text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import("//flutter/common/config.gni")
import("//flutter/impeller/tools/impeller.gni")
impeller_component("golden_playground_test") {
testonly = true
sources = [ "golden_playground_test.h" ]
deps = [
":digest",
"//flutter/fml",
"//flutter/impeller/aiks",
"//flutter/impeller/playground",
"//flutter/impeller/typographer/backends/skia:typographer_skia_backend",
"//flutter/testing:testing_lib",
]
if (is_mac) {
sources += [ "golden_playground_test_mac.cc" ]
deps += [
":metal_screenshot",
"//flutter/third_party/abseil-cpp/absl/base:no_destructor",
]
} else {
sources += [ "golden_playground_test_stub.cc" ]
}
}
impeller_component("digest") {
testonly = true
sources = [
"golden_digest.cc",
"golden_digest.h",
"working_directory.cc",
"working_directory.h",
]
deps = [ "//flutter/fml" ]
}
if (is_mac) {
impeller_component("metal_screenshot") {
testonly = true
sources = [
"metal_screenshot.h",
"metal_screenshot.mm",
"metal_screenshotter.h",
"metal_screenshotter.mm",
"screenshot.h",
"screenshotter.h",
"vulkan_screenshotter.h",
"vulkan_screenshotter.mm",
]
deps = [
"//flutter/impeller/aiks",
"//flutter/impeller/display_list",
"//flutter/impeller/playground",
"//flutter/impeller/renderer/backend/metal:metal",
"//flutter/impeller/renderer/backend/vulkan",
]
}
impeller_component("impeller_golden_tests") {
target_type = "executable"
testonly = true
sources = [
"golden_tests.cc",
"main.cc",
]
deps = [
":digest",
":metal_screenshot",
"//flutter/impeller/aiks",
"//flutter/impeller/aiks:aiks_unittests_golden",
"//flutter/impeller/fixtures",
"//flutter/third_party/angle:libEGL",
"//flutter/third_party/angle:libGLESv2",
"//flutter/third_party/googletest:gtest",
"//flutter/third_party/swiftshader",
]
}
}
| engine/impeller/golden_tests/BUILD.gn/0 | {
"file_path": "engine/impeller/golden_tests/BUILD.gn",
"repo_id": "engine",
"token_count": 976
} | 212 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/impeller/golden_tests/vulkan_screenshotter.h"
#include "flutter/fml/synchronization/waitable_event.h"
#include "flutter/impeller/golden_tests/metal_screenshot.h"
#define GLFW_INCLUDE_NONE
#include "third_party/glfw/include/GLFW/glfw3.h"
namespace impeller {
namespace testing {
namespace {
using CGContextPtr = std::unique_ptr<std::remove_pointer<CGContextRef>::type,
decltype(&CGContextRelease)>;
using CGImagePtr = std::unique_ptr<std::remove_pointer<CGImageRef>::type,
decltype(&CGImageRelease)>;
using CGColorSpacePtr =
std::unique_ptr<std::remove_pointer<CGColorSpaceRef>::type,
decltype(&CGColorSpaceRelease)>;
std::unique_ptr<Screenshot> ReadTexture(
const std::shared_ptr<Context>& surface_context,
const std::shared_ptr<Texture>& texture) {
DeviceBufferDescriptor buffer_desc;
buffer_desc.storage_mode = StorageMode::kHostVisible;
buffer_desc.size =
texture->GetTextureDescriptor().GetByteSizeOfBaseMipLevel();
buffer_desc.readback = true;
std::shared_ptr<DeviceBuffer> device_buffer =
surface_context->GetResourceAllocator()->CreateBuffer(buffer_desc);
FML_CHECK(device_buffer);
auto command_buffer = surface_context->CreateCommandBuffer();
auto blit_pass = command_buffer->CreateBlitPass();
bool success = blit_pass->AddCopy(texture, device_buffer);
FML_CHECK(success);
success = blit_pass->EncodeCommands(surface_context->GetResourceAllocator());
FML_CHECK(success);
fml::AutoResetWaitableEvent latch;
success =
surface_context->GetCommandQueue()
->Submit({command_buffer},
[&latch](CommandBuffer::Status status) {
FML_CHECK(status == CommandBuffer::Status::kCompleted);
latch.Signal();
})
.ok();
FML_CHECK(success);
latch.Wait();
device_buffer->Invalidate();
// TODO(gaaclarke): Replace CoreImage requirement with something
// crossplatform.
CGColorSpacePtr color_space(CGColorSpaceCreateDeviceRGB(),
&CGColorSpaceRelease);
CGBitmapInfo bitmap_info =
texture->GetTextureDescriptor().format == PixelFormat::kB8G8R8A8UNormInt
? kCGImageAlphaPremultipliedFirst | kCGBitmapByteOrder32Little
: kCGImageAlphaPremultipliedLast;
CGContextPtr context(
CGBitmapContextCreate(
device_buffer->OnGetContents(), texture->GetSize().width,
texture->GetSize().height,
/*bitsPerComponent=*/8,
/*bytesPerRow=*/texture->GetTextureDescriptor().GetBytesPerRow(),
color_space.get(), bitmap_info),
&CGContextRelease);
FML_CHECK(context);
CGImagePtr image(CGBitmapContextCreateImage(context.get()), &CGImageRelease);
FML_CHECK(image);
// TODO(https://github.com/flutter/flutter/issues/142641): Perform the flip at
// the blit stage to avoid this slow copy.
if (texture->GetYCoordScale() == -1) {
CGContextPtr flipped_context(
CGBitmapContextCreate(
nullptr, texture->GetSize().width, texture->GetSize().height,
/*bitsPerComponent=*/8,
/*bytesPerRow=*/0, color_space.get(), bitmap_info),
&CGContextRelease);
CGContextTranslateCTM(flipped_context.get(), 0, texture->GetSize().height);
CGContextScaleCTM(flipped_context.get(), 1.0, -1.0);
CGContextDrawImage(
flipped_context.get(),
CGRectMake(0, 0, texture->GetSize().width, texture->GetSize().height),
image.get());
CGImagePtr flipped_image(CGBitmapContextCreateImage(flipped_context.get()),
&CGImageRelease);
image.swap(flipped_image);
}
return std::make_unique<MetalScreenshot>(image.release());
}
} // namespace
VulkanScreenshotter::VulkanScreenshotter(
const std::unique_ptr<PlaygroundImpl>& playground)
: playground_(playground) {
FML_CHECK(playground_);
}
std::unique_ptr<Screenshot> VulkanScreenshotter::MakeScreenshot(
AiksContext& aiks_context,
const Picture& picture,
const ISize& size,
bool scale_content) {
Vector2 content_scale =
scale_content ? playground_->GetContentScale() : Vector2{1, 1};
std::shared_ptr<Image> image = picture.ToImage(
aiks_context,
ISize(size.width * content_scale.x, size.height * content_scale.y));
std::shared_ptr<Texture> texture = image->GetTexture();
return ReadTexture(aiks_context.GetContext(), texture);
}
} // namespace testing
} // namespace impeller
| engine/impeller/golden_tests/vulkan_screenshotter.mm/0 | {
"file_path": "engine/impeller/golden_tests/vulkan_screenshotter.mm",
"repo_id": "engine",
"token_count": 1822
} | 213 |
# The Impeller Image Library
Set of utilities for working with texture information. The library is indepenent
of the rendering subsystem.
| engine/impeller/playground/image/README.md/0 | {
"file_path": "engine/impeller/playground/image/README.md",
"repo_id": "engine",
"token_count": 32
} | 214 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/fml/time/time_point.h"
#include "impeller/base/timing.h"
#include "impeller/base/validation.h"
#include "impeller/playground/playground_test.h"
namespace impeller {
PlaygroundTest::PlaygroundTest()
: Playground(PlaygroundSwitches{flutter::testing::GetArgsForProcess()}) {}
PlaygroundTest::~PlaygroundTest() = default;
void PlaygroundTest::SetUp() {
if (!Playground::SupportsBackend(GetParam())) {
GTEST_SKIP_("Playground doesn't support this backend type.");
return;
}
if (!Playground::ShouldOpenNewPlaygrounds()) {
GTEST_SKIP_("Skipping due to user action.");
return;
}
ImpellerValidationErrorsSetFatal(true);
SetupContext(GetParam());
SetupWindow();
}
PlaygroundBackend PlaygroundTest::GetBackend() const {
return GetParam();
}
void PlaygroundTest::TearDown() {
TeardownWindow();
}
// |Playground|
std::unique_ptr<fml::Mapping> PlaygroundTest::OpenAssetAsMapping(
std::string asset_name) const {
return flutter::testing::OpenFixtureAsMapping(asset_name);
}
RuntimeStage::Map PlaygroundTest::OpenAssetAsRuntimeStage(
const char* asset_name) const {
const std::shared_ptr<fml::Mapping> fixture =
flutter::testing::OpenFixtureAsMapping(asset_name);
if (!fixture || fixture->GetSize() == 0) {
return {};
}
return RuntimeStage::DecodeRuntimeStages(fixture);
}
// |Playground|
std::string PlaygroundTest::GetWindowTitle() const {
std::stringstream stream;
stream << "Impeller Playground for '"
<< flutter::testing::GetCurrentTestName() << "' ";
switch (GetBackend()) {
case PlaygroundBackend::kMetal:
break;
case PlaygroundBackend::kOpenGLES:
if (switches_.use_angle) {
stream << " (Angle) ";
}
break;
case PlaygroundBackend::kVulkan:
if (switches_.use_swiftshader) {
stream << " (SwiftShader) ";
}
break;
}
stream << " (Press ESC to quit)";
return stream.str();
}
// |Playground|
bool PlaygroundTest::ShouldKeepRendering() const {
if (!switches_.timeout.has_value()) {
return true;
}
if (SecondsF{GetSecondsElapsed()} > switches_.timeout.value()) {
return false;
}
return true;
}
} // namespace impeller
| engine/impeller/playground/playground_test.cc/0 | {
"file_path": "engine/impeller/playground/playground_test.cc",
"repo_id": "engine",
"token_count": 858
} | 215 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_BUFFER_BINDINGS_GLES_H_
#define FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_BUFFER_BINDINGS_GLES_H_
#include <unordered_map>
#include <vector>
#include "impeller/core/shader_types.h"
#include "impeller/renderer/backend/gles/gles.h"
#include "impeller/renderer/backend/gles/proc_table_gles.h"
#include "impeller/renderer/command.h"
namespace impeller {
//------------------------------------------------------------------------------
/// @brief Sets up stage bindings for single draw call in the OpenGLES
/// backend.
///
class BufferBindingsGLES {
public:
BufferBindingsGLES();
~BufferBindingsGLES();
bool RegisterVertexStageInput(
const ProcTableGLES& gl,
const std::vector<ShaderStageIOSlot>& inputs,
const std::vector<ShaderStageBufferLayout>& layouts);
bool ReadUniformsBindings(const ProcTableGLES& gl, GLuint program);
bool BindVertexAttributes(const ProcTableGLES& gl,
size_t vertex_offset) const;
bool BindUniformData(const ProcTableGLES& gl,
Allocator& transients_allocator,
const Bindings& vertex_bindings,
const Bindings& fragment_bindings);
bool UnbindVertexAttributes(const ProcTableGLES& gl) const;
private:
//----------------------------------------------------------------------------
/// @brief The arguments to glVertexAttribPointer.
///
struct VertexAttribPointer {
GLuint index = 0u;
GLint size = 4;
GLenum type = GL_FLOAT;
GLenum normalized = GL_FALSE;
GLsizei stride = 0u;
GLsizei offset = 0u;
};
std::vector<VertexAttribPointer> vertex_attrib_arrays_;
std::unordered_map<std::string, GLint> uniform_locations_;
using BindingMap = std::unordered_map<std::string, std::vector<GLint>>;
BindingMap binding_map_ = {};
const std::vector<GLint>& ComputeUniformLocations(
const ShaderMetadata* metadata);
GLint ComputeTextureLocation(const ShaderMetadata* metadata);
bool BindUniformBuffer(const ProcTableGLES& gl,
Allocator& transients_allocator,
const BufferResource& buffer);
std::optional<size_t> BindTextures(const ProcTableGLES& gl,
const Bindings& bindings,
ShaderStage stage,
size_t unit_start_index = 0);
BufferBindingsGLES(const BufferBindingsGLES&) = delete;
BufferBindingsGLES& operator=(const BufferBindingsGLES&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_BUFFER_BINDINGS_GLES_H_
| engine/impeller/renderer/backend/gles/buffer_bindings_gles.h/0 | {
"file_path": "engine/impeller/renderer/backend/gles/buffer_bindings_gles.h",
"repo_id": "engine",
"token_count": 1133
} | 216 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/gles/handle_gles.h"
#include "flutter/fml/logging.h"
namespace impeller {
std::string HandleTypeToString(HandleType type) {
switch (type) {
case HandleType::kUnknown:
return "Unknown";
case HandleType::kTexture:
return "Texture";
case HandleType::kBuffer:
return "Buffer";
case HandleType::kProgram:
return "Program";
case HandleType::kRenderBuffer:
return "RenderBuffer";
case HandleType::kFrameBuffer:
return "Framebuffer";
}
FML_UNREACHABLE();
}
} // namespace impeller
| engine/impeller/renderer/backend/gles/handle_gles.cc/0 | {
"file_path": "engine/impeller/renderer/backend/gles/handle_gles.cc",
"repo_id": "engine",
"token_count": 262
} | 217 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/gles/shader_function_gles.h"
namespace impeller {
ShaderFunctionGLES::ShaderFunctionGLES(
UniqueID library_id,
ShaderStage stage,
std::string name,
std::shared_ptr<const fml::Mapping> mapping)
: ShaderFunction(library_id, std::move(name), stage),
mapping_(std::move(mapping)) {}
ShaderFunctionGLES::~ShaderFunctionGLES() = default;
const std::shared_ptr<const fml::Mapping>&
ShaderFunctionGLES::GetSourceMapping() const {
return mapping_;
}
} // namespace impeller
| engine/impeller/renderer/backend/gles/shader_function_gles.cc/0 | {
"file_path": "engine/impeller/renderer/backend/gles/shader_function_gles.cc",
"repo_id": "engine",
"token_count": 239
} | 218 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_TEXTURE_GLES_H_
#define FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_TEXTURE_GLES_H_
#include "impeller/base/backend_cast.h"
#include "impeller/core/texture.h"
#include "impeller/renderer/backend/gles/handle_gles.h"
#include "impeller/renderer/backend/gles/reactor_gles.h"
namespace impeller {
class TextureGLES final : public Texture,
public BackendCast<TextureGLES, Texture> {
public:
enum class Type {
kTexture,
kTextureMultisampled,
kRenderBuffer,
kRenderBufferMultisampled,
};
enum class IsWrapped {
kWrapped,
};
TextureGLES(ReactorGLES::Ref reactor, TextureDescriptor desc);
TextureGLES(ReactorGLES::Ref reactor,
TextureDescriptor desc,
IsWrapped wrapped);
// |Texture|
~TextureGLES() override;
std::optional<GLuint> GetGLHandle() const;
[[nodiscard]] bool Bind() const;
[[nodiscard]] bool GenerateMipmap();
enum class AttachmentType {
kColor0,
kDepth,
kStencil,
};
[[nodiscard]] bool SetAsFramebufferAttachment(
GLenum target,
AttachmentType attachment_type) const;
Type GetType() const;
bool IsWrapped() const { return is_wrapped_; }
private:
friend class AllocatorMTL;
ReactorGLES::Ref reactor_;
const Type type_;
HandleGLES handle_;
mutable bool contents_initialized_ = false;
const bool is_wrapped_;
bool is_valid_ = false;
TextureGLES(std::shared_ptr<ReactorGLES> reactor,
TextureDescriptor desc,
bool is_wrapped);
// |Texture|
void SetLabel(std::string_view label) override;
// |Texture|
bool OnSetContents(const uint8_t* contents,
size_t length,
size_t slice) override;
// |Texture|
bool OnSetContents(std::shared_ptr<const fml::Mapping> mapping,
size_t slice) override;
// |Texture|
bool IsValid() const override;
// |Texture|
ISize GetSize() const override;
// |Texture|
Scalar GetYCoordScale() const override;
void InitializeContentsIfNecessary() const;
TextureGLES(const TextureGLES&) = delete;
TextureGLES& operator=(const TextureGLES&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_RENDERER_BACKEND_GLES_TEXTURE_GLES_H_
| engine/impeller/renderer/backend/gles/texture_gles.h/0 | {
"file_path": "engine/impeller/renderer/backend/gles/texture_gles.h",
"repo_id": "engine",
"token_count": 963
} | 219 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_CONTEXT_MTL_H_
#define FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_CONTEXT_MTL_H_
#include <Metal/Metal.h>
#include <deque>
#include <string>
#include "flutter/fml/concurrent_message_loop.h"
#include "flutter/fml/macros.h"
#include "flutter/fml/synchronization/sync_switch.h"
#include "impeller/base/backend_cast.h"
#include "impeller/core/sampler.h"
#include "impeller/renderer/backend/metal/allocator_mtl.h"
#include "impeller/renderer/backend/metal/command_buffer_mtl.h"
#include "impeller/renderer/backend/metal/gpu_tracer_mtl.h"
#include "impeller/renderer/backend/metal/pipeline_library_mtl.h"
#include "impeller/renderer/backend/metal/shader_library_mtl.h"
#include "impeller/renderer/capabilities.h"
#include "impeller/renderer/command_queue.h"
#include "impeller/renderer/context.h"
#if TARGET_OS_SIMULATOR
#define IMPELLER_CA_METAL_LAYER_AVAILABLE API_AVAILABLE(macos(10.11), ios(13.0))
#else // TARGET_OS_SIMULATOR
#define IMPELLER_CA_METAL_LAYER_AVAILABLE API_AVAILABLE(macos(10.11), ios(8.0))
#endif // TARGET_OS_SIMULATOR
namespace impeller {
class ContextMTL final : public Context,
public BackendCast<ContextMTL, Context>,
public std::enable_shared_from_this<ContextMTL> {
public:
static std::shared_ptr<ContextMTL> Create(
const std::vector<std::string>& shader_library_paths,
std::shared_ptr<const fml::SyncSwitch> is_gpu_disabled_sync_switch);
static std::shared_ptr<ContextMTL> Create(
const std::vector<std::shared_ptr<fml::Mapping>>& shader_libraries_data,
std::shared_ptr<const fml::SyncSwitch> is_gpu_disabled_sync_switch,
const std::string& label);
static std::shared_ptr<ContextMTL> Create(
id<MTLDevice> device,
id<MTLCommandQueue> command_queue,
const std::vector<std::shared_ptr<fml::Mapping>>& shader_libraries_data,
std::shared_ptr<const fml::SyncSwitch> is_gpu_disabled_sync_switch,
const std::string& label);
// |Context|
~ContextMTL() override;
// |Context|
BackendType GetBackendType() const override;
id<MTLDevice> GetMTLDevice() const;
// |Context|
std::string DescribeGpuModel() const override;
// |Context|
bool IsValid() const override;
// |Context|
std::shared_ptr<Allocator> GetResourceAllocator() const override;
// |Context|
std::shared_ptr<ShaderLibrary> GetShaderLibrary() const override;
// |Context|
std::shared_ptr<SamplerLibrary> GetSamplerLibrary() const override;
// |Context|
std::shared_ptr<PipelineLibrary> GetPipelineLibrary() const override;
// |Context|
std::shared_ptr<CommandBuffer> CreateCommandBuffer() const override;
// |Context|
std::shared_ptr<CommandQueue> GetCommandQueue() const override;
// |Context|
const std::shared_ptr<const Capabilities>& GetCapabilities() const override;
void SetCapabilities(const std::shared_ptr<const Capabilities>& capabilities);
// |Context|
bool UpdateOffscreenLayerPixelFormat(PixelFormat format) override;
// |Context|
void Shutdown() override;
id<MTLCommandBuffer> CreateMTLCommandBuffer(const std::string& label) const;
std::shared_ptr<const fml::SyncSwitch> GetIsGpuDisabledSyncSwitch() const;
#ifdef IMPELLER_DEBUG
std::shared_ptr<GPUTracerMTL> GetGPUTracer() const;
#endif // IMPELLER_DEBUG
// |Context|
void StoreTaskForGPU(const std::function<void()>& task) override;
private:
class SyncSwitchObserver : public fml::SyncSwitch::Observer {
public:
explicit SyncSwitchObserver(ContextMTL& parent);
virtual ~SyncSwitchObserver() = default;
void OnSyncSwitchUpdate(bool new_value) override;
private:
ContextMTL& parent_;
};
id<MTLDevice> device_ = nullptr;
id<MTLCommandQueue> command_queue_ = nullptr;
std::shared_ptr<ShaderLibraryMTL> shader_library_;
std::shared_ptr<PipelineLibraryMTL> pipeline_library_;
std::shared_ptr<SamplerLibrary> sampler_library_;
std::shared_ptr<AllocatorMTL> resource_allocator_;
std::shared_ptr<const Capabilities> device_capabilities_;
std::shared_ptr<const fml::SyncSwitch> is_gpu_disabled_sync_switch_;
#ifdef IMPELLER_DEBUG
std::shared_ptr<GPUTracerMTL> gpu_tracer_;
#endif // IMPELLER_DEBUG
std::deque<std::function<void()>> tasks_awaiting_gpu_;
std::unique_ptr<SyncSwitchObserver> sync_switch_observer_;
std::shared_ptr<CommandQueue> command_queue_ip_;
bool is_valid_ = false;
ContextMTL(
id<MTLDevice> device,
id<MTLCommandQueue> command_queue,
NSArray<id<MTLLibrary>>* shader_libraries,
std::shared_ptr<const fml::SyncSwitch> is_gpu_disabled_sync_switch);
std::shared_ptr<CommandBuffer> CreateCommandBufferInQueue(
id<MTLCommandQueue> queue) const;
void FlushTasksAwaitingGPU();
ContextMTL(const ContextMTL&) = delete;
ContextMTL& operator=(const ContextMTL&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_CONTEXT_MTL_H_
| engine/impeller/renderer/backend/metal/context_mtl.h/0 | {
"file_path": "engine/impeller/renderer/backend/metal/context_mtl.h",
"repo_id": "engine",
"token_count": 1896
} | 220 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_RENDER_PASS_MTL_H_
#define FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_RENDER_PASS_MTL_H_
#include <Metal/Metal.h>
#include "flutter/fml/macros.h"
#include "impeller/renderer/backend/metal/pass_bindings_cache_mtl.h"
#include "impeller/renderer/render_pass.h"
#include "impeller/renderer/render_target.h"
namespace impeller {
class RenderPassMTL final : public RenderPass {
public:
// |RenderPass|
~RenderPassMTL() override;
private:
friend class CommandBufferMTL;
id<MTLCommandBuffer> buffer_ = nil;
id<MTLRenderCommandEncoder> encoder_ = nil;
MTLRenderPassDescriptor* desc_ = nil;
std::string label_;
bool is_metal_trace_active_ = false;
bool is_valid_ = false;
// Many parts of the codebase will start writing to a render pass but
// never submit them. This boolean is used to track if a submit happened
// so that in the dtor we can always ensure the render pass is finished.
mutable bool did_finish_encoding_ = false;
PassBindingsCacheMTL pass_bindings_;
// Per-command state
size_t instance_count_ = 1u;
size_t base_vertex_ = 0u;
size_t vertex_count_ = 0u;
bool has_valid_pipeline_ = false;
bool has_label_ = false;
BufferView index_buffer_ = {};
PrimitiveType primitive_type_ = {};
MTLIndexType index_type_ = {};
RenderPassMTL(std::shared_ptr<const Context> context,
const RenderTarget& target,
id<MTLCommandBuffer> buffer);
// |RenderPass|
void ReserveCommands(size_t command_count) override {}
// |RenderPass|
bool IsValid() const override;
// |RenderPass|
void OnSetLabel(std::string label) override;
// |RenderPass|
bool OnEncodeCommands(const Context& context) const override;
// |RenderPass|
void SetPipeline(
const std::shared_ptr<Pipeline<PipelineDescriptor>>& pipeline) override;
// |RenderPass|
void SetCommandLabel(std::string_view label) override;
// |RenderPass|
void SetStencilReference(uint32_t value) override;
// |RenderPass|
void SetBaseVertex(uint64_t value) override;
// |RenderPass|
void SetViewport(Viewport viewport) override;
// |RenderPass|
void SetScissor(IRect scissor) override;
// |RenderPass|
void SetInstanceCount(size_t count) override;
// |RenderPass|
bool SetVertexBuffer(VertexBuffer buffer) override;
// |RenderPass|
fml::Status Draw() override;
// |RenderPass|
bool BindResource(ShaderStage stage,
DescriptorType type,
const ShaderUniformSlot& slot,
const ShaderMetadata& metadata,
BufferView view) override;
// |RenderPass|
bool BindResource(ShaderStage stage,
DescriptorType type,
const ShaderUniformSlot& slot,
const std::shared_ptr<const ShaderMetadata>& metadata,
BufferView view) override;
// |RenderPass|
bool BindResource(ShaderStage stage,
DescriptorType type,
const SampledImageSlot& slot,
const ShaderMetadata& metadata,
std::shared_ptr<const Texture> texture,
const std::unique_ptr<const Sampler>& sampler) override;
RenderPassMTL(const RenderPassMTL&) = delete;
RenderPassMTL& operator=(const RenderPassMTL&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_RENDERER_BACKEND_METAL_RENDER_PASS_MTL_H_
| engine/impeller/renderer/backend/metal/render_pass_mtl.h/0 | {
"file_path": "engine/impeller/renderer/backend/metal/render_pass_mtl.h",
"repo_id": "engine",
"token_count": 1402
} | 221 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/metal/texture_wrapper_mtl.h"
#include <Metal/Metal.h>
#include "impeller/renderer/backend/metal/formats_mtl.h"
#include "impeller/renderer/backend/metal/texture_mtl.h"
namespace impeller {
std::shared_ptr<Texture> WrapTextureMTL(TextureDescriptor desc,
const void* mtl_texture,
std::function<void()> deletion_proc) {
auto texture = (__bridge id<MTLTexture>)mtl_texture;
desc.format = FromMTLPixelFormat(texture.pixelFormat);
return TextureMTL::Wrapper(desc, texture, std::move(deletion_proc));
}
} // namespace impeller
| engine/impeller/renderer/backend/metal/texture_wrapper_mtl.mm/0 | {
"file_path": "engine/impeller/renderer/backend/metal/texture_wrapper_mtl.mm",
"repo_id": "engine",
"token_count": 324
} | 222 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/vulkan/capabilities_vk.h"
#include <algorithm>
#include "impeller/base/validation.h"
#include "impeller/core/formats.h"
#include "impeller/renderer/backend/vulkan/vk.h"
namespace impeller {
static constexpr const char* kInstanceLayer = "ImpellerInstance";
CapabilitiesVK::CapabilitiesVK(bool enable_validations) {
auto extensions = vk::enumerateInstanceExtensionProperties();
auto layers = vk::enumerateInstanceLayerProperties();
if (extensions.result != vk::Result::eSuccess ||
layers.result != vk::Result::eSuccess) {
return;
}
for (const auto& ext : extensions.value) {
exts_[kInstanceLayer].insert(ext.extensionName);
}
for (const auto& layer : layers.value) {
const std::string layer_name = layer.layerName;
auto layer_exts = vk::enumerateInstanceExtensionProperties(layer_name);
if (layer_exts.result != vk::Result::eSuccess) {
return;
}
for (const auto& layer_ext : layer_exts.value) {
exts_[layer_name].insert(layer_ext.extensionName);
}
}
validations_enabled_ =
enable_validations && HasLayer("VK_LAYER_KHRONOS_validation");
if (enable_validations && !validations_enabled_) {
FML_LOG(ERROR)
<< "Requested Impeller context creation with validations but the "
"validation layers could not be found. Expect no Vulkan validation "
"checks!";
}
if (validations_enabled_) {
FML_LOG(INFO) << "Vulkan validations are enabled.";
}
is_valid_ = true;
}
CapabilitiesVK::~CapabilitiesVK() = default;
bool CapabilitiesVK::IsValid() const {
return is_valid_;
}
bool CapabilitiesVK::AreValidationsEnabled() const {
return validations_enabled_;
}
std::optional<std::vector<std::string>> CapabilitiesVK::GetEnabledLayers()
const {
std::vector<std::string> required;
if (validations_enabled_) {
// The presence of this layer is already checked in the ctor.
required.push_back("VK_LAYER_KHRONOS_validation");
}
return required;
}
std::optional<std::vector<std::string>>
CapabilitiesVK::GetEnabledInstanceExtensions() const {
std::vector<std::string> required;
if (!HasExtension("VK_KHR_surface")) {
// Swapchain support is required and this is a dependency of
// VK_KHR_swapchain.
VALIDATION_LOG << "Could not find the surface extension.";
return std::nullopt;
}
required.push_back("VK_KHR_surface");
auto has_wsi = false;
if (HasExtension("VK_MVK_macos_surface")) {
required.push_back("VK_MVK_macos_surface");
has_wsi = true;
}
if (HasExtension("VK_EXT_metal_surface")) {
required.push_back("VK_EXT_metal_surface");
has_wsi = true;
}
if (HasExtension("VK_KHR_portability_enumeration")) {
required.push_back("VK_KHR_portability_enumeration");
has_wsi = true;
}
if (HasExtension("VK_KHR_win32_surface")) {
required.push_back("VK_KHR_win32_surface");
has_wsi = true;
}
if (HasExtension("VK_KHR_android_surface")) {
required.push_back("VK_KHR_android_surface");
has_wsi = true;
}
if (HasExtension("VK_KHR_xcb_surface")) {
required.push_back("VK_KHR_xcb_surface");
has_wsi = true;
}
if (HasExtension("VK_KHR_xlib_surface")) {
required.push_back("VK_KHR_xlib_surface");
has_wsi = true;
}
if (HasExtension("VK_KHR_wayland_surface")) {
required.push_back("VK_KHR_wayland_surface");
has_wsi = true;
}
if (!has_wsi) {
// Don't really care which WSI extension there is as long there is at least
// one.
VALIDATION_LOG << "Could not find a WSI extension.";
return std::nullopt;
}
if (validations_enabled_) {
if (!HasExtension("VK_EXT_debug_utils")) {
VALIDATION_LOG << "Requested validations but could not find the "
"VK_EXT_debug_utils extension.";
return std::nullopt;
}
required.push_back("VK_EXT_debug_utils");
if (HasExtension("VK_EXT_validation_features")) {
// It's valid to not have `VK_EXT_validation_features` available. That's
// the case when using AGI as a frame debugger.
required.push_back("VK_EXT_validation_features");
}
}
return required;
}
static const char* GetExtensionName(RequiredCommonDeviceExtensionVK ext) {
switch (ext) {
case RequiredCommonDeviceExtensionVK::kKHRSwapchain:
return VK_KHR_SWAPCHAIN_EXTENSION_NAME;
case RequiredCommonDeviceExtensionVK::kLast:
return "Unknown";
}
FML_UNREACHABLE();
}
static const char* GetExtensionName(RequiredAndroidDeviceExtensionVK ext) {
switch (ext) {
case RequiredAndroidDeviceExtensionVK::
kANDROIDExternalMemoryAndroidHardwareBuffer:
return "VK_ANDROID_external_memory_android_hardware_buffer";
case RequiredAndroidDeviceExtensionVK::kKHRSamplerYcbcrConversion:
return VK_KHR_SAMPLER_YCBCR_CONVERSION_EXTENSION_NAME;
case RequiredAndroidDeviceExtensionVK::kKHRExternalMemory:
return VK_KHR_EXTERNAL_MEMORY_EXTENSION_NAME;
case RequiredAndroidDeviceExtensionVK::kEXTQueueFamilyForeign:
return VK_EXT_QUEUE_FAMILY_FOREIGN_EXTENSION_NAME;
case RequiredAndroidDeviceExtensionVK::kKHRDedicatedAllocation:
return VK_KHR_DEDICATED_ALLOCATION_EXTENSION_NAME;
case RequiredAndroidDeviceExtensionVK::kLast:
return "Unknown";
}
FML_UNREACHABLE();
}
static const char* GetExtensionName(OptionalDeviceExtensionVK ext) {
switch (ext) {
case OptionalDeviceExtensionVK::kEXTPipelineCreationFeedback:
return VK_EXT_PIPELINE_CREATION_FEEDBACK_EXTENSION_NAME;
case OptionalDeviceExtensionVK::kVKKHRPortabilitySubset:
return "VK_KHR_portability_subset";
case OptionalDeviceExtensionVK::kLast:
return "Unknown";
}
FML_UNREACHABLE();
}
template <class T>
static bool IterateExtensions(const std::function<bool(T)>& it) {
if (!it) {
return false;
}
for (size_t i = 0; i < static_cast<uint32_t>(T::kLast); i++) {
if (!it(static_cast<T>(i))) {
return false;
}
}
return true;
}
static std::optional<std::set<std::string>> GetSupportedDeviceExtensions(
const vk::PhysicalDevice& physical_device) {
auto device_extensions = physical_device.enumerateDeviceExtensionProperties();
if (device_extensions.result != vk::Result::eSuccess) {
return std::nullopt;
}
std::set<std::string> exts;
for (const auto& device_extension : device_extensions.value) {
exts.insert(device_extension.extensionName);
};
return exts;
}
std::optional<std::vector<std::string>>
CapabilitiesVK::GetEnabledDeviceExtensions(
const vk::PhysicalDevice& physical_device) const {
auto exts = GetSupportedDeviceExtensions(physical_device);
if (!exts.has_value()) {
return std::nullopt;
}
std::vector<std::string> enabled;
auto for_each_common_extension = [&](RequiredCommonDeviceExtensionVK ext) {
auto name = GetExtensionName(ext);
if (exts->find(name) == exts->end()) {
VALIDATION_LOG << "Device does not support required extension: " << name;
return false;
}
enabled.push_back(name);
return true;
};
auto for_each_android_extension = [&](RequiredAndroidDeviceExtensionVK ext) {
#ifdef FML_OS_ANDROID
auto name = GetExtensionName(ext);
if (exts->find(name) == exts->end()) {
VALIDATION_LOG << "Device does not support required Android extension: "
<< name;
return false;
}
enabled.push_back(name);
#endif // FML_OS_ANDROID
return true;
};
auto for_each_optional_extension = [&](OptionalDeviceExtensionVK ext) {
auto name = GetExtensionName(ext);
if (exts->find(name) != exts->end()) {
enabled.push_back(name);
}
return true;
};
const auto iterate_extensions =
IterateExtensions<RequiredCommonDeviceExtensionVK>(
for_each_common_extension) &&
IterateExtensions<RequiredAndroidDeviceExtensionVK>(
for_each_android_extension) &&
IterateExtensions<OptionalDeviceExtensionVK>(for_each_optional_extension);
if (!iterate_extensions) {
VALIDATION_LOG << "Device not suitable since required extensions are not "
"supported.";
return std::nullopt;
}
return enabled;
}
static bool HasSuitableColorFormat(const vk::PhysicalDevice& device,
vk::Format format) {
const auto props = device.getFormatProperties(format);
// This needs to be more comprehensive.
return !!(props.optimalTilingFeatures &
vk::FormatFeatureFlagBits::eColorAttachment);
}
static bool HasSuitableDepthStencilFormat(const vk::PhysicalDevice& device,
vk::Format format) {
const auto props = device.getFormatProperties(format);
return !!(props.optimalTilingFeatures &
vk::FormatFeatureFlagBits::eDepthStencilAttachment);
}
static bool PhysicalDeviceSupportsRequiredFormats(
const vk::PhysicalDevice& device) {
const auto has_color_format =
HasSuitableColorFormat(device, vk::Format::eB8G8R8A8Unorm);
const auto has_stencil_format =
HasSuitableDepthStencilFormat(device, vk::Format::eD32SfloatS8Uint) ||
HasSuitableDepthStencilFormat(device, vk::Format::eD24UnormS8Uint);
return has_color_format && has_stencil_format;
}
static bool HasRequiredProperties(const vk::PhysicalDevice& physical_device) {
auto properties = physical_device.getProperties();
if (!(properties.limits.framebufferColorSampleCounts &
(vk::SampleCountFlagBits::e1 | vk::SampleCountFlagBits::e4))) {
return false;
}
return true;
}
static bool HasRequiredQueues(const vk::PhysicalDevice& physical_device) {
auto queue_flags = vk::QueueFlags{};
for (const auto& queue : physical_device.getQueueFamilyProperties()) {
if (queue.queueCount == 0) {
continue;
}
queue_flags |= queue.queueFlags;
}
return static_cast<VkQueueFlags>(queue_flags &
(vk::QueueFlagBits::eGraphics |
vk::QueueFlagBits::eCompute |
vk::QueueFlagBits::eTransfer));
}
template <class ExtensionEnum>
static bool IsExtensionInList(const std::vector<std::string>& list,
ExtensionEnum ext) {
const std::string name = GetExtensionName(ext);
return std::find(list.begin(), list.end(), name) != list.end();
}
std::optional<CapabilitiesVK::PhysicalDeviceFeatures>
CapabilitiesVK::GetEnabledDeviceFeatures(
const vk::PhysicalDevice& device) const {
if (!PhysicalDeviceSupportsRequiredFormats(device)) {
VALIDATION_LOG << "Device doesn't support the required formats.";
return std::nullopt;
}
if (!HasRequiredProperties(device)) {
VALIDATION_LOG << "Device doesn't support the required properties.";
return std::nullopt;
}
if (!HasRequiredQueues(device)) {
VALIDATION_LOG << "Device doesn't support the required queues.";
return std::nullopt;
}
const auto enabled_extensions = GetEnabledDeviceExtensions(device);
if (!enabled_extensions.has_value()) {
VALIDATION_LOG << "Device doesn't support the required queues.";
return std::nullopt;
}
PhysicalDeviceFeatures supported_chain;
device.getFeatures2(&supported_chain.get());
PhysicalDeviceFeatures required_chain;
// Base features.
{
auto& required = required_chain.get().features;
const auto& supported = supported_chain.get().features;
// We require this for enabling wireframes in the playground. But its not
// necessarily a big deal if we don't have this feature.
required.fillModeNonSolid = supported.fillModeNonSolid;
}
// VK_KHR_sampler_ycbcr_conversion features.
if (IsExtensionInList(
enabled_extensions.value(),
RequiredAndroidDeviceExtensionVK::kKHRSamplerYcbcrConversion)) {
auto& required =
required_chain
.get<vk::PhysicalDeviceSamplerYcbcrConversionFeaturesKHR>();
const auto& supported =
supported_chain
.get<vk::PhysicalDeviceSamplerYcbcrConversionFeaturesKHR>();
required.samplerYcbcrConversion = supported.samplerYcbcrConversion;
}
return required_chain;
}
bool CapabilitiesVK::HasLayer(const std::string& layer) const {
for (const auto& [found_layer, exts] : exts_) {
if (found_layer == layer) {
return true;
}
}
return false;
}
bool CapabilitiesVK::HasExtension(const std::string& ext) const {
for (const auto& [layer, exts] : exts_) {
if (exts.find(ext) != exts.end()) {
return true;
}
}
return false;
}
void CapabilitiesVK::SetOffscreenFormat(PixelFormat pixel_format) const {
default_color_format_ = pixel_format;
}
bool CapabilitiesVK::SetPhysicalDevice(const vk::PhysicalDevice& device) {
if (HasSuitableDepthStencilFormat(device, vk::Format::eD32SfloatS8Uint)) {
default_depth_stencil_format_ = PixelFormat::kD32FloatS8UInt;
} else if (HasSuitableDepthStencilFormat(device,
vk::Format::eD24UnormS8Uint)) {
default_depth_stencil_format_ = PixelFormat::kD24UnormS8Uint;
} else {
default_depth_stencil_format_ = PixelFormat::kUnknown;
}
if (HasSuitableDepthStencilFormat(device, vk::Format::eS8Uint)) {
default_stencil_format_ = PixelFormat::kS8UInt;
} else if (default_depth_stencil_format_ != PixelFormat::kUnknown) {
default_stencil_format_ = default_depth_stencil_format_;
}
device_properties_ = device.getProperties();
auto physical_properties_2 =
device.getProperties2<vk::PhysicalDeviceProperties2,
vk::PhysicalDeviceSubgroupProperties>();
// Currently shaders only want access to arithmetic subgroup features.
// If that changes this needs to get updated, and so does Metal (which right
// now assumes it from compile time flags based on the MSL target version).
supports_compute_subgroups_ =
!!(physical_properties_2.get<vk::PhysicalDeviceSubgroupProperties>()
.supportedOperations &
vk::SubgroupFeatureFlagBits::eArithmetic);
{
// Query texture support.
// TODO(jonahwilliams):
// https://github.com/flutter/flutter/issues/129784
vk::PhysicalDeviceMemoryProperties memory_properties;
device.getMemoryProperties(&memory_properties);
for (auto i = 0u; i < memory_properties.memoryTypeCount; i++) {
if (memory_properties.memoryTypes[i].propertyFlags &
vk::MemoryPropertyFlagBits::eLazilyAllocated) {
supports_device_transient_textures_ = true;
}
}
}
// Determine the optional device extensions this physical device supports.
{
required_common_device_extensions_.clear();
required_android_device_extensions_.clear();
optional_device_extensions_.clear();
auto exts = GetSupportedDeviceExtensions(device);
if (!exts.has_value()) {
return false;
}
IterateExtensions<RequiredCommonDeviceExtensionVK>([&](auto ext) -> bool {
auto ext_name = GetExtensionName(ext);
if (exts->find(ext_name) != exts->end()) {
required_common_device_extensions_.insert(ext);
}
return true;
});
IterateExtensions<RequiredAndroidDeviceExtensionVK>([&](auto ext) -> bool {
auto ext_name = GetExtensionName(ext);
if (exts->find(ext_name) != exts->end()) {
required_android_device_extensions_.insert(ext);
}
return true;
});
IterateExtensions<OptionalDeviceExtensionVK>([&](auto ext) -> bool {
auto ext_name = GetExtensionName(ext);
if (exts->find(ext_name) != exts->end()) {
optional_device_extensions_.insert(ext);
}
return true;
});
}
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsOffscreenMSAA() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsImplicitResolvingMSAA() const {
return false;
}
// |Capabilities|
bool CapabilitiesVK::SupportsSSBO() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsBufferToTextureBlits() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsTextureToTextureBlits() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsFramebufferFetch() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsCompute() const {
// Vulkan 1.1 requires support for compute.
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsComputeSubgroups() const {
// Set by |SetPhysicalDevice|.
return supports_compute_subgroups_;
}
// |Capabilities|
bool CapabilitiesVK::SupportsReadFromResolve() const {
return false;
}
bool CapabilitiesVK::SupportsDecalSamplerAddressMode() const {
return true;
}
// |Capabilities|
bool CapabilitiesVK::SupportsDeviceTransientTextures() const {
return supports_device_transient_textures_;
}
// |Capabilities|
PixelFormat CapabilitiesVK::GetDefaultColorFormat() const {
return default_color_format_;
}
// |Capabilities|
PixelFormat CapabilitiesVK::GetDefaultStencilFormat() const {
return default_stencil_format_;
}
// |Capabilities|
PixelFormat CapabilitiesVK::GetDefaultDepthStencilFormat() const {
return default_depth_stencil_format_;
}
const vk::PhysicalDeviceProperties&
CapabilitiesVK::GetPhysicalDeviceProperties() const {
return device_properties_;
}
PixelFormat CapabilitiesVK::GetDefaultGlyphAtlasFormat() const {
return PixelFormat::kR8UNormInt;
}
bool CapabilitiesVK::HasExtension(RequiredCommonDeviceExtensionVK ext) const {
return required_common_device_extensions_.find(ext) !=
required_common_device_extensions_.end();
}
bool CapabilitiesVK::HasExtension(RequiredAndroidDeviceExtensionVK ext) const {
return required_android_device_extensions_.find(ext) !=
required_android_device_extensions_.end();
}
bool CapabilitiesVK::HasExtension(OptionalDeviceExtensionVK ext) const {
return optional_device_extensions_.find(ext) !=
optional_device_extensions_.end();
}
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/capabilities_vk.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/capabilities_vk.cc",
"repo_id": "engine",
"token_count": 6647
} | 223 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/vulkan/context_vk.h"
#include "fml/concurrent_message_loop.h"
#include "impeller/renderer/backend/vulkan/command_queue_vk.h"
#include "impeller/renderer/backend/vulkan/render_pass_builder_vk.h"
#include "impeller/renderer/render_target.h"
#ifdef FML_OS_ANDROID
#include <pthread.h>
#include <sys/resource.h>
#include <sys/time.h>
#endif // FML_OS_ANDROID
#include <map>
#include <memory>
#include <optional>
#include <string>
#include <vector>
#include "flutter/fml/cpu_affinity.h"
#include "flutter/fml/trace_event.h"
#include "impeller/base/validation.h"
#include "impeller/renderer/backend/vulkan/allocator_vk.h"
#include "impeller/renderer/backend/vulkan/capabilities_vk.h"
#include "impeller/renderer/backend/vulkan/command_buffer_vk.h"
#include "impeller/renderer/backend/vulkan/command_encoder_vk.h"
#include "impeller/renderer/backend/vulkan/command_pool_vk.h"
#include "impeller/renderer/backend/vulkan/command_queue_vk.h"
#include "impeller/renderer/backend/vulkan/debug_report_vk.h"
#include "impeller/renderer/backend/vulkan/fence_waiter_vk.h"
#include "impeller/renderer/backend/vulkan/gpu_tracer_vk.h"
#include "impeller/renderer/backend/vulkan/resource_manager_vk.h"
#include "impeller/renderer/backend/vulkan/surface_context_vk.h"
#include "impeller/renderer/backend/vulkan/yuv_conversion_library_vk.h"
#include "impeller/renderer/capabilities.h"
VULKAN_HPP_DEFAULT_DISPATCH_LOADER_DYNAMIC_STORAGE
namespace impeller {
// TODO(csg): Fix this after caps are reworked.
static bool gHasValidationLayers = false;
bool HasValidationLayers() {
return gHasValidationLayers;
}
static std::optional<vk::PhysicalDevice> PickPhysicalDevice(
const CapabilitiesVK& caps,
const vk::Instance& instance) {
for (const auto& device : instance.enumeratePhysicalDevices().value) {
if (caps.GetEnabledDeviceFeatures(device).has_value()) {
return device;
}
}
return std::nullopt;
}
static std::vector<vk::DeviceQueueCreateInfo> GetQueueCreateInfos(
std::initializer_list<QueueIndexVK> queues) {
std::map<size_t /* family */, size_t /* index */> family_index_map;
for (const auto& queue : queues) {
family_index_map[queue.family] = 0;
}
for (const auto& queue : queues) {
auto value = family_index_map[queue.family];
family_index_map[queue.family] = std::max(value, queue.index);
}
static float kQueuePriority = 1.0f;
std::vector<vk::DeviceQueueCreateInfo> infos;
for (const auto& item : family_index_map) {
vk::DeviceQueueCreateInfo info;
info.setQueueFamilyIndex(item.first);
info.setQueueCount(item.second + 1);
info.setQueuePriorities(kQueuePriority);
infos.push_back(info);
}
return infos;
}
static std::optional<QueueIndexVK> PickQueue(const vk::PhysicalDevice& device,
vk::QueueFlagBits flags) {
// This can be modified to ensure that dedicated queues are returned for each
// queue type depending on support.
const auto families = device.getQueueFamilyProperties();
for (size_t i = 0u; i < families.size(); i++) {
if (!(families[i].queueFlags & flags)) {
continue;
}
return QueueIndexVK{.family = i, .index = 0};
}
return std::nullopt;
}
std::shared_ptr<ContextVK> ContextVK::Create(Settings settings) {
auto context = std::shared_ptr<ContextVK>(new ContextVK());
context->Setup(std::move(settings));
if (!context->IsValid()) {
return nullptr;
}
return context;
}
// static
size_t ContextVK::ChooseThreadCountForWorkers(size_t hardware_concurrency) {
// Never create more than 4 worker threads. Attempt to use up to
// half of the available concurrency.
return std::clamp(hardware_concurrency / 2ull, /*lo=*/1ull, /*hi=*/4ull);
}
namespace {
thread_local uint64_t tls_context_count = 0;
uint64_t CalculateHash(void* ptr) {
// You could make a context once per nanosecond for 584 years on one thread
// before this overflows.
return ++tls_context_count;
}
} // namespace
ContextVK::ContextVK() : hash_(CalculateHash(this)) {}
ContextVK::~ContextVK() {
if (device_holder_ && device_holder_->device) {
[[maybe_unused]] auto result = device_holder_->device->waitIdle();
}
CommandPoolRecyclerVK::DestroyThreadLocalPools(this);
}
Context::BackendType ContextVK::GetBackendType() const {
return Context::BackendType::kVulkan;
}
void ContextVK::Setup(Settings settings) {
TRACE_EVENT0("impeller", "ContextVK::Setup");
if (!settings.proc_address_callback) {
return;
}
raster_message_loop_ = fml::ConcurrentMessageLoop::Create(
ChooseThreadCountForWorkers(std::thread::hardware_concurrency()));
raster_message_loop_->PostTaskToAllWorkers([]() {
// Currently we only use the worker task pool for small parts of a frame
// workload, if this changes this setting may need to be adjusted.
fml::RequestAffinity(fml::CpuAffinity::kNotPerformance);
#ifdef FML_OS_ANDROID
if (::setpriority(PRIO_PROCESS, gettid(), -5) != 0) {
FML_LOG(ERROR) << "Failed to set Workers task runner priority";
}
#endif // FML_OS_ANDROID
});
auto& dispatcher = VULKAN_HPP_DEFAULT_DISPATCHER;
dispatcher.init(settings.proc_address_callback);
// Enable Vulkan validation if either:
// 1. The user has explicitly enabled it.
// 2. We are in a combination of debug mode, and running on Android.
// (It's possible 2 is overly conservative and we can simplify this)
auto enable_validation = settings.enable_validation;
#if defined(FML_OS_ANDROID) && !defined(NDEBUG)
enable_validation = true;
#endif
auto caps =
std::shared_ptr<CapabilitiesVK>(new CapabilitiesVK(enable_validation));
if (!caps->IsValid()) {
VALIDATION_LOG << "Could not determine device capabilities.";
return;
}
gHasValidationLayers = caps->AreValidationsEnabled();
auto enabled_layers = caps->GetEnabledLayers();
auto enabled_extensions = caps->GetEnabledInstanceExtensions();
if (!enabled_layers.has_value() || !enabled_extensions.has_value()) {
VALIDATION_LOG << "Device has insufficient capabilities.";
return;
}
vk::InstanceCreateFlags instance_flags = {};
if (std::find(enabled_extensions.value().begin(),
enabled_extensions.value().end(),
"VK_KHR_portability_enumeration") !=
enabled_extensions.value().end()) {
instance_flags |= vk::InstanceCreateFlagBits::eEnumeratePortabilityKHR;
}
std::vector<const char*> enabled_layers_c;
std::vector<const char*> enabled_extensions_c;
for (const auto& layer : enabled_layers.value()) {
enabled_layers_c.push_back(layer.c_str());
}
for (const auto& ext : enabled_extensions.value()) {
enabled_extensions_c.push_back(ext.c_str());
}
vk::ApplicationInfo application_info;
application_info.setApplicationVersion(VK_API_VERSION_1_0);
application_info.setApiVersion(VK_API_VERSION_1_1);
application_info.setEngineVersion(VK_API_VERSION_1_0);
application_info.setPEngineName("Impeller");
application_info.setPApplicationName("Impeller");
vk::StructureChain<vk::InstanceCreateInfo, vk::ValidationFeaturesEXT>
instance_chain;
if (!caps->AreValidationsEnabled()) {
instance_chain.unlink<vk::ValidationFeaturesEXT>();
}
std::vector<vk::ValidationFeatureEnableEXT> enabled_validations = {
vk::ValidationFeatureEnableEXT::eSynchronizationValidation,
};
auto validation = instance_chain.get<vk::ValidationFeaturesEXT>();
validation.setEnabledValidationFeatures(enabled_validations);
auto instance_info = instance_chain.get<vk::InstanceCreateInfo>();
instance_info.setPEnabledLayerNames(enabled_layers_c);
instance_info.setPEnabledExtensionNames(enabled_extensions_c);
instance_info.setPApplicationInfo(&application_info);
instance_info.setFlags(instance_flags);
auto device_holder = std::make_shared<DeviceHolderImpl>();
{
auto instance = vk::createInstanceUnique(instance_info);
if (instance.result != vk::Result::eSuccess) {
VALIDATION_LOG << "Could not create Vulkan instance: "
<< vk::to_string(instance.result);
return;
}
device_holder->instance = std::move(instance.value);
}
dispatcher.init(device_holder->instance.get());
//----------------------------------------------------------------------------
/// Setup the debug report.
///
/// Do this as early as possible since we could use the debug report from
/// initialization issues.
///
auto debug_report =
std::make_unique<DebugReportVK>(*caps, device_holder->instance.get());
if (!debug_report->IsValid()) {
VALIDATION_LOG << "Could not set up debug report.";
return;
}
//----------------------------------------------------------------------------
/// Pick the physical device.
///
{
auto physical_device =
PickPhysicalDevice(*caps, device_holder->instance.get());
if (!physical_device.has_value()) {
VALIDATION_LOG << "No valid Vulkan device found.";
return;
}
device_holder->physical_device = physical_device.value();
}
//----------------------------------------------------------------------------
/// Pick device queues.
///
auto graphics_queue =
PickQueue(device_holder->physical_device, vk::QueueFlagBits::eGraphics);
auto transfer_queue =
PickQueue(device_holder->physical_device, vk::QueueFlagBits::eTransfer);
auto compute_queue =
PickQueue(device_holder->physical_device, vk::QueueFlagBits::eCompute);
if (!graphics_queue.has_value()) {
VALIDATION_LOG << "Could not pick graphics queue.";
return;
}
if (!transfer_queue.has_value()) {
FML_LOG(INFO) << "Dedicated transfer queue not avialable.";
transfer_queue = graphics_queue.value();
}
if (!compute_queue.has_value()) {
VALIDATION_LOG << "Could not pick compute queue.";
return;
}
//----------------------------------------------------------------------------
/// Create the logical device.
///
auto enabled_device_extensions =
caps->GetEnabledDeviceExtensions(device_holder->physical_device);
if (!enabled_device_extensions.has_value()) {
// This shouldn't happen since we already did device selection. But
// doesn't hurt to check again.
return;
}
std::vector<const char*> enabled_device_extensions_c;
for (const auto& ext : enabled_device_extensions.value()) {
enabled_device_extensions_c.push_back(ext.c_str());
}
const auto queue_create_infos = GetQueueCreateInfos(
{graphics_queue.value(), compute_queue.value(), transfer_queue.value()});
const auto enabled_features =
caps->GetEnabledDeviceFeatures(device_holder->physical_device);
if (!enabled_features.has_value()) {
// This shouldn't happen since the device can't be picked if this was not
// true. But doesn't hurt to check.
return;
}
vk::DeviceCreateInfo device_info;
device_info.setPNext(&enabled_features.value().get());
device_info.setQueueCreateInfos(queue_create_infos);
device_info.setPEnabledExtensionNames(enabled_device_extensions_c);
// Device layers are deprecated and ignored.
{
auto device_result =
device_holder->physical_device.createDeviceUnique(device_info);
if (device_result.result != vk::Result::eSuccess) {
VALIDATION_LOG << "Could not create logical device.";
return;
}
device_holder->device = std::move(device_result.value);
}
if (!caps->SetPhysicalDevice(device_holder->physical_device)) {
VALIDATION_LOG << "Capabilities could not be updated.";
return;
}
//----------------------------------------------------------------------------
/// Create the allocator.
///
auto allocator = std::shared_ptr<AllocatorVK>(new AllocatorVK(
weak_from_this(), //
application_info.apiVersion, //
device_holder->physical_device, //
device_holder, //
device_holder->instance.get(), //
*caps //
));
if (!allocator->IsValid()) {
VALIDATION_LOG << "Could not create memory allocator.";
return;
}
//----------------------------------------------------------------------------
/// Setup the pipeline library.
///
auto pipeline_library = std::shared_ptr<PipelineLibraryVK>(
new PipelineLibraryVK(device_holder, //
caps, //
std::move(settings.cache_directory), //
raster_message_loop_->GetTaskRunner() //
));
if (!pipeline_library->IsValid()) {
VALIDATION_LOG << "Could not create pipeline library.";
return;
}
auto sampler_library =
std::shared_ptr<SamplerLibraryVK>(new SamplerLibraryVK(device_holder));
auto shader_library = std::shared_ptr<ShaderLibraryVK>(
new ShaderLibraryVK(device_holder, //
settings.shader_libraries_data) //
);
if (!shader_library->IsValid()) {
VALIDATION_LOG << "Could not create shader library.";
return;
}
//----------------------------------------------------------------------------
/// Create the fence waiter.
///
auto fence_waiter =
std::shared_ptr<FenceWaiterVK>(new FenceWaiterVK(device_holder));
//----------------------------------------------------------------------------
/// Create the resource manager and command pool recycler.
///
auto resource_manager = ResourceManagerVK::Create();
if (!resource_manager) {
VALIDATION_LOG << "Could not create resource manager.";
return;
}
auto command_pool_recycler =
std::make_shared<CommandPoolRecyclerVK>(weak_from_this());
if (!command_pool_recycler) {
VALIDATION_LOG << "Could not create command pool recycler.";
return;
}
auto descriptor_pool_recycler =
std::make_shared<DescriptorPoolRecyclerVK>(weak_from_this());
if (!descriptor_pool_recycler) {
VALIDATION_LOG << "Could not create descriptor pool recycler.";
return;
}
//----------------------------------------------------------------------------
/// Fetch the queues.
///
QueuesVK queues(device_holder->device.get(), //
graphics_queue.value(), //
compute_queue.value(), //
transfer_queue.value() //
);
if (!queues.IsValid()) {
VALIDATION_LOG << "Could not fetch device queues.";
return;
}
VkPhysicalDeviceProperties physical_device_properties;
dispatcher.vkGetPhysicalDeviceProperties(device_holder->physical_device,
&physical_device_properties);
//----------------------------------------------------------------------------
/// All done!
///
device_holder_ = std::move(device_holder);
driver_info_ =
std::make_unique<DriverInfoVK>(device_holder_->physical_device);
debug_report_ = std::move(debug_report);
allocator_ = std::move(allocator);
shader_library_ = std::move(shader_library);
sampler_library_ = std::move(sampler_library);
pipeline_library_ = std::move(pipeline_library);
yuv_conversion_library_ = std::shared_ptr<YUVConversionLibraryVK>(
new YUVConversionLibraryVK(device_holder_));
queues_ = std::move(queues);
device_capabilities_ = std::move(caps);
fence_waiter_ = std::move(fence_waiter);
resource_manager_ = std::move(resource_manager);
command_pool_recycler_ = std::move(command_pool_recycler);
descriptor_pool_recycler_ = std::move(descriptor_pool_recycler);
device_name_ = std::string(physical_device_properties.deviceName);
command_queue_vk_ = std::make_shared<CommandQueueVK>(weak_from_this());
is_valid_ = true;
// Create the GPU Tracer later because it depends on state from
// the ContextVK.
gpu_tracer_ = std::make_shared<GPUTracerVK>(weak_from_this(),
settings.enable_gpu_tracing);
gpu_tracer_->InitializeQueryPool(*this);
//----------------------------------------------------------------------------
/// Label all the relevant objects. This happens after setup so that the
/// debug messengers have had a chance to be set up.
///
SetDebugName(GetDevice(), device_holder_->device.get(), "ImpellerDevice");
}
void ContextVK::SetOffscreenFormat(PixelFormat pixel_format) {
CapabilitiesVK::Cast(*device_capabilities_).SetOffscreenFormat(pixel_format);
}
// |Context|
std::string ContextVK::DescribeGpuModel() const {
return device_name_;
}
bool ContextVK::IsValid() const {
return is_valid_;
}
std::shared_ptr<Allocator> ContextVK::GetResourceAllocator() const {
return allocator_;
}
std::shared_ptr<ShaderLibrary> ContextVK::GetShaderLibrary() const {
return shader_library_;
}
std::shared_ptr<SamplerLibrary> ContextVK::GetSamplerLibrary() const {
return sampler_library_;
}
std::shared_ptr<PipelineLibrary> ContextVK::GetPipelineLibrary() const {
return pipeline_library_;
}
std::shared_ptr<CommandBuffer> ContextVK::CreateCommandBuffer() const {
return std::shared_ptr<CommandBufferVK>(
new CommandBufferVK(shared_from_this(), //
CreateGraphicsCommandEncoderFactory()) //
);
}
vk::Instance ContextVK::GetInstance() const {
return *device_holder_->instance;
}
const vk::Device& ContextVK::GetDevice() const {
return device_holder_->device.get();
}
const std::shared_ptr<fml::ConcurrentTaskRunner>
ContextVK::GetConcurrentWorkerTaskRunner() const {
return raster_message_loop_->GetTaskRunner();
}
void ContextVK::Shutdown() {
// There are multiple objects, for example |CommandPoolVK|, that in their
// destructors make a strong reference to |ContextVK|. Resetting these shared
// pointers ensures that cleanup happens in a correct order.
//
// tl;dr: Without it, we get thread::join failures on shutdown.
fence_waiter_.reset();
resource_manager_.reset();
raster_message_loop_->Terminate();
}
std::shared_ptr<SurfaceContextVK> ContextVK::CreateSurfaceContext() {
return std::make_shared<SurfaceContextVK>(shared_from_this());
}
const std::shared_ptr<const Capabilities>& ContextVK::GetCapabilities() const {
return device_capabilities_;
}
const std::shared_ptr<QueueVK>& ContextVK::GetGraphicsQueue() const {
return queues_.graphics_queue;
}
vk::PhysicalDevice ContextVK::GetPhysicalDevice() const {
return device_holder_->physical_device;
}
std::shared_ptr<FenceWaiterVK> ContextVK::GetFenceWaiter() const {
return fence_waiter_;
}
std::shared_ptr<ResourceManagerVK> ContextVK::GetResourceManager() const {
return resource_manager_;
}
std::shared_ptr<CommandPoolRecyclerVK> ContextVK::GetCommandPoolRecycler()
const {
return command_pool_recycler_;
}
std::unique_ptr<CommandEncoderFactoryVK>
ContextVK::CreateGraphicsCommandEncoderFactory() const {
return std::make_unique<CommandEncoderFactoryVK>(weak_from_this());
}
std::shared_ptr<GPUTracerVK> ContextVK::GetGPUTracer() const {
return gpu_tracer_;
}
std::shared_ptr<DescriptorPoolRecyclerVK> ContextVK::GetDescriptorPoolRecycler()
const {
return descriptor_pool_recycler_;
}
std::shared_ptr<CommandQueue> ContextVK::GetCommandQueue() const {
return command_queue_vk_;
}
// Creating a render pass is observed to take an additional 6ms on a Pixel 7
// device as the driver will lazily bootstrap and compile shaders to do so.
// The render pass does not need to be begun or executed.
void ContextVK::InitializeCommonlyUsedShadersIfNeeded() const {
RenderTargetAllocator rt_allocator(GetResourceAllocator());
RenderTarget render_target =
rt_allocator.CreateOffscreenMSAA(*this, {1, 1}, 1);
RenderPassBuilderVK builder;
for (const auto& [bind_point, color] : render_target.GetColorAttachments()) {
builder.SetColorAttachment(
bind_point, //
color.texture->GetTextureDescriptor().format, //
color.texture->GetTextureDescriptor().sample_count, //
color.load_action, //
color.store_action //
);
}
if (auto depth = render_target.GetDepthAttachment(); depth.has_value()) {
builder.SetDepthStencilAttachment(
depth->texture->GetTextureDescriptor().format, //
depth->texture->GetTextureDescriptor().sample_count, //
depth->load_action, //
depth->store_action //
);
} else if (auto stencil = render_target.GetStencilAttachment();
stencil.has_value()) {
builder.SetStencilAttachment(
stencil->texture->GetTextureDescriptor().format, //
stencil->texture->GetTextureDescriptor().sample_count, //
stencil->load_action, //
stencil->store_action //
);
}
auto pass = builder.Build(GetDevice());
}
const std::shared_ptr<YUVConversionLibraryVK>&
ContextVK::GetYUVConversionLibrary() const {
return yuv_conversion_library_;
}
const std::unique_ptr<DriverInfoVK>& ContextVK::GetDriverInfo() const {
return driver_info_;
}
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/context_vk.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/context_vk.cc",
"repo_id": "engine",
"token_count": 7629
} | 224 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "fml/synchronization/waitable_event.h"
#include "gtest/gtest.h" // IWYU pragma: keep
#include "impeller/renderer/backend/vulkan/fence_waiter_vk.h" // IWYU pragma: keep
#include "impeller/renderer/backend/vulkan/test/mock_vulkan.h"
namespace impeller {
namespace testing {
TEST(FenceWaiterVKTest, IgnoresNullFence) {
auto const context = MockVulkanContextBuilder().Build();
auto const waiter = context->GetFenceWaiter();
EXPECT_FALSE(waiter->AddFence(vk::UniqueFence(), []() {}));
}
TEST(FenceWaiterVKTest, IgnoresNullCallback) {
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
auto fence = device.createFenceUnique({}).value;
EXPECT_FALSE(waiter->AddFence(std::move(fence), nullptr));
}
TEST(FenceWaiterVKTest, ExecutesFenceCallback) {
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
auto signal = fml::ManualResetWaitableEvent();
auto fence = device.createFenceUnique({}).value;
waiter->AddFence(std::move(fence), [&signal]() { signal.Signal(); });
signal.Wait();
}
TEST(FenceWaiterVKTest, ExecutesFenceCallbackX2) {
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
auto signal = fml::ManualResetWaitableEvent();
auto fence = device.createFenceUnique({}).value;
waiter->AddFence(std::move(fence), [&signal]() { signal.Signal(); });
auto signal2 = fml::ManualResetWaitableEvent();
auto fence2 = device.createFenceUnique({}).value;
waiter->AddFence(std::move(fence2), [&signal2]() { signal2.Signal(); });
signal.Wait();
signal2.Wait();
}
TEST(FenceWaiterVKTest, ExecutesNewFenceThenOldFence) {
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
auto signal = fml::ManualResetWaitableEvent();
auto fence = device.createFenceUnique({}).value;
MockFence::SetStatus(fence, vk::Result::eNotReady);
auto raw_fence = MockFence::GetRawPointer(fence);
waiter->AddFence(std::move(fence), [&signal]() { signal.Signal(); });
// The easiest way to verify that the callback was _not_ called is to wait
// for a timeout, but that could introduce flakiness. Instead, we'll add a
// second fence that will signal immediately, and wait for that one instead.
{
auto signal2 = fml::ManualResetWaitableEvent();
auto fence2 = device.createFenceUnique({}).value;
MockFence::SetStatus(fence2, vk::Result::eSuccess);
waiter->AddFence(std::move(fence2), [&signal2]() { signal2.Signal(); });
signal2.Wait();
}
// Now, we'll signal the first fence, and wait for the callback to be called.
raw_fence->SetStatus(vk::Result::eSuccess);
// Now, we'll signal the first fence, and wait for the callback to be called.
signal.Wait();
}
TEST(FenceWaiterVKTest, AddFenceDoesNothingIfTerminating) {
auto signal = fml::ManualResetWaitableEvent();
{
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
waiter->Terminate();
auto fence = device.createFenceUnique({}).value;
waiter->AddFence(std::move(fence), [&signal]() { signal.Signal(); });
}
// Ensure the fence did _not_ signal.
EXPECT_TRUE(signal.WaitWithTimeout(fml::TimeDelta::FromMilliseconds(100)));
}
TEST(FenceWaiterVKTest, InProgressFencesStillWaitIfTerminated) {
MockFence* raw_fence = nullptr;
auto signal = fml::ManualResetWaitableEvent();
auto const context = MockVulkanContextBuilder().Build();
auto const device = context->GetDevice();
auto const waiter = context->GetFenceWaiter();
// Add a fence that isn't signalled yet.
auto fence = device.createFenceUnique({}).value;
// Even if the fence is eSuccess, it's not guaranteed to be called in time.
MockFence::SetStatus(fence, vk::Result::eNotReady);
raw_fence = MockFence::GetRawPointer(fence);
waiter->AddFence(std::move(fence), [&signal]() { signal.Signal(); });
// Terminate the waiter.
waiter->Terminate();
// Signal the fence.
raw_fence->SetStatus(vk::Result::eSuccess);
// This will hang if the fence was not signalled.
signal.Wait();
}
} // namespace testing
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/fence_waiter_vk_unittests.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/fence_waiter_vk_unittests.cc",
"repo_id": "engine",
"token_count": 1523
} | 225 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/testing/testing.h"
#include "gtest/gtest.h"
#include "impeller/playground/playground_test.h"
#include "impeller/renderer/backend/vulkan/texture_vk.h"
#include "impeller/renderer/render_target.h"
namespace impeller {
namespace testing {
using RendererTest = PlaygroundTest;
TEST_P(RendererTest, CachesRenderPassAndFramebuffer) {
if (GetBackend() != PlaygroundBackend::kVulkan) {
GTEST_SKIP() << "Test only applies to Vulkan";
}
auto allocator = std::make_shared<RenderTargetAllocator>(
GetContext()->GetResourceAllocator());
auto render_target =
allocator->CreateOffscreenMSAA(*GetContext(), {100, 100}, 1);
auto resolve_texture =
render_target.GetColorAttachments().find(0u)->second.resolve_texture;
auto& texture_vk = TextureVK::Cast(*resolve_texture);
EXPECT_EQ(texture_vk.GetCachedFramebuffer(), nullptr);
EXPECT_EQ(texture_vk.GetCachedRenderPass(), nullptr);
auto buffer = GetContext()->CreateCommandBuffer();
auto render_pass = buffer->CreateRenderPass(render_target);
EXPECT_NE(texture_vk.GetCachedFramebuffer(), nullptr);
EXPECT_NE(texture_vk.GetCachedRenderPass(), nullptr);
render_pass->EncodeCommands();
GetContext()->GetCommandQueue()->Submit({buffer});
// Can be reused without error.
auto buffer_2 = GetContext()->CreateCommandBuffer();
auto render_pass_2 = buffer_2->CreateRenderPass(render_target);
EXPECT_TRUE(render_pass_2->EncodeCommands());
EXPECT_TRUE(GetContext()->GetCommandQueue()->Submit({buffer_2}).ok());
}
} // namespace testing
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/render_pass_cache_unittests.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/render_pass_cache_unittests.cc",
"repo_id": "engine",
"token_count": 578
} | 226 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/backend/vulkan/surface_context_vk.h"
#include "flutter/fml/trace_event.h"
#include "impeller/renderer/backend/vulkan/command_pool_vk.h"
#include "impeller/renderer/backend/vulkan/context_vk.h"
#include "impeller/renderer/backend/vulkan/swapchain/khr/khr_swapchain_vk.h"
#include "impeller/renderer/surface.h"
namespace impeller {
SurfaceContextVK::SurfaceContextVK(const std::shared_ptr<ContextVK>& parent)
: parent_(parent) {}
SurfaceContextVK::~SurfaceContextVK() = default;
Context::BackendType SurfaceContextVK::GetBackendType() const {
return parent_->GetBackendType();
}
std::string SurfaceContextVK::DescribeGpuModel() const {
return parent_->DescribeGpuModel();
}
bool SurfaceContextVK::IsValid() const {
return parent_->IsValid();
}
std::shared_ptr<Allocator> SurfaceContextVK::GetResourceAllocator() const {
return parent_->GetResourceAllocator();
}
std::shared_ptr<ShaderLibrary> SurfaceContextVK::GetShaderLibrary() const {
return parent_->GetShaderLibrary();
}
std::shared_ptr<SamplerLibrary> SurfaceContextVK::GetSamplerLibrary() const {
return parent_->GetSamplerLibrary();
}
std::shared_ptr<PipelineLibrary> SurfaceContextVK::GetPipelineLibrary() const {
return parent_->GetPipelineLibrary();
}
std::shared_ptr<CommandBuffer> SurfaceContextVK::CreateCommandBuffer() const {
return parent_->CreateCommandBuffer();
}
std::shared_ptr<CommandQueue> SurfaceContextVK::GetCommandQueue() const {
return parent_->GetCommandQueue();
}
const std::shared_ptr<const Capabilities>& SurfaceContextVK::GetCapabilities()
const {
return parent_->GetCapabilities();
}
void SurfaceContextVK::Shutdown() {
parent_->Shutdown();
}
bool SurfaceContextVK::SetWindowSurface(vk::UniqueSurfaceKHR surface,
const ISize& size) {
auto swapchain = KHRSwapchainVK::Create(parent_, std::move(surface), size);
if (!swapchain) {
VALIDATION_LOG << "Could not create swapchain.";
return false;
}
if (!swapchain->IsValid()) {
VALIDATION_LOG << "Could not create valid swapchain.";
return false;
}
swapchain_ = std::move(swapchain);
return true;
}
std::unique_ptr<Surface> SurfaceContextVK::AcquireNextSurface() {
TRACE_EVENT0("impeller", __FUNCTION__);
auto surface = swapchain_ ? swapchain_->AcquireNextDrawable() : nullptr;
if (!surface) {
return nullptr;
}
if (auto pipeline_library = parent_->GetPipelineLibrary()) {
impeller::PipelineLibraryVK::Cast(*pipeline_library)
.DidAcquireSurfaceFrame();
}
parent_->GetCommandPoolRecycler()->Dispose();
parent_->GetResourceAllocator()->DebugTraceMemoryStatistics();
return surface;
}
void SurfaceContextVK::UpdateSurfaceSize(const ISize& size) const {
swapchain_->UpdateSurfaceSize(size);
}
#ifdef FML_OS_ANDROID
vk::UniqueSurfaceKHR SurfaceContextVK::CreateAndroidSurface(
ANativeWindow* window) const {
if (!parent_->GetInstance()) {
return vk::UniqueSurfaceKHR{VK_NULL_HANDLE};
}
auto create_info = vk::AndroidSurfaceCreateInfoKHR().setWindow(window);
auto surface_res =
parent_->GetInstance().createAndroidSurfaceKHRUnique(create_info);
if (surface_res.result != vk::Result::eSuccess) {
VALIDATION_LOG << "Could not create Android surface, error: "
<< vk::to_string(surface_res.result);
return vk::UniqueSurfaceKHR{VK_NULL_HANDLE};
}
return std::move(surface_res.value);
}
#endif // FML_OS_ANDROID
const vk::Device& SurfaceContextVK::GetDevice() const {
return parent_->GetDevice();
}
void SurfaceContextVK::InitializeCommonlyUsedShadersIfNeeded() const {
parent_->InitializeCommonlyUsedShadersIfNeeded();
}
const ContextVK& SurfaceContextVK::GetParent() const {
return *parent_;
}
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/surface_context_vk.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/surface_context_vk.cc",
"repo_id": "engine",
"token_count": 1371
} | 227 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/testing/testing.h" // IWYU pragma: keep
#include "gtest/gtest.h"
#include "impeller/renderer/backend/vulkan/swapchain/khr/khr_swapchain_vk.h"
#include "impeller/renderer/backend/vulkan/test/mock_vulkan.h"
#include "impeller/renderer/backend/vulkan/texture_vk.h"
#include "vulkan/vulkan_enums.hpp"
#include "vulkan/vulkan_handles.hpp"
namespace impeller {
namespace testing {
vk::UniqueSurfaceKHR CreateSurface(const ContextVK& context) {
#if FML_OS_DARWIN
impeller::vk::MetalSurfaceCreateInfoEXT createInfo = {};
auto [result, surface] =
context.GetInstance().createMetalSurfaceEXTUnique(createInfo);
FML_DCHECK(result == vk::Result::eSuccess);
return std::move(surface);
#else
return {};
#endif // FML_OS_DARWIN
}
TEST(SwapchainTest, CanCreateSwapchain) {
auto const context = MockVulkanContextBuilder().Build();
auto surface = CreateSurface(*context);
auto swapchain =
KHRSwapchainVK::Create(context, std::move(surface), ISize{1, 1});
EXPECT_TRUE(swapchain->IsValid());
}
TEST(SwapchainTest, RecreateSwapchainWhenSizeChanges) {
auto const context = MockVulkanContextBuilder().Build();
auto surface = CreateSurface(*context);
SetSwapchainImageSize(ISize{1, 1});
auto swapchain =
KHRSwapchainVK::Create(context, std::move(surface), ISize{1, 1},
/*enable_msaa=*/false);
auto image = swapchain->AcquireNextDrawable();
auto expected_size = ISize{1, 1};
EXPECT_EQ(image->GetSize(), expected_size);
SetSwapchainImageSize(ISize{100, 100});
swapchain->UpdateSurfaceSize(ISize{100, 100});
auto image_b = swapchain->AcquireNextDrawable();
expected_size = ISize{100, 100};
EXPECT_EQ(image_b->GetSize(), expected_size);
}
TEST(SwapchainTest, CachesRenderPassOnSwapchainImage) {
auto const context = MockVulkanContextBuilder().Build();
auto surface = CreateSurface(*context);
auto swapchain =
KHRSwapchainVK::Create(context, std::move(surface), ISize{1, 1});
EXPECT_TRUE(swapchain->IsValid());
// The mock swapchain will always create 3 images, verify each one starts
// out with the same MSAA and depth+stencil texture, and no cached
// framebuffer.
std::vector<std::shared_ptr<Texture>> msaa_textures;
std::vector<std::shared_ptr<Texture>> depth_stencil_textures;
for (auto i = 0u; i < 3u; i++) {
auto drawable = swapchain->AcquireNextDrawable();
RenderTarget render_target = drawable->GetTargetRenderPassDescriptor();
auto texture = render_target.GetRenderTargetTexture();
auto& texture_vk = TextureVK::Cast(*texture);
EXPECT_EQ(texture_vk.GetCachedFramebuffer(), nullptr);
EXPECT_EQ(texture_vk.GetCachedRenderPass(), nullptr);
auto command_buffer = context->CreateCommandBuffer();
auto render_pass = command_buffer->CreateRenderPass(render_target);
render_pass->EncodeCommands();
auto& depth = render_target.GetDepthAttachment();
depth_stencil_textures.push_back(depth.has_value() ? depth->texture
: nullptr);
msaa_textures.push_back(
render_target.GetColorAttachments().find(0u)->second.texture);
}
for (auto i = 1; i < 3; i++) {
EXPECT_EQ(msaa_textures[i - 1], msaa_textures[i]);
EXPECT_EQ(depth_stencil_textures[i - 1], depth_stencil_textures[i]);
}
// After each images has been acquired once and the render pass presented,
// each should have a cached framebuffer and render pass.
std::vector<SharedHandleVK<vk::Framebuffer>> framebuffers;
std::vector<SharedHandleVK<vk::RenderPass>> render_passes;
for (auto i = 0u; i < 3u; i++) {
auto drawable = swapchain->AcquireNextDrawable();
RenderTarget render_target = drawable->GetTargetRenderPassDescriptor();
auto texture = render_target.GetRenderTargetTexture();
auto& texture_vk = TextureVK::Cast(*texture);
EXPECT_NE(texture_vk.GetCachedFramebuffer(), nullptr);
EXPECT_NE(texture_vk.GetCachedRenderPass(), nullptr);
framebuffers.push_back(texture_vk.GetCachedFramebuffer());
render_passes.push_back(texture_vk.GetCachedRenderPass());
}
// Iterate through once more to verify render passes and framebuffers are
// unchanged.
for (auto i = 0u; i < 3u; i++) {
auto drawable = swapchain->AcquireNextDrawable();
RenderTarget render_target = drawable->GetTargetRenderPassDescriptor();
auto texture = render_target.GetRenderTargetTexture();
auto& texture_vk = TextureVK::Cast(*texture);
EXPECT_EQ(texture_vk.GetCachedFramebuffer(), framebuffers[i]);
EXPECT_EQ(texture_vk.GetCachedRenderPass(), render_passes[i]);
}
}
} // namespace testing
} // namespace impeller
| engine/impeller/renderer/backend/vulkan/test/swapchain_unittests.cc/0 | {
"file_path": "engine/impeller/renderer/backend/vulkan/test/swapchain_unittests.cc",
"repo_id": "engine",
"token_count": 1732
} | 228 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/compute_pipeline_builder.h"
namespace impeller {
//
} // namespace impeller
| engine/impeller/renderer/compute_pipeline_builder.cc/0 | {
"file_path": "engine/impeller/renderer/compute_pipeline_builder.cc",
"repo_id": "engine",
"token_count": 82
} | 229 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/renderer/pipeline_descriptor.h"
#include <utility>
#include "impeller/base/comparable.h"
#include "impeller/core/formats.h"
#include "impeller/renderer/shader_function.h"
#include "impeller/renderer/shader_library.h"
#include "impeller/renderer/vertex_descriptor.h"
namespace impeller {
PipelineDescriptor::PipelineDescriptor() = default;
PipelineDescriptor::~PipelineDescriptor() = default;
// Comparable<PipelineDescriptor>
std::size_t PipelineDescriptor::GetHash() const {
auto seed = fml::HashCombine();
fml::HashCombineSeed(seed, label_);
fml::HashCombineSeed(seed, sample_count_);
for (const auto& entry : entrypoints_) {
fml::HashCombineSeed(seed, entry.first);
if (auto second = entry.second) {
fml::HashCombineSeed(seed, second->GetHash());
}
}
for (const auto& des : color_attachment_descriptors_) {
fml::HashCombineSeed(seed, des.first);
fml::HashCombineSeed(seed, des.second.Hash());
}
if (vertex_descriptor_) {
fml::HashCombineSeed(seed, vertex_descriptor_->GetHash());
}
fml::HashCombineSeed(seed, depth_pixel_format_);
fml::HashCombineSeed(seed, stencil_pixel_format_);
fml::HashCombineSeed(seed, depth_attachment_descriptor_);
fml::HashCombineSeed(seed, front_stencil_attachment_descriptor_);
fml::HashCombineSeed(seed, back_stencil_attachment_descriptor_);
fml::HashCombineSeed(seed, winding_order_);
fml::HashCombineSeed(seed, cull_mode_);
fml::HashCombineSeed(seed, primitive_type_);
fml::HashCombineSeed(seed, polygon_mode_);
return seed;
}
// Comparable<PipelineDescriptor>
bool PipelineDescriptor::IsEqual(const PipelineDescriptor& other) const {
return label_ == other.label_ && sample_count_ == other.sample_count_ &&
DeepCompareMap(entrypoints_, other.entrypoints_) &&
color_attachment_descriptors_ == other.color_attachment_descriptors_ &&
DeepComparePointer(vertex_descriptor_, other.vertex_descriptor_) &&
stencil_pixel_format_ == other.stencil_pixel_format_ &&
depth_pixel_format_ == other.depth_pixel_format_ &&
depth_attachment_descriptor_ == other.depth_attachment_descriptor_ &&
front_stencil_attachment_descriptor_ ==
other.front_stencil_attachment_descriptor_ &&
back_stencil_attachment_descriptor_ ==
other.back_stencil_attachment_descriptor_ &&
winding_order_ == other.winding_order_ &&
cull_mode_ == other.cull_mode_ &&
primitive_type_ == other.primitive_type_ &&
polygon_mode_ == other.polygon_mode_ &&
specialization_constants_ == other.specialization_constants_;
}
PipelineDescriptor& PipelineDescriptor::SetLabel(std::string label) {
label_ = std::move(label);
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetSampleCount(SampleCount samples) {
sample_count_ = samples;
return *this;
}
PipelineDescriptor& PipelineDescriptor::AddStageEntrypoint(
std::shared_ptr<const ShaderFunction> function) {
if (!function) {
return *this;
}
if (function->GetStage() == ShaderStage::kUnknown) {
return *this;
}
entrypoints_[function->GetStage()] = std::move(function);
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetVertexDescriptor(
std::shared_ptr<VertexDescriptor> vertex_descriptor) {
vertex_descriptor_ = std::move(vertex_descriptor);
return *this;
}
size_t PipelineDescriptor::GetMaxColorAttacmentBindIndex() const {
size_t max = 0;
for (const auto& color : color_attachment_descriptors_) {
max = std::max(color.first, max);
}
return max;
}
PipelineDescriptor& PipelineDescriptor::SetColorAttachmentDescriptor(
size_t index,
ColorAttachmentDescriptor desc) {
color_attachment_descriptors_[index] = desc;
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetColorAttachmentDescriptors(
std::map<size_t /* index */, ColorAttachmentDescriptor> descriptors) {
color_attachment_descriptors_ = std::move(descriptors);
return *this;
}
const ColorAttachmentDescriptor*
PipelineDescriptor::GetColorAttachmentDescriptor(size_t index) const {
auto found = color_attachment_descriptors_.find(index);
return found == color_attachment_descriptors_.end() ? nullptr
: &found->second;
}
const ColorAttachmentDescriptor*
PipelineDescriptor::GetLegacyCompatibleColorAttachment() const {
// Legacy renderers may only render to a single color attachment at index 0u.
if (color_attachment_descriptors_.size() != 1u) {
return nullptr;
}
return GetColorAttachmentDescriptor(0u);
}
PipelineDescriptor& PipelineDescriptor::SetDepthPixelFormat(
PixelFormat format) {
depth_pixel_format_ = format;
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetStencilPixelFormat(
PixelFormat format) {
stencil_pixel_format_ = format;
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetDepthStencilAttachmentDescriptor(
std::optional<DepthAttachmentDescriptor> desc) {
depth_attachment_descriptor_ = desc;
return *this;
}
PipelineDescriptor& PipelineDescriptor::SetStencilAttachmentDescriptors(
std::optional<StencilAttachmentDescriptor> front_and_back) {
return SetStencilAttachmentDescriptors(front_and_back, front_and_back);
}
PipelineDescriptor& PipelineDescriptor::SetStencilAttachmentDescriptors(
std::optional<StencilAttachmentDescriptor> front,
std::optional<StencilAttachmentDescriptor> back) {
front_stencil_attachment_descriptor_ = front;
back_stencil_attachment_descriptor_ = back;
return *this;
}
void PipelineDescriptor::ClearStencilAttachments() {
back_stencil_attachment_descriptor_.reset();
front_stencil_attachment_descriptor_.reset();
SetStencilPixelFormat(impeller::PixelFormat::kUnknown);
}
void PipelineDescriptor::ClearDepthAttachment() {
depth_attachment_descriptor_.reset();
SetDepthPixelFormat(impeller::PixelFormat::kUnknown);
}
void PipelineDescriptor::ClearColorAttachment(size_t index) {
if (color_attachment_descriptors_.find(index) ==
color_attachment_descriptors_.end()) {
return;
}
color_attachment_descriptors_.erase(index);
}
void PipelineDescriptor::ResetAttachments() {
color_attachment_descriptors_.clear();
depth_attachment_descriptor_.reset();
front_stencil_attachment_descriptor_.reset();
back_stencil_attachment_descriptor_.reset();
}
PixelFormat PipelineDescriptor::GetStencilPixelFormat() const {
return stencil_pixel_format_;
}
std::optional<StencilAttachmentDescriptor>
PipelineDescriptor::GetFrontStencilAttachmentDescriptor() const {
return front_stencil_attachment_descriptor_;
}
std::optional<DepthAttachmentDescriptor>
PipelineDescriptor::GetDepthStencilAttachmentDescriptor() const {
return depth_attachment_descriptor_;
}
const std::map<size_t /* index */, ColorAttachmentDescriptor>&
PipelineDescriptor::GetColorAttachmentDescriptors() const {
return color_attachment_descriptors_;
}
const std::shared_ptr<VertexDescriptor>&
PipelineDescriptor::GetVertexDescriptor() const {
return vertex_descriptor_;
}
const std::map<ShaderStage, std::shared_ptr<const ShaderFunction>>&
PipelineDescriptor::GetStageEntrypoints() const {
return entrypoints_;
}
std::shared_ptr<const ShaderFunction> PipelineDescriptor::GetEntrypointForStage(
ShaderStage stage) const {
if (auto found = entrypoints_.find(stage); found != entrypoints_.end()) {
return found->second;
}
return nullptr;
}
const std::string& PipelineDescriptor::GetLabel() const {
return label_;
}
PixelFormat PipelineDescriptor::GetDepthPixelFormat() const {
return depth_pixel_format_;
}
std::optional<StencilAttachmentDescriptor>
PipelineDescriptor::GetBackStencilAttachmentDescriptor() const {
return back_stencil_attachment_descriptor_;
}
bool PipelineDescriptor::HasStencilAttachmentDescriptors() const {
return front_stencil_attachment_descriptor_.has_value() ||
back_stencil_attachment_descriptor_.has_value();
}
void PipelineDescriptor::SetCullMode(CullMode mode) {
cull_mode_ = mode;
}
CullMode PipelineDescriptor::GetCullMode() const {
return cull_mode_;
}
void PipelineDescriptor::SetWindingOrder(WindingOrder order) {
winding_order_ = order;
}
WindingOrder PipelineDescriptor::GetWindingOrder() const {
return winding_order_;
}
void PipelineDescriptor::SetPrimitiveType(PrimitiveType type) {
primitive_type_ = type;
}
PrimitiveType PipelineDescriptor::GetPrimitiveType() const {
return primitive_type_;
}
void PipelineDescriptor::SetPolygonMode(PolygonMode mode) {
polygon_mode_ = mode;
}
PolygonMode PipelineDescriptor::GetPolygonMode() const {
return polygon_mode_;
}
void PipelineDescriptor::SetSpecializationConstants(
std::vector<Scalar> values) {
specialization_constants_ = std::move(values);
}
const std::vector<Scalar>& PipelineDescriptor::GetSpecializationConstants()
const {
return specialization_constants_;
}
} // namespace impeller
| engine/impeller/renderer/pipeline_descriptor.cc/0 | {
"file_path": "engine/impeller/renderer/pipeline_descriptor.cc",
"repo_id": "engine",
"token_count": 3280
} | 230 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Size is passed in via specialization constant.
layout(local_size_x_id = 0) in;
layout(std430) buffer;
layout(binding = 1) writeonly buffer OutputData {
uint data[];
}
output_data;
void main() {
uint ident = gl_GlobalInvocationID.x;
output_data.data[ident] = ident;
}
| engine/impeller/renderer/threadgroup_sizing_test.comp/0 | {
"file_path": "engine/impeller/renderer/threadgroup_sizing_test.comp",
"repo_id": "engine",
"token_count": 138
} | 231 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_SCENE_ANIMATION_ANIMATION_H_
#define FLUTTER_IMPELLER_SCENE_ANIMATION_ANIMATION_H_
#include <memory>
#include <string>
#include <vector>
#include "flutter/fml/hash_combine.h"
#include "flutter/fml/macros.h"
#include "impeller/base/timing.h"
#include "impeller/geometry/quaternion.h"
#include "impeller/geometry/scalar.h"
#include "impeller/geometry/vector.h"
#include "impeller/scene/animation/property_resolver.h"
#include "impeller/scene/importer/scene_flatbuffers.h"
namespace impeller {
namespace scene {
class Node;
class Animation final {
public:
static std::shared_ptr<Animation> MakeFromFlatbuffer(
const fb::Animation& animation,
const std::vector<std::shared_ptr<Node>>& scene_nodes);
enum class Property {
kTranslation,
kRotation,
kScale,
};
struct BindKey {
std::string node_name;
Property property = Property::kTranslation;
struct Hash {
std::size_t operator()(const BindKey& o) const {
return fml::HashCombine(o.node_name, o.property);
}
};
struct Equal {
bool operator()(const BindKey& lhs, const BindKey& rhs) const {
return lhs.node_name == rhs.node_name && lhs.property == rhs.property;
}
};
};
struct Channel {
BindKey bind_target;
std::unique_ptr<PropertyResolver> resolver;
};
~Animation();
const std::string& GetName() const;
const std::vector<Channel>& GetChannels() const;
SecondsF GetEndTime() const;
private:
Animation();
std::string name_;
std::vector<Channel> channels_;
SecondsF end_time_;
Animation(const Animation&) = delete;
Animation& operator=(const Animation&) = delete;
};
} // namespace scene
} // namespace impeller
#endif // FLUTTER_IMPELLER_SCENE_ANIMATION_ANIMATION_H_
| engine/impeller/scene/animation/animation.h/0 | {
"file_path": "engine/impeller/scene/animation/animation.h",
"repo_id": "engine",
"token_count": 720
} | 232 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/scene/importer/importer.h"
#include <array>
#include <cstring>
#include <functional>
#include <iostream>
#include <iterator>
#include <memory>
#include <vector>
#include "flutter/fml/mapping.h"
#include "flutter/third_party/tinygltf/tiny_gltf.h"
#include "impeller/geometry/matrix.h"
#include "impeller/scene/importer/conversions.h"
#include "impeller/scene/importer/scene_flatbuffers.h"
#include "impeller/scene/importer/vertices_builder.h"
namespace impeller {
namespace scene {
namespace importer {
static const std::map<std::string, VerticesBuilder::AttributeType> kAttributes =
{{"POSITION", VerticesBuilder::AttributeType::kPosition},
{"NORMAL", VerticesBuilder::AttributeType::kNormal},
{"TANGENT", VerticesBuilder::AttributeType::kTangent},
{"TEXCOORD_0", VerticesBuilder::AttributeType::kTextureCoords},
{"COLOR_0", VerticesBuilder::AttributeType::kColor},
{"JOINTS_0", VerticesBuilder::AttributeType::kJoints},
{"WEIGHTS_0", VerticesBuilder::AttributeType::kWeights}};
static bool WithinRange(int index, size_t size) {
return index >= 0 && static_cast<size_t>(index) < size;
}
static bool MeshPrimitiveIsSkinned(const tinygltf::Primitive& primitive) {
return primitive.attributes.find("JOINTS_0") != primitive.attributes.end() &&
primitive.attributes.find("WEIGHTS_0") != primitive.attributes.end();
}
static void ProcessMaterial(const tinygltf::Model& gltf,
const tinygltf::Material& in_material,
fb::MaterialT& out_material) {
out_material.type = fb::MaterialType::kUnlit;
out_material.base_color_factor =
ToFBColor(in_material.pbrMetallicRoughness.baseColorFactor);
bool base_color_texture_valid =
in_material.pbrMetallicRoughness.baseColorTexture.texCoord == 0 &&
in_material.pbrMetallicRoughness.baseColorTexture.index >= 0 &&
in_material.pbrMetallicRoughness.baseColorTexture.index <
static_cast<int32_t>(gltf.textures.size());
out_material.base_color_texture =
base_color_texture_valid
// This is safe because every GLTF input texture is mapped to a
// `Scene->texture`.
? in_material.pbrMetallicRoughness.baseColorTexture.index
: -1;
}
static bool ProcessMeshPrimitive(const tinygltf::Model& gltf,
const tinygltf::Primitive& primitive,
fb::MeshPrimitiveT& mesh_primitive) {
//---------------------------------------------------------------------------
/// Vertices.
///
{
bool is_skinned = MeshPrimitiveIsSkinned(primitive);
std::unique_ptr<VerticesBuilder> builder =
is_skinned ? VerticesBuilder::MakeSkinned()
: VerticesBuilder::MakeUnskinned();
for (const auto& attribute : primitive.attributes) {
auto attribute_type = kAttributes.find(attribute.first);
if (attribute_type == kAttributes.end()) {
std::cerr << "Vertex attribute \"" << attribute.first
<< "\" not supported." << std::endl;
continue;
}
if (!is_skinned &&
(attribute_type->second == VerticesBuilder::AttributeType::kJoints ||
attribute_type->second ==
VerticesBuilder::AttributeType::kWeights)) {
// If the primitive doesn't have enough information to be skinned, skip
// skinning-related attributes.
continue;
}
const auto accessor = gltf.accessors[attribute.second];
const auto view = gltf.bufferViews[accessor.bufferView];
const auto buffer = gltf.buffers[view.buffer];
const unsigned char* source_start = &buffer.data[view.byteOffset];
VerticesBuilder::ComponentType type;
switch (accessor.componentType) {
case TINYGLTF_COMPONENT_TYPE_BYTE:
type = VerticesBuilder::ComponentType::kSignedByte;
break;
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_BYTE:
type = VerticesBuilder::ComponentType::kUnsignedByte;
break;
case TINYGLTF_COMPONENT_TYPE_SHORT:
type = VerticesBuilder::ComponentType::kSignedShort;
break;
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_SHORT:
type = VerticesBuilder::ComponentType::kUnsignedShort;
break;
case TINYGLTF_COMPONENT_TYPE_INT:
type = VerticesBuilder::ComponentType::kSignedInt;
break;
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_INT:
type = VerticesBuilder::ComponentType::kUnsignedInt;
break;
case TINYGLTF_COMPONENT_TYPE_FLOAT:
type = VerticesBuilder::ComponentType::kFloat;
break;
default:
std::cerr << "Skipping attribute \"" << attribute.first
<< "\" due to invalid component type." << std::endl;
continue;
}
builder->SetAttributeFromBuffer(
attribute_type->second, // attribute
type, // component_type
source_start, // buffer_start
accessor.ByteStride(view), // stride_bytes
accessor.count); // count
}
builder->WriteFBVertices(mesh_primitive);
}
//---------------------------------------------------------------------------
/// Indices.
///
{
if (!WithinRange(primitive.indices, gltf.accessors.size())) {
std::cerr << "Mesh primitive has no index buffer. Skipping." << std::endl;
return false;
}
auto index_accessor = gltf.accessors[primitive.indices];
auto index_view = gltf.bufferViews[index_accessor.bufferView];
auto indices = std::make_unique<fb::IndicesT>();
switch (index_accessor.componentType) {
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_SHORT:
indices->type = fb::IndexType::k16Bit;
break;
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_INT:
indices->type = fb::IndexType::k32Bit;
break;
default:
std::cerr << "Mesh primitive has unsupported index type "
<< index_accessor.componentType << ". Skipping.";
return false;
}
indices->count = index_accessor.count;
indices->data.resize(index_view.byteLength);
const auto* index_buffer =
&gltf.buffers[index_view.buffer].data[index_view.byteOffset];
std::memcpy(indices->data.data(), index_buffer, indices->data.size());
mesh_primitive.indices = std::move(indices);
}
//---------------------------------------------------------------------------
/// Material.
///
{
auto material = std::make_unique<fb::MaterialT>();
if (primitive.material >= 0 &&
primitive.material < static_cast<int>(gltf.materials.size())) {
ProcessMaterial(gltf, gltf.materials[primitive.material], *material);
} else {
material->type = fb::MaterialType::kUnlit;
}
mesh_primitive.material = std::move(material);
}
return true;
}
static void ProcessNode(const tinygltf::Model& gltf,
const tinygltf::Node& in_node,
fb::NodeT& out_node) {
out_node.name = in_node.name;
out_node.children = in_node.children;
//---------------------------------------------------------------------------
/// Transform.
///
Matrix transform;
if (in_node.scale.size() == 3) {
transform =
transform * Matrix::MakeScale({static_cast<Scalar>(in_node.scale[0]),
static_cast<Scalar>(in_node.scale[1]),
static_cast<Scalar>(in_node.scale[2])});
}
if (in_node.rotation.size() == 4) {
transform = transform * Matrix::MakeRotation(Quaternion(
in_node.rotation[0], in_node.rotation[1],
in_node.rotation[2], in_node.rotation[3]));
}
if (in_node.translation.size() == 3) {
transform = transform * Matrix::MakeTranslation(
{static_cast<Scalar>(in_node.translation[0]),
static_cast<Scalar>(in_node.translation[1]),
static_cast<Scalar>(in_node.translation[2])});
}
if (in_node.matrix.size() == 16) {
if (!transform.IsIdentity()) {
std::cerr << "The `matrix` attribute of node (name: " << in_node.name
<< ") is set in addition to one or more of the "
"`translation/rotation/scale` attributes. Using only the "
"`matrix` "
"attribute.";
}
transform = ToMatrix(in_node.matrix);
}
out_node.transform = ToFBMatrixUniquePtr(transform);
//---------------------------------------------------------------------------
/// Static meshes.
///
if (WithinRange(in_node.mesh, gltf.meshes.size())) {
auto& mesh = gltf.meshes[in_node.mesh];
for (const auto& primitive : mesh.primitives) {
auto mesh_primitive = std::make_unique<fb::MeshPrimitiveT>();
if (!ProcessMeshPrimitive(gltf, primitive, *mesh_primitive)) {
continue;
}
out_node.mesh_primitives.push_back(std::move(mesh_primitive));
}
}
//---------------------------------------------------------------------------
/// Skin.
///
if (WithinRange(in_node.skin, gltf.skins.size())) {
auto& skin = gltf.skins[in_node.skin];
auto ipskin = std::make_unique<fb::SkinT>();
ipskin->joints = skin.joints;
{
std::vector<fb::Matrix> matrices;
auto& matrix_accessor = gltf.accessors[skin.inverseBindMatrices];
auto& matrix_view = gltf.bufferViews[matrix_accessor.bufferView];
auto& matrix_buffer = gltf.buffers[matrix_view.buffer];
for (size_t matrix_i = 0; matrix_i < matrix_accessor.count; matrix_i++) {
auto* s = reinterpret_cast<const float*>(
matrix_buffer.data.data() + matrix_view.byteOffset +
matrix_accessor.ByteStride(matrix_view) * matrix_i);
Matrix m(s[0], s[1], s[2], s[3], //
s[4], s[5], s[6], s[7], //
s[8], s[9], s[10], s[11], //
s[12], s[13], s[14], s[15]);
matrices.push_back(ToFBMatrix(m));
}
ipskin->inverse_bind_matrices = std::move(matrices);
}
ipskin->skeleton = skin.skeleton;
out_node.skin = std::move(ipskin);
}
}
static void ProcessTexture(const tinygltf::Model& gltf,
const tinygltf::Texture& in_texture,
fb::TextureT& out_texture) {
if (!WithinRange(in_texture.source, gltf.images.size())) {
return;
}
auto& image = gltf.images[in_texture.source];
auto embedded = std::make_unique<fb::EmbeddedImageT>();
embedded->bytes = image.image;
size_t bytes_per_component = 0;
switch (image.pixel_type) {
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_BYTE:
embedded->component_type = fb::ComponentType::k8Bit;
bytes_per_component = 1;
break;
case TINYGLTF_COMPONENT_TYPE_UNSIGNED_SHORT:
embedded->component_type = fb::ComponentType::k16Bit;
bytes_per_component = 2;
break;
default:
std::cerr << "Texture component type " << image.pixel_type
<< " not supported." << std::endl;
return;
}
if (image.image.size() !=
bytes_per_component * image.component * image.width * image.height) {
std::cerr << "Decompressed texture had unexpected buffer size. Skipping."
<< std::endl;
return;
}
embedded->component_count = image.component;
embedded->width = image.width;
embedded->height = image.height;
out_texture.embedded_image = std::move(embedded);
out_texture.uri = image.uri;
}
static void ProcessAnimation(const tinygltf::Model& gltf,
const tinygltf::Animation& in_animation,
fb::AnimationT& out_animation) {
out_animation.name = in_animation.name;
// std::vector<impeller::fb::ChannelT> channels;
std::vector<impeller::fb::ChannelT> translation_channels;
std::vector<impeller::fb::ChannelT> rotation_channels;
std::vector<impeller::fb::ChannelT> scale_channels;
for (auto& in_channel : in_animation.channels) {
auto out_channel = fb::ChannelT();
out_channel.node = in_channel.target_node;
auto& sampler = in_animation.samplers[in_channel.sampler];
/// Keyframe times.
auto& times_accessor = gltf.accessors[sampler.input];
if (times_accessor.count <= 0) {
continue; // Nothing to record.
}
{
auto& times_bufferview = gltf.bufferViews[times_accessor.bufferView];
auto& times_buffer = gltf.buffers[times_bufferview.buffer];
if (times_accessor.componentType != TINYGLTF_COMPONENT_TYPE_FLOAT) {
std::cerr << "Unexpected component type \""
<< times_accessor.componentType
<< "\" for animation channel times accessor. Skipping."
<< std::endl;
continue;
}
if (times_accessor.type != TINYGLTF_TYPE_SCALAR) {
std::cerr << "Unexpected type \"" << times_accessor.type
<< "\" for animation channel times accessor. Skipping."
<< std::endl;
continue;
}
for (size_t time_i = 0; time_i < times_accessor.count; time_i++) {
const float* time_p = reinterpret_cast<const float*>(
times_buffer.data.data() + times_bufferview.byteOffset +
times_accessor.ByteStride(times_bufferview) * time_i);
out_channel.timeline.push_back(*time_p);
}
}
/// Keyframe values.
auto& values_accessor = gltf.accessors[sampler.output];
if (values_accessor.count != times_accessor.count) {
std::cerr << "Mismatch between time and value accessors for animation "
"channel. Skipping."
<< std::endl;
continue;
}
{
auto& values_bufferview = gltf.bufferViews[values_accessor.bufferView];
auto& values_buffer = gltf.buffers[values_bufferview.buffer];
if (values_accessor.componentType != TINYGLTF_COMPONENT_TYPE_FLOAT) {
std::cerr << "Unexpected component type \""
<< values_accessor.componentType
<< "\" for animation channel values accessor. Skipping."
<< std::endl;
continue;
}
if (in_channel.target_path == "translation") {
if (values_accessor.type != TINYGLTF_TYPE_VEC3) {
std::cerr << "Unexpected type \"" << values_accessor.type
<< "\" for animation channel \"translation\" accessor. "
"Skipping."
<< std::endl;
continue;
}
fb::TranslationKeyframesT keyframes;
for (size_t value_i = 0; value_i < values_accessor.count; value_i++) {
const float* value_p = reinterpret_cast<const float*>(
values_buffer.data.data() + values_bufferview.byteOffset +
values_accessor.ByteStride(values_bufferview) * value_i);
keyframes.values.push_back(
fb::Vec3(value_p[0], value_p[1], value_p[2]));
}
out_channel.keyframes.Set(std::move(keyframes));
translation_channels.push_back(std::move(out_channel));
} else if (in_channel.target_path == "rotation") {
if (values_accessor.type != TINYGLTF_TYPE_VEC4) {
std::cerr << "Unexpected type \"" << values_accessor.type
<< "\" for animation channel \"rotation\" accessor. "
"Skipping."
<< std::endl;
continue;
}
fb::RotationKeyframesT keyframes;
for (size_t value_i = 0; value_i < values_accessor.count; value_i++) {
const float* value_p = reinterpret_cast<const float*>(
values_buffer.data.data() + values_bufferview.byteOffset +
values_accessor.ByteStride(values_bufferview) * value_i);
keyframes.values.push_back(
fb::Vec4(value_p[0], value_p[1], value_p[2], value_p[3]));
}
out_channel.keyframes.Set(std::move(keyframes));
rotation_channels.push_back(std::move(out_channel));
} else if (in_channel.target_path == "scale") {
if (values_accessor.type != TINYGLTF_TYPE_VEC3) {
std::cerr << "Unexpected type \"" << values_accessor.type
<< "\" for animation channel \"scale\" accessor. "
"Skipping."
<< std::endl;
continue;
}
fb::ScaleKeyframesT keyframes;
for (size_t value_i = 0; value_i < values_accessor.count; value_i++) {
const float* value_p = reinterpret_cast<const float*>(
values_buffer.data.data() + values_bufferview.byteOffset +
values_accessor.ByteStride(values_bufferview) * value_i);
keyframes.values.push_back(
fb::Vec3(value_p[0], value_p[1], value_p[2]));
}
out_channel.keyframes.Set(std::move(keyframes));
scale_channels.push_back(std::move(out_channel));
} else {
std::cerr << "Unsupported animation channel target path \""
<< in_channel.target_path << "\". Skipping." << std::endl;
continue;
}
}
}
std::vector<std::unique_ptr<impeller::fb::ChannelT>> channels;
for (const auto& channel_list :
{translation_channels, rotation_channels, scale_channels}) {
for (const auto& channel : channel_list) {
channels.push_back(std::make_unique<fb::ChannelT>(channel));
}
}
out_animation.channels = std::move(channels);
}
bool ParseGLTF(const fml::Mapping& source_mapping, fb::SceneT& out_scene) {
tinygltf::Model gltf;
{
tinygltf::TinyGLTF loader;
std::string error;
std::string warning;
bool success = loader.LoadBinaryFromMemory(&gltf, &error, &warning,
source_mapping.GetMapping(),
source_mapping.GetSize());
if (!warning.empty()) {
std::cerr << "Warning while loading GLTF: " << warning << std::endl;
}
if (!error.empty()) {
std::cerr << "Error while loading GLTF: " << error << std::endl;
}
if (!success) {
return false;
}
}
const tinygltf::Scene& scene = gltf.scenes[gltf.defaultScene];
out_scene.children = scene.nodes;
out_scene.transform =
ToFBMatrixUniquePtr(Matrix::MakeScale(Vector3(1, 1, -1)));
for (size_t texture_i = 0; texture_i < gltf.textures.size(); texture_i++) {
auto texture = std::make_unique<fb::TextureT>();
ProcessTexture(gltf, gltf.textures[texture_i], *texture);
out_scene.textures.push_back(std::move(texture));
}
for (size_t node_i = 0; node_i < gltf.nodes.size(); node_i++) {
auto node = std::make_unique<fb::NodeT>();
ProcessNode(gltf, gltf.nodes[node_i], *node);
out_scene.nodes.push_back(std::move(node));
}
for (size_t animation_i = 0; animation_i < gltf.animations.size();
animation_i++) {
auto animation = std::make_unique<fb::AnimationT>();
ProcessAnimation(gltf, gltf.animations[animation_i], *animation);
out_scene.animations.push_back(std::move(animation));
}
return true;
}
} // namespace importer
} // namespace scene
} // namespace impeller
| engine/impeller/scene/importer/importer_gltf.cc/0 | {
"file_path": "engine/impeller/scene/importer/importer_gltf.cc",
"repo_id": "engine",
"token_count": 8454
} | 233 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/scene/scene.h"
#include <memory>
#include <utility>
#include "flutter/fml/logging.h"
#include "fml/closure.h"
#include "impeller/renderer/render_target.h"
#include "impeller/scene/scene_context.h"
#include "impeller/scene/scene_encoder.h"
namespace impeller {
namespace scene {
Scene::Scene(std::shared_ptr<SceneContext> scene_context)
: scene_context_(std::move(scene_context)) {
root_.is_root_ = true;
};
Scene::~Scene() {
for (auto& child : GetRoot().GetChildren()) {
child->parent_ = nullptr;
}
}
Node& Scene::GetRoot() {
return root_;
}
bool Scene::Render(const RenderTarget& render_target,
const Matrix& camera_transform) {
fml::ScopedCleanupClosure reset_state(
[context = scene_context_]() { context->GetTransientsBuffer().Reset(); });
// Collect the render commands from the scene.
SceneEncoder encoder;
if (!root_.Render(encoder,
*scene_context_->GetContext()->GetResourceAllocator(),
Matrix())) {
FML_LOG(ERROR) << "Failed to render frame.";
return false;
}
// Encode the commands.
std::shared_ptr<CommandBuffer> command_buffer =
encoder.BuildSceneCommandBuffer(*scene_context_, camera_transform,
render_target);
// TODO(bdero): Do post processing.
if (!scene_context_->GetContext()
->GetCommandQueue()
->Submit({command_buffer})
.ok()) {
FML_LOG(ERROR) << "Failed to submit command buffer.";
return false;
}
return true;
}
bool Scene::Render(const RenderTarget& render_target, const Camera& camera) {
return Render(render_target,
camera.GetTransform(render_target.GetRenderTargetSize()));
}
} // namespace scene
} // namespace impeller
| engine/impeller/scene/scene.cc/0 | {
"file_path": "engine/impeller/scene/scene.cc",
"repo_id": "engine",
"token_count": 744
} | 234 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_SHADER_ARCHIVE_MULTI_ARCH_SHADER_ARCHIVE_H_
#define FLUTTER_IMPELLER_SHADER_ARCHIVE_MULTI_ARCH_SHADER_ARCHIVE_H_
#include <map>
#include <memory>
#include "flutter/fml/macros.h"
#include "flutter/fml/mapping.h"
#include "impeller/shader_archive/shader_archive.h"
#include "impeller/shader_archive/shader_archive_types.h"
namespace impeller {
class MultiArchShaderArchive {
public:
static std::shared_ptr<ShaderArchive> CreateArchiveFromMapping(
const std::shared_ptr<const fml::Mapping>& mapping,
ArchiveRenderingBackend backend);
explicit MultiArchShaderArchive(
const std::shared_ptr<const fml::Mapping>& mapping);
~MultiArchShaderArchive();
std::shared_ptr<const fml::Mapping> GetArchive(
ArchiveRenderingBackend backend) const;
std::shared_ptr<ShaderArchive> GetShaderArchive(
ArchiveRenderingBackend backend) const;
bool IsValid() const;
private:
std::map<ArchiveRenderingBackend, std::shared_ptr<const fml::Mapping>>
backend_mappings_;
bool is_valid_ = false;
MultiArchShaderArchive(const MultiArchShaderArchive&) = delete;
MultiArchShaderArchive& operator=(const MultiArchShaderArchive&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_SHADER_ARCHIVE_MULTI_ARCH_SHADER_ARCHIVE_H_
| engine/impeller/shader_archive/multi_arch_shader_archive.h/0 | {
"file_path": "engine/impeller/shader_archive/multi_arch_shader_archive.h",
"repo_id": "engine",
"token_count": 538
} | 235 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: camel_case_types
import 'dart:ffi' as ffi;
import 'dart:io';
import 'dart:typed_data';
/// Determines the winding rule that decides how the interior of a Path is
/// calculated.
///
/// This enum is used by the [VerticesBuilder.tessellate] method.
// must match ordering in geometry/path.h
enum FillType {
/// The interior is defined by a non-zero sum of signed edge crossings.
nonZero,
/// The interior is defined by an odd number of edge crossings.
evenOdd,
}
/// Information about how to approximate points on a curved path segment.
///
/// In particular, the values in this object control how many vertices to
/// generate when approximating curves, and what tolerances to use when
/// calculating the sharpness of curves.
///
/// Used by [VerticesBuilder.tessellate].
class SmoothingApproximation {
/// Creates a new smoothing approximation instance with default values.
const SmoothingApproximation({
this.scale = 1.0,
this.angleTolerance = 0.0,
this.cuspLimit = 0.0,
});
/// The scaling coefficient to use when translating to screen coordinates.
///
/// Values approaching 0.0 will generate smoother looking curves with a
/// greater number of vertices, and will be more expensive to calculate.
final double scale;
/// The tolerance value in radians for calculating sharp angles.
///
/// Values approaching 0.0 will provide more accurate approximation of sharp
/// turns. A 0.0 value means angle conditions are not considered at all.
final double angleTolerance;
/// An angle in radians at which to introduce bevel cuts.
///
/// Values greater than zero will restirct the sharpness of bevel cuts on
/// turns.
final double cuspLimit;
}
/// Creates vertices from path commands.
///
/// First, build up the path contours with the [moveTo], [lineTo], [cubicTo],
/// and [close] methods. All methods expect absolute coordinates.
///
/// Then, use the [tessellate] method to create a [Float32List] of vertex pairs.
///
/// Finally, use the [dispose] method to clean up native resources. After
/// [dispose] has been called, this class must not be used again.
class VerticesBuilder {
/// Constructs a [VerticesBuilder] instance to which path commands can be
/// added.
VerticesBuilder() : _builder = _createPathFn();
ffi.Pointer<_PathBuilder>? _builder;
final List<ffi.Pointer<_Vertices>> _vertices = <ffi.Pointer<_Vertices>>[];
/// Adds a move verb to the absolute coordinates x,y.
void moveTo(double x, double y) {
assert(_builder != null);
_moveToFn(_builder!, x, y);
}
/// Adds a line verb to the absolute coordinates x,y.
void lineTo(double x, double y) {
assert(_builder != null);
_lineToFn(_builder!, x, y);
}
/// Adds a cubic Bezier curve with x1,y1 as the first control point, x2,y2 as
/// the second control point, and end point x3,y3.
void cubicTo(
double x1,
double y1,
double x2,
double y2,
double x3,
double y3,
) {
assert(_builder != null);
_cubicToFn(_builder!, x1, y1, x2, y2, x3, y3);
}
/// Adds a close command to the start of the current contour.
void close() {
assert(_builder != null);
_closeFn(_builder!, true);
}
/// Tessellates the path created by the previous method calls into a list of
/// vertices.
Float32List tessellate({
FillType fillType = FillType.nonZero,
SmoothingApproximation smoothing = const SmoothingApproximation(),
}) {
assert(_vertices.isEmpty);
assert(_builder != null);
final ffi.Pointer<_Vertices> vertices = _tessellateFn(
_builder!,
fillType.index,
smoothing.scale,
smoothing.angleTolerance,
smoothing.cuspLimit,
);
_vertices.add(vertices);
return vertices.ref.points.asTypedList(vertices.ref.size);
}
/// Releases native resources.
///
/// After calling dispose, this class must not be used again.
void dispose() {
assert(_builder != null);
_vertices.forEach(_destroyVerticesFn);
_destroyFn(_builder!);
_vertices.clear();
_builder = null;
}
}
// TODO(dnfield): Figure out where to put this.
// https://github.com/flutter/flutter/issues/99563
final ffi.DynamicLibrary _dylib = () {
if (Platform.isWindows) {
return ffi.DynamicLibrary.open('tessellator.dll');
} else if (Platform.isIOS || Platform.isMacOS) {
return ffi.DynamicLibrary.open('libtessellator.dylib');
} else if (Platform.isAndroid || Platform.isLinux) {
return ffi.DynamicLibrary.open('libtessellator.so');
}
throw UnsupportedError('Unknown platform: ${Platform.operatingSystem}');
}();
final class _Vertices extends ffi.Struct {
external ffi.Pointer<ffi.Float> points;
@ffi.Uint32()
external int size;
}
final class _PathBuilder extends ffi.Opaque {}
typedef _CreatePathBuilderType = ffi.Pointer<_PathBuilder> Function();
typedef _create_path_builder_type = ffi.Pointer<_PathBuilder> Function();
final _CreatePathBuilderType _createPathFn =
_dylib.lookupFunction<_create_path_builder_type, _CreatePathBuilderType>(
'CreatePathBuilder',
);
typedef _MoveToType = void Function(ffi.Pointer<_PathBuilder>, double, double);
typedef _move_to_type = ffi.Void Function(
ffi.Pointer<_PathBuilder>,
ffi.Float,
ffi.Float,
);
final _MoveToType _moveToFn = _dylib.lookupFunction<_move_to_type, _MoveToType>(
'MoveTo',
);
typedef _LineToType = void Function(ffi.Pointer<_PathBuilder>, double, double);
typedef _line_to_type = ffi.Void Function(
ffi.Pointer<_PathBuilder>,
ffi.Float,
ffi.Float,
);
final _LineToType _lineToFn = _dylib.lookupFunction<_line_to_type, _LineToType>(
'LineTo',
);
typedef _CubicToType = void Function(
ffi.Pointer<_PathBuilder>,
double,
double,
double,
double,
double,
double,
);
typedef _cubic_to_type = ffi.Void Function(
ffi.Pointer<_PathBuilder>,
ffi.Float,
ffi.Float,
ffi.Float,
ffi.Float,
ffi.Float,
ffi.Float,
);
final _CubicToType _cubicToFn =
_dylib.lookupFunction<_cubic_to_type, _CubicToType>('CubicTo');
typedef _CloseType = void Function(ffi.Pointer<_PathBuilder>, bool);
typedef _close_type = ffi.Void Function(ffi.Pointer<_PathBuilder>, ffi.Bool);
final _CloseType _closeFn =
_dylib.lookupFunction<_close_type, _CloseType>('Close');
typedef _TessellateType = ffi.Pointer<_Vertices> Function(
ffi.Pointer<_PathBuilder>,
int,
double,
double,
double,
);
typedef _tessellate_type = ffi.Pointer<_Vertices> Function(
ffi.Pointer<_PathBuilder>,
ffi.Int,
ffi.Float,
ffi.Float,
ffi.Float,
);
final _TessellateType _tessellateFn =
_dylib.lookupFunction<_tessellate_type, _TessellateType>('Tessellate');
typedef _DestroyType = void Function(ffi.Pointer<_PathBuilder>);
typedef _destroy_type = ffi.Void Function(ffi.Pointer<_PathBuilder>);
final _DestroyType _destroyFn =
_dylib.lookupFunction<_destroy_type, _DestroyType>(
'DestroyPathBuilder',
);
typedef _DestroyVerticesType = void Function(ffi.Pointer<_Vertices>);
typedef _destroy_vertices_type = ffi.Void Function(ffi.Pointer<_Vertices>);
final _DestroyVerticesType _destroyVerticesFn =
_dylib.lookupFunction<_destroy_vertices_type, _DestroyVerticesType>(
'DestroyVertices',
);
| engine/impeller/tessellator/dart/lib/tessellator.dart/0 | {
"file_path": "engine/impeller/tessellator/dart/lib/tessellator.dart",
"repo_id": "engine",
"token_count": 2526
} | 236 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_TOOLKIT_ANDROID_SURFACE_CONTROL_H_
#define FLUTTER_IMPELLER_TOOLKIT_ANDROID_SURFACE_CONTROL_H_
#include "flutter/fml/unique_object.h"
#include "impeller/toolkit/android/proc_table.h"
namespace impeller::android {
//------------------------------------------------------------------------------
/// @brief A wrapper for ASurfaceControl.
/// https://developer.android.com/ndk/reference/group/native-activity#asurfacecontrol
///
/// Instances of this class represent a node in the hierarchy of
/// surfaces sent to the system compositor for final composition.
///
/// This wrapper is only available on Android API 29 and above.
///
class SurfaceControl {
public:
//----------------------------------------------------------------------------
/// @return `true` if any surface controls can be created on this
/// platform.
///
static bool IsAvailableOnPlatform();
//----------------------------------------------------------------------------
/// @brief Creates a new surface control and adds it as a child of the
/// given window.
///
/// @param window The window
/// @param[in] debug_name A debug name. See it using
/// `adb shell dumpsys SurfaceFlinger` along with
/// other control properties. If no debug name is
/// specified, the value "Impeller Layer" is used.
///
explicit SurfaceControl(ANativeWindow* window,
const char* debug_name = nullptr);
//----------------------------------------------------------------------------
/// @brief Removes the surface control from the presentation hierarchy
/// managed by the system compositor and release the client side
/// reference to the control. At this point, it may be collected
/// when the compositor is also done using it.
///
~SurfaceControl();
SurfaceControl(const SurfaceControl&) = delete;
SurfaceControl& operator=(const SurfaceControl&) = delete;
bool IsValid() const;
ASurfaceControl* GetHandle() const;
//----------------------------------------------------------------------------
/// @brief Remove the surface control from the hierarchy of nodes
/// presented by the system compositor.
///
/// @return `true` If the control will be removed from the hierarchy of
/// nodes presented by the system compositor.
///
bool RemoveFromParent() const;
private:
struct UniqueASurfaceControlTraits {
static ASurfaceControl* InvalidValue() { return nullptr; }
static bool IsValid(ASurfaceControl* value) {
return value != InvalidValue();
}
static void Free(ASurfaceControl* value) {
GetProcTable().ASurfaceControl_release(value);
}
};
fml::UniqueObject<ASurfaceControl*, UniqueASurfaceControlTraits> control_;
};
} // namespace impeller::android
#endif // FLUTTER_IMPELLER_TOOLKIT_ANDROID_SURFACE_CONTROL_H_
| engine/impeller/toolkit/android/surface_control.h/0 | {
"file_path": "engine/impeller/toolkit/android/surface_control.h",
"repo_id": "engine",
"token_count": 1063
} | 237 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_TOOLKIT_EGL_SURFACE_H_
#define FLUTTER_IMPELLER_TOOLKIT_EGL_SURFACE_H_
#include "flutter/fml/macros.h"
#include "impeller/toolkit/egl/egl.h"
namespace impeller {
namespace egl {
class Surface {
public:
Surface(EGLDisplay display, EGLSurface surface);
~Surface();
bool IsValid() const;
const EGLSurface& GetHandle() const;
bool Present() const;
private:
EGLDisplay display_ = EGL_NO_DISPLAY;
EGLSurface surface_ = EGL_NO_SURFACE;
Surface(const Surface&) = delete;
Surface& operator=(const Surface&) = delete;
};
} // namespace egl
} // namespace impeller
#endif // FLUTTER_IMPELLER_TOOLKIT_EGL_SURFACE_H_
| engine/impeller/toolkit/egl/surface.h/0 | {
"file_path": "engine/impeller/toolkit/egl/surface.h",
"repo_id": "engine",
"token_count": 307
} | 238 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "impeller/typographer/backends/skia/glyph_atlas_context_skia.h"
#include "third_party/skia/include/core/SkBitmap.h"
namespace impeller {
GlyphAtlasContextSkia::GlyphAtlasContextSkia() = default;
GlyphAtlasContextSkia::~GlyphAtlasContextSkia() = default;
std::shared_ptr<SkBitmap> GlyphAtlasContextSkia::GetBitmap() const {
return bitmap_;
}
void GlyphAtlasContextSkia::UpdateBitmap(std::shared_ptr<SkBitmap> bitmap) {
bitmap_ = std::move(bitmap);
}
} // namespace impeller
| engine/impeller/typographer/backends/skia/glyph_atlas_context_skia.cc/0 | {
"file_path": "engine/impeller/typographer/backends/skia/glyph_atlas_context_skia.cc",
"repo_id": "engine",
"token_count": 235
} | 239 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_TYPOGRAPHER_BACKENDS_STB_TYPOGRAPHER_CONTEXT_STB_H_
#define FLUTTER_IMPELLER_TYPOGRAPHER_BACKENDS_STB_TYPOGRAPHER_CONTEXT_STB_H_
#include "impeller/typographer/typographer_context.h"
#include <memory>
#include "flutter/fml/macros.h"
namespace impeller {
class TypographerContextSTB : public TypographerContext {
public:
static std::unique_ptr<TypographerContext> Make();
TypographerContextSTB();
~TypographerContextSTB() override;
// |TypographerContext|
std::shared_ptr<GlyphAtlasContext> CreateGlyphAtlasContext() const override;
// |TypographerContext|
std::shared_ptr<GlyphAtlas> CreateGlyphAtlas(
Context& context,
GlyphAtlas::Type type,
const std::shared_ptr<GlyphAtlasContext>& atlas_context,
const FontGlyphMap& font_glyph_map) const override;
private:
TypographerContextSTB(const TypographerContextSTB&) = delete;
TypographerContextSTB& operator=(const TypographerContextSTB&) = delete;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_TYPOGRAPHER_BACKENDS_STB_TYPOGRAPHER_CONTEXT_STB_H_
| engine/impeller/typographer/backends/stb/typographer_context_stb.h/0 | {
"file_path": "engine/impeller/typographer/backends/stb/typographer_context_stb.h",
"repo_id": "engine",
"token_count": 451
} | 240 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_IMPELLER_TYPOGRAPHER_TEXT_RUN_H_
#define FLUTTER_IMPELLER_TYPOGRAPHER_TEXT_RUN_H_
#include <vector>
#include "impeller/geometry/matrix.h"
#include "impeller/typographer/font.h"
#include "impeller/typographer/glyph.h"
namespace impeller {
//------------------------------------------------------------------------------
/// @brief Represents a collection of positioned glyphs from a specific
/// font.
///
class TextRun {
public:
struct GlyphPosition {
Glyph glyph;
Point position;
GlyphPosition(Glyph p_glyph, Point p_position)
: glyph(p_glyph), position(p_position) {}
};
//----------------------------------------------------------------------------
/// @brief Create an empty text run with the specified font.
///
/// @param[in] font The font
///
explicit TextRun(const Font& font);
TextRun(const Font& font, std::vector<GlyphPosition>& glyphs);
~TextRun();
bool IsValid() const;
//----------------------------------------------------------------------------
/// @brief Add a glyph at the specified position to the run.
///
/// @param[in] glyph The glyph
/// @param[in] position The position
///
/// @return If the glyph could be added to the run.
///
bool AddGlyph(Glyph glyph, Point position);
//----------------------------------------------------------------------------
/// @brief Get the number of glyphs in the run.
///
/// @return The glyph count.
///
size_t GetGlyphCount() const;
//----------------------------------------------------------------------------
/// @brief Get a reference to all the glyph positions within the run.
///
/// @return The glyph positions.
///
const std::vector<GlyphPosition>& GetGlyphPositions() const;
//----------------------------------------------------------------------------
/// @brief Get the font for this run.
///
/// @return The font.
///
const Font& GetFont() const;
private:
Font font_;
std::vector<GlyphPosition> glyphs_;
bool is_valid_ = false;
};
} // namespace impeller
#endif // FLUTTER_IMPELLER_TYPOGRAPHER_TEXT_RUN_H_
| engine/impeller/typographer/text_run.h/0 | {
"file_path": "engine/impeller/typographer/text_run.h",
"repo_id": "engine",
"token_count": 720
} | 241 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/gpu/fixtures.h"
// These blobs are generated using xxd -i [myfile.iplr].
// This is a temporary hack to provision the shader library before the importer
// is done.
unsigned char kFlutterGPUUnlitVertIPLR[] = {
0x18, 0x00, 0x00, 0x00, 0x49, 0x50, 0x4c, 0x52, 0x00, 0x00, 0x0e, 0x00,
0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x08, 0x00, 0x0c, 0x00,
0x0e, 0x00, 0x00, 0x00, 0x18, 0x03, 0x00, 0x00, 0x80, 0x02, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x72, 0x02, 0x00, 0x00, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x6d, 0x65, 0x74, 0x61, 0x6c, 0x5f,
0x73, 0x74, 0x64, 0x6c, 0x69, 0x62, 0x3e, 0x0a, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x73, 0x69, 0x6d, 0x64, 0x2f, 0x73,
0x69, 0x6d, 0x64, 0x2e, 0x68, 0x3e, 0x0a, 0x0a, 0x75, 0x73, 0x69, 0x6e,
0x67, 0x20, 0x6e, 0x61, 0x6d, 0x65, 0x73, 0x70, 0x61, 0x63, 0x65, 0x20,
0x6d, 0x65, 0x74, 0x61, 0x6c, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75,
0x63, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x76, 0x65, 0x72,
0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74,
0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74,
0x34, 0x20, 0x76, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x5b, 0x5b,
0x75, 0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x30, 0x29, 0x5d,
0x5d, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74,
0x34, 0x20, 0x67, 0x6c, 0x5f, 0x50, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f,
0x6e, 0x20, 0x5b, 0x5b, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e,
0x5d, 0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75,
0x63, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x76, 0x65, 0x72,
0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x69, 0x6e, 0x0a,
0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x32,
0x20, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x20, 0x5b, 0x5b,
0x61, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x28, 0x30, 0x29,
0x5d, 0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x76, 0x65, 0x72, 0x74,
0x65, 0x78, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x76, 0x65, 0x72,
0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74,
0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75,
0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65,
0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x28, 0x66, 0x6c, 0x75, 0x74, 0x74,
0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74,
0x5f, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e,
0x5f, 0x69, 0x6e, 0x20, 0x69, 0x6e, 0x20, 0x5b, 0x5b, 0x73, 0x74, 0x61,
0x67, 0x65, 0x5f, 0x69, 0x6e, 0x5d, 0x5d, 0x2c, 0x20, 0x63, 0x6f, 0x6e,
0x73, 0x74, 0x61, 0x6e, 0x74, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x34,
0x26, 0x20, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x5b, 0x5b, 0x62, 0x75,
0x66, 0x66, 0x65, 0x72, 0x28, 0x30, 0x29, 0x5d, 0x5d, 0x2c, 0x20, 0x63,
0x6f, 0x6e, 0x73, 0x74, 0x61, 0x6e, 0x74, 0x20, 0x66, 0x6c, 0x6f, 0x61,
0x74, 0x34, 0x78, 0x34, 0x26, 0x20, 0x6d, 0x76, 0x70, 0x20, 0x5b, 0x5b,
0x62, 0x75, 0x66, 0x66, 0x65, 0x72, 0x28, 0x31, 0x29, 0x5d, 0x5d, 0x29,
0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74,
0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74,
0x5f, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e,
0x5f, 0x6f, 0x75, 0x74, 0x20, 0x6f, 0x75, 0x74, 0x20, 0x3d, 0x20, 0x7b,
0x7d, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f, 0x75, 0x74, 0x2e, 0x76,
0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x3d, 0x20, 0x63, 0x6f, 0x6c,
0x6f, 0x72, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f, 0x75, 0x74, 0x2e,
0x67, 0x6c, 0x5f, 0x50, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x20,
0x3d, 0x20, 0x6d, 0x76, 0x70, 0x20, 0x2a, 0x20, 0x66, 0x6c, 0x6f, 0x61,
0x74, 0x34, 0x28, 0x69, 0x6e, 0x2e, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69,
0x6f, 0x6e, 0x2c, 0x20, 0x30, 0x2e, 0x30, 0x2c, 0x20, 0x31, 0x2e, 0x30,
0x29, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x72, 0x65, 0x74, 0x75, 0x72,
0x6e, 0x20, 0x6f, 0x75, 0x74, 0x3b, 0x0a, 0x7d, 0x0a, 0x0a, 0x00, 0x00,
0x02, 0x00, 0x00, 0x00, 0x60, 0x00, 0x00, 0x00, 0x14, 0x00, 0x00, 0x00,
0x10, 0x00, 0x2c, 0x00, 0x04, 0x00, 0x0c, 0x00, 0x08, 0x00, 0x14, 0x00,
0x1c, 0x00, 0x24, 0x00, 0x10, 0x00, 0x00, 0x00, 0x28, 0x00, 0x00, 0x00,
0x0a, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x05, 0x00, 0x00, 0x00, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x00, 0x00, 0x00,
0x10, 0x00, 0x28, 0x00, 0x04, 0x00, 0x00, 0x00, 0x08, 0x00, 0x0c, 0x00,
0x14, 0x00, 0x1c, 0x00, 0x10, 0x00, 0x00, 0x00, 0x24, 0x00, 0x00, 0x00,
0x0a, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00,
0x6d, 0x76, 0x70, 0x00, 0x1d, 0x00, 0x00, 0x00, 0x66, 0x6c, 0x75, 0x74,
0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69,
0x74, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69,
0x6e, 0x00, 0x00, 0x00};
unsigned char kFlutterGPUUnlitFragIPLR[] = {
0x18, 0x00, 0x00, 0x00, 0x49, 0x50, 0x4c, 0x52, 0x00, 0x00, 0x0e, 0x00,
0x10, 0x00, 0x07, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x0c, 0x00,
0x0e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xe8, 0x01, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0xda, 0x01, 0x00, 0x00, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x6d, 0x65, 0x74, 0x61, 0x6c, 0x5f,
0x73, 0x74, 0x64, 0x6c, 0x69, 0x62, 0x3e, 0x0a, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x73, 0x69, 0x6d, 0x64, 0x2f, 0x73,
0x69, 0x6d, 0x64, 0x2e, 0x68, 0x3e, 0x0a, 0x0a, 0x75, 0x73, 0x69, 0x6e,
0x67, 0x20, 0x6e, 0x61, 0x6d, 0x65, 0x73, 0x70, 0x61, 0x63, 0x65, 0x20,
0x6d, 0x65, 0x74, 0x61, 0x6c, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75,
0x63, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x66, 0x72, 0x61,
0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f,
0x75, 0x74, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f,
0x61, 0x74, 0x34, 0x20, 0x66, 0x72, 0x61, 0x67, 0x5f, 0x63, 0x6f, 0x6c,
0x6f, 0x72, 0x20, 0x5b, 0x5b, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x28, 0x30,
0x29, 0x5d, 0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72,
0x75, 0x63, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f,
0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x66, 0x72,
0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f,
0x69, 0x6e, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f,
0x61, 0x74, 0x34, 0x20, 0x76, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20,
0x5b, 0x5b, 0x75, 0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x30,
0x29, 0x5d, 0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x66, 0x72, 0x61,
0x67, 0x6d, 0x65, 0x6e, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65,
0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f,
0x66, 0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69,
0x6e, 0x5f, 0x6f, 0x75, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65,
0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f,
0x66, 0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69,
0x6e, 0x28, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70,
0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x66, 0x72, 0x61, 0x67,
0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x69, 0x6e,
0x20, 0x69, 0x6e, 0x20, 0x5b, 0x5b, 0x73, 0x74, 0x61, 0x67, 0x65, 0x5f,
0x69, 0x6e, 0x5d, 0x5d, 0x29, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20,
0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f,
0x75, 0x6e, 0x6c, 0x69, 0x74, 0x5f, 0x66, 0x72, 0x61, 0x67, 0x6d, 0x65,
0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74, 0x20,
0x6f, 0x75, 0x74, 0x20, 0x3d, 0x20, 0x7b, 0x7d, 0x3b, 0x0a, 0x20, 0x20,
0x20, 0x20, 0x6f, 0x75, 0x74, 0x2e, 0x66, 0x72, 0x61, 0x67, 0x5f, 0x63,
0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x3d, 0x20, 0x69, 0x6e, 0x2e, 0x76, 0x5f,
0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x72,
0x65, 0x74, 0x75, 0x72, 0x6e, 0x20, 0x6f, 0x75, 0x74, 0x3b, 0x0a, 0x7d,
0x0a, 0x0a, 0x00, 0x00, 0x1f, 0x00, 0x00, 0x00, 0x66, 0x6c, 0x75, 0x74,
0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x75, 0x6e, 0x6c, 0x69,
0x74, 0x5f, 0x66, 0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d,
0x61, 0x69, 0x6e, 0x00};
unsigned char kFlutterGPUTextureVertIPLR[] = {
0x18, 0x00, 0x00, 0x00, 0x49, 0x50, 0x4c, 0x52, 0x00, 0x00, 0x0e, 0x00,
0x10, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x08, 0x00, 0x0c, 0x00,
0x0e, 0x00, 0x00, 0x00, 0x58, 0x03, 0x00, 0x00, 0x0c, 0x03, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x6d, 0x65, 0x74, 0x61, 0x6c, 0x5f,
0x73, 0x74, 0x64, 0x6c, 0x69, 0x62, 0x3e, 0x0a, 0x23, 0x69, 0x6e, 0x63,
0x6c, 0x75, 0x64, 0x65, 0x20, 0x3c, 0x73, 0x69, 0x6d, 0x64, 0x2f, 0x73,
0x69, 0x6d, 0x64, 0x2e, 0x68, 0x3e, 0x0a, 0x0a, 0x75, 0x73, 0x69, 0x6e,
0x67, 0x20, 0x6e, 0x61, 0x6d, 0x65, 0x73, 0x70, 0x61, 0x63, 0x65, 0x20,
0x6d, 0x65, 0x74, 0x61, 0x6c, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75,
0x63, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x76,
0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f,
0x75, 0x74, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f,
0x61, 0x74, 0x32, 0x20, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72,
0x65, 0x5f, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x73, 0x20, 0x5b, 0x5b, 0x75,
0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x30, 0x29, 0x5d, 0x5d,
0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x34,
0x20, 0x76, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x5b, 0x5b, 0x75,
0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x31, 0x29, 0x5d, 0x5d,
0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x34,
0x20, 0x67, 0x6c, 0x5f, 0x50, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e,
0x20, 0x5b, 0x5b, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x5d,
0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75, 0x63,
0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70,
0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x76, 0x65,
0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x69, 0x6e,
0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74,
0x33, 0x20, 0x70, 0x6f, 0x73, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x20, 0x5b,
0x5b, 0x61, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x28, 0x30,
0x29, 0x5d, 0x5d, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f,
0x61, 0x74, 0x32, 0x20, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f,
0x63, 0x6f, 0x6f, 0x72, 0x64, 0x73, 0x20, 0x5b, 0x5b, 0x61, 0x74, 0x74,
0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x28, 0x31, 0x29, 0x5d, 0x5d, 0x3b,
0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x34, 0x20,
0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x5b, 0x5b, 0x61, 0x74, 0x74, 0x72,
0x69, 0x62, 0x75, 0x74, 0x65, 0x28, 0x32, 0x29, 0x5d, 0x5d, 0x3b, 0x0a,
0x7d, 0x3b, 0x0a, 0x0a, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x20, 0x66,
0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74,
0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65,
0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74, 0x20, 0x66,
0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74,
0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65,
0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x28, 0x66, 0x6c, 0x75, 0x74, 0x74,
0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75,
0x72, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61,
0x69, 0x6e, 0x5f, 0x69, 0x6e, 0x20, 0x69, 0x6e, 0x20, 0x5b, 0x5b, 0x73,
0x74, 0x61, 0x67, 0x65, 0x5f, 0x69, 0x6e, 0x5d, 0x5d, 0x2c, 0x20, 0x63,
0x6f, 0x6e, 0x73, 0x74, 0x61, 0x6e, 0x74, 0x20, 0x66, 0x6c, 0x6f, 0x61,
0x74, 0x34, 0x78, 0x34, 0x26, 0x20, 0x6d, 0x76, 0x70, 0x20, 0x5b, 0x5b,
0x62, 0x75, 0x66, 0x66, 0x65, 0x72, 0x28, 0x30, 0x29, 0x5d, 0x5d, 0x29,
0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74,
0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75,
0x72, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x5f, 0x6d, 0x61,
0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74, 0x20, 0x6f, 0x75, 0x74, 0x20, 0x3d,
0x20, 0x7b, 0x7d, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f, 0x75, 0x74,
0x2e, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x63,
0x6f, 0x6f, 0x72, 0x64, 0x73, 0x20, 0x3d, 0x20, 0x69, 0x6e, 0x2e, 0x74,
0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x63, 0x6f, 0x6f, 0x72, 0x64,
0x73, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f, 0x75, 0x74, 0x2e, 0x76,
0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x3d, 0x20, 0x69, 0x6e, 0x2e,
0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f,
0x75, 0x74, 0x2e, 0x67, 0x6c, 0x5f, 0x50, 0x6f, 0x73, 0x69, 0x74, 0x69,
0x6f, 0x6e, 0x20, 0x3d, 0x20, 0x6d, 0x76, 0x70, 0x20, 0x2a, 0x20, 0x66,
0x6c, 0x6f, 0x61, 0x74, 0x34, 0x28, 0x69, 0x6e, 0x2e, 0x70, 0x6f, 0x73,
0x69, 0x74, 0x69, 0x6f, 0x6e, 0x2c, 0x20, 0x31, 0x2e, 0x30, 0x29, 0x3b,
0x0a, 0x20, 0x20, 0x20, 0x20, 0x72, 0x65, 0x74, 0x75, 0x72, 0x6e, 0x20,
0x6f, 0x75, 0x74, 0x3b, 0x0a, 0x7d, 0x0a, 0x0a, 0x01, 0x00, 0x00, 0x00,
0x14, 0x00, 0x00, 0x00, 0x10, 0x00, 0x28, 0x00, 0x04, 0x00, 0x00, 0x00,
0x08, 0x00, 0x0c, 0x00, 0x14, 0x00, 0x1c, 0x00, 0x10, 0x00, 0x00, 0x00,
0x24, 0x00, 0x00, 0x00, 0x0a, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x03, 0x00, 0x00, 0x00, 0x6d, 0x76, 0x70, 0x00, 0x1f, 0x00, 0x00, 0x00,
0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f,
0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x76, 0x65, 0x72, 0x74,
0x65, 0x78, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x00};
unsigned char kFlutterGPUTextureFragIPLR[] = {
0x1c, 0x00, 0x00, 0x00, 0x49, 0x50, 0x4c, 0x52, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x0e, 0x00, 0x14, 0x00, 0x07, 0x00, 0x00, 0x00, 0x08, 0x00,
0x0c, 0x00, 0x10, 0x00, 0x0e, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01,
0xd4, 0x02, 0x00, 0x00, 0x94, 0x02, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00,
0x85, 0x02, 0x00, 0x00, 0x23, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65,
0x20, 0x3c, 0x6d, 0x65, 0x74, 0x61, 0x6c, 0x5f, 0x73, 0x74, 0x64, 0x6c,
0x69, 0x62, 0x3e, 0x0a, 0x23, 0x69, 0x6e, 0x63, 0x6c, 0x75, 0x64, 0x65,
0x20, 0x3c, 0x73, 0x69, 0x6d, 0x64, 0x2f, 0x73, 0x69, 0x6d, 0x64, 0x2e,
0x68, 0x3e, 0x0a, 0x0a, 0x75, 0x73, 0x69, 0x6e, 0x67, 0x20, 0x6e, 0x61,
0x6d, 0x65, 0x73, 0x70, 0x61, 0x63, 0x65, 0x20, 0x6d, 0x65, 0x74, 0x61,
0x6c, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75, 0x63, 0x74, 0x20, 0x66,
0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74,
0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x66, 0x72, 0x61, 0x67, 0x6d,
0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f, 0x75, 0x74,
0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74,
0x34, 0x20, 0x66, 0x72, 0x61, 0x67, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72,
0x20, 0x5b, 0x5b, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x28, 0x30, 0x29, 0x5d,
0x5d, 0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x73, 0x74, 0x72, 0x75, 0x63,
0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70,
0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x66, 0x72,
0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f,
0x69, 0x6e, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f,
0x61, 0x74, 0x32, 0x20, 0x76, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72,
0x65, 0x5f, 0x63, 0x6f, 0x6f, 0x72, 0x64, 0x73, 0x20, 0x5b, 0x5b, 0x75,
0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x30, 0x29, 0x5d, 0x5d,
0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x66, 0x6c, 0x6f, 0x61, 0x74, 0x34,
0x20, 0x76, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x5b, 0x5b, 0x75,
0x73, 0x65, 0x72, 0x28, 0x6c, 0x6f, 0x63, 0x6e, 0x31, 0x29, 0x5d, 0x5d,
0x3b, 0x0a, 0x7d, 0x3b, 0x0a, 0x0a, 0x66, 0x72, 0x61, 0x67, 0x6d, 0x65,
0x6e, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x66,
0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e,
0x5f, 0x6f, 0x75, 0x74, 0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72,
0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65,
0x5f, 0x66, 0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61,
0x69, 0x6e, 0x28, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67,
0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x66,
0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e,
0x5f, 0x69, 0x6e, 0x20, 0x69, 0x6e, 0x20, 0x5b, 0x5b, 0x73, 0x74, 0x61,
0x67, 0x65, 0x5f, 0x69, 0x6e, 0x5d, 0x5d, 0x2c, 0x20, 0x74, 0x65, 0x78,
0x74, 0x75, 0x72, 0x65, 0x32, 0x64, 0x3c, 0x66, 0x6c, 0x6f, 0x61, 0x74,
0x3e, 0x20, 0x74, 0x65, 0x78, 0x20, 0x5b, 0x5b, 0x74, 0x65, 0x78, 0x74,
0x75, 0x72, 0x65, 0x28, 0x30, 0x29, 0x5d, 0x5d, 0x2c, 0x20, 0x73, 0x61,
0x6d, 0x70, 0x6c, 0x65, 0x72, 0x20, 0x74, 0x65, 0x78, 0x53, 0x6d, 0x70,
0x6c, 0x72, 0x20, 0x5b, 0x5b, 0x73, 0x61, 0x6d, 0x70, 0x6c, 0x65, 0x72,
0x28, 0x30, 0x29, 0x5d, 0x5d, 0x29, 0x0a, 0x7b, 0x0a, 0x20, 0x20, 0x20,
0x20, 0x66, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75,
0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x66, 0x72, 0x61,
0x67, 0x6d, 0x65, 0x6e, 0x74, 0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x5f, 0x6f,
0x75, 0x74, 0x20, 0x6f, 0x75, 0x74, 0x20, 0x3d, 0x20, 0x7b, 0x7d, 0x3b,
0x0a, 0x20, 0x20, 0x20, 0x20, 0x6f, 0x75, 0x74, 0x2e, 0x66, 0x72, 0x61,
0x67, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x3d, 0x20, 0x69, 0x6e,
0x2e, 0x76, 0x5f, 0x63, 0x6f, 0x6c, 0x6f, 0x72, 0x20, 0x2a, 0x20, 0x74,
0x65, 0x78, 0x2e, 0x73, 0x61, 0x6d, 0x70, 0x6c, 0x65, 0x28, 0x74, 0x65,
0x78, 0x53, 0x6d, 0x70, 0x6c, 0x72, 0x2c, 0x20, 0x69, 0x6e, 0x2e, 0x76,
0x5f, 0x74, 0x65, 0x78, 0x74, 0x75, 0x72, 0x65, 0x5f, 0x63, 0x6f, 0x6f,
0x72, 0x64, 0x73, 0x29, 0x3b, 0x0a, 0x20, 0x20, 0x20, 0x20, 0x72, 0x65,
0x74, 0x75, 0x72, 0x6e, 0x20, 0x6f, 0x75, 0x74, 0x3b, 0x0a, 0x7d, 0x0a,
0x0a, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x14, 0x00, 0x00, 0x00,
0x10, 0x00, 0x1c, 0x00, 0x04, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00,
0x0c, 0x00, 0x14, 0x00, 0x10, 0x00, 0x00, 0x00, 0x18, 0x00, 0x00, 0x00,
0x0c, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00,
0x74, 0x65, 0x78, 0x00, 0x21, 0x00, 0x00, 0x00, 0x66, 0x6c, 0x75, 0x74,
0x74, 0x65, 0x72, 0x5f, 0x67, 0x70, 0x75, 0x5f, 0x74, 0x65, 0x78, 0x74,
0x75, 0x72, 0x65, 0x5f, 0x66, 0x72, 0x61, 0x67, 0x6d, 0x65, 0x6e, 0x74,
0x5f, 0x6d, 0x61, 0x69, 0x6e, 0x00, 0x00, 0x00};
| engine/lib/gpu/fixtures.cc/0 | {
"file_path": "engine/lib/gpu/fixtures.cc",
"repo_id": "engine",
"token_count": 14714
} | 242 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
part of flutter_gpu;
base class Texture extends NativeFieldWrapperClass1 {
bool _valid = false;
get isValid {
return _valid;
}
/// Creates a new Texture.
Texture._initialize(
GpuContext gpuContext,
this.storageMode,
this.format,
this.width,
this.height,
this.sampleCount,
TextureCoordinateSystem coordinateSystem,
this.enableRenderTargetUsage,
this.enableShaderReadUsage,
this.enableShaderWriteUsage)
: _coordinateSystem = coordinateSystem {
if (sampleCount != 1 && sampleCount != 4) {
throw Exception("Only a sample count of 1 or 4 is currently supported");
}
_valid = _initialize(
gpuContext,
storageMode.index,
format.index,
width,
height,
sampleCount,
coordinateSystem.index,
enableRenderTargetUsage,
enableShaderReadUsage,
enableShaderWriteUsage);
}
final StorageMode storageMode;
final PixelFormat format;
final int width;
final int height;
final int sampleCount;
/// Enable using this texture as a render pass attachment.
final bool enableRenderTargetUsage;
/// Enable reading or sampling from this texture in a shader.
final bool enableShaderReadUsage;
/// Enable writing to the texture in a shader.
///
/// Note that this is distinct from [enableRenderTargetUsage].
final bool enableShaderWriteUsage;
TextureCoordinateSystem _coordinateSystem;
TextureCoordinateSystem get coordinateSystem {
return _coordinateSystem;
}
set coordinateSystem(TextureCoordinateSystem value) {
value;
_setCoordinateSystem(value.index);
}
int get bytesPerTexel {
return _bytesPerTexel();
}
int GetBaseMipLevelSizeInBytes() {
return bytesPerTexel * width * height;
}
/// Overwrite the entire base mipmap level of this [Texture].
///
/// This method can only be used if the [Texture] was created with
/// [StorageMode.hostVisible]. An exception will be thrown otherwise.
///
/// The length of [sourceBytes] must be exactly the size of the base mip
/// level, otherwise an exception will be thrown. The size of the base mip
/// level is always `width * height * bytesPerPixel`.
///
/// Returns [true] if the write was successful, or [false] if the write
/// failed due to an internal error.
bool overwrite(ByteData sourceBytes) {
if (storageMode != StorageMode.hostVisible) {
throw Exception(
'Texture.overwrite can only be used with Textures that are host visible');
}
int baseMipSize = GetBaseMipLevelSizeInBytes();
if (sourceBytes.lengthInBytes != baseMipSize) {
throw Exception(
'The length of sourceBytes (bytes: ${sourceBytes.lengthInBytes}) must exactly match the size of the base mip level (bytes: ${baseMipSize})');
}
return _overwrite(sourceBytes);
}
ui.Image asImage() {
if (!enableShaderReadUsage) {
throw Exception(
'Only shader readable Flutter GPU textures can be used as UI Images');
}
return _asImage();
}
/// Wrap with native counterpart.
@Native<
Bool Function(Handle, Pointer<Void>, Int, Int, Int, Int, Int, Int, Bool,
Bool, Bool)>(symbol: 'InternalFlutterGpu_Texture_Initialize')
external bool _initialize(
GpuContext gpuContext,
int storageMode,
int format,
int width,
int height,
int sampleCount,
int coordinateSystem,
bool enableRenderTargetUsage,
bool enableShaderReadUsage,
bool enableShaderWriteUsage);
@Native<Void Function(Handle, Int)>(
symbol: 'InternalFlutterGpu_Texture_SetCoordinateSystem')
external void _setCoordinateSystem(int coordinateSystem);
@Native<Int Function(Pointer<Void>)>(
symbol: 'InternalFlutterGpu_Texture_BytesPerTexel')
external int _bytesPerTexel();
@Native<Bool Function(Pointer<Void>, Handle)>(
symbol: 'InternalFlutterGpu_Texture_Overwrite')
external bool _overwrite(ByteData bytes);
@Native<Handle Function(Pointer<Void>)>(
symbol: 'InternalFlutterGpu_Texture_AsImage')
external ui.Image _asImage();
}
| engine/lib/gpu/lib/src/texture.dart/0 | {
"file_path": "engine/lib/gpu/lib/src/texture.dart",
"repo_id": "engine",
"token_count": 1468
} | 243 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_LIB_IO_DART_IO_H_
#define FLUTTER_LIB_IO_DART_IO_H_
#include <cstdint>
#include <string>
#include "flutter/fml/macros.h"
namespace flutter {
class DartIO {
public:
static void InitForIsolate(bool may_insecurely_connect_to_all_domains,
const std::string& domain_network_policy);
private:
FML_DISALLOW_IMPLICIT_CONSTRUCTORS(DartIO);
};
} // namespace flutter
#endif // FLUTTER_LIB_IO_DART_IO_H_
| engine/lib/io/dart_io.h/0 | {
"file_path": "engine/lib/io/dart_io.h",
"repo_id": "engine",
"token_count": 245
} | 244 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/compositing/scene_builder.h"
#include <memory>
#include "flutter/common/task_runners.h"
#include "flutter/fml/synchronization/waitable_event.h"
#include "flutter/runtime/dart_vm.h"
#include "flutter/shell/common/shell_test.h"
#include "flutter/shell/common/thread_host.h"
#include "flutter/testing/testing.h"
// CREATE_NATIVE_ENTRY is leaky by design
// NOLINTBEGIN(clang-analyzer-core.StackAddressEscape)
namespace flutter {
namespace testing {
TEST_F(ShellTest, SceneBuilderBuildAndSceneDisposeReleasesLayerStack) {
auto message_latch = std::make_shared<fml::AutoResetWaitableEvent>();
// prevent ClearDartWrapper() from deleting the scene builder.
SceneBuilder* retained_scene_builder;
Scene* retained_scene;
auto validate_builder_has_layers =
[&retained_scene_builder](Dart_NativeArguments args) {
auto handle = Dart_GetNativeArgument(args, 0);
intptr_t peer = 0;
Dart_Handle result = Dart_GetNativeInstanceField(
handle, tonic::DartWrappable::kPeerIndex, &peer);
ASSERT_FALSE(Dart_IsError(result));
retained_scene_builder = reinterpret_cast<SceneBuilder*>(peer);
retained_scene_builder->AddRef();
ASSERT_TRUE(retained_scene_builder);
ASSERT_EQ(retained_scene_builder->layer_stack().size(), 2ul);
};
auto validate_builder_has_no_layers =
[&retained_scene_builder](Dart_NativeArguments args) {
ASSERT_EQ(retained_scene_builder->layer_stack().size(), 0ul);
retained_scene_builder->Release();
retained_scene_builder = nullptr;
};
auto capture_scene = [&retained_scene](Dart_NativeArguments args) {
auto handle = Dart_GetNativeArgument(args, 0);
intptr_t peer = 0;
Dart_Handle result = Dart_GetNativeInstanceField(
handle, tonic::DartWrappable::kPeerIndex, &peer);
ASSERT_FALSE(Dart_IsError(result));
retained_scene = reinterpret_cast<Scene*>(peer);
retained_scene->AddRef();
ASSERT_TRUE(retained_scene);
};
auto validate_scene_has_no_layers =
[message_latch, &retained_scene](Dart_NativeArguments args) {
EXPECT_FALSE(retained_scene->takeLayerTree(100, 100));
retained_scene->Release();
retained_scene = nullptr;
message_latch->Signal();
};
Settings settings = CreateSettingsForFixture();
TaskRunners task_runners("test", // label
GetCurrentTaskRunner(), // platform
CreateNewThread(), // raster
CreateNewThread(), // ui
CreateNewThread() // io
);
AddNativeCallback("ValidateBuilderHasLayers",
CREATE_NATIVE_ENTRY(validate_builder_has_layers));
AddNativeCallback("ValidateBuilderHasNoLayers",
CREATE_NATIVE_ENTRY(validate_builder_has_no_layers));
AddNativeCallback("CaptureScene", CREATE_NATIVE_ENTRY(capture_scene));
AddNativeCallback("ValidateSceneHasNoLayers",
CREATE_NATIVE_ENTRY(validate_scene_has_no_layers));
std::unique_ptr<Shell> shell = CreateShell(settings, task_runners);
ASSERT_TRUE(shell->IsSetup());
auto configuration = RunConfiguration::InferFromSettings(settings);
configuration.SetEntrypoint("validateSceneBuilderAndScene");
shell->RunEngine(std::move(configuration), [](auto result) {
ASSERT_EQ(result, Engine::RunStatus::Success);
});
message_latch->Wait();
DestroyShell(std::move(shell), task_runners);
}
TEST_F(ShellTest, EngineLayerDisposeReleasesReference) {
auto message_latch = std::make_shared<fml::AutoResetWaitableEvent>();
std::shared_ptr<ContainerLayer> root_layer;
auto capture_root_layer = [&root_layer](Dart_NativeArguments args) {
auto handle = Dart_GetNativeArgument(args, 0);
intptr_t peer = 0;
Dart_Handle result = Dart_GetNativeInstanceField(
handle, tonic::DartWrappable::kPeerIndex, &peer);
ASSERT_FALSE(Dart_IsError(result));
SceneBuilder* scene_builder = reinterpret_cast<SceneBuilder*>(peer);
ASSERT_TRUE(scene_builder);
root_layer = scene_builder->layer_stack()[0];
ASSERT_TRUE(root_layer);
};
auto validate_layer_tree_counts = [&root_layer](Dart_NativeArguments args) {
// One for the EngineLayer, one for our pointer in the test.
EXPECT_EQ(root_layer->layers().front().use_count(), 2);
};
auto validate_engine_layer_dispose =
[message_latch, &root_layer](Dart_NativeArguments args) {
// Just one for our pointer now.
EXPECT_EQ(root_layer->layers().front().use_count(), 1);
root_layer.reset();
message_latch->Signal();
};
Settings settings = CreateSettingsForFixture();
TaskRunners task_runners("test", // label
GetCurrentTaskRunner(), // platform
CreateNewThread(), // raster
CreateNewThread(), // ui
CreateNewThread() // io
);
AddNativeCallback("CaptureRootLayer",
CREATE_NATIVE_ENTRY(capture_root_layer));
AddNativeCallback("ValidateLayerTreeCounts",
CREATE_NATIVE_ENTRY(validate_layer_tree_counts));
AddNativeCallback("ValidateEngineLayerDispose",
CREATE_NATIVE_ENTRY(validate_engine_layer_dispose));
std::unique_ptr<Shell> shell = CreateShell(settings, task_runners);
ASSERT_TRUE(shell->IsSetup());
auto configuration = RunConfiguration::InferFromSettings(settings);
configuration.SetEntrypoint("validateEngineLayerDispose");
shell->RunEngine(std::move(configuration), [](auto result) {
ASSERT_EQ(result, Engine::RunStatus::Success);
});
message_latch->Wait();
DestroyShell(std::move(shell), task_runners);
}
} // namespace testing
} // namespace flutter
// NOLINTEND(clang-analyzer-core.StackAddressEscape)
| engine/lib/ui/compositing/scene_builder_unittests.cc/0 | {
"file_path": "engine/lib/ui/compositing/scene_builder_unittests.cc",
"repo_id": "engine",
"token_count": 2402
} | 245 |
#version 320 es
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
precision highp float;
layout(location = 0) out vec4 fragColor;
layout(location = 0) uniform float a;
void main() {
fragColor = vec4(0.0,
// 57.2957795131 * pi / 180.0 = 1.0
float(radians(a * 57.2957795131)), 0.0, 1.0);
}
| engine/lib/ui/fixtures/shaders/supported_glsl_op_shaders/11_radians.frag/0 | {
"file_path": "engine/lib/ui/fixtures/shaders/supported_glsl_op_shaders/11_radians.frag",
"repo_id": "engine",
"token_count": 178
} | 246 |
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import("//build/compiled_action.gni")
import("//flutter/impeller/tools/impeller.gni")
import("//flutter/testing/testing.gni")
if (enable_unittests) {
test_shaders = [
"10_fract.frag",
"11_radians.frag",
"12_degrees.frag",
"13_sin.frag",
"14_cos.frag",
"15_tan.frag",
"16_asin.frag",
"17_acos.frag",
"18_atan.frag",
"25_atan2.frag",
"26_pow.frag",
"27_exp.frag",
"28_log.frag",
"29_exp2.frag",
"30_log2.frag",
"31_sqrt.frag",
"32_inversesqrt.frag",
"37_fmin.frag",
"40_fmax.frag",
"43_fclamp.frag",
"46_fmix.frag",
"48_step.frag",
"49_smoothstep.frag",
"4_abs.frag",
"66_length.frag",
"67_distance.frag",
"68_cross.frag",
"69_normalize.frag",
"6_sign.frag",
"70_faceforward.frag",
"71_reflect.frag",
"8_floor.frag",
"9_ceil.frag",
]
group("supported_glsl_op_shaders") {
testonly = true
deps = [ ":fixtures" ]
}
impellerc("compile_supported_glsl_shaders") {
shaders = test_shaders
shader_target_flags = [ "--sksl" ]
intermediates_subdir = "iplr"
sl_file_extension = "iplr"
iplr = true
}
test_fixtures("fixtures") {
deps = [ ":compile_supported_glsl_shaders" ]
fixtures = get_target_outputs(":compile_supported_glsl_shaders")
dest = "$root_gen_dir/flutter/lib/ui"
}
}
| engine/lib/ui/fixtures/shaders/supported_glsl_op_shaders/BUILD.gn/0 | {
"file_path": "engine/lib/ui/fixtures/shaders/supported_glsl_op_shaders/BUILD.gn",
"repo_id": "engine",
"token_count": 742
} | 247 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_LIB_UI_FLOATING_POINT_H_
#define FLUTTER_LIB_UI_FLOATING_POINT_H_
#include <algorithm>
#include <limits>
namespace flutter {
/// Converts a double to a float, truncating finite values that are larger than
/// FLT_MAX or smaller than FLT_MIN to those values.
static float SafeNarrow(double value) {
if (std::isinf(value) || std::isnan(value)) {
return static_cast<float>(value);
} else {
// Avoid truncation to inf/-inf.
return std::clamp(static_cast<float>(value),
std::numeric_limits<float>::lowest(),
std::numeric_limits<float>::max());
}
}
} // namespace flutter
#endif // FLUTTER_LIB_UI_FLOATING_POINT_H_
| engine/lib/ui/floating_point.h/0 | {
"file_path": "engine/lib/ui/floating_point.h",
"repo_id": "engine",
"token_count": 326
} | 248 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
part of dart.ui;
// Examples can assume:
// // (for the example in Color)
// // ignore_for_file: use_full_hex_values_for_flutter_colors
// late ui.Image _image;
// dynamic _cacheImage(dynamic _, [dynamic __]) { }
// dynamic _drawImage(dynamic _, [dynamic __]) { }
// Some methods in this file assert that their arguments are not null. These
// asserts are just to improve the error messages; they should only cover
// arguments that are either dereferenced _in Dart_, before being passed to the
// engine, or that the engine explicitly null-checks itself (after attempting to
// convert the argument to a native type). It should not be possible for a null
// or invalid value to be used by the engine even in release mode, since that
// would cause a crash. It is, however, acceptable for error messages to be much
// less useful or correct in release mode than in debug mode.
//
// Painting APIs will also warn about arguments representing NaN coordinates,
// which can not be rendered by Skia.
bool _rectIsValid(Rect rect) {
assert(!rect.hasNaN, 'Rect argument contained a NaN value.');
return true;
}
bool _rrectIsValid(RRect rrect) {
assert(!rrect.hasNaN, 'RRect argument contained a NaN value.');
return true;
}
bool _offsetIsValid(Offset offset) {
assert(!offset.dx.isNaN && !offset.dy.isNaN, 'Offset argument contained a NaN value.');
return true;
}
bool _matrix4IsValid(Float64List matrix4) {
assert(matrix4.length == 16, 'Matrix4 must have 16 entries.');
assert(matrix4.every((double value) => value.isFinite), 'Matrix4 entries must be finite.');
return true;
}
bool _radiusIsValid(Radius radius) {
assert(!radius.x.isNaN && !radius.y.isNaN, 'Radius argument contained a NaN value.');
return true;
}
Color _scaleAlpha(Color a, double factor) {
return a.withAlpha((a.alpha * factor).round().clamp(0, 255));
}
/// An immutable 32 bit color value in ARGB format.
///
/// Consider the light teal of the Flutter logo. It is fully opaque, with a red
/// channel value of 0x42 (66), a green channel value of 0xA5 (165), and a blue
/// channel value of 0xF5 (245). In the common "hash syntax" for color values,
/// it would be described as `#42A5F5`.
///
/// Here are some ways it could be constructed:
///
/// ```dart
/// Color c1 = const Color(0xFF42A5F5);
/// Color c2 = const Color.fromARGB(0xFF, 0x42, 0xA5, 0xF5);
/// Color c3 = const Color.fromARGB(255, 66, 165, 245);
/// Color c4 = const Color.fromRGBO(66, 165, 245, 1.0);
/// ```
///
/// If you are having a problem with `Color` wherein it seems your color is just
/// not painting, check to make sure you are specifying the full 8 hexadecimal
/// digits. If you only specify six, then the leading two digits are assumed to
/// be zero, which means fully-transparent:
///
/// ```dart
/// Color c1 = const Color(0xFFFFFF); // fully transparent white (invisible)
/// Color c2 = const Color(0xFFFFFFFF); // fully opaque white (visible)
/// ```
///
/// See also:
///
/// * [Colors](https://api.flutter.dev/flutter/material/Colors-class.html), which
/// defines the colors found in the Material Design specification.
class Color {
/// Construct a color from the lower 32 bits of an [int].
///
/// The bits are interpreted as follows:
///
/// * Bits 24-31 are the alpha value.
/// * Bits 16-23 are the red value.
/// * Bits 8-15 are the green value.
/// * Bits 0-7 are the blue value.
///
/// In other words, if AA is the alpha value in hex, RR the red value in hex,
/// GG the green value in hex, and BB the blue value in hex, a color can be
/// expressed as `const Color(0xAARRGGBB)`.
///
/// For example, to get a fully opaque orange, you would use `const
/// Color(0xFFFF9000)` (`FF` for the alpha, `FF` for the red, `90` for the
/// green, and `00` for the blue).
const Color(int value) : value = value & 0xFFFFFFFF;
/// Construct a color from the lower 8 bits of four integers.
///
/// * `a` is the alpha value, with 0 being transparent and 255 being fully
/// opaque.
/// * `r` is [red], from 0 to 255.
/// * `g` is [green], from 0 to 255.
/// * `b` is [blue], from 0 to 255.
///
/// Out of range values are brought into range using modulo 255.
///
/// See also [fromRGBO], which takes the alpha value as a floating point
/// value.
const Color.fromARGB(int a, int r, int g, int b) :
value = (((a & 0xff) << 24) |
((r & 0xff) << 16) |
((g & 0xff) << 8) |
((b & 0xff) << 0)) & 0xFFFFFFFF;
/// Create a color from red, green, blue, and opacity, similar to `rgba()` in CSS.
///
/// * `r` is [red], from 0 to 255.
/// * `g` is [green], from 0 to 255.
/// * `b` is [blue], from 0 to 255.
/// * `opacity` is alpha channel of this color as a double, with 0.0 being
/// transparent and 1.0 being fully opaque.
///
/// Out of range values are brought into range using modulo 255.
///
/// See also [fromARGB], which takes the opacity as an integer value.
const Color.fromRGBO(int r, int g, int b, double opacity) :
value = ((((opacity * 0xff ~/ 1) & 0xff) << 24) |
((r & 0xff) << 16) |
((g & 0xff) << 8) |
((b & 0xff) << 0)) & 0xFFFFFFFF;
/// A 32 bit value representing this color.
///
/// The bits are assigned as follows:
///
/// * Bits 24-31 are the alpha value.
/// * Bits 16-23 are the red value.
/// * Bits 8-15 are the green value.
/// * Bits 0-7 are the blue value.
final int value;
/// The alpha channel of this color in an 8 bit value.
///
/// A value of 0 means this color is fully transparent. A value of 255 means
/// this color is fully opaque.
int get alpha => (0xff000000 & value) >> 24;
/// The alpha channel of this color as a double.
///
/// A value of 0.0 means this color is fully transparent. A value of 1.0 means
/// this color is fully opaque.
double get opacity => alpha / 0xFF;
/// The red channel of this color in an 8 bit value.
int get red => (0x00ff0000 & value) >> 16;
/// The green channel of this color in an 8 bit value.
int get green => (0x0000ff00 & value) >> 8;
/// The blue channel of this color in an 8 bit value.
int get blue => (0x000000ff & value) >> 0;
/// Returns a new color that matches this color with the alpha channel
/// replaced with `a` (which ranges from 0 to 255).
///
/// Out of range values will have unexpected effects.
Color withAlpha(int a) {
return Color.fromARGB(a, red, green, blue);
}
/// Returns a new color that matches this color with the alpha channel
/// replaced with the given `opacity` (which ranges from 0.0 to 1.0).
///
/// Out of range values will have unexpected effects.
Color withOpacity(double opacity) {
assert(opacity >= 0.0 && opacity <= 1.0);
return withAlpha((255.0 * opacity).round());
}
/// Returns a new color that matches this color with the red channel replaced
/// with `r` (which ranges from 0 to 255).
///
/// Out of range values will have unexpected effects.
Color withRed(int r) {
return Color.fromARGB(alpha, r, green, blue);
}
/// Returns a new color that matches this color with the green channel
/// replaced with `g` (which ranges from 0 to 255).
///
/// Out of range values will have unexpected effects.
Color withGreen(int g) {
return Color.fromARGB(alpha, red, g, blue);
}
/// Returns a new color that matches this color with the blue channel replaced
/// with `b` (which ranges from 0 to 255).
///
/// Out of range values will have unexpected effects.
Color withBlue(int b) {
return Color.fromARGB(alpha, red, green, b);
}
// See <https://www.w3.org/TR/WCAG20/#relativeluminancedef>
static double _linearizeColorComponent(double component) {
if (component <= 0.03928) {
return component / 12.92;
}
return math.pow((component + 0.055) / 1.055, 2.4) as double;
}
/// Returns a brightness value between 0 for darkest and 1 for lightest.
///
/// Represents the relative luminance of the color. This value is computationally
/// expensive to calculate.
///
/// See <https://en.wikipedia.org/wiki/Relative_luminance>.
double computeLuminance() {
// See <https://www.w3.org/TR/WCAG20/#relativeluminancedef>
final double R = _linearizeColorComponent(red / 0xFF);
final double G = _linearizeColorComponent(green / 0xFF);
final double B = _linearizeColorComponent(blue / 0xFF);
return 0.2126 * R + 0.7152 * G + 0.0722 * B;
}
/// Linearly interpolate between two colors.
///
/// This is intended to be fast but as a result may be ugly. Consider
/// [HSVColor] or writing custom logic for interpolating colors.
///
/// If either color is null, this function linearly interpolates from a
/// transparent instance of the other color. This is usually preferable to
/// interpolating from [material.Colors.transparent] (`const
/// Color(0x00000000)`), which is specifically transparent _black_.
///
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
/// 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]). Each channel
/// will be clamped to the range 0 to 255.
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
static Color? lerp(Color? a, Color? b, double t) {
if (b == null) {
if (a == null) {
return null;
} else {
return _scaleAlpha(a, 1.0 - t);
}
} else {
if (a == null) {
return _scaleAlpha(b, t);
} else {
return Color.fromARGB(
_clampInt(_lerpInt(a.alpha, b.alpha, t).toInt(), 0, 255),
_clampInt(_lerpInt(a.red, b.red, t).toInt(), 0, 255),
_clampInt(_lerpInt(a.green, b.green, t).toInt(), 0, 255),
_clampInt(_lerpInt(a.blue, b.blue, t).toInt(), 0, 255),
);
}
}
}
/// Combine the foreground color as a transparent color over top
/// of a background color, and return the resulting combined color.
///
/// This uses standard alpha blending ("SRC over DST") rules to produce a
/// blended color from two colors. This can be used as a performance
/// enhancement when trying to avoid needless alpha blending compositing
/// operations for two things that are solid colors with the same shape, but
/// overlay each other: instead, just paint one with the combined color.
static Color alphaBlend(Color foreground, Color background) {
final int alpha = foreground.alpha;
if (alpha == 0x00) { // Foreground completely transparent.
return background;
}
final int invAlpha = 0xff - alpha;
int backAlpha = background.alpha;
if (backAlpha == 0xff) { // Opaque background case
return Color.fromARGB(
0xff,
(alpha * foreground.red + invAlpha * background.red) ~/ 0xff,
(alpha * foreground.green + invAlpha * background.green) ~/ 0xff,
(alpha * foreground.blue + invAlpha * background.blue) ~/ 0xff,
);
} else { // General case
backAlpha = (backAlpha * invAlpha) ~/ 0xff;
final int outAlpha = alpha + backAlpha;
assert(outAlpha != 0x00);
return Color.fromARGB(
outAlpha,
(foreground.red * alpha + background.red * backAlpha) ~/ outAlpha,
(foreground.green * alpha + background.green * backAlpha) ~/ outAlpha,
(foreground.blue * alpha + background.blue * backAlpha) ~/ outAlpha,
);
}
}
/// Returns an alpha value representative of the provided [opacity] value.
///
/// The [opacity] value may not be null.
static int getAlphaFromOpacity(double opacity) {
return (clampDouble(opacity, 0.0, 1.0) * 255).round();
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is Color
&& other.value == value;
}
@override
int get hashCode => value.hashCode;
@override
String toString() => 'Color(0x${value.toRadixString(16).padLeft(8, '0')})';
}
/// Algorithms to use when painting on the canvas.
///
/// When drawing a shape or image onto a canvas, different algorithms can be
/// used to blend the pixels. The different values of [BlendMode] specify
/// different such algorithms.
///
/// Each algorithm has two inputs, the _source_, which is the image being drawn,
/// and the _destination_, which is the image into which the source image is
/// being composited. The destination is often thought of as the _background_.
/// The source and destination both have four color channels, the red, green,
/// blue, and alpha channels. These are typically represented as numbers in the
/// range 0.0 to 1.0. The output of the algorithm also has these same four
/// channels, with values computed from the source and destination.
///
/// The documentation of each value below describes how the algorithm works. In
/// each case, an image shows the output of blending a source image with a
/// destination image. In the images below, the destination is represented by an
/// image with horizontal lines and an opaque landscape photograph, and the
/// source is represented by an image with vertical lines (the same lines but
/// rotated) and a bird clip-art image. The [src] mode shows only the source
/// image, and the [dst] mode shows only the destination image. In the
/// documentation below, the transparency is illustrated by a checkerboard
/// pattern. The [clear] mode drops both the source and destination, resulting
/// in an output that is entirely transparent (illustrated by a solid
/// checkerboard pattern).
///
/// The horizontal and vertical bars in these images show the red, green, and
/// blue channels with varying opacity levels, then all three color channels
/// together with those same varying opacity levels, then all three color
/// channels set to zero with those varying opacity levels, then two bars showing
/// a red/green/blue repeating gradient, the first with full opacity and the
/// second with partial opacity, and finally a bar with the three color channels
/// set to zero but the opacity varying in a repeating gradient.
///
/// ## Application to the [Canvas] API
///
/// When using [Canvas.saveLayer] and [Canvas.restore], the blend mode of the
/// [Paint] given to the [Canvas.saveLayer] will be applied when
/// [Canvas.restore] is called. Each call to [Canvas.saveLayer] introduces a new
/// layer onto which shapes and images are painted; when [Canvas.restore] is
/// called, that layer is then composited onto the parent layer, with the source
/// being the most-recently-drawn shapes and images, and the destination being
/// the parent layer. (For the first [Canvas.saveLayer] call, the parent layer
/// is the canvas itself.)
///
/// See also:
///
/// * [Paint.blendMode], which uses [BlendMode] to define the compositing
/// strategy.
enum BlendMode {
// This list comes from Skia's SkXfermode.h and the values (order) should be
// kept in sync.
// See: https://skia.org/docs/user/api/skpaint_overview/#SkXfermode
/// Drop both the source and destination images, leaving nothing.
///
/// This corresponds to the "clear" Porter-Duff operator.
///
/// 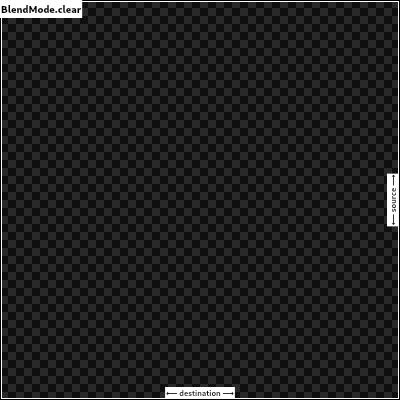
clear,
/// Drop the destination image, only paint the source image.
///
/// Conceptually, the destination is first cleared, then the source image is
/// painted.
///
/// This corresponds to the "Copy" Porter-Duff operator.
///
/// 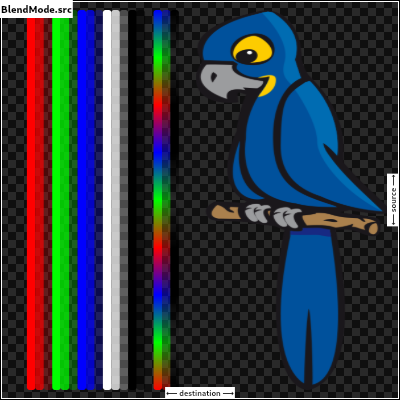
src,
/// Drop the source image, only paint the destination image.
///
/// Conceptually, the source image is discarded, leaving the destination
/// untouched.
///
/// This corresponds to the "Destination" Porter-Duff operator.
///
/// 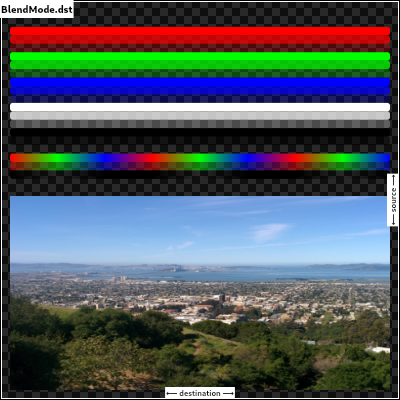
dst,
/// Composite the source image over the destination image.
///
/// This is the default value. It represents the most intuitive case, where
/// shapes are painted on top of what is below, with transparent areas showing
/// the destination layer.
///
/// This corresponds to the "Source over Destination" Porter-Duff operator,
/// also known as the Painter's Algorithm.
///
/// 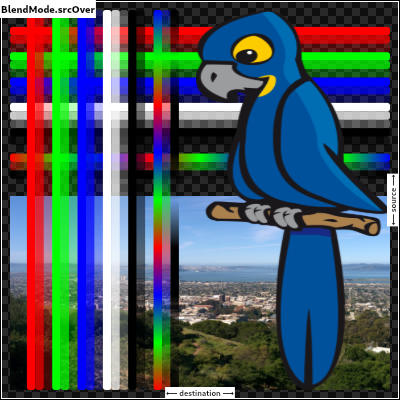
srcOver,
/// Composite the source image under the destination image.
///
/// This is the opposite of [srcOver].
///
/// This corresponds to the "Destination over Source" Porter-Duff operator.
///
/// 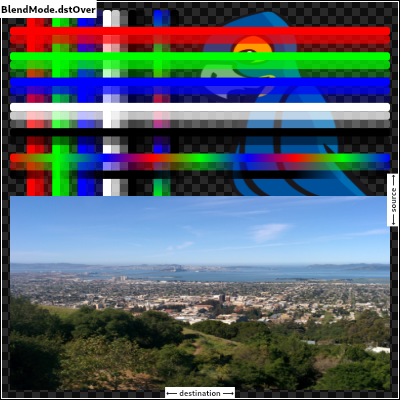
///
/// This is useful when the source image should have been painted before the
/// destination image, but could not be.
dstOver,
/// Show the source image, but only where the two images overlap. The
/// destination image is not rendered, it is treated merely as a mask. The
/// color channels of the destination are ignored, only the opacity has an
/// effect.
///
/// To show the destination image instead, consider [dstIn].
///
/// To reverse the semantic of the mask (only showing the source where the
/// destination is absent, rather than where it is present), consider
/// [srcOut].
///
/// This corresponds to the "Source in Destination" Porter-Duff operator.
///
/// 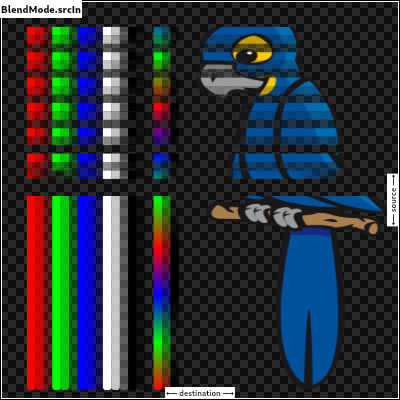
srcIn,
/// Show the destination image, but only where the two images overlap. The
/// source image is not rendered, it is treated merely as a mask. The color
/// channels of the source are ignored, only the opacity has an effect.
///
/// To show the source image instead, consider [srcIn].
///
/// To reverse the semantic of the mask (only showing the source where the
/// destination is present, rather than where it is absent), consider [dstOut].
///
/// This corresponds to the "Destination in Source" Porter-Duff operator.
///
/// 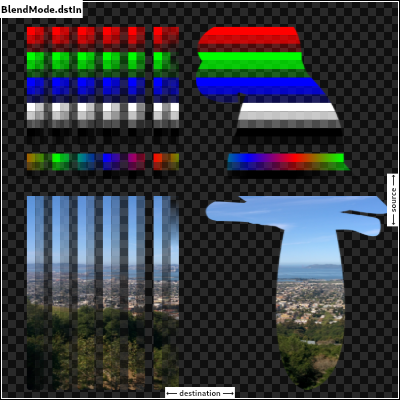
dstIn,
/// Show the source image, but only where the two images do not overlap. The
/// destination image is not rendered, it is treated merely as a mask. The color
/// channels of the destination are ignored, only the opacity has an effect.
///
/// To show the destination image instead, consider [dstOut].
///
/// To reverse the semantic of the mask (only showing the source where the
/// destination is present, rather than where it is absent), consider [srcIn].
///
/// This corresponds to the "Source out Destination" Porter-Duff operator.
///
/// 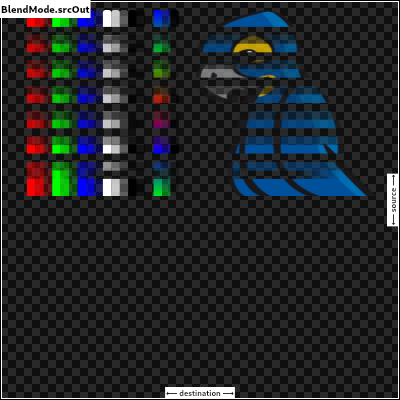
srcOut,
/// Show the destination image, but only where the two images do not overlap. The
/// source image is not rendered, it is treated merely as a mask. The color
/// channels of the source are ignored, only the opacity has an effect.
///
/// To show the source image instead, consider [srcOut].
///
/// To reverse the semantic of the mask (only showing the destination where the
/// source is present, rather than where it is absent), consider [dstIn].
///
/// This corresponds to the "Destination out Source" Porter-Duff operator.
///
/// 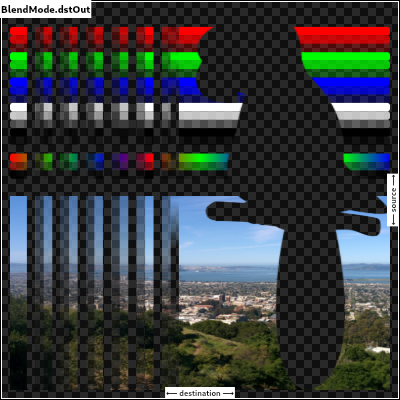
dstOut,
/// Composite the source image over the destination image, but only where it
/// overlaps the destination.
///
/// This corresponds to the "Source atop Destination" Porter-Duff operator.
///
/// This is essentially the [srcOver] operator, but with the output's opacity
/// channel being set to that of the destination image instead of being a
/// combination of both image's opacity channels.
///
/// For a variant with the destination on top instead of the source, see
/// [dstATop].
///
/// 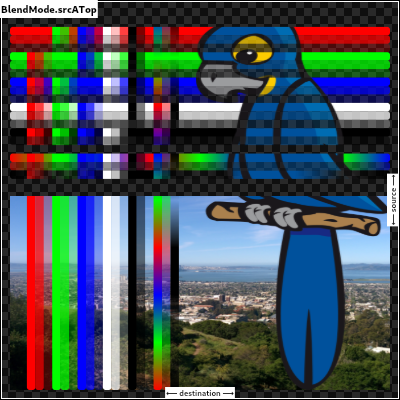
srcATop,
/// Composite the destination image over the source image, but only where it
/// overlaps the source.
///
/// This corresponds to the "Destination atop Source" Porter-Duff operator.
///
/// This is essentially the [dstOver] operator, but with the output's opacity
/// channel being set to that of the source image instead of being a
/// combination of both image's opacity channels.
///
/// For a variant with the source on top instead of the destination, see
/// [srcATop].
///
/// 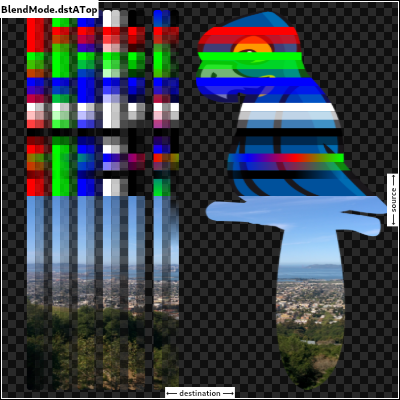
dstATop,
/// Apply a bitwise `xor` operator to the source and destination images. This
/// leaves transparency where they would overlap.
///
/// This corresponds to the "Source xor Destination" Porter-Duff operator.
///
/// 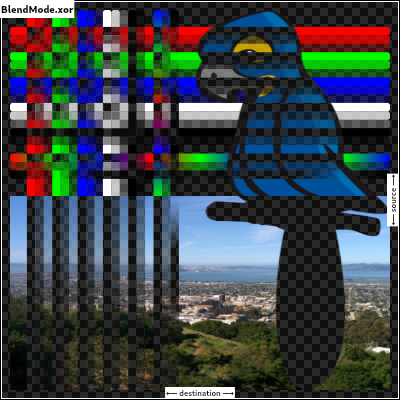
xor,
/// Sum the components of the source and destination images.
///
/// Transparency in a pixel of one of the images reduces the contribution of
/// that image to the corresponding output pixel, as if the color of that
/// pixel in that image was darker.
///
/// This corresponds to the "Source plus Destination" Porter-Duff operator.
///
/// This is the right blend mode for cross-fading between two images. Consider
/// two images A and B, and an interpolation time variable _t_ (from 0.0 to
/// 1.0). To cross fade between them, A should be drawn with opacity 1.0 - _t_
/// into a new layer using [BlendMode.srcOver], and B should be drawn on top
/// of it, at opacity _t_, into the same layer, using [BlendMode.plus].
///
/// 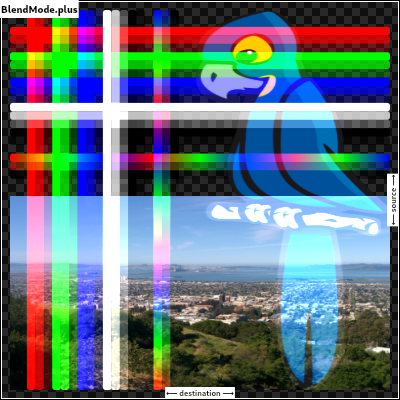
plus,
/// Multiply the color components of the source and destination images.
///
/// This can only result in the same or darker colors (multiplying by white,
/// 1.0, results in no change; multiplying by black, 0.0, results in black).
///
/// When compositing two opaque images, this has similar effect to overlapping
/// two transparencies on a projector.
///
/// For a variant that also multiplies the alpha channel, consider [multiply].
///
/// 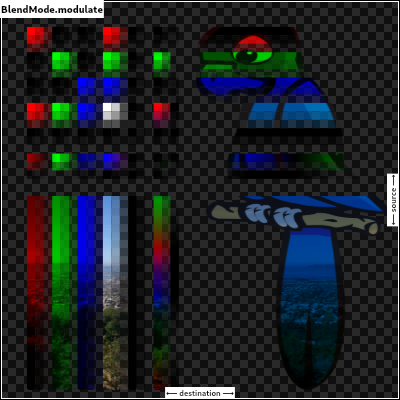
///
/// See also:
///
/// * [screen], which does a similar computation but inverted.
/// * [overlay], which combines [modulate] and [screen] to favor the
/// destination image.
/// * [hardLight], which combines [modulate] and [screen] to favor the
/// source image.
modulate,
// Following blend modes are defined in the CSS Compositing standard.
/// Multiply the inverse of the components of the source and destination
/// images, and inverse the result.
///
/// Inverting the components means that a fully saturated channel (opaque
/// white) is treated as the value 0.0, and values normally treated as 0.0
/// (black, transparent) are treated as 1.0.
///
/// This is essentially the same as [modulate] blend mode, but with the values
/// of the colors inverted before the multiplication and the result being
/// inverted back before rendering.
///
/// This can only result in the same or lighter colors (multiplying by black,
/// 1.0, results in no change; multiplying by white, 0.0, results in white).
/// Similarly, in the alpha channel, it can only result in more opaque colors.
///
/// This has similar effect to two projectors displaying their images on the
/// same screen simultaneously.
///
/// 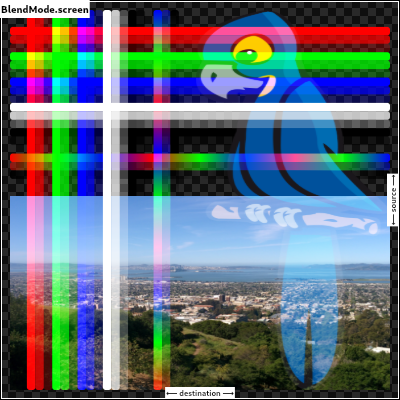
///
/// See also:
///
/// * [modulate], which does a similar computation but without inverting the
/// values.
/// * [overlay], which combines [modulate] and [screen] to favor the
/// destination image.
/// * [hardLight], which combines [modulate] and [screen] to favor the
/// source image.
screen, // The last coeff mode.
/// Multiply the components of the source and destination images after
/// adjusting them to favor the destination.
///
/// Specifically, if the destination value is smaller, this multiplies it with
/// the source value, whereas is the source value is smaller, it multiplies
/// the inverse of the source value with the inverse of the destination value,
/// then inverts the result.
///
/// Inverting the components means that a fully saturated channel (opaque
/// white) is treated as the value 0.0, and values normally treated as 0.0
/// (black, transparent) are treated as 1.0.
///
/// 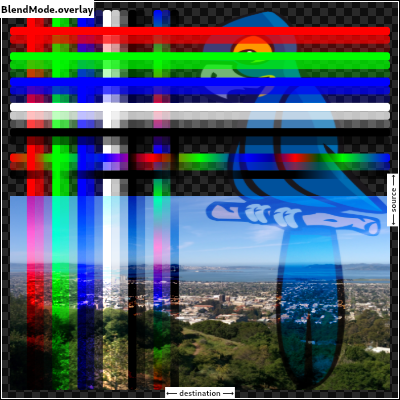
///
/// See also:
///
/// * [modulate], which always multiplies the values.
/// * [screen], which always multiplies the inverses of the values.
/// * [hardLight], which is similar to [overlay] but favors the source image
/// instead of the destination image.
overlay,
/// Composite the source and destination image by choosing the lowest value
/// from each color channel.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver].
///
/// 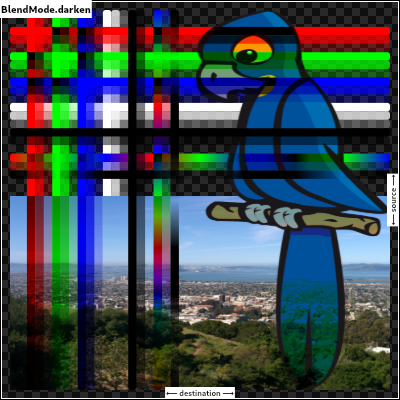
darken,
/// Composite the source and destination image by choosing the highest value
/// from each color channel.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver].
///
/// 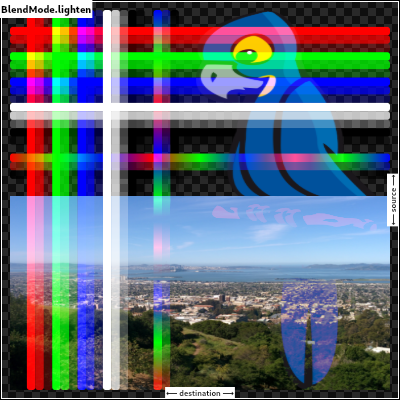
lighten,
/// Divide the destination by the inverse of the source.
///
/// Inverting the components means that a fully saturated channel (opaque
/// white) is treated as the value 0.0, and values normally treated as 0.0
/// (black, transparent) are treated as 1.0.
///
/// 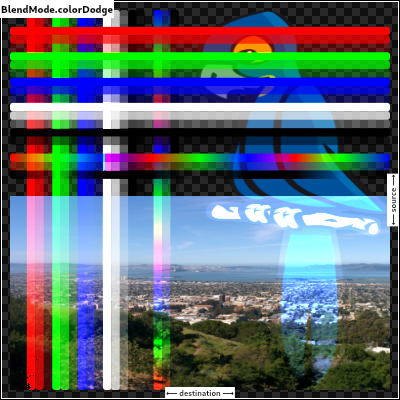
colorDodge,
/// Divide the inverse of the destination by the source, and inverse the result.
///
/// Inverting the components means that a fully saturated channel (opaque
/// white) is treated as the value 0.0, and values normally treated as 0.0
/// (black, transparent) are treated as 1.0.
///
/// 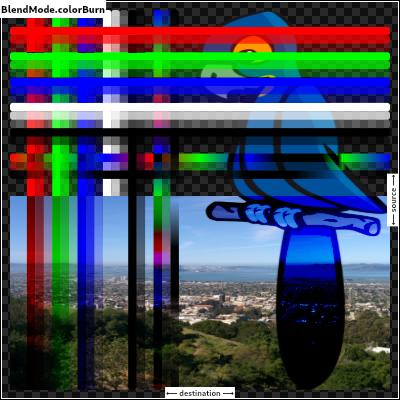
colorBurn,
/// Multiply the components of the source and destination images after
/// adjusting them to favor the source.
///
/// Specifically, if the source value is smaller, this multiplies it with the
/// destination value, whereas is the destination value is smaller, it
/// multiplies the inverse of the destination value with the inverse of the
/// source value, then inverts the result.
///
/// Inverting the components means that a fully saturated channel (opaque
/// white) is treated as the value 0.0, and values normally treated as 0.0
/// (black, transparent) are treated as 1.0.
///
/// 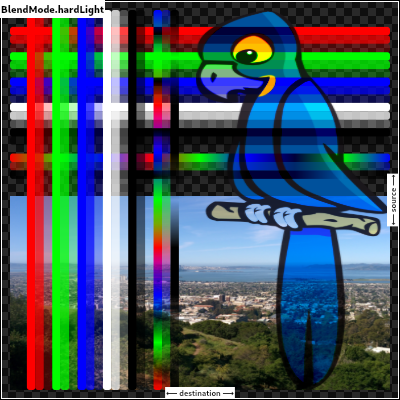
///
/// See also:
///
/// * [modulate], which always multiplies the values.
/// * [screen], which always multiplies the inverses of the values.
/// * [overlay], which is similar to [hardLight] but favors the destination
/// image instead of the source image.
hardLight,
/// Use [colorDodge] for source values below 0.5 and [colorBurn] for source
/// values above 0.5.
///
/// This results in a similar but softer effect than [overlay].
///
/// 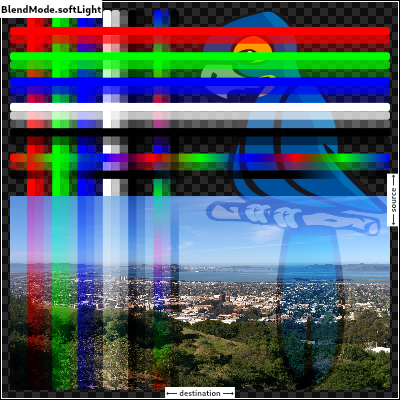
///
/// See also:
///
/// * [color], which is a more subtle tinting effect.
softLight,
/// Subtract the smaller value from the bigger value for each channel.
///
/// Compositing black has no effect; compositing white inverts the colors of
/// the other image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver].
///
/// The effect is similar to [exclusion] but harsher.
///
/// 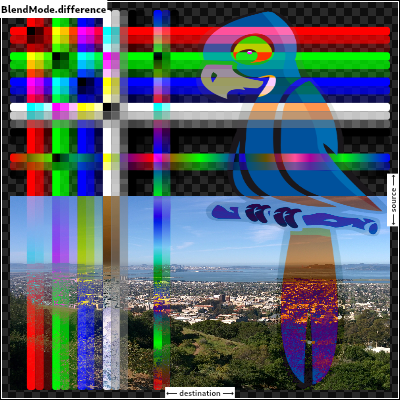
difference,
/// Subtract double the product of the two images from the sum of the two
/// images.
///
/// Compositing black has no effect; compositing white inverts the colors of
/// the other image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver].
///
/// The effect is similar to [difference] but softer.
///
/// 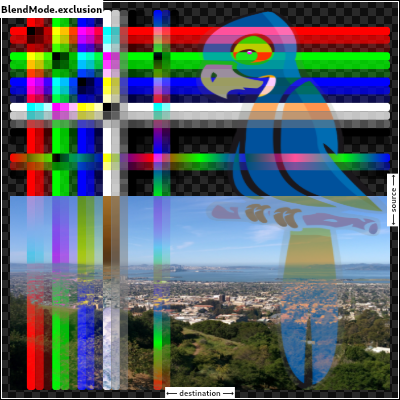
exclusion,
/// Multiply the components of the source and destination images, including
/// the alpha channel.
///
/// This can only result in the same or darker colors (multiplying by white,
/// 1.0, results in no change; multiplying by black, 0.0, results in black).
///
/// Since the alpha channel is also multiplied, a fully-transparent pixel
/// (opacity 0.0) in one image results in a fully transparent pixel in the
/// output. This is similar to [dstIn], but with the colors combined.
///
/// For a variant that multiplies the colors but does not multiply the alpha
/// channel, consider [modulate].
///
/// 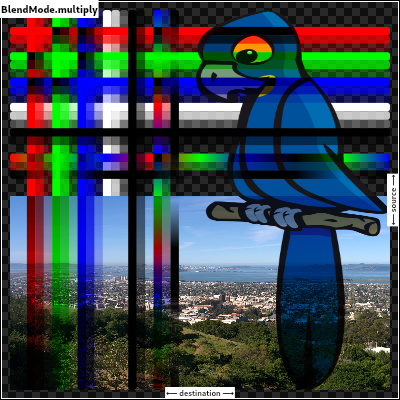
multiply, // The last separable mode.
/// Take the hue of the source image, and the saturation and luminosity of the
/// destination image.
///
/// The effect is to tint the destination image with the source image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver]. Regions that are entirely transparent in the source image take
/// their hue from the destination.
///
/// 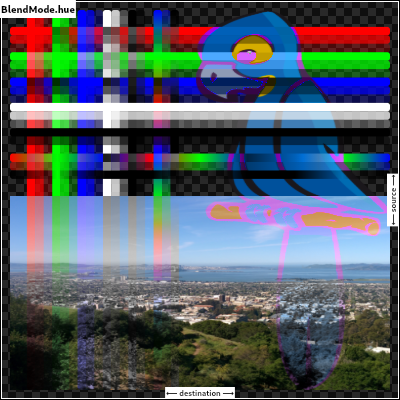
///
/// See also:
///
/// * [color], which is a similar but stronger effect as it also applies the
/// saturation of the source image.
/// * [HSVColor], which allows colors to be expressed using Hue rather than
/// the red/green/blue channels of [Color].
hue,
/// Take the saturation of the source image, and the hue and luminosity of the
/// destination image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver]. Regions that are entirely transparent in the source image take
/// their saturation from the destination.
///
/// 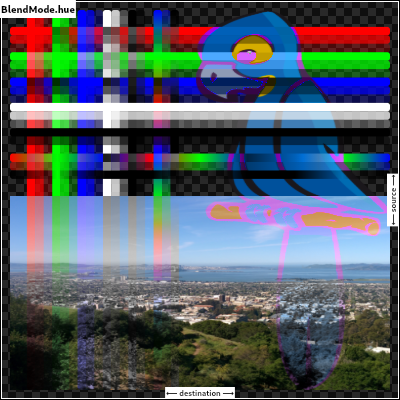
///
/// See also:
///
/// * [color], which also applies the hue of the source image.
/// * [luminosity], which applies the luminosity of the source image to the
/// destination.
saturation,
/// Take the hue and saturation of the source image, and the luminosity of the
/// destination image.
///
/// The effect is to tint the destination image with the source image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver]. Regions that are entirely transparent in the source image take
/// their hue and saturation from the destination.
///
/// 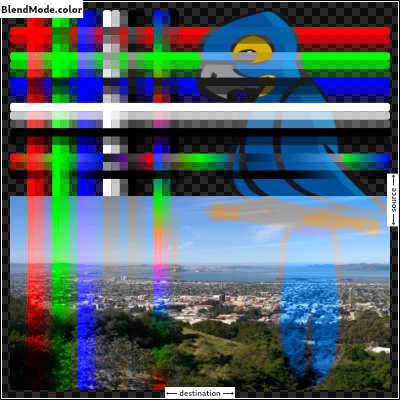
///
/// See also:
///
/// * [hue], which is a similar but weaker effect.
/// * [softLight], which is a similar tinting effect but also tints white.
/// * [saturation], which only applies the saturation of the source image.
color,
/// Take the luminosity of the source image, and the hue and saturation of the
/// destination image.
///
/// The opacity of the output image is computed in the same way as for
/// [srcOver]. Regions that are entirely transparent in the source image take
/// their luminosity from the destination.
///
/// 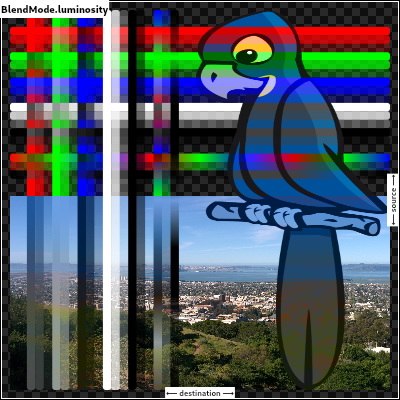
///
/// See also:
///
/// * [saturation], which applies the saturation of the source image to the
/// destination.
/// * [ImageFilter.blur], which can be used with [BackdropFilter] for a
/// related effect.
luminosity,
}
/// Quality levels for image sampling in [ImageFilter] and [Shader] objects that sample
/// images and for [Canvas] operations that render images.
///
/// When scaling up typically the quality is lowest at [none], higher at [low] and [medium],
/// and for very large scale factors (over 10x) the highest at [high].
///
/// When scaling down, [medium] provides the best quality especially when scaling an
/// image to less than half its size or for animating the scale factor between such
/// reductions. Otherwise, [low] and [high] provide similar effects for reductions of
/// between 50% and 100% but the image may lose detail and have dropouts below 50%.
///
/// To get high quality when scaling images up and down, or when the scale is
/// unknown, [medium] is typically a good balanced choice.
///
/// 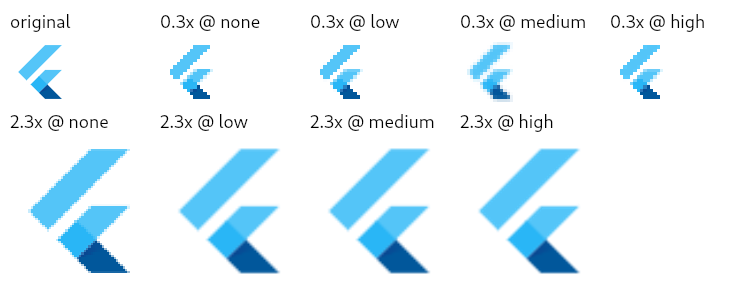
///
/// When building for the web using the `--web-renderer=html` option, filter
/// quality has no effect. All images are rendered using the respective
/// browser's default setting.
///
/// See also:
///
/// * [Paint.filterQuality], which is used to pass [FilterQuality] to the
/// engine while using drawImage calls on a [Canvas].
/// * [ImageShader].
/// * [ImageFilter.matrix].
/// * [Canvas.drawImage].
/// * [Canvas.drawImageRect].
/// * [Canvas.drawImageNine].
/// * [Canvas.drawAtlas].
enum FilterQuality {
// This list and the values (order) should be kept in sync with the equivalent list
// in lib/ui/painting/image_filter.cc
/// The fastest filtering method, albeit also the lowest quality.
///
/// This value results in a "Nearest Neighbor" algorithm which just
/// repeats or eliminates pixels as an image is scaled up or down.
none,
/// Better quality than [none], faster than [medium].
///
/// This value results in a "Bilinear" algorithm which smoothly
/// interpolates between pixels in an image.
low,
/// The best all around filtering method that is only worse than [high]
/// at extremely large scale factors.
///
/// This value improves upon the "Bilinear" algorithm specified by [low]
/// by utilizing a Mipmap that pre-computes high quality lower resolutions
/// of the image at half (and quarter and eighth, etc.) sizes and then
/// blends between those to prevent loss of detail at small scale sizes.
///
/// {@template dart.ui.filterQuality.seeAlso}
/// See also:
///
/// * [FilterQuality] class-level documentation that goes into detail about
/// relative qualities of the constant values.
/// {@endtemplate}
medium,
/// Best possible quality when scaling up images by scale factors larger than
/// 5-10x.
///
/// When images are scaled down, this can be worse than [medium] for scales
/// smaller than 0.5x, or when animating the scale factor.
///
/// This option is also the slowest.
///
/// This value results in a standard "Bicubic" algorithm which uses a 3rd order
/// equation to smooth the abrupt transitions between pixels while preserving
/// some of the sense of an edge and avoiding sharp peaks in the result.
///
/// {@macro dart.ui.filterQuality.seeAlso}
high,
}
/// Styles to use for line endings.
///
/// See also:
///
/// * [Paint.strokeCap] for how this value is used.
/// * [StrokeJoin] for the different kinds of line segment joins.
// These enum values must be kept in sync with DlStrokeCap.
enum StrokeCap {
/// Begin and end contours with a flat edge and no extension.
///
/// 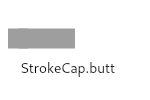
///
/// Compare to the [square] cap, which has the same shape, but extends past
/// the end of the line by half a stroke width.
butt,
/// Begin and end contours with a semi-circle extension.
///
/// 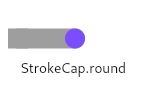
///
/// The cap is colored in the diagram above to highlight it: in normal use it
/// is the same color as the line.
round,
/// Begin and end contours with a half square extension. This is
/// similar to extending each contour by half the stroke width (as
/// given by [Paint.strokeWidth]).
///
/// 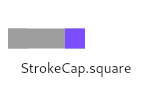
///
/// The cap is colored in the diagram above to highlight it: in normal use it
/// is the same color as the line.
///
/// Compare to the [butt] cap, which has the same shape, but doesn't extend
/// past the end of the line.
square,
}
/// Styles to use for line segment joins.
///
/// This only affects line joins for polygons drawn by [Canvas.drawPath] and
/// rectangles, not points drawn as lines with [Canvas.drawPoints].
///
/// See also:
///
/// * [Paint.strokeJoin] and [Paint.strokeMiterLimit] for how this value is
/// used.
/// * [StrokeCap] for the different kinds of line endings.
// These enum values must be kept in sync with DlStrokeJoin.
enum StrokeJoin {
/// Joins between line segments form sharp corners.
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/miter_4_join.mp4}
///
/// The center of the line segment is colored in the diagram above to
/// highlight the join, but in normal usage the join is the same color as the
/// line.
///
/// See also:
///
/// * [Paint.strokeJoin], used to set the line segment join style to this
/// value.
/// * [Paint.strokeMiterLimit], used to define when a miter is drawn instead
/// of a bevel when the join is set to this value.
miter,
/// Joins between line segments are semi-circular.
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/round_join.mp4}
///
/// The center of the line segment is colored in the diagram above to
/// highlight the join, but in normal usage the join is the same color as the
/// line.
///
/// See also:
///
/// * [Paint.strokeJoin], used to set the line segment join style to this
/// value.
round,
/// Joins between line segments connect the corners of the butt ends of the
/// line segments to give a beveled appearance.
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/bevel_join.mp4}
///
/// The center of the line segment is colored in the diagram above to
/// highlight the join, but in normal usage the join is the same color as the
/// line.
///
/// See also:
///
/// * [Paint.strokeJoin], used to set the line segment join style to this
/// value.
bevel,
}
/// Strategies for painting shapes and paths on a canvas.
///
/// See [Paint.style].
// These enum values must be kept in sync with DlDrawStyle.
enum PaintingStyle {
// This list comes from dl_paint.h and the values (order) should be kept
// in sync.
/// Apply the [Paint] to the inside of the shape. For example, when
/// applied to the [Canvas.drawCircle] call, this results in a disc
/// of the given size being painted.
fill,
/// Apply the [Paint] to the edge of the shape. For example, when
/// applied to the [Canvas.drawCircle] call, this results is a hoop
/// of the given size being painted. The line drawn on the edge will
/// be the width given by the [Paint.strokeWidth] property.
stroke,
}
/// Different ways to clip a widget's content.
enum Clip {
/// No clip at all.
///
/// This is the default option for most widgets: if the content does not
/// overflow the widget boundary, don't pay any performance cost for clipping.
///
/// If the content does overflow, please explicitly specify the following
/// [Clip] options:
/// * [hardEdge], which is the fastest clipping, but with lower fidelity.
/// * [antiAlias], which is a little slower than [hardEdge], but with smoothed edges.
/// * [antiAliasWithSaveLayer], which is much slower than [antiAlias], and should
/// rarely be used.
none,
/// Clip, but do not apply anti-aliasing.
///
/// This mode enables clipping, but curves and non-axis-aligned straight lines will be
/// jagged as no effort is made to anti-alias.
///
/// Faster than other clipping modes, but slower than [none].
///
/// This is a reasonable choice when clipping is needed, if the container is an axis-
/// aligned rectangle or an axis-aligned rounded rectangle with very small corner radii.
///
/// See also:
///
/// * [antiAlias], which is more reasonable when clipping is needed and the shape is not
/// an axis-aligned rectangle.
hardEdge,
/// Clip with anti-aliasing.
///
/// This mode has anti-aliased clipping edges to achieve a smoother look.
///
/// It' s much faster than [antiAliasWithSaveLayer], but slower than [hardEdge].
///
/// This will be the common case when dealing with circles and arcs.
///
/// Different from [hardEdge] and [antiAliasWithSaveLayer], this clipping may have
/// bleeding edge artifacts.
/// (See https://fiddle.skia.org/c/21cb4c2b2515996b537f36e7819288ae for an example.)
///
/// See also:
///
/// * [hardEdge], which is a little faster, but with lower fidelity.
/// * [antiAliasWithSaveLayer], which is much slower, but can avoid the
/// bleeding edges if there's no other way.
/// * [Paint.isAntiAlias], which is the anti-aliasing switch for general draw operations.
antiAlias,
/// Clip with anti-aliasing and saveLayer immediately following the clip.
///
/// This mode not only clips with anti-aliasing, but also allocates an offscreen
/// buffer. All subsequent paints are carried out on that buffer before finally
/// being clipped and composited back.
///
/// This is very slow. It has no bleeding edge artifacts (that [antiAlias] has)
/// but it changes the semantics as an offscreen buffer is now introduced.
/// (See https://github.com/flutter/flutter/issues/18057#issuecomment-394197336
/// for a difference between paint without saveLayer and paint with saveLayer.)
///
/// This will be only rarely needed. One case where you might need this is if
/// you have an image overlaid on a very different background color. In these
/// cases, consider whether you can avoid overlaying multiple colors in one
/// spot (e.g. by having the background color only present where the image is
/// absent). If you can, [antiAlias] would be fine and much faster.
///
/// See also:
///
/// * [antiAlias], which is much faster, and has similar clipping results.
antiAliasWithSaveLayer,
}
/// A description of the style to use when drawing on a [Canvas].
///
/// Most APIs on [Canvas] take a [Paint] object to describe the style
/// to use for that operation.
final class Paint {
/// Constructs an empty [Paint] object with all fields initialized to
/// their defaults.
Paint();
// Paint objects are encoded in two buffers:
//
// * _data is binary data in four-byte fields, each of which is either a
// uint32_t or a float. The default value for each field is encoded as
// zero to make initialization trivial. Most values already have a default
// value of zero, but some, such as color, have a non-zero default value.
// To encode or decode these values, XOR the value with the default value.
//
// * _objects is a list of unencodable objects, typically wrappers for native
// objects. The objects are simply stored in the list without any additional
// encoding.
//
// The binary format must match the deserialization code in paint.cc.
// C++ unit tests access this.
@pragma('vm:entry-point')
final ByteData _data = ByteData(_kDataByteCount);
static const int _kIsAntiAliasIndex = 0;
static const int _kColorIndex = 1;
static const int _kBlendModeIndex = 2;
static const int _kStyleIndex = 3;
static const int _kStrokeWidthIndex = 4;
static const int _kStrokeCapIndex = 5;
static const int _kStrokeJoinIndex = 6;
static const int _kStrokeMiterLimitIndex = 7;
static const int _kFilterQualityIndex = 8;
static const int _kMaskFilterIndex = 9;
static const int _kMaskFilterBlurStyleIndex = 10;
static const int _kMaskFilterSigmaIndex = 11;
static const int _kInvertColorIndex = 12;
static const int _kIsAntiAliasOffset = _kIsAntiAliasIndex << 2;
static const int _kColorOffset = _kColorIndex << 2;
static const int _kBlendModeOffset = _kBlendModeIndex << 2;
static const int _kStyleOffset = _kStyleIndex << 2;
static const int _kStrokeWidthOffset = _kStrokeWidthIndex << 2;
static const int _kStrokeCapOffset = _kStrokeCapIndex << 2;
static const int _kStrokeJoinOffset = _kStrokeJoinIndex << 2;
static const int _kStrokeMiterLimitOffset = _kStrokeMiterLimitIndex << 2;
static const int _kFilterQualityOffset = _kFilterQualityIndex << 2;
static const int _kMaskFilterOffset = _kMaskFilterIndex << 2;
static const int _kMaskFilterBlurStyleOffset = _kMaskFilterBlurStyleIndex << 2;
static const int _kMaskFilterSigmaOffset = _kMaskFilterSigmaIndex << 2;
static const int _kInvertColorOffset = _kInvertColorIndex << 2;
// If you add more fields, remember to update _kDataByteCount.
static const int _kDataByteCount = 52; // 4 * (last index + 1).
// Binary format must match the deserialization code in paint.cc.
// C++ unit tests access this.
@pragma('vm:entry-point')
List<Object?>? _objects;
List<Object?> _ensureObjectsInitialized() {
return _objects ??= List<Object?>.filled(_kObjectCount, null);
}
static const int _kShaderIndex = 0;
static const int _kColorFilterIndex = 1;
static const int _kImageFilterIndex = 2;
static const int _kObjectCount = 3; // Must be one larger than the largest index.
/// Whether to apply anti-aliasing to lines and images drawn on the
/// canvas.
///
/// Defaults to true.
bool get isAntiAlias {
return _data.getInt32(_kIsAntiAliasOffset, _kFakeHostEndian) == 0;
}
set isAntiAlias(bool value) {
// We encode true as zero and false as one because the default value, which
// we always encode as zero, is true.
final int encoded = value ? 0 : 1;
_data.setInt32(_kIsAntiAliasOffset, encoded, _kFakeHostEndian);
}
// Must be kept in sync with the default in paint.cc.
static const int _kColorDefault = 0xFF000000;
/// The color to use when stroking or filling a shape.
///
/// Defaults to opaque black.
///
/// See also:
///
/// * [style], which controls whether to stroke or fill (or both).
/// * [colorFilter], which overrides [color].
/// * [shader], which overrides [color] with more elaborate effects.
///
/// This color is not used when compositing. To colorize a layer, use
/// [colorFilter].
Color get color {
final int encoded = _data.getInt32(_kColorOffset, _kFakeHostEndian);
return Color(encoded ^ _kColorDefault);
}
set color(Color value) {
final int encoded = value.value ^ _kColorDefault;
_data.setInt32(_kColorOffset, encoded, _kFakeHostEndian);
}
// Must be kept in sync with the default in paint.cc.
static final int _kBlendModeDefault = BlendMode.srcOver.index;
/// A blend mode to apply when a shape is drawn or a layer is composited.
///
/// The source colors are from the shape being drawn (e.g. from
/// [Canvas.drawPath]) or layer being composited (the graphics that were drawn
/// between the [Canvas.saveLayer] and [Canvas.restore] calls), after applying
/// the [colorFilter], if any.
///
/// The destination colors are from the background onto which the shape or
/// layer is being composited.
///
/// Defaults to [BlendMode.srcOver].
///
/// See also:
///
/// * [Canvas.saveLayer], which uses its [Paint]'s [blendMode] to composite
/// the layer when [Canvas.restore] is called.
/// * [BlendMode], which discusses the user of [Canvas.saveLayer] with
/// [blendMode].
BlendMode get blendMode {
final int encoded = _data.getInt32(_kBlendModeOffset, _kFakeHostEndian);
return BlendMode.values[encoded ^ _kBlendModeDefault];
}
set blendMode(BlendMode value) {
final int encoded = value.index ^ _kBlendModeDefault;
_data.setInt32(_kBlendModeOffset, encoded, _kFakeHostEndian);
}
/// Whether to paint inside shapes, the edges of shapes, or both.
///
/// Defaults to [PaintingStyle.fill].
PaintingStyle get style {
return PaintingStyle.values[_data.getInt32(_kStyleOffset, _kFakeHostEndian)];
}
set style(PaintingStyle value) {
final int encoded = value.index;
_data.setInt32(_kStyleOffset, encoded, _kFakeHostEndian);
}
/// How wide to make edges drawn when [style] is set to
/// [PaintingStyle.stroke]. The width is given in logical pixels measured in
/// the direction orthogonal to the direction of the path.
///
/// Defaults to 0.0, which correspond to a hairline width.
double get strokeWidth {
return _data.getFloat32(_kStrokeWidthOffset, _kFakeHostEndian);
}
set strokeWidth(double value) {
final double encoded = value;
_data.setFloat32(_kStrokeWidthOffset, encoded, _kFakeHostEndian);
}
/// The kind of finish to place on the end of lines drawn when
/// [style] is set to [PaintingStyle.stroke].
///
/// Defaults to [StrokeCap.butt], i.e. no caps.
StrokeCap get strokeCap {
return StrokeCap.values[_data.getInt32(_kStrokeCapOffset, _kFakeHostEndian)];
}
set strokeCap(StrokeCap value) {
final int encoded = value.index;
_data.setInt32(_kStrokeCapOffset, encoded, _kFakeHostEndian);
}
/// The kind of finish to place on the joins between segments.
///
/// This applies to paths drawn when [style] is set to [PaintingStyle.stroke],
/// It does not apply to points drawn as lines with [Canvas.drawPoints].
///
/// Defaults to [StrokeJoin.miter], i.e. sharp corners.
///
/// Some examples of joins:
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/miter_4_join.mp4}
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/round_join.mp4}
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/bevel_join.mp4}
///
/// The centers of the line segments are colored in the diagrams above to
/// highlight the joins, but in normal usage the join is the same color as the
/// line.
///
/// See also:
///
/// * [strokeMiterLimit] to control when miters are replaced by bevels when
/// this is set to [StrokeJoin.miter].
/// * [strokeCap] to control what is drawn at the ends of the stroke.
/// * [StrokeJoin] for the definitive list of stroke joins.
StrokeJoin get strokeJoin {
return StrokeJoin.values[_data.getInt32(_kStrokeJoinOffset, _kFakeHostEndian)];
}
set strokeJoin(StrokeJoin value) {
final int encoded = value.index;
_data.setInt32(_kStrokeJoinOffset, encoded, _kFakeHostEndian);
}
// Must be kept in sync with the default in paint.cc.
static const double _kStrokeMiterLimitDefault = 4.0;
/// The limit for miters to be drawn on segments when the join is set to
/// [StrokeJoin.miter] and the [style] is set to [PaintingStyle.stroke]. If
/// this limit is exceeded, then a [StrokeJoin.bevel] join will be drawn
/// instead. This may cause some 'popping' of the corners of a path if the
/// angle between line segments is animated, as seen in the diagrams below.
///
/// This limit is expressed as a limit on the length of the miter.
///
/// Defaults to 4.0. Using zero as a limit will cause a [StrokeJoin.bevel]
/// join to be used all the time.
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/miter_0_join.mp4}
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/miter_4_join.mp4}
///
/// {@animation 300 300 https://flutter.github.io/assets-for-api-docs/assets/dart-ui/miter_6_join.mp4}
///
/// The centers of the line segments are colored in the diagrams above to
/// highlight the joins, but in normal usage the join is the same color as the
/// line.
///
/// See also:
///
/// * [strokeJoin] to control the kind of finish to place on the joins
/// between segments.
/// * [strokeCap] to control what is drawn at the ends of the stroke.
double get strokeMiterLimit {
return _data.getFloat32(_kStrokeMiterLimitOffset, _kFakeHostEndian);
}
set strokeMiterLimit(double value) {
final double encoded = value - _kStrokeMiterLimitDefault;
_data.setFloat32(_kStrokeMiterLimitOffset, encoded, _kFakeHostEndian);
}
/// A mask filter (for example, a blur) to apply to a shape after it has been
/// drawn but before it has been composited into the image.
///
/// See [MaskFilter] for details.
MaskFilter? get maskFilter {
switch (_data.getInt32(_kMaskFilterOffset, _kFakeHostEndian)) {
case MaskFilter._TypeNone:
return null;
case MaskFilter._TypeBlur:
return MaskFilter.blur(
BlurStyle.values[_data.getInt32(_kMaskFilterBlurStyleOffset, _kFakeHostEndian)],
_data.getFloat32(_kMaskFilterSigmaOffset, _kFakeHostEndian),
);
}
return null;
}
set maskFilter(MaskFilter? value) {
if (value == null) {
_data.setInt32(_kMaskFilterOffset, MaskFilter._TypeNone, _kFakeHostEndian);
_data.setInt32(_kMaskFilterBlurStyleOffset, 0, _kFakeHostEndian);
_data.setFloat32(_kMaskFilterSigmaOffset, 0.0, _kFakeHostEndian);
} else {
// For now we only support one kind of MaskFilter, so we don't need to
// check what the type is if it's not null.
_data.setInt32(_kMaskFilterOffset, MaskFilter._TypeBlur, _kFakeHostEndian);
_data.setInt32(_kMaskFilterBlurStyleOffset, value._style.index, _kFakeHostEndian);
_data.setFloat32(_kMaskFilterSigmaOffset, value._sigma, _kFakeHostEndian);
}
}
/// Controls the performance vs quality trade-off to use when sampling bitmaps,
/// as with an [ImageShader], or when drawing images, as with [Canvas.drawImage],
/// [Canvas.drawImageRect], [Canvas.drawImageNine] or [Canvas.drawAtlas].
///
/// Defaults to [FilterQuality.none].
// TODO(ianh): verify that the image drawing methods actually respect this
FilterQuality get filterQuality {
return FilterQuality.values[_data.getInt32(_kFilterQualityOffset, _kFakeHostEndian)];
}
set filterQuality(FilterQuality value) {
final int encoded = value.index;
_data.setInt32(_kFilterQualityOffset, encoded, _kFakeHostEndian);
}
/// The shader to use when stroking or filling a shape.
///
/// When this is null, the [color] is used instead.
///
/// See also:
///
/// * [Gradient], a shader that paints a color gradient.
/// * [ImageShader], a shader that tiles an [Image].
/// * [colorFilter], which overrides [shader].
/// * [color], which is used if [shader] and [colorFilter] are null.
Shader? get shader {
return _objects?[_kShaderIndex] as Shader?;
}
set shader(Shader? value) {
assert(() {
assert(
value == null || !value.debugDisposed,
'Attempted to set a disposed shader to $this',
);
return true;
}());
assert(() {
if (value is FragmentShader) {
if (!value._validateSamplers()) {
throw Exception('Invalid FragmentShader ${value._debugName ?? ''}: missing sampler');
}
}
return true;
}());
_ensureObjectsInitialized()[_kShaderIndex] = value;
}
/// A color filter to apply when a shape is drawn or when a layer is
/// composited.
///
/// See [ColorFilter] for details.
///
/// When a shape is being drawn, [colorFilter] overrides [color] and [shader].
ColorFilter? get colorFilter {
final _ColorFilter? nativeFilter = _objects?[_kColorFilterIndex] as _ColorFilter?;
return nativeFilter?.creator;
}
set colorFilter(ColorFilter? value) {
final _ColorFilter? nativeFilter = value?._toNativeColorFilter();
if (nativeFilter == null) {
if (_objects != null) {
_objects![_kColorFilterIndex] = null;
}
} else {
_ensureObjectsInitialized()[_kColorFilterIndex] = nativeFilter;
}
}
/// The [ImageFilter] to use when drawing raster images.
///
/// For example, to blur an image using [Canvas.drawImage], apply an
/// [ImageFilter.blur]:
///
/// ```dart
/// void paint(Canvas canvas, Size size) {
/// canvas.drawImage(
/// _image,
/// ui.Offset.zero,
/// Paint()..imageFilter = ui.ImageFilter.blur(sigmaX: 0.5, sigmaY: 0.5),
/// );
/// }
/// ```
///
/// See also:
///
/// * [MaskFilter], which is used for drawing geometry.
ImageFilter? get imageFilter {
final _ImageFilter? nativeFilter = _objects?[_kImageFilterIndex] as _ImageFilter?;
return nativeFilter?.creator;
}
set imageFilter(ImageFilter? value) {
if (value == null) {
if (_objects != null) {
_objects![_kImageFilterIndex] = null;
}
} else {
final List<Object?> objects = _ensureObjectsInitialized();
final _ImageFilter? imageFilter = objects[_kImageFilterIndex] as _ImageFilter?;
if (imageFilter?.creator != value) {
objects[_kImageFilterIndex] = value._toNativeImageFilter();
}
}
}
/// Whether the colors of the image are inverted when drawn.
///
/// Inverting the colors of an image applies a new color filter that will
/// be composed with any user provided color filters. This is primarily
/// used for implementing smart invert on iOS.
bool get invertColors {
return _data.getInt32(_kInvertColorOffset, _kFakeHostEndian) == 1;
}
set invertColors(bool value) {
_data.setInt32(_kInvertColorOffset, value ? 1 : 0, _kFakeHostEndian);
}
@override
String toString() {
if (const bool.fromEnvironment('dart.vm.product')) {
return super.toString();
}
final StringBuffer result = StringBuffer();
String semicolon = '';
result.write('Paint(');
if (style == PaintingStyle.stroke) {
result.write('$style');
if (strokeWidth != 0.0) {
result.write(' ${strokeWidth.toStringAsFixed(1)}');
} else {
result.write(' hairline');
}
if (strokeCap != StrokeCap.butt) {
result.write(' $strokeCap');
}
if (strokeJoin == StrokeJoin.miter) {
if (strokeMiterLimit != _kStrokeMiterLimitDefault) {
result.write(' $strokeJoin up to ${strokeMiterLimit.toStringAsFixed(1)}');
}
} else {
result.write(' $strokeJoin');
}
semicolon = '; ';
}
if (!isAntiAlias) {
result.write('${semicolon}antialias off');
semicolon = '; ';
}
if (color != const Color(_kColorDefault)) {
result.write('$semicolon$color');
semicolon = '; ';
}
if (blendMode.index != _kBlendModeDefault) {
result.write('$semicolon$blendMode');
semicolon = '; ';
}
if (colorFilter != null) {
result.write('${semicolon}colorFilter: $colorFilter');
semicolon = '; ';
}
if (maskFilter != null) {
result.write('${semicolon}maskFilter: $maskFilter');
semicolon = '; ';
}
if (filterQuality != FilterQuality.none) {
result.write('${semicolon}filterQuality: $filterQuality');
semicolon = '; ';
}
if (shader != null) {
result.write('${semicolon}shader: $shader');
semicolon = '; ';
}
if (imageFilter != null) {
result.write('${semicolon}imageFilter: $imageFilter');
semicolon = '; ';
}
if (invertColors) {
result.write('${semicolon}invert: $invertColors');
}
result.write(')');
return result.toString();
}
}
/// The color space describes the colors that are available to an [Image].
///
/// This value can help decide which [ImageByteFormat] to use with
/// [Image.toByteData]. Images that are in the [extendedSRGB] color space
/// should use something like [ImageByteFormat.rawExtendedRgba128] so that
/// colors outside of the sRGB gamut aren't lost.
///
/// This is also the result of [Image.colorSpace].
///
/// See also: https://en.wikipedia.org/wiki/Color_space
enum ColorSpace {
/// The sRGB color space.
///
/// You may know this as the standard color space for the web or the color
/// space of non-wide-gamut Flutter apps.
///
/// See also: https://en.wikipedia.org/wiki/SRGB
sRGB,
/// A color space that is backwards compatible with sRGB but can represent
/// colors outside of that gamut with values outside of [0..1]. In order to
/// see the extended values an [ImageByteFormat] like
/// [ImageByteFormat.rawExtendedRgba128] must be used.
extendedSRGB,
}
/// The format in which image bytes should be returned when using
/// [Image.toByteData].
// We do not expect to add more encoding formats to the ImageByteFormat enum,
// considering the binary size of the engine after LTO optimization. You can
// use the third-party pure dart image library to encode other formats.
// See: https://github.com/flutter/flutter/issues/16635 for more details.
enum ImageByteFormat {
/// Raw RGBA format.
///
/// Unencoded bytes, in RGBA row-primary form with premultiplied alpha, 8 bits per channel.
rawRgba,
/// Raw straight RGBA format.
///
/// Unencoded bytes, in RGBA row-primary form with straight alpha, 8 bits per channel.
rawStraightRgba,
/// Raw unmodified format.
///
/// Unencoded bytes, in the image's existing format. For example, a grayscale
/// image may use a single 8-bit channel for each pixel.
rawUnmodified,
/// Raw extended range RGBA format.
///
/// Unencoded bytes, in RGBA row-primary form with straight alpha, 32 bit
/// float (IEEE 754 binary32) per channel.
///
/// Example usage:
///
/// ```dart
/// import 'dart:ui' as ui;
/// import 'dart:typed_data';
///
/// Future<Map<String, double>> getFirstPixel(ui.Image image) async {
/// final ByteData data =
/// (await image.toByteData(format: ui.ImageByteFormat.rawExtendedRgba128))!;
/// final Float32List floats = Float32List.view(data.buffer);
/// return <String, double>{
/// 'r': floats[0],
/// 'g': floats[1],
/// 'b': floats[2],
/// 'a': floats[3],
/// };
/// }
/// ```
rawExtendedRgba128,
/// PNG format.
///
/// A loss-less compression format for images. This format is well suited for
/// images with hard edges, such as screenshots or sprites, and images with
/// text. Transparency is supported. The PNG format supports images up to
/// 2,147,483,647 pixels in either dimension, though in practice available
/// memory provides a more immediate limitation on maximum image size.
///
/// PNG images normally use the `.png` file extension and the `image/png` MIME
/// type.
///
/// See also:
///
/// * <https://en.wikipedia.org/wiki/Portable_Network_Graphics>, the Wikipedia page on PNG.
/// * <https://tools.ietf.org/rfc/rfc2083.txt>, the PNG standard.
png,
}
/// The format of pixel data given to [decodeImageFromPixels].
enum PixelFormat {
/// Each pixel is 32 bits, with the highest 8 bits encoding red, the next 8
/// bits encoding green, the next 8 bits encoding blue, and the lowest 8 bits
/// encoding alpha. Premultiplied alpha is used.
rgba8888,
/// Each pixel is 32 bits, with the highest 8 bits encoding blue, the next 8
/// bits encoding green, the next 8 bits encoding red, and the lowest 8 bits
/// encoding alpha. Premultiplied alpha is used.
bgra8888,
/// Each pixel is 128 bits, where each color component is a 32 bit float that
/// is normalized across the sRGB gamut. The first float is the red
/// component, followed by: green, blue and alpha. Premultiplied alpha isn't
/// used, matching [ImageByteFormat.rawExtendedRgba128].
rgbaFloat32,
}
/// Signature for [Image] lifecycle events.
typedef ImageEventCallback = void Function(Image image);
/// Opaque handle to raw decoded image data (pixels).
///
/// To obtain an [Image] object, use the [ImageDescriptor] API.
///
/// To draw an [Image], use one of the methods on the [Canvas] class, such as
/// [Canvas.drawImage].
///
/// A class or method that receives an image object must call [dispose] on the
/// handle when it is no longer needed. To create a shareable reference to the
/// underlying image, call [clone]. The method or object that receives
/// the new instance will then be responsible for disposing it, and the
/// underlying image itself will be disposed when all outstanding handles are
/// disposed.
///
/// If `dart:ui` passes an `Image` object and the recipient wishes to share
/// that handle with other callers, [clone] must be called _before_ [dispose].
/// A handle that has been disposed cannot create new handles anymore.
///
/// See also:
///
/// * [Image](https://api.flutter.dev/flutter/widgets/Image-class.html), the class in the [widgets] library.
/// * [ImageDescriptor], which allows reading information about the image and
/// creating a codec to decode it.
/// * [instantiateImageCodec], a utility method that wraps [ImageDescriptor].
class Image {
Image._(this._image, this.width, this.height) {
assert(() {
_debugStack = StackTrace.current;
return true;
}());
_image._handles.add(this);
onCreate?.call(this);
}
// C++ unit tests access this.
@pragma('vm:entry-point')
final _Image _image;
/// A callback that is invoked to report an image creation.
///
/// It's preferred to use [MemoryAllocations] in flutter/foundation.dart
/// than to use [onCreate] directly because [MemoryAllocations]
/// allows multiple callbacks.
static ImageEventCallback? onCreate;
/// A callback that is invoked to report the image disposal.
///
/// It's preferred to use [MemoryAllocations] in flutter/foundation.dart
/// than to use [onDispose] directly because [MemoryAllocations]
/// allows multiple callbacks.
static ImageEventCallback? onDispose;
StackTrace? _debugStack;
/// The number of image pixels along the image's horizontal axis.
final int width;
/// The number of image pixels along the image's vertical axis.
final int height;
bool _disposed = false;
/// Release this handle's claim on the underlying Image. This handle is no
/// longer usable after this method is called.
///
/// Once all outstanding handles have been disposed, the underlying image will
/// be disposed as well.
///
/// In debug mode, [debugGetOpenHandleStackTraces] will return a list of
/// [StackTrace] objects from all open handles' creation points. This is
/// useful when trying to determine what parts of the program are keeping an
/// image resident in memory.
void dispose() {
onDispose?.call(this);
assert(!_disposed && !_image._disposed);
assert(_image._handles.contains(this));
_disposed = true;
final bool removed = _image._handles.remove(this);
assert(removed);
if (_image._handles.isEmpty) {
_image.dispose();
}
}
/// Whether this reference to the underlying image is [dispose]d.
///
/// This only returns a valid value if asserts are enabled, and must not be
/// used otherwise.
bool get debugDisposed {
bool? disposed;
assert(() {
disposed = _disposed;
return true;
}());
return disposed ?? (throw StateError('Image.debugDisposed is only available when asserts are enabled.'));
}
/// Converts the [Image] object into a byte array.
///
/// The [format] argument specifies the format in which the bytes will be
/// returned.
///
/// Using [ImageByteFormat.rawRgba] on an image in the color space
/// [ColorSpace.extendedSRGB] will result in the gamut being squished to fit
/// into the sRGB gamut, resulting in the loss of wide-gamut colors.
///
/// Returns a future that completes with the binary image data or an error
/// if encoding fails.
// We do not expect to add more encoding formats to the ImageByteFormat enum,
// considering the binary size of the engine after LTO optimization. You can
// use the third-party pure dart image library to encode other formats.
// See: https://github.com/flutter/flutter/issues/16635 for more details.
Future<ByteData?> toByteData({ImageByteFormat format = ImageByteFormat.rawRgba}) {
assert(!_disposed && !_image._disposed);
return _image.toByteData(format: format);
}
/// The color space that is used by the [Image]'s colors.
///
/// This value is a consequence of how the [Image] has been created. For
/// example, loading a PNG that is in the Display P3 color space will result
/// in a [ColorSpace.extendedSRGB] image.
///
/// On rendering backends that don't support wide gamut colors (anything but
/// iOS impeller), wide gamut images will still report [ColorSpace.sRGB] if
/// rendering wide gamut colors isn't supported.
// Note: The docstring will become outdated as new platforms support wide
// gamut color, please keep it up to date.
ColorSpace get colorSpace {
final int colorSpaceValue = _image.colorSpace;
switch (colorSpaceValue) {
case 0:
return ColorSpace.sRGB;
case 1:
return ColorSpace.extendedSRGB;
default:
throw UnsupportedError('Unrecognized color space: $colorSpaceValue');
}
}
/// If asserts are enabled, returns the [StackTrace]s of each open handle from
/// [clone], in creation order.
///
/// If asserts are disabled, this method always returns null.
List<StackTrace>? debugGetOpenHandleStackTraces() {
List<StackTrace>? stacks;
assert(() {
stacks = _image._handles.map((Image handle) => handle._debugStack!).toList();
return true;
}());
return stacks;
}
/// Creates a disposable handle to this image.
///
/// Holders of an [Image] must dispose of the image when they no longer need
/// to access it or draw it. However, once the underlying image is disposed,
/// it is no longer possible to use it. If a holder of an image needs to share
/// access to that image with another object or method, [clone] creates a
/// duplicate handle. The underlying image will only be disposed once all
/// outstanding handles are disposed. This allows for safe sharing of image
/// references while still disposing of the underlying resources when all
/// consumers are finished.
///
/// It is safe to pass an [Image] handle to another object or method if the
/// current holder no longer needs it.
///
/// To check whether two [Image] references are referring to the same
/// underlying image memory, use [isCloneOf] rather than the equality operator
/// or [identical].
///
/// The following example demonstrates valid usage.
///
/// ```dart
/// import 'dart:async';
/// import 'dart:typed_data';
/// import 'dart:ui';
///
/// Future<Image> _loadImage(int width, int height) {
/// final Completer<Image> completer = Completer<Image>();
/// decodeImageFromPixels(
/// Uint8List.fromList(List<int>.filled(width * height * 4, 0xFF)),
/// width,
/// height,
/// PixelFormat.rgba8888,
/// // Don't worry about disposing or cloning this image - responsibility
/// // is transferred to the caller, and that is safe since this method
/// // will not touch it again.
/// (Image image) => completer.complete(image),
/// );
/// return completer.future;
/// }
///
/// Future<void> main() async {
/// final Image image = await _loadImage(5, 5);
/// // Make sure to clone the image, because MyHolder might dispose it
/// // and we need to access it again.
/// final MyImageHolder holder = MyImageHolder(image.clone());
/// final MyImageHolder holder2 = MyImageHolder(image.clone());
/// // Now we dispose it because we won't need it again.
/// image.dispose();
///
/// final PictureRecorder recorder = PictureRecorder();
/// final Canvas canvas = Canvas(recorder);
///
/// holder.draw(canvas);
/// holder.dispose();
///
/// canvas.translate(50, 50);
/// holder2.draw(canvas);
/// holder2.dispose();
/// }
///
/// class MyImageHolder {
/// MyImageHolder(this.image);
///
/// final Image image;
///
/// void draw(Canvas canvas) {
/// canvas.drawImage(image, Offset.zero, Paint());
/// }
///
/// void dispose() => image.dispose();
/// }
/// ```
///
/// The returned object behaves identically to this image. Calling
/// [dispose] on it will only dispose the underlying native resources if it
/// is the last remaining handle.
Image clone() {
if (_disposed) {
throw StateError(
'Cannot clone a disposed image.\n'
'The clone() method of a previously-disposed Image was called. Once an '
'Image object has been disposed, it can no longer be used to create '
'handles, as the underlying data may have been released.'
);
}
assert(!_image._disposed);
return Image._(_image, width, height);
}
/// Returns true if `other` is a [clone] of this and thus shares the same
/// underlying image memory, even if this or `other` is [dispose]d.
///
/// This method may return false for two images that were decoded from the
/// same underlying asset, if they are not sharing the same memory. For
/// example, if the same file is decoded using [instantiateImageCodec] twice,
/// or the same bytes are decoded using [decodeImageFromPixels] twice, there
/// will be two distinct [Image]s that render the same but do not share
/// underlying memory, and so will not be treated as clones of each other.
bool isCloneOf(Image other) => other._image == _image;
@override
String toString() => _image.toString();
}
@pragma('vm:entry-point')
base class _Image extends NativeFieldWrapperClass1 {
// This class is created by the engine, and should not be instantiated
// or extended directly.
//
// _Images are always handed out wrapped in [Image]s. To create an [Image],
// use the ImageDescriptor API.
@pragma('vm:entry-point')
_Image._();
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Image::width', isLeaf: true)
external int get width;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Image::height', isLeaf: true)
external int get height;
Future<ByteData?> toByteData({ImageByteFormat format = ImageByteFormat.rawRgba}) {
return _futurizeWithError((_CallbackWithError<ByteData?> callback) {
return _toByteData(format.index, (Uint8List? encoded, String? error) {
if (error == null && encoded != null) {
callback(encoded.buffer.asByteData(), null);
} else {
callback(null, error);
}
});
});
}
/// Returns an error message on failure, null on success.
@Native<Handle Function(Pointer<Void>, Int32, Handle)>(symbol: 'Image::toByteData')
external String? _toByteData(int format, void Function(Uint8List?, String?) callback);
bool _disposed = false;
void dispose() {
assert(!_disposed);
assert(
_handles.isEmpty,
'Attempted to dispose of an Image object that has ${_handles.length} '
'open handles.\n'
'If you see this, it is a bug in dart:ui. Please file an issue at '
'https://github.com/flutter/flutter/issues/new.',
);
_disposed = true;
_dispose();
}
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
@Native<Void Function(Pointer<Void>)>(symbol: 'Image::dispose')
external void _dispose();
final Set<Image> _handles = <Image>{};
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Image::colorSpace')
external int get colorSpace;
@override
String toString() => '[$width\u00D7$height]';
}
@pragma('vm:entry-point')
Image _wrapImage(_Image image) {
return Image._(image, image.width, image.height);
}
/// Callback signature for [decodeImageFromList].
typedef ImageDecoderCallback = void Function(Image result);
/// Information for a single frame of an animation.
///
/// To obtain an instance of the [FrameInfo] interface, see
/// [Codec.getNextFrame].
///
/// The recipient of an instance of this class is responsible for calling
/// [Image.dispose] on [image]. To share the image with other interested
/// parties, use [Image.clone]. If the [FrameInfo] object itself is passed to
/// another method or object, that method or object must assume it is
/// responsible for disposing the image when done, and the passer must not
/// access the [image] after that point.
///
/// For example, the following code sample is incorrect:
///
/// ```dart
/// /// BAD
/// Future<void> nextFrameRoutine(ui.Codec codec) async {
/// final ui.FrameInfo frameInfo = await codec.getNextFrame();
/// _cacheImage(frameInfo);
/// // ERROR - _cacheImage is now responsible for disposing the image, and
/// // the image may not be available any more for this drawing routine.
/// _drawImage(frameInfo);
/// // ERROR again - the previous methods might or might not have created
/// // handles to the image.
/// frameInfo.image.dispose();
/// }
/// ```
///
/// Correct usage is:
///
/// ```dart
/// /// GOOD
/// Future<void> nextFrameRoutine(ui.Codec codec) async {
/// final ui.FrameInfo frameInfo = await codec.getNextFrame();
/// _cacheImage(frameInfo.image.clone(), frameInfo.duration);
/// _drawImage(frameInfo.image.clone(), frameInfo.duration);
/// // This method is done with its handle, and has passed handles to its
/// // clients already.
/// // The image will live until those clients dispose of their handles, and
/// // this one must not be disposed since it will not be used again.
/// frameInfo.image.dispose();
/// }
/// ```
class FrameInfo {
/// This class is created by the engine, and should not be instantiated
/// or extended directly.
///
/// To obtain an instance of the [FrameInfo] interface, see
/// [Codec.getNextFrame].
FrameInfo._({required this.duration, required this.image});
/// The duration this frame should be shown.
///
/// A zero duration indicates that the frame should be shown indefinitely.
final Duration duration;
/// The [Image] object for this frame.
///
/// This object must be disposed by the recipient of this frame info.
///
/// To share this image with other interested parties, use [Image.clone].
final Image image;
}
/// A handle to an image codec.
///
/// This class is created by the engine, and should not be instantiated
/// or extended directly.
///
/// To obtain an instance of the [Codec] interface, see
/// [instantiateImageCodec].
abstract class Codec {
/// Number of frames in this image.
int get frameCount;
/// Number of times to repeat the animation.
///
/// * 0 when the animation should be played once.
/// * -1 for infinity repetitions.
int get repetitionCount;
/// Fetches the next animation frame.
///
/// Wraps back to the first frame after returning the last frame.
///
/// The returned future can complete with an error if the decoding has failed.
///
/// The caller of this method is responsible for disposing the
/// [FrameInfo.image] on the returned object.
Future<FrameInfo> getNextFrame();
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
void dispose();
}
base class _NativeCodec extends NativeFieldWrapperClass1 implements Codec {
//
// This class is created by the engine, and should not be instantiated
// or extended directly.
//
// To obtain an instance of the [Codec] interface, see
// [instantiateImageCodec].
_NativeCodec._();
int? _cachedFrameCount;
@override
int get frameCount => _cachedFrameCount ??= _frameCount;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Codec::frameCount', isLeaf: true)
external int get _frameCount;
int? _cachedRepetitionCount;
@override
int get repetitionCount => _cachedRepetitionCount ??= _repetitionCount;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Codec::repetitionCount', isLeaf: true)
external int get _repetitionCount;
@override
Future<FrameInfo> getNextFrame() async {
final Completer<FrameInfo> completer = Completer<FrameInfo>.sync();
final String? error = _getNextFrame((_Image? image, int durationMilliseconds, String decodeError) {
if (image == null) {
if (decodeError.isEmpty) {
decodeError = 'Codec failed to produce an image, possibly due to invalid image data.';
}
completer.completeError(Exception(decodeError));
} else {
completer.complete(FrameInfo._(
image: Image._(image, image.width, image.height),
duration: Duration(milliseconds: durationMilliseconds),
));
}
});
if (error != null) {
throw Exception(error);
}
return completer.future;
}
/// Returns an error message on failure, null on success.
@Native<Handle Function(Pointer<Void>, Handle)>(symbol: 'Codec::getNextFrame')
external String? _getNextFrame(void Function(_Image?, int, String) callback);
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'Codec::dispose')
external void dispose();
@override
String toString() => 'Codec(${_cachedFrameCount == null ? "" : "$_cachedFrameCount frames"})';
}
/// Instantiates an image [Codec].
///
/// This method is a convenience wrapper around the [ImageDescriptor] API, and
/// using [ImageDescriptor] directly is preferred since it allows the caller to
/// make better determinations about how and whether to use the `targetWidth`
/// and `targetHeight` parameters.
///
/// The `list` parameter is the binary image data (e.g a PNG or GIF binary data).
/// The data can be for either static or animated images. The following image
/// formats are supported:
// Update this list when changing the list of supported codecs.
/// {@template dart.ui.imageFormats}
/// JPEG, PNG, GIF, Animated GIF, WebP, Animated WebP, BMP, and WBMP. Additional
/// formats may be supported by the underlying platform. Flutter will
/// attempt to call platform API to decode unrecognized formats, and if the
/// platform API supports decoding the image Flutter will be able to render it.
/// {@endtemplate}
///
/// The `targetWidth` and `targetHeight` arguments specify the size of the
/// output image, in image pixels. If they are not equal to the intrinsic
/// dimensions of the image, then the image will be scaled after being decoded.
/// If the `allowUpscaling` parameter is not set to true, both dimensions will
/// be capped at the intrinsic dimensions of the image, even if only one of
/// them would have exceeded those intrinsic dimensions. If exactly one of these
/// two arguments is specified, then the aspect ratio will be maintained while
/// forcing the image to match the other given dimension. If neither is
/// specified, then the image maintains its intrinsic size.
///
/// Scaling the image to larger than its intrinsic size should usually be
/// avoided, since it causes the image to use more memory than necessary.
/// Instead, prefer scaling the [Canvas] transform. If the image must be scaled
/// up, the `allowUpscaling` parameter must be set to true.
///
/// The returned future can complete with an error if the image decoding has
/// failed.
Future<Codec> instantiateImageCodec(
Uint8List list, {
int? targetWidth,
int? targetHeight,
bool allowUpscaling = true,
}) async {
final ImmutableBuffer buffer = await ImmutableBuffer.fromUint8List(list);
return instantiateImageCodecFromBuffer(
buffer,
targetWidth: targetWidth,
targetHeight: targetHeight,
allowUpscaling: allowUpscaling,
);
}
/// Instantiates an image [Codec].
///
/// This method is a convenience wrapper around the [ImageDescriptor] API, and
/// using [ImageDescriptor] directly is preferred since it allows the caller to
/// make better determinations about how and whether to use the `targetWidth`
/// and `targetHeight` parameters.
///
/// The [buffer] parameter is the binary image data (e.g a PNG or GIF binary data).
/// The data can be for either static or animated images. The following image
/// formats are supported: {@macro dart.ui.imageFormats}
///
/// The [buffer] will be disposed by this method once the codec has been created,
/// so the caller must relinquish ownership of the [buffer] when they call this
/// method.
///
/// The [targetWidth] and [targetHeight] arguments specify the size of the
/// output image, in image pixels. If they are not equal to the intrinsic
/// dimensions of the image, then the image will be scaled after being decoded.
/// If the `allowUpscaling` parameter is not set to true, both dimensions will
/// be capped at the intrinsic dimensions of the image, even if only one of
/// them would have exceeded those intrinsic dimensions. If exactly one of these
/// two arguments is specified, then the aspect ratio will be maintained while
/// forcing the image to match the other given dimension. If neither is
/// specified, then the image maintains its intrinsic size.
///
/// Scaling the image to larger than its intrinsic size should usually be
/// avoided, since it causes the image to use more memory than necessary.
/// Instead, prefer scaling the [Canvas] transform. If the image must be scaled
/// up, the `allowUpscaling` parameter must be set to true.
///
/// The returned future can complete with an error if the image decoding has
/// failed.
///
/// ## Compatibility note on the web
///
/// When running Flutter on the web, only the CanvasKit renderer supports image
/// resizing capabilities (not the HTML renderer). So if image resizing is
/// critical to your use case, and you're deploying to the web, you should
/// build using the CanvasKit renderer.
Future<Codec> instantiateImageCodecFromBuffer(
ImmutableBuffer buffer, {
int? targetWidth,
int? targetHeight,
bool allowUpscaling = true,
}) {
return instantiateImageCodecWithSize(
buffer,
getTargetSize: (int intrinsicWidth, int intrinsicHeight) {
if (!allowUpscaling) {
if (targetWidth != null && targetWidth! > intrinsicWidth) {
targetWidth = intrinsicWidth;
}
if (targetHeight != null && targetHeight! > intrinsicHeight) {
targetHeight = intrinsicHeight;
}
}
return TargetImageSize(width: targetWidth, height: targetHeight);
},
);
}
/// Instantiates an image [Codec].
///
/// This method is a convenience wrapper around the [ImageDescriptor] API.
///
/// The [buffer] parameter is the binary image data (e.g a PNG or GIF binary
/// data). The data can be for either static or animated images. The following
/// image formats are supported: {@macro dart.ui.imageFormats}
///
/// The [buffer] will be disposed by this method once the codec has been
/// created, so the caller must relinquish ownership of the [buffer] when they
/// call this method.
///
/// The [getTargetSize] parameter, when specified, will be invoked and passed
/// the image's intrinsic size to determine the size to decode the image to.
/// The width and the height of the size it returns must be positive values
/// greater than or equal to 1, or null. It is valid to return a
/// [TargetImageSize] that specifies only one of `width` and `height` with the
/// other remaining null, in which case the omitted dimension will be scaled to
/// maintain the aspect ratio of the original dimensions. When both are null or
/// omitted, the image will be decoded at its native resolution (as will be the
/// case if the [getTargetSize] parameter is omitted).
///
/// Scaling the image to larger than its intrinsic size should usually be
/// avoided, since it causes the image to use more memory than necessary.
/// Instead, prefer scaling the [Canvas] transform.
///
/// The returned future can complete with an error if the image decoding has
/// failed.
///
/// ## Compatibility note on the web
///
/// When running Flutter on the web, only the CanvasKit renderer supports image
/// resizing capabilities (not the HTML renderer). So if image resizing is
/// critical to your use case, and you're deploying to the web, you should
/// build using the CanvasKit renderer.
Future<Codec> instantiateImageCodecWithSize(
ImmutableBuffer buffer, {
TargetImageSizeCallback? getTargetSize,
}) async {
getTargetSize ??= _getDefaultImageSize;
final ImageDescriptor descriptor = await ImageDescriptor.encoded(buffer);
try {
final TargetImageSize targetSize = getTargetSize(descriptor.width, descriptor.height);
assert(targetSize.width == null || targetSize.width! > 0);
assert(targetSize.height == null || targetSize.height! > 0);
return descriptor.instantiateCodec(
targetWidth: targetSize.width,
targetHeight: targetSize.height,
);
} finally {
buffer.dispose();
}
}
TargetImageSize _getDefaultImageSize(int intrinsicWidth, int intrinsicHeight) {
return const TargetImageSize();
}
/// Signature for a callback that determines the size to which an image should
/// be decoded given its intrinsic size.
///
/// See also:
///
/// * [instantiateImageCodecWithSize], which used this signature for its
/// `getTargetSize` argument.
typedef TargetImageSizeCallback = TargetImageSize Function(
int intrinsicWidth,
int intrinsicHeight,
);
/// A specification of the size to which an image should be decoded.
///
/// See also:
///
/// * [TargetImageSizeCallback], a callback that returns instances of this
/// class when consulted by image decoding methods such as
/// [instantiateImageCodecWithSize].
class TargetImageSize {
/// Creates a new instance of this class.
///
/// The `width` and `height` may both be null, but if they're non-null, they
/// must be positive.
const TargetImageSize({this.width, this.height})
: assert(width == null || width > 0),
assert(height == null || height > 0);
/// The width into which to load the image.
///
/// If this is non-null, the image will be decoded into the specified width.
/// If this is null and [height] is also null, the image will be decoded into
/// its intrinsic size. If this is null and [height] is non-null, the image
/// will be decoded into a width that maintains its intrinsic aspect ratio
/// while respecting the [height] value.
///
/// If this value is non-null, it must be positive.
final int? width;
/// The height into which to load the image.
///
/// If this is non-null, the image will be decoded into the specified height.
/// If this is null and [width] is also null, the image will be decoded into
/// its intrinsic size. If this is null and [width] is non-null, the image
/// will be decoded into a height that maintains its intrinsic aspect ratio
/// while respecting the [width] value.
///
/// If this value is non-null, it must be positive.
final int? height;
@override
String toString() => 'TargetImageSize($width x $height)';
}
/// Loads a single image frame from a byte array into an [Image] object.
///
/// This is a convenience wrapper around [instantiateImageCodec]. Prefer using
/// [instantiateImageCodec] which also supports multi frame images and offers
/// better error handling. This function swallows asynchronous errors.
void decodeImageFromList(Uint8List list, ImageDecoderCallback callback) {
_decodeImageFromListAsync(list, callback);
}
Future<void> _decodeImageFromListAsync(Uint8List list, ImageDecoderCallback callback) async {
final Codec codec = await instantiateImageCodec(list);
final FrameInfo frameInfo = await codec.getNextFrame();
callback(frameInfo.image);
}
/// Convert an array of pixel values into an [Image] object.
///
/// The `pixels` parameter is the pixel data. They are packed in bytes in the
/// order described by `format`, then grouped in rows, from left to right,
/// then top to bottom.
///
/// The `rowBytes` parameter is the number of bytes consumed by each row of
/// pixels in the data buffer. If unspecified, it defaults to `width` multiplied
/// by the number of bytes per pixel in the provided `format`.
///
/// The `targetWidth` and `targetHeight` arguments specify the size of the
/// output image, in image pixels. If they are not equal to the intrinsic
/// dimensions of the image, then the image will be scaled after being decoded.
/// If the `allowUpscaling` parameter is not set to true, both dimensions will
/// be capped at the intrinsic dimensions of the image, even if only one of
/// them would have exceeded those intrinsic dimensions. If exactly one of these
/// two arguments is specified, then the aspect ratio will be maintained while
/// forcing the image to match the other given dimension. If neither is
/// specified, then the image maintains its intrinsic size.
///
/// Scaling the image to larger than its intrinsic size should usually be
/// avoided, since it causes the image to use more memory than necessary.
/// Instead, prefer scaling the [Canvas] transform. If the image must be scaled
/// up, the `allowUpscaling` parameter must be set to true.
void decodeImageFromPixels(
Uint8List pixels,
int width,
int height,
PixelFormat format,
ImageDecoderCallback callback, {
int? rowBytes,
int? targetWidth,
int? targetHeight,
bool allowUpscaling = true,
}) {
if (targetWidth != null) {
assert(allowUpscaling || targetWidth <= width);
}
if (targetHeight != null) {
assert(allowUpscaling || targetHeight <= height);
}
ImmutableBuffer.fromUint8List(pixels)
.then((ImmutableBuffer buffer) {
final ImageDescriptor descriptor = ImageDescriptor.raw(
buffer,
width: width,
height: height,
rowBytes: rowBytes,
pixelFormat: format,
);
if (!allowUpscaling) {
if (targetWidth != null && targetWidth! > descriptor.width) {
targetWidth = descriptor.width;
}
if (targetHeight != null && targetHeight! > descriptor.height) {
targetHeight = descriptor.height;
}
}
descriptor
.instantiateCodec(
targetWidth: targetWidth,
targetHeight: targetHeight,
)
.then((Codec codec) {
final Future<FrameInfo> frameInfo = codec.getNextFrame();
codec.dispose();
return frameInfo;
})
.then((FrameInfo frameInfo) {
buffer.dispose();
descriptor.dispose();
return callback(frameInfo.image);
});
});
}
/// Determines the winding rule that decides how the interior of a [Path] is
/// calculated.
///
/// This enum is used by the [Path.fillType] property.
enum PathFillType {
/// The interior is defined by a non-zero sum of signed edge crossings.
///
/// For a given point, the point is considered to be on the inside of the path
/// if a line drawn from the point to infinity crosses lines going clockwise
/// around the point a different number of times than it crosses lines going
/// counter-clockwise around that point.
///
/// See: <https://en.wikipedia.org/wiki/Nonzero-rule>
nonZero,
/// The interior is defined by an odd number of edge crossings.
///
/// For a given point, the point is considered to be on the inside of the path
/// if a line drawn from the point to infinity crosses an odd number of lines.
///
/// See: <https://en.wikipedia.org/wiki/Even-odd_rule>
evenOdd,
}
/// Strategies for combining paths.
///
/// See also:
///
/// * [Path.combine], which uses this enum to decide how to combine two paths.
// Must be kept in sync with SkPathOp
enum PathOperation {
/// Subtract the second path from the first path.
///
/// For example, if the two paths are overlapping circles of equal diameter
/// but differing centers, the result would be a crescent portion of the
/// first circle that was not overlapped by the second circle.
///
/// See also:
///
/// * [reverseDifference], which is the same but subtracting the first path
/// from the second.
difference,
/// Create a new path that is the intersection of the two paths, leaving the
/// overlapping pieces of the path.
///
/// For example, if the two paths are overlapping circles of equal diameter
/// but differing centers, the result would be only the overlapping portion
/// of the two circles.
///
/// See also:
/// * [xor], which is the inverse of this operation
intersect,
/// Create a new path that is the union (inclusive-or) of the two paths.
///
/// For example, if the two paths are overlapping circles of equal diameter
/// but differing centers, the result would be a figure-eight like shape
/// matching the outer boundaries of both circles.
union,
/// Create a new path that is the exclusive-or of the two paths, leaving
/// everything but the overlapping pieces of the path.
///
/// For example, if the two paths are overlapping circles of equal diameter
/// but differing centers, the figure-eight like shape less the overlapping parts
///
/// See also:
/// * [intersect], which is the inverse of this operation
xor,
/// Subtract the first path from the second path.
///
/// For example, if the two paths are overlapping circles of equal diameter
/// but differing centers, the result would be a crescent portion of the
/// second circle that was not overlapped by the first circle.
///
/// See also:
///
/// * [difference], which is the same but subtracting the second path
/// from the first.
reverseDifference,
}
/// A handle for the framework to hold and retain an engine layer across frames.
abstract class EngineLayer {
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// EngineLayers indirectly retain platform specific graphics resources. Some
/// of these resources, such as images, may be memory intensive. It is
/// important to dispose of EngineLayer objects that will no longer be used as
/// soon as possible to avoid retaining these resources until the next
/// garbage collection.
///
/// Once this EngineLayer is disposed, it is no longer eligible for use as a
/// retained layer, and must not be passed as an `oldLayer` to any of the
/// [SceneBuilder] methods which accept that parameter.
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
void dispose();
}
base class _NativeEngineLayer extends NativeFieldWrapperClass1 implements EngineLayer {
/// This class is created by the engine, and should not be instantiated
/// or extended directly.
_NativeEngineLayer._();
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'EngineLayer::dispose')
external void dispose();
}
/// A complex, one-dimensional subset of a plane.
///
/// A path consists of a number of sub-paths, and a _current point_.
///
/// Sub-paths consist of segments of various types, such as lines,
/// arcs, or beziers. Sub-paths can be open or closed, and can
/// self-intersect.
///
/// Closed sub-paths enclose a (possibly discontiguous) region of the
/// plane based on the current [fillType].
///
/// The _current point_ is initially at the origin. After each
/// operation adding a segment to a sub-path, the current point is
/// updated to the end of that segment.
///
/// Paths can be drawn on canvases using [Canvas.drawPath], and can
/// used to create clip regions using [Canvas.clipPath].
abstract class Path {
factory Path() = _NativePath;
/// Creates a copy of another [Path].
///
/// This copy is fast and does not require additional memory unless either
/// the `source` path or the path returned by this constructor are modified.
factory Path.from(Path source) {
final _NativePath clonedPath = _NativePath._();
(source as _NativePath)._clone(clonedPath);
return clonedPath;
}
/// Determines how the interior of this path is calculated.
///
/// Defaults to the non-zero winding rule, [PathFillType.nonZero].
PathFillType get fillType;
set fillType(PathFillType value);
/// Starts a new sub-path at the given coordinate.
void moveTo(double x, double y);
/// Starts a new sub-path at the given offset from the current point.
void relativeMoveTo(double dx, double dy);
/// Adds a straight line segment from the current point to the given
/// point.
void lineTo(double x, double y);
/// Adds a straight line segment from the current point to the point
/// at the given offset from the current point.
void relativeLineTo(double dx, double dy);
/// Adds a quadratic bezier segment that curves from the current
/// point to the given point (x2,y2), using the control point
/// (x1,y1).
///
/// 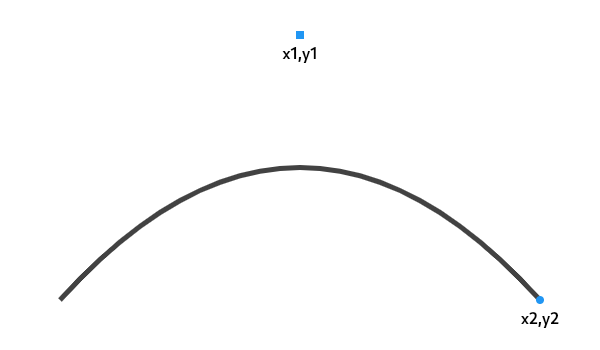
/// 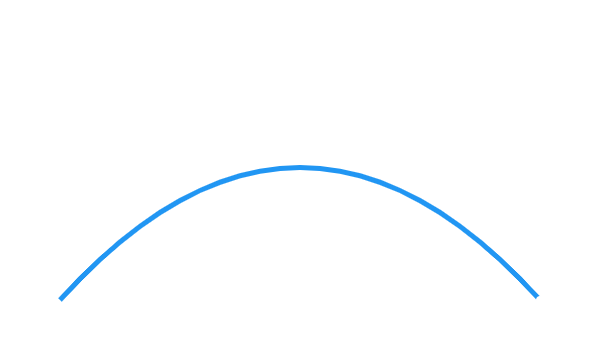
void quadraticBezierTo(double x1, double y1, double x2, double y2);
/// Adds a quadratic bezier segment that curves from the current
/// point to the point at the offset (x2,y2) from the current point,
/// using the control point at the offset (x1,y1) from the current
/// point.
void relativeQuadraticBezierTo(double x1, double y1, double x2, double y2);
/// Adds a cubic bezier segment that curves from the current point
/// to the given point (x3,y3), using the control points (x1,y1) and
/// (x2,y2).
///
/// 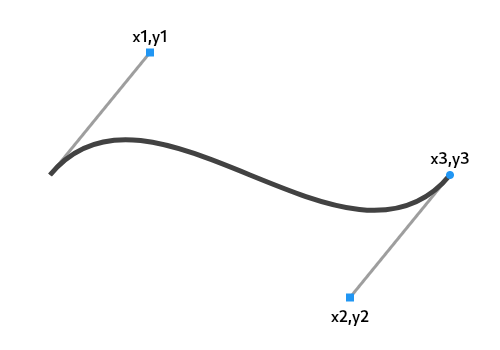
/// 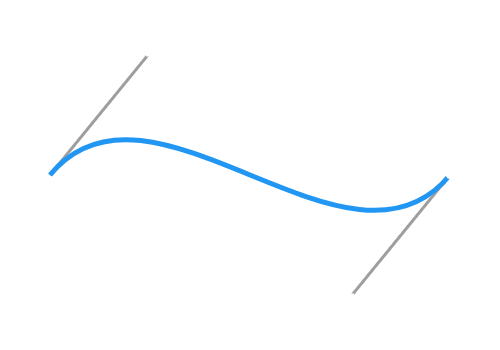
void cubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
/// Adds a cubic bezier segment that curves from the current point
/// to the point at the offset (x3,y3) from the current point, using
/// the control points at the offsets (x1,y1) and (x2,y2) from the
/// current point.
void relativeCubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
/// Adds a bezier segment that curves from the current point to the
/// given point (x2,y2), using the control points (x1,y1) and the
/// weight w. If the weight is greater than 1, then the curve is a
/// hyperbola; if the weight equals 1, it's a parabola; and if it is
/// less than 1, it is an ellipse.
///
/// 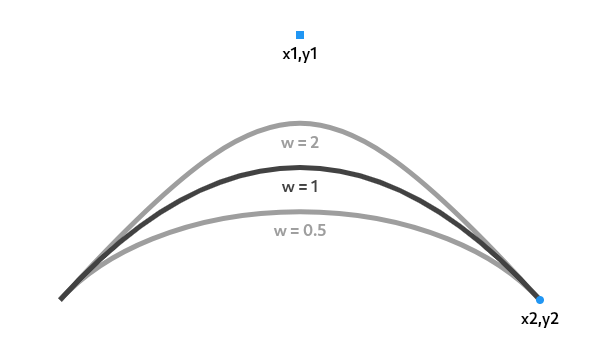
/// 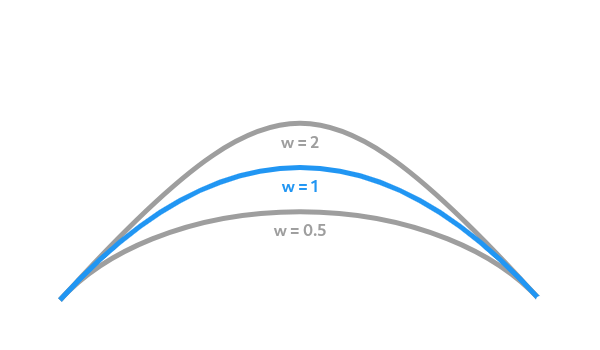
void conicTo(double x1, double y1, double x2, double y2, double w);
/// Adds a bezier segment that curves from the current point to the
/// point at the offset (x2,y2) from the current point, using the
/// control point at the offset (x1,y1) from the current point and
/// the weight w. If the weight is greater than 1, then the curve is
/// a hyperbola; if the weight equals 1, it's a parabola; and if it
/// is less than 1, it is an ellipse.
void relativeConicTo(double x1, double y1, double x2, double y2, double w);
/// If the `forceMoveTo` argument is false, adds a straight line
/// segment and an arc segment.
///
/// If the `forceMoveTo` argument is true, starts a new sub-path
/// consisting of an arc segment.
///
/// In either case, the arc segment consists of the arc that follows
/// the edge of the oval bounded by the given rectangle, from
/// startAngle radians around the oval up to startAngle + sweepAngle
/// radians around the oval, with zero radians being the point on
/// the right hand side of the oval that crosses the horizontal line
/// that intersects the center of the rectangle and with positive
/// angles going clockwise around the oval.
///
/// The line segment added if `forceMoveTo` is false starts at the
/// current point and ends at the start of the arc.
void arcTo(Rect rect, double startAngle, double sweepAngle, bool forceMoveTo);
/// Appends up to four conic curves weighted to describe an oval of `radius`
/// and rotated by `rotation` (measured in degrees and clockwise).
///
/// The first curve begins from the last point in the path and the last ends
/// at `arcEnd`. The curves follow a path in a direction determined by
/// `clockwise` and `largeArc` in such a way that the sweep angle
/// is always less than 360 degrees.
///
/// A simple line is appended if either radii are zero or the last
/// point in the path is `arcEnd`. The radii are scaled to fit the last path
/// point if both are greater than zero but too small to describe an arc.
///
void arcToPoint(Offset arcEnd, {
Radius radius = Radius.zero,
double rotation = 0.0,
bool largeArc = false,
bool clockwise = true,
});
/// Appends up to four conic curves weighted to describe an oval of `radius`
/// and rotated by `rotation` (measured in degrees and clockwise).
///
/// The last path point is described by (px, py).
///
/// The first curve begins from the last point in the path and the last ends
/// at `arcEndDelta.dx + px` and `arcEndDelta.dy + py`. The curves follow a
/// path in a direction determined by `clockwise` and `largeArc`
/// in such a way that the sweep angle is always less than 360 degrees.
///
/// A simple line is appended if either radii are zero, or, both
/// `arcEndDelta.dx` and `arcEndDelta.dy` are zero. The radii are scaled to
/// fit the last path point if both are greater than zero but too small to
/// describe an arc.
void relativeArcToPoint(
Offset arcEndDelta, {
Radius radius = Radius.zero,
double rotation = 0.0,
bool largeArc = false,
bool clockwise = true,
});
/// Adds a new sub-path that consists of four lines that outline the
/// given rectangle.
void addRect(Rect rect);
/// Adds a new sub-path that consists of a curve that forms the
/// ellipse that fills the given rectangle.
///
/// To add a circle, pass an appropriate rectangle as `oval`. [Rect.fromCircle]
/// can be used to easily describe the circle's center [Offset] and radius.
void addOval(Rect oval);
/// Adds a new sub-path with one arc segment that consists of the arc
/// that follows the edge of the oval bounded by the given
/// rectangle, from startAngle radians around the oval up to
/// startAngle + sweepAngle radians around the oval, with zero
/// radians being the point on the right hand side of the oval that
/// crosses the horizontal line that intersects the center of the
/// rectangle and with positive angles going clockwise around the
/// oval.
///
/// 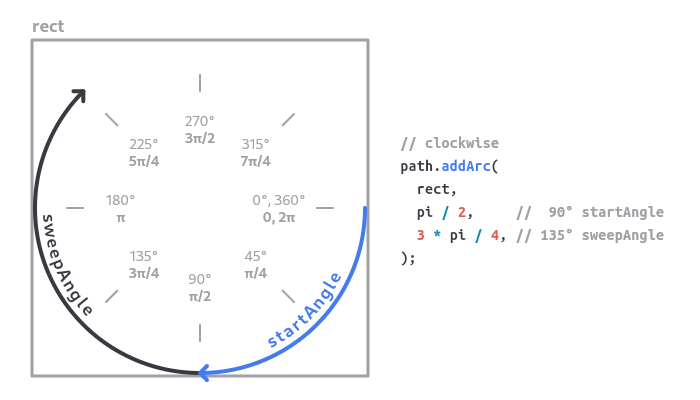
/// 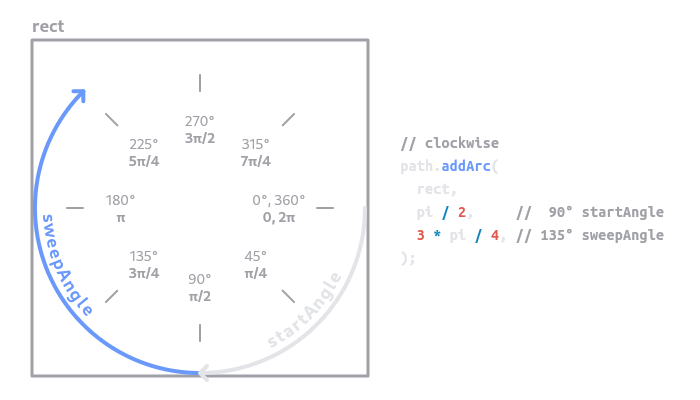
///
/// 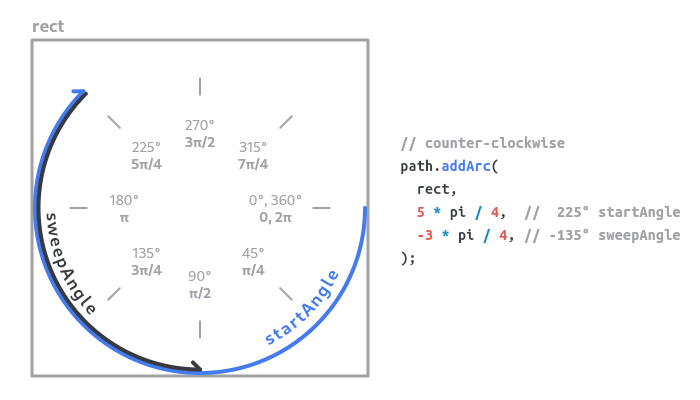
/// 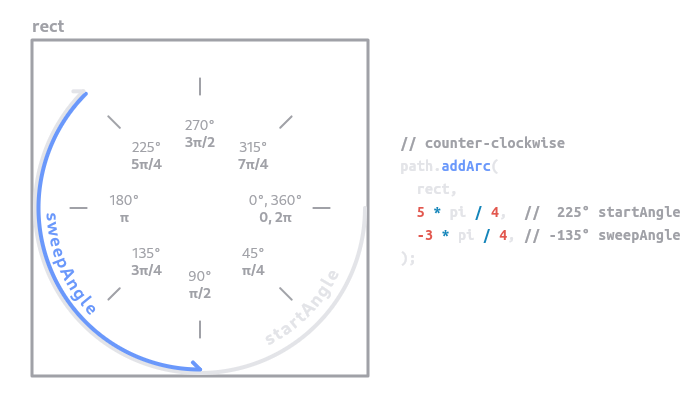
void addArc(Rect oval, double startAngle, double sweepAngle);
/// Adds a new sub-path with a sequence of line segments that connect the given
/// points.
///
/// If `close` is true, a final line segment will be added that connects the
/// last point to the first point.
///
/// The `points` argument is interpreted as offsets from the origin.
void addPolygon(List<Offset> points, bool close);
/// Adds a new sub-path that consists of the straight lines and
/// curves needed to form the rounded rectangle described by the
/// argument.
void addRRect(RRect rrect);
/// Adds the sub-paths of `path`, offset by `offset`, to this path.
///
/// If `matrix4` is specified, the path will be transformed by this matrix
/// after the matrix is translated by the given offset. The matrix is a 4x4
/// matrix stored in column major order.
void addPath(Path path, Offset offset, {Float64List? matrix4});
/// Adds the sub-paths of `path`, offset by `offset`, to this path.
/// The current sub-path is extended with the first sub-path
/// of `path`, connecting them with a lineTo if necessary.
///
/// If `matrix4` is specified, the path will be transformed by this matrix
/// after the matrix is translated by the given `offset`. The matrix is a 4x4
/// matrix stored in column major order.
void extendWithPath(Path path, Offset offset, {Float64List? matrix4});
/// Closes the last sub-path, as if a straight line had been drawn
/// from the current point to the first point of the sub-path.
void close();
/// Clears the [Path] object of all sub-paths, returning it to the
/// same state it had when it was created. The _current point_ is
/// reset to the origin.
void reset();
/// Tests to see if the given point is within the path. (That is, whether the
/// point would be in the visible portion of the path if the path was used
/// with [Canvas.clipPath].)
///
/// The `point` argument is interpreted as an offset from the origin.
///
/// Returns true if the point is in the path, and false otherwise.
bool contains(Offset point);
/// Returns a copy of the path with all the segments of every
/// sub-path translated by the given offset.
Path shift(Offset offset);
/// Returns a copy of the path with all the segments of every
/// sub-path transformed by the given matrix.
Path transform(Float64List matrix4);
/// Computes the bounding rectangle for this path.
///
/// A path containing only axis-aligned points on the same straight line will
/// have no area, and therefore `Rect.isEmpty` will return true for such a
/// path. Consider checking `rect.width + rect.height > 0.0` instead, or
/// using the [computeMetrics] API to check the path length.
///
/// For many more elaborate paths, the bounds may be inaccurate. For example,
/// when a path contains a circle, the points used to compute the bounds are
/// the circle's implied control points, which form a square around the circle;
/// if the circle has a transformation applied using [transform] then that
/// square is rotated, and the (axis-aligned, non-rotated) bounding box
/// therefore ends up grossly overestimating the actual area covered by the
/// circle.
// see https://skia.org/user/api/SkPath_Reference#SkPath_getBounds
Rect getBounds();
/// Combines the two paths according to the manner specified by the given
/// `operation`.
///
/// The resulting path will be constructed from non-overlapping contours. The
/// curve order is reduced where possible so that cubics may be turned into
/// quadratics, and quadratics maybe turned into lines.
static Path combine(PathOperation operation, Path path1, Path path2) {
final _NativePath path = _NativePath();
if (path._op(path1 as _NativePath, path2 as _NativePath, operation.index)) {
return path;
}
throw StateError('Path.combine() failed. This may be due an invalid path; in particular, check for NaN values.');
}
/// Creates a [PathMetrics] object for this path, which can describe various
/// properties about the contours of the path.
///
/// A [Path] is made up of zero or more contours. A contour is made up of
/// connected curves and segments, created via methods like [lineTo],
/// [cubicTo], [arcTo], [quadraticBezierTo], their relative counterparts, as
/// well as the add* methods such as [addRect]. Creating a new [Path] starts
/// a new contour once it has any drawing instructions, and another new
/// contour is started for each [moveTo] instruction.
///
/// A [PathMetric] object describes properties of an individual contour,
/// such as its length, whether it is closed, what the tangent vector of a
/// particular offset along the path is. It also provides a method for
/// creating sub-paths: [PathMetric.extractPath].
///
/// Calculating [PathMetric] objects is not trivial. The [PathMetrics] object
/// returned by this method is a lazy [Iterable], meaning it only performs
/// calculations when the iterator is moved to the next [PathMetric]. Callers
/// that wish to memoize this iterable can easily do so by using
/// [Iterable.toList] on the result of this method. In particular, callers
/// looking for information about how many contours are in the path should
/// either store the result of `path.computeMetrics().length`, or should use
/// `path.computeMetrics().toList()` so they can repeatedly check the length,
/// since calling `Iterable.length` causes traversal of the entire iterable.
///
/// In particular, callers should be aware that [PathMetrics.length] is the
/// number of contours, **not the length of the path**. To get the length of
/// a contour in a path, use [PathMetric.length].
///
/// If `forceClosed` is set to true, the contours of the path will be measured
/// as if they had been closed, even if they were not explicitly closed.
PathMetrics computeMetrics({bool forceClosed = false});
}
base class _NativePath extends NativeFieldWrapperClass1 implements Path {
/// Create a new empty [Path] object.
_NativePath() { _constructor(); }
/// Avoids creating a new native backing for the path for methods that will
/// create it later, such as [Path.from], [shift] and [transform].
_NativePath._();
@Native<Void Function(Handle)>(symbol: 'Path::Create')
external void _constructor();
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Path::clone')
external void _clone(Path outPath);
@override
PathFillType get fillType => PathFillType.values[_getFillType()];
@override
set fillType(PathFillType value) => _setFillType(value.index);
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Path::getFillType', isLeaf: true)
external int _getFillType();
@Native<Void Function(Pointer<Void>, Int32)>(symbol: 'Path::setFillType', isLeaf: true)
external void _setFillType(int fillType);
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Path::moveTo', isLeaf: true)
external void moveTo(double x, double y);
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Path::relativeMoveTo', isLeaf: true)
external void relativeMoveTo(double dx, double dy);
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Path::lineTo', isLeaf: true)
external void lineTo(double x, double y);
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Path::relativeLineTo', isLeaf: true)
external void relativeLineTo(double dx, double dy);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Path::quadraticBezierTo', isLeaf: true)
external void quadraticBezierTo(double x1, double y1, double x2, double y2);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Path::relativeQuadraticBezierTo', isLeaf: true)
external void relativeQuadraticBezierTo(
double x1, double y1, double x2, double y2);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double)>(symbol: 'Path::cubicTo', isLeaf: true)
external void cubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double)>(symbol: 'Path::relativeCubicTo', isLeaf: true)
external void relativeCubicTo(double x1, double y1, double x2, double y2, double x3, double y3);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double)>(symbol: 'Path::conicTo', isLeaf: true)
external void conicTo(double x1, double y1, double x2, double y2, double w);
@override
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double)>(symbol: 'Path::relativeConicTo', isLeaf: true)
external void relativeConicTo(double x1, double y1, double x2, double y2, double w);
@override
void arcTo(Rect rect, double startAngle, double sweepAngle, bool forceMoveTo) {
assert(_rectIsValid(rect));
_arcTo(rect.left, rect.top, rect.right, rect.bottom, startAngle, sweepAngle, forceMoveTo);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Bool)>(symbol: 'Path::arcTo', isLeaf: true)
external void _arcTo(double left, double top, double right, double bottom, double startAngle, double sweepAngle, bool forceMoveTo);
@override
void arcToPoint(Offset arcEnd, {
Radius radius = Radius.zero,
double rotation = 0.0,
bool largeArc = false,
bool clockwise = true,
}) {
assert(_offsetIsValid(arcEnd));
assert(_radiusIsValid(radius));
_arcToPoint(arcEnd.dx, arcEnd.dy, radius.x, radius.y, rotation, largeArc, clockwise);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Bool, Bool)>(symbol: 'Path::arcToPoint', isLeaf: true)
external void _arcToPoint(double arcEndX, double arcEndY, double radiusX, double radiusY, double rotation, bool largeArc, bool clockwise);
@override
void relativeArcToPoint(
Offset arcEndDelta, {
Radius radius = Radius.zero,
double rotation = 0.0,
bool largeArc = false,
bool clockwise = true,
}) {
assert(_offsetIsValid(arcEndDelta));
assert(_radiusIsValid(radius));
_relativeArcToPoint(arcEndDelta.dx, arcEndDelta.dy, radius.x, radius.y, rotation, largeArc, clockwise);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Bool, Bool)>(symbol: 'Path::relativeArcToPoint', isLeaf: true)
external void _relativeArcToPoint(
double arcEndX,
double arcEndY,
double radiusX,
double radiusY,
double rotation,
bool largeArc,
bool clockwise);
@override
void addRect(Rect rect) {
assert(_rectIsValid(rect));
_addRect(rect.left, rect.top, rect.right, rect.bottom);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Path::addRect', isLeaf: true)
external void _addRect(double left, double top, double right, double bottom);
@override
void addOval(Rect oval) {
assert(_rectIsValid(oval));
_addOval(oval.left, oval.top, oval.right, oval.bottom);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Path::addOval', isLeaf: true)
external void _addOval(double left, double top, double right, double bottom);
@override
void addArc(Rect oval, double startAngle, double sweepAngle) {
assert(_rectIsValid(oval));
_addArc(oval.left, oval.top, oval.right, oval.bottom, startAngle, sweepAngle);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double)>(symbol: 'Path::addArc', isLeaf: true)
external void _addArc(double left, double top, double right, double bottom, double startAngle, double sweepAngle);
@override
void addPolygon(List<Offset> points, bool close) {
_addPolygon(_encodePointList(points), close);
}
@Native<Void Function(Pointer<Void>, Handle, Bool)>(symbol: 'Path::addPolygon')
external void _addPolygon(Float32List points, bool close);
@override
void addRRect(RRect rrect) {
assert(_rrectIsValid(rrect));
_addRRect(rrect._getValue32());
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Path::addRRect')
external void _addRRect(Float32List rrect);
@override
void addPath(Path path, Offset offset, {Float64List? matrix4}) {
assert(_offsetIsValid(offset));
if (matrix4 != null) {
assert(_matrix4IsValid(matrix4));
_addPathWithMatrix(path as _NativePath, offset.dx, offset.dy, matrix4);
} else {
_addPath(path as _NativePath, offset.dx, offset.dy);
}
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>(symbol: 'Path::addPath')
external void _addPath(_NativePath path, double dx, double dy);
@Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>(symbol: 'Path::addPathWithMatrix')
external void _addPathWithMatrix(_NativePath path, double dx, double dy, Float64List matrix);
@override
void extendWithPath(Path path, Offset offset, {Float64List? matrix4}) {
assert(_offsetIsValid(offset));
if (matrix4 != null) {
assert(_matrix4IsValid(matrix4));
_extendWithPathAndMatrix(path as _NativePath, offset.dx, offset.dy, matrix4);
} else {
_extendWithPath(path as _NativePath, offset.dx, offset.dy);
}
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double)>(symbol: 'Path::extendWithPath')
external void _extendWithPath(_NativePath path, double dx, double dy);
@Native<Void Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle)>(symbol: 'Path::extendWithPathAndMatrix')
external void _extendWithPathAndMatrix(_NativePath path, double dx, double dy, Float64List matrix);
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'Path::close', isLeaf: true)
external void close();
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'Path::reset', isLeaf: true)
external void reset();
@override
bool contains(Offset point) {
assert(_offsetIsValid(point));
return _contains(point.dx, point.dy);
}
@Native<Bool Function(Pointer<Void>, Double, Double)>(symbol: 'Path::contains', isLeaf: true)
external bool _contains(double x, double y);
@override
Path shift(Offset offset) {
assert(_offsetIsValid(offset));
final _NativePath path = _NativePath._();
_shift(path, offset.dx, offset.dy);
return path;
}
@Native<Void Function(Pointer<Void>, Handle, Double, Double)>(symbol: 'Path::shift')
external void _shift(Path outPath, double dx, double dy);
@override
Path transform(Float64List matrix4) {
assert(_matrix4IsValid(matrix4));
final _NativePath path = _NativePath._();
_transform(path, matrix4);
return path;
}
@Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Path::transform')
external void _transform(Path outPath, Float64List matrix4);
@override
Rect getBounds() {
final Float32List rect = _getBounds();
return Rect.fromLTRB(rect[0], rect[1], rect[2], rect[3]);
}
@Native<Handle Function(Pointer<Void>)>(symbol: 'Path::getBounds')
external Float32List _getBounds();
@Native<Bool Function(Pointer<Void>, Pointer<Void>, Pointer<Void>, Int32)>(symbol: 'Path::op')
external bool _op(_NativePath path1, _NativePath path2, int operation);
@override
PathMetrics computeMetrics({bool forceClosed = false}) {
return PathMetrics._(this, forceClosed);
}
@override
String toString() => 'Path';
}
/// The geometric description of a tangent: the angle at a point.
///
/// See also:
/// * [PathMetric.getTangentForOffset], which returns the tangent of an offset along a path.
class Tangent {
/// Creates a [Tangent] with the given values.
///
/// The arguments must not be null.
const Tangent(this.position, this.vector);
/// Creates a [Tangent] based on the angle rather than the vector.
///
/// The [vector] is computed to be the unit vector at the given angle, interpreted
/// as clockwise radians from the x axis.
factory Tangent.fromAngle(Offset position, double angle) {
return Tangent(position, Offset(math.cos(angle), math.sin(angle)));
}
/// Position of the tangent.
///
/// When used with [PathMetric.getTangentForOffset], this represents the precise
/// position that the given offset along the path corresponds to.
final Offset position;
/// The vector of the curve at [position].
///
/// When used with [PathMetric.getTangentForOffset], this is the vector of the
/// curve that is at the given offset along the path (i.e. the direction of the
/// curve at [position]).
final Offset vector;
/// The direction of the curve at [position].
///
/// When used with [PathMetric.getTangentForOffset], this is the angle of the
/// curve that is the given offset along the path (i.e. the direction of the
/// curve at [position]).
///
/// This value is in radians, with 0.0 meaning pointing along the x axis in
/// the positive x-axis direction, positive numbers pointing downward toward
/// the negative y-axis, i.e. in a clockwise direction, and negative numbers
/// pointing upward toward the positive y-axis, i.e. in a counter-clockwise
/// direction.
// flip the sign to be consistent with [Path.arcTo]'s `sweepAngle`
double get angle => -math.atan2(vector.dy, vector.dx);
}
/// An iterable collection of [PathMetric] objects describing a [Path].
///
/// A [PathMetrics] object is created by using the [Path.computeMetrics] method,
/// and represents the path as it stood at the time of the call. Subsequent
/// modifications of the path do not affect the [PathMetrics] object.
///
/// Each path metric corresponds to a segment, or contour, of a path.
///
/// For example, a path consisting of a [Path.lineTo], a [Path.moveTo], and
/// another [Path.lineTo] will contain two contours and thus be represented by
/// two [PathMetric] objects.
///
/// This iterable does not memoize. Callers who need to traverse the list
/// multiple times, or who need to randomly access elements of the list, should
/// use [toList] on this object.
class PathMetrics extends collection.IterableBase<PathMetric> {
PathMetrics._(Path path, bool forceClosed) :
_iterator = PathMetricIterator._(_PathMeasure(path as _NativePath, forceClosed));
final Iterator<PathMetric> _iterator;
@override
Iterator<PathMetric> get iterator => _iterator;
}
/// Used by [PathMetrics] to track iteration from one segment of a path to the
/// next for measurement.
class PathMetricIterator implements Iterator<PathMetric> {
PathMetricIterator._(this._pathMeasure);
PathMetric? _pathMetric;
final _PathMeasure _pathMeasure;
@override
PathMetric get current {
final PathMetric? currentMetric = _pathMetric;
if (currentMetric == null) {
throw RangeError(
'PathMetricIterator is not pointing to a PathMetric. This can happen in two situations:\n'
'- The iteration has not started yet. If so, call "moveNext" to start iteration.\n'
'- The iterator ran out of elements. If so, check that "moveNext" returns true prior to calling "current".'
);
}
return currentMetric;
}
@override
bool moveNext() {
if (_pathMeasure._nextContour()) {
_pathMetric = PathMetric._(_pathMeasure);
return true;
}
_pathMetric = null;
return false;
}
}
/// Utilities for measuring a [Path] and extracting sub-paths.
///
/// Iterate over the object returned by [Path.computeMetrics] to obtain
/// [PathMetric] objects. Callers that want to randomly access elements or
/// iterate multiple times should use `path.computeMetrics().toList()`, since
/// [PathMetrics] does not memoize.
///
/// Once created, the metrics are only valid for the path as it was specified
/// when [Path.computeMetrics] was called. If additional contours are added or
/// any contours are updated, the metrics need to be recomputed. Previously
/// created metrics will still refer to a snapshot of the path at the time they
/// were computed, rather than to the actual metrics for the new mutations to
/// the path.
class PathMetric {
PathMetric._(this._measure)
: length = _measure.length(_measure.currentContourIndex),
isClosed = _measure.isClosed(_measure.currentContourIndex),
contourIndex = _measure.currentContourIndex;
/// Return the total length of the current contour.
///
/// The length may be calculated from an approximation of the geometry
/// originally added. For this reason, it is not recommended to rely on
/// this property for mathematically correct lengths of common shapes.
final double length;
/// Whether the contour is closed.
///
/// Returns true if the contour ends with a call to [Path.close] (which may
/// have been implied when using methods like [Path.addRect]) or if
/// `forceClosed` was specified as true in the call to [Path.computeMetrics].
/// Returns false otherwise.
final bool isClosed;
/// The zero-based index of the contour.
///
/// [Path] objects are made up of zero or more contours. The first contour is
/// created once a drawing command (e.g. [Path.lineTo]) is issued. A
/// [Path.moveTo] command after a drawing command may create a new contour,
/// although it may not if optimizations are applied that determine the move
/// command did not actually result in moving the pen.
///
/// This property is only valid with reference to its original iterator and
/// the contours of the path at the time the path's metrics were computed. If
/// additional contours were added or existing contours updated, this metric
/// will be invalid for the current state of the path.
final int contourIndex;
final _PathMeasure _measure;
/// Computes the position of the current contour at the given offset, and the
/// angle of the path at that point.
///
/// For example, calling this method with a distance of 1.41 for a line from
/// 0.0,0.0 to 2.0,2.0 would give a point 1.0,1.0 and the angle 45 degrees
/// (but in radians).
///
/// Returns null if the contour has zero [length].
///
/// The distance is clamped to the [length] of the current contour.
Tangent? getTangentForOffset(double distance) {
return _measure.getTangentForOffset(contourIndex, distance);
}
/// Given a start and end distance, return the intervening segment(s).
///
/// `start` and `end` are clamped to legal values (0..[length])
/// Begin the segment with a moveTo if `startWithMoveTo` is true.
Path extractPath(double start, double end, {bool startWithMoveTo = true}) {
return _measure.extractPath(contourIndex, start, end, startWithMoveTo: startWithMoveTo);
}
@override
String toString() => 'PathMetric(length: $length, isClosed: $isClosed, contourIndex: $contourIndex)';
}
base class _PathMeasure extends NativeFieldWrapperClass1 {
_PathMeasure(_NativePath path, bool forceClosed) {
_constructor(path, forceClosed);
}
@Native<Void Function(Handle, Pointer<Void>, Bool)>(symbol: 'PathMeasure::Create')
external void _constructor(_NativePath path, bool forceClosed);
double length(int contourIndex) {
assert(contourIndex <= currentContourIndex, 'Iterator must be advanced before index $contourIndex can be used.');
return _length(contourIndex);
}
@Native<Double Function(Pointer<Void>, Int32)>(symbol: 'PathMeasure::getLength', isLeaf: true)
external double _length(int contourIndex);
Tangent? getTangentForOffset(int contourIndex, double distance) {
assert(contourIndex <= currentContourIndex,
'Iterator must be advanced before index $contourIndex can be used.');
final Float32List posTan = _getPosTan(contourIndex, distance);
// first entry == 0 indicates that Skia returned false
if (posTan[0] == 0.0) {
return null;
} else {
return Tangent(
Offset(posTan[1], posTan[2]),
Offset(posTan[3], posTan[4])
);
}
}
@Native<Handle Function(Pointer<Void>, Int32, Double)>(symbol: 'PathMeasure::getPosTan')
external Float32List _getPosTan(int contourIndex, double distance);
Path extractPath(int contourIndex, double start, double end,
{bool startWithMoveTo = true}) {
assert(contourIndex <= currentContourIndex, 'Iterator must be advanced before index $contourIndex can be used.');
final _NativePath path = _NativePath._();
_extractPath(path, contourIndex, start, end, startWithMoveTo);
return path;
}
@Native<Void Function(Pointer<Void>, Handle, Int32, Double, Double, Bool)>(symbol: 'PathMeasure::getSegment')
external void _extractPath(Path outPath, int contourIndex, double start, double end, bool startWithMoveTo);
bool isClosed(int contourIndex) {
assert(contourIndex <= currentContourIndex, 'Iterator must be advanced before index $contourIndex can be used.');
return _isClosed(contourIndex);
}
@Native<Bool Function(Pointer<Void>, Int32)>(symbol: 'PathMeasure::isClosed', isLeaf: true)
external bool _isClosed(int contourIndex);
// Move to the next contour in the path.
//
// A path can have a next contour if [Path.moveTo] was called after drawing began.
// Return true if one exists, or false.
bool _nextContour() {
final bool next = _nativeNextContour();
if (next) {
currentContourIndex++;
}
return next;
}
@Native<Bool Function(Pointer<Void>)>(symbol: 'PathMeasure::nextContour', isLeaf: true)
external bool _nativeNextContour();
/// The index of the current contour in the list of contours in the path.
///
/// [nextContour] will increment this to the zero based index.
int currentContourIndex = -1;
}
/// Styles to use for blurs in [MaskFilter] objects.
// These enum values must be kept in sync with DlBlurStyle.
enum BlurStyle {
// These mirror DlBlurStyle and must be kept in sync.
/// Fuzzy inside and outside. This is useful for painting shadows that are
/// offset from the shape that ostensibly is casting the shadow.
normal,
/// Solid inside, fuzzy outside. This corresponds to drawing the shape, and
/// additionally drawing the blur. This can make objects appear brighter,
/// maybe even as if they were fluorescent.
solid,
/// Nothing inside, fuzzy outside. This is useful for painting shadows for
/// partially transparent shapes, when they are painted separately but without
/// an offset, so that the shadow doesn't paint below the shape.
outer,
/// Fuzzy inside, nothing outside. This can make shapes appear to be lit from
/// within.
inner,
}
/// A mask filter to apply to shapes as they are painted. A mask filter is a
/// function that takes a bitmap of color pixels, and returns another bitmap of
/// color pixels.
///
/// Instances of this class are used with [Paint.maskFilter] on [Paint] objects.
class MaskFilter {
/// Creates a mask filter that takes the shape being drawn and blurs it.
///
/// This is commonly used to approximate shadows.
///
/// The `style` argument controls the kind of effect to draw; see [BlurStyle].
///
/// The `sigma` argument controls the size of the effect. It is the standard
/// deviation of the Gaussian blur to apply. The value must be greater than
/// zero. The sigma corresponds to very roughly half the radius of the effect
/// in pixels.
///
/// A blur is an expensive operation and should therefore be used sparingly.
///
/// The arguments must not be null.
///
/// See also:
///
/// * [Canvas.drawShadow], which is a more efficient way to draw shadows.
const MaskFilter.blur(
this._style,
this._sigma,
);
final BlurStyle _style;
final double _sigma;
// The type of MaskFilter class to create for flutter::DisplayList.
// These constants must be kept in sync with MaskFilterType in paint.cc.
static const int _TypeNone = 0; // null
static const int _TypeBlur = 1; // DlBlurMaskFilter
@override
bool operator ==(Object other) {
return other is MaskFilter
&& other._style == _style
&& other._sigma == _sigma;
}
@override
int get hashCode => Object.hash(_style, _sigma);
@override
String toString() => 'MaskFilter.blur($_style, ${_sigma.toStringAsFixed(1)})';
}
/// A description of a color filter to apply when drawing a shape or compositing
/// a layer with a particular [Paint]. A color filter is a function that takes
/// two colors, and outputs one color. When applied during compositing, it is
/// independently applied to each pixel of the layer being drawn before the
/// entire layer is merged with the destination.
///
/// Instances of this class are used with [Paint.colorFilter] on [Paint]
/// objects.
class ColorFilter implements ImageFilter {
/// Creates a color filter that applies the blend mode given as the second
/// argument. The source color is the one given as the first argument, and the
/// destination color is the one from the layer being composited.
///
/// The output of this filter is then composited into the background according
/// to the [Paint.blendMode], using the output of this filter as the source
/// and the background as the destination.
const ColorFilter.mode(Color color, BlendMode blendMode)
: _color = color,
_blendMode = blendMode,
_matrix = null,
_type = _kTypeMode;
/// Construct a color filter from a 4x5 row-major matrix. The matrix is
/// interpreted as a 5x5 matrix, where the fifth row is the identity
/// configuration.
///
/// Every pixel's color value, represented as an `[R, G, B, A]`, is matrix
/// multiplied to create a new color:
///
/// | R' | | a00 a01 a02 a03 a04 | | R |
/// | G' | | a10 a11 a12 a13 a14 | | G |
/// | B' | = | a20 a21 a22 a23 a24 | * | B |
/// | A' | | a30 a31 a32 a33 a34 | | A |
/// | 1 | | 0 0 0 0 1 | | 1 |
///
/// The matrix is in row-major order and the translation column is specified
/// in unnormalized, 0...255, space. For example, the identity matrix is:
///
/// ```dart
/// const ColorFilter identity = ColorFilter.matrix(<double>[
/// 1, 0, 0, 0, 0,
/// 0, 1, 0, 0, 0,
/// 0, 0, 1, 0, 0,
/// 0, 0, 0, 1, 0,
/// ]);
/// ```
///
/// ## Examples
///
/// An inversion color matrix:
///
/// ```dart
/// const ColorFilter invert = ColorFilter.matrix(<double>[
/// -1, 0, 0, 0, 255,
/// 0, -1, 0, 0, 255,
/// 0, 0, -1, 0, 255,
/// 0, 0, 0, 1, 0,
/// ]);
/// ```
///
/// A sepia-toned color matrix (values based on the [Filter Effects Spec](https://www.w3.org/TR/filter-effects-1/#sepiaEquivalent)):
///
/// ```dart
/// const ColorFilter sepia = ColorFilter.matrix(<double>[
/// 0.393, 0.769, 0.189, 0, 0,
/// 0.349, 0.686, 0.168, 0, 0,
/// 0.272, 0.534, 0.131, 0, 0,
/// 0, 0, 0, 1, 0,
/// ]);
/// ```
///
/// A greyscale color filter (values based on the [Filter Effects Spec](https://www.w3.org/TR/filter-effects-1/#grayscaleEquivalent)):
///
/// ```dart
/// const ColorFilter greyscale = ColorFilter.matrix(<double>[
/// 0.2126, 0.7152, 0.0722, 0, 0,
/// 0.2126, 0.7152, 0.0722, 0, 0,
/// 0.2126, 0.7152, 0.0722, 0, 0,
/// 0, 0, 0, 1, 0,
/// ]);
/// ```
const ColorFilter.matrix(List<double> matrix)
: _color = null,
_blendMode = null,
_matrix = matrix,
_type = _kTypeMatrix;
/// Construct a color filter that applies the sRGB gamma curve to the RGB
/// channels.
const ColorFilter.linearToSrgbGamma()
: _color = null,
_blendMode = null,
_matrix = null,
_type = _kTypeLinearToSrgbGamma;
/// Creates a color filter that applies the inverse of the sRGB gamma curve
/// to the RGB channels.
const ColorFilter.srgbToLinearGamma()
: _color = null,
_blendMode = null,
_matrix = null,
_type = _kTypeSrgbToLinearGamma;
final Color? _color;
final BlendMode? _blendMode;
final List<double>? _matrix;
final int _type;
// The type of DlColorFilter class to create.
static const int _kTypeMode = 1; // MakeModeFilter
static const int _kTypeMatrix = 2; // MakeMatrixFilterRowMajor255
static const int _kTypeLinearToSrgbGamma = 3; // MakeLinearToSRGBGamma
static const int _kTypeSrgbToLinearGamma = 4; // MakeSRGBToLinearGamma
// DlColorImageFilter
@override
_ImageFilter _toNativeImageFilter() => _ImageFilter.fromColorFilter(this);
_ColorFilter? _toNativeColorFilter() {
switch (_type) {
case _kTypeMode:
if (_color == null || _blendMode == null) {
return null;
}
return _ColorFilter.mode(this);
case _kTypeMatrix:
final List<double>? matrix = _matrix;
if (matrix == null) {
return null;
}
assert(matrix.length == 20, 'Color Matrix must have 20 entries.');
return _ColorFilter.matrix(this);
case _kTypeLinearToSrgbGamma:
return _ColorFilter.linearToSrgbGamma(this);
case _kTypeSrgbToLinearGamma:
return _ColorFilter.srgbToLinearGamma(this);
default:
throw StateError('Unknown mode $_type for ColorFilter.');
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ColorFilter
&& other._type == _type
&& _listEquals<double>(other._matrix, _matrix)
&& other._color == _color
&& other._blendMode == _blendMode;
}
@override
int get hashCode {
final List<double>? matrix = _matrix;
return Object.hash(_color, _blendMode, matrix == null ? null : Object.hashAll(matrix), _type);
}
@override
String get _shortDescription {
switch (_type) {
case _kTypeMode:
return 'ColorFilter.mode($_color, $_blendMode)';
case _kTypeMatrix:
return 'ColorFilter.matrix($_matrix)';
case _kTypeLinearToSrgbGamma:
return 'ColorFilter.linearToSrgbGamma()';
case _kTypeSrgbToLinearGamma:
return 'ColorFilter.srgbToLinearGamma()';
default:
return 'unknow ColorFilter';
}
}
@override
String toString() {
switch (_type) {
case _kTypeMode:
return 'ColorFilter.mode($_color, $_blendMode)';
case _kTypeMatrix:
return 'ColorFilter.matrix($_matrix)';
case _kTypeLinearToSrgbGamma:
return 'ColorFilter.linearToSrgbGamma()';
case _kTypeSrgbToLinearGamma:
return 'ColorFilter.srgbToLinearGamma()';
default:
return "Unknown ColorFilter type. This is an error. If you're seeing this, please file an issue at https://github.com/flutter/flutter/issues/new.";
}
}
}
/// A [ColorFilter] that is backed by a native DlColorFilter.
///
/// This is a private class, rather than being the implementation of the public
/// ColorFilter, because we want ColorFilter to be const constructible and
/// efficiently comparable, so that widgets can check for ColorFilter equality to
/// avoid repainting.
base class _ColorFilter extends NativeFieldWrapperClass1 {
_ColorFilter.mode(this.creator)
: assert(creator._type == ColorFilter._kTypeMode) {
_constructor();
_initMode(creator._color!.value, creator._blendMode!.index);
}
_ColorFilter.matrix(this.creator)
: assert(creator._type == ColorFilter._kTypeMatrix) {
_constructor();
_initMatrix(Float32List.fromList(creator._matrix!));
}
_ColorFilter.linearToSrgbGamma(this.creator)
: assert(creator._type == ColorFilter._kTypeLinearToSrgbGamma) {
_constructor();
_initLinearToSrgbGamma();
}
_ColorFilter.srgbToLinearGamma(this.creator)
: assert(creator._type == ColorFilter._kTypeSrgbToLinearGamma) {
_constructor();
_initSrgbToLinearGamma();
}
/// The original Dart object that created the native wrapper, which retains
/// the values used for the filter.
final ColorFilter creator;
@Native<Void Function(Handle)>(symbol: 'ColorFilter::Create')
external void _constructor();
@Native<Void Function(Pointer<Void>, Int32, Int32)>(symbol: 'ColorFilter::initMode', isLeaf: true)
external void _initMode(int color, int blendMode);
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'ColorFilter::initMatrix')
external void _initMatrix(Float32List matrix);
@Native<Void Function(Pointer<Void>)>(symbol: 'ColorFilter::initLinearToSrgbGamma', isLeaf: true)
external void _initLinearToSrgbGamma();
@Native<Void Function(Pointer<Void>)>(symbol: 'ColorFilter::initSrgbToLinearGamma', isLeaf: true)
external void _initSrgbToLinearGamma();
}
/// A filter operation to apply to a raster image.
///
/// See also:
///
/// * [BackdropFilter], a widget that applies [ImageFilter] to its rendering.
/// * [ImageFiltered], a widget that applies [ImageFilter] to its children.
/// * [SceneBuilder.pushBackdropFilter], which is the low-level API for using
/// this class as a backdrop filter.
/// * [SceneBuilder.pushImageFilter], which is the low-level API for using
/// this class as a child layer filter.
abstract class ImageFilter {
// This class is not meant to be extended; this constructor prevents extension.
ImageFilter._(); // ignore: unused_element
/// Creates an image filter that applies a Gaussian blur.
factory ImageFilter.blur({ double sigmaX = 0.0, double sigmaY = 0.0, TileMode tileMode = TileMode.clamp }) {
return _GaussianBlurImageFilter(sigmaX: sigmaX, sigmaY: sigmaY, tileMode: tileMode);
}
/// Creates an image filter that dilates each input pixel's channel values
/// to the max value within the given radii along the x and y axes.
factory ImageFilter.dilate({ double radiusX = 0.0, double radiusY = 0.0 }) {
return _DilateImageFilter(radiusX: radiusX, radiusY: radiusY);
}
/// Create a filter that erodes each input pixel's channel values
/// to the minimum channel value within the given radii along the x and y axes.
factory ImageFilter.erode({ double radiusX = 0.0, double radiusY = 0.0 }) {
return _ErodeImageFilter(radiusX: radiusX, radiusY: radiusY);
}
/// Creates an image filter that applies a matrix transformation.
///
/// For example, applying a positive scale matrix (see [Matrix4.diagonal3])
/// when used with [BackdropFilter] would magnify the background image.
factory ImageFilter.matrix(Float64List matrix4,
{ FilterQuality filterQuality = FilterQuality.low }) {
if (matrix4.length != 16) {
throw ArgumentError('"matrix4" must have 16 entries.');
}
return _MatrixImageFilter(data: Float64List.fromList(matrix4), filterQuality: filterQuality);
}
/// Composes the `inner` filter with `outer`, to combine their effects.
///
/// Creates a single [ImageFilter] that when applied, has the same effect as
/// subsequently applying `inner` and `outer`, i.e.,
/// result = outer(inner(source)).
factory ImageFilter.compose({ required ImageFilter outer, required ImageFilter inner }) {
return _ComposeImageFilter(innerFilter: inner, outerFilter: outer);
}
// Converts this to a native DlImageFilter. See the comments of this method in
// subclasses for the exact type of DlImageFilter this method converts to.
_ImageFilter _toNativeImageFilter();
// The description text to show when the filter is part of a composite
// [ImageFilter] created using [ImageFilter.compose].
String get _shortDescription;
}
class _MatrixImageFilter implements ImageFilter {
_MatrixImageFilter({ required this.data, required this.filterQuality });
final Float64List data;
final FilterQuality filterQuality;
// MakeMatrixFilterRowMajor255
late final _ImageFilter nativeFilter = _ImageFilter.matrix(this);
@override
_ImageFilter _toNativeImageFilter() => nativeFilter;
@override
String get _shortDescription => 'matrix($data, $filterQuality)';
@override
String toString() => 'ImageFilter.matrix($data, $filterQuality)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _MatrixImageFilter
&& other.filterQuality == filterQuality
&& _listEquals<double>(other.data, data);
}
@override
int get hashCode => Object.hash(filterQuality, Object.hashAll(data));
}
class _GaussianBlurImageFilter implements ImageFilter {
_GaussianBlurImageFilter({ required this.sigmaX, required this.sigmaY, required this.tileMode });
final double sigmaX;
final double sigmaY;
final TileMode tileMode;
// MakeBlurFilter
late final _ImageFilter nativeFilter = _ImageFilter.blur(this);
@override
_ImageFilter _toNativeImageFilter() => nativeFilter;
String get _modeString {
switch (tileMode) {
case TileMode.clamp: return 'clamp';
case TileMode.mirror: return 'mirror';
case TileMode.repeated: return 'repeated';
case TileMode.decal: return 'decal';
}
}
@override
String get _shortDescription => 'blur($sigmaX, $sigmaY, $_modeString)';
@override
String toString() => 'ImageFilter.blur($sigmaX, $sigmaY, $_modeString)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _GaussianBlurImageFilter
&& other.sigmaX == sigmaX
&& other.sigmaY == sigmaY
&& other.tileMode == tileMode;
}
@override
int get hashCode => Object.hash(sigmaX, sigmaY);
}
class _DilateImageFilter implements ImageFilter {
_DilateImageFilter({ required this.radiusX, required this.radiusY });
final double radiusX;
final double radiusY;
late final _ImageFilter nativeFilter = _ImageFilter.dilate(this);
@override
_ImageFilter _toNativeImageFilter() => nativeFilter;
@override
String get _shortDescription => 'dilate($radiusX, $radiusY)';
@override
String toString() => 'ImageFilter.dilate($radiusX, $radiusY)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _DilateImageFilter
&& other.radiusX == radiusX
&& other.radiusY == radiusY;
}
@override
int get hashCode => Object.hash(radiusX, radiusY);
}
class _ErodeImageFilter implements ImageFilter {
_ErodeImageFilter({ required this.radiusX, required this.radiusY });
final double radiusX;
final double radiusY;
late final _ImageFilter nativeFilter = _ImageFilter.erode(this);
@override
_ImageFilter _toNativeImageFilter() => nativeFilter;
@override
String get _shortDescription => 'erode($radiusX, $radiusY)';
@override
String toString() => 'ImageFilter.erode($radiusX, $radiusY)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _ErodeImageFilter
&& other.radiusX == radiusX
&& other.radiusY == radiusY;
}
@override
int get hashCode => Object.hash(radiusX, radiusY);
}
class _ComposeImageFilter implements ImageFilter {
_ComposeImageFilter({ required this.innerFilter, required this.outerFilter });
final ImageFilter innerFilter;
final ImageFilter outerFilter;
// DlComposeImageFilter
late final _ImageFilter nativeFilter = _ImageFilter.composed(this);
@override
_ImageFilter _toNativeImageFilter() => nativeFilter;
@override
String get _shortDescription => '${innerFilter._shortDescription} -> ${outerFilter._shortDescription}';
@override
String toString() => 'ImageFilter.compose(source -> $_shortDescription -> result)';
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _ComposeImageFilter
&& other.innerFilter == innerFilter
&& other.outerFilter == outerFilter;
}
@override
int get hashCode => Object.hash(innerFilter, outerFilter);
}
/// An [ImageFilter] that is backed by a native DlImageFilter.
///
/// This is a private class, rather than being the implementation of the public
/// ImageFilter, because we want ImageFilter to be efficiently comparable, so that
/// widgets can check for ImageFilter equality to avoid repainting.
base class _ImageFilter extends NativeFieldWrapperClass1 {
/// Creates an image filter that applies a Gaussian blur.
_ImageFilter.blur(_GaussianBlurImageFilter filter)
: creator = filter {
_constructor();
_initBlur(filter.sigmaX, filter.sigmaY, filter.tileMode.index);
}
/// Creates an image filter that dilates each input pixel's channel values
/// to the max value within the given radii along the x and y axes.
_ImageFilter.dilate(_DilateImageFilter filter)
: creator = filter {
_constructor();
_initDilate(filter.radiusX, filter.radiusY);
}
/// Create a filter that erodes each input pixel's channel values
/// to the minimum channel value within the given radii along the x and y axes.
_ImageFilter.erode(_ErodeImageFilter filter)
: creator = filter {
_constructor();
_initErode(filter.radiusX, filter.radiusY);
}
/// Creates an image filter that applies a matrix transformation.
///
/// For example, applying a positive scale matrix (see [Matrix4.diagonal3])
/// when used with [BackdropFilter] would magnify the background image.
_ImageFilter.matrix(_MatrixImageFilter filter)
: creator = filter {
if (filter.data.length != 16) {
throw ArgumentError('"matrix4" must have 16 entries.');
}
_constructor();
_initMatrix(filter.data, filter.filterQuality.index);
}
/// Converts a color filter to an image filter.
_ImageFilter.fromColorFilter(ColorFilter filter)
: creator = filter {
_constructor();
final _ColorFilter? nativeFilter = filter._toNativeColorFilter();
_initColorFilter(nativeFilter);
}
/// Composes `_innerFilter` with `_outerFilter`.
_ImageFilter.composed(_ComposeImageFilter filter)
: creator = filter {
_constructor();
final _ImageFilter nativeFilterInner = filter.innerFilter._toNativeImageFilter();
final _ImageFilter nativeFilterOuter = filter.outerFilter._toNativeImageFilter();
_initComposed(nativeFilterOuter, nativeFilterInner);
}
@Native<Void Function(Handle)>(symbol: 'ImageFilter::Create')
external void _constructor();
@Native<Void Function(Pointer<Void>, Double, Double, Int32)>(symbol: 'ImageFilter::initBlur', isLeaf: true)
external void _initBlur(double sigmaX, double sigmaY, int tileMode);
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'ImageFilter::initDilate', isLeaf: true)
external void _initDilate(double radiusX, double radiusY);
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'ImageFilter::initErode', isLeaf: true)
external void _initErode(double radiusX, double radiusY);
@Native<Void Function(Pointer<Void>, Handle, Int32)>(symbol: 'ImageFilter::initMatrix')
external void _initMatrix(Float64List matrix4, int filterQuality);
@Native<Void Function(Pointer<Void>, Pointer<Void>)>(symbol: 'ImageFilter::initColorFilter')
external void _initColorFilter(_ColorFilter? colorFilter);
@Native<Void Function(Pointer<Void>, Pointer<Void>, Pointer<Void>)>(symbol: 'ImageFilter::initComposeFilter')
external void _initComposed(_ImageFilter outerFilter, _ImageFilter innerFilter);
/// The original Dart object that created the native wrapper, which retains
/// the values used for the filter.
final ImageFilter creator;
}
/// Base class for objects such as [Gradient] and [ImageShader] which
/// correspond to shaders as used by [Paint.shader].
base class Shader extends NativeFieldWrapperClass1 {
/// This class is created by the engine, and should not be instantiated
/// or extended directly.
@pragma('vm:entry-point')
Shader._();
bool _debugDisposed = false;
/// Whether [dispose] has been called.
///
/// This must only be used when asserts are enabled. Otherwise, it will throw.
bool get debugDisposed {
late bool disposed;
assert(() {
disposed = _debugDisposed;
return true;
}());
return disposed;
}
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// The underlying memory allocated by this object will be retained beyond
/// this call if it is still needed by another object that has not been
/// disposed. For example, a [Picture] that has not been disposed that
/// refers to an [ImageShader] may keep its underlying resources alive.
///
/// Classes that override this method must call `super.dispose()`.
void dispose() {
assert(() {
assert(!_debugDisposed);
_debugDisposed = true;
return true;
}());
}
}
/// Defines what happens at the edge of a gradient or the sampling of a source image
/// in an [ImageFilter].
///
/// A gradient is defined along a finite inner area. In the case of a linear
/// gradient, it's between the parallel lines that are orthogonal to the line
/// drawn between two points. In the case of radial gradients, it's the disc
/// that covers the circle centered on a particular point up to a given radius.
///
/// An image filter reads source samples from a source image and performs operations
/// on those samples to produce a result image. An image defines color samples only
/// for pixels within the bounds of the image but some filter operations, such as a blur
/// filter, read samples over a wide area to compute the output for a given pixel. Such
/// a filter would need to combine samples from inside the image with hypothetical
/// color values from outside the image.
///
/// This enum is used to define how the gradient or image filter should treat the regions
/// outside that defined inner area.
///
/// See also:
///
/// * [painting.Gradient], the superclass for [LinearGradient] and
/// [RadialGradient], as used by [BoxDecoration] et al, which works in
/// relative coordinates and can create a [Shader] representing the gradient
/// for a particular [Rect] on demand.
/// * [dart:ui.Gradient], the low-level class used when dealing with the
/// [Paint.shader] property directly, with its [Gradient.linear] and
/// [Gradient.radial] constructors.
/// * [dart:ui.ImageFilter.blur], an ImageFilter that may sometimes need to
/// read samples from outside an image to combine with the pixels near the
/// edge of the image.
// These enum values must be kept in sync with DlTileMode.
enum TileMode {
/// Samples beyond the edge are clamped to the nearest color in the defined inner area.
///
/// A gradient will paint all the regions outside the inner area with the
/// color at the end of the color stop list closest to that region.
///
/// An image filter will substitute the nearest edge pixel for any samples taken from
/// outside its source image.
///
/// 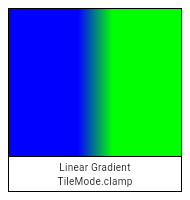
/// 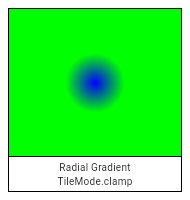
/// 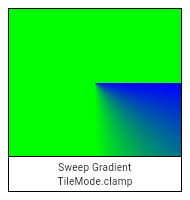
clamp,
/// Samples beyond the edge are repeated from the far end of the defined area.
///
/// For a gradient, this technique is as if the stop points from 0.0 to 1.0 were then
/// repeated from 1.0 to 2.0, 2.0 to 3.0, and so forth (and for linear gradients, similarly
/// from -1.0 to 0.0, -2.0 to -1.0, etc).
///
/// An image filter will treat its source image as if it were tiled across the enlarged
/// sample space from which it reads, each tile in the same orientation as the base image.
///
/// 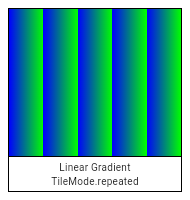
/// 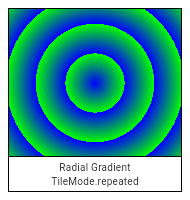
/// 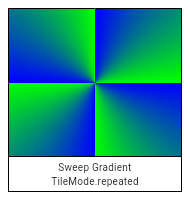
repeated,
/// Samples beyond the edge are mirrored back and forth across the defined area.
///
/// For a gradient, this technique is as if the stop points from 0.0 to 1.0 were then
/// repeated backwards from 2.0 to 1.0, then forwards from 2.0 to 3.0, then backwards
/// again from 4.0 to 3.0, and so forth (and for linear gradients, similarly in the
/// negative direction).
///
/// An image filter will treat its source image as tiled in an alternating forwards and
/// backwards or upwards and downwards direction across the sample space from which
/// it is reading.
///
/// 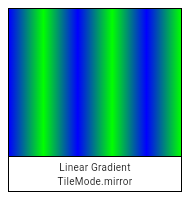
/// 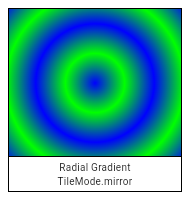
/// 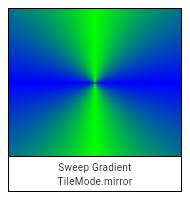
mirror,
/// Samples beyond the edge are treated as transparent black.
///
/// A gradient will render transparency over any region that is outside the circle of a
/// radial gradient or outside the parallel lines that define the inner area of a linear
/// gradient.
///
/// An image filter will substitute transparent black for any sample it must read from
/// outside its source image.
///
/// 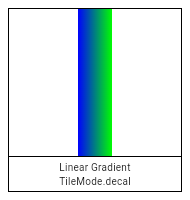
/// 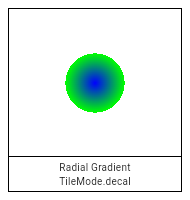
/// 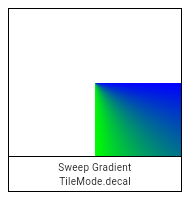
decal,
}
Int32List _encodeColorList(List<Color> colors) {
final int colorCount = colors.length;
final Int32List result = Int32List(colorCount);
for (int i = 0; i < colorCount; ++i) {
result[i] = colors[i].value;
}
return result;
}
Float32List _encodePointList(List<Offset> points) {
final int pointCount = points.length;
final Float32List result = Float32List(pointCount * 2);
for (int i = 0; i < pointCount; ++i) {
final int xIndex = i * 2;
final int yIndex = xIndex + 1;
final Offset point = points[i];
assert(_offsetIsValid(point));
result[xIndex] = point.dx;
result[yIndex] = point.dy;
}
return result;
}
Float32List _encodeTwoPoints(Offset pointA, Offset pointB) {
assert(_offsetIsValid(pointA));
assert(_offsetIsValid(pointB));
final Float32List result = Float32List(4);
result[0] = pointA.dx;
result[1] = pointA.dy;
result[2] = pointB.dx;
result[3] = pointB.dy;
return result;
}
/// A shader (as used by [Paint.shader]) that renders a color gradient.
///
/// There are several types of gradients, represented by the various constructors
/// on this class.
///
/// See also:
///
/// * [Gradient](https://api.flutter.dev/flutter/painting/Gradient-class.html), the class in the [painting] library.
///
base class Gradient extends Shader {
/// Creates a linear gradient from `from` to `to`.
///
/// If `colorStops` is provided, `colorStops[i]` is a number from 0.0 to 1.0
/// that specifies where `color[i]` begins in the gradient. If `colorStops` is
/// not provided, then only two stops, at 0.0 and 1.0, are implied (and
/// `color` must therefore only have two entries). Stop values less than 0.0
/// will be rounded up to 0.0 and stop values greater than 1.0 will be rounded
/// down to 1.0. Each stop value must be greater than or equal to the previous
/// stop value. Stop values that do not meet this criteria will be rounded up
/// to the previous stop value.
///
/// The behavior before `from` and after `to` is described by the `tileMode`
/// argument. For details, see the [TileMode] enum.
///
/// 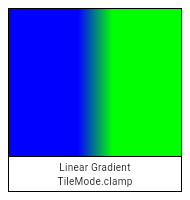
/// 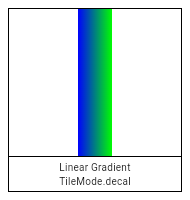
/// 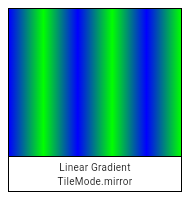
/// 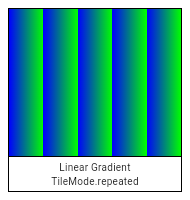
///
/// If `from`, `to`, `colors`, or `tileMode` are null, or if `colors` or
/// `colorStops` contain null values, this constructor will throw a
/// [NoSuchMethodError].
///
/// If `matrix4` is provided, the gradient fill will be transformed by the
/// specified 4x4 matrix relative to the local coordinate system. `matrix4` must
/// be a column-major matrix packed into a list of 16 values.
Gradient.linear(
Offset from,
Offset to,
List<Color> colors, [
List<double>? colorStops,
TileMode tileMode = TileMode.clamp,
Float64List? matrix4,
]) : assert(_offsetIsValid(from)),
assert(_offsetIsValid(to)),
assert(matrix4 == null || _matrix4IsValid(matrix4)),
super._() {
_validateColorStops(colors, colorStops);
final Float32List endPointsBuffer = _encodeTwoPoints(from, to);
final Int32List colorsBuffer = _encodeColorList(colors);
final Float32List? colorStopsBuffer = colorStops == null ? null : Float32List.fromList(colorStops);
_constructor();
_initLinear(endPointsBuffer, colorsBuffer, colorStopsBuffer, tileMode.index, matrix4);
}
/// Creates a radial gradient centered at `center` that ends at `radius`
/// distance from the center.
///
/// If `colorStops` is provided, `colorStops[i]` is a number from 0.0 to 1.0
/// that specifies where `color[i]` begins in the gradient. If `colorStops` is
/// not provided, then only two stops, at 0.0 and 1.0, are implied (and
/// `color` must therefore only have two entries). Stop values less than 0.0
/// will be rounded up to 0.0 and stop values greater than 1.0 will be rounded
/// down to 1.0. Each stop value must be greater than or equal to the previous
/// stop value. Stop values that do not meet this criteria will be rounded up
/// to the previous stop value.
///
/// The behavior before and after the radius is described by the `tileMode`
/// argument. For details, see the [TileMode] enum.
///
/// 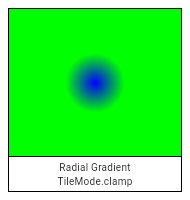
/// 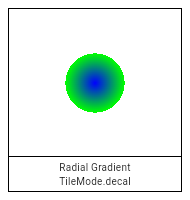
/// 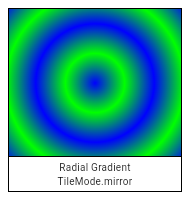
/// 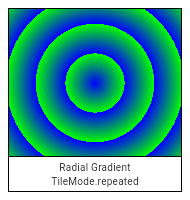
///
/// If `center`, `radius`, `colors`, or `tileMode` are null, or if `colors` or
/// `colorStops` contain null values, this constructor will throw a
/// [NoSuchMethodError].
///
/// If `matrix4` is provided, the gradient fill will be transformed by the
/// specified 4x4 matrix relative to the local coordinate system. `matrix4` must
/// be a column-major matrix packed into a list of 16 values.
///
/// If `focal` is provided and not equal to `center` and `focalRadius` is
/// provided and not equal to 0.0, the generated shader will be a two point
/// conical radial gradient, with `focal` being the center of the focal
/// circle and `focalRadius` being the radius of that circle. If `focal` is
/// provided and not equal to `center`, at least one of the two offsets must
/// not be equal to [Offset.zero].
Gradient.radial(
Offset center,
double radius,
List<Color> colors, [
List<double>? colorStops,
TileMode tileMode = TileMode.clamp,
Float64List? matrix4,
Offset? focal,
double focalRadius = 0.0
]) : assert(_offsetIsValid(center)),
assert(matrix4 == null || _matrix4IsValid(matrix4)),
super._() {
_validateColorStops(colors, colorStops);
final Int32List colorsBuffer = _encodeColorList(colors);
final Float32List? colorStopsBuffer = colorStops == null ? null : Float32List.fromList(colorStops);
// If focal is null or focal radius is null, this should be treated as a regular radial gradient
// If focal == center and the focal radius is 0.0, it's still a regular radial gradient
if (focal == null || (focal == center && focalRadius == 0.0)) {
_constructor();
_initRadial(center.dx, center.dy, radius, colorsBuffer, colorStopsBuffer, tileMode.index, matrix4);
} else {
assert(center != Offset.zero || focal != Offset.zero); // will result in exception(s) in Skia side
_constructor();
_initConical(focal.dx, focal.dy, focalRadius, center.dx, center.dy, radius, colorsBuffer, colorStopsBuffer, tileMode.index, matrix4);
}
}
/// Creates a sweep gradient centered at `center` that starts at `startAngle`
/// and ends at `endAngle`.
///
/// `startAngle` and `endAngle` should be provided in radians, with zero
/// radians being the horizontal line to the right of the `center` and with
/// positive angles going clockwise around the `center`.
///
/// If `colorStops` is provided, `colorStops[i]` is a number from 0.0 to 1.0
/// that specifies where `color[i]` begins in the gradient. If `colorStops` is
/// not provided, then only two stops, at 0.0 and 1.0, are implied (and
/// `color` must therefore only have two entries). Stop values less than 0.0
/// will be rounded up to 0.0 and stop values greater than 1.0 will be rounded
/// down to 1.0. Each stop value must be greater than or equal to the previous
/// stop value. Stop values that do not meet this criteria will be rounded up
/// to the previous stop value.
///
/// The behavior before `startAngle` and after `endAngle` is described by the
/// `tileMode` argument. For details, see the [TileMode] enum.
///
/// 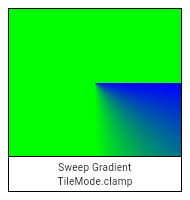
/// 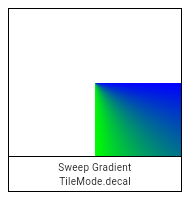
/// 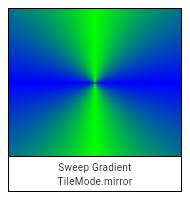
/// 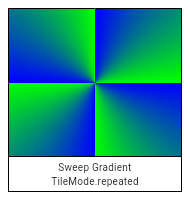
///
/// If `center`, `colors`, `tileMode`, `startAngle`, or `endAngle` are null,
/// or if `colors` or `colorStops` contain null values, this constructor will
/// throw a [NoSuchMethodError].
///
/// If `matrix4` is provided, the gradient fill will be transformed by the
/// specified 4x4 matrix relative to the local coordinate system. `matrix4` must
/// be a column-major matrix packed into a list of 16 values.
Gradient.sweep(
Offset center,
List<Color> colors, [
List<double>? colorStops,
TileMode tileMode = TileMode.clamp,
double startAngle = 0.0,
double endAngle = math.pi * 2,
Float64List? matrix4,
]) : assert(_offsetIsValid(center)),
assert(startAngle < endAngle),
assert(matrix4 == null || _matrix4IsValid(matrix4)),
super._() {
_validateColorStops(colors, colorStops);
final Int32List colorsBuffer = _encodeColorList(colors);
final Float32List? colorStopsBuffer = colorStops == null ? null : Float32List.fromList(colorStops);
_constructor();
_initSweep(center.dx, center.dy, colorsBuffer, colorStopsBuffer, tileMode.index, startAngle, endAngle, matrix4);
}
@Native<Void Function(Handle)>(symbol: 'Gradient::Create')
external void _constructor();
@Native<Void Function(Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initLinear')
external void _initLinear(Float32List endPoints, Int32List colors, Float32List? colorStops, int tileMode, Float64List? matrix4);
@Native<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initRadial')
external void _initRadial(
double centerX,
double centerY,
double radius,
Int32List colors,
Float32List? colorStops,
int tileMode,
Float64List? matrix4);
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32, Handle)>(symbol: 'Gradient::initTwoPointConical')
external void _initConical(
double startX,
double startY,
double startRadius,
double endX,
double endY,
double endRadius,
Int32List colors,
Float32List? colorStops,
int tileMode,
Float64List? matrix4);
@Native<Void Function(Pointer<Void>, Double, Double, Handle, Handle, Int32, Double, Double, Handle)>(symbol: 'Gradient::initSweep')
external void _initSweep(
double centerX,
double centerY,
Int32List colors,
Float32List? colorStops,
int tileMode,
double startAngle,
double endAngle,
Float64List? matrix);
static void _validateColorStops(
List<Color> colors, List<double>? colorStops) {
if (colorStops == null) {
if (colors.length != 2) {
throw ArgumentError('"colors" must have length 2 if "colorStops" is omitted.');
}
} else {
if (colors.length != colorStops.length) {
throw ArgumentError('"colors" and "colorStops" arguments must have equal length.');
}
}
}
}
/// A shader (as used by [Paint.shader]) that tiles an image.
base class ImageShader extends Shader {
/// Creates an image-tiling shader.
///
/// The first argument specifies the image to render. The
/// [decodeImageFromList] function can be used to decode an image from bytes
/// into the form expected here. (In production code, starting from
/// [instantiateImageCodec] may be preferable.)
///
/// The second and third arguments specify the [TileMode] for the x direction
/// and y direction respectively. [TileMode.repeated] can be used for tiling
/// images.
///
/// The fourth argument gives the matrix to apply to the effect. The
/// expression `Matrix4.identity().storage` creates a [Float64List]
/// prepopulated with the identity matrix.
///
/// All the arguments are required and must not be null, except for
/// [filterQuality]. If [filterQuality] is not specified at construction time
/// it will be deduced from the environment where it is used, such as from
/// [Paint.filterQuality].
@pragma('vm:entry-point')
ImageShader(Image image, TileMode tmx, TileMode tmy, Float64List matrix4, {
FilterQuality? filterQuality,
}) :
assert(!image.debugDisposed),
super._() {
if (matrix4.length != 16) {
throw ArgumentError('"matrix4" must have 16 entries.');
}
_constructor();
final String? error = _initWithImage(image._image, tmx.index, tmy.index, filterQuality?.index ?? -1, matrix4);
if (error != null) {
throw Exception(error);
}
}
@override
void dispose() {
super.dispose();
_dispose();
}
@Native<Void Function(Handle)>(symbol: 'ImageShader::Create')
external void _constructor();
@Native<Handle Function(Pointer<Void>, Pointer<Void>, Int32, Int32, Int32, Handle)>(symbol: 'ImageShader::initWithImage')
external String? _initWithImage(_Image image, int tmx, int tmy, int filterQualityIndex, Float64List matrix4);
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
@Native<Void Function(Pointer<Void>)>(symbol: 'ImageShader::dispose')
external void _dispose();
}
/// An instance of [FragmentProgram] creates [Shader] objects (as used by
/// [Paint.shader]).
///
/// For more information, see the website
/// [documentation]( https://docs.flutter.dev/development/ui/advanced/shaders).
base class FragmentProgram extends NativeFieldWrapperClass1 {
@pragma('vm:entry-point')
FragmentProgram._fromAsset(String assetKey) {
_constructor();
final String result = _initFromAsset(assetKey);
if (result.isNotEmpty) {
throw Exception(result);
}
assert(() {
_debugName = assetKey;
return true;
}());
}
String? _debugName;
/// Creates a fragment program from the asset with key [assetKey].
///
/// The asset must be a file produced as the output of the `impellerc`
/// compiler. The constructed object should then be reused via the
/// [fragmentShader] method to create [Shader] objects that can be used by
/// [Paint.shader].
static Future<FragmentProgram> fromAsset(String assetKey) {
// The flutter tool converts all asset keys with spaces into URI
// encoded paths (replacing ' ' with '%20', for example). We perform
// the same encoding here so that users can load assets with the same
// key they have written in the pubspec.
final String encodedKey = Uri(path: Uri.encodeFull(assetKey)).path;
final FragmentProgram? program = _shaderRegistry[encodedKey];
if (program != null) {
return Future<FragmentProgram>.value(program);
}
return Future<FragmentProgram>.microtask(() {
final FragmentProgram program = FragmentProgram._fromAsset(encodedKey);
_shaderRegistry[encodedKey] = program;
return program;
});
}
// This is a cache of shaders that have been loaded by
// FragmentProgram.fromAsset. It holds a strong reference to theFragmentPrograms
// The native engine will retain the resources associated with this shader
// program (PSO variants) until shutdown, so maintaining a strong reference
// here ensures we do not perform extra work if the dart object is continually
// re-initialized.
static final Map<String, FragmentProgram> _shaderRegistry =
<String, FragmentProgram>{};
static void _reinitializeShader(String assetKey) {
// If a shader for the asset isn't already registered, then there's no
// need to reinitialize it. The new shader will be loaded and initialized
// the next time the program access it.
final FragmentProgram? program = _shaderRegistry[assetKey];
if (program == null) {
return;
}
final String result = program._initFromAsset(assetKey);
if (result.isNotEmpty) {
throw result; // ignore: only_throw_errors
}
}
@pragma('vm:entry-point')
late int _uniformFloatCount;
@pragma('vm:entry-point')
late int _samplerCount;
@Native<Void Function(Handle)>(symbol: 'FragmentProgram::Create')
external void _constructor();
@Native<Handle Function(Pointer<Void>, Handle)>(symbol: 'FragmentProgram::initFromAsset')
external String _initFromAsset(String assetKey);
/// Returns a fresh instance of [FragmentShader].
FragmentShader fragmentShader() => FragmentShader._(this, debugName: _debugName);
}
/// A [Shader] generated from a [FragmentProgram].
///
/// Instances of this class can be obtained from the
/// [FragmentProgram.fragmentShader] method. The float uniforms list is
/// initialized to the size expected by the shader and is zero-filled. Uniforms
/// of float type can then be set by calling [setFloat]. Sampler uniforms are
/// set by calling [setImageSampler].
///
/// A [FragmentShader] can be re-used, and this is an efficient way to avoid
/// allocating and re-initializing the uniform buffer and samplers. However,
/// if two [FragmentShader] objects with different float uniforms or samplers
/// are required to exist simultaneously, they must be obtained from two
/// different calls to [FragmentProgram.fragmentShader].
base class FragmentShader extends Shader {
FragmentShader._(FragmentProgram program, { String? debugName }) : _debugName = debugName, super._() {
_floats = _constructor(
program,
program._uniformFloatCount,
program._samplerCount,
);
}
final String? _debugName;
static final Float32List _kEmptyFloat32List = Float32List(0);
Float32List _floats = _kEmptyFloat32List;
/// Sets the float uniform at [index] to [value].
///
/// All uniforms defined in a fragment shader that are not samplers must be
/// set through this method. This includes floats and vec2, vec3, and vec4.
/// The correct index for each uniform is determined by the order of the
/// uniforms as defined in the fragment program, ignoring any samplers. For
/// data types that are composed of multiple floats such as a vec4, more than
/// one call to [setFloat] is required.
///
/// For example, given the following uniforms in a fragment program:
///
/// ```glsl
/// uniform float uScale;
/// uniform sampler2D uTexture;
/// uniform vec2 uMagnitude;
/// uniform vec4 uColor;
/// ```
///
/// Then the corresponding Dart code to correctly initialize these uniforms
/// is:
///
/// ```dart
/// void updateShader(ui.FragmentShader shader, Color color, ui.Image image) {
/// shader.setFloat(0, 23); // uScale
/// shader.setFloat(1, 114); // uMagnitude x
/// shader.setFloat(2, 83); // uMagnitude y
///
/// // Convert color to premultiplied opacity.
/// shader.setFloat(3, color.red / 255 * color.opacity); // uColor r
/// shader.setFloat(4, color.green / 255 * color.opacity); // uColor g
/// shader.setFloat(5, color.blue / 255 * color.opacity); // uColor b
/// shader.setFloat(6, color.opacity); // uColor a
///
/// // initialize sampler uniform.
/// shader.setImageSampler(0, image);
/// }
/// ```
///
/// Note how the indexes used does not count the `sampler2D` uniform. This
/// uniform will be set separately with [setImageSampler], with the index starting
/// over at 0.
///
/// Any float uniforms that are left uninitialized will default to `0`.
void setFloat(int index, double value) {
assert(!debugDisposed, 'Tried to accesss uniforms on a disposed Shader: $this');
_floats[index] = value;
}
/// Sets the sampler uniform at [index] to [image].
///
/// The index provided to setImageSampler is the index of the sampler uniform defined
/// in the fragment program, excluding all non-sampler uniforms.
///
/// All the sampler uniforms that a shader expects must be provided or the
/// results will be undefined.
void setImageSampler(int index, Image image) {
assert(!debugDisposed, 'Tried to access uniforms on a disposed Shader: $this');
_setImageSampler(index, image._image);
}
/// Releases the native resources held by the [FragmentShader].
///
/// After this method is called, calling methods on the shader, or attaching
/// it to a [Paint] object will fail with an exception. Calling [dispose]
/// twice will also result in an exception being thrown.
@override
void dispose() {
super.dispose();
_floats = _kEmptyFloat32List;
_dispose();
}
@Native<Handle Function(Handle, Handle, Handle, Handle)>(symbol: 'ReusableFragmentShader::Create')
external Float32List _constructor(FragmentProgram program, int floatUniforms, int samplerUniforms);
@Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'ReusableFragmentShader::SetImageSampler')
external void _setImageSampler(int index, _Image sampler);
@Native<Bool Function(Pointer<Void>)>(symbol: 'ReusableFragmentShader::ValidateSamplers')
external bool _validateSamplers();
@Native<Void Function(Pointer<Void>)>(symbol: 'ReusableFragmentShader::Dispose')
external void _dispose();
}
/// Defines how a list of points is interpreted when drawing a set of triangles.
///
/// Used by [Canvas.drawVertices].
// These enum values must be kept in sync with DlVertexMode.
enum VertexMode {
/// Draw each sequence of three points as the vertices of a triangle.
triangles,
/// Draw each sliding window of three points as the vertices of a triangle.
triangleStrip,
/// Draw the first point and each sliding window of two points as the vertices
/// of a triangle.
///
/// This mode is not natively supported by most backends, and is instead
/// implemented by unrolling the points into the equivalent
/// [VertexMode.triangles], which is generally more efficient.
triangleFan,
}
/// A set of vertex data used by [Canvas.drawVertices].
///
/// Vertex data consists of a series of points in the canvas coordinate space.
/// Based on the [VertexMode], these points are interpreted either as
/// independent triangles ([VertexMode.triangles]), as a sliding window of
/// points forming a chain of triangles each sharing one side with the next
/// ([VertexMode.triangleStrip]), or as a fan of triangles with a single shared
/// point ([VertexMode.triangleFan]).
///
/// Each point can be associated with a color. Each triangle is painted as a
/// gradient that blends between the three colors at the three points of that
/// triangle. If no colors are specified, transparent black is assumed for all
/// the points.
///
/// These colors are then blended with the [Paint] specified in the call to
/// [Canvas.drawVertices]. This paint is either a solid color ([Paint.color]),
/// or a bitmap, specified using a shader ([Paint.shader]), typically either a
/// gradient ([Gradient]) or image ([ImageFilter]). The bitmap uses the same
/// coordinate space as the canvas (in the case of an [ImageFilter], this is
/// notably different than the coordinate space of the source image; the source
/// image is tiled according to the filter's configuration, and the image that
/// is sampled when painting the triangles is the infinite one after all the
/// repeating is applied.)
///
/// Each point in the [Vertices] is associated with a specific point on this
/// image. Each triangle is painted by sampling points from this image by
/// interpolating between the three points of the image corresponding to the
/// three points of the triangle.
///
/// The [Vertices.new] constructor configures all this using lists of [Offset]
/// and [Color] objects. The [Vertices.raw] constructor instead uses
/// [Float32List], [Int32List], and [Uint16List] objects, which more closely
/// corresponds to the data format used internally and therefore reduces some of
/// the conversion overhead. The raw constructor is useful if the data is coming
/// from another source (e.g. a file) and can therefore be parsed directly into
/// the underlying representation.
base class Vertices extends NativeFieldWrapperClass1 {
/// Creates a set of vertex data for use with [Canvas.drawVertices].
///
/// The `mode` parameter describes how the points should be interpreted: as
/// independent triangles ([VertexMode.triangles]), as a sliding window of
/// points forming a chain of triangles each sharing one side with the next
/// ([VertexMode.triangleStrip]), or as a fan of triangles with a single
/// shared point ([VertexMode.triangleFan]).
///
/// The `positions` parameter provides the points in the canvas space that
/// will be use to draw the triangles.
///
/// The `colors` parameter, if specified, provides the color for each point in
/// `positions`. Each triangle is painted as a gradient that blends between
/// the three colors at the three points of that triangle. (These colors are
/// then blended with the [Paint] specified in the call to
/// [Canvas.drawVertices].)
///
/// The `textureCoordinates` parameter, if specified, provides the points in
/// the [Paint] image to sample for the corresponding points in `positions`.
///
/// If the `colors` or `textureCoordinates` parameters are specified, they must
/// be the same length as `positions`.
///
/// The `indices` parameter specifies the order in which the points should be
/// painted. If it is omitted (or present but empty), the points are processed
/// in the order they are given in `positions`, as if the `indices` was a list
/// from 0 to n-1, where _n_ is the number of entries in `positions`. The
/// `indices` parameter, if present and non-empty, must have at least three
/// entries, but may be of any length beyond this. Indicies may refer to
/// offsets in the positions array multiple times, or may skip positions
/// entirely.
///
/// If the `indices` parameter is specified, all values in the list must be
/// valid index values for `positions`.
///
/// The `mode` and `positions` parameters must not be null.
///
/// This constructor converts its parameters into [dart:typed_data] lists
/// (e.g. using [Float32List]s for the coordinates) before sending them to the
/// Flutter engine. If the data provided to this constructor is not already in
/// [List] form, consider using the [Vertices.raw] constructor instead to
/// avoid converting the data twice.
Vertices(
VertexMode mode,
List<Offset> positions, {
List<Color>? colors,
List<Offset>? textureCoordinates,
List<int>? indices,
}) {
if (colors != null && colors.length != positions.length) {
throw ArgumentError('"positions" and "colors" lengths must match.');
}
if (textureCoordinates != null && textureCoordinates.length != positions.length) {
throw ArgumentError('"positions" and "textureCoordinates" lengths must match.');
}
if (indices != null) {
for (int index = 0; index < indices.length; index += 1) {
if (indices[index] >= positions.length) {
throw ArgumentError(
'"indices" values must be valid indices in the positions list '
'(i.e. numbers in the range 0..${positions.length - 1}), '
'but indices[$index] is ${indices[index]}, which is too big.',
);
}
}
}
final Float32List encodedPositions = _encodePointList(positions);
final Float32List? encodedTextureCoordinates = (textureCoordinates != null)
? _encodePointList(textureCoordinates)
: null;
final Int32List? encodedColors = colors != null
? _encodeColorList(colors)
: null;
final Uint16List? encodedIndices = indices != null
? Uint16List.fromList(indices)
: null;
if (!_init(this, mode.index, encodedPositions, encodedTextureCoordinates, encodedColors, encodedIndices)) {
throw ArgumentError('Invalid configuration for vertices.');
}
}
/// Creates a set of vertex data for use with [Canvas.drawVertices], using the
/// encoding expected by the Flutter engine.
///
/// The `mode` parameter describes how the points should be interpreted: as
/// independent triangles ([VertexMode.triangles]), as a sliding window of
/// points forming a chain of triangles each sharing one side with the next
/// ([VertexMode.triangleStrip]), or as a fan of triangles with a single
/// shared point ([VertexMode.triangleFan]).
///
/// The `positions` parameter provides the points in the canvas space that
/// will be use to draw the triangles. Each point is represented as two
/// numbers in the list, the first giving the x coordinate and the second
/// giving the y coordinate. (As a result, the list must have an even number
/// of entries.)
///
/// The `colors` parameter, if specified, provides the color for each point in
/// `positions`. Each color is represented as ARGB with 8 bit color channels
/// (like [Color.value]'s internal representation), and the list, if
/// specified, must therefore be half the length of `positions`. Each triangle
/// is painted as a gradient that blends between the three colors at the three
/// points of that triangle. (These colors are then blended with the [Paint]
/// specified in the call to [Canvas.drawVertices].)
///
/// The `textureCoordinates` parameter, if specified, provides the points in
/// the [Paint] image to sample for the corresponding points in `positions`.
/// Each point is represented as two numbers in the list, the first giving the
/// x coordinate and the second giving the y coordinate. This list, if
/// specified, must be the same length as `positions`.
///
/// The `indices` parameter specifies the order in which the points should be
/// painted. If it is omitted (or present but empty), the points are processed
/// in the order they are given in `positions`, as if the `indices` was a list
/// from 0 to n-2, where _n_ is the number of pairs in `positions` (i.e. half
/// the length of `positions`). The `indices` parameter, if present and
/// non-empty, must have at least three entries, but may be of any length
/// beyond this. Indicies may refer to offsets in the positions array multiple
/// times, or may skip positions entirely.
///
/// If the `indices` parameter is specified, all values in the list must be
/// valid index values for pairs in `positions`. For example, if there are 12
/// numbers in `positions` (representing 6 coordinates), the `indicies` must
/// be numbers in the range 0..5 inclusive.
///
/// The `mode` and `positions` parameters must not be null.
Vertices.raw(
VertexMode mode,
Float32List positions, {
Int32List? colors,
Float32List? textureCoordinates,
Uint16List? indices,
}) {
if (positions.length % 2 != 0) {
throw ArgumentError('"positions" must have an even number of entries (each coordinate is an x,y pair).');
}
if (colors != null && colors.length * 2 != positions.length) {
throw ArgumentError('"positions" and "colors" lengths must match.');
}
if (textureCoordinates != null && textureCoordinates.length != positions.length) {
throw ArgumentError('"positions" and "textureCoordinates" lengths must match.');
}
if (indices != null) {
for (int index = 0; index < indices.length; index += 1) {
if (indices[index] * 2 >= positions.length) {
throw ArgumentError(
'"indices" values must be valid indices in the positions list '
'(i.e. numbers in the range 0..${positions.length ~/ 2 - 1}), '
'but indices[$index] is ${indices[index]}, which is too big.',
);
}
}
}
if (!_init(this, mode.index, positions, textureCoordinates, colors, indices)) {
throw ArgumentError('Invalid configuration for vertices.');
}
}
@Native<Bool Function(Handle, Int32, Handle, Handle, Handle, Handle)>(symbol: 'Vertices::init')
external static bool _init(Vertices outVertices,
int mode,
Float32List positions,
Float32List? textureCoordinates,
Int32List? colors,
Uint16List? indices);
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
void dispose() {
assert(!_disposed);
assert(() {
_disposed = true;
return true;
}());
_dispose();
}
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
@Native<Void Function(Pointer<Void>)>(symbol: 'Vertices::dispose')
external void _dispose();
bool _disposed = false;
/// Whether this reference to the underlying vertex data is [dispose]d.
///
/// This only returns a valid value if asserts are enabled, and must not be
/// used otherwise.
bool get debugDisposed {
bool? disposed;
assert(() {
disposed = _disposed;
return true;
}());
return disposed ?? (throw StateError('Vertices.debugDisposed is only available when asserts are enabled.'));
}
}
/// Defines how a list of points is interpreted when drawing a set of points.
///
/// Used by [Canvas.drawPoints] and [Canvas.drawRawPoints].
// These enum values must be kept in sync with DlCanvas::PointMode.
enum PointMode {
/// Draw each point separately.
///
/// If the [Paint.strokeCap] is [StrokeCap.round], then each point is drawn
/// as a circle with the diameter of the [Paint.strokeWidth], filled as
/// described by the [Paint] (ignoring [Paint.style]).
///
/// Otherwise, each point is drawn as an axis-aligned square with sides of
/// length [Paint.strokeWidth], filled as described by the [Paint] (ignoring
/// [Paint.style]).
points,
/// Draw each sequence of two points as a line segment.
///
/// If the number of points is odd, then the last point is ignored.
///
/// The lines are stroked as described by the [Paint] (ignoring
/// [Paint.style]).
lines,
/// Draw the entire sequence of points as one line.
///
/// The lines are stroked as described by the [Paint] (ignoring
/// [Paint.style]).
polygon,
}
/// Defines how a new clip region should be merged with the existing clip
/// region.
///
/// Used by [Canvas.clipRect].
enum ClipOp {
/// Subtract the new region from the existing region.
difference,
/// Intersect the new region from the existing region.
intersect,
}
/// An interface for recording graphical operations.
///
/// [Canvas] objects are used in creating [Picture] objects, which can
/// themselves be used with a [SceneBuilder] to build a [Scene]. In
/// normal usage, however, this is all handled by the framework.
///
/// A canvas has a current transformation matrix which is applied to all
/// operations. Initially, the transformation matrix is the identity transform.
/// It can be modified using the [translate], [scale], [rotate], [skew],
/// and [transform] methods.
///
/// A canvas also has a current clip region which is applied to all operations.
/// Initially, the clip region is infinite. It can be modified using the
/// [clipRect], [clipRRect], and [clipPath] methods.
///
/// The current transform and clip can be saved and restored using the stack
/// managed by the [save], [saveLayer], and [restore] methods.
abstract class Canvas {
/// Creates a canvas for recording graphical operations into the
/// given picture recorder.
///
/// Graphical operations that affect pixels entirely outside the given
/// `cullRect` might be discarded by the implementation. However, the
/// implementation might draw outside these bounds if, for example, a command
/// draws partially inside and outside the `cullRect`. To ensure that pixels
/// outside a given region are discarded, consider using a [clipRect]. The
/// `cullRect` is optional; by default, all operations are kept.
///
/// To end the recording, call [PictureRecorder.endRecording] on the
/// given recorder.
factory Canvas(PictureRecorder recorder, [ Rect? cullRect ]) = _NativeCanvas;
/// Saves a copy of the current transform and clip on the save stack.
///
/// Call [restore] to pop the save stack.
///
/// See also:
///
/// * [saveLayer], which does the same thing but additionally also groups the
/// commands done until the matching [restore].
void save();
/// Saves a copy of the current transform and clip on the save stack, and then
/// creates a new group which subsequent calls will become a part of. When the
/// save stack is later popped, the group will be flattened into a layer and
/// have the given `paint`'s [Paint.colorFilter] and [Paint.blendMode]
/// applied.
///
/// This lets you create composite effects, for example making a group of
/// drawing commands semi-transparent. Without using [saveLayer], each part of
/// the group would be painted individually, so where they overlap would be
/// darker than where they do not. By using [saveLayer] to group them
/// together, they can be drawn with an opaque color at first, and then the
/// entire group can be made transparent using the [saveLayer]'s paint.
///
/// Call [restore] to pop the save stack and apply the paint to the group.
///
/// ## Using saveLayer with clips
///
/// When a rectangular clip operation (from [clipRect]) is not axis-aligned
/// with the raster buffer, or when the clip operation is not rectilinear
/// (e.g. because it is a rounded rectangle clip created by [clipRRect] or an
/// arbitrarily complicated path clip created by [clipPath]), the edge of the
/// clip needs to be anti-aliased.
///
/// If two draw calls overlap at the edge of such a clipped region, without
/// using [saveLayer], the first drawing will be anti-aliased with the
/// background first, and then the second will be anti-aliased with the result
/// of blending the first drawing and the background. On the other hand, if
/// [saveLayer] is used immediately after establishing the clip, the second
/// drawing will cover the first in the layer, and thus the second alone will
/// be anti-aliased with the background when the layer is clipped and
/// composited (when [restore] is called).
///
/// For example, this [CustomPainter.paint] method paints a clean white
/// rounded rectangle:
///
/// ```dart
/// void paint(Canvas canvas, Size size) {
/// Rect rect = Offset.zero & size;
/// canvas.save();
/// canvas.clipRRect(RRect.fromRectXY(rect, 100.0, 100.0));
/// canvas.saveLayer(rect, Paint());
/// canvas.drawPaint(Paint()..color = Colors.red);
/// canvas.drawPaint(Paint()..color = Colors.white);
/// canvas.restore();
/// canvas.restore();
/// }
/// ```
///
/// On the other hand, this one renders a red outline, the result of the red
/// paint being anti-aliased with the background at the clip edge, then the
/// white paint being similarly anti-aliased with the background _including
/// the clipped red paint_:
///
/// ```dart
/// void paint(Canvas canvas, Size size) {
/// // (this example renders poorly, prefer the example above)
/// Rect rect = Offset.zero & size;
/// canvas.save();
/// canvas.clipRRect(RRect.fromRectXY(rect, 100.0, 100.0));
/// canvas.drawPaint(Paint()..color = Colors.red);
/// canvas.drawPaint(Paint()..color = Colors.white);
/// canvas.restore();
/// }
/// ```
///
/// This point is moot if the clip only clips one draw operation. For example,
/// the following paint method paints a pair of clean white rounded
/// rectangles, even though the clips are not done on a separate layer:
///
/// ```dart
/// void paint(Canvas canvas, Size size) {
/// canvas.save();
/// canvas.clipRRect(RRect.fromRectXY(Offset.zero & (size / 2.0), 50.0, 50.0));
/// canvas.drawPaint(Paint()..color = Colors.white);
/// canvas.restore();
/// canvas.save();
/// canvas.clipRRect(RRect.fromRectXY(size.center(Offset.zero) & (size / 2.0), 50.0, 50.0));
/// canvas.drawPaint(Paint()..color = Colors.white);
/// canvas.restore();
/// }
/// ```
///
/// (Incidentally, rather than using [clipRRect] and [drawPaint] to draw
/// rounded rectangles like this, prefer the [drawRRect] method. These
/// examples are using [drawPaint] as a proxy for "complicated draw operations
/// that will get clipped", to illustrate the point.)
///
/// ## Performance considerations
///
/// Generally speaking, [saveLayer] is relatively expensive.
///
/// There are a several different hardware architectures for GPUs (graphics
/// processing units, the hardware that handles graphics), but most of them
/// involve batching commands and reordering them for performance. When layers
/// are used, they cause the rendering pipeline to have to switch render
/// target (from one layer to another). Render target switches can flush the
/// GPU's command buffer, which typically means that optimizations that one
/// could get with larger batching are lost. Render target switches also
/// generate a lot of memory churn because the GPU needs to copy out the
/// current frame buffer contents from the part of memory that's optimized for
/// writing, and then needs to copy it back in once the previous render target
/// (layer) is restored.
///
/// See also:
///
/// * [save], which saves the current state, but does not create a new layer
/// for subsequent commands.
/// * [BlendMode], which discusses the use of [Paint.blendMode] with
/// [saveLayer].
void saveLayer(Rect? bounds, Paint paint);
/// Pops the current save stack, if there is anything to pop.
/// Otherwise, does nothing.
///
/// Use [save] and [saveLayer] to push state onto the stack.
///
/// If the state was pushed with [saveLayer], then this call will also
/// cause the new layer to be composited into the previous layer.
void restore();
/// Restores the save stack to a previous level as might be obtained from [getSaveCount].
/// If [count] is less than 1, the stack is restored to its initial state.
/// If [count] is greater than the current [getSaveCount] then nothing happens.
///
/// Use [save] and [saveLayer] to push state onto the stack.
///
/// If any of the state stack levels restored by this call were pushed with
/// [saveLayer], then this call will also cause those layers to be composited
/// into their previous layers.
void restoreToCount(int count);
/// Returns the number of items on the save stack, including the
/// initial state. This means it returns 1 for a clean canvas, and
/// that each call to [save] and [saveLayer] increments it, and that
/// each matching call to [restore] decrements it.
///
/// This number cannot go below 1.
int getSaveCount();
/// Add a translation to the current transform, shifting the coordinate space
/// horizontally by the first argument and vertically by the second argument.
void translate(double dx, double dy);
/// Add an axis-aligned scale to the current transform, scaling by the first
/// argument in the horizontal direction and the second in the vertical
/// direction.
///
/// If [sy] is unspecified, [sx] will be used for the scale in both
/// directions.
void scale(double sx, [double? sy]);
/// Add a rotation to the current transform. The argument is in radians clockwise.
void rotate(double radians);
/// Add an axis-aligned skew to the current transform, with the first argument
/// being the horizontal skew in rise over run units clockwise around the
/// origin, and the second argument being the vertical skew in rise over run
/// units clockwise around the origin.
void skew(double sx, double sy);
/// Multiply the current transform by the specified 4⨉4 transformation matrix
/// specified as a list of values in column-major order.
void transform(Float64List matrix4);
/// Returns the current transform including the combined result of all transform
/// methods executed since the creation of this [Canvas] object, and respecting the
/// save/restore history.
///
/// Methods that can change the current transform include [translate], [scale],
/// [rotate], [skew], and [transform]. The [restore] method can also modify
/// the current transform by restoring it to the same value it had before its
/// associated [save] or [saveLayer] call.
Float64List getTransform();
/// Reduces the clip region to the intersection of the current clip and the
/// given rectangle.
///
/// 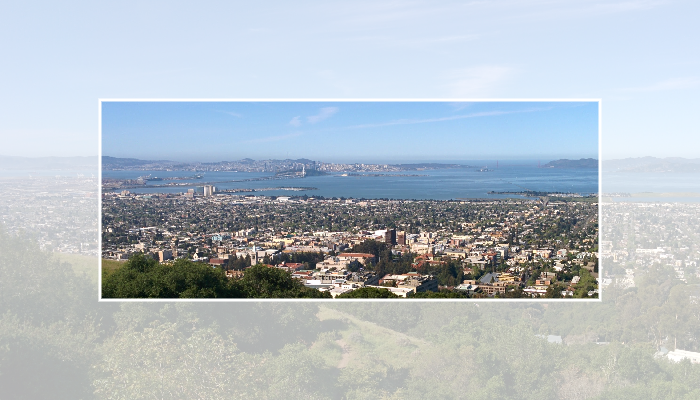
///
/// If [doAntiAlias] is true, then the clip will be anti-aliased.
///
/// If multiple draw commands intersect with the clip boundary, this can result
/// in incorrect blending at the clip boundary. See [saveLayer] for a
/// discussion of how to address that.
///
/// Use [ClipOp.difference] to subtract the provided rectangle from the
/// current clip.
void clipRect(Rect rect, { ClipOp clipOp = ClipOp.intersect, bool doAntiAlias = true });
/// Reduces the clip region to the intersection of the current clip and the
/// given rounded rectangle.
///
/// 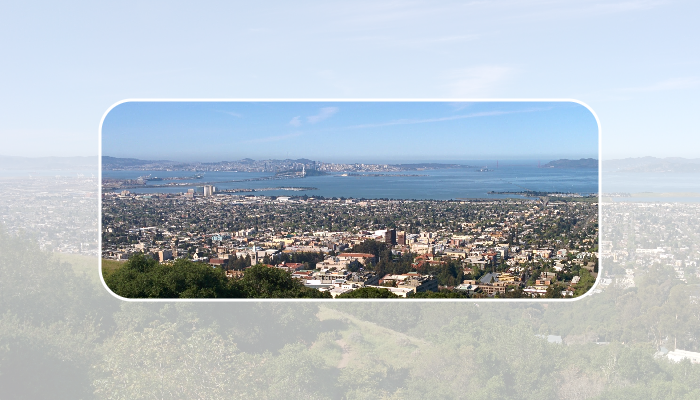
///
/// If [doAntiAlias] is true, then the clip will be anti-aliased.
///
/// If multiple draw commands intersect with the clip boundary, this can result
/// in incorrect blending at the clip boundary. See [saveLayer] for a
/// discussion of how to address that and some examples of using [clipRRect].
void clipRRect(RRect rrect, {bool doAntiAlias = true});
/// Reduces the clip region to the intersection of the current clip and the
/// given [Path].
///
/// 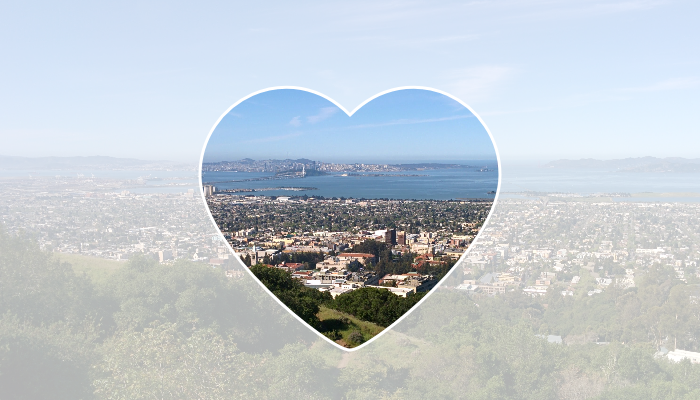
///
/// If [doAntiAlias] is true, then the clip will be anti-aliased.
///
/// If multiple draw commands intersect with the clip boundary, this can result
/// in incorrect blending at the clip boundary. See [saveLayer] for a
/// discussion of how to address that.
void clipPath(Path path, {bool doAntiAlias = true});
/// Returns the conservative bounds of the combined result of all clip methods
/// executed within the current save stack of this [Canvas] object, as measured
/// in the local coordinate space under which rendering operations are currently
/// performed.
///
/// The combined clip results are rounded out to an integer pixel boundary before
/// they are transformed back into the local coordinate space which accounts for
/// the pixel roundoff in rendering operations, particularly when antialiasing.
/// Because the [Picture] may eventually be rendered into a scene within the
/// context of transforming widgets or layers, the result may thus be overly
/// conservative due to premature rounding. Using the [getDestinationClipBounds]
/// method combined with the external transforms and rounding in the true device
/// coordinate system will produce more accurate results, but this value may
/// provide a more convenient approximation to compare rendering operations to
/// the established clip.
///
/// {@template dart.ui.canvas.conservativeClipBounds}
/// The conservative estimate of the bounds is based on intersecting the bounds
/// of each clip method that was executed with [ClipOp.intersect] and potentially
/// ignoring any clip method that was executed with [ClipOp.difference]. The
/// [ClipOp] argument is only present on the [clipRect] method.
///
/// To understand how the bounds estimate can be conservative, consider the
/// following two clip method calls:
///
/// ```dart
/// void draw(Canvas canvas) {
/// canvas.clipPath(Path()
/// ..addRect(const Rect.fromLTRB(10, 10, 20, 20))
/// ..addRect(const Rect.fromLTRB(80, 80, 100, 100)));
/// canvas.clipPath(Path()
/// ..addRect(const Rect.fromLTRB(80, 10, 100, 20))
/// ..addRect(const Rect.fromLTRB(10, 80, 20, 100)));
/// // ...
/// }
/// ```
///
/// After executing both of those calls there is no area left in which to draw
/// because the two paths have no overlapping regions. But, in this case,
/// [getLocalClipBounds] would return a rectangle from `10, 10` to `100, 100` because it
/// only intersects the bounds of the two path objects to obtain its conservative
/// estimate.
///
/// The clip bounds are not affected by the bounds of any enclosing
/// [saveLayer] call as the engine does not currently guarantee the strict
/// enforcement of those bounds during rendering.
///
/// Methods that can change the current clip include [clipRect], [clipRRect],
/// and [clipPath]. The [restore] method can also modify the current clip by
/// restoring it to the same value it had before its associated [save] or
/// [saveLayer] call.
/// {@endtemplate}
Rect getLocalClipBounds();
/// Returns the conservative bounds of the combined result of all clip methods
/// executed within the current save stack of this [Canvas] object, as measured
/// in the destination coordinate space in which the [Picture] will be rendered.
///
/// Unlike [getLocalClipBounds], the bounds are not rounded out to an integer
/// pixel boundary as the Destination coordinate space may not represent pixels
/// if the [Picture] being constructed will be further transformed when it is
/// rendered or added to a scene. In order to determine the true pixels being
/// affected, those external transforms should be applied first before rounding
/// out the result to integer pixel boundaries. Most typically, [Picture] objects
/// are rendered in a scene with a scale transform representing the Device Pixel
/// Ratio.
///
/// {@macro dart.ui.canvas.conservativeClipBounds}
Rect getDestinationClipBounds();
/// Paints the given [Color] onto the canvas, applying the given
/// [BlendMode], with the given color being the source and the background
/// being the destination.
void drawColor(Color color, BlendMode blendMode);
/// Draws a line between the given points using the given paint. The line is
/// stroked, the value of the [Paint.style] is ignored for this call.
///
/// The `p1` and `p2` arguments are interpreted as offsets from the origin.
///
/// 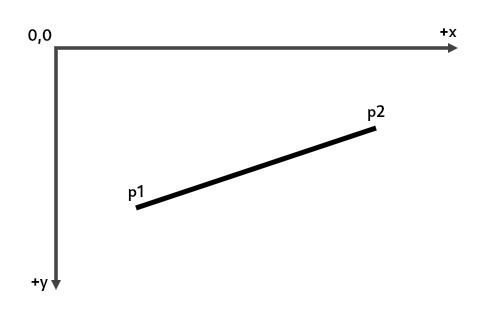
/// 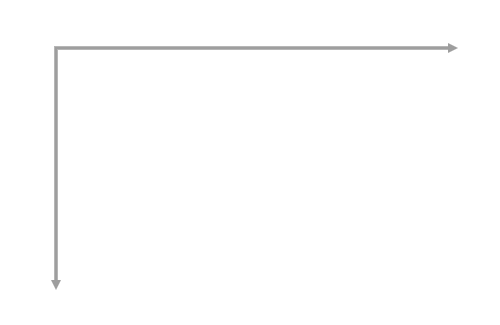
void drawLine(Offset p1, Offset p2, Paint paint);
/// Fills the canvas with the given [Paint].
///
/// To fill the canvas with a solid color and blend mode, consider
/// [drawColor] instead.
void drawPaint(Paint paint);
/// Draws a rectangle with the given [Paint]. Whether the rectangle is filled
/// or stroked (or both) is controlled by [Paint.style].
///
/// 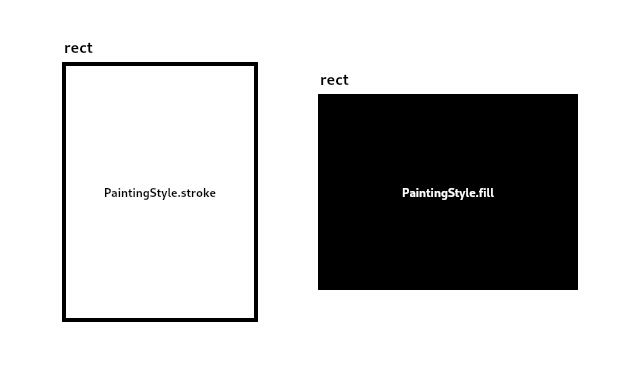
/// 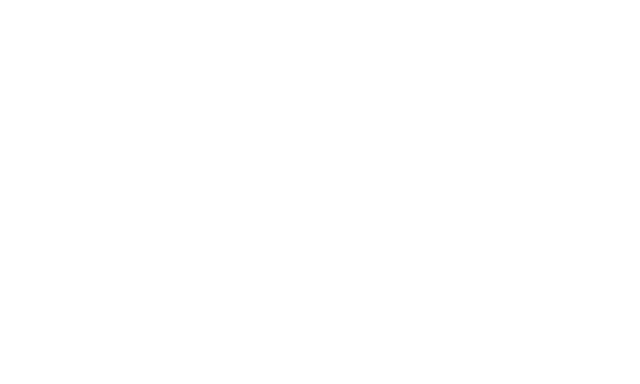
void drawRect(Rect rect, Paint paint);
/// Draws a rounded rectangle with the given [Paint]. Whether the rectangle is
/// filled or stroked (or both) is controlled by [Paint.style].
///
/// 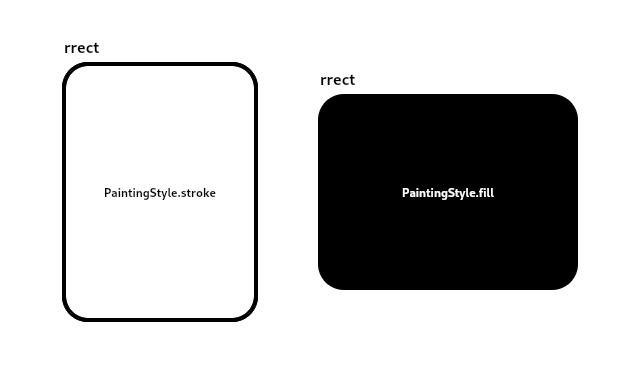
/// 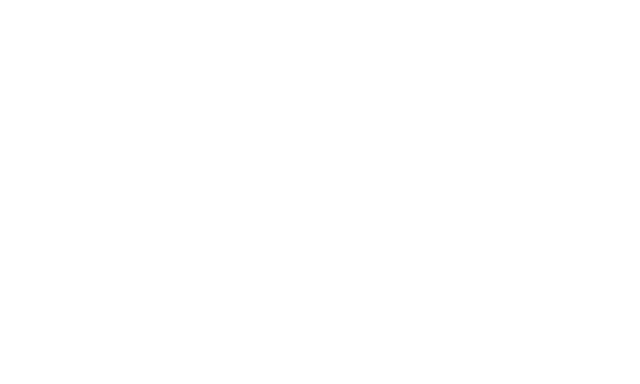
void drawRRect(RRect rrect, Paint paint);
/// Draws a shape consisting of the difference between two rounded rectangles
/// with the given [Paint]. Whether this shape is filled or stroked (or both)
/// is controlled by [Paint.style].
///
/// This shape is almost but not quite entirely unlike an annulus.
void drawDRRect(RRect outer, RRect inner, Paint paint);
/// Draws an axis-aligned oval that fills the given axis-aligned rectangle
/// with the given [Paint]. Whether the oval is filled or stroked (or both) is
/// controlled by [Paint.style].
///
/// 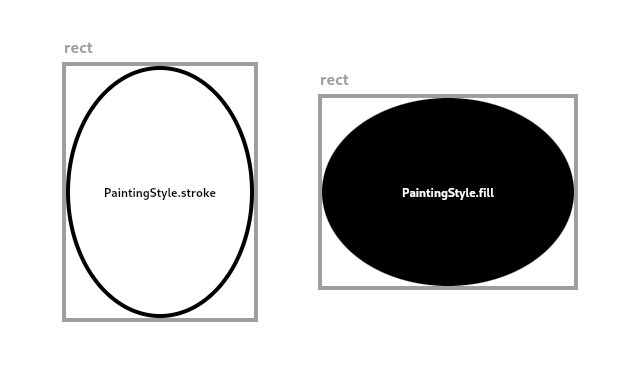
/// 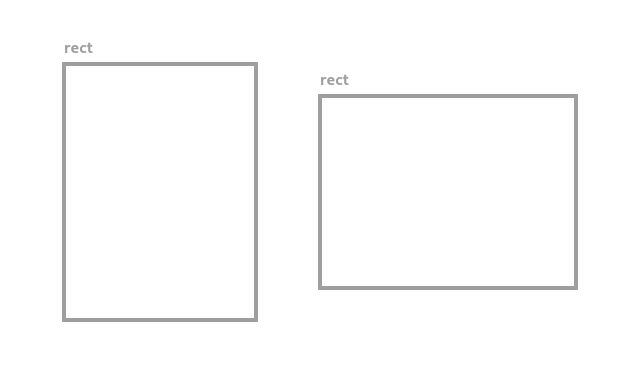
void drawOval(Rect rect, Paint paint);
/// Draws a circle centered at the point given by the first argument and
/// that has the radius given by the second argument, with the [Paint] given in
/// the third argument. Whether the circle is filled or stroked (or both) is
/// controlled by [Paint.style].
///
/// 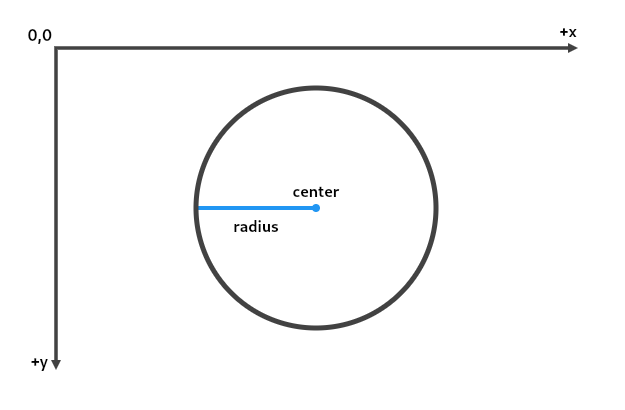
/// 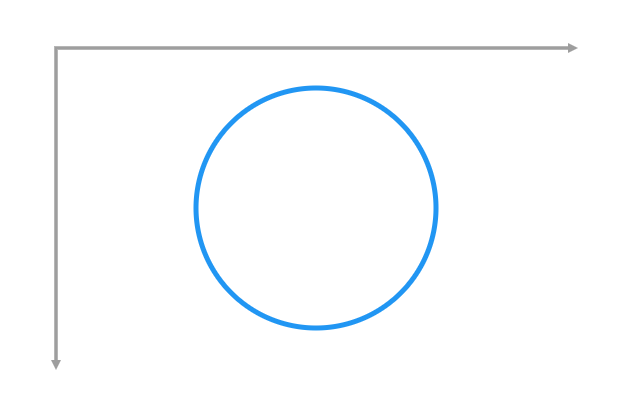
void drawCircle(Offset c, double radius, Paint paint);
/// Draw an arc scaled to fit inside the given rectangle.
///
/// It starts from `startAngle` radians around the oval up to
/// `startAngle` + `sweepAngle` radians around the oval, with zero radians
/// being the point on the right hand side of the oval that crosses the
/// horizontal line that intersects the center of the rectangle and with positive
/// angles going clockwise around the oval. If `useCenter` is true, the arc is
/// closed back to the center, forming a circle sector. Otherwise, the arc is
/// not closed, forming a circle segment.
///
/// 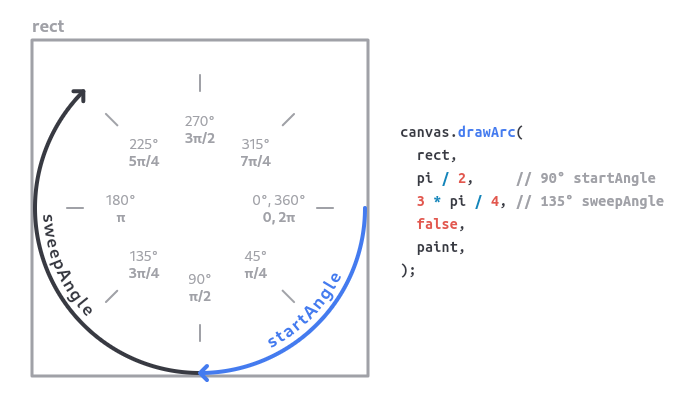
/// 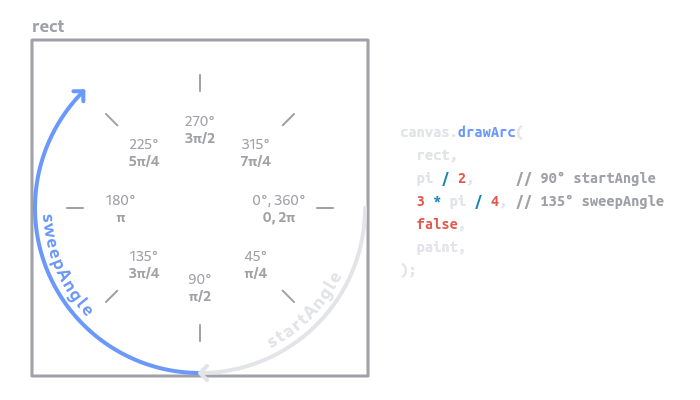
///
/// This method is optimized for drawing arcs and should be faster than [Path.arcTo].
void drawArc(Rect rect, double startAngle, double sweepAngle, bool useCenter, Paint paint);
/// Draws the given [Path] with the given [Paint].
///
/// Whether this shape is filled or stroked (or both) is controlled by
/// [Paint.style]. If the path is filled, then sub-paths within it are
/// implicitly closed (see [Path.close]).
void drawPath(Path path, Paint paint);
/// Draws the given [Image] into the canvas with its top-left corner at the
/// given [Offset]. The image is composited into the canvas using the given [Paint].
void drawImage(Image image, Offset offset, Paint paint);
/// Draws the subset of the given image described by the `src` argument into
/// the canvas in the axis-aligned rectangle given by the `dst` argument.
///
/// This might sample from outside the `src` rect by up to half the width of
/// an applied filter.
///
/// Multiple calls to this method with different arguments (from the same
/// image) can be batched into a single call to [drawAtlas] to improve
/// performance.
void drawImageRect(Image image, Rect src, Rect dst, Paint paint);
/// Draws the given [Image] into the canvas using the given [Paint].
///
/// The image is drawn in nine portions described by splitting the image by
/// drawing two horizontal lines and two vertical lines, where the `center`
/// argument describes the rectangle formed by the four points where these
/// four lines intersect each other. (This forms a 3-by-3 grid of regions,
/// the center region being described by the `center` argument.)
///
/// The four regions in the corners are drawn, without scaling, in the four
/// corners of the destination rectangle described by `dst`. The remaining
/// five regions are drawn by stretching them to fit such that they exactly
/// cover the destination rectangle while maintaining their relative
/// positions.
void drawImageNine(Image image, Rect center, Rect dst, Paint paint);
/// Draw the given picture onto the canvas. To create a picture, see
/// [PictureRecorder].
void drawPicture(Picture picture);
/// Draws the text in the given [Paragraph] into this canvas at the given
/// [Offset].
///
/// The [Paragraph] object must have had [Paragraph.layout] called on it
/// first.
///
/// To align the text, set the `textAlign` on the [ParagraphStyle] object
/// passed to the [ParagraphBuilder.new] constructor. For more details see
/// [TextAlign] and the discussion at [ParagraphStyle.new].
///
/// If the text is left aligned or justified, the left margin will be at the
/// position specified by the `offset` argument's [Offset.dx] coordinate.
///
/// If the text is right aligned or justified, the right margin will be at the
/// position described by adding the [ParagraphConstraints.width] given to
/// [Paragraph.layout], to the `offset` argument's [Offset.dx] coordinate.
///
/// If the text is centered, the centering axis will be at the position
/// described by adding half of the [ParagraphConstraints.width] given to
/// [Paragraph.layout], to the `offset` argument's [Offset.dx] coordinate.
void drawParagraph(Paragraph paragraph, Offset offset);
/// Draws a sequence of points according to the given [PointMode].
///
/// The `points` argument is interpreted as offsets from the origin.
///
/// The `paint` is used for each point ([PointMode.points]) or line
/// ([PointMode.lines] or [PointMode.polygon]), ignoring [Paint.style].
///
/// See also:
///
/// * [drawRawPoints], which takes `points` as a [Float32List] rather than a
/// [List<Offset>].
void drawPoints(PointMode pointMode, List<Offset> points, Paint paint);
/// Draws a sequence of points according to the given [PointMode].
///
/// The `points` argument is interpreted as a list of pairs of floating point
/// numbers, where each pair represents an x and y offset from the origin.
///
/// The `paint` is used for each point ([PointMode.points]) or line
/// ([PointMode.lines] or [PointMode.polygon]), ignoring [Paint.style].
///
/// See also:
///
/// * [drawPoints], which takes `points` as a [List<Offset>] rather than a
/// [List<Float32List>].
void drawRawPoints(PointMode pointMode, Float32List points, Paint paint);
/// Draws a set of [Vertices] onto the canvas as one or more triangles.
///
/// The [Paint.color] property specifies the default color to use for the
/// triangles.
///
/// The [Paint.shader] property, if set, overrides the color entirely,
/// replacing it with the colors from the specified [ImageShader], [Gradient],
/// or other shader.
///
/// The `blendMode` parameter is used to control how the colors in the
/// `vertices` are combined with the colors in the `paint`. If there are no
/// colors specified in `vertices` then the `blendMode` has no effect. If
/// there are colors in the `vertices`, then the color taken from the
/// [Paint.shader] or [Paint.color] in the `paint` is blended with the colors
/// specified in the `vertices` using the `blendMode` parameter. For the
/// purposes of this blending, the colors from the `paint` parameter are
/// considered the source, and the colors from the `vertices` are considered
/// the destination. [BlendMode.dst] ignores the `paint` and uses only the
/// colors of the `vertices`; [BlendMode.src] ignores the colors of the
/// `vertices` and uses only the colors in the `paint`.
///
/// All parameters must not be null.
///
/// See also:
/// * [Vertices.new], which creates a set of vertices to draw on the canvas.
/// * [Vertices.raw], which creates the vertices using typed data lists
/// rather than unencoded lists.
/// * [paint], Image shaders can be used to draw images on a triangular mesh.
void drawVertices(Vertices vertices, BlendMode blendMode, Paint paint);
/// Draws many parts of an image - the [atlas] - onto the canvas.
///
/// This method allows for optimization when you want to draw many parts of an
/// image onto the canvas, such as when using sprites or zooming. It is more efficient
/// than using multiple calls to [drawImageRect] and provides more functionality
/// to individually transform each image part by a separate rotation or scale and
/// blend or modulate those parts with a solid color.
///
/// The method takes a list of [Rect] objects that each define a piece of the
/// [atlas] image to be drawn independently. Each [Rect] is associated with an
/// [RSTransform] entry in the [transforms] list which defines the location,
/// rotation, and (uniform) scale with which to draw that portion of the image.
/// Each [Rect] can also be associated with an optional [Color] which will be
/// composed with the associated image part using the [blendMode] before blending
/// the result onto the canvas. The full operation can be broken down as:
///
/// - Blend each rectangular portion of the image specified by an entry in the
/// [rects] argument with its associated entry in the [colors] list using the
/// [blendMode] argument (if a color is specified). In this part of the operation,
/// the image part will be considered the source of the operation and the associated
/// color will be considered the destination.
/// - Blend the result from the first step onto the canvas using the translation,
/// rotation, and scale properties expressed in the associated entry in the
/// [transforms] list using the properties of the [Paint] object.
///
/// If the first stage of the operation which blends each part of the image with
/// a color is needed, then both the [colors] and [blendMode] arguments must
/// not be null and there must be an entry in the [colors] list for each
/// image part. If that stage is not needed, then the [colors] argument can
/// be either null or an empty list and the [blendMode] argument may also be null.
///
/// The optional [cullRect] argument can provide an estimate of the bounds of the
/// coordinates rendered by all components of the atlas to be compared against
/// the clip to quickly reject the operation if it does not intersect.
///
/// An example usage to render many sprites from a single sprite atlas with no
/// rotations or scales:
///
/// ```dart
/// class Sprite {
/// Sprite(this.index, this.center);
/// int index;
/// Offset center;
/// }
///
/// class MyPainter extends CustomPainter {
/// MyPainter(this.spriteAtlas, this.allSprites);
///
/// // assume spriteAtlas contains N 10x10 sprites side by side in a (N*10)x10 image
/// ui.Image spriteAtlas;
/// List<Sprite> allSprites;
///
/// @override
/// void paint(Canvas canvas, Size size) {
/// Paint paint = Paint();
/// canvas.drawAtlas(spriteAtlas, <RSTransform>[
/// for (final Sprite sprite in allSprites)
/// RSTransform.fromComponents(
/// rotation: 0.0,
/// scale: 1.0,
/// // Center of the sprite relative to its rect
/// anchorX: 5.0,
/// anchorY: 5.0,
/// // Location at which to draw the center of the sprite
/// translateX: sprite.center.dx,
/// translateY: sprite.center.dy,
/// ),
/// ], <Rect>[
/// for (final Sprite sprite in allSprites)
/// Rect.fromLTWH(sprite.index * 10.0, 0.0, 10.0, 10.0),
/// ], null, null, null, paint);
/// }
///
/// // ...
/// }
/// ```
///
/// Another example usage which renders sprites with an optional opacity and rotation:
///
/// ```dart
/// class Sprite {
/// Sprite(this.index, this.center, this.alpha, this.rotation);
/// int index;
/// Offset center;
/// int alpha;
/// double rotation;
/// }
///
/// class MyPainter extends CustomPainter {
/// MyPainter(this.spriteAtlas, this.allSprites);
///
/// // assume spriteAtlas contains N 10x10 sprites side by side in a (N*10)x10 image
/// ui.Image spriteAtlas;
/// List<Sprite> allSprites;
///
/// @override
/// void paint(Canvas canvas, Size size) {
/// Paint paint = Paint();
/// canvas.drawAtlas(spriteAtlas, <RSTransform>[
/// for (final Sprite sprite in allSprites)
/// RSTransform.fromComponents(
/// rotation: sprite.rotation,
/// scale: 1.0,
/// // Center of the sprite relative to its rect
/// anchorX: 5.0,
/// anchorY: 5.0,
/// // Location at which to draw the center of the sprite
/// translateX: sprite.center.dx,
/// translateY: sprite.center.dy,
/// ),
/// ], <Rect>[
/// for (final Sprite sprite in allSprites)
/// Rect.fromLTWH(sprite.index * 10.0, 0.0, 10.0, 10.0),
/// ], <Color>[
/// for (final Sprite sprite in allSprites)
/// Colors.white.withAlpha(sprite.alpha),
/// ], BlendMode.srcIn, null, paint);
/// }
///
/// // ...
/// }
/// ```
///
/// The length of the [transforms] and [rects] lists must be equal and
/// if the [colors] argument is not null then it must either be empty or
/// have the same length as the other two lists.
///
/// See also:
///
/// * [drawRawAtlas], which takes its arguments as typed data lists rather
/// than objects.
void drawAtlas(Image atlas,
List<RSTransform> transforms,
List<Rect> rects,
List<Color>? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint);
/// Draws many parts of an image - the [atlas] - onto the canvas.
///
/// This method allows for optimization when you want to draw many parts of an
/// image onto the canvas, such as when using sprites or zooming. It is more efficient
/// than using multiple calls to [drawImageRect] and provides more functionality
/// to individually transform each image part by a separate rotation or scale and
/// blend or modulate those parts with a solid color. It is also more efficient
/// than [drawAtlas] as the data in the arguments is already packed in a format
/// that can be directly used by the rendering code.
///
/// A full description of how this method uses its arguments to draw onto the
/// canvas can be found in the description of the [drawAtlas] method.
///
/// The [rstTransforms] argument is interpreted as a list of four-tuples, with
/// each tuple being ([RSTransform.scos], [RSTransform.ssin],
/// [RSTransform.tx], [RSTransform.ty]).
///
/// The [rects] argument is interpreted as a list of four-tuples, with each
/// tuple being ([Rect.left], [Rect.top], [Rect.right], [Rect.bottom]).
///
/// The [colors] argument, which can be null, is interpreted as a list of
/// 32-bit colors, with the same packing as [Color.value]. If the [colors]
/// argument is not null then the [blendMode] argument must also not be null.
///
/// An example usage to render many sprites from a single sprite atlas with no rotations
/// or scales:
///
/// ```dart
/// class Sprite {
/// Sprite(this.index, this.center);
/// int index;
/// Offset center;
/// }
///
/// class MyPainter extends CustomPainter {
/// MyPainter(this.spriteAtlas, this.allSprites);
///
/// // assume spriteAtlas contains N 10x10 sprites side by side in a (N*10)x10 image
/// ui.Image spriteAtlas;
/// List<Sprite> allSprites;
///
/// @override
/// void paint(Canvas canvas, Size size) {
/// // For best advantage, these lists should be cached and only specific
/// // entries updated when the sprite information changes. This code is
/// // illustrative of how to set up the data and not a recommendation for
/// // optimal usage.
/// Float32List rectList = Float32List(allSprites.length * 4);
/// Float32List transformList = Float32List(allSprites.length * 4);
/// for (int i = 0; i < allSprites.length; i++) {
/// Sprite sprite = allSprites[i];
/// final double rectX = sprite.index * 10.0;
/// rectList[i * 4 + 0] = rectX;
/// rectList[i * 4 + 1] = 0.0;
/// rectList[i * 4 + 2] = rectX + 10.0;
/// rectList[i * 4 + 3] = 10.0;
///
/// // This example sets the RSTransform values directly for a common case of no
/// // rotations or scales and just a translation to position the atlas entry. For
/// // more complicated transforms one could use the RSTransform class to compute
/// // the necessary values or do the same math directly.
/// transformList[i * 4 + 0] = 1.0;
/// transformList[i * 4 + 1] = 0.0;
/// transformList[i * 4 + 2] = sprite.center.dx - 5.0;
/// transformList[i * 4 + 3] = sprite.center.dy - 5.0;
/// }
/// Paint paint = Paint();
/// canvas.drawRawAtlas(spriteAtlas, transformList, rectList, null, null, null, paint);
/// }
///
/// // ...
/// }
/// ```
///
/// Another example usage which renders sprites with an optional opacity and rotation:
///
/// ```dart
/// class Sprite {
/// Sprite(this.index, this.center, this.alpha, this.rotation);
/// int index;
/// Offset center;
/// int alpha;
/// double rotation;
/// }
///
/// class MyPainter extends CustomPainter {
/// MyPainter(this.spriteAtlas, this.allSprites);
///
/// // assume spriteAtlas contains N 10x10 sprites side by side in a (N*10)x10 image
/// ui.Image spriteAtlas;
/// List<Sprite> allSprites;
///
/// @override
/// void paint(Canvas canvas, Size size) {
/// // For best advantage, these lists should be cached and only specific
/// // entries updated when the sprite information changes. This code is
/// // illustrative of how to set up the data and not a recommendation for
/// // optimal usage.
/// Float32List rectList = Float32List(allSprites.length * 4);
/// Float32List transformList = Float32List(allSprites.length * 4);
/// Int32List colorList = Int32List(allSprites.length);
/// for (int i = 0; i < allSprites.length; i++) {
/// Sprite sprite = allSprites[i];
/// final double rectX = sprite.index * 10.0;
/// rectList[i * 4 + 0] = rectX;
/// rectList[i * 4 + 1] = 0.0;
/// rectList[i * 4 + 2] = rectX + 10.0;
/// rectList[i * 4 + 3] = 10.0;
///
/// // This example uses an RSTransform object to compute the necessary values for
/// // the transform using a factory helper method because the sprites contain
/// // rotation values which are not trivial to work with. But if the math for the
/// // values falls out from other calculations on the sprites then the values could
/// // possibly be generated directly from the sprite update code.
/// final RSTransform transform = RSTransform.fromComponents(
/// rotation: sprite.rotation,
/// scale: 1.0,
/// // Center of the sprite relative to its rect
/// anchorX: 5.0,
/// anchorY: 5.0,
/// // Location at which to draw the center of the sprite
/// translateX: sprite.center.dx,
/// translateY: sprite.center.dy,
/// );
/// transformList[i * 4 + 0] = transform.scos;
/// transformList[i * 4 + 1] = transform.ssin;
/// transformList[i * 4 + 2] = transform.tx;
/// transformList[i * 4 + 3] = transform.ty;
///
/// // This example computes the color value directly, but one could also compute
/// // an actual Color object and use its Color.value getter for the same result.
/// // Since we are using BlendMode.srcIn, only the alpha component matters for
/// // these colors which makes this a simple shift operation.
/// colorList[i] = sprite.alpha << 24;
/// }
/// Paint paint = Paint();
/// canvas.drawRawAtlas(spriteAtlas, transformList, rectList, colorList, BlendMode.srcIn, null, paint);
/// }
///
/// // ...
/// }
/// ```
///
/// See also:
///
/// * [drawAtlas], which takes its arguments as objects rather than typed
/// data lists.
void drawRawAtlas(Image atlas,
Float32List rstTransforms,
Float32List rects,
Int32List? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint);
/// Draws a shadow for a [Path] representing the given material elevation.
///
/// The `transparentOccluder` argument should be true if the occluding object
/// is not opaque.
///
/// The arguments must not be null.
void drawShadow(Path path, Color color, double elevation, bool transparentOccluder);
}
base class _NativeCanvas extends NativeFieldWrapperClass1 implements Canvas {
_NativeCanvas(PictureRecorder recorder, [ Rect? cullRect ]) {
if (recorder.isRecording) {
throw ArgumentError('"recorder" must not already be associated with another Canvas.');
}
_recorder = recorder as _NativePictureRecorder;
_recorder!._canvas = this;
cullRect ??= Rect.largest;
_constructor(_recorder!, cullRect.left, cullRect.top, cullRect.right, cullRect.bottom);
}
@Native<Void Function(Handle, Pointer<Void>, Double, Double, Double, Double)>(symbol: 'Canvas::Create')
external void _constructor(_NativePictureRecorder recorder, double left, double top, double right, double bottom);
// The underlying DlCanvas is owned by the DisplayListBuilder used to create this Canvas.
// The Canvas holds a reference to the PictureRecorder to prevent the recorder from being
// garbage collected until PictureRecorder.endRecording is called.
_NativePictureRecorder? _recorder;
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'Canvas::save', isLeaf: true)
external void save();
static Rect _sorted(Rect rect) {
if (rect.isEmpty) {
rect = Rect.fromLTRB(
math.min(rect.left, rect.right),
math.min(rect.top, rect.bottom),
math.max(rect.left, rect.right),
math.max(rect.top, rect.bottom),
);
}
return rect;
}
@override
void saveLayer(Rect? bounds, Paint paint) {
if (bounds == null) {
_saveLayerWithoutBounds(paint._objects, paint._data);
} else {
assert(_rectIsValid(bounds));
_saveLayer(bounds.left, bounds.top, bounds.right, bounds.bottom, paint._objects, paint._data);
}
}
@Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::saveLayerWithoutBounds')
external void _saveLayerWithoutBounds(List<Object?>? paintObjects, ByteData paintData);
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::saveLayer')
external void _saveLayer(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'Canvas::restore', isLeaf: true)
external void restore();
@override
@Native<Void Function(Pointer<Void>, Int32)>(symbol: 'Canvas::restoreToCount', isLeaf: true)
external void restoreToCount(int count);
@override
@Native<Int32 Function(Pointer<Void>)>(symbol: 'Canvas::getSaveCount', isLeaf: true)
external int getSaveCount();
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::translate', isLeaf: true)
external void translate(double dx, double dy);
@override
void scale(double sx, [double? sy]) => _scale(sx, sy ?? sx);
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::scale', isLeaf: true)
external void _scale(double sx, double sy);
@override
@Native<Void Function(Pointer<Void>, Double)>(symbol: 'Canvas::rotate', isLeaf: true)
external void rotate(double radians);
@override
@Native<Void Function(Pointer<Void>, Double, Double)>(symbol: 'Canvas::skew', isLeaf: true)
external void skew(double sx, double sy);
@override
void transform(Float64List matrix4) {
if (matrix4.length != 16) {
throw ArgumentError('"matrix4" must have 16 entries.');
}
_transform(matrix4);
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::transform')
external void _transform(Float64List matrix4);
@override
Float64List getTransform() {
final Float64List matrix4 = Float64List(16);
_getTransform(matrix4);
return matrix4;
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getTransform')
external void _getTransform(Float64List matrix4);
@override
void clipRect(Rect rect, { ClipOp clipOp = ClipOp.intersect, bool doAntiAlias = true }) {
assert(_rectIsValid(rect));
rect = _sorted(rect);
// Even if rect is still empty - which implies it has a zero dimension -
// we still need to perform the clipRect operation as it will effectively
// nullify any further rendering until the next restore call.
_clipRect(rect.left, rect.top, rect.right, rect.bottom, clipOp.index, doAntiAlias);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Int32, Bool)>(symbol: 'Canvas::clipRect', isLeaf: true)
external void _clipRect(double left, double top, double right, double bottom, int clipOp, bool doAntiAlias);
@override
void clipRRect(RRect rrect, {bool doAntiAlias = true}) {
assert(_rrectIsValid(rrect));
_clipRRect(rrect._getValue32(), doAntiAlias);
}
@Native<Void Function(Pointer<Void>, Handle, Bool)>(symbol: 'Canvas::clipRRect')
external void _clipRRect(Float32List rrect, bool doAntiAlias);
@override
void clipPath(Path path, {bool doAntiAlias = true}) {
_clipPath(path as _NativePath, doAntiAlias);
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Bool)>(symbol: 'Canvas::clipPath')
external void _clipPath(_NativePath path, bool doAntiAlias);
@override
Rect getLocalClipBounds() {
final Float64List bounds = Float64List(4);
_getLocalClipBounds(bounds);
return Rect.fromLTRB(bounds[0], bounds[1], bounds[2], bounds[3]);
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getLocalClipBounds')
external void _getLocalClipBounds(Float64List bounds);
@override
Rect getDestinationClipBounds() {
final Float64List bounds = Float64List(4);
_getDestinationClipBounds(bounds);
return Rect.fromLTRB(bounds[0], bounds[1], bounds[2], bounds[3]);
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'Canvas::getDestinationClipBounds')
external void _getDestinationClipBounds(Float64List bounds);
@override
void drawColor(Color color, BlendMode blendMode) {
_drawColor(color.value, blendMode.index);
}
@Native<Void Function(Pointer<Void>, Uint32, Int32)>(symbol: 'Canvas::drawColor', isLeaf: true)
external void _drawColor(int color, int blendMode);
@override
void drawLine(Offset p1, Offset p2, Paint paint) {
assert(_offsetIsValid(p1));
assert(_offsetIsValid(p2));
_drawLine(p1.dx, p1.dy, p2.dx, p2.dy, paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawLine')
external void _drawLine(double x1, double y1, double x2, double y2, List<Object?>? paintObjects, ByteData paintData);
@override
void drawPaint(Paint paint) {
_drawPaint(paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::drawPaint')
external void _drawPaint(List<Object?>? paintObjects, ByteData paintData);
@override
void drawRect(Rect rect, Paint paint) {
assert(_rectIsValid(rect));
rect = _sorted(rect);
if (paint.style != PaintingStyle.fill || !rect.isEmpty) {
_drawRect(rect.left, rect.top, rect.right, rect.bottom, paint._objects, paint._data);
}
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawRect')
external void _drawRect(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
@override
void drawRRect(RRect rrect, Paint paint) {
assert(_rrectIsValid(rrect));
_drawRRect(rrect._getValue32(), paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Handle, Handle, Handle)>(symbol: 'Canvas::drawRRect')
external void _drawRRect(Float32List rrect, List<Object?>? paintObjects, ByteData paintData);
@override
void drawDRRect(RRect outer, RRect inner, Paint paint) {
assert(_rrectIsValid(outer));
assert(_rrectIsValid(inner));
_drawDRRect(outer._getValue32(), inner._getValue32(), paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Handle, Handle, Handle, Handle)>(symbol: 'Canvas::drawDRRect')
external void _drawDRRect(Float32List outer, Float32List inner, List<Object?>? paintObjects, ByteData paintData);
@override
void drawOval(Rect rect, Paint paint) {
assert(_rectIsValid(rect));
rect = _sorted(rect);
if (paint.style != PaintingStyle.fill || !rect.isEmpty) {
_drawOval(rect.left, rect.top, rect.right, rect.bottom, paint._objects, paint._data);
}
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawOval')
external void _drawOval(double left, double top, double right, double bottom, List<Object?>? paintObjects, ByteData paintData);
@override
void drawCircle(Offset c, double radius, Paint paint) {
assert(_offsetIsValid(c));
_drawCircle(c.dx, c.dy, radius, paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Handle, Handle)>(symbol: 'Canvas::drawCircle')
external void _drawCircle(double x, double y, double radius, List<Object?>? paintObjects, ByteData paintData);
@override
void drawArc(Rect rect, double startAngle, double sweepAngle, bool useCenter, Paint paint) {
assert(_rectIsValid(rect));
_drawArc(rect.left, rect.top, rect.right, rect.bottom, startAngle, sweepAngle, useCenter, paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Double, Double, Double, Double, Double, Double, Bool, Handle, Handle)>(symbol: 'Canvas::drawArc')
external void _drawArc(
double left,
double top,
double right,
double bottom,
double startAngle,
double sweepAngle,
bool useCenter,
List<Object?>? paintObjects,
ByteData paintData);
@override
void drawPath(Path path, Paint paint) {
_drawPath(path as _NativePath, paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Handle, Handle)>(symbol: 'Canvas::drawPath')
external void _drawPath(_NativePath path, List<Object?>? paintObjects, ByteData paintData);
@override
void drawImage(Image image, Offset offset, Paint paint) {
assert(!image.debugDisposed);
assert(_offsetIsValid(offset));
final String? error = _drawImage(image._image, offset.dx, offset.dy, paint._objects, paint._data, paint.filterQuality.index);
if (error != null) {
throw PictureRasterizationException._(error, stack: image._debugStack);
}
}
@Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImage')
external String? _drawImage(_Image image, double x, double y, List<Object?>? paintObjects, ByteData paintData, int filterQualityIndex);
@override
void drawImageRect(Image image, Rect src, Rect dst, Paint paint) {
assert(!image.debugDisposed);
assert(_rectIsValid(src));
assert(_rectIsValid(dst));
final String? error = _drawImageRect(image._image,
src.left,
src.top,
src.right,
src.bottom,
dst.left,
dst.top,
dst.right,
dst.bottom,
paint._objects,
paint._data,
paint.filterQuality.index);
if (error != null) {
throw PictureRasterizationException._(error, stack: image._debugStack);
}
}
@Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImageRect')
external String? _drawImageRect(
_Image image,
double srcLeft,
double srcTop,
double srcRight,
double srcBottom,
double dstLeft,
double dstTop,
double dstRight,
double dstBottom,
List<Object?>? paintObjects,
ByteData paintData,
int filterQualityIndex);
@override
void drawImageNine(Image image, Rect center, Rect dst, Paint paint) {
assert(!image.debugDisposed);
assert(_rectIsValid(center));
assert(_rectIsValid(dst));
final String? error = _drawImageNine(image._image,
center.left,
center.top,
center.right,
center.bottom,
dst.left,
dst.top,
dst.right,
dst.bottom,
paint._objects,
paint._data,
paint.filterQuality.index);
if (error != null) {
throw PictureRasterizationException._(error, stack: image._debugStack);
}
}
@Native<Handle Function(Pointer<Void>, Pointer<Void>, Double, Double, Double, Double, Double, Double, Double, Double, Handle, Handle, Int32)>(symbol: 'Canvas::drawImageNine')
external String? _drawImageNine(
_Image image,
double centerLeft,
double centerTop,
double centerRight,
double centerBottom,
double dstLeft,
double dstTop,
double dstRight,
double dstBottom,
List<Object?>? paintObjects,
ByteData paintData,
int filterQualityIndex);
@override
void drawPicture(Picture picture) {
assert(!picture.debugDisposed);
_drawPicture(picture as _NativePicture);
}
@Native<Void Function(Pointer<Void>, Pointer<Void>)>(symbol: 'Canvas::drawPicture')
external void _drawPicture(_NativePicture picture);
@override
void drawParagraph(Paragraph paragraph, Offset offset) {
final _NativeParagraph nativeParagraph = paragraph as _NativeParagraph;
assert(!nativeParagraph.debugDisposed);
assert(_offsetIsValid(offset));
assert(!nativeParagraph._needsLayout);
nativeParagraph._paint(this, offset.dx, offset.dy);
}
@override
void drawPoints(PointMode pointMode, List<Offset> points, Paint paint) {
_drawPoints(paint._objects, paint._data, pointMode.index, _encodePointList(points));
}
@override
void drawRawPoints(PointMode pointMode, Float32List points, Paint paint) {
if (points.length % 2 != 0) {
throw ArgumentError('"points" must have an even number of values.');
}
_drawPoints(paint._objects, paint._data, pointMode.index, points);
}
@Native<Void Function(Pointer<Void>, Handle, Handle, Int32, Handle)>(symbol: 'Canvas::drawPoints')
external void _drawPoints(List<Object?>? paintObjects, ByteData paintData, int pointMode, Float32List points);
@override
void drawVertices(Vertices vertices, BlendMode blendMode, Paint paint) {
assert(!vertices.debugDisposed);
_drawVertices(vertices, blendMode.index, paint._objects, paint._data);
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Int32, Handle, Handle)>(symbol: 'Canvas::drawVertices')
external void _drawVertices(Vertices vertices, int blendMode, List<Object?>? paintObjects, ByteData paintData);
@override
void drawAtlas(Image atlas,
List<RSTransform> transforms,
List<Rect> rects,
List<Color>? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint) {
assert(!atlas.debugDisposed);
assert(colors == null || colors.isEmpty || blendMode != null);
final int rectCount = rects.length;
if (transforms.length != rectCount) {
throw ArgumentError('"transforms" and "rects" lengths must match.');
}
if (colors != null && colors.isNotEmpty && colors.length != rectCount) {
throw ArgumentError('If non-null, "colors" length must match that of "transforms" and "rects".');
}
final Float32List rstTransformBuffer = Float32List(rectCount * 4);
final Float32List rectBuffer = Float32List(rectCount * 4);
for (int i = 0; i < rectCount; ++i) {
final int index0 = i * 4;
final int index1 = index0 + 1;
final int index2 = index0 + 2;
final int index3 = index0 + 3;
final RSTransform rstTransform = transforms[i];
final Rect rect = rects[i];
assert(_rectIsValid(rect));
rstTransformBuffer[index0] = rstTransform.scos;
rstTransformBuffer[index1] = rstTransform.ssin;
rstTransformBuffer[index2] = rstTransform.tx;
rstTransformBuffer[index3] = rstTransform.ty;
rectBuffer[index0] = rect.left;
rectBuffer[index1] = rect.top;
rectBuffer[index2] = rect.right;
rectBuffer[index3] = rect.bottom;
}
final Int32List? colorBuffer = (colors == null || colors.isEmpty) ? null : _encodeColorList(colors);
final Float32List? cullRectBuffer = cullRect?._getValue32();
final int qualityIndex = paint.filterQuality.index;
final String? error = _drawAtlas(
paint._objects, paint._data, qualityIndex, atlas._image, rstTransformBuffer, rectBuffer,
colorBuffer, (blendMode ?? BlendMode.src).index, cullRectBuffer
);
if (error != null) {
throw PictureRasterizationException._(error, stack: atlas._debugStack);
}
}
@override
void drawRawAtlas(Image atlas,
Float32List rstTransforms,
Float32List rects,
Int32List? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint) {
assert(colors == null || blendMode != null);
final int rectCount = rects.length;
if (rstTransforms.length != rectCount) {
throw ArgumentError('"rstTransforms" and "rects" lengths must match.');
}
if (rectCount % 4 != 0) {
throw ArgumentError('"rstTransforms" and "rects" lengths must be a multiple of four.');
}
if (colors != null && colors.length * 4 != rectCount) {
throw ArgumentError('If non-null, "colors" length must be one fourth the length of "rstTransforms" and "rects".');
}
final int qualityIndex = paint.filterQuality.index;
final String? error = _drawAtlas(
paint._objects, paint._data, qualityIndex, atlas._image, rstTransforms, rects,
colors, (blendMode ?? BlendMode.src).index, cullRect?._getValue32()
);
if (error != null) {
throw PictureRasterizationException._(error, stack: atlas._debugStack);
}
}
@Native<Handle Function(Pointer<Void>, Handle, Handle, Int32, Pointer<Void>, Handle, Handle, Handle, Int32, Handle)>(symbol: 'Canvas::drawAtlas')
external String? _drawAtlas(
List<Object?>? paintObjects,
ByteData paintData,
int filterQualityIndex,
_Image atlas,
Float32List rstTransforms,
Float32List rects,
Int32List? colors,
int blendMode,
Float32List? cullRect);
@override
void drawShadow(Path path, Color color, double elevation, bool transparentOccluder) {
_drawShadow(path as _NativePath, color.value, elevation, transparentOccluder);
}
@Native<Void Function(Pointer<Void>, Pointer<Void>, Uint32, Double, Bool)>(symbol: 'Canvas::drawShadow')
external void _drawShadow(_NativePath path, int color, double elevation, bool transparentOccluder);
@override
String toString() => 'Canvas(recording: ${_recorder != null})';
}
/// Signature for [Picture] lifecycle events.
typedef PictureEventCallback = void Function(Picture picture);
/// An object representing a sequence of recorded graphical operations.
///
/// To create a [Picture], use a [PictureRecorder].
///
/// A [Picture] can be placed in a [Scene] using a [SceneBuilder], via
/// the [SceneBuilder.addPicture] method. A [Picture] can also be
/// drawn into a [Canvas], using the [Canvas.drawPicture] method.
abstract class Picture {
/// A callback that is invoked to report a picture creation.
///
/// It's preferred to use [MemoryAllocations] in flutter/foundation.dart
/// than to use [onCreate] directly because [MemoryAllocations]
/// allows multiple callbacks.
static PictureEventCallback? onCreate;
/// A callback that is invoked to report the picture disposal.
///
/// It's preferred to use [MemoryAllocations] in flutter/foundation.dart
/// than to use [onDispose] directly because [MemoryAllocations]
/// allows multiple callbacks.
static PictureEventCallback? onDispose;
/// Creates an image from this picture.
///
/// The returned image will be `width` pixels wide and `height` pixels high.
/// The picture is rasterized within the 0 (left), 0 (top), `width` (right),
/// `height` (bottom) bounds. Content outside these bounds is clipped.
Future<Image> toImage(int width, int height);
/// Synchronously creates a handle to an image of this picture.
///
/// {@template dart.ui.painting.Picture.toImageSync}
/// The returned image will be `width` pixels wide and `height` pixels high.
/// The picture is rasterized within the 0 (left), 0 (top), `width` (right),
/// `height` (bottom) bounds. Content outside these bounds is clipped.
///
/// The image object is created and returned synchronously, but is rasterized
/// asynchronously. If the rasterization fails, an exception will be thrown
/// when the image is drawn to a [Canvas].
///
/// If a GPU context is available, this image will be created as GPU resident
/// and not copied back to the host. This means the image will be more
/// efficient to draw.
///
/// If no GPU context is available, the image will be rasterized on the CPU.
/// {@endtemplate}
Image toImageSync(int width, int height);
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
void dispose();
/// Whether this reference to the underlying picture is [dispose]d.
///
/// This only returns a valid value if asserts are enabled, and must not be
/// used otherwise.
bool get debugDisposed;
/// Returns the approximate number of bytes allocated for this object.
///
/// The actual size of this picture may be larger, particularly if it contains
/// references to image or other large objects.
int get approximateBytesUsed;
}
base class _NativePicture extends NativeFieldWrapperClass1 implements Picture {
/// This class is created by the engine, and should not be instantiated
/// or extended directly.
///
/// To create a [Picture], use a [PictureRecorder].
_NativePicture._();
@override
Future<Image> toImage(int width, int height) {
assert(!_disposed);
if (width <= 0 || height <= 0) {
throw Exception('Invalid image dimensions.');
}
return _futurize(
(_Callback<Image?> callback) => _toImage(width, height, (_Image? image) {
if (image == null) {
callback(null);
} else {
callback(Image._(image, image.width, image.height));
}
}),
);
}
@Native<Handle Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Picture::toImage')
external String? _toImage(int width, int height, void Function(_Image?) callback);
@override
Image toImageSync(int width, int height) {
assert(!_disposed);
if (width <= 0 || height <= 0) {
throw Exception('Invalid image dimensions.');
}
final _Image image = _Image._();
_toImageSync(width, height, image);
return Image._(image, image.width, image.height);
}
@Native<Void Function(Pointer<Void>, Uint32, Uint32, Handle)>(symbol: 'Picture::toImageSync')
external void _toImageSync(int width, int height, _Image outImage);
@override
void dispose() {
assert(!_disposed);
assert(() {
_disposed = true;
return true;
}());
Picture.onDispose?.call(this);
_dispose();
}
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
@Native<Void Function(Pointer<Void>)>(symbol: 'Picture::dispose')
external void _dispose();
bool _disposed = false;
@override
bool get debugDisposed {
bool? disposed;
assert(() {
disposed = _disposed;
return true;
}());
return disposed ?? (throw StateError('Picture.debugDisposed is only available when asserts are enabled.'));
}
@override
@Native<Uint64 Function(Pointer<Void>)>(symbol: 'Picture::GetAllocationSize', isLeaf: true)
external int get approximateBytesUsed;
@override
String toString() => 'Picture';
}
/// Records a [Picture] containing a sequence of graphical operations.
///
/// To begin recording, construct a [Canvas] to record the commands.
/// To end recording, use the [PictureRecorder.endRecording] method.
abstract class PictureRecorder {
/// Creates a new idle PictureRecorder. To associate it with a
/// [Canvas] and begin recording, pass this [PictureRecorder] to the
/// [Canvas] constructor.
factory PictureRecorder() = _NativePictureRecorder;
/// Whether this object is currently recording commands.
///
/// Specifically, this returns true if a [Canvas] object has been
/// created to record commands and recording has not yet ended via a
/// call to [endRecording], and false if either this
/// [PictureRecorder] has not yet been associated with a [Canvas],
/// or the [endRecording] method has already been called.
bool get isRecording;
/// Finishes recording graphical operations.
///
/// Returns a picture containing the graphical operations that have been
/// recorded thus far. After calling this function, both the picture recorder
/// and the canvas objects are invalid and cannot be used further.
Picture endRecording();
}
base class _NativePictureRecorder extends NativeFieldWrapperClass1 implements PictureRecorder {
_NativePictureRecorder() { _constructor(); }
@Native<Void Function(Handle)>(symbol: 'PictureRecorder::Create')
external void _constructor();
@override
bool get isRecording => _canvas != null;
@override
Picture endRecording() {
if (_canvas == null) {
throw StateError('PictureRecorder did not start recording.');
}
final _NativePicture picture = _NativePicture._();
_endRecording(picture);
_canvas!._recorder = null;
_canvas = null;
// We invoke the handler here, not in the Picture constructor, because we want
// [picture.approximateBytesUsed] to be available for the handler.
Picture.onCreate?.call(picture);
return picture;
}
@Native<Void Function(Pointer<Void>, Handle)>(symbol: 'PictureRecorder::endRecording')
external void _endRecording(_NativePicture outPicture);
_NativeCanvas? _canvas;
@override
String toString() => 'PictureRecorder(recording: $isRecording)';
}
/// A single shadow.
///
/// Multiple shadows are stacked together in a [TextStyle].
class Shadow {
/// Construct a shadow.
///
/// The default shadow is a black shadow with zero offset and zero blur.
/// Default shadows should be completely covered by the casting element,
/// and not be visible.
///
/// Transparency should be adjusted through the [color] alpha.
///
/// Shadow order matters due to compositing multiple translucent objects not
/// being commutative.
const Shadow({
this.color = const Color(_kColorDefault),
this.offset = Offset.zero,
this.blurRadius = 0.0,
}) : assert(blurRadius >= 0.0, 'Text shadow blur radius should be non-negative.');
static const int _kColorDefault = 0xFF000000;
// Constants for shadow encoding.
static const int _kBytesPerShadow = 16;
static const int _kColorOffset = 0 << 2;
static const int _kXOffset = 1 << 2;
static const int _kYOffset = 2 << 2;
static const int _kBlurOffset = 3 << 2;
/// Color that the shadow will be drawn with.
///
/// The shadows are shapes composited directly over the base canvas, and do not
/// represent optical occlusion.
final Color color;
/// The displacement of the shadow from the casting element.
///
/// Positive x/y offsets will shift the shadow to the right and down, while
/// negative offsets shift the shadow to the left and up. The offsets are
/// relative to the position of the element that is casting it.
final Offset offset;
/// The standard deviation of the Gaussian to convolve with the shadow's shape.
final double blurRadius;
/// Converts a blur radius in pixels to sigmas.
///
/// See the sigma argument to [MaskFilter.blur].
///
// See SkBlurMask::ConvertRadiusToSigma().
// <https://github.com/google/skia/blob/bb5b77db51d2e149ee66db284903572a5aac09be/src/effects/SkBlurMask.cpp#L23>
static double convertRadiusToSigma(double radius) {
return radius > 0 ? radius * 0.57735 + 0.5 : 0;
}
/// The [blurRadius] in sigmas instead of logical pixels.
///
/// See the sigma argument to [MaskFilter.blur].
double get blurSigma => convertRadiusToSigma(blurRadius);
/// Create the [Paint] object that corresponds to this shadow description.
///
/// The [offset] is not represented in the [Paint] object.
/// To honor this as well, the shape should be translated by [offset] before
/// being filled using this [Paint].
///
/// This class does not provide a way to disable shadows to avoid
/// inconsistencies in shadow blur rendering, primarily as a method of
/// reducing test flakiness. [toPaint] should be overridden in subclasses to
/// provide this functionality.
Paint toPaint() {
return Paint()
..color = color
..maskFilter = MaskFilter.blur(BlurStyle.normal, blurSigma);
}
/// Returns a new shadow with its [offset] and [blurRadius] scaled by the given
/// factor.
Shadow scale(double factor) {
return Shadow(
color: color,
offset: offset * factor,
blurRadius: blurRadius * factor,
);
}
/// Linearly interpolate between two shadows.
///
/// If either shadow is null, this function linearly interpolates from
/// a shadow that matches the other shadow in color but has a zero
/// offset and a zero blurRadius.
///
/// {@template dart.ui.shadow.lerp}
/// The `t` argument represents position on the timeline, with 0.0 meaning
/// that the interpolation has not started, returning `a` (or something
/// equivalent to `a`), 1.0 meaning that the interpolation has finished,
/// returning `b` (or something equivalent to `b`), and values in between
/// meaning that the interpolation is at the relevant point on the timeline
/// between `a` and `b`. The interpolation can be extrapolated beyond 0.0 and
/// 1.0, so negative values and values greater than 1.0 are valid (and can
/// easily be generated by curves such as [Curves.elasticInOut]).
///
/// Values for `t` are usually obtained from an [Animation<double>], such as
/// an [AnimationController].
/// {@endtemplate}
static Shadow? lerp(Shadow? a, Shadow? b, double t) {
if (b == null) {
if (a == null) {
return null;
} else {
return a.scale(1.0 - t);
}
} else {
if (a == null) {
return b.scale(t);
} else {
return Shadow(
color: Color.lerp(a.color, b.color, t)!,
offset: Offset.lerp(a.offset, b.offset, t)!,
blurRadius: _lerpDouble(a.blurRadius, b.blurRadius, t),
);
}
}
}
/// Linearly interpolate between two lists of shadows.
///
/// If the lists differ in length, excess items are lerped with null.
///
/// {@macro dart.ui.shadow.lerp}
static List<Shadow>? lerpList(List<Shadow>? a, List<Shadow>? b, double t) {
if (a == null && b == null) {
return null;
}
a ??= <Shadow>[];
b ??= <Shadow>[];
final List<Shadow> result = <Shadow>[];
final int commonLength = math.min(a.length, b.length);
for (int i = 0; i < commonLength; i += 1) {
result.add(Shadow.lerp(a[i], b[i], t)!);
}
for (int i = commonLength; i < a.length; i += 1) {
result.add(a[i].scale(1.0 - t));
}
for (int i = commonLength; i < b.length; i += 1) {
result.add(b[i].scale(t));
}
return result;
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
return other is Shadow
&& other.color == color
&& other.offset == offset
&& other.blurRadius == blurRadius;
}
@override
int get hashCode => Object.hash(color, offset, blurRadius);
// Serialize [shadows] into ByteData. The format is a single uint_32_t at
// the beginning indicating the number of shadows, followed by _kBytesPerShadow
// bytes for each shadow.
static ByteData _encodeShadows(List<Shadow>? shadows) {
if (shadows == null) {
return ByteData(0);
}
final int byteCount = shadows.length * _kBytesPerShadow;
final ByteData shadowsData = ByteData(byteCount);
int shadowOffset = 0;
for (int shadowIndex = 0; shadowIndex < shadows.length; ++shadowIndex) {
final Shadow shadow = shadows[shadowIndex];
shadowOffset = shadowIndex * _kBytesPerShadow;
shadowsData.setInt32(_kColorOffset + shadowOffset,
shadow.color.value ^ Shadow._kColorDefault, _kFakeHostEndian);
shadowsData.setFloat32(_kXOffset + shadowOffset,
shadow.offset.dx, _kFakeHostEndian);
shadowsData.setFloat32(_kYOffset + shadowOffset,
shadow.offset.dy, _kFakeHostEndian);
final double blurSigma = Shadow.convertRadiusToSigma(shadow.blurRadius);
shadowsData.setFloat32(_kBlurOffset + shadowOffset,
blurSigma, _kFakeHostEndian);
}
return shadowsData;
}
@override
String toString() => 'TextShadow($color, $offset, $blurRadius)';
}
/// A handle to a read-only byte buffer that is managed by the engine.
///
/// The creator of this object is responsible for calling [dispose] when it is
/// no longer needed.
base class ImmutableBuffer extends NativeFieldWrapperClass1 {
ImmutableBuffer._(this._length);
/// Creates a copy of the data from a [Uint8List] suitable for internal use
/// in the engine.
static Future<ImmutableBuffer> fromUint8List(Uint8List list) {
final ImmutableBuffer instance = ImmutableBuffer._(list.length);
return _futurize((_Callback<void> callback) {
return instance._init(list, callback);
}).then((_) => instance);
}
/// Create a buffer from the asset with key [assetKey].
///
/// Throws an [Exception] if the asset does not exist.
static Future<ImmutableBuffer> fromAsset(String assetKey) {
// The flutter tool converts all asset keys with spaces into URI
// encoded paths (replacing ' ' with '%20', for example). We perform
// the same encoding here so that users can load assets with the same
// key they have written in the pubspec.
final String encodedKey = Uri(path: Uri.encodeFull(assetKey)).path;
final ImmutableBuffer instance = ImmutableBuffer._(0);
return _futurize((_Callback<int> callback) {
return instance._initFromAsset(encodedKey, callback);
}).then((int length) {
if (length == -1) {
throw Exception('Asset not found');
}
return instance.._length = length;
});
}
/// Create a buffer from the file with [path].
///
/// Throws an [Exception] if the asset does not exist.
static Future<ImmutableBuffer> fromFilePath(String path) {
final ImmutableBuffer instance = ImmutableBuffer._(0);
return _futurize((_Callback<int> callback) {
return instance._initFromFile(path, callback);
}).then((int length) {
if (length == -1) {
throw Exception('Could not load file at $path.');
}
return instance.._length = length;
});
}
@Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::init')
external String? _init(Uint8List list, _Callback<void> callback);
@Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::initFromAsset')
external String? _initFromAsset(String assetKey, _Callback<int> callback);
@Native<Handle Function(Handle, Handle, Handle)>(symbol: 'ImmutableBuffer::initFromFile')
external String? _initFromFile(String assetKey, _Callback<int> callback);
/// The length, in bytes, of the underlying data.
int get length => _length;
int _length;
bool _debugDisposed = false;
/// Whether [dispose] has been called.
///
/// This must only be used when asserts are enabled. Otherwise, it will throw.
bool get debugDisposed {
late bool disposed;
assert(() {
disposed = _debugDisposed;
return true;
}());
return disposed;
}
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// The underlying memory allocated by this object will be retained beyond
/// this call if it is still needed by another object that has not been
/// disposed. For example, an [ImageDescriptor] that has not been disposed
/// may still retain a reference to the memory from this buffer even if it
/// has been disposed. Freeing that memory requires disposing all resources
/// that may still hold it.
void dispose() {
assert(() {
assert(!_debugDisposed);
_debugDisposed = true;
return true;
}());
_dispose();
}
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
@Native<Void Function(Pointer<Void>)>(symbol: 'ImmutableBuffer::dispose')
external void _dispose();
}
/// A descriptor of data that can be turned into an [Image] via a [Codec].
///
/// Use this class to determine the height, width, and byte size of image data
/// before decoding it.
abstract class ImageDescriptor {
/// Creates an image descriptor from raw image pixels.
///
/// The `pixels` parameter is the pixel data. They are packed in bytes in the
/// order described by `pixelFormat`, then grouped in rows, from left to right,
/// then top to bottom.
///
/// The `rowBytes` parameter is the number of bytes consumed by each row of
/// pixels in the data buffer. If unspecified, it defaults to `width` multiplied
/// by the number of bytes per pixel in the provided `format`.
// Not async because there's no expensive work to do here.
factory ImageDescriptor.raw(
ImmutableBuffer buffer, {
required int width,
required int height,
int? rowBytes,
required PixelFormat pixelFormat,
}) = _NativeImageDescriptor.raw;
/// Creates an image descriptor from encoded data in a supported format.
static Future<ImageDescriptor> encoded(ImmutableBuffer buffer) {
final _NativeImageDescriptor descriptor = _NativeImageDescriptor._();
return _futurize((_Callback<void> callback) {
return descriptor._initEncoded(buffer, callback);
}).then((_) => descriptor);
}
/// The width, in pixels, of the image.
///
/// On the Web, this is only supported for [raw] images.
int get width;
/// The height, in pixels, of the image.
///
/// On the Web, this is only supported for [raw] images.
int get height;
/// The number of bytes per pixel in the image.
///
/// On web, this is only supported for [raw] images.
int get bytesPerPixel;
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// This can't be a leaf call because the native function calls Dart API
/// (Dart_SetNativeInstanceField).
void dispose();
/// Creates a [Codec] object which is suitable for decoding the data in the
/// buffer to an [Image].
///
/// If only one of targetWidth or targetHeight are specified, the other
/// dimension will be scaled according to the aspect ratio of the supplied
/// dimension.
///
/// If either targetWidth or targetHeight is less than or equal to zero, it
/// will be treated as if it is null.
Future<Codec> instantiateCodec({int? targetWidth, int? targetHeight});
}
base class _NativeImageDescriptor extends NativeFieldWrapperClass1 implements ImageDescriptor {
_NativeImageDescriptor._();
/// Creates an image descriptor from raw image pixels.
///
/// The `pixels` parameter is the pixel data. They are packed in bytes in the
/// order described by `pixelFormat`, then grouped in rows, from left to right,
/// then top to bottom.
///
/// The `rowBytes` parameter is the number of bytes consumed by each row of
/// pixels in the data buffer. If unspecified, it defaults to `width` multiplied
/// by the number of bytes per pixel in the provided `format`.
// Not async because there's no expensive work to do here.
_NativeImageDescriptor.raw(
ImmutableBuffer buffer, {
required int width,
required int height,
int? rowBytes,
required PixelFormat pixelFormat,
}) {
_width = width;
_height = height;
// We only support 4 byte pixel formats in the PixelFormat enum.
_bytesPerPixel = 4;
_initRaw(this, buffer, width, height, rowBytes ?? -1, pixelFormat.index);
}
@Native<Handle Function(Handle, Pointer<Void>, Handle)>(symbol: 'ImageDescriptor::initEncoded')
external String? _initEncoded(ImmutableBuffer buffer, _Callback<void> callback);
@Native<Void Function(Handle, Handle, Int32, Int32, Int32, Int32)>(symbol: 'ImageDescriptor::initRaw')
external static void _initRaw(ImageDescriptor outDescriptor, ImmutableBuffer buffer, int width, int height, int rowBytes, int pixelFormat);
int? _width;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::width', isLeaf: true)
external int _getWidth();
@override
int get width => _width ??= _getWidth();
int? _height;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::height', isLeaf: true)
external int _getHeight();
@override
int get height => _height ??= _getHeight();
int? _bytesPerPixel;
@Native<Int32 Function(Pointer<Void>)>(symbol: 'ImageDescriptor::bytesPerPixel', isLeaf: true)
external int _getBytesPerPixel();
@override
int get bytesPerPixel => _bytesPerPixel ??= _getBytesPerPixel();
@override
@Native<Void Function(Pointer<Void>)>(symbol: 'ImageDescriptor::dispose')
external void dispose();
@override
Future<Codec> instantiateCodec({int? targetWidth, int? targetHeight}) async {
if (targetWidth != null && targetWidth <= 0) {
targetWidth = null;
}
if (targetHeight != null && targetHeight <= 0) {
targetHeight = null;
}
if (targetWidth == null && targetHeight == null) {
targetWidth = width;
targetHeight = height;
} else if (targetWidth == null && targetHeight != null) {
targetWidth = (targetHeight * (width / height)).round();
} else if (targetHeight == null && targetWidth != null) {
targetHeight = targetWidth ~/ (width / height);
}
assert(targetWidth != null);
assert(targetHeight != null);
final Codec codec = _NativeCodec._();
_instantiateCodec(codec, targetWidth!, targetHeight!);
return codec;
}
@Native<Void Function(Pointer<Void>, Handle, Int32, Int32)>(symbol: 'ImageDescriptor::instantiateCodec')
external void _instantiateCodec(Codec outCodec, int targetWidth, int targetHeight);
@override
String toString() => 'ImageDescriptor(width: ${_width ?? '?'}, height: ${_height ?? '?'}, bytes per pixel: ${_bytesPerPixel ?? '?'})';
}
/// Generic callback signature, used by [_futurize].
typedef _Callback<T> = void Function(T result);
/// Generic callback signature, used by [_futurizeWithError].
typedef _CallbackWithError<T> = void Function(T result, String? error);
/// Signature for a method that receives a [_Callback].
///
/// Return value should be null on success, and a string error message on
/// failure.
typedef _Callbacker<T> = String? Function(_Callback<T?> callback);
/// Signature for a method that receives a [_CallbackWithError].
/// See also: [_Callbacker]
typedef _CallbackerWithError<T> = String? Function(_CallbackWithError<T?> callback);
// Converts a method that receives a value-returning callback to a method that
// returns a Future.
//
// Return a [String] to cause an [Exception] to be synchronously thrown with
// that string as a message.
//
// If the callback is called with null, the future completes with an error.
//
// Example usage:
//
// ```dart
// typedef IntCallback = void Function(int result);
//
// String? _doSomethingAndCallback(IntCallback callback) {
// Timer(const Duration(seconds: 1), () { callback(1); });
// }
//
// Future<int> doSomething() {
// return _futurize(_doSomethingAndCallback);
// }
// ```
//
// This function is private and so not directly tested. Instead, an exact copy of it
// has been inlined into the test at lib/ui/fixtures/ui_test.dart. if you change this
// function, then you must update the test.
//
// TODO(ianh): We should either automate the code duplication or just make it public.
Future<T> _futurize<T>(_Callbacker<T> callbacker) {
final Completer<T> completer = Completer<T>.sync();
// If the callback synchronously throws an error, then synchronously
// rethrow that error instead of adding it to the completer. This
// prevents the Zone from receiving an uncaught exception.
bool isSync = true;
final String? error = callbacker((T? t) {
if (t == null) {
if (isSync) {
throw Exception('operation failed');
} else {
completer.completeError(Exception('operation failed'));
}
} else {
completer.complete(t);
}
});
isSync = false;
if (error != null) {
throw Exception(error);
}
return completer.future;
}
/// A variant of `_futurize` that can communicate specific errors.
Future<T> _futurizeWithError<T>(_CallbackerWithError<T> callbacker) {
final Completer<T> completer = Completer<T>.sync();
// If the callback synchronously throws an error, then synchronously
// rethrow that error instead of adding it to the completer. This
// prevents the Zone from receiving an uncaught exception.
bool isSync = true;
final String? error = callbacker((T? t, String? error) {
if (t != null) {
completer.complete(t);
} else {
if (isSync) {
throw Exception(error ?? 'operation failed');
} else {
completer.completeError(Exception(error ?? 'operation failed'));
}
}
});
isSync = false;
if (error != null) {
throw Exception(error);
}
return completer.future;
}
/// An exception thrown by [Canvas.drawImage] and related methods when drawing
/// an [Image] created via [Picture.toImageSync] that is in an invalid state.
///
/// This exception may be thrown if the requested image dimensions exceeded the
/// maximum 2D texture size allowed by the GPU, or if no GPU surface or context
/// was available for rasterization at request time.
class PictureRasterizationException implements Exception {
const PictureRasterizationException._(this.message, {this.stack});
/// A string containing details about the failure.
final String message;
/// If available, the stack trace at the time [Picture.toImageSync] was called.
final StackTrace? stack;
@override
String toString() {
final StringBuffer buffer = StringBuffer('Failed to rasterize a picture: $message.');
if (stack != null) {
buffer.writeln();
buffer.writeln('The callstack when the image was created was:');
buffer.writeln(stack!.toString());
}
return buffer.toString();
}
}
| engine/lib/ui/painting.dart/0 | {
"file_path": "engine/lib/ui/painting.dart",
"repo_id": "engine",
"token_count": 88979
} | 249 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_LIB_UI_PAINTING_FRAGMENT_PROGRAM_H_
#define FLUTTER_LIB_UI_PAINTING_FRAGMENT_PROGRAM_H_
#include "flutter/display_list/effects/dl_runtime_effect.h"
#include "flutter/lib/ui/dart_wrapper.h"
#include "flutter/lib/ui/painting/shader.h"
#include "third_party/tonic/dart_library_natives.h"
#include "third_party/tonic/typed_data/typed_list.h"
#include <memory>
#include <string>
#include <vector>
namespace flutter {
class FragmentShader;
class FragmentProgram : public RefCountedDartWrappable<FragmentProgram> {
DEFINE_WRAPPERTYPEINFO();
FML_FRIEND_MAKE_REF_COUNTED(FragmentProgram);
public:
~FragmentProgram() override;
static void Create(Dart_Handle wrapper);
std::string initFromAsset(const std::string& asset_name);
fml::RefPtr<FragmentShader> shader(Dart_Handle shader,
Dart_Handle uniforms_handle,
Dart_Handle samplers);
std::shared_ptr<DlColorSource> MakeDlColorSource(
std::shared_ptr<std::vector<uint8_t>> float_uniforms,
const std::vector<std::shared_ptr<DlColorSource>>& children);
private:
FragmentProgram();
sk_sp<DlRuntimeEffect> runtime_effect_;
};
} // namespace flutter
#endif // FLUTTER_LIB_UI_PAINTING_FRAGMENT_PROGRAM_H_
| engine/lib/ui/painting/fragment_program.h/0 | {
"file_path": "engine/lib/ui/painting/fragment_program.h",
"repo_id": "engine",
"token_count": 571
} | 250 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/painting/image_descriptor.h"
#include "flutter/fml/build_config.h"
#include "flutter/fml/logging.h"
#include "flutter/fml/trace_event.h"
#include "flutter/lib/ui/painting/multi_frame_codec.h"
#include "flutter/lib/ui/painting/single_frame_codec.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "third_party/tonic/dart_binding_macros.h"
#include "third_party/tonic/logging/dart_invoke.h"
namespace flutter {
IMPLEMENT_WRAPPERTYPEINFO(ui, ImageDescriptor);
const SkImageInfo ImageDescriptor::CreateImageInfo() const {
FML_DCHECK(generator_);
return generator_->GetInfo();
}
ImageDescriptor::ImageDescriptor(sk_sp<SkData> buffer,
const SkImageInfo& image_info,
std::optional<size_t> row_bytes)
: buffer_(std::move(buffer)),
generator_(nullptr),
image_info_(image_info),
row_bytes_(row_bytes) {}
ImageDescriptor::ImageDescriptor(sk_sp<SkData> buffer,
std::shared_ptr<ImageGenerator> generator)
: buffer_(std::move(buffer)),
generator_(std::move(generator)),
image_info_(CreateImageInfo()),
row_bytes_(std::nullopt) {}
Dart_Handle ImageDescriptor::initEncoded(Dart_Handle descriptor_handle,
ImmutableBuffer* immutable_buffer,
Dart_Handle callback_handle) {
if (!Dart_IsClosure(callback_handle)) {
return tonic::ToDart("Callback must be a function");
}
if (!immutable_buffer) {
return tonic::ToDart("Buffer parameter must not be null");
}
// This has to be valid because this method is called from Dart.
auto dart_state = UIDartState::Current();
auto registry = dart_state->GetImageGeneratorRegistry();
if (!registry) {
return tonic::ToDart(
"Failed to access the internal image decoder "
"registry on this isolate. Please file a bug on "
"https://github.com/flutter/flutter/issues.");
}
auto generator =
registry->CreateCompatibleGenerator(immutable_buffer->data());
if (!generator) {
// No compatible image decoder was found.
return tonic::ToDart("Invalid image data");
}
auto descriptor = fml::MakeRefCounted<ImageDescriptor>(
immutable_buffer->data(), std::move(generator));
FML_DCHECK(descriptor);
descriptor->AssociateWithDartWrapper(descriptor_handle);
tonic::DartInvoke(callback_handle, {Dart_TypeVoid()});
return Dart_Null();
}
void ImageDescriptor::initRaw(Dart_Handle descriptor_handle,
const fml::RefPtr<ImmutableBuffer>& data,
int width,
int height,
int row_bytes,
PixelFormat pixel_format) {
SkColorType color_type = kUnknown_SkColorType;
SkAlphaType alpha_type = kPremul_SkAlphaType;
switch (pixel_format) {
case PixelFormat::kRGBA8888:
color_type = kRGBA_8888_SkColorType;
break;
case PixelFormat::kBGRA8888:
color_type = kBGRA_8888_SkColorType;
break;
case PixelFormat::kRGBAFloat32:
// `PixelFormat.rgbaFloat32` is documented to not use premultiplied alpha.
color_type = kRGBA_F32_SkColorType;
alpha_type = kUnpremul_SkAlphaType;
break;
}
FML_DCHECK(color_type != kUnknown_SkColorType);
auto image_info = SkImageInfo::Make(width, height, color_type, alpha_type);
auto descriptor = fml::MakeRefCounted<ImageDescriptor>(
data->data(), std::move(image_info),
row_bytes == -1 ? std::nullopt : std::optional<size_t>(row_bytes));
descriptor->AssociateWithDartWrapper(descriptor_handle);
}
void ImageDescriptor::instantiateCodec(Dart_Handle codec_handle,
int target_width,
int target_height) {
fml::RefPtr<Codec> ui_codec;
if (!generator_ || generator_->GetFrameCount() == 1) {
ui_codec = fml::MakeRefCounted<SingleFrameCodec>(
static_cast<fml::RefPtr<ImageDescriptor>>(this), target_width,
target_height);
} else {
ui_codec = fml::MakeRefCounted<MultiFrameCodec>(generator_);
}
ui_codec->AssociateWithDartWrapper(codec_handle);
}
sk_sp<SkImage> ImageDescriptor::image() const {
return generator_->GetImage();
}
bool ImageDescriptor::get_pixels(const SkPixmap& pixmap) const {
FML_DCHECK(generator_);
return generator_->GetPixels(pixmap.info(), pixmap.writable_addr(),
pixmap.rowBytes());
}
} // namespace flutter
| engine/lib/ui/painting/image_descriptor.cc/0 | {
"file_path": "engine/lib/ui/painting/image_descriptor.cc",
"repo_id": "engine",
"token_count": 2010
} | 251 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_LIB_UI_PAINTING_IMAGE_GENERATOR_APNG_H_
#define FLUTTER_LIB_UI_PAINTING_IMAGE_GENERATOR_APNG_H_
#include "image_generator.h"
#include "flutter/fml/endianness.h"
#include "flutter/fml/logging.h"
#define PNG_FIELD(T, name) \
private: \
T name; \
\
public: \
T get_##name() const { \
return fml::BigEndianToArch<T>(name); \
} \
void set_##name(T n) { \
name = fml::BigEndianToArch<T>(n); \
}
namespace flutter {
class APNGImageGenerator : public ImageGenerator {
public:
~APNGImageGenerator();
// |ImageGenerator|
const SkImageInfo& GetInfo() override;
// |ImageGenerator|
unsigned int GetFrameCount() const override;
// |ImageGenerator|
unsigned int GetPlayCount() const override;
// |ImageGenerator|
const ImageGenerator::FrameInfo GetFrameInfo(
unsigned int frame_index) override;
// |ImageGenerator|
SkISize GetScaledDimensions(float desired_scale) override;
// |ImageGenerator|
bool GetPixels(const SkImageInfo& info,
void* pixels,
size_t row_bytes,
unsigned int frame_index,
std::optional<unsigned int> prior_frame) override;
static std::unique_ptr<ImageGenerator> MakeFromData(sk_sp<SkData> data);
private:
static constexpr uint8_t kPngSignature[8] = {137, 80, 78, 71, 13, 10, 26, 10};
static constexpr size_t kChunkCrcSize = 4;
enum ChunkType {
kImageHeaderChunkType = 'IHDR',
kAnimationControlChunkType = 'acTL',
kImageDataChunkType = 'IDAT',
kFrameControlChunkType = 'fcTL',
kFrameDataChunkType = 'fdAT',
kImageTrailerChunkType = 'IEND',
};
class __attribute__((packed, aligned(1))) ChunkHeader {
PNG_FIELD(uint32_t, data_length)
PNG_FIELD(ChunkType, type)
public:
void UpdateChunkCrc32();
private:
uint32_t ComputeChunkCrc32();
};
class __attribute__((packed, aligned(1))) ImageHeaderChunkData {
PNG_FIELD(uint32_t, width)
PNG_FIELD(uint32_t, height)
PNG_FIELD(uint8_t, bit_depth)
PNG_FIELD(uint8_t, color_type)
PNG_FIELD(uint8_t, compression_method)
PNG_FIELD(uint8_t, filter_method)
PNG_FIELD(uint8_t, interlace_method)
};
class __attribute__((packed, aligned(1))) AnimationControlChunkData {
PNG_FIELD(uint32_t, num_frames)
PNG_FIELD(uint32_t, num_plays)
};
class __attribute__((packed, aligned(1))) FrameControlChunkData {
PNG_FIELD(uint32_t, sequence_number)
PNG_FIELD(uint32_t, width)
PNG_FIELD(uint32_t, height)
PNG_FIELD(uint32_t, x_offset)
PNG_FIELD(uint32_t, y_offset)
PNG_FIELD(uint16_t, delay_num)
PNG_FIELD(uint16_t, delay_den)
PNG_FIELD(uint8_t, dispose_op)
PNG_FIELD(uint8_t, blend_op)
};
/// @brief The first PNG frame is always the "default" PNG frame. Absence of
/// `frame_info` is only possible on the "default" PNG frame.
/// Each frame goes through two decoding stages:
/// 1. Demuxing stage: An individual PNG codec is created for a frame
/// while walking through the APNG chunk stream -- this is placed
/// in the `codec` field.
/// 2. Decoding stage: When a frame is requested for the first time,
/// the decoded image is requested from the `SkCodec` and then
/// (depending on the `frame_info`) composited with a previous
/// frame. The final "canvas" frame is placed in the
/// `composited_image` field. At this point, the `codec` is freed
/// and the `composited_image` is handed to the caller for drawing.
struct APNGImage {
std::unique_ptr<SkCodec> codec;
// The rendered frame pixels.
std::vector<uint8_t> pixels;
// Absence of frame info is possible on the "default" image.
std::optional<ImageGenerator::FrameInfo> frame_info;
// X offset of this image when composited. Only applicable to frames.
unsigned int x_offset;
// Y offset of this image when composited. Only applicable to frames.
unsigned int y_offset;
};
APNGImageGenerator(sk_sp<SkData>& data,
SkImageInfo& image_info,
APNGImage&& default_image,
unsigned int frame_count,
unsigned int play_count,
const void* next_chunk_p,
const std::vector<uint8_t>& header);
static bool IsValidChunkHeader(const void* buffer,
size_t size,
const ChunkHeader* chunk);
static const ChunkHeader* GetNextChunk(const void* buffer,
size_t size,
const ChunkHeader* current_chunk);
/// @brief This is a utility template for casting a png buffer pointer to a
/// chunk header. Its primary purpose is to statically insert runtime
/// debug checks that detect invalid decoding behavior.
template <typename T>
static constexpr const T* CastChunkData(const ChunkHeader* chunk) {
if constexpr (std::is_same_v<T, ImageHeaderChunkData>) {
FML_DCHECK(chunk->get_type() == kImageHeaderChunkType);
} else if constexpr (std::is_same_v<T, AnimationControlChunkData>) {
FML_DCHECK(chunk->get_type() == kAnimationControlChunkType);
} else if constexpr (std::is_same_v<T, FrameControlChunkData>) {
FML_DCHECK(chunk->get_type() == kFrameControlChunkType);
} else {
static_assert(!sizeof(T), "Invalid chunk struct");
}
return reinterpret_cast<const T*>(reinterpret_cast<const uint8_t*>(chunk) +
sizeof(ChunkHeader));
}
static constexpr size_t GetChunkSize(const ChunkHeader* chunk) {
return sizeof(ChunkHeader) + chunk->get_data_length() + kChunkCrcSize;
}
static constexpr bool IsChunkCopySafe(const ChunkHeader* chunk) {
// The safe-to-copy bit is the 5th bit of the chunk name's 4th byte. This is
// the same as checking that the 4th byte is lowercase.
return (chunk->get_type() & 0x20) != 0;
}
/// @brief Extract a header that's safe to use for both the "default" image
/// and individual PNG frames. Strip the animation control chunk.
static std::pair<std::optional<std::vector<uint8_t>>, const void*>
ExtractHeader(const void* buffer_p, size_t buffer_size);
/// @brief Takes a chunk pointer to a chunk and demuxes/interprets the next
/// image in the APNG sequence. It also provides the next `chunk_p`
/// to use.
/// @see `APNGImage`
static std::pair<std::optional<APNGImage>, const void*> DemuxNextImage(
const void* buffer_p,
size_t buffer_size,
const std::vector<uint8_t>& header,
const void* chunk_p);
bool DemuxNextImageInternal();
bool DemuxToImageIndex(unsigned int image_index);
bool RenderDefaultImage(const SkImageInfo& info,
void* pixels,
size_t row_bytes);
FML_DISALLOW_COPY_ASSIGN_AND_MOVE(APNGImageGenerator);
sk_sp<SkData> data_;
SkImageInfo image_info_;
unsigned int frame_count_;
unsigned int play_count_;
// The first image is always the default image, which may or may not be a
// frame. All subsequent images are guaranteed to have frame data.
std::vector<APNGImage> images_;
unsigned int first_frame_index_;
const void* next_chunk_p_;
std::vector<uint8_t> header_;
};
} // namespace flutter
#endif // FLUTTER_LIB_UI_PAINTING_IMAGE_GENERATOR_APNG_H_
| engine/lib/ui/painting/image_generator_apng.h/0 | {
"file_path": "engine/lib/ui/painting/image_generator_apng.h",
"repo_id": "engine",
"token_count": 3290
} | 252 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_LIB_UI_PAINTING_PATH_H_
#define FLUTTER_LIB_UI_PAINTING_PATH_H_
#include "flutter/lib/ui/dart_wrapper.h"
#include "flutter/lib/ui/painting/rrect.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "flutter/lib/ui/volatile_path_tracker.h"
#include "third_party/skia/include/core/SkPath.h"
#include "third_party/skia/include/pathops/SkPathOps.h"
#include "third_party/tonic/typed_data/typed_list.h"
namespace flutter {
class CanvasPath : public RefCountedDartWrappable<CanvasPath> {
DEFINE_WRAPPERTYPEINFO();
FML_FRIEND_MAKE_REF_COUNTED(CanvasPath);
public:
~CanvasPath() override;
static void CreateFrom(Dart_Handle path_handle, const SkPath& src) {
auto path = fml::MakeRefCounted<CanvasPath>();
path->AssociateWithDartWrapper(path_handle);
path->tracked_path_->path = src;
}
static fml::RefPtr<CanvasPath> Create(Dart_Handle wrapper) {
UIDartState::ThrowIfUIOperationsProhibited();
auto res = fml::MakeRefCounted<CanvasPath>();
res->AssociateWithDartWrapper(wrapper);
return res;
}
int getFillType();
void setFillType(int fill_type);
void moveTo(double x, double y);
void relativeMoveTo(double x, double y);
void lineTo(double x, double y);
void relativeLineTo(double x, double y);
void quadraticBezierTo(double x1, double y1, double x2, double y2);
void relativeQuadraticBezierTo(double x1, double y1, double x2, double y2);
void cubicTo(double x1,
double y1,
double x2,
double y2,
double x3,
double y3);
void relativeCubicTo(double x1,
double y1,
double x2,
double y2,
double x3,
double y3);
void conicTo(double x1, double y1, double x2, double y2, double w);
void relativeConicTo(double x1, double y1, double x2, double y2, double w);
void arcTo(double left,
double top,
double right,
double bottom,
double startAngle,
double sweepAngle,
bool forceMoveTo);
void arcToPoint(double arcEndX,
double arcEndY,
double radiusX,
double radiusY,
double xAxisRotation,
bool isLargeArc,
bool isClockwiseDirection);
void relativeArcToPoint(double arcEndDeltaX,
double arcEndDeltaY,
double radiusX,
double radiusY,
double xAxisRotation,
bool isLargeArc,
bool isClockwiseDirection);
void addRect(double left, double top, double right, double bottom);
void addOval(double left, double top, double right, double bottom);
void addArc(double left,
double top,
double right,
double bottom,
double startAngle,
double sweepAngle);
void addPolygon(const tonic::Float32List& points, bool close);
void addRRect(const RRect& rrect);
void addPath(CanvasPath* path, double dx, double dy);
void addPathWithMatrix(CanvasPath* path,
double dx,
double dy,
Dart_Handle matrix4_handle);
void extendWithPath(CanvasPath* path, double dx, double dy);
void extendWithPathAndMatrix(CanvasPath* path,
double dx,
double dy,
Dart_Handle matrix4_handle);
void close();
void reset();
bool contains(double x, double y);
void shift(Dart_Handle path_handle, double dx, double dy);
void transform(Dart_Handle path_handle, Dart_Handle matrix4_handle);
tonic::Float32List getBounds();
bool op(CanvasPath* path1, CanvasPath* path2, int operation);
void clone(Dart_Handle path_handle);
const SkPath& path() const { return tracked_path_->path; }
private:
CanvasPath();
std::shared_ptr<VolatilePathTracker> path_tracker_;
std::shared_ptr<VolatilePathTracker::TrackedPath> tracked_path_;
// Must be called whenever the path is created or mutated.
void resetVolatility();
SkPath& mutable_path() { return tracked_path_->path; }
};
} // namespace flutter
#endif // FLUTTER_LIB_UI_PAINTING_PATH_H_
| engine/lib/ui/painting/path.h/0 | {
"file_path": "engine/lib/ui/painting/path.h",
"repo_id": "engine",
"token_count": 2039
} | 253 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/painting/single_frame_codec.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "third_party/tonic/logging/dart_invoke.h"
namespace flutter {
SingleFrameCodec::SingleFrameCodec(
const fml::RefPtr<ImageDescriptor>& descriptor,
uint32_t target_width,
uint32_t target_height)
: descriptor_(descriptor),
target_width_(target_width),
target_height_(target_height) {}
SingleFrameCodec::~SingleFrameCodec() = default;
int SingleFrameCodec::frameCount() const {
return 1;
}
int SingleFrameCodec::repetitionCount() const {
return 0;
}
Dart_Handle SingleFrameCodec::getNextFrame(Dart_Handle callback_handle) {
if (!Dart_IsClosure(callback_handle)) {
return tonic::ToDart("Callback must be a function");
}
if (status_ == Status::kComplete) {
if (!cached_image_->image()) {
return tonic::ToDart("Decoded image has been disposed");
}
tonic::DartInvoke(callback_handle, {tonic::ToDart(cached_image_),
tonic::ToDart(0), tonic::ToDart("")});
return Dart_Null();
}
// This has to be valid because this method is called from Dart.
auto dart_state = UIDartState::Current();
pending_callbacks_.emplace_back(dart_state, callback_handle);
if (status_ == Status::kInProgress) {
// Another call to getNextFrame is in progress and will invoke the
// pending callbacks when decoding completes.
return Dart_Null();
}
auto decoder = dart_state->GetImageDecoder();
if (!decoder) {
return tonic::ToDart(
"Failed to access the internal image decoder "
"registry on this isolate. Please file a bug on "
"https://github.com/flutter/flutter/issues.");
}
// The SingleFrameCodec must be deleted on the UI thread. Allocate a RefPtr
// on the heap to ensure that the SingleFrameCodec remains alive until the
// decoder callback is invoked on the UI thread. The callback can then
// drop the reference.
fml::RefPtr<SingleFrameCodec>* raw_codec_ref =
new fml::RefPtr<SingleFrameCodec>(this);
decoder->Decode(
descriptor_, target_width_, target_height_,
[raw_codec_ref](auto image, auto decode_error) {
std::unique_ptr<fml::RefPtr<SingleFrameCodec>> codec_ref(raw_codec_ref);
fml::RefPtr<SingleFrameCodec> codec(std::move(*codec_ref));
auto state = codec->pending_callbacks_.front().dart_state().lock();
if (!state) {
// This is probably because the isolate has been terminated before the
// image could be decoded.
return;
}
tonic::DartState::Scope scope(state.get());
if (image) {
auto canvas_image = fml::MakeRefCounted<CanvasImage>();
canvas_image->set_image(std::move(image));
codec->cached_image_ = std::move(canvas_image);
}
// The cached frame is now available and should be returned to any
// future callers.
codec->status_ = Status::kComplete;
// Invoke any callbacks that were provided before the frame was decoded.
for (const tonic::DartPersistentValue& callback :
codec->pending_callbacks_) {
tonic::DartInvoke(callback.value(),
{tonic::ToDart(codec->cached_image_),
tonic::ToDart(0), tonic::ToDart(decode_error)});
}
codec->pending_callbacks_.clear();
});
// The encoded data is no longer needed now that it has been handed off
// to the decoder.
descriptor_ = nullptr;
status_ = Status::kInProgress;
return Dart_Null();
}
} // namespace flutter
| engine/lib/ui/painting/single_frame_codec.cc/0 | {
"file_path": "engine/lib/ui/painting/single_frame_codec.cc",
"repo_id": "engine",
"token_count": 1468
} | 254 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/semantics/semantics_update.h"
#include <memory>
#include "flutter/lib/ui/painting/matrix.h"
#include "flutter/lib/ui/semantics/semantics_update_builder.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "third_party/tonic/converter/dart_converter.h"
#include "third_party/tonic/dart_args.h"
#include "third_party/tonic/dart_binding_macros.h"
#include "third_party/tonic/dart_library_natives.h"
namespace flutter {
IMPLEMENT_WRAPPERTYPEINFO(ui, SemanticsUpdate);
void SemanticsUpdate::create(Dart_Handle semantics_update_handle,
SemanticsNodeUpdates nodes,
CustomAccessibilityActionUpdates actions) {
auto semantics_update = fml::MakeRefCounted<SemanticsUpdate>(
std::move(nodes), std::move(actions));
semantics_update->AssociateWithDartWrapper(semantics_update_handle);
}
SemanticsUpdate::SemanticsUpdate(SemanticsNodeUpdates nodes,
CustomAccessibilityActionUpdates actions)
: nodes_(std::move(nodes)), actions_(std::move(actions)) {}
SemanticsUpdate::~SemanticsUpdate() = default;
SemanticsNodeUpdates SemanticsUpdate::takeNodes() {
return std::move(nodes_);
}
CustomAccessibilityActionUpdates SemanticsUpdate::takeActions() {
return std::move(actions_);
}
void SemanticsUpdate::dispose() {
ClearDartWrapper();
}
} // namespace flutter
| engine/lib/ui/semantics/semantics_update.cc/0 | {
"file_path": "engine/lib/ui/semantics/semantics_update.cc",
"repo_id": "engine",
"token_count": 578
} | 255 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/text/paragraph_builder.h"
#include <cstring>
#include "flutter/common/settings.h"
#include "flutter/common/task_runners.h"
#include "flutter/fml/logging.h"
#include "flutter/fml/task_runner.h"
#include "flutter/lib/ui/text/font_collection.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "flutter/lib/ui/window/platform_configuration.h"
#include "flutter/third_party/txt/src/txt/font_style.h"
#include "flutter/third_party/txt/src/txt/font_weight.h"
#include "flutter/third_party/txt/src/txt/paragraph_style.h"
#include "flutter/third_party/txt/src/txt/text_baseline.h"
#include "flutter/third_party/txt/src/txt/text_decoration.h"
#include "flutter/third_party/txt/src/txt/text_style.h"
#include "third_party/icu/source/common/unicode/ustring.h"
#include "third_party/skia/include/core/SkColor.h"
#include "third_party/tonic/converter/dart_converter.h"
#include "third_party/tonic/dart_args.h"
#include "third_party/tonic/dart_binding_macros.h"
#include "third_party/tonic/dart_library_natives.h"
#include "third_party/tonic/typed_data/dart_byte_data.h"
namespace flutter {
namespace {
// TextStyle
const int kTSLeadingDistributionIndex = 0;
const int kTSColorIndex = 1;
const int kTSTextDecorationIndex = 2;
const int kTSTextDecorationColorIndex = 3;
const int kTSTextDecorationStyleIndex = 4;
const int kTSFontWeightIndex = 5;
const int kTSFontStyleIndex = 6;
const int kTSTextBaselineIndex = 7;
const int kTSTextDecorationThicknessIndex = 8;
const int kTSFontFamilyIndex = 9;
const int kTSFontSizeIndex = 10;
const int kTSLetterSpacingIndex = 11;
const int kTSWordSpacingIndex = 12;
const int kTSHeightIndex = 13;
const int kTSLocaleIndex = 14;
const int kTSBackgroundIndex = 15;
const int kTSForegroundIndex = 16;
const int kTSTextShadowsIndex = 17;
const int kTSFontFeaturesIndex = 18;
const int kTSFontVariationsIndex = 19;
const int kTSLeadingDistributionMask = 1 << kTSLeadingDistributionIndex;
const int kTSColorMask = 1 << kTSColorIndex;
const int kTSTextDecorationMask = 1 << kTSTextDecorationIndex;
const int kTSTextDecorationColorMask = 1 << kTSTextDecorationColorIndex;
const int kTSTextDecorationStyleMask = 1 << kTSTextDecorationStyleIndex;
const int kTSTextDecorationThicknessMask = 1 << kTSTextDecorationThicknessIndex;
const int kTSFontWeightMask = 1 << kTSFontWeightIndex;
const int kTSFontStyleMask = 1 << kTSFontStyleIndex;
const int kTSTextBaselineMask = 1 << kTSTextBaselineIndex;
const int kTSFontFamilyMask = 1 << kTSFontFamilyIndex;
const int kTSFontSizeMask = 1 << kTSFontSizeIndex;
const int kTSLetterSpacingMask = 1 << kTSLetterSpacingIndex;
const int kTSWordSpacingMask = 1 << kTSWordSpacingIndex;
const int kTSHeightMask = 1 << kTSHeightIndex;
const int kTSLocaleMask = 1 << kTSLocaleIndex;
const int kTSBackgroundMask = 1 << kTSBackgroundIndex;
const int kTSForegroundMask = 1 << kTSForegroundIndex;
const int kTSTextShadowsMask = 1 << kTSTextShadowsIndex;
const int kTSFontFeaturesMask = 1 << kTSFontFeaturesIndex;
const int kTSFontVariationsMask = 1 << kTSFontVariationsIndex;
// ParagraphStyle
const int kPSTextAlignIndex = 1;
const int kPSTextDirectionIndex = 2;
const int kPSFontWeightIndex = 3;
const int kPSFontStyleIndex = 4;
const int kPSMaxLinesIndex = 5;
const int kPSTextHeightBehaviorIndex = 6;
const int kPSFontFamilyIndex = 7;
const int kPSFontSizeIndex = 8;
const int kPSHeightIndex = 9;
const int kPSStrutStyleIndex = 10;
const int kPSEllipsisIndex = 11;
const int kPSLocaleIndex = 12;
const int kPSTextAlignMask = 1 << kPSTextAlignIndex;
const int kPSTextDirectionMask = 1 << kPSTextDirectionIndex;
const int kPSFontWeightMask = 1 << kPSFontWeightIndex;
const int kPSFontStyleMask = 1 << kPSFontStyleIndex;
const int kPSMaxLinesMask = 1 << kPSMaxLinesIndex;
const int kPSFontFamilyMask = 1 << kPSFontFamilyIndex;
const int kPSFontSizeMask = 1 << kPSFontSizeIndex;
const int kPSHeightMask = 1 << kPSHeightIndex;
const int kPSTextHeightBehaviorMask = 1 << kPSTextHeightBehaviorIndex;
const int kPSStrutStyleMask = 1 << kPSStrutStyleIndex;
const int kPSEllipsisMask = 1 << kPSEllipsisIndex;
const int kPSLocaleMask = 1 << kPSLocaleIndex;
// TextShadows decoding
constexpr uint32_t kColorDefault = 0xFF000000;
constexpr uint32_t kBytesPerShadow = 16;
constexpr uint32_t kShadowPropertiesCount = 4;
constexpr uint32_t kColorOffset = 0;
constexpr uint32_t kXOffset = 1;
constexpr uint32_t kYOffset = 2;
constexpr uint32_t kBlurOffset = 3;
// FontFeature decoding
constexpr uint32_t kBytesPerFontFeature = 8;
constexpr uint32_t kFontFeatureTagLength = 4;
// FontVariation decoding
constexpr uint32_t kBytesPerFontVariation = 8;
constexpr uint32_t kFontVariationTagLength = 4;
// Strut decoding
const int kSFontWeightIndex = 0;
const int kSFontStyleIndex = 1;
const int kSFontFamilyIndex = 2;
const int kSLeadingDistributionIndex = 3;
const int kSFontSizeIndex = 4;
const int kSHeightIndex = 5;
const int kSLeadingIndex = 6;
const int kSForceStrutHeightIndex = 7;
const int kSFontWeightMask = 1 << kSFontWeightIndex;
const int kSFontStyleMask = 1 << kSFontStyleIndex;
const int kSFontFamilyMask = 1 << kSFontFamilyIndex;
const int kSLeadingDistributionMask = 1 << kSLeadingDistributionIndex;
const int kSFontSizeMask = 1 << kSFontSizeIndex;
const int kSHeightMask = 1 << kSHeightIndex;
const int kSLeadingMask = 1 << kSLeadingIndex;
const int kSForceStrutHeightMask = 1 << kSForceStrutHeightIndex;
} // namespace
IMPLEMENT_WRAPPERTYPEINFO(ui, ParagraphBuilder);
void ParagraphBuilder::Create(Dart_Handle wrapper,
Dart_Handle encoded_handle,
Dart_Handle strutData,
const std::string& fontFamily,
const std::vector<std::string>& strutFontFamilies,
double fontSize,
double height,
const std::u16string& ellipsis,
const std::string& locale) {
UIDartState::ThrowIfUIOperationsProhibited();
auto res = fml::MakeRefCounted<ParagraphBuilder>(
encoded_handle, strutData, fontFamily, strutFontFamilies, fontSize,
height, ellipsis, locale);
res->AssociateWithDartWrapper(wrapper);
}
// returns true if there is a font family defined. Font family is the only
// parameter passed directly.
void decodeStrut(Dart_Handle strut_data,
const std::vector<std::string>& strut_font_families,
txt::ParagraphStyle& paragraph_style) { // NOLINT
if (strut_data == Dart_Null()) {
return;
}
tonic::DartByteData byte_data(strut_data);
if (byte_data.length_in_bytes() == 0) {
return;
}
paragraph_style.strut_enabled = true;
const uint8_t* uint8_data = static_cast<const uint8_t*>(byte_data.data());
uint8_t mask = uint8_data[0];
// Data is stored in order of increasing size, eg, 8 bit ints will be before
// any 32 bit ints. In addition, the order of decoding is the same order
// as it is encoded, and the order is used to maintain consistency.
size_t byte_count = 1;
if (mask & kSFontWeightMask) {
paragraph_style.strut_font_weight =
static_cast<txt::FontWeight>(uint8_data[byte_count++]);
}
if (mask & kSFontStyleMask) {
paragraph_style.strut_font_style =
static_cast<txt::FontStyle>(uint8_data[byte_count++]);
}
paragraph_style.strut_half_leading = mask & kSLeadingDistributionMask;
std::vector<float> float_data;
float_data.resize((byte_data.length_in_bytes() - byte_count) / 4);
memcpy(float_data.data(),
static_cast<const char*>(byte_data.data()) + byte_count,
byte_data.length_in_bytes() - byte_count);
size_t float_count = 0;
if (mask & kSFontSizeMask) {
paragraph_style.strut_font_size = float_data[float_count++];
}
if (mask & kSHeightMask) {
paragraph_style.strut_height = float_data[float_count++];
paragraph_style.strut_has_height_override = true;
}
if (mask & kSLeadingMask) {
paragraph_style.strut_leading = float_data[float_count++];
}
// The boolean is stored as the last bit in the bitmask, as null
// and false have the same behavior.
paragraph_style.force_strut_height = mask & kSForceStrutHeightMask;
if (mask & kSFontFamilyMask) {
paragraph_style.strut_font_families = strut_font_families;
} else {
// Provide an empty font name so that the platform default font will be
// used.
paragraph_style.strut_font_families.push_back("");
}
}
ParagraphBuilder::ParagraphBuilder(
Dart_Handle encoded_data,
Dart_Handle strutData,
const std::string& fontFamily,
const std::vector<std::string>& strutFontFamilies,
double fontSize,
double height,
const std::u16string& ellipsis,
const std::string& locale) {
int32_t mask = 0;
txt::ParagraphStyle style;
{
tonic::Int32List encoded(encoded_data);
mask = encoded[0];
if (mask & kPSTextAlignMask) {
style.text_align =
static_cast<txt::TextAlign>(encoded[kPSTextAlignIndex]);
}
if (mask & kPSTextDirectionMask) {
style.text_direction =
static_cast<txt::TextDirection>(encoded[kPSTextDirectionIndex]);
}
if (mask & kPSFontWeightMask) {
style.font_weight =
static_cast<txt::FontWeight>(encoded[kPSFontWeightIndex]);
}
if (mask & kPSFontStyleMask) {
style.font_style =
static_cast<txt::FontStyle>(encoded[kPSFontStyleIndex]);
}
if (mask & kPSFontFamilyMask) {
style.font_family = fontFamily;
}
if (mask & kPSFontSizeMask) {
style.font_size = fontSize;
}
if (mask & kPSHeightMask) {
style.height = height;
style.has_height_override = true;
}
if (mask & kPSTextHeightBehaviorMask) {
style.text_height_behavior = encoded[kPSTextHeightBehaviorIndex];
}
if (mask & kPSMaxLinesMask) {
style.max_lines = encoded[kPSMaxLinesIndex];
}
}
if (mask & kPSStrutStyleMask) {
decodeStrut(strutData, strutFontFamilies, style);
}
if (mask & kPSEllipsisMask) {
style.ellipsis = ellipsis;
}
if (mask & kPSLocaleMask) {
style.locale = locale;
}
FontCollection& font_collection = UIDartState::Current()
->platform_configuration()
->client()
->GetFontCollection();
auto impeller_enabled = UIDartState::Current()->IsImpellerEnabled();
m_paragraph_builder_ = txt::ParagraphBuilder::CreateSkiaBuilder(
style, font_collection.GetFontCollection(), impeller_enabled);
}
ParagraphBuilder::~ParagraphBuilder() = default;
void decodeTextShadows(
Dart_Handle shadows_data,
std::vector<txt::TextShadow>& decoded_shadows) { // NOLINT
decoded_shadows.clear();
tonic::DartByteData byte_data(shadows_data);
FML_CHECK(byte_data.length_in_bytes() % kBytesPerShadow == 0);
const uint32_t* uint_data = static_cast<const uint32_t*>(byte_data.data());
const float* float_data = static_cast<const float*>(byte_data.data());
size_t shadow_count = byte_data.length_in_bytes() / kBytesPerShadow;
size_t shadow_count_offset = 0;
for (size_t shadow_index = 0; shadow_index < shadow_count; ++shadow_index) {
shadow_count_offset = shadow_index * kShadowPropertiesCount;
SkColor color =
uint_data[shadow_count_offset + kColorOffset] ^ kColorDefault;
decoded_shadows.emplace_back(
color,
SkPoint::Make(float_data[shadow_count_offset + kXOffset],
float_data[shadow_count_offset + kYOffset]),
float_data[shadow_count_offset + kBlurOffset]);
}
}
void decodeFontFeatures(Dart_Handle font_features_data,
txt::FontFeatures& font_features) { // NOLINT
tonic::DartByteData byte_data(font_features_data);
FML_CHECK(byte_data.length_in_bytes() % kBytesPerFontFeature == 0);
size_t feature_count = byte_data.length_in_bytes() / kBytesPerFontFeature;
for (size_t feature_index = 0; feature_index < feature_count;
++feature_index) {
size_t feature_offset = feature_index * kBytesPerFontFeature;
const char* feature_bytes =
static_cast<const char*>(byte_data.data()) + feature_offset;
std::string tag(feature_bytes, kFontFeatureTagLength);
int32_t value = *(reinterpret_cast<const int32_t*>(feature_bytes +
kFontFeatureTagLength));
font_features.SetFeature(tag, value);
}
}
void decodeFontVariations(Dart_Handle font_variations_data,
txt::FontVariations& font_variations) { // NOLINT
tonic::DartByteData byte_data(font_variations_data);
FML_CHECK(byte_data.length_in_bytes() % kBytesPerFontVariation == 0);
size_t variation_count = byte_data.length_in_bytes() / kBytesPerFontVariation;
for (size_t variation_index = 0; variation_index < variation_count;
++variation_index) {
size_t variation_offset = variation_index * kBytesPerFontVariation;
const char* variation_bytes =
static_cast<const char*>(byte_data.data()) + variation_offset;
std::string tag(variation_bytes, kFontVariationTagLength);
float value = *(reinterpret_cast<const float*>(variation_bytes +
kFontVariationTagLength));
font_variations.SetAxisValue(tag, value);
}
}
void ParagraphBuilder::pushStyle(const tonic::Int32List& encoded,
const std::vector<std::string>& fontFamilies,
double fontSize,
double letterSpacing,
double wordSpacing,
double height,
double decorationThickness,
const std::string& locale,
Dart_Handle background_objects,
Dart_Handle background_data,
Dart_Handle foreground_objects,
Dart_Handle foreground_data,
Dart_Handle shadows_data,
Dart_Handle font_features_data,
Dart_Handle font_variations_data) {
FML_DCHECK(encoded.num_elements() == 9);
int32_t mask = encoded[0];
// Set to use the properties of the previous style if the property is not
// explicitly given.
txt::TextStyle style = m_paragraph_builder_->PeekStyle();
style.half_leading = mask & kTSLeadingDistributionMask;
// Only change the style property from the previous value if a new explicitly
// set value is available
if (mask & kTSColorMask) {
style.color = encoded[kTSColorIndex];
}
if (mask & kTSTextDecorationMask) {
style.decoration =
static_cast<txt::TextDecoration>(encoded[kTSTextDecorationIndex]);
}
if (mask & kTSTextDecorationColorMask) {
style.decoration_color = encoded[kTSTextDecorationColorIndex];
}
if (mask & kTSTextDecorationStyleMask) {
style.decoration_style = static_cast<txt::TextDecorationStyle>(
encoded[kTSTextDecorationStyleIndex]);
}
if (mask & kTSTextDecorationThicknessMask) {
style.decoration_thickness_multiplier = decorationThickness;
}
if (mask & kTSTextBaselineMask) {
// TODO(abarth): Implement TextBaseline. The CSS version of this
// property wasn't wired up either.
}
if (mask & (kTSFontWeightMask | kTSFontStyleMask | kTSFontSizeMask |
kTSLetterSpacingMask | kTSWordSpacingMask)) {
if (mask & kTSFontWeightMask) {
style.font_weight =
static_cast<txt::FontWeight>(encoded[kTSFontWeightIndex]);
}
if (mask & kTSFontStyleMask) {
style.font_style =
static_cast<txt::FontStyle>(encoded[kTSFontStyleIndex]);
}
if (mask & kTSFontSizeMask) {
style.font_size = fontSize;
}
if (mask & kTSLetterSpacingMask) {
style.letter_spacing = letterSpacing;
}
if (mask & kTSWordSpacingMask) {
style.word_spacing = wordSpacing;
}
}
if (mask & kTSHeightMask) {
style.height = height;
style.has_height_override = true;
}
if (mask & kTSLocaleMask) {
style.locale = locale;
}
if (mask & kTSBackgroundMask) {
Paint background(background_objects, background_data);
if (background.isNotNull()) {
DlPaint dl_paint;
background.toDlPaint(dl_paint);
style.background = dl_paint;
}
}
if (mask & kTSForegroundMask) {
Paint foreground(foreground_objects, foreground_data);
if (foreground.isNotNull()) {
DlPaint dl_paint;
foreground.toDlPaint(dl_paint);
style.foreground = dl_paint;
}
}
if (mask & kTSTextShadowsMask) {
decodeTextShadows(shadows_data, style.text_shadows);
}
if (mask & kTSFontFamilyMask) {
// The child style's font families override the parent's font families.
// If the child's fonts are not available, then the font collection will
// use the system fallback fonts (not the parent's fonts).
style.font_families = fontFamilies;
}
if (mask & kTSFontFeaturesMask) {
decodeFontFeatures(font_features_data, style.font_features);
}
if (mask & kTSFontVariationsMask) {
decodeFontVariations(font_variations_data, style.font_variations);
}
m_paragraph_builder_->PushStyle(style);
}
void ParagraphBuilder::pop() {
m_paragraph_builder_->Pop();
}
Dart_Handle ParagraphBuilder::addText(const std::u16string& text) {
if (text.empty()) {
return Dart_Null();
}
// Use ICU to validate the UTF-16 input. Calling u_strToUTF8 with a null
// output buffer will return U_BUFFER_OVERFLOW_ERROR if the input is well
// formed.
const UChar* text_ptr = reinterpret_cast<const UChar*>(text.data());
UErrorCode error_code = U_ZERO_ERROR;
u_strToUTF8(nullptr, 0, nullptr, text_ptr, text.size(), &error_code);
if (error_code != U_BUFFER_OVERFLOW_ERROR) {
return tonic::ToDart("string is not well-formed UTF-16");
}
m_paragraph_builder_->AddText(text);
return Dart_Null();
}
void ParagraphBuilder::addPlaceholder(double width,
double height,
unsigned alignment,
double baseline_offset,
unsigned baseline) {
txt::PlaceholderRun placeholder_run(
width, height, static_cast<txt::PlaceholderAlignment>(alignment),
static_cast<txt::TextBaseline>(baseline), baseline_offset);
m_paragraph_builder_->AddPlaceholder(placeholder_run);
}
void ParagraphBuilder::build(Dart_Handle paragraph_handle) {
Paragraph::Create(paragraph_handle, m_paragraph_builder_->Build());
m_paragraph_builder_.reset();
ClearDartWrapper();
}
} // namespace flutter
| engine/lib/ui/text/paragraph_builder.cc/0 | {
"file_path": "engine/lib/ui/text/paragraph_builder.cc",
"repo_id": "engine",
"token_count": 7519
} | 256 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include <iostream>
#include <thread>
#include "flutter/fml/macros.h"
#include "flutter/fml/task_runner.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "flutter/lib/ui/window/platform_isolate.h"
#include "third_party/tonic/converter/dart_converter.h"
namespace flutter {
void PlatformIsolateNativeApi::Spawn(Dart_Handle entry_point) {
UIDartState* current_state = UIDartState::Current();
FML_DCHECK(current_state != nullptr);
if (!current_state->IsRootIsolate()) {
// TODO(flutter/flutter#136314): Remove this restriction.
Dart_EnterScope();
Dart_ThrowException(tonic::ToDart(
"PlatformIsolates can only be spawned on the root isolate."));
}
char* error = nullptr;
current_state->CreatePlatformIsolate(entry_point, &error);
if (error) {
Dart_EnterScope();
Dart_Handle error_handle = tonic::ToDart<const char*>(error);
::free(error);
Dart_ThrowException(error_handle);
}
}
bool PlatformIsolateNativeApi::IsRunningOnPlatformThread() {
UIDartState* current_state = UIDartState::Current();
FML_DCHECK(current_state != nullptr);
fml::RefPtr<fml::TaskRunner> platform_task_runner =
current_state->GetTaskRunners().GetPlatformTaskRunner();
if (!platform_task_runner) {
return false;
}
return platform_task_runner->RunsTasksOnCurrentThread();
}
} // namespace flutter
| engine/lib/ui/window/platform_isolate.cc/0 | {
"file_path": "engine/lib/ui/window/platform_isolate.cc",
"repo_id": "engine",
"token_count": 523
} | 257 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/lib/ui/window/pointer_data_packet_converter.h"
#include <cmath>
#include <cstring>
#include "flutter/fml/logging.h"
namespace flutter {
PointerDataPacketConverter::PointerDataPacketConverter() {}
PointerDataPacketConverter::~PointerDataPacketConverter() = default;
std::unique_ptr<PointerDataPacket> PointerDataPacketConverter::Convert(
std::unique_ptr<PointerDataPacket> packet) {
std::vector<PointerData> converted_pointers;
// Converts each pointer data in the buffer and stores it in the
// converted_pointers.
for (size_t i = 0; i < packet->GetLength(); i++) {
PointerData pointer_data = packet->GetPointerData(i);
ConvertPointerData(pointer_data, converted_pointers);
}
// Writes converted_pointers into converted_packet.
auto converted_packet =
std::make_unique<flutter::PointerDataPacket>(converted_pointers.size());
size_t count = 0;
for (auto& converted_pointer : converted_pointers) {
converted_packet->SetPointerData(count++, converted_pointer);
}
return converted_packet;
}
void PointerDataPacketConverter::ConvertPointerData(
PointerData pointer_data,
std::vector<PointerData>& converted_pointers) {
if (pointer_data.signal_kind == PointerData::SignalKind::kNone) {
switch (pointer_data.change) {
case PointerData::Change::kCancel: {
// Android's three finger gesture will send a cancel event
// to a non-existing pointer. Drops the cancel if pointer
// is not previously added.
// https://github.com/flutter/flutter/issues/20517
auto iter = states_.find(pointer_data.device);
if (iter != states_.end()) {
PointerState state = iter->second;
FML_DCHECK(state.is_down);
UpdatePointerIdentifier(pointer_data, state, false);
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes a move event if the location does not match.
PointerData synthesized_move_event = pointer_data;
synthesized_move_event.change = PointerData::Change::kMove;
synthesized_move_event.synthesized = 1;
UpdateDeltaAndState(synthesized_move_event, state);
converted_pointers.push_back(synthesized_move_event);
}
state.is_down = false;
states_[pointer_data.device] = state;
converted_pointers.push_back(pointer_data);
}
break;
}
case PointerData::Change::kAdd: {
FML_DCHECK(states_.find(pointer_data.device) == states_.end());
EnsurePointerState(pointer_data);
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kRemove: {
// Makes sure we have an existing pointer
auto iter = states_.find(pointer_data.device);
FML_DCHECK(iter != states_.end());
PointerState state = iter->second;
if (state.is_down) {
// Synthesizes cancel event if the pointer is down.
PointerData synthesized_cancel_event = pointer_data;
synthesized_cancel_event.change = PointerData::Change::kCancel;
synthesized_cancel_event.synthesized = 1;
UpdatePointerIdentifier(synthesized_cancel_event, state, false);
state.is_down = false;
states_[synthesized_cancel_event.device] = state;
converted_pointers.push_back(synthesized_cancel_event);
}
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes a hover event if the location does not match.
PointerData synthesized_hover_event = pointer_data;
synthesized_hover_event.change = PointerData::Change::kHover;
synthesized_hover_event.synthesized = 1;
UpdateDeltaAndState(synthesized_hover_event, state);
converted_pointers.push_back(synthesized_hover_event);
}
states_.erase(pointer_data.device);
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kHover: {
auto iter = states_.find(pointer_data.device);
PointerState state;
if (iter == states_.end()) {
// Synthesizes add event if the pointer is not previously added.
PointerData synthesized_add_event = pointer_data;
synthesized_add_event.change = PointerData::Change::kAdd;
synthesized_add_event.synthesized = 1;
synthesized_add_event.buttons = 0;
state = EnsurePointerState(synthesized_add_event);
converted_pointers.push_back(synthesized_add_event);
} else {
state = iter->second;
}
FML_DCHECK(!state.is_down);
state.buttons = pointer_data.buttons;
if (LocationNeedsUpdate(pointer_data, state)) {
UpdateDeltaAndState(pointer_data, state);
converted_pointers.push_back(pointer_data);
}
break;
}
case PointerData::Change::kDown: {
auto iter = states_.find(pointer_data.device);
PointerState state;
if (iter == states_.end()) {
// Synthesizes a add event if the pointer is not previously added.
PointerData synthesized_add_event = pointer_data;
synthesized_add_event.change = PointerData::Change::kAdd;
synthesized_add_event.synthesized = 1;
synthesized_add_event.buttons = 0;
state = EnsurePointerState(synthesized_add_event);
converted_pointers.push_back(synthesized_add_event);
} else {
state = iter->second;
}
FML_DCHECK(!state.is_down);
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes a hover event if the location does not match.
PointerData synthesized_hover_event = pointer_data;
synthesized_hover_event.change = PointerData::Change::kHover;
synthesized_hover_event.synthesized = 1;
synthesized_hover_event.buttons = 0;
UpdateDeltaAndState(synthesized_hover_event, state);
converted_pointers.push_back(synthesized_hover_event);
}
UpdatePointerIdentifier(pointer_data, state, true);
state.is_down = true;
state.buttons = pointer_data.buttons;
states_[pointer_data.device] = state;
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kMove: {
// Makes sure we have an existing pointer in down state
auto iter = states_.find(pointer_data.device);
FML_DCHECK(iter != states_.end());
PointerState state = iter->second;
FML_DCHECK(state.is_down);
UpdatePointerIdentifier(pointer_data, state, false);
UpdateDeltaAndState(pointer_data, state);
state.buttons = pointer_data.buttons;
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kUp: {
// Makes sure we have an existing pointer in down state
auto iter = states_.find(pointer_data.device);
FML_DCHECK(iter != states_.end());
PointerState state = iter->second;
FML_DCHECK(state.is_down);
UpdatePointerIdentifier(pointer_data, state, false);
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes a move event if the location does not match.
PointerData synthesized_move_event = pointer_data;
synthesized_move_event.change = PointerData::Change::kMove;
synthesized_move_event.buttons = state.buttons;
synthesized_move_event.synthesized = 1;
UpdateDeltaAndState(synthesized_move_event, state);
converted_pointers.push_back(synthesized_move_event);
}
state.is_down = false;
state.buttons = pointer_data.buttons;
states_[pointer_data.device] = state;
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kPanZoomStart: {
// Makes sure we have an existing pointer
auto iter = states_.find(pointer_data.device);
PointerState state;
if (iter == states_.end()) {
// Synthesizes add event if the pointer is not previously added.
PointerData synthesized_add_event = pointer_data;
synthesized_add_event.change = PointerData::Change::kAdd;
synthesized_add_event.synthesized = 1;
synthesized_add_event.buttons = 0;
state = EnsurePointerState(synthesized_add_event);
converted_pointers.push_back(synthesized_add_event);
} else {
state = iter->second;
}
FML_DCHECK(!state.is_down);
FML_DCHECK(!state.is_pan_zoom_active);
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes a hover event if the location does not match.
PointerData synthesized_hover_event = pointer_data;
synthesized_hover_event.change = PointerData::Change::kHover;
synthesized_hover_event.synthesized = 1;
synthesized_hover_event.buttons = 0;
UpdateDeltaAndState(synthesized_hover_event, state);
converted_pointers.push_back(synthesized_hover_event);
}
UpdatePointerIdentifier(pointer_data, state, true);
state.is_pan_zoom_active = true;
state.pan_x = 0;
state.pan_y = 0;
state.scale = 1;
state.rotation = 0;
states_[pointer_data.device] = state;
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kPanZoomUpdate: {
// Makes sure we have an existing pointer in pan_zoom_active state
auto iter = states_.find(pointer_data.device);
FML_DCHECK(iter != states_.end());
PointerState state = iter->second;
FML_DCHECK(!state.is_down);
FML_DCHECK(state.is_pan_zoom_active);
UpdatePointerIdentifier(pointer_data, state, false);
UpdateDeltaAndState(pointer_data, state);
converted_pointers.push_back(pointer_data);
break;
}
case PointerData::Change::kPanZoomEnd: {
// Makes sure we have an existing pointer in pan_zoom_active state
auto iter = states_.find(pointer_data.device);
FML_DCHECK(iter != states_.end());
PointerState state = iter->second;
FML_DCHECK(state.is_pan_zoom_active);
UpdatePointerIdentifier(pointer_data, state, false);
if (LocationNeedsUpdate(pointer_data, state)) {
// Synthesizes an update event if the location does not match.
PointerData synthesized_move_event = pointer_data;
synthesized_move_event.change = PointerData::Change::kPanZoomUpdate;
synthesized_move_event.pan_x = state.pan_x;
synthesized_move_event.pan_y = state.pan_y;
synthesized_move_event.pan_delta_x = 0;
synthesized_move_event.pan_delta_y = 0;
synthesized_move_event.scale = state.scale;
synthesized_move_event.rotation = state.rotation;
synthesized_move_event.synthesized = 1;
UpdateDeltaAndState(synthesized_move_event, state);
converted_pointers.push_back(synthesized_move_event);
}
state.is_pan_zoom_active = false;
states_[pointer_data.device] = state;
converted_pointers.push_back(pointer_data);
break;
}
default: {
converted_pointers.push_back(pointer_data);
break;
}
}
} else {
switch (pointer_data.signal_kind) {
case PointerData::SignalKind::kScroll:
case PointerData::SignalKind::kScrollInertiaCancel:
case PointerData::SignalKind::kScale: {
// Makes sure we have an existing pointer
auto iter = states_.find(pointer_data.device);
PointerState state;
if (iter == states_.end()) {
// Synthesizes a add event if the pointer is not previously added.
PointerData synthesized_add_event = pointer_data;
synthesized_add_event.signal_kind = PointerData::SignalKind::kNone;
synthesized_add_event.change = PointerData::Change::kAdd;
synthesized_add_event.synthesized = 1;
synthesized_add_event.buttons = 0;
state = EnsurePointerState(synthesized_add_event);
converted_pointers.push_back(synthesized_add_event);
} else {
state = iter->second;
}
if (LocationNeedsUpdate(pointer_data, state)) {
if (state.is_down) {
// Synthesizes a move event if the pointer is down.
PointerData synthesized_move_event = pointer_data;
synthesized_move_event.signal_kind = PointerData::SignalKind::kNone;
synthesized_move_event.change = PointerData::Change::kMove;
synthesized_move_event.buttons = state.buttons;
synthesized_move_event.synthesized = 1;
UpdateDeltaAndState(synthesized_move_event, state);
converted_pointers.push_back(synthesized_move_event);
} else {
// Synthesizes a hover event if the pointer is up.
PointerData synthesized_hover_event = pointer_data;
synthesized_hover_event.signal_kind =
PointerData::SignalKind::kNone;
synthesized_hover_event.change = PointerData::Change::kHover;
synthesized_hover_event.buttons = 0;
synthesized_hover_event.synthesized = 1;
UpdateDeltaAndState(synthesized_hover_event, state);
converted_pointers.push_back(synthesized_hover_event);
}
}
converted_pointers.push_back(pointer_data);
break;
}
default: {
// Ignores unknown signal kind.
break;
}
}
}
}
PointerState PointerDataPacketConverter::EnsurePointerState(
PointerData pointer_data) {
PointerState state;
state.pointer_identifier = 0;
state.is_down = false;
state.is_pan_zoom_active = false;
state.physical_x = pointer_data.physical_x;
state.physical_y = pointer_data.physical_y;
state.pan_x = 0;
state.pan_y = 0;
states_[pointer_data.device] = state;
return state;
}
void PointerDataPacketConverter::UpdateDeltaAndState(PointerData& pointer_data,
PointerState& state) {
pointer_data.physical_delta_x = pointer_data.physical_x - state.physical_x;
pointer_data.physical_delta_y = pointer_data.physical_y - state.physical_y;
pointer_data.pan_delta_x = pointer_data.pan_x - state.pan_x;
pointer_data.pan_delta_y = pointer_data.pan_y - state.pan_y;
state.physical_x = pointer_data.physical_x;
state.physical_y = pointer_data.physical_y;
state.pan_x = pointer_data.pan_x;
state.pan_y = pointer_data.pan_y;
state.scale = pointer_data.scale;
state.rotation = pointer_data.rotation;
states_[pointer_data.device] = state;
}
bool PointerDataPacketConverter::LocationNeedsUpdate(
const PointerData pointer_data,
const PointerState state) {
return state.physical_x != pointer_data.physical_x ||
state.physical_y != pointer_data.physical_y;
}
void PointerDataPacketConverter::UpdatePointerIdentifier(
PointerData& pointer_data,
PointerState& state,
bool start_new_pointer) {
if (start_new_pointer) {
state.pointer_identifier = ++pointer_;
states_[pointer_data.device] = state;
}
pointer_data.pointer_identifier = state.pointer_identifier;
}
} // namespace flutter
| engine/lib/ui/window/pointer_data_packet_converter.cc/0 | {
"file_path": "engine/lib/ui/window/pointer_data_packet_converter.cc",
"repo_id": "engine",
"token_count": 6540
} | 258 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:stack_trace/stack_trace.dart';
import 'package:typed_data/typed_buffers.dart';
class BrowserProcess {
/// Creates a new browser.
///
/// Clients pass in [startBrowser], which asynchronously returns the browser
/// process. Any errors in [startBrowser] (even those raised asynchronously
/// after it returns) are piped to [onExit] and will cause the browser to be
/// killed.
BrowserProcess(Future<Process> Function() startBrowser) {
// Don't return a Future here because there's no need for the caller to wait
// for the process to actually start. They should just wait for the HTTP
// request instead.
runZonedGuarded(() async {
final Process process = await startBrowser();
_processCompleter.complete(process);
final Uint8Buffer output = Uint8Buffer();
void drainOutput(Stream<List<int>> stream) {
try {
_ioSubscriptions
.add(stream.listen(output.addAll, cancelOnError: true));
} on StateError catch (_) {}
}
// If we don't drain the stdout and stderr the process can hang.
drainOutput(process.stdout);
drainOutput(process.stderr);
final int exitCode = await process.exitCode;
// This hack dodges an otherwise intractable race condition. When the user
// presses Control-C, the signal is sent to the browser and the test
// runner at the same time. It's possible for the browser to exit before
// the [Browser.close] is called, which would trigger the error below.
//
// A negative exit code signals that the process exited due to a signal.
// However, it's possible that this signal didn't come from the user's
// Control-C, in which case we do want to throw the error. The only way to
// resolve the ambiguity is to wait a brief amount of time and see if this
// browser is actually closed.
if (!_closed && exitCode < 0) {
await Future<void>.delayed(const Duration(milliseconds: 200));
}
if (!_closed && exitCode != 0) {
final String outputString = utf8.decode(output);
String message = 'Browser process failed with exit code $exitCode.';
if (outputString.isNotEmpty) {
message += '\nStandard output:\n$outputString';
}
throw Exception(message);
}
_onExitCompleter.complete();
}, (dynamic error, StackTrace? stackTrace) {
// Ignore any errors after the browser has been closed.
if (_closed) {
return;
}
// Make sure the process dies even if the error wasn't fatal.
_process.then((Process process) => process.kill());
stackTrace ??= Trace.current();
if (_onExitCompleter.isCompleted) {
return;
}
_onExitCompleter.completeError(
Exception('Failed to run browser process: $error.'),
stackTrace,
);
});
}
/// The underlying process.
///
/// This will fire once the process has started successfully.
Future<Process> get _process => _processCompleter.future;
final Completer<Process> _processCompleter = Completer<Process>();
/// Whether [close] has been called.
bool _closed = false;
/// A future that completes when the browser exits.
///
/// If there's a problem starting or running the browser, this will complete
/// with an error.
Future<void> get onExit => _onExitCompleter.future;
final Completer<void> _onExitCompleter = Completer<void>();
/// Standard IO streams for the underlying browser process.
final List<StreamSubscription<void>> _ioSubscriptions = <StreamSubscription<void>>[];
/// Kills the browser process.
///
/// Returns the same [Future] as [onExit], except that it won't emit
/// exceptions.
Future<void> close() async {
_closed = true;
// If we don't manually close the stream the test runner can hang.
// For example this happens with Chrome Headless.
// See SDK issue: https://github.com/dart-lang/sdk/issues/31264
for (final StreamSubscription<void> stream in _ioSubscriptions) {
unawaited(stream.cancel());
}
(await _process).kill();
// Swallow exceptions. The user should explicitly use [onExit] for these.
return onExit.catchError((dynamic _) {});
}
}
| engine/lib/web_ui/dev/browser_process.dart/0 | {
"file_path": "engine/lib/web_ui/dev/browser_process.dart",
"repo_id": "engine",
"token_count": 1504
} | 259 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' as io;
import 'package:http/http.dart';
import 'package:path/path.dart' as path;
import 'common.dart';
import 'environment.dart';
import 'exceptions.dart';
const String _firefoxExecutableVar = 'FIREFOX_EXECUTABLE';
/// Returns the installation of Firefox, installing it if necessary.
///
/// If [requestedVersion] is null, uses the version specified on the
/// command-line. If not specified on the command-line, uses the version
/// specified in the "browser_lock.yaml" file.
///
/// If [requestedVersion] is not null, installs that version. The value
/// may be "latest" (the latest stable Firefox version), "system"
/// (manually installed Firefox on the current operating system), or an
/// exact version number such as 69.0.3. Versions of Firefox can be found here:
///
/// https://download-installer.cdn.mozilla.net/pub/firefox/releases/
Future<BrowserInstallation> getOrInstallFirefox(
String requestedVersion, {
StringSink? infoLog,
}) async {
// These tests are aimed to run only on the Linux containers in Cirrus.
// Therefore Firefox installation is implemented only for Linux now.
if (!io.Platform.isLinux && !io.Platform.isMacOS) {
throw UnimplementedError('Firefox Installer is only supported on Linux '
'and Mac operating systems');
}
infoLog ??= io.stdout;
// When running on LUCI, if we specify the "firefox" dependency, then the
// bot will download Firefox from CIPD and place it in a cache and set the
// environment variable FIREFOX_EXECUTABLE.
if (io.Platform.environment.containsKey(_firefoxExecutableVar)) {
infoLog.writeln('Using Firefox from $_firefoxExecutableVar variable: '
'${io.Platform.environment[_firefoxExecutableVar]}');
return BrowserInstallation(
version: 'cipd',
executable: io.Platform.environment[_firefoxExecutableVar]!,
);
}
if (requestedVersion == 'system') {
return BrowserInstallation(
version: 'system',
executable: await _findSystemFirefoxExecutable(),
);
}
FirefoxInstaller? installer;
try {
installer = requestedVersion == 'latest'
? await FirefoxInstaller.latest()
: FirefoxInstaller(version: requestedVersion);
if (installer.isInstalled) {
infoLog.writeln(
'Installation was skipped because Firefox version ${installer.version} is already installed.');
} else {
infoLog.writeln('Installing Firefox version: ${installer.version}');
await installer.install();
final BrowserInstallation installation = installer.getInstallation()!;
infoLog.writeln(
'Installations complete. To launch it run ${installation.executable}');
}
return installer.getInstallation()!;
} finally {
installer?.close();
}
}
/// Manages the installation of a particular [version] of Firefox.
class FirefoxInstaller {
factory FirefoxInstaller({
required String version,
}) {
if (version == 'system') {
throw BrowserInstallerException(
'Cannot install system version of Firefox. System Firefox must be installed manually.');
}
if (version == 'latest') {
throw BrowserInstallerException(
'Expected a concrete Firefox version, but got $version. Maybe use FirefoxInstaller.latest()?');
}
final io.Directory firefoxInstallationDir = io.Directory(
path.join(environment.webUiDartToolDir.path, 'firefox'),
);
final io.Directory versionDir = io.Directory(
path.join(firefoxInstallationDir.path, version),
);
return FirefoxInstaller._(
version: version,
firefoxInstallationDir: firefoxInstallationDir,
versionDir: versionDir,
);
}
FirefoxInstaller._({
required this.version,
required this.firefoxInstallationDir,
required this.versionDir,
});
static Future<FirefoxInstaller> latest() async {
final String latestVersion = io.Platform.isLinux
? await fetchLatestFirefoxVersionLinux()
: await fetchLatestFirefoxVersionMacOS();
return FirefoxInstaller(version: latestVersion);
}
/// Firefox version managed by this installer.
final String version;
/// HTTP client used to download Firefox.
final Client client = Client();
/// Root directory that contains Firefox versions.
final io.Directory firefoxInstallationDir;
/// Installation directory for Firefox of the requested [version].
final io.Directory versionDir;
bool get isInstalled {
return versionDir.existsSync();
}
BrowserInstallation? getInstallation() {
if (!isInstalled) {
return null;
}
return BrowserInstallation(
version: version,
executable: PlatformBinding.instance.getFirefoxExecutablePath(versionDir),
);
}
/// Install the browser by downloading from the web.
Future<void> install() async {
final io.File downloadedFile = await _download();
if (io.Platform.isLinux) {
await _uncompress(downloadedFile);
} else if (io.Platform.isMacOS) {
await _mountDmgAndCopy(downloadedFile);
}
downloadedFile.deleteSync();
}
/// Downloads the browser version from web into a target file.
/// See [version].
Future<io.File> _download() async {
if (versionDir.existsSync()) {
versionDir.deleteSync(recursive: true);
}
versionDir.createSync(recursive: true);
final String url = PlatformBinding.instance.getFirefoxDownloadUrl(version);
final StreamedResponse download = await client.send(Request(
'GET',
Uri.parse(url),
));
final io.File downloadedFile =
io.File(path.join(versionDir.path, PlatformBinding.instance.getFirefoxDownloadFilename(version)));
final io.IOSink sink = downloadedFile.openWrite();
await download.stream.pipe(sink);
await sink.flush();
await sink.close();
return downloadedFile;
}
/// Uncompress the downloaded browser files for operating systems that
/// use a zip archive.
/// See [version].
Future<void> _uncompress(io.File downloadedFile) async {
final io.ProcessResult unzipResult = await io.Process.run('tar', <String>[
'-x',
'-f',
downloadedFile.path,
'-C',
versionDir.path,
]);
if (unzipResult.exitCode != 0) {
throw BrowserInstallerException(
'Failed to unzip the downloaded Firefox archive ${downloadedFile.path}.\n'
'The unzip process exited with code ${unzipResult.exitCode}.');
}
}
/// Mounts the dmg file using hdiutil, copies content of the volume to
/// target path and then unmounts dmg ready for deletion.
Future<void> _mountDmgAndCopy(io.File dmgFile) async {
final String volumeName = await _hdiUtilMount(dmgFile);
final String sourcePath = '$volumeName/Firefox.app';
final String targetPath = path.dirname(dmgFile.path);
try {
final io.ProcessResult installResult = await io.Process.run('cp', <String>[
'-r',
sourcePath,
targetPath,
]);
if (installResult.exitCode != 0) {
throw BrowserInstallerException(
'Failed to copy Firefox disk image contents from '
'$sourcePath to $targetPath.\n'
'Exit code ${installResult.exitCode}.\n'
'${installResult.stderr}');
}
} finally {
await _hdiUtilUnmount(volumeName);
}
}
Future<String> _hdiUtilMount(io.File dmgFile) async {
final io.ProcessResult mountResult = await io.Process.run('hdiutil', <String>[
'attach',
'-readonly',
dmgFile.path,
]);
if (mountResult.exitCode != 0) {
throw BrowserInstallerException(
'Failed to mount Firefox disk image ${dmgFile.path}.\n'
'Exit code ${mountResult.exitCode}.\n${mountResult.stderr}');
}
final List<String> processOutput = (mountResult.stdout as String).split('\n');
final String? volumePath = _volumeFromMountResult(processOutput);
if (volumePath == null) {
throw BrowserInstallerException(
'Failed to parse mount dmg result ${processOutput.join('\n')}.\n'
'Expected /Volumes/{volume name}');
}
return volumePath;
}
// Parses volume from mount result.
// Output is of form: {devicename} /Volumes/{name}.
String? _volumeFromMountResult(List<String> lines) {
for (final String line in lines) {
final int pos = line.indexOf('/Volumes');
if (pos != -1) {
return line.substring(pos);
}
}
return null;
}
Future<void> _hdiUtilUnmount(String volumeName) async {
final io.ProcessResult unmountResult = await io.Process.run('hdiutil', <String>[
'unmount',
volumeName,
]);
if (unmountResult.exitCode != 0) {
throw BrowserInstallerException(
'Failed to unmount Firefox disk image $volumeName.\n'
'Exit code ${unmountResult.exitCode}. ${unmountResult.stderr}');
}
}
void close() {
client.close();
}
}
Future<String> _findSystemFirefoxExecutable() async {
final io.ProcessResult which =
await io.Process.run('which', <String>['firefox']);
final bool found = which.exitCode != 0;
const String fireFoxDefaultInstallPath =
'/Applications/Firefox.app/Contents/MacOS/firefox';
if (!found) {
if (io.Platform.isMacOS &&
io.File(fireFoxDefaultInstallPath).existsSync()) {
return Future<String>.value(fireFoxDefaultInstallPath);
}
throw BrowserInstallerException(
'Failed to locate system Firefox installation.');
}
return which.stdout as String;
}
/// Fetches the latest available Firefox build version on Linux.
Future<String> fetchLatestFirefoxVersionLinux() async {
final RegExp forFirefoxVersion = RegExp('firefox-[0-9.]+[0-9]');
final io.HttpClientRequest request = await io.HttpClient()
.getUrl(Uri.parse(PlatformBinding.instance.getFirefoxLatestVersionUrl()));
request.followRedirects = false;
// We will parse the HttpHeaders to find the redirect location.
final io.HttpClientResponse response = await request.close();
final String location = response.headers.value('location')!;
final String version = forFirefoxVersion.stringMatch(location)!;
return version.substring(version.lastIndexOf('-') + 1);
}
/// Fetches the latest available Firefox build version on Mac OS.
Future<String> fetchLatestFirefoxVersionMacOS() async {
final RegExp forFirefoxVersion = RegExp('firefox/releases/[0-9.]+[0-9]');
final io.HttpClientRequest request = await io.HttpClient()
.getUrl(Uri.parse(PlatformBinding.instance.getFirefoxLatestVersionUrl()));
request.followRedirects = false;
// We will parse the HttpHeaders to find the redirect location.
final io.HttpClientResponse response = await request.close();
final String location = response.headers.value('location')!;
final String version = forFirefoxVersion.stringMatch(location)!;
return version.substring(version.lastIndexOf('/') + 1);
}
Future<BrowserInstallation> getInstaller({String requestedVersion = 'latest'}) async {
FirefoxInstaller? installer;
try {
installer = requestedVersion == 'latest'
? await FirefoxInstaller.latest()
: FirefoxInstaller(version: requestedVersion);
if (installer.isInstalled) {
print('Installation was skipped because Firefox version '
'${installer.version} is already installed.');
} else {
print('Installing Firefox version: ${installer.version}');
await installer.install();
final BrowserInstallation installation = installer.getInstallation()!;
print(
'Installations complete. To launch it run ${installation.executable}');
}
return installer.getInstallation()!;
} finally {
installer?.close();
}
}
| engine/lib/web_ui/dev/firefox_installer.dart/0 | {
"file_path": "engine/lib/web_ui/dev/firefox_installer.dart",
"repo_id": "engine",
"token_count": 3965
} | 260 |
#!/bin/bash
set -e
set -x
# web_analysis: a command-line utility for running 'dart analyze' on Flutter Web
# Engine. Used/Called by LUCI recipes:
#
# See: https://flutter.googlesource.com/recipes/+/refs/heads/main/recipes/web_engine.py
echo "Engine path $ENGINE_PATH"
WEB_UI_DIR="$ENGINE_PATH/src/flutter/lib/web_ui"
DART_SDK_DIR="${ENGINE_PATH}/src/out/host_debug_unopt/dart-sdk"
DART_PATH="$DART_SDK_DIR/bin/dart"
echo "Running \`dart pub get\` in 'engine/src/flutter/lib/web_ui'"
(cd "$WEB_UI_DIR"; $DART_PATH pub --suppress-analytics get)
echo "Running \`dart analyze\` in 'engine/src/flutter/lib/web_ui'"
(cd "$WEB_UI_DIR"; $DART_PATH analyze --suppress-analytics --fatal-infos)
| engine/lib/web_ui/dev/web_engine_analysis.sh/0 | {
"file_path": "engine/lib/web_ui/dev/web_engine_analysis.sh",
"repo_id": "engine",
"token_count": 288
} | 261 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
part of ui;
enum PointMode {
points,
lines,
polygon,
}
enum ClipOp {
difference,
intersect,
}
enum VertexMode {
triangles,
triangleStrip,
triangleFan,
}
abstract class Vertices {
factory Vertices(
VertexMode mode,
List<Offset> positions, {
List<Color>? colors,
List<Offset>? textureCoordinates,
List<int>? indices,
}) {
return engine.renderer.createVertices(mode,
positions,
textureCoordinates: textureCoordinates,
colors: colors,
indices: indices);
}
factory Vertices.raw(
VertexMode mode,
Float32List positions, {
Int32List? colors,
Float32List? textureCoordinates,
Uint16List? indices,
}) {
return engine.renderer.createVerticesRaw(mode,
positions,
textureCoordinates: textureCoordinates,
colors: colors,
indices: indices);
}
void dispose();
bool get debugDisposed;
}
abstract class PictureRecorder {
factory PictureRecorder() => engine.renderer.createPictureRecorder();
bool get isRecording;
Picture endRecording();
}
abstract class Canvas {
factory Canvas(PictureRecorder recorder, [Rect? cullRect]) =>
engine.renderer.createCanvas(recorder, cullRect);
void save();
void saveLayer(Rect? bounds, Paint paint);
void restore();
int getSaveCount();
void restoreToCount(int count);
void translate(double dx, double dy);
void scale(double sx, [double? sy]);
void rotate(double radians);
void skew(double sx, double sy);
void transform(Float64List matrix4);
Float64List getTransform();
void clipRect(Rect rect,
{ClipOp clipOp = ClipOp.intersect, bool doAntiAlias = true});
void clipRRect(RRect rrect, {bool doAntiAlias = true});
void clipPath(Path path, {bool doAntiAlias = true});
Rect getLocalClipBounds();
Rect getDestinationClipBounds();
void drawColor(Color color, BlendMode blendMode);
void drawLine(Offset p1, Offset p2, Paint paint);
void drawPaint(Paint paint);
void drawRect(Rect rect, Paint paint);
void drawRRect(RRect rrect, Paint paint);
void drawDRRect(RRect outer, RRect inner, Paint paint);
void drawOval(Rect rect, Paint paint);
void drawCircle(Offset c, double radius, Paint paint);
void drawArc(Rect rect, double startAngle, double sweepAngle, bool useCenter,
Paint paint);
void drawPath(Path path, Paint paint);
void drawImage(Image image, Offset offset, Paint paint);
void drawImageRect(Image image, Rect src, Rect dst, Paint paint);
void drawImageNine(Image image, Rect center, Rect dst, Paint paint);
void drawPicture(Picture picture);
void drawParagraph(Paragraph paragraph, Offset offset);
void drawPoints(PointMode pointMode, List<Offset> points, Paint paint);
void drawRawPoints(PointMode pointMode, Float32List points, Paint paint);
void drawVertices(Vertices vertices, BlendMode blendMode, Paint paint);
void drawAtlas(
Image atlas,
List<RSTransform> transforms,
List<Rect> rects,
List<Color>? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint,
);
void drawRawAtlas(
Image atlas,
Float32List rstTransforms,
Float32List rects,
Int32List? colors,
BlendMode? blendMode,
Rect? cullRect,
Paint paint,
);
void drawShadow(
Path path,
Color color,
double elevation,
bool transparentOccluder,
);
}
typedef PictureEventCallback = void Function(Picture picture);
abstract class Picture {
static PictureEventCallback? onCreate;
static PictureEventCallback? onDispose;
Future<Image> toImage(int width, int height);
Image toImageSync(int width, int height);
void dispose();
bool get debugDisposed;
int get approximateBytesUsed;
}
enum PathFillType {
nonZero,
evenOdd,
}
// Must be kept in sync with SkPathOp
enum PathOperation {
difference,
intersect,
union,
xor,
reverseDifference,
}
abstract class PictureRasterizationException implements Exception {
String get message;
StackTrace? stack;
}
| engine/lib/web_ui/lib/canvas.dart/0 | {
"file_path": "engine/lib/web_ui/lib/canvas.dart",
"repo_id": "engine",
"token_count": 1359
} | 262 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
part of ui;
class SemanticsAction {
const SemanticsAction._(this.index, this.name);
final int index;
final String name;
static const int _kTapIndex = 1 << 0;
static const int _kLongPressIndex = 1 << 1;
static const int _kScrollLeftIndex = 1 << 2;
static const int _kScrollRightIndex = 1 << 3;
static const int _kScrollUpIndex = 1 << 4;
static const int _kScrollDownIndex = 1 << 5;
static const int _kIncreaseIndex = 1 << 6;
static const int _kDecreaseIndex = 1 << 7;
static const int _kShowOnScreenIndex = 1 << 8;
static const int _kMoveCursorForwardByCharacterIndex = 1 << 9;
static const int _kMoveCursorBackwardByCharacterIndex = 1 << 10;
static const int _kSetSelectionIndex = 1 << 11;
static const int _kCopyIndex = 1 << 12;
static const int _kCutIndex = 1 << 13;
static const int _kPasteIndex = 1 << 14;
static const int _kDidGainAccessibilityFocusIndex = 1 << 15;
static const int _kDidLoseAccessibilityFocusIndex = 1 << 16;
static const int _kCustomActionIndex = 1 << 17;
static const int _kDismissIndex = 1 << 18;
static const int _kMoveCursorForwardByWordIndex = 1 << 19;
static const int _kMoveCursorBackwardByWordIndex = 1 << 20;
static const int _kSetTextIndex = 1 << 21;
static const SemanticsAction tap = SemanticsAction._(_kTapIndex, 'tap');
static const SemanticsAction longPress = SemanticsAction._(_kLongPressIndex, 'longPress');
static const SemanticsAction scrollLeft = SemanticsAction._(_kScrollLeftIndex, 'scrollLeft');
static const SemanticsAction scrollRight = SemanticsAction._(_kScrollRightIndex, 'scrollRight');
static const SemanticsAction scrollUp = SemanticsAction._(_kScrollUpIndex, 'scrollUp');
static const SemanticsAction scrollDown = SemanticsAction._(_kScrollDownIndex, 'scrollDown');
static const SemanticsAction increase = SemanticsAction._(_kIncreaseIndex, 'increase');
static const SemanticsAction decrease = SemanticsAction._(_kDecreaseIndex, 'decrease');
static const SemanticsAction showOnScreen = SemanticsAction._(_kShowOnScreenIndex, 'showOnScreen');
static const SemanticsAction moveCursorForwardByCharacter = SemanticsAction._(_kMoveCursorForwardByCharacterIndex, 'moveCursorForwardByCharacter');
static const SemanticsAction moveCursorBackwardByCharacter = SemanticsAction._(_kMoveCursorBackwardByCharacterIndex, 'moveCursorBackwardByCharacter');
static const SemanticsAction setText = SemanticsAction._(_kSetTextIndex, 'setText');
static const SemanticsAction setSelection = SemanticsAction._(_kSetSelectionIndex, 'setSelection');
static const SemanticsAction copy = SemanticsAction._(_kCopyIndex, 'copy');
static const SemanticsAction cut = SemanticsAction._(_kCutIndex, 'cut');
static const SemanticsAction paste = SemanticsAction._(_kPasteIndex, 'paste');
static const SemanticsAction didGainAccessibilityFocus = SemanticsAction._(_kDidGainAccessibilityFocusIndex, 'didGainAccessibilityFocus');
static const SemanticsAction didLoseAccessibilityFocus = SemanticsAction._(_kDidLoseAccessibilityFocusIndex, 'didLoseAccessibilityFocus');
static const SemanticsAction customAction = SemanticsAction._(_kCustomActionIndex, 'customAction');
static const SemanticsAction dismiss = SemanticsAction._(_kDismissIndex, 'dismiss');
static const SemanticsAction moveCursorForwardByWord = SemanticsAction._(_kMoveCursorForwardByWordIndex, 'moveCursorForwardByWord');
static const SemanticsAction moveCursorBackwardByWord = SemanticsAction._(_kMoveCursorBackwardByWordIndex, 'moveCursorBackwardByWord');
static const Map<int, SemanticsAction> _kActionById = <int, SemanticsAction>{
_kTapIndex: tap,
_kLongPressIndex: longPress,
_kScrollLeftIndex: scrollLeft,
_kScrollRightIndex: scrollRight,
_kScrollUpIndex: scrollUp,
_kScrollDownIndex: scrollDown,
_kIncreaseIndex: increase,
_kDecreaseIndex: decrease,
_kShowOnScreenIndex: showOnScreen,
_kMoveCursorForwardByCharacterIndex: moveCursorForwardByCharacter,
_kMoveCursorBackwardByCharacterIndex: moveCursorBackwardByCharacter,
_kSetSelectionIndex: setSelection,
_kCopyIndex: copy,
_kCutIndex: cut,
_kPasteIndex: paste,
_kDidGainAccessibilityFocusIndex: didGainAccessibilityFocus,
_kDidLoseAccessibilityFocusIndex: didLoseAccessibilityFocus,
_kCustomActionIndex: customAction,
_kDismissIndex: dismiss,
_kMoveCursorForwardByWordIndex: moveCursorForwardByWord,
_kMoveCursorBackwardByWordIndex: moveCursorBackwardByWord,
_kSetTextIndex: setText,
};
static List<SemanticsAction> get values => _kActionById.values.toList(growable: false);
static SemanticsAction? fromIndex(int index) => _kActionById[index];
@override
String toString() => 'SemanticsAction.$name';
}
class SemanticsFlag {
const SemanticsFlag._(this.index, this.name);
final int index;
final String name;
static const int _kHasCheckedStateIndex = 1 << 0;
static const int _kIsCheckedIndex = 1 << 1;
static const int _kIsSelectedIndex = 1 << 2;
static const int _kIsButtonIndex = 1 << 3;
static const int _kIsTextFieldIndex = 1 << 4;
static const int _kIsFocusedIndex = 1 << 5;
static const int _kHasEnabledStateIndex = 1 << 6;
static const int _kIsEnabledIndex = 1 << 7;
static const int _kIsInMutuallyExclusiveGroupIndex = 1 << 8;
static const int _kIsHeaderIndex = 1 << 9;
static const int _kIsObscuredIndex = 1 << 10;
static const int _kScopesRouteIndex = 1 << 11;
static const int _kNamesRouteIndex = 1 << 12;
static const int _kIsHiddenIndex = 1 << 13;
static const int _kIsImageIndex = 1 << 14;
static const int _kIsLiveRegionIndex = 1 << 15;
static const int _kHasToggledStateIndex = 1 << 16;
static const int _kIsToggledIndex = 1 << 17;
static const int _kHasImplicitScrollingIndex = 1 << 18;
static const int _kIsMultilineIndex = 1 << 19;
static const int _kIsReadOnlyIndex = 1 << 20;
static const int _kIsFocusableIndex = 1 << 21;
static const int _kIsLinkIndex = 1 << 22;
static const int _kIsSliderIndex = 1 << 23;
static const int _kIsKeyboardKeyIndex = 1 << 24;
static const int _kIsCheckStateMixedIndex = 1 << 25;
static const int _kHasExpandedStateIndex = 1 << 26;
static const int _kIsExpandedIndex = 1 << 27;
static const SemanticsFlag hasCheckedState = SemanticsFlag._(_kHasCheckedStateIndex, 'hasCheckedState');
static const SemanticsFlag isChecked = SemanticsFlag._(_kIsCheckedIndex, 'isChecked');
static const SemanticsFlag isSelected = SemanticsFlag._(_kIsSelectedIndex, 'isSelected');
static const SemanticsFlag isButton = SemanticsFlag._(_kIsButtonIndex, 'isButton');
static const SemanticsFlag isTextField = SemanticsFlag._(_kIsTextFieldIndex, 'isTextField');
static const SemanticsFlag isSlider = SemanticsFlag._(_kIsSliderIndex, 'isSlider');
static const SemanticsFlag isKeyboardKey = SemanticsFlag._(_kIsKeyboardKeyIndex, 'isKeyboardKey');
static const SemanticsFlag isReadOnly = SemanticsFlag._(_kIsReadOnlyIndex, 'isReadOnly');
static const SemanticsFlag isLink = SemanticsFlag._(_kIsLinkIndex, 'isLink');
static const SemanticsFlag isFocusable = SemanticsFlag._(_kIsFocusableIndex, 'isFocusable');
static const SemanticsFlag isFocused = SemanticsFlag._(_kIsFocusedIndex, 'isFocused');
static const SemanticsFlag hasEnabledState = SemanticsFlag._(_kHasEnabledStateIndex, 'hasEnabledState');
static const SemanticsFlag isEnabled = SemanticsFlag._(_kIsEnabledIndex, 'isEnabled');
static const SemanticsFlag isInMutuallyExclusiveGroup = SemanticsFlag._(_kIsInMutuallyExclusiveGroupIndex, 'isInMutuallyExclusiveGroup');
static const SemanticsFlag isHeader = SemanticsFlag._(_kIsHeaderIndex, 'isHeader');
static const SemanticsFlag isObscured = SemanticsFlag._(_kIsObscuredIndex, 'isObscured');
static const SemanticsFlag isMultiline = SemanticsFlag._(_kIsMultilineIndex, 'isMultiline');
static const SemanticsFlag scopesRoute = SemanticsFlag._(_kScopesRouteIndex, 'scopesRoute');
static const SemanticsFlag namesRoute = SemanticsFlag._(_kNamesRouteIndex, 'namesRoute');
static const SemanticsFlag isHidden = SemanticsFlag._(_kIsHiddenIndex, 'isHidden');
static const SemanticsFlag isImage = SemanticsFlag._(_kIsImageIndex, 'isImage');
static const SemanticsFlag isLiveRegion = SemanticsFlag._(_kIsLiveRegionIndex, 'isLiveRegion');
static const SemanticsFlag hasToggledState = SemanticsFlag._(_kHasToggledStateIndex, 'hasToggledState');
static const SemanticsFlag isToggled = SemanticsFlag._(_kIsToggledIndex, 'isToggled');
static const SemanticsFlag hasImplicitScrolling = SemanticsFlag._(_kHasImplicitScrollingIndex, 'hasImplicitScrolling');
static const SemanticsFlag isCheckStateMixed = SemanticsFlag._(_kIsCheckStateMixedIndex, 'isCheckStateMixed');
static const SemanticsFlag hasExpandedState = SemanticsFlag._(_kHasExpandedStateIndex, 'hasExpandedState');
static const SemanticsFlag isExpanded = SemanticsFlag._(_kIsExpandedIndex, 'isExpanded');
static const Map<int, SemanticsFlag> _kFlagById = <int, SemanticsFlag>{
_kHasCheckedStateIndex: hasCheckedState,
_kIsCheckedIndex: isChecked,
_kIsSelectedIndex: isSelected,
_kIsButtonIndex: isButton,
_kIsTextFieldIndex: isTextField,
_kIsFocusedIndex: isFocused,
_kHasEnabledStateIndex: hasEnabledState,
_kIsEnabledIndex: isEnabled,
_kIsInMutuallyExclusiveGroupIndex: isInMutuallyExclusiveGroup,
_kIsHeaderIndex: isHeader,
_kIsObscuredIndex: isObscured,
_kScopesRouteIndex: scopesRoute,
_kNamesRouteIndex: namesRoute,
_kIsHiddenIndex: isHidden,
_kIsImageIndex: isImage,
_kIsLiveRegionIndex: isLiveRegion,
_kHasToggledStateIndex: hasToggledState,
_kIsToggledIndex: isToggled,
_kHasImplicitScrollingIndex: hasImplicitScrolling,
_kIsMultilineIndex: isMultiline,
_kIsReadOnlyIndex: isReadOnly,
_kIsFocusableIndex: isFocusable,
_kIsLinkIndex: isLink,
_kIsSliderIndex: isSlider,
_kIsKeyboardKeyIndex: isKeyboardKey,
_kIsCheckStateMixedIndex: isCheckStateMixed,
_kHasExpandedStateIndex: hasExpandedState,
_kIsExpandedIndex: isExpanded,
};
static List<SemanticsFlag> get values => _kFlagById.values.toList(growable: false);
static SemanticsFlag? fromIndex(int index) => _kFlagById[index];
@override
String toString() => 'SemanticsFlag.$name';
}
// When adding a new StringAttributeType, the classes in these file must be
// updated as well.
// * engine/src/flutter/lib/ui/semantics.dart
// * engine/src/flutter/lib/ui/semantics/string_attribute.h
// * engine/src/flutter/shell/platform/android/io/flutter/view/AccessibilityBridge.java
// * engine/src/flutter/lib/web_ui/test/engine/semantics/semantics_api_test.dart
// * engine/src/flutter/testing/dart/semantics_test.dart
abstract class StringAttribute {
StringAttribute._({
required this.range,
});
final TextRange range;
StringAttribute copy({required TextRange range});
}
class SpellOutStringAttribute extends StringAttribute {
SpellOutStringAttribute({
required super.range,
}) : super._();
@override
StringAttribute copy({required TextRange range}) {
return SpellOutStringAttribute(range: range);
}
@override
String toString() {
return 'SpellOutStringAttribute($range)';
}
}
class LocaleStringAttribute extends StringAttribute {
LocaleStringAttribute({
required super.range,
required this.locale,
}) : super._();
final Locale locale;
@override
StringAttribute copy({required TextRange range}) {
return LocaleStringAttribute(range: range, locale: locale);
}
@override
String toString() {
return 'LocaleStringAttribute($range, ${locale.toLanguageTag()})';
}
}
class SemanticsUpdateBuilder {
SemanticsUpdateBuilder();
final List<engine.SemanticsNodeUpdate> _nodeUpdates = <engine.SemanticsNodeUpdate>[];
void updateNode({
required int id,
required int flags,
required int actions,
required int maxValueLength,
required int currentValueLength,
required int textSelectionBase,
required int textSelectionExtent,
required int platformViewId,
required int scrollChildren,
required int scrollIndex,
required double scrollPosition,
required double scrollExtentMax,
required double scrollExtentMin,
required double elevation,
required double thickness,
required Rect rect,
required String identifier,
required String label,
required List<StringAttribute> labelAttributes,
required String value,
required List<StringAttribute> valueAttributes,
required String increasedValue,
required List<StringAttribute> increasedValueAttributes,
required String decreasedValue,
required List<StringAttribute> decreasedValueAttributes,
required String hint,
required List<StringAttribute> hintAttributes,
String? tooltip,
TextDirection? textDirection,
required Float64List transform,
required Int32List childrenInTraversalOrder,
required Int32List childrenInHitTestOrder,
required Int32List additionalActions,
}) {
if (transform.length != 16) {
throw ArgumentError('transform argument must have 16 entries.');
}
_nodeUpdates.add(engine.SemanticsNodeUpdate(
id: id,
flags: flags,
actions: actions,
maxValueLength: maxValueLength,
currentValueLength: currentValueLength,
textSelectionBase: textSelectionBase,
textSelectionExtent: textSelectionExtent,
scrollChildren: scrollChildren,
scrollIndex: scrollIndex,
scrollPosition: scrollPosition,
scrollExtentMax: scrollExtentMax,
scrollExtentMin: scrollExtentMin,
rect: rect,
identifier: identifier,
label: label,
labelAttributes: labelAttributes,
value: value,
valueAttributes: valueAttributes,
increasedValue: increasedValue,
increasedValueAttributes: increasedValueAttributes,
decreasedValue: decreasedValue,
decreasedValueAttributes: decreasedValueAttributes,
hint: hint,
hintAttributes: hintAttributes,
tooltip: tooltip,
textDirection: textDirection,
transform: engine.toMatrix32(transform),
elevation: elevation,
thickness: thickness,
childrenInTraversalOrder: childrenInTraversalOrder,
childrenInHitTestOrder: childrenInHitTestOrder,
additionalActions: additionalActions,
platformViewId: platformViewId,
));
}
void updateCustomAction({
required int id,
String? label,
String? hint,
int overrideId = -1,
}) {
// TODO(yjbanov): implement.
}
SemanticsUpdate build() {
return SemanticsUpdate._(
nodeUpdates: _nodeUpdates,
);
}
}
abstract class SemanticsUpdate {
factory SemanticsUpdate._({List<engine.SemanticsNodeUpdate>? nodeUpdates}) =
engine.SemanticsUpdate;
void dispose();
}
| engine/lib/web_ui/lib/semantics.dart/0 | {
"file_path": "engine/lib/web_ui/lib/semantics.dart",
"repo_id": "engine",
"token_count": 4734
} | 263 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Uses the `ImageDecoder` class supplied by the browser.
//
// See also:
//
// * `image_wasm_codecs.dart`, which uses codecs supplied by the CanvasKit WASM bundle.
import 'dart:async';
import 'dart:convert' show base64;
import 'dart:js_interop';
import 'dart:math' as math;
import 'dart:typed_data';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
/// Image decoder backed by the browser's `ImageDecoder`.
class CkBrowserImageDecoder extends BrowserImageDecoder {
CkBrowserImageDecoder._({
required super.contentType,
required super.dataSource,
required super.debugSource,
});
static Future<CkBrowserImageDecoder> create({
required Uint8List data,
required String debugSource,
}) async {
// ImageDecoder does not detect image type automatically. It requires us to
// tell it what the image type is.
final String? contentType = detectContentType(data);
if (contentType == null) {
final String fileHeader;
if (data.isNotEmpty) {
fileHeader = '[${bytesToHexString(data.sublist(0, math.min(10, data.length)))}]';
} else {
fileHeader = 'empty';
}
throw ImageCodecException(
'Failed to detect image file format using the file header.\n'
'File header was $fileHeader.\n'
'Image source: $debugSource'
);
}
final CkBrowserImageDecoder decoder = CkBrowserImageDecoder._(
contentType: contentType,
dataSource: data.toJS,
debugSource: debugSource,
);
// Call once to initialize the decoder and populate late fields.
await decoder.initialize();
return decoder;
}
@override
ui.Image generateImageFromVideoFrame(VideoFrame frame) {
final SkImage? skImage = canvasKit.MakeLazyImageFromTextureSourceWithInfo(
frame,
SkPartialImageInfo(
alphaType: canvasKit.AlphaType.Premul,
colorType: canvasKit.ColorType.RGBA_8888,
colorSpace: SkColorSpaceSRGB,
width: frame.displayWidth,
height: frame.displayHeight,
),
);
if (skImage == null) {
throw ImageCodecException(
"Failed to create image from pixel data decoded using the browser's ImageDecoder.",
);
}
return CkImage(skImage, videoFrame: frame);
}
}
Future<ByteData> readPixelsFromVideoFrame(VideoFrame videoFrame, ui.ImageByteFormat format) async {
if (format == ui.ImageByteFormat.png) {
final Uint8List png = await encodeVideoFrameAsPng(videoFrame);
return png.buffer.asByteData();
}
final ByteBuffer pixels = await readVideoFramePixelsUnmodified(videoFrame);
// Check if the pixels are already in the right format and if so, return the
// original pixels without modification.
if (_shouldReadPixelsUnmodified(videoFrame, format)) {
return pixels.asByteData();
}
// At this point we know we want to read unencoded pixels, and that the video
// frame is _not_ using the same format as the requested one.
final bool isBgrFrame = videoFrame.format == 'BGRA' || videoFrame.format == 'BGRX';
if (format == ui.ImageByteFormat.rawRgba && isBgrFrame) {
_bgrToRgba(pixels);
return pixels.asByteData();
}
// Last resort, just return the original pixels.
return pixels.asByteData();
}
/// Mutates the [pixels], converting them from BGRX/BGRA to RGBA.
void _bgrToRgba(ByteBuffer pixels) {
final int pixelCount = pixels.lengthInBytes ~/ 4;
final Uint8List pixelBytes = pixels.asUint8List();
for (int i = 0; i < pixelCount; i += 4) {
// It seems even in little-endian machines the BGR_ pixels are encoded as
// big-endian, i.e. the blue byte is written into the lowest byte in the
// memory address space.
final int b = pixelBytes[i];
final int r = pixelBytes[i + 2];
// So far the codec has reported 255 for the X component, so there's no
// special treatment for alpha. This may need to change if we ever face
// codecs that do something different.
pixelBytes[i] = r;
pixelBytes[i + 2] = b;
}
}
bool _shouldReadPixelsUnmodified(VideoFrame videoFrame, ui.ImageByteFormat format) {
if (format == ui.ImageByteFormat.rawUnmodified) {
return true;
}
// Do not convert if the requested format is RGBA and the video frame is
// encoded as either RGBA or RGBX.
final bool isRgbFrame = videoFrame.format == 'RGBA' || videoFrame.format == 'RGBX';
return format == ui.ImageByteFormat.rawRgba && isRgbFrame;
}
Future<ByteBuffer> readVideoFramePixelsUnmodified(VideoFrame videoFrame) async {
final int size = videoFrame.allocationSize().toInt();
// In dart2wasm, Uint8List is not the same as a JS Uint8Array. So we
// explicitly construct the JS object here.
final JSUint8Array destination = createUint8ArrayFromLength(size);
final JSPromise<JSAny?> copyPromise = videoFrame.copyTo(destination);
await promiseToFuture<void>(copyPromise);
// In dart2wasm, `toDart` incurs a copy here. On JS backends, this is a
// no-op.
return destination.toDart.buffer;
}
Future<Uint8List> encodeVideoFrameAsPng(VideoFrame videoFrame) async {
final int width = videoFrame.displayWidth.toInt();
final int height = videoFrame.displayHeight.toInt();
final DomCanvasElement canvas = createDomCanvasElement(width: width, height:
height);
final DomCanvasRenderingContext2D ctx = canvas.context2D;
ctx.drawImage(videoFrame, 0, 0);
final String pngBase64 = canvas.toDataURL().substring('data:image/png;base64,'.length);
return base64.decode(pngBase64);
}
| engine/lib/web_ui/lib/src/engine/canvaskit/image_web_codecs.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/canvaskit/image_web_codecs.dart",
"repo_id": "engine",
"token_count": 1932
} | 264 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:ui/ui.dart' as ui;
import '../vector_math.dart';
import 'canvas.dart';
/// A cache of [Picture]s that have already been rasterized.
///
/// In the case of a [Picture] with a lot of complex drawing commands, it can
/// be faster to rasterize that [Picture] into it's own canvas and composite
/// that canvas into the scene rather than replay the drawing commands for that
/// picture into the overall scene.
///
/// This class is responsible for deciding if a [Picture] should be cached and
/// for creating the cached [Picture]s that can be drawn directly into the
/// canvas.
class RasterCache {
/// Make a decision on whether to cache [picture] under transform [matrix].
///
/// This is based on heuristics such as how many times [picture] has been
/// drawn before and the complexity of the drawing commands in [picture].
///
/// We also take into account the current transform [matrix], because, for
/// example, a picture may be rasterized with the identity transform, but
/// when it is used, the transform is a 3x scale. In this case, compositing
/// the rendered picture would result in pixelation. So, we must use both
/// the picture and the transform as a cache key.
///
/// The flag [isComplex] is a hint to the raster cache that this picture
/// contains complex drawing commands, and as such should be more strongly
/// considered for caching.
///
/// The flag [willChange] is a hint to the raster cache that this picture
/// will change, and so should be less likely to be cached.
void prepare(
ui.Picture picture, Matrix4 matrix, bool isComplex, bool willChange) {}
/// Gets a raster cache result for the [picture] at transform [matrix].
RasterCacheResult get(ui.Picture picture, Matrix4 matrix) =>
RasterCacheResult();
}
/// A cache entry for a given picture and matrix.
class RasterCacheResult {
/// Whether or not this result represents a rasterized picture that can be
/// drawn into the scene.
bool get isValid => false;
/// Draws the rasterized picture into the [canvas].
void draw(CkCanvas canvas) {}
}
| engine/lib/web_ui/lib/src/engine/canvaskit/raster_cache.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/canvaskit/raster_cache.dart",
"repo_id": "engine",
"token_count": 608
} | 265 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:typed_data';
import 'dom.dart';
import 'platform_dispatcher.dart';
import 'services.dart';
final ByteData? _fontChangeMessage =
const JSONMessageCodec().encodeMessage(
<String, dynamic>{'type': 'fontsChange'});
// Font load callbacks will typically arrive in sequence, we want to prevent
// sendFontChangeMessage of causing multiple synchronous rebuilds.
// This flag ensures we properly schedule a single call to framework.
bool _fontChangeScheduled = false;
FutureOr<void> sendFontChangeMessage() async {
if (!_fontChangeScheduled) {
_fontChangeScheduled = true;
// Batch updates into next animationframe.
domWindow.requestAnimationFrame((_) {
_fontChangeScheduled = false;
EnginePlatformDispatcher.instance.invokeOnPlatformMessage(
'flutter/system',
_fontChangeMessage,
(_) {},
);
});
}
}
| engine/lib/web_ui/lib/src/engine/font_change_util.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/font_change_util.dart",
"repo_id": "engine",
"token_count": 351
} | 266 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:ui/ui.dart' as ui;
import '../color_filter.dart';
import '../util.dart';
/// Implementation of [ui.Paint] used by the HTML rendering backend.
class SurfacePaint implements ui.Paint {
SurfacePaintData _paintData = SurfacePaintData();
@override
ui.BlendMode get blendMode => _paintData.blendMode ?? ui.BlendMode.srcOver;
@override
set blendMode(ui.BlendMode value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.blendMode = value;
}
@override
ui.PaintingStyle get style => _paintData.style ?? ui.PaintingStyle.fill;
@override
set style(ui.PaintingStyle value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.style = value;
}
@override
double get strokeWidth => _paintData.strokeWidth ?? 0.0;
@override
set strokeWidth(double value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.strokeWidth = value;
}
@override
ui.StrokeCap get strokeCap => _paintData.strokeCap ?? ui.StrokeCap.butt;
@override
set strokeCap(ui.StrokeCap value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.strokeCap = value;
}
@override
ui.StrokeJoin get strokeJoin => _paintData.strokeJoin ?? ui.StrokeJoin.miter;
@override
set strokeJoin(ui.StrokeJoin value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.strokeJoin = value;
}
@override
bool get isAntiAlias => _paintData.isAntiAlias;
@override
set isAntiAlias(bool value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.isAntiAlias = value;
}
@override
ui.Color get color => ui.Color(_paintData.color);
@override
set color(ui.Color value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.color = value.value;
}
@override
bool invertColors = false;
@override
ui.Shader? get shader => _paintData.shader;
@override
set shader(ui.Shader? value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.shader = value;
}
@override
ui.MaskFilter? get maskFilter => _paintData.maskFilter;
@override
set maskFilter(ui.MaskFilter? value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.maskFilter = value;
}
@override
ui.FilterQuality get filterQuality => _paintData.filterQuality ?? ui.FilterQuality.none;
@override
set filterQuality(ui.FilterQuality value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.filterQuality = value;
}
@override
ui.ColorFilter? get colorFilter => _paintData.colorFilter;
@override
set colorFilter(ui.ColorFilter? value) {
if (_frozen) {
_paintData = _paintData.clone();
_frozen = false;
}
_paintData.colorFilter = value as EngineColorFilter?;
}
// TODO(ferhat): see https://github.com/flutter/flutter/issues/33605
@override
double strokeMiterLimit = 0;
// TODO(ferhat): Implement ImageFilter, flutter/flutter#35156.
@override
ui.ImageFilter? imageFilter;
// True if Paint instance has used in RecordingCanvas.
bool _frozen = false;
// Marks this paint object as previously used.
SurfacePaintData get paintData {
// Flip bit so next time object gets mutated we create a clone of
// current paint data.
_frozen = true;
return _paintData;
}
// Must be kept in sync with the default in paint.cc.
static const double _kStrokeMiterLimitDefault = 4.0;
// Must be kept in sync with the default in paint.cc.
static const int _kColorDefault = 0xFF000000;
// Must be kept in sync with the default in paint.cc.
static final int _kBlendModeDefault = ui.BlendMode.srcOver.index;
@override
String toString() {
String resultString = 'Paint()';
assert(() {
final StringBuffer result = StringBuffer();
String semicolon = '';
result.write('Paint(');
if (style == ui.PaintingStyle.stroke) {
result.write('$style');
if (strokeWidth != 0.0) {
result.write(' ${strokeWidth.toStringAsFixed(1)}');
} else {
result.write(' hairline');
}
if (strokeCap != ui.StrokeCap.butt) {
result.write(' $strokeCap');
}
if (strokeJoin == ui.StrokeJoin.miter) {
if (strokeMiterLimit != _kStrokeMiterLimitDefault) {
result.write(' $strokeJoin up to ${strokeMiterLimit.toStringAsFixed(1)}');
}
} else {
result.write(' $strokeJoin');
}
semicolon = '; ';
}
if (!isAntiAlias) {
result.write('${semicolon}antialias off');
semicolon = '; ';
}
if (color != const ui.Color(_kColorDefault)) {
result.write('$semicolon$color');
semicolon = '; ';
}
if (blendMode.index != _kBlendModeDefault) {
result.write('$semicolon$blendMode');
semicolon = '; ';
}
if (colorFilter != null) {
result.write('${semicolon}colorFilter: $colorFilter');
semicolon = '; ';
}
if (maskFilter != null) {
result.write('${semicolon}maskFilter: $maskFilter');
semicolon = '; ';
}
if (filterQuality != ui.FilterQuality.none) {
result.write('${semicolon}filterQuality: $filterQuality');
semicolon = '; ';
}
if (shader != null) {
result.write('${semicolon}shader: $shader');
semicolon = '; ';
}
if (imageFilter != null) {
result.write('${semicolon}imageFilter: $imageFilter');
semicolon = '; ';
}
if (invertColors) {
result.write('${semicolon}invert: $invertColors');
}
result.write(')');
resultString = result.toString();
return true;
}());
return resultString;
}
}
/// Private Paint context data used for recording canvas commands allowing
/// Paint to be mutated post canvas draw operations.
class SurfacePaintData {
ui.BlendMode? blendMode;
ui.PaintingStyle? style;
double? strokeWidth;
ui.StrokeCap? strokeCap;
ui.StrokeJoin? strokeJoin;
bool isAntiAlias = true;
int color = 0xFF000000;
ui.Shader? shader;
ui.MaskFilter? maskFilter;
ui.FilterQuality? filterQuality;
EngineColorFilter? colorFilter;
// Internal for recording canvas use.
SurfacePaintData clone() {
return SurfacePaintData()
..blendMode = blendMode
..filterQuality = filterQuality
..maskFilter = maskFilter
..shader = shader
..isAntiAlias = isAntiAlias
..color = color
..colorFilter = colorFilter
..strokeWidth = strokeWidth
..style = style
..strokeJoin = strokeJoin
..strokeCap = strokeCap;
}
@override
String toString() {
String result = super.toString();
assert(() {
final StringBuffer buffer = StringBuffer('SurfacePaintData(');
if (blendMode != null) {
buffer.write('blendMode = $blendMode; ');
}
if (style != null) {
buffer.write('style = $style; ');
}
if (strokeWidth != null) {
buffer.write('strokeWidth = $strokeWidth; ');
}
if (strokeCap != null) {
buffer.write('strokeCap = $strokeCap; ');
}
if (strokeJoin != null) {
buffer.write('strokeJoin = $strokeJoin; ');
}
buffer.write('color = ${ui.Color(color).toCssString()}; ');
if (shader != null) {
buffer.write('shader = $shader; ');
}
if (maskFilter != null) {
buffer.write('maskFilter = $maskFilter; ');
}
if (filterQuality != null) {
buffer.write('filterQuality = $filterQuality; ');
}
if (colorFilter != null) {
buffer.write('colorFilter = $colorFilter; ');
}
buffer.write('isAntiAlias = $isAntiAlias)');
result = buffer.toString();
return true;
}());
return result;
}
}
class HtmlFragmentProgram implements ui.FragmentProgram {
@override
ui.FragmentShader fragmentShader() {
throw UnsupportedError('FragmentProgram is not supported for the HTML renderer.');
}
}
class HtmlFragmentShader implements ui.FragmentShader {
@override
void setFloat(int index, double value) {
throw UnsupportedError('FragmentShader is not supported for the HTML renderer.');
}
@override
void setImageSampler(int index, ui.Image image) {
throw UnsupportedError('FragmentShader is not supported for the HTML renderer.');
}
@override
void dispose() {
throw UnsupportedError('FragmentShader is not supported for the HTML renderer.');
}
@override
bool get debugDisposed {
throw UnsupportedError('FragmentShader is not supported for the HTML renderer.');
}
}
| engine/lib/web_ui/lib/src/engine/html/painting.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/html/painting.dart",
"repo_id": "engine",
"token_count": 3702
} | 267 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:js_interop';
import 'dart:math' as math;
import 'dart:typed_data';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
import 'package:ui/ui_web/src/ui_web.dart' as ui_web;
class HtmlRenderer implements Renderer {
static HtmlRenderer get instance => _instance;
static late HtmlRenderer _instance;
@override
String get rendererTag => 'html';
late final HtmlFontCollection _fontCollection = HtmlFontCollection();
@override
HtmlFontCollection get fontCollection => _fontCollection;
@override
void initialize() {
scheduleMicrotask(() {
// Access [lineLookup] to force the lazy unpacking of line break data
// now. Removing this line won't break anything. It's just an optimization
// to make the unpacking happen while we are waiting for network requests.
lineLookup;
});
_instance = this;
}
@override
ui.Paint createPaint() => SurfacePaint();
@override
ui.Vertices createVertices(
ui.VertexMode mode,
List<ui.Offset> positions, {
List<ui.Offset>? textureCoordinates,
List<ui.Color>? colors,
List<int>? indices,
}) => SurfaceVertices(
mode,
positions,
colors: colors,
indices: indices);
@override
ui.Vertices createVerticesRaw(
ui.VertexMode mode,
Float32List positions, {
Float32List? textureCoordinates,
Int32List? colors,
Uint16List? indices,
}) => SurfaceVertices.raw(
mode,
positions,
colors: colors,
indices: indices);
@override
ui.Canvas createCanvas(ui.PictureRecorder recorder, [ui.Rect? cullRect]) =>
SurfaceCanvas(recorder as EnginePictureRecorder, cullRect);
@override
ui.Gradient createLinearGradient(
ui.Offset from,
ui.Offset to,
List<ui.Color> colors, [
List<double>? colorStops,
ui.TileMode tileMode = ui.TileMode.clamp,
Float32List? matrix4
]) => GradientLinear(from, to, colors, colorStops, tileMode, matrix4);
@override
ui.Gradient createRadialGradient(
ui.Offset center,
double radius,
List<ui.Color> colors, [
List<double>? colorStops,
ui.TileMode tileMode = ui.TileMode.clamp,
Float32List? matrix4,
]) => GradientRadial(center, radius, colors, colorStops, tileMode, matrix4);
@override
ui.Gradient createConicalGradient(
ui.Offset focal,
double focalRadius,
ui.Offset center,
double radius,
List<ui.Color> colors,
[List<double>? colorStops,
ui.TileMode tileMode = ui.TileMode.clamp,
Float32List? matrix
]) => GradientConical(
focal,
focalRadius,
center,
radius,
colors,
colorStops,
tileMode,
matrix);
@override
ui.Gradient createSweepGradient(
ui.Offset center,
List<ui.Color> colors, [
List<double>? colorStops,
ui.TileMode tileMode = ui.TileMode.clamp,
double startAngle = 0.0,
double endAngle = math.pi * 2,
Float32List? matrix4
]) => GradientSweep(center, colors, colorStops, tileMode, startAngle, endAngle, matrix4);
@override
ui.PictureRecorder createPictureRecorder() => EnginePictureRecorder();
@override
ui.SceneBuilder createSceneBuilder() => SurfaceSceneBuilder();
// TODO(ferhat): implement TileMode.
@override
ui.ImageFilter createBlurImageFilter({
double sigmaX = 0.0,
double sigmaY = 0.0,
ui.TileMode tileMode = ui.TileMode.clamp
}) => EngineImageFilter.blur(sigmaX: sigmaX, sigmaY: sigmaY, tileMode: tileMode);
@override
ui.ImageFilter createDilateImageFilter({double radiusX = 0.0, double radiusY = 0.0}) {
// TODO(fzyzcjy): implement dilate. https://github.com/flutter/flutter/issues/101085
throw UnimplementedError('ImageFilter.dilate not implemented for HTML renderer.');
}
@override
ui.ImageFilter createErodeImageFilter({double radiusX = 0.0, double radiusY = 0.0}) {
// TODO(fzyzcjy): implement erode. https://github.com/flutter/flutter/issues/101085
throw UnimplementedError('ImageFilter.erode not implemented for HTML renderer.');
}
@override
ui.ImageFilter createMatrixImageFilter(
Float64List matrix4, {
ui.FilterQuality filterQuality = ui.FilterQuality.low
}) => EngineImageFilter.matrix(matrix: matrix4, filterQuality: filterQuality);
@override
ui.ImageFilter composeImageFilters({required ui.ImageFilter outer, required ui.ImageFilter inner}) {
// TODO(ferhat): add implementation and remove the "ignore".
// ignore: avoid_unused_constructor_parameters
throw UnimplementedError('ImageFilter.erode not implemented for HTML renderer.');
}
@override
Future<ui.Codec> instantiateImageCodec(
Uint8List list, {
int? targetWidth,
int? targetHeight,
bool allowUpscaling = true}) async {
final DomBlob blob = createDomBlob(<dynamic>[list.buffer]);
return HtmlBlobCodec(blob);
}
@override
Future<ui.Codec> instantiateImageCodecFromUrl(
Uri uri, {
ui_web.ImageCodecChunkCallback? chunkCallback,
}) async {
return HtmlCodec(uri.toString(), chunkCallback: chunkCallback);
}
@override
void decodeImageFromPixels(
Uint8List pixels,
int width,
int height,
ui.PixelFormat format,
ui.ImageDecoderCallback callback, {
int? rowBytes,
int? targetWidth,
int? targetHeight,
bool allowUpscaling = true
}) {
void executeCallback(ui.Codec codec) {
codec.getNextFrame().then((ui.FrameInfo frameInfo) {
callback(frameInfo.image);
});
}
ui.createBmp(pixels, width, height, rowBytes ?? width, format).then(
executeCallback);
}
@override
ui.ImageShader createImageShader(
ui.Image image,
ui.TileMode tmx,
ui.TileMode tmy,
Float64List matrix4,
ui.FilterQuality? filterQuality
) => EngineImageShader(image, tmx, tmy, matrix4, filterQuality);
@override
ui.Path createPath() => SurfacePath();
@override
ui.Path copyPath(ui.Path src) => SurfacePath.from(src as SurfacePath);
@override
ui.Path combinePaths(ui.PathOperation op, ui.Path path1, ui.Path path2) {
throw UnimplementedError('combinePaths not implemented in HTML renderer.');
}
@override
ui.TextStyle createTextStyle({
ui.Color? color,
ui.TextDecoration? decoration,
ui.Color? decorationColor,
ui.TextDecorationStyle? decorationStyle,
double? decorationThickness,
ui.FontWeight? fontWeight,
ui.FontStyle? fontStyle,
ui.TextBaseline? textBaseline,
String? fontFamily,
List<String>? fontFamilyFallback,
double? fontSize,
double? letterSpacing,
double? wordSpacing,
double? height,
ui.TextLeadingDistribution? leadingDistribution,
ui.Locale? locale,
ui.Paint? background,
ui.Paint? foreground,
List<ui.Shadow>? shadows,
List<ui.FontFeature>? fontFeatures,
List<ui.FontVariation>? fontVariations
}) => EngineTextStyle(
color: color,
decoration: decoration,
decorationColor: decorationColor,
decorationStyle: decorationStyle,
decorationThickness: decorationThickness,
fontWeight: fontWeight,
fontStyle: fontStyle,
textBaseline: textBaseline,
fontFamily: fontFamily,
fontFamilyFallback: fontFamilyFallback,
fontSize: fontSize,
letterSpacing: letterSpacing,
wordSpacing: wordSpacing,
height: height,
leadingDistribution: leadingDistribution,
locale: locale,
background: background,
foreground: foreground,
shadows: shadows,
fontFeatures: fontFeatures,
fontVariations: fontVariations,
);
@override
ui.ParagraphStyle createParagraphStyle({
ui.TextAlign? textAlign,
ui.TextDirection? textDirection,
int? maxLines,
String? fontFamily,
double? fontSize,
double? height,
ui.TextHeightBehavior? textHeightBehavior,
ui.FontWeight? fontWeight,
ui.FontStyle? fontStyle,
ui.StrutStyle? strutStyle,
String? ellipsis,
ui.Locale? locale
}) => EngineParagraphStyle(
textAlign: textAlign,
textDirection: textDirection,
maxLines: maxLines,
fontFamily: fontFamily,
fontSize: fontSize,
height: height,
textHeightBehavior: textHeightBehavior,
fontWeight: fontWeight,
fontStyle: fontStyle,
strutStyle: strutStyle,
ellipsis: ellipsis,
locale: locale,
);
@override
ui.StrutStyle createStrutStyle({
String? fontFamily,
List<String>? fontFamilyFallback,
double? fontSize,
double? height,
ui.TextLeadingDistribution? leadingDistribution,
double? leading,
ui.FontWeight? fontWeight,
ui.FontStyle? fontStyle,
bool? forceStrutHeight
}) => EngineStrutStyle(
fontFamily: fontFamily,
fontFamilyFallback: fontFamilyFallback,
fontSize: fontSize,
height: height,
leadingDistribution: leadingDistribution,
leading: leading,
fontWeight: fontWeight,
fontStyle: fontStyle,
forceStrutHeight: forceStrutHeight,
);
@override
ui.ParagraphBuilder createParagraphBuilder(ui.ParagraphStyle style) =>
CanvasParagraphBuilder(style as EngineParagraphStyle);
@override
Future<void> renderScene(ui.Scene scene, ui.FlutterView view) async {
final EngineFlutterView implicitView = EnginePlatformDispatcher.instance.implicitView!;
scene as SurfaceScene;
implicitView.dom.setScene(scene.webOnlyRootElement!);
final FrameTimingRecorder? recorder = scene.timingRecorder;
recorder?.recordRasterFinish();
recorder?.submitTimings();
}
@override
void clearFragmentProgramCache() { }
@override
Future<ui.FragmentProgram> createFragmentProgram(String assetKey) {
return Future<HtmlFragmentProgram>.value(HtmlFragmentProgram());
}
@override
ui.LineMetrics createLineMetrics({
required bool hardBreak,
required double ascent,
required double descent,
required double unscaledAscent,
required double height,
required double width,
required double left,
required double baseline,
required int lineNumber
}) => EngineLineMetrics(
hardBreak: hardBreak,
ascent: ascent,
descent: descent,
unscaledAscent: unscaledAscent,
height: height,
width: width,
left: left,
baseline: baseline,
lineNumber: lineNumber
);
@override
Future<ui.Image> createImageFromImageBitmap(DomImageBitmap imageSource) async {
final int width = imageSource.width.toDartInt;
final int height = imageSource.height.toDartInt;
final OffScreenCanvas canvas = OffScreenCanvas(width, height);
final DomCanvasRenderingContextBitmapRenderer context = canvas.getBitmapRendererContext()!;
context.transferFromImageBitmap(imageSource);
final DomHTMLImageElement imageElement = createDomHTMLImageElement();
late final DomEventListener loadListener;
late final DomEventListener errorListener;
final Completer<HtmlImage> completer = Completer<HtmlImage>();
loadListener = createDomEventListener((DomEvent event) {
completer.complete(HtmlImage(imageElement, width, height));
imageElement.removeEventListener('load', loadListener);
imageElement.removeEventListener('error', errorListener);
});
errorListener = createDomEventListener((DomEvent event) {
completer.completeError(Exception('Failed to create image from image bitmap.'));
imageElement.removeEventListener('load', loadListener);
imageElement.removeEventListener('error', errorListener);
});
imageElement.addEventListener('load', loadListener);
imageElement.addEventListener('error', errorListener);
imageElement.src = await canvas.toDataUrl();
return completer.future;
}
}
| engine/lib/web_ui/lib/src/engine/html/renderer.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/html/renderer.dart",
"repo_id": "engine",
"token_count": 4197
} | 268 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@JS()
library js_app;
import 'dart:js_interop';
import 'package:ui/src/engine.dart';
/// The JS bindings for the configuration object passed to [FlutterApp.addView].
@JS()
@anonymous
@staticInterop
class JsFlutterViewOptions {
external factory JsFlutterViewOptions();
}
/// The attributes of the [JsFlutterViewOptions] object.
extension JsFlutterViewOptionsExtension on JsFlutterViewOptions {
@JS('hostElement')
external DomElement? get _hostElement;
DomElement get hostElement {
assert (_hostElement != null, '`hostElement` passed to addView cannot be null.');
return _hostElement!;
}
@JS('viewConstraints')
external JsViewConstraints? get _viewConstraints;
JsViewConstraints? get viewConstraints {
return _viewConstraints;
}
external JSAny? get initialData;
}
/// The JS bindings for a [ViewConstraints] object.
@JS()
@anonymous
@staticInterop
class JsViewConstraints {
external factory JsViewConstraints({
double? minWidth,
double? maxWidth,
double? minHeight,
double? maxHeight,
});
}
/// The attributes of a [JsViewConstraints] object.
///
/// These attributes are expressed in *logical* pixels.
extension JsViewConstraintsExtension on JsViewConstraints {
external double? get maxHeight;
external double? get maxWidth;
external double? get minHeight;
external double? get minWidth;
}
/// The public JS API of a running Flutter Web App.
@JS()
@anonymous
@staticInterop
abstract class FlutterApp {
factory FlutterApp({
required AddFlutterViewFn addView,
required RemoveFlutterViewFn removeView,
}) =>
FlutterApp._(
addView: addView.toJS,
removeView: ((JSNumber id) => removeView(id.toDartInt)).toJS,
);
external factory FlutterApp._({
required JSFunction addView,
required JSFunction removeView,
});
}
/// Typedef for the function that adds a new view to the app.
///
/// Returns the ID of the newly created view.
typedef AddFlutterViewFn = int Function(JsFlutterViewOptions);
/// Typedef for the function that removes a view from the app.
///
/// Returns the configuration used to create the view.
typedef RemoveFlutterViewFn = JsFlutterViewOptions? Function(int);
| engine/lib/web_ui/lib/src/engine/js_interop/js_app.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/js_interop/js_app.dart",
"repo_id": "engine",
"token_count": 758
} | 269 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:meta/meta.dart';
import 'package:ui/ui.dart' as ui;
import '../../engine.dart';
/// Signature of functions added as a listener to [ui.AppLifecycleState] changes
typedef AppLifecycleStateListener = void Function(ui.AppLifecycleState state);
/// Determines the [ui.AppLifecycleState].
abstract class AppLifecycleState {
static final AppLifecycleState instance = _BrowserAppLifecycleState();
ui.AppLifecycleState get appLifecycleState => _appLifecycleState;
ui.AppLifecycleState _appLifecycleState = ui.AppLifecycleState.resumed;
final List<AppLifecycleStateListener> _listeners =
<AppLifecycleStateListener>[];
void addListener(AppLifecycleStateListener listener) {
if (_listeners.isEmpty) {
activate();
}
_listeners.add(listener);
listener(_appLifecycleState);
}
void removeListener(AppLifecycleStateListener listener) {
_listeners.remove(listener);
if (_listeners.isEmpty) {
deactivate();
}
}
@protected
void activate();
@protected
void deactivate();
@visibleForTesting
void onAppLifecycleStateChange(ui.AppLifecycleState newState) {
if (newState != _appLifecycleState) {
_appLifecycleState = newState;
for (final AppLifecycleStateListener listener in _listeners) {
listener(newState);
}
}
}
}
/// Manages [ui.AppLifecycleState] within a web context by monitoring specific
/// browser events.
///
/// This class listens to:
/// - 'beforeunload' on [DomWindow] to detect detachment,
/// - 'visibilitychange' on [DomHTMLDocument] to observe visibility changes,
/// - 'focus' and 'blur' on [DomWindow] to track application focus shifts.
class _BrowserAppLifecycleState extends AppLifecycleState {
@override
void activate() {
domWindow.addEventListener('focus', _focusListener);
domWindow.addEventListener('blur', _blurListener);
// TODO(web): Register 'beforeunload' only if lifecycle listeners exist, to improve efficiency: https://developer.mozilla.org/en-US/docs/Web/API/Window/beforeunload_event#usage_notes
domWindow.addEventListener('beforeunload', _beforeUnloadListener);
domDocument.addEventListener('visibilitychange', _visibilityChangeListener);
}
@override
void deactivate() {
domWindow.removeEventListener('focus', _focusListener);
domWindow.removeEventListener('blur', _blurListener);
domWindow.removeEventListener('beforeunload', _beforeUnloadListener);
domDocument.removeEventListener(
'visibilitychange',
_visibilityChangeListener,
);
}
late final DomEventListener _focusListener =
createDomEventListener((DomEvent event) {
onAppLifecycleStateChange(ui.AppLifecycleState.resumed);
});
late final DomEventListener _blurListener =
createDomEventListener((DomEvent event) {
onAppLifecycleStateChange(ui.AppLifecycleState.inactive);
});
late final DomEventListener _beforeUnloadListener =
createDomEventListener((DomEvent event) {
onAppLifecycleStateChange(ui.AppLifecycleState.detached);
});
late final DomEventListener _visibilityChangeListener =
createDomEventListener((DomEvent event) {
if (domDocument.visibilityState == 'visible') {
onAppLifecycleStateChange(ui.AppLifecycleState.resumed);
} else if (domDocument.visibilityState == 'hidden') {
onAppLifecycleStateChange(ui.AppLifecycleState.hidden);
}
});
}
| engine/lib/web_ui/lib/src/engine/platform_dispatcher/app_lifecycle_state.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/platform_dispatcher/app_lifecycle_state.dart",
"repo_id": "engine",
"token_count": 1169
} | 270 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:ui/ui.dart' as ui;
// These are additional APIs that are not part of the `dart:ui` interface that
// are needed internally to properly implement a `SceneBuilder` on top of the
// generic Canvas/Picture api.
abstract class SceneCanvas implements ui.Canvas {
// This is the same as a normal `saveLayer` call, but we can pass a backdrop image filter.
void saveLayerWithFilter(ui.Rect? bounds, ui.Paint paint, ui.ImageFilter backdropFilter);
}
abstract class ScenePicture implements ui.Picture {
// This is a conservative bounding box of all the drawing primitives in this picture.
ui.Rect get cullRect;
}
abstract class SceneImageFilter implements ui.ImageFilter {
// Since some image filters affect the actual drawing bounds of a given picture, this
// gives the maximum draw boundary for a picture with the given input bounds after it
// has been processed by the filter.
ui.Rect filterBounds(ui.Rect inputBounds);
}
| engine/lib/web_ui/lib/src/engine/scene_painting.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/scene_painting.dart",
"repo_id": "engine",
"token_count": 300
} | 271 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
/// Sets the "button" ARIA role.
class Button extends PrimaryRoleManager {
Button(SemanticsObject semanticsObject) : super.withBasics(
PrimaryRole.button,
semanticsObject,
labelRepresentation: LeafLabelRepresentation.domText,
) {
addTappable();
setAriaRole('button');
}
@override
bool focusAsRouteDefault() => focusable?.focusAsRouteDefault() ?? false;
@override
void update() {
super.update();
if (semanticsObject.enabledState() == EnabledState.disabled) {
setAttribute('aria-disabled', 'true');
} else {
removeAttribute('aria-disabled');
}
}
}
/// Listens to HTML "click" gestures detected by the browser.
///
/// This gestures is different from the click and tap gestures detected by the
/// framework from raw pointer events. When an assistive technology is enabled
/// the browser may not send us pointer events. In that mode we forward HTML
/// click as [ui.SemanticsAction.tap].
class Tappable extends RoleManager {
Tappable(SemanticsObject semanticsObject, PrimaryRoleManager owner)
: super(Role.tappable, semanticsObject, owner) {
_clickListener = createDomEventListener((DomEvent click) {
PointerBinding.clickDebouncer.onClick(
click,
semanticsObject.id,
_isListening,
);
});
owner.element.addEventListener('click', _clickListener);
}
DomEventListener? _clickListener;
bool _isListening = false;
@override
void update() {
final bool wasListening = _isListening;
_isListening = semanticsObject.enabledState() != EnabledState.disabled && semanticsObject.isTappable;
if (wasListening != _isListening) {
_updateAttribute();
}
}
void _updateAttribute() {
// The `flt-tappable` attribute marks the element for the ClickDebouncer to
// to know that it should debounce click events on this element. The
// contract is that the element that has this attribute is also the element
// that receives pointer and "click" events.
if (_isListening) {
owner.element.setAttribute('flt-tappable', '');
} else {
owner.element.removeAttribute('flt-tappable');
}
}
@override
void dispose() {
owner.removeEventListener('click', _clickListener);
_clickListener = null;
super.dispose();
}
}
| engine/lib/web_ui/lib/src/engine/semantics/tappable.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/semantics/tappable.dart",
"repo_id": "engine",
"token_count": 822
} | 272 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ffi';
import 'dart:js_interop';
import 'package:ui/src/engine.dart';
class SkwasmObjectWrapper<T extends NativeType> {
SkwasmObjectWrapper(this.handle, this.registry) {
registry.register(this);
}
final SkwasmFinalizationRegistry<T> registry;
final Pointer<T> handle;
bool _isDisposed = false;
void dispose() {
assert(!_isDisposed);
registry.evict(this);
_isDisposed = true;
}
bool get debugDisposed => _isDisposed;
}
typedef DisposeFunction<T extends NativeType> = void Function(Pointer<T>);
class SkwasmFinalizationRegistry<T extends NativeType> {
SkwasmFinalizationRegistry(this.dispose)
: registry = DomFinalizationRegistry(((JSNumber address) =>
dispose(Pointer<T>.fromAddress(address.toDartDouble.toInt()))
).toJS);
final DomFinalizationRegistry registry;
final DisposeFunction<T> dispose;
void register(SkwasmObjectWrapper<T> wrapper) {
final JSAny jsWrapper = wrapper.toJSWrapper;
registry.registerWithToken(
jsWrapper, wrapper.handle.address.toJS, jsWrapper);
}
void evict(SkwasmObjectWrapper<T> wrapper) {
final JSAny jsWrapper = wrapper.toJSWrapper;
registry.unregister(jsWrapper);
dispose(wrapper.handle);
}
}
| engine/lib/web_ui/lib/src/engine/skwasm/skwasm_impl/memory.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/skwasm/skwasm_impl/memory.dart",
"repo_id": "engine",
"token_count": 487
} | 273 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@DefaultAsset('skwasm')
library skwasm_impl;
import 'dart:ffi';
import 'package:ui/src/engine/skwasm/skwasm_impl.dart';
final class RawShader extends Opaque {}
typedef ShaderHandle = Pointer<RawShader>;
final class RawRuntimeEffect extends Opaque {}
typedef RuntimeEffectHandle = Pointer<RawRuntimeEffect>;
@Native<ShaderHandle Function(
RawPointArray,
RawColorArray,
Pointer<Float>,
Int,
Int,
RawMatrix33,
)>(symbol: 'shader_createLinearGradient', isLeaf: true)
external ShaderHandle shaderCreateLinearGradient(
RawPointArray endPoints, // two points
RawColorArray colors,
Pointer<Float> stops, // Can be nullptr
int count, // Number of stops/colors
int tileMode,
RawMatrix33 matrix, // Can be nullptr
);
@Native<ShaderHandle Function(
Float,
Float,
Float,
RawColorArray,
Pointer<Float>,
Int,
Int,
RawMatrix33,
)>(symbol: 'shader_createRadialGradient', isLeaf: true)
external ShaderHandle shaderCreateRadialGradient(
double centerX,
double centerY,
double radius,
RawColorArray colors,
Pointer<Float> stops,
int count,
int tileMode,
RawMatrix33 localMatrix,
);
@Native<ShaderHandle Function(
RawPointArray,
Float,
Float,
RawColorArray,
Pointer<Float>,
Int,
Int,
RawMatrix33,
)>(symbol: 'shader_createConicalGradient', isLeaf: true)
external ShaderHandle shaderCreateConicalGradient(
RawPointArray endPoints, // Two points,
double startRadius,
double endRadius,
RawColorArray colors,
Pointer<Float> stops,
int count,
int tileMode,
RawMatrix33 localMatrix,
);
@Native<ShaderHandle Function(
Float,
Float,
RawColorArray,
Pointer<Float>,
Int,
Int,
Float,
Float,
RawMatrix33,
)>(symbol: 'shader_createSweepGradient', isLeaf: true)
external ShaderHandle shaderCreateSweepGradient(
double centerX,
double centerY,
RawColorArray colors,
Pointer<Float> stops,
int count,
int tileMode,
double startAngle,
double endAngle,
RawMatrix33 localMatrix
);
@Native<Void Function(ShaderHandle)>(symbol: 'shader_dispose', isLeaf: true)
external void shaderDispose(ShaderHandle handle);
@Native<RuntimeEffectHandle Function(SkStringHandle)>(symbol: 'runtimeEffect_create', isLeaf: true)
external RuntimeEffectHandle runtimeEffectCreate(SkStringHandle source);
@Native<Void Function(RuntimeEffectHandle)>(symbol: 'runtimeEffect_dispose', isLeaf: true)
external void runtimeEffectDispose(RuntimeEffectHandle handle);
@Native<Size Function(RuntimeEffectHandle)>(symbol: 'runtimeEffect_getUniformSize', isLeaf: true)
external int runtimeEffectGetUniformSize(RuntimeEffectHandle handle);
@Native<ShaderHandle Function(
RuntimeEffectHandle,
SkDataHandle,
Pointer<ShaderHandle>,
Size
)>(symbol: 'shader_createRuntimeEffectShader', isLeaf: true)
external ShaderHandle shaderCreateRuntimeEffectShader(
RuntimeEffectHandle runtimeEffect,
SkDataHandle uniforms,
Pointer<ShaderHandle> childShaders,
int childCount
);
@Native<ShaderHandle Function(
ImageHandle,
Int,
Int,
Int,
RawMatrix33,
)>(symbol: 'shader_createFromImage', isLeaf: true)
external ShaderHandle shaderCreateFromImage(
ImageHandle handle,
int tileModeX,
int tileModeY,
int quality,
RawMatrix33 localMatrix,
);
| engine/lib/web_ui/lib/src/engine/skwasm/skwasm_impl/raw/raw_shaders.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/skwasm/skwasm_impl/raw/raw_shaders.dart",
"repo_id": "engine",
"token_count": 1099
} | 274 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// The web_sdk/sdk_rewriter.dart uses this directive.
// ignore: unnecessary_library_directive
library skwasm_stub;
export 'skwasm_stub/dart_js_conversion.dart';
export 'skwasm_stub/renderer.dart';
| engine/lib/web_ui/lib/src/engine/skwasm/skwasm_stub.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/skwasm/skwasm_stub.dart",
"repo_id": "engine",
"token_count": 119
} | 275 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:ui/ui.dart' as ui;
import 'fragmenter.dart';
import 'unicode_range.dart';
enum FragmentFlow {
/// The fragment flows from left to right regardless of its surroundings.
ltr,
/// The fragment flows from right to left regardless of its surroundings.
rtl,
/// The fragment flows the same as the previous fragment.
///
/// If it's the first fragment in a line, then it flows the same as the
/// paragraph direction.
///
/// E.g. digits.
previous,
/// If the previous and next fragments flow in the same direction, then this
/// fragment flows in that same direction. Otherwise, it flows the same as the
/// paragraph direction.
///
/// E.g. spaces, symbols.
sandwich,
}
/// Splits [text] into fragments based on directionality.
class BidiFragmenter extends TextFragmenter {
const BidiFragmenter(super.text);
@override
List<BidiFragment> fragment() {
return _computeBidiFragments(text);
}
}
class BidiFragment extends TextFragment {
const BidiFragment(super.start, super.end, this.textDirection, this.fragmentFlow);
final ui.TextDirection? textDirection;
final FragmentFlow fragmentFlow;
@override
int get hashCode => Object.hash(start, end, textDirection, fragmentFlow);
@override
bool operator ==(Object other) {
return other is BidiFragment &&
other.start == start &&
other.end == end &&
other.textDirection == textDirection &&
other.fragmentFlow == fragmentFlow;
}
@override
String toString() {
return 'BidiFragment($start, $end, $textDirection)';
}
}
// This data was taken from the source code of the Closure library:
//
// - https://github.com/google/closure-library/blob/9d24a6c1809a671c2e54c328897ebeae15a6d172/closure/goog/i18n/bidi.js#L203-L234
final UnicodePropertyLookup<ui.TextDirection?> _textDirectionLookup = UnicodePropertyLookup<ui.TextDirection?>(
<UnicodeRange<ui.TextDirection>>[
// LTR
const UnicodeRange<ui.TextDirection>(kChar_A, kChar_Z, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(kChar_a, kChar_z, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0x00C0, 0x00D6, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0x00D8, 0x00F6, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0x00F8, 0x02B8, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0x0300, 0x0590, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0x0591, 0x06EF, ui.TextDirection.rtl),
const UnicodeRange<ui.TextDirection>(0x06FA, 0x08FF, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0x0900, 0x1FFF, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0x200E, 0x200E, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0x200F, 0x200F, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0x2C00, 0xD801, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0xD802, 0xD803, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0xD804, 0xD839, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0xD83A, 0xD83B, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0xD83C, 0xDBFF, ui.TextDirection.ltr),
const UnicodeRange<ui.TextDirection>(0xF900, 0xFB1C, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0xFB1D, 0xFDFF, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0xFE00, 0xFE6F, ui.TextDirection.ltr),
// RTL
const UnicodeRange<ui.TextDirection>(0xFE70, 0xFEFC, ui.TextDirection.rtl),
// LTR
const UnicodeRange<ui.TextDirection>(0xFEFD, 0xFFFF, ui.TextDirection.ltr),
],
null,
);
List<BidiFragment> _computeBidiFragments(String text) {
final List<BidiFragment> fragments = <BidiFragment>[];
if (text.isEmpty) {
fragments.add(const BidiFragment(0, 0, null, FragmentFlow.previous));
return fragments;
}
int fragmentStart = 0;
ui.TextDirection? textDirection = _getTextDirection(text, 0);
FragmentFlow fragmentFlow = _getFragmentFlow(text, 0);
for (int i = 1; i < text.length; i++) {
final ui.TextDirection? charTextDirection = _getTextDirection(text, i);
if (charTextDirection != textDirection) {
// We've reached the end of a text direction fragment.
fragments.add(BidiFragment(fragmentStart, i, textDirection, fragmentFlow));
fragmentStart = i;
textDirection = charTextDirection;
fragmentFlow = _getFragmentFlow(text, i);
} else {
// This code handles the case of a sequence of digits followed by a sequence
// of LTR characters with no space in between.
if (fragmentFlow == FragmentFlow.previous) {
fragmentFlow = _getFragmentFlow(text, i);
}
}
}
fragments.add(BidiFragment(fragmentStart, text.length, textDirection, fragmentFlow));
return fragments;
}
ui.TextDirection? _getTextDirection(String text, int i) {
final int codePoint = getCodePoint(text, i)!;
if (_isDigit(codePoint) || _isMashriqiDigit(codePoint)) {
// A sequence of regular digits or Mashriqi digits always goes from left to
// regardless of their fragment flow direction.
return ui.TextDirection.ltr;
}
final ui.TextDirection? textDirection = _textDirectionLookup.findForChar(codePoint);
if (textDirection != null) {
return textDirection;
}
return null;
}
FragmentFlow _getFragmentFlow(String text, int i) {
final int codePoint = getCodePoint(text, i)!;
if (_isDigit(codePoint)) {
return FragmentFlow.previous;
}
if (_isMashriqiDigit(codePoint)) {
return FragmentFlow.rtl;
}
final ui.TextDirection? textDirection = _textDirectionLookup.findForChar(codePoint);
switch (textDirection) {
case ui.TextDirection.ltr:
return FragmentFlow.ltr;
case ui.TextDirection.rtl:
return FragmentFlow.rtl;
case null:
return FragmentFlow.sandwich;
}
}
bool _isDigit(int codePoint) {
return codePoint >= kChar_0 && codePoint <= kChar_9;
}
bool _isMashriqiDigit(int codePoint) {
return codePoint >= kMashriqi_0 && codePoint <= kMashriqi_9;
}
| engine/lib/web_ui/lib/src/engine/text/text_direction.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/text/text_direction.dart",
"repo_id": "engine",
"token_count": 2394
} | 276 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:js_interop';
import 'package:meta/meta.dart';
import 'package:ui/src/engine/display.dart';
import 'package:ui/src/engine/dom.dart';
import 'package:ui/ui.dart' as ui show Display;
/// Provides a stream of `devicePixelRatio` changes for the given display.
///
/// Note that until the Window Management API is generally available, this class
/// only monitors the global `devicePixelRatio` property, provided by the default
/// [EngineFlutterDisplay.instance].
///
/// See: https://developer.mozilla.org/en-US/docs/Web/API/Window_Management_API
class DisplayDprStream {
DisplayDprStream(
this._display, {
@visibleForTesting DebugDisplayDprStreamOverrides? overrides,
}) : _currentDpr = _display.devicePixelRatio,
_debugOverrides = overrides {
// Start listening to DPR changes.
_subscribeToMediaQuery();
}
/// A singleton instance of DisplayDprStream.
static DisplayDprStream instance =
DisplayDprStream(EngineFlutterDisplay.instance);
// The display object that will provide the DPR information.
final ui.Display _display;
// Last reported value of DPR.
double _currentDpr;
// Controls the [dprChanged] broadcast Stream.
final StreamController<double> _dprStreamController =
StreamController<double>.broadcast();
// Object that fires a `change` event for the `_currentDpr`.
late DomEventTarget _dprMediaQuery;
// Creates the media query for the latest known DPR value, and adds a change listener to it.
void _subscribeToMediaQuery() {
if (_debugOverrides?.getMediaQuery != null) {
_dprMediaQuery = _debugOverrides!.getMediaQuery!(_currentDpr);
} else {
_dprMediaQuery = domWindow.matchMedia('(resolution: ${_currentDpr}dppx)');
}
_dprMediaQuery.addEventListenerWithOptions(
'change',
createDomEventListener(_onDprMediaQueryChange),
<String, Object>{
// We only listen `once` because this event only triggers once when the
// DPR changes from `_currentDpr`. Once that happens, we need a new
// `_dprMediaQuery` that is watching the new `_currentDpr`.
//
// By using `once`, we don't need to worry about detaching the event
// listener from the old mediaQuery after we're done with it.
'once': true,
'passive': true,
},
);
}
// Handler of the _dprMediaQuery 'change' event.
//
// This calls subscribe again because events are listened to with `once: true`.
JSVoid _onDprMediaQueryChange(DomEvent _) {
_currentDpr = _display.devicePixelRatio;
_dprStreamController.add(_currentDpr);
// Re-subscribe...
_subscribeToMediaQuery();
}
/// A stream that emits the latest value of [EngineFlutterDisplay.instance.devicePixelRatio].
Stream<double> get dprChanged => _dprStreamController.stream;
// The overrides object that is used for testing.
final DebugDisplayDprStreamOverrides? _debugOverrides;
}
@visibleForTesting
class DebugDisplayDprStreamOverrides {
DebugDisplayDprStreamOverrides({
this.getMediaQuery,
});
final DomEventTarget Function(double currentValue)? getMediaQuery;
}
| engine/lib/web_ui/lib/src/engine/view_embedder/display_dpr_stream.dart/0 | {
"file_path": "engine/lib/web_ui/lib/src/engine/view_embedder/display_dpr_stream.dart",
"repo_id": "engine",
"token_count": 1075
} | 277 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:js_interop';
import 'package:ui/src/engine.dart';
/// Exposes web-only functionality for this app's `FlutterView`s objects.
final FlutterViewManagerProxy views = FlutterViewManagerProxy(
viewManager: EnginePlatformDispatcher.instance.viewManager,
);
/// This class exposes web-only attributes for the registered views in a multi-view app.
class FlutterViewManagerProxy {
FlutterViewManagerProxy({
required FlutterViewManager viewManager,
}) : _viewManager = viewManager;
// The proxied viewManager instance.
final FlutterViewManager _viewManager;
/// Returns the `hostElement` configuration value passed from JS when `viewId` was added.
///
/// This is useful for plugins and apps to have a safe DOM Element where they
/// can add their own custom HTML elements (for example: file inputs for the
/// file_selector plugin).
JSAny? getHostElement(int viewId) {
return _viewManager.getOptions(viewId)?.hostElement as JSAny?;
}
/// Returns the `initialData` configuration value passed from JS when `viewId` was added.
///
/// Developers can access the initial data from Dart in two ways:
/// * Defining their own `staticInterop` class that describes what you're
/// passing to your views, to retain type safety on Dart (preferred).
/// * Calling [NullableUndefineableJSAnyExtension.dartify] and accessing the
/// returned object as if it were a [Map] (not recommended).
JSAny? getInitialData(int viewId) {
return _viewManager.getOptions(viewId)?.initialData;
}
}
| engine/lib/web_ui/lib/ui_web/src/ui_web/flutter_views_proxy.dart/0 | {
"file_path": "engine/lib/web_ui/lib/ui_web/src/ui_web/flutter_views_proxy.dart",
"repo_id": "engine",
"token_count": 479
} | 278 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "export.h"
#include "third_party/skia/include/core/SkFontMgr.h"
#include "third_party/skia/include/ports/SkFontMgr_empty.h"
#include "third_party/skia/modules/skparagraph/include/FontCollection.h"
#include "third_party/skia/modules/skparagraph/include/TypefaceFontProvider.h"
#include "wrappers.h"
#include <memory>
using namespace skia::textlayout;
using namespace Skwasm;
SKWASM_EXPORT FlutterFontCollection* fontCollection_create() {
auto collection = sk_make_sp<FontCollection>();
auto provider = sk_make_sp<TypefaceFontProvider>();
collection->enableFontFallback();
collection->setDefaultFontManager(provider, "Roboto");
return new FlutterFontCollection{
std::move(collection),
std::move(provider),
};
}
SKWASM_EXPORT void fontCollection_dispose(FlutterFontCollection* collection) {
delete collection;
}
static sk_sp<SkFontMgr> default_fontmgr() {
static sk_sp<SkFontMgr> mgr = SkFontMgr_New_Custom_Empty();
return mgr;
}
SKWASM_EXPORT SkTypeface* typeface_create(SkData* fontData) {
auto typeface = default_fontmgr()->makeFromData(sk_ref_sp<SkData>(fontData));
return typeface.release();
}
SKWASM_EXPORT void typeface_dispose(SkTypeface* typeface) {
typeface->unref();
}
// Calculates the code points that are not covered by the specified typefaces.
// This function mutates the `codePoints` buffer in place and returns the count
// of code points that are not covered by the fonts.
SKWASM_EXPORT int typefaces_filterCoveredCodePoints(SkTypeface** typefaces,
int typefaceCount,
SkUnichar* codePoints,
int codePointCount) {
std::unique_ptr<SkGlyphID[]> glyphBuffer =
std::make_unique<SkGlyphID[]>(codePointCount);
SkGlyphID* glyphPointer = glyphBuffer.get();
int remainingCodePointCount = codePointCount;
for (int typefaceIndex = 0; typefaceIndex < typefaceCount; typefaceIndex++) {
typefaces[typefaceIndex]->unicharsToGlyphs(
codePoints, remainingCodePointCount, glyphPointer);
int outputIndex = 0;
for (int inputIndex = 0; inputIndex < remainingCodePointCount;
inputIndex++) {
if (glyphPointer[inputIndex] == 0) {
if (outputIndex != inputIndex) {
codePoints[outputIndex] = codePoints[inputIndex];
}
outputIndex++;
}
}
if (outputIndex == 0) {
return 0;
} else {
remainingCodePointCount = outputIndex;
}
}
return remainingCodePointCount;
}
SKWASM_EXPORT void fontCollection_registerTypeface(
FlutterFontCollection* collection,
SkTypeface* typeface,
SkString* fontName) {
if (fontName) {
SkString alias = *fontName;
collection->provider->registerTypeface(sk_ref_sp<SkTypeface>(typeface),
alias);
} else {
collection->provider->registerTypeface(sk_ref_sp<SkTypeface>(typeface));
}
}
SKWASM_EXPORT void fontCollection_clearCaches(
FlutterFontCollection* collection) {
collection->collection->clearCaches();
}
| engine/lib/web_ui/skwasm/fonts.cpp/0 | {
"file_path": "engine/lib/web_ui/skwasm/fonts.cpp",
"repo_id": "engine",
"token_count": 1272
} | 279 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "../export.h"
#include "third_party/skia/modules/skparagraph/include/Paragraph.h"
using namespace skia::textlayout;
SKWASM_EXPORT StrutStyle* strutStyle_create() {
auto style = new StrutStyle();
style->setStrutEnabled(true);
return style;
}
SKWASM_EXPORT void strutStyle_dispose(StrutStyle* style) {
delete style;
}
SKWASM_EXPORT void strutStyle_setFontFamilies(StrutStyle* style,
SkString** fontFamilies,
int count) {
std::vector<SkString> families;
families.reserve(count);
for (int i = 0; i < count; i++) {
families.push_back(*fontFamilies[i]);
}
style->setFontFamilies(std::move(families));
}
SKWASM_EXPORT void strutStyle_setFontSize(StrutStyle* style,
SkScalar fontSize) {
style->setFontSize(fontSize);
}
SKWASM_EXPORT void strutStyle_setHeight(StrutStyle* style, SkScalar height) {
style->setHeight(height);
style->setHeightOverride(true);
}
SKWASM_EXPORT void strutStyle_setHalfLeading(StrutStyle* style,
bool halfLeading) {
style->setHalfLeading(halfLeading);
}
SKWASM_EXPORT void strutStyle_setLeading(StrutStyle* style, SkScalar leading) {
style->setLeading(leading);
}
SKWASM_EXPORT void strutStyle_setFontStyle(StrutStyle* style,
int weight,
SkFontStyle::Slant slant) {
style->setFontStyle(SkFontStyle(weight, SkFontStyle::kNormal_Width, slant));
}
SKWASM_EXPORT void strutStyle_setForceStrutHeight(StrutStyle* style,
bool forceStrutHeight) {
style->setForceStrutHeight(forceStrutHeight);
}
| engine/lib/web_ui/skwasm/text/strut_style.cpp/0 | {
"file_path": "engine/lib/web_ui/skwasm/text/strut_style.cpp",
"repo_id": "engine",
"token_count": 880
} | 280 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:js_interop';
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'common.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
void testMain() {
group('CanvasKit', () {
setUpCanvasKitTest();
setUp(() async {
EngineFlutterDisplay.instance.debugOverrideDevicePixelRatio(1.0);
});
Future<DomImageBitmap> newBitmap(int width, int height) async {
return createImageBitmap(
createBlankDomImageData(width, height) as JSAny, (
x: 0,
y: 0,
width: width,
height: height,
));
}
// Regression test for https://github.com/flutter/flutter/issues/75286
test('updates canvas logical size when device-pixel ratio changes',
() async {
final RenderCanvas canvas = RenderCanvas();
canvas.render(await newBitmap(10, 16));
expect(canvas.canvasElement.width, 10);
expect(canvas.canvasElement.height, 16);
expect(canvas.canvasElement.style.width, '10px');
expect(canvas.canvasElement.style.height, '16px');
// Increase device-pixel ratio: this makes CSS pixels bigger, so we need
// fewer of them to cover the browser window.
EngineFlutterDisplay.instance.debugOverrideDevicePixelRatio(2.0);
canvas.render(await newBitmap(10, 16));
expect(canvas.canvasElement.width, 10);
expect(canvas.canvasElement.height, 16);
expect(canvas.canvasElement.style.width, '5px');
expect(canvas.canvasElement.style.height, '8px');
// Decrease device-pixel ratio: this makes CSS pixels smaller, so we need
// more of them to cover the browser window.
EngineFlutterDisplay.instance.debugOverrideDevicePixelRatio(0.5);
canvas.render(await newBitmap(10, 16));
expect(canvas.canvasElement.width, 10);
expect(canvas.canvasElement.height, 16);
expect(canvas.canvasElement.style.width, '20px');
expect(canvas.canvasElement.style.height, '32px');
});
});
}
| engine/lib/web_ui/test/canvaskit/render_canvas_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/canvaskit/render_canvas_test.dart",
"repo_id": "engine",
"token_count": 829
} | 281 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
// The scene that will be rendered in the next call to `onDrawFrame`.
ui.Scene? _sceneToRender;
// Completer that will complete when the call to render completes.
Completer<void>? _sceneCompleter;
/// Sets up rendering so that `onDrawFrame` will render the last requested
/// scene.
void setUpRenderingForTests() {
// Set `onDrawFrame` to call `renderer.renderScene`.
EnginePlatformDispatcher.instance.onDrawFrame = () {
if (_sceneToRender != null) {
EnginePlatformDispatcher.instance.render(_sceneToRender!).then<void>((_) {
_sceneCompleter?.complete();
}).catchError((Object error) {
_sceneCompleter?.completeError(error);
});
_sceneToRender = null;
}
};
}
/// Render the given [scene] in an `onDrawFrame` scope.
Future<void> renderScene(ui.Scene scene) {
_sceneToRender = scene;
_sceneCompleter = Completer<void>();
EnginePlatformDispatcher.instance.invokeOnDrawFrame();
return _sceneCompleter!.future;
}
| engine/lib/web_ui/test/common/rendering.dart/0 | {
"file_path": "engine/lib/web_ui/test/common/rendering.dart",
"repo_id": "engine",
"token_count": 416
} | 282 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
import 'package:ui/ui_web/src/ui_web.dart' as ui_web;
void main() {
internalBootstrapBrowserTest(() => testMain);
}
Future<void> testMain() async {
String? getCssThemeColor() {
final DomHTMLMetaElement? theme =
domDocument.querySelector('#flutterweb-theme') as DomHTMLMetaElement?;
return theme?.content;
}
const MethodCodec codec = JSONMethodCodec();
group('Title and Primary Color/Theme meta', () {
test('is set on the document by platform message', () {
// Run the unit test without emulating Flutter tester environment.
ui_web.debugEmulateFlutterTesterEnvironment = false;
expect(domDocument.title, '');
expect(getCssThemeColor(), isNull);
ui.PlatformDispatcher.instance.sendPlatformMessage(
'flutter/platform',
codec.encodeMethodCall(const MethodCall(
'SystemChrome.setApplicationSwitcherDescription',
<String, dynamic>{
'label': 'Title Test',
'primaryColor': 0xFF00FF00,
},
)),
null,
);
const ui.Color expectedPrimaryColor = ui.Color(0xFF00FF00);
expect(domDocument.title, 'Title Test');
expect(getCssThemeColor(), expectedPrimaryColor.toCssString());
ui.PlatformDispatcher.instance.sendPlatformMessage(
'flutter/platform',
codec.encodeMethodCall(const MethodCall(
'SystemChrome.setApplicationSwitcherDescription',
<String, dynamic>{
'label': 'Different title',
'primaryColor': 0xFFFABADA,
},
)),
null,
);
const ui.Color expectedNewPrimaryColor = ui.Color(0xFFFABADA);
expect(domDocument.title, 'Different title');
expect(getCssThemeColor(), expectedNewPrimaryColor.toCssString());
});
test('supports null title and primaryColor', () {
// Run the unit test without emulating Flutter tester environment.
ui_web.debugEmulateFlutterTesterEnvironment = false;
const ui.Color expectedNullColor = ui.Color(0xFF000000);
// TODO(yjbanov): https://github.com/flutter/flutter/issues/39159
domDocument.title = 'Something Else';
expect(domDocument.title, 'Something Else');
ui.PlatformDispatcher.instance.sendPlatformMessage(
'flutter/platform',
codec.encodeMethodCall(const MethodCall(
'SystemChrome.setApplicationSwitcherDescription',
<String, dynamic>{
'label': null,
'primaryColor': null,
},
)),
null,
);
expect(domDocument.title, '');
expect(getCssThemeColor(), expectedNullColor.toCssString());
domDocument.title = 'Something Else';
expect(domDocument.title, 'Something Else');
ui.PlatformDispatcher.instance.sendPlatformMessage(
'flutter/platform',
codec.encodeMethodCall(const MethodCall(
'SystemChrome.setApplicationSwitcherDescription',
<String, dynamic>{},
)),
null,
);
expect(domDocument.title, '');
expect(getCssThemeColor(), expectedNullColor.toCssString());
});
});
}
| engine/lib/web_ui/test/engine/platform_dispatcher/application_switcher_description_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/engine/platform_dispatcher/application_switcher_description_test.dart",
"repo_id": "engine",
"token_count": 1366
} | 283 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:typed_data';
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
const StandardMessageCodec codec = StandardMessageCodec();
void main() {
internalBootstrapBrowserTest(() => testMain);
}
void testMain() {
late AccessibilityAnnouncements accessibilityAnnouncements;
setUp(() {
final DomElement announcementsHost = createDomElement('flt-announcement-host');
accessibilityAnnouncements = AccessibilityAnnouncements(hostElement: announcementsHost);
setLiveMessageDurationForTest(const Duration(milliseconds: 10));
expect(
announcementsHost.querySelector('flt-announcement-polite'),
accessibilityAnnouncements.ariaLiveElementFor(Assertiveness.polite),
);
expect(
announcementsHost.querySelector('flt-announcement-assertive'),
accessibilityAnnouncements.ariaLiveElementFor(Assertiveness.assertive),
);
});
tearDown(() async {
await Future<void>.delayed(liveMessageDuration * 2);
});
group('$AccessibilityAnnouncements', () {
ByteData? encodeMessageOnly({required String message}) {
return codec.encodeMessage(<dynamic, dynamic>{
'data': <dynamic, dynamic>{'message': message},
});
}
void sendAnnouncementMessage({required String message, int? assertiveness}) {
accessibilityAnnouncements.handleMessage(codec, codec.encodeMessage(<dynamic, dynamic>{
'data': <dynamic, dynamic>{
'message': message,
'assertiveness': assertiveness,
},
}));
}
void expectMessages({String polite = '', String assertive = ''}) {
expect(accessibilityAnnouncements.ariaLiveElementFor(Assertiveness.polite).text, polite);
expect(accessibilityAnnouncements.ariaLiveElementFor(Assertiveness.assertive).text, assertive);
}
void expectNoMessages() => expectMessages();
test('Default value of aria-live is polite when assertiveness is not specified', () async {
accessibilityAnnouncements.handleMessage(codec, encodeMessageOnly(message: 'polite message'));
expectMessages(polite: 'polite message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('aria-live is assertive when assertiveness is set to 1', () async {
sendAnnouncementMessage(message: 'assertive message', assertiveness: 1);
expectMessages(assertive: 'assertive message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('aria-live is polite when assertiveness is null', () async {
sendAnnouncementMessage(message: 'polite message');
expectMessages(polite: 'polite message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('aria-live is polite when assertiveness is set to 0', () async {
sendAnnouncementMessage(message: 'polite message', assertiveness: 0);
expectMessages(polite: 'polite message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('Rapid-fire messages are each announced', () async {
sendAnnouncementMessage(message: 'Hello');
expectMessages(polite: 'Hello');
await Future<void>.delayed(liveMessageDuration * 0.5);
sendAnnouncementMessage(message: 'There');
expectMessages(polite: 'HelloThere\u00A0');
await Future<void>.delayed(liveMessageDuration * 0.6);
expectMessages(polite: 'There\u00A0');
await Future<void>.delayed(liveMessageDuration * 0.5);
expectNoMessages();
});
test('Repeated announcements are modified to ensure screen readers announce them', () async {
sendAnnouncementMessage(message: 'Hello');
expectMessages(polite: 'Hello');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
sendAnnouncementMessage(message: 'Hello');
expectMessages(polite: 'Hello\u00A0');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
sendAnnouncementMessage(message: 'Hello');
expectMessages(polite: 'Hello');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('announce() polite', () async {
accessibilityAnnouncements.announce('polite message', Assertiveness.polite);
expectMessages(polite: 'polite message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
test('announce() assertive', () async {
accessibilityAnnouncements.announce('assertive message', Assertiveness.assertive);
expectMessages(assertive: 'assertive message');
await Future<void>.delayed(liveMessageDuration);
expectNoMessages();
});
});
}
| engine/lib/web_ui/test/engine/semantics/semantics_announcement_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/engine/semantics/semantics_announcement_test.dart",
"repo_id": "engine",
"token_count": 1677
} | 284 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine/html/shaders/normalized_gradient.dart';
import 'package:ui/ui.dart' as ui hide window;
void main() {
internalBootstrapBrowserTest(() => testMain);
}
void testMain() {
group('Shader Normalized Gradient', () {
test('3 stop at start', () {
final NormalizedGradient gradient = NormalizedGradient(const <ui.Color>[
ui.Color(0xFF000000), ui.Color(0xFFFF7f3f)
], stops: <double>[0.0, 0.5]);
int res = _computeColorAt(gradient, 0.0);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.25);
assert(res == 0xFF7f3f1f);
res = _computeColorAt(gradient, 0.5);
assert(res == 0xFFFF7f3f);
res = _computeColorAt(gradient, 0.7);
assert(res == 0xFFFF7f3f);
res = _computeColorAt(gradient, 1.0);
assert(res == 0xFFFF7f3f);
});
test('3 stop at end', () {
final NormalizedGradient gradient = NormalizedGradient(const <ui.Color>[
ui.Color(0xFF000000), ui.Color(0xFFFF7f3f)
], stops: <double>[0.5, 1.0]);
int res = _computeColorAt(gradient, 0.0);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.25);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.5);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.75);
assert(res == 0xFF7f3f1f);
res = _computeColorAt(gradient, 1.0);
assert(res == 0xFFFF7f3f);
});
test('4 stop', () {
final NormalizedGradient gradient = NormalizedGradient(const <ui.Color>[
ui.Color(0xFF000000), ui.Color(0xFFFF7f3f)
], stops: <double>[0.25, 0.5]);
int res = _computeColorAt(gradient, 0.0);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.25);
assert(res == 0xFF000000);
res = _computeColorAt(gradient, 0.4);
assert(res == 0xFF994c25);
res = _computeColorAt(gradient, 0.5);
assert(res == 0xFFFF7f3f);
res = _computeColorAt(gradient, 0.75);
assert(res == 0xFFFF7f3f);
res = _computeColorAt(gradient, 1.0);
assert(res == 0xFFFF7f3f);
});
test('5 stop', () {
final NormalizedGradient gradient = NormalizedGradient(const <ui.Color>[
ui.Color(0x10000000), ui.Color(0x20FF0000),
ui.Color(0x4000FF00), ui.Color(0x800000FF),
ui.Color(0xFFFFFFFF)
], stops: <double>[0.0, 0.1, 0.2, 0.5, 1.0]);
int res = _computeColorAt(gradient, 0.0);
assert(res == 0x10000000);
res = _computeColorAt(gradient, 0.05);
assert(res == 0x187f0000);
res = _computeColorAt(gradient, 0.1);
assert(res == 0x20ff0000);
res = _computeColorAt(gradient, 0.15);
assert(res == 0x307f7f00);
res = _computeColorAt(gradient, 0.2);
assert(res == 0x4000ff00);
res = _computeColorAt(gradient, 0.4);
assert(res == 0x6a0054a9);
res = _computeColorAt(gradient, 0.5);
assert(res == 0x800000fe);
res = _computeColorAt(gradient, 0.9);
assert(res == 0xe5ccccff);
res = _computeColorAt(gradient, 1.0);
assert(res == 0xffffffff);
});
test('2 stops at ends', () {
final NormalizedGradient gradient = NormalizedGradient(const <ui.Color>[
ui.Color(0x00000000), ui.Color(0xFFFFFFFF)
]);
int res = _computeColorAt(gradient, 0.0);
assert(res == 0);
res = _computeColorAt(gradient, 1.0);
assert(res == 0xFFFFFFFF);
res = _computeColorAt(gradient, 0.5);
assert(res == 0x7f7f7f7f);
});
});
}
int _computeColorAt(NormalizedGradient gradient, double t) {
int i = 0;
while (t > gradient.thresholdAt(i + 1)) {
++i;
}
final double r = t * gradient.scaleAt(i * 4) + gradient.biasAt(i * 4);
final double g = t * gradient.scaleAt(i * 4 + 1) + gradient.biasAt(i * 4 + 1);
final double b = t * gradient.scaleAt(i * 4 + 2) + gradient.biasAt(i * 4 + 2);
final double a = t * gradient.scaleAt(i * 4 + 3) + gradient.biasAt(i * 4 + 3);
int val = 0;
val |= (a * 0xFF).toInt() & 0xFF;
val<<=8;
val |= (r * 0xFF).toInt() & 0xFF;
val<<=8;
val |= (g * 0xFF).toInt() & 0xFF;
val<<=8;
val |= (b * 0xFF).toInt() & 0xFF;
return val;
}
| engine/lib/web_ui/test/engine/surface/shaders/normalized_gradient_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/engine/surface/shaders/normalized_gradient_test.dart",
"repo_id": "engine",
"token_count": 1986
} | 285 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:js_interop';
import 'dart:math' as math;
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui_web/src/ui_web.dart';
void main() {
internalBootstrapBrowserTest(() => doTests);
}
Future<void> doTests() async {
group('FlutterViewManagerProxy', () {
final EnginePlatformDispatcher platformDispatcher =
EnginePlatformDispatcher.instance;
final FlutterViewManager viewManager =
FlutterViewManager(platformDispatcher);
final FlutterViewManagerProxy views =
FlutterViewManagerProxy(viewManager: viewManager);
late EngineFlutterView view;
late int viewId;
late DomElement hostElement;
int registerViewWithOptions(Map<String, Object?> options) {
final JsFlutterViewOptions jsOptions =
options.toJSAnyDeep as JsFlutterViewOptions;
viewManager.registerView(view, jsViewOptions: jsOptions);
return viewId;
}
setUp(() {
view = EngineFlutterView(platformDispatcher, createDomElement('div'));
viewId = view.viewId;
hostElement = createDomElement('div');
});
tearDown(() {
viewManager.unregisterView(viewId);
});
group('getHostElement', () {
test('null when viewId is unknown', () {
final JSAny? element = views.getHostElement(33930);
expect(element, isNull);
});
test('can retrieve hostElement for a known view', () {
final int viewId = registerViewWithOptions(<String, Object?>{
'hostElement': hostElement,
});
final JSAny? element = views.getHostElement(viewId);
expect(element, hostElement);
});
});
group('getInitialData', () {
test('null when viewId is unknown', () {
final JSAny? element = views.getInitialData(33930);
expect(element, isNull);
});
test('can retrieve initialData for a known view', () {
final int viewId = registerViewWithOptions(<String, Object?>{
'hostElement': hostElement,
'initialData': <String, Object?>{
'someInt': 42,
'someString': 'A String',
'decimals': <double>[math.pi, math.e],
},
});
final InitialData? element =
views.getInitialData(viewId) as InitialData?;
expect(element, isNotNull);
expect(element!.someInt, 42);
expect(element.someString, 'A String');
expect(element.decimals, <double>[math.pi, math.e]);
});
});
});
}
// The JS-interop definition of the `initialData` object passed to the views of this app.
@JS()
@staticInterop
class InitialData {}
/// The attributes of the [InitialData] object.
extension InitialDataExtension on InitialData {
external int get someInt;
external String? get someString;
@JS('decimals')
external JSArray<JSNumber> get _decimals;
List<double> get decimals =>
_decimals.toDart.map((JSNumber e) => e.toDartDouble).toList();
}
| engine/lib/web_ui/test/engine/view_embedder/flutter_views_proxy_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/engine/view_embedder/flutter_views_proxy_test.dart",
"repo_id": "engine",
"token_count": 1218
} | 286 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' hide TextStyle;
import '../screenshot.dart';
import '../testimage.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
SurfacePaint makePaint() => Paint() as SurfacePaint;
Future<void> testMain() async {
const double screenWidth = 100.0;
const double screenHeight = 100.0;
const Rect region = Rect.fromLTWH(0, 0, screenWidth, screenHeight);
// Regression test for https://github.com/flutter/flutter/issues/76966
test('Draws image with dstATop color filter', () async {
final RecordingCanvas canvas = RecordingCanvas(region);
canvas.drawImage(
createFlutterLogoTestImage(),
const Offset(10, 10),
makePaint()
..colorFilter =
const EngineColorFilter.mode(Color(0x40000000), BlendMode.dstATop));
await canvasScreenshot(canvas, 'image_color_fiter_dstatop', region: region);
});
test('Draws image with matrix color filter', () async {
final RecordingCanvas canvas = RecordingCanvas(region);
canvas.drawImage(
createFlutterLogoTestImage(),
const Offset(10, 10),
makePaint()
..colorFilter = const EngineColorFilter.matrix(<double>[
0.2126, 0.7152, 0.0722, 0, 0, //
0.2126, 0.7152, 0.0722, 0, 0, //
0.2126, 0.7152, 0.0722, 0, 0, //
0, 0, 0, 1, 0, //
]));
await canvasScreenshot(canvas, 'image_matrix_color_fiter', region: region);
});
}
| engine/lib/web_ui/test/html/compositing/canvas_image_filter_golden_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/html/compositing/canvas_image_filter_golden_test.dart",
"repo_id": "engine",
"token_count": 675
} | 287 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart';
import 'package:web_engine_tester/golden_tester.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
Future<void> testMain() async {
test('rect stroke with clip', () async {
const Rect region = Rect.fromLTWH(0, 0, 250, 250);
// Set `hasParagraphs` to true to force DOM rendering.
final BitmapCanvas canvas =
BitmapCanvas(region, RenderStrategy()..hasParagraphs = true);
const Rect rect = Rect.fromLTWH(0, 0, 150, 150);
canvas.clipRect(rect.inflate(10.0), ClipOp.intersect);
canvas.drawRect(
rect,
SurfacePaintData()
..color = 0x6fff0000
..strokeWidth = 20.0
..style = PaintingStyle.stroke,
);
canvas.drawRect(
rect,
SurfacePaintData()
..color = 0x6f0000ff
..strokeWidth = 10.0
..style = PaintingStyle.stroke,
);
canvas.drawRect(
rect,
SurfacePaintData()
..color = 0xff000000
..strokeWidth = 1.0
..style = PaintingStyle.stroke,
);
domDocument.body!.style.margin = '0px';
domDocument.body!.append(canvas.rootElement);
await matchGoldenFile('rect_clip_strokes_dom.png', region: region);
canvas.rootElement.remove();
});
test('rrect stroke with clip', () async {
const Rect region = Rect.fromLTWH(0, 0, 250, 250);
// Set `hasParagraphs` to true to force DOM rendering.
final BitmapCanvas canvas =
BitmapCanvas(region, RenderStrategy()..hasParagraphs = true);
final RRect rrect = RRect.fromRectAndRadius(
const Rect.fromLTWH(0, 0, 150, 150),
const Radius.circular(20),
);
canvas.clipRect(rrect.outerRect.inflate(10.0), ClipOp.intersect);
canvas.drawRRect(
rrect,
SurfacePaintData()
..color = 0x6fff0000
..strokeWidth = 20.0
..style = PaintingStyle.stroke,
);
canvas.drawRRect(
rrect,
SurfacePaintData()
..color = 0x6f0000ff
..strokeWidth = 10.0
..style = PaintingStyle.stroke,
);
canvas.drawRRect(
rrect,
SurfacePaintData()
..color = 0xff000000
..strokeWidth = 1.0
..style = PaintingStyle.stroke,
);
domDocument.body!.style.margin = '0px';
domDocument.body!.append(canvas.rootElement);
await matchGoldenFile('rrect_clip_strokes_dom.png', region: region);
canvas.rootElement.remove();
});
test('circle stroke with clip', () async {
const Rect region = Rect.fromLTWH(0, 0, 250, 250);
// Set `hasParagraphs` to true to force DOM rendering.
final BitmapCanvas canvas =
BitmapCanvas(region, RenderStrategy()..hasParagraphs = true);
const Rect rect = Rect.fromLTWH(0, 0, 150, 150);
canvas.clipRect(rect.inflate(10.0), ClipOp.intersect);
canvas.drawCircle(
rect.center,
rect.width / 2,
SurfacePaintData()
..color = 0x6fff0000
..strokeWidth = 20.0
..style = PaintingStyle.stroke,
);
canvas.drawCircle(
rect.center,
rect.width / 2,
SurfacePaintData()
..color = 0x6f0000ff
..strokeWidth = 10.0
..style = PaintingStyle.stroke,
);
canvas.drawCircle(
rect.center,
rect.width / 2,
SurfacePaintData()
..color = 0xff000000
..strokeWidth = 1.0
..style = PaintingStyle.stroke,
);
domDocument.body!.style.margin = '0px';
domDocument.body!.append(canvas.rootElement);
await matchGoldenFile('circle_clip_strokes_dom.png', region: region);
canvas.rootElement.remove();
});
}
| engine/lib/web_ui/test/html/drawing/dom_clip_stroke_golden_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/html/drawing/dom_clip_stroke_golden_test.dart",
"repo_id": "engine",
"token_count": 1592
} | 288 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:js_util' as js_util;
import 'dart:typed_data';
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' hide window;
import '../common/matchers.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
void testMain() {
group('Path', () {
test('Should have no subpaths when created', () {
final SurfacePath path = SurfacePath();
expect(path.isEmpty, isTrue);
});
test('LineTo should add command', () {
final SurfacePath path = SurfacePath();
path.moveTo(5.0, 10.0);
path.lineTo(20.0, 40.0);
path.lineTo(30.0, 50.0);
expect(path.pathRef.countPoints(), 3);
expect(path.pathRef.atPoint(2).dx, 30.0);
expect(path.pathRef.atPoint(2).dy, 50.0);
});
test('LineTo should add moveTo 0,0 when first call to Path API', () {
final SurfacePath path = SurfacePath();
path.lineTo(20.0, 40.0);
expect(path.pathRef.countPoints(), 2);
expect(path.pathRef.atPoint(0).dx, 0);
expect(path.pathRef.atPoint(0).dy, 0);
expect(path.pathRef.atPoint(1).dx, 20.0);
expect(path.pathRef.atPoint(1).dy, 40.0);
});
test('relativeLineTo should increments currentX', () {
final SurfacePath path = SurfacePath();
path.moveTo(5.0, 10.0);
path.lineTo(20.0, 40.0);
path.relativeLineTo(5.0, 5.0);
expect(path.pathRef.countPoints(), 3);
expect(path.pathRef.atPoint(2).dx, 25.0);
expect(path.pathRef.atPoint(2).dy, 45.0);
});
test('Should allow calling relativeLineTo before moveTo', () {
final SurfacePath path = SurfacePath();
path.relativeLineTo(5.0, 5.0);
path.moveTo(5.0, 10.0);
expect(path.pathRef.countPoints(), 3);
expect(path.pathRef.atPoint(1).dx, 5.0);
expect(path.pathRef.atPoint(1).dy, 5.0);
expect(path.pathRef.atPoint(2).dx, 5.0);
expect(path.pathRef.atPoint(2).dy, 10.0);
});
test('Should allow relativeLineTo after reset', () {
final SurfacePath path = SurfacePath();
final Path subPath = Path();
subPath.moveTo(50.0, 60.0);
subPath.lineTo(200.0, 200.0);
path.extendWithPath(subPath, Offset.zero);
path.reset();
path.relativeLineTo(5.0, 5.0);
expect(path.pathRef.countPoints(), 2);
expect(path.pathRef.atPoint(0).dx, 0);
expect(path.pathRef.atPoint(0).dy, 0);
expect(path.pathRef.atPoint(1).dx, 5.0);
});
test('Should detect rectangular path', () {
final SurfacePath path = SurfacePath();
path.addRect(const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0));
expect(path.toRect(), const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0));
});
test('Should detect horizontal line path', () {
SurfacePath path = SurfacePath();
path.moveTo(10, 20);
path.lineTo(100, 0);
expect(path.toStraightLine(), null);
path = SurfacePath();
path.moveTo(10, 20);
path.lineTo(200, 20);
final Rect r = path.toStraightLine()!;
expect(r, equals(const Rect.fromLTRB(10, 20, 200, 20)));
});
test('Should detect vertical line path', () {
final SurfacePath path = SurfacePath();
path.moveTo(10, 20);
path.lineTo(10, 200);
final Rect r = path.toStraightLine()!;
expect(r, equals(const Rect.fromLTRB(10, 20, 10, 200)));
});
test('Should detect non rectangular path if empty', () {
final SurfacePath path = SurfacePath();
expect(path.toRect(), null);
});
test('Should detect non rectangular path if there are multiple subpaths',
() {
final SurfacePath path = SurfacePath();
path.addRect(const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0));
path.addRect(const Rect.fromLTWH(5.0, 6.0, 7.0, 8.0));
expect(path.toRect(), null);
});
test('Should detect rounded rectangular path', () {
final SurfacePath path = SurfacePath();
path.addRRect(RRect.fromRectAndRadius(
const Rect.fromLTRB(1.0, 2.0, 30.0, 40.0),
const Radius.circular(2.0)));
expect(
path.toRoundedRect(),
RRect.fromRectAndRadius(const Rect.fromLTRB(1.0, 2.0, 30.0, 40.0),
const Radius.circular(2.0)));
});
test('Should detect non rounded rectangular path if empty', () {
final SurfacePath path = SurfacePath();
expect(path.toRoundedRect(), null);
});
test('Should detect rectangular path is not round', () {
final SurfacePath path = SurfacePath();
path.addRect(const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0));
expect(path.toRoundedRect(), null);
});
test(
'Should detect non rounded rectangular path if there are '
'multiple subpaths', () {
final SurfacePath path = SurfacePath();
path.addRRect(RRect.fromRectAndRadius(
const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0), const Radius.circular(2.0)));
path.addRRect(RRect.fromRectAndRadius(
const Rect.fromLTWH(1.0, 2.0, 3.0, 4.0), const Radius.circular(2.0)));
expect(path.toRoundedRect(), null);
});
test('Should compute bounds as empty for empty and moveTo only path', () {
final Path emptyPath = Path();
expect(emptyPath.getBounds(), Rect.zero);
final SurfacePath path = SurfacePath();
path.moveTo(50, 60);
expect(path.getBounds(), const Rect.fromLTRB(50, 60, 50, 60));
});
test('Should compute bounds for multiple addRect calls', () {
final Path emptyPath = Path();
expect(emptyPath.getBounds(), Rect.zero);
final SurfacePath path = SurfacePath();
path.addRect(const Rect.fromLTWH(0, 0, 270, 45));
path.addRect(const Rect.fromLTWH(134.5, 0, 1, 45));
expect(path.getBounds(), const Rect.fromLTRB(0, 0, 270, 45));
});
test('Should compute bounds for addRRect', () {
SurfacePath path = SurfacePath();
const Rect bounds = Rect.fromLTRB(30, 40, 400, 300);
RRect rrect = RRect.fromRectAndCorners(bounds,
topLeft: const Radius.elliptical(1, 2),
topRight: const Radius.elliptical(3, 4),
bottomLeft: const Radius.elliptical(5, 6),
bottomRight: const Radius.elliptical(7, 8));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
path = SurfacePath();
rrect = RRect.fromRectAndCorners(bounds,
topLeft: const Radius.elliptical(0, 2),
topRight: const Radius.elliptical(3, 4),
bottomLeft: const Radius.elliptical(5, 6),
bottomRight: const Radius.elliptical(7, 8));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
path = SurfacePath();
rrect = RRect.fromRectAndCorners(bounds,
topRight: const Radius.elliptical(3, 4),
bottomLeft: const Radius.elliptical(5, 6),
bottomRight: const Radius.elliptical(7, 8));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
path = SurfacePath();
rrect = RRect.fromRectAndCorners(bounds,
topLeft: const Radius.elliptical(1, 2),
bottomLeft: const Radius.elliptical(5, 6),
bottomRight: const Radius.elliptical(7, 8));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
path = SurfacePath();
rrect = RRect.fromRectAndCorners(bounds,
topLeft: const Radius.elliptical(1, 2),
topRight: const Radius.elliptical(3, 4),
bottomRight: const Radius.elliptical(7, 8));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
path = SurfacePath();
rrect = RRect.fromRectAndCorners(bounds,
topLeft: const Radius.elliptical(1, 2),
topRight: const Radius.elliptical(3, 4),
bottomLeft: const Radius.elliptical(5, 6));
path.addRRect(rrect);
expect(path.getBounds(), bounds);
expect(path.toRoundedRect(), rrect);
});
test('Should compute bounds for lines', () {
final SurfacePath path = SurfacePath();
path.moveTo(25, 30);
path.lineTo(100, 200);
expect(path.getBounds(), const Rect.fromLTRB(25, 30, 100, 200));
final SurfacePath path2 = SurfacePath();
path2.moveTo(250, 300);
path2.lineTo(50, 60);
expect(path2.getBounds(), const Rect.fromLTRB(50, 60, 250, 300));
});
test('Should compute bounds for polygon', () {
final SurfacePath path = SurfacePath();
path.addPolygon(const <Offset>[
Offset(50, 100),
Offset(250, 100),
Offset(152, 180),
Offset(159, 200),
Offset(151, 190)
], true);
expect(path.getBounds(), const Rect.fromLTRB(50, 100, 250, 200));
});
test('Should compute bounds for quadraticBezierTo', () {
final SurfacePath path1 = SurfacePath();
path1.moveTo(285.2, 682.1);
path1.quadraticBezierTo(432.0, 431.4, 594.9, 681.2);
expect(
path1.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(285.2, 556.5, 594.9, 682.1)));
// Control point below start , end.
final SurfacePath path2 = SurfacePath();
path2.moveTo(285.2, 682.1);
path2.quadraticBezierTo(447.4, 946.8, 594.9, 681.2);
expect(
path2.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(285.2, 681.2, 594.9, 814.2)));
// Control point to the right of end point.
final SurfacePath path3 = SurfacePath();
path3.moveTo(468.3, 685.6);
path3.quadraticBezierTo(644.7, 555.2, 594.9, 681.2);
expect(
path3.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(468.3, 619.3, 605.9, 685.6)));
});
test('Should compute bounds for cubicTo', () {
final SurfacePath path1 = SurfacePath();
path1.moveTo(220, 300);
path1.cubicTo(230, 120, 400, 125, 410, 280);
expect(
path1.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 164.3, 410.0, 300.0)));
// control point 1 to the right of control point 2
final SurfacePath path2 = SurfacePath();
path2.moveTo(220, 300);
path2.cubicTo(564.2, 13.7, 400.0, 125.0, 410.0, 280.0);
expect(
path2.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 122.8, 440.5, 300.0)));
// control point 1 to the right of control point 2 inflection
final SurfacePath path3 = SurfacePath();
path3.moveTo(220, 300);
path3.cubicTo(839.8, 67.9, 400.0, 125.0, 410.0, 280.0);
expect(
path3.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 144.5, 552.1, 300.0)));
// control point 1 below and between start and end points
final SurfacePath path4 = SurfacePath();
path4.moveTo(220.0, 300.0);
path4.cubicTo(354.8, 388.3, 400.0, 125.0, 410.0, 280.0);
expect(
path4.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 230.0, 410.0, 318.6)));
// control points inverted below
final SurfacePath path5 = SurfacePath();
path5.moveTo(220.0, 300.0);
path5.cubicTo(366.5, 487.3, 256.4, 489.9, 410.0, 280.0);
expect(
path5.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 280.0, 410.0, 439.0)));
// control points inverted below wide
final SurfacePath path6 = SurfacePath();
path6.moveTo(220.0, 300.0);
path6.cubicTo(496.1, 485.5, 121.4, 491.6, 410.0, 280.0);
expect(
path6.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 280.0, 410.0, 439.0)));
// control point 2 and end point swapped
final SurfacePath path7 = SurfacePath();
path7.moveTo(220.0, 300.0);
path7.cubicTo(230.0, 120.0, 394.5, 296.1, 382.3, 124.1);
expect(
path7.getBounds(),
within<Rect>(
distance: 0.1,
from: const Rect.fromLTRB(220.0, 124.1, 382.9, 300.0)));
});
// Regression test for https://github.com/flutter/flutter/issues/46813.
test('Should deep copy path', () {
final SurfacePath path = SurfacePath();
path.moveTo(25, 30);
path.lineTo(100, 200);
expect(path.getBounds(), const Rect.fromLTRB(25, 30, 100, 200));
final SurfacePath path2 = SurfacePath.from(path);
path2.lineTo(250, 300);
expect(path2.getBounds(), const Rect.fromLTRB(25, 30, 250, 300));
// Expect original path to stay the same.
expect(path.getBounds(), const Rect.fromLTRB(25, 30, 100, 200));
});
test('Should handle contains inclusive right,bottom coordinates', () {
final Path path = Path();
path.moveTo(50, 60);
path.lineTo(110, 60);
path.lineTo(110, 190);
path.lineTo(50, 190);
path.close();
expect(path.contains(const Offset(80, 190)), isTrue);
expect(path.contains(const Offset(110, 80)), isTrue);
expect(path.contains(const Offset(110, 190)), isTrue);
expect(path.contains(const Offset(110, 191)), isFalse);
});
test('Should not contain top-left of beveled border', () {
final Path path = Path();
path.moveTo(10, 25);
path.lineTo(15, 20);
path.lineTo(25, 20);
path.lineTo(30, 25);
path.lineTo(30, 35);
path.lineTo(25, 40);
path.lineTo(15, 40);
path.lineTo(10, 35);
path.close();
expect(path.contains(const Offset(10, 20)), isFalse);
});
test('Computes contains for cubic curves', () {
final Path path = Path();
path.moveTo(10, 25);
path.cubicTo(10, 20, 10, 20, 20, 15);
path.lineTo(25, 20);
path.cubicTo(30, 20, 30, 20, 30, 25);
path.lineTo(30, 35);
path.cubicTo(30, 40, 30, 40, 25, 40);
path.lineTo(15, 40);
path.cubicTo(10, 40, 10, 40, 10, 35);
path.close();
expect(path.contains(const Offset(10, 20)), isFalse);
expect(path.contains(const Offset(30, 40)), isFalse);
});
// Regression test for https://github.com/flutter/flutter/issues/44470
test('Should handle contains for devicepixelratio != 1.0', () {
js_util.setProperty(domWindow, 'devicePixelRatio', 4.0);
EngineFlutterDisplay.instance.debugOverrideDevicePixelRatio(4.0);
final Path path = Path()
..moveTo(50, 0)
..lineTo(100, 100)
..lineTo(0, 100)
..lineTo(50, 0)
..close();
expect(path.contains(const Offset(50, 50)), isTrue);
js_util.setProperty(domWindow, 'devicePixelRatio', 1.0);
EngineFlutterDisplay.instance.debugOverrideDevicePixelRatio(1.0);
// TODO(ferhat): Investigate failure on CI. Locally this passes.
// [Exception... "Failure" nsresult: "0x80004005 (NS_ERROR_FAILURE)"
}, skip: browserEngine == BrowserEngine.firefox);
// Path contains should handle case where invalid RRect with large
// corner radius is used for hit test. Use case is a RenderPhysicalShape
// with a clipper that contains RRect of width/height 50 but corner radius
// of 100.
//
// Regression test for https://github.com/flutter/flutter/issues/48887
test('Should hit test correctly for malformed rrect', () {
// Correctly formed rrect.
final Path path1 = Path()
..addRRect(RRect.fromLTRBR(50, 50, 100, 100, const Radius.circular(20)));
expect(path1.contains(const Offset(75, 75)), isTrue);
expect(path1.contains(const Offset(52, 75)), isTrue);
expect(path1.contains(const Offset(50, 50)), isFalse);
expect(path1.contains(const Offset(100, 50)), isFalse);
expect(path1.contains(const Offset(100, 100)), isFalse);
expect(path1.contains(const Offset(50, 100)), isFalse);
final Path path2 = Path()
..addRRect(RRect.fromLTRBR(50, 50, 100, 100, const Radius.circular(100)));
expect(path2.contains(const Offset(75, 75)), isTrue);
expect(path2.contains(const Offset(52, 75)), isTrue);
expect(path2.contains(const Offset(50, 50)), isFalse);
expect(path2.contains(const Offset(100, 50)), isFalse);
expect(path2.contains(const Offset(100, 100)), isFalse);
expect(path2.contains(const Offset(50, 100)), isFalse);
});
test('Should set segment masks', () {
final SurfacePath path = SurfacePath();
path.pathRef.computeSegmentMask();
expect(path.pathRef.segmentMasks, 0);
path.moveTo(20, 40);
path.pathRef.computeSegmentMask();
expect(path.pathRef.segmentMasks, 0);
path.lineTo(200, 40);
path.pathRef.computeSegmentMask();
expect(
path.pathRef.segmentMasks, SPathSegmentMask.kLine_SkPathSegmentMask);
});
test('Should convert conic to quad when approximation error is small', () {
final Conic conic = Conic(120.0, 20.0, 160.99470420829266, 20.0,
190.19301120261332, 34.38770865870253, 0.9252691032413082);
expect(conic.toQuads().length, 3);
});
test('Should be able to construct from empty path', () {
final SurfacePath path = SurfacePath();
expect(path.isEmpty, isTrue);
final SurfacePath path2 = SurfacePath.from(path);
expect(path2.isEmpty, isTrue);
});
});
group('PathRef', () {
test('Should return empty when created', () {
final PathRef pathRef = PathRef();
expect(pathRef.isEmpty, isTrue);
});
test('Should return non-empty when mutated', () {
final PathRef pathRef = PathRef();
pathRef.growForVerb(SPath.kMoveVerb, 0);
expect(pathRef.isEmpty, isFalse);
});
});
group('PathRefIterator', () {
test('Should iterate through empty path', () {
final Float32List points = Float32List(20);
final PathRef pathRef = PathRef();
final PathRefIterator iter = PathRefIterator(pathRef);
expect(iter.next(points), SPath.kDoneVerb);
});
test('Should iterate through verbs', () {
final Float32List points = Float32List(20);
final PathRef pathRef = PathRef();
pathRef.growForVerb(SPath.kMoveVerb, 0);
pathRef.growForVerb(SPath.kLineVerb, 0);
pathRef.growForVerb(SPath.kQuadVerb, 0);
pathRef.growForVerb(SPath.kCubicVerb, 0);
pathRef.growForVerb(SPath.kConicVerb, 0.8);
pathRef.growForVerb(SPath.kLineVerb, 0.8);
final PathRefIterator iter = PathRefIterator(pathRef);
expect(iter.next(points), SPath.kMoveVerb);
expect(iter.next(points), SPath.kLineVerb);
expect(iter.next(points), SPath.kQuadVerb);
expect(iter.next(points), SPath.kCubicVerb);
expect(iter.next(points), SPath.kConicVerb);
expect(iter.next(points), SPath.kLineVerb);
expect(iter.next(points), SPath.kDoneVerb);
});
test('Should iterate by index through empty path', () {
final PathRef pathRef = PathRef();
final PathRefIterator iter = PathRefIterator(pathRef);
expect(iter.nextIndex(), SPath.kDoneVerb);
});
test('Should iterate through contours', () {
final PathRef pathRef = PathRef();
pathRef.growForVerb(SPath.kMoveVerb, 0);
pathRef.growForVerb(SPath.kLineVerb, 0);
pathRef.growForVerb(SPath.kQuadVerb, 0);
pathRef.growForVerb(SPath.kCubicVerb, 0);
pathRef.growForVerb(SPath.kMoveVerb, 0);
pathRef.growForVerb(SPath.kConicVerb, 0.8);
pathRef.growForVerb(SPath.kLineVerb, 0.8);
pathRef.growForVerb(SPath.kCloseVerb, 0.8);
pathRef.growForVerb(SPath.kMoveVerb, 0);
pathRef.growForVerb(SPath.kLineVerb, 0);
pathRef.growForVerb(SPath.kLineVerb, 0);
final PathRefIterator iter = PathRefIterator(pathRef);
int start = iter.pointIndex;
int end = iter.skipToNextContour();
expect(end - start, 7);
start = end;
end = iter.skipToNextContour();
expect(end - start, 4);
start = end;
end = iter.skipToNextContour();
expect(end - start, 3);
start = end;
end = iter.skipToNextContour();
expect(start, end);
});
/// Regression test for https://github.com/flutter/flutter/issues/68702.
test('Path should return correct bounds after transform', () {
final Path path1 = Path()
..moveTo(100, 100)
..lineTo(200, 100)
..lineTo(150, 200)
..close();
final SurfacePath path2 = Path.from(path1) as SurfacePath;
final Rect bounds = path2.pathRef.getBounds();
final SurfacePath transformedPath = path2.transform(
Matrix4.identity().scaled(0.5, 0.5).toFloat64());
expect(transformedPath.pathRef.getBounds(), isNot(bounds));
});
});
}
| engine/lib/web_ui/test/html/path_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/html/path_test.dart",
"repo_id": "engine",
"token_count": 9315
} | 289 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import '../../common/fake_asset_manager.dart';
import '../../common/test_initialization.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
void testMain() {
group('$HtmlFontCollection', () {
setUpUnitTests();
const String testFontUrl = '/assets/fonts/ahem.ttf';
late FakeAssetScope testScope;
setUp(() {
testScope = fakeAssetManager.pushAssetScope();
testScope.setAssetPassthrough(testFontUrl);
// Clear the fonts before the test begins to wipe out the fonts from the
// test initialization.
domDocument.fonts!.clear();
});
tearDown(() {
fakeAssetManager.popAssetScope(testScope);
});
group('regular special characters', () {
test('Register Asset with no special characters', () async {
const String testFontFamily = 'Ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, 'Ahem');
});
test('Register Asset with white space in the family name', () async {
const String testFontFamily = 'Ahem ahem ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, 'Ahem ahem ahem');
},
// TODO(mdebbar): https://github.com/flutter/flutter/issues/51142
skip: browserEngine == BrowserEngine.webkit);
test('Register Asset with capital case letters', () async {
const String testFontFamily = 'AhEm';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, 'AhEm');
});
test('Register Asset with descriptor', () async {
const String testFontFamily = 'Ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{
'weight': 'bold'
})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
expect(f.weight, 'bold');
expect(f2.weight, 'bold');
fontFamilyList.add(f.family!);
});
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, 'Ahem');
});
});
group('fonts with special characters', () {
test('Register Asset twice with special character slash', () async {
const String testFontFamily = '/Ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
if (browserEngine != BrowserEngine.firefox) {
expect(fontFamilyList.length, equals(2));
expect(fontFamilyList, contains("'/Ahem'"));
expect(fontFamilyList, contains('/Ahem'));
} else {
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, '"/Ahem"');
}
},
// TODO(mdebbar): https://github.com/flutter/flutter/issues/51142
skip: browserEngine == BrowserEngine.webkit);
test('Register Asset twice with exclamation mark', () async {
const String testFontFamily = 'Ahem!!ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
if (browserEngine != BrowserEngine.firefox) {
expect(fontFamilyList.length, equals(2));
expect(fontFamilyList, contains("'Ahem!!ahem'"));
expect(fontFamilyList, contains('Ahem!!ahem'));
} else {
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, '"Ahem!!ahem"');
}
},
// TODO(mdebbar): https://github.com/flutter/flutter/issues/51142
skip: browserEngine == BrowserEngine.webkit);
test('Register Asset twice with comma', () async {
const String testFontFamily = 'Ahem ,ahem';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
if (browserEngine != BrowserEngine.firefox) {
expect(fontFamilyList.length, equals(2));
expect(fontFamilyList, contains("'Ahem ,ahem'"));
expect(fontFamilyList, contains('Ahem ,ahem'));
} else {
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, '"Ahem ,ahem"');
}
},
// TODO(mdebbar): https://github.com/flutter/flutter/issues/51142
skip: browserEngine == BrowserEngine.webkit);
test('Register Asset twice with a digit at the start of a token',
() async {
const String testFontFamily = 'Ahem 1998';
final List<String> fontFamilyList = <String>[];
final HtmlFontCollection collection = HtmlFontCollection();
await collection.loadAssetFonts(FontManifest(<FontFamily>[
FontFamily(testFontFamily, <FontAsset>[
FontAsset(testFontUrl, <String, String>{})
])
]));
domDocument.fonts!
.forEach((DomFontFace f, DomFontFace f2, DomFontFaceSet s) {
fontFamilyList.add(f.family!);
});
if (browserEngine != BrowserEngine.firefox) {
expect(fontFamilyList.length, equals(2));
expect(fontFamilyList, contains('Ahem 1998'));
expect(fontFamilyList, contains("'Ahem 1998'"));
} else {
expect(fontFamilyList.length, equals(1));
expect(fontFamilyList.first, '"Ahem 1998"');
}
},
// TODO(mdebbar): https://github.com/flutter/flutter/issues/51142
skip: browserEngine == BrowserEngine.webkit);
});
});
}
| engine/lib/web_ui/test/html/text/font_collection_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/html/text/font_collection_test.dart",
"repo_id": "engine",
"token_count": 3573
} | 290 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'dart:typed_data';
import 'package:test/bootstrap/browser.dart';
import 'package:test/test.dart';
import 'package:ui/src/engine.dart';
import 'package:ui/ui.dart' as ui;
import '../common/matchers.dart';
import '../common/test_initialization.dart';
void main() {
internalBootstrapBrowserTest(() => testMain);
}
Future<void> testMain() async {
setUpUnitTests(
setUpTestViewDimensions: false,
);
final bool deviceClipRoundsOut = renderer is! HtmlRenderer;
runCanvasTests(deviceClipRoundsOut: deviceClipRoundsOut);
}
void runCanvasTests({required bool deviceClipRoundsOut}) {
setUp(() {
EngineSemantics.debugResetSemantics();
});
group('ui.Canvas transform tests', () {
void transformsClose(Float64List value, Float64List expected) {
expect(expected.length, equals(16));
expect(value.length, equals(16));
for (int r = 0; r < 4; r++) {
for (int c = 0; c < 4; c++) {
expect(value[r*4 + c], within(from: expected[r*4 + c]));
}
}
}
void transformsNotClose(Float64List value, Float64List expected) {
// We check the lengths here even though [transformsClose] will
// check them so that the [TestFailure] we catch below can only
// be due to a difference in matrix values.
expect(expected.length, equals(16));
expect(value.length, equals(16));
try {
transformsClose(value, expected);
} on TestFailure {
return;
}
throw TestFailure('transforms were too close to equal'); // ignore: only_throw_errors
}
test('ui.Canvas.translate affects canvas.getTransform', () {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.translate(12, 14.5);
final Float64List matrix = Matrix4.translationValues(12, 14.5, 0).toFloat64();
final Float64List curMatrix = canvas.getTransform();
transformsClose(curMatrix, matrix);
canvas.translate(10, 10);
final Float64List newCurMatrix = canvas.getTransform();
transformsNotClose(newCurMatrix, matrix);
transformsClose(curMatrix, matrix);
});
test('ui.Canvas.scale affects canvas.getTransform', () {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.scale(12, 14.5);
final Float64List matrix = Matrix4.diagonal3Values(12, 14.5, 1).toFloat64();
final Float64List curMatrix = canvas.getTransform();
transformsClose(curMatrix, matrix);
canvas.scale(10, 10);
final Float64List newCurMatrix = canvas.getTransform();
transformsNotClose(newCurMatrix, matrix);
transformsClose(curMatrix, matrix);
});
test('Canvas.rotate affects canvas.getTransform', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.rotate(pi);
final Float64List matrix = Matrix4.rotationZ(pi).toFloat64();
final Float64List curMatrix = canvas.getTransform();
transformsClose(curMatrix, matrix);
canvas.rotate(pi / 2);
final Float64List newCurMatrix = canvas.getTransform();
transformsNotClose(newCurMatrix, matrix);
transformsClose(curMatrix, matrix);
});
test('Canvas.skew affects canvas.getTransform', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.skew(12, 14.5);
final Float64List matrix = (Matrix4.identity()..setEntry(0, 1, 12)..setEntry(1, 0, 14.5)).toFloat64();
final Float64List curMatrix = canvas.getTransform();
transformsClose(curMatrix, matrix);
canvas.skew(10, 10);
final Float64List newCurMatrix = canvas.getTransform();
transformsNotClose(newCurMatrix, matrix);
transformsClose(curMatrix, matrix);
});
test('Canvas.transform affects canvas.getTransform', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
final Float64List matrix = (Matrix4.identity()..translate(12.0, 14.5)..scale(12.0, 14.5)).toFloat64();
canvas.transform(matrix);
final Float64List curMatrix = canvas.getTransform();
transformsClose(curMatrix, matrix);
canvas.translate(10, 10);
final Float64List newCurMatrix = canvas.getTransform();
transformsNotClose(newCurMatrix, matrix);
transformsClose(curMatrix, matrix);
});
});
void rectsClose(ui.Rect value, ui.Rect expected) {
expect(value.left, closeTo(expected.left, 1e-6));
expect(value.top, closeTo(expected.top, 1e-6));
expect(value.right, closeTo(expected.right, 1e-6));
expect(value.bottom, closeTo(expected.bottom, 1e-6));
}
void rectsNotClose(ui.Rect value, ui.Rect expected) {
try {
rectsClose(value, expected);
} on TestFailure {
return;
}
throw TestFailure('transforms were too close to equal'); // ignore: only_throw_errors
}
group('ui.Canvas clip tests', () {
test('Canvas.clipRect affects canvas.getClipBounds', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder, const ui.Rect.fromLTRB(0, 0, 100, 100));
const ui.Rect clipRawBounds = ui.Rect.fromLTRB(10.2, 11.3, 20.4, 25.7);
const ui.Rect clipExpandedBounds = ui.Rect.fromLTRB(10, 11, 21, 26);
final ui.Rect clipDestBounds = deviceClipRoundsOut ? clipExpandedBounds : clipRawBounds;
canvas.clipRect(clipRawBounds);
// Save initial return values for testing restored values
final ui.Rect initialLocalBounds = canvas.getLocalClipBounds();
final ui.Rect initialDestinationBounds = canvas.getDestinationClipBounds();
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.save();
canvas.clipRect(const ui.Rect.fromLTRB(0, 0, 15, 15));
// Both clip bounds have changed
rectsNotClose(canvas.getLocalClipBounds(), clipExpandedBounds);
rectsNotClose(canvas.getDestinationClipBounds(), clipDestBounds);
// Previous return values have not changed
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
canvas.save();
canvas.scale(2, 2);
const ui.Rect scaledExpandedBounds = ui.Rect.fromLTRB(5, 5.5, 10.5, 13);
rectsClose(canvas.getLocalClipBounds(), scaledExpandedBounds);
// Destination bounds are unaffected by transform
rectsClose(canvas.getDestinationClipBounds(), clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
});
test('Canvas.clipRRect affects canvas.getClipBounds', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder, const ui.Rect.fromLTRB(0, 0, 100, 100));
const ui.Rect clipRawBounds = ui.Rect.fromLTRB(10.2, 11.3, 20.4, 25.7);
const ui.Rect clipExpandedBounds = ui.Rect.fromLTRB(10, 11, 21, 26);
final ui.Rect clipDestBounds = deviceClipRoundsOut ? clipExpandedBounds : clipRawBounds;
final ui.RRect clip = ui.RRect.fromRectAndRadius(clipRawBounds, const ui.Radius.circular(3));
canvas.clipRRect(clip);
// Save initial return values for testing restored values
final ui.Rect initialLocalBounds = canvas.getLocalClipBounds();
final ui.Rect initialDestinationBounds = canvas.getDestinationClipBounds();
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.save();
canvas.clipRect(const ui.Rect.fromLTRB(0, 0, 15, 15));
// Both clip bounds have changed
rectsNotClose(canvas.getLocalClipBounds(), clipExpandedBounds);
rectsNotClose(canvas.getDestinationClipBounds(), clipDestBounds);
// Previous return values have not changed
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
canvas.save();
canvas.scale(2, 2);
const ui.Rect scaledExpandedBounds = ui.Rect.fromLTRB(5, 5.5, 10.5, 13);
rectsClose(canvas.getLocalClipBounds(), scaledExpandedBounds);
// Destination bounds are unaffected by transform
rectsClose(canvas.getDestinationClipBounds(), clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
});
test('Canvas.clipPath affects canvas.getClipBounds', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder, const ui.Rect.fromLTRB(0, 0, 100, 100));
const ui.Rect clipRawBounds = ui.Rect.fromLTRB(10.2, 11.3, 20.4, 25.7);
const ui.Rect clipExpandedBounds = ui.Rect.fromLTRB(10, 11, 21, 26);
final ui.Rect clipDestBounds = deviceClipRoundsOut ? clipExpandedBounds : clipRawBounds;
final ui.Path clip = ui.Path()..addRect(clipRawBounds)..addOval(clipRawBounds);
canvas.clipPath(clip);
// Save initial return values for testing restored values
final ui.Rect initialLocalBounds = canvas.getLocalClipBounds();
final ui.Rect initialDestinationBounds = canvas.getDestinationClipBounds();
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.save();
canvas.clipRect(const ui.Rect.fromLTRB(0, 0, 15, 15));
// Both clip bounds have changed
rectsNotClose(canvas.getLocalClipBounds(), clipExpandedBounds);
rectsNotClose(canvas.getDestinationClipBounds(), clipDestBounds);
// Previous return values have not changed
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
canvas.save();
canvas.scale(2, 2);
const ui.Rect scaledExpandedBounds = ui.Rect.fromLTRB(5, 5.5, 10.5, 13);
rectsClose(canvas.getLocalClipBounds(), scaledExpandedBounds);
// Destination bounds are unaffected by transform
rectsClose(canvas.getDestinationClipBounds(), clipDestBounds);
canvas.restore();
// save/restore returned the values to their original values
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
});
test('Canvas.clipRect(diff) does not affect canvas.getClipBounds', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder, const ui.Rect.fromLTRB(0, 0, 100, 100));
const ui.Rect clipRawBounds = ui.Rect.fromLTRB(10.2, 11.3, 20.4, 25.7);
const ui.Rect clipExpandedBounds = ui.Rect.fromLTRB(10, 11, 21, 26);
final ui.Rect clipDestBounds = deviceClipRoundsOut ? clipExpandedBounds : clipRawBounds;
canvas.clipRect(clipRawBounds);
// Save initial return values for testing restored values
final ui.Rect initialLocalBounds = canvas.getLocalClipBounds();
final ui.Rect initialDestinationBounds = canvas.getDestinationClipBounds();
rectsClose(initialLocalBounds, clipExpandedBounds);
rectsClose(initialDestinationBounds, clipDestBounds);
canvas.clipRect(const ui.Rect.fromLTRB(0, 0, 15, 15), clipOp: ui.ClipOp.difference);
expect(canvas.getLocalClipBounds(), initialLocalBounds);
expect(canvas.getDestinationClipBounds(), initialDestinationBounds);
});
});
group('RestoreToCount function tests', () {
test('RestoreToCount can work', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.save();
canvas.save();
canvas.save();
canvas.save();
canvas.save();
expect(canvas.getSaveCount(), 6);
canvas.restoreToCount(2);
expect(canvas.getSaveCount(), 2);
canvas.restore();
expect(canvas.getSaveCount(), 1);
});
test('RestoreToCount count less than 1, the stack should be reset', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.save();
canvas.save();
canvas.save();
canvas.save();
canvas.save();
expect(canvas.getSaveCount(), equals(6));
canvas.restoreToCount(0);
expect(canvas.getSaveCount(), equals(1));
});
test('RestoreToCount count greater than current [getSaveCount]', () async {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder);
canvas.save();
canvas.save();
canvas.save();
canvas.save();
canvas.save();
expect(canvas.getSaveCount(), equals(6));
canvas.restoreToCount(canvas.getSaveCount() + 1);
expect(canvas.getSaveCount(), equals(6));
});
});
}
| engine/lib/web_ui/test/ui/canvas_test.dart/0 | {
"file_path": "engine/lib/web_ui/test/ui/canvas_test.dart",
"repo_id": "engine",
"token_count": 5342
} | 291 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_RUNTIME_DART_ISOLATE_H_
#define FLUTTER_RUNTIME_DART_ISOLATE_H_
#include <memory>
#include <optional>
#include <set>
#include <string>
#include <unordered_set>
#include "flutter/common/task_runners.h"
#include "flutter/fml/compiler_specific.h"
#include "flutter/fml/macros.h"
#include "flutter/fml/mapping.h"
#include "flutter/lib/ui/io_manager.h"
#include "flutter/lib/ui/snapshot_delegate.h"
#include "flutter/lib/ui/ui_dart_state.h"
#include "flutter/lib/ui/window/platform_configuration.h"
#include "flutter/runtime/dart_snapshot.h"
#include "flutter/runtime/isolate_configuration.h"
#include "third_party/dart/runtime/include/dart_api.h"
#include "third_party/tonic/dart_state.h"
namespace flutter {
class DartVM;
class DartIsolateGroupData;
class IsolateConfiguration;
//------------------------------------------------------------------------------
/// @brief Represents an instance of a live isolate. An isolate is a
/// separate Dart execution context. Different Dart isolates don't
/// share memory and can be scheduled concurrently by the Dart VM on
/// one of the Dart VM managed worker pool threads.
///
/// The entire lifecycle of a Dart isolate is controlled by the Dart
/// VM. Because of this, the engine never holds a strong pointer to
/// the Dart VM for extended periods of time. This allows the VM (or
/// the isolates themselves) to terminate Dart execution without
/// consulting the engine.
///
/// The isolate that the engine creates to act as the host for the
/// Flutter application code with UI bindings is called the root
/// isolate.
///
/// The root isolate is special in the following ways:
/// * The root isolate forms a new isolate group. Child isolates are
/// added to their parents groups. When the root isolate dies, all
/// isolates in its group are terminated.
/// * Only root isolates get UI bindings.
/// * Root isolates execute their code on engine managed threads.
/// All other isolates run their Dart code on Dart VM managed
/// thread pool workers that the engine has no control over.
/// * Since the engine does not know the thread on which non-root
/// isolates are run, the engine has no opportunity to get a
/// reference to non-root isolates. Such isolates can only be
/// terminated if they terminate themselves or their isolate group
/// is torn down.
///
class DartIsolate : public UIDartState {
public:
class Flags {
public:
Flags();
explicit Flags(const Dart_IsolateFlags* flags);
~Flags();
void SetNullSafetyEnabled(bool enabled);
void SetIsDontNeedSafe(bool value);
Dart_IsolateFlags Get() const;
private:
Dart_IsolateFlags flags_;
};
//----------------------------------------------------------------------------
/// @brief The engine represents all dart isolates as being in one of the
/// known phases. By invoking various methods on the Dart isolate,
/// the engine transition the Dart isolate from one phase to the
/// next. The Dart isolate will only move from one phase to the
/// next in the order specified in the `DartIsolate::Phase` enum.
/// That is, once the isolate has moved out of a particular phase,
/// it can never transition back to that phase in the future.
/// There is no error recovery mechanism and callers that find
/// their isolates in an undesirable phase must discard the
/// isolate and start over.
///
enum class Phase {
// NOLINTBEGIN(readability-identifier-naming)
//--------------------------------------------------------------------------
/// The initial phase of all Dart isolates. This is an internal phase and
/// callers can never get a reference to a Dart isolate in this phase.
///
Unknown,
//--------------------------------------------------------------------------
/// The Dart isolate has been created but none of the library tag or message
/// handers have been set yet. The is an internal phase and callers can
/// never get a reference to a Dart isolate in this phase.
///
Uninitialized,
//--------------------------------------------------------------------------
/// The Dart isolate has been fully initialized but none of the
/// libraries referenced by that isolate have been loaded yet. This is an
/// internal phase and callers can never get a reference to a Dart isolate
/// in this phase.
///
Initialized,
//--------------------------------------------------------------------------
/// The isolate has been fully initialized and is waiting for the caller to
/// associate isolate snapshots with the same. The isolate will only be
/// ready to execute Dart code once one of the `Prepare` calls are
/// successfully made.
///
LibrariesSetup,
//--------------------------------------------------------------------------
/// The isolate is fully ready to start running Dart code. Callers can
/// transition the isolate to the next state by calling the `Run` or
/// `RunFromLibrary` methods.
///
Ready,
//--------------------------------------------------------------------------
/// The isolate is currently running Dart code.
///
Running,
//--------------------------------------------------------------------------
/// The isolate is no longer running Dart code and is in the middle of being
/// collected. This is in internal phase and callers can never get a
/// reference to a Dart isolate in this phase.
///
Shutdown,
// NOLINTEND(readability-identifier-naming)
};
//----------------------------------------------------------------------------
/// @brief Creates an instance of a root isolate and returns a weak
/// pointer to the same. The isolate instance may only be used
/// safely on the engine thread on which it was created. In the
/// shell, this is the UI thread and task runner. Using the
/// isolate on any other thread is user error.
///
/// The isolate that the engine creates to act as the host for the
/// Flutter application code with UI bindings is called the root
/// isolate.
///
/// The root isolate is special in the following ways:
/// * The root isolate forms a new isolate group. Child isolates
/// are added to their parents groups. When the root isolate
/// dies, all isolates in its group are terminated.
/// * Only root isolates get UI bindings.
/// * Root isolates execute their code on engine managed threads.
/// All other isolates run their Dart code on Dart VM managed
/// thread pool workers that the engine has no control over.
/// * Since the engine does not know the thread on which non-root
/// isolates are run, the engine has no opportunity to get a
/// reference to non-root isolates. Such isolates can only be
/// terminated if they terminate themselves or their isolate
/// group is torn down.
///
/// @param[in] settings The settings used to create the
/// isolate.
/// @param[in] platform_configuration The platform configuration for
/// handling communication with the
/// framework.
/// @param[in] flags The Dart isolate flags for this
/// isolate instance.
/// @param[in] dart_entrypoint The name of the dart entrypoint
/// function to invoke.
/// @param[in] dart_entrypoint_library The name of the dart library
/// containing the entrypoint.
/// @param[in] dart_entrypoint_args Arguments passed as a List<String>
/// to Dart's entrypoint function.
/// @param[in] isolate_configuration The isolate configuration used to
/// configure the isolate before
/// invoking the entrypoint.
/// @param[in] root_isolate_create_callback A callback called after the root
/// isolate is created, _without_
/// isolate scope. This gives the
/// caller a chance to finish any
/// setup before running the Dart
/// program, and after any embedder
/// callbacks in the settings object.
/// @param[in] isolate_create_callback The isolate create callback. This
/// will be called when the before the
/// main Dart entrypoint is invoked in
/// the root isolate. The isolate is
/// already in the running state at
/// this point and an isolate scope is
/// current.
/// @param[in] isolate_shutdown_callback The isolate shutdown callback.
/// This will be called before the
/// isolate is about to transition
/// into the Shutdown phase. The
/// isolate is still running at this
/// point and an isolate scope is
/// current.
/// @param[in] context Engine-owned state which is
/// accessed by the root dart isolate.
/// @param[in] spawning_isolate The isolate that is spawning the
/// new isolate.
/// @return A weak pointer to the root Dart isolate. The caller must
/// ensure that the isolate is not referenced for long periods of
/// time as it prevents isolate collection when the isolate
/// terminates itself. The caller may also only use the isolate on
/// the thread on which the isolate was created.
///
static std::weak_ptr<DartIsolate> CreateRunningRootIsolate(
const Settings& settings,
const fml::RefPtr<const DartSnapshot>& isolate_snapshot,
std::unique_ptr<PlatformConfiguration> platform_configuration,
Flags flags,
const fml::closure& root_isolate_create_callback,
const fml::closure& isolate_create_callback,
const fml::closure& isolate_shutdown_callback,
std::optional<std::string> dart_entrypoint,
std::optional<std::string> dart_entrypoint_library,
const std::vector<std::string>& dart_entrypoint_args,
std::unique_ptr<IsolateConfiguration> isolate_configuration,
const UIDartState::Context& context,
const DartIsolate* spawning_isolate = nullptr);
// |UIDartState|
~DartIsolate() override;
//----------------------------------------------------------------------------
/// @brief The current phase of the isolate. The engine represents all
/// dart isolates as being in one of the known phases. By invoking
/// various methods on the Dart isolate, the engine transitions
/// the Dart isolate from one phase to the next. The Dart isolate
/// will only move from one phase to the next in the order
/// specified in the `DartIsolate::Phase` enum. That is, the once
/// the isolate has moved out of a particular phase, it can never
/// transition back to that phase in the future. There is no error
/// recovery mechanism and callers that find their isolates in an
/// undesirable phase must discard the isolate and start over.
///
/// @return The current isolate phase.
///
Phase GetPhase() const;
//----------------------------------------------------------------------------
/// @brief Returns the ID for an isolate which is used to query the
/// service protocol.
///
/// @return The service identifier for this isolate.
///
std::string GetServiceId();
//----------------------------------------------------------------------------
/// @brief Prepare the isolate for running for a precompiled code bundle.
/// The Dart VM must be configured for running precompiled code.
///
/// The isolate must already be in the `Phase::LibrariesSetup`
/// phase. After a successful call to this method, the isolate
/// will transition to the `Phase::Ready` phase.
///
/// @return Whether the isolate was prepared and the described phase
/// transition made.
///
[[nodiscard]] bool PrepareForRunningFromPrecompiledCode();
//----------------------------------------------------------------------------
/// @brief Prepare the isolate for running for a a list of kernel files.
///
/// The Dart VM must be configured for running from kernel
/// snapshots.
///
/// The isolate must already be in the `Phase::LibrariesSetup`
/// phase. This call can be made multiple times. After a series of
/// successful calls to this method, the caller can specify the
/// last kernel file mapping by specifying `last_piece` to `true`.
/// On success, the isolate will transition to the `Phase::Ready`
/// phase.
///
/// @param[in] kernel The kernel mapping.
/// @param[in] last_piece Indicates if this is the last kernel mapping
/// expected. After this point, the isolate will
/// attempt a transition to the `Phase::Ready` phase.
///
/// @return If the kernel mapping supplied was successfully used to
/// prepare the isolate.
///
[[nodiscard]] bool PrepareForRunningFromKernel(
const std::shared_ptr<const fml::Mapping>& kernel,
bool child_isolate,
bool last_piece);
//----------------------------------------------------------------------------
/// @brief Prepare the isolate for running for a a list of kernel files.
///
/// The Dart VM must be configured for running from kernel
/// snapshots.
///
/// The isolate must already be in the `Phase::LibrariesSetup`
/// phase. After a successful call to this method, the isolate
/// will transition to the `Phase::Ready` phase.
///
/// @param[in] kernels The kernels
///
/// @return If the kernel mappings supplied were successfully used to
/// prepare the isolate.
///
[[nodiscard]] bool PrepareForRunningFromKernels(
std::vector<std::shared_ptr<const fml::Mapping>> kernels);
//----------------------------------------------------------------------------
/// @brief Prepare the isolate for running for a a list of kernel files.
///
/// The Dart VM must be configured for running from kernel
/// snapshots.
///
/// The isolate must already be in the `Phase::LibrariesSetup`
/// phase. After a successful call to this method, the isolate
/// will transition to the `Phase::Ready` phase.
///
/// @param[in] kernels The kernels
///
/// @return If the kernel mappings supplied were successfully used to
/// prepare the isolate.
///
[[nodiscard]] bool PrepareForRunningFromKernels(
std::vector<std::unique_ptr<const fml::Mapping>> kernels);
//----------------------------------------------------------------------------
/// @brief Transition the root isolate to the `Phase::Running` phase and
/// invoke the main entrypoint (the "main" method) in the
/// specified library. The isolate must already be in the
/// `Phase::Ready` phase.
///
/// @param[in] library_name The name of the library in which to invoke the
/// supplied entrypoint.
/// @param[in] entrypoint The entrypoint in `library_name`
/// @param[in] args A list of string arguments to the entrypoint.
///
/// @return If the isolate successfully transitioned to the running phase
/// and the main entrypoint was invoked.
///
[[nodiscard]] bool RunFromLibrary(std::optional<std::string> library_name,
std::optional<std::string> entrypoint,
const std::vector<std::string>& args);
//----------------------------------------------------------------------------
/// @brief Transition the isolate to the `Phase::Shutdown` phase. The
/// only thing left to do is to collect the isolate.
///
/// @return If the isolate successfully transitioned to the shutdown
/// phase.
///
[[nodiscard]] bool Shutdown();
//----------------------------------------------------------------------------
/// @brief Registers a callback that will be invoked in isolate scope
/// just before the isolate transitions to the `Phase::Shutdown`
/// phase.
///
/// @param[in] closure The callback to invoke on isolate shutdown.
///
void AddIsolateShutdownCallback(const fml::closure& closure);
//----------------------------------------------------------------------------
/// @brief A weak pointer to the Dart isolate instance. This instance may
/// only be used on the task runner that created the root isolate.
///
/// @return The weak isolate pointer.
///
std::weak_ptr<DartIsolate> GetWeakIsolatePtr();
//----------------------------------------------------------------------------
/// @brief The task runner on which the Dart code for the root isolate is
/// running. For the root isolate, this is the UI task runner for
/// the shell that owns the root isolate.
///
/// @return The message handling task runner.
///
fml::RefPtr<fml::TaskRunner> GetMessageHandlingTaskRunner() const;
//----------------------------------------------------------------------------
/// @brief Creates a new isolate in the same group as this isolate, which
/// runs on the platform thread. This method can only be invoked
/// on the root isolate.
///
/// @param[in] entry_point The entrypoint to invoke once the isolate is
/// spawned. Will be run on the platform thread.
/// @param[out] error If spawning fails inside the Dart VM, this is
/// set to the error string. The error should be
/// reported to the user. Otherwise it is set to
/// null. It's possible for spawning to fail, but
/// this error still be null. In that case the
/// failure should not be reported to the user.
///
/// @return The newly created isolate, or null if spawning failed.
///
Dart_Isolate CreatePlatformIsolate(Dart_Handle entry_point,
char** error) override;
bool LoadLoadingUnit(
intptr_t loading_unit_id,
std::unique_ptr<const fml::Mapping> snapshot_data,
std::unique_ptr<const fml::Mapping> snapshot_instructions);
void LoadLoadingUnitError(intptr_t loading_unit_id,
const std::string& error_message,
bool transient);
DartIsolateGroupData& GetIsolateGroupData();
const DartIsolateGroupData& GetIsolateGroupData() const;
/// Returns the "main" entrypoint of the library contained in the kernel
/// data in `mapping`.
static Dart_Handle LoadLibraryFromKernel(
const std::shared_ptr<const fml::Mapping>& mapping);
private:
friend class IsolateConfiguration;
class AutoFireClosure {
public:
explicit AutoFireClosure(const fml::closure& closure);
~AutoFireClosure();
private:
fml::closure closure_;
FML_DISALLOW_COPY_AND_ASSIGN(AutoFireClosure);
};
friend class DartVM;
Phase phase_ = Phase::Unknown;
std::vector<std::unique_ptr<AutoFireClosure>> shutdown_callbacks_;
std::unordered_set<fml::RefPtr<DartSnapshot>> loading_unit_snapshots_;
fml::RefPtr<fml::TaskRunner> message_handling_task_runner_;
const bool may_insecurely_connect_to_all_domains_;
const bool is_platform_isolate_;
const bool is_spawning_in_group_;
std::string domain_network_policy_;
std::shared_ptr<PlatformIsolateManager> platform_isolate_manager_;
static std::weak_ptr<DartIsolate> CreateRootIsolate(
const Settings& settings,
fml::RefPtr<const DartSnapshot> isolate_snapshot,
std::unique_ptr<PlatformConfiguration> platform_configuration,
const Flags& flags,
const fml::closure& isolate_create_callback,
const fml::closure& isolate_shutdown_callback,
const UIDartState::Context& context,
const DartIsolate* spawning_isolate = nullptr);
DartIsolate(const Settings& settings,
bool is_root_isolate,
const UIDartState::Context& context,
bool is_spawning_in_group = false);
DartIsolate(const Settings& settings,
const UIDartState::Context& context,
std::shared_ptr<PlatformIsolateManager> platform_isolate_manager);
//----------------------------------------------------------------------------
/// @brief Initializes the given (current) isolate.
///
/// @param[in] dart_isolate The current isolate that is to be initialized.
///
/// @return Whether the initialization succeeded. Irrespective of whether
/// the initialization suceeded, the current isolate will still be
/// active.
///
[[nodiscard]] bool Initialize(Dart_Isolate dart_isolate);
void SetMessageHandlingTaskRunner(const fml::RefPtr<fml::TaskRunner>& runner);
bool LoadKernel(const std::shared_ptr<const fml::Mapping>& mapping,
bool last_piece);
[[nodiscard]] bool LoadLibraries();
bool UpdateThreadPoolNames() const;
[[nodiscard]] bool MarkIsolateRunnable();
void OnShutdownCallback();
// |Dart_IsolateGroupCreateCallback|
static Dart_Isolate DartIsolateGroupCreateCallback(
const char* advisory_script_uri,
const char* advisory_script_entrypoint,
const char* package_root,
const char* package_config,
Dart_IsolateFlags* flags,
std::shared_ptr<DartIsolate>* parent_isolate_group,
char** error);
// |Dart_IsolateInitializeCallback|
static bool DartIsolateInitializeCallback(void** child_callback_data,
char** error);
static Dart_Isolate DartCreateAndStartServiceIsolate(
const char* package_root,
const char* package_config,
Dart_IsolateFlags* flags,
char** error);
typedef std::function<Dart_Isolate(std::shared_ptr<DartIsolateGroupData>*,
std::shared_ptr<DartIsolate>*,
Dart_IsolateFlags*,
char**)>
IsolateMaker;
static Dart_Isolate CreateDartIsolateGroup(
std::unique_ptr<std::shared_ptr<DartIsolateGroupData>> isolate_group_data,
std::unique_ptr<std::shared_ptr<DartIsolate>> isolate_data,
Dart_IsolateFlags* flags,
char** error,
const IsolateMaker& make_isolate);
static bool InitializeIsolate(
const std::shared_ptr<DartIsolate>& embedder_isolate,
Dart_Isolate isolate,
char** error);
// |Dart_IsolateShutdownCallback|
static void DartIsolateShutdownCallback(
std::shared_ptr<DartIsolateGroupData>* isolate_group_data,
std::shared_ptr<DartIsolate>* isolate_data);
// |Dart_IsolateCleanupCallback|
static void DartIsolateCleanupCallback(
std::shared_ptr<DartIsolateGroupData>* isolate_group_data,
std::shared_ptr<DartIsolate>* isolate_data);
// |Dart_IsolateGroupCleanupCallback|
static void DartIsolateGroupCleanupCallback(
std::shared_ptr<DartIsolateGroupData>* isolate_group_data);
// |Dart_DeferredLoadHandler|
static Dart_Handle OnDartLoadLibrary(intptr_t loading_unit_id);
static void SpawnIsolateShutdownCallback(
std::shared_ptr<DartIsolateGroupData>* isolate_group_data,
std::shared_ptr<DartIsolate>* isolate_data);
FML_DISALLOW_COPY_AND_ASSIGN(DartIsolate);
};
} // namespace flutter
#endif // FLUTTER_RUNTIME_DART_ISOLATE_H_
| engine/runtime/dart_isolate.h/0 | {
"file_path": "engine/runtime/dart_isolate.h",
"repo_id": "engine",
"token_count": 9488
} | 292 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_RUNTIME_DART_VM_H_
#define FLUTTER_RUNTIME_DART_VM_H_
#include <memory>
#include <string>
#include "flutter/common/settings.h"
#include "flutter/fml/build_config.h"
#include "flutter/fml/closure.h"
#include "flutter/fml/macros.h"
#include "flutter/fml/memory/ref_counted.h"
#include "flutter/fml/memory/ref_ptr.h"
#include "flutter/fml/memory/weak_ptr.h"
#include "flutter/fml/message_loop.h"
#include "flutter/lib/ui/isolate_name_server/isolate_name_server.h"
#include "flutter/runtime/dart_isolate.h"
#include "flutter/runtime/dart_snapshot.h"
#include "flutter/runtime/dart_vm_data.h"
#include "flutter/runtime/service_protocol.h"
#include "flutter/runtime/skia_concurrent_executor.h"
#include "third_party/dart/runtime/include/dart_api.h"
namespace flutter {
//------------------------------------------------------------------------------
/// @brief Describes a running instance of the Dart VM. There may only be
/// one running instance of the Dart VM in the process at any given
/// time. The Dart VM may be created and destroyed on any thread.
/// Typically, the first Flutter shell instance running in the
/// process bootstraps the Dart VM in the process as it starts up.
/// This cost is borne on the platform task runner of that first
/// Flutter shell. When the last Flutter shell instance is
/// destroyed, the VM is destroyed as well if all shell instances
/// were launched with the `Settings::leak_vm` flag set to false. If
/// there is any shell launch in the process with `leak_vm` set to
/// true, the VM is never shut down in the process. When the VM is
/// shutdown, the cost of the shutdown is borne on the platform task
/// runner of the last shell instance to be shut down.
///
/// Due to threading considerations, callers may never create an
/// instance of the DartVM directly. All constructors to the DartVM
/// are private. Instead, all callers that need a running VM
/// reference need to access it via the `DartVMRef::Create` call.
/// This call returns a strong reference to the running VM if one
/// exists in the process already. If a running VM instance is not
/// available in the process, a new instance is created and a strong
/// reference returned to the callers. The DartVMRef::Create call
/// ensures that there are no data races during the creation or
/// shutdown of a Dart VM (since a VM may be created and destroyed
/// on any thread). Due to this behavior, all callers needing a
/// running VM instance must provide snapshots and VM settings
/// necessary to create a VM (even if they end up not being used).
///
/// In a running VM instance, the service isolate is launched by
/// default if the VM is configured to do so. All root isolates must
/// be launched and referenced explicitly.
class DartVM {
public:
~DartVM();
//----------------------------------------------------------------------------
/// @brief Checks if VM instances in the process can run precompiled
/// code. This call can be made at any time and does not depend on
/// a running VM instance. There are no threading restrictions.
///
/// @return If VM instances in the process run precompiled code.
///
static bool IsRunningPrecompiledCode();
//----------------------------------------------------------------------------
/// @brief The number of times the VM has been launched in the process.
/// This call is inherently racy because the VM could be in the
/// process of starting up on another thread between the time the
/// caller makes this call and uses to result. For this purpose,
/// this call is only meant to be used as a debugging aid and
/// primarily only used in tests where the threading model is
/// consistent.
///
/// @return The number of times the VM has been launched.
///
static size_t GetVMLaunchCount();
//----------------------------------------------------------------------------
/// @brief The settings used to launch the running VM instance.
///
/// @attention Even though all callers that need to acquire a strong
/// reference to a VM need to provide a valid settings object, the
/// VM will only reference the settings used by the first caller
/// that bootstraps the VM in the process.
///
/// @return A valid setting object.
///
const Settings& GetSettings() const;
//----------------------------------------------------------------------------
/// @brief The VM and isolate snapshots used by this running Dart VM
/// instance.
///
/// @return A valid VM data instance.
///
std::shared_ptr<const DartVMData> GetVMData() const;
//----------------------------------------------------------------------------
/// @brief The service protocol instance associated with this running
/// Dart VM instance. This object manages native handlers for
/// engine vended service protocol methods.
///
/// @return The service protocol for this Dart VM instance.
///
std::shared_ptr<ServiceProtocol> GetServiceProtocol() const;
//----------------------------------------------------------------------------
/// @brief The isolate name server for this running VM instance. The
/// isolate name server maps names (strings) to Dart ports.
/// Running isolates can discover and communicate with each other
/// by advertising and resolving ports at well known names.
///
/// @return The isolate name server.
///
std::shared_ptr<IsolateNameServer> GetIsolateNameServer() const;
//----------------------------------------------------------------------------
/// @brief The task runner whose tasks may be executed concurrently on a
/// pool of worker threads. All subsystems within a running shell
/// instance use this worker pool for their concurrent tasks. This
/// also means that the concurrent worker pool may service tasks
/// from multiple shell instances. The number of workers in a
/// concurrent worker pool depends on the hardware concurrency
/// of the target device (usually equal to the number of logical
/// CPU cores).
///
///
/// @attention Even though concurrent task queue is associated with a running
/// Dart VM instance, the worker pool used by the Flutter engine
/// is NOT shared with the Dart VM internal worker pool. The
/// presence of this worker pool as member of the Dart VM is
/// merely to utilize the strong thread safety guarantees around
/// Dart VM lifecycle for the lifecycle of the concurrent worker
/// pool as well.
///
/// @return The task runner for the concurrent worker thread pool.
///
std::shared_ptr<fml::ConcurrentTaskRunner> GetConcurrentWorkerTaskRunner()
const;
//----------------------------------------------------------------------------
/// @brief The concurrent message loop hosts threads that are used by the
/// engine to perform tasks long running background tasks.
/// Typically, to post tasks to this message loop, the
/// `GetConcurrentWorkerTaskRunner` method may be used.
///
/// @see GetConcurrentWorkerTaskRunner
///
/// @return The concurrent message loop used by this running Dart VM
/// instance.
///
std::shared_ptr<fml::ConcurrentMessageLoop> GetConcurrentMessageLoop();
private:
const Settings settings_;
std::shared_ptr<fml::ConcurrentMessageLoop> concurrent_message_loop_;
SkiaConcurrentExecutor skia_concurrent_executor_;
std::shared_ptr<const DartVMData> vm_data_;
const std::shared_ptr<IsolateNameServer> isolate_name_server_;
const std::shared_ptr<ServiceProtocol> service_protocol_;
friend class DartVMRef;
friend class DartIsolate;
static std::shared_ptr<DartVM> Create(
const Settings& settings,
fml::RefPtr<const DartSnapshot> vm_snapshot,
fml::RefPtr<const DartSnapshot> isolate_snapshot,
std::shared_ptr<IsolateNameServer> isolate_name_server);
DartVM(const std::shared_ptr<const DartVMData>& data,
std::shared_ptr<IsolateNameServer> isolate_name_server);
FML_DISALLOW_COPY_AND_ASSIGN(DartVM);
};
} // namespace flutter
#endif // FLUTTER_RUNTIME_DART_VM_H_
| engine/runtime/dart_vm.h/0 | {
"file_path": "engine/runtime/dart_vm.h",
"repo_id": "engine",
"token_count": 2946
} | 293 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/runtime/dart_isolate.h"
#include "flutter/fml/paths.h"
#include "flutter/runtime/dart_vm.h"
#include "flutter/runtime/dart_vm_lifecycle.h"
#include "flutter/testing/dart_isolate_runner.h"
#include "flutter/testing/fixture_test.h"
#include "flutter/testing/testing.h"
// CREATE_NATIVE_ENTRY is leaky by design
// NOLINTBEGIN(clang-analyzer-core.StackAddressEscape)
namespace flutter {
namespace testing {
const std::string kKernelFileName = "no_plugin_registrant_kernel_blob.bin";
const std::string kElfFileName = "no_plugin_registrant_app_elf_snapshot.so";
class DartIsolateTest : public FixtureTest {
public:
DartIsolateTest() : FixtureTest(kKernelFileName, kElfFileName, "") {}
};
TEST_F(DartIsolateTest, DartPluginRegistrantIsNotPresent) {
ASSERT_FALSE(DartVMRef::IsInstanceRunning());
std::vector<std::string> messages;
fml::AutoResetWaitableEvent latch;
AddNativeCallback(
"PassMessage",
CREATE_NATIVE_ENTRY(([&latch, &messages](Dart_NativeArguments args) {
auto message = tonic::DartConverter<std::string>::FromDart(
Dart_GetNativeArgument(args, 0));
messages.push_back(message);
latch.Signal();
})));
auto settings = CreateSettingsForFixture();
auto did_throw_exception = false;
settings.unhandled_exception_callback = [&](const std::string& error,
const std::string& stack_trace) {
did_throw_exception = true;
return true;
};
auto vm_ref = DartVMRef::Create(settings);
auto thread = CreateNewThread();
TaskRunners task_runners(GetCurrentTestName(), //
thread, //
thread, //
thread, //
thread //
);
auto kernel_path =
fml::paths::JoinPaths({GetFixturesPath(), kKernelFileName});
auto isolate = RunDartCodeInIsolate(vm_ref, settings, task_runners, "main",
{}, kernel_path);
ASSERT_TRUE(isolate);
ASSERT_EQ(isolate->get()->GetPhase(), DartIsolate::Phase::Running);
latch.Wait();
ASSERT_EQ(messages.size(), 1u);
ASSERT_EQ(messages[0], "main() was called");
ASSERT_FALSE(did_throw_exception);
}
} // namespace testing
} // namespace flutter
// NOLINTEND(clang-analyzer-core.StackAddressEscape)
| engine/runtime/no_dart_plugin_registrant_unittests.cc/0 | {
"file_path": "engine/runtime/no_dart_plugin_registrant_unittests.cc",
"repo_id": "engine",
"token_count": 1089
} | 294 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/runtime/test_font_data.h"
// License for the Ahem font embedded below is from:
// https://www.w3.org/Style/CSS/Test/Fonts/Ahem/COPYING
//
// The Ahem font in this directory belongs to the public domain. In
// jurisdictions that do not recognize public domain ownership of these
// files, the following Creative Commons Zero declaration applies:
//
// <http://labs.creativecommons.org/licenses/zero-waive/1.0/us/legalcode>
//
// which is quoted below:
//
// The person who has associated a work with this document (the "Work")
// affirms that he or she (the "Affirmer") is the/an author or owner of
// the Work. The Work may be any work of authorship, including a
// database.
//
// The Affirmer hereby fully, permanently and irrevocably waives and
// relinquishes all of her or his copyright and related or neighboring
// legal rights in the Work available under any federal or state law,
// treaty or contract, including but not limited to moral rights,
// publicity and privacy rights, rights protecting against unfair
// competition and any rights protecting the extraction, dissemination
// and reuse of data, whether such rights are present or future, vested
// or contingent (the "Waiver"). The Affirmer makes the Waiver for the
// benefit of the public at large and to the detriment of the Affirmer's
// heirs or successors.
//
// The Affirmer understands and intends that the Waiver has the effect
// of eliminating and entirely removing from the Affirmer's control all
// the copyright and related or neighboring legal rights previously held
// by the Affirmer in the Work, to that extent making the Work freely
// available to the public for any and all uses and purposes without
// restriction of any kind, including commercial use and uses in media
// and formats or by methods that have not yet been invented or
// conceived. Should the Waiver for any reason be judged legally
// ineffective in any jurisdiction, the Affirmer hereby grants a free,
// full, permanent, irrevocable, nonexclusive and worldwide license for
// all her or his copyright and related or neighboring legal rights in
// the Work.
#if EMBED_TEST_FONT_DATA
#include "third_party/skia/include/core/SkFontMgr.h"
#include "third_party/skia/include/core/SkTypeface.h"
#include "txt/platform.h"
static const unsigned char kAhemFont[] = {
0x00, 0x01, 0x00, 0x00, 0x00, 0x0b, 0x00, 0x80, 0x00, 0x03, 0x00, 0x30,
0x4f, 0x53, 0x2f, 0x32, 0x77, 0x60, 0xf9, 0x6f, 0x00, 0x00, 0x01, 0x38,
0x00, 0x00, 0x00, 0x60, 0x63, 0x6d, 0x61, 0x70, 0xa3, 0x7c, 0xaa, 0xc8,
0x00, 0x00, 0x05, 0x9c, 0x00, 0x00, 0x06, 0x82, 0x67, 0x61, 0x73, 0x70,
0x00, 0x17, 0x00, 0x09, 0x00, 0x00, 0x36, 0x2c, 0x00, 0x00, 0x00, 0x10,
0x67, 0x6c, 0x79, 0x66, 0x49, 0xb3, 0x74, 0xda, 0x00, 0x00, 0x0e, 0x24,
0x00, 0x00, 0x1a, 0x64, 0x68, 0x65, 0x61, 0x64, 0xdb, 0x52, 0x80, 0xe7,
0x00, 0x00, 0x00, 0xbc, 0x00, 0x00, 0x00, 0x36, 0x68, 0x68, 0x65, 0x61,
0x07, 0x0a, 0x04, 0x22, 0x00, 0x00, 0x00, 0xf4, 0x00, 0x00, 0x00, 0x24,
0x68, 0x6d, 0x74, 0x78, 0xc6, 0xfe, 0x00, 0x7d, 0x00, 0x00, 0x01, 0x98,
0x00, 0x00, 0x04, 0x04, 0x6c, 0x6f, 0x63, 0x61, 0x6f, 0xa1, 0x76, 0x4e,
0x00, 0x00, 0x0c, 0x20, 0x00, 0x00, 0x02, 0x04, 0x6d, 0x61, 0x78, 0x70,
0x01, 0x04, 0x00, 0x09, 0x00, 0x00, 0x01, 0x18, 0x00, 0x00, 0x00, 0x20,
0x6e, 0x61, 0x6d, 0x65, 0x6a, 0xf3, 0x71, 0xa1, 0x00, 0x00, 0x28, 0x88,
0x00, 0x00, 0x0a, 0xf4, 0x70, 0x6f, 0x73, 0x74, 0xe7, 0xba, 0xe4, 0xf2,
0x00, 0x00, 0x33, 0x7c, 0x00, 0x00, 0x02, 0xad, 0x00, 0x01, 0x00, 0x00,
0x00, 0x01, 0x33, 0x33, 0x9b, 0x88, 0x75, 0x76, 0x5f, 0x0f, 0x3c, 0xf5,
0x00, 0x09, 0x03, 0xe8, 0x00, 0x00, 0x00, 0x00, 0xb3, 0x6f, 0x5f, 0x59,
0x00, 0x00, 0x00, 0x00, 0xc4, 0xdd, 0xab, 0x23, 0x00, 0x00, 0xff, 0x38,
0x03, 0xe8, 0x03, 0x20, 0x00, 0x00, 0x00, 0x03, 0x00, 0x02, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x03, 0x20, 0xff, 0x38,
0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x01, 0x01, 0x00, 0x01, 0x00, 0x00, 0x01, 0x01, 0x00, 0x08,
0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x03, 0x03, 0xd6, 0x01, 0x90, 0x00, 0x05, 0x00, 0x00, 0x02, 0xbc,
0x02, 0x8a, 0x00, 0x00, 0x00, 0x8f, 0x02, 0xbc, 0x02, 0x8a, 0x00, 0x00,
0x01, 0xc5, 0x00, 0x32, 0x01, 0x03, 0x00, 0x00, 0x02, 0x00, 0x04, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0xaf, 0x10, 0x00,
0x20, 0x48, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x57, 0x33,
0x43, 0x20, 0x00, 0x40, 0x00, 0x20, 0xfe, 0xff, 0x03, 0x20, 0xff, 0x38,
0x00, 0x00, 0x03, 0x20, 0x00, 0xc8, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x03, 0x20, 0x03, 0x20, 0x00, 0x00, 0x00, 0x20, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x7d, 0x00, 0x00, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x03, 0xe8, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x01, 0xf4, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00, 0x01, 0x4d, 0x00, 0x00,
0x00, 0xfa, 0x00, 0x00, 0x00, 0xa7, 0x00, 0x00, 0x00, 0xc8, 0x00, 0x00,
0x00, 0x64, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0xe8, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x04, 0x34, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x00, 0x1c, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x01, 0xe6,
0x00, 0x06, 0x01, 0xca, 0x00, 0x00, 0x00, 0x20, 0x00, 0xe0, 0x00, 0x03,
0x00, 0x04, 0x00, 0x05, 0x00, 0x06, 0x00, 0x07, 0x00, 0x08, 0x00, 0x09,
0x00, 0x00, 0x00, 0x0a, 0x00, 0x0b, 0x00, 0x0c, 0x00, 0x0d, 0x00, 0x0e,
0x00, 0x0f, 0x00, 0x10, 0x00, 0x11, 0x00, 0x12, 0x00, 0x13, 0x00, 0x14,
0x00, 0x15, 0x00, 0x16, 0x00, 0x17, 0x00, 0x18, 0x00, 0x19, 0x00, 0x1a,
0x00, 0x1b, 0x00, 0x1c, 0x00, 0x1d, 0x00, 0x1e, 0x00, 0x1f, 0x00, 0x20,
0x00, 0x21, 0x00, 0x22, 0x00, 0x23, 0x00, 0x24, 0x00, 0x25, 0x00, 0x26,
0x00, 0x27, 0x00, 0x28, 0x00, 0x29, 0x00, 0x2a, 0x00, 0x2b, 0x00, 0x2c,
0x00, 0x2d, 0x00, 0x2e, 0x00, 0x2f, 0x00, 0x30, 0x00, 0x31, 0x00, 0x32,
0x00, 0x33, 0x00, 0x34, 0x00, 0x35, 0x00, 0x36, 0x00, 0x37, 0x00, 0x38,
0x00, 0x39, 0x00, 0x3a, 0x00, 0x3b, 0x00, 0x3c, 0x00, 0x3d, 0x00, 0x3e,
0x00, 0x3f, 0x00, 0x40, 0x00, 0x41, 0x00, 0x42, 0x00, 0x43, 0x00, 0x44,
0x00, 0x45, 0x00, 0x46, 0x00, 0x47, 0x00, 0x48, 0x00, 0x49, 0x00, 0x4a,
0x00, 0x4b, 0x00, 0x4c, 0x00, 0x4d, 0x00, 0x4e, 0x00, 0x4f, 0x00, 0x50,
0x00, 0x51, 0x00, 0x52, 0x00, 0x53, 0x00, 0x54, 0x00, 0x55, 0x00, 0x56,
0x00, 0x57, 0x00, 0x58, 0x00, 0x59, 0x00, 0x5a, 0x00, 0x5b, 0x00, 0x5c,
0x00, 0x5d, 0x00, 0x5e, 0x00, 0x5f, 0x00, 0x60, 0x00, 0x00, 0x00, 0x61,
0x00, 0x62, 0x00, 0x63, 0x00, 0x64, 0x00, 0x65, 0x00, 0x66, 0x00, 0x67,
0x00, 0x68, 0x00, 0x69, 0x00, 0x6a, 0x00, 0x6b, 0x00, 0x6c, 0x00, 0x6d,
0x00, 0x6e, 0x00, 0x6f, 0x00, 0x70, 0x00, 0x71, 0x00, 0x72, 0x00, 0x73,
0x00, 0x74, 0x00, 0x75, 0x00, 0x76, 0x00, 0x77, 0x00, 0x78, 0x00, 0x79,
0x00, 0x7a, 0x00, 0x7b, 0x00, 0x7c, 0x00, 0x7d, 0x00, 0x7e, 0x00, 0x7f,
0x00, 0x80, 0x00, 0xdb, 0x00, 0x81, 0x00, 0x82, 0x00, 0x83, 0x00, 0x84,
0x00, 0xdd, 0x00, 0x85, 0x00, 0x86, 0x00, 0x87, 0x00, 0x88, 0x00, 0xe3,
0x00, 0x89, 0x00, 0x8a, 0x00, 0xea, 0x00, 0x8b, 0x00, 0x8c, 0x00, 0xe8,
0x00, 0x8d, 0x00, 0xeb, 0x00, 0xec, 0x00, 0x8e, 0x00, 0x8f, 0x00, 0xe4,
0x00, 0xe6, 0x00, 0xe5, 0x00, 0xd4, 0x00, 0xe9, 0x00, 0x90, 0x00, 0x91,
0x00, 0xd3, 0x00, 0x92, 0x00, 0x93, 0x00, 0x94, 0x00, 0x95, 0x00, 0x96,
0x00, 0xe7, 0x00, 0xd1, 0x00, 0xed, 0x00, 0xd2, 0x00, 0x97, 0x00, 0x98,
0x00, 0xde, 0x00, 0x99, 0x00, 0x9a, 0x00, 0x9b, 0x00, 0x9c, 0x00, 0xce,
0x00, 0xcf, 0x00, 0xd5, 0x00, 0xd6, 0x00, 0xd8, 0x00, 0xd9, 0x00, 0x9d,
0x00, 0x9e, 0x00, 0x9f, 0x00, 0xee, 0x00, 0xa0, 0x00, 0xd0, 0x00, 0xe2,
0x00, 0x00, 0x00, 0xe0, 0x00, 0xe1, 0x00, 0x00, 0x00, 0x00, 0x00, 0xdc,
0x00, 0xa2, 0x00, 0xd7, 0x00, 0xda, 0x00, 0xdf, 0x00, 0xa3, 0x00, 0xa4,
0x00, 0xa5, 0x00, 0xa6, 0x00, 0xa7, 0x00, 0xa8, 0x00, 0xa9, 0x00, 0xaa,
0x00, 0xab, 0x00, 0xac, 0x00, 0xad, 0x00, 0x00, 0x00, 0xae, 0x00, 0xaf,
0x00, 0xb0, 0x00, 0xb1, 0x00, 0xb2, 0x00, 0xb3, 0x00, 0xb4, 0x00, 0xb5,
0x00, 0xb6, 0x00, 0xb7, 0x00, 0xb8, 0x00, 0xb9, 0x00, 0xba, 0x00, 0xbb,
0x00, 0xbc, 0x00, 0x04, 0x02, 0x4e, 0x00, 0x00, 0x00, 0x58, 0x00, 0x40,
0x00, 0x05, 0x00, 0x18, 0x00, 0x26, 0x00, 0x7e, 0x00, 0xff, 0x01, 0x31,
0x01, 0x53, 0x01, 0x78, 0x01, 0x92, 0x02, 0xc7, 0x02, 0xc9, 0x02, 0xdd,
0x03, 0x94, 0x03, 0xa9, 0x03, 0xbc, 0x03, 0xc0, 0x20, 0x06, 0x20, 0x0b,
0x20, 0x0d, 0x20, 0x10, 0x20, 0x14, 0x20, 0x1a, 0x20, 0x1e, 0x20, 0x22,
0x20, 0x26, 0x20, 0x30, 0x20, 0x3a, 0x20, 0x44, 0x21, 0x22, 0x21, 0x26,
0x22, 0x02, 0x22, 0x06, 0x22, 0x0f, 0x22, 0x12, 0x22, 0x1a, 0x22, 0x1e,
0x22, 0x2b, 0x22, 0x48, 0x22, 0x60, 0x22, 0x65, 0x22, 0xf2, 0x25, 0xca,
0x30, 0x00, 0xf0, 0x02, 0xfe, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x20,
0x00, 0x28, 0x00, 0xa0, 0x01, 0x31, 0x01, 0x52, 0x01, 0x78, 0x01, 0x92,
0x02, 0xc6, 0x02, 0xc9, 0x02, 0xd8, 0x03, 0x94, 0x03, 0xa9, 0x03, 0xbc,
0x03, 0xc0, 0x20, 0x02, 0x20, 0x09, 0x20, 0x0c, 0x20, 0x10, 0x20, 0x13,
0x20, 0x18, 0x20, 0x1c, 0x20, 0x20, 0x20, 0x26, 0x20, 0x30, 0x20, 0x39,
0x20, 0x44, 0x21, 0x22, 0x21, 0x26, 0x22, 0x02, 0x22, 0x06, 0x22, 0x0f,
0x22, 0x11, 0x22, 0x19, 0x22, 0x1e, 0x22, 0x2b, 0x22, 0x48, 0x22, 0x60,
0x22, 0x64, 0x22, 0xf2, 0x25, 0xca, 0x30, 0x00, 0xf0, 0x00, 0xfe, 0xff,
0xff, 0xff, 0xff, 0xe3, 0xff, 0xe2, 0x00, 0x00, 0xff, 0x81, 0xff, 0x7c,
0xff, 0x58, 0xff, 0x3f, 0x00, 0x00, 0xfd, 0xec, 0x00, 0x00, 0xfd, 0x3e,
0xfd, 0x2a, 0xfc, 0xd3, 0xfd, 0x14, 0xe0, 0xf4, 0xe0, 0xf2, 0xe0, 0xf3,
0xdf, 0xff, 0xe0, 0xc2, 0x00, 0x00, 0xe0, 0xbc, 0xe0, 0xbb, 0xe0, 0xb8,
0xe0, 0xaf, 0xe0, 0xa7, 0xe0, 0x9e, 0xdf, 0xc1, 0xdf, 0xad, 0xde, 0xe2,
0xde, 0xcc, 0xde, 0xd6, 0x00, 0x00, 0x00, 0x00, 0xde, 0xca, 0xde, 0xbe,
0xde, 0xa5, 0xde, 0x8a, 0xde, 0x87, 0xdd, 0xfb, 0xdb, 0x24, 0xd0, 0xfe,
0x10, 0xef, 0x01, 0xf6, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x54,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x0a, 0x00, 0x00,
0x01, 0x0a, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x02, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xf0, 0x00, 0xf2,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x99,
0x00, 0x95, 0x00, 0x82, 0x00, 0x83, 0x00, 0xa1, 0x00, 0x8e, 0x00, 0xbd,
0x00, 0x84, 0x00, 0x8a, 0x00, 0x88, 0x00, 0x90, 0x00, 0x97, 0x00, 0x96,
0x00, 0xc4, 0x00, 0x87, 0x00, 0xb5, 0x00, 0x81, 0x00, 0x8d, 0x00, 0xc7,
0x00, 0xc8, 0x00, 0x89, 0x00, 0x8f, 0x00, 0x85, 0x00, 0xa2, 0x00, 0xb9,
0x00, 0xc6, 0x00, 0x91, 0x00, 0x98, 0x00, 0xca, 0x00, 0xc9, 0x00, 0xcb,
0x00, 0x94, 0x00, 0x9a, 0x00, 0xa5, 0x00, 0xa3, 0x00, 0x9b, 0x00, 0x61,
0x00, 0x62, 0x00, 0x8b, 0x00, 0x63, 0x00, 0xa7, 0x00, 0x64, 0x00, 0xa4,
0x00, 0xa6, 0x00, 0xab, 0x00, 0xa8, 0x00, 0xa9, 0x00, 0xaa, 0x00, 0xbe,
0x00, 0x65, 0x00, 0xae, 0x00, 0xac, 0x00, 0xad, 0x00, 0x9c, 0x00, 0x66,
0x00, 0xc5, 0x00, 0x8c, 0x00, 0xb1, 0x00, 0xaf, 0x00, 0xb0, 0x00, 0x67,
0x00, 0xc0, 0x00, 0xc2, 0x00, 0x86, 0x00, 0x69, 0x00, 0x68, 0x00, 0x6a,
0x00, 0x6c, 0x00, 0x6b, 0x00, 0x6d, 0x00, 0x92, 0x00, 0x6e, 0x00, 0x70,
0x00, 0x6f, 0x00, 0x71, 0x00, 0x72, 0x00, 0x74, 0x00, 0x73, 0x00, 0x75,
0x00, 0x76, 0x00, 0xbf, 0x00, 0x77, 0x00, 0x79, 0x00, 0x78, 0x00, 0x7a,
0x00, 0x7c, 0x00, 0x7b, 0x00, 0x9f, 0x00, 0x93, 0x00, 0x7e, 0x00, 0x7d,
0x00, 0x7f, 0x00, 0x80, 0x00, 0xc1, 0x00, 0xc3, 0x00, 0xa0, 0x00, 0xb3,
0x00, 0xbc, 0x00, 0xb6, 0x00, 0xb7, 0x00, 0xb8, 0x00, 0xbb, 0x00, 0xb4,
0x00, 0xba, 0x00, 0x9d, 0x00, 0x9e, 0x00, 0xd7, 0x00, 0xe6, 0x00, 0xc4,
0x00, 0xa2, 0x00, 0xe7, 0x00, 0x04, 0x02, 0x4e, 0x00, 0x00, 0x00, 0x58,
0x00, 0x40, 0x00, 0x05, 0x00, 0x18, 0x00, 0x26, 0x00, 0x7e, 0x00, 0xff,
0x01, 0x31, 0x01, 0x53, 0x01, 0x78, 0x01, 0x92, 0x02, 0xc7, 0x02, 0xc9,
0x02, 0xdd, 0x03, 0x94, 0x03, 0xa9, 0x03, 0xbc, 0x03, 0xc0, 0x20, 0x06,
0x20, 0x0b, 0x20, 0x0d, 0x20, 0x10, 0x20, 0x14, 0x20, 0x1a, 0x20, 0x1e,
0x20, 0x22, 0x20, 0x26, 0x20, 0x30, 0x20, 0x3a, 0x20, 0x44, 0x21, 0x22,
0x21, 0x26, 0x22, 0x02, 0x22, 0x06, 0x22, 0x0f, 0x22, 0x12, 0x22, 0x1a,
0x22, 0x1e, 0x22, 0x2b, 0x22, 0x48, 0x22, 0x60, 0x22, 0x65, 0x22, 0xf2,
0x25, 0xca, 0x30, 0x00, 0xf0, 0x02, 0xfe, 0xff, 0xff, 0xff, 0x00, 0x00,
0x00, 0x20, 0x00, 0x28, 0x00, 0xa0, 0x01, 0x31, 0x01, 0x52, 0x01, 0x78,
0x01, 0x92, 0x02, 0xc6, 0x02, 0xc9, 0x02, 0xd8, 0x03, 0x94, 0x03, 0xa9,
0x03, 0xbc, 0x03, 0xc0, 0x20, 0x02, 0x20, 0x09, 0x20, 0x0c, 0x20, 0x10,
0x20, 0x13, 0x20, 0x18, 0x20, 0x1c, 0x20, 0x20, 0x20, 0x26, 0x20, 0x30,
0x20, 0x39, 0x20, 0x44, 0x21, 0x22, 0x21, 0x26, 0x22, 0x02, 0x22, 0x06,
0x22, 0x0f, 0x22, 0x11, 0x22, 0x19, 0x22, 0x1e, 0x22, 0x2b, 0x22, 0x48,
0x22, 0x60, 0x22, 0x64, 0x22, 0xf2, 0x25, 0xca, 0x30, 0x00, 0xf0, 0x00,
0xfe, 0xff, 0xff, 0xff, 0xff, 0xe3, 0xff, 0xe2, 0x00, 0x00, 0xff, 0x81,
0xff, 0x7c, 0xff, 0x58, 0xff, 0x3f, 0x00, 0x00, 0xfd, 0xec, 0x00, 0x00,
0xfd, 0x3e, 0xfd, 0x2a, 0xfc, 0xd3, 0xfd, 0x14, 0xe0, 0xf4, 0xe0, 0xf2,
0xe0, 0xf3, 0xdf, 0xff, 0xe0, 0xc2, 0x00, 0x00, 0xe0, 0xbc, 0xe0, 0xbb,
0xe0, 0xb8, 0xe0, 0xaf, 0xe0, 0xa7, 0xe0, 0x9e, 0xdf, 0xc1, 0xdf, 0xad,
0xde, 0xe2, 0xde, 0xcc, 0xde, 0xd6, 0x00, 0x00, 0x00, 0x00, 0xde, 0xca,
0xde, 0xbe, 0xde, 0xa5, 0xde, 0x8a, 0xde, 0x87, 0xdd, 0xfb, 0xdb, 0x24,
0xd0, 0xfe, 0x10, 0xef, 0x01, 0xf6, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00,
0x00, 0x54, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x0a,
0x00, 0x00, 0x01, 0x0a, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x02,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xf0,
0x00, 0xf2, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x99, 0x00, 0x95, 0x00, 0x82, 0x00, 0x83, 0x00, 0xa1, 0x00, 0x8e,
0x00, 0xbd, 0x00, 0x84, 0x00, 0x8a, 0x00, 0x88, 0x00, 0x90, 0x00, 0x97,
0x00, 0x96, 0x00, 0xc4, 0x00, 0x87, 0x00, 0xb5, 0x00, 0x81, 0x00, 0x8d,
0x00, 0xc7, 0x00, 0xc8, 0x00, 0x89, 0x00, 0x8f, 0x00, 0x85, 0x00, 0xa2,
0x00, 0xb9, 0x00, 0xc6, 0x00, 0x91, 0x00, 0x98, 0x00, 0xca, 0x00, 0xc9,
0x00, 0xcb, 0x00, 0x94, 0x00, 0x9a, 0x00, 0xa5, 0x00, 0xa3, 0x00, 0x9b,
0x00, 0x61, 0x00, 0x62, 0x00, 0x8b, 0x00, 0x63, 0x00, 0xa7, 0x00, 0x64,
0x00, 0xa4, 0x00, 0xa6, 0x00, 0xab, 0x00, 0xa8, 0x00, 0xa9, 0x00, 0xaa,
0x00, 0xbe, 0x00, 0x65, 0x00, 0xae, 0x00, 0xac, 0x00, 0xad, 0x00, 0x9c,
0x00, 0x66, 0x00, 0xc5, 0x00, 0x8c, 0x00, 0xb1, 0x00, 0xaf, 0x00, 0xb0,
0x00, 0x67, 0x00, 0xc0, 0x00, 0xc2, 0x00, 0x86, 0x00, 0x69, 0x00, 0x68,
0x00, 0x6a, 0x00, 0x6c, 0x00, 0x6b, 0x00, 0x6d, 0x00, 0x92, 0x00, 0x6e,
0x00, 0x70, 0x00, 0x6f, 0x00, 0x71, 0x00, 0x72, 0x00, 0x74, 0x00, 0x73,
0x00, 0x75, 0x00, 0x76, 0x00, 0xbf, 0x00, 0x77, 0x00, 0x79, 0x00, 0x78,
0x00, 0x7a, 0x00, 0x7c, 0x00, 0x7b, 0x00, 0x9f, 0x00, 0x93, 0x00, 0x7e,
0x00, 0x7d, 0x00, 0x7f, 0x00, 0x80, 0x00, 0xc1, 0x00, 0xc3, 0x00, 0xa0,
0x00, 0xb3, 0x00, 0xbc, 0x00, 0xb6, 0x00, 0xb7, 0x00, 0xb8, 0x00, 0xbb,
0x00, 0xb4, 0x00, 0xba, 0x00, 0x9d, 0x00, 0x9e, 0x00, 0xd7, 0x00, 0xe6,
0x00, 0xc4, 0x00, 0xa2, 0x00, 0xe7, 0x00, 0x00, 0x00, 0x00, 0x00, 0x14,
0x00, 0x14, 0x00, 0x14, 0x00, 0x14, 0x00, 0x22, 0x00, 0x30, 0x00, 0x3e,
0x00, 0x4c, 0x00, 0x5a, 0x00, 0x68, 0x00, 0x76, 0x00, 0x84, 0x00, 0x92,
0x00, 0xa0, 0x00, 0xae, 0x00, 0xbc, 0x00, 0xca, 0x00, 0xd8, 0x00, 0xe6,
0x00, 0xf4, 0x01, 0x02, 0x01, 0x10, 0x01, 0x1e, 0x01, 0x2c, 0x01, 0x3a,
0x01, 0x48, 0x01, 0x56, 0x01, 0x64, 0x01, 0x72, 0x01, 0x80, 0x01, 0x8e,
0x01, 0x9c, 0x01, 0xaa, 0x01, 0xb8, 0x01, 0xc6, 0x01, 0xd4, 0x01, 0xe2,
0x01, 0xf0, 0x01, 0xfe, 0x02, 0x0c, 0x02, 0x1a, 0x02, 0x28, 0x02, 0x36,
0x02, 0x44, 0x02, 0x52, 0x02, 0x60, 0x02, 0x6e, 0x02, 0x7c, 0x02, 0x8a,
0x02, 0x98, 0x02, 0xa6, 0x02, 0xb4, 0x02, 0xc2, 0x02, 0xd0, 0x02, 0xde,
0x02, 0xec, 0x02, 0xfa, 0x03, 0x08, 0x03, 0x16, 0x03, 0x24, 0x03, 0x32,
0x03, 0x40, 0x03, 0x4e, 0x03, 0x5c, 0x03, 0x6a, 0x03, 0x78, 0x03, 0x86,
0x03, 0x94, 0x03, 0xa2, 0x03, 0xb0, 0x03, 0xbe, 0x03, 0xcc, 0x03, 0xda,
0x03, 0xe8, 0x03, 0xf6, 0x04, 0x04, 0x04, 0x12, 0x04, 0x20, 0x04, 0x2e,
0x04, 0x3c, 0x04, 0x4a, 0x04, 0x58, 0x04, 0x64, 0x04, 0x72, 0x04, 0x80,
0x04, 0x8e, 0x04, 0x9c, 0x04, 0xaa, 0x04, 0xb8, 0x04, 0xc6, 0x04, 0xd4,
0x04, 0xe2, 0x04, 0xf0, 0x04, 0xfe, 0x05, 0x0c, 0x05, 0x1a, 0x05, 0x28,
0x05, 0x36, 0x05, 0x44, 0x05, 0x52, 0x05, 0x60, 0x05, 0x6e, 0x05, 0x7c,
0x05, 0x8a, 0x05, 0x98, 0x05, 0xa6, 0x05, 0xb4, 0x05, 0xc2, 0x05, 0xd0,
0x05, 0xde, 0x05, 0xec, 0x05, 0xfa, 0x06, 0x08, 0x06, 0x16, 0x06, 0x24,
0x06, 0x32, 0x06, 0x40, 0x06, 0x4e, 0x06, 0x5c, 0x06, 0x6a, 0x06, 0x78,
0x06, 0x86, 0x06, 0x94, 0x06, 0xa2, 0x06, 0xb0, 0x06, 0xbe, 0x06, 0xcc,
0x06, 0xda, 0x06, 0xe8, 0x06, 0xf6, 0x07, 0x04, 0x07, 0x12, 0x07, 0x20,
0x07, 0x2e, 0x07, 0x3c, 0x07, 0x4a, 0x07, 0x58, 0x07, 0x66, 0x07, 0x74,
0x07, 0x82, 0x07, 0x90, 0x07, 0x9e, 0x07, 0xac, 0x07, 0xba, 0x07, 0xc8,
0x07, 0xd6, 0x07, 0xe4, 0x07, 0xf2, 0x08, 0x00, 0x08, 0x0e, 0x08, 0x1c,
0x08, 0x2a, 0x08, 0x38, 0x08, 0x38, 0x08, 0x46, 0x08, 0x54, 0x08, 0x62,
0x08, 0x70, 0x08, 0x7e, 0x08, 0x8c, 0x08, 0x9a, 0x08, 0xa8, 0x08, 0xb6,
0x08, 0xc4, 0x08, 0xd2, 0x08, 0xe0, 0x08, 0xee, 0x08, 0xfc, 0x09, 0x0a,
0x09, 0x18, 0x09, 0x26, 0x09, 0x34, 0x09, 0x42, 0x09, 0x50, 0x09, 0x5e,
0x09, 0x6c, 0x09, 0x7a, 0x09, 0x88, 0x09, 0x96, 0x09, 0xa4, 0x09, 0xb2,
0x09, 0xc0, 0x09, 0xce, 0x09, 0xdc, 0x09, 0xea, 0x09, 0xf8, 0x0a, 0x06,
0x0a, 0x14, 0x0a, 0x22, 0x0a, 0x30, 0x0a, 0x3e, 0x0a, 0x4c, 0x0a, 0x5a,
0x0a, 0x68, 0x0a, 0x76, 0x0a, 0x84, 0x0a, 0x92, 0x0a, 0xa0, 0x0a, 0xae,
0x0a, 0xbc, 0x0a, 0xca, 0x0a, 0xd8, 0x0a, 0xe6, 0x0a, 0xf4, 0x0b, 0x02,
0x0b, 0x10, 0x0b, 0x1e, 0x0b, 0x2c, 0x0b, 0x3a, 0x0b, 0x48, 0x0b, 0x56,
0x0b, 0x64, 0x0b, 0x72, 0x0b, 0x80, 0x0b, 0x8e, 0x0b, 0x9c, 0x0b, 0xaa,
0x0b, 0xb8, 0x0b, 0xc6, 0x0b, 0xd4, 0x0b, 0xe2, 0x0b, 0xf0, 0x0b, 0xfe,
0x0c, 0x0c, 0x0c, 0x1a, 0x0c, 0x28, 0x0c, 0x36, 0x0c, 0x44, 0x0c, 0x52,
0x0c, 0x60, 0x0c, 0x6e, 0x0c, 0x7c, 0x0c, 0x8a, 0x0c, 0x98, 0x0c, 0xa6,
0x0c, 0xb4, 0x0c, 0xc2, 0x0c, 0xd0, 0x0c, 0xde, 0x0c, 0xec, 0x0c, 0xfa,
0x0d, 0x08, 0x0d, 0x16, 0x0d, 0x24, 0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32,
0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32,
0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32, 0x0d, 0x32, 0x00, 0x02, 0x00, 0x7d,
0x00, 0x00, 0x03, 0x6b, 0x03, 0x20, 0x00, 0x03, 0x00, 0x07, 0x00, 0x00,
0x33, 0x11, 0x21, 0x11, 0x25, 0x21, 0x11, 0x21, 0x7d, 0x02, 0xee, 0xfd,
0x8f, 0x01, 0xf4, 0xfe, 0x0c, 0x03, 0x20, 0xfc, 0xe0, 0x7d, 0x02, 0x26,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x00, 0x00, 0x00, 0x03,
0x00, 0x00, 0x31, 0x21, 0x15, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0xc8, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0xe0, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21,
0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8, 0x03, 0x20, 0x00, 0x03,
0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8, 0xfc, 0x18, 0x03, 0x20,
0xfc, 0x18, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x38, 0x03, 0xe8,
0x03, 0x20, 0x00, 0x03, 0x00, 0x00, 0x11, 0x21, 0x11, 0x21, 0x03, 0xe8,
0xfc, 0x18, 0x03, 0x20, 0xfc, 0x18, 0x00, 0x00, 0x00, 0x00, 0x00, 0x24,
0x01, 0xb6, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0xf0,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x08,
0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x0e,
0x01, 0xf0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x22,
0x01, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x08,
0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x05, 0x00, 0x18,
0x01, 0xfe, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x08,
0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0a, 0x03, 0x94,
0x02, 0x20, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0b, 0x00, 0x24,
0x05, 0xb4, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x52,
0x05, 0xd8, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0xf8,
0x06, 0x2a, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x04,
0x06, 0x2e, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x07,
0x07, 0x22, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x11,
0x07, 0x29, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x04,
0x06, 0x2e, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x05, 0x00, 0x0c,
0x07, 0x29, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x04,
0x06, 0x2e, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0a, 0x01, 0xca,
0x07, 0x3a, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0b, 0x00, 0x12,
0x09, 0x04, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x28,
0x09, 0x16, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x10, 0x00, 0x04,
0x06, 0x2e, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x11, 0x00, 0x07,
0x07, 0x22, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x12, 0x00, 0x04,
0x06, 0x2e, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x00, 0x01, 0xf0,
0x00, 0x00, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x01, 0x00, 0x08,
0x00, 0x08, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x02, 0x00, 0x0e,
0x01, 0xf0, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x03, 0x00, 0x22,
0x01, 0xfe, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x04, 0x00, 0x08,
0x00, 0x08, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x05, 0x00, 0x18,
0x01, 0xfe, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x06, 0x00, 0x08,
0x00, 0x08, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x0a, 0x03, 0x94,
0x02, 0x20, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x0b, 0x00, 0x24,
0x05, 0xb4, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x0e, 0x00, 0x50,
0x05, 0xd8, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x10, 0x00, 0x08,
0x00, 0x08, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x11, 0x00, 0x0e,
0x01, 0xf0, 0x00, 0x03, 0x00, 0x01, 0x04, 0x09, 0x00, 0x12, 0x00, 0x08,
0x00, 0x08, 0x00, 0x54, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20, 0x00, 0x41,
0x00, 0x68, 0x00, 0x65, 0x00, 0x6d, 0x00, 0x20, 0x00, 0x66, 0x00, 0x6f,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x62, 0x00, 0x65, 0x00, 0x6c,
0x00, 0x6f, 0x00, 0x6e, 0x00, 0x67, 0x00, 0x73, 0x00, 0x20, 0x00, 0x74,
0x00, 0x6f, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20,
0x00, 0x70, 0x00, 0x75, 0x00, 0x62, 0x00, 0x6c, 0x00, 0x69, 0x00, 0x63,
0x00, 0x20, 0x00, 0x64, 0x00, 0x6f, 0x00, 0x6d, 0x00, 0x61, 0x00, 0x69,
0x00, 0x6e, 0x00, 0x2e, 0x00, 0x20, 0x00, 0x49, 0x00, 0x6e, 0x00, 0x20,
0x00, 0x6a, 0x00, 0x75, 0x00, 0x72, 0x00, 0x69, 0x00, 0x73, 0x00, 0x64,
0x00, 0x69, 0x00, 0x63, 0x00, 0x74, 0x00, 0x69, 0x00, 0x6f, 0x00, 0x6e,
0x00, 0x73, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x61, 0x00, 0x74,
0x00, 0x20, 0x00, 0x64, 0x00, 0x6f, 0x00, 0x20, 0x00, 0x6e, 0x00, 0x6f,
0x00, 0x74, 0x00, 0x20, 0x00, 0x72, 0x00, 0x65, 0x00, 0x63, 0x00, 0x6f,
0x00, 0x67, 0x00, 0x6e, 0x00, 0x69, 0x00, 0x7a, 0x00, 0x65, 0x00, 0x20,
0x00, 0x70, 0x00, 0x75, 0x00, 0x62, 0x00, 0x6c, 0x00, 0x69, 0x00, 0x63,
0x00, 0x20, 0x00, 0x64, 0x00, 0x6f, 0x00, 0x6d, 0x00, 0x61, 0x00, 0x69,
0x00, 0x6e, 0x00, 0x20, 0x00, 0x6f, 0x00, 0x77, 0x00, 0x6e, 0x00, 0x65,
0x00, 0x72, 0x00, 0x73, 0x00, 0x68, 0x00, 0x69, 0x00, 0x70, 0x00, 0x20,
0x00, 0x6f, 0x00, 0x66, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65,
0x00, 0x73, 0x00, 0x65, 0x00, 0x20, 0x00, 0x66, 0x00, 0x69, 0x00, 0x6c,
0x00, 0x65, 0x00, 0x73, 0x00, 0x2c, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68,
0x00, 0x65, 0x00, 0x20, 0x00, 0x66, 0x00, 0x6f, 0x00, 0x6c, 0x00, 0x6c,
0x00, 0x6f, 0x00, 0x77, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x67, 0x00, 0x20,
0x00, 0x43, 0x00, 0x72, 0x00, 0x65, 0x00, 0x61, 0x00, 0x74, 0x00, 0x69,
0x00, 0x76, 0x00, 0x65, 0x00, 0x20, 0x00, 0x43, 0x00, 0x6f, 0x00, 0x6d,
0x00, 0x6d, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x73, 0x00, 0x20, 0x00, 0x5a,
0x00, 0x65, 0x00, 0x72, 0x00, 0x6f, 0x00, 0x20, 0x00, 0x64, 0x00, 0x65,
0x00, 0x63, 0x00, 0x6c, 0x00, 0x61, 0x00, 0x72, 0x00, 0x61, 0x00, 0x74,
0x00, 0x69, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x20, 0x00, 0x61, 0x00, 0x70,
0x00, 0x70, 0x00, 0x6c, 0x00, 0x69, 0x00, 0x65, 0x00, 0x73, 0x00, 0x3a,
0x00, 0x20, 0x00, 0x68, 0x00, 0x74, 0x00, 0x74, 0x00, 0x70, 0x00, 0x3a,
0x00, 0x2f, 0x00, 0x2f, 0x00, 0x6c, 0x00, 0x61, 0x00, 0x62, 0x00, 0x73,
0x00, 0x2e, 0x00, 0x63, 0x00, 0x72, 0x00, 0x65, 0x00, 0x61, 0x00, 0x74,
0x00, 0x69, 0x00, 0x76, 0x00, 0x65, 0x00, 0x63, 0x00, 0x6f, 0x00, 0x6d,
0x00, 0x6d, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x73, 0x00, 0x2e, 0x00, 0x6f,
0x00, 0x72, 0x00, 0x67, 0x00, 0x2f, 0x00, 0x6c, 0x00, 0x69, 0x00, 0x63,
0x00, 0x65, 0x00, 0x6e, 0x00, 0x73, 0x00, 0x65, 0x00, 0x73, 0x00, 0x2f,
0x00, 0x7a, 0x00, 0x65, 0x00, 0x72, 0x00, 0x6f, 0x00, 0x2d, 0x00, 0x77,
0x00, 0x61, 0x00, 0x69, 0x00, 0x76, 0x00, 0x65, 0x00, 0x2f, 0x00, 0x31,
0x00, 0x2e, 0x00, 0x30, 0x00, 0x2f, 0x00, 0x75, 0x00, 0x73, 0x00, 0x2f,
0x00, 0x6c, 0x00, 0x65, 0x00, 0x67, 0x00, 0x61, 0x00, 0x6c, 0x00, 0x63,
0x00, 0x6f, 0x00, 0x64, 0x00, 0x65, 0x00, 0x52, 0x00, 0x65, 0x00, 0x67,
0x00, 0x75, 0x00, 0x6c, 0x00, 0x61, 0x00, 0x72, 0x00, 0x56, 0x00, 0x65,
0x00, 0x72, 0x00, 0x73, 0x00, 0x69, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x20,
0x00, 0x31, 0x00, 0x2e, 0x00, 0x32, 0x00, 0x30, 0x00, 0x20, 0x00, 0x41,
0x00, 0x68, 0x00, 0x65, 0x00, 0x6d, 0x00, 0x54, 0x00, 0x68, 0x00, 0x65,
0x00, 0x20, 0x00, 0x41, 0x00, 0x68, 0x00, 0x65, 0x00, 0x6d, 0x00, 0x20,
0x00, 0x66, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x77,
0x00, 0x61, 0x00, 0x73, 0x00, 0x20, 0x00, 0x64, 0x00, 0x65, 0x00, 0x76,
0x00, 0x65, 0x00, 0x6c, 0x00, 0x6f, 0x00, 0x70, 0x00, 0x65, 0x00, 0x64,
0x00, 0x20, 0x00, 0x62, 0x00, 0x79, 0x00, 0x20, 0x00, 0x54, 0x00, 0x6f,
0x00, 0x64, 0x00, 0x64, 0x00, 0x20, 0x00, 0x46, 0x00, 0x61, 0x00, 0x68,
0x00, 0x72, 0x00, 0x6e, 0x00, 0x65, 0x00, 0x72, 0x00, 0x20, 0x00, 0x74,
0x00, 0x6f, 0x00, 0x20, 0x00, 0x68, 0x00, 0x65, 0x00, 0x6c, 0x00, 0x70,
0x00, 0x20, 0x00, 0x74, 0x00, 0x65, 0x00, 0x73, 0x00, 0x74, 0x00, 0x20,
0x00, 0x77, 0x00, 0x72, 0x00, 0x69, 0x00, 0x74, 0x00, 0x65, 0x00, 0x72,
0x00, 0x73, 0x00, 0x20, 0x00, 0x20, 0x00, 0x64, 0x00, 0x65, 0x00, 0x76,
0x00, 0x65, 0x00, 0x6c, 0x00, 0x6f, 0x00, 0x70, 0x00, 0x20, 0x00, 0x70,
0x00, 0x72, 0x00, 0x65, 0x00, 0x64, 0x00, 0x69, 0x00, 0x63, 0x00, 0x74,
0x00, 0x61, 0x00, 0x62, 0x00, 0x6c, 0x00, 0x65, 0x00, 0x20, 0x00, 0x74,
0x00, 0x65, 0x00, 0x73, 0x00, 0x74, 0x00, 0x73, 0x00, 0x2e, 0x00, 0x20,
0x00, 0x54, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20, 0x00, 0x66, 0x00, 0x6f,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x27, 0x00, 0x73, 0x00, 0x20, 0x00, 0x65,
0x00, 0x6d, 0x00, 0x20, 0x00, 0x73, 0x00, 0x71, 0x00, 0x75, 0x00, 0x61,
0x00, 0x72, 0x00, 0x65, 0x00, 0x20, 0x00, 0x69, 0x00, 0x73, 0x00, 0x20,
0x00, 0x65, 0x00, 0x78, 0x00, 0x61, 0x00, 0x63, 0x00, 0x74, 0x00, 0x6c,
0x00, 0x79, 0x00, 0x20, 0x00, 0x73, 0x00, 0x71, 0x00, 0x75, 0x00, 0x61,
0x00, 0x72, 0x00, 0x65, 0x00, 0x2e, 0x00, 0x20, 0x00, 0x49, 0x00, 0x74,
0x00, 0x73, 0x00, 0x20, 0x00, 0x61, 0x00, 0x73, 0x00, 0x63, 0x00, 0x65,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x61, 0x00, 0x6e, 0x00, 0x64,
0x00, 0x20, 0x00, 0x64, 0x00, 0x65, 0x00, 0x73, 0x00, 0x63, 0x00, 0x65,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x69, 0x00, 0x73, 0x00, 0x20,
0x00, 0x65, 0x00, 0x78, 0x00, 0x61, 0x00, 0x63, 0x00, 0x74, 0x00, 0x6c,
0x00, 0x79, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20,
0x00, 0x73, 0x00, 0x69, 0x00, 0x7a, 0x00, 0x65, 0x00, 0x20, 0x00, 0x6f,
0x00, 0x66, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20,
0x00, 0x65, 0x00, 0x6d, 0x00, 0x20, 0x00, 0x73, 0x00, 0x71, 0x00, 0x75,
0x00, 0x61, 0x00, 0x72, 0x00, 0x65, 0x00, 0x2e, 0x00, 0x20, 0x00, 0x54,
0x00, 0x68, 0x00, 0x69, 0x00, 0x73, 0x00, 0x20, 0x00, 0x6d, 0x00, 0x65,
0x00, 0x61, 0x00, 0x6e, 0x00, 0x73, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68,
0x00, 0x61, 0x00, 0x74, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65,
0x00, 0x20, 0x00, 0x66, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x74, 0x00, 0x27,
0x00, 0x73, 0x00, 0x20, 0x00, 0x65, 0x00, 0x78, 0x00, 0x74, 0x00, 0x65,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x69, 0x00, 0x73, 0x00, 0x20,
0x00, 0x65, 0x00, 0x78, 0x00, 0x61, 0x00, 0x63, 0x00, 0x74, 0x00, 0x6c,
0x00, 0x79, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x65, 0x00, 0x20,
0x00, 0x73, 0x00, 0x61, 0x00, 0x6d, 0x00, 0x65, 0x00, 0x20, 0x00, 0x61,
0x00, 0x73, 0x00, 0x20, 0x00, 0x69, 0x00, 0x74, 0x00, 0x73, 0x00, 0x20,
0x00, 0x6c, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x65, 0x00, 0x2d, 0x00, 0x68,
0x00, 0x65, 0x00, 0x69, 0x00, 0x67, 0x00, 0x68, 0x00, 0x74, 0x00, 0x2c,
0x00, 0x20, 0x00, 0x6d, 0x00, 0x65, 0x00, 0x61, 0x00, 0x6e, 0x00, 0x69,
0x00, 0x6e, 0x00, 0x67, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68, 0x00, 0x61,
0x00, 0x74, 0x00, 0x20, 0x00, 0x69, 0x00, 0x74, 0x00, 0x20, 0x00, 0x63,
0x00, 0x61, 0x00, 0x6e, 0x00, 0x20, 0x00, 0x62, 0x00, 0x65, 0x00, 0x20,
0x00, 0x65, 0x00, 0x78, 0x00, 0x61, 0x00, 0x63, 0x00, 0x74, 0x00, 0x6c,
0x00, 0x79, 0x00, 0x20, 0x00, 0x61, 0x00, 0x6c, 0x00, 0x69, 0x00, 0x67,
0x00, 0x6e, 0x00, 0x65, 0x00, 0x64, 0x00, 0x20, 0x00, 0x77, 0x00, 0x69,
0x00, 0x74, 0x00, 0x68, 0x00, 0x20, 0x00, 0x70, 0x00, 0x61, 0x00, 0x64,
0x00, 0x64, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x67, 0x00, 0x2c, 0x00, 0x20,
0x00, 0x62, 0x00, 0x6f, 0x00, 0x72, 0x00, 0x64, 0x00, 0x65, 0x00, 0x72,
0x00, 0x73, 0x00, 0x2c, 0x00, 0x20, 0x00, 0x6d, 0x00, 0x61, 0x00, 0x72,
0x00, 0x67, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x73, 0x00, 0x2c, 0x00, 0x20,
0x00, 0x61, 0x00, 0x6e, 0x00, 0x64, 0x00, 0x20, 0x00, 0x73, 0x00, 0x6f,
0x00, 0x20, 0x00, 0x66, 0x00, 0x6f, 0x00, 0x72, 0x00, 0x74, 0x00, 0x68,
0x00, 0x2e, 0x00, 0x20, 0x00, 0x4d, 0x00, 0x6f, 0x00, 0x73, 0x00, 0x74,
0x00, 0x20, 0x00, 0x63, 0x00, 0x68, 0x00, 0x61, 0x00, 0x72, 0x00, 0x61,
0x00, 0x63, 0x00, 0x74, 0x00, 0x65, 0x00, 0x72, 0x00, 0x73, 0x00, 0x20,
0x00, 0x61, 0x00, 0x72, 0x00, 0x65, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68,
0x00, 0x65, 0x00, 0x20, 0x00, 0x73, 0x00, 0x6f, 0x00, 0x6c, 0x00, 0x69,
0x00, 0x64, 0x00, 0x20, 0x00, 0x65, 0x00, 0x6d, 0x00, 0x20, 0x00, 0x73,
0x00, 0x71, 0x00, 0x75, 0x00, 0x61, 0x00, 0x72, 0x00, 0x65, 0x00, 0x2c,
0x00, 0x20, 0x00, 0x65, 0x00, 0x78, 0x00, 0x63, 0x00, 0x65, 0x00, 0x70,
0x00, 0x74, 0x00, 0x20, 0x00, 0x22, 0x00, 0xc9, 0x00, 0x22, 0x00, 0x20,
0x00, 0x61, 0x00, 0x6e, 0x00, 0x64, 0x00, 0x20, 0x00, 0x22, 0x00, 0x70,
0x00, 0x22, 0x00, 0x2c, 0x00, 0x20, 0x00, 0x77, 0x00, 0x68, 0x00, 0x69,
0x00, 0x63, 0x00, 0x68, 0x00, 0x20, 0x00, 0x73, 0x00, 0x68, 0x00, 0x6f,
0x00, 0x77, 0x00, 0x20, 0x00, 0x61, 0x00, 0x73, 0x00, 0x63, 0x00, 0x65,
0x00, 0x6e, 0x00, 0x74, 0x00, 0x2f, 0x00, 0x64, 0x00, 0x65, 0x00, 0x73,
0x00, 0x63, 0x00, 0x65, 0x00, 0x6e, 0x00, 0x74, 0x00, 0x20, 0x00, 0x66,
0x00, 0x72, 0x00, 0x6f, 0x00, 0x6d, 0x00, 0x20, 0x00, 0x74, 0x00, 0x68,
0x00, 0x65, 0x00, 0x20, 0x00, 0x62, 0x00, 0x61, 0x00, 0x73, 0x00, 0x65,
0x00, 0x6c, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x65, 0x00, 0x2e, 0x00, 0x68,
0x00, 0x74, 0x00, 0x74, 0x00, 0x70, 0x00, 0x3a, 0x00, 0x2f, 0x00, 0x2f,
0x00, 0x77, 0x00, 0x77, 0x00, 0x77, 0x00, 0x2e, 0x00, 0x77, 0x00, 0x33,
0x00, 0x63, 0x00, 0x2e, 0x00, 0x6f, 0x00, 0x72, 0x00, 0x67, 0x00, 0x68,
0x00, 0x74, 0x00, 0x74, 0x00, 0x70, 0x00, 0x3a, 0x00, 0x2f, 0x00, 0x2f,
0x00, 0x64, 0x00, 0x65, 0x00, 0x76, 0x00, 0x2e, 0x00, 0x77, 0x00, 0x33,
0x00, 0x2e, 0x00, 0x6f, 0x00, 0x72, 0x00, 0x67, 0x00, 0x2f, 0x00, 0x43,
0x00, 0x53, 0x00, 0x53, 0x00, 0x2f, 0x00, 0x66, 0x00, 0x6f, 0x00, 0x6e,
0x00, 0x74, 0x00, 0x73, 0x00, 0x2f, 0x00, 0x61, 0x00, 0x68, 0x00, 0x65,
0x00, 0x6d, 0x00, 0x2f, 0x00, 0x43, 0x00, 0x4f, 0x00, 0x50, 0x00, 0x59,
0x00, 0x49, 0x00, 0x4e, 0x00, 0x47, 0x00, 0x0d, 0x54, 0x68, 0x65, 0x20,
0x41, 0x68, 0x65, 0x6d, 0x20, 0x66, 0x6f, 0x6e, 0x74, 0x20, 0x62, 0x65,
0x6c, 0x6f, 0x6e, 0x67, 0x73, 0x20, 0x74, 0x6f, 0x20, 0x74, 0x68, 0x65,
0x20, 0x70, 0x75, 0x62, 0x6c, 0x69, 0x63, 0x20, 0x64, 0x6f, 0x6d, 0x61,
0x69, 0x6e, 0x2e, 0x20, 0x49, 0x6e, 0x20, 0x6a, 0x75, 0x72, 0x69, 0x73,
0x64, 0x69, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x20, 0x74, 0x68, 0x61,
0x74, 0x20, 0x64, 0x6f, 0x20, 0x6e, 0x6f, 0x74, 0x20, 0x72, 0x65, 0x63,
0x6f, 0x67, 0x6e, 0x69, 0x7a, 0x65, 0x20, 0x70, 0x75, 0x62, 0x6c, 0x69,
0x63, 0x20, 0x64, 0x6f, 0x6d, 0x61, 0x69, 0x6e, 0x20, 0x6f, 0x77, 0x6e,
0x65, 0x72, 0x73, 0x68, 0x69, 0x70, 0x20, 0x6f, 0x66, 0x20, 0x74, 0x68,
0x65, 0x73, 0x65, 0x20, 0x66, 0x69, 0x6c, 0x65, 0x73, 0x2c, 0x20, 0x74,
0x68, 0x65, 0x20, 0x66, 0x6f, 0x6c, 0x6c, 0x6f, 0x77, 0x69, 0x6e, 0x67,
0x20, 0x43, 0x72, 0x65, 0x61, 0x74, 0x69, 0x76, 0x65, 0x20, 0x43, 0x6f,
0x6d, 0x6d, 0x6f, 0x6e, 0x73, 0x20, 0x5a, 0x65, 0x72, 0x6f, 0x20, 0x64,
0x65, 0x63, 0x6c, 0x61, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x20, 0x61,
0x70, 0x70, 0x6c, 0x69, 0x65, 0x73, 0x3a, 0x20, 0x68, 0x74, 0x74, 0x70,
0x3a, 0x2f, 0x2f, 0x6c, 0x61, 0x62, 0x73, 0x2e, 0x63, 0x72, 0x65, 0x61,
0x74, 0x69, 0x76, 0x65, 0x63, 0x6f, 0x6d, 0x6d, 0x6f, 0x6e, 0x73, 0x2e,
0x6f, 0x72, 0x67, 0x2f, 0x6c, 0x69, 0x63, 0x65, 0x6e, 0x73, 0x65, 0x73,
0x2f, 0x7a, 0x65, 0x72, 0x6f, 0x2d, 0x77, 0x61, 0x69, 0x76, 0x65, 0x2f,
0x31, 0x2e, 0x30, 0x2f, 0x75, 0x73, 0x2f, 0x6c, 0x65, 0x67, 0x61, 0x6c,
0x63, 0x6f, 0x64, 0x65, 0x52, 0x65, 0x67, 0x75, 0x6c, 0x61, 0x72, 0x56,
0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x20, 0x31, 0x2e, 0x32, 0x30, 0x20,
0x41, 0x68, 0x65, 0x6d, 0x54, 0x68, 0x65, 0x20, 0x41, 0x68, 0x65, 0x6d,
0x20, 0x66, 0x6f, 0x6e, 0x74, 0x20, 0x77, 0x61, 0x73, 0x20, 0x64, 0x65,
0x76, 0x65, 0x6c, 0x6f, 0x70, 0x65, 0x64, 0x20, 0x62, 0x79, 0x20, 0x54,
0x6f, 0x64, 0x64, 0x20, 0x46, 0x61, 0x68, 0x72, 0x6e, 0x65, 0x72, 0x20,
0x74, 0x6f, 0x20, 0x68, 0x65, 0x6c, 0x70, 0x20, 0x74, 0x65, 0x73, 0x74,
0x20, 0x77, 0x72, 0x69, 0x74, 0x65, 0x72, 0x73, 0x20, 0x20, 0x64, 0x65,
0x76, 0x65, 0x6c, 0x6f, 0x70, 0x20, 0x70, 0x72, 0x65, 0x64, 0x69, 0x63,
0x74, 0x61, 0x62, 0x6c, 0x65, 0x20, 0x74, 0x65, 0x73, 0x74, 0x73, 0x2e,
0x20, 0x54, 0x68, 0x65, 0x20, 0x66, 0x6f, 0x6e, 0x74, 0x27, 0x73, 0x20,
0x65, 0x6d, 0x20, 0x73, 0x71, 0x75, 0x61, 0x72, 0x65, 0x20, 0x69, 0x73,
0x20, 0x65, 0x78, 0x61, 0x63, 0x74, 0x6c, 0x79, 0x20, 0x73, 0x71, 0x75,
0x61, 0x72, 0x65, 0x2e, 0x20, 0x49, 0x74, 0x73, 0x20, 0x61, 0x73, 0x63,
0x65, 0x6e, 0x74, 0x20, 0x61, 0x6e, 0x64, 0x20, 0x64, 0x65, 0x73, 0x63,
0x65, 0x6e, 0x74, 0x20, 0x69, 0x73, 0x20, 0x65, 0x78, 0x61, 0x63, 0x74,
0x6c, 0x79, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x69, 0x7a, 0x65, 0x20,
0x6f, 0x66, 0x20, 0x74, 0x68, 0x65, 0x20, 0x65, 0x6d, 0x20, 0x73, 0x71,
0x75, 0x61, 0x72, 0x65, 0x2e, 0x20, 0x54, 0x68, 0x69, 0x73, 0x20, 0x6d,
0x65, 0x61, 0x6e, 0x73, 0x20, 0x74, 0x68, 0x61, 0x74, 0x20, 0x74, 0x68,
0x65, 0x20, 0x66, 0x6f, 0x6e, 0x74, 0x27, 0x73, 0x20, 0x65, 0x78, 0x74,
0x65, 0x6e, 0x74, 0x20, 0x69, 0x73, 0x20, 0x65, 0x78, 0x61, 0x63, 0x74,
0x6c, 0x79, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x61, 0x6d, 0x65, 0x20,
0x61, 0x73, 0x20, 0x69, 0x74, 0x73, 0x20, 0x6c, 0x69, 0x6e, 0x65, 0x2d,
0x68, 0x65, 0x69, 0x67, 0x68, 0x74, 0x2c, 0x20, 0x6d, 0x65, 0x61, 0x6e,
0x69, 0x6e, 0x67, 0x20, 0x74, 0x68, 0x61, 0x74, 0x20, 0x69, 0x74, 0x20,
0x63, 0x61, 0x6e, 0x20, 0x62, 0x65, 0x20, 0x65, 0x78, 0x61, 0x63, 0x74,
0x6c, 0x79, 0x20, 0x61, 0x6c, 0x69, 0x67, 0x6e, 0x65, 0x64, 0x20, 0x77,
0x69, 0x74, 0x68, 0x20, 0x70, 0x61, 0x64, 0x64, 0x69, 0x6e, 0x67, 0x2c,
0x20, 0x62, 0x6f, 0x72, 0x64, 0x65, 0x72, 0x73, 0x2c, 0x20, 0x6d, 0x61,
0x72, 0x67, 0x69, 0x6e, 0x73, 0x2c, 0x20, 0x61, 0x6e, 0x64, 0x20, 0x73,
0x6f, 0x20, 0x66, 0x6f, 0x72, 0x74, 0x68, 0x2e, 0x20, 0x4d, 0x6f, 0x73,
0x74, 0x20, 0x63, 0x68, 0x61, 0x72, 0x61, 0x63, 0x74, 0x65, 0x72, 0x73,
0x20, 0x61, 0x72, 0x65, 0x20, 0x74, 0x68, 0x65, 0x20, 0x73, 0x6f, 0x6c,
0x69, 0x64, 0x20, 0x65, 0x6d, 0x20, 0x73, 0x71, 0x75, 0x61, 0x72, 0x65,
0x2c, 0x20, 0x65, 0x78, 0x63, 0x65, 0x70, 0x74, 0x20, 0x22, 0x83, 0x22,
0x20, 0x61, 0x6e, 0x64, 0x20, 0x22, 0x70, 0x22, 0x2c, 0x20, 0x77, 0x68,
0x69, 0x63, 0x68, 0x20, 0x73, 0x68, 0x6f, 0x77, 0x20, 0x61, 0x73, 0x63,
0x65, 0x6e, 0x74, 0x2f, 0x64, 0x65, 0x73, 0x63, 0x65, 0x6e, 0x74, 0x20,
0x66, 0x72, 0x6f, 0x6d, 0x20, 0x74, 0x68, 0x65, 0x20, 0x62, 0x61, 0x73,
0x65, 0x6c, 0x69, 0x6e, 0x65, 0x2e, 0x68, 0x74, 0x74, 0x70, 0x3a, 0x2f,
0x2f, 0x77, 0x77, 0x77, 0x2e, 0x77, 0x33, 0x63, 0x2e, 0x6f, 0x72, 0x67,
0x68, 0x74, 0x74, 0x70, 0x3a, 0x2f, 0x2f, 0x64, 0x65, 0x76, 0x2e, 0x77,
0x33, 0x2e, 0x6f, 0x72, 0x67, 0x2f, 0x43, 0x53, 0x53, 0x2f, 0x66, 0x6f,
0x6e, 0x74, 0x73, 0x2f, 0x61, 0x68, 0x65, 0x6d, 0x2f, 0x43, 0x4f, 0x50,
0x59, 0x49, 0x4e, 0x47, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0xff, 0x7b, 0x00, 0x14, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x01, 0x01, 0x00, 0x00, 0x01, 0x02, 0x01, 0x03, 0x00, 0x03, 0x00, 0x04,
0x00, 0x05, 0x00, 0x06, 0x00, 0x07, 0x00, 0x08, 0x00, 0x09, 0x00, 0x0b,
0x00, 0x0c, 0x00, 0x0d, 0x00, 0x0e, 0x00, 0x0f, 0x00, 0x10, 0x00, 0x11,
0x00, 0x12, 0x00, 0x13, 0x00, 0x14, 0x00, 0x15, 0x00, 0x16, 0x00, 0x17,
0x00, 0x18, 0x00, 0x19, 0x00, 0x1a, 0x00, 0x1b, 0x00, 0x1c, 0x00, 0x1d,
0x00, 0x1e, 0x00, 0x1f, 0x00, 0x20, 0x00, 0x21, 0x00, 0x22, 0x00, 0x23,
0x00, 0x24, 0x00, 0x25, 0x00, 0x26, 0x00, 0x27, 0x00, 0x28, 0x00, 0x29,
0x00, 0x2a, 0x00, 0x2b, 0x00, 0x2c, 0x00, 0x2d, 0x00, 0x2e, 0x00, 0x2f,
0x00, 0x30, 0x00, 0x31, 0x00, 0x32, 0x00, 0x33, 0x00, 0x34, 0x00, 0x35,
0x00, 0x36, 0x00, 0x37, 0x00, 0x38, 0x00, 0x39, 0x00, 0x3a, 0x00, 0x3b,
0x00, 0x3c, 0x00, 0x3d, 0x00, 0x3e, 0x00, 0x3f, 0x00, 0x40, 0x00, 0x41,
0x00, 0x42, 0x00, 0x43, 0x00, 0x44, 0x00, 0x45, 0x00, 0x46, 0x00, 0x47,
0x00, 0x48, 0x00, 0x49, 0x00, 0x4a, 0x00, 0x4b, 0x00, 0x4c, 0x00, 0x4d,
0x00, 0x4e, 0x00, 0x4f, 0x00, 0x50, 0x00, 0x51, 0x00, 0x52, 0x00, 0x53,
0x00, 0x54, 0x00, 0x55, 0x00, 0x56, 0x00, 0x57, 0x00, 0x58, 0x00, 0x59,
0x00, 0x5a, 0x00, 0x5b, 0x00, 0x5c, 0x00, 0x5d, 0x00, 0x5e, 0x00, 0x5f,
0x00, 0x60, 0x00, 0x61, 0x00, 0x62, 0x00, 0x63, 0x00, 0x64, 0x00, 0x65,
0x00, 0x66, 0x00, 0x67, 0x00, 0x68, 0x00, 0x69, 0x00, 0x6a, 0x00, 0x6b,
0x00, 0x6c, 0x00, 0x6d, 0x00, 0x6e, 0x00, 0x6f, 0x00, 0x70, 0x00, 0x71,
0x00, 0x72, 0x00, 0x73, 0x00, 0x74, 0x00, 0x75, 0x00, 0x76, 0x00, 0x77,
0x00, 0x78, 0x00, 0x79, 0x00, 0x7a, 0x00, 0x7b, 0x00, 0x7c, 0x00, 0x7d,
0x00, 0x7e, 0x00, 0x7f, 0x00, 0x80, 0x00, 0x81, 0x00, 0x83, 0x00, 0x84,
0x00, 0x85, 0x00, 0x86, 0x00, 0x88, 0x00, 0x89, 0x00, 0x8a, 0x00, 0x8b,
0x00, 0x8d, 0x00, 0x8e, 0x00, 0x90, 0x00, 0x91, 0x00, 0x93, 0x00, 0x96,
0x00, 0x97, 0x00, 0x9d, 0x00, 0x9e, 0x00, 0xa0, 0x00, 0xa1, 0x00, 0xa2,
0x00, 0xa3, 0x00, 0xa4, 0x00, 0xa9, 0x00, 0xaa, 0x00, 0xac, 0x00, 0xad,
0x00, 0xae, 0x00, 0xaf, 0x00, 0xb6, 0x00, 0xb7, 0x00, 0xb8, 0x00, 0xba,
0x00, 0xbd, 0x00, 0xc3, 0x00, 0xc7, 0x00, 0xc8, 0x00, 0xc9, 0x00, 0xca,
0x00, 0xcb, 0x00, 0xcc, 0x00, 0xcd, 0x00, 0xce, 0x00, 0xcf, 0x00, 0xd0,
0x00, 0xd1, 0x00, 0xd3, 0x00, 0xd4, 0x00, 0xd5, 0x00, 0xd6, 0x00, 0xd7,
0x00, 0xd8, 0x00, 0xd9, 0x00, 0xda, 0x00, 0xdb, 0x00, 0xdc, 0x00, 0xdd,
0x00, 0xde, 0x00, 0xdf, 0x00, 0xe0, 0x00, 0xe1, 0x00, 0xe8, 0x00, 0xe9,
0x00, 0xea, 0x00, 0xeb, 0x00, 0xec, 0x00, 0xed, 0x00, 0xee, 0x00, 0xef,
0x00, 0xf0, 0x00, 0xf1, 0x00, 0xf2, 0x00, 0xf3, 0x00, 0xf4, 0x00, 0xf5,
0x00, 0xf6, 0x01, 0x04, 0x01, 0x05, 0x00, 0xb0, 0x00, 0xb1, 0x00, 0xbb,
0x00, 0xa6, 0x00, 0xa8, 0x00, 0x9f, 0x00, 0x9b, 0x00, 0xb2, 0x00, 0xb3,
0x00, 0xc4, 0x00, 0xb4, 0x00, 0xb5, 0x00, 0xc5, 0x00, 0x82, 0x00, 0xc2,
0x00, 0x87, 0x00, 0xab, 0x00, 0xc6, 0x00, 0xbe, 0x00, 0xbf, 0x00, 0xbc,
0x00, 0x8c, 0x00, 0x98, 0x00, 0x9a, 0x00, 0x99, 0x00, 0xa5, 0x00, 0x92,
0x00, 0x9c, 0x00, 0x8f, 0x00, 0x94, 0x00, 0x95, 0x00, 0xa7, 0x00, 0xb9,
0x00, 0xd2, 0x00, 0xc0, 0x00, 0xc1, 0x01, 0x06, 0x00, 0x02, 0x01, 0x07,
0x01, 0x08, 0x01, 0x09, 0x01, 0x0a, 0x01, 0x0b, 0x01, 0x0c, 0x01, 0x0d,
0x01, 0x0e, 0x01, 0x0f, 0x01, 0x10, 0x01, 0x11, 0x01, 0x12, 0x01, 0x13,
0x04, 0x4e, 0x55, 0x4c, 0x4c, 0x08, 0x67, 0x6c, 0x79, 0x70, 0x68, 0x32,
0x34, 0x33, 0x08, 0x67, 0x6c, 0x79, 0x70, 0x68, 0x32, 0x30, 0x34, 0x08,
0x67, 0x6c, 0x79, 0x70, 0x68, 0x32, 0x30, 0x35, 0x02, 0x48, 0x54, 0x03,
0x44, 0x45, 0x4c, 0x07, 0x75, 0x6e, 0x69, 0x46, 0x45, 0x46, 0x46, 0x07,
0x75, 0x6e, 0x69, 0x32, 0x30, 0x30, 0x32, 0x07, 0x75, 0x6e, 0x69, 0x32,
0x30, 0x30, 0x33, 0x07, 0x75, 0x6e, 0x69, 0x32, 0x30, 0x30, 0x34, 0x07,
0x75, 0x6e, 0x69, 0x32, 0x30, 0x30, 0x35, 0x07, 0x75, 0x6e, 0x69, 0x32,
0x30, 0x30, 0x36, 0x07, 0x75, 0x6e, 0x69, 0x32, 0x30, 0x30, 0x39, 0x07,
0x75, 0x6e, 0x69, 0x32, 0x30, 0x30, 0x41, 0x07, 0x75, 0x6e, 0x69, 0x32,
0x30, 0x30, 0x42, 0x07, 0x75, 0x6e, 0x69, 0x33, 0x30, 0x30, 0x30, 0x09,
0x61, 0x66, 0x69, 0x69, 0x36, 0x31, 0x36, 0x36, 0x34, 0x07, 0x61, 0x66,
0x69, 0x69, 0x33, 0x30, 0x31, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x08, 0x00, 0x02, 0x00, 0x10, 0x00, 0x01, 0xff, 0xff, 0x00, 0x03};
static const unsigned int kAhemFontLength = 13884;
// "Cough" font is Flutter's custom test font and may expand to cover
// font-dependent testing specific features that "Ahem" cannot cover.
//
// Features and description of Cough:
//
// * EM square size of 1000. This is atypical of a power of 2 EM size, but
// is included to make it easier to tell the final value a metric is meant
// to be. For example, with fontSize 100, 10 units = 1 pixel.
// * The EM square has an ascent of 800 and a descent of 200.
// * The HHead, typo and win metrics are identical, making this font platform
// agnostic.
// * The ASCII glyphs of "A", "a", and "g" are included. This will likely expand
// in the future to include representative CJK glyphs as well as hanging
// glyphs. These glyphs are meant for testing purposes only, and only vaguely
// look like the character in order to minimize the size of the font.
// * Cap height is 800.
// * X height is 500.
// * Underline height is -100.
static const unsigned char kCoughFont[] = {
0x00, 0x01, 0x00, 0x00, 0x00, 0x0d, 0x00, 0x80, 0x00, 0x03, 0x00, 0x50,
0x46, 0x46, 0x54, 0x4d, 0x89, 0xba, 0x1a, 0x0b, 0x00, 0x00, 0x06, 0x10,
0x00, 0x00, 0x00, 0x1c, 0x47, 0x44, 0x45, 0x46, 0x00, 0x27, 0x00, 0x2d,
0x00, 0x00, 0x05, 0xe8, 0x00, 0x00, 0x00, 0x26, 0x4f, 0x53, 0x2f, 0x32,
0x7d, 0x51, 0x4a, 0x11, 0x00, 0x00, 0x01, 0x58, 0x00, 0x00, 0x00, 0x60,
0x63, 0x6d, 0x61, 0x70, 0x01, 0x14, 0x07, 0x66, 0x00, 0x00, 0x01, 0xd0,
0x00, 0x00, 0x01, 0x6a, 0x67, 0x61, 0x73, 0x70, 0xff, 0xff, 0x00, 0x03,
0x00, 0x00, 0x05, 0xe0, 0x00, 0x00, 0x00, 0x08, 0x67, 0x6c, 0x79, 0x66,
0x53, 0x7d, 0x78, 0xff, 0x00, 0x00, 0x03, 0x4c, 0x00, 0x00, 0x01, 0x08,
0x68, 0x65, 0x61, 0x64, 0x15, 0x1b, 0x17, 0x3d, 0x00, 0x00, 0x00, 0xdc,
0x00, 0x00, 0x00, 0x36, 0x68, 0x68, 0x65, 0x61, 0x05, 0x84, 0x01, 0x3b,
0x00, 0x00, 0x01, 0x14, 0x00, 0x00, 0x00, 0x24, 0x68, 0x6d, 0x74, 0x78,
0x05, 0x51, 0xff, 0xfd, 0x00, 0x00, 0x01, 0xb8, 0x00, 0x00, 0x00, 0x18,
0x6c, 0x6f, 0x63, 0x61, 0x00, 0x8c, 0x00, 0xea, 0x00, 0x00, 0x03, 0x3c,
0x00, 0x00, 0x00, 0x10, 0x6d, 0x61, 0x78, 0x70, 0x00, 0x0b, 0x00, 0x1d,
0x00, 0x00, 0x01, 0x38, 0x00, 0x00, 0x00, 0x20, 0x6e, 0x61, 0x6d, 0x65,
0x3f, 0x88, 0x53, 0x13, 0x00, 0x00, 0x04, 0x54, 0x00, 0x00, 0x01, 0x59,
0x70, 0x6f, 0x73, 0x74, 0xff, 0xed, 0x00, 0x71, 0x00, 0x00, 0x05, 0xb0,
0x00, 0x00, 0x00, 0x30, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x39, 0x28, 0xcc, 0xe3, 0x5f, 0x0f, 0x3c, 0xf5, 0x00, 0x0b, 0x03, 0xe8,
0x00, 0x00, 0x00, 0x00, 0xd9, 0xe6, 0x56, 0x09, 0x00, 0x00, 0x00, 0x00,
0xd9, 0xe6, 0x7e, 0x49, 0xff, 0xfe, 0xff, 0x37, 0x02, 0x31, 0x02, 0xcf,
0x00, 0x00, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0x03, 0x52, 0xff, 0x06, 0x00, 0x00, 0x02, 0x30,
0xff, 0xfe, 0xff, 0xff, 0x02, 0x31, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x05,
0x00, 0x01, 0x00, 0x00, 0x00, 0x07, 0x00, 0x1c, 0x00, 0x03, 0x00, 0x00,
0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x01, 0xe7,
0x01, 0x90, 0x00, 0x05, 0x00, 0x00, 0x00, 0x28, 0x00, 0x28, 0x00, 0x28,
0x00, 0x28, 0x00, 0x28, 0x00, 0x28, 0x00, 0x28, 0x00, 0x28, 0x00, 0x28,
0x00, 0xc8, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x42, 0x69, 0x72, 0x64, 0x00, 0x40,
0x00, 0x00, 0x00, 0x67, 0x03, 0x52, 0xff, 0x06, 0x00, 0x64, 0x03, 0x52,
0x00, 0xfa, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x01, 0xf4,
0x03, 0x20, 0x00, 0x00, 0x00, 0x20, 0x00, 0x01, 0x02, 0x12, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x0e, 0x00, 0x00,
0x02, 0x30, 0xff, 0xfe, 0x00, 0x00, 0xff, 0xff, 0x00, 0x00, 0x00, 0x03,
0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x1c, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x00, 0x64, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x1c,
0x00, 0x04, 0x00, 0x48, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x08, 0x00, 0x02,
0x00, 0x06, 0x00, 0x00, 0x00, 0x0d, 0x00, 0x20, 0x00, 0x41, 0x00, 0x61,
0x00, 0x67, 0xff, 0xff, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0d, 0x00, 0x20,
0x00, 0x41, 0x00, 0x61, 0x00, 0x67, 0xff, 0xff, 0x00, 0x01, 0xff, 0xf5,
0xff, 0xe3, 0xff, 0xc3, 0xff, 0xa4, 0xff, 0x9f, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x01, 0x06, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x01, 0x02, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01,
0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x05, 0x00, 0x00, 0x00, 0x00,
0x00, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x1c, 0x00, 0x1c, 0x00, 0x1c, 0x00, 0x1c, 0x00, 0x2e,
0x00, 0x54, 0x00, 0x84, 0x00, 0x02, 0x00, 0x00, 0x00, 0x32, 0x01, 0x90,
0x02, 0x9e, 0x00, 0x07, 0x00, 0x0f, 0x00, 0x00, 0x37, 0x32, 0x33, 0x34,
0x11, 0x22, 0x23, 0x14, 0x27, 0x32, 0x21, 0x14, 0x11, 0x22, 0x21, 0x34,
0x32, 0x64, 0xc8, 0x64, 0xc8, 0x32, 0x85, 0x01, 0x0b, 0x85, 0xfe, 0xf5,
0x64, 0xad, 0x01, 0x5b, 0xad, 0xdf, 0xcf, 0xfe, 0x63, 0xcf, 0x00, 0x00,
0x00, 0x01, 0xff, 0xfe, 0xff, 0xff, 0x02, 0x30, 0x02, 0xcf, 0x00, 0x05,
0x00, 0x00, 0x05, 0x22, 0x25, 0x36, 0x13, 0x16, 0x02, 0x30, 0x8d, 0xfe,
0x5b, 0x48, 0xd9, 0x44, 0x01, 0x01, 0xb4, 0x02, 0x1b, 0xb4, 0x00, 0x00,
0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x02, 0x31, 0x02, 0x30, 0x00, 0x0b,
0x00, 0x15, 0x00, 0x00, 0x31, 0x30, 0x31, 0x32, 0x37, 0x36, 0x37, 0x16,
0x13, 0x06, 0x27, 0x26, 0x01, 0x06, 0x07, 0x36, 0x37, 0x36, 0x23, 0x22,
0x27, 0x26, 0x02, 0x6b, 0x6e, 0x48, 0x44, 0xca, 0x8c, 0xd2, 0xd3, 0x01,
0x17, 0x18, 0x49, 0x34, 0x4e, 0x4e, 0x01, 0x02, 0x28, 0x29, 0xd0, 0xd4,
0x8c, 0x8c, 0xfe, 0x5e, 0x01, 0x01, 0x01, 0x01, 0x1b, 0x2e, 0x88, 0x01,
0x02, 0x02, 0x42, 0x43, 0x00, 0x03, 0xff, 0xff, 0xff, 0x37, 0x02, 0x30,
0x02, 0x30, 0x00, 0x07, 0x00, 0x11, 0x00, 0x1b, 0x00, 0x00, 0x03, 0x32,
0x21, 0x06, 0x11, 0x22, 0x05, 0x34, 0x13, 0x06, 0x03, 0x36, 0x25, 0x34,
0x27, 0x34, 0x31, 0x06, 0x01, 0x14, 0x15, 0x16, 0x17, 0x30, 0x17, 0x34,
0x35, 0x26, 0x01, 0x8c, 0x01, 0xa5, 0x01, 0x8c, 0xfe, 0x5d, 0x55, 0x01,
0x03, 0x67, 0x01, 0x33, 0x01, 0x65, 0xfe, 0xcb, 0x85, 0x85, 0xa0, 0xe6,
0x02, 0x30, 0xbe, 0xfd, 0xc6, 0x01, 0xbe, 0x01, 0xe4, 0x65, 0xfe, 0xd2,
0x01, 0x01, 0x65, 0x98, 0x97, 0x01, 0xfd, 0xfb, 0x31, 0x31, 0x02, 0x01,
0x01, 0x19, 0x4a, 0x01, 0x00, 0x00, 0x00, 0x0e, 0x00, 0xae, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x08, 0x00, 0x15, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x07, 0x00, 0x2e, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x08, 0x00, 0x48, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x08, 0x00, 0x63, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x05, 0x00, 0x0b, 0x00, 0x84, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x08, 0x00, 0xa2, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x01, 0x00, 0x10, 0x00, 0x03, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x02, 0x00, 0x0e, 0x00, 0x1e, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x03, 0x00, 0x10, 0x00, 0x36, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x04, 0x00, 0x10, 0x00, 0x51, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x05, 0x00, 0x16, 0x00, 0x6c, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x06, 0x00, 0x10, 0x00, 0x90, 0x00, 0x00,
0x00, 0x00, 0x54, 0x00, 0x79, 0x00, 0x70, 0x00, 0x65, 0x00, 0x66, 0x00,
0x61, 0x00, 0x63, 0x00, 0x65, 0x00, 0x00, 0x54, 0x79, 0x70, 0x65, 0x66,
0x61, 0x63, 0x65, 0x00, 0x00, 0x52, 0x00, 0x65, 0x00, 0x67, 0x00, 0x75,
0x00, 0x6c, 0x00, 0x61, 0x00, 0x72, 0x00, 0x00, 0x52, 0x65, 0x67, 0x75,
0x6c, 0x61, 0x72, 0x00, 0x00, 0x54, 0x00, 0x79, 0x00, 0x70, 0x00, 0x65,
0x00, 0x66, 0x00, 0x61, 0x00, 0x63, 0x00, 0x65, 0x00, 0x00, 0x54, 0x79,
0x70, 0x65, 0x66, 0x61, 0x63, 0x65, 0x00, 0x00, 0x54, 0x00, 0x79, 0x00,
0x70, 0x00, 0x65, 0x00, 0x66, 0x00, 0x61, 0x00, 0x63, 0x00, 0x65, 0x00,
0x00, 0x54, 0x79, 0x70, 0x65, 0x66, 0x61, 0x63, 0x65, 0x00, 0x00, 0x56,
0x00, 0x65, 0x00, 0x72, 0x00, 0x73, 0x00, 0x69, 0x00, 0x6f, 0x00, 0x6e,
0x00, 0x20, 0x00, 0x31, 0x00, 0x2e, 0x00, 0x30, 0x00, 0x00, 0x56, 0x65,
0x72, 0x73, 0x69, 0x6f, 0x6e, 0x20, 0x31, 0x2e, 0x30, 0x00, 0x00, 0x54,
0x00, 0x79, 0x00, 0x70, 0x00, 0x65, 0x00, 0x66, 0x00, 0x61, 0x00, 0x63,
0x00, 0x65, 0x00, 0x00, 0x54, 0x79, 0x70, 0x65, 0x66, 0x61, 0x63, 0x65,
0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0xff, 0x9c, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x07, 0x00, 0x00, 0x00, 0x01, 0x00, 0x02, 0x00, 0x03, 0x00, 0x24,
0x00, 0x44, 0x00, 0x4a, 0x00, 0x00, 0x00, 0x01, 0xff, 0xff, 0x00, 0x02,
0x00, 0x01, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x00, 0x00, 0x16, 0x00, 0x1e,
0x00, 0x02, 0x00, 0x01, 0x00, 0x01, 0x00, 0x06, 0x00, 0x01, 0x00, 0x04,
0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00,
0xd5, 0xed, 0x45, 0xb8, 0x00, 0x00, 0x00, 0x00, 0xd9, 0xe6, 0x56, 0x09,
0x00, 0x00, 0x00, 0x00, 0xd9, 0xe6, 0x7e, 0x49};
static const unsigned int kCoughFontLength = 1576;
static const unsigned char kFlutterTestFont[] = {
0x00, 0x01, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x80, 0x00, 0x03, 0x00, 0x60,
0x42, 0x41, 0x53, 0x45, 0x8a, 0x4b, 0x87, 0x01, 0x00, 0x00, 0x0b, 0x24,
0x00, 0x00, 0x00, 0x82, 0x47, 0x44, 0x45, 0x46, 0x00, 0x29, 0x00, 0x14,
0x00, 0x00, 0x0b, 0x04, 0x00, 0x00, 0x00, 0x1e, 0x4f, 0x53, 0x2f, 0x32,
0x13, 0xfd, 0x90, 0xf2, 0x00, 0x00, 0x01, 0x68, 0x00, 0x00, 0x00, 0x60,
0x63, 0x6d, 0x61, 0x70, 0xec, 0x11, 0x06, 0x0f, 0x00, 0x00, 0x02, 0x00,
0x00, 0x00, 0x05, 0x04, 0x63, 0x76, 0x74, 0x20, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x07, 0x18, 0x00, 0x00, 0x00, 0x02, 0x67, 0x6c, 0x79, 0x66,
0x09, 0x85, 0x89, 0xf8, 0x00, 0x00, 0x07, 0x3c, 0x00, 0x00, 0x00, 0xd8,
0x68, 0x65, 0x61, 0x64, 0x81, 0x1b, 0xef, 0xfd, 0x00, 0x00, 0x00, 0xec,
0x00, 0x00, 0x00, 0x36, 0x68, 0x68, 0x65, 0x61, 0x07, 0x02, 0x03, 0x0f,
0x00, 0x00, 0x01, 0x24, 0x00, 0x00, 0x00, 0x24, 0x68, 0x6d, 0x74, 0x78,
0x1b, 0x86, 0x00, 0x80, 0x00, 0x00, 0x01, 0xc8, 0x00, 0x00, 0x00, 0x38,
0x6c, 0x6f, 0x63, 0x61, 0x02, 0x7e, 0x02, 0x50, 0x00, 0x00, 0x07, 0x1c,
0x00, 0x00, 0x00, 0x1e, 0x6d, 0x61, 0x78, 0x70, 0x00, 0x52, 0x00, 0x2d,
0x00, 0x00, 0x01, 0x48, 0x00, 0x00, 0x00, 0x20, 0x6e, 0x61, 0x6d, 0x65,
0x1a, 0xef, 0x7a, 0x5b, 0x00, 0x00, 0x08, 0x14, 0x00, 0x00, 0x02, 0x25,
0x70, 0x6f, 0x73, 0x74, 0x89, 0x84, 0x28, 0x37, 0x00, 0x00, 0x0a, 0x3c,
0x00, 0x00, 0x00, 0xc7, 0x70, 0x72, 0x65, 0x70, 0x6f, 0x48, 0x68, 0x25,
0x00, 0x00, 0x07, 0x04, 0x00, 0x00, 0x00, 0x11, 0x00, 0x01, 0x00, 0x00,
0x00, 0x01, 0x00, 0x00, 0xab, 0xb4, 0x91, 0x15, 0x5f, 0x0f, 0x3c, 0xf5,
0x00, 0x1f, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x8e, 0xf4, 0x56, 0x80,
0x00, 0x00, 0x00, 0x00, 0x8e, 0xf4, 0x56, 0x80, 0x00, 0x00, 0xff, 0x00,
0x04, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x02, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0x03, 0x00, 0xff, 0x00,
0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x0e, 0x00, 0x01, 0x00, 0x00, 0x00, 0x0e, 0x00, 0x08,
0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x01,
0x00, 0x01, 0x00, 0x00, 0x00, 0x40, 0x00, 0x22, 0x00, 0x00, 0x00, 0x00,
0x00, 0x04, 0x02, 0x05, 0x01, 0x90, 0x00, 0x05, 0x00, 0x00, 0x02, 0x99,
0x02, 0xcc, 0x00, 0x00, 0x00, 0x8f, 0x02, 0x99, 0x02, 0xcc, 0x00, 0x00,
0x01, 0xeb, 0x00, 0x33, 0x01, 0x09, 0x00, 0x00, 0x02, 0x00, 0x05, 0x03,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x80, 0x00, 0x00, 0x03, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x80, 0x00, 0x20, 0xfe, 0xff, 0x03, 0x00, 0xff, 0x00,
0x00, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x20, 0x00, 0x01,
0x04, 0x00, 0x00, 0x80, 0x00, 0x00, 0x00, 0x00, 0x01, 0x55, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00, 0x04, 0x00, 0x00, 0x00,
0x04, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x01, 0x55, 0x00, 0x00,
0x01, 0x00, 0x00, 0x00, 0x00, 0xaa, 0x00, 0x00, 0x00, 0xcc, 0x00, 0x00,
0x00, 0x66, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x00, 0x00, 0x03, 0x00, 0x00, 0x00, 0x1c, 0x00, 0x01, 0x00, 0x00,
0x00, 0x00, 0x03, 0xfe, 0x00, 0x03, 0x00, 0x01, 0x00, 0x00, 0x00, 0x1c,
0x00, 0x04, 0x03, 0xe2, 0x00, 0x00, 0x00, 0x64, 0x00, 0x40, 0x00, 0x05,
0x00, 0x24, 0x00, 0x7e, 0x00, 0xff, 0x01, 0x31, 0x01, 0x53, 0x01, 0x78,
0x01, 0x92, 0x02, 0xc9, 0x02, 0xdd, 0x03, 0x94, 0x03, 0xa9, 0x03, 0xc0,
0x20, 0x0a, 0x20, 0x26, 0x20, 0x30, 0x20, 0x3a, 0x20, 0x44, 0x21, 0x26,
0x22, 0x06, 0x22, 0x12, 0x22, 0x1e, 0x22, 0x2b, 0x22, 0x48, 0x22, 0x65,
0x22, 0xf2, 0x25, 0xca, 0x30, 0x07, 0x4e, 0x03, 0x4e, 0x09, 0x4e, 0x2d,
0x4e, 0x5d, 0x4e, 0x8c, 0x4e, 0x94, 0x51, 0x6d, 0x53, 0x41, 0x54, 0x26,
0x56, 0xdb, 0x57, 0x1f, 0x65, 0x87, 0x66, 0x2f, 0x67, 0x2c, 0x6b, 0x63,
0x6c, 0x34, 0x6d, 0x4b, 0x70, 0x6b, 0x78, 0x6e, 0x8b, 0xd5, 0x91, 0xd1,
0xf0, 0x02, 0xfe, 0xff, 0xff, 0xff, 0x00, 0x00, 0x00, 0x20, 0x00, 0xa1,
0x01, 0x31, 0x01, 0x52, 0x01, 0x78, 0x01, 0x92, 0x02, 0xc6, 0x02, 0xd8,
0x03, 0x94, 0x03, 0xa5, 0x03, 0xbc, 0x20, 0x02, 0x20, 0x13, 0x20, 0x30,
0x20, 0x39, 0x20, 0x44, 0x21, 0x22, 0x22, 0x02, 0x22, 0x0f, 0x22, 0x19,
0x22, 0x2b, 0x22, 0x48, 0x22, 0x60, 0x22, 0xf2, 0x25, 0xca, 0x30, 0x07,
0x4e, 0x00, 0x4e, 0x09, 0x4e, 0x2d, 0x4e, 0x5d, 0x4e, 0x8c, 0x4e, 0x94,
0x51, 0x6b, 0x53, 0x41, 0x54, 0x26, 0x56, 0xd7, 0x57, 0x1f, 0x65, 0x87,
0x66, 0x2f, 0x67, 0x28, 0x6b, 0x63, 0x6c, 0x34, 0x6d, 0x4b, 0x70, 0x6b,
0x78, 0x6e, 0x8b, 0xd5, 0x91, 0xd1, 0xf0, 0x00, 0xfe, 0xff, 0xff, 0xff,
0x00, 0x00, 0x00, 0x00, 0xfe, 0xd2, 0x00, 0x00, 0xfe, 0x8b, 0xfe, 0x71,
0x00, 0x00, 0x00, 0x00, 0xfc, 0x6f, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0xdf, 0xd3, 0x00, 0x00, 0xdf, 0xbf, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0xdd, 0xd8, 0xdd, 0xbb, 0x00, 0x00, 0xdd, 0x11,
0xda, 0x39, 0xcf, 0xfc, 0x00, 0x00, 0xb1, 0xfa, 0xb1, 0xd6, 0xb1, 0xa6,
0xb1, 0x77, 0xb1, 0x6f, 0x00, 0x00, 0xac, 0xc2, 0xab, 0xdd, 0x00, 0x00,
0xa8, 0xe4, 0x9a, 0x7c, 0x99, 0xd4, 0x00, 0x00, 0x94, 0xa0, 0x93, 0xcf,
0x92, 0xb8, 0x8f, 0x98, 0x87, 0x95, 0x74, 0x2e, 0x6e, 0x32, 0x00, 0x00,
0x01, 0x0e, 0x00, 0x01, 0x00, 0x64, 0x01, 0x20, 0x00, 0x00, 0x01, 0xda,
0x00, 0x00, 0x00, 0x00, 0x01, 0xd8, 0x01, 0xde, 0x00, 0x00, 0x01, 0xe6,
0x01, 0xee, 0x01, 0xf6, 0x02, 0x06, 0x00, 0x00, 0x02, 0x2a, 0x00, 0x00,
0x02, 0x2a, 0x02, 0x32, 0x02, 0x3a, 0x02, 0x40, 0x00, 0x00, 0x00, 0x00,
0x02, 0x46, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x4a, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x46, 0x00, 0x00,
0x00, 0x00, 0x02, 0x46, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x48,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x02, 0x42, 0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x04, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x05, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x03, 0x00, 0x00,
0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x07, 0x00, 0x00, 0x00, 0x08, 0x00, 0x09, 0x00, 0x0a, 0x00, 0x00,
0x00, 0x00, 0x00, 0x0b, 0x00, 0x0c, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03,
0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03,
0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03,
0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00,
0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x03, 0x00, 0x00,
0x01, 0x06, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x01, 0x02, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x06, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x00, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x00,
0x03, 0x03, 0x03, 0x05, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x00, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x00, 0x03, 0x03, 0x00, 0x00,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x00, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03,
0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x03, 0x18, 0xb8, 0x00, 0x00,
0x4c, 0xb8, 0x00, 0xc0, 0x63, 0xb8, 0x00, 0x04, 0x62, 0x20, 0x67, 0x61,
0x44, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x26,
0x00, 0x26, 0x00, 0x26, 0x00, 0x3c, 0x00, 0x54, 0x00, 0x6c, 0x00, 0x6c,
0x00, 0x6c, 0x00, 0x6c, 0x00, 0x6c, 0x00, 0x6c, 0x00, 0x6c, 0x00, 0x6c,
0x00, 0x6c, 0x00, 0x00, 0x00, 0x02, 0x00, 0x80, 0x00, 0x00, 0x03, 0x80,
0x03, 0x00, 0x00, 0x03, 0x00, 0x07, 0x00, 0x22, 0x00, 0xb3, 0x00, 0x00,
0x00, 0x00, 0x16, 0xe0, 0x12, 0xb2, 0x01, 0x01, 0x01, 0x15, 0x36, 0x16,
0xb0, 0x00, 0x2f, 0xb0, 0x01, 0x2f, 0xb0, 0x02, 0x2f, 0xb0, 0x03, 0x2f,
0xb0, 0x04, 0x2f, 0xb0, 0x07, 0x2f, 0x25, 0x21, 0x11, 0x21, 0x03, 0x11,
0x21, 0x11, 0x01, 0x00, 0x02, 0x00, 0xfe, 0x00, 0x80, 0x03, 0x00, 0x80,
0x02, 0x00, 0xfd, 0x80, 0x03, 0x00, 0xfd, 0x00, 0x00, 0x01, 0x00, 0x00,
0xff, 0x00, 0x04, 0x00, 0x03, 0x00, 0x00, 0x03, 0x00, 0x11, 0x00, 0xb3,
0x00, 0x00, 0x00, 0x00, 0x16, 0xe0, 0x12, 0xb2, 0x01, 0x01, 0x01, 0x15,
0x36, 0x16, 0x00, 0x19, 0x01, 0x21, 0x11, 0x04, 0x00, 0xff, 0x00, 0x04,
0x00, 0xfc, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00, 0xff, 0x00, 0x04, 0x00,
0x00, 0x00, 0x00, 0x03, 0x00, 0x16, 0x00, 0xb3, 0x00, 0x00, 0x00, 0x00,
0x16, 0xe0, 0x12, 0xb2, 0x01, 0x01, 0x01, 0x15, 0x36, 0x16, 0xb0, 0x01,
0x2f, 0xb0, 0x02, 0x2f, 0x19, 0x01, 0x21, 0x11, 0x04, 0x00, 0xff, 0x00,
0x01, 0x00, 0xff, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00,
0x03, 0x00, 0x00, 0x03, 0x00, 0x16, 0x00, 0xb3, 0x00, 0x00, 0x00, 0x00,
0x16, 0xe0, 0x12, 0xb2, 0x01, 0x01, 0x01, 0x15, 0x36, 0x16, 0xb0, 0x00,
0x2f, 0xb0, 0x03, 0x2f, 0x31, 0x11, 0x21, 0x11, 0x04, 0x00, 0x03, 0x00,
0xfd, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x0e, 0x00, 0xae, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1d, 0x00, 0x3c, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x07, 0x00, 0x6a, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x07, 0x00, 0x82, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x03, 0x00, 0x26, 0x00, 0xd8, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x04, 0x00, 0x0b, 0x01, 0x17, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x05, 0x00, 0x0f, 0x01, 0x43, 0x00, 0x01,
0x00, 0x00, 0x00, 0x00, 0x00, 0x06, 0x00, 0x0b, 0x01, 0x6b, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x00, 0x00, 0x3a, 0x00, 0x00, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x01, 0x00, 0x0e, 0x00, 0x5a, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x02, 0x00, 0x0e, 0x00, 0x72, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x03, 0x00, 0x4c, 0x00, 0x8a, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x04, 0x00, 0x16, 0x00, 0xff, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x05, 0x00, 0x1e, 0x01, 0x23, 0x00, 0x03,
0x00, 0x01, 0x04, 0x09, 0x00, 0x06, 0x00, 0x16, 0x01, 0x53, 0x00, 0x43,
0x00, 0x6f, 0x00, 0x70, 0x00, 0x79, 0x00, 0x72, 0x00, 0x69, 0x00, 0x67,
0x00, 0x68, 0x00, 0x74, 0x00, 0x20, 0x00, 0x28, 0x00, 0x63, 0x00, 0x29,
0x00, 0x20, 0x00, 0x31, 0x00, 0x39, 0x00, 0x38, 0x00, 0x30, 0x00, 0x2c,
0x00, 0x20, 0x00, 0x41, 0x00, 0x6e, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x79,
0x00, 0x6d, 0x00, 0x6f, 0x00, 0x75, 0x00, 0x73, 0x00, 0x00, 0x43, 0x6f,
0x70, 0x79, 0x72, 0x69, 0x67, 0x68, 0x74, 0x20, 0x28, 0x63, 0x29, 0x20,
0x31, 0x39, 0x38, 0x30, 0x2c, 0x20, 0x41, 0x6e, 0x6f, 0x6e, 0x79, 0x6d,
0x6f, 0x75, 0x73, 0x00, 0x00, 0x4d, 0x00, 0x69, 0x00, 0x6e, 0x00, 0x67,
0x00, 0x4c, 0x00, 0x69, 0x00, 0x55, 0x00, 0x00, 0x4d, 0x69, 0x6e, 0x67,
0x4c, 0x69, 0x55, 0x00, 0x00, 0x52, 0x00, 0x65, 0x00, 0x67, 0x00, 0x75,
0x00, 0x6c, 0x00, 0x61, 0x00, 0x72, 0x00, 0x00, 0x52, 0x65, 0x67, 0x75,
0x6c, 0x61, 0x72, 0x00, 0x00, 0x46, 0x00, 0x6f, 0x00, 0x6e, 0x00, 0x74,
0x00, 0x46, 0x00, 0x6f, 0x00, 0x72, 0x00, 0x67, 0x00, 0x65, 0x00, 0x20,
0x00, 0x32, 0x00, 0x2e, 0x00, 0x30, 0x00, 0x20, 0x00, 0x3a, 0x00, 0x20,
0x00, 0x46, 0x00, 0x6c, 0x00, 0x75, 0x00, 0x74, 0x00, 0x74, 0x00, 0x65,
0x00, 0x72, 0x00, 0x54, 0x00, 0x65, 0x00, 0x73, 0x00, 0x74, 0x00, 0x20,
0x00, 0x3a, 0x00, 0x20, 0x00, 0x31, 0x00, 0x2d, 0x00, 0x31, 0x00, 0x2d,
0x00, 0x31, 0x00, 0x39, 0x00, 0x38, 0x00, 0x30, 0x00, 0x00, 0x46, 0x6f,
0x6e, 0x74, 0x46, 0x6f, 0x72, 0x67, 0x65, 0x20, 0x32, 0x2e, 0x30, 0x20,
0x3a, 0x20, 0x46, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x54, 0x65, 0x73,
0x74, 0x20, 0x3a, 0x20, 0x31, 0x2d, 0x31, 0x2d, 0x31, 0x39, 0x38, 0x30,
0x00, 0x00, 0x46, 0x00, 0x6c, 0x00, 0x75, 0x00, 0x74, 0x00, 0x74, 0x00,
0x65, 0x00, 0x72, 0x00, 0x54, 0x00, 0x65, 0x00, 0x73, 0x00, 0x74, 0x00,
0x00, 0x46, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x54, 0x65, 0x73, 0x74,
0x00, 0x00, 0x56, 0x00, 0x65, 0x00, 0x72, 0x00, 0x73, 0x00, 0x69, 0x00,
0x6f, 0x00, 0x6e, 0x00, 0x20, 0x00, 0x30, 0x00, 0x30, 0x00, 0x31, 0x00,
0x2e, 0x00, 0x30, 0x00, 0x30, 0x00, 0x30, 0x00, 0x00, 0x56, 0x65, 0x72,
0x73, 0x69, 0x6f, 0x6e, 0x20, 0x30, 0x30, 0x31, 0x2e, 0x30, 0x30, 0x30,
0x00, 0x00, 0x46, 0x00, 0x6c, 0x00, 0x75, 0x00, 0x74, 0x00, 0x74, 0x00,
0x65, 0x00, 0x72, 0x00, 0x54, 0x00, 0x65, 0x00, 0x73, 0x00, 0x74, 0x00,
0x00, 0x46, 0x6c, 0x75, 0x74, 0x74, 0x65, 0x72, 0x54, 0x65, 0x73, 0x74,
0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0xff, 0x7e, 0x00, 0x13, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
0x00, 0x0e, 0x00, 0x00, 0x00, 0x01, 0x00, 0x02, 0x01, 0x02, 0x01, 0x03,
0x01, 0x04, 0x01, 0x05, 0x01, 0x06, 0x01, 0x07, 0x01, 0x08, 0x01, 0x09,
0x01, 0x0a, 0x01, 0x0b, 0x01, 0x0c, 0x06, 0x53, 0x71, 0x75, 0x61, 0x72,
0x65, 0x0e, 0x41, 0x73, 0x63, 0x65, 0x6e, 0x74, 0x20, 0x46, 0x6c, 0x75,
0x73, 0x68, 0x65, 0x64, 0x0f, 0x44, 0x65, 0x73, 0x63, 0x65, 0x6e, 0x74,
0x20, 0x46, 0x6c, 0x75, 0x73, 0x68, 0x65, 0x64, 0x0c, 0x46, 0x75, 0x6c,
0x6c, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0b, 0x31, 0x2f,
0x32, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0b, 0x31, 0x2f,
0x33, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0b, 0x31, 0x2f,
0x34, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0b, 0x31, 0x2f,
0x36, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0b, 0x31, 0x2f,
0x35, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0c, 0x31, 0x2f,
0x31, 0x30, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x0c, 0x5a,
0x65, 0x72, 0x6f, 0x20, 0x41, 0x64, 0x76, 0x61, 0x6e, 0x63, 0x65, 0x00,
0x00, 0x01, 0x00, 0x00, 0x00, 0x0c, 0x00, 0x00, 0x00, 0x16, 0x00, 0x00,
0x00, 0x02, 0x00, 0x01, 0x00, 0x03, 0x00, 0x0d, 0x00, 0x01, 0x00, 0x04,
0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x00,
0x00, 0x08, 0x00, 0x00, 0x00, 0x04, 0x00, 0x12, 0x00, 0x03, 0x68, 0x61,
0x6e, 0x67, 0x69, 0x64, 0x65, 0x6f, 0x72, 0x6f, 0x6d, 0x6e, 0x00, 0x03,
0x67, 0x72, 0x65, 0x6b, 0x00, 0x14, 0x68, 0x61, 0x6e, 0x69, 0x00, 0x30,
0x6c, 0x61, 0x74, 0x6e, 0x00, 0x4c, 0x00, 0x06, 0x00, 0x00, 0x00, 0x00,
0x00, 0x02, 0x00, 0x03, 0x00, 0x0a, 0x00, 0x0e, 0x00, 0x12, 0x00, 0x01,
0x03, 0x00, 0x00, 0x01, 0xff, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x06,
0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x00, 0x03, 0x00, 0x0a, 0x00, 0x0e,
0x00, 0x12, 0x00, 0x01, 0x03, 0x00, 0x00, 0x01, 0xff, 0x00, 0x00, 0x01,
0x00, 0x00, 0x00, 0x06, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x03,
0x00, 0x0a, 0x00, 0x0e, 0x00, 0x12, 0x00, 0x01, 0x03, 0x00, 0x00, 0x01,
0xff, 0x00, 0x00, 0x01, 0x00, 0x00, 0x00, 0x00};
static const unsigned int kFlutterTestFontLength = 2984;
#endif // EMBED_TEST_FONT_DATA
namespace flutter {
std::vector<sk_sp<SkTypeface>> GetTestFontData() {
std::vector<sk_sp<SkTypeface>> typefaces;
#if EMBED_TEST_FONT_DATA
sk_sp<SkFontMgr> font_mgr = txt::GetDefaultFontManager();
typefaces.push_back(font_mgr->makeFromStream(
SkMemoryStream::MakeDirect(kFlutterTestFont, kFlutterTestFontLength)));
typefaces.push_back(font_mgr->makeFromStream(
SkMemoryStream::MakeDirect(kAhemFont, kAhemFontLength)));
typefaces.push_back(font_mgr->makeFromStream(
SkMemoryStream::MakeDirect(kCoughFont, kCoughFontLength)));
#endif // EMBED_TEST_FONT_DATA
return typefaces;
}
std::vector<std::string> GetTestFontFamilyNames() {
std::vector<std::string> names;
#if EMBED_TEST_FONT_DATA
names = {"FlutterTest", "Ahem", "Cough"};
#endif // EMBED_TEST_FONT_DATA
return names;
}
} // namespace flutter
| engine/runtime/test_font_data.cc/0 | {
"file_path": "engine/runtime/test_font_data.cc",
"repo_id": "engine",
"token_count": 82100
} | 295 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_SHELL_COMMON_DISPLAY_H_
#define FLUTTER_SHELL_COMMON_DISPLAY_H_
#include <optional>
#include "flutter/fml/macros.h"
#include "flutter/shell/common/variable_refresh_rate_reporter.h"
namespace flutter {
/// Unique ID per display that is stable until the Flutter application restarts.
/// See also: `flutter::Display`
typedef size_t DisplayId;
/// To be used when the display refresh rate is unknown.
static constexpr double kUnknownDisplayRefreshRate = 0;
/// The POD of a |Display|. This data is for a point in time and suitable
/// for copying.
struct DisplayData {
DisplayId id;
double width;
double height;
double pixel_ratio;
double refresh_rate;
};
/// Display refers to a graphics hardware system consisting of a framebuffer,
/// typically a monitor or a screen. This class holds the various display
/// settings.
class Display {
public:
//------------------------------------------------------------------------------
/// @brief Construct a new Display object in case where the display id of the
/// display is known.
Display(DisplayId display_id,
double refresh_rate,
double width,
double height,
double device_pixel_ratio)
: display_id_(display_id),
refresh_rate_(refresh_rate),
width_(width),
height_(height),
device_pixel_ratio_(device_pixel_ratio) {}
virtual ~Display() = default;
// Get the display's maximum refresh rate in the unit of frame per second.
// Return `kUnknownDisplayRefreshRate` if the refresh rate is unknown.
virtual double GetRefreshRate() const;
/// Returns the `DisplayId` of the display.
DisplayId GetDisplayId() const { return display_id_; }
/// The width of the display in physical pixels.
virtual double GetWidth() const { return width_; }
/// The height of the display in physical pixels.
virtual double GetHeight() const { return height_; }
/// The device pixel ratio of the display.
virtual double GetDevicePixelRatio() const { return device_pixel_ratio_; }
DisplayData GetDisplayData() {
return DisplayData{
.id = GetDisplayId(),
.width = GetWidth(),
.height = GetHeight(),
.pixel_ratio = GetDevicePixelRatio(),
.refresh_rate = GetRefreshRate(),
};
}
private:
DisplayId display_id_;
double refresh_rate_;
double width_;
double height_;
double device_pixel_ratio_;
FML_DISALLOW_COPY_AND_ASSIGN(Display);
};
} // namespace flutter
#endif // FLUTTER_SHELL_COMMON_DISPLAY_H_
| engine/shell/common/display.h/0 | {
"file_path": "engine/shell/common/display.h",
"repo_id": "engine",
"token_count": 857
} | 296 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_SHELL_COMMON_PIPELINE_H_
#define FLUTTER_SHELL_COMMON_PIPELINE_H_
#include <deque>
#include <memory>
#include <mutex>
#include "flutter/flow/frame_timings.h"
#include "flutter/flow/layers/layer_tree.h"
#include "flutter/fml/macros.h"
#include "flutter/fml/memory/ref_counted.h"
#include "flutter/fml/synchronization/semaphore.h"
#include "flutter/fml/trace_event.h"
namespace flutter {
struct PipelineProduceResult {
// Whether the item was successfully pushed into the pipeline.
bool success = false;
// Whether it is the first item of the pipeline. Only valid when 'success' is
// 'true'.
bool is_first_item = false;
};
enum class PipelineConsumeResult {
// NOLINTBEGIN(readability-identifier-naming)
NoneAvailable,
Done,
MoreAvailable,
// NOLINTEND(readability-identifier-naming)
};
size_t GetNextPipelineTraceID();
/// A thread-safe queue of resources for a single consumer and a single
/// producer, with a maximum queue depth.
///
/// Pipelines support two key operations: produce and consume.
///
/// The consumer calls |Consume| to wait for a resource to be produced and
/// consume it when ready.
///
/// The producer calls |Produce| to generate a `ProducerContinuation` which
/// provides a means to enqueue a resource in the pipeline, if the pipeline is
/// below its maximum depth. When the resource has been prepared, the producer
/// calls `Complete` on the continuation, which enqueues the resource and
/// signals the waiting consumer.
///
/// Pipelines generate the following tracing information:
/// * PipelineItem: async flow tracking time taken from the time a producer
/// calls |Produce| to the time a consumer consumes calls |Consume|.
/// * PipelineProduce: async flow tracking time taken from the time a producer
/// calls |Produce| to the time they complete the `ProducerContinuation` with
/// a resource.
/// * Pipeline Depth: counter of inflight resource producers.
///
/// The primary use of this class is as the frame pipeline used in Flutter's
/// animator/rasterizer.
template <class R>
class Pipeline {
public:
using Resource = R;
using ResourcePtr = std::unique_ptr<Resource>;
/// Denotes a spot in the pipeline reserved for the producer to finish
/// preparing a completed pipeline resource.
class ProducerContinuation {
public:
ProducerContinuation() : trace_id_(0) {}
ProducerContinuation(ProducerContinuation&& other)
: continuation_(other.continuation_), trace_id_(other.trace_id_) {
other.continuation_ = nullptr;
other.trace_id_ = 0;
}
ProducerContinuation& operator=(ProducerContinuation&& other) {
std::swap(continuation_, other.continuation_);
std::swap(trace_id_, other.trace_id_);
return *this;
}
~ProducerContinuation() {
if (continuation_) {
continuation_(nullptr, trace_id_);
TRACE_EVENT_ASYNC_END0("flutter", "PipelineProduce", trace_id_);
// The continuation is being dropped on the floor. End the flow.
TRACE_FLOW_END("flutter", "PipelineItem", trace_id_);
TRACE_EVENT_ASYNC_END0("flutter", "PipelineItem", trace_id_);
}
}
/// Completes the continuation with the specified resource.
[[nodiscard]] PipelineProduceResult Complete(ResourcePtr resource) {
PipelineProduceResult result;
if (continuation_) {
result = continuation_(std::move(resource), trace_id_);
continuation_ = nullptr;
TRACE_EVENT_ASYNC_END0("flutter", "PipelineProduce", trace_id_);
TRACE_FLOW_STEP("flutter", "PipelineItem", trace_id_);
}
return result;
}
explicit operator bool() const { return continuation_ != nullptr; }
private:
friend class Pipeline;
using Continuation =
std::function<PipelineProduceResult(ResourcePtr, size_t)>;
Continuation continuation_;
uint64_t trace_id_;
ProducerContinuation(const Continuation& continuation, uint64_t trace_id)
: continuation_(continuation), trace_id_(trace_id) {
TRACE_EVENT_ASYNC_BEGIN0_WITH_FLOW_IDS("flutter", "PipelineItem",
trace_id_, /*flow_id_count=*/1,
/*flow_ids=*/&trace_id);
TRACE_FLOW_BEGIN("flutter", "PipelineItem", trace_id_);
TRACE_EVENT_ASYNC_BEGIN0("flutter", "PipelineProduce", trace_id_);
}
FML_DISALLOW_COPY_AND_ASSIGN(ProducerContinuation);
};
explicit Pipeline(uint32_t depth)
: empty_(depth), available_(0), inflight_(0) {}
~Pipeline() = default;
bool IsValid() const { return empty_.IsValid() && available_.IsValid(); }
/// Creates a `ProducerContinuation` that a producer can use to add a
/// resource to the queue.
///
/// If the queue is already at its maximum depth, the `ProducerContinuation`
/// is returned with success = false.
ProducerContinuation Produce() {
if (!empty_.TryWait()) {
return {};
}
++inflight_;
FML_TRACE_COUNTER("flutter", "Pipeline Depth",
reinterpret_cast<int64_t>(this), //
"frames in flight", inflight_.load() //
);
return ProducerContinuation{
std::bind(&Pipeline::ProducerCommit, this, std::placeholders::_1,
std::placeholders::_2), // continuation
GetNextPipelineTraceID()}; // trace id
}
/// Creates a `ProducerContinuation` that will only push the task if the
/// queue is empty.
///
/// Prefer using |Produce|. ProducerContinuation returned by this method
/// doesn't guarantee that the frame will be rendered.
ProducerContinuation ProduceIfEmpty() {
if (!empty_.TryWait()) {
return {};
}
++inflight_;
FML_TRACE_COUNTER("flutter", "Pipeline Depth",
reinterpret_cast<int64_t>(this), //
"frames in flight", inflight_.load() //
);
return ProducerContinuation{
std::bind(&Pipeline::ProducerCommitIfEmpty, this, std::placeholders::_1,
std::placeholders::_2), // continuation
GetNextPipelineTraceID()}; // trace id
}
using Consumer = std::function<void(ResourcePtr)>;
/// @note Procedure doesn't copy all closures.
[[nodiscard]] PipelineConsumeResult Consume(const Consumer& consumer) {
if (consumer == nullptr) {
return PipelineConsumeResult::NoneAvailable;
}
if (!available_.TryWait()) {
return PipelineConsumeResult::NoneAvailable;
}
ResourcePtr resource;
size_t trace_id = 0;
size_t items_count = 0;
{
std::scoped_lock lock(queue_mutex_);
std::tie(resource, trace_id) = std::move(queue_.front());
queue_.pop_front();
items_count = queue_.size();
}
consumer(std::move(resource));
empty_.Signal();
--inflight_;
TRACE_FLOW_END("flutter", "PipelineItem", trace_id);
TRACE_EVENT_ASYNC_END0("flutter", "PipelineItem", trace_id);
return items_count > 0 ? PipelineConsumeResult::MoreAvailable
: PipelineConsumeResult::Done;
}
private:
fml::Semaphore empty_;
fml::Semaphore available_;
std::atomic<int> inflight_;
std::mutex queue_mutex_;
std::deque<std::pair<ResourcePtr, size_t>> queue_;
/// Commits a produced resource to the queue and signals the consumer that a
/// resource is available.
PipelineProduceResult ProducerCommit(ResourcePtr resource, size_t trace_id) {
bool is_first_item = false;
{
std::scoped_lock lock(queue_mutex_);
is_first_item = queue_.empty();
queue_.emplace_back(std::move(resource), trace_id);
}
// Ensure the queue mutex is not held as that would be a pessimization.
available_.Signal();
return {.success = true, .is_first_item = is_first_item};
}
PipelineProduceResult ProducerCommitIfEmpty(ResourcePtr resource,
size_t trace_id) {
{
std::scoped_lock lock(queue_mutex_);
if (!queue_.empty()) {
// Bail if the queue is not empty, opens up spaces to produce other
// frames.
empty_.Signal();
return {.success = false, .is_first_item = false};
}
queue_.emplace_back(std::move(resource), trace_id);
}
// Ensure the queue mutex is not held as that would be a pessimization.
available_.Signal();
return {.success = true, .is_first_item = true};
}
FML_DISALLOW_COPY_AND_ASSIGN(Pipeline);
};
} // namespace flutter
#endif // FLUTTER_SHELL_COMMON_PIPELINE_H_
| engine/shell/common/pipeline.h/0 | {
"file_path": "engine/shell/common/pipeline.h",
"repo_id": "engine",
"token_count": 3249
} | 297 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_SHELL_COMMON_SERIALIZATION_CALLBACKS_H_
#define FLUTTER_SHELL_COMMON_SERIALIZATION_CALLBACKS_H_
#include "flutter/fml/logging.h"
#include "third_party/skia/include/core/SkImage.h"
#include "third_party/skia/include/core/SkTypeface.h"
namespace flutter {
sk_sp<SkData> SerializeTypefaceWithoutData(SkTypeface* typeface, void* ctx);
sk_sp<SkData> SerializeTypefaceWithData(SkTypeface* typeface, void* ctx);
sk_sp<SkTypeface> DeserializeTypefaceWithoutData(const void* data,
size_t length,
void* ctx);
// Serializes only the metadata of the image and not the underlying pixel data.
sk_sp<SkData> SerializeImageWithoutData(SkImage* image, void* ctx);
sk_sp<SkImage> DeserializeImageWithoutData(const void* data,
size_t length,
void* ctx);
} // namespace flutter
#endif // FLUTTER_SHELL_COMMON_SERIALIZATION_CALLBACKS_H_
| engine/shell/common/serialization_callbacks.h/0 | {
"file_path": "engine/shell/common/serialization_callbacks.h",
"repo_id": "engine",
"token_count": 536
} | 298 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_SHELL_COMMON_SHELL_TEST_PLATFORM_VIEW_METAL_H_
#define FLUTTER_SHELL_COMMON_SHELL_TEST_PLATFORM_VIEW_METAL_H_
#include "flutter/fml/macros.h"
#include "flutter/shell/common/shell_test_platform_view.h"
#include "flutter/shell/gpu/gpu_surface_metal_delegate.h"
namespace flutter {
namespace testing {
class DarwinContextMetal;
class ShellTestPlatformViewMetal final : public ShellTestPlatformView,
public GPUSurfaceMetalDelegate {
public:
ShellTestPlatformViewMetal(PlatformView::Delegate& delegate,
const TaskRunners& task_runners,
std::shared_ptr<ShellTestVsyncClock> vsync_clock,
CreateVsyncWaiter create_vsync_waiter,
std::shared_ptr<ShellTestExternalViewEmbedder>
shell_test_external_view_embedder,
const std::shared_ptr<const fml::SyncSwitch>&
is_gpu_disabled_sync_switch);
// |ShellTestPlatformView|
virtual ~ShellTestPlatformViewMetal() override;
private:
const std::unique_ptr<DarwinContextMetal> metal_context_;
const CreateVsyncWaiter create_vsync_waiter_;
const std::shared_ptr<ShellTestVsyncClock> vsync_clock_;
const std::shared_ptr<ShellTestExternalViewEmbedder>
shell_test_external_view_embedder_;
// |ShellTestPlatformView|
virtual void SimulateVSync() override;
// |PlatformView|
std::unique_ptr<VsyncWaiter> CreateVSyncWaiter() override;
// |PlatformView|
std::shared_ptr<ExternalViewEmbedder> CreateExternalViewEmbedder() override;
// |PlatformView|
PointerDataDispatcherMaker GetDispatcherMaker() override;
// |PlatformView|
std::unique_ptr<Surface> CreateRenderingSurface() override;
// |PlatformView|
std::shared_ptr<impeller::Context> GetImpellerContext() const override;
// |GPUSurfaceMetalDelegate|
GPUCAMetalLayerHandle GetCAMetalLayer(
const SkISize& frame_info) const override;
// |GPUSurfaceMetalDelegate|
bool PresentDrawable(GrMTLHandle drawable) const override;
// |GPUSurfaceMetalDelegate|
GPUMTLTextureInfo GetMTLTexture(const SkISize& frame_info) const override;
// |GPUSurfaceMetalDelegate|
bool PresentTexture(GPUMTLTextureInfo texture) const override;
FML_DISALLOW_COPY_AND_ASSIGN(ShellTestPlatformViewMetal);
};
} // namespace testing
} // namespace flutter
#endif // FLUTTER_SHELL_COMMON_SHELL_TEST_PLATFORM_VIEW_METAL_H_
| engine/shell/common/shell_test_platform_view_metal.h/0 | {
"file_path": "engine/shell/common/shell_test_platform_view_metal.h",
"repo_id": "engine",
"token_count": 1052
} | 299 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include <initializer_list>
#include "flutter/common/settings.h"
#include "flutter/fml/command_line.h"
#include "flutter/shell/common/switches.h"
#include "gtest/gtest.h"
// TODO(zanderso): https://github.com/flutter/flutter/issues/127701
// NOLINTBEGIN(bugprone-unchecked-optional-access)
namespace flutter {
namespace testing {
TEST(SwitchesTest, SkiaTraceAllowlistFlag) {
fml::CommandLine command_line =
fml::CommandLineFromInitializerList({"command"});
Settings settings = SettingsFromCommandLine(command_line);
#if !FLUTTER_RELEASE
EXPECT_TRUE(settings.trace_skia);
EXPECT_TRUE(settings.trace_skia_allowlist.has_value());
EXPECT_EQ(settings.trace_skia_allowlist->size(), 1ul);
#else
EXPECT_FALSE(settings.trace_skia);
#endif
command_line =
fml::CommandLineFromInitializerList({"command", "--trace-skia"});
settings = SettingsFromCommandLine(command_line);
#if !FLUTTER_RELEASE
EXPECT_TRUE(settings.trace_skia);
EXPECT_FALSE(settings.trace_skia_allowlist.has_value());
#else
EXPECT_FALSE(settings.trace_skia);
#endif
command_line = fml::CommandLineFromInitializerList(
{"command", "--trace-skia-allowlist=aaa,bbb,ccc"});
settings = SettingsFromCommandLine(command_line);
#if !FLUTTER_RELEASE
EXPECT_TRUE(settings.trace_skia);
EXPECT_TRUE(settings.trace_skia_allowlist.has_value());
EXPECT_EQ(settings.trace_skia_allowlist->size(), 3ul);
EXPECT_EQ(settings.trace_skia_allowlist->back(), "ccc");
#else
EXPECT_FALSE(settings.trace_skia);
#endif
}
TEST(SwitchesTest, TraceToFile) {
fml::CommandLine command_line = fml::CommandLineFromInitializerList(
{"command", "--trace-to-file=trace.binpb"});
EXPECT_TRUE(command_line.HasOption("trace-to-file"));
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.trace_to_file, "trace.binpb");
}
TEST(SwitchesTest, RouteParsedFlag) {
fml::CommandLine command_line =
fml::CommandLineFromInitializerList({"command", "--route=/animation"});
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.route, "/animation");
command_line = fml::CommandLineFromInitializerList({"command", "--route"});
settings = SettingsFromCommandLine(command_line);
EXPECT_TRUE(settings.route.empty());
}
TEST(SwitchesTest, MsaaSamples) {
for (int samples : {0, 1, 2, 4, 8, 16}) {
fml::CommandLine command_line = fml::CommandLineFromInitializerList(
{"command", ("--msaa-samples=" + std::to_string(samples)).c_str()});
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.msaa_samples, samples);
}
fml::CommandLine command_line =
fml::CommandLineFromInitializerList({"command", "--msaa-samples=3"});
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.msaa_samples, 0);
command_line =
fml::CommandLineFromInitializerList({"command", "--msaa-samples=foobar"});
settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.msaa_samples, 0);
}
TEST(SwitchesTest, EnableEmbedderAPI) {
{
// enable
fml::CommandLine command_line = fml::CommandLineFromInitializerList(
{"command", "--enable-embedder-api"});
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.enable_embedder_api, true);
}
{
// default
fml::CommandLine command_line =
fml::CommandLineFromInitializerList({"command"});
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.enable_embedder_api, false);
}
}
TEST(SwitchesTest, NoEnableImpeller) {
{
// enable
fml::CommandLine command_line =
fml::CommandLineFromInitializerList({"command", "--enable-impeller"});
EXPECT_TRUE(command_line.HasOption("enable-impeller"));
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.enable_impeller, true);
}
{
// disable
fml::CommandLine command_line = fml::CommandLineFromInitializerList(
{"command", "--enable-impeller=false"});
EXPECT_TRUE(command_line.HasOption("enable-impeller"));
Settings settings = SettingsFromCommandLine(command_line);
EXPECT_EQ(settings.enable_impeller, false);
}
}
} // namespace testing
} // namespace flutter
// NOLINTEND(bugprone-unchecked-optional-access)
| engine/shell/common/switches_unittests.cc/0 | {
"file_path": "engine/shell/common/switches_unittests.cc",
"repo_id": "engine",
"token_count": 1615
} | 300 |
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import("//flutter/shell/config.gni")
template("shell_gpu_configuration") {
assert(defined(invoker.enable_software),
"Caller must declare if the Software backend must be enabled.")
assert(defined(invoker.enable_vulkan),
"Caller must declare if the Vulkan backend must be enabled.")
assert(defined(invoker.enable_gl),
"Caller must declare if the Open GL backend must be enabled.")
assert(defined(invoker.enable_metal),
"Caller must declare if the Metal backend must be enabled.")
group(target_name) {
public_deps = []
if (invoker.enable_software) {
public_deps += [ "//flutter/shell/gpu:gpu_surface_software" ]
}
if (invoker.enable_gl) {
public_deps += [ "//flutter/shell/gpu:gpu_surface_gl" ]
}
if (invoker.enable_vulkan) {
public_deps += [ "//flutter/shell/gpu:gpu_surface_vulkan" ]
}
if (invoker.enable_metal) {
public_deps += [ "//flutter/shell/gpu:gpu_surface_metal" ]
}
}
config("${target_name}_config") {
defines = []
if (invoker.enable_software) {
defines += [ "SHELL_ENABLE_SOFTWARE" ]
}
if (invoker.enable_gl) {
defines += [ "SHELL_ENABLE_GL" ]
}
if (invoker.enable_vulkan) {
defines += [ "SHELL_ENABLE_VULKAN" ]
}
if (invoker.enable_metal) {
defines += [ "SHELL_ENABLE_METAL" ]
}
}
}
| engine/shell/gpu/gpu.gni/0 | {
"file_path": "engine/shell/gpu/gpu.gni",
"repo_id": "engine",
"token_count": 605
} | 301 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter/shell/platform/android/android_context_gl_skia.h"
#include <utility>
#include "flutter/fml/trace_event.h"
#include "flutter/shell/platform/android/android_egl_surface.h"
namespace flutter {
template <class T>
using EGLResult = std::pair<bool, T>;
static EGLResult<EGLContext> CreateContext(EGLDisplay display,
EGLConfig config,
EGLContext share = EGL_NO_CONTEXT) {
EGLint attributes[] = {EGL_CONTEXT_CLIENT_VERSION, 2, EGL_NONE};
EGLContext context = eglCreateContext(display, config, share, attributes);
return {context != EGL_NO_CONTEXT, context};
}
static EGLResult<EGLConfig> ChooseEGLConfiguration(EGLDisplay display,
uint8_t msaa_samples) {
EGLint sample_buffers = msaa_samples > 1 ? 1 : 0;
EGLint attributes[] = {
// clang-format off
EGL_RENDERABLE_TYPE, EGL_OPENGL_ES2_BIT,
EGL_SURFACE_TYPE, EGL_WINDOW_BIT,
EGL_RED_SIZE, 8,
EGL_GREEN_SIZE, 8,
EGL_BLUE_SIZE, 8,
EGL_ALPHA_SIZE, 8,
EGL_DEPTH_SIZE, 0,
EGL_STENCIL_SIZE, 0,
EGL_SAMPLES, static_cast<EGLint>(msaa_samples),
EGL_SAMPLE_BUFFERS, sample_buffers,
EGL_NONE, // termination sentinel
// clang-format on
};
EGLint config_count = 0;
EGLConfig egl_config = nullptr;
if (eglChooseConfig(display, attributes, &egl_config, 1, &config_count) !=
EGL_TRUE) {
return {false, nullptr};
}
bool success = config_count > 0 && egl_config != nullptr;
return {success, success ? egl_config : nullptr};
}
static bool TeardownContext(EGLDisplay display, EGLContext context) {
if (context != EGL_NO_CONTEXT) {
return eglDestroyContext(display, context) == EGL_TRUE;
}
return true;
}
AndroidContextGLSkia::AndroidContextGLSkia(
fml::RefPtr<AndroidEnvironmentGL> environment,
const TaskRunners& task_runners,
uint8_t msaa_samples)
: AndroidContext(AndroidRenderingAPI::kSkiaOpenGLES),
environment_(std::move(environment)),
task_runners_(task_runners) {
if (!environment_->IsValid()) {
FML_LOG(ERROR) << "Could not create an Android GL environment.";
return;
}
bool success = false;
// Choose a valid configuration.
std::tie(success, config_) =
ChooseEGLConfiguration(environment_->Display(), msaa_samples);
if (!success) {
FML_LOG(ERROR) << "Could not choose an EGL configuration.";
LogLastEGLError();
return;
}
// Create a context for the configuration.
std::tie(success, context_) =
CreateContext(environment_->Display(), config_, EGL_NO_CONTEXT);
if (!success) {
FML_LOG(ERROR) << "Could not create an EGL context";
LogLastEGLError();
return;
}
std::tie(success, resource_context_) =
CreateContext(environment_->Display(), config_, context_);
if (!success) {
FML_LOG(ERROR) << "Could not create an EGL resource context";
LogLastEGLError();
return;
}
// All done!
valid_ = true;
}
AndroidContextGLSkia::~AndroidContextGLSkia() {
FML_DCHECK(task_runners_.GetPlatformTaskRunner()->RunsTasksOnCurrentThread());
sk_sp<GrDirectContext> main_context = GetMainSkiaContext();
SetMainSkiaContext(nullptr);
fml::AutoResetWaitableEvent latch;
// This context needs to be deallocated from the raster thread in order to
// keep a coherent usage of egl from a single thread.
fml::TaskRunner::RunNowOrPostTask(task_runners_.GetRasterTaskRunner(), [&] {
if (main_context) {
std::unique_ptr<AndroidEGLSurface> pbuffer_surface =
CreatePbufferSurface();
auto status = pbuffer_surface->MakeCurrent();
if (status != AndroidEGLSurfaceMakeCurrentStatus::kFailure) {
main_context->releaseResourcesAndAbandonContext();
main_context.reset();
ClearCurrent();
}
}
latch.Signal();
});
latch.Wait();
if (!TeardownContext(environment_->Display(), context_)) {
FML_LOG(ERROR)
<< "Could not tear down the EGL context. Possible resource leak.";
LogLastEGLError();
}
if (!TeardownContext(environment_->Display(), resource_context_)) {
FML_LOG(ERROR) << "Could not tear down the EGL resource context. Possible "
"resource leak.";
LogLastEGLError();
}
}
std::unique_ptr<AndroidEGLSurface> AndroidContextGLSkia::CreateOnscreenSurface(
const fml::RefPtr<AndroidNativeWindow>& window) const {
if (window->IsFakeWindow()) {
return CreatePbufferSurface();
} else {
EGLDisplay display = environment_->Display();
const EGLint attribs[] = {EGL_NONE};
EGLSurface surface = eglCreateWindowSurface(
display, config_,
reinterpret_cast<EGLNativeWindowType>(window->handle()), attribs);
return std::make_unique<AndroidEGLSurface>(surface, display, context_);
}
}
std::unique_ptr<AndroidEGLSurface>
AndroidContextGLSkia::CreateOffscreenSurface() const {
// We only ever create pbuffer surfaces for background resource loading
// contexts. We never bind the pbuffer to anything.
EGLDisplay display = environment_->Display();
const EGLint attribs[] = {EGL_WIDTH, 1, EGL_HEIGHT, 1, EGL_NONE};
EGLSurface surface = eglCreatePbufferSurface(display, config_, attribs);
return std::make_unique<AndroidEGLSurface>(surface, display,
resource_context_);
}
std::unique_ptr<AndroidEGLSurface> AndroidContextGLSkia::CreatePbufferSurface()
const {
EGLDisplay display = environment_->Display();
const EGLint attribs[] = {EGL_WIDTH, 1, EGL_HEIGHT, 1, EGL_NONE};
EGLSurface surface = eglCreatePbufferSurface(display, config_, attribs);
return std::make_unique<AndroidEGLSurface>(surface, display, context_);
}
fml::RefPtr<AndroidEnvironmentGL> AndroidContextGLSkia::Environment() const {
return environment_;
}
bool AndroidContextGLSkia::IsValid() const {
return valid_;
}
bool AndroidContextGLSkia::ClearCurrent() const {
if (eglGetCurrentContext() != context_) {
return true;
}
if (eglMakeCurrent(environment_->Display(), EGL_NO_SURFACE, EGL_NO_SURFACE,
EGL_NO_CONTEXT) != EGL_TRUE) {
FML_LOG(ERROR) << "Could not clear the current context";
LogLastEGLError();
return false;
}
return true;
}
EGLContext AndroidContextGLSkia::GetEGLContext() const {
return context_;
}
EGLDisplay AndroidContextGLSkia::GetEGLDisplay() const {
return environment_->Display();
}
EGLContext AndroidContextGLSkia::CreateNewContext() const {
bool success;
EGLContext context;
std::tie(success, context) =
CreateContext(environment_->Display(), config_, EGL_NO_CONTEXT);
return success ? context : EGL_NO_CONTEXT;
}
} // namespace flutter
| engine/shell/platform/android/android_context_gl_skia.cc/0 | {
"file_path": "engine/shell/platform/android/android_context_gl_skia.cc",
"repo_id": "engine",
"token_count": 2703
} | 302 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include <memory>
#include "flutter/shell/platform/android/android_shell_holder.h"
#include "gmock/gmock.h"
#include "gtest/gtest.h"
#include "shell/platform/android/jni/platform_view_android_jni.h"
namespace flutter {
namespace testing {
namespace {
class MockPlatformViewAndroidJNI : public PlatformViewAndroidJNI {
public:
MOCK_METHOD(void,
FlutterViewHandlePlatformMessage,
(std::unique_ptr<flutter::PlatformMessage> message,
int responseId),
(override));
MOCK_METHOD(void,
FlutterViewHandlePlatformMessageResponse,
(int responseId, std::unique_ptr<fml::Mapping> data),
(override));
MOCK_METHOD(void,
FlutterViewUpdateSemantics,
(std::vector<uint8_t> buffer,
std::vector<std::string> strings,
std::vector<std::vector<uint8_t>> string_attribute_args),
(override));
MOCK_METHOD(void,
FlutterViewUpdateCustomAccessibilityActions,
(std::vector<uint8_t> actions_buffer,
std::vector<std::string> strings),
(override));
MOCK_METHOD(void, FlutterViewOnFirstFrame, (), (override));
MOCK_METHOD(void, FlutterViewOnPreEngineRestart, (), (override));
MOCK_METHOD(void,
SurfaceTextureAttachToGLContext,
(JavaLocalRef surface_texture, int textureId),
(override));
MOCK_METHOD(bool,
SurfaceTextureShouldUpdate,
(JavaLocalRef surface_texture),
(override));
MOCK_METHOD(void,
SurfaceTextureUpdateTexImage,
(JavaLocalRef surface_texture),
(override));
MOCK_METHOD(void,
SurfaceTextureGetTransformMatrix,
(JavaLocalRef surface_texture, SkMatrix& transform),
(override));
MOCK_METHOD(void,
SurfaceTextureDetachFromGLContext,
(JavaLocalRef surface_texture),
(override));
MOCK_METHOD(JavaLocalRef,
ImageProducerTextureEntryAcquireLatestImage,
(JavaLocalRef image_texture_entry),
(override));
MOCK_METHOD(JavaLocalRef,
ImageGetHardwareBuffer,
(JavaLocalRef image),
(override));
MOCK_METHOD(void, ImageClose, (JavaLocalRef image), (override));
MOCK_METHOD(void,
HardwareBufferClose,
(JavaLocalRef hardware_buffer),
(override));
MOCK_METHOD(void,
FlutterViewOnDisplayPlatformView,
(int view_id,
int x,
int y,
int width,
int height,
int viewWidth,
int viewHeight,
MutatorsStack mutators_stack),
(override));
MOCK_METHOD(void,
FlutterViewDisplayOverlaySurface,
(int surface_id, int x, int y, int width, int height),
(override));
MOCK_METHOD(void, FlutterViewBeginFrame, (), (override));
MOCK_METHOD(void, FlutterViewEndFrame, (), (override));
MOCK_METHOD(std::unique_ptr<PlatformViewAndroidJNI::OverlayMetadata>,
FlutterViewCreateOverlaySurface,
(),
(override));
MOCK_METHOD(void, FlutterViewDestroyOverlaySurfaces, (), (override));
MOCK_METHOD(std::unique_ptr<std::vector<std::string>>,
FlutterViewComputePlatformResolvedLocale,
(std::vector<std::string> supported_locales_data),
(override));
MOCK_METHOD(double, GetDisplayRefreshRate, (), (override));
MOCK_METHOD(double, GetDisplayWidth, (), (override));
MOCK_METHOD(double, GetDisplayHeight, (), (override));
MOCK_METHOD(double, GetDisplayDensity, (), (override));
MOCK_METHOD(bool,
RequestDartDeferredLibrary,
(int loading_unit_id),
(override));
MOCK_METHOD(double,
FlutterViewGetScaledFontSize,
(double font_size, int configuration_id),
(const, override));
};
class MockPlatformMessageResponse : public PlatformMessageResponse {
public:
static fml::RefPtr<MockPlatformMessageResponse> Create() {
return fml::AdoptRef(new MockPlatformMessageResponse());
}
MOCK_METHOD(void, Complete, (std::unique_ptr<fml::Mapping> data), (override));
MOCK_METHOD(void, CompleteEmpty, (), (override));
};
} // namespace
TEST(AndroidShellHolder, Create) {
Settings settings;
settings.enable_software_rendering = false;
auto jni = std::make_shared<MockPlatformViewAndroidJNI>();
auto holder = std::make_unique<AndroidShellHolder>(settings, jni);
EXPECT_NE(holder.get(), nullptr);
EXPECT_TRUE(holder->IsValid());
EXPECT_NE(holder->GetPlatformView().get(), nullptr);
auto window = fml::MakeRefCounted<AndroidNativeWindow>(
nullptr, /*is_fake_window=*/true);
holder->GetPlatformView()->NotifyCreated(window);
}
TEST(AndroidShellHolder, HandlePlatformMessage) {
Settings settings;
settings.enable_software_rendering = false;
auto jni = std::make_shared<MockPlatformViewAndroidJNI>();
auto holder = std::make_unique<AndroidShellHolder>(settings, jni);
EXPECT_NE(holder.get(), nullptr);
EXPECT_TRUE(holder->IsValid());
EXPECT_NE(holder->GetPlatformView().get(), nullptr);
auto window = fml::MakeRefCounted<AndroidNativeWindow>(
nullptr, /*is_fake_window=*/true);
holder->GetPlatformView()->NotifyCreated(window);
EXPECT_TRUE(holder->GetPlatformMessageHandler());
size_t data_size = 4;
fml::MallocMapping bytes =
fml::MallocMapping(static_cast<uint8_t*>(malloc(data_size)), data_size);
fml::RefPtr<MockPlatformMessageResponse> response =
MockPlatformMessageResponse::Create();
auto message = std::make_unique<PlatformMessage>(
/*channel=*/"foo", /*data=*/std::move(bytes), /*response=*/response);
int response_id = 1;
EXPECT_CALL(*jni,
FlutterViewHandlePlatformMessage(::testing::_, response_id));
EXPECT_CALL(*response, CompleteEmpty());
holder->GetPlatformMessageHandler()->HandlePlatformMessage(
std::move(message));
holder->GetPlatformMessageHandler()
->InvokePlatformMessageEmptyResponseCallback(response_id);
}
} // namespace testing
} // namespace flutter
| engine/shell/platform/android/android_shell_holder_unittests.cc/0 | {
"file_path": "engine/shell/platform/android/android_shell_holder_unittests.cc",
"repo_id": "engine",
"token_count": 2671
} | 303 |
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import("//flutter/common/config.gni")
import("//flutter/testing/testing.gni")
source_set("external_view_embedder") {
sources = [
"external_view_embedder.cc",
"external_view_embedder.h",
"surface_pool.cc",
"surface_pool.h",
]
public_configs = [ "//flutter:config" ]
deps = [
"//flutter/common",
"//flutter/flow",
"//flutter/fml",
"//flutter/shell/platform/android/context",
"//flutter/shell/platform/android/jni",
"//flutter/shell/platform/android/surface",
"//flutter/skia",
]
}
test_fixtures("external_view_embedder_fixtures") {
fixtures = []
}
executable("android_external_view_embedder_unittests") {
testonly = true
sources = [
"external_view_embedder_unittests.cc",
"surface_pool_unittests.cc",
]
deps = [
":external_view_embedder",
":external_view_embedder_fixtures",
"//flutter/flow",
"//flutter/shell/gpu:gpu_surface_gl",
"//flutter/shell/platform/android/jni:jni_mock",
"//flutter/shell/platform/android/surface",
"//flutter/shell/platform/android/surface:surface_mock",
"//flutter/testing",
"//flutter/testing:dart",
"//flutter/testing:skia",
]
}
| engine/shell/platform/android/external_view_embedder/BUILD.gn/0 | {
"file_path": "engine/shell/platform/android/external_view_embedder/BUILD.gn",
"repo_id": "engine",
"token_count": 529
} | 304 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_SHELL_PLATFORM_ANDROID_IMAGE_EXTERNAL_TEXTURE_VK_H_
#define FLUTTER_SHELL_PLATFORM_ANDROID_IMAGE_EXTERNAL_TEXTURE_VK_H_
#include <cstdint>
#include <utility>
#include "flutter/shell/platform/android/image_external_texture.h"
#include "flutter/impeller/renderer/backend/vulkan/android/ahb_texture_source_vk.h"
#include "flutter/impeller/renderer/backend/vulkan/context_vk.h"
#include "flutter/impeller/renderer/backend/vulkan/vk.h"
#include "flutter/shell/platform/android/android_context_vulkan_impeller.h"
namespace flutter {
class ImageExternalTextureVK : public ImageExternalTexture {
public:
ImageExternalTextureVK(
const std::shared_ptr<impeller::ContextVK>& impeller_context,
int64_t id,
const fml::jni::ScopedJavaGlobalRef<jobject>&
hardware_buffer_texture_entry,
const std::shared_ptr<PlatformViewAndroidJNI>& jni_facade);
~ImageExternalTextureVK() override;
private:
void Attach(PaintContext& context) override;
void ProcessFrame(PaintContext& context, const SkRect& bounds) override;
void Detach() override;
const std::shared_ptr<impeller::ContextVK> impeller_context_;
};
} // namespace flutter
#endif // FLUTTER_SHELL_PLATFORM_ANDROID_IMAGE_EXTERNAL_TEXTURE_VK_H_
| engine/shell/platform/android/image_external_texture_vk.h/0 | {
"file_path": "engine/shell/platform/android/image_external_texture_vk.h",
"repo_id": "engine",
"token_count": 512
} | 305 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.embedding.android;
import androidx.annotation.NonNull;
/**
* An Android App Component exclusively attached to a {@link
* io.flutter.embedding.engine.FlutterEngine}.
*
* <p>An exclusive App Component's {@link #detachFromFlutterEngine} is invoked when another App
* Component is becoming attached to the {@link io.flutter.embedding.engine.FlutterEngine}.
*
* <p>The term "App Component" refer to the 4 component types: Activity, Service, Broadcast
* Receiver, and Content Provider, as defined in
* https://developer.android.com/guide/components/fundamentals.
*
* @param <T> The App Component behind this exclusive App Component.
*/
public interface ExclusiveAppComponent<T> {
/**
* Called when another App Component is about to become attached to the {@link
* io.flutter.embedding.engine.FlutterEngine} this App Component is currently attached to.
*
* <p>This App Component's connections to the {@link io.flutter.embedding.engine.FlutterEngine}
* are still valid at the moment of this call.
*/
void detachFromFlutterEngine();
/**
* Retrieve the App Component behind this exclusive App Component.
*
* @return The app component.
*/
@NonNull
T getAppComponent();
}
| engine/shell/platform/android/io/flutter/embedding/android/ExclusiveAppComponent.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/android/ExclusiveAppComponent.java",
"repo_id": "engine",
"token_count": 397
} | 306 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.embedding.android;
import android.view.KeyCharacterMap;
import android.view.KeyEvent;
import androidx.annotation.NonNull;
import io.flutter.Log;
import io.flutter.embedding.engine.systemchannels.KeyEventChannel;
import io.flutter.embedding.engine.systemchannels.KeyboardChannel;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.editing.InputConnectionAdaptor;
import io.flutter.plugin.editing.TextInputPlugin;
import java.util.HashSet;
import java.util.Map;
/**
* Processes keyboard events and cooperate with {@link TextInputPlugin}.
*
* <p>Flutter uses asynchronous event handling to avoid blocking the UI thread, but Android requires
* that events are handled synchronously. So when the Android system sends new @{link KeyEvent} to
* Flutter, Flutter responds synchronously that the key has been handled so that it won't propagate
* to other components. It then uses "delayed event synthesis", where it sends the event to the
* framework, and if the framework responds that it has not handled the event, then this class
* synthesizes a new event to send to Android, without handling it this time.
*
* <p>Flutter processes an Android {@link KeyEvent} with several components, each can choose whether
* to handled the event, and only unhandled events can move to the next section.
*
* <ul>
* <li><b>Keyboard</b>: Dispatch to the {@link KeyboardManager.Responder}s simultaneously. After
* all responders have responded (asynchronously), the event is considered handled if any
* responders decide to handle.
* <li><b>Text input</b>: Events are sent to {@link TextInputPlugin}, processed synchronously with
* a result of whether it is handled.
* <li><b>"Redispatch"</b>: If there's no currently focused text field in {@link TextInputPlugin},
* or the text field does not handle the {@link KeyEvent} either, the {@link KeyEvent} will be
* sent back to the top of the activity's view hierachy, allowing it to be "redispatched". The
* {@link KeyboardManager} will remember this event and skip the identical event at the next
* encounter.
* </ul>
*/
public class KeyboardManager
implements InputConnectionAdaptor.KeyboardDelegate, KeyboardChannel.KeyboardMethodHandler {
private static final String TAG = "KeyboardManager";
/**
* Applies the given Unicode character from {@link KeyEvent#getUnicodeChar()} to a previously
* entered Unicode combining character and returns the combination of these characters if a
* combination exists.
*
* <p>This class is not used by {@link KeyboardManager}, but by its responders.
*/
public static class CharacterCombiner {
private int combiningCharacter = 0;
public CharacterCombiner() {}
/**
* This method mutates {@link #combiningCharacter} over time to combine characters.
*
* <p>One of the following things happens in this method:
*
* <ul>
* <li>If no previous {@link #combiningCharacter} exists and the {@code newCharacterCodePoint}
* is not a combining character, then {@code newCharacterCodePoint} is returned.
* <li>If no previous {@link #combiningCharacter} exists and the {@code newCharacterCodePoint}
* is a combining character, then {@code newCharacterCodePoint} is saved as the {@link
* #combiningCharacter} and null is returned.
* <li>If a previous {@link #combiningCharacter} exists and the {@code newCharacterCodePoint}
* is also a combining character, then the {@code newCharacterCodePoint} is combined with
* the existing {@link #combiningCharacter} and null is returned.
* <li>If a previous {@link #combiningCharacter} exists and the {@code newCharacterCodePoint}
* is not a combining character, then the {@link #combiningCharacter} is applied to the
* regular {@code newCharacterCodePoint} and the resulting complex character is returned.
* The {@link #combiningCharacter} is cleared.
* </ul>
*
* <p>The following reference explains the concept of a "combining character":
* https://en.wikipedia.org/wiki/Combining_character
*/
Character applyCombiningCharacterToBaseCharacter(int newCharacterCodePoint) {
char complexCharacter = (char) newCharacterCodePoint;
boolean isNewCodePointACombiningCharacter =
(newCharacterCodePoint & KeyCharacterMap.COMBINING_ACCENT) != 0;
if (isNewCodePointACombiningCharacter) {
// If a combining character was entered before, combine this one with that one.
int plainCodePoint = newCharacterCodePoint & KeyCharacterMap.COMBINING_ACCENT_MASK;
if (combiningCharacter != 0) {
combiningCharacter = KeyCharacterMap.getDeadChar(combiningCharacter, plainCodePoint);
} else {
combiningCharacter = plainCodePoint;
}
} else {
// The new character is a regular character. Apply combiningCharacter to it, if
// it exists.
if (combiningCharacter != 0) {
int combinedChar = KeyCharacterMap.getDeadChar(combiningCharacter, newCharacterCodePoint);
if (combinedChar > 0) {
complexCharacter = (char) combinedChar;
}
combiningCharacter = 0;
}
}
return complexCharacter;
}
}
/**
* Construct a {@link KeyboardManager}.
*
* @param viewDelegate provides a set of interfaces that the keyboard manager needs to interact
* with other components and the platform, and is typically implements by {@link FlutterView}.
*/
public KeyboardManager(@NonNull ViewDelegate viewDelegate) {
this.viewDelegate = viewDelegate;
this.responders =
new Responder[] {
new KeyEmbedderResponder(viewDelegate.getBinaryMessenger()),
new KeyChannelResponder(new KeyEventChannel(viewDelegate.getBinaryMessenger())),
};
final KeyboardChannel keyboardChannel = new KeyboardChannel(viewDelegate.getBinaryMessenger());
keyboardChannel.setKeyboardMethodHandler(this);
}
/**
* The interface for responding to a {@link KeyEvent} asynchronously.
*
* <p>Implementers of this interface should be owned by a {@link KeyboardManager}, in order to
* receive key events.
*
* <p>After receiving a {@link KeyEvent}, the {@link Responder} must call the supplied {@link
* OnKeyEventHandledCallback} exactly once, to inform the {@link KeyboardManager} whether it
* wishes to handle the {@link KeyEvent}. The {@link KeyEvent} will not be propagated to the
* {@link TextInputPlugin} or be redispatched to the view hierachy if any key responders answered
* yes.
*
* <p>If a {@link Responder} fails to call the {@link OnKeyEventHandledCallback} callback, the
* {@link KeyEvent} will never be sent to the {@link TextInputPlugin}, and the {@link
* KeyboardManager} class can't detect such errors as there is no timeout.
*/
public interface Responder {
interface OnKeyEventHandledCallback {
void onKeyEventHandled(boolean canHandleEvent);
}
/**
* Informs this {@link Responder} that a new {@link KeyEvent} needs processing.
*
* @param keyEvent the new {@link KeyEvent} this {@link Responder} may be interested in.
* @param onKeyEventHandledCallback the method to call when this {@link Responder} has decided
* whether to handle the {@link KeyEvent}.
*/
void handleEvent(
@NonNull KeyEvent keyEvent, @NonNull OnKeyEventHandledCallback onKeyEventHandledCallback);
}
/**
* A set of interfaces that the {@link KeyboardManager} needs to interact with other components
* and the platform, and is typically implements by {@link FlutterView}.
*/
public interface ViewDelegate {
/** Returns a {@link BinaryMessenger} to send platform messages with. */
public BinaryMessenger getBinaryMessenger();
/**
* Send a {@link KeyEvent} that is not handled by the keyboard responders to the text input
* system.
*
* @param keyEvent the {@link KeyEvent} that should be processed by the text input system. It
* must not be null.
* @return Whether the text input handles the key event.
*/
public boolean onTextInputKeyEvent(@NonNull KeyEvent keyEvent);
/** Send a {@link KeyEvent} that is not handled by Flutter back to the platform. */
public void redispatch(@NonNull KeyEvent keyEvent);
}
private class PerEventCallbackBuilder {
private class Callback implements Responder.OnKeyEventHandledCallback {
boolean isCalled = false;
@Override
public void onKeyEventHandled(boolean canHandleEvent) {
if (isCalled) {
throw new IllegalStateException(
"The onKeyEventHandledCallback should be called exactly once.");
}
isCalled = true;
unrepliedCount -= 1;
isEventHandled |= canHandleEvent;
if (unrepliedCount == 0 && !isEventHandled) {
onUnhandled(keyEvent);
}
}
}
PerEventCallbackBuilder(@NonNull KeyEvent keyEvent) {
this.keyEvent = keyEvent;
}
final KeyEvent keyEvent;
int unrepliedCount = responders.length;
boolean isEventHandled = false;
public Responder.OnKeyEventHandledCallback buildCallback() {
return new Callback();
}
}
protected final Responder[] responders;
private final HashSet<KeyEvent> redispatchedEvents = new HashSet<>();
private final ViewDelegate viewDelegate;
@Override
public boolean handleEvent(@NonNull KeyEvent keyEvent) {
final boolean isRedispatchedEvent = redispatchedEvents.remove(keyEvent);
if (isRedispatchedEvent) {
return false;
}
if (responders.length > 0) {
final PerEventCallbackBuilder callbackBuilder = new PerEventCallbackBuilder(keyEvent);
for (final Responder primaryResponder : responders) {
primaryResponder.handleEvent(keyEvent, callbackBuilder.buildCallback());
}
} else {
onUnhandled(keyEvent);
}
return true;
}
public void destroy() {
final int remainingRedispatchCount = redispatchedEvents.size();
if (remainingRedispatchCount > 0) {
Log.w(
TAG,
"A KeyboardManager was destroyed with "
+ String.valueOf(remainingRedispatchCount)
+ " unhandled redispatch event(s).");
}
}
private void onUnhandled(@NonNull KeyEvent keyEvent) {
if (viewDelegate == null || viewDelegate.onTextInputKeyEvent(keyEvent)) {
return;
}
redispatchedEvents.add(keyEvent);
viewDelegate.redispatch(keyEvent);
if (redispatchedEvents.remove(keyEvent)) {
Log.w(TAG, "A redispatched key event was consumed before reaching KeyboardManager");
}
}
/**
* Returns an unmodifiable view of the pressed state.
*
* @return A map whose keys are physical keyboard key IDs and values are the corresponding logical
* keyboard key IDs.
*/
public Map<Long, Long> getKeyboardState() {
KeyEmbedderResponder embedderResponder = (KeyEmbedderResponder) responders[0];
return embedderResponder.getPressedState();
}
}
| engine/shell/platform/android/io/flutter/embedding/android/KeyboardManager.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/android/KeyboardManager.java",
"repo_id": "engine",
"token_count": 3716
} | 307 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.embedding.engine.dart;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import java.nio.ByteBuffer;
/** Handler that receives messages from Dart code. */
public interface PlatformMessageHandler {
/** Called from any thread. */
void handleMessageFromDart(
@NonNull final String channel,
@Nullable ByteBuffer message,
final int replyId,
long messageData);
void handlePlatformMessageResponse(int replyId, @Nullable ByteBuffer reply);
}
| engine/shell/platform/android/io/flutter/embedding/engine/dart/PlatformMessageHandler.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/engine/dart/PlatformMessageHandler.java",
"repo_id": "engine",
"token_count": 195
} | 308 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.embedding.engine.plugins.broadcastreceiver;
import android.content.BroadcastReceiver;
import androidx.annotation.NonNull;
import androidx.lifecycle.Lifecycle;
/**
* Control surface through which a {@link BroadcastReceiver} attaches to a {@link
* io.flutter.embedding.engine.FlutterEngine}.
*
* <p>A {@link BroadcastReceiver} that contains a {@link io.flutter.embedding.engine.FlutterEngine}
* should coordinate itself with the {@link io.flutter.embedding.engine.FlutterEngine}'s {@code
* BroadcastReceiverControlSurface}.
*/
public interface BroadcastReceiverControlSurface {
/**
* Call this method from the {@link BroadcastReceiver} that is running the {@link
* io.flutter.embedding.engine.FlutterEngine} that is associated with this {@code
* BroadcastReceiverControlSurface}.
*
* <p>Once a {@link BroadcastReceiver} is created, and its associated {@link
* io.flutter.embedding.engine.FlutterEngine} is executing Dart code, the {@link
* BroadcastReceiver} should invoke this method. At that point the {@link
* io.flutter.embedding.engine.FlutterEngine} is considered "attached" to the {@link
* BroadcastReceiver} and all {@link BroadcastReceiverAware} plugins are given access to the
* {@link BroadcastReceiver}.
*/
void attachToBroadcastReceiver(
@NonNull BroadcastReceiver broadcastReceiver, @NonNull Lifecycle lifecycle);
/**
* Call this method from the {@link BroadcastReceiver} that is attached to this {@code
* BroadcastReceiverControlSurfaces}'s {@link io.flutter.embedding.engine.FlutterEngine} when the
* {@link BroadcastReceiver} is about to be destroyed.
*
* <p>This method gives each {@link BroadcastReceiverAware} plugin an opportunity to clean up its
* references before the {@link BroadcastReceiver is destroyed}.
*/
void detachFromBroadcastReceiver();
}
| engine/shell/platform/android/io/flutter/embedding/engine/plugins/broadcastreceiver/BroadcastReceiverControlSurface.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/engine/plugins/broadcastreceiver/BroadcastReceiverControlSurface.java",
"repo_id": "engine",
"token_count": 601
} | 309 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.embedding.engine.renderer;
import android.graphics.SurfaceTexture;
import androidx.annotation.Keep;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
/**
* A wrapper for a SurfaceTexture that tracks whether the texture has been released.
*
* <p>The engine calls {@code SurfaceTexture.release} on the platform thread, but {@code
* updateTexImage} is called on the raster thread. This wrapper will prevent {@code updateTexImage}
* calls on an abandoned texture.
*/
@Keep
public class SurfaceTextureWrapper {
private SurfaceTexture surfaceTexture;
private boolean released;
private boolean attached;
private Runnable onFrameConsumed;
private boolean newFrameAvailable = false;
public SurfaceTextureWrapper(@NonNull SurfaceTexture surfaceTexture) {
this(surfaceTexture, null);
}
/**
* A wrapper for a SurfaceTexture.
*
* <p>The provided {@code onFrameConsumed} callback must be invoked when the most recent image was
* consumed.
*
* @param onFrameConsumed The callback after the {@code updateTexImage} is called.
*/
public SurfaceTextureWrapper(
@NonNull SurfaceTexture surfaceTexture, @Nullable Runnable onFrameConsumed) {
this.surfaceTexture = surfaceTexture;
this.released = false;
this.onFrameConsumed = onFrameConsumed;
}
@NonNull
public SurfaceTexture surfaceTexture() {
return surfaceTexture;
}
public void markDirty() {
synchronized (this) {
newFrameAvailable = true;
}
}
public boolean shouldUpdate() {
synchronized (this) {
return newFrameAvailable;
}
}
// Called by native.
@SuppressWarnings("unused")
public void updateTexImage() {
synchronized (this) {
newFrameAvailable = false;
if (!released) {
surfaceTexture.updateTexImage();
if (onFrameConsumed != null) {
onFrameConsumed.run();
}
}
}
}
public void release() {
synchronized (this) {
if (!released) {
surfaceTexture.release();
released = true;
attached = false;
}
}
}
// Called by native.
@SuppressWarnings("unused")
public void attachToGLContext(int texName) {
synchronized (this) {
if (released) {
return;
}
// When the rasterizer tasks run on a different thread, the GrContext is re-created.
// This causes the texture to be in an uninitialized state.
// This should *not* be an issue once platform views are always rendered as TextureLayers
// since thread merging will be always disabled on Android.
// For more see: SurfaceTextureExternalTextureGL::OnGrContextCreated in
// surface_texture_external_texture_gl.cc, and
// https://github.com/flutter/flutter/issues/98155
if (attached) {
surfaceTexture.detachFromGLContext();
}
surfaceTexture.attachToGLContext(texName);
attached = true;
}
}
// Called by native.
@SuppressWarnings("unused")
public void detachFromGLContext() {
synchronized (this) {
if (attached && !released) {
surfaceTexture.detachFromGLContext();
attached = false;
}
}
}
// Called by native.
@SuppressWarnings("unused")
public void getTransformMatrix(@NonNull float[] mtx) {
surfaceTexture.getTransformMatrix(mtx);
}
}
| engine/shell/platform/android/io/flutter/embedding/engine/renderer/SurfaceTextureWrapper.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/engine/renderer/SurfaceTextureWrapper.java",
"repo_id": "engine",
"token_count": 1182
} | 310 |
package io.flutter.embedding.engine.systemchannels;
import static io.flutter.Build.API_LEVELS;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.view.inputmethod.EditorInfo;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.VisibleForTesting;
import io.flutter.Log;
import io.flutter.embedding.engine.dart.DartExecutor;
import io.flutter.plugin.common.JSONMethodCodec;
import io.flutter.plugin.common.MethodCall;
import io.flutter.plugin.common.MethodChannel;
import io.flutter.plugin.editing.TextEditingDelta;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
/**
* {@link TextInputChannel} is a platform channel between Android and Flutter that is used to
* communicate information about the user's text input.
*
* <p>When the user presses an action button like "done" or "next", that action is sent from Android
* to Flutter through this {@link TextInputChannel}.
*
* <p>When an input system in the Flutter app wants to show the keyboard, or hide it, or configure
* editing state, etc. a message is sent from Flutter to Android through this {@link
* TextInputChannel}.
*
* <p>{@link TextInputChannel} comes with a default {@link
* io.flutter.plugin.common.MethodChannel.MethodCallHandler} that parses incoming messages from
* Flutter. Register a {@link TextInputMethodHandler} to respond to standard Flutter text input
* messages.
*/
public class TextInputChannel {
private static final String TAG = "TextInputChannel";
@NonNull public final MethodChannel channel;
@Nullable private TextInputMethodHandler textInputMethodHandler;
@NonNull @VisibleForTesting
final MethodChannel.MethodCallHandler parsingMethodHandler =
new MethodChannel.MethodCallHandler() {
@Override
public void onMethodCall(@NonNull MethodCall call, @NonNull MethodChannel.Result result) {
if (textInputMethodHandler == null) {
// If no explicit TextInputMethodHandler has been registered then we don't
// need to forward this call to an API. Return.
return;
}
String method = call.method;
Object args = call.arguments;
Log.v(TAG, "Received '" + method + "' message.");
switch (method) {
case "TextInput.show":
textInputMethodHandler.show();
result.success(null);
break;
case "TextInput.hide":
textInputMethodHandler.hide();
result.success(null);
break;
case "TextInput.setClient":
try {
final JSONArray argumentList = (JSONArray) args;
final int textInputClientId = argumentList.getInt(0);
final JSONObject jsonConfiguration = argumentList.getJSONObject(1);
textInputMethodHandler.setClient(
textInputClientId, Configuration.fromJson(jsonConfiguration));
result.success(null);
} catch (JSONException | NoSuchFieldException exception) {
// JSONException: missing keys or bad value types.
// NoSuchFieldException: one or more values were invalid.
result.error("error", exception.getMessage(), null);
}
break;
case "TextInput.requestAutofill":
textInputMethodHandler.requestAutofill();
result.success(null);
break;
case "TextInput.setPlatformViewClient":
try {
final JSONObject arguments = (JSONObject) args;
final int platformViewId = arguments.getInt("platformViewId");
final boolean usesVirtualDisplay =
arguments.optBoolean("usesVirtualDisplay", false);
textInputMethodHandler.setPlatformViewClient(platformViewId, usesVirtualDisplay);
result.success(null);
} catch (JSONException exception) {
result.error("error", exception.getMessage(), null);
}
break;
case "TextInput.setEditingState":
try {
final JSONObject editingState = (JSONObject) args;
textInputMethodHandler.setEditingState(TextEditState.fromJson(editingState));
result.success(null);
} catch (JSONException exception) {
result.error("error", exception.getMessage(), null);
}
break;
case "TextInput.setEditableSizeAndTransform":
try {
final JSONObject arguments = (JSONObject) args;
final double width = arguments.getDouble("width");
final double height = arguments.getDouble("height");
final JSONArray jsonMatrix = arguments.getJSONArray("transform");
final double[] matrix = new double[16];
for (int i = 0; i < 16; i++) {
matrix[i] = jsonMatrix.getDouble(i);
}
textInputMethodHandler.setEditableSizeAndTransform(width, height, matrix);
result.success(null);
} catch (JSONException exception) {
result.error("error", exception.getMessage(), null);
}
break;
case "TextInput.clearClient":
textInputMethodHandler.clearClient();
result.success(null);
break;
case "TextInput.sendAppPrivateCommand":
try {
final JSONObject arguments = (JSONObject) args;
final String action = arguments.getString("action");
final String data = arguments.getString("data");
Bundle bundle = null;
if (data != null && !data.isEmpty()) {
bundle = new Bundle();
bundle.putString("data", data);
}
textInputMethodHandler.sendAppPrivateCommand(action, bundle);
result.success(null);
} catch (JSONException exception) {
result.error("error", exception.getMessage(), null);
}
break;
case "TextInput.finishAutofillContext":
textInputMethodHandler.finishAutofillContext((boolean) args);
result.success(null);
break;
default:
result.notImplemented();
break;
}
}
};
/**
* Constructs a {@code TextInputChannel} that connects Android to the Dart code running in {@code
* dartExecutor}.
*
* <p>The given {@code dartExecutor} is permitted to be idle or executing code.
*
* <p>See {@link DartExecutor}.
*/
public TextInputChannel(@NonNull DartExecutor dartExecutor) {
this.channel = new MethodChannel(dartExecutor, "flutter/textinput", JSONMethodCodec.INSTANCE);
channel.setMethodCallHandler(parsingMethodHandler);
}
/**
* Instructs Flutter to reattach the last active text input client, if any.
*
* <p>This is necessary when the view hierarchy has been detached and reattached to a {@link
* io.flutter.embedding.engine.FlutterEngine}, as the engine may have kept alive a text editing
* client on the Dart side.
*/
public void requestExistingInputState() {
channel.invokeMethod("TextInputClient.requestExistingInputState", null);
}
private static HashMap<Object, Object> createEditingStateJSON(
String text, int selectionStart, int selectionEnd, int composingStart, int composingEnd) {
HashMap<Object, Object> state = new HashMap<>();
state.put("text", text);
state.put("selectionBase", selectionStart);
state.put("selectionExtent", selectionEnd);
state.put("composingBase", composingStart);
state.put("composingExtent", composingEnd);
return state;
}
private static HashMap<Object, Object> createEditingDeltaJSON(
ArrayList<TextEditingDelta> batchDeltas) {
HashMap<Object, Object> state = new HashMap<>();
JSONArray deltas = new JSONArray();
for (TextEditingDelta delta : batchDeltas) {
deltas.put(delta.toJSON());
}
state.put("deltas", deltas);
return state;
}
/**
* Instructs Flutter to update its text input editing state to reflect the given configuration.
*/
public void updateEditingState(
int inputClientId,
@NonNull String text,
int selectionStart,
int selectionEnd,
int composingStart,
int composingEnd) {
Log.v(
TAG,
"Sending message to update editing state: \n"
+ "Text: "
+ text
+ "\n"
+ "Selection start: "
+ selectionStart
+ "\n"
+ "Selection end: "
+ selectionEnd
+ "\n"
+ "Composing start: "
+ composingStart
+ "\n"
+ "Composing end: "
+ composingEnd);
final HashMap<Object, Object> state =
createEditingStateJSON(text, selectionStart, selectionEnd, composingStart, composingEnd);
channel.invokeMethod("TextInputClient.updateEditingState", Arrays.asList(inputClientId, state));
}
public void updateEditingStateWithDeltas(
int inputClientId, @NonNull ArrayList<TextEditingDelta> batchDeltas) {
Log.v(
TAG,
"Sending message to update editing state with deltas: \n"
+ "Number of deltas: "
+ batchDeltas.size());
final HashMap<Object, Object> state = createEditingDeltaJSON(batchDeltas);
channel.invokeMethod(
"TextInputClient.updateEditingStateWithDeltas", Arrays.asList(inputClientId, state));
}
public void updateEditingStateWithTag(
int inputClientId, @NonNull HashMap<String, TextEditState> editStates) {
Log.v(
TAG,
"Sending message to update editing state for "
+ String.valueOf(editStates.size())
+ " field(s).");
final HashMap<String, HashMap<Object, Object>> json = new HashMap<>();
for (Map.Entry<String, TextEditState> element : editStates.entrySet()) {
final TextEditState state = element.getValue();
json.put(
element.getKey(),
createEditingStateJSON(state.text, state.selectionStart, state.selectionEnd, -1, -1));
}
channel.invokeMethod(
"TextInputClient.updateEditingStateWithTag", Arrays.asList(inputClientId, json));
}
/** Instructs Flutter to execute a "newline" action. */
public void newline(int inputClientId) {
Log.v(TAG, "Sending 'newline' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.newline"));
}
/** Instructs Flutter to execute a "go" action. */
public void go(int inputClientId) {
Log.v(TAG, "Sending 'go' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.go"));
}
/** Instructs Flutter to execute a "search" action. */
public void search(int inputClientId) {
Log.v(TAG, "Sending 'search' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.search"));
}
/** Instructs Flutter to execute a "send" action. */
public void send(int inputClientId) {
Log.v(TAG, "Sending 'send' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.send"));
}
/** Instructs Flutter to execute a "done" action. */
public void done(int inputClientId) {
Log.v(TAG, "Sending 'done' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.done"));
}
/** Instructs Flutter to execute a "next" action. */
public void next(int inputClientId) {
Log.v(TAG, "Sending 'next' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.next"));
}
/** Instructs Flutter to execute a "previous" action. */
public void previous(int inputClientId) {
Log.v(TAG, "Sending 'previous' message.");
channel.invokeMethod(
"TextInputClient.performAction", Arrays.asList(inputClientId, "TextInputAction.previous"));
}
/** Instructs Flutter to execute an "unspecified" action. */
public void unspecifiedAction(int inputClientId) {
Log.v(TAG, "Sending 'unspecified' message.");
channel.invokeMethod(
"TextInputClient.performAction",
Arrays.asList(inputClientId, "TextInputAction.unspecified"));
}
/** Instructs Flutter to commit inserted content back to the text channel. */
public void commitContent(int inputClientId, Map<String, Object> content) {
Log.v(TAG, "Sending 'commitContent' message.");
channel.invokeMethod(
"TextInputClient.performAction",
Arrays.asList(inputClientId, "TextInputAction.commitContent", content));
}
public void performPrivateCommand(
int inputClientId, @NonNull String action, @NonNull Bundle data) {
HashMap<Object, Object> json = new HashMap<>();
json.put("action", action);
if (data != null) {
HashMap<String, Object> dataMap = new HashMap<>();
Set<String> keySet = data.keySet();
for (String key : keySet) {
Object value = data.get(key);
if (value instanceof byte[]) {
dataMap.put(key, data.getByteArray(key));
} else if (value instanceof Byte) {
dataMap.put(key, data.getByte(key));
} else if (value instanceof char[]) {
dataMap.put(key, data.getCharArray(key));
} else if (value instanceof Character) {
dataMap.put(key, data.getChar(key));
} else if (value instanceof CharSequence[]) {
dataMap.put(key, data.getCharSequenceArray(key));
} else if (value instanceof CharSequence) {
dataMap.put(key, data.getCharSequence(key));
} else if (value instanceof float[]) {
dataMap.put(key, data.getFloatArray(key));
} else if (value instanceof Float) {
dataMap.put(key, data.getFloat(key));
}
}
json.put("data", dataMap);
}
channel.invokeMethod(
"TextInputClient.performPrivateCommand", Arrays.asList(inputClientId, json));
}
/**
* Sets the {@link TextInputMethodHandler} which receives all events and requests that are parsed
* from the underlying platform channel.
*/
public void setTextInputMethodHandler(@Nullable TextInputMethodHandler textInputMethodHandler) {
this.textInputMethodHandler = textInputMethodHandler;
}
public interface TextInputMethodHandler {
// TODO(mattcarroll): javadoc
void show();
// TODO(mattcarroll): javadoc
void hide();
/**
* Requests that the autofill dropdown menu appear for the current client.
*
* <p>Has no effect if the current client does not support autofill.
*/
void requestAutofill();
/**
* Requests that the {@link android.view.autofill.AutofillManager} cancel or commit the current
* autofill context.
*
* <p>The method calls {@link android.view.autofill.AutofillManager#commit()} when {@code
* shouldSave} is true, and calls {@link android.view.autofill.AutofillManager#cancel()}
* otherwise.
*
* @param shouldSave whether the active autofill service should save the current user input for
* future use.
*/
void finishAutofillContext(boolean shouldSave);
// TODO(mattcarroll): javadoc
void setClient(int textInputClientId, @NonNull Configuration configuration);
/**
* Sets a platform view as the text input client.
*
* <p>Subsequent calls to createInputConnection will be delegated to the platform view until a
* different client is set.
*
* @param id the ID of the platform view to be set as a text input client.
* @param usesVirtualDisplay True if the platform view uses a virtual display, false if it uses
* hybrid composition.
*/
void setPlatformViewClient(int id, boolean usesVirtualDisplay);
/**
* Sets the size and the transform matrix of the current text input client.
*
* @param width the width of text input client. Must be finite.
* @param height the height of text input client. Must be finite.
* @param transform a 4x4 matrix that maps the local paint coordinate system to coordinate
* system of the FlutterView that owns the current client.
*/
void setEditableSizeAndTransform(double width, double height, @NonNull double[] transform);
// TODO(mattcarroll): javadoc
void setEditingState(@NonNull TextEditState editingState);
// TODO(mattcarroll): javadoc
void clearClient();
/**
* Sends client app private command to the current text input client(input method). The app
* private command result will be informed through {@code performPrivateCommand}.
*
* @param action Name of the command to be performed. This must be a scoped name. i.e. prefixed
* with a package name you own, so that different developers will not create conflicting
* commands.
* @param data Any data to include with the command.
*/
void sendAppPrivateCommand(@NonNull String action, @NonNull Bundle data);
}
/** A text editing configuration. */
public static class Configuration {
@NonNull
public static Configuration fromJson(@NonNull JSONObject json)
throws JSONException, NoSuchFieldException {
final String inputActionName = json.getString("inputAction");
if (inputActionName == null) {
throw new JSONException("Configuration JSON missing 'inputAction' property.");
}
Configuration[] fields = null;
if (!json.isNull("fields")) {
final JSONArray jsonFields = json.getJSONArray("fields");
fields = new Configuration[jsonFields.length()];
for (int i = 0; i < fields.length; i++) {
fields[i] = Configuration.fromJson(jsonFields.getJSONObject(i));
}
}
final Integer inputAction = inputActionFromTextInputAction(inputActionName);
// Build list of content commit mime types from the data in the JSON list.
List<String> contentList = new ArrayList<String>();
JSONArray contentCommitMimeTypes =
json.isNull("contentCommitMimeTypes")
? null
: json.getJSONArray("contentCommitMimeTypes");
if (contentCommitMimeTypes != null) {
for (int i = 0; i < contentCommitMimeTypes.length(); i++) {
contentList.add(contentCommitMimeTypes.optString(i));
}
}
return new Configuration(
json.optBoolean("obscureText"),
json.optBoolean("autocorrect", true),
json.optBoolean("enableSuggestions"),
json.optBoolean("enableIMEPersonalizedLearning"),
json.optBoolean("enableDeltaModel"),
TextCapitalization.fromValue(json.getString("textCapitalization")),
InputType.fromJson(json.getJSONObject("inputType")),
inputAction,
json.isNull("actionLabel") ? null : json.getString("actionLabel"),
json.isNull("autofill") ? null : Autofill.fromJson(json.getJSONObject("autofill")),
contentList.toArray(new String[contentList.size()]),
fields);
}
@NonNull
private static Integer inputActionFromTextInputAction(@NonNull String inputAction) {
switch (inputAction) {
case "TextInputAction.newline":
return EditorInfo.IME_ACTION_NONE;
case "TextInputAction.none":
return EditorInfo.IME_ACTION_NONE;
case "TextInputAction.unspecified":
return EditorInfo.IME_ACTION_UNSPECIFIED;
case "TextInputAction.done":
return EditorInfo.IME_ACTION_DONE;
case "TextInputAction.go":
return EditorInfo.IME_ACTION_GO;
case "TextInputAction.search":
return EditorInfo.IME_ACTION_SEARCH;
case "TextInputAction.send":
return EditorInfo.IME_ACTION_SEND;
case "TextInputAction.next":
return EditorInfo.IME_ACTION_NEXT;
case "TextInputAction.previous":
return EditorInfo.IME_ACTION_PREVIOUS;
default:
// Present default key if bad input type is given.
return EditorInfo.IME_ACTION_UNSPECIFIED;
}
}
public static class Autofill {
@NonNull
public static Autofill fromJson(@NonNull JSONObject json)
throws JSONException, NoSuchFieldException {
final String uniqueIdentifier = json.getString("uniqueIdentifier");
final JSONArray hints = json.getJSONArray("hints");
final String hintText = json.isNull("hintText") ? null : json.getString("hintText");
final JSONObject editingState = json.getJSONObject("editingValue");
final String[] autofillHints = new String[hints.length()];
for (int i = 0; i < hints.length(); i++) {
autofillHints[i] = translateAutofillHint(hints.getString(i));
}
return new Autofill(
uniqueIdentifier, autofillHints, hintText, TextEditState.fromJson(editingState));
}
public final String uniqueIdentifier;
public final String[] hints;
public final TextEditState editState;
public final String hintText;
@NonNull
private static String translateAutofillHint(@NonNull String hint) {
if (Build.VERSION.SDK_INT < API_LEVELS.API_26) {
return hint;
}
switch (hint) {
case "addressCity":
return "addressLocality";
case "addressState":
return "addressRegion";
case "birthday":
return "birthDateFull";
case "birthdayDay":
return "birthDateDay";
case "birthdayMonth":
return "birthDateMonth";
case "birthdayYear":
return "birthDateYear";
case "countryName":
return "addressCountry";
case "creditCardExpirationDate":
return View.AUTOFILL_HINT_CREDIT_CARD_EXPIRATION_DATE;
case "creditCardExpirationDay":
return View.AUTOFILL_HINT_CREDIT_CARD_EXPIRATION_DAY;
case "creditCardExpirationMonth":
return View.AUTOFILL_HINT_CREDIT_CARD_EXPIRATION_MONTH;
case "creditCardExpirationYear":
return View.AUTOFILL_HINT_CREDIT_CARD_EXPIRATION_YEAR;
case "creditCardNumber":
return View.AUTOFILL_HINT_CREDIT_CARD_NUMBER;
case "creditCardSecurityCode":
return View.AUTOFILL_HINT_CREDIT_CARD_SECURITY_CODE;
case "email":
return View.AUTOFILL_HINT_EMAIL_ADDRESS;
case "familyName":
return "personFamilyName";
case "fullStreetAddress":
return "streetAddress";
case "gender":
return "gender";
case "givenName":
return "personGivenName";
case "middleInitial":
return "personMiddleInitial";
case "middleName":
return "personMiddleName";
case "name":
return "personName";
case "namePrefix":
return "personNamePrefix";
case "nameSuffix":
return "personNameSuffix";
case "newPassword":
return "newPassword";
case "newUsername":
return "newUsername";
case "oneTimeCode":
return "smsOTPCode";
case "password":
return View.AUTOFILL_HINT_PASSWORD;
case "postalAddress":
return View.AUTOFILL_HINT_POSTAL_ADDRESS;
case "postalAddressExtended":
return "extendedAddress";
case "postalAddressExtendedPostalCode":
return "extendedPostalCode";
case "postalCode":
return View.AUTOFILL_HINT_POSTAL_CODE;
case "telephoneNumber":
return "phoneNumber";
case "telephoneNumberCountryCode":
return "phoneCountryCode";
case "telephoneNumberDevice":
return "phoneNumberDevice";
case "telephoneNumberNational":
return "phoneNational";
case "username":
return View.AUTOFILL_HINT_USERNAME;
default:
return hint;
}
}
public Autofill(
@NonNull String uniqueIdentifier,
@NonNull String[] hints,
@Nullable String hintText,
@NonNull TextEditState editingState) {
this.uniqueIdentifier = uniqueIdentifier;
this.hints = hints;
this.hintText = hintText;
this.editState = editingState;
}
}
public final boolean obscureText;
public final boolean autocorrect;
public final boolean enableSuggestions;
public final boolean enableIMEPersonalizedLearning;
public final boolean enableDeltaModel;
@NonNull public final TextCapitalization textCapitalization;
@NonNull public final InputType inputType;
@Nullable public final Integer inputAction;
@Nullable public final String actionLabel;
@Nullable public final Autofill autofill;
@Nullable public final String[] contentCommitMimeTypes;
@Nullable public final Configuration[] fields;
public Configuration(
boolean obscureText,
boolean autocorrect,
boolean enableSuggestions,
boolean enableIMEPersonalizedLearning,
boolean enableDeltaModel,
@NonNull TextCapitalization textCapitalization,
@NonNull InputType inputType,
@Nullable Integer inputAction,
@Nullable String actionLabel,
@Nullable Autofill autofill,
@Nullable String[] contentCommitMimeTypes,
@Nullable Configuration[] fields) {
this.obscureText = obscureText;
this.autocorrect = autocorrect;
this.enableSuggestions = enableSuggestions;
this.enableIMEPersonalizedLearning = enableIMEPersonalizedLearning;
this.enableDeltaModel = enableDeltaModel;
this.textCapitalization = textCapitalization;
this.inputType = inputType;
this.inputAction = inputAction;
this.actionLabel = actionLabel;
this.autofill = autofill;
this.contentCommitMimeTypes = contentCommitMimeTypes;
this.fields = fields;
}
}
/**
* A text input type.
*
* <p>If the {@link #type} is {@link TextInputType#NUMBER}, this {@code InputType} also reports
* whether that number {@link #isSigned} and {@link #isDecimal}.
*/
public static class InputType {
@NonNull
public static InputType fromJson(@NonNull JSONObject json)
throws JSONException, NoSuchFieldException {
return new InputType(
TextInputType.fromValue(json.getString("name")),
json.optBoolean("signed", false),
json.optBoolean("decimal", false));
}
@NonNull public final TextInputType type;
public final boolean isSigned;
public final boolean isDecimal;
public InputType(@NonNull TextInputType type, boolean isSigned, boolean isDecimal) {
this.type = type;
this.isSigned = isSigned;
this.isDecimal = isDecimal;
}
}
/** Types of text input. */
public enum TextInputType {
TEXT("TextInputType.text"),
DATETIME("TextInputType.datetime"),
NAME("TextInputType.name"),
POSTAL_ADDRESS("TextInputType.address"),
NUMBER("TextInputType.number"),
PHONE("TextInputType.phone"),
MULTILINE("TextInputType.multiline"),
EMAIL_ADDRESS("TextInputType.emailAddress"),
URL("TextInputType.url"),
VISIBLE_PASSWORD("TextInputType.visiblePassword"),
NONE("TextInputType.none");
static TextInputType fromValue(@NonNull String encodedName) throws NoSuchFieldException {
for (TextInputType textInputType : TextInputType.values()) {
if (textInputType.encodedName.equals(encodedName)) {
return textInputType;
}
}
throw new NoSuchFieldException("No such TextInputType: " + encodedName);
}
@NonNull private final String encodedName;
TextInputType(@NonNull String encodedName) {
this.encodedName = encodedName;
}
}
/** Text capitalization schemes. */
public enum TextCapitalization {
CHARACTERS("TextCapitalization.characters"),
WORDS("TextCapitalization.words"),
SENTENCES("TextCapitalization.sentences"),
NONE("TextCapitalization.none");
static TextCapitalization fromValue(@NonNull String encodedName) throws NoSuchFieldException {
for (TextCapitalization textCapitalization : TextCapitalization.values()) {
if (textCapitalization.encodedName.equals(encodedName)) {
return textCapitalization;
}
}
throw new NoSuchFieldException("No such TextCapitalization: " + encodedName);
}
@NonNull private final String encodedName;
TextCapitalization(@NonNull String encodedName) {
this.encodedName = encodedName;
}
}
/** State of an on-going text editing session. */
public static class TextEditState {
@NonNull
public static TextEditState fromJson(@NonNull JSONObject textEditState) throws JSONException {
return new TextEditState(
textEditState.getString("text"),
textEditState.getInt("selectionBase"),
textEditState.getInt("selectionExtent"),
textEditState.getInt("composingBase"),
textEditState.getInt("composingExtent"));
}
@NonNull public final String text;
public final int selectionStart;
public final int selectionEnd;
public final int composingStart;
public final int composingEnd;
public TextEditState(
@NonNull String text,
int selectionStart,
int selectionEnd,
int composingStart,
int composingEnd)
throws IndexOutOfBoundsException {
if ((selectionStart != -1 || selectionEnd != -1)
&& (selectionStart < 0 || selectionEnd < 0)) {
throw new IndexOutOfBoundsException(
"invalid selection: ("
+ String.valueOf(selectionStart)
+ ", "
+ String.valueOf(selectionEnd)
+ ")");
}
if ((composingStart != -1 || composingEnd != -1)
&& (composingStart < 0 || composingStart > composingEnd)) {
throw new IndexOutOfBoundsException(
"invalid composing range: ("
+ String.valueOf(composingStart)
+ ", "
+ String.valueOf(composingEnd)
+ ")");
}
if (composingEnd > text.length()) {
throw new IndexOutOfBoundsException(
"invalid composing start: " + String.valueOf(composingStart));
}
if (selectionStart > text.length()) {
throw new IndexOutOfBoundsException(
"invalid selection start: " + String.valueOf(selectionStart));
}
if (selectionEnd > text.length()) {
throw new IndexOutOfBoundsException(
"invalid selection end: " + String.valueOf(selectionEnd));
}
this.text = text;
this.selectionStart = selectionStart;
this.selectionEnd = selectionEnd;
this.composingStart = composingStart;
this.composingEnd = composingEnd;
}
public boolean hasSelection() {
// When selectionStart == -1, it's guaranteed that selectionEnd will also
// be -1.
return selectionStart >= 0;
}
public boolean hasComposing() {
return composingStart >= 0 && composingEnd > composingStart;
}
}
}
| engine/shell/platform/android/io/flutter/embedding/engine/systemchannels/TextInputChannel.java/0 | {
"file_path": "engine/shell/platform/android/io/flutter/embedding/engine/systemchannels/TextInputChannel.java",
"repo_id": "engine",
"token_count": 12645
} | 311 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.