text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [WidgetsApp].
void main() => runApp(const WidgetsAppExampleApp());
class WidgetsAppExampleApp extends StatelessWidget {
const WidgetsAppExampleApp({super.key});
@override
Widget build(BuildContext context) {
return WidgetsApp(
title: 'Example',
color: const Color(0xFF000000),
home: const Center(child: Text('Hello World')),
pageRouteBuilder: <T>(RouteSettings settings, WidgetBuilder builder) => PageRouteBuilder<T>(
settings: settings,
pageBuilder: (BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) =>
builder(context),
),
);
}
}
| flutter/examples/api/lib/widgets/app/widgets_app.widgets_app.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/app/widgets_app.widgets_app.0.dart",
"repo_id": "flutter",
"token_count": 286
} | 571 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [CustomMultiChildLayout].
void main() => runApp(const CustomMultiChildLayoutApp());
class CustomMultiChildLayoutApp extends StatelessWidget {
const CustomMultiChildLayoutApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: Directionality(
// TRY THIS: Try changing the direction here and hot-reloading to
// see the layout change.
textDirection: TextDirection.ltr,
child: Scaffold(
body: CustomMultiChildLayoutExample(),
),
),
);
}
}
/// Lays out the children in a cascade, where the top corner of the next child
/// is a little above (`overlap`) the lower end corner of the previous child.
///
/// Will relayout if the text direction changes.
class _CascadeLayoutDelegate extends MultiChildLayoutDelegate {
_CascadeLayoutDelegate({
required this.colors,
required this.overlap,
required this.textDirection,
});
final Map<String, Color> colors;
final double overlap;
final TextDirection textDirection;
// Perform layout will be called when re-layout is needed.
@override
void performLayout(Size size) {
final double columnWidth = size.width / colors.length;
Offset childPosition = Offset.zero;
switch (textDirection) {
case TextDirection.rtl:
childPosition += Offset(size.width, 0);
case TextDirection.ltr:
break;
}
for (final String color in colors.keys) {
// layoutChild must be called exactly once for each child.
final Size currentSize = layoutChild(
color,
BoxConstraints(maxHeight: size.height, maxWidth: columnWidth),
);
// positionChild must be called to change the position of a child from
// what it was in the previous layout. Each child starts at (0, 0) for the
// first layout.
switch (textDirection) {
case TextDirection.rtl:
positionChild(color, childPosition - Offset(currentSize.width, 0));
childPosition += Offset(-currentSize.width, currentSize.height - overlap);
case TextDirection.ltr:
positionChild(color, childPosition);
childPosition += Offset(currentSize.width, currentSize.height - overlap);
}
}
}
// shouldRelayout is called to see if the delegate has changed and requires a
// layout to occur. Should only return true if the delegate state itself
// changes: changes in the CustomMultiChildLayout attributes will
// automatically cause a relayout, like any other widget.
@override
bool shouldRelayout(_CascadeLayoutDelegate oldDelegate) {
return oldDelegate.textDirection != textDirection || oldDelegate.overlap != overlap;
}
}
class CustomMultiChildLayoutExample extends StatelessWidget {
const CustomMultiChildLayoutExample({super.key});
static const Map<String, Color> _colors = <String, Color>{
'Red': Colors.red,
'Green': Colors.green,
'Blue': Colors.blue,
'Cyan': Colors.cyan,
};
@override
Widget build(BuildContext context) {
return CustomMultiChildLayout(
delegate: _CascadeLayoutDelegate(
colors: _colors,
overlap: 30.0,
textDirection: Directionality.of(context),
),
children: <Widget>[
// Create all of the colored boxes in the colors map.
for (final MapEntry<String, Color> entry in _colors.entries)
// The "id" can be any Object, not just a String.
LayoutId(
id: entry.key,
child: Container(
color: entry.value,
width: 100.0,
height: 100.0,
alignment: Alignment.center,
child: Text(entry.key),
),
),
],
);
}
}
| flutter/examples/api/lib/widgets/basic/custom_multi_child_layout.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/custom_multi_child_layout.0.dart",
"repo_id": "flutter",
"token_count": 1425
} | 572 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ColorFiltered].
void main() => runApp(const ColorFilteredExampleApp());
class ColorFilteredExampleApp extends StatelessWidget {
const ColorFilteredExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ColorFiltered Sample')),
body: const ColorFilteredExample(),
),
);
}
}
class ColorFilteredExample extends StatelessWidget {
const ColorFilteredExample({super.key});
@override
Widget build(BuildContext context) {
return SingleChildScrollView(
child: Column(
children: <Widget>[
ColorFiltered(
colorFilter: const ColorFilter.mode(
Colors.red,
BlendMode.modulate,
),
child: Image.network(
'https://flutter.github.io/assets-for-api-docs/assets/widgets/owl-2.jpg',
),
),
ColorFiltered(
colorFilter: const ColorFilter.mode(
Colors.grey,
BlendMode.saturation,
),
child: Image.network(
'https://flutter.github.io/assets-for-api-docs/assets/widgets/owl.jpg',
),
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/color_filter/color_filtered.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/color_filter/color_filtered.0.dart",
"repo_id": "flutter",
"token_count": 643
} | 573 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Form].
void main() => runApp(const FormExampleApp());
class FormExampleApp extends StatelessWidget {
const FormExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Form Sample')),
body: const FormExample(),
),
);
}
}
class FormExample extends StatefulWidget {
const FormExample({super.key});
@override
State<FormExample> createState() => _FormExampleState();
}
class _FormExampleState extends State<FormExample> {
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
TextFormField(
decoration: const InputDecoration(
hintText: 'Enter your email',
),
validator: (String? value) {
if (value == null || value.isEmpty) {
return 'Please enter some text';
}
return null;
},
),
Padding(
padding: const EdgeInsets.symmetric(vertical: 16.0),
child: ElevatedButton(
onPressed: () {
// Validate will return true if the form is valid, or false if
// the form is invalid.
if (_formKey.currentState!.validate()) {
// Process data.
}
},
child: const Text('Submit'),
),
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/form/form.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/form/form.0.dart",
"repo_id": "flutter",
"token_count": 830
} | 574 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AnimatedPadding].
void main() => runApp(const AnimatedPaddingExampleApp());
class AnimatedPaddingExampleApp extends StatelessWidget {
const AnimatedPaddingExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('AnimatedPadding Sample')),
body: const AnimatedPaddingExample(),
),
);
}
}
class AnimatedPaddingExample extends StatefulWidget {
const AnimatedPaddingExample({super.key});
@override
State<AnimatedPaddingExample> createState() => _AnimatedPaddingExampleState();
}
class _AnimatedPaddingExampleState extends State<AnimatedPaddingExample> {
double padValue = 0.0;
void _updatePadding(double value) {
setState(() {
padValue = value;
});
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
AnimatedPadding(
padding: EdgeInsets.all(padValue),
duration: const Duration(seconds: 2),
curve: Curves.easeInOut,
child: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height / 5,
color: Colors.blue,
),
),
Text('Padding: $padValue'),
ElevatedButton(
child: const Text('Change padding'),
onPressed: () {
_updatePadding(padValue == 0.0 ? 100.0 : 0.0);
}),
],
);
}
}
| flutter/examples/api/lib/widgets/implicit_animations/animated_padding.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/implicit_animations/animated_padding.0.dart",
"repo_id": "flutter",
"token_count": 691
} | 575 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Navigator.restorablePushAndRemoveUntil].
void main() => runApp(const RestorablePushAndRemoveUntilExampleApp());
class RestorablePushAndRemoveUntilExampleApp extends StatelessWidget {
const RestorablePushAndRemoveUntilExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: RestorablePushAndRemoveUntilExample(),
);
}
}
class RestorablePushAndRemoveUntilExample extends StatefulWidget {
const RestorablePushAndRemoveUntilExample({super.key});
@override
State<RestorablePushAndRemoveUntilExample> createState() => _RestorablePushAndRemoveUntilExampleState();
}
class _RestorablePushAndRemoveUntilExampleState extends State<RestorablePushAndRemoveUntilExample> {
@pragma('vm:entry-point')
static Route<void> _myRouteBuilder(BuildContext context, Object? arguments) {
return MaterialPageRoute<void>(
builder: (BuildContext context) => const RestorablePushAndRemoveUntilExample(),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Sample Code'),
),
floatingActionButton: FloatingActionButton(
onPressed: () => Navigator.restorablePushAndRemoveUntil(
context,
_myRouteBuilder,
ModalRoute.withName('/'),
),
tooltip: 'Increment Counter',
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/api/lib/widgets/navigator/navigator.restorable_push_and_remove_until.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/navigator/navigator.restorable_push_and_remove_until.0.dart",
"repo_id": "flutter",
"token_count": 542
} | 576 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [GlowingOverscrollIndicator].
void main() => runApp(const GlowingOverscrollIndicatorExampleApp());
class GlowingOverscrollIndicatorExampleApp extends StatelessWidget {
const GlowingOverscrollIndicatorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('GlowingOverscrollIndicator Sample')),
body: const GlowingOverscrollIndicatorExample(),
),
);
}
}
class GlowingOverscrollIndicatorExample extends StatelessWidget {
const GlowingOverscrollIndicatorExample({super.key});
@override
Widget build(BuildContext context) {
final double leadingPaintOffset = MediaQuery.of(context).padding.top + AppBar().preferredSize.height;
return NotificationListener<OverscrollIndicatorNotification>(
onNotification: (OverscrollIndicatorNotification notification) {
if (notification.leading) {
notification.paintOffset = leadingPaintOffset;
}
return false;
},
child: CustomScrollView(
slivers: <Widget>[
const SliverAppBar(title: Text('Custom PaintOffset')),
SliverToBoxAdapter(
child: Container(
color: Colors.amberAccent,
height: 100,
child: const Center(child: Text('Glow all day!')),
),
),
const SliverFillRemaining(child: FlutterLogo()),
],
),
);
}
}
| flutter/examples/api/lib/widgets/overscroll_indicator/glowing_overscroll_indicator.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/overscroll_indicator/glowing_overscroll_indicator.0.dart",
"repo_id": "flutter",
"token_count": 646
} | 577 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [CustomScrollView].
void main() => runApp(const CustomScrollViewExampleApp());
class CustomScrollViewExampleApp extends StatelessWidget {
const CustomScrollViewExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: CustomScrollViewExample(),
);
}
}
class CustomScrollViewExample extends StatefulWidget {
const CustomScrollViewExample({super.key});
@override
State<CustomScrollViewExample> createState() => _CustomScrollViewExampleState();
}
class _CustomScrollViewExampleState extends State<CustomScrollViewExample> {
List<int> top = <int>[];
List<int> bottom = <int>[0];
@override
Widget build(BuildContext context) {
const Key centerKey = ValueKey<String>('bottom-sliver-list');
return Scaffold(
appBar: AppBar(
title: const Text('Press on the plus to add items above and below'),
leading: IconButton(
icon: const Icon(Icons.add),
onPressed: () {
setState(() {
top.add(-top.length - 1);
bottom.add(bottom.length);
});
},
),
),
body: CustomScrollView(
center: centerKey,
slivers: <Widget>[
SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return Container(
alignment: Alignment.center,
color: Colors.blue[200 + top[index] % 4 * 100],
height: 100 + top[index] % 4 * 20.0,
child: Text('Item: ${top[index]}'),
);
},
childCount: top.length,
),
),
SliverList(
key: centerKey,
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return Container(
alignment: Alignment.center,
color: Colors.blue[200 + bottom[index] % 4 * 100],
height: 100 + bottom[index] % 4 * 20.0,
child: Text('Item: ${bottom[index]}'),
);
},
childCount: bottom.length,
),
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/scroll_view/custom_scroll_view.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scroll_view/custom_scroll_view.1.dart",
"repo_id": "flutter",
"token_count": 1123
} | 578 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [SingleActivator.SingleActivator].
void main() => runApp(const SingleActivatorExampleApp());
class SingleActivatorExampleApp extends StatelessWidget {
const SingleActivatorExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('SingleActivator Sample')),
body: const Center(
child: SingleActivatorExample(),
),
),
);
}
}
class IncrementIntent extends Intent {
const IncrementIntent();
}
class SingleActivatorExample extends StatefulWidget {
const SingleActivatorExample({super.key});
@override
State<SingleActivatorExample> createState() => _SingleActivatorExampleState();
}
class _SingleActivatorExampleState extends State<SingleActivatorExample> {
int count = 0;
@override
Widget build(BuildContext context) {
return Shortcuts(
shortcuts: const <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.keyC, control: true): IncrementIntent(),
},
child: Actions(
actions: <Type, Action<Intent>>{
IncrementIntent: CallbackAction<IncrementIntent>(
onInvoke: (IncrementIntent intent) => setState(() {
count = count + 1;
}),
),
},
child: Focus(
autofocus: true,
child: Column(
children: <Widget>[
const Text('Add to the counter by pressing Ctrl+C'),
Text('count: $count'),
],
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/shortcuts/single_activator.single_activator.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/shortcuts/single_activator.single_activator.0.dart",
"repo_id": "flutter",
"token_count": 712
} | 579 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
void main() => runApp(const TextMagnifierExampleApp(text: 'Hello world!'));
class TextMagnifierExampleApp extends StatelessWidget {
const TextMagnifierExampleApp({
super.key,
this.textDirection = TextDirection.ltr,
required this.text,
});
final TextDirection textDirection;
final String text;
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
body: Padding(
padding: const EdgeInsets.symmetric(horizontal: 48.0),
child: Center(
child: TextField(
textDirection: textDirection,
// Create a custom magnifier configuration that
// this `TextField` will use to build a magnifier with.
magnifierConfiguration: TextMagnifierConfiguration(
magnifierBuilder: (_, __, ValueNotifier<MagnifierInfo> magnifierInfo) => CustomMagnifier(
magnifierInfo: magnifierInfo,
),
),
controller: TextEditingController(text: text),
),
),
),
),
);
}
}
class CustomMagnifier extends StatelessWidget {
const CustomMagnifier({super.key, required this.magnifierInfo});
static const Size magnifierSize = Size(200, 200);
// This magnifier will consume some text data and position itself
// based on the info in the magnifier.
final ValueNotifier<MagnifierInfo> magnifierInfo;
@override
Widget build(BuildContext context) {
// Use a value listenable builder because we want to rebuild
// every time the text selection info changes.
// `CustomMagnifier` could also be a `StatefulWidget` and call `setState`
// when `magnifierInfo` updates. This would be useful for more complex
// positioning cases.
return ValueListenableBuilder<MagnifierInfo>(
valueListenable: magnifierInfo,
builder: (BuildContext context, MagnifierInfo currentMagnifierInfo, _) {
// We want to position the magnifier at the global position of the gesture.
Offset magnifierPosition = currentMagnifierInfo.globalGesturePosition;
// You may use the `MagnifierInfo` however you'd like:
// In this case, we make sure the magnifier never goes out of the current line bounds.
magnifierPosition = Offset(
clampDouble(
magnifierPosition.dx,
currentMagnifierInfo.currentLineBoundaries.left,
currentMagnifierInfo.currentLineBoundaries.right,
),
clampDouble(
magnifierPosition.dy,
currentMagnifierInfo.currentLineBoundaries.top,
currentMagnifierInfo.currentLineBoundaries.bottom,
),
);
// Finally, align the magnifier to the bottom center. The initial anchor is
// the top left, so subtract bottom center alignment.
magnifierPosition -= Alignment.bottomCenter.alongSize(magnifierSize);
return Positioned(
left: magnifierPosition.dx,
top: magnifierPosition.dy,
child: RawMagnifier(
magnificationScale: 2,
// The focal point starts at the center of the magnifier.
// We probably want to point below the magnifier, so
// offset the focal point by half the magnifier height.
focalPointOffset: Offset(0, magnifierSize.height / 2),
// Decorate it however we'd like!
decoration: const MagnifierDecoration(
shape: StarBorder(
side: BorderSide(
color: Colors.green,
width: 2,
),
),
),
size: magnifierSize,
),
);
});
}
}
| flutter/examples/api/lib/widgets/text_magnifier/text_magnifier.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/text_magnifier/text_magnifier.0.dart",
"repo_id": "flutter",
"token_count": 1663
} | 580 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SizeTransition].
void main() => runApp(const SizeTransitionExampleApp());
class SizeTransitionExampleApp extends StatelessWidget {
const SizeTransitionExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: SizeTransitionExample(),
);
}
}
class SizeTransitionExample extends StatefulWidget {
const SizeTransitionExample({super.key});
@override
State<SizeTransitionExample> createState() => _SizeTransitionExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _SizeTransitionExampleState extends State<SizeTransitionExample> with TickerProviderStateMixin {
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 3),
vsync: this,
)..repeat();
late final Animation<double> _animation = CurvedAnimation(
parent: _controller,
curve: Curves.fastOutSlowIn,
);
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: SizeTransition(
sizeFactor: _animation,
axis: Axis.horizontal,
axisAlignment: -1,
child: const Center(
child: FlutterLogo(size: 200.0),
),
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/size_transition.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/size_transition.0.dart",
"repo_id": "flutter",
"token_count": 522
} | 581 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| flutter/examples/api/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "flutter/examples/api/macos/Runner/Configs/Release.xcconfig",
"repo_id": "flutter",
"token_count": 32
} | 582 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/dialog/cupertino_alert_dialog.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Perform an action on CupertinoAlertDialog', (WidgetTester tester) async {
const String actionText = 'Yes';
await tester.pumpWidget(
const example.AlertDialogApp(),
);
// Launch the CupertinoAlertDialog.
await tester.tap(find.byType(CupertinoButton));
await tester.pump();
await tester.pumpAndSettle();
expect(find.text(actionText), findsOneWidget);
// Tap on an action to close the CupertinoAlertDialog.
await tester.tap(find.text(actionText));
await tester.pumpAndSettle();
expect(find.text(actionText), findsNothing);
});
}
| flutter/examples/api/test/cupertino/dialog/cupertino_alert_dialog.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/dialog/cupertino_alert_dialog.0_test.dart",
"repo_id": "flutter",
"token_count": 332
} | 583 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/search_field/cupertino_search_field.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Value changed callback updates entered text', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SearchTextFieldApp(),
);
expect(find.byType(CupertinoSearchTextField), findsOneWidget);
await tester.enterText(find.byType(CupertinoSearchTextField), 'photos');
await tester.pump();
expect(find.text('The text has changed to: photos'), findsOneWidget);
await tester.enterText(find.byType(CupertinoSearchTextField), 'photos from vacation');
await tester.showKeyboard(find.byType(CupertinoTextField));
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pump();
expect(find.text('Submitted text: photos from vacation'), findsOneWidget);
});
}
| flutter/examples/api/test/cupertino/search_field/cupertino_search_field.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/search_field/cupertino_search_field.1_test.dart",
"repo_id": "flutter",
"token_count": 364
} | 584 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/animated_icon/animated_icons_data.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Show all the animated icons', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AnimatedIconApp(),
);
// Check if the total number of AnimatedIcons matches the icons list.
expect(find.byType(AnimatedIcon, skipOffstage: false), findsNWidgets(example.iconsList.length));
// Test the AnimatedIcon size.
final Size iconSize = tester.getSize(find.byType(AnimatedIcon).first);
expect(iconSize.width, 72.0);
expect(iconSize.height, 72.0);
// Check if AnimatedIcon is animating.
await tester.pump(const Duration(milliseconds: 500));
AnimatedIcon animatedIcon = tester.widget(find.byType(AnimatedIcon).first);
expect(animatedIcon.progress.value, 0.25);
// Check if animation is completed.
await tester.pump(const Duration(milliseconds: 1500));
animatedIcon = tester.widget(find.byType(AnimatedIcon).first);
expect(animatedIcon.progress.value, 1.0);
});
}
| flutter/examples/api/test/material/animated_icon/animated_icons_data.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/animated_icon/animated_icons_data.0_test.dart",
"repo_id": "flutter",
"token_count": 443
} | 585 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/bottom_sheet/show_modal_bottom_sheet.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('BottomSheet can be opened and closed', (WidgetTester tester) async {
const String titleText = 'Modal BottomSheet';
const String closeText = 'Close BottomSheet';
await tester.pumpWidget(
const example.BottomSheetApp(),
);
expect(find.text(titleText), findsNothing);
expect(find.text(closeText), findsNothing);
// Open the bottom sheet.
await tester.tap(find.widgetWithText(ElevatedButton, 'showModalBottomSheet'));
await tester.pumpAndSettle();
// Verify that the bottom sheet is open.
expect(find.text(titleText), findsOneWidget);
expect(find.text(closeText), findsOneWidget);
});
}
| flutter/examples/api/test/material/bottom_sheet/show_modal_bottom_sheet.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/bottom_sheet/show_modal_bottom_sheet.0_test.dart",
"repo_id": "flutter",
"token_count": 340
} | 586 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/material/context_menu/context_menu_controller.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('showing and hiding the custom context menu in the whole app', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ContextMenuControllerExampleApp(),
);
expect(BrowserContextMenu.enabled, !kIsWeb);
expect(find.byType(AdaptiveTextSelectionToolbar), findsNothing);
// Right clicking the middle of the app shows the custom context menu.
final Offset center = tester.getCenter(find.byType(Scaffold));
final TestGesture gesture = await tester.startGesture(
center,
kind: PointerDeviceKind.mouse,
buttons: kSecondaryMouseButton,
);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
expect(find.byType(AdaptiveTextSelectionToolbar), findsOneWidget);
expect(find.text('Print'), findsOneWidget);
// Tap to dismiss.
await tester.tapAt(center);
await tester.pumpAndSettle();
expect(find.byType(AdaptiveTextSelectionToolbar), findsNothing);
// Long pressing also shows the custom context menu.
await tester.longPressAt(center);
expect(find.byType(AdaptiveTextSelectionToolbar), findsOneWidget);
expect(find.text('Print'), findsOneWidget);
});
}
| flutter/examples/api/test/material/context_menu/context_menu_controller.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/context_menu/context_menu_controller.0_test.dart",
"repo_id": "flutter",
"token_count": 559
} | 587 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/filled_button/filled_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('FilledButton Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FilledButtonApp(),
);
expect(find.widgetWithText(AppBar, 'FilledButton Sample'), findsOneWidget);
final Finder disabledButton = find.widgetWithText(FilledButton, 'Disabled');
expect(disabledButton, findsNWidgets(2));
expect(tester.widget<FilledButton>(disabledButton.first).onPressed.runtimeType, Null);
expect(tester.widget<FilledButton>(disabledButton.last).onPressed.runtimeType, Null);
final Finder enabledButton = find.widgetWithText(FilledButton, 'Enabled');
expect(enabledButton, findsNWidgets(2));
expect(tester.widget<FilledButton>(enabledButton.first).onPressed.runtimeType, VoidCallback);
expect(tester.widget<FilledButton>(enabledButton.last).onPressed.runtimeType, VoidCallback);
});
}
| flutter/examples/api/test/material/filled_button/filled_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/filled_button/filled_button.0_test.dart",
"repo_id": "flutter",
"token_count": 388
} | 588 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/input_decorator/input_decoration.prefix_icon.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('InputDecorator prefixIcon alignment', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PrefixIconExampleApp(),
);
expect(tester.getCenter(find.byIcon(Icons.person)).dy, 28.0);
});
}
| flutter/examples/api/test/material/input_decorator/input_decoration.prefix_icon.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/input_decorator/input_decoration.prefix_icon.0_test.dart",
"repo_id": "flutter",
"token_count": 206
} | 589 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/navigation_bar/navigation_bar.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Navigation bar updates destination on tap',
(WidgetTester tester) async {
await tester.pumpWidget(
const example.NavigationBarApp(),
);
final NavigationBar navigationBarWidget = tester.firstWidget(find.byType(NavigationBar));
/// NavigationDestinations must be rendered
expect(find.text('Home'), findsOneWidget);
expect(find.text('Notifications'), findsOneWidget);
expect(find.text('Messages'), findsOneWidget);
/// Test notification badge.
final Badge notificationBadge = tester.firstWidget(find.ancestor(
of: find.byIcon(Icons.notifications_sharp),
matching: find.byType(Badge),
));
expect(notificationBadge.label, null);
/// Test messages badge.
final Badge messagesBadge = tester.firstWidget(find.ancestor(
of: find.byIcon(Icons.messenger_sharp),
matching: find.byType(Badge),
));
expect(messagesBadge.label, isNotNull);
/// Initial index must be zero
expect(navigationBarWidget.selectedIndex, 0);
expect(find.text('Home page'), findsOneWidget);
/// Switch to second tab
await tester.tap(find.text('Notifications'));
await tester.pumpAndSettle();
expect(find.text('This is a notification'), findsNWidgets(2));
/// Switch to third tab
await tester.tap(find.text('Messages'));
await tester.pumpAndSettle();
expect(find.text('Hi!'), findsOneWidget);
expect(find.text('Hello'), findsOneWidget);
});
}
| flutter/examples/api/test/material/navigation_bar/navigation_bar.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/navigation_bar/navigation_bar.0_test.dart",
"repo_id": "flutter",
"token_count": 627
} | 590 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_api_samples/material/progress_indicator/linear_progress_indicator.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Finds LinearProgressIndicator', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ProgressIndicatorApp(),
);
expect(
find.bySemanticsLabel('Linear progress indicator'),
findsOneWidget,
);
// Test if LinearProgressIndicator is animating.
await tester.pump(const Duration(seconds: 2));
expect(tester.hasRunningAnimations, isTrue);
});
}
| flutter/examples/api/test/material/progress_indicator/linear_progress_indicator.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/progress_indicator/linear_progress_indicator.0_test.dart",
"repo_id": "flutter",
"token_count": 253
} | 591 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/scaffold/scaffold_messenger_state.show_snack_bar.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ScaffoldMessenger showSnackBar animation can be customized using AnimationStyle',
(WidgetTester tester) async {
await tester.pumpWidget(
const example.SnackBarApp(),
);
// Tap the button to show the SnackBar with default animation style.
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
await tester.pump(const Duration(milliseconds: 125)); // Advance the animation by 125ms.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(576.7, 0.1));
await tester.pump(const Duration(milliseconds: 125)); // Advance the animation by 125ms.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(566, 0.1));
// Tap the close button to dismiss the SnackBar.
await tester.tap(find.byType(IconButton));
await tester.pump();
await tester.pump(const Duration(milliseconds: 250)); // Advance the animation by 250ms.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(614, 0.1));
// Select custom animation style.
await tester.tap(find.text('Custom'));
await tester.pumpAndSettle();
// Tap the button to show the SnackBar with custom animation style.
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
await tester.pump(const Duration(milliseconds: 1500)); // Advance the animation by 125ms.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(576.7, 0.1));
await tester.pump(const Duration(milliseconds: 1500)); // Advance the animation by 125ms.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(566, 0.1));
// Tap the close button to dismiss the SnackBar.
await tester.tap(find.byType(IconButton));
await tester.pump();
await tester.pump(const Duration(seconds: 1)); // Advance the animation by 1sec.
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(614, 0.1));
// Select no animation style.
await tester.tap(find.text('None'));
await tester.pumpAndSettle();
// Tap the button to show the SnackBar with no animation style.
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
expect(tester.getTopLeft(find.text('I am a snack bar.')).dy, closeTo(566, 0.1));
// Tap the close button to dismiss the SnackBar.
await tester.tap(find.byType(IconButton));
await tester.pump();
expect(find.text('I am a snack bar.'), findsNothing);
});
}
| flutter/examples/api/test/material/scaffold/scaffold_messenger_state.show_snack_bar.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/scaffold/scaffold_messenger_state.show_snack_bar.2_test.dart",
"repo_id": "flutter",
"token_count": 1082
} | 592 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/switch/switch.4.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Show adaptive switch theme', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SwitchApp(),
);
// Default is material style switches
expect(find.text('Show cupertino style'), findsOneWidget);
expect(find.text('Show material style'), findsNothing);
Finder adaptiveSwitch = find.byType(Switch).first;
expect(
adaptiveSwitch,
paints
..rrect(color: const Color(0xff6750a4)) // M3 primary color.
..rrect()
..rrect(color: Colors.white), // Thumb color
);
await tester.tap(find.widgetWithText(OutlinedButton, 'Add customization'));
await tester.pumpAndSettle();
// Theme adaptation does not affect material-style switch.
adaptiveSwitch = find.byType(Switch).first;
expect(
adaptiveSwitch,
paints
..rrect(color: const Color(0xff6750a4)) // M3 primary color.
..rrect()
..rrect(color: Colors.white), // Thumb color
);
await tester.tap(find.widgetWithText(OutlinedButton, 'Show cupertino style'));
await tester.pumpAndSettle();
expect(
adaptiveSwitch,
paints
..rrect(color: const Color(0xff795548)) // Customized track color only for cupertino.
..rrect()..rrect()..rrect()..rrect()
..rrect(color: const Color(0xffffeb3b)), // Customized thumb color only for cupertino.
);
await tester.tap(find.widgetWithText(OutlinedButton, 'Remove customization'));
await tester.pumpAndSettle();
expect(
adaptiveSwitch,
paints
..rrect(color: const Color(0xff34c759)) // Cupertino system green.
..rrect()..rrect()..rrect()..rrect()
..rrect(color: Colors.white), // Thumb color
);
});
}
| flutter/examples/api/test/material/switch/switch.4_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/switch/switch.4_test.dart",
"repo_id": "flutter",
"token_count": 781
} | 593 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/tooltip/tooltip.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tooltip wait and show duration', (WidgetTester tester) async {
const String tooltipText = 'I am a Tooltip';
await tester.pumpWidget(
const example.TooltipExampleApp(),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
addTearDown(() async {
return gesture.removePointer();
});
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
expect(find.text(tooltipText), findsNothing);
// Move the mouse over the text and wait for the tooltip to appear.
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(tester.getCenter(tooltip));
// Wait half a second and the tooltip should still not be visible.
await tester.pump(const Duration(milliseconds: 500));
expect(find.text(tooltipText), findsNothing);
// Wait another half a second and the tooltip should be visible now.
await tester.pump(const Duration(milliseconds: 500));
expect(find.text(tooltipText), findsOneWidget);
// Move the mouse away and wait for the tooltip to disappear.
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
// Wait another second and the tooltip should be gone.
await tester.pump(const Duration(seconds: 1));
await tester.pumpAndSettle();
expect(find.text(tooltipText), findsNothing);
});
}
| flutter/examples/api/test/material/tooltip/tooltip.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/tooltip/tooltip.1_test.dart",
"repo_id": "flutter",
"token_count": 596
} | 594 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/services/keyboard_key/physical_keyboard_key.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Responds to key', (WidgetTester tester) async {
await tester.pumpWidget(
const example.KeyExampleApp(),
);
await tester.tap(find.text('Click to focus'));
await tester.pumpAndSettle();
expect(find.text('Press a key'), findsOneWidget);
await tester.sendKeyEvent(LogicalKeyboardKey.keyQ, physicalKey: PhysicalKeyboardKey.keyA);
await tester.pumpAndSettle();
expect(find.text('Pressed the key next to CAPS LOCK!'), findsOneWidget);
await tester.sendKeyEvent(LogicalKeyboardKey.keyB, physicalKey: PhysicalKeyboardKey.keyB);
await tester.pumpAndSettle();
expect(find.text('Not the key next to CAPS LOCK: Pressed Key B'), findsOneWidget);
});
}
| flutter/examples/api/test/services/keyboard_key/physical_keyboard_key.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/services/keyboard_key/physical_keyboard_key.0_test.dart",
"repo_id": "flutter",
"token_count": 368
} | 595 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/animated_grid/animated_grid.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AnimatedGrid example', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AnimatedGridSample(),
);
expect(find.text('1'), findsOneWidget);
expect(find.text('2'), findsOneWidget);
expect(find.text('3'), findsOneWidget);
expect(find.text('4'), findsOneWidget);
expect(find.text('5'), findsOneWidget);
expect(find.text('6'), findsOneWidget);
expect(find.text('7'), findsNothing);
await tester.tap(find.byIcon(Icons.add_circle));
await tester.pumpAndSettle();
expect(find.text('7'), findsOneWidget);
await tester.tap(find.byIcon(Icons.remove_circle));
await tester.pumpAndSettle();
expect(find.text('7'), findsNothing);
await tester.tap(find.text('2'));
await tester.pumpAndSettle();
await tester.tap(find.byIcon(Icons.remove_circle));
await tester.pumpAndSettle();
expect(find.text('2'), findsNothing);
expect(find.text('6'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/animated_grid/animated_grid.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/animated_grid/animated_grid.0_test.dart",
"repo_id": "flutter",
"token_count": 486
} | 596 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/flow.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Clicking on the menu icon opens the Flow menu', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FlowApp(),
);
// The menu icon is in the top left corner of the screen.
Offset menuIcon = tester.getCenter(find.byIcon(Icons.menu));
expect(menuIcon, const Offset(80.0, 144.0));
// The home icon is also in the top left corner of the screen.
Offset homeIcon = tester.getCenter(find.byIcon(Icons.home));
expect(homeIcon, const Offset(80.0, 144.0));
// Tap the menu icon to open the flow menu.
await tester.tapAt(menuIcon);
await tester.pumpAndSettle();
// The home icon is still in the top left corner of the screen.
homeIcon = tester.getCenter(find.byIcon(Icons.home));
expect(homeIcon, const Offset(80.0, 144.0));
// The menu icon is now in the top right corner of the screen.
menuIcon = tester.getCenter(find.byIcon(Icons.menu));
expect(menuIcon, const Offset(720.0, 144.0));
});
}
| flutter/examples/api/test/widgets/basic/flow.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/flow.0_test.dart",
"repo_id": "flutter",
"token_count": 466
} | 597 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/form/form.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can go back when form is clean', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FormApp(),
);
expect(find.text('Are you sure?'), findsNothing);
await tester.tap(find.text('Go back'));
await tester.pumpAndSettle();
expect(find.text('Are you sure?'), findsNothing);
});
testWidgets('Cannot go back when form is dirty', (WidgetTester tester) async {
await tester.pumpWidget(
const example.FormApp(),
);
expect(find.text('Are you sure?'), findsNothing);
await tester.enterText(find.byType(TextFormField), 'some new text');
await tester.tap(find.text('Go back'));
await tester.pumpAndSettle();
expect(find.text('Are you sure?'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/form/form.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/form/form.1_test.dart",
"repo_id": "flutter",
"token_count": 384
} | 598 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/page_view/page_view.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets(
'tapping Reverse button should reverse PageView',
(WidgetTester tester) async {
await tester.pumpWidget(const example.PageViewExampleApp());
final Finder pageView = find.byType(PageView);
final Finder reverseFinder = find.text('Reverse items');
final Finder firstItemFinder = find.byKey(const ValueKey<String>('1'));
final Finder lastItemFinder = find.byKey(const ValueKey<String>('5'));
expect(pageView, findsOneWidget);
expect(reverseFinder, findsOneWidget);
expect(firstItemFinder, findsOneWidget);
expect(lastItemFinder, findsNothing);
await tester.tap(reverseFinder);
await tester.pump();
expect(firstItemFinder, findsNothing);
expect(lastItemFinder, findsOneWidget);
},
);
}
| flutter/examples/api/test/widgets/page_view/page_view.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/page_view/page_view.1_test.dart",
"repo_id": "flutter",
"token_count": 403
} | 599 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/sliver/sliver_opacity.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('SliverOpacity example', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SliverOpacityExampleApp(),
);
final Finder button = find.byType(FloatingActionButton);
final Finder opacity = find.byType(SliverOpacity);
expect((tester.widget(opacity) as SliverOpacity).opacity, 1.0);
await tester.tap(button);
await tester.pump();
expect((tester.widget(opacity) as SliverOpacity).opacity, 0.0);
});
}
| flutter/examples/api/test/widgets/sliver/sliver_opacity.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/sliver/sliver_opacity.1_test.dart",
"repo_id": "flutter",
"token_count": 289
} | 600 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/hello_world/macos/Flutter/Flutter-Release.xcconfig/0 | {
"file_path": "flutter/examples/hello_world/macos/Flutter/Flutter-Release.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 601 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/image_list/macos/Flutter/Flutter-Debug.xcconfig/0 | {
"file_path": "flutter/examples/image_list/macos/Flutter/Flutter-Debug.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 602 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
class LifecycleWatcher extends StatefulWidget {
const LifecycleWatcher({ super.key });
@override
State<LifecycleWatcher> createState() => _LifecycleWatcherState();
}
class _LifecycleWatcherState extends State<LifecycleWatcher>
with WidgetsBindingObserver {
AppLifecycleState? _lastLifecycleState;
@override
void initState() {
super.initState();
WidgetsBinding.instance.addObserver(this);
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
super.dispose();
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
setState(() {
_lastLifecycleState = state;
});
}
@override
Widget build(BuildContext context) {
if (_lastLifecycleState == null) {
return const Text('This widget has not observed any lifecycle changes.');
}
return Text('The most recent lifecycle state this widget observed was: $_lastLifecycleState.');
}
}
void main() {
runApp(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: LifecycleWatcher(),
),
),
);
}
| flutter/examples/layers/services/lifecycle.dart/0 | {
"file_path": "flutter/examples/layers/services/lifecycle.dart",
"repo_id": "flutter",
"token_count": 483
} | 603 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
class SpinningSquare extends StatefulWidget {
const SpinningSquare({super.key});
@override
State<SpinningSquare> createState() => _SpinningSquareState();
}
class _SpinningSquareState extends State<SpinningSquare> with SingleTickerProviderStateMixin {
// We use 3600 milliseconds instead of 1800 milliseconds because 0.0 -> 1.0
// represents an entire turn of the square whereas in the other examples
// we used 0.0 -> math.pi, which is only half a turn.
late final AnimationController _animation = AnimationController(
duration: const Duration(milliseconds: 3600),
vsync: this,
)..repeat();
@override
void dispose() {
_animation.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return RotationTransition(
turns: _animation,
child: Container(
width: 200.0,
height: 200.0,
color: const Color(0xFF00FF00),
),
);
}
}
void main() {
runApp(const Center(child: SpinningSquare()));
}
| flutter/examples/layers/widgets/spinning_square.dart/0 | {
"file_path": "flutter/examples/layers/widgets/spinning_square.dart",
"repo_id": "flutter",
"token_count": 396
} | 604 |
# Example of calling platform services from Flutter
This project demonstrates how to connect a Flutter app to platform-specific services.
You can read more about
[accessing platform and third-party services in Flutter](https://flutter.dev/platform-channels/).
## iOS
You can use the commands `flutter build` and `flutter run` from the app's root
directory to build/run the app or you can open `ios/Runner.xcworkspace` in Xcode
and build/run the project as usual.
## Android
You can use the commands `flutter build` and `flutter run` from the app's root
directory to build/run the app or to build with Android Studio, open the
`android` folder in Android Studio and build the project as usual.
## Windows
You can use the commands `flutter build` and `flutter run` from the app's root
directory to build/run the app or you can build once then open
`build\windows\platform_channel.sln` in Visual Studio to build and run.
| flutter/examples/platform_channel/README.md/0 | {
"file_path": "flutter/examples/platform_channel/README.md",
"repo_id": "flutter",
"token_count": 243
} | 605 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "my_application.h"
#include <flutter_linux/flutter_linux.h>
#include <math.h>
#include <upower.h>
#ifdef GDK_WINDOWING_X11
#include <gdk/gdkx.h>
#endif
#include "flutter/generated_plugin_registrant.h"
struct _MyApplication {
GtkApplication parent_instance;
char** dart_entrypoint_arguments;
FlView* view;
// Connection to UPower.
UpClient* up_client;
GPtrArray* battery_devices;
// Channel for Dart code to request the battery information.
FlMethodChannel* battery_channel;
// Channel to send updates to Dart code about battery charging state.
FlEventChannel* charging_channel;
gchar* last_charge_event;
bool emit_charge_events;
};
G_DEFINE_TYPE(MyApplication, my_application, GTK_TYPE_APPLICATION)
// Checks the charging state and emits an event if necessary.
static void update_charging_state(MyApplication* self) {
if (!self->emit_charge_events) {
return;
}
const gchar* charge_event = "discharging";
for (guint i = 0; i < self->battery_devices->len; i++) {
UpDevice* device =
static_cast<UpDevice*>(g_ptr_array_index(self->battery_devices, i));
guint state;
g_object_get(device, "state", &state, nullptr);
if (state == UP_DEVICE_STATE_CHARGING ||
state == UP_DEVICE_STATE_FULLY_CHARGED) {
charge_event = "charging";
}
}
if (g_strcmp0(charge_event, self->last_charge_event) != 0) {
g_autoptr(GError) error = nullptr;
g_autoptr(FlValue) value = fl_value_new_string(charge_event);
if (!fl_event_channel_send(self->charging_channel, value, nullptr,
&error)) {
g_warning("Failed to send charging event: %s", error->message);
return;
}
g_free(self->last_charge_event);
self->last_charge_event = g_strdup(charge_event);
}
}
// Called when a UPower device changes state.
static void up_device_state_changed_cb(MyApplication* self, GParamSpec* pspec,
UpDevice* device) {
update_charging_state(self);
}
// Called when UPower devices are added.
static void up_device_added_cb(MyApplication* self, UpDevice* device) {
// Listen for state changes from battery_devices.
guint kind;
g_object_get(device, "kind", &kind, nullptr);
if (kind == UP_DEVICE_KIND_BATTERY) {
g_ptr_array_add(self->battery_devices, g_object_ref(device));
g_signal_connect_swapped(device, "notify::state",
G_CALLBACK(up_device_state_changed_cb), self);
up_device_state_changed_cb(self, nullptr, device);
}
}
// Called when UPower devices are removed.
static void up_device_removed_cb(MyApplication* self, UpDevice* device) {
g_ptr_array_remove(self->battery_devices, device);
g_signal_handlers_disconnect_matched(
device, G_SIGNAL_MATCH_FUNC, 0, 0, nullptr,
reinterpret_cast<GClosure*>(up_device_state_changed_cb), nullptr);
}
// Gets the current battery level.
static FlMethodResponse* get_battery_level(MyApplication* self) {
// Find the first available battery and use that.
for (guint i = 0; i < self->battery_devices->len; i++) {
UpDevice* device =
static_cast<UpDevice*>(g_ptr_array_index(self->battery_devices, i));
double percentage;
g_object_get(device, "percentage", &percentage, nullptr);
g_autoptr(FlValue) result =
fl_value_new_int(static_cast<int64_t>(round(percentage)));
return FL_METHOD_RESPONSE(fl_method_success_response_new(result));
}
return FL_METHOD_RESPONSE(fl_method_error_response_new(
"NO_BATTERY", "Device does not have a battery.", nullptr));
}
// Called when the Dart code requests battery information.
static void battery_method_call_cb(FlMethodChannel* channel,
FlMethodCall* method_call,
gpointer user_data) {
MyApplication* self = static_cast<MyApplication*>(user_data);
g_autoptr(FlMethodResponse) response = nullptr;
if (strcmp(fl_method_call_get_name(method_call), "getBatteryLevel") == 0) {
response = get_battery_level(self);
} else {
response = FL_METHOD_RESPONSE(fl_method_not_implemented_response_new());
}
g_autoptr(GError) error = nullptr;
if (!fl_method_call_respond(method_call, response, &error)) {
g_warning("Failed to send response: %s", error->message);
}
}
// Called when the Dart code starts listening for charging events.
static FlMethodErrorResponse* charging_listen_cb(FlEventChannel* channel,
FlValue* args,
gpointer user_data) {
MyApplication* self = static_cast<MyApplication*>(user_data);
self->emit_charge_events = true;
update_charging_state(self);
return nullptr;
}
// Called when the Dart code stops listening for charging events.
static FlMethodErrorResponse* charging_cancel_cb(FlEventChannel* channel,
FlValue* args,
gpointer user_data) {
MyApplication* self = static_cast<MyApplication*>(user_data);
self->emit_charge_events = false;
return nullptr;
}
// Creates the platform channels this application provides.
static void create_channels(MyApplication* self) {
FlEngine* engine = fl_view_get_engine(self->view);
FlBinaryMessenger* messenger = fl_engine_get_binary_messenger(engine);
g_autoptr(FlStandardMethodCodec) codec = fl_standard_method_codec_new();
self->battery_channel = fl_method_channel_new(
messenger, "samples.flutter.io/battery", FL_METHOD_CODEC(codec));
fl_method_channel_set_method_call_handler(
self->battery_channel, battery_method_call_cb, self, nullptr);
self->charging_channel = fl_event_channel_new(
messenger, "samples.flutter.io/charging", FL_METHOD_CODEC(codec));
fl_event_channel_set_stream_handlers(self->charging_channel,
charging_listen_cb, charging_cancel_cb,
self, nullptr);
}
// Implements GApplication::activate.
static void my_application_activate(GApplication* application) {
MyApplication* self = MY_APPLICATION(application);
GtkWindow* window =
GTK_WINDOW(gtk_application_window_new(GTK_APPLICATION(application)));
// Use a header bar when running in GNOME as this is the common style used
// by applications and is the setup most users will be using (e.g. Ubuntu
// desktop).
// If running on X and not using GNOME then just use a traditional title bar
// in case the window manager does more exotic layout, e.g. tiling.
// If running on Wayland assume the header bar will work (may need changing
// if future cases occur).
gboolean use_header_bar = TRUE;
#ifdef GDK_WINDOWING_X11
GdkScreen* screen = gtk_window_get_screen(window);
if (GDK_IS_X11_SCREEN(screen)) {
const gchar* wm_name = gdk_x11_screen_get_window_manager_name(screen);
if (g_strcmp0(wm_name, "GNOME Shell") != 0) {
use_header_bar = FALSE;
}
}
#endif
if (use_header_bar) {
GtkHeaderBar* header_bar = GTK_HEADER_BAR(gtk_header_bar_new());
gtk_widget_show(GTK_WIDGET(header_bar));
gtk_header_bar_set_title(header_bar, "platform_channel");
gtk_header_bar_set_show_close_button(header_bar, TRUE);
gtk_window_set_titlebar(window, GTK_WIDGET(header_bar));
} else {
gtk_window_set_title(window, "platform_channel");
}
gtk_window_set_default_size(window, 1280, 720);
gtk_widget_show(GTK_WIDGET(window));
g_autoptr(FlDartProject) project = fl_dart_project_new();
fl_dart_project_set_dart_entrypoint_arguments(
project, self->dart_entrypoint_arguments);
// Connect to UPower.
self->up_client = up_client_new();
g_signal_connect_swapped(self->up_client, "device-added",
G_CALLBACK(up_device_added_cb), self);
g_signal_connect_swapped(self->up_client, "device-removed",
G_CALLBACK(up_device_removed_cb), self);
#if UP_CHECK_VERSION(0, 99, 8)
// up_client_get_devices was deprecated and replaced with
// up_client_get_devices2 in libupower 0.99.8.
g_autoptr(GPtrArray) devices = up_client_get_devices2(self->up_client);
#else
g_autoptr(GPtrArray) devices = up_client_get_devices(self->up_client);
#endif
for (guint i = 0; i < devices->len; i++) {
g_autoptr(UpDevice) device =
static_cast<UpDevice*>(g_ptr_array_index(devices, i));
up_device_added_cb(self, device);
}
self->view = fl_view_new(project);
gtk_widget_show(GTK_WIDGET(self->view));
gtk_container_add(GTK_CONTAINER(window), GTK_WIDGET(self->view));
fl_register_plugins(FL_PLUGIN_REGISTRY(self->view));
// Create application specific platform channels.
create_channels(self);
gtk_widget_grab_focus(GTK_WIDGET(self->view));
}
// Implements GApplication::local_command_line.
static gboolean my_application_local_command_line(GApplication* application,
gchar*** arguments,
int* exit_status) {
MyApplication* self = MY_APPLICATION(application);
// Strip out the first argument as it is the binary name.
self->dart_entrypoint_arguments = g_strdupv(*arguments + 1);
g_autoptr(GError) error = nullptr;
if (!g_application_register(application, nullptr, &error)) {
g_warning("Failed to register: %s", error->message);
*exit_status = 1;
return TRUE;
}
g_application_activate(application);
*exit_status = 0;
return TRUE;
}
// Implements GObject::dispose.
static void my_application_dispose(GObject* object) {
MyApplication* self = MY_APPLICATION(object);
for (guint i = 0; i < self->battery_devices->len; i++) {
UpDevice* device =
static_cast<UpDevice*>(g_ptr_array_index(self->battery_devices, i));
g_signal_handlers_disconnect_matched(device, G_SIGNAL_MATCH_DATA, 0, 0,
nullptr, nullptr, self);
}
g_signal_handlers_disconnect_matched(self->up_client, G_SIGNAL_MATCH_DATA, 0,
0, nullptr, nullptr, self);
g_clear_pointer(&self->dart_entrypoint_arguments, g_strfreev);
g_clear_object(&self->up_client);
g_clear_object(&self->battery_devices);
g_clear_object(&self->battery_channel);
g_clear_object(&self->charging_channel);
g_clear_pointer(&self->last_charge_event, g_free);
G_OBJECT_CLASS(my_application_parent_class)->dispose(object);
}
static void my_application_class_init(MyApplicationClass* klass) {
G_APPLICATION_CLASS(klass)->activate = my_application_activate;
G_APPLICATION_CLASS(klass)->local_command_line =
my_application_local_command_line;
G_OBJECT_CLASS(klass)->dispose = my_application_dispose;
}
static void my_application_init(MyApplication* self) {
self->battery_devices = g_ptr_array_new_with_free_func(g_object_unref);
}
MyApplication* my_application_new() {
return MY_APPLICATION(g_object_new(my_application_get_type(),
"application-id", APPLICATION_ID, "flags",
G_APPLICATION_NON_UNIQUE, nullptr));
}
| flutter/examples/platform_channel/linux/my_application.cc/0 | {
"file_path": "flutter/examples/platform_channel/linux/my_application.cc",
"repo_id": "flutter",
"token_count": 4521
} | 606 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import UIKit
import Flutter
enum ChannelName {
static let battery = "samples.flutter.io/battery"
static let charging = "samples.flutter.io/charging"
}
enum BatteryState {
static let charging = "charging"
static let discharging = "discharging"
}
enum MyFlutterErrorCode {
static let unavailable = "UNAVAILABLE"
}
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate, FlutterStreamHandler {
private var eventSink: FlutterEventSink?
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
GeneratedPluginRegistrant.register(with: self)
guard let controller = window?.rootViewController as? FlutterViewController else {
fatalError("rootViewController is not type FlutterViewController")
}
let batteryChannel = FlutterMethodChannel(name: ChannelName.battery,
binaryMessenger: controller.binaryMessenger)
batteryChannel.setMethodCallHandler({
[weak self] (call: FlutterMethodCall, result: FlutterResult) -> Void in
guard call.method == "getBatteryLevel" else {
result(FlutterMethodNotImplemented)
return
}
self?.receiveBatteryLevel(result: result)
})
let chargingChannel = FlutterEventChannel(name: ChannelName.charging,
binaryMessenger: controller.binaryMessenger)
chargingChannel.setStreamHandler(self)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
private func receiveBatteryLevel(result: FlutterResult) {
let device = UIDevice.current
device.isBatteryMonitoringEnabled = true
guard device.batteryState != .unknown else {
result(FlutterError(code: MyFlutterErrorCode.unavailable,
message: "Battery info unavailable",
details: nil))
return
}
result(Int(device.batteryLevel * 100))
}
public func onListen(withArguments arguments: Any?,
eventSink: @escaping FlutterEventSink) -> FlutterError? {
self.eventSink = eventSink
UIDevice.current.isBatteryMonitoringEnabled = true
sendBatteryStateEvent()
NotificationCenter.default.addObserver(
self,
selector: #selector(AppDelegate.onBatteryStateDidChange),
name: UIDevice.batteryStateDidChangeNotification,
object: nil)
return nil
}
@objc private func onBatteryStateDidChange(notification: NSNotification) {
sendBatteryStateEvent()
}
private func sendBatteryStateEvent() {
guard let eventSink = eventSink else {
return
}
switch UIDevice.current.batteryState {
case .full:
eventSink(BatteryState.charging)
case .charging:
eventSink(BatteryState.charging)
case .unplugged:
eventSink(BatteryState.discharging)
default:
eventSink(FlutterError(code: MyFlutterErrorCode.unavailable,
message: "Charging status unavailable",
details: nil))
}
}
public func onCancel(withArguments arguments: Any?) -> FlutterError? {
NotificationCenter.default.removeObserver(self)
eventSink = nil
return nil
}
}
| flutter/examples/platform_channel_swift/ios/Runner/AppDelegate.swift/0 | {
"file_path": "flutter/examples/platform_channel_swift/ios/Runner/AppDelegate.swift",
"repo_id": "flutter",
"token_count": 1275
} | 607 |
<!-- Copyright 2014 The Flutter Authors. All rights reserved.
Use of this source code is governed by a BSD-style license that can be
found in the LICENSE file. -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:theme="@style/Theme.AppCompat">
<androidx.appcompat.widget.Toolbar
android:id="@+id/my_toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
android:background="?attr/colorPrimary"
android:elevation="4dp"
android:theme="@style/ThemeOverlay.AppCompat.ActionBar"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light"/>
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/grey">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_weight="1"
android:gravity="center">
<TextView
android:id="@+id/button_tap"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/button_tap"
android:padding="18dp"
android:textSize="@dimen/font_size" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:theme="@style/MyButton"
android:text="@string/switch_view"
android:textAllCaps="false" />
</LinearLayout>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="@dimen/edge_margin"
android:background="@color/grey"
android:text="@string/android"
android:textSize="@dimen/platform_label_font_size" />
</LinearLayout>
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|right"
android:layout_margin="@dimen/edge_margin"
android:clickable="true"
android:elevation="@dimen/fab_elevation_resting"
android:src="@drawable/ic_add_black_24dp"
app:backgroundTint="@color/white"
app:fabSize="normal"
app:pressedTranslationZ="@dimen/fab_elevation_pressed"
app:rippleColor="@color/grey" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
</LinearLayout>
| flutter/examples/platform_view/android/app/src/main/res/layout/android_full_screen_layout.xml/0 | {
"file_path": "flutter/examples/platform_view/android/app/src/main/res/layout/android_full_screen_layout.xml",
"repo_id": "flutter",
"token_count": 1553
} | 608 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class TexturePage extends StatefulWidget {
const TexturePage({super.key});
@override
State<TexturePage> createState() => _TexturePageState();
}
class _TexturePageState extends State<TexturePage> {
static const int textureWidth = 300;
static const int textureHeight = 300;
static const MethodChannel channel =
MethodChannel('samples.flutter.io/texture');
final Future<int?> textureId =
channel.invokeMethod('create', <int>[textureWidth, textureHeight]);
// Set the color of the texture.
Future<void> setColor(int r, int g, int b) async {
await channel.invokeMethod('setColor', <int>[r, g, b]);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: const Text('Texture Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
FutureBuilder<int?>(
future: textureId,
builder: (BuildContext context, AsyncSnapshot<int?> snapshot) {
if (snapshot.hasData) {
if (snapshot.data != null) {
return SizedBox(
width: textureWidth.toDouble(),
height: textureHeight.toDouble(),
child: Texture(textureId: snapshot.data!),
);
} else {
return const Text('Error creating texture');
}
} else {
return const Text('Creating texture...');
}
},
),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Flutter Navy'),
onPressed: () => setColor(0x04, 0x2b, 0x59)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Flutter Blue'),
onPressed: () => setColor(0x05, 0x53, 0xb1)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Flutter Sky'),
onPressed: () => setColor(0x02, 0x7d, 0xfd)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Red'),
onPressed: () => setColor(0xf2, 0x5d, 0x50)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Yellow'),
onPressed: () => setColor(0xff, 0xf2, 0x75)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Purple'),
onPressed: () => setColor(0x62, 0x00, 0xee)),
const SizedBox(height: 10),
OutlinedButton(
child: const Text('Green'),
onPressed: () => setColor(0x1c, 0xda, 0xc5)),
],
),
),
);
}
}
void main() {
runApp(const MaterialApp(home: TexturePage()));
}
| flutter/examples/texture/lib/main.dart/0 | {
"file_path": "flutter/examples/texture/lib/main.dart",
"repo_id": "flutter",
"token_count": 1574
} | 609 |
tags:
# This tag tells the test framework to run these tagged files as part of a
# reduced test set.
reduced-test-set:
# This tag tells the test framework to not shuffle the test order according to
# the --test-randomize-ordering-seed for the suites that have this tag.
no-shuffle:
allow_test_randomization: false
| flutter/packages/flutter/dart_test.yaml/0 | {
"file_path": "flutter/packages/flutter/dart_test.yaml",
"repo_id": "flutter",
"token_count": 93
} | 610 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are from the Rendering library. *
version: 1
transforms:
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'rendering.dart' ]
constructor: ''
inClass: 'RenderParagraph'
changes:
- kind: 'addParameter'
index: 5
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Change made in https://github.com/flutter/flutter/pull/128522
- title: "Migrate to 'textScaler'"
date: 2023-06-09
element:
uris: [ 'rendering.dart' ]
constructor: ''
inClass: 'RenderEditable'
changes:
- kind: 'addParameter'
index: 15
name: 'textScaler'
style: optional_named
argumentValue:
expression: 'TextScaler.linear({% textScaleFactor %})'
requiredIf: "textScaleFactor != ''"
- kind: 'removeParameter'
name: 'textScaleFactor'
variables:
textScaleFactor:
kind: 'fragment'
value: 'arguments[textScaleFactor]'
# Changes made in https://github.com/flutter/flutter/pull/66305
- title: "Migrate to 'clipBehavior'"
date: 2020-09-22
element:
uris: [ 'rendering.dart' ]
field: 'overflow'
inClass: 'RenderStack'
changes:
- kind: 'rename'
newName: 'clipBehavior'
# Changes made in https://github.com/flutter/flutter/pull/66305
- title: "Migrate to 'clipBehavior'"
date: 2020-09-22
element:
uris: [ 'rendering.dart' ]
constructor: ''
inClass: 'RenderStack'
oneOf:
- if: "overflow == 'Overflow.clip'"
changes:
- kind: 'addParameter'
index: 0
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "overflow == 'Overflow.clip'"
- kind: 'removeParameter'
name: 'overflow'
- if: "overflow == 'Overflow.visible'"
changes:
- kind: 'addParameter'
index: 0
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "overflow == 'Overflow.visible'"
- kind: 'removeParameter'
name: 'overflow'
variables:
overflow:
kind: 'fragment'
value: 'arguments[overflow]'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'rendering.dart' ]
field: 'clipToSize'
inClass: 'RenderListWheelViewport'
changes:
- kind: 'rename'
newName: 'clipBehavior'
# Changes made in https://flutter.dev/docs/release/breaking-changes/clip-behavior
- title: "Migrate to 'clipBehavior'"
date: 2020-08-20
element:
uris: [ 'rendering.dart' ]
constructor: ''
inClass: 'RenderListWheelViewport'
oneOf:
- if: "clipToSize == 'true'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.hardEdge'
requiredIf: "clipToSize == 'true'"
- kind: 'removeParameter'
name: 'clipToSize'
- if: "clipToSize == 'false'"
changes:
- kind: 'addParameter'
index: 13
name: 'clipBehavior'
style: optional_named
argumentValue:
expression: 'Clip.none'
requiredIf: "clipToSize == 'false'"
- kind: 'removeParameter'
name: 'clipToSize'
variables:
clipToSize:
kind: 'fragment'
value: 'arguments[clipToSize]'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_rendering.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_rendering.yaml",
"repo_id": "flutter",
"token_count": 2110
} | 611 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Simple one-dimensional physics simulations, such as springs, friction, and
/// gravity, for use in user interface animations.
///
/// To use, import `package:flutter/physics.dart`.
library physics;
export 'src/physics/clamped_simulation.dart';
export 'src/physics/friction_simulation.dart';
export 'src/physics/gravity_simulation.dart';
export 'src/physics/simulation.dart';
export 'src/physics/spring_simulation.dart';
export 'src/physics/tolerance.dart';
export 'src/physics/utils.dart';
| flutter/packages/flutter/lib/physics.dart/0 | {
"file_path": "flutter/packages/flutter/lib/physics.dart",
"repo_id": "flutter",
"token_count": 201
} | 612 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show ImageFilter;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'localizations.dart';
import 'theme.dart';
// Standard iOS 10 tab bar height.
const double _kTabBarHeight = 50.0;
const Color _kDefaultTabBarBorderColor = CupertinoDynamicColor.withBrightness(
color: Color(0x4D000000),
darkColor: Color(0x29000000),
);
const Color _kDefaultTabBarInactiveColor = CupertinoColors.inactiveGray;
/// An iOS-styled bottom navigation tab bar.
///
/// Displays multiple tabs using [BottomNavigationBarItem] with one tab being
/// active, the first tab by default.
///
/// This [StatelessWidget] doesn't store the active tab itself. You must
/// listen to the [onTap] callbacks and call `setState` with a new [currentIndex]
/// for the new selection to reflect. This can also be done automatically
/// by wrapping this with a [CupertinoTabScaffold].
///
/// Tab changes typically trigger a switch between [Navigator]s, each with its
/// own navigation stack, per standard iOS design. This can be done by using
/// [CupertinoTabView]s inside each tab builder in [CupertinoTabScaffold].
///
/// If the given [backgroundColor]'s opacity is not 1.0 (which is the case by
/// default), it will produce a blurring effect to the content behind it.
///
/// When used as [CupertinoTabScaffold.tabBar], by default [CupertinoTabBar]
/// disables text scaling to match the native iOS behavior. To override
/// this behavior, wrap each of the `navigationBar`'s components inside a
/// [MediaQuery] with the desired [TextScaler].
///
/// {@tool dartpad}
/// This example shows a [CupertinoTabBar] placed in a [CupertinoTabScaffold].
///
/// ** See code in examples/api/lib/cupertino/bottom_tab_bar/cupertino_tab_bar.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoTabScaffold], which hosts the [CupertinoTabBar] at the bottom.
/// * [BottomNavigationBarItem], an item in a [CupertinoTabBar].
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/bars/tab-bars/>
class CupertinoTabBar extends StatelessWidget implements PreferredSizeWidget {
/// Creates a tab bar in the iOS style.
const CupertinoTabBar({
super.key,
required this.items,
this.onTap,
this.currentIndex = 0,
this.backgroundColor,
this.activeColor,
this.inactiveColor = _kDefaultTabBarInactiveColor,
this.iconSize = 30.0,
this.height = _kTabBarHeight,
this.border = const Border(
top: BorderSide(
color: _kDefaultTabBarBorderColor,
width: 0.0, // 0.0 means one physical pixel
),
),
}) : assert(
items.length >= 2,
"Tabs need at least 2 items to conform to Apple's HIG",
),
assert(0 <= currentIndex && currentIndex < items.length),
assert(height >= 0.0);
/// The interactive items laid out within the bottom navigation bar.
final List<BottomNavigationBarItem> items;
/// The callback that is called when a item is tapped.
///
/// The widget creating the bottom navigation bar needs to keep track of the
/// current index and call `setState` to rebuild it with the newly provided
/// index.
final ValueChanged<int>? onTap;
/// The index into [items] of the current active item.
///
/// Must be between 0 and the number of tabs minus 1, inclusive.
final int currentIndex;
/// The background color of the tab bar. If it contains transparency, the
/// tab bar will automatically produce a blurring effect to the content
/// behind it.
///
/// Defaults to [CupertinoTheme]'s `barBackgroundColor` when null.
final Color? backgroundColor;
/// The foreground color of the icon and title for the [BottomNavigationBarItem]
/// of the selected tab.
///
/// Defaults to [CupertinoTheme]'s `primaryColor` if null.
final Color? activeColor;
/// The foreground color of the icon and title for the [BottomNavigationBarItem]s
/// in the unselected state.
///
/// Defaults to a [CupertinoDynamicColor] that matches the disabled foreground
/// color of the native `UITabBar` component.
final Color inactiveColor;
/// The size of all of the [BottomNavigationBarItem] icons.
///
/// This value is used to configure the [IconTheme] for the navigation bar.
/// When a [BottomNavigationBarItem.icon] widget is not an [Icon] the widget
/// should configure itself to match the icon theme's size and color.
final double iconSize;
/// The height of the [CupertinoTabBar].
///
/// Defaults to 50.
final double height;
/// The border of the [CupertinoTabBar].
///
/// The default value is a one physical pixel top border with grey color.
final Border? border;
@override
Size get preferredSize => Size.fromHeight(height);
/// Indicates whether the tab bar is fully opaque or can have contents behind
/// it show through it.
bool opaque(BuildContext context) {
final Color backgroundColor =
this.backgroundColor ?? CupertinoTheme.of(context).barBackgroundColor;
return CupertinoDynamicColor.resolve(backgroundColor, context).alpha == 0xFF;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
final double bottomPadding = MediaQuery.viewPaddingOf(context).bottom;
final Color backgroundColor = CupertinoDynamicColor.resolve(
this.backgroundColor ?? CupertinoTheme.of(context).barBackgroundColor,
context,
);
BorderSide resolveBorderSide(BorderSide side) {
return side == BorderSide.none
? side
: side.copyWith(color: CupertinoDynamicColor.resolve(side.color, context));
}
// Return the border as is when it's a subclass.
final Border? resolvedBorder = border == null || border.runtimeType != Border
? border
: Border(
top: resolveBorderSide(border!.top),
left: resolveBorderSide(border!.left),
bottom: resolveBorderSide(border!.bottom),
right: resolveBorderSide(border!.right),
);
final Color inactive = CupertinoDynamicColor.resolve(inactiveColor, context);
Widget result = DecoratedBox(
decoration: BoxDecoration(
border: resolvedBorder,
color: backgroundColor,
),
child: SizedBox(
height: height + bottomPadding,
child: IconTheme.merge( // Default with the inactive state.
data: IconThemeData(color: inactive, size: iconSize),
child: DefaultTextStyle( // Default with the inactive state.
style: CupertinoTheme.of(context).textTheme.tabLabelTextStyle.copyWith(color: inactive),
child: Padding(
padding: EdgeInsets.only(bottom: bottomPadding),
child: Semantics(
explicitChildNodes: true,
child: Row(
// Align bottom since we want the labels to be aligned.
crossAxisAlignment: CrossAxisAlignment.end,
children: _buildTabItems(context),
),
),
),
),
),
),
);
if (!opaque(context)) {
// For non-opaque backgrounds, apply a blur effect.
result = ClipRect(
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: 10.0, sigmaY: 10.0),
child: result,
),
);
}
return result;
}
List<Widget> _buildTabItems(BuildContext context) {
final List<Widget> result = <Widget>[];
final CupertinoLocalizations localizations = CupertinoLocalizations.of(context);
for (int index = 0; index < items.length; index += 1) {
final bool active = index == currentIndex;
result.add(
_wrapActiveItem(
context,
Expanded(
// Make tab items part of the EditableText tap region so that
// switching tabs doesn't unfocus text fields.
child: TextFieldTapRegion(
child: Semantics(
selected: active,
hint: localizations.tabSemanticsLabel(
tabIndex: index + 1,
tabCount: items.length,
),
child: MouseRegion(
cursor: kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
child: GestureDetector(
behavior: HitTestBehavior.opaque,
onTap: onTap == null ? null : () { onTap!(index); },
child: Padding(
padding: const EdgeInsets.only(bottom: 4.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.end,
children: _buildSingleTabItem(items[index], active),
),
),
),
),
),
),
),
active: active,
),
);
}
return result;
}
List<Widget> _buildSingleTabItem(BottomNavigationBarItem item, bool active) {
return <Widget>[
Expanded(
child: Center(child: active ? item.activeIcon : item.icon),
),
if (item.label != null) Text(item.label!),
];
}
/// Change the active tab item's icon and title colors to active.
Widget _wrapActiveItem(BuildContext context, Widget item, { required bool active }) {
if (!active) {
return item;
}
final Color activeColor = CupertinoDynamicColor.resolve(
this.activeColor ?? CupertinoTheme.of(context).primaryColor,
context,
);
return IconTheme.merge(
data: IconThemeData(color: activeColor),
child: DefaultTextStyle.merge(
style: TextStyle(color: activeColor),
child: item,
),
);
}
/// Create a clone of the current [CupertinoTabBar] but with provided
/// parameters overridden.
CupertinoTabBar copyWith({
Key? key,
List<BottomNavigationBarItem>? items,
Color? backgroundColor,
Color? activeColor,
Color? inactiveColor,
double? iconSize,
double? height,
Border? border,
int? currentIndex,
ValueChanged<int>? onTap,
}) {
return CupertinoTabBar(
key: key ?? this.key,
items: items ?? this.items,
backgroundColor: backgroundColor ?? this.backgroundColor,
activeColor: activeColor ?? this.activeColor,
inactiveColor: inactiveColor ?? this.inactiveColor,
iconSize: iconSize ?? this.iconSize,
height: height ?? this.height,
border: border ?? this.border,
currentIndex: currentIndex ?? this.currentIndex,
onTap: onTap ?? this.onTap,
);
}
}
| flutter/packages/flutter/lib/src/cupertino/bottom_tab_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/bottom_tab_bar.dart",
"repo_id": "flutter",
"token_count": 3967
} | 613 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/physics.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
// Extracted from https://developer.apple.com/design/resources/.
// Minimum padding from edges of the segmented control to edges of
// encompassing widget.
const EdgeInsetsGeometry _kHorizontalItemPadding = EdgeInsets.symmetric(vertical: 2, horizontal: 3);
// The corner radius of the thumb.
const Radius _kThumbRadius = Radius.circular(6.93);
// The amount of space by which to expand the thumb from the size of the currently
// selected child.
const EdgeInsets _kThumbInsets = EdgeInsets.symmetric(horizontal: 1);
// Minimum height of the segmented control.
const double _kMinSegmentedControlHeight = 28.0;
const Color _kSeparatorColor = Color(0x4D8E8E93);
const CupertinoDynamicColor _kThumbColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFFFFFFF),
darkColor: Color(0xFF636366),
);
// The amount of space by which to inset each separator.
const EdgeInsets _kSeparatorInset = EdgeInsets.symmetric(vertical: 6);
const double _kSeparatorWidth = 1;
const Radius _kSeparatorRadius = Radius.circular(_kSeparatorWidth/2);
// The minimum scale factor of the thumb, when being pressed on for a sufficient
// amount of time.
const double _kMinThumbScale = 0.95;
// The minimum horizontal distance between the edges of the separator and the
// closest child.
const double _kSegmentMinPadding = 9.25;
// The threshold value used in hasDraggedTooFar, for checking against the square
// L2 distance from the location of the current drag pointer, to the closest
// vertex of the CupertinoSlidingSegmentedControl's Rect.
//
// Both the mechanism and the value are speculated.
const double _kTouchYDistanceThreshold = 50.0 * 50.0;
// The corner radius of the segmented control.
//
// Inspected from iOS 13.2 simulator.
const double _kCornerRadius = 8;
// The minimum opacity of an unselected segment, when the user presses on the
// segment and it starts to fadeout.
//
// Inspected from iOS 13.2 simulator.
const double _kContentPressedMinOpacity = 0.2;
// The spring animation used when the thumb changes its rect.
final SpringSimulation _kThumbSpringAnimationSimulation = SpringSimulation(
const SpringDescription(mass: 1, stiffness: 503.551, damping: 44.8799),
0,
1,
0, // Every time a new spring animation starts the previous animation stops.
);
const Duration _kSpringAnimationDuration = Duration(milliseconds: 412);
const Duration _kOpacityAnimationDuration = Duration(milliseconds: 470);
const Duration _kHighlightAnimationDuration = Duration(milliseconds: 200);
class _Segment<T> extends StatefulWidget {
const _Segment({
required ValueKey<T> key,
required this.child,
required this.pressed,
required this.highlighted,
required this.isDragging,
}) : super(key: key);
final Widget child;
final bool pressed;
final bool highlighted;
// Whether the thumb of the parent widget (CupertinoSlidingSegmentedControl)
// is currently being dragged.
final bool isDragging;
bool get shouldFadeoutContent => pressed && !highlighted;
bool get shouldScaleContent => pressed && highlighted && isDragging;
@override
_SegmentState<T> createState() => _SegmentState<T>();
}
class _SegmentState<T> extends State<_Segment<T>> with TickerProviderStateMixin<_Segment<T>> {
late final AnimationController highlightPressScaleController;
late Animation<double> highlightPressScaleAnimation;
@override
void initState() {
super.initState();
highlightPressScaleController = AnimationController(
duration: _kOpacityAnimationDuration,
value: widget.shouldScaleContent ? 1 : 0,
vsync: this,
);
highlightPressScaleAnimation = highlightPressScaleController.drive(
Tween<double>(begin: 1.0, end: _kMinThumbScale),
);
}
@override
void didUpdateWidget(_Segment<T> oldWidget) {
super.didUpdateWidget(oldWidget);
assert(oldWidget.key == widget.key);
if (oldWidget.shouldScaleContent != widget.shouldScaleContent) {
highlightPressScaleAnimation = highlightPressScaleController.drive(
Tween<double>(
begin: highlightPressScaleAnimation.value,
end: widget.shouldScaleContent ? _kMinThumbScale : 1.0,
),
);
highlightPressScaleController.animateWith(_kThumbSpringAnimationSimulation);
}
}
@override
void dispose() {
highlightPressScaleController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return MetaData(
// Expand the hitTest area of this widget.
behavior: HitTestBehavior.opaque,
child: IndexedStack(
alignment: Alignment.center,
children: <Widget>[
AnimatedOpacity(
opacity: widget.shouldFadeoutContent ? _kContentPressedMinOpacity : 1,
duration: _kOpacityAnimationDuration,
curve: Curves.ease,
child: AnimatedDefaultTextStyle(
style: DefaultTextStyle.of(context)
.style
.merge(TextStyle(fontWeight: widget.highlighted ? FontWeight.w500 : FontWeight.normal)),
duration: _kHighlightAnimationDuration,
curve: Curves.ease,
child: ScaleTransition(
scale: highlightPressScaleAnimation,
child: widget.child,
),
),
),
// The entire widget will assume the size of this widget, so when a
// segment's "highlight" animation plays the size of the parent stays
// the same and will always be greater than equal to that of the
// visible child (at index 0), to keep the size of the entire
// SegmentedControl widget consistent throughout the animation.
Offstage(
child: DefaultTextStyle.merge(
style: const TextStyle(fontWeight: FontWeight.w500),
child: widget.child,
),
),
],
),
);
}
}
// Fadeout the separator when either adjacent segment is highlighted.
class _SegmentSeparator extends StatefulWidget {
const _SegmentSeparator({
required ValueKey<int> key,
required this.highlighted,
}) : super(key: key);
final bool highlighted;
@override
_SegmentSeparatorState createState() => _SegmentSeparatorState();
}
class _SegmentSeparatorState extends State<_SegmentSeparator> with TickerProviderStateMixin<_SegmentSeparator> {
late final AnimationController separatorOpacityController;
@override
void initState() {
super.initState();
separatorOpacityController = AnimationController(
duration: _kSpringAnimationDuration,
value: widget.highlighted ? 0 : 1,
vsync: this,
);
}
@override
void didUpdateWidget(_SegmentSeparator oldWidget) {
super.didUpdateWidget(oldWidget);
assert(oldWidget.key == widget.key);
if (oldWidget.highlighted != widget.highlighted) {
separatorOpacityController.animateTo(
widget.highlighted ? 0 : 1,
duration: _kSpringAnimationDuration,
curve: Curves.ease,
);
}
}
@override
void dispose() {
separatorOpacityController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: separatorOpacityController,
child: const SizedBox(width: _kSeparatorWidth),
builder: (BuildContext context, Widget? child) {
return Padding(
padding: _kSeparatorInset,
child: DecoratedBox(
decoration: BoxDecoration(
color: _kSeparatorColor.withOpacity(_kSeparatorColor.opacity * separatorOpacityController.value),
borderRadius: const BorderRadius.all(_kSeparatorRadius),
),
child: child,
),
);
},
);
}
}
/// An iOS 13 style segmented control.
///
/// Displays the widgets provided in the [Map] of [children] in a horizontal list.
/// It allows the user to select between a number of mutually exclusive options,
/// by tapping or dragging within the segmented control.
///
/// A segmented control can feature any [Widget] as one of the values in its
/// [Map] of [children]. The type T is the type of the [Map] keys used to identify
/// each widget and determine which widget is selected. As required by the [Map]
/// class, keys must be of consistent types and must be comparable. The [children]
/// argument must be an ordered [Map] such as a [LinkedHashMap], the ordering of
/// the keys will determine the order of the widgets in the segmented control.
///
/// The widget calls the [onValueChanged] callback *when a valid user gesture
/// completes on an unselected segment*. The map key associated with the newly
/// selected widget is returned in the [onValueChanged] callback. Typically,
/// widgets that use a segmented control will listen for the [onValueChanged]
/// callback and rebuild the segmented control with a new [groupValue] to update
/// which option is currently selected.
///
/// The [children] will be displayed in the order of the keys in the [Map],
/// along the current [TextDirection]. Each child widget will have the same size.
/// The height of the segmented control is determined by the height of the
/// tallest child widget. The width of each child will be the intrinsic width of
/// the widest child, or the available horizontal space divided by the number of
/// [children], which ever is smaller.
///
/// A segmented control may optionally be created with custom colors. The
/// [thumbColor], [backgroundColor] arguments can be used to override the
/// segmented control's colors from its defaults.
///
/// {@tool dartpad}
/// This example shows a [CupertinoSlidingSegmentedControl] with an enum type.
///
/// The callback provided to [onValueChanged] should update the state of
/// the parent [StatefulWidget] using the [State.setState] method, so that
/// the parent gets rebuilt; for example:
///
/// ** See code in examples/api/lib/cupertino/segmented_control/cupertino_sliding_segmented_control.0.dart **
/// {@end-tool}
/// See also:
///
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/controls/segmented-controls/>
class CupertinoSlidingSegmentedControl<T> extends StatefulWidget {
/// Creates an iOS-style segmented control bar.
///
/// The [children] argument must be an ordered [Map] such as a
/// [LinkedHashMap]. Further, the length of the [children] list must be
/// greater than one.
///
/// Each widget value in the map of [children] must have an associated key
/// that uniquely identifies this widget. This key is what will be returned
/// in the [onValueChanged] callback when a new value from the [children] map
/// is selected.
///
/// The [groupValue] is the currently selected value for the segmented control.
/// If no [groupValue] is provided, or the [groupValue] is null, no widget will
/// appear as selected. The [groupValue] must be either null or one of the keys
/// in the [children] map.
CupertinoSlidingSegmentedControl({
super.key,
required this.children,
required this.onValueChanged,
this.groupValue,
this.thumbColor = _kThumbColor,
this.padding = _kHorizontalItemPadding,
this.backgroundColor = CupertinoColors.tertiarySystemFill,
}) : assert(children.length >= 2),
assert(
groupValue == null || children.keys.contains(groupValue),
'The groupValue must be either null or one of the keys in the children map.',
);
/// The identifying keys and corresponding widget values in the
/// segmented control.
///
/// This attribute must be an ordered [Map] such as a [LinkedHashMap]. Each
/// widget is typically a single-line [Text] widget or an [Icon] widget.
///
/// The map must have more than one entry.
final Map<T, Widget> children;
/// The identifier of the widget that is currently selected.
///
/// This must be one of the keys in the [Map] of [children].
/// If this attribute is null, no widget will be initially selected.
final T? groupValue;
/// The callback that is called when a new option is tapped.
///
/// The segmented control passes the newly selected widget's associated key
/// to the callback but does not actually change state until the parent
/// widget rebuilds the segmented control with the new [groupValue].
///
/// The callback provided to [onValueChanged] should update the state of
/// the parent [StatefulWidget] using the [State.setState] method, so that
/// the parent gets rebuilt; for example:
///
/// {@tool snippet}
///
/// ```dart
/// class SegmentedControlExample extends StatefulWidget {
/// const SegmentedControlExample({super.key});
///
/// @override
/// State createState() => SegmentedControlExampleState();
/// }
///
/// class SegmentedControlExampleState extends State<SegmentedControlExample> {
/// final Map<int, Widget> children = const <int, Widget>{
/// 0: Text('Child 1'),
/// 1: Text('Child 2'),
/// };
///
/// int? currentValue;
///
/// @override
/// Widget build(BuildContext context) {
/// return CupertinoSlidingSegmentedControl<int>(
/// children: children,
/// onValueChanged: (int? newValue) {
/// setState(() {
/// currentValue = newValue;
/// });
/// },
/// groupValue: currentValue,
/// );
/// }
/// }
/// ```
/// {@end-tool}
final ValueChanged<T?> onValueChanged;
/// The color used to paint the rounded rect behind the [children] and the separators.
///
/// The default value is [CupertinoColors.tertiarySystemFill]. The background
/// will not be painted if null is specified.
final Color backgroundColor;
/// The color used to paint the interior of the thumb that appears behind the
/// currently selected item.
///
/// The default value is a [CupertinoDynamicColor] that appears white in light
/// mode and becomes a gray color in dark mode.
final Color thumbColor;
/// The amount of space by which to inset the [children].
///
/// Defaults to `EdgeInsets.symmetric(vertical: 2, horizontal: 3)`.
final EdgeInsetsGeometry padding;
@override
State<CupertinoSlidingSegmentedControl<T>> createState() => _SegmentedControlState<T>();
}
class _SegmentedControlState<T> extends State<CupertinoSlidingSegmentedControl<T>>
with TickerProviderStateMixin<CupertinoSlidingSegmentedControl<T>> {
late final AnimationController thumbController = AnimationController(duration: _kSpringAnimationDuration, value: 0, vsync: this);
Animatable<Rect?>? thumbAnimatable;
late final AnimationController thumbScaleController = AnimationController(duration: _kSpringAnimationDuration, value: 0, vsync: this);
late Animation<double> thumbScaleAnimation = thumbScaleController.drive(Tween<double>(begin: 1, end: _kMinThumbScale));
final TapGestureRecognizer tap = TapGestureRecognizer();
final HorizontalDragGestureRecognizer drag = HorizontalDragGestureRecognizer();
final LongPressGestureRecognizer longPress = LongPressGestureRecognizer();
@override
void initState() {
super.initState();
// If the long press or horizontal drag recognizer gets accepted, we know for
// sure the gesture is meant for the segmented control. Hand everything to
// the drag gesture recognizer.
final GestureArenaTeam team = GestureArenaTeam();
longPress.team = team;
drag.team = team;
team.captain = drag;
drag
..onDown = onDown
..onUpdate = onUpdate
..onEnd = onEnd
..onCancel = onCancel;
tap.onTapUp = onTapUp;
// Empty callback to enable the long press recognizer.
longPress.onLongPress = () { };
highlighted = widget.groupValue;
}
@override
void didUpdateWidget(CupertinoSlidingSegmentedControl<T> oldWidget) {
super.didUpdateWidget(oldWidget);
// Temporarily ignore highlight changes from the widget when the thumb is
// being dragged. When the drag gesture finishes the widget will be forced
// to build (see the onEnd method), and didUpdateWidget will be called again.
if (!isThumbDragging && highlighted != widget.groupValue) {
thumbController.animateWith(_kThumbSpringAnimationSimulation);
thumbAnimatable = null;
highlighted = widget.groupValue;
}
}
@override
void dispose() {
thumbScaleController.dispose();
thumbController.dispose();
drag.dispose();
tap.dispose();
longPress.dispose();
super.dispose();
}
// Whether the current drag gesture started on a selected segment. When this
// flag is false, the `onUpdate` method does not update `highlighted`.
// Otherwise the thumb can be dragged around in an ongoing drag gesture.
bool? _startedOnSelectedSegment;
// Whether an ongoing horizontal drag gesture that started on the thumb is
// present. When true, defer/ignore changes to the `highlighted` variable
// from other sources (except for semantics) until the gesture ends, preventing
// them from interfering with the active drag gesture.
bool get isThumbDragging => _startedOnSelectedSegment ?? false;
// Converts local coordinate to segments. This method assumes each segment has
// the same width.
T segmentForXPosition(double dx) {
final RenderBox renderBox = context.findRenderObject()! as RenderBox;
final int numOfChildren = widget.children.length;
assert(renderBox.hasSize);
assert(numOfChildren >= 2);
int index = (dx ~/ (renderBox.size.width / numOfChildren)).clamp(0, numOfChildren - 1);
switch (Directionality.of(context)) {
case TextDirection.ltr:
break;
case TextDirection.rtl:
index = numOfChildren - 1 - index;
}
return widget.children.keys.elementAt(index);
}
bool _hasDraggedTooFar(DragUpdateDetails details) {
final RenderBox renderBox = context.findRenderObject()! as RenderBox;
assert(renderBox.hasSize);
final Size size = renderBox.size;
final Offset offCenter = details.localPosition - Offset(size.width/2, size.height/2);
final double l2 = math.pow(math.max(0.0, offCenter.dx.abs() - size.width/2), 2)
+ math.pow(math.max(0.0, offCenter.dy.abs() - size.height/2), 2) as double;
return l2 > _kTouchYDistanceThreshold;
}
// The thumb shrinks when the user presses on it, and starts expanding when
// the user lets go.
// This animation must be synced with the segment scale animation (see the
// _Segment widget) to make the overall animation look natural when the thumb
// is not sliding.
void _playThumbScaleAnimation({ required bool isExpanding }) {
thumbScaleAnimation = thumbScaleController.drive(
Tween<double>(
begin: thumbScaleAnimation.value,
end: isExpanding ? 1 : _kMinThumbScale,
),
);
thumbScaleController.animateWith(_kThumbSpringAnimationSimulation);
}
void onHighlightChangedByGesture(T newValue) {
if (highlighted == newValue) {
return;
}
setState(() { highlighted = newValue; });
// Additionally, start the thumb animation if the highlighted segment
// changes. If the thumbController is already running, the render object's
// paint method will create a new tween to drive the animation with.
// TODO(LongCatIsLooong): https://github.com/flutter/flutter/issues/74356:
// the current thumb will be painted at the same location twice (before and
// after the new animation starts).
thumbController.animateWith(_kThumbSpringAnimationSimulation);
thumbAnimatable = null;
}
void onPressedChangedByGesture(T? newValue) {
if (pressed != newValue) {
setState(() { pressed = newValue; });
}
}
void onTapUp(TapUpDetails details) {
// No gesture should interfere with an ongoing thumb drag.
if (isThumbDragging) {
return;
}
final T segment = segmentForXPosition(details.localPosition.dx);
onPressedChangedByGesture(null);
if (segment != widget.groupValue) {
widget.onValueChanged(segment);
}
}
void onDown(DragDownDetails details) {
final T touchDownSegment = segmentForXPosition(details.localPosition.dx);
_startedOnSelectedSegment = touchDownSegment == highlighted;
onPressedChangedByGesture(touchDownSegment);
if (isThumbDragging) {
_playThumbScaleAnimation(isExpanding: false);
}
}
void onUpdate(DragUpdateDetails details) {
if (isThumbDragging) {
final T segment = segmentForXPosition(details.localPosition.dx);
onPressedChangedByGesture(segment);
onHighlightChangedByGesture(segment);
} else {
final T? segment = _hasDraggedTooFar(details)
? null
: segmentForXPosition(details.localPosition.dx);
onPressedChangedByGesture(segment);
}
}
void onEnd(DragEndDetails details) {
final T? pressed = this.pressed;
if (isThumbDragging) {
_playThumbScaleAnimation(isExpanding: true);
if (highlighted != widget.groupValue) {
widget.onValueChanged(highlighted);
}
} else if (pressed != null) {
onHighlightChangedByGesture(pressed);
assert(pressed == highlighted);
if (highlighted != widget.groupValue) {
widget.onValueChanged(highlighted);
}
}
onPressedChangedByGesture(null);
_startedOnSelectedSegment = null;
}
void onCancel() {
if (isThumbDragging) {
_playThumbScaleAnimation(isExpanding: true);
}
onPressedChangedByGesture(null);
_startedOnSelectedSegment = null;
}
// The segment the sliding thumb is currently located at, or animating to. It
// may have a different value from widget.groupValue, since this widget does
// not report a selection change via `onValueChanged` until the user stops
// interacting with the widget (onTapUp). For example, the user can drag the
// thumb around, and the `onValueChanged` callback will not be invoked until
// the thumb is let go.
T? highlighted;
// The segment the user is currently pressing.
T? pressed;
@override
Widget build(BuildContext context) {
assert(widget.children.length >= 2);
List<Widget> children = <Widget>[];
bool isPreviousSegmentHighlighted = false;
int index = 0;
int? highlightedIndex;
for (final MapEntry<T, Widget> entry in widget.children.entries) {
final bool isHighlighted = highlighted == entry.key;
if (isHighlighted) {
highlightedIndex = index;
}
if (index != 0) {
children.add(
_SegmentSeparator(
// Let separators be TextDirection-invariant. If the TextDirection
// changes, the separators should mostly stay where they were.
key: ValueKey<int>(index),
highlighted: isPreviousSegmentHighlighted || isHighlighted,
),
);
}
children.add(
Semantics(
button: true,
onTap: () { widget.onValueChanged(entry.key); },
inMutuallyExclusiveGroup: true,
selected: widget.groupValue == entry.key,
child: MouseRegion(
cursor: kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
child: _Segment<T>(
key: ValueKey<T>(entry.key),
highlighted: isHighlighted,
pressed: pressed == entry.key,
isDragging: isThumbDragging,
child: entry.value,
),
),
),
);
index += 1;
isPreviousSegmentHighlighted = isHighlighted;
}
assert((highlightedIndex == null) == (highlighted == null));
switch (Directionality.of(context)) {
case TextDirection.ltr:
break;
case TextDirection.rtl:
children = children.reversed.toList(growable: false);
if (highlightedIndex != null) {
highlightedIndex = index - 1 - highlightedIndex;
}
}
return UnconstrainedBox(
constrainedAxis: Axis.horizontal,
child: Container(
padding: widget.padding.resolve(Directionality.of(context)),
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(_kCornerRadius)),
color: CupertinoDynamicColor.resolve(widget.backgroundColor, context),
),
child: AnimatedBuilder(
animation: thumbScaleAnimation,
builder: (BuildContext context, Widget? child) {
return _SegmentedControlRenderWidget<T>(
highlightedIndex: highlightedIndex,
thumbColor: CupertinoDynamicColor.resolve(widget.thumbColor, context),
thumbScale: thumbScaleAnimation.value,
state: this,
children: children,
);
},
),
),
);
}
}
class _SegmentedControlRenderWidget<T> extends MultiChildRenderObjectWidget {
const _SegmentedControlRenderWidget({
super.key,
super.children,
required this.highlightedIndex,
required this.thumbColor,
required this.thumbScale,
required this.state,
});
final int? highlightedIndex;
final Color thumbColor;
final double thumbScale;
final _SegmentedControlState<T> state;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderSegmentedControl<T>(
highlightedIndex: highlightedIndex,
thumbColor: thumbColor,
thumbScale: thumbScale,
state: state,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSegmentedControl<T> renderObject) {
assert(renderObject.state == state);
renderObject
..thumbColor = thumbColor
..thumbScale = thumbScale
..highlightedIndex = highlightedIndex;
}
}
class _SegmentedControlContainerBoxParentData extends ContainerBoxParentData<RenderBox> { }
// The behavior of a UISegmentedControl as observed on iOS 13.1:
//
// 1. Tap up inside events will set the current selected index to the index of the
// segment at the tap up location instantaneously (there might be animation but
// the index change seems to happen before animation finishes), unless the tap
// down event from the same touch event didn't happen within the segmented
// control, in which case the touch event will be ignored entirely (will be
// referring to these touch events as invalid touch events below).
//
// 2. A valid tap up event will also trigger the sliding CASpringAnimation (even
// when it lands on the current segment), starting from the current `frame`
// of the thumb. The previous sliding animation, if still playing, will be
// removed and its velocity reset to 0. The sliding animation has a fixed
// duration, regardless of the distance or transform.
//
// 3. When the sliding animation plays two other animations take place. In one animation
// the content of the current segment gradually becomes "highlighted", turning the
// font weight to semibold (CABasicAnimation, timingFunction = default, duration = 0.2).
// The other is the separator fadein/fadeout animation (duration = 0.41).
//
// 4. A tap down event on the segment pointed to by the current selected
// index will trigger a CABasicAnimation that shrinks the thumb to 95% of its
// original size, even if the sliding animation is still playing. The
/// corresponding tap up event inverts the process (eyeballed).
//
// 5. A tap down event on other segments will trigger a CABasicAnimation
// (timingFunction = default, duration = 0.47.) that fades out the content
// from its current alpha, eventually reducing the alpha of that segment to
// 20% unless interrupted by a tap up event or the pointer moves out of the
// region (either outside of the segmented control's vicinity or to a
// different segment). The reverse animation has the same duration and timing
// function.
class _RenderSegmentedControl<T> extends RenderBox
with ContainerRenderObjectMixin<RenderBox, ContainerBoxParentData<RenderBox>>,
RenderBoxContainerDefaultsMixin<RenderBox, ContainerBoxParentData<RenderBox>> {
_RenderSegmentedControl({
required int? highlightedIndex,
required Color thumbColor,
required double thumbScale,
required this.state,
}) : _highlightedIndex = highlightedIndex,
_thumbColor = thumbColor,
_thumbScale = thumbScale;
final _SegmentedControlState<T> state;
// The current **Unscaled** Thumb Rect in this RenderBox's coordinate space.
Rect? currentThumbRect;
@override
void attach(PipelineOwner owner) {
super.attach(owner);
state.thumbController.addListener(markNeedsPaint);
}
@override
void detach() {
state.thumbController.removeListener(markNeedsPaint);
super.detach();
}
double get thumbScale => _thumbScale;
double _thumbScale;
set thumbScale(double value) {
if (_thumbScale == value) {
return;
}
_thumbScale = value;
if (state.highlighted != null) {
markNeedsPaint();
}
}
int? get highlightedIndex => _highlightedIndex;
int? _highlightedIndex;
set highlightedIndex(int? value) {
if (_highlightedIndex == value) {
return;
}
_highlightedIndex = value;
markNeedsPaint();
}
Color get thumbColor => _thumbColor;
Color _thumbColor;
set thumbColor(Color value) {
if (_thumbColor == value) {
return;
}
_thumbColor = value;
markNeedsPaint();
}
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
assert(debugHandleEvent(event, entry));
// No gesture should interfere with an ongoing thumb drag.
if (event is PointerDownEvent && !state.isThumbDragging) {
state.tap.addPointer(event);
state.longPress.addPointer(event);
state.drag.addPointer(event);
}
}
// Intrinsic Dimensions
double get totalSeparatorWidth => (_kSeparatorInset.horizontal + _kSeparatorWidth) * (childCount ~/ 2);
RenderBox? nonSeparatorChildAfter(RenderBox child) {
final RenderBox? nextChild = childAfter(child);
return nextChild == null ? null : childAfter(nextChild);
}
@override
double computeMinIntrinsicWidth(double height) {
final int childCount = this.childCount ~/ 2 + 1;
RenderBox? child = firstChild;
double maxMinChildWidth = 0;
while (child != null) {
final double childWidth = child.getMinIntrinsicWidth(height);
maxMinChildWidth = math.max(maxMinChildWidth, childWidth);
child = nonSeparatorChildAfter(child);
}
return (maxMinChildWidth + 2 * _kSegmentMinPadding) * childCount + totalSeparatorWidth;
}
@override
double computeMaxIntrinsicWidth(double height) {
final int childCount = this.childCount ~/ 2 + 1;
RenderBox? child = firstChild;
double maxMaxChildWidth = 0;
while (child != null) {
final double childWidth = child.getMaxIntrinsicWidth(height);
maxMaxChildWidth = math.max(maxMaxChildWidth, childWidth);
child = nonSeparatorChildAfter(child);
}
return (maxMaxChildWidth + 2 * _kSegmentMinPadding) * childCount + totalSeparatorWidth;
}
@override
double computeMinIntrinsicHeight(double width) {
RenderBox? child = firstChild;
double maxMinChildHeight = _kMinSegmentedControlHeight;
while (child != null) {
final double childHeight = child.getMinIntrinsicHeight(width);
maxMinChildHeight = math.max(maxMinChildHeight, childHeight);
child = nonSeparatorChildAfter(child);
}
return maxMinChildHeight;
}
@override
double computeMaxIntrinsicHeight(double width) {
RenderBox? child = firstChild;
double maxMaxChildHeight = _kMinSegmentedControlHeight;
while (child != null) {
final double childHeight = child.getMaxIntrinsicHeight(width);
maxMaxChildHeight = math.max(maxMaxChildHeight, childHeight);
child = nonSeparatorChildAfter(child);
}
return maxMaxChildHeight;
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
return defaultComputeDistanceToHighestActualBaseline(baseline);
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! _SegmentedControlContainerBoxParentData) {
child.parentData = _SegmentedControlContainerBoxParentData();
}
}
Size _calculateChildSize(BoxConstraints constraints) {
final int childCount = this.childCount ~/ 2 + 1;
double childWidth = (constraints.minWidth - totalSeparatorWidth) / childCount;
double maxHeight = _kMinSegmentedControlHeight;
RenderBox? child = firstChild;
while (child != null) {
childWidth = math.max(childWidth, child.getMaxIntrinsicWidth(double.infinity) + 2 * _kSegmentMinPadding);
child = nonSeparatorChildAfter(child);
}
childWidth = math.min(
childWidth,
(constraints.maxWidth - totalSeparatorWidth) / childCount,
);
child = firstChild;
while (child != null) {
final double boxHeight = child.getMaxIntrinsicHeight(childWidth);
maxHeight = math.max(maxHeight, boxHeight);
child = nonSeparatorChildAfter(child);
}
return Size(childWidth, maxHeight);
}
Size _computeOverallSizeFromChildSize(Size childSize, BoxConstraints constraints) {
final int childCount = this.childCount ~/ 2 + 1;
return constraints.constrain(Size(childSize.width * childCount + totalSeparatorWidth, childSize.height));
}
@override
Size computeDryLayout(BoxConstraints constraints) {
final Size childSize = _calculateChildSize(constraints);
return _computeOverallSizeFromChildSize(childSize, constraints);
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
final Size childSize = _calculateChildSize(constraints);
final BoxConstraints childConstraints = BoxConstraints.tight(childSize);
final BoxConstraints separatorConstraints = childConstraints.heightConstraints();
RenderBox? child = firstChild;
int index = 0;
double start = 0;
while (child != null) {
child.layout(index.isEven ? childConstraints : separatorConstraints, parentUsesSize: true);
final _SegmentedControlContainerBoxParentData childParentData = child.parentData! as _SegmentedControlContainerBoxParentData;
final Offset childOffset = Offset(start, 0);
childParentData.offset = childOffset;
start += child.size.width;
assert(
index.isEven || child.size.width == _kSeparatorWidth + _kSeparatorInset.horizontal,
'${child.size.width} != ${_kSeparatorWidth + _kSeparatorInset.horizontal}',
);
child = childAfter(child);
index += 1;
}
size = _computeOverallSizeFromChildSize(childSize, constraints);
}
// This method is used to convert the original unscaled thumb rect painted in
// the previous frame, to a Rect that is within the valid boundary defined by
// the child segments.
//
// The overall size does not include that of the thumb. That is, if the thumb
// is located at the first or the last segment, the thumb can get cut off if
// one of the values in _kThumbInsets is positive.
Rect? moveThumbRectInBound(Rect? thumbRect, List<RenderBox> children) {
assert(hasSize);
assert(children.length >= 2);
if (thumbRect == null) {
return null;
}
final Offset firstChildOffset = (children.first.parentData! as _SegmentedControlContainerBoxParentData).offset;
final double leftMost = firstChildOffset.dx;
final double rightMost = (children.last.parentData! as _SegmentedControlContainerBoxParentData).offset.dx + children.last.size.width;
assert(rightMost > leftMost);
// Ignore the horizontal position and the height of `thumbRect`, and
// calculates them from `children`.
return Rect.fromLTRB(
math.max(thumbRect.left, leftMost - _kThumbInsets.left),
firstChildOffset.dy - _kThumbInsets.top,
math.min(thumbRect.right, rightMost + _kThumbInsets.right),
firstChildOffset.dy + children.first.size.height + _kThumbInsets.bottom,
);
}
@override
void paint(PaintingContext context, Offset offset) {
final List<RenderBox> children = getChildrenAsList();
for (int index = 1; index < childCount; index += 2) {
_paintSeparator(context, offset, children[index]);
}
final int? highlightedChildIndex = highlightedIndex;
// Paint thumb if there's a highlighted segment.
if (highlightedChildIndex != null) {
final RenderBox selectedChild = children[highlightedChildIndex * 2];
final _SegmentedControlContainerBoxParentData childParentData = selectedChild.parentData! as _SegmentedControlContainerBoxParentData;
final Rect newThumbRect = _kThumbInsets.inflateRect(childParentData.offset & selectedChild.size);
// Update thumb animation's tween, in case the end rect changed (e.g., a
// new segment is added during the animation).
if (state.thumbController.isAnimating) {
final Animatable<Rect?>? thumbTween = state.thumbAnimatable;
if (thumbTween == null) {
// This is the first frame of the animation.
final Rect startingRect = moveThumbRectInBound(currentThumbRect, children) ?? newThumbRect;
state.thumbAnimatable = RectTween(begin: startingRect, end: newThumbRect);
} else if (newThumbRect != thumbTween.transform(1)) {
// The thumbTween of the running sliding animation needs updating,
// without restarting the animation.
final Rect startingRect = moveThumbRectInBound(currentThumbRect, children) ?? newThumbRect;
state.thumbAnimatable = RectTween(begin: startingRect, end: newThumbRect)
.chain(CurveTween(curve: Interval(state.thumbController.value, 1)));
}
} else {
state.thumbAnimatable = null;
}
final Rect unscaledThumbRect = state.thumbAnimatable?.evaluate(state.thumbController) ?? newThumbRect;
currentThumbRect = unscaledThumbRect;
final Rect thumbRect = Rect.fromCenter(
center: unscaledThumbRect.center,
width: unscaledThumbRect.width * thumbScale,
height: unscaledThumbRect.height * thumbScale,
);
_paintThumb(context, offset, thumbRect);
} else {
currentThumbRect = null;
}
for (int index = 0; index < children.length; index += 2) {
_paintChild(context, offset, children[index]);
}
}
// Paint the separator to the right of the given child.
final Paint separatorPaint = Paint();
void _paintSeparator(PaintingContext context, Offset offset, RenderBox child) {
final _SegmentedControlContainerBoxParentData childParentData = child.parentData! as _SegmentedControlContainerBoxParentData;
context.paintChild(child, offset + childParentData.offset);
}
void _paintChild(PaintingContext context, Offset offset, RenderBox child) {
final _SegmentedControlContainerBoxParentData childParentData = child.parentData! as _SegmentedControlContainerBoxParentData;
context.paintChild(child, childParentData.offset + offset);
}
void _paintThumb(PaintingContext context, Offset offset, Rect thumbRect) {
// Colors extracted from https://developer.apple.com/design/resources/.
const List<BoxShadow> thumbShadow = <BoxShadow> [
BoxShadow(
color: Color(0x1F000000),
offset: Offset(0, 3),
blurRadius: 8,
),
BoxShadow(
color: Color(0x0A000000),
offset: Offset(0, 3),
blurRadius: 1,
),
];
final RRect thumbRRect = RRect.fromRectAndRadius(thumbRect.shift(offset), _kThumbRadius);
for (final BoxShadow shadow in thumbShadow) {
context.canvas.drawRRect(thumbRRect.shift(shadow.offset), shadow.toPaint());
}
context.canvas.drawRRect(
thumbRRect.inflate(0.5),
Paint()..color = const Color(0x0A000000),
);
context.canvas.drawRRect(
thumbRRect,
Paint()..color = thumbColor,
);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
RenderBox? child = lastChild;
while (child != null) {
final _SegmentedControlContainerBoxParentData childParentData =
child.parentData! as _SegmentedControlContainerBoxParentData;
if ((childParentData.offset & child.size).contains(position)) {
return result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset localOffset) {
assert(localOffset == position - childParentData.offset);
return child!.hitTest(result, position: localOffset);
},
);
}
child = childParentData.previousSibling;
}
return false;
}
}
| flutter/packages/flutter/lib/src/cupertino/sliding_segmented_control.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/sliding_segmented_control.dart",
"repo_id": "flutter",
"token_count": 13873
} | 614 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'bitfield.dart' as bitfield;
/// The dart:io implementation of [bitfield.kMaxUnsignedSMI].
const int kMaxUnsignedSMI = 0x3FFFFFFFFFFFFFFF; // ignore: avoid_js_rounded_ints, (VM-only code)
/// The dart:io implementation of [bitfield.Bitfield].
class BitField<T extends dynamic> implements bitfield.BitField<T> {
/// The dart:io implementation of [bitfield.Bitfield()].
BitField(this._length)
: assert(_length <= _smiBits),
_bits = _allZeros;
/// The dart:io implementation of [bitfield.Bitfield.filled].
BitField.filled(this._length, bool value)
: assert(_length <= _smiBits),
_bits = value ? _allOnes : _allZeros;
final int _length;
int _bits;
static const int _smiBits = 62; // see https://www.dartlang.org/articles/numeric-computation/#smis-and-mints
static const int _allZeros = 0;
static const int _allOnes = kMaxUnsignedSMI; // 2^(_kSMIBits+1)-1
@override
bool operator [](T index) {
final int intIndex = index.index as int;
assert(intIndex < _length);
return (_bits & 1 << intIndex) > 0;
}
@override
void operator []=(T index, bool value) {
final int intIndex = index.index as int;
assert(intIndex < _length);
if (value) {
_bits = _bits | (1 << intIndex);
} else {
_bits = _bits & ~(1 << intIndex);
}
}
@override
void reset([ bool value = false ]) {
_bits = value ? _allOnes : _allZeros;
}
}
| flutter/packages/flutter/lib/src/foundation/_bitfield_io.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/_bitfield_io.dart",
"repo_id": "flutter",
"token_count": 572
} | 615 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show VoidCallback;
import 'package:meta/meta.dart';
import 'assertions.dart';
import 'diagnostics.dart';
import 'memory_allocations.dart';
export 'dart:ui' show VoidCallback;
/// An object that maintains a list of listeners.
///
/// The listeners are typically used to notify clients that the object has been
/// updated.
///
/// There are two variants of this interface:
///
/// * [ValueListenable], an interface that augments the [Listenable] interface
/// with the concept of a _current value_.
///
/// * [Animation], an interface that augments the [ValueListenable] interface
/// to add the concept of direction (forward or reverse).
///
/// Many classes in the Flutter API use or implement these interfaces. The
/// following subclasses are especially relevant:
///
/// * [ChangeNotifier], which can be subclassed or mixed in to create objects
/// that implement the [Listenable] interface.
///
/// * [ValueNotifier], which implements the [ValueListenable] interface with
/// a mutable value that triggers the notifications when modified.
///
/// The terms "notify clients", "send notifications", "trigger notifications",
/// and "fire notifications" are used interchangeably.
///
/// See also:
///
/// * [AnimatedBuilder], a widget that uses a builder callback to rebuild
/// whenever a given [Listenable] triggers its notifications. This widget is
/// commonly used with [Animation] subclasses, hence its name, but is by no
/// means limited to animations, as it can be used with any [Listenable]. It
/// is a subclass of [AnimatedWidget], which can be used to create widgets
/// that are driven from a [Listenable].
/// * [ValueListenableBuilder], a widget that uses a builder callback to
/// rebuild whenever a [ValueListenable] object triggers its notifications,
/// providing the builder with the value of the object.
/// * [InheritedNotifier], an abstract superclass for widgets that use a
/// [Listenable]'s notifications to trigger rebuilds in descendant widgets
/// that declare a dependency on them, using the [InheritedWidget] mechanism.
/// * [Listenable.merge], which creates a [Listenable] that triggers
/// notifications whenever any of a list of other [Listenable]s trigger their
/// notifications.
abstract class Listenable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const Listenable();
/// Return a [Listenable] that triggers when any of the given [Listenable]s
/// themselves trigger.
///
/// Once the factory is called, items must not be added or removed from the iterable.
/// Doing so will lead to memory leaks or exceptions.
///
/// The iterable may contain nulls; they are ignored.
factory Listenable.merge(Iterable<Listenable?> listenables) = _MergingListenable;
/// Register a closure to be called when the object notifies its listeners.
void addListener(VoidCallback listener);
/// Remove a previously registered closure from the list of closures that the
/// object notifies.
void removeListener(VoidCallback listener);
}
/// An interface for subclasses of [Listenable] that expose a [value].
///
/// This interface is implemented by [ValueNotifier<T>] and [Animation<T>], and
/// allows other APIs to accept either of those implementations interchangeably.
///
/// See also:
///
/// * [ValueListenableBuilder], a widget that uses a builder callback to
/// rebuild whenever a [ValueListenable] object triggers its notifications,
/// providing the builder with the value of the object.
abstract class ValueListenable<T> extends Listenable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const ValueListenable();
/// The current value of the object. When the value changes, the callbacks
/// registered with [addListener] will be invoked.
T get value;
}
const String _flutterFoundationLibrary = 'package:flutter/foundation.dart';
/// A class that can be extended or mixed in that provides a change notification
/// API using [VoidCallback] for notifications.
///
/// It is O(1) for adding listeners and O(N) for removing listeners and dispatching
/// notifications (where N is the number of listeners).
///
/// ## Using ChangeNotifier subclasses for data models
///
/// A data structure can extend or mix in [ChangeNotifier] to implement the
/// [Listenable] interface and thus become usable with widgets that listen for
/// changes to [Listenable]s, such as [ListenableBuilder].
///
/// {@tool dartpad}
/// The following example implements a simple counter that utilizes a
/// [ListenableBuilder] to limit rebuilds to only the [Text] widget containing
/// the count. The current count is stored in a [ChangeNotifier] subclass, which
/// rebuilds the [ListenableBuilder]'s contents when its value is changed.
///
/// ** See code in examples/api/lib/widgets/transitions/listenable_builder.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// In this case, the [ChangeNotifier] subclass encapsulates a list, and notifies
/// the clients any time an item is added to the list. This example only supports
/// adding items; as an exercise, consider adding buttons to remove items from
/// the list as well.
///
/// ** See code in examples/api/lib/widgets/transitions/listenable_builder.3.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ValueNotifier], which is a [ChangeNotifier] that wraps a single value.
mixin class ChangeNotifier implements Listenable {
int _count = 0;
// The _listeners is intentionally set to a fixed-length _GrowableList instead
// of const [].
//
// The const [] creates an instance of _ImmutableList which would be
// different from fixed-length _GrowableList used elsewhere in this class.
// keeping runtime type the same during the lifetime of this class lets the
// compiler to infer concrete type for this property, and thus improves
// performance.
static final List<VoidCallback?> _emptyListeners = List<VoidCallback?>.filled(0, null);
List<VoidCallback?> _listeners = _emptyListeners;
int _notificationCallStackDepth = 0;
int _reentrantlyRemovedListeners = 0;
bool _debugDisposed = false;
/// If true, the event [ObjectCreated] for this instance was dispatched to
/// [FlutterMemoryAllocations].
///
/// As [ChangedNotifier] is used as mixin, it does not have constructor,
/// so we use [addListener] to dispatch the event.
bool _creationDispatched = false;
/// Used by subclasses to assert that the [ChangeNotifier] has not yet been
/// disposed.
///
/// {@tool snippet}
/// The [debugAssertNotDisposed] function should only be called inside of an
/// assert, as in this example.
///
/// ```dart
/// class MyNotifier with ChangeNotifier {
/// void doUpdate() {
/// assert(ChangeNotifier.debugAssertNotDisposed(this));
/// // ...
/// }
/// }
/// ```
/// {@end-tool}
// This is static and not an instance method because too many people try to
// implement ChangeNotifier instead of extending it (and so it is too breaking
// to add a method, especially for debug).
static bool debugAssertNotDisposed(ChangeNotifier notifier) {
assert(() {
if (notifier._debugDisposed) {
throw FlutterError(
'A ${notifier.runtimeType} was used after being disposed.\n'
'Once you have called dispose() on a ${notifier.runtimeType}, it '
'can no longer be used.',
);
}
return true;
}());
return true;
}
/// Whether any listeners are currently registered.
///
/// Clients should not depend on this value for their behavior, because having
/// one listener's logic change when another listener happens to start or stop
/// listening will lead to extremely hard-to-track bugs. Subclasses might use
/// this information to determine whether to do any work when there are no
/// listeners, however; for example, resuming a [Stream] when a listener is
/// added and pausing it when a listener is removed.
///
/// Typically this is used by overriding [addListener], checking if
/// [hasListeners] is false before calling `super.addListener()`, and if so,
/// starting whatever work is needed to determine when to call
/// [notifyListeners]; and similarly, by overriding [removeListener], checking
/// if [hasListeners] is false after calling `super.removeListener()`, and if
/// so, stopping that same work.
///
/// This method returns false if [dispose] has been called.
@protected
bool get hasListeners => _count > 0;
/// Dispatches event of the [object] creation to [FlutterMemoryAllocations.instance].
///
/// If the event was already dispatched or [kFlutterMemoryAllocationsEnabled]
/// is false, the method is noop.
///
/// Tools like leak_tracker use the event of object creation to help
/// developers identify the owner of the object, for troubleshooting purposes,
/// by taking stack trace at the moment of the event.
///
/// But, as [ChangeNotifier] is mixin, it does not have its own constructor. So, it
/// communicates object creation in first `addListener`, that results
/// in the stack trace pointing to `addListener`, not to constructor.
///
/// To make debugging easier, invoke [ChangeNotifier.maybeDispatchObjectCreation]
/// in constructor of the class. It will help
/// to identify the owner.
///
/// Make sure to invoke it with condition `if (kFlutterMemoryAllocationsEnabled) ...`
/// so that the method is tree-shaken away when the flag is false.
@protected
static void maybeDispatchObjectCreation(ChangeNotifier object) {
// Tree shaker does not include this method and the class MemoryAllocations
// if kFlutterMemoryAllocationsEnabled is false.
if (kFlutterMemoryAllocationsEnabled && !object._creationDispatched) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterFoundationLibrary,
className: '$ChangeNotifier',
object: object,
);
object._creationDispatched = true;
}
}
/// Register a closure to be called when the object changes.
///
/// If the given closure is already registered, an additional instance is
/// added, and must be removed the same number of times it is added before it
/// will stop being called.
///
/// This method must not be called after [dispose] has been called.
///
/// {@template flutter.foundation.ChangeNotifier.addListener}
/// If a listener is added twice, and is removed once during an iteration
/// (e.g. in response to a notification), it will still be called again. If,
/// on the other hand, it is removed as many times as it was registered, then
/// it will no longer be called. This odd behavior is the result of the
/// [ChangeNotifier] not being able to determine which listener is being
/// removed, since they are identical, therefore it will conservatively still
/// call all the listeners when it knows that any are still registered.
///
/// This surprising behavior can be unexpectedly observed when registering a
/// listener on two separate objects which are both forwarding all
/// registrations to a common upstream object.
/// {@endtemplate}
///
/// See also:
///
/// * [removeListener], which removes a previously registered closure from
/// the list of closures that are notified when the object changes.
@override
void addListener(VoidCallback listener) {
assert(ChangeNotifier.debugAssertNotDisposed(this));
if (kFlutterMemoryAllocationsEnabled) {
maybeDispatchObjectCreation(this);
}
if (_count == _listeners.length) {
if (_count == 0) {
_listeners = List<VoidCallback?>.filled(1, null);
} else {
final List<VoidCallback?> newListeners =
List<VoidCallback?>.filled(_listeners.length * 2, null);
for (int i = 0; i < _count; i++) {
newListeners[i] = _listeners[i];
}
_listeners = newListeners;
}
}
_listeners[_count++] = listener;
}
void _removeAt(int index) {
// The list holding the listeners is not growable for performances reasons.
// We still want to shrink this list if a lot of listeners have been added
// and then removed outside a notifyListeners iteration.
// We do this only when the real number of listeners is half the length
// of our list.
_count -= 1;
if (_count * 2 <= _listeners.length) {
final List<VoidCallback?> newListeners = List<VoidCallback?>.filled(_count, null);
// Listeners before the index are at the same place.
for (int i = 0; i < index; i++) {
newListeners[i] = _listeners[i];
}
// Listeners after the index move towards the start of the list.
for (int i = index; i < _count; i++) {
newListeners[i] = _listeners[i + 1];
}
_listeners = newListeners;
} else {
// When there are more listeners than half the length of the list, we only
// shift our listeners, so that we avoid to reallocate memory for the
// whole list.
for (int i = index; i < _count; i++) {
_listeners[i] = _listeners[i + 1];
}
_listeners[_count] = null;
}
}
/// Remove a previously registered closure from the list of closures that are
/// notified when the object changes.
///
/// If the given listener is not registered, the call is ignored.
///
/// This method returns immediately if [dispose] has been called.
///
/// {@macro flutter.foundation.ChangeNotifier.addListener}
///
/// See also:
///
/// * [addListener], which registers a closure to be called when the object
/// changes.
@override
void removeListener(VoidCallback listener) {
// This method is allowed to be called on disposed instances for usability
// reasons. Due to how our frame scheduling logic between render objects and
// overlays, it is common that the owner of this instance would be disposed a
// frame earlier than the listeners. Allowing calls to this method after it
// is disposed makes it easier for listeners to properly clean up.
for (int i = 0; i < _count; i++) {
final VoidCallback? listenerAtIndex = _listeners[i];
if (listenerAtIndex == listener) {
if (_notificationCallStackDepth > 0) {
// We don't resize the list during notifyListeners iterations
// but we set to null, the listeners we want to remove. We will
// effectively resize the list at the end of all notifyListeners
// iterations.
_listeners[i] = null;
_reentrantlyRemovedListeners++;
} else {
// When we are outside the notifyListeners iterations we can
// effectively shrink the list.
_removeAt(i);
}
break;
}
}
}
/// Discards any resources used by the object. After this is called, the
/// object is not in a usable state and should be discarded (calls to
/// [addListener] will throw after the object is disposed).
///
/// This method should only be called by the object's owner.
///
/// This method does not notify listeners, and clears the listener list once
/// it is called. Consumers of this class must decide on whether to notify
/// listeners or not immediately before disposal.
@mustCallSuper
void dispose() {
assert(ChangeNotifier.debugAssertNotDisposed(this));
assert(
_notificationCallStackDepth == 0,
'The "dispose()" method on $this was called during the call to '
'"notifyListeners()". This is likely to cause errors since it modifies '
'the list of listeners while the list is being used.',
);
assert(() {
_debugDisposed = true;
return true;
}());
if (kFlutterMemoryAllocationsEnabled && _creationDispatched) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_listeners = _emptyListeners;
_count = 0;
}
/// Call all the registered listeners.
///
/// Call this method whenever the object changes, to notify any clients the
/// object may have changed. Listeners that are added during this iteration
/// will not be visited. Listeners that are removed during this iteration will
/// not be visited after they are removed.
///
/// Exceptions thrown by listeners will be caught and reported using
/// [FlutterError.reportError].
///
/// This method must not be called after [dispose] has been called.
///
/// Surprising behavior can result when reentrantly removing a listener (e.g.
/// in response to a notification) that has been registered multiple times.
/// See the discussion at [removeListener].
@protected
@visibleForTesting
@pragma('vm:notify-debugger-on-exception')
void notifyListeners() {
assert(ChangeNotifier.debugAssertNotDisposed(this));
if (_count == 0) {
return;
}
// To make sure that listeners removed during this iteration are not called,
// we set them to null, but we don't shrink the list right away.
// By doing this, we can continue to iterate on our list until it reaches
// the last listener added before the call to this method.
// To allow potential listeners to recursively call notifyListener, we track
// the number of times this method is called in _notificationCallStackDepth.
// Once every recursive iteration is finished (i.e. when _notificationCallStackDepth == 0),
// we can safely shrink our list so that it will only contain not null
// listeners.
_notificationCallStackDepth++;
final int end = _count;
for (int i = 0; i < end; i++) {
try {
_listeners[i]?.call();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'foundation library',
context: ErrorDescription('while dispatching notifications for $runtimeType'),
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<ChangeNotifier>(
'The $runtimeType sending notification was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
],
));
}
}
_notificationCallStackDepth--;
if (_notificationCallStackDepth == 0 && _reentrantlyRemovedListeners > 0) {
// We really remove the listeners when all notifications are done.
final int newLength = _count - _reentrantlyRemovedListeners;
if (newLength * 2 <= _listeners.length) {
// As in _removeAt, we only shrink the list when the real number of
// listeners is half the length of our list.
final List<VoidCallback?> newListeners = List<VoidCallback?>.filled(newLength, null);
int newIndex = 0;
for (int i = 0; i < _count; i++) {
final VoidCallback? listener = _listeners[i];
if (listener != null) {
newListeners[newIndex++] = listener;
}
}
_listeners = newListeners;
} else {
// Otherwise we put all the null references at the end.
for (int i = 0; i < newLength; i += 1) {
if (_listeners[i] == null) {
// We swap this item with the next not null item.
int swapIndex = i + 1;
while (_listeners[swapIndex] == null) {
swapIndex += 1;
}
_listeners[i] = _listeners[swapIndex];
_listeners[swapIndex] = null;
}
}
}
_reentrantlyRemovedListeners = 0;
_count = newLength;
}
}
}
class _MergingListenable extends Listenable {
_MergingListenable(this._children);
final Iterable<Listenable?> _children;
@override
void addListener(VoidCallback listener) {
for (final Listenable? child in _children) {
child?.addListener(listener);
}
}
@override
void removeListener(VoidCallback listener) {
for (final Listenable? child in _children) {
child?.removeListener(listener);
}
}
@override
String toString() {
return 'Listenable.merge([${_children.join(", ")}])';
}
}
/// A [ChangeNotifier] that holds a single value.
///
/// When [value] is replaced with something that is not equal to the old
/// value as evaluated by the equality operator ==, this class notifies its
/// listeners.
///
/// ## Limitations
///
/// Because this class only notifies listeners when the [value]'s _identity_
/// changes, listeners will not be notified when mutable state within the
/// value itself changes.
///
/// For example, a `ValueNotifier<List<int>>` will not notify its listeners
/// when the _contents_ of the list are changed.
///
/// As a result, this class is best used with only immutable data types.
///
/// For mutable data types, consider extending [ChangeNotifier] directly.
class ValueNotifier<T> extends ChangeNotifier implements ValueListenable<T> {
/// Creates a [ChangeNotifier] that wraps this value.
ValueNotifier(this._value) {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// The current value stored in this notifier.
///
/// When the value is replaced with something that is not equal to the old
/// value as evaluated by the equality operator ==, this class notifies its
/// listeners.
@override
T get value => _value;
T _value;
set value(T newValue) {
if (_value == newValue) {
return;
}
_value = newValue;
notifyListeners();
}
@override
String toString() => '${describeIdentity(this)}($value)';
}
| flutter/packages/flutter/lib/src/foundation/change_notifier.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/change_notifier.dart",
"repo_id": "flutter",
"token_count": 6685
} | 616 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
export 'dart:ui' show FlutterView;
/// The device specific gesture settings scaled into logical pixels.
///
/// This configuration can be retrieved from the window, or more commonly from a
/// [MediaQuery] widget.
///
/// See also:
///
/// * [ui.GestureSettings], the configuration that this is derived from.
@immutable
class DeviceGestureSettings {
/// Create a new [DeviceGestureSettings] with configured settings in logical
/// pixels.
const DeviceGestureSettings({
this.touchSlop,
});
/// Create a new [DeviceGestureSettings] from the provided [view].
factory DeviceGestureSettings.fromView(ui.FlutterView view) {
final double? physicalTouchSlop = view.gestureSettings.physicalTouchSlop;
return DeviceGestureSettings(
touchSlop: physicalTouchSlop == null ? null : physicalTouchSlop / view.devicePixelRatio
);
}
/// The touch slop value in logical pixels, or `null` if it was not set.
final double? touchSlop;
/// The touch slop value for pan gestures, in logical pixels, or `null` if it
/// was not set.
double? get panSlop => touchSlop != null ? (touchSlop! * 2) : null;
@override
int get hashCode => Object.hash(touchSlop, 23);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is DeviceGestureSettings
&& other.touchSlop == touchSlop;
}
@override
String toString() => 'DeviceGestureSettings(touchSlop: $touchSlop)';
}
| flutter/packages/flutter/lib/src/gestures/gesture_settings.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/gesture_settings.dart",
"repo_id": "flutter",
"token_count": 533
} | 617 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:developer' show Flow, Timeline;
import 'dart:io' show Platform;
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart' hide Flow;
import 'app_bar.dart';
import 'back_button.dart';
import 'card.dart';
import 'constants.dart';
import 'debug.dart';
import 'dialog.dart';
import 'divider.dart';
import 'floating_action_button_location.dart';
import 'ink_decoration.dart';
import 'list_tile.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'page.dart';
import 'page_transitions_theme.dart';
import 'progress_indicator.dart';
import 'scaffold.dart';
import 'scrollbar.dart';
import 'text_button.dart';
import 'text_theme.dart';
import 'theme.dart';
// Examples can assume:
// BuildContext context;
/// A [ListTile] that shows an about box.
///
/// This widget is often added to an app's [Drawer]. When tapped it shows
/// an about box dialog with [showAboutDialog].
///
/// The about box will include a button that shows licenses for software used by
/// the application. The licenses shown are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
///
/// If your application does not have a [Drawer], you should provide an
/// affordance to call [showAboutDialog] or (at least) [showLicensePage].
///
/// {@tool dartpad}
/// This sample shows two ways to open [AboutDialog]. The first one
/// uses an [AboutListTile], and the second uses the [showAboutDialog] function.
///
/// ** See code in examples/api/lib/material/about/about_list_tile.0.dart **
/// {@end-tool}
class AboutListTile extends StatelessWidget {
/// Creates a list tile for showing an about box.
///
/// The arguments are all optional. The application name, if omitted, will be
/// derived from the nearest [Title] widget. The version, icon, and legalese
/// values default to the empty string.
const AboutListTile({
super.key,
this.icon,
this.child,
this.applicationName,
this.applicationVersion,
this.applicationIcon,
this.applicationLegalese,
this.aboutBoxChildren,
this.dense,
});
/// The icon to show for this drawer item.
///
/// By default no icon is shown.
///
/// This is not necessarily the same as the image shown in the dialog box
/// itself; which is controlled by the [applicationIcon] property.
final Widget? icon;
/// The label to show on this drawer item.
///
/// Defaults to a text widget that says "About Foo" where "Foo" is the
/// application name specified by [applicationName].
final Widget? child;
/// The name of the application.
///
/// This string is used in the default label for this drawer item (see
/// [child]) and as the caption of the [AboutDialog] that is shown.
///
/// Defaults to the value of [Title.title], if a [Title] widget can be found.
/// Otherwise, defaults to [Platform.resolvedExecutable].
final String? applicationName;
/// The version of this build of the application.
///
/// This string is shown under the application name in the [AboutDialog].
///
/// Defaults to the empty string.
final String? applicationVersion;
/// The icon to show next to the application name in the [AboutDialog].
///
/// By default no icon is shown.
///
/// Typically this will be an [ImageIcon] widget. It should honor the
/// [IconTheme]'s [IconThemeData.size].
///
/// This is not necessarily the same as the icon shown on the drawer item
/// itself, which is controlled by the [icon] property.
final Widget? applicationIcon;
/// A string to show in small print in the [AboutDialog].
///
/// Typically this is a copyright notice.
///
/// Defaults to the empty string.
final String? applicationLegalese;
/// Widgets to add to the [AboutDialog] after the name, version, and legalese.
///
/// This could include a link to a Web site, some descriptive text, credits,
/// or other information to show in the about box.
///
/// Defaults to nothing.
final List<Widget>? aboutBoxChildren;
/// Whether this list tile is part of a vertically dense list.
///
/// If this property is null, then its value is based on [ListTileThemeData.dense].
///
/// Dense list tiles default to a smaller height.
final bool? dense;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
assert(debugCheckHasMaterialLocalizations(context));
return ListTile(
leading: icon,
title: child ?? Text(MaterialLocalizations.of(context).aboutListTileTitle(
applicationName ?? _defaultApplicationName(context),
)),
dense: dense,
onTap: () {
showAboutDialog(
context: context,
applicationName: applicationName,
applicationVersion: applicationVersion,
applicationIcon: applicationIcon,
applicationLegalese: applicationLegalese,
children: aboutBoxChildren,
);
},
);
}
}
/// Displays an [AboutDialog], which describes the application and provides a
/// button to show licenses for software used by the application.
///
/// The arguments correspond to the properties on [AboutDialog].
///
/// If the application has a [Drawer], consider using [AboutListTile] instead
/// of calling this directly.
///
/// If you do not need an about box in your application, you should at least
/// provide an affordance to call [showLicensePage].
///
/// The licenses shown on the [LicensePage] are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
///
/// The [context], [barrierDismissible], [barrierColor], [barrierLabel],
/// [useRootNavigator], [routeSettings] and [anchorPoint] arguments are
/// passed to [showDialog], the documentation for which discusses how it is used.
void showAboutDialog({
required BuildContext context,
String? applicationName,
String? applicationVersion,
Widget? applicationIcon,
String? applicationLegalese,
List<Widget>? children,
bool barrierDismissible = true,
Color? barrierColor,
String? barrierLabel,
bool useRootNavigator = true,
RouteSettings? routeSettings,
Offset? anchorPoint,
}) {
showDialog<void>(
context: context,
barrierDismissible: barrierDismissible,
barrierColor: barrierColor,
barrierLabel: barrierLabel,
useRootNavigator: useRootNavigator,
builder: (BuildContext context) {
return AboutDialog(
applicationName: applicationName,
applicationVersion: applicationVersion,
applicationIcon: applicationIcon,
applicationLegalese: applicationLegalese,
children: children,
);
},
routeSettings: routeSettings,
anchorPoint: anchorPoint,
);
}
/// Displays a [LicensePage], which shows licenses for software used by the
/// application.
///
/// The application arguments correspond to the properties on [LicensePage].
///
/// The `context` argument is used to look up the [Navigator] for the page.
///
/// The `useRootNavigator` argument is used to determine whether to push the
/// page to the [Navigator] furthest from or nearest to the given `context`. It
/// is `false` by default.
///
/// If the application has a [Drawer], consider using [AboutListTile] instead
/// of calling this directly.
///
/// The [AboutDialog] shown by [showAboutDialog] includes a button that calls
/// [showLicensePage].
///
/// The licenses shown on the [LicensePage] are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
void showLicensePage({
required BuildContext context,
String? applicationName,
String? applicationVersion,
Widget? applicationIcon,
String? applicationLegalese,
bool useRootNavigator = false,
}) {
Navigator.of(context, rootNavigator: useRootNavigator).push(MaterialPageRoute<void>(
builder: (BuildContext context) => LicensePage(
applicationName: applicationName,
applicationVersion: applicationVersion,
applicationIcon: applicationIcon,
applicationLegalese: applicationLegalese,
),
));
}
/// The amount of vertical space to separate chunks of text.
const double _textVerticalSeparation = 18.0;
/// An about box. This is a dialog box with the application's icon, name,
/// version number, and copyright, plus a button to show licenses for software
/// used by the application.
///
/// To show an [AboutDialog], use [showAboutDialog].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=YFCSODyFxbE}
///
/// If the application has a [Drawer], the [AboutListTile] widget can make the
/// process of showing an about dialog simpler.
///
/// The [AboutDialog] shown by [showAboutDialog] includes a button that calls
/// [showLicensePage].
///
/// The licenses shown on the [LicensePage] are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
class AboutDialog extends StatelessWidget {
/// Creates an about box.
///
/// The arguments are all optional. The application name, if omitted, will be
/// derived from the nearest [Title] widget. The version, icon, and legalese
/// values default to the empty string.
const AboutDialog({
super.key,
this.applicationName,
this.applicationVersion,
this.applicationIcon,
this.applicationLegalese,
this.children,
});
/// The name of the application.
///
/// Defaults to the value of [Title.title], if a [Title] widget can be found.
/// Otherwise, defaults to [Platform.resolvedExecutable].
final String? applicationName;
/// The version of this build of the application.
///
/// This string is shown under the application name.
///
/// Defaults to the empty string.
final String? applicationVersion;
/// The icon to show next to the application name.
///
/// By default no icon is shown.
///
/// Typically this will be an [ImageIcon] widget. It should honor the
/// [IconTheme]'s [IconThemeData.size].
final Widget? applicationIcon;
/// A string to show in small print.
///
/// Typically this is a copyright notice.
///
/// Defaults to the empty string.
final String? applicationLegalese;
/// Widgets to add to the dialog box after the name, version, and legalese.
///
/// This could include a link to a Web site, some descriptive text, credits,
/// or other information to show in the about box.
///
/// Defaults to nothing.
final List<Widget>? children;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final String name = applicationName ?? _defaultApplicationName(context);
final String version = applicationVersion ?? _defaultApplicationVersion(context);
final Widget? icon = applicationIcon ?? _defaultApplicationIcon(context);
final ThemeData themeData = Theme.of(context);
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
return AlertDialog(
content: ListBody(
children: <Widget>[
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
if (icon != null) IconTheme(data: themeData.iconTheme, child: icon),
Expanded(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 24.0),
child: ListBody(
children: <Widget>[
Text(name, style: themeData.textTheme.headlineSmall),
Text(version, style: themeData.textTheme.bodyMedium),
const SizedBox(height: _textVerticalSeparation),
Text(applicationLegalese ?? '', style: themeData.textTheme.bodySmall),
],
),
),
),
],
),
...?children,
],
),
actions: <Widget>[
TextButton(
child: Text(
themeData.useMaterial3
? localizations.viewLicensesButtonLabel
: localizations.viewLicensesButtonLabel.toUpperCase()
),
onPressed: () {
showLicensePage(
context: context,
applicationName: applicationName,
applicationVersion: applicationVersion,
applicationIcon: applicationIcon,
applicationLegalese: applicationLegalese,
);
},
),
TextButton(
child: Text(
themeData.useMaterial3
? localizations.closeButtonLabel
: localizations.closeButtonLabel.toUpperCase()
),
onPressed: () {
Navigator.pop(context);
},
),
],
scrollable: true,
);
}
}
/// A page that shows licenses for software used by the application.
///
/// To show a [LicensePage], use [showLicensePage].
///
/// The [AboutDialog] shown by [showAboutDialog] and [AboutListTile] includes
/// a button that calls [showLicensePage].
///
/// The licenses shown on the [LicensePage] are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
class LicensePage extends StatefulWidget {
/// Creates a page that shows licenses for software used by the application.
///
/// The arguments are all optional. The application name, if omitted, will be
/// derived from the nearest [Title] widget. The version and legalese values
/// default to the empty string.
///
/// The licenses shown on the [LicensePage] are those returned by the
/// [LicenseRegistry] API, which can be used to add more licenses to the list.
const LicensePage({
super.key,
this.applicationName,
this.applicationVersion,
this.applicationIcon,
this.applicationLegalese,
});
/// The name of the application.
///
/// Defaults to the value of [Title.title], if a [Title] widget can be found.
/// Otherwise, defaults to [Platform.resolvedExecutable].
final String? applicationName;
/// The version of this build of the application.
///
/// This string is shown under the application name.
///
/// Defaults to the empty string.
final String? applicationVersion;
/// The icon to show below the application name.
///
/// By default no icon is shown.
///
/// Typically this will be an [ImageIcon] widget. It should honor the
/// [IconTheme]'s [IconThemeData.size].
final Widget? applicationIcon;
/// A string to show in small print.
///
/// Typically this is a copyright notice.
///
/// Defaults to the empty string.
final String? applicationLegalese;
@override
State<LicensePage> createState() => _LicensePageState();
}
class _LicensePageState extends State<LicensePage> {
final ValueNotifier<int?> selectedId = ValueNotifier<int?>(null);
@override
void dispose() {
selectedId.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return _MasterDetailFlow(
detailPageFABlessGutterWidth: _getGutterSize(context),
title: Text(MaterialLocalizations.of(context).licensesPageTitle),
detailPageBuilder: _packageLicensePage,
masterViewBuilder: _packagesView,
);
}
Widget _packageLicensePage(BuildContext _, Object? args, ScrollController? scrollController) {
assert(args is _DetailArguments);
final _DetailArguments detailArguments = args! as _DetailArguments;
return _PackageLicensePage(
packageName: detailArguments.packageName,
licenseEntries: detailArguments.licenseEntries,
scrollController: scrollController,
);
}
Widget _packagesView(final BuildContext _, final bool isLateral) {
final Widget about = _AboutProgram(
name: widget.applicationName ?? _defaultApplicationName(context),
icon: widget.applicationIcon ?? _defaultApplicationIcon(context),
version: widget.applicationVersion ?? _defaultApplicationVersion(context),
legalese: widget.applicationLegalese,
);
return _PackagesView(
about: about,
isLateral: isLateral,
selectedId: selectedId,
);
}
}
class _AboutProgram extends StatelessWidget {
const _AboutProgram({
required this.name,
required this.version,
this.icon,
this.legalese,
});
final String name;
final String version;
final Widget? icon;
final String? legalese;
@override
Widget build(BuildContext context) {
return Padding(
padding: EdgeInsets.symmetric(
horizontal: _getGutterSize(context),
vertical: 24.0,
),
child: Column(
children: <Widget>[
Text(
name,
style: Theme.of(context).textTheme.headlineSmall,
textAlign: TextAlign.center,
),
if (icon != null)
IconTheme(data: Theme.of(context).iconTheme, child: icon!),
if (version != '')
Padding(
padding: const EdgeInsets.only(bottom: _textVerticalSeparation),
child: Text(
version,
style: Theme.of(context).textTheme.bodyMedium,
textAlign: TextAlign.center,
),
),
if (legalese != null && legalese != '')
Text(
legalese!,
style: Theme.of(context).textTheme.bodySmall,
textAlign: TextAlign.center,
),
const SizedBox(height: _textVerticalSeparation),
Text(
'Powered by Flutter',
style: Theme.of(context).textTheme.bodyMedium,
textAlign: TextAlign.center,
),
],
),
);
}
}
class _PackagesView extends StatefulWidget {
const _PackagesView({
required this.about,
required this.isLateral,
required this.selectedId,
});
final Widget about;
final bool isLateral;
final ValueNotifier<int?> selectedId;
@override
_PackagesViewState createState() => _PackagesViewState();
}
class _PackagesViewState extends State<_PackagesView> {
final Future<_LicenseData> licenses = LicenseRegistry.licenses
.fold<_LicenseData>(
_LicenseData(),
(_LicenseData prev, LicenseEntry license) => prev..addLicense(license),
)
.then((_LicenseData licenseData) => licenseData..sortPackages());
@override
Widget build(BuildContext context) {
return FutureBuilder<_LicenseData>(
future: licenses,
builder: (BuildContext context, AsyncSnapshot<_LicenseData> snapshot) {
return LayoutBuilder(
key: ValueKey<ConnectionState>(snapshot.connectionState),
builder: (BuildContext context, BoxConstraints constraints) {
switch (snapshot.connectionState) {
case ConnectionState.done:
if (snapshot.hasError) {
assert(() {
FlutterError.reportError(FlutterErrorDetails(
exception: snapshot.error!,
stack: snapshot.stackTrace,
context: ErrorDescription('while decoding the license file'),
));
return true;
}());
return Center(child: Text(snapshot.error.toString()));
}
_initDefaultDetailPage(snapshot.data!, context);
return ValueListenableBuilder<int?>(
valueListenable: widget.selectedId,
builder: (BuildContext context, int? selectedId, Widget? _) {
return Center(
child: Material(
color: Theme.of(context).cardColor,
elevation: 4.0,
child: Container(
constraints: BoxConstraints.loose(const Size.fromWidth(600.0)),
child: _packagesList(context, selectedId, snapshot.data!, widget.isLateral),
),
),
);
},
);
case ConnectionState.none:
case ConnectionState.active:
case ConnectionState.waiting:
return Material(
color: Theme.of(context).cardColor,
child: Column(
children: <Widget>[
widget.about,
const Center(child: CircularProgressIndicator()),
],
),
);
}
},
);
},
);
}
void _initDefaultDetailPage(_LicenseData data, BuildContext context) {
if (data.packages.isEmpty) {
return;
}
final String packageName = data.packages[widget.selectedId.value ?? 0];
final List<int> bindings = data.packageLicenseBindings[packageName]!;
_MasterDetailFlow.of(context).setInitialDetailPage(
_DetailArguments(
packageName,
bindings.map((int i) => data.licenses[i]).toList(growable: false),
),
);
}
Widget _packagesList(
final BuildContext context,
final int? selectedId,
final _LicenseData data,
final bool drawSelection,
) {
return ListView.builder(
itemCount: data.packages.length + 1,
itemBuilder: (BuildContext context, int index) {
if (index == 0) {
return widget.about;
}
final int packageIndex = index - 1;
final String packageName = data.packages[packageIndex];
final List<int> bindings = data.packageLicenseBindings[packageName]!;
return _PackageListTile(
packageName: packageName,
index: packageIndex,
isSelected: drawSelection && packageIndex == (selectedId ?? 0),
numberLicenses: bindings.length,
onTap: () {
widget.selectedId.value = packageIndex;
_MasterDetailFlow.of(context).openDetailPage(_DetailArguments(
packageName,
bindings.map((int i) => data.licenses[i]).toList(growable: false),
));
},
);
},
);
}
}
class _PackageListTile extends StatelessWidget {
const _PackageListTile({
required this.packageName,
this.index,
required this.isSelected,
required this.numberLicenses,
this.onTap,
});
final String packageName;
final int? index;
final bool isSelected;
final int numberLicenses;
final GestureTapCallback? onTap;
@override
Widget build(BuildContext context) {
return Ink(
color: isSelected ? Theme.of(context).highlightColor : Theme.of(context).cardColor,
child: ListTile(
title: Text(packageName),
subtitle: Text(MaterialLocalizations.of(context).licensesPackageDetailText(numberLicenses)),
selected: isSelected,
onTap: onTap,
),
);
}
}
/// This is a collection of licenses and the packages to which they apply.
/// [packageLicenseBindings] records the m+:n+ relationship between the license
/// and packages as a map of package names to license indexes.
class _LicenseData {
final List<LicenseEntry> licenses = <LicenseEntry>[];
final Map<String, List<int>> packageLicenseBindings = <String, List<int>>{};
final List<String> packages = <String>[];
// Special treatment for the first package since it should be the package
// for delivered application.
String? firstPackage;
void addLicense(LicenseEntry entry) {
// Before the license can be added, we must first record the packages to
// which it belongs.
for (final String package in entry.packages) {
_addPackage(package);
// Bind this license to the package using the next index value. This
// creates a contract that this license must be inserted at this same
// index value.
packageLicenseBindings[package]!.add(licenses.length);
}
licenses.add(entry); // Completion of the contract above.
}
/// Add a package and initialize package license binding. This is a no-op if
/// the package has been seen before.
void _addPackage(String package) {
if (!packageLicenseBindings.containsKey(package)) {
packageLicenseBindings[package] = <int>[];
firstPackage ??= package;
packages.add(package);
}
}
/// Sort the packages using some comparison method, or by the default manner,
/// which is to put the application package first, followed by every other
/// package in case-insensitive alphabetical order.
void sortPackages([int Function(String a, String b)? compare]) {
packages.sort(compare ?? (String a, String b) {
// Based on how LicenseRegistry currently behaves, the first package
// returned is the end user application license. This should be
// presented first in the list. So here we make sure that first package
// remains at the front regardless of alphabetical sorting.
if (a == firstPackage) {
return -1;
}
if (b == firstPackage) {
return 1;
}
return a.toLowerCase().compareTo(b.toLowerCase());
});
}
}
@immutable
class _DetailArguments {
const _DetailArguments(this.packageName, this.licenseEntries);
final String packageName;
final List<LicenseEntry> licenseEntries;
@override
bool operator ==(final Object other) {
if (other is _DetailArguments) {
return other.packageName == packageName;
}
return other == this;
}
@override
int get hashCode => Object.hash(packageName, Object.hashAll(licenseEntries));
}
class _PackageLicensePage extends StatefulWidget {
const _PackageLicensePage({
required this.packageName,
required this.licenseEntries,
required this.scrollController,
});
final String packageName;
final List<LicenseEntry> licenseEntries;
final ScrollController? scrollController;
@override
_PackageLicensePageState createState() => _PackageLicensePageState();
}
class _PackageLicensePageState extends State<_PackageLicensePage> {
@override
void initState() {
super.initState();
_initLicenses();
}
final List<Widget> _licenses = <Widget>[];
bool _loaded = false;
Future<void> _initLicenses() async {
int debugFlowId = -1;
assert(() {
final Flow flow = Flow.begin();
Timeline.timeSync('_initLicenses()', () { }, flow: flow);
debugFlowId = flow.id;
return true;
}());
for (final LicenseEntry license in widget.licenseEntries) {
if (!mounted) {
return;
}
assert(() {
Timeline.timeSync('_initLicenses()', () { }, flow: Flow.step(debugFlowId));
return true;
}());
final List<LicenseParagraph> paragraphs =
await SchedulerBinding.instance.scheduleTask<List<LicenseParagraph>>(
license.paragraphs.toList,
Priority.animation,
debugLabel: 'License',
);
if (!mounted) {
return;
}
setState(() {
_licenses.add(const Padding(
padding: EdgeInsets.all(18.0),
child: Divider(),
));
for (final LicenseParagraph paragraph in paragraphs) {
if (paragraph.indent == LicenseParagraph.centeredIndent) {
_licenses.add(Padding(
padding: const EdgeInsets.only(top: 16.0),
child: Text(
paragraph.text,
style: const TextStyle(fontWeight: FontWeight.bold),
textAlign: TextAlign.center,
),
));
} else {
assert(paragraph.indent >= 0);
_licenses.add(Padding(
padding: EdgeInsetsDirectional.only(top: 8.0, start: 16.0 * paragraph.indent),
child: Text(paragraph.text),
));
}
}
});
}
setState(() {
_loaded = true;
});
assert(() {
Timeline.timeSync('Build scheduled', () { }, flow: Flow.end(debugFlowId));
return true;
}());
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final ThemeData theme = Theme.of(context);
final String title = widget.packageName;
final String subtitle = localizations.licensesPackageDetailText(widget.licenseEntries.length);
final double pad = _getGutterSize(context);
final EdgeInsets padding = EdgeInsets.only(left: pad, right: pad, bottom: pad);
final List<Widget> listWidgets = <Widget>[
..._licenses,
if (!_loaded)
const Padding(
padding: EdgeInsets.symmetric(vertical: 24.0),
child: Center(
child: CircularProgressIndicator(),
),
),
];
final Widget page;
if (widget.scrollController == null) {
page = Scaffold(
appBar: AppBar(
title: _PackageLicensePageTitle(
title: title,
subtitle: subtitle,
theme: theme.useMaterial3 ? theme.textTheme : theme.primaryTextTheme,
titleTextStyle: theme.appBarTheme.titleTextStyle,
foregroundColor: theme.appBarTheme.foregroundColor,
),
),
body: Center(
child: Material(
color: theme.cardColor,
elevation: 4.0,
child: Container(
constraints: BoxConstraints.loose(const Size.fromWidth(600.0)),
child: Localizations.override(
locale: const Locale('en', 'US'),
context: context,
child: ScrollConfiguration(
// A Scrollbar is built-in below.
behavior: ScrollConfiguration.of(context).copyWith(scrollbars: false),
child: Scrollbar(
child: ListView(
primary: true,
padding: padding,
children: listWidgets,
),
),
),
),
),
),
),
);
} else {
page = CustomScrollView(
controller: widget.scrollController,
slivers: <Widget>[
SliverAppBar(
automaticallyImplyLeading: false,
pinned: true,
backgroundColor: theme.cardColor,
title: _PackageLicensePageTitle(
title: title,
subtitle: subtitle,
theme: theme.textTheme,
titleTextStyle: theme.textTheme.titleLarge,
),
),
SliverPadding(
padding: padding,
sliver: SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) => Localizations.override(
locale: const Locale('en', 'US'),
context: context,
child: listWidgets[index],
),
childCount: listWidgets.length,
),
),
),
],
);
}
return DefaultTextStyle(
style: theme.textTheme.bodySmall!,
child: page,
);
}
}
class _PackageLicensePageTitle extends StatelessWidget {
const _PackageLicensePageTitle({
required this.title,
required this.subtitle,
required this.theme,
this.titleTextStyle,
this.foregroundColor,
});
final String title;
final String subtitle;
final TextTheme theme;
final TextStyle? titleTextStyle;
final Color? foregroundColor;
@override
Widget build(BuildContext context) {
final TextStyle? effectiveTitleTextStyle = titleTextStyle ?? theme.titleLarge;
return Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(title, style: effectiveTitleTextStyle?.copyWith(color: foregroundColor)),
Text(subtitle, style: theme.titleSmall?.copyWith(color: foregroundColor)),
],
);
}
}
String _defaultApplicationName(BuildContext context) {
// This doesn't handle the case of the application's title dynamically
// changing. In theory, we should make Title expose the current application
// title using an InheritedWidget, and so forth. However, in practice, if
// someone really wants their application title to change dynamically, they
// can provide an explicit applicationName to the widgets defined in this
// file, instead of relying on the default.
final Title? ancestorTitle = context.findAncestorWidgetOfExactType<Title>();
return ancestorTitle?.title ?? Platform.resolvedExecutable.split(Platform.pathSeparator).last;
}
String _defaultApplicationVersion(BuildContext context) {
// TODO(ianh): Get this from the embedder somehow.
return '';
}
Widget? _defaultApplicationIcon(BuildContext context) {
// TODO(ianh): Get this from the embedder somehow.
return null;
}
const int _materialGutterThreshold = 720;
const double _wideGutterSize = 24.0;
const double _narrowGutterSize = 12.0;
double _getGutterSize(BuildContext context) =>
MediaQuery.sizeOf(context).width >= _materialGutterThreshold ? _wideGutterSize : _narrowGutterSize;
/// Signature for the builder callback used by [_MasterDetailFlow].
typedef _MasterViewBuilder = Widget Function(BuildContext context, bool isLateralUI);
/// Signature for the builder callback used by [_MasterDetailFlow.detailPageBuilder].
///
/// scrollController is provided when the page destination is the draggable
/// sheet in the lateral UI. Otherwise, it is null.
typedef _DetailPageBuilder = Widget Function(BuildContext context, Object? arguments, ScrollController? scrollController);
/// Signature for the builder callback used by [_MasterDetailFlow.actionBuilder].
///
/// Builds the actions that go in the app bars constructed for the master and
/// lateral UI pages. actionLevel indicates the intended destination of the
/// return actions.
typedef _ActionBuilder = List<Widget> Function(BuildContext context, _ActionLevel actionLevel);
/// Describes which type of app bar the actions are intended for.
enum _ActionLevel {
/// Indicates the top app bar in the lateral UI.
top,
/// Indicates the master view app bar in the lateral UI.
view,
}
/// Describes which layout will be used by [_MasterDetailFlow].
enum _LayoutMode {
/// Always use a lateral layout.
lateral,
/// Always use a nested layout.
nested,
}
const String _navMaster = 'master';
const String _navDetail = 'detail';
enum _Focus { master, detail }
/// A Master Detail Flow widget. Depending on screen width it builds either a
/// lateral or nested navigation flow between a master view and a detail page.
/// bloc pattern.
///
/// If focus is on detail view, then switching to nested navigation will
/// populate the navigation history with the master page and the detail page on
/// top. Otherwise the focus is on the master view and just the master page
/// is shown.
class _MasterDetailFlow extends StatefulWidget {
/// Creates a master detail navigation flow which is either nested or
/// lateral depending on screen width.
const _MasterDetailFlow({
required this.detailPageBuilder,
required this.masterViewBuilder,
this.detailPageFABlessGutterWidth,
this.title,
});
/// Builder for the master view for lateral navigation.
///
/// If [masterPageBuilder] is not supplied the master page required for nested navigation, also
/// builds the master view inside a [Scaffold] with an [AppBar].
final _MasterViewBuilder masterViewBuilder;
/// Builder for the detail page.
///
/// If scrollController == null, the page is intended for nested navigation. The lateral detail
/// page is inside a [DraggableScrollableSheet] and should have a scrollable element that uses
/// the [ScrollController] provided. In fact, it is strongly recommended the entire lateral
/// page is scrollable.
final _DetailPageBuilder detailPageBuilder;
/// Override the width of the gutter when there is no floating action button.
final double? detailPageFABlessGutterWidth;
/// The title for the lateral UI [AppBar].
///
/// See [AppBar.title].
final Widget? title;
@override
_MasterDetailFlowState createState() => _MasterDetailFlowState();
// The master detail flow proxy from the closest instance of this class that encloses the given
// context.
//
// Typical usage is as follows:
//
// ```dart
// _MasterDetailFlow.of(context).openDetailPage(arguments);
// ```
static _MasterDetailFlowProxy of(BuildContext context) {
_PageOpener? pageOpener = context.findAncestorStateOfType<_MasterDetailScaffoldState>();
pageOpener ??= context.findAncestorStateOfType<_MasterDetailFlowState>();
assert(() {
if (pageOpener == null) {
throw FlutterError(
'Master Detail operation requested with a context that does not include a Master Detail '
'Flow.\nThe context used to open a detail page from the Master Detail Flow must be '
'that of a widget that is a descendant of a Master Detail Flow widget.',
);
}
return true;
}());
return _MasterDetailFlowProxy._(pageOpener!);
}
}
/// Interface for interacting with the [_MasterDetailFlow].
class _MasterDetailFlowProxy implements _PageOpener {
_MasterDetailFlowProxy._(this._pageOpener);
final _PageOpener _pageOpener;
/// Open detail page with arguments.
@override
void openDetailPage(Object arguments) =>
_pageOpener.openDetailPage(arguments);
/// Set the initial page to be open for the lateral layout. This can be set at any time, but
/// will have no effect after any calls to openDetailPage.
@override
void setInitialDetailPage(Object arguments) =>
_pageOpener.setInitialDetailPage(arguments);
}
abstract class _PageOpener {
void openDetailPage(Object arguments);
void setInitialDetailPage(Object arguments);
}
const int _materialWideDisplayThreshold = 840;
class _MasterDetailFlowState extends State<_MasterDetailFlow> implements _PageOpener {
/// Tracks whether focus is on the detail or master views. Determines behavior when switching
/// from lateral to nested navigation.
_Focus focus = _Focus.master;
/// Cache of arguments passed when opening a detail page. Used when rebuilding.
Object? _cachedDetailArguments;
/// Record of the layout that was built.
_LayoutMode? _builtLayout;
/// Key to access navigator in the nested layout.
final GlobalKey<NavigatorState> _navigatorKey = GlobalKey<NavigatorState>();
@override
void openDetailPage(Object arguments) {
_cachedDetailArguments = arguments;
switch (_builtLayout) {
case _LayoutMode.nested:
_navigatorKey.currentState!.pushNamed(_navDetail, arguments: arguments);
case _LayoutMode.lateral || null:
focus = _Focus.detail;
}
}
@override
void setInitialDetailPage(Object arguments) {
_cachedDetailArguments = arguments;
}
@override
Widget build(BuildContext context) {
return LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
final double availableWidth = constraints.maxWidth;
if (availableWidth >= _materialWideDisplayThreshold) {
return _lateralUI(context);
}
return _nestedUI(context);
});
}
Widget _nestedUI(BuildContext context) {
_builtLayout = _LayoutMode.nested;
final MaterialPageRoute<void> masterPageRoute = _masterPageRoute(context);
return NavigatorPopHandler(
onPop: () {
_navigatorKey.currentState!.maybePop();
},
child: Navigator(
key: _navigatorKey,
initialRoute: 'initial',
onGenerateInitialRoutes: (NavigatorState navigator, String initialRoute) {
return switch (focus) {
_Focus.master => <Route<void>>[masterPageRoute],
_Focus.detail => <Route<void>>[masterPageRoute, _detailPageRoute(_cachedDetailArguments)],
};
},
onGenerateRoute: (RouteSettings settings) {
switch (settings.name) {
case _navMaster:
// Matching state to navigation event.
focus = _Focus.master;
return masterPageRoute;
case _navDetail:
// Matching state to navigation event.
focus = _Focus.detail;
// Cache detail page settings.
_cachedDetailArguments = settings.arguments;
return _detailPageRoute(_cachedDetailArguments);
default:
throw Exception('Unknown route ${settings.name}');
}
},
),
);
}
MaterialPageRoute<void> _masterPageRoute(BuildContext context) {
return MaterialPageRoute<dynamic>(
builder: (BuildContext c) {
return BlockSemantics(
child: _MasterPage(
leading: Navigator.of(context).canPop()
? BackButton(onPressed: () { Navigator.of(context).pop(); })
: null,
title: widget.title,
masterViewBuilder: widget.masterViewBuilder,
),
);
},
);
}
MaterialPageRoute<void> _detailPageRoute(Object? arguments) {
return MaterialPageRoute<dynamic>(builder: (BuildContext context) {
return PopScope(
onPopInvoked: (bool didPop) {
// No need for setState() as rebuild happens on navigation pop.
focus = _Focus.master;
},
child: BlockSemantics(child: widget.detailPageBuilder(context, arguments, null)),
);
});
}
Widget _lateralUI(BuildContext context) {
_builtLayout = _LayoutMode.lateral;
return _MasterDetailScaffold(
actionBuilder: (_, __) => const<Widget>[],
detailPageBuilder: (BuildContext context, Object? args, ScrollController? scrollController) =>
widget.detailPageBuilder(context, args ?? _cachedDetailArguments, scrollController),
detailPageFABlessGutterWidth: widget.detailPageFABlessGutterWidth,
initialArguments: _cachedDetailArguments,
masterViewBuilder: (BuildContext context, bool isLateral) => widget.masterViewBuilder(context, isLateral),
title: widget.title,
);
}
}
class _MasterPage extends StatelessWidget {
const _MasterPage({
this.leading,
this.title,
this.masterViewBuilder,
});
final _MasterViewBuilder? masterViewBuilder;
final Widget? title;
final Widget? leading;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: title,
leading: leading,
actions: const <Widget>[],
),
body: masterViewBuilder!(context, false),
);
}
}
const double _kCardElevation = 4.0;
const double _kMasterViewWidth = 320.0;
const double _kDetailPageFABlessGutterWidth = 40.0;
const double _kDetailPageFABGutterWidth = 84.0;
class _MasterDetailScaffold extends StatefulWidget {
const _MasterDetailScaffold({
required this.detailPageBuilder,
required this.masterViewBuilder,
this.actionBuilder,
this.initialArguments,
this.title,
this.detailPageFABlessGutterWidth,
});
final _MasterViewBuilder masterViewBuilder;
/// Builder for the detail page.
///
/// The detail page is inside a [DraggableScrollableSheet] and should have a scrollable element
/// that uses the [ScrollController] provided. In fact, it is strongly recommended the entire
/// lateral page is scrollable.
final _DetailPageBuilder detailPageBuilder;
final _ActionBuilder? actionBuilder;
final Object? initialArguments;
final Widget? title;
final double? detailPageFABlessGutterWidth;
@override
_MasterDetailScaffoldState createState() => _MasterDetailScaffoldState();
}
class _MasterDetailScaffoldState extends State<_MasterDetailScaffold>
implements _PageOpener {
late FloatingActionButtonLocation floatingActionButtonLocation;
late double detailPageFABGutterWidth;
late double detailPageFABlessGutterWidth;
late double masterViewWidth;
final ValueNotifier<Object?> _detailArguments = ValueNotifier<Object?>(null);
@override
void initState() {
super.initState();
detailPageFABlessGutterWidth = widget.detailPageFABlessGutterWidth ?? _kDetailPageFABlessGutterWidth;
detailPageFABGutterWidth = _kDetailPageFABGutterWidth;
masterViewWidth = _kMasterViewWidth;
floatingActionButtonLocation = FloatingActionButtonLocation.endTop;
}
@override
void dispose() {
_detailArguments.dispose();
super.dispose();
}
@override
void openDetailPage(Object arguments) {
SchedulerBinding.instance.addPostFrameCallback((_) => _detailArguments.value = arguments);
_MasterDetailFlow.of(context).openDetailPage(arguments);
}
@override
void setInitialDetailPage(Object arguments) {
SchedulerBinding.instance.addPostFrameCallback((_) => _detailArguments.value = arguments);
_MasterDetailFlow.of(context).setInitialDetailPage(arguments);
}
@override
Widget build(BuildContext context) {
return Stack(
children: <Widget>[
Scaffold(
floatingActionButtonLocation: floatingActionButtonLocation,
appBar: AppBar(
title: widget.title,
actions: widget.actionBuilder!(context, _ActionLevel.top),
bottom: PreferredSize(
preferredSize: const Size.fromHeight(kToolbarHeight),
child: Row(
children: <Widget>[
ConstrainedBox(
constraints: BoxConstraints.tightFor(width: masterViewWidth),
child: IconTheme(
data: Theme.of(context).primaryIconTheme,
child: Container(
alignment: AlignmentDirectional.centerEnd,
padding: const EdgeInsets.all(8),
child: OverflowBar(
spacing: 8,
overflowAlignment: OverflowBarAlignment.end,
children: widget.actionBuilder!(context, _ActionLevel.view),
),
),
),
),
],
),
),
),
body: Align(
alignment: AlignmentDirectional.centerStart,
child: _masterPanel(context),
),
),
// Detail view stacked above main scaffold and master view.
SafeArea(
child: Padding(
padding: EdgeInsetsDirectional.only(
start: masterViewWidth - _kCardElevation,
end: detailPageFABlessGutterWidth,
),
child: ValueListenableBuilder<Object?>(
valueListenable: _detailArguments,
builder: (BuildContext context, Object? value, Widget? child) {
return AnimatedSwitcher(
transitionBuilder: (Widget child, Animation<double> animation) =>
const FadeUpwardsPageTransitionsBuilder().buildTransitions<void>(
null,
null,
animation,
null,
child,
),
duration: const Duration(milliseconds: 500),
child: Container(
key: ValueKey<Object?>(value ?? widget.initialArguments),
constraints: const BoxConstraints.expand(),
child: _DetailView(
builder: widget.detailPageBuilder,
arguments: value ?? widget.initialArguments,
),
),
);
},
),
),
),
],
);
}
ConstrainedBox _masterPanel(BuildContext context, {bool needsScaffold = false}) {
return ConstrainedBox(
constraints: BoxConstraints(maxWidth: masterViewWidth),
child: needsScaffold
? Scaffold(
appBar: AppBar(
title: widget.title,
actions: widget.actionBuilder!(context, _ActionLevel.top),
),
body: widget.masterViewBuilder(context, true),
)
: widget.masterViewBuilder(context, true),
);
}
}
class _DetailView extends StatelessWidget {
const _DetailView({
required _DetailPageBuilder builder,
Object? arguments,
}) : _builder = builder,
_arguments = arguments;
final _DetailPageBuilder _builder;
final Object? _arguments;
@override
Widget build(BuildContext context) {
if (_arguments == null) {
return const SizedBox.shrink();
}
final double screenHeight = MediaQuery.sizeOf(context).height;
final double minHeight = (screenHeight - kToolbarHeight) / screenHeight;
return DraggableScrollableSheet(
initialChildSize: minHeight,
minChildSize: minHeight,
expand: false,
builder: (BuildContext context, ScrollController controller) {
return MouseRegion(
// TODO(TonicArtos): Remove MouseRegion workaround for pointer hover events passing through DraggableScrollableSheet once https://github.com/flutter/flutter/issues/59741 is resolved.
child: Card(
color: Theme.of(context).cardColor,
elevation: _kCardElevation,
clipBehavior: Clip.antiAlias,
margin: const EdgeInsets.fromLTRB(_kCardElevation, 0.0, _kCardElevation, 0.0),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(top: Radius.circular(3.0)),
),
child: _builder(
context,
_arguments,
controller,
),
),
);
},
);
}
}
| flutter/packages/flutter/lib/src/material/about.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/about.dart",
"repo_id": "flutter",
"token_count": 18636
} | 618 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$menu_close = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 26.0),
Offset(6.667958372815065, 25.652081003354123),
Offset(8.330956385969174, 24.584197933972426),
Offset(10.795082531480682, 22.920903618043887),
Offset(14.118850428921743, 21.151292868049936),
Offset(18.25264983114299, 20.14945205026408),
Offset(21.311663261847183, 21.835975547204264),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 26.0),
Offset(6.667958372815065, 25.652081003354123),
Offset(8.330956385969174, 24.584197933972426),
Offset(10.795082531480682, 22.920903618043887),
Offset(14.118850428921743, 21.151292868049936),
Offset(18.25264983114299, 20.14945205026408),
Offset(21.311663261847183, 21.835975547204264),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
<Offset>[
Offset(42.0, 26.0),
Offset(41.25166967016726, 26.34711145869683),
Offset(39.30700496104292, 27.399384375173792),
Offset(36.28104377724833, 28.970941368922745),
Offset(32.04675383085589, 30.403280561808284),
Offset(26.655308355431437, 30.38812108642142),
Offset(22.838515792338228, 27.24978625225603),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
<Offset>[
Offset(42.0, 26.0),
Offset(41.25166967016726, 26.34711145869683),
Offset(39.30700496104292, 27.399384375173792),
Offset(36.28104377724833, 28.970941368922745),
Offset(32.04675383085589, 30.403280561808284),
Offset(26.655308355431437, 30.38812108642142),
Offset(22.838515792338228, 27.24978625225603),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 26.0),
Offset(41.25166967016726, 26.34711145869683),
Offset(39.30700496104292, 27.399384375173792),
Offset(36.28104377724833, 28.970941368922745),
Offset(32.04675383085589, 30.403280561808284),
Offset(26.655308355431437, 30.38812108642142),
Offset(22.838515792338228, 27.24978625225603),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
<Offset>[
Offset(42.0, 22.0),
Offset(41.332041627184935, 22.347918996645877),
Offset(39.669043614130416, 23.415802066036626),
Offset(37.20491746851932, 25.079096381956113),
Offset(33.88114957098939, 26.848707131904206),
Offset(29.747350168882384, 27.85054794976684),
Offset(26.688336738152817, 26.164024452795736),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
<Offset>[
Offset(42.0, 22.0),
Offset(41.332041627184935, 22.347918996645877),
Offset(39.669043614130416, 23.415802066036626),
Offset(37.20491746851932, 25.079096381956113),
Offset(33.88114957098939, 26.848707131904206),
Offset(29.747350168882384, 27.85054794976684),
Offset(26.688336738152817, 26.164024452795736),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 22.0),
Offset(41.332041627184935, 22.347918996645877),
Offset(39.669043614130416, 23.415802066036626),
Offset(37.20491746851932, 25.079096381956113),
Offset(33.88114957098939, 26.848707131904206),
Offset(29.747350168882384, 27.85054794976684),
Offset(26.688336738152817, 26.164024452795736),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
<Offset>[
Offset(6.0, 22.0),
Offset(6.74833032983274, 21.65288854130317),
Offset(8.692995039056669, 20.60061562483526),
Offset(11.718956222751673, 19.029058631077255),
Offset(15.953246169055248, 17.596719438145858),
Offset(21.344691644593937, 17.6118789136095),
Offset(25.161484207661772, 20.75021374774397),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
<Offset>[
Offset(6.0, 22.0),
Offset(6.74833032983274, 21.65288854130317),
Offset(8.692995039056669, 20.60061562483526),
Offset(11.718956222751673, 19.029058631077255),
Offset(15.953246169055248, 17.596719438145858),
Offset(21.344691644593937, 17.6118789136095),
Offset(25.161484207661772, 20.75021374774397),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 22.0),
Offset(6.74833032983274, 21.65288854130317),
Offset(8.692995039056669, 20.60061562483526),
Offset(11.718956222751673, 19.029058631077255),
Offset(15.953246169055248, 17.596719438145858),
Offset(21.344691644593937, 17.6118789136095),
Offset(25.161484207661772, 20.75021374774397),
Offset(25.982330778947503, 24.265263421597062),
Offset(25.77497547903557, 24.921662654566433),
Offset(25.464524005437774, 25.362046048229097),
Offset(25.148607506117536, 25.637284580300705),
Offset(24.8667640070585, 25.802420637938855),
Offset(24.630478422058573, 25.898024488598217),
Offset(24.43972594011363, 25.95106153095979),
Offset(24.290385908577957, 25.97880671721605),
Offset(24.177354699003402, 25.992120807265813),
Offset(24.09573805889554, 25.997707241834732),
Offset(24.041261664485504, 25.999574323460838),
Offset(24.010339588711656, 25.999973273047736),
Offset(24.000021633565517, 25.999999999883),
Offset(23.999999999999996, 26.000000000000004),
],
<Offset>[
Offset(6.0, 26.0),
Offset(6.667958372815065, 25.652081003354123),
Offset(8.330956385969174, 24.584197933972426),
Offset(10.795082531480682, 22.920903618043887),
Offset(14.118850428921743, 21.151292868049936),
Offset(18.25264983114299, 20.14945205026408),
Offset(21.311663261847183, 21.835975547204264),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
<Offset>[
Offset(6.0, 26.0),
Offset(6.667958372815065, 25.652081003354123),
Offset(8.330956385969174, 24.584197933972426),
Offset(10.795082531480682, 22.920903618043887),
Offset(14.118850428921743, 21.151292868049936),
Offset(18.25264983114299, 20.14945205026408),
Offset(21.311663261847183, 21.835975547204264),
Offset(22.017669221052497, 23.734736578402938),
Offset(22.22502452096443, 23.078337345433567),
Offset(22.535475994562226, 22.637953951770903),
Offset(22.851392493882464, 22.362715419699295),
Offset(23.1332359929415, 22.197579362061152),
Offset(23.369521577941427, 22.101975511401783),
Offset(23.560274059886364, 22.048938469040202),
Offset(23.709614091422043, 22.02119328278395),
Offset(23.822645300996605, 22.00787919273418),
Offset(23.90426194110445, 22.002292758165275),
Offset(23.958738335514504, 22.000425676539155),
Offset(23.989660411288344, 22.000026726952264),
Offset(23.999978366434483, 22.000000000117),
Offset(23.999999999999996, 22.000000000000004),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 36.0),
Offset(5.755802622931704, 35.48132577125743),
Offset(5.010307637171476, 33.62392385238556),
Offset(4.045724406149144, 29.753229622188503),
Offset(4.0861862642629525, 23.09758660034176),
Offset(8.564534830281378, 13.380886967716135),
Offset(17.231202711318005, 7.518259082609372),
Offset(27.314036258810987, 7.072010923819601),
Offset(34.88234825995056, 10.868941008448914),
Offset(39.083273856489825, 15.969526394266794),
Offset(40.919225828875916, 20.778998598927743),
Offset(41.3739602310385, 24.78219833097991),
Offset(41.11406980357167, 27.929167557007872),
Offset(40.542525130544135, 30.325075504900447),
Offset(39.89012097509991, 32.10612260851932),
Offset(39.28321027503917, 33.39611499843784),
Offset(38.78633478113526, 34.295159061960966),
Offset(38.427830072911185, 34.87959495005215),
Offset(38.21486700558917, 35.20562675712161),
Offset(38.14228859445484, 35.31348285156429),
Offset(38.14213562373095, 35.31370849898477),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 36.0),
Offset(5.755802622931704, 35.48132577125743),
Offset(5.010307637171476, 33.62392385238556),
Offset(4.045724406149144, 29.753229622188503),
Offset(4.0861862642629525, 23.09758660034176),
Offset(8.564534830281378, 13.380886967716135),
Offset(17.231202711318005, 7.518259082609372),
Offset(27.314036258810987, 7.072010923819601),
Offset(34.88234825995056, 10.868941008448914),
Offset(39.083273856489825, 15.969526394266794),
Offset(40.919225828875916, 20.778998598927743),
Offset(41.3739602310385, 24.78219833097991),
Offset(41.11406980357167, 27.929167557007872),
Offset(40.542525130544135, 30.325075504900447),
Offset(39.89012097509991, 32.10612260851932),
Offset(39.28321027503917, 33.39611499843784),
Offset(38.78633478113526, 34.295159061960966),
Offset(38.427830072911185, 34.87959495005215),
Offset(38.21486700558917, 35.20562675712161),
Offset(38.14228859445484, 35.31348285156429),
Offset(38.14213562373095, 35.31370849898477),
],
<Offset>[
Offset(42.0, 36.0),
Offset(41.74444683546158, 36.38547605961641),
Offset(40.779522756565214, 37.69372111300368),
Offset(38.528666977308376, 40.09376498715554),
Offset(33.888986943996294, 43.29147358735062),
Offset(24.750542697847216, 45.53696181075469),
Offset(15.464766435530956, 43.474895505995576),
Offset(8.222494539070887, 37.592709388360404),
Offset(4.758504180127748, 30.580714593150105),
Offset(4.129263053465191, 24.58446277139909),
Offset(4.926943149181838, 20.033621174401183),
Offset(6.282139476517855, 16.74701842430737),
Offset(7.742736159475957, 14.425312891970705),
Offset(9.093991024465053, 12.803953891864928),
Offset(10.244527895379168, 11.682146233885181),
Offset(11.164395063408941, 10.916519114743828),
Offset(11.853227009710306, 10.407762682849047),
Offset(12.32400850869499, 10.088659111654252),
Offset(12.595052974338124, 9.914815599625843),
Offset(12.68610028619067, 9.857982919050801),
Offset(12.68629150101523, 9.85786437626906),
],
<Offset>[
Offset(42.0, 36.0),
Offset(41.74444683546158, 36.38547605961641),
Offset(40.779522756565214, 37.69372111300368),
Offset(38.528666977308376, 40.09376498715554),
Offset(33.888986943996294, 43.29147358735062),
Offset(24.750542697847216, 45.53696181075469),
Offset(15.464766435530956, 43.474895505995576),
Offset(8.222494539070887, 37.592709388360404),
Offset(4.758504180127748, 30.580714593150105),
Offset(4.129263053465191, 24.58446277139909),
Offset(4.926943149181838, 20.033621174401183),
Offset(6.282139476517855, 16.74701842430737),
Offset(7.742736159475957, 14.425312891970705),
Offset(9.093991024465053, 12.803953891864928),
Offset(10.244527895379168, 11.682146233885181),
Offset(11.164395063408941, 10.916519114743828),
Offset(11.853227009710306, 10.407762682849047),
Offset(12.32400850869499, 10.088659111654252),
Offset(12.595052974338124, 9.914815599625843),
Offset(12.68610028619067, 9.857982919050801),
Offset(12.68629150101523, 9.85786437626906),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 36.0),
Offset(41.74444683546158, 36.38547605961641),
Offset(40.779522756565214, 37.69372111300368),
Offset(38.528666977308376, 40.09376498715554),
Offset(33.888986943996294, 43.29147358735062),
Offset(24.750542697847216, 45.53696181075469),
Offset(15.464766435530956, 43.474895505995576),
Offset(8.222494539070887, 37.592709388360404),
Offset(4.758504180127748, 30.580714593150105),
Offset(4.129263053465191, 24.58446277139909),
Offset(4.926943149181838, 20.033621174401183),
Offset(6.282139476517855, 16.74701842430737),
Offset(7.742736159475957, 14.425312891970705),
Offset(9.093991024465053, 12.803953891864928),
Offset(10.244527895379168, 11.682146233885181),
Offset(11.164395063408941, 10.916519114743828),
Offset(11.853227009710306, 10.407762682849047),
Offset(12.32400850869499, 10.088659111654252),
Offset(12.595052974338124, 9.914815599625843),
Offset(12.68610028619067, 9.857982919050801),
Offset(12.68629150101523, 9.85786437626906),
],
<Offset>[
Offset(42.0, 32.0),
Offset(41.84490797861258, 32.38673781377975),
Offset(41.231722452189445, 33.71936387751549),
Offset(39.67761535119361, 36.262326923693394),
Offset(36.132752164775056, 39.98005128960247),
Offset(28.323439902629275, 43.73851649213626),
Offset(19.459948260351645, 43.67116620330525),
Offset(11.613683257353195, 39.71399180166486),
Offset(6.948701245094547, 33.927808379797085),
Offset(5.086478206479892, 28.468241749512934),
Offset(4.844123435345551, 24.032763694367194),
Offset(5.389341709109795, 20.646109619254112),
Offset(6.242307863360715, 18.133238852425784),
Offset(7.147199734127774, 16.29823545920705),
Offset(7.975197187086486, 14.976101020520819),
Offset(8.66666218744294, 14.040831916036076),
Offset(9.199071856475648, 13.400330213007376),
Offset(9.569460082206334, 12.989083729900493),
Offset(9.784962845727483, 12.76146160309818),
Offset(9.857711404800284, 12.68644828663571),
Offset(9.857864376269042, 12.686291501015248),
],
<Offset>[
Offset(42.0, 32.0),
Offset(41.84490797861258, 32.38673781377975),
Offset(41.231722452189445, 33.71936387751549),
Offset(39.67761535119361, 36.262326923693394),
Offset(36.132752164775056, 39.98005128960247),
Offset(28.323439902629275, 43.73851649213626),
Offset(19.459948260351645, 43.67116620330525),
Offset(11.613683257353195, 39.71399180166486),
Offset(6.948701245094547, 33.927808379797085),
Offset(5.086478206479892, 28.468241749512934),
Offset(4.844123435345551, 24.032763694367194),
Offset(5.389341709109795, 20.646109619254112),
Offset(6.242307863360715, 18.133238852425784),
Offset(7.147199734127774, 16.29823545920705),
Offset(7.975197187086486, 14.976101020520819),
Offset(8.66666218744294, 14.040831916036076),
Offset(9.199071856475648, 13.400330213007376),
Offset(9.569460082206334, 12.989083729900493),
Offset(9.784962845727483, 12.76146160309818),
Offset(9.857711404800284, 12.68644828663571),
Offset(9.857864376269042, 12.686291501015248),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 32.0),
Offset(41.84490797861258, 32.38673781377975),
Offset(41.231722452189445, 33.71936387751549),
Offset(39.67761535119361, 36.262326923693394),
Offset(36.132752164775056, 39.98005128960247),
Offset(28.323439902629275, 43.73851649213626),
Offset(19.459948260351645, 43.67116620330525),
Offset(11.613683257353195, 39.71399180166486),
Offset(6.948701245094547, 33.927808379797085),
Offset(5.086478206479892, 28.468241749512934),
Offset(4.844123435345551, 24.032763694367194),
Offset(5.389341709109795, 20.646109619254112),
Offset(6.242307863360715, 18.133238852425784),
Offset(7.147199734127774, 16.29823545920705),
Offset(7.975197187086486, 14.976101020520819),
Offset(8.66666218744294, 14.040831916036076),
Offset(9.199071856475648, 13.400330213007376),
Offset(9.569460082206334, 12.989083729900493),
Offset(9.784962845727483, 12.76146160309818),
Offset(9.857711404800284, 12.68644828663571),
Offset(9.857864376269042, 12.686291501015248),
],
<Offset>[
Offset(6.0, 32.0),
Offset(5.8562637660827015, 31.482587525420783),
Offset(5.462507332795713, 29.649566616897364),
Offset(5.19467278003437, 25.921791558726365),
Offset(6.329951485041715, 19.786164302593612),
Offset(12.137432035063437, 11.58244164909771),
Offset(21.226384536138692, 7.714529779919044),
Offset(30.7052249770933, 9.193293337124057),
Offset(37.072545324917364, 14.216034795095894),
Offset(40.040489009504526, 19.85330537238064),
Offset(40.83640611503963, 24.77814111889375),
Offset(40.48116246363044, 28.68128952592665),
Offset(39.61364150745642, 31.63709351746295),
Offset(38.59573384020686, 33.81935707224257),
Offset(37.620790266807234, 35.40007739515496),
Offset(36.78547739907316, 36.520427799730086),
Offset(36.1321796279006, 37.28772659211929),
Offset(35.67328164642253, 37.78001956829839),
Offset(35.40477687697853, 38.05227276059395),
Offset(35.31389971306446, 38.1419482191492),
Offset(35.31370849898476, 38.14213562373095),
],
<Offset>[
Offset(6.0, 32.0),
Offset(5.8562637660827015, 31.482587525420783),
Offset(5.462507332795713, 29.649566616897364),
Offset(5.19467278003437, 25.921791558726365),
Offset(6.329951485041715, 19.786164302593612),
Offset(12.137432035063437, 11.58244164909771),
Offset(21.226384536138692, 7.714529779919044),
Offset(30.7052249770933, 9.193293337124057),
Offset(37.072545324917364, 14.216034795095894),
Offset(40.040489009504526, 19.85330537238064),
Offset(40.83640611503963, 24.77814111889375),
Offset(40.48116246363044, 28.68128952592665),
Offset(39.61364150745642, 31.63709351746295),
Offset(38.59573384020686, 33.81935707224257),
Offset(37.620790266807234, 35.40007739515496),
Offset(36.78547739907316, 36.520427799730086),
Offset(36.1321796279006, 37.28772659211929),
Offset(35.67328164642253, 37.78001956829839),
Offset(35.40477687697853, 38.05227276059395),
Offset(35.31389971306446, 38.1419482191492),
Offset(35.31370849898476, 38.14213562373095),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 32.0),
Offset(5.8562637660827015, 31.482587525420783),
Offset(5.462507332795713, 29.649566616897364),
Offset(5.19467278003437, 25.921791558726365),
Offset(6.329951485041715, 19.786164302593612),
Offset(12.137432035063437, 11.58244164909771),
Offset(21.226384536138692, 7.714529779919044),
Offset(30.7052249770933, 9.193293337124057),
Offset(37.072545324917364, 14.216034795095894),
Offset(40.040489009504526, 19.85330537238064),
Offset(40.83640611503963, 24.77814111889375),
Offset(40.48116246363044, 28.68128952592665),
Offset(39.61364150745642, 31.63709351746295),
Offset(38.59573384020686, 33.81935707224257),
Offset(37.620790266807234, 35.40007739515496),
Offset(36.78547739907316, 36.520427799730086),
Offset(36.1321796279006, 37.28772659211929),
Offset(35.67328164642253, 37.78001956829839),
Offset(35.40477687697853, 38.05227276059395),
Offset(35.31389971306446, 38.1419482191492),
Offset(35.31370849898476, 38.14213562373095),
],
<Offset>[
Offset(6.0, 36.0),
Offset(5.755802622931704, 35.48132577125743),
Offset(5.010307637171476, 33.62392385238556),
Offset(4.045724406149144, 29.753229622188503),
Offset(4.0861862642629525, 23.09758660034176),
Offset(8.564534830281378, 13.380886967716135),
Offset(17.231202711318005, 7.518259082609372),
Offset(27.314036258810987, 7.072010923819601),
Offset(34.88234825995056, 10.868941008448914),
Offset(39.083273856489825, 15.969526394266794),
Offset(40.919225828875916, 20.778998598927743),
Offset(41.3739602310385, 24.78219833097991),
Offset(41.11406980357167, 27.929167557007872),
Offset(40.542525130544135, 30.325075504900447),
Offset(39.89012097509991, 32.10612260851932),
Offset(39.28321027503917, 33.39611499843784),
Offset(38.78633478113526, 34.295159061960966),
Offset(38.427830072911185, 34.87959495005215),
Offset(38.21486700558917, 35.20562675712161),
Offset(38.14228859445484, 35.31348285156429),
Offset(38.14213562373095, 35.31370849898477),
],
<Offset>[
Offset(6.0, 36.0),
Offset(5.755802622931704, 35.48132577125743),
Offset(5.010307637171476, 33.62392385238556),
Offset(4.045724406149144, 29.753229622188503),
Offset(4.0861862642629525, 23.09758660034176),
Offset(8.564534830281378, 13.380886967716135),
Offset(17.231202711318005, 7.518259082609372),
Offset(27.314036258810987, 7.072010923819601),
Offset(34.88234825995056, 10.868941008448914),
Offset(39.083273856489825, 15.969526394266794),
Offset(40.919225828875916, 20.778998598927743),
Offset(41.3739602310385, 24.78219833097991),
Offset(41.11406980357167, 27.929167557007872),
Offset(40.542525130544135, 30.325075504900447),
Offset(39.89012097509991, 32.10612260851932),
Offset(39.28321027503917, 33.39611499843784),
Offset(38.78633478113526, 34.295159061960966),
Offset(38.427830072911185, 34.87959495005215),
Offset(38.21486700558917, 35.20562675712161),
Offset(38.14228859445484, 35.31348285156429),
Offset(38.14213562373095, 35.31370849898477),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(6.0, 16.0),
Offset(6.1715484384586965, 15.794477742439435),
Offset(6.7847088111550455, 15.101124417731686),
Offset(8.064809036741185, 13.831258504138926),
Offset(10.327896232258308, 12.039205529321242),
Offset(14.135313755104503, 9.942822494875724),
Offset(17.85576112924513, 8.665143896025008),
Offset(21.423658706813853, 7.951290714362276),
Offset(24.4827045503675, 7.678712896347676),
Offset(26.97423123596107, 7.701340160804744),
Offset(28.998592463240865, 7.903908926143316),
Offset(30.63345298306288, 8.203149386677556),
Offset(31.940891049382852, 8.538653103313674),
Offset(32.972454180204764, 8.86889674774221),
Offset(33.77116819246568, 9.167199716087978),
Offset(34.37258718307626, 9.41785407630459),
Offset(34.80589641289028, 9.612989774645834),
Offset(35.09487909270652, 9.750154330042164),
Offset(35.25887447203158, 9.830559256807962),
Offset(35.31359376965362, 9.857807024169409),
Offset(35.313708498984745, 9.85786437626905),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 16.0),
Offset(6.1715484384586965, 15.794477742439435),
Offset(6.7847088111550455, 15.101124417731686),
Offset(8.064809036741185, 13.831258504138926),
Offset(10.327896232258308, 12.039205529321242),
Offset(14.135313755104503, 9.942822494875724),
Offset(17.85576112924513, 8.665143896025008),
Offset(21.423658706813853, 7.951290714362276),
Offset(24.4827045503675, 7.678712896347676),
Offset(26.97423123596107, 7.701340160804744),
Offset(28.998592463240865, 7.903908926143316),
Offset(30.63345298306288, 8.203149386677556),
Offset(31.940891049382852, 8.538653103313674),
Offset(32.972454180204764, 8.86889674774221),
Offset(33.77116819246568, 9.167199716087978),
Offset(34.37258718307626, 9.41785407630459),
Offset(34.80589641289028, 9.612989774645834),
Offset(35.09487909270652, 9.750154330042164),
Offset(35.25887447203158, 9.830559256807962),
Offset(35.31359376965362, 9.857807024169409),
Offset(35.313708498984745, 9.85786437626905),
],
<Offset>[
Offset(42.0, 16.0),
Offset(42.16746021740808, 16.33700442573998),
Offset(42.70156935314198, 17.546350134810247),
Offset(43.51618803296806, 20.092227060671057),
Offset(44.054130843957765, 24.630515982034453),
Offset(42.51578514966233, 32.09115975577829),
Offset(38.14918656898573, 38.40025586692044),
Offset(31.819015610164328, 42.4177462160811),
Offset(25.688490276712407, 43.658513907222414),
Offset(20.913117319647185, 43.18743459360287),
Offset(17.449482120110062, 42.00109429799166),
Offset(15.000094691649505, 40.63150285293139),
Offset(13.281945592346325, 39.325724325359715),
Offset(12.08031575221366, 38.18644357392904),
Offset(11.243480256299911, 37.247500217520134),
Offset(10.667161603617046, 36.51126283372602),
Offset(10.280531326105294, 35.9664803851875),
Offset(10.035976293649936, 35.59684445677665),
Offset(9.901923001772541, 35.38491333072124),
Offset(9.857956160571883, 35.31385765884373),
Offset(9.857864376269035, 35.31370849898477),
],
<Offset>[
Offset(42.0, 16.0),
Offset(42.16746021740808, 16.33700442573998),
Offset(42.70156935314198, 17.546350134810247),
Offset(43.51618803296806, 20.092227060671057),
Offset(44.054130843957765, 24.630515982034453),
Offset(42.51578514966233, 32.09115975577829),
Offset(38.14918656898573, 38.40025586692044),
Offset(31.819015610164328, 42.4177462160811),
Offset(25.688490276712407, 43.658513907222414),
Offset(20.913117319647185, 43.18743459360287),
Offset(17.449482120110062, 42.00109429799166),
Offset(15.000094691649505, 40.63150285293139),
Offset(13.281945592346325, 39.325724325359715),
Offset(12.08031575221366, 38.18644357392904),
Offset(11.243480256299911, 37.247500217520134),
Offset(10.667161603617046, 36.51126283372602),
Offset(10.280531326105294, 35.9664803851875),
Offset(10.035976293649936, 35.59684445677665),
Offset(9.901923001772541, 35.38491333072124),
Offset(9.857956160571883, 35.31385765884373),
Offset(9.857864376269035, 35.31370849898477),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 16.0),
Offset(42.16746021740808, 16.33700442573998),
Offset(42.70156935314198, 17.546350134810247),
Offset(43.51618803296806, 20.092227060671057),
Offset(44.054130843957765, 24.630515982034453),
Offset(42.51578514966233, 32.09115975577829),
Offset(38.14918656898573, 38.40025586692044),
Offset(31.819015610164328, 42.4177462160811),
Offset(25.688490276712407, 43.658513907222414),
Offset(20.913117319647185, 43.18743459360287),
Offset(17.449482120110062, 42.00109429799166),
Offset(15.000094691649505, 40.63150285293139),
Offset(13.281945592346325, 39.325724325359715),
Offset(12.08031575221366, 38.18644357392904),
Offset(11.243480256299911, 37.247500217520134),
Offset(10.667161603617046, 36.51126283372602),
Offset(10.280531326105294, 35.9664803851875),
Offset(10.035976293649936, 35.59684445677665),
Offset(9.901923001772541, 35.38491333072124),
Offset(9.857956160571883, 35.31385765884373),
Offset(9.857864376269035, 35.31370849898477),
],
<Offset>[
Offset(42.0, 12.0),
Offset(42.227740959997035, 12.33745867252338),
Offset(42.973261099484034, 13.555587852367255),
Offset(44.211851205916076, 16.153184949979185),
Offset(45.453165338703684, 20.883156580734514),
Offset(44.97671151198483, 28.937774045271865),
Offset(41.453087899085226, 36.14543081806037),
Offset(35.64862177702197, 41.26270656015326),
Offset(29.68624594458738, 43.52453771540631),
Offset(24.8560167010692, 43.86089169541553),
Offset(21.238058272537653, 43.28432878056175),
Offset(18.60324507678882, 42.368542663088434),
Offset(16.702731283684777, 41.398940487252666),
Offset(15.33782095512331, 40.50779228815028),
Offset(14.363513645347927, 39.750576654871885),
Offset(13.67754035444165, 39.145199009221486),
Offset(13.208696949498814, 38.691520950385836),
Offset(12.90783075217599, 38.38116699000516),
Offset(12.741295676651793, 38.202352382972244),
Offset(12.68640623109125, 38.14226183763059),
Offset(12.686291501015226, 38.14213562373095),
],
<Offset>[
Offset(42.0, 12.0),
Offset(42.227740959997035, 12.33745867252338),
Offset(42.973261099484034, 13.555587852367255),
Offset(44.211851205916076, 16.153184949979185),
Offset(45.453165338703684, 20.883156580734514),
Offset(44.97671151198483, 28.937774045271865),
Offset(41.453087899085226, 36.14543081806037),
Offset(35.64862177702197, 41.26270656015326),
Offset(29.68624594458738, 43.52453771540631),
Offset(24.8560167010692, 43.86089169541553),
Offset(21.238058272537653, 43.28432878056175),
Offset(18.60324507678882, 42.368542663088434),
Offset(16.702731283684777, 41.398940487252666),
Offset(15.33782095512331, 40.50779228815028),
Offset(14.363513645347927, 39.750576654871885),
Offset(13.67754035444165, 39.145199009221486),
Offset(13.208696949498814, 38.691520950385836),
Offset(12.90783075217599, 38.38116699000516),
Offset(12.741295676651793, 38.202352382972244),
Offset(12.68640623109125, 38.14226183763059),
Offset(12.686291501015226, 38.14213562373095),
],
),
_PathCubicTo(
<Offset>[
Offset(42.0, 12.0),
Offset(42.227740959997035, 12.33745867252338),
Offset(42.973261099484034, 13.555587852367255),
Offset(44.211851205916076, 16.153184949979185),
Offset(45.453165338703684, 20.883156580734514),
Offset(44.97671151198483, 28.937774045271865),
Offset(41.453087899085226, 36.14543081806037),
Offset(35.64862177702197, 41.26270656015326),
Offset(29.68624594458738, 43.52453771540631),
Offset(24.8560167010692, 43.86089169541553),
Offset(21.238058272537653, 43.28432878056175),
Offset(18.60324507678882, 42.368542663088434),
Offset(16.702731283684777, 41.398940487252666),
Offset(15.33782095512331, 40.50779228815028),
Offset(14.363513645347927, 39.750576654871885),
Offset(13.67754035444165, 39.145199009221486),
Offset(13.208696949498814, 38.691520950385836),
Offset(12.90783075217599, 38.38116699000516),
Offset(12.741295676651793, 38.202352382972244),
Offset(12.68640623109125, 38.14226183763059),
Offset(12.686291501015226, 38.14213562373095),
],
<Offset>[
Offset(6.0, 12.0),
Offset(6.231829181047647, 11.794931989222837),
Offset(7.056400557497106, 11.110362135288694),
Offset(8.7604722096892, 9.89221639344705),
Offset(11.726930727004222, 8.291846128021302),
Offset(16.596240117427012, 6.7894367843693),
Offset(21.159662459344624, 6.4103188471649375),
Offset(25.253264873671498, 6.796251058434446),
Offset(28.48046021824247, 7.5447367045315765),
Offset(30.917130617383087, 8.374797262617399),
Offset(32.78716861566846, 9.187143408713407),
Offset(34.236603368202196, 9.940189196834599),
Offset(35.3616767407213, 10.611869265206622),
Offset(36.22995938311441, 11.190245461963444),
Offset(36.8912015815137, 11.67027615343973),
Offset(37.38296593390086, 12.051790251800059),
Offset(37.734062036283795, 12.338030339844167),
Offset(37.96673355123257, 12.534476863270674),
Offset(38.09824714691083, 12.647998309058966),
Offset(38.14204384017299, 12.686211202956269),
Offset(38.14213562373094, 12.68629150101524),
],
<Offset>[
Offset(6.0, 12.0),
Offset(6.231829181047647, 11.794931989222837),
Offset(7.056400557497106, 11.110362135288694),
Offset(8.7604722096892, 9.89221639344705),
Offset(11.726930727004222, 8.291846128021302),
Offset(16.596240117427012, 6.7894367843693),
Offset(21.159662459344624, 6.4103188471649375),
Offset(25.253264873671498, 6.796251058434446),
Offset(28.48046021824247, 7.5447367045315765),
Offset(30.917130617383087, 8.374797262617399),
Offset(32.78716861566846, 9.187143408713407),
Offset(34.236603368202196, 9.940189196834599),
Offset(35.3616767407213, 10.611869265206622),
Offset(36.22995938311441, 11.190245461963444),
Offset(36.8912015815137, 11.67027615343973),
Offset(37.38296593390086, 12.051790251800059),
Offset(37.734062036283795, 12.338030339844167),
Offset(37.96673355123257, 12.534476863270674),
Offset(38.09824714691083, 12.647998309058966),
Offset(38.14204384017299, 12.686211202956269),
Offset(38.14213562373094, 12.68629150101524),
],
),
_PathCubicTo(
<Offset>[
Offset(6.0, 12.0),
Offset(6.231829181047647, 11.794931989222837),
Offset(7.056400557497106, 11.110362135288694),
Offset(8.7604722096892, 9.89221639344705),
Offset(11.726930727004222, 8.291846128021302),
Offset(16.596240117427012, 6.7894367843693),
Offset(21.159662459344624, 6.4103188471649375),
Offset(25.253264873671498, 6.796251058434446),
Offset(28.48046021824247, 7.5447367045315765),
Offset(30.917130617383087, 8.374797262617399),
Offset(32.78716861566846, 9.187143408713407),
Offset(34.236603368202196, 9.940189196834599),
Offset(35.3616767407213, 10.611869265206622),
Offset(36.22995938311441, 11.190245461963444),
Offset(36.8912015815137, 11.67027615343973),
Offset(37.38296593390086, 12.051790251800059),
Offset(37.734062036283795, 12.338030339844167),
Offset(37.96673355123257, 12.534476863270674),
Offset(38.09824714691083, 12.647998309058966),
Offset(38.14204384017299, 12.686211202956269),
Offset(38.14213562373094, 12.68629150101524),
],
<Offset>[
Offset(6.0, 16.0),
Offset(6.1715484384586965, 15.794477742439435),
Offset(6.7847088111550455, 15.101124417731686),
Offset(8.064809036741185, 13.831258504138926),
Offset(10.327896232258308, 12.039205529321242),
Offset(14.135313755104503, 9.942822494875724),
Offset(17.85576112924513, 8.665143896025008),
Offset(21.423658706813853, 7.951290714362276),
Offset(24.4827045503675, 7.678712896347676),
Offset(26.97423123596107, 7.701340160804744),
Offset(28.998592463240865, 7.903908926143316),
Offset(30.63345298306288, 8.203149386677556),
Offset(31.940891049382852, 8.538653103313674),
Offset(32.972454180204764, 8.86889674774221),
Offset(33.77116819246568, 9.167199716087978),
Offset(34.37258718307626, 9.41785407630459),
Offset(34.80589641289028, 9.612989774645834),
Offset(35.09487909270652, 9.750154330042164),
Offset(35.25887447203158, 9.830559256807962),
Offset(35.31359376965362, 9.857807024169409),
Offset(35.313708498984745, 9.85786437626905),
],
<Offset>[
Offset(6.0, 16.0),
Offset(6.1715484384586965, 15.794477742439435),
Offset(6.7847088111550455, 15.101124417731686),
Offset(8.064809036741185, 13.831258504138926),
Offset(10.327896232258308, 12.039205529321242),
Offset(14.135313755104503, 9.942822494875724),
Offset(17.85576112924513, 8.665143896025008),
Offset(21.423658706813853, 7.951290714362276),
Offset(24.4827045503675, 7.678712896347676),
Offset(26.97423123596107, 7.701340160804744),
Offset(28.998592463240865, 7.903908926143316),
Offset(30.63345298306288, 8.203149386677556),
Offset(31.940891049382852, 8.538653103313674),
Offset(32.972454180204764, 8.86889674774221),
Offset(33.77116819246568, 9.167199716087978),
Offset(34.37258718307626, 9.41785407630459),
Offset(34.80589641289028, 9.612989774645834),
Offset(35.09487909270652, 9.750154330042164),
Offset(35.25887447203158, 9.830559256807962),
Offset(35.31359376965362, 9.857807024169409),
Offset(35.313708498984745, 9.85786437626905),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/menu_close.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/menu_close.g.dart",
"repo_id": "flutter",
"token_count": 29428
} | 619 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'bottom_app_bar_theme.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'elevation_overlay.dart';
import 'material.dart';
import 'scaffold.dart';
import 'theme.dart';
// Examples can assume:
// late Widget bottomAppBarContents;
/// A container that is typically used with [Scaffold.bottomNavigationBar].
///
/// Typically used with a [Scaffold] and a [FloatingActionButton].
///
/// {@tool snippet}
/// ```dart
/// Scaffold(
/// bottomNavigationBar: BottomAppBar(
/// color: Colors.white,
/// child: bottomAppBarContents,
/// ),
/// floatingActionButton: const FloatingActionButton(onPressed: null),
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows the [BottomAppBar], which can be configured to have a notch using the
/// [BottomAppBar.shape] property. This also includes an optional [FloatingActionButton], which illustrates
/// the [FloatingActionButtonLocation]s in relation to the [BottomAppBar].
///
/// ** See code in examples/api/lib/material/bottom_app_bar/bottom_app_bar.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows Material 3 [BottomAppBar] with its expected look and behaviors.
///
/// This also includes an optional [FloatingActionButton], which illustrates
/// the [FloatingActionButtonLocation.endContained].
///
/// ** See code in examples/api/lib/material/bottom_app_bar/bottom_app_bar.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [NotchedShape] which calculates the notch for a notched [BottomAppBar].
/// * [FloatingActionButton] which the [BottomAppBar] makes a notch for.
/// * [AppBar] for a toolbar that is shown at the top of the screen.
class BottomAppBar extends StatefulWidget {
/// Creates a bottom application bar.
///
/// The [clipBehavior] argument defaults to [Clip.none].
/// Additionally, [elevation] must be non-negative.
///
/// If [color], [elevation], or [shape] are null, their [BottomAppBarTheme] values will be used.
/// If the corresponding [BottomAppBarTheme] property is null, then the default
/// specified in the property's documentation will be used.
const BottomAppBar({
super.key,
this.color,
this.elevation,
this.shape,
this.clipBehavior = Clip.none,
this.notchMargin = 4.0,
this.child,
this.padding,
this.surfaceTintColor,
this.shadowColor,
this.height,
}) : assert(elevation == null || elevation >= 0.0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
///
/// Typically the child will be a [Row] whose first child
/// is an [IconButton] with the [Icons.menu] icon.
final Widget? child;
/// The amount of space to surround the child inside the bounds of the [BottomAppBar].
///
/// In Material 3 the padding will default to `EdgeInsets.symmetric(vertical: 12.0, horizontal: 16.0)`
/// Otherwise the value will default to EdgeInsets.zero.
final EdgeInsetsGeometry? padding;
/// The bottom app bar's background color.
///
/// If this property is null then [BottomAppBarTheme.color] of
/// [ThemeData.bottomAppBarTheme] is used. If that's null and [ThemeData.useMaterial3]
/// is true, the default value is [ColorScheme.surface]; if [ThemeData.useMaterial3]
/// is false, then the default value is `Color(0xFF424242)` in dark theme and
/// [Colors.white] in light theme.
final Color? color;
/// The z-coordinate at which to place this bottom app bar relative to its
/// parent.
///
/// This controls the size of the shadow below the bottom app bar. The
/// value is non-negative.
///
/// If this property is null then [BottomAppBarTheme.elevation] of
/// [ThemeData.bottomAppBarTheme] is used. If that's null and
/// [ThemeData.useMaterial3] is true, than the default value is 3 else is 8.
final double? elevation;
/// The notch that is made for the floating action button.
///
/// If this property is null then [BottomAppBarTheme.shape] of
/// [ThemeData.bottomAppBarTheme] is used. If that's null then the shape will
/// be rectangular with no notch.
final NotchedShape? shape;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The margin between the [FloatingActionButton] and the [BottomAppBar]'s
/// notch.
///
/// Not used if [shape] is null.
final double notchMargin;
/// A custom color for the Material 3 surface-tint elevation effect.
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// If this property is null, then [BottomAppBarTheme.surfaceTintColor]
/// of [ThemeData.bottomAppBarTheme] is used. If that is also null, the default
/// value is [Colors.transparent].
///
/// Ignored if [ThemeData.useMaterial3] is false.
///
/// See [Material.surfaceTintColor] for more details on how this overlay is applied.
final Color? surfaceTintColor;
/// The color of the shadow below the app bar.
///
/// If this property is null, then [BottomAppBarTheme.shadowColor] of
/// [ThemeData.bottomAppBarTheme] is used. If that is also null, the default value
/// is fully opaque black for Material 2, and transparent for Material 3.
///
/// See also:
///
/// * [elevation], which defines the size of the shadow below the app bar.
/// * [shape], which defines the shape of the app bar and its shadow.
final Color? shadowColor;
/// The double value used to indicate the height of the [BottomAppBar].
///
/// If this is null, the default value is the minimum in relation to the content,
/// unless [ThemeData.useMaterial3] is true, in which case it defaults to 80.0.
final double? height;
@override
State createState() => _BottomAppBarState();
}
class _BottomAppBarState extends State<BottomAppBar> {
late ValueListenable<ScaffoldGeometry> geometryListenable;
final GlobalKey materialKey = GlobalKey();
@override
void didChangeDependencies() {
super.didChangeDependencies();
geometryListenable = Scaffold.geometryOf(context);
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final bool isMaterial3 = theme.useMaterial3;
final BottomAppBarTheme babTheme = BottomAppBarTheme.of(context);
final BottomAppBarTheme defaults = isMaterial3 ? _BottomAppBarDefaultsM3(context) : _BottomAppBarDefaultsM2(context);
final bool hasFab = Scaffold.of(context).hasFloatingActionButton;
final NotchedShape? notchedShape = widget.shape ?? babTheme.shape ?? defaults.shape;
final CustomClipper<Path> clipper = notchedShape != null && hasFab
? _BottomAppBarClipper(
geometry: geometryListenable,
shape: notchedShape,
materialKey: materialKey,
notchMargin: widget.notchMargin,
)
: const ShapeBorderClipper(shape: RoundedRectangleBorder());
final double elevation = widget.elevation ?? babTheme.elevation ?? defaults.elevation!;
final double? height = widget.height ?? babTheme.height ?? defaults.height;
final Color color = widget.color ?? babTheme.color ?? defaults.color!;
final Color surfaceTintColor = widget.surfaceTintColor ?? babTheme.surfaceTintColor ?? defaults.surfaceTintColor!;
final Color effectiveColor = isMaterial3
? ElevationOverlay.applySurfaceTint(color, surfaceTintColor, elevation)
: ElevationOverlay.applyOverlay(context, color, elevation);
final Color shadowColor = widget.shadowColor ?? babTheme.shadowColor ?? defaults.shadowColor!;
final Widget child = SizedBox(
height: height,
child: Padding(
padding: widget.padding ?? babTheme.padding ?? (isMaterial3 ? const EdgeInsets.symmetric(vertical: 12.0, horizontal: 16.0) : EdgeInsets.zero),
child: widget.child,
),
);
final Material material = Material(
key: materialKey,
type: MaterialType.transparency,
child: SafeArea(child: child),
);
return PhysicalShape(
clipper: clipper,
elevation: elevation,
shadowColor: shadowColor,
color: effectiveColor,
clipBehavior: widget.clipBehavior,
child: material,
);
}
}
class _BottomAppBarClipper extends CustomClipper<Path> {
const _BottomAppBarClipper({
required this.geometry,
required this.shape,
required this.materialKey,
required this.notchMargin,
}) : super(reclip: geometry);
final ValueListenable<ScaffoldGeometry> geometry;
final NotchedShape shape;
final GlobalKey materialKey;
final double notchMargin;
// Returns the top of the BottomAppBar in global coordinates.
//
// If the Scaffold's bottomNavigationBar was specified, then we can use its
// geometry value, otherwise we compute the location based on the AppBar's
// Material widget.
double get bottomNavigationBarTop {
final double? bottomNavigationBarTop = geometry.value.bottomNavigationBarTop;
if (bottomNavigationBarTop != null) {
return bottomNavigationBarTop;
}
final RenderBox? box = materialKey.currentContext?.findRenderObject() as RenderBox?;
return box?.localToGlobal(Offset.zero).dy ?? 0;
}
@override
Path getClip(Size size) {
// button is the floating action button's bounding rectangle in the
// coordinate system whose origin is at the appBar's top left corner,
// or null if there is no floating action button.
final Rect? button = geometry.value.floatingActionButtonArea?.translate(0.0, bottomNavigationBarTop * -1.0);
return shape.getOuterPath(Offset.zero & size, button?.inflate(notchMargin));
}
@override
bool shouldReclip(_BottomAppBarClipper oldClipper) {
return oldClipper.geometry != geometry
|| oldClipper.shape != shape
|| oldClipper.notchMargin != notchMargin;
}
}
class _BottomAppBarDefaultsM2 extends BottomAppBarTheme {
const _BottomAppBarDefaultsM2(this.context)
: super(
elevation: 8.0,
);
final BuildContext context;
@override
Color? get color => Theme.of(context).brightness == Brightness.dark ? Colors.grey[800]! : Colors.white;
@override
Color? get surfaceTintColor => Theme.of(context).colorScheme.surfaceTint;
@override
Color get shadowColor => const Color(0xFF000000);
}
// BEGIN GENERATED TOKEN PROPERTIES - BottomAppBar
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _BottomAppBarDefaultsM3 extends BottomAppBarTheme {
_BottomAppBarDefaultsM3(this.context)
: super(
elevation: 3.0,
height: 80.0,
shape: const AutomaticNotchedShape(RoundedRectangleBorder()),
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color? get color => _colors.surfaceContainer;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
Color? get shadowColor => Colors.transparent;
}
// END GENERATED TOKEN PROPERTIES - BottomAppBar
| flutter/packages/flutter/lib/src/material/bottom_app_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/bottom_app_bar.dart",
"repo_id": "flutter",
"token_count": 3658
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'checkbox.dart';
import 'checkbox_theme.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// late bool? _throwShotAway;
// void setState(VoidCallback fn) { }
enum _CheckboxType { material, adaptive }
/// A [ListTile] with a [Checkbox]. In other words, a checkbox with a label.
///
/// The entire list tile is interactive: tapping anywhere in the tile toggles
/// the checkbox.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=RkSqPAn9szs}
///
/// The [value], [onChanged], [activeColor] and [checkColor] properties of this widget are
/// identical to the similarly-named properties on the [Checkbox] widget.
///
/// The [title], [subtitle], [isThreeLine], [dense], and [contentPadding] properties are like
/// those of the same name on [ListTile].
///
/// The [selected] property on this widget is similar to the [ListTile.selected]
/// property. This tile's [activeColor] is used for the selected item's text color, or
/// the theme's [CheckboxThemeData.overlayColor] if [activeColor] is null.
///
/// This widget does not coordinate the [selected] state and the [value] state; to have the list tile
/// appear selected when the checkbox is checked, pass the same value to both.
///
/// The checkbox is shown on the right by default in left-to-right languages
/// (i.e. the trailing edge). This can be changed using [controlAffinity]. The
/// [secondary] widget is placed on the opposite side. This maps to the
/// [ListTile.leading] and [ListTile.trailing] properties of [ListTile].
///
/// This widget requires a [Material] widget ancestor in the tree to paint
/// itself on, which is typically provided by the app's [Scaffold].
/// The [tileColor], and [selectedTileColor] are not painted by the
/// [CheckboxListTile] itself but by the [Material] widget ancestor.
/// In this case, one can wrap a [Material] widget around the [CheckboxListTile],
/// e.g.:
///
/// {@tool snippet}
/// ```dart
/// ColoredBox(
/// color: Colors.green,
/// child: Material(
/// child: CheckboxListTile(
/// tileColor: Colors.red,
/// title: const Text('CheckboxListTile with red background'),
/// value: true,
/// onChanged:(bool? value) { },
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Performance considerations when wrapping [CheckboxListTile] with [Material]
///
/// Wrapping a large number of [CheckboxListTile]s individually with [Material]s
/// is expensive. Consider only wrapping the [CheckboxListTile]s that require it
/// or include a common [Material] ancestor where possible.
///
/// To show the [CheckboxListTile] as disabled, pass null as the [onChanged]
/// callback.
///
/// {@tool dartpad}
/// 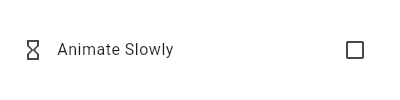
///
/// This widget shows a checkbox that, when checked, slows down all animations
/// (including the animation of the checkbox itself getting checked!).
///
/// This sample requires that you also import 'package:flutter/scheduler.dart',
/// so that you can reference [timeDilation].
///
/// ** See code in examples/api/lib/material/checkbox_list_tile/checkbox_list_tile.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample demonstrates how [CheckboxListTile] positions the checkbox widget
/// relative to the text in different configurations.
///
/// ** See code in examples/api/lib/material/checkbox_list_tile/checkbox_list_tile.1.dart **
/// {@end-tool}
///
/// ## Semantics in CheckboxListTile
///
/// Since the entirety of the CheckboxListTile is interactive, it should represent
/// itself as a single interactive entity.
///
/// To do so, a CheckboxListTile widget wraps its children with a [MergeSemantics]
/// widget. [MergeSemantics] will attempt to merge its descendant [Semantics]
/// nodes into one node in the semantics tree. Therefore, CheckboxListTile will
/// throw an error if any of its children requires its own [Semantics] node.
///
/// For example, you cannot nest a [RichText] widget as a descendant of
/// CheckboxListTile. [RichText] has an embedded gesture recognizer that
/// requires its own [Semantics] node, which directly conflicts with
/// CheckboxListTile's desire to merge all its descendants' semantic nodes
/// into one. Therefore, it may be necessary to create a custom radio tile
/// widget to accommodate similar use cases.
///
/// {@tool dartpad}
/// 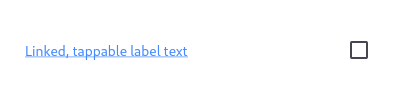
///
/// Here is an example of a custom labeled checkbox widget, called
/// LinkedLabelCheckbox, that includes an interactive [RichText] widget that
/// handles tap gestures.
///
/// ** See code in examples/api/lib/material/checkbox_list_tile/custom_labeled_checkbox.0.dart **
/// {@end-tool}
///
/// ## CheckboxListTile isn't exactly what I want
///
/// If the way CheckboxListTile pads and positions its elements isn't quite
/// what you're looking for, you can create custom labeled checkbox widgets by
/// combining [Checkbox] with other widgets, such as [Text], [Padding] and
/// [InkWell].
///
/// {@tool dartpad}
/// 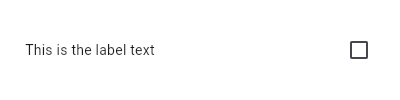
///
/// Here is an example of a custom LabeledCheckbox widget, but you can easily
/// make your own configurable widget.
///
/// ** See code in examples/api/lib/material/checkbox_list_tile/custom_labeled_checkbox.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListTileTheme], which can be used to affect the style of list tiles,
/// including checkbox list tiles.
/// * [RadioListTile], a similar widget for radio buttons.
/// * [SwitchListTile], a similar widget for switches.
/// * [ListTile] and [Checkbox], the widgets from which this widget is made.
class CheckboxListTile extends StatelessWidget {
/// Creates a combination of a list tile and a checkbox.
///
/// The checkbox tile itself does not maintain any state. Instead, when the
/// state of the checkbox changes, the widget calls the [onChanged] callback.
/// Most widgets that use a checkbox will listen for the [onChanged] callback
/// and rebuild the checkbox tile with a new [value] to update the visual
/// appearance of the checkbox.
///
/// The following arguments are required:
///
/// * [value], which determines whether the checkbox is checked. The [value]
/// can only be null if [tristate] is true.
/// * [onChanged], which is called when the value of the checkbox should
/// change. It can be set to null to disable the checkbox.
const CheckboxListTile({
super.key,
required this.value,
required this.onChanged,
this.mouseCursor,
this.activeColor,
this.fillColor,
this.checkColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
this.shape,
this.side,
this.isError = false,
this.enabled,
this.tileColor,
this.title,
this.subtitle,
this.isThreeLine = false,
this.dense,
this.secondary,
this.selected = false,
this.controlAffinity = ListTileControlAffinity.platform,
this.contentPadding,
this.tristate = false,
this.checkboxShape,
this.selectedTileColor,
this.onFocusChange,
this.enableFeedback,
this.checkboxSemanticLabel,
}) : _checkboxType = _CheckboxType.material,
assert(tristate || value != null),
assert(!isThreeLine || subtitle != null);
/// Creates a combination of a list tile and a platform adaptive checkbox.
///
/// The checkbox uses [Checkbox.adaptive] to show a [CupertinoCheckbox] for
/// iOS platforms, or [Checkbox] for all others.
///
/// All other properties are the same as [CheckboxListTile].
const CheckboxListTile.adaptive({
super.key,
required this.value,
required this.onChanged,
this.mouseCursor,
this.activeColor,
this.fillColor,
this.checkColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
this.shape,
this.side,
this.isError = false,
this.enabled,
this.tileColor,
this.title,
this.subtitle,
this.isThreeLine = false,
this.dense,
this.secondary,
this.selected = false,
this.controlAffinity = ListTileControlAffinity.platform,
this.contentPadding,
this.tristate = false,
this.checkboxShape,
this.selectedTileColor,
this.onFocusChange,
this.enableFeedback,
this.checkboxSemanticLabel,
}) : _checkboxType = _CheckboxType.adaptive,
assert(tristate || value != null),
assert(!isThreeLine || subtitle != null);
/// Whether this checkbox is checked.
final bool? value;
/// Called when the value of the checkbox should change.
///
/// The checkbox passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the checkbox tile with the
/// new value.
///
/// If null, the checkbox will be displayed as disabled.
///
/// {@tool snippet}
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// ```dart
/// CheckboxListTile(
/// value: _throwShotAway,
/// onChanged: (bool? newValue) {
/// setState(() {
/// _throwShotAway = newValue;
/// });
/// },
/// title: const Text('Throw away your shot'),
/// )
/// ```
/// {@end-tool}
final ValueChanged<bool?>? onChanged;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [CheckboxThemeData.mouseCursor] is used. If
/// that is also null, then [MaterialStateMouseCursor.clickable] is used.
final MouseCursor? mouseCursor;
/// The color to use when this checkbox is checked.
///
/// Defaults to [ColorScheme.secondary] of the current [Theme].
final Color? activeColor;
/// The color that fills the checkbox.
///
/// Resolves in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [activeColor] is used in the selected
/// state. If that is also null, the value of [CheckboxThemeData.fillColor]
/// is used. If that is also null, then the default value is used.
final MaterialStateProperty<Color?>? fillColor;
/// The color to use for the check icon when this checkbox is checked.
///
/// Defaults to Color(0xFFFFFFFF).
final Color? checkColor;
/// {@macro flutter.material.checkbox.hoverColor}
final Color? hoverColor;
/// The color for the checkbox's [Material].
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
///
/// If null, then the value of [activeColor] with alpha [kRadialReactionAlpha]
/// and [hoverColor] is used in the pressed and hovered state. If that is also null,
/// the value of [CheckboxThemeData.overlayColor] is used. If that is also null,
/// then the default value is used in the pressed and hovered state.
final MaterialStateProperty<Color?>? overlayColor;
/// {@macro flutter.material.checkbox.splashRadius}
///
/// If null, then the value of [CheckboxThemeData.splashRadius] is used. If
/// that is also null, then [kRadialReactionRadius] is used.
final double? splashRadius;
/// {@macro flutter.material.checkbox.materialTapTargetSize}
///
/// Defaults to [MaterialTapTargetSize.shrinkWrap].
final MaterialTapTargetSize? materialTapTargetSize;
/// Defines how compact the list tile's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
final VisualDensity? visualDensity;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.material.ListTile.shape}
final ShapeBorder? shape;
/// {@macro flutter.material.checkbox.side}
///
/// The given value is passed directly to [Checkbox.side].
///
/// If this property is null, then [CheckboxThemeData.side] of
/// [ThemeData.checkboxTheme] is used. If that is also null, then the side
/// will be width 2.
final BorderSide? side;
/// {@macro flutter.material.checkbox.isError}
///
/// Defaults to false.
final bool isError;
/// {@macro flutter.material.ListTile.tileColor}
final Color? tileColor;
/// The primary content of the list tile.
///
/// Typically a [Text] widget.
final Widget? title;
/// Additional content displayed below the title.
///
/// Typically a [Text] widget.
final Widget? subtitle;
/// A widget to display on the opposite side of the tile from the checkbox.
///
/// Typically an [Icon] widget.
final Widget? secondary;
/// Whether this list tile is intended to display three lines of text.
///
/// If false, the list tile is treated as having one line if the subtitle is
/// null and treated as having two lines if the subtitle is non-null.
final bool isThreeLine;
/// Whether this list tile is part of a vertically dense list.
///
/// If this property is null then its value is based on [ListTileThemeData.dense].
final bool? dense;
/// Whether to render icons and text in the [activeColor].
///
/// No effort is made to automatically coordinate the [selected] state and the
/// [value] state. To have the list tile appear selected when the checkbox is
/// checked, pass the same value to both.
///
/// Normally, this property is left to its default value, false.
final bool selected;
/// Where to place the control relative to the text.
final ListTileControlAffinity controlAffinity;
/// Defines insets surrounding the tile's contents.
///
/// This value will surround the [Checkbox], [title], [subtitle], and [secondary]
/// widgets in [CheckboxListTile].
///
/// When the value is null, the [contentPadding] is `EdgeInsets.symmetric(horizontal: 16.0)`.
final EdgeInsetsGeometry? contentPadding;
/// If true the checkbox's [value] can be true, false, or null.
///
/// Checkbox displays a dash when its value is null.
///
/// When a tri-state checkbox ([tristate] is true) is tapped, its [onChanged]
/// callback will be applied to true if the current value is false, to null if
/// value is true, and to false if value is null (i.e. it cycles through false
/// => true => null => false when tapped).
///
/// If tristate is false (the default), [value] must not be null.
final bool tristate;
/// {@macro flutter.material.checkbox.shape}
///
/// If this property is null then [CheckboxThemeData.shape] of [ThemeData.checkboxTheme]
/// is used. If that's null then the shape will be a [RoundedRectangleBorder]
/// with a circular corner radius of 1.0.
final OutlinedBorder? checkboxShape;
/// If non-null, defines the background color when [CheckboxListTile.selected] is true.
final Color? selectedTileColor;
/// {@macro flutter.material.inkwell.onFocusChange}
final ValueChanged<bool>? onFocusChange;
/// {@macro flutter.material.ListTile.enableFeedback}
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// Whether the CheckboxListTile is interactive.
///
/// If false, this list tile is styled with the disabled color from the
/// current [Theme] and the [ListTile.onTap] callback is
/// inoperative.
final bool? enabled;
/// {@macro flutter.material.checkbox.semanticLabel}
final String? checkboxSemanticLabel;
final _CheckboxType _checkboxType;
void _handleValueChange() {
assert(onChanged != null);
switch (value) {
case false:
onChanged!(true);
case true:
onChanged!(tristate ? null : false);
case null:
onChanged!(false);
}
}
@override
Widget build(BuildContext context) {
final Widget control;
switch (_checkboxType) {
case _CheckboxType.material:
control = ExcludeFocus(
child: Checkbox(
value: value,
onChanged: enabled ?? true ? onChanged : null,
mouseCursor: mouseCursor,
activeColor: activeColor,
fillColor: fillColor,
checkColor: checkColor,
hoverColor: hoverColor,
overlayColor: overlayColor,
splashRadius: splashRadius,
materialTapTargetSize: materialTapTargetSize ?? MaterialTapTargetSize.shrinkWrap,
autofocus: autofocus,
tristate: tristate,
shape: checkboxShape,
side: side,
isError: isError,
semanticLabel: checkboxSemanticLabel,
),
);
case _CheckboxType.adaptive:
control = ExcludeFocus(
child: Checkbox.adaptive(
value: value,
onChanged: enabled ?? true ? onChanged : null,
mouseCursor: mouseCursor,
activeColor: activeColor,
fillColor: fillColor,
checkColor: checkColor,
hoverColor: hoverColor,
overlayColor: overlayColor,
splashRadius: splashRadius,
materialTapTargetSize: materialTapTargetSize ?? MaterialTapTargetSize.shrinkWrap,
autofocus: autofocus,
tristate: tristate,
shape: checkboxShape,
side: side,
isError: isError,
semanticLabel: checkboxSemanticLabel,
),
);
}
final (Widget? leading, Widget? trailing) = switch (controlAffinity) {
ListTileControlAffinity.leading => (control, secondary),
ListTileControlAffinity.trailing || ListTileControlAffinity.platform => (secondary, control),
};
final ThemeData theme = Theme.of(context);
final CheckboxThemeData checkboxTheme = CheckboxTheme.of(context);
final Set<MaterialState> states = <MaterialState>{
if (selected) MaterialState.selected,
};
final Color effectiveActiveColor = activeColor
?? checkboxTheme.fillColor?.resolve(states)
?? theme.colorScheme.secondary;
return MergeSemantics(
child: ListTile(
selectedColor: effectiveActiveColor,
leading: leading,
title: title,
subtitle: subtitle,
trailing: trailing,
isThreeLine: isThreeLine,
dense: dense,
enabled: enabled ?? onChanged != null,
onTap: onChanged != null ? _handleValueChange : null,
selected: selected,
autofocus: autofocus,
contentPadding: contentPadding,
shape: shape,
selectedTileColor: selectedTileColor,
tileColor: tileColor,
visualDensity: visualDensity,
focusNode: focusNode,
onFocusChange: onFocusChange,
enableFeedback: enableFeedback,
),
);
}
}
| flutter/packages/flutter/lib/src/material/checkbox_list_tile.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/checkbox_list_tile.dart",
"repo_id": "flutter",
"token_count": 6456
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'scaffold.dart' show Scaffold, ScaffoldMessenger;
// Examples can assume:
// late BuildContext context;
/// Asserts that the given context has a [Material] ancestor within the closest
/// [LookupBoundary].
///
/// Used by many Material Design widgets to make sure that they are
/// only used in contexts where they can print ink onto some material.
///
/// To call this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasMaterial(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This method can be expensive (it walks the element tree).
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasMaterial(BuildContext context) {
assert(() {
if (LookupBoundary.findAncestorWidgetOfExactType<Material>(context) == null) {
final bool hiddenByBoundary = LookupBoundary.debugIsHidingAncestorWidgetOfExactType<Material>(context);
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Material widget found${hiddenByBoundary ? ' within the closest LookupBoundary' : ''}.'),
if (hiddenByBoundary)
ErrorDescription(
'There is an ancestor Material widget, but it is hidden by a LookupBoundary.'
),
ErrorDescription(
'${context.widget.runtimeType} widgets require a Material '
'widget ancestor within the closest LookupBoundary.\n'
'In Material Design, most widgets are conceptually "printed" on '
"a sheet of material. In Flutter's material library, that "
'material is represented by the Material widget. It is the '
'Material widget that renders ink splashes, for instance. '
'Because of this, many material library widgets require that '
'there be a Material widget in the tree above them.',
),
ErrorHint(
'To introduce a Material widget, you can either directly '
'include one, or use a widget that contains Material itself, '
'such as a Card, Dialog, Drawer, or Scaffold.',
),
...context.describeMissingAncestor(expectedAncestorType: Material),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has a [Localizations] ancestor that contains
/// a [MaterialLocalizations] delegate.
///
/// Used by many Material Design widgets to make sure that they are
/// only used in contexts where they have access to localizations.
///
/// To call this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasMaterialLocalizations(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This function has the side-effect of establishing an inheritance
/// relationship with the nearest [Localizations] widget (see
/// [BuildContext.dependOnInheritedWidgetOfExactType]). This is ok if the caller
/// always also calls [Localizations.of] or [Localizations.localeOf].
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasMaterialLocalizations(BuildContext context) {
assert(() {
if (Localizations.of<MaterialLocalizations>(context, MaterialLocalizations) == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No MaterialLocalizations found.'),
ErrorDescription(
'${context.widget.runtimeType} widgets require MaterialLocalizations '
'to be provided by a Localizations widget ancestor.',
),
ErrorDescription(
'The material library uses Localizations to generate messages, '
'labels, and abbreviations.',
),
ErrorHint(
'To introduce a MaterialLocalizations, either use a '
'MaterialApp at the root of your application to include them '
'automatically, or add a Localization widget with a '
'MaterialLocalizations delegate.',
),
...context.describeMissingAncestor(expectedAncestorType: MaterialLocalizations),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has a [Scaffold] ancestor.
///
/// Used by various widgets to make sure that they are only used in an
/// appropriate context.
///
/// To invoke this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasScaffold(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This method can be expensive (it walks the element tree).
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasScaffold(BuildContext context) {
assert(() {
if (context.widget is! Scaffold && context.findAncestorWidgetOfExactType<Scaffold>() == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No Scaffold widget found.'),
ErrorDescription('${context.widget.runtimeType} widgets require a Scaffold widget ancestor.'),
...context.describeMissingAncestor(expectedAncestorType: Scaffold),
ErrorHint(
'Typically, the Scaffold widget is introduced by the MaterialApp or '
'WidgetsApp widget at the top of your application widget tree.',
),
]);
}
return true;
}());
return true;
}
/// Asserts that the given context has a [ScaffoldMessenger] ancestor.
///
/// Used by various widgets to make sure that they are only used in an
/// appropriate context.
///
/// To invoke this function, use the following pattern, typically in the
/// relevant Widget's build method:
///
/// ```dart
/// assert(debugCheckHasScaffoldMessenger(context));
/// ```
///
/// Always place this before any early returns, so that the invariant is checked
/// in all cases. This prevents bugs from hiding until a particular codepath is
/// hit.
///
/// This method can be expensive (it walks the element tree).
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugCheckHasScaffoldMessenger(BuildContext context) {
assert(() {
if (context.findAncestorWidgetOfExactType<ScaffoldMessenger>() == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('No ScaffoldMessenger widget found.'),
ErrorDescription('${context.widget.runtimeType} widgets require a ScaffoldMessenger widget ancestor.'),
...context.describeMissingAncestor(expectedAncestorType: ScaffoldMessenger),
ErrorHint(
'Typically, the ScaffoldMessenger widget is introduced by the MaterialApp '
'at the top of your application widget tree.',
),
]);
}
return true;
}());
return true;
}
| flutter/packages/flutter/lib/src/material/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/debug.dart",
"repo_id": "flutter",
"token_count": 2315
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'theme.dart';
/// A utility class for dealing with the overlay color needed
/// to indicate elevation of surfaces.
abstract final class ElevationOverlay {
/// Applies a surface tint color to a given container color to indicate
/// the level of its elevation.
///
/// With Material Design 3, some components will use a "surface tint" color
/// overlay with an opacity applied to their base color to indicate they are
/// elevated. The amount of opacity will vary with the elevation as described
/// in: https://m3.material.io/styles/color/the-color-system/color-roles.
///
/// If [surfaceTint] is not null and not completely transparent ([Color.alpha]
/// is 0), then the returned color will be the given [color] with the
/// [surfaceTint] of the appropriate opacity applied to it. Otherwise it will
/// just return [color] unmodified.
static Color applySurfaceTint(Color color, Color? surfaceTint, double elevation) {
if (surfaceTint != null && surfaceTint != Colors.transparent) {
return Color.alphaBlend(surfaceTint.withOpacity(_surfaceTintOpacityForElevation(elevation)), color);
}
return color;
}
// Calculates the opacity of the surface tint color from the elevation by
// looking it up in the token generated table of opacities, interpolating
// between values as needed. If the elevation is outside the range of values
// in the table it will clamp to the smallest or largest opacity.
static double _surfaceTintOpacityForElevation(double elevation) {
if (elevation < _surfaceTintElevationOpacities[0].elevation) {
// Elevation less than the first entry, so just clamp it to the first one.
return _surfaceTintElevationOpacities[0].opacity;
}
// Walk the opacity list and find the closest match(es) for the elevation.
int index = 0;
while (elevation >= _surfaceTintElevationOpacities[index].elevation) {
// If we found it exactly or walked off the end of the list just return it.
if (elevation == _surfaceTintElevationOpacities[index].elevation ||
index + 1 == _surfaceTintElevationOpacities.length) {
return _surfaceTintElevationOpacities[index].opacity;
}
index += 1;
}
// Interpolate between the two opacity values
final _ElevationOpacity lower = _surfaceTintElevationOpacities[index - 1];
final _ElevationOpacity upper = _surfaceTintElevationOpacities[index];
final double t = (elevation - lower.elevation) / (upper.elevation - lower.elevation);
return lower.opacity + t * (upper.opacity - lower.opacity);
}
/// Applies an overlay color to a surface color to indicate
/// the level of its elevation in a dark theme.
///
/// If using Material Design 3, this type of color overlay is no longer used.
/// Instead a "surface tint" overlay is used instead. See [applySurfaceTint],
/// [ThemeData.useMaterial3] for more information.
///
/// Material drop shadows can be difficult to see in a dark theme, so the
/// elevation of a surface should be portrayed with an "overlay" in addition
/// to the shadow. As the elevation of the component increases, the
/// overlay increases in opacity. This function computes and applies this
/// overlay to a given color as needed.
///
/// If the ambient theme is dark ([ThemeData.brightness] is [Brightness.dark]),
/// and [ThemeData.applyElevationOverlayColor] is true, and the given
/// [color] is [ColorScheme.surface] then this will return a version of
/// the [color] with a semi-transparent [ColorScheme.onSurface] overlaid
/// on top of it. The opacity of the overlay is computed based on the
/// [elevation].
///
/// Otherwise it will just return the [color] unmodified.
///
/// See also:
///
/// * [ThemeData.applyElevationOverlayColor] which controls the whether
/// an overlay color will be applied to indicate elevation.
/// * [overlayColor] which computes the needed overlay color.
/// * [Material] which uses this to apply an elevation overlay to its surface.
/// * <https://material.io/design/color/dark-theme.html>, which specifies how
/// the overlay should be applied.
static Color applyOverlay(BuildContext context, Color color, double elevation) {
final ThemeData theme = Theme.of(context);
if (elevation > 0.0 &&
theme.applyElevationOverlayColor &&
theme.brightness == Brightness.dark &&
color.withOpacity(1.0) == theme.colorScheme.surface.withOpacity(1.0)) {
return colorWithOverlay(color, theme.colorScheme.onSurface, elevation);
}
return color;
}
/// Computes the appropriate overlay color used to indicate elevation in
/// dark themes.
///
/// If using Material Design 3, this type of color overlay is no longer used.
/// Instead a "surface tint" overlay is used instead. See [applySurfaceTint],
/// [ThemeData.useMaterial3] for more information.
///
/// See also:
///
/// * https://material.io/design/color/dark-theme.html#properties which
/// specifies the exact overlay values for a given elevation.
static Color overlayColor(BuildContext context, double elevation) {
final ThemeData theme = Theme.of(context);
return _overlayColor(theme.colorScheme.onSurface, elevation);
}
/// Returns a color blended by laying a semi-transparent overlay (using the
/// [overlay] color) on top of a surface (using the [surface] color).
///
/// If using Material Design 3, this type of color overlay is no longer used.
/// Instead a "surface tint" overlay is used instead. See [applySurfaceTint],
/// [ThemeData.useMaterial3] for more information.
///
/// The opacity of the overlay depends on [elevation]. As [elevation]
/// increases, the opacity will also increase.
///
/// See https://material.io/design/color/dark-theme.html#properties.
static Color colorWithOverlay(Color surface, Color overlay, double elevation) {
return Color.alphaBlend(_overlayColor(overlay, elevation), surface);
}
/// Applies an opacity to [color] based on [elevation].
static Color _overlayColor(Color color, double elevation) {
// Compute the opacity for the given elevation
// This formula matches the values in the spec:
// https://material.io/design/color/dark-theme.html#properties
final double opacity = (4.5 * math.log(elevation + 1) + 2) / 100.0;
return color.withOpacity(opacity);
}
}
// A data class to hold the opacity at a given elevation.
class _ElevationOpacity {
const _ElevationOpacity(this.elevation, this.opacity);
final double elevation;
final double opacity;
}
// BEGIN GENERATED TOKEN PROPERTIES - SurfaceTint
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
// Surface tint opacities based on elevations according to the
// Material Design 3 specification:
// https://m3.material.io/styles/color/the-color-system/color-roles
// Ordered by increasing elevation.
const List<_ElevationOpacity> _surfaceTintElevationOpacities = <_ElevationOpacity>[
_ElevationOpacity(0.0, 0.0), // Elevation level 0
_ElevationOpacity(1.0, 0.05), // Elevation level 1
_ElevationOpacity(3.0, 0.08), // Elevation level 2
_ElevationOpacity(6.0, 0.11), // Elevation level 3
_ElevationOpacity(8.0, 0.12), // Elevation level 4
_ElevationOpacity(12.0, 0.14), // Elevation level 5
];
// END GENERATED TOKEN PROPERTIES - SurfaceTint
| flutter/packages/flutter/lib/src/material/elevation_overlay.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/elevation_overlay.dart",
"repo_id": "flutter",
"token_count": 2372
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'button_style_button.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'icon_button_theme.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'tooltip.dart';
// Examples can assume:
// late BuildContext context;
// Minimum logical pixel size of the IconButton.
// See: <https://material.io/design/usability/accessibility.html#layout-typography>.
const double _kMinButtonSize = kMinInteractiveDimension;
enum _IconButtonVariant { standard, filled, filledTonal, outlined }
/// A Material Design icon button.
///
/// An icon button is a picture printed on a [Material] widget that reacts to
/// touches by filling with color (ink).
///
/// Icon buttons are commonly used in the [AppBar.actions] field, but they can
/// be used in many other places as well.
///
/// If the [onPressed] callback is null, then the button will be disabled and
/// will not react to touch.
///
/// Requires one of its ancestors to be a [Material] widget. In Material Design 3,
/// this requirement no longer exists because this widget builds a subclass of
/// [ButtonStyleButton].
///
/// The hit region of an icon button will, if possible, be at least
/// kMinInteractiveDimension pixels in size, regardless of the actual
/// [iconSize], to satisfy the [touch target size](https://material.io/design/layout/spacing-methods.html#touch-targets)
/// requirements in the Material Design specification. The [alignment] controls
/// how the icon itself is positioned within the hit region.
///
/// {@tool dartpad}
/// This sample shows an [IconButton] that uses the Material icon "volume_up" to
/// increase the volume.
///
/// 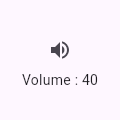
///
/// ** See code in examples/api/lib/material/icon_button/icon_button.0.dart **
/// {@end-tool}
///
/// ### Icon sizes
///
/// When creating an icon button with an [Icon], do not override the
/// icon's size with its [Icon.size] parameter, use the icon button's
/// [iconSize] parameter instead. For example do this:
///
/// ```dart
/// IconButton(
/// iconSize: 72,
/// icon: const Icon(Icons.favorite),
/// onPressed: () {
/// // ...
/// },
/// ),
/// ```
///
/// Avoid doing this:
///
/// ```dart
/// IconButton(
/// icon: const Icon(Icons.favorite, size: 72),
/// onPressed: () {
/// // ...
/// },
/// ),
/// ```
///
/// If you do, the button's size will be based on the default icon
/// size, not 72, which may produce unexpected layouts and clipping
/// issues.
///
/// ### Adding a filled background
///
/// Icon buttons don't support specifying a background color or other
/// background decoration because typically the icon is just displayed
/// on top of the parent widget's background. Icon buttons that appear
/// in [AppBar.actions] are an example of this.
///
/// It's easy enough to create an icon button with a filled background
/// using the [Ink] widget. The [Ink] widget renders a decoration on
/// the underlying [Material] along with the splash and highlight
/// [InkResponse] contributed by descendant widgets.
///
/// {@tool dartpad}
/// In this sample the icon button's background color is defined with an [Ink]
/// widget whose child is an [IconButton]. The icon button's filled background
/// is a light shade of blue, it's a filled circle, and it's as big as the
/// button is.
///
/// 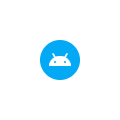
///
/// ** See code in examples/api/lib/material/icon_button/icon_button.1.dart **
/// {@end-tool}
///
/// Material Design 3 introduced new types (standard and contained) of [IconButton]s.
/// The default [IconButton] is the standard type. To create a filled icon button,
/// use [IconButton.filled]; to create a filled tonal icon button, use [IconButton.filledTonal];
/// to create a outlined icon button, use [IconButton.outlined].
///
/// Material Design 3 also treats [IconButton]s as toggle buttons. In order
/// to not break existing apps, the toggle feature can be optionally controlled
/// by the [isSelected] property.
///
/// If [isSelected] is null it will behave as a normal button. If [isSelected] is not
/// null then it will behave as a toggle button. If [isSelected] is true then it will
/// show [selectedIcon], if it false it will show the normal [icon].
///
/// In Material Design 3, both [IconTheme] and [IconButtonTheme] are used to override the default style
/// of [IconButton]. If both themes exist, the [IconButtonTheme] will override [IconTheme] no matter
/// which is closer to the [IconButton]. Each [IconButton]'s property is resolved by the order of
/// precedence: widget property, [IconButtonTheme] property, [IconTheme] property and
/// internal default property value.
///
/// In Material Design 3, the [IconButton.visualDensity] defaults to [VisualDensity.standard]
/// for all platforms; otherwise the button will have a rounded rectangle shape if
/// the [IconButton.visualDensity] is set to [VisualDensity.compact]. Users can
/// customize it by using [IconButtonTheme], [IconButton.style] or [IconButton.visualDensity].
///
/// {@tool dartpad}
/// This sample shows creation of [IconButton] widgets for standard, filled,
/// filled tonal and outlined types, as described in: https://m3.material.io/components/icon-buttons/overview
///
/// ** See code in examples/api/lib/material/icon_button/icon_button.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows creation of [IconButton] widgets with toggle feature for
/// standard, filled, filled tonal and outlined types, as described
/// in: https://m3.material.io/components/icon-buttons/overview
///
/// ** See code in examples/api/lib/material/icon_button/icon_button.3.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Icons], the library of Material Icons.
/// * [BackButton], an icon button for a "back" affordance which adapts to the
/// current platform's conventions.
/// * [CloseButton], an icon button for closing pages.
/// * [AppBar], to show a toolbar at the top of an application.
/// * [TextButton], [ElevatedButton], [OutlinedButton], for buttons with text labels and an optional icon.
/// * [InkResponse] and [InkWell], for the ink splash effect itself.
class IconButton extends StatelessWidget {
/// Creates an icon button.
///
/// Icon buttons are commonly used in the [AppBar.actions] field, but they can
/// be used in many other places as well.
///
/// Requires one of its ancestors to be a [Material] widget. This requirement
/// no longer exists if [ThemeData.useMaterial3] is set to true.
///
/// The [icon] argument must be specified, and is typically either an [Icon]
/// or an [ImageIcon].
const IconButton({
super.key,
this.iconSize,
this.visualDensity,
this.padding,
this.alignment,
this.splashRadius,
this.color,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.splashColor,
this.disabledColor,
required this.onPressed,
this.mouseCursor,
this.focusNode,
this.autofocus = false,
this.tooltip,
this.enableFeedback,
this.constraints,
this.style,
this.isSelected,
this.selectedIcon,
required this.icon,
}) : assert(splashRadius == null || splashRadius > 0),
_variant = _IconButtonVariant.standard;
/// Create a filled variant of IconButton.
///
/// Filled icon buttons have higher visual impact and should be used for
/// high emphasis actions, such as turning off a microphone or camera.
const IconButton.filled({
super.key,
this.iconSize,
this.visualDensity,
this.padding,
this.alignment,
this.splashRadius,
this.color,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.splashColor,
this.disabledColor,
required this.onPressed,
this.mouseCursor,
this.focusNode,
this.autofocus = false,
this.tooltip,
this.enableFeedback,
this.constraints,
this.style,
this.isSelected,
this.selectedIcon,
required this.icon,
}) : assert(splashRadius == null || splashRadius > 0),
_variant = _IconButtonVariant.filled;
/// Create a filled tonal variant of IconButton.
///
/// Filled tonal icon buttons are a middle ground between filled and outlined
/// icon buttons. They’re useful in contexts where the button requires slightly
/// more emphasis than an outline would give, such as a secondary action paired
/// with a high emphasis action.
const IconButton.filledTonal({
super.key,
this.iconSize,
this.visualDensity,
this.padding,
this.alignment,
this.splashRadius,
this.color,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.splashColor,
this.disabledColor,
required this.onPressed,
this.mouseCursor,
this.focusNode,
this.autofocus = false,
this.tooltip,
this.enableFeedback,
this.constraints,
this.style,
this.isSelected,
this.selectedIcon,
required this.icon,
}) : assert(splashRadius == null || splashRadius > 0),
_variant = _IconButtonVariant.filledTonal;
/// Create a filled tonal variant of IconButton.
///
/// Outlined icon buttons are medium-emphasis buttons. They’re useful when an
/// icon button needs more emphasis than a standard icon button but less than
/// a filled or filled tonal icon button.
const IconButton.outlined({
super.key,
this.iconSize,
this.visualDensity,
this.padding,
this.alignment,
this.splashRadius,
this.color,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.splashColor,
this.disabledColor,
required this.onPressed,
this.mouseCursor,
this.focusNode,
this.autofocus = false,
this.tooltip,
this.enableFeedback,
this.constraints,
this.style,
this.isSelected,
this.selectedIcon,
required this.icon,
}) : assert(splashRadius == null || splashRadius > 0),
_variant = _IconButtonVariant.outlined;
/// The size of the icon inside the button.
///
/// If null, uses [IconThemeData.size]. If it is also null, the default size
/// is 24.0.
///
/// The size given here is passed down to the widget in the [icon] property
/// via an [IconTheme]. Setting the size here instead of in, for example, the
/// [Icon.size] property allows the [IconButton] to size the splash area to
/// fit the [Icon]. If you were to set the size of the [Icon] using
/// [Icon.size] instead, then the [IconButton] would default to 24.0 and then
/// the [Icon] itself would likely get clipped.
///
/// This property is only used when [icon] is or contains an [Icon] widget. It will be
/// ignored if other widgets are used, such as an [Image].
///
/// If [ThemeData.useMaterial3] is set to true and this is null, the size of the
/// [IconButton] would default to 24.0. The size given here is passed down to the
/// [ButtonStyle.iconSize] property.
final double? iconSize;
/// Defines how compact the icon button's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
///
/// This property can be null. If null, it defaults to [VisualDensity.standard]
/// in Material Design 3 to make sure the button will be circular on all platforms.
///
/// See also:
///
/// * [ThemeData.visualDensity], which specifies the [visualDensity] for all
/// widgets within a [Theme].
final VisualDensity? visualDensity;
/// The padding around the button's icon. The entire padded icon will react
/// to input gestures.
///
/// This property can be null. If null, it defaults to 8.0 padding on all sides.
final EdgeInsetsGeometry? padding;
/// Defines how the icon is positioned within the IconButton.
///
/// This property can be null. If null, it defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry? alignment;
/// The splash radius.
///
/// If [ThemeData.useMaterial3] is set to true, this will not be used.
///
/// If null, default splash radius of [Material.defaultSplashRadius] is used.
final double? splashRadius;
/// The icon to display inside the button.
///
/// The [Icon.size] and [Icon.color] of the icon is configured automatically
/// based on the [iconSize] and [color] properties of _this_ widget using an
/// [IconTheme] and therefore should not be explicitly given in the icon
/// widget.
///
/// See [Icon], [ImageIcon].
final Widget icon;
/// The color for the button when it has the input focus.
///
/// If [ThemeData.useMaterial3] is set to true, this [focusColor] will be mapped
/// to be the [ButtonStyle.overlayColor] in focused state, which paints on top of
/// the button, as an overlay. Therefore, using a color with some transparency
/// is recommended. For example, one could customize the [focusColor] below:
///
/// ```dart
/// IconButton(
/// focusColor: Colors.orange.withOpacity(0.3),
/// icon: const Icon(Icons.sunny),
/// onPressed: () {
/// // ...
/// },
/// )
/// ```
///
/// Defaults to [ThemeData.focusColor] of the ambient theme.
final Color? focusColor;
/// The color for the button when a pointer is hovering over it.
///
/// If [ThemeData.useMaterial3] is set to true, this [hoverColor] will be mapped
/// to be the [ButtonStyle.overlayColor] in hovered state, which paints on top of
/// the button, as an overlay. Therefore, using a color with some transparency
/// is recommended. For example, one could customize the [hoverColor] below:
///
/// ```dart
/// IconButton(
/// hoverColor: Colors.orange.withOpacity(0.3),
/// icon: const Icon(Icons.ac_unit),
/// onPressed: () {
/// // ...
/// },
/// )
/// ```
///
/// Defaults to [ThemeData.hoverColor] of the ambient theme.
final Color? hoverColor;
/// The color to use for the icon inside the button, if the icon is enabled.
/// Defaults to leaving this up to the [icon] widget.
///
/// The icon is enabled if [onPressed] is not null.
///
/// ```dart
/// IconButton(
/// color: Colors.blue,
/// icon: const Icon(Icons.sunny_snowing),
/// onPressed: () {
/// // ...
/// },
/// )
/// ```
final Color? color;
/// The primary color of the button when the button is in the down (pressed) state.
/// The splash is represented as a circular overlay that appears above the
/// [highlightColor] overlay. The splash overlay has a center point that matches
/// the hit point of the user touch event. The splash overlay will expand to
/// fill the button area if the touch is held for long enough time. If the splash
/// color has transparency then the highlight and button color will show through.
///
/// If [ThemeData.useMaterial3] is set to true, this will not be used. Use
/// [highlightColor] instead to show the overlay color of the button when the button
/// is in the pressed state.
///
/// Defaults to the Theme's splash color, [ThemeData.splashColor].
final Color? splashColor;
/// The secondary color of the button when the button is in the down (pressed)
/// state. The highlight color is represented as a solid color that is overlaid over the
/// button color (if any). If the highlight color has transparency, the button color
/// will show through. The highlight fades in quickly as the button is held down.
///
/// If [ThemeData.useMaterial3] is set to true, this [highlightColor] will be mapped
/// to be the [ButtonStyle.overlayColor] in pressed state, which paints on top
/// of the button, as an overlay. Therefore, using a color with some transparency
/// is recommended. For example, one could customize the [highlightColor] below:
///
/// ```dart
/// IconButton(
/// highlightColor: Colors.orange.withOpacity(0.3),
/// icon: const Icon(Icons.question_mark),
/// onPressed: () {
/// // ...
/// },
/// )
/// ```
///
/// Defaults to the Theme's highlight color, [ThemeData.highlightColor].
final Color? highlightColor;
/// The color to use for the icon inside the button, if the icon is disabled.
/// Defaults to the [ThemeData.disabledColor] of the current [Theme].
///
/// The icon is disabled if [onPressed] is null.
final Color? disabledColor;
/// The callback that is called when the button is tapped or otherwise activated.
///
/// If this is set to null, the button will be disabled.
final VoidCallback? onPressed;
/// {@macro flutter.material.RawMaterialButton.mouseCursor}
///
/// If set to null, will default to
/// - [SystemMouseCursors.basic], if [onPressed] is null
/// - [SystemMouseCursors.click], otherwise
final MouseCursor? mouseCursor;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// Text that describes the action that will occur when the button is pressed.
///
/// This text is displayed when the user long-presses on the button and is
/// used for accessibility.
final String? tooltip;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// Optional size constraints for the button.
///
/// When unspecified, defaults to:
/// ```dart
/// const BoxConstraints(
/// minWidth: kMinInteractiveDimension,
/// minHeight: kMinInteractiveDimension,
/// )
/// ```
/// where [kMinInteractiveDimension] is 48.0, and then with visual density
/// applied.
///
/// The default constraints ensure that the button is accessible.
/// Specifying this parameter enables creation of buttons smaller than
/// the minimum size, but it is not recommended.
///
/// The visual density uses the [visualDensity] parameter if specified,
/// and `Theme.of(context).visualDensity` otherwise.
final BoxConstraints? constraints;
/// Customizes this button's appearance.
///
/// Non-null properties of this style override the corresponding
/// properties in [_IconButtonM3.themeStyleOf] and [_IconButtonM3.defaultStyleOf].
/// [MaterialStateProperty]s that resolve to non-null values will similarly
/// override the corresponding [MaterialStateProperty]s in [_IconButtonM3.themeStyleOf]
/// and [_IconButtonM3.defaultStyleOf].
///
/// The [style] is only used for Material 3 [IconButton]. If [ThemeData.useMaterial3]
/// is set to true, [style] is preferred for icon button customization, and any
/// parameters defined in [style] will override the same parameters in [IconButton].
///
/// For example, if [IconButton]'s [visualDensity] is set to [VisualDensity.standard]
/// and [style]'s [visualDensity] is set to [VisualDensity.compact],
/// the icon button will have [VisualDensity.compact] to define the button's layout.
///
/// Null by default.
final ButtonStyle? style;
/// The optional selection state of the icon button.
///
/// If this property is null, the button will behave as a normal push button,
/// otherwise, the button will toggle between showing [icon] and [selectedIcon]
/// based on the value of [isSelected]. If true, it will show [selectedIcon],
/// if false it will show [icon].
///
/// This property is only used if [ThemeData.useMaterial3] is true.
final bool? isSelected;
/// The icon to display inside the button when [isSelected] is true. This property
/// can be null. The original [icon] will be used for both selected and unselected
/// status if it is null.
///
/// The [Icon.size] and [Icon.color] of the icon is configured automatically
/// based on the [iconSize] and [color] properties using an [IconTheme] and
/// therefore should not be explicitly configured in the icon widget.
///
/// This property is only used if [ThemeData.useMaterial3] is true.
///
/// See also:
///
/// * [Icon], for icons based on glyphs from fonts instead of images.
/// * [ImageIcon], for showing icons from [AssetImage]s or other [ImageProvider]s.
final Widget? selectedIcon;
final _IconButtonVariant _variant;
/// A static convenience method that constructs an icon button
/// [ButtonStyle] given simple values. This method is only used for Material 3.
///
/// The [foregroundColor] color is used to create a [MaterialStateProperty]
/// [ButtonStyle.foregroundColor] value. Specify a value for [foregroundColor]
/// to specify the color of the button's icons. The [hoverColor], [focusColor]
/// and [highlightColor] colors are used to indicate the hover, focus,
/// and pressed states. Use [backgroundColor] for the button's background
/// fill color. Use [disabledForegroundColor] and [disabledBackgroundColor]
/// to specify the button's disabled icon and fill color.
///
/// Similarly, the [enabledMouseCursor] and [disabledMouseCursor]
/// parameters are used to construct [ButtonStyle].mouseCursor.
///
/// All of the other parameters are either used directly or used to
/// create a [MaterialStateProperty] with a single value for all
/// states.
///
/// All parameters default to null, by default this method returns
/// a [ButtonStyle] that doesn't override anything.
///
/// For example, to override the default icon color for a
/// [IconButton], as well as its overlay color, with all of the
/// standard opacity adjustments for the pressed, focused, and
/// hovered states, one could write:
///
/// ```dart
/// IconButton(
/// icon: const Icon(Icons.pets),
/// style: IconButton.styleFrom(foregroundColor: Colors.green),
/// onPressed: () {
/// // ...
/// },
/// ),
/// ```
static ButtonStyle styleFrom({
Color? foregroundColor,
Color? backgroundColor,
Color? disabledForegroundColor,
Color? disabledBackgroundColor,
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
Color? shadowColor,
Color? surfaceTintColor,
double? elevation,
Size? minimumSize,
Size? fixedSize,
Size? maximumSize,
double? iconSize,
BorderSide? side,
OutlinedBorder? shape,
EdgeInsetsGeometry? padding,
MouseCursor? enabledMouseCursor,
MouseCursor? disabledMouseCursor,
VisualDensity? visualDensity,
MaterialTapTargetSize? tapTargetSize,
Duration? animationDuration,
bool? enableFeedback,
AlignmentGeometry? alignment,
InteractiveInkFeatureFactory? splashFactory,
}) {
final MaterialStateProperty<Color?>? buttonBackgroundColor = (backgroundColor == null && disabledBackgroundColor == null)
? null
: _IconButtonDefaultBackground(backgroundColor, disabledBackgroundColor);
final MaterialStateProperty<Color?>? buttonForegroundColor = (foregroundColor == null && disabledForegroundColor == null)
? null
: _IconButtonDefaultForeground(foregroundColor, disabledForegroundColor);
final MaterialStateProperty<Color?>? overlayColor = (foregroundColor == null && hoverColor == null && focusColor == null && highlightColor == null)
? null
: _IconButtonDefaultOverlay(foregroundColor, focusColor, hoverColor, highlightColor);
final MaterialStateProperty<MouseCursor?> mouseCursor = _IconButtonDefaultMouseCursor(enabledMouseCursor, disabledMouseCursor);
return ButtonStyle(
backgroundColor: buttonBackgroundColor,
foregroundColor: buttonForegroundColor,
overlayColor: overlayColor,
shadowColor: ButtonStyleButton.allOrNull<Color>(shadowColor),
surfaceTintColor: ButtonStyleButton.allOrNull<Color>(surfaceTintColor),
elevation: ButtonStyleButton.allOrNull<double>(elevation),
padding: ButtonStyleButton.allOrNull<EdgeInsetsGeometry>(padding),
minimumSize: ButtonStyleButton.allOrNull<Size>(minimumSize),
fixedSize: ButtonStyleButton.allOrNull<Size>(fixedSize),
maximumSize: ButtonStyleButton.allOrNull<Size>(maximumSize),
iconSize: ButtonStyleButton.allOrNull<double>(iconSize),
side: ButtonStyleButton.allOrNull<BorderSide>(side),
shape: ButtonStyleButton.allOrNull<OutlinedBorder>(shape),
mouseCursor: mouseCursor,
visualDensity: visualDensity,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
splashFactory: splashFactory,
);
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
if (theme.useMaterial3) {
final Size? minSize = constraints == null
? null
: Size(constraints!.minWidth, constraints!.minHeight);
final Size? maxSize = constraints == null
? null
: Size(constraints!.maxWidth, constraints!.maxHeight);
ButtonStyle adjustedStyle = styleFrom(
visualDensity: visualDensity,
foregroundColor: color,
disabledForegroundColor: disabledColor,
focusColor: focusColor,
hoverColor: hoverColor,
highlightColor: highlightColor,
padding: padding,
minimumSize: minSize,
maximumSize: maxSize,
iconSize: iconSize,
alignment: alignment,
enabledMouseCursor: mouseCursor,
disabledMouseCursor: mouseCursor,
enableFeedback: enableFeedback,
);
if (style != null) {
adjustedStyle = style!.merge(adjustedStyle);
}
Widget effectiveIcon = icon;
if ((isSelected ?? false) && selectedIcon != null) {
effectiveIcon = selectedIcon!;
}
Widget iconButton = effectiveIcon;
if (tooltip != null) {
iconButton = Tooltip(
message: tooltip,
child: effectiveIcon,
);
}
return _SelectableIconButton(
style: adjustedStyle,
onPressed: onPressed,
autofocus: autofocus,
focusNode: focusNode,
isSelected: isSelected,
variant: _variant,
child: iconButton,
);
}
assert(debugCheckHasMaterial(context));
Color? currentColor;
if (onPressed != null) {
currentColor = color;
} else {
currentColor = disabledColor ?? theme.disabledColor;
}
final VisualDensity effectiveVisualDensity = visualDensity ?? theme.visualDensity;
final BoxConstraints unadjustedConstraints = constraints ?? const BoxConstraints(
minWidth: _kMinButtonSize,
minHeight: _kMinButtonSize,
);
final BoxConstraints adjustedConstraints = effectiveVisualDensity.effectiveConstraints(unadjustedConstraints);
final double effectiveIconSize = iconSize ?? IconTheme.of(context).size ?? 24.0;
final EdgeInsetsGeometry effectivePadding = padding ?? const EdgeInsets.all(8.0);
final AlignmentGeometry effectiveAlignment = alignment ?? Alignment.center;
final bool effectiveEnableFeedback = enableFeedback ?? true;
Widget result = ConstrainedBox(
constraints: adjustedConstraints,
child: Padding(
padding: effectivePadding,
child: SizedBox(
height: effectiveIconSize,
width: effectiveIconSize,
child: Align(
alignment: effectiveAlignment,
child: IconTheme.merge(
data: IconThemeData(
size: effectiveIconSize,
color: currentColor,
),
child: icon,
),
),
),
),
);
if (tooltip != null) {
result = Tooltip(
message: tooltip,
child: result,
);
}
return Semantics(
button: true,
enabled: onPressed != null,
child: InkResponse(
focusNode: focusNode,
autofocus: autofocus,
canRequestFocus: onPressed != null,
onTap: onPressed,
mouseCursor: mouseCursor ?? (onPressed == null ? SystemMouseCursors.basic : SystemMouseCursors.click),
enableFeedback: effectiveEnableFeedback,
focusColor: focusColor ?? theme.focusColor,
hoverColor: hoverColor ?? theme.hoverColor,
highlightColor: highlightColor ?? theme.highlightColor,
splashColor: splashColor ?? theme.splashColor,
radius: splashRadius ?? math.max(
Material.defaultSplashRadius,
(effectiveIconSize + math.min(effectivePadding.horizontal, effectivePadding.vertical)) * 0.7,
// x 0.5 for diameter -> radius and + 40% overflow derived from other Material apps.
),
child: result,
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('tooltip', tooltip, defaultValue: null, quoted: false));
properties.add(ObjectFlagProperty<VoidCallback>('onPressed', onPressed, ifNull: 'disabled'));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(ColorProperty('disabledColor', disabledColor, defaultValue: null));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: null));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: null));
properties.add(ColorProperty('highlightColor', highlightColor, defaultValue: null));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode, defaultValue: null));
}
}
class _SelectableIconButton extends StatefulWidget {
const _SelectableIconButton({
this.isSelected,
this.style,
this.focusNode,
required this.variant,
required this.autofocus,
required this.onPressed,
required this.child,
});
final bool? isSelected;
final ButtonStyle? style;
final FocusNode? focusNode;
final _IconButtonVariant variant;
final bool autofocus;
final VoidCallback? onPressed;
final Widget child;
@override
State<_SelectableIconButton> createState() => _SelectableIconButtonState();
}
class _SelectableIconButtonState extends State<_SelectableIconButton> {
late final MaterialStatesController statesController;
@override
void initState() {
super.initState();
if (widget.isSelected == null) {
statesController = MaterialStatesController();
} else {
statesController = MaterialStatesController(<MaterialState>{
if (widget.isSelected!) MaterialState.selected
});
}
}
@override
void didUpdateWidget(_SelectableIconButton oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.isSelected == null) {
if (statesController.value.contains(MaterialState.selected)) {
statesController.update(MaterialState.selected, false);
}
return;
}
if (widget.isSelected != oldWidget.isSelected) {
statesController.update(MaterialState.selected, widget.isSelected!);
}
}
@override
Widget build(BuildContext context) {
final bool toggleable = widget.isSelected != null;
return _IconButtonM3(
statesController: statesController,
style: widget.style,
autofocus: widget.autofocus,
focusNode: widget.focusNode,
onPressed: widget.onPressed,
variant: widget.variant,
toggleable: toggleable,
child: Semantics(
selected: widget.isSelected,
child: widget.child,
),
);
}
@override
void dispose() {
statesController.dispose();
super.dispose();
}
}
class _IconButtonM3 extends ButtonStyleButton {
const _IconButtonM3({
required super.onPressed,
super.style,
super.focusNode,
super.autofocus = false,
super.statesController,
required this.variant,
required this.toggleable,
required Widget super.child,
}) : super(
onLongPress: null,
onHover: null,
onFocusChange: null,
clipBehavior: Clip.none);
final _IconButtonVariant variant;
final bool toggleable;
/// ## Material 3 defaults
///
/// If [ThemeData.useMaterial3] is set to true the following defaults will
/// be used:
///
/// * `textStyle` - null
/// * `backgroundColor` - transparent
/// * `foregroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.38)
/// * selected - Theme.colorScheme.primary
/// * others - Theme.colorScheme.onSurfaceVariant
/// * `overlayColor`
/// * selected
/// * hovered - Theme.colorScheme.primary(0.08)
/// * focused or pressed - Theme.colorScheme.primary(0.1)
/// * hovered - Theme.colorScheme.onSurfaceVariant(0.08)
/// * pressed or focused - Theme.colorScheme.onSurfaceVariant(0.1)
/// * others - null
/// * `shadowColor` - null
/// * `surfaceTintColor` - null
/// * `elevation` - 0
/// * `padding` - all(8)
/// * `minimumSize` - Size(40, 40)
/// * `fixedSize` - null
/// * `maximumSize` - Size.infinite
/// * `iconSize` - 24
/// * `side` - null
/// * `shape` - StadiumBorder()
/// * `mouseCursor`
/// * disabled - SystemMouseCursors.basic
/// * others - SystemMouseCursors.click
/// * `visualDensity` - VisualDensity.standard
/// * `tapTargetSize` - theme.materialTapTargetSize
/// * `animationDuration` - kThemeChangeDuration
/// * `enableFeedback` - true
/// * `alignment` - Alignment.center
/// * `splashFactory` - Theme.splashFactory
@override
ButtonStyle defaultStyleOf(BuildContext context) {
return switch (variant) {
_IconButtonVariant.filled => _FilledIconButtonDefaultsM3(context, toggleable),
_IconButtonVariant.filledTonal => _FilledTonalIconButtonDefaultsM3(context, toggleable),
_IconButtonVariant.outlined => _OutlinedIconButtonDefaultsM3(context, toggleable),
_IconButtonVariant.standard => _IconButtonDefaultsM3(context, toggleable),
};
}
/// Returns the [IconButtonThemeData.style] of the closest [IconButtonTheme] ancestor.
/// The color and icon size can also be configured by the [IconTheme] if the same property
/// has a null value in [IconButtonTheme]. However, if any of the properties exist
/// in both [IconButtonTheme] and [IconTheme], [IconTheme] will be overridden.
@override
ButtonStyle? themeStyleOf(BuildContext context) {
final IconThemeData iconTheme = IconTheme.of(context);
final bool isDark = Theme.of(context).brightness == Brightness.dark;
bool isIconThemeDefault(Color? color) {
if (isDark) {
return identical(color, kDefaultIconLightColor);
}
return identical(color, kDefaultIconDarkColor);
}
final bool isDefaultColor = isIconThemeDefault(iconTheme.color);
final bool isDefaultSize = iconTheme.size == const IconThemeData.fallback().size;
final ButtonStyle iconThemeStyle = IconButton.styleFrom(
foregroundColor: isDefaultColor ? null : iconTheme.color,
iconSize: isDefaultSize ? null : iconTheme.size
);
return IconButtonTheme.of(context).style?.merge(iconThemeStyle) ?? iconThemeStyle;
}
}
@immutable
class _IconButtonDefaultBackground extends MaterialStateProperty<Color?> {
_IconButtonDefaultBackground(this.background, this.disabledBackground);
final Color? background;
final Color? disabledBackground;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledBackground;
}
return background;
}
@override
String toString() {
return '{disabled: $disabledBackground, otherwise: $background}';
}
}
@immutable
class _IconButtonDefaultForeground extends MaterialStateProperty<Color?> {
_IconButtonDefaultForeground(this.foregroundColor, this.disabledForegroundColor);
final Color? foregroundColor;
final Color? disabledForegroundColor;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledForegroundColor;
}
return foregroundColor;
}
@override
String toString() {
return '{disabled: $disabledForegroundColor, otherwise: $foregroundColor}';
}
}
@immutable
class _IconButtonDefaultOverlay extends MaterialStateProperty<Color?> {
_IconButtonDefaultOverlay(this.foregroundColor, this.focusColor, this.hoverColor, this.highlightColor);
final Color? foregroundColor;
final Color? focusColor;
final Color? hoverColor;
final Color? highlightColor;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return highlightColor ?? foregroundColor?.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return hoverColor ?? foregroundColor?.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return focusColor ?? foregroundColor?.withOpacity(0.1);
}
}
if (states.contains(MaterialState.pressed)) {
return highlightColor ?? foregroundColor?.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return hoverColor ?? foregroundColor?.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return focusColor ?? foregroundColor?.withOpacity(0.1);
}
return null;
}
@override
String toString() {
return '{hovered: $hoverColor, focused: $focusColor, pressed: $highlightColor, otherwise: null}';
}
}
@immutable
class _IconButtonDefaultMouseCursor extends MaterialStateProperty<MouseCursor?> with Diagnosticable {
_IconButtonDefaultMouseCursor(this.enabledCursor, this.disabledCursor);
final MouseCursor? enabledCursor;
final MouseCursor? disabledCursor;
@override
MouseCursor? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledCursor;
}
return enabledCursor;
}
}
// BEGIN GENERATED TOKEN PROPERTIES - IconButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _IconButtonDefaultsM3 extends ButtonStyle {
_IconButtonDefaultsM3(this.context, this.toggleable)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
final bool toggleable;
late final ColorScheme _colors = Theme.of(context).colorScheme;
// No default text style
@override
MaterialStateProperty<Color?>? get backgroundColor =>
const MaterialStatePropertyAll<Color?>(Colors.transparent);
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
return _colors.primary;
}
return _colors.onSurfaceVariant;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
return Colors.transparent;
});
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(0.0);
@override
MaterialStateProperty<Color>? get shadowColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.all(8.0));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(40.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
@override
MaterialStateProperty<double>? get iconSize =>
const MaterialStatePropertyAll<double>(24.0);
@override
MaterialStateProperty<BorderSide?>? get side => null;
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => VisualDensity.standard;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - IconButton
// BEGIN GENERATED TOKEN PROPERTIES - FilledIconButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FilledIconButtonDefaultsM3 extends ButtonStyle {
_FilledIconButtonDefaultsM3(this.context, this.toggleable)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
final bool toggleable;
late final ColorScheme _colors = Theme.of(context).colorScheme;
// No default text style
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
if (states.contains(MaterialState.selected)) {
return _colors.primary;
}
if (toggleable) { // toggleable but unselected case
return _colors.surfaceContainerHighest;
}
return _colors.primary;
});
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
return _colors.onPrimary;
}
if (toggleable) { // toggleable but unselected case
return _colors.primary;
}
return _colors.onPrimary;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.1);
}
}
if (toggleable) { // toggleable but unselected case
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
}
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.1);
}
return Colors.transparent;
});
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(0.0);
@override
MaterialStateProperty<Color>? get shadowColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.all(8.0));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(40.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
@override
MaterialStateProperty<double>? get iconSize =>
const MaterialStatePropertyAll<double>(24.0);
@override
MaterialStateProperty<BorderSide?>? get side => null;
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => VisualDensity.standard;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - FilledIconButton
// BEGIN GENERATED TOKEN PROPERTIES - FilledTonalIconButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FilledTonalIconButtonDefaultsM3 extends ButtonStyle {
_FilledTonalIconButtonDefaultsM3(this.context, this.toggleable)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
final bool toggleable;
late final ColorScheme _colors = Theme.of(context).colorScheme;
// No default text style
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
if (states.contains(MaterialState.selected)) {
return _colors.secondaryContainer;
}
if (toggleable) { // toggleable but unselected case
return _colors.surfaceContainerHighest;
}
return _colors.secondaryContainer;
});
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
return _colors.onSecondaryContainer;
}
if (toggleable) { // toggleable but unselected case
return _colors.onSurfaceVariant;
}
return _colors.onSecondaryContainer;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSecondaryContainer.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
}
if (toggleable) { // toggleable but unselected case
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.1);
}
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSecondaryContainer.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
return Colors.transparent;
});
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(0.0);
@override
MaterialStateProperty<Color>? get shadowColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.all(8.0));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(40.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
@override
MaterialStateProperty<double>? get iconSize =>
const MaterialStatePropertyAll<double>(24.0);
@override
MaterialStateProperty<BorderSide?>? get side => null;
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => VisualDensity.standard;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - FilledTonalIconButton
// BEGIN GENERATED TOKEN PROPERTIES - OutlinedIconButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _OutlinedIconButtonDefaultsM3 extends ButtonStyle {
_OutlinedIconButtonDefaultsM3(this.context, this.toggleable)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
final bool toggleable;
late final ColorScheme _colors = Theme.of(context).colorScheme;
// No default text style
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.onSurface.withOpacity(0.12);
}
return Colors.transparent;
}
if (states.contains(MaterialState.selected)) {
return _colors.inverseSurface;
}
return Colors.transparent;
});
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
return _colors.onInverseSurface;
}
return _colors.onSurfaceVariant;
});
@override
MaterialStateProperty<Color?>? get overlayColor => MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onInverseSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onInverseSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onInverseSurface.withOpacity(0.08);
}
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant.withOpacity(0.08);
}
return Colors.transparent;
});
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(0.0);
@override
MaterialStateProperty<Color>? get shadowColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.all(8.0));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(40.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
@override
MaterialStateProperty<double>? get iconSize =>
const MaterialStatePropertyAll<double>(24.0);
@override
MaterialStateProperty<BorderSide?>? get side =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return null;
} else {
if (states.contains(MaterialState.disabled)) {
return BorderSide(color: _colors.onSurface.withOpacity(0.12));
}
return BorderSide(color: _colors.outline);
}
});
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => VisualDensity.standard;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - OutlinedIconButton
| flutter/packages/flutter/lib/src/material/icon_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/icon_button.dart",
"repo_id": "flutter",
"token_count": 18949
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'constants.dart';
import 'elevation_overlay.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Signature for the callback used by ink effects to obtain the rectangle for the effect.
///
/// Used by [InkHighlight] and [InkSplash], for example.
typedef RectCallback = Rect Function();
/// The various kinds of material in Material Design. Used to
/// configure the default behavior of [Material] widgets.
///
/// See also:
///
/// * [Material], in particular [Material.type].
/// * [kMaterialEdges]
enum MaterialType {
/// Rectangle using default theme canvas color.
canvas,
/// Rounded edges, card theme color.
card,
/// A circle, no color by default (used for floating action buttons).
circle,
/// Rounded edges, no color by default (used for [MaterialButton] buttons).
button,
/// A transparent piece of material that draws ink splashes and highlights.
///
/// While the material metaphor describes child widgets as printed on the
/// material itself and do not hide ink effects, in practice the [Material]
/// widget draws child widgets on top of the ink effects.
/// A [Material] with type transparency can be placed on top of opaque widgets
/// to show ink effects on top of them.
///
/// Prefer using the [Ink] widget for showing ink effects on top of opaque
/// widgets.
transparency
}
/// The border radii used by the various kinds of material in Material Design.
///
/// See also:
///
/// * [MaterialType]
/// * [Material]
const Map<MaterialType, BorderRadius?> kMaterialEdges = <MaterialType, BorderRadius?>{
MaterialType.canvas: null,
MaterialType.card: BorderRadius.all(Radius.circular(2.0)),
MaterialType.circle: null,
MaterialType.button: BorderRadius.all(Radius.circular(2.0)),
MaterialType.transparency: null,
};
/// An interface for creating [InkSplash]s and [InkHighlight]s on a [Material].
///
/// Typically obtained via [Material.of].
abstract class MaterialInkController {
/// The color of the material.
Color? get color;
/// The ticker provider used by the controller.
///
/// Ink features that are added to this controller with [addInkFeature] should
/// use this vsync to drive their animations.
TickerProvider get vsync;
/// Add an [InkFeature], such as an [InkSplash] or an [InkHighlight].
///
/// The ink feature will paint as part of this controller.
void addInkFeature(InkFeature feature);
/// Notifies the controller that one of its ink features needs to repaint.
void markNeedsPaint();
}
/// A piece of material.
///
/// The Material widget is responsible for:
///
/// 1. Clipping: If [clipBehavior] is not [Clip.none], Material clips its widget
/// sub-tree to the shape specified by [shape], [type], and [borderRadius].
/// By default, [clipBehavior] is [Clip.none] for performance considerations.
/// See [Ink] for an example of how this affects clipping [Ink] widgets.
/// 2. Elevation: Material elevates its widget sub-tree on the Z axis by
/// [elevation] pixels, and draws the appropriate shadow.
/// 3. Ink effects: Material shows ink effects implemented by [InkFeature]s
/// like [InkSplash] and [InkHighlight] below its children.
///
/// ## The Material Metaphor
///
/// Material is the central metaphor in Material Design. Each piece of material
/// exists at a given elevation, which influences how that piece of material
/// visually relates to other pieces of material and how that material casts
/// shadows.
///
/// Most user interface elements are either conceptually printed on a piece of
/// material or themselves made of material. Material reacts to user input using
/// [InkSplash] and [InkHighlight] effects. To trigger a reaction on the
/// material, use a [MaterialInkController] obtained via [Material.of].
///
/// In general, the features of a [Material] should not change over time (e.g. a
/// [Material] should not change its [color], [shadowColor] or [type]).
/// Changes to [elevation], [shadowColor] and [surfaceTintColor] are animated
/// for [animationDuration]. Changes to [shape] are animated if [type] is
/// not [MaterialType.transparency] and [ShapeBorder.lerp] between the previous
/// and next [shape] values is supported. Shape changes are also animated
/// for [animationDuration].
///
/// ## Shape
///
/// The shape for material is determined by [shape], [type], and [borderRadius].
///
/// - If [shape] is non null, it determines the shape.
/// - If [shape] is null and [borderRadius] is non null, the shape is a
/// rounded rectangle, with corners specified by [borderRadius].
/// - If [shape] and [borderRadius] are null, [type] determines the
/// shape as follows:
/// - [MaterialType.canvas]: the default material shape is a rectangle.
/// - [MaterialType.card]: the default material shape is a rectangle with
/// rounded edges. The edge radii is specified by [kMaterialEdges].
/// - [MaterialType.circle]: the default material shape is a circle.
/// - [MaterialType.button]: the default material shape is a rectangle with
/// rounded edges. The edge radii is specified by [kMaterialEdges].
/// - [MaterialType.transparency]: the default material shape is a rectangle.
///
/// ## Border
///
/// If [shape] is not null, then its border will also be painted (if any).
///
/// ## Layout change notifications
///
/// If the layout changes (e.g. because there's a list on the material, and it's
/// been scrolled), a [LayoutChangedNotification] must be dispatched at the
/// relevant subtree. This in particular means that transitions (e.g.
/// [SlideTransition]) should not be placed inside [Material] widgets so as to
/// move subtrees that contain [InkResponse]s, [InkWell]s, [Ink]s, or other
/// widgets that use the [InkFeature] mechanism. Otherwise, in-progress ink
/// features (e.g., ink splashes and ink highlights) won't move to account for
/// the new layout.
///
/// ## Painting over the material
///
/// Material widgets will often trigger reactions on their nearest material
/// ancestor. For example, [ListTile.hoverColor] triggers a reaction on the
/// tile's material when a pointer is hovering over it. These reactions will be
/// obscured if any widget in between them and the material paints in such a
/// way as to obscure the material (such as setting a [BoxDecoration.color] on
/// a [DecoratedBox]). To avoid this behavior, use [InkDecoration] to decorate
/// the material itself.
///
/// See also:
///
/// * [MergeableMaterial], a piece of material that can split and re-merge.
/// * [Card], a wrapper for a [Material] of [type] [MaterialType.card].
/// * <https://material.io/design/>
/// * <https://m3.material.io/styles/color/the-color-system/color-roles>
class Material extends StatefulWidget {
/// Creates a piece of material.
///
/// The [elevation] must be non-negative.
///
/// If a [shape] is specified, then the [borderRadius] property must be
/// null and the [type] property must not be [MaterialType.circle]. If the
/// [borderRadius] is specified, then the [type] property must not be
/// [MaterialType.circle]. In both cases, these restrictions are intended to
/// catch likely errors.
const Material({
super.key,
this.type = MaterialType.canvas,
this.elevation = 0.0,
this.color,
this.shadowColor,
this.surfaceTintColor,
this.textStyle,
this.borderRadius,
this.shape,
this.borderOnForeground = true,
this.clipBehavior = Clip.none,
this.animationDuration = kThemeChangeDuration,
this.child,
}) : assert(elevation >= 0.0),
assert(!(shape != null && borderRadius != null)),
assert(!(identical(type, MaterialType.circle) && (borderRadius != null || shape != null)));
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The kind of material to show (e.g., card or canvas). This
/// affects the shape of the widget, the roundness of its corners if
/// the shape is rectangular, and the default color.
final MaterialType type;
/// {@template flutter.material.material.elevation}
/// The z-coordinate at which to place this material relative to its parent.
///
/// This controls the size of the shadow below the material and the opacity
/// of the elevation overlay color if it is applied.
///
/// If this is non-zero, the contents of the material are clipped, because the
/// widget conceptually defines an independent printed piece of material.
///
/// Defaults to 0. Changing this value will cause the shadow and the elevation
/// overlay or surface tint to animate over [Material.animationDuration].
///
/// The value is non-negative.
///
/// See also:
///
/// * [ThemeData.useMaterial3] which defines whether a surface tint or
/// elevation overlay is used to indicate elevation.
/// * [ThemeData.applyElevationOverlayColor] which controls the whether
/// an overlay color will be applied to indicate elevation.
/// * [Material.color] which may have an elevation overlay applied.
/// * [Material.shadowColor] which will be used for the color of a drop shadow.
/// * [Material.surfaceTintColor] which will be used as the overlay tint to
/// show elevation.
/// {@endtemplate}
final double elevation;
/// The color to paint the material.
///
/// Must be opaque. To create a transparent piece of material, use
/// [MaterialType.transparency].
///
/// If [ThemeData.useMaterial3] is true then an optional [surfaceTintColor]
/// overlay may be applied on top of this color to indicate elevation.
///
/// If [ThemeData.useMaterial3] is false and [ThemeData.applyElevationOverlayColor]
/// is true and [ThemeData.brightness] is [Brightness.dark] then a
/// semi-transparent overlay color will be composited on top of this
/// color to indicate the elevation. This is no longer needed for Material
/// Design 3, which uses [surfaceTintColor].
///
/// By default, the color is derived from the [type] of material.
final Color? color;
/// The color to paint the shadow below the material.
///
/// {@template flutter.material.material.shadowColor}
/// If null and [ThemeData.useMaterial3] is true then [ThemeData]'s
/// [ColorScheme.shadow] will be used. If [ThemeData.useMaterial3] is false
/// then [ThemeData.shadowColor] will be used.
///
/// To remove the drop shadow when [elevation] is greater than 0, set
/// [shadowColor] to [Colors.transparent].
///
/// See also:
/// * [ThemeData.useMaterial3], which determines the default value for this
/// property if it is null.
/// * [ThemeData.applyElevationOverlayColor], which turns elevation overlay
/// on or off for dark themes.
/// {@endtemplate}
final Color? shadowColor;
/// The color of the surface tint overlay applied to the material color
/// to indicate elevation.
///
/// {@template flutter.material.material.surfaceTintColor}
/// Material Design 3 introduced a new way for some components to indicate
/// their elevation by using a surface tint color overlay on top of the
/// base material [color]. This overlay is painted with an opacity that is
/// related to the [elevation] of the material.
///
/// If [ThemeData.useMaterial3] is false, then this property is not used.
///
/// If [ThemeData.useMaterial3] is true and [surfaceTintColor] is not null and
/// not [Colors.transparent], then it will be used to overlay the base [color]
/// with an opacity based on the [elevation].
///
/// Otherwise, no surface tint will be applied.
///
/// See also:
///
/// * [ThemeData.useMaterial3], which turns this feature on.
/// * [ElevationOverlay.applySurfaceTint], which is used to implement the
/// tint.
/// * https://m3.material.io/styles/color/the-color-system/color-roles
/// which specifies how the overlay is applied.
/// {@endtemplate}
final Color? surfaceTintColor;
/// The typographical style to use for text within this material.
final TextStyle? textStyle;
/// Defines the material's shape as well its shadow.
///
/// {@template flutter.material.material.shape}
/// If shape is non null, the [borderRadius] is ignored and the material's
/// clip boundary and shadow are defined by the shape.
///
/// A shadow is only displayed if the [elevation] is greater than
/// zero.
/// {@endtemplate}
final ShapeBorder? shape;
/// Whether to paint the [shape] border in front of the [child].
///
/// The default value is true.
/// If false, the border will be painted behind the [child].
final bool borderOnForeground;
/// {@template flutter.material.Material.clipBehavior}
/// The content will be clipped (or not) according to this option.
///
/// See the enum [Clip] for details of all possible options and their common
/// use cases.
/// {@endtemplate}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// Defines the duration of animated changes for [shape], [elevation],
/// [shadowColor], [surfaceTintColor] and the elevation overlay if it is applied.
///
/// The default value is [kThemeChangeDuration].
final Duration animationDuration;
/// If non-null, the corners of this box are rounded by this
/// [BorderRadiusGeometry] value.
///
/// Otherwise, the corners specified for the current [type] of material are
/// used.
///
/// If [shape] is non null then the border radius is ignored.
///
/// Must be null if [type] is [MaterialType.circle].
final BorderRadiusGeometry? borderRadius;
/// The ink controller from the closest instance of this class that
/// encloses the given context within the closest [LookupBoundary].
///
/// Typical usage is as follows:
///
/// ```dart
/// MaterialInkController? inkController = Material.maybeOf(context);
/// ```
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [Material.of], which is similar to this method, but asserts if
/// no [Material] ancestor is found.
static MaterialInkController? maybeOf(BuildContext context) {
return LookupBoundary.findAncestorRenderObjectOfType<_RenderInkFeatures>(context);
}
/// The ink controller from the closest instance of [Material] that encloses
/// the given context within the closest [LookupBoundary].
///
/// If no [Material] widget ancestor can be found then this method will assert
/// in debug mode, and throw an exception in release mode.
///
/// Typical usage is as follows:
///
/// ```dart
/// MaterialInkController inkController = Material.of(context);
/// ```
///
/// This method can be expensive (it walks the element tree).
///
/// See also:
///
/// * [Material.maybeOf], which is similar to this method, but returns null if
/// no [Material] ancestor is found.
static MaterialInkController of(BuildContext context) {
final MaterialInkController? controller = maybeOf(context);
assert(() {
if (controller == null) {
if (LookupBoundary.debugIsHidingAncestorRenderObjectOfType<_RenderInkFeatures>(context)) {
throw FlutterError(
'Material.of() was called with a context that does not have access to a Material widget.\n'
'The context provided to Material.of() does have a Material widget ancestor, but it is '
'hidden by a LookupBoundary. This can happen because you are using a widget that looks '
'for a Material ancestor, but no such ancestor exists within the closest LookupBoundary.\n'
'The context used was:\n'
' $context',
);
}
throw FlutterError(
'Material.of() was called with a context that does not contain a Material widget.\n'
'No Material widget ancestor could be found starting from the context that was passed to '
'Material.of(). This can happen because you are using a widget that looks for a Material '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return controller!;
}
@override
State<Material> createState() => _MaterialState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<MaterialType>('type', type));
properties.add(DoubleProperty('elevation', elevation, defaultValue: 0.0));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
textStyle?.debugFillProperties(properties, prefix: 'textStyle.');
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('borderOnForeground', borderOnForeground, defaultValue: true));
properties.add(DiagnosticsProperty<BorderRadiusGeometry>('borderRadius', borderRadius, defaultValue: null));
}
/// The default radius of an ink splash in logical pixels.
static const double defaultSplashRadius = 35.0;
}
class _MaterialState extends State<Material> with TickerProviderStateMixin {
final GlobalKey _inkFeatureRenderer = GlobalKey(debugLabel: 'ink renderer');
Color? _getBackgroundColor(BuildContext context) {
final ThemeData theme = Theme.of(context);
Color? color = widget.color;
if (color == null) {
switch (widget.type) {
case MaterialType.canvas:
color = theme.canvasColor;
case MaterialType.card:
color = theme.cardColor;
case MaterialType.button:
case MaterialType.circle:
case MaterialType.transparency:
break;
}
}
return color;
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final Color? backgroundColor = _getBackgroundColor(context);
final Color modelShadowColor = widget.shadowColor ?? (theme.useMaterial3 ? theme.colorScheme.shadow : theme.shadowColor);
// If no shadow color is specified, use 0 for elevation in the model so a drop shadow won't be painted.
final double modelElevation = widget.elevation;
assert(
backgroundColor != null || widget.type == MaterialType.transparency,
'If Material type is not MaterialType.transparency, a color must '
'either be passed in through the `color` property, or be defined '
'in the theme (ex. canvasColor != null if type is set to '
'MaterialType.canvas)',
);
Widget? contents = widget.child;
if (contents != null) {
contents = AnimatedDefaultTextStyle(
style: widget.textStyle ?? Theme.of(context).textTheme.bodyMedium!,
duration: widget.animationDuration,
child: contents,
);
}
contents = NotificationListener<LayoutChangedNotification>(
onNotification: (LayoutChangedNotification notification) {
final _RenderInkFeatures renderer = _inkFeatureRenderer.currentContext!.findRenderObject()! as _RenderInkFeatures;
renderer._didChangeLayout();
return false;
},
child: _InkFeatures(
key: _inkFeatureRenderer,
absorbHitTest: widget.type != MaterialType.transparency,
color: backgroundColor,
vsync: this,
child: contents,
),
);
// PhysicalModel has a temporary workaround for a performance issue that
// speeds up rectangular non transparent material (the workaround is to
// skip the call to ui.Canvas.saveLayer if the border radius is 0).
// Until the saveLayer performance issue is resolved, we're keeping this
// special case here for canvas material type that is using the default
// shape (rectangle). We could go down this fast path for explicitly
// specified rectangles (e.g shape RoundedRectangleBorder with radius 0, but
// we choose not to as we want the change from the fast-path to the
// slow-path to be noticeable in the construction site of Material.
if (widget.type == MaterialType.canvas && widget.shape == null && widget.borderRadius == null) {
final Color color = Theme.of(context).useMaterial3
? ElevationOverlay.applySurfaceTint(backgroundColor!, widget.surfaceTintColor, widget.elevation)
: ElevationOverlay.applyOverlay(context, backgroundColor!, widget.elevation);
return AnimatedPhysicalModel(
curve: Curves.fastOutSlowIn,
duration: widget.animationDuration,
shape: BoxShape.rectangle,
clipBehavior: widget.clipBehavior,
elevation: modelElevation,
color: color,
shadowColor: modelShadowColor,
animateColor: false,
child: contents,
);
}
final ShapeBorder shape = _getShape();
if (widget.type == MaterialType.transparency) {
return _transparentInterior(
context: context,
shape: shape,
clipBehavior: widget.clipBehavior,
contents: contents,
);
}
return _MaterialInterior(
curve: Curves.fastOutSlowIn,
duration: widget.animationDuration,
shape: shape,
borderOnForeground: widget.borderOnForeground,
clipBehavior: widget.clipBehavior,
elevation: widget.elevation,
color: backgroundColor!,
shadowColor: modelShadowColor,
surfaceTintColor: widget.surfaceTintColor,
child: contents,
);
}
static Widget _transparentInterior({
required BuildContext context,
required ShapeBorder shape,
required Clip clipBehavior,
required Widget contents,
}) {
final _ShapeBorderPaint child = _ShapeBorderPaint(
shape: shape,
child: contents,
);
return ClipPath(
clipper: ShapeBorderClipper(
shape: shape,
textDirection: Directionality.maybeOf(context),
),
clipBehavior: clipBehavior,
child: child,
);
}
// Determines the shape for this Material.
//
// If a shape was specified, it will determine the shape.
// If a borderRadius was specified, the shape is a rounded
// rectangle.
// Otherwise, the shape is determined by the widget type as described in the
// Material class documentation.
ShapeBorder _getShape() {
if (widget.shape != null) {
return widget.shape!;
}
if (widget.borderRadius != null) {
return RoundedRectangleBorder(borderRadius: widget.borderRadius!);
}
switch (widget.type) {
case MaterialType.canvas:
case MaterialType.transparency:
return const RoundedRectangleBorder();
case MaterialType.card:
case MaterialType.button:
return RoundedRectangleBorder(
borderRadius: widget.borderRadius ?? kMaterialEdges[widget.type]!,
);
case MaterialType.circle:
return const CircleBorder();
}
}
}
class _RenderInkFeatures extends RenderProxyBox implements MaterialInkController {
_RenderInkFeatures({
RenderBox? child,
required this.vsync,
required this.absorbHitTest,
this.color,
}) : super(child);
// This class should exist in a 1:1 relationship with a MaterialState object,
// since there's no current support for dynamically changing the ticker
// provider.
@override
final TickerProvider vsync;
// This is here to satisfy the MaterialInkController contract.
// The actual painting of this color is done by a Container in the
// MaterialState build method.
@override
Color? color;
bool absorbHitTest;
@visibleForTesting
List<InkFeature>? get debugInkFeatures {
if (kDebugMode) {
return _inkFeatures;
}
return null;
}
List<InkFeature>? _inkFeatures;
@override
void addInkFeature(InkFeature feature) {
assert(!feature._debugDisposed);
assert(feature._controller == this);
_inkFeatures ??= <InkFeature>[];
assert(!_inkFeatures!.contains(feature));
_inkFeatures!.add(feature);
markNeedsPaint();
}
void _removeFeature(InkFeature feature) {
assert(_inkFeatures != null);
_inkFeatures!.remove(feature);
markNeedsPaint();
}
void _didChangeLayout() {
if (_inkFeatures?.isNotEmpty ?? false) {
markNeedsPaint();
}
}
@override
bool hitTestSelf(Offset position) => absorbHitTest;
@override
void paint(PaintingContext context, Offset offset) {
final List<InkFeature>? inkFeatures = _inkFeatures;
if (inkFeatures != null && inkFeatures.isNotEmpty) {
final Canvas canvas = context.canvas;
canvas.save();
canvas.translate(offset.dx, offset.dy);
canvas.clipRect(Offset.zero & size);
for (final InkFeature inkFeature in inkFeatures) {
inkFeature._paint(canvas);
}
canvas.restore();
}
assert(inkFeatures == _inkFeatures);
super.paint(context, offset);
}
}
class _InkFeatures extends SingleChildRenderObjectWidget {
const _InkFeatures({
super.key,
this.color,
required this.vsync,
required this.absorbHitTest,
super.child,
});
// This widget must be owned by a MaterialState, which must be provided as the vsync.
// This relationship must be 1:1 and cannot change for the lifetime of the MaterialState.
final Color? color;
final TickerProvider vsync;
final bool absorbHitTest;
@override
_RenderInkFeatures createRenderObject(BuildContext context) {
return _RenderInkFeatures(
color: color,
absorbHitTest: absorbHitTest,
vsync: vsync,
);
}
@override
void updateRenderObject(BuildContext context, _RenderInkFeatures renderObject) {
renderObject..color = color
..absorbHitTest = absorbHitTest;
assert(vsync == renderObject.vsync);
}
}
/// A visual reaction on a piece of [Material].
///
/// To add an ink feature to a piece of [Material], obtain the
/// [MaterialInkController] via [Material.of] and call
/// [MaterialInkController.addInkFeature].
abstract class InkFeature {
/// Initializes fields for subclasses.
InkFeature({
required MaterialInkController controller,
required this.referenceBox,
this.onRemoved,
}) : _controller = controller as _RenderInkFeatures {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/material.dart',
className: '$InkFeature',
object: this,
);
}
}
/// The [MaterialInkController] associated with this [InkFeature].
///
/// Typically used by subclasses to call
/// [MaterialInkController.markNeedsPaint] when they need to repaint.
MaterialInkController get controller => _controller;
final _RenderInkFeatures _controller;
/// The render box whose visual position defines the frame of reference for this ink feature.
final RenderBox referenceBox;
/// Called when the ink feature is no longer visible on the material.
final VoidCallback? onRemoved;
bool _debugDisposed = false;
/// Free up the resources associated with this ink feature.
@mustCallSuper
void dispose() {
assert(!_debugDisposed);
assert(() {
_debugDisposed = true;
return true;
}());
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_controller._removeFeature(this);
onRemoved?.call();
}
// Returns the paint transform that allows `fromRenderObject` to perform paint
// in `toRenderObject`'s coordinate space.
//
// Returns null if either `fromRenderObject` or `toRenderObject` is not in the
// same render tree, or either of them is in an offscreen subtree (see
// RenderObject.paintsChild).
static Matrix4? _getPaintTransform(
RenderObject fromRenderObject,
RenderObject toRenderObject,
) {
// The paths to fromRenderObject and toRenderObject's common ancestor.
final List<RenderObject> fromPath = <RenderObject>[fromRenderObject];
final List<RenderObject> toPath = <RenderObject>[toRenderObject];
RenderObject from = fromRenderObject;
RenderObject to = toRenderObject;
while (!identical(from, to)) {
final int fromDepth = from.depth;
final int toDepth = to.depth;
if (fromDepth >= toDepth) {
final RenderObject? fromParent = from.parent;
// Return early if the 2 render objects are not in the same render tree,
// or either of them is offscreen and thus won't get painted.
if (fromParent is! RenderObject || !fromParent.paintsChild(from)) {
return null;
}
fromPath.add(fromParent);
from = fromParent;
}
if (fromDepth <= toDepth) {
final RenderObject? toParent = to.parent;
if (toParent is! RenderObject || !toParent.paintsChild(to)) {
return null;
}
toPath.add(toParent);
to = toParent;
}
}
assert(identical(from, to));
final Matrix4 transform = Matrix4.identity();
final Matrix4 inverseTransform = Matrix4.identity();
for (int index = toPath.length - 1; index > 0; index -= 1) {
toPath[index].applyPaintTransform(toPath[index - 1], transform);
}
for (int index = fromPath.length - 1; index > 0; index -= 1) {
fromPath[index].applyPaintTransform(fromPath[index - 1], inverseTransform);
}
final double det = inverseTransform.invert();
return det != 0 ? (inverseTransform..multiply(transform)) : null;
}
void _paint(Canvas canvas) {
assert(referenceBox.attached);
assert(!_debugDisposed);
// determine the transform that gets our coordinate system to be like theirs
final Matrix4? transform = _getPaintTransform(_controller, referenceBox);
if (transform != null) {
paintFeature(canvas, transform);
}
}
/// Override this method to paint the ink feature.
///
/// The transform argument gives the coordinate conversion from the coordinate
/// system of the canvas to the coordinate system of the [referenceBox].
@protected
void paintFeature(Canvas canvas, Matrix4 transform);
@override
String toString() => describeIdentity(this);
}
/// An interpolation between two [ShapeBorder]s.
///
/// This class specializes the interpolation of [Tween] to use [ShapeBorder.lerp].
class ShapeBorderTween extends Tween<ShapeBorder?> {
/// Creates a [ShapeBorder] tween.
///
/// the [begin] and [end] properties may be null; see [ShapeBorder.lerp] for
/// the null handling semantics.
ShapeBorderTween({super.begin, super.end});
/// Returns the value this tween has at the given animation clock value.
@override
ShapeBorder? lerp(double t) {
return ShapeBorder.lerp(begin, end, t);
}
}
/// The interior of non-transparent material.
///
/// Animates [elevation], [shadowColor], and [shape].
class _MaterialInterior extends ImplicitlyAnimatedWidget {
/// Creates a const instance of [_MaterialInterior].
///
/// The [elevation] must be specified and greater than or equal to zero.
const _MaterialInterior({
required this.child,
required this.shape,
this.borderOnForeground = true,
this.clipBehavior = Clip.none,
required this.elevation,
required this.color,
required this.shadowColor,
required this.surfaceTintColor,
super.curve,
required super.duration,
}) : assert(elevation >= 0.0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The border of the widget.
///
/// This border will be painted, and in addition the outer path of the border
/// determines the physical shape.
final ShapeBorder shape;
/// Whether to paint the border in front of the child.
///
/// The default value is true.
/// If false, the border will be painted behind the child.
final bool borderOnForeground;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The target z-coordinate at which to place this physical object relative
/// to its parent.
///
/// The value is non-negative.
final double elevation;
/// The target background color.
final Color color;
/// The target shadow color.
final Color? shadowColor;
/// The target surface tint color.
final Color? surfaceTintColor;
@override
_MaterialInteriorState createState() => _MaterialInteriorState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<ShapeBorder>('shape', shape));
description.add(DoubleProperty('elevation', elevation));
description.add(ColorProperty('color', color));
description.add(ColorProperty('shadowColor', shadowColor));
}
}
class _MaterialInteriorState extends AnimatedWidgetBaseState<_MaterialInterior> {
Tween<double>? _elevation;
ColorTween? _surfaceTintColor;
ColorTween? _shadowColor;
ShapeBorderTween? _border;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_elevation = visitor(
_elevation,
widget.elevation,
(dynamic value) => Tween<double>(begin: value as double),
) as Tween<double>?;
_shadowColor = widget.shadowColor != null
? visitor(
_shadowColor,
widget.shadowColor,
(dynamic value) => ColorTween(begin: value as Color),
) as ColorTween?
: null;
_surfaceTintColor = widget.surfaceTintColor != null
? visitor(
_surfaceTintColor,
widget.surfaceTintColor,
(dynamic value) => ColorTween(begin: value as Color),
) as ColorTween?
: null;
_border = visitor(
_border,
widget.shape,
(dynamic value) => ShapeBorderTween(begin: value as ShapeBorder),
) as ShapeBorderTween?;
}
@override
Widget build(BuildContext context) {
final ShapeBorder shape = _border!.evaluate(animation)!;
final double elevation = _elevation!.evaluate(animation);
final Color color = Theme.of(context).useMaterial3
? ElevationOverlay.applySurfaceTint(widget.color, _surfaceTintColor?.evaluate(animation), elevation)
: ElevationOverlay.applyOverlay(context, widget.color, elevation);
// If no shadow color is specified, use 0 for elevation in the model so a drop shadow won't be painted.
final double modelElevation = widget.shadowColor != null ? elevation : 0;
final Color shadowColor = _shadowColor?.evaluate(animation) ?? const Color(0x00000000);
return PhysicalShape(
clipper: ShapeBorderClipper(
shape: shape,
textDirection: Directionality.maybeOf(context),
),
clipBehavior: widget.clipBehavior,
elevation: modelElevation,
color: color,
shadowColor: shadowColor,
child: _ShapeBorderPaint(
shape: shape,
borderOnForeground: widget.borderOnForeground,
child: widget.child,
),
);
}
}
class _ShapeBorderPaint extends StatelessWidget {
const _ShapeBorderPaint({
required this.child,
required this.shape,
this.borderOnForeground = true,
});
final Widget child;
final ShapeBorder shape;
final bool borderOnForeground;
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: borderOnForeground ? null : _ShapeBorderPainter(shape, Directionality.maybeOf(context)),
foregroundPainter: borderOnForeground ? _ShapeBorderPainter(shape, Directionality.maybeOf(context)) : null,
child: child,
);
}
}
class _ShapeBorderPainter extends CustomPainter {
_ShapeBorderPainter(this.border, this.textDirection);
final ShapeBorder border;
final TextDirection? textDirection;
@override
void paint(Canvas canvas, Size size) {
border.paint(canvas, Offset.zero & size, textDirection: textDirection);
}
@override
bool shouldRepaint(_ShapeBorderPainter oldDelegate) {
return oldDelegate.border != border;
}
}
| flutter/packages/flutter/lib/src/material/material.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/material.dart",
"repo_id": "flutter",
"token_count": 11553
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'navigation_bar.dart';
import 'navigation_rail_theme.dart';
import 'text_theme.dart';
import 'theme.dart';
const double _kCircularIndicatorDiameter = 56;
const double _kIndicatorHeight = 32;
/// A Material Design widget that is meant to be displayed at the left or right of an
/// app to navigate between a small number of views, typically between three and
/// five.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=y9xchtVTtqQ}
///
/// The navigation rail is meant for layouts with wide viewports, such as a
/// desktop web or tablet landscape layout. For smaller layouts, like mobile
/// portrait, a [BottomNavigationBar] should be used instead.
///
/// A navigation rail is usually used as the first or last element of a [Row]
/// which defines the app's [Scaffold] body.
///
/// The appearance of all of the [NavigationRail]s within an app can be
/// specified with [NavigationRailTheme]. The default values for null theme
/// properties are based on the [Theme]'s [ThemeData.textTheme],
/// [ThemeData.iconTheme], and [ThemeData.colorScheme].
///
/// Adaptive layouts can build different instances of the [Scaffold] in order to
/// have a navigation rail for more horizontal layouts and a bottom navigation
/// bar for more vertical layouts. See
/// [the adaptive_scaffold.dart sample](https://github.com/flutter/samples/blob/main/experimental/web_dashboard/lib/src/widgets/third_party/adaptive_scaffold.dart)
/// for an example.
///
/// {@tool dartpad}
/// This example shows a [NavigationRail] used within a Scaffold with 3
/// [NavigationRailDestination]s. The main content is separated by a divider
/// (although elevation on the navigation rail can be used instead). The
/// `_selectedIndex` is updated by the `onDestinationSelected` callback.
///
/// ** See code in examples/api/lib/material/navigation_rail/navigation_rail.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of [NavigationRail] widget used within a Scaffold with 3
/// [NavigationRailDestination]s, as described in: https://m3.material.io/components/navigation-rail/overview
///
/// ** See code in examples/api/lib/material/navigation_rail/navigation_rail.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Scaffold], which can display the navigation rail within a [Row] of the
/// [Scaffold.body] slot.
/// * [NavigationRailDestination], which is used as a model to create tappable
/// destinations in the navigation rail.
/// * [BottomNavigationBar], which is a similar navigation widget that's laid
/// out horizontally.
/// * <https://material.io/components/navigation-rail/>
/// * <https://m3.material.io/components/navigation-rail>
class NavigationRail extends StatefulWidget {
/// Creates a Material Design navigation rail.
///
/// The value of [destinations] must be a list of two or more
/// [NavigationRailDestination] values.
///
/// If [elevation] is specified, it must be non-negative.
///
/// If [minWidth] is specified, it must be non-negative, and if
/// [minExtendedWidth] is specified, it must be non-negative and greater than
/// [minWidth].
///
/// The [extended] argument can only be set to true when the [labelType] is
/// null or [NavigationRailLabelType.none].
///
/// If [backgroundColor], [elevation], [groupAlignment], [labelType],
/// [unselectedLabelTextStyle], [selectedLabelTextStyle],
/// [unselectedIconTheme], or [selectedIconTheme] are null, then their
/// [NavigationRailThemeData] values will be used. If the corresponding
/// [NavigationRailThemeData] property is null, then the navigation rail
/// defaults are used. See the individual properties for more information.
///
/// Typically used within a [Row] that defines the [Scaffold.body] property.
const NavigationRail({
super.key,
this.backgroundColor,
this.extended = false,
this.leading,
this.trailing,
required this.destinations,
required this.selectedIndex,
this.onDestinationSelected,
this.elevation,
this.groupAlignment,
this.labelType,
this.unselectedLabelTextStyle,
this.selectedLabelTextStyle,
this.unselectedIconTheme,
this.selectedIconTheme,
this.minWidth,
this.minExtendedWidth,
this.useIndicator,
this.indicatorColor,
this.indicatorShape,
}) : assert(destinations.length >= 2),
assert(selectedIndex == null || (0 <= selectedIndex && selectedIndex < destinations.length)),
assert(elevation == null || elevation > 0),
assert(minWidth == null || minWidth > 0),
assert(minExtendedWidth == null || minExtendedWidth > 0),
assert((minWidth == null || minExtendedWidth == null) || minExtendedWidth >= minWidth),
assert(!extended || (labelType == null || labelType == NavigationRailLabelType.none));
/// Sets the color of the Container that holds all of the [NavigationRail]'s
/// contents.
///
/// The default value is [NavigationRailThemeData.backgroundColor]. If
/// [NavigationRailThemeData.backgroundColor] is null, then the default value
/// is based on [ColorScheme.surface] of [ThemeData.colorScheme].
final Color? backgroundColor;
/// Indicates that the [NavigationRail] should be in the extended state.
///
/// The extended state has a wider rail container, and the labels are
/// positioned next to the icons. [minExtendedWidth] can be used to set the
/// minimum width of the rail when it is in this state.
///
/// The rail will implicitly animate between the extended and normal state.
///
/// If the rail is going to be in the extended state, then the [labelType]
/// must be set to [NavigationRailLabelType.none].
///
/// The default value is false.
final bool extended;
/// The leading widget in the rail that is placed above the destinations.
///
/// It is placed at the top of the rail, above the [destinations]. Its
/// location is not affected by [groupAlignment].
///
/// This is commonly a [FloatingActionButton], but may also be a non-button,
/// such as a logo.
///
/// The default value is null.
final Widget? leading;
/// The trailing widget in the rail that is placed below the destinations.
///
/// The trailing widget is placed below the last [NavigationRailDestination].
/// It's location is affected by [groupAlignment].
///
/// This is commonly a list of additional options or destinations that is
/// usually only rendered when [extended] is true.
///
/// The default value is null.
final Widget? trailing;
/// Defines the appearance of the button items that are arrayed within the
/// navigation rail.
///
/// The value must be a list of two or more [NavigationRailDestination]
/// values.
final List<NavigationRailDestination> destinations;
/// The index into [destinations] for the current selected
/// [NavigationRailDestination] or null if no destination is selected.
final int? selectedIndex;
/// Called when one of the [destinations] is selected.
///
/// The stateful widget that creates the navigation rail needs to keep
/// track of the index of the selected [NavigationRailDestination] and call
/// `setState` to rebuild the navigation rail with the new [selectedIndex].
final ValueChanged<int>? onDestinationSelected;
/// The rail's elevation or z-coordinate.
///
/// If [Directionality] is [intl.TextDirection.LTR], the inner side is the
/// right side, and if [Directionality] is [intl.TextDirection.RTL], it is
/// the left side.
///
/// The default value is 0.
final double? elevation;
/// The vertical alignment for the group of [destinations] within the rail.
///
/// The [NavigationRailDestination]s are grouped together with the [trailing]
/// widget, between the [leading] widget and the bottom of the rail.
///
/// The value must be between -1.0 and 1.0.
///
/// If [groupAlignment] is -1.0, then the items are aligned to the top. If
/// [groupAlignment] is 0.0, then the items are aligned to the center. If
/// [groupAlignment] is 1.0, then the items are aligned to the bottom.
///
/// The default is -1.0.
///
/// See also:
/// * [Alignment.y]
///
final double? groupAlignment;
/// Defines the layout and behavior of the labels for the default, unextended
/// [NavigationRail].
///
/// When a navigation rail is [extended], the labels are always shown.
///
/// The default value is [NavigationRailThemeData.labelType]. If
/// [NavigationRailThemeData.labelType] is null, then the default value is
/// [NavigationRailLabelType.none].
///
/// See also:
///
/// * [NavigationRailLabelType] for information on the meaning of different
/// types.
final NavigationRailLabelType? labelType;
/// The [TextStyle] of a destination's label when it is unselected.
///
/// When one of the [destinations] is selected the [selectedLabelTextStyle]
/// will be used instead.
///
/// The default value is based on the [Theme]'s [TextTheme.bodyLarge]. The
/// default color is based on the [Theme]'s [ColorScheme.onSurface].
///
/// Properties from this text style, or
/// [NavigationRailThemeData.unselectedLabelTextStyle] if this is null, are
/// merged into the defaults.
final TextStyle? unselectedLabelTextStyle;
/// The [TextStyle] of a destination's label when it is selected.
///
/// When a [NavigationRailDestination] is not selected,
/// [unselectedLabelTextStyle] will be used.
///
/// The default value is based on the [TextTheme.bodyLarge] of
/// [ThemeData.textTheme]. The default color is based on the [Theme]'s
/// [ColorScheme.primary].
///
/// Properties from this text style,
/// or [NavigationRailThemeData.selectedLabelTextStyle] if this is null, are
/// merged into the defaults.
final TextStyle? selectedLabelTextStyle;
/// The visual properties of the icon in the unselected destination.
///
/// If this field is not provided, or provided with any null properties, then
/// a copy of the [IconThemeData.fallback] with a custom [NavigationRail]
/// specific color will be used.
///
/// The default value is the [Theme]'s [ThemeData.iconTheme] with a color
/// of the [Theme]'s [ColorScheme.onSurface] with an opacity of 0.64.
/// Properties from this icon theme, or
/// [NavigationRailThemeData.unselectedIconTheme] if this is null, are
/// merged into the defaults.
final IconThemeData? unselectedIconTheme;
/// The visual properties of the icon in the selected destination.
///
/// When a [NavigationRailDestination] is not selected,
/// [unselectedIconTheme] will be used.
///
/// The default value is the [Theme]'s [ThemeData.iconTheme] with a color
/// of the [Theme]'s [ColorScheme.primary]. Properties from this icon theme,
/// or [NavigationRailThemeData.selectedIconTheme] if this is null, are
/// merged into the defaults.
final IconThemeData? selectedIconTheme;
/// The smallest possible width for the rail regardless of the destination's
/// icon or label size.
///
/// The default is 72.
///
/// This value also defines the min width and min height of the destinations.
///
/// To make a compact rail, set this to 56 and use
/// [NavigationRailLabelType.none].
final double? minWidth;
/// The final width when the animation is complete for setting [extended] to
/// true.
///
/// This is only used when [extended] is set to true.
///
/// The default value is 256.
final double? minExtendedWidth;
/// If `true`, adds a rounded [NavigationIndicator] behind the selected
/// destination's icon.
///
/// The indicator's shape will be circular if [labelType] is
/// [NavigationRailLabelType.none], or a [StadiumBorder] if [labelType] is
/// [NavigationRailLabelType.all] or [NavigationRailLabelType.selected].
///
/// If `null`, defaults to [NavigationRailThemeData.useIndicator]. If that is
/// `null`, defaults to [ThemeData.useMaterial3].
final bool? useIndicator;
/// Overrides the default value of [NavigationRail]'s selection indicator color,
/// when [useIndicator] is true.
///
/// If this is null, [NavigationRailThemeData.indicatorColor] is used. If
/// that is null, defaults to [ColorScheme.secondaryContainer].
final Color? indicatorColor;
/// Overrides the default value of [NavigationRail]'s selection indicator shape,
/// when [useIndicator] is true.
///
/// If this is null, [NavigationRailThemeData.indicatorShape] is used. If
/// that is null, defaults to [StadiumBorder].
final ShapeBorder? indicatorShape;
/// Returns the animation that controls the [NavigationRail.extended] state.
///
/// This can be used to synchronize animations in the [leading] or [trailing]
/// widget, such as an animated menu or a [FloatingActionButton] animation.
///
/// {@tool dartpad}
/// This example shows how to use this animation to create a [FloatingActionButton]
/// that animates itself between the normal and extended states of the
/// [NavigationRail].
///
/// An instance of `MyNavigationRailFab` is created for [NavigationRail.leading].
/// Pressing the FAB button toggles the "extended" state of the [NavigationRail].
///
/// ** See code in examples/api/lib/material/navigation_rail/navigation_rail.extended_animation.0.dart **
/// {@end-tool}
static Animation<double> extendedAnimation(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<_ExtendedNavigationRailAnimation>()!.animation;
}
@override
State<NavigationRail> createState() => _NavigationRailState();
}
class _NavigationRailState extends State<NavigationRail> with TickerProviderStateMixin {
late List<AnimationController> _destinationControllers;
late List<Animation<double>> _destinationAnimations;
late AnimationController _extendedController;
late Animation<double> _extendedAnimation;
@override
void initState() {
super.initState();
_initControllers();
}
@override
void dispose() {
_disposeControllers();
super.dispose();
}
@override
void didUpdateWidget(NavigationRail oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.extended != oldWidget.extended) {
if (widget.extended) {
_extendedController.forward();
} else {
_extendedController.reverse();
}
}
// No animated segue if the length of the items list changes.
if (widget.destinations.length != oldWidget.destinations.length) {
_resetState();
return;
}
if (widget.selectedIndex != oldWidget.selectedIndex) {
if (oldWidget.selectedIndex != null) {
_destinationControllers[oldWidget.selectedIndex!].reverse();
}
if (widget.selectedIndex != null) {
_destinationControllers[widget.selectedIndex!].forward();
}
return;
}
}
@override
Widget build(BuildContext context) {
final NavigationRailThemeData navigationRailTheme = NavigationRailTheme.of(context);
final NavigationRailThemeData defaults = Theme.of(context).useMaterial3 ? _NavigationRailDefaultsM3(context) : _NavigationRailDefaultsM2(context);
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final Color backgroundColor = widget.backgroundColor ?? navigationRailTheme.backgroundColor ?? defaults.backgroundColor!;
final double elevation = widget.elevation ?? navigationRailTheme.elevation ?? defaults.elevation!;
final double minWidth = widget.minWidth ?? navigationRailTheme.minWidth ?? defaults.minWidth!;
final double minExtendedWidth = widget.minExtendedWidth ?? navigationRailTheme.minExtendedWidth ?? defaults.minExtendedWidth!;
final TextStyle unselectedLabelTextStyle = widget.unselectedLabelTextStyle ?? navigationRailTheme.unselectedLabelTextStyle ?? defaults.unselectedLabelTextStyle!;
final TextStyle selectedLabelTextStyle = widget.selectedLabelTextStyle ?? navigationRailTheme.selectedLabelTextStyle ?? defaults.selectedLabelTextStyle!;
final IconThemeData unselectedIconTheme = widget.unselectedIconTheme ?? navigationRailTheme.unselectedIconTheme ?? defaults.unselectedIconTheme!;
final IconThemeData selectedIconTheme = widget.selectedIconTheme ?? navigationRailTheme.selectedIconTheme ?? defaults.selectedIconTheme!;
final double groupAlignment = widget.groupAlignment ?? navigationRailTheme.groupAlignment ?? defaults.groupAlignment!;
final NavigationRailLabelType labelType = widget.labelType ?? navigationRailTheme.labelType ?? defaults.labelType!;
final bool useIndicator = widget.useIndicator ?? navigationRailTheme.useIndicator ?? defaults.useIndicator!;
final Color? indicatorColor = widget.indicatorColor ?? navigationRailTheme.indicatorColor ?? defaults.indicatorColor;
final ShapeBorder? indicatorShape = widget.indicatorShape ?? navigationRailTheme.indicatorShape ?? defaults.indicatorShape;
// For backwards compatibility, in M2 the opacity of the unselected icons needs
// to be set to the default if it isn't in the given theme. This can be removed
// when Material 3 is the default.
final IconThemeData effectiveUnselectedIconTheme = Theme.of(context).useMaterial3
? unselectedIconTheme
: unselectedIconTheme.copyWith(opacity: unselectedIconTheme.opacity ?? defaults.unselectedIconTheme!.opacity);
final bool isRTLDirection = Directionality.of(context) == TextDirection.rtl;
return _ExtendedNavigationRailAnimation(
animation: _extendedAnimation,
child: Semantics(
explicitChildNodes: true,
child: Material(
elevation: elevation,
color: backgroundColor,
child: SafeArea(
right: isRTLDirection,
left: !isRTLDirection,
child: Column(
children: <Widget>[
_verticalSpacer,
if (widget.leading != null)
...<Widget>[
widget.leading!,
_verticalSpacer,
],
Expanded(
child: Align(
alignment: Alignment(0, groupAlignment),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
for (int i = 0; i < widget.destinations.length; i += 1)
_RailDestination(
minWidth: minWidth,
minExtendedWidth: minExtendedWidth,
extendedTransitionAnimation: _extendedAnimation,
selected: widget.selectedIndex == i,
icon: widget.selectedIndex == i ? widget.destinations[i].selectedIcon : widget.destinations[i].icon,
label: widget.destinations[i].label,
destinationAnimation: _destinationAnimations[i],
labelType: labelType,
iconTheme: widget.selectedIndex == i ? selectedIconTheme : effectiveUnselectedIconTheme,
labelTextStyle: widget.selectedIndex == i ? selectedLabelTextStyle : unselectedLabelTextStyle,
padding: widget.destinations[i].padding,
useIndicator: useIndicator,
indicatorColor: useIndicator ? indicatorColor : null,
indicatorShape: useIndicator ? indicatorShape : null,
onTap: () {
if (widget.onDestinationSelected != null) {
widget.onDestinationSelected!(i);
}
},
indexLabel: localizations.tabLabel(
tabIndex: i + 1,
tabCount: widget.destinations.length,
),
disabled: widget.destinations[i].disabled,
),
if (widget.trailing != null)
widget.trailing!,
],
),
),
),
],
),
),
),
),
);
}
void _disposeControllers() {
for (final AnimationController controller in _destinationControllers) {
controller.dispose();
}
_extendedController.dispose();
}
void _initControllers() {
_destinationControllers = List<AnimationController>.generate(widget.destinations.length, (int index) {
return AnimationController(
duration: kThemeAnimationDuration,
vsync: this,
)..addListener(_rebuild);
});
_destinationAnimations = _destinationControllers.map((AnimationController controller) => controller.view).toList();
if (widget.selectedIndex != null) {
_destinationControllers[widget.selectedIndex!].value = 1.0;
}
_extendedController = AnimationController(
duration: kThemeAnimationDuration,
vsync: this,
value: widget.extended ? 1.0 : 0.0,
);
_extendedAnimation = CurvedAnimation(
parent: _extendedController,
curve: Curves.easeInOut,
);
_extendedController.addListener(() {
_rebuild();
});
}
void _resetState() {
_disposeControllers();
_initControllers();
}
void _rebuild() {
setState(() {
// Rebuilding when any of the controllers tick, i.e. when the items are
// animating.
});
}
}
class _RailDestination extends StatelessWidget {
_RailDestination({
required this.minWidth,
required this.minExtendedWidth,
required this.icon,
required this.label,
required this.destinationAnimation,
required this.extendedTransitionAnimation,
required this.labelType,
required this.selected,
required this.iconTheme,
required this.labelTextStyle,
required this.onTap,
required this.indexLabel,
this.padding,
required this.useIndicator,
this.indicatorColor,
this.indicatorShape,
this.disabled = false,
}) : _positionAnimation = CurvedAnimation(
parent: ReverseAnimation(destinationAnimation),
curve: Curves.easeInOut,
reverseCurve: Curves.easeInOut.flipped,
);
final double minWidth;
final double minExtendedWidth;
final Widget icon;
final Widget label;
final Animation<double> destinationAnimation;
final NavigationRailLabelType labelType;
final bool selected;
final Animation<double> extendedTransitionAnimation;
final IconThemeData iconTheme;
final TextStyle labelTextStyle;
final VoidCallback onTap;
final String indexLabel;
final EdgeInsetsGeometry? padding;
final bool useIndicator;
final Color? indicatorColor;
final ShapeBorder? indicatorShape;
final bool disabled;
final Animation<double> _positionAnimation;
@override
Widget build(BuildContext context) {
assert(
useIndicator || indicatorColor == null,
'[NavigationRail.indicatorColor] does not have an effect when [NavigationRail.useIndicator] is false',
);
final ThemeData theme = Theme.of(context);
final TextDirection textDirection = Directionality.of(context);
final bool material3 = theme.useMaterial3;
final EdgeInsets destinationPadding = (padding ?? EdgeInsets.zero).resolve(textDirection);
Offset indicatorOffset;
bool applyXOffset = false;
final Widget themedIcon = IconTheme(
data: disabled
? iconTheme.copyWith(color: theme.colorScheme.onSurface.withOpacity(0.38))
: iconTheme,
child: icon,
);
final Widget styledLabel = DefaultTextStyle(
style: disabled
? labelTextStyle.copyWith(color: theme.colorScheme.onSurface.withOpacity(0.38))
: labelTextStyle,
child: label,
);
Widget content;
// The indicator height is fixed and equal to _kIndicatorHeight.
// When the icon height is larger than the indicator height the indicator
// vertical offset is used to vertically center the indicator.
final bool isLargeIconSize = iconTheme.size != null && iconTheme.size! > _kIndicatorHeight;
final double indicatorVerticalOffset = isLargeIconSize ? (iconTheme.size! - _kIndicatorHeight) / 2 : 0;
switch (labelType) {
case NavigationRailLabelType.none:
// Split the destination spacing across the top and bottom to keep the icon centered.
final Widget? spacing = material3 ? const SizedBox(height: _verticalDestinationSpacingM3 / 2) : null;
indicatorOffset = Offset(
minWidth / 2 + destinationPadding.left,
_verticalDestinationSpacingM3 / 2 + destinationPadding.top + indicatorVerticalOffset,
);
final Widget iconPart = Column(
children: <Widget>[
if (spacing != null) spacing,
SizedBox(
width: minWidth,
height: material3 ? null : minWidth,
child: Center(
child: _AddIndicator(
addIndicator: useIndicator,
indicatorColor: indicatorColor,
indicatorShape: indicatorShape,
isCircular: !material3,
indicatorAnimation: destinationAnimation,
child: themedIcon,
),
),
),
if (spacing != null) spacing,
],
);
if (extendedTransitionAnimation.value == 0) {
content = Padding(
padding: padding ?? EdgeInsets.zero,
child: Stack(
children: <Widget>[
iconPart,
// For semantics when label is not showing,
SizedBox.shrink(
child: Visibility.maintain(
visible: false,
child: label,
),
),
],
),
);
} else {
final Animation<double> labelFadeAnimation = extendedTransitionAnimation.drive(CurveTween(curve: const Interval(0.0, 0.25)));
applyXOffset = true;
content = Padding(
padding: padding ?? EdgeInsets.zero,
child: ConstrainedBox(
constraints: BoxConstraints(
minWidth: lerpDouble(minWidth, minExtendedWidth, extendedTransitionAnimation.value)!,
),
child: ClipRect(
child: Row(
children: <Widget>[
iconPart,
Align(
heightFactor: 1.0,
widthFactor: extendedTransitionAnimation.value,
alignment: AlignmentDirectional.centerStart,
child: FadeTransition(
alwaysIncludeSemantics: true,
opacity: labelFadeAnimation,
child: styledLabel,
),
),
SizedBox(width: _horizontalDestinationPadding * extendedTransitionAnimation.value),
],
),
),
),
);
}
case NavigationRailLabelType.selected:
final double appearingAnimationValue = 1 - _positionAnimation.value;
final double verticalPadding = lerpDouble(_verticalDestinationPaddingNoLabel, _verticalDestinationPaddingWithLabel, appearingAnimationValue)!;
final Interval interval = selected ? const Interval(0.25, 0.75) : const Interval(0.75, 1.0);
final Animation<double> labelFadeAnimation = destinationAnimation.drive(CurveTween(curve: interval));
final double minHeight = material3 ? 0 : minWidth;
final Widget topSpacing = SizedBox(height: material3 ? 0 : verticalPadding);
final Widget labelSpacing = SizedBox(height: material3 ? lerpDouble(0, _verticalIconLabelSpacingM3, appearingAnimationValue)! : 0);
final Widget bottomSpacing = SizedBox(height: material3 ? _verticalDestinationSpacingM3 : verticalPadding);
final double indicatorHorizontalPadding = (destinationPadding.left / 2) - (destinationPadding.right / 2);
final double indicatorVerticalPadding = destinationPadding.top;
indicatorOffset = Offset(
minWidth / 2 + indicatorHorizontalPadding,
indicatorVerticalPadding + indicatorVerticalOffset,
);
if (minWidth < _NavigationRailDefaultsM2(context).minWidth!) {
indicatorOffset = Offset(
minWidth / 2 + _horizontalDestinationSpacingM3,
indicatorVerticalPadding + indicatorVerticalOffset,
);
}
content = Container(
constraints: BoxConstraints(
minWidth: minWidth,
minHeight: minHeight,
),
padding: padding ?? const EdgeInsets.symmetric(horizontal: _horizontalDestinationPadding),
child: ClipRect(
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
topSpacing,
_AddIndicator(
addIndicator: useIndicator,
indicatorColor: indicatorColor,
indicatorShape: indicatorShape,
isCircular: false,
indicatorAnimation: destinationAnimation,
child: themedIcon,
),
labelSpacing,
Align(
alignment: Alignment.topCenter,
heightFactor: appearingAnimationValue,
widthFactor: 1.0,
child: FadeTransition(
alwaysIncludeSemantics: true,
opacity: labelFadeAnimation,
child: styledLabel,
),
),
bottomSpacing,
],
),
),
);
case NavigationRailLabelType.all:
final double minHeight = material3 ? 0 : minWidth;
final Widget topSpacing = SizedBox(height: material3 ? 0 : _verticalDestinationPaddingWithLabel);
final Widget labelSpacing = SizedBox(height: material3 ? _verticalIconLabelSpacingM3 : 0);
final Widget bottomSpacing = SizedBox(height: material3 ? _verticalDestinationSpacingM3 : _verticalDestinationPaddingWithLabel);
final double indicatorHorizontalPadding = (destinationPadding.left / 2) - (destinationPadding.right / 2);
final double indicatorVerticalPadding = destinationPadding.top;
indicatorOffset = Offset(
minWidth / 2 + indicatorHorizontalPadding,
indicatorVerticalPadding + indicatorVerticalOffset,
);
if (minWidth < _NavigationRailDefaultsM2(context).minWidth!) {
indicatorOffset = Offset(
minWidth / 2 + _horizontalDestinationSpacingM3,
indicatorVerticalPadding + indicatorVerticalOffset,
);
}
content = Container(
constraints: BoxConstraints(
minWidth: minWidth,
minHeight: minHeight,
),
padding: padding ?? const EdgeInsets.symmetric(horizontal: _horizontalDestinationPadding),
child: Column(
children: <Widget>[
topSpacing,
_AddIndicator(
addIndicator: useIndicator,
indicatorColor: indicatorColor,
indicatorShape: indicatorShape,
isCircular: false,
indicatorAnimation: destinationAnimation,
child: themedIcon,
),
labelSpacing,
styledLabel,
bottomSpacing,
],
),
);
}
final ColorScheme colors = Theme.of(context).colorScheme;
final bool primaryColorAlphaModified = colors.primary.alpha < 255.0;
final Color effectiveSplashColor = primaryColorAlphaModified
? colors.primary
: colors.primary.withOpacity(0.12);
final Color effectiveHoverColor = primaryColorAlphaModified
? colors.primary
: colors.primary.withOpacity(0.04);
return Semantics(
container: true,
selected: selected,
child: Stack(
children: <Widget>[
Material(
type: MaterialType.transparency,
child: _IndicatorInkWell(
onTap: disabled ? null : onTap,
borderRadius: BorderRadius.all(Radius.circular(minWidth / 2.0)),
customBorder: indicatorShape,
splashColor: effectiveSplashColor,
hoverColor: effectiveHoverColor,
useMaterial3: material3,
indicatorOffset: indicatorOffset,
applyXOffset: applyXOffset,
textDirection: textDirection,
child: content,
),
),
Semantics(
label: indexLabel,
),
],
),
);
}
}
class _IndicatorInkWell extends InkResponse {
const _IndicatorInkWell({
super.child,
super.onTap,
ShapeBorder? customBorder,
BorderRadius? borderRadius,
super.splashColor,
super.hoverColor,
required this.useMaterial3,
required this.indicatorOffset,
required this.applyXOffset,
required this.textDirection,
}) : super(
containedInkWell: true,
highlightShape: BoxShape.rectangle,
borderRadius: useMaterial3 ? null : borderRadius,
customBorder: useMaterial3 ? customBorder : null,
);
final bool useMaterial3;
// The offset used to position Ink highlight.
final Offset indicatorOffset;
// Whether the horizontal offset from indicatorOffset should be used to position Ink highlight.
// If true, Ink highlight uses the indicator horizontal offset. If false, Ink highlight is centered horizontally.
final bool applyXOffset;
// The text direction used to adjust the indicator horizontal offset.
final TextDirection textDirection;
@override
RectCallback? getRectCallback(RenderBox referenceBox) {
if (useMaterial3) {
final double boxWidth = referenceBox.size.width;
double indicatorHorizontalCenter = applyXOffset ? indicatorOffset.dx : boxWidth / 2;
if (textDirection == TextDirection.rtl) {
indicatorHorizontalCenter = boxWidth - indicatorHorizontalCenter;
}
return () {
return Rect.fromLTWH(
indicatorHorizontalCenter - (_kCircularIndicatorDiameter / 2),
indicatorOffset.dy,
_kCircularIndicatorDiameter,
_kIndicatorHeight,
);
};
}
return null;
}
}
/// When [addIndicator] is `true`, puts [child] center aligned in a [Stack] with
/// a [NavigationIndicator] behind it, otherwise returns [child].
///
/// When [isCircular] is true, the indicator will be a circle, otherwise the
/// indicator will be a stadium shape.
class _AddIndicator extends StatelessWidget {
const _AddIndicator({
required this.addIndicator,
required this.isCircular,
required this.indicatorColor,
required this.indicatorShape,
required this.indicatorAnimation,
required this.child,
});
final bool addIndicator;
final bool isCircular;
final Color? indicatorColor;
final ShapeBorder? indicatorShape;
final Animation<double> indicatorAnimation;
final Widget child;
@override
Widget build(BuildContext context) {
if (!addIndicator) {
return child;
}
late final Widget indicator;
if (isCircular) {
indicator = NavigationIndicator(
animation: indicatorAnimation,
height: _kCircularIndicatorDiameter,
width: _kCircularIndicatorDiameter,
borderRadius: BorderRadius.circular(_kCircularIndicatorDiameter / 2),
color: indicatorColor,
);
} else {
indicator = NavigationIndicator(
animation: indicatorAnimation,
width: _kCircularIndicatorDiameter,
shape: indicatorShape,
color: indicatorColor,
);
}
return Stack(
alignment: Alignment.center,
children: <Widget>[
indicator,
child,
],
);
}
}
/// Defines the behavior of the labels of a [NavigationRail].
///
/// See also:
///
/// * [NavigationRail]
enum NavigationRailLabelType {
/// Only the [NavigationRailDestination]s are shown.
none,
/// Only the selected [NavigationRailDestination] will show its label.
///
/// The label will animate in and out as new [NavigationRailDestination]s are
/// selected.
selected,
/// All [NavigationRailDestination]s will show their label.
all,
}
/// Defines a [NavigationRail] button that represents one "destination" view.
///
/// See also:
///
/// * [NavigationRail]
class NavigationRailDestination {
/// Creates a destination that is used with [NavigationRail.destinations].
///
/// When the [NavigationRail.labelType] is [NavigationRailLabelType.none], the
/// label is still used for semantics, and may still be used if
/// [NavigationRail.extended] is true.
const NavigationRailDestination({
required this.icon,
Widget? selectedIcon,
this.indicatorColor,
this.indicatorShape,
required this.label,
this.padding,
this.disabled = false,
}) : selectedIcon = selectedIcon ?? icon;
/// The icon of the destination.
///
/// Typically the icon is an [Icon] or an [ImageIcon] widget. If another type
/// of widget is provided then it should configure itself to match the current
/// [IconTheme] size and color.
///
/// If [selectedIcon] is provided, this will only be displayed when the
/// destination is not selected.
///
/// To make the [NavigationRail] more accessible, consider choosing an
/// icon with a stroked and filled version, such as [Icons.cloud] and
/// [Icons.cloud_queue]. The [icon] should be set to the stroked version and
/// [selectedIcon] to the filled version.
final Widget icon;
/// An alternative icon displayed when this destination is selected.
///
/// If this icon is not provided, the [NavigationRail] will display [icon] in
/// either state. The size, color, and opacity of the
/// [NavigationRail.selectedIconTheme] will still apply.
///
/// See also:
///
/// * [NavigationRailDestination.icon], for a description of how to pair
/// icons.
final Widget selectedIcon;
/// The color of the [indicatorShape] when this destination is selected.
final Color? indicatorColor;
/// The shape of the selection indicator.
final ShapeBorder? indicatorShape;
/// The label for the destination.
///
/// The label must be provided when used with the [NavigationRail]. When the
/// [NavigationRail.labelType] is [NavigationRailLabelType.none], the label is
/// still used for semantics, and may still be used if
/// [NavigationRail.extended] is true.
final Widget label;
/// The amount of space to inset the destination item.
final EdgeInsetsGeometry? padding;
/// Indicates that this destination is inaccessible.
final bool disabled;
}
class _ExtendedNavigationRailAnimation extends InheritedWidget {
const _ExtendedNavigationRailAnimation({
required this.animation,
required super.child,
});
final Animation<double> animation;
@override
bool updateShouldNotify(_ExtendedNavigationRailAnimation old) => animation != old.animation;
}
// There don't appear to be tokens for these values, but they are
// shown in the spec.
const double _horizontalDestinationPadding = 8.0;
const double _verticalDestinationPaddingNoLabel = 24.0;
const double _verticalDestinationPaddingWithLabel = 16.0;
const Widget _verticalSpacer = SizedBox(height: 8.0);
const double _verticalIconLabelSpacingM3 = 4.0;
const double _verticalDestinationSpacingM3 = 12.0;
const double _horizontalDestinationSpacingM3 = 12.0;
// Hand coded defaults based on Material Design 2.
class _NavigationRailDefaultsM2 extends NavigationRailThemeData {
_NavigationRailDefaultsM2(BuildContext context)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme,
super(
elevation: 0,
groupAlignment: -1,
labelType: NavigationRailLabelType.none,
useIndicator: false,
minWidth: 72.0,
minExtendedWidth: 256,
);
final ThemeData _theme;
final ColorScheme _colors;
@override Color? get backgroundColor => _colors.surface;
@override TextStyle? get unselectedLabelTextStyle {
return _theme.textTheme.bodyLarge!.copyWith(color: _colors.onSurface.withOpacity(0.64));
}
@override TextStyle? get selectedLabelTextStyle {
return _theme.textTheme.bodyLarge!.copyWith(color: _colors.primary);
}
@override IconThemeData? get unselectedIconTheme {
return IconThemeData(
size: 24.0,
color: _colors.onSurface,
opacity: 0.64,
);
}
@override IconThemeData? get selectedIconTheme {
return IconThemeData(
size: 24.0,
color: _colors.primary,
opacity: 1.0,
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - NavigationRail
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _NavigationRailDefaultsM3 extends NavigationRailThemeData {
_NavigationRailDefaultsM3(this.context)
: super(
elevation: 0.0,
groupAlignment: -1,
labelType: NavigationRailLabelType.none,
useIndicator: true,
minWidth: 80.0,
minExtendedWidth: 256,
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override Color? get backgroundColor => _colors.surface;
@override TextStyle? get unselectedLabelTextStyle {
return _textTheme.labelMedium!.copyWith(color: _colors.onSurface);
}
@override TextStyle? get selectedLabelTextStyle {
return _textTheme.labelMedium!.copyWith(color: _colors.onSurface);
}
@override IconThemeData? get unselectedIconTheme {
return IconThemeData(
size: 24.0,
color: _colors.onSurfaceVariant,
);
}
@override IconThemeData? get selectedIconTheme {
return IconThemeData(
size: 24.0,
color: _colors.onSecondaryContainer,
);
}
@override Color? get indicatorColor => _colors.secondaryContainer;
@override ShapeBorder? get indicatorShape => const StadiumBorder();
}
// END GENERATED TOKEN PROPERTIES - NavigationRail
| flutter/packages/flutter/lib/src/material/navigation_rail.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/navigation_rail.dart",
"repo_id": "flutter",
"token_count": 15524
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart' show clampDouble;
import 'debug.dart';
import 'material_localizations.dart';
import 'progress_indicator.dart';
import 'theme.dart';
// The over-scroll distance that moves the indicator to its maximum
// displacement, as a percentage of the scrollable's container extent.
const double _kDragContainerExtentPercentage = 0.25;
// How much the scroll's drag gesture can overshoot the RefreshIndicator's
// displacement; max displacement = _kDragSizeFactorLimit * displacement.
const double _kDragSizeFactorLimit = 1.5;
// When the scroll ends, the duration of the refresh indicator's animation
// to the RefreshIndicator's displacement.
const Duration _kIndicatorSnapDuration = Duration(milliseconds: 150);
// The duration of the ScaleTransition that starts when the refresh action
// has completed.
const Duration _kIndicatorScaleDuration = Duration(milliseconds: 200);
/// The signature for a function that's called when the user has dragged a
/// [RefreshIndicator] far enough to demonstrate that they want the app to
/// refresh. The returned [Future] must complete when the refresh operation is
/// finished.
///
/// Used by [RefreshIndicator.onRefresh].
typedef RefreshCallback = Future<void> Function();
// The state machine moves through these modes only when the scrollable
// identified by scrollableKey has been scrolled to its min or max limit.
enum _RefreshIndicatorMode {
drag, // Pointer is down.
armed, // Dragged far enough that an up event will run the onRefresh callback.
snap, // Animating to the indicator's final "displacement".
refresh, // Running the refresh callback.
done, // Animating the indicator's fade-out after refreshing.
canceled, // Animating the indicator's fade-out after not arming.
}
/// Used to configure how [RefreshIndicator] can be triggered.
enum RefreshIndicatorTriggerMode {
/// The indicator can be triggered regardless of the scroll position
/// of the [Scrollable] when the drag starts.
anywhere,
/// The indicator can only be triggered if the [Scrollable] is at the edge
/// when the drag starts.
onEdge,
}
enum _IndicatorType { material, adaptive }
/// A widget that supports the Material "swipe to refresh" idiom.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=ORApMlzwMdM}
///
/// When the child's [Scrollable] descendant overscrolls, an animated circular
/// progress indicator is faded into view. When the scroll ends, if the
/// indicator has been dragged far enough for it to become completely opaque,
/// the [onRefresh] callback is called. The callback is expected to update the
/// scrollable's contents and then complete the [Future] it returns. The refresh
/// indicator disappears after the callback's [Future] has completed.
///
/// The trigger mode is configured by [RefreshIndicator.triggerMode].
///
/// {@tool dartpad}
/// This example shows how [RefreshIndicator] can be triggered in different ways.
///
/// ** See code in examples/api/lib/material/refresh_indicator/refresh_indicator.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to trigger [RefreshIndicator] in a nested scroll view using
/// the [notificationPredicate] property.
///
/// ** See code in examples/api/lib/material/refresh_indicator/refresh_indicator.1.dart **
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Refresh indicator does not show up
///
/// The [RefreshIndicator] will appear if its scrollable descendant can be
/// overscrolled, i.e. if the scrollable's content is bigger than its viewport.
/// To ensure that the [RefreshIndicator] will always appear, even if the
/// scrollable's content fits within its viewport, set the scrollable's
/// [Scrollable.physics] property to [AlwaysScrollableScrollPhysics]:
///
/// ```dart
/// ListView(
/// physics: const AlwaysScrollableScrollPhysics(),
/// // ...
/// )
/// ```
///
/// A [RefreshIndicator] can only be used with a vertical scroll view.
///
/// See also:
///
/// * <https://material.io/design/platform-guidance/android-swipe-to-refresh.html>
/// * [RefreshIndicatorState], can be used to programmatically show the refresh indicator.
/// * [RefreshProgressIndicator], widget used by [RefreshIndicator] to show
/// the inner circular progress spinner during refreshes.
/// * [CupertinoSliverRefreshControl], an iOS equivalent of the pull-to-refresh pattern.
/// Must be used as a sliver inside a [CustomScrollView] instead of wrapping
/// around a [ScrollView] because it's a part of the scrollable instead of
/// being overlaid on top of it.
class RefreshIndicator extends StatefulWidget {
/// Creates a refresh indicator.
///
/// The [onRefresh], [child], and [notificationPredicate] arguments must be
/// non-null. The default
/// [displacement] is 40.0 logical pixels.
///
/// The [semanticsLabel] is used to specify an accessibility label for this widget.
/// If it is null, it will be defaulted to [MaterialLocalizations.refreshIndicatorSemanticLabel].
/// An empty string may be passed to avoid having anything read by screen reading software.
/// The [semanticsValue] may be used to specify progress on the widget.
const RefreshIndicator({
super.key,
required this.child,
this.displacement = 40.0,
this.edgeOffset = 0.0,
required this.onRefresh,
this.color,
this.backgroundColor,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.semanticsLabel,
this.semanticsValue,
this.strokeWidth = RefreshProgressIndicator.defaultStrokeWidth,
this.triggerMode = RefreshIndicatorTriggerMode.onEdge,
}) : _indicatorType = _IndicatorType.material;
/// Creates an adaptive [RefreshIndicator] based on whether the target
/// platform is iOS or macOS, following Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// When the descendant overscrolls, a different spinning progress indicator
/// is shown depending on platform. On iOS and macOS,
/// [CupertinoActivityIndicator] is shown, but on all other platforms,
/// [CircularProgressIndicator] appears.
///
/// If a [CupertinoActivityIndicator] is shown, the following parameters are ignored:
/// [backgroundColor], [semanticsLabel], [semanticsValue], [strokeWidth].
///
/// The target platform is based on the current [Theme]: [ThemeData.platform].
///
/// Notably the scrollable widget itself will have slightly different behavior
/// from [CupertinoSliverRefreshControl], due to a difference in structure.
const RefreshIndicator.adaptive({
super.key,
required this.child,
this.displacement = 40.0,
this.edgeOffset = 0.0,
required this.onRefresh,
this.color,
this.backgroundColor,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.semanticsLabel,
this.semanticsValue,
this.strokeWidth = RefreshProgressIndicator.defaultStrokeWidth,
this.triggerMode = RefreshIndicatorTriggerMode.onEdge,
}) : _indicatorType = _IndicatorType.adaptive;
/// The widget below this widget in the tree.
///
/// The refresh indicator will be stacked on top of this child. The indicator
/// will appear when child's Scrollable descendant is over-scrolled.
///
/// Typically a [ListView] or [CustomScrollView].
final Widget child;
/// The distance from the child's top or bottom [edgeOffset] where
/// the refresh indicator will settle. During the drag that exposes the refresh
/// indicator, its actual displacement may significantly exceed this value.
///
/// In most cases, [displacement] distance starts counting from the parent's
/// edges. However, if [edgeOffset] is larger than zero then the [displacement]
/// value is calculated from that offset instead of the parent's edge.
final double displacement;
/// The offset where [RefreshProgressIndicator] starts to appear on drag start.
///
/// Depending whether the indicator is showing on the top or bottom, the value
/// of this variable controls how far from the parent's edge the progress
/// indicator starts to appear. This may come in handy when, for example, the
/// UI contains a top [Widget] which covers the parent's edge where the progress
/// indicator would otherwise appear.
///
/// By default, the edge offset is set to 0.
///
/// See also:
///
/// * [displacement], can be used to change the distance from the edge that
/// the indicator settles.
final double edgeOffset;
/// A function that's called when the user has dragged the refresh indicator
/// far enough to demonstrate that they want the app to refresh. The returned
/// [Future] must complete when the refresh operation is finished.
final RefreshCallback onRefresh;
/// The progress indicator's foreground color. The current theme's
/// [ColorScheme.primary] by default.
final Color? color;
/// The progress indicator's background color. The current theme's
/// [ThemeData.canvasColor] by default.
final Color? backgroundColor;
/// A check that specifies whether a [ScrollNotification] should be
/// handled by this widget.
///
/// By default, checks whether `notification.depth == 0`. Set it to something
/// else for more complicated layouts.
final ScrollNotificationPredicate notificationPredicate;
/// {@macro flutter.progress_indicator.ProgressIndicator.semanticsLabel}
///
/// This will be defaulted to [MaterialLocalizations.refreshIndicatorSemanticLabel]
/// if it is null.
final String? semanticsLabel;
/// {@macro flutter.progress_indicator.ProgressIndicator.semanticsValue}
final String? semanticsValue;
/// Defines [strokeWidth] for `RefreshIndicator`.
///
/// By default, the value of [strokeWidth] is 2.0 pixels.
final double strokeWidth;
final _IndicatorType _indicatorType;
/// Defines how this [RefreshIndicator] can be triggered when users overscroll.
///
/// The [RefreshIndicator] can be pulled out in two cases,
/// 1, Keep dragging if the scrollable widget at the edge with zero scroll position
/// when the drag starts.
/// 2, Keep dragging after overscroll occurs if the scrollable widget has
/// a non-zero scroll position when the drag starts.
///
/// If this is [RefreshIndicatorTriggerMode.anywhere], both of the cases above can be triggered.
///
/// If this is [RefreshIndicatorTriggerMode.onEdge], only case 1 can be triggered.
///
/// Defaults to [RefreshIndicatorTriggerMode.onEdge].
final RefreshIndicatorTriggerMode triggerMode;
@override
RefreshIndicatorState createState() => RefreshIndicatorState();
}
/// Contains the state for a [RefreshIndicator]. This class can be used to
/// programmatically show the refresh indicator, see the [show] method.
class RefreshIndicatorState extends State<RefreshIndicator> with TickerProviderStateMixin<RefreshIndicator> {
late AnimationController _positionController;
late AnimationController _scaleController;
late Animation<double> _positionFactor;
late Animation<double> _scaleFactor;
late Animation<double> _value;
late Animation<Color?> _valueColor;
_RefreshIndicatorMode? _mode;
late Future<void> _pendingRefreshFuture;
bool? _isIndicatorAtTop;
double? _dragOffset;
late Color _effectiveValueColor = widget.color ?? Theme.of(context).colorScheme.primary;
static final Animatable<double> _threeQuarterTween = Tween<double>(begin: 0.0, end: 0.75);
static final Animatable<double> _kDragSizeFactorLimitTween = Tween<double>(begin: 0.0, end: _kDragSizeFactorLimit);
static final Animatable<double> _oneToZeroTween = Tween<double>(begin: 1.0, end: 0.0);
@override
void initState() {
super.initState();
_positionController = AnimationController(vsync: this);
_positionFactor = _positionController.drive(_kDragSizeFactorLimitTween);
_value = _positionController.drive(_threeQuarterTween); // The "value" of the circular progress indicator during a drag.
_scaleController = AnimationController(vsync: this);
_scaleFactor = _scaleController.drive(_oneToZeroTween);
}
@override
void didChangeDependencies() {
_setupColorTween();
super.didChangeDependencies();
}
@override
void didUpdateWidget(covariant RefreshIndicator oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.color != widget.color) {
_setupColorTween();
}
}
@override
void dispose() {
_positionController.dispose();
_scaleController.dispose();
super.dispose();
}
void _setupColorTween() {
// Reset the current value color.
_effectiveValueColor = widget.color ?? Theme.of(context).colorScheme.primary;
final Color color = _effectiveValueColor;
if (color.alpha == 0x00) {
// Set an always stopped animation instead of a driven tween.
_valueColor = AlwaysStoppedAnimation<Color>(color);
} else {
// Respect the alpha of the given color.
_valueColor = _positionController.drive(
ColorTween(
begin: color.withAlpha(0),
end: color.withAlpha(color.alpha),
).chain(
CurveTween(
curve: const Interval(0.0, 1.0 / _kDragSizeFactorLimit),
),
),
);
}
}
bool _shouldStart(ScrollNotification notification) {
// If the notification.dragDetails is null, this scroll is not triggered by
// user dragging. It may be a result of ScrollController.jumpTo or ballistic scroll.
// In this case, we don't want to trigger the refresh indicator.
return ((notification is ScrollStartNotification && notification.dragDetails != null)
|| (notification is ScrollUpdateNotification && notification.dragDetails != null && widget.triggerMode == RefreshIndicatorTriggerMode.anywhere))
&& (( notification.metrics.axisDirection == AxisDirection.up && notification.metrics.extentAfter == 0.0)
|| (notification.metrics.axisDirection == AxisDirection.down && notification.metrics.extentBefore == 0.0))
&& _mode == null
&& _start(notification.metrics.axisDirection);
}
bool _handleScrollNotification(ScrollNotification notification) {
if (!widget.notificationPredicate(notification)) {
return false;
}
if (_shouldStart(notification)) {
setState(() {
_mode = _RefreshIndicatorMode.drag;
});
return false;
}
final bool? indicatorAtTopNow = switch (notification.metrics.axisDirection) {
AxisDirection.down || AxisDirection.up => true,
AxisDirection.left || AxisDirection.right => null,
};
if (indicatorAtTopNow != _isIndicatorAtTop) {
if (_mode == _RefreshIndicatorMode.drag || _mode == _RefreshIndicatorMode.armed) {
_dismiss(_RefreshIndicatorMode.canceled);
}
} else if (notification is ScrollUpdateNotification) {
if (_mode == _RefreshIndicatorMode.drag || _mode == _RefreshIndicatorMode.armed) {
if (notification.metrics.axisDirection == AxisDirection.down) {
_dragOffset = _dragOffset! - notification.scrollDelta!;
} else if (notification.metrics.axisDirection == AxisDirection.up) {
_dragOffset = _dragOffset! + notification.scrollDelta!;
}
_checkDragOffset(notification.metrics.viewportDimension);
}
if (_mode == _RefreshIndicatorMode.armed && notification.dragDetails == null) {
// On iOS start the refresh when the Scrollable bounces back from the
// overscroll (ScrollNotification indicating this don't have dragDetails
// because the scroll activity is not directly triggered by a drag).
_show();
}
} else if (notification is OverscrollNotification) {
if (_mode == _RefreshIndicatorMode.drag || _mode == _RefreshIndicatorMode.armed) {
if (notification.metrics.axisDirection == AxisDirection.down) {
_dragOffset = _dragOffset! - notification.overscroll;
} else if (notification.metrics.axisDirection == AxisDirection.up) {
_dragOffset = _dragOffset! + notification.overscroll;
}
_checkDragOffset(notification.metrics.viewportDimension);
}
} else if (notification is ScrollEndNotification) {
switch (_mode) {
case _RefreshIndicatorMode.armed:
if (_positionController.value < 1.0) {
_dismiss(_RefreshIndicatorMode.canceled);
} else {
_show();
}
case _RefreshIndicatorMode.drag:
_dismiss(_RefreshIndicatorMode.canceled);
case _RefreshIndicatorMode.canceled:
case _RefreshIndicatorMode.done:
case _RefreshIndicatorMode.refresh:
case _RefreshIndicatorMode.snap:
case null:
// do nothing
break;
}
}
return false;
}
bool _handleIndicatorNotification(OverscrollIndicatorNotification notification) {
if (notification.depth != 0 || !notification.leading) {
return false;
}
if (_mode == _RefreshIndicatorMode.drag) {
notification.disallowIndicator();
return true;
}
return false;
}
bool _start(AxisDirection direction) {
assert(_mode == null);
assert(_isIndicatorAtTop == null);
assert(_dragOffset == null);
switch (direction) {
case AxisDirection.down:
case AxisDirection.up:
_isIndicatorAtTop = true;
case AxisDirection.left:
case AxisDirection.right:
_isIndicatorAtTop = null;
// we do not support horizontal scroll views.
return false;
}
_dragOffset = 0.0;
_scaleController.value = 0.0;
_positionController.value = 0.0;
return true;
}
void _checkDragOffset(double containerExtent) {
assert(_mode == _RefreshIndicatorMode.drag || _mode == _RefreshIndicatorMode.armed);
double newValue = _dragOffset! / (containerExtent * _kDragContainerExtentPercentage);
if (_mode == _RefreshIndicatorMode.armed) {
newValue = math.max(newValue, 1.0 / _kDragSizeFactorLimit);
}
_positionController.value = clampDouble(newValue, 0.0, 1.0); // this triggers various rebuilds
if (_mode == _RefreshIndicatorMode.drag && _valueColor.value!.alpha == _effectiveValueColor.alpha) {
_mode = _RefreshIndicatorMode.armed;
}
}
// Stop showing the refresh indicator.
Future<void> _dismiss(_RefreshIndicatorMode newMode) async {
await Future<void>.value();
// This can only be called from _show() when refreshing and
// _handleScrollNotification in response to a ScrollEndNotification or
// direction change.
assert(newMode == _RefreshIndicatorMode.canceled || newMode == _RefreshIndicatorMode.done);
setState(() {
_mode = newMode;
});
switch (_mode!) {
case _RefreshIndicatorMode.done:
await _scaleController.animateTo(1.0, duration: _kIndicatorScaleDuration);
case _RefreshIndicatorMode.canceled:
await _positionController.animateTo(0.0, duration: _kIndicatorScaleDuration);
case _RefreshIndicatorMode.armed:
case _RefreshIndicatorMode.drag:
case _RefreshIndicatorMode.refresh:
case _RefreshIndicatorMode.snap:
assert(false);
}
if (mounted && _mode == newMode) {
_dragOffset = null;
_isIndicatorAtTop = null;
setState(() {
_mode = null;
});
}
}
void _show() {
assert(_mode != _RefreshIndicatorMode.refresh);
assert(_mode != _RefreshIndicatorMode.snap);
final Completer<void> completer = Completer<void>();
_pendingRefreshFuture = completer.future;
_mode = _RefreshIndicatorMode.snap;
_positionController
.animateTo(1.0 / _kDragSizeFactorLimit, duration: _kIndicatorSnapDuration)
.then<void>((void value) {
if (mounted && _mode == _RefreshIndicatorMode.snap) {
setState(() {
// Show the indeterminate progress indicator.
_mode = _RefreshIndicatorMode.refresh;
});
final Future<void> refreshResult = widget.onRefresh();
refreshResult.whenComplete(() {
if (mounted && _mode == _RefreshIndicatorMode.refresh) {
completer.complete();
_dismiss(_RefreshIndicatorMode.done);
}
});
}
});
}
/// Show the refresh indicator and run the refresh callback as if it had
/// been started interactively. If this method is called while the refresh
/// callback is running, it quietly does nothing.
///
/// Creating the [RefreshIndicator] with a [GlobalKey<RefreshIndicatorState>]
/// makes it possible to refer to the [RefreshIndicatorState].
///
/// The future returned from this method completes when the
/// [RefreshIndicator.onRefresh] callback's future completes.
///
/// If you await the future returned by this function from a [State], you
/// should check that the state is still [mounted] before calling [setState].
///
/// When initiated in this manner, the refresh indicator is independent of any
/// actual scroll view. It defaults to showing the indicator at the top. To
/// show it at the bottom, set `atTop` to false.
Future<void> show({ bool atTop = true }) {
if (_mode != _RefreshIndicatorMode.refresh &&
_mode != _RefreshIndicatorMode.snap) {
if (_mode == null) {
_start(atTop ? AxisDirection.down : AxisDirection.up);
}
_show();
}
return _pendingRefreshFuture;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final Widget child = NotificationListener<ScrollNotification>(
onNotification: _handleScrollNotification,
child: NotificationListener<OverscrollIndicatorNotification>(
onNotification: _handleIndicatorNotification,
child: widget.child,
),
);
assert(() {
if (_mode == null) {
assert(_dragOffset == null);
assert(_isIndicatorAtTop == null);
} else {
assert(_dragOffset != null);
assert(_isIndicatorAtTop != null);
}
return true;
}());
final bool showIndeterminateIndicator =
_mode == _RefreshIndicatorMode.refresh || _mode == _RefreshIndicatorMode.done;
return Stack(
children: <Widget>[
child,
if (_mode != null) Positioned(
top: _isIndicatorAtTop! ? widget.edgeOffset : null,
bottom: !_isIndicatorAtTop! ? widget.edgeOffset : null,
left: 0.0,
right: 0.0,
child: SizeTransition(
axisAlignment: _isIndicatorAtTop! ? 1.0 : -1.0,
sizeFactor: _positionFactor, // this is what brings it down
child: Container(
padding: _isIndicatorAtTop!
? EdgeInsets.only(top: widget.displacement)
: EdgeInsets.only(bottom: widget.displacement),
alignment: _isIndicatorAtTop!
? Alignment.topCenter
: Alignment.bottomCenter,
child: ScaleTransition(
scale: _scaleFactor,
child: AnimatedBuilder(
animation: _positionController,
builder: (BuildContext context, Widget? child) {
final Widget materialIndicator = RefreshProgressIndicator(
semanticsLabel: widget.semanticsLabel ?? MaterialLocalizations.of(context).refreshIndicatorSemanticLabel,
semanticsValue: widget.semanticsValue,
value: showIndeterminateIndicator ? null : _value.value,
valueColor: _valueColor,
backgroundColor: widget.backgroundColor,
strokeWidth: widget.strokeWidth,
);
final Widget cupertinoIndicator = CupertinoActivityIndicator(
color: widget.color,
);
switch (widget._indicatorType) {
case _IndicatorType.material:
return materialIndicator;
case _IndicatorType.adaptive: {
final ThemeData theme = Theme.of(context);
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return materialIndicator;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return cupertinoIndicator;
}
}
}
},
),
),
),
),
),
],
);
}
}
| flutter/packages/flutter/lib/src/material/refresh_indicator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/refresh_indicator.dart",
"repo_id": "flutter",
"token_count": 8673
} | 627 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show Path, lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'material_state.dart';
import 'slider.dart';
import 'theme.dart';
/// Applies a slider theme to descendant [Slider] widgets.
///
/// A slider theme describes the colors and shape choices of the slider
/// components.
///
/// Descendant widgets obtain the current theme's [SliderThemeData] object using
/// [SliderTheme.of]. When a widget uses [SliderTheme.of], it is automatically
/// rebuilt if the theme later changes.
///
/// The slider is as big as the largest of
/// the [SliderComponentShape.getPreferredSize] of the thumb shape,
/// the [SliderComponentShape.getPreferredSize] of the overlay shape,
/// and the [SliderTickMarkShape.getPreferredSize] of the tick mark shape.
///
/// See also:
///
/// * [SliderThemeData], which describes the actual configuration of a slider
/// theme.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
/// * [SliderTrackShape], which can be used to create custom shapes for the
/// [Slider]'s track.
/// * [SliderTickMarkShape], which can be used to create custom shapes for the
/// [Slider]'s tick marks.
/// * [RangeSliderThumbShape], which can be used to create custom shapes for
/// the [RangeSlider]'s thumb.
/// * [RangeSliderValueIndicatorShape], which can be used to create custom
/// shapes for the [RangeSlider]'s value indicator.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RangeSliderTickMarkShape], which can be used to create custom shapes for
/// the [RangeSlider]'s tick marks.
class SliderTheme extends InheritedTheme {
/// Applies the given theme [data] to [child].
const SliderTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the color and shape values for descendant slider widgets.
final SliderThemeData data;
/// Returns the data from the closest [SliderTheme] instance that encloses
/// the given context.
///
/// Defaults to the ambient [ThemeData.sliderTheme] if there is no
/// [SliderTheme] in the given build context.
///
/// {@tool snippet}
///
/// ```dart
/// class Launch extends StatefulWidget {
/// const Launch({super.key});
///
/// @override
/// State createState() => LaunchState();
/// }
///
/// class LaunchState extends State<Launch> {
/// double _rocketThrust = 0;
///
/// @override
/// Widget build(BuildContext context) {
/// return SliderTheme(
/// data: SliderTheme.of(context).copyWith(activeTrackColor: const Color(0xff804040)),
/// child: Slider(
/// onChanged: (double value) { setState(() { _rocketThrust = value; }); },
/// value: _rocketThrust,
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [SliderThemeData], which describes the actual configuration of a slider
/// theme.
static SliderThemeData of(BuildContext context) {
final SliderTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<SliderTheme>();
return inheritedTheme != null ? inheritedTheme.data : Theme.of(context).sliderTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return SliderTheme(data: data, child: child);
}
@override
bool updateShouldNotify(SliderTheme oldWidget) => data != oldWidget.data;
}
/// Describes the conditions under which the value indicator on a [Slider]
/// will be shown. Used with [SliderThemeData.showValueIndicator].
///
/// See also:
///
/// * [Slider], a Material Design slider widget.
/// * [SliderThemeData], which describes the actual configuration of a slider
/// theme.
enum ShowValueIndicator {
/// The value indicator will only be shown for discrete sliders (sliders
/// where [Slider.divisions] is non-null).
onlyForDiscrete,
/// The value indicator will only be shown for continuous sliders (sliders
/// where [Slider.divisions] is null).
onlyForContinuous,
/// The value indicator will be shown for all types of sliders.
always,
/// The value indicator will never be shown.
never,
}
/// Identifier for a thumb.
///
/// There are 2 thumbs in a [RangeSlider], [start] and [end].
///
/// For [TextDirection.ltr], the [start] thumb is the left-most thumb and the
/// [end] thumb is the right-most thumb. For [TextDirection.rtl] the [start]
/// thumb is the right-most thumb, and the [end] thumb is the left-most thumb.
enum Thumb {
/// Left-most thumb for [TextDirection.ltr], otherwise, right-most thumb.
start,
/// Right-most thumb for [TextDirection.ltr], otherwise, left-most thumb.
end,
}
/// Holds the color, shape, and typography values for a Material Design slider
/// theme.
///
/// Use this class to configure a [SliderTheme] widget, or to set the
/// [ThemeData.sliderTheme] for a [Theme] widget.
///
/// To obtain the current ambient slider theme, use [SliderTheme.of].
///
/// This theme is for both the [Slider] and the [RangeSlider]. The properties
/// that are only for the [Slider] are: [tickMarkShape], [thumbShape],
/// [trackShape], and [valueIndicatorShape]. The properties that are only for
/// the [RangeSlider] are [rangeTickMarkShape], [rangeThumbShape],
/// [rangeTrackShape], [rangeValueIndicatorShape],
/// [overlappingShapeStrokeColor], [minThumbSeparation], and [thumbSelector].
/// All other properties are used by both the [Slider] and the [RangeSlider].
///
/// The parts of a slider are:
///
/// * The "thumb", which is a shape that slides horizontally when the user
/// drags it.
/// * The "track", which is the line that the slider thumb slides along.
/// * The "tick marks", which are regularly spaced marks that are drawn when
/// using discrete divisions.
/// * The "value indicator", which appears when the user is dragging the thumb
/// to indicate the value being selected.
/// * The "overlay", which appears around the thumb, and is shown when the
/// thumb is pressed, focused, or hovered. It is painted underneath the
/// thumb, so it must extend beyond the bounds of the thumb itself to
/// actually be visible.
/// * The "active" side of the slider is the side between the thumb and the
/// minimum value.
/// * The "inactive" side of the slider is the side between the thumb and the
/// maximum value.
/// * The [Slider] is disabled when it is not accepting user input. See
/// [Slider] for details on when this happens.
///
/// The thumb, track, tick marks, value indicator, and overlay can be customized
/// by creating subclasses of [SliderTrackShape],
/// [SliderComponentShape], and/or [SliderTickMarkShape]. See
/// [RoundSliderThumbShape], [RectangularSliderTrackShape],
/// [RoundSliderTickMarkShape], [RectangularSliderValueIndicatorShape], and
/// [RoundSliderOverlayShape] for examples.
///
/// The track painting can be skipped by specifying 0 for [trackHeight].
/// The thumb painting can be skipped by specifying
/// [SliderComponentShape.noThumb] for [SliderThemeData.thumbShape].
/// The overlay painting can be skipped by specifying
/// [SliderComponentShape.noOverlay] for [SliderThemeData.overlayShape].
/// The tick mark painting can be skipped by specifying
/// [SliderTickMarkShape.noTickMark] for [SliderThemeData.tickMarkShape].
/// The value indicator painting can be skipped by specifying the
/// appropriate [ShowValueIndicator] for [SliderThemeData.showValueIndicator].
///
/// See also:
///
/// * [SliderTheme] widget, which can override the slider theme of its
/// children.
/// * [Theme] widget, which performs a similar function to [SliderTheme],
/// but for overall themes.
/// * [ThemeData], which has a default [SliderThemeData].
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
/// * [SliderTrackShape], which can be used to create custom shapes for the
/// [Slider]'s track.
/// * [SliderTickMarkShape], which can be used to create custom shapes for the
/// [Slider]'s tick marks.
/// * [RangeSliderThumbShape], which can be used to create custom shapes for
/// the [RangeSlider]'s thumb.
/// * [RangeSliderValueIndicatorShape], which can be used to create custom
/// shapes for the [RangeSlider]'s value indicator.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RangeSliderTickMarkShape], which can be used to create custom shapes for
/// the [RangeSlider]'s tick marks.
@immutable
class SliderThemeData with Diagnosticable {
/// Create a [SliderThemeData] given a set of exact values.
///
/// This will rarely be used directly. It is used by [lerp] to
/// create intermediate themes based on two themes.
///
/// The simplest way to create a SliderThemeData is to use
/// [copyWith] on the one you get from [SliderTheme.of], or create an
/// entirely new one with [SliderThemeData.fromPrimaryColors].
///
/// {@tool snippet}
///
/// ```dart
/// class Blissful extends StatefulWidget {
/// const Blissful({super.key});
///
/// @override
/// State createState() => BlissfulState();
/// }
///
/// class BlissfulState extends State<Blissful> {
/// double _bliss = 0;
///
/// @override
/// Widget build(BuildContext context) {
/// return SliderTheme(
/// data: SliderTheme.of(context).copyWith(activeTrackColor: const Color(0xff404080)),
/// child: Slider(
/// onChanged: (double value) { setState(() { _bliss = value; }); },
/// value: _bliss,
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
const SliderThemeData({
this.trackHeight,
this.activeTrackColor,
this.inactiveTrackColor,
this.secondaryActiveTrackColor,
this.disabledActiveTrackColor,
this.disabledInactiveTrackColor,
this.disabledSecondaryActiveTrackColor,
this.activeTickMarkColor,
this.inactiveTickMarkColor,
this.disabledActiveTickMarkColor,
this.disabledInactiveTickMarkColor,
this.thumbColor,
this.overlappingShapeStrokeColor,
this.disabledThumbColor,
this.overlayColor,
this.valueIndicatorColor,
this.valueIndicatorStrokeColor,
this.overlayShape,
this.tickMarkShape,
this.thumbShape,
this.trackShape,
this.valueIndicatorShape,
this.rangeTickMarkShape,
this.rangeThumbShape,
this.rangeTrackShape,
this.rangeValueIndicatorShape,
this.showValueIndicator,
this.valueIndicatorTextStyle,
this.minThumbSeparation,
this.thumbSelector,
this.mouseCursor,
this.allowedInteraction,
});
/// Generates a SliderThemeData from three main colors.
///
/// Usually these are the primary, dark and light colors from
/// a [ThemeData].
///
/// The opacities of these colors will be overridden with the Material Design
/// defaults when assigning them to the slider theme component colors.
///
/// This is used to generate the default slider theme for a [ThemeData].
factory SliderThemeData.fromPrimaryColors({
required Color primaryColor,
required Color primaryColorDark,
required Color primaryColorLight,
required TextStyle valueIndicatorTextStyle,
}) {
// These are Material Design defaults, and are used to derive
// component Colors (with opacity) from base colors.
const int activeTrackAlpha = 0xff;
const int inactiveTrackAlpha = 0x3d; // 24% opacity
const int secondaryActiveTrackAlpha = 0x8a; // 54% opacity
const int disabledActiveTrackAlpha = 0x52; // 32% opacity
const int disabledInactiveTrackAlpha = 0x1f; // 12% opacity
const int disabledSecondaryActiveTrackAlpha = 0x1f; // 12% opacity
const int activeTickMarkAlpha = 0x8a; // 54% opacity
const int inactiveTickMarkAlpha = 0x8a; // 54% opacity
const int disabledActiveTickMarkAlpha = 0x1f; // 12% opacity
const int disabledInactiveTickMarkAlpha = 0x1f; // 12% opacity
const int thumbAlpha = 0xff;
const int disabledThumbAlpha = 0x52; // 32% opacity
const int overlayAlpha = 0x1f; // 12% opacity
const int valueIndicatorAlpha = 0xff;
return SliderThemeData(
trackHeight: 2.0,
activeTrackColor: primaryColor.withAlpha(activeTrackAlpha),
inactiveTrackColor: primaryColor.withAlpha(inactiveTrackAlpha),
secondaryActiveTrackColor: primaryColor.withAlpha(secondaryActiveTrackAlpha),
disabledActiveTrackColor: primaryColorDark.withAlpha(disabledActiveTrackAlpha),
disabledInactiveTrackColor: primaryColorDark.withAlpha(disabledInactiveTrackAlpha),
disabledSecondaryActiveTrackColor: primaryColorDark.withAlpha(disabledSecondaryActiveTrackAlpha),
activeTickMarkColor: primaryColorLight.withAlpha(activeTickMarkAlpha),
inactiveTickMarkColor: primaryColor.withAlpha(inactiveTickMarkAlpha),
disabledActiveTickMarkColor: primaryColorLight.withAlpha(disabledActiveTickMarkAlpha),
disabledInactiveTickMarkColor: primaryColorDark.withAlpha(disabledInactiveTickMarkAlpha),
thumbColor: primaryColor.withAlpha(thumbAlpha),
overlappingShapeStrokeColor: Colors.white,
disabledThumbColor: primaryColorDark.withAlpha(disabledThumbAlpha),
overlayColor: primaryColor.withAlpha(overlayAlpha),
valueIndicatorColor: primaryColor.withAlpha(valueIndicatorAlpha),
valueIndicatorStrokeColor: primaryColor.withAlpha(valueIndicatorAlpha),
overlayShape: const RoundSliderOverlayShape(),
tickMarkShape: const RoundSliderTickMarkShape(),
thumbShape: const RoundSliderThumbShape(),
trackShape: const RoundedRectSliderTrackShape(),
valueIndicatorShape: const PaddleSliderValueIndicatorShape(),
rangeTickMarkShape: const RoundRangeSliderTickMarkShape(),
rangeThumbShape: const RoundRangeSliderThumbShape(),
rangeTrackShape: const RoundedRectRangeSliderTrackShape(),
rangeValueIndicatorShape: const PaddleRangeSliderValueIndicatorShape(),
valueIndicatorTextStyle: valueIndicatorTextStyle,
showValueIndicator: ShowValueIndicator.onlyForDiscrete,
);
}
/// The height of the [Slider] track.
final double? trackHeight;
/// The color of the [Slider] track between the [Slider.min] position and the
/// current thumb position.
final Color? activeTrackColor;
/// The color of the [Slider] track between the current thumb position and the
/// [Slider.max] position.
final Color? inactiveTrackColor;
/// The color of the [Slider] track between the current thumb position and the
/// [Slider.secondaryTrackValue] position.
final Color? secondaryActiveTrackColor;
/// The color of the [Slider] track between the [Slider.min] position and the
/// current thumb position when the [Slider] is disabled.
final Color? disabledActiveTrackColor;
/// The color of the [Slider] track between the current thumb position and the
/// [Slider.secondaryTrackValue] position when the [Slider] is disabled.
final Color? disabledSecondaryActiveTrackColor;
/// The color of the [Slider] track between the current thumb position and the
/// [Slider.max] position when the [Slider] is disabled.
final Color? disabledInactiveTrackColor;
/// The color of the track's tick marks that are drawn between the [Slider.min]
/// position and the current thumb position.
final Color? activeTickMarkColor;
/// The color of the track's tick marks that are drawn between the current
/// thumb position and the [Slider.max] position.
final Color? inactiveTickMarkColor;
/// The color of the track's tick marks that are drawn between the [Slider.min]
/// position and the current thumb position when the [Slider] is disabled.
final Color? disabledActiveTickMarkColor;
/// The color of the track's tick marks that are drawn between the current
/// thumb position and the [Slider.max] position when the [Slider] is
/// disabled.
final Color? disabledInactiveTickMarkColor;
/// The color given to the [thumbShape] to draw itself with.
final Color? thumbColor;
/// The color given to the perimeter of the top [rangeThumbShape] when the
/// thumbs are overlapping and the top [rangeValueIndicatorShape] when the
/// value indicators are overlapping.
final Color? overlappingShapeStrokeColor;
/// The color given to the [thumbShape] to draw itself with when the
/// [Slider] is disabled.
final Color? disabledThumbColor;
/// The color of the overlay drawn around the slider thumb when it is
/// pressed, focused, or hovered.
///
/// This is typically a semi-transparent color.
final Color? overlayColor;
/// The color given to the [valueIndicatorShape] to draw itself with.
final Color? valueIndicatorColor;
/// The color given to the [valueIndicatorShape] stroke.
final Color? valueIndicatorStrokeColor;
/// The shape that will be used to draw the [Slider]'s overlay.
///
/// Both the [overlayColor] and a non default [overlayShape] may be specified.
/// The default [overlayShape] refers to the [overlayColor].
///
/// The default value is [RoundSliderOverlayShape].
final SliderComponentShape? overlayShape;
/// The shape that will be used to draw the [Slider]'s tick marks.
///
/// The [SliderTickMarkShape.getPreferredSize] is used to help determine the
/// location of each tick mark on the track. The slider's minimum size will
/// be at least this big.
///
/// The default value is [RoundSliderTickMarkShape].
///
/// See also:
///
/// * [RoundRangeSliderTickMarkShape], which is the default tick mark
/// shape for the range slider.
final SliderTickMarkShape? tickMarkShape;
/// The shape that will be used to draw the [Slider]'s thumb.
///
/// The default value is [RoundSliderThumbShape].
///
/// See also:
///
/// * [RoundRangeSliderThumbShape], which is the default thumb shape for
/// the [RangeSlider].
final SliderComponentShape? thumbShape;
/// The shape that will be used to draw the [Slider]'s track.
///
/// The [SliderTrackShape.getPreferredRect] method is used to map
/// slider-relative gesture coordinates to the correct thumb position on the
/// track. It is also used to horizontally position tick marks, when the
/// slider is discrete.
///
/// The default value is [RoundedRectSliderTrackShape].
///
/// See also:
///
/// * [RoundedRectRangeSliderTrackShape], which is the default track
/// shape for the [RangeSlider].
final SliderTrackShape? trackShape;
/// The shape that will be used to draw the [Slider]'s value
/// indicator.
///
/// The default value is [PaddleSliderValueIndicatorShape].
///
/// See also:
///
/// * [PaddleRangeSliderValueIndicatorShape], which is the default value
/// indicator shape for the [RangeSlider].
final SliderComponentShape? valueIndicatorShape;
/// The shape that will be used to draw the [RangeSlider]'s tick marks.
///
/// The [RangeSliderTickMarkShape.getPreferredSize] is used to help determine
/// the location of each tick mark on the track. The slider's minimum size
/// will be at least this big.
///
/// The default value is [RoundRangeSliderTickMarkShape].
///
/// See also:
///
/// * [RoundSliderTickMarkShape], which is the default tick mark shape
/// for the [Slider].
final RangeSliderTickMarkShape? rangeTickMarkShape;
/// The shape that will be used for the [RangeSlider]'s thumbs.
///
/// By default the same shape is used for both thumbs, but strokes the top
/// thumb when it overlaps the bottom thumb. The top thumb is always the last
/// selected thumb.
///
/// The default value is [RoundRangeSliderThumbShape].
///
/// See also:
///
/// * [RoundSliderThumbShape], which is the default thumb shape for the
/// [Slider].
final RangeSliderThumbShape? rangeThumbShape;
/// The shape that will be used to draw the [RangeSlider]'s track.
///
/// The [SliderTrackShape.getPreferredRect] method is used to map
/// slider-relative gesture coordinates to the correct thumb position on the
/// track. It is also used to horizontally position the tick marks, when the
/// slider is discrete.
///
/// The default value is [RoundedRectRangeSliderTrackShape].
///
/// See also:
///
/// * [RoundedRectSliderTrackShape], which is the default track
/// shape for the [Slider].
final RangeSliderTrackShape? rangeTrackShape;
/// The shape that will be used for the [RangeSlider]'s value indicators.
///
/// The default shape uses the same value indicator for each thumb, but
/// strokes the top value indicator when it overlaps the bottom value
/// indicator. The top indicator corresponds to the top thumb, which is always
/// the most recently selected thumb.
///
/// The default value is [PaddleRangeSliderValueIndicatorShape].
///
/// See also:
///
/// * [PaddleSliderValueIndicatorShape], which is the default value
/// indicator shape for the [Slider].
final RangeSliderValueIndicatorShape? rangeValueIndicatorShape;
/// Whether the value indicator should be shown for different types of
/// sliders.
///
/// By default, [showValueIndicator] is set to
/// [ShowValueIndicator.onlyForDiscrete]. The value indicator is only shown
/// when the thumb is being touched.
final ShowValueIndicator? showValueIndicator;
/// The text style for the text on the value indicator.
final TextStyle? valueIndicatorTextStyle;
/// Limits the thumb's separation distance.
///
/// Use this only if you want to control the visual appearance of the thumbs
/// in terms of a logical pixel value. This can be done when you want a
/// specific look for thumbs when they are close together. To limit with the
/// real values, rather than logical pixels, the values can be restricted by
/// the parent.
final double? minThumbSeparation;
/// Determines which thumb should be selected when the slider is interacted
/// with.
///
/// If null, the default thumb selector finds the closest thumb, excluding
/// taps that are between the thumbs and not within any one touch target.
/// When the selection is within the touch target bounds of both thumbs, no
/// thumb is selected until the selection is moved.
///
/// Override this for custom thumb selection.
final RangeThumbSelector? thumbSelector;
/// {@macro flutter.material.slider.mouseCursor}
///
/// If specified, overrides the default value of [Slider.mouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// Allowed way for the user to interact with the [Slider].
///
/// If specified, overrides the default value of [Slider.allowedInteraction].
final SliderInteraction? allowedInteraction;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
SliderThemeData copyWith({
double? trackHeight,
Color? activeTrackColor,
Color? inactiveTrackColor,
Color? secondaryActiveTrackColor,
Color? disabledActiveTrackColor,
Color? disabledInactiveTrackColor,
Color? disabledSecondaryActiveTrackColor,
Color? activeTickMarkColor,
Color? inactiveTickMarkColor,
Color? disabledActiveTickMarkColor,
Color? disabledInactiveTickMarkColor,
Color? thumbColor,
Color? overlappingShapeStrokeColor,
Color? disabledThumbColor,
Color? overlayColor,
Color? valueIndicatorColor,
Color? valueIndicatorStrokeColor,
SliderComponentShape? overlayShape,
SliderTickMarkShape? tickMarkShape,
SliderComponentShape? thumbShape,
SliderTrackShape? trackShape,
SliderComponentShape? valueIndicatorShape,
RangeSliderTickMarkShape? rangeTickMarkShape,
RangeSliderThumbShape? rangeThumbShape,
RangeSliderTrackShape? rangeTrackShape,
RangeSliderValueIndicatorShape? rangeValueIndicatorShape,
ShowValueIndicator? showValueIndicator,
TextStyle? valueIndicatorTextStyle,
double? minThumbSeparation,
RangeThumbSelector? thumbSelector,
MaterialStateProperty<MouseCursor?>? mouseCursor,
SliderInteraction? allowedInteraction,
}) {
return SliderThemeData(
trackHeight: trackHeight ?? this.trackHeight,
activeTrackColor: activeTrackColor ?? this.activeTrackColor,
inactiveTrackColor: inactiveTrackColor ?? this.inactiveTrackColor,
secondaryActiveTrackColor: secondaryActiveTrackColor ?? this.secondaryActiveTrackColor,
disabledActiveTrackColor: disabledActiveTrackColor ?? this.disabledActiveTrackColor,
disabledInactiveTrackColor: disabledInactiveTrackColor ?? this.disabledInactiveTrackColor,
disabledSecondaryActiveTrackColor: disabledSecondaryActiveTrackColor ?? this.disabledSecondaryActiveTrackColor,
activeTickMarkColor: activeTickMarkColor ?? this.activeTickMarkColor,
inactiveTickMarkColor: inactiveTickMarkColor ?? this.inactiveTickMarkColor,
disabledActiveTickMarkColor: disabledActiveTickMarkColor ?? this.disabledActiveTickMarkColor,
disabledInactiveTickMarkColor: disabledInactiveTickMarkColor ?? this.disabledInactiveTickMarkColor,
thumbColor: thumbColor ?? this.thumbColor,
overlappingShapeStrokeColor: overlappingShapeStrokeColor ?? this.overlappingShapeStrokeColor,
disabledThumbColor: disabledThumbColor ?? this.disabledThumbColor,
overlayColor: overlayColor ?? this.overlayColor,
valueIndicatorColor: valueIndicatorColor ?? this.valueIndicatorColor,
valueIndicatorStrokeColor: valueIndicatorStrokeColor ?? this.valueIndicatorStrokeColor,
overlayShape: overlayShape ?? this.overlayShape,
tickMarkShape: tickMarkShape ?? this.tickMarkShape,
thumbShape: thumbShape ?? this.thumbShape,
trackShape: trackShape ?? this.trackShape,
valueIndicatorShape: valueIndicatorShape ?? this.valueIndicatorShape,
rangeTickMarkShape: rangeTickMarkShape ?? this.rangeTickMarkShape,
rangeThumbShape: rangeThumbShape ?? this.rangeThumbShape,
rangeTrackShape: rangeTrackShape ?? this.rangeTrackShape,
rangeValueIndicatorShape: rangeValueIndicatorShape ?? this.rangeValueIndicatorShape,
showValueIndicator: showValueIndicator ?? this.showValueIndicator,
valueIndicatorTextStyle: valueIndicatorTextStyle ?? this.valueIndicatorTextStyle,
minThumbSeparation: minThumbSeparation ?? this.minThumbSeparation,
thumbSelector: thumbSelector ?? this.thumbSelector,
mouseCursor: mouseCursor ?? this.mouseCursor,
allowedInteraction: allowedInteraction ?? this.allowedInteraction,
);
}
/// Linearly interpolate between two slider themes.
///
/// {@macro dart.ui.shadow.lerp}
static SliderThemeData lerp(SliderThemeData a, SliderThemeData b, double t) {
if (identical(a, b)) {
return a;
}
return SliderThemeData(
trackHeight: lerpDouble(a.trackHeight, b.trackHeight, t),
activeTrackColor: Color.lerp(a.activeTrackColor, b.activeTrackColor, t),
inactiveTrackColor: Color.lerp(a.inactiveTrackColor, b.inactiveTrackColor, t),
secondaryActiveTrackColor: Color.lerp(a.secondaryActiveTrackColor, b.secondaryActiveTrackColor, t),
disabledActiveTrackColor: Color.lerp(a.disabledActiveTrackColor, b.disabledActiveTrackColor, t),
disabledInactiveTrackColor: Color.lerp(a.disabledInactiveTrackColor, b.disabledInactiveTrackColor, t),
disabledSecondaryActiveTrackColor: Color.lerp(a.disabledSecondaryActiveTrackColor, b.disabledSecondaryActiveTrackColor, t),
activeTickMarkColor: Color.lerp(a.activeTickMarkColor, b.activeTickMarkColor, t),
inactiveTickMarkColor: Color.lerp(a.inactiveTickMarkColor, b.inactiveTickMarkColor, t),
disabledActiveTickMarkColor: Color.lerp(a.disabledActiveTickMarkColor, b.disabledActiveTickMarkColor, t),
disabledInactiveTickMarkColor: Color.lerp(a.disabledInactiveTickMarkColor, b.disabledInactiveTickMarkColor, t),
thumbColor: Color.lerp(a.thumbColor, b.thumbColor, t),
overlappingShapeStrokeColor: Color.lerp(a.overlappingShapeStrokeColor, b.overlappingShapeStrokeColor, t),
disabledThumbColor: Color.lerp(a.disabledThumbColor, b.disabledThumbColor, t),
overlayColor: Color.lerp(a.overlayColor, b.overlayColor, t),
valueIndicatorColor: Color.lerp(a.valueIndicatorColor, b.valueIndicatorColor, t),
valueIndicatorStrokeColor: Color.lerp(a.valueIndicatorStrokeColor, b.valueIndicatorStrokeColor, t),
overlayShape: t < 0.5 ? a.overlayShape : b.overlayShape,
tickMarkShape: t < 0.5 ? a.tickMarkShape : b.tickMarkShape,
thumbShape: t < 0.5 ? a.thumbShape : b.thumbShape,
trackShape: t < 0.5 ? a.trackShape : b.trackShape,
valueIndicatorShape: t < 0.5 ? a.valueIndicatorShape : b.valueIndicatorShape,
rangeTickMarkShape: t < 0.5 ? a.rangeTickMarkShape : b.rangeTickMarkShape,
rangeThumbShape: t < 0.5 ? a.rangeThumbShape : b.rangeThumbShape,
rangeTrackShape: t < 0.5 ? a.rangeTrackShape : b.rangeTrackShape,
rangeValueIndicatorShape: t < 0.5 ? a.rangeValueIndicatorShape : b.rangeValueIndicatorShape,
showValueIndicator: t < 0.5 ? a.showValueIndicator : b.showValueIndicator,
valueIndicatorTextStyle: TextStyle.lerp(a.valueIndicatorTextStyle, b.valueIndicatorTextStyle, t),
minThumbSeparation: lerpDouble(a.minThumbSeparation, b.minThumbSeparation, t),
thumbSelector: t < 0.5 ? a.thumbSelector : b.thumbSelector,
mouseCursor: t < 0.5 ? a.mouseCursor : b.mouseCursor,
allowedInteraction: t < 0.5 ? a.allowedInteraction : b.allowedInteraction,
);
}
@override
int get hashCode => Object.hash(
trackHeight,
activeTrackColor,
inactiveTrackColor,
secondaryActiveTrackColor,
disabledActiveTrackColor,
disabledInactiveTrackColor,
disabledSecondaryActiveTrackColor,
activeTickMarkColor,
inactiveTickMarkColor,
disabledActiveTickMarkColor,
disabledInactiveTickMarkColor,
thumbColor,
overlappingShapeStrokeColor,
disabledThumbColor,
overlayColor,
valueIndicatorColor,
overlayShape,
tickMarkShape,
thumbShape,
Object.hash(
trackShape,
valueIndicatorShape,
rangeTickMarkShape,
rangeThumbShape,
rangeTrackShape,
rangeValueIndicatorShape,
showValueIndicator,
valueIndicatorTextStyle,
minThumbSeparation,
thumbSelector,
mouseCursor,
allowedInteraction,
),
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SliderThemeData
&& other.trackHeight == trackHeight
&& other.activeTrackColor == activeTrackColor
&& other.inactiveTrackColor == inactiveTrackColor
&& other.secondaryActiveTrackColor == secondaryActiveTrackColor
&& other.disabledActiveTrackColor == disabledActiveTrackColor
&& other.disabledInactiveTrackColor == disabledInactiveTrackColor
&& other.disabledSecondaryActiveTrackColor == disabledSecondaryActiveTrackColor
&& other.activeTickMarkColor == activeTickMarkColor
&& other.inactiveTickMarkColor == inactiveTickMarkColor
&& other.disabledActiveTickMarkColor == disabledActiveTickMarkColor
&& other.disabledInactiveTickMarkColor == disabledInactiveTickMarkColor
&& other.thumbColor == thumbColor
&& other.overlappingShapeStrokeColor == overlappingShapeStrokeColor
&& other.disabledThumbColor == disabledThumbColor
&& other.overlayColor == overlayColor
&& other.valueIndicatorColor == valueIndicatorColor
&& other.valueIndicatorStrokeColor == valueIndicatorStrokeColor
&& other.overlayShape == overlayShape
&& other.tickMarkShape == tickMarkShape
&& other.thumbShape == thumbShape
&& other.trackShape == trackShape
&& other.valueIndicatorShape == valueIndicatorShape
&& other.rangeTickMarkShape == rangeTickMarkShape
&& other.rangeThumbShape == rangeThumbShape
&& other.rangeTrackShape == rangeTrackShape
&& other.rangeValueIndicatorShape == rangeValueIndicatorShape
&& other.showValueIndicator == showValueIndicator
&& other.valueIndicatorTextStyle == valueIndicatorTextStyle
&& other.minThumbSeparation == minThumbSeparation
&& other.thumbSelector == thumbSelector
&& other.mouseCursor == mouseCursor
&& other.allowedInteraction == allowedInteraction;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
const SliderThemeData defaultData = SliderThemeData();
properties.add(DoubleProperty('trackHeight', trackHeight, defaultValue: defaultData.trackHeight));
properties.add(ColorProperty('activeTrackColor', activeTrackColor, defaultValue: defaultData.activeTrackColor));
properties.add(ColorProperty('inactiveTrackColor', inactiveTrackColor, defaultValue: defaultData.inactiveTrackColor));
properties.add(ColorProperty('secondaryActiveTrackColor', secondaryActiveTrackColor, defaultValue: defaultData.secondaryActiveTrackColor));
properties.add(ColorProperty('disabledActiveTrackColor', disabledActiveTrackColor, defaultValue: defaultData.disabledActiveTrackColor));
properties.add(ColorProperty('disabledInactiveTrackColor', disabledInactiveTrackColor, defaultValue: defaultData.disabledInactiveTrackColor));
properties.add(ColorProperty('disabledSecondaryActiveTrackColor', disabledSecondaryActiveTrackColor, defaultValue: defaultData.disabledSecondaryActiveTrackColor));
properties.add(ColorProperty('activeTickMarkColor', activeTickMarkColor, defaultValue: defaultData.activeTickMarkColor));
properties.add(ColorProperty('inactiveTickMarkColor', inactiveTickMarkColor, defaultValue: defaultData.inactiveTickMarkColor));
properties.add(ColorProperty('disabledActiveTickMarkColor', disabledActiveTickMarkColor, defaultValue: defaultData.disabledActiveTickMarkColor));
properties.add(ColorProperty('disabledInactiveTickMarkColor', disabledInactiveTickMarkColor, defaultValue: defaultData.disabledInactiveTickMarkColor));
properties.add(ColorProperty('thumbColor', thumbColor, defaultValue: defaultData.thumbColor));
properties.add(ColorProperty('overlappingShapeStrokeColor', overlappingShapeStrokeColor, defaultValue: defaultData.overlappingShapeStrokeColor));
properties.add(ColorProperty('disabledThumbColor', disabledThumbColor, defaultValue: defaultData.disabledThumbColor));
properties.add(ColorProperty('overlayColor', overlayColor, defaultValue: defaultData.overlayColor));
properties.add(ColorProperty('valueIndicatorColor', valueIndicatorColor, defaultValue: defaultData.valueIndicatorColor));
properties.add(ColorProperty('valueIndicatorStrokeColor', valueIndicatorStrokeColor, defaultValue: defaultData.valueIndicatorStrokeColor));
properties.add(DiagnosticsProperty<SliderComponentShape>('overlayShape', overlayShape, defaultValue: defaultData.overlayShape));
properties.add(DiagnosticsProperty<SliderTickMarkShape>('tickMarkShape', tickMarkShape, defaultValue: defaultData.tickMarkShape));
properties.add(DiagnosticsProperty<SliderComponentShape>('thumbShape', thumbShape, defaultValue: defaultData.thumbShape));
properties.add(DiagnosticsProperty<SliderTrackShape>('trackShape', trackShape, defaultValue: defaultData.trackShape));
properties.add(DiagnosticsProperty<SliderComponentShape>('valueIndicatorShape', valueIndicatorShape, defaultValue: defaultData.valueIndicatorShape));
properties.add(DiagnosticsProperty<RangeSliderTickMarkShape>('rangeTickMarkShape', rangeTickMarkShape, defaultValue: defaultData.rangeTickMarkShape));
properties.add(DiagnosticsProperty<RangeSliderThumbShape>('rangeThumbShape', rangeThumbShape, defaultValue: defaultData.rangeThumbShape));
properties.add(DiagnosticsProperty<RangeSliderTrackShape>('rangeTrackShape', rangeTrackShape, defaultValue: defaultData.rangeTrackShape));
properties.add(DiagnosticsProperty<RangeSliderValueIndicatorShape>('rangeValueIndicatorShape', rangeValueIndicatorShape, defaultValue: defaultData.rangeValueIndicatorShape));
properties.add(EnumProperty<ShowValueIndicator>('showValueIndicator', showValueIndicator, defaultValue: defaultData.showValueIndicator));
properties.add(DiagnosticsProperty<TextStyle>('valueIndicatorTextStyle', valueIndicatorTextStyle, defaultValue: defaultData.valueIndicatorTextStyle));
properties.add(DoubleProperty('minThumbSeparation', minThumbSeparation, defaultValue: defaultData.minThumbSeparation));
properties.add(DiagnosticsProperty<RangeThumbSelector>('thumbSelector', thumbSelector, defaultValue: defaultData.thumbSelector));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>>('mouseCursor', mouseCursor, defaultValue: defaultData.mouseCursor));
properties.add(EnumProperty<SliderInteraction>('allowedInteraction', allowedInteraction, defaultValue: defaultData.allowedInteraction));
}
}
/// Base class for slider thumb, thumb overlay, and value indicator shapes.
///
/// Create a subclass of this if you would like a custom shape.
///
/// All shapes are painted to the same canvas and ordering is important.
/// The overlay is painted first, then the value indicator, then the thumb.
///
/// The thumb painting can be skipped by specifying [noThumb] for
/// [SliderThemeData.thumbShape].
///
/// The overlay painting can be skipped by specifying [noOverlay] for
/// [SliderThemeData.overlayShape].
///
/// See also:
///
/// * [RoundSliderThumbShape], which is the default [Slider]'s thumb shape that
/// paints a solid circle.
/// * [RoundSliderOverlayShape], which is the default [Slider] and
/// [RangeSlider]'s overlay shape that paints a transparent circle.
/// * [PaddleSliderValueIndicatorShape], which is the default [Slider]'s value
/// indicator shape that paints a custom path with text in it.
abstract class SliderComponentShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliderComponentShape();
/// Returns the preferred size of the shape, based on the given conditions.
Size getPreferredSize(bool isEnabled, bool isDiscrete);
/// Paints the shape, taking into account the state passed to it.
///
/// {@template flutter.material.SliderComponentShape.paint.context}
/// The `context` argument is the same as the one that includes the [Slider]'s
/// render box.
/// {@endtemplate}
///
/// {@template flutter.material.SliderComponentShape.paint.center}
/// The `center` argument is the offset for where this shape's center should be
/// painted. This offset is relative to the origin of the [context] canvas.
/// {@endtemplate}
///
/// The `activationAnimation` argument is an animation triggered when the user
/// begins to interact with the slider. It reverses when the user stops interacting
/// with the slider.
///
/// {@template flutter.material.SliderComponentShape.paint.enableAnimation}
/// The `enableAnimation` argument is an animation triggered when the [Slider]
/// is enabled, and it reverses when the slider is disabled. The [Slider] is
/// enabled when [Slider.onChanged] is not null.Use this to paint intermediate
/// frames for this shape when the slider changes enabled state.
/// {@endtemplate}
///
/// {@template flutter.material.SliderComponentShape.paint.isDiscrete}
/// The `isDiscrete` argument is true if [Slider.divisions] is non-null. When
/// true, the slider will render tick marks on top of the track.
/// {@endtemplate}
///
/// If the `labelPainter` argument is non-null, then [TextPainter.paint]
/// should be called on the `labelPainter` with the location that the label
/// should appear. If the `labelPainter` argument is null, then no label was
/// supplied to the [Slider].
///
/// {@template flutter.material.SliderComponentShape.paint.parentBox}
/// The `parentBox` argument is the [RenderBox] of the [Slider]. Its attributes,
/// such as size, can be used to assist in painting this shape.
/// {@endtemplate}
///
/// {@template flutter.material.SliderComponentShape.paint.sliderTheme}
/// the `sliderTheme` argument is the theme assigned to the [Slider] that this
/// shape belongs to.
/// {@endtemplate}
///
/// The `textDirection` argument can be used to determine how any extra text
/// or graphics (besides the text painted by the `labelPainter`) should be
/// positioned. The `labelPainter` already has the [textDirection] set.
///
/// The `value` argument is the current parametric value (from 0.0 to 1.0) of
/// the slider.
///
/// {@template flutter.material.SliderComponentShape.paint.textScaleFactor}
/// The `textScaleFactor` argument can be used to determine whether the
/// component should paint larger or smaller, depending on whether
/// [textScaleFactor] is greater than 1 for larger, and between 0 and 1 for
/// smaller. It's usually computed from [MediaQueryData.textScaler].
/// {@endtemplate}
///
/// {@template flutter.material.SliderComponentShape.paint.sizeWithOverflow}
/// The `sizeWithOverflow` argument can be used to determine the bounds the
/// drawing of the components that are outside of the regular slider bounds.
/// It's the size of the box, whose center is aligned with the slider's
/// bounds, that the value indicators must be drawn within. Typically, it is
/// bigger than the slider.
/// {@endtemplate}
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
});
/// Special instance of [SliderComponentShape] to skip the thumb drawing.
///
/// See also:
///
/// * [SliderThemeData.thumbShape], which is the shape that the [Slider]
/// uses when painting the thumb.
static final SliderComponentShape noThumb = _EmptySliderComponentShape();
/// Special instance of [SliderComponentShape] to skip the overlay drawing.
///
/// See also:
///
/// * [SliderThemeData.overlayShape], which is the shape that the [Slider]
/// uses when painting the overlay.
static final SliderComponentShape noOverlay = _EmptySliderComponentShape();
}
/// Base class for [Slider] tick mark shapes.
///
/// Create a subclass of this if you would like a custom slider tick mark shape.
///
/// The tick mark painting can be skipped by specifying [noTickMark] for
/// [SliderThemeData.tickMarkShape].
///
/// See also:
///
/// * [RoundSliderTickMarkShape], which is the default [Slider]'s tick mark
/// shape that paints a solid circle.
/// * [SliderTrackShape], which can be used to create custom shapes for the
/// [Slider]'s track.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class SliderTickMarkShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliderTickMarkShape();
/// Returns the preferred size of the shape.
///
/// It is used to help position the tick marks within the slider.
///
/// {@macro flutter.material.SliderComponentShape.paint.sliderTheme}
///
/// {@template flutter.material.SliderTickMarkShape.getPreferredSize.isEnabled}
/// The `isEnabled` argument is false when [Slider.onChanged] is null and true
/// otherwise. When true, the slider will respond to input.
/// {@endtemplate}
Size getPreferredSize({
required SliderThemeData sliderTheme,
required bool isEnabled,
});
/// Paints the slider track.
///
/// {@macro flutter.material.SliderComponentShape.paint.context}
///
/// {@macro flutter.material.SliderComponentShape.paint.center}
///
/// {@macro flutter.material.SliderComponentShape.paint.parentBox}
///
/// {@macro flutter.material.SliderComponentShape.paint.sliderTheme}
///
/// {@macro flutter.material.SliderComponentShape.paint.enableAnimation}
///
/// {@macro flutter.material.SliderTickMarkShape.getPreferredSize.isEnabled}
///
/// The `textDirection` argument can be used to determine how the tick marks
/// are painting depending on whether they are on an active track segment or
/// not. The track segment between the start of the slider and the thumb is
/// the active track segment. The track segment between the thumb and the end
/// of the slider is the inactive track segment. In LTR text direction, the
/// start of the slider is on the left, and in RTL text direction, the start
/// of the slider is on the right.
void paint(
PaintingContext context,
Offset center, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset thumbCenter,
required bool isEnabled,
required TextDirection textDirection,
});
/// Special instance of [SliderTickMarkShape] to skip the tick mark painting.
///
/// See also:
///
/// * [SliderThemeData.tickMarkShape], which is the shape that the [Slider]
/// uses when painting tick marks.
static final SliderTickMarkShape noTickMark = _EmptySliderTickMarkShape();
}
/// Base class for slider track shapes.
///
/// The slider's thumb moves along the track. A discrete slider's tick marks
/// are drawn after the track, but before the thumb, and are aligned with the
/// track.
///
/// The [getPreferredRect] helps position the slider thumb and tick marks
/// relative to the track.
///
/// See also:
///
/// * [RoundedRectSliderTrackShape] for the default [Slider]'s track shape that
/// paints a stadium-like track.
/// * [SliderTickMarkShape], which can be used to create custom shapes for the
/// [Slider]'s tick marks.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class SliderTrackShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliderTrackShape();
/// Returns the preferred bounds of the shape.
///
/// It is used to provide horizontal boundaries for the thumb's position, and
/// to help position the slider thumb and tick marks relative to the track.
///
/// The `parentBox` argument can be used to help determine the preferredRect relative to
/// attributes of the render box of the slider itself, such as size.
///
/// The `offset` argument is relative to the caller's bounding box. It can be used to
/// convert gesture coordinates from global to slider-relative coordinates.
///
/// {@macro flutter.material.SliderComponentShape.paint.sliderTheme}
///
/// {@macro flutter.material.SliderTickMarkShape.getPreferredSize.isEnabled}
///
/// {@macro flutter.material.SliderComponentShape.paint.isDiscrete}
Rect getPreferredRect({
required RenderBox parentBox,
Offset offset = Offset.zero,
required SliderThemeData sliderTheme,
bool isEnabled,
bool isDiscrete,
});
/// Paints the track shape based on the state passed to it.
///
/// {@macro flutter.material.SliderComponentShape.paint.context}
///
/// The `offset` argument the offset of the origin of the `parentBox` to the
/// origin of its `context` canvas. This shape must be painted relative to
/// this offset. See [PaintingContextCallback].
///
/// {@macro flutter.material.SliderComponentShape.paint.parentBox}
///
/// {@macro flutter.material.SliderComponentShape.paint.sliderTheme}
///
/// {@macro flutter.material.SliderComponentShape.paint.enableAnimation}
///
/// The `thumbCenter` argument is the offset of the center of the thumb
/// relative to the origin of the [PaintingContext.canvas]. It can be used as
/// the point that divides the track into 2 segments.
///
/// The `secondaryOffset` argument is the offset of the secondary value
/// relative to the origin of the [PaintingContext.canvas].
///
/// If not null, the track is divided into 3 segments.
///
/// {@macro flutter.material.SliderTickMarkShape.getPreferredSize.isEnabled}
///
/// {@macro flutter.material.SliderComponentShape.paint.isDiscrete}
///
/// The `textDirection` argument can be used to determine how the track
/// segments are painted depending on whether they are active or not.
///
/// {@template flutter.material.SliderTrackShape.paint.trackSegment}
/// The track segment between the start of the slider and the thumb is the
/// active track segment. The track segment between the thumb and the end of the
/// slider is the inactive track segment. In [TextDirection.ltr], the start of
/// the slider is on the left, and in [TextDirection.rtl], the start of the
/// slider is on the right.
/// {@endtemplate}
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset thumbCenter,
Offset? secondaryOffset,
bool isEnabled,
bool isDiscrete,
required TextDirection textDirection,
});
}
/// Base class for [RangeSlider] thumb shapes.
///
/// See also:
///
/// * [RoundRangeSliderThumbShape] for the default [RangeSlider]'s thumb shape
/// that paints a solid circle.
/// * [RangeSliderTickMarkShape], which can be used to create custom shapes for
/// the [RangeSlider]'s tick marks.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RangeSliderValueIndicatorShape], which can be used to create custom
/// shapes for the [RangeSlider]'s value indicator.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class RangeSliderThumbShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RangeSliderThumbShape();
/// Returns the preferred size of the shape, based on the given conditions.
///
/// {@template flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
/// The `isDiscrete` argument is true if [RangeSlider.divisions] is non-null.
/// When true, the slider will render tick marks on top of the track.
/// {@endtemplate}
///
/// {@template flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
/// The `isEnabled` argument is false when [RangeSlider.onChanged] is null and
/// true otherwise. When true, the slider will respond to input.
/// {@endtemplate}
Size getPreferredSize(bool isEnabled, bool isDiscrete);
/// Paints the thumb shape based on the state passed to it.
///
/// {@template flutter.material.RangeSliderThumbShape.paint.context}
/// The `context` argument represents the [RangeSlider]'s render box.
/// {@endtemplate}
///
/// {@macro flutter.material.SliderComponentShape.paint.center}
///
/// {@template flutter.material.RangeSliderThumbShape.paint.activationAnimation}
/// The `activationAnimation` argument is an animation triggered when the user
/// begins to interact with the [RangeSlider]. It reverses when the user stops
/// interacting with the slider.
/// {@endtemplate}
///
/// {@template flutter.material.RangeSliderThumbShape.paint.enableAnimation}
/// The `enableAnimation` argument is an animation triggered when the
/// [RangeSlider] is enabled, and it reverses when the slider is disabled. The
/// [RangeSlider] is enabled when [RangeSlider.onChanged] is not null. Use
/// this to paint intermediate frames for this shape when the slider changes
/// enabled state.
/// {@endtemplate}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
///
/// If the `isOnTop` argument is true, this thumb is painted on top of the
/// other slider thumb because this thumb is the one that was most recently
/// selected.
///
/// {@template flutter.material.RangeSliderThumbShape.paint.sliderTheme}
/// The `sliderTheme` argument is the theme assigned to the [RangeSlider] that
/// this shape belongs to.
/// {@endtemplate}
///
/// The `textDirection` argument can be used to determine how the orientation
/// of either slider thumb should be changed, such as drawing different
/// shapes for the left and right thumb.
///
/// {@template flutter.material.RangeSliderThumbShape.paint.thumb}
/// The `thumb` argument is the specifier for which of the two thumbs this
/// method should paint (start or end).
/// {@endtemplate}
///
/// The `isPressed` argument can be used to give the selected thumb
/// additional selected or pressed state visual feedback, such as a larger
/// shadow.
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
bool isDiscrete,
bool isEnabled,
bool isOnTop,
TextDirection textDirection,
required SliderThemeData sliderTheme,
Thumb thumb,
bool isPressed,
});
}
/// Base class for [RangeSlider] value indicator shapes.
///
/// See also:
///
/// * [PaddleRangeSliderValueIndicatorShape] for the default [RangeSlider]'s
/// value indicator shape that paints a custom path with text in it.
/// * [RangeSliderTickMarkShape], which can be used to create custom shapes for
/// the [RangeSlider]'s tick marks.
/// * [RangeSliderThumbShape], which can be used to create custom shapes for
/// the [RangeSlider]'s thumb.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class RangeSliderValueIndicatorShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RangeSliderValueIndicatorShape();
/// Returns the preferred size of the shape, based on the given conditions.
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
///
/// The `labelPainter` argument helps determine the width of the shape. It is
/// variable width because it is derived from a formatted string.
///
/// {@macro flutter.material.SliderComponentShape.paint.textScaleFactor}
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
required TextPainter labelPainter,
required double textScaleFactor,
});
/// Determines the best offset to keep this shape on the screen.
///
/// Override this method when the center of the value indicator should be
/// shifted from the vertical center of the thumb.
double getHorizontalShift({
RenderBox? parentBox,
Offset? center,
TextPainter? labelPainter,
Animation<double>? activationAnimation,
double? textScaleFactor,
Size? sizeWithOverflow,
}) {
return 0;
}
/// Paints the value indicator shape based on the state passed to it.
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.context}
///
/// {@macro flutter.material.SliderComponentShape.paint.center}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.activationAnimation}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.enableAnimation}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
///
/// The `isOnTop` argument is the top-most value indicator between the two value
/// indicators, which is always the indicator for the most recently selected thumb. In
/// the default case, this is used to paint a stroke around the top indicator
/// for better visibility between the two indicators.
///
/// {@macro flutter.material.SliderComponentShape.paint.textScaleFactor}
///
/// {@macro flutter.material.SliderComponentShape.paint.sizeWithOverflow}
///
/// {@template flutter.material.RangeSliderValueIndicatorShape.paint.parentBox}
/// The `parentBox` argument is the [RenderBox] of the [RangeSlider]. Its
/// attributes, such as size, can be used to assist in painting this shape.
/// {@endtemplate}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.sliderTheme}
///
/// The `textDirection` argument can be used to determine how any extra text
/// or graphics, besides the text painted by the [labelPainter] should be
/// positioned. The `labelPainter` argument already has the `textDirection`
/// set.
///
/// The `value` argument is the current parametric value (from 0.0 to 1.0) of
/// the slider.
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.thumb}
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
bool isDiscrete,
bool isOnTop,
required TextPainter labelPainter,
double textScaleFactor,
Size sizeWithOverflow,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
TextDirection textDirection,
double value,
Thumb thumb,
});
}
/// Base class for [RangeSlider] tick mark shapes.
///
/// This is a simplified version of [SliderComponentShape] with a
/// [SliderThemeData] passed when getting the preferred size.
///
/// See also:
///
/// * [RoundRangeSliderTickMarkShape] for the default [RangeSlider]'s tick mark
/// shape that paints a solid circle.
/// * [RangeSliderThumbShape], which can be used to create custom shapes for
/// the [RangeSlider]'s thumb.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RangeSliderValueIndicatorShape], which can be used to create custom
/// shapes for the [RangeSlider]'s value indicator.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class RangeSliderTickMarkShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RangeSliderTickMarkShape();
/// Returns the preferred size of the shape.
///
/// It is used to help position the tick marks within the slider.
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.sliderTheme}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
Size getPreferredSize({
required SliderThemeData sliderTheme,
bool isEnabled,
});
/// Paints the slider track.
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.context}
///
/// {@macro flutter.material.SliderComponentShape.paint.center}
///
/// {@macro flutter.material.RangeSliderValueIndicatorShape.paint.parentBox}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.sliderTheme}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.enableAnimation}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
///
/// The `textDirection` argument can be used to determine how the tick marks
/// are painted depending on whether they are on an active track segment or not.
///
/// {@template flutter.material.RangeSliderTickMarkShape.paint.trackSegment}
/// The track segment between the two thumbs is the active track segment. The
/// track segments between the thumb and each end of the slider are the inactive
/// track segments. In [TextDirection.ltr], the start of the slider is on the
/// left, and in [TextDirection.rtl], the start of the slider is on the right.
/// {@endtemplate}
void paint(
PaintingContext context,
Offset center, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset startThumbCenter,
required Offset endThumbCenter,
bool isEnabled,
required TextDirection textDirection,
});
}
/// Base class for [RangeSlider] track shapes.
///
/// The slider's thumbs move along the track. A discrete slider's tick marks
/// are drawn after the track, but before the thumb, and are aligned with the
/// track.
///
/// The [getPreferredRect] helps position the slider thumbs and tick marks
/// relative to the track.
///
/// See also:
///
/// * [RoundedRectRangeSliderTrackShape] for the default [RangeSlider]'s track
/// shape that paints a stadium-like track.
/// * [RangeSliderTickMarkShape], which can be used to create custom shapes for
/// the [RangeSlider]'s tick marks.
/// * [RangeSliderThumbShape], which can be used to create custom shapes for
/// the [RangeSlider]'s thumb.
/// * [RangeSliderValueIndicatorShape], which can be used to create custom
/// shapes for the [RangeSlider]'s value indicator.
/// * [SliderComponentShape], which can be used to create custom shapes for
/// the [Slider]'s thumb, overlay, and value indicator and the
/// [RangeSlider]'s overlay.
abstract class RangeSliderTrackShape {
/// This abstract const constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const RangeSliderTrackShape();
/// Returns the preferred bounds of the shape.
///
/// It is used to provide horizontal boundaries for the position of the
/// thumbs, and to help position the slider thumbs and tick marks relative to
/// the track.
///
/// The `parentBox` argument can be used to help determine the preferredRect
/// relative to attributes of the render box of the slider itself, such as
/// size.
///
/// The `offset` argument is relative to the caller's bounding box. It can be
/// used to convert gesture coordinates from global to slider-relative
/// coordinates.
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.sliderTheme}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
Rect getPreferredRect({
required RenderBox parentBox,
Offset offset = Offset.zero,
required SliderThemeData sliderTheme,
bool isEnabled,
bool isDiscrete,
});
/// Paints the track shape based on the state passed to it.
///
/// {@macro flutter.material.SliderComponentShape.paint.context}
///
/// The `offset` argument is the offset of the origin of the `parentBox` to
/// the origin of its `context` canvas. This shape must be painted relative
/// to this offset. See [PaintingContextCallback].
///
/// {@macro flutter.material.RangeSliderValueIndicatorShape.paint.parentBox}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.sliderTheme}
///
/// {@macro flutter.material.RangeSliderThumbShape.paint.enableAnimation}
///
/// The `startThumbCenter` argument is the offset of the center of the start
/// thumb relative to the origin of the [PaintingContext.canvas]. It can be
/// used as one point that divides the track between inactive and active.
///
/// The `endThumbCenter` argument is the offset of the center of the end
/// thumb relative to the origin of the [PaintingContext.canvas]. It can be
/// used as one point that divides the track between inactive and active.
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isEnabled}
///
/// {@macro flutter.material.RangeSliderThumbShape.getPreferredSize.isDiscrete}
///
/// The `textDirection` argument can be used to determine how the track
/// segments are painted depending on whether they are on an active track
/// segment or not.
///
/// {@macro flutter.material.RangeSliderTickMarkShape.paint.trackSegment}
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset startThumbCenter,
required Offset endThumbCenter,
bool isEnabled = false,
bool isDiscrete = false,
required TextDirection textDirection,
});
}
/// Base track shape that provides an implementation of [getPreferredRect] for
/// default sizing.
///
/// The height is set from [SliderThemeData.trackHeight] and the width of the
/// parent box less the larger of the widths of [SliderThemeData.thumbShape] and
/// [SliderThemeData.overlayShape].
///
/// See also:
///
/// * [RectangularSliderTrackShape], which is a track shape with sharp
/// rectangular edges
/// * [RoundedRectSliderTrackShape], which is a track shape with round
/// stadium-like edges.
mixin BaseSliderTrackShape {
/// Returns a rect that represents the track bounds that fits within the
/// [Slider].
///
/// The width is the width of the [Slider] or [RangeSlider], but padded by
/// the max of the overlay and thumb radius. The height is defined by the
/// [SliderThemeData.trackHeight].
///
/// The [Rect] is centered both horizontally and vertically within the slider
/// bounds.
Rect getPreferredRect({
required RenderBox parentBox,
Offset offset = Offset.zero,
required SliderThemeData sliderTheme,
bool isEnabled = false,
bool isDiscrete = false,
}) {
final double thumbWidth = sliderTheme.thumbShape!.getPreferredSize(isEnabled, isDiscrete).width;
final double overlayWidth = sliderTheme.overlayShape!.getPreferredSize(isEnabled, isDiscrete).width;
final double trackHeight = sliderTheme.trackHeight!;
assert(overlayWidth >= 0);
assert(trackHeight >= 0);
final double trackLeft = offset.dx + math.max(overlayWidth / 2, thumbWidth / 2);
final double trackTop = offset.dy + (parentBox.size.height - trackHeight) / 2;
final double trackRight = trackLeft + parentBox.size.width - math.max(thumbWidth, overlayWidth);
final double trackBottom = trackTop + trackHeight;
// If the parentBox's size less than slider's size the trackRight will be less than trackLeft, so switch them.
return Rect.fromLTRB(math.min(trackLeft, trackRight), trackTop, math.max(trackLeft, trackRight), trackBottom);
}
}
/// A [Slider] track that's a simple rectangle.
///
/// It paints a solid colored rectangle, vertically centered in the
/// `parentBox`. The track rectangle extends to the bounds of the `parentBox`,
/// but is padded by the [RoundSliderOverlayShape] radius. The height is defined
/// by the [SliderThemeData.trackHeight]. The color is determined by the
/// [Slider]'s enabled state and the track segment's active state which are
/// defined by:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// {@macro flutter.material.SliderTrackShape.paint.trackSegment}
///
/// ![A slider widget, consisting of 5 divisions and showing the rectangular slider track shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_slider_track_shape.png)
///
/// See also:
///
/// * [Slider], for the component that is meant to display this shape.
/// * [SliderThemeData], where an instance of this class is set to inform the
/// slider of the visual details of the its track.
/// * [SliderTrackShape], which can be used to create custom shapes for the
/// [Slider]'s track.
/// * [RoundedRectSliderTrackShape], for a similar track with rounded edges.
class RectangularSliderTrackShape extends SliderTrackShape with BaseSliderTrackShape {
/// Creates a slider track that draws 2 rectangles.
const RectangularSliderTrackShape();
@override
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required TextDirection textDirection,
required Offset thumbCenter,
Offset? secondaryOffset,
bool isDiscrete = false,
bool isEnabled = false,
}) {
assert(sliderTheme.disabledActiveTrackColor != null);
assert(sliderTheme.disabledInactiveTrackColor != null);
assert(sliderTheme.activeTrackColor != null);
assert(sliderTheme.inactiveTrackColor != null);
assert(sliderTheme.thumbShape != null);
// If the slider [SliderThemeData.trackHeight] is less than or equal to 0,
// then it makes no difference whether the track is painted or not,
// therefore the painting can be a no-op.
if (sliderTheme.trackHeight! <= 0) {
return;
}
// Assign the track segment paints, which are left: active, right: inactive,
// but reversed for right to left text.
final ColorTween activeTrackColorTween = ColorTween(begin: sliderTheme.disabledActiveTrackColor, end: sliderTheme.activeTrackColor);
final ColorTween inactiveTrackColorTween = ColorTween(begin: sliderTheme.disabledInactiveTrackColor, end: sliderTheme.inactiveTrackColor);
final Paint activePaint = Paint()..color = activeTrackColorTween.evaluate(enableAnimation)!;
final Paint inactivePaint = Paint()..color = inactiveTrackColorTween.evaluate(enableAnimation)!;
final (Paint leftTrackPaint, Paint rightTrackPaint) = switch (textDirection) {
TextDirection.ltr => (activePaint, inactivePaint),
TextDirection.rtl => (inactivePaint, activePaint),
};
final Rect trackRect = getPreferredRect(
parentBox: parentBox,
offset: offset,
sliderTheme: sliderTheme,
isEnabled: isEnabled,
isDiscrete: isDiscrete,
);
final Rect leftTrackSegment = Rect.fromLTRB(trackRect.left, trackRect.top, thumbCenter.dx, trackRect.bottom);
if (!leftTrackSegment.isEmpty) {
context.canvas.drawRect(leftTrackSegment, leftTrackPaint);
}
final Rect rightTrackSegment = Rect.fromLTRB(thumbCenter.dx, trackRect.top, trackRect.right, trackRect.bottom);
if (!rightTrackSegment.isEmpty) {
context.canvas.drawRect(rightTrackSegment, rightTrackPaint);
}
final bool showSecondaryTrack = (secondaryOffset != null) &&
((textDirection == TextDirection.ltr)
? (secondaryOffset.dx > thumbCenter.dx)
: (secondaryOffset.dx < thumbCenter.dx));
if (showSecondaryTrack) {
final ColorTween secondaryTrackColorTween = ColorTween(begin: sliderTheme.disabledSecondaryActiveTrackColor, end: sliderTheme.secondaryActiveTrackColor);
final Paint secondaryTrackPaint = Paint()..color = secondaryTrackColorTween.evaluate(enableAnimation)!;
final Rect secondaryTrackSegment = Rect.fromLTRB(
(textDirection == TextDirection.ltr) ? thumbCenter.dx : secondaryOffset.dx,
trackRect.top,
(textDirection == TextDirection.ltr) ? secondaryOffset.dx : thumbCenter.dx,
trackRect.bottom,
);
if (!secondaryTrackSegment.isEmpty) {
context.canvas.drawRect(secondaryTrackSegment, secondaryTrackPaint);
}
}
}
}
/// The default shape of a [Slider]'s track.
///
/// It paints a solid colored rectangle with rounded edges, vertically centered
/// in the `parentBox`. The track rectangle extends to the bounds of the
/// `parentBox`, but is padded by the larger of [RoundSliderOverlayShape]'s
/// radius and [RoundSliderThumbShape]'s radius. The height is defined by the
/// [SliderThemeData.trackHeight]. The color is determined by the [Slider]'s
/// enabled state and the track segment's active state which are defined by:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// {@macro flutter.material.SliderTrackShape.paint.trackSegment}
///
/// ![A slider widget, consisting of 5 divisions and showing the rounded rect slider track shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_rect_slider_track_shape.png)
///
/// See also:
///
/// * [Slider], for the component that is meant to display this shape.
/// * [SliderThemeData], where an instance of this class is set to inform the
/// slider of the visual details of the its track.
/// * [SliderTrackShape], which can be used to create custom shapes for the
/// [Slider]'s track.
/// * [RectangularSliderTrackShape], for a similar track with sharp edges.
class RoundedRectSliderTrackShape extends SliderTrackShape with BaseSliderTrackShape {
/// Create a slider track that draws two rectangles with rounded outer edges.
const RoundedRectSliderTrackShape();
@override
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required TextDirection textDirection,
required Offset thumbCenter,
Offset? secondaryOffset,
bool isDiscrete = false,
bool isEnabled = false,
double additionalActiveTrackHeight = 2,
}) {
assert(sliderTheme.disabledActiveTrackColor != null);
assert(sliderTheme.disabledInactiveTrackColor != null);
assert(sliderTheme.activeTrackColor != null);
assert(sliderTheme.inactiveTrackColor != null);
assert(sliderTheme.thumbShape != null);
// If the slider [SliderThemeData.trackHeight] is less than or equal to 0,
// then it makes no difference whether the track is painted or not,
// therefore the painting can be a no-op.
if (sliderTheme.trackHeight == null || sliderTheme.trackHeight! <= 0) {
return;
}
// Assign the track segment paints, which are leading: active and
// trailing: inactive.
final ColorTween activeTrackColorTween = ColorTween(begin: sliderTheme.disabledActiveTrackColor, end: sliderTheme.activeTrackColor);
final ColorTween inactiveTrackColorTween = ColorTween(begin: sliderTheme.disabledInactiveTrackColor, end: sliderTheme.inactiveTrackColor);
final Paint activePaint = Paint()..color = activeTrackColorTween.evaluate(enableAnimation)!;
final Paint inactivePaint = Paint()..color = inactiveTrackColorTween.evaluate(enableAnimation)!;
final (Paint leftTrackPaint, Paint rightTrackPaint) = switch (textDirection) {
TextDirection.ltr => (activePaint, inactivePaint),
TextDirection.rtl => (inactivePaint, activePaint),
};
final Rect trackRect = getPreferredRect(
parentBox: parentBox,
offset: offset,
sliderTheme: sliderTheme,
isEnabled: isEnabled,
isDiscrete: isDiscrete,
);
final Radius trackRadius = Radius.circular(trackRect.height / 2);
final Radius activeTrackRadius = Radius.circular((trackRect.height + additionalActiveTrackHeight) / 2);
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
trackRect.left,
(textDirection == TextDirection.ltr) ? trackRect.top - (additionalActiveTrackHeight / 2): trackRect.top,
thumbCenter.dx,
(textDirection == TextDirection.ltr) ? trackRect.bottom + (additionalActiveTrackHeight / 2) : trackRect.bottom,
topLeft: (textDirection == TextDirection.ltr) ? activeTrackRadius : trackRadius,
bottomLeft: (textDirection == TextDirection.ltr) ? activeTrackRadius: trackRadius,
),
leftTrackPaint,
);
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
thumbCenter.dx,
(textDirection == TextDirection.rtl) ? trackRect.top - (additionalActiveTrackHeight / 2) : trackRect.top,
trackRect.right,
(textDirection == TextDirection.rtl) ? trackRect.bottom + (additionalActiveTrackHeight / 2) : trackRect.bottom,
topRight: (textDirection == TextDirection.rtl) ? activeTrackRadius : trackRadius,
bottomRight: (textDirection == TextDirection.rtl) ? activeTrackRadius : trackRadius,
),
rightTrackPaint,
);
final bool showSecondaryTrack = (secondaryOffset != null) &&
((textDirection == TextDirection.ltr)
? (secondaryOffset.dx > thumbCenter.dx)
: (secondaryOffset.dx < thumbCenter.dx));
if (showSecondaryTrack) {
final ColorTween secondaryTrackColorTween = ColorTween(begin: sliderTheme.disabledSecondaryActiveTrackColor, end: sliderTheme.secondaryActiveTrackColor);
final Paint secondaryTrackPaint = Paint()..color = secondaryTrackColorTween.evaluate(enableAnimation)!;
if (textDirection == TextDirection.ltr) {
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
thumbCenter.dx,
trackRect.top,
secondaryOffset.dx,
trackRect.bottom,
topRight: trackRadius,
bottomRight: trackRadius,
),
secondaryTrackPaint,
);
} else {
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
secondaryOffset.dx,
trackRect.top,
thumbCenter.dx,
trackRect.bottom,
topLeft: trackRadius,
bottomLeft: trackRadius,
),
secondaryTrackPaint,
);
}
}
}
}
/// Base range slider track shape that provides an implementation of [getPreferredRect] for
/// default sizing.
///
/// The height is set from [SliderThemeData.trackHeight] and the width of the
/// parent box less the larger of the widths of [SliderThemeData.rangeThumbShape] and
/// [SliderThemeData.overlayShape].
///
/// See also:
///
/// * [RectangularRangeSliderTrackShape], which is a track shape with sharp
/// rectangular edges
mixin BaseRangeSliderTrackShape {
/// Returns a rect that represents the track bounds that fits within the
/// [Slider].
///
/// The width is the width of the [RangeSlider], but padded by the max
/// of the overlay and thumb radius. The height is defined by the [SliderThemeData.trackHeight].
///
/// The [Rect] is centered both horizontally and vertically within the slider
/// bounds.
Rect getPreferredRect({
required RenderBox parentBox,
Offset offset = Offset.zero,
required SliderThemeData sliderTheme,
bool isEnabled = false,
bool isDiscrete = false,
}) {
assert(sliderTheme.rangeThumbShape != null);
assert(sliderTheme.overlayShape != null);
assert(sliderTheme.trackHeight != null);
final double thumbWidth = sliderTheme.rangeThumbShape!.getPreferredSize(isEnabled, isDiscrete).width;
final double overlayWidth = sliderTheme.overlayShape!.getPreferredSize(isEnabled, isDiscrete).width;
final double trackHeight = sliderTheme.trackHeight!;
assert(overlayWidth >= 0);
assert(trackHeight >= 0);
final double trackLeft = offset.dx + math.max(overlayWidth / 2, thumbWidth / 2);
final double trackTop = offset.dy + (parentBox.size.height - trackHeight) / 2;
final double trackRight = trackLeft + parentBox.size.width - math.max(thumbWidth, overlayWidth);
final double trackBottom = trackTop + trackHeight;
// If the parentBox's size less than slider's size the trackRight will be less than trackLeft, so switch them.
return Rect.fromLTRB(math.min(trackLeft, trackRight), trackTop, math.max(trackLeft, trackRight), trackBottom);
}
}
/// A [RangeSlider] track that's a simple rectangle.
///
/// It paints a solid colored rectangle, vertically centered in the
/// `parentBox`. The track rectangle extends to the bounds of the `parentBox`,
/// but is padded by the [RoundSliderOverlayShape] radius. The height is
/// defined by the [SliderThemeData.trackHeight]. The color is determined by the
/// [Slider]'s enabled state and the track segment's active state which are
/// defined by:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// {@macro flutter.material.RangeSliderTickMarkShape.paint.trackSegment}
///
/// ![A range slider widget, consisting of 5 divisions and showing the rectangular range slider track shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_range_slider_track_shape.png)
///
/// See also:
///
/// * [RangeSlider], for the component that is meant to display this shape.
/// * [SliderThemeData], where an instance of this class is set to inform the
/// slider of the visual details of the its track.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RoundedRectRangeSliderTrackShape], for a similar track with rounded
/// edges.
class RectangularRangeSliderTrackShape extends RangeSliderTrackShape with BaseRangeSliderTrackShape {
/// Create a slider track with rectangular outer edges.
///
/// The middle track segment is the selected range and is active, and the two
/// outer track segments are inactive.
const RectangularRangeSliderTrackShape();
@override
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double>? enableAnimation,
required Offset startThumbCenter,
required Offset endThumbCenter,
bool isEnabled = false,
bool isDiscrete = false,
required TextDirection textDirection,
}) {
assert(sliderTheme.disabledActiveTrackColor != null);
assert(sliderTheme.disabledInactiveTrackColor != null);
assert(sliderTheme.activeTrackColor != null);
assert(sliderTheme.inactiveTrackColor != null);
assert(sliderTheme.rangeThumbShape != null);
assert(enableAnimation != null);
// Assign the track segment paints, which are left: active, right: inactive,
// but reversed for right to left text.
final ColorTween activeTrackColorTween = ColorTween(begin: sliderTheme.disabledActiveTrackColor, end: sliderTheme.activeTrackColor);
final ColorTween inactiveTrackColorTween = ColorTween(begin: sliderTheme.disabledInactiveTrackColor, end: sliderTheme.inactiveTrackColor);
final Paint activePaint = Paint()..color = activeTrackColorTween.evaluate(enableAnimation!)!;
final Paint inactivePaint = Paint()..color = inactiveTrackColorTween.evaluate(enableAnimation)!;
final (Offset leftThumbOffset, Offset rightThumbOffset) = switch (textDirection) {
TextDirection.ltr => (startThumbCenter, endThumbCenter),
TextDirection.rtl => (endThumbCenter, startThumbCenter),
};
final Rect trackRect = getPreferredRect(
parentBox: parentBox,
offset: offset,
sliderTheme: sliderTheme,
isEnabled: isEnabled,
isDiscrete: isDiscrete,
);
final Rect leftTrackSegment = Rect.fromLTRB(trackRect.left, trackRect.top, leftThumbOffset.dx, trackRect.bottom);
if (!leftTrackSegment.isEmpty) {
context.canvas.drawRect(leftTrackSegment, inactivePaint);
}
final Rect middleTrackSegment = Rect.fromLTRB(leftThumbOffset.dx, trackRect.top, rightThumbOffset.dx, trackRect.bottom);
if (!middleTrackSegment.isEmpty) {
context.canvas.drawRect(middleTrackSegment, activePaint);
}
final Rect rightTrackSegment = Rect.fromLTRB(rightThumbOffset.dx, trackRect.top, trackRect.right, trackRect.bottom);
if (!rightTrackSegment.isEmpty) {
context.canvas.drawRect(rightTrackSegment, inactivePaint);
}
}
}
/// The default shape of a [RangeSlider]'s track.
///
/// It paints a solid colored rectangle with rounded edges, vertically centered
/// in the `parentBox`. The track rectangle extends to the bounds of the
/// `parentBox`, but is padded by the larger of [RoundSliderOverlayShape]'s
/// radius and [RoundRangeSliderThumbShape]'s radius. The height is defined by
/// the [SliderThemeData.trackHeight]. The color is determined by the
/// [RangeSlider]'s enabled state and the track segment's active state which are
/// defined by:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// {@macro flutter.material.RangeSliderTickMarkShape.paint.trackSegment}
///
/// ![A range slider widget, consisting of 5 divisions and showing the rounded rect range slider track shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_rect_range_slider_track_shape.png)
///
/// See also:
///
/// * [RangeSlider], for the component that is meant to display this shape.
/// * [SliderThemeData], where an instance of this class is set to inform the
/// slider of the visual details of the its track.
/// * [RangeSliderTrackShape], which can be used to create custom shapes for
/// the [RangeSlider]'s track.
/// * [RectangularRangeSliderTrackShape], for a similar track with sharp edges.
class RoundedRectRangeSliderTrackShape extends RangeSliderTrackShape with BaseRangeSliderTrackShape {
/// Create a slider track with rounded outer edges.
///
/// The middle track segment is the selected range and is active, and the two
/// outer track segments are inactive.
const RoundedRectRangeSliderTrackShape();
@override
void paint(
PaintingContext context,
Offset offset, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset startThumbCenter,
required Offset endThumbCenter,
bool isEnabled = false,
bool isDiscrete = false,
required TextDirection textDirection,
double additionalActiveTrackHeight = 2,
}) {
assert(sliderTheme.disabledActiveTrackColor != null);
assert(sliderTheme.disabledInactiveTrackColor != null);
assert(sliderTheme.activeTrackColor != null);
assert(sliderTheme.inactiveTrackColor != null);
assert(sliderTheme.rangeThumbShape != null);
if (sliderTheme.trackHeight == null || sliderTheme.trackHeight! <= 0) {
return;
}
// Assign the track segment paints, which are left: active, right: inactive,
// but reversed for right to left text.
final ColorTween activeTrackColorTween = ColorTween(
begin: sliderTheme.disabledActiveTrackColor,
end: sliderTheme.activeTrackColor,
);
final ColorTween inactiveTrackColorTween = ColorTween(
begin: sliderTheme.disabledInactiveTrackColor,
end: sliderTheme.inactiveTrackColor,
);
final Paint activePaint = Paint()
..color = activeTrackColorTween.evaluate(enableAnimation)!;
final Paint inactivePaint = Paint()
..color = inactiveTrackColorTween.evaluate(enableAnimation)!;
final (Offset leftThumbOffset, Offset rightThumbOffset) = switch (textDirection) {
TextDirection.ltr => (startThumbCenter, endThumbCenter),
TextDirection.rtl => (endThumbCenter, startThumbCenter),
};
final Size thumbSize = sliderTheme.rangeThumbShape!.getPreferredSize(isEnabled, isDiscrete);
final double thumbRadius = thumbSize.width / 2;
assert(thumbRadius > 0);
final Rect trackRect = getPreferredRect(
parentBox: parentBox,
offset: offset,
sliderTheme: sliderTheme,
isEnabled: isEnabled,
isDiscrete: isDiscrete,
);
final Radius trackRadius = Radius.circular(trackRect.height / 2);
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
trackRect.left,
trackRect.top,
leftThumbOffset.dx,
trackRect.bottom,
topLeft: trackRadius,
bottomLeft: trackRadius,
),
inactivePaint,
);
context.canvas.drawRect(
Rect.fromLTRB(
leftThumbOffset.dx,
trackRect.top - (additionalActiveTrackHeight / 2),
rightThumbOffset.dx,
trackRect.bottom + (additionalActiveTrackHeight / 2),
),
activePaint,
);
context.canvas.drawRRect(
RRect.fromLTRBAndCorners(
rightThumbOffset.dx,
trackRect.top,
trackRect.right,
trackRect.bottom,
topRight: trackRadius,
bottomRight: trackRadius,
),
inactivePaint,
);
}
}
/// The default shape of each [Slider] tick mark.
///
/// Tick marks are only displayed if the slider is discrete, which can be done
/// by setting the [Slider.divisions] to an integer value.
///
/// It paints a solid circle, centered in the on the track.
/// The color is determined by the [Slider]'s enabled state and track's active
/// states. These colors are defined in:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// ![A slider widget, consisting of 5 divisions and showing the round slider tick mark shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_slider_tick_mark_shape.png)
///
/// See also:
///
/// * [Slider], which includes tick marks defined by this shape.
/// * [SliderTheme], which can be used to configure the tick mark shape of all
/// sliders in a widget subtree.
class RoundSliderTickMarkShape extends SliderTickMarkShape {
/// Create a slider tick mark that draws a circle.
const RoundSliderTickMarkShape({
this.tickMarkRadius,
});
/// The preferred radius of the round tick mark.
///
/// If it is not provided, then 1/4 of the [SliderThemeData.trackHeight] is used.
final double? tickMarkRadius;
@override
Size getPreferredSize({
required SliderThemeData sliderTheme,
required bool isEnabled,
}) {
assert(sliderTheme.trackHeight != null);
// The tick marks are tiny circles. If no radius is provided, then the
// radius is defaulted to be a fraction of the
// [SliderThemeData.trackHeight]. The fraction is 1/4.
return Size.fromRadius(tickMarkRadius ?? sliderTheme.trackHeight! / 4);
}
@override
void paint(
PaintingContext context,
Offset center, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required TextDirection textDirection,
required Offset thumbCenter,
required bool isEnabled,
}) {
assert(sliderTheme.disabledActiveTickMarkColor != null);
assert(sliderTheme.disabledInactiveTickMarkColor != null);
assert(sliderTheme.activeTickMarkColor != null);
assert(sliderTheme.inactiveTickMarkColor != null);
// The paint color of the tick mark depends on its position relative
// to the thumb and the text direction.
final double xOffset = center.dx - thumbCenter.dx;
final (Color? begin, Color? end) = switch (textDirection) {
TextDirection.ltr when xOffset > 0 => (sliderTheme.disabledInactiveTickMarkColor, sliderTheme.inactiveTickMarkColor),
TextDirection.rtl when xOffset < 0 => (sliderTheme.disabledInactiveTickMarkColor, sliderTheme.inactiveTickMarkColor),
TextDirection.ltr || TextDirection.rtl => (sliderTheme.disabledActiveTickMarkColor, sliderTheme.activeTickMarkColor),
};
final Paint paint = Paint()..color = ColorTween(begin: begin, end: end).evaluate(enableAnimation)!;
// The tick marks are tiny circles that are the same height as the track.
final double tickMarkRadius = getPreferredSize(
isEnabled: isEnabled,
sliderTheme: sliderTheme,
).width / 2;
if (tickMarkRadius > 0) {
context.canvas.drawCircle(center, tickMarkRadius, paint);
}
}
}
/// The default shape of each [RangeSlider] tick mark.
///
/// Tick marks are only displayed if the slider is discrete, which can be done
/// by setting the [RangeSlider.divisions] to an integer value.
///
/// It paints a solid circle, centered on the track.
/// The color is determined by the [Slider]'s enabled state and track's active
/// states. These colors are defined in:
/// [SliderThemeData.activeTrackColor],
/// [SliderThemeData.inactiveTrackColor],
/// [SliderThemeData.disabledActiveTrackColor],
/// [SliderThemeData.disabledInactiveTrackColor].
///
/// ![A slider widget, consisting of 5 divisions and showing the round range slider tick mark shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_range_slider_tick_mark_shape.png )
///
/// See also:
///
/// * [RangeSlider], which includes tick marks defined by this shape.
/// * [SliderTheme], which can be used to configure the tick mark shape of all
/// sliders in a widget subtree.
class RoundRangeSliderTickMarkShape extends RangeSliderTickMarkShape {
/// Create a range slider tick mark that draws a circle.
const RoundRangeSliderTickMarkShape({
this.tickMarkRadius,
});
/// The preferred radius of the round tick mark.
///
/// If it is not provided, then 1/4 of the [SliderThemeData.trackHeight] is used.
final double? tickMarkRadius;
@override
Size getPreferredSize({
required SliderThemeData sliderTheme,
bool isEnabled = false,
}) {
assert(sliderTheme.trackHeight != null);
return Size.fromRadius(tickMarkRadius ?? sliderTheme.trackHeight! / 4);
}
@override
void paint(
PaintingContext context,
Offset center, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset startThumbCenter,
required Offset endThumbCenter,
bool isEnabled = false,
required TextDirection textDirection,
}) {
assert(sliderTheme.disabledActiveTickMarkColor != null);
assert(sliderTheme.disabledInactiveTickMarkColor != null);
assert(sliderTheme.activeTickMarkColor != null);
assert(sliderTheme.inactiveTickMarkColor != null);
final bool isBetweenThumbs = switch (textDirection) {
TextDirection.ltr => startThumbCenter.dx < center.dx && center.dx < endThumbCenter.dx,
TextDirection.rtl => endThumbCenter.dx < center.dx && center.dx < startThumbCenter.dx,
};
final Color? begin = isBetweenThumbs ? sliderTheme.disabledActiveTickMarkColor : sliderTheme.disabledInactiveTickMarkColor;
final Color? end = isBetweenThumbs ? sliderTheme.activeTickMarkColor : sliderTheme.inactiveTickMarkColor;
final Paint paint = Paint()..color = ColorTween(begin: begin, end: end).evaluate(enableAnimation)!;
// The tick marks are tiny circles that are the same height as the track.
final double tickMarkRadius = getPreferredSize(
isEnabled: isEnabled,
sliderTheme: sliderTheme,
).width / 2;
if (tickMarkRadius > 0) {
context.canvas.drawCircle(center, tickMarkRadius, paint);
}
}
}
/// A special version of [SliderTickMarkShape] that has a zero size and paints
/// nothing.
///
/// This class is used to create a special instance of a [SliderTickMarkShape]
/// that will not paint any tick mark shape. A static reference is stored in
/// [SliderTickMarkShape.noTickMark]. When this value is specified for
/// [SliderThemeData.tickMarkShape], the tick mark painting is skipped.
class _EmptySliderTickMarkShape extends SliderTickMarkShape {
@override
Size getPreferredSize({
required SliderThemeData sliderTheme,
required bool isEnabled,
}) {
return Size.zero;
}
@override
void paint(
PaintingContext context,
Offset center, {
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required Animation<double> enableAnimation,
required Offset thumbCenter,
required bool isEnabled,
required TextDirection textDirection,
}) {
// no-op.
}
}
/// A special version of [SliderComponentShape] that has a zero size and paints
/// nothing.
///
/// This class is used to create a special instance of a [SliderComponentShape]
/// that will not paint any component shape. A static reference is stored in
/// [SliderTickMarkShape.noThumb] and [SliderTickMarkShape.noOverlay]. When this value
/// is specified for [SliderThemeData.thumbShape], the thumb painting is
/// skipped. When this value is specified for [SliderThemeData.overlayShape],
/// the overlay painting is skipped.
class _EmptySliderComponentShape extends SliderComponentShape {
@override
Size getPreferredSize(bool isEnabled, bool isDiscrete) => Size.zero;
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
// no-op.
}
}
/// The default shape of a [Slider]'s thumb.
///
/// There is a shadow for the resting, pressed, hovered, and focused state.
///
/// ![A slider widget, consisting of 5 divisions and showing the round slider thumb shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_slider_thumb_shape.png)
///
/// See also:
///
/// * [Slider], which includes a thumb defined by this shape.
/// * [SliderTheme], which can be used to configure the thumb shape of all
/// sliders in a widget subtree.
class RoundSliderThumbShape extends SliderComponentShape {
/// Create a slider thumb that draws a circle.
const RoundSliderThumbShape({
this.enabledThumbRadius = 10.0,
this.disabledThumbRadius,
this.elevation = 1.0,
this.pressedElevation = 6.0,
});
/// The preferred radius of the round thumb shape when the slider is enabled.
///
/// If it is not provided, then the Material Design default of 10 is used.
final double enabledThumbRadius;
/// The preferred radius of the round thumb shape when the slider is disabled.
///
/// If no disabledRadius is provided, then it is equal to the
/// [enabledThumbRadius]
final double? disabledThumbRadius;
double get _disabledThumbRadius => disabledThumbRadius ?? enabledThumbRadius;
/// The resting elevation adds shadow to the unpressed thumb.
///
/// The default is 1.
///
/// Use 0 for no shadow. The higher the value, the larger the shadow. For
/// example, a value of 12 will create a very large shadow.
///
final double elevation;
/// The pressed elevation adds shadow to the pressed thumb.
///
/// The default is 6.
///
/// Use 0 for no shadow. The higher the value, the larger the shadow. For
/// example, a value of 12 will create a very large shadow.
final double pressedElevation;
@override
Size getPreferredSize(bool isEnabled, bool isDiscrete) {
return Size.fromRadius(isEnabled ? enabledThumbRadius : _disabledThumbRadius);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
assert(sliderTheme.disabledThumbColor != null);
assert(sliderTheme.thumbColor != null);
final Canvas canvas = context.canvas;
final Tween<double> radiusTween = Tween<double>(
begin: _disabledThumbRadius,
end: enabledThumbRadius,
);
final ColorTween colorTween = ColorTween(
begin: sliderTheme.disabledThumbColor,
end: sliderTheme.thumbColor,
);
final Color color = colorTween.evaluate(enableAnimation)!;
final double radius = radiusTween.evaluate(enableAnimation);
final Tween<double> elevationTween = Tween<double>(
begin: elevation,
end: pressedElevation,
);
final double evaluatedElevation = elevationTween.evaluate(activationAnimation);
final Path path = Path()
..addArc(Rect.fromCenter(center: center, width: 2 * radius, height: 2 * radius), 0, math.pi * 2);
bool paintShadows = true;
assert(() {
if (debugDisableShadows) {
_debugDrawShadow(canvas, path, evaluatedElevation);
paintShadows = false;
}
return true;
}());
if (paintShadows) {
canvas.drawShadow(path, Colors.black, evaluatedElevation, true);
}
canvas.drawCircle(
center,
radius,
Paint()..color = color,
);
}
}
/// The default shape of a [RangeSlider]'s thumbs.
///
/// There is a shadow for the resting and pressed state.
///
/// ![A slider widget, consisting of 5 divisions and showing the round range slider thumb shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_range_slider_thumb_shape.png)
///
/// See also:
///
/// * [RangeSlider], which includes thumbs defined by this shape.
/// * [SliderTheme], which can be used to configure the thumb shapes of all
/// range sliders in a widget subtree.
class RoundRangeSliderThumbShape extends RangeSliderThumbShape {
/// Create a slider thumb that draws a circle.
const RoundRangeSliderThumbShape({
this.enabledThumbRadius = 10.0,
this.disabledThumbRadius,
this.elevation = 1.0,
this.pressedElevation = 6.0,
});
/// The preferred radius of the round thumb shape when the slider is enabled.
///
/// If it is not provided, then the Material Design default of 10 is used.
final double enabledThumbRadius;
/// The preferred radius of the round thumb shape when the slider is disabled.
///
/// If no disabledRadius is provided, then it is equal to the
/// [enabledThumbRadius].
final double? disabledThumbRadius;
double get _disabledThumbRadius => disabledThumbRadius ?? enabledThumbRadius;
/// The resting elevation adds shadow to the unpressed thumb.
///
/// The default is 1.
final double elevation;
/// The pressed elevation adds shadow to the pressed thumb.
///
/// The default is 6.
final double pressedElevation;
@override
Size getPreferredSize(bool isEnabled, bool isDiscrete) {
return Size.fromRadius(isEnabled ? enabledThumbRadius : _disabledThumbRadius);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
bool isDiscrete = false,
bool isEnabled = false,
bool? isOnTop,
required SliderThemeData sliderTheme,
TextDirection? textDirection,
Thumb? thumb,
bool? isPressed,
}) {
assert(sliderTheme.showValueIndicator != null);
assert(sliderTheme.overlappingShapeStrokeColor != null);
final Canvas canvas = context.canvas;
final Tween<double> radiusTween = Tween<double>(
begin: _disabledThumbRadius,
end: enabledThumbRadius,
);
final ColorTween colorTween = ColorTween(
begin: sliderTheme.disabledThumbColor,
end: sliderTheme.thumbColor,
);
final double radius = radiusTween.evaluate(enableAnimation);
final Tween<double> elevationTween = Tween<double>(
begin: elevation,
end: pressedElevation,
);
// Add a stroke of 1dp around the circle if this thumb would overlap
// the other thumb.
if (isOnTop ?? false) {
final Paint strokePaint = Paint()
..color = sliderTheme.overlappingShapeStrokeColor!
..strokeWidth = 1.0
..style = PaintingStyle.stroke;
canvas.drawCircle(center, radius, strokePaint);
}
final Color color = colorTween.evaluate(enableAnimation)!;
final double evaluatedElevation = isPressed! ? elevationTween.evaluate(activationAnimation) : elevation;
final Path shadowPath = Path()
..addArc(Rect.fromCenter(center: center, width: 2 * radius, height: 2 * radius), 0, math.pi * 2);
bool paintShadows = true;
assert(() {
if (debugDisableShadows) {
_debugDrawShadow(canvas, shadowPath, evaluatedElevation);
paintShadows = false;
}
return true;
}());
if (paintShadows) {
canvas.drawShadow(shadowPath, Colors.black, evaluatedElevation, true);
}
canvas.drawCircle(
center,
radius,
Paint()..color = color,
);
}
}
/// The default shape of a [Slider]'s thumb overlay.
///
/// The shape of the overlay is a circle with the same center as the thumb, but
/// with a larger radius. It animates to full size when the thumb is pressed,
/// and animates back down to size 0 when it is released. It is painted behind
/// the thumb, and is expected to extend beyond the bounds of the thumb so that
/// it is visible.
///
/// The overlay color is defined by [SliderThemeData.overlayColor].
///
/// See also:
///
/// * [Slider], which includes an overlay defined by this shape.
/// * [SliderTheme], which can be used to configure the overlay shape of all
/// sliders in a widget subtree.
class RoundSliderOverlayShape extends SliderComponentShape {
/// Create a slider thumb overlay that draws a circle.
const RoundSliderOverlayShape({ this.overlayRadius = 24.0 });
/// The preferred radius of the round thumb shape when enabled.
///
/// If it is not provided, then half of the [SliderThemeData.trackHeight] is
/// used.
final double overlayRadius;
@override
Size getPreferredSize(bool isEnabled, bool isDiscrete) {
return Size.fromRadius(overlayRadius);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
final Canvas canvas = context.canvas;
final Tween<double> radiusTween = Tween<double>(
begin: 0.0,
end: overlayRadius,
);
canvas.drawCircle(
center,
radiusTween.evaluate(activationAnimation),
Paint()..color = sliderTheme.overlayColor!,
);
}
}
/// The default shape of a [Slider]'s value indicator.
///
/// ![A slider widget, consisting of 5 divisions and showing the rectangular slider value indicator shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_slider_value_indicator_shape.png)
///
/// See also:
///
/// * [Slider], which includes a value indicator defined by this shape.
/// * [SliderTheme], which can be used to configure the slider value indicator
/// of all sliders in a widget subtree.
class RectangularSliderValueIndicatorShape extends SliderComponentShape {
/// Create a slider value indicator that resembles a rectangular tooltip.
const RectangularSliderValueIndicatorShape();
static const _RectangularSliderValueIndicatorPathPainter _pathPainter = _RectangularSliderValueIndicatorPathPainter();
@override
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
TextPainter? labelPainter,
double? textScaleFactor,
}) {
assert(labelPainter != null);
assert(textScaleFactor != null && textScaleFactor >= 0);
return _pathPainter.getPreferredSize(labelPainter!, textScaleFactor!);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
final Canvas canvas = context.canvas;
final double scale = activationAnimation.value;
_pathPainter.paint(
parentBox: parentBox,
canvas: canvas,
center: center,
scale: scale,
labelPainter: labelPainter,
textScaleFactor: textScaleFactor,
sizeWithOverflow: sizeWithOverflow,
backgroundPaintColor: sliderTheme.valueIndicatorColor!,
strokePaintColor: sliderTheme.valueIndicatorStrokeColor,
);
}
}
/// The default shape of a [RangeSlider]'s value indicators.
///
/// ![A slider widget, consisting of 5 divisions and showing the rectangular range slider value indicator shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_range_slider_value_indicator_shape.png)
///
/// See also:
///
/// * [RangeSlider], which includes value indicators defined by this shape.
/// * [SliderTheme], which can be used to configure the range slider value
/// indicator of all sliders in a widget subtree.
class RectangularRangeSliderValueIndicatorShape
extends RangeSliderValueIndicatorShape {
/// Create a range slider value indicator that resembles a rectangular tooltip.
const RectangularRangeSliderValueIndicatorShape();
static const _RectangularSliderValueIndicatorPathPainter _pathPainter = _RectangularSliderValueIndicatorPathPainter();
@override
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
required TextPainter labelPainter,
required double textScaleFactor,
}) {
assert(textScaleFactor >= 0);
return _pathPainter.getPreferredSize(labelPainter, textScaleFactor);
}
@override
double getHorizontalShift({
RenderBox? parentBox,
Offset? center,
TextPainter? labelPainter,
Animation<double>? activationAnimation,
double? textScaleFactor,
Size? sizeWithOverflow,
}) {
return _pathPainter.getHorizontalShift(
parentBox: parentBox!,
center: center!,
labelPainter: labelPainter!,
textScaleFactor: textScaleFactor!,
sizeWithOverflow: sizeWithOverflow!,
scale: activationAnimation!.value,
);
}
@override
void paint(
PaintingContext context,
Offset center, {
Animation<double>? activationAnimation,
Animation<double>? enableAnimation,
bool? isDiscrete,
bool? isOnTop,
TextPainter? labelPainter,
double? textScaleFactor,
Size? sizeWithOverflow,
RenderBox? parentBox,
SliderThemeData? sliderTheme,
TextDirection? textDirection,
double? value,
Thumb? thumb,
}) {
final Canvas canvas = context.canvas;
final double scale = activationAnimation!.value;
_pathPainter.paint(
parentBox: parentBox!,
canvas: canvas,
center: center,
scale: scale,
labelPainter: labelPainter!,
textScaleFactor: textScaleFactor!,
sizeWithOverflow: sizeWithOverflow!,
backgroundPaintColor: sliderTheme!.valueIndicatorColor!,
strokePaintColor: isOnTop! ? sliderTheme.overlappingShapeStrokeColor : sliderTheme.valueIndicatorStrokeColor,
);
}
}
class _RectangularSliderValueIndicatorPathPainter {
const _RectangularSliderValueIndicatorPathPainter();
static const double _triangleHeight = 8.0;
static const double _labelPadding = 16.0;
static const double _preferredHeight = 32.0;
static const double _minLabelWidth = 16.0;
static const double _bottomTipYOffset = 14.0;
static const double _preferredHalfHeight = _preferredHeight / 2;
static const double _upperRectRadius = 4;
Size getPreferredSize(
TextPainter labelPainter,
double textScaleFactor,
) {
return Size(
_upperRectangleWidth(labelPainter, 1, textScaleFactor),
labelPainter.height + _labelPadding,
);
}
double getHorizontalShift({
required RenderBox parentBox,
required Offset center,
required TextPainter labelPainter,
required double textScaleFactor,
required Size sizeWithOverflow,
required double scale,
}) {
assert(!sizeWithOverflow.isEmpty);
const double edgePadding = 8.0;
final double rectangleWidth = _upperRectangleWidth(labelPainter, scale, textScaleFactor);
/// Value indicator draws on the Overlay and by using the global Offset
/// we are making sure we use the bounds of the Overlay instead of the Slider.
final Offset globalCenter = parentBox.localToGlobal(center);
// The rectangle must be shifted towards the center so that it minimizes the
// chance of it rendering outside the bounds of the render box. If the shift
// is negative, then the lobe is shifted from right to left, and if it is
// positive, then the lobe is shifted from left to right.
final double overflowLeft = math.max(0, rectangleWidth / 2 - globalCenter.dx + edgePadding);
final double overflowRight = math.max(0, rectangleWidth / 2 - (sizeWithOverflow.width - globalCenter.dx - edgePadding));
if (rectangleWidth < sizeWithOverflow.width) {
return overflowLeft - overflowRight;
} else if (overflowLeft - overflowRight > 0) {
return overflowLeft - (edgePadding * textScaleFactor);
} else {
return -overflowRight + (edgePadding * textScaleFactor);
}
}
double _upperRectangleWidth(TextPainter labelPainter, double scale, double textScaleFactor) {
final double unscaledWidth = math.max(_minLabelWidth * textScaleFactor, labelPainter.width) + _labelPadding * 2;
return unscaledWidth * scale;
}
void paint({
required RenderBox parentBox,
required Canvas canvas,
required Offset center,
required double scale,
required TextPainter labelPainter,
required double textScaleFactor,
required Size sizeWithOverflow,
required Color backgroundPaintColor,
Color? strokePaintColor,
}) {
if (scale == 0.0) {
// Zero scale essentially means "do not draw anything", so it's safe to just return.
return;
}
assert(!sizeWithOverflow.isEmpty);
final double rectangleWidth = _upperRectangleWidth(labelPainter, scale, textScaleFactor);
final double horizontalShift = getHorizontalShift(
parentBox: parentBox,
center: center,
labelPainter: labelPainter,
textScaleFactor: textScaleFactor,
sizeWithOverflow: sizeWithOverflow,
scale: scale,
);
final double rectHeight = labelPainter.height + _labelPadding;
final Rect upperRect = Rect.fromLTWH(
-rectangleWidth / 2 + horizontalShift,
-_triangleHeight - rectHeight,
rectangleWidth,
rectHeight,
);
final Path trianglePath = Path()
..lineTo(-_triangleHeight, -_triangleHeight)
..lineTo(_triangleHeight, -_triangleHeight)
..close();
final Paint fillPaint = Paint()..color = backgroundPaintColor;
final RRect upperRRect = RRect.fromRectAndRadius(upperRect, const Radius.circular(_upperRectRadius));
trianglePath.addRRect(upperRRect);
canvas.save();
// Prepare the canvas for the base of the tooltip, which is relative to the
// center of the thumb.
canvas.translate(center.dx, center.dy - _bottomTipYOffset);
canvas.scale(scale, scale);
if (strokePaintColor != null) {
final Paint strokePaint = Paint()
..color = strokePaintColor
..strokeWidth = 1.0
..style = PaintingStyle.stroke;
canvas.drawPath(trianglePath, strokePaint);
}
canvas.drawPath(trianglePath, fillPaint);
// The label text is centered within the value indicator.
final double bottomTipToUpperRectTranslateY = -_preferredHalfHeight / 2 - upperRect.height;
canvas.translate(0, bottomTipToUpperRectTranslateY);
final Offset boxCenter = Offset(horizontalShift, upperRect.height / 2);
final Offset halfLabelPainterOffset = Offset(labelPainter.width / 2, labelPainter.height / 2);
final Offset labelOffset = boxCenter - halfLabelPainterOffset;
labelPainter.paint(canvas, labelOffset);
canvas.restore();
}
}
/// A variant shape of a [Slider]'s value indicator . The value indicator is in
/// the shape of an upside-down pear.
///
/// ![A slider widget, consisting of 5 divisions and showing the paddle slider value indicator shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/paddle_slider_value_indicator_shape.png)
///
/// See also:
///
/// * [Slider], which includes a value indicator defined by this shape.
/// * [SliderTheme], which can be used to configure the slider value indicator
/// of all sliders in a widget subtree.
class PaddleSliderValueIndicatorShape extends SliderComponentShape {
/// Create a slider value indicator in the shape of an upside-down pear.
const PaddleSliderValueIndicatorShape();
static const _PaddleSliderValueIndicatorPathPainter _pathPainter = _PaddleSliderValueIndicatorPathPainter();
@override
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
TextPainter? labelPainter,
double? textScaleFactor,
}) {
assert(labelPainter != null);
assert(textScaleFactor != null && textScaleFactor >= 0);
return _pathPainter.getPreferredSize(labelPainter!, textScaleFactor!);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
assert(!sizeWithOverflow.isEmpty);
final ColorTween enableColor = ColorTween(
begin: sliderTheme.disabledThumbColor,
end: sliderTheme.valueIndicatorColor,
);
_pathPainter.paint(
context.canvas,
center,
Paint()..color = enableColor.evaluate(enableAnimation)!,
activationAnimation.value,
labelPainter,
textScaleFactor,
sizeWithOverflow,
sliderTheme.valueIndicatorStrokeColor,
);
}
}
/// A variant shape of a [RangeSlider]'s value indicators. The value indicator
/// is in the shape of an upside-down pear.
///
/// ![A slider widget, consisting of 5 divisions and showing the paddle range slider value indicator shape.]
/// (https://flutter.github.io/assets-for-api-docs/assets/material/paddle_range_slider_value_indicator_shape.png)
///
/// See also:
///
/// * [RangeSlider], which includes value indicators defined by this shape.
/// * [SliderTheme], which can be used to configure the range slider value
/// indicator of all sliders in a widget subtree.
class PaddleRangeSliderValueIndicatorShape extends RangeSliderValueIndicatorShape {
/// Create a slider value indicator in the shape of an upside-down pear.
const PaddleRangeSliderValueIndicatorShape();
static const _PaddleSliderValueIndicatorPathPainter _pathPainter = _PaddleSliderValueIndicatorPathPainter();
@override
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
required TextPainter labelPainter,
required double textScaleFactor,
}) {
assert(textScaleFactor >= 0);
return _pathPainter.getPreferredSize(labelPainter, textScaleFactor);
}
@override
double getHorizontalShift({
RenderBox? parentBox,
Offset? center,
TextPainter? labelPainter,
Animation<double>? activationAnimation,
double? textScaleFactor,
Size? sizeWithOverflow,
}) {
return _pathPainter.getHorizontalShift(
center: center!,
labelPainter: labelPainter!,
scale: activationAnimation!.value,
textScaleFactor: textScaleFactor!,
sizeWithOverflow: sizeWithOverflow!,
);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
bool? isDiscrete,
bool isOnTop = false,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
TextDirection? textDirection,
Thumb? thumb,
double? value,
double? textScaleFactor,
Size? sizeWithOverflow,
}) {
assert(!sizeWithOverflow!.isEmpty);
final ColorTween enableColor = ColorTween(
begin: sliderTheme.disabledThumbColor,
end: sliderTheme.valueIndicatorColor,
);
// Add a stroke of 1dp around the top paddle.
_pathPainter.paint(
context.canvas,
center,
Paint()..color = enableColor.evaluate(enableAnimation)!,
activationAnimation.value,
labelPainter,
textScaleFactor!,
sizeWithOverflow!,
isOnTop ? sliderTheme.overlappingShapeStrokeColor : sliderTheme.valueIndicatorStrokeColor,
);
}
}
class _PaddleSliderValueIndicatorPathPainter {
const _PaddleSliderValueIndicatorPathPainter();
// These constants define the shape of the default value indicator.
// The value indicator changes shape based on the size of
// the label: The top lobe spreads horizontally, and the
// top arc on the neck moves down to keep it merging smoothly
// with the top lobe as it expands.
// Radius of the top lobe of the value indicator.
static const double _topLobeRadius = 16.0;
static const double _minLabelWidth = 16.0;
// Radius of the bottom lobe of the value indicator.
static const double _bottomLobeRadius = 10.0;
static const double _labelPadding = 8.0;
static const double _distanceBetweenTopBottomCenters = 40.0;
static const double _middleNeckWidth = 3.0;
static const double _bottomNeckRadius = 4.5;
// The base of the triangle between the top lobe center and the centers of
// the two top neck arcs.
static const double _neckTriangleBase = _topNeckRadius + _middleNeckWidth / 2;
static const double _rightBottomNeckCenterX = _middleNeckWidth / 2 + _bottomNeckRadius;
static const double _rightBottomNeckAngleStart = math.pi;
static const Offset _topLobeCenter = Offset(0.0, -_distanceBetweenTopBottomCenters);
static const double _topNeckRadius = 13.0;
// The length of the hypotenuse of the triangle formed by the center
// of the left top lobe arc and the center of the top left neck arc.
// Used to calculate the position of the center of the arc.
static const double _neckTriangleHypotenuse = _topLobeRadius + _topNeckRadius;
// Some convenience values to help readability.
static const double _twoSeventyDegrees = 3.0 * math.pi / 2.0;
static const double _ninetyDegrees = math.pi / 2.0;
static const double _thirtyDegrees = math.pi / 6.0;
static const double _preferredHeight = _distanceBetweenTopBottomCenters + _topLobeRadius + _bottomLobeRadius;
// Set to true if you want a rectangle to be drawn around the label bubble.
// This helps with building tests that check that the label draws in the right
// place (because it prints the rect in the failed test output). It should not
// be checked in while set to "true".
static const bool _debuggingLabelLocation = false;
Size getPreferredSize(
TextPainter labelPainter,
double textScaleFactor,
) {
assert(textScaleFactor >= 0);
final double width = math.max(_minLabelWidth * textScaleFactor, labelPainter.width) + _labelPadding * 2 * textScaleFactor;
return Size(width, _preferredHeight * textScaleFactor);
}
// Adds an arc to the path that has the attributes passed in. This is
// a convenience to make adding arcs have less boilerplate.
static void _addArc(Path path, Offset center, double radius, double startAngle, double endAngle) {
assert(center.isFinite);
final Rect arcRect = Rect.fromCircle(center: center, radius: radius);
path.arcTo(arcRect, startAngle, endAngle - startAngle, false);
}
double getHorizontalShift({
required Offset center,
required TextPainter labelPainter,
required double scale,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
assert(!sizeWithOverflow.isEmpty);
final double inverseTextScale = textScaleFactor != 0 ? 1.0 / textScaleFactor : 0.0;
final double labelHalfWidth = labelPainter.width / 2.0;
final double halfWidthNeeded = math.max(
0.0,
inverseTextScale * labelHalfWidth - (_topLobeRadius - _labelPadding),
);
final double shift = _getIdealOffset(halfWidthNeeded, textScaleFactor * scale, center, sizeWithOverflow.width);
return shift * textScaleFactor;
}
// Determines the "best" offset to keep the bubble within the slider. The
// calling code will bound that with the available movement in the paddle shape.
double _getIdealOffset(
double halfWidthNeeded,
double scale,
Offset center,
double widthWithOverflow,
) {
const double edgeMargin = 8.0;
final Rect topLobeRect = Rect.fromLTWH(
-_topLobeRadius - halfWidthNeeded,
-_topLobeRadius - _distanceBetweenTopBottomCenters,
2.0 * (_topLobeRadius + halfWidthNeeded),
2.0 * _topLobeRadius,
);
// We can just multiply by scale instead of a transform, since we're scaling
// around (0, 0).
final Offset topLeft = (topLobeRect.topLeft * scale) + center;
final Offset bottomRight = (topLobeRect.bottomRight * scale) + center;
double shift = 0.0;
if (topLeft.dx < edgeMargin) {
shift = edgeMargin - topLeft.dx;
}
final double endGlobal = widthWithOverflow;
if (bottomRight.dx > endGlobal - edgeMargin) {
shift = endGlobal - edgeMargin - bottomRight.dx;
}
shift = scale == 0.0 ? 0.0 : shift / scale;
if (shift < 0.0) {
// Shifting to the left.
shift = math.max(shift, -halfWidthNeeded);
} else {
// Shifting to the right.
shift = math.min(shift, halfWidthNeeded);
}
return shift;
}
void paint(
Canvas canvas,
Offset center,
Paint paint,
double scale,
TextPainter labelPainter,
double textScaleFactor,
Size sizeWithOverflow,
Color? strokePaintColor,
) {
if (scale == 0.0) {
// Zero scale essentially means "do not draw anything", so it's safe to just return. Otherwise,
// our math below will attempt to divide by zero and send needless NaNs to the engine.
return;
}
assert(!sizeWithOverflow.isEmpty);
// The entire value indicator should scale with the size of the label,
// to keep it large enough to encompass the label text.
final double overallScale = scale * textScaleFactor;
final double inverseTextScale = textScaleFactor != 0 ? 1.0 / textScaleFactor : 0.0;
final double labelHalfWidth = labelPainter.width / 2.0;
canvas.save();
canvas.translate(center.dx, center.dy);
canvas.scale(overallScale, overallScale);
final double bottomNeckTriangleHypotenuse = _bottomNeckRadius + _bottomLobeRadius / overallScale;
final double rightBottomNeckCenterY = -math.sqrt(math.pow(bottomNeckTriangleHypotenuse, 2) - math.pow(_rightBottomNeckCenterX, 2));
final double rightBottomNeckAngleEnd = math.pi + math.atan(rightBottomNeckCenterY / _rightBottomNeckCenterX);
final Path path = Path()..moveTo(_middleNeckWidth / 2, rightBottomNeckCenterY);
_addArc(
path,
Offset(_rightBottomNeckCenterX, rightBottomNeckCenterY),
_bottomNeckRadius,
_rightBottomNeckAngleStart,
rightBottomNeckAngleEnd,
);
_addArc(
path,
Offset.zero,
_bottomLobeRadius / overallScale,
rightBottomNeckAngleEnd - math.pi,
2 * math.pi - rightBottomNeckAngleEnd,
);
_addArc(
path,
Offset(-_rightBottomNeckCenterX, rightBottomNeckCenterY),
_bottomNeckRadius,
math.pi - rightBottomNeckAngleEnd,
0,
);
// This is the needed extra width for the label. It is only positive when
// the label exceeds the minimum size contained by the round top lobe.
final double halfWidthNeeded = math.max(
0.0,
inverseTextScale * labelHalfWidth - (_topLobeRadius - _labelPadding),
);
final double shift = _getIdealOffset( halfWidthNeeded, overallScale, center, sizeWithOverflow.width);
final double leftWidthNeeded = halfWidthNeeded - shift;
final double rightWidthNeeded = halfWidthNeeded + shift;
// The parameter that describes how far along the transition from round to
// stretched we are.
final double leftAmount = math.max(0.0, math.min(1.0, leftWidthNeeded / _neckTriangleBase));
final double rightAmount = math.max(0.0, math.min(1.0, rightWidthNeeded / _neckTriangleBase));
// The angle between the top neck arc's center and the top lobe's center
// and vertical. The base amount is chosen so that the neck is smooth,
// even when the lobe is shifted due to its size.
final double leftTheta = (1.0 - leftAmount) * _thirtyDegrees;
final double rightTheta = (1.0 - rightAmount) * _thirtyDegrees;
// The center of the top left neck arc.
final Offset leftTopNeckCenter = Offset(
-_neckTriangleBase,
_topLobeCenter.dy + math.cos(leftTheta) * _neckTriangleHypotenuse,
);
final Offset neckRightCenter = Offset(
_neckTriangleBase,
_topLobeCenter.dy + math.cos(rightTheta) * _neckTriangleHypotenuse,
);
final double leftNeckArcAngle = _ninetyDegrees - leftTheta;
final double rightNeckArcAngle = math.pi + _ninetyDegrees - rightTheta;
// The distance between the end of the bottom neck arc and the beginning of
// the top neck arc. We use this to shrink/expand it based on the scale
// factor of the value indicator.
final double neckStretchBaseline = math.max(0.0, rightBottomNeckCenterY - math.max(leftTopNeckCenter.dy, neckRightCenter.dy));
final double t = math.pow(inverseTextScale, 3.0) as double;
final double stretch = clampDouble(neckStretchBaseline * t, 0.0, 10.0 * neckStretchBaseline);
final Offset neckStretch = Offset(0.0, neckStretchBaseline - stretch);
assert(!_debuggingLabelLocation || () {
final Offset leftCenter = _topLobeCenter - Offset(leftWidthNeeded, 0.0) + neckStretch;
final Offset rightCenter = _topLobeCenter + Offset(rightWidthNeeded, 0.0) + neckStretch;
final Rect valueRect = Rect.fromLTRB(
leftCenter.dx - _topLobeRadius,
leftCenter.dy - _topLobeRadius,
rightCenter.dx + _topLobeRadius,
rightCenter.dy + _topLobeRadius,
);
final Paint outlinePaint = Paint()
..color = const Color(0xffff0000)
..style = PaintingStyle.stroke
..strokeWidth = 1.0;
canvas.drawRect(valueRect, outlinePaint);
return true;
}());
_addArc(
path,
leftTopNeckCenter + neckStretch,
_topNeckRadius,
0.0,
-leftNeckArcAngle,
);
_addArc(
path,
_topLobeCenter - Offset(leftWidthNeeded, 0.0) + neckStretch,
_topLobeRadius,
_ninetyDegrees + leftTheta,
_twoSeventyDegrees,
);
_addArc(
path,
_topLobeCenter + Offset(rightWidthNeeded, 0.0) + neckStretch,
_topLobeRadius,
_twoSeventyDegrees,
_twoSeventyDegrees + math.pi - rightTheta,
);
_addArc(
path,
neckRightCenter + neckStretch,
_topNeckRadius,
rightNeckArcAngle,
math.pi,
);
if (strokePaintColor != null) {
final Paint strokePaint = Paint()
..color = strokePaintColor
..strokeWidth = 1.0
..style = PaintingStyle.stroke;
canvas.drawPath(path, strokePaint);
}
canvas.drawPath(path, paint);
// Draw the label.
canvas.save();
canvas.translate(shift, -_distanceBetweenTopBottomCenters + neckStretch.dy);
canvas.scale(inverseTextScale, inverseTextScale);
labelPainter.paint(canvas, Offset.zero - Offset(labelHalfWidth, labelPainter.height / 2.0));
canvas.restore();
canvas.restore();
}
}
/// A callback that formats a numeric value from a [Slider] or [RangeSlider] widget.
///
/// See also:
///
/// * [Slider.semanticFormatterCallback], which shows an example use case.
/// * [RangeSlider.semanticFormatterCallback], which shows an example use case.
typedef SemanticFormatterCallback = String Function(double value);
/// Decides which thumbs (if any) should be selected.
///
/// The default finds the closest thumb, but if the thumbs are close to each
/// other, it waits for movement defined by [dx] to determine the selected
/// thumb.
///
/// Override [SliderThemeData.thumbSelector] for custom thumb selection.
typedef RangeThumbSelector = Thumb? Function(
TextDirection textDirection,
RangeValues values,
double tapValue,
Size thumbSize,
Size trackSize,
double dx,
);
/// Object for representing range slider thumb values.
///
/// This object is passed into [RangeSlider.values] to set its values, and it
/// is emitted in [RangeSlider.onChanged], [RangeSlider.onChangeStart], and
/// [RangeSlider.onChangeEnd] when the values change.
@immutable
class RangeValues {
/// Creates pair of start and end values.
const RangeValues(this.start, this.end);
/// The value of the start thumb.
///
/// For LTR text direction, the start is the left thumb, and for RTL text
/// direction, the start is the right thumb.
final double start;
/// The value of the end thumb.
///
/// For LTR text direction, the end is the right thumb, and for RTL text
/// direction, the end is the left thumb.
final double end;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is RangeValues
&& other.start == start
&& other.end == end;
}
@override
int get hashCode => Object.hash(start, end);
@override
String toString() {
return '${objectRuntimeType(this, 'RangeValues')}($start, $end)';
}
}
/// Object for setting range slider label values that appear in the value
/// indicator for each thumb.
///
/// Used in combination with [SliderThemeData.showValueIndicator] to display
/// labels above the thumbs.
@immutable
class RangeLabels {
/// Creates pair of start and end labels.
const RangeLabels(this.start, this.end);
/// The label of the start thumb.
///
/// For LTR text direction, the start is the left thumb, and for RTL text
/// direction, the start is the right thumb.
final String start;
/// The label of the end thumb.
///
/// For LTR text direction, the end is the right thumb, and for RTL text
/// direction, the end is the left thumb.
final String end;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is RangeLabels
&& other.start == start
&& other.end == end;
}
@override
int get hashCode => Object.hash(start, end);
@override
String toString() {
return '${objectRuntimeType(this, 'RangeLabels')}($start, $end)';
}
}
void _debugDrawShadow(Canvas canvas, Path path, double elevation) {
if (elevation > 0.0) {
canvas.drawPath(
path,
Paint()
..color = Colors.black
..style = PaintingStyle.stroke
..strokeWidth = elevation * 2.0,
);
}
}
/// The default shape of a Material 3 [Slider]'s value indicator.
///
/// See also:
///
/// * [Slider], which includes a value indicator defined by this shape.
/// * [SliderTheme], which can be used to configure the slider value indicator
/// of all sliders in a widget subtree.
class DropSliderValueIndicatorShape extends SliderComponentShape {
/// Create a slider value indicator that resembles a drop shape.
const DropSliderValueIndicatorShape();
static const _DropSliderValueIndicatorPathPainter _pathPainter = _DropSliderValueIndicatorPathPainter();
@override
Size getPreferredSize(
bool isEnabled,
bool isDiscrete, {
TextPainter? labelPainter,
double? textScaleFactor,
}) {
assert(labelPainter != null);
assert(textScaleFactor != null && textScaleFactor >= 0);
return _pathPainter.getPreferredSize(labelPainter!, textScaleFactor!);
}
@override
void paint(
PaintingContext context,
Offset center, {
required Animation<double> activationAnimation,
required Animation<double> enableAnimation,
required bool isDiscrete,
required TextPainter labelPainter,
required RenderBox parentBox,
required SliderThemeData sliderTheme,
required TextDirection textDirection,
required double value,
required double textScaleFactor,
required Size sizeWithOverflow,
}) {
final Canvas canvas = context.canvas;
final double scale = activationAnimation.value;
_pathPainter.paint(
parentBox: parentBox,
canvas: canvas,
center: center,
scale: scale,
labelPainter: labelPainter,
textScaleFactor: textScaleFactor,
sizeWithOverflow: sizeWithOverflow,
backgroundPaintColor: sliderTheme.valueIndicatorColor!,
strokePaintColor: sliderTheme.valueIndicatorStrokeColor,
);
}
}
class _DropSliderValueIndicatorPathPainter {
const _DropSliderValueIndicatorPathPainter();
static const double _triangleHeight = 10.0;
static const double _labelPadding = 8.0;
static const double _preferredHeight = 32.0;
static const double _minLabelWidth = 20.0;
static const double _minRectHeight = 28.0;
static const double _rectYOffset = 6.0;
static const double _bottomTipYOffset = 16.0;
static const double _preferredHalfHeight = _preferredHeight / 2;
static const double _upperRectRadius = 4;
Size getPreferredSize(
TextPainter labelPainter,
double textScaleFactor,
) {
final double width = math.max(_minLabelWidth, labelPainter.width) + _labelPadding * 2 * textScaleFactor;
return Size(width, _preferredHeight * textScaleFactor);
}
double getHorizontalShift({
required RenderBox parentBox,
required Offset center,
required TextPainter labelPainter,
required double textScaleFactor,
required Size sizeWithOverflow,
required double scale,
}) {
assert(!sizeWithOverflow.isEmpty);
const double edgePadding = 8.0;
final double rectangleWidth = _upperRectangleWidth(labelPainter, scale);
/// Value indicator draws on the Overlay and by using the global Offset
/// we are making sure we use the bounds of the Overlay instead of the Slider.
final Offset globalCenter = parentBox.localToGlobal(center);
// The rectangle must be shifted towards the center so that it minimizes the
// chance of it rendering outside the bounds of the render box. If the shift
// is negative, then the lobe is shifted from right to left, and if it is
// positive, then the lobe is shifted from left to right.
final double overflowLeft = math.max(0, rectangleWidth / 2 - globalCenter.dx + edgePadding);
final double overflowRight = math.max(0, rectangleWidth / 2 - (sizeWithOverflow.width - globalCenter.dx - edgePadding));
if (rectangleWidth < sizeWithOverflow.width) {
return overflowLeft - overflowRight;
} else if (overflowLeft - overflowRight > 0) {
return overflowLeft - (edgePadding * textScaleFactor);
} else {
return -overflowRight + (edgePadding * textScaleFactor);
}
}
double _upperRectangleWidth(TextPainter labelPainter, double scale) {
final double unscaledWidth = math.max(_minLabelWidth, labelPainter.width) + _labelPadding;
return unscaledWidth * scale;
}
BorderRadius _adjustBorderRadius(Rect rect) {
const double rectness = 0.0;
return BorderRadius.lerp(
BorderRadius.circular(_upperRectRadius),
BorderRadius.all(Radius.circular(rect.shortestSide / 2.0)),
1.0 - rectness,
)!;
}
void paint({
required RenderBox parentBox,
required Canvas canvas,
required Offset center,
required double scale,
required TextPainter labelPainter,
required double textScaleFactor,
required Size sizeWithOverflow,
required Color backgroundPaintColor,
Color? strokePaintColor,
}) {
if (scale == 0.0) {
// Zero scale essentially means "do not draw anything", so it's safe to just return.
return;
}
assert(!sizeWithOverflow.isEmpty);
final double rectangleWidth = _upperRectangleWidth(labelPainter, scale);
final double horizontalShift = getHorizontalShift(
parentBox: parentBox,
center: center,
labelPainter: labelPainter,
textScaleFactor: textScaleFactor,
sizeWithOverflow: sizeWithOverflow,
scale: scale,
);
final Rect upperRect = Rect.fromLTWH(
-rectangleWidth / 2 + horizontalShift,
-_rectYOffset - _minRectHeight,
rectangleWidth,
_minRectHeight,
);
final Paint fillPaint = Paint()..color = backgroundPaintColor;
canvas.save();
canvas.translate(center.dx, center.dy - _bottomTipYOffset);
canvas.scale(scale, scale);
final BorderRadius adjustedBorderRadius = _adjustBorderRadius(upperRect);
final RRect borderRect = adjustedBorderRadius.resolve(labelPainter.textDirection).toRRect(upperRect);
final Path trianglePath = Path()
..lineTo(-_triangleHeight, -_triangleHeight)
..lineTo(_triangleHeight, -_triangleHeight)
..close();
trianglePath.addRRect(borderRect);
if (strokePaintColor != null) {
final Paint strokePaint = Paint()
..color = strokePaintColor
..strokeWidth = 1.0
..style = PaintingStyle.stroke;
canvas.drawPath(trianglePath, strokePaint);
}
canvas.drawPath(trianglePath, fillPaint);
// The label text is centered within the value indicator.
final double bottomTipToUpperRectTranslateY = -_preferredHalfHeight / 2 - upperRect.height;
canvas.translate(0, bottomTipToUpperRectTranslateY);
final Offset boxCenter = Offset(horizontalShift, upperRect.height / 1.75);
final Offset halfLabelPainterOffset = Offset(labelPainter.width / 2, labelPainter.height / 2);
final Offset labelOffset = boxCenter - halfLabelPainterOffset;
labelPainter.paint(canvas, labelOffset);
canvas.restore();
}
}
| flutter/packages/flutter/lib/src/material/slider_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/slider_theme.dart",
"repo_id": "flutter",
"token_count": 44867
} | 628 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle;
import 'package:flutter/gestures.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'adaptive_text_selection_toolbar.dart';
import 'input_decorator.dart';
import 'material_state.dart';
import 'text_field.dart';
import 'theme.dart';
export 'package:flutter/services.dart' show SmartDashesType, SmartQuotesType;
/// A [FormField] that contains a [TextField].
///
/// This is a convenience widget that wraps a [TextField] widget in a
/// [FormField].
///
/// A [Form] ancestor is not required. The [Form] allows one to
/// save, reset, or validate multiple fields at once. To use without a [Form],
/// pass a `GlobalKey<FormFieldState>` (see [GlobalKey]) to the constructor and use
/// [GlobalKey.currentState] to save or reset the form field.
///
/// When a [controller] is specified, its [TextEditingController.text]
/// defines the [initialValue]. If this [FormField] is part of a scrolling
/// container that lazily constructs its children, like a [ListView] or a
/// [CustomScrollView], then a [controller] should be specified.
/// The controller's lifetime should be managed by a stateful widget ancestor
/// of the scrolling container.
///
/// If a [controller] is not specified, [initialValue] can be used to give
/// the automatically generated controller an initial value.
///
/// {@macro flutter.material.textfield.wantKeepAlive}
///
/// Remember to call [TextEditingController.dispose] of the [TextEditingController]
/// when it is no longer needed. This will ensure any resources used by the object
/// are discarded.
///
/// By default, `decoration` will apply the [ThemeData.inputDecorationTheme] for
/// the current context to the [InputDecoration], see
/// [InputDecoration.applyDefaults].
///
/// For a documentation about the various parameters, see [TextField].
///
/// {@tool snippet}
///
/// Creates a [TextFormField] with an [InputDecoration] and validator function.
///
/// 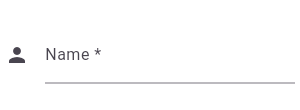
///
/// 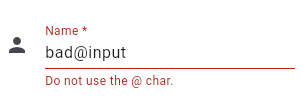
///
/// ```dart
/// TextFormField(
/// decoration: const InputDecoration(
/// icon: Icon(Icons.person),
/// hintText: 'What do people call you?',
/// labelText: 'Name *',
/// ),
/// onSaved: (String? value) {
/// // This optional block of code can be used to run
/// // code when the user saves the form.
/// },
/// validator: (String? value) {
/// return (value != null && value.contains('@')) ? 'Do not use the @ char.' : null;
/// },
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to move the focus to the next field when the user
/// presses the SPACE key.
///
/// ** See code in examples/api/lib/material/text_form_field/text_form_field.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * <https://material.io/design/components/text-fields.html>
/// * [TextField], which is the underlying text field without the [Form]
/// integration.
/// * [InputDecorator], which shows the labels and other visual elements that
/// surround the actual text editing widget.
/// * Learn how to use a [TextEditingController] in one of our [cookbook recipes](https://flutter.dev/docs/cookbook/forms/text-field-changes#2-use-a-texteditingcontroller).
class TextFormField extends FormField<String> {
/// Creates a [FormField] that contains a [TextField].
///
/// When a [controller] is specified, [initialValue] must be null (the
/// default). If [controller] is null, then a [TextEditingController]
/// will be constructed automatically and its `text` will be initialized
/// to [initialValue] or the empty string.
///
/// For documentation about the various parameters, see the [TextField] class
/// and [TextField.new], the constructor.
TextFormField({
super.key,
this.controller,
String? initialValue,
FocusNode? focusNode,
InputDecoration? decoration = const InputDecoration(),
TextInputType? keyboardType,
TextCapitalization textCapitalization = TextCapitalization.none,
TextInputAction? textInputAction,
TextStyle? style,
StrutStyle? strutStyle,
TextDirection? textDirection,
TextAlign textAlign = TextAlign.start,
TextAlignVertical? textAlignVertical,
bool autofocus = false,
bool readOnly = false,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
ToolbarOptions? toolbarOptions,
bool? showCursor,
String obscuringCharacter = '•',
bool obscureText = false,
bool autocorrect = true,
SmartDashesType? smartDashesType,
SmartQuotesType? smartQuotesType,
bool enableSuggestions = true,
MaxLengthEnforcement? maxLengthEnforcement,
int? maxLines = 1,
int? minLines,
bool expands = false,
int? maxLength,
this.onChanged,
GestureTapCallback? onTap,
bool onTapAlwaysCalled = false,
TapRegionCallback? onTapOutside,
VoidCallback? onEditingComplete,
ValueChanged<String>? onFieldSubmitted,
super.onSaved,
super.validator,
List<TextInputFormatter>? inputFormatters,
bool? enabled,
bool? ignorePointers,
double cursorWidth = 2.0,
double? cursorHeight,
Radius? cursorRadius,
Color? cursorColor,
Color? cursorErrorColor,
Brightness? keyboardAppearance,
EdgeInsets scrollPadding = const EdgeInsets.all(20.0),
bool? enableInteractiveSelection,
TextSelectionControls? selectionControls,
InputCounterWidgetBuilder? buildCounter,
ScrollPhysics? scrollPhysics,
Iterable<String>? autofillHints,
AutovalidateMode? autovalidateMode,
ScrollController? scrollController,
super.restorationId,
bool enableIMEPersonalizedLearning = true,
MouseCursor? mouseCursor,
EditableTextContextMenuBuilder? contextMenuBuilder = _defaultContextMenuBuilder,
SpellCheckConfiguration? spellCheckConfiguration,
TextMagnifierConfiguration? magnifierConfiguration,
UndoHistoryController? undoController,
AppPrivateCommandCallback? onAppPrivateCommand,
bool? cursorOpacityAnimates,
ui.BoxHeightStyle selectionHeightStyle = ui.BoxHeightStyle.tight,
ui.BoxWidthStyle selectionWidthStyle = ui.BoxWidthStyle.tight,
DragStartBehavior dragStartBehavior = DragStartBehavior.start,
ContentInsertionConfiguration? contentInsertionConfiguration,
MaterialStatesController? statesController,
Clip clipBehavior = Clip.hardEdge,
bool scribbleEnabled = true,
bool canRequestFocus = true,
}) : assert(initialValue == null || controller == null),
assert(obscuringCharacter.length == 1),
assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
!expands || (maxLines == null && minLines == null),
'minLines and maxLines must be null when expands is true.',
),
assert(!obscureText || maxLines == 1, 'Obscured fields cannot be multiline.'),
assert(maxLength == null || maxLength == TextField.noMaxLength || maxLength > 0),
super(
initialValue: controller != null ? controller.text : (initialValue ?? ''),
enabled: enabled ?? decoration?.enabled ?? true,
autovalidateMode: autovalidateMode ?? AutovalidateMode.disabled,
builder: (FormFieldState<String> field) {
final _TextFormFieldState state = field as _TextFormFieldState;
final InputDecoration effectiveDecoration = (decoration ?? const InputDecoration())
.applyDefaults(Theme.of(field.context).inputDecorationTheme);
void onChangedHandler(String value) {
field.didChange(value);
onChanged?.call(value);
}
return UnmanagedRestorationScope(
bucket: field.bucket,
child: TextField(
restorationId: restorationId,
controller: state._effectiveController,
focusNode: focusNode,
decoration: effectiveDecoration.copyWith(errorText: field.errorText),
keyboardType: keyboardType,
textInputAction: textInputAction,
style: style,
strutStyle: strutStyle,
textAlign: textAlign,
textAlignVertical: textAlignVertical,
textDirection: textDirection,
textCapitalization: textCapitalization,
autofocus: autofocus,
statesController: statesController,
toolbarOptions: toolbarOptions,
readOnly: readOnly,
showCursor: showCursor,
obscuringCharacter: obscuringCharacter,
obscureText: obscureText,
autocorrect: autocorrect,
smartDashesType: smartDashesType ?? (obscureText ? SmartDashesType.disabled : SmartDashesType.enabled),
smartQuotesType: smartQuotesType ?? (obscureText ? SmartQuotesType.disabled : SmartQuotesType.enabled),
enableSuggestions: enableSuggestions,
maxLengthEnforcement: maxLengthEnforcement,
maxLines: maxLines,
minLines: minLines,
expands: expands,
maxLength: maxLength,
onChanged: onChangedHandler,
onTap: onTap,
onTapAlwaysCalled: onTapAlwaysCalled,
onTapOutside: onTapOutside,
onEditingComplete: onEditingComplete,
onSubmitted: onFieldSubmitted,
inputFormatters: inputFormatters,
enabled: enabled ?? decoration?.enabled ?? true,
ignorePointers: ignorePointers,
cursorWidth: cursorWidth,
cursorHeight: cursorHeight,
cursorRadius: cursorRadius,
cursorColor: cursorColor,
cursorErrorColor: cursorErrorColor,
scrollPadding: scrollPadding,
scrollPhysics: scrollPhysics,
keyboardAppearance: keyboardAppearance,
enableInteractiveSelection: enableInteractiveSelection ?? (!obscureText || !readOnly),
selectionControls: selectionControls,
buildCounter: buildCounter,
autofillHints: autofillHints,
scrollController: scrollController,
enableIMEPersonalizedLearning: enableIMEPersonalizedLearning,
mouseCursor: mouseCursor,
contextMenuBuilder: contextMenuBuilder,
spellCheckConfiguration: spellCheckConfiguration,
magnifierConfiguration: magnifierConfiguration,
undoController: undoController,
onAppPrivateCommand: onAppPrivateCommand,
cursorOpacityAnimates: cursorOpacityAnimates,
selectionHeightStyle: selectionHeightStyle,
selectionWidthStyle: selectionWidthStyle,
dragStartBehavior: dragStartBehavior,
contentInsertionConfiguration: contentInsertionConfiguration,
clipBehavior: clipBehavior,
scribbleEnabled: scribbleEnabled,
canRequestFocus: canRequestFocus,
),
);
},
);
/// Controls the text being edited.
///
/// If null, this widget will create its own [TextEditingController] and
/// initialize its [TextEditingController.text] with [initialValue].
final TextEditingController? controller;
/// {@template flutter.material.TextFormField.onChanged}
/// Called when the user initiates a change to the TextField's
/// value: when they have inserted or deleted text or reset the form.
/// {@endtemplate}
final ValueChanged<String>? onChanged;
static Widget _defaultContextMenuBuilder(BuildContext context, EditableTextState editableTextState) {
return AdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
}
@override
FormFieldState<String> createState() => _TextFormFieldState();
}
class _TextFormFieldState extends FormFieldState<String> {
RestorableTextEditingController? _controller;
TextEditingController get _effectiveController => _textFormField.controller ?? _controller!.value;
TextFormField get _textFormField => super.widget as TextFormField;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
super.restoreState(oldBucket, initialRestore);
if (_controller != null) {
_registerController();
}
// Make sure to update the internal [FormFieldState] value to sync up with
// text editing controller value.
setValue(_effectiveController.text);
}
void _registerController() {
assert(_controller != null);
registerForRestoration(_controller!, 'controller');
}
void _createLocalController([TextEditingValue? value]) {
assert(_controller == null);
_controller = value == null
? RestorableTextEditingController()
: RestorableTextEditingController.fromValue(value);
if (!restorePending) {
_registerController();
}
}
@override
void initState() {
super.initState();
if (_textFormField.controller == null) {
_createLocalController(widget.initialValue != null ? TextEditingValue(text: widget.initialValue!) : null);
} else {
_textFormField.controller!.addListener(_handleControllerChanged);
}
}
@override
void didUpdateWidget(TextFormField oldWidget) {
super.didUpdateWidget(oldWidget);
if (_textFormField.controller != oldWidget.controller) {
oldWidget.controller?.removeListener(_handleControllerChanged);
_textFormField.controller?.addListener(_handleControllerChanged);
if (oldWidget.controller != null && _textFormField.controller == null) {
_createLocalController(oldWidget.controller!.value);
}
if (_textFormField.controller != null) {
setValue(_textFormField.controller!.text);
if (oldWidget.controller == null) {
unregisterFromRestoration(_controller!);
_controller!.dispose();
_controller = null;
}
}
}
}
@override
void dispose() {
_textFormField.controller?.removeListener(_handleControllerChanged);
_controller?.dispose();
super.dispose();
}
@override
void didChange(String? value) {
super.didChange(value);
if (_effectiveController.text != value) {
_effectiveController.text = value ?? '';
}
}
@override
void reset() {
// Set the controller value before calling super.reset() to let
// _handleControllerChanged suppress the change.
_effectiveController.text = widget.initialValue ?? '';
super.reset();
_textFormField.onChanged?.call(_effectiveController.text);
}
void _handleControllerChanged() {
// Suppress changes that originated from within this class.
//
// In the case where a controller has been passed in to this widget, we
// register this change listener. In these cases, we'll also receive change
// notifications for changes originating from within this class -- for
// example, the reset() method. In such cases, the FormField value will
// already have been set.
if (_effectiveController.text != value) {
didChange(_effectiveController.text);
}
}
}
| flutter/packages/flutter/lib/src/material/text_form_field.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/text_form_field.dart",
"repo_id": "flutter",
"token_count": 5658
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
class _TooltipVisibilityScope extends InheritedWidget {
const _TooltipVisibilityScope({
required super.child,
required this.visible,
});
final bool visible;
@override
bool updateShouldNotify(_TooltipVisibilityScope old) {
return old.visible != visible;
}
}
/// Overrides the visibility of descendant [Tooltip] widgets.
///
/// If disabled, the descendant [Tooltip] widgets will not display a tooltip
/// when tapped, long-pressed, hovered by the mouse, or when
/// `ensureTooltipVisible` is called. This only visually disables tooltips but
/// continues to provide any semantic information that is provided.
class TooltipVisibility extends StatelessWidget {
/// Creates a widget that configures the visibility of [Tooltip].
const TooltipVisibility({
super.key,
required this.visible,
required this.child,
});
/// The widget below this widget in the tree.
///
/// The entire app can be wrapped in this widget to globally control [Tooltip]
/// visibility.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Determines the visibility of [Tooltip] widgets that inherit from this widget.
final bool visible;
/// The [visible] of the closest instance of this class that encloses the
/// given context. Defaults to `true` if none are found.
static bool of(BuildContext context) {
final _TooltipVisibilityScope? visibility = context.dependOnInheritedWidgetOfExactType<_TooltipVisibilityScope>();
return visibility?.visible ?? true;
}
@override
Widget build(BuildContext context) {
return _TooltipVisibilityScope(
visible: visible,
child: child,
);
}
}
| flutter/packages/flutter/lib/src/material/tooltip_visibility.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/tooltip_visibility.dart",
"repo_id": "flutter",
"token_count": 541
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show Canvas, Clip, Paint, Path, RRect, Rect, VoidCallback;
/// Clip utilities used by [PaintingContext].
abstract class ClipContext {
/// The canvas on which to paint.
Canvas get canvas;
void _clipAndPaint(void Function(bool doAntiAlias) canvasClipCall, Clip clipBehavior, Rect bounds, VoidCallback painter) {
canvas.save();
switch (clipBehavior) {
case Clip.none:
break;
case Clip.hardEdge:
canvasClipCall(false);
case Clip.antiAlias:
canvasClipCall(true);
case Clip.antiAliasWithSaveLayer:
canvasClipCall(true);
canvas.saveLayer(bounds, Paint());
}
painter();
if (clipBehavior == Clip.antiAliasWithSaveLayer) {
canvas.restore();
}
canvas.restore();
}
/// Clip [canvas] with [Path] according to [Clip] and then paint. [canvas] is
/// restored to the pre-clip status afterwards.
///
/// `bounds` is the saveLayer bounds used for [Clip.antiAliasWithSaveLayer].
void clipPathAndPaint(Path path, Clip clipBehavior, Rect bounds, VoidCallback painter) {
_clipAndPaint((bool doAntiAlias) => canvas.clipPath(path, doAntiAlias: doAntiAlias), clipBehavior, bounds, painter);
}
/// Clip [canvas] with [Path] according to `rrect` and then paint. [canvas] is
/// restored to the pre-clip status afterwards.
///
/// `bounds` is the saveLayer bounds used for [Clip.antiAliasWithSaveLayer].
void clipRRectAndPaint(RRect rrect, Clip clipBehavior, Rect bounds, VoidCallback painter) {
_clipAndPaint((bool doAntiAlias) => canvas.clipRRect(rrect, doAntiAlias: doAntiAlias), clipBehavior, bounds, painter);
}
/// Clip [canvas] with [Path] according to `rect` and then paint. [canvas] is
/// restored to the pre-clip status afterwards.
///
/// `bounds` is the saveLayer bounds used for [Clip.antiAliasWithSaveLayer].
void clipRectAndPaint(Rect rect, Clip clipBehavior, Rect bounds, VoidCallback painter) {
_clipAndPaint((bool doAntiAlias) => canvas.clipRect(rect, doAntiAlias: doAntiAlias), clipBehavior, bounds, painter);
}
}
| flutter/packages/flutter/lib/src/painting/clip.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/clip.dart",
"repo_id": "flutter",
"token_count": 744
} | 631 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show ParagraphBuilder, StringAttribute;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'basic_types.dart';
import 'text_painter.dart';
import 'text_scaler.dart';
import 'text_span.dart';
import 'text_style.dart';
// Examples can assume:
// late InlineSpan myInlineSpan;
/// Mutable wrapper of an integer that can be passed by reference to track a
/// value across a recursive stack.
class Accumulator {
/// [Accumulator] may be initialized with a specified value, otherwise, it will
/// initialize to zero.
Accumulator([this._value = 0]);
/// The integer stored in this [Accumulator].
int get value => _value;
int _value;
/// Increases the [value] by the `addend`.
void increment(int addend) {
assert(addend >= 0);
_value += addend;
}
}
/// Called on each span as [InlineSpan.visitChildren] walks the [InlineSpan] tree.
///
/// Returns true when the walk should continue, and false to stop visiting further
/// [InlineSpan]s.
typedef InlineSpanVisitor = bool Function(InlineSpan span);
/// The textual and semantic label information for an [InlineSpan].
///
/// For [PlaceholderSpan]s, [InlineSpanSemanticsInformation.placeholder] is used by default.
///
/// See also:
///
/// * [InlineSpan.getSemanticsInformation]
@immutable
class InlineSpanSemanticsInformation {
/// Constructs an object that holds the text and semantics label values of an
/// [InlineSpan].
///
/// Use [InlineSpanSemanticsInformation.placeholder] instead of directly setting
/// [isPlaceholder].
const InlineSpanSemanticsInformation(
this.text, {
this.isPlaceholder = false,
this.semanticsLabel,
this.stringAttributes = const <ui.StringAttribute>[],
this.recognizer,
}) : assert(!isPlaceholder || (text == '\uFFFC' && semanticsLabel == null && recognizer == null)),
requiresOwnNode = isPlaceholder || recognizer != null;
/// The text info for a [PlaceholderSpan].
static const InlineSpanSemanticsInformation placeholder = InlineSpanSemanticsInformation('\uFFFC', isPlaceholder: true);
/// The text value, if any. For [PlaceholderSpan]s, this will be the unicode
/// placeholder value.
final String text;
/// The semanticsLabel, if any.
final String? semanticsLabel;
/// The gesture recognizer, if any, for this span.
final GestureRecognizer? recognizer;
/// Whether this is for a placeholder span.
final bool isPlaceholder;
/// True if this configuration should get its own semantics node.
///
/// This will be the case of the [recognizer] is not null, of if
/// [isPlaceholder] is true.
final bool requiresOwnNode;
/// The string attributes attached to this semantics information
final List<ui.StringAttribute> stringAttributes;
@override
bool operator ==(Object other) {
return other is InlineSpanSemanticsInformation
&& other.text == text
&& other.semanticsLabel == semanticsLabel
&& other.recognizer == recognizer
&& other.isPlaceholder == isPlaceholder
&& listEquals<ui.StringAttribute>(other.stringAttributes, stringAttributes);
}
@override
int get hashCode => Object.hash(text, semanticsLabel, recognizer, isPlaceholder);
@override
String toString() => '${objectRuntimeType(this, 'InlineSpanSemanticsInformation')}{text: $text, semanticsLabel: $semanticsLabel, recognizer: $recognizer}';
}
/// Combines _semanticsInfo entries where permissible.
///
/// Consecutive inline spans can be combined if their
/// [InlineSpanSemanticsInformation.requiresOwnNode] return false.
List<InlineSpanSemanticsInformation> combineSemanticsInfo(List<InlineSpanSemanticsInformation> infoList) {
final List<InlineSpanSemanticsInformation> combined = <InlineSpanSemanticsInformation>[];
String workingText = '';
String workingLabel = '';
List<ui.StringAttribute> workingAttributes = <ui.StringAttribute>[];
for (final InlineSpanSemanticsInformation info in infoList) {
if (info.requiresOwnNode) {
combined.add(InlineSpanSemanticsInformation(
workingText,
semanticsLabel: workingLabel,
stringAttributes: workingAttributes,
));
workingText = '';
workingLabel = '';
workingAttributes = <ui.StringAttribute>[];
combined.add(info);
} else {
workingText += info.text;
final String effectiveLabel = info.semanticsLabel ?? info.text;
for (final ui.StringAttribute infoAttribute in info.stringAttributes) {
workingAttributes.add(
infoAttribute.copy(
range: TextRange(
start: infoAttribute.range.start + workingLabel.length,
end: infoAttribute.range.end + workingLabel.length,
),
),
);
}
workingLabel += effectiveLabel;
}
}
combined.add(InlineSpanSemanticsInformation(
workingText,
semanticsLabel: workingLabel,
stringAttributes: workingAttributes,
));
return combined;
}
/// An immutable span of inline content which forms part of a paragraph.
///
/// * The subclass [TextSpan] specifies text and may contain child [InlineSpan]s.
/// * The subclass [PlaceholderSpan] represents a placeholder that may be
/// filled with non-text content. [PlaceholderSpan] itself defines a
/// [ui.PlaceholderAlignment] and a [TextBaseline]. To be useful,
/// [PlaceholderSpan] must be extended to define content. An instance of
/// this is the [WidgetSpan] class in the widgets library.
/// * The subclass [WidgetSpan] specifies embedded inline widgets.
///
/// {@tool snippet}
///
/// This example shows a tree of [InlineSpan]s that make a query asking for a
/// name with a [TextField] embedded inline.
///
/// ```dart
/// Text.rich(
/// TextSpan(
/// text: 'My name is ',
/// style: const TextStyle(color: Colors.black),
/// children: <InlineSpan>[
/// WidgetSpan(
/// alignment: PlaceholderAlignment.baseline,
/// baseline: TextBaseline.alphabetic,
/// child: ConstrainedBox(
/// constraints: const BoxConstraints(maxWidth: 100),
/// child: const TextField(),
/// )
/// ),
/// const TextSpan(
/// text: '.',
/// ),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Text], a widget for showing uniformly-styled text.
/// * [RichText], a widget for finer control of text rendering.
/// * [TextPainter], a class for painting [InlineSpan] objects on a [Canvas].
@immutable
abstract class InlineSpan extends DiagnosticableTree {
/// Creates an [InlineSpan] with the given values.
const InlineSpan({
this.style,
});
/// The [TextStyle] to apply to this span.
///
/// The [style] is also applied to any child spans when this is an instance
/// of [TextSpan].
final TextStyle? style;
/// Apply the properties of this object to the given [ParagraphBuilder], from
/// which a [Paragraph] can be obtained.
///
/// The `textScaler` parameter specifies a [TextScaler] that the text and
/// placeholders will be scaled by. The scaling is performed before layout,
/// so the text will be laid out with the scaled glyphs and placeholders.
///
/// The `dimensions` parameter specifies the sizes of the placeholders.
/// Each [PlaceholderSpan] must be paired with a [PlaceholderDimensions]
/// in the same order as defined in the [InlineSpan] tree.
///
/// [Paragraph] objects can be drawn on [Canvas] objects.
void build(ui.ParagraphBuilder builder, {
TextScaler textScaler = TextScaler.noScaling,
List<PlaceholderDimensions>? dimensions,
});
/// Walks this [InlineSpan] and any descendants in pre-order and calls `visitor`
/// for each span that has content.
///
/// When `visitor` returns true, the walk will continue. When `visitor` returns
/// false, then the walk will end.
///
/// See also:
///
/// * [visitDirectChildren], which preforms `build`-order traversal on the
/// immediate children of this [InlineSpan], regardless of whether they
/// have content.
bool visitChildren(InlineSpanVisitor visitor);
/// Calls `visitor` for each immediate child of this [InlineSpan].
///
/// The immediate children are visited in the same order they are added to
/// a [ui.ParagraphBuilder] in the [build] method, which is also the logical
/// order of the child [InlineSpan]s in the text.
///
/// The traversal stops when all immediate children are visited, or when the
/// `visitor` callback returns `false` on an immediate child. This method
/// itself returns a `bool` indicating whether the visitor callback returned
/// `true` on all immediate children.
///
/// See also:
///
/// * [visitChildren], which performs preorder traversal on this [InlineSpan]
/// if it has content, and all its descendants with content.
bool visitDirectChildren(InlineSpanVisitor visitor);
/// Returns the [InlineSpan] that contains the given position in the text.
InlineSpan? getSpanForPosition(TextPosition position) {
assert(debugAssertIsValid());
final Accumulator offset = Accumulator();
InlineSpan? result;
visitChildren((InlineSpan span) {
result = span.getSpanForPositionVisitor(position, offset);
return result == null;
});
return result;
}
/// Performs the check at each [InlineSpan] for if the `position` falls within the range
/// of the span and returns the span if it does.
///
/// The `offset` parameter tracks the current index offset in the text buffer formed
/// if the contents of the [InlineSpan] tree were concatenated together starting
/// from the root [InlineSpan].
///
/// This method should not be directly called. Use [getSpanForPosition] instead.
@protected
InlineSpan? getSpanForPositionVisitor(TextPosition position, Accumulator offset);
/// Flattens the [InlineSpan] tree into a single string.
///
/// Styles are not honored in this process. If `includeSemanticsLabels` is
/// true, then the text returned will include the [TextSpan.semanticsLabel]s
/// instead of the text contents for [TextSpan]s.
///
/// When `includePlaceholders` is true, [PlaceholderSpan]s in the tree will be
/// represented as a 0xFFFC 'object replacement character'.
String toPlainText({bool includeSemanticsLabels = true, bool includePlaceholders = true}) {
final StringBuffer buffer = StringBuffer();
computeToPlainText(buffer, includeSemanticsLabels: includeSemanticsLabels, includePlaceholders: includePlaceholders);
return buffer.toString();
}
/// Flattens the [InlineSpan] tree to a list of
/// [InlineSpanSemanticsInformation] objects.
///
/// [PlaceholderSpan]s in the tree will be represented with a
/// [InlineSpanSemanticsInformation.placeholder] value.
List<InlineSpanSemanticsInformation> getSemanticsInformation() {
final List<InlineSpanSemanticsInformation> collector = <InlineSpanSemanticsInformation>[];
computeSemanticsInformation(collector);
return collector;
}
/// Walks the [InlineSpan] tree and accumulates a list of
/// [InlineSpanSemanticsInformation] objects.
///
/// This method should not be directly called. Use
/// [getSemanticsInformation] instead.
///
/// [PlaceholderSpan]s in the tree will be represented with a
/// [InlineSpanSemanticsInformation.placeholder] value.
@protected
void computeSemanticsInformation(List<InlineSpanSemanticsInformation> collector);
/// Walks the [InlineSpan] tree and writes the plain text representation to `buffer`.
///
/// This method should not be directly called. Use [toPlainText] instead.
///
/// Styles are not honored in this process. If `includeSemanticsLabels` is
/// true, then the text returned will include the [TextSpan.semanticsLabel]s
/// instead of the text contents for [TextSpan]s.
///
/// When `includePlaceholders` is true, [PlaceholderSpan]s in the tree will be
/// represented as a 0xFFFC 'object replacement character'.
///
/// The plain-text representation of this [InlineSpan] is written into the `buffer`.
/// This method will then recursively call [computeToPlainText] on its children
/// [InlineSpan]s if available.
@protected
void computeToPlainText(StringBuffer buffer, {bool includeSemanticsLabels = true, bool includePlaceholders = true});
/// Returns the UTF-16 code unit at the given `index` in the flattened string.
///
/// This only accounts for the [TextSpan.text] values and ignores [PlaceholderSpan]s.
///
/// Returns null if the `index` is out of bounds.
int? codeUnitAt(int index) {
if (index < 0) {
return null;
}
final Accumulator offset = Accumulator();
int? result;
visitChildren((InlineSpan span) {
result = span.codeUnitAtVisitor(index, offset);
return result == null;
});
return result;
}
/// Performs the check at each [InlineSpan] for if the `index` falls within the range
/// of the span and returns the corresponding code unit. Returns null otherwise.
///
/// The `offset` parameter tracks the current index offset in the text buffer formed
/// if the contents of the [InlineSpan] tree were concatenated together starting
/// from the root [InlineSpan].
///
/// This method should not be directly called. Use [codeUnitAt] instead.
@protected
int? codeUnitAtVisitor(int index, Accumulator offset);
/// In debug mode, throws an exception if the object is not in a
/// valid configuration. Otherwise, returns true.
///
/// This is intended to be used as follows:
///
/// ```dart
/// assert(myInlineSpan.debugAssertIsValid());
/// ```
bool debugAssertIsValid() => true;
/// Describe the difference between this span and another, in terms of
/// how much damage it will make to the rendering. The comparison is deep.
///
/// Comparing [InlineSpan] objects of different types, for example, comparing
/// a [TextSpan] to a [WidgetSpan], always results in [RenderComparison.layout].
///
/// See also:
///
/// * [TextStyle.compareTo], which does the same thing for [TextStyle]s.
RenderComparison compareTo(InlineSpan other);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is InlineSpan
&& other.style == style;
}
@override
int get hashCode => style.hashCode;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.defaultDiagnosticsTreeStyle = DiagnosticsTreeStyle.whitespace;
style?.debugFillProperties(properties);
}
}
| flutter/packages/flutter/lib/src/painting/inline_span.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/inline_span.dart",
"repo_id": "flutter",
"token_count": 4594
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:ui' as ui show
ParagraphStyle,
StrutStyle,
TextStyle,
lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'colors.dart';
import 'strut_style.dart';
import 'text_painter.dart';
import 'text_scaler.dart';
const String _kDefaultDebugLabel = 'unknown';
const String _kColorForegroundWarning = 'Cannot provide both a color and a foreground\n'
'The color argument is just a shorthand for "foreground: Paint()..color = color".';
const String _kColorBackgroundWarning = 'Cannot provide both a backgroundColor and a background\n'
'The backgroundColor argument is just a shorthand for "background: Paint()..color = color".';
// Examples can assume:
// late BuildContext context;
/// An immutable style describing how to format and paint text.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=1z6YP7YmvwA}
///
/// ### Bold
///
/// {@tool snippet}
/// Here, a single line of text in a [Text] widget is given a specific style
/// override. The style is mixed with the ambient [DefaultTextStyle] by the
/// [Text] widget.
///
/// 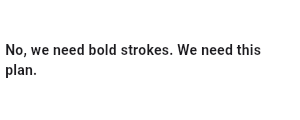
///
/// ```dart
/// const Text(
/// 'No, we need bold strokes. We need this plan.',
/// style: TextStyle(fontWeight: FontWeight.bold),
/// )
/// ```
/// {@end-tool}
///
/// ### Italics
///
/// {@tool snippet}
/// As in the previous example, the [Text] widget is given a specific style
/// override which is implicitly mixed with the ambient [DefaultTextStyle].
///
/// 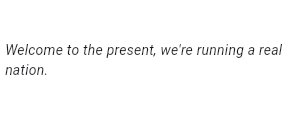
///
/// ```dart
/// const Text(
/// "Welcome to the present, we're running a real nation.",
/// style: TextStyle(fontStyle: FontStyle.italic),
/// )
/// ```
/// {@end-tool}
///
/// ### Opacity and Color
///
/// Each line here is progressively more opaque. The base color is
/// [material.Colors.black], and [Color.withOpacity] is used to create a
/// derivative color with the desired opacity. The root [TextSpan] for this
/// [RichText] widget is explicitly given the ambient [DefaultTextStyle], since
/// [RichText] does not do that automatically. The inner [TextStyle] objects are
/// implicitly mixed with the parent [TextSpan]'s [TextSpan.style].
///
/// If [color] is specified, [foreground] must be null and vice versa. [color] is
/// treated as a shorthand for `Paint()..color = color`.
///
/// If [backgroundColor] is specified, [background] must be null and vice versa.
/// The [backgroundColor] is treated as a shorthand for
/// `background: Paint()..color = backgroundColor`.
///
/// 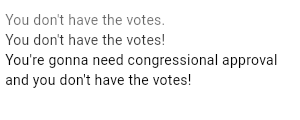
///
/// ```dart
/// RichText(
/// text: TextSpan(
/// style: DefaultTextStyle.of(context).style,
/// children: <TextSpan>[
/// TextSpan(
/// text: "You don't have the votes.\n",
/// style: TextStyle(color: Colors.black.withOpacity(0.6)),
/// ),
/// TextSpan(
/// text: "You don't have the votes!\n",
/// style: TextStyle(color: Colors.black.withOpacity(0.8)),
/// ),
/// TextSpan(
/// text: "You're gonna need congressional approval and you don't have the votes!\n",
/// style: TextStyle(color: Colors.black.withOpacity(1.0)),
/// ),
/// ],
/// ),
/// )
/// ```
///
/// ### Size
///
/// {@tool snippet}
/// In this example, the ambient [DefaultTextStyle] is explicitly manipulated to
/// obtain a [TextStyle] that doubles the default font size.
///
/// 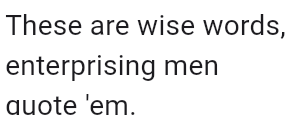
///
/// ```dart
/// Text(
/// "These are wise words, enterprising men quote 'em.",
/// style: DefaultTextStyle.of(context).style.apply(fontSizeFactor: 2.0),
/// )
/// ```
/// {@end-tool}
///
/// ### Line height
///
/// By default, text will layout with line height as defined by the font.
/// Font-metrics defined line height may be taller or shorter than the font size.
/// The [height] property allows manual adjustment of the height of the line as
/// a multiple of [fontSize]. For most fonts, setting [height] to 1.0 is not
/// the same as omitting or setting height to null. The following diagram
/// illustrates the difference between the font-metrics-defined line height and
/// the line height produced with `height: 1.0` (also known as the EM-square):
///
/// 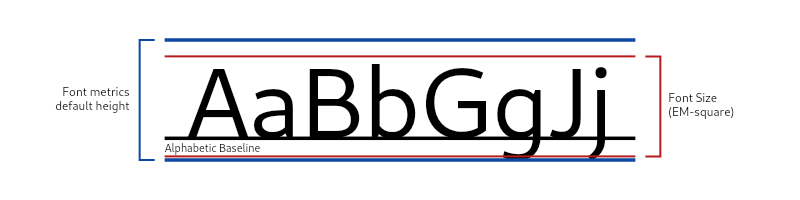
///
/// {@tool snippet}
/// The [height] property can be used to change the line height. Here, the line
/// height is set to 5 times the font size, so that the text is very spaced out.
/// Since the `fontSize` is set to 10, the final height of the line is
/// 50 pixels.
///
/// ```dart
/// const Text(
/// 'Ladies and gentlemen, you coulda been anywhere in the world tonight, but you’re here with us in New York City.',
/// style: TextStyle(height: 5, fontSize: 10),
/// )
/// ```
/// {@end-tool}
///
/// Examples of the resulting heights from different values of `TextStyle.height`:
///
/// 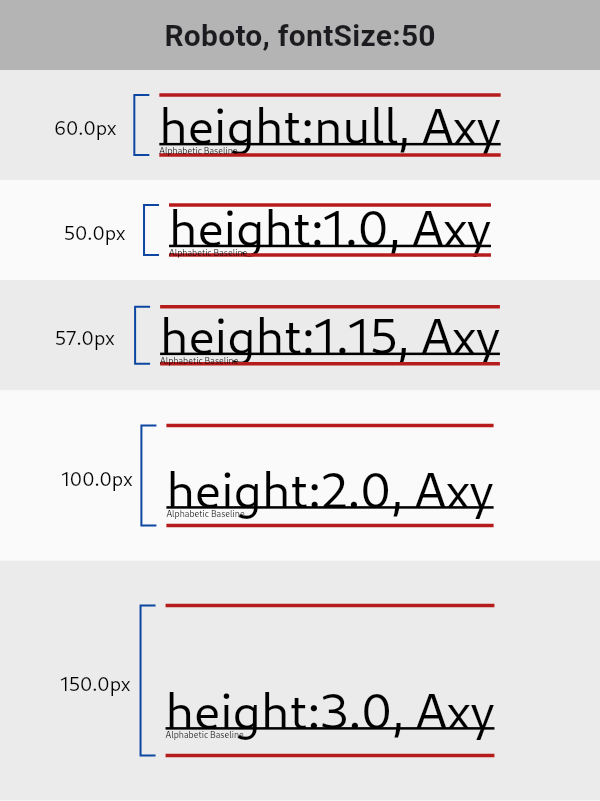
///
/// See [StrutStyle] for further control of line height at the paragraph level.
///
/// ### Leading Distribution and Trimming
///
/// [Leading](https://en.wikipedia.org/wiki/Leading) is the vertical space
/// between glyphs from adjacent lines. Quantitatively, it is the line height
/// (see the previous section) subtracted by the font's ascent and descent.
/// It's possible to have a negative `Leading` if [height] is sufficiently
/// small.
///
/// When the [height] multiplier is null, `leading` and how it is distributed
/// is up to the font's
/// [metrics](https://en.wikipedia.org/wiki/Typeface#Font_metrics).
/// When the [height] multiplier is specified, the exact behavior can be
/// configured via [leadingDistribution] and [TextPainter.textHeightBehavior].
///
/// 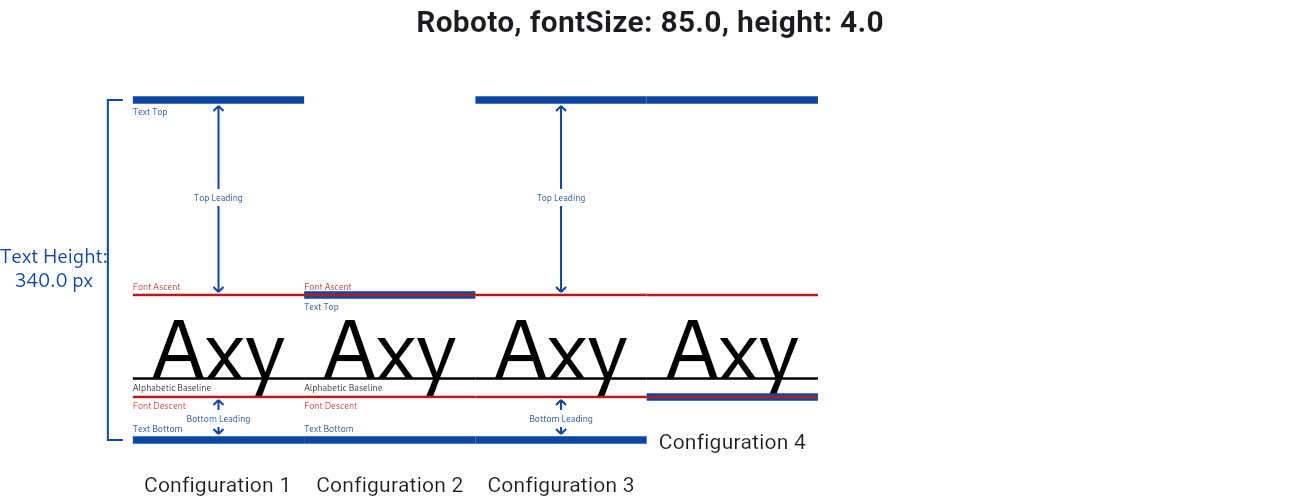
///
/// Above is a side-by-side comparison of different [leadingDistribution] and
/// [TextPainter.textHeightBehavior] combinations.
///
/// * Configuration 1: The default. [leadingDistribution] is set to [TextLeadingDistribution.proportional].
/// * Configuration 2: same as Configuration 1, except [TextHeightBehavior.applyHeightToFirstAscent] is set to false.
/// * Configuration 3: [leadingDistribution] is set to [TextLeadingDistribution.even].
/// * Configuration 4: same as Configuration 3, except [TextHeightBehavior.applyHeightToLastDescent] is set to false.
///
/// The [leadingDistribution] property controls how leading is distributed over
/// and under the text. With [TextLeadingDistribution.proportional]
/// (Configuration 1), `Top Leading : Bottom Leading = Font Ascent : Font
/// Descent`, which also means the alphabetic baseline divides the line height
/// into 2 parts proportional to the font's ascent and descent. With
/// [TextLeadingDistribution.even] (Configuration 3), `Top Leading` equals
/// `Bottom Leading`, and the glyphs are roughly centered within the allotted
/// line height.
///
/// The [TextPainter.textHeightBehavior] is a property that controls leading at
/// the paragraph level. The `applyHeightToFirstAscent` property is applied
/// **after** [height] and [leadingDistribution]. Setting it to false trims the
/// "Top Leading" of the text box to match the font's ascent if it's on the
/// first line (see Configuration 2). Similarly setting
/// `applyHeightToLastDescent` to false reduces "Bottom Leading" to 0 for the
/// last line of text (Configuration 4).
///
/// ### Wavy red underline with black text
///
/// {@tool snippet}
/// Styles can be combined. In this example, the misspelled word is drawn in
/// black text and underlined with a wavy red line to indicate a spelling error.
/// (The remainder is styled according to the Flutter default text styles, not
/// the ambient [DefaultTextStyle], since no explicit style is given and
/// [RichText] does not automatically use the ambient [DefaultTextStyle].)
///
/// 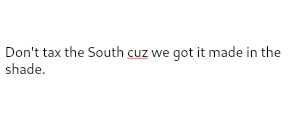
///
/// ```dart
/// RichText(
/// text: const TextSpan(
/// text: "Don't tax the South ",
/// children: <TextSpan>[
/// TextSpan(
/// text: 'cuz',
/// style: TextStyle(
/// color: Colors.black,
/// decoration: TextDecoration.underline,
/// decorationColor: Colors.red,
/// decorationStyle: TextDecorationStyle.wavy,
/// ),
/// ),
/// TextSpan(
/// text: ' we got it made in the shade',
/// ),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ### Borders and stroke (Foreground)
///
/// {@tool snippet}
/// To create bordered text, a [Paint] with [Paint.style] set to [PaintingStyle.stroke]
/// should be provided as a [foreground] paint. The following example uses a [Stack]
/// to produce a stroke and fill effect.
///
/// 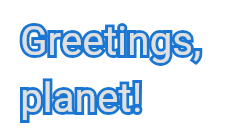
///
/// ```dart
/// Stack(
/// children: <Widget>[
/// // Stroked text as border.
/// Text(
/// 'Greetings, planet!',
/// style: TextStyle(
/// fontSize: 40,
/// foreground: Paint()
/// ..style = PaintingStyle.stroke
/// ..strokeWidth = 6
/// ..color = Colors.blue[700]!,
/// ),
/// ),
/// // Solid text as fill.
/// Text(
/// 'Greetings, planet!',
/// style: TextStyle(
/// fontSize: 40,
/// color: Colors.grey[300],
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// ### Gradients (Foreground)
///
/// {@tool snippet}
/// The [foreground] property also allows effects such as gradients to be
/// applied to the text. Here we provide a [Paint] with a [ui.Gradient]
/// shader.
///
/// 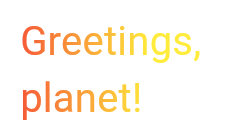
///
/// ```dart
/// Text(
/// 'Greetings, planet!',
/// style: TextStyle(
/// fontSize: 40,
/// foreground: Paint()
/// ..shader = ui.Gradient.linear(
/// const Offset(0, 20),
/// const Offset(150, 20),
/// <Color>[
/// Colors.red,
/// Colors.yellow,
/// ],
/// )
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ### Custom Fonts
///
/// Custom fonts can be declared in the `pubspec.yaml` file as shown below:
///
/// ```yaml
/// flutter:
/// fonts:
/// - family: Raleway
/// fonts:
/// - asset: fonts/Raleway-Regular.ttf
/// - asset: fonts/Raleway-Medium.ttf
/// weight: 500
/// - asset: assets/fonts/Raleway-SemiBold.ttf
/// weight: 600
/// - family: Schyler
/// fonts:
/// - asset: fonts/Schyler-Regular.ttf
/// - asset: fonts/Schyler-Italic.ttf
/// style: italic
/// ```
///
/// The `family` property determines the name of the font, which you can use in
/// the [fontFamily] argument. The `asset` property is a path to the font file,
/// relative to the `pubspec.yaml` file. The `weight` property specifies the
/// weight of the glyph outlines in the file as an integer multiple of 100
/// between 100 and 900. This corresponds to the [FontWeight] class and can be
/// used in the [fontWeight] argument. The `style` property specifies whether the
/// outlines in the file are `italic` or `normal`. These values correspond to
/// the [FontStyle] class and can be used in the [fontStyle] argument.
///
/// To select a custom font, create [TextStyle] using the [fontFamily]
/// argument as shown in the example below:
///
/// {@tool snippet}
/// 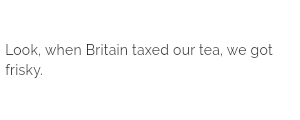
///
/// ```dart
/// const TextStyle(fontFamily: 'Raleway')
/// ```
/// {@end-tool}
///
/// To use a font family defined in a package, the `package` argument must be
/// provided. For instance, suppose the font declaration above is in the
/// `pubspec.yaml` of a package named `my_package` which the app depends on.
/// Then creating the TextStyle is done as follows:
///
/// ```dart
/// const TextStyle(fontFamily: 'Raleway', package: 'my_package')
/// ```
///
/// If the package internally uses the font it defines, it should still specify
/// the `package` argument when creating the text style as in the example above.
///
/// A package can also provide font files without declaring a font in its
/// `pubspec.yaml`. These files should then be in the `lib/` folder of the
/// package. The font files will not automatically be bundled in the app, instead
/// the app can use these selectively when declaring a font. Suppose a package
/// named `my_package` has:
///
/// lib/fonts/Raleway-Medium.ttf
///
/// Then the app can declare a font like in the example below:
///
/// ```yaml
/// flutter:
/// fonts:
/// - family: Raleway
/// fonts:
/// - asset: assets/fonts/Raleway-Regular.ttf
/// - asset: packages/my_package/fonts/Raleway-Medium.ttf
/// weight: 500
/// ```
///
/// The `lib/` is implied, so it should not be included in the asset path.
///
/// In this case, since the app locally defines the font, the TextStyle is
/// created without the `package` argument:
///
/// {@tool snippet}
/// ```dart
/// const TextStyle(fontFamily: 'Raleway')
/// ```
/// {@end-tool}
///
/// #### Supported font formats
///
/// Font formats currently supported by Flutter:
///
/// * `.ttc`
/// * `.ttf`
/// * `.otf`
///
/// Flutter does not support `.woff` and `.woff2` fonts for all platforms.
///
/// ### Custom Font Fallback
///
/// A custom [fontFamilyFallback] list can be provided. The list should be an
/// ordered list of strings of font family names in the order they will be attempted.
///
/// The fonts in [fontFamilyFallback] will be used only if the requested glyph is
/// not present in the [fontFamily].
///
/// The fallback order is:
///
/// * [fontFamily]
/// * [fontFamilyFallback] in order of first to last.
/// * System fallback fonts which will vary depending on platform.
///
/// The glyph used will always be the first matching version in fallback order.
///
/// The [fontFamilyFallback] property is commonly used to specify different font
/// families for multilingual text spans as well as separate fonts for glyphs such
/// as emojis.
///
/// {@tool snippet}
/// In the following example, any glyphs not present in the font `Raleway` will be attempted
/// to be resolved with `Noto Sans CJK SC`, and then with `Noto Color Emoji`:
///
/// ```dart
/// const TextStyle(
/// fontFamily: 'Raleway',
/// fontFamilyFallback: <String>[
/// 'Noto Sans CJK SC',
/// 'Noto Color Emoji',
/// ],
/// )
/// ```
/// {@end-tool}
///
/// If all custom fallback font families are exhausted and no match was found
/// or no custom fallback was provided, the platform font fallback will be used.
///
/// ### Inconsistent platform fonts
///
/// By default, fonts differ depending on the platform.
///
/// * The default font-family for `Android`,`Fuchsia` and `Linux` is `Roboto`.
/// * The default font-family for `iOS` is `SF Pro Display`/`SF Pro Text`.
/// * The default font-family for `MacOS` is `.AppleSystemUIFont`.
/// * The default font-family for `Windows` is `Segoe UI`.
//
// The implementation of these defaults can be found in:
// /packages/flutter/lib/src/material/typography.dart
///
/// Since Flutter's font discovery for default fonts depends on the fonts present
/// on the device, it is not safe to assume all default fonts will be available or
/// consistent across devices.
///
/// A known example of this is that Samsung devices ship with a CJK font that has
/// smaller line spacing than the Android default. This results in Samsung devices
/// displaying more tightly spaced text than on other Android devices when no
/// custom font is specified.
///
/// To avoid this, a custom font should be specified if absolute font consistency
/// is required for your application.
///
/// See also:
///
/// * [Text], the widget for showing text in a single style.
/// * [DefaultTextStyle], the widget that specifies the default text styles for
/// [Text] widgets, configured using a [TextStyle].
/// * [RichText], the widget for showing a paragraph of mix-style text.
/// * [TextSpan], the class that wraps a [TextStyle] for the purposes of
/// passing it to a [RichText].
/// * [TextStyle](https://api.flutter.dev/flutter/dart-ui/TextStyle-class.html), the class in the [dart:ui] library.
/// * Cookbook: [Use a custom font](https://flutter.dev/docs/cookbook/design/fonts)
/// * Cookbook: [Use themes to share colors and font styles](https://flutter.dev/docs/cookbook/design/themes)
@immutable
class TextStyle with Diagnosticable {
/// Creates a text style.
///
/// The `package` argument must be non-null if the font family is defined in a
/// package. It is combined with the `fontFamily` argument to set the
/// [fontFamily] property.
///
/// On Apple devices the strings 'CupertinoSystemText' and
/// 'CupertinoSystemDisplay' are used in [fontFamily] as proxies for the
/// Apple system fonts. They currently redirect to the equivalent of SF Pro
/// Text and SF Pro Display respectively. 'CupertinoSystemText' is designed
/// for fonts below 20 point size, and 'CupertinoSystemDisplay' is recommended
/// for sizes 20 and above. When used on non-Apple platforms, these strings
/// will return the regular fallback font family instead.
const TextStyle({
this.inherit = true,
this.color,
this.backgroundColor,
this.fontSize,
this.fontWeight,
this.fontStyle,
this.letterSpacing,
this.wordSpacing,
this.textBaseline,
this.height,
this.leadingDistribution,
this.locale,
this.foreground,
this.background,
this.shadows,
this.fontFeatures,
this.fontVariations,
this.decoration,
this.decorationColor,
this.decorationStyle,
this.decorationThickness,
this.debugLabel,
String? fontFamily,
List<String>? fontFamilyFallback,
String? package,
this.overflow,
}) : fontFamily = package == null ? fontFamily : 'packages/$package/$fontFamily',
_fontFamilyFallback = fontFamilyFallback,
_package = package,
assert(color == null || foreground == null, _kColorForegroundWarning),
assert(backgroundColor == null || background == null, _kColorBackgroundWarning);
/// Whether null values in this [TextStyle] can be replaced with their value
/// in another [TextStyle] using [merge].
///
/// The [merge] operation is not commutative: the [inherit] value of the
/// method argument decides whether the two [TextStyle]s can be combined
/// together. If it is false, the method argument [TextStyle] will be returned.
/// Otherwise, the combining is allowed, and the returned [TextStyle] inherits
/// the [inherit] value from the method receiver.
///
/// This property does not affect the text style inheritance in an [InlineSpan]
/// tree: an [InlineSpan]'s text style is merged with that of an ancestor
/// [InlineSpan] if it has unspecified fields, regardless of its [inherit]
/// value.
///
/// Properties that don't have explicit values or other default values to fall
/// back to will revert to the defaults: white in color, a font size of 14
/// pixels, in a sans-serif font face.
///
/// See also:
/// * [TextStyle.merge], which can be used to combine properties from two
/// [TextStyle]s.
final bool inherit;
/// The color to use when painting the text.
///
/// If [foreground] is specified, this value must be null. The [color] property
/// is shorthand for `Paint()..color = color`.
///
/// In [merge], [apply], and [lerp], conflicts between [color] and [foreground]
/// specification are resolved in [foreground]'s favor - i.e. if [foreground] is
/// specified in one place, it will dominate [color] in another.
final Color? color;
/// The color to use as the background for the text.
///
/// If [background] is specified, this value must be null. The
/// [backgroundColor] property is shorthand for
/// `background: Paint()..color = backgroundColor`.
///
/// In [merge], [apply], and [lerp], conflicts between [backgroundColor] and [background]
/// specification are resolved in [background]'s favor - i.e. if [background] is
/// specified in one place, it will dominate [color] in another.
final Color? backgroundColor;
/// The name of the font to use when painting the text (e.g., Roboto).
///
/// If the font is defined in a package, this will be prefixed with
/// 'packages/package_name/' (e.g. 'packages/cool_fonts/Roboto'). The
/// prefixing is done by the constructor when the `package` argument is
/// provided.
///
/// The value provided in [fontFamily] will act as the preferred/first font
/// family that glyphs are looked for in, followed in order by the font families
/// in [fontFamilyFallback]. When [fontFamily] is null or not provided, the
/// first value in [fontFamilyFallback] acts as the preferred/first font
/// family. When neither is provided, then the default platform font will
/// be used.
///
/// When running on Apple devices, the strings 'CupertinoSystemText' and
/// 'CupertinoSystemDisplay' are used as proxies for the Apple system fonts.
/// They currently redirect to the equivalent of SF Pro Text and SF Pro Display
/// respectively. 'CupertinoSystemText' is designed for fonts below 20 point
/// size, and 'CupertinoSystemDisplay' is recommended for sizes 20 and above.
/// When used on non-Apple platforms, these strings will return the regular
/// fallback font family instead.
final String? fontFamily;
/// The ordered list of font families to fall back on when a glyph cannot be
/// found in a higher priority font family.
///
/// The value provided in [fontFamily] will act as the preferred/first font
/// family that glyphs are looked for in, followed in order by the font families
/// in [fontFamilyFallback]. If all font families are exhausted and no match
/// was found, the default platform font family will be used instead.
///
/// When [fontFamily] is null or not provided, the first value in [fontFamilyFallback]
/// acts as the preferred/first font family. When neither is provided, then
/// the default platform font will be used. Providing an empty list or null
/// for this property is the same as omitting it.
///
/// For example, if a glyph is not found in [fontFamily], then each font family
/// in [fontFamilyFallback] will be searched in order until it is found. If it
/// is not found, then a box will be drawn in its place.
///
/// If the font is defined in a package, each font family in the list will be
/// prefixed with 'packages/package_name/' (e.g. 'packages/cool_fonts/Roboto').
/// The package name should be provided by the `package` argument in the
/// constructor.
List<String>? get fontFamilyFallback => _package == null ? _fontFamilyFallback : _fontFamilyFallback?.map((String str) => 'packages/$_package/$str').toList();
final List<String>? _fontFamilyFallback;
// This is stored in order to prefix the fontFamilies in _fontFamilyFallback
// in the [fontFamilyFallback] getter.
final String? _package;
/// The size of fonts (in logical pixels) to use when painting the text.
///
/// The value specified matches the dimension of the
/// [em square](https://fonts.google.com/knowledge/glossary/em) of the
/// underlying font, and more often then not isn't exactly the height or the
/// width of glyphs in the font.
///
/// During painting, the [fontSize] is multiplied by the current
/// `textScaleFactor` to let users make it easier to read text by increasing
/// its size.
///
/// The [getParagraphStyle] method defaults to 14 logical pixels if [fontSize]
/// is set to null.
final double? fontSize;
/// The typeface thickness to use when painting the text (e.g., bold).
final FontWeight? fontWeight;
/// The typeface variant to use when drawing the letters (e.g., italics).
final FontStyle? fontStyle;
/// The amount of space (in logical pixels) to add between each letter.
/// A negative value can be used to bring the letters closer.
final double? letterSpacing;
/// The amount of space (in logical pixels) to add at each sequence of
/// white-space (i.e. between each word). A negative value can be used to
/// bring the words closer.
final double? wordSpacing;
/// The common baseline that should be aligned between this text span and its
/// parent text span, or, for the root text spans, with the line box.
final TextBaseline? textBaseline;
/// The height of this text span, as a multiple of the font size.
///
/// When [height] is null or omitted, the line height will be determined
/// by the font's metrics directly, which may differ from the fontSize.
/// When [height] is non-null, the line height of the span of text will be a
/// multiple of [fontSize] and be exactly `fontSize * height` logical pixels
/// tall.
///
/// For most fonts, setting [height] to 1.0 is not the same as omitting or
/// setting height to null because the [fontSize] sets the height of the EM-square,
/// which is different than the font provided metrics for line height. The
/// following diagram illustrates the difference between the font-metrics
/// defined line height and the line height produced with `height: 1.0`
/// (which forms the upper and lower edges of the EM-square):
///
/// 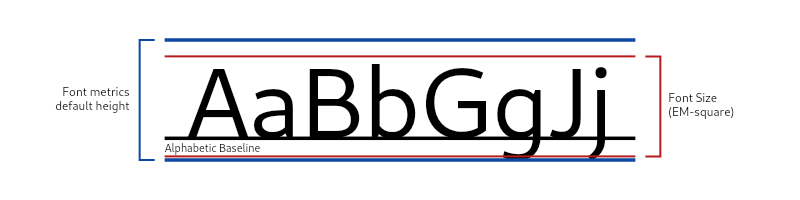
///
/// Examples of the resulting line heights from different values of `TextStyle.height`:
///
/// 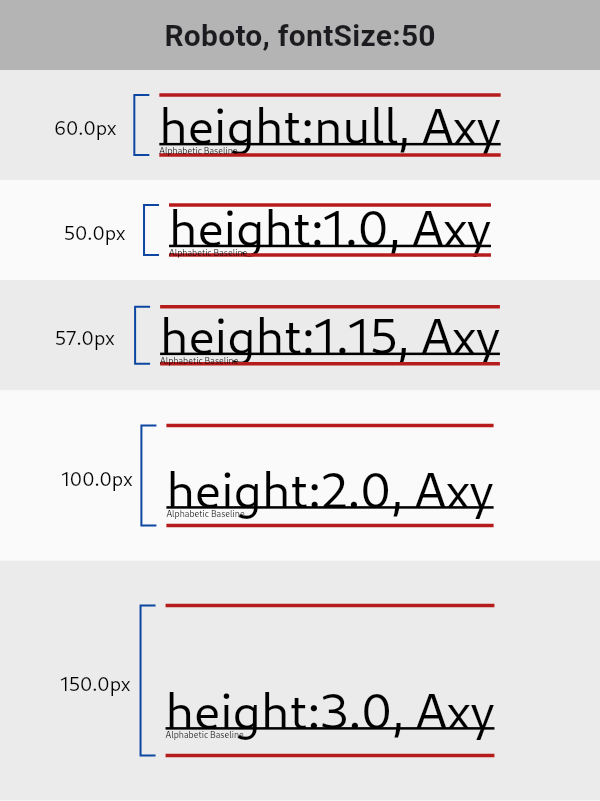
///
/// See [StrutStyle] and [TextHeightBehavior] for further control of line
/// height at the paragraph level.
final double? height;
/// How the vertical space added by the [height] multiplier should be
/// distributed over and under the text.
///
/// When a non-null [height] is specified, after accommodating the glyphs of
/// the text, the remaining vertical space from the allotted line height will
/// be distributed over and under the text, according to the
/// [leadingDistribution] property. See the [TextStyle] class's documentation
/// for an example.
///
/// When [height] is null, [leadingDistribution] does not affect the text
/// layout.
///
/// Defaults to null, which defers to the paragraph's
/// `ParagraphStyle.textHeightBehavior`'s [leadingDistribution].
final TextLeadingDistribution? leadingDistribution;
/// The locale used to select region-specific glyphs.
///
/// This property is rarely set. Typically the locale used to select
/// region-specific glyphs is defined by the text widget's [BuildContext]
/// using `Localizations.localeOf(context)`. For example [RichText] defines
/// its locale this way. However, a rich text widget's [TextSpan]s could
/// specify text styles with different explicit locales in order to select
/// different region-specific glyphs for each text span.
final Locale? locale;
/// The paint drawn as a foreground for the text.
///
/// The value should ideally be cached and reused each time if multiple text
/// styles are created with the same paint settings. Otherwise, each time it
/// will appear like the style changed, which will result in unnecessary
/// updates all the way through the framework.
///
/// If [color] is specified, this value must be null. The [color] property
/// is shorthand for `Paint()..color = color`.
///
/// In [merge], [apply], and [lerp], conflicts between [color] and [foreground]
/// specification are resolved in [foreground]'s favor - i.e. if [foreground] is
/// specified in one place, it will dominate [color] in another.
final Paint? foreground;
/// The paint drawn as a background for the text.
///
/// The value should ideally be cached and reused each time if multiple text
/// styles are created with the same paint settings. Otherwise, each time it
/// will appear like the style changed, which will result in unnecessary
/// updates all the way through the framework.
///
/// If [backgroundColor] is specified, this value must be null. The
/// [backgroundColor] property is shorthand for
/// `background: Paint()..color = backgroundColor`.
///
/// In [merge], [apply], and [lerp], conflicts between [backgroundColor] and
/// [background] specification are resolved in [background]'s favor - i.e. if
/// [background] is specified in one place, it will dominate [backgroundColor]
/// in another.
final Paint? background;
/// The decorations to paint near the text (e.g., an underline).
///
/// Multiple decorations can be applied using [TextDecoration.combine].
final TextDecoration? decoration;
/// The color in which to paint the text decorations.
final Color? decorationColor;
/// The style in which to paint the text decorations (e.g., dashed).
final TextDecorationStyle? decorationStyle;
/// The thickness of the decoration stroke as a multiplier of the thickness
/// defined by the font.
///
/// The font provides a base stroke width for [decoration]s which scales off
/// of the [fontSize]. This property may be used to achieve a thinner or
/// thicker decoration stroke, without changing the [fontSize]. For example,
/// a [decorationThickness] of 2.0 will draw a decoration twice as thick as
/// the font defined decoration thickness.
///
/// {@tool snippet}
/// To achieve a bolded strike-through, we can apply a thicker stroke for the
/// decoration.
///
/// ```dart
/// const Text(
/// 'This has a very BOLD strike through!',
/// style: TextStyle(
/// decoration: TextDecoration.lineThrough,
/// decorationThickness: 2.85,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// We can apply a very thin and subtle wavy underline (perhaps, when words
/// are misspelled) by using a [decorationThickness] < 1.0.
///
/// ```dart
/// const Text(
/// 'oopsIforgottousespaces!',
/// style: TextStyle(
/// decoration: TextDecoration.underline,
/// decorationStyle: TextDecorationStyle.wavy,
/// decorationColor: Colors.red,
/// decorationThickness: 0.5,
/// ),
/// )
/// ```
/// {@end-tool}
///
/// The default [decorationThickness] is 1.0, which will use the font's base
/// stroke thickness/width.
final double? decorationThickness;
/// A human-readable description of this text style.
///
/// This property is maintained only in debug builds.
///
/// When merging ([merge]), copying ([copyWith]), modifying using [apply], or
/// interpolating ([lerp]), the label of the resulting style is marked with
/// the debug labels of the original styles. This helps figuring out where a
/// particular text style came from.
///
/// This property is not considered when comparing text styles using `==` or
/// [compareTo], and it does not affect [hashCode].
final String? debugLabel;
/// A list of [Shadow]s that will be painted underneath the text.
///
/// Multiple shadows are supported to replicate lighting from multiple light
/// sources.
///
/// Shadows must be in the same order for [TextStyle] to be considered as
/// equivalent as order produces differing transparency.
final List<Shadow>? shadows;
/// A list of [FontFeature]s that affect how the font selects glyphs.
///
/// Some fonts support multiple variants of how a given character can be
/// rendered. For example, a font might provide both proportional and
/// tabular numbers, or it might offer versions of the zero digit with
/// and without slashes. [FontFeature]s can be used to select which of
/// these variants will be used for rendering.
///
/// Font features are not interpolated by [lerp].
///
/// See also:
///
/// * [fontVariations], for font features that have continuous parameters.
final List<FontFeature>? fontFeatures;
/// A list of [FontVariation]s that affect how a variable font is rendered.
///
/// Some fonts are variable fonts that can generate multiple font faces based
/// on the values of customizable attributes. For example, a variable font
/// may have a weight axis that can be set to a value between 1 and 1000.
/// [FontVariation]s can be used to select the values of these design axes.
///
/// For example, to control the weight axis of the Roboto Slab variable font
/// (https://fonts.google.com/specimen/Roboto+Slab):
/// ```dart
/// const TextStyle(
/// fontFamily: 'RobotoSlab',
/// fontVariations: <FontVariation>[FontVariation('wght', 900.0)]
/// )
/// ```
///
/// Font variations can be interpolated via [lerp]. This is fastest when the
/// same font variation axes are specified, in the same order, in both
/// [TextStyle] objects. See [lerpFontVariations].
///
/// See also:
///
/// * [fontFeatures], for font variations that have discrete values.
final List<FontVariation>? fontVariations;
/// How visual text overflow should be handled.
final TextOverflow? overflow;
// Return the original value of fontFamily, without the additional
// "packages/$_package/" prefix.
String? get _fontFamily {
if (_package != null) {
final String fontFamilyPrefix = 'packages/$_package/';
assert(fontFamily?.startsWith(fontFamilyPrefix) ?? true);
return fontFamily?.substring(fontFamilyPrefix.length);
}
return fontFamily;
}
/// Creates a copy of this text style but with the given fields replaced with
/// the new values.
///
/// One of [color] or [foreground] must be null, and if this has [foreground]
/// specified it will be given preference over any color parameter.
///
/// One of [backgroundColor] or [background] must be null, and if this has
/// [background] specified it will be given preference over any
/// backgroundColor parameter.
TextStyle copyWith({
bool? inherit,
Color? color,
Color? backgroundColor,
double? fontSize,
FontWeight? fontWeight,
FontStyle? fontStyle,
double? letterSpacing,
double? wordSpacing,
TextBaseline? textBaseline,
double? height,
TextLeadingDistribution? leadingDistribution,
Locale? locale,
Paint? foreground,
Paint? background,
List<Shadow>? shadows,
List<FontFeature>? fontFeatures,
List<FontVariation>? fontVariations,
TextDecoration? decoration,
Color? decorationColor,
TextDecorationStyle? decorationStyle,
double? decorationThickness,
String? debugLabel,
String? fontFamily,
List<String>? fontFamilyFallback,
String? package,
TextOverflow? overflow,
}) {
assert(color == null || foreground == null, _kColorForegroundWarning);
assert(backgroundColor == null || background == null, _kColorBackgroundWarning);
String? newDebugLabel;
assert(() {
if (debugLabel != null) {
newDebugLabel = debugLabel;
} else if (this.debugLabel != null) {
newDebugLabel = '(${this.debugLabel}).copyWith';
}
return true;
}());
return TextStyle(
inherit: inherit ?? this.inherit,
color: this.foreground == null && foreground == null ? color ?? this.color : null,
backgroundColor: this.background == null && background == null ? backgroundColor ?? this.backgroundColor : null,
fontSize: fontSize ?? this.fontSize,
fontWeight: fontWeight ?? this.fontWeight,
fontStyle: fontStyle ?? this.fontStyle,
letterSpacing: letterSpacing ?? this.letterSpacing,
wordSpacing: wordSpacing ?? this.wordSpacing,
textBaseline: textBaseline ?? this.textBaseline,
height: height ?? this.height,
leadingDistribution: leadingDistribution ?? this.leadingDistribution,
locale: locale ?? this.locale,
foreground: foreground ?? this.foreground,
background: background ?? this.background,
shadows: shadows ?? this.shadows,
fontFeatures: fontFeatures ?? this.fontFeatures,
fontVariations: fontVariations ?? this.fontVariations,
decoration: decoration ?? this.decoration,
decorationColor: decorationColor ?? this.decorationColor,
decorationStyle: decorationStyle ?? this.decorationStyle,
decorationThickness: decorationThickness ?? this.decorationThickness,
debugLabel: newDebugLabel,
fontFamily: fontFamily ?? _fontFamily,
fontFamilyFallback: fontFamilyFallback ?? _fontFamilyFallback,
package: package ?? _package,
overflow: overflow ?? this.overflow,
);
}
/// Creates a copy of this text style replacing or altering the specified
/// properties.
///
/// The non-numeric properties [color], [fontFamily], [decoration],
/// [decorationColor] and [decorationStyle] are replaced with the new values.
///
/// [foreground] will be given preference over [color] if it is not null and
/// [background] will be given preference over [backgroundColor] if it is not
/// null.
///
/// The numeric properties are multiplied by the given factors and then
/// incremented by the given deltas.
///
/// For example, `style.apply(fontSizeFactor: 2.0, fontSizeDelta: 1.0)` would
/// return a [TextStyle] whose [fontSize] is `style.fontSize * 2.0 + 1.0`.
///
/// For the [fontWeight], the delta is applied to the [FontWeight] enum index
/// values, so that for instance `style.apply(fontWeightDelta: -2)` when
/// applied to a `style` whose [fontWeight] is [FontWeight.w500] will return a
/// [TextStyle] with a [FontWeight.w300].
///
/// If the underlying values are null, then the corresponding factors and/or
/// deltas must not be specified.
///
/// If [foreground] is specified on this object, then applying [color] here
/// will have no effect and if [background] is specified on this object, then
/// applying [backgroundColor] here will have no effect either.
TextStyle apply({
Color? color,
Color? backgroundColor,
TextDecoration? decoration,
Color? decorationColor,
TextDecorationStyle? decorationStyle,
double decorationThicknessFactor = 1.0,
double decorationThicknessDelta = 0.0,
String? fontFamily,
List<String>? fontFamilyFallback,
double fontSizeFactor = 1.0,
double fontSizeDelta = 0.0,
int fontWeightDelta = 0,
FontStyle? fontStyle,
double letterSpacingFactor = 1.0,
double letterSpacingDelta = 0.0,
double wordSpacingFactor = 1.0,
double wordSpacingDelta = 0.0,
double heightFactor = 1.0,
double heightDelta = 0.0,
TextBaseline? textBaseline,
TextLeadingDistribution? leadingDistribution,
Locale? locale,
List<Shadow>? shadows,
List<FontFeature>? fontFeatures,
List<FontVariation>? fontVariations,
String? package,
TextOverflow? overflow,
}) {
assert(fontSize != null || (fontSizeFactor == 1.0 && fontSizeDelta == 0.0));
assert(fontWeight != null || fontWeightDelta == 0.0);
assert(letterSpacing != null || (letterSpacingFactor == 1.0 && letterSpacingDelta == 0.0));
assert(wordSpacing != null || (wordSpacingFactor == 1.0 && wordSpacingDelta == 0.0));
assert(decorationThickness != null || (decorationThicknessFactor == 1.0 && decorationThicknessDelta == 0.0));
String? modifiedDebugLabel;
assert(() {
if (debugLabel != null) {
modifiedDebugLabel = '($debugLabel).apply';
}
return true;
}());
return TextStyle(
inherit: inherit,
color: foreground == null ? color ?? this.color : null,
backgroundColor: background == null ? backgroundColor ?? this.backgroundColor : null,
fontFamily: fontFamily ?? _fontFamily,
fontFamilyFallback: fontFamilyFallback ?? _fontFamilyFallback,
fontSize: fontSize == null ? null : fontSize! * fontSizeFactor + fontSizeDelta,
fontWeight: fontWeight == null ? null : FontWeight.values[(fontWeight!.index + fontWeightDelta).clamp(0, FontWeight.values.length - 1)],
fontStyle: fontStyle ?? this.fontStyle,
letterSpacing: letterSpacing == null ? null : letterSpacing! * letterSpacingFactor + letterSpacingDelta,
wordSpacing: wordSpacing == null ? null : wordSpacing! * wordSpacingFactor + wordSpacingDelta,
textBaseline: textBaseline ?? this.textBaseline,
height: height == null ? null : height! * heightFactor + heightDelta,
leadingDistribution: leadingDistribution ?? this.leadingDistribution,
locale: locale ?? this.locale,
foreground: foreground,
background: background,
shadows: shadows ?? this.shadows,
fontFeatures: fontFeatures ?? this.fontFeatures,
fontVariations: fontVariations ?? this.fontVariations,
decoration: decoration ?? this.decoration,
decorationColor: decorationColor ?? this.decorationColor,
decorationStyle: decorationStyle ?? this.decorationStyle,
decorationThickness: decorationThickness == null ? null : decorationThickness! * decorationThicknessFactor + decorationThicknessDelta,
overflow: overflow ?? this.overflow,
package: package ?? _package,
debugLabel: modifiedDebugLabel,
);
}
/// Returns a new text style that is a combination of this style and the given
/// [other] style.
///
/// If the given [other] text style has its [TextStyle.inherit] set to true,
/// its null properties are replaced with the non-null properties of this text
/// style. The [other] style _inherits_ the properties of this style. Another
/// way to think of it is that the "missing" properties of the [other] style
/// are _filled_ by the properties of this style.
///
/// If the given [other] text style has its [TextStyle.inherit] set to false,
/// returns the given [other] style unchanged. The [other] style does not
/// inherit properties of this style.
///
/// If the given text style is null, returns this text style.
///
/// One of [color] or [foreground] must be null, and if this or `other` has
/// [foreground] specified it will be given preference over any color parameter.
///
/// Similarly, one of [backgroundColor] or [background] must be null, and if
/// this or `other` has [background] specified it will be given preference
/// over any backgroundColor parameter.
TextStyle merge(TextStyle? other) {
if (other == null) {
return this;
}
if (!other.inherit) {
return other;
}
String? mergedDebugLabel;
assert(() {
if (other.debugLabel != null || debugLabel != null) {
mergedDebugLabel = '(${debugLabel ?? _kDefaultDebugLabel}).merge(${other.debugLabel ?? _kDefaultDebugLabel})';
}
return true;
}());
return copyWith(
color: other.color,
backgroundColor: other.backgroundColor,
fontSize: other.fontSize,
fontWeight: other.fontWeight,
fontStyle: other.fontStyle,
letterSpacing: other.letterSpacing,
wordSpacing: other.wordSpacing,
textBaseline: other.textBaseline,
height: other.height,
leadingDistribution: other.leadingDistribution,
locale: other.locale,
foreground: other.foreground,
background: other.background,
shadows: other.shadows,
fontFeatures: other.fontFeatures,
fontVariations: other.fontVariations,
decoration: other.decoration,
decorationColor: other.decorationColor,
decorationStyle: other.decorationStyle,
decorationThickness: other.decorationThickness,
debugLabel: mergedDebugLabel,
fontFamily: other._fontFamily,
fontFamilyFallback: other._fontFamilyFallback,
package: other._package,
overflow: other.overflow,
);
}
/// Interpolate between two text styles for animated transitions.
///
/// Interpolation will not work well if the styles don't specify the same fields.
/// When this happens, to keep the interpolated transition smooth, the
/// implementation uses the non-null value throughout the transition for
/// lerpable fields such as colors (for example, if one [TextStyle] specified
/// `fontSize` but the other didn't, the returned [TextStyle] will use the
/// `fontSize` from the [TextStyle] that specified it, regardless of the `t`
/// value).
///
/// This method throws when the given [TextStyle]s don't have the same
/// [inherit] value and a lerpable field is missing from both [TextStyle]s,
/// as that could result in jumpy transitions.
///
/// {@macro dart.ui.shadow.lerp}
///
/// If [foreground] is specified on either of `a` or `b`, both will be treated
/// as if they have a [foreground] paint (creating a new [Paint] if necessary
/// based on the [color] property).
///
/// If [background] is specified on either of `a` or `b`, both will be treated
/// as if they have a [background] paint (creating a new [Paint] if necessary
/// based on the [backgroundColor] property).
static TextStyle? lerp(TextStyle? a, TextStyle? b, double t) {
if (identical(a, b)) {
return a;
}
String? lerpDebugLabel;
assert(() {
lerpDebugLabel = 'lerp(${a?.debugLabel ?? _kDefaultDebugLabel} ⎯${t.toStringAsFixed(1)}→ ${b?.debugLabel ?? _kDefaultDebugLabel})';
return true;
}());
if (a == null) {
return TextStyle(
inherit: b!.inherit,
color: Color.lerp(null, b.color, t),
backgroundColor: Color.lerp(null, b.backgroundColor, t),
fontSize: t < 0.5 ? null : b.fontSize,
fontWeight: FontWeight.lerp(null, b.fontWeight, t),
fontStyle: t < 0.5 ? null : b.fontStyle,
letterSpacing: t < 0.5 ? null : b.letterSpacing,
wordSpacing: t < 0.5 ? null : b.wordSpacing,
textBaseline: t < 0.5 ? null : b.textBaseline,
height: t < 0.5 ? null : b.height,
leadingDistribution: t < 0.5 ? null : b.leadingDistribution,
locale: t < 0.5 ? null : b.locale,
foreground: t < 0.5 ? null : b.foreground,
background: t < 0.5 ? null : b.background,
shadows: t < 0.5 ? null : b.shadows,
fontFeatures: t < 0.5 ? null : b.fontFeatures,
fontVariations: lerpFontVariations(null, b.fontVariations, t),
decoration: t < 0.5 ? null : b.decoration,
decorationColor: Color.lerp(null, b.decorationColor, t),
decorationStyle: t < 0.5 ? null : b.decorationStyle,
decorationThickness: t < 0.5 ? null : b.decorationThickness,
debugLabel: lerpDebugLabel,
fontFamily: t < 0.5 ? null : b._fontFamily,
fontFamilyFallback: t < 0.5 ? null : b._fontFamilyFallback,
package: t < 0.5 ? null : b._package,
overflow: t < 0.5 ? null : b.overflow,
);
}
if (b == null) {
return TextStyle(
inherit: a.inherit,
color: Color.lerp(a.color, null, t),
backgroundColor: Color.lerp(null, a.backgroundColor, t),
fontSize: t < 0.5 ? a.fontSize : null,
fontWeight: FontWeight.lerp(a.fontWeight, null, t),
fontStyle: t < 0.5 ? a.fontStyle : null,
letterSpacing: t < 0.5 ? a.letterSpacing : null,
wordSpacing: t < 0.5 ? a.wordSpacing : null,
textBaseline: t < 0.5 ? a.textBaseline : null,
height: t < 0.5 ? a.height : null,
leadingDistribution: t < 0.5 ? a.leadingDistribution : null,
locale: t < 0.5 ? a.locale : null,
foreground: t < 0.5 ? a.foreground : null,
background: t < 0.5 ? a.background : null,
shadows: t < 0.5 ? a.shadows : null,
fontFeatures: t < 0.5 ? a.fontFeatures : null,
fontVariations: lerpFontVariations(a.fontVariations, null, t),
decoration: t < 0.5 ? a.decoration : null,
decorationColor: Color.lerp(a.decorationColor, null, t),
decorationStyle: t < 0.5 ? a.decorationStyle : null,
decorationThickness: t < 0.5 ? a.decorationThickness : null,
debugLabel: lerpDebugLabel,
fontFamily: t < 0.5 ? a._fontFamily : null,
fontFamilyFallback: t < 0.5 ? a._fontFamilyFallback : null,
package: t < 0.5 ? a._package : null,
overflow: t < 0.5 ? a.overflow : null,
);
}
assert(() {
if (a.inherit == b.inherit) {
return true;
}
final List<String> nullFields = <String>[
if (a.foreground == null && b.foreground == null && a.color == null && b.color == null) 'color',
if (a.background == null && b.background == null && a.backgroundColor == null && b.backgroundColor == null) 'backgroundColor',
if (a.fontSize == null && b.fontSize == null) 'fontSize',
if (a.letterSpacing == null && b.letterSpacing == null) 'letterSpacing',
if (a.wordSpacing == null && b.wordSpacing == null) 'wordSpacing',
if (a.height == null && b.height == null) 'height',
if (a.decorationColor == null && b.decorationColor == null) 'decorationColor',
if (a.decorationThickness == null && b.decorationThickness == null) 'decorationThickness',
];
if (nullFields.isEmpty) {
return true;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('Failed to interpolate TextStyles with different inherit values.'),
ErrorSpacer(),
ErrorDescription('The TextStyles being interpolated were:'),
a.toDiagnosticsNode(name: 'from', style: DiagnosticsTreeStyle.singleLine),
b.toDiagnosticsNode(name: 'to', style: DiagnosticsTreeStyle.singleLine),
ErrorDescription(
'The following fields are unspecified in both TextStyles:\n'
'${nullFields.map((String name) => '"$name"').join(', ')}.\n'
'When "inherit" changes during the transition, these fields may '
'observe abrupt value changes as a result, causing "jump"s in the '
'transition.'
),
ErrorSpacer(),
ErrorHint(
'In general, TextStyle.lerp only works well when both TextStyles have '
'the same "inherit" value, and specify the same fields.',
),
ErrorHint(
'If the TextStyles were directly created by you, consider bringing '
'them to parity to ensure a smooth transition.'
),
ErrorSpacer(),
ErrorHint(
'If one of the TextStyles being lerped is significantly more elaborate '
'than the other, and has "inherited" set to false, it is often because '
'it is merged with another TextStyle before being lerped. Comparing '
'the "debugLabel"s of the two TextStyles may help identify if that was '
'the case.'
),
ErrorHint(
'For example, you may see this error message when trying to lerp '
'between "ThemeData()" and "Theme.of(context)". This is because '
'TextStyles from "Theme.of(context)" are merged with TextStyles from '
'another theme and thus are more elaborate than the TextStyles from '
'"ThemeData()" (which is reflected in their "debugLabel"s -- '
'TextStyles from "Theme.of(context)" should have labels in the form of '
'"(<A TextStyle>).merge(<Another TextStyle>)"). It is recommended to '
'only lerp ThemeData with matching TextStyles.'
),
]);
}());
return TextStyle(
inherit: t < 0.5 ? a.inherit : b.inherit,
color: a.foreground == null && b.foreground == null ? Color.lerp(a.color, b.color, t) : null,
backgroundColor: a.background == null && b.background == null ? Color.lerp(a.backgroundColor, b.backgroundColor, t) : null,
fontSize: ui.lerpDouble(a.fontSize ?? b.fontSize, b.fontSize ?? a.fontSize, t),
fontWeight: FontWeight.lerp(a.fontWeight, b.fontWeight, t),
fontStyle: t < 0.5 ? a.fontStyle : b.fontStyle,
letterSpacing: ui.lerpDouble(a.letterSpacing ?? b.letterSpacing, b.letterSpacing ?? a.letterSpacing, t),
wordSpacing: ui.lerpDouble(a.wordSpacing ?? b.wordSpacing, b.wordSpacing ?? a.wordSpacing, t),
textBaseline: t < 0.5 ? a.textBaseline : b.textBaseline,
height: ui.lerpDouble(a.height ?? b.height, b.height ?? a.height, t),
leadingDistribution: t < 0.5 ? a.leadingDistribution : b.leadingDistribution,
locale: t < 0.5 ? a.locale : b.locale,
foreground: (a.foreground != null || b.foreground != null)
? t < 0.5
? a.foreground ?? (Paint()..color = a.color!)
: b.foreground ?? (Paint()..color = b.color!)
: null,
background: (a.background != null || b.background != null)
? t < 0.5
? a.background ?? (Paint()..color = a.backgroundColor!)
: b.background ?? (Paint()..color = b.backgroundColor!)
: null,
shadows: t < 0.5 ? a.shadows : b.shadows,
fontFeatures: t < 0.5 ? a.fontFeatures : b.fontFeatures,
fontVariations: lerpFontVariations(a.fontVariations, b.fontVariations, t),
decoration: t < 0.5 ? a.decoration : b.decoration,
decorationColor: Color.lerp(a.decorationColor, b.decorationColor, t),
decorationStyle: t < 0.5 ? a.decorationStyle : b.decorationStyle,
decorationThickness: ui.lerpDouble(a.decorationThickness ?? b.decorationThickness, b.decorationThickness ?? a.decorationThickness, t),
debugLabel: lerpDebugLabel,
fontFamily: t < 0.5 ? a._fontFamily : b._fontFamily,
fontFamilyFallback: t < 0.5 ? a._fontFamilyFallback : b._fontFamilyFallback,
package: t < 0.5 ? a._package : b._package,
overflow: t < 0.5 ? a.overflow : b.overflow,
);
}
/// The style information for text runs, encoded for use by `dart:ui`.
ui.TextStyle getTextStyle({
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double textScaleFactor = 1.0,
TextScaler textScaler = TextScaler.noScaling,
}) {
assert(
identical(textScaler, TextScaler.noScaling) || textScaleFactor == 1.0,
'textScaleFactor is deprecated and cannot be specified when textScaler is specified.',
);
final double? fontSize = switch (this.fontSize) {
null => null,
final double size when textScaler == TextScaler.noScaling => size * textScaleFactor,
final double size => textScaler.scale(size),
};
return ui.TextStyle(
color: color,
decoration: decoration,
decorationColor: decorationColor,
decorationStyle: decorationStyle,
decorationThickness: decorationThickness,
fontWeight: fontWeight,
fontStyle: fontStyle,
textBaseline: textBaseline,
leadingDistribution: leadingDistribution,
fontFamily: fontFamily,
fontFamilyFallback: fontFamilyFallback,
fontSize: fontSize,
letterSpacing: letterSpacing,
wordSpacing: wordSpacing,
height: height,
locale: locale,
foreground: foreground,
background: switch ((background, backgroundColor)) {
(final Paint paint, _) => paint,
(_, final Color color) => Paint()..color = color,
_ => null,
},
shadows: shadows,
fontFeatures: fontFeatures,
fontVariations: fontVariations,
);
}
/// The style information for paragraphs, encoded for use by `dart:ui`.
///
/// If the `textScaleFactor` argument is omitted, it defaults to one. The
/// other arguments may be null. The `maxLines` argument, if specified and
/// non-null, must be greater than zero.
///
/// If the font size on this style isn't set, it will default to 14 logical
/// pixels.
ui.ParagraphStyle getParagraphStyle({
TextAlign? textAlign,
TextDirection? textDirection,
TextScaler textScaler = TextScaler.noScaling,
String? ellipsis,
int? maxLines,
TextHeightBehavior? textHeightBehavior,
Locale? locale,
String? fontFamily,
double? fontSize,
FontWeight? fontWeight,
FontStyle? fontStyle,
double? height,
StrutStyle? strutStyle,
}) {
assert(maxLines == null || maxLines > 0);
final TextLeadingDistribution? leadingDistribution = this.leadingDistribution;
final TextHeightBehavior? effectiveTextHeightBehavior = textHeightBehavior
?? (leadingDistribution == null ? null : TextHeightBehavior(leadingDistribution: leadingDistribution));
return ui.ParagraphStyle(
textAlign: textAlign,
textDirection: textDirection,
// Here, we establish the contents of this TextStyle as the paragraph's default font
// unless an override is passed in.
fontWeight: fontWeight ?? this.fontWeight,
fontStyle: fontStyle ?? this.fontStyle,
fontFamily: fontFamily ?? this.fontFamily,
fontSize: textScaler.scale(fontSize ?? this.fontSize ?? kDefaultFontSize),
height: height ?? this.height,
textHeightBehavior: effectiveTextHeightBehavior,
strutStyle: strutStyle == null ? null : ui.StrutStyle(
fontFamily: strutStyle.fontFamily,
fontFamilyFallback: strutStyle.fontFamilyFallback,
fontSize: switch (strutStyle.fontSize) {
null => null,
final double unscaled => textScaler.scale(unscaled),
},
height: strutStyle.height,
leading: strutStyle.leading,
leadingDistribution: strutStyle.leadingDistribution,
fontWeight: strutStyle.fontWeight,
fontStyle: strutStyle.fontStyle,
forceStrutHeight: strutStyle.forceStrutHeight,
),
maxLines: maxLines,
ellipsis: ellipsis,
locale: locale,
);
}
/// Describe the difference between this style and another, in terms of how
/// much damage it will make to the rendering.
///
/// See also:
///
/// * [TextSpan.compareTo], which does the same thing for entire [TextSpan]s.
RenderComparison compareTo(TextStyle other) {
if (identical(this, other)) {
return RenderComparison.identical;
}
if (inherit != other.inherit ||
fontFamily != other.fontFamily ||
fontSize != other.fontSize ||
fontWeight != other.fontWeight ||
fontStyle != other.fontStyle ||
letterSpacing != other.letterSpacing ||
wordSpacing != other.wordSpacing ||
textBaseline != other.textBaseline ||
height != other.height ||
leadingDistribution != other.leadingDistribution ||
locale != other.locale ||
foreground != other.foreground ||
background != other.background ||
!listEquals(shadows, other.shadows) ||
!listEquals(fontFeatures, other.fontFeatures) ||
!listEquals(fontVariations, other.fontVariations) ||
!listEquals(fontFamilyFallback, other.fontFamilyFallback) ||
overflow != other.overflow) {
return RenderComparison.layout;
}
if (color != other.color ||
backgroundColor != other.backgroundColor ||
decoration != other.decoration ||
decorationColor != other.decorationColor ||
decorationStyle != other.decorationStyle ||
decorationThickness != other.decorationThickness) {
return RenderComparison.paint;
}
return RenderComparison.identical;
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is TextStyle
&& other.inherit == inherit
&& other.color == color
&& other.backgroundColor == backgroundColor
&& other.fontSize == fontSize
&& other.fontWeight == fontWeight
&& other.fontStyle == fontStyle
&& other.letterSpacing == letterSpacing
&& other.wordSpacing == wordSpacing
&& other.textBaseline == textBaseline
&& other.height == height
&& other.leadingDistribution == leadingDistribution
&& other.locale == locale
&& other.foreground == foreground
&& other.background == background
&& listEquals(other.shadows, shadows)
&& listEquals(other.fontFeatures, fontFeatures)
&& listEquals(other.fontVariations, fontVariations)
&& other.decoration == decoration
&& other.decorationColor == decorationColor
&& other.decorationStyle == decorationStyle
&& other.decorationThickness == decorationThickness
&& other.fontFamily == fontFamily
&& listEquals(other.fontFamilyFallback, fontFamilyFallback)
&& other._package == _package
&& other.overflow == overflow;
}
@override
int get hashCode {
final List<String>? fontFamilyFallback = this.fontFamilyFallback;
final int fontHash = Object.hash(
decorationStyle,
decorationThickness,
fontFamily,
fontFamilyFallback == null ? null : Object.hashAll(fontFamilyFallback),
_package,
overflow,
);
final List<Shadow>? shadows = this.shadows;
final List<FontFeature>? fontFeatures = this.fontFeatures;
final List<FontVariation>? fontVariations = this.fontVariations;
return Object.hash(
inherit,
color,
backgroundColor,
fontSize,
fontWeight,
fontStyle,
letterSpacing,
wordSpacing,
textBaseline,
height,
leadingDistribution,
locale,
foreground,
background,
shadows == null ? null : Object.hashAll(shadows),
fontFeatures == null ? null : Object.hashAll(fontFeatures),
fontVariations == null ? null : Object.hashAll(fontVariations),
decoration,
decorationColor,
fontHash,
);
}
@override
String toStringShort() => objectRuntimeType(this, 'TextStyle');
/// Adds all properties prefixing property names with the optional `prefix`.
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties, { String prefix = '' }) {
super.debugFillProperties(properties);
if (debugLabel != null) {
properties.add(MessageProperty('${prefix}debugLabel', debugLabel!));
}
final List<DiagnosticsNode> styles = <DiagnosticsNode>[
ColorProperty('${prefix}color', color, defaultValue: null),
ColorProperty('${prefix}backgroundColor', backgroundColor, defaultValue: null),
StringProperty('${prefix}family', fontFamily, defaultValue: null, quoted: false),
IterableProperty<String>('${prefix}familyFallback', fontFamilyFallback, defaultValue: null),
DoubleProperty('${prefix}size', fontSize, defaultValue: null),
];
String? weightDescription;
if (fontWeight != null) {
weightDescription = '${fontWeight!.index + 1}00';
}
// TODO(jacobr): switch this to use enumProperty which will either cause the
// weight description to change to w600 from 600 or require existing
// enumProperty to handle this special case.
styles.add(DiagnosticsProperty<FontWeight>(
'${prefix}weight',
fontWeight,
description: weightDescription,
defaultValue: null,
));
styles.add(EnumProperty<FontStyle>('${prefix}style', fontStyle, defaultValue: null));
styles.add(DoubleProperty('${prefix}letterSpacing', letterSpacing, defaultValue: null));
styles.add(DoubleProperty('${prefix}wordSpacing', wordSpacing, defaultValue: null));
styles.add(EnumProperty<TextBaseline>('${prefix}baseline', textBaseline, defaultValue: null));
styles.add(DoubleProperty('${prefix}height', height, unit: 'x', defaultValue: null));
styles.add(EnumProperty<TextLeadingDistribution>('${prefix}leadingDistribution', leadingDistribution, defaultValue: null));
styles.add(DiagnosticsProperty<Locale>('${prefix}locale', locale, defaultValue: null));
styles.add(DiagnosticsProperty<Paint>('${prefix}foreground', foreground, defaultValue: null));
styles.add(DiagnosticsProperty<Paint>('${prefix}background', background, defaultValue: null));
if (decoration != null || decorationColor != null || decorationStyle != null || decorationThickness != null) {
final List<String> decorationDescription = <String>[];
if (decorationStyle != null) {
decorationDescription.add(decorationStyle!.name);
}
// Hide decorationColor from the default text view as it is shown in the
// terse decoration summary as well.
styles.add(ColorProperty('${prefix}decorationColor', decorationColor, defaultValue: null, level: DiagnosticLevel.fine));
if (decorationColor != null) {
decorationDescription.add('$decorationColor');
}
// Intentionally collide with the property 'decoration' added below.
// Tools that show hidden properties could choose the first property
// matching the name to disambiguate.
styles.add(DiagnosticsProperty<TextDecoration>('${prefix}decoration', decoration, defaultValue: null, level: DiagnosticLevel.hidden));
if (decoration != null) {
decorationDescription.add('$decoration');
}
assert(decorationDescription.isNotEmpty);
styles.add(MessageProperty('${prefix}decoration', decorationDescription.join(' ')));
styles.add(DoubleProperty('${prefix}decorationThickness', decorationThickness, unit: 'x', defaultValue: null));
}
final bool styleSpecified = styles.any((DiagnosticsNode n) => !n.isFiltered(DiagnosticLevel.info));
properties.add(DiagnosticsProperty<bool>('${prefix}inherit', inherit, level: (!styleSpecified && inherit) ? DiagnosticLevel.fine : DiagnosticLevel.info));
styles.forEach(properties.add);
if (!styleSpecified) {
properties.add(FlagProperty('inherit', value: inherit, ifTrue: '$prefix<all styles inherited>', ifFalse: '$prefix<no style specified>'));
}
styles.add(EnumProperty<TextOverflow>('${prefix}overflow', overflow, defaultValue: null));
}
}
/// Interpolate between two lists of [FontVariation] objects.
///
/// Variations are paired by axis, and interpolated using [FontVariation.lerp].
///
/// Entries that are only present in one list are animated using a step-function
/// at t=0.5 that enables or disables the variation. This can be jarring and
/// largely defeats the point of animating font variations. For best results,
/// specify the same axes in both lists, and for best performance, specify them
/// in the same order.
///
/// ## Performance details
///
/// This algorithm is O(N), but the constant factor varies based on the input,
/// and that is probably more important (because typically N is going to be
/// tiny, like 1 or 2; at the time of writing, there are only about five defined
/// axes that fonts typically use!).
///
/// It is fastest when the lists contain the same axes ([FontVariation.axis]) in
/// the same order. The result is again in the same order, and no attempt is
/// made to detect or remove duplicates in this process. This is, by far, the
/// recommended way to use this algorithm.
///
/// When the order of items in the two input lists vary, the constant factor
/// increases substantially, as it involves creating two maps and a set,
/// inserting every variation in both lists into the maps and the set, and then
/// iterating over them to recreate the list.
///
/// In this case, the resulting order is arbitrary. Duplicates are dropped; in
/// each list, the last [FontVariation] for any particular axis is the one used
/// to compute the value for that axis. Values that only appear on one side are
/// interpolated using [FontVariation.lerp] against a null value, and resulting
/// null values are omitted from the resulting list.
///
/// When the lists begin with matching pairs of axes, the fast algorithm is used
/// up to the point where the lists diverge, and the more expensive algorithm
/// is used on the remaining entries.
///
/// See also:
///
/// * [TextStyle.lerp], which uses this function to handle
/// [TextStyle.fontVariations].
List<FontVariation>? lerpFontVariations(List<FontVariation>? a, List<FontVariation>? b, double t) {
if (t == 0.0) {
return a;
}
if (t == 1.0) {
return b;
}
if (a == null || a.isEmpty || b == null || b.isEmpty) {
// If one side is empty, that means anything on the other
// side will use the null-to-something lerp, which is to
// say, a step function at t=0.5.
return t < 0.5 ? a : b;
}
assert(a.isNotEmpty && b.isNotEmpty);
final List<FontVariation> result = <FontVariation>[];
// First, try the efficient O(N) solution in the event that
// the lists are compatible.
int index = 0;
final int minLength = a.length < b.length ? a.length : b.length;
for (; index < minLength; index += 1) {
if (a[index].axis != b[index].axis) {
// The lists aren't compatible.
break;
}
result.add(FontVariation.lerp(a[index], b[index], t)!);
}
final int maxLength = a.length > b.length ? a.length : b.length;
if (index < maxLength) {
// If we get here, we have found some case where we cannot
// use the efficient approach.
final Set<String> axes = HashSet<String>();
final Map<String, FontVariation> aVariations = HashMap<String, FontVariation>();
for (int indexA = index; indexA < a.length; indexA += 1) {
aVariations[a[indexA].axis] = a[indexA];
axes.add(a[indexA].axis);
}
final Map<String, FontVariation> bVariations = HashMap<String, FontVariation>();
for (int indexB = index; indexB < b.length; indexB += 1) {
bVariations[b[indexB].axis] = b[indexB];
axes.add(b[indexB].axis);
}
for (final String axis in axes) {
final FontVariation? variation = FontVariation.lerp(aVariations[axis], bVariations[axis], t);
if (variation != null) {
result.add(variation);
}
}
}
return result;
}
| flutter/packages/flutter/lib/src/painting/text_style.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/text_style.dart",
"repo_id": "flutter",
"token_count": 23267
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'dart:math' as math;
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle, LineMetrics, PlaceholderAlignment, TextBox;
import 'package:characters/characters.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/semantics.dart';
import 'package:flutter/services.dart';
import 'box.dart';
import 'custom_paint.dart';
import 'layer.dart';
import 'layout_helper.dart';
import 'object.dart';
import 'paragraph.dart';
import 'viewport_offset.dart';
const double _kCaretGap = 1.0; // pixels
const double _kCaretHeightOffset = 2.0; // pixels
// The additional size on the x and y axis with which to expand the prototype
// cursor to render the floating cursor in pixels.
const EdgeInsets _kFloatingCursorSizeIncrease = EdgeInsets.symmetric(horizontal: 0.5, vertical: 1.0);
// The corner radius of the floating cursor in pixels.
const Radius _kFloatingCursorRadius = Radius.circular(1.0);
// This constant represents the shortest squared distance required between the floating cursor
// and the regular cursor when both are present in the text field.
// If the squared distance between the two cursors is less than this value,
// it's not necessary to display both cursors at the same time.
// This behavior is consistent with the one observed in iOS UITextField.
const double _kShortestDistanceSquaredWithFloatingAndRegularCursors = 15.0 * 15.0;
/// Represents the coordinates of the point in a selection, and the text
/// direction at that point, relative to top left of the [RenderEditable] that
/// holds the selection.
@immutable
class TextSelectionPoint {
/// Creates a description of a point in a text selection.
const TextSelectionPoint(this.point, this.direction);
/// Coordinates of the lower left or lower right corner of the selection,
/// relative to the top left of the [RenderEditable] object.
final Offset point;
/// Direction of the text at this edge of the selection.
final TextDirection? direction;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is TextSelectionPoint
&& other.point == point
&& other.direction == direction;
}
@override
String toString() {
return switch (direction) {
TextDirection.ltr => '$point-ltr',
TextDirection.rtl => '$point-rtl',
null => '$point',
};
}
@override
int get hashCode => Object.hash(point, direction);
}
/// The consecutive sequence of [TextPosition]s that the caret should move to
/// when the user navigates the paragraph using the upward arrow key or the
/// downward arrow key.
///
/// {@template flutter.rendering.RenderEditable.verticalArrowKeyMovement}
/// When the user presses the upward arrow key or the downward arrow key, on
/// many platforms (macOS for instance), the caret will move to the previous
/// line or the next line, while maintaining its original horizontal location.
/// When it encounters a shorter line, the caret moves to the closest horizontal
/// location within that line, and restores the original horizontal location
/// when a long enough line is encountered.
///
/// Additionally, the caret will move to the beginning of the document if the
/// upward arrow key is pressed and the caret is already on the first line. If
/// the downward arrow key is pressed next, the caret will restore its original
/// horizontal location and move to the second line. Similarly the caret moves
/// to the end of the document if the downward arrow key is pressed when it's
/// already on the last line.
///
/// Consider a left-aligned paragraph:
/// aa|
/// a
/// aaa
/// where the caret was initially placed at the end of the first line. Pressing
/// the downward arrow key once will move the caret to the end of the second
/// line, and twice the arrow key moves to the third line after the second "a"
/// on that line. Pressing the downward arrow key again, the caret will move to
/// the end of the third line (the end of the document). Pressing the upward
/// arrow key in this state will result in the caret moving to the end of the
/// second line.
///
/// Vertical caret runs are typically interrupted when the layout of the text
/// changes (including when the text itself changes), or when the selection is
/// changed by other input events or programmatically (for example, when the
/// user pressed the left arrow key).
/// {@endtemplate}
///
/// The [movePrevious] method moves the caret location (which is
/// [VerticalCaretMovementRun.current]) to the previous line, and in case
/// the caret is already on the first line, the method does nothing and returns
/// false. Similarly the [moveNext] method moves the caret to the next line, and
/// returns false if the caret is already on the last line.
///
/// The [moveByOffset] method takes a pixel offset from the current position to move
/// the caret up or down.
///
/// If the underlying paragraph's layout changes, [isValid] becomes false and
/// the [VerticalCaretMovementRun] must not be used. The [isValid] property must
/// be checked before calling [movePrevious], [moveNext] and [moveByOffset],
/// or accessing [current].
class VerticalCaretMovementRun implements Iterator<TextPosition> {
VerticalCaretMovementRun._(
this._editable,
this._lineMetrics,
this._currentTextPosition,
this._currentLine,
this._currentOffset,
);
Offset _currentOffset;
int _currentLine;
TextPosition _currentTextPosition;
final List<ui.LineMetrics> _lineMetrics;
final RenderEditable _editable;
bool _isValid = true;
/// Whether this [VerticalCaretMovementRun] can still continue.
///
/// A [VerticalCaretMovementRun] run is valid if the underlying text layout
/// hasn't changed.
///
/// The [current] value and the [movePrevious], [moveNext] and [moveByOffset]
/// methods must not be accessed when [isValid] is false.
bool get isValid {
if (!_isValid) {
return false;
}
final List<ui.LineMetrics> newLineMetrics = _editable._textPainter.computeLineMetrics();
// Use the implementation detail of the computeLineMetrics method to figure
// out if the current text layout has been invalidated.
if (!identical(newLineMetrics, _lineMetrics)) {
_isValid = false;
}
return _isValid;
}
final Map<int, MapEntry<Offset, TextPosition>> _positionCache = <int, MapEntry<Offset, TextPosition>>{};
MapEntry<Offset, TextPosition> _getTextPositionForLine(int lineNumber) {
assert(isValid);
assert(lineNumber >= 0);
final MapEntry<Offset, TextPosition>? cachedPosition = _positionCache[lineNumber];
if (cachedPosition != null) {
return cachedPosition;
}
assert(lineNumber != _currentLine);
final Offset newOffset = Offset(_currentOffset.dx, _lineMetrics[lineNumber].baseline);
final TextPosition closestPosition = _editable._textPainter.getPositionForOffset(newOffset);
final MapEntry<Offset, TextPosition> position = MapEntry<Offset, TextPosition>(newOffset, closestPosition);
_positionCache[lineNumber] = position;
return position;
}
@override
TextPosition get current {
assert(isValid);
return _currentTextPosition;
}
@override
bool moveNext() {
assert(isValid);
if (_currentLine + 1 >= _lineMetrics.length) {
return false;
}
final MapEntry<Offset, TextPosition> position = _getTextPositionForLine(_currentLine + 1);
_currentLine += 1;
_currentOffset = position.key;
_currentTextPosition = position.value;
return true;
}
/// Move back to the previous element.
///
/// Returns true and updates [current] if successful.
bool movePrevious() {
assert(isValid);
if (_currentLine <= 0) {
return false;
}
final MapEntry<Offset, TextPosition> position = _getTextPositionForLine(_currentLine - 1);
_currentLine -= 1;
_currentOffset = position.key;
_currentTextPosition = position.value;
return true;
}
/// Move forward or backward by a number of elements determined
/// by pixel [offset].
///
/// If [offset] is negative, move backward; otherwise move forward.
///
/// Returns true and updates [current] if successful.
bool moveByOffset(double offset) {
final Offset initialOffset = _currentOffset;
if (offset >= 0.0) {
while (_currentOffset.dy < initialOffset.dy + offset) {
if (!moveNext()) {
break;
}
}
} else {
while (_currentOffset.dy > initialOffset.dy + offset) {
if (!movePrevious()) {
break;
}
}
}
return initialOffset != _currentOffset;
}
}
/// Displays some text in a scrollable container with a potentially blinking
/// cursor and with gesture recognizers.
///
/// This is the renderer for an editable text field. It does not directly
/// provide affordances for editing the text, but it does handle text selection
/// and manipulation of the text cursor.
///
/// The [text] is displayed, scrolled by the given [offset], aligned according
/// to [textAlign]. The [maxLines] property controls whether the text displays
/// on one line or many. The [selection], if it is not collapsed, is painted in
/// the [selectionColor]. If it _is_ collapsed, then it represents the cursor
/// position. The cursor is shown while [showCursor] is true. It is painted in
/// the [cursorColor].
///
/// Keyboard handling, IME handling, scrolling, toggling the [showCursor] value
/// to actually blink the cursor, and other features not mentioned above are the
/// responsibility of higher layers and not handled by this object.
class RenderEditable extends RenderBox with RelayoutWhenSystemFontsChangeMixin, ContainerRenderObjectMixin<RenderBox, TextParentData>, RenderInlineChildrenContainerDefaults implements TextLayoutMetrics {
/// Creates a render object that implements the visual aspects of a text field.
///
/// The [textAlign] argument defaults to [TextAlign.start].
///
/// If [showCursor] is not specified, then it defaults to hiding the cursor.
///
/// The [maxLines] property can be set to null to remove the restriction on
/// the number of lines. By default, it is 1, meaning this is a single-line
/// text field. If it is not null, it must be greater than zero.
///
/// Use [ViewportOffset.zero] for the [offset] if there is no need for
/// scrolling.
RenderEditable({
InlineSpan? text,
required TextDirection textDirection,
TextAlign textAlign = TextAlign.start,
Color? cursorColor,
Color? backgroundCursorColor,
ValueNotifier<bool>? showCursor,
bool? hasFocus,
required LayerLink startHandleLayerLink,
required LayerLink endHandleLayerLink,
int? maxLines = 1,
int? minLines,
bool expands = false,
StrutStyle? strutStyle,
Color? selectionColor,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double textScaleFactor = 1.0,
TextScaler textScaler = TextScaler.noScaling,
TextSelection? selection,
required ViewportOffset offset,
this.ignorePointer = false,
bool readOnly = false,
bool forceLine = true,
TextHeightBehavior? textHeightBehavior,
TextWidthBasis textWidthBasis = TextWidthBasis.parent,
String obscuringCharacter = '•',
bool obscureText = false,
Locale? locale,
double cursorWidth = 1.0,
double? cursorHeight,
Radius? cursorRadius,
bool paintCursorAboveText = false,
Offset cursorOffset = Offset.zero,
double devicePixelRatio = 1.0,
ui.BoxHeightStyle selectionHeightStyle = ui.BoxHeightStyle.tight,
ui.BoxWidthStyle selectionWidthStyle = ui.BoxWidthStyle.tight,
bool? enableInteractiveSelection,
this.floatingCursorAddedMargin = const EdgeInsets.fromLTRB(4, 4, 4, 5),
TextRange? promptRectRange,
Color? promptRectColor,
Clip clipBehavior = Clip.hardEdge,
required this.textSelectionDelegate,
RenderEditablePainter? painter,
RenderEditablePainter? foregroundPainter,
List<RenderBox>? children,
}) : assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
!expands || (maxLines == null && minLines == null),
'minLines and maxLines must be null when expands is true.',
),
assert(
identical(textScaler, TextScaler.noScaling) || textScaleFactor == 1.0,
'textScaleFactor is deprecated and cannot be specified when textScaler is specified.',
),
assert(obscuringCharacter.characters.length == 1),
assert(cursorWidth >= 0.0),
assert(cursorHeight == null || cursorHeight >= 0.0),
_textPainter = TextPainter(
text: text,
textAlign: textAlign,
textDirection: textDirection,
textScaler: textScaler == TextScaler.noScaling ? TextScaler.linear(textScaleFactor) : textScaler,
locale: locale,
maxLines: maxLines == 1 ? 1 : null,
strutStyle: strutStyle,
textHeightBehavior: textHeightBehavior,
textWidthBasis: textWidthBasis,
),
_showCursor = showCursor ?? ValueNotifier<bool>(false),
_maxLines = maxLines,
_minLines = minLines,
_expands = expands,
_selection = selection,
_offset = offset,
_cursorWidth = cursorWidth,
_cursorHeight = cursorHeight,
_paintCursorOnTop = paintCursorAboveText,
_enableInteractiveSelection = enableInteractiveSelection,
_devicePixelRatio = devicePixelRatio,
_startHandleLayerLink = startHandleLayerLink,
_endHandleLayerLink = endHandleLayerLink,
_obscuringCharacter = obscuringCharacter,
_obscureText = obscureText,
_readOnly = readOnly,
_forceLine = forceLine,
_clipBehavior = clipBehavior,
_hasFocus = hasFocus ?? false,
_disposeShowCursor = showCursor == null {
assert(!_showCursor.value || cursorColor != null);
_selectionPainter.highlightColor = selectionColor;
_selectionPainter.highlightedRange = selection;
_selectionPainter.selectionHeightStyle = selectionHeightStyle;
_selectionPainter.selectionWidthStyle = selectionWidthStyle;
_autocorrectHighlightPainter.highlightColor = promptRectColor;
_autocorrectHighlightPainter.highlightedRange = promptRectRange;
_caretPainter.caretColor = cursorColor;
_caretPainter.cursorRadius = cursorRadius;
_caretPainter.cursorOffset = cursorOffset;
_caretPainter.backgroundCursorColor = backgroundCursorColor;
_updateForegroundPainter(foregroundPainter);
_updatePainter(painter);
addAll(children);
}
/// Child render objects
_RenderEditableCustomPaint? _foregroundRenderObject;
_RenderEditableCustomPaint? _backgroundRenderObject;
@override
void dispose() {
_leaderLayerHandler.layer = null;
_foregroundRenderObject?.dispose();
_foregroundRenderObject = null;
_backgroundRenderObject?.dispose();
_backgroundRenderObject = null;
_clipRectLayer.layer = null;
_cachedBuiltInForegroundPainters?.dispose();
_cachedBuiltInPainters?.dispose();
_selectionStartInViewport.dispose();
_selectionEndInViewport.dispose();
_autocorrectHighlightPainter.dispose();
_selectionPainter.dispose();
_caretPainter.dispose();
_textPainter.dispose();
_textIntrinsicsCache?.dispose();
if (_disposeShowCursor) {
_showCursor.dispose();
_disposeShowCursor = false;
}
super.dispose();
}
void _updateForegroundPainter(RenderEditablePainter? newPainter) {
final _CompositeRenderEditablePainter effectivePainter = newPainter == null
? _builtInForegroundPainters
: _CompositeRenderEditablePainter(painters: <RenderEditablePainter>[
_builtInForegroundPainters,
newPainter,
]);
if (_foregroundRenderObject == null) {
final _RenderEditableCustomPaint foregroundRenderObject = _RenderEditableCustomPaint(painter: effectivePainter);
adoptChild(foregroundRenderObject);
_foregroundRenderObject = foregroundRenderObject;
} else {
_foregroundRenderObject?.painter = effectivePainter;
}
_foregroundPainter = newPainter;
}
/// The [RenderEditablePainter] to use for painting above this
/// [RenderEditable]'s text content.
///
/// The new [RenderEditablePainter] will replace the previously specified
/// foreground painter, and schedule a repaint if the new painter's
/// `shouldRepaint` method returns true.
RenderEditablePainter? get foregroundPainter => _foregroundPainter;
RenderEditablePainter? _foregroundPainter;
set foregroundPainter(RenderEditablePainter? newPainter) {
if (newPainter == _foregroundPainter) {
return;
}
_updateForegroundPainter(newPainter);
}
void _updatePainter(RenderEditablePainter? newPainter) {
final _CompositeRenderEditablePainter effectivePainter = newPainter == null
? _builtInPainters
: _CompositeRenderEditablePainter(painters: <RenderEditablePainter>[_builtInPainters, newPainter]);
if (_backgroundRenderObject == null) {
final _RenderEditableCustomPaint backgroundRenderObject = _RenderEditableCustomPaint(painter: effectivePainter);
adoptChild(backgroundRenderObject);
_backgroundRenderObject = backgroundRenderObject;
} else {
_backgroundRenderObject?.painter = effectivePainter;
}
_painter = newPainter;
}
/// Sets the [RenderEditablePainter] to use for painting beneath this
/// [RenderEditable]'s text content.
///
/// The new [RenderEditablePainter] will replace the previously specified
/// painter, and schedule a repaint if the new painter's `shouldRepaint`
/// method returns true.
RenderEditablePainter? get painter => _painter;
RenderEditablePainter? _painter;
set painter(RenderEditablePainter? newPainter) {
if (newPainter == _painter) {
return;
}
_updatePainter(newPainter);
}
// Caret Painters:
// A single painter for both the regular caret and the floating cursor.
late final _CaretPainter _caretPainter = _CaretPainter();
// Text Highlight painters:
final _TextHighlightPainter _selectionPainter = _TextHighlightPainter();
final _TextHighlightPainter _autocorrectHighlightPainter = _TextHighlightPainter();
_CompositeRenderEditablePainter get _builtInForegroundPainters => _cachedBuiltInForegroundPainters ??= _createBuiltInForegroundPainters();
_CompositeRenderEditablePainter? _cachedBuiltInForegroundPainters;
_CompositeRenderEditablePainter _createBuiltInForegroundPainters() {
return _CompositeRenderEditablePainter(
painters: <RenderEditablePainter>[
if (paintCursorAboveText) _caretPainter,
],
);
}
_CompositeRenderEditablePainter get _builtInPainters => _cachedBuiltInPainters ??= _createBuiltInPainters();
_CompositeRenderEditablePainter? _cachedBuiltInPainters;
_CompositeRenderEditablePainter _createBuiltInPainters() {
return _CompositeRenderEditablePainter(
painters: <RenderEditablePainter>[
_autocorrectHighlightPainter,
_selectionPainter,
if (!paintCursorAboveText) _caretPainter,
],
);
}
/// Whether the [handleEvent] will propagate pointer events to selection
/// handlers.
///
/// If this property is true, the [handleEvent] assumes that this renderer
/// will be notified of input gestures via [handleTapDown], [handleTap],
/// [handleDoubleTap], and [handleLongPress].
///
/// If there are any gesture recognizers in the text span, the [handleEvent]
/// will still propagate pointer events to those recognizers.
///
/// The default value of this property is false.
bool ignorePointer;
/// {@macro dart.ui.textHeightBehavior}
TextHeightBehavior? get textHeightBehavior => _textPainter.textHeightBehavior;
set textHeightBehavior(TextHeightBehavior? value) {
if (_textPainter.textHeightBehavior == value) {
return;
}
_textPainter.textHeightBehavior = value;
markNeedsLayout();
}
/// {@macro flutter.painting.textPainter.textWidthBasis}
TextWidthBasis get textWidthBasis => _textPainter.textWidthBasis;
set textWidthBasis(TextWidthBasis value) {
if (_textPainter.textWidthBasis == value) {
return;
}
_textPainter.textWidthBasis = value;
markNeedsLayout();
}
/// The pixel ratio of the current device.
///
/// Should be obtained by querying MediaQuery for the devicePixelRatio.
double get devicePixelRatio => _devicePixelRatio;
double _devicePixelRatio;
set devicePixelRatio(double value) {
if (devicePixelRatio == value) {
return;
}
_devicePixelRatio = value;
markNeedsLayout();
}
/// Character used for obscuring text if [obscureText] is true.
///
/// Must have a length of exactly one.
String get obscuringCharacter => _obscuringCharacter;
String _obscuringCharacter;
set obscuringCharacter(String value) {
if (_obscuringCharacter == value) {
return;
}
assert(value.characters.length == 1);
_obscuringCharacter = value;
markNeedsLayout();
}
/// Whether to hide the text being edited (e.g., for passwords).
bool get obscureText => _obscureText;
bool _obscureText;
set obscureText(bool value) {
if (_obscureText == value) {
return;
}
_obscureText = value;
_cachedAttributedValue = null;
markNeedsSemanticsUpdate();
}
/// Controls how tall the selection highlight boxes are computed to be.
///
/// See [ui.BoxHeightStyle] for details on available styles.
ui.BoxHeightStyle get selectionHeightStyle => _selectionPainter.selectionHeightStyle;
set selectionHeightStyle(ui.BoxHeightStyle value) {
_selectionPainter.selectionHeightStyle = value;
}
/// Controls how wide the selection highlight boxes are computed to be.
///
/// See [ui.BoxWidthStyle] for details on available styles.
ui.BoxWidthStyle get selectionWidthStyle => _selectionPainter.selectionWidthStyle;
set selectionWidthStyle(ui.BoxWidthStyle value) {
_selectionPainter.selectionWidthStyle = value;
}
/// The object that controls the text selection, used by this render object
/// for implementing cut, copy, and paste keyboard shortcuts.
///
/// It will make cut, copy and paste functionality work with the most recently
/// set [TextSelectionDelegate].
TextSelectionDelegate textSelectionDelegate;
/// Track whether position of the start of the selected text is within the viewport.
///
/// For example, if the text contains "Hello World", and the user selects
/// "Hello", then scrolls so only "World" is visible, this will become false.
/// If the user scrolls back so that the "H" is visible again, this will
/// become true.
///
/// This bool indicates whether the text is scrolled so that the handle is
/// inside the text field viewport, as opposed to whether it is actually
/// visible on the screen.
ValueListenable<bool> get selectionStartInViewport => _selectionStartInViewport;
final ValueNotifier<bool> _selectionStartInViewport = ValueNotifier<bool>(true);
/// Track whether position of the end of the selected text is within the viewport.
///
/// For example, if the text contains "Hello World", and the user selects
/// "World", then scrolls so only "Hello" is visible, this will become
/// 'false'. If the user scrolls back so that the "d" is visible again, this
/// will become 'true'.
///
/// This bool indicates whether the text is scrolled so that the handle is
/// inside the text field viewport, as opposed to whether it is actually
/// visible on the screen.
ValueListenable<bool> get selectionEndInViewport => _selectionEndInViewport;
final ValueNotifier<bool> _selectionEndInViewport = ValueNotifier<bool>(true);
/// Returns the TextPosition above or below the given offset.
TextPosition _getTextPositionVertical(TextPosition position, double verticalOffset) {
final Offset caretOffset = _textPainter.getOffsetForCaret(position, _caretPrototype);
final Offset caretOffsetTranslated = caretOffset.translate(0.0, verticalOffset);
return _textPainter.getPositionForOffset(caretOffsetTranslated);
}
// Start TextLayoutMetrics.
/// {@macro flutter.services.TextLayoutMetrics.getLineAtOffset}
@override
TextSelection getLineAtOffset(TextPosition position) {
final TextRange line = _textPainter.getLineBoundary(position);
// If text is obscured, the entire string should be treated as one line.
if (obscureText) {
return TextSelection(baseOffset: 0, extentOffset: plainText.length);
}
return TextSelection(baseOffset: line.start, extentOffset: line.end);
}
/// {@macro flutter.painting.TextPainter.getWordBoundary}
@override
TextRange getWordBoundary(TextPosition position) {
return _textPainter.getWordBoundary(position);
}
/// {@macro flutter.services.TextLayoutMetrics.getTextPositionAbove}
@override
TextPosition getTextPositionAbove(TextPosition position) {
// The caret offset gives a location in the upper left hand corner of
// the caret so the middle of the line above is a half line above that
// point and the line below is 1.5 lines below that point.
final double preferredLineHeight = _textPainter.preferredLineHeight;
final double verticalOffset = -0.5 * preferredLineHeight;
return _getTextPositionVertical(position, verticalOffset);
}
/// {@macro flutter.services.TextLayoutMetrics.getTextPositionBelow}
@override
TextPosition getTextPositionBelow(TextPosition position) {
// The caret offset gives a location in the upper left hand corner of
// the caret so the middle of the line above is a half line above that
// point and the line below is 1.5 lines below that point.
final double preferredLineHeight = _textPainter.preferredLineHeight;
final double verticalOffset = 1.5 * preferredLineHeight;
return _getTextPositionVertical(position, verticalOffset);
}
// End TextLayoutMetrics.
void _updateSelectionExtentsVisibility(Offset effectiveOffset) {
assert(selection != null);
if (!selection!.isValid) {
_selectionStartInViewport.value = false;
_selectionEndInViewport.value = false;
return;
}
final Rect visibleRegion = Offset.zero & size;
final Offset startOffset = _textPainter.getOffsetForCaret(
TextPosition(offset: selection!.start, affinity: selection!.affinity),
_caretPrototype,
);
// Check if the selection is visible with an approximation because a
// difference between rounded and unrounded values causes the caret to be
// reported as having a slightly (< 0.5) negative y offset. This rounding
// happens in paragraph.cc's layout and TextPainter's
// _applyFloatingPointHack. Ideally, the rounding mismatch will be fixed and
// this can be changed to be a strict check instead of an approximation.
const double visibleRegionSlop = 0.5;
_selectionStartInViewport.value = visibleRegion
.inflate(visibleRegionSlop)
.contains(startOffset + effectiveOffset);
final Offset endOffset = _textPainter.getOffsetForCaret(
TextPosition(offset: selection!.end, affinity: selection!.affinity),
_caretPrototype,
);
_selectionEndInViewport.value = visibleRegion
.inflate(visibleRegionSlop)
.contains(endOffset + effectiveOffset);
}
void _setTextEditingValue(TextEditingValue newValue, SelectionChangedCause cause) {
textSelectionDelegate.userUpdateTextEditingValue(newValue, cause);
}
void _setSelection(TextSelection nextSelection, SelectionChangedCause cause) {
if (nextSelection.isValid) {
// The nextSelection is calculated based on plainText, which can be out
// of sync with the textSelectionDelegate.textEditingValue by one frame.
// This is due to the render editable and editable text handle pointer
// event separately. If the editable text changes the text during the
// event handler, the render editable will use the outdated text stored in
// the plainText when handling the pointer event.
//
// If this happens, we need to make sure the new selection is still valid.
final int textLength = textSelectionDelegate.textEditingValue.text.length;
nextSelection = nextSelection.copyWith(
baseOffset: math.min(nextSelection.baseOffset, textLength),
extentOffset: math.min(nextSelection.extentOffset, textLength),
);
}
_setTextEditingValue(
textSelectionDelegate.textEditingValue.copyWith(selection: nextSelection),
cause,
);
}
@override
void markNeedsPaint() {
super.markNeedsPaint();
// Tell the painters to repaint since text layout may have changed.
_foregroundRenderObject?.markNeedsPaint();
_backgroundRenderObject?.markNeedsPaint();
}
@override
void systemFontsDidChange() {
super.systemFontsDidChange();
_textPainter.markNeedsLayout();
}
/// Returns a plain text version of the text in [TextPainter].
///
/// If [obscureText] is true, returns the obscured text. See
/// [obscureText] and [obscuringCharacter].
/// In order to get the styled text as an [InlineSpan] tree, use [text].
String get plainText => _textPainter.plainText;
/// The text to paint in the form of a tree of [InlineSpan]s.
///
/// In order to get the plain text representation, use [plainText].
InlineSpan? get text => _textPainter.text;
final TextPainter _textPainter;
AttributedString? _cachedAttributedValue;
List<InlineSpanSemanticsInformation>? _cachedCombinedSemanticsInfos;
set text(InlineSpan? value) {
if (_textPainter.text == value) {
return;
}
_cachedLineBreakCount = null;
_textPainter.text = value;
_cachedAttributedValue = null;
_cachedCombinedSemanticsInfos = null;
_canComputeIntrinsicsCached = null;
markNeedsLayout();
markNeedsSemanticsUpdate();
}
TextPainter? _textIntrinsicsCache;
TextPainter get _textIntrinsics {
return (_textIntrinsicsCache ??= TextPainter())
..text = _textPainter.text
..textAlign = _textPainter.textAlign
..textDirection = _textPainter.textDirection
..textScaler = _textPainter.textScaler
..maxLines = _textPainter.maxLines
..ellipsis = _textPainter.ellipsis
..locale = _textPainter.locale
..strutStyle = _textPainter.strutStyle
..textWidthBasis = _textPainter.textWidthBasis
..textHeightBehavior = _textPainter.textHeightBehavior;
}
/// How the text should be aligned horizontally.
TextAlign get textAlign => _textPainter.textAlign;
set textAlign(TextAlign value) {
if (_textPainter.textAlign == value) {
return;
}
_textPainter.textAlign = value;
markNeedsLayout();
}
/// The directionality of the text.
///
/// This decides how the [TextAlign.start], [TextAlign.end], and
/// [TextAlign.justify] values of [textAlign] are interpreted.
///
/// This is also used to disambiguate how to render bidirectional text. For
/// example, if the [text] is an English phrase followed by a Hebrew phrase,
/// in a [TextDirection.ltr] context the English phrase will be on the left
/// and the Hebrew phrase to its right, while in a [TextDirection.rtl]
/// context, the English phrase will be on the right and the Hebrew phrase on
/// its left.
// TextPainter.textDirection is nullable, but it is set to a
// non-null value in the RenderEditable constructor and we refuse to
// set it to null here, so _textPainter.textDirection cannot be null.
TextDirection get textDirection => _textPainter.textDirection!;
set textDirection(TextDirection value) {
if (_textPainter.textDirection == value) {
return;
}
_textPainter.textDirection = value;
markNeedsLayout();
markNeedsSemanticsUpdate();
}
/// Used by this renderer's internal [TextPainter] to select a locale-specific
/// font.
///
/// In some cases the same Unicode character may be rendered differently depending
/// on the locale. For example the '骨' character is rendered differently in
/// the Chinese and Japanese locales. In these cases the [locale] may be used
/// to select a locale-specific font.
///
/// If this value is null, a system-dependent algorithm is used to select
/// the font.
Locale? get locale => _textPainter.locale;
set locale(Locale? value) {
if (_textPainter.locale == value) {
return;
}
_textPainter.locale = value;
markNeedsLayout();
}
/// The [StrutStyle] used by the renderer's internal [TextPainter] to
/// determine the strut to use.
StrutStyle? get strutStyle => _textPainter.strutStyle;
set strutStyle(StrutStyle? value) {
if (_textPainter.strutStyle == value) {
return;
}
_textPainter.strutStyle = value;
markNeedsLayout();
}
/// The color to use when painting the cursor.
Color? get cursorColor => _caretPainter.caretColor;
set cursorColor(Color? value) {
_caretPainter.caretColor = value;
}
/// The color to use when painting the cursor aligned to the text while
/// rendering the floating cursor.
///
/// Typically this would be set to [CupertinoColors.inactiveGray].
///
/// If this is null, the background cursor is not painted.
///
/// See also:
///
/// * [FloatingCursorDragState], which explains the floating cursor feature
/// in detail.
Color? get backgroundCursorColor => _caretPainter.backgroundCursorColor;
set backgroundCursorColor(Color? value) {
_caretPainter.backgroundCursorColor = value;
}
bool _disposeShowCursor;
/// Whether to paint the cursor.
ValueNotifier<bool> get showCursor => _showCursor;
ValueNotifier<bool> _showCursor;
set showCursor(ValueNotifier<bool> value) {
if (_showCursor == value) {
return;
}
if (attached) {
_showCursor.removeListener(_showHideCursor);
}
if (_disposeShowCursor) {
_showCursor.dispose();
_disposeShowCursor = false;
}
_showCursor = value;
if (attached) {
_showHideCursor();
_showCursor.addListener(_showHideCursor);
}
}
void _showHideCursor() {
_caretPainter.shouldPaint = showCursor.value;
}
/// Whether the editable is currently focused.
bool get hasFocus => _hasFocus;
bool _hasFocus = false;
set hasFocus(bool value) {
if (_hasFocus == value) {
return;
}
_hasFocus = value;
markNeedsSemanticsUpdate();
}
/// Whether this rendering object will take a full line regardless the text width.
bool get forceLine => _forceLine;
bool _forceLine = false;
set forceLine(bool value) {
if (_forceLine == value) {
return;
}
_forceLine = value;
markNeedsLayout();
}
/// Whether this rendering object is read only.
bool get readOnly => _readOnly;
bool _readOnly = false;
set readOnly(bool value) {
if (_readOnly == value) {
return;
}
_readOnly = value;
markNeedsSemanticsUpdate();
}
/// The maximum number of lines for the text to span, wrapping if necessary.
///
/// If this is 1 (the default), the text will not wrap, but will extend
/// indefinitely instead.
///
/// If this is null, there is no limit to the number of lines.
///
/// When this is not null, the intrinsic height of the render object is the
/// height of one line of text multiplied by this value. In other words, this
/// also controls the height of the actual editing widget.
int? get maxLines => _maxLines;
int? _maxLines;
/// The value may be null. If it is not null, then it must be greater than zero.
set maxLines(int? value) {
assert(value == null || value > 0);
if (maxLines == value) {
return;
}
_maxLines = value;
// Special case maxLines == 1 to keep only the first line so we can get the
// height of the first line in case there are hard line breaks in the text.
// See the `_preferredHeight` method.
_textPainter.maxLines = value == 1 ? 1 : null;
markNeedsLayout();
}
/// {@macro flutter.widgets.editableText.minLines}
int? get minLines => _minLines;
int? _minLines;
/// The value may be null. If it is not null, then it must be greater than zero.
set minLines(int? value) {
assert(value == null || value > 0);
if (minLines == value) {
return;
}
_minLines = value;
markNeedsLayout();
}
/// {@macro flutter.widgets.editableText.expands}
bool get expands => _expands;
bool _expands;
set expands(bool value) {
if (expands == value) {
return;
}
_expands = value;
markNeedsLayout();
}
/// The color to use when painting the selection.
Color? get selectionColor => _selectionPainter.highlightColor;
set selectionColor(Color? value) {
_selectionPainter.highlightColor = value;
}
/// Deprecated. Will be removed in a future version of Flutter. Use
/// [textScaler] instead.
///
/// The number of font pixels for each logical pixel.
///
/// For example, if the text scale factor is 1.5, text will be 50% larger than
/// the specified font size.
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double get textScaleFactor => _textPainter.textScaleFactor;
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
set textScaleFactor(double value) {
textScaler = TextScaler.linear(value);
}
/// {@macro flutter.painting.textPainter.textScaler}
TextScaler get textScaler => _textPainter.textScaler;
set textScaler(TextScaler value) {
if (_textPainter.textScaler == value) {
return;
}
_textPainter.textScaler = value;
markNeedsLayout();
}
/// The region of text that is selected, if any.
///
/// The caret position is represented by a collapsed selection.
///
/// If [selection] is null, there is no selection and attempts to
/// manipulate the selection will throw.
TextSelection? get selection => _selection;
TextSelection? _selection;
set selection(TextSelection? value) {
if (_selection == value) {
return;
}
_selection = value;
_selectionPainter.highlightedRange = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
/// The offset at which the text should be painted.
///
/// If the text content is larger than the editable line itself, the editable
/// line clips the text. This property controls which part of the text is
/// visible by shifting the text by the given offset before clipping.
ViewportOffset get offset => _offset;
ViewportOffset _offset;
set offset(ViewportOffset value) {
if (_offset == value) {
return;
}
if (attached) {
_offset.removeListener(markNeedsPaint);
}
_offset = value;
if (attached) {
_offset.addListener(markNeedsPaint);
}
markNeedsLayout();
}
/// How thick the cursor will be.
double get cursorWidth => _cursorWidth;
double _cursorWidth = 1.0;
set cursorWidth(double value) {
if (_cursorWidth == value) {
return;
}
_cursorWidth = value;
markNeedsLayout();
}
/// How tall the cursor will be.
///
/// This can be null, in which case the getter will actually return [preferredLineHeight].
///
/// Setting this to itself fixes the value to the current [preferredLineHeight]. Setting
/// this to null returns the behavior of deferring to [preferredLineHeight].
// TODO(ianh): This is a confusing API. We should have a separate getter for the effective cursor height.
double get cursorHeight => _cursorHeight ?? preferredLineHeight;
double? _cursorHeight;
set cursorHeight(double? value) {
if (_cursorHeight == value) {
return;
}
_cursorHeight = value;
markNeedsLayout();
}
/// {@template flutter.rendering.RenderEditable.paintCursorAboveText}
/// If the cursor should be painted on top of the text or underneath it.
///
/// By default, the cursor should be painted on top for iOS platforms and
/// underneath for Android platforms.
/// {@endtemplate}
bool get paintCursorAboveText => _paintCursorOnTop;
bool _paintCursorOnTop;
set paintCursorAboveText(bool value) {
if (_paintCursorOnTop == value) {
return;
}
_paintCursorOnTop = value;
// Clear cached built-in painters and reconfigure painters.
_cachedBuiltInForegroundPainters = null;
_cachedBuiltInPainters = null;
// Call update methods to rebuild and set the effective painters.
_updateForegroundPainter(_foregroundPainter);
_updatePainter(_painter);
}
/// {@template flutter.rendering.RenderEditable.cursorOffset}
/// The offset that is used, in pixels, when painting the cursor on screen.
///
/// By default, the cursor position should be set to an offset of
/// (-[cursorWidth] * 0.5, 0.0) on iOS platforms and (0, 0) on Android
/// platforms. The origin from where the offset is applied to is the arbitrary
/// location where the cursor ends up being rendered from by default.
/// {@endtemplate}
Offset get cursorOffset => _caretPainter.cursorOffset;
set cursorOffset(Offset value) {
_caretPainter.cursorOffset = value;
}
/// How rounded the corners of the cursor should be.
///
/// A null value is the same as [Radius.zero].
Radius? get cursorRadius => _caretPainter.cursorRadius;
set cursorRadius(Radius? value) {
_caretPainter.cursorRadius = value;
}
/// The [LayerLink] of start selection handle.
///
/// [RenderEditable] is responsible for calculating the [Offset] of this
/// [LayerLink], which will be used as [CompositedTransformTarget] of start handle.
LayerLink get startHandleLayerLink => _startHandleLayerLink;
LayerLink _startHandleLayerLink;
set startHandleLayerLink(LayerLink value) {
if (_startHandleLayerLink == value) {
return;
}
_startHandleLayerLink = value;
markNeedsPaint();
}
/// The [LayerLink] of end selection handle.
///
/// [RenderEditable] is responsible for calculating the [Offset] of this
/// [LayerLink], which will be used as [CompositedTransformTarget] of end handle.
LayerLink get endHandleLayerLink => _endHandleLayerLink;
LayerLink _endHandleLayerLink;
set endHandleLayerLink(LayerLink value) {
if (_endHandleLayerLink == value) {
return;
}
_endHandleLayerLink = value;
markNeedsPaint();
}
/// The padding applied to text field. Used to determine the bounds when
/// moving the floating cursor.
///
/// Defaults to a padding with left, top and right set to 4, bottom to 5.
///
/// See also:
///
/// * [FloatingCursorDragState], which explains the floating cursor feature
/// in detail.
EdgeInsets floatingCursorAddedMargin;
/// Returns true if the floating cursor is visible, false otherwise.
bool get floatingCursorOn => _floatingCursorOn;
bool _floatingCursorOn = false;
late TextPosition _floatingCursorTextPosition;
/// Whether to allow the user to change the selection.
///
/// Since [RenderEditable] does not handle selection manipulation
/// itself, this actually only affects whether the accessibility
/// hints provided to the system (via
/// [describeSemanticsConfiguration]) will enable selection
/// manipulation. It's the responsibility of this object's owner
/// to provide selection manipulation affordances.
///
/// This field is used by [selectionEnabled] (which then controls
/// the accessibility hints mentioned above). When null,
/// [obscureText] is used to determine the value of
/// [selectionEnabled] instead.
bool? get enableInteractiveSelection => _enableInteractiveSelection;
bool? _enableInteractiveSelection;
set enableInteractiveSelection(bool? value) {
if (_enableInteractiveSelection == value) {
return;
}
_enableInteractiveSelection = value;
markNeedsLayout();
markNeedsSemanticsUpdate();
}
/// Whether interactive selection are enabled based on the values of
/// [enableInteractiveSelection] and [obscureText].
///
/// Since [RenderEditable] does not handle selection manipulation
/// itself, this actually only affects whether the accessibility
/// hints provided to the system (via
/// [describeSemanticsConfiguration]) will enable selection
/// manipulation. It's the responsibility of this object's owner
/// to provide selection manipulation affordances.
///
/// By default, [enableInteractiveSelection] is null, [obscureText] is false,
/// and this getter returns true.
///
/// If [enableInteractiveSelection] is null and [obscureText] is true, then this
/// getter returns false. This is the common case for password fields.
///
/// If [enableInteractiveSelection] is non-null then its value is
/// returned. An application might [enableInteractiveSelection] to
/// true to enable interactive selection for a password field, or to
/// false to unconditionally disable interactive selection.
bool get selectionEnabled {
return enableInteractiveSelection ?? !obscureText;
}
/// The color used to paint the prompt rectangle.
///
/// The prompt rectangle will only be requested on non-web iOS applications.
// TODO(ianh): We should change the getter to return null when _promptRectRange is null
// (otherwise, if you set it to null and then get it, you get back non-null).
// Alternatively, we could stop supporting setting this to null.
Color? get promptRectColor => _autocorrectHighlightPainter.highlightColor;
set promptRectColor(Color? newValue) {
_autocorrectHighlightPainter.highlightColor = newValue;
}
/// Dismisses the currently displayed prompt rectangle and displays a new prompt rectangle
/// over [newRange] in the given color [promptRectColor].
///
/// The prompt rectangle will only be requested on non-web iOS applications.
///
/// When set to null, the currently displayed prompt rectangle (if any) will be dismissed.
// ignore: use_setters_to_change_properties, (API predates enforcing the lint)
void setPromptRectRange(TextRange? newRange) {
_autocorrectHighlightPainter.highlightedRange = newRange;
}
/// The maximum amount the text is allowed to scroll.
///
/// This value is only valid after layout and can change as additional
/// text is entered or removed in order to accommodate expanding when
/// [expands] is set to true.
double get maxScrollExtent => _maxScrollExtent;
double _maxScrollExtent = 0;
double get _caretMargin => _kCaretGap + cursorWidth;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
/// Collected during [describeSemanticsConfiguration], used by
/// [assembleSemanticsNode] and [_combineSemanticsInfo].
List<InlineSpanSemanticsInformation>? _semanticsInfo;
// Caches [SemanticsNode]s created during [assembleSemanticsNode] so they
// can be re-used when [assembleSemanticsNode] is called again. This ensures
// stable ids for the [SemanticsNode]s of [TextSpan]s across
// [assembleSemanticsNode] invocations.
LinkedHashMap<Key, SemanticsNode>? _cachedChildNodes;
/// Returns a list of rects that bound the given selection, and the text
/// direction. The text direction is used by the engine to calculate
/// the closest position to a given point.
///
/// See [TextPainter.getBoxesForSelection] for more details.
List<TextBox> getBoxesForSelection(TextSelection selection) {
_computeTextMetricsIfNeeded();
return _textPainter.getBoxesForSelection(selection)
.map((TextBox textBox) => TextBox.fromLTRBD(
textBox.left + _paintOffset.dx,
textBox.top + _paintOffset.dy,
textBox.right + _paintOffset.dx,
textBox.bottom + _paintOffset.dy,
textBox.direction
)).toList();
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
_semanticsInfo = _textPainter.text!.getSemanticsInformation();
// TODO(chunhtai): the macOS does not provide a public API to support text
// selections across multiple semantics nodes. Remove this platform check
// once we can support it.
// https://github.com/flutter/flutter/issues/77957
if (_semanticsInfo!.any((InlineSpanSemanticsInformation info) => info.recognizer != null) &&
defaultTargetPlatform != TargetPlatform.macOS) {
assert(readOnly && !obscureText);
// For Selectable rich text with recognizer, we need to create a semantics
// node for each text fragment.
config
..isSemanticBoundary = true
..explicitChildNodes = true;
return;
}
if (_cachedAttributedValue == null) {
if (obscureText) {
_cachedAttributedValue = AttributedString(obscuringCharacter * plainText.length);
} else {
final StringBuffer buffer = StringBuffer();
int offset = 0;
final List<StringAttribute> attributes = <StringAttribute>[];
for (final InlineSpanSemanticsInformation info in _semanticsInfo!) {
final String label = info.semanticsLabel ?? info.text;
for (final StringAttribute infoAttribute in info.stringAttributes) {
final TextRange originalRange = infoAttribute.range;
attributes.add(
infoAttribute.copy(
range: TextRange(start: offset + originalRange.start, end: offset + originalRange.end),
),
);
}
buffer.write(label);
offset += label.length;
}
_cachedAttributedValue = AttributedString(buffer.toString(), attributes: attributes);
}
}
config
..attributedValue = _cachedAttributedValue!
..isObscured = obscureText
..isMultiline = _isMultiline
..textDirection = textDirection
..isFocused = hasFocus
..isTextField = true
..isReadOnly = readOnly;
if (hasFocus && selectionEnabled) {
config.onSetSelection = _handleSetSelection;
}
if (hasFocus && !readOnly) {
config.onSetText = _handleSetText;
}
if (selectionEnabled && (selection?.isValid ?? false)) {
config.textSelection = selection;
if (_textPainter.getOffsetBefore(selection!.extentOffset) != null) {
config
..onMoveCursorBackwardByWord = _handleMoveCursorBackwardByWord
..onMoveCursorBackwardByCharacter = _handleMoveCursorBackwardByCharacter;
}
if (_textPainter.getOffsetAfter(selection!.extentOffset) != null) {
config
..onMoveCursorForwardByWord = _handleMoveCursorForwardByWord
..onMoveCursorForwardByCharacter = _handleMoveCursorForwardByCharacter;
}
}
}
void _handleSetText(String text) {
textSelectionDelegate.userUpdateTextEditingValue(
TextEditingValue(
text: text,
selection: TextSelection.collapsed(offset: text.length),
),
SelectionChangedCause.keyboard,
);
}
@override
void assembleSemanticsNode(SemanticsNode node, SemanticsConfiguration config, Iterable<SemanticsNode> children) {
assert(_semanticsInfo != null && _semanticsInfo!.isNotEmpty);
final List<SemanticsNode> newChildren = <SemanticsNode>[];
TextDirection currentDirection = textDirection;
Rect currentRect;
double ordinal = 0.0;
int start = 0;
int placeholderIndex = 0;
int childIndex = 0;
RenderBox? child = firstChild;
final LinkedHashMap<Key, SemanticsNode> newChildCache = LinkedHashMap<Key, SemanticsNode>();
_cachedCombinedSemanticsInfos ??= combineSemanticsInfo(_semanticsInfo!);
for (final InlineSpanSemanticsInformation info in _cachedCombinedSemanticsInfos!) {
final TextSelection selection = TextSelection(
baseOffset: start,
extentOffset: start + info.text.length,
);
start += info.text.length;
if (info.isPlaceholder) {
// A placeholder span may have 0 to multiple semantics nodes, we need
// to annotate all of the semantics nodes belong to this span.
while (children.length > childIndex &&
children.elementAt(childIndex).isTagged(PlaceholderSpanIndexSemanticsTag(placeholderIndex))) {
final SemanticsNode childNode = children.elementAt(childIndex);
final TextParentData parentData = child!.parentData! as TextParentData;
assert(parentData.offset != null);
newChildren.add(childNode);
childIndex += 1;
}
child = childAfter(child!);
placeholderIndex += 1;
} else {
final TextDirection initialDirection = currentDirection;
final List<ui.TextBox> rects = _textPainter.getBoxesForSelection(selection);
if (rects.isEmpty) {
continue;
}
Rect rect = rects.first.toRect();
currentDirection = rects.first.direction;
for (final ui.TextBox textBox in rects.skip(1)) {
rect = rect.expandToInclude(textBox.toRect());
currentDirection = textBox.direction;
}
// Any of the text boxes may have had infinite dimensions.
// We shouldn't pass infinite dimensions up to the bridges.
rect = Rect.fromLTWH(
math.max(0.0, rect.left),
math.max(0.0, rect.top),
math.min(rect.width, constraints.maxWidth),
math.min(rect.height, constraints.maxHeight),
);
// Round the current rectangle to make this API testable and add some
// padding so that the accessibility rects do not overlap with the text.
currentRect = Rect.fromLTRB(
rect.left.floorToDouble() - 4.0,
rect.top.floorToDouble() - 4.0,
rect.right.ceilToDouble() + 4.0,
rect.bottom.ceilToDouble() + 4.0,
);
final SemanticsConfiguration configuration = SemanticsConfiguration()
..sortKey = OrdinalSortKey(ordinal++)
..textDirection = initialDirection
..attributedLabel = AttributedString(info.semanticsLabel ?? info.text, attributes: info.stringAttributes);
final GestureRecognizer? recognizer = info.recognizer;
if (recognizer != null) {
if (recognizer is TapGestureRecognizer) {
if (recognizer.onTap != null) {
configuration.onTap = recognizer.onTap;
configuration.isLink = true;
}
} else if (recognizer is DoubleTapGestureRecognizer) {
if (recognizer.onDoubleTap != null) {
configuration.onTap = recognizer.onDoubleTap;
configuration.isLink = true;
}
} else if (recognizer is LongPressGestureRecognizer) {
if (recognizer.onLongPress != null) {
configuration.onLongPress = recognizer.onLongPress;
}
} else {
assert(false, '${recognizer.runtimeType} is not supported.');
}
}
if (node.parentPaintClipRect != null) {
final Rect paintRect = node.parentPaintClipRect!.intersect(currentRect);
configuration.isHidden = paintRect.isEmpty && !currentRect.isEmpty;
}
late final SemanticsNode newChild;
if (_cachedChildNodes?.isNotEmpty ?? false) {
newChild = _cachedChildNodes!.remove(_cachedChildNodes!.keys.first)!;
} else {
final UniqueKey key = UniqueKey();
newChild = SemanticsNode(
key: key,
showOnScreen: _createShowOnScreenFor(key),
);
}
newChild
..updateWith(config: configuration)
..rect = currentRect;
newChildCache[newChild.key!] = newChild;
newChildren.add(newChild);
}
}
_cachedChildNodes = newChildCache;
node.updateWith(config: config, childrenInInversePaintOrder: newChildren);
}
VoidCallback? _createShowOnScreenFor(Key key) {
return () {
final SemanticsNode node = _cachedChildNodes![key]!;
showOnScreen(descendant: this, rect: node.rect);
};
}
// TODO(ianh): in theory, [selection] could become null between when
// we last called describeSemanticsConfiguration and when the
// callbacks are invoked, in which case the callbacks will crash...
void _handleSetSelection(TextSelection selection) {
_setSelection(selection, SelectionChangedCause.keyboard);
}
void _handleMoveCursorForwardByCharacter(bool extendSelection) {
assert(selection != null);
final int? extentOffset = _textPainter.getOffsetAfter(selection!.extentOffset);
if (extentOffset == null) {
return;
}
final int baseOffset = !extendSelection ? extentOffset : selection!.baseOffset;
_setSelection(
TextSelection(baseOffset: baseOffset, extentOffset: extentOffset),
SelectionChangedCause.keyboard,
);
}
void _handleMoveCursorBackwardByCharacter(bool extendSelection) {
assert(selection != null);
final int? extentOffset = _textPainter.getOffsetBefore(selection!.extentOffset);
if (extentOffset == null) {
return;
}
final int baseOffset = !extendSelection ? extentOffset : selection!.baseOffset;
_setSelection(
TextSelection(baseOffset: baseOffset, extentOffset: extentOffset),
SelectionChangedCause.keyboard,
);
}
void _handleMoveCursorForwardByWord(bool extendSelection) {
assert(selection != null);
final TextRange currentWord = _textPainter.getWordBoundary(selection!.extent);
final TextRange? nextWord = _getNextWord(currentWord.end);
if (nextWord == null) {
return;
}
final int baseOffset = extendSelection ? selection!.baseOffset : nextWord.start;
_setSelection(
TextSelection(
baseOffset: baseOffset,
extentOffset: nextWord.start,
),
SelectionChangedCause.keyboard,
);
}
void _handleMoveCursorBackwardByWord(bool extendSelection) {
assert(selection != null);
final TextRange currentWord = _textPainter.getWordBoundary(selection!.extent);
final TextRange? previousWord = _getPreviousWord(currentWord.start - 1);
if (previousWord == null) {
return;
}
final int baseOffset = extendSelection ? selection!.baseOffset : previousWord.start;
_setSelection(
TextSelection(
baseOffset: baseOffset,
extentOffset: previousWord.start,
),
SelectionChangedCause.keyboard,
);
}
TextRange? _getNextWord(int offset) {
while (true) {
final TextRange range = _textPainter.getWordBoundary(TextPosition(offset: offset));
if (!range.isValid || range.isCollapsed) {
return null;
}
if (!_onlyWhitespace(range)) {
return range;
}
offset = range.end;
}
}
TextRange? _getPreviousWord(int offset) {
while (offset >= 0) {
final TextRange range = _textPainter.getWordBoundary(TextPosition(offset: offset));
if (!range.isValid || range.isCollapsed) {
return null;
}
if (!_onlyWhitespace(range)) {
return range;
}
offset = range.start - 1;
}
return null;
}
// Check if the given text range only contains white space or separator
// characters.
//
// Includes newline characters from ASCII and separators from the
// [unicode separator category](https://www.compart.com/en/unicode/category/Zs)
// TODO(zanderso): replace when we expose this ICU information.
bool _onlyWhitespace(TextRange range) {
for (int i = range.start; i < range.end; i++) {
final int codeUnit = text!.codeUnitAt(i)!;
if (!TextLayoutMetrics.isWhitespace(codeUnit)) {
return false;
}
}
return true;
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_foregroundRenderObject?.attach(owner);
_backgroundRenderObject?.attach(owner);
_tap = TapGestureRecognizer(debugOwner: this)
..onTapDown = _handleTapDown
..onTap = _handleTap;
_longPress = LongPressGestureRecognizer(debugOwner: this)..onLongPress = _handleLongPress;
_offset.addListener(markNeedsPaint);
_showHideCursor();
_showCursor.addListener(_showHideCursor);
}
@override
void detach() {
_tap.dispose();
_longPress.dispose();
_offset.removeListener(markNeedsPaint);
_showCursor.removeListener(_showHideCursor);
super.detach();
_foregroundRenderObject?.detach();
_backgroundRenderObject?.detach();
}
@override
void redepthChildren() {
final RenderObject? foregroundChild = _foregroundRenderObject;
final RenderObject? backgroundChild = _backgroundRenderObject;
if (foregroundChild != null) {
redepthChild(foregroundChild);
}
if (backgroundChild != null) {
redepthChild(backgroundChild);
}
super.redepthChildren();
}
@override
void visitChildren(RenderObjectVisitor visitor) {
final RenderObject? foregroundChild = _foregroundRenderObject;
final RenderObject? backgroundChild = _backgroundRenderObject;
if (foregroundChild != null) {
visitor(foregroundChild);
}
if (backgroundChild != null) {
visitor(backgroundChild);
}
super.visitChildren(visitor);
}
bool get _isMultiline => maxLines != 1;
Axis get _viewportAxis => _isMultiline ? Axis.vertical : Axis.horizontal;
Offset get _paintOffset {
return switch (_viewportAxis) {
Axis.horizontal => Offset(-offset.pixels, 0.0),
Axis.vertical => Offset(0.0, -offset.pixels),
};
}
double get _viewportExtent {
assert(hasSize);
return switch (_viewportAxis) {
Axis.horizontal => size.width,
Axis.vertical => size.height,
};
}
double _getMaxScrollExtent(Size contentSize) {
assert(hasSize);
return switch (_viewportAxis) {
Axis.horizontal => math.max(0.0, contentSize.width - size.width),
Axis.vertical => math.max(0.0, contentSize.height - size.height),
};
}
// We need to check the paint offset here because during animation, the start of
// the text may position outside the visible region even when the text fits.
bool get _hasVisualOverflow => _maxScrollExtent > 0 || _paintOffset != Offset.zero;
/// Returns the local coordinates of the endpoints of the given selection.
///
/// If the selection is collapsed (and therefore occupies a single point), the
/// returned list is of length one. Otherwise, the selection is not collapsed
/// and the returned list is of length two. In this case, however, the two
/// points might actually be co-located (e.g., because of a bidirectional
/// selection that contains some text but whose ends meet in the middle).
///
/// See also:
///
/// * [getLocalRectForCaret], which is the equivalent but for
/// a [TextPosition] rather than a [TextSelection].
List<TextSelectionPoint> getEndpointsForSelection(TextSelection selection) {
_computeTextMetricsIfNeeded();
final Offset paintOffset = _paintOffset;
final List<ui.TextBox> boxes = selection.isCollapsed ?
<ui.TextBox>[] : _textPainter.getBoxesForSelection(selection, boxHeightStyle: selectionHeightStyle, boxWidthStyle: selectionWidthStyle);
if (boxes.isEmpty) {
// TODO(mpcomplete): This doesn't work well at an RTL/LTR boundary.
final Offset caretOffset = _textPainter.getOffsetForCaret(selection.extent, _caretPrototype);
final Offset start = Offset(0.0, preferredLineHeight) + caretOffset + paintOffset;
return <TextSelectionPoint>[TextSelectionPoint(start, null)];
} else {
final Offset start = Offset(clampDouble(boxes.first.start, 0, _textPainter.size.width), boxes.first.bottom) + paintOffset;
final Offset end = Offset(clampDouble(boxes.last.end, 0, _textPainter.size.width), boxes.last.bottom) + paintOffset;
return <TextSelectionPoint>[
TextSelectionPoint(start, boxes.first.direction),
TextSelectionPoint(end, boxes.last.direction),
];
}
}
/// Returns the smallest [Rect], in the local coordinate system, that covers
/// the text within the [TextRange] specified.
///
/// This method is used to calculate the approximate position of the IME bar
/// on iOS.
///
/// Returns null if [TextRange.isValid] is false for the given `range`, or the
/// given `range` is collapsed.
Rect? getRectForComposingRange(TextRange range) {
if (!range.isValid || range.isCollapsed) {
return null;
}
_computeTextMetricsIfNeeded();
final List<ui.TextBox> boxes = _textPainter.getBoxesForSelection(
TextSelection(baseOffset: range.start, extentOffset: range.end),
boxHeightStyle: selectionHeightStyle,
boxWidthStyle: selectionWidthStyle,
);
return boxes.fold(
null,
(Rect? accum, TextBox incoming) => accum?.expandToInclude(incoming.toRect()) ?? incoming.toRect(),
)?.shift(_paintOffset);
}
/// Returns the position in the text for the given global coordinate.
///
/// See also:
///
/// * [getLocalRectForCaret], which is the reverse operation, taking
/// a [TextPosition] and returning a [Rect].
/// * [TextPainter.getPositionForOffset], which is the equivalent method
/// for a [TextPainter] object.
TextPosition getPositionForPoint(Offset globalPosition) {
_computeTextMetricsIfNeeded();
globalPosition += -_paintOffset;
return _textPainter.getPositionForOffset(globalToLocal(globalPosition));
}
/// Returns the [Rect] in local coordinates for the caret at the given text
/// position.
///
/// See also:
///
/// * [getPositionForPoint], which is the reverse operation, taking
/// an [Offset] in global coordinates and returning a [TextPosition].
/// * [getEndpointsForSelection], which is the equivalent but for
/// a selection rather than a particular text position.
/// * [TextPainter.getOffsetForCaret], the equivalent method for a
/// [TextPainter] object.
Rect getLocalRectForCaret(TextPosition caretPosition) {
_computeTextMetricsIfNeeded();
final Rect caretPrototype = _caretPrototype;
final Offset caretOffset = _textPainter.getOffsetForCaret(caretPosition, caretPrototype);
Rect caretRect = caretPrototype.shift(caretOffset + cursorOffset);
final double scrollableWidth = math.max(_textPainter.width + _caretMargin, size.width);
final double caretX = clampDouble(caretRect.left, 0, math.max(scrollableWidth - _caretMargin, 0));
caretRect = Offset(caretX, caretRect.top) & caretRect.size;
final double caretHeight = cursorHeight;
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
final double fullHeight = _textPainter.getFullHeightForCaret(caretPosition, caretPrototype) ?? _textPainter.preferredLineHeight;
final double heightDiff = fullHeight - caretRect.height;
// Center the caret vertically along the text.
caretRect = Rect.fromLTWH(
caretRect.left,
caretRect.top + heightDiff / 2,
caretRect.width,
caretRect.height,
);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
// Override the height to take the full height of the glyph at the TextPosition
// when not on iOS. iOS has special handling that creates a taller caret.
// TODO(garyq): See the TODO for _computeCaretPrototype().
caretRect = Rect.fromLTWH(
caretRect.left,
caretRect.top - _kCaretHeightOffset,
caretRect.width,
caretHeight,
);
}
caretRect = caretRect.shift(_paintOffset);
return caretRect.shift(_snapToPhysicalPixel(caretRect.topLeft));
}
@override
double computeMinIntrinsicWidth(double height) {
if (!_canComputeIntrinsics) {
return 0.0;
}
final List<PlaceholderDimensions> placeholderDimensions = layoutInlineChildren(double.infinity, (RenderBox child, BoxConstraints constraints) => Size(child.getMinIntrinsicWidth(double.infinity), 0.0));
final (double minWidth, double maxWidth) = _adjustConstraints();
return (_textIntrinsics
..setPlaceholderDimensions(placeholderDimensions)
..layout(minWidth: minWidth, maxWidth: maxWidth))
.minIntrinsicWidth;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (!_canComputeIntrinsics) {
return 0.0;
}
final List<PlaceholderDimensions> placeholderDimensions = layoutInlineChildren(
double.infinity,
// Height and baseline is irrelevant as all text will be laid
// out in a single line. Therefore, using 0.0 as a dummy for the height.
(RenderBox child, BoxConstraints constraints) => Size(child.getMaxIntrinsicWidth(double.infinity), 0.0),
);
final (double minWidth, double maxWidth) = _adjustConstraints();
return (_textIntrinsics
..setPlaceholderDimensions(placeholderDimensions)
..layout(minWidth: minWidth, maxWidth: maxWidth))
.maxIntrinsicWidth + _caretMargin;
}
/// An estimate of the height of a line in the text. See [TextPainter.preferredLineHeight].
/// This does not require the layout to be updated.
double get preferredLineHeight => _textPainter.preferredLineHeight;
int? _cachedLineBreakCount;
int _countHardLineBreaks(String text) {
final int? cachedValue = _cachedLineBreakCount;
if (cachedValue != null) {
return cachedValue;
}
int count = 0;
for (int index = 0; index < text.length; index += 1) {
switch (text.codeUnitAt(index)) {
case 0x000A: // LF
case 0x0085: // NEL
case 0x000B: // VT
case 0x000C: // FF, treating it as a regular line separator
case 0x2028: // LS
case 0x2029: // PS
count += 1;
}
}
return _cachedLineBreakCount = count;
}
double _preferredHeight(double width) {
final int? maxLines = this.maxLines;
final int? minLines = this.minLines ?? maxLines;
final double minHeight = preferredLineHeight * (minLines ?? 0);
assert(maxLines != 1 || _textIntrinsics.maxLines == 1);
if (maxLines == null) {
final double estimatedHeight;
if (width == double.infinity) {
estimatedHeight = preferredLineHeight * (_countHardLineBreaks(plainText) + 1);
} else {
final (double minWidth, double maxWidth) = _adjustConstraints(maxWidth: width);
estimatedHeight = (_textIntrinsics..layout(minWidth: minWidth, maxWidth: maxWidth)).height;
}
return math.max(estimatedHeight, minHeight);
}
// Special case maxLines == 1 since it forces the scrollable direction
// to be horizontal. Report the real height to prevent the text from being
// clipped.
if (maxLines == 1) {
// The _layoutText call lays out the paragraph using infinite width when
// maxLines == 1. Also _textPainter.maxLines will be set to 1 so should
// there be any line breaks only the first line is shown.
final (double minWidth, double maxWidth) = _adjustConstraints(maxWidth: width);
return (_textIntrinsics..layout(minWidth: minWidth, maxWidth: maxWidth)).height;
}
if (minLines == maxLines) {
return minHeight;
}
final double maxHeight = preferredLineHeight * maxLines;
final (double minWidth, double maxWidth) = _adjustConstraints(maxWidth: width);
return clampDouble(
(_textIntrinsics..layout(minWidth: minWidth, maxWidth: maxWidth)).height,
minHeight,
maxHeight,
);
}
@override
double computeMinIntrinsicHeight(double width) => computeMaxIntrinsicHeight(width);
@override
double computeMaxIntrinsicHeight(double width) {
if (!_canComputeIntrinsics) {
return 0.0;
}
_textIntrinsics.setPlaceholderDimensions(layoutInlineChildren(width, ChildLayoutHelper.dryLayoutChild));
return _preferredHeight(width);
}
@override
double computeDistanceToActualBaseline(TextBaseline baseline) {
_computeTextMetricsIfNeeded();
return _textPainter.computeDistanceToActualBaseline(baseline);
}
@override
bool hitTestSelf(Offset position) => true;
@override
@protected
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final Offset effectivePosition = position - _paintOffset;
final GlyphInfo? glyph = _textPainter.getClosestGlyphForOffset(effectivePosition);
// The hit-test can't fall through the horizontal gaps between visually
// adjacent characters on the same line, even with a large letter-spacing or
// text justification, as graphemeClusterLayoutBounds.width is the advance
// width to the next character, so there's no gap between their
// graphemeClusterLayoutBounds rects.
final InlineSpan? spanHit = glyph != null && glyph.graphemeClusterLayoutBounds.contains(effectivePosition)
? _textPainter.text!.getSpanForPosition(TextPosition(offset: glyph.graphemeClusterCodeUnitRange.start))
: null;
switch (spanHit) {
case final HitTestTarget span:
result.add(HitTestEntry(span));
return true;
case _:
return hitTestInlineChildren(result, effectivePosition);
}
}
late TapGestureRecognizer _tap;
late LongPressGestureRecognizer _longPress;
@override
void handleEvent(PointerEvent event, BoxHitTestEntry entry) {
assert(debugHandleEvent(event, entry));
if (event is PointerDownEvent) {
assert(!debugNeedsLayout);
if (!ignorePointer) {
// Propagates the pointer event to selection handlers.
_tap.addPointer(event);
_longPress.addPointer(event);
}
}
}
Offset? _lastTapDownPosition;
Offset? _lastSecondaryTapDownPosition;
/// {@template flutter.rendering.RenderEditable.lastSecondaryTapDownPosition}
/// The position of the most recent secondary tap down event on this text
/// input.
/// {@endtemplate}
Offset? get lastSecondaryTapDownPosition => _lastSecondaryTapDownPosition;
/// Tracks the position of a secondary tap event.
///
/// Should be called before attempting to change the selection based on the
/// position of a secondary tap.
void handleSecondaryTapDown(TapDownDetails details) {
_lastTapDownPosition = details.globalPosition;
_lastSecondaryTapDownPosition = details.globalPosition;
}
/// If [ignorePointer] is false (the default) then this method is called by
/// the internal gesture recognizer's [TapGestureRecognizer.onTapDown]
/// callback.
///
/// When [ignorePointer] is true, an ancestor widget must respond to tap
/// down events by calling this method.
void handleTapDown(TapDownDetails details) {
_lastTapDownPosition = details.globalPosition;
}
void _handleTapDown(TapDownDetails details) {
assert(!ignorePointer);
handleTapDown(details);
}
/// If [ignorePointer] is false (the default) then this method is called by
/// the internal gesture recognizer's [TapGestureRecognizer.onTap]
/// callback.
///
/// When [ignorePointer] is true, an ancestor widget must respond to tap
/// events by calling this method.
void handleTap() {
selectPosition(cause: SelectionChangedCause.tap);
}
void _handleTap() {
assert(!ignorePointer);
handleTap();
}
/// If [ignorePointer] is false (the default) then this method is called by
/// the internal gesture recognizer's [DoubleTapGestureRecognizer.onDoubleTap]
/// callback.
///
/// When [ignorePointer] is true, an ancestor widget must respond to double
/// tap events by calling this method.
void handleDoubleTap() {
selectWord(cause: SelectionChangedCause.doubleTap);
}
/// If [ignorePointer] is false (the default) then this method is called by
/// the internal gesture recognizer's [LongPressGestureRecognizer.onLongPress]
/// callback.
///
/// When [ignorePointer] is true, an ancestor widget must respond to long
/// press events by calling this method.
void handleLongPress() {
selectWord(cause: SelectionChangedCause.longPress);
}
void _handleLongPress() {
assert(!ignorePointer);
handleLongPress();
}
/// Move selection to the location of the last tap down.
///
/// {@template flutter.rendering.RenderEditable.selectPosition}
/// This method is mainly used to translate user inputs in global positions
/// into a [TextSelection]. When used in conjunction with a [EditableText],
/// the selection change is fed back into [TextEditingController.selection].
///
/// If you have a [TextEditingController], it's generally easier to
/// programmatically manipulate its `value` or `selection` directly.
/// {@endtemplate}
void selectPosition({ required SelectionChangedCause cause }) {
selectPositionAt(from: _lastTapDownPosition!, cause: cause);
}
/// Select text between the global positions [from] and [to].
///
/// [from] corresponds to the [TextSelection.baseOffset], and [to] corresponds
/// to the [TextSelection.extentOffset].
void selectPositionAt({ required Offset from, Offset? to, required SelectionChangedCause cause }) {
_computeTextMetricsIfNeeded();
final TextPosition fromPosition = _textPainter.getPositionForOffset(globalToLocal(from - _paintOffset));
final TextPosition? toPosition = to == null
? null
: _textPainter.getPositionForOffset(globalToLocal(to - _paintOffset));
final int baseOffset = fromPosition.offset;
final int extentOffset = toPosition?.offset ?? fromPosition.offset;
final TextSelection newSelection = TextSelection(
baseOffset: baseOffset,
extentOffset: extentOffset,
affinity: fromPosition.affinity,
);
_setSelection(newSelection, cause);
}
/// {@macro flutter.painting.TextPainter.wordBoundaries}
WordBoundary get wordBoundaries => _textPainter.wordBoundaries;
/// Select a word around the location of the last tap down.
///
/// {@macro flutter.rendering.RenderEditable.selectPosition}
void selectWord({ required SelectionChangedCause cause }) {
selectWordsInRange(from: _lastTapDownPosition!, cause: cause);
}
/// Selects the set words of a paragraph that intersect a given range of global positions.
///
/// The set of words selected are not strictly bounded by the range of global positions.
///
/// The first and last endpoints of the selection will always be at the
/// beginning and end of a word respectively.
///
/// {@macro flutter.rendering.RenderEditable.selectPosition}
void selectWordsInRange({ required Offset from, Offset? to, required SelectionChangedCause cause }) {
_computeTextMetricsIfNeeded();
final TextPosition fromPosition = _textPainter.getPositionForOffset(globalToLocal(from - _paintOffset));
final TextSelection fromWord = getWordAtOffset(fromPosition);
final TextPosition toPosition = to == null ? fromPosition : _textPainter.getPositionForOffset(globalToLocal(to - _paintOffset));
final TextSelection toWord = toPosition == fromPosition ? fromWord : getWordAtOffset(toPosition);
final bool isFromWordBeforeToWord = fromWord.start < toWord.end;
_setSelection(
TextSelection(
baseOffset: isFromWordBeforeToWord ? fromWord.base.offset : fromWord.extent.offset,
extentOffset: isFromWordBeforeToWord ? toWord.extent.offset : toWord.base.offset,
affinity: fromWord.affinity,
),
cause,
);
}
/// Move the selection to the beginning or end of a word.
///
/// {@macro flutter.rendering.RenderEditable.selectPosition}
void selectWordEdge({ required SelectionChangedCause cause }) {
_computeTextMetricsIfNeeded();
assert(_lastTapDownPosition != null);
final TextPosition position = _textPainter.getPositionForOffset(globalToLocal(_lastTapDownPosition! - _paintOffset));
final TextRange word = _textPainter.getWordBoundary(position);
late TextSelection newSelection;
if (position.offset <= word.start) {
newSelection = TextSelection.collapsed(offset: word.start);
} else {
newSelection = TextSelection.collapsed(offset: word.end, affinity: TextAffinity.upstream);
}
_setSelection(newSelection, cause);
}
/// Returns a [TextSelection] that encompasses the word at the given
/// [TextPosition].
@visibleForTesting
TextSelection getWordAtOffset(TextPosition position) {
// When long-pressing past the end of the text, we want a collapsed cursor.
if (position.offset >= plainText.length) {
return TextSelection.fromPosition(
TextPosition(offset: plainText.length, affinity: TextAffinity.upstream)
);
}
// If text is obscured, the entire sentence should be treated as one word.
if (obscureText) {
return TextSelection(baseOffset: 0, extentOffset: plainText.length);
}
final TextRange word = _textPainter.getWordBoundary(position);
final int effectiveOffset;
switch (position.affinity) {
case TextAffinity.upstream:
// upstream affinity is effectively -1 in text position.
effectiveOffset = position.offset - 1;
case TextAffinity.downstream:
effectiveOffset = position.offset;
}
assert(effectiveOffset >= 0);
// On iOS, select the previous word if there is a previous word, or select
// to the end of the next word if there is a next word. Select nothing if
// there is neither a previous word nor a next word.
//
// If the platform is Android and the text is read only, try to select the
// previous word if there is one; otherwise, select the single whitespace at
// the position.
if (effectiveOffset > 0
&& TextLayoutMetrics.isWhitespace(plainText.codeUnitAt(effectiveOffset))) {
final TextRange? previousWord = _getPreviousWord(word.start);
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
if (previousWord == null) {
final TextRange? nextWord = _getNextWord(word.start);
if (nextWord == null) {
return TextSelection.collapsed(offset: position.offset);
}
return TextSelection(
baseOffset: position.offset,
extentOffset: nextWord.end,
);
}
return TextSelection(
baseOffset: previousWord.start,
extentOffset: position.offset,
);
case TargetPlatform.android:
if (readOnly) {
if (previousWord == null) {
return TextSelection(
baseOffset: position.offset,
extentOffset: position.offset + 1,
);
}
return TextSelection(
baseOffset: previousWord.start,
extentOffset: position.offset,
);
}
case TargetPlatform.fuchsia:
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
}
}
return TextSelection(baseOffset: word.start, extentOffset: word.end);
}
// Placeholder dimensions representing the sizes of child inline widgets.
//
// These need to be cached because the text painter's placeholder dimensions
// will be overwritten during intrinsic width/height calculations and must be
// restored to the original values before final layout and painting.
List<PlaceholderDimensions>? _placeholderDimensions;
(double minWidth, double maxWidth) _adjustConstraints({ double minWidth = 0.0, double maxWidth = double.infinity }) {
final double availableMaxWidth = math.max(0.0, maxWidth - _caretMargin);
final double availableMinWidth = math.min(minWidth, availableMaxWidth);
return (
forceLine ? availableMaxWidth : availableMinWidth,
_isMultiline ? availableMaxWidth : double.infinity,
);
}
// Computes the text metrics if `_textPainter`'s layout information was marked
// as dirty.
//
// This method must be called in `RenderEditable`'s public methods that expose
// `_textPainter`'s metrics. For instance, `systemFontsDidChange` sets
// _textPainter._paragraph to null, so accessing _textPainter's metrics
// immediately after `systemFontsDidChange` without first calling this method
// may crash.
//
// This method is also called in various paint methods (`RenderEditable.paint`
// as well as its foreground/background painters' `paint`). It's needed
// because invisible render objects kept in the tree by `KeepAlive` may not
// get a chance to do layout but can still paint.
// See https://github.com/flutter/flutter/issues/84896.
//
// This method only re-computes layout if the underlying `_textPainter`'s
// layout cache is invalidated (by calling `TextPainter.markNeedsLayout`), or
// the constraints used to layout the `_textPainter` is different. See
// `TextPainter.layout`.
void _computeTextMetricsIfNeeded() {
final (double minWidth, double maxWidth) = _adjustConstraints(minWidth: constraints.minWidth, maxWidth: constraints.maxWidth);
_textPainter.layout(minWidth: minWidth, maxWidth: maxWidth);
}
late Rect _caretPrototype;
// TODO(LongCatIsLooong): https://github.com/flutter/flutter/issues/120836
//
/// On iOS, the cursor is taller than the cursor on Android. The height
/// of the cursor for iOS is approximate and obtained through an eyeball
/// comparison.
void _computeCaretPrototype() {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
_caretPrototype = Rect.fromLTWH(0.0, 0.0, cursorWidth, cursorHeight + 2);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
_caretPrototype = Rect.fromLTWH(0.0, _kCaretHeightOffset, cursorWidth, cursorHeight - 2.0 * _kCaretHeightOffset);
}
}
// Computes the offset to apply to the given [sourceOffset] so it perfectly
// snaps to physical pixels.
Offset _snapToPhysicalPixel(Offset sourceOffset) {
final Offset globalOffset = localToGlobal(sourceOffset);
final double pixelMultiple = 1.0 / _devicePixelRatio;
return Offset(
globalOffset.dx.isFinite
? (globalOffset.dx / pixelMultiple).round() * pixelMultiple - globalOffset.dx
: 0,
globalOffset.dy.isFinite
? (globalOffset.dy / pixelMultiple).round() * pixelMultiple - globalOffset.dy
: 0,
);
}
bool _canComputeDryLayoutForInlineWidgets() {
return text?.visitChildren((InlineSpan span) {
return (span is! PlaceholderSpan) || switch (span.alignment) {
ui.PlaceholderAlignment.baseline ||
ui.PlaceholderAlignment.aboveBaseline ||
ui.PlaceholderAlignment.belowBaseline => false,
ui.PlaceholderAlignment.top ||
ui.PlaceholderAlignment.middle ||
ui.PlaceholderAlignment.bottom => true,
};
}) ?? true;
}
bool? _canComputeIntrinsicsCached;
bool get _canComputeIntrinsics => _canComputeIntrinsicsCached ??= _canComputeDryLayoutForInlineWidgets();
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (!_canComputeIntrinsics) {
assert(debugCannotComputeDryLayout(
reason: 'Dry layout not available for alignments that require baseline.',
));
return Size.zero;
}
final (double minWidth, double maxWidth) = _adjustConstraints(minWidth: constraints.minWidth, maxWidth: constraints.maxWidth);
_textIntrinsics
..setPlaceholderDimensions(layoutInlineChildren(constraints.maxWidth, ChildLayoutHelper.dryLayoutChild))
..layout(minWidth: minWidth, maxWidth: maxWidth);
final double width = forceLine
? constraints.maxWidth
: constraints.constrainWidth(_textIntrinsics.size.width + _caretMargin);
return Size(width, constraints.constrainHeight(_preferredHeight(constraints.maxWidth)));
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
_placeholderDimensions = layoutInlineChildren(constraints.maxWidth, ChildLayoutHelper.layoutChild);
final (double minWidth, double maxWidth) = _adjustConstraints(minWidth: constraints.minWidth, maxWidth: constraints.maxWidth);
_textPainter
..setPlaceholderDimensions(_placeholderDimensions)
..layout(minWidth: minWidth, maxWidth: maxWidth);
positionInlineChildren(_textPainter.inlinePlaceholderBoxes!);
_computeCaretPrototype();
// We grab _textPainter.size here because assigning to `size` on the next
// line will trigger us to validate our intrinsic sizes, which will change
// _textPainter's layout because the intrinsic size calculations are
// destructive, which would mean we would get different results if we later
// used properties on _textPainter in this method.
// Other _textPainter state like didExceedMaxLines will also be affected,
// though we currently don't use those here.
// See also RenderParagraph which has a similar issue.
final Size textPainterSize = _textPainter.size;
final double width = forceLine
? constraints.maxWidth
: constraints.constrainWidth(_textPainter.size.width + _caretMargin);
assert(maxLines != 1 || _textPainter.maxLines == 1);
final double preferredHeight = switch (maxLines) {
null => math.max(_textPainter.height, preferredLineHeight * (minLines ?? 0)),
1 => _textPainter.height,
final int maxLines => clampDouble(
_textPainter.height,
preferredLineHeight * (minLines ?? maxLines),
preferredLineHeight * maxLines,
),
};
size = Size(width, constraints.constrainHeight(preferredHeight));
final Size contentSize = Size(textPainterSize.width + _caretMargin, textPainterSize.height);
final BoxConstraints painterConstraints = BoxConstraints.tight(contentSize);
_foregroundRenderObject?.layout(painterConstraints);
_backgroundRenderObject?.layout(painterConstraints);
_maxScrollExtent = _getMaxScrollExtent(contentSize);
offset.applyViewportDimension(_viewportExtent);
offset.applyContentDimensions(0.0, _maxScrollExtent);
}
// The relative origin in relation to the distance the user has theoretically
// dragged the floating cursor offscreen. This value is used to account for the
// difference in the rendering position and the raw offset value.
Offset _relativeOrigin = Offset.zero;
Offset? _previousOffset;
bool _shouldResetOrigin = true;
bool _resetOriginOnLeft = false;
bool _resetOriginOnRight = false;
bool _resetOriginOnTop = false;
bool _resetOriginOnBottom = false;
double? _resetFloatingCursorAnimationValue;
static Offset _calculateAdjustedCursorOffset(Offset offset, Rect boundingRects) {
final double adjustedX = clampDouble(offset.dx, boundingRects.left, boundingRects.right);
final double adjustedY = clampDouble(offset.dy, boundingRects.top, boundingRects.bottom);
return Offset(adjustedX, adjustedY);
}
/// Returns the position within the text field closest to the raw cursor offset.
///
/// See also:
///
/// * [FloatingCursorDragState], which explains the floating cursor feature
/// in detail.
Offset calculateBoundedFloatingCursorOffset(Offset rawCursorOffset, {bool? shouldResetOrigin}) {
Offset deltaPosition = Offset.zero;
final double topBound = -floatingCursorAddedMargin.top;
final double bottomBound = math.min(size.height, _textPainter.height) - preferredLineHeight + floatingCursorAddedMargin.bottom;
final double leftBound = -floatingCursorAddedMargin.left;
final double rightBound = math.min(size.width, _textPainter.width) + floatingCursorAddedMargin.right;
final Rect boundingRects = Rect.fromLTRB(leftBound, topBound, rightBound, bottomBound);
if (shouldResetOrigin != null) {
_shouldResetOrigin = shouldResetOrigin;
}
if (!_shouldResetOrigin) {
return _calculateAdjustedCursorOffset(rawCursorOffset, boundingRects);
}
if (_previousOffset != null) {
deltaPosition = rawCursorOffset - _previousOffset!;
}
// If the raw cursor offset has gone off an edge, we want to reset the relative
// origin of the dragging when the user drags back into the field.
if (_resetOriginOnLeft && deltaPosition.dx > 0) {
_relativeOrigin = Offset(rawCursorOffset.dx - boundingRects.left, _relativeOrigin.dy);
_resetOriginOnLeft = false;
} else if (_resetOriginOnRight && deltaPosition.dx < 0) {
_relativeOrigin = Offset(rawCursorOffset.dx - boundingRects.right, _relativeOrigin.dy);
_resetOriginOnRight = false;
}
if (_resetOriginOnTop && deltaPosition.dy > 0) {
_relativeOrigin = Offset(_relativeOrigin.dx, rawCursorOffset.dy - boundingRects.top);
_resetOriginOnTop = false;
} else if (_resetOriginOnBottom && deltaPosition.dy < 0) {
_relativeOrigin = Offset(_relativeOrigin.dx, rawCursorOffset.dy - boundingRects.bottom);
_resetOriginOnBottom = false;
}
final double currentX = rawCursorOffset.dx - _relativeOrigin.dx;
final double currentY = rawCursorOffset.dy - _relativeOrigin.dy;
final Offset adjustedOffset = _calculateAdjustedCursorOffset(Offset(currentX, currentY), boundingRects);
if (currentX < boundingRects.left && deltaPosition.dx < 0) {
_resetOriginOnLeft = true;
} else if (currentX > boundingRects.right && deltaPosition.dx > 0) {
_resetOriginOnRight = true;
}
if (currentY < boundingRects.top && deltaPosition.dy < 0) {
_resetOriginOnTop = true;
} else if (currentY > boundingRects.bottom && deltaPosition.dy > 0) {
_resetOriginOnBottom = true;
}
_previousOffset = rawCursorOffset;
return adjustedOffset;
}
/// Sets the screen position of the floating cursor and the text position
/// closest to the cursor.
///
/// See also:
///
/// * [FloatingCursorDragState], which explains the floating cursor feature
/// in detail.
void setFloatingCursor(FloatingCursorDragState state, Offset boundedOffset, TextPosition lastTextPosition, { double? resetLerpValue }) {
if (state == FloatingCursorDragState.End) {
_relativeOrigin = Offset.zero;
_previousOffset = null;
_shouldResetOrigin = true;
_resetOriginOnBottom = false;
_resetOriginOnTop = false;
_resetOriginOnRight = false;
_resetOriginOnBottom = false;
}
_floatingCursorOn = state != FloatingCursorDragState.End;
_resetFloatingCursorAnimationValue = resetLerpValue;
if (_floatingCursorOn) {
_floatingCursorTextPosition = lastTextPosition;
final double? animationValue = _resetFloatingCursorAnimationValue;
final EdgeInsets sizeAdjustment = animationValue != null
? EdgeInsets.lerp(_kFloatingCursorSizeIncrease, EdgeInsets.zero, animationValue)!
: _kFloatingCursorSizeIncrease;
_caretPainter.floatingCursorRect = sizeAdjustment.inflateRect(_caretPrototype).shift(boundedOffset);
} else {
_caretPainter.floatingCursorRect = null;
}
_caretPainter.showRegularCaret = _resetFloatingCursorAnimationValue == null;
}
MapEntry<int, Offset> _lineNumberFor(TextPosition startPosition, List<ui.LineMetrics> metrics) {
// TODO(LongCatIsLooong): include line boundaries information in
// ui.LineMetrics, then we can get rid of this.
final Offset offset = _textPainter.getOffsetForCaret(startPosition, Rect.zero);
for (final ui.LineMetrics lineMetrics in metrics) {
if (lineMetrics.baseline > offset.dy) {
return MapEntry<int, Offset>(lineMetrics.lineNumber, Offset(offset.dx, lineMetrics.baseline));
}
}
assert(startPosition.offset == 0, 'unable to find the line for $startPosition');
return MapEntry<int, Offset>(
math.max(0, metrics.length - 1),
Offset(offset.dx, metrics.isNotEmpty ? metrics.last.baseline + metrics.last.descent : 0.0),
);
}
/// Starts a [VerticalCaretMovementRun] at the given location in the text, for
/// handling consecutive vertical caret movements.
///
/// This can be used to handle consecutive upward/downward arrow key movements
/// in an input field.
///
/// {@macro flutter.rendering.RenderEditable.verticalArrowKeyMovement}
///
/// The [VerticalCaretMovementRun.isValid] property indicates whether the text
/// layout has changed and the vertical caret run is invalidated.
///
/// The caller should typically discard a [VerticalCaretMovementRun] when
/// its [VerticalCaretMovementRun.isValid] becomes false, or on other
/// occasions where the vertical caret run should be interrupted.
VerticalCaretMovementRun startVerticalCaretMovement(TextPosition startPosition) {
final List<ui.LineMetrics> metrics = _textPainter.computeLineMetrics();
final MapEntry<int, Offset> currentLine = _lineNumberFor(startPosition, metrics);
return VerticalCaretMovementRun._(
this,
metrics,
startPosition,
currentLine.key,
currentLine.value,
);
}
void _paintContents(PaintingContext context, Offset offset) {
final Offset effectiveOffset = offset + _paintOffset;
if (selection != null && !_floatingCursorOn) {
_updateSelectionExtentsVisibility(effectiveOffset);
}
final RenderBox? foregroundChild = _foregroundRenderObject;
final RenderBox? backgroundChild = _backgroundRenderObject;
// The painters paint in the viewport's coordinate space, since the
// textPainter's coordinate space is not known to high level widgets.
if (backgroundChild != null) {
context.paintChild(backgroundChild, offset);
}
_textPainter.paint(context.canvas, effectiveOffset);
paintInlineChildren(context, effectiveOffset);
if (foregroundChild != null) {
context.paintChild(foregroundChild, offset);
}
}
final LayerHandle<LeaderLayer> _leaderLayerHandler = LayerHandle<LeaderLayer>();
void _paintHandleLayers(PaintingContext context, List<TextSelectionPoint> endpoints, Offset offset) {
Offset startPoint = endpoints[0].point;
startPoint = Offset(
clampDouble(startPoint.dx, 0.0, size.width),
clampDouble(startPoint.dy, 0.0, size.height),
);
_leaderLayerHandler.layer = LeaderLayer(link: startHandleLayerLink, offset: startPoint + offset);
context.pushLayer(
_leaderLayerHandler.layer!,
super.paint,
Offset.zero,
);
if (endpoints.length == 2) {
Offset endPoint = endpoints[1].point;
endPoint = Offset(
clampDouble(endPoint.dx, 0.0, size.width),
clampDouble(endPoint.dy, 0.0, size.height),
);
context.pushLayer(
LeaderLayer(link: endHandleLayerLink, offset: endPoint + offset),
super.paint,
Offset.zero,
);
}
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
if (child == _foregroundRenderObject || child == _backgroundRenderObject) {
return;
}
defaultApplyPaintTransform(child, transform);
}
@override
void paint(PaintingContext context, Offset offset) {
_computeTextMetricsIfNeeded();
if (_hasVisualOverflow && clipBehavior != Clip.none) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
_paintContents,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
_paintContents(context, offset);
}
final TextSelection? selection = this.selection;
if (selection != null && selection.isValid) {
_paintHandleLayers(context, getEndpointsForSelection(selection), offset);
}
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
Rect? describeApproximatePaintClip(RenderObject child) {
switch (clipBehavior) {
case Clip.none:
return null;
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
return _hasVisualOverflow ? Offset.zero & size : null;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('cursorColor', cursorColor));
properties.add(DiagnosticsProperty<ValueNotifier<bool>>('showCursor', showCursor));
properties.add(IntProperty('maxLines', maxLines));
properties.add(IntProperty('minLines', minLines));
properties.add(DiagnosticsProperty<bool>('expands', expands, defaultValue: false));
properties.add(ColorProperty('selectionColor', selectionColor));
properties.add(DiagnosticsProperty<TextScaler>('textScaler', textScaler, defaultValue: TextScaler.noScaling));
properties.add(DiagnosticsProperty<Locale>('locale', locale, defaultValue: null));
properties.add(DiagnosticsProperty<TextSelection>('selection', selection));
properties.add(DiagnosticsProperty<ViewportOffset>('offset', offset));
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
return <DiagnosticsNode>[
if (text != null)
text!.toDiagnosticsNode(
name: 'text',
style: DiagnosticsTreeStyle.transition,
),
];
}
}
class _RenderEditableCustomPaint extends RenderBox {
_RenderEditableCustomPaint({
RenderEditablePainter? painter,
}) : _painter = painter,
super();
@override
RenderEditable? get parent => super.parent as RenderEditable?;
@override
bool get isRepaintBoundary => true;
@override
bool get sizedByParent => true;
RenderEditablePainter? get painter => _painter;
RenderEditablePainter? _painter;
set painter(RenderEditablePainter? newValue) {
if (newValue == painter) {
return;
}
final RenderEditablePainter? oldPainter = painter;
_painter = newValue;
if (newValue?.shouldRepaint(oldPainter) ?? true) {
markNeedsPaint();
}
if (attached) {
oldPainter?.removeListener(markNeedsPaint);
newValue?.addListener(markNeedsPaint);
}
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderEditable? parent = this.parent;
assert(parent != null);
final RenderEditablePainter? painter = this.painter;
if (painter != null && parent != null) {
parent._computeTextMetricsIfNeeded();
painter.paint(context.canvas, size, parent);
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_painter?.addListener(markNeedsPaint);
}
@override
void detach() {
_painter?.removeListener(markNeedsPaint);
super.detach();
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) => constraints.biggest;
}
/// An interface that paints within a [RenderEditable]'s bounds, above or
/// beneath its text content.
///
/// This painter is typically used for painting auxiliary content that depends
/// on text layout metrics (for instance, for painting carets and text highlight
/// blocks). It can paint independently from its [RenderEditable], allowing it
/// to repaint without triggering a repaint on the entire [RenderEditable] stack
/// when only auxiliary content changes (e.g. a blinking cursor) are present. It
/// will be scheduled to repaint when:
///
/// * It's assigned to a new [RenderEditable] (replacing a prior
/// [RenderEditablePainter]) and the [shouldRepaint] method returns true.
/// * Any of the [RenderEditable]s it is attached to repaints.
/// * The [notifyListeners] method is called, which typically happens when the
/// painter's attributes change.
///
/// See also:
///
/// * [RenderEditable.foregroundPainter], which takes a [RenderEditablePainter]
/// and sets it as the foreground painter of the [RenderEditable].
/// * [RenderEditable.painter], which takes a [RenderEditablePainter]
/// and sets it as the background painter of the [RenderEditable].
/// * [CustomPainter], a similar class which paints within a [RenderCustomPaint].
abstract class RenderEditablePainter extends ChangeNotifier {
/// Determines whether repaint is needed when a new [RenderEditablePainter]
/// is provided to a [RenderEditable].
///
/// If the new instance represents different information than the old
/// instance, then the method should return true, otherwise it should return
/// false. When [oldDelegate] is null, this method should always return true
/// unless the new painter initially does not paint anything.
///
/// If the method returns false, then the [paint] call might be optimized
/// away. However, the [paint] method will get called whenever the
/// [RenderEditable]s it attaches to repaint, even if [shouldRepaint] returns
/// false.
bool shouldRepaint(RenderEditablePainter? oldDelegate);
/// Paints within the bounds of a [RenderEditable].
///
/// The given [Canvas] has the same coordinate space as the [RenderEditable],
/// which may be different from the coordinate space the [RenderEditable]'s
/// [TextPainter] uses, when the text moves inside the [RenderEditable].
///
/// Paint operations performed outside of the region defined by the [canvas]'s
/// origin and the [size] parameter may get clipped, when [RenderEditable]'s
/// [RenderEditable.clipBehavior] is not [Clip.none].
void paint(Canvas canvas, Size size, RenderEditable renderEditable);
}
class _TextHighlightPainter extends RenderEditablePainter {
_TextHighlightPainter({
TextRange? highlightedRange,
Color? highlightColor,
}) : _highlightedRange = highlightedRange,
_highlightColor = highlightColor;
final Paint highlightPaint = Paint();
Color? get highlightColor => _highlightColor;
Color? _highlightColor;
set highlightColor(Color? newValue) {
if (newValue == _highlightColor) {
return;
}
_highlightColor = newValue;
notifyListeners();
}
TextRange? get highlightedRange => _highlightedRange;
TextRange? _highlightedRange;
set highlightedRange(TextRange? newValue) {
if (newValue == _highlightedRange) {
return;
}
_highlightedRange = newValue;
notifyListeners();
}
/// Controls how tall the selection highlight boxes are computed to be.
///
/// See [ui.BoxHeightStyle] for details on available styles.
ui.BoxHeightStyle get selectionHeightStyle => _selectionHeightStyle;
ui.BoxHeightStyle _selectionHeightStyle = ui.BoxHeightStyle.tight;
set selectionHeightStyle(ui.BoxHeightStyle value) {
if (_selectionHeightStyle == value) {
return;
}
_selectionHeightStyle = value;
notifyListeners();
}
/// Controls how wide the selection highlight boxes are computed to be.
///
/// See [ui.BoxWidthStyle] for details on available styles.
ui.BoxWidthStyle get selectionWidthStyle => _selectionWidthStyle;
ui.BoxWidthStyle _selectionWidthStyle = ui.BoxWidthStyle.tight;
set selectionWidthStyle(ui.BoxWidthStyle value) {
if (_selectionWidthStyle == value) {
return;
}
_selectionWidthStyle = value;
notifyListeners();
}
@override
void paint(Canvas canvas, Size size, RenderEditable renderEditable) {
final TextRange? range = highlightedRange;
final Color? color = highlightColor;
if (range == null || color == null || range.isCollapsed) {
return;
}
highlightPaint.color = color;
final TextPainter textPainter = renderEditable._textPainter;
final List<TextBox> boxes = textPainter.getBoxesForSelection(
TextSelection(baseOffset: range.start, extentOffset: range.end),
boxHeightStyle: selectionHeightStyle,
boxWidthStyle: selectionWidthStyle,
);
for (final TextBox box in boxes) {
canvas.drawRect(
box.toRect().shift(renderEditable._paintOffset)
.intersect(Rect.fromLTWH(0, 0, textPainter.width, textPainter.height)),
highlightPaint,
);
}
}
@override
bool shouldRepaint(RenderEditablePainter? oldDelegate) {
if (identical(oldDelegate, this)) {
return false;
}
if (oldDelegate == null) {
return highlightColor != null && highlightedRange != null;
}
return oldDelegate is! _TextHighlightPainter
|| oldDelegate.highlightColor != highlightColor
|| oldDelegate.highlightedRange != highlightedRange
|| oldDelegate.selectionHeightStyle != selectionHeightStyle
|| oldDelegate.selectionWidthStyle != selectionWidthStyle;
}
}
class _CaretPainter extends RenderEditablePainter {
_CaretPainter();
bool get shouldPaint => _shouldPaint;
bool _shouldPaint = true;
set shouldPaint(bool value) {
if (shouldPaint == value) {
return;
}
_shouldPaint = value;
notifyListeners();
}
// This is directly manipulated by the RenderEditable during
// setFloatingCursor.
//
// When changing this value, the caller is responsible for ensuring that
// listeners are notified.
bool showRegularCaret = false;
final Paint caretPaint = Paint();
late final Paint floatingCursorPaint = Paint();
Color? get caretColor => _caretColor;
Color? _caretColor;
set caretColor(Color? value) {
if (caretColor?.value == value?.value) {
return;
}
_caretColor = value;
notifyListeners();
}
Radius? get cursorRadius => _cursorRadius;
Radius? _cursorRadius;
set cursorRadius(Radius? value) {
if (_cursorRadius == value) {
return;
}
_cursorRadius = value;
notifyListeners();
}
Offset get cursorOffset => _cursorOffset;
Offset _cursorOffset = Offset.zero;
set cursorOffset(Offset value) {
if (_cursorOffset == value) {
return;
}
_cursorOffset = value;
notifyListeners();
}
Color? get backgroundCursorColor => _backgroundCursorColor;
Color? _backgroundCursorColor;
set backgroundCursorColor(Color? value) {
if (backgroundCursorColor?.value == value?.value) {
return;
}
_backgroundCursorColor = value;
if (showRegularCaret) {
notifyListeners();
}
}
Rect? get floatingCursorRect => _floatingCursorRect;
Rect? _floatingCursorRect;
set floatingCursorRect(Rect? value) {
if (_floatingCursorRect == value) {
return;
}
_floatingCursorRect = value;
notifyListeners();
}
void paintRegularCursor(Canvas canvas, RenderEditable renderEditable, Color caretColor, TextPosition textPosition) {
final Rect integralRect = renderEditable.getLocalRectForCaret(textPosition);
if (shouldPaint) {
if (floatingCursorRect != null) {
final double distanceSquared = (floatingCursorRect!.center - integralRect.center).distanceSquared;
if (distanceSquared < _kShortestDistanceSquaredWithFloatingAndRegularCursors) {
return;
}
}
final Radius? radius = cursorRadius;
caretPaint.color = caretColor;
if (radius == null) {
canvas.drawRect(integralRect, caretPaint);
} else {
final RRect caretRRect = RRect.fromRectAndRadius(integralRect, radius);
canvas.drawRRect(caretRRect, caretPaint);
}
}
}
@override
void paint(Canvas canvas, Size size, RenderEditable renderEditable) {
// Compute the caret location even when `shouldPaint` is false.
final TextSelection? selection = renderEditable.selection;
if (selection == null || !selection.isCollapsed || !selection.isValid) {
return;
}
final Rect? floatingCursorRect = this.floatingCursorRect;
final Color? caretColor = floatingCursorRect == null
? this.caretColor
: showRegularCaret ? backgroundCursorColor : null;
final TextPosition caretTextPosition = floatingCursorRect == null
? selection.extent
: renderEditable._floatingCursorTextPosition;
if (caretColor != null) {
paintRegularCursor(canvas, renderEditable, caretColor, caretTextPosition);
}
final Color? floatingCursorColor = this.caretColor?.withOpacity(0.75);
// Floating Cursor.
if (floatingCursorRect == null || floatingCursorColor == null || !shouldPaint) {
return;
}
canvas.drawRRect(
RRect.fromRectAndRadius(floatingCursorRect, _kFloatingCursorRadius),
floatingCursorPaint..color = floatingCursorColor,
);
}
@override
bool shouldRepaint(RenderEditablePainter? oldDelegate) {
if (identical(this, oldDelegate)) {
return false;
}
if (oldDelegate == null) {
return shouldPaint;
}
return oldDelegate is! _CaretPainter
|| oldDelegate.shouldPaint != shouldPaint
|| oldDelegate.showRegularCaret != showRegularCaret
|| oldDelegate.caretColor != caretColor
|| oldDelegate.cursorRadius != cursorRadius
|| oldDelegate.cursorOffset != cursorOffset
|| oldDelegate.backgroundCursorColor != backgroundCursorColor
|| oldDelegate.floatingCursorRect != floatingCursorRect;
}
}
class _CompositeRenderEditablePainter extends RenderEditablePainter {
_CompositeRenderEditablePainter({ required this.painters });
final List<RenderEditablePainter> painters;
@override
void addListener(VoidCallback listener) {
for (final RenderEditablePainter painter in painters) {
painter.addListener(listener);
}
}
@override
void removeListener(VoidCallback listener) {
for (final RenderEditablePainter painter in painters) {
painter.removeListener(listener);
}
}
@override
void paint(Canvas canvas, Size size, RenderEditable renderEditable) {
for (final RenderEditablePainter painter in painters) {
painter.paint(canvas, size, renderEditable);
}
}
@override
bool shouldRepaint(RenderEditablePainter? oldDelegate) {
if (identical(oldDelegate, this)) {
return false;
}
if (oldDelegate is! _CompositeRenderEditablePainter || oldDelegate.painters.length != painters.length) {
return true;
}
final Iterator<RenderEditablePainter> oldPainters = oldDelegate.painters.iterator;
final Iterator<RenderEditablePainter> newPainters = painters.iterator;
while (oldPainters.moveNext() && newPainters.moveNext()) {
if (newPainters.current.shouldRepaint(oldPainters.current)) {
return true;
}
}
return false;
}
}
| flutter/packages/flutter/lib/src/rendering/editable.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/editable.dart",
"repo_id": "flutter",
"token_count": 38676
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart';
import 'box.dart';
import 'layer.dart';
import 'object.dart';
const double _kQuarterTurnsInRadians = math.pi / 2.0;
/// Rotates its child by a integral number of quarter turns.
///
/// Unlike [RenderTransform], which applies a transform just prior to painting,
/// this object applies its rotation prior to layout, which means the entire
/// rotated box consumes only as much space as required by the rotated child.
class RenderRotatedBox extends RenderBox with RenderObjectWithChildMixin<RenderBox> {
/// Creates a rotated render box.
RenderRotatedBox({
required int quarterTurns,
RenderBox? child,
}) : _quarterTurns = quarterTurns {
this.child = child;
}
/// The number of clockwise quarter turns the child should be rotated.
int get quarterTurns => _quarterTurns;
int _quarterTurns;
set quarterTurns(int value) {
if (_quarterTurns == value) {
return;
}
_quarterTurns = value;
markNeedsLayout();
}
bool get _isVertical => quarterTurns.isOdd;
@override
double computeMinIntrinsicWidth(double height) {
if (child == null) {
return 0.0;
}
return _isVertical ? child!.getMinIntrinsicHeight(height) : child!.getMinIntrinsicWidth(height);
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child == null) {
return 0.0;
}
return _isVertical ? child!.getMaxIntrinsicHeight(height) : child!.getMaxIntrinsicWidth(height);
}
@override
double computeMinIntrinsicHeight(double width) {
if (child == null) {
return 0.0;
}
return _isVertical ? child!.getMinIntrinsicWidth(width) : child!.getMinIntrinsicHeight(width);
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child == null) {
return 0.0;
}
return _isVertical ? child!.getMaxIntrinsicWidth(width) : child!.getMaxIntrinsicHeight(width);
}
Matrix4? _paintTransform;
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (child == null) {
return constraints.smallest;
}
final Size childSize = child!.getDryLayout(_isVertical ? constraints.flipped : constraints);
return _isVertical ? Size(childSize.height, childSize.width) : childSize;
}
@override
void performLayout() {
_paintTransform = null;
if (child != null) {
child!.layout(_isVertical ? constraints.flipped : constraints, parentUsesSize: true);
size = _isVertical ? Size(child!.size.height, child!.size.width) : child!.size;
_paintTransform = Matrix4.identity()
..translate(size.width / 2.0, size.height / 2.0)
..rotateZ(_kQuarterTurnsInRadians * (quarterTurns % 4))
..translate(-child!.size.width / 2.0, -child!.size.height / 2.0);
} else {
size = constraints.smallest;
}
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
assert(_paintTransform != null || debugNeedsLayout || child == null);
if (child == null || _paintTransform == null) {
return false;
}
return result.addWithPaintTransform(
transform: _paintTransform,
position: position,
hitTest: (BoxHitTestResult result, Offset position) {
return child!.hitTest(result, position: position);
},
);
}
void _paintChild(PaintingContext context, Offset offset) {
context.paintChild(child!, offset);
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null) {
_transformLayer.layer = context.pushTransform(
needsCompositing,
offset,
_paintTransform!,
_paintChild,
oldLayer: _transformLayer.layer,
);
} else {
_transformLayer.layer = null;
}
}
final LayerHandle<TransformLayer> _transformLayer = LayerHandle<TransformLayer>();
@override
void dispose() {
_transformLayer.layer = null;
super.dispose();
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
if (_paintTransform != null) {
transform.multiply(_paintTransform!);
}
super.applyPaintTransform(child, transform);
}
}
| flutter/packages/flutter/lib/src/rendering/rotated_box.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/rotated_box.dart",
"repo_id": "flutter",
"token_count": 1571
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'layer.dart';
import 'object.dart';
/// A rectangle upon which a backend texture is mapped.
///
/// Backend textures are images that can be applied (mapped) to an area of the
/// Flutter view. They are created, managed, and updated using a
/// platform-specific texture registry. This is typically done by a plugin
/// that integrates with host platform video player, camera, or OpenGL APIs,
/// or similar image sources.
///
/// A texture box refers to its backend texture using an integer ID. Texture
/// IDs are obtained from the texture registry and are scoped to the Flutter
/// view. Texture IDs may be reused after deregistration, at the discretion
/// of the registry. The use of texture IDs currently unknown to the registry
/// will silently result in a blank rectangle.
///
/// Texture boxes are repainted autonomously as dictated by the backend (e.g. on
/// arrival of a video frame). Such repainting generally does not involve
/// executing Dart code.
///
/// The size of the rectangle is determined by the parent, and the texture is
/// automatically scaled to fit.
///
/// See also:
///
/// * [TextureRegistry](/javadoc/io/flutter/view/TextureRegistry.html)
/// for how to create and manage backend textures on Android.
/// * [TextureRegistry Protocol](/ios-embedder/protocol_flutter_texture_registry-p.html)
/// for how to create and manage backend textures on iOS.
class TextureBox extends RenderBox {
/// Creates a box backed by the texture identified by [textureId], and use
/// [filterQuality] to set texture's [FilterQuality].
TextureBox({
required int textureId,
bool freeze = false,
FilterQuality filterQuality = FilterQuality.low,
}) : _textureId = textureId,
_freeze = freeze,
_filterQuality = filterQuality;
/// The identity of the backend texture.
int get textureId => _textureId;
int _textureId;
set textureId(int value) {
if (value != _textureId) {
_textureId = value;
markNeedsPaint();
}
}
/// When true the texture will not be updated with new frames.
bool get freeze => _freeze;
bool _freeze;
set freeze(bool value) {
if (value != _freeze) {
_freeze = value;
markNeedsPaint();
}
}
/// {@macro flutter.widgets.Texture.filterQuality}
FilterQuality get filterQuality => _filterQuality;
FilterQuality _filterQuality;
set filterQuality(FilterQuality value) {
if (value != _filterQuality) {
_filterQuality = value;
markNeedsPaint();
}
}
@override
bool get sizedByParent => true;
@override
bool get alwaysNeedsCompositing => true;
@override
bool get isRepaintBoundary => true;
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return constraints.biggest;
}
@override
bool hitTestSelf(Offset position) => true;
@override
void paint(PaintingContext context, Offset offset) {
context.addLayer(TextureLayer(
rect: Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height),
textureId: _textureId,
freeze: freeze,
filterQuality: _filterQuality,
));
}
}
| flutter/packages/flutter/lib/src/rendering/texture.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/texture.dart",
"repo_id": "flutter",
"token_count": 1012
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async' show Completer;
import 'dart:isolate' show ReceivePort;
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'binary_messenger.dart';
import 'binding.dart';
/// A [BinaryMessenger] for use on background (non-root) isolates.
class BackgroundIsolateBinaryMessenger extends BinaryMessenger {
BackgroundIsolateBinaryMessenger._();
final ReceivePort _receivePort = ReceivePort();
final Map<int, Completer<ByteData?>> _completers =
<int, Completer<ByteData?>>{};
int _messageCount = 0;
/// The existing instance of this class, if any.
///
/// Throws if [ensureInitialized] has not been called at least once.
static BinaryMessenger get instance {
if (_instance == null) {
throw StateError(
'The BackgroundIsolateBinaryMessenger.instance value is invalid '
'until BackgroundIsolateBinaryMessenger.ensureInitialized is '
'executed.');
}
return _instance!;
}
static BinaryMessenger? _instance;
/// Ensures that [BackgroundIsolateBinaryMessenger.instance] has been initialized.
///
/// The argument should be the value obtained from [ServicesBinding.rootIsolateToken]
/// on the root isolate.
///
/// This function is idempotent (calling it multiple times is harmless but has no effect).
static void ensureInitialized(ui.RootIsolateToken token) {
if (_instance == null) {
ui.PlatformDispatcher.instance.registerBackgroundIsolate(token);
final BackgroundIsolateBinaryMessenger portBinaryMessenger =
BackgroundIsolateBinaryMessenger._();
_instance = portBinaryMessenger;
portBinaryMessenger._receivePort.listen((dynamic message) {
try {
final List<dynamic> args = message as List<dynamic>;
final int identifier = args[0] as int;
final Uint8List bytes = args[1] as Uint8List;
final ByteData byteData = ByteData.sublistView(bytes);
portBinaryMessenger._completers
.remove(identifier)!
.complete(byteData);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context:
ErrorDescription('during a platform message response callback'),
));
}
});
}
}
@override
Future<void> handlePlatformMessage(String channel, ByteData? data,
ui.PlatformMessageResponseCallback? callback) {
throw UnimplementedError('handlePlatformMessage is deprecated.');
}
@override
Future<ByteData?>? send(String channel, ByteData? message) {
final Completer<ByteData?> completer = Completer<ByteData?>();
_messageCount += 1;
final int messageIdentifier = _messageCount;
_completers[messageIdentifier] = completer;
ui.PlatformDispatcher.instance.sendPortPlatformMessage(
channel,
message,
messageIdentifier,
_receivePort.sendPort,
);
return completer.future;
}
@override
void setMessageHandler(String channel, MessageHandler? handler) {
throw UnsupportedError(
'Background isolates do not support setMessageHandler(). Messages from the host platform always go to the root isolate.');
}
}
| flutter/packages/flutter/lib/src/services/_background_isolate_binary_messenger_io.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/_background_isolate_binary_messenger_io.dart",
"repo_id": "flutter",
"token_count": 1216
} | 637 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
// DO NOT EDIT -- DO NOT EDIT -- DO NOT EDIT
// This file is generated by dev/tools/gen_keycodes/bin/gen_keycodes.dart and
// should not be edited directly.
//
// Edit the template dev/tools/gen_keycodes/data/keyboard_key.tmpl instead.
// See dev/tools/gen_keycodes/README.md for more information.
/// A base class for all keyboard key types.
///
/// See also:
///
/// * [PhysicalKeyboardKey], a class with static values that describe the keys
/// that are returned from [RawKeyEvent.physicalKey].
/// * [LogicalKeyboardKey], a class with static values that describe the keys
/// that are returned from [RawKeyEvent.logicalKey].
abstract class KeyboardKey with Diagnosticable {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const KeyboardKey();
}
/// A class with static values that describe the keys that are returned from
/// [RawKeyEvent.logicalKey].
///
/// These represent *logical* keys, which are keys which are interpreted in the
/// context of any modifiers, modes, or keyboard layouts which may be in effect.
///
/// This is contrast to [PhysicalKeyboardKey], which represents a physical key
/// in a particular location on the keyboard, without regard for the modifier
/// state, mode, or keyboard layout.
///
/// As an example, if you wanted to implement an app where the "Q" key "quit"
/// something, you'd want to look at the logical key to detect this, since you
/// would like to have it match the key with "Q" on it, instead of always
/// looking for "the key next to the TAB key", since on a French keyboard,
/// the key next to the TAB key has an "A" on it.
///
/// Conversely, if you wanted a game where the key next to the CAPS LOCK (the
/// "A" key on a QWERTY keyboard) moved the player to the left, you'd want to
/// look at the physical key to make sure that regardless of the character the
/// key produces, you got the key that is in that location on the keyboard.
///
/// {@tool dartpad}
/// This example shows how to detect if the user has selected the logical "Q"
/// key and handle the key if they have.
///
/// ** See code in examples/api/lib/services/keyboard_key/logical_keyboard_key.0.dart **
/// {@end-tool}
/// See also:
///
/// * [RawKeyEvent], the keyboard event object received by widgets that listen
/// to keyboard events.
/// * [Focus.onKey], the handler on a widget that lets you handle key events.
/// * [RawKeyboardListener], a widget used to listen to keyboard events (but
/// not handle them).
@immutable
class LogicalKeyboardKey extends KeyboardKey {
/// Creates a new LogicalKeyboardKey object for a key ID.
const LogicalKeyboardKey(this.keyId);
/// A unique code representing this key.
///
/// This is an opaque code. It should not be unpacked to derive information
/// from it, as the representation of the code could change at any time.
final int keyId;
// Returns the bits that are not included in [valueMask], shifted to the
// right.
//
// For example, if the input is 0x12abcdabcd, then the result is 0x12.
//
// This is mostly equivalent to a right shift, resolving the problem that
// JavaScript only support 32-bit bitwise operation and needs to use division
// instead.
static int _nonValueBits(int n) {
// `n >> valueMaskWidth` is equivalent to `n / divisorForValueMask`.
const int divisorForValueMask = valueMask + 1;
const int valueMaskWidth = 32;
// Equivalent to assert(divisorForValueMask == (1 << valueMaskWidth)).
const int firstDivisorWidth = 28;
assert(divisorForValueMask ==
(1 << firstDivisorWidth) * (1 << (valueMaskWidth - firstDivisorWidth)));
// JS only supports up to 2^53 - 1, therefore non-value bits can only
// contain (maxSafeIntegerWidth - valueMaskWidth) bits.
const int maxSafeIntegerWidth = 52;
const int nonValueMask = (1 << (maxSafeIntegerWidth - valueMaskWidth)) - 1;
if (kIsWeb) {
return (n / divisorForValueMask).floor() & nonValueMask;
} else {
return (n >> valueMaskWidth) & nonValueMask;
}
}
static String? _unicodeKeyLabel(int keyId) {
if (_nonValueBits(keyId) == 0) {
return String.fromCharCode(keyId).toUpperCase();
}
return null;
}
/// A description representing the character produced by a [RawKeyEvent].
///
/// This value is useful for providing readable strings for keys or keyboard
/// shortcuts. Do not use this value to compare equality of keys; compare
/// [keyId] instead.
///
/// For printable keys, this is usually the printable character in upper case
/// ignoring modifiers or combining keys, such as 'A', '1', or '/'. This
/// might also return accented letters (such as 'Ù') for keys labeled as so,
/// but not if such character is a result from preceding combining keys ('`̀'
/// followed by key U).
///
/// For other keys, [keyLabel] looks up the full key name from a predefined
/// map, such as 'F1', 'Shift Left', or 'Media Down'. This value is an empty
/// string if there's no key label data for a key.
///
/// For the printable representation that takes into consideration the
/// modifiers and combining keys, see [RawKeyEvent.character].
///
/// {@macro flutter.services.RawKeyEventData.keyLabel}
String get keyLabel {
return _unicodeKeyLabel(keyId)
?? _keyLabels[keyId]
?? '';
}
/// The debug string to print for this keyboard key, which will be null in
/// release mode.
///
/// For printable keys, this is usually a more descriptive name related to
/// [keyLabel], such as 'Key A', 'Digit 1', 'Backslash'. This might
/// also return accented letters (such as 'Key Ù') for keys labeled as so.
///
/// For other keys, this looks up the full key name from a predefined map (the
/// same value as [keyLabel]), such as 'F1', 'Shift Left', or 'Media Down'. If
/// there's no key label data for a key, this returns a name that explains the
/// ID (such as 'Key with ID 0x00100012345').
String? get debugName {
String? result;
assert(() {
result = _keyLabels[keyId];
if (result == null) {
final String? unicodeKeyLabel = _unicodeKeyLabel(keyId);
if (unicodeKeyLabel != null) {
result = 'Key $unicodeKeyLabel';
} else {
result = 'Key with ID 0x${keyId.toRadixString(16).padLeft(11, '0')}';
}
}
return true;
}());
return result;
}
@override
int get hashCode => keyId.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is LogicalKeyboardKey
&& other.keyId == keyId;
}
/// Returns the [LogicalKeyboardKey] constant that matches the given ID, or
/// null, if not found.
static LogicalKeyboardKey? findKeyByKeyId(int keyId) => _knownLogicalKeys[keyId];
/// Returns true if the given label represents a Unicode control character.
///
/// Examples of control characters are characters like "U+000A LINE FEED (LF)"
/// or "U+001B ESCAPE (ESC)".
///
/// See <https://en.wikipedia.org/wiki/Unicode_control_characters> for more
/// information.
///
/// Used by [RawKeyEvent] subclasses to help construct IDs.
static bool isControlCharacter(String label) {
if (label.length != 1) {
return false;
}
final int codeUnit = label.codeUnitAt(0);
return (codeUnit <= 0x1f && codeUnit >= 0x00) || (codeUnit >= 0x7f && codeUnit <= 0x9f);
}
/// Returns true if the [keyId] of this object is one that is auto-generated by
/// Flutter.
///
/// Auto-generated key IDs are generated in response to platform key codes
/// which Flutter doesn't recognize, and their IDs shouldn't be used in a
/// persistent way.
///
/// Auto-generated IDs should be a rare occurrence: Flutter supports most keys.
///
/// Keys that generate Unicode characters (even if unknown to Flutter) will
/// not return true for [isAutogenerated], since they will be assigned a
/// Unicode-based code that will remain stable.
///
/// If Flutter adds support for a previously unsupported key code, the ID it
/// reports will change, but the ID will remain stable on the platform it is
/// produced on until Flutter adds support for recognizing it.
///
/// So, hypothetically, if Android added a new key code of 0xffff,
/// representing a new "do what I mean" key, then the auto-generated code
/// would be 0x1020000ffff, but once Flutter added the "doWhatIMean" key to
/// the definitions below, the new code would be 0x0020000ffff for all
/// platforms that had a "do what I mean" key from then on.
bool get isAutogenerated => (keyId & planeMask) >= startOfPlatformPlanes;
/// Returns a set of pseudo-key synonyms for the given `key`.
///
/// This allows finding the pseudo-keys that also represent a concrete `key`
/// so that a class with a key map can match pseudo-keys as well as the actual
/// generated keys.
///
/// Pseudo-keys returned in the set are typically used to represent keys which
/// appear in multiple places on the keyboard, such as the [shift], [alt],
/// [control], and [meta] keys. Pseudo-keys in the returned set won't ever be
/// generated directly, but if a more specific key event is received, then
/// this set can be used to find the more general pseudo-key. For example, if
/// this is a [shiftLeft] key, this accessor will return the set
/// `<LogicalKeyboardKey>{ shift }`.
Set<LogicalKeyboardKey> get synonyms => _synonyms[this] ?? <LogicalKeyboardKey>{};
/// Takes a set of keys, and returns the same set, but with any keys that have
/// synonyms replaced.
///
/// It is used, for example, to take sets of keys with members like
/// [controlRight] and [controlLeft] and convert that set to contain just
/// [control], so that the question "is any control key down?" can be asked.
static Set<LogicalKeyboardKey> collapseSynonyms(Set<LogicalKeyboardKey> input) {
return input.expand((LogicalKeyboardKey element) {
return _synonyms[element] ?? <LogicalKeyboardKey>{element};
}).toSet();
}
/// Returns the given set with any pseudo-keys expanded into their synonyms.
///
/// It is used, for example, to take sets of keys with members like [control]
/// and [shift] and convert that set to contain [controlLeft], [controlRight],
/// [shiftLeft], and [shiftRight].
static Set<LogicalKeyboardKey> expandSynonyms(Set<LogicalKeyboardKey> input) {
return input.expand((LogicalKeyboardKey element) {
return _reverseSynonyms[element] ?? <LogicalKeyboardKey>{element};
}).toSet();
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('keyId', '0x${keyId.toRadixString(16).padLeft(8, '0')}'));
properties.add(StringProperty('keyLabel', keyLabel));
properties.add(StringProperty('debugName', debugName, defaultValue: null));
}
/// Mask for the 32-bit value portion of the key code.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int valueMask = 0x000ffffffff;
/// Mask for the plane prefix portion of the key code.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int planeMask = 0x0ff00000000;
/// The plane value for keys which have a Unicode representation.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int unicodePlane = 0x00000000000;
/// The plane value for keys defined by Chromium and does not have a Unicode
/// representation.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int unprintablePlane = 0x00100000000;
/// The plane value for keys defined by Flutter.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int flutterPlane = 0x00200000000;
/// The platform plane with the lowest mask value, beyond which the keys are
/// considered autogenerated.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int startOfPlatformPlanes = 0x01100000000;
/// The plane value for the private keys defined by the Android embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int androidPlane = 0x01100000000;
/// The plane value for the private keys defined by the Fuchsia embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int fuchsiaPlane = 0x01200000000;
/// The plane value for the private keys defined by the iOS embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int iosPlane = 0x01300000000;
/// The plane value for the private keys defined by the macOS embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int macosPlane = 0x01400000000;
/// The plane value for the private keys defined by the Gtk embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int gtkPlane = 0x01500000000;
/// The plane value for the private keys defined by the Windows embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int windowsPlane = 0x01600000000;
/// The plane value for the private keys defined by the Web embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int webPlane = 0x01700000000;
/// The plane value for the private keys defined by the GLFW embedding.
///
/// This is used by platform-specific code to generate Flutter key codes.
static const int glfwPlane = 0x01800000000;
/// Represents the logical "Space" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey space = LogicalKeyboardKey(0x00000000020);
/// Represents the logical "Exclamation" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey exclamation = LogicalKeyboardKey(0x00000000021);
/// Represents the logical "Quote" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey quote = LogicalKeyboardKey(0x00000000022);
/// Represents the logical "Number Sign" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numberSign = LogicalKeyboardKey(0x00000000023);
/// Represents the logical "Dollar" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey dollar = LogicalKeyboardKey(0x00000000024);
/// Represents the logical "Percent" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey percent = LogicalKeyboardKey(0x00000000025);
/// Represents the logical "Ampersand" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey ampersand = LogicalKeyboardKey(0x00000000026);
/// Represents the logical "Quote Single" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey quoteSingle = LogicalKeyboardKey(0x00000000027);
/// Represents the logical "Parenthesis Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey parenthesisLeft = LogicalKeyboardKey(0x00000000028);
/// Represents the logical "Parenthesis Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey parenthesisRight = LogicalKeyboardKey(0x00000000029);
/// Represents the logical "Asterisk" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey asterisk = LogicalKeyboardKey(0x0000000002a);
/// Represents the logical "Add" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey add = LogicalKeyboardKey(0x0000000002b);
/// Represents the logical "Comma" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey comma = LogicalKeyboardKey(0x0000000002c);
/// Represents the logical "Minus" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey minus = LogicalKeyboardKey(0x0000000002d);
/// Represents the logical "Period" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey period = LogicalKeyboardKey(0x0000000002e);
/// Represents the logical "Slash" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey slash = LogicalKeyboardKey(0x0000000002f);
/// Represents the logical "Digit 0" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit0 = LogicalKeyboardKey(0x00000000030);
/// Represents the logical "Digit 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit1 = LogicalKeyboardKey(0x00000000031);
/// Represents the logical "Digit 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit2 = LogicalKeyboardKey(0x00000000032);
/// Represents the logical "Digit 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit3 = LogicalKeyboardKey(0x00000000033);
/// Represents the logical "Digit 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit4 = LogicalKeyboardKey(0x00000000034);
/// Represents the logical "Digit 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit5 = LogicalKeyboardKey(0x00000000035);
/// Represents the logical "Digit 6" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit6 = LogicalKeyboardKey(0x00000000036);
/// Represents the logical "Digit 7" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit7 = LogicalKeyboardKey(0x00000000037);
/// Represents the logical "Digit 8" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit8 = LogicalKeyboardKey(0x00000000038);
/// Represents the logical "Digit 9" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey digit9 = LogicalKeyboardKey(0x00000000039);
/// Represents the logical "Colon" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colon = LogicalKeyboardKey(0x0000000003a);
/// Represents the logical "Semicolon" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey semicolon = LogicalKeyboardKey(0x0000000003b);
/// Represents the logical "Less" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey less = LogicalKeyboardKey(0x0000000003c);
/// Represents the logical "Equal" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey equal = LogicalKeyboardKey(0x0000000003d);
/// Represents the logical "Greater" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey greater = LogicalKeyboardKey(0x0000000003e);
/// Represents the logical "Question" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey question = LogicalKeyboardKey(0x0000000003f);
/// Represents the logical "At" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey at = LogicalKeyboardKey(0x00000000040);
/// Represents the logical "Bracket Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey bracketLeft = LogicalKeyboardKey(0x0000000005b);
/// Represents the logical "Backslash" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey backslash = LogicalKeyboardKey(0x0000000005c);
/// Represents the logical "Bracket Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey bracketRight = LogicalKeyboardKey(0x0000000005d);
/// Represents the logical "Caret" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey caret = LogicalKeyboardKey(0x0000000005e);
/// Represents the logical "Underscore" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey underscore = LogicalKeyboardKey(0x0000000005f);
/// Represents the logical "Backquote" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey backquote = LogicalKeyboardKey(0x00000000060);
/// Represents the logical "Key A" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyA = LogicalKeyboardKey(0x00000000061);
/// Represents the logical "Key B" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyB = LogicalKeyboardKey(0x00000000062);
/// Represents the logical "Key C" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyC = LogicalKeyboardKey(0x00000000063);
/// Represents the logical "Key D" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyD = LogicalKeyboardKey(0x00000000064);
/// Represents the logical "Key E" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyE = LogicalKeyboardKey(0x00000000065);
/// Represents the logical "Key F" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyF = LogicalKeyboardKey(0x00000000066);
/// Represents the logical "Key G" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyG = LogicalKeyboardKey(0x00000000067);
/// Represents the logical "Key H" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyH = LogicalKeyboardKey(0x00000000068);
/// Represents the logical "Key I" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyI = LogicalKeyboardKey(0x00000000069);
/// Represents the logical "Key J" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyJ = LogicalKeyboardKey(0x0000000006a);
/// Represents the logical "Key K" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyK = LogicalKeyboardKey(0x0000000006b);
/// Represents the logical "Key L" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyL = LogicalKeyboardKey(0x0000000006c);
/// Represents the logical "Key M" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyM = LogicalKeyboardKey(0x0000000006d);
/// Represents the logical "Key N" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyN = LogicalKeyboardKey(0x0000000006e);
/// Represents the logical "Key O" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyO = LogicalKeyboardKey(0x0000000006f);
/// Represents the logical "Key P" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyP = LogicalKeyboardKey(0x00000000070);
/// Represents the logical "Key Q" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyQ = LogicalKeyboardKey(0x00000000071);
/// Represents the logical "Key R" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyR = LogicalKeyboardKey(0x00000000072);
/// Represents the logical "Key S" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyS = LogicalKeyboardKey(0x00000000073);
/// Represents the logical "Key T" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyT = LogicalKeyboardKey(0x00000000074);
/// Represents the logical "Key U" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyU = LogicalKeyboardKey(0x00000000075);
/// Represents the logical "Key V" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyV = LogicalKeyboardKey(0x00000000076);
/// Represents the logical "Key W" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyW = LogicalKeyboardKey(0x00000000077);
/// Represents the logical "Key X" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyX = LogicalKeyboardKey(0x00000000078);
/// Represents the logical "Key Y" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyY = LogicalKeyboardKey(0x00000000079);
/// Represents the logical "Key Z" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey keyZ = LogicalKeyboardKey(0x0000000007a);
/// Represents the logical "Brace Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey braceLeft = LogicalKeyboardKey(0x0000000007b);
/// Represents the logical "Bar" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey bar = LogicalKeyboardKey(0x0000000007c);
/// Represents the logical "Brace Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey braceRight = LogicalKeyboardKey(0x0000000007d);
/// Represents the logical "Tilde" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tilde = LogicalKeyboardKey(0x0000000007e);
/// Represents the logical "Unidentified" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey unidentified = LogicalKeyboardKey(0x00100000001);
/// Represents the logical "Backspace" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey backspace = LogicalKeyboardKey(0x00100000008);
/// Represents the logical "Tab" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tab = LogicalKeyboardKey(0x00100000009);
/// Represents the logical "Enter" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey enter = LogicalKeyboardKey(0x0010000000d);
/// Represents the logical "Escape" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey escape = LogicalKeyboardKey(0x0010000001b);
/// Represents the logical "Delete" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey delete = LogicalKeyboardKey(0x0010000007f);
/// Represents the logical "Accel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey accel = LogicalKeyboardKey(0x00100000101);
/// Represents the logical "Alt Graph" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey altGraph = LogicalKeyboardKey(0x00100000103);
/// Represents the logical "Caps Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey capsLock = LogicalKeyboardKey(0x00100000104);
/// Represents the logical "Fn" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey fn = LogicalKeyboardKey(0x00100000106);
/// Represents the logical "Fn Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey fnLock = LogicalKeyboardKey(0x00100000107);
/// Represents the logical "Hyper" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hyper = LogicalKeyboardKey(0x00100000108);
/// Represents the logical "Num Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numLock = LogicalKeyboardKey(0x0010000010a);
/// Represents the logical "Scroll Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey scrollLock = LogicalKeyboardKey(0x0010000010c);
/// Represents the logical "Super" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey superKey = LogicalKeyboardKey(0x0010000010e);
/// Represents the logical "Symbol" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey symbol = LogicalKeyboardKey(0x0010000010f);
/// Represents the logical "Symbol Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey symbolLock = LogicalKeyboardKey(0x00100000110);
/// Represents the logical "Shift Level 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey shiftLevel5 = LogicalKeyboardKey(0x00100000111);
/// Represents the logical "Arrow Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey arrowDown = LogicalKeyboardKey(0x00100000301);
/// Represents the logical "Arrow Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey arrowLeft = LogicalKeyboardKey(0x00100000302);
/// Represents the logical "Arrow Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey arrowRight = LogicalKeyboardKey(0x00100000303);
/// Represents the logical "Arrow Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey arrowUp = LogicalKeyboardKey(0x00100000304);
/// Represents the logical "End" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey end = LogicalKeyboardKey(0x00100000305);
/// Represents the logical "Home" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey home = LogicalKeyboardKey(0x00100000306);
/// Represents the logical "Page Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pageDown = LogicalKeyboardKey(0x00100000307);
/// Represents the logical "Page Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pageUp = LogicalKeyboardKey(0x00100000308);
/// Represents the logical "Clear" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey clear = LogicalKeyboardKey(0x00100000401);
/// Represents the logical "Copy" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey copy = LogicalKeyboardKey(0x00100000402);
/// Represents the logical "Cr Sel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey crSel = LogicalKeyboardKey(0x00100000403);
/// Represents the logical "Cut" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey cut = LogicalKeyboardKey(0x00100000404);
/// Represents the logical "Erase Eof" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey eraseEof = LogicalKeyboardKey(0x00100000405);
/// Represents the logical "Ex Sel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey exSel = LogicalKeyboardKey(0x00100000406);
/// Represents the logical "Insert" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey insert = LogicalKeyboardKey(0x00100000407);
/// Represents the logical "Paste" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey paste = LogicalKeyboardKey(0x00100000408);
/// Represents the logical "Redo" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey redo = LogicalKeyboardKey(0x00100000409);
/// Represents the logical "Undo" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey undo = LogicalKeyboardKey(0x0010000040a);
/// Represents the logical "Accept" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey accept = LogicalKeyboardKey(0x00100000501);
/// Represents the logical "Again" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey again = LogicalKeyboardKey(0x00100000502);
/// Represents the logical "Attn" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey attn = LogicalKeyboardKey(0x00100000503);
/// Represents the logical "Cancel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey cancel = LogicalKeyboardKey(0x00100000504);
/// Represents the logical "Context Menu" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey contextMenu = LogicalKeyboardKey(0x00100000505);
/// Represents the logical "Execute" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey execute = LogicalKeyboardKey(0x00100000506);
/// Represents the logical "Find" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey find = LogicalKeyboardKey(0x00100000507);
/// Represents the logical "Help" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey help = LogicalKeyboardKey(0x00100000508);
/// Represents the logical "Pause" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pause = LogicalKeyboardKey(0x00100000509);
/// Represents the logical "Play" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey play = LogicalKeyboardKey(0x0010000050a);
/// Represents the logical "Props" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey props = LogicalKeyboardKey(0x0010000050b);
/// Represents the logical "Select" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey select = LogicalKeyboardKey(0x0010000050c);
/// Represents the logical "Zoom In" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey zoomIn = LogicalKeyboardKey(0x0010000050d);
/// Represents the logical "Zoom Out" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey zoomOut = LogicalKeyboardKey(0x0010000050e);
/// Represents the logical "Brightness Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey brightnessDown = LogicalKeyboardKey(0x00100000601);
/// Represents the logical "Brightness Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey brightnessUp = LogicalKeyboardKey(0x00100000602);
/// Represents the logical "Camera" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey camera = LogicalKeyboardKey(0x00100000603);
/// Represents the logical "Eject" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey eject = LogicalKeyboardKey(0x00100000604);
/// Represents the logical "Log Off" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey logOff = LogicalKeyboardKey(0x00100000605);
/// Represents the logical "Power" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey power = LogicalKeyboardKey(0x00100000606);
/// Represents the logical "Power Off" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey powerOff = LogicalKeyboardKey(0x00100000607);
/// Represents the logical "Print Screen" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey printScreen = LogicalKeyboardKey(0x00100000608);
/// Represents the logical "Hibernate" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hibernate = LogicalKeyboardKey(0x00100000609);
/// Represents the logical "Standby" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey standby = LogicalKeyboardKey(0x0010000060a);
/// Represents the logical "Wake Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey wakeUp = LogicalKeyboardKey(0x0010000060b);
/// Represents the logical "All Candidates" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey allCandidates = LogicalKeyboardKey(0x00100000701);
/// Represents the logical "Alphanumeric" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey alphanumeric = LogicalKeyboardKey(0x00100000702);
/// Represents the logical "Code Input" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey codeInput = LogicalKeyboardKey(0x00100000703);
/// Represents the logical "Compose" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey compose = LogicalKeyboardKey(0x00100000704);
/// Represents the logical "Convert" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey convert = LogicalKeyboardKey(0x00100000705);
/// Represents the logical "Final Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey finalMode = LogicalKeyboardKey(0x00100000706);
/// Represents the logical "Group First" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey groupFirst = LogicalKeyboardKey(0x00100000707);
/// Represents the logical "Group Last" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey groupLast = LogicalKeyboardKey(0x00100000708);
/// Represents the logical "Group Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey groupNext = LogicalKeyboardKey(0x00100000709);
/// Represents the logical "Group Previous" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey groupPrevious = LogicalKeyboardKey(0x0010000070a);
/// Represents the logical "Mode Change" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey modeChange = LogicalKeyboardKey(0x0010000070b);
/// Represents the logical "Next Candidate" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey nextCandidate = LogicalKeyboardKey(0x0010000070c);
/// Represents the logical "Non Convert" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey nonConvert = LogicalKeyboardKey(0x0010000070d);
/// Represents the logical "Previous Candidate" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey previousCandidate = LogicalKeyboardKey(0x0010000070e);
/// Represents the logical "Process" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey process = LogicalKeyboardKey(0x0010000070f);
/// Represents the logical "Single Candidate" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey singleCandidate = LogicalKeyboardKey(0x00100000710);
/// Represents the logical "Hangul Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hangulMode = LogicalKeyboardKey(0x00100000711);
/// Represents the logical "Hanja Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hanjaMode = LogicalKeyboardKey(0x00100000712);
/// Represents the logical "Junja Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey junjaMode = LogicalKeyboardKey(0x00100000713);
/// Represents the logical "Eisu" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey eisu = LogicalKeyboardKey(0x00100000714);
/// Represents the logical "Hankaku" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hankaku = LogicalKeyboardKey(0x00100000715);
/// Represents the logical "Hiragana" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hiragana = LogicalKeyboardKey(0x00100000716);
/// Represents the logical "Hiragana Katakana" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey hiraganaKatakana = LogicalKeyboardKey(0x00100000717);
/// Represents the logical "Kana Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey kanaMode = LogicalKeyboardKey(0x00100000718);
/// Represents the logical "Kanji Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey kanjiMode = LogicalKeyboardKey(0x00100000719);
/// Represents the logical "Katakana" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey katakana = LogicalKeyboardKey(0x0010000071a);
/// Represents the logical "Romaji" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey romaji = LogicalKeyboardKey(0x0010000071b);
/// Represents the logical "Zenkaku" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey zenkaku = LogicalKeyboardKey(0x0010000071c);
/// Represents the logical "Zenkaku Hankaku" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey zenkakuHankaku = LogicalKeyboardKey(0x0010000071d);
/// Represents the logical "F1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f1 = LogicalKeyboardKey(0x00100000801);
/// Represents the logical "F2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f2 = LogicalKeyboardKey(0x00100000802);
/// Represents the logical "F3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f3 = LogicalKeyboardKey(0x00100000803);
/// Represents the logical "F4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f4 = LogicalKeyboardKey(0x00100000804);
/// Represents the logical "F5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f5 = LogicalKeyboardKey(0x00100000805);
/// Represents the logical "F6" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f6 = LogicalKeyboardKey(0x00100000806);
/// Represents the logical "F7" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f7 = LogicalKeyboardKey(0x00100000807);
/// Represents the logical "F8" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f8 = LogicalKeyboardKey(0x00100000808);
/// Represents the logical "F9" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f9 = LogicalKeyboardKey(0x00100000809);
/// Represents the logical "F10" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f10 = LogicalKeyboardKey(0x0010000080a);
/// Represents the logical "F11" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f11 = LogicalKeyboardKey(0x0010000080b);
/// Represents the logical "F12" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f12 = LogicalKeyboardKey(0x0010000080c);
/// Represents the logical "F13" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f13 = LogicalKeyboardKey(0x0010000080d);
/// Represents the logical "F14" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f14 = LogicalKeyboardKey(0x0010000080e);
/// Represents the logical "F15" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f15 = LogicalKeyboardKey(0x0010000080f);
/// Represents the logical "F16" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f16 = LogicalKeyboardKey(0x00100000810);
/// Represents the logical "F17" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f17 = LogicalKeyboardKey(0x00100000811);
/// Represents the logical "F18" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f18 = LogicalKeyboardKey(0x00100000812);
/// Represents the logical "F19" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f19 = LogicalKeyboardKey(0x00100000813);
/// Represents the logical "F20" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f20 = LogicalKeyboardKey(0x00100000814);
/// Represents the logical "F21" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f21 = LogicalKeyboardKey(0x00100000815);
/// Represents the logical "F22" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f22 = LogicalKeyboardKey(0x00100000816);
/// Represents the logical "F23" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f23 = LogicalKeyboardKey(0x00100000817);
/// Represents the logical "F24" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey f24 = LogicalKeyboardKey(0x00100000818);
/// Represents the logical "Soft 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft1 = LogicalKeyboardKey(0x00100000901);
/// Represents the logical "Soft 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft2 = LogicalKeyboardKey(0x00100000902);
/// Represents the logical "Soft 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft3 = LogicalKeyboardKey(0x00100000903);
/// Represents the logical "Soft 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft4 = LogicalKeyboardKey(0x00100000904);
/// Represents the logical "Soft 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft5 = LogicalKeyboardKey(0x00100000905);
/// Represents the logical "Soft 6" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft6 = LogicalKeyboardKey(0x00100000906);
/// Represents the logical "Soft 7" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft7 = LogicalKeyboardKey(0x00100000907);
/// Represents the logical "Soft 8" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey soft8 = LogicalKeyboardKey(0x00100000908);
/// Represents the logical "Close" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey close = LogicalKeyboardKey(0x00100000a01);
/// Represents the logical "Mail Forward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mailForward = LogicalKeyboardKey(0x00100000a02);
/// Represents the logical "Mail Reply" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mailReply = LogicalKeyboardKey(0x00100000a03);
/// Represents the logical "Mail Send" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mailSend = LogicalKeyboardKey(0x00100000a04);
/// Represents the logical "Media Play Pause" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaPlayPause = LogicalKeyboardKey(0x00100000a05);
/// Represents the logical "Media Stop" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaStop = LogicalKeyboardKey(0x00100000a07);
/// Represents the logical "Media Track Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaTrackNext = LogicalKeyboardKey(0x00100000a08);
/// Represents the logical "Media Track Previous" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaTrackPrevious = LogicalKeyboardKey(0x00100000a09);
/// Represents the logical "New" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey newKey = LogicalKeyboardKey(0x00100000a0a);
/// Represents the logical "Open" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey open = LogicalKeyboardKey(0x00100000a0b);
/// Represents the logical "Print" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey print = LogicalKeyboardKey(0x00100000a0c);
/// Represents the logical "Save" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey save = LogicalKeyboardKey(0x00100000a0d);
/// Represents the logical "Spell Check" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey spellCheck = LogicalKeyboardKey(0x00100000a0e);
/// Represents the logical "Audio Volume Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioVolumeDown = LogicalKeyboardKey(0x00100000a0f);
/// Represents the logical "Audio Volume Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioVolumeUp = LogicalKeyboardKey(0x00100000a10);
/// Represents the logical "Audio Volume Mute" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioVolumeMute = LogicalKeyboardKey(0x00100000a11);
/// Represents the logical "Launch Application 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchApplication2 = LogicalKeyboardKey(0x00100000b01);
/// Represents the logical "Launch Calendar" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchCalendar = LogicalKeyboardKey(0x00100000b02);
/// Represents the logical "Launch Mail" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchMail = LogicalKeyboardKey(0x00100000b03);
/// Represents the logical "Launch Media Player" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchMediaPlayer = LogicalKeyboardKey(0x00100000b04);
/// Represents the logical "Launch Music Player" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchMusicPlayer = LogicalKeyboardKey(0x00100000b05);
/// Represents the logical "Launch Application 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchApplication1 = LogicalKeyboardKey(0x00100000b06);
/// Represents the logical "Launch Screen Saver" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchScreenSaver = LogicalKeyboardKey(0x00100000b07);
/// Represents the logical "Launch Spreadsheet" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchSpreadsheet = LogicalKeyboardKey(0x00100000b08);
/// Represents the logical "Launch Web Browser" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchWebBrowser = LogicalKeyboardKey(0x00100000b09);
/// Represents the logical "Launch Web Cam" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchWebCam = LogicalKeyboardKey(0x00100000b0a);
/// Represents the logical "Launch Word Processor" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchWordProcessor = LogicalKeyboardKey(0x00100000b0b);
/// Represents the logical "Launch Contacts" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchContacts = LogicalKeyboardKey(0x00100000b0c);
/// Represents the logical "Launch Phone" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchPhone = LogicalKeyboardKey(0x00100000b0d);
/// Represents the logical "Launch Assistant" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchAssistant = LogicalKeyboardKey(0x00100000b0e);
/// Represents the logical "Launch Control Panel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey launchControlPanel = LogicalKeyboardKey(0x00100000b0f);
/// Represents the logical "Browser Back" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserBack = LogicalKeyboardKey(0x00100000c01);
/// Represents the logical "Browser Favorites" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserFavorites = LogicalKeyboardKey(0x00100000c02);
/// Represents the logical "Browser Forward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserForward = LogicalKeyboardKey(0x00100000c03);
/// Represents the logical "Browser Home" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserHome = LogicalKeyboardKey(0x00100000c04);
/// Represents the logical "Browser Refresh" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserRefresh = LogicalKeyboardKey(0x00100000c05);
/// Represents the logical "Browser Search" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserSearch = LogicalKeyboardKey(0x00100000c06);
/// Represents the logical "Browser Stop" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey browserStop = LogicalKeyboardKey(0x00100000c07);
/// Represents the logical "Audio Balance Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioBalanceLeft = LogicalKeyboardKey(0x00100000d01);
/// Represents the logical "Audio Balance Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioBalanceRight = LogicalKeyboardKey(0x00100000d02);
/// Represents the logical "Audio Bass Boost Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioBassBoostDown = LogicalKeyboardKey(0x00100000d03);
/// Represents the logical "Audio Bass Boost Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioBassBoostUp = LogicalKeyboardKey(0x00100000d04);
/// Represents the logical "Audio Fader Front" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioFaderFront = LogicalKeyboardKey(0x00100000d05);
/// Represents the logical "Audio Fader Rear" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioFaderRear = LogicalKeyboardKey(0x00100000d06);
/// Represents the logical "Audio Surround Mode Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioSurroundModeNext = LogicalKeyboardKey(0x00100000d07);
/// Represents the logical "AVR Input" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey avrInput = LogicalKeyboardKey(0x00100000d08);
/// Represents the logical "AVR Power" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey avrPower = LogicalKeyboardKey(0x00100000d09);
/// Represents the logical "Channel Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey channelDown = LogicalKeyboardKey(0x00100000d0a);
/// Represents the logical "Channel Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey channelUp = LogicalKeyboardKey(0x00100000d0b);
/// Represents the logical "Color F0 Red" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF0Red = LogicalKeyboardKey(0x00100000d0c);
/// Represents the logical "Color F1 Green" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF1Green = LogicalKeyboardKey(0x00100000d0d);
/// Represents the logical "Color F2 Yellow" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF2Yellow = LogicalKeyboardKey(0x00100000d0e);
/// Represents the logical "Color F3 Blue" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF3Blue = LogicalKeyboardKey(0x00100000d0f);
/// Represents the logical "Color F4 Grey" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF4Grey = LogicalKeyboardKey(0x00100000d10);
/// Represents the logical "Color F5 Brown" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey colorF5Brown = LogicalKeyboardKey(0x00100000d11);
/// Represents the logical "Closed Caption Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey closedCaptionToggle = LogicalKeyboardKey(0x00100000d12);
/// Represents the logical "Dimmer" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey dimmer = LogicalKeyboardKey(0x00100000d13);
/// Represents the logical "Display Swap" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey displaySwap = LogicalKeyboardKey(0x00100000d14);
/// Represents the logical "Exit" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey exit = LogicalKeyboardKey(0x00100000d15);
/// Represents the logical "Favorite Clear 0" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteClear0 = LogicalKeyboardKey(0x00100000d16);
/// Represents the logical "Favorite Clear 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteClear1 = LogicalKeyboardKey(0x00100000d17);
/// Represents the logical "Favorite Clear 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteClear2 = LogicalKeyboardKey(0x00100000d18);
/// Represents the logical "Favorite Clear 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteClear3 = LogicalKeyboardKey(0x00100000d19);
/// Represents the logical "Favorite Recall 0" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteRecall0 = LogicalKeyboardKey(0x00100000d1a);
/// Represents the logical "Favorite Recall 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteRecall1 = LogicalKeyboardKey(0x00100000d1b);
/// Represents the logical "Favorite Recall 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteRecall2 = LogicalKeyboardKey(0x00100000d1c);
/// Represents the logical "Favorite Recall 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteRecall3 = LogicalKeyboardKey(0x00100000d1d);
/// Represents the logical "Favorite Store 0" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteStore0 = LogicalKeyboardKey(0x00100000d1e);
/// Represents the logical "Favorite Store 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteStore1 = LogicalKeyboardKey(0x00100000d1f);
/// Represents the logical "Favorite Store 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteStore2 = LogicalKeyboardKey(0x00100000d20);
/// Represents the logical "Favorite Store 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey favoriteStore3 = LogicalKeyboardKey(0x00100000d21);
/// Represents the logical "Guide" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey guide = LogicalKeyboardKey(0x00100000d22);
/// Represents the logical "Guide Next Day" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey guideNextDay = LogicalKeyboardKey(0x00100000d23);
/// Represents the logical "Guide Previous Day" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey guidePreviousDay = LogicalKeyboardKey(0x00100000d24);
/// Represents the logical "Info" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey info = LogicalKeyboardKey(0x00100000d25);
/// Represents the logical "Instant Replay" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey instantReplay = LogicalKeyboardKey(0x00100000d26);
/// Represents the logical "Link" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey link = LogicalKeyboardKey(0x00100000d27);
/// Represents the logical "List Program" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey listProgram = LogicalKeyboardKey(0x00100000d28);
/// Represents the logical "Live Content" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey liveContent = LogicalKeyboardKey(0x00100000d29);
/// Represents the logical "Lock" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lock = LogicalKeyboardKey(0x00100000d2a);
/// Represents the logical "Media Apps" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaApps = LogicalKeyboardKey(0x00100000d2b);
/// Represents the logical "Media Fast Forward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaFastForward = LogicalKeyboardKey(0x00100000d2c);
/// Represents the logical "Media Last" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaLast = LogicalKeyboardKey(0x00100000d2d);
/// Represents the logical "Media Pause" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaPause = LogicalKeyboardKey(0x00100000d2e);
/// Represents the logical "Media Play" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaPlay = LogicalKeyboardKey(0x00100000d2f);
/// Represents the logical "Media Record" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaRecord = LogicalKeyboardKey(0x00100000d30);
/// Represents the logical "Media Rewind" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaRewind = LogicalKeyboardKey(0x00100000d31);
/// Represents the logical "Media Skip" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaSkip = LogicalKeyboardKey(0x00100000d32);
/// Represents the logical "Next Favorite Channel" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey nextFavoriteChannel = LogicalKeyboardKey(0x00100000d33);
/// Represents the logical "Next User Profile" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey nextUserProfile = LogicalKeyboardKey(0x00100000d34);
/// Represents the logical "On Demand" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey onDemand = LogicalKeyboardKey(0x00100000d35);
/// Represents the logical "P In P Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pInPDown = LogicalKeyboardKey(0x00100000d36);
/// Represents the logical "P In P Move" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pInPMove = LogicalKeyboardKey(0x00100000d37);
/// Represents the logical "P In P Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pInPToggle = LogicalKeyboardKey(0x00100000d38);
/// Represents the logical "P In P Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pInPUp = LogicalKeyboardKey(0x00100000d39);
/// Represents the logical "Play Speed Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey playSpeedDown = LogicalKeyboardKey(0x00100000d3a);
/// Represents the logical "Play Speed Reset" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey playSpeedReset = LogicalKeyboardKey(0x00100000d3b);
/// Represents the logical "Play Speed Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey playSpeedUp = LogicalKeyboardKey(0x00100000d3c);
/// Represents the logical "Random Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey randomToggle = LogicalKeyboardKey(0x00100000d3d);
/// Represents the logical "Rc Low Battery" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey rcLowBattery = LogicalKeyboardKey(0x00100000d3e);
/// Represents the logical "Record Speed Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey recordSpeedNext = LogicalKeyboardKey(0x00100000d3f);
/// Represents the logical "Rf Bypass" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey rfBypass = LogicalKeyboardKey(0x00100000d40);
/// Represents the logical "Scan Channels Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey scanChannelsToggle = LogicalKeyboardKey(0x00100000d41);
/// Represents the logical "Screen Mode Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey screenModeNext = LogicalKeyboardKey(0x00100000d42);
/// Represents the logical "Settings" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey settings = LogicalKeyboardKey(0x00100000d43);
/// Represents the logical "Split Screen Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey splitScreenToggle = LogicalKeyboardKey(0x00100000d44);
/// Represents the logical "STB Input" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey stbInput = LogicalKeyboardKey(0x00100000d45);
/// Represents the logical "STB Power" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey stbPower = LogicalKeyboardKey(0x00100000d46);
/// Represents the logical "Subtitle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey subtitle = LogicalKeyboardKey(0x00100000d47);
/// Represents the logical "Teletext" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey teletext = LogicalKeyboardKey(0x00100000d48);
/// Represents the logical "TV" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tv = LogicalKeyboardKey(0x00100000d49);
/// Represents the logical "TV Input" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInput = LogicalKeyboardKey(0x00100000d4a);
/// Represents the logical "TV Power" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvPower = LogicalKeyboardKey(0x00100000d4b);
/// Represents the logical "Video Mode Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey videoModeNext = LogicalKeyboardKey(0x00100000d4c);
/// Represents the logical "Wink" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey wink = LogicalKeyboardKey(0x00100000d4d);
/// Represents the logical "Zoom Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey zoomToggle = LogicalKeyboardKey(0x00100000d4e);
/// Represents the logical "DVR" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey dvr = LogicalKeyboardKey(0x00100000d4f);
/// Represents the logical "Media Audio Track" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaAudioTrack = LogicalKeyboardKey(0x00100000d50);
/// Represents the logical "Media Skip Backward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaSkipBackward = LogicalKeyboardKey(0x00100000d51);
/// Represents the logical "Media Skip Forward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaSkipForward = LogicalKeyboardKey(0x00100000d52);
/// Represents the logical "Media Step Backward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaStepBackward = LogicalKeyboardKey(0x00100000d53);
/// Represents the logical "Media Step Forward" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaStepForward = LogicalKeyboardKey(0x00100000d54);
/// Represents the logical "Media Top Menu" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaTopMenu = LogicalKeyboardKey(0x00100000d55);
/// Represents the logical "Navigate In" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey navigateIn = LogicalKeyboardKey(0x00100000d56);
/// Represents the logical "Navigate Next" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey navigateNext = LogicalKeyboardKey(0x00100000d57);
/// Represents the logical "Navigate Out" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey navigateOut = LogicalKeyboardKey(0x00100000d58);
/// Represents the logical "Navigate Previous" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey navigatePrevious = LogicalKeyboardKey(0x00100000d59);
/// Represents the logical "Pairing" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey pairing = LogicalKeyboardKey(0x00100000d5a);
/// Represents the logical "Media Close" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mediaClose = LogicalKeyboardKey(0x00100000d5b);
/// Represents the logical "Audio Bass Boost Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioBassBoostToggle = LogicalKeyboardKey(0x00100000e02);
/// Represents the logical "Audio Treble Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioTrebleDown = LogicalKeyboardKey(0x00100000e04);
/// Represents the logical "Audio Treble Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey audioTrebleUp = LogicalKeyboardKey(0x00100000e05);
/// Represents the logical "Microphone Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey microphoneToggle = LogicalKeyboardKey(0x00100000e06);
/// Represents the logical "Microphone Volume Down" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey microphoneVolumeDown = LogicalKeyboardKey(0x00100000e07);
/// Represents the logical "Microphone Volume Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey microphoneVolumeUp = LogicalKeyboardKey(0x00100000e08);
/// Represents the logical "Microphone Volume Mute" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey microphoneVolumeMute = LogicalKeyboardKey(0x00100000e09);
/// Represents the logical "Speech Correction List" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey speechCorrectionList = LogicalKeyboardKey(0x00100000f01);
/// Represents the logical "Speech Input Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey speechInputToggle = LogicalKeyboardKey(0x00100000f02);
/// Represents the logical "App Switch" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey appSwitch = LogicalKeyboardKey(0x00100001001);
/// Represents the logical "Call" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey call = LogicalKeyboardKey(0x00100001002);
/// Represents the logical "Camera Focus" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey cameraFocus = LogicalKeyboardKey(0x00100001003);
/// Represents the logical "End Call" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey endCall = LogicalKeyboardKey(0x00100001004);
/// Represents the logical "Go Back" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey goBack = LogicalKeyboardKey(0x00100001005);
/// Represents the logical "Go Home" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey goHome = LogicalKeyboardKey(0x00100001006);
/// Represents the logical "Headset Hook" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey headsetHook = LogicalKeyboardKey(0x00100001007);
/// Represents the logical "Last Number Redial" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lastNumberRedial = LogicalKeyboardKey(0x00100001008);
/// Represents the logical "Notification" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey notification = LogicalKeyboardKey(0x00100001009);
/// Represents the logical "Manner Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey mannerMode = LogicalKeyboardKey(0x0010000100a);
/// Represents the logical "Voice Dial" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey voiceDial = LogicalKeyboardKey(0x0010000100b);
/// Represents the logical "TV 3 D Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tv3DMode = LogicalKeyboardKey(0x00100001101);
/// Represents the logical "TV Antenna Cable" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvAntennaCable = LogicalKeyboardKey(0x00100001102);
/// Represents the logical "TV Audio Description" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvAudioDescription = LogicalKeyboardKey(0x00100001103);
/// Represents the logical "TV Audio Description Mix Down" key on the
/// keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvAudioDescriptionMixDown = LogicalKeyboardKey(0x00100001104);
/// Represents the logical "TV Audio Description Mix Up" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvAudioDescriptionMixUp = LogicalKeyboardKey(0x00100001105);
/// Represents the logical "TV Contents Menu" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvContentsMenu = LogicalKeyboardKey(0x00100001106);
/// Represents the logical "TV Data Service" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvDataService = LogicalKeyboardKey(0x00100001107);
/// Represents the logical "TV Input Component 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputComponent1 = LogicalKeyboardKey(0x00100001108);
/// Represents the logical "TV Input Component 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputComponent2 = LogicalKeyboardKey(0x00100001109);
/// Represents the logical "TV Input Composite 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputComposite1 = LogicalKeyboardKey(0x0010000110a);
/// Represents the logical "TV Input Composite 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputComposite2 = LogicalKeyboardKey(0x0010000110b);
/// Represents the logical "TV Input HDMI 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputHDMI1 = LogicalKeyboardKey(0x0010000110c);
/// Represents the logical "TV Input HDMI 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputHDMI2 = LogicalKeyboardKey(0x0010000110d);
/// Represents the logical "TV Input HDMI 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputHDMI3 = LogicalKeyboardKey(0x0010000110e);
/// Represents the logical "TV Input HDMI 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputHDMI4 = LogicalKeyboardKey(0x0010000110f);
/// Represents the logical "TV Input VGA 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvInputVGA1 = LogicalKeyboardKey(0x00100001110);
/// Represents the logical "TV Media Context" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvMediaContext = LogicalKeyboardKey(0x00100001111);
/// Represents the logical "TV Network" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvNetwork = LogicalKeyboardKey(0x00100001112);
/// Represents the logical "TV Number Entry" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvNumberEntry = LogicalKeyboardKey(0x00100001113);
/// Represents the logical "TV Radio Service" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvRadioService = LogicalKeyboardKey(0x00100001114);
/// Represents the logical "TV Satellite" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvSatellite = LogicalKeyboardKey(0x00100001115);
/// Represents the logical "TV Satellite BS" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvSatelliteBS = LogicalKeyboardKey(0x00100001116);
/// Represents the logical "TV Satellite CS" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvSatelliteCS = LogicalKeyboardKey(0x00100001117);
/// Represents the logical "TV Satellite Toggle" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvSatelliteToggle = LogicalKeyboardKey(0x00100001118);
/// Represents the logical "TV Terrestrial Analog" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvTerrestrialAnalog = LogicalKeyboardKey(0x00100001119);
/// Represents the logical "TV Terrestrial Digital" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvTerrestrialDigital = LogicalKeyboardKey(0x0010000111a);
/// Represents the logical "TV Timer" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey tvTimer = LogicalKeyboardKey(0x0010000111b);
/// Represents the logical "Key 11" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey key11 = LogicalKeyboardKey(0x00100001201);
/// Represents the logical "Key 12" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey key12 = LogicalKeyboardKey(0x00100001202);
/// Represents the logical "Suspend" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey suspend = LogicalKeyboardKey(0x00200000000);
/// Represents the logical "Resume" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey resume = LogicalKeyboardKey(0x00200000001);
/// Represents the logical "Sleep" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey sleep = LogicalKeyboardKey(0x00200000002);
/// Represents the logical "Abort" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey abort = LogicalKeyboardKey(0x00200000003);
/// Represents the logical "Lang 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lang1 = LogicalKeyboardKey(0x00200000010);
/// Represents the logical "Lang 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lang2 = LogicalKeyboardKey(0x00200000011);
/// Represents the logical "Lang 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lang3 = LogicalKeyboardKey(0x00200000012);
/// Represents the logical "Lang 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lang4 = LogicalKeyboardKey(0x00200000013);
/// Represents the logical "Lang 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey lang5 = LogicalKeyboardKey(0x00200000014);
/// Represents the logical "Intl Backslash" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey intlBackslash = LogicalKeyboardKey(0x00200000020);
/// Represents the logical "Intl Ro" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey intlRo = LogicalKeyboardKey(0x00200000021);
/// Represents the logical "Intl Yen" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey intlYen = LogicalKeyboardKey(0x00200000022);
/// Represents the logical "Control Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey controlLeft = LogicalKeyboardKey(0x00200000100);
/// Represents the logical "Control Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey controlRight = LogicalKeyboardKey(0x00200000101);
/// Represents the logical "Shift Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey shiftLeft = LogicalKeyboardKey(0x00200000102);
/// Represents the logical "Shift Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey shiftRight = LogicalKeyboardKey(0x00200000103);
/// Represents the logical "Alt Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey altLeft = LogicalKeyboardKey(0x00200000104);
/// Represents the logical "Alt Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey altRight = LogicalKeyboardKey(0x00200000105);
/// Represents the logical "Meta Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey metaLeft = LogicalKeyboardKey(0x00200000106);
/// Represents the logical "Meta Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey metaRight = LogicalKeyboardKey(0x00200000107);
/// Represents the logical "Control" key on the keyboard.
///
/// This key represents the union of the keys {controlLeft, controlRight} when
/// comparing keys. This key will never be generated directly, its main use is
/// in defining key maps.
static const LogicalKeyboardKey control = LogicalKeyboardKey(0x002000001f0);
/// Represents the logical "Shift" key on the keyboard.
///
/// This key represents the union of the keys {shiftLeft, shiftRight} when
/// comparing keys. This key will never be generated directly, its main use is
/// in defining key maps.
static const LogicalKeyboardKey shift = LogicalKeyboardKey(0x002000001f2);
/// Represents the logical "Alt" key on the keyboard.
///
/// This key represents the union of the keys {altLeft, altRight} when
/// comparing keys. This key will never be generated directly, its main use is
/// in defining key maps.
static const LogicalKeyboardKey alt = LogicalKeyboardKey(0x002000001f4);
/// Represents the logical "Meta" key on the keyboard.
///
/// This key represents the union of the keys {metaLeft, metaRight} when
/// comparing keys. This key will never be generated directly, its main use is
/// in defining key maps.
static const LogicalKeyboardKey meta = LogicalKeyboardKey(0x002000001f6);
/// Represents the logical "Numpad Enter" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadEnter = LogicalKeyboardKey(0x0020000020d);
/// Represents the logical "Numpad Paren Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadParenLeft = LogicalKeyboardKey(0x00200000228);
/// Represents the logical "Numpad Paren Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadParenRight = LogicalKeyboardKey(0x00200000229);
/// Represents the logical "Numpad Multiply" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadMultiply = LogicalKeyboardKey(0x0020000022a);
/// Represents the logical "Numpad Add" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadAdd = LogicalKeyboardKey(0x0020000022b);
/// Represents the logical "Numpad Comma" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadComma = LogicalKeyboardKey(0x0020000022c);
/// Represents the logical "Numpad Subtract" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadSubtract = LogicalKeyboardKey(0x0020000022d);
/// Represents the logical "Numpad Decimal" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadDecimal = LogicalKeyboardKey(0x0020000022e);
/// Represents the logical "Numpad Divide" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadDivide = LogicalKeyboardKey(0x0020000022f);
/// Represents the logical "Numpad 0" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad0 = LogicalKeyboardKey(0x00200000230);
/// Represents the logical "Numpad 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad1 = LogicalKeyboardKey(0x00200000231);
/// Represents the logical "Numpad 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad2 = LogicalKeyboardKey(0x00200000232);
/// Represents the logical "Numpad 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad3 = LogicalKeyboardKey(0x00200000233);
/// Represents the logical "Numpad 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad4 = LogicalKeyboardKey(0x00200000234);
/// Represents the logical "Numpad 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad5 = LogicalKeyboardKey(0x00200000235);
/// Represents the logical "Numpad 6" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad6 = LogicalKeyboardKey(0x00200000236);
/// Represents the logical "Numpad 7" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad7 = LogicalKeyboardKey(0x00200000237);
/// Represents the logical "Numpad 8" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad8 = LogicalKeyboardKey(0x00200000238);
/// Represents the logical "Numpad 9" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpad9 = LogicalKeyboardKey(0x00200000239);
/// Represents the logical "Numpad Equal" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey numpadEqual = LogicalKeyboardKey(0x0020000023d);
/// Represents the logical "Game Button 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton1 = LogicalKeyboardKey(0x00200000301);
/// Represents the logical "Game Button 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton2 = LogicalKeyboardKey(0x00200000302);
/// Represents the logical "Game Button 3" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton3 = LogicalKeyboardKey(0x00200000303);
/// Represents the logical "Game Button 4" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton4 = LogicalKeyboardKey(0x00200000304);
/// Represents the logical "Game Button 5" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton5 = LogicalKeyboardKey(0x00200000305);
/// Represents the logical "Game Button 6" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton6 = LogicalKeyboardKey(0x00200000306);
/// Represents the logical "Game Button 7" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton7 = LogicalKeyboardKey(0x00200000307);
/// Represents the logical "Game Button 8" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton8 = LogicalKeyboardKey(0x00200000308);
/// Represents the logical "Game Button 9" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton9 = LogicalKeyboardKey(0x00200000309);
/// Represents the logical "Game Button 10" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton10 = LogicalKeyboardKey(0x0020000030a);
/// Represents the logical "Game Button 11" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton11 = LogicalKeyboardKey(0x0020000030b);
/// Represents the logical "Game Button 12" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton12 = LogicalKeyboardKey(0x0020000030c);
/// Represents the logical "Game Button 13" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton13 = LogicalKeyboardKey(0x0020000030d);
/// Represents the logical "Game Button 14" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton14 = LogicalKeyboardKey(0x0020000030e);
/// Represents the logical "Game Button 15" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton15 = LogicalKeyboardKey(0x0020000030f);
/// Represents the logical "Game Button 16" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButton16 = LogicalKeyboardKey(0x00200000310);
/// Represents the logical "Game Button A" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonA = LogicalKeyboardKey(0x00200000311);
/// Represents the logical "Game Button B" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonB = LogicalKeyboardKey(0x00200000312);
/// Represents the logical "Game Button C" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonC = LogicalKeyboardKey(0x00200000313);
/// Represents the logical "Game Button Left 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonLeft1 = LogicalKeyboardKey(0x00200000314);
/// Represents the logical "Game Button Left 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonLeft2 = LogicalKeyboardKey(0x00200000315);
/// Represents the logical "Game Button Mode" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonMode = LogicalKeyboardKey(0x00200000316);
/// Represents the logical "Game Button Right 1" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonRight1 = LogicalKeyboardKey(0x00200000317);
/// Represents the logical "Game Button Right 2" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonRight2 = LogicalKeyboardKey(0x00200000318);
/// Represents the logical "Game Button Select" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonSelect = LogicalKeyboardKey(0x00200000319);
/// Represents the logical "Game Button Start" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonStart = LogicalKeyboardKey(0x0020000031a);
/// Represents the logical "Game Button Thumb Left" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonThumbLeft = LogicalKeyboardKey(0x0020000031b);
/// Represents the logical "Game Button Thumb Right" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonThumbRight = LogicalKeyboardKey(0x0020000031c);
/// Represents the logical "Game Button X" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonX = LogicalKeyboardKey(0x0020000031d);
/// Represents the logical "Game Button Y" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonY = LogicalKeyboardKey(0x0020000031e);
/// Represents the logical "Game Button Z" key on the keyboard.
///
/// See the function [RawKeyEvent.logicalKey] for more information.
static const LogicalKeyboardKey gameButtonZ = LogicalKeyboardKey(0x0020000031f);
/// A list of all predefined constant [LogicalKeyboardKey]s.
static Iterable<LogicalKeyboardKey> get knownLogicalKeys => _knownLogicalKeys.values;
// A list of all predefined constant LogicalKeyboardKeys so they can be
// searched.
static const Map<int, LogicalKeyboardKey> _knownLogicalKeys = <int, LogicalKeyboardKey>{
0x00000000020: space,
0x00000000021: exclamation,
0x00000000022: quote,
0x00000000023: numberSign,
0x00000000024: dollar,
0x00000000025: percent,
0x00000000026: ampersand,
0x00000000027: quoteSingle,
0x00000000028: parenthesisLeft,
0x00000000029: parenthesisRight,
0x0000000002a: asterisk,
0x0000000002b: add,
0x0000000002c: comma,
0x0000000002d: minus,
0x0000000002e: period,
0x0000000002f: slash,
0x00000000030: digit0,
0x00000000031: digit1,
0x00000000032: digit2,
0x00000000033: digit3,
0x00000000034: digit4,
0x00000000035: digit5,
0x00000000036: digit6,
0x00000000037: digit7,
0x00000000038: digit8,
0x00000000039: digit9,
0x0000000003a: colon,
0x0000000003b: semicolon,
0x0000000003c: less,
0x0000000003d: equal,
0x0000000003e: greater,
0x0000000003f: question,
0x00000000040: at,
0x0000000005b: bracketLeft,
0x0000000005c: backslash,
0x0000000005d: bracketRight,
0x0000000005e: caret,
0x0000000005f: underscore,
0x00000000060: backquote,
0x00000000061: keyA,
0x00000000062: keyB,
0x00000000063: keyC,
0x00000000064: keyD,
0x00000000065: keyE,
0x00000000066: keyF,
0x00000000067: keyG,
0x00000000068: keyH,
0x00000000069: keyI,
0x0000000006a: keyJ,
0x0000000006b: keyK,
0x0000000006c: keyL,
0x0000000006d: keyM,
0x0000000006e: keyN,
0x0000000006f: keyO,
0x00000000070: keyP,
0x00000000071: keyQ,
0x00000000072: keyR,
0x00000000073: keyS,
0x00000000074: keyT,
0x00000000075: keyU,
0x00000000076: keyV,
0x00000000077: keyW,
0x00000000078: keyX,
0x00000000079: keyY,
0x0000000007a: keyZ,
0x0000000007b: braceLeft,
0x0000000007c: bar,
0x0000000007d: braceRight,
0x0000000007e: tilde,
0x00100000001: unidentified,
0x00100000008: backspace,
0x00100000009: tab,
0x0010000000d: enter,
0x0010000001b: escape,
0x0010000007f: delete,
0x00100000101: accel,
0x00100000103: altGraph,
0x00100000104: capsLock,
0x00100000106: fn,
0x00100000107: fnLock,
0x00100000108: hyper,
0x0010000010a: numLock,
0x0010000010c: scrollLock,
0x0010000010e: superKey,
0x0010000010f: symbol,
0x00100000110: symbolLock,
0x00100000111: shiftLevel5,
0x00100000301: arrowDown,
0x00100000302: arrowLeft,
0x00100000303: arrowRight,
0x00100000304: arrowUp,
0x00100000305: end,
0x00100000306: home,
0x00100000307: pageDown,
0x00100000308: pageUp,
0x00100000401: clear,
0x00100000402: copy,
0x00100000403: crSel,
0x00100000404: cut,
0x00100000405: eraseEof,
0x00100000406: exSel,
0x00100000407: insert,
0x00100000408: paste,
0x00100000409: redo,
0x0010000040a: undo,
0x00100000501: accept,
0x00100000502: again,
0x00100000503: attn,
0x00100000504: cancel,
0x00100000505: contextMenu,
0x00100000506: execute,
0x00100000507: find,
0x00100000508: help,
0x00100000509: pause,
0x0010000050a: play,
0x0010000050b: props,
0x0010000050c: select,
0x0010000050d: zoomIn,
0x0010000050e: zoomOut,
0x00100000601: brightnessDown,
0x00100000602: brightnessUp,
0x00100000603: camera,
0x00100000604: eject,
0x00100000605: logOff,
0x00100000606: power,
0x00100000607: powerOff,
0x00100000608: printScreen,
0x00100000609: hibernate,
0x0010000060a: standby,
0x0010000060b: wakeUp,
0x00100000701: allCandidates,
0x00100000702: alphanumeric,
0x00100000703: codeInput,
0x00100000704: compose,
0x00100000705: convert,
0x00100000706: finalMode,
0x00100000707: groupFirst,
0x00100000708: groupLast,
0x00100000709: groupNext,
0x0010000070a: groupPrevious,
0x0010000070b: modeChange,
0x0010000070c: nextCandidate,
0x0010000070d: nonConvert,
0x0010000070e: previousCandidate,
0x0010000070f: process,
0x00100000710: singleCandidate,
0x00100000711: hangulMode,
0x00100000712: hanjaMode,
0x00100000713: junjaMode,
0x00100000714: eisu,
0x00100000715: hankaku,
0x00100000716: hiragana,
0x00100000717: hiraganaKatakana,
0x00100000718: kanaMode,
0x00100000719: kanjiMode,
0x0010000071a: katakana,
0x0010000071b: romaji,
0x0010000071c: zenkaku,
0x0010000071d: zenkakuHankaku,
0x00100000801: f1,
0x00100000802: f2,
0x00100000803: f3,
0x00100000804: f4,
0x00100000805: f5,
0x00100000806: f6,
0x00100000807: f7,
0x00100000808: f8,
0x00100000809: f9,
0x0010000080a: f10,
0x0010000080b: f11,
0x0010000080c: f12,
0x0010000080d: f13,
0x0010000080e: f14,
0x0010000080f: f15,
0x00100000810: f16,
0x00100000811: f17,
0x00100000812: f18,
0x00100000813: f19,
0x00100000814: f20,
0x00100000815: f21,
0x00100000816: f22,
0x00100000817: f23,
0x00100000818: f24,
0x00100000901: soft1,
0x00100000902: soft2,
0x00100000903: soft3,
0x00100000904: soft4,
0x00100000905: soft5,
0x00100000906: soft6,
0x00100000907: soft7,
0x00100000908: soft8,
0x00100000a01: close,
0x00100000a02: mailForward,
0x00100000a03: mailReply,
0x00100000a04: mailSend,
0x00100000a05: mediaPlayPause,
0x00100000a07: mediaStop,
0x00100000a08: mediaTrackNext,
0x00100000a09: mediaTrackPrevious,
0x00100000a0a: newKey,
0x00100000a0b: open,
0x00100000a0c: print,
0x00100000a0d: save,
0x00100000a0e: spellCheck,
0x00100000a0f: audioVolumeDown,
0x00100000a10: audioVolumeUp,
0x00100000a11: audioVolumeMute,
0x00100000b01: launchApplication2,
0x00100000b02: launchCalendar,
0x00100000b03: launchMail,
0x00100000b04: launchMediaPlayer,
0x00100000b05: launchMusicPlayer,
0x00100000b06: launchApplication1,
0x00100000b07: launchScreenSaver,
0x00100000b08: launchSpreadsheet,
0x00100000b09: launchWebBrowser,
0x00100000b0a: launchWebCam,
0x00100000b0b: launchWordProcessor,
0x00100000b0c: launchContacts,
0x00100000b0d: launchPhone,
0x00100000b0e: launchAssistant,
0x00100000b0f: launchControlPanel,
0x00100000c01: browserBack,
0x00100000c02: browserFavorites,
0x00100000c03: browserForward,
0x00100000c04: browserHome,
0x00100000c05: browserRefresh,
0x00100000c06: browserSearch,
0x00100000c07: browserStop,
0x00100000d01: audioBalanceLeft,
0x00100000d02: audioBalanceRight,
0x00100000d03: audioBassBoostDown,
0x00100000d04: audioBassBoostUp,
0x00100000d05: audioFaderFront,
0x00100000d06: audioFaderRear,
0x00100000d07: audioSurroundModeNext,
0x00100000d08: avrInput,
0x00100000d09: avrPower,
0x00100000d0a: channelDown,
0x00100000d0b: channelUp,
0x00100000d0c: colorF0Red,
0x00100000d0d: colorF1Green,
0x00100000d0e: colorF2Yellow,
0x00100000d0f: colorF3Blue,
0x00100000d10: colorF4Grey,
0x00100000d11: colorF5Brown,
0x00100000d12: closedCaptionToggle,
0x00100000d13: dimmer,
0x00100000d14: displaySwap,
0x00100000d15: exit,
0x00100000d16: favoriteClear0,
0x00100000d17: favoriteClear1,
0x00100000d18: favoriteClear2,
0x00100000d19: favoriteClear3,
0x00100000d1a: favoriteRecall0,
0x00100000d1b: favoriteRecall1,
0x00100000d1c: favoriteRecall2,
0x00100000d1d: favoriteRecall3,
0x00100000d1e: favoriteStore0,
0x00100000d1f: favoriteStore1,
0x00100000d20: favoriteStore2,
0x00100000d21: favoriteStore3,
0x00100000d22: guide,
0x00100000d23: guideNextDay,
0x00100000d24: guidePreviousDay,
0x00100000d25: info,
0x00100000d26: instantReplay,
0x00100000d27: link,
0x00100000d28: listProgram,
0x00100000d29: liveContent,
0x00100000d2a: lock,
0x00100000d2b: mediaApps,
0x00100000d2c: mediaFastForward,
0x00100000d2d: mediaLast,
0x00100000d2e: mediaPause,
0x00100000d2f: mediaPlay,
0x00100000d30: mediaRecord,
0x00100000d31: mediaRewind,
0x00100000d32: mediaSkip,
0x00100000d33: nextFavoriteChannel,
0x00100000d34: nextUserProfile,
0x00100000d35: onDemand,
0x00100000d36: pInPDown,
0x00100000d37: pInPMove,
0x00100000d38: pInPToggle,
0x00100000d39: pInPUp,
0x00100000d3a: playSpeedDown,
0x00100000d3b: playSpeedReset,
0x00100000d3c: playSpeedUp,
0x00100000d3d: randomToggle,
0x00100000d3e: rcLowBattery,
0x00100000d3f: recordSpeedNext,
0x00100000d40: rfBypass,
0x00100000d41: scanChannelsToggle,
0x00100000d42: screenModeNext,
0x00100000d43: settings,
0x00100000d44: splitScreenToggle,
0x00100000d45: stbInput,
0x00100000d46: stbPower,
0x00100000d47: subtitle,
0x00100000d48: teletext,
0x00100000d49: tv,
0x00100000d4a: tvInput,
0x00100000d4b: tvPower,
0x00100000d4c: videoModeNext,
0x00100000d4d: wink,
0x00100000d4e: zoomToggle,
0x00100000d4f: dvr,
0x00100000d50: mediaAudioTrack,
0x00100000d51: mediaSkipBackward,
0x00100000d52: mediaSkipForward,
0x00100000d53: mediaStepBackward,
0x00100000d54: mediaStepForward,
0x00100000d55: mediaTopMenu,
0x00100000d56: navigateIn,
0x00100000d57: navigateNext,
0x00100000d58: navigateOut,
0x00100000d59: navigatePrevious,
0x00100000d5a: pairing,
0x00100000d5b: mediaClose,
0x00100000e02: audioBassBoostToggle,
0x00100000e04: audioTrebleDown,
0x00100000e05: audioTrebleUp,
0x00100000e06: microphoneToggle,
0x00100000e07: microphoneVolumeDown,
0x00100000e08: microphoneVolumeUp,
0x00100000e09: microphoneVolumeMute,
0x00100000f01: speechCorrectionList,
0x00100000f02: speechInputToggle,
0x00100001001: appSwitch,
0x00100001002: call,
0x00100001003: cameraFocus,
0x00100001004: endCall,
0x00100001005: goBack,
0x00100001006: goHome,
0x00100001007: headsetHook,
0x00100001008: lastNumberRedial,
0x00100001009: notification,
0x0010000100a: mannerMode,
0x0010000100b: voiceDial,
0x00100001101: tv3DMode,
0x00100001102: tvAntennaCable,
0x00100001103: tvAudioDescription,
0x00100001104: tvAudioDescriptionMixDown,
0x00100001105: tvAudioDescriptionMixUp,
0x00100001106: tvContentsMenu,
0x00100001107: tvDataService,
0x00100001108: tvInputComponent1,
0x00100001109: tvInputComponent2,
0x0010000110a: tvInputComposite1,
0x0010000110b: tvInputComposite2,
0x0010000110c: tvInputHDMI1,
0x0010000110d: tvInputHDMI2,
0x0010000110e: tvInputHDMI3,
0x0010000110f: tvInputHDMI4,
0x00100001110: tvInputVGA1,
0x00100001111: tvMediaContext,
0x00100001112: tvNetwork,
0x00100001113: tvNumberEntry,
0x00100001114: tvRadioService,
0x00100001115: tvSatellite,
0x00100001116: tvSatelliteBS,
0x00100001117: tvSatelliteCS,
0x00100001118: tvSatelliteToggle,
0x00100001119: tvTerrestrialAnalog,
0x0010000111a: tvTerrestrialDigital,
0x0010000111b: tvTimer,
0x00100001201: key11,
0x00100001202: key12,
0x00200000000: suspend,
0x00200000001: resume,
0x00200000002: sleep,
0x00200000003: abort,
0x00200000010: lang1,
0x00200000011: lang2,
0x00200000012: lang3,
0x00200000013: lang4,
0x00200000014: lang5,
0x00200000020: intlBackslash,
0x00200000021: intlRo,
0x00200000022: intlYen,
0x00200000100: controlLeft,
0x00200000101: controlRight,
0x00200000102: shiftLeft,
0x00200000103: shiftRight,
0x00200000104: altLeft,
0x00200000105: altRight,
0x00200000106: metaLeft,
0x00200000107: metaRight,
0x002000001f0: control,
0x002000001f2: shift,
0x002000001f4: alt,
0x002000001f6: meta,
0x0020000020d: numpadEnter,
0x00200000228: numpadParenLeft,
0x00200000229: numpadParenRight,
0x0020000022a: numpadMultiply,
0x0020000022b: numpadAdd,
0x0020000022c: numpadComma,
0x0020000022d: numpadSubtract,
0x0020000022e: numpadDecimal,
0x0020000022f: numpadDivide,
0x00200000230: numpad0,
0x00200000231: numpad1,
0x00200000232: numpad2,
0x00200000233: numpad3,
0x00200000234: numpad4,
0x00200000235: numpad5,
0x00200000236: numpad6,
0x00200000237: numpad7,
0x00200000238: numpad8,
0x00200000239: numpad9,
0x0020000023d: numpadEqual,
0x00200000301: gameButton1,
0x00200000302: gameButton2,
0x00200000303: gameButton3,
0x00200000304: gameButton4,
0x00200000305: gameButton5,
0x00200000306: gameButton6,
0x00200000307: gameButton7,
0x00200000308: gameButton8,
0x00200000309: gameButton9,
0x0020000030a: gameButton10,
0x0020000030b: gameButton11,
0x0020000030c: gameButton12,
0x0020000030d: gameButton13,
0x0020000030e: gameButton14,
0x0020000030f: gameButton15,
0x00200000310: gameButton16,
0x00200000311: gameButtonA,
0x00200000312: gameButtonB,
0x00200000313: gameButtonC,
0x00200000314: gameButtonLeft1,
0x00200000315: gameButtonLeft2,
0x00200000316: gameButtonMode,
0x00200000317: gameButtonRight1,
0x00200000318: gameButtonRight2,
0x00200000319: gameButtonSelect,
0x0020000031a: gameButtonStart,
0x0020000031b: gameButtonThumbLeft,
0x0020000031c: gameButtonThumbRight,
0x0020000031d: gameButtonX,
0x0020000031e: gameButtonY,
0x0020000031f: gameButtonZ,
};
// A map of keys to the pseudo-key synonym for that key.
static final Map<LogicalKeyboardKey, Set<LogicalKeyboardKey>> _synonyms = <LogicalKeyboardKey, Set<LogicalKeyboardKey>>{
shiftLeft: <LogicalKeyboardKey>{shift},
shiftRight: <LogicalKeyboardKey>{shift},
metaLeft: <LogicalKeyboardKey>{meta},
metaRight: <LogicalKeyboardKey>{meta},
altLeft: <LogicalKeyboardKey>{alt},
altRight: <LogicalKeyboardKey>{alt},
controlLeft: <LogicalKeyboardKey>{control},
controlRight: <LogicalKeyboardKey>{control},
};
// A map of pseudo-key to the set of keys that are synonyms for that pseudo-key.
static final Map<LogicalKeyboardKey, Set<LogicalKeyboardKey>> _reverseSynonyms = <LogicalKeyboardKey, Set<LogicalKeyboardKey>>{
shift: <LogicalKeyboardKey>{shiftLeft, shiftRight},
meta: <LogicalKeyboardKey>{metaLeft, metaRight},
alt: <LogicalKeyboardKey>{altLeft, altRight},
control: <LogicalKeyboardKey>{controlLeft, controlRight},
};
static const Map<int, String> _keyLabels = <int, String>{
0x00000000020: 'Space',
0x00000000021: 'Exclamation',
0x00000000022: 'Quote',
0x00000000023: 'Number Sign',
0x00000000024: 'Dollar',
0x00000000025: 'Percent',
0x00000000026: 'Ampersand',
0x00000000027: 'Quote Single',
0x00000000028: 'Parenthesis Left',
0x00000000029: 'Parenthesis Right',
0x0000000002a: 'Asterisk',
0x0000000002b: 'Add',
0x0000000002c: 'Comma',
0x0000000002d: 'Minus',
0x0000000002e: 'Period',
0x0000000002f: 'Slash',
0x00000000030: 'Digit 0',
0x00000000031: 'Digit 1',
0x00000000032: 'Digit 2',
0x00000000033: 'Digit 3',
0x00000000034: 'Digit 4',
0x00000000035: 'Digit 5',
0x00000000036: 'Digit 6',
0x00000000037: 'Digit 7',
0x00000000038: 'Digit 8',
0x00000000039: 'Digit 9',
0x0000000003a: 'Colon',
0x0000000003b: 'Semicolon',
0x0000000003c: 'Less',
0x0000000003d: 'Equal',
0x0000000003e: 'Greater',
0x0000000003f: 'Question',
0x00000000040: 'At',
0x0000000005b: 'Bracket Left',
0x0000000005c: 'Backslash',
0x0000000005d: 'Bracket Right',
0x0000000005e: 'Caret',
0x0000000005f: 'Underscore',
0x00000000060: 'Backquote',
0x00000000061: 'Key A',
0x00000000062: 'Key B',
0x00000000063: 'Key C',
0x00000000064: 'Key D',
0x00000000065: 'Key E',
0x00000000066: 'Key F',
0x00000000067: 'Key G',
0x00000000068: 'Key H',
0x00000000069: 'Key I',
0x0000000006a: 'Key J',
0x0000000006b: 'Key K',
0x0000000006c: 'Key L',
0x0000000006d: 'Key M',
0x0000000006e: 'Key N',
0x0000000006f: 'Key O',
0x00000000070: 'Key P',
0x00000000071: 'Key Q',
0x00000000072: 'Key R',
0x00000000073: 'Key S',
0x00000000074: 'Key T',
0x00000000075: 'Key U',
0x00000000076: 'Key V',
0x00000000077: 'Key W',
0x00000000078: 'Key X',
0x00000000079: 'Key Y',
0x0000000007a: 'Key Z',
0x0000000007b: 'Brace Left',
0x0000000007c: 'Bar',
0x0000000007d: 'Brace Right',
0x0000000007e: 'Tilde',
0x00100000001: 'Unidentified',
0x00100000008: 'Backspace',
0x00100000009: 'Tab',
0x0010000000d: 'Enter',
0x0010000001b: 'Escape',
0x0010000007f: 'Delete',
0x00100000101: 'Accel',
0x00100000103: 'Alt Graph',
0x00100000104: 'Caps Lock',
0x00100000106: 'Fn',
0x00100000107: 'Fn Lock',
0x00100000108: 'Hyper',
0x0010000010a: 'Num Lock',
0x0010000010c: 'Scroll Lock',
0x0010000010e: 'Super',
0x0010000010f: 'Symbol',
0x00100000110: 'Symbol Lock',
0x00100000111: 'Shift Level 5',
0x00100000301: 'Arrow Down',
0x00100000302: 'Arrow Left',
0x00100000303: 'Arrow Right',
0x00100000304: 'Arrow Up',
0x00100000305: 'End',
0x00100000306: 'Home',
0x00100000307: 'Page Down',
0x00100000308: 'Page Up',
0x00100000401: 'Clear',
0x00100000402: 'Copy',
0x00100000403: 'Cr Sel',
0x00100000404: 'Cut',
0x00100000405: 'Erase Eof',
0x00100000406: 'Ex Sel',
0x00100000407: 'Insert',
0x00100000408: 'Paste',
0x00100000409: 'Redo',
0x0010000040a: 'Undo',
0x00100000501: 'Accept',
0x00100000502: 'Again',
0x00100000503: 'Attn',
0x00100000504: 'Cancel',
0x00100000505: 'Context Menu',
0x00100000506: 'Execute',
0x00100000507: 'Find',
0x00100000508: 'Help',
0x00100000509: 'Pause',
0x0010000050a: 'Play',
0x0010000050b: 'Props',
0x0010000050c: 'Select',
0x0010000050d: 'Zoom In',
0x0010000050e: 'Zoom Out',
0x00100000601: 'Brightness Down',
0x00100000602: 'Brightness Up',
0x00100000603: 'Camera',
0x00100000604: 'Eject',
0x00100000605: 'Log Off',
0x00100000606: 'Power',
0x00100000607: 'Power Off',
0x00100000608: 'Print Screen',
0x00100000609: 'Hibernate',
0x0010000060a: 'Standby',
0x0010000060b: 'Wake Up',
0x00100000701: 'All Candidates',
0x00100000702: 'Alphanumeric',
0x00100000703: 'Code Input',
0x00100000704: 'Compose',
0x00100000705: 'Convert',
0x00100000706: 'Final Mode',
0x00100000707: 'Group First',
0x00100000708: 'Group Last',
0x00100000709: 'Group Next',
0x0010000070a: 'Group Previous',
0x0010000070b: 'Mode Change',
0x0010000070c: 'Next Candidate',
0x0010000070d: 'Non Convert',
0x0010000070e: 'Previous Candidate',
0x0010000070f: 'Process',
0x00100000710: 'Single Candidate',
0x00100000711: 'Hangul Mode',
0x00100000712: 'Hanja Mode',
0x00100000713: 'Junja Mode',
0x00100000714: 'Eisu',
0x00100000715: 'Hankaku',
0x00100000716: 'Hiragana',
0x00100000717: 'Hiragana Katakana',
0x00100000718: 'Kana Mode',
0x00100000719: 'Kanji Mode',
0x0010000071a: 'Katakana',
0x0010000071b: 'Romaji',
0x0010000071c: 'Zenkaku',
0x0010000071d: 'Zenkaku Hankaku',
0x00100000801: 'F1',
0x00100000802: 'F2',
0x00100000803: 'F3',
0x00100000804: 'F4',
0x00100000805: 'F5',
0x00100000806: 'F6',
0x00100000807: 'F7',
0x00100000808: 'F8',
0x00100000809: 'F9',
0x0010000080a: 'F10',
0x0010000080b: 'F11',
0x0010000080c: 'F12',
0x0010000080d: 'F13',
0x0010000080e: 'F14',
0x0010000080f: 'F15',
0x00100000810: 'F16',
0x00100000811: 'F17',
0x00100000812: 'F18',
0x00100000813: 'F19',
0x00100000814: 'F20',
0x00100000815: 'F21',
0x00100000816: 'F22',
0x00100000817: 'F23',
0x00100000818: 'F24',
0x00100000901: 'Soft 1',
0x00100000902: 'Soft 2',
0x00100000903: 'Soft 3',
0x00100000904: 'Soft 4',
0x00100000905: 'Soft 5',
0x00100000906: 'Soft 6',
0x00100000907: 'Soft 7',
0x00100000908: 'Soft 8',
0x00100000a01: 'Close',
0x00100000a02: 'Mail Forward',
0x00100000a03: 'Mail Reply',
0x00100000a04: 'Mail Send',
0x00100000a05: 'Media Play Pause',
0x00100000a07: 'Media Stop',
0x00100000a08: 'Media Track Next',
0x00100000a09: 'Media Track Previous',
0x00100000a0a: 'New',
0x00100000a0b: 'Open',
0x00100000a0c: 'Print',
0x00100000a0d: 'Save',
0x00100000a0e: 'Spell Check',
0x00100000a0f: 'Audio Volume Down',
0x00100000a10: 'Audio Volume Up',
0x00100000a11: 'Audio Volume Mute',
0x00100000b01: 'Launch Application 2',
0x00100000b02: 'Launch Calendar',
0x00100000b03: 'Launch Mail',
0x00100000b04: 'Launch Media Player',
0x00100000b05: 'Launch Music Player',
0x00100000b06: 'Launch Application 1',
0x00100000b07: 'Launch Screen Saver',
0x00100000b08: 'Launch Spreadsheet',
0x00100000b09: 'Launch Web Browser',
0x00100000b0a: 'Launch Web Cam',
0x00100000b0b: 'Launch Word Processor',
0x00100000b0c: 'Launch Contacts',
0x00100000b0d: 'Launch Phone',
0x00100000b0e: 'Launch Assistant',
0x00100000b0f: 'Launch Control Panel',
0x00100000c01: 'Browser Back',
0x00100000c02: 'Browser Favorites',
0x00100000c03: 'Browser Forward',
0x00100000c04: 'Browser Home',
0x00100000c05: 'Browser Refresh',
0x00100000c06: 'Browser Search',
0x00100000c07: 'Browser Stop',
0x00100000d01: 'Audio Balance Left',
0x00100000d02: 'Audio Balance Right',
0x00100000d03: 'Audio Bass Boost Down',
0x00100000d04: 'Audio Bass Boost Up',
0x00100000d05: 'Audio Fader Front',
0x00100000d06: 'Audio Fader Rear',
0x00100000d07: 'Audio Surround Mode Next',
0x00100000d08: 'AVR Input',
0x00100000d09: 'AVR Power',
0x00100000d0a: 'Channel Down',
0x00100000d0b: 'Channel Up',
0x00100000d0c: 'Color F0 Red',
0x00100000d0d: 'Color F1 Green',
0x00100000d0e: 'Color F2 Yellow',
0x00100000d0f: 'Color F3 Blue',
0x00100000d10: 'Color F4 Grey',
0x00100000d11: 'Color F5 Brown',
0x00100000d12: 'Closed Caption Toggle',
0x00100000d13: 'Dimmer',
0x00100000d14: 'Display Swap',
0x00100000d15: 'Exit',
0x00100000d16: 'Favorite Clear 0',
0x00100000d17: 'Favorite Clear 1',
0x00100000d18: 'Favorite Clear 2',
0x00100000d19: 'Favorite Clear 3',
0x00100000d1a: 'Favorite Recall 0',
0x00100000d1b: 'Favorite Recall 1',
0x00100000d1c: 'Favorite Recall 2',
0x00100000d1d: 'Favorite Recall 3',
0x00100000d1e: 'Favorite Store 0',
0x00100000d1f: 'Favorite Store 1',
0x00100000d20: 'Favorite Store 2',
0x00100000d21: 'Favorite Store 3',
0x00100000d22: 'Guide',
0x00100000d23: 'Guide Next Day',
0x00100000d24: 'Guide Previous Day',
0x00100000d25: 'Info',
0x00100000d26: 'Instant Replay',
0x00100000d27: 'Link',
0x00100000d28: 'List Program',
0x00100000d29: 'Live Content',
0x00100000d2a: 'Lock',
0x00100000d2b: 'Media Apps',
0x00100000d2c: 'Media Fast Forward',
0x00100000d2d: 'Media Last',
0x00100000d2e: 'Media Pause',
0x00100000d2f: 'Media Play',
0x00100000d30: 'Media Record',
0x00100000d31: 'Media Rewind',
0x00100000d32: 'Media Skip',
0x00100000d33: 'Next Favorite Channel',
0x00100000d34: 'Next User Profile',
0x00100000d35: 'On Demand',
0x00100000d36: 'P In P Down',
0x00100000d37: 'P In P Move',
0x00100000d38: 'P In P Toggle',
0x00100000d39: 'P In P Up',
0x00100000d3a: 'Play Speed Down',
0x00100000d3b: 'Play Speed Reset',
0x00100000d3c: 'Play Speed Up',
0x00100000d3d: 'Random Toggle',
0x00100000d3e: 'Rc Low Battery',
0x00100000d3f: 'Record Speed Next',
0x00100000d40: 'Rf Bypass',
0x00100000d41: 'Scan Channels Toggle',
0x00100000d42: 'Screen Mode Next',
0x00100000d43: 'Settings',
0x00100000d44: 'Split Screen Toggle',
0x00100000d45: 'STB Input',
0x00100000d46: 'STB Power',
0x00100000d47: 'Subtitle',
0x00100000d48: 'Teletext',
0x00100000d49: 'TV',
0x00100000d4a: 'TV Input',
0x00100000d4b: 'TV Power',
0x00100000d4c: 'Video Mode Next',
0x00100000d4d: 'Wink',
0x00100000d4e: 'Zoom Toggle',
0x00100000d4f: 'DVR',
0x00100000d50: 'Media Audio Track',
0x00100000d51: 'Media Skip Backward',
0x00100000d52: 'Media Skip Forward',
0x00100000d53: 'Media Step Backward',
0x00100000d54: 'Media Step Forward',
0x00100000d55: 'Media Top Menu',
0x00100000d56: 'Navigate In',
0x00100000d57: 'Navigate Next',
0x00100000d58: 'Navigate Out',
0x00100000d59: 'Navigate Previous',
0x00100000d5a: 'Pairing',
0x00100000d5b: 'Media Close',
0x00100000e02: 'Audio Bass Boost Toggle',
0x00100000e04: 'Audio Treble Down',
0x00100000e05: 'Audio Treble Up',
0x00100000e06: 'Microphone Toggle',
0x00100000e07: 'Microphone Volume Down',
0x00100000e08: 'Microphone Volume Up',
0x00100000e09: 'Microphone Volume Mute',
0x00100000f01: 'Speech Correction List',
0x00100000f02: 'Speech Input Toggle',
0x00100001001: 'App Switch',
0x00100001002: 'Call',
0x00100001003: 'Camera Focus',
0x00100001004: 'End Call',
0x00100001005: 'Go Back',
0x00100001006: 'Go Home',
0x00100001007: 'Headset Hook',
0x00100001008: 'Last Number Redial',
0x00100001009: 'Notification',
0x0010000100a: 'Manner Mode',
0x0010000100b: 'Voice Dial',
0x00100001101: 'TV 3 D Mode',
0x00100001102: 'TV Antenna Cable',
0x00100001103: 'TV Audio Description',
0x00100001104: 'TV Audio Description Mix Down',
0x00100001105: 'TV Audio Description Mix Up',
0x00100001106: 'TV Contents Menu',
0x00100001107: 'TV Data Service',
0x00100001108: 'TV Input Component 1',
0x00100001109: 'TV Input Component 2',
0x0010000110a: 'TV Input Composite 1',
0x0010000110b: 'TV Input Composite 2',
0x0010000110c: 'TV Input HDMI 1',
0x0010000110d: 'TV Input HDMI 2',
0x0010000110e: 'TV Input HDMI 3',
0x0010000110f: 'TV Input HDMI 4',
0x00100001110: 'TV Input VGA 1',
0x00100001111: 'TV Media Context',
0x00100001112: 'TV Network',
0x00100001113: 'TV Number Entry',
0x00100001114: 'TV Radio Service',
0x00100001115: 'TV Satellite',
0x00100001116: 'TV Satellite BS',
0x00100001117: 'TV Satellite CS',
0x00100001118: 'TV Satellite Toggle',
0x00100001119: 'TV Terrestrial Analog',
0x0010000111a: 'TV Terrestrial Digital',
0x0010000111b: 'TV Timer',
0x00100001201: 'Key 11',
0x00100001202: 'Key 12',
0x00200000000: 'Suspend',
0x00200000001: 'Resume',
0x00200000002: 'Sleep',
0x00200000003: 'Abort',
0x00200000010: 'Lang 1',
0x00200000011: 'Lang 2',
0x00200000012: 'Lang 3',
0x00200000013: 'Lang 4',
0x00200000014: 'Lang 5',
0x00200000020: 'Intl Backslash',
0x00200000021: 'Intl Ro',
0x00200000022: 'Intl Yen',
0x00200000100: 'Control Left',
0x00200000101: 'Control Right',
0x00200000102: 'Shift Left',
0x00200000103: 'Shift Right',
0x00200000104: 'Alt Left',
0x00200000105: 'Alt Right',
0x00200000106: 'Meta Left',
0x00200000107: 'Meta Right',
0x002000001f0: 'Control',
0x002000001f2: 'Shift',
0x002000001f4: 'Alt',
0x002000001f6: 'Meta',
0x0020000020d: 'Numpad Enter',
0x00200000228: 'Numpad Paren Left',
0x00200000229: 'Numpad Paren Right',
0x0020000022a: 'Numpad Multiply',
0x0020000022b: 'Numpad Add',
0x0020000022c: 'Numpad Comma',
0x0020000022d: 'Numpad Subtract',
0x0020000022e: 'Numpad Decimal',
0x0020000022f: 'Numpad Divide',
0x00200000230: 'Numpad 0',
0x00200000231: 'Numpad 1',
0x00200000232: 'Numpad 2',
0x00200000233: 'Numpad 3',
0x00200000234: 'Numpad 4',
0x00200000235: 'Numpad 5',
0x00200000236: 'Numpad 6',
0x00200000237: 'Numpad 7',
0x00200000238: 'Numpad 8',
0x00200000239: 'Numpad 9',
0x0020000023d: 'Numpad Equal',
0x00200000301: 'Game Button 1',
0x00200000302: 'Game Button 2',
0x00200000303: 'Game Button 3',
0x00200000304: 'Game Button 4',
0x00200000305: 'Game Button 5',
0x00200000306: 'Game Button 6',
0x00200000307: 'Game Button 7',
0x00200000308: 'Game Button 8',
0x00200000309: 'Game Button 9',
0x0020000030a: 'Game Button 10',
0x0020000030b: 'Game Button 11',
0x0020000030c: 'Game Button 12',
0x0020000030d: 'Game Button 13',
0x0020000030e: 'Game Button 14',
0x0020000030f: 'Game Button 15',
0x00200000310: 'Game Button 16',
0x00200000311: 'Game Button A',
0x00200000312: 'Game Button B',
0x00200000313: 'Game Button C',
0x00200000314: 'Game Button Left 1',
0x00200000315: 'Game Button Left 2',
0x00200000316: 'Game Button Mode',
0x00200000317: 'Game Button Right 1',
0x00200000318: 'Game Button Right 2',
0x00200000319: 'Game Button Select',
0x0020000031a: 'Game Button Start',
0x0020000031b: 'Game Button Thumb Left',
0x0020000031c: 'Game Button Thumb Right',
0x0020000031d: 'Game Button X',
0x0020000031e: 'Game Button Y',
0x0020000031f: 'Game Button Z',
};
}
/// A class with static values that describe the keys that are returned from
/// [RawKeyEvent.physicalKey].
///
/// These represent *physical* keys, which are keys which represent a particular
/// key location on a QWERTY keyboard. It ignores any modifiers, modes, or
/// keyboard layouts which may be in effect. This is contrast to
/// [LogicalKeyboardKey], which represents a logical key interpreted in the
/// context of modifiers, modes, and/or keyboard layouts.
///
/// As an example, if you wanted a game where the key next to the CAPS LOCK (the
/// "A" key on a QWERTY keyboard) moved the player to the left, you'd want to
/// look at the physical key to make sure that regardless of the character the
/// key produces, you got the key that is in that location on the keyboard.
///
/// Conversely, if you wanted to implement an app where the "Q" key "quit"
/// something, you'd want to look at the logical key to detect this, since you
/// would like to have it match the key with "Q" on it, instead of always
/// looking for "the key next to the TAB key", since on a French keyboard,
/// the key next to the TAB key has an "A" on it.
///
/// {@tool dartpad}
/// This example shows how to detect if the user has selected the physical key
/// to the right of the CAPS LOCK key.
///
/// ** See code in examples/api/lib/services/keyboard_key/physical_keyboard_key.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RawKeyEvent], the keyboard event object received by widgets that listen
/// to keyboard events.
/// * [Focus.onKey], the handler on a widget that lets you handle key events.
/// * [RawKeyboardListener], a widget used to listen to keyboard events (but
/// not handle them).
@immutable
class PhysicalKeyboardKey extends KeyboardKey {
/// Creates a new PhysicalKeyboardKey object for a USB HID usage.
const PhysicalKeyboardKey(this.usbHidUsage);
/// The unique USB HID usage ID of this physical key on the keyboard.
///
/// Due to the variations in platform APIs, this may not be the actual HID
/// usage code from the hardware, but a value derived from available
/// information on the platform.
///
/// See <https://www.usb.org/sites/default/files/documents/hut1_12v2.pdf>
/// for the HID usage values and their meanings.
final int usbHidUsage;
/// The debug string to print for this keyboard key, which will be null in
/// release mode.
String? get debugName {
String? result;
assert(() {
result = _debugNames[usbHidUsage] ??
'Key with ID 0x${usbHidUsage.toRadixString(16).padLeft(8, '0')}';
return true;
}());
return result;
}
@override
int get hashCode => usbHidUsage.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is PhysicalKeyboardKey
&& other.usbHidUsage == usbHidUsage;
}
/// Finds a known [PhysicalKeyboardKey] that matches the given USB HID usage
/// code.
static PhysicalKeyboardKey? findKeyByCode(int usageCode) => _knownPhysicalKeys[usageCode];
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(StringProperty('usbHidUsage', '0x${usbHidUsage.toRadixString(16).padLeft(8, '0')}'));
properties.add(StringProperty('debugName', debugName, defaultValue: null));
}
// Key constants for all keyboard keys in the USB HID specification at the
// time Flutter was built.
/// Represents the location of the "Hyper" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey hyper = PhysicalKeyboardKey(0x00000010);
/// Represents the location of the "Super Key" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey superKey = PhysicalKeyboardKey(0x00000011);
/// Represents the location of the "Fn" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey fn = PhysicalKeyboardKey(0x00000012);
/// Represents the location of the "Fn Lock" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey fnLock = PhysicalKeyboardKey(0x00000013);
/// Represents the location of the "Suspend" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey suspend = PhysicalKeyboardKey(0x00000014);
/// Represents the location of the "Resume" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey resume = PhysicalKeyboardKey(0x00000015);
/// Represents the location of the "Turbo" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey turbo = PhysicalKeyboardKey(0x00000016);
/// Represents the location of the "Privacy Screen Toggle" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey privacyScreenToggle = PhysicalKeyboardKey(0x00000017);
/// Represents the location of the "Microphone Mute Toggle" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey microphoneMuteToggle = PhysicalKeyboardKey(0x00000018);
/// Represents the location of the "Sleep" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey sleep = PhysicalKeyboardKey(0x00010082);
/// Represents the location of the "Wake Up" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey wakeUp = PhysicalKeyboardKey(0x00010083);
/// Represents the location of the "Display Toggle Int Ext" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey displayToggleIntExt = PhysicalKeyboardKey(0x000100b5);
/// Represents the location of the "Game Button 1" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton1 = PhysicalKeyboardKey(0x0005ff01);
/// Represents the location of the "Game Button 2" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton2 = PhysicalKeyboardKey(0x0005ff02);
/// Represents the location of the "Game Button 3" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton3 = PhysicalKeyboardKey(0x0005ff03);
/// Represents the location of the "Game Button 4" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton4 = PhysicalKeyboardKey(0x0005ff04);
/// Represents the location of the "Game Button 5" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton5 = PhysicalKeyboardKey(0x0005ff05);
/// Represents the location of the "Game Button 6" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton6 = PhysicalKeyboardKey(0x0005ff06);
/// Represents the location of the "Game Button 7" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton7 = PhysicalKeyboardKey(0x0005ff07);
/// Represents the location of the "Game Button 8" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton8 = PhysicalKeyboardKey(0x0005ff08);
/// Represents the location of the "Game Button 9" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton9 = PhysicalKeyboardKey(0x0005ff09);
/// Represents the location of the "Game Button 10" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton10 = PhysicalKeyboardKey(0x0005ff0a);
/// Represents the location of the "Game Button 11" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton11 = PhysicalKeyboardKey(0x0005ff0b);
/// Represents the location of the "Game Button 12" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton12 = PhysicalKeyboardKey(0x0005ff0c);
/// Represents the location of the "Game Button 13" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton13 = PhysicalKeyboardKey(0x0005ff0d);
/// Represents the location of the "Game Button 14" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton14 = PhysicalKeyboardKey(0x0005ff0e);
/// Represents the location of the "Game Button 15" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton15 = PhysicalKeyboardKey(0x0005ff0f);
/// Represents the location of the "Game Button 16" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButton16 = PhysicalKeyboardKey(0x0005ff10);
/// Represents the location of the "Game Button A" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonA = PhysicalKeyboardKey(0x0005ff11);
/// Represents the location of the "Game Button B" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonB = PhysicalKeyboardKey(0x0005ff12);
/// Represents the location of the "Game Button C" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonC = PhysicalKeyboardKey(0x0005ff13);
/// Represents the location of the "Game Button Left 1" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonLeft1 = PhysicalKeyboardKey(0x0005ff14);
/// Represents the location of the "Game Button Left 2" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonLeft2 = PhysicalKeyboardKey(0x0005ff15);
/// Represents the location of the "Game Button Mode" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonMode = PhysicalKeyboardKey(0x0005ff16);
/// Represents the location of the "Game Button Right 1" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonRight1 = PhysicalKeyboardKey(0x0005ff17);
/// Represents the location of the "Game Button Right 2" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonRight2 = PhysicalKeyboardKey(0x0005ff18);
/// Represents the location of the "Game Button Select" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonSelect = PhysicalKeyboardKey(0x0005ff19);
/// Represents the location of the "Game Button Start" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonStart = PhysicalKeyboardKey(0x0005ff1a);
/// Represents the location of the "Game Button Thumb Left" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonThumbLeft = PhysicalKeyboardKey(0x0005ff1b);
/// Represents the location of the "Game Button Thumb Right" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonThumbRight = PhysicalKeyboardKey(0x0005ff1c);
/// Represents the location of the "Game Button X" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonX = PhysicalKeyboardKey(0x0005ff1d);
/// Represents the location of the "Game Button Y" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonY = PhysicalKeyboardKey(0x0005ff1e);
/// Represents the location of the "Game Button Z" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey gameButtonZ = PhysicalKeyboardKey(0x0005ff1f);
/// Represents the location of the "Usb Reserved" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey usbReserved = PhysicalKeyboardKey(0x00070000);
/// Represents the location of the "Usb Error Roll Over" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey usbErrorRollOver = PhysicalKeyboardKey(0x00070001);
/// Represents the location of the "Usb Post Fail" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey usbPostFail = PhysicalKeyboardKey(0x00070002);
/// Represents the location of the "Usb Error Undefined" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey usbErrorUndefined = PhysicalKeyboardKey(0x00070003);
/// Represents the location of the "Key A" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyA = PhysicalKeyboardKey(0x00070004);
/// Represents the location of the "Key B" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyB = PhysicalKeyboardKey(0x00070005);
/// Represents the location of the "Key C" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyC = PhysicalKeyboardKey(0x00070006);
/// Represents the location of the "Key D" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyD = PhysicalKeyboardKey(0x00070007);
/// Represents the location of the "Key E" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyE = PhysicalKeyboardKey(0x00070008);
/// Represents the location of the "Key F" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyF = PhysicalKeyboardKey(0x00070009);
/// Represents the location of the "Key G" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyG = PhysicalKeyboardKey(0x0007000a);
/// Represents the location of the "Key H" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyH = PhysicalKeyboardKey(0x0007000b);
/// Represents the location of the "Key I" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyI = PhysicalKeyboardKey(0x0007000c);
/// Represents the location of the "Key J" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyJ = PhysicalKeyboardKey(0x0007000d);
/// Represents the location of the "Key K" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyK = PhysicalKeyboardKey(0x0007000e);
/// Represents the location of the "Key L" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyL = PhysicalKeyboardKey(0x0007000f);
/// Represents the location of the "Key M" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyM = PhysicalKeyboardKey(0x00070010);
/// Represents the location of the "Key N" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyN = PhysicalKeyboardKey(0x00070011);
/// Represents the location of the "Key O" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyO = PhysicalKeyboardKey(0x00070012);
/// Represents the location of the "Key P" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyP = PhysicalKeyboardKey(0x00070013);
/// Represents the location of the "Key Q" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyQ = PhysicalKeyboardKey(0x00070014);
/// Represents the location of the "Key R" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyR = PhysicalKeyboardKey(0x00070015);
/// Represents the location of the "Key S" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyS = PhysicalKeyboardKey(0x00070016);
/// Represents the location of the "Key T" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyT = PhysicalKeyboardKey(0x00070017);
/// Represents the location of the "Key U" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyU = PhysicalKeyboardKey(0x00070018);
/// Represents the location of the "Key V" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyV = PhysicalKeyboardKey(0x00070019);
/// Represents the location of the "Key W" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyW = PhysicalKeyboardKey(0x0007001a);
/// Represents the location of the "Key X" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyX = PhysicalKeyboardKey(0x0007001b);
/// Represents the location of the "Key Y" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyY = PhysicalKeyboardKey(0x0007001c);
/// Represents the location of the "Key Z" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyZ = PhysicalKeyboardKey(0x0007001d);
/// Represents the location of the "Digit 1" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit1 = PhysicalKeyboardKey(0x0007001e);
/// Represents the location of the "Digit 2" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit2 = PhysicalKeyboardKey(0x0007001f);
/// Represents the location of the "Digit 3" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit3 = PhysicalKeyboardKey(0x00070020);
/// Represents the location of the "Digit 4" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit4 = PhysicalKeyboardKey(0x00070021);
/// Represents the location of the "Digit 5" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit5 = PhysicalKeyboardKey(0x00070022);
/// Represents the location of the "Digit 6" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit6 = PhysicalKeyboardKey(0x00070023);
/// Represents the location of the "Digit 7" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit7 = PhysicalKeyboardKey(0x00070024);
/// Represents the location of the "Digit 8" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit8 = PhysicalKeyboardKey(0x00070025);
/// Represents the location of the "Digit 9" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit9 = PhysicalKeyboardKey(0x00070026);
/// Represents the location of the "Digit 0" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey digit0 = PhysicalKeyboardKey(0x00070027);
/// Represents the location of the "Enter" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey enter = PhysicalKeyboardKey(0x00070028);
/// Represents the location of the "Escape" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey escape = PhysicalKeyboardKey(0x00070029);
/// Represents the location of the "Backspace" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey backspace = PhysicalKeyboardKey(0x0007002a);
/// Represents the location of the "Tab" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey tab = PhysicalKeyboardKey(0x0007002b);
/// Represents the location of the "Space" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey space = PhysicalKeyboardKey(0x0007002c);
/// Represents the location of the "Minus" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey minus = PhysicalKeyboardKey(0x0007002d);
/// Represents the location of the "Equal" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey equal = PhysicalKeyboardKey(0x0007002e);
/// Represents the location of the "Bracket Left" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey bracketLeft = PhysicalKeyboardKey(0x0007002f);
/// Represents the location of the "Bracket Right" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey bracketRight = PhysicalKeyboardKey(0x00070030);
/// Represents the location of the "Backslash" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey backslash = PhysicalKeyboardKey(0x00070031);
/// Represents the location of the "Semicolon" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey semicolon = PhysicalKeyboardKey(0x00070033);
/// Represents the location of the "Quote" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey quote = PhysicalKeyboardKey(0x00070034);
/// Represents the location of the "Backquote" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey backquote = PhysicalKeyboardKey(0x00070035);
/// Represents the location of the "Comma" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey comma = PhysicalKeyboardKey(0x00070036);
/// Represents the location of the "Period" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey period = PhysicalKeyboardKey(0x00070037);
/// Represents the location of the "Slash" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey slash = PhysicalKeyboardKey(0x00070038);
/// Represents the location of the "Caps Lock" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey capsLock = PhysicalKeyboardKey(0x00070039);
/// Represents the location of the "F1" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f1 = PhysicalKeyboardKey(0x0007003a);
/// Represents the location of the "F2" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f2 = PhysicalKeyboardKey(0x0007003b);
/// Represents the location of the "F3" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f3 = PhysicalKeyboardKey(0x0007003c);
/// Represents the location of the "F4" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f4 = PhysicalKeyboardKey(0x0007003d);
/// Represents the location of the "F5" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f5 = PhysicalKeyboardKey(0x0007003e);
/// Represents the location of the "F6" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f6 = PhysicalKeyboardKey(0x0007003f);
/// Represents the location of the "F7" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f7 = PhysicalKeyboardKey(0x00070040);
/// Represents the location of the "F8" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f8 = PhysicalKeyboardKey(0x00070041);
/// Represents the location of the "F9" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f9 = PhysicalKeyboardKey(0x00070042);
/// Represents the location of the "F10" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f10 = PhysicalKeyboardKey(0x00070043);
/// Represents the location of the "F11" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f11 = PhysicalKeyboardKey(0x00070044);
/// Represents the location of the "F12" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f12 = PhysicalKeyboardKey(0x00070045);
/// Represents the location of the "Print Screen" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey printScreen = PhysicalKeyboardKey(0x00070046);
/// Represents the location of the "Scroll Lock" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey scrollLock = PhysicalKeyboardKey(0x00070047);
/// Represents the location of the "Pause" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey pause = PhysicalKeyboardKey(0x00070048);
/// Represents the location of the "Insert" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey insert = PhysicalKeyboardKey(0x00070049);
/// Represents the location of the "Home" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey home = PhysicalKeyboardKey(0x0007004a);
/// Represents the location of the "Page Up" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey pageUp = PhysicalKeyboardKey(0x0007004b);
/// Represents the location of the "Delete" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey delete = PhysicalKeyboardKey(0x0007004c);
/// Represents the location of the "End" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey end = PhysicalKeyboardKey(0x0007004d);
/// Represents the location of the "Page Down" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey pageDown = PhysicalKeyboardKey(0x0007004e);
/// Represents the location of the "Arrow Right" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey arrowRight = PhysicalKeyboardKey(0x0007004f);
/// Represents the location of the "Arrow Left" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey arrowLeft = PhysicalKeyboardKey(0x00070050);
/// Represents the location of the "Arrow Down" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey arrowDown = PhysicalKeyboardKey(0x00070051);
/// Represents the location of the "Arrow Up" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey arrowUp = PhysicalKeyboardKey(0x00070052);
/// Represents the location of the "Num Lock" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numLock = PhysicalKeyboardKey(0x00070053);
/// Represents the location of the "Numpad Divide" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadDivide = PhysicalKeyboardKey(0x00070054);
/// Represents the location of the "Numpad Multiply" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMultiply = PhysicalKeyboardKey(0x00070055);
/// Represents the location of the "Numpad Subtract" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadSubtract = PhysicalKeyboardKey(0x00070056);
/// Represents the location of the "Numpad Add" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadAdd = PhysicalKeyboardKey(0x00070057);
/// Represents the location of the "Numpad Enter" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadEnter = PhysicalKeyboardKey(0x00070058);
/// Represents the location of the "Numpad 1" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad1 = PhysicalKeyboardKey(0x00070059);
/// Represents the location of the "Numpad 2" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad2 = PhysicalKeyboardKey(0x0007005a);
/// Represents the location of the "Numpad 3" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad3 = PhysicalKeyboardKey(0x0007005b);
/// Represents the location of the "Numpad 4" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad4 = PhysicalKeyboardKey(0x0007005c);
/// Represents the location of the "Numpad 5" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad5 = PhysicalKeyboardKey(0x0007005d);
/// Represents the location of the "Numpad 6" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad6 = PhysicalKeyboardKey(0x0007005e);
/// Represents the location of the "Numpad 7" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad7 = PhysicalKeyboardKey(0x0007005f);
/// Represents the location of the "Numpad 8" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad8 = PhysicalKeyboardKey(0x00070060);
/// Represents the location of the "Numpad 9" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad9 = PhysicalKeyboardKey(0x00070061);
/// Represents the location of the "Numpad 0" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpad0 = PhysicalKeyboardKey(0x00070062);
/// Represents the location of the "Numpad Decimal" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadDecimal = PhysicalKeyboardKey(0x00070063);
/// Represents the location of the "Intl Backslash" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey intlBackslash = PhysicalKeyboardKey(0x00070064);
/// Represents the location of the "Context Menu" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey contextMenu = PhysicalKeyboardKey(0x00070065);
/// Represents the location of the "Power" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey power = PhysicalKeyboardKey(0x00070066);
/// Represents the location of the "Numpad Equal" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadEqual = PhysicalKeyboardKey(0x00070067);
/// Represents the location of the "F13" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f13 = PhysicalKeyboardKey(0x00070068);
/// Represents the location of the "F14" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f14 = PhysicalKeyboardKey(0x00070069);
/// Represents the location of the "F15" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f15 = PhysicalKeyboardKey(0x0007006a);
/// Represents the location of the "F16" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f16 = PhysicalKeyboardKey(0x0007006b);
/// Represents the location of the "F17" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f17 = PhysicalKeyboardKey(0x0007006c);
/// Represents the location of the "F18" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f18 = PhysicalKeyboardKey(0x0007006d);
/// Represents the location of the "F19" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f19 = PhysicalKeyboardKey(0x0007006e);
/// Represents the location of the "F20" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f20 = PhysicalKeyboardKey(0x0007006f);
/// Represents the location of the "F21" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f21 = PhysicalKeyboardKey(0x00070070);
/// Represents the location of the "F22" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f22 = PhysicalKeyboardKey(0x00070071);
/// Represents the location of the "F23" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f23 = PhysicalKeyboardKey(0x00070072);
/// Represents the location of the "F24" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey f24 = PhysicalKeyboardKey(0x00070073);
/// Represents the location of the "Open" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey open = PhysicalKeyboardKey(0x00070074);
/// Represents the location of the "Help" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey help = PhysicalKeyboardKey(0x00070075);
/// Represents the location of the "Select" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey select = PhysicalKeyboardKey(0x00070077);
/// Represents the location of the "Again" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey again = PhysicalKeyboardKey(0x00070079);
/// Represents the location of the "Undo" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey undo = PhysicalKeyboardKey(0x0007007a);
/// Represents the location of the "Cut" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey cut = PhysicalKeyboardKey(0x0007007b);
/// Represents the location of the "Copy" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey copy = PhysicalKeyboardKey(0x0007007c);
/// Represents the location of the "Paste" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey paste = PhysicalKeyboardKey(0x0007007d);
/// Represents the location of the "Find" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey find = PhysicalKeyboardKey(0x0007007e);
/// Represents the location of the "Audio Volume Mute" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey audioVolumeMute = PhysicalKeyboardKey(0x0007007f);
/// Represents the location of the "Audio Volume Up" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey audioVolumeUp = PhysicalKeyboardKey(0x00070080);
/// Represents the location of the "Audio Volume Down" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey audioVolumeDown = PhysicalKeyboardKey(0x00070081);
/// Represents the location of the "Numpad Comma" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadComma = PhysicalKeyboardKey(0x00070085);
/// Represents the location of the "Intl Ro" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey intlRo = PhysicalKeyboardKey(0x00070087);
/// Represents the location of the "Kana Mode" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey kanaMode = PhysicalKeyboardKey(0x00070088);
/// Represents the location of the "Intl Yen" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey intlYen = PhysicalKeyboardKey(0x00070089);
/// Represents the location of the "Convert" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey convert = PhysicalKeyboardKey(0x0007008a);
/// Represents the location of the "Non Convert" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey nonConvert = PhysicalKeyboardKey(0x0007008b);
/// Represents the location of the "Lang 1" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lang1 = PhysicalKeyboardKey(0x00070090);
/// Represents the location of the "Lang 2" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lang2 = PhysicalKeyboardKey(0x00070091);
/// Represents the location of the "Lang 3" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lang3 = PhysicalKeyboardKey(0x00070092);
/// Represents the location of the "Lang 4" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lang4 = PhysicalKeyboardKey(0x00070093);
/// Represents the location of the "Lang 5" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lang5 = PhysicalKeyboardKey(0x00070094);
/// Represents the location of the "Abort" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey abort = PhysicalKeyboardKey(0x0007009b);
/// Represents the location of the "Props" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey props = PhysicalKeyboardKey(0x000700a3);
/// Represents the location of the "Numpad Paren Left" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadParenLeft = PhysicalKeyboardKey(0x000700b6);
/// Represents the location of the "Numpad Paren Right" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadParenRight = PhysicalKeyboardKey(0x000700b7);
/// Represents the location of the "Numpad Backspace" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadBackspace = PhysicalKeyboardKey(0x000700bb);
/// Represents the location of the "Numpad Memory Store" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMemoryStore = PhysicalKeyboardKey(0x000700d0);
/// Represents the location of the "Numpad Memory Recall" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMemoryRecall = PhysicalKeyboardKey(0x000700d1);
/// Represents the location of the "Numpad Memory Clear" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMemoryClear = PhysicalKeyboardKey(0x000700d2);
/// Represents the location of the "Numpad Memory Add" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMemoryAdd = PhysicalKeyboardKey(0x000700d3);
/// Represents the location of the "Numpad Memory Subtract" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadMemorySubtract = PhysicalKeyboardKey(0x000700d4);
/// Represents the location of the "Numpad Sign Change" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadSignChange = PhysicalKeyboardKey(0x000700d7);
/// Represents the location of the "Numpad Clear" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadClear = PhysicalKeyboardKey(0x000700d8);
/// Represents the location of the "Numpad Clear Entry" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey numpadClearEntry = PhysicalKeyboardKey(0x000700d9);
/// Represents the location of the "Control Left" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey controlLeft = PhysicalKeyboardKey(0x000700e0);
/// Represents the location of the "Shift Left" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey shiftLeft = PhysicalKeyboardKey(0x000700e1);
/// Represents the location of the "Alt Left" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey altLeft = PhysicalKeyboardKey(0x000700e2);
/// Represents the location of the "Meta Left" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey metaLeft = PhysicalKeyboardKey(0x000700e3);
/// Represents the location of the "Control Right" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey controlRight = PhysicalKeyboardKey(0x000700e4);
/// Represents the location of the "Shift Right" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey shiftRight = PhysicalKeyboardKey(0x000700e5);
/// Represents the location of the "Alt Right" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey altRight = PhysicalKeyboardKey(0x000700e6);
/// Represents the location of the "Meta Right" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey metaRight = PhysicalKeyboardKey(0x000700e7);
/// Represents the location of the "Info" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey info = PhysicalKeyboardKey(0x000c0060);
/// Represents the location of the "Closed Caption Toggle" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey closedCaptionToggle = PhysicalKeyboardKey(0x000c0061);
/// Represents the location of the "Brightness Up" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessUp = PhysicalKeyboardKey(0x000c006f);
/// Represents the location of the "Brightness Down" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessDown = PhysicalKeyboardKey(0x000c0070);
/// Represents the location of the "Brightness Toggle" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessToggle = PhysicalKeyboardKey(0x000c0072);
/// Represents the location of the "Brightness Minimum" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessMinimum = PhysicalKeyboardKey(0x000c0073);
/// Represents the location of the "Brightness Maximum" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessMaximum = PhysicalKeyboardKey(0x000c0074);
/// Represents the location of the "Brightness Auto" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey brightnessAuto = PhysicalKeyboardKey(0x000c0075);
/// Represents the location of the "Kbd Illum Up" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey kbdIllumUp = PhysicalKeyboardKey(0x000c0079);
/// Represents the location of the "Kbd Illum Down" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey kbdIllumDown = PhysicalKeyboardKey(0x000c007a);
/// Represents the location of the "Media Last" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaLast = PhysicalKeyboardKey(0x000c0083);
/// Represents the location of the "Launch Phone" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchPhone = PhysicalKeyboardKey(0x000c008c);
/// Represents the location of the "Program Guide" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey programGuide = PhysicalKeyboardKey(0x000c008d);
/// Represents the location of the "Exit" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey exit = PhysicalKeyboardKey(0x000c0094);
/// Represents the location of the "Channel Up" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey channelUp = PhysicalKeyboardKey(0x000c009c);
/// Represents the location of the "Channel Down" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey channelDown = PhysicalKeyboardKey(0x000c009d);
/// Represents the location of the "Media Play" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaPlay = PhysicalKeyboardKey(0x000c00b0);
/// Represents the location of the "Media Pause" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaPause = PhysicalKeyboardKey(0x000c00b1);
/// Represents the location of the "Media Record" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaRecord = PhysicalKeyboardKey(0x000c00b2);
/// Represents the location of the "Media Fast Forward" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaFastForward = PhysicalKeyboardKey(0x000c00b3);
/// Represents the location of the "Media Rewind" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaRewind = PhysicalKeyboardKey(0x000c00b4);
/// Represents the location of the "Media Track Next" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaTrackNext = PhysicalKeyboardKey(0x000c00b5);
/// Represents the location of the "Media Track Previous" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaTrackPrevious = PhysicalKeyboardKey(0x000c00b6);
/// Represents the location of the "Media Stop" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaStop = PhysicalKeyboardKey(0x000c00b7);
/// Represents the location of the "Eject" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey eject = PhysicalKeyboardKey(0x000c00b8);
/// Represents the location of the "Media Play Pause" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaPlayPause = PhysicalKeyboardKey(0x000c00cd);
/// Represents the location of the "Speech Input Toggle" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey speechInputToggle = PhysicalKeyboardKey(0x000c00cf);
/// Represents the location of the "Bass Boost" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey bassBoost = PhysicalKeyboardKey(0x000c00e5);
/// Represents the location of the "Media Select" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mediaSelect = PhysicalKeyboardKey(0x000c0183);
/// Represents the location of the "Launch Word Processor" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchWordProcessor = PhysicalKeyboardKey(0x000c0184);
/// Represents the location of the "Launch Spreadsheet" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchSpreadsheet = PhysicalKeyboardKey(0x000c0186);
/// Represents the location of the "Launch Mail" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchMail = PhysicalKeyboardKey(0x000c018a);
/// Represents the location of the "Launch Contacts" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchContacts = PhysicalKeyboardKey(0x000c018d);
/// Represents the location of the "Launch Calendar" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchCalendar = PhysicalKeyboardKey(0x000c018e);
/// Represents the location of the "Launch App2" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchApp2 = PhysicalKeyboardKey(0x000c0192);
/// Represents the location of the "Launch App1" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchApp1 = PhysicalKeyboardKey(0x000c0194);
/// Represents the location of the "Launch Internet Browser" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchInternetBrowser = PhysicalKeyboardKey(0x000c0196);
/// Represents the location of the "Log Off" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey logOff = PhysicalKeyboardKey(0x000c019c);
/// Represents the location of the "Lock Screen" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey lockScreen = PhysicalKeyboardKey(0x000c019e);
/// Represents the location of the "Launch Control Panel" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchControlPanel = PhysicalKeyboardKey(0x000c019f);
/// Represents the location of the "Select Task" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey selectTask = PhysicalKeyboardKey(0x000c01a2);
/// Represents the location of the "Launch Documents" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchDocuments = PhysicalKeyboardKey(0x000c01a7);
/// Represents the location of the "Spell Check" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey spellCheck = PhysicalKeyboardKey(0x000c01ab);
/// Represents the location of the "Launch Keyboard Layout" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchKeyboardLayout = PhysicalKeyboardKey(0x000c01ae);
/// Represents the location of the "Launch Screen Saver" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchScreenSaver = PhysicalKeyboardKey(0x000c01b1);
/// Represents the location of the "Launch Audio Browser" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchAudioBrowser = PhysicalKeyboardKey(0x000c01b7);
/// Represents the location of the "Launch Assistant" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey launchAssistant = PhysicalKeyboardKey(0x000c01cb);
/// Represents the location of the "New Key" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey newKey = PhysicalKeyboardKey(0x000c0201);
/// Represents the location of the "Close" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey close = PhysicalKeyboardKey(0x000c0203);
/// Represents the location of the "Save" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey save = PhysicalKeyboardKey(0x000c0207);
/// Represents the location of the "Print" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey print = PhysicalKeyboardKey(0x000c0208);
/// Represents the location of the "Browser Search" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserSearch = PhysicalKeyboardKey(0x000c0221);
/// Represents the location of the "Browser Home" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserHome = PhysicalKeyboardKey(0x000c0223);
/// Represents the location of the "Browser Back" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserBack = PhysicalKeyboardKey(0x000c0224);
/// Represents the location of the "Browser Forward" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserForward = PhysicalKeyboardKey(0x000c0225);
/// Represents the location of the "Browser Stop" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserStop = PhysicalKeyboardKey(0x000c0226);
/// Represents the location of the "Browser Refresh" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserRefresh = PhysicalKeyboardKey(0x000c0227);
/// Represents the location of the "Browser Favorites" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey browserFavorites = PhysicalKeyboardKey(0x000c022a);
/// Represents the location of the "Zoom In" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey zoomIn = PhysicalKeyboardKey(0x000c022d);
/// Represents the location of the "Zoom Out" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey zoomOut = PhysicalKeyboardKey(0x000c022e);
/// Represents the location of the "Zoom Toggle" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey zoomToggle = PhysicalKeyboardKey(0x000c0232);
/// Represents the location of the "Redo" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey redo = PhysicalKeyboardKey(0x000c0279);
/// Represents the location of the "Mail Reply" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mailReply = PhysicalKeyboardKey(0x000c0289);
/// Represents the location of the "Mail Forward" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mailForward = PhysicalKeyboardKey(0x000c028b);
/// Represents the location of the "Mail Send" key on a generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey mailSend = PhysicalKeyboardKey(0x000c028c);
/// Represents the location of the "Keyboard Layout Select" key on a
/// generalized keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey keyboardLayoutSelect = PhysicalKeyboardKey(0x000c029d);
/// Represents the location of the "Show All Windows" key on a generalized
/// keyboard.
///
/// See the function [RawKeyEvent.physicalKey] for more information.
static const PhysicalKeyboardKey showAllWindows = PhysicalKeyboardKey(0x000c029f);
/// A list of all predefined constant [PhysicalKeyboardKey]s.
static Iterable<PhysicalKeyboardKey> get knownPhysicalKeys => _knownPhysicalKeys.values;
// A list of all the predefined constant PhysicalKeyboardKeys so that they
// can be searched.
static const Map<int, PhysicalKeyboardKey> _knownPhysicalKeys = <int, PhysicalKeyboardKey>{
0x00000010: hyper,
0x00000011: superKey,
0x00000012: fn,
0x00000013: fnLock,
0x00000014: suspend,
0x00000015: resume,
0x00000016: turbo,
0x00000017: privacyScreenToggle,
0x00000018: microphoneMuteToggle,
0x00010082: sleep,
0x00010083: wakeUp,
0x000100b5: displayToggleIntExt,
0x0005ff01: gameButton1,
0x0005ff02: gameButton2,
0x0005ff03: gameButton3,
0x0005ff04: gameButton4,
0x0005ff05: gameButton5,
0x0005ff06: gameButton6,
0x0005ff07: gameButton7,
0x0005ff08: gameButton8,
0x0005ff09: gameButton9,
0x0005ff0a: gameButton10,
0x0005ff0b: gameButton11,
0x0005ff0c: gameButton12,
0x0005ff0d: gameButton13,
0x0005ff0e: gameButton14,
0x0005ff0f: gameButton15,
0x0005ff10: gameButton16,
0x0005ff11: gameButtonA,
0x0005ff12: gameButtonB,
0x0005ff13: gameButtonC,
0x0005ff14: gameButtonLeft1,
0x0005ff15: gameButtonLeft2,
0x0005ff16: gameButtonMode,
0x0005ff17: gameButtonRight1,
0x0005ff18: gameButtonRight2,
0x0005ff19: gameButtonSelect,
0x0005ff1a: gameButtonStart,
0x0005ff1b: gameButtonThumbLeft,
0x0005ff1c: gameButtonThumbRight,
0x0005ff1d: gameButtonX,
0x0005ff1e: gameButtonY,
0x0005ff1f: gameButtonZ,
0x00070000: usbReserved,
0x00070001: usbErrorRollOver,
0x00070002: usbPostFail,
0x00070003: usbErrorUndefined,
0x00070004: keyA,
0x00070005: keyB,
0x00070006: keyC,
0x00070007: keyD,
0x00070008: keyE,
0x00070009: keyF,
0x0007000a: keyG,
0x0007000b: keyH,
0x0007000c: keyI,
0x0007000d: keyJ,
0x0007000e: keyK,
0x0007000f: keyL,
0x00070010: keyM,
0x00070011: keyN,
0x00070012: keyO,
0x00070013: keyP,
0x00070014: keyQ,
0x00070015: keyR,
0x00070016: keyS,
0x00070017: keyT,
0x00070018: keyU,
0x00070019: keyV,
0x0007001a: keyW,
0x0007001b: keyX,
0x0007001c: keyY,
0x0007001d: keyZ,
0x0007001e: digit1,
0x0007001f: digit2,
0x00070020: digit3,
0x00070021: digit4,
0x00070022: digit5,
0x00070023: digit6,
0x00070024: digit7,
0x00070025: digit8,
0x00070026: digit9,
0x00070027: digit0,
0x00070028: enter,
0x00070029: escape,
0x0007002a: backspace,
0x0007002b: tab,
0x0007002c: space,
0x0007002d: minus,
0x0007002e: equal,
0x0007002f: bracketLeft,
0x00070030: bracketRight,
0x00070031: backslash,
0x00070033: semicolon,
0x00070034: quote,
0x00070035: backquote,
0x00070036: comma,
0x00070037: period,
0x00070038: slash,
0x00070039: capsLock,
0x0007003a: f1,
0x0007003b: f2,
0x0007003c: f3,
0x0007003d: f4,
0x0007003e: f5,
0x0007003f: f6,
0x00070040: f7,
0x00070041: f8,
0x00070042: f9,
0x00070043: f10,
0x00070044: f11,
0x00070045: f12,
0x00070046: printScreen,
0x00070047: scrollLock,
0x00070048: pause,
0x00070049: insert,
0x0007004a: home,
0x0007004b: pageUp,
0x0007004c: delete,
0x0007004d: end,
0x0007004e: pageDown,
0x0007004f: arrowRight,
0x00070050: arrowLeft,
0x00070051: arrowDown,
0x00070052: arrowUp,
0x00070053: numLock,
0x00070054: numpadDivide,
0x00070055: numpadMultiply,
0x00070056: numpadSubtract,
0x00070057: numpadAdd,
0x00070058: numpadEnter,
0x00070059: numpad1,
0x0007005a: numpad2,
0x0007005b: numpad3,
0x0007005c: numpad4,
0x0007005d: numpad5,
0x0007005e: numpad6,
0x0007005f: numpad7,
0x00070060: numpad8,
0x00070061: numpad9,
0x00070062: numpad0,
0x00070063: numpadDecimal,
0x00070064: intlBackslash,
0x00070065: contextMenu,
0x00070066: power,
0x00070067: numpadEqual,
0x00070068: f13,
0x00070069: f14,
0x0007006a: f15,
0x0007006b: f16,
0x0007006c: f17,
0x0007006d: f18,
0x0007006e: f19,
0x0007006f: f20,
0x00070070: f21,
0x00070071: f22,
0x00070072: f23,
0x00070073: f24,
0x00070074: open,
0x00070075: help,
0x00070077: select,
0x00070079: again,
0x0007007a: undo,
0x0007007b: cut,
0x0007007c: copy,
0x0007007d: paste,
0x0007007e: find,
0x0007007f: audioVolumeMute,
0x00070080: audioVolumeUp,
0x00070081: audioVolumeDown,
0x00070085: numpadComma,
0x00070087: intlRo,
0x00070088: kanaMode,
0x00070089: intlYen,
0x0007008a: convert,
0x0007008b: nonConvert,
0x00070090: lang1,
0x00070091: lang2,
0x00070092: lang3,
0x00070093: lang4,
0x00070094: lang5,
0x0007009b: abort,
0x000700a3: props,
0x000700b6: numpadParenLeft,
0x000700b7: numpadParenRight,
0x000700bb: numpadBackspace,
0x000700d0: numpadMemoryStore,
0x000700d1: numpadMemoryRecall,
0x000700d2: numpadMemoryClear,
0x000700d3: numpadMemoryAdd,
0x000700d4: numpadMemorySubtract,
0x000700d7: numpadSignChange,
0x000700d8: numpadClear,
0x000700d9: numpadClearEntry,
0x000700e0: controlLeft,
0x000700e1: shiftLeft,
0x000700e2: altLeft,
0x000700e3: metaLeft,
0x000700e4: controlRight,
0x000700e5: shiftRight,
0x000700e6: altRight,
0x000700e7: metaRight,
0x000c0060: info,
0x000c0061: closedCaptionToggle,
0x000c006f: brightnessUp,
0x000c0070: brightnessDown,
0x000c0072: brightnessToggle,
0x000c0073: brightnessMinimum,
0x000c0074: brightnessMaximum,
0x000c0075: brightnessAuto,
0x000c0079: kbdIllumUp,
0x000c007a: kbdIllumDown,
0x000c0083: mediaLast,
0x000c008c: launchPhone,
0x000c008d: programGuide,
0x000c0094: exit,
0x000c009c: channelUp,
0x000c009d: channelDown,
0x000c00b0: mediaPlay,
0x000c00b1: mediaPause,
0x000c00b2: mediaRecord,
0x000c00b3: mediaFastForward,
0x000c00b4: mediaRewind,
0x000c00b5: mediaTrackNext,
0x000c00b6: mediaTrackPrevious,
0x000c00b7: mediaStop,
0x000c00b8: eject,
0x000c00cd: mediaPlayPause,
0x000c00cf: speechInputToggle,
0x000c00e5: bassBoost,
0x000c0183: mediaSelect,
0x000c0184: launchWordProcessor,
0x000c0186: launchSpreadsheet,
0x000c018a: launchMail,
0x000c018d: launchContacts,
0x000c018e: launchCalendar,
0x000c0192: launchApp2,
0x000c0194: launchApp1,
0x000c0196: launchInternetBrowser,
0x000c019c: logOff,
0x000c019e: lockScreen,
0x000c019f: launchControlPanel,
0x000c01a2: selectTask,
0x000c01a7: launchDocuments,
0x000c01ab: spellCheck,
0x000c01ae: launchKeyboardLayout,
0x000c01b1: launchScreenSaver,
0x000c01b7: launchAudioBrowser,
0x000c01cb: launchAssistant,
0x000c0201: newKey,
0x000c0203: close,
0x000c0207: save,
0x000c0208: print,
0x000c0221: browserSearch,
0x000c0223: browserHome,
0x000c0224: browserBack,
0x000c0225: browserForward,
0x000c0226: browserStop,
0x000c0227: browserRefresh,
0x000c022a: browserFavorites,
0x000c022d: zoomIn,
0x000c022e: zoomOut,
0x000c0232: zoomToggle,
0x000c0279: redo,
0x000c0289: mailReply,
0x000c028b: mailForward,
0x000c028c: mailSend,
0x000c029d: keyboardLayoutSelect,
0x000c029f: showAllWindows,
};
static const Map<int, String> _debugNames = kReleaseMode ?
<int, String>{} :
<int, String>{
0x00000010: 'Hyper',
0x00000011: 'Super Key',
0x00000012: 'Fn',
0x00000013: 'Fn Lock',
0x00000014: 'Suspend',
0x00000015: 'Resume',
0x00000016: 'Turbo',
0x00000017: 'Privacy Screen Toggle',
0x00000018: 'Microphone Mute Toggle',
0x00010082: 'Sleep',
0x00010083: 'Wake Up',
0x000100b5: 'Display Toggle Int Ext',
0x0005ff01: 'Game Button 1',
0x0005ff02: 'Game Button 2',
0x0005ff03: 'Game Button 3',
0x0005ff04: 'Game Button 4',
0x0005ff05: 'Game Button 5',
0x0005ff06: 'Game Button 6',
0x0005ff07: 'Game Button 7',
0x0005ff08: 'Game Button 8',
0x0005ff09: 'Game Button 9',
0x0005ff0a: 'Game Button 10',
0x0005ff0b: 'Game Button 11',
0x0005ff0c: 'Game Button 12',
0x0005ff0d: 'Game Button 13',
0x0005ff0e: 'Game Button 14',
0x0005ff0f: 'Game Button 15',
0x0005ff10: 'Game Button 16',
0x0005ff11: 'Game Button A',
0x0005ff12: 'Game Button B',
0x0005ff13: 'Game Button C',
0x0005ff14: 'Game Button Left 1',
0x0005ff15: 'Game Button Left 2',
0x0005ff16: 'Game Button Mode',
0x0005ff17: 'Game Button Right 1',
0x0005ff18: 'Game Button Right 2',
0x0005ff19: 'Game Button Select',
0x0005ff1a: 'Game Button Start',
0x0005ff1b: 'Game Button Thumb Left',
0x0005ff1c: 'Game Button Thumb Right',
0x0005ff1d: 'Game Button X',
0x0005ff1e: 'Game Button Y',
0x0005ff1f: 'Game Button Z',
0x00070000: 'Usb Reserved',
0x00070001: 'Usb Error Roll Over',
0x00070002: 'Usb Post Fail',
0x00070003: 'Usb Error Undefined',
0x00070004: 'Key A',
0x00070005: 'Key B',
0x00070006: 'Key C',
0x00070007: 'Key D',
0x00070008: 'Key E',
0x00070009: 'Key F',
0x0007000a: 'Key G',
0x0007000b: 'Key H',
0x0007000c: 'Key I',
0x0007000d: 'Key J',
0x0007000e: 'Key K',
0x0007000f: 'Key L',
0x00070010: 'Key M',
0x00070011: 'Key N',
0x00070012: 'Key O',
0x00070013: 'Key P',
0x00070014: 'Key Q',
0x00070015: 'Key R',
0x00070016: 'Key S',
0x00070017: 'Key T',
0x00070018: 'Key U',
0x00070019: 'Key V',
0x0007001a: 'Key W',
0x0007001b: 'Key X',
0x0007001c: 'Key Y',
0x0007001d: 'Key Z',
0x0007001e: 'Digit 1',
0x0007001f: 'Digit 2',
0x00070020: 'Digit 3',
0x00070021: 'Digit 4',
0x00070022: 'Digit 5',
0x00070023: 'Digit 6',
0x00070024: 'Digit 7',
0x00070025: 'Digit 8',
0x00070026: 'Digit 9',
0x00070027: 'Digit 0',
0x00070028: 'Enter',
0x00070029: 'Escape',
0x0007002a: 'Backspace',
0x0007002b: 'Tab',
0x0007002c: 'Space',
0x0007002d: 'Minus',
0x0007002e: 'Equal',
0x0007002f: 'Bracket Left',
0x00070030: 'Bracket Right',
0x00070031: 'Backslash',
0x00070033: 'Semicolon',
0x00070034: 'Quote',
0x00070035: 'Backquote',
0x00070036: 'Comma',
0x00070037: 'Period',
0x00070038: 'Slash',
0x00070039: 'Caps Lock',
0x0007003a: 'F1',
0x0007003b: 'F2',
0x0007003c: 'F3',
0x0007003d: 'F4',
0x0007003e: 'F5',
0x0007003f: 'F6',
0x00070040: 'F7',
0x00070041: 'F8',
0x00070042: 'F9',
0x00070043: 'F10',
0x00070044: 'F11',
0x00070045: 'F12',
0x00070046: 'Print Screen',
0x00070047: 'Scroll Lock',
0x00070048: 'Pause',
0x00070049: 'Insert',
0x0007004a: 'Home',
0x0007004b: 'Page Up',
0x0007004c: 'Delete',
0x0007004d: 'End',
0x0007004e: 'Page Down',
0x0007004f: 'Arrow Right',
0x00070050: 'Arrow Left',
0x00070051: 'Arrow Down',
0x00070052: 'Arrow Up',
0x00070053: 'Num Lock',
0x00070054: 'Numpad Divide',
0x00070055: 'Numpad Multiply',
0x00070056: 'Numpad Subtract',
0x00070057: 'Numpad Add',
0x00070058: 'Numpad Enter',
0x00070059: 'Numpad 1',
0x0007005a: 'Numpad 2',
0x0007005b: 'Numpad 3',
0x0007005c: 'Numpad 4',
0x0007005d: 'Numpad 5',
0x0007005e: 'Numpad 6',
0x0007005f: 'Numpad 7',
0x00070060: 'Numpad 8',
0x00070061: 'Numpad 9',
0x00070062: 'Numpad 0',
0x00070063: 'Numpad Decimal',
0x00070064: 'Intl Backslash',
0x00070065: 'Context Menu',
0x00070066: 'Power',
0x00070067: 'Numpad Equal',
0x00070068: 'F13',
0x00070069: 'F14',
0x0007006a: 'F15',
0x0007006b: 'F16',
0x0007006c: 'F17',
0x0007006d: 'F18',
0x0007006e: 'F19',
0x0007006f: 'F20',
0x00070070: 'F21',
0x00070071: 'F22',
0x00070072: 'F23',
0x00070073: 'F24',
0x00070074: 'Open',
0x00070075: 'Help',
0x00070077: 'Select',
0x00070079: 'Again',
0x0007007a: 'Undo',
0x0007007b: 'Cut',
0x0007007c: 'Copy',
0x0007007d: 'Paste',
0x0007007e: 'Find',
0x0007007f: 'Audio Volume Mute',
0x00070080: 'Audio Volume Up',
0x00070081: 'Audio Volume Down',
0x00070085: 'Numpad Comma',
0x00070087: 'Intl Ro',
0x00070088: 'Kana Mode',
0x00070089: 'Intl Yen',
0x0007008a: 'Convert',
0x0007008b: 'Non Convert',
0x00070090: 'Lang 1',
0x00070091: 'Lang 2',
0x00070092: 'Lang 3',
0x00070093: 'Lang 4',
0x00070094: 'Lang 5',
0x0007009b: 'Abort',
0x000700a3: 'Props',
0x000700b6: 'Numpad Paren Left',
0x000700b7: 'Numpad Paren Right',
0x000700bb: 'Numpad Backspace',
0x000700d0: 'Numpad Memory Store',
0x000700d1: 'Numpad Memory Recall',
0x000700d2: 'Numpad Memory Clear',
0x000700d3: 'Numpad Memory Add',
0x000700d4: 'Numpad Memory Subtract',
0x000700d7: 'Numpad Sign Change',
0x000700d8: 'Numpad Clear',
0x000700d9: 'Numpad Clear Entry',
0x000700e0: 'Control Left',
0x000700e1: 'Shift Left',
0x000700e2: 'Alt Left',
0x000700e3: 'Meta Left',
0x000700e4: 'Control Right',
0x000700e5: 'Shift Right',
0x000700e6: 'Alt Right',
0x000700e7: 'Meta Right',
0x000c0060: 'Info',
0x000c0061: 'Closed Caption Toggle',
0x000c006f: 'Brightness Up',
0x000c0070: 'Brightness Down',
0x000c0072: 'Brightness Toggle',
0x000c0073: 'Brightness Minimum',
0x000c0074: 'Brightness Maximum',
0x000c0075: 'Brightness Auto',
0x000c0079: 'Kbd Illum Up',
0x000c007a: 'Kbd Illum Down',
0x000c0083: 'Media Last',
0x000c008c: 'Launch Phone',
0x000c008d: 'Program Guide',
0x000c0094: 'Exit',
0x000c009c: 'Channel Up',
0x000c009d: 'Channel Down',
0x000c00b0: 'Media Play',
0x000c00b1: 'Media Pause',
0x000c00b2: 'Media Record',
0x000c00b3: 'Media Fast Forward',
0x000c00b4: 'Media Rewind',
0x000c00b5: 'Media Track Next',
0x000c00b6: 'Media Track Previous',
0x000c00b7: 'Media Stop',
0x000c00b8: 'Eject',
0x000c00cd: 'Media Play Pause',
0x000c00cf: 'Speech Input Toggle',
0x000c00e5: 'Bass Boost',
0x000c0183: 'Media Select',
0x000c0184: 'Launch Word Processor',
0x000c0186: 'Launch Spreadsheet',
0x000c018a: 'Launch Mail',
0x000c018d: 'Launch Contacts',
0x000c018e: 'Launch Calendar',
0x000c0192: 'Launch App2',
0x000c0194: 'Launch App1',
0x000c0196: 'Launch Internet Browser',
0x000c019c: 'Log Off',
0x000c019e: 'Lock Screen',
0x000c019f: 'Launch Control Panel',
0x000c01a2: 'Select Task',
0x000c01a7: 'Launch Documents',
0x000c01ab: 'Spell Check',
0x000c01ae: 'Launch Keyboard Layout',
0x000c01b1: 'Launch Screen Saver',
0x000c01b7: 'Launch Audio Browser',
0x000c01cb: 'Launch Assistant',
0x000c0201: 'New Key',
0x000c0203: 'Close',
0x000c0207: 'Save',
0x000c0208: 'Print',
0x000c0221: 'Browser Search',
0x000c0223: 'Browser Home',
0x000c0224: 'Browser Back',
0x000c0225: 'Browser Forward',
0x000c0226: 'Browser Stop',
0x000c0227: 'Browser Refresh',
0x000c022a: 'Browser Favorites',
0x000c022d: 'Zoom In',
0x000c022e: 'Zoom Out',
0x000c0232: 'Zoom Toggle',
0x000c0279: 'Redo',
0x000c0289: 'Mail Reply',
0x000c028b: 'Mail Forward',
0x000c028c: 'Mail Send',
0x000c029d: 'Keyboard Layout Select',
0x000c029f: 'Show All Windows',
};
}
| flutter/packages/flutter/lib/src/services/keyboard_key.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/keyboard_key.g.dart",
"repo_id": "flutter",
"token_count": 74244
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'keyboard_maps.g.dart';
import 'raw_keyboard.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'keyboard_key.g.dart' show LogicalKeyboardKey, PhysicalKeyboardKey;
String? _unicodeChar(String key) {
if (key.length == 1) {
return key.substring(0, 1);
}
return null;
}
/// Platform-specific key event data for Web.
///
/// This class is DEPRECATED. Platform specific key event data will no longer
/// available. See [KeyEvent] for what is available.
///
/// See also:
///
/// * [RawKeyboard], which uses this interface to expose key data.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
@immutable
class RawKeyEventDataWeb extends RawKeyEventData {
/// Creates a key event data structure specific for Web.
@Deprecated(
'Platform specific key event data is no longer available. See KeyEvent for what is available. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
const RawKeyEventDataWeb({
required this.code,
required this.key,
this.location = 0,
this.metaState = modifierNone,
this.keyCode = 0,
});
/// The `KeyboardEvent.code` corresponding to this event.
///
/// The [code] represents a physical key on the keyboard, a value that isn't
/// altered by keyboard layout or the state of the modifier keys.
///
/// See <https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/code>
/// for more information.
final String code;
/// The `KeyboardEvent.key` corresponding to this event.
///
/// The [key] represents the key pressed by the user, taking into
/// consideration the state of modifier keys such as Shift as well as the
/// keyboard locale and layout.
///
/// See <https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/key>
/// for more information.
final String key;
/// The `KeyboardEvent.location` corresponding to this event.
///
/// The [location] represents the location of the key on the keyboard or other
/// input device, such as left or right modifier keys, or Numpad keys.
///
/// See <https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/location>
/// for more information.
final int location;
/// The modifiers that were present when the key event occurred.
///
/// See `lib/src/engine/keyboard.dart` in the web engine for the numerical
/// values of the [metaState]. These constants are also replicated as static
/// constants in this class.
///
/// See also:
///
/// * [modifiersPressed], which returns a Map of currently pressed modifiers
/// and their keyboard side.
/// * [isModifierPressed], to see if a specific modifier is pressed.
/// * [isControlPressed], to see if a CTRL key is pressed.
/// * [isShiftPressed], to see if a SHIFT key is pressed.
/// * [isAltPressed], to see if an ALT key is pressed.
/// * [isMetaPressed], to see if a META key is pressed.
final int metaState;
/// The `KeyboardEvent.keyCode` corresponding to this event.
///
/// See <https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/keyCode>
/// for more information.
final int keyCode;
@override
String get keyLabel => key == 'Unidentified' ? '' : _unicodeChar(key) ?? '';
@override
PhysicalKeyboardKey get physicalKey {
return kWebToPhysicalKey[code] ?? PhysicalKeyboardKey(LogicalKeyboardKey.webPlane + code.hashCode);
}
@override
LogicalKeyboardKey get logicalKey {
// Look to see if the keyCode is a key based on location. Typically they are
// numpad keys (versus main area keys) and left/right modifiers.
final LogicalKeyboardKey? maybeLocationKey = kWebLocationMap[key]?[location];
if (maybeLocationKey != null) {
return maybeLocationKey;
}
// Look to see if the [key] is one we know about and have a mapping for.
final LogicalKeyboardKey? newKey = kWebToLogicalKey[key];
if (newKey != null) {
return newKey;
}
final bool isPrintable = key.length == 1;
if (isPrintable) {
return LogicalKeyboardKey(key.toLowerCase().codeUnitAt(0));
}
// This is a non-printable key that we don't know about, so we mint a new
// key from `code`. Don't mint with `key`, because the `key` will always be
// "Unidentified" .
return LogicalKeyboardKey(code.hashCode + LogicalKeyboardKey.webPlane);
}
@override
bool isModifierPressed(ModifierKey key, {KeyboardSide side = KeyboardSide.any}) {
return switch (key) {
ModifierKey.controlModifier => metaState & modifierControl != 0,
ModifierKey.shiftModifier => metaState & modifierShift != 0,
ModifierKey.altModifier => metaState & modifierAlt != 0,
ModifierKey.metaModifier => metaState & modifierMeta != 0,
ModifierKey.numLockModifier => metaState & modifierNumLock != 0,
ModifierKey.capsLockModifier => metaState & modifierCapsLock != 0,
ModifierKey.scrollLockModifier => metaState & modifierScrollLock != 0,
// On Web, the browser doesn't report the state of the FN and SYM modifiers.
ModifierKey.functionModifier || ModifierKey.symbolModifier => false,
};
}
@override
KeyboardSide getModifierSide(ModifierKey key) {
// On Web, we don't distinguish the sides of modifier keys. Both left shift
// and right shift, for example, are reported as the "Shift" modifier.
//
// See <https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/getModifierState>
// for more information.
return KeyboardSide.any;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<String>('code', code));
properties.add(DiagnosticsProperty<String>('key', key));
properties.add(DiagnosticsProperty<int>('location', location));
properties.add(DiagnosticsProperty<int>('metaState', metaState));
properties.add(DiagnosticsProperty<int>('keyCode', keyCode));
}
@override
bool operator==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is RawKeyEventDataWeb
&& other.code == code
&& other.key == key
&& other.location == location
&& other.metaState == metaState
&& other.keyCode == keyCode;
}
@override
int get hashCode => Object.hash(
code,
key,
location,
metaState,
keyCode,
);
// Modifier key masks.
/// No modifier keys are pressed in the [metaState] field.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierNone = 0;
/// This mask is used to check the [metaState] field to test whether one of
/// the SHIFT modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierShift = 0x01;
/// This mask is used to check the [metaState] field to test whether one of
/// the ALT modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierAlt = 0x02;
/// This mask is used to check the [metaState] field to test whether one of
/// the CTRL modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierControl = 0x04;
/// This mask is used to check the [metaState] field to test whether one of
/// the META modifier keys is pressed.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierMeta = 0x08;
/// This mask is used to check the [metaState] field to test whether the NUM
/// LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierNumLock = 0x10;
/// This mask is used to check the [metaState] field to test whether the CAPS
/// LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierCapsLock = 0x20;
/// This mask is used to check the [metaState] field to test whether the
/// SCROLL LOCK modifier key is on.
///
/// Use this value if you need to decode the [metaState] field yourself, but
/// it's much easier to use [isModifierPressed] if you just want to know if
/// a modifier is pressed.
static const int modifierScrollLock = 0x40;
}
| flutter/packages/flutter/lib/src/services/raw_keyboard_web.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/raw_keyboard_web.dart",
"repo_id": "flutter",
"token_count": 3023
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This code is copied from `package:web` which still needs its own
// documentation for public members. Since this is a shim that users should not
// use, we ignore this lint for this file.
// ignore_for_file: public_member_api_docs
/// A stripped down version of `package:web` to avoid pinning that repo in
/// Flutter as a dependency.
///
/// These are manually copied over from `package:web` as needed, and should stay
/// in sync with the latest package version as much as possible.
///
/// If missing members are needed, copy them over into the corresponding
/// extension or interface. If missing interfaces/types are needed, copy them
/// over while excluding unnecessary inheritance to make the copy minimal. These
/// types are erased at runtime, so excluding supertypes is safe. If a member is
/// needed that belongs to a supertype, then add the necessary `implements`
/// clause to the subtype when you add that supertype. Keep extensions next to
/// the interface they extend.
library;
import 'dart:js_interop';
typedef EventListener = JSFunction;
typedef XMLHttpRequestResponseType = String;
@JS()
external Document get document;
@JS()
external Window get window;
extension type CSSStyleDeclaration._(JSObject _) implements JSObject {
external set backgroundColor(String value);
external String get backgroundColor;
external set border(String value);
external String get border;
external set height(String value);
external String get height;
external set width(String value);
external String get width;
}
extension type CSSStyleSheet._(JSObject _) implements JSObject {
external int insertRule(
String rule, [
int index,
]);
}
extension type Document._(JSObject _) implements JSObject {
external Element createElement(
String localName, [
JSAny options,
]);
external Range createRange();
external HTMLHeadElement? get head;
}
extension type DOMTokenList._(JSObject _) implements JSObject {
external void add(String tokens);
}
extension type Element._(JSObject _) implements Node, JSObject {
external DOMTokenList get classList;
external void append(JSAny nodes);
}
extension type Event._(JSObject _) implements JSObject {}
extension type EventTarget._(JSObject _) implements JSObject {
external void addEventListener(
String type,
EventListener? callback, [
JSAny options,
]);
}
extension type HTMLElement._(JSObject _) implements Element, JSObject {
external String get innerText;
external set innerText(String value);
external CSSStyleDeclaration get style;
}
extension type HTMLHeadElement._(JSObject _) implements HTMLElement, JSObject {}
extension type HTMLStyleElement._(JSObject _) implements HTMLElement, JSObject {
external CSSStyleSheet? get sheet;
}
extension type MediaQueryList._(JSObject _) implements EventTarget, JSObject {
external bool get matches;
}
extension type MouseEvent._(JSObject _) implements JSObject {
external num get offsetX;
external num get offsetY;
external int get button;
}
extension type Navigator._(JSObject _) implements JSObject {
external String get platform;
}
extension type Node._(JSObject _) implements EventTarget, JSObject {}
extension type Range._(JSObject _) implements JSObject {
external void selectNode(Node node);
}
extension type Selection._(JSObject _) implements JSObject {
external void addRange(Range range);
external void removeAllRanges();
}
extension type Window._(JSObject _) implements EventTarget, JSObject {
external Navigator get navigator;
external MediaQueryList matchMedia(String query);
external Selection? getSelection();
}
extension type XMLHttpRequest._(JSObject _)
implements XMLHttpRequestEventTarget, JSObject {
external factory XMLHttpRequest();
external void open(
String method,
String url, [
bool async,
String? username,
String? password,
]);
external void setRequestHeader(
String name,
String value,
);
external void send([JSAny? body]);
external int get status;
external set responseType(XMLHttpRequestResponseType value);
external XMLHttpRequestResponseType get responseType;
external JSAny? get response;
}
extension type XMLHttpRequestEventTarget._(JSObject _)
implements EventTarget, JSObject {}
| flutter/packages/flutter/lib/src/web.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/web.dart",
"repo_id": "flutter",
"token_count": 1210
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'framework.dart';
export 'package:flutter/services.dart' show AutofillHints;
/// Predefined autofill context clean up actions.
enum AutofillContextAction {
/// Destroys the current autofill context after informing the platform to save
/// the user input from it.
///
/// Corresponds to calling [TextInput.finishAutofillContext] with
/// `shouldSave == true`.
commit,
/// Destroys the current autofill context without saving the user input.
///
/// Corresponds to calling [TextInput.finishAutofillContext] with
/// `shouldSave == false`.
cancel,
}
/// An [AutofillScope] widget that groups [AutofillClient]s together.
///
/// [AutofillClient]s that share the same closest [AutofillGroup] ancestor must
/// be built together, and they be will be autofilled together.
///
/// {@macro flutter.services.AutofillScope}
///
/// The [AutofillGroup] widget only knows about [AutofillClient]s registered to
/// it using the [AutofillGroupState.register] API. Typically, [AutofillGroup]
/// will not pick up [AutofillClient]s that are not mounted, for example, an
/// [AutofillClient] within a [Scrollable] that has never been scrolled into the
/// viewport. To workaround this problem, ensure clients in the same
/// [AutofillGroup] are built together.
///
/// The topmost [AutofillGroup] widgets (the ones that are closest to the root
/// widget) can be used to clean up the current autofill context when the
/// current autofill context is no longer relevant.
///
/// {@macro flutter.services.TextInput.finishAutofillContext}
///
/// By default, [onDisposeAction] is set to [AutofillContextAction.commit], in
/// which case when any of the topmost [AutofillGroup]s is being disposed, the
/// platform will be informed to save the user input from the current autofill
/// context, then the current autofill context will be destroyed, to free
/// resources. You can, for example, wrap a route that contains a [Form] full of
/// autofillable input fields in an [AutofillGroup], so the user input of the
/// [Form] can be saved for future autofill by the platform.
///
/// {@tool dartpad}
/// An example form with autofillable fields grouped into different
/// [AutofillGroup]s.
///
/// ** See code in examples/api/lib/widgets/autofill/autofill_group.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AutofillContextAction], an enum that contains predefined autofill context
/// clean up actions to be run when a topmost [AutofillGroup] is disposed.
class AutofillGroup extends StatefulWidget {
/// Creates a scope for autofillable input fields.
const AutofillGroup({
super.key,
required this.child,
this.onDisposeAction = AutofillContextAction.commit,
});
/// Returns the [AutofillGroupState] of the closest [AutofillGroup] widget
/// which encloses the given context, or null if one cannot be found.
///
/// Calling this method will create a dependency on the closest
/// [AutofillGroup] in the [context], if there is one.
///
/// {@macro flutter.widgets.AutofillGroupState}
///
/// See also:
///
/// * [AutofillGroup.of], which is similar to this method, but asserts if an
/// [AutofillGroup] cannot be found.
/// * [EditableTextState], where this method is used to retrieve the closest
/// [AutofillGroupState].
static AutofillGroupState? maybeOf(BuildContext context) {
final _AutofillScope? scope = context.dependOnInheritedWidgetOfExactType<_AutofillScope>();
return scope?._scope;
}
/// Returns the [AutofillGroupState] of the closest [AutofillGroup] widget
/// which encloses the given context.
///
/// If no instance is found, this method will assert in debug mode and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest
/// [AutofillGroup] in the [context].
///
/// {@macro flutter.widgets.AutofillGroupState}
///
/// See also:
///
/// * [AutofillGroup.maybeOf], which is similar to this method, but returns
/// null if an [AutofillGroup] cannot be found.
/// * [EditableTextState], where this method is used to retrieve the closest
/// [AutofillGroupState].
static AutofillGroupState of(BuildContext context) {
final AutofillGroupState? groupState = maybeOf(context);
assert(() {
if (groupState == null) {
throw FlutterError(
'AutofillGroup.of() was called with a context that does not contain an '
'AutofillGroup widget.\n'
'No AutofillGroup widget ancestor could be found starting from the '
'context that was passed to AutofillGroup.of(). This can happen '
'because you are using a widget that looks for an AutofillGroup '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return groupState!;
}
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The [AutofillContextAction] to be run when this [AutofillGroup] is the
/// topmost [AutofillGroup] and it's being disposed, in order to clean up the
/// current autofill context.
///
/// {@macro flutter.services.TextInput.finishAutofillContext}
///
/// Defaults to [AutofillContextAction.commit], which prompts the platform to
/// save the user input and destroy the current autofill context.
final AutofillContextAction onDisposeAction;
@override
AutofillGroupState createState() => AutofillGroupState();
}
/// State associated with an [AutofillGroup] widget.
///
/// {@template flutter.widgets.AutofillGroupState}
/// An [AutofillGroupState] can be used to register an [AutofillClient] when it
/// enters this [AutofillGroup] (for example, when an [EditableText] is mounted or
/// reparented onto the [AutofillGroup]'s subtree), and unregister an
/// [AutofillClient] when it exits (for example, when an [EditableText] gets
/// unmounted or reparented out of the [AutofillGroup]'s subtree).
///
/// The [AutofillGroupState] class also provides an [AutofillGroupState.attach]
/// method that can be called by [TextInputClient]s that support autofill,
/// instead of [TextInput.attach], to create a [TextInputConnection] to interact
/// with the platform's text input system.
/// {@endtemplate}
///
/// Typically obtained using [AutofillGroup.of].
class AutofillGroupState extends State<AutofillGroup> with AutofillScopeMixin {
final Map<String, AutofillClient> _clients = <String, AutofillClient>{};
// Whether this AutofillGroup widget is the topmost AutofillGroup (i.e., it
// has no AutofillGroup ancestor). Each topmost AutofillGroup runs its
// `AutofillGroup.onDisposeAction` when it gets disposed.
bool _isTopmostAutofillGroup = false;
@override
AutofillClient? getAutofillClient(String autofillId) => _clients[autofillId];
@override
Iterable<AutofillClient> get autofillClients {
return _clients.values
.where((AutofillClient client) => client.textInputConfiguration.autofillConfiguration.enabled);
}
/// Adds the [AutofillClient] to this [AutofillGroup].
///
/// Typically, this is called by [TextInputClient]s that support autofill (for
/// example, [EditableTextState]) in [State.didChangeDependencies], when the
/// input field should be registered to a new [AutofillGroup].
///
/// See also:
///
/// * [EditableTextState.didChangeDependencies], where this method is called
/// to update the current [AutofillScope] when needed.
void register(AutofillClient client) {
_clients.putIfAbsent(client.autofillId, () => client);
}
/// Removes an [AutofillClient] with the given `autofillId` from this
/// [AutofillGroup].
///
/// Typically, this should be called by a text field when it's being disposed,
/// or before it's registered with a different [AutofillGroup].
///
/// See also:
///
/// * [EditableTextState.didChangeDependencies], where this method is called
/// to unregister from the previous [AutofillScope].
/// * [EditableTextState.dispose], where this method is called to unregister
/// from the current [AutofillScope] when the widget is about to be removed
/// from the tree.
void unregister(String autofillId) {
assert(_clients.containsKey(autofillId));
_clients.remove(autofillId);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_isTopmostAutofillGroup = AutofillGroup.maybeOf(context) == null;
}
@override
Widget build(BuildContext context) {
return _AutofillScope(
autofillScopeState: this,
child: widget.child,
);
}
@override
void dispose() {
super.dispose();
if (!_isTopmostAutofillGroup) {
return;
}
switch (widget.onDisposeAction) {
case AutofillContextAction.cancel:
TextInput.finishAutofillContext(shouldSave: false);
case AutofillContextAction.commit:
TextInput.finishAutofillContext();
}
}
}
class _AutofillScope extends InheritedWidget {
const _AutofillScope({
required super.child,
AutofillGroupState? autofillScopeState,
}) : _scope = autofillScopeState;
final AutofillGroupState? _scope;
AutofillGroup get client => _scope!.widget;
@override
bool updateShouldNotify(_AutofillScope old) => _scope != old._scope;
}
| flutter/packages/flutter/lib/src/widgets/autofill.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/autofill.dart",
"repo_id": "flutter",
"token_count": 2995
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'automatic_keep_alive.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
const Curve _kResizeTimeCurve = Interval(0.4, 1.0, curve: Curves.ease);
const double _kMinFlingVelocity = 700.0;
const double _kMinFlingVelocityDelta = 400.0;
const double _kFlingVelocityScale = 1.0 / 300.0;
const double _kDismissThreshold = 0.4;
/// Signature used by [Dismissible] to indicate that it has been dismissed in
/// the given `direction`.
///
/// Used by [Dismissible.onDismissed].
typedef DismissDirectionCallback = void Function(DismissDirection direction);
/// Signature used by [Dismissible] to give the application an opportunity to
/// confirm or veto a dismiss gesture.
///
/// Used by [Dismissible.confirmDismiss].
typedef ConfirmDismissCallback = Future<bool?> Function(DismissDirection direction);
/// Signature used by [Dismissible] to indicate that the dismissible has been dragged.
///
/// Used by [Dismissible.onUpdate].
typedef DismissUpdateCallback = void Function(DismissUpdateDetails details);
/// The direction in which a [Dismissible] can be dismissed.
enum DismissDirection {
/// The [Dismissible] can be dismissed by dragging either up or down.
vertical,
/// The [Dismissible] can be dismissed by dragging either left or right.
horizontal,
/// The [Dismissible] can be dismissed by dragging in the reverse of the
/// reading direction (e.g., from right to left in left-to-right languages).
endToStart,
/// The [Dismissible] can be dismissed by dragging in the reading direction
/// (e.g., from left to right in left-to-right languages).
startToEnd,
/// The [Dismissible] can be dismissed by dragging up only.
up,
/// The [Dismissible] can be dismissed by dragging down only.
down,
/// The [Dismissible] cannot be dismissed by dragging.
none
}
/// A widget that can be dismissed by dragging in the indicated [direction].
///
/// Dragging or flinging this widget in the [DismissDirection] causes the child
/// to slide out of view. Following the slide animation, if [resizeDuration] is
/// non-null, the Dismissible widget animates its height (or width, whichever is
/// perpendicular to the dismiss direction) to zero over the [resizeDuration].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=iEMgjrfuc58}
///
/// {@tool dartpad}
/// This sample shows how you can use the [Dismissible] widget to
/// remove list items using swipe gestures. Swipe any of the list
/// tiles to the left or right to dismiss them from the [ListView].
///
/// ** See code in examples/api/lib/widgets/dismissible/dismissible.0.dart **
/// {@end-tool}
///
/// Backgrounds can be used to implement the "leave-behind" idiom. If a background
/// is specified it is stacked behind the Dismissible's child and is exposed when
/// the child moves.
///
/// The widget calls the [onDismissed] callback either after its size has
/// collapsed to zero (if [resizeDuration] is non-null) or immediately after
/// the slide animation (if [resizeDuration] is null). If the Dismissible is a
/// list item, it must have a key that distinguishes it from the other items and
/// its [onDismissed] callback must remove the item from the list.
class Dismissible extends StatefulWidget {
/// Creates a widget that can be dismissed.
///
/// The [key] argument is required because [Dismissible]s are commonly used in
/// lists and removed from the list when dismissed. Without keys, the default
/// behavior is to sync widgets based on their index in the list, which means
/// the item after the dismissed item would be synced with the state of the
/// dismissed item. Using keys causes the widgets to sync according to their
/// keys and avoids this pitfall.
const Dismissible({
required Key key,
required this.child,
this.background,
this.secondaryBackground,
this.confirmDismiss,
this.onResize,
this.onUpdate,
this.onDismissed,
this.direction = DismissDirection.horizontal,
this.resizeDuration = const Duration(milliseconds: 300),
this.dismissThresholds = const <DismissDirection, double>{},
this.movementDuration = const Duration(milliseconds: 200),
this.crossAxisEndOffset = 0.0,
this.dragStartBehavior = DragStartBehavior.start,
this.behavior = HitTestBehavior.opaque,
}) : assert(secondaryBackground == null || background != null),
super(key: key);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// A widget that is stacked behind the child. If secondaryBackground is also
/// specified then this widget only appears when the child has been dragged
/// down or to the right.
final Widget? background;
/// A widget that is stacked behind the child and is exposed when the child
/// has been dragged up or to the left. It may only be specified when background
/// has also been specified.
final Widget? secondaryBackground;
/// Gives the app an opportunity to confirm or veto a pending dismissal.
///
/// The widget cannot be dragged again until the returned future resolves.
///
/// If the returned Future<bool> completes true, then this widget will be
/// dismissed, otherwise it will be moved back to its original location.
///
/// If the returned Future<bool?> completes to false or null the [onResize]
/// and [onDismissed] callbacks will not run.
final ConfirmDismissCallback? confirmDismiss;
/// Called when the widget changes size (i.e., when contracting before being dismissed).
final VoidCallback? onResize;
/// Called when the widget has been dismissed, after finishing resizing.
final DismissDirectionCallback? onDismissed;
/// The direction in which the widget can be dismissed.
final DismissDirection direction;
/// The amount of time the widget will spend contracting before [onDismissed] is called.
///
/// If null, the widget will not contract and [onDismissed] will be called
/// immediately after the widget is dismissed.
final Duration? resizeDuration;
/// The offset threshold the item has to be dragged in order to be considered
/// dismissed.
///
/// Represented as a fraction, e.g. if it is 0.4 (the default), then the item
/// has to be dragged at least 40% towards one direction to be considered
/// dismissed. Clients can define different thresholds for each dismiss
/// direction.
///
/// Flinging is treated as being equivalent to dragging almost to 1.0, so
/// flinging can dismiss an item past any threshold less than 1.0.
///
/// Setting a threshold of 1.0 (or greater) prevents a drag in the given
/// [DismissDirection] even if it would be allowed by the [direction]
/// property.
///
/// See also:
///
/// * [direction], which controls the directions in which the items can
/// be dismissed.
final Map<DismissDirection, double> dismissThresholds;
/// Defines the duration for card to dismiss or to come back to original position if not dismissed.
final Duration movementDuration;
/// Defines the end offset across the main axis after the card is dismissed.
///
/// If non-zero value is given then widget moves in cross direction depending on whether
/// it is positive or negative.
final double crossAxisEndOffset;
/// Determines the way that drag start behavior is handled.
///
/// If set to [DragStartBehavior.start], the drag gesture used to dismiss a
/// dismissible will begin at the position where the drag gesture won the arena.
/// If set to [DragStartBehavior.down] it will begin at the position where
/// a down event is first detected.
///
/// In general, setting this to [DragStartBehavior.start] will make drag
/// animation smoother and setting it to [DragStartBehavior.down] will make
/// drag behavior feel slightly more reactive.
///
/// By default, the drag start behavior is [DragStartBehavior.start].
///
/// See also:
///
/// * [DragGestureRecognizer.dragStartBehavior], which gives an example for the different behaviors.
final DragStartBehavior dragStartBehavior;
/// How to behave during hit tests.
///
/// This defaults to [HitTestBehavior.opaque].
final HitTestBehavior behavior;
/// Called when the dismissible widget has been dragged.
///
/// If [onUpdate] is not null, then it will be invoked for every pointer event
/// to dispatch the latest state of the drag. For example, this callback
/// can be used to for example change the color of the background widget
/// depending on whether the dismiss threshold is currently reached.
final DismissUpdateCallback? onUpdate;
@override
State<Dismissible> createState() => _DismissibleState();
}
/// Details for [DismissUpdateCallback].
///
/// See also:
///
/// * [Dismissible.onUpdate], which receives this information.
class DismissUpdateDetails {
/// Create a new instance of [DismissUpdateDetails].
DismissUpdateDetails({
this.direction = DismissDirection.horizontal,
this.reached = false,
this.previousReached = false,
this.progress = 0.0,
});
/// The direction that the dismissible is being dragged.
final DismissDirection direction;
/// Whether the dismiss threshold is currently reached.
final bool reached;
/// Whether the dismiss threshold was reached the last time this callback was invoked.
///
/// This can be used in conjunction with [DismissUpdateDetails.reached] to catch the moment
/// that the [Dismissible] is dragged across the threshold.
final bool previousReached;
/// The offset ratio of the dismissible in its parent container.
///
/// A value of 0.0 represents the normal position and 1.0 means the child is
/// completely outside its parent.
///
/// This can be used to synchronize other elements to what the dismissible is doing on screen,
/// e.g. using this value to set the opacity thereby fading dismissible as it's dragged offscreen.
final double progress;
}
class _DismissibleClipper extends CustomClipper<Rect> {
_DismissibleClipper({
required this.axis,
required this.moveAnimation,
}) : super(reclip: moveAnimation);
final Axis axis;
final Animation<Offset> moveAnimation;
@override
Rect getClip(Size size) {
switch (axis) {
case Axis.horizontal:
final double offset = moveAnimation.value.dx * size.width;
if (offset < 0) {
return Rect.fromLTRB(size.width + offset, 0.0, size.width, size.height);
}
return Rect.fromLTRB(0.0, 0.0, offset, size.height);
case Axis.vertical:
final double offset = moveAnimation.value.dy * size.height;
if (offset < 0) {
return Rect.fromLTRB(0.0, size.height + offset, size.width, size.height);
}
return Rect.fromLTRB(0.0, 0.0, size.width, offset);
}
}
@override
Rect getApproximateClipRect(Size size) => getClip(size);
@override
bool shouldReclip(_DismissibleClipper oldClipper) {
return oldClipper.axis != axis
|| oldClipper.moveAnimation.value != moveAnimation.value;
}
}
enum _FlingGestureKind { none, forward, reverse }
class _DismissibleState extends State<Dismissible> with TickerProviderStateMixin, AutomaticKeepAliveClientMixin {
@override
void initState() {
super.initState();
_moveController = AnimationController(duration: widget.movementDuration, vsync: this)
..addStatusListener(_handleDismissStatusChanged)
..addListener(_handleDismissUpdateValueChanged);
_updateMoveAnimation();
}
AnimationController? _moveController;
late Animation<Offset> _moveAnimation;
AnimationController? _resizeController;
Animation<double>? _resizeAnimation;
double _dragExtent = 0.0;
bool _confirming = false;
bool _dragUnderway = false;
Size? _sizePriorToCollapse;
bool _dismissThresholdReached = false;
final GlobalKey _contentKey = GlobalKey();
@override
bool get wantKeepAlive => (_moveController?.isAnimating ?? false) || (_resizeController?.isAnimating ?? false);
@override
void dispose() {
_moveController!.dispose();
_resizeController?.dispose();
super.dispose();
}
bool get _directionIsXAxis {
return widget.direction == DismissDirection.horizontal
|| widget.direction == DismissDirection.endToStart
|| widget.direction == DismissDirection.startToEnd;
}
DismissDirection _extentToDirection(double extent) {
if (extent == 0.0) {
return DismissDirection.none;
}
if (_directionIsXAxis) {
return switch (Directionality.of(context)) {
TextDirection.rtl when extent < 0 => DismissDirection.startToEnd,
TextDirection.ltr when extent > 0 => DismissDirection.startToEnd,
TextDirection.rtl || TextDirection.ltr => DismissDirection.endToStart,
};
}
return extent > 0 ? DismissDirection.down : DismissDirection.up;
}
DismissDirection get _dismissDirection => _extentToDirection(_dragExtent);
bool get _isActive {
return _dragUnderway || _moveController!.isAnimating;
}
double get _overallDragAxisExtent {
final Size size = context.size!;
return _directionIsXAxis ? size.width : size.height;
}
void _handleDragStart(DragStartDetails details) {
if (_confirming) {
return;
}
_dragUnderway = true;
if (_moveController!.isAnimating) {
_dragExtent = _moveController!.value * _overallDragAxisExtent * _dragExtent.sign;
_moveController!.stop();
} else {
_dragExtent = 0.0;
_moveController!.value = 0.0;
}
setState(() {
_updateMoveAnimation();
});
}
void _handleDragUpdate(DragUpdateDetails details) {
if (!_isActive || _moveController!.isAnimating) {
return;
}
final double delta = details.primaryDelta!;
final double oldDragExtent = _dragExtent;
switch (widget.direction) {
case DismissDirection.horizontal:
case DismissDirection.vertical:
_dragExtent += delta;
case DismissDirection.up:
if (_dragExtent + delta < 0) {
_dragExtent += delta;
}
case DismissDirection.down:
if (_dragExtent + delta > 0) {
_dragExtent += delta;
}
case DismissDirection.endToStart:
switch (Directionality.of(context)) {
case TextDirection.rtl:
if (_dragExtent + delta > 0) {
_dragExtent += delta;
}
case TextDirection.ltr:
if (_dragExtent + delta < 0) {
_dragExtent += delta;
}
}
case DismissDirection.startToEnd:
switch (Directionality.of(context)) {
case TextDirection.rtl:
if (_dragExtent + delta < 0) {
_dragExtent += delta;
}
case TextDirection.ltr:
if (_dragExtent + delta > 0) {
_dragExtent += delta;
}
}
case DismissDirection.none:
_dragExtent = 0;
}
if (oldDragExtent.sign != _dragExtent.sign) {
setState(() {
_updateMoveAnimation();
});
}
if (!_moveController!.isAnimating) {
_moveController!.value = _dragExtent.abs() / _overallDragAxisExtent;
}
}
void _handleDismissUpdateValueChanged() {
if (widget.onUpdate != null) {
final bool oldDismissThresholdReached = _dismissThresholdReached;
_dismissThresholdReached = _moveController!.value > (widget.dismissThresholds[_dismissDirection] ?? _kDismissThreshold);
final DismissUpdateDetails details = DismissUpdateDetails(
direction: _dismissDirection,
reached: _dismissThresholdReached,
previousReached: oldDismissThresholdReached,
progress: _moveController!.value,
);
widget.onUpdate!(details);
}
}
void _updateMoveAnimation() {
final double end = _dragExtent.sign;
_moveAnimation = _moveController!.drive(
Tween<Offset>(
begin: Offset.zero,
end: _directionIsXAxis
? Offset(end, widget.crossAxisEndOffset)
: Offset(widget.crossAxisEndOffset, end),
),
);
}
_FlingGestureKind _describeFlingGesture(Velocity velocity) {
if (_dragExtent == 0.0) {
// If it was a fling, then it was a fling that was let loose at the exact
// middle of the range (i.e. when there's no displacement). In that case,
// we assume that the user meant to fling it back to the center, as
// opposed to having wanted to drag it out one way, then fling it past the
// center and into and out the other side.
return _FlingGestureKind.none;
}
final double vx = velocity.pixelsPerSecond.dx;
final double vy = velocity.pixelsPerSecond.dy;
DismissDirection flingDirection;
// Verify that the fling is in the generally right direction and fast enough.
if (_directionIsXAxis) {
if (vx.abs() - vy.abs() < _kMinFlingVelocityDelta || vx.abs() < _kMinFlingVelocity) {
return _FlingGestureKind.none;
}
assert(vx != 0.0);
flingDirection = _extentToDirection(vx);
} else {
if (vy.abs() - vx.abs() < _kMinFlingVelocityDelta || vy.abs() < _kMinFlingVelocity) {
return _FlingGestureKind.none;
}
assert(vy != 0.0);
flingDirection = _extentToDirection(vy);
}
if (flingDirection == _dismissDirection) {
return _FlingGestureKind.forward;
}
return _FlingGestureKind.reverse;
}
void _handleDragEnd(DragEndDetails details) {
if (!_isActive || _moveController!.isAnimating) {
return;
}
_dragUnderway = false;
if (_moveController!.isCompleted) {
_handleMoveCompleted();
return;
}
final double flingVelocity = _directionIsXAxis ? details.velocity.pixelsPerSecond.dx : details.velocity.pixelsPerSecond.dy;
switch (_describeFlingGesture(details.velocity)) {
case _FlingGestureKind.forward:
assert(_dragExtent != 0.0);
assert(!_moveController!.isDismissed);
if ((widget.dismissThresholds[_dismissDirection] ?? _kDismissThreshold) >= 1.0) {
_moveController!.reverse();
break;
}
_dragExtent = flingVelocity.sign;
_moveController!.fling(velocity: flingVelocity.abs() * _kFlingVelocityScale);
case _FlingGestureKind.reverse:
assert(_dragExtent != 0.0);
assert(!_moveController!.isDismissed);
_dragExtent = flingVelocity.sign;
_moveController!.fling(velocity: -flingVelocity.abs() * _kFlingVelocityScale);
case _FlingGestureKind.none:
if (!_moveController!.isDismissed) { // we already know it's not completed, we check that above
if (_moveController!.value > (widget.dismissThresholds[_dismissDirection] ?? _kDismissThreshold)) {
_moveController!.forward();
} else {
_moveController!.reverse();
}
}
}
}
Future<void> _handleDismissStatusChanged(AnimationStatus status) async {
if (status == AnimationStatus.completed && !_dragUnderway) {
await _handleMoveCompleted();
}
if (mounted) {
updateKeepAlive();
}
}
Future<void> _handleMoveCompleted() async {
if ((widget.dismissThresholds[_dismissDirection] ?? _kDismissThreshold) >= 1.0) {
_moveController!.reverse();
return;
}
final bool result = await _confirmStartResizeAnimation();
if (mounted) {
if (result) {
_startResizeAnimation();
} else {
_moveController!.reverse();
}
}
}
Future<bool> _confirmStartResizeAnimation() async {
if (widget.confirmDismiss != null) {
_confirming = true;
final DismissDirection direction = _dismissDirection;
try {
return await widget.confirmDismiss!(direction) ?? false;
} finally {
_confirming = false;
}
}
return true;
}
void _startResizeAnimation() {
assert(_moveController!.isCompleted);
assert(_resizeController == null);
assert(_sizePriorToCollapse == null);
if (widget.resizeDuration == null) {
if (widget.onDismissed != null) {
final DismissDirection direction = _dismissDirection;
widget.onDismissed!(direction);
}
} else {
_resizeController = AnimationController(duration: widget.resizeDuration, vsync: this)
..addListener(_handleResizeProgressChanged)
..addStatusListener((AnimationStatus status) => updateKeepAlive());
_resizeController!.forward();
setState(() {
_sizePriorToCollapse = context.size;
_resizeAnimation = _resizeController!.drive(
CurveTween(
curve: _kResizeTimeCurve,
),
).drive(
Tween<double>(
begin: 1.0,
end: 0.0,
),
);
});
}
}
void _handleResizeProgressChanged() {
if (_resizeController!.isCompleted) {
widget.onDismissed?.call(_dismissDirection);
} else {
widget.onResize?.call();
}
}
@override
Widget build(BuildContext context) {
super.build(context); // See AutomaticKeepAliveClientMixin.
assert(!_directionIsXAxis || debugCheckHasDirectionality(context));
Widget? background = widget.background;
if (widget.secondaryBackground != null) {
final DismissDirection direction = _dismissDirection;
if (direction == DismissDirection.endToStart || direction == DismissDirection.up) {
background = widget.secondaryBackground;
}
}
if (_resizeAnimation != null) {
// we've been dragged aside, and are now resizing.
assert(() {
if (_resizeAnimation!.status != AnimationStatus.forward) {
assert(_resizeAnimation!.status == AnimationStatus.completed);
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('A dismissed Dismissible widget is still part of the tree.'),
ErrorHint(
'Make sure to implement the onDismissed handler and to immediately remove the Dismissible '
'widget from the application once that handler has fired.',
),
]);
}
return true;
}());
return SizeTransition(
sizeFactor: _resizeAnimation!,
axis: _directionIsXAxis ? Axis.vertical : Axis.horizontal,
child: SizedBox(
width: _sizePriorToCollapse!.width,
height: _sizePriorToCollapse!.height,
child: background,
),
);
}
Widget content = SlideTransition(
position: _moveAnimation,
child: KeyedSubtree(key: _contentKey, child: widget.child),
);
if (background != null) {
content = Stack(children: <Widget>[
if (!_moveAnimation.isDismissed)
Positioned.fill(
child: ClipRect(
clipper: _DismissibleClipper(
axis: _directionIsXAxis ? Axis.horizontal : Axis.vertical,
moveAnimation: _moveAnimation,
),
child: background,
),
),
content,
]);
}
// If the DismissDirection is none, we do not add drag gestures because the content
// cannot be dragged.
if (widget.direction == DismissDirection.none) {
return content;
}
// We are not resizing but we may be being dragging in widget.direction.
return GestureDetector(
onHorizontalDragStart: _directionIsXAxis ? _handleDragStart : null,
onHorizontalDragUpdate: _directionIsXAxis ? _handleDragUpdate : null,
onHorizontalDragEnd: _directionIsXAxis ? _handleDragEnd : null,
onVerticalDragStart: _directionIsXAxis ? null : _handleDragStart,
onVerticalDragUpdate: _directionIsXAxis ? null : _handleDragUpdate,
onVerticalDragEnd: _directionIsXAxis ? null : _handleDragEnd,
behavior: widget.behavior,
dragStartBehavior: widget.dragStartBehavior,
child: content,
);
}
}
| flutter/packages/flutter/lib/src/widgets/dismissible.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/dismissible.dart",
"repo_id": "flutter",
"token_count": 8591
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
import 'icon_data.dart';
import 'icon_theme.dart';
import 'icon_theme_data.dart';
import 'media_query.dart';
/// A graphical icon widget drawn with a glyph from a font described in
/// an [IconData] such as material's predefined [IconData]s in [Icons].
///
/// Icons are not interactive. For an interactive icon, consider material's
/// [IconButton].
///
/// There must be an ambient [Directionality] widget when using [Icon].
/// Typically this is introduced automatically by the [WidgetsApp] or
/// [MaterialApp].
///
/// This widget assumes that the rendered icon is squared. Non-squared icons may
/// render incorrectly.
///
/// {@tool snippet}
///
/// This example shows how to create a [Row] of [Icon]s in different colors and
/// sizes. The first [Icon] uses a [semanticLabel] to announce in accessibility
/// modes like TalkBack and VoiceOver.
///
/// 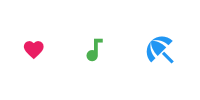
///
/// ```dart
/// const Row(
/// mainAxisAlignment: MainAxisAlignment.spaceAround,
/// children: <Widget>[
/// Icon(
/// Icons.favorite,
/// color: Colors.pink,
/// size: 24.0,
/// semanticLabel: 'Text to announce in accessibility modes',
/// ),
/// Icon(
/// Icons.audiotrack,
/// color: Colors.green,
/// size: 30.0,
/// ),
/// Icon(
/// Icons.beach_access,
/// color: Colors.blue,
/// size: 36.0,
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [IconButton], for interactive icons.
/// * [Icons], for the list of available Material Icons for use with this class.
/// * [IconTheme], which provides ambient configuration for icons.
/// * [ImageIcon], for showing icons from [AssetImage]s or other [ImageProvider]s.
class Icon extends StatelessWidget {
/// Creates an icon.
const Icon(
this.icon, {
super.key,
this.size,
this.fill,
this.weight,
this.grade,
this.opticalSize,
this.color,
this.shadows,
this.semanticLabel,
this.textDirection,
this.applyTextScaling,
}) : assert(fill == null || (0.0 <= fill && fill <= 1.0)),
assert(weight == null || (0.0 < weight)),
assert(opticalSize == null || (0.0 < opticalSize));
/// The icon to display. The available icons are described in [Icons].
///
/// The icon can be null, in which case the widget will render as an empty
/// space of the specified [size].
final IconData? icon;
/// The size of the icon in logical pixels.
///
/// Icons occupy a square with width and height equal to size.
///
/// Defaults to the nearest [IconTheme]'s [IconThemeData.size].
///
/// If this [Icon] is being placed inside an [IconButton], then use
/// [IconButton.iconSize] instead, so that the [IconButton] can make the splash
/// area the appropriate size as well. The [IconButton] uses an [IconTheme] to
/// pass down the size to the [Icon].
final double? size;
/// The fill for drawing the icon.
///
/// Requires the underlying icon font to support the `FILL` [FontVariation]
/// axis, otherwise has no effect. Variable font filenames often indicate
/// the supported axes. Must be between 0.0 (unfilled) and 1.0 (filled),
/// inclusive.
///
/// Can be used to convey a state transition for animation or interaction.
///
/// Defaults to nearest [IconTheme]'s [IconThemeData.fill].
///
/// See also:
/// * [weight], for controlling stroke weight.
/// * [grade], for controlling stroke weight in a more granular way.
/// * [opticalSize], for controlling optical size.
final double? fill;
/// The stroke weight for drawing the icon.
///
/// Requires the underlying icon font to support the `wght` [FontVariation]
/// axis, otherwise has no effect. Variable font filenames often indicate
/// the supported axes. Must be greater than 0.
///
/// Defaults to nearest [IconTheme]'s [IconThemeData.weight].
///
/// See also:
/// * [fill], for controlling fill.
/// * [grade], for controlling stroke weight in a more granular way.
/// * [opticalSize], for controlling optical size.
/// * https://fonts.google.com/knowledge/glossary/weight_axis
final double? weight;
/// The grade (granular stroke weight) for drawing the icon.
///
/// Requires the underlying icon font to support the `GRAD` [FontVariation]
/// axis, otherwise has no effect. Variable font filenames often indicate
/// the supported axes. Can be negative.
///
/// Grade and [weight] both affect a symbol's stroke weight (thickness), but
/// grade has a smaller impact on the size of the symbol.
///
/// Grade is also available in some text fonts. One can match grade levels
/// between text and symbols for a harmonious visual effect. For example, if
/// the text font has a -25 grade value, the symbols can match it with a
/// suitable value, say -25.
///
/// Defaults to nearest [IconTheme]'s [IconThemeData.grade].
///
/// See also:
/// * [fill], for controlling fill.
/// * [weight], for controlling stroke weight in a less granular way.
/// * [opticalSize], for controlling optical size.
/// * https://fonts.google.com/knowledge/glossary/grade_axis
final double? grade;
/// The optical size for drawing the icon.
///
/// Requires the underlying icon font to support the `opsz` [FontVariation]
/// axis, otherwise has no effect. Variable font filenames often indicate
/// the supported axes. Must be greater than 0.
///
/// For an icon to look the same at different sizes, the stroke weight
/// (thickness) must change as the icon size scales. Optical size offers a way
/// to automatically adjust the stroke weight as icon size changes.
///
/// Defaults to nearest [IconTheme]'s [IconThemeData.opticalSize].
///
/// See also:
/// * [fill], for controlling fill.
/// * [weight], for controlling stroke weight.
/// * [grade], for controlling stroke weight in a more granular way.
/// * https://fonts.google.com/knowledge/glossary/optical_size_axis
final double? opticalSize;
/// The color to use when drawing the icon.
///
/// Defaults to the nearest [IconTheme]'s [IconThemeData.color].
///
/// The color (whether specified explicitly here or obtained from the
/// [IconTheme]) will be further adjusted by the nearest [IconTheme]'s
/// [IconThemeData.opacity].
///
/// {@tool snippet}
/// Typically, a Material Design color will be used, as follows:
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.blue.shade400,
/// )
/// ```
/// {@end-tool}
final Color? color;
/// A list of [Shadow]s that will be painted underneath the icon.
///
/// Multiple shadows are supported to replicate lighting from multiple light
/// sources.
///
/// Shadows must be in the same order for [Icon] to be considered as
/// equivalent as order produces differing transparency.
///
/// Defaults to the nearest [IconTheme]'s [IconThemeData.shadows].
final List<Shadow>? shadows;
/// Semantic label for the icon.
///
/// Announced in accessibility modes (e.g TalkBack/VoiceOver).
/// This label does not show in the UI.
///
/// * [SemanticsProperties.label], which is set to [semanticLabel] in the
/// underlying [Semantics] widget.
final String? semanticLabel;
/// The text direction to use for rendering the icon.
///
/// If this is null, the ambient [Directionality] is used instead.
///
/// Some icons follow the reading direction. For example, "back" buttons point
/// left in left-to-right environments and right in right-to-left
/// environments. Such icons have their [IconData.matchTextDirection] field
/// set to true, and the [Icon] widget uses the [textDirection] to determine
/// the orientation in which to draw the icon.
///
/// This property has no effect if the [icon]'s [IconData.matchTextDirection]
/// field is false, but for consistency a text direction value must always be
/// specified, either directly using this property or using [Directionality].
final TextDirection? textDirection;
/// Whether to scale the size of this widget using the ambient [MediaQuery]'s [TextScaler].
///
/// This is specially useful when you have an icon associated with a text, as
/// scaling the text without scaling the icon would result in a confusing
/// interface.
///
/// Defaults to the nearest [IconTheme]'s
/// [IconThemeData.applyTextScaling].
final bool? applyTextScaling;
@override
Widget build(BuildContext context) {
assert(this.textDirection != null || debugCheckHasDirectionality(context));
final TextDirection textDirection = this.textDirection ?? Directionality.of(context);
final IconThemeData iconTheme = IconTheme.of(context);
final bool applyTextScaling = this.applyTextScaling ?? iconTheme.applyTextScaling ?? false;
final double tentativeIconSize = size ?? iconTheme.size ?? kDefaultFontSize;
final double iconSize = applyTextScaling ? MediaQuery.textScalerOf(context).scale(tentativeIconSize) : tentativeIconSize;
final double? iconFill = fill ?? iconTheme.fill;
final double? iconWeight = weight ?? iconTheme.weight;
final double? iconGrade = grade ?? iconTheme.grade;
final double? iconOpticalSize = opticalSize ?? iconTheme.opticalSize;
final List<Shadow>? iconShadows = shadows ?? iconTheme.shadows;
final IconData? icon = this.icon;
if (icon == null) {
return Semantics(
label: semanticLabel,
child: SizedBox(width: iconSize, height: iconSize),
);
}
final double iconOpacity = iconTheme.opacity ?? 1.0;
Color iconColor = color ?? iconTheme.color!;
if (iconOpacity != 1.0) {
iconColor = iconColor.withOpacity(iconColor.opacity * iconOpacity);
}
final TextStyle fontStyle = TextStyle(
fontVariations: <FontVariation>[
if (iconFill != null) FontVariation('FILL', iconFill),
if (iconWeight != null) FontVariation('wght', iconWeight),
if (iconGrade != null) FontVariation('GRAD', iconGrade),
if (iconOpticalSize != null) FontVariation('opsz', iconOpticalSize),
],
inherit: false,
color: iconColor,
fontSize: iconSize,
fontFamily: icon.fontFamily,
package: icon.fontPackage,
fontFamilyFallback: icon.fontFamilyFallback,
shadows: iconShadows,
height: 1.0, // Makes sure the font's body is vertically centered within the iconSize x iconSize square.
leadingDistribution: TextLeadingDistribution.even,
);
Widget iconWidget = RichText(
overflow: TextOverflow.visible, // Never clip.
textDirection: textDirection, // Since we already fetched it for the assert...
text: TextSpan(
text: String.fromCharCode(icon.codePoint),
style: fontStyle,
),
);
if (icon.matchTextDirection) {
switch (textDirection) {
case TextDirection.rtl:
iconWidget = Transform(
transform: Matrix4.identity()..scale(-1.0, 1.0, 1.0),
alignment: Alignment.center,
transformHitTests: false,
child: iconWidget,
);
case TextDirection.ltr:
break;
}
}
return Semantics(
label: semanticLabel,
child: ExcludeSemantics(
child: SizedBox(
width: iconSize,
height: iconSize,
child: Center(
child: iconWidget,
),
),
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IconDataProperty('icon', icon, ifNull: '<empty>', showName: false));
properties.add(DoubleProperty('size', size, defaultValue: null));
properties.add(DoubleProperty('fill', fill, defaultValue: null));
properties.add(DoubleProperty('weight', weight, defaultValue: null));
properties.add(DoubleProperty('grade', grade, defaultValue: null));
properties.add(DoubleProperty('opticalSize', opticalSize, defaultValue: null));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(IterableProperty<Shadow>('shadows', shadows, defaultValue: null));
properties.add(StringProperty('semanticLabel', semanticLabel, defaultValue: null));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('applyTextScaling', applyTextScaling, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/icon.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/icon.dart",
"repo_id": "flutter",
"token_count": 4132
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'framework.dart';
// Examples can assume:
// class MyWidget extends StatelessWidget { const MyWidget({super.key, required this.child}); final Widget child; @override Widget build(BuildContext context) => child; }
/// A lookup boundary controls what entities are visible to descendants of the
/// boundary via the static lookup methods provided by the boundary.
///
/// The static lookup methods of the boundary mirror the lookup methods by the
/// same name exposed on [BuildContext] and they can be used as direct
/// replacements. Unlike the methods on [BuildContext], these methods do not
/// find any ancestor entities of the closest [LookupBoundary] surrounding the
/// provided [BuildContext]. The root of the tree is an implicit lookup boundary.
///
/// {@tool snippet}
/// In the example below, the [LookupBoundary.findAncestorWidgetOfExactType]
/// call returns null because the [LookupBoundary] "hides" `MyWidget` from the
/// [BuildContext] that was queried.
///
/// ```dart
/// MyWidget(
/// child: LookupBoundary(
/// child: Builder(
/// builder: (BuildContext context) {
/// MyWidget? widget = LookupBoundary.findAncestorWidgetOfExactType<MyWidget>(context);
/// return Text('$widget'); // "null"
/// },
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// A [LookupBoundary] only affects the behavior of the static lookup methods
/// defined on the boundary. It does not affect the behavior of the lookup
/// methods defined on [BuildContext].
///
/// A [LookupBoundary] is rarely instantiated directly. They are inserted at
/// locations of the widget tree where the render tree diverges from the element
/// tree, which is rather uncommon. Such anomalies are created by
/// [RenderObjectElement]s that don't attach their [RenderObject] to the closest
/// ancestor [RenderObjectElement], e.g. because they bootstrap a separate
/// stand-alone render tree.
// TODO(goderbauer): Reference the View widget here once available.
/// This behavior breaks the assumption some widgets have about the structure of
/// the render tree: These widgets may try to reach out to an ancestor widget,
/// assuming that their associated [RenderObject]s are also ancestors, which due
/// to the anomaly may not be the case. At the point where the divergence in the
/// two trees is introduced, a [LookupBoundary] can be used to hide that ancestor
/// from the querying widget.
///
/// As an example, [Material.of] relies on lookup boundaries to hide the
/// [Material] widget from certain descendant button widget. Buttons reach out
/// to their [Material] ancestor to draw ink splashes on its associated render
/// object. This only produces the desired effect if the button render object
/// is a descendant of the [Material] render object. If the element tree and
/// the render tree are not in sync due to anomalies described above, this may
/// not be the case. To avoid incorrect visuals, the [Material] relies on
/// lookup boundaries to hide itself from descendants in subtrees with such
/// anomalies. Those subtrees are expected to introduce their own [Material]
/// widget that buttons there can utilize without crossing a lookup boundary.
class LookupBoundary extends InheritedWidget {
/// Creates a [LookupBoundary].
///
/// A none-null [child] widget must be provided.
const LookupBoundary({super.key, required super.child});
/// Obtains the nearest widget of the given type `T` within the current
/// [LookupBoundary] of `context`, which must be the type of a concrete
/// [InheritedWidget] subclass, and registers the provided build `context`
/// with that widget such that when that widget changes (or a new widget of
/// that type is introduced, or the widget goes away), the build context is
/// rebuilt so that it can obtain new values from that widget.
///
/// This method behaves exactly like
/// [BuildContext.dependOnInheritedWidgetOfExactType], except it only
/// considers [InheritedWidget]s of the specified type `T` between the
/// provided [BuildContext] and its closest [LookupBoundary] ancestor.
/// [InheritedWidget]s past that [LookupBoundary] are invisible to this
/// method. The root of the tree is treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.dependOnInheritedWidgetOfExactType}
static T? dependOnInheritedWidgetOfExactType<T extends InheritedWidget>(BuildContext context, { Object? aspect }) {
// The following call makes sure that context depends on something so
// Element.didChangeDependencies is called when context moves in the tree
// even when requested dependency remains unfulfilled (i.e. null is
// returned).
context.dependOnInheritedWidgetOfExactType<LookupBoundary>();
final InheritedElement? candidate = getElementForInheritedWidgetOfExactType<T>(context);
if (candidate == null) {
return null;
}
context.dependOnInheritedElement(candidate, aspect: aspect);
return candidate.widget as T;
}
/// Obtains the element corresponding to the nearest widget of the given type
/// `T` within the current [LookupBoundary] of `context`.
///
/// `T` must be the type of a concrete [InheritedWidget] subclass. Returns
/// null if no such element is found.
///
/// This method behaves exactly like
/// [BuildContext.getElementForInheritedWidgetOfExactType], except it only
/// considers [InheritedWidget]s of the specified type `T` between the
/// provided [BuildContext] and its closest [LookupBoundary] ancestor.
/// [InheritedWidget]s past that [LookupBoundary] are invisible to this
/// method. The root of the tree is treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.getElementForInheritedWidgetOfExactType}
static InheritedElement? getElementForInheritedWidgetOfExactType<T extends InheritedWidget>(BuildContext context) {
final InheritedElement? candidate = context.getElementForInheritedWidgetOfExactType<T>();
if (candidate == null) {
return null;
}
final Element? boundary = context.getElementForInheritedWidgetOfExactType<LookupBoundary>();
if (boundary != null && boundary.depth > candidate.depth) {
return null;
}
return candidate;
}
/// Returns the nearest ancestor widget of the given type `T` within the
/// current [LookupBoundary] of `context`.
///
/// `T` must be the type of a concrete [Widget] subclass.
///
/// This method behaves exactly like
/// [BuildContext.findAncestorWidgetOfExactType], except it only considers
/// [Widget]s of the specified type `T` between the provided [BuildContext]
/// and its closest [LookupBoundary] ancestor. [Widget]s past that
/// [LookupBoundary] are invisible to this method. The root of the tree is
/// treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.findAncestorWidgetOfExactType}
static T? findAncestorWidgetOfExactType<T extends Widget>(BuildContext context) {
Element? target;
context.visitAncestorElements((Element ancestor) {
if (ancestor.widget.runtimeType == T) {
target = ancestor;
return false;
}
return ancestor.widget.runtimeType != LookupBoundary;
});
return target?.widget as T?;
}
/// Returns the [State] object of the nearest ancestor [StatefulWidget] widget
/// within the current [LookupBoundary] of `context` that is an instance of
/// the given type `T`.
///
/// This method behaves exactly like
/// [BuildContext.findAncestorWidgetOfExactType], except it only considers
/// [State] objects of the specified type `T` between the provided
/// [BuildContext] and its closest [LookupBoundary] ancestor. [State] objects
/// past that [LookupBoundary] are invisible to this method. The root of the
/// tree is treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.findAncestorStateOfType}
static T? findAncestorStateOfType<T extends State>(BuildContext context) {
StatefulElement? target;
context.visitAncestorElements((Element ancestor) {
if (ancestor is StatefulElement && ancestor.state is T) {
target = ancestor;
return false;
}
return ancestor.widget.runtimeType != LookupBoundary;
});
return target?.state as T?;
}
/// Returns the [State] object of the furthest ancestor [StatefulWidget]
/// widget within the current [LookupBoundary] of `context` that is an
/// instance of the given type `T`.
///
/// This method behaves exactly like
/// [BuildContext.findRootAncestorStateOfType], except it considers the
/// closest [LookupBoundary] ancestor of `context` to be the root. [State]
/// objects past that [LookupBoundary] are invisible to this method. The root
/// of the tree is treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.findRootAncestorStateOfType}
static T? findRootAncestorStateOfType<T extends State>(BuildContext context) {
StatefulElement? target;
context.visitAncestorElements((Element ancestor) {
if (ancestor is StatefulElement && ancestor.state is T) {
target = ancestor;
}
return ancestor.widget.runtimeType != LookupBoundary;
});
return target?.state as T?;
}
/// Returns the [RenderObject] object of the nearest ancestor
/// [RenderObjectWidget] widget within the current [LookupBoundary] of
/// `context` that is an instance of the given type `T`.
///
/// This method behaves exactly like
/// [BuildContext.findAncestorRenderObjectOfType], except it only considers
/// [RenderObject]s of the specified type `T` between the provided
/// [BuildContext] and its closest [LookupBoundary] ancestor. [RenderObject]s
/// past that [LookupBoundary] are invisible to this method. The root of the
/// tree is treated as an implicit lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.findAncestorRenderObjectOfType}
static T? findAncestorRenderObjectOfType<T extends RenderObject>(BuildContext context) {
Element? target;
context.visitAncestorElements((Element ancestor) {
if (ancestor is RenderObjectElement && ancestor.renderObject is T) {
target = ancestor;
return false;
}
return ancestor.widget.runtimeType != LookupBoundary;
});
return target?.renderObject as T?;
}
/// Walks the ancestor chain, starting with the parent of the build context's
/// widget, invoking the argument for each ancestor until a [LookupBoundary]
/// or the root is reached.
///
/// This method behaves exactly like [BuildContext.visitAncestorElements],
/// except it only walks the tree up to the closest [LookupBoundary] ancestor
/// of the provided context. The root of the tree is treated as an implicit
/// lookup boundary.
///
/// {@macro flutter.widgets.BuildContext.visitAncestorElements}
static void visitAncestorElements(BuildContext context, ConditionalElementVisitor visitor) {
context.visitAncestorElements((Element ancestor) {
return visitor(ancestor) && ancestor.widget.runtimeType != LookupBoundary;
});
}
/// Walks the non-[LookupBoundary] child [Element]s of the provided
/// `context`.
///
/// This method behaves exactly like [BuildContext.visitChildElements],
/// except it only visits children that are not a [LookupBoundary].
///
/// {@macro flutter.widgets.BuildContext.visitChildElements}
static void visitChildElements(BuildContext context, ElementVisitor visitor) {
context.visitChildElements((Element child) {
if (child.widget.runtimeType != LookupBoundary) {
visitor(child);
}
});
}
/// Returns true if a [LookupBoundary] is hiding the nearest
/// [Widget] of the specified type `T` from the provided [BuildContext].
///
/// This method throws when asserts are disabled.
static bool debugIsHidingAncestorWidgetOfExactType<T extends Widget>(BuildContext context) {
bool? result;
assert(() {
bool hiddenByBoundary = false;
bool ancestorFound = false;
context.visitAncestorElements((Element ancestor) {
if (ancestor.widget.runtimeType == T) {
ancestorFound = true;
return false;
}
hiddenByBoundary = hiddenByBoundary || ancestor.widget.runtimeType == LookupBoundary;
return true;
});
result = ancestorFound & hiddenByBoundary;
return true;
} ());
return result!;
}
/// Returns true if a [LookupBoundary] is hiding the nearest [StatefulWidget]
/// with a [State] of the specified type `T` from the provided [BuildContext].
///
/// This method throws when asserts are disabled.
static bool debugIsHidingAncestorStateOfType<T extends State>(BuildContext context) {
bool? result;
assert(() {
bool hiddenByBoundary = false;
bool ancestorFound = false;
context.visitAncestorElements((Element ancestor) {
if (ancestor is StatefulElement && ancestor.state is T) {
ancestorFound = true;
return false;
}
hiddenByBoundary = hiddenByBoundary || ancestor.widget.runtimeType == LookupBoundary;
return true;
});
result = ancestorFound & hiddenByBoundary;
return true;
} ());
return result!;
}
/// Returns true if a [LookupBoundary] is hiding the nearest
/// [RenderObjectWidget] with a [RenderObject] of the specified type `T`
/// from the provided [BuildContext].
///
/// This method throws when asserts are disabled.
static bool debugIsHidingAncestorRenderObjectOfType<T extends RenderObject>(BuildContext context) {
bool? result;
assert(() {
bool hiddenByBoundary = false;
bool ancestorFound = false;
context.visitAncestorElements((Element ancestor) {
if (ancestor is RenderObjectElement && ancestor.renderObject is T) {
ancestorFound = true;
return false;
}
hiddenByBoundary = hiddenByBoundary || ancestor.widget.runtimeType == LookupBoundary;
return true;
});
result = ancestorFound & hiddenByBoundary;
return true;
} ());
return result!;
}
@override
bool updateShouldNotify(covariant InheritedWidget oldWidget) => false;
}
| flutter/packages/flutter/lib/src/widgets/lookup_boundary.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/lookup_boundary.dart",
"repo_id": "flutter",
"token_count": 4308
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
/// Displays performance statistics.
///
/// The overlay shows two time series. The first shows how much time was
/// required on this thread to produce each frame. The second shows how much
/// time was required on the raster thread (formerly known as the GPU thread)
/// to produce each frame. Ideally, both these values would be less than
/// the total frame budget for the hardware on which the app is running.
/// For example, if the hardware has a screen that updates at 60 Hz, each
/// thread should ideally spend less than 16ms producing each frame.
/// This ideal condition is indicated by a green vertical line for each thread.
/// Otherwise, the performance overlay shows a red vertical line.
///
/// The simplest way to show the performance overlay is to set
/// [MaterialApp.showPerformanceOverlay] or [WidgetsApp.showPerformanceOverlay]
/// to true.
class PerformanceOverlay extends LeafRenderObjectWidget {
// TODO(abarth): We should have a page on the web site with a screenshot and
// an explanation of all the various readouts.
/// Create a performance overlay that only displays specific statistics. The
/// mask is created by shifting 1 by the index of the specific
/// [PerformanceOverlayOption] to enable.
const PerformanceOverlay({
super.key,
this.optionsMask = 0,
this.rasterizerThreshold = 0,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
});
/// Create a performance overlay that displays all available statistics.
PerformanceOverlay.allEnabled({
super.key,
this.rasterizerThreshold = 0,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
}) : optionsMask =
1 << PerformanceOverlayOption.displayRasterizerStatistics.index |
1 << PerformanceOverlayOption.visualizeRasterizerStatistics.index |
1 << PerformanceOverlayOption.displayEngineStatistics.index |
1 << PerformanceOverlayOption.visualizeEngineStatistics.index;
/// The mask is created by shifting 1 by the index of the specific
/// [PerformanceOverlayOption] to enable.
final int optionsMask;
/// The rasterizer threshold is an integer specifying the number of frame
/// intervals that the rasterizer must miss before it decides that the frame
/// is suitable for capturing an SkPicture trace for further analysis.
///
/// For example, if you want a trace of all pictures that could not be
/// rendered by the rasterizer within the frame boundary (and hence caused
/// jank), specify 1. Specifying 2 will trace all pictures that took more
/// than 2 frame intervals to render. Adjust this value to only capture
/// the particularly expensive pictures while skipping the others. Specifying
/// 0 disables all capture.
///
/// Captured traces are placed on your device in the application documents
/// directory in this form "trace_<collection_time>.skp". These can
/// be viewed in the Skia debugger.
///
/// Notes:
/// The rasterizer only takes into account the time it took to render
/// the already constructed picture. This include the Skia calls (which is
/// also why an SkPicture trace is generated) but not any of the time spent in
/// dart to construct that picture. To profile that part of your code, use
/// the instrumentation available in observatory.
///
/// To decide what threshold interval to use, count the number of horizontal
/// lines displayed in the performance overlay for the rasterizer (not the
/// engine). That should give an idea of how often frames are skipped (and by
/// how many frame intervals).
final int rasterizerThreshold;
/// Whether the raster cache should checkerboard cached entries.
///
/// The compositor can sometimes decide to cache certain portions of the
/// widget hierarchy. Such portions typically don't change often from frame to
/// frame and are expensive to render. This can speed up overall rendering. However,
/// there is certain upfront cost to constructing these cache entries. And, if
/// the cache entries are not used very often, this cost may not be worth the
/// speedup in rendering of subsequent frames. If the developer wants to be certain
/// that populating the raster cache is not causing stutters, this option can be
/// set. Depending on the observations made, hints can be provided to the compositor
/// that aid it in making better decisions about caching.
final bool checkerboardRasterCacheImages;
/// Whether the compositor should checkerboard layers that are rendered to offscreen
/// bitmaps. This can be useful for debugging rendering performance.
///
/// Render target switches are caused by using opacity layers (via a [FadeTransition] or
/// [Opacity] widget), clips, shader mask layers, etc. Selecting a new render target
/// and merging it with the rest of the scene has a performance cost. This can sometimes
/// be avoided by using equivalent widgets that do not require these layers (for example,
/// replacing an [Opacity] widget with an [widgets.Image] using a [BlendMode]).
final bool checkerboardOffscreenLayers;
@override
RenderPerformanceOverlay createRenderObject(BuildContext context) => RenderPerformanceOverlay(
optionsMask: optionsMask,
rasterizerThreshold: rasterizerThreshold,
checkerboardRasterCacheImages: checkerboardRasterCacheImages,
checkerboardOffscreenLayers: checkerboardOffscreenLayers,
);
@override
void updateRenderObject(BuildContext context, RenderPerformanceOverlay renderObject) {
renderObject
..optionsMask = optionsMask
..rasterizerThreshold = rasterizerThreshold
..checkerboardRasterCacheImages = checkerboardRasterCacheImages
..checkerboardOffscreenLayers = checkerboardOffscreenLayers;
}
}
| flutter/packages/flutter/lib/src/widgets/performance_overlay.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/performance_overlay.dart",
"repo_id": "flutter",
"token_count": 1534
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/painting.dart';
import 'package:flutter/scheduler.dart';
import 'disposable_build_context.dart';
import 'framework.dart';
import 'scrollable.dart';
/// An [ImageProvider] that makes use of
/// [Scrollable.recommendDeferredLoadingForContext] to avoid loading images when
/// rapidly scrolling.
///
/// This provider assumes that its wrapped [imageProvider] correctly uses the
/// [ImageCache], and does not attempt to re-acquire or decode images in the
/// cache.
///
/// Calling [resolve] on this provider will cause it to obtain the image key
/// and then check the following:
///
/// 1. If the returned [ImageStream] has been completed, end. This can happen
/// if the caller sets the completer on the stream.
/// 2. If the [ImageCache] has a completer for the key for this image, ask the
/// wrapped provider to resolve.
/// This can happen if the image was precached, or another [ImageProvider]
/// already resolved the same image.
/// 3. If the [context] has been disposed, end. This can happen if the caller
/// has been disposed and is no longer interested in resolving the image.
/// 4. If the widget is scrolling with high velocity at this point in time,
/// wait until the beginning of the next frame and go back to step 1.
/// 5. Delegate loading the image to the wrapped provider and finish.
///
/// If the cycle ends at steps 1 or 3, the [ImageStream] will never be marked as
/// complete and listeners will not be notified.
///
/// The [Image] widget wraps its incoming providers with this provider to avoid
/// overutilization of resources for images that would never appear on screen or
/// only be visible for a very brief period.
@optionalTypeArgs
class ScrollAwareImageProvider<T extends Object> extends ImageProvider<T> {
/// Creates a [ScrollAwareImageProvider].
///
/// The [context] object is the [BuildContext] of the [State] using this
/// provider. It is used to determine scrolling velocity during [resolve].
///
/// The [imageProvider] is used to create a key and load the image. It must
/// not be null, and is assumed to interact with the cache in the normal way
/// that [ImageProvider.resolveStreamForKey] does.
const ScrollAwareImageProvider({
required this.context,
required this.imageProvider,
});
/// The context that may or may not be enclosed by a [Scrollable].
///
/// Once [DisposableBuildContext.dispose] is called on this context,
/// the provider will stop trying to resolve the image if it has not already
/// been resolved.
final DisposableBuildContext context;
/// The wrapped image provider to delegate [obtainKey] and [loadImage] to.
final ImageProvider<T> imageProvider;
@override
void resolveStreamForKey(
ImageConfiguration configuration,
ImageStream stream,
T key,
ImageErrorListener handleError,
) {
// Something managed to complete the stream, or it's already in the image
// cache. Notify the wrapped provider and expect it to behave by not
// reloading the image since it's already resolved.
// Do this even if the context has gone out of the tree, since it will
// update LRU information about the cache. Even though we never showed the
// image, it was still touched more recently.
// Do this before checking scrolling, so that if the bytes are available we
// render them even though we're scrolling fast - there's no additional
// allocations to do for texture memory, it's already there.
if (stream.completer != null || PaintingBinding.instance.imageCache.containsKey(key)) {
imageProvider.resolveStreamForKey(configuration, stream, key, handleError);
return;
}
// The context has gone out of the tree - ignore it.
if (context.context == null) {
return;
}
// Something still wants this image, but check if the context is scrolling
// too fast before scheduling work that might never show on screen.
// Try to get to end of the frame callbacks of the next frame, and then
// check again.
if (Scrollable.recommendDeferredLoadingForContext(context.context!)) {
SchedulerBinding.instance.scheduleFrameCallback((_) {
scheduleMicrotask(() => resolveStreamForKey(configuration, stream, key, handleError));
});
return;
}
// We are in the tree, we're not scrolling too fast, the cache doesn't
// have our image, and no one has otherwise completed the stream. Go.
imageProvider.resolveStreamForKey(configuration, stream, key, handleError);
}
@override
ImageStreamCompleter loadBuffer(T key, DecoderBufferCallback decode) => imageProvider.loadBuffer(key, decode);
@override
ImageStreamCompleter loadImage(T key, ImageDecoderCallback decode) => imageProvider.loadImage(key, decode);
@override
Future<T> obtainKey(ImageConfiguration configuration) => imageProvider.obtainKey(configuration);
}
| flutter/packages/flutter/lib/src/widgets/scroll_aware_image_provider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_aware_image_provider.dart",
"repo_id": "flutter",
"token_count": 1437
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'package:vector_math/vector_math_64.dart';
import 'actions.dart';
import 'basic.dart';
import 'context_menu_button_item.dart';
import 'debug.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'magnifier.dart';
import 'media_query.dart';
import 'overlay.dart';
import 'platform_selectable_region_context_menu.dart';
import 'selection_container.dart';
import 'text_editing_intents.dart';
import 'text_selection.dart';
import 'text_selection_toolbar_anchors.dart';
// Examples can assume:
// FocusNode _focusNode = FocusNode();
// late GlobalKey key;
const Set<PointerDeviceKind> _kLongPressSelectionDevices = <PointerDeviceKind>{
PointerDeviceKind.touch,
PointerDeviceKind.stylus,
PointerDeviceKind.invertedStylus,
};
// In practice some selectables like widgetspan shift several pixels. So when
// the vertical position diff is within the threshold, compare the horizontal
// position to make the compareScreenOrder function more robust.
const double _kSelectableVerticalComparingThreshold = 3.0;
/// A widget that introduces an area for user selections.
///
/// Flutter widgets are not selectable by default. Wrapping a widget subtree
/// with a [SelectableRegion] widget enables selection within that subtree (for
/// example, [Text] widgets automatically look for selectable regions to enable
/// selection). The wrapped subtree can be selected by users using mouse or
/// touch gestures, e.g. users can select widgets by holding the mouse
/// left-click and dragging across widgets, or they can use long press gestures
/// to select words on touch devices.
///
/// A [SelectableRegion] widget requires configuration; in particular specific
/// [selectionControls] must be provided.
///
/// The [SelectionArea] widget from the [material] library configures a
/// [SelectableRegion] in a platform-specific manner (e.g. using a Material
/// toolbar on Android, a Cupertino toolbar on iOS), and it may therefore be
/// simpler to use that widget rather than using [SelectableRegion] directly.
///
/// ## An overview of the selection system.
///
/// Every [Selectable] under the [SelectableRegion] can be selected. They form a
/// selection tree structure to handle the selection.
///
/// The [SelectableRegion] is a wrapper over [SelectionContainer]. It listens to
/// user gestures and sends corresponding [SelectionEvent]s to the
/// [SelectionContainer] it creates.
///
/// A [SelectionContainer] is a single [Selectable] that handles
/// [SelectionEvent]s on behalf of child [Selectable]s in the subtree. It
/// creates a [SelectionRegistrarScope] with its [SelectionContainer.delegate]
/// to collect child [Selectable]s and sends the [SelectionEvent]s it receives
/// from the parent [SelectionRegistrar] to the appropriate child [Selectable]s.
/// It creates an abstraction for the parent [SelectionRegistrar] as if it is
/// interacting with a single [Selectable].
///
/// The [SelectionContainer] created by [SelectableRegion] is the root node of a
/// selection tree. Each non-leaf node in the tree is a [SelectionContainer],
/// and the leaf node is a leaf widget whose render object implements
/// [Selectable]. They are connected through [SelectionRegistrarScope]s created
/// by [SelectionContainer]s.
///
/// Both [SelectionContainer]s and the leaf [Selectable]s need to register
/// themselves to the [SelectionRegistrar] from the
/// [SelectionContainer.maybeOf] if they want to participate in the
/// selection.
///
/// An example selection tree will look like:
///
/// {@tool snippet}
///
/// ```dart
/// MaterialApp(
/// home: SelectableRegion(
/// selectionControls: materialTextSelectionControls,
/// focusNode: _focusNode, // initialized to FocusNode()
/// child: Scaffold(
/// appBar: AppBar(title: const Text('Flutter Code Sample')),
/// body: ListView(
/// children: const <Widget>[
/// Text('Item 0', style: TextStyle(fontSize: 50.0)),
/// Text('Item 1', style: TextStyle(fontSize: 50.0)),
/// ],
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
///
/// SelectionContainer
/// (SelectableRegion)
/// / \
/// / \
/// / \
/// Selectable \
/// ("Flutter Code Sample") \
/// \
/// SelectionContainer
/// (ListView)
/// / \
/// / \
/// / \
/// Selectable Selectable
/// ("Item 0") ("Item 1")
///
///
/// ## Making a widget selectable
///
/// Some leaf widgets, such as [Text], have all of the selection logic wired up
/// automatically and can be selected as long as they are under a
/// [SelectableRegion].
///
/// To make a custom selectable widget, its render object needs to mix in
/// [Selectable] and implement the required APIs to handle [SelectionEvent]s
/// as well as paint appropriate selection highlights.
///
/// The render object also needs to register itself to a [SelectionRegistrar].
/// For the most cases, one can use [SelectionRegistrant] to auto-register
/// itself with the register returned from [SelectionContainer.maybeOf] as
/// seen in the example below.
///
/// {@tool dartpad}
/// This sample demonstrates how to create an adapter widget that makes any
/// child widget selectable.
///
/// ** See code in examples/api/lib/material/selectable_region/selectable_region.0.dart **
/// {@end-tool}
///
/// ## Complex layout
///
/// By default, the screen order is used as the selection order. If a group of
/// [Selectable]s needs to select differently, consider wrapping them with a
/// [SelectionContainer] to customize its selection behavior.
///
/// {@tool dartpad}
/// This sample demonstrates how to create a [SelectionContainer] that only
/// allows selecting everything or nothing with no partial selection.
///
/// ** See code in examples/api/lib/material/selection_container/selection_container.0.dart **
/// {@end-tool}
///
/// In the case where a group of widgets should be excluded from selection under
/// a [SelectableRegion], consider wrapping that group of widgets using
/// [SelectionContainer.disabled].
///
/// {@tool dartpad}
/// This sample demonstrates how to disable selection for a Text in a Column.
///
/// ** See code in examples/api/lib/material/selection_container/selection_container_disabled.0.dart **
/// {@end-tool}
///
/// To create a separate selection system from its parent selection area,
/// wrap part of the subtree with another [SelectableRegion]. The selection of the
/// child selection area can not extend past its subtree, and the selection of
/// the parent selection area can not extend inside the child selection area.
///
/// ## Tests
///
/// In a test, a region can be selected either by faking drag events (e.g. using
/// [WidgetTester.dragFrom]) or by sending intents to a widget inside the region
/// that has been given a [GlobalKey], e.g.:
///
/// ```dart
/// Actions.invoke(key.currentContext!, const SelectAllTextIntent(SelectionChangedCause.keyboard));
/// ```
///
/// See also:
///
/// * [SelectionArea], which creates a [SelectableRegion] with
/// platform-adaptive selection controls.
/// * [SelectableText], which enables selection on a single run of text.
/// * [SelectionHandler], which contains APIs to handle selection events from the
/// [SelectableRegion].
/// * [Selectable], which provides API to participate in the selection system.
/// * [SelectionRegistrar], which [Selectable] needs to subscribe to receive
/// selection events.
/// * [SelectionContainer], which collects selectable widgets in the subtree
/// and provides api to dispatch selection event to the collected widget.
class SelectableRegion extends StatefulWidget {
/// Create a new [SelectableRegion] widget.
///
/// The [selectionControls] are used for building the selection handles and
/// toolbar for mobile devices.
const SelectableRegion({
super.key,
this.contextMenuBuilder,
required this.focusNode,
required this.selectionControls,
required this.child,
this.magnifierConfiguration = TextMagnifierConfiguration.disabled,
this.onSelectionChanged,
});
/// The configuration for the magnifier used with selections in this region.
///
/// By default, [SelectableRegion]'s [TextMagnifierConfiguration] is disabled.
/// For a version of [SelectableRegion] that adapts automatically to the
/// current platform, consider [SelectionArea].
///
/// {@macro flutter.widgets.magnifier.intro}
final TextMagnifierConfiguration magnifierConfiguration;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode focusNode;
/// The child widget this selection area applies to.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// {@macro flutter.widgets.EditableText.contextMenuBuilder}
final SelectableRegionContextMenuBuilder? contextMenuBuilder;
/// The delegate to build the selection handles and toolbar for mobile
/// devices.
///
/// The [emptyTextSelectionControls] global variable provides a default
/// [TextSelectionControls] implementation with no controls.
final TextSelectionControls selectionControls;
/// Called when the selected content changes.
final ValueChanged<SelectedContent?>? onSelectionChanged;
/// Returns the [ContextMenuButtonItem]s representing the buttons in this
/// platform's default selection menu.
///
/// For example, [SelectableRegion] uses this to generate the default buttons
/// for its context menu.
///
/// See also:
///
/// * [SelectableRegionState.contextMenuButtonItems], which gives the
/// [ContextMenuButtonItem]s for a specific SelectableRegion.
/// * [EditableText.getEditableButtonItems], which performs a similar role but
/// for content that is both selectable and editable.
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar itself, and can
/// take a list of [ContextMenuButtonItem]s with
/// [AdaptiveTextSelectionToolbar.buttonItems].
/// * [AdaptiveTextSelectionToolbar.getAdaptiveButtons], which builds the button
/// Widgets for the current platform given [ContextMenuButtonItem]s.
static List<ContextMenuButtonItem> getSelectableButtonItems({
required final SelectionGeometry selectionGeometry,
required final VoidCallback onCopy,
required final VoidCallback onSelectAll,
required final VoidCallback? onShare,
}) {
final bool canCopy = selectionGeometry.status == SelectionStatus.uncollapsed;
final bool canSelectAll = selectionGeometry.hasContent;
final bool platformCanShare = switch (defaultTargetPlatform) {
TargetPlatform.android
=> selectionGeometry.status == SelectionStatus.uncollapsed,
TargetPlatform.macOS
|| TargetPlatform.fuchsia
|| TargetPlatform.linux
|| TargetPlatform.windows
=> false,
// TODO(bleroux): the share button should be shown on iOS but the share
// functionality requires some changes on the engine side because, on iPad,
// it needs an anchor for the popup.
// See: https://github.com/flutter/flutter/issues/141775.
TargetPlatform.iOS
=> false,
};
final bool canShare = onShare != null && platformCanShare;
// On Android, the share button is before the select all button.
final bool showShareBeforeSelectAll = defaultTargetPlatform == TargetPlatform.android;
// Determine which buttons will appear so that the order and total number is
// known. A button's position in the menu can slightly affect its
// appearance.
return <ContextMenuButtonItem>[
if (canCopy)
ContextMenuButtonItem(
onPressed: onCopy,
type: ContextMenuButtonType.copy,
),
if (canShare && showShareBeforeSelectAll)
ContextMenuButtonItem(
onPressed: onShare,
type: ContextMenuButtonType.share,
),
if (canSelectAll)
ContextMenuButtonItem(
onPressed: onSelectAll,
type: ContextMenuButtonType.selectAll,
),
if (canShare && !showShareBeforeSelectAll)
ContextMenuButtonItem(
onPressed: onShare,
type: ContextMenuButtonType.share,
),
];
}
@override
State<StatefulWidget> createState() => SelectableRegionState();
}
/// State for a [SelectableRegion].
class SelectableRegionState extends State<SelectableRegion> with TextSelectionDelegate implements SelectionRegistrar {
late final Map<Type, Action<Intent>> _actions = <Type, Action<Intent>>{
SelectAllTextIntent: _makeOverridable(_SelectAllAction(this)),
CopySelectionTextIntent: _makeOverridable(_CopySelectionAction(this)),
ExtendSelectionToNextWordBoundaryOrCaretLocationIntent: _makeOverridable(_GranularlyExtendSelectionAction<ExtendSelectionToNextWordBoundaryOrCaretLocationIntent>(this, granularity: TextGranularity.word)),
ExpandSelectionToDocumentBoundaryIntent: _makeOverridable(_GranularlyExtendSelectionAction<ExpandSelectionToDocumentBoundaryIntent>(this, granularity: TextGranularity.document)),
ExpandSelectionToLineBreakIntent: _makeOverridable(_GranularlyExtendSelectionAction<ExpandSelectionToLineBreakIntent>(this, granularity: TextGranularity.line)),
ExtendSelectionByCharacterIntent: _makeOverridable(_GranularlyExtendCaretSelectionAction<ExtendSelectionByCharacterIntent>(this, granularity: TextGranularity.character)),
ExtendSelectionToNextWordBoundaryIntent: _makeOverridable(_GranularlyExtendCaretSelectionAction<ExtendSelectionToNextWordBoundaryIntent>(this, granularity: TextGranularity.word)),
ExtendSelectionToLineBreakIntent: _makeOverridable(_GranularlyExtendCaretSelectionAction<ExtendSelectionToLineBreakIntent>(this, granularity: TextGranularity.line)),
ExtendSelectionVerticallyToAdjacentLineIntent: _makeOverridable(_DirectionallyExtendCaretSelectionAction<ExtendSelectionVerticallyToAdjacentLineIntent>(this)),
ExtendSelectionToDocumentBoundaryIntent: _makeOverridable(_GranularlyExtendCaretSelectionAction<ExtendSelectionToDocumentBoundaryIntent>(this, granularity: TextGranularity.document)),
};
final Map<Type, GestureRecognizerFactory> _gestureRecognizers = <Type, GestureRecognizerFactory>{};
SelectionOverlay? _selectionOverlay;
final LayerLink _startHandleLayerLink = LayerLink();
final LayerLink _endHandleLayerLink = LayerLink();
final LayerLink _toolbarLayerLink = LayerLink();
final _SelectableRegionContainerDelegate _selectionDelegate = _SelectableRegionContainerDelegate();
// there should only ever be one selectable, which is the SelectionContainer.
Selectable? _selectable;
bool get _hasSelectionOverlayGeometry => _selectionDelegate.value.startSelectionPoint != null
|| _selectionDelegate.value.endSelectionPoint != null;
Orientation? _lastOrientation;
SelectedContent? _lastSelectedContent;
/// {@macro flutter.rendering.RenderEditable.lastSecondaryTapDownPosition}
Offset? lastSecondaryTapDownPosition;
/// The [SelectionOverlay] that is currently visible on the screen.
///
/// Can be null if there is no visible [SelectionOverlay].
@visibleForTesting
SelectionOverlay? get selectionOverlay => _selectionOverlay;
/// The text processing service used to retrieve the native text processing actions.
final ProcessTextService _processTextService = DefaultProcessTextService();
/// The list of native text processing actions provided by the engine.
final List<ProcessTextAction> _processTextActions = <ProcessTextAction>[];
@override
void initState() {
super.initState();
widget.focusNode.addListener(_handleFocusChanged);
_initMouseGestureRecognizer();
_initTouchGestureRecognizer();
// Taps and right clicks.
_gestureRecognizers[TapGestureRecognizer] = GestureRecognizerFactoryWithHandlers<TapGestureRecognizer>(
() => TapGestureRecognizer(debugOwner: this),
(TapGestureRecognizer instance) {
instance.onTapUp = (TapUpDetails details) {
if (defaultTargetPlatform == TargetPlatform.iOS && _positionIsOnActiveSelection(globalPosition: details.globalPosition)) {
// On iOS when the tap occurs on the previous selection, instead of
// moving the selection, the context menu will be toggled.
final bool toolbarIsVisible = _selectionOverlay?.toolbarIsVisible ?? false;
if (toolbarIsVisible) {
hideToolbar(false);
} else {
_showToolbar(location: details.globalPosition);
}
} else {
hideToolbar();
_collapseSelectionAt(offset: details.globalPosition);
}
};
instance.onSecondaryTapDown = _handleRightClickDown;
},
);
_initProcessTextActions();
}
/// Query the engine to initialize the list of text processing actions to show
/// in the text selection toolbar.
Future<void> _initProcessTextActions() async {
_processTextActions.clear();
_processTextActions.addAll(await _processTextService.queryTextActions());
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
break;
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return;
}
// Hide the text selection toolbar on mobile when orientation changes.
final Orientation orientation = MediaQuery.orientationOf(context);
if (_lastOrientation == null) {
_lastOrientation = orientation;
return;
}
if (orientation != _lastOrientation) {
_lastOrientation = orientation;
hideToolbar(defaultTargetPlatform == TargetPlatform.android);
}
}
@override
void didUpdateWidget(SelectableRegion oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.focusNode != oldWidget.focusNode) {
oldWidget.focusNode.removeListener(_handleFocusChanged);
widget.focusNode.addListener(_handleFocusChanged);
if (widget.focusNode.hasFocus != oldWidget.focusNode.hasFocus) {
_handleFocusChanged();
}
}
}
Action<T> _makeOverridable<T extends Intent>(Action<T> defaultAction) {
return Action<T>.overridable(context: context, defaultAction: defaultAction);
}
void _handleFocusChanged() {
if (!widget.focusNode.hasFocus) {
if (kIsWeb) {
PlatformSelectableRegionContextMenu.detach(_selectionDelegate);
}
_clearSelection();
}
if (kIsWeb) {
PlatformSelectableRegionContextMenu.attach(_selectionDelegate);
}
}
void _updateSelectionStatus() {
final SelectionGeometry geometry = _selectionDelegate.value;
final TextSelection selection = switch (geometry.status) {
SelectionStatus.uncollapsed || SelectionStatus.collapsed => const TextSelection(baseOffset: 0, extentOffset: 1),
SelectionStatus.none => const TextSelection.collapsed(offset: 1),
};
textEditingValue = TextEditingValue(text: '__', selection: selection);
if (_hasSelectionOverlayGeometry) {
_updateSelectionOverlay();
} else {
_selectionOverlay?.dispose();
_selectionOverlay = null;
}
}
// gestures.
// Converts the details.consecutiveTapCount from a TapAndDrag*Details object,
// which can grow to be infinitely large, to a value between 1 and the supported
// max consecutive tap count. The value that the raw count is converted to is
// based on the default observed behavior on the native platforms.
//
// This method should be used in all instances when details.consecutiveTapCount
// would be used.
static int _getEffectiveConsecutiveTapCount(int rawCount) {
const int maxConsecutiveTap = 2;
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
// From observation, these platforms reset their tap count to 0 when
// the number of consecutive taps exceeds the max consecutive tap supported.
// For example on Debian Linux with GTK, when going past a triple click,
// on the fourth click the selection is moved to the precise click
// position, on the fifth click the word at the position is selected, and
// on the sixth click the paragraph at the position is selected.
return rawCount <= maxConsecutiveTap ? rawCount : (rawCount % maxConsecutiveTap == 0 ? maxConsecutiveTap : rawCount % maxConsecutiveTap);
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.windows:
// From observation, these platforms either hold their tap count at the max
// consecutive tap supported. For example on macOS, when going past a triple
// click, the selection should be retained at the paragraph that was first
// selected on triple click.
return min(rawCount, maxConsecutiveTap);
}
}
void _initMouseGestureRecognizer() {
_gestureRecognizers[TapAndPanGestureRecognizer] = GestureRecognizerFactoryWithHandlers<TapAndPanGestureRecognizer>(
() => TapAndPanGestureRecognizer(debugOwner:this, supportedDevices: <PointerDeviceKind>{ PointerDeviceKind.mouse }),
(TapAndPanGestureRecognizer instance) {
instance
..onTapDown = _startNewMouseSelectionGesture
..onTapUp = _handleMouseTapUp
..onDragStart = _handleMouseDragStart
..onDragUpdate = _handleMouseDragUpdate
..onDragEnd = _handleMouseDragEnd
..onCancel = _clearSelection
..dragStartBehavior = DragStartBehavior.down;
},
);
}
void _initTouchGestureRecognizer() {
_gestureRecognizers[LongPressGestureRecognizer] = GestureRecognizerFactoryWithHandlers<LongPressGestureRecognizer>(
() => LongPressGestureRecognizer(debugOwner: this, supportedDevices: _kLongPressSelectionDevices),
(LongPressGestureRecognizer instance) {
instance
..onLongPressStart = _handleTouchLongPressStart
..onLongPressMoveUpdate = _handleTouchLongPressMoveUpdate
..onLongPressEnd = _handleTouchLongPressEnd;
},
);
}
void _startNewMouseSelectionGesture(TapDragDownDetails details) {
switch (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount)) {
case 1:
widget.focusNode.requestFocus();
hideToolbar();
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
// On mobile platforms the selection is set on tap up.
break;
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
_collapseSelectionAt(offset: details.globalPosition);
}
case 2:
_selectWordAt(offset: details.globalPosition);
}
_updateSelectedContentIfNeeded();
}
void _handleMouseDragStart(TapDragStartDetails details) {
switch (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount)) {
case 1:
_selectStartTo(offset: details.globalPosition);
}
_updateSelectedContentIfNeeded();
}
void _handleMouseDragUpdate(TapDragUpdateDetails details) {
switch (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount)) {
case 1:
_selectEndTo(offset: details.globalPosition, continuous: true);
case 2:
_selectEndTo(offset: details.globalPosition, continuous: true, textGranularity: TextGranularity.word);
}
_updateSelectedContentIfNeeded();
}
void _handleMouseDragEnd(TapDragEndDetails details) {
_finalizeSelection();
_updateSelectedContentIfNeeded();
}
void _handleMouseTapUp(TapDragUpDetails details) {
switch (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount)) {
case 1:
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
_collapseSelectionAt(offset: details.globalPosition);
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
// On desktop platforms the selection is set on tap down.
break;
}
}
_updateSelectedContentIfNeeded();
}
void _updateSelectedContentIfNeeded() {
if (_lastSelectedContent?.plainText != _selectable?.getSelectedContent()?.plainText) {
_lastSelectedContent = _selectable?.getSelectedContent();
widget.onSelectionChanged?.call(_lastSelectedContent);
}
}
void _handleTouchLongPressStart(LongPressStartDetails details) {
HapticFeedback.selectionClick();
widget.focusNode.requestFocus();
_selectWordAt(offset: details.globalPosition);
// Platforms besides Android will show the text selection handles when
// the long press is initiated. Android shows the text selection handles when
// the long press has ended, usually after a pointer up event is received.
if (defaultTargetPlatform != TargetPlatform.android) {
_showHandles();
}
_updateSelectedContentIfNeeded();
}
void _handleTouchLongPressMoveUpdate(LongPressMoveUpdateDetails details) {
_selectEndTo(offset: details.globalPosition, textGranularity: TextGranularity.word);
_updateSelectedContentIfNeeded();
}
void _handleTouchLongPressEnd(LongPressEndDetails details) {
_finalizeSelection();
_updateSelectedContentIfNeeded();
_showToolbar();
if (defaultTargetPlatform == TargetPlatform.android) {
_showHandles();
}
}
bool _positionIsOnActiveSelection({required Offset globalPosition}) {
for (final Rect selectionRect in _selectionDelegate.value.selectionRects) {
final Matrix4 transform = _selectable!.getTransformTo(null);
final Rect globalRect = MatrixUtils.transformRect(transform, selectionRect);
if (globalRect.contains(globalPosition)) {
return true;
}
}
return false;
}
void _handleRightClickDown(TapDownDetails details) {
final Offset? previousSecondaryTapDownPosition = lastSecondaryTapDownPosition;
final bool toolbarIsVisible = _selectionOverlay?.toolbarIsVisible ?? false;
lastSecondaryTapDownPosition = details.globalPosition;
widget.focusNode.requestFocus();
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.windows:
// If lastSecondaryTapDownPosition is within the current selection then
// keep the current selection, if not then collapse it.
final bool lastSecondaryTapDownPositionWasOnActiveSelection = _positionIsOnActiveSelection(globalPosition: details.globalPosition);
if (!lastSecondaryTapDownPositionWasOnActiveSelection) {
_collapseSelectionAt(offset: lastSecondaryTapDownPosition!);
}
_showHandles();
_showToolbar(location: lastSecondaryTapDownPosition);
case TargetPlatform.iOS:
_selectWordAt(offset: lastSecondaryTapDownPosition!);
_showHandles();
_showToolbar(location: lastSecondaryTapDownPosition);
case TargetPlatform.macOS:
if (previousSecondaryTapDownPosition == lastSecondaryTapDownPosition && toolbarIsVisible) {
hideToolbar();
return;
}
_selectWordAt(offset: lastSecondaryTapDownPosition!);
_showHandles();
_showToolbar(location: lastSecondaryTapDownPosition);
case TargetPlatform.linux:
if (toolbarIsVisible) {
hideToolbar();
return;
}
// If lastSecondaryTapDownPosition is within the current selection then
// keep the current selection, if not then collapse it.
final bool lastSecondaryTapDownPositionWasOnActiveSelection = _positionIsOnActiveSelection(globalPosition: details.globalPosition);
if (!lastSecondaryTapDownPositionWasOnActiveSelection) {
_collapseSelectionAt(offset: lastSecondaryTapDownPosition!);
}
_showHandles();
_showToolbar(location: lastSecondaryTapDownPosition);
}
_updateSelectedContentIfNeeded();
}
// Selection update helper methods.
Offset? _selectionEndPosition;
bool get _userDraggingSelectionEnd => _selectionEndPosition != null;
bool _scheduledSelectionEndEdgeUpdate = false;
/// Sends end [SelectionEdgeUpdateEvent] to the selectable subtree.
///
/// If the selectable subtree returns a [SelectionResult.pending], this method
/// continues to send [SelectionEdgeUpdateEvent]s every frame until the result
/// is not pending or users end their gestures.
void _triggerSelectionEndEdgeUpdate({TextGranularity? textGranularity}) {
// This method can be called when the drag is not in progress. This can
// happen if the child scrollable returns SelectionResult.pending, and
// the selection area scheduled a selection update for the next frame, but
// the drag is lifted before the scheduled selection update is run.
if (_scheduledSelectionEndEdgeUpdate || !_userDraggingSelectionEnd) {
return;
}
if (_selectable?.dispatchSelectionEvent(
SelectionEdgeUpdateEvent.forEnd(globalPosition: _selectionEndPosition!, granularity: textGranularity)) == SelectionResult.pending) {
_scheduledSelectionEndEdgeUpdate = true;
SchedulerBinding.instance.addPostFrameCallback((Duration timeStamp) {
if (!_scheduledSelectionEndEdgeUpdate) {
return;
}
_scheduledSelectionEndEdgeUpdate = false;
_triggerSelectionEndEdgeUpdate(textGranularity: textGranularity);
}, debugLabel: 'SelectableRegion.endEdgeUpdate');
return;
}
}
void _onAnyDragEnd(DragEndDetails details) {
if (widget.selectionControls is! TextSelectionHandleControls) {
_selectionOverlay!.hideMagnifier();
_selectionOverlay!.showToolbar();
} else {
_selectionOverlay!.hideMagnifier();
_selectionOverlay!.showToolbar(
context: context,
contextMenuBuilder: (BuildContext context) {
return widget.contextMenuBuilder!(context, this);
},
);
}
_stopSelectionStartEdgeUpdate();
_stopSelectionEndEdgeUpdate();
_updateSelectedContentIfNeeded();
}
void _stopSelectionEndEdgeUpdate() {
_scheduledSelectionEndEdgeUpdate = false;
_selectionEndPosition = null;
}
Offset? _selectionStartPosition;
bool get _userDraggingSelectionStart => _selectionStartPosition != null;
bool _scheduledSelectionStartEdgeUpdate = false;
/// Sends start [SelectionEdgeUpdateEvent] to the selectable subtree.
///
/// If the selectable subtree returns a [SelectionResult.pending], this method
/// continues to send [SelectionEdgeUpdateEvent]s every frame until the result
/// is not pending or users end their gestures.
void _triggerSelectionStartEdgeUpdate({TextGranularity? textGranularity}) {
// This method can be called when the drag is not in progress. This can
// happen if the child scrollable returns SelectionResult.pending, and
// the selection area scheduled a selection update for the next frame, but
// the drag is lifted before the scheduled selection update is run.
if (_scheduledSelectionStartEdgeUpdate || !_userDraggingSelectionStart) {
return;
}
if (_selectable?.dispatchSelectionEvent(
SelectionEdgeUpdateEvent.forStart(globalPosition: _selectionStartPosition!, granularity: textGranularity)) == SelectionResult.pending) {
_scheduledSelectionStartEdgeUpdate = true;
SchedulerBinding.instance.addPostFrameCallback((Duration timeStamp) {
if (!_scheduledSelectionStartEdgeUpdate) {
return;
}
_scheduledSelectionStartEdgeUpdate = false;
_triggerSelectionStartEdgeUpdate(textGranularity: textGranularity);
}, debugLabel: 'SelectableRegion.startEdgeUpdate');
return;
}
}
void _stopSelectionStartEdgeUpdate() {
_scheduledSelectionStartEdgeUpdate = false;
_selectionEndPosition = null;
}
// SelectionOverlay helper methods.
late Offset _selectionStartHandleDragPosition;
late Offset _selectionEndHandleDragPosition;
void _handleSelectionStartHandleDragStart(DragStartDetails details) {
assert(_selectionDelegate.value.startSelectionPoint != null);
final Offset localPosition = _selectionDelegate.value.startSelectionPoint!.localPosition;
final Matrix4 globalTransform = _selectable!.getTransformTo(null);
_selectionStartHandleDragPosition = MatrixUtils.transformPoint(globalTransform, localPosition);
_selectionOverlay!.showMagnifier(_buildInfoForMagnifier(
details.globalPosition,
_selectionDelegate.value.startSelectionPoint!,
));
_updateSelectedContentIfNeeded();
}
void _handleSelectionStartHandleDragUpdate(DragUpdateDetails details) {
_selectionStartHandleDragPosition = _selectionStartHandleDragPosition + details.delta;
// The value corresponds to the paint origin of the selection handle.
// Offset it to the center of the line to make it feel more natural.
_selectionStartPosition = _selectionStartHandleDragPosition - Offset(0, _selectionDelegate.value.startSelectionPoint!.lineHeight / 2);
_triggerSelectionStartEdgeUpdate();
_selectionOverlay!.updateMagnifier(_buildInfoForMagnifier(
details.globalPosition,
_selectionDelegate.value.startSelectionPoint!,
));
_updateSelectedContentIfNeeded();
}
void _handleSelectionEndHandleDragStart(DragStartDetails details) {
assert(_selectionDelegate.value.endSelectionPoint != null);
final Offset localPosition = _selectionDelegate.value.endSelectionPoint!.localPosition;
final Matrix4 globalTransform = _selectable!.getTransformTo(null);
_selectionEndHandleDragPosition = MatrixUtils.transformPoint(globalTransform, localPosition);
_selectionOverlay!.showMagnifier(_buildInfoForMagnifier(
details.globalPosition,
_selectionDelegate.value.endSelectionPoint!,
));
_updateSelectedContentIfNeeded();
}
void _handleSelectionEndHandleDragUpdate(DragUpdateDetails details) {
_selectionEndHandleDragPosition = _selectionEndHandleDragPosition + details.delta;
// The value corresponds to the paint origin of the selection handle.
// Offset it to the center of the line to make it feel more natural.
_selectionEndPosition = _selectionEndHandleDragPosition - Offset(0, _selectionDelegate.value.endSelectionPoint!.lineHeight / 2);
_triggerSelectionEndEdgeUpdate();
_selectionOverlay!.updateMagnifier(_buildInfoForMagnifier(
details.globalPosition,
_selectionDelegate.value.endSelectionPoint!,
));
_updateSelectedContentIfNeeded();
}
MagnifierInfo _buildInfoForMagnifier(Offset globalGesturePosition, SelectionPoint selectionPoint) {
final Vector3 globalTransform = _selectable!.getTransformTo(null).getTranslation();
final Offset globalTransformAsOffset = Offset(globalTransform.x, globalTransform.y);
final Offset globalSelectionPointPosition = selectionPoint.localPosition + globalTransformAsOffset;
final Rect caretRect = Rect.fromLTWH(
globalSelectionPointPosition.dx,
globalSelectionPointPosition.dy - selectionPoint.lineHeight,
0,
selectionPoint.lineHeight
);
return MagnifierInfo(
globalGesturePosition: globalGesturePosition,
caretRect: caretRect,
fieldBounds: globalTransformAsOffset & _selectable!.size,
currentLineBoundaries: globalTransformAsOffset & _selectable!.size,
);
}
void _createSelectionOverlay() {
assert(_hasSelectionOverlayGeometry);
if (_selectionOverlay != null) {
return;
}
final SelectionPoint? start = _selectionDelegate.value.startSelectionPoint;
final SelectionPoint? end = _selectionDelegate.value.endSelectionPoint;
_selectionOverlay = SelectionOverlay(
context: context,
debugRequiredFor: widget,
startHandleType: start?.handleType ?? TextSelectionHandleType.left,
lineHeightAtStart: start?.lineHeight ?? end!.lineHeight,
onStartHandleDragStart: _handleSelectionStartHandleDragStart,
onStartHandleDragUpdate: _handleSelectionStartHandleDragUpdate,
onStartHandleDragEnd: _onAnyDragEnd,
endHandleType: end?.handleType ?? TextSelectionHandleType.right,
lineHeightAtEnd: end?.lineHeight ?? start!.lineHeight,
onEndHandleDragStart: _handleSelectionEndHandleDragStart,
onEndHandleDragUpdate: _handleSelectionEndHandleDragUpdate,
onEndHandleDragEnd: _onAnyDragEnd,
selectionEndpoints: selectionEndpoints,
selectionControls: widget.selectionControls,
selectionDelegate: this,
clipboardStatus: null,
startHandleLayerLink: _startHandleLayerLink,
endHandleLayerLink: _endHandleLayerLink,
toolbarLayerLink: _toolbarLayerLink,
magnifierConfiguration: widget.magnifierConfiguration
);
}
void _updateSelectionOverlay() {
if (_selectionOverlay == null) {
return;
}
assert(_hasSelectionOverlayGeometry);
final SelectionPoint? start = _selectionDelegate.value.startSelectionPoint;
final SelectionPoint? end = _selectionDelegate.value.endSelectionPoint;
_selectionOverlay!
..startHandleType = start?.handleType ?? TextSelectionHandleType.left
..lineHeightAtStart = start?.lineHeight ?? end!.lineHeight
..endHandleType = end?.handleType ?? TextSelectionHandleType.right
..lineHeightAtEnd = end?.lineHeight ?? start!.lineHeight
..selectionEndpoints = selectionEndpoints;
}
/// Shows the selection handles.
///
/// Returns true if the handles are shown, false if the handles can't be
/// shown.
bool _showHandles() {
if (_selectionOverlay != null) {
_selectionOverlay!.showHandles();
return true;
}
if (!_hasSelectionOverlayGeometry) {
return false;
}
_createSelectionOverlay();
_selectionOverlay!.showHandles();
return true;
}
/// Shows the text selection toolbar.
///
/// If the parameter `location` is set, the toolbar will be shown at the
/// location. Otherwise, the toolbar location will be calculated based on the
/// handles' locations. The `location` is in the coordinates system of the
/// [Overlay].
///
/// Returns true if the toolbar is shown, false if the toolbar can't be shown.
bool _showToolbar({Offset? location}) {
if (!_hasSelectionOverlayGeometry && _selectionOverlay == null) {
return false;
}
// Web is using native dom elements to enable clipboard functionality of the
// context menu: copy, paste, select, cut. It might also provide additional
// functionality depending on the browser (such as translate). Due to this,
// we should not show a Flutter toolbar for the editable text elements
// unless the browser's context menu is explicitly disabled.
if (kIsWeb && BrowserContextMenu.enabled) {
return false;
}
if (_selectionOverlay == null) {
_createSelectionOverlay();
}
_selectionOverlay!.toolbarLocation = location;
if (widget.selectionControls is! TextSelectionHandleControls) {
_selectionOverlay!.showToolbar();
return true;
}
_selectionOverlay!.hideToolbar();
_selectionOverlay!.showToolbar(
context: context,
contextMenuBuilder: (BuildContext context) {
return widget.contextMenuBuilder!(context, this);
},
);
return true;
}
/// Sets or updates selection end edge to the `offset` location.
///
/// A selection always contains a select start edge and selection end edge.
/// They can be created by calling both [_selectStartTo] and [_selectEndTo], or
/// use other selection APIs, such as [_selectWordAt] or [selectAll].
///
/// This method sets or updates the selection end edge by sending
/// [SelectionEdgeUpdateEvent]s to the child [Selectable]s.
///
/// If `continuous` is set to true and the update causes scrolling, the
/// method will continue sending the same [SelectionEdgeUpdateEvent]s to the
/// child [Selectable]s every frame until the scrolling finishes or a
/// [_finalizeSelection] is called.
///
/// The `continuous` argument defaults to false.
///
/// The `offset` is in global coordinates.
///
/// Provide the `textGranularity` if the selection should not move by the default
/// [TextGranularity.character]. Only [TextGranularity.character] and
/// [TextGranularity.word] are currently supported.
///
/// See also:
/// * [_selectStartTo], which sets or updates selection start edge.
/// * [_finalizeSelection], which stops the `continuous` updates.
/// * [_clearSelection], which clears the ongoing selection.
/// * [_selectWordAt], which selects a whole word at the location.
/// * [_collapseSelectionAt], which collapses the selection at the location.
/// * [selectAll], which selects the entire content.
void _selectEndTo({required Offset offset, bool continuous = false, TextGranularity? textGranularity}) {
if (!continuous) {
_selectable?.dispatchSelectionEvent(SelectionEdgeUpdateEvent.forEnd(globalPosition: offset, granularity: textGranularity));
return;
}
if (_selectionEndPosition != offset) {
_selectionEndPosition = offset;
_triggerSelectionEndEdgeUpdate(textGranularity: textGranularity);
}
}
/// Sets or updates selection start edge to the `offset` location.
///
/// A selection always contains a select start edge and selection end edge.
/// They can be created by calling both [_selectStartTo] and [_selectEndTo], or
/// use other selection APIs, such as [_selectWordAt] or [selectAll].
///
/// This method sets or updates the selection start edge by sending
/// [SelectionEdgeUpdateEvent]s to the child [Selectable]s.
///
/// If `continuous` is set to true and the update causes scrolling, the
/// method will continue sending the same [SelectionEdgeUpdateEvent]s to the
/// child [Selectable]s every frame until the scrolling finishes or a
/// [_finalizeSelection] is called.
///
/// The `continuous` argument defaults to false.
///
/// The `offset` is in global coordinates.
///
/// Provide the `textGranularity` if the selection should not move by the default
/// [TextGranularity.character]. Only [TextGranularity.character] and
/// [TextGranularity.word] are currently supported.
///
/// See also:
/// * [_selectEndTo], which sets or updates selection end edge.
/// * [_finalizeSelection], which stops the `continuous` updates.
/// * [_clearSelection], which clears the ongoing selection.
/// * [_selectWordAt], which selects a whole word at the location.
/// * [_collapseSelectionAt], which collapses the selection at the location.
/// * [selectAll], which selects the entire content.
void _selectStartTo({required Offset offset, bool continuous = false, TextGranularity? textGranularity}) {
if (!continuous) {
_selectable?.dispatchSelectionEvent(SelectionEdgeUpdateEvent.forStart(globalPosition: offset, granularity: textGranularity));
return;
}
if (_selectionStartPosition != offset) {
_selectionStartPosition = offset;
_triggerSelectionStartEdgeUpdate(textGranularity: textGranularity);
}
}
/// Collapses the selection at the given `offset` location.
///
/// See also:
/// * [_selectStartTo], which sets or updates selection start edge.
/// * [_selectEndTo], which sets or updates selection end edge.
/// * [_finalizeSelection], which stops the `continuous` updates.
/// * [_clearSelection], which clears the ongoing selection.
/// * [_selectWordAt], which selects a whole word at the location.
/// * [selectAll], which selects the entire content.
void _collapseSelectionAt({required Offset offset}) {
_selectStartTo(offset: offset);
_selectEndTo(offset: offset);
}
/// Selects a whole word at the `offset` location.
///
/// If the whole word is already in the current selection, selection won't
/// change. One call [_clearSelection] first if the selection needs to be
/// updated even if the word is already covered by the current selection.
///
/// One can also use [_selectEndTo] or [_selectStartTo] to adjust the selection
/// edges after calling this method.
///
/// See also:
/// * [_selectStartTo], which sets or updates selection start edge.
/// * [_selectEndTo], which sets or updates selection end edge.
/// * [_finalizeSelection], which stops the `continuous` updates.
/// * [_clearSelection], which clears the ongoing selection.
/// * [_collapseSelectionAt], which collapses the selection at the location.
/// * [selectAll], which selects the entire content.
void _selectWordAt({required Offset offset}) {
// There may be other selection ongoing.
_finalizeSelection();
_selectable?.dispatchSelectionEvent(SelectWordSelectionEvent(globalPosition: offset));
}
/// Stops any ongoing selection updates.
///
/// This method is different from [_clearSelection] that it does not remove
/// the current selection. It only stops the continuous updates.
///
/// A continuous update can happen as result of calling [_selectStartTo] or
/// [_selectEndTo] with `continuous` sets to true which causes a [Selectable]
/// to scroll. Calling this method will stop the update as well as the
/// scrolling.
void _finalizeSelection() {
_stopSelectionEndEdgeUpdate();
_stopSelectionStartEdgeUpdate();
}
/// Removes the ongoing selection.
void _clearSelection() {
_finalizeSelection();
_directionalHorizontalBaseline = null;
_adjustingSelectionEnd = null;
_selectable?.dispatchSelectionEvent(const ClearSelectionEvent());
_updateSelectedContentIfNeeded();
}
Future<void> _copy() async {
final SelectedContent? data = _selectable?.getSelectedContent();
if (data == null) {
return;
}
await Clipboard.setData(ClipboardData(text: data.plainText));
}
Future<void> _share() async {
final SelectedContent? data = _selectable?.getSelectedContent();
if (data == null) {
return;
}
await SystemChannels.platform.invokeMethod('Share.invoke', data.plainText);
}
/// {@macro flutter.widgets.EditableText.getAnchors}
///
/// See also:
///
/// * [contextMenuButtonItems], which provides the [ContextMenuButtonItem]s
/// for the default context menu buttons.
TextSelectionToolbarAnchors get contextMenuAnchors {
if (lastSecondaryTapDownPosition != null) {
return TextSelectionToolbarAnchors(
primaryAnchor: lastSecondaryTapDownPosition!,
);
}
final RenderBox renderBox = context.findRenderObject()! as RenderBox;
return TextSelectionToolbarAnchors.fromSelection(
renderBox: renderBox,
startGlyphHeight: startGlyphHeight,
endGlyphHeight: endGlyphHeight,
selectionEndpoints: selectionEndpoints,
);
}
bool? _adjustingSelectionEnd;
bool _determineIsAdjustingSelectionEnd(bool forward) {
if (_adjustingSelectionEnd != null) {
return _adjustingSelectionEnd!;
}
final bool isReversed;
final SelectionPoint start = _selectionDelegate.value
.startSelectionPoint!;
final SelectionPoint end = _selectionDelegate.value.endSelectionPoint!;
if (start.localPosition.dy > end.localPosition.dy) {
isReversed = true;
} else if (start.localPosition.dy < end.localPosition.dy) {
isReversed = false;
} else {
isReversed = start.localPosition.dx > end.localPosition.dx;
}
// Always move the selection edge that increases the selection range.
return _adjustingSelectionEnd = forward != isReversed;
}
void _granularlyExtendSelection(TextGranularity granularity, bool forward) {
_directionalHorizontalBaseline = null;
if (!_selectionDelegate.value.hasSelection) {
return;
}
_selectable?.dispatchSelectionEvent(
GranularlyExtendSelectionEvent(
forward: forward,
isEnd: _determineIsAdjustingSelectionEnd(forward),
granularity: granularity,
),
);
_updateSelectedContentIfNeeded();
}
double? _directionalHorizontalBaseline;
void _directionallyExtendSelection(bool forward) {
if (!_selectionDelegate.value.hasSelection) {
return;
}
final bool adjustingSelectionExtend = _determineIsAdjustingSelectionEnd(forward);
final SelectionPoint baseLinePoint = adjustingSelectionExtend
? _selectionDelegate.value.endSelectionPoint!
: _selectionDelegate.value.startSelectionPoint!;
_directionalHorizontalBaseline ??= baseLinePoint.localPosition.dx;
final Offset globalSelectionPointOffset = MatrixUtils.transformPoint(context.findRenderObject()!.getTransformTo(null), Offset(_directionalHorizontalBaseline!, 0));
_selectable?.dispatchSelectionEvent(
DirectionallyExtendSelectionEvent(
isEnd: _adjustingSelectionEnd!,
direction: forward ? SelectionExtendDirection.nextLine : SelectionExtendDirection.previousLine,
dx: globalSelectionPointOffset.dx,
),
);
_updateSelectedContentIfNeeded();
}
// [TextSelectionDelegate] overrides.
/// Returns the [ContextMenuButtonItem]s representing the buttons in this
/// platform's default selection menu.
///
/// See also:
///
/// * [SelectableRegion.getSelectableButtonItems], which performs a similar role,
/// but for any selectable text, not just specifically SelectableRegion.
/// * [EditableTextState.contextMenuButtonItems], which performs a similar role
/// but for content that is not just selectable but also editable.
/// * [contextMenuAnchors], which provides the anchor points for the default
/// context menu.
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar itself, and can
/// take a list of [ContextMenuButtonItem]s with
/// [AdaptiveTextSelectionToolbar.buttonItems].
/// * [AdaptiveTextSelectionToolbar.getAdaptiveButtons], which builds the
/// button Widgets for the current platform given [ContextMenuButtonItem]s.
List<ContextMenuButtonItem> get contextMenuButtonItems {
return SelectableRegion.getSelectableButtonItems(
selectionGeometry: _selectionDelegate.value,
onCopy: () {
_copy();
// On Android copy should clear the selection.
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
_clearSelection();
case TargetPlatform.iOS:
hideToolbar(false);
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
hideToolbar();
}
},
onSelectAll: () {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
selectAll(SelectionChangedCause.toolbar);
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
selectAll();
hideToolbar();
}
},
onShare: () {
_share();
// On Android, share should clear the selection.
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
_clearSelection();
case TargetPlatform.iOS:
hideToolbar(false);
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
hideToolbar();
}
},
)..addAll(_textProcessingActionButtonItems);
}
List<ContextMenuButtonItem> get _textProcessingActionButtonItems {
final List<ContextMenuButtonItem> buttonItems = <ContextMenuButtonItem>[];
final SelectedContent? data = _selectable?.getSelectedContent();
if (data == null) {
return buttonItems;
}
for (final ProcessTextAction action in _processTextActions) {
buttonItems.add(ContextMenuButtonItem(
label: action.label,
onPressed: () async {
final String selectedText = data.plainText;
if (selectedText.isNotEmpty) {
await _processTextService.processTextAction(action.id, selectedText, true);
hideToolbar();
}
},
));
}
return buttonItems;
}
/// The line height at the start of the current selection.
double get startGlyphHeight {
return _selectionDelegate.value.startSelectionPoint!.lineHeight;
}
/// The line height at the end of the current selection.
double get endGlyphHeight {
return _selectionDelegate.value.endSelectionPoint!.lineHeight;
}
/// Returns the local coordinates of the endpoints of the current selection.
List<TextSelectionPoint> get selectionEndpoints {
final SelectionPoint? start = _selectionDelegate.value.startSelectionPoint;
final SelectionPoint? end = _selectionDelegate.value.endSelectionPoint;
late List<TextSelectionPoint> points;
final Offset startLocalPosition = start?.localPosition ?? end!.localPosition;
final Offset endLocalPosition = end?.localPosition ?? start!.localPosition;
if (startLocalPosition.dy > endLocalPosition.dy) {
points = <TextSelectionPoint>[
TextSelectionPoint(endLocalPosition, TextDirection.ltr),
TextSelectionPoint(startLocalPosition, TextDirection.ltr),
];
} else {
points = <TextSelectionPoint>[
TextSelectionPoint(startLocalPosition, TextDirection.ltr),
TextSelectionPoint(endLocalPosition, TextDirection.ltr),
];
}
return points;
}
// [TextSelectionDelegate] overrides.
// TODO(justinmc): After deprecations have been removed, remove
// TextSelectionDelegate from this class.
// https://github.com/flutter/flutter/issues/111213
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
bool get cutEnabled => false;
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
bool get pasteEnabled => false;
@override
void hideToolbar([bool hideHandles = true]) {
_selectionOverlay?.hideToolbar();
if (hideHandles) {
_selectionOverlay?.hideHandles();
}
}
@override
void selectAll([SelectionChangedCause? cause]) {
_clearSelection();
_selectable?.dispatchSelectionEvent(const SelectAllSelectionEvent());
if (cause == SelectionChangedCause.toolbar) {
_showToolbar();
_showHandles();
}
_updateSelectedContentIfNeeded();
}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
void copySelection(SelectionChangedCause cause) {
_copy();
_clearSelection();
}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
TextEditingValue textEditingValue = const TextEditingValue(text: '_');
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
void bringIntoView(TextPosition position) {/* SelectableRegion must be in view at this point. */}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
void cutSelection(SelectionChangedCause cause) {
assert(false);
}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
void userUpdateTextEditingValue(TextEditingValue value, SelectionChangedCause cause) {/* SelectableRegion maintains its own state */}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
Future<void> pasteText(SelectionChangedCause cause) async {
assert(false);
}
// [SelectionRegistrar] override.
@override
void add(Selectable selectable) {
assert(_selectable == null);
_selectable = selectable;
_selectable!.addListener(_updateSelectionStatus);
_selectable!.pushHandleLayers(_startHandleLayerLink, _endHandleLayerLink);
}
@override
void remove(Selectable selectable) {
assert(_selectable == selectable);
_selectable!.removeListener(_updateSelectionStatus);
_selectable!.pushHandleLayers(null, null);
_selectable = null;
}
@override
void dispose() {
_selectable?.removeListener(_updateSelectionStatus);
_selectable?.pushHandleLayers(null, null);
_selectionDelegate.dispose();
// In case dispose was triggered before gesture end, remove the magnifier
// so it doesn't remain stuck in the overlay forever.
_selectionOverlay?.hideMagnifier();
_selectionOverlay?.dispose();
_selectionOverlay = null;
super.dispose();
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasOverlay(context));
Widget result = SelectionContainer(
registrar: this,
delegate: _selectionDelegate,
child: widget.child,
);
if (kIsWeb) {
result = PlatformSelectableRegionContextMenu(
child: result,
);
}
return CompositedTransformTarget(
link: _toolbarLayerLink,
child: RawGestureDetector(
gestures: _gestureRecognizers,
behavior: HitTestBehavior.translucent,
excludeFromSemantics: true,
child: Actions(
actions: _actions,
child: Focus(
includeSemantics: false,
focusNode: widget.focusNode,
child: result,
),
),
),
);
}
}
/// An action that does not override any [Action.overridable] in the subtree.
///
/// If this action is invoked by an [Action.overridable], it will immediately
/// invoke the [Action.overridable] and do nothing else. Otherwise, it will call
/// [invokeAction].
abstract class _NonOverrideAction<T extends Intent> extends ContextAction<T> {
Object? invokeAction(T intent, [BuildContext? context]);
@override
Object? invoke(T intent, [BuildContext? context]) {
if (callingAction != null) {
return callingAction!.invoke(intent);
}
return invokeAction(intent, context);
}
}
class _SelectAllAction extends _NonOverrideAction<SelectAllTextIntent> {
_SelectAllAction(this.state);
final SelectableRegionState state;
@override
void invokeAction(SelectAllTextIntent intent, [BuildContext? context]) {
state.selectAll(SelectionChangedCause.keyboard);
}
}
class _CopySelectionAction extends _NonOverrideAction<CopySelectionTextIntent> {
_CopySelectionAction(this.state);
final SelectableRegionState state;
@override
void invokeAction(CopySelectionTextIntent intent, [BuildContext? context]) {
state._copy();
}
}
class _GranularlyExtendSelectionAction<T extends DirectionalTextEditingIntent> extends _NonOverrideAction<T> {
_GranularlyExtendSelectionAction(this.state, {required this.granularity});
final SelectableRegionState state;
final TextGranularity granularity;
@override
void invokeAction(T intent, [BuildContext? context]) {
state._granularlyExtendSelection(granularity, intent.forward);
}
}
class _GranularlyExtendCaretSelectionAction<T extends DirectionalCaretMovementIntent> extends _NonOverrideAction<T> {
_GranularlyExtendCaretSelectionAction(this.state, {required this.granularity});
final SelectableRegionState state;
final TextGranularity granularity;
@override
void invokeAction(T intent, [BuildContext? context]) {
if (intent.collapseSelection) {
// Selectable region never collapses selection.
return;
}
state._granularlyExtendSelection(granularity, intent.forward);
}
}
class _DirectionallyExtendCaretSelectionAction<T extends DirectionalCaretMovementIntent> extends _NonOverrideAction<T> {
_DirectionallyExtendCaretSelectionAction(this.state);
final SelectableRegionState state;
@override
void invokeAction(T intent, [BuildContext? context]) {
if (intent.collapseSelection) {
// Selectable region never collapses selection.
return;
}
state._directionallyExtendSelection(intent.forward);
}
}
class _SelectableRegionContainerDelegate extends MultiSelectableSelectionContainerDelegate {
final Set<Selectable> _hasReceivedStartEvent = <Selectable>{};
final Set<Selectable> _hasReceivedEndEvent = <Selectable>{};
Offset? _lastStartEdgeUpdateGlobalPosition;
Offset? _lastEndEdgeUpdateGlobalPosition;
@override
void remove(Selectable selectable) {
_hasReceivedStartEvent.remove(selectable);
_hasReceivedEndEvent.remove(selectable);
super.remove(selectable);
}
void _updateLastEdgeEventsFromGeometries() {
if (currentSelectionStartIndex != -1 && selectables[currentSelectionStartIndex].value.hasSelection) {
final Selectable start = selectables[currentSelectionStartIndex];
final Offset localStartEdge = start.value.startSelectionPoint!.localPosition +
Offset(0, - start.value.startSelectionPoint!.lineHeight / 2);
_lastStartEdgeUpdateGlobalPosition = MatrixUtils.transformPoint(start.getTransformTo(null), localStartEdge);
}
if (currentSelectionEndIndex != -1 && selectables[currentSelectionEndIndex].value.hasSelection) {
final Selectable end = selectables[currentSelectionEndIndex];
final Offset localEndEdge = end.value.endSelectionPoint!.localPosition +
Offset(0, -end.value.endSelectionPoint!.lineHeight / 2);
_lastEndEdgeUpdateGlobalPosition = MatrixUtils.transformPoint(end.getTransformTo(null), localEndEdge);
}
}
@override
SelectionResult handleSelectAll(SelectAllSelectionEvent event) {
final SelectionResult result = super.handleSelectAll(event);
for (final Selectable selectable in selectables) {
_hasReceivedStartEvent.add(selectable);
_hasReceivedEndEvent.add(selectable);
}
// Synthesize last update event so the edge updates continue to work.
_updateLastEdgeEventsFromGeometries();
return result;
}
/// Selects a word in a selectable at the location
/// [SelectWordSelectionEvent.globalPosition].
@override
SelectionResult handleSelectWord(SelectWordSelectionEvent event) {
final SelectionResult result = super.handleSelectWord(event);
if (currentSelectionStartIndex != -1) {
_hasReceivedStartEvent.add(selectables[currentSelectionStartIndex]);
}
if (currentSelectionEndIndex != -1) {
_hasReceivedEndEvent.add(selectables[currentSelectionEndIndex]);
}
_updateLastEdgeEventsFromGeometries();
return result;
}
@override
SelectionResult handleClearSelection(ClearSelectionEvent event) {
final SelectionResult result = super.handleClearSelection(event);
_hasReceivedStartEvent.clear();
_hasReceivedEndEvent.clear();
_lastStartEdgeUpdateGlobalPosition = null;
_lastEndEdgeUpdateGlobalPosition = null;
return result;
}
@override
SelectionResult handleSelectionEdgeUpdate(SelectionEdgeUpdateEvent event) {
if (event.type == SelectionEventType.endEdgeUpdate) {
_lastEndEdgeUpdateGlobalPosition = event.globalPosition;
} else {
_lastStartEdgeUpdateGlobalPosition = event.globalPosition;
}
return super.handleSelectionEdgeUpdate(event);
}
@override
void dispose() {
_hasReceivedStartEvent.clear();
_hasReceivedEndEvent.clear();
super.dispose();
}
@override
SelectionResult dispatchSelectionEventToChild(Selectable selectable, SelectionEvent event) {
switch (event.type) {
case SelectionEventType.startEdgeUpdate:
_hasReceivedStartEvent.add(selectable);
ensureChildUpdated(selectable);
case SelectionEventType.endEdgeUpdate:
_hasReceivedEndEvent.add(selectable);
ensureChildUpdated(selectable);
case SelectionEventType.clear:
_hasReceivedStartEvent.remove(selectable);
_hasReceivedEndEvent.remove(selectable);
case SelectionEventType.selectAll:
case SelectionEventType.selectWord:
break;
case SelectionEventType.granularlyExtendSelection:
case SelectionEventType.directionallyExtendSelection:
_hasReceivedStartEvent.add(selectable);
_hasReceivedEndEvent.add(selectable);
ensureChildUpdated(selectable);
}
return super.dispatchSelectionEventToChild(selectable, event);
}
@override
void ensureChildUpdated(Selectable selectable) {
if (_lastEndEdgeUpdateGlobalPosition != null && _hasReceivedEndEvent.add(selectable)) {
final SelectionEdgeUpdateEvent synthesizedEvent = SelectionEdgeUpdateEvent.forEnd(
globalPosition: _lastEndEdgeUpdateGlobalPosition!,
);
if (currentSelectionEndIndex == -1) {
handleSelectionEdgeUpdate(synthesizedEvent);
}
selectable.dispatchSelectionEvent(synthesizedEvent);
}
if (_lastStartEdgeUpdateGlobalPosition != null && _hasReceivedStartEvent.add(selectable)) {
final SelectionEdgeUpdateEvent synthesizedEvent = SelectionEdgeUpdateEvent.forStart(
globalPosition: _lastStartEdgeUpdateGlobalPosition!,
);
if (currentSelectionStartIndex == -1) {
handleSelectionEdgeUpdate(synthesizedEvent);
}
selectable.dispatchSelectionEvent(synthesizedEvent);
}
}
@override
void didChangeSelectables() {
if (_lastEndEdgeUpdateGlobalPosition != null) {
handleSelectionEdgeUpdate(
SelectionEdgeUpdateEvent.forEnd(
globalPosition: _lastEndEdgeUpdateGlobalPosition!,
),
);
}
if (_lastStartEdgeUpdateGlobalPosition != null) {
handleSelectionEdgeUpdate(
SelectionEdgeUpdateEvent.forStart(
globalPosition: _lastStartEdgeUpdateGlobalPosition!,
),
);
}
final Set<Selectable> selectableSet = selectables.toSet();
_hasReceivedEndEvent.removeWhere((Selectable selectable) => !selectableSet.contains(selectable));
_hasReceivedStartEvent.removeWhere((Selectable selectable) => !selectableSet.contains(selectable));
super.didChangeSelectables();
}
}
/// An abstract base class for updating multiple selectable children.
///
/// This class provide basic [SelectionEvent] handling and child [Selectable]
/// updating. The subclass needs to implement [ensureChildUpdated] to ensure
/// child [Selectable] is updated properly.
///
/// This class optimize the selection update by keeping track of the
/// [Selectable]s that currently contain the selection edges.
abstract class MultiSelectableSelectionContainerDelegate extends SelectionContainerDelegate with ChangeNotifier {
/// Creates an instance of [MultiSelectableSelectionContainerDelegate].
MultiSelectableSelectionContainerDelegate() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// Gets the list of selectables this delegate is managing.
List<Selectable> selectables = <Selectable>[];
/// The number of additional pixels added to the selection handle drawable
/// area.
///
/// Selection handles that are outside of the drawable area will be hidden.
/// That logic prevents handles that get scrolled off the viewport from being
/// drawn on the screen.
///
/// The drawable area = current rectangle of [SelectionContainer] +
/// _kSelectionHandleDrawableAreaPadding on each side.
///
/// This was an eyeballed value to create smooth user experiences.
static const double _kSelectionHandleDrawableAreaPadding = 5.0;
/// The current selectable that contains the selection end edge.
@protected
int currentSelectionEndIndex = -1;
/// The current selectable that contains the selection start edge.
@protected
int currentSelectionStartIndex = -1;
LayerLink? _startHandleLayer;
Selectable? _startHandleLayerOwner;
LayerLink? _endHandleLayer;
Selectable? _endHandleLayerOwner;
bool _isHandlingSelectionEvent = false;
bool _scheduledSelectableUpdate = false;
bool _selectionInProgress = false;
Set<Selectable> _additions = <Selectable>{};
bool _extendSelectionInProgress = false;
@override
void add(Selectable selectable) {
assert(!selectables.contains(selectable));
_additions.add(selectable);
_scheduleSelectableUpdate();
}
@override
void remove(Selectable selectable) {
if (_additions.remove(selectable)) {
// The same selectable was added in the same frame and is not yet
// incorporated into the selectables.
//
// Removing such selectable doesn't require selection geometry update.
return;
}
_removeSelectable(selectable);
_scheduleSelectableUpdate();
}
/// Notifies this delegate that layout of the container has changed.
void layoutDidChange() {
_updateSelectionGeometry();
}
void _scheduleSelectableUpdate() {
if (!_scheduledSelectableUpdate) {
_scheduledSelectableUpdate = true;
void runScheduledTask([Duration? duration]) {
if (!_scheduledSelectableUpdate) {
return;
}
_scheduledSelectableUpdate = false;
_updateSelectables();
}
if (SchedulerBinding.instance.schedulerPhase == SchedulerPhase.postFrameCallbacks) {
// A new task can be scheduled as a result of running the scheduled task
// from another MultiSelectableSelectionContainerDelegate. This can
// happen if nesting two SelectionContainers. The selectable can be
// safely updated in the same frame in this case.
scheduleMicrotask(runScheduledTask);
} else {
SchedulerBinding.instance.addPostFrameCallback(
runScheduledTask,
debugLabel: 'SelectionContainer.runScheduledTask',
);
}
}
}
void _updateSelectables() {
// Remove offScreen selectable.
if (_additions.isNotEmpty) {
_flushAdditions();
}
didChangeSelectables();
}
void _flushAdditions() {
final List<Selectable> mergingSelectables = _additions.toList()..sort(compareOrder);
final List<Selectable> existingSelectables = selectables;
selectables = <Selectable>[];
int mergingIndex = 0;
int existingIndex = 0;
int selectionStartIndex = currentSelectionStartIndex;
int selectionEndIndex = currentSelectionEndIndex;
// Merge two sorted lists.
while (mergingIndex < mergingSelectables.length || existingIndex < existingSelectables.length) {
if (mergingIndex >= mergingSelectables.length ||
(existingIndex < existingSelectables.length &&
compareOrder(existingSelectables[existingIndex], mergingSelectables[mergingIndex]) < 0)) {
if (existingIndex == currentSelectionStartIndex) {
selectionStartIndex = selectables.length;
}
if (existingIndex == currentSelectionEndIndex) {
selectionEndIndex = selectables.length;
}
selectables.add(existingSelectables[existingIndex]);
existingIndex += 1;
continue;
}
// If the merging selectable falls in the selection range, their selection
// needs to be updated.
final Selectable mergingSelectable = mergingSelectables[mergingIndex];
if (existingIndex < max(currentSelectionStartIndex, currentSelectionEndIndex) &&
existingIndex > min(currentSelectionStartIndex, currentSelectionEndIndex)) {
ensureChildUpdated(mergingSelectable);
}
mergingSelectable.addListener(_handleSelectableGeometryChange);
selectables.add(mergingSelectable);
mergingIndex += 1;
}
assert(mergingIndex == mergingSelectables.length &&
existingIndex == existingSelectables.length &&
selectables.length == existingIndex + mergingIndex);
assert(selectionStartIndex >= -1 || selectionStartIndex < selectables.length);
assert(selectionEndIndex >= -1 || selectionEndIndex < selectables.length);
// selection indices should not be set to -1 unless they originally were.
assert((currentSelectionStartIndex == -1) == (selectionStartIndex == -1));
assert((currentSelectionEndIndex == -1) == (selectionEndIndex == -1));
currentSelectionEndIndex = selectionEndIndex;
currentSelectionStartIndex = selectionStartIndex;
_additions = <Selectable>{};
}
void _removeSelectable(Selectable selectable) {
assert(selectables.contains(selectable), 'The selectable is not in this registrar.');
final int index = selectables.indexOf(selectable);
selectables.removeAt(index);
if (index <= currentSelectionEndIndex) {
currentSelectionEndIndex -= 1;
}
if (index <= currentSelectionStartIndex) {
currentSelectionStartIndex -= 1;
}
selectable.removeListener(_handleSelectableGeometryChange);
}
/// Called when this delegate finishes updating the selectables.
@protected
@mustCallSuper
void didChangeSelectables() {
_updateSelectionGeometry();
}
@override
SelectionGeometry get value => _selectionGeometry;
SelectionGeometry _selectionGeometry = const SelectionGeometry(
hasContent: false,
status: SelectionStatus.none,
);
/// Updates the [value] in this class and notifies listeners if necessary.
void _updateSelectionGeometry() {
final SelectionGeometry newValue = getSelectionGeometry();
if (_selectionGeometry != newValue) {
_selectionGeometry = newValue;
notifyListeners();
}
_updateHandleLayersAndOwners();
}
Rect _getBoundingBox(Selectable selectable) {
Rect result = selectable.boundingBoxes.first;
for (int index = 1; index < selectable.boundingBoxes.length; index += 1) {
result = result.expandToInclude(selectable.boundingBoxes[index]);
}
return result;
}
/// The compare function this delegate used for determining the selection
/// order of the selectables.
///
/// Defaults to screen order.
@protected
Comparator<Selectable> get compareOrder => _compareScreenOrder;
int _compareScreenOrder(Selectable a, Selectable b) {
final Rect rectA = MatrixUtils.transformRect(
a.getTransformTo(null),
_getBoundingBox(a),
);
final Rect rectB = MatrixUtils.transformRect(
b.getTransformTo(null),
_getBoundingBox(b),
);
final int result = _compareVertically(rectA, rectB);
if (result != 0) {
return result;
}
return _compareHorizontally(rectA, rectB);
}
/// Compares two rectangles in the screen order solely by their vertical
/// positions.
///
/// Returns positive if a is lower, negative if a is higher, 0 if their
/// order can't be determine solely by their vertical position.
static int _compareVertically(Rect a, Rect b) {
// The rectangles overlap so defer to horizontal comparison.
if ((a.top - b.top < _kSelectableVerticalComparingThreshold && a.bottom - b.bottom > - _kSelectableVerticalComparingThreshold) ||
(b.top - a.top < _kSelectableVerticalComparingThreshold && b.bottom - a.bottom > - _kSelectableVerticalComparingThreshold)) {
return 0;
}
if ((a.top - b.top).abs() > _kSelectableVerticalComparingThreshold) {
return a.top > b.top ? 1 : -1;
}
return a.bottom > b.bottom ? 1 : -1;
}
/// Compares two rectangles in the screen order by their horizontal positions
/// assuming one of the rectangles enclose the other rect vertically.
///
/// Returns positive if a is lower, negative if a is higher.
static int _compareHorizontally(Rect a, Rect b) {
// a encloses b.
if (a.left - b.left < precisionErrorTolerance && a.right - b.right > - precisionErrorTolerance) {
return -1;
}
// b encloses a.
if (b.left - a.left < precisionErrorTolerance && b.right - a.right > - precisionErrorTolerance) {
return 1;
}
if ((a.left - b.left).abs() > precisionErrorTolerance) {
return a.left > b.left ? 1 : -1;
}
return a.right > b.right ? 1 : -1;
}
void _handleSelectableGeometryChange() {
// Geometries of selectable children may change multiple times when handling
// selection events. Ignore these updates since the selection geometry of
// this delegate will be updated after handling the selection events.
if (_isHandlingSelectionEvent) {
return;
}
_updateSelectionGeometry();
}
/// Gets the combined selection geometry for child selectables.
@protected
SelectionGeometry getSelectionGeometry() {
if (currentSelectionEndIndex == -1 ||
currentSelectionStartIndex == -1 ||
selectables.isEmpty) {
// There is no valid selection.
return SelectionGeometry(
status: SelectionStatus.none,
hasContent: selectables.isNotEmpty,
);
}
if (!_extendSelectionInProgress) {
currentSelectionStartIndex = _adjustSelectionIndexBasedOnSelectionGeometry(
currentSelectionStartIndex,
currentSelectionEndIndex,
);
currentSelectionEndIndex = _adjustSelectionIndexBasedOnSelectionGeometry(
currentSelectionEndIndex,
currentSelectionStartIndex,
);
}
// Need to find the non-null start selection point.
SelectionGeometry startGeometry = selectables[currentSelectionStartIndex].value;
final bool forwardSelection = currentSelectionEndIndex >= currentSelectionStartIndex;
int startIndexWalker = currentSelectionStartIndex;
while (startIndexWalker != currentSelectionEndIndex && startGeometry.startSelectionPoint == null) {
startIndexWalker += forwardSelection ? 1 : -1;
startGeometry = selectables[startIndexWalker].value;
}
SelectionPoint? startPoint;
if (startGeometry.startSelectionPoint != null) {
final Matrix4 startTransform = getTransformFrom(selectables[startIndexWalker]);
final Offset start = MatrixUtils.transformPoint(startTransform, startGeometry.startSelectionPoint!.localPosition);
// It can be NaN if it is detached or off-screen.
if (start.isFinite) {
startPoint = SelectionPoint(
localPosition: start,
lineHeight: startGeometry.startSelectionPoint!.lineHeight,
handleType: startGeometry.startSelectionPoint!.handleType,
);
}
}
// Need to find the non-null end selection point.
SelectionGeometry endGeometry = selectables[currentSelectionEndIndex].value;
int endIndexWalker = currentSelectionEndIndex;
while (endIndexWalker != currentSelectionStartIndex && endGeometry.endSelectionPoint == null) {
endIndexWalker += forwardSelection ? -1 : 1;
endGeometry = selectables[endIndexWalker].value;
}
SelectionPoint? endPoint;
if (endGeometry.endSelectionPoint != null) {
final Matrix4 endTransform = getTransformFrom(selectables[endIndexWalker]);
final Offset end = MatrixUtils.transformPoint(endTransform, endGeometry.endSelectionPoint!.localPosition);
// It can be NaN if it is detached or off-screen.
if (end.isFinite) {
endPoint = SelectionPoint(
localPosition: end,
lineHeight: endGeometry.endSelectionPoint!.lineHeight,
handleType: endGeometry.endSelectionPoint!.handleType,
);
}
}
// Need to collect selection rects from selectables ranging from the
// currentSelectionStartIndex to the currentSelectionEndIndex.
final List<Rect> selectionRects = <Rect>[];
final Rect? drawableArea = hasSize ? Rect
.fromLTWH(0, 0, containerSize.width, containerSize.height) : null;
for (int index = currentSelectionStartIndex; index <= currentSelectionEndIndex; index++) {
final List<Rect> currSelectableSelectionRects = selectables[index].value.selectionRects;
final List<Rect> selectionRectsWithinDrawableArea = currSelectableSelectionRects.map((Rect selectionRect) {
final Matrix4 transform = getTransformFrom(selectables[index]);
final Rect localRect = MatrixUtils.transformRect(transform, selectionRect);
return drawableArea?.intersect(localRect) ?? localRect;
}).where((Rect selectionRect) {
return selectionRect.isFinite && !selectionRect.isEmpty;
}).toList();
selectionRects.addAll(selectionRectsWithinDrawableArea);
}
return SelectionGeometry(
startSelectionPoint: startPoint,
endSelectionPoint: endPoint,
selectionRects: selectionRects,
status: startGeometry != endGeometry
? SelectionStatus.uncollapsed
: startGeometry.status,
// Would have at least one selectable child.
hasContent: true,
);
}
// The currentSelectionStartIndex or currentSelectionEndIndex may not be
// the current index that contains selection edges. This can happen if the
// selection edge is in between two selectables. One of the selectable will
// have its selection collapsed at the index 0 or contentLength depends on
// whether the selection is reversed or not. The current selection index can
// be point to either one.
//
// This method adjusts the index to point to selectable with valid selection.
int _adjustSelectionIndexBasedOnSelectionGeometry(int currentIndex, int towardIndex) {
final bool forward = towardIndex > currentIndex;
while (currentIndex != towardIndex &&
selectables[currentIndex].value.status != SelectionStatus.uncollapsed) {
currentIndex += forward ? 1 : -1;
}
return currentIndex;
}
@override
void pushHandleLayers(LayerLink? startHandle, LayerLink? endHandle) {
if (_startHandleLayer == startHandle && _endHandleLayer == endHandle) {
return;
}
_startHandleLayer = startHandle;
_endHandleLayer = endHandle;
_updateHandleLayersAndOwners();
}
/// Pushes both handle layers to the selectables that contain selection edges.
///
/// This method needs to be called every time the selectables that contain the
/// selection edges change, i.e. [currentSelectionStartIndex] or
/// [currentSelectionEndIndex] changes. Otherwise, the handle may be painted
/// in the wrong place.
void _updateHandleLayersAndOwners() {
LayerLink? effectiveStartHandle = _startHandleLayer;
LayerLink? effectiveEndHandle = _endHandleLayer;
if (effectiveStartHandle != null || effectiveEndHandle != null) {
final Rect? drawableArea = hasSize ? Rect
.fromLTWH(0, 0, containerSize.width, containerSize.height)
.inflate(_kSelectionHandleDrawableAreaPadding) : null;
final bool hideStartHandle = value.startSelectionPoint == null || drawableArea == null || !drawableArea.contains(value.startSelectionPoint!.localPosition);
final bool hideEndHandle = value.endSelectionPoint == null || drawableArea == null|| !drawableArea.contains(value.endSelectionPoint!.localPosition);
effectiveStartHandle = hideStartHandle ? null : _startHandleLayer;
effectiveEndHandle = hideEndHandle ? null : _endHandleLayer;
}
if (currentSelectionStartIndex == -1 || currentSelectionEndIndex == -1) {
// No valid selection.
if (_startHandleLayerOwner != null) {
_startHandleLayerOwner!.pushHandleLayers(null, null);
_startHandleLayerOwner = null;
}
if (_endHandleLayerOwner != null) {
_endHandleLayerOwner!.pushHandleLayers(null, null);
_endHandleLayerOwner = null;
}
return;
}
if (selectables[currentSelectionStartIndex] != _startHandleLayerOwner) {
_startHandleLayerOwner?.pushHandleLayers(null, null);
}
if (selectables[currentSelectionEndIndex] != _endHandleLayerOwner) {
_endHandleLayerOwner?.pushHandleLayers(null, null);
}
_startHandleLayerOwner = selectables[currentSelectionStartIndex];
if (currentSelectionStartIndex == currentSelectionEndIndex) {
// Selection edges is on the same selectable.
_endHandleLayerOwner = _startHandleLayerOwner;
_startHandleLayerOwner!.pushHandleLayers(effectiveStartHandle, effectiveEndHandle);
return;
}
_startHandleLayerOwner!.pushHandleLayers(effectiveStartHandle, null);
_endHandleLayerOwner = selectables[currentSelectionEndIndex];
_endHandleLayerOwner!.pushHandleLayers(null, effectiveEndHandle);
}
/// Copies the selected contents of all selectables.
@override
SelectedContent? getSelectedContent() {
final List<SelectedContent> selections = <SelectedContent>[];
for (final Selectable selectable in selectables) {
final SelectedContent? data = selectable.getSelectedContent();
if (data != null) {
selections.add(data);
}
}
if (selections.isEmpty) {
return null;
}
final StringBuffer buffer = StringBuffer();
for (final SelectedContent selection in selections) {
buffer.write(selection.plainText);
}
return SelectedContent(
plainText: buffer.toString(),
);
}
// Clears the selection on all selectables not in the range of
// currentSelectionStartIndex..currentSelectionEndIndex.
//
// If one of the edges does not exist, then this method will clear the selection
// in all selectables except the existing edge.
//
// If neither of the edges exist this method immediately returns.
void _flushInactiveSelections() {
if (currentSelectionStartIndex == -1 && currentSelectionEndIndex == -1) {
return;
}
if (currentSelectionStartIndex == -1 || currentSelectionEndIndex == -1) {
final int skipIndex = currentSelectionStartIndex == -1 ? currentSelectionEndIndex : currentSelectionStartIndex;
selectables
.where((Selectable target) => target != selectables[skipIndex])
.forEach((Selectable target) => dispatchSelectionEventToChild(target, const ClearSelectionEvent()));
return;
}
final int skipStart = min(currentSelectionStartIndex, currentSelectionEndIndex);
final int skipEnd = max(currentSelectionStartIndex, currentSelectionEndIndex);
for (int index = 0; index < selectables.length; index += 1) {
if (index >= skipStart && index <= skipEnd) {
continue;
}
dispatchSelectionEventToChild(selectables[index], const ClearSelectionEvent());
}
}
/// Selects all contents of all selectables.
@protected
SelectionResult handleSelectAll(SelectAllSelectionEvent event) {
for (final Selectable selectable in selectables) {
dispatchSelectionEventToChild(selectable, event);
}
currentSelectionStartIndex = 0;
currentSelectionEndIndex = selectables.length - 1;
return SelectionResult.none;
}
/// Selects a word in a selectable at the location
/// [SelectWordSelectionEvent.globalPosition].
@protected
SelectionResult handleSelectWord(SelectWordSelectionEvent event) {
SelectionResult? lastSelectionResult;
for (int index = 0; index < selectables.length; index += 1) {
bool globalRectsContainsPosition = false;
if (selectables[index].boundingBoxes.isNotEmpty) {
for (final Rect rect in selectables[index].boundingBoxes) {
final Rect globalRect = MatrixUtils.transformRect(selectables[index].getTransformTo(null), rect);
if (globalRect.contains(event.globalPosition)) {
globalRectsContainsPosition = true;
break;
}
}
}
if (globalRectsContainsPosition) {
final SelectionGeometry existingGeometry = selectables[index].value;
lastSelectionResult = dispatchSelectionEventToChild(selectables[index], event);
if (index == selectables.length - 1 && lastSelectionResult == SelectionResult.next) {
return SelectionResult.next;
}
if (lastSelectionResult == SelectionResult.next) {
continue;
}
if (index == 0 && lastSelectionResult == SelectionResult.previous) {
return SelectionResult.previous;
}
if (selectables[index].value != existingGeometry) {
// Geometry has changed as a result of select word, need to clear the
// selection of other selectables to keep selection in sync.
selectables
.where((Selectable target) => target != selectables[index])
.forEach((Selectable target) => dispatchSelectionEventToChild(target, const ClearSelectionEvent()));
currentSelectionStartIndex = currentSelectionEndIndex = index;
}
return SelectionResult.end;
} else {
if (lastSelectionResult == SelectionResult.next) {
currentSelectionStartIndex = currentSelectionEndIndex = index - 1;
return SelectionResult.end;
}
}
}
assert(lastSelectionResult == null);
return SelectionResult.end;
}
/// Removes the selection of all selectables this delegate manages.
@protected
SelectionResult handleClearSelection(ClearSelectionEvent event) {
for (final Selectable selectable in selectables) {
dispatchSelectionEventToChild(selectable, event);
}
currentSelectionEndIndex = -1;
currentSelectionStartIndex = -1;
return SelectionResult.none;
}
/// Extend current selection in a certain text granularity.
@protected
SelectionResult handleGranularlyExtendSelection(GranularlyExtendSelectionEvent event) {
assert((currentSelectionStartIndex == -1) == (currentSelectionEndIndex == -1));
if (currentSelectionStartIndex == -1) {
if (event.forward) {
currentSelectionStartIndex = currentSelectionEndIndex = 0;
} else {
currentSelectionStartIndex = currentSelectionEndIndex = selectables.length;
}
}
int targetIndex = event.isEnd ? currentSelectionEndIndex : currentSelectionStartIndex;
SelectionResult result = dispatchSelectionEventToChild(selectables[targetIndex], event);
if (event.forward) {
assert(result != SelectionResult.previous);
while (targetIndex < selectables.length - 1 && result == SelectionResult.next) {
targetIndex += 1;
result = dispatchSelectionEventToChild(selectables[targetIndex], event);
assert(result != SelectionResult.previous);
}
} else {
assert(result != SelectionResult.next);
while (targetIndex > 0 && result == SelectionResult.previous) {
targetIndex -= 1;
result = dispatchSelectionEventToChild(selectables[targetIndex], event);
assert(result != SelectionResult.next);
}
}
if (event.isEnd) {
currentSelectionEndIndex = targetIndex;
} else {
currentSelectionStartIndex = targetIndex;
}
return result;
}
/// Extend current selection in a certain text granularity.
@protected
SelectionResult handleDirectionallyExtendSelection(DirectionallyExtendSelectionEvent event) {
assert((currentSelectionStartIndex == -1) == (currentSelectionEndIndex == -1));
if (currentSelectionStartIndex == -1) {
currentSelectionStartIndex = currentSelectionEndIndex = switch (event.direction) {
SelectionExtendDirection.previousLine || SelectionExtendDirection.backward => selectables.length,
SelectionExtendDirection.nextLine || SelectionExtendDirection.forward => 0,
};
}
int targetIndex = event.isEnd ? currentSelectionEndIndex : currentSelectionStartIndex;
SelectionResult result = dispatchSelectionEventToChild(selectables[targetIndex], event);
switch (event.direction) {
case SelectionExtendDirection.previousLine:
assert(result == SelectionResult.end || result == SelectionResult.previous);
if (result == SelectionResult.previous) {
if (targetIndex > 0) {
targetIndex -= 1;
result = dispatchSelectionEventToChild(
selectables[targetIndex],
event.copyWith(direction: SelectionExtendDirection.backward),
);
assert(result == SelectionResult.end);
}
}
case SelectionExtendDirection.nextLine:
assert(result == SelectionResult.end || result == SelectionResult.next);
if (result == SelectionResult.next) {
if (targetIndex < selectables.length - 1) {
targetIndex += 1;
result = dispatchSelectionEventToChild(
selectables[targetIndex],
event.copyWith(direction: SelectionExtendDirection.forward),
);
assert(result == SelectionResult.end);
}
}
case SelectionExtendDirection.forward:
case SelectionExtendDirection.backward:
assert(result == SelectionResult.end);
}
if (event.isEnd) {
currentSelectionEndIndex = targetIndex;
} else {
currentSelectionStartIndex = targetIndex;
}
return result;
}
/// Updates the selection edges.
@protected
SelectionResult handleSelectionEdgeUpdate(SelectionEdgeUpdateEvent event) {
if (event.type == SelectionEventType.endEdgeUpdate) {
return currentSelectionEndIndex == -1 ? _initSelection(event, isEnd: true) : _adjustSelection(event, isEnd: true);
}
return currentSelectionStartIndex == -1 ? _initSelection(event, isEnd: false) : _adjustSelection(event, isEnd: false);
}
@override
SelectionResult dispatchSelectionEvent(SelectionEvent event) {
final bool selectionWillBeInProgress = event is! ClearSelectionEvent;
if (!_selectionInProgress && selectionWillBeInProgress) {
// Sort the selectable every time a selection start.
selectables.sort(compareOrder);
}
_selectionInProgress = selectionWillBeInProgress;
_isHandlingSelectionEvent = true;
late SelectionResult result;
switch (event.type) {
case SelectionEventType.startEdgeUpdate:
case SelectionEventType.endEdgeUpdate:
_extendSelectionInProgress = false;
result = handleSelectionEdgeUpdate(event as SelectionEdgeUpdateEvent);
case SelectionEventType.clear:
_extendSelectionInProgress = false;
result = handleClearSelection(event as ClearSelectionEvent);
case SelectionEventType.selectAll:
_extendSelectionInProgress = false;
result = handleSelectAll(event as SelectAllSelectionEvent);
case SelectionEventType.selectWord:
_extendSelectionInProgress = false;
result = handleSelectWord(event as SelectWordSelectionEvent);
case SelectionEventType.granularlyExtendSelection:
_extendSelectionInProgress = true;
result = handleGranularlyExtendSelection(event as GranularlyExtendSelectionEvent);
case SelectionEventType.directionallyExtendSelection:
_extendSelectionInProgress = true;
result = handleDirectionallyExtendSelection(event as DirectionallyExtendSelectionEvent);
}
_isHandlingSelectionEvent = false;
_updateSelectionGeometry();
return result;
}
@override
void dispose() {
for (final Selectable selectable in selectables) {
selectable.removeListener(_handleSelectableGeometryChange);
}
selectables = const <Selectable>[];
_scheduledSelectableUpdate = false;
super.dispose();
}
/// Ensures the selectable child has received up to date selection event.
///
/// This method is called when a new [Selectable] is added to the delegate,
/// and its screen location falls into the previous selection.
///
/// Subclasses are responsible for updating the selection of this newly added
/// [Selectable].
@protected
void ensureChildUpdated(Selectable selectable);
/// Dispatches a selection event to a specific selectable.
///
/// Override this method if subclasses need to generate additional events or
/// treatments prior to sending the selection events.
@protected
SelectionResult dispatchSelectionEventToChild(Selectable selectable, SelectionEvent event) {
return selectable.dispatchSelectionEvent(event);
}
/// Initializes the selection of the selectable children.
///
/// The goal is to find the selectable child that contains the selection edge.
/// Returns [SelectionResult.end] if the selection edge ends on any of the
/// children. Otherwise, it returns [SelectionResult.previous] if the selection
/// does not reach any of its children. Returns [SelectionResult.next]
/// if the selection reaches the end of its children.
///
/// Ideally, this method should only be called twice at the beginning of the
/// drag selection, once for start edge update event, once for end edge update
/// event.
SelectionResult _initSelection(SelectionEdgeUpdateEvent event, {required bool isEnd}) {
assert((isEnd && currentSelectionEndIndex == -1) || (!isEnd && currentSelectionStartIndex == -1));
int newIndex = -1;
bool hasFoundEdgeIndex = false;
SelectionResult? result;
for (int index = 0; index < selectables.length && !hasFoundEdgeIndex; index += 1) {
final Selectable child = selectables[index];
final SelectionResult childResult = dispatchSelectionEventToChild(child, event);
switch (childResult) {
case SelectionResult.next:
case SelectionResult.none:
newIndex = index;
case SelectionResult.end:
newIndex = index;
result = SelectionResult.end;
hasFoundEdgeIndex = true;
case SelectionResult.previous:
hasFoundEdgeIndex = true;
if (index == 0) {
newIndex = 0;
result = SelectionResult.previous;
}
result ??= SelectionResult.end;
case SelectionResult.pending:
newIndex = index;
result = SelectionResult.pending;
hasFoundEdgeIndex = true;
}
}
if (newIndex == -1) {
assert(selectables.isEmpty);
return SelectionResult.none;
}
if (isEnd) {
currentSelectionEndIndex = newIndex;
} else {
currentSelectionStartIndex = newIndex;
}
_flushInactiveSelections();
// The result can only be null if the loop went through the entire list
// without any of the selection returned end or previous. In this case, the
// caller of this method needs to find the next selectable in their list.
return result ?? SelectionResult.next;
}
/// Adjusts the selection based on the drag selection update event if there
/// is already a selectable child that contains the selection edge.
///
/// This method starts by sending the selection event to the current
/// selectable that contains the selection edge, and finds forward or backward
/// if that selectable no longer contains the selection edge.
SelectionResult _adjustSelection(SelectionEdgeUpdateEvent event, {required bool isEnd}) {
assert(() {
if (isEnd) {
assert(currentSelectionEndIndex < selectables.length && currentSelectionEndIndex >= 0);
return true;
}
assert(currentSelectionStartIndex < selectables.length && currentSelectionStartIndex >= 0);
return true;
}());
SelectionResult? finalResult;
// Determines if the edge being adjusted is within the current viewport.
// - If so, we begin the search for the new selection edge position at the
// currentSelectionEndIndex/currentSelectionStartIndex.
// - If not, we attempt to locate the new selection edge starting from
// the opposite end.
// - If neither edge is in the current viewport, the search for the new
// selection edge position begins at 0.
//
// This can happen when there is a scrollable child and the edge being adjusted
// has been scrolled out of view.
final bool isCurrentEdgeWithinViewport = isEnd ? _selectionGeometry.endSelectionPoint != null : _selectionGeometry.startSelectionPoint != null;
final bool isOppositeEdgeWithinViewport = isEnd ? _selectionGeometry.startSelectionPoint != null : _selectionGeometry.endSelectionPoint != null;
int newIndex = switch ((isEnd, isCurrentEdgeWithinViewport, isOppositeEdgeWithinViewport)) {
(true, true, true) => currentSelectionEndIndex,
(true, true, false) => currentSelectionEndIndex,
(true, false, true) => currentSelectionStartIndex,
(true, false, false) => 0,
(false, true, true) => currentSelectionStartIndex,
(false, true, false) => currentSelectionStartIndex,
(false, false, true) => currentSelectionEndIndex,
(false, false, false) => 0,
};
bool? forward;
late SelectionResult currentSelectableResult;
// This loop sends the selection event to one of the following to determine
// the direction of the search.
// - currentSelectionEndIndex/currentSelectionStartIndex if the current edge
// is in the current viewport.
// - The opposite edge index if the current edge is not in the current viewport.
// - Index 0 if neither edge is in the current viewport.
//
// If the result is `SelectionResult.next`, this loop look backward.
// Otherwise, it looks forward.
//
// The terminate condition are:
// 1. the selectable returns end, pending, none.
// 2. the selectable returns previous when looking forward.
// 2. the selectable returns next when looking backward.
while (newIndex < selectables.length && newIndex >= 0 && finalResult == null) {
currentSelectableResult = dispatchSelectionEventToChild(selectables[newIndex], event);
switch (currentSelectableResult) {
case SelectionResult.end:
case SelectionResult.pending:
case SelectionResult.none:
finalResult = currentSelectableResult;
case SelectionResult.next:
if (forward == false) {
newIndex += 1;
finalResult = SelectionResult.end;
} else if (newIndex == selectables.length - 1) {
finalResult = currentSelectableResult;
} else {
forward = true;
newIndex += 1;
}
case SelectionResult.previous:
if (forward ?? false) {
newIndex -= 1;
finalResult = SelectionResult.end;
} else if (newIndex == 0) {
finalResult = currentSelectableResult;
} else {
forward = false;
newIndex -= 1;
}
}
}
if (isEnd) {
currentSelectionEndIndex = newIndex;
} else {
currentSelectionStartIndex = newIndex;
}
_flushInactiveSelections();
return finalResult!;
}
}
/// Signature for a widget builder that builds a context menu for the given
/// [SelectableRegionState].
///
/// See also:
///
/// * [EditableTextContextMenuBuilder], which performs the same role for
/// [EditableText].
typedef SelectableRegionContextMenuBuilder = Widget Function(
BuildContext context,
SelectableRegionState selectableRegionState,
);
| flutter/packages/flutter/lib/src/widgets/selectable_region.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/selectable_region.dart",
"repo_id": "flutter",
"token_count": 34163
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'basic.dart';
import 'framework.dart';
/// Spacer creates an adjustable, empty spacer that can be used to tune the
/// spacing between widgets in a [Flex] container, like [Row] or [Column].
///
/// The [Spacer] widget will take up any available space, so setting the
/// [Flex.mainAxisAlignment] on a flex container that contains a [Spacer] to
/// [MainAxisAlignment.spaceAround], [MainAxisAlignment.spaceBetween], or
/// [MainAxisAlignment.spaceEvenly] will not have any visible effect: the
/// [Spacer] has taken up all of the additional space, therefore there is none
/// left to redistribute.
///
/// {@tool snippet}
///
/// ```dart
/// const Row(
/// children: <Widget>[
/// Text('Begin'),
/// Spacer(), // Defaults to a flex of one.
/// Text('Middle'),
/// // Gives twice the space between Middle and End than Begin and Middle.
/// Spacer(flex: 2),
/// Text('End'),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=7FJgd7QN1zI}
///
/// See also:
///
/// * [Row] and [Column], which are the most common containers to use a Spacer
/// in.
/// * [SizedBox], to create a box with a specific size and an optional child.
class Spacer extends StatelessWidget {
/// Creates a flexible space to insert into a [Flexible] widget.
///
/// The [flex] parameter may not be null or less than one.
const Spacer({super.key, this.flex = 1})
: assert(flex > 0);
/// The flex factor to use in determining how much space to take up.
///
/// The amount of space the [Spacer] can occupy in the main axis is determined
/// by dividing the free space proportionately, after placing the inflexible
/// children, according to the flex factors of the flexible children.
///
/// Defaults to one.
final int flex;
@override
Widget build(BuildContext context) {
return Expanded(
flex: flex,
child: const SizedBox.shrink(),
);
}
}
| flutter/packages/flutter/lib/src/widgets/spacer.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/spacer.dart",
"repo_id": "flutter",
"token_count": 661
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/animation.dart';
import 'package:flutter/rendering.dart';
import 'framework.dart';
import 'scroll_delegate.dart';
import 'scroll_notification.dart';
import 'scroll_position.dart';
export 'package:flutter/rendering.dart' show AxisDirection;
// Examples can assume:
// late final RenderBox child;
// late final BoxConstraints constraints;
// class RenderSimpleTwoDimensionalViewport extends RenderTwoDimensionalViewport {
// RenderSimpleTwoDimensionalViewport({
// required super.horizontalOffset,
// required super.horizontalAxisDirection,
// required super.verticalOffset,
// required super.verticalAxisDirection,
// required super.delegate,
// required super.mainAxis,
// required super.childManager,
// super.cacheExtent,
// super.clipBehavior = Clip.hardEdge,
// });
// @override
// void layoutChildSequence() { }
// }
/// Signature for a function that creates a widget for a given [ChildVicinity],
/// e.g., in a [TwoDimensionalScrollView], but may return null.
///
/// Used by [TwoDimensionalChildBuilderDelegate.builder] and other APIs that
/// use lazily-generated widgets where the child count may not be known
/// ahead of time.
///
/// Unlike most builders, this callback can return null, indicating the
/// [ChildVicinity.xIndex] or [ChildVicinity.yIndex] is out of range. Whether
/// and when this is valid depends on the semantics of the builder. For example,
/// [TwoDimensionalChildBuilderDelegate.builder] returns
/// null when one or both of the indices is out of range, where the range is
/// defined by the [TwoDimensionalChildBuilderDelegate.maxXIndex] or
/// [TwoDimensionalChildBuilderDelegate.maxYIndex]; so in that case the
/// vicinity values may determine whether returning null is valid or not.
///
/// See also:
///
/// * [WidgetBuilder], which is similar but only takes a [BuildContext].
/// * [NullableIndexedWidgetBuilder], which is similar but may return null.
/// * [IndexedWidgetBuilder], which is similar but not nullable.
typedef TwoDimensionalIndexedWidgetBuilder = Widget? Function(BuildContext context, ChildVicinity vicinity);
/// A widget through which a portion of larger content can be viewed, typically
/// in combination with a [TwoDimensionalScrollable].
///
/// [TwoDimensionalViewport] is the visual workhorse of the two dimensional
/// scrolling machinery. It displays a subset of its children according to its
/// own dimensions and the given [horizontalOffset] an [verticalOffset]. As the
/// offsets vary, different children are visible through the viewport.
///
/// Subclasses must implement [createRenderObject] and [updateRenderObject].
/// Both of these methods require the render object to be a subclass of
/// [RenderTwoDimensionalViewport]. This class will create its own
/// [RenderObjectElement] which already implements the
/// [TwoDimensionalChildManager], which means subclasses should cast the
/// [BuildContext] to provide as the child manager to the
/// [RenderTwoDimensionalViewport].
///
/// {@tool snippet}
/// This is an example of a subclass implementation of [TwoDimensionalViewport],
/// `SimpleTwoDimensionalViewport`. The `RenderSimpleTwoDimensionalViewport` is
/// a subclass of [RenderTwoDimensionalViewport].
///
/// ```dart
/// class SimpleTwoDimensionalViewport extends TwoDimensionalViewport {
/// const SimpleTwoDimensionalViewport({
/// super.key,
/// required super.verticalOffset,
/// required super.verticalAxisDirection,
/// required super.horizontalOffset,
/// required super.horizontalAxisDirection,
/// required super.delegate,
/// required super.mainAxis,
/// super.cacheExtent,
/// super.clipBehavior = Clip.hardEdge,
/// });
///
/// @override
/// RenderSimpleTwoDimensionalViewport createRenderObject(BuildContext context) {
/// return RenderSimpleTwoDimensionalViewport(
/// horizontalOffset: horizontalOffset,
/// horizontalAxisDirection: horizontalAxisDirection,
/// verticalOffset: verticalOffset,
/// verticalAxisDirection: verticalAxisDirection,
/// mainAxis: mainAxis,
/// delegate: delegate,
/// childManager: context as TwoDimensionalChildManager,
/// cacheExtent: cacheExtent,
/// clipBehavior: clipBehavior,
/// );
/// }
///
/// @override
/// void updateRenderObject(BuildContext context, RenderSimpleTwoDimensionalViewport renderObject) {
/// renderObject
/// ..horizontalOffset = horizontalOffset
/// ..horizontalAxisDirection = horizontalAxisDirection
/// ..verticalOffset = verticalOffset
/// ..verticalAxisDirection = verticalAxisDirection
/// ..mainAxis = mainAxis
/// ..delegate = delegate
/// ..cacheExtent = cacheExtent
/// ..clipBehavior = clipBehavior;
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Viewport], the equivalent of this widget that scrolls in only one
/// dimension.
abstract class TwoDimensionalViewport extends RenderObjectWidget {
/// Creates a viewport for [RenderBox] objects that extend and scroll in both
/// horizontal and vertical dimensions.
///
/// The viewport listens to the [horizontalOffset] and [verticalOffset], which
/// means this widget does not need to be rebuilt when the offsets change.
const TwoDimensionalViewport({
super.key,
required this.verticalOffset,
required this.verticalAxisDirection,
required this.horizontalOffset,
required this.horizontalAxisDirection,
required this.delegate,
required this.mainAxis,
this.cacheExtent,
this.clipBehavior = Clip.hardEdge,
}) : assert(
verticalAxisDirection == AxisDirection.down || verticalAxisDirection == AxisDirection.up,
'TwoDimensionalViewport.verticalAxisDirection is not Axis.vertical.'
),
assert(
horizontalAxisDirection == AxisDirection.left || horizontalAxisDirection == AxisDirection.right,
'TwoDimensionalViewport.horizontalAxisDirection is not Axis.horizontal.'
);
/// Which part of the content inside the viewport should be visible in the
/// vertical axis.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport vertically, this value changes, which changes the
/// content that is displayed.
///
/// Typically a [ScrollPosition].
final ViewportOffset verticalOffset;
/// The direction in which the [verticalOffset]'s [ViewportOffset.pixels]
/// increases.
///
/// For example, if the axis direction is [AxisDirection.down], a scroll
/// offset of zero is at the top of the viewport and increases towards the
/// bottom of the viewport.
///
/// Must be either [AxisDirection.down] or [AxisDirection.up] in correlation
/// with an [Axis.vertical].
final AxisDirection verticalAxisDirection;
/// Which part of the content inside the viewport should be visible in the
/// horizontal axis.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport horizontally, this value changes, which changes the
/// content that is displayed.
///
/// Typically a [ScrollPosition].
final ViewportOffset horizontalOffset;
/// The direction in which the [horizontalOffset]'s [ViewportOffset.pixels]
/// increases.
///
/// For example, if the axis direction is [AxisDirection.right], a scroll
/// offset of zero is at the left of the viewport and increases towards the
/// right of the viewport.
///
/// Must be either [AxisDirection.left] or [AxisDirection.right] in correlation
/// with an [Axis.horizontal].
final AxisDirection horizontalAxisDirection;
/// The main axis of the two.
///
/// Used to determine the paint order of the children of the viewport. When
/// the main axis is [Axis.vertical], children will be painted in row major
/// order, according to their associated [ChildVicinity]. When the main axis
/// is [Axis.horizontal], the children will be painted in column major order.
final Axis mainAxis;
/// {@macro flutter.rendering.RenderViewportBase.cacheExtent}
final double? cacheExtent;
/// {@macro flutter.material.Material.clipBehavior}
final Clip clipBehavior;
/// A delegate that provides the children for the [TwoDimensionalViewport].
final TwoDimensionalChildDelegate delegate;
@override
RenderObjectElement createElement() => _TwoDimensionalViewportElement(this);
@override
RenderTwoDimensionalViewport createRenderObject(BuildContext context);
@override
void updateRenderObject(BuildContext context, RenderTwoDimensionalViewport renderObject);
}
class _TwoDimensionalViewportElement extends RenderObjectElement
with NotifiableElementMixin, ViewportElementMixin implements TwoDimensionalChildManager {
_TwoDimensionalViewportElement(super.widget);
@override
RenderTwoDimensionalViewport get renderObject => super.renderObject as RenderTwoDimensionalViewport;
// Contains all children, including those that are keyed.
Map<ChildVicinity, Element> _vicinityToChild = <ChildVicinity, Element>{};
Map<Key, Element> _keyToChild = <Key, Element>{};
// Used between _startLayout() & _endLayout() to compute the new values for
// _vicinityToChild and _keyToChild.
Map<ChildVicinity, Element>? _newVicinityToChild;
Map<Key, Element>? _newKeyToChild;
@override
void performRebuild() {
super.performRebuild();
// Children list is updated during layout since we only know during layout
// which children will be visible.
renderObject.markNeedsLayout(withDelegateRebuild: true);
}
@override
void forgetChild(Element child) {
assert(!_debugIsDoingLayout);
super.forgetChild(child);
_vicinityToChild.remove(child.slot);
if (child.widget.key != null) {
_keyToChild.remove(child.widget.key);
}
}
@override
void insertRenderObjectChild(RenderBox child, ChildVicinity slot) {
renderObject._insertChild(child, slot);
}
@override
void moveRenderObjectChild(RenderBox child, ChildVicinity oldSlot, ChildVicinity newSlot) {
renderObject._moveChild(child, from: oldSlot, to: newSlot);
}
@override
void removeRenderObjectChild(RenderBox child, ChildVicinity slot) {
renderObject._removeChild(child, slot);
}
@override
void visitChildren(ElementVisitor visitor) {
_vicinityToChild.values.forEach(visitor);
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<Element> children = _vicinityToChild.values.toList()..sort(_compareChildren);
return <DiagnosticsNode>[
for (final Element child in children)
child.toDiagnosticsNode(name: child.slot.toString())
];
}
static int _compareChildren(Element a, Element b) {
final ChildVicinity aSlot = a.slot! as ChildVicinity;
final ChildVicinity bSlot = b.slot! as ChildVicinity;
return aSlot.compareTo(bSlot);
}
// ---- ChildManager implementation ----
bool get _debugIsDoingLayout => _newKeyToChild != null && _newVicinityToChild != null;
@override
void _startLayout() {
assert(!_debugIsDoingLayout);
_newVicinityToChild = <ChildVicinity, Element>{};
_newKeyToChild = <Key, Element>{};
}
@override
void _buildChild(ChildVicinity vicinity) {
assert(_debugIsDoingLayout);
owner!.buildScope(this, () {
final Widget? newWidget = (widget as TwoDimensionalViewport).delegate.build(this, vicinity);
if (newWidget == null) {
return;
}
final Element? oldElement = _retrieveOldElement(newWidget, vicinity);
final Element? newChild = updateChild(oldElement, newWidget, vicinity);
assert(newChild != null);
// Ensure we are not overwriting an existing child.
assert(_newVicinityToChild![vicinity] == null);
_newVicinityToChild![vicinity] = newChild!;
if (newWidget.key != null) {
// Ensure we are not overwriting an existing key
assert(_newKeyToChild![newWidget.key!] == null);
_newKeyToChild![newWidget.key!] = newChild;
}
});
}
Element? _retrieveOldElement(Widget newWidget, ChildVicinity vicinity) {
if (newWidget.key != null) {
final Element? result = _keyToChild.remove(newWidget.key);
if (result != null) {
_vicinityToChild.remove(result.slot);
}
return result;
}
final Element? potentialOldElement = _vicinityToChild[vicinity];
if (potentialOldElement != null && potentialOldElement.widget.key == null) {
return _vicinityToChild.remove(vicinity);
}
return null;
}
@override
void _reuseChild(ChildVicinity vicinity) {
assert(_debugIsDoingLayout);
final Element? elementToReuse = _vicinityToChild.remove(vicinity);
assert(
elementToReuse != null,
'Expected to re-use an element at $vicinity, but none was found.'
);
_newVicinityToChild![vicinity] = elementToReuse!;
if (elementToReuse.widget.key != null) {
assert(_keyToChild.containsKey(elementToReuse.widget.key));
assert(_keyToChild[elementToReuse.widget.key] == elementToReuse);
_newKeyToChild![elementToReuse.widget.key!] = _keyToChild.remove(elementToReuse.widget.key)!;
}
}
@override
void _endLayout() {
assert(_debugIsDoingLayout);
// Unmount all elements that have not been reused in the layout cycle.
for (final Element element in _vicinityToChild.values) {
if (element.widget.key == null) {
// If it has a key, we handle it below.
updateChild(element, null, null);
} else {
assert(_keyToChild.containsValue(element));
}
}
for (final Element element in _keyToChild.values) {
assert(element.widget.key != null);
updateChild(element, null, null);
}
_vicinityToChild = _newVicinityToChild!;
_keyToChild = _newKeyToChild!;
_newVicinityToChild = null;
_newKeyToChild = null;
assert(!_debugIsDoingLayout);
}
}
/// Parent data structure used by [RenderTwoDimensionalViewport].
///
/// The parent data primarily describes where a child is in the viewport. The
/// [layoutOffset] must be set by subclasses of [RenderTwoDimensionalViewport],
/// during [RenderTwoDimensionalViewport.layoutChildSequence] which represents
/// the position of the child in the viewport.
///
/// The [paintOffset] is computed by [RenderTwoDimensionalViewport] after
/// [RenderTwoDimensionalViewport.layoutChildSequence]. If subclasses of
/// RenderTwoDimensionalViewport override the paint method, the [paintOffset]
/// should be used to position the child in the viewport in order to account for
/// a reversed [AxisDirection] in one or both dimensions.
class TwoDimensionalViewportParentData extends ParentData with KeepAliveParentDataMixin {
/// The offset at which to paint the child in the parent's coordinate system.
///
/// This [Offset] represents the top left corner of the child of the
/// [TwoDimensionalViewport].
///
/// This value must be set by implementors during
/// [RenderTwoDimensionalViewport.layoutChildSequence]. After the method is
/// complete, the [RenderTwoDimensionalViewport] will compute the
/// [paintOffset] based on this value to account for the [AxisDirection].
Offset? layoutOffset;
/// The logical positioning of children in two dimensions.
///
/// While children may not be strictly laid out in rows and columns, the
/// relative positioning determines traversal of
/// children in row or column major format.
///
/// This is set in the [RenderTwoDimensionalViewport.buildOrObtainChildFor].
ChildVicinity vicinity = ChildVicinity.invalid;
/// Whether or not the child is actually visible within the viewport.
///
/// For example, if a child is contained within the
/// [RenderTwoDimensionalViewport.cacheExtent] and out of view.
///
/// This is used during [RenderTwoDimensionalViewport.paint] in order to skip
/// painting children that cannot be seen.
bool get isVisible {
assert(() {
if (_paintExtent == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('The paint extent of the child has not been determined yet.'),
ErrorDescription(
'The paint extent, and therefore the visibility, of a child of a '
'RenderTwoDimensionalViewport is computed after '
'RenderTwoDimensionalViewport.layoutChildSequence.'
),
]);
}
return true;
}());
return _paintExtent != Size.zero || _paintExtent!.height != 0.0 || _paintExtent!.width != 0.0;
}
/// Represents the extent in both dimensions of the child that is actually
/// visible.
///
/// For example, if a child [RenderBox] had a height of 100 pixels, and a
/// width of 100 pixels, but was scrolled to positions such that only 50
/// pixels of both width and height were visible, the paintExtent would be
/// represented as `Size(50.0, 50.0)`.
///
/// This is set in [RenderTwoDimensionalViewport.updateChildPaintData].
Size? _paintExtent;
/// The previous sibling in the parent's child list according to the traversal
/// order specified by [RenderTwoDimensionalViewport.mainAxis].
RenderBox? _previousSibling;
/// The next sibling in the parent's child list according to the traversal
/// order specified by [RenderTwoDimensionalViewport.mainAxis].
RenderBox? _nextSibling;
/// The position of the child relative to the bounds and [AxisDirection] of
/// the viewport.
///
/// This is the distance from the top left visible corner of the parent to the
/// top left visible corner of the child. When the [AxisDirection]s are
/// [AxisDirection.down] or [AxisDirection.right], this value is the same as
/// the [layoutOffset]. This value deviates when scrolling in the reverse
/// directions of [AxisDirection.up] and [AxisDirection.left] to reposition
/// the children correctly.
///
/// This is set in [RenderTwoDimensionalViewport.updateChildPaintData], after
/// [RenderTwoDimensionalViewport.layoutChildSequence].
///
/// If overriding [RenderTwoDimensionalViewport.paint], use this value to
/// position the children instead of [layoutOffset].
Offset? paintOffset;
@override
bool get keptAlive => keepAlive && !isVisible;
@override
String toString() {
return 'vicinity=$vicinity; '
'layoutOffset=$layoutOffset; '
'paintOffset=$paintOffset; '
'${_paintExtent == null
? 'not visible; '
: '${!isVisible ? 'not ' : ''}visible - paintExtent=$_paintExtent; '}'
'${keepAlive ? "keepAlive; " : ""}';
}
}
/// A base class for viewing render objects that scroll in two dimensions.
///
/// The viewport listens to two [ViewportOffset]s, which determines the
/// visible content.
///
/// Subclasses must implement [layoutChildSequence], calling on
/// [buildOrObtainChildFor] to manage the children of the viewport.
///
/// Subclasses should not override [performLayout], as it handles housekeeping
/// on either side of the call to [layoutChildSequence].
abstract class RenderTwoDimensionalViewport extends RenderBox implements RenderAbstractViewport {
/// Initializes fields for subclasses.
///
/// The [cacheExtent], if null, defaults to
/// [RenderAbstractViewport.defaultCacheExtent].
RenderTwoDimensionalViewport({
required ViewportOffset horizontalOffset,
required AxisDirection horizontalAxisDirection,
required ViewportOffset verticalOffset,
required AxisDirection verticalAxisDirection,
required TwoDimensionalChildDelegate delegate,
required Axis mainAxis,
required TwoDimensionalChildManager childManager,
double? cacheExtent,
Clip clipBehavior = Clip.hardEdge,
}) : assert(
verticalAxisDirection == AxisDirection.down || verticalAxisDirection == AxisDirection.up,
'TwoDimensionalViewport.verticalAxisDirection is not Axis.vertical.'
),
assert(
horizontalAxisDirection == AxisDirection.left || horizontalAxisDirection == AxisDirection.right,
'TwoDimensionalViewport.horizontalAxisDirection is not Axis.horizontal.'
),
_childManager = childManager,
_horizontalOffset = horizontalOffset,
_horizontalAxisDirection = horizontalAxisDirection,
_verticalOffset = verticalOffset,
_verticalAxisDirection = verticalAxisDirection,
_delegate = delegate,
_mainAxis = mainAxis,
_cacheExtent = cacheExtent ?? RenderAbstractViewport.defaultCacheExtent,
_clipBehavior = clipBehavior {
assert(() {
_debugDanglingKeepAlives = <RenderBox>[];
return true;
}());
}
/// Which part of the content inside the viewport should be visible in the
/// horizontal axis.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport horizontally, this value changes, which changes the
/// content that is displayed.
///
/// Typically a [ScrollPosition].
ViewportOffset get horizontalOffset => _horizontalOffset;
ViewportOffset _horizontalOffset;
set horizontalOffset(ViewportOffset value) {
if (_horizontalOffset == value) {
return;
}
if (attached) {
_horizontalOffset.removeListener(markNeedsLayout);
}
_horizontalOffset = value;
if (attached) {
_horizontalOffset.addListener(markNeedsLayout);
}
markNeedsLayout();
}
/// The direction in which the [horizontalOffset] increases.
///
/// For example, if the axis direction is [AxisDirection.right], a scroll
/// offset of zero is at the left of the viewport and increases towards the
/// right of the viewport.
AxisDirection get horizontalAxisDirection => _horizontalAxisDirection;
AxisDirection _horizontalAxisDirection;
set horizontalAxisDirection(AxisDirection value) {
if (_horizontalAxisDirection == value) {
return;
}
_horizontalAxisDirection = value;
markNeedsLayout();
}
/// Which part of the content inside the viewport should be visible in the
/// vertical axis.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport vertically, this value changes, which changes the
/// content that is displayed.
///
/// Typically a [ScrollPosition].
ViewportOffset get verticalOffset => _verticalOffset;
ViewportOffset _verticalOffset;
set verticalOffset(ViewportOffset value) {
if (_verticalOffset == value) {
return;
}
if (attached) {
_verticalOffset.removeListener(markNeedsLayout);
}
_verticalOffset = value;
if (attached) {
_verticalOffset.addListener(markNeedsLayout);
}
markNeedsLayout();
}
/// The direction in which the [verticalOffset] increases.
///
/// For example, if the axis direction is [AxisDirection.down], a scroll
/// offset of zero is at the top the viewport and increases towards the
/// bottom of the viewport.
AxisDirection get verticalAxisDirection => _verticalAxisDirection;
AxisDirection _verticalAxisDirection;
set verticalAxisDirection(AxisDirection value) {
if (_verticalAxisDirection == value) {
return;
}
_verticalAxisDirection = value;
markNeedsLayout();
}
/// Supplies children for layout in the viewport.
TwoDimensionalChildDelegate get delegate => _delegate;
TwoDimensionalChildDelegate _delegate;
set delegate(covariant TwoDimensionalChildDelegate value) {
if (_delegate == value) {
return;
}
if (attached) {
_delegate.removeListener(_handleDelegateNotification);
}
final TwoDimensionalChildDelegate oldDelegate = _delegate;
_delegate = value;
if (attached) {
_delegate.addListener(_handleDelegateNotification);
}
if (_delegate.runtimeType != oldDelegate.runtimeType || _delegate.shouldRebuild(oldDelegate)) {
_handleDelegateNotification();
}
}
/// The major axis of the two dimensions.
///
/// This is can be used by subclasses to determine paint order,
/// visitor patterns like row and column major ordering, or hit test
/// precedence.
///
/// See also:
///
/// * [TwoDimensionalScrollView], which assigns the [PrimaryScrollController]
/// to the [TwoDimensionalScrollView.mainAxis] and shares this value.
Axis get mainAxis => _mainAxis;
Axis _mainAxis;
set mainAxis(Axis value) {
if (_mainAxis == value) {
return;
}
_mainAxis = value;
// Child order needs to be resorted, which happens in performLayout.
markNeedsLayout();
}
/// {@macro flutter.rendering.RenderViewportBase.cacheExtent}
double get cacheExtent => _cacheExtent ?? RenderAbstractViewport.defaultCacheExtent;
double? _cacheExtent;
set cacheExtent(double? value) {
if (_cacheExtent == value) {
return;
}
_cacheExtent = value;
markNeedsLayout();
}
/// {@macro flutter.material.Material.clipBehavior}
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior;
set clipBehavior(Clip value) {
if (_clipBehavior == value) {
return;
}
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
final TwoDimensionalChildManager _childManager;
final Map<ChildVicinity, RenderBox> _children = <ChildVicinity, RenderBox>{};
/// Children that have been laid out (or re-used) during the course of
/// performLayout, used to update the keep alive bucket at the end of
/// performLayout.
final Map<ChildVicinity, RenderBox> _activeChildrenForLayoutPass = <ChildVicinity, RenderBox>{};
/// The nodes being kept alive despite not being visible.
final Map<ChildVicinity, RenderBox> _keepAliveBucket = <ChildVicinity, RenderBox>{};
late List<RenderBox> _debugDanglingKeepAlives;
bool _hasVisualOverflow = false;
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
bool get isRepaintBoundary => true;
@override
bool get sizedByParent => true;
// Keeps track of the upper and lower bounds of ChildVicinity indices when
// subclasses call buildOrObtainChildFor during layoutChildSequence. These
// values are used to sort children in accordance with the mainAxis for
// paint order.
int? _leadingXIndex;
int? _trailingXIndex;
int? _leadingYIndex;
int? _trailingYIndex;
/// The first child of the viewport according to the traversal order of the
/// [mainAxis].
///
/// {@template flutter.rendering.twoDimensionalViewport.paintOrder}
/// The [mainAxis] correlates with the [ChildVicinity] of each child to paint
/// the children in a row or column major order.
///
/// By default, the [mainAxis] is [Axis.vertical], which would result in a
/// row major paint order, visiting children in the horizontal indices before
/// advancing to the next vertical index.
/// {@endtemplate}
///
/// This value is null during [layoutChildSequence] as children are reified
/// into the correct order after layout is completed. This can be used when
/// overriding [paint] in order to paint the children in the correct order.
RenderBox? get firstChild => _firstChild;
RenderBox? _firstChild;
/// The last child in the viewport according to the traversal order of the
/// [mainAxis].
///
/// {@macro flutter.rendering.twoDimensionalViewport.paintOrder}
///
/// This value is null during [layoutChildSequence] as children are reified
/// into the correct order after layout is completed. This can be used when
/// overriding [paint] in order to paint the children in the correct order.
RenderBox? get lastChild => _lastChild;
RenderBox? _lastChild;
/// The previous child before the given child in the child list according to
/// the traversal order of the [mainAxis].
///
/// {@macro flutter.rendering.twoDimensionalViewport.paintOrder}
///
/// This method is useful when overriding [paint] in order to paint children
/// in the correct order.
RenderBox? childBefore(RenderBox child) {
assert(child.parent == this);
return parentDataOf(child)._previousSibling;
}
/// The next child after the given child in the child list according to
/// the traversal order of the [mainAxis].
///
/// {@macro flutter.rendering.twoDimensionalViewport.paintOrder}
///
/// This method is useful when overriding [paint] in order to paint children
/// in the correct order.
RenderBox? childAfter(RenderBox child) {
assert(child.parent == this);
return parentDataOf(child)._nextSibling;
}
void _handleDelegateNotification() {
return markNeedsLayout(withDelegateRebuild: true);
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! TwoDimensionalViewportParentData) {
child.parentData = TwoDimensionalViewportParentData();
}
}
/// Convenience method for retrieving and casting the [ParentData] of the
/// viewport's children.
///
/// Children must have a [ParentData] of type
/// [TwoDimensionalViewportParentData], or a subclass thereof.
@protected
@mustCallSuper
TwoDimensionalViewportParentData parentDataOf(RenderBox child) {
assert(_children.containsValue(child) ||
_keepAliveBucket.containsValue(child) ||
_debugOrphans!.contains(child));
return child.parentData! as TwoDimensionalViewportParentData;
}
/// Returns the active child located at the provided [ChildVicinity], if there
/// is one.
///
/// This can be used by subclasses to access currently active children to make
/// use of their size or [TwoDimensionalViewportParentData], such as when
/// overriding the [paint] method.
///
/// Returns null if there is no active child for the given [ChildVicinity].
@protected
RenderBox? getChildFor(covariant ChildVicinity vicinity) => _children[vicinity];
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_horizontalOffset.addListener(markNeedsLayout);
_verticalOffset.addListener(markNeedsLayout);
_delegate.addListener(_handleDelegateNotification);
for (final RenderBox child in _children.values) {
child.attach(owner);
}
for (final RenderBox child in _keepAliveBucket.values) {
child.attach(owner);
}
}
@override
void detach() {
super.detach();
_horizontalOffset.removeListener(markNeedsLayout);
_verticalOffset.removeListener(markNeedsLayout);
_delegate.removeListener(_handleDelegateNotification);
for (final RenderBox child in _children.values) {
child.detach();
}
for (final RenderBox child in _keepAliveBucket.values) {
child.detach();
}
}
@override
void redepthChildren() {
for (final RenderBox child in _children.values) {
child.redepthChildren();
}
_keepAliveBucket.values.forEach(redepthChild);
}
@override
void visitChildren(RenderObjectVisitor visitor) {
RenderBox? child = _firstChild;
while (child != null) {
visitor(child);
child = parentDataOf(child)._nextSibling;
}
_keepAliveBucket.values.forEach(visitor);
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
// Only children that are visible should be visited, and they must be in
// paint order.
RenderBox? child = _firstChild;
while (child != null) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
visitor(child);
child = childParentData._nextSibling;
}
// Do not visit children in [_keepAliveBucket].
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> debugChildren = <DiagnosticsNode>[
..._children.keys.map<DiagnosticsNode>((ChildVicinity vicinity) {
return _children[vicinity]!.toDiagnosticsNode(name: vicinity.toString());
})
];
return debugChildren;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
assert(debugCheckHasBoundedAxis(Axis.vertical, constraints));
assert(debugCheckHasBoundedAxis(Axis.horizontal, constraints));
return constraints.biggest;
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
for (final RenderBox child in _children.values) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
if (!childParentData.isVisible) {
// Can't hit a child that is not visible.
continue;
}
final bool isHit = result.addWithPaintOffset(
offset: childParentData.paintOffset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.paintOffset!);
return child.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
}
return false;
}
/// The dimensions of the viewport.
///
/// This [Size] represents the width and height of the visible area.
Size get viewportDimension {
assert(hasSize);
return size;
}
@override
void performResize() {
final Size? oldSize = hasSize ? size : null;
super.performResize();
// Ignoring return value since we are doing a layout either way
// (performLayout will be invoked next).
horizontalOffset.applyViewportDimension(size.width);
verticalOffset.applyViewportDimension(size.height);
if (oldSize != size) {
// Specs can depend on viewport size.
_didResize = true;
}
}
@protected
@override
RevealedOffset getOffsetToReveal(
RenderObject target,
double alignment, {
Rect? rect,
Axis? axis,
}) {
// If an axis has not been specified, use the mainAxis.
axis ??= mainAxis;
final (double offset, AxisDirection axisDirection) = switch (axis) {
Axis.vertical => (verticalOffset.pixels, verticalAxisDirection),
Axis.horizontal => (horizontalOffset.pixels, horizontalAxisDirection),
};
rect ??= target.paintBounds;
// `child` will be the last RenderObject before the viewport when walking
// up from `target`.
RenderObject child = target;
while (child.parent != this) {
child = child.parent!;
}
assert(child.parent == this);
final RenderBox box = child as RenderBox;
final Rect rectLocal = MatrixUtils.transformRect(target.getTransformTo(child), rect);
double leadingScrollOffset = offset;
// The scroll offset of `rect` within `child`.
leadingScrollOffset += switch (axisDirection) {
AxisDirection.up => child.size.height - rectLocal.bottom,
AxisDirection.left => child.size.width - rectLocal.right,
AxisDirection.right => rectLocal.left,
AxisDirection.down => rectLocal.top,
};
// The scroll offset in the viewport to `rect`.
final Offset paintOffset = parentDataOf(box).paintOffset!;
leadingScrollOffset += switch (axisDirection) {
AxisDirection.up => viewportDimension.height - paintOffset.dy - box.size.height,
AxisDirection.left => viewportDimension.width - paintOffset.dx - box.size.width,
AxisDirection.right => paintOffset.dx,
AxisDirection.down => paintOffset.dy,
};
// This step assumes the viewport's layout is up-to-date, i.e., if
// the position is changed after the last performLayout, the new scroll
// position will not be accounted for.
final Matrix4 transform = target.getTransformTo(this);
Rect targetRect = MatrixUtils.transformRect(transform, rect);
final double mainAxisExtentDifference = switch (axis) {
Axis.horizontal => viewportDimension.width - rectLocal.width,
Axis.vertical => viewportDimension.height - rectLocal.height,
};
final double targetOffset = leadingScrollOffset - mainAxisExtentDifference * alignment;
final double offsetDifference = switch (axis) {
Axis.horizontal => horizontalOffset.pixels - targetOffset,
Axis.vertical => verticalOffset.pixels - targetOffset,
};
targetRect = switch (axisDirection) {
AxisDirection.up => targetRect.translate(0.0, -offsetDifference),
AxisDirection.down => targetRect.translate(0.0, offsetDifference),
AxisDirection.left => targetRect.translate(-offsetDifference, 0.0),
AxisDirection.right => targetRect.translate( offsetDifference, 0.0),
};
final RevealedOffset revealedOffset = RevealedOffset(
offset: targetOffset,
rect: targetRect,
);
return revealedOffset;
}
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
// It is possible for one and not both axes to allow for implicit scrolling,
// so handling is split between the options for allowed implicit scrolling.
final bool allowHorizontal = horizontalOffset.allowImplicitScrolling;
final bool allowVertical = verticalOffset.allowImplicitScrolling;
AxisDirection? axisDirection;
switch ((allowHorizontal, allowVertical)) {
case (true, true):
// Both allow implicit scrolling.
break;
case (false, true):
// Only the vertical Axis allows implicit scrolling.
axisDirection = verticalAxisDirection;
case (true, false):
// Only the horizontal Axis allows implicit scrolling.
axisDirection = horizontalAxisDirection;
case (false, false):
// Neither axis allows for implicit scrolling.
return super.showOnScreen(
descendant: descendant,
rect: rect,
duration: duration,
curve: curve,
);
}
final Rect? newRect = RenderTwoDimensionalViewport.showInViewport(
descendant: descendant,
viewport: this,
axisDirection: axisDirection,
rect: rect,
duration: duration,
curve: curve,
);
super.showOnScreen(
rect: newRect,
duration: duration,
curve: curve,
);
}
/// Make (a portion of) the given `descendant` of the given `viewport` fully
/// visible in one or both dimensions of the `viewport` by manipulating the
/// [ViewportOffset]s.
///
/// The `axisDirection` determines from which axes the `descendant` will be
/// revealed. When the `axisDirection` is null, both axes will be updated to
/// reveal the descendant.
///
/// The optional `rect` parameter describes which area of the `descendant`
/// should be shown in the viewport. If `rect` is null, the entire
/// `descendant` will be revealed. The `rect` parameter is interpreted
/// relative to the coordinate system of `descendant`.
///
/// The returned [Rect] describes the new location of `descendant` or `rect`
/// in the viewport after it has been revealed. See [RevealedOffset.rect]
/// for a full definition of this [Rect].
///
/// The parameter `viewport` is required and cannot be null. If `descendant`
/// is null, this is a no-op and `rect` is returned.
///
/// If both `descendant` and `rect` are null, null is returned because there
/// is nothing to be shown in the viewport.
///
/// The `duration` parameter can be set to a non-zero value to animate the
/// target object into the viewport with an animation defined by `curve`.
///
/// See also:
///
/// * [RenderObject.showOnScreen], overridden by
/// [RenderTwoDimensionalViewport] to delegate to this method.
static Rect? showInViewport({
RenderObject? descendant,
Rect? rect,
required RenderTwoDimensionalViewport viewport,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
AxisDirection? axisDirection,
}) {
if (descendant == null) {
return rect;
}
Rect? showVertical(Rect? rect) {
return RenderTwoDimensionalViewport._showInViewportForAxisDirection(
descendant: descendant,
viewport: viewport,
axis: Axis.vertical,
rect: rect,
duration: duration,
curve: curve,
);
}
Rect? showHorizontal(Rect? rect) {
return RenderTwoDimensionalViewport._showInViewportForAxisDirection(
descendant: descendant,
viewport: viewport,
axis: Axis.horizontal,
rect: rect,
duration: duration,
curve: curve,
);
}
switch (axisDirection) {
case AxisDirection.left:
case AxisDirection.right:
return showHorizontal(rect);
case AxisDirection.up:
case AxisDirection.down:
return showVertical(rect);
case null:
// Update rect after revealing in one axis before revealing in the next.
rect = showHorizontal(rect) ?? rect;
// We only return the final rect after both have been revealed.
rect = showVertical(rect);
if (rect == null) {
// `descendant` is between leading and trailing edge and hence already
// fully shown on screen.
assert(viewport.parent != null);
final Matrix4 transform = descendant.getTransformTo(viewport.parent);
return MatrixUtils.transformRect(
transform,
rect ?? descendant.paintBounds,
);
}
return rect;
}
}
static Rect? _showInViewportForAxisDirection({
required RenderObject descendant,
Rect? rect,
required RenderTwoDimensionalViewport viewport,
required Axis axis,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
final ViewportOffset offset = switch (axis) {
Axis.vertical => viewport.verticalOffset,
Axis.horizontal => viewport.horizontalOffset,
};
final RevealedOffset leadingEdgeOffset = viewport.getOffsetToReveal(
descendant,
0.0,
rect: rect,
axis: axis,
);
final RevealedOffset trailingEdgeOffset = viewport.getOffsetToReveal(
descendant,
1.0,
rect: rect,
axis: axis,
);
final double currentOffset = offset.pixels;
final RevealedOffset? targetOffset = RevealedOffset.clampOffset(
leadingEdgeOffset: leadingEdgeOffset,
trailingEdgeOffset: trailingEdgeOffset,
currentOffset: currentOffset,
);
if (targetOffset == null) {
// Already visible in this axis.
return null;
}
offset.moveTo(targetOffset.offset, duration: duration, curve: curve);
return targetOffset.rect;
}
/// Should be used by subclasses to invalidate any cached metrics for the
/// viewport.
///
/// This is set to true when the viewport has been resized, indicating that
/// any cached dimensions are invalid.
///
/// After performLayout, the value is set to false until the viewport
/// dimensions are changed again in [performResize].
///
/// Subclasses are not required to use this value, but it can be used to
/// safely cache layout information in between layout calls.
bool get didResize => _didResize;
bool _didResize = true;
/// Should be used by subclasses to invalidate any cached data from the
/// [delegate].
///
/// This value is set to false after [layoutChildSequence]. If
/// [markNeedsLayout] is called `withDelegateRebuild` set to true, then this
/// value will be updated to true, signifying any cached delegate information
/// needs to be updated in the next call to [layoutChildSequence].
///
/// Subclasses are not required to use this value, but it can be used to
/// safely cache layout information in between layout calls.
@protected
bool get needsDelegateRebuild => _needsDelegateRebuild;
bool _needsDelegateRebuild = true;
@override
void markNeedsLayout({ bool withDelegateRebuild = false }) {
_needsDelegateRebuild = _needsDelegateRebuild || withDelegateRebuild;
super.markNeedsLayout();
}
/// Primary work horse of [performLayout].
///
/// Subclasses must implement this method to layout the children of the
/// viewport. The [TwoDimensionalViewportParentData.layoutOffset] must be set
/// during this method in order for the children to be positioned during paint.
/// Further, children of the viewport must be laid out with the expectation
/// that the parent (this viewport) will use their size.
///
/// ```dart
/// child.layout(constraints, parentUsesSize: true);
/// ```
///
/// The primary methods used for creating and obtaining children is
/// [buildOrObtainChildFor], which takes a [ChildVicinity] that is used by the
/// [TwoDimensionalChildDelegate]. If a child is not provided by the delegate
/// for the provided vicinity, the method will return null, otherwise, it will
/// return the [RenderBox] of the child.
///
/// After [layoutChildSequence] is completed, any remaining children that were
/// not obtained will be disposed.
void layoutChildSequence();
@override
void performLayout() {
_firstChild = null;
_lastChild = null;
_activeChildrenForLayoutPass.clear();
_childManager._startLayout();
// Subclass lays out children.
layoutChildSequence();
assert(_debugCheckContentDimensions());
_didResize = false;
_needsDelegateRebuild = false;
_cacheKeepAlives();
invokeLayoutCallback<BoxConstraints>((BoxConstraints _) {
_childManager._endLayout();
assert(_debugOrphans?.isEmpty ?? true);
assert(_debugDanglingKeepAlives.isEmpty);
// Ensure we are not keeping anything alive that should not be any longer.
assert(_keepAliveBucket.values.where((RenderBox child) {
return !parentDataOf(child).keepAlive;
}).isEmpty);
// Organize children in paint order and complete parent data after
// un-used children are disposed of by the childManager.
_reifyChildren();
});
}
void _cacheKeepAlives() {
final List<RenderBox> remainingChildren = _children.values.toSet().difference(
_activeChildrenForLayoutPass.values.toSet()
).toList();
for (final RenderBox child in remainingChildren) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
if (childParentData.keepAlive) {
_keepAliveBucket[childParentData.vicinity] = child;
// Let the child manager know we intend to keep this.
_childManager._reuseChild(childParentData.vicinity);
}
}
}
// Ensures all children have a layoutOffset, sets paintExtent & paintOffset,
// and arranges children in paint order.
void _reifyChildren() {
assert(_leadingXIndex != null);
assert(_trailingXIndex != null);
assert(_leadingYIndex != null);
assert(_trailingYIndex != null);
assert(_firstChild == null);
assert(_lastChild == null);
RenderBox? previousChild;
switch (mainAxis) {
case Axis.vertical:
// Row major traversal.
// This seems backwards, but the vertical axis is the typical default
// axis for scrolling in Flutter, while Row-major ordering is the
// typical default for matrices, which is why the inverse follows
// through in the horizontal case below.
// Minor
for (int minorIndex = _leadingYIndex!; minorIndex <= _trailingYIndex!; minorIndex++) {
// Major
for (int majorIndex = _leadingXIndex!; majorIndex <= _trailingXIndex!; majorIndex++) {
final ChildVicinity vicinity = ChildVicinity(xIndex: majorIndex, yIndex: minorIndex);
previousChild = _completeChildParentData(
vicinity,
previousChild: previousChild,
) ?? previousChild;
}
}
case Axis.horizontal:
// Column major traversal
// Minor
for (int minorIndex = _leadingXIndex!; minorIndex <= _trailingXIndex!; minorIndex++) {
// Major
for (int majorIndex = _leadingYIndex!; majorIndex <= _trailingYIndex!; majorIndex++) {
final ChildVicinity vicinity = ChildVicinity(xIndex: minorIndex, yIndex: majorIndex);
previousChild = _completeChildParentData(
vicinity,
previousChild: previousChild,
) ?? previousChild;
}
}
}
_lastChild = previousChild;
parentDataOf(_lastChild!)._nextSibling = null;
// Reset for next layout pass.
_leadingXIndex = null;
_trailingXIndex = null;
_leadingYIndex = null;
_trailingYIndex = null;
}
RenderBox? _completeChildParentData(ChildVicinity vicinity, { RenderBox? previousChild }) {
assert(vicinity != ChildVicinity.invalid);
// It is possible and valid for a vicinity to be skipped.
// For example, a table can have merged cells, spanning multiple
// indices, but only represented by one RenderBox and ChildVicinity.
if (_children.containsKey(vicinity)) {
final RenderBox child = _children[vicinity]!;
assert(parentDataOf(child).vicinity == vicinity);
updateChildPaintData(child);
if (previousChild == null) {
// _firstChild is only set once.
assert(_firstChild == null);
_firstChild = child;
} else {
parentDataOf(previousChild)._nextSibling = child;
parentDataOf(child)._previousSibling = previousChild;
}
return child;
}
return null;
}
bool _debugCheckContentDimensions() {
const String hint = 'Subclasses should call applyContentDimensions on the '
'verticalOffset and horizontalOffset to set the min and max scroll offset. '
'If the contents exceed one or both sides of the viewportDimension, '
'ensure the viewportDimension height or width is subtracted in that axis '
'for the correct extent.';
assert(() {
if (!(verticalOffset as ScrollPosition).hasContentDimensions) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The verticalOffset was not given content dimensions during '
'layoutChildSequence.'
),
ErrorHint(hint),
]);
}
return true;
}());
assert(() {
if (!(horizontalOffset as ScrollPosition).hasContentDimensions) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The horizontalOffset was not given content dimensions during '
'layoutChildSequence.'
),
ErrorHint(hint),
]);
}
return true;
}());
return true;
}
/// Returns the child for a given [ChildVicinity], should be called during
/// [layoutChildSequence] in order to instantiate or retrieve children.
///
/// This method will build the child if it has not been already, or will reuse
/// it if it already exists, whether it was part of the previous frame or kept
/// alive.
///
/// Children for the given [ChildVicinity] will be inserted into the active
/// children list, and so should be visible, or contained within the
/// [cacheExtent].
RenderBox? buildOrObtainChildFor(ChildVicinity vicinity) {
assert(vicinity != ChildVicinity.invalid);
// This should only be called during layout.
assert(debugDoingThisLayout);
if (_leadingXIndex == null || _trailingXIndex == null || _leadingXIndex == null || _trailingYIndex == null) {
// First child of this layout pass. Set leading and trailing trackers.
_leadingXIndex = vicinity.xIndex;
_trailingXIndex = vicinity.xIndex;
_leadingYIndex = vicinity.yIndex;
_trailingYIndex = vicinity.yIndex;
} else {
// If any of these are still null, we missed a child.
assert(_leadingXIndex != null);
assert(_trailingXIndex != null);
assert(_leadingYIndex != null);
assert(_trailingYIndex != null);
// Update as we go.
_leadingXIndex = math.min(vicinity.xIndex, _leadingXIndex!);
_trailingXIndex = math.max(vicinity.xIndex, _trailingXIndex!);
_leadingYIndex = math.min(vicinity.yIndex, _leadingYIndex!);
_trailingYIndex = math.max(vicinity.yIndex, _trailingYIndex!);
}
if (_needsDelegateRebuild || (!_children.containsKey(vicinity) && !_keepAliveBucket.containsKey(vicinity))) {
invokeLayoutCallback<BoxConstraints>((BoxConstraints _) {
_childManager._buildChild(vicinity);
});
} else {
_keepAliveBucket.remove(vicinity);
_childManager._reuseChild(vicinity);
}
if (!_children.containsKey(vicinity)) {
// There is no child for this vicinity, we may have reached the end of the
// children in one or both of the x/y indices.
return null;
}
assert(_children.containsKey(vicinity));
final RenderBox child = _children[vicinity]!;
_activeChildrenForLayoutPass[vicinity] = child;
parentDataOf(child).vicinity = vicinity;
return child;
}
/// Called after [layoutChildSequence] to compute the
/// [TwoDimensionalViewportParentData.paintOffset] and
/// [TwoDimensionalViewportParentData._paintExtent] of the child.
void updateChildPaintData(RenderBox child) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
assert(
childParentData.layoutOffset != null,
'The child with ChildVicinity(xIndex: ${childParentData.vicinity.xIndex}, '
'yIndex: ${childParentData.vicinity.yIndex}) was not provided a '
'layoutOffset. This should be set during layoutChildSequence, '
'representing the position of the child.'
);
assert(child.hasSize); // Child must have been laid out by now.
// Set paintExtent (and visibility)
childParentData._paintExtent = computeChildPaintExtent(
childParentData.layoutOffset!,
child.size,
);
// Set paintOffset
childParentData.paintOffset = computeAbsolutePaintOffsetFor(
child,
layoutOffset: childParentData.layoutOffset!,
);
// If the child is partially visible, or not visible at all, there is
// visual overflow.
_hasVisualOverflow = _hasVisualOverflow
|| childParentData.layoutOffset != childParentData._paintExtent
|| !childParentData.isVisible;
}
/// Computes the portion of the child that is visible, assuming that only the
/// region from the [ViewportOffset.pixels] of both dimensions to the
/// [cacheExtent] is visible, and that the relationship between scroll offsets
/// and paint offsets is linear.
///
/// For example, if the [ViewportOffset]s each have a scroll offset of 100 and
/// the arguments to this method describe a child with [layoutOffset] of
/// `Offset(50.0, 50.0)`, with a size of `Size(200.0, 200.0)`, then the
/// returned value would be `Size(150.0, 150.0)`, representing the visible
/// extent of the child.
Size computeChildPaintExtent(Offset layoutOffset, Size childSize) {
if (childSize == Size.zero || childSize.height == 0.0 || childSize.width == 0.0) {
return Size.zero;
}
// Horizontal extent
final double width;
if (layoutOffset.dx < 0.0) {
// The child is positioned beyond the leading edge of the viewport.
if (layoutOffset.dx + childSize.width <= 0.0) {
// The child does not extend into the viewable area, it is not visible.
return Size.zero;
}
// If the child is positioned starting at -50, then the paint extent is
// the width + (-50).
width = layoutOffset.dx + childSize.width;
} else if (layoutOffset.dx >= viewportDimension.width) {
// The child is positioned after the trailing edge of the viewport, also
// not visible.
return Size.zero;
} else {
// The child is positioned within the viewport bounds, but may extend
// beyond it.
assert(layoutOffset.dx >= 0 && layoutOffset.dx < viewportDimension.width);
if (layoutOffset.dx + childSize.width > viewportDimension.width) {
width = viewportDimension.width - layoutOffset.dx;
} else {
assert(layoutOffset.dx + childSize.width <= viewportDimension.width);
width = childSize.width;
}
}
// Vertical extent
final double height;
if (layoutOffset.dy < 0.0) {
// The child is positioned beyond the leading edge of the viewport.
if (layoutOffset.dy + childSize.height <= 0.0) {
// The child does not extend into the viewable area, it is not visible.
return Size.zero;
}
// If the child is positioned starting at -50, then the paint extent is
// the width + (-50).
height = layoutOffset.dy + childSize.height;
} else if (layoutOffset.dy >= viewportDimension.height) {
// The child is positioned after the trailing edge of the viewport, also
// not visible.
return Size.zero;
} else {
// The child is positioned within the viewport bounds, but may extend
// beyond it.
assert(layoutOffset.dy >= 0 && layoutOffset.dy < viewportDimension.height);
if (layoutOffset.dy + childSize.height > viewportDimension.height) {
height = viewportDimension.height - layoutOffset.dy;
} else {
assert(layoutOffset.dy + childSize.height <= viewportDimension.height);
height = childSize.height;
}
}
return Size(width, height);
}
/// The offset at which the given `child` should be painted.
///
/// The returned offset is from the top left corner of the inside of the
/// viewport to the top left corner of the paint coordinate system of the
/// `child`.
///
/// This is useful when the one or both of the axes of the viewport are
/// reversed. The normalized layout offset of the child is used to compute
/// the paint offset in relation to the [verticalAxisDirection] and
/// [horizontalAxisDirection].
@protected
Offset computeAbsolutePaintOffsetFor(
RenderBox child, {
required Offset layoutOffset,
}) {
// This is only usable once we have sizes.
assert(hasSize);
assert(child.hasSize);
final double xOffset = switch (horizontalAxisDirection) {
AxisDirection.right => layoutOffset.dx,
AxisDirection.left => viewportDimension.width - (layoutOffset.dx + child.size.width),
AxisDirection.up || AxisDirection.down => throw Exception('This should not happen'),
};
final double yOffset = switch (verticalAxisDirection) {
AxisDirection.up => viewportDimension.height - (layoutOffset.dy + child.size.height),
AxisDirection.down => layoutOffset.dy,
AxisDirection.right || AxisDirection.left => throw Exception('This should not happen'),
};
return Offset(xOffset, yOffset);
}
@override
void paint(PaintingContext context, Offset offset) {
if (_children.isEmpty) {
return;
}
if (_hasVisualOverflow && clipBehavior != Clip.none) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & viewportDimension,
_paintChildren,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
_paintChildren(context, offset);
}
}
void _paintChildren(PaintingContext context, Offset offset) {
RenderBox? child = _firstChild;
while (child != null) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
if (childParentData.isVisible) {
context.paintChild(child, offset + childParentData.paintOffset!);
}
child = childParentData._nextSibling;
}
}
// ---- Called from _TwoDimensionalViewportElement ----
void _insertChild(RenderBox child, ChildVicinity slot) {
assert(_debugTrackOrphans(newOrphan: _children[slot]));
assert(!_keepAliveBucket.containsValue(child));
_children[slot] = child;
adoptChild(child);
}
void _moveChild(RenderBox child, {required ChildVicinity from, required ChildVicinity to}) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
if (!childParentData.keptAlive) {
if (_children[from] == child) {
_children.remove(from);
}
assert(_debugTrackOrphans(newOrphan: _children[to], noLongerOrphan: child));
_children[to] = child;
return;
}
// If the child in the bucket is not current child, that means someone has
// already moved and replaced current child, and we cannot remove this
// child.
if (_keepAliveBucket[childParentData.vicinity] == child) {
_keepAliveBucket.remove(childParentData.vicinity);
}
assert(() {
_debugDanglingKeepAlives.remove(child);
return true;
}());
// If there is an existing child in the new slot, that mean that child
// will be moved to other index. In other cases, the existing child should
// have been removed by _removeChild. Thus, it is ok to overwrite it.
assert(() {
if (_keepAliveBucket.containsKey(childParentData.vicinity)) {
_debugDanglingKeepAlives.add(_keepAliveBucket[childParentData.vicinity]!);
}
return true;
}());
_keepAliveBucket[childParentData.vicinity] = child;
}
void _removeChild(RenderBox child, ChildVicinity slot) {
final TwoDimensionalViewportParentData childParentData = parentDataOf(child);
if (!childParentData.keptAlive) {
if (_children[slot] == child) {
_children.remove(slot);
}
assert(_debugTrackOrphans(noLongerOrphan: child));
dropChild(child);
return;
}
assert(_keepAliveBucket[childParentData.vicinity] == child);
assert(() {
_debugDanglingKeepAlives.remove(child);
return true;
}());
_keepAliveBucket.remove(childParentData.vicinity);
dropChild(child);
}
List<RenderBox>? _debugOrphans;
// When a child is inserted into a slot currently occupied by another child,
// it becomes an orphan until it is either moved to another slot or removed.
bool _debugTrackOrphans({RenderBox? newOrphan, RenderBox? noLongerOrphan}) {
assert(() {
_debugOrphans ??= <RenderBox>[];
if (newOrphan != null) {
_debugOrphans!.add(newOrphan);
}
if (noLongerOrphan != null) {
_debugOrphans!.remove(noLongerOrphan);
}
return true;
}());
return true;
}
/// Throws an exception saying that the object does not support returning
/// intrinsic dimensions if, in debug mode, we are not in the
/// [RenderObject.debugCheckingIntrinsics] mode.
///
/// This is used by [computeMinIntrinsicWidth] et al because viewports do not
/// generally support returning intrinsic dimensions. See the discussion at
/// [computeMinIntrinsicWidth].
@protected
bool debugThrowIfNotCheckingIntrinsics() {
assert(() {
if (!RenderObject.debugCheckingIntrinsics) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType does not support returning intrinsic dimensions.'),
ErrorDescription(
'Calculating the intrinsic dimensions would require instantiating every child of '
'the viewport, which defeats the point of viewports being lazy.',
),
]);
}
return true;
}());
return true;
}
@override
double computeMinIntrinsicWidth(double height) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
final Offset paintOffset = parentDataOf(child).paintOffset!;
transform.translate(paintOffset.dx, paintOffset.dy);
}
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
}
/// A delegate used by [RenderTwoDimensionalViewport] to manage its children.
///
/// [RenderTwoDimensionalViewport] objects reify their children lazily to avoid
/// spending resources on children that are not visible in the viewport. This
/// delegate lets these objects create, reuse and remove children.
abstract class TwoDimensionalChildManager {
void _startLayout();
void _buildChild(ChildVicinity vicinity);
void _reuseChild(ChildVicinity vicinity);
void _endLayout();
}
/// The relative position of a child in a [TwoDimensionalViewport] in relation
/// to other children of the viewport.
///
/// While children can be plotted arbitrarily in two dimensional space, the
/// [ChildVicinity] is used to disambiguate their positions, determining how to
/// traverse the children of the space.
///
/// Combined with the [RenderTwoDimensionalViewport.mainAxis], each child's
/// vicinity determines its paint order among all of the children.
@immutable
class ChildVicinity implements Comparable<ChildVicinity> {
/// Creates a reference to a child in a two dimensional plane, with the
/// [xIndex] and [yIndex] being relative to other children in the viewport.
const ChildVicinity({
required this.xIndex,
required this.yIndex,
}) : assert(xIndex >= -1),
assert(yIndex >= -1);
/// Represents an unassigned child position. The given child may be in the
/// process of moving from one position to another.
static const ChildVicinity invalid = ChildVicinity(xIndex: -1, yIndex: -1);
/// The index of the child in the horizontal axis, relative to neighboring
/// children.
///
/// While children's offset and positioning may not be strictly defined in
/// terms of rows and columns, like a table, [ChildVicinity.xIndex] and
/// [ChildVicinity.yIndex] represents order of traversal in row or column
/// major format.
final int xIndex;
/// The index of the child in the vertical axis, relative to neighboring
/// children.
///
/// While children's offset and positioning may not be strictly defined in
/// terms of rows and columns, like a table, [ChildVicinity.xIndex] and
/// [ChildVicinity.yIndex] represents order of traversal in row or column
/// major format.
final int yIndex;
@override
bool operator ==(Object other) {
return other is ChildVicinity
&& other.xIndex == xIndex
&& other.yIndex == yIndex;
}
@override
int get hashCode => Object.hash(xIndex, yIndex);
@override
int compareTo(ChildVicinity other) {
if (xIndex == other.xIndex) {
return yIndex - other.yIndex;
}
return xIndex - other.xIndex;
}
@override
String toString() {
return '(xIndex: $xIndex, yIndex: $yIndex)';
}
}
| flutter/packages/flutter/lib/src/widgets/two_dimensional_viewport.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/two_dimensional_viewport.dart",
"repo_id": "flutter",
"token_count": 22094
} | 649 |
include: ../analysis_options.yaml
linter:
rules:
# Tests try to throw and catch things in exciting ways all the time, so
# we disable these lints for the tests.
only_throw_errors: false
avoid_catches_without_on_clauses: false
avoid_catching_errors: false
| flutter/packages/flutter/test/analysis_options.yaml/0 | {
"file_path": "flutter/packages/flutter/test/analysis_options.yaml",
"repo_id": "flutter",
"token_count": 94
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/clipboard_utils.dart';
import '../widgets/live_text_utils.dart';
import '../widgets/text_selection_toolbar_utils.dart';
void main() {
final MockClipboard mockClipboard = MockClipboard();
setUp(() async {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(
SystemChannels.platform,
mockClipboard.handleMethodCall,
);
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
});
tearDown(() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(
SystemChannels.platform,
null,
);
});
testWidgets('Builds the right toolbar on each platform, including web, and shows buttonItems', (WidgetTester tester) async {
const String buttonText = 'Click me';
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoAdaptiveTextSelectionToolbar.buttonItems(
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
buttonItems: <ContextMenuButtonItem>[
ContextMenuButtonItem(
label: buttonText,
onPressed: () {
},
),
],
),
),
),
);
expect(find.text(buttonText), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbar), findsOneWidget);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsNothing);
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(CupertinoTextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsOneWidget);
}
},
variant: TargetPlatformVariant.all(),
skip: isBrowser, // [intended] see https://github.com/flutter/flutter/issues/108382
);
testWidgets('Can build children directly as well', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoAdaptiveTextSelectionToolbar(
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
children: <Widget>[
Container(key: key),
],
),
),
),
);
expect(find.byKey(key), findsOneWidget);
},
skip: isBrowser, // [intended] see https://github.com/flutter/flutter/issues/108382
);
testWidgets('Can build from EditableTextState', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(CupertinoApp(
home: Align(
alignment: Alignment.topLeft,
child: SizedBox(
width: 400,
child: EditableText(
controller: controller,
backgroundCursorColor: const Color(0xff00ffff),
focusNode: focusNode,
style: const TextStyle(),
cursorColor: const Color(0xff00ffff),
selectionControls: cupertinoTextSelectionHandleControls,
contextMenuBuilder: (
BuildContext context,
EditableTextState editableTextState,
) {
return CupertinoAdaptiveTextSelectionToolbar.editableText(
key: key,
editableTextState: editableTextState,
);
},
),
),
),
));
await tester.pump(); // Wait for autofocus to take effect.
expect(find.byKey(key), findsNothing);
// Long-press to bring up the context menu.
final Finder textFinder = find.byType(EditableText);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
expect(find.byKey(key), findsOneWidget);
expect(find.text('Copy'), findsNothing);
expect(find.text('Cut'), findsNothing);
expect(find.text('Select all'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsOneWidget);
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsOneWidget);
}
},
skip: kIsWeb, // [intended] on web the browser handles the context menu.
variant: TargetPlatformVariant.all(),
);
testWidgets('Can build for editable text from raw parameters', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(CupertinoApp(
home: Center(
child: CupertinoAdaptiveTextSelectionToolbar.editable(
key: key,
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
clipboardStatus: ClipboardStatus.pasteable,
onCopy: () {},
onCut: () {},
onPaste: () {},
onSelectAll: () {},
onLiveTextInput: () {},
onLookUp: () {},
onSearchWeb: () {},
onShare: () {},
),
),
));
expect(find.byKey(key), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(6));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(find.text('Share...'), findsOneWidget);
expect(findCupertinoOverflowNextButton(), findsOneWidget);
await tapCupertinoOverflowNextButton(tester);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(4));
expect(findCupertinoOverflowBackButton(), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(6));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(findCupertinoOverflowNextButton(), findsOneWidget);
await tapCupertinoOverflowNextButton(tester);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(4));
expect(findCupertinoOverflowBackButton(), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share...'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNWidgets(8));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(find.text('Share...'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
}
},
skip: kIsWeb, // [intended] on web the browser handles the context menu.
variant: TargetPlatformVariant.all(),
);
testWidgets('Builds the correct button per-platform', (WidgetTester tester) async {
const String buttonText = 'Click me';
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: Builder(
builder: (BuildContext context) {
return Column(
children: CupertinoAdaptiveTextSelectionToolbar.getAdaptiveButtons(
context,
<ContextMenuButtonItem>[
ContextMenuButtonItem(
label: buttonText,
onPressed: () {
},
),
],
).toList(),
);
},
),
),
),
);
expect(find.text(buttonText), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsOneWidget);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNothing);
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsOneWidget);
}
},
variant: TargetPlatformVariant.all(),
);
}
| flutter/packages/flutter/test/cupertino/adaptive_text_selection_toolbar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/adaptive_text_selection_toolbar_test.dart",
"repo_id": "flutter",
"token_count": 4101
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('shows header', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
header: const Text('Header'),
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
expect(find.text('Header'), findsOneWidget);
});
testWidgets('shows footer', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
footer: const Text('Footer'),
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
expect(find.text('Footer'), findsOneWidget);
});
testWidgets('shows long dividers in edge-to-edge section part 1', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
// Since the children list is reconstructed with dividers in it, the column
// retrieved should have 3 items for an input [children] param with 1 child.
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
expect(childrenColumn.children.length, 3);
});
testWidgets('shows long dividers in edge-to-edge section part 2', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
// Since the children list is reconstructed with dividers in it, the column
// retrieved should have 5 items for an input [children] param with 2
// children. Two long dividers, two rows, and one short divider.
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
expect(childrenColumn.children.length, 5);
});
testWidgets('does not show long dividers in insetGrouped section part 1', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection.insetGrouped(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
// Since the children list is reconstructed without long dividers in it, the
// column retrieved should have 1 item for an input [children] param with 1
// child.
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
expect(childrenColumn.children.length, 1);
});
testWidgets('does not show long dividers in insetGrouped section part 2', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection.insetGrouped(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
// Since the children list is reconstructed with short dividers in it, the
// column retrieved should have 3 items for an input [children] param with 2
// children. Two long dividers, two rows, and one short divider.
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
expect(childrenColumn.children.length, 3);
});
testWidgets('sets background color for section', (WidgetTester tester) async {
const Color backgroundColor = CupertinoColors.systemBlue;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(),
child: CupertinoListSection(
backgroundColor: backgroundColor,
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
final DecoratedBox decoratedBox = tester.widget(find.byType(DecoratedBox).first);
final BoxDecoration boxDecoration = decoratedBox.decoration as BoxDecoration;
expect(boxDecoration.color, backgroundColor);
});
testWidgets('setting clipBehavior clips children section', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
clipBehavior: Clip.antiAlias,
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
expect(find.byType(ClipRRect), findsOneWidget);
});
testWidgets('not setting clipBehavior does not clip children section', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
expect(find.byType(ClipRRect), findsNothing);
});
testWidgets('CupertinoListSection respects separatorColor', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
separatorColor: const Color.fromARGB(255, 143, 193, 51),
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
for (final Widget e in childrenColumn.children) {
if (e is Container) {
expect(e.color, const Color.fromARGB(255, 143, 193, 51));
}
}
});
testWidgets('CupertinoListSection.separatorColor defaults CupertinoColors.separator', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
children: const <Widget>[
CupertinoListTile(title: Text('CupertinoListTile')),
CupertinoListTile(title: Text('CupertinoListTile')),
],
),
),
),
);
final BuildContext context = tester.element(find.byType(CupertinoListSection));
final Column childrenColumn = tester.widget(find.byType(Column).at(1));
for (final Widget e in childrenColumn.children) {
if (e is Container) {
expect(e.color, CupertinoColors.separator.resolveFrom(context));
}
}
});
testWidgets('does not show margin by default', (WidgetTester tester) async {
const Widget child = CupertinoListTile(title: Text('CupertinoListTile'));
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
header: const Text('Header'),
children: const <Widget>[
child,
],
),
),
),
);
expect(tester.getTopLeft(find.byWidget(child)), offsetMoreOrLessEquals(const Offset(0, 41), epsilon: 1));
});
testWidgets('shows custom margin', (WidgetTester tester) async {
const Widget child = CupertinoListTile(title: Text('CupertinoListTile'));
const double margin = 10;
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoListSection(
header: const Text('Header'),
margin: const EdgeInsets.all(margin),
children: const <Widget>[
child,
],
),
),
),
);
expect(tester.getTopLeft(find.byWidget(child)), offsetMoreOrLessEquals(const Offset(margin, 41 + margin), epsilon: 1));
});
}
| flutter/packages/flutter/test/cupertino/list_section_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/list_section_test.dart",
"repo_id": "flutter",
"token_count": 3565
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets(
'default search field has a border radius',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(),
),
),
);
final BoxDecoration decoration = tester
.widget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoSearchTextField),
matching: find.byType(DecoratedBox),
),
)
.decoration as BoxDecoration;
expect(
decoration.borderRadius,
const BorderRadius.all(Radius.circular(9)),
);
},
);
testWidgets(
'decoration overrides default background color',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
decoration: BoxDecoration(color: Color.fromARGB(1, 1, 1, 1)),
),
),
),
);
final BoxDecoration decoration = tester
.widget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoSearchTextField),
matching: find.byType(DecoratedBox),
),
)
.decoration as BoxDecoration;
expect(
decoration.color,
const Color.fromARGB(1, 1, 1, 1),
);
},
);
testWidgets(
'decoration overrides default border radius',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
decoration: BoxDecoration(borderRadius: BorderRadius.zero),
),
),
),
);
final BoxDecoration decoration = tester
.widget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoSearchTextField),
matching: find.byType(DecoratedBox),
),
)
.decoration as BoxDecoration;
expect(
decoration.borderRadius,
BorderRadius.zero,
);
},
);
testWidgets(
'text entries are padded by default',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'initial');
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
),
),
),
);
expect(
tester.getTopLeft(find.text('initial')) -
tester.getTopLeft(find.byType(CupertinoSearchTextField)),
const Offset(31.5, 8.0),
);
},
);
testWidgets('can change keyboard type', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
keyboardType: TextInputType.number,
),
),
),
);
await tester.tap(find.byType(CupertinoSearchTextField));
await tester.showKeyboard(find.byType(CupertinoSearchTextField));
expect((tester.testTextInput.setClientArgs!['inputType'] as Map<String, dynamic>)['name'], equals('TextInputType.number'));
},
);
testWidgets(
'can control text content via controller',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
),
),
),
);
controller.text = 'controller text';
await tester.pump();
expect(find.text('controller text'), findsOneWidget);
controller.text = '';
await tester.pump();
expect(find.text('controller text'), findsNothing);
},
);
testWidgets('placeholder color', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.dark),
home: Center(
child: CupertinoSearchTextField(),
),
),
);
Text placeholder = tester.widget(find.text('Search'));
expect(placeholder.style!.color!.value, CupertinoColors.systemGrey.darkColor.value);
await tester.pumpAndSettle();
await tester.pumpWidget(
const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: Center(
child: CupertinoSearchTextField(),
),
),
);
placeholder = tester.widget(find.text('Search'));
expect(placeholder.style!.color!.value, CupertinoColors.systemGrey.color.value);
});
testWidgets(
"placeholderStyle modifies placeholder's style and doesn't affect text's style",
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
placeholder: 'placeholder',
style: TextStyle(
color: Color(0x00FFFFFF),
fontWeight: FontWeight.w300,
),
placeholderStyle: TextStyle(
color: Color(0xAAFFFFFF),
fontWeight: FontWeight.w600,
),
),
),
),
);
final Text placeholder = tester.widget(find.text('placeholder'));
expect(placeholder.style!.color, const Color(0xAAFFFFFF));
expect(placeholder.style!.fontWeight, FontWeight.w600);
await tester.enterText(find.byType(CupertinoSearchTextField), 'input');
await tester.pump();
final EditableText inputText = tester.widget(find.text('input'));
expect(inputText.style.color, const Color(0x00FFFFFF));
expect(inputText.style.fontWeight, FontWeight.w300);
},
);
testWidgets(
'prefix widget is in front of the text',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'input');
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
),
),
),
);
expect(
tester.getTopRight(find.byIcon(CupertinoIcons.search)).dx + 5.5,
tester.getTopLeft(find.byType(EditableText)).dx,
);
expect(
tester.getTopLeft(find.byType(EditableText)).dx,
tester.getTopLeft(find.byType(CupertinoSearchTextField)).dx +
tester.getSize(find.byIcon(CupertinoIcons.search)).width +
11.5,
);
},
);
testWidgets(
'suffix widget is after the text',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'Hi');
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
),
),
),
);
expect(
tester.getTopRight(find.byType(EditableText)).dx + 5.5,
tester.getTopLeft(find.byIcon(CupertinoIcons.xmark_circle_fill)).dx,
);
expect(
tester.getTopRight(find.byType(EditableText)).dx,
tester.getTopRight(find.byType(CupertinoSearchTextField)).dx -
tester
.getSize(find.byIcon(CupertinoIcons.xmark_circle_fill))
.width -
10.5,
);
},
);
testWidgets('prefix widget visibility', (WidgetTester tester) async {
const Key prefixIcon = Key('prefix');
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
prefixIcon: SizedBox(
key: prefixIcon,
width: 50,
height: 50,
),
),
),
),
);
expect(find.byIcon(CupertinoIcons.search), findsNothing);
expect(find.byKey(prefixIcon), findsOneWidget);
await tester.enterText(
find.byType(CupertinoSearchTextField), 'text input');
await tester.pump();
expect(find.text('text input'), findsOneWidget);
expect(find.byIcon(CupertinoIcons.search), findsNothing);
expect(find.byKey(prefixIcon), findsOneWidget);
});
testWidgets(
'suffix widget respects visibility mode',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
suffixMode: OverlayVisibilityMode.notEditing,
),
),
),
);
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsOneWidget);
await tester.enterText(find.byType(CupertinoSearchTextField), 'text input');
await tester.pump();
expect(find.text('text input'), findsOneWidget);
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsNothing);
},
);
testWidgets(
'clear button shows with right visibility mode',
(WidgetTester tester) async {
TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
placeholder: 'placeholder does not affect clear button',
),
),
),
);
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsNothing);
await tester.enterText(find.byType(CupertinoSearchTextField), 'text input');
await tester.pump();
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsOneWidget);
expect(find.text('text input'), findsOneWidget);
controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
placeholder: 'placeholder does not affect clear button',
suffixMode: OverlayVisibilityMode.notEditing,
),
),
),
);
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsOneWidget);
controller.text = 'input';
await tester.pump();
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsNothing);
},
);
testWidgets(
'clear button removes text',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
),
),
),
);
controller.text = 'text entry';
await tester.pump();
await tester.tap(find.byIcon(CupertinoIcons.xmark_circle_fill));
await tester.pump();
expect(controller.text, '');
expect(find.text('Search'), findsOneWidget);
expect(find.text('text entry'), findsNothing);
expect(find.byIcon(CupertinoIcons.xmark_circle_fill), findsNothing);
},
);
testWidgets(
'tapping clear button also calls onChanged when text not empty',
(WidgetTester tester) async {
String value = 'text entry';
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
placeholder: 'placeholder',
onChanged: (String newValue) => value = newValue,
),
),
),
);
controller.text = value;
await tester.pump();
await tester.tap(find.byIcon(CupertinoIcons.xmark_circle_fill));
await tester.pump();
expect(controller.text, isEmpty);
expect(find.text('text entry'), findsNothing);
expect(value, isEmpty);
},
);
testWidgets(
'RTL puts attachments to the right places',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: CupertinoSearchTextField(
suffixMode: OverlayVisibilityMode.always,
),
),
),
),
);
expect(
tester.getTopLeft(find.byIcon(CupertinoIcons.search)).dx,
800.0 - 26.0,
);
expect(
tester.getTopRight(find.byIcon(CupertinoIcons.xmark_circle_fill)).dx,
25.0,
);
},
);
testWidgets(
'Can modify prefix and suffix insets',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
suffixMode: OverlayVisibilityMode.always,
prefixInsets: EdgeInsets.zero,
suffixInsets: EdgeInsets.zero,
),
),
),
);
expect(
tester.getTopLeft(find.byIcon(CupertinoIcons.search)).dx,
0.0,
);
expect(
tester.getTopRight(find.byIcon(CupertinoIcons.xmark_circle_fill)).dx,
800.0,
);
},
);
testWidgets(
'custom suffix onTap overrides default clearing behavior',
(WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'Text');
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
controller: controller,
onSuffixTap: () {},
),
),
),
);
await tester.pump();
await tester.tap(find.byIcon(CupertinoIcons.xmark_circle_fill));
await tester.pump();
expect(controller.text, isNotEmpty);
expect(find.text('Text'), findsOneWidget);
},
);
testWidgets('onTap is properly forwarded to the inner text field', (WidgetTester tester) async {
int onTapCallCount = 0;
// onTap can be null.
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(),
),
),
);
// onTap callback is called if not null.
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
onTap: () {
onTapCallCount++;
},
),
),
),
);
expect(onTapCallCount, 0);
await tester.tap(find.byType(CupertinoTextField));
expect(onTapCallCount, 1);
});
testWidgets('autocorrect is properly forwarded to the inner text field', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
autocorrect: false,
),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.autocorrect, false);
});
testWidgets('enabled is properly forwarded to the inner text field', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
enabled: false,
),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.enabled, false);
});
testWidgets('textInputAction is set to TextInputAction.search by default', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.textInputAction, TextInputAction.search);
});
testWidgets('autofocus:true gives focus to the widget', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
focusNode: focusNode,
autofocus: true,
),
),
),
);
expect(focusNode.hasFocus, isTrue);
});
testWidgets('smartQuotesType is properly forwarded to the inner text field', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
smartQuotesType: SmartQuotesType.disabled,
),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.smartQuotesType, SmartQuotesType.disabled);
});
testWidgets('smartDashesType is properly forwarded to the inner text field', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
smartDashesType: SmartDashesType.disabled,
),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.smartDashesType, SmartDashesType.disabled);
});
testWidgets(
'enableIMEPersonalizedLearning is properly forwarded to the inner text field', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Center(
child: CupertinoSearchTextField(
enableIMEPersonalizedLearning: false,
),
),
),
);
final CupertinoTextField textField = tester.widget(find.byType(CupertinoTextField));
expect(textField.enableIMEPersonalizedLearning, false);
});
}
| flutter/packages/flutter/test/cupertino/search_field_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/search_field_test.dart",
"repo_id": "flutter",
"token_count": 8296
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
int buildCount = 0;
CupertinoThemeData? actualTheme;
IconThemeData? actualIconTheme;
final Widget singletonThemeSubtree = Builder(
builder: (BuildContext context) {
buildCount++;
actualTheme = CupertinoTheme.of(context);
actualIconTheme = IconTheme.of(context);
return const Placeholder();
},
);
Future<CupertinoThemeData> testTheme(WidgetTester tester, CupertinoThemeData theme) async {
await tester.pumpWidget(
CupertinoTheme(
data: theme,
child: singletonThemeSubtree,
),
);
return actualTheme!;
}
Future<IconThemeData> testIconTheme(WidgetTester tester, CupertinoThemeData theme) async {
await tester.pumpWidget(
CupertinoTheme(
data: theme,
child: singletonThemeSubtree,
),
);
return actualIconTheme!;
}
void main() {
setUp(() {
buildCount = 0;
actualTheme = null;
actualIconTheme = null;
});
testWidgets('Default theme has defaults', (WidgetTester tester) async {
final CupertinoThemeData theme = await testTheme(tester, const CupertinoThemeData());
expect(theme.brightness, isNull);
expect(theme.primaryColor, CupertinoColors.activeBlue);
expect(theme.textTheme.textStyle.fontSize, 17.0);
expect(theme.applyThemeToAll, false);
});
testWidgets('Theme attributes cascade', (WidgetTester tester) async {
final CupertinoThemeData theme = await testTheme(tester, const CupertinoThemeData(
primaryColor: CupertinoColors.systemRed,
));
expect(theme.textTheme.actionTextStyle.color, isSameColorAs(CupertinoColors.systemRed.color));
});
testWidgets('Dependent attribute can be overridden from cascaded value', (WidgetTester tester) async {
final CupertinoThemeData theme = await testTheme(tester, const CupertinoThemeData(
brightness: Brightness.dark,
textTheme: CupertinoTextThemeData(
textStyle: TextStyle(color: CupertinoColors.black),
),
));
// The brightness still cascaded down to the background color.
expect(theme.scaffoldBackgroundColor, isSameColorAs(CupertinoColors.black));
// But not to the font color which we overrode.
expect(theme.textTheme.textStyle.color, isSameColorAs(CupertinoColors.black));
});
testWidgets(
'Reading themes creates dependencies',
(WidgetTester tester) async {
// Reading the theme creates a dependency.
CupertinoThemeData theme = await testTheme(tester, const CupertinoThemeData(
// Default brightness is light,
barBackgroundColor: Color(0x11223344),
textTheme: CupertinoTextThemeData(
textStyle: TextStyle(fontFamily: 'Skeuomorphic'),
),
));
expect(buildCount, 1);
expect(theme.textTheme.textStyle.fontFamily, 'Skeuomorphic');
// Changing another property also triggers a rebuild.
theme = await testTheme(tester, const CupertinoThemeData(
brightness: Brightness.light,
barBackgroundColor: Color(0x11223344),
textTheme: CupertinoTextThemeData(
textStyle: TextStyle(fontFamily: 'Skeuomorphic'),
),
));
expect(buildCount, 2);
// Re-reading the same value doesn't change anything.
expect(theme.textTheme.textStyle.fontFamily, 'Skeuomorphic');
theme = await testTheme(tester, const CupertinoThemeData(
brightness: Brightness.light,
barBackgroundColor: Color(0x11223344),
textTheme: CupertinoTextThemeData(
textStyle: TextStyle(fontFamily: 'Flat'),
),
));
expect(buildCount, 3);
expect(theme.textTheme.textStyle.fontFamily, 'Flat');
},
);
testWidgets(
'copyWith works',
(WidgetTester tester) async {
const CupertinoThemeData originalTheme = CupertinoThemeData(
brightness: Brightness.dark,
applyThemeToAll: true,
);
final CupertinoThemeData theme = await testTheme(tester, originalTheme.copyWith(
primaryColor: CupertinoColors.systemGreen,
applyThemeToAll: false,
));
expect(theme.brightness, Brightness.dark);
expect(theme.primaryColor, isSameColorAs(CupertinoColors.systemGreen.darkColor));
// Now check calculated derivatives.
expect(theme.textTheme.actionTextStyle.color, isSameColorAs(CupertinoColors.systemGreen.darkColor));
expect(theme.scaffoldBackgroundColor, isSameColorAs(CupertinoColors.black));
expect(theme.applyThemeToAll, false);
},
);
testWidgets("Theme has default IconThemeData, which is derived from the theme's primary color", (WidgetTester tester) async {
const CupertinoDynamicColor primaryColor = CupertinoColors.systemRed;
const CupertinoThemeData themeData = CupertinoThemeData(primaryColor: primaryColor);
final IconThemeData resultingIconTheme = await testIconTheme(tester, themeData);
expect(resultingIconTheme.color, isSameColorAs(primaryColor));
// Works in dark mode if primaryColor is a CupertinoDynamicColor.
final Color darkColor = (await testIconTheme(
tester,
themeData.copyWith(brightness: Brightness.dark),
)).color!;
expect(darkColor, isSameColorAs(primaryColor.darkColor));
});
testWidgets('IconTheme.of creates a dependency on iconTheme', (WidgetTester tester) async {
IconThemeData iconTheme = await testIconTheme(tester, const CupertinoThemeData(primaryColor: CupertinoColors.destructiveRed));
expect(buildCount, 1);
expect(iconTheme.color, CupertinoColors.destructiveRed);
iconTheme = await testIconTheme(tester, const CupertinoThemeData(primaryColor: CupertinoColors.activeOrange));
expect(buildCount, 2);
expect(iconTheme.color, CupertinoColors.activeOrange);
});
testWidgets('CupertinoTheme diagnostics', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const CupertinoThemeData().debugFillProperties(builder);
final Set<String> description = builder.properties
.map((DiagnosticsNode node) => node.name.toString())
.toSet();
expect(
setEquals(
description,
<String>{
'brightness',
'primaryColor',
'primaryContrastingColor',
'barBackgroundColor',
'scaffoldBackgroundColor',
'applyThemeToAll',
'textStyle',
'actionTextStyle',
'tabLabelTextStyle',
'navTitleTextStyle',
'navLargeTitleTextStyle',
'navActionTextStyle',
'pickerTextStyle',
'dateTimePickerTextStyle',
},
),
isTrue,
);
});
testWidgets('CupertinoTheme.toStringDeep uses single-line style', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/47651.
expect(
const CupertinoTheme(
data: CupertinoThemeData(primaryColor: Color(0x00000000)),
child: SizedBox(),
).toStringDeep().trimRight(),
isNot(contains('\n')),
);
});
testWidgets('CupertinoThemeData equality', (WidgetTester tester) async {
const CupertinoThemeData a = CupertinoThemeData(brightness: Brightness.dark);
final CupertinoThemeData b = a.copyWith();
final CupertinoThemeData c = a.copyWith(brightness: Brightness.light);
expect(a, equals(b));
expect(b, equals(a));
expect(a, isNot(equals(c)));
expect(c, isNot(equals(a)));
expect(b, isNot(equals(c)));
expect(c, isNot(equals(b)));
});
late Brightness currentBrightness;
void colorMatches(Color? componentColor, CupertinoDynamicColor expectedDynamicColor) {
switch (currentBrightness) {
case Brightness.light:
expect(componentColor, isSameColorAs(expectedDynamicColor.color));
case Brightness.dark:
expect(componentColor, isSameColorAs(expectedDynamicColor.darkColor));
}
}
void dynamicColorsTestGroup() {
testWidgets('CupertinoTheme.of resolves colors', (WidgetTester tester) async {
final CupertinoThemeData data = CupertinoThemeData(brightness: currentBrightness, primaryColor: CupertinoColors.systemRed);
final CupertinoThemeData theme = await testTheme(tester, data);
expect(data.primaryColor, isSameColorAs(CupertinoColors.systemRed));
colorMatches(theme.primaryColor, CupertinoColors.systemRed);
});
testWidgets('CupertinoTheme.of resolves default values', (WidgetTester tester) async {
const CupertinoDynamicColor primaryColor = CupertinoColors.systemRed;
final CupertinoThemeData data = CupertinoThemeData(brightness: currentBrightness, primaryColor: primaryColor);
const CupertinoDynamicColor barBackgroundColor = CupertinoDynamicColor.withBrightness(
color: Color(0xF0F9F9F9),
darkColor: Color(0xF01D1D1D),
);
final CupertinoThemeData theme = await testTheme(tester, data);
colorMatches(theme.primaryContrastingColor, CupertinoColors.systemBackground);
colorMatches(theme.barBackgroundColor, barBackgroundColor);
colorMatches(theme.scaffoldBackgroundColor, CupertinoColors.systemBackground);
colorMatches(theme.textTheme.textStyle.color, CupertinoColors.label);
colorMatches(theme.textTheme.actionTextStyle.color, primaryColor);
colorMatches(theme.textTheme.tabLabelTextStyle.color, CupertinoColors.inactiveGray);
colorMatches(theme.textTheme.navTitleTextStyle.color, CupertinoColors.label);
colorMatches(theme.textTheme.navLargeTitleTextStyle.color, CupertinoColors.label);
colorMatches(theme.textTheme.navActionTextStyle.color, primaryColor);
colorMatches(theme.textTheme.pickerTextStyle.color, CupertinoColors.label);
colorMatches(theme.textTheme.dateTimePickerTextStyle.color, CupertinoColors.label);
});
}
currentBrightness = Brightness.light;
group('light colors', dynamicColorsTestGroup);
currentBrightness = Brightness.dark;
group('dark colors', dynamicColorsTestGroup);
}
| flutter/packages/flutter/test/cupertino/theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/theme_test.dart",
"repo_id": "flutter",
"token_count": 3650
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
class TestNotifier extends ChangeNotifier {
void notify() {
notifyListeners();
}
bool get isListenedTo => hasListeners;
}
class HasListenersTester<T> extends ValueNotifier<T> {
HasListenersTester(super.value);
bool get testHasListeners => hasListeners;
}
class A {
bool result = false;
void test() {
result = true;
}
}
class B extends A with ChangeNotifier {
B() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
@override
void test() {
notifyListeners();
super.test();
}
}
class Counter with ChangeNotifier {
Counter() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
int get value => _value;
int _value = 0;
set value(int value) {
if (_value != value) {
_value = value;
notifyListeners();
}
}
void notify() {
notifyListeners();
}
}
void main() {
testWidgets('ChangeNotifier can not dispose in callback', (WidgetTester tester) async {
final TestNotifier test = TestNotifier();
bool callbackDidFinish = false;
void foo() {
test.dispose();
callbackDidFinish = true;
}
test.addListener(foo);
test.notify();
final AssertionError error = tester.takeException() as AssertionError;
expect(error.toString().contains('dispose()'), isTrue);
// Make sure it crashes during dispose call.
expect(callbackDidFinish, isFalse);
test.dispose();
});
testWidgets('ChangeNotifier', (WidgetTester tester) async {
final List<String> log = <String>[];
void listener() {
log.add('listener');
}
void listener1() {
log.add('listener1');
}
void listener2() {
log.add('listener2');
}
void badListener() {
log.add('badListener');
throw ArgumentError();
}
final TestNotifier test = TestNotifier();
test.addListener(listener);
test.addListener(listener);
test.notify();
expect(log, <String>['listener', 'listener']);
log.clear();
test.removeListener(listener);
test.notify();
expect(log, <String>['listener']);
log.clear();
test.removeListener(listener);
test.notify();
expect(log, <String>[]);
log.clear();
test.removeListener(listener);
test.notify();
expect(log, <String>[]);
log.clear();
test.addListener(listener);
test.notify();
expect(log, <String>['listener']);
log.clear();
test.addListener(listener1);
test.notify();
expect(log, <String>['listener', 'listener1']);
log.clear();
test.addListener(listener2);
test.notify();
expect(log, <String>['listener', 'listener1', 'listener2']);
log.clear();
test.removeListener(listener1);
test.notify();
expect(log, <String>['listener', 'listener2']);
log.clear();
test.addListener(listener1);
test.notify();
expect(log, <String>['listener', 'listener2', 'listener1']);
log.clear();
test.addListener(badListener);
test.notify();
expect(log, <String>['listener', 'listener2', 'listener1', 'badListener']);
expect(tester.takeException(), isArgumentError);
log.clear();
test.addListener(listener1);
test.removeListener(listener);
test.removeListener(listener1);
test.removeListener(listener2);
test.addListener(listener2);
test.notify();
expect(log, <String>['badListener', 'listener1', 'listener2']);
expect(tester.takeException(), isArgumentError);
log.clear();
test.dispose();
});
test('ChangeNotifier with mutating listener', () {
final TestNotifier test = TestNotifier();
final List<String> log = <String>[];
void listener1() {
log.add('listener1');
}
void listener3() {
log.add('listener3');
}
void listener4() {
log.add('listener4');
}
void listener2() {
log.add('listener2');
test.removeListener(listener1);
test.removeListener(listener3);
test.addListener(listener4);
}
test.addListener(listener1);
test.addListener(listener2);
test.addListener(listener3);
test.notify();
expect(log, <String>['listener1', 'listener2']);
log.clear();
test.notify();
expect(log, <String>['listener2', 'listener4']);
log.clear();
test.notify();
expect(log, <String>['listener2', 'listener4', 'listener4']);
log.clear();
});
test('During notifyListeners, a listener was added and removed immediately', () {
final TestNotifier source = TestNotifier();
final List<String> log = <String>[];
void listener3() {
log.add('listener3');
}
void listener2() {
log.add('listener2');
}
void listener1() {
log.add('listener1');
source.addListener(listener2);
source.removeListener(listener2);
source.addListener(listener3);
}
source.addListener(listener1);
source.notify();
expect(log, <String>['listener1']);
});
test(
'If a listener in the middle of the list of listeners removes itself, '
'notifyListeners still notifies all listeners',
() {
final TestNotifier source = TestNotifier();
final List<String> log = <String>[];
void selfRemovingListener() {
log.add('selfRemovingListener');
source.removeListener(selfRemovingListener);
}
void listener1() {
log.add('listener1');
}
source.addListener(listener1);
source.addListener(selfRemovingListener);
source.addListener(listener1);
source.notify();
expect(log, <String>['listener1', 'selfRemovingListener', 'listener1']);
},
);
test('If the first listener removes itself, notifyListeners still notify all listeners', () {
final TestNotifier source = TestNotifier();
final List<String> log = <String>[];
void selfRemovingListener() {
log.add('selfRemovingListener');
source.removeListener(selfRemovingListener);
}
void listener1() {
log.add('listener1');
}
source.addListener(selfRemovingListener);
source.addListener(listener1);
source.notifyListeners();
expect(log, <String>['selfRemovingListener', 'listener1']);
});
test('Merging change notifiers', () {
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
final TestNotifier source3 = TestNotifier();
final List<String> log = <String>[];
final Listenable merged = Listenable.merge(<Listenable>[source1, source2]);
void listener1() {
log.add('listener1');
}
void listener2() {
log.add('listener2');
}
merged.addListener(listener1);
source1.notify();
source2.notify();
source3.notify();
expect(log, <String>['listener1', 'listener1']);
log.clear();
merged.removeListener(listener1);
source1.notify();
source2.notify();
source3.notify();
expect(log, isEmpty);
log.clear();
merged.addListener(listener1);
merged.addListener(listener2);
source1.notify();
source2.notify();
source3.notify();
expect(log, <String>['listener1', 'listener2', 'listener1', 'listener2']);
log.clear();
});
test('Merging change notifiers supports any iterable', () {
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
final List<String> log = <String>[];
final Listenable merged = Listenable.merge(<Listenable?>{source1, source2});
void listener() => log.add('listener');
merged.addListener(listener);
source1.notify();
source2.notify();
expect(log, <String>['listener', 'listener']);
log.clear();
});
test('Merging change notifiers ignores null', () {
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
final List<String> log = <String>[];
final Listenable merged =
Listenable.merge(<Listenable?>[null, source1, null, source2, null]);
void listener() {
log.add('listener');
}
merged.addListener(listener);
source1.notify();
source2.notify();
expect(log, <String>['listener', 'listener']);
log.clear();
});
test('Can remove from merged notifier', () {
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
final List<String> log = <String>[];
final Listenable merged = Listenable.merge(<Listenable>[source1, source2]);
void listener() {
log.add('listener');
}
merged.addListener(listener);
source1.notify();
source2.notify();
expect(log, <String>['listener', 'listener']);
log.clear();
merged.removeListener(listener);
source1.notify();
source2.notify();
expect(log, isEmpty);
});
test('Cannot use a disposed ChangeNotifier except for remove listener', () {
final TestNotifier source = TestNotifier();
source.dispose();
expect(() {
source.addListener(() {});
}, throwsFlutterError);
expect(() {
source.dispose();
}, throwsFlutterError);
expect(() {
source.notify();
}, throwsFlutterError);
});
test('Can remove listener on a disposed ChangeNotifier', () {
final TestNotifier source = TestNotifier();
FlutterError? error;
try {
source.dispose();
source.removeListener(() {});
} on FlutterError catch (e) {
error = e;
}
expect(error, isNull);
});
test('Can check hasListener on a disposed ChangeNotifier', () {
final HasListenersTester<int> source = HasListenersTester<int>(0);
source.addListener(() { });
expect(source.testHasListeners, isTrue);
FlutterError? error;
try {
source.dispose();
expect(source.testHasListeners, isFalse);
} on FlutterError catch (e) {
error = e;
}
expect(error, isNull);
});
test('Value notifier', () {
final ValueNotifier<double> notifier = ValueNotifier<double>(2.0);
final List<double> log = <double>[];
void listener() {
log.add(notifier.value);
}
notifier.addListener(listener);
notifier.value = 3.0;
expect(log, equals(<double>[3.0]));
log.clear();
notifier.value = 3.0;
expect(log, isEmpty);
});
test('Listenable.merge toString', () {
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
Listenable listenableUnderTest = Listenable.merge(<Listenable>[]);
expect(listenableUnderTest.toString(), 'Listenable.merge([])');
listenableUnderTest = Listenable.merge(<Listenable?>[null]);
expect(listenableUnderTest.toString(), 'Listenable.merge([null])');
listenableUnderTest = Listenable.merge(<Listenable>[source1]);
expect(
listenableUnderTest.toString(),
"Listenable.merge([Instance of 'TestNotifier'])",
);
listenableUnderTest = Listenable.merge(<Listenable>[source1, source2]);
expect(
listenableUnderTest.toString(),
"Listenable.merge([Instance of 'TestNotifier', Instance of 'TestNotifier'])",
);
listenableUnderTest = Listenable.merge(<Listenable?>[null, source2]);
expect(
listenableUnderTest.toString(),
"Listenable.merge([null, Instance of 'TestNotifier'])",
);
});
test('Listenable.merge does not leak', () {
// Regression test for https://github.com/flutter/flutter/issues/25163.
final TestNotifier source1 = TestNotifier();
final TestNotifier source2 = TestNotifier();
void fakeListener() {}
final Listenable listenableUnderTest =
Listenable.merge(<Listenable>[source1, source2]);
expect(source1.isListenedTo, isFalse);
expect(source2.isListenedTo, isFalse);
listenableUnderTest.addListener(fakeListener);
expect(source1.isListenedTo, isTrue);
expect(source2.isListenedTo, isTrue);
listenableUnderTest.removeListener(fakeListener);
expect(source1.isListenedTo, isFalse);
expect(source2.isListenedTo, isFalse);
});
test('hasListeners', () {
final HasListenersTester<bool> notifier = HasListenersTester<bool>(true);
expect(notifier.testHasListeners, isFalse);
void test1() {}
void test2() {}
notifier.addListener(test1);
expect(notifier.testHasListeners, isTrue);
notifier.addListener(test1);
expect(notifier.testHasListeners, isTrue);
notifier.removeListener(test1);
expect(notifier.testHasListeners, isTrue);
notifier.removeListener(test1);
expect(notifier.testHasListeners, isFalse);
notifier.addListener(test1);
expect(notifier.testHasListeners, isTrue);
notifier.addListener(test2);
expect(notifier.testHasListeners, isTrue);
notifier.removeListener(test1);
expect(notifier.testHasListeners, isTrue);
notifier.removeListener(test2);
expect(notifier.testHasListeners, isFalse);
});
test('ChangeNotifier as a mixin', () {
// We document that this is a valid way to use this class.
final B b = B();
int notifications = 0;
b.addListener(() {
notifications += 1;
});
expect(b.result, isFalse);
expect(notifications, 0);
b.test();
expect(b.result, isTrue);
expect(notifications, 1);
});
test('Throws FlutterError when disposed and called', () {
final TestNotifier testNotifier = TestNotifier();
testNotifier.dispose();
FlutterError? error;
try {
testNotifier.dispose();
} on FlutterError catch (e) {
error = e;
}
expect(error, isNotNull);
expect(error, isFlutterError);
expect(
error!.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' A TestNotifier was used after being disposed.\n'
' Once you have called dispose() on a TestNotifier, it can no\n'
' longer be used.\n',
),
);
});
test('Calling debugAssertNotDisposed works as intended', () {
final TestNotifier testNotifier = TestNotifier();
expect(ChangeNotifier.debugAssertNotDisposed(testNotifier), isTrue);
testNotifier.dispose();
FlutterError? error;
try {
ChangeNotifier.debugAssertNotDisposed(testNotifier);
} on FlutterError catch (e) {
error = e;
}
expect(error, isNotNull);
expect(error, isFlutterError);
expect(
error!.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' A TestNotifier was used after being disposed.\n'
' Once you have called dispose() on a TestNotifier, it can no\n'
' longer be used.\n',
),
);
});
test('notifyListener can be called recursively', () {
final Counter counter = Counter();
final List<String> log = <String>[];
void listener1() {
log.add('listener1');
if (counter.value < 0) {
counter.value = 0;
}
}
counter.addListener(listener1);
counter.notify();
expect(log, <String>['listener1']);
log.clear();
counter.value = 3;
expect(log, <String>['listener1']);
log.clear();
counter.value = -2;
expect(log, <String>['listener1', 'listener1']);
log.clear();
});
test('Remove Listeners while notifying on a list which will not resize', () {
final TestNotifier test = TestNotifier();
final List<String> log = <String>[];
final List<VoidCallback> listeners = <VoidCallback>[];
void autoRemove() {
// We remove 4 listeners.
// We will end up with (13-4 = 9) listeners.
test.removeListener(listeners[1]);
test.removeListener(listeners[3]);
test.removeListener(listeners[4]);
test.removeListener(autoRemove);
}
test.addListener(autoRemove);
// We add 12 more listeners.
for (int i = 0; i < 12; i++) {
void listener() {
log.add('listener$i');
}
listeners.add(listener);
test.addListener(listener);
}
final List<int> remainingListenerIndexes = <int>[
0,
2,
5,
6,
7,
8,
9,
10,
11,
];
final List<String> expectedLog =
remainingListenerIndexes.map((int i) => 'listener$i').toList();
test.notify();
expect(log, expectedLog);
log.clear();
// We expect to have the same result after the removal of previous listeners.
test.notify();
expect(log, expectedLog);
// We remove all other listeners.
for (int i = 0; i < remainingListenerIndexes.length; i++) {
test.removeListener(listeners[remainingListenerIndexes[i]]);
}
log.clear();
test.notify();
expect(log, <String>[]);
});
}
| flutter/packages/flutter/test/foundation/change_notifier_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/change_notifier_test.dart",
"repo_id": "flutter",
"token_count": 6424
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:fake_async/fake_async.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
import 'capture_output.dart';
void main() {
test('debugPrint', () {
expect(
captureOutput(() { debugPrintSynchronously('Hello, world'); }),
equals(<String>['Hello, world']),
);
expect(
captureOutput(() { debugPrintSynchronously('Hello, world', wrapWidth: 10); }),
equals(<String>['Hello,\nworld']),
);
for (int i = 0; i < 14; ++i) {
expect(
captureOutput(() { debugPrintSynchronously('Hello, world', wrapWidth: i); }),
equals(<String>['Hello,\nworld']),
);
}
expect(
captureOutput(() { debugPrintThrottled('Hello, world'); }),
equals(<String>['Hello, world']),
);
expect(
captureOutput(() { debugPrintThrottled('Hello, world', wrapWidth: 10); }),
equals(<String>['Hello,', 'world']),
);
});
test('debugPrint throttling', () {
FakeAsync().run((FakeAsync async) {
List<String> log = captureOutput(() {
debugPrintThrottled('${'A' * (22 * 1024)}\nB');
});
expect(log.length, 1);
async.elapse(const Duration(seconds: 2));
expect(log.length, 2);
log = captureOutput(() {
debugPrintThrottled('C' * (22 * 1024));
debugPrintThrottled('D');
});
expect(log.length, 1);
async.elapse(const Duration(seconds: 2));
expect(log.length, 2);
});
});
test('debugPrint can print null', () {
expect(
captureOutput(() { debugPrintThrottled(null); }),
equals(<String>['null']),
);
expect(
captureOutput(() { debugPrintThrottled(null, wrapWidth: 80); }),
equals(<String>['null']),
);
});
}
| flutter/packages/flutter/test/foundation/print_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/print_test.dart",
"repo_id": "flutter",
"token_count": 789
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
typedef HandleEventCallback = void Function(PointerEvent event);
class TestGestureFlutterBinding extends BindingBase with GestureBinding, SchedulerBinding {
@override
void initInstances() {
super.initInstances();
_instance = this;
}
/// The singleton instance of this object.
///
/// Provides access to the features exposed by this class. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [TestGestureFlutterBinding.ensureInitialized].
static TestGestureFlutterBinding get instance => BindingBase.checkInstance(_instance);
static TestGestureFlutterBinding? _instance;
/// Returns an instance of the [TestGestureFlutterBinding], creating and
/// initializing it if necessary.
static TestGestureFlutterBinding ensureInitialized() {
if (_instance == null) {
TestGestureFlutterBinding();
}
return _instance!;
}
HandleEventCallback? onHandlePointerEvent;
@override
void handlePointerEvent(PointerEvent event) {
onHandlePointerEvent?.call(event);
super.handlePointerEvent(event);
}
HandleEventCallback? onHandleEvent;
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
super.handleEvent(event, entry);
onHandleEvent?.call(event);
}
}
void main() {
final TestGestureFlutterBinding binding = TestGestureFlutterBinding.ensureInitialized();
test('Pointer tap events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.down),
ui.PointerData(change: ui.PointerChange.up),
],
);
final List<PointerEvent> events = <PointerEvent>[];
TestGestureFlutterBinding.instance.onHandleEvent = events.add;
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 2);
expect(events[0], isA<PointerDownEvent>());
expect(events[1], isA<PointerUpEvent>());
});
test('Pointer move events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.down),
ui.PointerData(change: ui.PointerChange.move),
ui.PointerData(change: ui.PointerChange.up),
],
);
final List<PointerEvent> events = <PointerEvent>[];
binding.onHandleEvent = events.add;
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 3);
expect(events[0], isA<PointerDownEvent>());
expect(events[1], isA<PointerMoveEvent>());
expect(events[2], isA<PointerUpEvent>());
});
test('Pointer hover events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add),
ui.PointerData(change: ui.PointerChange.hover),
ui.PointerData(change: ui.PointerChange.hover),
ui.PointerData(change: ui.PointerChange.remove),
ui.PointerData(change: ui.PointerChange.add),
ui.PointerData(change: ui.PointerChange.hover),
],
);
final List<PointerEvent> pointerRouterEvents = <PointerEvent>[];
GestureBinding.instance.pointerRouter.addGlobalRoute(pointerRouterEvents.add);
final List<PointerEvent> events = <PointerEvent>[];
binding.onHandleEvent = events.add;
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 3);
expect(events[0], isA<PointerHoverEvent>());
expect(events[1], isA<PointerHoverEvent>());
expect(events[2], isA<PointerHoverEvent>());
expect(pointerRouterEvents.length, 6, reason: 'pointerRouterEvents contains: $pointerRouterEvents');
expect(pointerRouterEvents[0], isA<PointerAddedEvent>());
expect(pointerRouterEvents[1], isA<PointerHoverEvent>());
expect(pointerRouterEvents[2], isA<PointerHoverEvent>());
expect(pointerRouterEvents[3], isA<PointerRemovedEvent>());
expect(pointerRouterEvents[4], isA<PointerAddedEvent>());
expect(pointerRouterEvents[5], isA<PointerHoverEvent>());
});
test('Pointer cancel events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.down),
ui.PointerData(),
],
);
final List<PointerEvent> events = <PointerEvent>[];
binding.onHandleEvent = events.add;
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 2);
expect(events[0], isA<PointerDownEvent>());
expect(events[1], isA<PointerCancelEvent>());
});
test('Can cancel pointers', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.down),
ui.PointerData(change: ui.PointerChange.up),
],
);
final List<PointerEvent> events = <PointerEvent>[];
binding.onHandleEvent = (PointerEvent event) {
events.add(event);
if (event is PointerDownEvent) {
binding.cancelPointer(event.pointer);
}
};
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 2);
expect(events[0], isA<PointerDownEvent>());
expect(events[1], isA<PointerCancelEvent>());
});
const double devicePixelRatio = 2.5;
test('Can expand add and hover pointers', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, device: 24),
ui.PointerData(change: ui.PointerChange.hover, device: 24),
ui.PointerData(change: ui.PointerChange.remove, device: 24),
ui.PointerData(change: ui.PointerChange.add, device: 24),
ui.PointerData(change: ui.PointerChange.hover, device: 24),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[1], isA<PointerHoverEvent>());
expect(events[2], isA<PointerRemovedEvent>());
expect(events[3], isA<PointerAddedEvent>());
expect(events[4], isA<PointerHoverEvent>());
});
test('Can handle malformed scrolling event.', () {
ui.PointerDataPacket packet = const ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, device: 24),
],
);
List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 1);
expect(events[0], isA<PointerAddedEvent>());
// Send packet contains malformed scroll events.
packet = const ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaX: double.infinity, scrollDeltaY: 10),
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaX: double.nan, scrollDeltaY: 10),
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaX: double.negativeInfinity, scrollDeltaY: 10),
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaY: double.infinity, scrollDeltaX: 10),
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaY: double.nan, scrollDeltaX: 10),
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaY: double.negativeInfinity, scrollDeltaX: 10),
],
);
events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 0);
// Send packet with a valid scroll event.
packet = const ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(signalKind: ui.PointerSignalKind.scroll, device: 24, scrollDeltaX: 10, scrollDeltaY: 10),
],
);
// Make sure PointerEventConverter can expand when device pixel ratio is valid.
events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 1);
expect(events[0], isA<PointerScrollEvent>());
// Make sure PointerEventConverter returns none when device pixel ratio is invalid.
events = PointerEventConverter.expand(packet.data, (int viewId) => 0).toList();
expect(events.length, 0);
});
test('Can expand pointer scroll events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add),
ui.PointerData(change: ui.PointerChange.hover, signalKind: ui.PointerSignalKind.scroll),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 2);
expect(events[0], isA<PointerAddedEvent>());
expect(events[1], isA<PointerScrollEvent>());
});
test('Should synthesize kPrimaryButton for touch when no button is set', () {
final Offset location = const Offset(10.0, 10.0) * devicePixelRatio;
final ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.hover, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.down, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.move, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.up, physicalX: location.dx, physicalY: location.dy),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[0].buttons, equals(0));
expect(events[1], isA<PointerHoverEvent>());
expect(events[1].buttons, equals(0));
expect(events[2], isA<PointerDownEvent>());
expect(events[2].buttons, equals(kPrimaryButton));
expect(events[3], isA<PointerMoveEvent>());
expect(events[3].buttons, equals(kPrimaryButton));
expect(events[4], isA<PointerUpEvent>());
expect(events[4].buttons, equals(0));
});
test('Should not synthesize kPrimaryButton for touch when a button is set', () {
final Offset location = const Offset(10.0, 10.0) * devicePixelRatio;
final ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.hover, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.down, buttons: kSecondaryButton, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.move, buttons: kSecondaryButton, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.up, physicalX: location.dx, physicalY: location.dy),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[0].buttons, equals(0));
expect(events[1], isA<PointerHoverEvent>());
expect(events[1].buttons, equals(0));
expect(events[2], isA<PointerDownEvent>());
expect(events[2].buttons, equals(kSecondaryButton));
expect(events[3], isA<PointerMoveEvent>());
expect(events[3].buttons, equals(kSecondaryButton));
expect(events[4], isA<PointerUpEvent>());
expect(events[4].buttons, equals(0));
});
test('Should synthesize kPrimaryButton for stylus when no button is set', () {
final Offset location = const Offset(10.0, 10.0) * devicePixelRatio;
for (final PointerDeviceKind kind in <PointerDeviceKind>[
PointerDeviceKind.stylus,
PointerDeviceKind.invertedStylus,
]) {
final ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.hover, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.down, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.move, buttons: kSecondaryStylusButton, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.up, kind: kind, physicalX: location.dx, physicalY: location.dy),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[0].buttons, equals(0));
expect(events[1], isA<PointerHoverEvent>());
expect(events[1].buttons, equals(0));
expect(events[2], isA<PointerDownEvent>());
expect(events[2].buttons, equals(kPrimaryButton));
expect(events[3], isA<PointerMoveEvent>());
expect(events[3].buttons, equals(kSecondaryStylusButton));
expect(events[4], isA<PointerUpEvent>());
expect(events[4].buttons, equals(0));
}
});
test('Should synthesize kPrimaryButton for unknown devices when no button is set', () {
final Offset location = const Offset(10.0, 10.0) * devicePixelRatio;
const PointerDeviceKind kind = PointerDeviceKind.unknown;
final ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.hover, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.down, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.move, buttons: kSecondaryButton, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.up, kind: kind, physicalX: location.dx, physicalY: location.dy),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[0].buttons, equals(0));
expect(events[1], isA<PointerHoverEvent>());
expect(events[1].buttons, equals(0));
expect(events[2], isA<PointerDownEvent>());
expect(events[2].buttons, equals(kPrimaryButton));
expect(events[3], isA<PointerMoveEvent>());
expect(events[3].buttons, equals(kSecondaryButton));
expect(events[4], isA<PointerUpEvent>());
expect(events[4].buttons, equals(0));
});
test('Should not synthesize kPrimaryButton for mouse', () {
final Offset location = const Offset(10.0, 10.0) * devicePixelRatio;
for (final PointerDeviceKind kind in <PointerDeviceKind>[
PointerDeviceKind.mouse,
]) {
final ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.add, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.hover, kind: kind, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.down, kind: kind, buttons: kMiddleMouseButton, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.move, kind: kind, buttons: kMiddleMouseButton | kSecondaryMouseButton, physicalX: location.dx, physicalY: location.dy),
ui.PointerData(change: ui.PointerChange.up, kind: kind, physicalX: location.dx, physicalY: location.dy),
],
);
final List<PointerEvent> events = PointerEventConverter.expand(packet.data, (int viewId) => devicePixelRatio).toList();
expect(events.length, 5);
expect(events[0], isA<PointerAddedEvent>());
expect(events[0].buttons, equals(0));
expect(events[1], isA<PointerHoverEvent>());
expect(events[1].buttons, equals(0));
expect(events[2], isA<PointerDownEvent>());
expect(events[2].buttons, equals(kMiddleMouseButton));
expect(events[3], isA<PointerMoveEvent>());
expect(events[3].buttons, equals(kMiddleMouseButton | kSecondaryMouseButton));
expect(events[4], isA<PointerUpEvent>());
expect(events[4].buttons, equals(0));
}
});
test('Pointer pan/zoom events', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.panZoomStart),
ui.PointerData(change: ui.PointerChange.panZoomUpdate),
ui.PointerData(change: ui.PointerChange.panZoomEnd),
],
);
final List<PointerEvent> events = <PointerEvent>[];
binding.onHandleEvent = events.add;
binding.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 3);
expect(events[0], isA<PointerPanZoomStartEvent>());
expect(events[1], isA<PointerPanZoomUpdateEvent>());
expect(events[2], isA<PointerPanZoomEndEvent>());
});
test('Error handling', () {
const ui.PointerDataPacket packet = ui.PointerDataPacket(
data: <ui.PointerData>[
ui.PointerData(change: ui.PointerChange.down),
ui.PointerData(change: ui.PointerChange.up),
],
);
final List<String> events = <String>[];
binding.onHandlePointerEvent = (PointerEvent event) { throw Exception('zipzapzooey $event'); };
FlutterError.onError = (FlutterErrorDetails details) { events.add(details.toString()); };
try {
GestureBinding.instance.platformDispatcher.onPointerDataPacket?.call(packet);
expect(events.length, 1);
expect(events[0], contains('while handling a pointer data\npacket')); // The default stringifying behavior uses 65 character wrapWidth.
expect(events[0], contains('zipzapzooey'));
expect(events[0], contains('PointerDownEvent'));
expect(events[0], isNot(contains('PointerUpEvent'))); // Failure happens on the first message, remaining messages aren't processed.
} finally {
binding.onHandlePointerEvent = null;
FlutterError.onError = FlutterError.presentError;
}
});
test('PointerEventConverter processes view IDs', () {
const int startID = 987654;
const List<ui.PointerData> data = <ui.PointerData>[
ui.PointerData(viewId: startID + 0, change: ui.PointerChange.cancel), // ignore: avoid_redundant_argument_values
ui.PointerData(viewId: startID + 1, change: ui.PointerChange.add),
ui.PointerData(viewId: startID + 2, change: ui.PointerChange.remove),
ui.PointerData(viewId: startID + 3, change: ui.PointerChange.hover),
ui.PointerData(viewId: startID + 4, change: ui.PointerChange.down),
ui.PointerData(viewId: startID + 5, change: ui.PointerChange.move),
ui.PointerData(viewId: startID + 6, change: ui.PointerChange.up),
ui.PointerData(viewId: startID + 7, change: ui.PointerChange.panZoomStart),
ui.PointerData(viewId: startID + 8, change: ui.PointerChange.panZoomUpdate),
ui.PointerData(viewId: startID + 9, change: ui.PointerChange.panZoomEnd),
];
final List<int> viewIds = <int>[];
double devicePixelRatioGetter(int viewId) {
viewIds.add(viewId);
return viewId / 10.0;
}
final List<PointerEvent> events = PointerEventConverter.expand(data, devicePixelRatioGetter).toList();
final List<int> expectedViewIds = List<int>.generate(10, (int index) => startID + index);
expect(viewIds, expectedViewIds);
expect(events, hasLength(10));
expect(events.map((PointerEvent event) => event.viewId), expectedViewIds);
});
}
| flutter/packages/flutter/test/gestures/gesture_binding_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/gesture_binding_test.dart",
"repo_id": "flutter",
"token_count": 7899
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import '../gestures/gesture_tester.dart';
// Anything longer than [kDoubleTapTimeout] will reset the consecutive tap count.
final Duration kConsecutiveTapDelay = kDoubleTapTimeout ~/ 2;
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
late List<String> events;
late BaseTapAndDragGestureRecognizer tapAndDrag;
void setUpTapAndPanGestureRecognizer() {
tapAndDrag = TapAndPanGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..maxConsecutiveTap = 3
..onTapDown = (TapDragDownDetails details) {
events.add('down#${details.consecutiveTapCount}');
}
..onTapUp = (TapDragUpDetails details) {
events.add('up#${details.consecutiveTapCount}');
}
..onDragStart = (TapDragStartDetails details) {
events.add('panstart#${details.consecutiveTapCount}');
}
..onDragUpdate = (TapDragUpdateDetails details) {
events.add('panupdate#${details.consecutiveTapCount}');
}
..onDragEnd = (TapDragEndDetails details) {
events.add('panend#${details.consecutiveTapCount}');
}
..onCancel = () {
events.add('cancel');
};
addTearDown(tapAndDrag.dispose);
}
void setUpTapAndHorizontalDragGestureRecognizer() {
tapAndDrag = TapAndHorizontalDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..maxConsecutiveTap = 3
..onTapDown = (TapDragDownDetails details) {
events.add('down#${details.consecutiveTapCount}');
}
..onTapUp = (TapDragUpDetails details) {
events.add('up#${details.consecutiveTapCount}');
}
..onDragStart = (TapDragStartDetails details) {
events.add('horizontaldragstart#${details.consecutiveTapCount}');
}
..onDragUpdate = (TapDragUpdateDetails details) {
events.add('horizontaldragupdate#${details.consecutiveTapCount}');
}
..onDragEnd = (TapDragEndDetails details) {
events.add('horizontaldragend#${details.consecutiveTapCount}');
}
..onCancel = () {
events.add('cancel');
};
addTearDown(tapAndDrag.dispose);
}
setUp(() {
events = <String>[];
});
// Down/up pair 1: normal tap sequence
const PointerDownEvent down1 = PointerDownEvent(
pointer: 1,
position: Offset(10.0, 10.0),
);
const PointerUpEvent up1 = PointerUpEvent(
pointer: 1,
position: Offset(11.0, 9.0),
);
const PointerCancelEvent cancel1 = PointerCancelEvent(
pointer: 1,
);
// Down/up pair 2: normal tap sequence close to pair 1
const PointerDownEvent down2 = PointerDownEvent(
pointer: 2,
position: Offset(12.0, 12.0),
);
const PointerUpEvent up2 = PointerUpEvent(
pointer: 2,
position: Offset(13.0, 11.0),
);
// Down/up pair 3: normal tap sequence close to pair 1
const PointerDownEvent down3 = PointerDownEvent(
pointer: 3,
position: Offset(12.0, 12.0),
);
const PointerUpEvent up3 = PointerUpEvent(
pointer: 3,
position: Offset(13.0, 11.0),
);
// Down/up pair 4: normal tap sequence far away from pair 1
const PointerDownEvent down4 = PointerDownEvent(
pointer: 4,
position: Offset(130.0, 130.0),
);
const PointerUpEvent up4 = PointerUpEvent(
pointer: 4,
position: Offset(131.0, 129.0),
);
// Down/move/up sequence 5: intervening motion
const PointerDownEvent down5 = PointerDownEvent(
pointer: 5,
position: Offset(10.0, 10.0),
);
const PointerMoveEvent move5 = PointerMoveEvent(
pointer: 5,
position: Offset(25.0, 25.0),
);
const PointerUpEvent up5 = PointerUpEvent(
pointer: 5,
position: Offset(25.0, 25.0),
);
// Mouse Down/move/up sequence 6: intervening motion - kPrecisePointerPanSlop
const PointerDownEvent down6 = PointerDownEvent(
kind: PointerDeviceKind.mouse,
pointer: 6,
position: Offset(10.0, 10.0),
);
const PointerMoveEvent move6 = PointerMoveEvent(
kind: PointerDeviceKind.mouse,
pointer: 6,
position: Offset(15.0, 15.0),
delta: Offset(5.0, 5.0),
);
const PointerUpEvent up6 = PointerUpEvent(
kind: PointerDeviceKind.mouse,
pointer: 6,
position: Offset(15.0, 15.0),
);
testGesture('Recognizes consecutive taps', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
events.clear();
tester.async.elapse(kConsecutiveTapDelay);
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#2', 'up#2']);
events.clear();
tester.async.elapse(kConsecutiveTapDelay);
tapAndDrag.addPointer(down3);
tester.closeArena(3);
tester.route(down3);
tester.route(up3);
GestureBinding.instance.gestureArena.sweep(3);
expect(events, <String>['down#3', 'up#3']);
});
testGesture('Resets if times out in between taps', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
events.clear();
tester.async.elapse(const Duration(milliseconds: 1000));
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Resets if taps are far apart', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
events.clear();
tester.async.elapse(const Duration(milliseconds: 100));
tapAndDrag.addPointer(down4);
tester.closeArena(4);
tester.route(down4);
tester.route(up4);
GestureBinding.instance.gestureArena.sweep(4);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Resets if consecutiveTapCount reaches maxConsecutiveTap', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
// First tap.
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
// Second tap.
events.clear();
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#2', 'up#2']);
// Third tap.
events.clear();
tapAndDrag.addPointer(down3);
tester.closeArena(3);
tester.route(down3);
tester.route(up3);
GestureBinding.instance.gestureArena.sweep(3);
expect(events, <String>['down#3', 'up#3']);
// Fourth tap. Here we arrived at the `maxConsecutiveTap` for `consecutiveTapCount`
// so our count should reset and our new count should be `1`.
events.clear();
tapAndDrag.addPointer(down3);
tester.closeArena(3);
tester.route(down3);
tester.route(up3);
GestureBinding.instance.gestureArena.sweep(3);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Should recognize drag', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final TestPointer pointer = TestPointer(5);
final PointerDownEvent down = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(down);
tester.closeArena(5);
tester.route(down);
tester.route(pointer.move(const Offset(40.0, 45.0)));
tester.route(pointer.up());
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['down#1', 'panstart#1', 'panupdate#1', 'panend#1']);
});
testGesture('Recognizes consecutive taps + drag', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downA = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downA);
tester.closeArena(5);
tester.route(downA);
tester.route(pointer.up());
GestureBinding.instance.gestureArena.sweep(5);
tester.async.elapse(kConsecutiveTapDelay);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.up());
GestureBinding.instance.gestureArena.sweep(5);
tester.async.elapse(kConsecutiveTapDelay);
final PointerDownEvent downC = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downC);
tester.closeArena(5);
tester.route(downC);
tester.route(pointer.move(const Offset(40.0, 45.0)));
tester.route(pointer.up());
expect(events, <String>[
'down#1',
'up#1',
'down#2',
'up#2',
'down#3',
'panstart#3',
'panupdate#3',
'panend#3']);
});
testGesture('Recognizer rejects pointer that is not the primary one (FIFO) - before acceptance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tapAndDrag.addPointer(down2);
tester.closeArena(1);
tester.route(down1);
tester.closeArena(2);
tester.route(down2);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Calls tap up when the recognizer accepts before handleEvent is called', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
GestureBinding.instance.gestureArena.sweep(1);
tester.route(down1);
tester.route(up1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Recognizer rejects pointer that is not the primary one (FILO) - before acceptance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tapAndDrag.addPointer(down2);
tester.closeArena(1);
tester.route(down1);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Recognizer rejects pointer that is not the primary one (FIFO) - after acceptance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Recognizer rejects pointer that is not the primary one (FILO) - after acceptance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Recognizer detects tap gesture when pointer does not move past tap tolerance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
// In this test the tap has not travelled past the tap tolerance defined by
// [kDoubleTapTouchSlop]. It is expected for the recognizer to detect a tap
// and fire drag cancel.
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Recognizer detects drag gesture when pointer moves past tap tolerance but not the drag minimum', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
// In this test, the pointer has moved past the tap tolerance but it has
// not reached the distance travelled to be considered a drag gesture. In
// this case it is expected for the recognizer to detect a drag and fire tap cancel.
tapAndDrag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
tester.route(move5);
tester.route(up5);
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['down#1', 'panstart#1', 'panend#1']);
});
testGesture('Beats TapGestureRecognizer when mouse pointer moves past kPrecisePointerPanSlop', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
// This is a regression test for https://github.com/flutter/flutter/issues/122141.
final TapGestureRecognizer taps = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
events.add('tapdown');
}
..onTapUp = (TapUpDetails details) {
events.add('tapup');
}
..onTapCancel = () {
events.add('tapscancel');
};
addTearDown(taps.dispose);
tapAndDrag.addPointer(down6);
taps.addPointer(down6);
tester.closeArena(6);
tester.route(down6);
tester.route(move6);
tester.route(up6);
GestureBinding.instance.gestureArena.sweep(6);
expect(events, <String>['down#1', 'panstart#1', 'panupdate#1', 'panend#1']);
});
testGesture('Recognizer declares self-victory in a non-empty arena when pointer travels minimum distance to be considered a drag', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final PanGestureRecognizer pans = PanGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('panstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('panupdate');
}
..onEnd = (DragEndDetails details) {
events.add('panend');
}
..onCancel = () {
events.add('pancancel');
};
addTearDown(pans.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
// When competing against another [DragGestureRecognizer], the recognizer
// that first in the arena will win after sweep is called.
tapAndDrag.addPointer(downB);
pans.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(40.0, 45.0)));
tester.route(pointer.up());
expect(events, <String>[
'pancancel',
'down#1',
'panstart#1',
'panupdate#1',
'panend#1']);
});
testGesture('TapAndHorizontalDragGestureRecognizer accepts drag on a pan when the arena has already been won by the primary pointer', (GestureTester tester) {
setUpTapAndHorizontalDragGestureRecognizer();
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(25.0, 45.0)));
tester.route(pointer.up());
expect(events, <String>[
'down#1',
'horizontaldragstart#1',
'horizontaldragupdate#1',
'horizontaldragend#1']);
});
testGesture('TapAndHorizontalDragGestureRecognizer loses to VerticalDragGestureRecognizer on a vertical drag', (GestureTester tester) {
setUpTapAndHorizontalDragGestureRecognizer();
final VerticalDragGestureRecognizer verticalDrag = VerticalDragGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('verticalstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('verticalupdate');
}
..onEnd = (DragEndDetails details) {
events.add('verticalend');
}
..onCancel = () {
events.add('verticalcancel');
};
addTearDown(verticalDrag.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
verticalDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(10.0, 45.0)));
tester.route(pointer.move(const Offset(10.0, 100.0)));
tester.route(pointer.up());
expect(events, <String>[
'verticalstart',
'verticalupdate',
'verticalend']);
});
testGesture('TapAndPanGestureRecognizer loses to VerticalDragGestureRecognizer on a vertical drag', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final VerticalDragGestureRecognizer verticalDrag = VerticalDragGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('verticalstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('verticalupdate');
}
..onEnd = (DragEndDetails details) {
events.add('verticalend');
}
..onCancel = () {
events.add('verticalcancel');
};
addTearDown(verticalDrag.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
verticalDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(10.0, 45.0)));
tester.route(pointer.move(const Offset(10.0, 100.0)));
tester.route(pointer.up());
expect(events, <String>[
'verticalstart',
'verticalupdate',
'verticalend']);
});
testGesture('TapAndHorizontalDragGestureRecognizer beats VerticalDragGestureRecognizer on a horizontal drag', (GestureTester tester) {
setUpTapAndHorizontalDragGestureRecognizer();
final VerticalDragGestureRecognizer verticalDrag = VerticalDragGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('verticalstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('verticalupdate');
}
..onEnd = (DragEndDetails details) {
events.add('verticalend');
}
..onCancel = () {
events.add('verticalcancel');
};
addTearDown(verticalDrag.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
verticalDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(45.0, 10.0)));
tester.route(pointer.up());
expect(events, <String>[
'verticalcancel',
'down#1',
'horizontaldragstart#1',
'horizontaldragupdate#1',
'horizontaldragend#1']);
});
testGesture('TapAndPanGestureRecognizer beats VerticalDragGestureRecognizer on a horizontal pan', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final VerticalDragGestureRecognizer verticalDrag = VerticalDragGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('verticalstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('verticalupdate');
}
..onEnd = (DragEndDetails details) {
events.add('verticalend');
}
..onCancel = () {
events.add('verticalcancel');
};
addTearDown(verticalDrag.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
verticalDrag.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.route(pointer.move(const Offset(45.0, 25.0)));
tester.route(pointer.up());
expect(events, <String>[
'verticalcancel',
'down#1',
'panstart#1',
'panupdate#1',
'panend#1']);
});
testGesture('Beats LongPressGestureRecognizer on a consecutive tap greater than one', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final LongPressGestureRecognizer longpress = LongPressGestureRecognizer()
..onLongPressStart = (LongPressStartDetails details) {
events.add('longpressstart');
}
..onLongPressMoveUpdate = (LongPressMoveUpdateDetails details) {
events.add('longpressmoveupdate');
}
..onLongPressEnd = (LongPressEndDetails details) {
events.add('longpressend');
}
..onLongPressCancel = () {
events.add('longpresscancel');
};
addTearDown(longpress.dispose);
final TestPointer pointer = TestPointer(5);
final PointerDownEvent downA = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downA);
longpress.addPointer(downA);
tester.closeArena(5);
tester.route(downA);
tester.route(pointer.up());
GestureBinding.instance.gestureArena.sweep(5);
tester.async.elapse(kConsecutiveTapDelay);
final PointerDownEvent downB = pointer.down(const Offset(10.0, 10.0));
tapAndDrag.addPointer(downB);
longpress.addPointer(downB);
tester.closeArena(5);
tester.route(downB);
tester.async.elapse(const Duration(milliseconds: 500));
tester.route(pointer.move(const Offset(40.0, 45.0)));
tester.route(pointer.up());
expect(events, <String>[
'longpresscancel',
'down#1',
'up#1',
'down#2',
'panstart#2',
'panupdate#2',
'panend#2']);
});
// This is a regression test for https://github.com/flutter/flutter/issues/129161.
testGesture('Beats TapGestureRecognizer and DoubleTapGestureRecognizer when the pointer has not moved and this recognizer is the first in the arena', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final TapGestureRecognizer taps = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
events.add('tapdown');
}
..onTapUp = (TapUpDetails details) {
events.add('tapup');
}
..onTapCancel = () {
events.add('tapscancel');
};
addTearDown(taps.dispose);
final DoubleTapGestureRecognizer doubleTaps = DoubleTapGestureRecognizer()
..onDoubleTapDown = (TapDownDetails details) {
events.add('doubletapdown');
}
..onDoubleTap = () {
events.add('doubletapup');
}
..onDoubleTapCancel = () {
events.add('doubletapcancel');
};
addTearDown(doubleTaps.dispose);
tapAndDrag.addPointer(down1);
taps.addPointer(down1);
doubleTaps.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
// Wait for GestureArena to resolve itself.
tester.async.elapse(kDoubleTapTimeout);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Beats TapGestureRecognizer when the pointer has not moved and this recognizer is the first in the arena', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final TapGestureRecognizer taps = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
events.add('tapdown');
}
..onTapUp = (TapUpDetails details) {
events.add('tapup');
}
..onTapCancel = () {
events.add('tapscancel');
};
addTearDown(taps.dispose);
tapAndDrag.addPointer(down1);
taps.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Beats TapGestureRecognizer when the pointer has exceeded the slop tolerance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final TapGestureRecognizer taps = TapGestureRecognizer()
..onTapDown = (TapDownDetails details) {
events.add('tapdown');
}
..onTapUp = (TapUpDetails details) {
events.add('tapup');
}
..onTapCancel = () {
events.add('tapscancel');
};
addTearDown(taps.dispose);
tapAndDrag.addPointer(down5);
taps.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
tester.route(move5);
tester.route(up5);
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['down#1', 'panstart#1', 'panend#1']);
events.clear();
tester.async.elapse(const Duration(milliseconds: 1000));
taps.addPointer(down1);
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['tapdown', 'tapup']);
});
testGesture('Ties with PanGestureRecognizer when pointer has not met sufficient global distance to be a drag', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
final PanGestureRecognizer pans = PanGestureRecognizer()
..onStart = (DragStartDetails details) {
events.add('panstart');
}
..onUpdate = (DragUpdateDetails details) {
events.add('panupdate');
}
..onEnd = (DragEndDetails details) {
events.add('panend');
}
..onCancel = () {
events.add('pancancel');
};
addTearDown(pans.dispose);
tapAndDrag.addPointer(down5);
pans.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
tester.route(move5);
tester.route(up5);
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['pancancel']);
});
testGesture('Defaults to drag when pointer dragged past slop tolerance', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
tester.route(move5);
tester.route(up5);
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['down#1', 'panstart#1', 'panend#1']);
events.clear();
tester.async.elapse(const Duration(milliseconds: 1000));
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'up#1']);
});
testGesture('Fires cancel and resets for PointerCancelEvent', (GestureTester tester) {
setUpTapAndPanGestureRecognizer();
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(cancel1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1', 'cancel']);
events.clear();
tester.async.elapse(const Duration(milliseconds: 100));
tapAndDrag.addPointer(down2);
tester.closeArena(2);
tester.route(down2);
tester.route(up2);
GestureBinding.instance.gestureArena.sweep(2);
expect(events, <String>['down#1', 'up#1']);
});
// This is a regression test for https://github.com/flutter/flutter/issues/102084.
testGesture('Does not call onDragEnd if not provided', (GestureTester tester) {
tapAndDrag = TapAndDragGestureRecognizer()
..dragStartBehavior = DragStartBehavior.down
..maxConsecutiveTap = 3
..onTapDown = (TapDragDownDetails details) {
events.add('down#${details.consecutiveTapCount}');
};
addTearDown(tapAndDrag.dispose);
FlutterErrorDetails? errorDetails;
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails details) {
errorDetails = details;
};
addTearDown(() {
FlutterError.onError = oldHandler;
});
tapAndDrag.addPointer(down5);
tester.closeArena(5);
tester.route(down5);
tester.route(move5);
tester.route(up5);
GestureBinding.instance.gestureArena.sweep(5);
expect(events, <String>['down#1']);
expect(errorDetails, isNull);
events.clear();
tester.async.elapse(const Duration(milliseconds: 1000));
tapAndDrag.addPointer(down1);
tester.closeArena(1);
tester.route(down1);
tester.route(up1);
GestureBinding.instance.gestureArena.sweep(1);
expect(events, <String>['down#1']);
});
}
| flutter/packages/flutter/test/gestures/tap_and_drag_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/tap_and_drag_test.dart",
"repo_id": "flutter",
"token_count": 11852
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/clipboard_utils.dart';
import '../widgets/editable_text_utils.dart';
import '../widgets/live_text_utils.dart';
import '../widgets/text_selection_toolbar_utils.dart';
void main() {
final MockClipboard mockClipboard = MockClipboard();
setUp(() async {
TestWidgetsFlutterBinding.ensureInitialized();
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(
SystemChannels.platform,
mockClipboard.handleMethodCall,
);
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
});
testWidgets('Builds the right toolbar on each platform, including web, and shows buttonItems', (WidgetTester tester) async {
const String buttonText = 'Click me';
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: AdaptiveTextSelectionToolbar.buttonItems(
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
buttonItems: <ContextMenuButtonItem>[
ContextMenuButtonItem(
label: buttonText,
onPressed: () {
},
),
],
),
),
),
),
);
expect(find.text(buttonText), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
expect(find.byType(TextSelectionToolbar), findsOneWidget);
expect(find.byType(CupertinoTextSelectionToolbar), findsNothing);
expect(find.byType(DesktopTextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsNothing);
case TargetPlatform.iOS:
expect(find.byType(TextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbar), findsOneWidget);
expect(find.byType(DesktopTextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsNothing);
case TargetPlatform.macOS:
expect(find.byType(TextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbar), findsNothing);
expect(find.byType(DesktopTextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsOneWidget);
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(TextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbar), findsNothing);
expect(find.byType(DesktopTextSelectionToolbar), findsOneWidget);
expect(find.byType(CupertinoDesktopTextSelectionToolbar), findsNothing);
}
},
variant: TargetPlatformVariant.all(),
skip: isBrowser, // [intended] see https://github.com/flutter/flutter/issues/108382
);
testWidgets('Can build children directly as well', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: AdaptiveTextSelectionToolbar(
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
children: <Widget>[
Container(key: key),
],
),
),
),
),
);
expect(find.byKey(key), findsOneWidget);
});
testWidgets('Can build from EditableTextState', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
final TextEditingController controller = TextEditingController();
final FocusNode focusNode = FocusNode();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: SizedBox(
width: 400,
child: EditableText(
controller: controller,
backgroundCursorColor: const Color(0xff00ffff),
focusNode: focusNode,
style: const TextStyle(),
cursorColor: const Color(0xff00ffff),
selectionControls: materialTextSelectionHandleControls,
contextMenuBuilder: (
BuildContext context,
EditableTextState editableTextState,
) {
return AdaptiveTextSelectionToolbar.editableText(
key: key,
editableTextState: editableTextState,
);
},
),
),
),
),
),
);
await tester.pump(); // Wait for autofocus to take effect.
expect(find.byKey(key), findsNothing);
// Long-press to bring up the context menu.
final Finder textFinder = find.byType(EditableText);
await tester.longPress(textFinder);
tester.state<EditableTextState>(textFinder).showToolbar();
await tester.pumpAndSettle();
expect(find.byKey(key), findsOneWidget);
expect(find.text('Copy'), findsNothing);
expect(find.text('Cut'), findsNothing);
expect(find.text('Select all'), findsNothing);
expect(find.text('Paste'), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
expect(find.byType(TextSelectionToolbarTextButton), findsOneWidget);
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsOneWidget);
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(DesktopTextSelectionToolbarButton), findsOneWidget);
case TargetPlatform.macOS:
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsOneWidget);
}
controller.dispose();
focusNode.dispose();
},
skip: kIsWeb, // [intended] on web the browser handles the context menu.
variant: TargetPlatformVariant.all(),
);
testWidgets('Can build for editable text from raw parameters', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: AdaptiveTextSelectionToolbar.editable(
key: key,
anchors: const TextSelectionToolbarAnchors(
primaryAnchor: Offset.zero,
),
clipboardStatus: ClipboardStatus.pasteable,
onCopy: () {},
onCut: () {},
onPaste: () {},
onSelectAll: () {},
onLiveTextInput: () {},
onLookUp: () {},
onSearchWeb: () {},
onShare: () {},
),
),
),
),
);
expect(find.byKey(key), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.android:
expect(find.byType(TextSelectionToolbarTextButton), findsNWidgets(6));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Share'), findsOneWidget);
expect(find.text('Select all'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(findMaterialOverflowNextButton(), findsOneWidget); // Material overflow buttons are not TextSelectionToolbarTextButton.
await tapMaterialOverflowNextButton(tester);
expect(find.byType(TextSelectionToolbarTextButton), findsNWidgets(2));
expect(find.text('Search Web'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
expect(findMaterialOverflowBackButton(), findsOneWidget); // Material overflow buttons are not TextSelectionToolbarTextButton.
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(6));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(findCupertinoOverflowNextButton(), findsOneWidget);
await tapCupertinoOverflowNextButton(tester);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNWidgets(4));
expect(findCupertinoOverflowBackButton(), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share...'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
case TargetPlatform.fuchsia:
expect(find.byType(TextSelectionToolbarTextButton), findsNWidgets(8));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select all'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share'), findsOneWidget);
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(DesktopTextSelectionToolbarButton), findsNWidgets(8));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select all'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
case TargetPlatform.macOS:
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNWidgets(8));
expect(find.text('Cut'), findsOneWidget);
expect(find.text('Copy'), findsOneWidget);
expect(find.text('Paste'), findsOneWidget);
expect(find.text('Select All'), findsOneWidget);
expect(find.text('Look Up'), findsOneWidget);
expect(find.text('Search Web'), findsOneWidget);
expect(find.text('Share...'), findsOneWidget);
expect(findLiveTextButton(), findsOneWidget);
}
},
skip: kIsWeb, // [intended] on web the browser handles the context menu.
variant: TargetPlatformVariant.all(),
);
group('buttonItems', () {
testWidgets('getEditableTextButtonItems builds the correct button items per-platform', (WidgetTester tester) async {
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
Set<ContextMenuButtonType> buttonTypes = <ContextMenuButtonType>{};
final TextEditingController controller = TextEditingController();
final FocusNode focusNode = FocusNode();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: EditableText(
controller: controller,
backgroundCursorColor: Colors.grey,
focusNode: focusNode,
style: const TextStyle(),
cursorColor: Colors.red,
selectionControls: materialTextSelectionHandleControls,
contextMenuBuilder: (
BuildContext context,
EditableTextState editableTextState,
) {
buttonTypes = editableTextState.contextMenuButtonItems
.map((ContextMenuButtonItem buttonItem) => buttonItem.type)
.toSet();
return const SizedBox.shrink();
},
),
),
),
),
);
final EditableTextState state =
tester.state<EditableTextState>(find.byType(EditableText));
// With no text in the field.
await tester.tapAt(textOffsetToPosition(tester, 0));
await tester.pump();
expect(state.showToolbar(), true);
await tester.pump();
expect(buttonTypes, isNot(contains(ContextMenuButtonType.cut)));
expect(buttonTypes, isNot(contains(ContextMenuButtonType.copy)));
expect(buttonTypes, contains(ContextMenuButtonType.paste));
expect(buttonTypes, isNot(contains(ContextMenuButtonType.selectAll)));
// With text but no selection.
controller.text = 'lorem ipsum';
await tester.pump();
expect(buttonTypes, isNot(contains(ContextMenuButtonType.cut)));
expect(buttonTypes, isNot(contains(ContextMenuButtonType.copy)));
expect(buttonTypes, contains(ContextMenuButtonType.paste));
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(buttonTypes, contains(ContextMenuButtonType.selectAll));
case TargetPlatform.macOS:
expect(buttonTypes, isNot(contains(ContextMenuButtonType.selectAll)));
}
// With text and selection.
controller.value = controller.value.copyWith(
selection: const TextSelection(
baseOffset: 0,
extentOffset: 'lorem'.length,
),
);
await tester.pump();
expect(buttonTypes, contains(ContextMenuButtonType.cut));
expect(buttonTypes, contains(ContextMenuButtonType.copy));
expect(buttonTypes, contains(ContextMenuButtonType.paste));
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(buttonTypes, contains(ContextMenuButtonType.selectAll));
case TargetPlatform.iOS:
case TargetPlatform.macOS:
expect(buttonTypes, isNot(contains(ContextMenuButtonType.selectAll)));
}
focusNode.dispose();
controller.dispose();
},
variant: TargetPlatformVariant.all(),
skip: kIsWeb, // [intended]
);
testWidgets('getAdaptiveButtons builds the correct button widgets per-platform', (WidgetTester tester) async {
const String buttonText = 'Click me';
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: Builder(
builder: (BuildContext context) {
final List<ContextMenuButtonItem> buttonItems = <ContextMenuButtonItem>[
ContextMenuButtonItem(
label: buttonText,
onPressed: () {
},
),
];
return ListView(
children: AdaptiveTextSelectionToolbar.getAdaptiveButtons(
context,
buttonItems,
).toList(),
);
},
),
),
),
),
);
expect(find.text(buttonText), findsOneWidget);
switch (defaultTargetPlatform) {
case TargetPlatform.fuchsia:
case TargetPlatform.android:
expect(find.byType(TextSelectionToolbarTextButton), findsOneWidget);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.byType(DesktopTextSelectionToolbarButton), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNothing);
case TargetPlatform.iOS:
expect(find.byType(TextSelectionToolbarTextButton), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsOneWidget);
expect(find.byType(DesktopTextSelectionToolbarButton), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNothing);
case TargetPlatform.macOS:
expect(find.byType(TextSelectionToolbarTextButton), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.byType(DesktopTextSelectionToolbarButton), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsOneWidget);
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(TextSelectionToolbarTextButton), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.byType(DesktopTextSelectionToolbarButton), findsOneWidget);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNothing);
}
},
variant: TargetPlatformVariant.all(),
);
});
}
| flutter/packages/flutter/test/material/adaptive_text_selection_toolbar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/adaptive_text_selection_toolbar_test.dart",
"repo_id": "flutter",
"token_count": 7108
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('BottomAppBarTheme lerp special cases', () {
expect(BottomAppBarTheme.lerp(null, null, 0), const BottomAppBarTheme());
const BottomAppBarTheme data = BottomAppBarTheme();
expect(identical(BottomAppBarTheme.lerp(data, data, 0.5), data), true);
});
group('Material 2 tests', () {
testWidgets('Material2 - BAB theme overrides color', (WidgetTester tester) async {
const Color themedColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(color: themedColor);
await tester.pumpWidget(_withTheme(theme, useMaterial3: false));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, themedColor);
});
testWidgets('Material2 - BAB color - Widget', (WidgetTester tester) async {
const Color babThemeColor = Colors.black87;
const Color babColor = Colors.pink;
const BottomAppBarTheme theme = BottomAppBarTheme(color: babThemeColor);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: false,
bottomAppBarTheme: theme,
),
home: const Scaffold(body: BottomAppBar(color: babColor)),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, babColor);
});
testWidgets('Material2 - BAB color - BabTheme', (WidgetTester tester) async {
const Color babThemeColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(color: babThemeColor);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: false,
bottomAppBarTheme: theme,
),
home: const Scaffold(body: BottomAppBar()),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, babThemeColor);
});
testWidgets('Material2 - BAB color - Theme', (WidgetTester tester) async {
const Color themeColor = Colors.white10;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false, bottomAppBarTheme: const BottomAppBarTheme(color: themeColor)),
home: const Scaffold(body: BottomAppBar()),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, themeColor);
});
testWidgets('Material2 - BAB color - Default', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(body: BottomAppBar()),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, Colors.white);
});
testWidgets('Material2 - BAB theme customizes shape', (WidgetTester tester) async {
const BottomAppBarTheme theme = BottomAppBarTheme(
color: Colors.white30,
shape: CircularNotchedRectangle(),
elevation: 1.0,
);
await tester.pumpWidget(_withTheme(theme, useMaterial3: false));
await expectLater(
find.byKey(_painterKey),
matchesGoldenFile('bottom_app_bar_theme.custom_shape.png'),
);
});
testWidgets('Material2 - BAB theme does not affect defaults', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(body: BottomAppBar()),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, Colors.white);
expect(widget.elevation, equals(8.0));
});
});
group('Material 3 tests', () {
testWidgets('Material3 - BAB theme overrides color', (WidgetTester tester) async {
const Color themedColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(
color: themedColor,
elevation: 0
);
await tester.pumpWidget(_withTheme(theme, useMaterial3: true));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, themedColor);
});
testWidgets('Material3 - BAB color - Widget', (WidgetTester tester) async {
const Color babThemeColor = Colors.black87;
const Color babColor = Colors.pink;
const BottomAppBarTheme theme = BottomAppBarTheme(color: babThemeColor);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
bottomAppBarTheme: theme,
),
home: const Scaffold(body: BottomAppBar(color: babColor, surfaceTintColor: Colors.transparent)),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, babColor);
});
testWidgets('Material3 - BAB color - BabTheme', (WidgetTester tester) async {
const Color babThemeColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(color: babThemeColor);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
bottomAppBarTheme: theme,
),
home: const Scaffold(body: BottomAppBar(surfaceTintColor: Colors.transparent)),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, babThemeColor);
});
testWidgets('Material3 - BAB theme does not affect defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
await tester.pumpWidget(MaterialApp(
theme: theme,
home: const Scaffold(body: BottomAppBar(surfaceTintColor: Colors.transparent)),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, theme.colorScheme.surfaceContainer);
expect(widget.elevation, equals(3.0));
});
testWidgets('Material3 - BAB theme overrides surfaceTintColor', (WidgetTester tester) async {
const Color color = Colors.blue; // base color that the surface tint will be applied to
const Color babThemeSurfaceTintColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(
color: color, surfaceTintColor: babThemeSurfaceTintColor, elevation: 0,
);
await tester.pumpWidget(_withTheme(theme, useMaterial3: true));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, ElevationOverlay.applySurfaceTint(color, babThemeSurfaceTintColor, 0));
});
testWidgets('Material3 - BAB theme overrides shadowColor', (WidgetTester tester) async {
const Color babThemeShadowColor = Colors.yellow;
const BottomAppBarTheme theme = BottomAppBarTheme(
shadowColor: babThemeShadowColor, elevation: 0
);
await tester.pumpWidget(_withTheme(theme, useMaterial3: true));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.shadowColor, babThemeShadowColor);
});
testWidgets('Material3 - BAB surfaceTintColor - Widget', (WidgetTester tester) async {
const Color color = Colors.white10; // base color that the surface tint will be applied to
const Color babThemeSurfaceTintColor = Colors.black87;
const Color babSurfaceTintColor = Colors.pink;
const BottomAppBarTheme theme = BottomAppBarTheme(
surfaceTintColor: babThemeSurfaceTintColor
);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
bottomAppBarTheme: theme,
),
home: const Scaffold(
body: BottomAppBar(color: color, surfaceTintColor: babSurfaceTintColor)
),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, ElevationOverlay.applySurfaceTint(color, babSurfaceTintColor, 3.0));
});
testWidgets('Material3 - BAB surfaceTintColor - BabTheme', (WidgetTester tester) async {
const Color color = Colors.blue; // base color that the surface tint will be applied to
const Color babThemeColor = Colors.black87;
const BottomAppBarTheme theme = BottomAppBarTheme(
surfaceTintColor: babThemeColor
);
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
bottomAppBarTheme: theme,
),
home: const Scaffold(body: BottomAppBar(color: color)),
));
final PhysicalShape widget = _getBabRenderObject(tester);
expect(widget.color, ElevationOverlay.applySurfaceTint(color, babThemeColor, 3.0));
});
});
}
PhysicalShape _getBabRenderObject(WidgetTester tester) {
return tester.widget<PhysicalShape>(
find.descendant(
of: find.byType(BottomAppBar),
matching: find.byType(PhysicalShape),
),
);
}
final Key _painterKey = UniqueKey();
Widget _withTheme(BottomAppBarTheme theme, {required bool useMaterial3}) {
return MaterialApp(
theme: ThemeData(useMaterial3: useMaterial3, bottomAppBarTheme: theme),
home: Scaffold(
floatingActionButton: const FloatingActionButton(onPressed: null),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: RepaintBoundary(
key: _painterKey,
child: const BottomAppBar(
child: Row(
children: <Widget>[
Icon(Icons.add),
Expanded(child: SizedBox()),
Icon(Icons.add),
],
),
),
),
),
);
}
| flutter/packages/flutter/test/material/bottom_app_bar_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/bottom_app_bar_theme_test.dart",
"repo_id": "flutter",
"token_count": 3655
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
RenderBox getMaterialBox(WidgetTester tester) {
return tester.firstRenderObject<RenderBox>(
find.descendant(
of: find.byType(RawChip),
matching: find.byType(CustomPaint),
),
);
}
Material getMaterial(WidgetTester tester) {
return tester.widget<Material>(
find.descendant(
of: find.byType(RawChip),
matching: find.byType(Material),
),
);
}
IconThemeData getIconData(WidgetTester tester) {
final IconTheme iconTheme = tester.firstWidget(
find.descendant(
of: find.byType(RawChip),
matching: find.byType(IconTheme),
),
);
return iconTheme.data;
}
DefaultTextStyle getLabelStyle(WidgetTester tester) {
return tester.widget(
find.descendant(
of: find.byType(RawChip),
matching: find.byType(DefaultTextStyle),
).last,
);
}
void main() {
test('ChipThemeData copyWith, ==, hashCode basics', () {
expect(const ChipThemeData(), const ChipThemeData().copyWith());
expect(const ChipThemeData().hashCode, const ChipThemeData().copyWith().hashCode);
});
test('ChipThemeData lerp special cases', () {
expect(ChipThemeData.lerp(null, null, 0), null);
const ChipThemeData data = ChipThemeData();
expect(identical(ChipThemeData.lerp(data, data, 0.5), data), true);
});
test('ChipThemeData defaults', () {
const ChipThemeData themeData = ChipThemeData();
expect(themeData.color, null);
expect(themeData.backgroundColor, null);
expect(themeData.deleteIconColor, null);
expect(themeData.disabledColor, null);
expect(themeData.selectedColor, null);
expect(themeData.secondarySelectedColor, null);
expect(themeData.shadowColor, null);
expect(themeData.surfaceTintColor, null);
expect(themeData.selectedShadowColor, null);
expect(themeData.showCheckmark, null);
expect(themeData.checkmarkColor, null);
expect(themeData.labelPadding, null);
expect(themeData.padding, null);
expect(themeData.side, null);
expect(themeData.shape, null);
expect(themeData.labelStyle, null);
expect(themeData.secondaryLabelStyle, null);
expect(themeData.brightness, null);
expect(themeData.elevation, null);
expect(themeData.pressElevation, null);
expect(themeData.iconTheme, null);
expect(themeData.avatarBoxConstraints, null);
expect(themeData.deleteIconBoxConstraints, null);
});
testWidgets('Default ChipThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const ChipThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('ChipThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const ChipThemeData(
color: MaterialStatePropertyAll<Color>(Color(0xfffffff0)),
backgroundColor: Color(0xfffffff1),
deleteIconColor: Color(0xfffffff2),
disabledColor: Color(0xfffffff3),
selectedColor: Color(0xfffffff4),
secondarySelectedColor: Color(0xfffffff5),
shadowColor: Color(0xfffffff6),
surfaceTintColor: Color(0xfffffff7),
selectedShadowColor: Color(0xfffffff8),
showCheckmark: true,
checkmarkColor: Color(0xfffffff9),
labelPadding: EdgeInsets.all(1),
padding: EdgeInsets.all(2),
side: BorderSide(width: 10),
shape: RoundedRectangleBorder(),
labelStyle: TextStyle(fontSize: 10),
secondaryLabelStyle: TextStyle(fontSize: 20),
brightness: Brightness.dark,
elevation: 5,
pressElevation: 6,
iconTheme: IconThemeData(color: Color(0xffffff10)),
avatarBoxConstraints: BoxConstraints.tightForFinite(),
deleteIconBoxConstraints: BoxConstraints.tightForFinite(),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, equalsIgnoringHashCodes(<String>[
'color: WidgetStatePropertyAll(Color(0xfffffff0))',
'backgroundColor: Color(0xfffffff1)',
'deleteIconColor: Color(0xfffffff2)',
'disabledColor: Color(0xfffffff3)',
'selectedColor: Color(0xfffffff4)',
'secondarySelectedColor: Color(0xfffffff5)',
'shadowColor: Color(0xfffffff6)',
'surfaceTintColor: Color(0xfffffff7)',
'selectedShadowColor: Color(0xfffffff8)',
'showCheckmark: true',
'checkMarkColor: Color(0xfffffff9)',
'labelPadding: EdgeInsets.all(1.0)',
'padding: EdgeInsets.all(2.0)',
'side: BorderSide(width: 10.0)',
'shape: RoundedRectangleBorder(BorderSide(width: 0.0, style: none), BorderRadius.zero)',
'labelStyle: TextStyle(inherit: true, size: 10.0)',
'secondaryLabelStyle: TextStyle(inherit: true, size: 20.0)',
'brightness: dark',
'elevation: 5.0',
'pressElevation: 6.0',
'iconTheme: IconThemeData#00000(color: Color(0xffffff10))',
'avatarBoxConstraints: BoxConstraints(unconstrained)',
'deleteIconBoxConstraints: BoxConstraints(unconstrained)',
]));
});
testWidgets('Material3 - Chip uses ThemeData chip theme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
backgroundColor: Color(0xff112233),
elevation: 4,
padding: EdgeInsets.all(50),
labelPadding: EdgeInsets.all(25),
shape: RoundedRectangleBorder(),
labelStyle: TextStyle(fontSize: 32),
iconTheme: IconThemeData(color: Color(0xff332211)),
);
final ThemeData theme = ThemeData(chipTheme: chipTheme);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(chipTheme: chipTheme),
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..rect(color: chipTheme.backgroundColor));
expect(getMaterial(tester).elevation, chipTheme.elevation);
expect(tester.getSize(find.byType(RawChip)), const Size(402, 252)); // label + padding + labelPadding
expect(
getMaterial(tester).shape,
chipTheme.shape?.copyWith(side: BorderSide(color: theme.colorScheme.outline)),
);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, chipTheme.iconTheme!.color);
});
testWidgets('Material2 - Chip uses ThemeData chip theme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
backgroundColor: Color(0xff112233),
elevation: 4,
padding: EdgeInsets.all(50),
labelPadding: EdgeInsets.all(25),
shape: RoundedRectangleBorder(),
labelStyle: TextStyle(fontSize: 32),
iconTheme: IconThemeData(color: Color(0xff332211)),
);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(chipTheme: chipTheme, useMaterial3: false),
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..rect(color: chipTheme.backgroundColor));
expect(getMaterial(tester).elevation, chipTheme.elevation);
expect(tester.getSize(find.byType(RawChip)), const Size(400, 250)); // label + padding + labelPadding
expect(getMaterial(tester).shape, chipTheme.shape);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, chipTheme.iconTheme!.color);
});
testWidgets('Material3 - Chip uses local ChipTheme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
backgroundColor: Color(0xff112233),
elevation: 4,
padding: EdgeInsets.all(50),
labelPadding: EdgeInsets.all(25),
labelStyle: TextStyle(fontSize: 32),
shape: RoundedRectangleBorder(),
iconTheme: IconThemeData(color: Color(0xff332211)),
);
final ThemeData theme = ThemeData(chipTheme: const ChipThemeData());
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: ChipTheme(
data: chipTheme,
child: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
);
},
),
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..rect(color: chipTheme.backgroundColor));
expect(tester.getSize(find.byType(RawChip)), const Size(402, 252)); // label + padding + labelPadding
expect(getMaterial(tester).elevation, chipTheme.elevation);
expect(
getMaterial(tester).shape,
chipTheme.shape?.copyWith(side: BorderSide(color: theme.colorScheme.outline)),
);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, chipTheme.iconTheme!.color);
});
testWidgets('Material2 - Chip uses local ChipTheme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
backgroundColor: Color(0xff112233),
elevation: 4,
padding: EdgeInsets.all(50),
labelPadding: EdgeInsets.all(25),
labelStyle: TextStyle(fontSize: 32),
shape: RoundedRectangleBorder(),
iconTheme: IconThemeData(color: Color(0xff332211)),
);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(chipTheme: const ChipThemeData(), useMaterial3: false),
home: ChipTheme(
data: chipTheme,
child: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
);
},
),
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..rect(color: chipTheme.backgroundColor));
expect(tester.getSize(find.byType(RawChip)), const Size(400, 250)); // label + padding + labelPadding
expect(getMaterial(tester).elevation, chipTheme.elevation);
expect(getMaterial(tester).shape, chipTheme.shape);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, chipTheme.iconTheme!.color);
});
testWidgets('Chip properties overrides ChipTheme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
backgroundColor: Color(0xff112233),
elevation: 4,
padding: EdgeInsets.all(50),
labelPadding: EdgeInsets.all(25),
labelStyle: TextStyle(fontSize: 32),
shape: RoundedRectangleBorder(),
iconTheme: IconThemeData(color: Color(0xff332211)),
);
const Color backgroundColor = Color(0xff000000);
const double elevation = 6.0;
const EdgeInsets padding = EdgeInsets.all(10);
const EdgeInsets labelPadding = EdgeInsets.all(5);
const TextStyle labelStyle = TextStyle(fontSize: 20);
const RoundedRectangleBorder shape = RoundedRectangleBorder(side: BorderSide(color: Color(0xff0000ff)));
const IconThemeData iconTheme = IconThemeData(color: Color(0xff00ff00));
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(chipTheme: chipTheme),
home: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
backgroundColor: backgroundColor,
elevation: elevation,
padding: padding,
labelPadding: labelPadding,
labelStyle: labelStyle,
shape: shape,
iconTheme: iconTheme,
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
);
},
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..rect(color: backgroundColor));
expect(tester.getSize(find.byType(RawChip)), const Size(242, 132)); // label + padding + labelPadding
expect(getMaterial(tester).elevation, elevation);
expect(getMaterial(tester).shape, shape);
expect(getLabelStyle(tester).style.fontSize, labelStyle.fontSize);
expect(getIconData(tester).color, iconTheme.color);
});
testWidgets('Material3 - Chip uses constructor parameters', (WidgetTester tester) async {
const Color backgroundColor = Color(0xff332211);
const double elevation = 3;
const double fontSize = 32;
const OutlinedBorder shape = CircleBorder(side: BorderSide(color: Color(0xff0000ff)));
const IconThemeData iconTheme = IconThemeData(color: Color(0xff443322));
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
backgroundColor: backgroundColor,
elevation: elevation,
padding: const EdgeInsets.all(50),
labelPadding:const EdgeInsets.all(25),
labelStyle: const TextStyle(fontSize: fontSize),
shape: shape,
iconTheme: iconTheme,
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
);
},
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..circle(color: backgroundColor));
expect(tester.getSize(find.byType(RawChip)), const Size(402, 252)); // label + padding + labelPadding
expect(getMaterial(tester).elevation, elevation);
expect(getMaterial(tester).shape, shape);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, iconTheme.color);
});
testWidgets('Material2 - Chip uses constructor parameters', (WidgetTester tester) async {
const Color backgroundColor = Color(0xff332211);
const double elevation = 3;
const double fontSize = 32;
const OutlinedBorder shape = CircleBorder();
const IconThemeData iconTheme = IconThemeData(color: Color(0xff443322));
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
backgroundColor: backgroundColor,
elevation: elevation,
padding: const EdgeInsets.all(50),
labelPadding:const EdgeInsets.all(25),
labelStyle: const TextStyle(fontSize: fontSize),
shape: shape,
iconTheme: iconTheme,
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
);
},
),
),
);
final RenderBox materialBox = getMaterialBox(tester);
expect(materialBox, paints..circle(color: backgroundColor));
expect(tester.getSize(find.byType(RawChip)), const Size(400, 250)); // label + padding + labelPadding
expect(getMaterial(tester).elevation, elevation);
expect(getMaterial(tester).shape, shape);
expect(getLabelStyle(tester).style.fontSize, 32);
expect(getIconData(tester).color, iconTheme.color);
});
testWidgets('ChipTheme.fromDefaults', (WidgetTester tester) async {
const TextStyle labelStyle = TextStyle();
ChipThemeData chipTheme = ChipThemeData.fromDefaults(
brightness: Brightness.light,
secondaryColor: Colors.red,
labelStyle: labelStyle,
);
expect(chipTheme.backgroundColor, Colors.black.withAlpha(0x1f));
expect(chipTheme.deleteIconColor, Colors.black.withAlpha(0xde));
expect(chipTheme.disabledColor, Colors.black.withAlpha(0x0c));
expect(chipTheme.selectedColor, Colors.black.withAlpha(0x3d));
expect(chipTheme.secondarySelectedColor, Colors.red.withAlpha(0x3d));
expect(chipTheme.shadowColor, Colors.black);
expect(chipTheme.surfaceTintColor, null);
expect(chipTheme.selectedShadowColor, Colors.black);
expect(chipTheme.showCheckmark, true);
expect(chipTheme.checkmarkColor, null);
expect(chipTheme.labelPadding, null);
expect(chipTheme.padding, const EdgeInsets.all(4.0));
expect(chipTheme.side, null);
expect(chipTheme.shape, null);
expect(chipTheme.labelStyle, labelStyle.copyWith(color: Colors.black.withAlpha(0xde)));
expect(chipTheme.secondaryLabelStyle, labelStyle.copyWith(color: Colors.red.withAlpha(0xde)));
expect(chipTheme.brightness, Brightness.light);
expect(chipTheme.elevation, 0.0);
expect(chipTheme.pressElevation, 8.0);
chipTheme = ChipThemeData.fromDefaults(
brightness: Brightness.dark,
secondaryColor: Colors.tealAccent[200]!,
labelStyle: const TextStyle(),
);
expect(chipTheme.backgroundColor, Colors.white.withAlpha(0x1f));
expect(chipTheme.deleteIconColor, Colors.white.withAlpha(0xde));
expect(chipTheme.disabledColor, Colors.white.withAlpha(0x0c));
expect(chipTheme.selectedColor, Colors.white.withAlpha(0x3d));
expect(chipTheme.secondarySelectedColor, Colors.tealAccent[200]!.withAlpha(0x3d));
expect(chipTheme.shadowColor, Colors.black);
expect(chipTheme.selectedShadowColor, Colors.black);
expect(chipTheme.showCheckmark, true);
expect(chipTheme.checkmarkColor, null);
expect(chipTheme.labelPadding, null);
expect(chipTheme.padding, const EdgeInsets.all(4.0));
expect(chipTheme.side, null);
expect(chipTheme.shape, null);
expect(chipTheme.labelStyle, labelStyle.copyWith(color: Colors.white.withAlpha(0xde)));
expect(chipTheme.secondaryLabelStyle, labelStyle.copyWith(color: Colors.tealAccent[200]!.withAlpha(0xde)));
expect(chipTheme.brightness, Brightness.dark);
expect(chipTheme.elevation, 0.0);
expect(chipTheme.pressElevation, 8.0);
});
testWidgets('ChipThemeData generates correct opacities for defaults', (WidgetTester tester) async {
const Color customColor1 = Color(0xcafefeed);
const Color customColor2 = Color(0xdeadbeef);
final TextStyle customStyle = ThemeData.fallback().textTheme.bodyLarge!.copyWith(color: customColor2);
final ChipThemeData lightTheme = ChipThemeData.fromDefaults(
secondaryColor: customColor1,
brightness: Brightness.light,
labelStyle: customStyle,
);
expect(lightTheme.backgroundColor, equals(Colors.black.withAlpha(0x1f)));
expect(lightTheme.deleteIconColor, equals(Colors.black.withAlpha(0xde)));
expect(lightTheme.disabledColor, equals(Colors.black.withAlpha(0x0c)));
expect(lightTheme.selectedColor, equals(Colors.black.withAlpha(0x3d)));
expect(lightTheme.secondarySelectedColor, equals(customColor1.withAlpha(0x3d)));
expect(lightTheme.labelPadding, isNull);
expect(lightTheme.padding, equals(const EdgeInsets.all(4.0)));
expect(lightTheme.side, isNull);
expect(lightTheme.shape, isNull);
expect(lightTheme.labelStyle?.color, equals(Colors.black.withAlpha(0xde)));
expect(lightTheme.secondaryLabelStyle?.color, equals(customColor1.withAlpha(0xde)));
expect(lightTheme.brightness, equals(Brightness.light));
final ChipThemeData darkTheme = ChipThemeData.fromDefaults(
secondaryColor: customColor1,
brightness: Brightness.dark,
labelStyle: customStyle,
);
expect(darkTheme.backgroundColor, equals(Colors.white.withAlpha(0x1f)));
expect(darkTheme.deleteIconColor, equals(Colors.white.withAlpha(0xde)));
expect(darkTheme.disabledColor, equals(Colors.white.withAlpha(0x0c)));
expect(darkTheme.selectedColor, equals(Colors.white.withAlpha(0x3d)));
expect(darkTheme.secondarySelectedColor, equals(customColor1.withAlpha(0x3d)));
expect(darkTheme.labelPadding, isNull);
expect(darkTheme.padding, equals(const EdgeInsets.all(4.0)));
expect(darkTheme.side, isNull);
expect(darkTheme.shape, isNull);
expect(darkTheme.labelStyle?.color, equals(Colors.white.withAlpha(0xde)));
expect(darkTheme.secondaryLabelStyle?.color, equals(customColor1.withAlpha(0xde)));
expect(darkTheme.brightness, equals(Brightness.dark));
final ChipThemeData customTheme = ChipThemeData.fromDefaults(
primaryColor: customColor1,
secondaryColor: customColor2,
labelStyle: customStyle,
);
//expect(customTheme.backgroundColor, equals(customColor1.withAlpha(0x1f)));
expect(customTheme.deleteIconColor, equals(customColor1.withAlpha(0xde)));
expect(customTheme.disabledColor, equals(customColor1.withAlpha(0x0c)));
expect(customTheme.selectedColor, equals(customColor1.withAlpha(0x3d)));
expect(customTheme.secondarySelectedColor, equals(customColor2.withAlpha(0x3d)));
expect(customTheme.labelPadding, isNull);
expect(customTheme.padding, equals(const EdgeInsets.all(4.0)));
expect(customTheme.side, isNull);
expect(customTheme.shape, isNull);
expect(customTheme.labelStyle?.color, equals(customColor1.withAlpha(0xde)));
expect(customTheme.secondaryLabelStyle?.color, equals(customColor2.withAlpha(0xde)));
expect(customTheme.brightness, equals(Brightness.light));
});
testWidgets('ChipThemeData lerps correctly', (WidgetTester tester) async {
final ChipThemeData chipThemeBlack = ChipThemeData.fromDefaults(
secondaryColor: Colors.black,
brightness: Brightness.dark,
labelStyle: ThemeData.fallback().textTheme.bodyLarge!.copyWith(color: Colors.black),
).copyWith(
elevation: 1.0,
labelPadding: const EdgeInsets.symmetric(horizontal: 8.0),
shape: const StadiumBorder(),
side: const BorderSide(),
pressElevation: 4.0,
shadowColor: Colors.black,
surfaceTintColor: Colors.black,
selectedShadowColor: Colors.black,
showCheckmark: false,
checkmarkColor: Colors.black,
);
final ChipThemeData chipThemeWhite = ChipThemeData.fromDefaults(
secondaryColor: Colors.white,
brightness: Brightness.light,
labelStyle: ThemeData.fallback().textTheme.bodyLarge!.copyWith(color: Colors.white),
).copyWith(
padding: const EdgeInsets.all(2.0),
labelPadding: const EdgeInsets.only(top: 8.0, bottom: 8.0),
shape: const BeveledRectangleBorder(),
side: const BorderSide(color: Colors.white),
elevation: 5.0,
pressElevation: 10.0,
shadowColor: Colors.white,
surfaceTintColor: Colors.white,
selectedShadowColor: Colors.white,
showCheckmark: true,
checkmarkColor: Colors.white,
);
final ChipThemeData lerp = ChipThemeData.lerp(chipThemeBlack, chipThemeWhite, 0.5)!;
const Color middleGrey = Color(0xff7f7f7f);
expect(lerp.backgroundColor, equals(middleGrey.withAlpha(0x1f)));
expect(lerp.deleteIconColor, equals(middleGrey.withAlpha(0xde)));
expect(lerp.disabledColor, equals(middleGrey.withAlpha(0x0c)));
expect(lerp.selectedColor, equals(middleGrey.withAlpha(0x3d)));
expect(lerp.secondarySelectedColor, equals(middleGrey.withAlpha(0x3d)));
expect(lerp.shadowColor, equals(middleGrey));
expect(lerp.surfaceTintColor, equals(middleGrey));
expect(lerp.selectedShadowColor, equals(middleGrey));
expect(lerp.showCheckmark, equals(true));
expect(lerp.labelPadding, equals(const EdgeInsets.all(4.0)));
expect(lerp.padding, equals(const EdgeInsets.all(3.0)));
expect(lerp.side!.color, equals(middleGrey));
expect(lerp.shape, isA<BeveledRectangleBorder>());
expect(lerp.labelStyle?.color, equals(middleGrey.withAlpha(0xde)));
expect(lerp.secondaryLabelStyle?.color, equals(middleGrey.withAlpha(0xde)));
expect(lerp.brightness, equals(Brightness.light));
expect(lerp.elevation, 3.0);
expect(lerp.pressElevation, 7.0);
expect(lerp.checkmarkColor, equals(middleGrey));
expect(lerp.iconTheme, isNull);
expect(ChipThemeData.lerp(null, null, 0.25), isNull);
final ChipThemeData lerpANull25 = ChipThemeData.lerp(null, chipThemeWhite, 0.25)!;
expect(lerpANull25.backgroundColor, equals(Colors.black.withAlpha(0x08)));
expect(lerpANull25.deleteIconColor, equals(Colors.black.withAlpha(0x38)));
expect(lerpANull25.disabledColor, equals(Colors.black.withAlpha(0x03)));
expect(lerpANull25.selectedColor, equals(Colors.black.withAlpha(0x0f)));
expect(lerpANull25.secondarySelectedColor, equals(Colors.white.withAlpha(0x0f)));
expect(lerpANull25.shadowColor, equals(Colors.white.withAlpha(0x40)));
expect(lerpANull25.surfaceTintColor, equals(Colors.white.withAlpha(0x40)));
expect(lerpANull25.selectedShadowColor, equals(Colors.white.withAlpha(0x40)));
expect(lerpANull25.showCheckmark, equals(true));
expect(lerpANull25.labelPadding, equals(const EdgeInsets.only(top: 2.0, bottom: 2.0)));
expect(lerpANull25.padding, equals(const EdgeInsets.all(0.5)));
expect(lerpANull25.side!.color, equals(Colors.white.withAlpha(0x3f)));
expect(lerpANull25.shape, isA<BeveledRectangleBorder>());
expect(lerpANull25.labelStyle?.color, equals(Colors.black.withAlpha(0x38)));
expect(lerpANull25.secondaryLabelStyle?.color, equals(Colors.white.withAlpha(0x38)));
expect(lerpANull25.brightness, equals(Brightness.light));
expect(lerpANull25.elevation, 1.25);
expect(lerpANull25.pressElevation, 2.5);
expect(lerpANull25.checkmarkColor, equals(Colors.white.withAlpha(0x40)));
expect(lerp.iconTheme, isNull);
final ChipThemeData lerpANull75 = ChipThemeData.lerp(null, chipThemeWhite, 0.75)!;
expect(lerpANull75.backgroundColor, equals(Colors.black.withAlpha(0x17)));
expect(lerpANull75.deleteIconColor, equals(Colors.black.withAlpha(0xa7)));
expect(lerpANull75.disabledColor, equals(Colors.black.withAlpha(0x09)));
expect(lerpANull75.selectedColor, equals(Colors.black.withAlpha(0x2e)));
expect(lerpANull75.secondarySelectedColor, equals(Colors.white.withAlpha(0x2e)));
expect(lerpANull75.shadowColor, equals(Colors.white.withAlpha(0xbf)));
expect(lerpANull75.surfaceTintColor, equals(Colors.white.withAlpha(0xbf)));
expect(lerpANull75.selectedShadowColor, equals(Colors.white.withAlpha(0xbf)));
expect(lerpANull75.showCheckmark, equals(true));
expect(lerpANull75.labelPadding, equals(const EdgeInsets.only(top: 6.0, bottom: 6.0)));
expect(lerpANull75.padding, equals(const EdgeInsets.all(1.5)));
expect(lerpANull75.side!.color, equals(Colors.white.withAlpha(0xbf)));
expect(lerpANull75.shape, isA<BeveledRectangleBorder>());
expect(lerpANull75.labelStyle?.color, equals(Colors.black.withAlpha(0xa7)));
expect(lerpANull75.secondaryLabelStyle?.color, equals(Colors.white.withAlpha(0xa7)));
expect(lerpANull75.brightness, equals(Brightness.light));
expect(lerpANull75.elevation, 3.75);
expect(lerpANull75.pressElevation, 7.5);
expect(lerpANull75.checkmarkColor, equals(Colors.white.withAlpha(0xbf)));
final ChipThemeData lerpBNull25 = ChipThemeData.lerp(chipThemeBlack, null, 0.25)!;
expect(lerpBNull25.backgroundColor, equals(Colors.white.withAlpha(0x17)));
expect(lerpBNull25.deleteIconColor, equals(Colors.white.withAlpha(0xa7)));
expect(lerpBNull25.disabledColor, equals(Colors.white.withAlpha(0x09)));
expect(lerpBNull25.selectedColor, equals(Colors.white.withAlpha(0x2e)));
expect(lerpBNull25.secondarySelectedColor, equals(Colors.black.withAlpha(0x2e)));
expect(lerpBNull25.shadowColor, equals(Colors.black.withAlpha(0xbf)));
expect(lerpBNull25.surfaceTintColor, equals(Colors.black.withAlpha(0xbf)));
expect(lerpBNull25.selectedShadowColor, equals(Colors.black.withAlpha(0xbf)));
expect(lerpBNull25.showCheckmark, equals(false));
expect(lerpBNull25.labelPadding, equals(const EdgeInsets.only(left: 6.0, right: 6.0)));
expect(lerpBNull25.padding, equals(const EdgeInsets.all(3.0)));
expect(lerpBNull25.side!.color, equals(Colors.black.withAlpha(0x3f)));
expect(lerpBNull25.shape, isA<StadiumBorder>());
expect(lerpBNull25.labelStyle?.color, equals(Colors.white.withAlpha(0xa7)));
expect(lerpBNull25.secondaryLabelStyle?.color, equals(Colors.black.withAlpha(0xa7)));
expect(lerpBNull25.brightness, equals(Brightness.dark));
expect(lerpBNull25.elevation, 0.75);
expect(lerpBNull25.pressElevation, 3.0);
expect(lerpBNull25.checkmarkColor, equals(Colors.black.withAlpha(0xbf)));
expect(lerp.iconTheme, isNull);
final ChipThemeData lerpBNull75 = ChipThemeData.lerp(chipThemeBlack, null, 0.75)!;
expect(lerpBNull75.backgroundColor, equals(Colors.white.withAlpha(0x08)));
expect(lerpBNull75.deleteIconColor, equals(Colors.white.withAlpha(0x38)));
expect(lerpBNull75.disabledColor, equals(Colors.white.withAlpha(0x03)));
expect(lerpBNull75.selectedColor, equals(Colors.white.withAlpha(0x0f)));
expect(lerpBNull75.secondarySelectedColor, equals(Colors.black.withAlpha(0x0f)));
expect(lerpBNull75.shadowColor, equals(Colors.black.withAlpha(0x40)));
expect(lerpBNull75.surfaceTintColor, equals(Colors.black.withAlpha(0x40)));
expect(lerpBNull75.selectedShadowColor, equals(Colors.black.withAlpha(0x40)));
expect(lerpBNull75.showCheckmark, equals(true));
expect(lerpBNull75.labelPadding, equals(const EdgeInsets.only(left: 2.0, right: 2.0)));
expect(lerpBNull75.padding, equals(const EdgeInsets.all(1.0)));
expect(lerpBNull75.side!.color, equals(Colors.black.withAlpha(0xbf)));
expect(lerpBNull75.shape, isA<StadiumBorder>());
expect(lerpBNull75.labelStyle?.color, equals(Colors.white.withAlpha(0x38)));
expect(lerpBNull75.secondaryLabelStyle?.color, equals(Colors.black.withAlpha(0x38)));
expect(lerpBNull75.brightness, equals(Brightness.light));
expect(lerpBNull75.elevation, 0.25);
expect(lerpBNull75.pressElevation, 1.0);
expect(lerpBNull75.checkmarkColor, equals(Colors.black.withAlpha(0x40)));
expect(lerp.iconTheme, isNull);
});
testWidgets('Chip uses stateful color from chip theme', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
const Color pressedColor = Color(0x00000001);
const Color hoverColor = Color(0x00000002);
const Color focusedColor = Color(0x00000003);
const Color defaultColor = Color(0x00000004);
const Color selectedColor = Color(0x00000005);
const Color disabledColor = Color(0x00000006);
Color getTextColor(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledColor;
}
if (states.contains(MaterialState.pressed)) {
return pressedColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverColor;
}
if (states.contains(MaterialState.focused)) {
return focusedColor;
}
if (states.contains(MaterialState.selected)) {
return selectedColor;
}
return defaultColor;
}
final TextStyle labelStyle = TextStyle(
color: MaterialStateColor.resolveWith(getTextColor),
);
Widget chipWidget({ bool enabled = true, bool selected = false }) {
return MaterialApp(
theme: ThemeData(
chipTheme: ThemeData().chipTheme.copyWith(
labelStyle: labelStyle,
secondaryLabelStyle: labelStyle,
),
),
home: Scaffold(
body: Focus(
focusNode: focusNode,
child: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: enabled ? (_) {} : null,
),
),
),
);
}
Color textColor() {
return tester.renderObject<RenderParagraph>(find.text('Chip')).text.style!.color!;
}
// Default, not disabled.
await tester.pumpWidget(chipWidget());
expect(textColor(), equals(defaultColor));
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(textColor(), selectedColor);
// Focused.
final FocusNode chipFocusNode = focusNode.children.first;
chipFocusNode.requestFocus();
await tester.pumpAndSettle();
expect(textColor(), focusedColor);
// Hovered.
final Offset center = tester.getCenter(find.byType(ChoiceChip));
final TestGesture gesture = await tester.createGesture(
kind: PointerDeviceKind.mouse,
);
await gesture.addPointer();
await gesture.moveTo(center);
await tester.pumpAndSettle();
expect(textColor(), hoverColor);
// Pressed.
await gesture.down(center);
await tester.pumpAndSettle();
expect(textColor(), pressedColor);
// Disabled.
await tester.pumpWidget(chipWidget(enabled: false));
await tester.pumpAndSettle();
expect(textColor(), disabledColor);
focusNode.dispose();
});
testWidgets('Material2 - Chip uses stateful border side from resolveWith pattern', (WidgetTester tester) async {
const Color selectedColor = Color(0x00000001);
const Color defaultColor = Color(0x00000002);
BorderSide getBorderSide(Set<MaterialState> states) {
Color color = defaultColor;
if (states.contains(MaterialState.selected)) {
color = selectedColor;
}
return BorderSide(color: color);
}
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(
useMaterial3: false,
chipTheme: ThemeData.light().chipTheme.copyWith(
side: MaterialStateBorderSide.resolveWith(getBorderSide),
),
),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(find.byType(RawChip), paints..rrect()..rrect(color: defaultColor));
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(find.byType(RawChip), paints..rrect()..rrect(color: selectedColor));
});
testWidgets('Material3 - Chip uses stateful border side from resolveWith pattern', (WidgetTester tester) async {
const Color selectedColor = Color(0x00000001);
const Color defaultColor = Color(0x00000002);
BorderSide getBorderSide(Set<MaterialState> states) {
Color color = defaultColor;
if (states.contains(MaterialState.selected)) {
color = selectedColor;
}
return BorderSide(color: color);
}
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(
chipTheme: ChipThemeData(
side: MaterialStateBorderSide.resolveWith(getBorderSide),
),
),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(find.byType(RawChip), paints..drrect(color: defaultColor));
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(find.byType(RawChip), paints..drrect(color: selectedColor));
});
testWidgets('Material2 - Chip uses stateful border side from chip theme', (WidgetTester tester) async {
const Color selectedColor = Color(0x00000001);
const Color defaultColor = Color(0x00000002);
BorderSide getBorderSide(Set<MaterialState> states) {
Color color = defaultColor;
if (states.contains(MaterialState.selected)) {
color = selectedColor;
}
return BorderSide(color: color);
}
final ChipThemeData chipTheme = ChipThemeData.fromDefaults(
brightness: Brightness.light,
secondaryColor: Colors.blue,
labelStyle: const TextStyle(),
).copyWith(
side: _MaterialStateBorderSide(getBorderSide),
);
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(useMaterial3: false, chipTheme: chipTheme),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(find.byType(RawChip), paints..rrect()..rrect(color: defaultColor));
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(find.byType(RawChip), paints..rrect()..rrect(color: selectedColor));
});
testWidgets('Material3 - Chip uses stateful border side from chip theme', (WidgetTester tester) async {
const Color selectedColor = Color(0x00000001);
const Color defaultColor = Color(0x00000002);
BorderSide getBorderSide(Set<MaterialState> states) {
Color color = defaultColor;
if (states.contains(MaterialState.selected)) {
color = selectedColor;
}
return BorderSide(color: color);
}
final ChipThemeData chipTheme = ChipThemeData(
side: _MaterialStateBorderSide(getBorderSide),
);
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(chipTheme: chipTheme),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(find.byType(RawChip), paints..drrect(color: defaultColor));
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(find.byType(RawChip), paints..drrect(color: selectedColor));
});
testWidgets('Material2 - Chip uses stateful shape from chip theme', (WidgetTester tester) async {
OutlinedBorder? getShape(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const RoundedRectangleBorder();
}
return null;
}
final ChipThemeData chipTheme = ChipThemeData.fromDefaults(
brightness: Brightness.light,
secondaryColor: Colors.blue,
labelStyle: const TextStyle(),
).copyWith(
shape: _MaterialStateOutlinedBorder(getShape),
);
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(useMaterial3: false, chipTheme: chipTheme),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(getMaterial(tester).shape, isA<StadiumBorder>());
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(getMaterial(tester).shape, isA<RoundedRectangleBorder>());
});
testWidgets('Material3 - Chip uses stateful shape from chip theme', (WidgetTester tester) async {
OutlinedBorder? getShape(Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const StadiumBorder();
}
return null;
}
final ChipThemeData chipTheme = ChipThemeData(
shape: _MaterialStateOutlinedBorder(getShape),
);
Widget chipWidget({ bool selected = false }) {
return MaterialApp(
theme: ThemeData(chipTheme: chipTheme),
home: Scaffold(
body: ChoiceChip(
label: const Text('Chip'),
selected: selected,
onSelected: (_) {},
),
),
);
}
// Default.
await tester.pumpWidget(chipWidget());
expect(getMaterial(tester).shape, isA<RoundedRectangleBorder>());
// Selected.
await tester.pumpWidget(chipWidget(selected: true));
expect(getMaterial(tester).shape, isA<StadiumBorder>());
});
testWidgets('RawChip uses material state color from ChipTheme', (WidgetTester tester) async {
const Color disabledSelectedColor = Color(0xffffff00);
const Color disabledColor = Color(0xff00ff00);
const Color backgroundColor = Color(0xff0000ff);
const Color selectedColor = Color(0xffff0000);
Widget buildApp({ required bool enabled, required bool selected }) {
return MaterialApp(
theme: ThemeData(
chipTheme: ChipThemeData(
color: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)
&& states.contains(MaterialState.selected)) {
return disabledSelectedColor;
}
if (states.contains(MaterialState.disabled)) {
return disabledColor;
}
if (states.contains(MaterialState.selected)) {
return selectedColor;
}
return backgroundColor;
}),
),
),
home: Material(
child: RawChip(
isEnabled: enabled,
selected: selected,
label: const Text('RawChip'),
),
),
);
}
// Check theme color for enabled chip.
await tester.pumpWidget(buildApp(enabled: true, selected: false));
await tester.pumpAndSettle();
// Enabled chip should have the provided backgroundColor.
expect(getMaterialBox(tester), paints..rrect(color: backgroundColor));
// Check theme color for disabled chip.
await tester.pumpWidget(buildApp(enabled: false, selected: false));
await tester.pumpAndSettle();
// Disabled chip should have the provided disabledColor.
expect(getMaterialBox(tester),paints..rrect(color: disabledColor));
// Check theme color for enabled and selected chip.
await tester.pumpWidget(buildApp(enabled: true, selected: true));
await tester.pumpAndSettle();
// Enabled & selected chip should have the provided selectedColor.
expect(getMaterialBox(tester), paints..rrect(color: selectedColor));
// Check theme color for disabled & selected chip.
await tester.pumpWidget(buildApp(enabled: false, selected: true));
await tester.pumpAndSettle();
// Disabled & selected chip should have the provided disabledSelectedColor.
expect(getMaterialBox(tester), paints..rrect(color: disabledSelectedColor));
});
testWidgets('RawChip uses state colors from ChipTheme', (WidgetTester tester) async {
const ChipThemeData chipTheme = ChipThemeData(
disabledColor: Color(0xadfefafe),
backgroundColor: Color(0xcafefeed),
selectedColor: Color(0xbeefcafe),
);
Widget buildApp({ required bool enabled, required bool selected }) {
return MaterialApp(
theme: ThemeData(chipTheme: chipTheme),
home: Material(
child: RawChip(
isEnabled: enabled,
selected: selected,
label: const Text('RawChip'),
),
),
);
}
// Check theme color for enabled chip.
await tester.pumpWidget(buildApp(enabled: true, selected: false));
await tester.pumpAndSettle();
// Enabled chip should have the provided backgroundColor.
expect(getMaterialBox(tester), paints..rrect(color: chipTheme.backgroundColor));
// Check theme color for disabled chip.
await tester.pumpWidget(buildApp(enabled: false, selected: false));
await tester.pumpAndSettle();
// Disabled chip should have the provided disabledColor.
expect(getMaterialBox(tester),paints..rrect(color: chipTheme.disabledColor));
// Check theme color for enabled and selected chip.
await tester.pumpWidget(buildApp(enabled: true, selected: true));
await tester.pumpAndSettle();
// Enabled & selected chip should have the provided selectedColor.
expect(getMaterialBox(tester), paints..rrect(color: chipTheme.selectedColor));
});
// This is a regression test for https://github.com/flutter/flutter/issues/119163.
testWidgets('RawChip respects checkmark properties from ChipTheme', (WidgetTester tester) async {
Widget buildRawChip({ChipThemeData? chipTheme}) {
return MaterialApp(
theme: ThemeData(
chipTheme: chipTheme,
),
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
selected: true,
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
),
);
}
// Test that the checkmark is painted.
await tester.pumpWidget(buildRawChip(
chipTheme: const ChipThemeData(
checkmarkColor: Color(0xffff0000),
),
));
RenderBox materialBox = getMaterialBox(tester);
expect(
materialBox,
paints..path(
color: const Color(0xffff0000),
style: PaintingStyle.stroke,
),
);
// Test that the checkmark is not painted when ChipThemeData.showCheckmark is false.
await tester.pumpWidget(buildRawChip(
chipTheme: const ChipThemeData(
showCheckmark: false,
checkmarkColor: Color(0xffff0000),
),
));
await tester.pumpAndSettle();
materialBox = getMaterialBox(tester);
expect(
materialBox,
isNot(paints..path(
color: const Color(0xffff0000),
style: PaintingStyle.stroke,
)),
);
});
testWidgets("Material3 - RawChip.shape's side is used when provided", (WidgetTester tester) async {
Widget buildChip({ OutlinedBorder? shape, BorderSide? side }) {
return MaterialApp(
theme: ThemeData(
chipTheme: ChipThemeData(
shape: shape,
side: side,
),
),
home: const Material(
child: Center(
child: RawChip(
label: Text('RawChip'),
),
),
),
);
}
// Test [RawChip.shape] with a side.
await tester.pumpWidget(buildChip(
shape: const RoundedRectangleBorder(
side: BorderSide(color: Color(0xffff00ff)),
borderRadius: BorderRadius.all(Radius.circular(7.0)),
)),
);
// Chip should have the provided shape and the side from [RawChip.shape].
expect(
getMaterial(tester).shape,
const RoundedRectangleBorder(
side: BorderSide(color: Color(0xffff00ff)),
borderRadius: BorderRadius.all(Radius.circular(7.0)),
),
);
// Test [RawChip.shape] with a side and [RawChip.side].
await tester.pumpWidget(buildChip(
shape: const RoundedRectangleBorder(
side: BorderSide(color: Color(0xffff00ff)),
borderRadius: BorderRadius.all(Radius.circular(7.0)),
),
side: const BorderSide(color: Color(0xfffff000))),
);
await tester.pumpAndSettle();
// Chip use shape from [RawChip.shape] and the side from [RawChip.side].
// [RawChip.shape]'s side should be ignored.
expect(
getMaterial(tester).shape,
const RoundedRectangleBorder(
side: BorderSide(color: Color(0xfffff000)),
borderRadius: BorderRadius.all(Radius.circular(7.0)),
),
);
});
testWidgets('Material3 - ChipThemeData.iconTheme respects default iconTheme.size', (WidgetTester tester) async {
Widget buildChip({ IconThemeData? iconTheme }) {
return MaterialApp(
theme: ThemeData(chipTheme: ChipThemeData(iconTheme: iconTheme)),
home: Directionality(
textDirection: TextDirection.ltr,
child: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.add),
label: const SizedBox(width: 100, height: 100),
onSelected: (bool newValue) { },
),
),
),
),
);
}
await tester.pumpWidget(buildChip(iconTheme: const IconThemeData(color: Color(0xff332211))));
// Icon should have the default chip iconSize.
expect(getIconData(tester).size, 18.0);
expect(getIconData(tester).color, const Color(0xff332211));
// Icon should have the provided iconSize.
await tester.pumpWidget(buildChip(iconTheme: const IconThemeData(color: Color(0xff112233), size: 23.0)));
await tester.pumpAndSettle();
expect(getIconData(tester).size, 23.0);
expect(getIconData(tester).color, const Color(0xff112233));
});
testWidgets('ChipThemeData.avatarBoxConstraints updates avatar size constraints', (WidgetTester tester) async {
const double border = 1.0;
const double iconSize = 18.0;
const double labelPadding = 8.0;
const double padding = 8.0;
const Size labelSize = Size(75, 75);
// Test default avatar layout constraints.
await tester.pumpWidget(MaterialApp(
theme: ThemeData(chipTheme: const ChipThemeData(
avatarBoxConstraints: BoxConstraints.tightForFinite(),
)),
home: Material(
child: Center(
child: RawChip(
avatar: const Icon(Icons.favorite),
label: Container(
width: labelSize.width,
height: labelSize.width,
color: const Color(0xFFFF0000),
),
),
),
),
));
expect(tester.getSize(find.byType(RawChip)).width, equals(127.0));
expect(tester.getSize(find.byType(RawChip)).height, equals(93.0));
// Calculate the distance between avatar and chip edges.
final Offset chipTopLeft = tester.getTopLeft(find.byWidget(getMaterial(tester)));
final Offset avatarCenter = tester.getCenter(find.byIcon(Icons.favorite));
expect(chipTopLeft.dx, avatarCenter.dx - (iconSize / 2) - padding - border);
expect(chipTopLeft.dy, avatarCenter.dy - (labelSize.width / 2) - padding - border);
// Calculate the distance between avatar and label.
final Offset labelTopLeft = tester.getTopLeft(find.byType(Container));
expect(labelTopLeft.dx, avatarCenter.dx + (iconSize / 2) + labelPadding);
});
testWidgets('ChipThemeData.deleteIconBoxConstraints updates delete icon size constraints', (WidgetTester tester) async {
const double border = 1.0;
const double iconSize = 18.0;
const double labelPadding = 8.0;
const double padding = 8.0;
const Size labelSize = Size(75, 75);
// Test custom delete layout constraints.
await tester.pumpWidget(MaterialApp(
theme: ThemeData(chipTheme: const ChipThemeData(
deleteIconBoxConstraints: BoxConstraints.tightForFinite(),
)),
home: Material(
child: Center(
child: RawChip(
onDeleted: () { },
label: Container(
width: labelSize.width,
height: labelSize.width,
color: const Color(0xFFFF0000),
),
),
),
),
));
expect(tester.getSize(find.byType(RawChip)).width, equals(127.0));
expect(tester.getSize(find.byType(RawChip)).height, equals(93.0));
// Calculate the distance between delete icon and chip edges.
final Offset chipTopRight = tester.getTopRight(find.byWidget(getMaterial(tester)));
final Offset deleteIconCenter = tester.getCenter(find.byIcon(Icons.cancel));
expect(chipTopRight.dx, deleteIconCenter.dx + (iconSize / 2) + padding + border);
expect(chipTopRight.dy, deleteIconCenter.dy - (labelSize.width / 2) - padding - border);
// Calculate the distance between delete icon and label.
final Offset labelTopRight = tester.getTopRight(find.byType(Container));
expect(labelTopRight.dx, deleteIconCenter.dx - (iconSize / 2) - labelPadding);
});
}
class _MaterialStateOutlinedBorder extends StadiumBorder implements MaterialStateOutlinedBorder {
const _MaterialStateOutlinedBorder(this.resolver);
final MaterialPropertyResolver<OutlinedBorder?> resolver;
@override
OutlinedBorder? resolve(Set<MaterialState> states) => resolver(states);
}
class _MaterialStateBorderSide extends MaterialStateBorderSide {
const _MaterialStateBorderSide(this.resolver);
final MaterialPropertyResolver<BorderSide?> resolver;
@override
BorderSide? resolve(Set<MaterialState> states) => resolver(states);
}
| flutter/packages/flutter/test/material/chip_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/chip_theme_test.dart",
"repo_id": "flutter",
"token_count": 21741
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Material3 - Divider control test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Center(child: Divider()),
),
);
final RenderBox box = tester.firstRenderObject(find.byType(Divider));
expect(box.size.height, 16.0);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
expect(decoration.border!.bottom.width, 1.0);
});
testWidgets('Material2 - Divider control test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Center(child: Divider()),
),
);
final RenderBox box = tester.firstRenderObject(find.byType(Divider));
expect(box.size.height, 16.0);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
expect(decoration.border!.bottom.width, 0.0);
});
testWidgets('Divider custom thickness', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: Divider(thickness: 5.0)),
),
);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
expect(decoration.border!.bottom.width, 5.0);
});
testWidgets('Horizontal divider custom indentation', (WidgetTester tester) async {
const double customIndent = 10.0;
Rect dividerRect;
Rect lineRect;
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: Divider(indent: customIndent)),
),
);
// The divider line is drawn with a DecoratedBox with a border
dividerRect = tester.getRect(find.byType(Divider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.left, dividerRect.left + customIndent);
expect(lineRect.right, dividerRect.right);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: Divider(endIndent: customIndent)),
),
);
dividerRect = tester.getRect(find.byType(Divider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.left, dividerRect.left);
expect(lineRect.right, dividerRect.right - customIndent);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: Divider(
indent: customIndent,
endIndent: customIndent,
),
),
),
);
dividerRect = tester.getRect(find.byType(Divider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.left, dividerRect.left + customIndent);
expect(lineRect.right, dividerRect.right - customIndent);
});
testWidgets('Material3 - Vertical Divider Test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Center(child: VerticalDivider()),
),
);
final RenderBox box = tester.firstRenderObject(find.byType(VerticalDivider));
expect(box.size.width, 16.0);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
final Border border = decoration.border! as Border;
expect(border.left.width, 1.0);
});
testWidgets('Material2 - Vertical Divider Test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Center(child: VerticalDivider()),
),
);
final RenderBox box = tester.firstRenderObject(find.byType(VerticalDivider));
expect(box.size.width, 16.0);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
final Border border = decoration.border! as Border;
expect(border.left.width, 0.0);
});
testWidgets('Divider custom thickness', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: VerticalDivider(thickness: 5.0)),
),
);
final Container container = tester.widget(find.byType(Container));
final BoxDecoration decoration = container.decoration! as BoxDecoration;
final Border border = decoration.border! as Border;
expect(border.left.width, 5.0);
});
testWidgets('Vertical Divider Test 2', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Material(
child: SizedBox(
height: 24.0,
child: Row(
children: <Widget>[
Text('Hey.'),
VerticalDivider(),
],
),
),
),
),
);
final RenderBox box = tester.firstRenderObject(find.byType(VerticalDivider));
final RenderBox containerBox = tester.firstRenderObject(find.byType(Container).last);
expect(box.size.width, 16.0);
expect(containerBox.size.height, 600.0);
expect(find.byType(VerticalDivider), paints..path(strokeWidth: 0.0));
});
testWidgets('Vertical divider custom indentation', (WidgetTester tester) async {
const double customIndent = 10.0;
Rect dividerRect;
Rect lineRect;
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: VerticalDivider(indent: customIndent)),
),
);
// The divider line is drawn with a DecoratedBox with a border
dividerRect = tester.getRect(find.byType(VerticalDivider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.top, dividerRect.top + customIndent);
expect(lineRect.bottom, dividerRect.bottom);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(child: VerticalDivider(endIndent: customIndent)),
),
);
dividerRect = tester.getRect(find.byType(VerticalDivider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.top, dividerRect.top);
expect(lineRect.bottom, dividerRect.bottom - customIndent);
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: VerticalDivider(
indent: customIndent,
endIndent: customIndent,
),
),
),
);
dividerRect = tester.getRect(find.byType(VerticalDivider));
lineRect = tester.getRect(find.byType(DecoratedBox));
expect(lineRect.top, dividerRect.top + customIndent);
expect(lineRect.bottom, dividerRect.bottom - customIndent);
});
// Regression test for https://github.com/flutter/flutter/issues/39533
testWidgets('createBorderSide does not throw exception with null context', (WidgetTester tester) async {
// Passing a null context used to throw an exception but no longer does.
expect(() => Divider.createBorderSide(null), isNot(throwsAssertionError));
expect(() => Divider.createBorderSide(null), isNot(throwsNoSuchMethodError));
});
}
| flutter/packages/flutter/test/material/divider_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/divider_test.dart",
"repo_id": "flutter",
"token_count": 2978
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
import 'feedback_tester.dart';
void main () {
const Duration kWaitDuration = Duration(seconds: 1);
late FeedbackTester feedback;
setUp(() {
feedback = FeedbackTester();
});
tearDown(() {
feedback.dispose();
});
group('Feedback on Android', () {
late List<Map<String, Object>> semanticEvents;
setUp(() {
semanticEvents = <Map<String, Object>>[];
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, (dynamic message) async {
final Map<dynamic, dynamic> typedMessage = message as Map<dynamic, dynamic>;
semanticEvents.add(typedMessage.cast<String, Object>());
});
});
tearDown(() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, null);
});
testWidgets('forTap', (WidgetTester tester) async {
final SemanticsTester semanticsTester = SemanticsTester(tester);
await tester.pumpWidget(TestWidget(
tapHandler: (BuildContext context) {
return () => Feedback.forTap(context);
},
));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
expect(semanticEvents, isEmpty);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
final RenderObject object = tester.firstRenderObject(find.byType(GestureDetector));
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 1);
expect(semanticEvents.single, <String, dynamic>{
'type': 'tap',
'nodeId': object.debugSemantics!.id,
'data': <String, dynamic>{},
});
expect(object.debugSemantics!.getSemanticsData().hasAction(SemanticsAction.tap), true);
semanticsTester.dispose();
});
testWidgets('forTap Wrapper', (WidgetTester tester) async {
final SemanticsTester semanticsTester = SemanticsTester(tester);
int callbackCount = 0;
void callback() {
callbackCount++;
}
await tester.pumpWidget(TestWidget(
tapHandler: (BuildContext context) {
return Feedback.wrapForTap(callback, context)!;
},
));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
expect(callbackCount, 0);
await tester.tap(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
final RenderObject object = tester.firstRenderObject(find.byType(GestureDetector));
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 1);
expect(callbackCount, 1);
expect(semanticEvents.single, <String, dynamic>{
'type': 'tap',
'nodeId': object.debugSemantics!.id,
'data': <String, dynamic>{},
});
expect(object.debugSemantics!.getSemanticsData().hasAction(SemanticsAction.tap), true);
semanticsTester.dispose();
});
testWidgets('forLongPress', (WidgetTester tester) async {
final SemanticsTester semanticsTester = SemanticsTester(tester);
await tester.pumpWidget(TestWidget(
longPressHandler: (BuildContext context) {
return () => Feedback.forLongPress(context);
},
));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
await tester.longPress(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
final RenderObject object = tester.firstRenderObject(find.byType(GestureDetector));
expect(feedback.hapticCount, 1);
expect(feedback.clickSoundCount, 0);
expect(semanticEvents.single, <String, dynamic>{
'type': 'longPress',
'nodeId': object.debugSemantics!.id,
'data': <String, dynamic>{},
});
expect(object.debugSemantics!.getSemanticsData().hasAction(SemanticsAction.longPress), true);
semanticsTester.dispose();
});
testWidgets('forLongPress Wrapper', (WidgetTester tester) async {
final SemanticsTester semanticsTester = SemanticsTester(tester);
int callbackCount = 0;
void callback() {
callbackCount++;
}
await tester.pumpWidget(TestWidget(
longPressHandler: (BuildContext context) {
return Feedback.wrapForLongPress(callback, context)!;
},
));
await tester.pumpAndSettle(kWaitDuration);
final RenderObject object = tester.firstRenderObject(find.byType(GestureDetector));
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
expect(callbackCount, 0);
await tester.longPress(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 1);
expect(feedback.clickSoundCount, 0);
expect(callbackCount, 1);
expect(semanticEvents.single, <String, dynamic>{
'type': 'longPress',
'nodeId': object.debugSemantics!.id,
'data': <String, dynamic>{},
});
expect(object.debugSemantics!.getSemanticsData().hasAction(SemanticsAction.longPress), true);
semanticsTester.dispose();
});
});
group('Feedback on iOS', () {
testWidgets('forTap', (WidgetTester tester) async {
await tester.pumpWidget(Theme(
data: ThemeData(platform: TargetPlatform.iOS),
child: TestWidget(
tapHandler: (BuildContext context) {
return () => Feedback.forTap(context);
},
),
));
await tester.tap(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
});
testWidgets('forLongPress', (WidgetTester tester) async {
await tester.pumpWidget(Theme(
data: ThemeData(platform: TargetPlatform.iOS),
child: TestWidget(
longPressHandler: (BuildContext context) {
return () => Feedback.forLongPress(context);
},
),
));
await tester.longPress(find.text('X'));
await tester.pumpAndSettle(kWaitDuration);
expect(feedback.hapticCount, 0);
expect(feedback.clickSoundCount, 0);
});
});
}
class TestWidget extends StatelessWidget {
const TestWidget({
super.key,
this.tapHandler = nullHandler,
this.longPressHandler = nullHandler,
});
final HandlerCreator tapHandler;
final HandlerCreator longPressHandler;
static VoidCallback? nullHandler(BuildContext context) => null;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: tapHandler(context),
onLongPress: longPressHandler(context),
child: const Text('X', textDirection: TextDirection.ltr),
);
}
}
typedef HandlerCreator = VoidCallback? Function(BuildContext context);
| flutter/packages/flutter/test/material/feedback_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/feedback_test.dart",
"repo_id": "flutter",
"token_count": 2816
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Theme.wrap()', (WidgetTester tester) async {
const Color primaryColor = Color(0xFF00FF00);
final Key primaryContainerKey = UniqueKey();
// Effectively the same as a StatelessWidget subclass.
final Widget primaryBox = Builder(
builder: (BuildContext context) {
return Container(
key: primaryContainerKey,
color: Theme.of(context).primaryColor,
);
},
);
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Builder( // Introduce a context so the app's Theme is visible.
builder: (BuildContext context) {
navigatorContext = context;
return Theme(
data: Theme.of(context).copyWith(primaryColor: primaryColor),
child: Builder( // Introduce a context so the shadow Theme is visible to captureAll().
builder: (BuildContext context) {
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The primaryBox will see the default Theme when built.
builder: (BuildContext _) => primaryBox,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow Theme.
builder: (BuildContext _) => InheritedTheme.captureAll(context, primaryBox),
),
);
},
),
],
),
);
},
),
);
},
),
),
);
}
Color containerColor() {
return tester.widget<Container>(find.byKey(primaryContainerKey)).color!;
}
await tester.pumpWidget(buildFrame());
// Show the route which contains primaryBox which was wrapped with
// InheritedTheme.captureAll().
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
expect(containerColor(), primaryColor);
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
// Show the route which contains primaryBox
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
expect(containerColor(), isNot(primaryColor));
});
testWidgets('Material2 - PopupMenuTheme.wrap()', (WidgetTester tester) async {
const double menuFontSize = 24;
const Color menuTextColor = Color(0xFF0000FF);
Widget buildFrame() {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: PopupMenuTheme(
data: const PopupMenuThemeData(
// The menu route's elevation, shape, and color are defined by the
// current context, so they're not affected by ThemeData.captureAll().
textStyle: TextStyle(fontSize: menuFontSize, color: menuTextColor),
),
child: Center(
child: PopupMenuButton<int>(
// The appearance of the menu items' text is defined by the
// PopupMenuTheme defined above. Popup menus use
// InheritedTheme.captureAll() by default.
child: const Text('show popupmenu'),
onSelected: (int result) { },
itemBuilder: (BuildContext context) {
return const <PopupMenuEntry<int>>[
PopupMenuItem<int>(value: 1, child: Text('One')),
PopupMenuItem<int>(value: 2, child: Text('Two')),
];
},
),
),
),
),
);
}
TextStyle itemTextStyle(String text) {
return tester.widget<RichText>(
find.descendant(of: find.text(text), matching: find.byType(RichText)),
).text.style!;
}
await tester.pumpWidget(buildFrame());
await tester.tap(find.text('show popupmenu'));
await tester.pumpAndSettle(); // menu route animation
expect(itemTextStyle('One').fontSize, menuFontSize);
expect(itemTextStyle('One').color, menuTextColor);
expect(itemTextStyle('Two').fontSize, menuFontSize);
expect(itemTextStyle('Two').color, menuTextColor);
// Dismiss the menu
await tester.tap(find.text('One'));
await tester.pumpAndSettle(); // menu route animation
});
testWidgets('Material3 - PopupMenuTheme.wrap()', (WidgetTester tester) async {
const TextStyle textStyle = TextStyle(fontSize: 24.0, color: Color(0xFF0000FF));
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: PopupMenuTheme(
data: const PopupMenuThemeData(
// The menu route's elevation, shape, and color are defined by the
// current context, so they're not affected by ThemeData.captureAll().
labelTextStyle: MaterialStatePropertyAll<TextStyle>(textStyle),
),
child: Center(
child: PopupMenuButton<int>(
// The appearance of the menu items' text is defined by the
// PopupMenuTheme defined above. Popup menus use
// InheritedTheme.captureAll() by default.
child: const Text('show popupmenu'),
onSelected: (int result) { },
itemBuilder: (BuildContext context) {
return const <PopupMenuEntry<int>>[
PopupMenuItem<int>(value: 1, child: Text('One')),
PopupMenuItem<int>(value: 2, child: Text('Two')),
];
},
),
),
),
),
);
}
TextStyle itemTextStyle(String text) {
return tester.widget<RichText>(
find.descendant(of: find.text(text), matching: find.byType(RichText)),
).text.style!;
}
await tester.pumpWidget(buildFrame());
await tester.tap(find.text('show popupmenu'));
await tester.pumpAndSettle(); // menu route animation
expect(itemTextStyle('One').fontSize, textStyle.fontSize);
expect(itemTextStyle('One').color, textStyle.color);
expect(itemTextStyle('Two').fontSize, textStyle.fontSize);
expect(itemTextStyle('Two').color, textStyle.color);
// Dismiss the menu
await tester.tap(find.text('One'));
await tester.pumpAndSettle(); // menu route animation
});
testWidgets('BannerTheme.wrap()', (WidgetTester tester) async {
const Color bannerBackgroundColor = Color(0xFF0000FF);
const double bannerFontSize = 48;
const Color bannerTextColor = Color(0xFF00FF00);
final Widget banner = MaterialBanner(
content: const Text('hello'),
actions: <Widget>[
TextButton(
child: const Text('action'),
onPressed: () { },
),
],
);
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: MaterialBannerTheme(
data: const MaterialBannerThemeData(
backgroundColor: bannerBackgroundColor,
contentTextStyle: TextStyle(fontSize: bannerFontSize, color: bannerTextColor),
),
child: Builder( // Introduce a context so the shadow BannerTheme is visible to captureAll().
builder: (BuildContext context) {
navigatorContext = context;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The Banner will see the default BannerTheme when built.
builder: (BuildContext _) => banner,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow BannerTheme.
builder: (BuildContext _) => InheritedTheme.captureAll(context, banner),
),
);
},
),
],
),
);
},
),
),
),
);
}
Color bannerColor() {
return tester.widget<Material>(
find.descendant(of: find.byType(MaterialBanner), matching: find.byType(Material)).first,
).color!;
}
TextStyle getTextStyle(String text) {
return tester.widget<RichText>(
find.descendant(
of: find.text(text),
matching: find.byType(RichText),
),
).text.style!;
}
await tester.pumpWidget(buildFrame());
// Show the route which contains the banner.
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
expect(bannerColor(), bannerBackgroundColor);
expect(getTextStyle('hello').fontSize, bannerFontSize);
expect(getTextStyle('hello').color, bannerTextColor);
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
expect(bannerColor(), isNot(bannerBackgroundColor));
expect(getTextStyle('hello').fontSize, isNot(bannerFontSize));
expect(getTextStyle('hello').color, isNot(bannerTextColor));
});
testWidgets('DividerTheme.wrap()', (WidgetTester tester) async {
const Color dividerColor = Color(0xFF0000FF);
const double dividerSpace = 13;
const double dividerThickness = 7;
const Widget divider = Center(child: Divider());
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: DividerTheme(
data: const DividerThemeData(
color: dividerColor,
space: dividerSpace,
thickness: dividerThickness,
),
child: Builder( // Introduce a context so the shadow DividerTheme is visible to captureAll().
builder: (BuildContext context) {
navigatorContext = context;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The Banner will see the default BannerTheme when built.
builder: (BuildContext _) => divider,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow BannerTheme.
builder: (BuildContext _) => InheritedTheme.captureAll(context, divider),
),
);
},
),
],
),
);
},
),
),
),
);
}
BorderSide dividerBorder() {
final BoxDecoration decoration = tester.widget<Container>(
find.descendant(of: find.byType(Divider), matching: find.byType(Container)).first,
).decoration! as BoxDecoration;
return decoration.border!.bottom;
}
await tester.pumpWidget(buildFrame());
// Show a route which contains a divider.
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
expect(tester.getSize(find.byType(Divider)).height, dividerSpace);
expect(dividerBorder().color, dividerColor);
expect(dividerBorder().width, dividerThickness);
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
expect(tester.getSize(find.byType(Divider)).height, isNot(dividerSpace));
expect(dividerBorder().color, isNot(dividerColor));
expect(dividerBorder().width, isNot(dividerThickness));
});
testWidgets('ListTileTheme.wrap()', (WidgetTester tester) async {
const Color tileSelectedColor = Color(0xFF00FF00);
const Color tileIconColor = Color(0xFF0000FF);
const Color tileTextColor = Color(0xFFFF0000);
final Key selectedIconKey = UniqueKey();
final Key unselectedIconKey = UniqueKey();
final Widget listTiles = Scaffold(
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ListTile(
leading: Icon(Icons.computer, key: selectedIconKey),
title: const Text('selected'),
selected: true,
),
ListTile(
leading: Icon(Icons.add, key: unselectedIconKey),
title: const Text('unselected'),
),
],
),
),
);
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: ListTileTheme(
selectedColor: tileSelectedColor,
textColor: tileTextColor,
iconColor: tileIconColor,
child: Builder( // Introduce a context so the shadow ListTileTheme is visible to captureAll().
builder: (BuildContext context) {
navigatorContext = context;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The Banner will see the default BannerTheme when built.
builder: (BuildContext _) => listTiles,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow BannerTheme.
builder: (BuildContext _) => InheritedTheme.captureAll(context, listTiles),
),
);
},
),
],
),
);
},
),
),
),
);
}
TextStyle getTextStyle(String text) {
return tester.widget<RichText>(
find.descendant(of: find.text(text), matching: find.byType(RichText)),
).text.style!;
}
TextStyle getIconStyle(Key key) {
return tester.widget<RichText>(
find.descendant(
of: find.byKey(key),
matching: find.byType(RichText),
),
).text.style!;
}
await tester.pumpWidget(buildFrame());
// Show a route which contains listTiles.
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
expect(getTextStyle('unselected').color, tileTextColor);
expect(getTextStyle('selected').color, tileSelectedColor);
expect(getIconStyle(selectedIconKey).color, tileSelectedColor);
expect(getIconStyle(unselectedIconKey).color, tileIconColor);
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
expect(getTextStyle('unselected').color, isNot(tileTextColor));
expect(getTextStyle('selected').color, isNot(tileSelectedColor));
expect(getIconStyle(selectedIconKey).color, isNot(tileSelectedColor));
expect(getIconStyle(unselectedIconKey).color, isNot(tileIconColor));
});
testWidgets('SliderTheme.wrap()', (WidgetTester tester) async {
const Color activeTrackColor = Color(0xFF00FF00);
const Color inactiveTrackColor = Color(0xFF0000FF);
const Color thumbColor = Color(0xFFFF0000);
final Widget slider = Scaffold(
body: Center(
child: Slider(
value: 0.5,
onChanged: (double value) { },
),
),
);
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: SliderTheme(
data: const SliderThemeData(
activeTrackColor: activeTrackColor,
inactiveTrackColor: inactiveTrackColor,
thumbColor: thumbColor,
),
child: Builder( // Introduce a context so the shadow SliderTheme is visible to captureAll().
builder: (BuildContext context) {
navigatorContext = context;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The slider will see the default SliderTheme when built.
builder: (BuildContext _) => slider,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow SliderTheme.
builder: (BuildContext _) => InheritedTheme.captureAll(context, slider),
),
);
},
),
],
),
);
},
),
),
),
);
}
await tester.pumpWidget(buildFrame());
// Show a route which contains listTiles.
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
RenderBox sliderBox = tester.firstRenderObject<RenderBox>(find.byType(Slider));
expect(sliderBox, paints..rrect(color: activeTrackColor)..rrect(color: inactiveTrackColor));
expect(sliderBox, paints..circle(color: thumbColor));
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
sliderBox = tester.firstRenderObject<RenderBox>(find.byType(Slider));
expect(sliderBox, isNot(paints..rrect(color: activeTrackColor)..rrect(color: inactiveTrackColor)));
expect(sliderBox, isNot(paints..circle(color: thumbColor)));
});
testWidgets('ToggleButtonsTheme.wrap()', (WidgetTester tester) async {
const Color buttonColor = Color(0xFF00FF00);
const Color selectedButtonColor = Color(0xFFFF0000);
final Widget toggleButtons = Scaffold(
body: Center(
child: ToggleButtons(
isSelected: const <bool>[true, false],
children: const <Widget>[
Text('selected'),
Text('unselected'),
],
onPressed: (int index) { },
),
),
);
late BuildContext navigatorContext;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: ToggleButtonsTheme(
data: const ToggleButtonsThemeData(
color: buttonColor,
selectedColor: selectedButtonColor,
),
child: Builder( // Introduce a context so the shadow ToggleButtonsTheme is visible to captureAll().
builder: (BuildContext context) {
navigatorContext = context;
return Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
child: const Text('push unwrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// The slider will see the default ToggleButtonsTheme when built.
builder: (BuildContext _) => toggleButtons,
),
);
},
),
ElevatedButton(
child: const Text('push wrapped'),
onPressed: () {
Navigator.of(context).push<void>(
MaterialPageRoute<void>(
// Capture the shadow toggleButtons.
builder: (BuildContext _) => InheritedTheme.captureAll(context, toggleButtons),
),
);
},
),
],
),
);
},
),
),
),
);
}
Color getTextColor(String text) {
return tester.widget<RichText>(
find.descendant(of: find.text(text), matching: find.byType(RichText)),
).text.style!.color!;
}
await tester.pumpWidget(buildFrame());
// Show a route which contains toggleButtons.
await tester.tap(find.text('push wrapped'));
await tester.pumpAndSettle(); // route animation
expect(getTextColor('selected'), selectedButtonColor);
expect(getTextColor('unselected'), buttonColor);
Navigator.of(navigatorContext).pop();
await tester.pumpAndSettle(); // route animation
await tester.tap(find.text('push unwrapped'));
await tester.pumpAndSettle(); // route animation
expect(getTextColor('selected'), isNot(selectedButtonColor));
expect(getTextColor('unselected'), isNot(buttonColor));
});
}
| flutter/packages/flutter/test/material/inherited_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/inherited_theme_test.dart",
"repo_id": "flutter",
"token_count": 12110
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
import '../widgets/test_border.dart' show TestBorder;
class NotifyMaterial extends StatelessWidget {
const NotifyMaterial({ super.key });
@override
Widget build(BuildContext context) {
const LayoutChangedNotification().dispatch(context);
return Container();
}
}
Widget buildMaterial({
double elevation = 0.0,
Color shadowColor = const Color(0xFF00FF00),
Color? surfaceTintColor,
Color color = const Color(0xFF0000FF),
}) {
return Center(
child: SizedBox(
height: 100.0,
width: 100.0,
child: Material(
color: color,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
elevation: elevation,
shape: const CircleBorder(),
),
),
);
}
RenderPhysicalShape getModel(WidgetTester tester) {
return tester.renderObject(find.byType(PhysicalShape));
}
class PaintRecorder extends CustomPainter {
PaintRecorder(this.log);
final List<Size> log;
@override
void paint(Canvas canvas, Size size) {
log.add(size);
final Paint paint = Paint()..color = const Color(0xFF0000FF);
canvas.drawRect(Offset.zero & size, paint);
}
@override
bool shouldRepaint(PaintRecorder oldDelegate) => false;
}
class ElevationColor {
const ElevationColor(this.elevation, this.color);
final double elevation;
final Color color;
}
void main() {
// Regression test for https://github.com/flutter/flutter/issues/81504
testWidgets('MaterialApp.home nullable and update test', (WidgetTester tester) async {
// _WidgetsAppState._usesNavigator == true
await tester.pumpWidget(const MaterialApp(home: SizedBox.shrink()));
// _WidgetsAppState._usesNavigator == false
await tester.pumpWidget(const MaterialApp()); // Do not crash!
// _WidgetsAppState._usesNavigator == true
await tester.pumpWidget(const MaterialApp(home: SizedBox.shrink())); // Do not crash!
expect(tester.takeException(), null);
});
testWidgets('default Material debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const Material().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>['type: canvas']);
});
testWidgets('Material implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const Material(
color: Color(0xFFFFFFFF),
shadowColor: Color(0xffff0000),
surfaceTintColor: Color(0xff0000ff),
textStyle: TextStyle(color: Color(0xff00ff00)),
borderRadius: BorderRadiusDirectional.all(Radius.circular(10)),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'type: canvas',
'color: Color(0xffffffff)',
'shadowColor: Color(0xffff0000)',
'surfaceTintColor: Color(0xff0000ff)',
'textStyle.inherit: true',
'textStyle.color: Color(0xff00ff00)',
'borderRadius: BorderRadiusDirectional.circular(10.0)',
]);
});
testWidgets('LayoutChangedNotification test', (WidgetTester tester) async {
await tester.pumpWidget(
const Material(
child: NotifyMaterial(),
),
);
});
testWidgets('ListView scroll does not repaint', (WidgetTester tester) async {
final List<Size> log = <Size>[];
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Column(
children: <Widget>[
SizedBox(
width: 150.0,
height: 150.0,
child: CustomPaint(
painter: PaintRecorder(log),
),
),
Expanded(
child: Material(
child: Column(
children: <Widget>[
Expanded(
child: ListView(
children: <Widget>[
Container(
height: 2000.0,
color: const Color(0xFF00FF00),
),
],
),
),
SizedBox(
width: 100.0,
height: 100.0,
child: CustomPaint(
painter: PaintRecorder(log),
),
),
],
),
),
),
],
),
),
);
// We paint twice because we have two CustomPaint widgets in the tree above
// to test repainting both inside and outside the Material widget.
expect(log, equals(<Size>[
const Size(150.0, 150.0),
const Size(100.0, 100.0),
]));
log.clear();
await tester.drag(find.byType(ListView), const Offset(0.0, -300.0));
await tester.pump();
expect(log, isEmpty);
});
testWidgets('Shadow color defaults', (WidgetTester tester) async {
Widget buildWithShadow(Color? shadowColor) {
return Center(
child: SizedBox(
height: 100.0,
width: 100.0,
child: Material(
shadowColor: shadowColor,
elevation: 10,
shape: const CircleBorder(),
),
)
);
}
// Default M2 shadow color
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
),
child: buildWithShadow(null),
)
);
await tester.pumpAndSettle();
expect(getModel(tester).shadowColor, ThemeData().shadowColor);
// Default M3 shadow color
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: true,
),
child: buildWithShadow(null),
)
);
await tester.pumpAndSettle();
expect(getModel(tester).shadowColor, ThemeData().colorScheme.shadow);
// Drop shadow can be turned off with a transparent color.
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: true,
),
child: buildWithShadow(Colors.transparent),
)
);
await tester.pumpAndSettle();
expect(getModel(tester).shadowColor, Colors.transparent);
});
testWidgets('Shadows animate smoothly', (WidgetTester tester) async {
// This code verifies that the PhysicalModel's elevation animates over
// a kThemeChangeDuration time interval.
await tester.pumpWidget(buildMaterial());
final RenderPhysicalShape modelA = getModel(tester);
expect(modelA.elevation, equals(0.0));
await tester.pumpWidget(buildMaterial(elevation: 9.0));
final RenderPhysicalShape modelB = getModel(tester);
expect(modelB.elevation, equals(0.0));
await tester.pump(const Duration(milliseconds: 1));
final RenderPhysicalShape modelC = getModel(tester);
expect(modelC.elevation, moreOrLessEquals(0.0, epsilon: 0.001));
await tester.pump(kThemeChangeDuration ~/ 2);
final RenderPhysicalShape modelD = getModel(tester);
expect(modelD.elevation, isNot(moreOrLessEquals(0.0, epsilon: 0.001)));
await tester.pump(kThemeChangeDuration);
final RenderPhysicalShape modelE = getModel(tester);
expect(modelE.elevation, equals(9.0));
});
testWidgets('Shadow colors animate smoothly', (WidgetTester tester) async {
// This code verifies that the PhysicalModel's shadowColor animates over
// a kThemeChangeDuration time interval.
await tester.pumpWidget(buildMaterial());
final RenderPhysicalShape modelA = getModel(tester);
expect(modelA.shadowColor, equals(const Color(0xFF00FF00)));
await tester.pumpWidget(buildMaterial(shadowColor: const Color(0xFFFF0000)));
final RenderPhysicalShape modelB = getModel(tester);
expect(modelB.shadowColor, equals(const Color(0xFF00FF00)));
await tester.pump(const Duration(milliseconds: 1));
final RenderPhysicalShape modelC = getModel(tester);
expect(modelC.shadowColor, within<Color>(distance: 1, from: const Color(0xFF00FF00)));
await tester.pump(kThemeChangeDuration ~/ 2);
final RenderPhysicalShape modelD = getModel(tester);
expect(modelD.shadowColor, isNot(within<Color>(distance: 1, from: const Color(0xFF00FF00))));
await tester.pump(kThemeChangeDuration);
final RenderPhysicalShape modelE = getModel(tester);
expect(modelE.shadowColor, equals(const Color(0xFFFF0000)));
});
testWidgets('Transparent material widget does not absorb hit test', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/58665.
bool pressed = false;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Stack(
children: <Widget>[
ElevatedButton(
onPressed: () {
pressed = true;
},
child: null,
),
const Material(
type: MaterialType.transparency,
child: SizedBox(
width: 400.0,
height: 500.0,
),
),
],
),
),
),
);
await tester.tap(find.byType(ElevatedButton));
expect(pressed, isTrue);
});
group('Surface Tint Overlay', () {
testWidgets('applyElevationOverlayColor does not effect anything with useMaterial3 set to true', (WidgetTester tester) async {
const Color surfaceColor = Color(0xFF121212);
await tester.pumpWidget(Theme(
data: ThemeData(
useMaterial3: true,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.dark().copyWith(surface: surfaceColor),
),
child: buildMaterial(color: surfaceColor, elevation: 8.0),
));
final RenderPhysicalShape model = getModel(tester);
expect(model.color, equals(surfaceColor));
});
testWidgets('surfaceTintColor is used to as an overlay to indicate elevation', (WidgetTester tester) async {
const Color baseColor = Color(0xFF121212);
const Color surfaceTintColor = Color(0xff44CCFF);
// With no surfaceTintColor specified, it should not apply an overlay
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: true,
),
child: buildMaterial(
color: baseColor,
elevation: 12.0,
),
),
);
await tester.pumpAndSettle();
final RenderPhysicalShape noTintModel = getModel(tester);
expect(noTintModel.color, equals(baseColor));
// With transparent surfaceTintColor, it should not apply an overlay
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: true,
),
child: buildMaterial(
color: baseColor,
surfaceTintColor: Colors.transparent,
elevation: 12.0,
),
),
);
await tester.pumpAndSettle();
final RenderPhysicalShape transparentTintModel = getModel(tester);
expect(transparentTintModel.color, equals(baseColor));
// With surfaceTintColor specified, it should not apply an overlay based
// on the elevation.
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: true,
),
child: buildMaterial(
color: baseColor,
surfaceTintColor: surfaceTintColor,
elevation: 12.0,
),
),
);
await tester.pumpAndSettle();
final RenderPhysicalShape tintModel = getModel(tester);
// Final color should be the base with a tint of 0.14 opacity or 0xff192c33
expect(tintModel.color, equals(const Color(0xff192c33)));
});
}); // Surface Tint Overlay group
group('Elevation Overlay M2', () {
// These tests only apply to the Material 2 overlay mechanism. This group
// can be removed after migration to Material 3 is complete.
testWidgets('applyElevationOverlayColor set to false does not change surface color', (WidgetTester tester) async {
const Color surfaceColor = Color(0xFF121212);
await tester.pumpWidget(Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: false,
colorScheme: const ColorScheme.dark().copyWith(surface: surfaceColor),
),
child: buildMaterial(color: surfaceColor, elevation: 8.0),
));
final RenderPhysicalShape model = getModel(tester);
expect(model.color, equals(surfaceColor));
});
testWidgets('applyElevationOverlayColor set to true applies a semi-transparent onSurface color to the surface color', (WidgetTester tester) async {
const Color surfaceColor = Color(0xFF121212);
const Color onSurfaceColor = Colors.greenAccent;
// The colors we should get with a base surface color of 0xFF121212 for
// and a given elevation
const List<ElevationColor> elevationColors = <ElevationColor>[
ElevationColor(0.0, Color(0xFF121212)),
ElevationColor(1.0, Color(0xFF161D19)),
ElevationColor(2.0, Color(0xFF18211D)),
ElevationColor(3.0, Color(0xFF19241E)),
ElevationColor(4.0, Color(0xFF1A2620)),
ElevationColor(6.0, Color(0xFF1B2922)),
ElevationColor(8.0, Color(0xFF1C2C24)),
ElevationColor(12.0, Color(0xFF1D3027)),
ElevationColor(16.0, Color(0xFF1E3329)),
ElevationColor(24.0, Color(0xFF20362B)),
];
for (final ElevationColor test in elevationColors) {
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.dark().copyWith(
surface: surfaceColor,
onSurface: onSurfaceColor,
),
),
child: buildMaterial(
color: surfaceColor,
elevation: test.elevation,
),
),
);
await tester.pumpAndSettle(); // wait for the elevation animation to finish
final RenderPhysicalShape model = getModel(tester);
expect(model.color, equals(test.color));
}
});
testWidgets('overlay will not apply to materials using a non-surface color', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.dark(),
),
child: buildMaterial(
color: Colors.cyan,
elevation: 8.0,
),
),
);
final RenderPhysicalShape model = getModel(tester);
// Shouldn't change, as it is not using a ColorScheme.surface color
expect(model.color, equals(Colors.cyan));
});
testWidgets('overlay will not apply to materials using a light theme', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.light(),
),
child: buildMaterial(
color: Colors.cyan,
elevation: 8.0,
),
),
);
final RenderPhysicalShape model = getModel(tester);
// Shouldn't change, as it was under a light color scheme.
expect(model.color, equals(Colors.cyan));
});
testWidgets('overlay will apply to materials with a non-opaque surface color', (WidgetTester tester) async {
const Color surfaceColor = Color(0xFF121212);
const Color surfaceColorWithOverlay = Color(0xC6353535);
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.dark(),
),
child: buildMaterial(
color: surfaceColor.withOpacity(.75),
elevation: 8.0,
),
),
);
final RenderPhysicalShape model = getModel(tester);
expect(model.color, equals(surfaceColorWithOverlay));
expect(model.color, isNot(equals(surfaceColor)));
});
testWidgets('Expected overlay color can be computed using colorWithOverlay', (WidgetTester tester) async {
const Color surfaceColor = Color(0xFF123456);
const Color onSurfaceColor = Color(0xFF654321);
const double elevation = 8.0;
final Color surfaceColorWithOverlay =
ElevationOverlay.colorWithOverlay(surfaceColor, onSurfaceColor, elevation);
await tester.pumpWidget(
Theme(
data: ThemeData(
useMaterial3: false,
applyElevationOverlayColor: true,
colorScheme: const ColorScheme.dark(
surface: surfaceColor,
onSurface: onSurfaceColor,
),
),
child: buildMaterial(
color: surfaceColor,
elevation: elevation,
),
),
);
final RenderPhysicalShape model = getModel(tester);
expect(model.color, equals(surfaceColorWithOverlay));
expect(model.color, isNot(equals(surfaceColor)));
});
}); // Elevation Overlay M2 group
group('Transparency clipping', () {
testWidgets('No clip by default', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
final RenderClipPath renderClip = tester.allRenderObjects.whereType<RenderClipPath>().first;
expect(renderClip.clipBehavior, equals(Clip.none));
});
testWidgets('clips to bounding rect by default given Clip.antiAlias', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
clipBehavior: Clip.antiAlias,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), clipsWithBoundingRect);
});
testWidgets('clips to rounded rect when borderRadius provided given Clip.antiAlias', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
borderRadius: const BorderRadius.all(Radius.circular(10.0)),
clipBehavior: Clip.antiAlias,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(
find.byKey(materialKey),
clipsWithBoundingRRect(
borderRadius: const BorderRadius.all(Radius.circular(10.0)),
),
);
});
testWidgets('clips to shape when provided given Clip.antiAlias', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
shape: const StadiumBorder(),
clipBehavior: Clip.antiAlias,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(
find.byKey(materialKey),
clipsWithShapeBorder(
shape: const StadiumBorder(),
),
);
});
testWidgets('supports directional clips', (WidgetTester tester) async {
final List<String> logs = <String>[];
final ShapeBorder shape = TestBorder((String message) { logs.add(message); });
Widget buildMaterial() {
return Material(
type: MaterialType.transparency,
shape: shape,
clipBehavior: Clip.antiAlias,
child: const SizedBox(width: 100.0, height: 100.0),
);
}
final Widget material = buildMaterial();
// verify that a regular clip works as one would expect
logs.add('--0');
await tester.pumpWidget(material);
// verify that pumping again doesn't recompute the clip
// even though the widget itself is new (the shape doesn't change identity)
logs.add('--1');
await tester.pumpWidget(buildMaterial());
// verify that Material passes the TextDirection on to its shape when it's transparent
logs.add('--2');
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: material,
));
// verify that changing the text direction from LTR to RTL has an effect
// even though the widget itself is identical
logs.add('--3');
await tester.pumpWidget(Directionality(
textDirection: TextDirection.rtl,
child: material,
));
// verify that pumping again with a text direction has no effect
logs.add('--4');
await tester.pumpWidget(Directionality(
textDirection: TextDirection.rtl,
child: buildMaterial(),
));
logs.add('--5');
// verify that changing the text direction and the widget at the same time
// works as expected
await tester.pumpWidget(Directionality(
textDirection: TextDirection.ltr,
child: material,
));
expect(logs, <String>[
'--0',
'getOuterPath Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) null',
'paint Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) null',
'--1',
'--2',
'getOuterPath Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.ltr',
'paint Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.ltr',
'--3',
'getOuterPath Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.rtl',
'paint Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.rtl',
'--4',
'--5',
'getOuterPath Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.ltr',
'paint Rect.fromLTRB(0.0, 0.0, 800.0, 600.0) TextDirection.ltr',
]);
});
});
group('PhysicalModels', () {
testWidgets('canvas', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: BorderRadius.zero,
elevation: 0.0,
));
});
testWidgets('canvas with borderRadius and elevation', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
borderRadius: const BorderRadius.all(Radius.circular(5.0)),
elevation: 1.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: const BorderRadius.all(Radius.circular(5.0)),
elevation: 1.0,
));
});
testWidgets('canvas with shape and elevation', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
shape: const StadiumBorder(),
elevation: 1.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalShape(
shape: const StadiumBorder(),
elevation: 1.0,
));
});
testWidgets('card', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.card,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: const BorderRadius.all(Radius.circular(2.0)),
elevation: 0.0,
));
});
testWidgets('card with borderRadius and elevation', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.card,
borderRadius: const BorderRadius.all(Radius.circular(5.0)),
elevation: 5.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: const BorderRadius.all(Radius.circular(5.0)),
elevation: 5.0,
));
});
testWidgets('card with shape and elevation', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.card,
shape: const StadiumBorder(),
elevation: 5.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalShape(
shape: const StadiumBorder(),
elevation: 5.0,
));
});
testWidgets('circle', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.circle,
color: const Color(0xFF0000FF),
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.circle,
elevation: 0.0,
));
});
testWidgets('button', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.button,
color: const Color(0xFF0000FF),
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: const BorderRadius.all(Radius.circular(2.0)),
elevation: 0.0,
));
});
testWidgets('button with elevation and borderRadius', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.button,
color: const Color(0xFF0000FF),
borderRadius: const BorderRadius.all(Radius.circular(6.0)),
elevation: 4.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalModel(
shape: BoxShape.rectangle,
borderRadius: const BorderRadius.all(Radius.circular(6.0)),
elevation: 4.0,
));
});
testWidgets('button with elevation and shape', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.button,
color: const Color(0xFF0000FF),
shape: const StadiumBorder(),
elevation: 4.0,
child: const SizedBox(width: 100.0, height: 100.0),
),
);
expect(find.byKey(materialKey), rendersOnPhysicalShape(
shape: const StadiumBorder(),
elevation: 4.0,
));
});
});
group('Border painting', () {
testWidgets('border is painted on physical layers', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.button,
color: const Color(0xFF0000FF),
shape: const CircleBorder(
side: BorderSide(
width: 2.0,
color: Color(0xFF0000FF),
),
),
child: const SizedBox(width: 100.0, height: 100.0),
),
);
final RenderBox box = tester.renderObject(find.byKey(materialKey));
expect(box, paints..circle());
});
testWidgets('border is painted for transparent material', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
shape: const CircleBorder(
side: BorderSide(
width: 2.0,
color: Color(0xFF0000FF),
),
),
child: const SizedBox(width: 100.0, height: 100.0),
),
);
final RenderBox box = tester.renderObject(find.byKey(materialKey));
expect(box, paints..circle());
});
testWidgets('border is not painted for when border side is none', (WidgetTester tester) async {
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(
Material(
key: materialKey,
type: MaterialType.transparency,
shape: const CircleBorder(),
child: const SizedBox(width: 100.0, height: 100.0),
),
);
final RenderBox box = tester.renderObject(find.byKey(materialKey));
expect(box, isNot(paints..circle()));
});
testWidgets('Material2 - border is painted above child by default', (WidgetTester tester) async {
final Key painterKey = UniqueKey();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: RepaintBoundary(
key: painterKey,
child: Card(
child: SizedBox(
width: 200,
height: 300,
child: Material(
clipBehavior: Clip.hardEdge,
shape: const RoundedRectangleBorder(
side: BorderSide(color: Colors.grey, width: 6),
borderRadius: BorderRadius.all(Radius.circular(8)),
),
child: Column(
children: <Widget>[
Container(
color: Colors.green,
height: 150,
),
],
),
),
),
),
),
),
));
await expectLater(
find.byKey(painterKey),
matchesGoldenFile('m2_material.border_paint_above.png'),
);
});
testWidgets('Material3 - border is painted above child by default', (WidgetTester tester) async {
final Key painterKey = UniqueKey();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
body: RepaintBoundary(
key: painterKey,
child: Card(
child: SizedBox(
width: 200,
height: 300,
child: Material(
clipBehavior: Clip.hardEdge,
shape: const RoundedRectangleBorder(
side: BorderSide(color: Colors.grey, width: 6),
borderRadius: BorderRadius.all(Radius.circular(8)),
),
child: Column(
children: <Widget>[
Container(
color: Colors.green,
height: 150,
),
],
),
),
),
),
),
),
));
await expectLater(
find.byKey(painterKey),
matchesGoldenFile('m3_material.border_paint_above.png'),
);
});
testWidgets('Material2 - border is painted below child when specified', (WidgetTester tester) async {
final Key painterKey = UniqueKey();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: RepaintBoundary(
key: painterKey,
child: Card(
child: SizedBox(
width: 200,
height: 300,
child: Material(
clipBehavior: Clip.hardEdge,
shape: const RoundedRectangleBorder(
side: BorderSide(color: Colors.grey, width: 6),
borderRadius: BorderRadius.all(Radius.circular(8)),
),
borderOnForeground: false,
child: Column(
children: <Widget>[
Container(
color: Colors.green,
height: 150,
),
],
),
),
),
),
),
),
));
await expectLater(
find.byKey(painterKey),
matchesGoldenFile('m2_material.border_paint_below.png'),
);
});
testWidgets('Material3 - border is painted below child when specified', (WidgetTester tester) async {
final Key painterKey = UniqueKey();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
body: RepaintBoundary(
key: painterKey,
child: Card(
child: SizedBox(
width: 200,
height: 300,
child: Material(
clipBehavior: Clip.hardEdge,
shape: const RoundedRectangleBorder(
side: BorderSide(color: Colors.grey, width: 6),
borderRadius: BorderRadius.all(Radius.circular(8)),
),
borderOnForeground: false,
child: Column(
children: <Widget>[
Container(
color: Colors.green,
height: 150,
),
],
),
),
),
),
),
),
));
await expectLater(
find.byKey(painterKey),
matchesGoldenFile('m3_material.border_paint_below.png'),
);
});
});
testWidgets('InkFeature skips painting if intermediate node skips', (WidgetTester tester) async {
final GlobalKey sizedBoxKey = GlobalKey();
final GlobalKey materialKey = GlobalKey();
await tester.pumpWidget(Material(
key: materialKey,
child: Offstage(
child: SizedBox(key: sizedBoxKey, width: 20, height: 20),
),
));
final MaterialInkController controller = Material.of(sizedBoxKey.currentContext!);
final TrackPaintInkFeature tracker = TrackPaintInkFeature(
controller: controller,
referenceBox: sizedBoxKey.currentContext!.findRenderObject()! as RenderBox,
);
controller.addInkFeature(tracker);
expect(tracker.paintCount, 0);
final ContainerLayer layer1 = ContainerLayer();
addTearDown(layer1.dispose);
// Force a repaint. Since it's offstage, the ink feature should not get painted.
materialKey.currentContext!.findRenderObject()!.paint(PaintingContext(layer1, Rect.largest), Offset.zero);
expect(tracker.paintCount, 0);
await tester.pumpWidget(Material(
key: materialKey,
child: Offstage(
offstage: false,
child: SizedBox(key: sizedBoxKey, width: 20, height: 20),
),
));
// Gets a paint because the global keys have reused the elements and it is
// now onstage.
expect(tracker.paintCount, 1);
final ContainerLayer layer2 = ContainerLayer();
addTearDown(layer2.dispose);
// Force a repaint again. This time, it gets repainted because it is onstage.
materialKey.currentContext!.findRenderObject()!.paint(PaintingContext(layer2, Rect.largest), Offset.zero);
expect(tracker.paintCount, 2);
tracker.dispose();
});
testWidgets('$InkFeature dispatches memory events', (WidgetTester tester) async {
await tester.pumpWidget(
const Material(
child: SizedBox(width: 20, height: 20),
),
);
final Element element = tester.element(find.byType(SizedBox));
final MaterialInkController controller = Material.of(element);
final RenderBox referenceBox = element.findRenderObject()! as RenderBox;
await expectLater(
await memoryEvents(
() => _InkFeature(
controller: controller,
referenceBox: referenceBox,
).dispose(),
_InkFeature,
),
areCreateAndDispose,
);
});
group('LookupBoundary', () {
testWidgets('hides Material from Material.maybeOf', (WidgetTester tester) async {
MaterialInkController? material;
await tester.pumpWidget(
Material(
child: LookupBoundary(
child: Builder(
builder: (BuildContext context) {
material = Material.maybeOf(context);
return Container();
},
),
),
),
);
expect(material, isNull);
});
testWidgets('hides Material from Material.of', (WidgetTester tester) async {
await tester.pumpWidget(
Material(
child: LookupBoundary(
child: Builder(
builder: (BuildContext context) {
Material.of(context);
return Container();
},
),
),
),
);
final Object? exception = tester.takeException();
expect(exception, isFlutterError);
final FlutterError error = exception! as FlutterError;
expect(
error.toStringDeep(),
'FlutterError\n'
' Material.of() was called with a context that does not have access\n'
' to a Material widget.\n'
' The context provided to Material.of() does have a Material widget\n'
' ancestor, but it is hidden by a LookupBoundary. This can happen\n'
' because you are using a widget that looks for a Material\n'
' ancestor, but no such ancestor exists within the closest\n'
' LookupBoundary.\n'
' The context used was:\n'
' Builder(dirty)\n'
);
});
testWidgets('hides Material from debugCheckHasMaterial', (WidgetTester tester) async {
await tester.pumpWidget(
Material(
child: LookupBoundary(
child: Builder(
builder: (BuildContext context) {
debugCheckHasMaterial(context);
return Container();
},
),
),
),
);
final Object? exception = tester.takeException();
expect(exception, isFlutterError);
final FlutterError error = exception! as FlutterError;
expect(
error.toStringDeep(), startsWith(
'FlutterError\n'
' No Material widget found within the closest LookupBoundary.\n'
' There is an ancestor Material widget, but it is hidden by a\n'
' LookupBoundary.\n'
' Builder widgets require a Material widget ancestor within the\n'
' closest LookupBoundary.\n'
' In Material Design, most widgets are conceptually "printed" on a\n'
" sheet of material. In Flutter's material library, that material\n"
' is represented by the Material widget. It is the Material widget\n'
' that renders ink splashes, for instance. Because of this, many\n'
' material library widgets require that there be a Material widget\n'
' in the tree above them.\n'
' To introduce a Material widget, you can either directly include\n'
' one, or use a widget that contains Material itself, such as a\n'
' Card, Dialog, Drawer, or Scaffold.\n'
' The specific widget that could not find a Material ancestor was:\n'
' Builder\n'
' The ancestors of this widget were:\n'
' LookupBoundary\n'
),
);
});
});
}
class TrackPaintInkFeature extends InkFeature {
TrackPaintInkFeature({required super.controller, required super.referenceBox});
int paintCount = 0;
@override
void paintFeature(Canvas canvas, Matrix4 transform) {
paintCount += 1;
}
}
class _InkFeature extends InkFeature {
_InkFeature({
required super.controller,
required super.referenceBox,
}) {
controller.addInkFeature(this);
}
@override
void paintFeature(Canvas canvas, Matrix4 transform) {}
}
| flutter/packages/flutter/test/material/material_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/material_test.dart",
"repo_id": "flutter",
"token_count": 18667
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@Tags(<String>['reduced-test-set'])
library;
import 'dart:ui' as ui;
import 'package:flutter/cupertino.dart' show CupertinoPageRoute;
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('test page transition (_FadeUpwardsPageTransition)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Page 1')),
theme: ThemeData(
pageTransitionsTheme: const PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
TargetPlatform.android: FadeUpwardsPageTransitionsBuilder(),
},
),
),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(child: Text('Page 2'));
},
},
),
);
final Offset widget1TopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 1));
FadeTransition widget2Opacity =
tester.element(find.text('Page 2')).findAncestorWidgetOfExactType<FadeTransition>()!;
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
final Size widget2Size = tester.getSize(find.text('Page 2'));
// Android transition is vertical only.
expect(widget1TopLeft.dx == widget2TopLeft.dx, true);
// Page 1 is above page 2 mid-transition.
expect(widget1TopLeft.dy < widget2TopLeft.dy, true);
// Animation begins 3/4 of the way up the page.
expect(widget2TopLeft.dy < widget2Size.height / 4.0, true);
// Animation starts with page 2 being near transparent.
expect(widget2Opacity.opacity.value < 0.01, true);
await tester.pump(const Duration(milliseconds: 300));
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 1));
widget2Opacity =
tester.element(find.text('Page 2')).findAncestorWidgetOfExactType<FadeTransition>()!;
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 2 starts to move down.
expect(widget1TopLeft.dy < widget2TopLeft.dy, true);
// Page 2 starts to lose opacity.
expect(widget2Opacity.opacity.value < 1.0, true);
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
}, variant: TargetPlatformVariant.only(TargetPlatform.android));
testWidgets('test page transition (CupertinoPageTransition)', (WidgetTester tester) async {
final Key page2Key = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Page 1')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return Material(
key: page2Key,
child: const Text('Page 2'),
);
},
},
),
);
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 150));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
final RenderDecoratedBox box = tester.element(find.byKey(page2Key))
.findAncestorRenderObjectOfType<RenderDecoratedBox>()!;
// Page 1 is moving to the left.
expect(widget1TransientTopLeft.dx < widget1InitialTopLeft.dx, true);
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy == widget1InitialTopLeft.dy, true);
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy == widget2TopLeft.dy, true);
// Page 2 is coming in from the right.
expect(widget2TopLeft.dx > widget1InitialTopLeft.dx, true);
// As explained in _CupertinoEdgeShadowPainter.paint the shadow is drawn
// as a bunch of rects. The rects are covering an area to the left of
// where the page 2 box is and a width of 5% of the page 2 box width.
// `paints` tests relative to the painter's given canvas
// rather than relative to the screen so assert that the shadow starts at
// offset.dx = 0.
final PaintPattern paintsShadow = paints;
for (int i = 0; i < 0.05 * 800; i += 1) {
paintsShadow.rect(rect: Rect.fromLTWH(-i.toDouble() - 1.0 , 0.0, 1.0, 600));
}
expect(box, paintsShadow);
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is coming back from the left.
expect(widget1TransientTopLeft.dx < widget1InitialTopLeft.dx, true);
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy == widget1InitialTopLeft.dy, true);
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy == widget2TopLeft.dy, true);
// Page 2 is leaving towards the right.
expect(widget2TopLeft.dx > widget1InitialTopLeft.dx, true);
await tester.pumpAndSettle();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft == widget1TransientTopLeft, true);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('test page transition (_ZoomPageTransition) without rasterization', (WidgetTester tester) async {
Iterable<Layer> findLayers(Finder of) {
return tester.layerListOf(
find.ancestor(of: of, matching: find.byType(SnapshotWidget)).first,
);
}
OpacityLayer findForwardFadeTransition(Finder of) {
return findLayers(of).whereType<OpacityLayer>().first;
}
TransformLayer findForwardScaleTransition(Finder of) {
return findLayers(of).whereType<TransformLayer>().first;
}
await tester.pumpWidget(
MaterialApp(
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
allowSnapshotting: false,
builder: (BuildContext context) {
if (settings.name == '/') {
return const Material(child: Text('Page 1'));
}
return const Material(child: Text('Page 2'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
TransformLayer widget1Scale = findForwardScaleTransition(find.text('Page 1'));
TransformLayer widget2Scale = findForwardScaleTransition(find.text('Page 2'));
OpacityLayer widget2Opacity = findForwardFadeTransition(find.text('Page 2'));
double getScale(TransformLayer layer) {
return layer.transform!.storage[0];
}
// Page 1 is enlarging, starts from 1.0.
expect(getScale(widget1Scale), greaterThan(1.0));
// Page 2 is enlarging from the value less than 1.0.
expect(getScale(widget2Scale), lessThan(1.0));
// Page 2 is becoming none transparent.
expect(widget2Opacity.alpha, lessThan(255));
await tester.pump(const Duration(milliseconds: 250));
await tester.pump(const Duration(milliseconds: 1));
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1Scale = findForwardScaleTransition(find.text('Page 1'));
widget2Scale = findForwardScaleTransition(find.text('Page 2'));
widget2Opacity = findForwardFadeTransition(find.text('Page 2'));
// Page 1 is narrowing down, but still larger than 1.0.
expect(getScale(widget1Scale), greaterThan(1.0));
// Page 2 is smaller than 1.0.
expect(getScale(widget2Scale), lessThan(1.0));
// Page 2 is becoming transparent.
expect(widget2Opacity.alpha, lessThan(255));
await tester.pump(const Duration(milliseconds: 200));
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
}, variant: TargetPlatformVariant.only(TargetPlatform.android));
testWidgets('Material2 - test page transition (_ZoomPageTransition) with rasterization re-rasterizes when view insets change', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(1000, 1000);
tester.view.viewInsets = FakeViewPadding.zero;
// Intentionally use nested scaffolds to simulate the view insets being
// consumed.
final Key key = GlobalKey();
await tester.pumpWidget(
RepaintBoundary(
key: key,
child: MaterialApp(
theme: ThemeData(useMaterial3: false),
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(body: Scaffold(
body: Material(child: SizedBox.shrink())
));
},
);
},
),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
await expectLater(find.byKey(key), matchesGoldenFile('m2_zoom_page_transition.small.png'));
// Change the view insets.
tester.view.viewInsets = const FakeViewPadding(bottom: 500);
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
await expectLater(find.byKey(key), matchesGoldenFile('m2_zoom_page_transition.big.png'));
}, variant: TargetPlatformVariant.only(TargetPlatform.android), skip: kIsWeb); // [intended] rasterization is not used on the web.
testWidgets('Material3 - test page transition (_ZoomPageTransition) with rasterization re-rasterizes when view insets change', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(1000, 1000);
tester.view.viewInsets = FakeViewPadding.zero;
// Intentionally use nested scaffolds to simulate the view insets being
// consumed.
final Key key = GlobalKey();
await tester.pumpWidget(
RepaintBoundary(
key: key,
child: MaterialApp(
debugShowCheckedModeBanner: false, // https://github.com/flutter/flutter/issues/143616
theme: ThemeData(useMaterial3: true),
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(body: Scaffold(
body: Material(child: SizedBox.shrink())
));
},
);
},
),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
await expectLater(find.byKey(key), matchesGoldenFile('m3_zoom_page_transition.small.png'));
// Change the view insets.
tester.view.viewInsets = const FakeViewPadding(bottom: 500);
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
await expectLater(find.byKey(key), matchesGoldenFile('m3_zoom_page_transition.big.png'));
}, variant: TargetPlatformVariant.only(TargetPlatform.android), skip: kIsWeb); // [intended] rasterization is not used on the web.
testWidgets(
'test page transition (_ZoomPageTransition) with rasterization disables snapshotting for enter route',
(WidgetTester tester) async {
Iterable<Layer> findLayers(Finder of) {
return tester.layerListOf(
find.ancestor(of: of, matching: find.byType(SnapshotWidget)).first,
);
}
bool isTransitioningWithoutSnapshotting(Finder of) {
// When snapshotting is off, the OpacityLayer and TransformLayer will be
// applied directly.
final Iterable<Layer> layers = findLayers(of);
return layers.whereType<OpacityLayer>().length == 1 &&
layers.whereType<TransformLayer>().length == 1;
}
bool isSnapshotted(Finder of) {
final Iterable<Layer> layers = findLayers(of);
// The scrim and the snapshot image are the only two layers.
return layers.length == 2 &&
layers.whereType<OffsetLayer>().length == 1 &&
layers.whereType<PictureLayer>().length == 1;
}
await tester.pumpWidget(
MaterialApp(
routes: <String, WidgetBuilder>{
'/1': (_) => const Material(child: Text('Page 1')),
'/2': (_) => const Material(child: Text('Page 2')),
},
initialRoute: '/1',
builder: (BuildContext context, Widget? child) {
final ThemeData themeData = Theme.of(context);
return Theme(
data: themeData.copyWith(
pageTransitionsTheme: PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
...themeData.pageTransitionsTheme.builders,
TargetPlatform.android: const ZoomPageTransitionsBuilder(
allowEnterRouteSnapshotting: false,
),
},
),
),
child: Builder(builder: (_) => child!),
);
},
),
);
final Finder page1Finder = find.text('Page 1');
final Finder page2Finder = find.text('Page 2');
// Page 1 on top.
expect(isSnapshotted(page1Finder), isFalse);
// Transitioning from page 1 to page 2.
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/2');
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
expect(isSnapshotted(page1Finder), isTrue);
expect(isTransitioningWithoutSnapshotting(page2Finder), isTrue);
// Page 2 on top.
await tester.pumpAndSettle();
expect(isSnapshotted(page2Finder), isFalse);
// Transitioning back from page 2 to page 1.
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
expect(isTransitioningWithoutSnapshotting(page1Finder), isTrue);
expect(isSnapshotted(page2Finder), isTrue);
// Page 1 on top.
await tester.pumpAndSettle();
expect(isSnapshotted(page1Finder), isFalse);
}, variant: TargetPlatformVariant.only(TargetPlatform.android), skip: kIsWeb); // [intended] rasterization is not used on the web.
testWidgets('test fullscreen dialog transition', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(child: Text('Page 1')),
),
);
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).push(MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Material(child: Text('Page 2'));
},
fullscreenDialog: true,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 doesn't move.
expect(widget1TransientTopLeft == widget1InitialTopLeft, true);
// Fullscreen dialogs transitions vertically only.
expect(widget1InitialTopLeft.dx == widget2TopLeft.dx, true);
// Page 2 is coming in from the bottom.
expect(widget2TopLeft.dy > widget1InitialTopLeft.dy, true);
await tester.pumpAndSettle();
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 doesn't move.
expect(widget1TransientTopLeft == widget1InitialTopLeft, true);
// Fullscreen dialogs transitions vertically only.
expect(widget1InitialTopLeft.dx == widget2TopLeft.dx, true);
// Page 2 is leaving towards the bottom.
expect(widget2TopLeft.dy > widget1InitialTopLeft.dy, true);
await tester.pumpAndSettle();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft == widget1TransientTopLeft, true);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('test no back gesture on Android', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: const Scaffold(body: Text('Page 1')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Scaffold(body: Text('Page 2'));
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from left edge to invoke the gesture.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 100.0));
await gesture.moveBy(const Offset(400.0, 0.0));
await tester.pump();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Page 2 didn't move.
expect(tester.getTopLeft(find.text('Page 2')), Offset.zero);
}, variant: TargetPlatformVariant.only(TargetPlatform.android));
testWidgets('test back gesture', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: const Scaffold(body: Text('Page 1')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Scaffold(body: Text('Page 2'));
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from left edge to invoke the gesture.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 100.0));
await gesture.moveBy(const Offset(400.0, 0.0));
await tester.pump();
// Page 1 is now visible.
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), isOnstage);
// The route widget position needs to track the finger position very exactly.
expect(tester.getTopLeft(find.text('Page 2')), const Offset(400.0, 0.0));
await gesture.moveBy(const Offset(-200.0, 0.0));
await tester.pump();
expect(tester.getTopLeft(find.text('Page 2')), const Offset(200.0, 0.0));
await gesture.moveBy(const Offset(-100.0, 200.0));
await tester.pump();
expect(tester.getTopLeft(find.text('Page 2')), const Offset(100.0, 0.0));
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('back gesture while OS changes', (WidgetTester tester) async {
final Map<String, WidgetBuilder> routes = <String, WidgetBuilder>{
'/': (BuildContext context) => Material(
child: TextButton(
child: const Text('PUSH'),
onPressed: () { Navigator.of(context).pushNamed('/b'); },
),
),
'/b': (BuildContext context) => const Text('HELLO'),
};
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
routes: routes,
),
);
await tester.tap(find.text('PUSH'));
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 2);
expect(find.text('PUSH'), findsNothing);
expect(find.text('HELLO'), findsOneWidget);
final Offset helloPosition1 = tester.getCenter(find.text('HELLO'));
final TestGesture gesture = await tester.startGesture(const Offset(2.5, 300.0));
await tester.pump(const Duration(milliseconds: 20));
await gesture.moveBy(const Offset(100.0, 0.0));
expect(find.text('PUSH'), findsNothing);
expect(find.text('HELLO'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 20));
expect(find.text('PUSH'), findsOneWidget);
expect(find.text('HELLO'), findsOneWidget);
final Offset helloPosition2 = tester.getCenter(find.text('HELLO'));
expect(helloPosition1.dx, lessThan(helloPosition2.dx));
expect(helloPosition1.dy, helloPosition2.dy);
expect(Theme.of(tester.element(find.text('HELLO'))).platform, TargetPlatform.iOS);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.android),
routes: routes,
),
);
// Now we have to let the theme animation run through.
// This takes three frames (including the first one above):
// 1. Start the Theme animation. It's at t=0 so everything else is identical.
// 2. Start any animations that are informed by the Theme, for example, the
// DefaultTextStyle, on the first frame that the theme is not at t=0. In
// this case, it's at t=1.0 of the theme animation, so this is also the
// frame in which the theme animation ends.
// 3. End all the other animations.
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 2);
expect(Theme.of(tester.element(find.text('HELLO'))).platform, TargetPlatform.android);
final Offset helloPosition3 = tester.getCenter(find.text('HELLO'));
expect(helloPosition3, helloPosition2);
expect(find.text('PUSH'), findsOneWidget);
expect(find.text('HELLO'), findsOneWidget);
await gesture.moveBy(const Offset(100.0, 0.0));
await tester.pump(const Duration(milliseconds: 20));
expect(find.text('PUSH'), findsOneWidget);
expect(find.text('HELLO'), findsOneWidget);
final Offset helloPosition4 = tester.getCenter(find.text('HELLO'));
expect(helloPosition3.dx, lessThan(helloPosition4.dx));
expect(helloPosition3.dy, helloPosition4.dy);
await gesture.moveBy(const Offset(500.0, 0.0));
await gesture.up();
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 3);
expect(find.text('PUSH'), findsOneWidget);
expect(find.text('HELLO'), findsNothing);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.macOS),
routes: routes,
),
);
await tester.tap(find.text('PUSH'));
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 2);
expect(find.text('PUSH'), findsNothing);
expect(find.text('HELLO'), findsOneWidget);
final Offset helloPosition5 = tester.getCenter(find.text('HELLO'));
await gesture.down(const Offset(2.5, 300.0));
await tester.pump(const Duration(milliseconds: 20));
await gesture.moveBy(const Offset(100.0, 0.0));
expect(find.text('PUSH'), findsNothing);
expect(find.text('HELLO'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 20));
expect(find.text('PUSH'), findsOneWidget);
expect(find.text('HELLO'), findsOneWidget);
final Offset helloPosition6 = tester.getCenter(find.text('HELLO'));
expect(helloPosition5.dx, lessThan(helloPosition6.dx));
expect(helloPosition5.dy, helloPosition6.dy);
expect(Theme.of(tester.element(find.text('HELLO'))).platform, TargetPlatform.macOS);
});
testWidgets('test no back gesture on fullscreen dialogs', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(body: Text('Page 1')),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(body: Text('Page 2'));
},
fullscreenDialog: true,
));
await tester.pumpAndSettle();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Drag from left edge to invoke the gesture.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 100.0));
await gesture.moveBy(const Offset(400.0, 0.0));
await tester.pump();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Page 2 didn't move.
expect(tester.getTopLeft(find.text('Page 2')), Offset.zero);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('test adaptable transitions switch during execution', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
platform: TargetPlatform.android,
pageTransitionsTheme: const PageTransitionsTheme(
builders: <TargetPlatform, PageTransitionsBuilder>{
TargetPlatform.android: FadeUpwardsPageTransitionsBuilder(),
},
),
),
home: const Material(child: Text('Page 1')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(child: Text('Page 2'));
},
},
),
);
final Offset widget1InitialTopLeft = tester.getTopLeft(find.text('Page 1'));
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
Offset widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
final Size widget2Size = tester.getSize(find.text('Page 2'));
// Android transition is vertical only.
expect(widget1InitialTopLeft.dx == widget2TopLeft.dx, true);
// Page 1 is above page 2 mid-transition.
expect(widget1InitialTopLeft.dy < widget2TopLeft.dy, true);
// Animation begins from the top of the page.
expect(widget2TopLeft.dy < widget2Size.height, true);
await tester.pump(const Duration(milliseconds: 300));
// Page 2 covers page 1.
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
// Re-pump the same app but with iOS instead of Android.
await tester.pumpWidget(
MaterialApp(
home: const Material(child: Text('Page 1')),
routes: <String, WidgetBuilder>{
'/next': (BuildContext context) {
return const Material(child: Text('Page 2'));
},
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
Offset widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
widget2TopLeft = tester.getTopLeft(find.text('Page 2'));
// Page 1 is coming back from the left.
expect(widget1TransientTopLeft.dx < widget1InitialTopLeft.dx, true);
// Page 1 isn't moving vertically.
expect(widget1TransientTopLeft.dy == widget1InitialTopLeft.dy, true);
// iOS transition is horizontal only.
expect(widget1InitialTopLeft.dy == widget2TopLeft.dy, true);
// Page 2 is leaving towards the right.
expect(widget2TopLeft.dx > widget1InitialTopLeft.dx, true);
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
widget1TransientTopLeft = tester.getTopLeft(find.text('Page 1'));
// Page 1 is back where it started.
expect(widget1InitialTopLeft == widget1TransientTopLeft, true);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('test edge swipe then drop back at starting point works', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
final TestGesture gesture = await tester.startGesture(const Offset(5, 200));
await gesture.moveBy(const Offset(300, 0));
await tester.pump();
// Bring it exactly back such that there's nothing to animate when releasing.
await gesture.moveBy(const Offset(-300, 0));
await gesture.up();
await tester.pump();
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('test edge swipe then drop back at ending point works', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
final String pageNumber = settings.name == '/' ? '1' : '2';
return Center(child: Text('Page $pageNumber'));
},
);
},
),
);
tester.state<NavigatorState>(find.byType(Navigator)).pushNamed('/next');
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), isOnstage);
final TestGesture gesture = await tester.startGesture(const Offset(5, 200));
// The width of the page.
await gesture.moveBy(const Offset(800, 0));
await gesture.up();
await tester.pump();
expect(find.text('Page 1'), isOnstage);
expect(find.text('Page 2'), findsNothing);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Back swipe dismiss interrupted by route push', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/28728
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(
body: Center(child: Text('route')),
);
},
));
},
child: const Text('push'),
),
),
),
),
);
// Check the basic iOS back-swipe dismiss transition. Dragging the pushed
// route halfway across the screen will trigger the iOS dismiss animation.
await tester.tap(find.text('push'));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0));
await gesture.up();
await tester.pump();
expect( // The 'route' route has been dragged to the right, halfway across the screen.
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(Scaffold))),
const Offset(400, 0),
);
expect( // The 'push' route is sliding in from the left.
tester.getTopLeft(find.ancestor(of: find.text('push'), matching: find.byType(Scaffold))).dx,
lessThan(0),
);
await tester.pumpAndSettle();
expect(find.text('push'), findsOneWidget);
expect(
tester.getTopLeft(find.ancestor(of: find.text('push'), matching: find.byType(Scaffold))),
Offset.zero,
);
expect(find.text('route'), findsNothing);
// Run the dismiss animation 60%, which exposes the route "push" button,
// and then press the button. A drag dropped animation is 400ms when dropped
// exactly halfway. It follows a curve that is very steep initially.
await tester.tap(find.text('push'));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0)); // Drag halfway.
await gesture.up();
await tester.pump(); // Trigger the dropped snapping animation.
expect(
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(Scaffold))),
const Offset(400, 0),
);
// Let the dismissing snapping animation go 60%.
await tester.pump(const Duration(milliseconds: 240));
expect(
tester.getTopLeft(find.ancestor(of: find.text('route'), matching: find.byType(Scaffold))).dx,
moreOrLessEquals(798, epsilon: 1),
);
// Use the navigator to push a route instead of tapping the 'push' button.
// The topmost route (the one that's animating away), ignores input while
// the pop is underway because route.navigator.userGestureInProgress.
Navigator.push<void>(scaffoldKey.currentContext!, MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(
body: Center(child: Text('route')),
);
},
));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('During back swipe the route ignores input', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/39989
final GlobalKey homeScaffoldKey = GlobalKey();
final GlobalKey pageScaffoldKey = GlobalKey();
int homeTapCount = 0;
int pageTapCount = 0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: homeScaffoldKey,
body: GestureDetector(
onTap: () {
homeTapCount += 1;
},
),
),
),
);
await tester.tap(find.byKey(homeScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 0);
Navigator.push<void>(homeScaffoldKey.currentContext!, MaterialPageRoute<void>(
builder: (BuildContext context) {
return Scaffold(
key: pageScaffoldKey,
appBar: AppBar(title: const Text('Page')),
body: Padding(
padding: const EdgeInsets.all(16),
child: GestureDetector(
onTap: () {
pageTapCount += 1;
},
),
),
);
},
));
await tester.pumpAndSettle();
await tester.tap(find.byKey(pageScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 1);
// Start the basic iOS back-swipe dismiss transition. Drag the pushed
// "page" route halfway across the screen. The underlying "home" will
// start sliding in from the left.
final TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(400, 0));
await tester.pump();
expect(tester.getTopLeft(find.byKey(pageScaffoldKey)), const Offset(400, 0));
expect(tester.getTopLeft(find.byKey(homeScaffoldKey)).dx, lessThan(0));
// Tapping on the "page" route doesn't trigger the GestureDetector because
// it's being dragged.
await tester.tap(find.byKey(pageScaffoldKey), warnIfMissed: false);
expect(homeTapCount, 1);
expect(pageTapCount, 1);
// Tapping the "page" route's back button doesn't do anything either.
await tester.tap(find.byTooltip('Back'), warnIfMissed: false);
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.byKey(pageScaffoldKey)), const Offset(400, 0));
expect(tester.getTopLeft(find.byKey(homeScaffoldKey)).dx, lessThan(0));
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('After a pop caused by a back-swipe, input reaches the exposed route', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/41024
final GlobalKey homeScaffoldKey = GlobalKey();
final GlobalKey pageScaffoldKey = GlobalKey();
int homeTapCount = 0;
int pageTapCount = 0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: homeScaffoldKey,
body: GestureDetector(
onTap: () {
homeTapCount += 1;
},
),
),
),
);
await tester.tap(find.byKey(homeScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 0);
final ValueNotifier<bool> notifier = Navigator.of(homeScaffoldKey.currentContext!).userGestureInProgressNotifier;
expect(notifier.value, false);
Navigator.push<void>(homeScaffoldKey.currentContext!, MaterialPageRoute<void>(
builder: (BuildContext context) {
return Scaffold(
key: pageScaffoldKey,
appBar: AppBar(title: const Text('Page')),
body: Padding(
padding: const EdgeInsets.all(16),
child: GestureDetector(
onTap: () {
pageTapCount += 1;
},
),
),
);
},
));
await tester.pumpAndSettle();
await tester.tap(find.byKey(pageScaffoldKey));
expect(homeTapCount, 1);
expect(pageTapCount, 1);
// Trigger the basic iOS back-swipe dismiss transition. Drag the pushed
// "page" route more than halfway across the screen and then release it.
final TestGesture gesture = await tester.startGesture(const Offset(5, 300));
await gesture.moveBy(const Offset(500, 0));
await tester.pump();
expect(tester.getTopLeft(find.byKey(pageScaffoldKey)), const Offset(500, 0));
expect(tester.getTopLeft(find.byKey(homeScaffoldKey)).dx, lessThan(0));
expect(notifier.value, true);
await gesture.up();
await tester.pumpAndSettle();
expect(notifier.value, false);
expect(find.byKey(pageScaffoldKey), findsNothing);
// The back-swipe dismiss pop transition has finished and input on the
// home page still works.
await tester.tap(find.byKey(homeScaffoldKey));
expect(homeTapCount, 2);
expect(pageTapCount, 1);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('A MaterialPageRoute should slide out with CupertinoPageTransition when a compatible PageRoute is pushed on top of it', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/44864.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
home: Scaffold(
appBar: AppBar(title: const Text('Title')),
),
),
);
final Offset titleInitialTopLeft = tester.getTopLeft(find.text('Title'));
tester.state<NavigatorState>(find.byType(Navigator)).push<void>(
CupertinoPageRoute<void>(builder: (BuildContext context) => const Placeholder()),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 150));
final Offset titleTransientTopLeft = tester.getTopLeft(find.text('Title'));
// Title of the first route slides to the left.
expect(titleInitialTopLeft.dy, equals(titleTransientTopLeft.dy));
expect(titleInitialTopLeft.dx, greaterThan(titleTransientTopLeft.dx));
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('MaterialPage works', (WidgetTester tester) async {
final LocalKey pageKey = UniqueKey();
final TransitionDetector detector = TransitionDetector();
List<Page<void>> myPages = <Page<void>>[
MaterialPage<void>(key: pageKey, child: const Text('first')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test should never execute this.
return true;
},
transitionDelegate: detector,
),
);
expect(detector.hasTransition, isFalse);
expect(find.text('first'), findsOneWidget);
myPages = <Page<void>>[
MaterialPage<void>(key: pageKey, child: const Text('second')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test should never execute this.
return true;
},
transitionDelegate: detector,
),
);
// There should be no transition because the page has the same key.
expect(detector.hasTransition, isFalse);
// The content does update.
expect(find.text('first'), findsNothing);
expect(find.text('second'), findsOneWidget);
});
testWidgets('MaterialPage can toggle MaintainState', (WidgetTester tester) async {
final LocalKey pageKeyOne = UniqueKey();
final LocalKey pageKeyTwo = UniqueKey();
final TransitionDetector detector = TransitionDetector();
List<Page<void>> myPages = <Page<void>>[
MaterialPage<void>(key: pageKeyOne, maintainState: false, child: const Text('first')),
MaterialPage<void>(key: pageKeyTwo, child: const Text('second')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test should never execute this.
return true;
},
transitionDelegate: detector,
),
);
expect(detector.hasTransition, isFalse);
// Page one does not maintain state.
expect(find.text('first', skipOffstage: false), findsNothing);
expect(find.text('second'), findsOneWidget);
myPages = <Page<void>>[
MaterialPage<void>(key: pageKeyOne, child: const Text('first')),
MaterialPage<void>(key: pageKeyTwo, child: const Text('second')),
];
await tester.pumpWidget(
buildNavigator(
view: tester.view,
pages: myPages,
onPopPage: (Route<dynamic> route, dynamic result) {
assert(false); // The test should never execute this.
return true;
},
transitionDelegate: detector,
),
);
// There should be no transition because the page has the same key.
expect(detector.hasTransition, isFalse);
// Page one sets the maintain state to be true, its widget tree should be
// built.
expect(find.text('first', skipOffstage: false), findsOneWidget);
expect(find.text('second'), findsOneWidget);
});
testWidgets('MaterialPage does not lose its state when transitioning out', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigator = GlobalKey<NavigatorState>();
await tester.pumpWidget(KeepsStateTestWidget(navigatorKey: navigator));
expect(find.text('subpage'), findsOneWidget);
expect(find.text('home'), findsNothing);
navigator.currentState!.pop();
await tester.pump();
expect(find.text('subpage'), findsOneWidget);
expect(find.text('home'), findsOneWidget);
});
testWidgets('MaterialPage restores its state', (WidgetTester tester) async {
await tester.pumpWidget(
RootRestorationScope(
restorationId: 'root',
child: TestDependencies(
child: Navigator(
onPopPage: (Route<dynamic> route, dynamic result) { return false; },
pages: const <Page<Object?>>[
MaterialPage<void>(
restorationId: 'p1',
child: TestRestorableWidget(restorationId: 'p1'),
),
],
restorationScopeId: 'nav',
onGenerateRoute: (RouteSettings settings) {
return MaterialPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return TestRestorableWidget(restorationId: settings.name!);
},
);
},
),
),
),
);
expect(find.text('p1'), findsOneWidget);
expect(find.text('count: 0'), findsOneWidget);
await tester.tap(find.text('increment'));
await tester.pump();
expect(find.text('count: 1'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).restorablePushNamed('p2');
await tester.pumpAndSettle();
expect(find.text('p1'), findsNothing);
expect(find.text('p2'), findsOneWidget);
await tester.tap(find.text('increment'));
await tester.pump();
await tester.tap(find.text('increment'));
await tester.pump();
expect(find.text('count: 2'), findsOneWidget);
await tester.restartAndRestore();
expect(find.text('p2'), findsOneWidget);
expect(find.text('count: 2'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pumpAndSettle();
expect(find.text('p1'), findsOneWidget);
expect(find.text('count: 1'), findsOneWidget);
});
testWidgets('MaterialPageRoute can be dismissed with escape keyboard shortcut', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/132138.
final GlobalKey scaffoldKey = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push<void>(scaffoldKey.currentContext!, MaterialPageRoute<void>(
builder: (BuildContext context) {
return const Scaffold(
body: Center(child: Text('route')),
);
},
barrierDismissible: true,
));
},
child: const Text('push'),
),
),
),
),
);
await tester.tap(find.text('push'));
await tester.pumpAndSettle();
expect(find.text('route'), findsOneWidget);
expect(find.text('push'), findsNothing);
// Try to dismiss the route with the escape key.
await tester.sendKeyEvent(LogicalKeyboardKey.escape);
await tester.pumpAndSettle();
expect(find.text('route'), findsNothing);
});
}
class TransitionDetector extends DefaultTransitionDelegate<void> {
bool hasTransition = false;
@override
Iterable<RouteTransitionRecord> resolve({
required List<RouteTransitionRecord> newPageRouteHistory,
required Map<RouteTransitionRecord?, RouteTransitionRecord> locationToExitingPageRoute,
required Map<RouteTransitionRecord?, List<RouteTransitionRecord>> pageRouteToPagelessRoutes,
}) {
hasTransition = true;
return super.resolve(
newPageRouteHistory: newPageRouteHistory,
locationToExitingPageRoute: locationToExitingPageRoute,
pageRouteToPagelessRoutes: pageRouteToPagelessRoutes,
);
}
}
Widget buildNavigator({
required List<Page<dynamic>> pages,
required PopPageCallback onPopPage,
required ui.FlutterView view,
GlobalKey<NavigatorState>? key,
TransitionDelegate<dynamic>? transitionDelegate,
}) {
return MediaQuery(
data: MediaQueryData.fromView(view),
child: Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: Navigator(
key: key,
pages: pages,
onPopPage: onPopPage,
transitionDelegate: transitionDelegate ?? const DefaultTransitionDelegate<dynamic>(),
),
),
),
);
}
class KeepsStateTestWidget extends StatefulWidget {
const KeepsStateTestWidget({super.key, this.navigatorKey});
final Key? navigatorKey;
@override
State<KeepsStateTestWidget> createState() => _KeepsStateTestWidgetState();
}
class _KeepsStateTestWidgetState extends State<KeepsStateTestWidget> {
String? _subpage = 'subpage';
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Navigator(
key: widget.navigatorKey,
pages: <Page<void>>[
const MaterialPage<void>(child: Text('home')),
if (_subpage != null) MaterialPage<void>(child: Text(_subpage!)),
],
onPopPage: (Route<dynamic> route, dynamic result) {
if (!route.didPop(result)) {
return false;
}
setState(() {
_subpage = null;
});
return true;
},
),
);
}
}
class TestRestorableWidget extends StatefulWidget {
const TestRestorableWidget({super.key, required this.restorationId});
final String restorationId;
@override
State<StatefulWidget> createState() => _TestRestorableWidgetState();
}
class _TestRestorableWidgetState extends State<TestRestorableWidget> with RestorationMixin {
@override
String? get restorationId => widget.restorationId;
final RestorableInt counter = RestorableInt(0);
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(counter, 'counter');
}
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
Text(widget.restorationId),
Text('count: ${counter.value}'),
ElevatedButton(
onPressed: () {
setState(() {
counter.value++;
});
},
child: const Text('increment'),
),
],
);
}
@override
void dispose() {
counter.dispose();
super.dispose();
}
}
class TestDependencies extends StatelessWidget {
const TestDependencies({required this.child, super.key});
final Widget child;
@override
Widget build(BuildContext context) {
return Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: MediaQueryData.fromView(View.of(context)),
child: child,
),
);
}
}
| flutter/packages/flutter/test/material/page_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/page_test.dart",
"repo_id": "flutter",
"token_count": 19951
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
const Color _kAndroidThumbIdleColor = Color(0xffbcbcbc);
Widget _buildSingleChildScrollViewWithScrollbar({
TextDirection textDirection = TextDirection.ltr,
EdgeInsets padding = EdgeInsets.zero,
Widget? child,
}) {
return Directionality(
textDirection: textDirection,
child: MediaQuery(
data: MediaQueryData(padding: padding),
child: Scrollbar(
child: SingleChildScrollView(child: child),
),
),
);
}
void main() {
testWidgets('Viewport basic test (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(_buildSingleChildScrollViewWithScrollbar(
child: const SizedBox(width: 4000.0, height: 4000.0),
));
expect(find.byType(Scrollbar), isNot(paints..rect()));
await tester.fling(find.byType(SingleChildScrollView), const Offset(0.0, -10.0), 10.0);
expect(
find.byType(Scrollbar),
paints
..rect(
rect: const Rect.fromLTRB(796.0, 0.0, 800.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(796.0, 0.0),
p2: const Offset(796.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(796.0, 1.5, 800.0, 91.5),
color: _kAndroidThumbIdleColor,
),
);
});
testWidgets('Viewport basic test (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(_buildSingleChildScrollViewWithScrollbar(
textDirection: TextDirection.rtl,
child: const SizedBox(width: 4000.0, height: 4000.0),
));
expect(find.byType(Scrollbar), isNot(paints..rect()));
await tester.fling(find.byType(SingleChildScrollView), const Offset(0.0, -10.0), 10.0);
expect(
find.byType(Scrollbar),
paints
..rect(
rect: const Rect.fromLTRB(0.0, 0.0, 4.0, 600.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(4.0, 0.0),
p2: const Offset(4.0, 600.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTRB(0.0, 1.5, 4.0, 91.5),
color: _kAndroidThumbIdleColor,
),
);
});
testWidgets('works with MaterialApp and Scaffold', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.fromLTRB(0, 20, 0, 34),
),
child: Scaffold(
appBar: AppBar(title: const Text('Title')),
body: Scrollbar(
child: ListView(
children: const <Widget>[SizedBox(width: 4000, height: 4000)],
),
),
),
),
));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(ListView)));
// On Android it should not overscroll.
await gesture.moveBy(const Offset(0, 100));
// Trigger fade in animation.
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(
find.byType(Scrollbar),
paints
..rect(
rect: const Rect.fromLTRB(796.0, 0.0, 800.0, 490.0),
color: const Color(0x00000000),
)
..line(
p1: const Offset(796.0, 0.0),
p2: const Offset(796.0, 490.0),
strokeWidth: 1.0,
color: const Color(0x00000000),
)
..rect(
rect: const Rect.fromLTWH(796.0, 0.0, 4.0, (600.0 - 56 - 34 - 20) / 4000 * (600 - 56 - 34 - 20)),
color: _kAndroidThumbIdleColor,
),
);
});
testWidgets("should not paint when there isn't enough space", (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.fromLTRB(0, 20, 0, 34),
),
child: Scaffold(
appBar: AppBar(title: const Text('Title')),
body: Scrollbar(
child: ListView(
children: const <Widget>[SizedBox(width: 40, height: 40)],
),
),
),
),
));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(ListView)));
// On Android it should not overscroll.
await gesture.moveBy(const Offset(0, 100));
// Trigger fade in animation.
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.byType(Scrollbar), isNot(paints..rect()));
});
}
| flutter/packages/flutter/test/material/scrollbar_paint_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/scrollbar_paint_test.dart",
"repo_id": "flutter",
"token_count": 2178
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Material3 has sentence case labels', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: Stepper(
onStepTapped: (int i) {},
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
expect(find.text('Continue'), findsWidgets);
expect(find.text('Cancel'), findsWidgets);
});
testWidgets('Stepper tap callback test', (WidgetTester tester) async {
int index = 0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
onStepTapped: (int i) {
index = i;
},
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
await tester.tap(find.text('Step 2'));
expect(index, 1);
});
testWidgets('Stepper expansion test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 200.0,
height: 200.0,
),
),
],
),
),
),
),
);
RenderBox box = tester.renderObject(find.byType(Stepper));
expect(box.size.height, 332.0);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
currentStep: 1,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 200.0,
height: 200.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
box = tester.renderObject(find.byType(Stepper));
expect(box.size.height, greaterThan(332.0));
await tester.pump(const Duration(milliseconds: 100));
box = tester.renderObject(find.byType(Stepper));
expect(box.size.height, 432.0);
});
testWidgets('Stepper horizontal size test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(Stepper));
expect(box.size.height, 600.0);
});
testWidgets('Stepper visibility test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: Text('A'),
),
Step(
title: Text('Step 2'),
content: Text('B'),
),
],
),
),
),
);
expect(find.text('A'), findsOneWidget);
expect(find.text('B'), findsNothing);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
currentStep: 1,
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: Text('A'),
),
Step(
title: Text('Step 2'),
content: Text('B'),
),
],
),
),
),
);
expect(find.text('A'), findsNothing);
expect(find.text('B'), findsOneWidget);
});
testWidgets('Material2 - Stepper button test', (WidgetTester tester) async {
bool continuePressed = false;
bool cancelPressed = false;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Material(
child: Stepper(
type: StepperType.horizontal,
onStepContinue: () {
continuePressed = true;
},
onStepCancel: () {
cancelPressed = true;
},
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 200.0,
height: 200.0,
),
),
],
),
),
),
);
await tester.tap(find.text('CONTINUE'));
await tester.tap(find.text('CANCEL'));
expect(continuePressed, isTrue);
expect(cancelPressed, isTrue);
});
testWidgets('Material3 - Stepper button test', (WidgetTester tester) async {
bool continuePressed = false;
bool cancelPressed = false;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Material(
child: Stepper(
type: StepperType.horizontal,
onStepContinue: () {
continuePressed = true;
},
onStepCancel: () {
cancelPressed = true;
},
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 200.0,
height: 200.0,
),
),
],
),
),
),
);
await tester.tap(find.text('Continue'));
await tester.tap(find.text('Cancel'));
expect(continuePressed, isTrue);
expect(cancelPressed, isTrue);
});
testWidgets('Stepper disabled step test', (WidgetTester tester) async {
int index = 0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
onStepTapped: (int i) {
index = i;
},
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('Step 2'),
state: StepState.disabled,
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
await tester.tap(find.text('Step 2'));
expect(index, 0);
});
testWidgets('Stepper scroll test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 300.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 100.0,
height: 300.0,
),
),
Step(
title: Text('Step 3'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
final ScrollableState scrollableState = tester.firstState(find.byType(Scrollable));
expect(scrollableState.position.pixels, 0.0);
await tester.tap(find.text('Step 3'));
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
currentStep: 2,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 300.0,
),
),
Step(
title: Text('Step 2'),
content: SizedBox(
width: 100.0,
height: 300.0,
),
),
Step(
title: Text('Step 3'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(scrollableState.position.pixels, greaterThan(0.0));
});
testWidgets('Stepper index test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.complete,
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('B'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
expect(find.text('1'), findsNothing);
expect(find.text('2'), findsOneWidget);
});
testWidgets('Stepper custom controls test', (WidgetTester tester) async {
bool continuePressed = false;
void setContinue() {
continuePressed = true;
}
bool canceledPressed = false;
void setCanceled() {
canceledPressed = true;
}
Widget builder(BuildContext context, ControlsDetails details) {
return Container(
margin: const EdgeInsets.only(top: 16.0),
child: ConstrainedBox(
constraints: const BoxConstraints.tightFor(height: 48.0),
child: Row(
children: <Widget>[
TextButton(
onPressed: details.onStepContinue,
child: const Text('Let us continue!'),
),
Container(
margin: const EdgeInsetsDirectional.only(start: 8.0),
child: TextButton(
onPressed: details.onStepCancel,
child: const Text('Cancel This!'),
),
),
],
),
),
);
}
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
controlsBuilder: builder,
onStepCancel: setCanceled,
onStepContinue: setContinue,
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.complete,
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('B'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
// 2 because stepper creates a set of controls for each step
expect(find.text('Let us continue!'), findsNWidgets(2));
expect(find.text('Cancel This!'), findsNWidgets(2));
await tester.tap(find.text('Cancel This!').first);
await tester.pumpAndSettle();
await tester.tap(find.text('Let us continue!').first);
await tester.pumpAndSettle();
expect(canceledPressed, isTrue);
expect(continuePressed, isTrue);
});
testWidgets('Stepper custom indexed controls test', (WidgetTester tester) async {
int currentStep = 0;
void setContinue() {
currentStep += 1;
}
void setCanceled() {
currentStep -= 1;
}
Widget builder(BuildContext context, ControlsDetails details) {
// For the purposes of testing, only render something for the active
// step.
if (!details.isActive) {
return Container();
}
return Container(
margin: const EdgeInsets.only(top: 16.0),
child: ConstrainedBox(
constraints: const BoxConstraints.tightFor(height: 48.0),
child: Row(
children: <Widget>[
TextButton(
onPressed: details.onStepContinue,
child: Text('Continue to ${details.stepIndex + 1}'),
),
Container(
margin: const EdgeInsetsDirectional.only(start: 8.0),
child: TextButton(
onPressed: details.onStepCancel,
child: Text('Return to ${details.stepIndex - 1}'),
),
),
],
),
),
);
}
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Stepper(
currentStep: currentStep,
controlsBuilder: builder,
onStepCancel: () => setState(setCanceled),
onStepContinue: () => setState(setContinue),
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.complete,
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
Step(
title: Text('C'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
);
},
),
),
),
),
);
// Never mind that there is no Step -1 or Step 2 -- actual build method
// implementations would make those checks.
expect(find.text('Return to -1'), findsNWidgets(1));
expect(find.text('Continue to 1'), findsNWidgets(1));
expect(find.text('Return to 0'), findsNWidgets(0));
expect(find.text('Continue to 2'), findsNWidgets(0));
await tester.tap(find.text('Continue to 1').first);
await tester.pumpAndSettle();
// Never mind that there is no Step -1 or Step 2 -- actual build method
// implementations would make those checks.
expect(find.text('Return to -1'), findsNWidgets(0));
expect(find.text('Continue to 1'), findsNWidgets(0));
expect(find.text('Return to 0'), findsNWidgets(1));
expect(find.text('Continue to 2'), findsNWidgets(1));
});
testWidgets('Stepper error test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.error,
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
expect(find.text('!'), findsOneWidget);
});
testWidgets('Nested stepper error test', (WidgetTester tester) async {
late FlutterErrorDetails errorDetails;
final FlutterExceptionHandler? oldHandler = FlutterError.onError;
FlutterError.onError = (FlutterErrorDetails details) {
errorDetails = details;
};
try {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
type: StepperType.horizontal,
steps: <Step>[
Step(
title: const Text('Step 2'),
content: Stepper(
steps: const <Step>[
Step(
title: Text('Nested step 1'),
content: Text('A'),
),
Step(
title: Text('Nested step 2'),
content: Text('A'),
),
],
),
),
const Step(
title: Text('Step 1'),
content: Text('A'),
),
],
),
),
),
);
} finally {
FlutterError.onError = oldHandler;
}
expect(errorDetails.stack, isNotNull);
// Check the ErrorDetails without the stack trace
final String fullErrorMessage = errorDetails.toString();
final List<String> lines = fullErrorMessage.split('\n');
// The lines in the middle of the error message contain the stack trace
// which will change depending on where the test is run.
final String errorMessage = lines.takeWhile(
(String line) => line != '',
).join('\n');
expect(errorMessage.length, lessThan(fullErrorMessage.length));
expect(errorMessage, startsWith(
'══╡ EXCEPTION CAUGHT BY WIDGETS LIBRARY ╞════════════════════════\n'
'The following assertion was thrown building Stepper(',
));
// The description string of the stepper looks slightly different depending
// on the platform and is omitted here.
expect(errorMessage, endsWith(
'):\n'
'Steppers must not be nested.\n'
'The material specification advises that one should avoid\n'
'embedding steppers within steppers.\n'
'https://material.io/archive/guidelines/components/steppers.html#steppers-usage',
));
});
///https://github.com/flutter/flutter/issues/16920
testWidgets('Stepper icons size test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.editing,
content: SizedBox(width: 100.0, height: 100.0),
),
Step(
title: Text('B'),
state: StepState.complete,
content: SizedBox(width: 100.0, height: 100.0),
),
],
),
),
),
);
RenderBox renderObject = tester.renderObject(find.byIcon(Icons.edit));
expect(renderObject.size, equals(const Size.square(18.0)));
renderObject = tester.renderObject(find.byIcon(Icons.check));
expect(renderObject.size, equals(const Size.square(18.0)));
});
testWidgets('Stepper physics scroll error test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: ListView(
children: <Widget>[
Stepper(
steps: const <Step>[
Step(title: Text('Step 1'), content: Text('Text 1')),
Step(title: Text('Step 2'), content: Text('Text 2')),
Step(title: Text('Step 3'), content: Text('Text 3')),
Step(title: Text('Step 4'), content: Text('Text 4')),
Step(title: Text('Step 5'), content: Text('Text 5')),
Step(title: Text('Step 6'), content: Text('Text 6')),
Step(title: Text('Step 7'), content: Text('Text 7')),
Step(title: Text('Step 8'), content: Text('Text 8')),
Step(title: Text('Step 9'), content: Text('Text 9')),
Step(title: Text('Step 10'), content: Text('Text 10')),
],
),
const Text('Text After Stepper'),
],
),
),
),
);
await tester.fling(find.byType(Stepper), const Offset(0.0, -100.0), 1000.0);
await tester.pumpAndSettle();
expect(find.text('Text After Stepper'), findsNothing);
});
testWidgets("Vertical Stepper can't be focused when disabled.", (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
steps: const <Step>[
Step(
title: Text('Step 0'),
state: StepState.disabled,
content: Text('Text 0'),
),
],
),
),
),
);
await tester.pump();
final FocusNode disabledNode = Focus.of(tester.element(find.text('Step 0')), scopeOk: true);
disabledNode.requestFocus();
await tester.pump();
expect(disabledNode.hasPrimaryFocus, isFalse);
});
testWidgets("Horizontal Stepper can't be focused when disabled.", (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Step 0'),
state: StepState.disabled,
content: Text('Text 0'),
),
],
),
),
),
);
await tester.pump();
final FocusNode disabledNode = Focus.of(tester.element(find.text('Step 0')), scopeOk: true);
disabledNode.requestFocus();
await tester.pump();
expect(disabledNode.hasPrimaryFocus, isFalse);
});
testWidgets('Stepper header title should not overflow', (WidgetTester tester) async {
const String longText =
'A long long long long long long long long long long long long text';
await tester.pumpWidget(
MaterialApp(
home: Material(
child: ListView(
children: <Widget>[
Stepper(
steps: const <Step>[
Step(
title: Text(longText),
content: Text('Text content'),
),
],
),
],
),
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('Stepper header subtitle should not overflow', (WidgetTester tester) async {
const String longText =
'A long long long long long long long long long long long long text';
await tester.pumpWidget(
MaterialApp(
home: Material(
child: ListView(
children: <Widget>[
Stepper(
steps: const <Step>[
Step(
title: Text('Regular title'),
subtitle: Text(longText),
content: Text('Text content'),
),
],
),
],
),
),
),
);
expect(tester.takeException(), isNull);
});
testWidgets('Material2 - Stepper enabled button styles', (WidgetTester tester) async {
Widget buildFrame(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Material(
child: Stepper(
type: StepperType.horizontal,
onStepCancel: () { },
onStepContinue: () { },
steps: const <Step>[
Step(
title: Text('step1'),
content: SizedBox(width: 100, height: 100),
),
],
),
),
);
}
Material buttonMaterial(String label) {
return tester.widget<Material>(
find.descendant(of: find.widgetWithText(TextButton, label), matching: find.byType(Material)),
);
}
const OutlinedBorder buttonShape = RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2)));
final ThemeData themeLight = ThemeData(useMaterial3: false);
await tester.pumpWidget(buildFrame(themeLight));
const String continueStr = 'CONTINUE';
const String cancelStr = 'CANCEL';
const Rect continueButtonRect = Rect.fromLTRB(24.0, 212.0, 168.0, 260.0);
const Rect cancelButtonRect = Rect.fromLTRB(176.0, 212.0, 292.0, 260.0);
expect(buttonMaterial(continueStr).color!.value, 0xff2196f3);
expect(buttonMaterial(continueStr).textStyle!.color!.value, 0xffffffff);
expect(buttonMaterial(continueStr).shape, buttonShape);
expect(tester.getRect(find.widgetWithText(TextButton, continueStr)), continueButtonRect);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0x8a000000);
expect(buttonMaterial(cancelStr).shape, buttonShape);
expect(tester.getRect(find.widgetWithText(TextButton, cancelStr)), cancelButtonRect);
final ThemeData themeDark = ThemeData.dark(useMaterial3: false);
await tester.pumpWidget(buildFrame(themeDark));
await tester.pumpAndSettle(); // Complete the theme animation.
expect(buttonMaterial(continueStr).color!.value, 0);
expect(buttonMaterial(continueStr).textStyle!.color!.value, 0xffffffff);
expect(buttonMaterial(continueStr).shape, buttonShape);
expect(tester.getRect(find.widgetWithText(TextButton, continueStr)), continueButtonRect);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0xb3ffffff);
expect(buttonMaterial(cancelStr).shape, buttonShape);
expect(tester.getRect(find.widgetWithText(TextButton, cancelStr)), cancelButtonRect);
});
testWidgets('Material3 - Stepper enabled button styles', (WidgetTester tester) async {
Widget buildFrame(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Material(
child: Stepper(
type: StepperType.horizontal,
onStepCancel: () { },
onStepContinue: () { },
steps: const <Step>[
Step(
title: Text('step1'),
content: SizedBox(width: 100, height: 100),
),
],
),
),
);
}
Material buttonMaterial(String label) {
return tester.widget<Material>(
find.descendant(of: find.widgetWithText(TextButton, label), matching: find.byType(Material)),
);
}
const OutlinedBorder buttonShape = RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(2)));
final ThemeData themeLight = ThemeData(useMaterial3: true);
await tester.pumpWidget(buildFrame(themeLight));
const String continueStr = 'Continue';
const String cancelStr = 'Cancel';
const Rect continueButtonRect = Rect.fromLTRB(24.0, 212.0, 168.8, 260.0);
const Rect cancelButtonRect = Rect.fromLTRB(176.8, 212.0, 293.4, 260.0);
expect(buttonMaterial(continueStr).color!.value, themeLight.colorScheme.primary.value);
expect(buttonMaterial(continueStr).textStyle!.color!.value, 0xffffffff);
expect(buttonMaterial(continueStr).shape, buttonShape);
expect(
tester.getRect(find.widgetWithText(TextButton, continueStr)),
rectMoreOrLessEquals(continueButtonRect, epsilon: 0.001),
);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0x8a000000);
expect(buttonMaterial(cancelStr).shape, buttonShape);
expect(
tester.getRect(find.widgetWithText(TextButton, cancelStr)),
rectMoreOrLessEquals(cancelButtonRect, epsilon: 0.001),
);
final ThemeData themeDark = ThemeData.dark(useMaterial3: true);
await tester.pumpWidget(buildFrame(themeDark));
await tester.pumpAndSettle(); // Complete the theme animation.
expect(buttonMaterial(continueStr).color!.value, 0);
expect(buttonMaterial(continueStr).textStyle!.color!.value, themeDark.colorScheme.onSurface.value);
expect(buttonMaterial(continueStr).shape, buttonShape);
expect(
tester.getRect(find.widgetWithText(TextButton, continueStr)),
rectMoreOrLessEquals(continueButtonRect, epsilon: 0.001),
);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0xb3ffffff);
expect(buttonMaterial(cancelStr).shape, buttonShape);
expect(
tester.getRect(find.widgetWithText(TextButton, cancelStr)),
rectMoreOrLessEquals(cancelButtonRect, epsilon: 0.001),
);
});
testWidgets('Material2 - Stepper disabled button styles', (WidgetTester tester) async {
Widget buildFrame(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Material(
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('step1'),
content: SizedBox(width: 100, height: 100),
),
],
),
),
);
}
Material buttonMaterial(String label) {
return tester.widget<Material>(
find.descendant(of: find.widgetWithText(TextButton, label), matching: find.byType(Material)),
);
}
final ThemeData themeLight = ThemeData(useMaterial3: false);
await tester.pumpWidget(buildFrame(themeLight));
const String continueStr = 'CONTINUE';
const String cancelStr = 'CANCEL';
expect(buttonMaterial(continueStr).color!.value, 0);
expect(buttonMaterial(continueStr).textStyle!.color!.value, 0x61000000);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0x61000000);
final ThemeData themeDark = ThemeData.dark(useMaterial3: false);
await tester.pumpWidget(buildFrame(themeDark));
await tester.pumpAndSettle(); // Complete the theme animation.
expect(buttonMaterial(continueStr).color!.value, 0);
expect(buttonMaterial(continueStr).textStyle!.color!.value, 0x61ffffff);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(buttonMaterial(cancelStr).textStyle!.color!.value, 0x61ffffff);
});
testWidgets('Material3 - Stepper disabled button styles', (WidgetTester tester) async {
Widget buildFrame(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Material(
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('step1'),
content: SizedBox(width: 100, height: 100),
),
],
),
),
);
}
Material buttonMaterial(String label) {
return tester.widget<Material>(
find.descendant(of: find.widgetWithText(TextButton, label), matching: find.byType(Material)),
);
}
final ThemeData themeLight = ThemeData(useMaterial3: true);
final ColorScheme colorsLight = themeLight.colorScheme;
await tester.pumpWidget(buildFrame(themeLight));
const String continueStr = 'Continue';
const String cancelStr = 'Cancel';
expect(buttonMaterial(continueStr).color!.value, 0);
expect(
buttonMaterial(continueStr).textStyle!.color!.value,
colorsLight.onSurface.withOpacity(0.38).value,
);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(
buttonMaterial(cancelStr).textStyle!.color!.value,
colorsLight.onSurface.withOpacity(0.38).value,
);
final ThemeData themeDark = ThemeData.dark(useMaterial3: true);
final ColorScheme colorsDark = themeDark.colorScheme;
await tester.pumpWidget(buildFrame(themeDark));
await tester.pumpAndSettle(); // Complete the theme animation.
expect(buttonMaterial(continueStr).color!.value, 0);
expect(
buttonMaterial(continueStr).textStyle!.color!.value,
colorsDark.onSurface.withOpacity(0.38).value,
);
expect(buttonMaterial(cancelStr).color!.value, 0);
expect(
buttonMaterial(cancelStr).textStyle!.color!.value,
colorsDark.onSurface.withOpacity(0.38).value,
);
});
testWidgets('Vertical and Horizontal Stepper physics test', (WidgetTester tester) async {
const ScrollPhysics physics = NeverScrollableScrollPhysics();
for (final StepperType type in StepperType.values) {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
physics: physics,
type: type,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
final ListView listView = tester.widget<ListView>(find.descendant(of: find.byType(Stepper), matching: find.byType(ListView)));
expect(listView.physics, physics);
}
});
testWidgets('ScrollController is passed to the stepper listview', (WidgetTester tester) async {
final ScrollController controller = ScrollController();
addTearDown(() => controller.dispose());
for (final StepperType type in StepperType.values) {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
controller: controller,
type: type,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
);
final ListView listView = tester.widget<ListView>(
find.descendant(of: find.byType(Stepper),
matching: find.byType(ListView),
));
expect(listView.controller, controller);
}
});
testWidgets('Stepper horizontal size test', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/pull/77732
Widget buildFrame({ bool isActive = true, Brightness? brightness }) {
return MaterialApp(
theme: brightness == Brightness.dark ? ThemeData.dark() : ThemeData.light(),
home: Scaffold(
body: Center(
child: Stepper(
type: StepperType.horizontal,
steps: <Step>[
Step(
title: const Text('step'),
content: const Text('content'),
isActive: isActive,
),
],
),
),
),
);
}
Color? circleFillColor() {
final Finder container = find.widgetWithText(AnimatedContainer, '1');
return (tester.widget<AnimatedContainer>(container).decoration as BoxDecoration?)?.color;
}
// Light theme
final ColorScheme light = ThemeData.light().colorScheme;
await tester.pumpWidget(buildFrame(brightness: Brightness.light));
expect(circleFillColor(), light.primary);
await tester.pumpWidget(buildFrame(isActive: false, brightness: Brightness.light));
await tester.pumpAndSettle();
expect(circleFillColor(), light.onSurface.withOpacity(0.38));
// Dark theme
final ColorScheme dark = ThemeData.dark().colorScheme;
await tester.pumpWidget(buildFrame(brightness: Brightness.dark));
await tester.pumpAndSettle();
expect(circleFillColor(), dark.secondary);
await tester.pumpWidget(buildFrame(isActive: false, brightness: Brightness.dark));
await tester.pumpAndSettle();
expect(circleFillColor(), dark.background);
});
testWidgets('Stepper custom elevation', (WidgetTester tester) async {
const double elevation = 4.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SizedBox(
width: 200,
height: 75,
child: Stepper(
type: StepperType.horizontal,
elevation: elevation,
steps: const <Step>[
Step(
title: Text('Regular title'),
content: Text('Text content'),
),
],
),
),
),
),
);
final Material material = tester.firstWidget<Material>(
find.descendant(
of: find.byType(Stepper),
matching: find.byType(Material),
),
);
expect(material.elevation, elevation);
});
testWidgets('Stepper with default elevation', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SizedBox(
width: 200,
height: 75,
child: Stepper(
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Regular title'),
content: Text('Text content')
),
],
),
),
),
),
);
final Material material = tester.firstWidget<Material>(
find.descendant(
of: find.byType(Stepper),
matching: find.byType(Material),
),
);
expect(material.elevation, 2.0);
});
testWidgets('Stepper horizontal preserves state', (WidgetTester tester) async {
const Color untappedColor = Colors.blue;
const Color tappedColor = Colors.red;
int index = 0;
Widget buildFrame() {
return MaterialApp(
home: Scaffold(
body: Center(
// Must break this out into its own widget purely to be able to call `setState()`
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Stepper(
onStepTapped: (int i) => setState(() => index = i),
currentStep: index,
type: StepperType.horizontal,
steps: const <Step>[
Step(
title: Text('Step 1'),
content: _TappableColorWidget(
key: Key('tappable-color'),
tappedColor: tappedColor,
untappedColor: untappedColor,
),
),
Step(
title: Text('Step 2'),
content: Text('Step 2 Content'),
),
],
);
},
),
),
),
);
}
final Widget widget = buildFrame();
await tester.pumpWidget(widget);
// Set up a getter to examine the MacGuffin's color
Color getColor() => tester.widget<ColoredBox>(
find.descendant(of: find.byKey(const Key('tappable-color')), matching: find.byType(ColoredBox)),
).color;
// We are on step 1
expect(find.text('Step 2 Content'), findsNothing);
expect(getColor(), untappedColor);
await tester.tap(find.byKey(const Key('tap-me')));
await tester.pumpAndSettle();
expect(getColor(), tappedColor);
// Now flip to step 2
await tester.tap(find.text('Step 2'));
await tester.pumpAndSettle();
// Confirm that we did in fact flip to step 2
expect(find.text('Step 2 Content'), findsOneWidget);
// Now go back to step 1
await tester.tap(find.text('Step 1'));
await tester.pumpAndSettle();
// Confirm that we flipped back to step 1
expect(find.text('Step 2 Content'), findsNothing);
// The color should still be `tappedColor`
expect(getColor(), tappedColor);
});
testWidgets('Stepper custom margin', (WidgetTester tester) async {
const EdgeInsetsGeometry margin = EdgeInsetsDirectional.only(
bottom: 20,
top: 20,
);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SizedBox(
width: 200,
height: 75,
child: Stepper(
margin: margin,
steps: const <Step>[
Step(
title: Text('Regular title'),
content: Text('Text content')
),
],
),
),
),
),
);
final Stepper material = tester.firstWidget<Stepper>(
find.descendant(
of: find.byType(Material),
matching: find.byType(Stepper),
),
);
expect(material.margin, equals(margin));
});
testWidgets('Stepper with Alternative Label', (WidgetTester tester) async {
int index = 0;
late TextStyle bodyLargeStyle;
late TextStyle bodyMediumStyle;
late TextStyle bodySmallStyle;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
bodyLargeStyle = Theme.of(context).textTheme.bodyText1!;
bodyMediumStyle = Theme.of(context).textTheme.bodyText2!;
bodySmallStyle = Theme.of(context).textTheme.caption!;
return Stepper(
type: StepperType.horizontal,
currentStep: index,
onStepTapped: (int i) {
setState(() {
index = i;
});
},
steps: <Step>[
Step(
title: const Text('Title 1'),
content: const Text('Content 1'),
label: Text('Label 1', style: Theme.of(context).textTheme.bodySmall),
),
Step(
title: const Text('Title 2'),
content: const Text('Content 2'),
label: Text('Label 2', style: Theme.of(context).textTheme.bodyLarge),
),
Step(
title: const Text('Title 3'),
content: const Text('Content 3'),
label: Text('Label 3', style: Theme.of(context).textTheme.bodyMedium),
),
],
);
}),
),
),
);
// Check Styles of Label Text Widgets before tapping steps
final Text label1TextWidget =
tester.widget<Text>(find.text('Label 1'));
final Text label3TextWidget =
tester.widget<Text>(find.text('Label 3'));
expect(bodySmallStyle, label1TextWidget.style);
expect(bodyMediumStyle, label3TextWidget.style);
late Text selectedLabelTextWidget;
late Text nextLabelTextWidget;
// Tap to Step1 Label then, `index` become 0
await tester.tap(find.text('Label 1'));
expect(index, 0);
// Check Styles of Selected Label Text Widgets and Another Label Text Widget
selectedLabelTextWidget =
tester.widget<Text>(find.text('Label ${index + 1}'));
expect(bodySmallStyle, selectedLabelTextWidget.style);
nextLabelTextWidget =
tester.widget<Text>(find.text('Label ${index + 2}'));
expect(bodyLargeStyle, nextLabelTextWidget.style);
// Tap to Step2 Label then, `index` become 1
await tester.tap(find.text('Label 2'));
expect(index, 1);
// Check Styles of Selected Label Text Widgets and Another Label Text Widget
selectedLabelTextWidget =
tester.widget<Text>(find.text('Label ${index + 1}'));
expect(bodyLargeStyle, selectedLabelTextWidget.style);
nextLabelTextWidget =
tester.widget<Text>(find.text('Label ${index + 2}'));
expect(bodyMediumStyle, nextLabelTextWidget.style);
});
testWidgets('Stepper Connector Style', (WidgetTester tester) async {
const Color selectedColor = Colors.black;
const Color disabledColor = Colors.white;
int index = 0;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Stepper(
type: StepperType.horizontal,
connectorColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) =>
states.contains(MaterialState.selected)
? selectedColor
: disabledColor),
onStepTapped: (int i) => setState(() => index = i),
currentStep: index,
steps: <Step>[
Step(
isActive: index >= 0,
title: const Text('step1'),
content: const Text('step1 content'),
),
Step(
isActive: index >= 1,
title: const Text('step2'),
content: const Text('step2 content'),
),
],
);
},
),
),
),
)
);
Color? circleColor(String circleText) => (tester.widget<AnimatedContainer>(
find.widgetWithText(AnimatedContainer, circleText),
).decoration as BoxDecoration?)?.color;
Color? lineColor(String keyStep) => tester.widget<Container>(find.byKey(Key(keyStep))).color;
// Step 1
// check if I'm in step 1
expect(find.text('step1 content'), findsOneWidget);
expect(find.text('step2 content'), findsNothing);
expect(circleColor('1'), selectedColor);
expect(circleColor('2'), disabledColor);
// in two steps case there will be single line
expect(lineColor('line0'), selectedColor);
// now hitting step two
await tester.tap(find.text('step2'));
await tester.pumpAndSettle();
// check if I'm in step 1
expect(find.text('step1 content'), findsNothing);
expect(find.text('step2 content'), findsOneWidget);
expect(circleColor('1'), selectedColor);
expect(circleColor('2'), selectedColor);
expect(lineColor('line0'), selectedColor);
});
testWidgets('Stepper stepIconBuilder test', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
stepIconBuilder: (int index, StepState state) {
if (state == StepState.complete) {
return const FlutterLogo(size: 18);
}
return null;
},
steps: const <Step>[
Step(
title: Text('A'),
state: StepState.complete,
content: SizedBox(width: 100.0, height: 100.0),
),
Step(
title: Text('B'),
state: StepState.editing,
content: SizedBox(width: 100.0, height: 100.0),
),
Step(
title: Text('C'),
state: StepState.error,
content: SizedBox(width: 100.0, height: 100.0),
),
],
),
),
),
);
/// Finds the overridden widget for StepState.complete
expect(find.byType(FlutterLogo), findsOneWidget);
/// StepState.editing and StepState.error should have a default icon
expect(find.byIcon(Icons.edit), findsOneWidget);
expect(find.text('!'), findsOneWidget);
});
testWidgets('StepperProperties test', (WidgetTester tester) async {
const Widget widget = SizedBox.shrink();
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
stepIconHeight: 24,
stepIconWidth: 24,
stepIconMargin: const EdgeInsets.all(8),
steps: List<Step>.generate(3, (int index) {
return Step(
title: Text('Step $index'),
content: widget,
);
}),
),
),
),
);
final Finder stepperFinder = find.byType(Stepper);
final Stepper stepper = tester.widget<Stepper>(stepperFinder);
expect(stepper.stepIconHeight, 24);
expect(stepper.stepIconWidth, 24);
expect(stepper.stepIconMargin, const EdgeInsets.all(8));
});
testWidgets('StepStyle test', (WidgetTester tester) async {
final StepStyle stepStyle = StepStyle(
color: Colors.white,
errorColor: Colors.orange,
connectorColor: Colors.red,
connectorThickness: 2,
border: Border.all(),
gradient: const LinearGradient(
colors: <Color>[Colors.red, Colors.blue],
),
indexStyle: const TextStyle(color: Colors.black),
);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Stepper(
steps: <Step>[
Step(
title: const Text('Regular title'),
content: const Text('Text content'),
stepStyle: stepStyle,
),
],
),
),
),
);
final Finder stepperFinder = find.byType(Stepper);
final Stepper stepper = tester.widget<Stepper>(stepperFinder);
final StepStyle? style = stepper.steps.first.stepStyle;
expect(style?.color, stepStyle.color);
expect(style?.errorColor, stepStyle.errorColor);
expect(style?.connectorColor, stepStyle.connectorColor);
expect(style?.connectorThickness, stepStyle.connectorThickness);
expect(style?.border, stepStyle.border);
expect(style?.gradient, stepStyle.gradient);
expect(style?.indexStyle, stepStyle.indexStyle);
//copyWith
final StepStyle newStyle = stepStyle.copyWith(
color: Colors.black,
errorColor: Colors.red,
connectorColor: Colors.blue,
connectorThickness: 3,
border: Border.all(),
gradient: const LinearGradient(
colors: <Color>[Colors.red, Colors.blue],
),
indexStyle: const TextStyle(color: Colors.black),
);
expect(newStyle.color, Colors.black);
expect(newStyle.errorColor, Colors.red);
expect(newStyle.connectorColor, Colors.blue);
expect(newStyle.connectorThickness, 3);
expect(newStyle.border, stepStyle.border);
expect(newStyle.gradient, stepStyle.gradient);
expect(newStyle.indexStyle, stepStyle.indexStyle);
//merge
final StepStyle mergedStyle = stepStyle.merge(newStyle);
expect(mergedStyle.color, Colors.black);
expect(mergedStyle.errorColor, Colors.red);
expect(mergedStyle.connectorColor, Colors.blue);
expect(mergedStyle.connectorThickness, 3);
expect(mergedStyle.border, stepStyle.border);
expect(mergedStyle.gradient, stepStyle.gradient);
expect(mergedStyle.indexStyle, stepStyle.indexStyle);
});
}
class _TappableColorWidget extends StatefulWidget {
const _TappableColorWidget({required this.tappedColor, required this.untappedColor, super.key,});
final Color tappedColor;
final Color untappedColor;
@override
State<StatefulWidget> createState() => _TappableColorWidgetState();
}
class _TappableColorWidgetState extends State<_TappableColorWidget> {
Color? color;
@override
void initState() {
super.initState();
color = widget.untappedColor;
}
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () {
setState((){
color = widget.tappedColor;
});
},
child: Container(
key: const Key('tap-me'),
height: 50,
width: 50,
color: color,
),
);
}
}
| flutter/packages/flutter/test/material/stepper_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/stepper_test.dart",
"repo_id": "flutter",
"token_count": 25977
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
const String tooltipText = 'TIP';
const double _customPaddingValue = 10.0;
void main() {
test('TooltipThemeData copyWith, ==, hashCode basics', () {
expect(const TooltipThemeData(), const TooltipThemeData().copyWith());
expect(const TooltipThemeData().hashCode, const TooltipThemeData().copyWith().hashCode);
});
test('TooltipThemeData lerp special cases', () {
expect(TooltipThemeData.lerp(null, null, 0), null);
const TooltipThemeData data = TooltipThemeData();
expect(identical(TooltipThemeData.lerp(data, data, 0.5), data), true);
});
test('TooltipThemeData defaults', () {
const TooltipThemeData theme = TooltipThemeData();
expect(theme.height, null);
expect(theme.padding, null);
expect(theme.verticalOffset, null);
expect(theme.preferBelow, null);
expect(theme.excludeFromSemantics, null);
expect(theme.decoration, null);
expect(theme.textStyle, null);
expect(theme.textAlign, null);
expect(theme.waitDuration, null);
expect(theme.showDuration, null);
expect(theme.exitDuration, null);
expect(theme.triggerMode, null);
expect(theme.enableFeedback, null);
});
testWidgets('Default TooltipThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const TooltipThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('TooltipThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const Duration wait = Duration(milliseconds: 100);
const Duration show = Duration(milliseconds: 200);
const Duration exit = Duration(milliseconds: 100);
const TooltipTriggerMode triggerMode = TooltipTriggerMode.longPress;
const bool enableFeedback = true;
const TooltipThemeData(
height: 15.0,
padding: EdgeInsets.all(20.0),
verticalOffset: 10.0,
preferBelow: false,
excludeFromSemantics: true,
decoration: BoxDecoration(color: Color(0xffffffff)),
textStyle: TextStyle(decoration: TextDecoration.underline),
textAlign: TextAlign.center,
waitDuration: wait,
showDuration: show,
exitDuration: exit,
triggerMode: triggerMode,
enableFeedback: enableFeedback,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'height: 15.0',
'padding: EdgeInsets.all(20.0)',
'vertical offset: 10.0',
'position: above',
'semantics: excluded',
'decoration: BoxDecoration(color: Color(0xffffffff))',
'textStyle: TextStyle(inherit: true, decoration: TextDecoration.underline)',
'textAlign: TextAlign.center',
'wait duration: $wait',
'show duration: $show',
'exit duration: $exit',
'triggerMode: $triggerMode',
'enableFeedback: true',
]);
});
testWidgets('Tooltip verticalOffset, preferBelow; center prefer above fits - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
height: 100.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: false,
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 300.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
/********************* 800x600 screen
* ___ * }- 10.0 margin
* |___| * }-100.0 height
* | * }-100.0 vertical offset
* o * y=300.0
* *
* *
* *
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(100.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(100.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(200.0));
});
testWidgets('Tooltip verticalOffset, preferBelow; center prefer above fits - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(
height: 100.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: false,
),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 300.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
/********************* 800x600 screen
* ___ * }- 10.0 margin
* |___| * }-100.0 height
* | * }-100.0 vertical offset
* o * y=300.0
* *
* *
* *
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(100.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(100.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(200.0));
});
testWidgets('Tooltip verticalOffset, preferBelow; center prefer above does not fit - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
height: 190.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: false,
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 299.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
// we try to put it here but it doesn't fit:
/********************* 800x600 screen
* ___ * }- 10.0 margin
* |___| * }-190.0 height (starts at y=9.0)
* | * }-100.0 vertical offset
* o * y=299.0
* *
* *
* *
*********************/
// so we put it here:
/********************* 800x600 screen
* *
* *
* o * y=299.0
* _|_ * }-100.0 vertical offset
* |___| * }-190.0 height
* * }- 10.0 margin
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(190.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(399.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(589.0));
});
testWidgets('Tooltip verticalOffset, preferBelow; center prefer above does not fit - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(
height: 190.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: false,
),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 299.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
// we try to put it here but it doesn't fit:
/********************* 800x600 screen
* ___ * }- 10.0 margin
* |___| * }-190.0 height (starts at y=9.0)
* | * }-100.0 vertical offset
* o * y=299.0
* *
* *
* *
*********************/
// so we put it here:
/********************* 800x600 screen
* *
* *
* o * y=299.0
* _|_ * }-100.0 vertical offset
* |___| * }-190.0 height
* * }- 10.0 margin
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(190.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(399.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(589.0));
});
testWidgets('Tooltip verticalOffset, preferBelow; center preferBelow fits - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
height: 190.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: true,
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 300.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pumpAndSettle(); // faded in, show timer started (and at 0.0)
/********************* 800x600 screen
* *
* *
* o * y=300.0
* _|_ * }-100.0 vertical offset
* |___| * }-190.0 height
* * }- 10.0 margin
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(190.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(400.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(590.0));
});
testWidgets('Tooltip verticalOffset, preferBelow; center prefer below fits - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(
height: 190.0,
padding: EdgeInsets.zero,
verticalOffset: 100.0,
preferBelow: true,
),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Stack(
children: <Widget>[
Positioned(
left: 400.0,
top: 300.0,
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
),
],
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pumpAndSettle(); // faded in, show timer started (and at 0.0)
/********************* 800x600 screen
* *
* *
* o * y=300.0
* _|_ * }-100.0 vertical offset
* |___| * }-190.0 height
* * }- 10.0 margin
*********************/
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent! as RenderBox;
expect(tip.size.height, equals(190.0));
expect(tip.localToGlobal(tip.size.topLeft(Offset.zero)).dy, equals(400.0));
expect(tip.localToGlobal(tip.size.bottomRight(Offset.zero)).dy, equals(590.0));
});
testWidgets('Tooltip margin - ThemeData', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Theme(
data: ThemeData(
tooltipTheme: const TooltipThemeData(
padding: EdgeInsets.zero,
margin: EdgeInsets.all(_customPaddingValue),
),
),
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent!.parent! as RenderBox;
final RenderBox tooltipContent = tester.renderObject(find.text(tooltipText));
final Offset topLeftTipInGlobal = tip.localToGlobal(tip.size.topLeft(Offset.zero));
final Offset topLeftTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.topLeft(Offset.zero));
expect(topLeftTooltipContentInGlobal.dx, topLeftTipInGlobal.dx + _customPaddingValue);
expect(topLeftTooltipContentInGlobal.dy, topLeftTipInGlobal.dy + _customPaddingValue);
final Offset topRightTipInGlobal = tip.localToGlobal(tip.size.topRight(Offset.zero));
final Offset topRightTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.topRight(Offset.zero));
expect(topRightTooltipContentInGlobal.dx, topRightTipInGlobal.dx - _customPaddingValue);
expect(topRightTooltipContentInGlobal.dy, topRightTipInGlobal.dy + _customPaddingValue);
final Offset bottomLeftTipInGlobal = tip.localToGlobal(tip.size.bottomLeft(Offset.zero));
final Offset bottomLeftTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.bottomLeft(Offset.zero));
expect(bottomLeftTooltipContentInGlobal.dx, bottomLeftTipInGlobal.dx + _customPaddingValue);
expect(bottomLeftTooltipContentInGlobal.dy, bottomLeftTipInGlobal.dy - _customPaddingValue);
final Offset bottomRightTipInGlobal = tip.localToGlobal(tip.size.bottomRight(Offset.zero));
final Offset bottomRightTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.bottomRight(Offset.zero));
expect(bottomRightTooltipContentInGlobal.dx, bottomRightTipInGlobal.dx - _customPaddingValue);
expect(bottomRightTooltipContentInGlobal.dy, bottomRightTipInGlobal.dy - _customPaddingValue);
});
testWidgets('Tooltip margin - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return TooltipTheme(
data: const TooltipThemeData(
padding: EdgeInsets.zero,
margin: EdgeInsets.all(_customPaddingValue),
),
child: Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
),
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent!.parent! as RenderBox;
final RenderBox tooltipContent = tester.renderObject(find.text(tooltipText));
final Offset topLeftTipInGlobal = tip.localToGlobal(tip.size.topLeft(Offset.zero));
final Offset topLeftTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.topLeft(Offset.zero));
expect(topLeftTooltipContentInGlobal.dx, topLeftTipInGlobal.dx + _customPaddingValue);
expect(topLeftTooltipContentInGlobal.dy, topLeftTipInGlobal.dy + _customPaddingValue);
final Offset topRightTipInGlobal = tip.localToGlobal(tip.size.topRight(Offset.zero));
final Offset topRightTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.topRight(Offset.zero));
expect(topRightTooltipContentInGlobal.dx, topRightTipInGlobal.dx - _customPaddingValue);
expect(topRightTooltipContentInGlobal.dy, topRightTipInGlobal.dy + _customPaddingValue);
final Offset bottomLeftTipInGlobal = tip.localToGlobal(tip.size.bottomLeft(Offset.zero));
final Offset bottomLeftTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.bottomLeft(Offset.zero));
expect(bottomLeftTooltipContentInGlobal.dx, bottomLeftTipInGlobal.dx + _customPaddingValue);
expect(bottomLeftTooltipContentInGlobal.dy, bottomLeftTipInGlobal.dy - _customPaddingValue);
final Offset bottomRightTipInGlobal = tip.localToGlobal(tip.size.bottomRight(Offset.zero));
final Offset bottomRightTooltipContentInGlobal = tooltipContent.localToGlobal(tooltipContent.size.bottomRight(Offset.zero));
expect(bottomRightTooltipContentInGlobal.dx, bottomRightTipInGlobal.dx - _customPaddingValue);
expect(bottomRightTooltipContentInGlobal.dy, bottomRightTipInGlobal.dy - _customPaddingValue);
});
testWidgets('Tooltip message textStyle - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
textStyle: TextStyle(
color: Colors.orange,
decoration: TextDecoration.underline,
),
),
),
home: Tooltip(
key: key,
message: tooltipText,
child: Container(
width: 100.0,
height: 100.0,
color: Colors.green[500],
),
),
));
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final TextStyle textStyle = tester.widget<Text>(find.text(tooltipText)).style!;
expect(textStyle.color, Colors.orange);
expect(textStyle.fontFamily, null);
expect(textStyle.decoration, TextDecoration.underline);
});
testWidgets('Tooltip message textStyle - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
await tester.pumpWidget(MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(),
child: Tooltip(
textStyle: const TextStyle(
color: Colors.orange,
decoration: TextDecoration.underline,
),
key: key,
message: tooltipText,
child: Container(
width: 100.0,
height: 100.0,
color: Colors.green[500],
),
),
),
));
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final TextStyle textStyle = tester.widget<Text>(find.text(tooltipText)).style!;
expect(textStyle.color, Colors.orange);
expect(textStyle.fontFamily, null);
expect(textStyle.decoration, TextDecoration.underline);
});
testWidgets('Tooltip message textAlign - TooltipTheme', (WidgetTester tester) async {
Future<void> pumpTooltipWithTextAlign({TextAlign? textAlign}) async {
final GlobalKey<TooltipState> tooltipKey = GlobalKey<TooltipState>();
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(
textAlign: textAlign,
),
child: Tooltip(
key: tooltipKey,
message: tooltipText,
child: Container(
width: 100.0,
height: 100.0,
color: Colors.green[500],
),
),
),
),
);
tooltipKey.currentState?.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
}
// Default value should be TextAlign.start
await pumpTooltipWithTextAlign();
TextAlign textAlign = tester.widget<Text>(find.text(tooltipText)).textAlign!;
expect(textAlign, TextAlign.start);
await pumpTooltipWithTextAlign(textAlign: TextAlign.center);
textAlign = tester.widget<Text>(find.text(tooltipText)).textAlign!;
expect(textAlign, TextAlign.center);
await pumpTooltipWithTextAlign(textAlign: TextAlign.end);
textAlign = tester.widget<Text>(find.text(tooltipText)).textAlign!;
expect(textAlign, TextAlign.end);
});
testWidgets('Material2 - Tooltip decoration - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const Decoration customDecoration = ShapeDecoration(
shape: StadiumBorder(),
color: Color(0x80800000),
);
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
useMaterial3: false,
tooltipTheme: const TooltipThemeData(
decoration: customDecoration,
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent! as RenderBox;
expect(tip.size.height, equals(32.0));
expect(tip.size.width, equals(74.0));
expect(tip, paints..rrect(color: const Color(0x80800000)));
});
testWidgets('Material3 - Tooltip decoration - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const Decoration customDecoration = ShapeDecoration(
shape: StadiumBorder(),
color: Color(0x80800000),
);
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
decoration: customDecoration,
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent! as RenderBox;
expect(tip.size.height, equals(32.0));
expect(tip.size.width, equals(74.75));
expect(tip, paints..rrect(color: const Color(0x80800000)));
});
testWidgets('Material2 - Tooltip decoration - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const Decoration customDecoration = ShapeDecoration(
shape: StadiumBorder(),
color: Color(0x80800000),
);
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: TooltipTheme(
data: const TooltipThemeData(decoration: customDecoration),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent! as RenderBox;
expect(tip.size.height, equals(32.0));
expect(tip.size.width, equals(74.0));
expect(tip, paints..rrect(color: const Color(0x80800000)));
});
testWidgets('Material3 - Tooltip decoration - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const Decoration customDecoration = ShapeDecoration(
shape: StadiumBorder(),
color: Color(0x80800000),
);
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(decoration: customDecoration),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
child: const SizedBox.shrink(),
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pump(const Duration(seconds: 2)); // faded in, show timer started (and at 0.0)
final RenderBox tip = tester.renderObject(find.text(tooltipText)).parent!.parent!.parent!.parent! as RenderBox;
expect(tip.size.height, equals(32.0));
expect(tip.size.width, equals(74.75));
expect(tip, paints..rrect(color: const Color(0x80800000)));
});
testWidgets('Tooltip height and padding - ThemeData.tooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const double customTooltipHeight = 100.0;
const double customPaddingVal = 20.0;
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
height: customTooltipHeight,
padding: EdgeInsets.all(customPaddingVal),
),
),
home: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
);
},
),
],
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pumpAndSettle();
final RenderBox tip = tester.renderObject(find.ancestor(
of: find.text(tooltipText),
matching: find.byType(Padding).first, // select [Tooltip.padding] instead of [Tooltip.margin]
));
final RenderBox content = tester.renderObject(find.ancestor(
of: find.text(tooltipText),
matching: find.byType(Center),
));
expect(tip.size.height, equals(customTooltipHeight));
expect(content.size.height, equals(customTooltipHeight - 2 * customPaddingVal));
expect(content.size.width, equals(tip.size.width - 2 * customPaddingVal));
});
testWidgets('Tooltip height and padding - TooltipTheme', (WidgetTester tester) async {
final GlobalKey<TooltipState> key = GlobalKey<TooltipState>();
const double customTooltipHeight = 100.0;
const double customPaddingValue = 20.0;
late final OverlayEntry entry;
addTearDown(() => entry..remove()..dispose());
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(
height: customTooltipHeight,
padding: EdgeInsets.all(customPaddingValue),
),
child: Overlay(
initialEntries: <OverlayEntry>[
entry = OverlayEntry(
builder: (BuildContext context) {
return Tooltip(
key: key,
message: tooltipText,
);
},
),
],
),
),
),
);
key.currentState!.ensureTooltipVisible();
await tester.pumpAndSettle();
final RenderBox tip = tester.renderObject(find.ancestor(
of: find.text(tooltipText),
matching: find.byType(Padding).first, // select [Tooltip.padding] instead of [Tooltip.margin]
));
final RenderBox content = tester.renderObject(find.ancestor(
of: find.text(tooltipText),
matching: find.byType(Center),
));
expect(tip.size.height, equals(customTooltipHeight));
expect(content.size.height, equals(customTooltipHeight - 2 * customPaddingValue));
expect(content.size.width, equals(tip.size.width - 2 * customPaddingValue));
});
testWidgets('Tooltip waitDuration - ThemeData.tooltipTheme', (WidgetTester tester) async {
const Duration customWaitDuration = Duration(milliseconds: 500);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
await gesture.moveTo(Offset.zero);
await tester.pumpWidget(
MaterialApp(
home: Theme(
data: ThemeData(
tooltipTheme: const TooltipThemeData(
waitDuration: customWaitDuration,
),
),
child: const Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(Offset.zero);
await tester.pump();
await gesture.moveTo(tester.getCenter(tooltip));
await tester.pump();
await tester.pump(const Duration(milliseconds: 250));
expect(find.text(tooltipText), findsNothing); // Should not appear yet
await tester.pump(const Duration(milliseconds: 250));
expect(find.text(tooltipText), findsOneWidget); // Should appear after customWaitDuration
await gesture.moveTo(Offset.zero);
await tester.pump();
// Wait for it to disappear.
await tester.pump(const Duration(milliseconds: 100)); // Should disappear after default exitDuration
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip waitDuration - TooltipTheme', (WidgetTester tester) async {
const Duration customWaitDuration = Duration(milliseconds: 500);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
await gesture.moveTo(Offset.zero);
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(waitDuration: customWaitDuration),
child: Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(Offset.zero);
await tester.pump();
await gesture.moveTo(tester.getCenter(tooltip));
await tester.pump();
await tester.pump(const Duration(milliseconds: 250));
expect(find.text(tooltipText), findsNothing); // Should not appear yet
await tester.pump(const Duration(milliseconds: 250));
expect(find.text(tooltipText), findsOneWidget); // Should appear after customWaitDuration
await gesture.moveTo(Offset.zero);
await tester.pump();
// Wait for it to disappear.
await tester.pump(const Duration(milliseconds: 100)); // Should disappear after default exitDuration
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip showDuration - ThemeData.tooltipTheme', (WidgetTester tester) async {
const Duration customShowDuration = Duration(milliseconds: 3000);
await tester.pumpWidget(
MaterialApp(
home: Theme(
data: ThemeData(
tooltipTheme: const TooltipThemeData(
showDuration: customShowDuration,
),
),
child: const Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
final TestGesture gesture = await tester.startGesture(tester.getCenter(tooltip));
await tester.pump();
await tester.pump(kLongPressTimeout);
await gesture.up();
expect(find.text(tooltipText), findsOneWidget);
await tester.pump();
await tester.pump(const Duration(milliseconds: 2000)); // Tooltip should remain
expect(find.text(tooltipText), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1000));
await tester.pumpAndSettle(); // Tooltip should fade out after
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip showDuration - TooltipTheme', (WidgetTester tester) async {
const Duration customShowDuration = Duration(milliseconds: 3000);
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(showDuration: customShowDuration),
child: Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
final TestGesture gesture = await tester.startGesture(tester.getCenter(tooltip));
await tester.pump();
await tester.pump(kLongPressTimeout);
await gesture.up();
expect(find.text(tooltipText), findsOneWidget);
await tester.pump();
await tester.pump(const Duration(milliseconds: 2000)); // Tooltip should remain
expect(find.text(tooltipText), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1000));
await tester.pumpAndSettle(); // Tooltip should fade out after
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip exitDuration - ThemeData.tooltipTheme', (WidgetTester tester) async {
const Duration customExitDuration = Duration(milliseconds: 500);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
await gesture.moveTo(Offset.zero);
await tester.pumpWidget(
MaterialApp(
home: Theme(
data: ThemeData(
tooltipTheme: const TooltipThemeData(
exitDuration: customExitDuration,
),
),
child: const Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(tester.getCenter(tooltip));
await tester.pump();
expect(find.text(tooltipText), findsOneWidget);
await gesture.moveTo(Offset.zero);
await tester.pump(const Duration(milliseconds: 200));
await tester.pumpAndSettle();
expect(find.text(tooltipText), findsOneWidget);
// Wait for it to disappear.
await tester.pump(customExitDuration);
await tester.pumpAndSettle();
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip exitDuration - TooltipTheme', (WidgetTester tester) async {
const Duration customExitDuration = Duration(milliseconds: 500);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(const Offset(1.0, 1.0));
await tester.pump();
await gesture.moveTo(Offset.zero);
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(exitDuration: customExitDuration),
child: Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
await gesture.moveTo(tester.getCenter(tooltip));
await tester.pump();
expect(find.text(tooltipText), findsOneWidget);
await gesture.moveTo(Offset.zero);
await tester.pump(const Duration(milliseconds: 200));
await tester.pumpAndSettle();
expect(find.text(tooltipText), findsOneWidget);
// Wait for it to disappear.
await tester.pump(customExitDuration);
await tester.pumpAndSettle();
expect(find.text(tooltipText), findsNothing);
});
testWidgets('Tooltip triggerMode - ThemeData.triggerMode', (WidgetTester tester) async {
const TooltipTriggerMode triggerMode = TooltipTriggerMode.tap;
await tester.pumpWidget(
MaterialApp(
home: Theme(
data: ThemeData(
tooltipTheme: const TooltipThemeData(triggerMode: triggerMode),
),
child: const Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(width: 100.0, height: 100.0),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
final TestGesture gesture = await tester.startGesture(tester.getCenter(tooltip));
await gesture.up();
await tester.pump();
expect(find.text(tooltipText), findsOneWidget); // Tooltip should show immediately after tap
});
testWidgets('Tooltip triggerMode - TooltipTheme', (WidgetTester tester) async {
const TooltipTriggerMode triggerMode = TooltipTriggerMode.tap;
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(triggerMode: triggerMode),
child: Center(
child: Tooltip(
message: tooltipText,
child: SizedBox(width: 100.0, height: 100.0),
),
),
),
),
);
final Finder tooltip = find.byType(Tooltip);
final TestGesture gesture = await tester.startGesture(tester.getCenter(tooltip));
await gesture.up();
await tester.pump();
expect(find.text(tooltipText), findsOneWidget); // Tooltip should show immediately after tap
});
testWidgets('Semantics included by default - ThemeData.tooltipTheme', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(),
home: const Center(
child: Tooltip(
message: 'Foo',
child: Text('Bar'),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
tooltip: 'Foo',
label: 'Bar',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
), ignoreRect: true, ignoreId: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('Semantics included by default - TooltipTheme', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(),
child: Center(
child: Tooltip(
message: 'Foo',
child: Text('Bar'),
),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
tooltip: 'Foo',
label: 'Bar',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
), ignoreRect: true, ignoreId: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('Semantics excluded - ThemeData.tooltipTheme', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
tooltipTheme: const TooltipThemeData(
excludeFromSemantics: true,
),
),
home: const Center(
child: Tooltip(
message: 'Foo',
child: Text('Bar'),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
label: 'Bar',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
), ignoreRect: true, ignoreId: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('Semantics excluded - TooltipTheme', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
const MaterialApp(
home: TooltipTheme(
data: TooltipThemeData(excludeFromSemantics: true),
child: Center(
child: Tooltip(
message: 'Foo',
child: Text('Bar'),
),
),
),
),
);
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
label: 'Bar',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
), ignoreRect: true, ignoreId: true, ignoreTransform: true));
semantics.dispose();
});
testWidgets('has semantic events by default - ThemeData.tooltipTheme', (WidgetTester tester) async {
final List<dynamic> semanticEvents = <dynamic>[];
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, (dynamic message) async {
semanticEvents.add(message);
});
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(),
home: Center(
child: Tooltip(
message: 'Foo',
child: Container(
width: 100.0,
height: 100.0,
color: Colors.green[500],
),
),
),
),
);
await tester.longPress(find.byType(Tooltip));
final RenderObject object = tester.firstRenderObject(find.byType(Tooltip));
expect(semanticEvents, unorderedEquals(<dynamic>[
<String, dynamic>{
'type': 'longPress',
'nodeId': findDebugSemantics(object).id,
'data': <String, dynamic>{},
},
<String, dynamic>{
'type': 'tooltip',
'data': <String, dynamic>{
'message': 'Foo',
},
},
]));
semantics.dispose();
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, null);
});
testWidgets('has semantic events by default - TooltipTheme', (WidgetTester tester) async {
final List<dynamic> semanticEvents = <dynamic>[];
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, (dynamic message) async {
semanticEvents.add(message);
});
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
home: TooltipTheme(
data: const TooltipThemeData(),
child: Center(
child: Tooltip(
message: 'Foo',
child: Container(
width: 100.0,
height: 100.0,
color: Colors.green[500],
),
),
),
),
),
);
await tester.longPress(find.byType(Tooltip));
final RenderObject object = tester.firstRenderObject(find.byType(Tooltip));
expect(semanticEvents, unorderedEquals(<dynamic>[
<String, dynamic>{
'type': 'longPress',
'nodeId': findDebugSemantics(object).id,
'data': <String, dynamic>{},
},
<String, dynamic>{
'type': 'tooltip',
'data': <String, dynamic>{
'message': 'Foo',
},
},
]));
semantics.dispose();
tester.binding.defaultBinaryMessenger.setMockDecodedMessageHandler<dynamic>(SystemChannels.accessibility, null);
});
testWidgets('default Tooltip debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const Tooltip(message: 'message').debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString()).toList();
expect(description, <String>[
'"message"',
]);
});
}
SemanticsNode findDebugSemantics(RenderObject object) {
return object.debugSemantics ?? findDebugSemantics(object.parent!);
}
| flutter/packages/flutter/test/material/tooltip_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/tooltip_theme_test.dart",
"repo_id": "flutter",
"token_count": 23897
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('BoxDecoration.lerp identical a,b', () {
expect(BoxDecoration.lerp(null, null, 0), null);
const BoxDecoration decoration = BoxDecoration();
expect(identical(BoxDecoration.lerp(decoration, decoration, 0.5), decoration), true);
});
test('BoxDecoration with BorderRadiusDirectional', () {
const BoxDecoration decoration = BoxDecoration(
color: Color(0xFF000000),
borderRadius: BorderRadiusDirectional.only(topStart: Radius.circular(100.0)),
);
final BoxPainter painter = decoration.createBoxPainter();
const Size size = Size(1000.0, 1000.0);
expect(
(Canvas canvas) {
painter.paint(
canvas,
Offset.zero,
const ImageConfiguration(size: size, textDirection: TextDirection.rtl),
);
},
paints
..rrect(rrect: RRect.fromRectAndCorners(Offset.zero & size, topRight: const Radius.circular(100.0))),
);
expect(decoration.hitTest(size, const Offset(10.0, 10.0), textDirection: TextDirection.rtl), isTrue);
expect(decoration.hitTest(size, const Offset(990.0, 10.0), textDirection: TextDirection.rtl), isFalse);
expect(
(Canvas canvas) {
painter.paint(
canvas,
Offset.zero,
const ImageConfiguration(size: size, textDirection: TextDirection.ltr),
);
},
paints
..rrect(rrect: RRect.fromRectAndCorners(Offset.zero & size, topLeft: const Radius.circular(100.0))),
);
expect(decoration.hitTest(size, const Offset(10.0, 10.0), textDirection: TextDirection.ltr), isFalse);
expect(decoration.hitTest(size, const Offset(990.0, 10.0), textDirection: TextDirection.ltr), isTrue);
});
test('BoxDecoration with LinearGradient using AlignmentDirectional', () {
const BoxDecoration decoration = BoxDecoration(
color: Color(0xFF000000),
gradient: LinearGradient(
begin: AlignmentDirectional.centerStart,
end: AlignmentDirectional.bottomEnd,
colors: <Color>[
Color(0xFF000000),
Color(0xFFFFFFFF),
],
),
);
final BoxPainter painter = decoration.createBoxPainter();
const Size size = Size(1000.0, 1000.0);
expect(
(Canvas canvas) {
painter.paint(
canvas,
Offset.zero,
const ImageConfiguration(size: size, textDirection: TextDirection.rtl),
);
},
paints..rect(rect: Offset.zero & size),
);
});
test('BoxDecoration.getClipPath with borderRadius', () {
const double radius = 10;
final BoxDecoration decoration = BoxDecoration(
borderRadius: BorderRadius.circular(radius),
);
const Rect rect = Rect.fromLTWH(0.0, 0.0, 100.0, 20.0);
final Path clipPath = decoration.getClipPath(rect, TextDirection.ltr);
final Matcher isLookLikeExpectedPath = isPathThat(
includes: const <Offset>[ Offset(30.0, 10.0), Offset(50.0, 10.0), ],
excludes: const <Offset>[ Offset(1.0, 1.0), Offset(99.0, 19.0), ],
);
expect(clipPath, isLookLikeExpectedPath);
});
test('BoxDecoration.getClipPath with shape BoxShape.circle', () {
const BoxDecoration decoration = BoxDecoration(
shape: BoxShape.circle,
);
const Rect rect = Rect.fromLTWH(0.0, 0.0, 100.0, 20.0);
final Path clipPath = decoration.getClipPath(rect, TextDirection.ltr);
final Matcher isLookLikeExpectedPath = isPathThat(
includes: const <Offset>[ Offset(50.0, 0.0), Offset(40.0, 10.0), ],
excludes: const <Offset>[ Offset(40.0, 0.0), Offset(10.0, 10.0), ],
);
expect(clipPath, isLookLikeExpectedPath);
});
test('BoxDecorations with different blendModes are not equal', () {
// Regression test for https://github.com/flutter/flutter/issues/100754.
const BoxDecoration one = BoxDecoration(
color: Color(0x00000000),
backgroundBlendMode: BlendMode.color,
);
const BoxDecoration two = BoxDecoration(
color: Color(0x00000000),
backgroundBlendMode: BlendMode.difference,
);
expect(one == two, isFalse);
});
}
| flutter/packages/flutter/test/painting/box_decoration_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/box_decoration_test.dart",
"repo_id": "flutter",
"token_count": 1710
} | 670 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.