha substitución",
"menuDismissLabel": "Pechar menú",
"lookUpButtonLabel": "Mirar cara arriba",
"searchWebButtonLabel": "Buscar na Web",
"shareButtonLabel": "Compartir…",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_gl.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_gl.arb",
"repo_id": "flutter",
"token_count": 448
} | 768 |
{
"datePickerHourSemanticsLabelOne": "$hour시 정각",
"datePickerHourSemanticsLabelOther": "$hour시 정각",
"datePickerMinuteSemanticsLabelOne": "1분",
"datePickerMinuteSemanticsLabelOther": "$minute분",
"datePickerDateOrder": "ymd",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "오전",
"postMeridiemAbbreviation": "오후",
"todayLabel": "오늘",
"alertDialogLabel": "알림",
"timerPickerHourLabelOne": "시간",
"timerPickerHourLabelOther": "시간",
"timerPickerMinuteLabelOne": "분",
"timerPickerMinuteLabelOther": "분",
"timerPickerSecondLabelOne": "초",
"timerPickerSecondLabelOther": "초",
"cutButtonLabel": "잘라냄",
"copyButtonLabel": "복사",
"pasteButtonLabel": "붙여넣기",
"selectAllButtonLabel": "전체 선택",
"tabSemanticsLabel": "탭 $tabCount개 중 $tabIndex번째",
"modalBarrierDismissLabel": "닫기",
"searchTextFieldPlaceholderLabel": "검색",
"noSpellCheckReplacementsLabel": "수정사항 없음",
"menuDismissLabel": "메뉴 닫기",
"lookUpButtonLabel": "찾기",
"searchWebButtonLabel": "웹 검색",
"shareButtonLabel": "공유...",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_ko.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_ko.arb",
"repo_id": "flutter",
"token_count": 542
} | 769 |
{
"datePickerHourSemanticsLabelOne": "$hour ਵਜੇ",
"datePickerHourSemanticsLabelOther": "$hour ਵਜੇ",
"datePickerMinuteSemanticsLabelOne": "1 ਮਿੰਟ",
"datePickerMinuteSemanticsLabelOther": "$minute ਮਿੰਟ",
"datePickerDateOrder": "dmy",
"datePickerDateTimeOrder": "date_time_dayPeriod",
"anteMeridiemAbbreviation": "AM",
"postMeridiemAbbreviation": "PM",
"todayLabel": "ਅੱਜ",
"alertDialogLabel": "ਸੁਚੇਤਨਾ",
"timerPickerHourLabelOne": "ਘੰਟਾ",
"timerPickerHourLabelOther": "ਘੰਟੇ",
"timerPickerMinuteLabelOne": "ਮਿੰ.",
"timerPickerMinuteLabelOther": "ਮਿੰ.",
"timerPickerSecondLabelOne": "ਸਕਿੰ.",
"timerPickerSecondLabelOther": "ਸਕਿੰ.",
"cutButtonLabel": "ਕੱਟ ਕਰੋ",
"copyButtonLabel": "ਕਾਪੀ ਕਰੋ",
"pasteButtonLabel": "ਪੇਸਟ ਕਰੋ",
"selectAllButtonLabel": "ਸਭ ਚੁਣੋ",
"tabSemanticsLabel": "$tabCount ਵਿੱਚੋਂ $tabIndex ਟੈਬ",
"modalBarrierDismissLabel": "ਖਾਰਜ ਕਰੋ",
"searchTextFieldPlaceholderLabel": "ਖੋਜੋ",
"noSpellCheckReplacementsLabel": "ਕੋਈ ਸੁਝਾਅ ਨਹੀਂ ਮਿਲਿਆ",
"menuDismissLabel": "ਮੀਨੂ ਖਾਰਜ ਕਰੋ",
"lookUpButtonLabel": "ਖੋਜੋ",
"searchWebButtonLabel": "ਵੈੱਬ 'ਤੇ ਖੋਜੋ",
"shareButtonLabel": "ਸਾਂਝਾ ਕਰੋ...",
"clearButtonLabel": "Clear"
}
| flutter/packages/flutter_localizations/lib/src/l10n/cupertino_pa.arb/0 | {
"file_path": "flutter/packages/flutter_localizations/lib/src/l10n/cupertino_pa.arb",
"repo_id": "flutter",
"token_count": 643
} | 770 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.