text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
/// The desired mode to launch a URL.
///
/// Support for these modes varies by platform. Platforms that do not support
/// the requested mode may substitute another mode.
enum PreferredLaunchMode {
/// Leaves the decision of how to launch the URL to the platform
/// implementation.
platformDefault,
/// Loads the URL in an in-app web view (e.g., Safari View Controller).
inAppWebView,
/// Passes the URL to the OS to be handled by another application.
externalApplication,
/// Passes the URL to the OS to be handled by another non-browser application.
externalNonBrowserApplication,
}
/// Additional configuration options for [PreferredLaunchMode.inAppWebView].
///
/// Not all options are supported on all platforms. This is a superset of
/// available options exposed across all implementations.
@immutable
class InAppWebViewConfiguration {
/// Creates a new WebViewConfiguration with the given settings.
const InAppWebViewConfiguration({
this.enableJavaScript = true,
this.enableDomStorage = true,
this.headers = const <String, String>{},
});
/// Whether or not JavaScript is enabled for the web content.
final bool enableJavaScript;
/// Whether or not DOM storage is enabled for the web content.
final bool enableDomStorage;
/// Additional headers to pass in the load request.
final Map<String, String> headers;
}
/// Options for [launchUrl].
@immutable
class LaunchOptions {
/// Creates a new parameter object with the given options.
const LaunchOptions({
this.mode = PreferredLaunchMode.platformDefault,
this.webViewConfiguration = const InAppWebViewConfiguration(),
this.webOnlyWindowName,
});
/// The requested launch mode.
final PreferredLaunchMode mode;
/// Configuration for the web view in [PreferredLaunchMode.inAppWebView] mode.
final InAppWebViewConfiguration webViewConfiguration;
/// A web-platform-specific option to set the link target.
///
/// Default behaviour when unset should be to open the url in a new tab.
final String? webOnlyWindowName;
}
| plugins/packages/url_launcher/url_launcher_platform_interface/lib/src/types.dart/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_platform_interface/lib/src/types.dart",
"repo_id": "plugins",
"token_count": 585
} | 1,171 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v5.0.1), do not edit directly.
// See also: https://pub.dev/packages/pigeon
// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, prefer_null_aware_operators, omit_local_variable_types, unused_shown_name, unnecessary_import
import 'dart:async';
import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;
import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;
import 'package:flutter/services.dart';
class UrlLauncherApi {
/// Constructor for [UrlLauncherApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
UrlLauncherApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<bool> canLaunchUrl(String arg_url) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UrlLauncherApi.canLaunchUrl', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_url]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
Future<void> launchUrl(String arg_url) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UrlLauncherApi.launchUrl', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_url]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
| plugins/packages/url_launcher/url_launcher_windows/lib/src/messages.g.dart/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_windows/lib/src/messages.g.dart",
"repo_id": "plugins",
"token_count": 1030
} | 1,172 |
targets:
$default:
sources:
include:
- lib/**
- android/app/src/main/**
# Some default includes that aren't really used here but will prevent
# false-negative warnings:
- $package$
- lib/$lib$
exclude:
- '**/.*/**'
- '**/build/**'
- 'android/app/src/main/res/**'
builders:
code_excerpter|code_excerpter:
enabled: true
generate_for:
- '**/*.dart'
- android/**/*.xml
| plugins/packages/video_player/video_player/example/build.excerpt.yaml/0 | {
"file_path": "plugins/packages/video_player/video_player/example/build.excerpt.yaml",
"repo_id": "plugins",
"token_count": 252
} | 1,173 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v2.0.1), do not edit directly.
// See also: https://pub.dev/packages/pigeon
package io.flutter.plugins.videoplayer;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import io.flutter.plugin.common.BasicMessageChannel;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.common.MessageCodec;
import io.flutter.plugin.common.StandardMessageCodec;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/** Generated class from Pigeon. */
@SuppressWarnings({"unused", "unchecked", "CodeBlock2Expr", "RedundantSuppression"})
public class Messages {
/** Generated class from Pigeon that represents data sent in messages. */
public static class TextureMessage {
private @NonNull Long textureId;
public @NonNull Long getTextureId() {
return textureId;
}
public void setTextureId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"textureId\" is null.");
}
this.textureId = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private TextureMessage() {}
public static class Builder {
private @Nullable Long textureId;
public @NonNull Builder setTextureId(@NonNull Long setterArg) {
this.textureId = setterArg;
return this;
}
public @NonNull TextureMessage build() {
TextureMessage pigeonReturn = new TextureMessage();
pigeonReturn.setTextureId(textureId);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("textureId", textureId);
return toMapResult;
}
static @NonNull TextureMessage fromMap(@NonNull Map<String, Object> map) {
TextureMessage pigeonResult = new TextureMessage();
Object textureId = map.get("textureId");
pigeonResult.setTextureId(
(textureId == null)
? null
: ((textureId instanceof Integer) ? (Integer) textureId : (Long) textureId));
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class LoopingMessage {
private @NonNull Long textureId;
public @NonNull Long getTextureId() {
return textureId;
}
public void setTextureId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"textureId\" is null.");
}
this.textureId = setterArg;
}
private @NonNull Boolean isLooping;
public @NonNull Boolean getIsLooping() {
return isLooping;
}
public void setIsLooping(@NonNull Boolean setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"isLooping\" is null.");
}
this.isLooping = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private LoopingMessage() {}
public static class Builder {
private @Nullable Long textureId;
public @NonNull Builder setTextureId(@NonNull Long setterArg) {
this.textureId = setterArg;
return this;
}
private @Nullable Boolean isLooping;
public @NonNull Builder setIsLooping(@NonNull Boolean setterArg) {
this.isLooping = setterArg;
return this;
}
public @NonNull LoopingMessage build() {
LoopingMessage pigeonReturn = new LoopingMessage();
pigeonReturn.setTextureId(textureId);
pigeonReturn.setIsLooping(isLooping);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("textureId", textureId);
toMapResult.put("isLooping", isLooping);
return toMapResult;
}
static @NonNull LoopingMessage fromMap(@NonNull Map<String, Object> map) {
LoopingMessage pigeonResult = new LoopingMessage();
Object textureId = map.get("textureId");
pigeonResult.setTextureId(
(textureId == null)
? null
: ((textureId instanceof Integer) ? (Integer) textureId : (Long) textureId));
Object isLooping = map.get("isLooping");
pigeonResult.setIsLooping((Boolean) isLooping);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class VolumeMessage {
private @NonNull Long textureId;
public @NonNull Long getTextureId() {
return textureId;
}
public void setTextureId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"textureId\" is null.");
}
this.textureId = setterArg;
}
private @NonNull Double volume;
public @NonNull Double getVolume() {
return volume;
}
public void setVolume(@NonNull Double setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"volume\" is null.");
}
this.volume = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private VolumeMessage() {}
public static class Builder {
private @Nullable Long textureId;
public @NonNull Builder setTextureId(@NonNull Long setterArg) {
this.textureId = setterArg;
return this;
}
private @Nullable Double volume;
public @NonNull Builder setVolume(@NonNull Double setterArg) {
this.volume = setterArg;
return this;
}
public @NonNull VolumeMessage build() {
VolumeMessage pigeonReturn = new VolumeMessage();
pigeonReturn.setTextureId(textureId);
pigeonReturn.setVolume(volume);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("textureId", textureId);
toMapResult.put("volume", volume);
return toMapResult;
}
static @NonNull VolumeMessage fromMap(@NonNull Map<String, Object> map) {
VolumeMessage pigeonResult = new VolumeMessage();
Object textureId = map.get("textureId");
pigeonResult.setTextureId(
(textureId == null)
? null
: ((textureId instanceof Integer) ? (Integer) textureId : (Long) textureId));
Object volume = map.get("volume");
pigeonResult.setVolume((Double) volume);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class PlaybackSpeedMessage {
private @NonNull Long textureId;
public @NonNull Long getTextureId() {
return textureId;
}
public void setTextureId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"textureId\" is null.");
}
this.textureId = setterArg;
}
private @NonNull Double speed;
public @NonNull Double getSpeed() {
return speed;
}
public void setSpeed(@NonNull Double setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"speed\" is null.");
}
this.speed = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private PlaybackSpeedMessage() {}
public static class Builder {
private @Nullable Long textureId;
public @NonNull Builder setTextureId(@NonNull Long setterArg) {
this.textureId = setterArg;
return this;
}
private @Nullable Double speed;
public @NonNull Builder setSpeed(@NonNull Double setterArg) {
this.speed = setterArg;
return this;
}
public @NonNull PlaybackSpeedMessage build() {
PlaybackSpeedMessage pigeonReturn = new PlaybackSpeedMessage();
pigeonReturn.setTextureId(textureId);
pigeonReturn.setSpeed(speed);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("textureId", textureId);
toMapResult.put("speed", speed);
return toMapResult;
}
static @NonNull PlaybackSpeedMessage fromMap(@NonNull Map<String, Object> map) {
PlaybackSpeedMessage pigeonResult = new PlaybackSpeedMessage();
Object textureId = map.get("textureId");
pigeonResult.setTextureId(
(textureId == null)
? null
: ((textureId instanceof Integer) ? (Integer) textureId : (Long) textureId));
Object speed = map.get("speed");
pigeonResult.setSpeed((Double) speed);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class PositionMessage {
private @NonNull Long textureId;
public @NonNull Long getTextureId() {
return textureId;
}
public void setTextureId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"textureId\" is null.");
}
this.textureId = setterArg;
}
private @NonNull Long position;
public @NonNull Long getPosition() {
return position;
}
public void setPosition(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"position\" is null.");
}
this.position = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private PositionMessage() {}
public static class Builder {
private @Nullable Long textureId;
public @NonNull Builder setTextureId(@NonNull Long setterArg) {
this.textureId = setterArg;
return this;
}
private @Nullable Long position;
public @NonNull Builder setPosition(@NonNull Long setterArg) {
this.position = setterArg;
return this;
}
public @NonNull PositionMessage build() {
PositionMessage pigeonReturn = new PositionMessage();
pigeonReturn.setTextureId(textureId);
pigeonReturn.setPosition(position);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("textureId", textureId);
toMapResult.put("position", position);
return toMapResult;
}
static @NonNull PositionMessage fromMap(@NonNull Map<String, Object> map) {
PositionMessage pigeonResult = new PositionMessage();
Object textureId = map.get("textureId");
pigeonResult.setTextureId(
(textureId == null)
? null
: ((textureId instanceof Integer) ? (Integer) textureId : (Long) textureId));
Object position = map.get("position");
pigeonResult.setPosition(
(position == null)
? null
: ((position instanceof Integer) ? (Integer) position : (Long) position));
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class CreateMessage {
private @Nullable String asset;
public @Nullable String getAsset() {
return asset;
}
public void setAsset(@Nullable String setterArg) {
this.asset = setterArg;
}
private @Nullable String uri;
public @Nullable String getUri() {
return uri;
}
public void setUri(@Nullable String setterArg) {
this.uri = setterArg;
}
private @Nullable String packageName;
public @Nullable String getPackageName() {
return packageName;
}
public void setPackageName(@Nullable String setterArg) {
this.packageName = setterArg;
}
private @Nullable String formatHint;
public @Nullable String getFormatHint() {
return formatHint;
}
public void setFormatHint(@Nullable String setterArg) {
this.formatHint = setterArg;
}
private @NonNull Map<String, String> httpHeaders;
public @NonNull Map<String, String> getHttpHeaders() {
return httpHeaders;
}
public void setHttpHeaders(@NonNull Map<String, String> setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"httpHeaders\" is null.");
}
this.httpHeaders = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private CreateMessage() {}
public static class Builder {
private @Nullable String asset;
public @NonNull Builder setAsset(@Nullable String setterArg) {
this.asset = setterArg;
return this;
}
private @Nullable String uri;
public @NonNull Builder setUri(@Nullable String setterArg) {
this.uri = setterArg;
return this;
}
private @Nullable String packageName;
public @NonNull Builder setPackageName(@Nullable String setterArg) {
this.packageName = setterArg;
return this;
}
private @Nullable String formatHint;
public @NonNull Builder setFormatHint(@Nullable String setterArg) {
this.formatHint = setterArg;
return this;
}
private @Nullable Map<String, String> httpHeaders;
public @NonNull Builder setHttpHeaders(@NonNull Map<String, String> setterArg) {
this.httpHeaders = setterArg;
return this;
}
public @NonNull CreateMessage build() {
CreateMessage pigeonReturn = new CreateMessage();
pigeonReturn.setAsset(asset);
pigeonReturn.setUri(uri);
pigeonReturn.setPackageName(packageName);
pigeonReturn.setFormatHint(formatHint);
pigeonReturn.setHttpHeaders(httpHeaders);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("asset", asset);
toMapResult.put("uri", uri);
toMapResult.put("packageName", packageName);
toMapResult.put("formatHint", formatHint);
toMapResult.put("httpHeaders", httpHeaders);
return toMapResult;
}
static @NonNull CreateMessage fromMap(@NonNull Map<String, Object> map) {
CreateMessage pigeonResult = new CreateMessage();
Object asset = map.get("asset");
pigeonResult.setAsset((String) asset);
Object uri = map.get("uri");
pigeonResult.setUri((String) uri);
Object packageName = map.get("packageName");
pigeonResult.setPackageName((String) packageName);
Object formatHint = map.get("formatHint");
pigeonResult.setFormatHint((String) formatHint);
Object httpHeaders = map.get("httpHeaders");
pigeonResult.setHttpHeaders((Map<String, String>) httpHeaders);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class MixWithOthersMessage {
private @NonNull Boolean mixWithOthers;
public @NonNull Boolean getMixWithOthers() {
return mixWithOthers;
}
public void setMixWithOthers(@NonNull Boolean setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"mixWithOthers\" is null.");
}
this.mixWithOthers = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private MixWithOthersMessage() {}
public static class Builder {
private @Nullable Boolean mixWithOthers;
public @NonNull Builder setMixWithOthers(@NonNull Boolean setterArg) {
this.mixWithOthers = setterArg;
return this;
}
public @NonNull MixWithOthersMessage build() {
MixWithOthersMessage pigeonReturn = new MixWithOthersMessage();
pigeonReturn.setMixWithOthers(mixWithOthers);
return pigeonReturn;
}
}
@NonNull
Map<String, Object> toMap() {
Map<String, Object> toMapResult = new HashMap<>();
toMapResult.put("mixWithOthers", mixWithOthers);
return toMapResult;
}
static @NonNull MixWithOthersMessage fromMap(@NonNull Map<String, Object> map) {
MixWithOthersMessage pigeonResult = new MixWithOthersMessage();
Object mixWithOthers = map.get("mixWithOthers");
pigeonResult.setMixWithOthers((Boolean) mixWithOthers);
return pigeonResult;
}
}
private static class AndroidVideoPlayerApiCodec extends StandardMessageCodec {
public static final AndroidVideoPlayerApiCodec INSTANCE = new AndroidVideoPlayerApiCodec();
private AndroidVideoPlayerApiCodec() {}
@Override
protected Object readValueOfType(byte type, ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return CreateMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 129:
return LoopingMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 130:
return MixWithOthersMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 131:
return PlaybackSpeedMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 132:
return PositionMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 133:
return TextureMessage.fromMap((Map<String, Object>) readValue(buffer));
case (byte) 134:
return VolumeMessage.fromMap((Map<String, Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(ByteArrayOutputStream stream, Object value) {
if (value instanceof CreateMessage) {
stream.write(128);
writeValue(stream, ((CreateMessage) value).toMap());
} else if (value instanceof LoopingMessage) {
stream.write(129);
writeValue(stream, ((LoopingMessage) value).toMap());
} else if (value instanceof MixWithOthersMessage) {
stream.write(130);
writeValue(stream, ((MixWithOthersMessage) value).toMap());
} else if (value instanceof PlaybackSpeedMessage) {
stream.write(131);
writeValue(stream, ((PlaybackSpeedMessage) value).toMap());
} else if (value instanceof PositionMessage) {
stream.write(132);
writeValue(stream, ((PositionMessage) value).toMap());
} else if (value instanceof TextureMessage) {
stream.write(133);
writeValue(stream, ((TextureMessage) value).toMap());
} else if (value instanceof VolumeMessage) {
stream.write(134);
writeValue(stream, ((VolumeMessage) value).toMap());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface AndroidVideoPlayerApi {
void initialize();
@NonNull
TextureMessage create(@NonNull CreateMessage msg);
void dispose(@NonNull TextureMessage msg);
void setLooping(@NonNull LoopingMessage msg);
void setVolume(@NonNull VolumeMessage msg);
void setPlaybackSpeed(@NonNull PlaybackSpeedMessage msg);
void play(@NonNull TextureMessage msg);
@NonNull
PositionMessage position(@NonNull TextureMessage msg);
void seekTo(@NonNull PositionMessage msg);
void pause(@NonNull TextureMessage msg);
void setMixWithOthers(@NonNull MixWithOthersMessage msg);
/** The codec used by AndroidVideoPlayerApi. */
static MessageCodec<Object> getCodec() {
return AndroidVideoPlayerApiCodec.INSTANCE;
}
/**
* Sets up an instance of `AndroidVideoPlayerApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, AndroidVideoPlayerApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.initialize", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
api.initialize();
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
CreateMessage msgArg = (CreateMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
TextureMessage output = api.create(msgArg);
wrapped.put("result", output);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.dispose", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
TextureMessage msgArg = (TextureMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.dispose(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.setLooping", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
LoopingMessage msgArg = (LoopingMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.setLooping(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.setVolume", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
VolumeMessage msgArg = (VolumeMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.setVolume(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.AndroidVideoPlayerApi.setPlaybackSpeed",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
PlaybackSpeedMessage msgArg = (PlaybackSpeedMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.setPlaybackSpeed(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.play", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
TextureMessage msgArg = (TextureMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.play(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.position", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
TextureMessage msgArg = (TextureMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
PositionMessage output = api.position(msgArg);
wrapped.put("result", output);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.seekTo", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
PositionMessage msgArg = (PositionMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.seekTo(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AndroidVideoPlayerApi.pause", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
TextureMessage msgArg = (TextureMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.pause(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.AndroidVideoPlayerApi.setMixWithOthers",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
Map<String, Object> wrapped = new HashMap<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
MixWithOthersMessage msgArg = (MixWithOthersMessage) args.get(0);
if (msgArg == null) {
throw new NullPointerException("msgArg unexpectedly null.");
}
api.setMixWithOthers(msgArg);
wrapped.put("result", null);
} catch (Error | RuntimeException exception) {
wrapped.put("error", wrapError(exception));
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static Map<String, Object> wrapError(Throwable exception) {
Map<String, Object> errorMap = new HashMap<>();
errorMap.put("message", exception.toString());
errorMap.put("code", exception.getClass().getSimpleName());
errorMap.put(
"details",
"Cause: " + exception.getCause() + ", Stacktrace: " + Log.getStackTraceString(exception));
return errorMap;
}
}
| plugins/packages/video_player/video_player_android/android/src/main/java/io/flutter/plugins/videoplayer/Messages.java/0 | {
"file_path": "plugins/packages/video_player/video_player_android/android/src/main/java/io/flutter/plugins/videoplayer/Messages.java",
"repo_id": "plugins",
"token_count": 13443
} | 1,174 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:html' as html;
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:video_player_platform_interface/video_player_platform_interface.dart';
import 'package:video_player_web/src/duration_utils.dart';
import 'package:video_player_web/src/video_player.dart';
import 'utils.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
group('VideoPlayer', () {
late html.VideoElement video;
setUp(() {
// Never set "src" on the video, so this test doesn't hit the network!
video = html.VideoElement()
..controls = true
..setAttribute('playsinline', 'false');
});
testWidgets('fixes critical video element config', (WidgetTester _) async {
VideoPlayer(videoElement: video).initialize();
expect(video.controls, isFalse,
reason: 'Video is controlled through code');
expect(video.getAttribute('autoplay'), 'false',
reason: 'Cannot autoplay on the web');
expect(video.getAttribute('playsinline'), 'true',
reason: 'Needed by safari iOS');
});
testWidgets('setVolume', (WidgetTester tester) async {
final VideoPlayer player = VideoPlayer(videoElement: video)..initialize();
player.setVolume(0);
expect(video.volume, isZero, reason: 'Volume should be zero');
expect(video.muted, isTrue, reason: 'muted attribute should be true');
expect(() {
player.setVolume(-0.0001);
}, throwsAssertionError, reason: 'Volume cannot be < 0');
expect(() {
player.setVolume(1.0001);
}, throwsAssertionError, reason: 'Volume cannot be > 1');
});
testWidgets('setPlaybackSpeed', (WidgetTester tester) async {
final VideoPlayer player = VideoPlayer(videoElement: video)..initialize();
expect(() {
player.setPlaybackSpeed(-1);
}, throwsAssertionError, reason: 'Playback speed cannot be < 0');
expect(() {
player.setPlaybackSpeed(0);
}, throwsAssertionError, reason: 'Playback speed cannot be == 0');
});
testWidgets('seekTo', (WidgetTester tester) async {
final VideoPlayer player = VideoPlayer(videoElement: video)..initialize();
expect(() {
player.seekTo(const Duration(seconds: -1));
}, throwsAssertionError, reason: 'Cannot seek into negative numbers');
});
// The events tested in this group do *not* represent the actual sequence
// of events from a real "video" element. They're crafted to test the
// behavior of the VideoPlayer in different states with different events.
group('events', () {
late StreamController<VideoEvent> streamController;
late VideoPlayer player;
late Stream<VideoEvent> timedStream;
final Set<VideoEventType> bufferingEvents = <VideoEventType>{
VideoEventType.bufferingStart,
VideoEventType.bufferingEnd,
};
setUp(() {
streamController = StreamController<VideoEvent>();
player =
VideoPlayer(videoElement: video, eventController: streamController)
..initialize();
// This stream will automatically close after 100 ms without seeing any events
timedStream = streamController.stream.timeout(
const Duration(milliseconds: 100),
onTimeout: (EventSink<VideoEvent> sink) {
sink.close();
},
);
});
testWidgets('buffering dispatches only when it changes',
(WidgetTester tester) async {
// Take all the "buffering" events that we see during the next few seconds
final Future<List<bool>> stream = timedStream
.where(
(VideoEvent event) => bufferingEvents.contains(event.eventType))
.map((VideoEvent event) =>
event.eventType == VideoEventType.bufferingStart)
.toList();
// Simulate some events coming from the player...
player.setBuffering(true);
player.setBuffering(true);
player.setBuffering(true);
player.setBuffering(false);
player.setBuffering(false);
player.setBuffering(true);
player.setBuffering(false);
player.setBuffering(true);
player.setBuffering(false);
final List<bool> events = await stream;
expect(events, hasLength(6));
expect(events, <bool>[true, false, true, false, true, false]);
});
testWidgets('canplay event does not change buffering state',
(WidgetTester tester) async {
// Take all the "buffering" events that we see during the next few seconds
final Future<List<bool>> stream = timedStream
.where(
(VideoEvent event) => bufferingEvents.contains(event.eventType))
.map((VideoEvent event) =>
event.eventType == VideoEventType.bufferingStart)
.toList();
player.setBuffering(true);
// Simulate "canplay" event...
video.dispatchEvent(html.Event('canplay'));
final List<bool> events = await stream;
expect(events, hasLength(1));
expect(events, <bool>[true]);
});
testWidgets('canplaythrough event does change buffering state',
(WidgetTester tester) async {
// Take all the "buffering" events that we see during the next few seconds
final Future<List<bool>> stream = timedStream
.where(
(VideoEvent event) => bufferingEvents.contains(event.eventType))
.map((VideoEvent event) =>
event.eventType == VideoEventType.bufferingStart)
.toList();
player.setBuffering(true);
// Simulate "canplaythrough" event...
video.dispatchEvent(html.Event('canplaythrough'));
final List<bool> events = await stream;
expect(events, hasLength(2));
expect(events, <bool>[true, false]);
});
testWidgets('initialized dispatches only once',
(WidgetTester tester) async {
// Dispatch some bogus "canplay" events from the video object
video.dispatchEvent(html.Event('canplay'));
video.dispatchEvent(html.Event('canplay'));
video.dispatchEvent(html.Event('canplay'));
// Take all the "initialized" events that we see during the next few seconds
final Future<List<VideoEvent>> stream = timedStream
.where((VideoEvent event) =>
event.eventType == VideoEventType.initialized)
.toList();
video.dispatchEvent(html.Event('canplay'));
video.dispatchEvent(html.Event('canplay'));
video.dispatchEvent(html.Event('canplay'));
final List<VideoEvent> events = await stream;
expect(events, hasLength(1));
expect(events[0].eventType, VideoEventType.initialized);
});
// Issue: https://github.com/flutter/flutter/issues/105649
testWidgets('supports `Infinity` duration', (WidgetTester _) async {
setInfinityDuration(video);
expect(video.duration.isInfinite, isTrue);
final Future<List<VideoEvent>> stream = timedStream
.where((VideoEvent event) =>
event.eventType == VideoEventType.initialized)
.toList();
video.dispatchEvent(html.Event('canplay'));
final List<VideoEvent> events = await stream;
expect(events, hasLength(1));
expect(events[0].eventType, VideoEventType.initialized);
expect(events[0].duration, equals(jsCompatibleTimeUnset));
});
});
});
}
| plugins/packages/video_player/video_player_web/example/integration_test/video_player_test.dart/0 | {
"file_path": "plugins/packages/video_player/video_player_web/example/integration_test/video_player_test.dart",
"repo_id": "plugins",
"token_count": 3013
} | 1,175 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// TODO(a14n): remove this import once Flutter 3.1 or later reaches stable (including flutter/flutter#104231)
// ignore: unnecessary_import
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:webview_flutter_platform_interface/webview_flutter_platform_interface.dart';
import 'navigation_delegate.dart';
import 'webview_widget.dart';
/// Controls a WebView provided by the host platform.
///
/// Pass this to a [WebViewWidget] to display the WebView.
///
/// A [WebViewController] can only be used by a single [WebViewWidget] at a
/// time.
///
/// ## Platform-Specific Features
/// This class contains an underlying implementation provided by the current
/// platform. Once a platform implementation is imported, the examples below
/// can be followed to use features provided by a platform's implementation.
///
/// {@macro webview_flutter.WebViewController.fromPlatformCreationParams}
///
/// Below is an example of accessing the platform-specific implementation for
/// iOS and Android:
///
/// ```dart
/// final WebViewController webViewController = WebViewController();
///
/// if (WebViewPlatform.instance is WebKitWebViewPlatform) {
/// final WebKitWebViewController webKitController =
/// webViewController.platform as WebKitWebViewController;
/// } else if (WebViewPlatform.instance is AndroidWebViewPlatform) {
/// final AndroidWebViewController androidController =
/// webViewController.platform as AndroidWebViewController;
/// }
/// ```
class WebViewController {
/// Constructs a [WebViewController].
///
/// See [WebViewController.fromPlatformCreationParams] for setting parameters
/// for a specific platform.
WebViewController()
: this.fromPlatformCreationParams(
const PlatformWebViewControllerCreationParams(),
);
/// Constructs a [WebViewController] from creation params for a specific
/// platform.
///
/// {@template webview_flutter.WebViewCookieManager.fromPlatformCreationParams}
/// Below is an example of setting platform-specific creation parameters for
/// iOS and Android:
///
/// ```dart
/// PlatformWebViewControllerCreationParams params =
/// const PlatformWebViewControllerCreationParams();
///
/// if (WebViewPlatform.instance is WebKitWebViewPlatform) {
/// params = WebKitWebViewControllerCreationParams
/// .fromPlatformWebViewControllerCreationParams(
/// params,
/// );
/// } else if (WebViewPlatform.instance is AndroidWebViewPlatform) {
/// params = AndroidWebViewControllerCreationParams
/// .fromPlatformWebViewControllerCreationParams(
/// params,
/// );
/// }
///
/// final WebViewController webViewController =
/// WebViewController.fromPlatformCreationParams(
/// params,
/// );
/// ```
/// {@endtemplate}
WebViewController.fromPlatformCreationParams(
PlatformWebViewControllerCreationParams params,
) : this.fromPlatform(PlatformWebViewController(params));
/// Constructs a [WebViewController] from a specific platform implementation.
WebViewController.fromPlatform(this.platform);
/// Implementation of [PlatformWebViewController] for the current platform.
final PlatformWebViewController platform;
/// Loads the file located on the specified [absoluteFilePath].
///
/// The [absoluteFilePath] parameter should contain the absolute path to the
/// file as it is stored on the device. For example:
/// `/Users/username/Documents/www/index.html`.
///
/// Throws a `PlatformException` if the [absoluteFilePath] does not exist.
Future<void> loadFile(String absoluteFilePath) {
return platform.loadFile(absoluteFilePath);
}
/// Loads the Flutter asset specified in the pubspec.yaml file.
///
/// Throws a `PlatformException` if [key] is not part of the specified assets
/// in the pubspec.yaml file.
Future<void> loadFlutterAsset(String key) {
assert(key.isNotEmpty);
return platform.loadFlutterAsset(key);
}
/// Loads the supplied HTML string.
///
/// The [baseUrl] parameter is used when resolving relative URLs within the
/// HTML string.
Future<void> loadHtmlString(String html, {String? baseUrl}) {
assert(html.isNotEmpty);
return platform.loadHtmlString(html, baseUrl: baseUrl);
}
/// Makes a specific HTTP request ands loads the response in the webview.
///
/// [method] must be one of the supported HTTP methods in [LoadRequestMethod].
///
/// If [headers] is not empty, its key-value pairs will be added as the
/// headers for the request.
///
/// If [body] is not null, it will be added as the body for the request.
///
/// Throws an ArgumentError if [uri] has an empty scheme.
Future<void> loadRequest(
Uri uri, {
LoadRequestMethod method = LoadRequestMethod.get,
Map<String, String> headers = const <String, String>{},
Uint8List? body,
}) {
if (uri.scheme.isEmpty) {
throw ArgumentError('Missing scheme in uri: $uri');
}
return platform.loadRequest(LoadRequestParams(
uri: uri,
method: method,
headers: headers,
body: body,
));
}
/// Returns the current URL that the WebView is displaying.
///
/// If no URL was ever loaded, returns `null`.
Future<String?> currentUrl() {
return platform.currentUrl();
}
/// Checks whether there's a back history item.
Future<bool> canGoBack() {
return platform.canGoBack();
}
/// Checks whether there's a forward history item.
Future<bool> canGoForward() {
return platform.canGoForward();
}
/// Goes back in the history of this WebView.
///
/// If there is no back history item this is a no-op.
Future<void> goBack() {
return platform.goBack();
}
/// Goes forward in the history of this WebView.
///
/// If there is no forward history item this is a no-op.
Future<void> goForward() {
return platform.goForward();
}
/// Reloads the current URL.
Future<void> reload() {
return platform.reload();
}
/// Sets the [NavigationDelegate] containing the callback methods that are
/// called during navigation events.
Future<void> setNavigationDelegate(NavigationDelegate delegate) {
return platform.setPlatformNavigationDelegate(delegate.platform);
}
/// Clears all caches used by the WebView.
///
/// The following caches are cleared:
/// 1. Browser HTTP Cache.
/// 2. [Cache API](https://developers.google.com/web/fundamentals/instant-and-offline/web-storage/cache-api)
/// caches. Service workers tend to use this cache.
/// 3. Application cache.
Future<void> clearCache() {
return platform.clearCache();
}
/// Clears the local storage used by the WebView.
Future<void> clearLocalStorage() {
return platform.clearLocalStorage();
}
/// Runs the given JavaScript in the context of the current page.
///
/// The Future completes with an error if a JavaScript error occurred.
Future<void> runJavaScript(String javaScript) {
return platform.runJavaScript(javaScript);
}
/// Runs the given JavaScript in the context of the current page, and returns
/// the result.
///
/// The Future completes with an error if a JavaScript error occurred, or if
/// the type the given expression evaluates to is unsupported. Unsupported
/// values include certain non-primitive types on iOS, as well as `undefined`
/// or `null` on iOS 14+.
Future<Object> runJavaScriptReturningResult(String javaScript) {
return platform.runJavaScriptReturningResult(javaScript);
}
/// Adds a new JavaScript channel to the set of enabled channels.
///
/// The JavaScript code can then call `postMessage` on that object to send a
/// message that will be passed to [onMessageReceived].
///
/// For example, after adding the following JavaScript channel:
///
/// ```dart
/// final WebViewController controller = WebViewController();
/// controller.addJavaScriptChannel(
/// name: 'Print',
/// onMessageReceived: (JavascriptMessage message) {
/// print(message.message);
/// },
/// );
/// ```
///
/// JavaScript code can call:
///
/// ```javascript
/// Print.postMessage('Hello');
/// ```
///
/// to asynchronously invoke the message handler which will print the message
/// to standard output.
///
/// Adding a new JavaScript channel only takes affect after the next page is
/// loaded.
///
/// A channel [name] cannot be the same for multiple channels.
Future<void> addJavaScriptChannel(
String name, {
required void Function(JavaScriptMessage) onMessageReceived,
}) {
assert(name.isNotEmpty);
return platform.addJavaScriptChannel(JavaScriptChannelParams(
name: name,
onMessageReceived: onMessageReceived,
));
}
/// Removes the JavaScript channel with the matching name from the set of
/// enabled channels.
///
/// This disables the channel with the matching name if it was previously
/// enabled through the [addJavaScriptChannel].
Future<void> removeJavaScriptChannel(String javaScriptChannelName) {
return platform.removeJavaScriptChannel(javaScriptChannelName);
}
/// The title of the currently loaded page.
Future<String?> getTitle() {
return platform.getTitle();
}
/// Sets the scrolled position of this view.
///
/// The parameters `x` and `y` specify the position to scroll to in WebView
/// pixels.
Future<void> scrollTo(int x, int y) {
return platform.scrollTo(x, y);
}
/// Moves the scrolled position of this view.
///
/// The parameters `x` and `y` specify the amount of WebView pixels to scroll
/// by.
Future<void> scrollBy(int x, int y) {
return platform.scrollBy(x, y);
}
/// Returns the current scroll position of this view.
///
/// Scroll position is measured from the top left.
Future<Offset> getScrollPosition() {
return platform.getScrollPosition();
}
/// Whether to support zooming using the on-screen zoom controls and gestures.
Future<void> enableZoom(bool enabled) {
return platform.enableZoom(enabled);
}
/// Sets the current background color of this view.
Future<void> setBackgroundColor(Color color) {
return platform.setBackgroundColor(color);
}
/// Sets the JavaScript execution mode to be used by the WebView.
Future<void> setJavaScriptMode(JavaScriptMode javaScriptMode) {
return platform.setJavaScriptMode(javaScriptMode);
}
/// Sets the value used for the HTTP `User-Agent:` request header.
Future<void> setUserAgent(String? userAgent) {
return platform.setUserAgent(userAgent);
}
}
| plugins/packages/webview_flutter/webview_flutter/lib/src/webview_controller.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter/lib/src/webview_controller.dart",
"repo_id": "plugins",
"token_count": 3143
} | 1,176 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v4.2.14), do not edit directly.
// See also: https://pub.dev/packages/pigeon
package io.flutter.plugins.webviewflutter;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import io.flutter.plugin.common.BasicMessageChannel;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.common.MessageCodec;
import io.flutter.plugin.common.StandardMessageCodec;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/** Generated class from Pigeon. */
@SuppressWarnings({"unused", "unchecked", "CodeBlock2Expr", "RedundantSuppression"})
public class GeneratedAndroidWebView {
/**
* Mode of how to select files for a file chooser.
*
* <p>See
* https://developer.android.com/reference/android/webkit/WebChromeClient.FileChooserParams.
*/
public enum FileChooserMode {
/**
* Open single file and requires that the file exists before allowing the user to pick it.
*
* <p>See
* https://developer.android.com/reference/android/webkit/WebChromeClient.FileChooserParams#MODE_OPEN.
*/
OPEN(0),
/**
* Similar to [open] but allows multiple files to be selected.
*
* <p>See
* https://developer.android.com/reference/android/webkit/WebChromeClient.FileChooserParams#MODE_OPEN_MULTIPLE.
*/
OPEN_MULTIPLE(1),
/**
* Allows picking a nonexistent file and saving it.
*
* <p>See
* https://developer.android.com/reference/android/webkit/WebChromeClient.FileChooserParams#MODE_SAVE.
*/
SAVE(2);
private final int index;
private FileChooserMode(final int index) {
this.index = index;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class FileChooserModeEnumData {
private @NonNull FileChooserMode value;
public @NonNull FileChooserMode getValue() {
return value;
}
public void setValue(@NonNull FileChooserMode setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"value\" is null.");
}
this.value = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private FileChooserModeEnumData() {}
public static final class Builder {
private @Nullable FileChooserMode value;
public @NonNull Builder setValue(@NonNull FileChooserMode setterArg) {
this.value = setterArg;
return this;
}
public @NonNull FileChooserModeEnumData build() {
FileChooserModeEnumData pigeonReturn = new FileChooserModeEnumData();
pigeonReturn.setValue(value);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(1);
toListResult.add(value == null ? null : value.index);
return toListResult;
}
static @NonNull FileChooserModeEnumData fromList(@NonNull ArrayList<Object> list) {
FileChooserModeEnumData pigeonResult = new FileChooserModeEnumData();
Object value = list.get(0);
pigeonResult.setValue(value == null ? null : FileChooserMode.values()[(int) value]);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class WebResourceRequestData {
private @NonNull String url;
public @NonNull String getUrl() {
return url;
}
public void setUrl(@NonNull String setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"url\" is null.");
}
this.url = setterArg;
}
private @NonNull Boolean isForMainFrame;
public @NonNull Boolean getIsForMainFrame() {
return isForMainFrame;
}
public void setIsForMainFrame(@NonNull Boolean setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"isForMainFrame\" is null.");
}
this.isForMainFrame = setterArg;
}
private @Nullable Boolean isRedirect;
public @Nullable Boolean getIsRedirect() {
return isRedirect;
}
public void setIsRedirect(@Nullable Boolean setterArg) {
this.isRedirect = setterArg;
}
private @NonNull Boolean hasGesture;
public @NonNull Boolean getHasGesture() {
return hasGesture;
}
public void setHasGesture(@NonNull Boolean setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"hasGesture\" is null.");
}
this.hasGesture = setterArg;
}
private @NonNull String method;
public @NonNull String getMethod() {
return method;
}
public void setMethod(@NonNull String setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"method\" is null.");
}
this.method = setterArg;
}
private @NonNull Map<String, String> requestHeaders;
public @NonNull Map<String, String> getRequestHeaders() {
return requestHeaders;
}
public void setRequestHeaders(@NonNull Map<String, String> setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"requestHeaders\" is null.");
}
this.requestHeaders = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private WebResourceRequestData() {}
public static final class Builder {
private @Nullable String url;
public @NonNull Builder setUrl(@NonNull String setterArg) {
this.url = setterArg;
return this;
}
private @Nullable Boolean isForMainFrame;
public @NonNull Builder setIsForMainFrame(@NonNull Boolean setterArg) {
this.isForMainFrame = setterArg;
return this;
}
private @Nullable Boolean isRedirect;
public @NonNull Builder setIsRedirect(@Nullable Boolean setterArg) {
this.isRedirect = setterArg;
return this;
}
private @Nullable Boolean hasGesture;
public @NonNull Builder setHasGesture(@NonNull Boolean setterArg) {
this.hasGesture = setterArg;
return this;
}
private @Nullable String method;
public @NonNull Builder setMethod(@NonNull String setterArg) {
this.method = setterArg;
return this;
}
private @Nullable Map<String, String> requestHeaders;
public @NonNull Builder setRequestHeaders(@NonNull Map<String, String> setterArg) {
this.requestHeaders = setterArg;
return this;
}
public @NonNull WebResourceRequestData build() {
WebResourceRequestData pigeonReturn = new WebResourceRequestData();
pigeonReturn.setUrl(url);
pigeonReturn.setIsForMainFrame(isForMainFrame);
pigeonReturn.setIsRedirect(isRedirect);
pigeonReturn.setHasGesture(hasGesture);
pigeonReturn.setMethod(method);
pigeonReturn.setRequestHeaders(requestHeaders);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(6);
toListResult.add(url);
toListResult.add(isForMainFrame);
toListResult.add(isRedirect);
toListResult.add(hasGesture);
toListResult.add(method);
toListResult.add(requestHeaders);
return toListResult;
}
static @NonNull WebResourceRequestData fromList(@NonNull ArrayList<Object> list) {
WebResourceRequestData pigeonResult = new WebResourceRequestData();
Object url = list.get(0);
pigeonResult.setUrl((String) url);
Object isForMainFrame = list.get(1);
pigeonResult.setIsForMainFrame((Boolean) isForMainFrame);
Object isRedirect = list.get(2);
pigeonResult.setIsRedirect((Boolean) isRedirect);
Object hasGesture = list.get(3);
pigeonResult.setHasGesture((Boolean) hasGesture);
Object method = list.get(4);
pigeonResult.setMethod((String) method);
Object requestHeaders = list.get(5);
pigeonResult.setRequestHeaders((Map<String, String>) requestHeaders);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class WebResourceErrorData {
private @NonNull Long errorCode;
public @NonNull Long getErrorCode() {
return errorCode;
}
public void setErrorCode(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"errorCode\" is null.");
}
this.errorCode = setterArg;
}
private @NonNull String description;
public @NonNull String getDescription() {
return description;
}
public void setDescription(@NonNull String setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"description\" is null.");
}
this.description = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private WebResourceErrorData() {}
public static final class Builder {
private @Nullable Long errorCode;
public @NonNull Builder setErrorCode(@NonNull Long setterArg) {
this.errorCode = setterArg;
return this;
}
private @Nullable String description;
public @NonNull Builder setDescription(@NonNull String setterArg) {
this.description = setterArg;
return this;
}
public @NonNull WebResourceErrorData build() {
WebResourceErrorData pigeonReturn = new WebResourceErrorData();
pigeonReturn.setErrorCode(errorCode);
pigeonReturn.setDescription(description);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(errorCode);
toListResult.add(description);
return toListResult;
}
static @NonNull WebResourceErrorData fromList(@NonNull ArrayList<Object> list) {
WebResourceErrorData pigeonResult = new WebResourceErrorData();
Object errorCode = list.get(0);
pigeonResult.setErrorCode(
(errorCode == null)
? null
: ((errorCode instanceof Integer) ? (Integer) errorCode : (Long) errorCode));
Object description = list.get(1);
pigeonResult.setDescription((String) description);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static class WebViewPoint {
private @NonNull Long x;
public @NonNull Long getX() {
return x;
}
public void setX(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"x\" is null.");
}
this.x = setterArg;
}
private @NonNull Long y;
public @NonNull Long getY() {
return y;
}
public void setY(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"y\" is null.");
}
this.y = setterArg;
}
/** Constructor is private to enforce null safety; use Builder. */
private WebViewPoint() {}
public static final class Builder {
private @Nullable Long x;
public @NonNull Builder setX(@NonNull Long setterArg) {
this.x = setterArg;
return this;
}
private @Nullable Long y;
public @NonNull Builder setY(@NonNull Long setterArg) {
this.y = setterArg;
return this;
}
public @NonNull WebViewPoint build() {
WebViewPoint pigeonReturn = new WebViewPoint();
pigeonReturn.setX(x);
pigeonReturn.setY(y);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(x);
toListResult.add(y);
return toListResult;
}
static @NonNull WebViewPoint fromList(@NonNull ArrayList<Object> list) {
WebViewPoint pigeonResult = new WebViewPoint();
Object x = list.get(0);
pigeonResult.setX((x == null) ? null : ((x instanceof Integer) ? (Integer) x : (Long) x));
Object y = list.get(1);
pigeonResult.setY((y == null) ? null : ((y instanceof Integer) ? (Integer) y : (Long) y));
return pigeonResult;
}
}
public interface Result<T> {
void success(T result);
void error(Throwable error);
}
/**
* Handles methods calls to the native Java Object class.
*
* <p>Also handles calls to remove the reference to an instance with `dispose`.
*
* <p>See https://docs.oracle.com/javase/7/docs/api/java/lang/Object.html.
*
* <p>Generated interface from Pigeon that represents a handler of messages from Flutter.
*/
public interface JavaObjectHostApi {
void dispose(@NonNull Long identifier);
/** The codec used by JavaObjectHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `JavaObjectHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, JavaObjectHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.JavaObjectHostApi.dispose", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number identifierArg = (Number) args.get(0);
if (identifierArg == null) {
throw new NullPointerException("identifierArg unexpectedly null.");
}
api.dispose((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/**
* Handles callbacks methods for the native Java Object class.
*
* <p>See https://docs.oracle.com/javase/7/docs/api/java/lang/Object.html.
*
* <p>Generated class from Pigeon that represents Flutter messages that can be called from Java.
*/
public static class JavaObjectFlutterApi {
private final BinaryMessenger binaryMessenger;
public JavaObjectFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by JavaObjectFlutterApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void dispose(@NonNull Long identifierArg, Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.JavaObjectFlutterApi.dispose", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> {
callback.reply(null);
});
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CookieManagerHostApi {
void clearCookies(Result<Boolean> result);
void setCookie(@NonNull String url, @NonNull String value);
/** The codec used by CookieManagerHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `CookieManagerHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, CookieManagerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CookieManagerHostApi.clearCookies",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
Result<Boolean> resultCallback =
new Result<Boolean>() {
public void success(Boolean result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.clearCookies(resultCallback);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
reply.reply(wrappedError);
}
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CookieManagerHostApi.setCookie", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
String urlArg = (String) args.get(0);
if (urlArg == null) {
throw new NullPointerException("urlArg unexpectedly null.");
}
String valueArg = (String) args.get(1);
if (valueArg == null) {
throw new NullPointerException("valueArg unexpectedly null.");
}
api.setCookie(urlArg, valueArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class WebViewHostApiCodec extends StandardMessageCodec {
public static final WebViewHostApiCodec INSTANCE = new WebViewHostApiCodec();
private WebViewHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return WebViewPoint.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof WebViewPoint) {
stream.write(128);
writeValue(stream, ((WebViewPoint) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface WebViewHostApi {
void create(@NonNull Long instanceId, @NonNull Boolean useHybridComposition);
void loadData(
@NonNull Long instanceId,
@NonNull String data,
@Nullable String mimeType,
@Nullable String encoding);
void loadDataWithBaseUrl(
@NonNull Long instanceId,
@Nullable String baseUrl,
@NonNull String data,
@Nullable String mimeType,
@Nullable String encoding,
@Nullable String historyUrl);
void loadUrl(
@NonNull Long instanceId, @NonNull String url, @NonNull Map<String, String> headers);
void postUrl(@NonNull Long instanceId, @NonNull String url, @NonNull byte[] data);
@Nullable
String getUrl(@NonNull Long instanceId);
@NonNull
Boolean canGoBack(@NonNull Long instanceId);
@NonNull
Boolean canGoForward(@NonNull Long instanceId);
void goBack(@NonNull Long instanceId);
void goForward(@NonNull Long instanceId);
void reload(@NonNull Long instanceId);
void clearCache(@NonNull Long instanceId, @NonNull Boolean includeDiskFiles);
void evaluateJavascript(
@NonNull Long instanceId, @NonNull String javascriptString, Result<String> result);
@Nullable
String getTitle(@NonNull Long instanceId);
void scrollTo(@NonNull Long instanceId, @NonNull Long x, @NonNull Long y);
void scrollBy(@NonNull Long instanceId, @NonNull Long x, @NonNull Long y);
@NonNull
Long getScrollX(@NonNull Long instanceId);
@NonNull
Long getScrollY(@NonNull Long instanceId);
@NonNull
WebViewPoint getScrollPosition(@NonNull Long instanceId);
void setWebContentsDebuggingEnabled(@NonNull Boolean enabled);
void setWebViewClient(@NonNull Long instanceId, @NonNull Long webViewClientInstanceId);
void addJavaScriptChannel(@NonNull Long instanceId, @NonNull Long javaScriptChannelInstanceId);
void removeJavaScriptChannel(
@NonNull Long instanceId, @NonNull Long javaScriptChannelInstanceId);
void setDownloadListener(@NonNull Long instanceId, @Nullable Long listenerInstanceId);
void setWebChromeClient(@NonNull Long instanceId, @Nullable Long clientInstanceId);
void setBackgroundColor(@NonNull Long instanceId, @NonNull Long color);
/** The codec used by WebViewHostApi. */
static MessageCodec<Object> getCodec() {
return WebViewHostApiCodec.INSTANCE;
}
/** Sets up an instance of `WebViewHostApi` to handle messages through the `binaryMessenger`. */
static void setup(BinaryMessenger binaryMessenger, WebViewHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean useHybridCompositionArg = (Boolean) args.get(1);
if (useHybridCompositionArg == null) {
throw new NullPointerException("useHybridCompositionArg unexpectedly null.");
}
api.create(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
useHybridCompositionArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.loadData", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String dataArg = (String) args.get(1);
if (dataArg == null) {
throw new NullPointerException("dataArg unexpectedly null.");
}
String mimeTypeArg = (String) args.get(2);
String encodingArg = (String) args.get(3);
api.loadData(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
dataArg,
mimeTypeArg,
encodingArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.loadDataWithBaseUrl",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String baseUrlArg = (String) args.get(1);
String dataArg = (String) args.get(2);
if (dataArg == null) {
throw new NullPointerException("dataArg unexpectedly null.");
}
String mimeTypeArg = (String) args.get(3);
String encodingArg = (String) args.get(4);
String historyUrlArg = (String) args.get(5);
api.loadDataWithBaseUrl(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
baseUrlArg,
dataArg,
mimeTypeArg,
encodingArg,
historyUrlArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.loadUrl", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String urlArg = (String) args.get(1);
if (urlArg == null) {
throw new NullPointerException("urlArg unexpectedly null.");
}
Map<String, String> headersArg = (Map<String, String>) args.get(2);
if (headersArg == null) {
throw new NullPointerException("headersArg unexpectedly null.");
}
api.loadUrl(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
urlArg,
headersArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.postUrl", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String urlArg = (String) args.get(1);
if (urlArg == null) {
throw new NullPointerException("urlArg unexpectedly null.");
}
byte[] dataArg = (byte[]) args.get(2);
if (dataArg == null) {
throw new NullPointerException("dataArg unexpectedly null.");
}
api.postUrl(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), urlArg, dataArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.getUrl", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String output =
api.getUrl((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.canGoBack", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean output =
api.canGoBack((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.canGoForward", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean output =
api.canGoForward((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.goBack", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.goBack((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.goForward", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.goForward((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.reload", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.reload((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.clearCache", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean includeDiskFilesArg = (Boolean) args.get(1);
if (includeDiskFilesArg == null) {
throw new NullPointerException("includeDiskFilesArg unexpectedly null.");
}
api.clearCache(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
includeDiskFilesArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.evaluateJavascript",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String javascriptStringArg = (String) args.get(1);
if (javascriptStringArg == null) {
throw new NullPointerException("javascriptStringArg unexpectedly null.");
}
Result<String> resultCallback =
new Result<String>() {
public void success(String result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.evaluateJavascript(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
javascriptStringArg,
resultCallback);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
reply.reply(wrappedError);
}
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.getTitle", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String output =
api.getTitle((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.scrollTo", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number xArg = (Number) args.get(1);
if (xArg == null) {
throw new NullPointerException("xArg unexpectedly null.");
}
Number yArg = (Number) args.get(2);
if (yArg == null) {
throw new NullPointerException("yArg unexpectedly null.");
}
api.scrollTo(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(xArg == null) ? null : xArg.longValue(),
(yArg == null) ? null : yArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.scrollBy", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number xArg = (Number) args.get(1);
if (xArg == null) {
throw new NullPointerException("xArg unexpectedly null.");
}
Number yArg = (Number) args.get(2);
if (yArg == null) {
throw new NullPointerException("yArg unexpectedly null.");
}
api.scrollBy(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(xArg == null) ? null : xArg.longValue(),
(yArg == null) ? null : yArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.getScrollX", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Long output =
api.getScrollX((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.getScrollY", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Long output =
api.getScrollY((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.getScrollPosition", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
WebViewPoint output =
api.getScrollPosition(
(instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.setWebContentsDebuggingEnabled",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Boolean enabledArg = (Boolean) args.get(0);
if (enabledArg == null) {
throw new NullPointerException("enabledArg unexpectedly null.");
}
api.setWebContentsDebuggingEnabled(enabledArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewHostApi.setWebViewClient", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number webViewClientInstanceIdArg = (Number) args.get(1);
if (webViewClientInstanceIdArg == null) {
throw new NullPointerException("webViewClientInstanceIdArg unexpectedly null.");
}
api.setWebViewClient(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(webViewClientInstanceIdArg == null)
? null
: webViewClientInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.addJavaScriptChannel",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number javaScriptChannelInstanceIdArg = (Number) args.get(1);
if (javaScriptChannelInstanceIdArg == null) {
throw new NullPointerException(
"javaScriptChannelInstanceIdArg unexpectedly null.");
}
api.addJavaScriptChannel(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(javaScriptChannelInstanceIdArg == null)
? null
: javaScriptChannelInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.removeJavaScriptChannel",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number javaScriptChannelInstanceIdArg = (Number) args.get(1);
if (javaScriptChannelInstanceIdArg == null) {
throw new NullPointerException(
"javaScriptChannelInstanceIdArg unexpectedly null.");
}
api.removeJavaScriptChannel(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(javaScriptChannelInstanceIdArg == null)
? null
: javaScriptChannelInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.setDownloadListener",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number listenerInstanceIdArg = (Number) args.get(1);
api.setDownloadListener(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(listenerInstanceIdArg == null) ? null : listenerInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.setWebChromeClient",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number clientInstanceIdArg = (Number) args.get(1);
api.setWebChromeClient(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(clientInstanceIdArg == null) ? null : clientInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewHostApi.setBackgroundColor",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number colorArg = (Number) args.get(1);
if (colorArg == null) {
throw new NullPointerException("colorArg unexpectedly null.");
}
api.setBackgroundColor(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(colorArg == null) ? null : colorArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface WebSettingsHostApi {
void create(@NonNull Long instanceId, @NonNull Long webViewInstanceId);
void setDomStorageEnabled(@NonNull Long instanceId, @NonNull Boolean flag);
void setJavaScriptCanOpenWindowsAutomatically(@NonNull Long instanceId, @NonNull Boolean flag);
void setSupportMultipleWindows(@NonNull Long instanceId, @NonNull Boolean support);
void setJavaScriptEnabled(@NonNull Long instanceId, @NonNull Boolean flag);
void setUserAgentString(@NonNull Long instanceId, @Nullable String userAgentString);
void setMediaPlaybackRequiresUserGesture(@NonNull Long instanceId, @NonNull Boolean require);
void setSupportZoom(@NonNull Long instanceId, @NonNull Boolean support);
void setLoadWithOverviewMode(@NonNull Long instanceId, @NonNull Boolean overview);
void setUseWideViewPort(@NonNull Long instanceId, @NonNull Boolean use);
void setDisplayZoomControls(@NonNull Long instanceId, @NonNull Boolean enabled);
void setBuiltInZoomControls(@NonNull Long instanceId, @NonNull Boolean enabled);
void setAllowFileAccess(@NonNull Long instanceId, @NonNull Boolean enabled);
/** The codec used by WebSettingsHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `WebSettingsHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, WebSettingsHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebSettingsHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Number webViewInstanceIdArg = (Number) args.get(1);
if (webViewInstanceIdArg == null) {
throw new NullPointerException("webViewInstanceIdArg unexpectedly null.");
}
api.create(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
(webViewInstanceIdArg == null) ? null : webViewInstanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setDomStorageEnabled",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean flagArg = (Boolean) args.get(1);
if (flagArg == null) {
throw new NullPointerException("flagArg unexpectedly null.");
}
api.setDomStorageEnabled(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), flagArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setJavaScriptCanOpenWindowsAutomatically",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean flagArg = (Boolean) args.get(1);
if (flagArg == null) {
throw new NullPointerException("flagArg unexpectedly null.");
}
api.setJavaScriptCanOpenWindowsAutomatically(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), flagArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setSupportMultipleWindows",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean supportArg = (Boolean) args.get(1);
if (supportArg == null) {
throw new NullPointerException("supportArg unexpectedly null.");
}
api.setSupportMultipleWindows(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), supportArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setJavaScriptEnabled",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean flagArg = (Boolean) args.get(1);
if (flagArg == null) {
throw new NullPointerException("flagArg unexpectedly null.");
}
api.setJavaScriptEnabled(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), flagArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setUserAgentString",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String userAgentStringArg = (String) args.get(1);
api.setUserAgentString(
(instanceIdArg == null) ? null : instanceIdArg.longValue(),
userAgentStringArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setMediaPlaybackRequiresUserGesture",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean requireArg = (Boolean) args.get(1);
if (requireArg == null) {
throw new NullPointerException("requireArg unexpectedly null.");
}
api.setMediaPlaybackRequiresUserGesture(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), requireArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setSupportZoom",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean supportArg = (Boolean) args.get(1);
if (supportArg == null) {
throw new NullPointerException("supportArg unexpectedly null.");
}
api.setSupportZoom(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), supportArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setLoadWithOverviewMode",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean overviewArg = (Boolean) args.get(1);
if (overviewArg == null) {
throw new NullPointerException("overviewArg unexpectedly null.");
}
api.setLoadWithOverviewMode(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), overviewArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setUseWideViewPort",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean useArg = (Boolean) args.get(1);
if (useArg == null) {
throw new NullPointerException("useArg unexpectedly null.");
}
api.setUseWideViewPort(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), useArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setDisplayZoomControls",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean enabledArg = (Boolean) args.get(1);
if (enabledArg == null) {
throw new NullPointerException("enabledArg unexpectedly null.");
}
api.setDisplayZoomControls(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), enabledArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setBuiltInZoomControls",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean enabledArg = (Boolean) args.get(1);
if (enabledArg == null) {
throw new NullPointerException("enabledArg unexpectedly null.");
}
api.setBuiltInZoomControls(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), enabledArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebSettingsHostApi.setAllowFileAccess",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean enabledArg = (Boolean) args.get(1);
if (enabledArg == null) {
throw new NullPointerException("enabledArg unexpectedly null.");
}
api.setAllowFileAccess(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), enabledArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface JavaScriptChannelHostApi {
void create(@NonNull Long instanceId, @NonNull String channelName);
/** The codec used by JavaScriptChannelHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `JavaScriptChannelHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, JavaScriptChannelHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.JavaScriptChannelHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
String channelNameArg = (String) args.get(1);
if (channelNameArg == null) {
throw new NullPointerException("channelNameArg unexpectedly null.");
}
api.create(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), channelNameArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class JavaScriptChannelFlutterApi {
private final BinaryMessenger binaryMessenger;
public JavaScriptChannelFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by JavaScriptChannelFlutterApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void postMessage(
@NonNull Long instanceIdArg, @NonNull String messageArg, Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.JavaScriptChannelFlutterApi.postMessage",
getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, messageArg)),
channelReply -> {
callback.reply(null);
});
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface WebViewClientHostApi {
void create(@NonNull Long instanceId);
void setSynchronousReturnValueForShouldOverrideUrlLoading(
@NonNull Long instanceId, @NonNull Boolean value);
/** The codec used by WebViewClientHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `WebViewClientHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, WebViewClientHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewClientHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.create((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientHostApi.setSynchronousReturnValueForShouldOverrideUrlLoading",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean valueArg = (Boolean) args.get(1);
if (valueArg == null) {
throw new NullPointerException("valueArg unexpectedly null.");
}
api.setSynchronousReturnValueForShouldOverrideUrlLoading(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), valueArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class WebViewClientFlutterApiCodec extends StandardMessageCodec {
public static final WebViewClientFlutterApiCodec INSTANCE = new WebViewClientFlutterApiCodec();
private WebViewClientFlutterApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return WebResourceErrorData.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 129:
return WebResourceRequestData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof WebResourceErrorData) {
stream.write(128);
writeValue(stream, ((WebResourceErrorData) value).toList());
} else if (value instanceof WebResourceRequestData) {
stream.write(129);
writeValue(stream, ((WebResourceRequestData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class WebViewClientFlutterApi {
private final BinaryMessenger binaryMessenger;
public WebViewClientFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by WebViewClientFlutterApi. */
static MessageCodec<Object> getCodec() {
return WebViewClientFlutterApiCodec.INSTANCE;
}
public void onPageStarted(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull String urlArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientFlutterApi.onPageStarted",
getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, webViewInstanceIdArg, urlArg)),
channelReply -> {
callback.reply(null);
});
}
public void onPageFinished(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull String urlArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientFlutterApi.onPageFinished",
getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, webViewInstanceIdArg, urlArg)),
channelReply -> {
callback.reply(null);
});
}
public void onReceivedRequestError(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull WebResourceRequestData requestArg,
@NonNull WebResourceErrorData errorArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientFlutterApi.onReceivedRequestError",
getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(instanceIdArg, webViewInstanceIdArg, requestArg, errorArg)),
channelReply -> {
callback.reply(null);
});
}
public void onReceivedError(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull Long errorCodeArg,
@NonNull String descriptionArg,
@NonNull String failingUrlArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientFlutterApi.onReceivedError",
getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(
instanceIdArg,
webViewInstanceIdArg,
errorCodeArg,
descriptionArg,
failingUrlArg)),
channelReply -> {
callback.reply(null);
});
}
public void requestLoading(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull WebResourceRequestData requestArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebViewClientFlutterApi.requestLoading",
getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, webViewInstanceIdArg, requestArg)),
channelReply -> {
callback.reply(null);
});
}
public void urlLoading(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull String urlArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebViewClientFlutterApi.urlLoading", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, webViewInstanceIdArg, urlArg)),
channelReply -> {
callback.reply(null);
});
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface DownloadListenerHostApi {
void create(@NonNull Long instanceId);
/** The codec used by DownloadListenerHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `DownloadListenerHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, DownloadListenerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.DownloadListenerHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.create((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class DownloadListenerFlutterApi {
private final BinaryMessenger binaryMessenger;
public DownloadListenerFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by DownloadListenerFlutterApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void onDownloadStart(
@NonNull Long instanceIdArg,
@NonNull String urlArg,
@NonNull String userAgentArg,
@NonNull String contentDispositionArg,
@NonNull String mimetypeArg,
@NonNull Long contentLengthArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.DownloadListenerFlutterApi.onDownloadStart",
getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(
instanceIdArg,
urlArg,
userAgentArg,
contentDispositionArg,
mimetypeArg,
contentLengthArg)),
channelReply -> {
callback.reply(null);
});
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface WebChromeClientHostApi {
void create(@NonNull Long instanceId);
void setSynchronousReturnValueForOnShowFileChooser(
@NonNull Long instanceId, @NonNull Boolean value);
/** The codec used by WebChromeClientHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `WebChromeClientHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, WebChromeClientHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebChromeClientHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.create((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebChromeClientHostApi.setSynchronousReturnValueForOnShowFileChooser",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
Boolean valueArg = (Boolean) args.get(1);
if (valueArg == null) {
throw new NullPointerException("valueArg unexpectedly null.");
}
api.setSynchronousReturnValueForOnShowFileChooser(
(instanceIdArg == null) ? null : instanceIdArg.longValue(), valueArg);
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface FlutterAssetManagerHostApi {
@NonNull
List<String> list(@NonNull String path);
@NonNull
String getAssetFilePathByName(@NonNull String name);
/** The codec used by FlutterAssetManagerHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `FlutterAssetManagerHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, FlutterAssetManagerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.FlutterAssetManagerHostApi.list", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
String pathArg = (String) args.get(0);
if (pathArg == null) {
throw new NullPointerException("pathArg unexpectedly null.");
}
List<String> output = api.list(pathArg);
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.FlutterAssetManagerHostApi.getAssetFilePathByName",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
String nameArg = (String) args.get(0);
if (nameArg == null) {
throw new NullPointerException("nameArg unexpectedly null.");
}
String output = api.getAssetFilePathByName(nameArg);
wrapped.add(0, output);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class WebChromeClientFlutterApi {
private final BinaryMessenger binaryMessenger;
public WebChromeClientFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by WebChromeClientFlutterApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void onProgressChanged(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull Long progressArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebChromeClientFlutterApi.onProgressChanged",
getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(instanceIdArg, webViewInstanceIdArg, progressArg)),
channelReply -> {
callback.reply(null);
});
}
public void onShowFileChooser(
@NonNull Long instanceIdArg,
@NonNull Long webViewInstanceIdArg,
@NonNull Long paramsInstanceIdArg,
Reply<List<String>> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.WebChromeClientFlutterApi.onShowFileChooser",
getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(instanceIdArg, webViewInstanceIdArg, paramsInstanceIdArg)),
channelReply -> {
@SuppressWarnings("ConstantConditions")
List<String> output = (List<String>) channelReply;
callback.reply(output);
});
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface WebStorageHostApi {
void create(@NonNull Long instanceId);
void deleteAllData(@NonNull Long instanceId);
/** The codec used by WebStorageHostApi. */
static MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `WebStorageHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(BinaryMessenger binaryMessenger, WebStorageHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebStorageHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.create((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.WebStorageHostApi.deleteAllData", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList wrapped = new ArrayList<>();
try {
ArrayList<Object> args = (ArrayList<Object>) message;
assert args != null;
Number instanceIdArg = (Number) args.get(0);
if (instanceIdArg == null) {
throw new NullPointerException("instanceIdArg unexpectedly null.");
}
api.deleteAllData((instanceIdArg == null) ? null : instanceIdArg.longValue());
wrapped.add(0, null);
} catch (Error | RuntimeException exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class FileChooserParamsFlutterApiCodec extends StandardMessageCodec {
public static final FileChooserParamsFlutterApiCodec INSTANCE =
new FileChooserParamsFlutterApiCodec();
private FileChooserParamsFlutterApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return FileChooserModeEnumData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof FileChooserModeEnumData) {
stream.write(128);
writeValue(stream, ((FileChooserModeEnumData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/**
* Handles callbacks methods for the native Java FileChooserParams class.
*
* <p>See
* https://developer.android.com/reference/android/webkit/WebChromeClient.FileChooserParams.
*
* <p>Generated class from Pigeon that represents Flutter messages that can be called from Java.
*/
public static class FileChooserParamsFlutterApi {
private final BinaryMessenger binaryMessenger;
public FileChooserParamsFlutterApi(BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by FileChooserParamsFlutterApi. */
static MessageCodec<Object> getCodec() {
return FileChooserParamsFlutterApiCodec.INSTANCE;
}
public void create(
@NonNull Long instanceIdArg,
@NonNull Boolean isCaptureEnabledArg,
@NonNull List<String> acceptTypesArg,
@NonNull FileChooserModeEnumData modeArg,
@Nullable String filenameHintArg,
Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.FileChooserParamsFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(
instanceIdArg, isCaptureEnabledArg, acceptTypesArg, modeArg, filenameHintArg)),
channelReply -> {
callback.reply(null);
});
}
}
@NonNull
private static ArrayList<Object> wrapError(@NonNull Throwable exception) {
ArrayList<Object> errorList = new ArrayList<>(3);
errorList.add(exception.toString());
errorList.add(exception.getClass().getSimpleName());
errorList.add(
"Cause: " + exception.getCause() + ", Stacktrace: " + Log.getStackTraceString(exception));
return errorList;
}
}
| plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/GeneratedAndroidWebView.java/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/GeneratedAndroidWebView.java",
"repo_id": "plugins",
"token_count": 52000
} | 1,177 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.webviewflutter;
import android.annotation.SuppressLint;
import android.content.Context;
import android.hardware.display.DisplayManager;
import android.view.View;
import android.webkit.DownloadListener;
import android.webkit.WebChromeClient;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.platform.PlatformView;
import io.flutter.plugins.webviewflutter.GeneratedAndroidWebView.WebViewHostApi;
import java.util.Map;
import java.util.Objects;
/**
* Host api implementation for {@link WebView}.
*
* <p>Handles creating {@link WebView}s that intercommunicate with a paired Dart object.
*/
public class WebViewHostApiImpl implements WebViewHostApi {
private final InstanceManager instanceManager;
private final WebViewProxy webViewProxy;
// Only used with WebView using virtual displays.
@Nullable private final View containerView;
private final BinaryMessenger binaryMessenger;
private Context context;
/** Handles creating and calling static methods for {@link WebView}s. */
public static class WebViewProxy {
/**
* Creates a {@link WebViewPlatformView}.
*
* @param context an Activity Context to access application assets
* @param binaryMessenger used to communicate with Dart over asynchronous messages
* @param instanceManager mangages instances used to communicate with the corresponding objects
* in Dart
* @return the created {@link WebViewPlatformView}
*/
public WebViewPlatformView createWebView(
Context context, BinaryMessenger binaryMessenger, InstanceManager instanceManager) {
return new WebViewPlatformView(context, binaryMessenger, instanceManager);
}
/**
* Creates a {@link InputAwareWebViewPlatformView}.
*
* @param context an Activity Context to access application assets
* @param containerView parent View of the WebView
* @return the created {@link InputAwareWebViewPlatformView}
*/
public InputAwareWebViewPlatformView createInputAwareWebView(
Context context,
BinaryMessenger binaryMessenger,
InstanceManager instanceManager,
@Nullable View containerView) {
return new InputAwareWebViewPlatformView(
context, binaryMessenger, instanceManager, containerView);
}
/**
* Forwards call to {@link WebView#setWebContentsDebuggingEnabled}.
*
* @param enabled whether debugging should be enabled
*/
public void setWebContentsDebuggingEnabled(boolean enabled) {
WebView.setWebContentsDebuggingEnabled(enabled);
}
}
/** Implementation of {@link WebView} that can be used as a Flutter {@link PlatformView}s. */
public static class WebViewPlatformView extends WebView implements PlatformView {
private WebViewClient currentWebViewClient;
private WebChromeClientHostApiImpl.SecureWebChromeClient currentWebChromeClient;
/**
* Creates a {@link WebViewPlatformView}.
*
* @param context an Activity Context to access application assets. This value cannot be null.
*/
public WebViewPlatformView(
Context context, BinaryMessenger binaryMessenger, InstanceManager instanceManager) {
super(context);
currentWebViewClient = new WebViewClient();
currentWebChromeClient = new WebChromeClientHostApiImpl.SecureWebChromeClient();
setWebViewClient(currentWebViewClient);
setWebChromeClient(currentWebChromeClient);
}
@Override
public View getView() {
return this;
}
@Override
public void dispose() {}
@Override
public void setWebViewClient(WebViewClient webViewClient) {
super.setWebViewClient(webViewClient);
currentWebViewClient = webViewClient;
currentWebChromeClient.setWebViewClient(webViewClient);
}
@Override
public void setWebChromeClient(WebChromeClient client) {
super.setWebChromeClient(client);
if (!(client instanceof WebChromeClientHostApiImpl.SecureWebChromeClient)) {
throw new AssertionError("Client must be a SecureWebChromeClient.");
}
currentWebChromeClient = (WebChromeClientHostApiImpl.SecureWebChromeClient) client;
currentWebChromeClient.setWebViewClient(currentWebViewClient);
}
// When running unit tests, the parent `WebView` class is replaced by a stub that returns null
// for every method. This is overridden so that this returns the current WebChromeClient during
// unit tests. This should only remain overridden as long as `setWebChromeClient` is overridden.
@Nullable
@Override
public WebChromeClient getWebChromeClient() {
return currentWebChromeClient;
}
}
/**
* Implementation of {@link InputAwareWebView} that can be used as a Flutter {@link
* PlatformView}s.
*/
@SuppressLint("ViewConstructor")
public static class InputAwareWebViewPlatformView extends InputAwareWebView
implements PlatformView {
private WebViewClient currentWebViewClient;
private WebChromeClientHostApiImpl.SecureWebChromeClient currentWebChromeClient;
/**
* Creates a {@link InputAwareWebViewPlatformView}.
*
* @param context an Activity Context to access application assets. This value cannot be null.
*/
public InputAwareWebViewPlatformView(
Context context,
BinaryMessenger binaryMessenger,
InstanceManager instanceManager,
View containerView) {
super(context, containerView);
currentWebViewClient = new WebViewClient();
currentWebChromeClient = new WebChromeClientHostApiImpl.SecureWebChromeClient();
setWebViewClient(currentWebViewClient);
setWebChromeClient(currentWebChromeClient);
}
@Override
public View getView() {
return this;
}
@Override
public void onFlutterViewAttached(@NonNull View flutterView) {
setContainerView(flutterView);
}
@Override
public void onFlutterViewDetached() {
setContainerView(null);
}
@Override
public void dispose() {
super.dispose();
destroy();
}
@Override
public void onInputConnectionLocked() {
lockInputConnection();
}
@Override
public void onInputConnectionUnlocked() {
unlockInputConnection();
}
@Override
public void setWebViewClient(WebViewClient webViewClient) {
super.setWebViewClient(webViewClient);
currentWebViewClient = webViewClient;
currentWebChromeClient.setWebViewClient(webViewClient);
}
@Override
public void setWebChromeClient(WebChromeClient client) {
super.setWebChromeClient(client);
if (!(client instanceof WebChromeClientHostApiImpl.SecureWebChromeClient)) {
throw new AssertionError("Client must be a SecureWebChromeClient.");
}
currentWebChromeClient = (WebChromeClientHostApiImpl.SecureWebChromeClient) client;
currentWebChromeClient.setWebViewClient(currentWebViewClient);
}
}
/**
* Creates a host API that handles creating {@link WebView}s and invoking its methods.
*
* @param instanceManager maintains instances stored to communicate with Dart objects
* @param binaryMessenger used to communicate with Dart over asynchronous messages
* @param webViewProxy handles creating {@link WebView}s and calling its static methods
* @param context an Activity Context to access application assets. This value cannot be null.
* @param containerView parent of the webView
*/
public WebViewHostApiImpl(
InstanceManager instanceManager,
BinaryMessenger binaryMessenger,
WebViewProxy webViewProxy,
Context context,
@Nullable View containerView) {
this.instanceManager = instanceManager;
this.binaryMessenger = binaryMessenger;
this.webViewProxy = webViewProxy;
this.context = context;
this.containerView = containerView;
}
/**
* Sets the context to construct {@link WebView}s.
*
* @param context the new context.
*/
public void setContext(Context context) {
this.context = context;
}
@Override
public void create(Long instanceId, Boolean useHybridComposition) {
DisplayListenerProxy displayListenerProxy = new DisplayListenerProxy();
DisplayManager displayManager =
(DisplayManager) context.getSystemService(Context.DISPLAY_SERVICE);
displayListenerProxy.onPreWebViewInitialization(displayManager);
final WebView webView =
useHybridComposition
? webViewProxy.createWebView(context, binaryMessenger, instanceManager)
: webViewProxy.createInputAwareWebView(
context, binaryMessenger, instanceManager, containerView);
displayListenerProxy.onPostWebViewInitialization(displayManager);
instanceManager.addDartCreatedInstance(webView, instanceId);
}
@Override
public void loadData(Long instanceId, String data, String mimeType, String encoding) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.loadData(data, mimeType, encoding);
}
@Override
public void loadDataWithBaseUrl(
Long instanceId,
String baseUrl,
String data,
String mimeType,
String encoding,
String historyUrl) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.loadDataWithBaseURL(baseUrl, data, mimeType, encoding, historyUrl);
}
@Override
public void loadUrl(Long instanceId, String url, Map<String, String> headers) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.loadUrl(url, headers);
}
@Override
public void postUrl(Long instanceId, String url, byte[] data) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.postUrl(url, data);
}
@Override
public String getUrl(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return webView.getUrl();
}
@Override
public Boolean canGoBack(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return webView.canGoBack();
}
@Override
public Boolean canGoForward(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return webView.canGoForward();
}
@Override
public void goBack(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.goBack();
}
@Override
public void goForward(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.goForward();
}
@Override
public void reload(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.reload();
}
@Override
public void clearCache(Long instanceId, Boolean includeDiskFiles) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.clearCache(includeDiskFiles);
}
@Override
public void evaluateJavascript(
Long instanceId, String javascriptString, GeneratedAndroidWebView.Result<String> result) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.evaluateJavascript(javascriptString, result::success);
}
@Override
public String getTitle(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return webView.getTitle();
}
@Override
public void scrollTo(Long instanceId, Long x, Long y) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.scrollTo(x.intValue(), y.intValue());
}
@Override
public void scrollBy(Long instanceId, Long x, Long y) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.scrollBy(x.intValue(), y.intValue());
}
@Override
public Long getScrollX(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return (long) webView.getScrollX();
}
@Override
public Long getScrollY(Long instanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
return (long) webView.getScrollY();
}
@NonNull
@Override
public GeneratedAndroidWebView.WebViewPoint getScrollPosition(@NonNull Long instanceId) {
final WebView webView = Objects.requireNonNull(instanceManager.getInstance(instanceId));
return new GeneratedAndroidWebView.WebViewPoint.Builder()
.setX((long) webView.getScrollX())
.setY((long) webView.getScrollY())
.build();
}
@Override
public void setWebContentsDebuggingEnabled(Boolean enabled) {
webViewProxy.setWebContentsDebuggingEnabled(enabled);
}
@Override
public void setWebViewClient(Long instanceId, Long webViewClientInstanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.setWebViewClient((WebViewClient) instanceManager.getInstance(webViewClientInstanceId));
}
@Override
public void addJavaScriptChannel(Long instanceId, Long javaScriptChannelInstanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
final JavaScriptChannel javaScriptChannel =
(JavaScriptChannel) instanceManager.getInstance(javaScriptChannelInstanceId);
webView.addJavascriptInterface(javaScriptChannel, javaScriptChannel.javaScriptChannelName);
}
@Override
public void removeJavaScriptChannel(Long instanceId, Long javaScriptChannelInstanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
final JavaScriptChannel javaScriptChannel =
(JavaScriptChannel) instanceManager.getInstance(javaScriptChannelInstanceId);
webView.removeJavascriptInterface(javaScriptChannel.javaScriptChannelName);
}
@Override
public void setDownloadListener(Long instanceId, Long listenerInstanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.setDownloadListener((DownloadListener) instanceManager.getInstance(listenerInstanceId));
}
@Override
public void setWebChromeClient(Long instanceId, Long clientInstanceId) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.setWebChromeClient((WebChromeClient) instanceManager.getInstance(clientInstanceId));
}
@Override
public void setBackgroundColor(Long instanceId, Long color) {
final WebView webView = (WebView) instanceManager.getInstance(instanceId);
webView.setBackgroundColor(color.intValue());
}
/** Maintains instances used to communicate with the corresponding WebView Dart object. */
public InstanceManager getInstanceManager() {
return instanceManager;
}
}
| plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/WebViewHostApiImpl.java/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/WebViewHostApiImpl.java",
"repo_id": "plugins",
"token_count": 4742
} | 1,178 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.webviewflutter;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
import android.content.Context;
import android.webkit.WebView;
import io.flutter.embedding.engine.FlutterEngine;
import io.flutter.embedding.engine.plugins.FlutterPlugin;
import io.flutter.embedding.engine.plugins.PluginRegistry;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.platform.PlatformViewRegistry;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
public class WebViewFlutterAndroidExternalApiTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock Context mockContext;
@Mock BinaryMessenger mockBinaryMessenger;
@Mock PlatformViewRegistry mockViewRegistry;
@Mock FlutterPlugin.FlutterPluginBinding mockPluginBinding;
@Test
public void getWebView() {
final WebViewFlutterPlugin webViewFlutterPlugin = new WebViewFlutterPlugin();
when(mockPluginBinding.getApplicationContext()).thenReturn(mockContext);
when(mockPluginBinding.getPlatformViewRegistry()).thenReturn(mockViewRegistry);
when(mockPluginBinding.getBinaryMessenger()).thenReturn(mockBinaryMessenger);
webViewFlutterPlugin.onAttachedToEngine(mockPluginBinding);
final InstanceManager instanceManager = webViewFlutterPlugin.getInstanceManager();
assertNotNull(instanceManager);
final WebView mockWebView = mock(WebView.class);
instanceManager.addDartCreatedInstance(mockWebView, 0);
final PluginRegistry mockPluginRegistry = mock(PluginRegistry.class);
when(mockPluginRegistry.get(WebViewFlutterPlugin.class)).thenReturn(webViewFlutterPlugin);
final FlutterEngine mockFlutterEngine = mock(FlutterEngine.class);
when(mockFlutterEngine.getPlugins()).thenReturn(mockPluginRegistry);
assertEquals(WebViewFlutterAndroidExternalApi.getWebView(mockFlutterEngine, 0), mockWebView);
webViewFlutterPlugin.onDetachedFromEngine(mockPluginBinding);
}
}
| plugins/packages/webview_flutter/webview_flutter_android/android/src/test/java/io/flutter/plugins/webviewflutter/WebViewFlutterAndroidExternalApiTest.java/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/android/src/test/java/io/flutter/plugins/webviewflutter/WebViewFlutterAndroidExternalApiTest.java",
"repo_id": "plugins",
"token_count": 739
} | 1,179 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:webview_flutter_android/src/legacy/webview_surface_android.dart';
import 'package:webview_flutter_platform_interface/src/webview_flutter_platform_interface_legacy.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('SurfaceAndroidWebView', () {
late List<MethodCall> log;
setUpAll(() {
_ambiguate(TestDefaultBinaryMessengerBinding.instance)!
.defaultBinaryMessenger
.setMockMethodCallHandler(
SystemChannels.platform_views,
(MethodCall call) async {
log.add(call);
if (call.method == 'resize') {
final Map<String, Object?> arguments =
(call.arguments as Map<Object?, Object?>)
.cast<String, Object?>();
return <String, Object?>{
'width': arguments['width'],
'height': arguments['height'],
};
}
return null;
},
);
});
tearDownAll(() {
_ambiguate(TestDefaultBinaryMessengerBinding.instance)!
.defaultBinaryMessenger
.setMockMethodCallHandler(SystemChannels.platform_views, null);
});
setUp(() {
log = <MethodCall>[];
});
testWidgets(
'uses hybrid composition when background color is not 100% opaque',
(WidgetTester tester) async {
await tester.pumpWidget(Builder(builder: (BuildContext context) {
return SurfaceAndroidWebView().build(
context: context,
creationParams: CreationParams(
backgroundColor: Colors.transparent,
webSettings: WebSettings(
userAgent: const WebSetting<String?>.absent(),
hasNavigationDelegate: false,
)),
javascriptChannelRegistry: JavascriptChannelRegistry(null),
webViewPlatformCallbacksHandler:
TestWebViewPlatformCallbacksHandler(),
);
}));
await tester.pumpAndSettle();
final MethodCall createMethodCall = log[0];
expect(createMethodCall.method, 'create');
expect(createMethodCall.arguments, containsPair('hybrid', true));
});
testWidgets('default text direction is ltr', (WidgetTester tester) async {
await tester.pumpWidget(Builder(builder: (BuildContext context) {
return SurfaceAndroidWebView().build(
context: context,
creationParams: CreationParams(
webSettings: WebSettings(
userAgent: const WebSetting<String?>.absent(),
hasNavigationDelegate: false,
)),
javascriptChannelRegistry: JavascriptChannelRegistry(null),
webViewPlatformCallbacksHandler:
TestWebViewPlatformCallbacksHandler(),
);
}));
await tester.pumpAndSettle();
final MethodCall createMethodCall = log[0];
expect(createMethodCall.method, 'create');
expect(
createMethodCall.arguments,
containsPair(
'direction',
AndroidViewController.kAndroidLayoutDirectionLtr,
),
);
});
});
}
class TestWebViewPlatformCallbacksHandler
implements WebViewPlatformCallbacksHandler {
@override
FutureOr<bool> onNavigationRequest({
required String url,
required bool isForMainFrame,
}) {
throw UnimplementedError();
}
@override
void onPageFinished(String url) {}
@override
void onPageStarted(String url) {}
@override
void onProgress(int progress) {}
@override
void onWebResourceError(WebResourceError error) {}
}
/// This allows a value of type T or T? to be treated as a value of type T?.
///
/// We use this so that APIs that have become non-nullable can still be used
/// with `!` and `?` on the stable branch.
T? _ambiguate<T>(T? value) => value;
| plugins/packages/webview_flutter/webview_flutter_android/test/legacy/surface_android_test.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/test/legacy/surface_android_test.dart",
"repo_id": "plugins",
"token_count": 1654
} | 1,180 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:webview_flutter_platform_interface/src/webview_flutter_platform_interface_legacy.dart';
void main() {
WebViewCookieManagerPlatform? cookieManager;
setUp(() {
cookieManager = TestWebViewCookieManagerPlatform();
});
test('clearCookies should throw UnimplementedError', () {
expect(() => cookieManager!.clearCookies(), throwsUnimplementedError);
});
test('setCookie should throw UnimplementedError', () {
const WebViewCookie cookie =
WebViewCookie(domain: 'flutter.dev', name: 'foo', value: 'bar');
expect(() => cookieManager!.setCookie(cookie), throwsUnimplementedError);
});
}
class TestWebViewCookieManagerPlatform extends WebViewCookieManagerPlatform {}
| plugins/packages/webview_flutter/webview_flutter_platform_interface/test/legacy/platform_interface/webview_cookie_manager_test.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_platform_interface/test/legacy/platform_interface/webview_cookie_manager_test.dart",
"repo_id": "plugins",
"token_count": 284
} | 1,181 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:html' as html;
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:webview_flutter_web_example/legacy/web_view.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
// URLs to navigate to in tests. These need to be URLs that we are confident will
// always be accessible, and won't do redirection. (E.g., just
// 'https://www.google.com/' will sometimes redirect traffic that looks
// like it's coming from a bot, which is true of these tests).
const String primaryUrl = 'https://flutter.dev/';
const String secondaryUrl = 'https://www.google.com/robots.txt';
testWidgets('initialUrl', (WidgetTester tester) async {
final Completer<WebViewController> controllerCompleter =
Completer<WebViewController>();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: WebView(
key: GlobalKey(),
initialUrl: primaryUrl,
onWebViewCreated: (WebViewController controller) {
controllerCompleter.complete(controller);
},
),
),
);
await controllerCompleter.future;
// Assert an iframe has been rendered to the DOM with the correct src attribute.
final html.IFrameElement? element =
html.document.querySelector('iframe') as html.IFrameElement?;
expect(element, isNotNull);
expect(element!.src, primaryUrl);
});
testWidgets('loadUrl', (WidgetTester tester) async {
final Completer<WebViewController> controllerCompleter =
Completer<WebViewController>();
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: WebView(
key: GlobalKey(),
initialUrl: primaryUrl,
onWebViewCreated: (WebViewController controller) {
controllerCompleter.complete(controller);
},
),
),
);
final WebViewController controller = await controllerCompleter.future;
await controller.loadUrl(secondaryUrl);
// Assert an iframe has been rendered to the DOM with the correct src attribute.
final html.IFrameElement? element =
html.document.querySelector('iframe') as html.IFrameElement?;
expect(element, isNotNull);
expect(element!.src, secondaryUrl);
});
}
| plugins/packages/webview_flutter/webview_flutter_web/example/integration_test/legacy/webview_flutter_test.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_web/example/integration_test/legacy/webview_flutter_test.dart",
"repo_id": "plugins",
"token_count": 936
} | 1,182 |
## 3.1.0
* Adds support to access native `WKWebView`.
## 3.0.5
* Renames Pigeon output files.
## 3.0.4
* Fixes bug that prevented the web view from being garbage collected.
## 3.0.3
* Updates example code for `use_build_context_synchronously` lint.
## 3.0.2
* Updates code for stricter lint checks.
## 3.0.1
* Adds support for retrieving navigation type with internal class.
* Updates README with details on contributing.
* Updates pigeon dev dependency to `4.2.13`.
## 3.0.0
* **BREAKING CHANGE** Updates platform implementation to `2.0.0` release of
`webview_flutter_platform_interface`. See
[webview_flutter](https://pub.dev/packages/webview_flutter/versions/4.0.0) for updated usage.
* Updates code for `no_leading_underscores_for_local_identifiers` lint.
## 2.9.5
* Updates imports for `prefer_relative_imports`.
## 2.9.4
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
* Fixes typo in an internal method name, from `setCookieForInsances` to `setCookieForInstances`.
## 2.9.3
* Updates `webview_flutter_platform_interface` constraint to the correct minimum
version.
## 2.9.2
* Fixes crash when an Objective-C object in `FWFInstanceManager` is released, but the dealloc
callback is no longer available.
## 2.9.1
* Fixes regression where the behavior for the `UIScrollView` insets were removed.
## 2.9.0
* Ignores unnecessary import warnings in preparation for [upcoming Flutter changes](https://github.com/flutter/flutter/pull/106316).
* Replaces platform implementation with WebKit API built with pigeon.
## 2.8.1
* Ignores unnecessary import warnings in preparation for [upcoming Flutter changes](https://github.com/flutter/flutter/pull/104231).
## 2.8.0
* Raises minimum Dart version to 2.17 and Flutter version to 3.0.0.
## 2.7.5
* Minor fixes for new analysis options.
## 2.7.4
* Removes unnecessary imports.
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 2.7.3
* Removes two occurrences of the compiler warning: "'RequiresUserActionForMediaPlayback' is deprecated: first deprecated in ios 10.0".
## 2.7.2
* Fixes an integration test race condition.
* Migrates deprecated `Scaffold.showSnackBar` to `ScaffoldMessenger` in example app.
## 2.7.1
* Fixes header import for cookie manager to be relative only.
## 2.7.0
* Adds implementation of the `loadFlutterAsset` method from the platform interface.
## 2.6.0
* Implements new cookie manager for setting cookies and providing initial cookies.
## 2.5.0
* Adds an option to set the background color of the webview.
* Migrates from `analysis_options_legacy.yaml` to `analysis_options.yaml`.
* Integration test fixes.
* Updates to webview_flutter_platform_interface version 1.5.2.
## 2.4.0
* Implemented new `loadFile` and `loadHtmlString` methods from the platform interface.
## 2.3.0
* Implemented new `loadRequest` method from platform interface.
## 2.2.0
* Implemented new `runJavascript` and `runJavascriptReturningResult` methods in platform interface.
## 2.1.0
* Add `zoomEnabled` functionality.
## 2.0.14
* Update example App so navigation menu loads immediatly but only becomes available when `WebViewController` is available (same behavior as example App in webview_flutter package).
## 2.0.13
* Extract WKWebView implementation from `webview_flutter`.
| plugins/packages/webview_flutter/webview_flutter_wkwebview/CHANGELOG.md/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_wkwebview/CHANGELOG.md",
"repo_id": "plugins",
"token_count": 1065
} | 1,183 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@import Flutter;
@import XCTest;
@import webview_flutter_wkwebview;
#import <OCMock/OCMock.h>
@interface FWFHTTPCookieStoreHostApiTests : XCTestCase
@end
@implementation FWFHTTPCookieStoreHostApiTests
- (void)testCreateFromWebsiteDataStoreWithIdentifier API_AVAILABLE(ios(11.0)) {
FWFInstanceManager *instanceManager = [[FWFInstanceManager alloc] init];
FWFHTTPCookieStoreHostApiImpl *hostAPI =
[[FWFHTTPCookieStoreHostApiImpl alloc] initWithInstanceManager:instanceManager];
WKWebsiteDataStore *mockDataStore = OCMClassMock([WKWebsiteDataStore class]);
OCMStub([mockDataStore httpCookieStore]).andReturn(OCMClassMock([WKHTTPCookieStore class]));
[instanceManager addDartCreatedInstance:mockDataStore withIdentifier:0];
FlutterError *error;
[hostAPI createFromWebsiteDataStoreWithIdentifier:@1 dataStoreIdentifier:@0 error:&error];
WKHTTPCookieStore *cookieStore = (WKHTTPCookieStore *)[instanceManager instanceForIdentifier:1];
XCTAssertTrue([cookieStore isKindOfClass:[WKHTTPCookieStore class]]);
XCTAssertNil(error);
}
- (void)testSetCookie API_AVAILABLE(ios(11.0)) {
WKHTTPCookieStore *mockHttpCookieStore = OCMClassMock([WKHTTPCookieStore class]);
FWFInstanceManager *instanceManager = [[FWFInstanceManager alloc] init];
[instanceManager addDartCreatedInstance:mockHttpCookieStore withIdentifier:0];
FWFHTTPCookieStoreHostApiImpl *hostAPI =
[[FWFHTTPCookieStoreHostApiImpl alloc] initWithInstanceManager:instanceManager];
FWFNSHttpCookieData *cookieData = [FWFNSHttpCookieData
makeWithPropertyKeys:@[ [FWFNSHttpCookiePropertyKeyEnumData
makeWithValue:FWFNSHttpCookiePropertyKeyEnumName] ]
propertyValues:@[ @"hello" ]];
FlutterError *__block blockError;
[hostAPI setCookieForStoreWithIdentifier:@0
cookie:cookieData
completion:^(FlutterError *error) {
blockError = error;
}];
OCMVerify([mockHttpCookieStore
setCookie:[NSHTTPCookie cookieWithProperties:@{NSHTTPCookieName : @"hello"}]
completionHandler:OCMOCK_ANY]);
XCTAssertNil(blockError);
}
@end
| plugins/packages/webview_flutter/webview_flutter_wkwebview/example/ios/RunnerTests/FWFHTTPCookieStoreHostApiTests.m/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_wkwebview/example/ios/RunnerTests/FWFHTTPCookieStoreHostApiTests.m",
"repo_id": "plugins",
"token_count": 934
} | 1,184 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v4.2.13), do not edit directly.
// See also: https://pub.dev/packages/pigeon
// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, prefer_null_aware_operators, omit_local_variable_types, unused_shown_name, unnecessary_import
import 'dart:async';
import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;
import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;
import 'package:flutter/services.dart';
/// Mirror of NSKeyValueObservingOptions.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvalueobservingoptions?language=objc.
enum NSKeyValueObservingOptionsEnum {
newValue,
oldValue,
initialValue,
priorNotification,
}
/// Mirror of NSKeyValueChange.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvaluechange?language=objc.
enum NSKeyValueChangeEnum {
setting,
insertion,
removal,
replacement,
}
/// Mirror of NSKeyValueChangeKey.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvaluechangekey?language=objc.
enum NSKeyValueChangeKeyEnum {
indexes,
kind,
newValue,
notificationIsPrior,
oldValue,
}
/// Mirror of WKUserScriptInjectionTime.
///
/// See https://developer.apple.com/documentation/webkit/wkuserscriptinjectiontime?language=objc.
enum WKUserScriptInjectionTimeEnum {
atDocumentStart,
atDocumentEnd,
}
/// Mirror of WKAudiovisualMediaTypes.
///
/// See [WKAudiovisualMediaTypes](https://developer.apple.com/documentation/webkit/wkaudiovisualmediatypes?language=objc).
enum WKAudiovisualMediaTypeEnum {
none,
audio,
video,
all,
}
/// Mirror of WKWebsiteDataTypes.
///
/// See https://developer.apple.com/documentation/webkit/wkwebsitedatarecord/data_store_record_types?language=objc.
enum WKWebsiteDataTypeEnum {
cookies,
memoryCache,
diskCache,
offlineWebApplicationCache,
localStorage,
sessionStorage,
webSQLDatabases,
indexedDBDatabases,
}
/// Mirror of WKNavigationActionPolicy.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationactionpolicy?language=objc.
enum WKNavigationActionPolicyEnum {
allow,
cancel,
}
/// Mirror of NSHTTPCookiePropertyKey.
///
/// See https://developer.apple.com/documentation/foundation/nshttpcookiepropertykey.
enum NSHttpCookiePropertyKeyEnum {
comment,
commentUrl,
discard,
domain,
expires,
maximumAge,
name,
originUrl,
path,
port,
sameSitePolicy,
secure,
value,
version,
}
/// An object that contains information about an action that causes navigation
/// to occur.
///
/// Wraps [WKNavigationType](https://developer.apple.com/documentation/webkit/wknavigationaction?language=objc).
enum WKNavigationType {
/// A link activation.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypelinkactivated?language=objc.
linkActivated,
/// A request to submit a form.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeformsubmitted?language=objc.
submitted,
/// A request for the frame’s next or previous item.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypebackforward?language=objc.
backForward,
/// A request to reload the webpage.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypereload?language=objc.
reload,
/// A request to resubmit a form.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeformresubmitted?language=objc.
formResubmitted,
/// A navigation request that originates for some other reason.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeother?language=objc.
other,
}
class NSKeyValueObservingOptionsEnumData {
NSKeyValueObservingOptionsEnumData({
required this.value,
});
NSKeyValueObservingOptionsEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static NSKeyValueObservingOptionsEnumData decode(Object result) {
result as List<Object?>;
return NSKeyValueObservingOptionsEnumData(
value: NSKeyValueObservingOptionsEnum.values[result[0]! as int],
);
}
}
class NSKeyValueChangeKeyEnumData {
NSKeyValueChangeKeyEnumData({
required this.value,
});
NSKeyValueChangeKeyEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static NSKeyValueChangeKeyEnumData decode(Object result) {
result as List<Object?>;
return NSKeyValueChangeKeyEnumData(
value: NSKeyValueChangeKeyEnum.values[result[0]! as int],
);
}
}
class WKUserScriptInjectionTimeEnumData {
WKUserScriptInjectionTimeEnumData({
required this.value,
});
WKUserScriptInjectionTimeEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static WKUserScriptInjectionTimeEnumData decode(Object result) {
result as List<Object?>;
return WKUserScriptInjectionTimeEnumData(
value: WKUserScriptInjectionTimeEnum.values[result[0]! as int],
);
}
}
class WKAudiovisualMediaTypeEnumData {
WKAudiovisualMediaTypeEnumData({
required this.value,
});
WKAudiovisualMediaTypeEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static WKAudiovisualMediaTypeEnumData decode(Object result) {
result as List<Object?>;
return WKAudiovisualMediaTypeEnumData(
value: WKAudiovisualMediaTypeEnum.values[result[0]! as int],
);
}
}
class WKWebsiteDataTypeEnumData {
WKWebsiteDataTypeEnumData({
required this.value,
});
WKWebsiteDataTypeEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static WKWebsiteDataTypeEnumData decode(Object result) {
result as List<Object?>;
return WKWebsiteDataTypeEnumData(
value: WKWebsiteDataTypeEnum.values[result[0]! as int],
);
}
}
class WKNavigationActionPolicyEnumData {
WKNavigationActionPolicyEnumData({
required this.value,
});
WKNavigationActionPolicyEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static WKNavigationActionPolicyEnumData decode(Object result) {
result as List<Object?>;
return WKNavigationActionPolicyEnumData(
value: WKNavigationActionPolicyEnum.values[result[0]! as int],
);
}
}
class NSHttpCookiePropertyKeyEnumData {
NSHttpCookiePropertyKeyEnumData({
required this.value,
});
NSHttpCookiePropertyKeyEnum value;
Object encode() {
return <Object?>[
value.index,
];
}
static NSHttpCookiePropertyKeyEnumData decode(Object result) {
result as List<Object?>;
return NSHttpCookiePropertyKeyEnumData(
value: NSHttpCookiePropertyKeyEnum.values[result[0]! as int],
);
}
}
/// Mirror of NSURLRequest.
///
/// See https://developer.apple.com/documentation/foundation/nsurlrequest?language=objc.
class NSUrlRequestData {
NSUrlRequestData({
required this.url,
this.httpMethod,
this.httpBody,
required this.allHttpHeaderFields,
});
String url;
String? httpMethod;
Uint8List? httpBody;
Map<String?, String?> allHttpHeaderFields;
Object encode() {
return <Object?>[
url,
httpMethod,
httpBody,
allHttpHeaderFields,
];
}
static NSUrlRequestData decode(Object result) {
result as List<Object?>;
return NSUrlRequestData(
url: result[0]! as String,
httpMethod: result[1] as String?,
httpBody: result[2] as Uint8List?,
allHttpHeaderFields:
(result[3] as Map<Object?, Object?>?)!.cast<String?, String?>(),
);
}
}
/// Mirror of WKUserScript.
///
/// See https://developer.apple.com/documentation/webkit/wkuserscript?language=objc.
class WKUserScriptData {
WKUserScriptData({
required this.source,
this.injectionTime,
required this.isMainFrameOnly,
});
String source;
WKUserScriptInjectionTimeEnumData? injectionTime;
bool isMainFrameOnly;
Object encode() {
return <Object?>[
source,
injectionTime?.encode(),
isMainFrameOnly,
];
}
static WKUserScriptData decode(Object result) {
result as List<Object?>;
return WKUserScriptData(
source: result[0]! as String,
injectionTime: result[1] != null
? WKUserScriptInjectionTimeEnumData.decode(
result[1]! as List<Object?>)
: null,
isMainFrameOnly: result[2]! as bool,
);
}
}
/// Mirror of WKNavigationAction.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationaction.
class WKNavigationActionData {
WKNavigationActionData({
required this.request,
required this.targetFrame,
required this.navigationType,
});
NSUrlRequestData request;
WKFrameInfoData targetFrame;
WKNavigationType navigationType;
Object encode() {
return <Object?>[
request.encode(),
targetFrame.encode(),
navigationType.index,
];
}
static WKNavigationActionData decode(Object result) {
result as List<Object?>;
return WKNavigationActionData(
request: NSUrlRequestData.decode(result[0]! as List<Object?>),
targetFrame: WKFrameInfoData.decode(result[1]! as List<Object?>),
navigationType: WKNavigationType.values[result[2]! as int],
);
}
}
/// Mirror of WKFrameInfo.
///
/// See https://developer.apple.com/documentation/webkit/wkframeinfo?language=objc.
class WKFrameInfoData {
WKFrameInfoData({
required this.isMainFrame,
});
bool isMainFrame;
Object encode() {
return <Object?>[
isMainFrame,
];
}
static WKFrameInfoData decode(Object result) {
result as List<Object?>;
return WKFrameInfoData(
isMainFrame: result[0]! as bool,
);
}
}
/// Mirror of NSError.
///
/// See https://developer.apple.com/documentation/foundation/nserror?language=objc.
class NSErrorData {
NSErrorData({
required this.code,
required this.domain,
required this.localizedDescription,
});
int code;
String domain;
String localizedDescription;
Object encode() {
return <Object?>[
code,
domain,
localizedDescription,
];
}
static NSErrorData decode(Object result) {
result as List<Object?>;
return NSErrorData(
code: result[0]! as int,
domain: result[1]! as String,
localizedDescription: result[2]! as String,
);
}
}
/// Mirror of WKScriptMessage.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessage?language=objc.
class WKScriptMessageData {
WKScriptMessageData({
required this.name,
this.body,
});
String name;
Object? body;
Object encode() {
return <Object?>[
name,
body,
];
}
static WKScriptMessageData decode(Object result) {
result as List<Object?>;
return WKScriptMessageData(
name: result[0]! as String,
body: result[1] as Object?,
);
}
}
/// Mirror of NSHttpCookieData.
///
/// See https://developer.apple.com/documentation/foundation/nshttpcookie?language=objc.
class NSHttpCookieData {
NSHttpCookieData({
required this.propertyKeys,
required this.propertyValues,
});
List<NSHttpCookiePropertyKeyEnumData?> propertyKeys;
List<Object?> propertyValues;
Object encode() {
return <Object?>[
propertyKeys,
propertyValues,
];
}
static NSHttpCookieData decode(Object result) {
result as List<Object?>;
return NSHttpCookieData(
propertyKeys: (result[0] as List<Object?>?)!
.cast<NSHttpCookiePropertyKeyEnumData?>(),
propertyValues: (result[1] as List<Object?>?)!.cast<Object?>(),
);
}
}
class _WKWebsiteDataStoreHostApiCodec extends StandardMessageCodec {
const _WKWebsiteDataStoreHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is WKWebsiteDataTypeEnumData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return WKWebsiteDataTypeEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of WKWebsiteDataStore.
///
/// See https://developer.apple.com/documentation/webkit/wkwebsitedatastore?language=objc.
class WKWebsiteDataStoreHostApi {
/// Constructor for [WKWebsiteDataStoreHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKWebsiteDataStoreHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _WKWebsiteDataStoreHostApiCodec();
Future<void> createFromWebViewConfiguration(
int arg_identifier, int arg_configurationIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebsiteDataStoreHostApi.createFromWebViewConfiguration',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_configurationIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> createDefaultDataStore(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebsiteDataStoreHostApi.createDefaultDataStore',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<bool> removeDataOfTypes(
int arg_identifier,
List<WKWebsiteDataTypeEnumData?> arg_dataTypes,
double arg_modificationTimeInSecondsSinceEpoch) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebsiteDataStoreHostApi.removeDataOfTypes', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_dataTypes,
arg_modificationTimeInSecondsSinceEpoch
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
}
/// Mirror of UIView.
///
/// See https://developer.apple.com/documentation/uikit/uiview?language=objc.
class UIViewHostApi {
/// Constructor for [UIViewHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
UIViewHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> setBackgroundColor(int arg_identifier, int? arg_value) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIViewHostApi.setBackgroundColor', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_value]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setOpaque(int arg_identifier, bool arg_opaque) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIViewHostApi.setOpaque', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_opaque]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
/// Mirror of UIScrollView.
///
/// See https://developer.apple.com/documentation/uikit/uiscrollview?language=objc.
class UIScrollViewHostApi {
/// Constructor for [UIScrollViewHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
UIScrollViewHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> createFromWebView(
int arg_identifier, int arg_webViewIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIScrollViewHostApi.createFromWebView', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_webViewIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<List<double?>> getContentOffset(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIScrollViewHostApi.getContentOffset', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as List<Object?>?)!.cast<double?>();
}
}
Future<void> scrollBy(int arg_identifier, double arg_x, double arg_y) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIScrollViewHostApi.scrollBy', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_x, arg_y]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setContentOffset(
int arg_identifier, double arg_x, double arg_y) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.UIScrollViewHostApi.setContentOffset', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_x, arg_y]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _WKWebViewConfigurationHostApiCodec extends StandardMessageCodec {
const _WKWebViewConfigurationHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is WKAudiovisualMediaTypeEnumData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return WKAudiovisualMediaTypeEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of WKWebViewConfiguration.
///
/// See https://developer.apple.com/documentation/webkit/wkwebviewconfiguration?language=objc.
class WKWebViewConfigurationHostApi {
/// Constructor for [WKWebViewConfigurationHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKWebViewConfigurationHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec =
_WKWebViewConfigurationHostApiCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewConfigurationHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> createFromWebView(
int arg_identifier, int arg_webViewIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewConfigurationHostApi.createFromWebView',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_webViewIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setAllowsInlineMediaPlayback(
int arg_identifier, bool arg_allow) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewConfigurationHostApi.setAllowsInlineMediaPlayback',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_allow]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setMediaTypesRequiringUserActionForPlayback(int arg_identifier,
List<WKAudiovisualMediaTypeEnumData?> arg_types) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewConfigurationHostApi.setMediaTypesRequiringUserActionForPlayback',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_types]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
/// Handles callbacks from an WKWebViewConfiguration instance.
///
/// See https://developer.apple.com/documentation/webkit/wkwebviewconfiguration?language=objc.
abstract class WKWebViewConfigurationFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(WKWebViewConfigurationFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewConfigurationFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKWebViewConfigurationFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKWebViewConfigurationFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class _WKUserContentControllerHostApiCodec extends StandardMessageCodec {
const _WKUserContentControllerHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is WKUserScriptData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is WKUserScriptInjectionTimeEnumData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return WKUserScriptData.decode(readValue(buffer)!);
case 129:
return WKUserScriptInjectionTimeEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of WKUserContentController.
///
/// See https://developer.apple.com/documentation/webkit/wkusercontentcontroller?language=objc.
class WKUserContentControllerHostApi {
/// Constructor for [WKUserContentControllerHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKUserContentControllerHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec =
_WKUserContentControllerHostApiCodec();
Future<void> createFromWebViewConfiguration(
int arg_identifier, int arg_configurationIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.createFromWebViewConfiguration',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_configurationIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> addScriptMessageHandler(
int arg_identifier, int arg_handlerIdentifier, String arg_name) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.addScriptMessageHandler',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_handlerIdentifier, arg_name])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> removeScriptMessageHandler(
int arg_identifier, String arg_name) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.removeScriptMessageHandler',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_name]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> removeAllScriptMessageHandlers(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.removeAllScriptMessageHandlers',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> addUserScript(
int arg_identifier, WKUserScriptData arg_userScript) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.addUserScript',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_userScript]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> removeAllUserScripts(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUserContentControllerHostApi.removeAllUserScripts',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
/// Mirror of WKUserPreferences.
///
/// See https://developer.apple.com/documentation/webkit/wkpreferences?language=objc.
class WKPreferencesHostApi {
/// Constructor for [WKPreferencesHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKPreferencesHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> createFromWebViewConfiguration(
int arg_identifier, int arg_configurationIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKPreferencesHostApi.createFromWebViewConfiguration',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_configurationIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setJavaScriptEnabled(
int arg_identifier, bool arg_enabled) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKPreferencesHostApi.setJavaScriptEnabled', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_enabled]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
/// Mirror of WKScriptMessageHandler.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessagehandler?language=objc.
class WKScriptMessageHandlerHostApi {
/// Constructor for [WKScriptMessageHandlerHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKScriptMessageHandlerHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKScriptMessageHandlerHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _WKScriptMessageHandlerFlutterApiCodec extends StandardMessageCodec {
const _WKScriptMessageHandlerFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is WKScriptMessageData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return WKScriptMessageData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Handles callbacks from an WKScriptMessageHandler instance.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessagehandler?language=objc.
abstract class WKScriptMessageHandlerFlutterApi {
static const MessageCodec<Object?> codec =
_WKScriptMessageHandlerFlutterApiCodec();
void didReceiveScriptMessage(int identifier,
int userContentControllerIdentifier, WKScriptMessageData message);
static void setup(WKScriptMessageHandlerFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKScriptMessageHandlerFlutterApi.didReceiveScriptMessage',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKScriptMessageHandlerFlutterApi.didReceiveScriptMessage was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKScriptMessageHandlerFlutterApi.didReceiveScriptMessage was null, expected non-null int.');
final int? arg_userContentControllerIdentifier = (args[1] as int?);
assert(arg_userContentControllerIdentifier != null,
'Argument for dev.flutter.pigeon.WKScriptMessageHandlerFlutterApi.didReceiveScriptMessage was null, expected non-null int.');
final WKScriptMessageData? arg_message =
(args[2] as WKScriptMessageData?);
assert(arg_message != null,
'Argument for dev.flutter.pigeon.WKScriptMessageHandlerFlutterApi.didReceiveScriptMessage was null, expected non-null WKScriptMessageData.');
api.didReceiveScriptMessage(arg_identifier!,
arg_userContentControllerIdentifier!, arg_message!);
return;
});
}
}
}
}
/// Mirror of WKNavigationDelegate.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationdelegate?language=objc.
class WKNavigationDelegateHostApi {
/// Constructor for [WKNavigationDelegateHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKNavigationDelegateHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _WKNavigationDelegateFlutterApiCodec extends StandardMessageCodec {
const _WKNavigationDelegateFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSErrorData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is NSUrlRequestData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is WKFrameInfoData) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionData) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionPolicyEnumData) {
buffer.putUint8(132);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSErrorData.decode(readValue(buffer)!);
case 129:
return NSUrlRequestData.decode(readValue(buffer)!);
case 130:
return WKFrameInfoData.decode(readValue(buffer)!);
case 131:
return WKNavigationActionData.decode(readValue(buffer)!);
case 132:
return WKNavigationActionPolicyEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Handles callbacks from an WKNavigationDelegate instance.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationdelegate?language=objc.
abstract class WKNavigationDelegateFlutterApi {
static const MessageCodec<Object?> codec =
_WKNavigationDelegateFlutterApiCodec();
void didFinishNavigation(int identifier, int webViewIdentifier, String? url);
void didStartProvisionalNavigation(
int identifier, int webViewIdentifier, String? url);
Future<WKNavigationActionPolicyEnumData> decidePolicyForNavigationAction(
int identifier,
int webViewIdentifier,
WKNavigationActionData navigationAction);
void didFailNavigation(
int identifier, int webViewIdentifier, NSErrorData error);
void didFailProvisionalNavigation(
int identifier, int webViewIdentifier, NSErrorData error);
void webViewWebContentProcessDidTerminate(
int identifier, int webViewIdentifier);
static void setup(WKNavigationDelegateFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFinishNavigation',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFinishNavigation was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFinishNavigation was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFinishNavigation was null, expected non-null int.');
final String? arg_url = (args[2] as String?);
api.didFinishNavigation(
arg_identifier!, arg_webViewIdentifier!, arg_url);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didStartProvisionalNavigation',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didStartProvisionalNavigation was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didStartProvisionalNavigation was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didStartProvisionalNavigation was null, expected non-null int.');
final String? arg_url = (args[2] as String?);
api.didStartProvisionalNavigation(
arg_identifier!, arg_webViewIdentifier!, arg_url);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.decidePolicyForNavigationAction',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.decidePolicyForNavigationAction was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.decidePolicyForNavigationAction was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.decidePolicyForNavigationAction was null, expected non-null int.');
final WKNavigationActionData? arg_navigationAction =
(args[2] as WKNavigationActionData?);
assert(arg_navigationAction != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.decidePolicyForNavigationAction was null, expected non-null WKNavigationActionData.');
final WKNavigationActionPolicyEnumData output =
await api.decidePolicyForNavigationAction(arg_identifier!,
arg_webViewIdentifier!, arg_navigationAction!);
return output;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailNavigation',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailNavigation was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailNavigation was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailNavigation was null, expected non-null int.');
final NSErrorData? arg_error = (args[2] as NSErrorData?);
assert(arg_error != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailNavigation was null, expected non-null NSErrorData.');
api.didFailNavigation(
arg_identifier!, arg_webViewIdentifier!, arg_error!);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailProvisionalNavigation',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailProvisionalNavigation was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailProvisionalNavigation was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailProvisionalNavigation was null, expected non-null int.');
final NSErrorData? arg_error = (args[2] as NSErrorData?);
assert(arg_error != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.didFailProvisionalNavigation was null, expected non-null NSErrorData.');
api.didFailProvisionalNavigation(
arg_identifier!, arg_webViewIdentifier!, arg_error!);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKNavigationDelegateFlutterApi.webViewWebContentProcessDidTerminate',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.webViewWebContentProcessDidTerminate was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.webViewWebContentProcessDidTerminate was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKNavigationDelegateFlutterApi.webViewWebContentProcessDidTerminate was null, expected non-null int.');
api.webViewWebContentProcessDidTerminate(
arg_identifier!, arg_webViewIdentifier!);
return;
});
}
}
}
}
class _NSObjectHostApiCodec extends StandardMessageCodec {
const _NSObjectHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSKeyValueObservingOptionsEnumData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSKeyValueObservingOptionsEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of NSObject.
///
/// See https://developer.apple.com/documentation/objectivec/nsobject.
class NSObjectHostApi {
/// Constructor for [NSObjectHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
NSObjectHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _NSObjectHostApiCodec();
Future<void> dispose(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.NSObjectHostApi.dispose', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> addObserver(
int arg_identifier,
int arg_observerIdentifier,
String arg_keyPath,
List<NSKeyValueObservingOptionsEnumData?> arg_options) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.NSObjectHostApi.addObserver', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_observerIdentifier,
arg_keyPath,
arg_options
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> removeObserver(int arg_identifier, int arg_observerIdentifier,
String arg_keyPath) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.NSObjectHostApi.removeObserver', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(
<Object?>[arg_identifier, arg_observerIdentifier, arg_keyPath])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _NSObjectFlutterApiCodec extends StandardMessageCodec {
const _NSObjectFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSErrorData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is NSHttpCookieData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is NSHttpCookiePropertyKeyEnumData) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is NSKeyValueChangeKeyEnumData) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else if (value is NSKeyValueObservingOptionsEnumData) {
buffer.putUint8(132);
writeValue(buffer, value.encode());
} else if (value is NSUrlRequestData) {
buffer.putUint8(133);
writeValue(buffer, value.encode());
} else if (value is WKAudiovisualMediaTypeEnumData) {
buffer.putUint8(134);
writeValue(buffer, value.encode());
} else if (value is WKFrameInfoData) {
buffer.putUint8(135);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionData) {
buffer.putUint8(136);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionPolicyEnumData) {
buffer.putUint8(137);
writeValue(buffer, value.encode());
} else if (value is WKScriptMessageData) {
buffer.putUint8(138);
writeValue(buffer, value.encode());
} else if (value is WKUserScriptData) {
buffer.putUint8(139);
writeValue(buffer, value.encode());
} else if (value is WKUserScriptInjectionTimeEnumData) {
buffer.putUint8(140);
writeValue(buffer, value.encode());
} else if (value is WKWebsiteDataTypeEnumData) {
buffer.putUint8(141);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSErrorData.decode(readValue(buffer)!);
case 129:
return NSHttpCookieData.decode(readValue(buffer)!);
case 130:
return NSHttpCookiePropertyKeyEnumData.decode(readValue(buffer)!);
case 131:
return NSKeyValueChangeKeyEnumData.decode(readValue(buffer)!);
case 132:
return NSKeyValueObservingOptionsEnumData.decode(readValue(buffer)!);
case 133:
return NSUrlRequestData.decode(readValue(buffer)!);
case 134:
return WKAudiovisualMediaTypeEnumData.decode(readValue(buffer)!);
case 135:
return WKFrameInfoData.decode(readValue(buffer)!);
case 136:
return WKNavigationActionData.decode(readValue(buffer)!);
case 137:
return WKNavigationActionPolicyEnumData.decode(readValue(buffer)!);
case 138:
return WKScriptMessageData.decode(readValue(buffer)!);
case 139:
return WKUserScriptData.decode(readValue(buffer)!);
case 140:
return WKUserScriptInjectionTimeEnumData.decode(readValue(buffer)!);
case 141:
return WKWebsiteDataTypeEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Handles callbacks from an NSObject instance.
///
/// See https://developer.apple.com/documentation/objectivec/nsobject.
abstract class NSObjectFlutterApi {
static const MessageCodec<Object?> codec = _NSObjectFlutterApiCodec();
void observeValue(
int identifier,
String keyPath,
int objectIdentifier,
List<NSKeyValueChangeKeyEnumData?> changeKeys,
List<Object?> changeValues);
void dispose(int identifier);
static void setup(NSObjectFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.NSObjectFlutterApi.observeValue', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null, expected non-null int.');
final String? arg_keyPath = (args[1] as String?);
assert(arg_keyPath != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null, expected non-null String.');
final int? arg_objectIdentifier = (args[2] as int?);
assert(arg_objectIdentifier != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null, expected non-null int.');
final List<NSKeyValueChangeKeyEnumData?>? arg_changeKeys =
(args[3] as List<Object?>?)?.cast<NSKeyValueChangeKeyEnumData?>();
assert(arg_changeKeys != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null, expected non-null List<NSKeyValueChangeKeyEnumData?>.');
final List<Object?>? arg_changeValues =
(args[4] as List<Object?>?)?.cast<Object?>();
assert(arg_changeValues != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.observeValue was null, expected non-null List<Object?>.');
api.observeValue(arg_identifier!, arg_keyPath!, arg_objectIdentifier!,
arg_changeKeys!, arg_changeValues!);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.NSObjectFlutterApi.dispose', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.dispose was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.NSObjectFlutterApi.dispose was null, expected non-null int.');
api.dispose(arg_identifier!);
return;
});
}
}
}
}
class _WKWebViewHostApiCodec extends StandardMessageCodec {
const _WKWebViewHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSErrorData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is NSHttpCookieData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is NSHttpCookiePropertyKeyEnumData) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is NSKeyValueChangeKeyEnumData) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else if (value is NSKeyValueObservingOptionsEnumData) {
buffer.putUint8(132);
writeValue(buffer, value.encode());
} else if (value is NSUrlRequestData) {
buffer.putUint8(133);
writeValue(buffer, value.encode());
} else if (value is WKAudiovisualMediaTypeEnumData) {
buffer.putUint8(134);
writeValue(buffer, value.encode());
} else if (value is WKFrameInfoData) {
buffer.putUint8(135);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionData) {
buffer.putUint8(136);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionPolicyEnumData) {
buffer.putUint8(137);
writeValue(buffer, value.encode());
} else if (value is WKScriptMessageData) {
buffer.putUint8(138);
writeValue(buffer, value.encode());
} else if (value is WKUserScriptData) {
buffer.putUint8(139);
writeValue(buffer, value.encode());
} else if (value is WKUserScriptInjectionTimeEnumData) {
buffer.putUint8(140);
writeValue(buffer, value.encode());
} else if (value is WKWebsiteDataTypeEnumData) {
buffer.putUint8(141);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSErrorData.decode(readValue(buffer)!);
case 129:
return NSHttpCookieData.decode(readValue(buffer)!);
case 130:
return NSHttpCookiePropertyKeyEnumData.decode(readValue(buffer)!);
case 131:
return NSKeyValueChangeKeyEnumData.decode(readValue(buffer)!);
case 132:
return NSKeyValueObservingOptionsEnumData.decode(readValue(buffer)!);
case 133:
return NSUrlRequestData.decode(readValue(buffer)!);
case 134:
return WKAudiovisualMediaTypeEnumData.decode(readValue(buffer)!);
case 135:
return WKFrameInfoData.decode(readValue(buffer)!);
case 136:
return WKNavigationActionData.decode(readValue(buffer)!);
case 137:
return WKNavigationActionPolicyEnumData.decode(readValue(buffer)!);
case 138:
return WKScriptMessageData.decode(readValue(buffer)!);
case 139:
return WKUserScriptData.decode(readValue(buffer)!);
case 140:
return WKUserScriptInjectionTimeEnumData.decode(readValue(buffer)!);
case 141:
return WKWebsiteDataTypeEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of WKWebView.
///
/// See https://developer.apple.com/documentation/webkit/wkwebview?language=objc.
class WKWebViewHostApi {
/// Constructor for [WKWebViewHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKWebViewHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _WKWebViewHostApiCodec();
Future<void> create(
int arg_identifier, int arg_configurationIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_configurationIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setUIDelegate(
int arg_identifier, int? arg_uiDelegateIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.setUIDelegate', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_uiDelegateIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setNavigationDelegate(
int arg_identifier, int? arg_navigationDelegateIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.setNavigationDelegate', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_navigationDelegateIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<String?> getUrl(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.getUrl', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as String?);
}
}
Future<double> getEstimatedProgress(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.getEstimatedProgress', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as double?)!;
}
}
Future<void> loadRequest(
int arg_identifier, NSUrlRequestData arg_request) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.loadRequest', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_request]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> loadHtmlString(
int arg_identifier, String arg_string, String? arg_baseUrl) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.loadHtmlString', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_string, arg_baseUrl])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> loadFileUrl(
int arg_identifier, String arg_url, String arg_readAccessUrl) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.loadFileUrl', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_url, arg_readAccessUrl])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> loadFlutterAsset(int arg_identifier, String arg_key) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.loadFlutterAsset', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_key]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<bool> canGoBack(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.canGoBack', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
Future<bool> canGoForward(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.canGoForward', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
Future<void> goBack(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.goBack', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> goForward(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.goForward', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> reload(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.reload', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<String?> getTitle(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.getTitle', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as String?);
}
}
Future<void> setAllowsBackForwardNavigationGestures(
int arg_identifier, bool arg_allow) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.setAllowsBackForwardNavigationGestures',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_allow]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setCustomUserAgent(
int arg_identifier, String? arg_userAgent) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.setCustomUserAgent', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_userAgent]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<Object?> evaluateJavaScript(
int arg_identifier, String arg_javaScriptString) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKWebViewHostApi.evaluateJavaScript', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_javaScriptString])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as Object?);
}
}
}
/// Mirror of WKUIDelegate.
///
/// See https://developer.apple.com/documentation/webkit/wkuidelegate?language=objc.
class WKUIDelegateHostApi {
/// Constructor for [WKUIDelegateHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKUIDelegateHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUIDelegateHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _WKUIDelegateFlutterApiCodec extends StandardMessageCodec {
const _WKUIDelegateFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSUrlRequestData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is WKFrameInfoData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is WKNavigationActionData) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSUrlRequestData.decode(readValue(buffer)!);
case 129:
return WKFrameInfoData.decode(readValue(buffer)!);
case 130:
return WKNavigationActionData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Handles callbacks from an WKUIDelegate instance.
///
/// See https://developer.apple.com/documentation/webkit/wkuidelegate?language=objc.
abstract class WKUIDelegateFlutterApi {
static const MessageCodec<Object?> codec = _WKUIDelegateFlutterApiCodec();
void onCreateWebView(int identifier, int webViewIdentifier,
int configurationIdentifier, WKNavigationActionData navigationAction);
static void setup(WKUIDelegateFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView was null, expected non-null int.');
final int? arg_webViewIdentifier = (args[1] as int?);
assert(arg_webViewIdentifier != null,
'Argument for dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView was null, expected non-null int.');
final int? arg_configurationIdentifier = (args[2] as int?);
assert(arg_configurationIdentifier != null,
'Argument for dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView was null, expected non-null int.');
final WKNavigationActionData? arg_navigationAction =
(args[3] as WKNavigationActionData?);
assert(arg_navigationAction != null,
'Argument for dev.flutter.pigeon.WKUIDelegateFlutterApi.onCreateWebView was null, expected non-null WKNavigationActionData.');
api.onCreateWebView(arg_identifier!, arg_webViewIdentifier!,
arg_configurationIdentifier!, arg_navigationAction!);
return;
});
}
}
}
}
class _WKHttpCookieStoreHostApiCodec extends StandardMessageCodec {
const _WKHttpCookieStoreHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is NSHttpCookieData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is NSHttpCookiePropertyKeyEnumData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return NSHttpCookieData.decode(readValue(buffer)!);
case 129:
return NSHttpCookiePropertyKeyEnumData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
/// Mirror of WKHttpCookieStore.
///
/// See https://developer.apple.com/documentation/webkit/wkhttpcookiestore?language=objc.
class WKHttpCookieStoreHostApi {
/// Constructor for [WKHttpCookieStoreHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
WKHttpCookieStoreHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _WKHttpCookieStoreHostApiCodec();
Future<void> createFromWebsiteDataStore(
int arg_identifier, int arg_websiteDataStoreIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKHttpCookieStoreHostApi.createFromWebsiteDataStore',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_websiteDataStoreIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setCookie(
int arg_identifier, NSHttpCookieData arg_cookie) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.WKHttpCookieStoreHostApi.setCookie', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_cookie]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
| plugins/packages/webview_flutter/webview_flutter_wkwebview/lib/src/common/web_kit.g.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_wkwebview/lib/src/common/web_kit.g.dart",
"repo_id": "plugins",
"token_count": 35220
} | 1,185 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:pigeon/pigeon.dart';
@ConfigurePigeon(
PigeonOptions(
dartOut: 'lib/src/common/web_kit.g.dart',
dartTestOut: 'test/src/common/test_web_kit.g.dart',
dartOptions: DartOptions(copyrightHeader: <String>[
'Copyright 2013 The Flutter Authors. All rights reserved.',
'Use of this source code is governed by a BSD-style license that can be',
'found in the LICENSE file.',
]),
objcHeaderOut: 'ios/Classes/FWFGeneratedWebKitApis.h',
objcSourceOut: 'ios/Classes/FWFGeneratedWebKitApis.m',
objcOptions: ObjcOptions(
header: 'ios/Classes/FWFGeneratedWebKitApis.h',
prefix: 'FWF',
copyrightHeader: <String>[
'Copyright 2013 The Flutter Authors. All rights reserved.',
'Use of this source code is governed by a BSD-style license that can be',
'found in the LICENSE file.',
],
),
),
)
/// Mirror of NSKeyValueObservingOptions.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvalueobservingoptions?language=objc.
enum NSKeyValueObservingOptionsEnum {
newValue,
oldValue,
initialValue,
priorNotification,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class NSKeyValueObservingOptionsEnumData {
late NSKeyValueObservingOptionsEnum value;
}
/// Mirror of NSKeyValueChange.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvaluechange?language=objc.
enum NSKeyValueChangeEnum {
setting,
insertion,
removal,
replacement,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class NSKeyValueChangeEnumData {
late NSKeyValueChangeEnum value;
}
/// Mirror of NSKeyValueChangeKey.
///
/// See https://developer.apple.com/documentation/foundation/nskeyvaluechangekey?language=objc.
enum NSKeyValueChangeKeyEnum {
indexes,
kind,
newValue,
notificationIsPrior,
oldValue,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class NSKeyValueChangeKeyEnumData {
late NSKeyValueChangeKeyEnum value;
}
/// Mirror of WKUserScriptInjectionTime.
///
/// See https://developer.apple.com/documentation/webkit/wkuserscriptinjectiontime?language=objc.
enum WKUserScriptInjectionTimeEnum {
atDocumentStart,
atDocumentEnd,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class WKUserScriptInjectionTimeEnumData {
late WKUserScriptInjectionTimeEnum value;
}
/// Mirror of WKAudiovisualMediaTypes.
///
/// See [WKAudiovisualMediaTypes](https://developer.apple.com/documentation/webkit/wkaudiovisualmediatypes?language=objc).
enum WKAudiovisualMediaTypeEnum {
none,
audio,
video,
all,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class WKAudiovisualMediaTypeEnumData {
late WKAudiovisualMediaTypeEnum value;
}
/// Mirror of WKWebsiteDataTypes.
///
/// See https://developer.apple.com/documentation/webkit/wkwebsitedatarecord/data_store_record_types?language=objc.
enum WKWebsiteDataTypeEnum {
cookies,
memoryCache,
diskCache,
offlineWebApplicationCache,
localStorage,
sessionStorage,
webSQLDatabases,
indexedDBDatabases,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class WKWebsiteDataTypeEnumData {
late WKWebsiteDataTypeEnum value;
}
/// Mirror of WKNavigationActionPolicy.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationactionpolicy?language=objc.
enum WKNavigationActionPolicyEnum {
allow,
cancel,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class WKNavigationActionPolicyEnumData {
late WKNavigationActionPolicyEnum value;
}
/// Mirror of NSHTTPCookiePropertyKey.
///
/// See https://developer.apple.com/documentation/foundation/nshttpcookiepropertykey.
enum NSHttpCookiePropertyKeyEnum {
comment,
commentUrl,
discard,
domain,
expires,
maximumAge,
name,
originUrl,
path,
port,
sameSitePolicy,
secure,
value,
version,
}
// TODO(bparrishMines): Enums need be wrapped in a data class because thay can't
// be used as primitive arguments. See https://github.com/flutter/flutter/issues/87307
class NSHttpCookiePropertyKeyEnumData {
late NSHttpCookiePropertyKeyEnum value;
}
/// An object that contains information about an action that causes navigation
/// to occur.
///
/// Wraps [WKNavigationType](https://developer.apple.com/documentation/webkit/wknavigationaction?language=objc).
enum WKNavigationType {
/// A link activation.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypelinkactivated?language=objc.
linkActivated,
/// A request to submit a form.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeformsubmitted?language=objc.
submitted,
/// A request for the frame’s next or previous item.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypebackforward?language=objc.
backForward,
/// A request to reload the webpage.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypereload?language=objc.
reload,
/// A request to resubmit a form.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeformresubmitted?language=objc.
formResubmitted,
/// A navigation request that originates for some other reason.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationtype/wknavigationtypeother?language=objc.
other,
}
/// Mirror of NSURLRequest.
///
/// See https://developer.apple.com/documentation/foundation/nsurlrequest?language=objc.
class NSUrlRequestData {
late String url;
late String? httpMethod;
late Uint8List? httpBody;
late Map<String?, String?> allHttpHeaderFields;
}
/// Mirror of WKUserScript.
///
/// See https://developer.apple.com/documentation/webkit/wkuserscript?language=objc.
class WKUserScriptData {
late String source;
late WKUserScriptInjectionTimeEnumData? injectionTime;
late bool isMainFrameOnly;
}
/// Mirror of WKNavigationAction.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationaction.
class WKNavigationActionData {
late NSUrlRequestData request;
late WKFrameInfoData targetFrame;
late WKNavigationType navigationType;
}
/// Mirror of WKFrameInfo.
///
/// See https://developer.apple.com/documentation/webkit/wkframeinfo?language=objc.
class WKFrameInfoData {
late bool isMainFrame;
}
/// Mirror of NSError.
///
/// See https://developer.apple.com/documentation/foundation/nserror?language=objc.
class NSErrorData {
late int code;
late String domain;
late String localizedDescription;
}
/// Mirror of WKScriptMessage.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessage?language=objc.
class WKScriptMessageData {
late String name;
late Object? body;
}
/// Mirror of NSHttpCookieData.
///
/// See https://developer.apple.com/documentation/foundation/nshttpcookie?language=objc.
class NSHttpCookieData {
// TODO(bparrishMines): Change to a map when Objective-C data classes conform
// to `NSCopying`. See https://github.com/flutter/flutter/issues/103383.
// `NSDictionary`s are unable to use data classes as keys because they don't
// conform to `NSCopying`. This splits the map of properties into a list of
// keys and values with the ordered maintained.
late List<NSHttpCookiePropertyKeyEnumData?> propertyKeys;
late List<Object?> propertyValues;
}
/// Mirror of WKWebsiteDataStore.
///
/// See https://developer.apple.com/documentation/webkit/wkwebsitedatastore?language=objc.
@HostApi(dartHostTestHandler: 'TestWKWebsiteDataStoreHostApi')
abstract class WKWebsiteDataStoreHostApi {
@ObjCSelector(
'createFromWebViewConfigurationWithIdentifier:configurationIdentifier:',
)
void createFromWebViewConfiguration(
int identifier,
int configurationIdentifier,
);
@ObjCSelector('createDefaultDataStoreWithIdentifier:')
void createDefaultDataStore(int identifier);
@ObjCSelector(
'removeDataFromDataStoreWithIdentifier:ofTypes:modifiedSince:',
)
@async
bool removeDataOfTypes(
int identifier,
List<WKWebsiteDataTypeEnumData> dataTypes,
double modificationTimeInSecondsSinceEpoch,
);
}
/// Mirror of UIView.
///
/// See https://developer.apple.com/documentation/uikit/uiview?language=objc.
@HostApi(dartHostTestHandler: 'TestUIViewHostApi')
abstract class UIViewHostApi {
@ObjCSelector('setBackgroundColorForViewWithIdentifier:toValue:')
void setBackgroundColor(int identifier, int? value);
@ObjCSelector('setOpaqueForViewWithIdentifier:isOpaque:')
void setOpaque(int identifier, bool opaque);
}
/// Mirror of UIScrollView.
///
/// See https://developer.apple.com/documentation/uikit/uiscrollview?language=objc.
@HostApi(dartHostTestHandler: 'TestUIScrollViewHostApi')
abstract class UIScrollViewHostApi {
@ObjCSelector('createFromWebViewWithIdentifier:webViewIdentifier:')
void createFromWebView(int identifier, int webViewIdentifier);
@ObjCSelector('contentOffsetForScrollViewWithIdentifier:')
List<double?> getContentOffset(int identifier);
@ObjCSelector('scrollByForScrollViewWithIdentifier:x:y:')
void scrollBy(int identifier, double x, double y);
@ObjCSelector('setContentOffsetForScrollViewWithIdentifier:toX:y:')
void setContentOffset(int identifier, double x, double y);
}
/// Mirror of WKWebViewConfiguration.
///
/// See https://developer.apple.com/documentation/webkit/wkwebviewconfiguration?language=objc.
@HostApi(dartHostTestHandler: 'TestWKWebViewConfigurationHostApi')
abstract class WKWebViewConfigurationHostApi {
@ObjCSelector('createWithIdentifier:')
void create(int identifier);
@ObjCSelector('createFromWebViewWithIdentifier:webViewIdentifier:')
void createFromWebView(int identifier, int webViewIdentifier);
@ObjCSelector(
'setAllowsInlineMediaPlaybackForConfigurationWithIdentifier:isAllowed:',
)
void setAllowsInlineMediaPlayback(int identifier, bool allow);
@ObjCSelector(
'setMediaTypesRequiresUserActionForConfigurationWithIdentifier:forTypes:',
)
void setMediaTypesRequiringUserActionForPlayback(
int identifier,
List<WKAudiovisualMediaTypeEnumData> types,
);
}
/// Handles callbacks from an WKWebViewConfiguration instance.
///
/// See https://developer.apple.com/documentation/webkit/wkwebviewconfiguration?language=objc.
@FlutterApi()
abstract class WKWebViewConfigurationFlutterApi {
@ObjCSelector('createWithIdentifier:')
void create(int identifier);
}
/// Mirror of WKUserContentController.
///
/// See https://developer.apple.com/documentation/webkit/wkusercontentcontroller?language=objc.
@HostApi(dartHostTestHandler: 'TestWKUserContentControllerHostApi')
abstract class WKUserContentControllerHostApi {
@ObjCSelector(
'createFromWebViewConfigurationWithIdentifier:configurationIdentifier:',
)
void createFromWebViewConfiguration(
int identifier,
int configurationIdentifier,
);
@ObjCSelector(
'addScriptMessageHandlerForControllerWithIdentifier:handlerIdentifier:ofName:',
)
void addScriptMessageHandler(
int identifier,
int handlerIdentifier,
String name,
);
@ObjCSelector('removeScriptMessageHandlerForControllerWithIdentifier:name:')
void removeScriptMessageHandler(int identifier, String name);
@ObjCSelector('removeAllScriptMessageHandlersForControllerWithIdentifier:')
void removeAllScriptMessageHandlers(int identifier);
@ObjCSelector('addUserScriptForControllerWithIdentifier:userScript:')
void addUserScript(int identifier, WKUserScriptData userScript);
@ObjCSelector('removeAllUserScriptsForControllerWithIdentifier:')
void removeAllUserScripts(int identifier);
}
/// Mirror of WKUserPreferences.
///
/// See https://developer.apple.com/documentation/webkit/wkpreferences?language=objc.
@HostApi(dartHostTestHandler: 'TestWKPreferencesHostApi')
abstract class WKPreferencesHostApi {
@ObjCSelector(
'createFromWebViewConfigurationWithIdentifier:configurationIdentifier:',
)
void createFromWebViewConfiguration(
int identifier,
int configurationIdentifier,
);
@ObjCSelector('setJavaScriptEnabledForPreferencesWithIdentifier:isEnabled:')
void setJavaScriptEnabled(int identifier, bool enabled);
}
/// Mirror of WKScriptMessageHandler.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessagehandler?language=objc.
@HostApi(dartHostTestHandler: 'TestWKScriptMessageHandlerHostApi')
abstract class WKScriptMessageHandlerHostApi {
@ObjCSelector('createWithIdentifier:')
void create(int identifier);
}
/// Handles callbacks from an WKScriptMessageHandler instance.
///
/// See https://developer.apple.com/documentation/webkit/wkscriptmessagehandler?language=objc.
@FlutterApi()
abstract class WKScriptMessageHandlerFlutterApi {
@ObjCSelector(
'didReceiveScriptMessageForHandlerWithIdentifier:userContentControllerIdentifier:message:',
)
void didReceiveScriptMessage(
int identifier,
int userContentControllerIdentifier,
WKScriptMessageData message,
);
}
/// Mirror of WKNavigationDelegate.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationdelegate?language=objc.
@HostApi(dartHostTestHandler: 'TestWKNavigationDelegateHostApi')
abstract class WKNavigationDelegateHostApi {
@ObjCSelector('createWithIdentifier:')
void create(int identifier);
}
/// Handles callbacks from an WKNavigationDelegate instance.
///
/// See https://developer.apple.com/documentation/webkit/wknavigationdelegate?language=objc.
@FlutterApi()
abstract class WKNavigationDelegateFlutterApi {
@ObjCSelector(
'didFinishNavigationForDelegateWithIdentifier:webViewIdentifier:URL:',
)
void didFinishNavigation(
int identifier,
int webViewIdentifier,
String? url,
);
@ObjCSelector(
'didStartProvisionalNavigationForDelegateWithIdentifier:webViewIdentifier:URL:',
)
void didStartProvisionalNavigation(
int identifier,
int webViewIdentifier,
String? url,
);
@ObjCSelector(
'decidePolicyForNavigationActionForDelegateWithIdentifier:webViewIdentifier:navigationAction:',
)
@async
WKNavigationActionPolicyEnumData decidePolicyForNavigationAction(
int identifier,
int webViewIdentifier,
WKNavigationActionData navigationAction,
);
@ObjCSelector(
'didFailNavigationForDelegateWithIdentifier:webViewIdentifier:error:',
)
void didFailNavigation(
int identifier,
int webViewIdentifier,
NSErrorData error,
);
@ObjCSelector(
'didFailProvisionalNavigationForDelegateWithIdentifier:webViewIdentifier:error:',
)
void didFailProvisionalNavigation(
int identifier,
int webViewIdentifier,
NSErrorData error,
);
@ObjCSelector(
'webViewWebContentProcessDidTerminateForDelegateWithIdentifier:webViewIdentifier:',
)
void webViewWebContentProcessDidTerminate(
int identifier,
int webViewIdentifier,
);
}
/// Mirror of NSObject.
///
/// See https://developer.apple.com/documentation/objectivec/nsobject.
@HostApi(dartHostTestHandler: 'TestNSObjectHostApi')
abstract class NSObjectHostApi {
@ObjCSelector('disposeObjectWithIdentifier:')
void dispose(int identifier);
@ObjCSelector(
'addObserverForObjectWithIdentifier:observerIdentifier:keyPath:options:',
)
void addObserver(
int identifier,
int observerIdentifier,
String keyPath,
List<NSKeyValueObservingOptionsEnumData> options,
);
@ObjCSelector(
'removeObserverForObjectWithIdentifier:observerIdentifier:keyPath:',
)
void removeObserver(int identifier, int observerIdentifier, String keyPath);
}
/// Handles callbacks from an NSObject instance.
///
/// See https://developer.apple.com/documentation/objectivec/nsobject.
@FlutterApi()
abstract class NSObjectFlutterApi {
@ObjCSelector(
'observeValueForObjectWithIdentifier:keyPath:objectIdentifier:changeKeys:changeValues:',
)
void observeValue(
int identifier,
String keyPath,
int objectIdentifier,
// TODO(bparrishMines): Change to a map when Objective-C data classes conform
// to `NSCopying`. See https://github.com/flutter/flutter/issues/103383.
// `NSDictionary`s are unable to use data classes as keys because they don't
// conform to `NSCopying`. This splits the map of properties into a list of
// keys and values with the ordered maintained.
List<NSKeyValueChangeKeyEnumData?> changeKeys,
List<Object?> changeValues,
);
@ObjCSelector('disposeObjectWithIdentifier:')
void dispose(int identifier);
}
/// Mirror of WKWebView.
///
/// See https://developer.apple.com/documentation/webkit/wkwebview?language=objc.
@HostApi(dartHostTestHandler: 'TestWKWebViewHostApi')
abstract class WKWebViewHostApi {
@ObjCSelector('createWithIdentifier:configurationIdentifier:')
void create(int identifier, int configurationIdentifier);
@ObjCSelector('setUIDelegateForWebViewWithIdentifier:delegateIdentifier:')
void setUIDelegate(int identifier, int? uiDelegateIdentifier);
@ObjCSelector(
'setNavigationDelegateForWebViewWithIdentifier:delegateIdentifier:',
)
void setNavigationDelegate(int identifier, int? navigationDelegateIdentifier);
@ObjCSelector('URLForWebViewWithIdentifier:')
String? getUrl(int identifier);
@ObjCSelector('estimatedProgressForWebViewWithIdentifier:')
double getEstimatedProgress(int identifier);
@ObjCSelector('loadRequestForWebViewWithIdentifier:request:')
void loadRequest(int identifier, NSUrlRequestData request);
@ObjCSelector('loadHTMLForWebViewWithIdentifier:HTMLString:baseURL:')
void loadHtmlString(int identifier, String string, String? baseUrl);
@ObjCSelector('loadFileForWebViewWithIdentifier:fileURL:readAccessURL:')
void loadFileUrl(int identifier, String url, String readAccessUrl);
@ObjCSelector('loadAssetForWebViewWithIdentifier:assetKey:')
void loadFlutterAsset(int identifier, String key);
@ObjCSelector('canGoBackForWebViewWithIdentifier:')
bool canGoBack(int identifier);
@ObjCSelector('canGoForwardForWebViewWithIdentifier:')
bool canGoForward(int identifier);
@ObjCSelector('goBackForWebViewWithIdentifier:')
void goBack(int identifier);
@ObjCSelector('goForwardForWebViewWithIdentifier:')
void goForward(int identifier);
@ObjCSelector('reloadWebViewWithIdentifier:')
void reload(int identifier);
@ObjCSelector('titleForWebViewWithIdentifier:')
String? getTitle(int identifier);
@ObjCSelector('setAllowsBackForwardForWebViewWithIdentifier:isAllowed:')
void setAllowsBackForwardNavigationGestures(int identifier, bool allow);
@ObjCSelector('setUserAgentForWebViewWithIdentifier:userAgent:')
void setCustomUserAgent(int identifier, String? userAgent);
@ObjCSelector('evaluateJavaScriptForWebViewWithIdentifier:javaScriptString:')
@async
Object? evaluateJavaScript(int identifier, String javaScriptString);
}
/// Mirror of WKUIDelegate.
///
/// See https://developer.apple.com/documentation/webkit/wkuidelegate?language=objc.
@HostApi(dartHostTestHandler: 'TestWKUIDelegateHostApi')
abstract class WKUIDelegateHostApi {
@ObjCSelector('createWithIdentifier:')
void create(int identifier);
}
/// Handles callbacks from an WKUIDelegate instance.
///
/// See https://developer.apple.com/documentation/webkit/wkuidelegate?language=objc.
@FlutterApi()
abstract class WKUIDelegateFlutterApi {
@ObjCSelector(
'onCreateWebViewForDelegateWithIdentifier:webViewIdentifier:configurationIdentifier:navigationAction:',
)
void onCreateWebView(
int identifier,
int webViewIdentifier,
int configurationIdentifier,
WKNavigationActionData navigationAction,
);
}
/// Mirror of WKHttpCookieStore.
///
/// See https://developer.apple.com/documentation/webkit/wkhttpcookiestore?language=objc.
@HostApi(dartHostTestHandler: 'TestWKHttpCookieStoreHostApi')
abstract class WKHttpCookieStoreHostApi {
@ObjCSelector('createFromWebsiteDataStoreWithIdentifier:dataStoreIdentifier:')
void createFromWebsiteDataStore(
int identifier,
int websiteDataStoreIdentifier,
);
@ObjCSelector('setCookieForStoreWithIdentifier:cookie:')
@async
void setCookie(int identifier, NSHttpCookieData cookie);
}
| plugins/packages/webview_flutter/webview_flutter_wkwebview/pigeons/web_kit.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_wkwebview/pigeons/web_kit.dart",
"repo_id": "plugins",
"token_count": 6790
} | 1,186 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
// TODO(a14n): remove this import once Flutter 3.1 or later reaches stable (including flutter/flutter#104231)
// ignore: unnecessary_import
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'package:webview_flutter_platform_interface/webview_flutter_platform_interface.dart';
import 'package:webview_flutter_wkwebview/src/common/instance_manager.dart';
import 'package:webview_flutter_wkwebview/src/foundation/foundation.dart';
import 'package:webview_flutter_wkwebview/src/ui_kit/ui_kit.dart';
import 'package:webview_flutter_wkwebview/src/web_kit/web_kit.dart';
import 'package:webview_flutter_wkwebview/src/webkit_proxy.dart';
import 'package:webview_flutter_wkwebview/webview_flutter_wkwebview.dart';
import 'webkit_webview_controller_test.mocks.dart';
@GenerateMocks(<Type>[
UIScrollView,
WKPreferences,
WKUserContentController,
WKWebsiteDataStore,
WKWebView,
WKWebViewConfiguration,
])
void main() {
WidgetsFlutterBinding.ensureInitialized();
group('WebKitWebViewController', () {
WebKitWebViewController createControllerWithMocks({
MockUIScrollView? mockScrollView,
MockWKPreferences? mockPreferences,
MockWKUserContentController? mockUserContentController,
MockWKWebsiteDataStore? mockWebsiteDataStore,
MockWKWebView Function(
WKWebViewConfiguration configuration, {
void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
)?
observeValue,
})?
createMockWebView,
MockWKWebViewConfiguration? mockWebViewConfiguration,
InstanceManager? instanceManager,
}) {
final MockWKWebViewConfiguration nonNullMockWebViewConfiguration =
mockWebViewConfiguration ?? MockWKWebViewConfiguration();
late final MockWKWebView nonNullMockWebView;
final PlatformWebViewControllerCreationParams controllerCreationParams =
WebKitWebViewControllerCreationParams(
webKitProxy: WebKitProxy(
createWebViewConfiguration: ({InstanceManager? instanceManager}) {
return nonNullMockWebViewConfiguration;
},
createWebView: (
_, {
void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
)?
observeValue,
InstanceManager? instanceManager,
}) {
nonNullMockWebView = createMockWebView == null
? MockWKWebView()
: createMockWebView(
nonNullMockWebViewConfiguration,
observeValue: observeValue,
);
return nonNullMockWebView;
},
),
instanceManager: instanceManager,
);
final WebKitWebViewController controller = WebKitWebViewController(
controllerCreationParams,
);
when(nonNullMockWebView.scrollView)
.thenReturn(mockScrollView ?? MockUIScrollView());
when(nonNullMockWebView.configuration)
.thenReturn(nonNullMockWebViewConfiguration);
when(nonNullMockWebViewConfiguration.preferences)
.thenReturn(mockPreferences ?? MockWKPreferences());
when(nonNullMockWebViewConfiguration.userContentController).thenReturn(
mockUserContentController ?? MockWKUserContentController());
when(nonNullMockWebViewConfiguration.websiteDataStore)
.thenReturn(mockWebsiteDataStore ?? MockWKWebsiteDataStore());
return controller;
}
group('WebKitWebViewControllerCreationParams', () {
test('allowsInlineMediaPlayback', () {
final MockWKWebViewConfiguration mockConfiguration =
MockWKWebViewConfiguration();
WebKitWebViewControllerCreationParams(
webKitProxy: WebKitProxy(
createWebViewConfiguration: ({InstanceManager? instanceManager}) {
return mockConfiguration;
},
),
allowsInlineMediaPlayback: true,
);
verify(
mockConfiguration.setAllowsInlineMediaPlayback(true),
);
});
test('mediaTypesRequiringUserAction', () {
final MockWKWebViewConfiguration mockConfiguration =
MockWKWebViewConfiguration();
WebKitWebViewControllerCreationParams(
webKitProxy: WebKitProxy(
createWebViewConfiguration: ({InstanceManager? instanceManager}) {
return mockConfiguration;
},
),
mediaTypesRequiringUserAction: const <PlaybackMediaTypes>{
PlaybackMediaTypes.video,
},
);
verify(
mockConfiguration.setMediaTypesRequiringUserActionForPlayback(
<WKAudiovisualMediaType>{
WKAudiovisualMediaType.video,
},
),
);
});
test('mediaTypesRequiringUserAction defaults to include audio and video',
() {
final MockWKWebViewConfiguration mockConfiguration =
MockWKWebViewConfiguration();
WebKitWebViewControllerCreationParams(
webKitProxy: WebKitProxy(
createWebViewConfiguration: ({InstanceManager? instanceManager}) {
return mockConfiguration;
},
),
);
verify(
mockConfiguration.setMediaTypesRequiringUserActionForPlayback(
<WKAudiovisualMediaType>{
WKAudiovisualMediaType.audio,
WKAudiovisualMediaType.video,
},
),
);
});
test('mediaTypesRequiringUserAction sets value to none if set is empty',
() {
final MockWKWebViewConfiguration mockConfiguration =
MockWKWebViewConfiguration();
WebKitWebViewControllerCreationParams(
webKitProxy: WebKitProxy(
createWebViewConfiguration: ({InstanceManager? instanceManager}) {
return mockConfiguration;
},
),
mediaTypesRequiringUserAction: const <PlaybackMediaTypes>{},
);
verify(
mockConfiguration.setMediaTypesRequiringUserActionForPlayback(
<WKAudiovisualMediaType>{WKAudiovisualMediaType.none},
),
);
});
});
test('loadFile', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadFile('/path/to/file.html');
verify(mockWebView.loadFileUrl(
'/path/to/file.html',
readAccessUrl: '/path/to',
));
});
test('loadFlutterAsset', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadFlutterAsset('test_assets/index.html');
verify(mockWebView.loadFlutterAsset('test_assets/index.html'));
});
test('loadHtmlString', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
const String htmlString = '<html><body>Test data.</body></html>';
await controller.loadHtmlString(htmlString, baseUrl: 'baseUrl');
verify(mockWebView.loadHtmlString(
'<html><body>Test data.</body></html>',
baseUrl: 'baseUrl',
));
});
group('loadRequest', () {
test('Throws ArgumentError for empty scheme', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
expect(
() async => controller.loadRequest(
LoadRequestParams(uri: Uri.parse('www.google.com')),
),
throwsA(isA<ArgumentError>()),
);
});
test('GET without headers', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadRequest(
LoadRequestParams(uri: Uri.parse('https://www.google.com')),
);
final NSUrlRequest request = verify(mockWebView.loadRequest(captureAny))
.captured
.single as NSUrlRequest;
expect(request.url, 'https://www.google.com');
expect(request.allHttpHeaderFields, <String, String>{});
expect(request.httpMethod, 'get');
});
test('GET with headers', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadRequest(
LoadRequestParams(
uri: Uri.parse('https://www.google.com'),
headers: const <String, String>{'a': 'header'},
),
);
final NSUrlRequest request = verify(mockWebView.loadRequest(captureAny))
.captured
.single as NSUrlRequest;
expect(request.url, 'https://www.google.com');
expect(request.allHttpHeaderFields, <String, String>{'a': 'header'});
expect(request.httpMethod, 'get');
});
test('POST without body', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadRequest(LoadRequestParams(
uri: Uri.parse('https://www.google.com'),
method: LoadRequestMethod.post,
));
final NSUrlRequest request = verify(mockWebView.loadRequest(captureAny))
.captured
.single as NSUrlRequest;
expect(request.url, 'https://www.google.com');
expect(request.httpMethod, 'post');
});
test('POST with body', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.loadRequest(LoadRequestParams(
uri: Uri.parse('https://www.google.com'),
method: LoadRequestMethod.post,
body: Uint8List.fromList('Test Body'.codeUnits),
));
final NSUrlRequest request = verify(mockWebView.loadRequest(captureAny))
.captured
.single as NSUrlRequest;
expect(request.url, 'https://www.google.com');
expect(request.httpMethod, 'post');
expect(
request.httpBody,
Uint8List.fromList('Test Body'.codeUnits),
);
});
});
test('canGoBack', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.canGoBack()).thenAnswer(
(_) => Future<bool>.value(false),
);
expect(controller.canGoBack(), completion(false));
});
test('canGoForward', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.canGoForward()).thenAnswer(
(_) => Future<bool>.value(true),
);
expect(controller.canGoForward(), completion(true));
});
test('goBack', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.goBack();
verify(mockWebView.goBack());
});
test('goForward', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.goForward();
verify(mockWebView.goForward());
});
test('reload', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.reload();
verify(mockWebView.reload());
});
test('setAllowsBackForwardNavigationGestures', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.setAllowsBackForwardNavigationGestures(true);
verify(mockWebView.setAllowsBackForwardNavigationGestures(true));
});
test('runJavaScriptReturningResult', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
final Object result = Object();
when(mockWebView.evaluateJavaScript('runJavaScript')).thenAnswer(
(_) => Future<Object>.value(result),
);
expect(
controller.runJavaScriptReturningResult('runJavaScript'),
completion(result),
);
});
test('runJavaScriptReturningResult throws error on null return value', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.evaluateJavaScript('runJavaScript')).thenAnswer(
(_) => Future<String?>.value(),
);
expect(
() => controller.runJavaScriptReturningResult('runJavaScript'),
throwsArgumentError,
);
});
test('runJavaScript', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.evaluateJavaScript('runJavaScript')).thenAnswer(
(_) => Future<String>.value('returnString'),
);
expect(
controller.runJavaScript('runJavaScript'),
completes,
);
});
test('runJavaScript ignores exception with unsupported javaScript type',
() {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.evaluateJavaScript('runJavaScript'))
.thenThrow(PlatformException(
code: '',
details: const NSError(
code: WKErrorCode.javaScriptResultTypeIsUnsupported,
domain: '',
localizedDescription: '',
),
));
expect(
controller.runJavaScript('runJavaScript'),
completes,
);
});
test('getTitle', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.getTitle())
.thenAnswer((_) => Future<String>.value('Web Title'));
expect(controller.getTitle(), completion('Web Title'));
});
test('currentUrl', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
when(mockWebView.getUrl())
.thenAnswer((_) => Future<String>.value('myUrl.com'));
expect(controller.currentUrl(), completion('myUrl.com'));
});
test('scrollTo', () async {
final MockUIScrollView mockScrollView = MockUIScrollView();
final WebKitWebViewController controller = createControllerWithMocks(
mockScrollView: mockScrollView,
);
await controller.scrollTo(2, 4);
verify(mockScrollView.setContentOffset(const Point<double>(2.0, 4.0)));
});
test('scrollBy', () async {
final MockUIScrollView mockScrollView = MockUIScrollView();
final WebKitWebViewController controller = createControllerWithMocks(
mockScrollView: mockScrollView,
);
await controller.scrollBy(2, 4);
verify(mockScrollView.scrollBy(const Point<double>(2.0, 4.0)));
});
test('getScrollPosition', () {
final MockUIScrollView mockScrollView = MockUIScrollView();
final WebKitWebViewController controller = createControllerWithMocks(
mockScrollView: mockScrollView,
);
when(mockScrollView.getContentOffset()).thenAnswer(
(_) => Future<Point<double>>.value(const Point<double>(8.0, 16.0)),
);
expect(
controller.getScrollPosition(),
completion(const Offset(8.0, 16.0)),
);
});
test('disable zoom', () async {
final MockWKUserContentController mockUserContentController =
MockWKUserContentController();
final WebKitWebViewController controller = createControllerWithMocks(
mockUserContentController: mockUserContentController,
);
await controller.enableZoom(false);
final WKUserScript zoomScript =
verify(mockUserContentController.addUserScript(captureAny))
.captured
.first as WKUserScript;
expect(zoomScript.isMainFrameOnly, isTrue);
expect(zoomScript.injectionTime, WKUserScriptInjectionTime.atDocumentEnd);
expect(
zoomScript.source,
"var meta = document.createElement('meta');\n"
"meta.name = 'viewport';\n"
"meta.content = 'width=device-width, initial-scale=1.0, maximum-scale=1.0, "
"user-scalable=no';\n"
"var head = document.getElementsByTagName('head')[0];head.appendChild(meta);",
);
});
test('setBackgroundColor', () async {
final MockWKWebView mockWebView = MockWKWebView();
final MockUIScrollView mockScrollView = MockUIScrollView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
mockScrollView: mockScrollView,
);
controller.setBackgroundColor(Colors.red);
// UIScrollView.setBackgroundColor must be called last.
verifyInOrder(<Object>[
mockWebView.setOpaque(false),
mockWebView.setBackgroundColor(Colors.transparent),
mockScrollView.setBackgroundColor(Colors.red),
]);
});
test('userAgent', () async {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
await controller.setUserAgent('MyUserAgent');
verify(mockWebView.setCustomUserAgent('MyUserAgent'));
});
test('enable JavaScript', () async {
final MockWKPreferences mockPreferences = MockWKPreferences();
final WebKitWebViewController controller = createControllerWithMocks(
mockPreferences: mockPreferences,
);
await controller.setJavaScriptMode(JavaScriptMode.unrestricted);
verify(mockPreferences.setJavaScriptEnabled(true));
});
test('disable JavaScript', () async {
final MockWKPreferences mockPreferences = MockWKPreferences();
final WebKitWebViewController controller = createControllerWithMocks(
mockPreferences: mockPreferences,
);
await controller.setJavaScriptMode(JavaScriptMode.disabled);
verify(mockPreferences.setJavaScriptEnabled(false));
});
test('clearCache', () {
final MockWKWebsiteDataStore mockWebsiteDataStore =
MockWKWebsiteDataStore();
final WebKitWebViewController controller = createControllerWithMocks(
mockWebsiteDataStore: mockWebsiteDataStore,
);
when(
mockWebsiteDataStore.removeDataOfTypes(
<WKWebsiteDataType>{
WKWebsiteDataType.memoryCache,
WKWebsiteDataType.diskCache,
WKWebsiteDataType.offlineWebApplicationCache,
},
DateTime.fromMillisecondsSinceEpoch(0),
),
).thenAnswer((_) => Future<bool>.value(false));
expect(controller.clearCache(), completes);
});
test('clearLocalStorage', () {
final MockWKWebsiteDataStore mockWebsiteDataStore =
MockWKWebsiteDataStore();
final WebKitWebViewController controller = createControllerWithMocks(
mockWebsiteDataStore: mockWebsiteDataStore,
);
when(
mockWebsiteDataStore.removeDataOfTypes(
<WKWebsiteDataType>{WKWebsiteDataType.localStorage},
DateTime.fromMillisecondsSinceEpoch(0),
),
).thenAnswer((_) => Future<bool>.value(false));
expect(controller.clearLocalStorage(), completes);
});
test('addJavaScriptChannel', () async {
final WebKitProxy webKitProxy = WebKitProxy(
createScriptMessageHandler: ({
required void Function(
WKUserContentController userContentController,
WKScriptMessage message,
)
didReceiveScriptMessage,
}) {
return WKScriptMessageHandler.detached(
didReceiveScriptMessage: didReceiveScriptMessage,
);
},
);
final WebKitJavaScriptChannelParams javaScriptChannelParams =
WebKitJavaScriptChannelParams(
name: 'name',
onMessageReceived: (JavaScriptMessage message) {},
webKitProxy: webKitProxy,
);
final MockWKUserContentController mockUserContentController =
MockWKUserContentController();
final WebKitWebViewController controller = createControllerWithMocks(
mockUserContentController: mockUserContentController,
);
await controller.addJavaScriptChannel(javaScriptChannelParams);
verify(mockUserContentController.addScriptMessageHandler(
argThat(isA<WKScriptMessageHandler>()),
'name',
));
final WKUserScript userScript =
verify(mockUserContentController.addUserScript(captureAny))
.captured
.single as WKUserScript;
expect(userScript.source, 'window.name = webkit.messageHandlers.name;');
expect(
userScript.injectionTime,
WKUserScriptInjectionTime.atDocumentStart,
);
});
test('removeJavaScriptChannel', () async {
final WebKitProxy webKitProxy = WebKitProxy(
createScriptMessageHandler: ({
required void Function(
WKUserContentController userContentController,
WKScriptMessage message,
)
didReceiveScriptMessage,
}) {
return WKScriptMessageHandler.detached(
didReceiveScriptMessage: didReceiveScriptMessage,
);
},
);
final WebKitJavaScriptChannelParams javaScriptChannelParams =
WebKitJavaScriptChannelParams(
name: 'name',
onMessageReceived: (JavaScriptMessage message) {},
webKitProxy: webKitProxy,
);
final MockWKUserContentController mockUserContentController =
MockWKUserContentController();
final WebKitWebViewController controller = createControllerWithMocks(
mockUserContentController: mockUserContentController,
);
await controller.addJavaScriptChannel(javaScriptChannelParams);
reset(mockUserContentController);
await controller.removeJavaScriptChannel('name');
verify(mockUserContentController.removeAllUserScripts());
verify(mockUserContentController.removeScriptMessageHandler('name'));
verifyNoMoreInteractions(mockUserContentController);
});
test('removeJavaScriptChannel with zoom disabled', () async {
final WebKitProxy webKitProxy = WebKitProxy(
createScriptMessageHandler: ({
required void Function(
WKUserContentController userContentController,
WKScriptMessage message,
)
didReceiveScriptMessage,
}) {
return WKScriptMessageHandler.detached(
didReceiveScriptMessage: didReceiveScriptMessage,
);
},
);
final WebKitJavaScriptChannelParams javaScriptChannelParams =
WebKitJavaScriptChannelParams(
name: 'name',
onMessageReceived: (JavaScriptMessage message) {},
webKitProxy: webKitProxy,
);
final MockWKUserContentController mockUserContentController =
MockWKUserContentController();
final WebKitWebViewController controller = createControllerWithMocks(
mockUserContentController: mockUserContentController,
);
await controller.enableZoom(false);
await controller.addJavaScriptChannel(javaScriptChannelParams);
clearInteractions(mockUserContentController);
await controller.removeJavaScriptChannel('name');
final WKUserScript zoomScript =
verify(mockUserContentController.addUserScript(captureAny))
.captured
.first as WKUserScript;
expect(zoomScript.isMainFrameOnly, isTrue);
expect(zoomScript.injectionTime, WKUserScriptInjectionTime.atDocumentEnd);
expect(
zoomScript.source,
"var meta = document.createElement('meta');\n"
"meta.name = 'viewport';\n"
"meta.content = 'width=device-width, initial-scale=1.0, maximum-scale=1.0, "
"user-scalable=no';\n"
"var head = document.getElementsByTagName('head')[0];head.appendChild(meta);",
);
});
test('setPlatformNavigationDelegate', () {
final MockWKWebView mockWebView = MockWKWebView();
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
);
final WebKitNavigationDelegate navigationDelegate =
WebKitNavigationDelegate(
const WebKitNavigationDelegateCreationParams(
webKitProxy: WebKitProxy(
createNavigationDelegate: CapturingNavigationDelegate.new,
createUIDelegate: CapturingUIDelegate.new,
),
),
);
controller.setPlatformNavigationDelegate(navigationDelegate);
verify(
mockWebView.setNavigationDelegate(
CapturingNavigationDelegate.lastCreatedDelegate,
),
);
verify(
mockWebView.setUIDelegate(
CapturingUIDelegate.lastCreatedDelegate,
),
);
});
test('setPlatformNavigationDelegate onProgress', () async {
final MockWKWebView mockWebView = MockWKWebView();
late final void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
) webViewObserveValue;
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (
_, {
void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
)?
observeValue,
}) {
webViewObserveValue = observeValue!;
return mockWebView;
},
);
verify(
mockWebView.addObserver(
mockWebView,
keyPath: 'estimatedProgress',
options: <NSKeyValueObservingOptions>{
NSKeyValueObservingOptions.newValue,
},
),
);
final WebKitNavigationDelegate navigationDelegate =
WebKitNavigationDelegate(
const WebKitNavigationDelegateCreationParams(
webKitProxy: WebKitProxy(
createNavigationDelegate: CapturingNavigationDelegate.new,
createUIDelegate: WKUIDelegate.detached,
),
),
);
late final int callbackProgress;
navigationDelegate.setOnProgress(
(int progress) => callbackProgress = progress,
);
await controller.setPlatformNavigationDelegate(navigationDelegate);
webViewObserveValue(
'estimatedProgress',
mockWebView,
<NSKeyValueChangeKey, Object?>{NSKeyValueChangeKey.newValue: 0.0},
);
expect(callbackProgress, 0);
});
test(
'setPlatformNavigationDelegate onProgress can be changed by the WebKitNavigationDelegage',
() async {
final MockWKWebView mockWebView = MockWKWebView();
late final void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
) webViewObserveValue;
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (
_, {
void Function(
String keyPath,
NSObject object,
Map<NSKeyValueChangeKey, Object?> change,
)?
observeValue,
}) {
webViewObserveValue = observeValue!;
return mockWebView;
},
);
final WebKitNavigationDelegate navigationDelegate =
WebKitNavigationDelegate(
const WebKitNavigationDelegateCreationParams(
webKitProxy: WebKitProxy(
createNavigationDelegate: CapturingNavigationDelegate.new,
createUIDelegate: WKUIDelegate.detached,
),
),
);
// First value of onProgress does nothing.
await navigationDelegate.setOnProgress((_) {});
await controller.setPlatformNavigationDelegate(navigationDelegate);
// Second value of onProgress sets `callbackProgress`.
late final int callbackProgress;
await navigationDelegate.setOnProgress(
(int progress) => callbackProgress = progress,
);
webViewObserveValue(
'estimatedProgress',
mockWebView,
<NSKeyValueChangeKey, Object?>{NSKeyValueChangeKey.newValue: 0.0},
);
expect(callbackProgress, 0);
});
test('webViewIdentifier', () {
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final MockWKWebView mockWebView = MockWKWebView();
when(mockWebView.copy()).thenReturn(MockWKWebView());
instanceManager.addHostCreatedInstance(mockWebView, 0);
final WebKitWebViewController controller = createControllerWithMocks(
createMockWebView: (_, {dynamic observeValue}) => mockWebView,
instanceManager: instanceManager,
);
expect(
controller.webViewIdentifier,
instanceManager.getIdentifier(mockWebView),
);
});
});
group('WebKitJavaScriptChannelParams', () {
test('onMessageReceived', () async {
late final WKScriptMessageHandler messageHandler;
final WebKitProxy webKitProxy = WebKitProxy(
createScriptMessageHandler: ({
required void Function(
WKUserContentController userContentController,
WKScriptMessage message,
)
didReceiveScriptMessage,
}) {
messageHandler = WKScriptMessageHandler.detached(
didReceiveScriptMessage: didReceiveScriptMessage,
);
return messageHandler;
},
);
late final String callbackMessage;
WebKitJavaScriptChannelParams(
name: 'name',
onMessageReceived: (JavaScriptMessage message) {
callbackMessage = message.message;
},
webKitProxy: webKitProxy,
);
messageHandler.didReceiveScriptMessage(
MockWKUserContentController(),
const WKScriptMessage(name: 'name', body: 'myMessage'),
);
expect(callbackMessage, 'myMessage');
});
});
}
// Records the last created instance of itself.
class CapturingNavigationDelegate extends WKNavigationDelegate {
CapturingNavigationDelegate({
super.didFinishNavigation,
super.didStartProvisionalNavigation,
super.didFailNavigation,
super.didFailProvisionalNavigation,
super.decidePolicyForNavigationAction,
super.webViewWebContentProcessDidTerminate,
}) : super.detached() {
lastCreatedDelegate = this;
}
static CapturingNavigationDelegate lastCreatedDelegate =
CapturingNavigationDelegate();
}
// Records the last created instance of itself.
class CapturingUIDelegate extends WKUIDelegate {
CapturingUIDelegate({super.onCreateWebView}) : super.detached() {
lastCreatedDelegate = this;
}
static CapturingUIDelegate lastCreatedDelegate = CapturingUIDelegate();
}
| plugins/packages/webview_flutter/webview_flutter_wkwebview/test/webkit_webview_controller_test.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_wkwebview/test/webkit_webview_controller_test.dart",
"repo_id": "plugins",
"token_count": 13538
} | 1,187 |
# Packages that have not yet adopted code-excerpt.
#
# This only exists to allow incrementally adopting the new requirement.
# Packages shoud never be added to this list.
# TODO(ecosystem): Remove everything from this list. See
# https://github.com/flutter/flutter/issues/102679
- camera_web
- espresso
- google_maps_flutter/google_maps_flutter
- google_sign_in/google_sign_in
- google_sign_in_web
- image_picker/image_picker
- image_picker_for_web
- in_app_purchase/in_app_purchase
- ios_platform_images
- path_provider/path_provider
- plugin_platform_interface
- quick_actions/quick_actions
- shared_preferences/shared_preferences
- webview_flutter_android
- webview_flutter_web
| plugins/script/configs/temp_exclude_excerpt.yaml/0 | {
"file_path": "plugins/script/configs/temp_exclude_excerpt.yaml",
"repo_id": "plugins",
"token_count": 226
} | 1,188 |
#!/bin/bash
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
set -e
# WARNING! Do not remove this script, or change its behavior, unless you have
# verified that it will not break the dart-lang analysis run of this
# repository: https://github.com/dart-lang/sdk/blob/main/tools/bots/flutter/analyze_flutter_plugins.sh
readonly SCRIPT_DIR="$(cd "$(dirname "${BASH_SOURCE[0]}")" >/dev/null && pwd)"
readonly REPO_DIR="$(dirname "$SCRIPT_DIR")"
# The tool expects to be run from the repo root.
# PACKAGE_SHARDING is (optionally) set from Cirrus. See .cirrus.yml
cd "$REPO_DIR"
# Ensure that the tooling has been activated.
.ci/scripts/prepare_tool.sh
dart pub global run flutter_plugin_tools "$@" \
--packages-for-branch \
--log-timing \
$PACKAGE_SHARDING
| plugins/script/tool_runner.sh/0 | {
"file_path": "plugins/script/tool_runner.sh",
"repo_id": "plugins",
"token_count": 297
} | 1,189 |
<?xml version="1.0" encoding="UTF-8"?>
<Workspace
version = "1.0">
<FileRef
location = "group:IOSFullScreen.xcodeproj">
</FileRef>
<FileRef
location = "group:Pods/Pods.xcodeproj">
</FileRef>
</Workspace>
| samples/add_to_app/fullscreen/ios_fullscreen/IOSFullScreen.xcworkspace/contents.xcworkspacedata/0 | {
"file_path": "samples/add_to_app/fullscreen/ios_fullscreen/IOSFullScreen.xcworkspace/contents.xcworkspacedata",
"repo_id": "samples",
"token_count": 106
} | 1,190 |
# multiple_flutters
This is a sample that shows how to use the Flutter Engine Groups API to embed
multiple instances of Flutter into an existing Android or iOS project.
## Getting Started
For iOS instructions, see:
[multiple_flutters_ios](./multiple_flutters_ios/README.md).
For Android instructions, see:
[multiple_flutters_android](./multiple_flutters_android/README.md).
## Requirements
* Flutter -- after Flutter v2
* Android
* Android Studio
* iOS
* Xcode
* Cocoapods
## Flutter Engine Group
These examples use the Flutter Engine Group APIs on the host platform which
allows engines to share memory and CPU intensive resources. This leads to easier
embedding of Flutter into an existing app since multiple entrypoints can be
maintained via a FlutterFragment on Android or a UIViewController on iOS.
Before FlutterEngineGroup, users had to juggle the usage of a small number of
engines judiciously.
More info on those API's can be found in the source
code:
- iOS -
[FlutterEngineGroup.h](https://github.com/flutter/engine/blob/master/shell/platform/darwin/ios/framework/Headers/FlutterEngineGroup.h)
- Android -
[FlutterEngineGroup.java](https://github.com/flutter/engine/blob/master/shell/platform/android/io/flutter/embedding/engine/FlutterEngineGroup.java)
## Important Files
### iOS
- [DataModel.swift](./multiple_flutters_ios/MultipleFluttersIos/HostViewController.swift)
— Observable data model that is the source of truth for the host platform and Flutter.
- [HostViewController.swift](./multiple_flutters_ios/MultipleFluttersIos/HostViewController.swift)
— A UIViewController that synchronizes to the DataModel.
- [main.dart](./multiple_flutters_module/lib/main.dart) — The Flutter source
code.
- [SingleFlutterViewController.swift](./multiple_flutters_ios/MultipleFluttersIos/SingleFlutterViewController.swift)
— A subclass of FlutterViewController that synchronizes with the DataModel.
- [DoubleFlutterViewController.swift](./multiple_flutters_ios/MultipleFluttersIos/DoubleFlutterViewController.swift)
— A UIViewController that embeds multiple Flutter instances.
### Android
## Data Synchronization Description
This sample code performs data synchronization to share data between the host
platform and multiple instances of Flutter by combining the
[Observer design pattern](https://en.wikipedia.org/wiki/Observer_pattern) and
[Flutter platform channels](https://flutter.dev/docs/development/platform-integration/platform-channels).
Here is how it works:
- The definitive source of truth for the data lives in the host platform data
model.
- Every host view displaying Flutter content maintains: a Flutter engine, a
bidirectional platform channel, and a subscription to the host data model.
- Flutter instances maintain a copy of the data they are interested in reading,
and this data is seeded by the host when the instance is first displayed.
- Mutations from Flutter code are sent to the host platform via the channel. The
host platform performs the mutations, and then notifies all host view
controllers and Flutter engines of the new value.
- Mutations from host code happen directly on the data model who notifies host
view controllers and Flutter engines of the new value.
This is just one possible way to synchronize the data between the host platform
and multiple Flutter instances. A more complete implementation is proposed in
the work of
[flutter/issues/72030](https://github.com/flutter/flutter/issues/72030).
| samples/add_to_app/multiple_flutters/README.md/0 | {
"file_path": "samples/add_to_app/multiple_flutters/README.md",
"repo_id": "samples",
"token_count": 930
} | 1,191 |
name: multiple_flutters_module
description: A module that is embedded in the multiple_flutters_ios and multiple_flutters_android sample code.
version: 1.0.0+1
environment:
sdk: ^3.2.0
dependencies:
flutter:
sdk: flutter
url_launcher: ^6.0.6
cupertino_icons: ^1.0.0
dev_dependencies:
analysis_defaults:
path: ../../../analysis_defaults
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
module:
androidX: true
androidPackage: com.example.multiple_flutters_module
iosBundleIdentifier: com.example.multipleFluttersModule
| samples/add_to_app/multiple_flutters/multiple_flutters_module/pubspec.yaml/0 | {
"file_path": "samples/add_to_app/multiple_flutters/multiple_flutters_module/pubspec.yaml",
"repo_id": "samples",
"token_count": 213
} | 1,192 |
<?xml version="1.0" encoding="UTF-8"?>
<Workspace
version = "1.0">
<FileRef
location = "group:IOSUsingPlugin.xcodeproj">
</FileRef>
<FileRef
location = "group:Pods/Pods.xcodeproj">
</FileRef>
</Workspace>
| samples/add_to_app/plugin/ios_using_plugin/IOSUsingPlugin.xcworkspace/contents.xcworkspacedata/0 | {
"file_path": "samples/add_to_app/plugin/ios_using_plugin/IOSUsingPlugin.xcworkspace/contents.xcworkspacedata",
"repo_id": "samples",
"token_count": 106
} | 1,193 |
# prebuild_module
Embeds a full screen instance of Flutter as a prebuilt library that can be
loaded into an existing iOS or Android app.
## Description
These apps are essentially identical to `android_fullscreen` and
`ios_fullscreen`, respectively, with one key difference. Rather than being set
up to compile the `flutter_module` from source each time the app is built, they
import a the module as a prebuilt `aar` (Android) or framework (iOS). This can
be useful for teams that don't want to require every developer working on the
app to have the Flutter toolchain installed on their local machines.
Prior to building either project for the first time, the `flutter_module` needs
to be built.
**Building for `android_using_prebuilt_module`**
To build `flutter_module` as an aar, run this command from the `flutter_module`
directory:
```bash
flutter build aar
```
It will produce `aar` files for debug, profile, and release mode. The Android
app is configured to import the appropriate `aar` based on its own build
configuration, so if you build a debug version of the app, it will look
for the debug `aar`, and so on.
If the `flutter_module` project is updated, the `aar` files must be rebuilt via
one of the commands above in order for those changes to appear in the app.
**Building for `ios_using_prebuilt_module`**
To build `flutter_module` as a set of frameworks, run this command from the
`flutter_module` directory:
```bash
flutter build ios-framework --xcframework --output=../ios_using_prebuilt_module/Flutter
```
This will output frameworks for debug, profile, and release modes into
`ios_using_prebuilt_module/Flutter`. The project file for
`ios_using_prebuilt_module` has been configured to find the frameworks there.
For more information on how to modify an existing iOS app to reference prebuilt
Flutter frameworks, see this article in the Flutter GitHub wiki:
https://flutter.dev/docs/development/add-to-app/ios/project-setup
## tl;dr
If you're just looking to get up and running quickly, these bash commands will
fetch packages and set up dependencies (note that the above commands assume
you're building for both iOS and Android, with both toolchains installed):
```bash
#!/bin/bash
set -e
cd flutter_module/
flutter pub get
# For Android builds:
flutter build aar
open -a "Android Studio" ../android_using_prebuilt_module/ # macOS only
# Or open the ../android_using_prebuilt_module folder in Android Studio for
# other platforms.
# For iOS builds:
flutter build ios-framework --xcframework --output=../ios_using_prebuilt_module/Flutter
open ../ios_using_prebuilt_module/IOSUsingPrebuiltModule.xcodeproj
```
## Requirements
* Flutter
* Android
* Android Studio
* iOS
* Xcode
* Cocoapods
## Questions/issues
See [add_to_app/README.md](../README.md) for further help.
| samples/add_to_app/prebuilt_module/README.md/0 | {
"file_path": "samples/add_to_app/prebuilt_module/README.md",
"repo_id": "samples",
"token_count": 809
} | 1,194 |
def localProperties = new Properties()
def localPropertiesFile = rootProject.file('local.properties')
if (localPropertiesFile.exists()) {
localPropertiesFile.withReader('UTF-8') { reader ->
localProperties.load(reader)
}
}
def flutterRoot = localProperties.getProperty('flutter.sdk')
if (flutterRoot == null) {
throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.")
}
def flutterVersionCode = localProperties.getProperty('flutter.versionCode')
if (flutterVersionCode == null) {
flutterVersionCode = '1'
}
def flutterVersionName = localProperties.getProperty('flutter.versionName')
if (flutterVersionName == null) {
flutterVersionName = '1.0'
}
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle"
android {
compileSdkVersion 31
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
// TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html).
applicationId "com.example.splash_screen_sample"
minSdkVersion 21
targetSdkVersion 30
versionCode flutterVersionCode.toInteger()
versionName flutterVersionName
}
buildTypes {
release {
// TODO: Add your own signing config for the release build.
// Signing with the debug keys for now, so `flutter run --release` works.
signingConfig signingConfigs.debug
}
}
}
flutter {
source '../..'
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
implementation "androidx.core:core-splashscreen:1.0.0-alpha02"
implementation "androidx.core:core:1.5.0-alpha05"
implementation "androidx.core:core-ktx:1.6.0"
implementation 'androidx.constraintlayout:constraintlayout:1.1.2'
implementation "androidx.core:core-animation:1.0.0-alpha02"
implementation "androidx.interpolator:interpolator:1.0.0"
def appcompat_version = "1.3.1"
implementation "androidx.appcompat:appcompat:$appcompat_version"
// For loading and tinting drawables on older versions of the platform
implementation "androidx.appcompat:appcompat-resources:$appcompat_version"
implementation "androidx.constraintlayout:constraintlayout:2.1.0"
}
| samples/android_splash_screen/android/app/build.gradle/0 | {
"file_path": "samples/android_splash_screen/android/app/build.gradle",
"repo_id": "samples",
"token_count": 889
} | 1,195 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' show Platform;
import 'package:flutter/foundation.dart' show kIsWeb;
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
import 'package:window_size/window_size.dart';
import 'src/basics/basics.dart';
import 'src/misc/misc.dart';
void main() {
setupWindow();
runApp(const AnimationSamples());
}
const double windowWidth = 480;
const double windowHeight = 854;
void setupWindow() {
if (!kIsWeb && (Platform.isWindows || Platform.isLinux || Platform.isMacOS)) {
WidgetsFlutterBinding.ensureInitialized();
setWindowTitle('Animation Samples');
setWindowMinSize(const Size(windowWidth, windowHeight));
setWindowMaxSize(const Size(windowWidth, windowHeight));
getCurrentScreen().then((screen) {
setWindowFrame(Rect.fromCenter(
center: screen!.frame.center,
width: windowWidth,
height: windowHeight,
));
});
}
}
class Demo {
final String name;
final String route;
final WidgetBuilder builder;
const Demo({
required this.name,
required this.route,
required this.builder,
});
}
final basicDemos = [
Demo(
name: 'AnimatedContainer',
route: AnimatedContainerDemo.routeName,
builder: (context) => const AnimatedContainerDemo(),
),
Demo(
name: 'PageRouteBuilder',
route: PageRouteBuilderDemo.routeName,
builder: (context) => const PageRouteBuilderDemo(),
),
Demo(
name: 'Animation Controller',
route: AnimationControllerDemo.routeName,
builder: (context) => const AnimationControllerDemo(),
),
Demo(
name: 'Tweens',
route: TweenDemo.routeName,
builder: (context) => const TweenDemo(),
),
Demo(
name: 'AnimatedBuilder',
route: AnimatedBuilderDemo.routeName,
builder: (context) => const AnimatedBuilderDemo(),
),
Demo(
name: 'Custom Tween',
route: CustomTweenDemo.routeName,
builder: (context) => const CustomTweenDemo(),
),
Demo(
name: 'Tween Sequences',
route: TweenSequenceDemo.routeName,
builder: (context) => const TweenSequenceDemo(),
),
Demo(
name: 'Fade Transition',
route: FadeTransitionDemo.routeName,
builder: (context) => const FadeTransitionDemo(),
),
];
final miscDemos = [
Demo(
name: 'Expandable Card',
route: ExpandCardDemo.routeName,
builder: (context) => const ExpandCardDemo(),
),
Demo(
name: 'Carousel',
route: CarouselDemo.routeName,
builder: (context) => CarouselDemo(),
),
Demo(
name: 'Focus Image',
route: FocusImageDemo.routeName,
builder: (context) => const FocusImageDemo(),
),
Demo(
name: 'Card Swipe',
route: CardSwipeDemo.routeName,
builder: (context) => const CardSwipeDemo(),
),
Demo(
name: 'Flutter Animate',
route: FlutterAnimateDemo.routeName,
builder: (context) => const FlutterAnimateDemo(),
),
Demo(
name: 'Repeating Animation',
route: RepeatingAnimationDemo.routeName,
builder: (context) => const RepeatingAnimationDemo(),
),
Demo(
name: 'Spring Physics',
route: PhysicsCardDragDemo.routeName,
builder: (context) => const PhysicsCardDragDemo(),
),
Demo(
name: 'AnimatedList',
route: AnimatedListDemo.routeName,
builder: (context) => const AnimatedListDemo(),
),
Demo(
name: 'AnimatedPositioned',
route: AnimatedPositionedDemo.routeName,
builder: (context) => const AnimatedPositionedDemo(),
),
Demo(
name: 'AnimatedSwitcher',
route: AnimatedSwitcherDemo.routeName,
builder: (context) => const AnimatedSwitcherDemo(),
),
Demo(
name: 'Hero Animation',
route: HeroAnimationDemo.routeName,
builder: (context) => const HeroAnimationDemo(),
),
Demo(
name: 'Curved Animation',
route: CurvedAnimationDemo.routeName,
builder: (context) => const CurvedAnimationDemo(),
),
];
final router = GoRouter(
routes: [
GoRoute(
path: '/',
builder: (context, state) => const HomePage(),
routes: [
for (final demo in basicDemos)
GoRoute(
path: demo.route,
builder: (context, state) => demo.builder(context),
),
for (final demo in miscDemos)
GoRoute(
path: demo.route,
builder: (context, state) => demo.builder(context),
),
],
),
],
);
class AnimationSamples extends StatelessWidget {
const AnimationSamples({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp.router(
title: 'Animation Samples',
theme: ThemeData(
colorSchemeSeed: Colors.deepPurple,
),
routerConfig: router,
);
}
}
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
final headerStyle = Theme.of(context).textTheme.titleLarge;
return Scaffold(
appBar: AppBar(
title: const Text('Animation Samples'),
),
body: ListView(
children: [
ListTile(title: Text('Basics', style: headerStyle)),
...basicDemos.map((d) => DemoTile(demo: d)),
ListTile(title: Text('Misc', style: headerStyle)),
...miscDemos.map((d) => DemoTile(demo: d)),
],
),
);
}
}
class DemoTile extends StatelessWidget {
final Demo demo;
const DemoTile({required this.demo, super.key});
@override
Widget build(BuildContext context) {
return ListTile(
title: Text(demo.name),
onTap: () {
context.go('/${demo.route}');
},
);
}
}
| samples/animations/lib/main.dart/0 | {
"file_path": "samples/animations/lib/main.dart",
"repo_id": "samples",
"token_count": 2178
} | 1,196 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class ExpandCardDemo extends StatelessWidget {
const ExpandCardDemo({super.key});
static const String routeName = 'misc/expand_card';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Expandable Card'),
),
body: const Center(
child: ExpandCard(),
),
);
}
}
class ExpandCard extends StatefulWidget {
const ExpandCard({super.key});
@override
State<ExpandCard> createState() => _ExpandCardState();
}
class _ExpandCardState extends State<ExpandCard>
with SingleTickerProviderStateMixin {
static const Duration duration = Duration(milliseconds: 300);
bool selected = false;
double get size => selected ? 256 : 128;
void toggleExpanded() {
setState(() {
selected = !selected;
});
}
@override
Widget build(context) {
return GestureDetector(
onTap: () => toggleExpanded(),
child: Card(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: AnimatedContainer(
duration: duration,
width: size,
height: size,
curve: Curves.ease,
child: AnimatedCrossFade(
duration: duration,
firstCurve: Curves.easeInOutCubic,
secondCurve: Curves.easeInOutCubic,
crossFadeState: selected
? CrossFadeState.showSecond
: CrossFadeState.showFirst,
// Use Positioned.fill() to pass the constraints to its children.
// This allows the Images to use BoxFit.cover to cover the correct
// size
layoutBuilder:
(topChild, topChildKey, bottomChild, bottomChildKey) {
return Stack(
children: [
Positioned.fill(
key: bottomChildKey,
child: bottomChild,
),
Positioned.fill(
key: topChildKey,
child: topChild,
),
],
);
},
firstChild: Image.asset(
'assets/eat_cape_town_sm.jpg',
fit: BoxFit.cover,
),
secondChild: Image.asset(
'assets/eat_new_orleans_sm.jpg',
fit: BoxFit.cover,
),
),
),
),
),
);
}
}
| samples/animations/lib/src/misc/expand_card.dart/0 | {
"file_path": "samples/animations/lib/src/misc/expand_card.dart",
"repo_id": "samples",
"token_count": 1322
} | 1,197 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:animations/src/misc/animated_positioned.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
Widget createAnimatedPositionedDemoScreen() => const MaterialApp(
home: AnimatedPositionedDemo(),
);
void main() {
group('AnimatedPositioned Tests', () {
testWidgets('Position of Button Changes on Tap', (tester) async {
await tester.pumpWidget(createAnimatedPositionedDemoScreen());
var button = find.byType(InkWell);
// Get initial position of the widget.
var initialPosition = tester.getTopLeft(button);
expect(initialPosition, isNotNull);
// Tap on the widget.
await tester.tap(button);
await tester.pumpAndSettle();
// The new position should not be equal to initial position.
var newPosition = tester.getTopLeft(button);
expect(newPosition, isNot(offsetMoreOrLessEquals(initialPosition)));
});
});
}
| samples/animations/test/misc/animated_positioned_test.dart/0 | {
"file_path": "samples/animations/test/misc/animated_positioned_test.dart",
"repo_id": "samples",
"token_count": 373
} | 1,198 |
include: package:analysis_defaults/flutter.yaml
| samples/background_isolate_channels/analysis_options.yaml/0 | {
"file_path": "samples/background_isolate_channels/analysis_options.yaml",
"repo_id": "samples",
"token_count": 15
} | 1,199 |
#include "Generated.xcconfig"
| samples/code_sharing/client/ios/Flutter/Debug.xcconfig/0 | {
"file_path": "samples/code_sharing/client/ios/Flutter/Debug.xcconfig",
"repo_id": "samples",
"token_count": 12
} | 1,200 |
#include "ephemeral/Flutter-Generated.xcconfig"
| samples/code_sharing/client/macos/Flutter/Flutter-Debug.xcconfig/0 | {
"file_path": "samples/code_sharing/client/macos/Flutter/Flutter-Debug.xcconfig",
"repo_id": "samples",
"token_count": 19
} | 1,201 |
## 1.0.0
- Initial version.
| samples/code_sharing/shared/CHANGELOG.md/0 | {
"file_path": "samples/code_sharing/shared/CHANGELOG.md",
"repo_id": "samples",
"token_count": 13
} | 1,202 |
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher.dart';
import 'constants.dart';
import 'platform_selector.dart';
class FieldTypesPage extends StatelessWidget {
FieldTypesPage({
super.key,
required this.onChangedPlatform,
});
static const String route = 'field-types';
static const String title = 'The Context Menu in Different Field Types';
static const String subtitle =
'How contextual menus work in TextField, CupertinoTextField, and EditableText';
static const String url = '$kCodeUrl/field_types_page.dart';
final PlatformCallback onChangedPlatform;
final TextEditingController _controller = TextEditingController(
text:
"Material text field shows the menu for any platform by default. You'll see the correct menu for your platform here.",
);
final TextEditingController _cupertinoController = TextEditingController(
text:
"CupertinoTextField can't show Material menus by default. On non-Apple platforms, you'll still see a Cupertino menu here.",
);
final TextEditingController _cupertinoControllerFixed = TextEditingController(
text:
"But CupertinoTextField can be made to adaptively show any menu. You'll see the correct menu for your platform here.",
);
final TextEditingController _cupertinoControllerForced =
TextEditingController(
text: 'Or forced to always show a specific menu (Material desktop menu).',
);
final TextEditingController _editableController = TextEditingController(
text:
"EditableText doesn't show any selection menu by itself, even when contextMenuBuilder is passed.",
);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(FieldTypesPage.title),
actions: <Widget>[
PlatformSelector(
onChangedPlatform: onChangedPlatform,
),
IconButton(
icon: const Icon(Icons.code),
onPressed: () async {
if (!await launchUrl(Uri.parse(url))) {
throw 'Could not launch $url';
}
},
),
],
),
body: Center(
child: SizedBox(
width: 400.0,
child: ListView(
children: <Widget>[
const SizedBox(height: 20.0),
TextField(
maxLines: 3,
controller: _controller,
),
const SizedBox(height: 60.0),
CupertinoTextField(
maxLines: 3,
controller: _cupertinoController,
),
const SizedBox(height: 20.0),
CupertinoTextField(
maxLines: 3,
controller: _cupertinoControllerFixed,
contextMenuBuilder: (context, editableTextState) {
return AdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
},
),
const SizedBox(height: 20.0),
CupertinoTextField(
maxLines: 3,
controller: _cupertinoControllerForced,
contextMenuBuilder: (context, editableTextState) {
return DesktopTextSelectionToolbar(
anchor: editableTextState.contextMenuAnchors.primaryAnchor,
children: AdaptiveTextSelectionToolbar.getAdaptiveButtons(
context,
editableTextState.contextMenuButtonItems,
).toList(),
);
},
),
const SizedBox(height: 60.0),
Container(
color: Colors.white,
child: EditableText(
maxLines: 3,
controller: _editableController,
focusNode: FocusNode(),
style: Typography.material2021().black.displayMedium!,
cursorColor: Colors.blue,
backgroundCursorColor: Colors.white,
// contextMenuBuilder doesn't do anything here!
// EditableText has no built-in gesture detection for
// selection. A wrapper would have to implement
// TextSelectionGestureDetectorBuilderDelegate, etc.
contextMenuBuilder: (context, editableTextState) {
return AdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
},
),
),
],
),
),
),
);
}
}
| samples/context_menus/lib/field_types_page.dart/0 | {
"file_path": "samples/context_menus/lib/field_types_page.dart",
"repo_id": "samples",
"token_count": 2207
} | 1,203 |
#include "Generated.xcconfig"
| samples/deeplink_store_example/ios/Flutter/Debug.xcconfig/0 | {
"file_path": "samples/deeplink_store_example/ios/Flutter/Debug.xcconfig",
"repo_id": "samples",
"token_count": 12
} | 1,204 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// GENERATED CODE - DO NOT MODIFY BY HAND
part of 'links.dart';
// **************************************************************************
// BuiltValueGenerator
// **************************************************************************
Serializer<Links> _$linksSerializer = new _$LinksSerializer();
class _$LinksSerializer implements StructuredSerializer<Links> {
@override
final Iterable<Type> types = const [Links, _$Links];
@override
final String wireName = 'Links';
@override
Iterable<Object?> serialize(Serializers serializers, Links object,
{FullType specifiedType = FullType.unspecified}) {
final result = <Object?>[];
Object? value;
value = object.self;
if (value != null) {
result
..add('self')
..add(serializers.serialize(value,
specifiedType: const FullType(String)));
}
value = object.html;
if (value != null) {
result
..add('html')
..add(serializers.serialize(value,
specifiedType: const FullType(String)));
}
value = object.download;
if (value != null) {
result
..add('download')
..add(serializers.serialize(value,
specifiedType: const FullType(String)));
}
value = object.downloadLocation;
if (value != null) {
result
..add('download_location')
..add(serializers.serialize(value,
specifiedType: const FullType(String)));
}
return result;
}
@override
Links deserialize(Serializers serializers, Iterable<Object?> serialized,
{FullType specifiedType = FullType.unspecified}) {
final result = new LinksBuilder();
final iterator = serialized.iterator;
while (iterator.moveNext()) {
final key = iterator.current! as String;
iterator.moveNext();
final Object? value = iterator.current;
switch (key) {
case 'self':
result.self = serializers.deserialize(value,
specifiedType: const FullType(String)) as String?;
break;
case 'html':
result.html = serializers.deserialize(value,
specifiedType: const FullType(String)) as String?;
break;
case 'download':
result.download = serializers.deserialize(value,
specifiedType: const FullType(String)) as String?;
break;
case 'download_location':
result.downloadLocation = serializers.deserialize(value,
specifiedType: const FullType(String)) as String?;
break;
}
}
return result.build();
}
}
class _$Links extends Links {
@override
final String? self;
@override
final String? html;
@override
final String? download;
@override
final String? downloadLocation;
factory _$Links([void Function(LinksBuilder)? updates]) =>
(new LinksBuilder()..update(updates))._build();
_$Links._({this.self, this.html, this.download, this.downloadLocation})
: super._();
@override
Links rebuild(void Function(LinksBuilder) updates) =>
(toBuilder()..update(updates)).build();
@override
LinksBuilder toBuilder() => new LinksBuilder()..replace(this);
@override
bool operator ==(Object other) {
if (identical(other, this)) return true;
return other is Links &&
self == other.self &&
html == other.html &&
download == other.download &&
downloadLocation == other.downloadLocation;
}
@override
int get hashCode {
var _$hash = 0;
_$hash = $jc(_$hash, self.hashCode);
_$hash = $jc(_$hash, html.hashCode);
_$hash = $jc(_$hash, download.hashCode);
_$hash = $jc(_$hash, downloadLocation.hashCode);
_$hash = $jf(_$hash);
return _$hash;
}
@override
String toString() {
return (newBuiltValueToStringHelper(r'Links')
..add('self', self)
..add('html', html)
..add('download', download)
..add('downloadLocation', downloadLocation))
.toString();
}
}
class LinksBuilder implements Builder<Links, LinksBuilder> {
_$Links? _$v;
String? _self;
String? get self => _$this._self;
set self(String? self) => _$this._self = self;
String? _html;
String? get html => _$this._html;
set html(String? html) => _$this._html = html;
String? _download;
String? get download => _$this._download;
set download(String? download) => _$this._download = download;
String? _downloadLocation;
String? get downloadLocation => _$this._downloadLocation;
set downloadLocation(String? downloadLocation) =>
_$this._downloadLocation = downloadLocation;
LinksBuilder();
LinksBuilder get _$this {
final $v = _$v;
if ($v != null) {
_self = $v.self;
_html = $v.html;
_download = $v.download;
_downloadLocation = $v.downloadLocation;
_$v = null;
}
return this;
}
@override
void replace(Links other) {
ArgumentError.checkNotNull(other, 'other');
_$v = other as _$Links;
}
@override
void update(void Function(LinksBuilder)? updates) {
if (updates != null) updates(this);
}
@override
Links build() => _build();
_$Links _build() {
final _$result = _$v ??
new _$Links._(
self: self,
html: html,
download: download,
downloadLocation: downloadLocation);
replace(_$result);
return _$result;
}
}
// ignore_for_file: deprecated_member_use_from_same_package,type=lint
| samples/desktop_photo_search/fluent_ui/lib/src/unsplash/links.g.dart/0 | {
"file_path": "samples/desktop_photo_search/fluent_ui/lib/src/unsplash/links.g.dart",
"repo_id": "samples",
"token_count": 2133
} | 1,205 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:fluent_ui/fluent_ui.dart' hide Card, FluentIcons;
import 'package:fluentui_system_icons/fluentui_system_icons.dart';
import 'package:flutter/material.dart' show Card, BorderSide;
import 'package:transparent_image/transparent_image.dart';
import 'package:url_launcher/link.dart';
import '../../unsplash_access_key.dart';
import '../unsplash/photo.dart';
final _unsplashHomepage = Uri.parse(
'https://unsplash.com/?utm_source=$unsplashAppName&utm_medium=referral');
typedef PhotoDetailsPhotoSaveCallback = void Function(Photo);
class PhotoDetails extends StatefulWidget {
const PhotoDetails({
required this.photo,
required this.onPhotoSave,
super.key,
});
final Photo photo;
final PhotoDetailsPhotoSaveCallback onPhotoSave;
@override
State<PhotoDetails> createState() => _PhotoDetailsState();
}
class _PhotoDetailsState extends State<PhotoDetails> {
Widget _buildPhotoAttribution(BuildContext context) {
return Row(
children: [
const Text('Photo by'),
Link(
uri: Uri.parse(
'https://unsplash.com/@${widget.photo.user!.username}?utm_source=$unsplashAppName&utm_medium=referral'),
builder: (context, followLink) => HyperlinkButton(
onPressed: followLink,
child: Text(widget.photo.user!.name),
),
),
const Text('on'),
Link(
uri: _unsplashHomepage,
builder: (context, followLink) => HyperlinkButton(
onPressed: followLink,
child: const Text('Unsplash'),
),
),
],
);
}
@override
Widget build(BuildContext context) {
return Scrollbar(
child: SingleChildScrollView(
primary: true,
child: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(height: 16),
Card(
shape: ContinuousRectangleBorder(
side: const BorderSide(color: Colors.black),
borderRadius: BorderRadius.circular(4),
),
child: AnimatedSize(
duration: const Duration(milliseconds: 750),
child: Padding(
padding: const EdgeInsets.fromLTRB(16, 16, 16, 40),
child: ConstrainedBox(
constraints: const BoxConstraints(
minWidth: 400,
minHeight: 400,
),
child: FadeInImage.memoryNetwork(
placeholder: kTransparentImage,
imageSemanticLabel: widget.photo.description,
image: widget.photo.urls!.small!,
),
),
),
),
),
const SizedBox(height: 8),
Padding(
padding: const EdgeInsets.only(left: 4),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
children: [
_buildPhotoAttribution(context),
const SizedBox(width: 8),
IconButton(
icon: const Icon(
FluentIcons.arrow_download_20_regular,
size: 20,
),
onPressed: () => widget.onPhotoSave(widget.photo),
),
],
),
),
const SizedBox(height: 48),
],
),
),
),
);
}
}
| samples/desktop_photo_search/fluent_ui/lib/src/widgets/photo_details.dart/0 | {
"file_path": "samples/desktop_photo_search/fluent_ui/lib/src/widgets/photo_details.dart",
"repo_id": "samples",
"token_count": 1997
} | 1,206 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:async/async.dart' show StreamGroup;
import 'package:grinder/grinder.dart';
void main(List<String> args) => grind(args);
@DefaultTask()
@Depends(pubGet, generateJsonBindings, analyzeSource, test)
void build() {}
@Task()
Future<void> pubGet() async => _logProcessOutput(
Process.start(
'flutter',
['pub', 'get'],
),
);
@Task()
Future<void> generateJsonBindings() async => _logProcessOutput(
Process.start(
'flutter',
['pub', 'run', 'build_runner', 'build', '--delete-conflicting-outputs'],
),
);
@Task()
Future<void> watch() async => _logProcessOutput(
Process.start(
'flutter',
['pub', 'run', 'build_runner', 'watch'],
),
);
@Task()
Future<void> analyzeSource() async => _logProcessOutput(
Process.start(
'flutter',
['analyze'],
),
);
@Task()
Future<void> test() async => _logProcessOutput(
Process.start(
'flutter',
['test'],
),
);
@Task()
Future<void> clean() => _logProcessOutput(
Process.start(
'flutter',
['clean'],
),
);
Future<void> _logProcessOutput(Future<Process> proc) async {
final process = await proc;
final output = StreamGroup.merge([process.stdout, process.stderr]);
await for (final message in output) {
log(utf8.decode(message));
}
}
| samples/desktop_photo_search/fluent_ui/tool/grind.dart/0 | {
"file_path": "samples/desktop_photo_search/fluent_ui/tool/grind.dart",
"repo_id": "samples",
"token_count": 658
} | 1,207 |
group 'dev.flutter.federated_plugin'
version '1.0-SNAPSHOT'
buildscript {
ext.kotlin_version = '1.3.50'
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:4.1.0'
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
rootProject.allprojects {
repositories {
google()
mavenCentral()
}
}
apply plugin: 'com.android.library'
apply plugin: 'kotlin-android'
android {
compileSdkVersion 30
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = '1.8'
}
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
minSdkVersion 16
}
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
}
| samples/experimental/federated_plugin/federated_plugin/android/build.gradle/0 | {
"file_path": "samples/experimental/federated_plugin/federated_plugin/android/build.gradle",
"repo_id": "samples",
"token_count": 427
} | 1,208 |
name: federated_plugin_example
description: Demonstrates how to use the federated_plugin plugin.
publish_to: 'none' # Remove this line if you wish to publish to pub.dev
environment:
sdk: ^3.2.0
dependencies:
flutter:
sdk: flutter
federated_plugin:
path: ../
cupertino_icons: ^1.0.2
dev_dependencies:
analysis_defaults:
path: ../../../../analysis_defaults
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
| samples/experimental/federated_plugin/federated_plugin/example/pubspec.yaml/0 | {
"file_path": "samples/experimental/federated_plugin/federated_plugin/example/pubspec.yaml",
"repo_id": "samples",
"token_count": 170
} | 1,209 |
#
# To learn more about a Podspec see http://guides.cocoapods.org/syntax/podspec.html.
# Run `pod lib lint federated_plugin.podspec` to validate before publishing.
#
Pod::Spec.new do |s|
s.name = 'federated_plugin'
s.version = '0.0.1'
s.summary = 'A new flutter plugin project.'
s.description = <<-DESC
A new flutter plugin project.
DESC
s.homepage = 'http://example.com'
s.license = { :file => '../LICENSE' }
s.author = { 'Your Company' => '[email protected]' }
s.source = { :path => '.' }
s.source_files = 'Classes/**/*'
s.dependency 'Flutter'
s.platform = :ios, '9.0'
# Flutter.framework does not contain a i386 slice.
s.pod_target_xcconfig = { 'DEFINES_MODULE' => 'YES', 'EXCLUDED_ARCHS[sdk=iphonesimulator*]' => 'i386' }
s.swift_version = '5.0'
end
| samples/experimental/federated_plugin/federated_plugin/ios/federated_plugin.podspec/0 | {
"file_path": "samples/experimental/federated_plugin/federated_plugin/ios/federated_plugin.podspec",
"repo_id": "samples",
"token_count": 402
} | 1,210 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:federated_plugin_platform_interface/battery_method_channel.dart';
import 'package:plugin_platform_interface/plugin_platform_interface.dart';
/// Interface which allows all the platform plugins to implement the same
/// functionality.
abstract class FederatedPluginInterface extends PlatformInterface {
FederatedPluginInterface() : super(token: _object);
static FederatedPluginInterface _federatedPluginInterface =
BatteryMethodChannel();
static final Object _object = Object();
/// Provides instance of [BatteryMethodChannel] to invoke platform calls.
static FederatedPluginInterface get instance => _federatedPluginInterface;
static set instance(FederatedPluginInterface instance) {
PlatformInterface.verifyToken(instance, _object);
_federatedPluginInterface = instance;
}
/// Returns the current battery level of device.
Future<int> getBatteryLevel() async {
throw UnimplementedError('getBatteryLevel() has not been implemented.');
}
}
| samples/experimental/federated_plugin/federated_plugin_platform_interface/lib/federated_plugin_platform_interface.dart/0 | {
"file_path": "samples/experimental/federated_plugin/federated_plugin_platform_interface/lib/federated_plugin_platform_interface.dart",
"repo_id": "samples",
"token_count": 294
} | 1,211 |
// The federated_plugin_windows uses the default BatteryMethodChannel used by
// federated_plugin_platform_interface to do platform calls.
| samples/experimental/federated_plugin/federated_plugin_windows/lib/federated_plugin_windows.dart/0 | {
"file_path": "samples/experimental/federated_plugin/federated_plugin_windows/lib/federated_plugin_windows.dart",
"repo_id": "samples",
"token_count": 32
} | 1,212 |
// Copyright 2021 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:developer';
import 'dart:io';
import 'dart:typed_data';
import 'package:file_selector/file_selector.dart' as file_selector;
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'package:json2yaml/json2yaml.dart';
import 'package:linting_tool/model/profile.dart';
import 'package:linting_tool/model/rule.dart';
import 'package:linting_tool/repository/hive_service.dart';
import 'package:linting_tool/repository/repository.dart';
import 'package:yaml/yaml.dart';
const _boxName = 'rules_profile';
class ProfilesStore extends ChangeNotifier {
final Repository repository;
ProfilesStore(http.Client httpClient) : repository = Repository(httpClient) {
fetchSavedProfiles();
}
bool _isLoading = true;
bool get isLoading => _isLoading;
List<RulesProfile> _savedProfiles = [];
List<RulesProfile> get savedProfiles => _savedProfiles;
String? _error;
String? get error => _error;
Future<void> fetchSavedProfiles() async {
if (!_isLoading) _isLoading = true;
notifyListeners();
try {
_savedProfiles = await HiveService.getBoxes<RulesProfile>(_boxName);
} on Exception catch (e) {
log(e.toString());
}
_isLoading = false;
notifyListeners();
}
Future<void> addToNewProfile(RulesProfile profile) async {
await HiveService.addBox<RulesProfile>(profile, _boxName);
await Future.delayed(const Duration(milliseconds: 100), () async {
await fetchSavedProfiles();
});
}
Future<void> addToExistingProfile(RulesProfile profile, Rule rule) async {
// ignore: todo
// TODO(abd99): Consider refactoring to LinkedHashSet/SplayTreeSet to avoid
// duplication automatically.
// ref: https://github.com/flutter/samples/pull/870#discussion_r685666792
final rules = profile.rules;
// If rule is already in profile, skip updating profile
if (rules.contains(rule)) {
return;
}
rules.add(rule);
final newProfile = RulesProfile(name: profile.name, rules: rules);
await HiveService.updateBox<RulesProfile>(profile, newProfile, _boxName);
await Future.delayed(const Duration(milliseconds: 100), () async {
await fetchSavedProfiles();
});
}
Future<void> updateProfile(
RulesProfile oldProfile, RulesProfile newProfile) async {
await HiveService.updateBox<RulesProfile>(oldProfile, newProfile, _boxName);
await Future.delayed(const Duration(milliseconds: 100), () async {
await fetchSavedProfiles();
});
}
Future<void> removeRuleFromProfile(RulesProfile profile, Rule rule) async {
final newProfile =
RulesProfile(name: profile.name, rules: profile.rules..remove(rule));
await updateProfile(profile, newProfile);
}
Future<void> deleteProfile(RulesProfile profile) async {
await HiveService.deleteBox<RulesProfile>(profile, _boxName);
await Future.delayed(const Duration(milliseconds: 100), () async {
await fetchSavedProfiles();
});
}
Future<bool> exportProfileFile(
RulesProfile profile, {
RulesStyle rulesStyle = RulesStyle.booleanMap,
}) async {
_isLoading = true;
notifyListeners();
var resultSaved = false;
try {
final templateFileData = await repository.getTemplateFile();
/// Fetch formatted data to create new YamlFile.
final newYamlFile =
_prepareYamlFile(profile, templateFileData, rulesStyle);
resultSaved = await _saveFileToDisk(newYamlFile);
} on SocketException catch (e) {
log(e.toString());
_error = 'Check internet connection.';
resultSaved = false;
} on Exception catch (e) {
log(e.toString());
}
_isLoading = false;
notifyListeners();
return resultSaved;
}
Future<bool> _saveFileToDisk(String newYamlFile) async {
const name = 'analysis_options.yaml';
/// Get file path using file picker.
final saveLocation = await file_selector.getSaveLocation(
suggestedName: name,
);
final data = Uint8List.fromList(newYamlFile.codeUnits);
final file = file_selector.XFile.fromData(data, name: name);
/// Save file to disk if path was provided.
if (saveLocation != null) {
await file.saveTo(saveLocation.path);
return true;
}
const errorMessage = 'File path not found.';
_error = errorMessage;
throw Exception(errorMessage);
}
String _prepareYamlFile(
RulesProfile profile, YamlMap templateFile, RulesStyle rulesStyle) {
final rules = profile.rules.map((e) => e.name);
final rulesData =
json.decode(json.encode(templateFile)) as Map<String, dynamic>;
/// Add rules to existing template according to formatting style.
if (rulesStyle == RulesStyle.booleanMap) {
final rulesMap = <String, bool>{for (final rule in rules) rule: true};
rulesData.update('linter', (dynamic value) => {'rules': rulesMap});
} else {
rulesData.update('linter', (dynamic value) => {'rules': rules});
}
return json2yaml(rulesData, yamlStyle: YamlStyle.pubspecYaml);
}
}
/// Formatting style for rules.
enum RulesStyle {
list,
booleanMap,
}
| samples/experimental/linting_tool/lib/model/profiles_store.dart/0 | {
"file_path": "samples/experimental/linting_tool/lib/model/profiles_store.dart",
"repo_id": "samples",
"token_count": 1849
} | 1,213 |
// Copyright 2021 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:linting_tool/model/profile.dart';
import 'package:linting_tool/model/profiles_store.dart';
import 'package:linting_tool/model/rule.dart';
import 'package:linting_tool/theme/app_theme.dart';
import 'package:linting_tool/theme/colors.dart';
import 'package:provider/provider.dart';
class LintExpansionTile extends StatefulWidget {
final Rule rule;
const LintExpansionTile({
required this.rule,
super.key,
});
@override
State<LintExpansionTile> createState() => _LintExpansionTileState();
}
class _LintExpansionTileState extends State<LintExpansionTile> {
var isExpanded = false;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
final textTheme = theme.textTheme;
final rule = widget.rule;
final incompatibleString =
rule.incompatible.isNotEmpty ? rule.incompatible.join(', ') : 'none';
final setsString = rule.sets.isNotEmpty ? rule.sets.join(', ') : 'none';
return ExpansionTile(
collapsedBackgroundColor: AppColors.white50,
title: Text(
rule.name,
style: textTheme.titleMedium!.copyWith(
fontWeight: FontWeight.w700,
),
),
subtitle: Text(
rule.description,
style: textTheme.bodySmall!,
),
initiallyExpanded: isExpanded,
onExpansionChanged: (value) {
setState(() {
isExpanded = value;
});
},
expandedAlignment: Alignment.centerLeft,
childrenPadding: const EdgeInsets.symmetric(
horizontal: 16.0,
vertical: 8.0,
),
backgroundColor: AppColors.white50,
maintainState: true,
expandedCrossAxisAlignment: CrossAxisAlignment.start,
children: [
Text.rich(
TextSpan(
children: [
TextSpan(
text: 'Group:',
style: textTheme.titleSmall,
),
TextSpan(
text: ' ${rule.group}',
),
],
),
textAlign: TextAlign.left,
),
Text.rich(
TextSpan(
children: [
TextSpan(
text: 'State:',
style: textTheme.titleSmall,
),
TextSpan(
text: ' ${rule.state}',
),
],
),
textAlign: TextAlign.left,
),
Text.rich(
TextSpan(
children: [
TextSpan(
text: 'Incompatible:',
style: textTheme.titleSmall,
),
TextSpan(
text: ' $incompatibleString',
),
],
),
textAlign: TextAlign.left,
),
Text.rich(
TextSpan(
children: [
TextSpan(
text: 'Sets:',
style: textTheme.titleSmall,
),
TextSpan(
text: ' $setsString',
),
],
),
textAlign: TextAlign.left,
),
const SizedBox(
height: 16.0,
),
MarkdownBody(
data: rule.details,
selectable: true,
styleSheet: AppTheme.buildMarkDownTheme(theme),
),
const SizedBox(
height: 8.0,
),
Align(
alignment: Alignment.centerRight,
child: ElevatedButton(
child: const Text('Add to profile'),
onPressed: () async {
ProfileType? destinationProfileType =
await showDialog<ProfileType>(
context: context,
builder: (context) {
return const _ProfileTypeDialog();
},
);
if (!context.mounted) return;
if (destinationProfileType == ProfileType.newProfile) {
await showDialog<String>(
context: context,
builder: (context) {
return NewProfileDialog(rule: rule);
},
);
} else if (destinationProfileType ==
ProfileType.existingProfile) {
await showDialog<String>(
context: context,
builder: (context) {
return ExistingProfileDialog(rule: rule);
},
);
}
},
),
),
const SizedBox(
height: 16.0,
),
],
);
}
}
enum ProfileType {
newProfile,
existingProfile,
}
class _ProfileTypeDialog extends StatelessWidget {
const _ProfileTypeDialog();
@override
Widget build(BuildContext context) {
var profilesStore = context.watch<ProfilesStore>();
return AlertDialog(
actionsPadding: const EdgeInsets.only(
left: 16.0,
right: 16.0,
bottom: 16.0,
),
title: const Text('Select Profile Type'),
actions: [
if (profilesStore.savedProfiles.isNotEmpty)
ElevatedButton(
onPressed: () {
Navigator.pop(context, ProfileType.existingProfile);
},
child: const Text('Existing'),
),
TextButton(
onPressed: () {
Navigator.pop(context, ProfileType.newProfile);
},
child: const Text('New'),
),
],
);
}
}
class NewProfileDialog extends StatefulWidget {
final Rule rule;
const NewProfileDialog({
required this.rule,
super.key,
});
@override
State<NewProfileDialog> createState() => _NewProfileDialogState();
}
class _NewProfileDialogState extends State<NewProfileDialog> {
@override
Widget build(BuildContext context) {
String name = '';
final formKey = GlobalKey<FormState>();
return AlertDialog(
title: const Text('Create new lint profile'),
content: Form(
key: formKey,
autovalidateMode: AutovalidateMode.onUserInteraction,
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text('Profile Name'),
TextFormField(
onChanged: (value) {
name = value;
},
validator: (value) {
if (value == null || value.isEmpty) {
return 'Name cannot be empty.';
}
return null;
},
),
],
),
),
actionsPadding: const EdgeInsets.all(16.0),
actions: [
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Cancel'),
),
ElevatedButton(
onPressed: () async {
if (formKey.currentState!.validate()) {
var newProfile = RulesProfile(
name: name,
rules: [widget.rule],
);
await Provider.of<ProfilesStore>(context, listen: false)
.addToNewProfile(newProfile);
if (!context.mounted) return;
Navigator.pop(context);
}
},
child: const Text('Save'),
),
],
);
}
}
class ExistingProfileDialog extends StatefulWidget {
const ExistingProfileDialog({
super.key,
required this.rule,
});
final Rule rule;
@override
State<ExistingProfileDialog> createState() => _ExistingProfileDialogState();
}
class _ExistingProfileDialogState extends State<ExistingProfileDialog> {
@override
Widget build(BuildContext context) {
final profilesStore = Provider.of<ProfilesStore>(context);
final savedProfiles = profilesStore.savedProfiles;
return AlertDialog(
title: const Text('Select a lint profile'),
content: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: List.generate(
savedProfiles.length,
(index) => ListTile(
title: Text(savedProfiles[index].name),
onTap: () async {
await profilesStore.addToExistingProfile(
savedProfiles[index], widget.rule);
if (!context.mounted) return;
Navigator.pop(context);
},
),
),
),
actionsPadding: const EdgeInsets.all(16.0),
actions: [
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('Cancel'),
),
],
);
}
}
| samples/experimental/linting_tool/lib/widgets/lint_expansion_tile.dart/0 | {
"file_path": "samples/experimental/linting_tool/lib/widgets/lint_expansion_tile.dart",
"repo_id": "samples",
"token_count": 4444
} | 1,214 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| samples/experimental/linting_tool/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "samples/experimental/linting_tool/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "samples",
"token_count": 32
} | 1,215 |
#include "../../src/dart-sdk/include/dart_api_dl.c"
| samples/experimental/pedometer/ios/Classes/dart_api_dl.c/0 | {
"file_path": "samples/experimental/pedometer/ios/Classes/dart_api_dl.c",
"repo_id": "samples",
"token_count": 25
} | 1,216 |
// Copyright (c) 2022, the Dart project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
#pragma once
// Note: include appropriate system jni.h as found by CMake, not third_party/jni.h.
#include <jni.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#if _WIN32
#include <windows.h>
#else
#include <pthread.h>
#include <unistd.h>
#endif
#if _WIN32
#define FFI_PLUGIN_EXPORT __declspec(dllexport)
#else
#define FFI_PLUGIN_EXPORT
#endif
#if defined _WIN32
#define thread_local __declspec(thread)
#else
#define thread_local __thread
#endif
#ifdef __ANDROID__
#include <android/log.h>
#endif
#ifdef __ANDROID__
#define __ENVP_CAST (JNIEnv**)
#else
#define __ENVP_CAST (void**)
#endif
/// Locking functions for windows and pthread.
#if defined _WIN32
#include <windows.h>
typedef CRITICAL_SECTION MutexLock;
typedef CONDITION_VARIABLE ConditionVariable;
static inline void init_lock(MutexLock* lock) {
InitializeCriticalSection(lock);
}
static inline void acquire_lock(MutexLock* lock) {
EnterCriticalSection(lock);
}
static inline void release_lock(MutexLock* lock) {
LeaveCriticalSection(lock);
}
static inline void destroy_lock(MutexLock* lock) {
DeleteCriticalSection(lock);
}
static inline void init_cond(ConditionVariable* cond) {
InitializeConditionVariable(cond);
}
static inline void signal_cond(ConditionVariable* cond) {
WakeConditionVariable(cond);
}
static inline void wait_for(ConditionVariable* cond, MutexLock* lock) {
SleepConditionVariableCS(cond, lock, INFINITE);
}
static inline void destroy_cond(ConditionVariable* cond) {
// Not available.
}
#elif defined __APPLE__ || defined __LINUX__ || defined __ANDROID__ || \
defined __GNUC__
#include <pthread.h>
typedef pthread_mutex_t MutexLock;
typedef pthread_cond_t ConditionVariable;
static inline void init_lock(MutexLock* lock) {
pthread_mutex_init(lock, NULL);
}
static inline void acquire_lock(MutexLock* lock) {
pthread_mutex_lock(lock);
}
static inline void release_lock(MutexLock* lock) {
pthread_mutex_unlock(lock);
}
static inline void destroy_lock(MutexLock* lock) {
pthread_mutex_destroy(lock);
}
static inline void init_cond(ConditionVariable* cond) {
pthread_cond_init(cond, NULL);
}
static inline void signal_cond(ConditionVariable* cond) {
pthread_cond_signal(cond);
}
static inline void wait_for(ConditionVariable* cond, MutexLock* lock) {
pthread_cond_wait(cond, lock);
}
static inline void destroy_cond(ConditionVariable* cond) {
pthread_cond_destroy(cond);
}
#else
#error "No locking/condition variable support; Possibly unsupported platform"
#endif
typedef struct CallbackResult {
MutexLock lock;
ConditionVariable cond;
int ready;
jobject object;
} CallbackResult;
typedef struct JniLocks {
MutexLock classLoadingLock;
MutexLock methodLoadingLock;
MutexLock fieldLoadingLock;
} JniLocks;
/// Represents the error when dart-jni layer has already spawned singleton VM.
#define DART_JNI_SINGLETON_EXISTS (-99);
/// Stores the global state of the JNI.
typedef struct JniContext {
JavaVM* jvm;
jobject classLoader;
jmethodID loadClassMethod;
jobject currentActivity;
jobject appContext;
JniLocks locks;
} JniContext;
// jniEnv for this thread, used by inline functions in this header,
// therefore declared as extern.
extern thread_local JNIEnv* jniEnv;
extern JniContext* jni;
/// Types used by JNI API to distinguish between primitive types.
enum JniType {
booleanType = 0,
byteType = 1,
shortType = 2,
charType = 3,
intType = 4,
longType = 5,
floatType = 6,
doubleType = 7,
objectType = 8,
voidType = 9,
};
/// Result type for use by JNI.
///
/// If [exception] is null, it means the result is valid.
/// It's assumed that the caller knows the expected type in [result].
typedef struct JniResult {
jvalue value;
jthrowable exception;
} JniResult;
/// Similar to [JniResult] but for class lookups.
typedef struct JniClassLookupResult {
jclass value;
jthrowable exception;
} JniClassLookupResult;
/// Similar to [JniResult] but for method/field ID lookups.
typedef struct JniPointerResult {
const void* value;
jthrowable exception;
} JniPointerResult;
/// JniExceptionDetails holds 2 jstring objects, one is the result of
/// calling `toString` on exception object, other is stack trace;
typedef struct JniExceptionDetails {
jstring message;
jstring stacktrace;
} JniExceptionDetails;
/// This struct contains functions which wrap method call / field access conveniently along with
/// exception checking.
///
/// Flutter embedding checks for pending JNI exceptions before an FFI transition, which requires us
/// to check for and clear the exception before returning to dart code, which requires these functions
/// to return result types.
typedef struct JniAccessorsStruct {
JniClassLookupResult (*getClass)(char* internalName);
JniPointerResult (*getFieldID)(jclass cls, char* fieldName, char* signature);
JniPointerResult (*getStaticFieldID)(jclass cls,
char* fieldName,
char* signature);
JniPointerResult (*getMethodID)(jclass cls,
char* methodName,
char* signature);
JniPointerResult (*getStaticMethodID)(jclass cls,
char* methodName,
char* signature);
JniResult (*newObject)(jclass cls, jmethodID ctor, jvalue* args);
JniResult (*newPrimitiveArray)(jsize length, int type);
JniResult (*newObjectArray)(jsize length,
jclass elementClass,
jobject initialElement);
JniResult (*getArrayElement)(jarray array, int index, int type);
JniResult (*callMethod)(jobject obj,
jmethodID methodID,
int callType,
jvalue* args);
JniResult (*callStaticMethod)(jclass cls,
jmethodID methodID,
int callType,
jvalue* args);
JniResult (*getField)(jobject obj, jfieldID fieldID, int callType);
JniResult (*getStaticField)(jclass cls, jfieldID fieldID, int callType);
JniExceptionDetails (*getExceptionDetails)(jthrowable exception);
} JniAccessorsStruct;
FFI_PLUGIN_EXPORT JniAccessorsStruct* GetAccessors();
FFI_PLUGIN_EXPORT JavaVM* GetJavaVM(void);
FFI_PLUGIN_EXPORT JNIEnv* GetJniEnv(void);
/// Spawn a JVM with given arguments.
///
/// Returns JNI_OK on success, and one of the documented JNI error codes on
/// failure. It returns DART_JNI_SINGLETON_EXISTS if an attempt to spawn multiple
/// JVMs is made, even if the underlying API potentially supports multiple VMs.
FFI_PLUGIN_EXPORT int SpawnJvm(JavaVMInitArgs* args);
/// Load class through platform-specific mechanism.
///
/// Currently uses application classloader on android,
/// and JNIEnv->FindClass on other platforms.
FFI_PLUGIN_EXPORT jclass FindClass(const char* name);
/// Returns Application classLoader (on Android),
/// which can be used to load application and platform classes.
///
/// On other platforms, NULL is returned.
FFI_PLUGIN_EXPORT jobject GetClassLoader(void);
/// Returns application context on Android.
///
/// On other platforms, NULL is returned.
FFI_PLUGIN_EXPORT jobject GetApplicationContext(void);
/// Returns current activity of the app on Android.
FFI_PLUGIN_EXPORT jobject GetCurrentActivity(void);
static inline void attach_thread() {
if (jniEnv == NULL) {
(*jni->jvm)->AttachCurrentThread(jni->jvm, __ENVP_CAST & jniEnv, NULL);
}
}
/// Load class into [cls] using platform specific mechanism
static inline void load_class_platform(jclass* cls, const char* name) {
#ifdef __ANDROID__
jstring className = (*jniEnv)->NewStringUTF(jniEnv, name);
*cls = (*jniEnv)->CallObjectMethod(jniEnv, jni->classLoader,
jni->loadClassMethod, className);
(*jniEnv)->DeleteLocalRef(jniEnv, className);
#else
*cls = (*jniEnv)->FindClass(jniEnv, name);
#endif
}
static inline void load_class_local_ref(jclass* cls, const char* name) {
if (*cls == NULL) {
acquire_lock(&jni->locks.classLoadingLock);
if (*cls == NULL) {
load_class_platform(cls, name);
}
release_lock(&jni->locks.classLoadingLock);
}
}
static inline void load_class_global_ref(jclass* cls, const char* name) {
if (*cls == NULL) {
jclass tmp = NULL;
acquire_lock(&jni->locks.classLoadingLock);
if (*cls == NULL) {
load_class_platform(&tmp, name);
if (!(*jniEnv)->ExceptionCheck(jniEnv)) {
*cls = (*jniEnv)->NewGlobalRef(jniEnv, tmp);
(*jniEnv)->DeleteLocalRef(jniEnv, tmp);
}
}
release_lock(&jni->locks.classLoadingLock);
}
}
static inline void load_method(jclass cls,
jmethodID* res,
const char* name,
const char* sig) {
if (*res == NULL) {
acquire_lock(&jni->locks.methodLoadingLock);
if (*res == NULL) {
*res = (*jniEnv)->GetMethodID(jniEnv, cls, name, sig);
}
release_lock(&jni->locks.methodLoadingLock);
}
}
static inline void load_static_method(jclass cls,
jmethodID* res,
const char* name,
const char* sig) {
if (*res == NULL) {
acquire_lock(&jni->locks.methodLoadingLock);
if (*res == NULL) {
*res = (*jniEnv)->GetStaticMethodID(jniEnv, cls, name, sig);
}
release_lock(&jni->locks.methodLoadingLock);
}
}
static inline void load_field(jclass cls,
jfieldID* res,
const char* name,
const char* sig) {
if (*res == NULL) {
acquire_lock(&jni->locks.fieldLoadingLock);
if (*res == NULL) {
*res = (*jniEnv)->GetFieldID(jniEnv, cls, name, sig);
}
release_lock(&jni->locks.fieldLoadingLock);
}
}
static inline void load_static_field(jclass cls,
jfieldID* res,
const char* name,
const char* sig) {
if (*res == NULL) {
acquire_lock(&jni->locks.fieldLoadingLock);
if (*res == NULL) {
*res = (*jniEnv)->GetStaticFieldID(jniEnv, cls, name, sig);
}
release_lock(&jni->locks.fieldLoadingLock);
}
}
static inline jobject to_global_ref(jobject ref) {
jobject g = (*jniEnv)->NewGlobalRef(jniEnv, ref);
(*jniEnv)->DeleteLocalRef(jniEnv, ref);
return g;
}
// These functions are useful for C+Dart bindings, and not required for pure dart bindings.
FFI_PLUGIN_EXPORT JniContext* GetJniContextPtr();
/// For use by jni_gen's generated code
/// don't use these.
// these 2 fn ptr vars will be defined by generated code library
extern JniContext* (*context_getter)(void);
extern JNIEnv* (*env_getter)(void);
// this function will be exported by generated code library
// it will set above 2 variables.
FFI_PLUGIN_EXPORT void setJniGetters(struct JniContext* (*cg)(void),
JNIEnv* (*eg)(void));
static inline void load_env() {
if (jniEnv == NULL) {
jni = context_getter();
jniEnv = env_getter();
}
}
static inline jthrowable check_exception() {
jthrowable exception = (*jniEnv)->ExceptionOccurred(jniEnv);
if (exception != NULL) (*jniEnv)->ExceptionClear(jniEnv);
if (exception == NULL) return NULL;
return to_global_ref(exception);
}
static inline JniResult to_global_ref_result(jobject ref) {
JniResult result;
result.exception = check_exception();
if (result.exception == NULL) {
result.value.l = to_global_ref(ref);
}
return result;
}
FFI_PLUGIN_EXPORT intptr_t InitDartApiDL(void* data);
FFI_PLUGIN_EXPORT
JniResult DartException__ctor(jstring message);
FFI_PLUGIN_EXPORT
JniResult PortContinuation__ctor(int64_t j);
FFI_PLUGIN_EXPORT
JniResult PortProxy__newInstance(jobject binaryName,
int64_t port,
int64_t functionPtr);
FFI_PLUGIN_EXPORT void resultFor(CallbackResult* result, jobject object);
| samples/experimental/pedometer/src/health_connect/dartjni.h/0 | {
"file_path": "samples/experimental/pedometer/src/health_connect/dartjni.h",
"repo_id": "samples",
"token_count": 4939
} | 1,217 |
// Copyright 2023 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'dart:ui' as ui;
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import '../model/puzzle_model.dart';
import '../page_content/pages_flow.dart';
import 'components.dart';
class RotatorPuzzle extends StatefulWidget {
final PageConfig pageConfig;
final int numTiles;
final int puzzleNum;
final Shader shader;
final int shaderDuration;
final String tileShadedString;
final double tileShadedStringSize;
final EdgeInsets tileShadedStringPadding;
final int tileShadedStringAnimDuration;
final List<WonkyAnimSetting> tileShadedStringAnimSettings;
final double tileScaleModifier;
const RotatorPuzzle({
super.key,
required this.pageConfig,
required this.numTiles,
required this.puzzleNum,
required this.shader,
required this.shaderDuration,
required this.tileShadedString,
required this.tileShadedStringSize,
required this.tileShadedStringPadding,
required this.tileShadedStringAnimDuration,
this.tileShadedStringAnimSettings = const [],
this.tileScaleModifier = 1.0,
});
@override
State<RotatorPuzzle> createState() => RotatorPuzzleState();
}
class RotatorPuzzleState extends State<RotatorPuzzle>
with TickerProviderStateMixin {
late PuzzleModel puzzleModel;
bool solved = false;
late final AnimationController animationController = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 1000),
);
late final CurvedAnimation animationCurve = CurvedAnimation(
parent: animationController,
curve: const Interval(
0.2,
0.45,
curve: Curves.easeOut,
),
);
late Animation<double> opacAnimation =
Tween<double>(begin: 0.4, end: 1.0).animate(animationCurve)
..addListener(() {
setState(() {});
});
List<GlobalKey<RotatorPuzzleTileState>> tileKeys = [];
GlobalKey<FragmentShadedState> shadedWidgetStackHackStateKey = GlobalKey();
GlobalKey shadedWidgetRepaintBoundaryKey = GlobalKey();
GlobalKey<WonkyCharState> tileBgWonkyCharKey = GlobalKey();
ui.Image? shadedImg;
@override
void initState() {
for (int i = 0; i < widget.numTiles; i++) {
tileKeys.add(GlobalKey<RotatorPuzzleTileState>());
}
puzzleModel = PuzzleModel(
dim: widget.numTiles,
); //TODO check if correct; correlate dim and numTiles; probably get rid of numTiles
generateTiles();
shuffle();
super.initState();
}
List<RotatorPuzzleTile> generateTiles() {
// TODO move to build?
List<RotatorPuzzleTile> tiles = [];
int dim = sqrt(widget.numTiles).round();
for (int i = 0; i < widget.numTiles; i++) {
RotatorPuzzleTile tile = RotatorPuzzleTile(
key: tileKeys[i],
tileID: i,
row: (i / dim).floor(),
col: i % dim,
parentState: this,
shader: widget.shader,
shaderDuration: widget.shaderDuration,
tileShadedString: widget.tileShadedString,
tileShadedStringSize: widget.tileShadedStringSize,
tileShadedStringPadding: widget.tileShadedStringPadding,
animationSettings: widget.tileShadedStringAnimSettings,
tileShadedStringAnimDuration: widget.tileShadedStringAnimDuration,
tileScaleModifier: widget.tileScaleModifier,
);
tiles.add(tile);
}
return tiles;
}
void handlePointerDown({required int tileID}) {
puzzleModel.rotateTile(tileID);
if (puzzleModel.allRotationsCorrect()) {
handleSolved();
}
}
void handleSolved() {
animationController.addStatusListener((status) {
solved = true;
for (GlobalKey<RotatorPuzzleTileState> k in tileKeys) {
if (null != k.currentState && k.currentState!.mounted) {
startDampening();
tileBgWonkyCharKey.currentState!.stopAnimation();
}
}
if (status == AnimationStatus.completed) {
Future.delayed(
const Duration(milliseconds: FragmentShaded.dampenDuration + 250),
() {
widget.pageConfig.pageController.nextPage(
duration:
const Duration(milliseconds: PagesFlow.pageScrollDuration),
curve: Curves.easeOut,
);
});
}
});
animationController.forward();
}
void shuffle() {
Random rng = Random(0xC00010FF);
for (int i = 0; i < widget.numTiles; i++) {
int rando = rng.nextInt(3);
puzzleModel.setTileStatus(i, rando);
if (puzzleModel.allRotationsCorrect()) {
// fallback to prevent starting on solved puzzle
puzzleModel.setTileStatus(0, 1);
}
}
}
double tileSize() {
return widget.pageConfig.puzzleSize / sqrt(widget.numTiles);
}
List<double> tileCoords({required int row, required int col}) {
return <double>[col * tileSize(), row * tileSize()];
}
void setImageFromRepaintBoundary(GlobalKey which) {
final BuildContext? context = which.currentContext;
if (null != context) {
final RenderRepaintBoundary boundary =
context.findRenderObject()! as RenderRepaintBoundary;
final ui.Image img = boundary.toImageSync();
if (mounted) {
setState(() {
shadedImg = img;
});
}
}
}
void startDampening() {
if (null != shadedWidgetStackHackStateKey.currentState &&
shadedWidgetStackHackStateKey.currentState!.mounted) {
shadedWidgetStackHackStateKey.currentState!.startDampening();
}
}
@override
Widget build(BuildContext context) {
// TODO fix widget implementation to remove the need for this hack
// to force a setState rebuild
WidgetsBinding.instance.addPostFrameCallback((_) {
if (mounted) {
setState(() {});
}
});
// end hack ----------------
setImageFromRepaintBoundary(shadedWidgetRepaintBoundaryKey);
return Center(
child: SizedBox(
width: widget.pageConfig.puzzleSize,
height: widget.pageConfig.puzzleSize,
child: Opacity(
opacity: opacAnimation.value,
child: Stack(
children: <Widget>[
Positioned(
left: -9999,
top: -9999,
child: RepaintBoundary(
key: shadedWidgetRepaintBoundaryKey,
child: SizedBox(
width: widget.pageConfig.puzzleSize * 4,
height: widget.pageConfig.puzzleSize * 4,
child: Center(
child: FragmentShaded(
key: shadedWidgetStackHackStateKey,
shader: widget.shader,
shaderDuration: widget.shaderDuration,
child: Padding(
padding: widget.tileShadedStringPadding,
child: WonkyChar(
key: tileBgWonkyCharKey,
text: widget.tileShadedString,
size: widget.tileShadedStringSize,
animDurationMillis:
widget.tileShadedStringAnimDuration,
animationSettings:
widget.tileShadedStringAnimSettings,
),
),
),
),
),
),
),
] +
generateTiles(),
),
),
),
);
}
}
////////////////////////////////////////////////////////
class RotatorPuzzleTile extends StatefulWidget {
final int tileID;
final RotatorPuzzleState parentState;
final Shader shader;
final int shaderDuration;
final String tileShadedString;
final double tileShadedStringSize;
final EdgeInsets tileShadedStringPadding;
final int tileShadedStringAnimDuration;
final List<WonkyAnimSetting> animationSettings;
final double tileScaleModifier;
// TODO get row/col out into model
final int row;
final int col;
RotatorPuzzleTile({
super.key,
required this.tileID,
required this.row,
required this.col,
required this.parentState,
required this.shader,
required this.shaderDuration,
required this.tileShadedString,
required this.tileShadedStringSize,
required this.tileShadedStringPadding,
required this.animationSettings,
required this.tileShadedStringAnimDuration,
required this.tileScaleModifier,
});
final State<RotatorPuzzleTile> tileState = RotatorPuzzleTileState();
@override
State<RotatorPuzzleTile> createState() => RotatorPuzzleTileState();
}
class RotatorPuzzleTileState extends State<RotatorPuzzleTile>
with TickerProviderStateMixin {
double touchedOpac = 0.0;
Duration touchedOpacDur = const Duration(milliseconds: 50);
late final AnimationController animationController = AnimationController(
vsync: this,
duration: const Duration(
milliseconds: 100,
),
);
late final CurvedAnimation animationCurve = CurvedAnimation(
parent: animationController,
curve: Curves.easeOut,
);
late Animation<double> animation;
@override
void initState() {
super.initState();
animation = Tween<double>(
// initialize animation to starting point
begin: currentStatus() * pi * 0.5,
end: currentStatus() * pi * 0.5,
).animate(animationController);
}
@override
Widget build(BuildContext context) {
// TODO fix widget implementation to remove the need for this hack
// to force a setState rebuild
WidgetsBinding.instance.addPostFrameCallback((_) {
if (mounted) {
setState(() {});
}
});
// end hack ------------------------------
List<double> coords =
widget.parentState.tileCoords(row: widget.row, col: widget.col);
double zeroPoint = widget.parentState.widget.pageConfig.puzzleSize * .5 -
widget.parentState.tileSize() * 0.5;
return Stack(
children: [
Stack(
children: [
Positioned(
left: coords[0],
top: coords[1],
child: Transform(
transform: Matrix4.rotationZ(animation.value),
alignment: Alignment.center,
child: GestureDetector(
onTap: handlePointerDown,
child: ClipRect(
child: SizedBox(
width: widget.parentState.tileSize(),
height: widget.parentState.tileSize(),
child: OverflowBox(
maxHeight:
widget.parentState.widget.pageConfig.puzzleSize,
maxWidth:
widget.parentState.widget.pageConfig.puzzleSize,
child: Transform.translate(
offset: Offset(
zeroPoint -
widget.col * widget.parentState.tileSize(),
zeroPoint -
widget.row * widget.parentState.tileSize(),
),
child: SizedBox(
width:
widget.parentState.widget.pageConfig.puzzleSize,
height:
widget.parentState.widget.pageConfig.puzzleSize,
child: Transform.scale(
scale: widget.tileScaleModifier,
child: RawImage(
image: widget.parentState.shadedImg,
),
),
),
),
),
),
),
),
),
),
// puzzle tile overlay fades in/out on tap, to indicate touched tile
Positioned(
left: coords[0],
top: coords[1],
child: IgnorePointer(
child: AnimatedOpacity(
opacity: touchedOpac,
duration: touchedOpacDur,
onEnd: () {
if (touchedOpac == 1.0) {
touchedOpac = 0.0;
touchedOpacDur = const Duration(milliseconds: 300);
setState(() {});
}
},
child: DecoratedBox(
decoration: const BoxDecoration(
color: Color.fromARGB(120, 0, 0, 0)),
child: SizedBox(
width: widget.parentState.tileSize(),
height: widget.parentState.tileSize(),
),
),
),
),
),
],
),
],
);
}
void handlePointerDown() {
if (!widget.parentState.solved) {
int oldStatus = currentStatus();
widget.parentState.handlePointerDown(tileID: widget.tileID);
touchedOpac = 1.0;
touchedOpacDur = const Duration(milliseconds: 100);
rotateTile(oldStatus: oldStatus);
setState(() {});
}
}
int currentStatus() {
return widget.parentState.puzzleModel.getTileStatus(widget.tileID);
}
void rotateTile({required int oldStatus}) {
animation = Tween<double>(
begin: oldStatus * pi * 0.5,
end: currentStatus() * pi * 0.5,
).animate(animationController)
..addListener(() {
setState(() {});
});
animationController.reset();
animationController.forward();
}
}
| samples/experimental/varfont_shader_puzzle/lib/components/rotator_puzzle.dart/0 | {
"file_path": "samples/experimental/varfont_shader_puzzle/lib/components/rotator_puzzle.dart",
"repo_id": "samples",
"token_count": 6470
} | 1,218 |
# Veggie Seasons
The [Veggie Seasons](https://github.com/flutter/samples/tree/main/veggieseasons) app has been moved out of the experimental
directory of this repository as it now works on stable channel of Flutter.
| samples/experimental/veggieseasons/README.md/0 | {
"file_path": "samples/experimental/veggieseasons/README.md",
"repo_id": "samples",
"token_count": 58
} | 1,219 |
// Copyright 2020, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart' as intl;
import 'package:provider/provider.dart';
import '../api/api.dart';
import '../app.dart';
import '../widgets/categories_dropdown.dart';
import '../widgets/dialogs.dart';
class EntriesPage extends StatefulWidget {
const EntriesPage({super.key});
@override
State<EntriesPage> createState() => _EntriesPageState();
}
class _EntriesPageState extends State<EntriesPage> {
Category? _selected;
@override
Widget build(BuildContext context) {
var appState = Provider.of<AppState>(context);
return Column(
children: [
CategoryDropdown(
api: appState.api!.categories,
onSelected: (category) => setState(() => _selected = category)),
Expanded(
child: _selected == null
? const Center(child: CircularProgressIndicator())
: EntriesList(
category: _selected,
api: appState.api!.entries,
),
),
],
);
}
}
class EntriesList extends StatefulWidget {
final Category? category;
final EntryApi api;
EntriesList({
this.category,
required this.api,
}) : super(key: ValueKey(category?.id));
@override
State<EntriesList> createState() => _EntriesListState();
}
class _EntriesListState extends State<EntriesList> {
@override
Widget build(BuildContext context) {
if (widget.category == null) {
return _buildLoadingIndicator();
}
return FutureBuilder<List<Entry>>(
future: widget.api.list(widget.category!.id!),
builder: (context, futureSnapshot) {
if (!futureSnapshot.hasData) {
return _buildLoadingIndicator();
}
return StreamBuilder<List<Entry>>(
initialData: futureSnapshot.data,
stream: widget.api.subscribe(widget.category!.id!),
builder: (context, snapshot) {
if (!snapshot.hasData) {
return _buildLoadingIndicator();
}
return ListView.builder(
itemBuilder: (context, index) {
return EntryTile(
category: widget.category,
entry: snapshot.data![index],
);
},
itemCount: snapshot.data!.length,
);
},
);
},
);
}
Widget _buildLoadingIndicator() {
return const Center(child: CircularProgressIndicator());
}
}
class EntryTile extends StatelessWidget {
final Category? category;
final Entry? entry;
const EntryTile({
this.category,
this.entry,
super.key,
});
@override
Widget build(BuildContext context) {
return ListTile(
title: Text(entry!.value.toString()),
subtitle: Text(intl.DateFormat('MM/dd/yy h:mm a').format(entry!.time)),
trailing: Row(
mainAxisSize: MainAxisSize.min,
children: [
TextButton(
child: const Text('Edit'),
onPressed: () {
showDialog<void>(
context: context,
builder: (context) {
return EditEntryDialog(category: category, entry: entry);
},
);
},
),
TextButton(
child: const Text('Delete'),
onPressed: () async {
final appState = Provider.of<AppState>(context, listen: false);
final scaffoldMessenger = ScaffoldMessenger.of(context);
final bool? shouldDelete = await showDialog<bool>(
context: context,
builder: (context) => AlertDialog(
title: const Text('Delete entry?'),
actions: [
TextButton(
child: const Text('Cancel'),
onPressed: () => Navigator.of(context).pop(false),
),
TextButton(
child: const Text('Delete'),
onPressed: () => Navigator.of(context).pop(true),
),
],
),
);
if (shouldDelete != null && shouldDelete) {
await appState.api!.entries.delete(category!.id!, entry!.id!);
scaffoldMessenger.showSnackBar(
const SnackBar(
content: Text('Entry deleted'),
),
);
}
},
),
],
),
);
}
}
| samples/experimental/web_dashboard/lib/src/pages/entries.dart/0 | {
"file_path": "samples/experimental/web_dashboard/lib/src/pages/entries.dart",
"repo_id": "samples",
"token_count": 2239
} | 1,220 |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>NSLocationWhenInUseUsageDescription</key>
<string>Finding Ice Cream stores near you</string>
<key>CFBundleDevelopmentRegion</key>
<string>$(DEVELOPMENT_LANGUAGE)</string>
<key>CFBundleDisplayName</key>
<string>Find Ice Cream</string>
<key>CFBundleExecutable</key>
<string>$(EXECUTABLE_NAME)</string>
<key>CFBundleIdentifier</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleInfoDictionaryVersion</key>
<string>6.0</string>
<key>CFBundleName</key>
<string>flutter_maps_firestore</string>
<key>CFBundlePackageType</key>
<string>APPL</string>
<key>CFBundleShortVersionString</key>
<string>$(FLUTTER_BUILD_NAME)</string>
<key>CFBundleSignature</key>
<string>????</string>
<key>CFBundleVersion</key>
<string>$(FLUTTER_BUILD_NUMBER)</string>
<key>LSRequiresIPhoneOS</key>
<true/>
<key>UILaunchStoryboardName</key>
<string>LaunchScreen</string>
<key>UIMainStoryboardFile</key>
<string>Main</string>
<key>UISupportedInterfaceOrientations</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>UISupportedInterfaceOrientations~ipad</key>
<array>
<string>UIInterfaceOrientationPortrait</string>
<string>UIInterfaceOrientationPortraitUpsideDown</string>
<string>UIInterfaceOrientationLandscapeLeft</string>
<string>UIInterfaceOrientationLandscapeRight</string>
</array>
<key>CADisableMinimumFrameDurationOnPhone</key>
<true/>
<key>UIApplicationSupportsIndirectInputEvents</key>
<true/>
</dict>
</plist>
| samples/flutter_maps_firestore/ios/Runner/Info.plist/0 | {
"file_path": "samples/flutter_maps_firestore/ios/Runner/Info.plist",
"repo_id": "samples",
"token_count": 681
} | 1,221 |
<?xml version="1.0" encoding="utf-8"?>
<!--
Google Play game services IDs.
TODO: When ready, update this file with actual IDs from Google Play.
You can download this file from Google Play Console,
in the Play Games Services section. The button is called "Get resources".
Details in README.txt.
-->
<resources>
<!--app_id-->
<string name="app_id" translatable="false">123456789012</string>
<!--package_name-->
<string name="package_name" translatable="false">name.of.your.game</string>
<!--leaderboard Something-->
<string name="leaderboard_highest_score" translatable="false">sOmEtHiNg</string>
</resources>
| samples/game_template/android/app/src/main/res/values/games-ids.xml/0 | {
"file_path": "samples/game_template/android/app/src/main/res/values/games-ids.xml",
"repo_id": "samples",
"token_count": 203
} | 1,222 |
// Copyright 2022, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
// Uncomment the following lines when enabling Firebase Crashlytics
// import 'dart:io';
// import 'package:firebase_core/firebase_core.dart';
// import 'package:firebase_crashlytics/firebase_crashlytics.dart';
// import 'package:flutter/foundation.dart';
// import 'firebase_options.dart';
import 'dart:developer' as dev;
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:go_router/go_router.dart';
import 'package:logging/logging.dart';
import 'package:provider/provider.dart';
import 'src/ads/ads_controller.dart';
import 'src/app_lifecycle/app_lifecycle.dart';
import 'src/audio/audio_controller.dart';
import 'src/games_services/games_services.dart';
import 'src/games_services/score.dart';
import 'src/in_app_purchase/in_app_purchase.dart';
import 'src/level_selection/level_selection_screen.dart';
import 'src/level_selection/levels.dart';
import 'src/main_menu/main_menu_screen.dart';
import 'src/play_session/play_session_screen.dart';
import 'src/player_progress/persistence/local_storage_player_progress_persistence.dart';
import 'src/player_progress/persistence/player_progress_persistence.dart';
import 'src/player_progress/player_progress.dart';
import 'src/settings/persistence/local_storage_settings_persistence.dart';
import 'src/settings/persistence/settings_persistence.dart';
import 'src/settings/settings.dart';
import 'src/settings/settings_screen.dart';
import 'src/style/my_transition.dart';
import 'src/style/palette.dart';
import 'src/style/snack_bar.dart';
import 'src/win_game/win_game_screen.dart';
Future<void> main() async {
// Subscribe to log messages.
Logger.root.onRecord.listen((record) {
dev.log(
record.message,
time: record.time,
level: record.level.value,
name: record.loggerName,
zone: record.zone,
error: record.error,
stackTrace: record.stackTrace,
);
});
WidgetsFlutterBinding.ensureInitialized();
// TODO: To enable Firebase Crashlytics, uncomment the following line.
// See the 'Crashlytics' section of the main README.md file for details.
// if (!kIsWeb && (Platform.isIOS || Platform.isAndroid)) {
// try {
// await Firebase.initializeApp(
// options: DefaultFirebaseOptions.currentPlatform,
// );
//
// FlutterError.onError = (errorDetails) {
// FirebaseCrashlytics.instance.recordFlutterFatalError(errorDetails);
// };
//
// // Pass all uncaught asynchronous errors
// // that aren't handled by the Flutter framework to Crashlytics.
// PlatformDispatcher.instance.onError = (error, stack) {
// FirebaseCrashlytics.instance.recordError(error, stack, fatal: true);
// return true;
// };
// } catch (e) {
// debugPrint("Firebase couldn't be initialized: $e");
// }
// }
_log.info('Going full screen');
SystemChrome.setEnabledSystemUIMode(
SystemUiMode.edgeToEdge,
);
// TODO: When ready, uncomment the following lines to enable integrations.
// Read the README for more info on each integration.
AdsController? adsController;
// if (!kIsWeb && (Platform.isIOS || Platform.isAndroid)) {
// /// Prepare the google_mobile_ads plugin so that the first ad loads
// /// faster. This can be done later or with a delay if startup
// /// experience suffers.
// adsController = AdsController(MobileAds.instance);
// adsController.initialize();
// }
GamesServicesController? gamesServicesController;
// if (!kIsWeb && (Platform.isIOS || Platform.isAndroid)) {
// gamesServicesController = GamesServicesController()
// // Attempt to log the player in.
// ..initialize();
// }
InAppPurchaseController? inAppPurchaseController;
// if (!kIsWeb && (Platform.isIOS || Platform.isAndroid)) {
// inAppPurchaseController = InAppPurchaseController(InAppPurchase.instance)
// // Subscribing to [InAppPurchase.instance.purchaseStream] as soon
// // as possible in order not to miss any updates.
// ..subscribe();
// // Ask the store what the player has bought already.
// inAppPurchaseController.restorePurchases();
// }
runApp(
MyApp(
settingsPersistence: LocalStorageSettingsPersistence(),
playerProgressPersistence: LocalStoragePlayerProgressPersistence(),
inAppPurchaseController: inAppPurchaseController,
adsController: adsController,
gamesServicesController: gamesServicesController,
),
);
}
Logger _log = Logger('main.dart');
class MyApp extends StatelessWidget {
static final _router = GoRouter(
routes: [
GoRoute(
path: '/',
builder: (context, state) =>
const MainMenuScreen(key: Key('main menu')),
routes: [
GoRoute(
path: 'play',
pageBuilder: (context, state) => buildMyTransition<void>(
key: ValueKey('play'),
child: const LevelSelectionScreen(
key: Key('level selection'),
),
color: context.watch<Palette>().backgroundLevelSelection,
),
routes: [
GoRoute(
path: 'session/:level',
pageBuilder: (context, state) {
final levelNumber =
int.parse(state.pathParameters['level']!);
final level = gameLevels
.singleWhere((e) => e.number == levelNumber);
return buildMyTransition<void>(
key: ValueKey('level'),
child: PlaySessionScreen(
level,
key: const Key('play session'),
),
color: context.watch<Palette>().backgroundPlaySession,
);
},
),
GoRoute(
path: 'won',
redirect: (context, state) {
if (state.extra == null) {
// Trying to navigate to a win screen without any data.
// Possibly by using the browser's back button.
return '/';
}
// Otherwise, do not redirect.
return null;
},
pageBuilder: (context, state) {
final map = state.extra! as Map<String, dynamic>;
final score = map['score'] as Score;
return buildMyTransition<void>(
key: ValueKey('won'),
child: WinGameScreen(
score: score,
key: const Key('win game'),
),
color: context.watch<Palette>().backgroundPlaySession,
);
},
)
]),
GoRoute(
path: 'settings',
builder: (context, state) =>
const SettingsScreen(key: Key('settings')),
),
]),
],
);
final PlayerProgressPersistence playerProgressPersistence;
final SettingsPersistence settingsPersistence;
final GamesServicesController? gamesServicesController;
final InAppPurchaseController? inAppPurchaseController;
final AdsController? adsController;
const MyApp({
required this.playerProgressPersistence,
required this.settingsPersistence,
required this.inAppPurchaseController,
required this.adsController,
required this.gamesServicesController,
super.key,
});
@override
Widget build(BuildContext context) {
return AppLifecycleObserver(
child: MultiProvider(
providers: [
ChangeNotifierProvider(
create: (context) {
var progress = PlayerProgress(playerProgressPersistence);
progress.getLatestFromStore();
return progress;
},
),
Provider<GamesServicesController?>.value(
value: gamesServicesController),
Provider<AdsController?>.value(value: adsController),
ChangeNotifierProvider<InAppPurchaseController?>.value(
value: inAppPurchaseController),
Provider<SettingsController>(
lazy: false,
create: (context) => SettingsController(
persistence: settingsPersistence,
)..loadStateFromPersistence(),
),
ProxyProvider2<SettingsController, ValueNotifier<AppLifecycleState>,
AudioController>(
// Ensures that the AudioController is created on startup,
// and not "only when it's needed", as is default behavior.
// This way, music starts immediately.
lazy: false,
create: (context) => AudioController()..initialize(),
update: (context, settings, lifecycleNotifier, audio) {
if (audio == null) throw ArgumentError.notNull();
audio.attachSettings(settings);
audio.attachLifecycleNotifier(lifecycleNotifier);
return audio;
},
dispose: (context, audio) => audio.dispose(),
),
Provider(
create: (context) => Palette(),
),
],
child: Builder(builder: (context) {
final palette = context.watch<Palette>();
return MaterialApp.router(
title: 'Flutter Demo',
theme: ThemeData.from(
colorScheme: ColorScheme.fromSeed(
seedColor: palette.darkPen,
background: palette.backgroundMain,
),
textTheme: TextTheme(
bodyMedium: TextStyle(
color: palette.ink,
),
),
),
routeInformationProvider: _router.routeInformationProvider,
routeInformationParser: _router.routeInformationParser,
routerDelegate: _router.routerDelegate,
scaffoldMessengerKey: scaffoldMessengerKey,
);
}),
),
);
}
}
| samples/game_template/lib/main.dart/0 | {
"file_path": "samples/game_template/lib/main.dart",
"repo_id": "samples",
"token_count": 4573
} | 1,223 |
// Copyright 2022, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
import 'package:logging/logging.dart' hide Level;
import 'package:provider/provider.dart';
import '../ads/ads_controller.dart';
import '../audio/audio_controller.dart';
import '../audio/sounds.dart';
import '../game_internals/level_state.dart';
import '../games_services/games_services.dart';
import '../games_services/score.dart';
import '../in_app_purchase/in_app_purchase.dart';
import '../level_selection/levels.dart';
import '../player_progress/player_progress.dart';
import '../style/confetti.dart';
import '../style/palette.dart';
class PlaySessionScreen extends StatefulWidget {
final GameLevel level;
const PlaySessionScreen(this.level, {super.key});
@override
State<PlaySessionScreen> createState() => _PlaySessionScreenState();
}
class _PlaySessionScreenState extends State<PlaySessionScreen> {
static final _log = Logger('PlaySessionScreen');
static const _celebrationDuration = Duration(milliseconds: 2000);
static const _preCelebrationDuration = Duration(milliseconds: 500);
bool _duringCelebration = false;
late DateTime _startOfPlay;
@override
Widget build(BuildContext context) {
final palette = context.watch<Palette>();
return MultiProvider(
providers: [
ChangeNotifierProvider(
create: (context) => LevelState(
goal: widget.level.difficulty,
onWin: _playerWon,
),
),
],
child: IgnorePointer(
ignoring: _duringCelebration,
child: Scaffold(
backgroundColor: palette.backgroundPlaySession,
body: Stack(
children: [
Center(
// This is the entirety of the "game".
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Align(
alignment: Alignment.centerRight,
child: InkResponse(
onTap: () => GoRouter.of(context).push('/settings'),
child: Image.asset(
'assets/images/settings.png',
semanticLabel: 'Settings',
),
),
),
const Spacer(),
Text('Drag the slider to ${widget.level.difficulty}%'
' or above!'),
Consumer<LevelState>(
builder: (context, levelState, child) => Slider(
label: 'Level Progress',
autofocus: true,
value: levelState.progress / 100,
onChanged: (value) =>
levelState.setProgress((value * 100).round()),
onChangeEnd: (value) => levelState.evaluate(),
),
),
const Spacer(),
Padding(
padding: const EdgeInsets.all(8.0),
child: SizedBox(
width: double.infinity,
child: FilledButton(
onPressed: () => GoRouter.of(context).go('/play'),
child: const Text('Back'),
),
),
),
],
),
),
SizedBox.expand(
child: Visibility(
visible: _duringCelebration,
child: IgnorePointer(
child: Confetti(
isStopped: !_duringCelebration,
),
),
),
),
],
),
),
),
);
}
@override
void initState() {
super.initState();
_startOfPlay = DateTime.now();
// Preload ad for the win screen.
final adsRemoved =
context.read<InAppPurchaseController?>()?.adRemoval.active ?? false;
if (!adsRemoved) {
final adsController = context.read<AdsController?>();
adsController?.preloadAd();
}
}
Future<void> _playerWon() async {
_log.info('Level ${widget.level.number} won');
final score = Score(
widget.level.number,
widget.level.difficulty,
DateTime.now().difference(_startOfPlay),
);
final playerProgress = context.read<PlayerProgress>();
playerProgress.setLevelReached(widget.level.number);
// Let the player see the game just after winning for a bit.
await Future<void>.delayed(_preCelebrationDuration);
if (!mounted) return;
setState(() {
_duringCelebration = true;
});
final audioController = context.read<AudioController>();
audioController.playSfx(SfxType.congrats);
final gamesServicesController = context.read<GamesServicesController?>();
if (gamesServicesController != null) {
// Award achievement.
if (widget.level.awardsAchievement) {
await gamesServicesController.awardAchievement(
android: widget.level.achievementIdAndroid!,
iOS: widget.level.achievementIdIOS!,
);
}
// Send score to leaderboard.
await gamesServicesController.submitLeaderboardScore(score);
}
/// Give the player some time to see the celebration animation.
await Future<void>.delayed(_celebrationDuration);
if (!mounted) return;
GoRouter.of(context).go('/play/won', extra: {'score': score});
}
}
| samples/game_template/lib/src/play_session/play_session_screen.dart/0 | {
"file_path": "samples/game_template/lib/src/play_session/play_session_screen.dart",
"repo_id": "samples",
"token_count": 2725
} | 1,224 |
// Copyright 2022, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
import 'package:provider/provider.dart';
import '../ads/ads_controller.dart';
import '../ads/banner_ad_widget.dart';
import '../games_services/score.dart';
import '../in_app_purchase/in_app_purchase.dart';
import '../style/palette.dart';
import '../style/responsive_screen.dart';
class WinGameScreen extends StatelessWidget {
final Score score;
const WinGameScreen({
super.key,
required this.score,
});
@override
Widget build(BuildContext context) {
final adsControllerAvailable = context.watch<AdsController?>() != null;
final adsRemoved =
context.watch<InAppPurchaseController?>()?.adRemoval.active ?? false;
final palette = context.watch<Palette>();
const gap = SizedBox(height: 10);
return Scaffold(
backgroundColor: palette.backgroundPlaySession,
body: ResponsiveScreen(
squarishMainArea: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
if (adsControllerAvailable && !adsRemoved) ...[
const Expanded(
child: Center(
child: BannerAdWidget(),
),
),
],
gap,
const Center(
child: Text(
'You won!',
style: TextStyle(fontFamily: 'Permanent Marker', fontSize: 50),
),
),
gap,
Center(
child: Text(
'Score: ${score.score}\n'
'Time: ${score.formattedTime}',
style: const TextStyle(
fontFamily: 'Permanent Marker', fontSize: 20),
),
),
],
),
rectangularMenuArea: FilledButton(
onPressed: () {
GoRouter.of(context).go('/play');
},
child: const Text('Continue'),
),
),
);
}
}
| samples/game_template/lib/src/win_game/win_game_screen.dart/0 | {
"file_path": "samples/game_template/lib/src/win_game/win_game_screen.dart",
"repo_id": "samples",
"token_count": 1003
} | 1,225 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| samples/game_template/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "samples/game_template/macos/Runner/Configs/Release.xcconfig",
"repo_id": "samples",
"token_count": 32
} | 1,226 |
#import "GeneratedPluginRegistrant.h"
| samples/google_maps/ios/Runner/Runner-Bridging-Header.h/0 | {
"file_path": "samples/google_maps/ios/Runner/Runner-Bridging-Header.h",
"repo_id": "samples",
"token_count": 13
} | 1,227 |
# infinite_list
A Flutter sample app that shows an implementation of the "infinite list" UX pattern. That is,
a list is shown to the user as if it was continuous although it is internally paginated.
This is a common feature of mobile apps, from shopping catalogs through search engines
to social media clients.
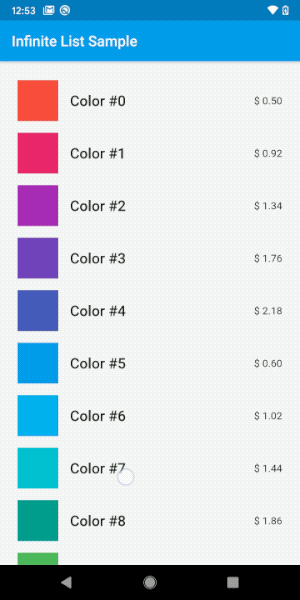
This particular sample uses the [Provider][] package but any other state management approach
would do.
[Provider]: https://pub.dev/packages/provider
## Goals for this sample
* Show how UI code can be "shielded" from complex asynchrony and pagination logic using
a `ChangeNotifier`.
* Illustrate use of `Selector` from the Provider package.
## Questions/issues
If you have a general question about Flutter, the best places to go are:
* [Flutter documentation](https://flutter.dev/)
* [StackOverflow](https://stackoverflow.com/questions/tagged/flutter)
If you run into an issue with the sample itself, please
[file an issue](https://github.com/flutter/samples/issues).
| samples/infinite_list/README.md/0 | {
"file_path": "samples/infinite_list/README.md",
"repo_id": "samples",
"token_count": 322
} | 1,228 |
// Copyright 2020 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class Item {
final Color color;
final int price;
final String name;
Item({
required this.color,
required this.name,
required this.price,
});
Item.loading() : this(color: Colors.grey, name: '...', price: 0);
bool get isLoading => name == '...';
}
| samples/infinite_list/lib/src/api/item.dart/0 | {
"file_path": "samples/infinite_list/lib/src/api/item.dart",
"repo_id": "samples",
"token_count": 150
} | 1,229 |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="17154" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" colorMatched="YES" initialViewController="BYZ-38-t0r">
<device id="retina6_1" orientation="portrait" appearance="light"/>
<dependencies>
<deployment identifier="iOS"/>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="17124"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--Flutter View Controller-->
<scene sceneID="tne-QT-ifu">
<objects>
<viewController id="BYZ-38-t0r" customClass="FlutterViewController" sceneMemberID="viewController">
<layoutGuides>
<viewControllerLayoutGuide type="top" id="y3c-jy-aDJ"/>
<viewControllerLayoutGuide type="bottom" id="wfy-db-euE"/>
</layoutGuides>
<view key="view" contentMode="scaleToFill" id="8bC-Xf-vdC">
<rect key="frame" x="0.0" y="0.0" width="414" height="896"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<color key="backgroundColor" red="1" green="1" blue="1" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
</view>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="dkx-z0-nzr" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="86" y="-22"/>
</scene>
</scenes>
</document>
| samples/ios_app_clip/ios/Runner/Base.lproj/Main.storyboard/0 | {
"file_path": "samples/ios_app_clip/ios/Runner/Base.lproj/Main.storyboard",
"repo_id": "samples",
"token_count": 820
} | 1,230 |
name: isolate_example
description: A Flutter sample to demonstrate isolates
version: 1.0.0+1
publish_to: none
environment:
sdk: ^3.2.0
dependencies:
flutter:
sdk: flutter
provider: ^6.0.2
window_size:
git:
url: https://github.com/google/flutter-desktop-embedding.git
path: plugins/window_size
dev_dependencies:
analysis_defaults:
path: ../analysis_defaults
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
| samples/isolate_example/pubspec.yaml/0 | {
"file_path": "samples/isolate_example/pubspec.yaml",
"repo_id": "samples",
"token_count": 184
} | 1,231 |
// Copyright 2021, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
import 'dart:io' show Platform;
import 'package:flutter/foundation.dart' show kIsWeb;
import 'package:flutter/material.dart';
import 'package:url_strategy/url_strategy.dart';
import 'package:window_size/window_size.dart';
import 'src/app.dart';
void main() {
// Use package:url_strategy until this pull request is released:
// https://github.com/flutter/flutter/pull/77103
// Use to setHashUrlStrategy() to use "/#/" in the address bar (default). Use
// setPathUrlStrategy() to use the path. You may need to configure your web
// server to redirect all paths to index.html.
//
// On mobile platforms, both functions are no-ops.
setHashUrlStrategy();
// setPathUrlStrategy();
setupWindow();
runApp(const Bookstore());
}
const double windowWidth = 480;
const double windowHeight = 854;
void setupWindow() {
if (!kIsWeb && (Platform.isWindows || Platform.isLinux || Platform.isMacOS)) {
WidgetsFlutterBinding.ensureInitialized();
setWindowTitle('Navigation and routing');
setWindowMinSize(const Size(windowWidth, windowHeight));
setWindowMaxSize(const Size(windowWidth, windowHeight));
getCurrentScreen().then((screen) {
setWindowFrame(Rect.fromCenter(
center: screen!.frame.center,
width: windowWidth,
height: windowHeight,
));
});
}
}
| samples/navigation_and_routing/lib/main.dart/0 | {
"file_path": "samples/navigation_and_routing/lib/main.dart",
"repo_id": "samples",
"token_count": 493
} | 1,232 |
// Copyright 2021, the Flutter project authors. Please see the AUTHORS file
// for details. All rights reserved. Use of this source code is governed by a
// BSD-style license that can be found in the LICENSE file.
import 'package:flutter/material.dart';
class FadeTransitionPage<T> extends Page<T> {
final Widget child;
final Duration duration;
const FadeTransitionPage({
super.key,
required this.child,
this.duration = const Duration(milliseconds: 300),
});
@override
Route<T> createRoute(BuildContext context) =>
PageBasedFadeTransitionRoute<T>(this);
}
class PageBasedFadeTransitionRoute<T> extends PageRoute<T> {
final FadeTransitionPage<T> _page;
PageBasedFadeTransitionRoute(this._page) : super(settings: _page);
@override
Color? get barrierColor => null;
@override
String? get barrierLabel => null;
@override
Duration get transitionDuration => _page.duration;
@override
bool get maintainState => true;
@override
Widget buildPage(BuildContext context, Animation<double> animation,
Animation<double> secondaryAnimation) {
var curveTween = CurveTween(curve: Curves.easeIn);
return FadeTransition(
opacity: animation.drive(curveTween),
child: (settings as FadeTransitionPage).child,
);
}
@override
Widget buildTransitions(BuildContext context, Animation<double> animation,
Animation<double> secondaryAnimation, Widget child) =>
child;
}
| samples/navigation_and_routing/lib/src/widgets/fade_transition_page.dart/0 | {
"file_path": "samples/navigation_and_routing/lib/src/widgets/fade_transition_page.dart",
"repo_id": "samples",
"token_count": 466
} | 1,233 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| samples/navigation_and_routing/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "samples/navigation_and_routing/macos/Runner/Configs/Release.xcconfig",
"repo_id": "samples",
"token_count": 32
} | 1,234 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package dev.flutter.platform_channels
import android.hardware.Sensor
import android.hardware.SensorEvent
import android.hardware.SensorEventListener
import android.hardware.SensorManager
import io.flutter.plugin.common.EventChannel
class AccelerometerStreamHandler(sManager: SensorManager, s: Sensor) : EventChannel.StreamHandler, SensorEventListener {
private val sensorManager: SensorManager = sManager
private val accelerometerSensor: Sensor = s
private lateinit var eventSink: EventChannel.EventSink
override fun onListen(arguments: Any?, events: EventChannel.EventSink?) {
if (events != null) {
eventSink = events
sensorManager.registerListener(this, accelerometerSensor, SensorManager.SENSOR_DELAY_UI)
}
}
override fun onCancel(arguments: Any?) {
sensorManager.unregisterListener(this)
}
override fun onAccuracyChanged(sensor: Sensor?, accuracy: Int) {}
override fun onSensorChanged(sensorEvent: SensorEvent?) {
if (sensorEvent != null) {
val axisValues = listOf(sensorEvent.values[0], sensorEvent.values[1], sensorEvent.values[2])
eventSink.success(axisValues)
} else {
eventSink.error("DATA_UNAVAILABLE", "Cannot get accelerometer data", null)
}
}
}
| samples/platform_channels/android/app/src/main/kotlin/dev/flutter/platform_channels/AccelerometerStreamHandler.kt/0 | {
"file_path": "samples/platform_channels/android/app/src/main/kotlin/dev/flutter/platform_channels/AccelerometerStreamHandler.kt",
"repo_id": "samples",
"token_count": 503
} | 1,235 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
/// This class includes implementation of two platform methods [increment],
/// and [decrement] which are used to increment and decrement value
/// of count respectively.
class Counter {
/// Creates a [MethodChannel] with the specified name to invoke platform method.
/// In order to communicate across platforms, the name of MethodChannel
/// should be same on native and dart side.
static MethodChannel methodChannel = const MethodChannel('methodChannelDemo');
/// This method is responsible to increment and return the value of count.
static Future<int> increment({required int counterValue}) async {
final result = await methodChannel
.invokeMethod<int>('increment', {'count': counterValue});
return result!;
}
/// This method is responsible to decrement and return the value of count.
static Future<int> decrement({required int counterValue}) async {
final result = await methodChannel
.invokeMethod<int>('decrement', {'count': counterValue});
return result!;
}
}
| samples/platform_channels/lib/src/counter_method_channel.dart/0 | {
"file_path": "samples/platform_channels/lib/src/counter_method_channel.dart",
"repo_id": "samples",
"token_count": 321
} | 1,236 |
# Platform Design
Instead of transliterating widgets one by one between Cupertino and Material,
Android and iOS apps often follow different information architecture patterns
that require some design decisions.
This sample project shows a Flutter app that maximizes application code reuse
while adhering to different design patterns on Android and iOS. On
Android, it uses Material's [lateral navigation](https://material.io/design/navigation/understanding-navigation.html#types-of-navigation)
based on a drawer and on iOS, it adheres to Apple Human Interface Guideline's
[flat navigation](https://developer.apple.com/design/human-interface-guidelines/ios/app-architecture/navigation/)
by using a bottom tab bar.
Visually, the app presents platform-agnostic content surrounded by
platform-specific 'chrome'.
# Preview

See https://youtu.be/svhbbFZg1IA for a longer non-gif format.
# Features
## Home
Defines the top level navigation structure of the app and shows the contents
of the songs tab on launch.
### Android
* Uses the drawer paradigm on the root page.
### iOS
* Uses bottom tab bars with parallel navigation stacks.
## Songs feed tab
Shows platform-agnostic cards that is tappable and that performs a hero
transition on top of the platform native page transitions.
Both platforms also show a button in their app/nav bar to toggle the platform.
### Android
* Android uses a static pull-to-refresh pattern with an additional refresh
button in the app bar.
* The song details page must be popped in order to change tabs on Android.
### iOS
* The iOS songs tab uses a scrollable iOS 11 large title style navigation bar.
* iOS uses an overscrolling pull-to-refresh pattern.
* On iOS, parallel tabs are always accessible and the songs tab's navigation
stack is preserved when changing tabs.
## News Tab
Shows platform-agnostic news boxes.
### Android
* The news tab always appears on top of the songs tab when summoned from the
drawer.
### iOS
* The news tab appears instead of the songs tab on iOS when switching tabs from
the tab bar.
## Profile Tab
Shows a number of user preferences.
### Android
* The profile tab appears on top of the songs tab on Android.
* Has tappable preference cards which shows a multiple-choice dialog on Android.
* The log out button shows a 2 button dialog on Android.
### iOS
* The profile tab appears instead of the songs tab on iOS.
* Has tappable preference cards which shows a picker on iOS.
* The log out button shows a 3 choice action sheet on iOS.
## Settings Tab
Shows a number of app settings via Material switches which auto adapt to the
platform.
### Android
* The settings is directly available in the drawer on Android since a Material
Design drawer can fit many tabs.
### iOS
* The settings is accessible from a button inside the profile tab's nav bar on
iOS. This is a common pattern since there are conventionally more items in the
drawer than there are tabs.
* On iOS, the settings page is shown as a full screen dialog instead of a tab
in the tab scaffold.
| samples/platform_design/README.md/0 | {
"file_path": "samples/platform_design/README.md",
"repo_id": "samples",
"token_count": 782
} | 1,237 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'settings_tab.dart';
import 'widgets.dart';
class ProfileTab extends StatelessWidget {
static const title = 'Profile';
static const androidIcon = Icon(Icons.person);
static const iosIcon = Icon(CupertinoIcons.profile_circled);
const ProfileTab({super.key});
Widget _buildBody(BuildContext context) {
return SafeArea(
child: Padding(
padding: const EdgeInsets.all(24.0),
child: Column(
children: [
const Padding(
padding: EdgeInsets.all(8),
child: Center(
child: Text(
'😼',
style: TextStyle(
fontSize: 80,
decoration: TextDecoration.none,
),
),
),
),
const PreferenceCard(
header: 'MY INTENSITY PREFERENCE',
content: '🔥',
preferenceChoices: [
'Super heavy',
'Dial it to 11',
"Head bangin'",
'1000W',
'My neighbor hates me',
],
),
const PreferenceCard(
header: 'CURRENT MOOD',
content: '🤘🏾🚀',
preferenceChoices: [
'Over the moon',
'Basking in sunlight',
'Hello fellow Martians',
'Into the darkness',
],
),
Expanded(
child: Container(),
),
const LogOutButton(),
],
),
),
);
}
// ===========================================================================
// Non-shared code below because on iOS, the settings tab is nested inside of
// the profile tab as a button in the nav bar.
// ===========================================================================
Widget _buildAndroid(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(title),
),
body: _buildBody(context),
);
}
Widget _buildIos(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
trailing: CupertinoButton(
padding: EdgeInsets.zero,
child: SettingsTab.iosIcon,
onPressed: () {
// This pushes the settings page as a full page modal dialog on top
// of the tab bar and everything.
Navigator.of(context, rootNavigator: true).push<void>(
CupertinoPageRoute(
title: SettingsTab.title,
fullscreenDialog: true,
builder: (context) => const SettingsTab(),
),
);
},
),
),
child: _buildBody(context),
);
}
@override
Widget build(context) {
return PlatformWidget(
androidBuilder: _buildAndroid,
iosBuilder: _buildIos,
);
}
}
class PreferenceCard extends StatelessWidget {
const PreferenceCard({
required this.header,
required this.content,
required this.preferenceChoices,
super.key,
});
final String header;
final String content;
final List<String> preferenceChoices;
@override
Widget build(context) {
return PressableCard(
color: Colors.green,
flattenAnimation: const AlwaysStoppedAnimation(0),
child: Stack(
children: [
SizedBox(
height: 120,
width: 250,
child: Padding(
padding: const EdgeInsets.only(top: 40),
child: Center(
child: Text(
content,
style: const TextStyle(fontSize: 48),
),
),
),
),
Positioned(
top: 0,
left: 0,
right: 0,
child: Container(
color: Colors.black12,
height: 40,
padding: const EdgeInsets.only(left: 12),
alignment: Alignment.centerLeft,
child: Text(
header,
style: const TextStyle(
color: Colors.white,
fontSize: 14,
fontWeight: FontWeight.w600,
),
),
),
),
],
),
onPressed: () {
showChoices(context, preferenceChoices);
},
);
}
}
class LogOutButton extends StatelessWidget {
static const _logoutMessage = Text(
"You can't actually log out! This is just a demo of how alerts work.");
const LogOutButton({super.key});
// ===========================================================================
// Non-shared code below because this tab shows different interfaces. On
// Android, it's showing an alert dialog with 2 buttons and on iOS,
// it's showing an action sheet with 3 choices.
//
// This is a design choice and you may want to do something different in your
// app.
// ===========================================================================
Widget _buildAndroid(BuildContext context) {
return ElevatedButton(
child: const Text('LOG OUT', style: TextStyle(color: Colors.red)),
onPressed: () {
// You should do something with the result of the dialog prompt in a
// real app but this is just a demo.
showDialog<void>(
context: context,
builder: (context) {
return AlertDialog(
title: const Text('Log out?'),
content: _logoutMessage,
actions: [
TextButton(
child: const Text('Got it'),
onPressed: () => Navigator.pop(context),
),
TextButton(
child: const Text('Cancel'),
onPressed: () => Navigator.pop(context),
),
],
);
},
);
},
);
}
Widget _buildIos(BuildContext context) {
return CupertinoButton(
color: CupertinoColors.destructiveRed,
child: const Text('Log out'),
onPressed: () {
// You should do something with the result of the action sheet prompt
// in a real app but this is just a demo.
showCupertinoModalPopup<void>(
context: context,
builder: (context) {
return CupertinoActionSheet(
title: const Text('Log out?'),
message: _logoutMessage,
actions: [
CupertinoActionSheetAction(
isDestructiveAction: true,
onPressed: () => Navigator.pop(context),
child: const Text('Reprogram the night man'),
),
CupertinoActionSheetAction(
child: const Text('Got it'),
onPressed: () => Navigator.pop(context),
),
],
cancelButton: CupertinoActionSheetAction(
isDefaultAction: true,
onPressed: () => Navigator.pop(context),
child: const Text('Cancel'),
),
);
},
);
},
);
}
@override
Widget build(context) {
return PlatformWidget(
androidBuilder: _buildAndroid,
iosBuilder: _buildIos,
);
}
}
| samples/platform_design/lib/profile_tab.dart/0 | {
"file_path": "samples/platform_design/lib/profile_tab.dart",
"repo_id": "samples",
"token_count": 3642
} | 1,238 |
org.gradle.jvmargs=-Xmx4G
android.useAndroidX=true
android.enableJetifier=true
| samples/provider_shopper/android/gradle.properties/0 | {
"file_path": "samples/provider_shopper/android/gradle.properties",
"repo_id": "samples",
"token_count": 30
} | 1,239 |
// Copyright 2019 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:provider_shopper/models/catalog.dart';
class CartModel extends ChangeNotifier {
/// The private field backing [catalog].
late CatalogModel _catalog;
/// Internal, private state of the cart. Stores the ids of each item.
final List<int> _itemIds = [];
/// The current catalog. Used to construct items from numeric ids.
CatalogModel get catalog => _catalog;
set catalog(CatalogModel newCatalog) {
_catalog = newCatalog;
// Notify listeners, in case the new catalog provides information
// different from the previous one. For example, availability of an item
// might have changed.
notifyListeners();
}
/// List of items in the cart.
List<Item> get items => _itemIds.map((id) => _catalog.getById(id)).toList();
/// The current total price of all items.
int get totalPrice =>
items.fold(0, (total, current) => total + current.price);
/// Adds [item] to cart. This is the only way to modify the cart from outside.
void add(Item item) {
_itemIds.add(item.id);
// This line tells [Model] that it should rebuild the widgets that
// depend on it.
notifyListeners();
}
void remove(Item item) {
_itemIds.remove(item.id);
// Don't forget to tell dependent widgets to rebuild _every time_
// you change the model.
notifyListeners();
}
}
| samples/provider_shopper/lib/models/cart.dart/0 | {
"file_path": "samples/provider_shopper/lib/models/cart.dart",
"repo_id": "samples",
"token_count": 472
} | 1,240 |
import 'package:flutter_test/flutter_test.dart';
import 'package:simple_shader/main.dart';
void main() {
testWidgets('Smoke test', (tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(const MyApp());
});
}
| samples/simple_shader/test/widget_test.dart/0 | {
"file_path": "samples/simple_shader/test/widget_test.dart",
"repo_id": "samples",
"token_count": 90
} | 1,241 |
name: simplistic_calculator
description: A new Flutter project.
publish_to: 'none'
version: 1.0.0+1
environment:
sdk: ^3.2.0
dependencies:
auto_size_text: ^3.0.0
cupertino_icons: ^1.0.2
fluent_ui: ^4.6.0
fluentui_system_icons: ^1.1.190
flutter:
sdk: flutter
flutter_layout_grid: ^2.0.1
flutter_riverpod: ^2.0.2
math_expressions: ^2.3.0
window_size:
git:
url: https://github.com/google/flutter-desktop-embedding
path: plugins/window_size
dev_dependencies:
analysis_defaults:
path: ../analysis_defaults
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
| samples/simplistic_calculator/pubspec.yaml/0 | {
"file_path": "samples/simplistic_calculator/pubspec.yaml",
"repo_id": "samples",
"token_count": 272
} | 1,242 |
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'app_state_manager.dart';
class TextEditingDeltaHistoryView extends StatelessWidget {
const TextEditingDeltaHistoryView({super.key});
List<Widget> _buildTextEditingDeltaHistoryViews(
List<TextEditingDelta> textEditingDeltas) {
List<Widget> textEditingDeltaViews = [];
for (final TextEditingDelta delta in textEditingDeltas) {
final TextEditingDeltaView deltaView;
if (delta is TextEditingDeltaInsertion) {
deltaView = TextEditingDeltaView(
deltaType: 'Insertion',
deltaText: delta.textInserted,
deltaRange: TextRange.collapsed(delta.insertionOffset),
newSelection: delta.selection,
newComposing: delta.composing,
);
} else if (delta is TextEditingDeltaDeletion) {
deltaView = TextEditingDeltaView(
deltaType: 'Deletion',
deltaText: delta.textDeleted,
deltaRange: delta.deletedRange,
newSelection: delta.selection,
newComposing: delta.composing,
);
} else if (delta is TextEditingDeltaReplacement) {
deltaView = TextEditingDeltaView(
deltaType: 'Replacement',
deltaText: delta.replacementText,
deltaRange: delta.replacedRange,
newSelection: delta.selection,
newComposing: delta.composing,
);
} else if (delta is TextEditingDeltaNonTextUpdate) {
deltaView = TextEditingDeltaView(
deltaType: 'NonTextUpdate',
deltaText: '',
deltaRange: TextRange.empty,
newSelection: delta.selection,
newComposing: delta.composing,
);
} else {
deltaView = const TextEditingDeltaView(
deltaType: 'Error',
deltaText: 'Error',
deltaRange: TextRange.empty,
newSelection: TextRange.empty,
newComposing: TextRange.empty,
);
}
textEditingDeltaViews.add(deltaView);
}
return textEditingDeltaViews.reversed.toList();
}
Widget _buildTextEditingDeltaViewHeader() {
return Padding(
padding: const EdgeInsets.symmetric(horizontal: 35.0, vertical: 10.0),
child: Row(
children: [
Expanded(
child: Tooltip(
message: 'The type of text input that is occurring.'
' Check out the documentation for TextEditingDelta for more information.',
child: _buildTextEditingDeltaViewHeading('Delta Type'),
),
),
Expanded(
child: Tooltip(
message: 'The text that is being inserted or deleted',
child: _buildTextEditingDeltaViewHeading('Delta Text'),
),
),
Expanded(
child: Tooltip(
message:
'The offset in the text where the text input is occurring.',
child: _buildTextEditingDeltaViewHeading('Delta Offset'),
),
),
Expanded(
child: Tooltip(
message:
'The new text selection range after the text input has occurred.',
child: _buildTextEditingDeltaViewHeading('New Selection'),
),
),
Expanded(
child: Tooltip(
message:
'The new composing range after the text input has occurred.',
child: _buildTextEditingDeltaViewHeading('New Composing'),
),
),
],
),
);
}
Widget _buildTextEditingDeltaViewHeading(String text) {
return Text(
text,
style: const TextStyle(
fontWeight: FontWeight.w600,
decoration: TextDecoration.underline,
),
);
}
@override
Widget build(BuildContext context) {
final AppStateManager manager = AppStateManager.of(context);
return Column(
children: [
_buildTextEditingDeltaViewHeader(),
Expanded(
child: ListView.separated(
padding: const EdgeInsets.symmetric(horizontal: 35.0),
itemBuilder: (context, index) {
return _buildTextEditingDeltaHistoryViews(
manager.appState.textEditingDeltaHistory)[index];
},
itemCount: manager.appState.textEditingDeltaHistory.length,
separatorBuilder: (context, index) {
return const SizedBox(height: 2.0);
},
),
),
const SizedBox(height: 10),
],
);
}
}
class TextEditingDeltaView extends StatelessWidget {
const TextEditingDeltaView({
super.key,
required this.deltaType,
required this.deltaText,
required this.deltaRange,
required this.newSelection,
required this.newComposing,
});
final String deltaType;
final String deltaText;
final TextRange deltaRange;
final TextRange newSelection;
final TextRange newComposing;
@override
Widget build(BuildContext context) {
late final Color rowColor;
switch (deltaType) {
case 'Insertion':
rowColor = Colors.greenAccent.shade100;
case 'Deletion':
rowColor = Colors.redAccent.shade100;
case 'Replacement':
rowColor = Colors.yellowAccent.shade100;
case 'NonTextUpdate':
rowColor = Colors.blueAccent.shade100;
default:
rowColor = Colors.white;
}
return Container(
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(4.0)),
color: rowColor,
),
padding: const EdgeInsets.only(top: 4.0, bottom: 4.0, left: 8.0),
child: Row(
children: [
Expanded(child: Text(deltaType)),
Expanded(child: Text(deltaText)),
Expanded(child: Text('(${deltaRange.start}, ${deltaRange.end})')),
Expanded(child: Text('(${newSelection.start}, ${newSelection.end})')),
Expanded(child: Text('(${newComposing.start}, ${newComposing.end})')),
],
),
);
}
}
| samples/simplistic_editor/lib/text_editing_delta_history_view.dart/0 | {
"file_path": "samples/simplistic_editor/lib/text_editing_delta_history_view.dart",
"repo_id": "samples",
"token_count": 2703
} | 1,243 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:provider/provider.dart';
import 'package:testing_app/models/favorites.dart';
import 'package:testing_app/screens/favorites.dart';
late Favorites favoritesList;
Widget createFavoritesScreen() => ChangeNotifierProvider<Favorites>(
create: (context) {
favoritesList = Favorites();
return favoritesList;
},
child: const MaterialApp(
home: FavoritesPage(),
),
);
void addItems() {
for (var i = 0; i < 5; i++) {
favoritesList.add(i);
}
}
void main() {
group('Favorites Page Widget Tests', () {
testWidgets('Test if Placeholder shows in case of empty list',
(tester) async {
await tester.pumpWidget(createFavoritesScreen());
// Verify if the placeholder text shows up.
expect(find.text('No favorites added.'), findsOneWidget);
});
testWidgets('Test if ListView shows up', (tester) async {
await tester.pumpWidget(createFavoritesScreen());
addItems();
await tester.pumpAndSettle();
// Verify if ListView shows up.
expect(find.byType(ListView), findsOneWidget);
});
testWidgets('Testing Remove Button', (tester) async {
await tester.pumpWidget(createFavoritesScreen());
addItems();
await tester.pumpAndSettle();
// Get the total number of items available.
final totalItems = tester.widgetList(find.byIcon(Icons.close)).length;
// Remove one item.
await tester.tap(find.byIcon(Icons.close).first);
await tester.pumpAndSettle();
// Check if removed properly.
expect(
tester.widgetList(find.byIcon(Icons.close)).length,
lessThan(totalItems),
);
// Verify if the appropriate message is shown.
expect(find.text('Removed from favorites.'), findsOneWidget);
});
});
}
| samples/testing_app/test/favorites_test.dart/0 | {
"file_path": "samples/testing_app/test/favorites_test.dart",
"repo_id": "samples",
"token_count": 766
} | 1,244 |
#!/bin/bash
set -e
DIR="${BASH_SOURCE%/*}"
source "$DIR/flutter_ci_script_shared.sh"
flutter doctor -v
declare -ar PROJECT_NAMES=(
"add_to_app/android_view/flutter_module_using_plugin"
"add_to_app/books/flutter_module_books"
"add_to_app/fullscreen/flutter_module"
"add_to_app/multiple_flutters/multiple_flutters_module"
"add_to_app/plugin/flutter_module_using_plugin"
"add_to_app/prebuilt_module/flutter_module"
"analysis_defaults"
"android_splash_screen"
"animations"
"background_isolate_channels"
"code_sharing/client"
"code_sharing/server"
"code_sharing/shared"
"context_menus"
"deeplink_store_example"
"desktop_photo_search/fluent_ui"
"desktop_photo_search/material"
"experimental/federated_plugin/federated_plugin"
"experimental/federated_plugin/federated_plugin/example"
"experimental/federated_plugin/federated_plugin_macos"
"experimental/federated_plugin/federated_plugin_platform_interface"
"experimental/federated_plugin/federated_plugin_web"
"experimental/federated_plugin/federated_plugin_windows"
"experimental/linting_tool"
"experimental/pedometer"
"experimental/pedometer/example"
"experimental/varfont_shader_puzzle"
"experimental/web_dashboard"
"flutter_maps_firestore"
"form_app"
"game_template"
"google_maps"
"infinite_list"
"ios_app_clip"
"isolate_example"
"material_3_demo"
"navigation_and_routing"
"place_tracker"
"platform_channels"
"platform_design"
"platform_view_swift"
"provider_counter"
"provider_shopper"
"simple_shader"
"simplistic_calculator"
"simplistic_editor"
"testing_app"
"veggieseasons"
"web_embedding/element_embedding_demo"
"web/_tool"
"web/samples_index"
)
ci_projects "stable" "${PROJECT_NAMES[@]}"
echo "-- Success --"
| samples/tool/flutter_ci_script_stable.sh/0 | {
"file_path": "samples/tool/flutter_ci_script_stable.sh",
"repo_id": "samples",
"token_count": 797
} | 1,245 |
// Copyright 2018 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/cupertino.dart';
import 'package:shared_preferences/shared_preferences.dart';
import 'package:veggieseasons/data/veggie.dart';
/// A model class that mirrors the options in [SettingsScreen] and stores data
/// in shared preferences.
class Preferences extends ChangeNotifier {
// Keys to use with shared preferences.
static const _caloriesKey = 'calories';
static const _preferredCategoriesKey = 'preferredCategories';
// Indicates whether a call to [_loadFromSharedPrefs] is in progress;
Future<void>? _loading;
int _desiredCalories = 2000;
final Set<VeggieCategory> _preferredCategories = <VeggieCategory>{};
Future<int> get desiredCalories async {
await _loading;
return _desiredCalories;
}
Future<Set<VeggieCategory>> get preferredCategories async {
await _loading;
return Set.from(_preferredCategories);
}
Future<void> addPreferredCategory(VeggieCategory category) async {
_preferredCategories.add(category);
await _saveToSharedPrefs();
notifyListeners();
}
Future<void> removePreferredCategory(VeggieCategory category) async {
_preferredCategories.remove(category);
await _saveToSharedPrefs();
notifyListeners();
}
Future<void> setDesiredCalories(int calories) async {
_desiredCalories = calories;
await _saveToSharedPrefs();
notifyListeners();
}
Future<void> restoreDefaults() async {
final prefs = await SharedPreferences.getInstance();
await prefs.clear();
load();
}
void load() {
_loading = _loadFromSharedPrefs();
}
Future<void> _saveToSharedPrefs() async {
final prefs = await SharedPreferences.getInstance();
await prefs.setInt(_caloriesKey, _desiredCalories);
// Store preferred categories as a comma-separated string containing their
// indices.
await prefs.setString(_preferredCategoriesKey,
_preferredCategories.map((c) => c.index.toString()).join(','));
}
Future<void> _loadFromSharedPrefs() async {
final prefs = await SharedPreferences.getInstance();
_desiredCalories = prefs.getInt(_caloriesKey) ?? 2000;
_preferredCategories.clear();
final names = prefs.getString(_preferredCategoriesKey);
if (names != null && names.isNotEmpty) {
for (final name in names.split(',')) {
final index = int.tryParse(name) ?? -1;
_preferredCategories.add(VeggieCategory.values[index]);
}
}
notifyListeners();
}
}
| samples/veggieseasons/lib/data/preferences.dart/0 | {
"file_path": "samples/veggieseasons/lib/data/preferences.dart",
"repo_id": "samples",
"token_count": 875
} | 1,246 |
// Copyright 2018 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:go_router/go_router.dart';
import 'package:veggieseasons/data/veggie.dart';
import 'package:veggieseasons/styles.dart';
class ZoomClipAssetImage extends StatelessWidget {
const ZoomClipAssetImage({
required this.zoom,
this.height,
this.width,
required this.imageAsset,
super.key,
});
final double zoom;
final double? height;
final double? width;
final String imageAsset;
@override
Widget build(BuildContext context) {
return Container(
height: height,
width: width,
alignment: Alignment.center,
child: ClipRRect(
borderRadius: BorderRadius.circular(10),
child: OverflowBox(
maxHeight: height! * zoom,
maxWidth: width! * zoom,
child: Image.asset(
imageAsset,
fit: BoxFit.fill,
),
),
),
);
}
}
class VeggieHeadline extends StatelessWidget {
final Veggie veggie;
const VeggieHeadline(this.veggie, {super.key});
List<Widget> _buildSeasonDots(List<Season> seasons) {
var widgets = <Widget>[];
for (var season in seasons) {
widgets.add(const SizedBox(width: 4));
widgets.add(
Container(
height: 10,
width: 10,
decoration: BoxDecoration(
color: Styles.seasonColors[season],
borderRadius: BorderRadius.circular(5),
),
),
);
}
return widgets;
}
@override
Widget build(BuildContext context) {
final themeData = CupertinoTheme.of(context);
return GestureDetector(
onTap: () {
// GoRouter does not support relative routes,
// so navigate to the absolute route, which can be either
// `/favorites/details/${veggie.id}` or `/search/details/${veggie.id}`
// see https://github.com/flutter/flutter/issues/108177
context.go('${GoRouter.of(context).location}/details/${veggie.id}');
},
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
ZoomClipAssetImage(
imageAsset: veggie.imageAssetPath,
zoom: 2.4,
height: 72,
width: 72,
),
const SizedBox(width: 8),
Flexible(
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Row(
children: [
Text(
veggie.name,
style: Styles.headlineName(themeData),
),
..._buildSeasonDots(veggie.seasons),
],
),
Text(
veggie.shortDescription,
style: themeData.textTheme.textStyle,
),
],
),
)
],
),
);
}
}
| samples/veggieseasons/lib/widgets/veggie_headline.dart/0 | {
"file_path": "samples/veggieseasons/lib/widgets/veggie_headline.dart",
"repo_id": "samples",
"token_count": 1506
} | 1,247 |
// Copyright 2020 The Flutter team. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file
import 'dart:io';
const ansiGreen = 32;
const ansiRed = 31;
const ansiMagenta = 35;
Future<bool> run(
String workingDir, String commandName, List<String> args) async {
var commandDescription = '`${([commandName, ...args]).join(' ')}`';
logWrapped(ansiMagenta, ' Running $commandDescription');
var proc = await Process.start(
commandName,
args,
workingDirectory: '${Directory.current.path}/$workingDir',
mode: ProcessStartMode.inheritStdio,
);
var exitCode = await proc.exitCode;
if (exitCode != 0) {
logWrapped(
ansiRed, ' Failed! ($exitCode) – $workingDir – $commandDescription');
return false;
} else {
logWrapped(ansiGreen, ' Success! – $workingDir – $commandDescription');
return true;
}
}
void logWrapped(int code, String message) {
print('\x1B[${code}m$message\x1B[0m');
}
Iterable<String> listPackageDirs(Directory dir) sync* {
if (File('${dir.path}/pubspec.yaml').existsSync()) {
yield dir.path;
} else {
for (var subDir in dir
.listSync(followLinks: true)
.whereType<Directory>()
.where((d) => !Uri.file(d.path).pathSegments.last.startsWith('.'))) {
yield* listPackageDirs(subDir);
}
}
}
| samples/web/_tool/common.dart/0 | {
"file_path": "samples/web/_tool/common.dart",
"repo_id": "samples",
"token_count": 513
} | 1,248 |
include: package:lints/recommended.yaml
analyzer:
exclude:
- lib/src/data.g.dart
language:
strict-casts: true
strict-inference: true
strict-raw-types: true
linter:
rules:
avoid_types_on_closure_parameters: true
avoid_void_async: true
cancel_subscriptions: true
close_sinks: true
directives_ordering: true
package_api_docs: true
package_prefixed_library_names: true
prefer_final_in_for_each: true
prefer_single_quotes: true
test_types_in_equals: true
throw_in_finally: true
unawaited_futures: true
unnecessary_statements: true
use_enums: true
use_super_parameters: true
| samples/web/samples_index/analysis_options.yaml/0 | {
"file_path": "samples/web/samples_index/analysis_options.yaml",
"repo_id": "samples",
"token_count": 260
} | 1,249 |
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
"version": 1,
"newProjectRoot": "projects",
"projects": {
"ng-flutter": {
"projectType": "application",
"schematics": {},
"root": "",
"sourceRoot": "src",
"prefix": "app",
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser",
"options": {
"outputPath": "dist/ng-flutter",
"index": "src/index.html",
"main": "src/main.ts",
"polyfills": [
"zone.js"
],
"tsConfig": "tsconfig.app.json",
"assets": [
"src/favicon.ico",
"src/assets",
{
"input": "flutter/build/web/",
"glob": "**/*",
"output": "/flutter/"
}
],
"styles": [
"@angular/material/prebuilt-themes/indigo-pink.css",
"src/styles.css"
],
"scripts": [
{
"input": "flutter/build/web/flutter.js",
"inject": true,
"bundleName": "flutter"
}
]
},
"configurations": {
"production": {
"budgets": [
{
"type": "initial",
"maximumWarning": "500kb",
"maximumError": "1mb"
},
{
"type": "anyComponentStyle",
"maximumWarning": "2kb",
"maximumError": "4kb"
}
],
"outputHashing": "all"
},
"development": {
"buildOptimizer": false,
"optimization": false,
"vendorChunk": true,
"extractLicenses": false,
"sourceMap": true,
"namedChunks": true
}
},
"defaultConfiguration": "production"
},
"serve": {
"builder": "@angular-devkit/build-angular:dev-server",
"configurations": {
"production": {
"buildTarget": "ng-flutter:build:production"
},
"development": {
"buildTarget": "ng-flutter:build:development"
}
},
"defaultConfiguration": "development"
},
"extract-i18n": {
"builder": "@angular-devkit/build-angular:extract-i18n",
"options": {
"buildTarget": "ng-flutter:build"
}
},
"test": {
"builder": "@angular-devkit/build-angular:karma",
"options": {
"polyfills": [
"zone.js",
"zone.js/testing"
],
"tsConfig": "tsconfig.spec.json",
"assets": [
"src/favicon.ico",
"src/assets"
],
"styles": [
"@angular/material/prebuilt-themes/indigo-pink.css",
"src/styles.css"
],
"scripts": []
}
}
}
}
},
"cli": {
"analytics": "0ff9b6e8-2034-4f87-9ac7-46dbd612ebad"
}
}
| samples/web_embedding/ng-flutter/angular.json/0 | {
"file_path": "samples/web_embedding/ng-flutter/angular.json",
"repo_id": "samples",
"token_count": 1960
} | 1,250 |
/* To learn more about this file see: https://angular.io/config/tsconfig. */
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": []
},
"files": [
"src/main.ts"
],
"include": [
"src/**/*.d.ts"
]
}
| samples/web_embedding/ng-flutter/tsconfig.app.json/0 | {
"file_path": "samples/web_embedding/ng-flutter/tsconfig.app.json",
"repo_id": "samples",
"token_count": 117
} | 1,251 |
FROM ruby:3.2.3-slim-bookworm@sha256:97fccffe954d1e0c7fa6634020379417d67435a7f9a7c10b6ef3f49e498307e6 as base
ENV TZ=US/Pacific
RUN apt-get update && apt-get install -yq --no-install-recommends \
apt-transport-https \
build-essential \
ca-certificates \
curl \
git \
gnupg \
lsof \
make \
rsync \
unzip \
xdg-user-dirs \
&& rm -rf /var/lib/apt/lists/*
WORKDIR /app
# ============== NODEJS INTSALL ==============
FROM base AS node
RUN mkdir -p /etc/apt/keyrings \
&& curl -fsSL https://deb.nodesource.com/gpgkey/nodesource-repo.gpg.key | gpg --dearmor -o /etc/apt/keyrings/nodesource.gpg \
&& echo "deb [signed-by=/etc/apt/keyrings/nodesource.gpg] https://deb.nodesource.com/node_20.x nodistro main" | tee /etc/apt/sources.list.d/nodesource.list \
&& apt-get update -yq \
&& apt-get install nodejs -yq \
&& npm install -g npm # Ensure latest npm
# ============== BUILD PROD JEKYLL SITE ==============
FROM node AS build
ENV JEKYLL_ENV=production
ENV RUBY_YJIT_ENABLE=1
RUN gem install bundler
COPY Gemfile Gemfile.lock ./
RUN bundle config set force_ruby_platform true
RUN BUNDLE_WITHOUT="test development" bundle install --jobs=4 --retry=2
ENV NODE_ENV=production
COPY package.json ./
RUN npm install
COPY ./ ./
RUN echo 'User-agent: *\nDisallow:\n\nSitemap: https://docs.flutter.dev/sitemap.xml' > src/robots.txt
ARG BUILD_CONFIGS=_config.yml
ENV BUILD_CONFIGS=$BUILD_CONFIGS
RUN bundle exec jekyll build --config $BUILD_CONFIGS
| website/Dockerfile/0 | {
"file_path": "website/Dockerfile",
"repo_id": "website",
"token_count": 672
} | 1,252 |
// Copyright 2017 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that
// can be found in the LICENSE file.
// A "radial transformation" as defined here:
// https://m1.material.io/motion/transforming-material.html#transforming-material-radial-transformation
// In this example, the destination route (which completely obscures
// the source route once the page transition has finished),
// displays the Hero image in a Card containing a column of two
// widgets: the image, and some descriptive text.
import 'dart:math' as math;
import 'package:flutter/material.dart';
import 'package:flutter/scheduler.dart' show timeDilation;
class Photo extends StatelessWidget {
const Photo({super.key, required this.photo, this.onTap});
final String photo;
final VoidCallback? onTap;
@override
Widget build(BuildContext context) {
return Material(
// Slightly opaque color appears where the image has transparency.
color: Theme.of(context).primaryColor.withOpacity(0.25),
child: InkWell(
onTap: onTap,
child: LayoutBuilder(
builder: (context, size) {
return Image.asset(
photo,
fit: BoxFit.contain,
);
},
),
),
);
}
}
class RadialExpansion extends StatelessWidget {
const RadialExpansion({
super.key,
required this.maxRadius,
this.child,
}) : clipRectSize = 2.0 * (maxRadius / math.sqrt2);
final double maxRadius;
final double clipRectSize;
final Widget? child;
@override
Widget build(BuildContext context) {
return ClipOval(
child: Center(
child: SizedBox(
width: clipRectSize,
height: clipRectSize,
child: ClipRect(
child: child,
),
),
),
);
}
}
class RadialExpansionDemo extends StatelessWidget {
const RadialExpansionDemo({super.key});
static double kMinRadius = 32.0;
static double kMaxRadius = 128.0;
static Interval opacityCurve =
const Interval(0.0, 0.75, curve: Curves.fastOutSlowIn);
static RectTween _createRectTween(Rect? begin, Rect? end) {
return MaterialRectCenterArcTween(begin: begin, end: end);
}
static Widget _buildPage(
BuildContext context, String imageName, String description) {
return Container(
color: Theme.of(context).canvasColor,
child: Center(
child: Card(
elevation: 8,
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(
width: kMaxRadius * 2.0,
height: kMaxRadius * 2.0,
child: Hero(
createRectTween: _createRectTween,
tag: imageName,
child: RadialExpansion(
maxRadius: kMaxRadius,
child: Photo(
photo: imageName,
onTap: () {
Navigator.of(context).pop();
},
),
),
),
),
Text(
description,
style: const TextStyle(fontWeight: FontWeight.bold),
textScaler: const TextScaler.linear(3),
),
const SizedBox(height: 16),
],
),
),
),
);
}
Widget _buildHero(
BuildContext context,
String imageName,
String description,
) {
return SizedBox(
width: kMinRadius * 2.0,
height: kMinRadius * 2.0,
child: Hero(
createRectTween: _createRectTween,
tag: imageName,
child: RadialExpansion(
maxRadius: kMaxRadius,
child: Photo(
photo: imageName,
onTap: () {
Navigator.of(context).push(
PageRouteBuilder<void>(
pageBuilder: (context, animation, secondaryAnimation) {
return AnimatedBuilder(
animation: animation,
builder: (context, child) {
return Opacity(
opacity: opacityCurve.transform(animation.value),
child: _buildPage(context, imageName, description),
);
},
);
},
),
);
},
),
),
),
);
}
@override
Widget build(BuildContext context) {
timeDilation = 5.0; // 1.0 is normal animation speed.
return Scaffold(
appBar: AppBar(
title: const Text('Radial Transition Demo'),
),
body: Container(
padding: const EdgeInsets.all(32),
alignment: FractionalOffset.bottomLeft,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
_buildHero(context, 'images/chair-alpha.png', 'Chair'),
_buildHero(context, 'images/binoculars-alpha.png', 'Binoculars'),
_buildHero(context, 'images/beachball-alpha.png', 'Beach ball'),
],
),
),
);
}
}
void main() {
runApp(
const MaterialApp(
home: RadialExpansionDemo(),
),
);
}
| website/examples/_animation/radial_hero_animation/lib/main.dart/0 | {
"file_path": "website/examples/_animation/radial_hero_animation/lib/main.dart",
"repo_id": "website",
"token_count": 2546
} | 1,253 |
import 'package:flutter/material.dart';
void main() => runApp(const LogoApp());
class LogoApp extends StatefulWidget {
const LogoApp({super.key});
@override
State<LogoApp> createState() => _LogoAppState();
}
class _LogoAppState extends State<LogoApp> with SingleTickerProviderStateMixin {
late Animation<double> animation;
late AnimationController controller;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 2), vsync: this);
// #docregion addListener
animation = Tween<double>(begin: 0, end: 300).animate(controller)
..addListener(() {
// #enddocregion addListener
setState(() {
// The state that has changed here is the animation object's value.
});
// #docregion addListener
});
// #enddocregion addListener
controller.forward();
}
@override
Widget build(BuildContext context) {
return Center(
child: Container(
margin: const EdgeInsets.symmetric(vertical: 10),
height: animation.value,
width: animation.value,
child: const FlutterLogo(),
),
);
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
}
| website/examples/animation/animate1/lib/main.dart/0 | {
"file_path": "website/examples/animation/animate1/lib/main.dart",
"repo_id": "website",
"token_count": 462
} | 1,254 |
import 'package:flutter/material.dart';
void main() {
runApp(const TabBarDemo());
}
class TabBarDemo extends StatelessWidget {
const TabBarDemo({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: DefaultTabController(
length: 3,
child: Scaffold(
appBar: AppBar(
bottom: const TabBar(
tabs: [
Tab(icon: Icon(Icons.directions_car)),
Tab(icon: Icon(Icons.directions_transit)),
Tab(icon: Icon(Icons.directions_bike)),
],
),
title: const Text('Tabs Demo'),
),
// #docregion TabBarView
body: const TabBarView(
children: [
Icon(Icons.directions_car),
Icon(Icons.directions_transit),
Icon(Icons.directions_bike),
],
),
// #enddocregion TabBarView
),
),
);
}
}
| website/examples/cookbook/design/tabs/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/design/tabs/lib/main.dart",
"repo_id": "website",
"token_count": 510
} | 1,255 |
import 'dart:math';
import 'dart:ui' as ui;
import 'package:flutter/material.dart';
void main() {
runApp(const App(home: ExampleGradientBubbles()));
}
@immutable
class App extends StatelessWidget {
const App({super.key, this.home});
final Widget? home;
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Chat',
theme: ThemeData.dark(useMaterial3: true),
home: home,
);
}
}
@immutable
class ExampleGradientBubbles extends StatefulWidget {
const ExampleGradientBubbles({super.key});
@override
State<ExampleGradientBubbles> createState() => _ExampleGradientBubblesState();
}
class _ExampleGradientBubblesState extends State<ExampleGradientBubbles> {
late final List<Message> data;
@override
void initState() {
super.initState();
data = MessageGenerator.generate(60, 1337);
}
@override
Widget build(BuildContext context) {
return Theme(
data: ThemeData(
brightness: Brightness.dark,
primaryColor: const Color(0xFF4F4F4F),
),
child: Scaffold(
appBar: AppBar(
title: const Text('Flutter Chat'),
),
body: ListView.builder(
padding: const EdgeInsets.symmetric(vertical: 16.0),
reverse: true,
itemCount: data.length,
itemBuilder: (context, index) {
final message = data[index];
return MessageBubble(
message: message,
child: Text(message.text),
);
},
),
),
);
}
}
@immutable
class MessageBubble extends StatelessWidget {
const MessageBubble({
super.key,
required this.message,
required this.child,
});
final Message message;
final Widget child;
@override
Widget build(BuildContext context) {
final messageAlignment =
message.isMine ? Alignment.topLeft : Alignment.topRight;
return FractionallySizedBox(
alignment: messageAlignment,
widthFactor: 0.8,
child: Align(
alignment: messageAlignment,
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 6.0, horizontal: 20.0),
child: ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(16.0)),
child: BubbleBackground(
colors: [
if (message.isMine) ...const [
Color(0xFF6C7689),
Color(0xFF3A364B),
] else ...const [
Color(0xFF19B7FF),
Color(0xFF491CCB),
],
],
child: DefaultTextStyle.merge(
style: const TextStyle(
fontSize: 18.0,
color: Colors.white,
),
child: Padding(
padding: const EdgeInsets.all(12.0),
child: child,
),
),
),
),
),
),
);
}
}
@immutable
class BubbleBackground extends StatelessWidget {
const BubbleBackground({
super.key,
required this.colors,
this.child,
});
final List<Color> colors;
final Widget? child;
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: BubblePainter(
scrollable: Scrollable.of(context),
bubbleContext: context,
colors: colors,
),
child: child,
);
}
}
class BubblePainter extends CustomPainter {
BubblePainter({
required ScrollableState scrollable,
required BuildContext bubbleContext,
required List<Color> colors,
}) : _scrollable = scrollable,
_bubbleContext = bubbleContext,
_colors = colors,
super(repaint: scrollable.position);
final ScrollableState _scrollable;
final BuildContext _bubbleContext;
final List<Color> _colors;
@override
void paint(Canvas canvas, Size size) {
final scrollableBox = _scrollable.context.findRenderObject() as RenderBox;
final scrollableRect = Offset.zero & scrollableBox.size;
final bubbleBox = _bubbleContext.findRenderObject() as RenderBox;
final origin =
bubbleBox.localToGlobal(Offset.zero, ancestor: scrollableBox);
final paint = Paint()
..shader = ui.Gradient.linear(
scrollableRect.topCenter,
scrollableRect.bottomCenter,
_colors,
[0.0, 1.0],
TileMode.clamp,
Matrix4.translationValues(-origin.dx, -origin.dy, 0.0).storage,
);
canvas.drawRect(Offset.zero & size, paint);
}
@override
bool shouldRepaint(BubblePainter oldDelegate) {
return oldDelegate._scrollable != _scrollable ||
oldDelegate._bubbleContext != _bubbleContext ||
oldDelegate._colors != _colors;
}
}
enum MessageOwner { myself, other }
@immutable
class Message {
const Message({
required this.owner,
required this.text,
});
final MessageOwner owner;
final String text;
bool get isMine => owner == MessageOwner.myself;
}
class MessageGenerator {
static List<Message> generate(int count, [int? seed]) {
final random = Random(seed);
return List.unmodifiable(List<Message>.generate(count, (index) {
return Message(
owner: random.nextBool() ? MessageOwner.myself : MessageOwner.other,
text: _exampleData[random.nextInt(_exampleData.length)],
);
}));
}
static final _exampleData = [
'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
'In tempus mauris at velit egestas, sed blandit felis ultrices.',
'Ut molestie mauris et ligula finibus iaculis.',
'Sed a tempor ligula.',
'Test',
'Phasellus ullamcorper, mi ut imperdiet consequat, nibh augue condimentum nunc, vitae molestie massa augue nec erat.',
'Donec scelerisque, erat vel placerat facilisis, eros turpis egestas nulla, a sodales elit nibh et enim.',
'Mauris quis dignissim neque. In a odio leo. Aliquam egestas egestas tempor. Etiam at tortor metus.',
'Quisque lacinia imperdiet faucibus.',
'Proin egestas arcu non nisl laoreet, vitae iaculis enim volutpat. In vehicula convallis magna.',
'Phasellus at diam a sapien laoreet gravida.',
'Fusce maximus fermentum sem a scelerisque.',
'Nam convallis sapien augue, malesuada aliquam dui bibendum nec.',
'Quisque dictum tincidunt ex non lobortis.',
'In hac habitasse platea dictumst.',
'Ut pharetra ligula libero, sit amet imperdiet lorem luctus sit amet.',
'Sed ex lorem, lacinia et varius vitae, sagittis eget libero.',
'Vestibulum scelerisque velit sed augue ultricies, ut vestibulum lorem luctus.',
'Pellentesque et risus pretium, egestas ipsum at, facilisis lectus.',
'Praesent id eleifend lacus.',
'Fusce convallis eu tortor sit amet mattis.',
'Vivamus lacinia magna ut urna feugiat tincidunt.',
'Sed in diam ut dolor imperdiet vehicula non ac turpis.',
'Praesent at est hendrerit, laoreet tortor sed, varius mi.',
'Nunc in odio leo.',
'Praesent placerat semper libero, ut aliquet dolor.',
'Vestibulum elementum leo metus, vitae auctor lorem tincidunt ut.',
];
}
| website/examples/cookbook/effects/gradient_bubbles/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/effects/gradient_bubbles/lib/main.dart",
"repo_id": "website",
"token_count": 3041
} | 1,256 |
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
const Color darkBlue = Color.fromARGB(255, 18, 32, 47);
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.dark().copyWith(scaffoldBackgroundColor: darkBlue),
debugShowCheckedModeBanner: false,
home: const Scaffold(
body: Center(
child: ExampleParallax(),
),
),
);
}
}
class ExampleParallax extends StatelessWidget {
const ExampleParallax({
super.key,
});
@override
Widget build(BuildContext context) {
return SingleChildScrollView(
child: Column(
children: [
for (final location in locations)
LocationListItem(
imageUrl: location.imageUrl,
name: location.name,
country: location.place,
),
],
),
);
}
}
class LocationListItem extends StatelessWidget {
LocationListItem({
super.key,
required this.imageUrl,
required this.name,
required this.country,
});
final String imageUrl;
final String name;
final String country;
final GlobalKey _backgroundImageKey = GlobalKey();
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(horizontal: 24, vertical: 16),
child: AspectRatio(
aspectRatio: 16 / 9,
child: ClipRRect(
borderRadius: BorderRadius.circular(16),
child: Stack(
children: [
_buildParallaxBackground(context),
_buildGradient(),
_buildTitleAndSubtitle(),
],
),
),
),
);
}
Widget _buildParallaxBackground(BuildContext context) {
return Flow(
delegate: ParallaxFlowDelegate(
scrollable: Scrollable.of(context),
listItemContext: context,
backgroundImageKey: _backgroundImageKey,
),
children: [
Image.network(
imageUrl,
key: _backgroundImageKey,
fit: BoxFit.cover,
),
],
);
}
Widget _buildGradient() {
return Positioned.fill(
child: DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.transparent, Colors.black.withOpacity(0.7)],
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
stops: const [0.6, 0.95],
),
),
),
);
}
Widget _buildTitleAndSubtitle() {
return Positioned(
left: 20,
bottom: 20,
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
name,
style: const TextStyle(
color: Colors.white,
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
Text(
country,
style: const TextStyle(
color: Colors.white,
fontSize: 14,
),
),
],
),
);
}
}
// #docregion SuperScrollPosition
class ParallaxFlowDelegate extends FlowDelegate {
ParallaxFlowDelegate({
required this.scrollable,
required this.listItemContext,
required this.backgroundImageKey,
}) : super(repaint: scrollable.position);
// #enddocregion SuperScrollPosition
final ScrollableState scrollable;
final BuildContext listItemContext;
final GlobalKey backgroundImageKey;
// #docregion TightWidth
@override
BoxConstraints getConstraintsForChild(int i, BoxConstraints constraints) {
return BoxConstraints.tightFor(
width: constraints.maxWidth,
);
}
// #enddocregion TightWidth
@override
void paintChildren(FlowPaintingContext context) {
// Calculate the position of this list item within the viewport.
final scrollableBox = scrollable.context.findRenderObject() as RenderBox;
final listItemBox = listItemContext.findRenderObject() as RenderBox;
final listItemOffset = listItemBox.localToGlobal(
listItemBox.size.centerLeft(Offset.zero),
ancestor: scrollableBox);
// Determine the percent position of this list item within the
// scrollable area.
final viewportDimension = scrollable.position.viewportDimension;
final scrollFraction =
(listItemOffset.dy / viewportDimension).clamp(0.0, 1.0);
// Calculate the vertical alignment of the background
// based on the scroll percent.
final verticalAlignment = Alignment(0.0, scrollFraction * 2 - 1);
// Convert the background alignment into a pixel offset for
// painting purposes.
final backgroundSize =
(backgroundImageKey.currentContext!.findRenderObject() as RenderBox)
.size;
final listItemSize = context.size;
final childRect =
verticalAlignment.inscribe(backgroundSize, Offset.zero & listItemSize);
// Paint the background.
context.paintChild(
0,
transform:
Transform.translate(offset: Offset(0.0, childRect.top)).transform,
);
}
// #docregion ShouldRepaint
@override
bool shouldRepaint(ParallaxFlowDelegate oldDelegate) {
return scrollable != oldDelegate.scrollable ||
listItemContext != oldDelegate.listItemContext ||
backgroundImageKey != oldDelegate.backgroundImageKey;
}
// #enddocregion ShouldRepaint
}
class Parallax extends SingleChildRenderObjectWidget {
const Parallax({
super.key,
required Widget background,
}) : super(child: background);
@override
RenderObject createRenderObject(BuildContext context) {
return RenderParallax(scrollable: Scrollable.of(context));
}
@override
void updateRenderObject(
BuildContext context, covariant RenderParallax renderObject) {
renderObject.scrollable = Scrollable.of(context);
}
}
class ParallaxParentData extends ContainerBoxParentData<RenderBox> {}
class RenderParallax extends RenderBox
with RenderObjectWithChildMixin<RenderBox>, RenderProxyBoxMixin {
RenderParallax({
required ScrollableState scrollable,
}) : _scrollable = scrollable;
ScrollableState _scrollable;
ScrollableState get scrollable => _scrollable;
set scrollable(ScrollableState value) {
if (value != _scrollable) {
if (attached) {
_scrollable.position.removeListener(markNeedsLayout);
}
_scrollable = value;
if (attached) {
_scrollable.position.addListener(markNeedsLayout);
}
}
}
@override
void attach(covariant PipelineOwner owner) {
super.attach(owner);
_scrollable.position.addListener(markNeedsLayout);
}
@override
void detach() {
_scrollable.position.removeListener(markNeedsLayout);
super.detach();
}
@override
void setupParentData(covariant RenderObject child) {
if (child.parentData is! ParallaxParentData) {
child.parentData = ParallaxParentData();
}
}
@override
void performLayout() {
size = constraints.biggest;
// Force the background to take up all available width
// and then scale its height based on the image's aspect ratio.
final background = child!;
final backgroundImageConstraints =
BoxConstraints.tightFor(width: size.width);
background.layout(backgroundImageConstraints, parentUsesSize: true);
// Set the background's local offset, which is zero.
(background.parentData as ParallaxParentData).offset = Offset.zero;
}
@override
void paint(PaintingContext context, Offset offset) {
// Get the size of the scrollable area.
final viewportDimension = scrollable.position.viewportDimension;
// Calculate the global position of this list item.
final scrollableBox = scrollable.context.findRenderObject() as RenderBox;
final backgroundOffset =
localToGlobal(size.centerLeft(Offset.zero), ancestor: scrollableBox);
// Determine the percent position of this list item within the
// scrollable area.
final scrollFraction =
(backgroundOffset.dy / viewportDimension).clamp(0.0, 1.0);
// Calculate the vertical alignment of the background
// based on the scroll percent.
final verticalAlignment = Alignment(0.0, scrollFraction * 2 - 1);
// Convert the background alignment into a pixel offset for
// painting purposes.
final background = child!;
final backgroundSize = background.size;
final listItemSize = size;
final childRect =
verticalAlignment.inscribe(backgroundSize, Offset.zero & listItemSize);
// Paint the background.
context.paintChild(
background,
(background.parentData as ParallaxParentData).offset +
offset +
Offset(0.0, childRect.top));
}
}
class Location {
const Location({
required this.name,
required this.place,
required this.imageUrl,
});
final String name;
final String place;
final String imageUrl;
}
const urlPrefix =
'https://docs.flutter.dev/cookbook/img-files/effects/parallax';
const locations = [
Location(
name: 'Mount Rushmore',
place: 'U.S.A',
imageUrl: '$urlPrefix/01-mount-rushmore.jpg',
),
Location(
name: 'Gardens By The Bay',
place: 'Singapore',
imageUrl: '$urlPrefix/02-singapore.jpg',
),
Location(
name: 'Machu Picchu',
place: 'Peru',
imageUrl: '$urlPrefix/03-machu-picchu.jpg',
),
Location(
name: 'Vitznau',
place: 'Switzerland',
imageUrl: '$urlPrefix/04-vitznau.jpg',
),
Location(
name: 'Bali',
place: 'Indonesia',
imageUrl: '$urlPrefix/05-bali.jpg',
),
Location(
name: 'Mexico City',
place: 'Mexico',
imageUrl: '$urlPrefix/06-mexico-city.jpg',
),
Location(
name: 'Cairo',
place: 'Egypt',
imageUrl: '$urlPrefix/07-cairo.jpg',
),
];
| website/examples/cookbook/effects/parallax_scrolling/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/effects/parallax_scrolling/lib/main.dart",
"repo_id": "website",
"token_count": 3834
} | 1,257 |
import 'package:flutter/material.dart';
class ShimmerLoading extends StatefulWidget {
const ShimmerLoading({
super.key,
required this.isLoading,
required this.child,
});
final bool isLoading;
final Widget child;
@override
State<ShimmerLoading> createState() => _ShimmerLoadingState();
}
// #docregion ShimmerLoadingStatePt2
class _ShimmerLoadingState extends State<ShimmerLoading> {
@override
Widget build(BuildContext context) {
if (!widget.isLoading) {
return widget.child;
}
// Collect ancestor shimmer information.
final shimmer = Shimmer.of(context)!;
if (!shimmer.isSized) {
// The ancestor Shimmer widget isn't laid
// out yet. Return an empty box.
return const SizedBox();
}
final shimmerSize = shimmer.size;
final gradient = shimmer.gradient;
final offsetWithinShimmer = shimmer.getDescendantOffset(
descendant: context.findRenderObject() as RenderBox,
);
return ShaderMask(
blendMode: BlendMode.srcATop,
shaderCallback: (bounds) {
return gradient.createShader(
Rect.fromLTWH(
-offsetWithinShimmer.dx,
-offsetWithinShimmer.dy,
shimmerSize.width,
shimmerSize.height,
),
);
},
child: widget.child,
);
}
}
// #enddocregion ShimmerLoadingStatePt2
class Shimmer extends StatefulWidget {
static ShimmerState? of(BuildContext context) {
return context.findAncestorStateOfType<ShimmerState>();
}
const Shimmer({
super.key,
required this.linearGradient,
this.child,
});
final LinearGradient linearGradient;
final Widget? child;
@override
ShimmerState createState() => ShimmerState();
}
class ShimmerState extends State<Shimmer> {
Gradient get gradient => LinearGradient(
colors: widget.linearGradient.colors,
stops: widget.linearGradient.stops,
begin: widget.linearGradient.begin,
end: widget.linearGradient.end,
);
bool get isSized => (context.findRenderObject() as RenderBox).hasSize;
Size get size => (context.findRenderObject() as RenderBox).size;
Offset getDescendantOffset({
required RenderBox descendant,
Offset offset = Offset.zero,
}) {
final shimmerBox = context.findRenderObject() as RenderBox;
return descendant.localToGlobal(offset, ancestor: shimmerBox);
}
@override
Widget build(BuildContext context) {
return widget.child ?? const SizedBox();
}
}
| website/examples/cookbook/effects/shimmer_loading/lib/shimmer_loading_state_pt2.dart/0 | {
"file_path": "website/examples/cookbook/effects/shimmer_loading/lib/shimmer_loading_state_pt2.dart",
"repo_id": "website",
"token_count": 903
} | 1,258 |
import 'dart:math';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
home: ExampleIsTyping(),
debugShowCheckedModeBanner: false,
),
);
}
const _backgroundColor = Color(0xFF333333);
class ExampleIsTyping extends StatefulWidget {
const ExampleIsTyping({
super.key,
});
@override
State<ExampleIsTyping> createState() => _ExampleIsTypingState();
}
class _ExampleIsTypingState extends State<ExampleIsTyping> {
bool _isSomeoneTyping = false;
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: _backgroundColor,
appBar: AppBar(
title: const Text('Typing Indicator'),
),
body: Column(
children: [
Expanded(
child: ListView.builder(
padding: const EdgeInsets.symmetric(vertical: 8),
itemCount: 25,
reverse: true,
itemBuilder: (context, index) {
return Padding(
padding: const EdgeInsets.only(left: 100),
child: FakeMessage(isBig: index.isOdd),
);
},
),
),
Align(
alignment: Alignment.bottomLeft,
child: TypingIndicator(
showIndicator: _isSomeoneTyping,
),
),
Container(
color: Colors.grey,
padding: const EdgeInsets.all(16),
child: Center(
child: CupertinoSwitch(
onChanged: (newValue) {
setState(() {
_isSomeoneTyping = newValue;
});
},
value: _isSomeoneTyping,
),
),
),
],
),
);
}
}
class TypingIndicator extends StatefulWidget {
const TypingIndicator({
super.key,
this.showIndicator = false,
this.bubbleColor = const Color(0xFF646b7f),
this.flashingCircleDarkColor = const Color(0xFF333333),
this.flashingCircleBrightColor = const Color(0xFFaec1dd),
});
final bool showIndicator;
final Color bubbleColor;
final Color flashingCircleDarkColor;
final Color flashingCircleBrightColor;
@override
State<TypingIndicator> createState() => _TypingIndicatorState();
}
class _TypingIndicatorState extends State<TypingIndicator>
with TickerProviderStateMixin {
late AnimationController _appearanceController;
late Animation<double> _indicatorSpaceAnimation;
late Animation<double> _smallBubbleAnimation;
late Animation<double> _mediumBubbleAnimation;
late Animation<double> _largeBubbleAnimation;
late AnimationController _repeatingController;
final List<Interval> _dotIntervals = const [
Interval(0.25, 0.8),
Interval(0.35, 0.9),
Interval(0.45, 1.0),
];
@override
void initState() {
super.initState();
_appearanceController = AnimationController(
vsync: this,
)..addListener(() {
setState(() {});
});
_indicatorSpaceAnimation = CurvedAnimation(
parent: _appearanceController,
curve: const Interval(0.0, 0.4, curve: Curves.easeOut),
reverseCurve: const Interval(0.0, 1.0, curve: Curves.easeOut),
).drive(Tween<double>(
begin: 0.0,
end: 60.0,
));
_smallBubbleAnimation = CurvedAnimation(
parent: _appearanceController,
curve: const Interval(0.0, 0.5, curve: Curves.elasticOut),
reverseCurve: const Interval(0.0, 0.3, curve: Curves.easeOut),
);
_mediumBubbleAnimation = CurvedAnimation(
parent: _appearanceController,
curve: const Interval(0.2, 0.7, curve: Curves.elasticOut),
reverseCurve: const Interval(0.2, 0.6, curve: Curves.easeOut),
);
_largeBubbleAnimation = CurvedAnimation(
parent: _appearanceController,
curve: const Interval(0.3, 1.0, curve: Curves.elasticOut),
reverseCurve: const Interval(0.5, 1.0, curve: Curves.easeOut),
);
_repeatingController = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 1500),
);
if (widget.showIndicator) {
_showIndicator();
}
}
@override
void didUpdateWidget(TypingIndicator oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.showIndicator != oldWidget.showIndicator) {
if (widget.showIndicator) {
_showIndicator();
} else {
_hideIndicator();
}
}
}
@override
void dispose() {
_appearanceController.dispose();
_repeatingController.dispose();
super.dispose();
}
void _showIndicator() {
_appearanceController
..duration = const Duration(milliseconds: 750)
..forward();
_repeatingController.repeat();
}
void _hideIndicator() {
_appearanceController
..duration = const Duration(milliseconds: 150)
..reverse();
_repeatingController.stop();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _indicatorSpaceAnimation,
builder: (context, child) {
return SizedBox(
height: _indicatorSpaceAnimation.value,
child: child,
);
},
child: Stack(
children: [
AnimatedBubble(
animation: _smallBubbleAnimation,
left: 8,
bottom: 8,
bubble: CircleBubble(
size: 8,
bubbleColor: widget.bubbleColor,
),
),
AnimatedBubble(
animation: _mediumBubbleAnimation,
left: 10,
bottom: 10,
bubble: CircleBubble(
size: 16,
bubbleColor: widget.bubbleColor,
),
),
AnimatedBubble(
animation: _largeBubbleAnimation,
left: 12,
bottom: 12,
bubble: StatusBubble(
repeatingController: _repeatingController,
dotIntervals: _dotIntervals,
flashingCircleDarkColor: widget.flashingCircleDarkColor,
flashingCircleBrightColor: widget.flashingCircleBrightColor,
bubbleColor: widget.bubbleColor,
),
),
],
),
);
}
}
class CircleBubble extends StatelessWidget {
const CircleBubble({
super.key,
required this.size,
required this.bubbleColor,
});
final double size;
final Color bubbleColor;
@override
Widget build(BuildContext context) {
return Container(
width: size,
height: size,
decoration: BoxDecoration(
shape: BoxShape.circle,
color: bubbleColor,
),
);
}
}
class AnimatedBubble extends StatelessWidget {
const AnimatedBubble({
super.key,
required this.animation,
required this.left,
required this.bottom,
required this.bubble,
});
final Animation<double> animation;
final double left;
final double bottom;
final Widget bubble;
@override
Widget build(BuildContext context) {
return Positioned(
left: left,
bottom: bottom,
child: AnimatedBuilder(
animation: animation,
builder: (context, child) {
return Transform.scale(
scale: animation.value,
alignment: Alignment.bottomLeft,
child: child,
);
},
child: bubble,
),
);
}
}
class StatusBubble extends StatelessWidget {
const StatusBubble({
super.key,
required this.repeatingController,
required this.dotIntervals,
required this.flashingCircleBrightColor,
required this.flashingCircleDarkColor,
required this.bubbleColor,
});
final AnimationController repeatingController;
final List<Interval> dotIntervals;
final Color flashingCircleDarkColor;
final Color flashingCircleBrightColor;
final Color bubbleColor;
@override
Widget build(BuildContext context) {
return Container(
width: 85,
height: 44,
padding: const EdgeInsets.symmetric(horizontal: 8),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(27),
color: bubbleColor,
),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
FlashingCircle(
index: 0,
repeatingController: repeatingController,
dotIntervals: dotIntervals,
flashingCircleDarkColor: flashingCircleDarkColor,
flashingCircleBrightColor: flashingCircleBrightColor,
),
FlashingCircle(
index: 1,
repeatingController: repeatingController,
dotIntervals: dotIntervals,
flashingCircleDarkColor: flashingCircleDarkColor,
flashingCircleBrightColor: flashingCircleBrightColor,
),
FlashingCircle(
index: 2,
repeatingController: repeatingController,
dotIntervals: dotIntervals,
flashingCircleDarkColor: flashingCircleDarkColor,
flashingCircleBrightColor: flashingCircleBrightColor,
),
],
),
);
}
}
class FlashingCircle extends StatelessWidget {
const FlashingCircle({
super.key,
required this.index,
required this.repeatingController,
required this.dotIntervals,
required this.flashingCircleBrightColor,
required this.flashingCircleDarkColor,
});
final int index;
final AnimationController repeatingController;
final List<Interval> dotIntervals;
final Color flashingCircleDarkColor;
final Color flashingCircleBrightColor;
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: repeatingController,
builder: (context, child) {
final circleFlashPercent = dotIntervals[index].transform(
repeatingController.value,
);
final circleColorPercent = sin(pi * circleFlashPercent);
return Container(
width: 12,
height: 12,
decoration: BoxDecoration(
shape: BoxShape.circle,
color: Color.lerp(
flashingCircleDarkColor,
flashingCircleBrightColor,
circleColorPercent,
),
),
);
},
);
}
}
class FakeMessage extends StatelessWidget {
const FakeMessage({
super.key,
required this.isBig,
});
final bool isBig;
@override
Widget build(BuildContext context) {
return Container(
margin: const EdgeInsets.symmetric(vertical: 8, horizontal: 24),
height: isBig ? 128 : 36,
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(8)),
color: Colors.grey.shade300,
),
);
}
}
| website/examples/cookbook/effects/typing_indicator/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/effects/typing_indicator/lib/main.dart",
"repo_id": "website",
"token_count": 4636
} | 1,259 |
import 'package:flutter/material.dart';
// #docregion Step1
// Define a custom Form widget.
class MyCustomForm extends StatefulWidget {
const MyCustomForm({super.key});
@override
State<MyCustomForm> createState() => _MyCustomFormState();
}
// Define a corresponding State class.
// This class holds data related to the Form.
class _MyCustomFormState extends State<MyCustomForm> {
// Create a text controller. Later, use it to retrieve the
// current value of the TextField.
final myController = TextEditingController();
@override
void dispose() {
// Clean up the controller when the widget is removed from the
// widget tree.
myController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
// Fill this out in the next step.
return Container();
}
}
// #enddocregion Step1
| website/examples/cookbook/forms/text_field_changes/lib/main_step1.dart/0 | {
"file_path": "website/examples/cookbook/forms/text_field_changes/lib/main_step1.dart",
"repo_id": "website",
"token_count": 254
} | 1,260 |
// This file has only enough in it to satisfy `firestore_controller.dart`.
import 'package:flutter/foundation.dart';
import 'playing_area.dart';
class BoardState {
final VoidCallback onWin;
final PlayingArea areaOne = PlayingArea();
final PlayingArea areaTwo = PlayingArea();
BoardState({required this.onWin});
void dispose() {
areaOne.dispose();
areaTwo.dispose();
}
}
| website/examples/cookbook/games/firestore_multiplayer/lib/game_internals/board_state.dart/0 | {
"file_path": "website/examples/cookbook/games/firestore_multiplayer/lib/game_internals/board_state.dart",
"repo_id": "website",
"token_count": 123
} | 1,261 |
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
const title = 'InkWell Demo';
return const MaterialApp(
title: title,
home: MyHomePage(title: title),
);
}
}
class MyHomePage extends StatelessWidget {
final String title;
const MyHomePage({super.key, required this.title});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
),
body: const Center(
child: MyButton(),
),
);
}
}
class MyButton extends StatelessWidget {
const MyButton({super.key});
@override
Widget build(BuildContext context) {
// #docregion InkWell
// The InkWell wraps the custom flat button widget.
return InkWell(
// When the user taps the button, show a snackbar.
onTap: () {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Tap'),
));
},
child: const Padding(
padding: EdgeInsets.all(12),
child: Text('Flat Button'),
),
);
// #enddocregion InkWell
}
}
| website/examples/cookbook/gestures/ripples/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/gestures/ripples/lib/main.dart",
"repo_id": "website",
"token_count": 485
} | 1,262 |
import 'package:flutter/material.dart';
void main() {
runApp(
MyApp(
// #docregion Items
items: List<ListItem>.generate(
1000,
(i) => i % 6 == 0
? HeadingItem('Heading $i')
: MessageItem('Sender $i', 'Message body $i'),
),
// #enddocregion Items
),
);
}
class MyApp extends StatelessWidget {
final List<ListItem> items;
const MyApp({super.key, required this.items});
@override
Widget build(BuildContext context) {
const title = 'Mixed List';
return MaterialApp(
title: title,
home: Scaffold(
appBar: AppBar(
title: const Text(title),
),
// #docregion builder
body: ListView.builder(
// Let the ListView know how many items it needs to build.
itemCount: items.length,
// Provide a builder function. This is where the magic happens.
// Convert each item into a widget based on the type of item it is.
itemBuilder: (context, index) {
final item = items[index];
return ListTile(
title: item.buildTitle(context),
subtitle: item.buildSubtitle(context),
);
},
),
// #enddocregion builder
),
);
}
}
// #docregion ListItem
/// The base class for the different types of items the list can contain.
abstract class ListItem {
/// The title line to show in a list item.
Widget buildTitle(BuildContext context);
/// The subtitle line, if any, to show in a list item.
Widget buildSubtitle(BuildContext context);
}
/// A ListItem that contains data to display a heading.
class HeadingItem implements ListItem {
final String heading;
HeadingItem(this.heading);
@override
Widget buildTitle(BuildContext context) {
return Text(
heading,
style: Theme.of(context).textTheme.headlineSmall,
);
}
@override
Widget buildSubtitle(BuildContext context) => const SizedBox.shrink();
}
/// A ListItem that contains data to display a message.
class MessageItem implements ListItem {
final String sender;
final String body;
MessageItem(this.sender, this.body);
@override
Widget buildTitle(BuildContext context) => Text(sender);
@override
Widget buildSubtitle(BuildContext context) => Text(body);
}
// #enddocregion ListItem
| website/examples/cookbook/lists/mixed_list/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/lists/mixed_list/lib/main.dart",
"repo_id": "website",
"token_count": 895
} | 1,263 |
import 'package:flutter/material.dart';
void main() {
runApp(
const MaterialApp(
title: 'Returning Data',
home: HomeScreen(),
),
);
}
class HomeScreen extends StatelessWidget {
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Returning Data Demo'),
),
body: const Center(
child: SelectionButton(),
),
);
}
}
class SelectionButton extends StatefulWidget {
const SelectionButton({super.key});
@override
State<SelectionButton> createState() => _SelectionButtonState();
}
class _SelectionButtonState extends State<SelectionButton> {
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: () {
_navigateAndDisplaySelection(context);
},
child: const Text('Pick an option, any option!'),
);
}
// #docregion navigateAndDisplay
// A method that launches the SelectionScreen and awaits the result from
// Navigator.pop.
Future<void> _navigateAndDisplaySelection(BuildContext context) async {
// Navigator.push returns a Future that completes after calling
// Navigator.pop on the Selection Screen.
final result = await Navigator.push(
context,
MaterialPageRoute(builder: (context) => const SelectionScreen()),
);
// When a BuildContext is used from a StatefulWidget, the mounted property
// must be checked after an asynchronous gap.
if (!context.mounted) return;
// After the Selection Screen returns a result, hide any previous snackbars
// and show the new result.
ScaffoldMessenger.of(context)
..removeCurrentSnackBar()
..showSnackBar(SnackBar(content: Text('$result')));
}
// #enddocregion navigateAndDisplay
}
class SelectionScreen extends StatelessWidget {
const SelectionScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Pick an option'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(8),
// #docregion Yep
child: ElevatedButton(
onPressed: () {
// Close the screen and return "Yep!" as the result.
Navigator.pop(context, 'Yep!');
},
child: const Text('Yep!'),
),
// #enddocregion Yep
),
Padding(
padding: const EdgeInsets.all(8),
// #docregion Nope
child: ElevatedButton(
onPressed: () {
// Close the screen and return "Nope." as the result.
Navigator.pop(context, 'Nope.');
},
child: const Text('Nope.'),
),
// #enddocregion Nope
)
],
),
),
);
}
}
| website/examples/cookbook/navigation/returning_data/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/navigation/returning_data/lib/main.dart",
"repo_id": "website",
"token_count": 1291
} | 1,264 |
import 'dart:async';
import 'dart:convert';
import 'package:flutter/material.dart';
// #docregion Http
import 'package:http/http.dart' as http;
// #enddocregion Http
// #docregion fetchAlbum
Future<Album> fetchAlbum() async {
final response = await http
.get(Uri.parse('https://jsonplaceholder.typicode.com/albums/1'));
if (response.statusCode == 200) {
// If the server did return a 200 OK response,
// then parse the JSON.
return Album.fromJson(jsonDecode(response.body) as Map<String, dynamic>);
} else {
// If the server did not return a 200 OK response,
// then throw an exception.
throw Exception('Failed to load album');
}
}
// #enddocregion fetchAlbum
// #docregion Album
class Album {
final int userId;
final int id;
final String title;
const Album({
required this.userId,
required this.id,
required this.title,
});
factory Album.fromJson(Map<String, dynamic> json) {
return switch (json) {
{
'userId': int userId,
'id': int id,
'title': String title,
} =>
Album(
userId: userId,
id: id,
title: title,
),
_ => throw const FormatException('Failed to load album.'),
};
}
}
// #enddocregion Album
void main() => runApp(const MyApp());
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
// #docregion State
class _MyAppState extends State<MyApp> {
late Future<Album> futureAlbum;
@override
void initState() {
super.initState();
futureAlbum = fetchAlbum();
}
// #enddocregion State
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Fetch Data Example',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
),
home: Scaffold(
appBar: AppBar(
title: const Text('Fetch Data Example'),
),
body: Center(
// #docregion FutureBuilder
child: FutureBuilder<Album>(
future: futureAlbum,
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text(snapshot.data!.title);
} else if (snapshot.hasError) {
return Text('${snapshot.error}');
}
// By default, show a loading spinner.
return const CircularProgressIndicator();
},
),
// #enddocregion FutureBuilder
),
),
);
}
// #docregion State
}
// #enddocregion State
| website/examples/cookbook/networking/fetch_data/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/networking/fetch_data/lib/main.dart",
"repo_id": "website",
"token_count": 1095
} | 1,265 |
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'Shared preferences demo',
home: MyHomePage(title: 'Shared preferences demo'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
@override
void initState() {
super.initState();
_loadCounter();
}
/// Load the initial counter value from persistent storage on start,
/// or fallback to 0 if it doesn't exist.
Future<void> _loadCounter() async {
final prefs = await SharedPreferences.getInstance();
setState(() {
_counter = prefs.getInt('counter') ?? 0;
});
}
/// After a click, increment the counter state and
/// asynchronously save it to persistent storage.
Future<void> _incrementCounter() async {
final prefs = await SharedPreferences.getInstance();
setState(() {
_counter = (prefs.getInt('counter') ?? 0) + 1;
prefs.setInt('counter', _counter);
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text(
'You have pushed the button this many times: ',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headlineMedium,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
}
| website/examples/cookbook/persistence/key_value/lib/main.dart/0 | {
"file_path": "website/examples/cookbook/persistence/key_value/lib/main.dart",
"repo_id": "website",
"token_count": 807
} | 1,266 |
name: google_mobile_ads_example
description: Google mobile ads
environment:
sdk: ^3.3.0
dependencies:
flutter:
sdk: flutter
google_mobile_ads: ^4.0.0
dev_dependencies:
example_utils:
path: ../../../example_utils
flutter:
uses-material-design: true
| website/examples/cookbook/plugins/google_mobile_ads/pubspec.yaml/0 | {
"file_path": "website/examples/cookbook/plugins/google_mobile_ads/pubspec.yaml",
"repo_id": "website",
"token_count": 104
} | 1,267 |
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
// #docregion TestWidgetStep2
testWidgets('Add and remove a todo', (tester) async {
// Build the widget
await tester.pumpWidget(const TodoList());
// Enter 'hi' into the TextField.
await tester.enterText(find.byType(TextField), 'hi');
});
// #enddocregion TestWidgetStep2
// #docregion TestWidgetStep3
testWidgets('Add and remove a todo', (tester) async {
// Enter text code...
// Tap the add button.
await tester.tap(find.byType(FloatingActionButton));
// Rebuild the widget after the state has changed.
await tester.pump();
// Expect to find the item on screen.
expect(find.text('hi'), findsOneWidget);
});
// #enddocregion TestWidgetStep3
// #docregion TestWidgetStep4
testWidgets('Add and remove a todo', (tester) async {
// Enter text and add the item...
// Swipe the item to dismiss it.
await tester.drag(find.byType(Dismissible), const Offset(500, 0));
// Build the widget until the dismiss animation ends.
await tester.pumpAndSettle();
// Ensure that the item is no longer on screen.
expect(find.text('hi'), findsNothing);
});
// #enddocregion TestWidgetStep4
}
class TodoList extends StatefulWidget {
const TodoList({super.key});
@override
State<TodoList> createState() => _TodoListState();
}
class _TodoListState extends State<TodoList> {
static const _appTitle = 'Todo List';
final todos = <String>[];
final controller = TextEditingController();
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _appTitle,
home: Scaffold(
appBar: AppBar(
title: const Text(_appTitle),
),
body: Column(
children: [
TextField(
controller: controller,
),
Expanded(
child: ListView.builder(
itemCount: todos.length,
itemBuilder: (context, index) {
final todo = todos[index];
return Dismissible(
key: Key('$todo$index'),
onDismissed: (direction) => todos.removeAt(index),
background: Container(color: Colors.red),
child: ListTile(title: Text(todo)),
);
},
),
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
todos.add(controller.text);
controller.clear();
});
},
child: const Icon(Icons.add),
),
),
);
}
}
| website/examples/cookbook/testing/widget/tap_drag/test/main_steps.dart/0 | {
"file_path": "website/examples/cookbook/testing/widget/tap_drag/test/main_steps.dart",
"repo_id": "website",
"token_count": 1203
} | 1,268 |
import 'address.dart';
import 'user.dart';
void exampleJson() {
// #docregion print
Address address = Address('My st.', 'New York');
User user = User('John', address);
print(user.toJson());
// #enddocregion print
}
| website/examples/development/data-and-backend/json/lib/nested/main.dart/0 | {
"file_path": "website/examples/development/data-and-backend/json/lib/nested/main.dart",
"repo_id": "website",
"token_count": 77
} | 1,269 |
name: platform_integration
description: Examples for documenting platform integration.
publish_to: none
version: 1.0.0+1
environment:
sdk: ^3.3.0
dependencies:
flutter:
sdk: flutter
pigeon: ^17.0.0
cupertino_icons: ^1.0.6
dev_dependencies:
example_utils:
path: ../../example_utils
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
| website/examples/development/platform_integration/pubspec.yaml/0 | {
"file_path": "website/examples/development/platform_integration/pubspec.yaml",
"repo_id": "website",
"token_count": 148
} | 1,270 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.