text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SearchAnchor.bar].
void main() => runApp(const SearchBarApp());
class SearchBarApp extends StatefulWidget {
const SearchBarApp({super.key});
@override
State<SearchBarApp> createState() => _SearchBarAppState();
}
class _SearchBarAppState extends State<SearchBarApp> {
Color? selectedColorSeed;
List<ColorLabel> searchHistory = <ColorLabel>[];
Iterable<Widget> getHistoryList(SearchController controller) {
return searchHistory.map(
(ColorLabel color) => ListTile(
leading: const Icon(Icons.history),
title: Text(color.label),
trailing: IconButton(
icon: const Icon(Icons.call_missed),
onPressed: () {
controller.text = color.label;
controller.selection = TextSelection.collapsed(offset: controller.text.length);
},
),
),
);
}
Iterable<Widget> getSuggestions(SearchController controller) {
final String input = controller.value.text;
return ColorLabel.values.where((ColorLabel color) => color.label.contains(input)).map(
(ColorLabel filteredColor) => ListTile(
leading: CircleAvatar(backgroundColor: filteredColor.color),
title: Text(filteredColor.label),
trailing: IconButton(
icon: const Icon(Icons.call_missed),
onPressed: () {
controller.text = filteredColor.label;
controller.selection = TextSelection.collapsed(offset: controller.text.length);
},
),
onTap: () {
controller.closeView(filteredColor.label);
handleSelection(filteredColor);
},
),
);
}
void handleSelection(ColorLabel selectedColor) {
setState(() {
selectedColorSeed = selectedColor.color;
if (searchHistory.length >= 5) {
searchHistory.removeLast();
}
searchHistory.insert(0, selectedColor);
});
}
@override
Widget build(BuildContext context) {
final ThemeData themeData = ThemeData(useMaterial3: true, colorSchemeSeed: selectedColorSeed);
final ColorScheme colors = themeData.colorScheme;
return MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(title: const Text('Search Bar Sample')),
body: Align(
alignment: Alignment.topCenter,
child: Column(
children: <Widget>[
SearchAnchor.bar(
barHintText: 'Search colors',
suggestionsBuilder: (BuildContext context, SearchController controller) {
if (controller.text.isEmpty) {
if (searchHistory.isNotEmpty) {
return getHistoryList(controller);
}
return <Widget>[Center(child: Text('No search history.', style: TextStyle(color: colors.outline)))];
}
return getSuggestions(controller);
},
),
cardSize,
Card(color: colors.primary, child: cardSize),
Card(color: colors.onPrimary, child: cardSize),
Card(color: colors.primaryContainer, child: cardSize),
Card(color: colors.onPrimaryContainer, child: cardSize),
Card(color: colors.secondary, child: cardSize),
Card(color: colors.onSecondary, child: cardSize),
],
),
),
),
);
}
}
SizedBox cardSize = const SizedBox(
width: 80,
height: 30,
);
enum ColorLabel {
red('red', Colors.red),
orange('orange', Colors.orange),
yellow('yellow', Colors.yellow),
green('green', Colors.green),
blue('blue', Colors.blue),
indigo('indigo', Colors.indigo),
violet('violet', Color(0xFF8F00FF)),
purple('purple', Colors.purple),
pink('pink', Colors.pink),
silver('silver', Color(0xFF808080)),
gold('gold', Color(0xFFFFD700)),
beige('beige', Color(0xFFF5F5DC)),
brown('brown', Colors.brown),
grey('grey', Colors.grey),
black('black', Colors.black),
white('white', Colors.white);
const ColorLabel(this.label, this.color);
final String label;
final Color color;
}
| flutter/examples/api/lib/material/search_anchor/search_anchor.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/search_anchor/search_anchor.0.dart",
"repo_id": "flutter",
"token_count": 1837
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SnackBar].
void main() => runApp(const SnackBarExampleApp());
class SnackBarExampleApp extends StatelessWidget {
const SnackBarExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('SnackBar Sample')),
body: const Center(
child: SnackBarExample(),
),
),
);
}
}
class SnackBarExample extends StatelessWidget {
const SnackBarExample({super.key});
@override
Widget build(BuildContext context) {
return ElevatedButton(
child: const Text('Show Snackbar'),
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
action: SnackBarAction(
label: 'Action',
onPressed: () {
// Code to execute.
},
),
content: const Text('Awesome SnackBar!'),
duration: const Duration(milliseconds: 1500),
width: 280.0, // Width of the SnackBar.
padding: const EdgeInsets.symmetric(
horizontal: 8.0, // Inner padding for SnackBar content.
),
behavior: SnackBarBehavior.floating,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10.0),
),
),
);
},
);
}
}
| flutter/examples/api/lib/material/snack_bar/snack_bar.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/snack_bar/snack_bar.1.dart",
"repo_id": "flutter",
"token_count": 707
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TabBar].
void main() => runApp(const TabBarApp());
class TabBarApp extends StatelessWidget {
const TabBarApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const TabBarExample(),
);
}
}
class TabBarExample extends StatefulWidget {
const TabBarExample({super.key});
@override
State<TabBarExample> createState() => _TabBarExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _TabBarExampleState extends State<TabBarExample> with TickerProviderStateMixin {
late final TabController _tabController;
@override
void initState() {
super.initState();
_tabController = TabController(length: 3, vsync: this);
}
@override
void dispose() {
_tabController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('TabBar Sample'),
bottom: TabBar(
controller: _tabController,
tabs: const <Widget>[
Tab(
icon: Icon(Icons.cloud_outlined),
),
Tab(
icon: Icon(Icons.beach_access_sharp),
),
Tab(
icon: Icon(Icons.brightness_5_sharp),
),
],
),
),
body: TabBarView(
controller: _tabController,
children: const <Widget>[
Center(
child: Text("It's cloudy here"),
),
Center(
child: Text("It's rainy here"),
),
Center(
child: Text("It's sunny here"),
),
],
),
);
}
}
| flutter/examples/api/lib/material/tabs/tab_bar.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/tabs/tab_bar.1.dart",
"repo_id": "flutter",
"token_count": 841
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Tooltip].
void main() => runApp(const TooltipExampleApp());
class TooltipExampleApp extends StatelessWidget {
const TooltipExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(tooltipTheme: const TooltipThemeData(preferBelow: false)),
home: const TooltipSample(title: 'Tooltip Sample'),
);
}
}
class TooltipSample extends StatelessWidget {
const TooltipSample({super.key, required this.title});
final String title;
@override
Widget build(BuildContext context) {
final GlobalKey<TooltipState> tooltipkey = GlobalKey<TooltipState>();
return Scaffold(
appBar: AppBar(title: Text(title)),
body: Center(
child: Tooltip(
// Provide a global key with the "TooltipState" type to show
// the tooltip manually when trigger mode is set to manual.
key: tooltipkey,
triggerMode: TooltipTriggerMode.manual,
showDuration: const Duration(seconds: 1),
message: 'I am a Tooltip',
child: const Text('Tap on the FAB'),
),
),
floatingActionButton: FloatingActionButton.extended(
onPressed: () {
// Show Tooltip programmatically on button tap.
tooltipkey.currentState?.ensureTooltipVisible();
},
label: const Text('Show Tooltip'),
),
);
}
}
| flutter/examples/api/lib/material/tooltip/tooltip.3.dart/0 | {
"file_path": "flutter/examples/api/lib/material/tooltip/tooltip.3.dart",
"repo_id": "flutter",
"token_count": 586
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [LogicalKeyboardKey].
void main() => runApp(const KeyExampleApp());
class KeyExampleApp extends StatelessWidget {
const KeyExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Key Handling Example')),
body: const MyKeyExample(),
),
);
}
}
class MyKeyExample extends StatefulWidget {
const MyKeyExample({super.key});
@override
State<MyKeyExample> createState() => _MyKeyExampleState();
}
class _MyKeyExampleState extends State<MyKeyExample> {
// The node used to request the keyboard focus.
final FocusNode _focusNode = FocusNode();
// The message to display.
String? _message;
// Focus nodes need to be disposed.
@override
void dispose() {
_focusNode.dispose();
super.dispose();
}
// Handles the key events from the Focus widget and updates the
// _message.
KeyEventResult _handleKeyEvent(FocusNode node, KeyEvent event) {
setState(() {
if (event.logicalKey == LogicalKeyboardKey.keyQ) {
_message = 'Pressed the "Q" key!';
} else {
if (kReleaseMode) {
_message = 'Not a Q: Pressed 0x${event.logicalKey.keyId.toRadixString(16)}';
} else {
// As the name implies, the debugName will only print useful
// information in debug mode.
_message = 'Not a Q: Pressed ${event.logicalKey.debugName}';
}
}
});
return event.logicalKey == LogicalKeyboardKey.keyQ ? KeyEventResult.handled : KeyEventResult.ignored;
}
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Container(
color: Colors.white,
alignment: Alignment.center,
child: DefaultTextStyle(
style: textTheme.headlineMedium!,
child: Focus(
focusNode: _focusNode,
onKeyEvent: _handleKeyEvent,
child: ListenableBuilder(
listenable: _focusNode,
builder: (BuildContext context, Widget? child) {
if (!_focusNode.hasFocus) {
return GestureDetector(
onTap: () {
FocusScope.of(context).requestFocus(_focusNode);
},
child: const Text('Click to focus'),
);
}
return Text(_message ?? 'Press a key');
},
),
),
),
);
}
}
| flutter/examples/api/lib/services/keyboard_key/logical_keyboard_key.0.dart/0 | {
"file_path": "flutter/examples/api/lib/services/keyboard_key/logical_keyboard_key.0.dart",
"repo_id": "flutter",
"token_count": 1124
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [Action.Action.overridable].
void main() {
runApp(
const MaterialApp(
home: Scaffold(
body: Center(child: VerificationCodeGenerator()),
),
),
);
}
const CopyTextIntent copyTextIntent = CopyTextIntent._();
class CopyTextIntent extends Intent {
const CopyTextIntent._();
}
class CopyableText extends StatelessWidget {
const CopyableText({super.key, required this.text});
final String text;
void _copy(CopyTextIntent intent) => Clipboard.setData(ClipboardData(text: text));
@override
Widget build(BuildContext context) {
final Action<CopyTextIntent> defaultCopyAction = CallbackAction<CopyTextIntent>(onInvoke: _copy);
return Shortcuts(
shortcuts: const <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.keyC, control: true): copyTextIntent
},
child: Actions(
actions: <Type, Action<Intent>>{
// The Action is made overridable so the VerificationCodeGenerator
// widget can override how copying is handled.
CopyTextIntent: Action<CopyTextIntent>.overridable(defaultAction: defaultCopyAction, context: context),
},
child: Focus(
autofocus: true,
child: DefaultTextStyle.merge(
style: const TextStyle(fontSize: 20, fontWeight: FontWeight.bold),
child: Text(text),
),
),
),
);
}
}
class VerificationCodeGenerator extends StatelessWidget {
const VerificationCodeGenerator({super.key});
void _copy(CopyTextIntent intent) {
debugPrint('Content copied');
Clipboard.setData(const ClipboardData(text: '111222333'));
}
@override
Widget build(BuildContext context) {
return Actions(
actions: <Type, Action<Intent>>{CopyTextIntent: CallbackAction<CopyTextIntent>(onInvoke: _copy)},
child: const Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Press Ctrl-C to Copy'),
SizedBox(height: 10),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
CopyableText(text: '111'),
SizedBox(width: 5),
CopyableText(text: '222'),
SizedBox(width: 5),
CopyableText(text: '333'),
],
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/actions/action.action_overridable.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/actions/action.action_overridable.0.dart",
"repo_id": "flutter",
"token_count": 1055
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RawAutocomplete].
void main() => runApp(const AutocompleteExampleApp());
class AutocompleteExampleApp extends StatelessWidget {
const AutocompleteExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('RawAutocomplete Custom Type'),
),
body: const Center(
child: AutocompleteCustomTypeExample(),
),
),
);
}
}
// An example of a type that someone might want to autocomplete a list of.
@immutable
class User {
const User({
required this.email,
required this.name,
});
final String email;
final String name;
@override
String toString() {
return '$name, $email';
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is User && other.name == name && other.email == email;
}
@override
int get hashCode => Object.hash(email, name);
}
class AutocompleteCustomTypeExample extends StatelessWidget {
const AutocompleteCustomTypeExample({super.key});
static const List<User> _userOptions = <User>[
User(name: 'Alice', email: '[email protected]'),
User(name: 'Bob', email: '[email protected]'),
User(name: 'Charlie', email: '[email protected]'),
];
static String _displayStringForOption(User option) => option.name;
@override
Widget build(BuildContext context) {
return RawAutocomplete<User>(
optionsBuilder: (TextEditingValue textEditingValue) {
return _userOptions.where((User option) {
// Search based on User.toString, which includes both name and
// email, even though the display string is just the name.
return option.toString().contains(textEditingValue.text.toLowerCase());
});
},
displayStringForOption: _displayStringForOption,
fieldViewBuilder: (
BuildContext context,
TextEditingController textEditingController,
FocusNode focusNode,
VoidCallback onFieldSubmitted,
) {
return TextFormField(
controller: textEditingController,
focusNode: focusNode,
onFieldSubmitted: (String value) {
onFieldSubmitted();
},
);
},
optionsViewBuilder: (BuildContext context, AutocompleteOnSelected<User> onSelected, Iterable<User> options) {
return Align(
alignment: Alignment.topLeft,
child: Material(
elevation: 4.0,
child: SizedBox(
height: 200.0,
child: ListView.builder(
padding: const EdgeInsets.all(8.0),
itemCount: options.length,
itemBuilder: (BuildContext context, int index) {
final User option = options.elementAt(index);
return GestureDetector(
onTap: () {
onSelected(option);
},
child: ListTile(
title: Text(_displayStringForOption(option)),
),
);
},
),
),
),
);
},
);
}
}
| flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.1.dart",
"repo_id": "flutter",
"token_count": 1469
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [IgnorePointer].
void main() => runApp(const IgnorePointerApp());
class IgnorePointerApp extends StatelessWidget {
const IgnorePointerApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text('IgnorePointer Sample'),
),
body: const Center(child: IgnorePointerExample()),
),
);
}
}
class IgnorePointerExample extends StatefulWidget {
const IgnorePointerExample({super.key});
@override
State<IgnorePointerExample> createState() => _IgnorePointerExampleState();
}
class _IgnorePointerExampleState extends State<IgnorePointerExample> {
bool ignoring = false;
void setIgnoring(bool newValue) {
setState(() {
ignoring = newValue;
});
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Text('Ignoring: $ignoring'),
IgnorePointer(
ignoring: ignoring,
child: ElevatedButton(
style: ElevatedButton.styleFrom(
padding: const EdgeInsets.all(24.0),
),
onPressed: () {},
child: const Text('Click me!'),
),
),
FilledButton(
onPressed: () {
setIgnoring(!ignoring);
},
child: Text(
ignoring ? 'Set ignoring to false' : 'Set ignoring to true',
),
),
],
);
}
}
| flutter/examples/api/lib/widgets/basic/ignore_pointer.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/ignore_pointer.0.dart",
"repo_id": "flutter",
"token_count": 734
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TextEditingController].
void main() => runApp(const TextEditingControllerExampleApp());
class TextEditingControllerExampleApp extends StatelessWidget {
const TextEditingControllerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: TextEditingControllerExample(),
);
}
}
class TextEditingControllerExample extends StatefulWidget {
const TextEditingControllerExample({super.key});
@override
State<TextEditingControllerExample> createState() => _TextEditingControllerExampleState();
}
class _TextEditingControllerExampleState extends State<TextEditingControllerExample> {
final TextEditingController _controller = TextEditingController();
@override
void initState() {
super.initState();
_controller.addListener(() {
final String text = _controller.text.toLowerCase();
_controller.value = _controller.value.copyWith(
text: text,
selection: TextSelection(baseOffset: text.length, extentOffset: text.length),
composing: TextRange.empty,
);
});
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(6),
child: TextFormField(
controller: _controller,
decoration: const InputDecoration(border: OutlineInputBorder()),
),
),
);
}
}
| flutter/examples/api/lib/widgets/editable_text/text_editing_controller.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/editable_text/text_editing_controller.0.dart",
"repo_id": "flutter",
"token_count": 576
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
/// Flutter code sample for [GestureDetector].
void main() {
debugPrintGestureArenaDiagnostics = true;
runApp(const NestedGestureDetectorsApp());
}
enum _OnTapWinner { none, yellow, green }
class NestedGestureDetectorsApp extends StatelessWidget {
const NestedGestureDetectorsApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Nested GestureDetectors')),
body: const NestedGestureDetectorsExample(),
),
);
}
}
class NestedGestureDetectorsExample extends StatefulWidget {
const NestedGestureDetectorsExample({super.key});
@override
State<NestedGestureDetectorsExample> createState() => _NestedGestureDetectorsExampleState();
}
class _NestedGestureDetectorsExampleState extends State<NestedGestureDetectorsExample> {
bool _isYellowTranslucent = false;
_OnTapWinner _winner = _OnTapWinner.none;
final Border highlightBorder = Border.all(color: Colors.red, width: 5);
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
Expanded(
child: GestureDetector(
onTap: () {
debugPrint('Green onTap');
setState(() {
_winner = _OnTapWinner.green;
});
},
onTapDown: (_) => debugPrint('Green onTapDown'),
onTapCancel: () => debugPrint('Green onTapCancel'),
child: Container(
alignment: Alignment.center,
decoration: BoxDecoration(
border: _winner == _OnTapWinner.green ? highlightBorder : null,
color: Colors.green,
),
child: GestureDetector(
// Setting behavior to transparent or opaque as no impact on
// parent-child hit testing. A tap on 'Yellow' is also in
// 'Green' bounds. Both enter the gesture arena, 'Yellow' wins
// because it is in front.
behavior: _isYellowTranslucent ? HitTestBehavior.translucent : HitTestBehavior.opaque,
onTap: () {
debugPrint('Yellow onTap');
setState(() {
_winner = _OnTapWinner.yellow;
});
},
child: Container(
alignment: Alignment.center,
decoration: BoxDecoration(
border: _winner == _OnTapWinner.yellow ? highlightBorder : null,
color: Colors.amber,
),
width: 200,
height: 200,
child: Text(
'HitTextBehavior.${_isYellowTranslucent ? 'translucent' : 'opaque'}',
textAlign: TextAlign.center,
),
),
),
),
),
),
Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
children: <Widget>[
ElevatedButton(
child: const Text('Reset'),
onPressed: () {
setState(() {
_isYellowTranslucent = false;
_winner = _OnTapWinner.none;
});
},
),
const SizedBox(width: 8),
ElevatedButton(
child: Text(
'Set Yellow behavior to ${_isYellowTranslucent ? 'opaque' : 'translucent'}',
),
onPressed: () {
setState(() => _isYellowTranslucent = !_isYellowTranslucent);
},
),
],
),
),
],
);
}
@override
void dispose() {
debugPrintGestureArenaDiagnostics = false;
super.dispose();
}
}
| flutter/examples/api/lib/widgets/gesture_detector/gesture_detector.2.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/gesture_detector/gesture_detector.2.dart",
"repo_id": "flutter",
"token_count": 2009
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InheritedTheme].
void main() {
runApp(const InheritedThemeExampleApp());
}
class MyAppBody extends StatelessWidget {
const MyAppBody({super.key});
@override
Widget build(BuildContext context) {
final NavigatorState navigator = Navigator.of(context);
// This InheritedTheme.capture() saves references to themes that are
// found above the context provided to this widget's build method
// excluding themes are found above the navigator. Those themes do
// not have to be captured, because they will already be visible from
// the new route pushed onto said navigator.
// Themes are captured outside of the route's builder because when the
// builder executes, the context may not be valid anymore.
final CapturedThemes themes = InheritedTheme.capture(from: context, to: navigator.context);
return GestureDetector(
onTap: () {
Navigator.of(context).push(
MaterialPageRoute<void>(
builder: (BuildContext _) {
// Wrap the actual child of the route in the previously
// captured themes.
return themes.wrap(
Container(
alignment: Alignment.center,
color: Colors.white,
child: const Text('Hello World'),
),
);
},
),
);
},
child: const Center(child: Text('Tap Here')),
);
}
}
class InheritedThemeExampleApp extends StatelessWidget {
const InheritedThemeExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: Scaffold(
// Override the DefaultTextStyle defined by the Scaffold.
// Descendant widgets will inherit this big blue text style.
body: DefaultTextStyle(
style: TextStyle(fontSize: 48, color: Colors.blue),
child: MyAppBody(),
),
),
);
}
}
| flutter/examples/api/lib/widgets/inherited_theme/inherited_theme.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/inherited_theme/inherited_theme.0.dart",
"repo_id": "flutter",
"token_count": 810
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// This sample demonstrates using [NavigatorPopHandler] to handle system back
/// gestures when there are nested [Navigator] widgets by delegating to the
/// current [Navigator].
void main() => runApp(const NavigatorPopHandlerApp());
class NavigatorPopHandlerApp extends StatelessWidget {
const NavigatorPopHandlerApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
initialRoute: '/',
routes: <String, WidgetBuilder>{
'/': (BuildContext context) => _HomePage(),
'/nested_navigators': (BuildContext context) => const NestedNavigatorsPage(),
},
);
}
}
class _HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Nested Navigators Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Home Page'),
const Text('A system back gesture here will exit the app.'),
const SizedBox(height: 20.0),
ListTile(
title: const Text('Nested Navigator route'),
subtitle: const Text('This route has another Navigator widget in addition to the one inside MaterialApp above.'),
onTap: () {
Navigator.of(context).pushNamed('/nested_navigators');
},
),
],
),
),
);
}
}
class NestedNavigatorsPage extends StatefulWidget {
const NestedNavigatorsPage({super.key});
@override
State<NestedNavigatorsPage> createState() => _NestedNavigatorsPageState();
}
class _NestedNavigatorsPageState extends State<NestedNavigatorsPage> {
final GlobalKey<NavigatorState> _nestedNavigatorKey = GlobalKey<NavigatorState>();
@override
Widget build(BuildContext context) {
return NavigatorPopHandler(
onPop: () {
_nestedNavigatorKey.currentState!.maybePop();
},
child: Navigator(
key: _nestedNavigatorKey,
initialRoute: 'nested_navigators/one',
onGenerateRoute: (RouteSettings settings) {
switch (settings.name) {
case 'nested_navigators/one':
final BuildContext rootContext = context;
return MaterialPageRoute<void>(
builder: (BuildContext context) => NestedNavigatorsPageOne(
onBack: () {
Navigator.of(rootContext).pop();
},
),
);
case 'nested_navigators/one/another_one':
return MaterialPageRoute<void>(
builder: (BuildContext context) => const NestedNavigatorsPageTwo(
),
);
default:
throw Exception('Invalid route: ${settings.name}');
}
},
),
);
}
}
class NestedNavigatorsPageOne extends StatelessWidget {
const NestedNavigatorsPageOne({
required this.onBack,
super.key,
});
final VoidCallback onBack;
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.grey,
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Nested Navigators Page One'),
const Text('A system back here returns to the home page.'),
TextButton(
onPressed: () {
Navigator.of(context).pushNamed('nested_navigators/one/another_one');
},
child: const Text('Go to another route in this nested Navigator'),
),
TextButton(
// Can't use Navigator.of(context).pop() because this is the root
// route, so it can't be popped. The Navigator above this needs to
// be popped.
onPressed: onBack,
child: const Text('Go back'),
),
],
),
),
);
}
}
class NestedNavigatorsPageTwo extends StatelessWidget {
const NestedNavigatorsPageTwo({
super.key,
});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.grey.withBlue(180),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Nested Navigators Page Two'),
const Text('A system back here will go back to Nested Navigators Page One'),
TextButton(
onPressed: () {
Navigator.of(context).pop();
},
child: const Text('Go back'),
),
],
),
),
);
}
}
| flutter/examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.0.dart",
"repo_id": "flutter",
"token_count": 2146
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [PreferredSize].
void main() => runApp(const PreferredSizeExampleApp());
class PreferredSizeExampleApp extends StatelessWidget {
const PreferredSizeExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: PreferredSizeExample(),
);
}
}
class AppBarContent extends StatelessWidget {
const AppBarContent({super.key});
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Padding(
padding: const EdgeInsets.symmetric(horizontal: 10),
child: Row(
children: <Widget>[
const Text(
'PreferredSize Sample',
style: TextStyle(color: Colors.white),
),
const Spacer(),
IconButton(
icon: const Icon(
Icons.search,
size: 20,
),
color: Colors.white,
onPressed: () {},
),
IconButton(
icon: const Icon(
Icons.more_vert,
size: 20,
),
color: Colors.white,
onPressed: () {},
),
],
),
),
],
);
}
}
class PreferredSizeExample extends StatelessWidget {
const PreferredSizeExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: PreferredSize(
preferredSize: const Size.fromHeight(80.0),
child: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: <Color>[Colors.blue, Colors.pink],
),
),
child: const AppBarContent(),
),
),
body: const Center(
child: Text('Content'),
),
);
}
}
| flutter/examples/api/lib/widgets/preferred_size/preferred_size.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/preferred_size/preferred_size.0.dart",
"repo_id": "flutter",
"token_count": 1024
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RawScrollbar].
void main() => runApp(const RawScrollbarExampleApp());
class RawScrollbarExampleApp extends StatelessWidget {
const RawScrollbarExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('RawScrollbar Sample')),
body: const RawScrollbarExample(),
),
);
}
}
class RawScrollbarExample extends StatefulWidget {
const RawScrollbarExample({super.key});
@override
State<RawScrollbarExample> createState() => _RawScrollbarExampleState();
}
class _RawScrollbarExampleState extends State<RawScrollbarExample> {
final ScrollController _controllerOne = ScrollController();
@override
Widget build(BuildContext context) {
return RawScrollbar(
controller: _controllerOne,
thumbVisibility: true,
child: GridView.builder(
controller: _controllerOne,
itemCount: 120,
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(crossAxisCount: 3),
itemBuilder: (BuildContext context, int index) {
return Center(
child: Text('item $index'),
);
},
),
);
}
}
| flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.2.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.2.dart",
"repo_id": "flutter",
"token_count": 496
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
void main() => runApp(const SliverMainAxisGroupExampleApp());
class SliverMainAxisGroupExampleApp extends StatelessWidget {
const SliverMainAxisGroupExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('SliverMainAxisGroup Sample')),
body: const SliverMainAxisGroupExample(),
),
);
}
}
class SliverMainAxisGroupExample extends StatelessWidget {
const SliverMainAxisGroupExample({super.key});
@override
Widget build(BuildContext context) {
return CustomScrollView(
slivers: <Widget>[
SliverMainAxisGroup(
slivers: <Widget>[
const SliverAppBar(
title: Text('Section Title'),
expandedHeight: 70.0,
pinned: true,
),
SliverList.builder(
itemBuilder: (BuildContext context, int index) {
return Container(
color: index.isEven ? Colors.amber[300] : Colors.blue[300],
height: 100.0,
child: Center(
child: Text(
'Item $index',
style: const TextStyle(fontSize: 24),
),
),
);
},
itemCount: 5,
),
SliverToBoxAdapter(
child: Container(
color: Colors.cyan,
height: 100,
child: const Center(
child: Text('Another sliver child', style: TextStyle(fontSize: 24)),
),
),
)
],
),
SliverToBoxAdapter(
child: Container(
height: 1000,
decoration: const BoxDecoration(color: Colors.greenAccent),
child: const Center(
child: Text('Hello World!', style: TextStyle(fontSize: 24)),
),
),
),
],
);
}
}
| flutter/examples/api/lib/widgets/sliver/sliver_main_axis_group.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/sliver/sliver_main_axis_group.0.dart",
"repo_id": "flutter",
"token_count": 1102
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [FadeTransition].
void main() => runApp(const FadeTransitionExampleApp());
class FadeTransitionExampleApp extends StatelessWidget {
const FadeTransitionExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: FadeTransitionExample(),
);
}
}
class FadeTransitionExample extends StatefulWidget {
const FadeTransitionExample({super.key});
@override
State<FadeTransitionExample> createState() => _FadeTransitionExampleState();
}
/// [AnimationController]s can be created with `vsync: this` because of
/// [TickerProviderStateMixin].
class _FadeTransitionExampleState extends State<FadeTransitionExample> with TickerProviderStateMixin {
late final AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true);
late final Animation<double> _animation = CurvedAnimation(
parent: _controller,
curve: Curves.easeIn,
);
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return ColoredBox(
color: Colors.white,
child: FadeTransition(
opacity: _animation,
child: const Padding(padding: EdgeInsets.all(8), child: FlutterLogo()),
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/fade_transition.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/fade_transition.0.dart",
"repo_id": "flutter",
"token_count": 505
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/page_scaffold/cupertino_page_scaffold.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can increment counter', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PageScaffoldApp(),
);
expect(find.byType(CupertinoPageScaffold), findsOneWidget);
expect(find.text('You have pressed the button 0 times.'), findsOneWidget);
await tester.tap(find.byType(CupertinoButton));
await tester.pumpAndSettle();
expect(find.text('You have pressed the button 1 times.'), findsOneWidget);
});
}
| flutter/examples/api/test/cupertino/page_scaffold/cupertino_page_scaffold.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/page_scaffold/cupertino_page_scaffold.0_test.dart",
"repo_id": "flutter",
"token_count": 282
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/tab_scaffold/cupertino_tab_scaffold.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can use CupertinoTabView as the root widget', (WidgetTester tester) async {
await tester.pumpWidget(
const example.TabScaffoldApp(),
);
expect(find.text('Page 1 of tab 0'), findsOneWidget);
await tester.tap(find.byIcon(CupertinoIcons.search_circle_fill));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsOneWidget);
await tester.tap(find.text('Next page'));
await tester.pumpAndSettle();
expect(find.text('Page 2 of tab 1'), findsOneWidget);
await tester.tap(find.text('Back'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsOneWidget);
});
}
| flutter/examples/api/test/cupertino/tab_scaffold/cupertino_tab_scaffold.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/tab_scaffold/cupertino_tab_scaffold.0_test.dart",
"repo_id": "flutter",
"token_count": 371
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/app_bar/sliver_app_bar.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
const Offset _kOffset = Offset(0.0, -200.0);
void main() {
testWidgets('SliverAppbar can be pinned', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AppBarApp(),
);
expect(find.widgetWithText(SliverAppBar, 'SliverAppBar'), findsOneWidget);
expect(tester.getBottomLeft(find.text('SliverAppBar')).dy, 144.0);
await tester.drag(find.text('0'), _kOffset, touchSlopY: 0, warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(tester.getBottomLeft(find.text('SliverAppBar')).dy, 40.0);
});
}
| flutter/examples/api/test/material/app_bar/sliver_app_bar.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/app_bar/sliver_app_bar.1_test.dart",
"repo_id": "flutter",
"token_count": 344
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/checkbox/checkbox.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Checkbox can be checked', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CheckboxExampleApp(),
);
Checkbox checkbox = tester.widget(find.byType(Checkbox));
expect(checkbox.value, isFalse);
await tester.tap(find.byType(Checkbox));
await tester.pump();
checkbox = tester.widget(find.byType(Checkbox));
expect(checkbox.value, isTrue);
await tester.tap(find.byType(Checkbox));
await tester.pump();
checkbox = tester.widget(find.byType(Checkbox));
expect(checkbox.value, isFalse);
});
testWidgets('Checkbox color can be changed', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CheckboxExampleApp(),
);
final Checkbox checkbox = tester.widget(find.byType(Checkbox));
expect(
checkbox.checkColor,
Colors.white,
);
expect(
checkbox.fillColor!.resolve(<MaterialState>{}),
Colors.red,
);
expect(
checkbox.fillColor!.resolve(<MaterialState>{MaterialState.pressed}),
Colors.blue,
);
expect(
checkbox.fillColor!.resolve(<MaterialState>{MaterialState.hovered}),
Colors.blue,
);
expect(
checkbox.fillColor!.resolve(<MaterialState>{MaterialState.focused}),
Colors.blue,
);
});
}
| flutter/examples/api/test/material/checkbox/checkbox.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/checkbox/checkbox.0_test.dart",
"repo_id": "flutter",
"token_count": 630
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/data_table/data_table.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('DataTable is scrollable', (WidgetTester tester) async {
await tester.pumpWidget(
const example.DataTableExampleApp(),
);
expect(find.byType(SingleChildScrollView), findsOneWidget);
expect(tester.getTopLeft(find.text('Row 5')), const Offset(66.0, 366.0));
await tester.drag(find.byType(SingleChildScrollView), const Offset(0.0, -200.0));
await tester.pumpAndSettle();
expect(tester.getTopLeft(find.text('Row 5')), const Offset(66.0, 186.0));
});
}
| flutter/examples/api/test/material/data_table/data_table.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/data_table/data_table.1_test.dart",
"repo_id": "flutter",
"token_count": 302
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/dropdown/dropdown_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Select an item from DropdownButton', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.DropdownButtonApp(),
),
),
);
expect(find.text('One'), findsOneWidget);
await tester.tap(find.text('One'));
await tester.pumpAndSettle();
await tester.tap(find.text('Two').last);
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
});
}
| flutter/examples/api/test/material/dropdown/dropdown_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/dropdown/dropdown_button.0_test.dart",
"repo_id": "flutter",
"token_count": 310
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/icon_button/icon_button.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('IconButton', (WidgetTester tester) async {
await tester.pumpWidget(
const example.IconButtonExampleApp(),
);
await tester.pumpAndSettle();
expect(find.byIcon(Icons.android), findsOneWidget);
final Ink ink = tester.widget<Ink>(
find.ancestor(
of: find.byIcon(Icons.android),
matching: find.byType(Ink),
),
);
final ShapeDecoration decoration = ink.decoration! as ShapeDecoration;
expect(decoration.color, Colors.lightBlue);
expect(decoration.shape, const CircleBorder());
final IconButton iconButton = ink.child! as IconButton;
expect(iconButton.color, Colors.white) ;
});
}
| flutter/examples/api/test/material/icon_button/icon_button.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/icon_button/icon_button.1_test.dart",
"repo_id": "flutter",
"token_count": 362
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/list_tile/list_tile.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ListTile leading and trailing widgets are aligned appropriately', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ListTileApp(),
);
expect(find.byType(ListTile), findsNWidgets(3));
Offset listTileTopLeft = tester.getTopLeft(find.byType(ListTile).at(0));
Offset leadingTopLeft = tester.getTopLeft(find.byType(CircleAvatar).at(0));
Offset trailingTopLeft = tester.getTopLeft(find.byType(Icon).at(0));
// The leading and trailing widgets are centered vertically with the text.
expect(leadingTopLeft - listTileTopLeft, const Offset(16.0, 16.0));
expect(trailingTopLeft - listTileTopLeft, const Offset(752.0, 24.0));
listTileTopLeft = tester.getTopLeft(find.byType(ListTile).at(1));
leadingTopLeft = tester.getTopLeft(find.byType(CircleAvatar).at(1));
trailingTopLeft = tester.getTopLeft(find.byType(Icon).at(1));
// The leading and trailing widgets are centered vertically with the text.
expect(leadingTopLeft - listTileTopLeft, const Offset(16.0, 30.0));
expect(trailingTopLeft - listTileTopLeft, const Offset(752.0, 38.0));
listTileTopLeft = tester.getTopLeft(find.byType(ListTile).at(2));
leadingTopLeft = tester.getTopLeft(find.byType(CircleAvatar).at(2));
trailingTopLeft = tester.getTopLeft(find.byType(Icon).at(2));
// The leading and trailing widgets are aligned to the top vertically with the text.
expect(leadingTopLeft - listTileTopLeft, const Offset(16.0, 8.0));
expect(trailingTopLeft - listTileTopLeft, const Offset(752.0, 8.0));
});
}
| flutter/examples/api/test/material/list_tile/list_tile.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/list_tile/list_tile.2_test.dart",
"repo_id": "flutter",
"token_count": 666
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/outlined_button/outlined_button.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('OutlinedButton Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.OutlinedButtonExampleApp(),
);
expect(find.widgetWithText(AppBar, 'OutlinedButton Sample'), findsOneWidget);
final Finder outlinedButton = find.widgetWithText(OutlinedButton, 'Click Me');
expect(outlinedButton, findsOneWidget);
final OutlinedButton outlinedButtonWidget = tester.widget<OutlinedButton>(outlinedButton);
expect(outlinedButtonWidget.onPressed.runtimeType, VoidCallback);
});
}
| flutter/examples/api/test/material/outlined_button/outlined_button.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/outlined_button/outlined_button.0_test.dart",
"repo_id": "flutter",
"token_count": 279
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/radio_list_tile/radio_list_tile.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Radio aligns appropriately', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RadioListTileApp(),
);
expect(find.byType(RadioListTile<example.Groceries>), findsNWidgets(3));
Offset tileTopLeft = tester.getTopLeft(find.byType(RadioListTile<example.Groceries>).at(0));
Offset radioTopLeft = tester.getTopLeft(find.byType(Radio<example.Groceries>).at(0));
// The radio is centered vertically with the text.
expect(radioTopLeft - tileTopLeft, const Offset(16.0, 16.0));
tileTopLeft = tester.getTopLeft(find.byType(RadioListTile<example.Groceries>).at(1));
radioTopLeft = tester.getTopLeft(find.byType(Radio<example.Groceries>).at(1));
// The radio is centered vertically with the text.
expect(radioTopLeft - tileTopLeft, const Offset(16.0, 30.0));
tileTopLeft = tester.getTopLeft(find.byType(RadioListTile<example.Groceries>).at(2));
radioTopLeft = tester.getTopLeft(find.byType(Radio<example.Groceries>).at(2));
// The radio is aligned to the top vertically with the text.
expect(radioTopLeft - tileTopLeft, const Offset(16.0, 8.0));
});
testWidgets('Radios can be checked', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RadioListTileApp(),
);
expect(find.byType(RadioListTile<example.Groceries>), findsNWidgets(3));
final Finder radioListTile = find.byType(RadioListTile<example.Groceries>);
// Initially the first radio is checked.
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(0)).groupValue,
example.Groceries.pickles,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(1)).groupValue,
example.Groceries.pickles,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(2)).groupValue,
example.Groceries.pickles,
);
// Tap the second radio.
await tester.tap(find.byType(Radio<example.Groceries>).at(1));
await tester.pumpAndSettle();
// The second radio is checked.
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(0)).groupValue,
example.Groceries.tomato,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(1)).groupValue,
example.Groceries.tomato,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(2)).groupValue,
example.Groceries.tomato,
);
// Tap the third radio.
await tester.tap(find.byType(Radio<example.Groceries>).at(2));
await tester.pumpAndSettle();
// The third radio is checked.
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(0)).groupValue,
example.Groceries.lettuce,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(1)).groupValue,
example.Groceries.lettuce,
);
expect(
tester.widget<RadioListTile<example.Groceries>>(radioListTile.at(2)).groupValue,
example.Groceries.lettuce,
);
});
}
| flutter/examples/api/test/material/radio_list_tile/radio_list_tile.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/radio_list_tile/radio_list_tile.1_test.dart",
"repo_id": "flutter",
"token_count": 1312
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/slider/slider.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Slider can change its value', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SliderApp(),
);
expect(find.byType(Slider), findsOneWidget);
final Finder sliderFinder = find.byType(Slider);
Slider slider = tester.widget(sliderFinder);
expect(slider.value, 20);
final Offset center = tester.getCenter(sliderFinder);
await tester.tapAt(Offset(center.dx + 100, center.dy));
await tester.pump();
slider = tester.widget(sliderFinder);
expect(slider.value, 60.0);
});
}
| flutter/examples/api/test/material/slider/slider.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/slider/slider.0_test.dart",
"repo_id": "flutter",
"token_count": 318
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/painting/linear_border/linear_border.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Smoke Test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ExampleApp(),
);
expect(find.byType(example.Home), findsOneWidget);
// Scroll the interpolation example into view
await tester.scrollUntilVisible(
find.byIcon(Icons.play_arrow),
500.0,
scrollable: find.byType(Scrollable),
);
expect(find.byIcon(Icons.play_arrow), findsOneWidget);
// Run the interpolation example
await tester.tap(find.byIcon(Icons.play_arrow));
await tester.pumpAndSettle();
await tester.tap(find.byIcon(Icons.play_arrow));
await tester.pumpAndSettle();
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Interpolation')));
await gesture.moveTo(tester.getCenter(find.text('Hover')));
await tester.pumpAndSettle();
await gesture.moveTo(tester.getCenter(find.text('Interpolation')));
await tester.pumpAndSettle();
});
}
| flutter/examples/api/test/painting/linear_border.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/painting/linear_border.0_test.dart",
"repo_id": "flutter",
"token_count": 479
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_api_samples/widgets/basic/absorb_pointer.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AbsorbPointer prevents hit testing on its child', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AbsorbPointerApp(),
);
// Get the center of the stack.
final Offset center = tester.getCenter(find.byType(Stack).first);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
// Add the point to the center of the stack where the AbsorbPointer is.
await gesture.addPointer(location: center);
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
// Move the pointer to the left of the stack where the AbsorbPointer is not.
await gesture.moveTo(center + const Offset(-100, 0));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
});
}
| flutter/examples/api/test/widgets/basic/absorb_pointer.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/absorb_pointer.0_test.dart",
"repo_id": "flutter",
"token_count": 417
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/mouse_region.on_exit.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('MouseRegion detects mouse hover', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(home: example.MouseRegionApp()),
);
Container container = tester.widget<Container>(find.byType(Container));
expect(container.decoration, const BoxDecoration(color: Colors.blue));
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Container)));
await tester.pump();
container = tester.widget<Container>(find.byType(Container));
expect(container.decoration, const BoxDecoration(color: Colors.yellow));
});
}
| flutter/examples/api/test/widgets/basic/mouse_region.on_exit.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/mouse_region.on_exit.0_test.dart",
"repo_id": "flutter",
"token_count": 350
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/inherited_model/inherited_model.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Rebuild widget using InheritedModel', (WidgetTester tester) async {
await tester.pumpWidget(
const example.InheritedModelApp(),
);
BoxDecoration? decoration = tester.widget<AnimatedContainer>(
find.byType(AnimatedContainer).first,
).decoration as BoxDecoration?;
expect(decoration!.color, Colors.blue);
await tester.tap(find.text('Update background'));
await tester.pumpAndSettle();
decoration = tester.widget<AnimatedContainer>(
find.byType(AnimatedContainer).first,
).decoration as BoxDecoration?;
expect(decoration!.color, Colors.red);
double? size = tester.widget<FlutterLogo>(find.byType(FlutterLogo)).size;
expect(size, 100.0);
await tester.tap(find.text('Resize Logo'));
await tester.pumpAndSettle();
size = tester.widget<FlutterLogo>(find.byType(FlutterLogo)).size;
expect(size, 200.0);
});
}
| flutter/examples/api/test/widgets/inherited_model/inherited_model.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/inherited_model/inherited_model.0_test.dart",
"repo_id": "flutter",
"token_count": 447
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/scroll_position/scroll_controller_notification.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can toggle between scroll notification types', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ScrollNotificationDemo(),
);
expect(find.byType(CustomScrollView), findsOneWidget);
expect(find.text('Last notification: Null'), findsNothing);
// Toggle to use NotificationListener
await tester.tap(
find.byWidgetPredicate((Widget widget) {
return widget is Radio<bool> && !widget.value;
})
);
await tester.pumpAndSettle();
expect(find.text('Last notification: Null'), findsOneWidget);
await tester.drag(find.byType(CustomScrollView), const Offset(20.0, 20.0));
await tester.pumpAndSettle();
expect(find.text('Last notification: UserScrollNotification'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/scroll_position/scroll_controller_notification.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/scroll_position/scroll_controller_notification.0_test.dart",
"repo_id": "flutter",
"token_count": 386
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/transitions/listenable_builder.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tapping FAB increments counter', (WidgetTester tester) async {
await tester.pumpWidget(const example.ListenableBuilderExample());
String getCount() => (tester.widget(find.descendant(of: find.byType(ListenableBuilder), matching: find.byType(Text))) as Text).data!;
expect(find.text('Current counter value:'), findsOneWidget);
expect(find.text('0'), findsOneWidget);
expect(find.byIcon(Icons.add), findsOneWidget);
expect(getCount(), equals('0'));
await tester.tap(find.byType(FloatingActionButton).first);
await tester.pumpAndSettle();
expect(getCount(), equals('1'));
});
}
| flutter/examples/api/test/widgets/transitions/listenable_builder.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/transitions/listenable_builder.1_test.dart",
"repo_id": "flutter",
"token_count": 324
} | 671 |
#include "Generated.xcconfig"
| flutter/examples/flutter_view/ios/Flutter/Debug.xcconfig/0 | {
"file_path": "flutter/examples/flutter_view/ios/Flutter/Debug.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 672 |
distributionBase=GRADLE_USER_HOME
distributionPath=wrapper/dists
zipStoreBase=GRADLE_USER_HOME
zipStorePath=wrapper/dists
distributionUrl=https\://services.gradle.org/distributions/gradle-8.2.1-all.zip
| flutter/examples/hello_world/android/gradle/wrapper/gradle-wrapper.properties/0 | {
"file_path": "flutter/examples/hello_world/android/gradle/wrapper/gradle-wrapper.properties",
"repo_id": "flutter",
"token_count": 73
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import Cocoa
import FlutterMacOS
class MainFlutterWindow: NSWindow {
override func awakeFromNib() {
let flutterViewController = FlutterViewController()
let windowFrame = self.frame
self.contentViewController = flutterViewController
self.setFrame(windowFrame, display: true)
RegisterGeneratedPlugins(registry: flutterViewController)
super.awakeFromNib()
}
}
| flutter/examples/hello_world/macos/Runner/MainFlutterWindow.swift/0 | {
"file_path": "flutter/examples/hello_world/macos/Runner/MainFlutterWindow.swift",
"repo_id": "flutter",
"token_count": 164
} | 674 |
{
"info" : {
"author" : "xcode",
"version" : 1
}
}
| flutter/examples/layers/macos/Runner/Assets.xcassets/Contents.json/0 | {
"file_path": "flutter/examples/layers/macos/Runner/Assets.xcassets/Contents.json",
"repo_id": "flutter",
"token_count": 35
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This example shows how to build a render tree with a non-cartesian coordinate
// system. Most of the guts of this examples are in src/sector_layout.dart.
import 'package:flutter/rendering.dart';
import 'src/binding.dart';
import 'src/sector_layout.dart';
RenderBox buildSectorExample() {
final RenderSectorRing rootCircle = RenderSectorRing(padding: 20.0);
rootCircle.add(RenderSolidColor(const Color(0xFF00FFFF), desiredDeltaTheta: kTwoPi * 0.15));
rootCircle.add(RenderSolidColor(const Color(0xFF0000FF), desiredDeltaTheta: kTwoPi * 0.4));
final RenderSectorSlice stack = RenderSectorSlice(padding: 2.0);
stack.add(RenderSolidColor(const Color(0xFFFFFF00), desiredDeltaRadius: 20.0));
stack.add(RenderSolidColor(const Color(0xFFFF9000), desiredDeltaRadius: 20.0));
stack.add(RenderSolidColor(const Color(0xFF00FF00)));
rootCircle.add(stack);
return RenderBoxToRenderSectorAdapter(innerRadius: 50.0, child: rootCircle);
}
void main() {
ViewRenderingFlutterBinding(root: buildSectorExample()).scheduleFrame();
}
| flutter/examples/layers/rendering/custom_coordinate_systems.dart/0 | {
"file_path": "flutter/examples/layers/rendering/custom_coordinate_systems.dart",
"repo_id": "flutter",
"token_count": 386
} | 676 |
{
"images" : [
{
"idiom" : "iphone",
"size" : "20x20",
"scale" : "2x"
},
{
"idiom" : "iphone",
"size" : "20x20",
"scale" : "3x"
},
{
"idiom" : "iphone",
"size" : "29x29",
"scale" : "2x"
},
{
"idiom" : "iphone",
"size" : "29x29",
"scale" : "3x"
},
{
"idiom" : "iphone",
"size" : "40x40",
"scale" : "2x"
},
{
"idiom" : "iphone",
"size" : "40x40",
"scale" : "3x"
},
{
"idiom" : "iphone",
"size" : "60x60",
"scale" : "2x"
},
{
"idiom" : "iphone",
"size" : "60x60",
"scale" : "3x"
},
{
"idiom" : "ipad",
"size" : "20x20",
"scale" : "1x"
},
{
"idiom" : "ipad",
"size" : "20x20",
"scale" : "2x"
},
{
"idiom" : "ipad",
"size" : "29x29",
"scale" : "1x"
},
{
"idiom" : "ipad",
"size" : "29x29",
"scale" : "2x"
},
{
"idiom" : "ipad",
"size" : "40x40",
"scale" : "1x"
},
{
"idiom" : "ipad",
"size" : "40x40",
"scale" : "2x"
},
{
"idiom" : "ipad",
"size" : "76x76",
"scale" : "1x"
},
{
"idiom" : "ipad",
"size" : "76x76",
"scale" : "2x"
},
{
"idiom" : "ipad",
"size" : "83.5x83.5",
"scale" : "2x"
}
],
"info" : {
"version" : 1,
"author" : "xcode"
}
} | flutter/examples/platform_channel/ios/Runner/Assets.xcassets/AppIcon.appiconset/Contents.json/0 | {
"file_path": "flutter/examples/platform_channel/ios/Runner/Assets.xcassets/AppIcon.appiconset/Contents.json",
"repo_id": "flutter",
"token_count": 967
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class PlatformChannel extends StatefulWidget {
const PlatformChannel({super.key});
@override
State<PlatformChannel> createState() => _PlatformChannelState();
}
class _PlatformChannelState extends State<PlatformChannel> {
static const MethodChannel methodChannel =
MethodChannel('samples.flutter.io/battery');
static const EventChannel eventChannel =
EventChannel('samples.flutter.io/charging');
String _batteryLevel = 'Battery level: unknown.';
String _chargingStatus = 'Battery status: unknown.';
Future<void> _getBatteryLevel() async {
String batteryLevel;
try {
final int? result = await methodChannel.invokeMethod('getBatteryLevel');
batteryLevel = 'Battery level: $result%.';
} on PlatformException {
batteryLevel = 'Failed to get battery level.';
}
setState(() {
_batteryLevel = batteryLevel;
});
}
@override
void initState() {
super.initState();
eventChannel.receiveBroadcastStream().listen(_onEvent, onError: _onError);
}
void _onEvent(Object? event) {
setState(() {
_chargingStatus =
"Battery status: ${event == 'charging' ? '' : 'dis'}charging.";
});
}
void _onError(Object error) {
setState(() {
_chargingStatus = 'Battery status: unknown.';
});
}
@override
Widget build(BuildContext context) {
return Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(_batteryLevel, key: const Key('Battery level label')),
Padding(
padding: const EdgeInsets.all(16.0),
child: ElevatedButton(
onPressed: _getBatteryLevel,
child: const Text('Refresh'),
),
),
],
),
Text(_chargingStatus),
],
),
);
}
}
void main() {
runApp(const MaterialApp(home: PlatformChannel()));
}
| flutter/examples/platform_channel_swift/lib/main.dart/0 | {
"file_path": "flutter/examples/platform_channel_swift/lib/main.dart",
"repo_id": "flutter",
"token_count": 911
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "my_texture.h"
// An object that generates a texture for Flutter.
struct _MyTexture {
FlPixelBufferTexture parent_instance;
// Dimensions of texture.
uint32_t width;
uint32_t height;
// Buffer used to store texture.
uint8_t* buffer;
};
G_DEFINE_TYPE(MyTexture, my_texture, fl_pixel_buffer_texture_get_type())
// Implements GObject::dispose.
static void my_texture_dispose(GObject* object) {
MyTexture* self = MY_TEXTURE(object);
free(self->buffer);
G_OBJECT_CLASS(my_texture_parent_class)->dispose(object);
}
// Implements FlPixelBufferTexture::copy_pixels.
static gboolean my_texture_copy_pixels(FlPixelBufferTexture* texture,
const uint8_t** out_buffer,
uint32_t* width, uint32_t* height,
GError** error) {
MyTexture* self = MY_TEXTURE(texture);
*out_buffer = self->buffer;
*width = self->width;
*height = self->height;
return TRUE;
}
static void my_texture_class_init(MyTextureClass* klass) {
G_OBJECT_CLASS(klass)->dispose = my_texture_dispose;
FL_PIXEL_BUFFER_TEXTURE_CLASS(klass)->copy_pixels = my_texture_copy_pixels;
}
static void my_texture_init(MyTexture* self) {}
MyTexture* my_texture_new(uint32_t width, uint32_t height, uint8_t r, uint8_t g,
uint8_t b) {
MyTexture* self = MY_TEXTURE(g_object_new(my_texture_get_type(), nullptr));
self->width = width;
self->height = height;
self->buffer = static_cast<uint8_t*>(malloc(self->width * self->height * 4));
my_texture_set_color(self, r, g, b);
return self;
}
// Draws the texture with the requested color.
void my_texture_set_color(MyTexture* self, uint8_t r, uint8_t g, uint8_t b) {
g_return_if_fail(MY_IS_TEXTURE(self));
for (size_t y = 0; y < self->height; y++) {
for (size_t x = 0; x < self->width; x++) {
uint8_t* pixel = self->buffer + (y * self->width * 4) + (x * 4);
pixel[0] = r;
pixel[1] = g;
pixel[2] = b;
pixel[3] = 255;
}
}
}
| flutter/examples/texture/linux/my_texture.cc/0 | {
"file_path": "flutter/examples/texture/linux/my_texture.cc",
"repo_id": "flutter",
"token_count": 919
} | 679 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are from the Gestures library. *
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/28602
- title: "Rename to 'fromMouseEvent'"
date: 2020-12-15
element:
uris: [ 'gestures.dart' ]
constructor: 'fromHoverEvent'
inClass: 'PointerEnterEvent'
changes:
- kind: 'rename'
newName: 'fromMouseEvent'
# Changes made in https://github.com/flutter/flutter/pull/28602
- title: "Rename to 'fromMouseEvent'"
date: 2020-12-15
element:
uris: [ 'gestures.dart' ]
constructor: 'fromHoverEvent'
inClass: 'PointerExitEvent'
changes:
- kind: 'rename'
newName: 'fromMouseEvent'
# Changes made in https://github.com/flutter/flutter/pull/66043
- title: "Use withKind"
date: 2020-09-17
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'VelocityTracker'
oneOf:
- if: "pointerDeviceKind == ''"
changes:
- kind: 'rename'
newName: 'withKind'
- kind: 'addParameter'
index: 0
name: 'kind'
style: required_positional
argumentValue:
expression: 'PointerDeviceKind.touch'
- if: "pointerDeviceKind != ''"
changes:
- kind: 'rename'
newName: 'withKind'
variables:
pointerDeviceKind:
kind: 'fragment'
value: 'arguments[0]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'ScaleGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'MultiTapGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 4
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'DoubleTapGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'DelayedMultiDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 4
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'VerticalMultiDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'HorizontalMultiDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'ImmediateMultiDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'MultiDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'LongPressGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 4
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'ForcePressGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 6
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'EagerGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 2
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'PrimaryPointerGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 6
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'OneSequenceGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'GestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'HorizontalDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'VerticalDragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 3
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Changes made in https://github.com/flutter/flutter/pull/81858
- title: "Migrate to 'supportedDevices'"
date: 2021-05-04
element:
uris: [ 'gestures.dart' ]
constructor: ''
inClass: 'DragGestureRecognizer'
oneOf:
- if: "kind != ''"
changes:
- kind: 'addParameter'
index: 5
name: 'supportedDevices'
style: optional_named
argumentValue:
expression: '<PointerDeviceKind>{{% kind %}}'
requiredIf: "kind != ''"
- kind: 'removeParameter'
name: 'kind'
variables:
kind:
kind: 'fragment'
value: 'arguments[kind]'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_gestures.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_gestures.yaml",
"repo_id": "flutter",
"token_count": 6734
} | 680 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for Element from the Widgets library. *
# For fixes to
# * Actions: fix_actions.yaml
# * BuildContext: fix_build_context.yaml
# * ListWheelScrollView: fix_list_wheel_scroll_view.yaml
# * Widgets (general): fix_widgets.yaml
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'dependOnInheritedElement'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'inheritFromElement'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'dependOnInheritedElement'
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'dependOnInheritedWidgetOfExactType'"
date: 2020-09-14
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'inheritFromWidgetOfExactType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'dependOnInheritedWidgetOfExactType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0]'
- kind: 'removeParameter'
index: 0
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'getElementForInheritedWidgetOfExactType'"
date: 2020-09-14
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'ancestorInheritedElementForWidgetOfExactType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'getElementForInheritedWidgetOfExactType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0]'
- kind: 'removeParameter'
index: 0
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'getElementForInheritedWidgetOfExactType'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'ancestorWidgetOfExactType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'findAncestorWidgetOfExactType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0]'
- kind: 'removeParameter'
index: 0
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'findAncestorStateOfType'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'ancestorStateOfType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'findAncestorStateOfType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0].typeArguments[0]'
- kind: 'removeParameter'
index: 0
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'findAncestorRenderObjectOfType'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'ancestorRenderObjectOfType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'findAncestorRenderObjectOfType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0].typeArguments[0]'
- kind: 'removeParameter'
index: 0
# Changes made in https://github.com/flutter/flutter/pull/44189.
- title: "Rename to 'findAncestorRenderObjectOfType'"
date: 2020-12-23
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'rootAncestorStateOfType'
inClass: 'Element'
changes:
- kind: 'rename'
newName: 'findRootAncestorStateOfType'
- kind: 'addTypeParameter'
index: 0
name: 'T'
argumentValue:
expression: '{% type %}'
variables:
type:
kind: 'fragment'
value: 'arguments[0].typeArguments[0]'
- kind: 'removeParameter'
index: 0
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_widgets/fix_element.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_widgets/fix_element.yaml",
"repo_id": "flutter",
"token_count": 2433
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/physics.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/semantics.dart';
import 'animation.dart';
import 'curves.dart';
import 'listener_helpers.dart';
export 'package:flutter/physics.dart' show Simulation, SpringDescription;
export 'package:flutter/scheduler.dart' show TickerFuture, TickerProvider;
export 'animation.dart' show Animation, AnimationStatus;
export 'curves.dart' show Curve;
const String _flutterAnimationLibrary = 'package:flutter/animation.dart';
// Examples can assume:
// late AnimationController _controller, fadeAnimationController, sizeAnimationController;
// late bool dismissed;
// void setState(VoidCallback fn) { }
/// The direction in which an animation is running.
enum _AnimationDirection {
/// The animation is running from beginning to end.
forward,
/// The animation is running backwards, from end to beginning.
reverse,
}
final SpringDescription _kFlingSpringDescription = SpringDescription.withDampingRatio(
mass: 1.0,
stiffness: 500.0,
);
const Tolerance _kFlingTolerance = Tolerance(
velocity: double.infinity,
distance: 0.01,
);
/// Configures how an [AnimationController] behaves when animations are
/// disabled.
///
/// When [AccessibilityFeatures.disableAnimations] is true, the device is asking
/// Flutter to reduce or disable animations as much as possible. To honor this,
/// we reduce the duration and the corresponding number of frames for
/// animations. This enum is used to allow certain [AnimationController]s to opt
/// out of this behavior.
///
/// For example, the [AnimationController] which controls the physics simulation
/// for a scrollable list will have [AnimationBehavior.preserve], so that when
/// a user attempts to scroll it does not jump to the end/beginning too quickly.
enum AnimationBehavior {
/// The [AnimationController] will reduce its duration when
/// [AccessibilityFeatures.disableAnimations] is true.
normal,
/// The [AnimationController] will preserve its behavior.
///
/// This is the default for repeating animations in order to prevent them from
/// flashing rapidly on the screen if the widget does not take the
/// [AccessibilityFeatures.disableAnimations] flag into account.
preserve,
}
/// A controller for an animation.
///
/// This class lets you perform tasks such as:
///
/// * Play an animation [forward] or in [reverse], or [stop] an animation.
/// * Set the animation to a specific [value].
/// * Define the [upperBound] and [lowerBound] values of an animation.
/// * Create a [fling] animation effect using a physics simulation.
///
/// By default, an [AnimationController] linearly produces values that range
/// from 0.0 to 1.0, during a given duration. The animation controller generates
/// a new value whenever the device running your app is ready to display a new
/// frame (typically, this rate is around 60 values per second).
///
/// ## Ticker providers
///
/// An [AnimationController] needs a [TickerProvider], which is configured using
/// the `vsync` argument on the constructor.
///
/// The [TickerProvider] interface describes a factory for [Ticker] objects. A
/// [Ticker] is an object that knows how to register itself with the
/// [SchedulerBinding] and fires a callback every frame. The
/// [AnimationController] class uses a [Ticker] to step through the animation
/// that it controls.
///
/// If an [AnimationController] is being created from a [State], then the State
/// can use the [TickerProviderStateMixin] and [SingleTickerProviderStateMixin]
/// classes to implement the [TickerProvider] interface. The
/// [TickerProviderStateMixin] class always works for this purpose; the
/// [SingleTickerProviderStateMixin] is slightly more efficient in the case of
/// the class only ever needing one [Ticker] (e.g. if the class creates only a
/// single [AnimationController] during its entire lifetime).
///
/// The widget test framework [WidgetTester] object can be used as a ticker
/// provider in the context of tests. In other contexts, you will have to either
/// pass a [TickerProvider] from a higher level (e.g. indirectly from a [State]
/// that mixes in [TickerProviderStateMixin]), or create a custom
/// [TickerProvider] subclass.
///
/// ## Life cycle
///
/// An [AnimationController] should be [dispose]d when it is no longer needed.
/// This reduces the likelihood of leaks. When used with a [StatefulWidget], it
/// is common for an [AnimationController] to be created in the
/// [State.initState] method and then disposed in the [State.dispose] method.
///
/// ## Using [Future]s with [AnimationController]
///
/// The methods that start animations return a [TickerFuture] object which
/// completes when the animation completes successfully, and never throws an
/// error; if the animation is canceled, the future never completes. This object
/// also has a [TickerFuture.orCancel] property which returns a future that
/// completes when the animation completes successfully, and completes with an
/// error when the animation is aborted.
///
/// This can be used to write code such as the `fadeOutAndUpdateState` method
/// below.
///
/// {@tool snippet}
///
/// Here is a stateful `Foo` widget. Its [State] uses the
/// [SingleTickerProviderStateMixin] to implement the necessary
/// [TickerProvider], creating its controller in the [State.initState] method
/// and disposing of it in the [State.dispose] method. The duration of the
/// controller is configured from a property in the `Foo` widget; as that
/// changes, the [State.didUpdateWidget] method is used to update the
/// controller.
///
/// ```dart
/// class Foo extends StatefulWidget {
/// const Foo({ super.key, required this.duration });
///
/// final Duration duration;
///
/// @override
/// State<Foo> createState() => _FooState();
/// }
///
/// class _FooState extends State<Foo> with SingleTickerProviderStateMixin {
/// late AnimationController _controller;
///
/// @override
/// void initState() {
/// super.initState();
/// _controller = AnimationController(
/// vsync: this, // the SingleTickerProviderStateMixin
/// duration: widget.duration,
/// );
/// }
///
/// @override
/// void didUpdateWidget(Foo oldWidget) {
/// super.didUpdateWidget(oldWidget);
/// _controller.duration = widget.duration;
/// }
///
/// @override
/// void dispose() {
/// _controller.dispose();
/// super.dispose();
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Container(); // ...
/// }
/// }
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// The following method (for a [State] subclass) drives two animation
/// controllers using Dart's asynchronous syntax for awaiting [Future] objects:
///
/// ```dart
/// Future<void> fadeOutAndUpdateState() async {
/// try {
/// await fadeAnimationController.forward().orCancel;
/// await sizeAnimationController.forward().orCancel;
/// setState(() {
/// dismissed = true;
/// });
/// } on TickerCanceled {
/// // the animation got canceled, probably because we were disposed
/// }
/// }
/// ```
/// {@end-tool}
///
/// The assumption in the code above is that the animation controllers are being
/// disposed in the [State] subclass' override of the [State.dispose] method.
/// Since disposing the controller cancels the animation (raising a
/// [TickerCanceled] exception), the code here can skip verifying whether
/// [State.mounted] is still true at each step. (Again, this assumes that the
/// controllers are created in [State.initState] and disposed in
/// [State.dispose], as described in the previous section.)
///
/// {@tool dartpad}
/// This example shows how to use [AnimationController] and
/// [SlideTransition] to create an animated digit like you might find
/// on an old pinball machine our your car's odometer. New digit
/// values slide into place from below, as the old value slides
/// upwards and out of view. Taps that occur while the controller is
/// already animating cause the controller's
/// [AnimationController.duration] to be reduced so that the visuals
/// don't fall behind.
///
/// ** See code in examples/api/lib/animation/animation_controller/animated_digit.0.dart **
/// {@end-tool}
/// See also:
///
/// * [Tween], the base class for converting an [AnimationController] to a
/// range of values of other types.
class AnimationController extends Animation<double>
with AnimationEagerListenerMixin, AnimationLocalListenersMixin, AnimationLocalStatusListenersMixin {
/// Creates an animation controller.
///
/// * `value` is the initial value of the animation. If defaults to the lower
/// bound.
///
/// * [duration] is the length of time this animation should last.
///
/// * [debugLabel] is a string to help identify this animation during
/// debugging (used by [toString]).
///
/// * [lowerBound] is the smallest value this animation can obtain and the
/// value at which this animation is deemed to be dismissed. It cannot be
/// null.
///
/// * [upperBound] is the largest value this animation can obtain and the
/// value at which this animation is deemed to be completed. It cannot be
/// null.
///
/// * `vsync` is the required [TickerProvider] for the current context. It can
/// be changed by calling [resync]. See [TickerProvider] for advice on
/// obtaining a ticker provider.
AnimationController({
double? value,
this.duration,
this.reverseDuration,
this.debugLabel,
this.lowerBound = 0.0,
this.upperBound = 1.0,
this.animationBehavior = AnimationBehavior.normal,
required TickerProvider vsync,
}) : assert(upperBound >= lowerBound),
_direction = _AnimationDirection.forward {
if (kFlutterMemoryAllocationsEnabled) {
_maybeDispatchObjectCreation();
}
_ticker = vsync.createTicker(_tick);
_internalSetValue(value ?? lowerBound);
}
/// Creates an animation controller with no upper or lower bound for its
/// value.
///
/// * [value] is the initial value of the animation.
///
/// * [duration] is the length of time this animation should last.
///
/// * [debugLabel] is a string to help identify this animation during
/// debugging (used by [toString]).
///
/// * `vsync` is the required [TickerProvider] for the current context. It can
/// be changed by calling [resync]. See [TickerProvider] for advice on
/// obtaining a ticker provider.
///
/// This constructor is most useful for animations that will be driven using a
/// physics simulation, especially when the physics simulation has no
/// pre-determined bounds.
AnimationController.unbounded({
double value = 0.0,
this.duration,
this.reverseDuration,
this.debugLabel,
required TickerProvider vsync,
this.animationBehavior = AnimationBehavior.preserve,
}) : lowerBound = double.negativeInfinity,
upperBound = double.infinity,
_direction = _AnimationDirection.forward {
if (kFlutterMemoryAllocationsEnabled) {
_maybeDispatchObjectCreation();
}
_ticker = vsync.createTicker(_tick);
_internalSetValue(value);
}
/// Dispatches event of object creation to [FlutterMemoryAllocations.instance].
void _maybeDispatchObjectCreation() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: _flutterAnimationLibrary,
className: '$AnimationController',
object: this,
);
}
}
/// The value at which this animation is deemed to be dismissed.
final double lowerBound;
/// The value at which this animation is deemed to be completed.
final double upperBound;
/// A label that is used in the [toString] output. Intended to aid with
/// identifying animation controller instances in debug output.
final String? debugLabel;
/// The behavior of the controller when [AccessibilityFeatures.disableAnimations]
/// is true.
///
/// Defaults to [AnimationBehavior.normal] for the [AnimationController.new]
/// constructor, and [AnimationBehavior.preserve] for the
/// [AnimationController.unbounded] constructor.
final AnimationBehavior animationBehavior;
/// Returns an [Animation<double>] for this animation controller, so that a
/// pointer to this object can be passed around without allowing users of that
/// pointer to mutate the [AnimationController] state.
Animation<double> get view => this;
/// The length of time this animation should last.
///
/// If [reverseDuration] is specified, then [duration] is only used when going
/// [forward]. Otherwise, it specifies the duration going in both directions.
Duration? duration;
/// The length of time this animation should last when going in [reverse].
///
/// The value of [duration] is used if [reverseDuration] is not specified or
/// set to null.
Duration? reverseDuration;
Ticker? _ticker;
/// Recreates the [Ticker] with the new [TickerProvider].
void resync(TickerProvider vsync) {
final Ticker oldTicker = _ticker!;
_ticker = vsync.createTicker(_tick);
_ticker!.absorbTicker(oldTicker);
}
Simulation? _simulation;
/// The current value of the animation.
///
/// Setting this value notifies all the listeners that the value
/// changed.
///
/// Setting this value also stops the controller if it is currently
/// running; if this happens, it also notifies all the status
/// listeners.
@override
double get value => _value;
late double _value;
/// Stops the animation controller and sets the current value of the
/// animation.
///
/// The new value is clamped to the range set by [lowerBound] and
/// [upperBound].
///
/// Value listeners are notified even if this does not change the value.
/// Status listeners are notified if the animation was previously playing.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// See also:
///
/// * [reset], which is equivalent to setting [value] to [lowerBound].
/// * [stop], which aborts the animation without changing its value or status
/// and without dispatching any notifications other than completing or
/// canceling the [TickerFuture].
/// * [forward], [reverse], [animateTo], [animateWith], [fling], and [repeat],
/// which start the animation controller.
set value(double newValue) {
stop();
_internalSetValue(newValue);
notifyListeners();
_checkStatusChanged();
}
/// Sets the controller's value to [lowerBound], stopping the animation (if
/// in progress), and resetting to its beginning point, or dismissed state.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// See also:
///
/// * [value], which can be explicitly set to a specific value as desired.
/// * [forward], which starts the animation in the forward direction.
/// * [stop], which aborts the animation without changing its value or status
/// and without dispatching any notifications other than completing or
/// canceling the [TickerFuture].
void reset() {
value = lowerBound;
}
/// The rate of change of [value] per second.
///
/// If [isAnimating] is false, then [value] is not changing and the rate of
/// change is zero.
double get velocity {
if (!isAnimating) {
return 0.0;
}
return _simulation!.dx(lastElapsedDuration!.inMicroseconds.toDouble() / Duration.microsecondsPerSecond);
}
void _internalSetValue(double newValue) {
_value = clampDouble(newValue, lowerBound, upperBound);
if (_value == lowerBound) {
_status = AnimationStatus.dismissed;
} else if (_value == upperBound) {
_status = AnimationStatus.completed;
} else {
_status = (_direction == _AnimationDirection.forward) ?
AnimationStatus.forward :
AnimationStatus.reverse;
}
}
/// The amount of time that has passed between the time the animation started
/// and the most recent tick of the animation.
///
/// If the controller is not animating, the last elapsed duration is null.
Duration? get lastElapsedDuration => _lastElapsedDuration;
Duration? _lastElapsedDuration;
/// Whether this animation is currently animating in either the forward or reverse direction.
///
/// This is separate from whether it is actively ticking. An animation
/// controller's ticker might get muted, in which case the animation
/// controller's callbacks will no longer fire even though time is continuing
/// to pass. See [Ticker.muted] and [TickerMode].
bool get isAnimating => _ticker != null && _ticker!.isActive;
_AnimationDirection _direction;
@override
AnimationStatus get status => _status;
late AnimationStatus _status;
/// Starts running this animation forwards (towards the end).
///
/// Returns a [TickerFuture] that completes when the animation is complete.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// During the animation, [status] is reported as [AnimationStatus.forward],
/// which switches to [AnimationStatus.completed] when [upperBound] is
/// reached at the end of the animation.
TickerFuture forward({ double? from }) {
assert(() {
if (duration == null) {
throw FlutterError(
'AnimationController.forward() called with no default duration.\n'
'The "duration" property should be set, either in the constructor or later, before '
'calling the forward() function.',
);
}
return true;
}());
assert(
_ticker != null,
'AnimationController.forward() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
_direction = _AnimationDirection.forward;
if (from != null) {
value = from;
}
return _animateToInternal(upperBound);
}
/// Starts running this animation in reverse (towards the beginning).
///
/// Returns a [TickerFuture] that completes when the animation is dismissed.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// During the animation, [status] is reported as [AnimationStatus.reverse],
/// which switches to [AnimationStatus.dismissed] when [lowerBound] is
/// reached at the end of the animation.
TickerFuture reverse({ double? from }) {
assert(() {
if (duration == null && reverseDuration == null) {
throw FlutterError(
'AnimationController.reverse() called with no default duration or reverseDuration.\n'
'The "duration" or "reverseDuration" property should be set, either in the constructor or later, before '
'calling the reverse() function.',
);
}
return true;
}());
assert(
_ticker != null,
'AnimationController.reverse() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
_direction = _AnimationDirection.reverse;
if (from != null) {
value = from;
}
return _animateToInternal(lowerBound);
}
/// Drives the animation from its current value to target.
///
/// Returns a [TickerFuture] that completes when the animation is complete.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// During the animation, [status] is reported as [AnimationStatus.forward]
/// regardless of whether `target` > [value] or not. At the end of the
/// animation, when `target` is reached, [status] is reported as
/// [AnimationStatus.completed].
///
/// If the `target` argument is the same as the current [value] of the
/// animation, then this won't animate, and the returned [TickerFuture] will
/// be already complete.
TickerFuture animateTo(double target, { Duration? duration, Curve curve = Curves.linear }) {
assert(() {
if (this.duration == null && duration == null) {
throw FlutterError(
'AnimationController.animateTo() called with no explicit duration and no default duration.\n'
'Either the "duration" argument to the animateTo() method should be provided, or the '
'"duration" property should be set, either in the constructor or later, before '
'calling the animateTo() function.',
);
}
return true;
}());
assert(
_ticker != null,
'AnimationController.animateTo() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
_direction = _AnimationDirection.forward;
return _animateToInternal(target, duration: duration, curve: curve);
}
/// Drives the animation from its current value to target.
///
/// Returns a [TickerFuture] that completes when the animation is complete.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// During the animation, [status] is reported as [AnimationStatus.reverse]
/// regardless of whether `target` < [value] or not. At the end of the
/// animation, when `target` is reached, [status] is reported as
/// [AnimationStatus.dismissed].
TickerFuture animateBack(double target, { Duration? duration, Curve curve = Curves.linear }) {
assert(() {
if (this.duration == null && reverseDuration == null && duration == null) {
throw FlutterError(
'AnimationController.animateBack() called with no explicit duration and no default duration or reverseDuration.\n'
'Either the "duration" argument to the animateBack() method should be provided, or the '
'"duration" or "reverseDuration" property should be set, either in the constructor or later, before '
'calling the animateBack() function.',
);
}
return true;
}());
assert(
_ticker != null,
'AnimationController.animateBack() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
_direction = _AnimationDirection.reverse;
return _animateToInternal(target, duration: duration, curve: curve);
}
TickerFuture _animateToInternal(double target, { Duration? duration, Curve curve = Curves.linear }) {
final double scale = switch (animationBehavior) {
// Since the framework cannot handle zero duration animations, we run it at 5% of the normal
// duration to limit most animations to a single frame.
// Ideally, the framework would be able to handle zero duration animations, however, the common
// pattern of an eternally repeating animation might cause an endless loop if it weren't delayed
// for at least one frame.
AnimationBehavior.normal when SemanticsBinding.instance.disableAnimations => 0.05,
AnimationBehavior.normal || AnimationBehavior.preserve => 1.0,
};
Duration? simulationDuration = duration;
if (simulationDuration == null) {
assert(!(this.duration == null && _direction == _AnimationDirection.forward));
assert(!(this.duration == null && _direction == _AnimationDirection.reverse && reverseDuration == null));
final double range = upperBound - lowerBound;
final double remainingFraction = range.isFinite ? (target - _value).abs() / range : 1.0;
final Duration directionDuration =
(_direction == _AnimationDirection.reverse && reverseDuration != null)
? reverseDuration!
: this.duration!;
simulationDuration = directionDuration * remainingFraction;
} else if (target == value) {
// Already at target, don't animate.
simulationDuration = Duration.zero;
}
stop();
if (simulationDuration == Duration.zero) {
if (value != target) {
_value = clampDouble(target, lowerBound, upperBound);
notifyListeners();
}
_status = (_direction == _AnimationDirection.forward) ?
AnimationStatus.completed :
AnimationStatus.dismissed;
_checkStatusChanged();
return TickerFuture.complete();
}
assert(simulationDuration > Duration.zero);
assert(!isAnimating);
return _startSimulation(_InterpolationSimulation(_value, target, simulationDuration, curve, scale));
}
/// Starts running this animation in the forward direction, and
/// restarts the animation when it completes.
///
/// Defaults to repeating between the [lowerBound] and [upperBound] of the
/// [AnimationController] when no explicit value is set for [min] and [max].
///
/// With [reverse] set to true, instead of always starting over at [min]
/// the starting value will alternate between [min] and [max] values on each
/// repeat. The [status] will be reported as [AnimationStatus.reverse] when
/// the animation runs from [max] to [min].
///
/// Each run of the animation will have a duration of `period`. If `period` is not
/// provided, [duration] will be used instead, which has to be set before [repeat] is
/// called either in the constructor or later by using the [duration] setter.
///
/// Returns a [TickerFuture] that never completes. The [TickerFuture.orCancel] future
/// completes with an error when the animation is stopped (e.g. with [stop]).
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
TickerFuture repeat({ double? min, double? max, bool reverse = false, Duration? period }) {
min ??= lowerBound;
max ??= upperBound;
period ??= duration;
assert(() {
if (period == null) {
throw FlutterError(
'AnimationController.repeat() called without an explicit period and with no default Duration.\n'
'Either the "period" argument to the repeat() method should be provided, or the '
'"duration" property should be set, either in the constructor or later, before '
'calling the repeat() function.',
);
}
return true;
}());
assert(max >= min);
assert(max <= upperBound && min >= lowerBound);
stop();
return _startSimulation(_RepeatingSimulation(_value, min, max, reverse, period!, _directionSetter));
}
void _directionSetter(_AnimationDirection direction) {
_direction = direction;
_status = (_direction == _AnimationDirection.forward) ?
AnimationStatus.forward :
AnimationStatus.reverse;
_checkStatusChanged();
}
/// Drives the animation with a spring (within [lowerBound] and [upperBound])
/// and initial velocity.
///
/// If velocity is positive, the animation will complete, otherwise it will
/// dismiss. The velocity is specified in units per second. If the
/// [SemanticsBinding.disableAnimations] flag is set, the velocity is somewhat
/// arbitrarily multiplied by 200.
///
/// The [springDescription] parameter can be used to specify a custom
/// [SpringType.criticallyDamped] or [SpringType.overDamped] spring with which
/// to drive the animation. By default, a [SpringType.criticallyDamped] spring
/// is used. See [SpringDescription.withDampingRatio] for how to create a
/// suitable [SpringDescription].
///
/// The resulting spring simulation cannot be of type [SpringType.underDamped];
/// such a spring would oscillate rather than fling.
///
/// Returns a [TickerFuture] that completes when the animation is complete.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
TickerFuture fling({ double velocity = 1.0, SpringDescription? springDescription, AnimationBehavior? animationBehavior }) {
springDescription ??= _kFlingSpringDescription;
_direction = velocity < 0.0 ? _AnimationDirection.reverse : _AnimationDirection.forward;
final double target = velocity < 0.0 ? lowerBound - _kFlingTolerance.distance
: upperBound + _kFlingTolerance.distance;
final AnimationBehavior behavior = animationBehavior ?? this.animationBehavior;
final double scale = switch (behavior) {
// This is arbitrary (it was chosen because it worked for the drawer widget).
AnimationBehavior.normal when SemanticsBinding.instance.disableAnimations => 200.0,
AnimationBehavior.normal || AnimationBehavior.preserve => 1.0,
};
final SpringSimulation simulation = SpringSimulation(springDescription, value, target, velocity * scale)
..tolerance = _kFlingTolerance;
assert(
simulation.type != SpringType.underDamped,
'The specified spring simulation is of type SpringType.underDamped.\n'
'An underdamped spring results in oscillation rather than a fling. '
'Consider specifying a different springDescription, or use animateWith() '
'with an explicit SpringSimulation if an underdamped spring is intentional.',
);
stop();
return _startSimulation(simulation);
}
/// Drives the animation according to the given simulation.
///
/// The values from the simulation are clamped to the [lowerBound] and
/// [upperBound]. To avoid this, consider creating the [AnimationController]
/// using the [AnimationController.unbounded] constructor.
///
/// Returns a [TickerFuture] that completes when the animation is complete.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
///
/// The [status] is always [AnimationStatus.forward] for the entire duration
/// of the simulation.
TickerFuture animateWith(Simulation simulation) {
assert(
_ticker != null,
'AnimationController.animateWith() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
stop();
_direction = _AnimationDirection.forward;
return _startSimulation(simulation);
}
TickerFuture _startSimulation(Simulation simulation) {
assert(!isAnimating);
_simulation = simulation;
_lastElapsedDuration = Duration.zero;
_value = clampDouble(simulation.x(0.0), lowerBound, upperBound);
final TickerFuture result = _ticker!.start();
_status = (_direction == _AnimationDirection.forward) ?
AnimationStatus.forward :
AnimationStatus.reverse;
_checkStatusChanged();
return result;
}
/// Stops running this animation.
///
/// This does not trigger any notifications. The animation stops in its
/// current state.
///
/// By default, the most recently returned [TickerFuture] is marked as having
/// been canceled, meaning the future never completes and its
/// [TickerFuture.orCancel] derivative future completes with a [TickerCanceled]
/// error. By passing the `canceled` argument with the value false, this is
/// reversed, and the futures complete successfully.
///
/// See also:
///
/// * [reset], which stops the animation and resets it to the [lowerBound],
/// and which does send notifications.
/// * [forward], [reverse], [animateTo], [animateWith], [fling], and [repeat],
/// which restart the animation controller.
void stop({ bool canceled = true }) {
assert(
_ticker != null,
'AnimationController.stop() called after AnimationController.dispose()\n'
'AnimationController methods should not be used after calling dispose.',
);
_simulation = null;
_lastElapsedDuration = null;
_ticker!.stop(canceled: canceled);
}
/// Release the resources used by this object. The object is no longer usable
/// after this method is called.
///
/// The most recently returned [TickerFuture], if any, is marked as having been
/// canceled, meaning the future never completes and its [TickerFuture.orCancel]
/// derivative future completes with a [TickerCanceled] error.
@override
void dispose() {
assert(() {
if (_ticker == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('AnimationController.dispose() called more than once.'),
ErrorDescription('A given $runtimeType cannot be disposed more than once.\n'),
DiagnosticsProperty<AnimationController>(
'The following $runtimeType object was disposed multiple times',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
]);
}
return true;
}());
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_ticker!.dispose();
_ticker = null;
clearStatusListeners();
clearListeners();
super.dispose();
}
AnimationStatus _lastReportedStatus = AnimationStatus.dismissed;
void _checkStatusChanged() {
final AnimationStatus newStatus = status;
if (_lastReportedStatus != newStatus) {
_lastReportedStatus = newStatus;
notifyStatusListeners(newStatus);
}
}
void _tick(Duration elapsed) {
_lastElapsedDuration = elapsed;
final double elapsedInSeconds = elapsed.inMicroseconds.toDouble() / Duration.microsecondsPerSecond;
assert(elapsedInSeconds >= 0.0);
_value = clampDouble(_simulation!.x(elapsedInSeconds), lowerBound, upperBound);
if (_simulation!.isDone(elapsedInSeconds)) {
_status = (_direction == _AnimationDirection.forward) ?
AnimationStatus.completed :
AnimationStatus.dismissed;
stop(canceled: false);
}
notifyListeners();
_checkStatusChanged();
}
@override
String toStringDetails() {
final String paused = isAnimating ? '' : '; paused';
final String ticker = _ticker == null ? '; DISPOSED' : (_ticker!.muted ? '; silenced' : '');
String label = '';
assert(() {
if (debugLabel != null) {
label = '; for $debugLabel';
}
return true;
}());
final String more = '${super.toStringDetails()} ${value.toStringAsFixed(3)}';
return '$more$paused$ticker$label';
}
}
class _InterpolationSimulation extends Simulation {
_InterpolationSimulation(this._begin, this._end, Duration duration, this._curve, double scale)
: assert(duration.inMicroseconds > 0),
_durationInSeconds = (duration.inMicroseconds * scale) / Duration.microsecondsPerSecond;
final double _durationInSeconds;
final double _begin;
final double _end;
final Curve _curve;
@override
double x(double timeInSeconds) {
final double t = clampDouble(timeInSeconds / _durationInSeconds, 0.0, 1.0);
if (t == 0.0) {
return _begin;
} else if (t == 1.0) {
return _end;
} else {
return _begin + (_end - _begin) * _curve.transform(t);
}
}
@override
double dx(double timeInSeconds) {
final double epsilon = tolerance.time;
return (x(timeInSeconds + epsilon) - x(timeInSeconds - epsilon)) / (2 * epsilon);
}
@override
bool isDone(double timeInSeconds) => timeInSeconds > _durationInSeconds;
}
typedef _DirectionSetter = void Function(_AnimationDirection direction);
class _RepeatingSimulation extends Simulation {
_RepeatingSimulation(double initialValue, this.min, this.max, this.reverse, Duration period, this.directionSetter)
: _periodInSeconds = period.inMicroseconds / Duration.microsecondsPerSecond,
_initialT = (max == min) ? 0.0 : ((clampDouble(initialValue, min, max) - min) / (max - min)) * (period.inMicroseconds / Duration.microsecondsPerSecond) {
assert(_periodInSeconds > 0.0);
assert(_initialT >= 0.0);
}
final double min;
final double max;
final bool reverse;
final _DirectionSetter directionSetter;
final double _periodInSeconds;
final double _initialT;
@override
double x(double timeInSeconds) {
assert(timeInSeconds >= 0.0);
final double totalTimeInSeconds = timeInSeconds + _initialT;
final double t = (totalTimeInSeconds / _periodInSeconds) % 1.0;
final bool isPlayingReverse = (totalTimeInSeconds ~/ _periodInSeconds).isOdd;
if (reverse && isPlayingReverse) {
directionSetter(_AnimationDirection.reverse);
return ui.lerpDouble(max, min, t)!;
} else {
directionSetter(_AnimationDirection.forward);
return ui.lerpDouble(min, max, t)!;
}
}
@override
double dx(double timeInSeconds) => (max - min) / _periodInSeconds;
@override
bool isDone(double timeInSeconds) => false;
}
| flutter/packages/flutter/lib/src/animation/animation_controller.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/animation/animation_controller.dart",
"repo_id": "flutter",
"token_count": 11342
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
/// A button in a _ContextMenuSheet.
///
/// A typical use case is to pass a [Text] as the [child] here, but be sure to
/// use [TextOverflow.ellipsis] for the [Text.overflow] field if the text may be
/// long, as without it the text will wrap to the next line.
class CupertinoContextMenuAction extends StatefulWidget {
/// Construct a CupertinoContextMenuAction.
const CupertinoContextMenuAction({
super.key,
required this.child,
this.isDefaultAction = false,
this.isDestructiveAction = false,
this.onPressed,
this.trailingIcon,
});
/// The widget that will be placed inside the action.
final Widget child;
/// Indicates whether this action should receive the style of an emphasized,
/// default action.
final bool isDefaultAction;
/// Indicates whether this action should receive the style of a destructive
/// action.
final bool isDestructiveAction;
/// Called when the action is pressed.
final VoidCallback? onPressed;
/// An optional icon to display to the right of the child.
///
/// Will be colored in the same way as the [TextStyle] used for [child] (for
/// example, if using [isDestructiveAction]).
final IconData? trailingIcon;
@override
State<CupertinoContextMenuAction> createState() => _CupertinoContextMenuActionState();
}
class _CupertinoContextMenuActionState extends State<CupertinoContextMenuAction> {
static const Color _kBackgroundColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFF1F1F1),
darkColor: Color(0xFF212122),
);
static const Color _kBackgroundColorPressed = CupertinoDynamicColor.withBrightness(
color: Color(0xFFDDDDDD),
darkColor: Color(0xFF3F3F40),
);
static const double _kButtonHeight = 43;
static const TextStyle _kActionSheetActionStyle = TextStyle(
fontFamily: 'CupertinoSystemText',
inherit: false,
fontSize: 16.0,
fontWeight: FontWeight.w400,
color: CupertinoColors.black,
textBaseline: TextBaseline.alphabetic,
);
final GlobalKey _globalKey = GlobalKey();
bool _isPressed = false;
void onTapDown(TapDownDetails details) {
setState(() {
_isPressed = true;
});
}
void onTapUp(TapUpDetails details) {
setState(() {
_isPressed = false;
});
}
void onTapCancel() {
setState(() {
_isPressed = false;
});
}
TextStyle get _textStyle {
if (widget.isDefaultAction) {
return _kActionSheetActionStyle.copyWith(
color: CupertinoDynamicColor.resolve(CupertinoColors.label, context),
fontWeight: FontWeight.w600,
);
}
if (widget.isDestructiveAction) {
return _kActionSheetActionStyle.copyWith(
color: CupertinoColors.destructiveRed,
);
}
return _kActionSheetActionStyle.copyWith(
color: CupertinoDynamicColor.resolve(CupertinoColors.label, context)
);
}
@override
Widget build(BuildContext context) {
return MouseRegion(
cursor: widget.onPressed != null && kIsWeb ? SystemMouseCursors.click : MouseCursor.defer,
child: GestureDetector(
key: _globalKey,
onTapDown: onTapDown,
onTapUp: onTapUp,
onTapCancel: onTapCancel,
onTap: widget.onPressed,
behavior: HitTestBehavior.opaque,
child: ConstrainedBox(
constraints: const BoxConstraints(
minHeight: _kButtonHeight,
),
child: Semantics(
button: true,
child: Container(
decoration: BoxDecoration(
color: _isPressed
? CupertinoDynamicColor.resolve(_kBackgroundColorPressed, context)
: CupertinoDynamicColor.resolve(_kBackgroundColor, context),
),
padding: const EdgeInsets.only(
top: 8,
bottom: 8,
left: 15.5,
right: 17.5,
),
child: DefaultTextStyle(
style: _textStyle,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Flexible(
child: widget.child,
),
if (widget.trailingIcon != null)
Icon(
widget.trailingIcon,
color: _textStyle.color,
size: 21.0,
),
],
),
),
),
),
),
),
);
}
}
| flutter/packages/flutter/lib/src/cupertino/context_menu_action.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/context_menu_action.dart",
"repo_id": "flutter",
"token_count": 2050
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' show ImageFilter;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'button.dart';
import 'colors.dart';
import 'constants.dart';
import 'icons.dart';
import 'page_scaffold.dart';
import 'route.dart';
import 'theme.dart';
/// Standard iOS navigation bar height without the status bar.
///
/// This height is constant and independent of accessibility as it is in iOS.
const double _kNavBarPersistentHeight = kMinInteractiveDimensionCupertino;
/// Size increase from expanding the navigation bar into an iOS-11-style large title
/// form in a [CustomScrollView].
const double _kNavBarLargeTitleHeightExtension = 52.0;
/// Number of logical pixels scrolled down before the title text is transferred
/// from the normal navigation bar to a big title below the navigation bar.
const double _kNavBarShowLargeTitleThreshold = 10.0;
const double _kNavBarEdgePadding = 16.0;
const double _kNavBarBottomPadding = 8.0;
const double _kNavBarBackButtonTapWidth = 50.0;
/// Title text transfer fade.
const Duration _kNavBarTitleFadeDuration = Duration(milliseconds: 150);
const Color _kDefaultNavBarBorderColor = Color(0x4D000000);
const Border _kDefaultNavBarBorder = Border(
bottom: BorderSide(
color: _kDefaultNavBarBorderColor,
width: 0.0, // 0.0 means one physical pixel
),
);
// There's a single tag for all instances of navigation bars because they can
// all transition between each other (per Navigator) via Hero transitions.
const _HeroTag _defaultHeroTag = _HeroTag(null);
@immutable
class _HeroTag {
const _HeroTag(this.navigator);
final NavigatorState? navigator;
// Let the Hero tag be described in tree dumps.
@override
String toString() => 'Default Hero tag for Cupertino navigation bars with navigator $navigator';
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is _HeroTag
&& other.navigator == navigator;
}
@override
int get hashCode => identityHashCode(navigator);
}
// An `AnimatedWidget` that imposes a fixed size on its child widget, and
// shifts the child widget in the parent stack, driven by its `offsetAnimation`
// property.
class _FixedSizeSlidingTransition extends AnimatedWidget {
const _FixedSizeSlidingTransition({
required this.isLTR,
required this.offsetAnimation,
required this.size,
required this.child,
}) : super(listenable: offsetAnimation);
// Whether the writing direction used in the navigation bar transition is
// left-to-right.
final bool isLTR;
// The fixed size to impose on `child`.
final Size size;
// The animated offset from the top-leading corner of the stack.
//
// When `isLTR` is true, the `Offset` is the position of the child widget in
// the stack render box's regular coordinate space.
//
// When `isLTR` is false, the coordinate system is flipped around the
// horizontal axis and the origin is set to the top right corner of the render
// boxes. In other words, this parameter describes the offset from the top
// right corner of the stack, to the top right corner of the child widget, and
// the x-axis runs right to left.
final Animation<Offset> offsetAnimation;
final Widget child;
@override
Widget build(BuildContext context) {
return Positioned(
top: offsetAnimation.value.dy,
left: isLTR ? offsetAnimation.value.dx : null,
right: isLTR ? null : offsetAnimation.value.dx,
width: size.width,
height: size.height,
child: child,
);
}
}
/// Returns `child` wrapped with background and a bottom border if background color
/// is opaque. Otherwise, also blur with [BackdropFilter].
///
/// When `updateSystemUiOverlay` is true, the nav bar will update the OS
/// status bar's color theme based on the background color of the nav bar.
Widget _wrapWithBackground({
Border? border,
required Color backgroundColor,
Brightness? brightness,
required Widget child,
bool updateSystemUiOverlay = true,
}) {
Widget result = child;
if (updateSystemUiOverlay) {
final bool isDark = backgroundColor.computeLuminance() < 0.179;
final Brightness newBrightness = brightness ?? (isDark ? Brightness.dark : Brightness.light);
final SystemUiOverlayStyle overlayStyle = switch (newBrightness) {
Brightness.dark => SystemUiOverlayStyle.light,
Brightness.light => SystemUiOverlayStyle.dark,
};
// [SystemUiOverlayStyle.light] and [SystemUiOverlayStyle.dark] set some system
// navigation bar properties,
// Before https://github.com/flutter/flutter/pull/104827 those properties
// had no effect, now they are used if there is no AnnotatedRegion on the
// bottom of the screen.
// For backward compatibility, create a `SystemUiOverlayStyle` without the
// system navigation bar properties.
result = AnnotatedRegion<SystemUiOverlayStyle>(
value: SystemUiOverlayStyle(
statusBarColor: overlayStyle.statusBarColor,
statusBarBrightness: overlayStyle.statusBarBrightness,
statusBarIconBrightness: overlayStyle.statusBarIconBrightness,
systemStatusBarContrastEnforced: overlayStyle.systemStatusBarContrastEnforced,
),
child: result,
);
}
final DecoratedBox childWithBackground = DecoratedBox(
decoration: BoxDecoration(
border: border,
color: backgroundColor,
),
child: result,
);
if (backgroundColor.alpha == 0xFF) {
return childWithBackground;
}
return ClipRect(
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: 10.0, sigmaY: 10.0),
child: childWithBackground,
),
);
}
// Whether the current route supports nav bar hero transitions from or to.
bool _isTransitionable(BuildContext context) {
final ModalRoute<dynamic>? route = ModalRoute.of(context);
// Fullscreen dialogs never transitions their nav bar with other push-style
// pages' nav bars or with other fullscreen dialog pages on the way in or on
// the way out.
return route is PageRoute && !route.fullscreenDialog;
}
/// An iOS-styled navigation bar.
///
/// The navigation bar is a toolbar that minimally consists of a widget, normally
/// a page title, in the [middle] of the toolbar.
///
/// It also supports a [leading] and [trailing] widget before and after the
/// [middle] widget while keeping the [middle] widget centered.
///
/// The [leading] widget will automatically be a back chevron icon button (or a
/// close button in case of a fullscreen dialog) to pop the current route if none
/// is provided and [automaticallyImplyLeading] is true (true by default).
///
/// The [middle] widget will automatically be a title text from the current
/// [CupertinoPageRoute] if none is provided and [automaticallyImplyMiddle] is
/// true (true by default).
///
/// It should be placed at top of the screen and automatically accounts for
/// the OS's status bar.
///
/// If the given [backgroundColor]'s opacity is not 1.0 (which is the case by
/// default), it will produce a blurring effect to the content behind it.
///
/// When [transitionBetweenRoutes] is true, this navigation bar will transition
/// on top of the routes instead of inside them if the route being transitioned
/// to also has a [CupertinoNavigationBar] or a [CupertinoSliverNavigationBar]
/// with [transitionBetweenRoutes] set to true. If [transitionBetweenRoutes] is
/// true, none of the [Widget] parameters can contain a key in its subtree since
/// that widget will exist in multiple places in the tree simultaneously.
///
/// By default, only one [CupertinoNavigationBar] or [CupertinoSliverNavigationBar]
/// should be present in each [PageRoute] to support the default transitions.
/// Use [transitionBetweenRoutes] or [heroTag] to customize the transition
/// behavior for multiple navigation bars per route.
///
/// When used in a [CupertinoPageScaffold], [CupertinoPageScaffold.navigationBar]
/// disables text scaling to match the native iOS behavior. To override
/// this behavior, wrap each of the `navigationBar`'s components inside a
/// [MediaQuery] with the desired [TextScaler].
///
/// {@tool dartpad}
/// This example shows a [CupertinoNavigationBar] placed in a [CupertinoPageScaffold].
/// Since [backgroundColor]'s opacity is not 1.0, there is a blur effect and
/// content slides underneath.
///
/// ** See code in examples/api/lib/cupertino/nav_bar/cupertino_navigation_bar.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoPageScaffold], a page layout helper typically hosting the
/// [CupertinoNavigationBar].
/// * [CupertinoSliverNavigationBar] for a navigation bar to be placed in a
/// scrolling list and that supports iOS-11-style large titles.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/bars/navigation-bars/>
class CupertinoNavigationBar extends StatefulWidget implements ObstructingPreferredSizeWidget {
/// Creates a navigation bar in the iOS style.
const CupertinoNavigationBar({
super.key,
this.leading,
this.automaticallyImplyLeading = true,
this.automaticallyImplyMiddle = true,
this.previousPageTitle,
this.middle,
this.trailing,
this.border = _kDefaultNavBarBorder,
this.backgroundColor,
this.brightness,
this.padding,
this.transitionBetweenRoutes = true,
this.heroTag = _defaultHeroTag,
}) : assert(
!transitionBetweenRoutes || identical(heroTag, _defaultHeroTag),
'Cannot specify a heroTag override if this navigation bar does not '
'transition due to transitionBetweenRoutes = false.',
);
/// {@template flutter.cupertino.CupertinoNavigationBar.leading}
/// Widget to place at the start of the navigation bar. Normally a back button
/// for a normal page or a cancel button for full page dialogs.
///
/// If null and [automaticallyImplyLeading] is true, an appropriate button
/// will be automatically created.
/// {@endtemplate}
final Widget? leading;
/// {@template flutter.cupertino.CupertinoNavigationBar.automaticallyImplyLeading}
/// Controls whether we should try to imply the leading widget if null.
///
/// If true and [leading] is null, automatically try to deduce what the [leading]
/// widget should be. If [leading] widget is not null, this parameter has no effect.
///
/// Specifically this navigation bar will:
///
/// 1. Show a 'Close' button if the current route is a `fullscreenDialog`.
/// 2. Show a back chevron with [previousPageTitle] if [previousPageTitle] is
/// not null.
/// 3. Show a back chevron with the previous route's `title` if the current
/// route is a [CupertinoPageRoute] and the previous route is also a
/// [CupertinoPageRoute].
/// {@endtemplate}
final bool automaticallyImplyLeading;
/// Controls whether we should try to imply the middle widget if null.
///
/// If true and [middle] is null, automatically fill in a [Text] widget with
/// the current route's `title` if the route is a [CupertinoPageRoute].
/// If [middle] widget is not null, this parameter has no effect.
final bool automaticallyImplyMiddle;
/// {@template flutter.cupertino.CupertinoNavigationBar.previousPageTitle}
/// Manually specify the previous route's title when automatically implying
/// the leading back button.
///
/// Overrides the text shown with the back chevron instead of automatically
/// showing the previous [CupertinoPageRoute]'s `title` when
/// [automaticallyImplyLeading] is true.
///
/// Has no effect when [leading] is not null or if [automaticallyImplyLeading]
/// is false.
/// {@endtemplate}
final String? previousPageTitle;
/// Widget to place in the middle of the navigation bar. Normally a title or
/// a segmented control.
///
/// If null and [automaticallyImplyMiddle] is true, an appropriate [Text]
/// title will be created if the current route is a [CupertinoPageRoute] and
/// has a `title`.
final Widget? middle;
/// {@template flutter.cupertino.CupertinoNavigationBar.trailing}
/// Widget to place at the end of the navigation bar. Normally additional actions
/// taken on the page such as a search or edit function.
/// {@endtemplate}
final Widget? trailing;
// TODO(xster): https://github.com/flutter/flutter/issues/10469 implement
// support for double row navigation bars.
/// {@template flutter.cupertino.CupertinoNavigationBar.backgroundColor}
/// The background color of the navigation bar. If it contains transparency, the
/// tab bar will automatically produce a blurring effect to the content
/// behind it.
///
/// Defaults to [CupertinoTheme]'s `barBackgroundColor` if null.
/// {@endtemplate}
final Color? backgroundColor;
/// {@template flutter.cupertino.CupertinoNavigationBar.brightness}
/// The brightness of the specified [backgroundColor].
///
/// Setting this value changes the style of the system status bar. Typically
/// used to increase the contrast ratio of the system status bar over
/// [backgroundColor].
///
/// If set to null, the value of the property will be inferred from the relative
/// luminance of [backgroundColor].
/// {@endtemplate}
final Brightness? brightness;
/// {@template flutter.cupertino.CupertinoNavigationBar.padding}
/// Padding for the contents of the navigation bar.
///
/// If null, the navigation bar will adopt the following defaults:
///
/// * Vertically, contents will be sized to the same height as the navigation
/// bar itself minus the status bar.
/// * Horizontally, padding will be 16 pixels according to iOS specifications
/// unless the leading widget is an automatically inserted back button, in
/// which case the padding will be 0.
///
/// Vertical padding won't change the height of the nav bar.
/// {@endtemplate}
final EdgeInsetsDirectional? padding;
/// {@template flutter.cupertino.CupertinoNavigationBar.border}
/// The border of the navigation bar. By default renders a single pixel bottom border side.
///
/// If a border is null, the navigation bar will not display a border.
/// {@endtemplate}
final Border? border;
/// {@template flutter.cupertino.CupertinoNavigationBar.transitionBetweenRoutes}
/// Whether to transition between navigation bars.
///
/// When [transitionBetweenRoutes] is true, this navigation bar will transition
/// on top of the routes instead of inside it if the route being transitioned
/// to also has a [CupertinoNavigationBar] or a [CupertinoSliverNavigationBar]
/// with [transitionBetweenRoutes] set to true.
///
/// This transition will also occur on edge back swipe gestures like on iOS
/// but only if the previous page below has `maintainState` set to true on the
/// [PageRoute].
///
/// When set to true, only one navigation bar can be present per route unless
/// [heroTag] is also set.
///
/// This value defaults to true.
/// {@endtemplate}
final bool transitionBetweenRoutes;
/// {@template flutter.cupertino.CupertinoNavigationBar.heroTag}
/// Tag for the navigation bar's Hero widget if [transitionBetweenRoutes] is true.
///
/// Defaults to a common tag between all [CupertinoNavigationBar] and
/// [CupertinoSliverNavigationBar] instances of the same [Navigator]. With the
/// default tag, all navigation bars of the same navigator can transition
/// between each other as long as there's only one navigation bar per route.
///
/// This [heroTag] can be overridden to manually handle having multiple
/// navigation bars per route or to transition between multiple
/// [Navigator]s.
///
/// To disable Hero transitions for this navigation bar, set
/// [transitionBetweenRoutes] to false.
/// {@endtemplate}
final Object heroTag;
/// True if the navigation bar's background color has no transparency.
@override
bool shouldFullyObstruct(BuildContext context) {
final Color backgroundColor = CupertinoDynamicColor.maybeResolve(this.backgroundColor, context)
?? CupertinoTheme.of(context).barBackgroundColor;
return backgroundColor.alpha == 0xFF;
}
@override
Size get preferredSize {
return const Size.fromHeight(_kNavBarPersistentHeight);
}
@override
State<CupertinoNavigationBar> createState() => _CupertinoNavigationBarState();
}
// A state class exists for the nav bar so that the keys of its sub-components
// don't change when rebuilding the nav bar, causing the sub-components to
// lose their own states.
class _CupertinoNavigationBarState extends State<CupertinoNavigationBar> {
late _NavigationBarStaticComponentsKeys keys;
@override
void initState() {
super.initState();
keys = _NavigationBarStaticComponentsKeys();
}
@override
Widget build(BuildContext context) {
final Color backgroundColor =
CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context) ?? CupertinoTheme.of(context).barBackgroundColor;
final _NavigationBarStaticComponents components = _NavigationBarStaticComponents(
keys: keys,
route: ModalRoute.of(context),
userLeading: widget.leading,
automaticallyImplyLeading: widget.automaticallyImplyLeading,
automaticallyImplyTitle: widget.automaticallyImplyMiddle,
previousPageTitle: widget.previousPageTitle,
userMiddle: widget.middle,
userTrailing: widget.trailing,
padding: widget.padding,
userLargeTitle: null,
large: false,
);
final Widget navBar = _wrapWithBackground(
border: widget.border,
backgroundColor: backgroundColor,
brightness: widget.brightness,
child: DefaultTextStyle(
style: CupertinoTheme.of(context).textTheme.textStyle,
child: _PersistentNavigationBar(
components: components,
padding: widget.padding,
),
),
);
if (!widget.transitionBetweenRoutes || !_isTransitionable(context)) {
// Lint ignore to maintain backward compatibility.
return navBar;
}
return Builder(
// Get the context that might have a possibly changed CupertinoTheme.
builder: (BuildContext context) {
return Hero(
tag: widget.heroTag == _defaultHeroTag
? _HeroTag(Navigator.of(context))
: widget.heroTag,
createRectTween: _linearTranslateWithLargestRectSizeTween,
placeholderBuilder: _navBarHeroLaunchPadBuilder,
flightShuttleBuilder: _navBarHeroFlightShuttleBuilder,
transitionOnUserGestures: true,
child: _TransitionableNavigationBar(
componentsKeys: keys,
backgroundColor: backgroundColor,
backButtonTextStyle: CupertinoTheme.of(context).textTheme.navActionTextStyle,
titleTextStyle: CupertinoTheme.of(context).textTheme.navTitleTextStyle,
largeTitleTextStyle: null,
border: widget.border,
hasUserMiddle: widget.middle != null,
largeExpanded: false,
child: navBar,
),
);
},
);
}
}
/// An iOS-styled navigation bar with iOS-11-style large titles using slivers.
///
/// The [CupertinoSliverNavigationBar] must be placed in a sliver group such
/// as the [CustomScrollView].
///
/// This navigation bar consists of two sections, a pinned static section on top
/// and a sliding section containing iOS-11-style large title below it.
///
/// It should be placed at top of the screen and automatically accounts for
/// the iOS status bar.
///
/// Minimally, a [largeTitle] widget will appear in the middle of the app bar
/// when the sliver is collapsed and transfer to the area below in larger font
/// when the sliver is expanded.
///
/// For advanced uses, an optional [middle] widget can be supplied to show a
/// different widget in the middle of the navigation bar when the sliver is collapsed.
///
/// Like [CupertinoNavigationBar], it also supports a [leading] and [trailing]
/// widget on the static section on top that remains while scrolling.
///
/// The [leading] widget will automatically be a back chevron icon button (or a
/// close button in case of a fullscreen dialog) to pop the current route if none
/// is provided and [automaticallyImplyLeading] is true (true by default).
///
/// The [largeTitle] widget will automatically be a title text from the current
/// [CupertinoPageRoute] if none is provided and [automaticallyImplyTitle] is
/// true (true by default).
///
/// When [transitionBetweenRoutes] is true, this navigation bar will transition
/// on top of the routes instead of inside them if the route being transitioned
/// to also has a [CupertinoNavigationBar] or a [CupertinoSliverNavigationBar]
/// with [transitionBetweenRoutes] set to true. If [transitionBetweenRoutes] is
/// true, none of the [Widget] parameters can contain any [GlobalKey]s in their
/// subtrees since those widgets will exist in multiple places in the tree
/// simultaneously.
///
/// By default, only one [CupertinoNavigationBar] or [CupertinoSliverNavigationBar]
/// should be present in each [PageRoute] to support the default transitions.
/// Use [transitionBetweenRoutes] or [heroTag] to customize the transition
/// behavior for multiple navigation bars per route.
///
/// [CupertinoSliverNavigationBar] by default disables text scaling to match the
/// native iOS behavior. To override this behavior, wrap each of the
/// [CupertinoSliverNavigationBar]'s components inside a [MediaQuery] with the
/// desired [TextScaler].
///
/// The [stretch] parameter determines whether the nav bar should stretch to
/// fill the over-scroll area. The nav bar can still expand and contract as the
/// user scrolls, but it will also stretch when the user over-scrolls if the
/// [stretch] value is `true`. Defaults to `false`.
///
/// {@tool dartpad}
/// This example shows [CupertinoSliverNavigationBar] in action inside a [CustomScrollView].
///
/// ** See code in examples/api/lib/cupertino/nav_bar/cupertino_sliver_nav_bar.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoNavigationBar], an iOS navigation bar for use on non-scrolling
/// pages.
/// * [CustomScrollView], a ScrollView that creates custom scroll effects using slivers.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/bars/navigation-bars/>
class CupertinoSliverNavigationBar extends StatefulWidget {
/// Creates a navigation bar for scrolling lists.
///
/// If [automaticallyImplyTitle] is false, then the [largeTitle] argument is
/// required.
const CupertinoSliverNavigationBar({
super.key,
this.largeTitle,
this.leading,
this.automaticallyImplyLeading = true,
this.automaticallyImplyTitle = true,
this.alwaysShowMiddle = true,
this.previousPageTitle,
this.middle,
this.trailing,
this.border = _kDefaultNavBarBorder,
this.backgroundColor,
this.brightness,
this.padding,
this.transitionBetweenRoutes = true,
this.heroTag = _defaultHeroTag,
this.stretch = false,
}) : assert(
automaticallyImplyTitle || largeTitle != null,
'No largeTitle has been provided but automaticallyImplyTitle is also '
'false. Either provide a largeTitle or set automaticallyImplyTitle to '
'true.',
);
/// The navigation bar's title.
///
/// This text will appear in the top static navigation bar when collapsed and
/// below the navigation bar, in a larger font, when expanded.
///
/// A suitable [DefaultTextStyle] is provided around this widget as it is
/// moved around, to change its font size.
///
/// If [middle] is null, then the [largeTitle] widget will be inserted into
/// the tree in two places when transitioning from the collapsed state to the
/// expanded state. It is therefore imperative that this subtree not contain
/// any [GlobalKey]s, and that it not rely on maintaining state (for example,
/// animations will not survive the transition from one location to the other,
/// and may in fact be visible in two places at once during the transition).
///
/// If null and [automaticallyImplyTitle] is true, an appropriate [Text]
/// title will be created if the current route is a [CupertinoPageRoute] and
/// has a `title`.
///
/// This parameter must either be non-null or the route must have a title
/// ([CupertinoPageRoute.title]) and [automaticallyImplyTitle] must be true.
final Widget? largeTitle;
/// {@macro flutter.cupertino.CupertinoNavigationBar.leading}
///
/// This widget is visible in both collapsed and expanded states.
final Widget? leading;
/// {@macro flutter.cupertino.CupertinoNavigationBar.automaticallyImplyLeading}
final bool automaticallyImplyLeading;
/// Controls whether we should try to imply the [largeTitle] widget if null.
///
/// If true and [largeTitle] is null, automatically fill in a [Text] widget
/// with the current route's `title` if the route is a [CupertinoPageRoute].
/// If [largeTitle] widget is not null, this parameter has no effect.
final bool automaticallyImplyTitle;
/// Controls whether [middle] widget should always be visible (even in
/// expanded state).
///
/// If true (default) and [middle] is not null, [middle] widget is always
/// visible. If false, [middle] widget is visible only in collapsed state if
/// it is provided.
///
/// This should be set to false if you only want to show [largeTitle] in
/// expanded state and [middle] in collapsed state.
final bool alwaysShowMiddle;
/// {@macro flutter.cupertino.CupertinoNavigationBar.previousPageTitle}
final String? previousPageTitle;
/// A widget to place in the middle of the static navigation bar instead of
/// the [largeTitle].
///
/// This widget is visible in both collapsed and expanded states if
/// [alwaysShowMiddle] is true, otherwise just in collapsed state. The text
/// supplied in [largeTitle] will no longer appear in collapsed state if a
/// [middle] widget is provided.
final Widget? middle;
/// {@macro flutter.cupertino.CupertinoNavigationBar.trailing}
///
/// This widget is visible in both collapsed and expanded states.
final Widget? trailing;
/// {@macro flutter.cupertino.CupertinoNavigationBar.backgroundColor}
final Color? backgroundColor;
/// {@macro flutter.cupertino.CupertinoNavigationBar.brightness}
final Brightness? brightness;
/// {@macro flutter.cupertino.CupertinoNavigationBar.padding}
final EdgeInsetsDirectional? padding;
/// {@macro flutter.cupertino.CupertinoNavigationBar.border}
final Border? border;
/// {@macro flutter.cupertino.CupertinoNavigationBar.transitionBetweenRoutes}
final bool transitionBetweenRoutes;
/// {@macro flutter.cupertino.CupertinoNavigationBar.heroTag}
final Object heroTag;
/// True if the navigation bar's background color has no transparency.
bool get opaque => backgroundColor?.alpha == 0xFF;
/// Whether the nav bar should stretch to fill the over-scroll area.
///
/// The nav bar can still expand and contract as the user scrolls, but it will
/// also stretch when the user over-scrolls if the [stretch] value is `true`.
///
/// When set to `true`, the nav bar will prevent subsequent slivers from
/// accessing overscrolls. This may be undesirable for using overscroll-based
/// widgets like the [CupertinoSliverRefreshControl].
///
/// Defaults to `false`.
final bool stretch;
@override
State<CupertinoSliverNavigationBar> createState() => _CupertinoSliverNavigationBarState();
}
// A state class exists for the nav bar so that the keys of its sub-components
// don't change when rebuilding the nav bar, causing the sub-components to
// lose their own states.
class _CupertinoSliverNavigationBarState extends State<CupertinoSliverNavigationBar> {
late _NavigationBarStaticComponentsKeys keys;
@override
void initState() {
super.initState();
keys = _NavigationBarStaticComponentsKeys();
}
@override
Widget build(BuildContext context) {
final _NavigationBarStaticComponents components = _NavigationBarStaticComponents(
keys: keys,
route: ModalRoute.of(context),
userLeading: widget.leading,
automaticallyImplyLeading: widget.automaticallyImplyLeading,
automaticallyImplyTitle: widget.automaticallyImplyTitle,
previousPageTitle: widget.previousPageTitle,
userMiddle: widget.middle,
userTrailing: widget.trailing,
userLargeTitle: widget.largeTitle,
padding: widget.padding,
large: true,
);
return MediaQuery.withNoTextScaling(
child: SliverPersistentHeader(
pinned: true, // iOS navigation bars are always pinned.
delegate: _LargeTitleNavigationBarSliverDelegate(
keys: keys,
components: components,
userMiddle: widget.middle,
backgroundColor: CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context) ?? CupertinoTheme.of(context).barBackgroundColor,
brightness: widget.brightness,
border: widget.border,
padding: widget.padding,
actionsForegroundColor: CupertinoTheme.of(context).primaryColor,
transitionBetweenRoutes: widget.transitionBetweenRoutes,
heroTag: widget.heroTag,
persistentHeight: _kNavBarPersistentHeight + MediaQuery.paddingOf(context).top,
alwaysShowMiddle: widget.alwaysShowMiddle && widget.middle != null,
stretchConfiguration: widget.stretch ? OverScrollHeaderStretchConfiguration() : null,
),
),
);
}
}
class _LargeTitleNavigationBarSliverDelegate
extends SliverPersistentHeaderDelegate with DiagnosticableTreeMixin {
_LargeTitleNavigationBarSliverDelegate({
required this.keys,
required this.components,
required this.userMiddle,
required this.backgroundColor,
required this.brightness,
required this.border,
required this.padding,
required this.actionsForegroundColor,
required this.transitionBetweenRoutes,
required this.heroTag,
required this.persistentHeight,
required this.alwaysShowMiddle,
required this.stretchConfiguration,
});
final _NavigationBarStaticComponentsKeys keys;
final _NavigationBarStaticComponents components;
final Widget? userMiddle;
final Color backgroundColor;
final Brightness? brightness;
final Border? border;
final EdgeInsetsDirectional? padding;
final Color actionsForegroundColor;
final bool transitionBetweenRoutes;
final Object heroTag;
final double persistentHeight;
final bool alwaysShowMiddle;
@override
double get minExtent => persistentHeight;
@override
double get maxExtent => persistentHeight + _kNavBarLargeTitleHeightExtension;
@override
OverScrollHeaderStretchConfiguration? stretchConfiguration;
@override
Widget build(BuildContext context, double shrinkOffset, bool overlapsContent) {
final bool showLargeTitle = shrinkOffset < maxExtent - minExtent - _kNavBarShowLargeTitleThreshold;
final _PersistentNavigationBar persistentNavigationBar =
_PersistentNavigationBar(
components: components,
padding: padding,
// If a user specified middle exists, always show it. Otherwise, show
// title when sliver is collapsed.
middleVisible: alwaysShowMiddle ? null : !showLargeTitle,
);
final Widget navBar = _wrapWithBackground(
border: border,
backgroundColor: CupertinoDynamicColor.resolve(backgroundColor, context),
brightness: brightness,
child: DefaultTextStyle(
style: CupertinoTheme.of(context).textTheme.textStyle,
child: Stack(
fit: StackFit.expand,
children: <Widget>[
Positioned(
top: persistentHeight,
left: 0.0,
right: 0.0,
bottom: 0.0,
child: ClipRect(
child: Padding(
padding: const EdgeInsetsDirectional.only(
start: _kNavBarEdgePadding,
bottom: _kNavBarBottomPadding
),
child: SafeArea(
top: false,
bottom: false,
child: AnimatedOpacity(
opacity: showLargeTitle ? 1.0 : 0.0,
duration: _kNavBarTitleFadeDuration,
child: Semantics(
header: true,
child: DefaultTextStyle(
style: CupertinoTheme.of(context)
.textTheme
.navLargeTitleTextStyle,
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: _LargeTitle(
child: components.largeTitle,
),
),
),
),
),
),
),
),
Positioned(
left: 0.0,
right: 0.0,
top: 0.0,
child: persistentNavigationBar,
),
],
),
),
);
if (!transitionBetweenRoutes || !_isTransitionable(context)) {
return navBar;
}
return Hero(
tag: heroTag == _defaultHeroTag
? _HeroTag(Navigator.of(context))
: heroTag,
createRectTween: _linearTranslateWithLargestRectSizeTween,
flightShuttleBuilder: _navBarHeroFlightShuttleBuilder,
placeholderBuilder: _navBarHeroLaunchPadBuilder,
transitionOnUserGestures: true,
// This is all the way down here instead of being at the top level of
// CupertinoSliverNavigationBar like CupertinoNavigationBar because it
// needs to wrap the top level RenderBox rather than a RenderSliver.
child: _TransitionableNavigationBar(
componentsKeys: keys,
backgroundColor: CupertinoDynamicColor.resolve(backgroundColor, context),
backButtonTextStyle: CupertinoTheme.of(context).textTheme.navActionTextStyle,
titleTextStyle: CupertinoTheme.of(context).textTheme.navTitleTextStyle,
largeTitleTextStyle: CupertinoTheme.of(context).textTheme.navLargeTitleTextStyle,
border: border,
hasUserMiddle: userMiddle != null && (alwaysShowMiddle || !showLargeTitle),
largeExpanded: showLargeTitle,
child: navBar,
),
);
}
@override
bool shouldRebuild(_LargeTitleNavigationBarSliverDelegate oldDelegate) {
return components != oldDelegate.components
|| userMiddle != oldDelegate.userMiddle
|| backgroundColor != oldDelegate.backgroundColor
|| border != oldDelegate.border
|| padding != oldDelegate.padding
|| actionsForegroundColor != oldDelegate.actionsForegroundColor
|| transitionBetweenRoutes != oldDelegate.transitionBetweenRoutes
|| persistentHeight != oldDelegate.persistentHeight
|| alwaysShowMiddle != oldDelegate.alwaysShowMiddle
|| heroTag != oldDelegate.heroTag;
}
}
/// The large title of the navigation bar.
///
/// Magnifies on over-scroll when [CupertinoSliverNavigationBar.stretch]
/// parameter is true.
class _LargeTitle extends SingleChildRenderObjectWidget {
const _LargeTitle({ super.child });
@override
_RenderLargeTitle createRenderObject(BuildContext context) {
return _RenderLargeTitle(alignment: AlignmentDirectional.bottomStart.resolve(Directionality.of(context)));
}
@override
void updateRenderObject(BuildContext context, _RenderLargeTitle renderObject) {
renderObject.alignment = AlignmentDirectional.bottomStart.resolve(Directionality.of(context));
}
}
class _RenderLargeTitle extends RenderShiftedBox {
_RenderLargeTitle({
required Alignment alignment,
}) : _alignment = alignment,
super(null);
Alignment get alignment => _alignment;
Alignment _alignment;
set alignment(Alignment value) {
if (_alignment == value) {
return;
}
_alignment = value;
markNeedsLayout();
}
double _scale = 1.0;
@override
void performLayout() {
final RenderBox? child = this.child;
Size childSize = Size.zero;
size = constraints.biggest;
if (child == null) {
return;
}
final BoxConstraints childConstraints = constraints.widthConstraints().loosen();
child.layout(childConstraints, parentUsesSize: true);
final double maxScale = child.size.width != 0.0
? clampDouble(constraints.maxWidth / child.size.width, 1.0, 1.1)
: 1.1;
_scale = clampDouble(
1.0 + (constraints.maxHeight - (_kNavBarLargeTitleHeightExtension - _kNavBarBottomPadding)) / (_kNavBarLargeTitleHeightExtension - _kNavBarBottomPadding) * 0.03,
1.0,
maxScale,
);
childSize = child.size * _scale;
final BoxParentData childParentData = child.parentData! as BoxParentData;
childParentData.offset = alignment.alongOffset(size - childSize as Offset);
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
assert(child == this.child);
super.applyPaintTransform(child, transform);
transform.scale(_scale, _scale);
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderBox? child = this.child;
if (child == null) {
layer = null;
} else {
final BoxParentData childParentData = child.parentData! as BoxParentData;
layer = context.pushTransform(
needsCompositing,
offset + childParentData.offset,
Matrix4.diagonal3Values(_scale, _scale, 1.0),
(PaintingContext context, Offset offset) => context.paintChild(child, offset),
oldLayer: layer as TransformLayer?,
);
}
}
@override
bool hitTestChildren(BoxHitTestResult result, {required Offset position}) {
final RenderBox? child = this.child;
if (child == null) {
return false;
}
final Offset childOffset = (child.parentData! as BoxParentData).offset;
final Matrix4 transform = Matrix4.identity()
..scale(1.0/_scale, 1.0/_scale, 1.0)
..translate(-childOffset.dx, -childOffset.dy);
return result.addWithRawTransform(
transform: transform,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
return child.hitTest(result, position: transformed);
}
);
}
}
/// The top part of the navigation bar that's never scrolled away.
///
/// Consists of the entire navigation bar without background and border when used
/// without large titles. With large titles, it's the top static half that
/// doesn't scroll.
class _PersistentNavigationBar extends StatelessWidget {
const _PersistentNavigationBar({
required this.components,
this.padding,
this.middleVisible,
});
final _NavigationBarStaticComponents components;
final EdgeInsetsDirectional? padding;
/// Whether the middle widget has a visible animated opacity. A null value
/// means the middle opacity will not be animated.
final bool? middleVisible;
@override
Widget build(BuildContext context) {
Widget? middle = components.middle;
if (middle != null) {
middle = DefaultTextStyle(
style: CupertinoTheme.of(context).textTheme.navTitleTextStyle,
child: Semantics(header: true, child: middle),
);
// When the middle's visibility can change on the fly like with large title
// slivers, wrap with animated opacity.
middle = middleVisible == null
? middle
: AnimatedOpacity(
opacity: middleVisible! ? 1.0 : 0.0,
duration: _kNavBarTitleFadeDuration,
child: middle,
);
}
Widget? leading = components.leading;
final Widget? backChevron = components.backChevron;
final Widget? backLabel = components.backLabel;
if (leading == null && backChevron != null && backLabel != null) {
leading = CupertinoNavigationBarBackButton._assemble(
backChevron,
backLabel,
);
}
Widget paddedToolbar = NavigationToolbar(
leading: leading,
middle: middle,
trailing: components.trailing,
middleSpacing: 6.0,
);
if (padding != null) {
paddedToolbar = Padding(
padding: EdgeInsets.only(
top: padding!.top,
bottom: padding!.bottom,
),
child: paddedToolbar,
);
}
return SizedBox(
height: _kNavBarPersistentHeight + MediaQuery.paddingOf(context).top,
child: SafeArea(
bottom: false,
child: paddedToolbar,
),
);
}
}
// A collection of keys always used when building static routes' nav bars's
// components with _NavigationBarStaticComponents and read in
// _NavigationBarTransition in Hero flights in order to reference the components'
// RenderBoxes for their positions.
//
// These keys should never re-appear inside the Hero flights.
@immutable
class _NavigationBarStaticComponentsKeys {
_NavigationBarStaticComponentsKeys()
: navBarBoxKey = GlobalKey(debugLabel: 'Navigation bar render box'),
leadingKey = GlobalKey(debugLabel: 'Leading'),
backChevronKey = GlobalKey(debugLabel: 'Back chevron'),
backLabelKey = GlobalKey(debugLabel: 'Back label'),
middleKey = GlobalKey(debugLabel: 'Middle'),
trailingKey = GlobalKey(debugLabel: 'Trailing'),
largeTitleKey = GlobalKey(debugLabel: 'Large title');
final GlobalKey navBarBoxKey;
final GlobalKey leadingKey;
final GlobalKey backChevronKey;
final GlobalKey backLabelKey;
final GlobalKey middleKey;
final GlobalKey trailingKey;
final GlobalKey largeTitleKey;
}
// Based on various user Widgets and other parameters, construct KeyedSubtree
// components that are used in common by the CupertinoNavigationBar and
// CupertinoSliverNavigationBar. The KeyedSubtrees are inserted into static
// routes and the KeyedSubtrees' child are reused in the Hero flights.
@immutable
class _NavigationBarStaticComponents {
_NavigationBarStaticComponents({
required _NavigationBarStaticComponentsKeys keys,
required ModalRoute<dynamic>? route,
required Widget? userLeading,
required bool automaticallyImplyLeading,
required bool automaticallyImplyTitle,
required String? previousPageTitle,
required Widget? userMiddle,
required Widget? userTrailing,
required Widget? userLargeTitle,
required EdgeInsetsDirectional? padding,
required bool large,
}) : leading = createLeading(
leadingKey: keys.leadingKey,
userLeading: userLeading,
route: route,
automaticallyImplyLeading: automaticallyImplyLeading,
padding: padding,
),
backChevron = createBackChevron(
backChevronKey: keys.backChevronKey,
userLeading: userLeading,
route: route,
automaticallyImplyLeading: automaticallyImplyLeading,
),
backLabel = createBackLabel(
backLabelKey: keys.backLabelKey,
userLeading: userLeading,
route: route,
previousPageTitle: previousPageTitle,
automaticallyImplyLeading: automaticallyImplyLeading,
),
middle = createMiddle(
middleKey: keys.middleKey,
userMiddle: userMiddle,
userLargeTitle: userLargeTitle,
route: route,
automaticallyImplyTitle: automaticallyImplyTitle,
large: large,
),
trailing = createTrailing(
trailingKey: keys.trailingKey,
userTrailing: userTrailing,
padding: padding,
),
largeTitle = createLargeTitle(
largeTitleKey: keys.largeTitleKey,
userLargeTitle: userLargeTitle,
route: route,
automaticImplyTitle: automaticallyImplyTitle,
large: large,
);
static Widget? _derivedTitle({
required bool automaticallyImplyTitle,
ModalRoute<dynamic>? currentRoute,
}) {
// Auto use the CupertinoPageRoute's title if middle not provided.
if (automaticallyImplyTitle &&
currentRoute is CupertinoRouteTransitionMixin &&
currentRoute.title != null) {
return Text(currentRoute.title!);
}
return null;
}
final KeyedSubtree? leading;
static KeyedSubtree? createLeading({
required GlobalKey leadingKey,
required Widget? userLeading,
required ModalRoute<dynamic>? route,
required bool automaticallyImplyLeading,
required EdgeInsetsDirectional? padding,
}) {
Widget? leadingContent;
if (userLeading != null) {
leadingContent = userLeading;
} else if (
automaticallyImplyLeading &&
route is PageRoute &&
route.canPop &&
route.fullscreenDialog
) {
leadingContent = CupertinoButton(
padding: EdgeInsets.zero,
onPressed: () { route.navigator!.maybePop(); },
child: const Text('Close'),
);
}
if (leadingContent == null) {
return null;
}
return KeyedSubtree(
key: leadingKey,
child: Padding(
padding: EdgeInsetsDirectional.only(
start: padding?.start ?? _kNavBarEdgePadding,
),
child: IconTheme.merge(
data: const IconThemeData(
size: 32.0,
),
child: leadingContent,
),
),
);
}
final KeyedSubtree? backChevron;
static KeyedSubtree? createBackChevron({
required GlobalKey backChevronKey,
required Widget? userLeading,
required ModalRoute<dynamic>? route,
required bool automaticallyImplyLeading,
}) {
if (
userLeading != null ||
!automaticallyImplyLeading ||
route == null ||
!route.canPop ||
(route is PageRoute && route.fullscreenDialog)
) {
return null;
}
return KeyedSubtree(key: backChevronKey, child: const _BackChevron());
}
/// This widget is not decorated with a font since the font style could
/// animate during transitions.
final KeyedSubtree? backLabel;
static KeyedSubtree? createBackLabel({
required GlobalKey backLabelKey,
required Widget? userLeading,
required ModalRoute<dynamic>? route,
required bool automaticallyImplyLeading,
required String? previousPageTitle,
}) {
if (
userLeading != null ||
!automaticallyImplyLeading ||
route == null ||
!route.canPop ||
(route is PageRoute && route.fullscreenDialog)
) {
return null;
}
return KeyedSubtree(
key: backLabelKey,
child: _BackLabel(
specifiedPreviousTitle: previousPageTitle,
route: route,
),
);
}
/// This widget is not decorated with a font since the font style could
/// animate during transitions.
final KeyedSubtree? middle;
static KeyedSubtree? createMiddle({
required GlobalKey middleKey,
required Widget? userMiddle,
required Widget? userLargeTitle,
required bool large,
required bool automaticallyImplyTitle,
required ModalRoute<dynamic>? route,
}) {
Widget? middleContent = userMiddle;
if (large) {
middleContent ??= userLargeTitle;
}
middleContent ??= _derivedTitle(
automaticallyImplyTitle: automaticallyImplyTitle,
currentRoute: route,
);
if (middleContent == null) {
return null;
}
return KeyedSubtree(
key: middleKey,
child: middleContent,
);
}
final KeyedSubtree? trailing;
static KeyedSubtree? createTrailing({
required GlobalKey trailingKey,
required Widget? userTrailing,
required EdgeInsetsDirectional? padding,
}) {
if (userTrailing == null) {
return null;
}
return KeyedSubtree(
key: trailingKey,
child: Padding(
padding: EdgeInsetsDirectional.only(
end: padding?.end ?? _kNavBarEdgePadding,
),
child: IconTheme.merge(
data: const IconThemeData(
size: 32.0,
),
child: userTrailing,
),
),
);
}
/// This widget is not decorated with a font since the font style could
/// animate during transitions.
final KeyedSubtree? largeTitle;
static KeyedSubtree? createLargeTitle({
required GlobalKey largeTitleKey,
required Widget? userLargeTitle,
required bool large,
required bool automaticImplyTitle,
required ModalRoute<dynamic>? route,
}) {
if (!large) {
return null;
}
final Widget? largeTitleContent = userLargeTitle ?? _derivedTitle(
automaticallyImplyTitle: automaticImplyTitle,
currentRoute: route,
);
assert(
largeTitleContent != null,
'largeTitle was not provided and there was no title from the route.',
);
return KeyedSubtree(
key: largeTitleKey,
child: largeTitleContent!,
);
}
}
/// A nav bar back button typically used in [CupertinoNavigationBar].
///
/// This is automatically inserted into [CupertinoNavigationBar] and
/// [CupertinoSliverNavigationBar]'s `leading` slot when
/// `automaticallyImplyLeading` is true.
///
/// When manually inserted, the [CupertinoNavigationBarBackButton] should only
/// be used in routes that can be popped unless a custom [onPressed] is
/// provided.
///
/// Shows a back chevron and the previous route's title when available from
/// the previous [CupertinoPageRoute.title]. If [previousPageTitle] is specified,
/// it will be shown instead.
class CupertinoNavigationBarBackButton extends StatelessWidget {
/// Construct a [CupertinoNavigationBarBackButton] that can be used to pop
/// the current route.
const CupertinoNavigationBarBackButton({
super.key,
this.color,
this.previousPageTitle,
this.onPressed,
}) : _backChevron = null,
_backLabel = null;
// Allow the back chevron and label to be separately created (and keyed)
// because they animate separately during page transitions.
const CupertinoNavigationBarBackButton._assemble(
this._backChevron,
this._backLabel,
) : previousPageTitle = null,
color = null,
onPressed = null;
/// The [Color] of the back button.
///
/// Can be used to override the color of the back button chevron and label.
///
/// Defaults to [CupertinoTheme]'s `primaryColor` if null.
final Color? color;
/// An override for showing the previous route's title. If null, it will be
/// automatically derived from [CupertinoPageRoute.title] if the current and
/// previous routes are both [CupertinoPageRoute]s.
final String? previousPageTitle;
/// An override callback to perform instead of the default behavior which is
/// to pop the [Navigator].
///
/// It can, for instance, be used to pop the platform's navigation stack
/// via [SystemNavigator] instead of Flutter's [Navigator] in add-to-app
/// situations.
///
/// Defaults to null.
final VoidCallback? onPressed;
final Widget? _backChevron;
final Widget? _backLabel;
@override
Widget build(BuildContext context) {
final ModalRoute<dynamic>? currentRoute = ModalRoute.of(context);
if (onPressed == null) {
assert(
currentRoute?.canPop ?? false,
'CupertinoNavigationBarBackButton should only be used in routes that can be popped',
);
}
TextStyle actionTextStyle = CupertinoTheme.of(context).textTheme.navActionTextStyle;
if (color != null) {
actionTextStyle = actionTextStyle.copyWith(color: CupertinoDynamicColor.maybeResolve(color, context));
}
return CupertinoButton(
padding: EdgeInsets.zero,
child: Semantics(
container: true,
excludeSemantics: true,
label: 'Back',
button: true,
child: DefaultTextStyle(
style: actionTextStyle,
child: ConstrainedBox(
constraints: const BoxConstraints(minWidth: _kNavBarBackButtonTapWidth),
child: Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const Padding(padding: EdgeInsetsDirectional.only(start: 8.0)),
_backChevron ?? const _BackChevron(),
const Padding(padding: EdgeInsetsDirectional.only(start: 6.0)),
Flexible(
child: _backLabel ?? _BackLabel(
specifiedPreviousTitle: previousPageTitle,
route: currentRoute,
),
),
],
),
),
),
),
onPressed: () {
if (onPressed != null) {
onPressed!();
} else {
Navigator.maybePop(context);
}
},
);
}
}
class _BackChevron extends StatelessWidget {
const _BackChevron();
@override
Widget build(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
final TextStyle textStyle = DefaultTextStyle.of(context).style;
// Replicate the Icon logic here to get a tightly sized icon and add
// custom non-square padding.
Widget iconWidget = Padding(
padding: const EdgeInsetsDirectional.only(start: 6, end: 2),
child: Text.rich(
TextSpan(
text: String.fromCharCode(CupertinoIcons.back.codePoint),
style: TextStyle(
inherit: false,
color: textStyle.color,
fontSize: 30.0,
fontFamily: CupertinoIcons.back.fontFamily,
package: CupertinoIcons.back.fontPackage,
),
),
),
);
switch (textDirection) {
case TextDirection.rtl:
iconWidget = Transform(
transform: Matrix4.identity()..scale(-1.0, 1.0, 1.0),
alignment: Alignment.center,
transformHitTests: false,
child: iconWidget,
);
case TextDirection.ltr:
break;
}
return iconWidget;
}
}
/// A widget that shows next to the back chevron when `automaticallyImplyLeading`
/// is true.
class _BackLabel extends StatelessWidget {
const _BackLabel({
required this.specifiedPreviousTitle,
required this.route,
});
final String? specifiedPreviousTitle;
final ModalRoute<dynamic>? route;
// `child` is never passed in into ValueListenableBuilder so it's always
// null here and unused.
Widget _buildPreviousTitleWidget(BuildContext context, String? previousTitle, Widget? child) {
if (previousTitle == null) {
return const SizedBox.shrink();
}
Text textWidget = Text(
previousTitle,
maxLines: 1,
overflow: TextOverflow.ellipsis,
);
if (previousTitle.length > 12) {
textWidget = const Text('Back');
}
return Align(
alignment: AlignmentDirectional.centerStart,
widthFactor: 1.0,
child: textWidget,
);
}
@override
Widget build(BuildContext context) {
if (specifiedPreviousTitle != null) {
return _buildPreviousTitleWidget(context, specifiedPreviousTitle, null);
} else if (route is CupertinoRouteTransitionMixin<dynamic> && !route!.isFirst) {
final CupertinoRouteTransitionMixin<dynamic> cupertinoRoute = route! as CupertinoRouteTransitionMixin<dynamic>;
// There is no timing issue because the previousTitle Listenable changes
// happen during route modifications before the ValueListenableBuilder
// is built.
return ValueListenableBuilder<String?>(
valueListenable: cupertinoRoute.previousTitle,
builder: _buildPreviousTitleWidget,
);
} else {
return const SizedBox.shrink();
}
}
}
/// This should always be the first child of Hero widgets.
///
/// This class helps each Hero transition obtain the start or end navigation
/// bar's box size and the inner components of the navigation bar that will
/// move around.
///
/// It should be wrapped around the biggest [RenderBox] of the static
/// navigation bar in each route.
class _TransitionableNavigationBar extends StatelessWidget {
_TransitionableNavigationBar({
required this.componentsKeys,
required this.backgroundColor,
required this.backButtonTextStyle,
required this.titleTextStyle,
required this.largeTitleTextStyle,
required this.border,
required this.hasUserMiddle,
required this.largeExpanded,
required this.child,
}) : assert(!largeExpanded || largeTitleTextStyle != null),
super(key: componentsKeys.navBarBoxKey);
final _NavigationBarStaticComponentsKeys componentsKeys;
final Color? backgroundColor;
final TextStyle backButtonTextStyle;
final TextStyle titleTextStyle;
final TextStyle? largeTitleTextStyle;
final Border? border;
final bool hasUserMiddle;
final bool largeExpanded;
final Widget child;
RenderBox get renderBox {
final RenderBox box = componentsKeys.navBarBoxKey.currentContext!.findRenderObject()! as RenderBox;
assert(
box.attached,
'_TransitionableNavigationBar.renderBox should be called when building '
'hero flight shuttles when the from and the to nav bar boxes are already '
'laid out and painted.',
);
return box;
}
@override
Widget build(BuildContext context) {
assert(() {
bool inHero = false;
context.visitAncestorElements((Element ancestor) {
if (ancestor is ComponentElement) {
assert(
ancestor.widget.runtimeType != _NavigationBarTransition,
'_TransitionableNavigationBar should never re-appear inside '
'_NavigationBarTransition. Keyed _TransitionableNavigationBar should '
'only serve as anchor points in routes rather than appearing inside '
'Hero flights themselves.',
);
if (ancestor.widget.runtimeType == Hero) {
inHero = true;
}
}
return true;
});
assert(
inHero,
'_TransitionableNavigationBar should only be added as the immediate '
'child of Hero widgets.',
);
return true;
}());
return child;
}
}
/// This class represents the widget that will be in the Hero flight instead of
/// the 2 static navigation bars by taking inner components from both.
///
/// The `topNavBar` parameter is the nav bar that was on top regardless of
/// push/pop direction.
///
/// Similarly, the `bottomNavBar` parameter is the nav bar that was at the
/// bottom regardless of the push/pop direction.
///
/// If [MediaQuery.padding] is still present in this widget's [BuildContext],
/// that padding will become part of the transitional navigation bar as well.
///
/// [MediaQuery.padding] should be consistent between the from/to routes and
/// the Hero overlay. Inconsistent [MediaQuery.padding] will produce undetermined
/// results.
class _NavigationBarTransition extends StatelessWidget {
_NavigationBarTransition({
required this.animation,
required this.topNavBar,
required this.bottomNavBar,
}) : heightTween = Tween<double>(
begin: bottomNavBar.renderBox.size.height,
end: topNavBar.renderBox.size.height,
),
backgroundTween = ColorTween(
begin: bottomNavBar.backgroundColor,
end: topNavBar.backgroundColor,
),
borderTween = BorderTween(
begin: bottomNavBar.border,
end: topNavBar.border,
);
final Animation<double> animation;
final _TransitionableNavigationBar topNavBar;
final _TransitionableNavigationBar bottomNavBar;
final Tween<double> heightTween;
final ColorTween backgroundTween;
final BorderTween borderTween;
@override
Widget build(BuildContext context) {
final _NavigationBarComponentsTransition componentsTransition = _NavigationBarComponentsTransition(
animation: animation,
bottomNavBar: bottomNavBar,
topNavBar: topNavBar,
directionality: Directionality.of(context),
);
final List<Widget> children = <Widget>[
// Draw an empty navigation bar box with changing shape behind all the
// moving components without any components inside it itself.
AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return _wrapWithBackground(
// Don't update the system status bar color mid-flight.
updateSystemUiOverlay: false,
backgroundColor: backgroundTween.evaluate(animation)!,
border: borderTween.evaluate(animation),
child: SizedBox(
height: heightTween.evaluate(animation),
width: double.infinity,
),
);
},
),
// Draw all the components on top of the empty bar box.
if (componentsTransition.bottomBackChevron != null) componentsTransition.bottomBackChevron!,
if (componentsTransition.bottomBackLabel != null) componentsTransition.bottomBackLabel!,
if (componentsTransition.bottomLeading != null) componentsTransition.bottomLeading!,
if (componentsTransition.bottomMiddle != null) componentsTransition.bottomMiddle!,
if (componentsTransition.bottomLargeTitle != null) componentsTransition.bottomLargeTitle!,
if (componentsTransition.bottomTrailing != null) componentsTransition.bottomTrailing!,
// Draw top components on top of the bottom components.
if (componentsTransition.topLeading != null) componentsTransition.topLeading!,
if (componentsTransition.topBackChevron != null) componentsTransition.topBackChevron!,
if (componentsTransition.topBackLabel != null) componentsTransition.topBackLabel!,
if (componentsTransition.topMiddle != null) componentsTransition.topMiddle!,
if (componentsTransition.topLargeTitle != null) componentsTransition.topLargeTitle!,
if (componentsTransition.topTrailing != null) componentsTransition.topTrailing!,
];
// The actual outer box is big enough to contain both the bottom and top
// navigation bars. It's not a direct Rect lerp because some components
// can actually be outside the linearly lerp'ed Rect in the middle of
// the animation, such as the topLargeTitle. The text scaling is disabled to
// avoid odd transitions between pages.
return MediaQuery.withNoTextScaling(
child: SizedBox(
height: math.max(heightTween.begin!, heightTween.end!) + MediaQuery.paddingOf(context).top,
width: double.infinity,
child: Stack(
children: children,
),
),
);
}
}
/// This class helps create widgets that are in transition based on static
/// components from the bottom and top navigation bars.
///
/// It animates these transitional components both in terms of position and
/// their appearance.
///
/// Instead of running the transitional components through their normal static
/// navigation bar layout logic, this creates transitional widgets that are based
/// on these widgets' existing render objects' layout and position.
///
/// This is possible because this widget is only used during Hero transitions
/// where both the from and to routes are already built and laid out.
///
/// The components' existing layout constraints and positions are then
/// replicated using [Positioned] or [PositionedTransition] wrappers.
///
/// This class should never return [KeyedSubtree]s created by
/// _NavigationBarStaticComponents directly. Since widgets from
/// _NavigationBarStaticComponents are still present in the widget tree during the
/// hero transitions, it would cause global key duplications. Instead, return
/// only the [KeyedSubtree]s' child.
@immutable
class _NavigationBarComponentsTransition {
_NavigationBarComponentsTransition({
required this.animation,
required _TransitionableNavigationBar bottomNavBar,
required _TransitionableNavigationBar topNavBar,
required TextDirection directionality,
}) : bottomComponents = bottomNavBar.componentsKeys,
topComponents = topNavBar.componentsKeys,
bottomNavBarBox = bottomNavBar.renderBox,
topNavBarBox = topNavBar.renderBox,
bottomBackButtonTextStyle = bottomNavBar.backButtonTextStyle,
topBackButtonTextStyle = topNavBar.backButtonTextStyle,
bottomTitleTextStyle = bottomNavBar.titleTextStyle,
topTitleTextStyle = topNavBar.titleTextStyle,
bottomLargeTitleTextStyle = bottomNavBar.largeTitleTextStyle,
topLargeTitleTextStyle = topNavBar.largeTitleTextStyle,
bottomHasUserMiddle = bottomNavBar.hasUserMiddle,
topHasUserMiddle = topNavBar.hasUserMiddle,
bottomLargeExpanded = bottomNavBar.largeExpanded,
topLargeExpanded = topNavBar.largeExpanded,
transitionBox =
// paintBounds are based on offset zero so it's ok to expand the Rects.
bottomNavBar.renderBox.paintBounds.expandToInclude(topNavBar.renderBox.paintBounds),
forwardDirection = directionality == TextDirection.ltr ? 1.0 : -1.0;
static final Animatable<double> fadeOut = Tween<double>(
begin: 1.0,
end: 0.0,
);
static final Animatable<double> fadeIn = Tween<double>(
begin: 0.0,
end: 1.0,
);
final Animation<double> animation;
final _NavigationBarStaticComponentsKeys bottomComponents;
final _NavigationBarStaticComponentsKeys topComponents;
// These render boxes that are the ancestors of all the bottom and top
// components are used to determine the components' relative positions inside
// their respective navigation bars.
final RenderBox bottomNavBarBox;
final RenderBox topNavBarBox;
final TextStyle bottomBackButtonTextStyle;
final TextStyle topBackButtonTextStyle;
final TextStyle bottomTitleTextStyle;
final TextStyle topTitleTextStyle;
final TextStyle? bottomLargeTitleTextStyle;
final TextStyle? topLargeTitleTextStyle;
final bool bottomHasUserMiddle;
final bool topHasUserMiddle;
final bool bottomLargeExpanded;
final bool topLargeExpanded;
// This is the outer box in which all the components will be fitted. The
// sizing component of RelativeRects will be based on this rect's size.
final Rect transitionBox;
// x-axis unity number representing the direction of growth for text.
final double forwardDirection;
// Take a widget it its original ancestor navigation bar render box and
// translate it into a RelativeBox in the transition navigation bar box.
RelativeRect positionInTransitionBox(
GlobalKey key, {
required RenderBox from,
}) {
final RenderBox componentBox = key.currentContext!.findRenderObject()! as RenderBox;
assert(componentBox.attached);
return RelativeRect.fromRect(
componentBox.localToGlobal(Offset.zero, ancestor: from) & componentBox.size,
transitionBox,
);
}
// Create an animated widget that moves the given child widget between its
// original position in its ancestor navigation bar to another widget's
// position in that widget's navigation bar.
//
// Anchor their positions based on the vertical middle of their respective
// render boxes' leading edge.
//
// This method assumes there's no other transforms other than translations
// when converting a rect from the original navigation bar's coordinate space
// to the other navigation bar's coordinate space, to avoid performing
// floating point operations on the size of the child widget, so that the
// incoming constraints used for sizing the child widget will be exactly the
// same.
_FixedSizeSlidingTransition slideFromLeadingEdge({
required GlobalKey fromKey,
required RenderBox fromNavBarBox,
required GlobalKey toKey,
required RenderBox toNavBarBox,
required Widget child,
}) {
final RenderBox fromBox = fromKey.currentContext!.findRenderObject()! as RenderBox;
final RenderBox toBox = toKey.currentContext!.findRenderObject()! as RenderBox;
final bool isLTR = forwardDirection > 0;
// The animation moves the fromBox so its anchor (left-center or right-center
// depending on the writing direction) aligns with toBox's anchor.
final Offset fromAnchorLocal = Offset(
isLTR ? 0 : fromBox.size.width,
fromBox.size.height / 2,
);
final Offset toAnchorLocal = Offset(
isLTR ? 0 : toBox.size.width,
toBox.size.height / 2,
);
final Offset fromAnchorInFromBox = fromBox.localToGlobal(fromAnchorLocal, ancestor: fromNavBarBox);
final Offset toAnchorInToBox = toBox.localToGlobal(toAnchorLocal, ancestor: toNavBarBox);
// We can't get ahold of the render box of the stack (i.e., `transitionBox`)
// we place components on yet, but we know the stack needs to be top-leading
// aligned with both fromNavBarBox and toNavBarBox to make the transition
// look smooth. Also use the top-leading point as the origin for ease of
// calculation.
// The offset to move fromAnchor to toAnchor, in transitionBox's top-leading
// coordinates.
final Offset translation = isLTR
? toAnchorInToBox - fromAnchorInFromBox
: Offset(toNavBarBox.size.width - toAnchorInToBox.dx, toAnchorInToBox.dy)
- Offset(fromNavBarBox.size.width - fromAnchorInFromBox.dx, fromAnchorInFromBox.dy);
final RelativeRect fromBoxMargin = positionInTransitionBox(fromKey, from: fromNavBarBox);
final Offset fromOriginInTransitionBox = Offset(
isLTR ? fromBoxMargin.left : fromBoxMargin.right,
fromBoxMargin.top,
);
final Tween<Offset> anchorMovementInTransitionBox = Tween<Offset>(
begin: fromOriginInTransitionBox,
end: fromOriginInTransitionBox + translation,
);
return _FixedSizeSlidingTransition(
isLTR: isLTR,
offsetAnimation: animation.drive(anchorMovementInTransitionBox),
size: fromBox.size,
child: child,
);
}
Animation<double> fadeInFrom(double t, { Curve curve = Curves.easeIn }) {
return animation.drive(fadeIn.chain(
CurveTween(curve: Interval(t, 1.0, curve: curve)),
));
}
Animation<double> fadeOutBy(double t, { Curve curve = Curves.easeOut }) {
return animation.drive(fadeOut.chain(
CurveTween(curve: Interval(0.0, t, curve: curve)),
));
}
Widget? get bottomLeading {
final KeyedSubtree? bottomLeading = bottomComponents.leadingKey.currentWidget as KeyedSubtree?;
if (bottomLeading == null) {
return null;
}
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(bottomComponents.leadingKey, from: bottomNavBarBox),
child: FadeTransition(
opacity: fadeOutBy(0.4),
child: bottomLeading.child,
),
);
}
Widget? get bottomBackChevron {
final KeyedSubtree? bottomBackChevron = bottomComponents.backChevronKey.currentWidget as KeyedSubtree?;
if (bottomBackChevron == null) {
return null;
}
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(bottomComponents.backChevronKey, from: bottomNavBarBox),
child: FadeTransition(
opacity: fadeOutBy(0.6),
child: DefaultTextStyle(
style: bottomBackButtonTextStyle,
child: bottomBackChevron.child,
),
),
);
}
Widget? get bottomBackLabel {
final KeyedSubtree? bottomBackLabel = bottomComponents.backLabelKey.currentWidget as KeyedSubtree?;
if (bottomBackLabel == null) {
return null;
}
final RelativeRect from = positionInTransitionBox(bottomComponents.backLabelKey, from: bottomNavBarBox);
// Transition away by sliding horizontally to the leading edge off of the screen.
final RelativeRectTween positionTween = RelativeRectTween(
begin: from,
end: from.shift(
Offset(
forwardDirection * (-bottomNavBarBox.size.width / 2.0),
0.0,
),
),
);
return PositionedTransition(
rect: animation.drive(positionTween),
child: FadeTransition(
opacity: fadeOutBy(0.2),
child: DefaultTextStyle(
style: bottomBackButtonTextStyle,
child: bottomBackLabel.child,
),
),
);
}
Widget? get bottomMiddle {
final KeyedSubtree? bottomMiddle = bottomComponents.middleKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? topBackLabel = topComponents.backLabelKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? topLeading = topComponents.leadingKey.currentWidget as KeyedSubtree?;
// The middle component is non-null when the nav bar is a large title
// nav bar but would be invisible when expanded, therefore don't show it here.
if (!bottomHasUserMiddle && bottomLargeExpanded) {
return null;
}
if (bottomMiddle != null && topBackLabel != null) {
// Move from current position to the top page's back label position.
return slideFromLeadingEdge(
fromKey: bottomComponents.middleKey,
fromNavBarBox: bottomNavBarBox,
toKey: topComponents.backLabelKey,
toNavBarBox: topNavBarBox,
child: FadeTransition(
// A custom middle widget like a segmented control fades away faster.
opacity: fadeOutBy(bottomHasUserMiddle ? 0.4 : 0.7),
child: Align(
// As the text shrinks, make sure it's still anchored to the leading
// edge of a constantly sized outer box.
alignment: AlignmentDirectional.centerStart,
child: DefaultTextStyleTransition(
style: animation.drive(TextStyleTween(
begin: bottomTitleTextStyle,
end: topBackButtonTextStyle,
)),
child: bottomMiddle.child,
),
),
),
);
}
// When the top page has a leading widget override (one of the few ways to
// not have a top back label), don't move the bottom middle widget and just
// fade.
if (bottomMiddle != null && topLeading != null) {
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(bottomComponents.middleKey, from: bottomNavBarBox),
child: FadeTransition(
opacity: fadeOutBy(bottomHasUserMiddle ? 0.4 : 0.7),
// Keep the font when transitioning into a non-back label leading.
child: DefaultTextStyle(
style: bottomTitleTextStyle,
child: bottomMiddle.child,
),
),
);
}
return null;
}
Widget? get bottomLargeTitle {
final KeyedSubtree? bottomLargeTitle = bottomComponents.largeTitleKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? topBackLabel = topComponents.backLabelKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? topLeading = topComponents.leadingKey.currentWidget as KeyedSubtree?;
if (bottomLargeTitle == null || !bottomLargeExpanded) {
return null;
}
if (topBackLabel != null) {
// Move from current position to the top page's back label position.
return slideFromLeadingEdge(
fromKey: bottomComponents.largeTitleKey,
fromNavBarBox: bottomNavBarBox,
toKey: topComponents.backLabelKey,
toNavBarBox: topNavBarBox,
child: FadeTransition(
opacity: fadeOutBy(0.6),
child: Align(
// As the text shrinks, make sure it's still anchored to the leading
// edge of a constantly sized outer box.
alignment: AlignmentDirectional.centerStart,
child: DefaultTextStyleTransition(
style: animation.drive(TextStyleTween(
begin: bottomLargeTitleTextStyle,
end: topBackButtonTextStyle,
)),
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: bottomLargeTitle.child,
),
),
),
);
}
if (topLeading != null) {
// Unlike bottom middle, the bottom large title moves when it can't
// transition to the top back label position.
final RelativeRect from = positionInTransitionBox(bottomComponents.largeTitleKey, from: bottomNavBarBox);
final RelativeRectTween positionTween = RelativeRectTween(
begin: from,
end: from.shift(
Offset(
forwardDirection * bottomNavBarBox.size.width / 4.0,
0.0,
),
),
);
// Just shift slightly towards the trailing edge instead of moving to the
// back label position.
return PositionedTransition(
rect: animation.drive(positionTween),
child: FadeTransition(
opacity: fadeOutBy(0.4),
// Keep the font when transitioning into a non-back-label leading.
child: DefaultTextStyle(
style: bottomLargeTitleTextStyle!,
child: bottomLargeTitle.child,
),
),
);
}
return null;
}
Widget? get bottomTrailing {
final KeyedSubtree? bottomTrailing = bottomComponents.trailingKey.currentWidget as KeyedSubtree?;
if (bottomTrailing == null) {
return null;
}
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(bottomComponents.trailingKey, from: bottomNavBarBox),
child: FadeTransition(
opacity: fadeOutBy(0.6),
child: bottomTrailing.child,
),
);
}
Widget? get topLeading {
final KeyedSubtree? topLeading = topComponents.leadingKey.currentWidget as KeyedSubtree?;
if (topLeading == null) {
return null;
}
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(topComponents.leadingKey, from: topNavBarBox),
child: FadeTransition(
opacity: fadeInFrom(0.6),
child: topLeading.child,
),
);
}
Widget? get topBackChevron {
final KeyedSubtree? topBackChevron = topComponents.backChevronKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? bottomBackChevron = bottomComponents.backChevronKey.currentWidget as KeyedSubtree?;
if (topBackChevron == null) {
return null;
}
final RelativeRect to = positionInTransitionBox(topComponents.backChevronKey, from: topNavBarBox);
RelativeRect from = to;
// If it's the first page with a back chevron, shift in slightly from the
// right.
if (bottomBackChevron == null) {
final RenderBox topBackChevronBox = topComponents.backChevronKey.currentContext!.findRenderObject()! as RenderBox;
from = to.shift(
Offset(
forwardDirection * topBackChevronBox.size.width * 2.0,
0.0,
),
);
}
final RelativeRectTween positionTween = RelativeRectTween(
begin: from,
end: to,
);
return PositionedTransition(
rect: animation.drive(positionTween),
child: FadeTransition(
opacity: fadeInFrom(bottomBackChevron == null ? 0.7 : 0.4),
child: DefaultTextStyle(
style: topBackButtonTextStyle,
child: topBackChevron.child,
),
),
);
}
Widget? get topBackLabel {
final KeyedSubtree? bottomMiddle = bottomComponents.middleKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? bottomLargeTitle = bottomComponents.largeTitleKey.currentWidget as KeyedSubtree?;
final KeyedSubtree? topBackLabel = topComponents.backLabelKey.currentWidget as KeyedSubtree?;
if (topBackLabel == null) {
return null;
}
final RenderAnimatedOpacity? topBackLabelOpacity =
topComponents.backLabelKey.currentContext?.findAncestorRenderObjectOfType<RenderAnimatedOpacity>();
Animation<double>? midClickOpacity;
if (topBackLabelOpacity != null && topBackLabelOpacity.opacity.value < 1.0) {
midClickOpacity = animation.drive(Tween<double>(
begin: 0.0,
end: topBackLabelOpacity.opacity.value,
));
}
// Pick up from an incoming transition from the large title. This is
// duplicated here from the bottomLargeTitle transition widget because the
// content text might be different. For instance, if the bottomLargeTitle
// text is too long, the topBackLabel will say 'Back' instead of the original
// text.
if (bottomLargeTitle != null &&
bottomLargeExpanded) {
return slideFromLeadingEdge(
fromKey: bottomComponents.largeTitleKey,
fromNavBarBox: bottomNavBarBox,
toKey: topComponents.backLabelKey,
toNavBarBox: topNavBarBox,
child: FadeTransition(
opacity: midClickOpacity ?? fadeInFrom(0.4),
child: DefaultTextStyleTransition(
style: animation.drive(TextStyleTween(
begin: bottomLargeTitleTextStyle,
end: topBackButtonTextStyle,
)),
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: topBackLabel.child,
),
),
);
}
// The topBackLabel always comes from the large title first if available
// and expanded instead of middle.
if (bottomMiddle != null) {
return slideFromLeadingEdge(
fromKey: bottomComponents.middleKey,
fromNavBarBox: bottomNavBarBox,
toKey: topComponents.backLabelKey,
toNavBarBox: topNavBarBox,
child: FadeTransition(
opacity: midClickOpacity ?? fadeInFrom(0.3),
child: DefaultTextStyleTransition(
style: animation.drive(TextStyleTween(
begin: bottomTitleTextStyle,
end: topBackButtonTextStyle,
)),
child: topBackLabel.child,
),
),
);
}
return null;
}
Widget? get topMiddle {
final KeyedSubtree? topMiddle = topComponents.middleKey.currentWidget as KeyedSubtree?;
if (topMiddle == null) {
return null;
}
// The middle component is non-null when the nav bar is a large title
// nav bar but would be invisible when expanded, therefore don't show it here.
if (!topHasUserMiddle && topLargeExpanded) {
return null;
}
final RelativeRect to = positionInTransitionBox(topComponents.middleKey, from: topNavBarBox);
final RenderBox toBox = topComponents.middleKey.currentContext!.findRenderObject()! as RenderBox;
final bool isLTR = forwardDirection > 0;
// Anchor is the top-leading point of toBox, in transition box's top-leading
// coordinate space.
final Offset toAnchorInTransitionBox = Offset(
isLTR ? to.left : to.right,
to.top,
);
// Shift in from the trailing edge of the screen.
final Tween<Offset> anchorMovementInTransitionBox = Tween<Offset>(
begin: Offset(
// the "width / 2" here makes the middle widget's horizontal center on
// the trailing edge of the top nav bar.
topNavBarBox.size.width - toBox.size.width / 2,
to.top,
),
end: toAnchorInTransitionBox,
);
return _FixedSizeSlidingTransition(
isLTR: isLTR,
offsetAnimation: animation.drive(anchorMovementInTransitionBox),
size: toBox.size,
child: FadeTransition(
opacity: fadeInFrom(0.25),
child: DefaultTextStyle(
style: topTitleTextStyle,
child: topMiddle.child,
),
),
);
}
Widget? get topTrailing {
final KeyedSubtree? topTrailing = topComponents.trailingKey.currentWidget as KeyedSubtree?;
if (topTrailing == null) {
return null;
}
return Positioned.fromRelativeRect(
rect: positionInTransitionBox(topComponents.trailingKey, from: topNavBarBox),
child: FadeTransition(
opacity: fadeInFrom(0.4),
child: topTrailing.child,
),
);
}
Widget? get topLargeTitle {
final KeyedSubtree? topLargeTitle = topComponents.largeTitleKey.currentWidget as KeyedSubtree?;
if (topLargeTitle == null || !topLargeExpanded) {
return null;
}
final RelativeRect to = positionInTransitionBox(topComponents.largeTitleKey, from: topNavBarBox);
// Shift in from the trailing edge of the screen.
final RelativeRectTween positionTween = RelativeRectTween(
begin: to.shift(
Offset(
forwardDirection * topNavBarBox.size.width,
0.0,
),
),
end: to,
);
return PositionedTransition(
rect: animation.drive(positionTween),
child: FadeTransition(
opacity: fadeInFrom(0.3),
child: DefaultTextStyle(
style: topLargeTitleTextStyle!,
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: topLargeTitle.child,
),
),
);
}
}
/// Navigation bars' hero rect tween that will move between the static bars
/// but keep a constant size that's the bigger of both navigation bars.
RectTween _linearTranslateWithLargestRectSizeTween(Rect? begin, Rect? end) {
final Size largestSize = Size(
math.max(begin!.size.width, end!.size.width),
math.max(begin.size.height, end.size.height),
);
return RectTween(
begin: begin.topLeft & largestSize,
end: end.topLeft & largestSize,
);
}
Widget _navBarHeroLaunchPadBuilder(
BuildContext context,
Size heroSize,
Widget child,
) {
assert(child is _TransitionableNavigationBar);
// Tree reshaping is fine here because the Heroes' child is always a
// _TransitionableNavigationBar which has a GlobalKey.
// Keeping the Hero subtree here is needed (instead of just swapping out the
// anchor nav bars for fixed size boxes during flights) because the nav bar
// and their specific component children may serve as anchor points again if
// another mid-transition flight diversion is triggered.
// This is ok performance-wise because static nav bars are generally cheap to
// build and layout but expensive to GPU render (due to clips and blurs) which
// we're skipping here.
return Visibility(
maintainSize: true,
maintainAnimation: true,
maintainState: true,
visible: false,
child: child,
);
}
/// Navigation bars' hero flight shuttle builder.
Widget _navBarHeroFlightShuttleBuilder(
BuildContext flightContext,
Animation<double> animation,
HeroFlightDirection flightDirection,
BuildContext fromHeroContext,
BuildContext toHeroContext,
) {
assert(fromHeroContext.widget is Hero);
assert(toHeroContext.widget is Hero);
final Hero fromHeroWidget = fromHeroContext.widget as Hero;
final Hero toHeroWidget = toHeroContext.widget as Hero;
assert(fromHeroWidget.child is _TransitionableNavigationBar);
assert(toHeroWidget.child is _TransitionableNavigationBar);
final _TransitionableNavigationBar fromNavBar = fromHeroWidget.child as _TransitionableNavigationBar;
final _TransitionableNavigationBar toNavBar = toHeroWidget.child as _TransitionableNavigationBar;
assert(
fromNavBar.componentsKeys.navBarBoxKey.currentContext!.owner != null,
'The from nav bar to Hero must have been mounted in the previous frame',
);
assert(
toNavBar.componentsKeys.navBarBoxKey.currentContext!.owner != null,
'The to nav bar to Hero must have been mounted in the previous frame',
);
switch (flightDirection) {
case HeroFlightDirection.push:
return _NavigationBarTransition(
animation: animation,
bottomNavBar: fromNavBar,
topNavBar: toNavBar,
);
case HeroFlightDirection.pop:
return _NavigationBarTransition(
animation: animation,
bottomNavBar: toNavBar,
topNavBar: fromNavBar,
);
}
}
| flutter/packages/flutter/lib/src/cupertino/nav_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/nav_bar.dart",
"repo_id": "flutter",
"token_count": 30297
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'adaptive_text_selection_toolbar.dart';
import 'colors.dart';
import 'form_row.dart';
import 'text_field.dart';
/// Creates a [CupertinoFormRow] containing a [FormField] that wraps
/// a [CupertinoTextField].
///
/// A [Form] ancestor is not required. The [Form] allows one to
/// save, reset, or validate multiple fields at once. To use without a [Form],
/// pass a [GlobalKey] to the constructor and use [GlobalKey.currentState] to
/// save or reset the form field.
///
/// When a [controller] is specified, its [TextEditingController.text]
/// defines the [initialValue]. If this [FormField] is part of a scrolling
/// container that lazily constructs its children, like a [ListView] or a
/// [CustomScrollView], then a [controller] should be specified.
/// The controller's lifetime should be managed by a stateful widget ancestor
/// of the scrolling container.
///
/// The [prefix] parameter is displayed at the start of the row. Standard iOS
/// guidelines encourage passing a [Text] widget to [prefix] to detail the
/// nature of the input.
///
/// The [padding] parameter is used to pad the contents of the row. It is
/// directly passed to [CupertinoFormRow]. If the [padding]
/// parameter is null, [CupertinoFormRow] constructs its own default
/// padding (which is the standard form row padding in iOS.) If no edge
/// insets are intended, explicitly pass [EdgeInsets.zero] to [padding].
///
/// If a [controller] is not specified, [initialValue] can be used to give
/// the automatically generated controller an initial value.
///
/// Consider calling [TextEditingController.dispose] of the [controller], if one
/// is specified, when it is no longer needed. This will ensure we discard any
/// resources used by the object.
///
/// For documentation about the various parameters, see the
/// [CupertinoTextField] class and [CupertinoTextField.borderless],
/// the constructor.
///
/// {@tool snippet}
///
/// Creates a [CupertinoTextFormFieldRow] with a leading text and validator
/// function.
///
/// If the user enters valid text, the CupertinoTextField appears normally
/// without any warnings to the user.
///
/// If the user enters invalid text, the error message returned from the
/// validator function is displayed in dark red underneath the input.
///
/// ```dart
/// CupertinoTextFormFieldRow(
/// prefix: const Text('Username'),
/// onSaved: (String? value) {
/// // This optional block of code can be used to run
/// // code when the user saves the form.
/// },
/// validator: (String? value) {
/// return (value != null && value.contains('@')) ? 'Do not use the @ char.' : null;
/// },
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to move the focus to the next field when the user
/// presses the SPACE key.
///
/// ** See code in examples/api/lib/cupertino/text_form_field_row/cupertino_text_form_field_row.1.dart **
/// {@end-tool}
class CupertinoTextFormFieldRow extends FormField<String> {
/// Creates a [CupertinoFormRow] containing a [FormField] that wraps
/// a [CupertinoTextField].
///
/// When a [controller] is specified, [initialValue] must be null (the
/// default). If [controller] is null, then a [TextEditingController]
/// will be constructed automatically and its `text` will be initialized
/// to [initialValue] or the empty string.
///
/// The [prefix] parameter is displayed at the start of the row. Standard iOS
/// guidelines encourage passing a [Text] widget to [prefix] to detail the
/// nature of the input.
///
/// The [padding] parameter is used to pad the contents of the row. It is
/// directly passed to [CupertinoFormRow]. If the [padding]
/// parameter is null, [CupertinoFormRow] constructs its own default
/// padding (which is the standard form row padding in iOS.) If no edge
/// insets are intended, explicitly pass [EdgeInsets.zero] to [padding].
///
/// For documentation about the various parameters, see the
/// [CupertinoTextField] class and [CupertinoTextField.borderless],
/// the constructor.
CupertinoTextFormFieldRow({
super.key,
this.prefix,
this.padding,
this.controller,
String? initialValue,
FocusNode? focusNode,
BoxDecoration? decoration,
TextInputType? keyboardType,
TextCapitalization textCapitalization = TextCapitalization.none,
TextInputAction? textInputAction,
TextStyle? style,
StrutStyle? strutStyle,
TextDirection? textDirection,
TextAlign textAlign = TextAlign.start,
TextAlignVertical? textAlignVertical,
bool autofocus = false,
bool readOnly = false,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
ToolbarOptions? toolbarOptions,
bool? showCursor,
String obscuringCharacter = '•',
bool obscureText = false,
bool autocorrect = true,
SmartDashesType? smartDashesType,
SmartQuotesType? smartQuotesType,
bool enableSuggestions = true,
int? maxLines = 1,
int? minLines,
bool expands = false,
int? maxLength,
this.onChanged,
GestureTapCallback? onTap,
VoidCallback? onEditingComplete,
ValueChanged<String>? onFieldSubmitted,
super.onSaved,
super.validator,
List<TextInputFormatter>? inputFormatters,
bool? enabled,
double cursorWidth = 2.0,
double? cursorHeight,
Color? cursorColor,
Brightness? keyboardAppearance,
EdgeInsets scrollPadding = const EdgeInsets.all(20.0),
bool enableInteractiveSelection = true,
TextSelectionControls? selectionControls,
ScrollPhysics? scrollPhysics,
Iterable<String>? autofillHints,
AutovalidateMode super.autovalidateMode = AutovalidateMode.disabled,
String? placeholder,
TextStyle? placeholderStyle = const TextStyle(
fontWeight: FontWeight.w400,
color: CupertinoColors.placeholderText,
),
EditableTextContextMenuBuilder? contextMenuBuilder = _defaultContextMenuBuilder,
super.restorationId,
}) : assert(initialValue == null || controller == null),
assert(obscuringCharacter.length == 1),
assert(maxLines == null || maxLines > 0),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
!expands || (maxLines == null && minLines == null),
'minLines and maxLines must be null when expands is true.',
),
assert(!obscureText || maxLines == 1, 'Obscured fields cannot be multiline.'),
assert(maxLength == null || maxLength > 0),
super(
initialValue: controller?.text ?? initialValue ?? '',
builder: (FormFieldState<String> field) {
final _CupertinoTextFormFieldRowState state =
field as _CupertinoTextFormFieldRowState;
void onChangedHandler(String value) {
field.didChange(value);
onChanged?.call(value);
}
return CupertinoFormRow(
prefix: prefix,
padding: padding,
error: (field.errorText == null) ? null : Text(field.errorText!),
child: UnmanagedRestorationScope(
bucket: field.bucket,
child: CupertinoTextField.borderless(
restorationId: restorationId,
controller: state._effectiveController,
focusNode: focusNode,
keyboardType: keyboardType,
decoration: decoration,
textInputAction: textInputAction,
style: style,
strutStyle: strutStyle,
textAlign: textAlign,
textAlignVertical: textAlignVertical,
textCapitalization: textCapitalization,
textDirection: textDirection,
autofocus: autofocus,
toolbarOptions: toolbarOptions,
readOnly: readOnly,
showCursor: showCursor,
obscuringCharacter: obscuringCharacter,
obscureText: obscureText,
autocorrect: autocorrect,
smartDashesType: smartDashesType,
smartQuotesType: smartQuotesType,
enableSuggestions: enableSuggestions,
maxLines: maxLines,
minLines: minLines,
expands: expands,
maxLength: maxLength,
onChanged: onChangedHandler,
onTap: onTap,
onEditingComplete: onEditingComplete,
onSubmitted: onFieldSubmitted,
inputFormatters: inputFormatters,
enabled: enabled ?? true,
cursorWidth: cursorWidth,
cursorHeight: cursorHeight,
cursorColor: cursorColor,
scrollPadding: scrollPadding,
scrollPhysics: scrollPhysics,
keyboardAppearance: keyboardAppearance,
enableInteractiveSelection: enableInteractiveSelection,
selectionControls: selectionControls,
autofillHints: autofillHints,
placeholder: placeholder,
placeholderStyle: placeholderStyle,
contextMenuBuilder: contextMenuBuilder,
),
),
);
},
);
/// A widget that is displayed at the start of the row.
///
/// The [prefix] widget is displayed at the start of the row. Standard iOS
/// guidelines encourage passing a [Text] widget to [prefix] to detail the
/// nature of the input.
final Widget? prefix;
/// Content padding for the row.
///
/// The [padding] widget is passed to [CupertinoFormRow]. If the [padding]
/// parameter is null, [CupertinoFormRow] constructs its own default
/// padding, which is the standard form row padding in iOS.
///
/// If no edge insets are intended, explicitly pass [EdgeInsets.zero] to
/// [padding].
final EdgeInsetsGeometry? padding;
/// Controls the text being edited.
///
/// If null, this widget will create its own [TextEditingController] and
/// initialize its [TextEditingController.text] with [initialValue].
final TextEditingController? controller;
/// {@macro flutter.material.TextFormField.onChanged}
final ValueChanged<String>? onChanged;
static Widget _defaultContextMenuBuilder(BuildContext context, EditableTextState editableTextState) {
return CupertinoAdaptiveTextSelectionToolbar.editableText(
editableTextState: editableTextState,
);
}
@override
FormFieldState<String> createState() => _CupertinoTextFormFieldRowState();
}
class _CupertinoTextFormFieldRowState extends FormFieldState<String> {
RestorableTextEditingController? _controller;
TextEditingController get _effectiveController =>
_cupertinoTextFormFieldRow.controller ?? _controller!.value;
CupertinoTextFormFieldRow get _cupertinoTextFormFieldRow =>
super.widget as CupertinoTextFormFieldRow;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
super.restoreState(oldBucket, initialRestore);
if (_controller != null) {
_registerController();
}
// This makes sure to update the internal [FormFieldState] value to sync up with
// text editing controller value.
setValue(_effectiveController.text);
}
void _registerController() {
assert(_controller != null);
registerForRestoration(_controller!, 'controller');
}
void _createLocalController([TextEditingValue? value]) {
assert(_controller == null);
_controller = value == null
? RestorableTextEditingController()
: RestorableTextEditingController.fromValue(value);
if (!restorePending) {
_registerController();
}
}
@override
void initState() {
super.initState();
if (_cupertinoTextFormFieldRow.controller == null) {
_createLocalController(widget.initialValue != null ? TextEditingValue(text: widget.initialValue!) : null);
} else {
_cupertinoTextFormFieldRow.controller!.addListener(_handleControllerChanged);
}
}
@override
void didUpdateWidget(CupertinoTextFormFieldRow oldWidget) {
super.didUpdateWidget(oldWidget);
if (_cupertinoTextFormFieldRow.controller != oldWidget.controller) {
oldWidget.controller?.removeListener(_handleControllerChanged);
_cupertinoTextFormFieldRow.controller?.addListener(_handleControllerChanged);
if (oldWidget.controller != null && _cupertinoTextFormFieldRow.controller == null) {
_createLocalController(oldWidget.controller!.value);
}
if (_cupertinoTextFormFieldRow.controller != null) {
setValue(_cupertinoTextFormFieldRow.controller!.text);
if (oldWidget.controller == null) {
unregisterFromRestoration(_controller!);
_controller!.dispose();
_controller = null;
}
}
}
}
@override
void dispose() {
_cupertinoTextFormFieldRow.controller?.removeListener(_handleControllerChanged);
_controller?.dispose();
super.dispose();
}
@override
void didChange(String? value) {
super.didChange(value);
if (value != null && _effectiveController.text != value) {
_effectiveController.text = value;
}
}
@override
void reset() {
// Set the controller value before calling super.reset() to let
// _handleControllerChanged suppress the change.
_effectiveController.text = widget.initialValue!;
super.reset();
_cupertinoTextFormFieldRow.onChanged?.call(_effectiveController.text);
}
void _handleControllerChanged() {
// Suppress changes that originated from within this class.
//
// In the case where a controller has been passed in to this widget, we
// register this change listener. In these cases, we'll also receive change
// notifications for changes originating from within this class -- for
// example, the reset() method. In such cases, the FormField value will
// already have been set.
if (_effectiveController.text != value) {
didChange(_effectiveController.text);
}
}
}
| flutter/packages/flutter/lib/src/cupertino/text_form_field_row.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/text_form_field_row.dart",
"repo_id": "flutter",
"token_count": 5211
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'assertions.dart';
import 'constants.dart';
import 'platform.dart' as platform;
export 'platform.dart' show TargetPlatform;
/// The dart:io implementation of [platform.defaultTargetPlatform].
@pragma('vm:platform-const-if', !kDebugMode)
platform.TargetPlatform get defaultTargetPlatform {
platform.TargetPlatform? result;
if (Platform.isAndroid) {
result = platform.TargetPlatform.android;
} else if (Platform.isIOS) {
result = platform.TargetPlatform.iOS;
} else if (Platform.isFuchsia) {
result = platform.TargetPlatform.fuchsia;
} else if (Platform.isLinux) {
result = platform.TargetPlatform.linux;
} else if (Platform.isMacOS) {
result = platform.TargetPlatform.macOS;
} else if (Platform.isWindows) {
result = platform.TargetPlatform.windows;
}
assert(() {
if (Platform.environment.containsKey('FLUTTER_TEST')) {
result = platform.TargetPlatform.android;
}
return true;
}());
if (kDebugMode && platform.debugDefaultTargetPlatformOverride != null) {
result = platform.debugDefaultTargetPlatformOverride;
}
if (result == null) {
throw FlutterError(
'Unknown platform.\n'
'${Platform.operatingSystem} was not recognized as a target platform. '
'Consider updating the list of TargetPlatforms to include this platform.',
);
}
return result!;
}
| flutter/packages/flutter/lib/src/foundation/_platform_io.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/_platform_io.dart",
"repo_id": "flutter",
"token_count": 491
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import '_isolates_io.dart'
if (dart.library.js_util) '_isolates_web.dart' as isolates;
/// Signature for the callback passed to [compute].
///
/// {@macro flutter.foundation.compute.callback}
///
typedef ComputeCallback<M, R> = FutureOr<R> Function(M message);
/// The signature of [compute], which spawns an isolate, runs `callback` on
/// that isolate, passes it `message`, and (eventually) returns the value
/// returned by `callback`.
typedef ComputeImpl = Future<R> Function<M, R>(ComputeCallback<M, R> callback, M message, { String? debugLabel });
/// Asynchronously runs the given [callback] - with the provided [message] -
/// in the background and completes with the result.
///
/// {@template flutter.foundation.compute.usecase}
/// This is useful for operations that take longer than a few milliseconds, and
/// which would therefore risk skipping frames. For tasks that will only take a
/// few milliseconds, consider [SchedulerBinding.scheduleTask] instead.
/// {@endtemplate}
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=5AxWC49ZMzs}
///
/// {@tool snippet}
/// The following code uses the [compute] function to check whether a given
/// integer is a prime number.
///
/// ```dart
/// Future<bool> isPrime(int value) {
/// return compute(_calculate, value);
/// }
///
/// bool _calculate(int value) {
/// if (value == 1) {
/// return false;
/// }
/// for (int i = 2; i < value; ++i) {
/// if (value % i == 0) {
/// return false;
/// }
/// }
/// return true;
/// }
/// ```
/// {@end-tool}
///
/// On web platforms this will run [callback] on the current eventloop.
/// On native platforms this will run [callback] in a separate isolate.
///
/// {@template flutter.foundation.compute.callback}
///
/// The `callback`, the `message` given to it as well as the result have to be
/// objects that can be sent across isolates (as they may be transitively copied
/// if needed). The majority of objects can be sent across isolates.
///
/// See [SendPort.send] for more information about exceptions as well as a note
/// of warning about sending closures, which can capture more state than needed.
///
/// {@endtemplate}
///
/// On native platforms `await compute(fun, message)` is equivalent to
/// `await Isolate.run(() => fun(message))`. See also [Isolate.run].
///
/// The `debugLabel` - if provided - is used as name for the isolate that
/// executes `callback`. [Timeline] events produced by that isolate will have
/// the name associated with them. This is useful when profiling an application.
Future<R> compute<M, R>(ComputeCallback<M, R> callback, M message, {String? debugLabel}) {
return isolates.compute<M, R>(callback, message, debugLabel: debugLabel);
}
| flutter/packages/flutter/lib/src/foundation/isolates.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/isolates.dart",
"repo_id": "flutter",
"token_count": 866
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'debug.dart';
/// Whether the gesture was accepted or rejected.
enum GestureDisposition {
/// This gesture was accepted as the interpretation of the user's input.
accepted,
/// This gesture was rejected as the interpretation of the user's input.
rejected,
}
/// Represents an object participating in an arena.
///
/// Receives callbacks from the GestureArena to notify the object when it wins
/// or loses a gesture negotiation. Exactly one of [acceptGesture] or
/// [rejectGesture] will be called for each arena this member was added to,
/// regardless of what caused the arena to be resolved. For example, if a
/// member resolves the arena itself, that member still receives an
/// [acceptGesture] callback.
abstract class GestureArenaMember {
/// Called when this member wins the arena for the given pointer id.
void acceptGesture(int pointer);
/// Called when this member loses the arena for the given pointer id.
void rejectGesture(int pointer);
}
/// An interface to pass information to an arena.
///
/// A given [GestureArenaMember] can have multiple entries in multiple arenas
/// with different pointer ids.
class GestureArenaEntry {
GestureArenaEntry._(this._arena, this._pointer, this._member);
final GestureArenaManager _arena;
final int _pointer;
final GestureArenaMember _member;
/// Call this member to claim victory (with accepted) or admit defeat (with rejected).
///
/// It's fine to attempt to resolve a gesture recognizer for an arena that is
/// already resolved.
void resolve(GestureDisposition disposition) {
_arena._resolve(_pointer, _member, disposition);
}
}
class _GestureArena {
final List<GestureArenaMember> members = <GestureArenaMember>[];
bool isOpen = true;
bool isHeld = false;
bool hasPendingSweep = false;
/// If a member attempts to win while the arena is still open, it becomes the
/// "eager winner". We look for an eager winner when closing the arena to new
/// participants, and if there is one, we resolve the arena in its favor at
/// that time.
GestureArenaMember? eagerWinner;
void add(GestureArenaMember member) {
assert(isOpen);
members.add(member);
}
@override
String toString() {
final StringBuffer buffer = StringBuffer();
if (members.isEmpty) {
buffer.write('<empty>');
} else {
buffer.write(members.map<String>((GestureArenaMember member) {
if (member == eagerWinner) {
return '$member (eager winner)';
}
return '$member';
}).join(', '));
}
if (isOpen) {
buffer.write(' [open]');
}
if (isHeld) {
buffer.write(' [held]');
}
if (hasPendingSweep) {
buffer.write(' [hasPendingSweep]');
}
return buffer.toString();
}
}
/// Used for disambiguating the meaning of sequences of pointer events.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=Q85LBtBdi0U}
///
/// The first member to accept or the last member to not reject wins.
///
/// See <https://flutter.dev/gestures/#gesture-disambiguation> for more
/// information about the role this class plays in the gesture system.
///
/// To debug problems with gestures, consider using
/// [debugPrintGestureArenaDiagnostics].
class GestureArenaManager {
final Map<int, _GestureArena> _arenas = <int, _GestureArena>{};
/// Adds a new member (e.g., gesture recognizer) to the arena.
GestureArenaEntry add(int pointer, GestureArenaMember member) {
final _GestureArena state = _arenas.putIfAbsent(pointer, () {
assert(_debugLogDiagnostic(pointer, '★ Opening new gesture arena.'));
return _GestureArena();
});
state.add(member);
assert(_debugLogDiagnostic(pointer, 'Adding: $member'));
return GestureArenaEntry._(this, pointer, member);
}
/// Prevents new members from entering the arena.
///
/// Called after the framework has finished dispatching the pointer down event.
void close(int pointer) {
final _GestureArena? state = _arenas[pointer];
if (state == null) {
return; // This arena either never existed or has been resolved.
}
state.isOpen = false;
assert(_debugLogDiagnostic(pointer, 'Closing', state));
_tryToResolveArena(pointer, state);
}
/// Forces resolution of the arena, giving the win to the first member.
///
/// Sweep is typically after all the other processing for a [PointerUpEvent]
/// have taken place. It ensures that multiple passive gestures do not cause a
/// stalemate that prevents the user from interacting with the app.
///
/// Recognizers that wish to delay resolving an arena past [PointerUpEvent]
/// should call [hold] to delay sweep until [release] is called.
///
/// See also:
///
/// * [hold]
/// * [release]
void sweep(int pointer) {
final _GestureArena? state = _arenas[pointer];
if (state == null) {
return; // This arena either never existed or has been resolved.
}
assert(!state.isOpen);
if (state.isHeld) {
state.hasPendingSweep = true;
assert(_debugLogDiagnostic(pointer, 'Delaying sweep', state));
return; // This arena is being held for a long-lived member.
}
assert(_debugLogDiagnostic(pointer, 'Sweeping', state));
_arenas.remove(pointer);
if (state.members.isNotEmpty) {
// First member wins.
assert(_debugLogDiagnostic(pointer, 'Winner: ${state.members.first}'));
state.members.first.acceptGesture(pointer);
// Give all the other members the bad news.
for (int i = 1; i < state.members.length; i++) {
state.members[i].rejectGesture(pointer);
}
}
}
/// Prevents the arena from being swept.
///
/// Typically, a winner is chosen in an arena after all the other
/// [PointerUpEvent] processing by [sweep]. If a recognizer wishes to delay
/// resolving an arena past [PointerUpEvent], the recognizer can [hold] the
/// arena open using this function. To release such a hold and let the arena
/// resolve, call [release].
///
/// See also:
///
/// * [sweep]
/// * [release]
void hold(int pointer) {
final _GestureArena? state = _arenas[pointer];
if (state == null) {
return; // This arena either never existed or has been resolved.
}
state.isHeld = true;
assert(_debugLogDiagnostic(pointer, 'Holding', state));
}
/// Releases a hold, allowing the arena to be swept.
///
/// If a sweep was attempted on a held arena, the sweep will be done
/// on release.
///
/// See also:
///
/// * [sweep]
/// * [hold]
void release(int pointer) {
final _GestureArena? state = _arenas[pointer];
if (state == null) {
return; // This arena either never existed or has been resolved.
}
state.isHeld = false;
assert(_debugLogDiagnostic(pointer, 'Releasing', state));
if (state.hasPendingSweep) {
sweep(pointer);
}
}
/// Reject or accept a gesture recognizer.
///
/// This is called by calling [GestureArenaEntry.resolve] on the object returned from [add].
void _resolve(int pointer, GestureArenaMember member, GestureDisposition disposition) {
final _GestureArena? state = _arenas[pointer];
if (state == null) {
return; // This arena has already resolved.
}
assert(state.members.contains(member));
switch (disposition) {
case GestureDisposition.accepted:
assert(_debugLogDiagnostic(pointer, 'Accepting: $member'));
if (state.isOpen) {
state.eagerWinner ??= member;
} else {
assert(_debugLogDiagnostic(pointer, 'Self-declared winner: $member'));
_resolveInFavorOf(pointer, state, member);
}
case GestureDisposition.rejected:
assert(_debugLogDiagnostic(pointer, 'Rejecting: $member'));
state.members.remove(member);
member.rejectGesture(pointer);
if (!state.isOpen) {
_tryToResolveArena(pointer, state);
}
}
}
void _tryToResolveArena(int pointer, _GestureArena state) {
assert(_arenas[pointer] == state);
assert(!state.isOpen);
if (state.members.length == 1) {
scheduleMicrotask(() => _resolveByDefault(pointer, state));
} else if (state.members.isEmpty) {
_arenas.remove(pointer);
assert(_debugLogDiagnostic(pointer, 'Arena empty.'));
} else if (state.eagerWinner != null) {
assert(_debugLogDiagnostic(pointer, 'Eager winner: ${state.eagerWinner}'));
_resolveInFavorOf(pointer, state, state.eagerWinner!);
}
}
void _resolveByDefault(int pointer, _GestureArena state) {
if (!_arenas.containsKey(pointer)) {
return; // This arena has already resolved.
}
assert(_arenas[pointer] == state);
assert(!state.isOpen);
final List<GestureArenaMember> members = state.members;
assert(members.length == 1);
_arenas.remove(pointer);
assert(_debugLogDiagnostic(pointer, 'Default winner: ${state.members.first}'));
state.members.first.acceptGesture(pointer);
}
void _resolveInFavorOf(int pointer, _GestureArena state, GestureArenaMember member) {
assert(state == _arenas[pointer]);
assert(state.eagerWinner == null || state.eagerWinner == member);
assert(!state.isOpen);
_arenas.remove(pointer);
for (final GestureArenaMember rejectedMember in state.members) {
if (rejectedMember != member) {
rejectedMember.rejectGesture(pointer);
}
}
member.acceptGesture(pointer);
}
bool _debugLogDiagnostic(int pointer, String message, [ _GestureArena? state ]) {
assert(() {
if (debugPrintGestureArenaDiagnostics) {
final int? count = state?.members.length;
final String s = count != 1 ? 's' : '';
debugPrint('Gesture arena ${pointer.toString().padRight(4)} ❙ $message${ count != null ? " with $count member$s." : ""}');
}
return true;
}());
return true;
}
}
| flutter/packages/flutter/lib/src/gestures/arena.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/arena.dart",
"repo_id": "flutter",
"token_count": 3482
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'arena.dart';
import 'binding.dart';
import 'constants.dart';
import 'events.dart';
import 'pointer_router.dart';
import 'recognizer.dart';
import 'tap.dart';
export 'dart:ui' show Offset, PointerDeviceKind;
export 'events.dart' show PointerDownEvent;
export 'tap.dart' show GestureTapCancelCallback, GestureTapDownCallback, TapDownDetails, TapUpDetails;
/// Signature for callback when the user has tapped the screen at the same
/// location twice in quick succession.
///
/// See also:
///
/// * [GestureDetector.onDoubleTap], which matches this signature.
typedef GestureDoubleTapCallback = void Function();
/// Signature used by [MultiTapGestureRecognizer] for when a pointer that might
/// cause a tap has contacted the screen at a particular location.
typedef GestureMultiTapDownCallback = void Function(int pointer, TapDownDetails details);
/// Signature used by [MultiTapGestureRecognizer] for when a pointer that will
/// trigger a tap has stopped contacting the screen at a particular location.
typedef GestureMultiTapUpCallback = void Function(int pointer, TapUpDetails details);
/// Signature used by [MultiTapGestureRecognizer] for when a tap has occurred.
typedef GestureMultiTapCallback = void Function(int pointer);
/// Signature for when the pointer that previously triggered a
/// [GestureMultiTapDownCallback] will not end up causing a tap.
typedef GestureMultiTapCancelCallback = void Function(int pointer);
/// CountdownZoned tracks whether the specified duration has elapsed since
/// creation, honoring [Zone].
class _CountdownZoned {
_CountdownZoned({ required Duration duration }) {
Timer(duration, _onTimeout);
}
bool _timeout = false;
bool get timeout => _timeout;
void _onTimeout() {
_timeout = true;
}
}
/// TapTracker helps track individual tap sequences as part of a
/// larger gesture.
class _TapTracker {
_TapTracker({
required PointerDownEvent event,
required this.entry,
required Duration doubleTapMinTime,
required this.gestureSettings,
}) : pointer = event.pointer,
_initialGlobalPosition = event.position,
initialButtons = event.buttons,
_doubleTapMinTimeCountdown = _CountdownZoned(duration: doubleTapMinTime);
final DeviceGestureSettings? gestureSettings;
final int pointer;
final GestureArenaEntry entry;
final Offset _initialGlobalPosition;
final int initialButtons;
final _CountdownZoned _doubleTapMinTimeCountdown;
bool _isTrackingPointer = false;
void startTrackingPointer(PointerRoute route, Matrix4? transform) {
if (!_isTrackingPointer) {
_isTrackingPointer = true;
GestureBinding.instance.pointerRouter.addRoute(pointer, route, transform);
}
}
void stopTrackingPointer(PointerRoute route) {
if (_isTrackingPointer) {
_isTrackingPointer = false;
GestureBinding.instance.pointerRouter.removeRoute(pointer, route);
}
}
bool isWithinGlobalTolerance(PointerEvent event, double tolerance) {
final Offset offset = event.position - _initialGlobalPosition;
return offset.distance <= tolerance;
}
bool hasElapsedMinTime() {
return _doubleTapMinTimeCountdown.timeout;
}
bool hasSameButton(PointerDownEvent event) {
return event.buttons == initialButtons;
}
}
/// Recognizes when the user has tapped the screen at the same location twice in
/// quick succession.
///
/// [DoubleTapGestureRecognizer] competes on pointer events when it
/// has a non-null callback. If it has no callbacks, it is a no-op.
///
class DoubleTapGestureRecognizer extends GestureRecognizer {
/// Create a gesture recognizer for double taps.
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
DoubleTapGestureRecognizer({
super.debugOwner,
super.supportedDevices,
AllowedButtonsFilter? allowedButtonsFilter,
}) : super(allowedButtonsFilter: allowedButtonsFilter ?? _defaultButtonAcceptBehavior);
// The default value for [allowedButtonsFilter].
// Accept the input if, and only if, [kPrimaryButton] is pressed.
static bool _defaultButtonAcceptBehavior(int buttons) => buttons == kPrimaryButton;
// Implementation notes:
//
// The double tap recognizer can be in one of four states. There's no
// explicit enum for the states, because they are already captured by
// the state of existing fields. Specifically:
//
// 1. Waiting on first tap: In this state, the _trackers list is empty, and
// _firstTap is null.
// 2. First tap in progress: In this state, the _trackers list contains all
// the states for taps that have begun but not completed. This list can
// have more than one entry if two pointers begin to tap.
// 3. Waiting on second tap: In this state, one of the in-progress taps has
// completed successfully. The _trackers list is again empty, and
// _firstTap records the successful tap.
// 4. Second tap in progress: Much like the "first tap in progress" state, but
// _firstTap is non-null. If a tap completes successfully while in this
// state, the callback is called and the state is reset.
//
// There are various other scenarios that cause the state to reset:
//
// - All in-progress taps are rejected (by time, distance, pointercancel, etc)
// - The long timer between taps expires
// - The gesture arena decides we have been rejected wholesale
/// A pointer has contacted the screen with a primary button at the same
/// location twice in quick succession, which might be the start of a double
/// tap.
///
/// This triggers immediately after the down event of the second tap.
///
/// If this recognizer doesn't win the arena, [onDoubleTapCancel] is called
/// next. Otherwise, [onDoubleTap] is called next.
///
/// See also:
///
/// * [allowedButtonsFilter], which decides which button will be allowed.
/// * [TapDownDetails], which is passed as an argument to this callback.
/// * [GestureDetector.onDoubleTapDown], which exposes this callback.
GestureTapDownCallback? onDoubleTapDown;
/// Called when the user has tapped the screen with a primary button at the
/// same location twice in quick succession.
///
/// This triggers when the pointer stops contacting the device after the
/// second tap.
///
/// See also:
///
/// * [allowedButtonsFilter], which decides which button will be allowed.
/// * [GestureDetector.onDoubleTap], which exposes this callback.
GestureDoubleTapCallback? onDoubleTap;
/// A pointer that previously triggered [onDoubleTapDown] will not end up
/// causing a double tap.
///
/// This triggers once the gesture loses the arena if [onDoubleTapDown] has
/// previously been triggered.
///
/// If this recognizer wins the arena, [onDoubleTap] is called instead.
///
/// See also:
///
/// * [allowedButtonsFilter], which decides which button will be allowed.
/// * [GestureDetector.onDoubleTapCancel], which exposes this callback.
GestureTapCancelCallback? onDoubleTapCancel;
Timer? _doubleTapTimer;
_TapTracker? _firstTap;
final Map<int, _TapTracker> _trackers = <int, _TapTracker>{};
@override
bool isPointerAllowed(PointerDownEvent event) {
if (_firstTap == null) {
if (onDoubleTapDown == null &&
onDoubleTap == null &&
onDoubleTapCancel == null) {
return false;
}
}
// If second tap is not allowed, reset the state.
final bool isPointerAllowed = super.isPointerAllowed(event);
if (!isPointerAllowed) {
_reset();
}
return isPointerAllowed;
}
@override
void addAllowedPointer(PointerDownEvent event) {
if (_firstTap != null) {
if (!_firstTap!.isWithinGlobalTolerance(event, kDoubleTapSlop)) {
// Ignore out-of-bounds second taps.
return;
} else if (!_firstTap!.hasElapsedMinTime() || !_firstTap!.hasSameButton(event)) {
// Restart when the second tap is too close to the first (touch screens
// often detect touches intermittently), or when buttons mismatch.
_reset();
return _trackTap(event);
} else if (onDoubleTapDown != null) {
final TapDownDetails details = TapDownDetails(
globalPosition: event.position,
localPosition: event.localPosition,
kind: getKindForPointer(event.pointer),
);
invokeCallback<void>('onDoubleTapDown', () => onDoubleTapDown!(details));
}
}
_trackTap(event);
}
void _trackTap(PointerDownEvent event) {
_stopDoubleTapTimer();
final _TapTracker tracker = _TapTracker(
event: event,
entry: GestureBinding.instance.gestureArena.add(event.pointer, this),
doubleTapMinTime: kDoubleTapMinTime,
gestureSettings: gestureSettings,
);
_trackers[event.pointer] = tracker;
tracker.startTrackingPointer(_handleEvent, event.transform);
}
void _handleEvent(PointerEvent event) {
final _TapTracker tracker = _trackers[event.pointer]!;
if (event is PointerUpEvent) {
if (_firstTap == null) {
_registerFirstTap(tracker);
} else {
_registerSecondTap(tracker);
}
} else if (event is PointerMoveEvent) {
if (!tracker.isWithinGlobalTolerance(event, kDoubleTapTouchSlop)) {
_reject(tracker);
}
} else if (event is PointerCancelEvent) {
_reject(tracker);
}
}
@override
void acceptGesture(int pointer) { }
@override
void rejectGesture(int pointer) {
_TapTracker? tracker = _trackers[pointer];
// If tracker isn't in the list, check if this is the first tap tracker
if (tracker == null &&
_firstTap != null &&
_firstTap!.pointer == pointer) {
tracker = _firstTap;
}
// If tracker is still null, we rejected ourselves already
if (tracker != null) {
_reject(tracker);
}
}
void _reject(_TapTracker tracker) {
_trackers.remove(tracker.pointer);
tracker.entry.resolve(GestureDisposition.rejected);
_freezeTracker(tracker);
if (_firstTap != null) {
if (tracker == _firstTap) {
_reset();
} else {
_checkCancel();
if (_trackers.isEmpty) {
_reset();
}
}
}
}
@override
void dispose() {
_reset();
super.dispose();
}
void _reset() {
_stopDoubleTapTimer();
if (_firstTap != null) {
if (_trackers.isNotEmpty) {
_checkCancel();
}
// Note, order is important below in order for the resolve -> reject logic
// to work properly.
final _TapTracker tracker = _firstTap!;
_firstTap = null;
_reject(tracker);
GestureBinding.instance.gestureArena.release(tracker.pointer);
}
_clearTrackers();
}
void _registerFirstTap(_TapTracker tracker) {
_startDoubleTapTimer();
GestureBinding.instance.gestureArena.hold(tracker.pointer);
// Note, order is important below in order for the clear -> reject logic to
// work properly.
_freezeTracker(tracker);
_trackers.remove(tracker.pointer);
_clearTrackers();
_firstTap = tracker;
}
void _registerSecondTap(_TapTracker tracker) {
_firstTap!.entry.resolve(GestureDisposition.accepted);
tracker.entry.resolve(GestureDisposition.accepted);
_freezeTracker(tracker);
_trackers.remove(tracker.pointer);
_checkUp(tracker.initialButtons);
_reset();
}
void _clearTrackers() {
_trackers.values.toList().forEach(_reject);
assert(_trackers.isEmpty);
}
void _freezeTracker(_TapTracker tracker) {
tracker.stopTrackingPointer(_handleEvent);
}
void _startDoubleTapTimer() {
_doubleTapTimer ??= Timer(kDoubleTapTimeout, _reset);
}
void _stopDoubleTapTimer() {
if (_doubleTapTimer != null) {
_doubleTapTimer!.cancel();
_doubleTapTimer = null;
}
}
void _checkUp(int buttons) {
if (onDoubleTap != null) {
invokeCallback<void>('onDoubleTap', onDoubleTap!);
}
}
void _checkCancel() {
if (onDoubleTapCancel != null) {
invokeCallback<void>('onDoubleTapCancel', onDoubleTapCancel!);
}
}
@override
String get debugDescription => 'double tap';
}
/// TapGesture represents a full gesture resulting from a single tap sequence,
/// as part of a [MultiTapGestureRecognizer]. Tap gestures are passive, meaning
/// that they will not preempt any other arena member in play.
class _TapGesture extends _TapTracker {
_TapGesture({
required this.gestureRecognizer,
required PointerEvent event,
required Duration longTapDelay,
required super.gestureSettings,
}) : _lastPosition = OffsetPair.fromEventPosition(event),
super(
event: event as PointerDownEvent,
entry: GestureBinding.instance.gestureArena.add(event.pointer, gestureRecognizer),
doubleTapMinTime: kDoubleTapMinTime,
) {
startTrackingPointer(handleEvent, event.transform);
if (longTapDelay > Duration.zero) {
_timer = Timer(longTapDelay, () {
_timer = null;
gestureRecognizer._dispatchLongTap(event.pointer, _lastPosition);
});
}
}
final MultiTapGestureRecognizer gestureRecognizer;
bool _wonArena = false;
Timer? _timer;
OffsetPair _lastPosition;
OffsetPair? _finalPosition;
void handleEvent(PointerEvent event) {
assert(event.pointer == pointer);
if (event is PointerMoveEvent) {
if (!isWithinGlobalTolerance(event, computeHitSlop(event.kind, gestureSettings))) {
cancel();
} else {
_lastPosition = OffsetPair.fromEventPosition(event);
}
} else if (event is PointerCancelEvent) {
cancel();
} else if (event is PointerUpEvent) {
stopTrackingPointer(handleEvent);
_finalPosition = OffsetPair.fromEventPosition(event);
_check();
}
}
@override
void stopTrackingPointer(PointerRoute route) {
_timer?.cancel();
_timer = null;
super.stopTrackingPointer(route);
}
void accept() {
_wonArena = true;
_check();
}
void reject() {
stopTrackingPointer(handleEvent);
gestureRecognizer._dispatchCancel(pointer);
}
void cancel() {
// If we won the arena already, then entry is resolved, so resolving
// again is a no-op. But we still need to clean up our own state.
if (_wonArena) {
reject();
} else {
entry.resolve(GestureDisposition.rejected); // eventually calls reject()
}
}
void _check() {
if (_wonArena && _finalPosition != null) {
gestureRecognizer._dispatchTap(pointer, _finalPosition!);
}
}
}
/// Recognizes taps on a per-pointer basis.
///
/// [MultiTapGestureRecognizer] considers each sequence of pointer events that
/// could constitute a tap independently of other pointers: For example, down-1,
/// down-2, up-1, up-2 produces two taps, on up-1 and up-2.
///
/// See also:
///
/// * [TapGestureRecognizer]
class MultiTapGestureRecognizer extends GestureRecognizer {
/// Creates a multi-tap gesture recognizer.
///
/// The [longTapDelay] defaults to [Duration.zero], which means
/// [onLongTapDown] is called immediately after [onTapDown].
///
/// {@macro flutter.gestures.GestureRecognizer.supportedDevices}
MultiTapGestureRecognizer({
this.longTapDelay = Duration.zero,
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
});
/// A pointer that might cause a tap has contacted the screen at a particular
/// location.
GestureMultiTapDownCallback? onTapDown;
/// A pointer that will trigger a tap has stopped contacting the screen at a
/// particular location.
GestureMultiTapUpCallback? onTapUp;
/// A tap has occurred.
GestureMultiTapCallback? onTap;
/// The pointer that previously triggered [onTapDown] will not end up causing
/// a tap.
GestureMultiTapCancelCallback? onTapCancel;
/// The amount of time between [onTapDown] and [onLongTapDown].
Duration longTapDelay;
/// A pointer that might cause a tap is still in contact with the screen at a
/// particular location after [longTapDelay].
GestureMultiTapDownCallback? onLongTapDown;
final Map<int, _TapGesture> _gestureMap = <int, _TapGesture>{};
@override
void addAllowedPointer(PointerDownEvent event) {
assert(!_gestureMap.containsKey(event.pointer));
_gestureMap[event.pointer] = _TapGesture(
gestureRecognizer: this,
event: event,
longTapDelay: longTapDelay,
gestureSettings: gestureSettings,
);
if (onTapDown != null) {
invokeCallback<void>('onTapDown', () {
onTapDown!(event.pointer, TapDownDetails(
globalPosition: event.position,
localPosition: event.localPosition,
kind: event.kind,
));
});
}
}
@override
void acceptGesture(int pointer) {
assert(_gestureMap.containsKey(pointer));
_gestureMap[pointer]!.accept();
}
@override
void rejectGesture(int pointer) {
assert(_gestureMap.containsKey(pointer));
_gestureMap[pointer]!.reject();
assert(!_gestureMap.containsKey(pointer));
}
void _dispatchCancel(int pointer) {
assert(_gestureMap.containsKey(pointer));
_gestureMap.remove(pointer);
if (onTapCancel != null) {
invokeCallback<void>('onTapCancel', () => onTapCancel!(pointer));
}
}
void _dispatchTap(int pointer, OffsetPair position) {
assert(_gestureMap.containsKey(pointer));
_gestureMap.remove(pointer);
if (onTapUp != null) {
invokeCallback<void>('onTapUp', () {
onTapUp!(pointer, TapUpDetails(
kind: getKindForPointer(pointer),
localPosition: position.local,
globalPosition: position.global,
));
});
}
if (onTap != null) {
invokeCallback<void>('onTap', () => onTap!(pointer));
}
}
void _dispatchLongTap(int pointer, OffsetPair lastPosition) {
assert(_gestureMap.containsKey(pointer));
if (onLongTapDown != null) {
invokeCallback<void>('onLongTapDown', () {
onLongTapDown!(
pointer,
TapDownDetails(
globalPosition: lastPosition.global,
localPosition: lastPosition.local,
kind: getKindForPointer(pointer),
),
);
});
}
}
@override
void dispose() {
final List<_TapGesture> localGestures = List<_TapGesture>.of(_gestureMap.values);
for (final _TapGesture gesture in localGestures) {
gesture.cancel();
}
// Rejection of each gesture should cause it to be removed from our map
assert(_gestureMap.isEmpty);
super.dispose();
}
@override
String get debugDescription => 'multitap';
}
/// Signature used by [SerialTapGestureRecognizer.onSerialTapDown] for when a
/// pointer that might cause a serial tap has contacted the screen at a
/// particular location.
typedef GestureSerialTapDownCallback = void Function(SerialTapDownDetails details);
/// Details for [GestureSerialTapDownCallback], such as the tap count within
/// the series.
///
/// See also:
///
/// * [SerialTapGestureRecognizer], which passes this information to its
/// [SerialTapGestureRecognizer.onSerialTapDown] callback.
class SerialTapDownDetails {
/// Creates details for a [GestureSerialTapDownCallback].
///
/// The `count` argument must be greater than zero.
SerialTapDownDetails({
this.globalPosition = Offset.zero,
Offset? localPosition,
required this.kind,
this.buttons = 0,
this.count = 1,
}) : assert(count > 0),
localPosition = localPosition ?? globalPosition;
/// The global position at which the pointer contacted the screen.
final Offset globalPosition;
/// The local position at which the pointer contacted the screen.
final Offset localPosition;
/// The kind of the device that initiated the event.
final PointerDeviceKind kind;
/// Which buttons were pressed when the pointer contacted the screen.
///
/// See also:
///
/// * [PointerEvent.buttons], which this field reflects.
final int buttons;
/// The number of consecutive taps that this "tap down" represents.
///
/// This value will always be greater than zero. When the first pointer in a
/// possible series contacts the screen, this value will be `1`, the second
/// tap in a double-tap will be `2`, and so on.
///
/// If a tap is determined to not be in the same series as the tap that
/// preceded it (e.g. because too much time elapsed between the two taps or
/// the two taps had too much distance between them), then this count will
/// reset back to `1`, and a new series will have begun.
final int count;
}
/// Signature used by [SerialTapGestureRecognizer.onSerialTapCancel] for when a
/// pointer that previously triggered a [GestureSerialTapDownCallback] will not
/// end up completing the serial tap.
typedef GestureSerialTapCancelCallback = void Function(SerialTapCancelDetails details);
/// Details for [GestureSerialTapCancelCallback], such as the tap count within
/// the series.
///
/// See also:
///
/// * [SerialTapGestureRecognizer], which passes this information to its
/// [SerialTapGestureRecognizer.onSerialTapCancel] callback.
class SerialTapCancelDetails {
/// Creates details for a [GestureSerialTapCancelCallback].
///
/// The `count` argument must be greater than zero.
SerialTapCancelDetails({
this.count = 1,
}) : assert(count > 0);
/// The number of consecutive taps that were in progress when the gesture was
/// interrupted.
///
/// This number will match the corresponding count that was specified in
/// [SerialTapDownDetails.count] for the tap that is being canceled. See
/// that field for more information on how this count is reported.
final int count;
}
/// Signature used by [SerialTapGestureRecognizer.onSerialTapUp] for when a
/// pointer that will trigger a serial tap has stopped contacting the screen.
typedef GestureSerialTapUpCallback = void Function(SerialTapUpDetails details);
/// Details for [GestureSerialTapUpCallback], such as the tap count within
/// the series.
///
/// See also:
///
/// * [SerialTapGestureRecognizer], which passes this information to its
/// [SerialTapGestureRecognizer.onSerialTapUp] callback.
class SerialTapUpDetails {
/// Creates details for a [GestureSerialTapUpCallback].
///
/// The `count` argument must be greater than zero.
SerialTapUpDetails({
this.globalPosition = Offset.zero,
Offset? localPosition,
this.kind,
this.count = 1,
}) : assert(count > 0),
localPosition = localPosition ?? globalPosition;
/// The global position at which the pointer contacted the screen.
final Offset globalPosition;
/// The local position at which the pointer contacted the screen.
final Offset localPosition;
/// The kind of the device that initiated the event.
final PointerDeviceKind? kind;
/// The number of consecutive taps that this tap represents.
///
/// This value will always be greater than zero. When the first pointer in a
/// possible series completes its tap, this value will be `1`, the second
/// tap in a double-tap will be `2`, and so on.
///
/// If a tap is determined to not be in the same series as the tap that
/// preceded it (e.g. because too much time elapsed between the two taps or
/// the two taps had too much distance between them), then this count will
/// reset back to `1`, and a new series will have begun.
final int count;
}
/// Recognizes serial taps (taps in a series).
///
/// A collection of taps are considered to be _in a series_ if they occur in
/// rapid succession in the same location (within a tolerance). The number of
/// taps in the series is its count. A double-tap, for instance, is a special
/// case of a tap series with a count of two.
///
/// ### Gesture arena behavior
///
/// [SerialTapGestureRecognizer] competes on all pointer events (regardless of
/// button). It will declare defeat if it determines that a gesture is not a
/// tap (e.g. if the pointer is dragged too far while it's contacting the
/// screen). It will immediately declare victory for every tap that it
/// recognizes.
///
/// Each time a pointer contacts the screen, this recognizer will enter that
/// gesture into the arena. This means that this recognizer will yield multiple
/// winning entries in the arena for a single tap series as the series
/// progresses.
///
/// If this recognizer loses the arena (either by declaring defeat or by
/// another recognizer declaring victory) while the pointer is contacting the
/// screen, it will fire [onSerialTapCancel], and [onSerialTapUp] will not
/// be fired.
///
/// ### Button behavior
///
/// A tap series is defined to have the same buttons across all taps. If a tap
/// with a different combination of buttons is delivered in the middle of a
/// series, it will "steal" the series and begin a new series, starting the
/// count over.
///
/// ### Interleaving tap behavior
///
/// A tap must be _completed_ in order for a subsequent tap to be considered
/// "in the same series" as that tap. Thus, if tap A is in-progress (the down
/// event has been received, but the corresponding up event has not yet been
/// received), and tap B begins (another pointer contacts the screen), tap A
/// will fire [onSerialTapCancel], and tap B will begin a new series (tap B's
/// [SerialTapDownDetails.count] will be 1).
///
/// ### Relation to `TapGestureRecognizer` and `DoubleTapGestureRecognizer`
///
/// [SerialTapGestureRecognizer] fires [onSerialTapDown] and [onSerialTapUp]
/// for every tap that it recognizes (passing the count in the details),
/// regardless of whether that tap is a single-tap, double-tap, etc. This
/// makes it especially useful when you want to respond to every tap in a
/// series. Contrast this with [DoubleTapGestureRecognizer], which only fires
/// if the user completes a double-tap, and [TapGestureRecognizer], which
/// _doesn't_ fire if the recognizer is competing with a
/// `DoubleTapGestureRecognizer`, and the user double-taps.
///
/// For example, consider a list item that should be _selected_ on the first
/// tap and _cause an edit dialog to open_ on a double-tap. If you use both
/// [TapGestureRecognizer] and [DoubleTapGestureRecognizer], there are a few
/// problems:
///
/// 1. If the user single-taps the list item, it will not select
/// the list item until after enough time has passed to rule out a
/// double-tap.
/// 2. If the user double-taps the list item, it will not select the list
/// item at all.
///
/// The solution is to use [SerialTapGestureRecognizer] and use the tap count
/// to either select the list item or open the edit dialog.
///
/// ### When competing with `TapGestureRecognizer` and `DoubleTapGestureRecognizer`
///
/// Unlike [TapGestureRecognizer] and [DoubleTapGestureRecognizer],
/// [SerialTapGestureRecognizer] aggressively declares victory when it detects
/// a tap, so when it is competing with those gesture recognizers, it will beat
/// them in the arena, regardless of which recognizer entered the arena first.
class SerialTapGestureRecognizer extends GestureRecognizer {
/// Creates a serial tap gesture recognizer.
SerialTapGestureRecognizer({
super.debugOwner,
super.supportedDevices,
super.allowedButtonsFilter,
});
/// A pointer has contacted the screen at a particular location, which might
/// be the start of a serial tap.
///
/// If this recognizer loses the arena before the serial tap is completed
/// (either because the gesture does not end up being a tap or because another
/// recognizer wins the arena), [onSerialTapCancel] is called next. Otherwise,
/// [onSerialTapUp] is called next.
///
/// The [SerialTapDownDetails.count] that is passed to this callback
/// specifies the series tap count.
GestureSerialTapDownCallback? onSerialTapDown;
/// A pointer that previously triggered [onSerialTapDown] will not end up
/// triggering the corresponding [onSerialTapUp].
///
/// If the user completes the serial tap, [onSerialTapUp] is called instead.
///
/// The [SerialTapCancelDetails.count] that is passed to this callback will
/// match the [SerialTapDownDetails.count] that was passed to the
/// [onSerialTapDown] callback.
GestureSerialTapCancelCallback? onSerialTapCancel;
/// A pointer has stopped contacting the screen at a particular location,
/// representing a serial tap.
///
/// If the user didn't complete the tap, or if another recognizer won the
/// arena, then [onSerialTapCancel] is called instead.
///
/// The [SerialTapUpDetails.count] that is passed to this callback specifies
/// the series tap count and will match the [SerialTapDownDetails.count] that
/// was passed to the [onSerialTapDown] callback.
GestureSerialTapUpCallback? onSerialTapUp;
Timer? _serialTapTimer;
final List<_TapTracker> _completedTaps = <_TapTracker>[];
final Map<int, GestureDisposition> _gestureResolutions = <int, GestureDisposition>{};
_TapTracker? _pendingTap;
/// Indicates whether this recognizer is currently tracking a pointer that's
/// in contact with the screen.
///
/// If this is true, it implies that [onSerialTapDown] has fired, but neither
/// [onSerialTapCancel] nor [onSerialTapUp] have yet fired.
bool get isTrackingPointer => _pendingTap != null;
@override
bool isPointerAllowed(PointerDownEvent event) {
if (onSerialTapDown == null &&
onSerialTapCancel == null &&
onSerialTapUp == null) {
return false;
}
return super.isPointerAllowed(event);
}
@override
void addAllowedPointer(PointerDownEvent event) {
if ((_completedTaps.isNotEmpty && !_representsSameSeries(_completedTaps.last, event))
|| _pendingTap != null) {
_reset();
}
_trackTap(event);
}
bool _representsSameSeries(_TapTracker tap, PointerDownEvent event) {
return tap.hasElapsedMinTime() // touch screens often detect touches intermittently
&& tap.hasSameButton(event)
&& tap.isWithinGlobalTolerance(event, kDoubleTapSlop);
}
void _trackTap(PointerDownEvent event) {
_stopSerialTapTimer();
if (onSerialTapDown != null) {
final SerialTapDownDetails details = SerialTapDownDetails(
globalPosition: event.position,
localPosition: event.localPosition,
kind: getKindForPointer(event.pointer),
buttons: event.buttons,
count: _completedTaps.length + 1,
);
invokeCallback<void>('onSerialTapDown', () => onSerialTapDown!(details));
}
final _TapTracker tracker = _TapTracker(
gestureSettings: gestureSettings,
event: event,
entry: GestureBinding.instance.gestureArena.add(event.pointer, this),
doubleTapMinTime: kDoubleTapMinTime,
);
assert(_pendingTap == null);
_pendingTap = tracker;
tracker.startTrackingPointer(_handleEvent, event.transform);
}
void _handleEvent(PointerEvent event) {
assert(_pendingTap != null);
assert(_pendingTap!.pointer == event.pointer);
final _TapTracker tracker = _pendingTap!;
if (event is PointerUpEvent) {
_registerTap(event, tracker);
} else if (event is PointerMoveEvent) {
if (!tracker.isWithinGlobalTolerance(event, kDoubleTapTouchSlop)) {
_reset();
}
} else if (event is PointerCancelEvent) {
_reset();
}
}
@override
void acceptGesture(int pointer) {
assert(_pendingTap != null);
assert(_pendingTap!.pointer == pointer);
_gestureResolutions[pointer] = GestureDisposition.accepted;
}
@override
void rejectGesture(int pointer) {
_gestureResolutions[pointer] = GestureDisposition.rejected;
_reset();
}
void _rejectPendingTap() {
assert(_pendingTap != null);
final _TapTracker tracker = _pendingTap!;
_pendingTap = null;
// Order is important here; the `resolve` call can yield a re-entrant
// `reset()`, so we need to check cancel here while we can trust the
// length of our _completedTaps list.
_checkCancel(_completedTaps.length + 1);
if (!_gestureResolutions.containsKey(tracker.pointer)) {
tracker.entry.resolve(GestureDisposition.rejected);
}
_stopTrackingPointer(tracker);
}
@override
void dispose() {
_reset();
super.dispose();
}
void _reset() {
if (_pendingTap != null) {
_rejectPendingTap();
}
_pendingTap = null;
_completedTaps.clear();
_gestureResolutions.clear();
_stopSerialTapTimer();
}
void _registerTap(PointerUpEvent event, _TapTracker tracker) {
assert(tracker == _pendingTap);
assert(tracker.pointer == event.pointer);
_startSerialTapTimer();
assert(_gestureResolutions[event.pointer] != GestureDisposition.rejected);
if (!_gestureResolutions.containsKey(event.pointer)) {
tracker.entry.resolve(GestureDisposition.accepted);
}
assert(_gestureResolutions[event.pointer] == GestureDisposition.accepted);
_stopTrackingPointer(tracker);
// Note, order is important below in order for the clear -> reject logic to
// work properly.
_pendingTap = null;
_checkUp(event, tracker);
_completedTaps.add(tracker);
}
void _stopTrackingPointer(_TapTracker tracker) {
tracker.stopTrackingPointer(_handleEvent);
}
void _startSerialTapTimer() {
_serialTapTimer ??= Timer(kDoubleTapTimeout, _reset);
}
void _stopSerialTapTimer() {
if (_serialTapTimer != null) {
_serialTapTimer!.cancel();
_serialTapTimer = null;
}
}
void _checkUp(PointerUpEvent event, _TapTracker tracker) {
if (onSerialTapUp != null) {
final SerialTapUpDetails details = SerialTapUpDetails(
globalPosition: event.position,
localPosition: event.localPosition,
kind: getKindForPointer(tracker.pointer),
count: _completedTaps.length + 1,
);
invokeCallback<void>('onSerialTapUp', () => onSerialTapUp!(details));
}
}
void _checkCancel(int count) {
if (onSerialTapCancel != null) {
final SerialTapCancelDetails details = SerialTapCancelDetails(
count: count,
);
invokeCallback<void>('onSerialTapCancel', () => onSerialTapCancel!(details));
}
}
@override
String get debugDescription => 'serial tap';
}
| flutter/packages/flutter/lib/src/gestures/multitap.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/multitap.dart",
"repo_id": "flutter",
"token_count": 11213
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// TODO(goderbauer): Clean up the part-of hack currently used for testing the private implementation.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
// The code for drawing animated icons is kept in a private API, as we are not
// yet ready for exposing a public API for (partial) vector graphics support.
// See: https://github.com/flutter/flutter/issues/1831 for details regarding
// generic vector graphics support in Flutter.
/// Shows an animated icon at a given animation [progress].
///
/// The available icons are specified in [AnimatedIcons].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=pJcbh8pbvJs}
///
/// {@tool dartpad}
/// This example shows how to create an animated icon. The icon is animated
/// forward and reverse in a loop.
///
/// ** See code in examples/api/lib/material/animated_icon/animated_icon.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Icons], for the list of available static Material Icons.
class AnimatedIcon extends StatelessWidget {
/// Creates an AnimatedIcon.
///
/// The [size] and [color] default to the value given by the current
/// [IconTheme].
const AnimatedIcon({
super.key,
required this.icon,
required this.progress,
this.color,
this.size,
this.semanticLabel,
this.textDirection,
});
/// The animation progress for the animated icon.
///
/// The value is clamped to be between 0 and 1.
///
/// This determines the actual frame that is displayed.
final Animation<double> progress;
/// The color to use when drawing the icon.
///
/// Defaults to the current [IconTheme] color, if any.
///
/// The given color will be adjusted by the opacity of the current
/// [IconTheme], if any.
///
/// In material apps, if there is a [Theme] without any [IconTheme]s
/// specified, icon colors default to white if the theme is dark
/// and black if the theme is light.
///
/// If no [IconTheme] and no [Theme] is specified, icons will default to black.
///
/// See [Theme] to set the current theme and [ThemeData.brightness]
/// for setting the current theme's brightness.
final Color? color;
/// The size of the icon in logical pixels.
///
/// Icons occupy a square with width and height equal to size.
///
/// Defaults to the current [IconTheme] size.
final double? size;
/// The icon to display. Available icons are listed in [AnimatedIcons].
final AnimatedIconData icon;
/// Semantic label for the icon.
///
/// Announced in accessibility modes (e.g TalkBack/VoiceOver).
/// This label does not show in the UI.
///
/// See also:
///
/// * [SemanticsProperties.label], which is set to [semanticLabel] in the
/// underlying [Semantics] widget.
final String? semanticLabel;
/// The text direction to use for rendering the icon.
///
/// If this is null, the ambient [Directionality] is used instead.
///
/// If the text direction is [TextDirection.rtl], the icon will be mirrored
/// horizontally (e.g back arrow will point right).
final TextDirection? textDirection;
static ui.Path _pathFactory() => ui.Path();
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
final _AnimatedIconData iconData = icon as _AnimatedIconData;
final IconThemeData iconTheme = IconTheme.of(context);
assert(iconTheme.isConcrete);
final double iconSize = size ?? iconTheme.size!;
final TextDirection textDirection = this.textDirection ?? Directionality.of(context);
final double iconOpacity = iconTheme.opacity!;
Color iconColor = color ?? iconTheme.color!;
if (iconOpacity != 1.0) {
iconColor = iconColor.withOpacity(iconColor.opacity * iconOpacity);
}
return Semantics(
label: semanticLabel,
child: CustomPaint(
size: Size(iconSize, iconSize),
painter: _AnimatedIconPainter(
paths: iconData.paths,
progress: progress,
color: iconColor,
scale: iconSize / iconData.size.width,
shouldMirror: textDirection == TextDirection.rtl && iconData.matchTextDirection,
uiPathFactory: _pathFactory,
),
),
);
}
}
typedef _UiPathFactory = ui.Path Function();
class _AnimatedIconPainter extends CustomPainter {
_AnimatedIconPainter({
required this.paths,
required this.progress,
required this.color,
required this.scale,
required this.shouldMirror,
required this.uiPathFactory,
}) : super(repaint: progress);
// This list is assumed to be immutable, changes to the contents of the list
// will not trigger a redraw as shouldRepaint will keep returning false.
final List<_PathFrames> paths;
final Animation<double> progress;
final Color color;
final double scale;
/// If this is true the image will be mirrored horizontally.
final bool shouldMirror;
final _UiPathFactory uiPathFactory;
@override
void paint(ui.Canvas canvas, Size size) {
// The RenderCustomPaint render object performs canvas.save before invoking
// this and canvas.restore after, so we don't need to do it here.
if (shouldMirror) {
canvas.rotate(math.pi);
canvas.translate(-size.width, -size.height);
}
canvas.scale(scale, scale);
final double clampedProgress = clampDouble(progress.value, 0.0, 1.0);
for (final _PathFrames path in paths) {
path.paint(canvas, color, uiPathFactory, clampedProgress);
}
}
@override
bool shouldRepaint(_AnimatedIconPainter oldDelegate) {
return oldDelegate.progress.value != progress.value
|| oldDelegate.color != color
// We are comparing the paths list by reference, assuming the list is
// treated as immutable to be more efficient.
|| oldDelegate.paths != paths
|| oldDelegate.scale != scale
|| oldDelegate.uiPathFactory != uiPathFactory;
}
@override
bool? hitTest(Offset position) => null;
@override
bool shouldRebuildSemantics(CustomPainter oldDelegate) => false;
@override
SemanticsBuilderCallback? get semanticsBuilder => null;
}
class _PathFrames {
const _PathFrames({
required this.commands,
required this.opacities,
});
final List<_PathCommand> commands;
final List<double> opacities;
void paint(ui.Canvas canvas, Color color, _UiPathFactory uiPathFactory, double progress) {
final double opacity = _interpolate<double?>(opacities, progress, ui.lerpDouble)!;
final ui.Paint paint = ui.Paint()
..style = PaintingStyle.fill
..color = color.withOpacity(color.opacity * opacity);
final ui.Path path = uiPathFactory();
for (final _PathCommand command in commands) {
command.apply(path, progress);
}
canvas.drawPath(path, paint);
}
}
/// Paths are being built by a set of commands e.g moveTo, lineTo, etc...
///
/// _PathCommand instances represents such a command, and can apply it to
/// a given Path.
abstract class _PathCommand {
const _PathCommand();
/// Applies the path command to [path].
///
/// For example if the object is a [_PathMoveTo] command it will invoke
/// [Path.moveTo] on [path].
void apply(ui.Path path, double progress);
}
class _PathMoveTo extends _PathCommand {
const _PathMoveTo(this.points);
final List<Offset> points;
@override
void apply(Path path, double progress) {
final Offset offset = _interpolate<Offset?>(points, progress, Offset.lerp)!;
path.moveTo(offset.dx, offset.dy);
}
}
class _PathCubicTo extends _PathCommand {
const _PathCubicTo(this.controlPoints1, this.controlPoints2, this.targetPoints);
final List<Offset> controlPoints2;
final List<Offset> controlPoints1;
final List<Offset> targetPoints;
@override
void apply(Path path, double progress) {
final Offset controlPoint1 = _interpolate<Offset?>(controlPoints1, progress, Offset.lerp)!;
final Offset controlPoint2 = _interpolate<Offset?>(controlPoints2, progress, Offset.lerp)!;
final Offset targetPoint = _interpolate<Offset?>(targetPoints, progress, Offset.lerp)!;
path.cubicTo(
controlPoint1.dx, controlPoint1.dy,
controlPoint2.dx, controlPoint2.dy,
targetPoint.dx, targetPoint.dy,
);
}
}
// ignore: unused_element
class _PathLineTo extends _PathCommand {
const _PathLineTo(this.points);
final List<Offset> points;
@override
void apply(Path path, double progress) {
final Offset point = _interpolate<Offset?>(points, progress, Offset.lerp)!;
path.lineTo(point.dx, point.dy);
}
}
class _PathClose extends _PathCommand {
const _PathClose();
@override
void apply(Path path, double progress) {
path.close();
}
}
/// Interpolates a value given a set of values equally spaced in time.
///
/// [interpolator] is the interpolation function used to interpolate between 2
/// points of type T.
///
/// This is currently done with linear interpolation between every 2 consecutive
/// points. Linear interpolation was smooth enough with the limited set of
/// animations we have tested, so we use it for simplicity. If we find this to
/// not be smooth enough we can try applying spline instead.
///
/// [progress] is expected to be between 0.0 and 1.0.
T _interpolate<T>(List<T> values, double progress, _Interpolator<T> interpolator) {
assert(progress <= 1.0);
assert(progress >= 0.0);
if (values.length == 1) {
return values[0];
}
final double targetIdx = ui.lerpDouble(0, values.length -1, progress)!;
final int lowIdx = targetIdx.floor();
final int highIdx = targetIdx.ceil();
final double t = targetIdx - lowIdx;
return interpolator(values[lowIdx], values[highIdx], t);
}
typedef _Interpolator<T> = T Function(T a, T b, double progress);
| flutter/packages/flutter/lib/src/material/animated_icons/animated_icons.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/animated_icons.dart",
"repo_id": "flutter",
"token_count": 3187
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'arc.dart';
import 'colors.dart';
import 'floating_action_button.dart';
import 'icons.dart';
import 'material_localizations.dart';
import 'page.dart';
import 'scaffold.dart' show ScaffoldMessenger, ScaffoldMessengerState;
import 'scrollbar.dart';
import 'theme.dart';
import 'tooltip.dart';
// Examples can assume:
// typedef GlobalWidgetsLocalizations = DefaultWidgetsLocalizations;
// typedef GlobalMaterialLocalizations = DefaultMaterialLocalizations;
/// [MaterialApp] uses this [TextStyle] as its [DefaultTextStyle] to encourage
/// developers to be intentional about their [DefaultTextStyle].
///
/// In Material Design, most [Text] widgets are contained in [Material] widgets,
/// which sets a specific [DefaultTextStyle]. If you're seeing text that uses
/// this text style, consider putting your text in a [Material] widget (or
/// another widget that sets a [DefaultTextStyle]).
const TextStyle _errorTextStyle = TextStyle(
color: Color(0xD0FF0000),
fontFamily: 'monospace',
fontSize: 48.0,
fontWeight: FontWeight.w900,
decoration: TextDecoration.underline,
decorationColor: Color(0xFFFFFF00),
decorationStyle: TextDecorationStyle.double,
debugLabel: 'fallback style; consider putting your text in a Material',
);
/// Describes which theme will be used by [MaterialApp].
enum ThemeMode {
/// Use either the light or dark theme based on what the user has selected in
/// the system settings.
system,
/// Always use the light mode regardless of system preference.
light,
/// Always use the dark mode (if available) regardless of system preference.
dark,
}
/// An application that uses Material Design.
///
/// A convenience widget that wraps a number of widgets that are commonly
/// required for Material Design applications. It builds upon a [WidgetsApp] by
/// adding material-design specific functionality, such as [AnimatedTheme] and
/// [GridPaper].
///
/// [MaterialApp] configures its [WidgetsApp.textStyle] with an ugly red/yellow
/// text style that's intended to warn the developer that their app hasn't defined
/// a default text style. Typically the app's [Scaffold] builds a [Material] widget
/// whose default [Material.textStyle] defines the text style for the entire scaffold.
///
/// The [MaterialApp] configures the top-level [Navigator] to search for routes
/// in the following order:
///
/// 1. For the `/` route, the [home] property, if non-null, is used.
///
/// 2. Otherwise, the [routes] table is used, if it has an entry for the route.
///
/// 3. Otherwise, [onGenerateRoute] is called, if provided. It should return a
/// non-null value for any _valid_ route not handled by [home] and [routes].
///
/// 4. Finally if all else fails [onUnknownRoute] is called.
///
/// If a [Navigator] is created, at least one of these options must handle the
/// `/` route, since it is used when an invalid [initialRoute] is specified on
/// startup (e.g. by another application launching this one with an intent on
/// Android; see [dart:ui.PlatformDispatcher.defaultRouteName]).
///
/// This widget also configures the observer of the top-level [Navigator] (if
/// any) to perform [Hero] animations.
///
/// {@template flutter.material.MaterialApp.defaultSelectionStyle}
/// The [MaterialApp] automatically creates a [DefaultSelectionStyle]. It uses
/// the colors in the [ThemeData.textSelectionTheme] if they are not null;
/// otherwise, the [MaterialApp] sets [DefaultSelectionStyle.selectionColor] to
/// [ColorScheme.primary] with 0.4 opacity and
/// [DefaultSelectionStyle.cursorColor] to [ColorScheme.primary].
/// {@endtemplate}
///
/// If [home], [routes], [onGenerateRoute], and [onUnknownRoute] are all null,
/// and [builder] is not null, then no [Navigator] is created.
///
/// {@tool snippet}
/// This example shows how to create a [MaterialApp] that disables the "debug"
/// banner with a [home] route that will be displayed when the app is launched.
///
/// 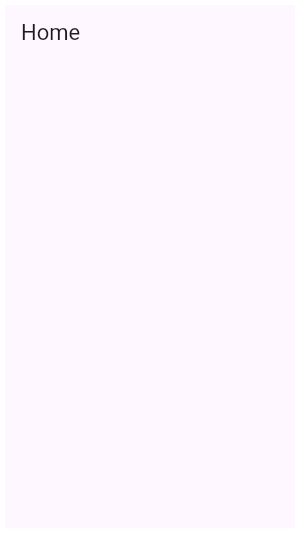
///
/// ```dart
/// MaterialApp(
/// home: Scaffold(
/// appBar: AppBar(
/// title: const Text('Home'),
/// ),
/// ),
/// debugShowCheckedModeBanner: false,
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example shows how to create a [MaterialApp] that uses the [routes]
/// `Map` to define the "home" route and an "about" route.
///
/// ```dart
/// MaterialApp(
/// routes: <String, WidgetBuilder>{
/// '/': (BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// title: const Text('Home Route'),
/// ),
/// );
/// },
/// '/about': (BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// title: const Text('About Route'),
/// ),
/// );
/// }
/// },
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// This example shows how to create a [MaterialApp] that defines a [theme] that
/// will be used for material widgets in the app.
///
/// 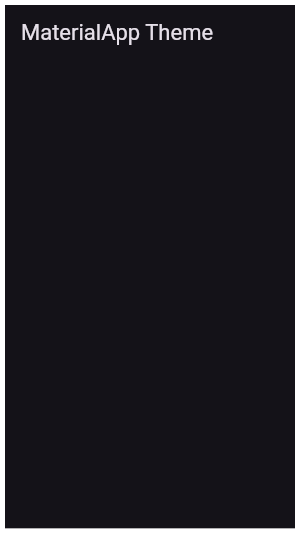
///
/// ```dart
/// MaterialApp(
/// theme: ThemeData(
/// brightness: Brightness.dark,
/// primaryColor: Colors.blueGrey
/// ),
/// home: Scaffold(
/// appBar: AppBar(
/// title: const Text('MaterialApp Theme'),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Why is my app's text red with yellow underlines?
///
/// [Text] widgets that lack a [Material] ancestor will be rendered with an ugly
/// red/yellow text style.
///
/// 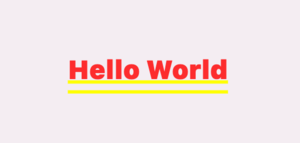
///
/// The typical fix is to give the widget a [Scaffold] ancestor. The [Scaffold] creates
/// a [Material] widget that defines its default text style.
///
/// ```dart
/// const MaterialApp(
/// title: 'Material App',
/// home: Scaffold(
/// body: Center(
/// child: Text('Hello World'),
/// ),
/// ),
/// )
/// ```
///
/// See also:
///
/// * [Scaffold], which provides standard app elements like an [AppBar] and a [Drawer].
/// * [Navigator], which is used to manage the app's stack of pages.
/// * [MaterialPageRoute], which defines an app page that transitions in a material-specific way.
/// * [WidgetsApp], which defines the basic app elements but does not depend on the material library.
/// * The Flutter Internationalization Tutorial,
/// <https://flutter.dev/tutorials/internationalization/>.
class MaterialApp extends StatefulWidget {
/// Creates a MaterialApp.
///
/// At least one of [home], [routes], [onGenerateRoute], or [builder] must be
/// non-null. If only [routes] is given, it must include an entry for the
/// [Navigator.defaultRouteName] (`/`), since that is the route used when the
/// application is launched with an intent that specifies an otherwise
/// unsupported route.
///
/// This class creates an instance of [WidgetsApp].
const MaterialApp({
super.key,
this.navigatorKey,
this.scaffoldMessengerKey,
this.home,
Map<String, WidgetBuilder> this.routes = const <String, WidgetBuilder>{},
this.initialRoute,
this.onGenerateRoute,
this.onGenerateInitialRoutes,
this.onUnknownRoute,
this.onNavigationNotification,
List<NavigatorObserver> this.navigatorObservers = const <NavigatorObserver>[],
this.builder,
this.title = '',
this.onGenerateTitle,
this.color,
this.theme,
this.darkTheme,
this.highContrastTheme,
this.highContrastDarkTheme,
this.themeMode = ThemeMode.system,
this.themeAnimationDuration = kThemeAnimationDuration,
this.themeAnimationCurve = Curves.linear,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.debugShowMaterialGrid = false,
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowCheckedModeBanner = true,
this.shortcuts,
this.actions,
this.restorationScopeId,
this.scrollBehavior,
@Deprecated(
'Remove this parameter as it is now ignored. '
'MaterialApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
this.themeAnimationStyle,
}) : routeInformationProvider = null,
routeInformationParser = null,
routerDelegate = null,
backButtonDispatcher = null,
routerConfig = null;
/// Creates a [MaterialApp] that uses the [Router] instead of a [Navigator].
///
/// {@macro flutter.widgets.WidgetsApp.router}
const MaterialApp.router({
super.key,
this.scaffoldMessengerKey,
this.routeInformationProvider,
this.routeInformationParser,
this.routerDelegate,
this.routerConfig,
this.backButtonDispatcher,
this.builder,
this.title = '',
this.onGenerateTitle,
this.onNavigationNotification,
this.color,
this.theme,
this.darkTheme,
this.highContrastTheme,
this.highContrastDarkTheme,
this.themeMode = ThemeMode.system,
this.themeAnimationDuration = kThemeAnimationDuration,
this.themeAnimationCurve = Curves.linear,
this.locale,
this.localizationsDelegates,
this.localeListResolutionCallback,
this.localeResolutionCallback,
this.supportedLocales = const <Locale>[Locale('en', 'US')],
this.debugShowMaterialGrid = false,
this.showPerformanceOverlay = false,
this.checkerboardRasterCacheImages = false,
this.checkerboardOffscreenLayers = false,
this.showSemanticsDebugger = false,
this.debugShowCheckedModeBanner = true,
this.shortcuts,
this.actions,
this.restorationScopeId,
this.scrollBehavior,
@Deprecated(
'Remove this parameter as it is now ignored. '
'MaterialApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
this.useInheritedMediaQuery = false,
this.themeAnimationStyle,
}) : assert(routerDelegate != null || routerConfig != null),
navigatorObservers = null,
navigatorKey = null,
onGenerateRoute = null,
home = null,
onGenerateInitialRoutes = null,
onUnknownRoute = null,
routes = null,
initialRoute = null;
/// {@macro flutter.widgets.widgetsApp.navigatorKey}
final GlobalKey<NavigatorState>? navigatorKey;
/// A key to use when building the [ScaffoldMessenger].
///
/// If a [scaffoldMessengerKey] is specified, the [ScaffoldMessenger] can be
/// directly manipulated without first obtaining it from a [BuildContext] via
/// [ScaffoldMessenger.of]: from the [scaffoldMessengerKey], use the
/// [GlobalKey.currentState] getter.
final GlobalKey<ScaffoldMessengerState>? scaffoldMessengerKey;
/// {@macro flutter.widgets.widgetsApp.home}
final Widget? home;
/// The application's top-level routing table.
///
/// When a named route is pushed with [Navigator.pushNamed], the route name is
/// looked up in this map. If the name is present, the associated
/// [widgets.WidgetBuilder] is used to construct a [MaterialPageRoute] that
/// performs an appropriate transition, including [Hero] animations, to the
/// new route.
///
/// {@macro flutter.widgets.widgetsApp.routes}
final Map<String, WidgetBuilder>? routes;
/// {@macro flutter.widgets.widgetsApp.initialRoute}
final String? initialRoute;
/// {@macro flutter.widgets.widgetsApp.onGenerateRoute}
final RouteFactory? onGenerateRoute;
/// {@macro flutter.widgets.widgetsApp.onGenerateInitialRoutes}
final InitialRouteListFactory? onGenerateInitialRoutes;
/// {@macro flutter.widgets.widgetsApp.onUnknownRoute}
final RouteFactory? onUnknownRoute;
/// {@macro flutter.widgets.widgetsApp.onNavigationNotification}
final NotificationListenerCallback<NavigationNotification>? onNavigationNotification;
/// {@macro flutter.widgets.widgetsApp.navigatorObservers}
final List<NavigatorObserver>? navigatorObservers;
/// {@macro flutter.widgets.widgetsApp.routeInformationProvider}
final RouteInformationProvider? routeInformationProvider;
/// {@macro flutter.widgets.widgetsApp.routeInformationParser}
final RouteInformationParser<Object>? routeInformationParser;
/// {@macro flutter.widgets.widgetsApp.routerDelegate}
final RouterDelegate<Object>? routerDelegate;
/// {@macro flutter.widgets.widgetsApp.backButtonDispatcher}
final BackButtonDispatcher? backButtonDispatcher;
/// {@macro flutter.widgets.widgetsApp.routerConfig}
final RouterConfig<Object>? routerConfig;
/// {@macro flutter.widgets.widgetsApp.builder}
///
/// Material specific features such as [showDialog] and [showMenu], and widgets
/// such as [Tooltip], [PopupMenuButton], also require a [Navigator] to properly
/// function.
final TransitionBuilder? builder;
/// {@macro flutter.widgets.widgetsApp.title}
///
/// This value is passed unmodified to [WidgetsApp.title].
final String title;
/// {@macro flutter.widgets.widgetsApp.onGenerateTitle}
///
/// This value is passed unmodified to [WidgetsApp.onGenerateTitle].
final GenerateAppTitle? onGenerateTitle;
/// Default visual properties, like colors fonts and shapes, for this app's
/// material widgets.
///
/// A second [darkTheme] [ThemeData] value, which is used to provide a dark
/// version of the user interface can also be specified. [themeMode] will
/// control which theme will be used if a [darkTheme] is provided.
///
/// The default value of this property is the value of [ThemeData.light()].
///
/// See also:
///
/// * [themeMode], which controls which theme to use.
/// * [MediaQueryData.platformBrightness], which indicates the platform's
/// desired brightness and is used to automatically toggle between [theme]
/// and [darkTheme] in [MaterialApp].
/// * [ThemeData.brightness], which indicates the [Brightness] of a theme's
/// colors.
final ThemeData? theme;
/// The [ThemeData] to use when a 'dark mode' is requested by the system.
///
/// Some host platforms allow the users to select a system-wide 'dark mode',
/// or the application may want to offer the user the ability to choose a
/// dark theme just for this application. This is theme that will be used for
/// such cases. [themeMode] will control which theme will be used.
///
/// This theme should have a [ThemeData.brightness] set to [Brightness.dark].
///
/// Uses [theme] instead when null. Defaults to the value of
/// [ThemeData.light()] when both [darkTheme] and [theme] are null.
///
/// See also:
///
/// * [themeMode], which controls which theme to use.
/// * [MediaQueryData.platformBrightness], which indicates the platform's
/// desired brightness and is used to automatically toggle between [theme]
/// and [darkTheme] in [MaterialApp].
/// * [ThemeData.brightness], which is typically set to the value of
/// [MediaQueryData.platformBrightness].
final ThemeData? darkTheme;
/// The [ThemeData] to use when 'high contrast' is requested by the system.
///
/// Some host platforms (for example, iOS) allow the users to increase
/// contrast through an accessibility setting.
///
/// Uses [theme] instead when null.
///
/// See also:
///
/// * [MediaQueryData.highContrast], which indicates the platform's
/// desire to increase contrast.
final ThemeData? highContrastTheme;
/// The [ThemeData] to use when a 'dark mode' and 'high contrast' is requested
/// by the system.
///
/// Some host platforms (for example, iOS) allow the users to increase
/// contrast through an accessibility setting.
///
/// This theme should have a [ThemeData.brightness] set to [Brightness.dark].
///
/// Uses [darkTheme] instead when null.
///
/// See also:
///
/// * [MediaQueryData.highContrast], which indicates the platform's
/// desire to increase contrast.
final ThemeData? highContrastDarkTheme;
/// Determines which theme will be used by the application if both [theme]
/// and [darkTheme] are provided.
///
/// If set to [ThemeMode.system], the choice of which theme to use will
/// be based on the user's system preferences. If the [MediaQuery.platformBrightnessOf]
/// is [Brightness.light], [theme] will be used. If it is [Brightness.dark],
/// [darkTheme] will be used (unless it is null, in which case [theme]
/// will be used.
///
/// If set to [ThemeMode.light] the [theme] will always be used,
/// regardless of the user's system preference.
///
/// If set to [ThemeMode.dark] the [darkTheme] will be used
/// regardless of the user's system preference. If [darkTheme] is null
/// then it will fallback to using [theme].
///
/// The default value is [ThemeMode.system].
///
/// See also:
///
/// * [theme], which is used when a light mode is selected.
/// * [darkTheme], which is used when a dark mode is selected.
/// * [ThemeData.brightness], which indicates to various parts of the
/// system what kind of theme is being used.
final ThemeMode? themeMode;
/// The duration of animated theme changes.
///
/// When the theme changes (either by the [theme], [darkTheme] or [themeMode]
/// parameters changing) it is animated to the new theme over time.
/// The [themeAnimationDuration] determines how long this animation takes.
///
/// To have the theme change immediately, you can set this to [Duration.zero].
///
/// The default is [kThemeAnimationDuration].
///
/// See also:
/// [themeAnimationCurve], which defines the curve used for the animation.
final Duration themeAnimationDuration;
/// The curve to apply when animating theme changes.
///
/// The default is [Curves.linear].
///
/// This is ignored if [themeAnimationDuration] is [Duration.zero].
///
/// See also:
/// [themeAnimationDuration], which defines how long the animation is.
final Curve themeAnimationCurve;
/// {@macro flutter.widgets.widgetsApp.color}
final Color? color;
/// {@macro flutter.widgets.widgetsApp.locale}
final Locale? locale;
/// {@macro flutter.widgets.widgetsApp.localizationsDelegates}
///
/// Internationalized apps that require translations for one of the locales
/// listed in [GlobalMaterialLocalizations] should specify this parameter
/// and list the [supportedLocales] that the application can handle.
///
/// ```dart
/// // The GlobalMaterialLocalizations and GlobalWidgetsLocalizations
/// // classes require the following import:
/// // import 'package:flutter_localizations/flutter_localizations.dart';
///
/// const MaterialApp(
/// localizationsDelegates: <LocalizationsDelegate<Object>>[
/// // ... app-specific localization delegate(s) here
/// GlobalMaterialLocalizations.delegate,
/// GlobalWidgetsLocalizations.delegate,
/// ],
/// supportedLocales: <Locale>[
/// Locale('en', 'US'), // English
/// Locale('he', 'IL'), // Hebrew
/// // ... other locales the app supports
/// ],
/// // ...
/// )
/// ```
///
/// ## Adding localizations for a new locale
///
/// The information that follows applies to the unusual case of an app
/// adding translations for a language not already supported by
/// [GlobalMaterialLocalizations].
///
/// Delegates that produce [WidgetsLocalizations] and [MaterialLocalizations]
/// are included automatically. Apps can provide their own versions of these
/// localizations by creating implementations of
/// [LocalizationsDelegate<WidgetsLocalizations>] or
/// [LocalizationsDelegate<MaterialLocalizations>] whose load methods return
/// custom versions of [WidgetsLocalizations] or [MaterialLocalizations].
///
/// For example: to add support to [MaterialLocalizations] for a locale it
/// doesn't already support, say `const Locale('foo', 'BR')`, one first
/// creates a subclass of [MaterialLocalizations] that provides the
/// translations:
///
/// ```dart
/// class FooLocalizations extends MaterialLocalizations {
/// FooLocalizations();
/// @override
/// String get okButtonLabel => 'foo';
/// // ...
/// // lots of other getters and methods to override!
/// }
/// ```
///
/// One must then create a [LocalizationsDelegate] subclass that can provide
/// an instance of the [MaterialLocalizations] subclass. In this case, this is
/// essentially just a method that constructs a `FooLocalizations` object. A
/// [SynchronousFuture] is used here because no asynchronous work takes place
/// upon "loading" the localizations object.
///
/// ```dart
/// // continuing from previous example...
/// class FooLocalizationsDelegate extends LocalizationsDelegate<MaterialLocalizations> {
/// const FooLocalizationsDelegate();
/// @override
/// bool isSupported(Locale locale) {
/// return locale == const Locale('foo', 'BR');
/// }
/// @override
/// Future<FooLocalizations> load(Locale locale) {
/// assert(locale == const Locale('foo', 'BR'));
/// return SynchronousFuture<FooLocalizations>(FooLocalizations());
/// }
/// @override
/// bool shouldReload(FooLocalizationsDelegate old) => false;
/// }
/// ```
///
/// Constructing a [MaterialApp] with a `FooLocalizationsDelegate` overrides
/// the automatically included delegate for [MaterialLocalizations] because
/// only the first delegate of each [LocalizationsDelegate.type] is used and
/// the automatically included delegates are added to the end of the app's
/// [localizationsDelegates] list.
///
/// ```dart
/// // continuing from previous example...
/// const MaterialApp(
/// localizationsDelegates: <LocalizationsDelegate<Object>>[
/// FooLocalizationsDelegate(),
/// ],
/// // ...
/// )
/// ```
/// See also:
///
/// * [supportedLocales], which must be specified along with
/// [localizationsDelegates].
/// * [GlobalMaterialLocalizations], a [localizationsDelegates] value
/// which provides material localizations for many languages.
/// * The Flutter Internationalization Tutorial,
/// <https://flutter.dev/tutorials/internationalization/>.
final Iterable<LocalizationsDelegate<dynamic>>? localizationsDelegates;
/// {@macro flutter.widgets.widgetsApp.localeListResolutionCallback}
///
/// This callback is passed along to the [WidgetsApp] built by this widget.
final LocaleListResolutionCallback? localeListResolutionCallback;
/// {@macro flutter.widgets.LocaleResolutionCallback}
///
/// This callback is passed along to the [WidgetsApp] built by this widget.
final LocaleResolutionCallback? localeResolutionCallback;
/// {@macro flutter.widgets.widgetsApp.supportedLocales}
///
/// It is passed along unmodified to the [WidgetsApp] built by this widget.
///
/// See also:
///
/// * [localizationsDelegates], which must be specified for localized
/// applications.
/// * [GlobalMaterialLocalizations], a [localizationsDelegates] value
/// which provides material localizations for many languages.
/// * The Flutter Internationalization Tutorial,
/// <https://flutter.dev/tutorials/internationalization/>.
final Iterable<Locale> supportedLocales;
/// Turns on a performance overlay.
///
/// See also:
///
/// * <https://flutter.dev/debugging/#performance-overlay>
final bool showPerformanceOverlay;
/// Turns on checkerboarding of raster cache images.
final bool checkerboardRasterCacheImages;
/// Turns on checkerboarding of layers rendered to offscreen bitmaps.
final bool checkerboardOffscreenLayers;
/// Turns on an overlay that shows the accessibility information
/// reported by the framework.
final bool showSemanticsDebugger;
/// {@macro flutter.widgets.widgetsApp.debugShowCheckedModeBanner}
final bool debugShowCheckedModeBanner;
/// {@macro flutter.widgets.widgetsApp.shortcuts}
/// {@tool snippet}
/// This example shows how to add a single shortcut for
/// [LogicalKeyboardKey.select] to the default shortcuts without needing to
/// add your own [Shortcuts] widget.
///
/// Alternatively, you could insert a [Shortcuts] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// shortcuts: <ShortcutActivator, Intent>{
/// ... WidgetsApp.defaultShortcuts,
/// const SingleActivator(LogicalKeyboardKey.select): const ActivateIntent(),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
/// {@macro flutter.widgets.widgetsApp.shortcuts.seeAlso}
final Map<ShortcutActivator, Intent>? shortcuts;
/// {@macro flutter.widgets.widgetsApp.actions}
/// {@tool snippet}
/// This example shows how to add a single action handling an
/// [ActivateAction] to the default actions without needing to
/// add your own [Actions] widget.
///
/// Alternatively, you could insert a [Actions] widget with just the mapping
/// you want to add between the [WidgetsApp] and its child and get the same
/// effect.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return WidgetsApp(
/// actions: <Type, Action<Intent>>{
/// ... WidgetsApp.defaultActions,
/// ActivateAction: CallbackAction<Intent>(
/// onInvoke: (Intent intent) {
/// // Do something here...
/// return null;
/// },
/// ),
/// },
/// color: const Color(0xFFFF0000),
/// builder: (BuildContext context, Widget? child) {
/// return const Placeholder();
/// },
/// );
/// }
/// ```
/// {@end-tool}
/// {@macro flutter.widgets.widgetsApp.actions.seeAlso}
final Map<Type, Action<Intent>>? actions;
/// {@macro flutter.widgets.widgetsApp.restorationScopeId}
final String? restorationScopeId;
/// {@template flutter.material.materialApp.scrollBehavior}
/// The default [ScrollBehavior] for the application.
///
/// [ScrollBehavior]s describe how [Scrollable] widgets behave. Providing
/// a [ScrollBehavior] can set the default [ScrollPhysics] across
/// an application, and manage [Scrollable] decorations like [Scrollbar]s and
/// [GlowingOverscrollIndicator]s.
/// {@endtemplate}
///
/// When null, defaults to [MaterialScrollBehavior].
///
/// See also:
///
/// * [ScrollConfiguration], which controls how [Scrollable] widgets behave
/// in a subtree.
final ScrollBehavior? scrollBehavior;
/// Turns on a [GridPaper] overlay that paints a baseline grid
/// Material apps.
///
/// Only available in debug mode.
///
/// See also:
///
/// * <https://material.io/design/layout/spacing-methods.html>
final bool debugShowMaterialGrid;
/// {@macro flutter.widgets.widgetsApp.useInheritedMediaQuery}
@Deprecated(
'This setting is now ignored. '
'MaterialApp never introduces its own MediaQuery; the View widget takes care of that. '
'This feature was deprecated after v3.7.0-29.0.pre.'
)
final bool useInheritedMediaQuery;
/// Used to override the theme animation curve and duration.
///
/// If [AnimationStyle.duration] is provided, it will be used to override
/// the theme animation duration in the underlying [AnimatedTheme] widget.
/// If it is null, then [themeAnimationDuration] will be used. Otherwise,
/// defaults to 200ms.
///
/// If [AnimationStyle.curve] is provided, it will be used to override
/// the theme animation curve in the underlying [AnimatedTheme] widget.
/// If it is null, then [themeAnimationCurve] will be used. Otherwise,
/// defaults to [Curves.linear].
///
/// To disable the theme animation, use [AnimationStyle.noAnimation].
///
/// {@tool dartpad}
/// This sample showcases how to override the theme animation curve and
/// duration in the [MaterialApp] widget using [AnimationStyle].
///
/// ** See code in examples/api/lib/material/app/app.0.dart **
/// {@end-tool}
final AnimationStyle? themeAnimationStyle;
@override
State<MaterialApp> createState() => _MaterialAppState();
/// The [HeroController] used for Material page transitions.
///
/// Used by the [MaterialApp].
static HeroController createMaterialHeroController() {
return HeroController(
createRectTween: (Rect? begin, Rect? end) {
return MaterialRectArcTween(begin: begin, end: end);
},
);
}
}
/// Describes how [Scrollable] widgets behave for [MaterialApp]s.
///
/// {@macro flutter.widgets.scrollBehavior}
///
/// Setting a [MaterialScrollBehavior] will apply a
/// [GlowingOverscrollIndicator] to [Scrollable] descendants when executing on
/// [TargetPlatform.android] and [TargetPlatform.fuchsia].
///
/// When using the desktop platform, if the [Scrollable] widget scrolls in the
/// [Axis.vertical], a [Scrollbar] is applied.
///
/// If the scroll direction is [Axis.horizontal] scroll views are less
/// discoverable, so consider adding a Scrollbar in these cases, either directly
/// or through the [buildScrollbar] method.
///
/// [ThemeData.useMaterial3] specifies the
/// overscroll indicator that is used on [TargetPlatform.android], which
/// defaults to true, resulting in a [StretchingOverscrollIndicator]. Setting
/// [ThemeData.useMaterial3] to false will instead use a
/// [GlowingOverscrollIndicator].
///
/// See also:
///
/// * [ScrollBehavior], the default scrolling behavior extended by this class.
class MaterialScrollBehavior extends ScrollBehavior {
/// Creates a MaterialScrollBehavior that decorates [Scrollable]s with
/// [StretchingOverscrollIndicator]s and [Scrollbar]s based on the current
/// platform and provided [ScrollableDetails].
///
/// [ThemeData.useMaterial3] specifies the
/// overscroll indicator that is used on [TargetPlatform.android], which
/// defaults to true, resulting in a [StretchingOverscrollIndicator]. Setting
/// [ThemeData.useMaterial3] to false will instead use a
/// [GlowingOverscrollIndicator].
const MaterialScrollBehavior();
@override
TargetPlatform getPlatform(BuildContext context) => Theme.of(context).platform;
@override
Widget buildScrollbar(BuildContext context, Widget child, ScrollableDetails details) {
// When modifying this function, consider modifying the implementation in
// the base class ScrollBehavior as well.
switch (axisDirectionToAxis(details.direction)) {
case Axis.horizontal:
return child;
case Axis.vertical:
switch (getPlatform(context)) {
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
assert(details.controller != null);
return Scrollbar(
controller: details.controller,
child: child,
);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
return child;
}
}
}
@override
Widget buildOverscrollIndicator(BuildContext context, Widget child, ScrollableDetails details) {
// When modifying this function, consider modifying the implementation in
// the base class ScrollBehavior as well.
final AndroidOverscrollIndicator indicator = Theme.of(context).useMaterial3
? AndroidOverscrollIndicator.stretch
: AndroidOverscrollIndicator.glow;
switch (getPlatform(context)) {
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
return child;
case TargetPlatform.android:
switch (indicator) {
case AndroidOverscrollIndicator.stretch:
return StretchingOverscrollIndicator(
axisDirection: details.direction,
clipBehavior: details.clipBehavior ?? Clip.hardEdge,
child: child,
);
case AndroidOverscrollIndicator.glow:
break;
}
case TargetPlatform.fuchsia:
break;
}
return GlowingOverscrollIndicator(
axisDirection: details.direction,
color: Theme.of(context).colorScheme.secondary,
child: child,
);
}
}
class _MaterialAppState extends State<MaterialApp> {
late HeroController _heroController;
bool get _usesRouter => widget.routerDelegate != null || widget.routerConfig != null;
@override
void initState() {
super.initState();
_heroController = MaterialApp.createMaterialHeroController();
}
@override
void dispose() {
_heroController.dispose();
super.dispose();
}
// Combine the Localizations for Material with the ones contributed
// by the localizationsDelegates parameter, if any. Only the first delegate
// of a particular LocalizationsDelegate.type is loaded so the
// localizationsDelegate parameter can be used to override
// _MaterialLocalizationsDelegate.
Iterable<LocalizationsDelegate<dynamic>> get _localizationsDelegates {
return <LocalizationsDelegate<dynamic>>[
if (widget.localizationsDelegates != null)
...widget.localizationsDelegates!,
DefaultMaterialLocalizations.delegate,
DefaultCupertinoLocalizations.delegate,
];
}
Widget _inspectorSelectButtonBuilder(BuildContext context, VoidCallback onPressed) {
return FloatingActionButton(
onPressed: onPressed,
mini: true,
child: const Icon(Icons.search),
);
}
ThemeData _themeBuilder(BuildContext context) {
ThemeData? theme;
// Resolve which theme to use based on brightness and high contrast.
final ThemeMode mode = widget.themeMode ?? ThemeMode.system;
final Brightness platformBrightness = MediaQuery.platformBrightnessOf(context);
final bool useDarkTheme = mode == ThemeMode.dark
|| (mode == ThemeMode.system && platformBrightness == ui.Brightness.dark);
final bool highContrast = MediaQuery.highContrastOf(context);
if (useDarkTheme && highContrast && widget.highContrastDarkTheme != null) {
theme = widget.highContrastDarkTheme;
} else if (useDarkTheme && widget.darkTheme != null) {
theme = widget.darkTheme;
} else if (highContrast && widget.highContrastTheme != null) {
theme = widget.highContrastTheme;
}
theme ??= widget.theme ?? ThemeData.light();
return theme;
}
Widget _materialBuilder(BuildContext context, Widget? child) {
final ThemeData theme = _themeBuilder(context);
final Color effectiveSelectionColor = theme.textSelectionTheme.selectionColor ?? theme.colorScheme.primary.withOpacity(0.40);
final Color effectiveCursorColor = theme.textSelectionTheme.cursorColor ?? theme.colorScheme.primary;
Widget childWidget = child ?? const SizedBox.shrink();
if (widget.themeAnimationStyle != AnimationStyle.noAnimation) {
if (widget.builder != null) {
childWidget = Builder(
builder: (BuildContext context) {
// Why are we surrounding a builder with a builder?
//
// The widget.builder may contain code that invokes
// Theme.of(), which should return the theme we selected
// above in AnimatedTheme. However, if we invoke
// widget.builder() directly as the child of AnimatedTheme
// then there is no Context separating them, and the
// widget.builder() will not find the theme. Therefore, we
// surround widget.builder with yet another builder so that
// a context separates them and Theme.of() correctly
// resolves to the theme we passed to AnimatedTheme.
return widget.builder!(context, child);
},
);
}
childWidget = AnimatedTheme(
data: theme,
duration: widget.themeAnimationStyle?.duration ?? widget.themeAnimationDuration,
curve: widget.themeAnimationStyle?.curve ?? widget.themeAnimationCurve,
child: childWidget,
);
} else {
childWidget = Theme(
data: theme,
child: childWidget,
);
}
return ScaffoldMessenger(
key: widget.scaffoldMessengerKey,
child: DefaultSelectionStyle(
selectionColor: effectiveSelectionColor,
cursorColor: effectiveCursorColor,
child: childWidget,
),
);
}
Widget _buildWidgetApp(BuildContext context) {
// The color property is always pulled from the light theme, even if dark
// mode is activated. This was done to simplify the technical details
// of switching themes and it was deemed acceptable because this color
// property is only used on old Android OSes to color the app bar in
// Android's switcher UI.
//
// blue is the primary color of the default theme.
final Color materialColor = widget.color ?? widget.theme?.primaryColor ?? Colors.blue;
if (_usesRouter) {
return WidgetsApp.router(
key: GlobalObjectKey(this),
routeInformationProvider: widget.routeInformationProvider,
routeInformationParser: widget.routeInformationParser,
routerDelegate: widget.routerDelegate,
routerConfig: widget.routerConfig,
backButtonDispatcher: widget.backButtonDispatcher,
onNavigationNotification: widget.onNavigationNotification,
builder: _materialBuilder,
title: widget.title,
onGenerateTitle: widget.onGenerateTitle,
textStyle: _errorTextStyle,
color: materialColor,
locale: widget.locale,
localizationsDelegates: _localizationsDelegates,
localeResolutionCallback: widget.localeResolutionCallback,
localeListResolutionCallback: widget.localeListResolutionCallback,
supportedLocales: widget.supportedLocales,
showPerformanceOverlay: widget.showPerformanceOverlay,
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
showSemanticsDebugger: widget.showSemanticsDebugger,
debugShowCheckedModeBanner: widget.debugShowCheckedModeBanner,
inspectorSelectButtonBuilder: _inspectorSelectButtonBuilder,
shortcuts: widget.shortcuts,
actions: widget.actions,
restorationScopeId: widget.restorationScopeId,
);
}
return WidgetsApp(
key: GlobalObjectKey(this),
navigatorKey: widget.navigatorKey,
navigatorObservers: widget.navigatorObservers!,
pageRouteBuilder: <T>(RouteSettings settings, WidgetBuilder builder) {
return MaterialPageRoute<T>(settings: settings, builder: builder);
},
home: widget.home,
routes: widget.routes!,
initialRoute: widget.initialRoute,
onGenerateRoute: widget.onGenerateRoute,
onGenerateInitialRoutes: widget.onGenerateInitialRoutes,
onUnknownRoute: widget.onUnknownRoute,
onNavigationNotification: widget.onNavigationNotification,
builder: _materialBuilder,
title: widget.title,
onGenerateTitle: widget.onGenerateTitle,
textStyle: _errorTextStyle,
color: materialColor,
locale: widget.locale,
localizationsDelegates: _localizationsDelegates,
localeResolutionCallback: widget.localeResolutionCallback,
localeListResolutionCallback: widget.localeListResolutionCallback,
supportedLocales: widget.supportedLocales,
showPerformanceOverlay: widget.showPerformanceOverlay,
checkerboardRasterCacheImages: widget.checkerboardRasterCacheImages,
checkerboardOffscreenLayers: widget.checkerboardOffscreenLayers,
showSemanticsDebugger: widget.showSemanticsDebugger,
debugShowCheckedModeBanner: widget.debugShowCheckedModeBanner,
inspectorSelectButtonBuilder: _inspectorSelectButtonBuilder,
shortcuts: widget.shortcuts,
actions: widget.actions,
restorationScopeId: widget.restorationScopeId,
);
}
@override
Widget build(BuildContext context) {
Widget result = _buildWidgetApp(context);
result = Focus(
canRequestFocus: false,
onKeyEvent: (FocusNode node, KeyEvent event) {
if ((event is! KeyDownEvent && event is! KeyRepeatEvent) ||
event.logicalKey != LogicalKeyboardKey.escape) {
return KeyEventResult.ignored;
}
return Tooltip.dismissAllToolTips() ? KeyEventResult.handled : KeyEventResult.ignored;
},
child: result,
);
assert(() {
if (widget.debugShowMaterialGrid) {
result = GridPaper(
color: const Color(0xE0F9BBE0),
interval: 8.0,
subdivisions: 1,
child: result,
);
}
return true;
}());
return ScrollConfiguration(
behavior: widget.scrollBehavior ?? const MaterialScrollBehavior(),
child: HeroControllerScope(
controller: _heroController,
child: result,
),
);
}
}
| flutter/packages/flutter/lib/src/material/app.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/app.dart",
"repo_id": "flutter",
"token_count": 13469
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button_theme.dart';
import 'constants.dart';
import 'ink_well.dart';
import 'material.dart';
import 'material_state.dart';
import 'material_state_mixin.dart';
import 'theme.dart';
import 'theme_data.dart';
/// Creates a button based on [Semantics], [Material], and [InkWell]
/// widgets.
///
/// This class does not use the current [Theme] or [ButtonTheme] to
/// compute default values for unspecified parameters. It's intended to
/// be used for custom Material buttons that optionally incorporate defaults
/// from the themes or from app-specific sources.
///
/// This class is planned to be deprecated in a future release, see
/// [ButtonStyleButton], the base class of [ElevatedButton], [FilledButton],
/// [OutlinedButton] and [TextButton].
///
/// See also:
///
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [FilledButton], a filled button that doesn't elevate when pressed.
/// * [FilledButton.tonal], a filled button variant that uses a secondary fill color.
/// * [OutlinedButton], a button with an outlined border and no fill color.
/// * [TextButton], a button with no outline or fill color.
@Category(<String>['Material', 'Button'])
class RawMaterialButton extends StatefulWidget {
/// Create a button based on [Semantics], [Material], and [InkWell] widgets.
///
/// The [elevation], [focusElevation], [hoverElevation], [highlightElevation],
/// and [disabledElevation] parameters must be non-negative.
const RawMaterialButton({
super.key,
required this.onPressed,
this.onLongPress,
this.onHighlightChanged,
this.mouseCursor,
this.textStyle,
this.fillColor,
this.focusColor,
this.hoverColor,
this.highlightColor,
this.splashColor,
this.elevation = 2.0,
this.focusElevation = 4.0,
this.hoverElevation = 4.0,
this.highlightElevation = 8.0,
this.disabledElevation = 0.0,
this.padding = EdgeInsets.zero,
this.visualDensity = VisualDensity.standard,
this.constraints = const BoxConstraints(minWidth: 88.0, minHeight: 36.0),
this.shape = const RoundedRectangleBorder(),
this.animationDuration = kThemeChangeDuration,
this.clipBehavior = Clip.none,
this.focusNode,
this.autofocus = false,
MaterialTapTargetSize? materialTapTargetSize,
this.child,
this.enableFeedback = true,
}) : materialTapTargetSize = materialTapTargetSize ?? MaterialTapTargetSize.padded,
assert(elevation >= 0.0),
assert(focusElevation >= 0.0),
assert(hoverElevation >= 0.0),
assert(highlightElevation >= 0.0),
assert(disabledElevation >= 0.0);
/// Called when the button is tapped or otherwise activated.
///
/// If this callback and [onLongPress] are null, then the button will be disabled.
///
/// See also:
///
/// * [enabled], which is true if the button is enabled.
final VoidCallback? onPressed;
/// Called when the button is long-pressed.
///
/// If this callback and [onPressed] are null, then the button will be disabled.
///
/// See also:
///
/// * [enabled], which is true if the button is enabled.
final VoidCallback? onLongPress;
/// Called by the underlying [InkWell] widget's [InkWell.onHighlightChanged]
/// callback.
///
/// If [onPressed] changes from null to non-null while a gesture is ongoing,
/// this can fire during the build phase (in which case calling
/// [State.setState] is not allowed).
final ValueChanged<bool>? onHighlightChanged;
/// {@template flutter.material.RawMaterialButton.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// button.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.pressed].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// If this property is null, [MaterialStateMouseCursor.clickable] will be used.
final MouseCursor? mouseCursor;
/// Defines the default text style, with [Material.textStyle], for the
/// button's [child].
///
/// If [TextStyle.color] is a [MaterialStateProperty<Color>], [MaterialStateProperty.resolve]
/// is used for the following [MaterialState]s:
///
/// * [MaterialState.pressed].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
final TextStyle? textStyle;
/// The color of the button's [Material].
final Color? fillColor;
/// The color for the button's [Material] when it has the input focus.
final Color? focusColor;
/// The color for the button's [Material] when a pointer is hovering over it.
final Color? hoverColor;
/// The highlight color for the button's [InkWell].
final Color? highlightColor;
/// The splash color for the button's [InkWell].
final Color? splashColor;
/// The elevation for the button's [Material] when the button
/// is [enabled] but not pressed.
///
/// Defaults to 2.0. The value is always non-negative.
///
/// See also:
///
/// * [highlightElevation], the default elevation.
/// * [hoverElevation], the elevation when a pointer is hovering over the
/// button.
/// * [focusElevation], the elevation when the button is focused.
/// * [disabledElevation], the elevation when the button is disabled.
final double elevation;
/// The elevation for the button's [Material] when the button
/// is [enabled] and a pointer is hovering over it.
///
/// Defaults to 4.0. The value is always non-negative.
///
/// If the button is [enabled], and being pressed (in the highlighted state),
/// then the [highlightElevation] take precedence over the [hoverElevation].
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [focusElevation], the elevation when the button is focused.
/// * [disabledElevation], the elevation when the button is disabled.
/// * [highlightElevation], the elevation when the button is pressed.
final double hoverElevation;
/// The elevation for the button's [Material] when the button
/// is [enabled] and has the input focus.
///
/// Defaults to 4.0. The value is always non-negative.
///
/// If the button is [enabled], and being pressed (in the highlighted state),
/// or a mouse cursor is hovering over the button, then the [hoverElevation]
/// and [highlightElevation] take precedence over the [focusElevation].
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [hoverElevation], the elevation when a pointer is hovering over the
/// button.
/// * [disabledElevation], the elevation when the button is disabled.
/// * [highlightElevation], the elevation when the button is pressed.
final double focusElevation;
/// The elevation for the button's [Material] when the button
/// is [enabled] and pressed.
///
/// Defaults to 8.0. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [hoverElevation], the elevation when a pointer is hovering over the
/// button.
/// * [focusElevation], the elevation when the button is focused.
/// * [disabledElevation], the elevation when the button is disabled.
final double highlightElevation;
/// The elevation for the button's [Material] when the button
/// is not [enabled].
///
/// Defaults to 0.0. The value is always non-negative.
///
/// See also:
///
/// * [elevation], the default elevation.
/// * [hoverElevation], the elevation when a pointer is hovering over the
/// button.
/// * [focusElevation], the elevation when the button is focused.
/// * [highlightElevation], the elevation when the button is pressed.
final double disabledElevation;
/// The internal padding for the button's [child].
final EdgeInsetsGeometry padding;
/// Defines how compact the button's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
///
/// See also:
///
/// * [ThemeData.visualDensity], which specifies the [visualDensity] for all widgets
/// within a [Theme].
final VisualDensity visualDensity;
/// Defines the button's size.
///
/// Typically used to constrain the button's minimum size.
final BoxConstraints constraints;
/// The shape of the button's [Material].
///
/// The button's highlight and splash are clipped to this shape. If the
/// button has an elevation, then its drop shadow is defined by this shape.
///
/// If [shape] is a [MaterialStateProperty<ShapeBorder>], [MaterialStateProperty.resolve]
/// is used for the following [MaterialState]s:
///
/// * [MaterialState.pressed].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
final ShapeBorder shape;
/// Defines the duration of animated changes for [shape] and [elevation].
///
/// The default value is [kThemeChangeDuration].
final Duration animationDuration;
/// Typically the button's label.
final Widget? child;
/// Whether the button is enabled or disabled.
///
/// Buttons are disabled by default. To enable a button, set its [onPressed]
/// or [onLongPress] properties to a non-null value.
bool get enabled => onPressed != null || onLongPress != null;
/// Configures the minimum size of the tap target.
///
/// Defaults to [MaterialTapTargetSize.padded].
///
/// See also:
///
/// * [MaterialTapTargetSize], for a description of how this affects tap targets.
final MaterialTapTargetSize materialTapTargetSize;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// Whether detected gestures should provide acoustic and/or haptic feedback.
///
/// For example, on Android a tap will produce a clicking sound and a
/// long-press will produce a short vibration, when feedback is enabled.
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool enableFeedback;
@override
State<RawMaterialButton> createState() => _RawMaterialButtonState();
}
class _RawMaterialButtonState extends State<RawMaterialButton> with MaterialStateMixin {
@override
void initState() {
super.initState();
setMaterialState(MaterialState.disabled, !widget.enabled);
}
@override
void didUpdateWidget(RawMaterialButton oldWidget) {
super.didUpdateWidget(oldWidget);
setMaterialState(MaterialState.disabled, !widget.enabled);
// If the button is disabled while a press gesture is currently ongoing,
// InkWell makes a call to handleHighlightChanged. This causes an exception
// because it calls setState in the middle of a build. To preempt this, we
// manually update pressed to false when this situation occurs.
if (isDisabled && isPressed) {
removeMaterialState(MaterialState.pressed);
}
}
double get _effectiveElevation {
// These conditionals are in order of precedence, so be careful about
// reorganizing them.
if (isDisabled) {
return widget.disabledElevation;
}
if (isPressed) {
return widget.highlightElevation;
}
if (isHovered) {
return widget.hoverElevation;
}
if (isFocused) {
return widget.focusElevation;
}
return widget.elevation;
}
@override
Widget build(BuildContext context) {
final Color? effectiveTextColor = MaterialStateProperty.resolveAs<Color?>(widget.textStyle?.color, materialStates);
final ShapeBorder? effectiveShape = MaterialStateProperty.resolveAs<ShapeBorder?>(widget.shape, materialStates);
final Offset densityAdjustment = widget.visualDensity.baseSizeAdjustment;
final BoxConstraints effectiveConstraints = widget.visualDensity.effectiveConstraints(widget.constraints);
final MouseCursor? effectiveMouseCursor = MaterialStateProperty.resolveAs<MouseCursor?>(
widget.mouseCursor ?? MaterialStateMouseCursor.clickable,
materialStates,
);
final EdgeInsetsGeometry padding = widget.padding.add(
EdgeInsets.only(
left: densityAdjustment.dx,
top: densityAdjustment.dy,
right: densityAdjustment.dx,
bottom: densityAdjustment.dy,
),
).clamp(EdgeInsets.zero, EdgeInsetsGeometry.infinity);
final Widget result = ConstrainedBox(
constraints: effectiveConstraints,
child: Material(
elevation: _effectiveElevation,
textStyle: widget.textStyle?.copyWith(color: effectiveTextColor),
shape: effectiveShape,
color: widget.fillColor,
// For compatibility during the M3 migration the default shadow needs to be passed.
shadowColor: Theme.of(context).useMaterial3 ? Theme.of(context).shadowColor : null,
type: widget.fillColor == null ? MaterialType.transparency : MaterialType.button,
animationDuration: widget.animationDuration,
clipBehavior: widget.clipBehavior,
child: InkWell(
focusNode: widget.focusNode,
canRequestFocus: widget.enabled,
onFocusChange: updateMaterialState(MaterialState.focused),
autofocus: widget.autofocus,
onHighlightChanged: updateMaterialState(MaterialState.pressed, onChanged: widget.onHighlightChanged),
splashColor: widget.splashColor,
highlightColor: widget.highlightColor,
focusColor: widget.focusColor,
hoverColor: widget.hoverColor,
onHover: updateMaterialState(MaterialState.hovered),
onTap: widget.onPressed,
onLongPress: widget.onLongPress,
enableFeedback: widget.enableFeedback,
customBorder: effectiveShape,
mouseCursor: effectiveMouseCursor,
child: IconTheme.merge(
data: IconThemeData(color: effectiveTextColor),
child: Container(
padding: padding,
child: Center(
widthFactor: 1.0,
heightFactor: 1.0,
child: widget.child,
),
),
),
),
),
);
final Size minSize;
switch (widget.materialTapTargetSize) {
case MaterialTapTargetSize.padded:
minSize = Size(
kMinInteractiveDimension + densityAdjustment.dx,
kMinInteractiveDimension + densityAdjustment.dy,
);
assert(minSize.width >= 0.0);
assert(minSize.height >= 0.0);
case MaterialTapTargetSize.shrinkWrap:
minSize = Size.zero;
}
return Semantics(
container: true,
button: true,
enabled: widget.enabled,
child: _InputPadding(
minSize: minSize,
child: result,
),
);
}
}
/// A widget to pad the area around a [MaterialButton]'s inner [Material].
///
/// Redirect taps that occur in the padded area around the child to the center
/// of the child. This increases the size of the button and the button's
/// "tap target", but not its material or its ink splashes.
class _InputPadding extends SingleChildRenderObjectWidget {
const _InputPadding({
super.child,
required this.minSize,
});
final Size minSize;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderInputPadding(minSize);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderInputPadding renderObject) {
renderObject.minSize = minSize;
}
}
class _RenderInputPadding extends RenderShiftedBox {
_RenderInputPadding(this._minSize, [RenderBox? child]) : super(child);
Size get minSize => _minSize;
Size _minSize;
set minSize(Size value) {
if (_minSize == value) {
return;
}
_minSize = value;
markNeedsLayout();
}
@override
double computeMinIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMinIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMinIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
if (child != null) {
return math.max(child!.getMaxIntrinsicWidth(height), minSize.width);
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
if (child != null) {
return math.max(child!.getMaxIntrinsicHeight(width), minSize.height);
}
return 0.0;
}
Size _computeSize({required BoxConstraints constraints, required ChildLayouter layoutChild}) {
if (child != null) {
final Size childSize = layoutChild(child!, constraints);
final double height = math.max(childSize.width, minSize.width);
final double width = math.max(childSize.height, minSize.height);
return constraints.constrain(Size(height, width));
}
return Size.zero;
}
@override
Size computeDryLayout(BoxConstraints constraints) {
return _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.dryLayoutChild,
);
}
@override
void performLayout() {
size = _computeSize(
constraints: constraints,
layoutChild: ChildLayoutHelper.layoutChild,
);
if (child != null) {
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Alignment.center.alongOffset(size - child!.size as Offset);
}
}
@override
bool hitTest(BoxHitTestResult result, { required Offset position }) {
if (super.hitTest(result, position: position)) {
return true;
}
final Offset center = child!.size.center(Offset.zero);
return result.addWithRawTransform(
transform: MatrixUtils.forceToPoint(center),
position: center,
hitTest: (BoxHitTestResult result, Offset position) {
assert(position == center);
return child!.hitTest(result, position: center);
},
);
}
}
| flutter/packages/flutter/lib/src/material/button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/button.dart",
"repo_id": "flutter",
"token_count": 6097
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'package:material_color_utilities/material_color_utilities.dart';
import 'colors.dart';
import 'theme_data.dart';
/// {@template flutter.material.color_scheme.ColorScheme}
/// A set of 45 colors based on the
/// [Material spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// that can be used to configure the color properties of most components.
/// {@endtemplate}
///
/// ### Colors in Material 3
///
/// {@macro flutter.material.colors.colorRoles}
///
/// The main accent color groups in the scheme are [primary], [secondary],
/// and [tertiary].
///
/// * Primary colors are used for key components across the UI, such as the FAB,
/// prominent buttons, and active states.
///
/// * Secondary colors are used for less prominent components in the UI, such as
/// filter chips, while expanding the opportunity for color expression.
///
/// * Tertiary colors are used for contrasting accents that can be used to
/// balance primary and secondary colors or bring heightened attention to
/// an element, such as an input field. The tertiary colors are left
/// for makers to use at their discretion and are intended to support
/// broader color expression in products.
///
/// Each accent color group (primary, secondary and tertiary) includes '-Fixed'
/// '-Dim' color roles, such as [primaryFixed] and [primaryFixedDim]. Fixed roles
/// are appropriate to use in places where Container roles are normally used,
/// but they stay the same color between light and dark themes. The '-Dim' roles
/// provide a stronger, more emphasized color with the same fixed behavior.
///
/// The remaining colors of the scheme are composed of neutral colors used for
/// backgrounds and surfaces, as well as specific colors for errors, dividers
/// and shadows. Surface colors are used for backgrounds and large, low-emphasis
/// areas of the screen.
///
/// Material 3 also introduces tone-based surfaces and surface containers.
/// They replace the old opacity-based model which applied a tinted overlay on
/// top of surfaces based on their elevation. These colors include: [surfaceBright],
/// [surfaceDim], [surfaceContainerLowest], [surfaceContainerLow], [surfaceContainer],
/// [surfaceContainerHigh], and [surfaceContainerHighest].
///
/// Many of the colors have matching 'on' colors, which are used for drawing
/// content on top of the matching color. For example, if something is using
/// [primary] for a background color, [onPrimary] would be used to paint text
/// and icons on top of it. For this reason, the 'on' colors should have a
/// contrast ratio with their matching colors of at least 4.5:1 in order to
/// be readable. On '-FixedVariant' roles, such as [onPrimaryFixedVariant],
/// also have the same color between light and dark themes, but compared
/// with on '-Fixed' roles, such as [onPrimaryFixed], they provide a
/// lower-emphasis option for text and icons.
///
/// {@tool dartpad}
/// This example shows all Material [ColorScheme] roles in light and dark
/// brightnesses.
///
/// ** See code in examples/api/lib/material/color_scheme/color_scheme.0.dart **
/// {@end-tool}
///
/// ### Setting Colors in Flutter
///
///{@macro flutter.material.colors.settingColors}
@immutable
class ColorScheme with Diagnosticable {
/// Create a ColorScheme instance from the given colors.
///
/// [ColorScheme.fromSeed] can be used as a simpler way to create a full
/// color scheme derived from a single seed color.
///
/// For the color parameters that are nullable, it is still recommended
/// that applications provide values for them. They are only nullable due
/// to backwards compatibility concerns.
///
/// If a color is not provided, the closest fallback color from the given
/// colors will be used for it (e.g. [primaryContainer] will default
/// to [primary]). Material Design 3 makes use of these colors for many
/// component defaults, so for the best results the application should
/// supply colors for all the parameters. An easy way to ensure this is to
/// use [ColorScheme.fromSeed] to generate a full set of colors.
///
/// During the migration to Material Design 3, if an app's
/// [ThemeData.useMaterial3] is false, then components will only
/// use the following colors for defaults:
///
/// * [primary]
/// * [onPrimary]
/// * [secondary]
/// * [onSecondary]
/// * [error]
/// * [onError]
/// * [surface]
/// * [onSurface]
/// DEPRECATED:
/// * [background]
/// * [onBackground]
const ColorScheme({
required this.brightness,
required this.primary,
required this.onPrimary,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
required this.secondary,
required this.onSecondary,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
required this.error,
required this.onError,
Color? errorContainer,
Color? onErrorContainer,
required this.surface,
required this.onSurface,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) : _primaryContainer = primaryContainer,
_onPrimaryContainer = onPrimaryContainer,
_primaryFixed = primaryFixed,
_primaryFixedDim = primaryFixedDim,
_onPrimaryFixed = onPrimaryFixed,
_onPrimaryFixedVariant = onPrimaryFixedVariant,
_secondaryContainer = secondaryContainer,
_onSecondaryContainer = onSecondaryContainer,
_secondaryFixed = secondaryFixed,
_secondaryFixedDim = secondaryFixedDim,
_onSecondaryFixed = onSecondaryFixed,
_onSecondaryFixedVariant = onSecondaryFixedVariant,
_tertiary = tertiary,
_onTertiary = onTertiary,
_tertiaryContainer = tertiaryContainer,
_onTertiaryContainer = onTertiaryContainer,
_tertiaryFixed = tertiaryFixed,
_tertiaryFixedDim = tertiaryFixedDim,
_onTertiaryFixed = onTertiaryFixed,
_onTertiaryFixedVariant = onTertiaryFixedVariant,
_errorContainer = errorContainer,
_onErrorContainer = onErrorContainer,
_surfaceDim = surfaceDim,
_surfaceBright = surfaceBright,
_surfaceContainerLowest = surfaceContainerLowest,
_surfaceContainerLow = surfaceContainerLow,
_surfaceContainer = surfaceContainer,
_surfaceContainerHigh = surfaceContainerHigh,
_surfaceContainerHighest = surfaceContainerHighest,
_onSurfaceVariant = onSurfaceVariant,
_outline = outline,
_outlineVariant = outlineVariant,
_shadow = shadow,
_scrim = scrim,
_inverseSurface = inverseSurface,
_onInverseSurface = onInverseSurface,
_inversePrimary = inversePrimary,
_surfaceTint = surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
_background = background,
_onBackground = onBackground,
_surfaceVariant = surfaceVariant;
/// Generate a [ColorScheme] derived from the given `seedColor`.
///
/// Using the seedColor as a starting point, a set of tonal palettes are
/// constructed. These tonal palettes are based on the Material 3 Color
/// system and provide all the needed colors for a [ColorScheme]. These
/// colors are designed to work well together and meet contrast
/// requirements for accessibility.
///
/// If any of the optional color parameters are non-null they will be
/// used in place of the generated colors for that field in the resulting
/// color scheme. This allows apps to override specific colors for their
/// needs.
///
/// Given the nature of the algorithm, the seedColor may not wind up as
/// one of the ColorScheme colors.
///
/// See also:
///
/// * <https://m3.material.io/styles/color/the-color-system/color-roles>, the
/// Material 3 Color system specification.
/// * <https://pub.dev/packages/material_color_utilities>, the package
/// used to generate the tonal palettes needed for the scheme.
factory ColorScheme.fromSeed({
required Color seedColor,
Brightness brightness = Brightness.light,
Color? primary,
Color? onPrimary,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
Color? secondary,
Color? onSecondary,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
Color? error,
Color? onError,
Color? errorContainer,
Color? onErrorContainer,
Color? outline,
Color? outlineVariant,
Color? surface,
Color? onSurface,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? shadow,
Color? scrim,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) {
final SchemeTonalSpot scheme;
switch (brightness) {
case Brightness.light:
scheme = SchemeTonalSpot(sourceColorHct: Hct.fromInt(seedColor.value), isDark: false, contrastLevel: 0.0);
case Brightness.dark:
scheme = SchemeTonalSpot(sourceColorHct: Hct.fromInt(seedColor.value), isDark: true, contrastLevel: 0.0);
}
return ColorScheme(
primary: primary ?? Color(MaterialDynamicColors.primary.getArgb(scheme)),
onPrimary: onPrimary ?? Color(MaterialDynamicColors.onPrimary.getArgb(scheme)),
primaryContainer: primaryContainer ?? Color(MaterialDynamicColors.primaryContainer.getArgb(scheme)),
onPrimaryContainer: onPrimaryContainer ?? Color(MaterialDynamicColors.onPrimaryContainer.getArgb(scheme)),
primaryFixed: primaryFixed ?? Color(MaterialDynamicColors.primaryFixed.getArgb(scheme)),
primaryFixedDim: primaryFixedDim ?? Color(MaterialDynamicColors.primaryFixedDim.getArgb(scheme)),
onPrimaryFixed: onPrimaryFixed ?? Color(MaterialDynamicColors.onPrimaryFixed.getArgb(scheme)),
onPrimaryFixedVariant: onPrimaryFixedVariant ?? Color(MaterialDynamicColors.onPrimaryFixedVariant.getArgb(scheme)),
secondary: secondary ?? Color(MaterialDynamicColors.secondary.getArgb(scheme)),
onSecondary: onSecondary ?? Color(MaterialDynamicColors.onSecondary.getArgb(scheme)),
secondaryContainer: secondaryContainer ?? Color(MaterialDynamicColors.secondaryContainer.getArgb(scheme)),
onSecondaryContainer: onSecondaryContainer ?? Color(MaterialDynamicColors.onSecondaryContainer.getArgb(scheme)),
secondaryFixed: secondaryFixed ?? Color(MaterialDynamicColors.secondaryFixed.getArgb(scheme)),
secondaryFixedDim: secondaryFixedDim ?? Color(MaterialDynamicColors.secondaryFixedDim.getArgb(scheme)),
onSecondaryFixed: onSecondaryFixed ?? Color(MaterialDynamicColors.onSecondaryFixed.getArgb(scheme)),
onSecondaryFixedVariant: onSecondaryFixedVariant ?? Color(MaterialDynamicColors.onSecondaryFixedVariant.getArgb(scheme)),
tertiary: tertiary ?? Color(MaterialDynamicColors.tertiary.getArgb(scheme)),
onTertiary: onTertiary ?? Color(MaterialDynamicColors.onTertiary.getArgb(scheme)),
tertiaryContainer: tertiaryContainer ?? Color(MaterialDynamicColors.tertiaryContainer.getArgb(scheme)),
onTertiaryContainer: onTertiaryContainer ?? Color(MaterialDynamicColors.onTertiaryContainer.getArgb(scheme)),
tertiaryFixed: tertiaryFixed ?? Color(MaterialDynamicColors.tertiaryFixed.getArgb(scheme)),
tertiaryFixedDim: tertiaryFixedDim ?? Color(MaterialDynamicColors.tertiaryFixedDim.getArgb(scheme)),
onTertiaryFixed: onTertiaryFixed ?? Color(MaterialDynamicColors.onTertiaryFixed.getArgb(scheme)),
onTertiaryFixedVariant: onTertiaryFixedVariant ?? Color(MaterialDynamicColors.onTertiaryFixedVariant.getArgb(scheme)),
error: error ?? Color(MaterialDynamicColors.error.getArgb(scheme)),
onError: onError ?? Color(MaterialDynamicColors.onError.getArgb(scheme)),
errorContainer: errorContainer ?? Color(MaterialDynamicColors.errorContainer.getArgb(scheme)),
onErrorContainer: onErrorContainer ?? Color(MaterialDynamicColors.onErrorContainer.getArgb(scheme)),
outline: outline ?? Color(MaterialDynamicColors.outline.getArgb(scheme)),
outlineVariant: outlineVariant ?? Color(MaterialDynamicColors.outlineVariant.getArgb(scheme)),
surface: surface ?? Color(MaterialDynamicColors.surface.getArgb(scheme)),
surfaceDim: surfaceDim ?? Color(MaterialDynamicColors.surfaceDim.getArgb(scheme)),
surfaceBright: surfaceBright ?? Color(MaterialDynamicColors.surfaceBright.getArgb(scheme)),
surfaceContainerLowest: surfaceContainerLowest ?? Color(MaterialDynamicColors.surfaceContainerLowest.getArgb(scheme)),
surfaceContainerLow: surfaceContainerLow ?? Color(MaterialDynamicColors.surfaceContainerLow.getArgb(scheme)),
surfaceContainer: surfaceContainer ?? Color(MaterialDynamicColors.surfaceContainer.getArgb(scheme)),
surfaceContainerHigh: surfaceContainerHigh ?? Color(MaterialDynamicColors.surfaceContainerHigh.getArgb(scheme)),
surfaceContainerHighest: surfaceContainerHighest ?? Color(MaterialDynamicColors.surfaceContainerHighest.getArgb(scheme)),
onSurface: onSurface ?? Color(MaterialDynamicColors.onSurface.getArgb(scheme)),
onSurfaceVariant: onSurfaceVariant ?? Color(MaterialDynamicColors.onSurfaceVariant.getArgb(scheme)),
inverseSurface: inverseSurface ?? Color(MaterialDynamicColors.inverseSurface.getArgb(scheme)),
onInverseSurface: onInverseSurface ?? Color(MaterialDynamicColors.inverseOnSurface.getArgb(scheme)),
inversePrimary: inversePrimary ?? Color(MaterialDynamicColors.inversePrimary.getArgb(scheme)),
shadow: shadow ?? Color(MaterialDynamicColors.shadow.getArgb(scheme)),
scrim: scrim ?? Color(MaterialDynamicColors.scrim.getArgb(scheme)),
surfaceTint: surfaceTint ?? Color(MaterialDynamicColors.primary.getArgb(scheme)),
brightness: brightness,
// DEPRECATED (newest deprecations at the bottom)
background: background ?? Color(MaterialDynamicColors.background.getArgb(scheme)),
onBackground: onBackground ?? Color(MaterialDynamicColors.onBackground.getArgb(scheme)),
surfaceVariant: surfaceVariant ?? Color(MaterialDynamicColors.surfaceVariant.getArgb(scheme)),
);
}
/// Create a light ColorScheme based on a purple primary color that matches the
/// [baseline Material 2 color scheme](https://material.io/design/color/the-color-system.html#color-theme-creation).
///
/// This constructor shouldn't be used to update the Material 3 color scheme.
///
/// For Material 3, use [ColorScheme.fromSeed] to create a color scheme
/// from a single seed color based on the Material 3 color system.
///
/// {@tool snippet}
/// This example demonstrates how to create a color scheme similar to [ColorScheme.light]
/// using the [ColorScheme.fromSeed] constructor:
///
/// ```dart
/// colorScheme: ColorScheme.fromSeed(seedColor: const Color(0xff6200ee)).copyWith(
/// primaryContainer: const Color(0xff6200ee),
/// onPrimaryContainer: Colors.white,
/// secondaryContainer: const Color(0xff03dac6),
/// onSecondaryContainer: Colors.black,
/// error: const Color(0xffb00020),
/// onError: Colors.white,
/// ),
/// ```
/// {@end-tool}
const ColorScheme.light({
this.brightness = Brightness.light,
this.primary = const Color(0xff6200ee),
this.onPrimary = Colors.white,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
this.secondary = const Color(0xff03dac6),
this.onSecondary = Colors.black,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
this.error = const Color(0xffb00020),
this.onError = Colors.white,
Color? errorContainer,
Color? onErrorContainer,
this.surface = Colors.white,
this.onSurface = Colors.black,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background = Colors.white,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground = Colors.black,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant
}) : _primaryContainer = primaryContainer,
_onPrimaryContainer = onPrimaryContainer,
_primaryFixed = primaryFixed,
_primaryFixedDim = primaryFixedDim,
_onPrimaryFixed = onPrimaryFixed,
_onPrimaryFixedVariant = onPrimaryFixedVariant,
_secondaryContainer = secondaryContainer,
_onSecondaryContainer = onSecondaryContainer,
_secondaryFixed = secondaryFixed,
_secondaryFixedDim = secondaryFixedDim,
_onSecondaryFixed = onSecondaryFixed,
_onSecondaryFixedVariant = onSecondaryFixedVariant,
_tertiary = tertiary,
_onTertiary = onTertiary,
_tertiaryContainer = tertiaryContainer,
_onTertiaryContainer = onTertiaryContainer,
_tertiaryFixed = tertiaryFixed,
_tertiaryFixedDim = tertiaryFixedDim,
_onTertiaryFixed = onTertiaryFixed,
_onTertiaryFixedVariant = onTertiaryFixedVariant,
_errorContainer = errorContainer,
_onErrorContainer = onErrorContainer,
_surfaceDim = surfaceDim,
_surfaceBright = surfaceBright,
_surfaceContainerLowest = surfaceContainerLowest,
_surfaceContainerLow = surfaceContainerLow,
_surfaceContainer = surfaceContainer,
_surfaceContainerHigh = surfaceContainerHigh,
_surfaceContainerHighest = surfaceContainerHighest,
_onSurfaceVariant = onSurfaceVariant,
_outline = outline,
_outlineVariant = outlineVariant,
_shadow = shadow,
_scrim = scrim,
_inverseSurface = inverseSurface,
_onInverseSurface = onInverseSurface,
_inversePrimary = inversePrimary,
_surfaceTint = surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
_background = background,
_onBackground = onBackground,
_surfaceVariant = surfaceVariant;
/// Create the dark color scheme that matches the
/// [baseline Material 2 color scheme](https://material.io/design/color/dark-theme.html#ui-application).
///
/// This constructor shouldn't be used to update the Material 3 color scheme.
///
/// For Material 3, use [ColorScheme.fromSeed] to create a color scheme
/// from a single seed color based on the Material 3 color system.
/// Override the `brightness` property of [ColorScheme.fromSeed] to create a
/// dark color scheme.
///
/// {@tool snippet}
/// This example demonstrates how to create a color scheme similar to [ColorScheme.dark]
/// using the [ColorScheme.fromSeed] constructor:
///
/// ```dart
/// colorScheme: ColorScheme.fromSeed(
/// seedColor: const Color(0xffbb86fc),
/// brightness: Brightness.dark,
/// ).copyWith(
/// primaryContainer: const Color(0xffbb86fc),
/// onPrimaryContainer: Colors.black,
/// secondaryContainer: const Color(0xff03dac6),
/// onSecondaryContainer: Colors.black,
/// error: const Color(0xffcf6679),
/// onError: Colors.black,
/// ),
/// ```
/// {@end-tool}
const ColorScheme.dark({
this.brightness = Brightness.dark,
this.primary = const Color(0xffbb86fc),
this.onPrimary = Colors.black,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
this.secondary = const Color(0xff03dac6),
this.onSecondary = Colors.black,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
this.error = const Color(0xffcf6679),
this.onError = Colors.black,
Color? errorContainer,
Color? onErrorContainer,
this.surface = const Color(0xff121212),
this.onSurface = Colors.white,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background = const Color(0xff121212),
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground = Colors.white,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) : _primaryContainer = primaryContainer,
_onPrimaryContainer = onPrimaryContainer,
_primaryFixed = primaryFixed,
_primaryFixedDim = primaryFixedDim,
_onPrimaryFixed = onPrimaryFixed,
_onPrimaryFixedVariant = onPrimaryFixedVariant,
_secondaryContainer = secondaryContainer,
_onSecondaryContainer = onSecondaryContainer,
_secondaryFixed = secondaryFixed,
_secondaryFixedDim = secondaryFixedDim,
_onSecondaryFixed = onSecondaryFixed,
_onSecondaryFixedVariant = onSecondaryFixedVariant,
_tertiary = tertiary,
_onTertiary = onTertiary,
_tertiaryContainer = tertiaryContainer,
_onTertiaryContainer = onTertiaryContainer,
_tertiaryFixed = tertiaryFixed,
_tertiaryFixedDim = tertiaryFixedDim,
_onTertiaryFixed = onTertiaryFixed,
_onTertiaryFixedVariant = onTertiaryFixedVariant,
_errorContainer = errorContainer,
_onErrorContainer = onErrorContainer,
_surfaceDim = surfaceDim,
_surfaceBright = surfaceBright,
_surfaceContainerLowest = surfaceContainerLowest,
_surfaceContainerLow = surfaceContainerLow,
_surfaceContainer = surfaceContainer,
_surfaceContainerHigh = surfaceContainerHigh,
_surfaceContainerHighest = surfaceContainerHighest,
_onSurfaceVariant = onSurfaceVariant,
_outline = outline,
_outlineVariant = outlineVariant,
_shadow = shadow,
_scrim = scrim,
_inverseSurface = inverseSurface,
_onInverseSurface = onInverseSurface,
_inversePrimary = inversePrimary,
_surfaceTint = surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
_background = background,
_onBackground = onBackground,
_surfaceVariant = surfaceVariant;
/// Create a high contrast ColorScheme based on a purple primary color that
/// matches the [baseline Material 2 color scheme](https://material.io/design/color/the-color-system.html#color-theme-creation).
///
/// This constructor shouldn't be used to update the Material 3 color scheme.
///
/// For Material 3, use [ColorScheme.fromSeed] to create a color scheme
/// from a single seed color based on the Material 3 color system.
///
/// {@tool snippet}
/// This example demonstrates how to create a color scheme similar to [ColorScheme.highContrastLight]
/// using the [ColorScheme.fromSeed] constructor:
///
/// ```dart
/// colorScheme: ColorScheme.fromSeed(seedColor: const Color(0xff0000ba)).copyWith(
/// primaryContainer: const Color(0xff0000ba),
/// onPrimaryContainer: Colors.white,
/// secondaryContainer: const Color(0xff66fff9),
/// onSecondaryContainer: Colors.black,
/// error: const Color(0xff790000),
/// onError: Colors.white,
/// ),
/// ```
/// {@end-tool}
const ColorScheme.highContrastLight({
this.brightness = Brightness.light,
this.primary = const Color(0xff0000ba),
this.onPrimary = Colors.white,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
this.secondary = const Color(0xff66fff9),
this.onSecondary = Colors.black,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
this.error = const Color(0xff790000),
this.onError = Colors.white,
Color? errorContainer,
Color? onErrorContainer,
this.surface = Colors.white,
this.onSurface = Colors.black,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background = Colors.white,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground = Colors.black,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) : _primaryContainer = primaryContainer,
_onPrimaryContainer = onPrimaryContainer,
_primaryFixed = primaryFixed,
_primaryFixedDim = primaryFixedDim,
_onPrimaryFixed = onPrimaryFixed,
_onPrimaryFixedVariant = onPrimaryFixedVariant,
_secondaryContainer = secondaryContainer,
_onSecondaryContainer = onSecondaryContainer,
_secondaryFixed = secondaryFixed,
_secondaryFixedDim = secondaryFixedDim,
_onSecondaryFixed = onSecondaryFixed,
_onSecondaryFixedVariant = onSecondaryFixedVariant,
_tertiary = tertiary,
_onTertiary = onTertiary,
_tertiaryContainer = tertiaryContainer,
_onTertiaryContainer = onTertiaryContainer,
_tertiaryFixed = tertiaryFixed,
_tertiaryFixedDim = tertiaryFixedDim,
_onTertiaryFixed = onTertiaryFixed,
_onTertiaryFixedVariant = onTertiaryFixedVariant,
_errorContainer = errorContainer,
_onErrorContainer = onErrorContainer,
_surfaceDim = surfaceDim,
_surfaceBright = surfaceBright,
_surfaceContainerLowest = surfaceContainerLowest,
_surfaceContainerLow = surfaceContainerLow,
_surfaceContainer = surfaceContainer,
_surfaceContainerHigh = surfaceContainerHigh,
_surfaceContainerHighest = surfaceContainerHighest,
_onSurfaceVariant = onSurfaceVariant,
_outline = outline,
_outlineVariant = outlineVariant,
_shadow = shadow,
_scrim = scrim,
_inverseSurface = inverseSurface,
_onInverseSurface = onInverseSurface,
_inversePrimary = inversePrimary,
_surfaceTint = surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
_background = background,
_onBackground = onBackground,
_surfaceVariant = surfaceVariant;
/// Create a high contrast ColorScheme based on the dark
/// [baseline Material 2 color scheme](https://material.io/design/color/dark-theme.html#ui-application).
///
/// This constructor shouldn't be used to update the Material 3 color scheme.
///
/// For Material 3, use [ColorScheme.fromSeed] to create a color scheme
/// from a single seed color based on the Material 3 color system.
/// Override the `brightness` property of [ColorScheme.fromSeed] to create a
/// dark color scheme.
///
/// {@tool snippet}
/// This example demonstrates how to create a color scheme similar to [ColorScheme.highContrastDark]
/// using the [ColorScheme.fromSeed] constructor:
///
/// ```dart
/// colorScheme: ColorScheme.fromSeed(
/// seedColor: const Color(0xffefb7ff),
/// brightness: Brightness.dark,
/// ).copyWith(
/// primaryContainer: const Color(0xffefb7ff),
/// onPrimaryContainer: Colors.black,
/// secondaryContainer: const Color(0xff66fff9),
/// onSecondaryContainer: Colors.black,
/// error: const Color(0xff9b374d),
/// onError: Colors.white,
/// ),
/// ```
/// {@end-tool}
const ColorScheme.highContrastDark({
this.brightness = Brightness.dark,
this.primary = const Color(0xffefb7ff),
this.onPrimary = Colors.black,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
this.secondary = const Color(0xff66fff9),
this.onSecondary = Colors.black,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
this.error = const Color(0xff9b374d),
this.onError = Colors.black,
Color? errorContainer,
Color? onErrorContainer,
this.surface = const Color(0xff121212),
this.onSurface = Colors.white,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background = const Color(0xff121212),
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground = Colors.white,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) : _primaryContainer = primaryContainer,
_onPrimaryContainer = onPrimaryContainer,
_primaryFixed = primaryFixed,
_primaryFixedDim = primaryFixedDim,
_onPrimaryFixed = onPrimaryFixed,
_onPrimaryFixedVariant = onPrimaryFixedVariant,
_secondaryContainer = secondaryContainer,
_onSecondaryContainer = onSecondaryContainer,
_secondaryFixed = secondaryFixed,
_secondaryFixedDim = secondaryFixedDim,
_onSecondaryFixed = onSecondaryFixed,
_onSecondaryFixedVariant = onSecondaryFixedVariant,
_tertiary = tertiary,
_onTertiary = onTertiary,
_tertiaryContainer = tertiaryContainer,
_onTertiaryContainer = onTertiaryContainer,
_tertiaryFixed = tertiaryFixed,
_tertiaryFixedDim = tertiaryFixedDim,
_onTertiaryFixed = onTertiaryFixed,
_onTertiaryFixedVariant = onTertiaryFixedVariant,
_errorContainer = errorContainer,
_onErrorContainer = onErrorContainer,
_surfaceDim = surfaceDim,
_surfaceBright = surfaceBright,
_surfaceContainerLowest = surfaceContainerLowest,
_surfaceContainerLow = surfaceContainerLow,
_surfaceContainer = surfaceContainer,
_surfaceContainerHigh = surfaceContainerHigh,
_surfaceContainerHighest = surfaceContainerHighest,
_onSurfaceVariant = onSurfaceVariant,
_outline = outline,
_outlineVariant = outlineVariant,
_shadow = shadow,
_scrim = scrim,
_inverseSurface = inverseSurface,
_onInverseSurface = onInverseSurface,
_inversePrimary = inversePrimary,
_surfaceTint = surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
_background = background,
_onBackground = onBackground,
_surfaceVariant = surfaceVariant;
/// Creates a color scheme from a [MaterialColor] swatch.
///
/// In Material 3, this constructor is ignored by [ThemeData] when creating
/// its default color scheme. Instead, [ThemeData] uses [ColorScheme.fromSeed]
/// to create its default color scheme. This constructor shouldn't be used
/// to update the Material 3 color scheme. It will be phased out gradually;
/// see https://github.com/flutter/flutter/issues/120064 for more details.
///
/// If [ThemeData.useMaterial3] is false, then this constructor is used by
/// [ThemeData] to create its default color scheme.
factory ColorScheme.fromSwatch({
MaterialColor primarySwatch = Colors.blue,
Color? accentColor,
Color? cardColor,
Color? backgroundColor,
Color? errorColor,
Brightness brightness = Brightness.light,
}) {
final bool isDark = brightness == Brightness.dark;
final bool primaryIsDark = _brightnessFor(primarySwatch) == Brightness.dark;
final Color secondary = accentColor ?? (isDark ? Colors.tealAccent[200]! : primarySwatch);
final bool secondaryIsDark = _brightnessFor(secondary) == Brightness.dark;
return ColorScheme(
primary: primarySwatch,
secondary: secondary,
surface: cardColor ?? (isDark ? Colors.grey[800]! : Colors.white),
error: errorColor ?? Colors.red[700]!,
onPrimary: primaryIsDark ? Colors.white : Colors.black,
onSecondary: secondaryIsDark ? Colors.white : Colors.black,
onSurface: isDark ? Colors.white : Colors.black,
onError: isDark ? Colors.black : Colors.white,
brightness: brightness,
// DEPRECATED (newest deprecations at the bottom)
background: backgroundColor ?? (isDark ? Colors.grey[700]! : primarySwatch[200]!),
onBackground: primaryIsDark ? Colors.white : Colors.black,
);
}
static Brightness _brightnessFor(Color color) => ThemeData.estimateBrightnessForColor(color);
/// The overall brightness of this color scheme.
final Brightness brightness;
/// The color displayed most frequently across your app’s screens and components.
final Color primary;
/// A color that's clearly legible when drawn on [primary].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [primary] and [onPrimary] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
final Color onPrimary;
final Color? _primaryContainer;
/// A color used for elements needing less emphasis than [primary].
Color get primaryContainer => _primaryContainer ?? primary;
final Color? _onPrimaryContainer;
/// A color that's clearly legible when drawn on [primaryContainer].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [primaryContainer] and [onPrimaryContainer] of at least 4.5:1
/// is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onPrimaryContainer => _onPrimaryContainer ?? onPrimary;
final Color? _primaryFixed;
/// A substitute for [primaryContainer] that's the same color for the dark
/// and light themes.
Color get primaryFixed => _primaryFixed ?? primary;
final Color? _primaryFixedDim;
/// A color used for elements needing more emphasis than [primaryFixed].
Color get primaryFixedDim => _primaryFixedDim ?? primary;
final Color? _onPrimaryFixed;
/// A color that is used for text and icons that exist on top of elements having
/// [primaryFixed] color.
Color get onPrimaryFixed => _onPrimaryFixed ?? onPrimary;
final Color? _onPrimaryFixedVariant;
/// A color that provides a lower-emphasis option for text and icons than
/// [onPrimaryFixed].
Color get onPrimaryFixedVariant => _onPrimaryFixedVariant ?? onPrimary;
/// An accent color used for less prominent components in the UI, such as
/// filter chips, while expanding the opportunity for color expression.
final Color secondary;
/// A color that's clearly legible when drawn on [secondary].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [secondary] and [onSecondary] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
final Color onSecondary;
final Color? _secondaryContainer;
/// A color used for elements needing less emphasis than [secondary].
Color get secondaryContainer => _secondaryContainer ?? secondary;
final Color? _onSecondaryContainer;
/// A color that's clearly legible when drawn on [secondaryContainer].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [secondaryContainer] and [onSecondaryContainer] of at least 4.5:1 is
/// recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onSecondaryContainer => _onSecondaryContainer ?? onSecondary;
final Color? _secondaryFixed;
/// A substitute for [secondaryContainer] that's the same color for the dark
/// and light themes.
Color get secondaryFixed => _secondaryFixed ?? secondary;
final Color? _secondaryFixedDim;
/// A color used for elements needing more emphasis than [secondaryFixed].
Color get secondaryFixedDim => _secondaryFixedDim ?? secondary;
final Color? _onSecondaryFixed;
/// A color that is used for text and icons that exist on top of elements having
/// [secondaryFixed] color.
Color get onSecondaryFixed => _onSecondaryFixed ?? onSecondary;
final Color? _onSecondaryFixedVariant;
/// A color that provides a lower-emphasis option for text and icons than
/// [onSecondaryFixed].
Color get onSecondaryFixedVariant => _onSecondaryFixedVariant ?? onSecondary;
final Color? _tertiary;
/// A color used as a contrasting accent that can balance [primary]
/// and [secondary] colors or bring heightened attention to an element,
/// such as an input field.
Color get tertiary => _tertiary ?? secondary;
final Color? _onTertiary;
/// A color that's clearly legible when drawn on [tertiary].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [tertiary] and [onTertiary] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onTertiary => _onTertiary ?? onSecondary;
final Color? _tertiaryContainer;
/// A color used for elements needing less emphasis than [tertiary].
Color get tertiaryContainer => _tertiaryContainer ?? tertiary;
final Color? _onTertiaryContainer;
/// A color that's clearly legible when drawn on [tertiaryContainer].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [tertiaryContainer] and [onTertiaryContainer] of at least 4.5:1 is
/// recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onTertiaryContainer => _onTertiaryContainer ?? onTertiary;
final Color? _tertiaryFixed;
/// A substitute for [tertiaryContainer] that's the same color for dark
/// and light themes.
Color get tertiaryFixed => _tertiaryFixed ?? tertiary;
final Color? _tertiaryFixedDim;
/// A color used for elements needing more emphasis than [tertiaryFixed].
Color get tertiaryFixedDim => _tertiaryFixedDim ?? tertiary;
final Color? _onTertiaryFixed;
/// A color that is used for text and icons that exist on top of elements having
/// [tertiaryFixed] color.
Color get onTertiaryFixed => _onTertiaryFixed ?? onTertiary;
final Color? _onTertiaryFixedVariant;
/// A color that provides a lower-emphasis option for text and icons than
/// [onTertiaryFixed].
Color get onTertiaryFixedVariant => _onTertiaryFixedVariant ?? onTertiary;
/// The color to use for input validation errors, e.g. for
/// [InputDecoration.errorText].
final Color error;
/// A color that's clearly legible when drawn on [error].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [error] and [onError] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
final Color onError;
final Color? _errorContainer;
/// A color used for error elements needing less emphasis than [error].
Color get errorContainer => _errorContainer ?? error;
final Color? _onErrorContainer;
/// A color that's clearly legible when drawn on [errorContainer].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [errorContainer] and [onErrorContainer] of at least 4.5:1 is
/// recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onErrorContainer => _onErrorContainer ?? onError;
/// The background color for widgets like [Card].
final Color surface;
/// A color that's clearly legible when drawn on [surface].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [surface] and [onSurface] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
final Color onSurface;
final Color? _surfaceVariant;
/// A color variant of [surface] that can be used for differentiation against
/// a component using [surface].
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color get surfaceVariant => _surfaceVariant ?? surface;
final Color? _surfaceDim;
/// A color that's always darkest in the dark or light theme.
Color get surfaceDim => _surfaceDim ?? surface;
final Color? _surfaceBright;
/// A color that's always the lightest in the dark or light theme.
Color get surfaceBright => _surfaceBright ?? surface;
final Color? _surfaceContainerLowest;
/// A surface container color with the lightest tone and the least emphasis
/// relative to the surface.
Color get surfaceContainerLowest => _surfaceContainerLowest ?? surface;
final Color? _surfaceContainerLow;
/// A surface container color with a lighter tone that creates less emphasis
/// than [surfaceContainer] but more emphasis than [surfaceContainerLowest].
Color get surfaceContainerLow => _surfaceContainerLow ?? surface;
final Color? _surfaceContainer;
/// A recommended color role for a distinct area within the surface.
///
/// Surface container color roles are independent of elevation. They replace the old
/// opacity-based model which applied a tinted overlay on top of
/// surfaces based on their elevation.
///
/// Surface container colors include [surfaceContainerLowest], [surfaceContainerLow],
/// [surfaceContainer], [surfaceContainerHigh] and [surfaceContainerHighest].
Color get surfaceContainer => _surfaceContainer ?? surface;
final Color? _surfaceContainerHigh;
/// A surface container color with a darker tone. It is used to create more
/// emphasis than [surfaceContainer] but less emphasis than [surfaceContainerHighest].
Color get surfaceContainerHigh => _surfaceContainerHigh ?? surface;
final Color? _surfaceContainerHighest;
/// A surface container color with the darkest tone. It is used to create the
/// most emphasis against the surface.
Color get surfaceContainerHighest => _surfaceContainerHighest ?? surface;
final Color? _onSurfaceVariant;
/// A color that's clearly legible when drawn on [surfaceVariant].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [surfaceVariant] and [onSurfaceVariant] of at least 4.5:1 is
/// recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onSurfaceVariant => _onSurfaceVariant ?? onSurface;
final Color? _outline;
/// A utility color that creates boundaries and emphasis to improve usability.
Color get outline => _outline ?? onBackground;
final Color? _outlineVariant;
/// A utility color that creates boundaries for decorative elements when a
/// 3:1 contrast isn’t required, such as for dividers or decorative elements.
Color get outlineVariant => _outlineVariant ?? onBackground;
final Color? _shadow;
/// A color use to paint the drop shadows of elevated components.
Color get shadow => _shadow ?? const Color(0xff000000);
final Color? _scrim;
/// A color use to paint the scrim around of modal components.
Color get scrim => _scrim ?? const Color(0xff000000);
final Color? _inverseSurface;
/// A surface color used for displaying the reverse of what’s seen in the
/// surrounding UI, for example in a SnackBar to bring attention to
/// an alert.
Color get inverseSurface => _inverseSurface ?? onSurface;
final Color? _onInverseSurface;
/// A color that's clearly legible when drawn on [inverseSurface].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [inverseSurface] and [onInverseSurface] of at least 4.5:1 is
/// recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
Color get onInverseSurface => _onInverseSurface ?? surface;
final Color? _inversePrimary;
/// An accent color used for displaying a highlight color on [inverseSurface]
/// backgrounds, like button text in a SnackBar.
Color get inversePrimary => _inversePrimary ?? onPrimary;
final Color? _surfaceTint;
/// A color used as an overlay on a surface color to indicate a component's
/// elevation.
Color get surfaceTint => _surfaceTint ?? primary;
final Color? _background;
/// A color that typically appears behind scrollable content.
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color get background => _background ?? surface;
final Color? _onBackground;
/// A color that's clearly legible when drawn on [background].
///
/// To ensure that an app is accessible, a contrast ratio between
/// [background] and [onBackground] of at least 4.5:1 is recommended. See
/// <https://www.w3.org/TR/UNDERSTANDING-WCAG20/visual-audio-contrast-contrast.html>.
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color get onBackground => _onBackground ?? onSurface;
/// Creates a copy of this color scheme with the given fields
/// replaced by the non-null parameter values.
ColorScheme copyWith({
Brightness? brightness,
Color? primary,
Color? onPrimary,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
Color? secondary,
Color? onSecondary,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
Color? error,
Color? onError,
Color? errorContainer,
Color? onErrorContainer,
Color? surface,
Color? onSurface,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? outline,
Color? outlineVariant,
Color? shadow,
Color? scrim,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) {
return ColorScheme(
brightness: brightness ?? this.brightness,
primary : primary ?? this.primary,
onPrimary : onPrimary ?? this.onPrimary,
primaryContainer : primaryContainer ?? this.primaryContainer,
onPrimaryContainer : onPrimaryContainer ?? this.onPrimaryContainer,
primaryFixed: primaryFixed ?? this.primaryFixed,
primaryFixedDim: primaryFixedDim ?? this.primaryFixedDim,
onPrimaryFixed: onPrimaryFixed ?? this.onPrimaryFixed,
onPrimaryFixedVariant: onPrimaryFixedVariant ?? this.onPrimaryFixedVariant,
secondary : secondary ?? this.secondary,
onSecondary : onSecondary ?? this.onSecondary,
secondaryContainer : secondaryContainer ?? this.secondaryContainer,
onSecondaryContainer : onSecondaryContainer ?? this.onSecondaryContainer,
secondaryFixed: secondaryFixed ?? this.secondaryFixed,
secondaryFixedDim: secondaryFixedDim ?? this.secondaryFixedDim,
onSecondaryFixed: onSecondaryFixed ?? this.onSecondaryFixed,
onSecondaryFixedVariant: onSecondaryFixedVariant ?? this.onSecondaryFixedVariant,
tertiary : tertiary ?? this.tertiary,
onTertiary : onTertiary ?? this.onTertiary,
tertiaryContainer : tertiaryContainer ?? this.tertiaryContainer,
onTertiaryContainer : onTertiaryContainer ?? this.onTertiaryContainer,
tertiaryFixed: tertiaryFixed ?? this.tertiaryFixed,
tertiaryFixedDim: tertiaryFixedDim ?? this.tertiaryFixedDim,
onTertiaryFixed: onTertiaryFixed ?? this.onTertiaryFixed,
onTertiaryFixedVariant: onTertiaryFixedVariant ?? this.onTertiaryFixedVariant,
error : error ?? this.error,
onError : onError ?? this.onError,
errorContainer : errorContainer ?? this.errorContainer,
onErrorContainer : onErrorContainer ?? this.onErrorContainer,
surface : surface ?? this.surface,
onSurface : onSurface ?? this.onSurface,
surfaceDim : surfaceDim ?? this.surfaceDim,
surfaceBright : surfaceBright ?? this.surfaceBright,
surfaceContainerLowest : surfaceContainerLowest ?? this.surfaceContainerLowest,
surfaceContainerLow : surfaceContainerLow ?? this.surfaceContainerLow,
surfaceContainer : surfaceContainer ?? this.surfaceContainer,
surfaceContainerHigh : surfaceContainerHigh ?? this.surfaceContainerHigh,
surfaceContainerHighest : surfaceContainerHighest ?? this.surfaceContainerHighest,
onSurfaceVariant : onSurfaceVariant ?? this.onSurfaceVariant,
outline : outline ?? this.outline,
outlineVariant : outlineVariant ?? this.outlineVariant,
shadow : shadow ?? this.shadow,
scrim : scrim ?? this.scrim,
inverseSurface : inverseSurface ?? this.inverseSurface,
onInverseSurface : onInverseSurface ?? this.onInverseSurface,
inversePrimary : inversePrimary ?? this.inversePrimary,
surfaceTint: surfaceTint ?? this.surfaceTint,
// DEPRECATED (newest deprecations at the bottom)
background : background ?? this.background,
onBackground : onBackground ?? this.onBackground,
surfaceVariant : surfaceVariant ?? this.surfaceVariant,
);
}
/// Linearly interpolate between two [ColorScheme] objects.
///
/// {@macro dart.ui.shadow.lerp}
static ColorScheme lerp(ColorScheme a, ColorScheme b, double t) {
if (identical(a, b)) {
return a;
}
return ColorScheme(
brightness: t < 0.5 ? a.brightness : b.brightness,
primary: Color.lerp(a.primary, b.primary, t)!,
onPrimary: Color.lerp(a.onPrimary, b.onPrimary, t)!,
primaryContainer: Color.lerp(a.primaryContainer, b.primaryContainer, t),
onPrimaryContainer: Color.lerp(a.onPrimaryContainer, b.onPrimaryContainer, t),
primaryFixed: Color.lerp(a.primaryFixed, b.primaryFixed, t),
primaryFixedDim: Color.lerp(a.primaryFixedDim, b.primaryFixedDim, t),
onPrimaryFixed: Color.lerp(a.onPrimaryFixed, b.onPrimaryFixed, t),
onPrimaryFixedVariant: Color.lerp(a.onPrimaryFixedVariant, b.onPrimaryFixedVariant, t),
secondary: Color.lerp(a.secondary, b.secondary, t)!,
onSecondary: Color.lerp(a.onSecondary, b.onSecondary, t)!,
secondaryContainer: Color.lerp(a.secondaryContainer, b.secondaryContainer, t),
onSecondaryContainer: Color.lerp(a.onSecondaryContainer, b.onSecondaryContainer, t),
secondaryFixed: Color.lerp(a.secondaryFixed, b.secondaryFixed, t),
secondaryFixedDim: Color.lerp(a.secondaryFixedDim, b.secondaryFixedDim, t),
onSecondaryFixed: Color.lerp(a.onSecondaryFixed, b.onSecondaryFixed, t),
onSecondaryFixedVariant: Color.lerp(a.onSecondaryFixedVariant, b.onSecondaryFixedVariant, t),
tertiary: Color.lerp(a.tertiary, b.tertiary, t),
onTertiary: Color.lerp(a.onTertiary, b.onTertiary, t),
tertiaryContainer: Color.lerp(a.tertiaryContainer, b.tertiaryContainer, t),
onTertiaryContainer: Color.lerp(a.onTertiaryContainer, b.onTertiaryContainer, t),
tertiaryFixed: Color.lerp(a.tertiaryFixed, b.tertiaryFixed, t),
tertiaryFixedDim: Color.lerp(a.tertiaryFixedDim, b.tertiaryFixedDim, t),
onTertiaryFixed: Color.lerp(a.onTertiaryFixed, b.onTertiaryFixed, t),
onTertiaryFixedVariant: Color.lerp(a.onTertiaryFixedVariant, b.onTertiaryFixedVariant, t),
error: Color.lerp(a.error, b.error, t)!,
onError: Color.lerp(a.onError, b.onError, t)!,
errorContainer: Color.lerp(a.errorContainer, b.errorContainer, t),
onErrorContainer: Color.lerp(a.onErrorContainer, b.onErrorContainer, t),
surface: Color.lerp(a.surface, b.surface, t)!,
onSurface: Color.lerp(a.onSurface, b.onSurface, t)!,
surfaceDim: Color.lerp(a.surfaceDim, b.surfaceDim, t),
surfaceBright: Color.lerp(a.surfaceBright, b.surfaceBright, t),
surfaceContainerLowest: Color.lerp(a.surfaceContainerLowest, b.surfaceContainerLowest, t),
surfaceContainerLow: Color.lerp(a.surfaceContainerLow, b.surfaceContainerLow, t),
surfaceContainer: Color.lerp(a.surfaceContainer, b.surfaceContainer, t),
surfaceContainerHigh: Color.lerp(a.surfaceContainerHigh, b.surfaceContainerHigh, t),
surfaceContainerHighest: Color.lerp(a.surfaceContainerHighest, b.surfaceContainerHighest, t),
onSurfaceVariant: Color.lerp(a.onSurfaceVariant, b.onSurfaceVariant, t),
outline: Color.lerp(a.outline, b.outline, t),
outlineVariant: Color.lerp(a.outlineVariant, b.outlineVariant, t),
shadow: Color.lerp(a.shadow, b.shadow, t),
scrim: Color.lerp(a.scrim, b.scrim, t),
inverseSurface: Color.lerp(a.inverseSurface, b.inverseSurface, t),
onInverseSurface: Color.lerp(a.onInverseSurface, b.onInverseSurface, t),
inversePrimary: Color.lerp(a.inversePrimary, b.inversePrimary, t),
surfaceTint: Color.lerp(a.surfaceTint, b.surfaceTint, t),
// DEPRECATED (newest deprecations at the bottom)
background: Color.lerp(a.background, b.background, t),
onBackground: Color.lerp(a.onBackground, b.onBackground, t),
surfaceVariant: Color.lerp(a.surfaceVariant, b.surfaceVariant, t),
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ColorScheme
&& other.brightness == brightness
&& other.primary == primary
&& other.onPrimary == onPrimary
&& other.primaryContainer == primaryContainer
&& other.onPrimaryContainer == onPrimaryContainer
&& other.primaryFixed == primaryFixed
&& other.primaryFixedDim == primaryFixedDim
&& other.onPrimaryFixed == onPrimaryFixed
&& other.onPrimaryFixedVariant == onPrimaryFixedVariant
&& other.secondary == secondary
&& other.onSecondary == onSecondary
&& other.secondaryContainer == secondaryContainer
&& other.onSecondaryContainer == onSecondaryContainer
&& other.secondaryFixed == secondaryFixed
&& other.secondaryFixedDim == secondaryFixedDim
&& other.onSecondaryFixed == onSecondaryFixed
&& other.onSecondaryFixedVariant == onSecondaryFixedVariant
&& other.tertiary == tertiary
&& other.onTertiary == onTertiary
&& other.tertiaryContainer == tertiaryContainer
&& other.onTertiaryContainer == onTertiaryContainer
&& other.tertiaryFixed == tertiaryFixed
&& other.tertiaryFixedDim == tertiaryFixedDim
&& other.onTertiaryFixed == onTertiaryFixed
&& other.onTertiaryFixedVariant == onTertiaryFixedVariant
&& other.error == error
&& other.onError == onError
&& other.errorContainer == errorContainer
&& other.onErrorContainer == onErrorContainer
&& other.surface == surface
&& other.onSurface == onSurface
&& other.surfaceDim == surfaceDim
&& other.surfaceBright == surfaceBright
&& other.surfaceContainerLowest == surfaceContainerLowest
&& other.surfaceContainerLow == surfaceContainerLow
&& other.surfaceContainer == surfaceContainer
&& other.surfaceContainerHigh == surfaceContainerHigh
&& other.surfaceContainerHighest == surfaceContainerHighest
&& other.onSurfaceVariant == onSurfaceVariant
&& other.outline == outline
&& other.outlineVariant == outlineVariant
&& other.shadow == shadow
&& other.scrim == scrim
&& other.inverseSurface == inverseSurface
&& other.onInverseSurface == onInverseSurface
&& other.inversePrimary == inversePrimary
&& other.surfaceTint == surfaceTint
// DEPRECATED (newest deprecations at the bottom)
&& other.background == background
&& other.onBackground == onBackground
&& other.surfaceVariant == surfaceVariant;
}
@override
int get hashCode => Object.hash(
brightness,
primary,
onPrimary,
primaryContainer,
onPrimaryContainer,
secondary,
onSecondary,
secondaryContainer,
onSecondaryContainer,
tertiary,
onTertiary,
tertiaryContainer,
onTertiaryContainer,
error,
onError,
errorContainer,
onErrorContainer,
Object.hash(
surface,
onSurface,
surfaceDim,
surfaceBright,
surfaceContainerLowest,
surfaceContainerLow,
surfaceContainer,
surfaceContainerHigh,
surfaceContainerHighest,
onSurfaceVariant,
outline,
outlineVariant,
shadow,
scrim,
inverseSurface,
onInverseSurface,
inversePrimary,
surfaceTint,
Object.hash(
primaryFixed,
primaryFixedDim,
onPrimaryFixed,
onPrimaryFixedVariant,
secondaryFixed,
secondaryFixedDim,
onSecondaryFixed,
onSecondaryFixedVariant,
tertiaryFixed,
tertiaryFixedDim,
onTertiaryFixed,
onTertiaryFixedVariant,
// DEPRECATED (newest deprecations at the bottom)
background,
onBackground,
surfaceVariant,
)
),
);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
const ColorScheme defaultScheme = ColorScheme.light();
properties.add(DiagnosticsProperty<Brightness>('brightness', brightness, defaultValue: defaultScheme.brightness));
properties.add(ColorProperty('primary', primary, defaultValue: defaultScheme.primary));
properties.add(ColorProperty('onPrimary', onPrimary, defaultValue: defaultScheme.onPrimary));
properties.add(ColorProperty('primaryContainer', primaryContainer, defaultValue: defaultScheme.primaryContainer));
properties.add(ColorProperty('onPrimaryContainer', onPrimaryContainer, defaultValue: defaultScheme.onPrimaryContainer));
properties.add(ColorProperty('primaryFixed', primaryFixed, defaultValue: defaultScheme.primaryFixed));
properties.add(ColorProperty('primaryFixedDim', primaryFixedDim, defaultValue: defaultScheme.primaryFixedDim));
properties.add(ColorProperty('onPrimaryFixed', onPrimaryFixed, defaultValue: defaultScheme.onPrimaryFixed));
properties.add(ColorProperty('onPrimaryFixedVariant', onPrimaryFixedVariant, defaultValue: defaultScheme.onPrimaryFixedVariant));
properties.add(ColorProperty('secondary', secondary, defaultValue: defaultScheme.secondary));
properties.add(ColorProperty('onSecondary', onSecondary, defaultValue: defaultScheme.onSecondary));
properties.add(ColorProperty('secondaryContainer', secondaryContainer, defaultValue: defaultScheme.secondaryContainer));
properties.add(ColorProperty('onSecondaryContainer', onSecondaryContainer, defaultValue: defaultScheme.onSecondaryContainer));
properties.add(ColorProperty('secondaryFixed', secondaryFixed, defaultValue: defaultScheme.secondaryFixed));
properties.add(ColorProperty('secondaryFixedDim', secondaryFixedDim, defaultValue: defaultScheme.secondaryFixedDim));
properties.add(ColorProperty('onSecondaryFixed', onSecondaryFixed, defaultValue: defaultScheme.onSecondaryFixed));
properties.add(ColorProperty('onSecondaryFixedVariant', onSecondaryFixedVariant, defaultValue: defaultScheme.onSecondaryFixedVariant));
properties.add(ColorProperty('tertiary', tertiary, defaultValue: defaultScheme.tertiary));
properties.add(ColorProperty('onTertiary', onTertiary, defaultValue: defaultScheme.onTertiary));
properties.add(ColorProperty('tertiaryContainer', tertiaryContainer, defaultValue: defaultScheme.tertiaryContainer));
properties.add(ColorProperty('onTertiaryContainer', onTertiaryContainer, defaultValue: defaultScheme.onTertiaryContainer));
properties.add(ColorProperty('tertiaryFixed', tertiaryFixed, defaultValue: defaultScheme.tertiaryFixed));
properties.add(ColorProperty('tertiaryFixedDim', tertiaryFixedDim, defaultValue: defaultScheme.tertiaryFixedDim));
properties.add(ColorProperty('onTertiaryFixed', onTertiaryFixed, defaultValue: defaultScheme.onTertiaryFixed));
properties.add(ColorProperty('onTertiaryFixedVariant', onTertiaryFixedVariant, defaultValue: defaultScheme.onTertiaryFixedVariant));
properties.add(ColorProperty('error', error, defaultValue: defaultScheme.error));
properties.add(ColorProperty('onError', onError, defaultValue: defaultScheme.onError));
properties.add(ColorProperty('errorContainer', errorContainer, defaultValue: defaultScheme.errorContainer));
properties.add(ColorProperty('onErrorContainer', onErrorContainer, defaultValue: defaultScheme.onErrorContainer));
properties.add(ColorProperty('surface', surface, defaultValue: defaultScheme.surface));
properties.add(ColorProperty('onSurface', onSurface, defaultValue: defaultScheme.onSurface));
properties.add(ColorProperty('surfaceDim', surfaceDim, defaultValue: defaultScheme.surfaceDim));
properties.add(ColorProperty('surfaceBright', surfaceBright, defaultValue: defaultScheme.surfaceBright));
properties.add(ColorProperty('surfaceContainerLowest', surfaceContainerLowest, defaultValue: defaultScheme.surfaceContainerLowest));
properties.add(ColorProperty('surfaceContainerLow', surfaceContainerLow, defaultValue: defaultScheme.surfaceContainerLow));
properties.add(ColorProperty('surfaceContainer', surfaceContainer, defaultValue: defaultScheme.surfaceContainer));
properties.add(ColorProperty('surfaceContainerHigh', surfaceContainerHigh, defaultValue: defaultScheme.surfaceContainerHigh));
properties.add(ColorProperty('surfaceContainerHighest', surfaceContainerHighest, defaultValue: defaultScheme.surfaceContainerHighest));
properties.add(ColorProperty('onSurfaceVariant', onSurfaceVariant, defaultValue: defaultScheme.onSurfaceVariant));
properties.add(ColorProperty('outline', outline, defaultValue: defaultScheme.outline));
properties.add(ColorProperty('outlineVariant', outlineVariant, defaultValue: defaultScheme.outlineVariant));
properties.add(ColorProperty('shadow', shadow, defaultValue: defaultScheme.shadow));
properties.add(ColorProperty('scrim', scrim, defaultValue: defaultScheme.scrim));
properties.add(ColorProperty('inverseSurface', inverseSurface, defaultValue: defaultScheme.inverseSurface));
properties.add(ColorProperty('onInverseSurface', onInverseSurface, defaultValue: defaultScheme.onInverseSurface));
properties.add(ColorProperty('inversePrimary', inversePrimary, defaultValue: defaultScheme.inversePrimary));
properties.add(ColorProperty('surfaceTint', surfaceTint, defaultValue: defaultScheme.surfaceTint));
// DEPRECATED (newest deprecations at the bottom)
properties.add(ColorProperty('background', background, defaultValue: defaultScheme.background));
properties.add(ColorProperty('onBackground', onBackground, defaultValue: defaultScheme.onBackground));
properties.add(ColorProperty('surfaceVariant', surfaceVariant, defaultValue: defaultScheme.surfaceVariant));
}
/// Generate a [ColorScheme] derived from the given `imageProvider`.
///
/// Material Color Utilities extracts the dominant color from the
/// supplied [ImageProvider]. Using this color, a [ColorScheme] is generated
/// with harmonious colors that meet contrast requirements for accessibility.
///
/// If any of the optional color parameters are non-null, they will be
/// used in place of the generated colors for that field in the resulting
/// [ColorScheme]. This allows apps to override specific colors for their
/// needs.
///
/// Given the nature of the algorithm, the most dominant color of the
/// `imageProvider` may not wind up as one of the [ColorScheme] colors.
///
/// The provided image will be scaled down to a maximum size of 112x112 pixels
/// during color extraction.
///
/// {@tool dartpad}
/// This sample shows how to use [ColorScheme.fromImageProvider] to create
/// content-based dynamic color schemes.
///
/// ** See code in examples/api/lib/material/color_scheme/dynamic_content_color.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [M3 Guidelines: Dynamic color from content](https://m3.material.io/styles/color/dynamic-color/user-generated-color#8af550b9-a19e-4e9f-bb0a-7f611fed5d0f)
/// * <https://pub.dev/packages/dynamic_color>, a package to create
/// [ColorScheme]s based on a platform's implementation of dynamic color.
/// * <https://m3.material.io/styles/color/the-color-system/color-roles>, the
/// Material 3 Color system specification.
/// * <https://pub.dev/packages/material_color_utilities>, the package
/// used to algorithmically determine the dominant color and to generate
/// the [ColorScheme].
static Future<ColorScheme> fromImageProvider({
required ImageProvider provider,
Brightness brightness = Brightness.light,
Color? primary,
Color? onPrimary,
Color? primaryContainer,
Color? onPrimaryContainer,
Color? primaryFixed,
Color? primaryFixedDim,
Color? onPrimaryFixed,
Color? onPrimaryFixedVariant,
Color? secondary,
Color? onSecondary,
Color? secondaryContainer,
Color? onSecondaryContainer,
Color? secondaryFixed,
Color? secondaryFixedDim,
Color? onSecondaryFixed,
Color? onSecondaryFixedVariant,
Color? tertiary,
Color? onTertiary,
Color? tertiaryContainer,
Color? onTertiaryContainer,
Color? tertiaryFixed,
Color? tertiaryFixedDim,
Color? onTertiaryFixed,
Color? onTertiaryFixedVariant,
Color? error,
Color? onError,
Color? errorContainer,
Color? onErrorContainer,
Color? outline,
Color? outlineVariant,
Color? surface,
Color? onSurface,
Color? surfaceDim,
Color? surfaceBright,
Color? surfaceContainerLowest,
Color? surfaceContainerLow,
Color? surfaceContainer,
Color? surfaceContainerHigh,
Color? surfaceContainerHighest,
Color? onSurfaceVariant,
Color? inverseSurface,
Color? onInverseSurface,
Color? inversePrimary,
Color? shadow,
Color? scrim,
Color? surfaceTint,
@Deprecated(
'Use surface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? background,
@Deprecated(
'Use onSurface instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? onBackground,
@Deprecated(
'Use surfaceContainerHighest instead. '
'This feature was deprecated after v3.18.0-0.1.pre.'
)
Color? surfaceVariant,
}) async {
// Extract dominant colors from image.
final QuantizerResult quantizerResult =
await _extractColorsFromImageProvider(provider);
final Map<int, int> colorToCount = quantizerResult.colorToCount.map(
(int key, int value) => MapEntry<int, int>(_getArgbFromAbgr(key), value),
);
// Score colors for color scheme suitability.
final List<int> scoredResults = Score.score(colorToCount, desired: 1);
final ui.Color baseColor = Color(scoredResults.first);
final SchemeTonalSpot scheme;
switch (brightness) {
case Brightness.light:
scheme = SchemeTonalSpot(sourceColorHct: Hct.fromInt(baseColor.value), isDark: false, contrastLevel: 0.0);
case Brightness.dark:
scheme = SchemeTonalSpot(sourceColorHct: Hct.fromInt(baseColor.value), isDark: true, contrastLevel: 0.0);
}
return ColorScheme(
primary: primary ?? Color(MaterialDynamicColors.primary.getArgb(scheme)),
onPrimary: onPrimary ?? Color(MaterialDynamicColors.onPrimary.getArgb(scheme)),
primaryContainer: primaryContainer ?? Color(MaterialDynamicColors.primaryContainer.getArgb(scheme)),
onPrimaryContainer: onPrimaryContainer ?? Color(MaterialDynamicColors.onPrimaryContainer.getArgb(scheme)),
primaryFixed: primaryFixed ?? Color(MaterialDynamicColors.primaryFixed.getArgb(scheme)),
primaryFixedDim: primaryFixedDim ?? Color(MaterialDynamicColors.primaryFixedDim.getArgb(scheme)),
onPrimaryFixed: onPrimaryFixed ?? Color(MaterialDynamicColors.onPrimaryFixed.getArgb(scheme)),
onPrimaryFixedVariant: onPrimaryFixedVariant ?? Color(MaterialDynamicColors.onPrimaryFixedVariant.getArgb(scheme)),
secondary: secondary ?? Color(MaterialDynamicColors.secondary.getArgb(scheme)),
onSecondary: onSecondary ?? Color(MaterialDynamicColors.onSecondary.getArgb(scheme)),
secondaryContainer: secondaryContainer ?? Color(MaterialDynamicColors.secondaryContainer.getArgb(scheme)),
onSecondaryContainer: onSecondaryContainer ?? Color(MaterialDynamicColors.onSecondaryContainer.getArgb(scheme)),
secondaryFixed: secondaryFixed ?? Color(MaterialDynamicColors.secondaryFixed.getArgb(scheme)),
secondaryFixedDim: secondaryFixedDim ?? Color(MaterialDynamicColors.secondaryFixedDim.getArgb(scheme)),
onSecondaryFixed: onSecondaryFixed ?? Color(MaterialDynamicColors.onSecondaryFixed.getArgb(scheme)),
onSecondaryFixedVariant: onSecondaryFixedVariant ?? Color(MaterialDynamicColors.onSecondaryFixedVariant.getArgb(scheme)),
tertiary: tertiary ?? Color(MaterialDynamicColors.tertiary.getArgb(scheme)),
onTertiary: onTertiary ?? Color(MaterialDynamicColors.onTertiary.getArgb(scheme)),
tertiaryContainer: tertiaryContainer ?? Color(MaterialDynamicColors.tertiaryContainer.getArgb(scheme)),
onTertiaryContainer: onTertiaryContainer ?? Color(MaterialDynamicColors.onTertiaryContainer.getArgb(scheme)),
tertiaryFixed: tertiaryFixed ?? Color(MaterialDynamicColors.tertiaryFixed.getArgb(scheme)),
tertiaryFixedDim: tertiaryFixedDim ?? Color(MaterialDynamicColors.tertiaryFixedDim.getArgb(scheme)),
onTertiaryFixed: onTertiaryFixed ?? Color(MaterialDynamicColors.onTertiaryFixed.getArgb(scheme)),
onTertiaryFixedVariant: onTertiaryFixedVariant ?? Color(MaterialDynamicColors.onTertiaryFixedVariant.getArgb(scheme)),
error: error ?? Color(MaterialDynamicColors.error.getArgb(scheme)),
onError: onError ?? Color(MaterialDynamicColors.onError.getArgb(scheme)),
errorContainer: errorContainer ?? Color(MaterialDynamicColors.errorContainer.getArgb(scheme)),
onErrorContainer: onErrorContainer ?? Color(MaterialDynamicColors.onErrorContainer.getArgb(scheme)),
outline: outline ?? Color(MaterialDynamicColors.outline.getArgb(scheme)),
outlineVariant: outlineVariant ?? Color(MaterialDynamicColors.outlineVariant.getArgb(scheme)),
surface: surface ?? Color(MaterialDynamicColors.surface.getArgb(scheme)),
surfaceDim: surfaceDim ?? Color(MaterialDynamicColors.surfaceDim.getArgb(scheme)),
surfaceBright: surfaceBright ?? Color(MaterialDynamicColors.surfaceBright.getArgb(scheme)),
surfaceContainerLowest: surfaceContainerLowest ?? Color(MaterialDynamicColors.surfaceContainerLowest.getArgb(scheme)),
surfaceContainerLow: surfaceContainerLow ?? Color(MaterialDynamicColors.surfaceContainerLow.getArgb(scheme)),
surfaceContainer: surfaceContainer ?? Color(MaterialDynamicColors.surfaceContainer.getArgb(scheme)),
surfaceContainerHigh: surfaceContainerHigh ?? Color(MaterialDynamicColors.surfaceContainerHigh.getArgb(scheme)),
surfaceContainerHighest: surfaceContainerHighest ?? Color(MaterialDynamicColors.surfaceContainerHighest.getArgb(scheme)),
onSurface: onSurface ?? Color(MaterialDynamicColors.onSurface.getArgb(scheme)),
onSurfaceVariant: onSurfaceVariant ?? Color(MaterialDynamicColors.onSurfaceVariant.getArgb(scheme)),
inverseSurface: inverseSurface ?? Color(MaterialDynamicColors.inverseSurface.getArgb(scheme)),
onInverseSurface: onInverseSurface ?? Color(MaterialDynamicColors.inverseOnSurface.getArgb(scheme)),
inversePrimary: inversePrimary ?? Color(MaterialDynamicColors.inversePrimary.getArgb(scheme)),
shadow: shadow ?? Color(MaterialDynamicColors.shadow.getArgb(scheme)),
scrim: scrim ?? Color(MaterialDynamicColors.scrim.getArgb(scheme)),
surfaceTint: surfaceTint ?? Color(MaterialDynamicColors.primary.getArgb(scheme)),
brightness: brightness,
// DEPRECATED (newest deprecations at the bottom)
background: background ?? Color(MaterialDynamicColors.background.getArgb(scheme)),
onBackground: onBackground ?? Color(MaterialDynamicColors.onBackground.getArgb(scheme)),
surfaceVariant: surfaceVariant ?? Color(MaterialDynamicColors.surfaceVariant.getArgb(scheme)),
);
}
// ColorScheme.fromImageProvider() utilities.
// Extracts bytes from an [ImageProvider] and returns a [QuantizerResult]
// containing the most dominant colors.
static Future<QuantizerResult> _extractColorsFromImageProvider(ImageProvider imageProvider) async {
final ui.Image scaledImage = await _imageProviderToScaled(imageProvider);
final ByteData? imageBytes = await scaledImage.toByteData();
final QuantizerResult quantizerResult = await QuantizerCelebi().quantize(
imageBytes!.buffer.asUint32List(),
128,
returnInputPixelToClusterPixel: true,
);
return quantizerResult;
}
// Scale image size down to reduce computation time of color extraction.
static Future<ui.Image> _imageProviderToScaled(ImageProvider imageProvider) async {
const double maxDimension = 112.0;
final ImageStream stream = imageProvider.resolve(
const ImageConfiguration(size: Size(maxDimension, maxDimension)));
final Completer<ui.Image> imageCompleter = Completer<ui.Image>();
late ImageStreamListener listener;
late ui.Image scaledImage;
Timer? loadFailureTimeout;
listener = ImageStreamListener((ImageInfo info, bool sync) async {
loadFailureTimeout?.cancel();
stream.removeListener(listener);
final ui.Image image = info.image;
final int width = image.width;
final int height = image.height;
double paintWidth = width.toDouble();
double paintHeight = height.toDouble();
assert(width > 0 && height > 0);
final bool rescale = width > maxDimension || height > maxDimension;
if (rescale) {
paintWidth = (width > height) ? maxDimension : (maxDimension / height) * width;
paintHeight = (height > width) ? maxDimension : (maxDimension / width) * height;
}
final ui.PictureRecorder pictureRecorder = ui.PictureRecorder();
final Canvas canvas = Canvas(pictureRecorder);
paintImage(
canvas: canvas,
rect: Rect.fromLTRB(0, 0, paintWidth, paintHeight),
image: image,
filterQuality: FilterQuality.none,
);
final ui.Picture picture = pictureRecorder.endRecording();
scaledImage = await picture.toImage(paintWidth.toInt(), paintHeight.toInt());
imageCompleter.complete(info.image);
}, onError: (Object exception, StackTrace? stackTrace) {
stream.removeListener(listener);
throw Exception('Failed to render image: $exception');
});
loadFailureTimeout = Timer(const Duration(seconds: 5), () {
stream.removeListener(listener);
imageCompleter.completeError(
TimeoutException('Timeout occurred trying to load image'));
});
stream.addListener(listener);
await imageCompleter.future;
return scaledImage;
}
// Converts AABBGGRR color int to AARRGGBB format.
static int _getArgbFromAbgr(int abgr) {
const int exceptRMask = 0xFF00FFFF;
const int onlyRMask = ~exceptRMask;
const int exceptBMask = 0xFFFFFF00;
const int onlyBMask = ~exceptBMask;
final int r = (abgr & onlyRMask) >> 16;
final int b = abgr & onlyBMask;
return (abgr & exceptRMask & exceptBMask) | (b << 16) | r;
}
}
| flutter/packages/flutter/lib/src/material/color_scheme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/color_scheme.dart",
"repo_id": "flutter",
"token_count": 26888
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'divider_theme.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// A thin horizontal line, with padding on either side.
///
/// In the Material Design language, this represents a divider. Dividers can be
/// used in lists, [Drawer]s, and elsewhere to separate content.
///
/// To create a divider between [ListTile] items, consider using
/// [ListTile.divideTiles], which is optimized for this case.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=_liUC641Nmk}
///
/// The box's total height is controlled by [height]. The appropriate
/// padding is automatically computed from the height.
///
/// {@tool dartpad}
/// This sample shows how to display a Divider between an orange and blue box
/// inside a column. The Divider is 20 logical pixels in height and contains a
/// vertically centered black line that is 5 logical pixels thick. The black
/// line is indented by 20 logical pixels.
///
/// 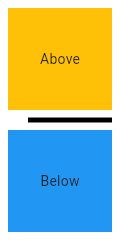
///
/// ** See code in examples/api/lib/material/divider/divider.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of [Divider] widget, as described in:
/// https://m3.material.io/components/divider/overview
///
/// ** See code in examples/api/lib/material/divider/divider.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [PopupMenuDivider], which is the equivalent but for popup menus.
/// * [ListTile.divideTiles], another approach to dividing widgets in a list.
/// * [VerticalDivider], which is the vertical analog of this widget.
/// * <https://material.io/design/components/dividers.html>
class Divider extends StatelessWidget {
/// Creates a Material Design divider.
///
/// The [height], [thickness], [indent], and [endIndent] must be null or
/// non-negative.
const Divider({
super.key,
this.height,
this.thickness,
this.indent,
this.endIndent,
this.color,
}) : assert(height == null || height >= 0.0),
assert(thickness == null || thickness >= 0.0),
assert(indent == null || indent >= 0.0),
assert(endIndent == null || endIndent >= 0.0);
/// The divider's height extent.
///
/// The divider itself is always drawn as a horizontal line that is centered
/// within the height specified by this value.
///
/// If this is null, then the [DividerThemeData.space] is used. If that is
/// also null, then this defaults to 16.0.
final double? height;
/// The thickness of the line drawn within the divider.
///
/// A divider with a [thickness] of 0.0 is always drawn as a line with a
/// height of exactly one device pixel.
///
/// If this is null, then the [DividerThemeData.thickness] is used. If
/// that is also null, then this defaults to 0.0.
final double? thickness;
/// The amount of empty space to the leading edge of the divider.
///
/// If this is null, then the [DividerThemeData.indent] is used. If that is
/// also null, then this defaults to 0.0.
final double? indent;
/// The amount of empty space to the trailing edge of the divider.
///
/// If this is null, then the [DividerThemeData.endIndent] is used. If that is
/// also null, then this defaults to 0.0.
final double? endIndent;
/// The color to use when painting the line.
///
/// If this is null, then the [DividerThemeData.color] is used. If that is
/// also null, then [ThemeData.dividerColor] is used.
///
/// {@tool snippet}
///
/// ```dart
/// const Divider(
/// color: Colors.deepOrange,
/// )
/// ```
/// {@end-tool}
final Color? color;
/// Computes the [BorderSide] that represents a divider.
///
/// If [color] is null, then [DividerThemeData.color] is used. If that is also
/// null, then if [ThemeData.useMaterial3] is true then it defaults to
/// [ThemeData.colorScheme]'s [ColorScheme.outlineVariant]. Otherwise
/// [ThemeData.dividerColor] is used.
///
/// If [width] is null, then [DividerThemeData.thickness] is used. If that is
/// also null, then this defaults to 0.0 (a hairline border).
///
/// If [context] is null, the default color of [BorderSide] is used and the
/// default width of 0.0 is used.
///
/// {@tool snippet}
///
/// This example uses this method to create a box that has a divider above and
/// below it. This is sometimes useful with lists, for instance, to separate a
/// scrollable section from the rest of the interface.
///
/// ```dart
/// DecoratedBox(
/// decoration: BoxDecoration(
/// border: Border(
/// top: Divider.createBorderSide(context),
/// bottom: Divider.createBorderSide(context),
/// ),
/// ),
/// // child: ...
/// )
/// ```
/// {@end-tool}
static BorderSide createBorderSide(BuildContext? context, { Color? color, double? width }) {
final DividerThemeData? dividerTheme = context != null ? DividerTheme.of(context) : null;
final DividerThemeData? defaults = context != null
? Theme.of(context).useMaterial3 ? _DividerDefaultsM3(context) : _DividerDefaultsM2(context)
: null;
final Color? effectiveColor = color ?? dividerTheme?.color ?? defaults?.color;
final double effectiveWidth = width ?? dividerTheme?.thickness ?? defaults?.thickness ?? 0.0;
// Prevent assertion since it is possible that context is null and no color
// is specified.
if (effectiveColor == null) {
return BorderSide(
width: effectiveWidth,
);
}
return BorderSide(
color: effectiveColor,
width: effectiveWidth,
);
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final DividerThemeData dividerTheme = DividerTheme.of(context);
final DividerThemeData defaults = theme.useMaterial3 ? _DividerDefaultsM3(context) : _DividerDefaultsM2(context);
final double height = this.height ?? dividerTheme.space ?? defaults.space!;
final double thickness = this.thickness ?? dividerTheme.thickness ?? defaults.thickness!;
final double indent = this.indent ?? dividerTheme.indent ?? defaults.indent!;
final double endIndent = this.endIndent ?? dividerTheme.endIndent ?? defaults.endIndent!;
return SizedBox(
height: height,
child: Center(
child: Container(
height: thickness,
margin: EdgeInsetsDirectional.only(start: indent, end: endIndent),
decoration: BoxDecoration(
border: Border(
bottom: createBorderSide(context, color: color, width: thickness),
),
),
),
),
);
}
}
/// A thin vertical line, with padding on either side.
///
/// In the Material Design language, this represents a divider. Vertical
/// dividers can be used in horizontally scrolling lists, such as a
/// [ListView] with [ListView.scrollDirection] set to [Axis.horizontal].
///
/// The box's total width is controlled by [width]. The appropriate
/// padding is automatically computed from the width.
///
/// {@tool dartpad}
/// This sample shows how to display a [VerticalDivider] between a purple and orange box
/// inside a [Row]. The [VerticalDivider] is 20 logical pixels in width and contains a
/// horizontally centered black line that is 1 logical pixels thick. The grey
/// line is indented by 20 logical pixels.
///
/// ** See code in examples/api/lib/material/divider/vertical_divider.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample shows the creation of [VerticalDivider] widget, as described in:
/// https://m3.material.io/components/divider/overview
///
/// ** See code in examples/api/lib/material/divider/vertical_divider.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListView.separated], which can be used to generate vertical dividers.
/// * [Divider], which is the horizontal analog of this widget.
/// * <https://material.io/design/components/dividers.html>
class VerticalDivider extends StatelessWidget {
/// Creates a Material Design vertical divider.
///
/// The [width], [thickness], [indent], and [endIndent] must be null or
/// non-negative.
const VerticalDivider({
super.key,
this.width,
this.thickness,
this.indent,
this.endIndent,
this.color,
}) : assert(width == null || width >= 0.0),
assert(thickness == null || thickness >= 0.0),
assert(indent == null || indent >= 0.0),
assert(endIndent == null || endIndent >= 0.0);
/// The divider's width.
///
/// The divider itself is always drawn as a vertical line that is centered
/// within the width specified by this value.
///
/// If this is null, then the [DividerThemeData.space] is used. If that is
/// also null, then this defaults to 16.0.
final double? width;
/// The thickness of the line drawn within the divider.
///
/// A divider with a [thickness] of 0.0 is always drawn as a line with a
/// width of exactly one device pixel.
///
/// If this is null, then the [DividerThemeData.thickness] is used which
/// defaults to 0.0.
final double? thickness;
/// The amount of empty space on top of the divider.
///
/// If this is null, then the [DividerThemeData.indent] is used. If that is
/// also null, then this defaults to 0.0.
final double? indent;
/// The amount of empty space under the divider.
///
/// If this is null, then the [DividerThemeData.endIndent] is used. If that is
/// also null, then this defaults to 0.0.
final double? endIndent;
/// The color to use when painting the line.
///
/// If this is null, then the [DividerThemeData.color] is used. If that is
/// also null, then [ThemeData.dividerColor] is used.
///
/// {@tool snippet}
///
/// ```dart
/// const Divider(
/// color: Colors.deepOrange,
/// )
/// ```
/// {@end-tool}
final Color? color;
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
final DividerThemeData dividerTheme = DividerTheme.of(context);
final DividerThemeData defaults = theme.useMaterial3 ? _DividerDefaultsM3(context) : _DividerDefaultsM2(context);
final double width = this.width ?? dividerTheme.space ?? defaults.space!;
final double thickness = this.thickness ?? dividerTheme.thickness ?? defaults.thickness!;
final double indent = this.indent ?? dividerTheme.indent ?? defaults.indent!;
final double endIndent = this.endIndent ?? dividerTheme.endIndent ?? defaults.endIndent!;
return SizedBox(
width: width,
child: Center(
child: Container(
width: thickness,
margin: EdgeInsetsDirectional.only(top: indent, bottom: endIndent),
decoration: BoxDecoration(
border: Border(
left: Divider.createBorderSide(context, color: color, width: thickness),
),
),
),
),
);
}
}
class _DividerDefaultsM2 extends DividerThemeData {
const _DividerDefaultsM2(this.context) : super(
space: 16,
thickness: 0,
indent: 0,
endIndent: 0,
);
final BuildContext context;
@override Color? get color => Theme.of(context).dividerColor;
}
// BEGIN GENERATED TOKEN PROPERTIES - Divider
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _DividerDefaultsM3 extends DividerThemeData {
const _DividerDefaultsM3(this.context) : super(
space: 16,
thickness: 1.0,
indent: 0,
endIndent: 0,
);
final BuildContext context;
@override Color? get color => Theme.of(context).colorScheme.outlineVariant;
}
// END GENERATED TOKEN PROPERTIES - Divider
| flutter/packages/flutter/lib/src/material/divider.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/divider.dart",
"repo_id": "flutter",
"token_count": 3956
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'button_style_button.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'filled_button_theme.dart';
import 'ink_well.dart';
import 'material_state.dart';
import 'theme.dart';
import 'theme_data.dart';
enum _FilledButtonVariant { filled, tonal }
/// A Material Design filled button.
///
/// Filled buttons have the most visual impact after the [FloatingActionButton],
/// and should be used for important, final actions that complete a flow,
/// like **Save**, **Join now**, or **Confirm**.
///
/// A filled button is a label [child] displayed on a [Material]
/// widget. The label's [Text] and [Icon] widgets are displayed in
/// [style]'s [ButtonStyle.foregroundColor] and the button's filled
/// background is the [ButtonStyle.backgroundColor].
///
/// The filled button's default style is defined by
/// [defaultStyleOf]. The style of this filled button can be
/// overridden with its [style] parameter. The style of all filled
/// buttons in a subtree can be overridden with the
/// [FilledButtonTheme], and the style of all of the filled
/// buttons in an app can be overridden with the [Theme]'s
/// [ThemeData.filledButtonTheme] property.
///
/// The static [styleFrom] method is a convenient way to create a
/// filled button [ButtonStyle] from simple values.
///
/// If [onPressed] and [onLongPress] callbacks are null, then the
/// button will be disabled.
///
/// To create a 'filled tonal' button, use [FilledButton.tonal].
///
/// {@tool dartpad}
/// This sample produces enabled and disabled filled and filled tonal
/// buttons.
///
/// ** See code in examples/api/lib/material/filled_button/filled_button.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [OutlinedButton], a button with an outlined border and no fill color.
/// * [TextButton], a button with no outline or fill color.
/// * <https://material.io/design/components/buttons.html>
/// * <https://m3.material.io/components/buttons>
class FilledButton extends ButtonStyleButton {
/// Create a FilledButton.
const FilledButton({
super.key,
required super.onPressed,
super.onLongPress,
super.onHover,
super.onFocusChange,
super.style,
super.focusNode,
super.autofocus = false,
super.clipBehavior = Clip.none,
super.statesController,
required super.child,
super.iconAlignment,
}) : _variant = _FilledButtonVariant.filled;
/// Create a filled button from [icon] and [label].
///
/// The icon and label are arranged in a row with padding at the start and end
/// and a gap between them.
///
/// If [icon] is null, will create a [FilledButton] instead.
///
/// {@macro flutter.material.ButtonStyleButton.iconAlignment}
///
factory FilledButton.icon({
Key? key,
required VoidCallback? onPressed,
VoidCallback? onLongPress,
ValueChanged<bool>? onHover,
ValueChanged<bool>? onFocusChange,
ButtonStyle? style,
FocusNode? focusNode,
bool? autofocus,
Clip? clipBehavior,
MaterialStatesController? statesController,
Widget? icon,
required Widget label,
IconAlignment iconAlignment = IconAlignment.start,
}) {
if (icon == null) {
return FilledButton(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
onHover: onHover,
onFocusChange: onFocusChange,
style: style,
focusNode: focusNode,
autofocus: autofocus ?? false,
clipBehavior: clipBehavior ?? Clip.none,
statesController: statesController,
child: label,
);
}
return _FilledButtonWithIcon(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
onHover: onHover,
onFocusChange: onFocusChange,
style: style,
focusNode: focusNode,
autofocus: autofocus ?? false,
clipBehavior: clipBehavior ?? Clip.none,
statesController: statesController,
icon: icon,
label: label,
iconAlignment: iconAlignment,
);
}
/// Create a tonal variant of FilledButton.
///
/// A filled tonal button is an alternative middle ground between
/// [FilledButton] and [OutlinedButton]. They’re useful in contexts where
/// a lower-priority button requires slightly more emphasis than an
/// outline would give, such as "Next" in an onboarding flow.
const FilledButton.tonal({
super.key,
required super.onPressed,
super.onLongPress,
super.onHover,
super.onFocusChange,
super.style,
super.focusNode,
super.autofocus = false,
super.clipBehavior = Clip.none,
super.statesController,
required super.child,
}) : _variant = _FilledButtonVariant.tonal;
/// Create a filled tonal button from [icon] and [label].
///
/// The [icon] and [label] are arranged in a row with padding at the start and
/// end and a gap between them.
///
/// If [icon] is null, will create a [FilledButton.tonal] instead.
factory FilledButton.tonalIcon({
Key? key,
required VoidCallback? onPressed,
VoidCallback? onLongPress,
ValueChanged<bool>? onHover,
ValueChanged<bool>? onFocusChange,
ButtonStyle? style,
FocusNode? focusNode,
bool? autofocus,
Clip? clipBehavior,
MaterialStatesController? statesController,
Widget? icon,
required Widget label,
IconAlignment iconAlignment = IconAlignment.start,
}) {
if (icon == null) {
return FilledButton.tonal(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
onHover: onHover,
onFocusChange: onFocusChange,
style: style,
focusNode: focusNode,
autofocus: autofocus ?? false,
clipBehavior: clipBehavior ?? Clip.none,
statesController: statesController,
child: label,
);
}
return _FilledButtonWithIcon.tonal(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
onHover: onHover,
onFocusChange: onFocusChange,
style: style,
focusNode: focusNode,
autofocus: autofocus,
clipBehavior: clipBehavior,
statesController: statesController,
icon: icon,
label: label,
iconAlignment: iconAlignment,
);
}
/// A static convenience method that constructs a filled button
/// [ButtonStyle] given simple values.
///
/// The [foregroundColor] and [disabledForegroundColor] colors are used
/// to create a [MaterialStateProperty] [ButtonStyle.foregroundColor], and
/// a derived [ButtonStyle.overlayColor] if [overlayColor] isn't specified.
///
/// If [overlayColor] is specified and its value is [Colors.transparent]
/// then the pressed/focused/hovered highlights are effectively defeated.
/// Otherwise a [MaterialStateProperty] with the same opacities as the
/// default is created.
///
/// Similarly, the [enabledMouseCursor] and [disabledMouseCursor]
/// parameters are used to construct [ButtonStyle.mouseCursor].
///
/// The button's elevations are defined relative to the [elevation]
/// parameter. The disabled elevation is the same as the parameter
/// value, [elevation] + 2 is used when the button is hovered
/// or focused, and elevation + 6 is used when the button is pressed.
///
/// All of the other parameters are either used directly or used to
/// create a [MaterialStateProperty] with a single value for all
/// states.
///
/// All parameters default to null, by default this method returns
/// a [ButtonStyle] that doesn't override anything.
///
/// For example, to override the default text and icon colors for a
/// [FilledButton], as well as its overlay color, with all of the
/// standard opacity adjustments for the pressed, focused, and
/// hovered states, one could write:
///
/// ```dart
/// FilledButton(
/// style: FilledButton.styleFrom(foregroundColor: Colors.green),
/// onPressed: () {},
/// child: const Text('Filled button'),
/// );
/// ```
///
/// or for a Filled tonal variant:
/// ```dart
/// FilledButton.tonal(
/// style: FilledButton.styleFrom(foregroundColor: Colors.green),
/// onPressed: () {},
/// child: const Text('Filled tonal button'),
/// );
/// ```
static ButtonStyle styleFrom({
Color? foregroundColor,
Color? backgroundColor,
Color? disabledForegroundColor,
Color? disabledBackgroundColor,
Color? shadowColor,
Color? surfaceTintColor,
Color? iconColor,
Color? disabledIconColor,
Color? overlayColor,
double? elevation,
TextStyle? textStyle,
EdgeInsetsGeometry? padding,
Size? minimumSize,
Size? fixedSize,
Size? maximumSize,
BorderSide? side,
OutlinedBorder? shape,
MouseCursor? enabledMouseCursor,
MouseCursor? disabledMouseCursor,
VisualDensity? visualDensity,
MaterialTapTargetSize? tapTargetSize,
Duration? animationDuration,
bool? enableFeedback,
AlignmentGeometry? alignment,
InteractiveInkFeatureFactory? splashFactory,
ButtonLayerBuilder? backgroundBuilder,
ButtonLayerBuilder? foregroundBuilder,
}) {
final MaterialStateProperty<Color?>? foregroundColorProp = switch ((foregroundColor, disabledForegroundColor)) {
(null, null) => null,
(_, _) => _FilledButtonDefaultColor(foregroundColor, disabledForegroundColor),
};
final MaterialStateProperty<Color?>? backgroundColorProp = switch ((backgroundColor, disabledBackgroundColor)) {
(null, null) => null,
(_, _) => _FilledButtonDefaultColor(backgroundColor, disabledBackgroundColor),
};
final MaterialStateProperty<Color?>? iconColorProp = switch ((iconColor, disabledIconColor)) {
(null, null) => null,
(_, _) => _FilledButtonDefaultColor(iconColor, disabledIconColor),
};
final MaterialStateProperty<Color?>? overlayColorProp = switch ((foregroundColor, overlayColor)) {
(null, null) => null,
(_, final Color overlayColor) when overlayColor.value == 0 => const MaterialStatePropertyAll<Color?>(Colors.transparent),
(_, _) => _FilledButtonDefaultOverlay((overlayColor ?? foregroundColor)!),
};
final MaterialStateProperty<MouseCursor?> mouseCursor = _FilledButtonDefaultMouseCursor(enabledMouseCursor, disabledMouseCursor);
return ButtonStyle(
textStyle: MaterialStatePropertyAll<TextStyle?>(textStyle),
backgroundColor: backgroundColorProp,
foregroundColor: foregroundColorProp,
overlayColor: overlayColorProp,
shadowColor: ButtonStyleButton.allOrNull<Color>(shadowColor),
surfaceTintColor: ButtonStyleButton.allOrNull<Color>(surfaceTintColor),
iconColor: iconColorProp,
elevation: ButtonStyleButton.allOrNull(elevation),
padding: ButtonStyleButton.allOrNull<EdgeInsetsGeometry>(padding),
minimumSize: ButtonStyleButton.allOrNull<Size>(minimumSize),
fixedSize: ButtonStyleButton.allOrNull<Size>(fixedSize),
maximumSize: ButtonStyleButton.allOrNull<Size>(maximumSize),
side: ButtonStyleButton.allOrNull<BorderSide>(side),
shape: ButtonStyleButton.allOrNull<OutlinedBorder>(shape),
mouseCursor: mouseCursor,
visualDensity: visualDensity,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
splashFactory: splashFactory,
backgroundBuilder: backgroundBuilder,
foregroundBuilder: foregroundBuilder,
);
}
final _FilledButtonVariant _variant;
/// Defines the button's default appearance.
///
/// The button [child]'s [Text] and [Icon] widgets are rendered with
/// the [ButtonStyle]'s foreground color. The button's [InkWell] adds
/// the style's overlay color when the button is focused, hovered
/// or pressed. The button's background color becomes its [Material]
/// color.
///
/// All of the ButtonStyle's defaults appear below. In this list
/// "Theme.foo" is shorthand for `Theme.of(context).foo`. Color
/// scheme values like "onSurface(0.38)" are shorthand for
/// `onSurface.withOpacity(0.38)`. [MaterialStateProperty] valued
/// properties that are not followed by a sublist have the same
/// value for all states, otherwise the values are as specified for
/// each state, and "others" means all other states.
///
/// {@macro flutter.material.elevated_button.default_font_size}
///
/// The color of the [ButtonStyle.textStyle] is not used, the
/// [ButtonStyle.foregroundColor] color is used instead.
///
/// * `textStyle` - Theme.textTheme.labelLarge
/// * `backgroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.12)
/// * others - Theme.colorScheme.secondaryContainer
/// * `foregroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.38)
/// * others - Theme.colorScheme.onSecondaryContainer
/// * `overlayColor`
/// * hovered - Theme.colorScheme.onSecondaryContainer(0.08)
/// * focused or pressed - Theme.colorScheme.onSecondaryContainer(0.12)
/// * `shadowColor` - Theme.colorScheme.shadow
/// * `surfaceTintColor` - null
/// * `elevation`
/// * disabled - 0
/// * default - 0
/// * hovered - 1
/// * focused or pressed - 0
/// * `padding`
/// * `default font size <= 14` - horizontal(16)
/// * `14 < default font size <= 28` - lerp(horizontal(16), horizontal(8))
/// * `28 < default font size <= 36` - lerp(horizontal(8), horizontal(4))
/// * `36 < default font size` - horizontal(4)
/// * `minimumSize` - Size(64, 40)
/// * `fixedSize` - null
/// * `maximumSize` - Size.infinite
/// * `side` - null
/// * `shape` - StadiumBorder()
/// * `mouseCursor`
/// * disabled - SystemMouseCursors.basic
/// * others - SystemMouseCursors.click
/// * `visualDensity` - Theme.visualDensity
/// * `tapTargetSize` - Theme.materialTapTargetSize
/// * `animationDuration` - kThemeChangeDuration
/// * `enableFeedback` - true
/// * `alignment` - Alignment.center
/// * `splashFactory` - Theme.splashFactory
///
/// The default padding values for the [FilledButton.icon] factory are slightly different:
///
/// * `padding`
/// * `default font size <= 14` - start(12) end(16)
/// * `14 < default font size <= 28` - lerp(start(12) end(16), horizontal(8))
/// * `28 < default font size <= 36` - lerp(horizontal(8), horizontal(4))
/// * `36 < default font size` - horizontal(4)
///
/// The default value for `side`, which defines the appearance of the button's
/// outline, is null. That means that the outline is defined by the button
/// shape's [OutlinedBorder.side]. Typically the default value of an
/// [OutlinedBorder]'s side is [BorderSide.none], so an outline is not drawn.
///
/// ## Material 3 defaults
///
/// If [ThemeData.useMaterial3] is set to true the following defaults will
/// be used:
///
/// * `textStyle` - Theme.textTheme.labelLarge
/// * `backgroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.12)
/// * others - Theme.colorScheme.secondaryContainer
/// * `foregroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.38)
/// * others - Theme.colorScheme.onSecondaryContainer
/// * `overlayColor`
/// * hovered - Theme.colorScheme.onSecondaryContainer(0.08)
/// * focused or pressed - Theme.colorScheme.onSecondaryContainer(0.1)
/// * `shadowColor` - Theme.colorScheme.shadow
/// * `surfaceTintColor` - Colors.transparent
/// * `elevation`
/// * disabled - 0
/// * default - 1
/// * hovered - 3
/// * focused or pressed - 1
/// * `padding`
/// * `default font size <= 14` - horizontal(24)
/// * `14 < default font size <= 28` - lerp(horizontal(24), horizontal(12))
/// * `28 < default font size <= 36` - lerp(horizontal(12), horizontal(6))
/// * `36 < default font size` - horizontal(6)
/// * `minimumSize` - Size(64, 40)
/// * `fixedSize` - null
/// * `maximumSize` - Size.infinite
/// * `side` - null
/// * `shape` - StadiumBorder()
/// * `mouseCursor`
/// * disabled - SystemMouseCursors.basic
/// * others - SystemMouseCursors.click
/// * `visualDensity` - Theme.visualDensity
/// * `tapTargetSize` - Theme.materialTapTargetSize
/// * `animationDuration` - kThemeChangeDuration
/// * `enableFeedback` - true
/// * `alignment` - Alignment.center
/// * `splashFactory` - Theme.splashFactory
///
/// For the [FilledButton.icon] factory, the start (generally the left) value of
/// [padding] is reduced from 24 to 16.
@override
ButtonStyle defaultStyleOf(BuildContext context) {
return switch (_variant) {
_FilledButtonVariant.filled => _FilledButtonDefaultsM3(context),
_FilledButtonVariant.tonal => _FilledTonalButtonDefaultsM3(context),
};
}
/// Returns the [FilledButtonThemeData.style] of the closest
/// [FilledButtonTheme] ancestor.
@override
ButtonStyle? themeStyleOf(BuildContext context) {
return FilledButtonTheme.of(context).style;
}
}
EdgeInsetsGeometry _scaledPadding(BuildContext context) {
final ThemeData theme = Theme.of(context);
final double defaultFontSize = theme.textTheme.labelLarge?.fontSize ?? 14.0;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0;
final double padding1x = theme.useMaterial3 ? 24.0 : 16.0;
return ButtonStyleButton.scaledPadding(
EdgeInsets.symmetric(horizontal: padding1x),
EdgeInsets.symmetric(horizontal: padding1x / 2),
EdgeInsets.symmetric(horizontal: padding1x / 2 / 2),
effectiveTextScale,
);
}
@immutable
class _FilledButtonDefaultColor extends MaterialStateProperty<Color?> with Diagnosticable {
_FilledButtonDefaultColor(this.color, this.disabled);
final Color? color;
final Color? disabled;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabled;
}
return color;
}
}
@immutable
class _FilledButtonDefaultOverlay extends MaterialStateProperty<Color?> with Diagnosticable {
_FilledButtonDefaultOverlay(this.overlay);
final Color overlay;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return overlay.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return overlay.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return overlay.withOpacity(0.1);
}
return null;
}
}
@immutable
class _FilledButtonDefaultMouseCursor extends MaterialStateProperty<MouseCursor?> with Diagnosticable {
_FilledButtonDefaultMouseCursor(this.enabledCursor, this.disabledCursor);
final MouseCursor? enabledCursor;
final MouseCursor? disabledCursor;
@override
MouseCursor? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledCursor;
}
return enabledCursor;
}
}
class _FilledButtonWithIcon extends FilledButton {
_FilledButtonWithIcon({
super.key,
required super.onPressed,
super.onLongPress,
super.onHover,
super.onFocusChange,
super.style,
super.focusNode,
bool? autofocus,
super.clipBehavior,
super.statesController,
required Widget icon,
required Widget label,
super.iconAlignment,
}) : super(
autofocus: autofocus ?? false,
child: _FilledButtonWithIconChild(
icon: icon,
label: label,
buttonStyle: style,
iconAlignment: iconAlignment,
),
);
_FilledButtonWithIcon.tonal({
super.key,
required super.onPressed,
super.onLongPress,
super.onHover,
super.onFocusChange,
super.style,
super.focusNode,
bool? autofocus,
super.clipBehavior,
super.statesController,
required Widget icon,
required Widget label,
required IconAlignment iconAlignment,
}) : super.tonal(
autofocus: autofocus ?? false,
child: _FilledButtonWithIconChild(
icon: icon,
label: label,
buttonStyle: style,
iconAlignment: iconAlignment,
),
);
@override
ButtonStyle defaultStyleOf(BuildContext context) {
final bool useMaterial3 = Theme.of(context).useMaterial3;
final ButtonStyle buttonStyle = super.defaultStyleOf(context);
final double defaultFontSize = buttonStyle.textStyle?.resolve(const <MaterialState>{})?.fontSize ?? 14.0;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0;
final EdgeInsetsGeometry scaledPadding = useMaterial3
? ButtonStyleButton.scaledPadding(
const EdgeInsetsDirectional.fromSTEB(16, 0, 24, 0),
const EdgeInsetsDirectional.fromSTEB(8, 0, 12, 0),
const EdgeInsetsDirectional.fromSTEB(4, 0, 6, 0),
effectiveTextScale,
) : ButtonStyleButton.scaledPadding(
const EdgeInsetsDirectional.fromSTEB(12, 0, 16, 0),
const EdgeInsets.symmetric(horizontal: 8),
const EdgeInsetsDirectional.fromSTEB(8, 0, 4, 0),
effectiveTextScale,
);
return buttonStyle.copyWith(
padding: MaterialStatePropertyAll<EdgeInsetsGeometry>(scaledPadding),
);
}
}
class _FilledButtonWithIconChild extends StatelessWidget {
const _FilledButtonWithIconChild({
required this.label,
required this.icon,
required this.buttonStyle,
required this.iconAlignment,
});
final Widget label;
final Widget icon;
final ButtonStyle? buttonStyle;
final IconAlignment iconAlignment;
@override
Widget build(BuildContext context) {
final double defaultFontSize = buttonStyle?.textStyle?.resolve(const <MaterialState>{})?.fontSize ?? 14.0;
final double scale = clampDouble(MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0, 1.0, 2.0) - 1.0;
// Adjust the gap based on the text scale factor. Start at 8, and lerp
// to 4 based on how large the text is.
final double gap = lerpDouble(8, 4, scale)!;
return Row(
mainAxisSize: MainAxisSize.min,
children: iconAlignment == IconAlignment.start
? <Widget>[icon, SizedBox(width: gap), Flexible(child: label)]
: <Widget>[Flexible(child: label), SizedBox(width: gap), icon],
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - FilledButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FilledButtonDefaultsM3 extends ButtonStyle {
_FilledButtonDefaultsM3(this.context)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
MaterialStateProperty<TextStyle?> get textStyle =>
MaterialStatePropertyAll<TextStyle?>(Theme.of(context).textTheme.labelLarge);
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
return _colors.primary;
});
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.onPrimary;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimary.withOpacity(0.1);
}
return null;
});
@override
MaterialStateProperty<Color>? get shadowColor =>
MaterialStatePropertyAll<Color>(_colors.shadow);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<double>? get elevation =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return 0.0;
}
if (states.contains(MaterialState.pressed)) {
return 0.0;
}
if (states.contains(MaterialState.hovered)) {
return 1.0;
}
if (states.contains(MaterialState.focused)) {
return 0.0;
}
return 0.0;
});
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
MaterialStatePropertyAll<EdgeInsetsGeometry>(_scaledPadding(context));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(64.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
// No default side
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => Theme.of(context).visualDensity;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - FilledButton
// BEGIN GENERATED TOKEN PROPERTIES - FilledTonalButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _FilledTonalButtonDefaultsM3 extends ButtonStyle {
_FilledTonalButtonDefaultsM3(this.context)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
MaterialStateProperty<TextStyle?> get textStyle =>
MaterialStatePropertyAll<TextStyle?>(Theme.of(context).textTheme.labelLarge);
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
return _colors.secondaryContainer;
});
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.onSecondaryContainer;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSecondaryContainer.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSecondaryContainer.withOpacity(0.1);
}
return null;
});
@override
MaterialStateProperty<Color>? get shadowColor =>
MaterialStatePropertyAll<Color>(_colors.shadow);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<double>? get elevation =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return 0.0;
}
if (states.contains(MaterialState.pressed)) {
return 0.0;
}
if (states.contains(MaterialState.hovered)) {
return 1.0;
}
if (states.contains(MaterialState.focused)) {
return 0.0;
}
return 0.0;
});
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
MaterialStatePropertyAll<EdgeInsetsGeometry>(_scaledPadding(context));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(64.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
// No default side
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => Theme.of(context).visualDensity;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - FilledTonalButton
| flutter/packages/flutter/lib/src/material/filled_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/filled_button.dart",
"repo_id": "flutter",
"token_count": 10402
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui;
import 'package:flutter/widgets.dart';
import 'package:vector_math/vector_math_64.dart';
import 'ink_well.dart';
import 'material.dart';
/// Begin a Material 3 ink sparkle ripple, centered at the tap or click position
/// relative to the [referenceBox].
///
/// This effect relies on a shader and therefore is unsupported on the Flutter
/// Web HTML backend.
///
/// To use this effect, pass an instance of [splashFactory] to the
/// `splashFactory` parameter of either the Material [ThemeData] or any
/// component that has a `splashFactory` parameter, such as buttons:
/// - [ElevatedButton]
/// - [TextButton]
/// - [OutlinedButton]
///
/// The [controller] argument is typically obtained via
/// `Material.of(context)`.
///
/// If [containedInkWell] is true, then the effect will be sized to fit
/// the well rectangle, and clipped to it when drawn. The well
/// rectangle is the box returned by [rectCallback], if provided, or
/// otherwise is the bounds of the [referenceBox].
///
/// If [containedInkWell] is false, then [rectCallback] should be null.
/// The ink ripple is clipped only to the edges of the [Material].
/// This is the default.
///
/// When the ripple is removed, [onRemoved] will be called.
///
/// {@tool snippet}
///
/// For typical use, pass the [InkSparkle.splashFactory] to the `splashFactory`
/// parameter of a button style or [ThemeData].
///
/// ```dart
/// ElevatedButton(
/// style: ElevatedButton.styleFrom(splashFactory: InkSparkle.splashFactory),
/// child: const Text('Sparkle!'),
/// onPressed: () { },
/// )
/// ```
/// {@end-tool}
class InkSparkle extends InteractiveInkFeature {
/// Begin a sparkly ripple effect, centered at [position] relative to
/// [referenceBox].
///
/// The [color] defines the color of the splash itself. The sparkles are
/// always white.
///
/// The [controller] argument is typically obtained via
/// `Material.of(context)`.
///
/// [textDirection] is used by [customBorder] if it is non-null. This allows
/// the [customBorder]'s path to be properly defined if it was the path was
/// expressed in terms of "start" and "end" instead of
/// "left" and "right".
///
/// If [containedInkWell] is true, then the ripple will be sized to fit
/// the well rectangle, then clipped to it when drawn. The well
/// rectangle is the box returned by [rectCallback], if provided, or
/// otherwise is the bounds of the [referenceBox].
///
/// If [containedInkWell] is false, then [rectCallback] should be null.
/// The ink ripple is clipped only to the edges of the [Material].
/// This is the default.
///
/// Clipping can happen in 3 different ways:
/// 1. If [customBorder] is provided, it is used to determine the path for
/// clipping.
/// 2. If [customBorder] is null, and [borderRadius] is provided, then the
/// canvas is clipped by an [RRect] created from [borderRadius].
/// 3. If [borderRadius] is the default [BorderRadius.zero], then the canvas
/// is clipped with [rectCallback].
/// When the ripple is removed, [onRemoved] will be called.
///
/// [turbulenceSeed] can be passed if a non random seed should be used for
/// the turbulence and sparkles. By default, the seed is a random number
/// between 0.0 and 1000.0.
///
/// Turbulence is an input to the shader and helps to provides a more natural,
/// non-circular, "splash" effect.
///
/// Sparkle randomization is also driven by the [turbulenceSeed]. Sparkles are
/// identified in the shader as "noise", and the sparkles are derived from
/// pseudorandom triangular noise.
InkSparkle({
required super.controller,
required super.referenceBox,
required super.color,
required Offset position,
required TextDirection textDirection,
bool containedInkWell = true,
RectCallback? rectCallback,
BorderRadius? borderRadius,
super.customBorder,
double? radius,
super.onRemoved,
double? turbulenceSeed,
}) : assert(containedInkWell || rectCallback == null),
_color = color,
_position = position,
_borderRadius = borderRadius ?? BorderRadius.zero,
_textDirection = textDirection,
_targetRadius = (radius ?? _getTargetRadius(
referenceBox,
containedInkWell,
rectCallback,
position,
)
) * _targetRadiusMultiplier,
_clipCallback = _getClipCallback(referenceBox, containedInkWell, rectCallback) {
// InkSparkle will not be painted until the async compilation completes.
_InkSparkleFactory.initializeShader();
controller.addInkFeature(this);
// Immediately begin animating the ink.
_animationController = AnimationController(
duration: _animationDuration,
vsync: controller.vsync,
)..addListener(controller.markNeedsPaint)
..addStatusListener(_handleStatusChanged)
..forward();
_radiusScale = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: CurveTween(curve: Curves.fastOutSlowIn),
weight: 75,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(1.0),
weight: 25,
),
],
).animate(_animationController);
// Functionally equivalent to Android 12's SkSL:
//`return mix(u_touch, u_resolution, saturate(in_radius_scale * 2.0))`
final Tween<Vector2> centerTween = Tween<Vector2>(
begin: Vector2.array(<double>[_position.dx, _position.dy]),
end: Vector2.array(<double>[referenceBox.size.width / 2, referenceBox.size.height / 2]),
);
final Animation<double> centerProgress = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.0, end: 1.0),
weight: 50,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(1.0),
weight: 50,
),
],
).animate(_radiusScale);
_center = centerTween.animate(centerProgress);
_alpha = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.0, end: 1.0),
weight: 13,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(1.0),
weight: 27,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 1.0, end: 0.0),
weight: 60,
),
],
).animate(_animationController);
_sparkleAlpha = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.0, end: 1.0),
weight: 13,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(1.0),
weight: 27,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 1.0, end: 0.0),
weight: 50,
),
],
).animate(_animationController);
// Creates an element of randomness so that ink emanating from the same
// pixel have slightly different rings and sparkles.
assert((){
// In tests, randomness can cause flakes. So if a seed has not
// already been specified (i.e. for the purpose of the test), set it to
// the constant turbulence seed.
turbulenceSeed ??= _InkSparkleFactory.constantSeed;
return true;
}());
_turbulenceSeed = turbulenceSeed ?? math.Random().nextDouble() * 1000.0;
}
void _handleStatusChanged(AnimationStatus status) {
if (status == AnimationStatus.completed) {
dispose();
}
}
static const Duration _animationDuration = Duration(milliseconds: 617);
static const double _targetRadiusMultiplier = 2.3;
static const double _rotateRight = math.pi * 0.0078125;
static const double _rotateLeft = -_rotateRight;
static const double _noiseDensity = 2.1;
late AnimationController _animationController;
// The Android 12 version has these values calculated in the GLSL. They are
// constant for every pixel in the animation, so the Flutter implementation
// computes these animation values in software in order to simplify the shader
// implementation and provide better performance on most devices.
late Animation<Vector2> _center;
late Animation<double> _radiusScale;
late Animation<double> _alpha;
late Animation<double> _sparkleAlpha;
late double _turbulenceSeed;
final Color _color;
final Offset _position;
final BorderRadius _borderRadius;
final double _targetRadius;
final RectCallback? _clipCallback;
final TextDirection _textDirection;
late final ui.FragmentShader _fragmentShader;
bool _fragmentShaderInitialized = false;
/// Used to specify this type of ink splash for an [InkWell], [InkResponse],
/// material [Theme], or [ButtonStyle].
///
/// Since no [turbulenceSeed] is passed, the effect will be random for
/// subsequent presses in the same position.
static const InteractiveInkFeatureFactory splashFactory = _InkSparkleFactory();
/// Used to specify this type of ink splash for an [InkWell], [InkResponse],
/// material [Theme], or [ButtonStyle].
///
/// Since a [turbulenceSeed] is passed, the effect will not be random for
/// subsequent presses in the same position. This can be used for testing.
static const InteractiveInkFeatureFactory constantTurbulenceSeedSplashFactory = _InkSparkleFactory.constantTurbulenceSeed();
@override
void dispose() {
_animationController.stop();
_animationController.dispose();
if (_fragmentShaderInitialized) {
_fragmentShader.dispose();
}
super.dispose();
}
@override
void paintFeature(Canvas canvas, Matrix4 transform) {
assert(_animationController.isAnimating);
// InkSparkle can only paint if its shader has been compiled.
if (_InkSparkleFactory._program == null) {
// Skipping paintFeature because the shader it relies on is not ready to
// be used. InkSparkleFactory.initializeShader must complete
// before InkSparkle can paint.
return;
}
if (!_fragmentShaderInitialized) {
_fragmentShader = _InkSparkleFactory._program!.fragmentShader();
_fragmentShaderInitialized = true;
}
canvas.save();
_transformCanvas(canvas: canvas, transform: transform);
if (_clipCallback != null) {
_clipCanvas(
canvas: canvas,
clipCallback: _clipCallback,
textDirection: _textDirection,
customBorder: customBorder,
borderRadius: _borderRadius,
);
}
_updateFragmentShader();
final Paint paint = Paint()..shader = _fragmentShader;
if (_clipCallback != null) {
canvas.drawRect(_clipCallback(), paint);
} else {
canvas.drawPaint(paint);
}
canvas.restore();
}
double get _width => referenceBox.size.width;
double get _height => referenceBox.size.height;
/// All double values for uniforms come from the Android 12 ripple
/// implementation from the following files:
/// - https://cs.android.com/android/platform/superproject/+/master:frameworks/base/graphics/java/android/graphics/drawable/RippleShader.java
/// - https://cs.android.com/android/platform/superproject/+/master:frameworks/base/graphics/java/android/graphics/drawable/RippleDrawable.java
/// - https://cs.android.com/android/platform/superproject/+/master:frameworks/base/graphics/java/android/graphics/drawable/RippleAnimationSession.java
void _updateFragmentShader() {
const double turbulenceScale = 1.5;
final double turbulencePhase = _turbulenceSeed + _radiusScale.value;
final double noisePhase = turbulencePhase;
final double rotation1 = turbulencePhase * _rotateRight + 1.7 * math.pi;
final double rotation2 = turbulencePhase * _rotateLeft + 2.0 * math.pi;
final double rotation3 = turbulencePhase * _rotateRight + 2.75 * math.pi;
_fragmentShader
// uColor
..setFloat(0, _color.red / 255.0)
..setFloat(1, _color.green / 255.0)
..setFloat(2, _color.blue / 255.0)
..setFloat(3, _color.alpha / 255.0)
// Composite 1 (u_alpha, u_sparkle_alpha, u_blur, u_radius_scale)
..setFloat(4, _alpha.value)
..setFloat(5, _sparkleAlpha.value)
..setFloat(6, 1.0)
..setFloat(7, _radiusScale.value)
// uCenter
..setFloat(8, _center.value.x)
..setFloat(9, _center.value.y)
// uMaxRadius
..setFloat(10, _targetRadius)
// uResolutionScale
..setFloat(11, 1.0 / _width)
..setFloat(12, 1.0 / _height)
// uNoiseScale
..setFloat(13, _noiseDensity / _width)
..setFloat(14, _noiseDensity / _height)
// uNoisePhase
..setFloat(15, noisePhase / 1000.0)
// uCircle1
..setFloat(16, turbulenceScale * 0.5 + (turbulencePhase * 0.01 * math.cos(turbulenceScale * 0.55)))
..setFloat(17, turbulenceScale * 0.5 + (turbulencePhase * 0.01 * math.sin(turbulenceScale * 0.55)))
// uCircle2
..setFloat(18, turbulenceScale * 0.2 + (turbulencePhase * -0.0066 * math.cos(turbulenceScale * 0.45)))
..setFloat(19, turbulenceScale * 0.2 + (turbulencePhase * -0.0066 * math.sin(turbulenceScale * 0.45)))
// uCircle3
..setFloat(20, turbulenceScale + (turbulencePhase * -0.0066 * math.cos(turbulenceScale * 0.35)))
..setFloat(21, turbulenceScale + (turbulencePhase * -0.0066 * math.sin(turbulenceScale * 0.35)))
// uRotation1
..setFloat(22, math.cos(rotation1))
..setFloat(23, math.sin(rotation1))
// uRotation2
..setFloat(24, math.cos(rotation2))
..setFloat(25, math.sin(rotation2))
// uRotation3
..setFloat(26, math.cos(rotation3))
..setFloat(27, math.sin(rotation3));
}
/// Transforms the canvas for an ink feature to be painted on the [canvas].
///
/// This should be called before painting ink features that do not use
/// [paintInkCircle].
///
/// The [transform] argument is the [Matrix4] transform that typically
/// shifts the coordinate space of the canvas to the space in which
/// the ink feature is to be painted.
///
/// For examples on how the function is used, see [InkSparkle] and [paintInkCircle].
void _transformCanvas({
required Canvas canvas,
required Matrix4 transform,
}) {
final Offset? originOffset = MatrixUtils.getAsTranslation(transform);
if (originOffset == null) {
canvas.transform(transform.storage);
} else {
canvas.translate(originOffset.dx, originOffset.dy);
}
}
/// Clips the canvas for an ink feature to be painted on the [canvas].
///
/// This should be called before painting ink features with [paintFeature]
/// that do not use [paintInkCircle].
///
/// The [clipCallback] is the callback used to obtain the [Rect] used for clipping
/// the ink effect.
///
/// If [clipCallback] is null, no clipping is performed on the ink circle.
///
/// The [textDirection] is used by [customBorder] if it is non-null. This
/// allows the [customBorder]'s path to be properly defined if the path was
/// expressed in terms of "start" and "end" instead of "left" and "right".
///
/// For examples on how the function is used, see [InkSparkle].
void _clipCanvas({
required Canvas canvas,
required RectCallback clipCallback,
TextDirection? textDirection,
ShapeBorder? customBorder,
BorderRadius borderRadius = BorderRadius.zero,
}) {
final Rect rect = clipCallback();
if (customBorder != null) {
canvas.clipPath(
customBorder.getOuterPath(rect, textDirection: textDirection));
} else if (borderRadius != BorderRadius.zero) {
canvas.clipRRect(RRect.fromRectAndCorners(
rect,
topLeft: borderRadius.topLeft,
topRight: borderRadius.topRight,
bottomLeft: borderRadius.bottomLeft,
bottomRight: borderRadius.bottomRight,
));
} else {
canvas.clipRect(rect);
}
}
}
class _InkSparkleFactory extends InteractiveInkFeatureFactory {
const _InkSparkleFactory() : turbulenceSeed = null;
const _InkSparkleFactory.constantTurbulenceSeed() : turbulenceSeed = _InkSparkleFactory.constantSeed;
static const double constantSeed = 1337.0;
static void initializeShader() {
if (!_initCalled) {
ui.FragmentProgram.fromAsset('shaders/ink_sparkle.frag').then(
(ui.FragmentProgram program) {
_program = program;
},
);
_initCalled = true;
}
}
static bool _initCalled = false;
static ui.FragmentProgram? _program;
final double? turbulenceSeed;
@override
InteractiveInkFeature create({
required MaterialInkController controller,
required RenderBox referenceBox,
required ui.Offset position,
required ui.Color color,
required ui.TextDirection textDirection,
bool containedInkWell = false,
RectCallback? rectCallback,
BorderRadius? borderRadius,
ShapeBorder? customBorder,
double? radius,
ui.VoidCallback? onRemoved,
}) {
return InkSparkle(
controller: controller,
referenceBox: referenceBox,
position: position,
color: color,
textDirection: textDirection,
containedInkWell: containedInkWell,
rectCallback: rectCallback,
borderRadius: borderRadius,
customBorder: customBorder,
radius: radius,
onRemoved: onRemoved,
turbulenceSeed: turbulenceSeed,
);
}
}
RectCallback? _getClipCallback(
RenderBox referenceBox,
bool containedInkWell,
RectCallback? rectCallback,
) {
if (rectCallback != null) {
assert(containedInkWell);
return rectCallback;
}
if (containedInkWell) {
return () => Offset.zero & referenceBox.size;
}
return null;
}
double _getTargetRadius(
RenderBox referenceBox,
bool containedInkWell,
RectCallback? rectCallback,
Offset position,
) {
final Size size = rectCallback != null ? rectCallback().size : referenceBox.size;
final double d1 = size.bottomRight(Offset.zero).distance;
final double d2 = (size.topRight(Offset.zero) - size.bottomLeft(Offset.zero)).distance;
return math.max(d1, d2) / 2.0;
}
| flutter/packages/flutter/lib/src/material/ink_sparkle.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/ink_sparkle.dart",
"repo_id": "flutter",
"token_count": 6666
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'menu_anchor.dart';
import 'menu_style.dart';
import 'menu_theme.dart';
import 'theme.dart';
// Examples can assume:
// late Widget child;
/// A data class that [MenuBarTheme] uses to define the visual properties of
/// [MenuBar] widgets.
///
/// This class defines the visual properties of [MenuBar] widgets themselves,
/// but not their submenus. Those properties are defined by [MenuThemeData] or
/// [MenuButtonThemeData] instead.
///
/// Descendant widgets obtain the current [MenuBarThemeData] object using
/// `MenuBarTheme.of(context)`.
///
/// Typically, a [MenuBarThemeData] is specified as part of the overall [Theme]
/// with [ThemeData.menuBarTheme]. Otherwise, [MenuTheme] can be used to
/// configure its own widget subtree.
///
/// All [MenuBarThemeData] properties are `null` by default. If any of these
/// properties are null, the menu bar will provide its own defaults.
///
/// See also:
///
/// * [MenuThemeData], which describes the theme for the submenus of a
/// [MenuBar].
/// * [MenuButtonThemeData], which describes the theme for the [MenuItemButton]s
/// in a menu.
/// * [ThemeData], which describes the overall theme for the application.
@immutable
class MenuBarThemeData extends MenuThemeData {
/// Creates a const set of properties used to configure [MenuTheme].
const MenuBarThemeData({super.style});
/// Linearly interpolate between two [MenuBar] themes.
static MenuBarThemeData? lerp(MenuBarThemeData? a, MenuBarThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return MenuBarThemeData(style: MenuStyle.lerp(a?.style, b?.style, t));
}
}
/// An inherited widget that defines the configuration for the [MenuBar] widgets
/// in this widget's descendants.
///
/// This class defines the visual properties of [MenuBar] widgets themselves,
/// but not their submenus. Those properties are defined by [MenuTheme] or
/// [MenuButtonTheme] instead.
///
/// Values specified here are used for [MenuBar]'s properties that are not given
/// an explicit non-null value.
///
/// See also:
/// * [MenuStyle], a configuration object that holds attributes of a menu, and
/// is used by this theme to define those attributes.
/// * [MenuTheme], which does the same thing for the menus created by a
/// [SubmenuButton] or [MenuAnchor].
/// * [MenuButtonTheme], which does the same thing for the [MenuItemButton]s
/// inside of the menus.
/// * [SubmenuButton], a button that manages a submenu that uses these
/// properties.
/// * [MenuBar], a widget that creates a menu bar that can use [SubmenuButton]s.
class MenuBarTheme extends InheritedTheme {
/// Creates a theme that controls the configurations for [MenuBar] and
/// [MenuItemButton] in its widget subtree.
const MenuBarTheme({
super.key,
required this.data,
required super.child,
});
/// The properties to set for [MenuBar] in this widget's descendants.
final MenuBarThemeData data;
/// Returns the closest instance of this class's [data] value that encloses
/// the given context. If there is no ancestor, it returns
/// [ThemeData.menuBarTheme].
///
/// Typical usage is as follows:
///
/// ```dart
/// Widget build(BuildContext context) {
/// return MenuTheme(
/// data: const MenuThemeData(
/// style: MenuStyle(
/// backgroundColor: MaterialStatePropertyAll<Color?>(Colors.red),
/// ),
/// ),
/// child: child,
/// );
/// }
/// ```
static MenuBarThemeData of(BuildContext context) {
final MenuBarTheme? menuBarTheme = context.dependOnInheritedWidgetOfExactType<MenuBarTheme>();
return menuBarTheme?.data ?? Theme.of(context).menuBarTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return MenuBarTheme(data: data, child: child);
}
@override
bool updateShouldNotify(MenuBarTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/menu_bar_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/menu_bar_theme.dart",
"repo_id": "flutter",
"token_count": 1220
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'colors.dart';
import 'theme.dart';
// Slides the page upwards and fades it in, starting from 1/4 screen
// below the top. The transition is intended to match the default for
// Android O.
class _FadeUpwardsPageTransition extends StatelessWidget {
_FadeUpwardsPageTransition({
required Animation<double> routeAnimation, // The route's linear 0.0 - 1.0 animation.
required this.child,
}) : _positionAnimation = routeAnimation.drive(_bottomUpTween.chain(_fastOutSlowInTween)),
_opacityAnimation = routeAnimation.drive(_easeInTween);
// Fractional offset from 1/4 screen below the top to fully on screen.
static final Tween<Offset> _bottomUpTween = Tween<Offset>(
begin: const Offset(0.0, 0.25),
end: Offset.zero,
);
static final Animatable<double> _fastOutSlowInTween = CurveTween(curve: Curves.fastOutSlowIn);
static final Animatable<double> _easeInTween = CurveTween(curve: Curves.easeIn);
final Animation<Offset> _positionAnimation;
final Animation<double> _opacityAnimation;
final Widget child;
@override
Widget build(BuildContext context) {
return SlideTransition(
position: _positionAnimation,
// TODO(ianh): tell the transform to be un-transformed for hit testing
child: FadeTransition(
opacity: _opacityAnimation,
child: child,
),
);
}
}
// This transition is intended to match the default for Android P.
class _OpenUpwardsPageTransition extends StatelessWidget {
const _OpenUpwardsPageTransition({
required this.animation,
required this.secondaryAnimation,
required this.child,
});
// The new page slides upwards just a little as its clip
// rectangle exposes the page from bottom to top.
static final Tween<Offset> _primaryTranslationTween = Tween<Offset>(
begin: const Offset(0.0, 0.05),
end: Offset.zero,
);
// The old page slides upwards a little as the new page appears.
static final Tween<Offset> _secondaryTranslationTween = Tween<Offset>(
begin: Offset.zero,
end: const Offset(0.0, -0.025),
);
// The scrim obscures the old page by becoming increasingly opaque.
static final Tween<double> _scrimOpacityTween = Tween<double>(
begin: 0.0,
end: 0.25,
);
// Used by all of the transition animations.
static const Curve _transitionCurve = Cubic(0.20, 0.00, 0.00, 1.00);
final Animation<double> animation;
final Animation<double> secondaryAnimation;
final Widget child;
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
final Size size = constraints.biggest;
final CurvedAnimation primaryAnimation = CurvedAnimation(
parent: animation,
curve: _transitionCurve,
reverseCurve: _transitionCurve.flipped,
);
// Gradually expose the new page from bottom to top.
final Animation<double> clipAnimation = Tween<double>(
begin: 0.0,
end: size.height,
).animate(primaryAnimation);
final Animation<double> opacityAnimation = _scrimOpacityTween.animate(primaryAnimation);
final Animation<Offset> primaryTranslationAnimation = _primaryTranslationTween.animate(primaryAnimation);
final Animation<Offset> secondaryTranslationAnimation = _secondaryTranslationTween.animate(
CurvedAnimation(
parent: secondaryAnimation,
curve: _transitionCurve,
reverseCurve: _transitionCurve.flipped,
),
);
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return Container(
color: Colors.black.withOpacity(opacityAnimation.value),
alignment: Alignment.bottomLeft,
child: ClipRect(
child: SizedBox(
height: clipAnimation.value,
child: OverflowBox(
alignment: Alignment.bottomLeft,
maxHeight: size.height,
child: child,
),
),
),
);
},
child: AnimatedBuilder(
animation: secondaryAnimation,
child: FractionalTranslation(
translation: primaryTranslationAnimation.value,
child: child,
),
builder: (BuildContext context, Widget? child) {
return FractionalTranslation(
translation: secondaryTranslationAnimation.value,
child: child,
);
},
),
);
},
);
}
}
// Zooms and fades a new page in, zooming out the previous page. This transition
// is designed to match the Android Q activity transition.
class _ZoomPageTransition extends StatelessWidget {
/// Creates a [_ZoomPageTransition].
///
/// The [animation] and [secondaryAnimation] arguments are required and must
/// not be null.
const _ZoomPageTransition({
required this.animation,
required this.secondaryAnimation,
required this.allowSnapshotting,
required this.allowEnterRouteSnapshotting,
this.child,
});
// A curve sequence that is similar to the 'fastOutExtraSlowIn' curve used in
// the native transition.
static final List<TweenSequenceItem<double>> fastOutExtraSlowInTweenSequenceItems = <TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.0, end: 0.4)
.chain(CurveTween(curve: const Cubic(0.05, 0.0, 0.133333, 0.06))),
weight: 0.166666,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 0.4, end: 1.0)
.chain(CurveTween(curve: const Cubic(0.208333, 0.82, 0.25, 1.0))),
weight: 1.0 - 0.166666,
),
];
static final TweenSequence<double> _scaleCurveSequence = TweenSequence<double>(fastOutExtraSlowInTweenSequenceItems);
/// The animation that drives the [child]'s entrance and exit.
///
/// See also:
///
/// * [TransitionRoute.animation], which is the value given to this property
/// when the [_ZoomPageTransition] is used as a page transition.
final Animation<double> animation;
/// The animation that transitions [child] when new content is pushed on top
/// of it.
///
/// See also:
///
/// * [TransitionRoute.secondaryAnimation], which is the value given to this
/// property when the [_ZoomPageTransition] is used as a page transition.
final Animation<double> secondaryAnimation;
/// Whether the [SnapshotWidget] will be used.
///
/// When this value is true, performance is improved by disabling animations
/// on both the outgoing and incoming route. This also implies that ink-splashes
/// or similar animations will not animate during the transition.
///
/// See also:
///
/// * [TransitionRoute.allowSnapshotting], which defines whether the route
/// transition will prefer to animate a snapshot of the entering and exiting
/// routes.
final bool allowSnapshotting;
/// The widget below this widget in the tree.
///
/// This widget will transition in and out as driven by [animation] and
/// [secondaryAnimation].
final Widget? child;
/// Whether to enable snapshotting on the entering route during the
/// transition animation.
///
/// If not specified, defaults to true.
/// If false, the route snapshotting will not be applied to the route being
/// animating into, e.g. when transitioning from route A to route B, B will
/// not be snapshotted.
final bool allowEnterRouteSnapshotting;
@override
Widget build(BuildContext context) {
return DualTransitionBuilder(
animation: animation,
forwardBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomEnterTransition(
animation: animation,
allowSnapshotting: allowSnapshotting && allowEnterRouteSnapshotting,
child: child,
);
},
reverseBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomExitTransition(
animation: animation,
allowSnapshotting: allowSnapshotting,
reverse: true,
child: child,
);
},
child: DualTransitionBuilder(
animation: ReverseAnimation(secondaryAnimation),
forwardBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomEnterTransition(
animation: animation,
allowSnapshotting: allowSnapshotting && allowEnterRouteSnapshotting ,
reverse: true,
child: child,
);
},
reverseBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomExitTransition(
animation: animation,
allowSnapshotting: allowSnapshotting,
child: child,
);
},
child: child,
),
);
}
}
class _ZoomEnterTransition extends StatefulWidget {
const _ZoomEnterTransition({
required this.animation,
this.reverse = false,
required this.allowSnapshotting,
this.child,
});
final Animation<double> animation;
final Widget? child;
final bool allowSnapshotting;
final bool reverse;
@override
State<_ZoomEnterTransition> createState() => _ZoomEnterTransitionState();
}
class _ZoomEnterTransitionState extends State<_ZoomEnterTransition> with _ZoomTransitionBase<_ZoomEnterTransition> {
// See SnapshotWidget doc comment, this is disabled on web because the HTML backend doesn't
// support this functionality and the canvaskit backend uses a single thread for UI and raster
// work which diminishes the impact of this performance improvement.
@override
bool get useSnapshot => !kIsWeb && widget.allowSnapshotting;
late _ZoomEnterTransitionPainter delegate;
static final Animatable<double> _fadeInTransition = Tween<double>(
begin: 0.0,
end: 1.00,
).chain(CurveTween(curve: const Interval(0.125, 0.250)));
static final Animatable<double> _scaleDownTransition = Tween<double>(
begin: 1.10,
end: 1.00,
).chain(_ZoomPageTransition._scaleCurveSequence);
static final Animatable<double> _scaleUpTransition = Tween<double>(
begin: 0.85,
end: 1.00,
).chain(_ZoomPageTransition._scaleCurveSequence);
static final Animatable<double?> _scrimOpacityTween = Tween<double?>(
begin: 0.0,
end: 0.60,
).chain(CurveTween(curve: const Interval(0.2075, 0.4175)));
void _updateAnimations() {
fadeTransition = widget.reverse
? kAlwaysCompleteAnimation
: _fadeInTransition.animate(widget.animation);
scaleTransition = (widget.reverse
? _scaleDownTransition
: _scaleUpTransition
).animate(widget.animation);
widget.animation.addListener(onAnimationValueChange);
widget.animation.addStatusListener(onAnimationStatusChange);
}
@override
void initState() {
_updateAnimations();
delegate = _ZoomEnterTransitionPainter(
reverse: widget.reverse,
fade: fadeTransition,
scale: scaleTransition,
animation: widget.animation,
);
super.initState();
}
@override
void didUpdateWidget(covariant _ZoomEnterTransition oldWidget) {
if (oldWidget.reverse != widget.reverse || oldWidget.animation != widget.animation) {
oldWidget.animation.removeListener(onAnimationValueChange);
oldWidget.animation.removeStatusListener(onAnimationStatusChange);
_updateAnimations();
delegate.dispose();
delegate = _ZoomEnterTransitionPainter(
reverse: widget.reverse,
fade: fadeTransition,
scale: scaleTransition,
animation: widget.animation,
);
}
super.didUpdateWidget(oldWidget);
}
@override
void dispose() {
widget.animation.removeListener(onAnimationValueChange);
widget.animation.removeStatusListener(onAnimationStatusChange);
delegate.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return SnapshotWidget(
painter: delegate,
controller: controller,
mode: SnapshotMode.permissive,
autoresize: true,
child: widget.child,
);
}
}
class _ZoomExitTransition extends StatefulWidget {
const _ZoomExitTransition({
required this.animation,
this.reverse = false,
required this.allowSnapshotting,
this.child,
});
final Animation<double> animation;
final bool allowSnapshotting;
final bool reverse;
final Widget? child;
@override
State<_ZoomExitTransition> createState() => _ZoomExitTransitionState();
}
class _ZoomExitTransitionState extends State<_ZoomExitTransition> with _ZoomTransitionBase<_ZoomExitTransition> {
late _ZoomExitTransitionPainter delegate;
// See SnapshotWidget doc comment, this is disabled on web because the HTML backend doesn't
// support this functionality and the canvaskit backend uses a single thread for UI and raster
// work which diminishes the impact of this performance improvement.
@override
bool get useSnapshot => !kIsWeb && widget.allowSnapshotting;
static final Animatable<double> _fadeOutTransition = Tween<double>(
begin: 1.0,
end: 0.0,
).chain(CurveTween(curve: const Interval(0.0825, 0.2075)));
static final Animatable<double> _scaleUpTransition = Tween<double>(
begin: 1.00,
end: 1.05,
).chain(_ZoomPageTransition._scaleCurveSequence);
static final Animatable<double> _scaleDownTransition = Tween<double>(
begin: 1.00,
end: 0.90,
).chain(_ZoomPageTransition._scaleCurveSequence);
void _updateAnimations() {
fadeTransition = widget.reverse
? _fadeOutTransition.animate(widget.animation)
: kAlwaysCompleteAnimation;
scaleTransition = (widget.reverse
? _scaleDownTransition
: _scaleUpTransition
).animate(widget.animation);
widget.animation.addListener(onAnimationValueChange);
widget.animation.addStatusListener(onAnimationStatusChange);
}
@override
void initState() {
_updateAnimations();
delegate = _ZoomExitTransitionPainter(
reverse: widget.reverse,
fade: fadeTransition,
scale: scaleTransition,
animation: widget.animation,
);
super.initState();
}
@override
void didUpdateWidget(covariant _ZoomExitTransition oldWidget) {
if (oldWidget.reverse != widget.reverse || oldWidget.animation != widget.animation) {
oldWidget.animation.removeListener(onAnimationValueChange);
oldWidget.animation.removeStatusListener(onAnimationStatusChange);
_updateAnimations();
delegate.dispose();
delegate = _ZoomExitTransitionPainter(
reverse: widget.reverse,
fade: fadeTransition,
scale: scaleTransition,
animation: widget.animation,
);
}
super.didUpdateWidget(oldWidget);
}
@override
void dispose() {
widget.animation.removeListener(onAnimationValueChange);
widget.animation.removeStatusListener(onAnimationStatusChange);
delegate.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return SnapshotWidget(
painter: delegate,
controller: controller,
mode: SnapshotMode.permissive,
autoresize: true,
child: widget.child,
);
}
}
/// Used by [PageTransitionsTheme] to define a [MaterialPageRoute] page
/// transition animation.
///
/// Apps can configure the map of builders for [ThemeData.pageTransitionsTheme]
/// to customize the default [MaterialPageRoute] page transition animation
/// for different platforms.
///
/// See also:
///
/// * [FadeUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android O.
/// * [OpenUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android P.
/// * [ZoomPageTransitionsBuilder], which defines the default page transition
/// that's similar to the one provided in Android Q.
/// * [CupertinoPageTransitionsBuilder], which defines a horizontal page
/// transition that matches native iOS page transitions.
abstract class PageTransitionsBuilder {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const PageTransitionsBuilder();
/// Wraps the child with one or more transition widgets which define how [route]
/// arrives on and leaves the screen.
///
/// The [MaterialPageRoute.buildTransitions] method looks up the
/// current [PageTransitionsTheme] with `Theme.of(context).pageTransitionsTheme`
/// and delegates to this method with a [PageTransitionsBuilder] based
/// on the theme's [ThemeData.platform].
Widget buildTransitions<T>(
PageRoute<T> route,
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
);
}
/// Used by [PageTransitionsTheme] to define a vertically fading
/// [MaterialPageRoute] page transition animation that looks like
/// the default page transition used on Android O.
///
/// The animation fades the new page in while translating it upwards,
/// starting from about 25% below the top of the screen.
///
/// See also:
///
/// * [OpenUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android P.
/// * [ZoomPageTransitionsBuilder], which defines the default page transition
/// that's similar to the one provided in Android Q.
/// * [CupertinoPageTransitionsBuilder], which defines a horizontal page
/// transition that matches native iOS page transitions.
class FadeUpwardsPageTransitionsBuilder extends PageTransitionsBuilder {
/// Constructs a page transition animation that slides the page up.
const FadeUpwardsPageTransitionsBuilder();
@override
Widget buildTransitions<T>(
PageRoute<T>? route,
BuildContext? context,
Animation<double> animation,
Animation<double>? secondaryAnimation,
Widget child,
) {
return _FadeUpwardsPageTransition(routeAnimation: animation, child: child);
}
}
/// Used by [PageTransitionsTheme] to define a vertical [MaterialPageRoute] page
/// transition animation that looks like the default page transition
/// used on Android P.
///
/// See also:
///
/// * [FadeUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android O.
/// * [ZoomPageTransitionsBuilder], which defines the default page transition
/// that's similar to the one provided in Android Q.
/// * [CupertinoPageTransitionsBuilder], which defines a horizontal page
/// transition that matches native iOS page transitions.
class OpenUpwardsPageTransitionsBuilder extends PageTransitionsBuilder {
/// Constructs a page transition animation that matches the transition used on
/// Android P.
const OpenUpwardsPageTransitionsBuilder();
@override
Widget buildTransitions<T>(
PageRoute<T>? route,
BuildContext? context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
return _OpenUpwardsPageTransition(
animation: animation,
secondaryAnimation: secondaryAnimation,
child: child,
);
}
}
/// Used by [PageTransitionsTheme] to define a zooming [MaterialPageRoute] page
/// transition animation that looks like the default page transition used on
/// Android Q.
///
/// See also:
///
/// * [FadeUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android O.
/// * [OpenUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android P.
/// * [CupertinoPageTransitionsBuilder], which defines a horizontal page
/// transition that matches native iOS page transitions.
class ZoomPageTransitionsBuilder extends PageTransitionsBuilder {
/// Constructs a page transition animation that matches the transition used on
/// Android Q.
const ZoomPageTransitionsBuilder({
this.allowSnapshotting = true,
this.allowEnterRouteSnapshotting = true,
});
/// Whether zoom page transitions will prefer to animate a snapshot of the entering
/// and exiting routes.
///
/// If not specified, defaults to true.
///
/// When this value is true, zoom page transitions will snapshot the entering and
/// exiting routes. These snapshots are then animated in place of the underlying
/// widgets to improve performance of the transition.
///
/// Generally this means that animations that occur on the entering/exiting route
/// while the route animation plays may appear frozen - unless they are a hero
/// animation or something that is drawn in a separate overlay.
///
/// {@tool dartpad}
/// This example shows a [MaterialApp] that disables snapshotting for the zoom
/// transitions on Android.
///
/// ** See code in examples/api/lib/material/page_transitions_theme/page_transitions_theme.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [PageRoute.allowSnapshotting], which enables or disables snapshotting
/// on a per route basis.
final bool allowSnapshotting;
/// Whether to enable snapshotting on the entering route during the
/// transition animation.
///
/// If not specified, defaults to true.
/// If false, the route snapshotting will not be applied to the route being
/// animating into, e.g. when transitioning from route A to route B, B will
/// not be snapshotted.
final bool allowEnterRouteSnapshotting;
// Allows devicelab benchmarks to force disable the snapshotting. This is
// intended to allow us to profile and fix the underlying performance issues
// for the Impeller backend.
static const bool _kProfileForceDisableSnapshotting = bool.fromEnvironment('flutter.benchmarks.force_disable_snapshot');
@override
Widget buildTransitions<T>(
PageRoute<T>? route,
BuildContext? context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget? child,
) {
if (_kProfileForceDisableSnapshotting) {
return _ZoomPageTransitionNoCache(
animation: animation,
secondaryAnimation: secondaryAnimation,
child: child,
);
}
return _ZoomPageTransition(
animation: animation,
secondaryAnimation: secondaryAnimation,
allowSnapshotting: allowSnapshotting && (route?.allowSnapshotting ?? true),
allowEnterRouteSnapshotting: allowEnterRouteSnapshotting,
child: child,
);
}
}
/// Used by [PageTransitionsTheme] to define a horizontal [MaterialPageRoute]
/// page transition animation that matches native iOS page transitions.
///
/// See also:
///
/// * [FadeUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android O.
/// * [OpenUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android P.
/// * [ZoomPageTransitionsBuilder], which defines the default page transition
/// that's similar to the one provided in Android Q.
class CupertinoPageTransitionsBuilder extends PageTransitionsBuilder {
/// Constructs a page transition animation that matches the iOS transition.
const CupertinoPageTransitionsBuilder();
@override
Widget buildTransitions<T>(
PageRoute<T> route,
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
return CupertinoRouteTransitionMixin.buildPageTransitions<T>(route, context, animation, secondaryAnimation, child);
}
}
/// Defines the page transition animations used by [MaterialPageRoute]
/// for different [TargetPlatform]s.
///
/// The [MaterialPageRoute.buildTransitions] method looks up the
/// current [PageTransitionsTheme] with `Theme.of(context).pageTransitionsTheme`
/// and delegates to [buildTransitions].
///
/// If a builder with a matching platform is not found, then the
/// [ZoomPageTransitionsBuilder] is used.
///
/// {@tool dartpad}
/// This example shows a [MaterialApp] that defines a custom [PageTransitionsTheme].
///
/// ** See code in examples/api/lib/material/page_transitions_theme/page_transitions_theme.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ThemeData.pageTransitionsTheme], which defines the default page
/// transitions for the overall theme.
/// * [FadeUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android O.
/// * [OpenUpwardsPageTransitionsBuilder], which defines a page transition
/// that's similar to the one provided by Android P.
/// * [ZoomPageTransitionsBuilder], which defines the default page transition
/// that's similar to the one provided by Android Q.
/// * [CupertinoPageTransitionsBuilder], which defines a horizontal page
/// transition that matches native iOS page transitions.
@immutable
class PageTransitionsTheme with Diagnosticable {
/// Constructs an object that selects a transition based on the platform.
///
/// By default the list of builders is: [ZoomPageTransitionsBuilder]
/// for [TargetPlatform.android], and [CupertinoPageTransitionsBuilder] for
/// [TargetPlatform.iOS] and [TargetPlatform.macOS].
const PageTransitionsTheme({ Map<TargetPlatform, PageTransitionsBuilder> builders = _defaultBuilders }) : _builders = builders;
static const Map<TargetPlatform, PageTransitionsBuilder> _defaultBuilders = <TargetPlatform, PageTransitionsBuilder>{
TargetPlatform.android: ZoomPageTransitionsBuilder(),
TargetPlatform.iOS: CupertinoPageTransitionsBuilder(),
TargetPlatform.macOS: CupertinoPageTransitionsBuilder(),
};
/// The [PageTransitionsBuilder]s supported by this theme.
Map<TargetPlatform, PageTransitionsBuilder> get builders => _builders;
final Map<TargetPlatform, PageTransitionsBuilder> _builders;
/// Delegates to the builder for the current [ThemeData.platform].
/// If a builder for the current platform is not found, then the
/// [ZoomPageTransitionsBuilder] is used.
///
/// [MaterialPageRoute.buildTransitions] delegates to this method.
Widget buildTransitions<T>(
PageRoute<T> route,
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
TargetPlatform platform = Theme.of(context).platform;
if (CupertinoRouteTransitionMixin.isPopGestureInProgress(route)) {
platform = TargetPlatform.iOS;
}
final PageTransitionsBuilder matchingBuilder = builders[platform] ?? switch (platform) {
TargetPlatform.iOS => const CupertinoPageTransitionsBuilder(),
TargetPlatform.android || TargetPlatform.fuchsia || TargetPlatform.windows || TargetPlatform.macOS || TargetPlatform.linux => const ZoomPageTransitionsBuilder(),
};
return matchingBuilder.buildTransitions<T>(route, context, animation, secondaryAnimation, child);
}
// Map the builders to a list with one PageTransitionsBuilder per platform for
// the operator == overload.
List<PageTransitionsBuilder?> _all(Map<TargetPlatform, PageTransitionsBuilder> builders) {
return TargetPlatform.values.map((TargetPlatform platform) => builders[platform]).toList();
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
if (other is PageTransitionsTheme && identical(builders, other.builders)) {
return true;
}
return other is PageTransitionsTheme
&& listEquals<PageTransitionsBuilder?>(_all(other.builders), _all(builders));
}
@override
int get hashCode => Object.hashAll(_all(builders));
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(
DiagnosticsProperty<Map<TargetPlatform, PageTransitionsBuilder>>(
'builders',
builders,
defaultValue: PageTransitionsTheme._defaultBuilders,
),
);
}
}
// Take an image and draw it centered and scaled. The image is already scaled by the [pixelRatio].
void _drawImageScaledAndCentered(PaintingContext context, ui.Image image, double scale, double opacity, double pixelRatio) {
if (scale <= 0.0 || opacity <= 0.0) {
return;
}
final Paint paint = Paint()
..filterQuality = ui.FilterQuality.low
..color = Color.fromRGBO(0, 0, 0, opacity);
final double logicalWidth = image.width / pixelRatio;
final double logicalHeight = image.height / pixelRatio;
final double scaledLogicalWidth = logicalWidth * scale;
final double scaledLogicalHeight = logicalHeight * scale;
final double left = (logicalWidth - scaledLogicalWidth) / 2;
final double top = (logicalHeight - scaledLogicalHeight) / 2;
final Rect dst = Rect.fromLTWH(left, top, scaledLogicalWidth, scaledLogicalHeight);
context.canvas.drawImageRect(image, Rect.fromLTWH(0, 0, image.width.toDouble(), image.height.toDouble()), dst, paint);
}
void _updateScaledTransform(Matrix4 transform, double scale, Size size) {
transform.setIdentity();
if (scale == 1.0) {
return;
}
transform.scale(scale, scale);
final double dx = ((size.width * scale) - size.width) / 2;
final double dy = ((size.height * scale) - size.height) / 2;
transform.translate(-dx, -dy);
}
mixin _ZoomTransitionBase<S extends StatefulWidget> on State<S> {
bool get useSnapshot;
// Don't rasterize if:
// 1. Rasterization is disabled by the platform.
// 2. The animation is paused/stopped.
// 3. The values of the scale/fade transition do not
// benefit from rasterization.
final SnapshotController controller = SnapshotController();
late Animation<double> fadeTransition;
late Animation<double> scaleTransition;
void onAnimationValueChange() {
if ((scaleTransition.value == 1.0) &&
(fadeTransition.value == 0.0 ||
fadeTransition.value == 1.0)) {
controller.allowSnapshotting = false;
} else {
controller.allowSnapshotting = useSnapshot;
}
}
void onAnimationStatusChange(AnimationStatus status) {
controller.allowSnapshotting = switch (status) {
AnimationStatus.dismissed || AnimationStatus.completed => false,
AnimationStatus.forward || AnimationStatus.reverse => useSnapshot,
};
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
}
class _ZoomEnterTransitionPainter extends SnapshotPainter {
_ZoomEnterTransitionPainter({
required this.reverse,
required this.scale,
required this.fade,
required this.animation,
}) {
animation.addListener(notifyListeners);
animation.addStatusListener(_onStatusChange);
scale.addListener(notifyListeners);
fade.addListener(notifyListeners);
}
void _onStatusChange(_) {
notifyListeners();
}
final bool reverse;
final Animation<double> animation;
final Animation<double> scale;
final Animation<double> fade;
final Matrix4 _transform = Matrix4.zero();
final LayerHandle<OpacityLayer> _opacityHandle = LayerHandle<OpacityLayer>();
final LayerHandle<TransformLayer> _transformHandler = LayerHandle<TransformLayer>();
void _drawScrim(PaintingContext context, Offset offset, Size size) {
double scrimOpacity = 0.0;
// The transition's scrim opacity only increases on the forward transition.
// In the reverse transition, the opacity should always be 0.0.
//
// Therefore, we need to only apply the scrim opacity animation when
// the transition is running forwards.
//
// The reason that we check that the animation's status is not `completed`
// instead of checking that it is `forward` is that this allows
// the interrupted reversal of the forward transition to smoothly fade
// the scrim away. This prevents a disjointed removal of the scrim.
if (!reverse && animation.status != AnimationStatus.completed) {
scrimOpacity = _ZoomEnterTransitionState._scrimOpacityTween.evaluate(animation)!;
}
assert(!reverse || scrimOpacity == 0.0);
if (scrimOpacity > 0.0) {
context.canvas.drawRect(
offset & size,
Paint()..color = Colors.black.withOpacity(scrimOpacity),
);
}
}
@override
void paint(PaintingContext context, ui.Offset offset, Size size, PaintingContextCallback painter) {
switch (animation.status) {
case AnimationStatus.completed:
case AnimationStatus.dismissed:
return painter(context, offset);
case AnimationStatus.forward:
case AnimationStatus.reverse:
}
_drawScrim(context, offset, size);
_updateScaledTransform(_transform, scale.value, size);
_transformHandler.layer = context.pushTransform(true, offset, _transform, (PaintingContext context, Offset offset) {
_opacityHandle.layer = context.pushOpacity(offset, (fade.value * 255).round(), painter, oldLayer: _opacityHandle.layer);
}, oldLayer: _transformHandler.layer);
}
@override
void paintSnapshot(PaintingContext context, Offset offset, Size size, ui.Image image, Size sourceSize, double pixelRatio) {
_drawScrim(context, offset, size);
_drawImageScaledAndCentered(context, image, scale.value, fade.value, pixelRatio);
}
@override
void dispose() {
animation.removeListener(notifyListeners);
animation.removeStatusListener(_onStatusChange);
scale.removeListener(notifyListeners);
fade.removeListener(notifyListeners);
_opacityHandle.layer = null;
_transformHandler.layer = null;
super.dispose();
}
@override
bool shouldRepaint(covariant _ZoomEnterTransitionPainter oldDelegate) {
return oldDelegate.reverse != reverse
|| oldDelegate.animation.value != animation.value
|| oldDelegate.scale.value != scale.value
|| oldDelegate.fade.value != fade.value;
}
}
class _ZoomExitTransitionPainter extends SnapshotPainter {
_ZoomExitTransitionPainter({
required this.reverse,
required this.scale,
required this.fade,
required this.animation,
}) {
scale.addListener(notifyListeners);
fade.addListener(notifyListeners);
animation.addStatusListener(_onStatusChange);
}
void _onStatusChange(_) {
notifyListeners();
}
final bool reverse;
final Animation<double> scale;
final Animation<double> fade;
final Animation<double> animation;
final Matrix4 _transform = Matrix4.zero();
final LayerHandle<OpacityLayer> _opacityHandle = LayerHandle<OpacityLayer>();
final LayerHandle<TransformLayer> _transformHandler = LayerHandle<TransformLayer>();
@override
void paintSnapshot(PaintingContext context, Offset offset, Size size, ui.Image image, Size sourceSize, double pixelRatio) {
_drawImageScaledAndCentered(context, image, scale.value, fade.value, pixelRatio);
}
@override
void paint(PaintingContext context, ui.Offset offset, Size size, PaintingContextCallback painter) {
switch (animation.status) {
case AnimationStatus.completed:
case AnimationStatus.dismissed:
return painter(context, offset);
case AnimationStatus.forward:
case AnimationStatus.reverse:
break;
}
_updateScaledTransform(_transform, scale.value, size);
_transformHandler.layer = context.pushTransform(true, offset, _transform, (PaintingContext context, Offset offset) {
_opacityHandle.layer = context.pushOpacity(offset, (fade.value * 255).round(), painter, oldLayer: _opacityHandle.layer);
}, oldLayer: _transformHandler.layer);
}
@override
bool shouldRepaint(covariant _ZoomExitTransitionPainter oldDelegate) {
return oldDelegate.reverse != reverse
|| oldDelegate.fade.value != fade.value
|| oldDelegate.scale.value != scale.value;
}
@override
void dispose() {
_opacityHandle.layer = null;
_transformHandler.layer = null;
scale.removeListener(notifyListeners);
fade.removeListener(notifyListeners);
animation.removeStatusListener(_onStatusChange);
super.dispose();
}
}
// Zooms and fades a new page in, zooming out the previous page. This transition
// is designed to match the Android Q activity transition.
//
// This was the historical implementation of the cacheless zoom page transition
// that was too slow to run on the Skia backend. This is being benchmarked on
// the Impeller backend so that we can improve performance enough to restore
// the default behavior.
class _ZoomPageTransitionNoCache extends StatelessWidget {
/// Creates a [_ZoomPageTransitionNoCache].
///
/// The [animation] and [secondaryAnimation] argument are required and must
/// not be null.
const _ZoomPageTransitionNoCache({
required this.animation,
required this.secondaryAnimation,
this.child,
});
/// The animation that drives the [child]'s entrance and exit.
///
/// See also:
///
/// * [TransitionRoute.animation], which is the value given to this property
/// when the [_ZoomPageTransition] is used as a page transition.
final Animation<double> animation;
/// The animation that transitions [child] when new content is pushed on top
/// of it.
///
/// See also:
///
/// * [TransitionRoute.secondaryAnimation], which is the value given to this
/// property when the [_ZoomPageTransition] is used as a page transition.
final Animation<double> secondaryAnimation;
/// The widget below this widget in the tree.
///
/// This widget will transition in and out as driven by [animation] and
/// [secondaryAnimation].
final Widget? child;
@override
Widget build(BuildContext context) {
return DualTransitionBuilder(
animation: animation,
forwardBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomEnterTransitionNoCache(
animation: animation,
child: child,
);
},
reverseBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomExitTransitionNoCache(
animation: animation,
reverse: true,
child: child,
);
},
child: DualTransitionBuilder(
animation: ReverseAnimation(secondaryAnimation),
forwardBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomEnterTransitionNoCache(
animation: animation,
reverse: true,
child: child,
);
},
reverseBuilder: (
BuildContext context,
Animation<double> animation,
Widget? child,
) {
return _ZoomExitTransitionNoCache(
animation: animation,
child: child,
);
},
child: child,
),
);
}
}
class _ZoomEnterTransitionNoCache extends StatelessWidget {
const _ZoomEnterTransitionNoCache({
required this.animation,
this.reverse = false,
this.child,
});
final Animation<double> animation;
final Widget? child;
final bool reverse;
@override
Widget build(BuildContext context) {
double opacity = 0;
// The transition's scrim opacity only increases on the forward transition.
// In the reverse transition, the opacity should always be 0.0.
//
// Therefore, we need to only apply the scrim opacity animation when
// the transition is running forwards.
//
// The reason that we check that the animation's status is not `completed`
// instead of checking that it is `forward` is that this allows
// the interrupted reversal of the forward transition to smoothly fade
// the scrim away. This prevents a disjointed removal of the scrim.
if (!reverse && animation.status != AnimationStatus.completed) {
opacity = _ZoomEnterTransitionState._scrimOpacityTween.evaluate(animation)!;
}
final Animation<double> fadeTransition = reverse
? kAlwaysCompleteAnimation
: _ZoomEnterTransitionState._fadeInTransition.animate(animation);
final Animation<double> scaleTransition = (reverse
? _ZoomEnterTransitionState._scaleDownTransition
: _ZoomEnterTransitionState._scaleUpTransition
).animate(animation);
return AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
return ColoredBox(
color: Colors.black.withOpacity(opacity),
child: child,
);
},
child: FadeTransition(
opacity: fadeTransition,
child: ScaleTransition(
scale: scaleTransition,
filterQuality: FilterQuality.none,
child: child,
),
),
);
}
}
class _ZoomExitTransitionNoCache extends StatelessWidget {
const _ZoomExitTransitionNoCache({
required this.animation,
this.reverse = false,
this.child,
});
final Animation<double> animation;
final bool reverse;
final Widget? child;
@override
Widget build(BuildContext context) {
final Animation<double> fadeTransition = reverse
? _ZoomExitTransitionState._fadeOutTransition.animate(animation)
: kAlwaysCompleteAnimation;
final Animation<double> scaleTransition = (reverse
? _ZoomExitTransitionState._scaleDownTransition
: _ZoomExitTransitionState._scaleUpTransition
).animate(animation);
return FadeTransition(
opacity: fadeTransition,
child: ScaleTransition(
scale: scaleTransition,
filterQuality: FilterQuality.none,
child: child,
),
);
}
}
| flutter/packages/flutter/lib/src/material/page_transitions_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/page_transitions_theme.dart",
"repo_id": "flutter",
"token_count": 13882
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'dart:ui';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'divider.dart';
import 'divider_theme.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'ink_well.dart';
import 'input_border.dart';
import 'input_decorator.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'search_bar_theme.dart';
import 'search_view_theme.dart';
import 'text_field.dart';
import 'text_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
const int _kOpenViewMilliseconds = 600;
const Duration _kOpenViewDuration = Duration(milliseconds: _kOpenViewMilliseconds);
const Duration _kAnchorFadeDuration = Duration(milliseconds: 150);
const Curve _kViewFadeOnInterval = Interval(0.0, 1/2);
const Curve _kViewIconsFadeOnInterval = Interval(1/6, 2/6);
const Curve _kViewDividerFadeOnInterval = Interval(0.0, 1/6);
const Curve _kViewListFadeOnInterval = Interval(133 / _kOpenViewMilliseconds, 233 / _kOpenViewMilliseconds);
const double _kDisableSearchBarOpacity = 0.38;
/// Signature for a function that creates a [Widget] which is used to open a search view.
///
/// The `controller` callback provided to [SearchAnchor.builder] can be used
/// to open the search view and control the editable field on the view.
typedef SearchAnchorChildBuilder = Widget Function(BuildContext context, SearchController controller);
/// Signature for a function that creates a [Widget] to build the suggestion list
/// based on the input in the search bar.
///
/// The `controller` callback provided to [SearchAnchor.suggestionsBuilder] can be used
/// to close the search view and control the editable field on the view.
typedef SuggestionsBuilder = FutureOr<Iterable<Widget>> Function(BuildContext context, SearchController controller);
/// Signature for a function that creates a [Widget] to layout the suggestion list.
///
/// Parameter `suggestions` is the content list that this function wants to lay out.
typedef ViewBuilder = Widget Function(Iterable<Widget> suggestions);
/// Manages a "search view" route that allows the user to select one of the
/// suggested completions for a search query.
///
/// The search view's route can either be shown by creating a [SearchController]
/// and then calling [SearchController.openView] or by tapping on an anchor.
/// When the anchor is tapped or [SearchController.openView] is called, the search view either
/// grows to a specific size, or grows to fill the entire screen. By default,
/// the search view only shows full screen on mobile platforms. Use [SearchAnchor.isFullScreen]
/// to override the default setting.
///
/// The search view is usually opened by a [SearchBar], an [IconButton] or an [Icon].
/// If [builder] returns an Icon, or any un-tappable widgets, we don't have
/// to explicitly call [SearchController.openView].
///
/// The search view route will be popped if the window size is changed and the
/// search view route is not in full-screen mode. However, if the search view route
/// is in full-screen mode, changing the window size, such as rotating a mobile
/// device from portrait mode to landscape mode, will not close the search view.
///
/// {@tool dartpad}
/// This example shows how to use an IconButton to open a search view in a [SearchAnchor].
/// It also shows how to use [SearchController] to open or close the search view route.
///
/// ** See code in examples/api/lib/material/search_anchor/search_anchor.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to set up a floating (or pinned) AppBar with a
/// [SearchAnchor] for a title.
///
/// ** See code in examples/api/lib/material/search_anchor/search_anchor.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to fetch the search suggestions from a remote API.
///
/// ** See code in examples/api/lib/material/search_anchor/search_anchor.3.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example demonstrates fetching the search suggestions asynchronously and
/// debouncing network calls.
///
/// ** See code in examples/api/lib/material/search_anchor/search_anchor.4.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SearchBar], a widget that defines a search bar.
/// * [SearchBarTheme], a widget that overrides the default configuration of a search bar.
/// * [SearchViewTheme], a widget that overrides the default configuration of a search view.
class SearchAnchor extends StatefulWidget {
/// Creates a const [SearchAnchor].
///
/// The [builder] and [suggestionsBuilder] arguments are required.
const SearchAnchor({
super.key,
this.isFullScreen,
this.searchController,
this.viewBuilder,
this.viewLeading,
this.viewTrailing,
this.viewHintText,
this.viewBackgroundColor,
this.viewElevation,
this.viewSurfaceTintColor,
this.viewSide,
this.viewShape,
this.headerHeight,
this.headerTextStyle,
this.headerHintStyle,
this.dividerColor,
this.viewConstraints,
this.textCapitalization,
this.viewOnChanged,
this.viewOnSubmitted,
required this.builder,
required this.suggestionsBuilder,
this.textInputAction,
this.keyboardType,
});
/// Create a [SearchAnchor] that has a [SearchBar] which opens a search view.
///
/// All the barX parameters are used to customize the anchor. Similarly, all the
/// viewX parameters are used to override the view's defaults.
///
/// {@tool dartpad}
/// This example shows how to use a [SearchAnchor.bar] which uses a default search
/// bar to open a search view route.
///
/// ** See code in examples/api/lib/material/search_anchor/search_anchor.0.dart **
/// {@end-tool}
factory SearchAnchor.bar({
Widget? barLeading,
Iterable<Widget>? barTrailing,
String? barHintText,
GestureTapCallback? onTap,
ValueChanged<String>? onSubmitted,
ValueChanged<String>? onChanged,
MaterialStateProperty<double?>? barElevation,
MaterialStateProperty<Color?>? barBackgroundColor,
MaterialStateProperty<Color?>? barOverlayColor,
MaterialStateProperty<BorderSide?>? barSide,
MaterialStateProperty<OutlinedBorder?>? barShape,
MaterialStateProperty<EdgeInsetsGeometry?>? barPadding,
MaterialStateProperty<TextStyle?>? barTextStyle,
MaterialStateProperty<TextStyle?>? barHintStyle,
Widget? viewLeading,
Iterable<Widget>? viewTrailing,
String? viewHintText,
Color? viewBackgroundColor,
double? viewElevation,
BorderSide? viewSide,
OutlinedBorder? viewShape,
double? viewHeaderHeight,
TextStyle? viewHeaderTextStyle,
TextStyle? viewHeaderHintStyle,
Color? dividerColor,
BoxConstraints? constraints,
BoxConstraints? viewConstraints,
bool? isFullScreen,
SearchController searchController,
TextCapitalization textCapitalization,
required SuggestionsBuilder suggestionsBuilder,
TextInputAction? textInputAction,
TextInputType? keyboardType,
}) = _SearchAnchorWithSearchBar;
/// Whether the search view grows to fill the entire screen when the
/// [SearchAnchor] is tapped.
///
/// By default, the search view is full-screen on mobile devices. On other
/// platforms, the search view only grows to a specific size that is determined
/// by the anchor and the default size.
final bool? isFullScreen;
/// An optional controller that allows opening and closing of the search view from
/// other widgets.
///
/// If this is null, one internal search controller is created automatically
/// and it is used to open the search view when the user taps on the anchor.
final SearchController? searchController;
/// Optional callback to obtain a widget to lay out the suggestion list of the
/// search view.
///
/// Default view uses a [ListView] with a vertical scroll direction.
final ViewBuilder? viewBuilder;
/// An optional widget to display before the text input field when the search
/// view is open.
///
/// Typically the [viewLeading] widget is an [Icon] or an [IconButton].
///
/// Defaults to a back button which pops the view.
final Widget? viewLeading;
/// An optional widget list to display after the text input field when the search
/// view is open.
///
/// Typically the [viewTrailing] widget list only has one or two widgets.
///
/// Defaults to an icon button which clears the text in the input field.
final Iterable<Widget>? viewTrailing;
/// Text that is displayed when the search bar's input field is empty.
final String? viewHintText;
/// The search view's background fill color.
///
/// If null, the value of [SearchViewThemeData.backgroundColor] will be used.
/// If this is also null, then the default value is [ColorScheme.surfaceContainerHigh].
final Color? viewBackgroundColor;
/// The elevation of the search view's [Material].
///
/// If null, the value of [SearchViewThemeData.elevation] will be used. If this
/// is also null, then default value is 6.0.
final double? viewElevation;
/// The surface tint color of the search view's [Material].
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// If null, the value of [SearchViewThemeData.surfaceTintColor] will be used.
/// If this is also null, then the default value is [ColorScheme.surfaceTint].
final Color? viewSurfaceTintColor;
/// The color and weight of the search view's outline.
///
/// This value is combined with [viewShape] to create a shape decorated
/// with an outline. This will be ignored if the view is full-screen.
///
/// If null, the value of [SearchViewThemeData.side] will be used. If this is
/// also null, the search view doesn't have a side by default.
final BorderSide? viewSide;
/// The shape of the search view's underlying [Material].
///
/// This shape is combined with [viewSide] to create a shape decorated
/// with an outline.
///
/// If null, the value of [SearchViewThemeData.shape] will be used.
/// If this is also null, then the default value is a rectangle shape for full-screen
/// mode and a [RoundedRectangleBorder] shape with a 28.0 radius otherwise.
final OutlinedBorder? viewShape;
/// The height of the search field on the search view.
///
/// If null, the value of [SearchViewThemeData.headerHeight] will be used. If
/// this is also null, the default value is 56.0.
final double? headerHeight;
/// The style to use for the text being edited on the search view.
///
/// If null, defaults to the `bodyLarge` text style from the current [Theme].
/// The default text color is [ColorScheme.onSurface].
final TextStyle? headerTextStyle;
/// The style to use for the [viewHintText] on the search view.
///
/// If null, the value of [SearchViewThemeData.headerHintStyle] will be used.
/// If this is also null, the value of [headerTextStyle] will be used. If this is also null,
/// defaults to the `bodyLarge` text style from the current [Theme]. The default
/// text color is [ColorScheme.onSurfaceVariant].
final TextStyle? headerHintStyle;
/// The color of the divider on the search view.
///
/// If this property is null, then [SearchViewThemeData.dividerColor] is used.
/// If that is also null, the default value is [ColorScheme.outline].
final Color? dividerColor;
/// Optional size constraints for the search view.
///
/// By default, the search view has the same width as the anchor and is 2/3
/// the height of the screen. If the width and height of the view are within
/// the [viewConstraints], the view will show its default size. Otherwise,
/// the size of the view will be constrained by this property.
///
/// If null, the value of [SearchViewThemeData.constraints] will be used. If
/// this is also null, then the constraints defaults to:
/// ```dart
/// const BoxConstraints(minWidth: 360.0, minHeight: 240.0)
/// ```
final BoxConstraints? viewConstraints;
/// {@macro flutter.widgets.editableText.textCapitalization}
final TextCapitalization? textCapitalization;
/// Called each time the user modifies the search view's text field.
///
/// See also:
///
/// * [viewOnSubmitted], which is called when the user indicates that they
/// are done editing the search view's text field.
final ValueChanged<String>? viewOnChanged;
/// Called when the user indicates that they are done editing the text in the
/// text field of a search view. Typically this is called when the user presses
/// the enter key.
///
/// See also:
///
/// * [viewOnChanged], which is called when the user modifies the text field
/// of the search view.
final ValueChanged<String>? viewOnSubmitted;
/// Called to create a widget which can open a search view route when it is tapped.
///
/// The widget returned by this builder is faded out when it is tapped.
/// At the same time a search view route is faded in.
final SearchAnchorChildBuilder builder;
/// Called to get the suggestion list for the search view.
///
/// By default, the list returned by this builder is laid out in a [ListView].
/// To get a different layout, use [viewBuilder] to override.
final SuggestionsBuilder suggestionsBuilder;
/// {@macro flutter.widgets.TextField.textInputAction}
final TextInputAction? textInputAction;
/// The type of action button to use for the keyboard.
///
/// Defaults to the default value specified in [TextField].
final TextInputType? keyboardType;
@override
State<SearchAnchor> createState() => _SearchAnchorState();
}
class _SearchAnchorState extends State<SearchAnchor> {
Size? _screenSize;
bool _anchorIsVisible = true;
final GlobalKey _anchorKey = GlobalKey();
bool get _viewIsOpen => !_anchorIsVisible;
SearchController? _internalSearchController;
SearchController get _searchController => widget.searchController ?? (_internalSearchController ??= SearchController());
@override
void initState() {
super.initState();
_searchController._attach(this);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
final Size updatedScreenSize = MediaQuery.of(context).size;
if (_screenSize != null && _screenSize != updatedScreenSize) {
if (_searchController.isOpen && !getShowFullScreenView()) {
_closeView(null);
}
}
_screenSize = updatedScreenSize;
}
@override
void didUpdateWidget(SearchAnchor oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.searchController != widget.searchController) {
oldWidget.searchController?._detach(this);
_searchController._attach(this);
}
}
@override
void dispose() {
super.dispose();
widget.searchController?._detach(this);
_internalSearchController?._detach(this);
_internalSearchController?.dispose();
}
void _openView() {
final NavigatorState navigator = Navigator.of(context);
navigator.push(_SearchViewRoute(
viewOnChanged: widget.viewOnChanged,
viewOnSubmitted: widget.viewOnSubmitted,
viewLeading: widget.viewLeading,
viewTrailing: widget.viewTrailing,
viewHintText: widget.viewHintText,
viewBackgroundColor: widget.viewBackgroundColor,
viewElevation: widget.viewElevation,
viewSurfaceTintColor: widget.viewSurfaceTintColor,
viewSide: widget.viewSide,
viewShape: widget.viewShape,
viewHeaderHeight: widget.headerHeight,
viewHeaderTextStyle: widget.headerTextStyle,
viewHeaderHintStyle: widget.headerHintStyle,
dividerColor: widget.dividerColor,
viewConstraints: widget.viewConstraints,
showFullScreenView: getShowFullScreenView(),
toggleVisibility: toggleVisibility,
textDirection: Directionality.of(context),
viewBuilder: widget.viewBuilder,
anchorKey: _anchorKey,
searchController: _searchController,
suggestionsBuilder: widget.suggestionsBuilder,
textCapitalization: widget.textCapitalization,
capturedThemes: InheritedTheme.capture(from: context, to: navigator.context),
textInputAction: widget.textInputAction,
keyboardType: widget.keyboardType,
));
}
void _closeView(String? selectedText) {
if (selectedText != null) {
_searchController.text = selectedText;
}
Navigator.of(context).pop();
}
bool toggleVisibility() {
setState(() {
_anchorIsVisible = !_anchorIsVisible;
});
return _anchorIsVisible;
}
bool getShowFullScreenView() {
return widget.isFullScreen ?? switch (Theme.of(context).platform) {
TargetPlatform.iOS || TargetPlatform.android || TargetPlatform.fuchsia => true,
TargetPlatform.macOS || TargetPlatform.linux || TargetPlatform.windows => false,
};
}
@override
Widget build(BuildContext context) {
return AnimatedOpacity(
key: _anchorKey,
opacity: _anchorIsVisible ? 1.0 : 0.0,
duration: _kAnchorFadeDuration,
child: GestureDetector(
onTap: _openView,
child: widget.builder(context, _searchController),
),
);
}
}
class _SearchViewRoute extends PopupRoute<_SearchViewRoute> {
_SearchViewRoute({
this.viewOnChanged,
this.viewOnSubmitted,
this.toggleVisibility,
this.textDirection,
this.viewBuilder,
this.viewLeading,
this.viewTrailing,
this.viewHintText,
this.viewBackgroundColor,
this.viewElevation,
this.viewSurfaceTintColor,
this.viewSide,
this.viewShape,
this.viewHeaderHeight,
this.viewHeaderTextStyle,
this.viewHeaderHintStyle,
this.dividerColor,
this.viewConstraints,
this.textCapitalization,
required this.showFullScreenView,
required this.anchorKey,
required this.searchController,
required this.suggestionsBuilder,
required this.capturedThemes,
this.textInputAction,
this.keyboardType,
});
final ValueChanged<String>? viewOnChanged;
final ValueChanged<String>? viewOnSubmitted;
final ValueGetter<bool>? toggleVisibility;
final TextDirection? textDirection;
final ViewBuilder? viewBuilder;
final Widget? viewLeading;
final Iterable<Widget>? viewTrailing;
final String? viewHintText;
final Color? viewBackgroundColor;
final double? viewElevation;
final Color? viewSurfaceTintColor;
final BorderSide? viewSide;
final OutlinedBorder? viewShape;
final double? viewHeaderHeight;
final TextStyle? viewHeaderTextStyle;
final TextStyle? viewHeaderHintStyle;
final Color? dividerColor;
final BoxConstraints? viewConstraints;
final TextCapitalization? textCapitalization;
final bool showFullScreenView;
final GlobalKey anchorKey;
final SearchController searchController;
final SuggestionsBuilder suggestionsBuilder;
final CapturedThemes capturedThemes;
final TextInputAction? textInputAction;
final TextInputType? keyboardType;
@override
Color? get barrierColor => Colors.transparent;
@override
bool get barrierDismissible => true;
@override
String? get barrierLabel => 'Dismiss';
late final SearchViewThemeData viewDefaults;
late final SearchViewThemeData viewTheme;
final RectTween _rectTween = RectTween();
Rect? getRect() {
final BuildContext? context = anchorKey.currentContext;
if (context != null) {
final RenderBox searchBarBox = context.findRenderObject()! as RenderBox;
final Size boxSize = searchBarBox.size;
final NavigatorState navigator = Navigator.of(context);
final Offset boxLocation = searchBarBox.localToGlobal(Offset.zero, ancestor: navigator.context.findRenderObject());
return boxLocation & boxSize;
}
return null;
}
@override
TickerFuture didPush() {
assert(anchorKey.currentContext != null);
updateViewConfig(anchorKey.currentContext!);
updateTweens(anchorKey.currentContext!);
toggleVisibility?.call();
return super.didPush();
}
@override
bool didPop(_SearchViewRoute? result) {
assert(anchorKey.currentContext != null);
updateTweens(anchorKey.currentContext!);
toggleVisibility?.call();
return super.didPop(result);
}
void updateViewConfig(BuildContext context) {
viewDefaults = _SearchViewDefaultsM3(context, isFullScreen: showFullScreenView);
viewTheme = SearchViewTheme.of(context);
}
void updateTweens(BuildContext context) {
final RenderBox navigator = Navigator.of(context).context.findRenderObject()! as RenderBox;
final Size screenSize = navigator.size;
final Rect anchorRect = getRect() ?? Rect.zero;
final BoxConstraints effectiveConstraints = viewConstraints ?? viewTheme.constraints ?? viewDefaults.constraints!;
_rectTween.begin = anchorRect;
final double viewWidth = clampDouble(anchorRect.width, effectiveConstraints.minWidth, effectiveConstraints.maxWidth);
final double viewHeight = clampDouble(screenSize.height * 2 / 3, effectiveConstraints.minHeight, effectiveConstraints.maxHeight);
switch (textDirection ?? TextDirection.ltr) {
case TextDirection.ltr:
final double viewLeftToScreenRight = screenSize.width - anchorRect.left;
final double viewTopToScreenBottom = screenSize.height - anchorRect.top;
// Make sure the search view doesn't go off the screen. If the search view
// doesn't fit, move the top-left corner of the view to fit the window.
// If the window is smaller than the view, then we resize the view to fit the window.
Offset topLeft = anchorRect.topLeft;
if (viewLeftToScreenRight < viewWidth) {
topLeft = Offset(screenSize.width - math.min(viewWidth, screenSize.width), topLeft.dy);
}
if (viewTopToScreenBottom < viewHeight) {
topLeft = Offset(topLeft.dx, screenSize.height - math.min(viewHeight, screenSize.height));
}
final Size endSize = Size(viewWidth, viewHeight);
_rectTween.end = showFullScreenView ? Offset.zero & screenSize : (topLeft & endSize);
return;
case TextDirection.rtl:
final double viewRightToScreenLeft = anchorRect.right;
final double viewTopToScreenBottom = screenSize.height - anchorRect.top;
// Make sure the search view doesn't go off the screen.
Offset topLeft = Offset(math.max(anchorRect.right - viewWidth, 0.0), anchorRect.top);
if (viewRightToScreenLeft < viewWidth) {
topLeft = Offset(0.0, topLeft.dy);
}
if (viewTopToScreenBottom < viewHeight) {
topLeft = Offset(topLeft.dx, screenSize.height - math.min(viewHeight, screenSize.height));
}
final Size endSize = Size(viewWidth, viewHeight);
_rectTween.end = showFullScreenView ? Offset.zero & screenSize : (topLeft & endSize);
}
}
@override
Widget buildPage(BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
return Directionality(
textDirection: textDirection ?? TextDirection.ltr,
child: AnimatedBuilder(
animation: animation,
builder: (BuildContext context, Widget? child) {
final Animation<double> curvedAnimation = CurvedAnimation(
parent: animation,
curve: Curves.easeInOutCubicEmphasized,
reverseCurve: Curves.easeInOutCubicEmphasized.flipped,
);
final Rect viewRect = _rectTween.evaluate(curvedAnimation)!;
final double topPadding = showFullScreenView
? lerpDouble(0.0, MediaQuery.paddingOf(context).top, curvedAnimation.value)!
: 0.0;
return FadeTransition(
opacity: CurvedAnimation(
parent: animation,
curve: _kViewFadeOnInterval,
reverseCurve: _kViewFadeOnInterval.flipped,
),
child: capturedThemes.wrap(
_ViewContent(
viewOnChanged: viewOnChanged,
viewOnSubmitted: viewOnSubmitted,
viewLeading: viewLeading,
viewTrailing: viewTrailing,
viewHintText: viewHintText,
viewBackgroundColor: viewBackgroundColor,
viewElevation: viewElevation,
viewSurfaceTintColor: viewSurfaceTintColor,
viewSide: viewSide,
viewShape: viewShape,
viewHeaderHeight: viewHeaderHeight,
viewHeaderTextStyle: viewHeaderTextStyle,
viewHeaderHintStyle: viewHeaderHintStyle,
dividerColor: dividerColor,
showFullScreenView: showFullScreenView,
animation: curvedAnimation,
topPadding: topPadding,
viewMaxWidth: _rectTween.end!.width,
viewRect: viewRect,
viewBuilder: viewBuilder,
searchController: searchController,
suggestionsBuilder: suggestionsBuilder,
textCapitalization: textCapitalization,
textInputAction: textInputAction,
keyboardType: keyboardType,
),
),
);
}
),
);
}
@override
Duration get transitionDuration => _kOpenViewDuration;
}
class _ViewContent extends StatefulWidget {
const _ViewContent({
this.viewOnChanged,
this.viewOnSubmitted,
this.viewBuilder,
this.viewLeading,
this.viewTrailing,
this.viewHintText,
this.viewBackgroundColor,
this.viewElevation,
this.viewSurfaceTintColor,
this.viewSide,
this.viewShape,
this.viewHeaderHeight,
this.viewHeaderTextStyle,
this.viewHeaderHintStyle,
this.dividerColor,
this.textCapitalization,
required this.showFullScreenView,
required this.topPadding,
required this.animation,
required this.viewMaxWidth,
required this.viewRect,
required this.searchController,
required this.suggestionsBuilder,
this.textInputAction,
this.keyboardType,
});
final ValueChanged<String>? viewOnChanged;
final ValueChanged<String>? viewOnSubmitted;
final ViewBuilder? viewBuilder;
final Widget? viewLeading;
final Iterable<Widget>? viewTrailing;
final String? viewHintText;
final Color? viewBackgroundColor;
final double? viewElevation;
final Color? viewSurfaceTintColor;
final BorderSide? viewSide;
final OutlinedBorder? viewShape;
final double? viewHeaderHeight;
final TextStyle? viewHeaderTextStyle;
final TextStyle? viewHeaderHintStyle;
final Color? dividerColor;
final TextCapitalization? textCapitalization;
final bool showFullScreenView;
final double topPadding;
final Animation<double> animation;
final double viewMaxWidth;
final Rect viewRect;
final SearchController searchController;
final SuggestionsBuilder suggestionsBuilder;
final TextInputAction? textInputAction;
final TextInputType? keyboardType;
@override
State<_ViewContent> createState() => _ViewContentState();
}
class _ViewContentState extends State<_ViewContent> {
Size? _screenSize;
late Rect _viewRect;
late final SearchController _controller;
Iterable<Widget> result = <Widget>[];
@override
void initState() {
super.initState();
_viewRect = widget.viewRect;
_controller = widget.searchController;
_controller.addListener(updateSuggestions);
}
@override
void didUpdateWidget(covariant _ViewContent oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.viewRect != oldWidget.viewRect) {
setState(() {
_viewRect = widget.viewRect;
});
}
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
final Size updatedScreenSize = MediaQuery.of(context).size;
if (_screenSize != updatedScreenSize) {
_screenSize = updatedScreenSize;
if (widget.showFullScreenView) {
_viewRect = Offset.zero & _screenSize!;
}
}
unawaited(updateSuggestions());
}
@override
void dispose() {
_controller.removeListener(updateSuggestions);
super.dispose();
}
Widget viewBuilder(Iterable<Widget> suggestions) {
if (widget.viewBuilder == null) {
return MediaQuery.removePadding(
context: context,
removeTop: true,
child: ListView(
children: suggestions.toList()
),
);
}
return widget.viewBuilder!(suggestions);
}
Future<void> updateSuggestions() async {
final Iterable<Widget> suggestions = await widget.suggestionsBuilder(context, _controller);
if (mounted) {
setState(() {
result = suggestions;
});
}
}
@override
Widget build(BuildContext context) {
final Widget defaultLeading = IconButton(
icon: const Icon(Icons.arrow_back),
tooltip: MaterialLocalizations.of(context).backButtonTooltip,
onPressed: () { Navigator.of(context).pop(); },
style: const ButtonStyle(tapTargetSize: MaterialTapTargetSize.shrinkWrap),
);
final List<Widget> defaultTrailing = <Widget>[
if (_controller.text.isNotEmpty) IconButton(
icon: const Icon(Icons.close),
tooltip: MaterialLocalizations.of(context).clearButtonTooltip,
onPressed: () {
_controller.clear();
},
),
];
final SearchViewThemeData viewDefaults = _SearchViewDefaultsM3(context, isFullScreen: widget.showFullScreenView);
final SearchViewThemeData viewTheme = SearchViewTheme.of(context);
final DividerThemeData dividerTheme = DividerTheme.of(context);
final Color effectiveBackgroundColor = widget.viewBackgroundColor
?? viewTheme.backgroundColor
?? viewDefaults.backgroundColor!;
final Color effectiveSurfaceTint = widget.viewSurfaceTintColor
?? viewTheme.surfaceTintColor
?? viewDefaults.surfaceTintColor!;
final double effectiveElevation = widget.viewElevation
?? viewTheme.elevation
?? viewDefaults.elevation!;
final BorderSide? effectiveSide = widget.viewSide
?? viewTheme.side
?? viewDefaults.side;
OutlinedBorder effectiveShape = widget.viewShape
?? viewTheme.shape
?? viewDefaults.shape!;
if (effectiveSide != null) {
effectiveShape = effectiveShape.copyWith(side: effectiveSide);
}
final Color effectiveDividerColor = widget.dividerColor
?? viewTheme.dividerColor
?? dividerTheme.color
?? viewDefaults.dividerColor!;
final double? effectiveHeaderHeight = widget.viewHeaderHeight ?? viewTheme.headerHeight;
final BoxConstraints? headerConstraints = effectiveHeaderHeight == null
? null
: BoxConstraints.tightFor(height: effectiveHeaderHeight);
final TextStyle? effectiveTextStyle = widget.viewHeaderTextStyle
?? viewTheme.headerTextStyle
?? viewDefaults.headerTextStyle;
final TextStyle? effectiveHintStyle = widget.viewHeaderHintStyle
?? viewTheme.headerHintStyle
?? widget.viewHeaderTextStyle
?? viewTheme.headerTextStyle
?? viewDefaults.headerHintStyle;
final Widget viewDivider = DividerTheme(
data: dividerTheme.copyWith(color: effectiveDividerColor),
child: const Divider(height: 1),
);
return Align(
alignment: Alignment.topLeft,
child: Transform.translate(
offset: _viewRect.topLeft,
child: SizedBox(
width: _viewRect.width,
height: _viewRect.height,
child: Material(
clipBehavior: Clip.antiAlias,
shape: effectiveShape,
color: effectiveBackgroundColor,
surfaceTintColor: effectiveSurfaceTint,
elevation: effectiveElevation,
child: ClipRect(
clipBehavior: Clip.antiAlias,
child: OverflowBox(
alignment: Alignment.topLeft,
maxWidth: math.min(widget.viewMaxWidth, _screenSize!.width),
minWidth: 0,
child: FadeTransition(
opacity: CurvedAnimation(
parent: widget.animation,
curve: _kViewIconsFadeOnInterval,
reverseCurve: _kViewIconsFadeOnInterval.flipped,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Padding(
padding: EdgeInsets.only(top: widget.topPadding),
child: SafeArea(
top: false,
bottom: false,
child: SearchBar(
autoFocus: true,
constraints: headerConstraints ?? (widget.showFullScreenView ? BoxConstraints(minHeight: _SearchViewDefaultsM3.fullScreenBarHeight) : null),
leading: widget.viewLeading ?? defaultLeading,
trailing: widget.viewTrailing ?? defaultTrailing,
hintText: widget.viewHintText,
backgroundColor: const MaterialStatePropertyAll<Color>(Colors.transparent),
overlayColor: const MaterialStatePropertyAll<Color>(Colors.transparent),
elevation: const MaterialStatePropertyAll<double>(0.0),
textStyle: MaterialStatePropertyAll<TextStyle?>(effectiveTextStyle),
hintStyle: MaterialStatePropertyAll<TextStyle?>(effectiveHintStyle),
controller: _controller,
onChanged: (String value) {
widget.viewOnChanged?.call(value);
updateSuggestions();
},
onSubmitted: widget.viewOnSubmitted,
textCapitalization: widget.textCapitalization,
textInputAction: widget.textInputAction,
keyboardType: widget.keyboardType,
),
),
),
FadeTransition(
opacity: CurvedAnimation(
parent: widget.animation,
curve: _kViewDividerFadeOnInterval,
reverseCurve: _kViewFadeOnInterval.flipped,
),
child: viewDivider),
Expanded(
child: FadeTransition(
opacity: CurvedAnimation(
parent: widget.animation,
curve: _kViewListFadeOnInterval,
reverseCurve: _kViewListFadeOnInterval.flipped,
),
child: viewBuilder(result),
),
),
],
),
),
),
),
),
),
),
);
}
}
class _SearchAnchorWithSearchBar extends SearchAnchor {
_SearchAnchorWithSearchBar({
Widget? barLeading,
Iterable<Widget>? barTrailing,
String? barHintText,
GestureTapCallback? onTap,
MaterialStateProperty<double?>? barElevation,
MaterialStateProperty<Color?>? barBackgroundColor,
MaterialStateProperty<Color?>? barOverlayColor,
MaterialStateProperty<BorderSide?>? barSide,
MaterialStateProperty<OutlinedBorder?>? barShape,
MaterialStateProperty<EdgeInsetsGeometry?>? barPadding,
MaterialStateProperty<TextStyle?>? barTextStyle,
MaterialStateProperty<TextStyle?>? barHintStyle,
super.viewLeading,
super.viewTrailing,
String? viewHintText,
super.viewBackgroundColor,
super.viewElevation,
super.viewSide,
super.viewShape,
double? viewHeaderHeight,
TextStyle? viewHeaderTextStyle,
TextStyle? viewHeaderHintStyle,
super.dividerColor,
BoxConstraints? constraints,
super.viewConstraints,
super.isFullScreen,
super.searchController,
super.textCapitalization,
ValueChanged<String>? onChanged,
ValueChanged<String>? onSubmitted,
required super.suggestionsBuilder,
super.textInputAction,
super.keyboardType,
}) : super(
viewHintText: viewHintText ?? barHintText,
headerHeight: viewHeaderHeight,
headerTextStyle: viewHeaderTextStyle,
headerHintStyle: viewHeaderHintStyle,
viewOnSubmitted: onSubmitted,
viewOnChanged: onChanged,
builder: (BuildContext context, SearchController controller) {
return SearchBar(
constraints: constraints,
controller: controller,
onTap: () {
controller.openView();
onTap?.call();
},
onChanged: (String value) {
controller.openView();
},
onSubmitted: onSubmitted,
hintText: barHintText,
hintStyle: barHintStyle,
textStyle: barTextStyle,
elevation: barElevation,
backgroundColor: barBackgroundColor,
overlayColor: barOverlayColor,
side: barSide,
shape: barShape,
padding: barPadding ?? const MaterialStatePropertyAll<EdgeInsets>(EdgeInsets.symmetric(horizontal: 16.0)),
leading: barLeading ?? const Icon(Icons.search),
trailing: barTrailing,
textCapitalization: textCapitalization,
textInputAction: textInputAction,
keyboardType: keyboardType,
);
}
);
}
/// A controller to manage a search view created by [SearchAnchor].
///
/// A [SearchController] is used to control a menu after it has been created,
/// with methods such as [openView] and [closeView]. It can also control the text in the
/// input field.
///
/// See also:
///
/// * [SearchAnchor], a widget that defines a region that opens a search view.
/// * [TextEditingController], A controller for an editable text field.
class SearchController extends TextEditingController {
// The anchor that this controller controls.
//
// This is set automatically when a [SearchController] is given to the anchor
// it controls.
_SearchAnchorState? _anchor;
/// Whether this controller has associated search anchor.
bool get isAttached => _anchor != null;
/// Whether or not the associated search view is currently open.
bool get isOpen {
assert(isAttached);
return _anchor!._viewIsOpen;
}
/// Opens the search view that this controller is associated with.
void openView() {
assert(isAttached);
_anchor!._openView();
}
/// Close the search view that this search controller is associated with.
///
/// If `selectedText` is given, then the text value of the controller is set to
/// `selectedText`.
void closeView(String? selectedText) {
assert(isAttached);
_anchor!._closeView(selectedText);
}
// ignore: use_setters_to_change_properties
void _attach(_SearchAnchorState anchor) {
_anchor = anchor;
}
void _detach(_SearchAnchorState anchor) {
if (_anchor == anchor) {
_anchor = null;
}
}
}
/// A Material Design search bar.
///
/// A [SearchBar] looks like a [TextField]. Tapping a SearchBar typically shows a
/// "search view" route: a route with the search bar at the top and a list of
/// suggested completions for the search bar's text below. [SearchBar]s are
/// usually created by a [SearchAnchor.builder]. The builder provides a
/// [SearchController] that's used by the search bar's [SearchBar.onTap] or
/// [SearchBar.onChanged] callbacks to show the search view and to hide it
/// when the user selects a suggestion.
///
/// For [TextDirection.ltr], the [leading] widget is on the left side of the bar.
/// It should contain either a navigational action (such as a menu or up-arrow)
/// or a non-functional search icon.
///
/// The [trailing] is an optional list that appears at the other end of
/// the search bar. Typically only one or two action icons are included.
/// These actions can represent additional modes of searching (like voice search),
/// a separate high-level action (such as current location) or an overflow menu.
///
/// {@tool dartpad}
/// This example demonstrates how to use a [SearchBar] as the return value of the
/// [SearchAnchor.builder] property. The [SearchBar] also includes a leading search
/// icon and a trailing action to toggle the brightness.
///
/// ** See code in examples/api/lib/material/search_anchor/search_bar.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SearchAnchor], a widget that typically uses an [IconButton] or a [SearchBar]
/// to manage a "search view" route.
/// * [SearchBarTheme], a widget that overrides the default configuration of a search bar.
/// * [SearchViewTheme], a widget that overrides the default configuration of a search view.
class SearchBar extends StatefulWidget {
/// Creates a Material Design search bar.
const SearchBar({
super.key,
this.controller,
this.focusNode,
this.hintText,
this.leading,
this.trailing,
this.onTap,
this.onChanged,
this.onSubmitted,
this.constraints,
this.elevation,
this.backgroundColor,
this.shadowColor,
this.surfaceTintColor,
this.overlayColor,
this.side,
this.shape,
this.padding,
this.textStyle,
this.hintStyle,
this.textCapitalization,
this.enabled = true,
this.autoFocus = false,
this.textInputAction,
this.keyboardType,
});
/// Controls the text being edited in the search bar's text field.
///
/// If null, this widget will create its own [TextEditingController].
final TextEditingController? controller;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// Text that suggests what sort of input the field accepts.
///
/// Displayed at the same location on the screen where text may be entered
/// when the input is empty.
///
/// Defaults to null.
final String? hintText;
/// A widget to display before the text input field.
///
/// Typically the [leading] widget is an [Icon] or an [IconButton].
final Widget? leading;
/// A list of Widgets to display in a row after the text field.
///
/// Typically these actions can represent additional modes of searching
/// (like voice search), an avatar, a separate high-level action (such as
/// current location) or an overflow menu. There should not be more than
/// two trailing actions.
final Iterable<Widget>? trailing;
/// Called when the user taps this search bar.
final GestureTapCallback? onTap;
/// Invoked upon user input.
final ValueChanged<String>? onChanged;
/// Called when the user indicates that they are done editing the text in the
/// field.
final ValueChanged<String>? onSubmitted;
/// Optional size constraints for the search bar.
///
/// If null, the value of [SearchBarThemeData.constraints] will be used. If
/// this is also null, then the constraints defaults to:
/// ```dart
/// const BoxConstraints(minWidth: 360.0, maxWidth: 800.0, minHeight: 56.0)
/// ```
final BoxConstraints? constraints;
/// The elevation of the search bar's [Material].
///
/// If null, the value of [SearchBarThemeData.elevation] will be used. If this
/// is also null, then default value is 6.0.
final MaterialStateProperty<double?>? elevation;
/// The search bar's background fill color.
///
/// If null, the value of [SearchBarThemeData.backgroundColor] will be used.
/// If this is also null, then the default value is [ColorScheme.surfaceContainerHigh].
final MaterialStateProperty<Color?>? backgroundColor;
/// The shadow color of the search bar's [Material].
///
/// If null, the value of [SearchBarThemeData.shadowColor] will be used.
/// If this is also null, then the default value is [ColorScheme.shadow].
final MaterialStateProperty<Color?>? shadowColor;
/// The surface tint color of the search bar's [Material].
///
/// This is not recommended for use. [Material 3 spec](https://m3.material.io/styles/color/the-color-system/color-roles)
/// introduced a set of tone-based surfaces and surface containers in its [ColorScheme],
/// which provide more flexibility. The intention is to eventually remove surface tint color from
/// the framework.
///
/// If null, the value of [SearchBarThemeData.surfaceTintColor] will be used.
/// If this is also null, then the default value is [Colors.transparent].
final MaterialStateProperty<Color?>? surfaceTintColor;
/// The highlight color that's typically used to indicate that
/// the search bar is focused, hovered, or pressed.
final MaterialStateProperty<Color?>? overlayColor;
/// The color and weight of the search bar's outline.
///
/// This value is combined with [shape] to create a shape decorated
/// with an outline.
///
/// If null, the value of [SearchBarThemeData.side] will be used. If this is
/// also null, the search bar doesn't have a side by default.
final MaterialStateProperty<BorderSide?>? side;
/// The shape of the search bar's underlying [Material].
///
/// This shape is combined with [side] to create a shape decorated
/// with an outline.
///
/// If null, the value of [SearchBarThemeData.shape] will be used.
/// If this is also null, defaults to [StadiumBorder].
final MaterialStateProperty<OutlinedBorder?>? shape;
/// The padding between the search bar's boundary and its contents.
///
/// If null, the value of [SearchBarThemeData.padding] will be used.
/// If this is also null, then the default value is 16.0 horizontally.
final MaterialStateProperty<EdgeInsetsGeometry?>? padding;
/// The style to use for the text being edited.
///
/// If null, defaults to the `bodyLarge` text style from the current [Theme].
/// The default text color is [ColorScheme.onSurface].
final MaterialStateProperty<TextStyle?>? textStyle;
/// The style to use for the [hintText].
///
/// If null, the value of [SearchBarThemeData.hintStyle] will be used. If this
/// is also null, the value of [textStyle] will be used. If this is also null,
/// defaults to the `bodyLarge` text style from the current [Theme].
/// The default text color is [ColorScheme.onSurfaceVariant].
final MaterialStateProperty<TextStyle?>? hintStyle;
/// {@macro flutter.widgets.editableText.textCapitalization}
final TextCapitalization? textCapitalization;
/// If false the text field is "disabled" so the SearchBar will ignore taps.
final bool enabled;
/// {@macro flutter.widgets.editableText.autofocus}
final bool autoFocus;
/// {@macro flutter.widgets.TextField.textInputAction}
final TextInputAction? textInputAction;
/// The type of action button to use for the keyboard.
///
/// Defaults to the default value specified in [TextField].
final TextInputType? keyboardType;
@override
State<SearchBar> createState() => _SearchBarState();
}
class _SearchBarState extends State<SearchBar> {
late final MaterialStatesController _internalStatesController;
FocusNode? _internalFocusNode;
FocusNode get _focusNode => widget.focusNode ?? (_internalFocusNode ??= FocusNode());
@override
void initState() {
super.initState();
_internalStatesController = MaterialStatesController();
_internalStatesController.addListener(() {
setState(() {});
});
}
@override
void dispose() {
_internalStatesController.dispose();
_internalFocusNode?.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final IconThemeData iconTheme = IconTheme.of(context);
final SearchBarThemeData searchBarTheme = SearchBarTheme.of(context);
final SearchBarThemeData defaults = _SearchBarDefaultsM3(context);
T? resolve<T>(
MaterialStateProperty<T>? widgetValue,
MaterialStateProperty<T>? themeValue,
MaterialStateProperty<T>? defaultValue,
) {
final Set<MaterialState> states = _internalStatesController.value;
return widgetValue?.resolve(states) ?? themeValue?.resolve(states) ?? defaultValue?.resolve(states);
}
final TextStyle? effectiveTextStyle = resolve<TextStyle?>(widget.textStyle, searchBarTheme.textStyle, defaults.textStyle);
final double? effectiveElevation = resolve<double?>(widget.elevation, searchBarTheme.elevation, defaults.elevation);
final Color? effectiveShadowColor = resolve<Color?>(widget.shadowColor, searchBarTheme.shadowColor, defaults.shadowColor);
final Color? effectiveBackgroundColor = resolve<Color?>(widget.backgroundColor, searchBarTheme.backgroundColor, defaults.backgroundColor);
final Color? effectiveSurfaceTintColor = resolve<Color?>(widget.surfaceTintColor, searchBarTheme.surfaceTintColor, defaults.surfaceTintColor);
final OutlinedBorder? effectiveShape = resolve<OutlinedBorder?>(widget.shape, searchBarTheme.shape, defaults.shape);
final BorderSide? effectiveSide = resolve<BorderSide?>(widget.side, searchBarTheme.side, defaults.side);
final EdgeInsetsGeometry? effectivePadding = resolve<EdgeInsetsGeometry?>(widget.padding, searchBarTheme.padding, defaults.padding);
final MaterialStateProperty<Color?>? effectiveOverlayColor = widget.overlayColor ?? searchBarTheme.overlayColor ?? defaults.overlayColor;
final TextCapitalization effectiveTextCapitalization = widget.textCapitalization ?? searchBarTheme.textCapitalization ?? defaults.textCapitalization!;
final Set<MaterialState> states = _internalStatesController.value;
final TextStyle? effectiveHintStyle = widget.hintStyle?.resolve(states)
?? searchBarTheme.hintStyle?.resolve(states)
?? widget.textStyle?.resolve(states)
?? searchBarTheme.textStyle?.resolve(states)
?? defaults.hintStyle?.resolve(states);
final bool isDark = Theme.of(context).brightness == Brightness.dark;
bool isIconThemeColorDefault(Color? color) {
if (isDark) {
return color == kDefaultIconLightColor;
}
return color == kDefaultIconDarkColor;
}
Widget? leading;
if (widget.leading != null) {
leading = IconTheme.merge(
data: isIconThemeColorDefault(iconTheme.color)
? IconThemeData(color: colorScheme.onSurface)
: iconTheme,
child: widget.leading!,
);
}
List<Widget>? trailing;
if (widget.trailing != null) {
trailing = widget.trailing?.map((Widget trailing) => IconTheme.merge(
data: isIconThemeColorDefault(iconTheme.color)
? IconThemeData(color: colorScheme.onSurfaceVariant)
: iconTheme,
child: trailing,
)).toList();
}
return ConstrainedBox(
constraints: widget.constraints ?? searchBarTheme.constraints ?? defaults.constraints!,
child: Opacity(
opacity: widget.enabled ? 1 : _kDisableSearchBarOpacity,
child: Material(
elevation: effectiveElevation!,
shadowColor: effectiveShadowColor,
color: effectiveBackgroundColor,
surfaceTintColor: effectiveSurfaceTintColor,
shape: effectiveShape?.copyWith(side: effectiveSide),
child: IgnorePointer(
ignoring: !widget.enabled,
child: InkWell(
onTap: () {
widget.onTap?.call();
if (!_focusNode.hasFocus) {
_focusNode.requestFocus();
}
},
overlayColor: effectiveOverlayColor,
customBorder: effectiveShape?.copyWith(side: effectiveSide),
statesController: _internalStatesController,
child: Padding(
padding: effectivePadding!,
child: Row(
textDirection: textDirection,
children: <Widget>[
if (leading != null) leading,
Expanded(
child: Padding(
padding: effectivePadding,
child: TextField(
autofocus: widget.autoFocus,
onTap: widget.onTap,
onTapAlwaysCalled: true,
focusNode: _focusNode,
onChanged: widget.onChanged,
onSubmitted: widget.onSubmitted,
controller: widget.controller,
style: effectiveTextStyle,
enabled: widget.enabled,
decoration: InputDecoration(
hintText: widget.hintText,
).applyDefaults(InputDecorationTheme(
hintStyle: effectiveHintStyle,
// The configuration below is to make sure that the text field
// in `SearchBar` will not be overridden by the overall `InputDecorationTheme`
enabledBorder: InputBorder.none,
border: InputBorder.none,
focusedBorder: InputBorder.none,
contentPadding: EdgeInsets.zero,
// Setting `isDense` to true to allow the text field height to be
// smaller than 48.0
isDense: true,
)),
textCapitalization: effectiveTextCapitalization,
textInputAction: widget.textInputAction,
keyboardType: widget.keyboardType,
),
),
),
if (trailing != null) ...trailing,
],
),
),
),
),
),
),
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - SearchBar
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _SearchBarDefaultsM3 extends SearchBarThemeData {
_SearchBarDefaultsM3(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
@override
MaterialStateProperty<Color?>? get backgroundColor =>
MaterialStatePropertyAll<Color>(_colors.surfaceContainerHigh);
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(6.0);
@override
MaterialStateProperty<Color>? get shadowColor =>
MaterialStatePropertyAll<Color>(_colors.shadow);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return Colors.transparent;
}
return Colors.transparent;
});
// No default side
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
const MaterialStatePropertyAll<EdgeInsetsGeometry>(EdgeInsets.symmetric(horizontal: 8.0));
@override
MaterialStateProperty<TextStyle?> get textStyle =>
MaterialStatePropertyAll<TextStyle?>(_textTheme.bodyLarge?.copyWith(color: _colors.onSurface));
@override
MaterialStateProperty<TextStyle?> get hintStyle =>
MaterialStatePropertyAll<TextStyle?>(_textTheme.bodyLarge?.copyWith(color: _colors.onSurfaceVariant));
@override
BoxConstraints get constraints =>
const BoxConstraints(minWidth: 360.0, maxWidth: 800.0, minHeight: 56.0);
@override
TextCapitalization get textCapitalization => TextCapitalization.none;
}
// END GENERATED TOKEN PROPERTIES - SearchBar
// BEGIN GENERATED TOKEN PROPERTIES - SearchView
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _SearchViewDefaultsM3 extends SearchViewThemeData {
_SearchViewDefaultsM3(this.context, {required this.isFullScreen});
final BuildContext context;
final bool isFullScreen;
late final ColorScheme _colors = Theme.of(context).colorScheme;
late final TextTheme _textTheme = Theme.of(context).textTheme;
static double fullScreenBarHeight = 72.0;
@override
Color? get backgroundColor => _colors.surfaceContainerHigh;
@override
double? get elevation => 6.0;
@override
Color? get surfaceTintColor => Colors.transparent;
// No default side
@override
OutlinedBorder? get shape => isFullScreen
? const RoundedRectangleBorder()
: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(28.0)));
@override
TextStyle? get headerTextStyle => _textTheme.bodyLarge?.copyWith(color: _colors.onSurface);
@override
TextStyle? get headerHintStyle => _textTheme.bodyLarge?.copyWith(color: _colors.onSurfaceVariant);
@override
BoxConstraints get constraints => const BoxConstraints(minWidth: 360.0, minHeight: 240.0);
@override
Color? get dividerColor => _colors.outline;
}
// END GENERATED TOKEN PROPERTIES - SearchView
| flutter/packages/flutter/lib/src/material/search_anchor.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/search_anchor.dart",
"repo_id": "flutter",
"token_count": 20730
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'material_state.dart';
import 'shadows.dart';
import 'switch_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
import 'toggleable.dart';
// Examples can assume:
// bool _giveVerse = true;
// late StateSetter setState;
const double _kSwitchMinSize = kMinInteractiveDimension - 8.0;
enum _SwitchType { material, adaptive }
/// A Material Design switch.
///
/// Used to toggle the on/off state of a single setting.
///
/// The switch itself does not maintain any state. Instead, when the state of
/// the switch changes, the widget calls the [onChanged] callback. Most widgets
/// that use a switch will listen for the [onChanged] callback and rebuild the
/// switch with a new [value] to update the visual appearance of the switch.
///
/// If the [onChanged] callback is null, then the switch will be disabled (it
/// will not respond to input). A disabled switch's thumb and track are rendered
/// in shades of grey by default. The default appearance of a disabled switch
/// can be overridden with [inactiveThumbColor] and [inactiveTrackColor].
///
/// Requires one of its ancestors to be a [Material] widget.
///
/// Material Design 3 provides the option to add icons on the thumb of the [Switch].
/// If [ThemeData.useMaterial3] is set to true, users can use [Switch.thumbIcon]
/// to add optional Icons based on the different [MaterialState]s of the [Switch].
///
/// {@tool dartpad}
/// This example shows a toggleable [Switch]. When the thumb slides to the other
/// side of the track, the switch is toggled between on/off.
///
/// ** See code in examples/api/lib/material/switch/switch.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to customize [Switch] using [MaterialStateProperty]
/// switch properties.
///
/// ** See code in examples/api/lib/material/switch/switch.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to add icons on the thumb of the [Switch] using the
/// [Switch.thumbIcon] property.
///
/// ** See code in examples/api/lib/material/switch/switch.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to use the ambient [CupertinoThemeData] to style all
/// widgets which would otherwise use iOS defaults.
///
/// ** See code in examples/api/lib/material/switch/switch.3.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SwitchListTile], which combines this widget with a [ListTile] so that
/// you can give the switch a label.
/// * [Checkbox], another widget with similar semantics.
/// * [Radio], for selecting among a set of explicit values.
/// * [Slider], for selecting a value in a range.
/// * [MaterialStateProperty], an interface for objects that "resolve" to
/// different values depending on a widget's material state.
/// * <https://material.io/design/components/selection-controls.html#switches>
class Switch extends StatelessWidget {
/// Creates a Material Design switch.
///
/// The switch itself does not maintain any state. Instead, when the state of
/// the switch changes, the widget calls the [onChanged] callback. Most widgets
/// that use a switch will listen for the [onChanged] callback and rebuild the
/// switch with a new [value] to update the visual appearance of the switch.
///
/// The following arguments are required:
///
/// * [value] determines whether this switch is on or off.
/// * [onChanged] is called when the user toggles the switch on or off.
const Switch({
super.key,
required this.value,
required this.onChanged,
this.activeColor,
this.activeTrackColor,
this.inactiveThumbColor,
this.inactiveTrackColor,
this.activeThumbImage,
this.onActiveThumbImageError,
this.inactiveThumbImage,
this.onInactiveThumbImageError,
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.trackOutlineWidth,
this.thumbIcon,
this.materialTapTargetSize,
this.dragStartBehavior = DragStartBehavior.start,
this.mouseCursor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.focusNode,
this.onFocusChange,
this.autofocus = false,
}) : _switchType = _SwitchType.material,
applyCupertinoTheme = false,
assert(activeThumbImage != null || onActiveThumbImageError == null),
assert(inactiveThumbImage != null || onInactiveThumbImageError == null);
/// Creates an adaptive [Switch] based on whether the target platform is iOS
/// or macOS, following Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// Creates a switch that looks and feels native when the [ThemeData.platform]
/// is iOS or macOS, otherwise a Material Design switch is created.
///
/// To provide a custom switch theme that's only used by this factory
/// constructor, add a custom `Adaptation<SwitchThemeData>` class to
/// [ThemeData.adaptations]. This can be useful in situations where you don't
/// want the overall [ThemeData.switchTheme] to apply when this adaptive
/// constructor is used.
///
/// {@tool dartpad}
/// This sample shows how to create and use subclasses of [Adaptation] that
/// define adaptive [SwitchThemeData]s.
///
/// ** See code in examples/api/lib/material/switch/switch.4.dart **
/// {@end-tool}
///
/// The target platform is based on the current [Theme]: [ThemeData.platform].
const Switch.adaptive({
super.key,
required this.value,
required this.onChanged,
this.activeColor,
this.activeTrackColor,
this.inactiveThumbColor,
this.inactiveTrackColor,
this.activeThumbImage,
this.onActiveThumbImageError,
this.inactiveThumbImage,
this.onInactiveThumbImageError,
this.materialTapTargetSize,
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.trackOutlineWidth,
this.thumbIcon,
this.dragStartBehavior = DragStartBehavior.start,
this.mouseCursor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.focusNode,
this.onFocusChange,
this.autofocus = false,
this.applyCupertinoTheme,
}) : assert(activeThumbImage != null || onActiveThumbImageError == null),
assert(inactiveThumbImage != null || onInactiveThumbImageError == null),
_switchType = _SwitchType.adaptive;
/// Whether this switch is on or off.
final bool value;
/// Called when the user toggles the switch on or off.
///
/// The switch passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the switch with the new
/// value.
///
/// If null, the switch will be displayed as disabled.
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// ```dart
/// Switch(
/// value: _giveVerse,
/// onChanged: (bool newValue) {
/// setState(() {
/// _giveVerse = newValue;
/// });
/// },
/// )
/// ```
final ValueChanged<bool>? onChanged;
/// {@template flutter.material.switch.activeColor}
/// The color to use when this switch is on.
/// {@endtemplate}
///
/// Defaults to [ColorScheme.secondary].
///
/// If [thumbColor] returns a non-null color in the [MaterialState.selected]
/// state, it will be used instead of this color.
final Color? activeColor;
/// {@template flutter.material.switch.activeTrackColor}
/// The color to use on the track when this switch is on.
/// {@endtemplate}
///
/// Defaults to [ColorScheme.secondary] with the opacity set at 50%.
///
/// If [trackColor] returns a non-null color in the [MaterialState.selected]
/// state, it will be used instead of this color.
final Color? activeTrackColor;
/// {@template flutter.material.switch.inactiveThumbColor}
/// The color to use on the thumb when this switch is off.
/// {@endtemplate}
///
/// Defaults to the colors described in the Material design specification.
///
/// If [thumbColor] returns a non-null color in the default state, it will be
/// used instead of this color.
final Color? inactiveThumbColor;
/// {@template flutter.material.switch.inactiveTrackColor}
/// The color to use on the track when this switch is off.
/// {@endtemplate}
///
/// Defaults to the colors described in the Material design specification.
///
/// If [trackColor] returns a non-null color in the default state, it will be
/// used instead of this color.
final Color? inactiveTrackColor;
/// {@template flutter.material.switch.activeThumbImage}
/// An image to use on the thumb of this switch when the switch is on.
/// {@endtemplate}
final ImageProvider? activeThumbImage;
/// {@template flutter.material.switch.onActiveThumbImageError}
/// An optional error callback for errors emitted when loading
/// [activeThumbImage].
/// {@endtemplate}
final ImageErrorListener? onActiveThumbImageError;
/// {@template flutter.material.switch.inactiveThumbImage}
/// An image to use on the thumb of this switch when the switch is off.
/// {@endtemplate}
final ImageProvider? inactiveThumbImage;
/// {@template flutter.material.switch.onInactiveThumbImageError}
/// An optional error callback for errors emitted when loading
/// [inactiveThumbImage].
/// {@endtemplate}
final ImageErrorListener? onInactiveThumbImageError;
/// {@template flutter.material.switch.thumbColor}
/// The color of this [Switch]'s thumb.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [thumbColor] based on the current
/// [MaterialState] of the [Switch], providing a different [Color] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Switch(
/// value: true,
/// onChanged: (bool value) { },
/// thumbColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return Colors.orange.withOpacity(.48);
/// }
/// return Colors.orange;
/// }),
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// If null, then the value of [activeColor] is used in the selected
/// state and [inactiveThumbColor] in the default state. If that is also null,
/// then the value of [SwitchThemeData.thumbColor] is used. If that is also
/// null, then the following colors are used:
///
/// | State | Light theme | Dark theme |
/// |----------|-----------------------------------|-----------------------------------|
/// | Default | `Colors.grey.shade50` | `Colors.grey.shade400` |
/// | Selected | [ColorScheme.secondary] | [ColorScheme.secondary] |
/// | Disabled | `Colors.grey.shade400` | `Colors.grey.shade800` |
final MaterialStateProperty<Color?>? thumbColor;
/// {@template flutter.material.switch.trackColor}
/// The color of this [Switch]'s track.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [trackColor] based on the current
/// [MaterialState] of the [Switch], providing a different [Color] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Switch(
/// value: true,
/// onChanged: (bool value) { },
/// thumbColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return Colors.orange.withOpacity(.48);
/// }
/// return Colors.orange;
/// }),
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// If null, then the value of [activeTrackColor] is used in the selected
/// state and [inactiveTrackColor] in the default state. If that is also null,
/// then the value of [SwitchThemeData.trackColor] is used. If that is also
/// null, then the following colors are used:
///
/// | State | Light theme | Dark theme |
/// |----------|---------------------------------|---------------------------------|
/// | Default | `Color(0x52000000)` | `Colors.white30` |
/// | Selected | [activeColor] with alpha `0x80` | [activeColor] with alpha `0x80` |
/// | Disabled | `Colors.black12` | `Colors.white10` |
final MaterialStateProperty<Color?>? trackColor;
/// {@template flutter.material.switch.trackOutlineColor}
/// The outline color of this [Switch]'s track.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [trackOutlineColor] based on the current
/// [MaterialState] of the [Switch], providing a different [Color] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Switch(
/// value: true,
/// onChanged: (bool value) { },
/// trackOutlineColor: MaterialStateProperty.resolveWith<Color?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return Colors.orange.withOpacity(.48);
/// }
/// return null; // Use the default color.
/// }),
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// In Material 3, the outline color defaults to transparent in the selected
/// state and [ColorScheme.outline] in the unselected state. In Material 2,
/// the [Switch] track has no outline by default.
final MaterialStateProperty<Color?>? trackOutlineColor;
/// {@template flutter.material.switch.trackOutlineWidth}
/// The outline width of this [Switch]'s track.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [trackOutlineWidth] based on the current
/// [MaterialState] of the [Switch], providing a different outline width when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Switch(
/// value: true,
/// onChanged: (bool value) { },
/// trackOutlineWidth: MaterialStateProperty.resolveWith<double?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return 5.0;
/// }
/// return null; // Use the default width.
/// }),
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// Defaults to 2.0.
final MaterialStateProperty<double?>? trackOutlineWidth;
/// {@template flutter.material.switch.thumbIcon}
/// The icon to use on the thumb of this switch
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
///
/// {@tool snippet}
/// This example resolves the [thumbIcon] based on the current
/// [MaterialState] of the [Switch], providing a different [Icon] when it is
/// [MaterialState.disabled].
///
/// ```dart
/// Switch(
/// value: true,
/// onChanged: (bool value) { },
/// thumbIcon: MaterialStateProperty.resolveWith<Icon?>((Set<MaterialState> states) {
/// if (states.contains(MaterialState.disabled)) {
/// return const Icon(Icons.close);
/// }
/// return null; // All other states will use the default thumbIcon.
/// }),
/// )
/// ```
/// {@end-tool}
/// {@endtemplate}
///
/// If null, then the value of [SwitchThemeData.thumbIcon] is used. If this is also null,
/// then the [Switch] does not have any icons on the thumb.
final MaterialStateProperty<Icon?>? thumbIcon;
/// {@template flutter.material.switch.materialTapTargetSize}
/// Configures the minimum size of the tap target.
/// {@endtemplate}
///
/// If null, then the value of [SwitchThemeData.materialTapTargetSize] is
/// used. If that is also null, then the value of
/// [ThemeData.materialTapTargetSize] is used.
///
/// See also:
///
/// * [MaterialTapTargetSize], for a description of how this affects tap targets.
final MaterialTapTargetSize? materialTapTargetSize;
final _SwitchType _switchType;
/// {@macro flutter.cupertino.CupertinoSwitch.applyTheme}
final bool? applyCupertinoTheme;
/// {@macro flutter.cupertino.CupertinoSwitch.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@template flutter.material.switch.mouseCursor}
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.disabled].
/// {@endtemplate}
///
/// If null, then the value of [SwitchThemeData.mouseCursor] is used. If that
/// is also null, then [MaterialStateMouseCursor.clickable] is used.
///
/// See also:
///
/// * [MaterialStateMouseCursor], a [MouseCursor] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept
/// either a [MouseCursor] or a [MaterialStateProperty<MouseCursor>].
final MouseCursor? mouseCursor;
/// The color for the button's [Material] when it has the input focus.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.focused]
/// state, it will be used instead.
///
/// If null, then the value of [SwitchThemeData.overlayColor] is used in the
/// focused state. If that is also null, then the value of
/// [ThemeData.focusColor] is used.
final Color? focusColor;
/// The color for the button's [Material] when a pointer is hovering over it.
///
/// If [overlayColor] returns a non-null color in the [MaterialState.hovered]
/// state, it will be used instead.
///
/// If null, then the value of [SwitchThemeData.overlayColor] is used in the
/// hovered state. If that is also null, then the value of
/// [ThemeData.hoverColor] is used.
final Color? hoverColor;
/// {@template flutter.material.switch.overlayColor}
/// The color for the switch's [Material].
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// {@endtemplate}
///
/// If null, then the value of [activeColor] with alpha
/// [kRadialReactionAlpha], [focusColor] and [hoverColor] is used in the
/// pressed, focused and hovered state. If that is also null,
/// the value of [SwitchThemeData.overlayColor] is used. If that is
/// also null, then the value of [ColorScheme.secondary] with alpha
/// [kRadialReactionAlpha], [ThemeData.focusColor] and [ThemeData.hoverColor]
/// is used in the pressed, focused and hovered state.
final MaterialStateProperty<Color?>? overlayColor;
/// {@template flutter.material.switch.splashRadius}
/// The splash radius of the circular [Material] ink response.
/// {@endtemplate}
///
/// If null, then the value of [SwitchThemeData.splashRadius] is used. If that
/// is also null, then [kRadialReactionRadius] is used.
final double? splashRadius;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.material.inkwell.onFocusChange}
final ValueChanged<bool>? onFocusChange;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
Size _getSwitchSize(BuildContext context) {
final ThemeData theme = Theme.of(context);
SwitchThemeData switchTheme = SwitchTheme.of(context);
if (_switchType == _SwitchType.adaptive) {
final Adaptation<SwitchThemeData> switchAdaptation = theme.getAdaptation<SwitchThemeData>()
?? const _SwitchThemeAdaptation();
switchTheme = switchAdaptation.adapt(theme, switchTheme);
}
final _SwitchConfig switchConfig = theme.useMaterial3 ? _SwitchConfigM3(context) : _SwitchConfigM2();
final MaterialTapTargetSize effectiveMaterialTapTargetSize = materialTapTargetSize
?? switchTheme.materialTapTargetSize
?? theme.materialTapTargetSize;
return switch (effectiveMaterialTapTargetSize) {
MaterialTapTargetSize.padded => Size(switchConfig.switchWidth, switchConfig.switchHeight),
MaterialTapTargetSize.shrinkWrap => Size(switchConfig.switchWidth, switchConfig.switchHeightCollapsed),
};
}
@override
Widget build(BuildContext context) {
Color? effectiveActiveThumbColor;
Color? effectiveActiveTrackColor;
switch (_switchType) {
case _SwitchType.material:
effectiveActiveThumbColor = activeColor;
case _SwitchType.adaptive:
switch (Theme.of(context).platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
effectiveActiveThumbColor = activeColor;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
effectiveActiveTrackColor = activeColor;
}
}
return _MaterialSwitch(
value: value,
onChanged: onChanged,
size: _getSwitchSize(context),
activeColor: effectiveActiveThumbColor,
activeTrackColor: activeTrackColor ?? effectiveActiveTrackColor,
inactiveThumbColor: inactiveThumbColor,
inactiveTrackColor: inactiveTrackColor,
activeThumbImage: activeThumbImage,
onActiveThumbImageError: onActiveThumbImageError,
inactiveThumbImage: inactiveThumbImage,
onInactiveThumbImageError: onInactiveThumbImageError,
thumbColor: thumbColor,
trackColor: trackColor,
trackOutlineColor: trackOutlineColor,
trackOutlineWidth: trackOutlineWidth,
thumbIcon: thumbIcon,
materialTapTargetSize: materialTapTargetSize,
dragStartBehavior: dragStartBehavior,
mouseCursor: mouseCursor,
focusColor: focusColor,
hoverColor: hoverColor,
overlayColor: overlayColor,
splashRadius: splashRadius,
focusNode: focusNode,
onFocusChange: onFocusChange,
autofocus: autofocus,
applyCupertinoTheme: applyCupertinoTheme,
switchType: _switchType,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('value', value: value, ifTrue: 'on', ifFalse: 'off', showName: true));
properties.add(ObjectFlagProperty<ValueChanged<bool>>('onChanged', onChanged, ifNull: 'disabled'));
}
}
class _MaterialSwitch extends StatefulWidget {
const _MaterialSwitch({
required this.value,
required this.onChanged,
required this.size,
required this.switchType,
this.activeColor,
this.activeTrackColor,
this.inactiveThumbColor,
this.inactiveTrackColor,
this.activeThumbImage,
this.onActiveThumbImageError,
this.inactiveThumbImage,
this.onInactiveThumbImageError,
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.trackOutlineWidth,
this.thumbIcon,
this.materialTapTargetSize,
this.dragStartBehavior = DragStartBehavior.start,
this.mouseCursor,
this.focusColor,
this.hoverColor,
this.overlayColor,
this.splashRadius,
this.focusNode,
this.onFocusChange,
this.autofocus = false,
this.applyCupertinoTheme,
}) : assert(activeThumbImage != null || onActiveThumbImageError == null),
assert(inactiveThumbImage != null || onInactiveThumbImageError == null);
final bool value;
final ValueChanged<bool>? onChanged;
final Color? activeColor;
final Color? activeTrackColor;
final Color? inactiveThumbColor;
final Color? inactiveTrackColor;
final ImageProvider? activeThumbImage;
final ImageErrorListener? onActiveThumbImageError;
final ImageProvider? inactiveThumbImage;
final ImageErrorListener? onInactiveThumbImageError;
final MaterialStateProperty<Color?>? thumbColor;
final MaterialStateProperty<Color?>? trackColor;
final MaterialStateProperty<Color?>? trackOutlineColor;
final MaterialStateProperty<double?>? trackOutlineWidth;
final MaterialStateProperty<Icon?>? thumbIcon;
final MaterialTapTargetSize? materialTapTargetSize;
final DragStartBehavior dragStartBehavior;
final MouseCursor? mouseCursor;
final Color? focusColor;
final Color? hoverColor;
final MaterialStateProperty<Color?>? overlayColor;
final double? splashRadius;
final FocusNode? focusNode;
final ValueChanged<bool>? onFocusChange;
final bool autofocus;
final Size size;
final bool? applyCupertinoTheme;
final _SwitchType switchType;
@override
State<StatefulWidget> createState() => _MaterialSwitchState();
}
class _MaterialSwitchState extends State<_MaterialSwitch> with TickerProviderStateMixin, ToggleableStateMixin {
final _SwitchPainter _painter = _SwitchPainter();
@override
void didUpdateWidget(_MaterialSwitch oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.value != widget.value) {
// During a drag we may have modified the curve, reset it if its possible
// to do without visual discontinuation.
if (position.value == 0.0 || position.value == 1.0) {
switch (widget.switchType) {
case _SwitchType.adaptive:
switch (Theme.of(context).platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
updateCurve();
case TargetPlatform.iOS:
case TargetPlatform.macOS:
position
..curve = Curves.linear
..reverseCurve = Curves.linear;
}
case _SwitchType.material:
updateCurve();
}
}
animateToValue();
}
}
@override
void dispose() {
_painter.dispose();
super.dispose();
}
@override
ValueChanged<bool?>? get onChanged => widget.onChanged != null ? _handleChanged : null;
@override
bool get tristate => false;
@override
bool? get value => widget.value;
void updateCurve() {
if (Theme.of(context).useMaterial3) {
position
..curve = Curves.easeOutBack
..reverseCurve = Curves.easeOutBack.flipped;
} else {
position
..curve = Curves.easeIn
..reverseCurve = Curves.easeOut;
}
}
MaterialStateProperty<Color?> get _widgetThumbColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return widget.inactiveThumbColor;
}
if (states.contains(MaterialState.selected)) {
return widget.activeColor;
}
return widget.inactiveThumbColor;
});
}
MaterialStateProperty<Color?> get _widgetTrackColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return widget.activeTrackColor;
}
return widget.inactiveTrackColor;
});
}
double get _trackInnerLength {
switch (widget.switchType) {
case _SwitchType.adaptive:
switch (Theme.of(context).platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return widget.size.width - _kSwitchMinSize;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
final _SwitchConfig config = _SwitchConfigCupertino(context);
final double trackInnerStart = config.trackHeight / 2.0;
final double trackInnerEnd = config.trackWidth - trackInnerStart;
final double trackInnerLength = trackInnerEnd - trackInnerStart;
return trackInnerLength;
}
case _SwitchType.material:
return widget.size.width - _kSwitchMinSize;
}
}
void _handleDragStart(DragStartDetails details) {
if (isInteractive) {
reactionController.forward();
}
}
void _handleDragUpdate(DragUpdateDetails details) {
if (isInteractive) {
position
..curve = Curves.linear
..reverseCurve = null;
final double delta = details.primaryDelta! / _trackInnerLength;
positionController.value += switch (Directionality.of(context)) {
TextDirection.rtl => -delta,
TextDirection.ltr => delta,
};
}
}
bool _needsPositionAnimation = false;
void _handleDragEnd(DragEndDetails details) {
if (position.value >= 0.5 != widget.value) {
widget.onChanged?.call(!widget.value);
// Wait with finishing the animation until widget.value has changed to
// !widget.value as part of the widget.onChanged call above.
setState(() {
_needsPositionAnimation = true;
});
} else {
animateToValue();
}
reactionController.reverse();
}
void _handleChanged(bool? value) {
assert(value != null);
assert(widget.onChanged != null);
widget.onChanged?.call(value!);
}
bool isCupertino = false;
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
if (_needsPositionAnimation) {
_needsPositionAnimation = false;
animateToValue();
}
final ThemeData theme = Theme.of(context);
SwitchThemeData switchTheme = SwitchTheme.of(context);
final Color cupertinoPrimaryColor = theme.cupertinoOverrideTheme?.primaryColor ?? theme.colorScheme.primary;
_SwitchConfig switchConfig;
SwitchThemeData defaults;
bool applyCupertinoTheme = false;
double disabledOpacity = 1;
switch (widget.switchType) {
case _SwitchType.material:
switchConfig = theme.useMaterial3 ? _SwitchConfigM3(context) : _SwitchConfigM2();
defaults = theme.useMaterial3 ? _SwitchDefaultsM3(context) : _SwitchDefaultsM2(context);
case _SwitchType.adaptive:
final Adaptation<SwitchThemeData> switchAdaptation = theme.getAdaptation<SwitchThemeData>()
?? const _SwitchThemeAdaptation();
switchTheme = switchAdaptation.adapt(theme, switchTheme);
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
switchConfig = theme.useMaterial3 ? _SwitchConfigM3(context) : _SwitchConfigM2();
defaults = theme.useMaterial3 ? _SwitchDefaultsM3(context) : _SwitchDefaultsM2(context);
case TargetPlatform.iOS:
case TargetPlatform.macOS:
isCupertino = true;
applyCupertinoTheme = widget.applyCupertinoTheme
?? theme.cupertinoOverrideTheme?.applyThemeToAll
?? false;
disabledOpacity = 0.5;
switchConfig = _SwitchConfigCupertino(context);
defaults = _SwitchDefaultsCupertino(context);
reactionController.duration = const Duration(milliseconds: 200);
}
}
positionController.duration = Duration(milliseconds: switchConfig.toggleDuration);
// Colors need to be resolved in selected and non selected states separately
// so that they can be lerped between.
final Set<MaterialState> activeStates = states..add(MaterialState.selected);
final Set<MaterialState> inactiveStates = states..remove(MaterialState.selected);
final Color? activeThumbColor = widget.thumbColor?.resolve(activeStates)
?? _widgetThumbColor.resolve(activeStates)
?? switchTheme.thumbColor?.resolve(activeStates);
final Color effectiveActiveThumbColor = activeThumbColor
?? defaults.thumbColor!.resolve(activeStates)!;
final Color? inactiveThumbColor = widget.thumbColor?.resolve(inactiveStates)
?? _widgetThumbColor.resolve(inactiveStates)
?? switchTheme.thumbColor?.resolve(inactiveStates);
final Color effectiveInactiveThumbColor = inactiveThumbColor
?? defaults.thumbColor!.resolve(inactiveStates)!;
final Color effectiveActiveTrackColor = widget.trackColor?.resolve(activeStates)
?? _widgetTrackColor.resolve(activeStates)
?? (applyCupertinoTheme ? cupertinoPrimaryColor : switchTheme.trackColor?.resolve(activeStates))
?? _widgetThumbColor.resolve(activeStates)?.withAlpha(0x80)
?? defaults.trackColor!.resolve(activeStates)!;
final Color? effectiveActiveTrackOutlineColor = widget.trackOutlineColor?.resolve(activeStates)
?? switchTheme.trackOutlineColor?.resolve(activeStates)
?? defaults.trackOutlineColor!.resolve(activeStates);
final double? effectiveActiveTrackOutlineWidth = widget.trackOutlineWidth?.resolve(activeStates)
?? switchTheme.trackOutlineWidth?.resolve(activeStates)
?? defaults.trackOutlineWidth?.resolve(activeStates);
final Color effectiveInactiveTrackColor = widget.trackColor?.resolve(inactiveStates)
?? _widgetTrackColor.resolve(inactiveStates)
?? switchTheme.trackColor?.resolve(inactiveStates)
?? defaults.trackColor!.resolve(inactiveStates)!;
final Color? effectiveInactiveTrackOutlineColor = widget.trackOutlineColor?.resolve(inactiveStates)
?? switchTheme.trackOutlineColor?.resolve(inactiveStates)
?? defaults.trackOutlineColor?.resolve(inactiveStates);
final double? effectiveInactiveTrackOutlineWidth = widget.trackOutlineWidth?.resolve(inactiveStates)
?? switchTheme.trackOutlineWidth?.resolve(inactiveStates)
?? defaults.trackOutlineWidth?.resolve(inactiveStates);
final Icon? effectiveActiveIcon = widget.thumbIcon?.resolve(activeStates)
?? switchTheme.thumbIcon?.resolve(activeStates);
final Icon? effectiveInactiveIcon = widget.thumbIcon?.resolve(inactiveStates)
?? switchTheme.thumbIcon?.resolve(inactiveStates);
final Color effectiveActiveIconColor = effectiveActiveIcon?.color ?? switchConfig.iconColor.resolve(activeStates);
final Color effectiveInactiveIconColor = effectiveInactiveIcon?.color ?? switchConfig.iconColor.resolve(inactiveStates);
final Set<MaterialState> focusedStates = states..add(MaterialState.focused);
final Color effectiveFocusOverlayColor = widget.overlayColor?.resolve(focusedStates)
?? widget.focusColor
?? switchTheme.overlayColor?.resolve(focusedStates)
?? (applyCupertinoTheme
? HSLColor
.fromColor(cupertinoPrimaryColor.withOpacity(0.80))
.withLightness(0.69).withSaturation(0.835)
.toColor()
: null)
?? defaults.overlayColor!.resolve(focusedStates)!;
final Set<MaterialState> hoveredStates = states..add(MaterialState.hovered);
final Color effectiveHoverOverlayColor = widget.overlayColor?.resolve(hoveredStates)
?? widget.hoverColor
?? switchTheme.overlayColor?.resolve(hoveredStates)
?? defaults.overlayColor!.resolve(hoveredStates)!;
final Set<MaterialState> activePressedStates = activeStates..add(MaterialState.pressed);
final Color effectiveActivePressedThumbColor = widget.thumbColor?.resolve(activePressedStates)
?? _widgetThumbColor.resolve(activePressedStates)
?? switchTheme.thumbColor?.resolve(activePressedStates)
?? defaults.thumbColor!.resolve(activePressedStates)!;
final Color effectiveActivePressedOverlayColor = widget.overlayColor?.resolve(activePressedStates)
?? switchTheme.overlayColor?.resolve(activePressedStates)
?? activeThumbColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(activePressedStates)!;
final Set<MaterialState> inactivePressedStates = inactiveStates..add(MaterialState.pressed);
final Color effectiveInactivePressedThumbColor = widget.thumbColor?.resolve(inactivePressedStates)
?? _widgetThumbColor.resolve(inactivePressedStates)
?? switchTheme.thumbColor?.resolve(inactivePressedStates)
?? defaults.thumbColor!.resolve(inactivePressedStates)!;
final Color effectiveInactivePressedOverlayColor = widget.overlayColor?.resolve(inactivePressedStates)
?? switchTheme.overlayColor?.resolve(inactivePressedStates)
?? inactiveThumbColor?.withAlpha(kRadialReactionAlpha)
?? defaults.overlayColor!.resolve(inactivePressedStates)!;
final MaterialStateProperty<MouseCursor> effectiveMouseCursor = MaterialStateProperty.resolveWith<MouseCursor>((Set<MaterialState> states) {
return MaterialStateProperty.resolveAs<MouseCursor?>(widget.mouseCursor, states)
?? switchTheme.mouseCursor?.resolve(states)
?? defaults.mouseCursor!.resolve(states)!;
});
final double effectiveActiveThumbRadius = effectiveActiveIcon == null ? switchConfig.activeThumbRadius : switchConfig.thumbRadiusWithIcon;
final double effectiveInactiveThumbRadius = effectiveInactiveIcon == null && widget.inactiveThumbImage == null
? switchConfig.inactiveThumbRadius : switchConfig.thumbRadiusWithIcon;
final double effectiveSplashRadius = widget.splashRadius ?? switchTheme.splashRadius ?? defaults.splashRadius!;
return Semantics(
toggled: widget.value,
child: GestureDetector(
excludeFromSemantics: true,
onHorizontalDragStart: _handleDragStart,
onHorizontalDragUpdate: _handleDragUpdate,
onHorizontalDragEnd: _handleDragEnd,
dragStartBehavior: widget.dragStartBehavior,
child: Opacity(
opacity: onChanged == null ? disabledOpacity : 1,
child: buildToggleable(
mouseCursor: effectiveMouseCursor,
focusNode: widget.focusNode,
onFocusChange: widget.onFocusChange,
autofocus: widget.autofocus,
size: widget.size,
painter: _painter
..position = position
..reaction = reaction
..reactionFocusFade = reactionFocusFade
..reactionHoverFade = reactionHoverFade
..inactiveReactionColor = effectiveInactivePressedOverlayColor
..reactionColor = effectiveActivePressedOverlayColor
..hoverColor = effectiveHoverOverlayColor
..focusColor = effectiveFocusOverlayColor
..splashRadius = effectiveSplashRadius
..downPosition = downPosition
..isFocused = states.contains(MaterialState.focused)
..isHovered = states.contains(MaterialState.hovered)
..activeColor = effectiveActiveThumbColor
..inactiveColor = effectiveInactiveThumbColor
..activePressedColor = effectiveActivePressedThumbColor
..inactivePressedColor = effectiveInactivePressedThumbColor
..activeThumbImage = widget.activeThumbImage
..onActiveThumbImageError = widget.onActiveThumbImageError
..inactiveThumbImage = widget.inactiveThumbImage
..onInactiveThumbImageError = widget.onInactiveThumbImageError
..activeTrackColor = effectiveActiveTrackColor
..activeTrackOutlineColor = effectiveActiveTrackOutlineColor
..activeTrackOutlineWidth = effectiveActiveTrackOutlineWidth
..inactiveTrackColor = effectiveInactiveTrackColor
..inactiveTrackOutlineColor = effectiveInactiveTrackOutlineColor
..inactiveTrackOutlineWidth = effectiveInactiveTrackOutlineWidth
..configuration = createLocalImageConfiguration(context)
..isInteractive = isInteractive
..trackInnerLength = _trackInnerLength
..textDirection = Directionality.of(context)
..surfaceColor = theme.colorScheme.surface
..inactiveThumbRadius = effectiveInactiveThumbRadius
..activeThumbRadius = effectiveActiveThumbRadius
..pressedThumbRadius = switchConfig.pressedThumbRadius
..thumbOffset = switchConfig.thumbOffset
..trackHeight = switchConfig.trackHeight
..trackWidth = switchConfig.trackWidth
..activeIconColor = effectiveActiveIconColor
..inactiveIconColor = effectiveInactiveIconColor
..activeIcon = effectiveActiveIcon
..inactiveIcon = effectiveInactiveIcon
..iconTheme = IconTheme.of(context)
..thumbShadow = switchConfig.thumbShadow
..transitionalThumbSize = switchConfig.transitionalThumbSize
..positionController = positionController
..isCupertino = isCupertino,
),
),
),
);
}
}
class _SwitchPainter extends ToggleablePainter {
AnimationController get positionController => _positionController!;
AnimationController? _positionController;
set positionController(AnimationController? value) {
assert(value != null);
if (value == _positionController) {
return;
}
_positionController = value;
notifyListeners();
}
Icon? get activeIcon => _activeIcon;
Icon? _activeIcon;
set activeIcon(Icon? value) {
if (value == _activeIcon) {
return;
}
_activeIcon = value;
notifyListeners();
}
Icon? get inactiveIcon => _inactiveIcon;
Icon? _inactiveIcon;
set inactiveIcon(Icon? value) {
if (value == _inactiveIcon) {
return;
}
_inactiveIcon = value;
notifyListeners();
}
IconThemeData? get iconTheme => _iconTheme;
IconThemeData? _iconTheme;
set iconTheme(IconThemeData? value) {
if (value == _iconTheme) {
return;
}
_iconTheme = value;
notifyListeners();
}
Color get activeIconColor => _activeIconColor!;
Color? _activeIconColor;
set activeIconColor(Color value) {
if (value == _activeIconColor) {
return;
}
_activeIconColor = value;
notifyListeners();
}
Color get inactiveIconColor => _inactiveIconColor!;
Color? _inactiveIconColor;
set inactiveIconColor(Color value) {
if (value == _inactiveIconColor) {
return;
}
_inactiveIconColor = value;
notifyListeners();
}
Color get activePressedColor => _activePressedColor!;
Color? _activePressedColor;
set activePressedColor(Color? value) {
assert(value != null);
if (value == _activePressedColor) {
return;
}
_activePressedColor = value;
notifyListeners();
}
Color get inactivePressedColor => _inactivePressedColor!;
Color? _inactivePressedColor;
set inactivePressedColor(Color? value) {
assert(value != null);
if (value == _inactivePressedColor) {
return;
}
_inactivePressedColor = value;
notifyListeners();
}
double get activeThumbRadius => _activeThumbRadius!;
double? _activeThumbRadius;
set activeThumbRadius(double value) {
if (value == _activeThumbRadius) {
return;
}
_activeThumbRadius = value;
notifyListeners();
}
double get inactiveThumbRadius => _inactiveThumbRadius!;
double? _inactiveThumbRadius;
set inactiveThumbRadius(double value) {
if (value == _inactiveThumbRadius) {
return;
}
_inactiveThumbRadius = value;
notifyListeners();
}
double get pressedThumbRadius => _pressedThumbRadius!;
double? _pressedThumbRadius;
set pressedThumbRadius(double value) {
if (value == _pressedThumbRadius) {
return;
}
_pressedThumbRadius = value;
notifyListeners();
}
double? get thumbOffset => _thumbOffset;
double? _thumbOffset;
set thumbOffset(double? value) {
if (value == _thumbOffset) {
return;
}
_thumbOffset = value;
notifyListeners();
}
Size get transitionalThumbSize => _transitionalThumbSize!;
Size? _transitionalThumbSize;
set transitionalThumbSize(Size value) {
if (value == _transitionalThumbSize) {
return;
}
_transitionalThumbSize = value;
notifyListeners();
}
double get trackHeight => _trackHeight!;
double? _trackHeight;
set trackHeight(double value) {
if (value == _trackHeight) {
return;
}
_trackHeight = value;
notifyListeners();
}
double get trackWidth => _trackWidth!;
double? _trackWidth;
set trackWidth(double value) {
if (value == _trackWidth) {
return;
}
_trackWidth = value;
notifyListeners();
}
ImageProvider? get activeThumbImage => _activeThumbImage;
ImageProvider? _activeThumbImage;
set activeThumbImage(ImageProvider? value) {
if (value == _activeThumbImage) {
return;
}
_activeThumbImage = value;
notifyListeners();
}
ImageErrorListener? get onActiveThumbImageError => _onActiveThumbImageError;
ImageErrorListener? _onActiveThumbImageError;
set onActiveThumbImageError(ImageErrorListener? value) {
if (value == _onActiveThumbImageError) {
return;
}
_onActiveThumbImageError = value;
notifyListeners();
}
ImageProvider? get inactiveThumbImage => _inactiveThumbImage;
ImageProvider? _inactiveThumbImage;
set inactiveThumbImage(ImageProvider? value) {
if (value == _inactiveThumbImage) {
return;
}
_inactiveThumbImage = value;
notifyListeners();
}
ImageErrorListener? get onInactiveThumbImageError => _onInactiveThumbImageError;
ImageErrorListener? _onInactiveThumbImageError;
set onInactiveThumbImageError(ImageErrorListener? value) {
if (value == _onInactiveThumbImageError) {
return;
}
_onInactiveThumbImageError = value;
notifyListeners();
}
Color get activeTrackColor => _activeTrackColor!;
Color? _activeTrackColor;
set activeTrackColor(Color value) {
if (value == _activeTrackColor) {
return;
}
_activeTrackColor = value;
notifyListeners();
}
Color? get activeTrackOutlineColor => _activeTrackOutlineColor;
Color? _activeTrackOutlineColor;
set activeTrackOutlineColor(Color? value) {
if (value == _activeTrackOutlineColor) {
return;
}
_activeTrackOutlineColor = value;
notifyListeners();
}
Color? get inactiveTrackOutlineColor => _inactiveTrackOutlineColor;
Color? _inactiveTrackOutlineColor;
set inactiveTrackOutlineColor(Color? value) {
if (value == _inactiveTrackOutlineColor) {
return;
}
_inactiveTrackOutlineColor = value;
notifyListeners();
}
double? get activeTrackOutlineWidth => _activeTrackOutlineWidth;
double? _activeTrackOutlineWidth;
set activeTrackOutlineWidth(double? value) {
if (value == _activeTrackOutlineWidth) {
return;
}
_activeTrackOutlineWidth = value;
notifyListeners();
}
double? get inactiveTrackOutlineWidth => _inactiveTrackOutlineWidth;
double? _inactiveTrackOutlineWidth;
set inactiveTrackOutlineWidth(double? value) {
if (value == _inactiveTrackOutlineWidth) {
return;
}
_inactiveTrackOutlineWidth = value;
notifyListeners();
}
Color get inactiveTrackColor => _inactiveTrackColor!;
Color? _inactiveTrackColor;
set inactiveTrackColor(Color value) {
if (value == _inactiveTrackColor) {
return;
}
_inactiveTrackColor = value;
notifyListeners();
}
ImageConfiguration get configuration => _configuration!;
ImageConfiguration? _configuration;
set configuration(ImageConfiguration value) {
if (value == _configuration) {
return;
}
_configuration = value;
notifyListeners();
}
TextDirection get textDirection => _textDirection!;
TextDirection? _textDirection;
set textDirection(TextDirection value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
notifyListeners();
}
Color get surfaceColor => _surfaceColor!;
Color? _surfaceColor;
set surfaceColor(Color value) {
if (value == _surfaceColor) {
return;
}
_surfaceColor = value;
notifyListeners();
}
bool get isInteractive => _isInteractive!;
bool? _isInteractive;
set isInteractive(bool value) {
if (value == _isInteractive) {
return;
}
_isInteractive = value;
notifyListeners();
}
double get trackInnerLength => _trackInnerLength!;
double? _trackInnerLength;
set trackInnerLength(double value) {
if (value == _trackInnerLength) {
return;
}
_trackInnerLength = value;
notifyListeners();
}
bool get isCupertino => _isCupertino!;
bool? _isCupertino;
set isCupertino(bool? value) {
assert(value != null);
if (value == _isCupertino) {
return;
}
_isCupertino = value;
notifyListeners();
}
List<BoxShadow>? get thumbShadow => _thumbShadow;
List<BoxShadow>? _thumbShadow;
set thumbShadow(List<BoxShadow>? value) {
if (value == _thumbShadow) {
return;
}
_thumbShadow = value;
notifyListeners();
}
final TextPainter _textPainter = TextPainter();
Color? _cachedThumbColor;
ImageProvider? _cachedThumbImage;
ImageErrorListener? _cachedThumbErrorListener;
BoxPainter? _cachedThumbPainter;
ShapeDecoration _createDefaultThumbDecoration(Color color, ImageProvider? image, ImageErrorListener? errorListener) {
return ShapeDecoration(
color: color,
image: image == null ? null : DecorationImage(image: image, onError: errorListener),
shape: const StadiumBorder(),
shadows: isCupertino ? null : thumbShadow,
);
}
bool _isPainting = false;
void _handleDecorationChanged() {
// If the image decoration is available synchronously, we'll get called here
// during paint. There's no reason to mark ourselves as needing paint if we
// are already in the middle of painting. (In fact, doing so would trigger
// an assert).
if (!_isPainting) {
notifyListeners();
}
}
bool _stopPressAnimation = false;
double? _pressedInactiveThumbRadius;
double? _pressedActiveThumbRadius;
late double? _pressedThumbExtension;
@override
void paint(Canvas canvas, Size size) {
final double currentValue = position.value;
final double visualPosition = switch (textDirection) {
TextDirection.rtl => 1.0 - currentValue,
TextDirection.ltr => currentValue,
};
if (reaction.status == AnimationStatus.reverse && !_stopPressAnimation) {
_stopPressAnimation = true;
} else {
_stopPressAnimation = false;
}
// To get the thumb radius when the press ends, the value can be any number
// between activeThumbRadius/inactiveThumbRadius and pressedThumbRadius.
if (!_stopPressAnimation) {
_pressedThumbExtension = isCupertino ? reaction.value * 7 : 0;
if (reaction.isCompleted) {
// This happens when the thumb is dragged instead of being tapped.
_pressedInactiveThumbRadius = lerpDouble(inactiveThumbRadius, pressedThumbRadius, reaction.value);
_pressedActiveThumbRadius = lerpDouble(activeThumbRadius, pressedThumbRadius, reaction.value);
}
if (currentValue == 0) {
_pressedInactiveThumbRadius = lerpDouble(inactiveThumbRadius, pressedThumbRadius, reaction.value);
_pressedActiveThumbRadius = activeThumbRadius;
}
if (currentValue == 1) {
_pressedActiveThumbRadius = lerpDouble(activeThumbRadius, pressedThumbRadius, reaction.value);
_pressedInactiveThumbRadius = inactiveThumbRadius;
}
}
final Size inactiveThumbSize = isCupertino ? Size(_pressedInactiveThumbRadius! * 2 + _pressedThumbExtension!, _pressedInactiveThumbRadius! * 2) : Size.fromRadius(_pressedInactiveThumbRadius ?? inactiveThumbRadius);
final Size activeThumbSize = isCupertino ? Size(_pressedActiveThumbRadius! * 2 + _pressedThumbExtension!, _pressedActiveThumbRadius! * 2) : Size.fromRadius(_pressedActiveThumbRadius ?? activeThumbRadius);
Animation<Size> thumbSizeAnimation(bool isForward) {
List<TweenSequenceItem<Size>> thumbSizeSequence;
if (isForward) {
thumbSizeSequence = <TweenSequenceItem<Size>>[
TweenSequenceItem<Size>(
tween: Tween<Size>(begin: inactiveThumbSize, end: transitionalThumbSize)
.chain(CurveTween(curve: const Cubic(0.31, 0.00, 0.56, 1.00))),
weight: 11,
),
TweenSequenceItem<Size>(
tween: Tween<Size>(begin: transitionalThumbSize, end: activeThumbSize)
.chain(CurveTween(curve: const Cubic(0.20, 0.00, 0.00, 1.00))),
weight: 72,
),
TweenSequenceItem<Size>(
tween: ConstantTween<Size>(activeThumbSize),
weight: 17,
)
];
} else {
thumbSizeSequence = <TweenSequenceItem<Size>>[
TweenSequenceItem<Size>(
tween: ConstantTween<Size>(inactiveThumbSize),
weight: 17,
),
TweenSequenceItem<Size>(
tween: Tween<Size>(begin: inactiveThumbSize, end: transitionalThumbSize)
.chain(CurveTween(curve: const Cubic(0.20, 0.00, 0.00, 1.00).flipped)),
weight: 72,
),
TweenSequenceItem<Size>(
tween: Tween<Size>(begin: transitionalThumbSize, end: activeThumbSize)
.chain(CurveTween(curve: const Cubic(0.31, 0.00, 0.56, 1.00).flipped)),
weight: 11,
),
];
}
return TweenSequence<Size>(thumbSizeSequence).animate(positionController);
}
Size? thumbSize;
if (isCupertino) {
if (reaction.isCompleted) {
thumbSize = Size(_pressedInactiveThumbRadius! * 2 + _pressedThumbExtension!, _pressedInactiveThumbRadius! * 2);
} else {
if (position.isDismissed || position.status == AnimationStatus.forward) {
thumbSize = Size.lerp(inactiveThumbSize, activeThumbSize, position.value);
} else {
thumbSize = Size.lerp(inactiveThumbSize, activeThumbSize, position.value);
}
}
} else {
if (reaction.isCompleted) {
thumbSize = Size.fromRadius(pressedThumbRadius);
} else {
if (position.isDismissed || position.status == AnimationStatus.forward) {
thumbSize = thumbSizeAnimation(true).value;
} else {
thumbSize = thumbSizeAnimation(false).value;
}
}
}
// The thumb contracts slightly during the animation in Material 2.
final double inset = thumbOffset == null ? 0 : 1.0 - (currentValue - thumbOffset!).abs() * 2.0;
thumbSize = Size(thumbSize!.width - inset, thumbSize.height - inset);
final double colorValue = CurvedAnimation(parent: positionController, curve: Curves.easeOut, reverseCurve: Curves.easeIn).value;
final Color trackColor = Color.lerp(inactiveTrackColor, activeTrackColor, colorValue)!;
final Color? trackOutlineColor = inactiveTrackOutlineColor == null || activeTrackOutlineColor == null ? null
: Color.lerp(inactiveTrackOutlineColor, activeTrackOutlineColor, colorValue);
final double? trackOutlineWidth = lerpDouble(inactiveTrackOutlineWidth, activeTrackOutlineWidth, colorValue);
Color lerpedThumbColor;
if (!reaction.isDismissed) {
lerpedThumbColor = Color.lerp(inactivePressedColor, activePressedColor, colorValue)!;
} else if (positionController.status == AnimationStatus.forward) {
lerpedThumbColor = Color.lerp(inactivePressedColor, activeColor, colorValue)!;
} else if (positionController.status == AnimationStatus.reverse) {
lerpedThumbColor = Color.lerp(inactiveColor, activePressedColor, colorValue)!;
} else {
lerpedThumbColor = Color.lerp(inactiveColor, activeColor, colorValue)!;
}
// Blend the thumb color against a `surfaceColor` background in case the
// thumbColor is not opaque. This way we do not see through the thumb to the
// track underneath.
final Color thumbColor = Color.alphaBlend(lerpedThumbColor, surfaceColor);
final Icon? thumbIcon = currentValue < 0.5 ? inactiveIcon : activeIcon;
final ImageProvider? thumbImage = currentValue < 0.5 ? inactiveThumbImage : activeThumbImage;
final ImageErrorListener? thumbErrorListener = currentValue < 0.5 ? onInactiveThumbImageError : onActiveThumbImageError;
final Paint paint = Paint()
..color = trackColor;
final Offset trackPaintOffset = _computeTrackPaintOffset(size, trackWidth, trackHeight);
final Offset thumbPaintOffset = _computeThumbPaintOffset(trackPaintOffset, thumbSize, visualPosition);
final Offset radialReactionOrigin = Offset(thumbPaintOffset.dx + thumbSize.height / 2, size.height / 2);
_paintTrackWith(canvas, paint, trackPaintOffset, trackOutlineColor, trackOutlineWidth);
paintRadialReaction(canvas: canvas, origin: radialReactionOrigin);
_paintThumbWith(
thumbPaintOffset,
canvas,
colorValue,
thumbColor,
thumbImage,
thumbErrorListener,
thumbIcon,
thumbSize,
inset,
);
}
/// Computes canvas offset for track's upper left corner
Offset _computeTrackPaintOffset(Size canvasSize, double trackWidth, double trackHeight) {
final double horizontalOffset = (canvasSize.width - trackWidth) / 2.0;
final double verticalOffset = (canvasSize.height - trackHeight) / 2.0;
return Offset(horizontalOffset, verticalOffset);
}
/// Computes canvas offset for thumb's upper left corner as if it were a
/// square
Offset _computeThumbPaintOffset(Offset trackPaintOffset, Size thumbSize, double visualPosition) {
// How much thumb radius extends beyond the track
final double trackRadius = trackHeight / 2;
final double additionalThumbRadius = thumbSize.height / 2 - trackRadius;
final double horizontalProgress = visualPosition * (trackInnerLength - _pressedThumbExtension!);
final double thumbHorizontalOffset = trackPaintOffset.dx + trackRadius + (_pressedThumbExtension! / 2) - thumbSize.width / 2 + horizontalProgress;
final double thumbVerticalOffset = trackPaintOffset.dy - additionalThumbRadius;
return Offset(thumbHorizontalOffset, thumbVerticalOffset);
}
void _paintTrackWith(Canvas canvas, Paint paint, Offset trackPaintOffset, Color? trackOutlineColor, double? trackOutlineWidth) {
final Rect trackRect = Rect.fromLTWH(
trackPaintOffset.dx,
trackPaintOffset.dy,
trackWidth,
trackHeight,
);
final double trackRadius = trackHeight / 2;
final RRect trackRRect = RRect.fromRectAndRadius(
trackRect,
Radius.circular(trackRadius),
);
canvas.drawRRect(trackRRect, paint);
// paint track outline
if (trackOutlineColor != null) {
final Rect outlineTrackRect = Rect.fromLTWH(
trackPaintOffset.dx + 1,
trackPaintOffset.dy + 1,
trackWidth - 2,
trackHeight - 2,
);
final RRect outlineTrackRRect = RRect.fromRectAndRadius(
outlineTrackRect,
Radius.circular(trackRadius),
);
final Paint outlinePaint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = trackOutlineWidth ?? 2.0
..color = trackOutlineColor;
canvas.drawRRect(outlineTrackRRect, outlinePaint);
}
if (isCupertino) {
if (isFocused) {
final RRect focusedOutline = trackRRect.inflate(1.75);
final Paint focusedPaint = Paint()
..style = PaintingStyle.stroke
..color = focusColor
..strokeWidth = _kCupertinoFocusTrackOutline;
canvas.drawRRect(focusedOutline, focusedPaint);
}
canvas.clipRRect(trackRRect);
}
}
void _paintThumbWith(
Offset thumbPaintOffset,
Canvas canvas,
double currentValue,
Color thumbColor,
ImageProvider? thumbImage,
ImageErrorListener? thumbErrorListener,
Icon? thumbIcon,
Size thumbSize,
double inset,
) {
try {
_isPainting = true;
if (_cachedThumbPainter == null || thumbColor != _cachedThumbColor || thumbImage != _cachedThumbImage || thumbErrorListener != _cachedThumbErrorListener) {
_cachedThumbColor = thumbColor;
_cachedThumbImage = thumbImage;
_cachedThumbErrorListener = thumbErrorListener;
_cachedThumbPainter?.dispose();
_cachedThumbPainter = _createDefaultThumbDecoration(thumbColor, thumbImage, thumbErrorListener).createBoxPainter(_handleDecorationChanged);
}
final BoxPainter thumbPainter = _cachedThumbPainter!;
if (isCupertino) {
_paintCupertinoThumbShadowAndBorder(canvas, thumbPaintOffset, thumbSize);
}
thumbPainter.paint(
canvas,
thumbPaintOffset,
configuration.copyWith(size: thumbSize),
);
if (thumbIcon != null && thumbIcon.icon != null) {
final Color iconColor = Color.lerp(inactiveIconColor, activeIconColor, currentValue)!;
final double iconSize = thumbIcon.size ?? _SwitchConfigM3.iconSize;
final IconData iconData = thumbIcon.icon!;
final double? iconWeight = thumbIcon.weight ?? iconTheme?.weight;
final double? iconFill = thumbIcon.fill ?? iconTheme?.fill;
final double? iconGrade = thumbIcon.grade ?? iconTheme?.grade;
final double? iconOpticalSize = thumbIcon.opticalSize ?? iconTheme?.opticalSize;
final List<Shadow>? iconShadows = thumbIcon.shadows ?? iconTheme?.shadows;
final TextSpan textSpan = TextSpan(
text: String.fromCharCode(iconData.codePoint),
style: TextStyle(
fontVariations: <FontVariation>[
if (iconFill != null) FontVariation('FILL', iconFill),
if (iconWeight != null) FontVariation('wght', iconWeight),
if (iconGrade != null) FontVariation('GRAD', iconGrade),
if (iconOpticalSize != null) FontVariation('opsz', iconOpticalSize),
],
color: iconColor,
fontSize: iconSize,
inherit: false,
fontFamily: iconData.fontFamily,
package: iconData.fontPackage,
shadows: iconShadows,
),
);
_textPainter
..textDirection = textDirection
..text = textSpan;
_textPainter.layout();
final double additionalHorizontalOffset = (thumbSize.width - iconSize) / 2;
final double additionalVerticalOffset = (thumbSize.height - iconSize) / 2;
final Offset offset = thumbPaintOffset + Offset(additionalHorizontalOffset, additionalVerticalOffset);
_textPainter.paint(canvas, offset);
}
} finally {
_isPainting = false;
}
}
void _paintCupertinoThumbShadowAndBorder(Canvas canvas, Offset thumbPaintOffset, Size thumbSize,) {
final RRect thumbBounds = RRect.fromLTRBR(
thumbPaintOffset.dx,
thumbPaintOffset.dy,
thumbPaintOffset.dx + thumbSize.width,
thumbPaintOffset.dy + thumbSize.height,
Radius.circular(thumbSize.height / 2.0),
);
if (thumbShadow != null) {
for (final BoxShadow shadow in thumbShadow!) {
canvas.drawRRect(thumbBounds.shift(shadow.offset), shadow.toPaint());
}
}
canvas.drawRRect(
thumbBounds.inflate(0.5),
Paint()..color = const Color(0x0A000000),
);
}
@override
void dispose() {
_textPainter.dispose();
_cachedThumbPainter?.dispose();
_cachedThumbPainter = null;
_cachedThumbColor = null;
_cachedThumbImage = null;
_cachedThumbErrorListener = null;
super.dispose();
}
}
class _SwitchThemeAdaptation extends Adaptation<SwitchThemeData> {
const _SwitchThemeAdaptation();
@override
SwitchThemeData adapt(ThemeData theme, SwitchThemeData defaultValue) {
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return defaultValue;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return const SwitchThemeData();
}
}
}
mixin _SwitchConfig {
double get trackHeight;
double get trackWidth;
double get switchWidth;
double get switchHeight;
double get switchHeightCollapsed;
double get activeThumbRadius;
double get inactiveThumbRadius;
double get pressedThumbRadius;
double get thumbRadiusWithIcon;
List<BoxShadow>? get thumbShadow;
MaterialStateProperty<Color> get iconColor;
double? get thumbOffset;
Size get transitionalThumbSize;
int get toggleDuration;
}
// Hand coded defaults for iOS/macOS Switch
class _SwitchDefaultsCupertino extends SwitchThemeData {
const _SwitchDefaultsCupertino(this.context);
final BuildContext context;
@override
MaterialStateProperty<MouseCursor?> get mouseCursor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic;
});
}
@override
MaterialStateProperty<Color> get thumbColor => const MaterialStatePropertyAll<Color>(Colors.white);
@override
MaterialStateProperty<Color> get trackColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return CupertinoDynamicColor.resolve(CupertinoColors.systemGreen, context);
}
return CupertinoDynamicColor.resolve(CupertinoColors.secondarySystemFill, context);
});
}
@override
MaterialStateProperty<Color?> get trackOutlineColor => const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return HSLColor
.fromColor(CupertinoDynamicColor.resolve(CupertinoColors.systemGreen, context).withOpacity(0.80))
.withLightness(0.69).withSaturation(0.835)
.toColor();
}
return Colors.transparent;
});
}
@override
double get splashRadius => 0.0;
}
const double _kCupertinoFocusTrackOutline = 3.5;
class _SwitchConfigCupertino with _SwitchConfig {
_SwitchConfigCupertino(this.context)
: _colors = Theme.of(context).colorScheme;
BuildContext context;
final ColorScheme _colors;
@override
MaterialStateProperty<Color> get iconColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.onPrimaryContainer;
});
}
@override
double get activeThumbRadius => 14.0;
@override
double get inactiveThumbRadius => 14.0;
@override
double get pressedThumbRadius => 14.0;
@override
double get switchHeight => _kSwitchMinSize + 8.0;
@override
double get switchHeightCollapsed => _kSwitchMinSize;
@override
double get switchWidth => 60.0;
@override
double get thumbRadiusWithIcon => 14.0;
@override
List<BoxShadow>? get thumbShadow => const <BoxShadow> [
BoxShadow(
color: Color(0x26000000),
offset: Offset(0, 3),
blurRadius: 8.0,
),
BoxShadow(
color: Color(0x0F000000),
offset: Offset(0, 3),
blurRadius: 1.0,
),
];
@override
double get trackHeight => 31.0;
@override
double get trackWidth => 51.0;
// The thumb size at the middle of the track. Hand coded default based on the animation specs.
@override
Size get transitionalThumbSize => const Size(28.0, 28.0);
// Hand coded default by comparing with [CupertinoSwitch].
@override
int get toggleDuration => 140;
// Hand coded default based on the animation specs.
@override
double? get thumbOffset => null;
}
// Hand coded defaults based on Material Design 2.
class _SwitchConfigM2 with _SwitchConfig {
_SwitchConfigM2();
@override
double get activeThumbRadius => 10.0;
@override
MaterialStateProperty<Color> get iconColor => MaterialStateProperty.all<Color>(Colors.transparent);
@override
double get inactiveThumbRadius => 10.0;
@override
double get pressedThumbRadius => 10.0;
@override
double get switchHeight => _kSwitchMinSize + 8.0;
@override
double get switchHeightCollapsed => _kSwitchMinSize;
@override
double get switchWidth => trackWidth - 2 * (trackHeight / 2.0) + _kSwitchMinSize;
@override
double get thumbRadiusWithIcon => 10.0;
@override
List<BoxShadow>? get thumbShadow => kElevationToShadow[1];
@override
double get trackHeight => 14.0;
@override
double get trackWidth => 33.0;
@override
double get thumbOffset => 0.5;
@override
Size get transitionalThumbSize => const Size(20, 20);
@override
int get toggleDuration => 200;
}
class _SwitchDefaultsM2 extends SwitchThemeData {
_SwitchDefaultsM2(BuildContext context)
: _theme = Theme.of(context),
_colors = Theme.of(context).colorScheme;
final ThemeData _theme;
final ColorScheme _colors;
@override
MaterialStateProperty<Color> get thumbColor {
final bool isDark = _theme.brightness == Brightness.dark;
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return isDark ? Colors.grey.shade800 : Colors.grey.shade400;
}
if (states.contains(MaterialState.selected)) {
return _colors.secondary;
}
return isDark ? Colors.grey.shade400 : Colors.grey.shade50;
});
}
@override
MaterialStateProperty<Color> get trackColor {
final bool isDark = _theme.brightness == Brightness.dark;
const Color black32 = Color(0x52000000); // Black with 32% opacity
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return isDark ? Colors.white10 : Colors.black12;
}
if (states.contains(MaterialState.selected)) {
final Color activeColor = _colors.secondary;
return activeColor.withAlpha(0x80);
}
return isDark ? Colors.white30 : black32;
});
}
@override
MaterialStateProperty<Color?>? get trackOutlineColor => const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialTapTargetSize get materialTapTargetSize => _theme.materialTapTargetSize;
@override
MaterialStateProperty<MouseCursor> get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) => MaterialStateMouseCursor.clickable.resolve(states));
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return thumbColor.resolve(states).withAlpha(kRadialReactionAlpha);
}
if (states.contains(MaterialState.hovered)) {
return _theme.hoverColor;
}
if (states.contains(MaterialState.focused)) {
return _theme.focusColor;
}
return null;
});
}
@override
double get splashRadius => kRadialReactionRadius;
}
// BEGIN GENERATED TOKEN PROPERTIES - Switch
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _SwitchDefaultsM3 extends SwitchThemeData {
_SwitchDefaultsM3(this.context);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
MaterialStateProperty<Color> get thumbColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.surface.withOpacity(1.0);
}
return _colors.onSurface.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.primaryContainer;
}
if (states.contains(MaterialState.hovered)) {
return _colors.primaryContainer;
}
if (states.contains(MaterialState.focused)) {
return _colors.primaryContainer;
}
return _colors.onPrimary;
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurfaceVariant;
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurfaceVariant;
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurfaceVariant;
}
return _colors.outline;
});
}
@override
MaterialStateProperty<Color> get trackColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.onSurface.withOpacity(0.12);
}
return _colors.surfaceContainerHighest.withOpacity(0.12);
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.primary;
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary;
}
if (states.contains(MaterialState.focused)) {
return _colors.primary;
}
return _colors.primary;
}
if (states.contains(MaterialState.pressed)) {
return _colors.surfaceContainerHighest;
}
if (states.contains(MaterialState.hovered)) {
return _colors.surfaceContainerHighest;
}
if (states.contains(MaterialState.focused)) {
return _colors.surfaceContainerHighest;
}
return _colors.surfaceContainerHighest;
});
}
@override
MaterialStateProperty<Color?> get trackOutlineColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return Colors.transparent;
}
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.12);
}
return _colors.outline;
});
}
@override
MaterialStateProperty<Color?> get overlayColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return null;
}
if (states.contains(MaterialState.pressed)) {
return _colors.onSurface.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.onSurface.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.onSurface.withOpacity(0.1);
}
return null;
});
}
@override
MaterialStateProperty<MouseCursor> get mouseCursor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states)
=> MaterialStateMouseCursor.clickable.resolve(states));
}
@override
MaterialStatePropertyAll<double> get trackOutlineWidth => const MaterialStatePropertyAll<double>(2.0);
@override
double get splashRadius => 40.0 / 2;
}
class _SwitchConfigM3 with _SwitchConfig {
_SwitchConfigM3(this.context)
: _colors = Theme.of(context).colorScheme;
BuildContext context;
final ColorScheme _colors;
static const double iconSize = 16.0;
@override
double get activeThumbRadius => 24.0 / 2;
@override
MaterialStateProperty<Color> get iconColor {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
if (states.contains(MaterialState.selected)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.surfaceContainerHighest.withOpacity(0.38);
}
if (states.contains(MaterialState.selected)) {
if (states.contains(MaterialState.pressed)) {
return _colors.onPrimaryContainer;
}
if (states.contains(MaterialState.hovered)) {
return _colors.onPrimaryContainer;
}
if (states.contains(MaterialState.focused)) {
return _colors.onPrimaryContainer;
}
return _colors.onPrimaryContainer;
}
if (states.contains(MaterialState.pressed)) {
return _colors.surfaceContainerHighest;
}
if (states.contains(MaterialState.hovered)) {
return _colors.surfaceContainerHighest;
}
if (states.contains(MaterialState.focused)) {
return _colors.surfaceContainerHighest;
}
return _colors.surfaceContainerHighest;
});
}
@override
double get inactiveThumbRadius => 16.0 / 2;
@override
double get pressedThumbRadius => 28.0 / 2;
@override
double get switchHeight => _kSwitchMinSize + 8.0;
@override
double get switchHeightCollapsed => _kSwitchMinSize;
@override
double get switchWidth => trackWidth - 2 * (trackHeight / 2.0) + _kSwitchMinSize;
@override
double get thumbRadiusWithIcon => 24.0 / 2;
@override
List<BoxShadow>? get thumbShadow => kElevationToShadow[0];
@override
double get trackHeight => 32.0;
@override
double get trackWidth => 52.0;
// The thumb size at the middle of the track. Hand coded default based on the animation specs.
@override
Size get transitionalThumbSize => const Size(34, 22);
// Hand coded default based on the animation specs.
@override
int get toggleDuration => 300;
// Hand coded default based on the animation specs.
@override
double? get thumbOffset => null;
}
// END GENERATED TOKEN PROPERTIES - Switch
| flutter/packages/flutter/lib/src/material/switch.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/switch.dart",
"repo_id": "flutter",
"token_count": 28117
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'material_localizations.dart';
import 'theme_data.dart';
import 'typography.dart';
export 'theme_data.dart' show Brightness, ThemeData;
/// The duration over which theme changes animate by default.
const Duration kThemeAnimationDuration = Duration(milliseconds: 200);
/// Applies a theme to descendant widgets.
///
/// A theme describes the colors and typographic choices of an application.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=oTvQDJOBXmM}
///
/// Descendant widgets obtain the current theme's [ThemeData] object using
/// [Theme.of]. When a widget uses [Theme.of], it is automatically rebuilt if
/// the theme later changes, so that the changes can be applied.
///
/// The [Theme] widget implies an [IconTheme] widget, set to the value of the
/// [ThemeData.iconTheme] of the [data] for the [Theme].
///
/// See also:
///
/// * [ThemeData], which describes the actual configuration of a theme.
/// * [AnimatedTheme], which animates the [ThemeData] when it changes rather
/// than changing the theme all at once.
/// * [MaterialApp], which includes an [AnimatedTheme] widget configured via
/// the [MaterialApp.theme] argument.
class Theme extends StatelessWidget {
/// Applies the given theme [data] to [child].
const Theme({
super.key,
required this.data,
required this.child,
});
/// Specifies the color and typography values for descendant widgets.
final ThemeData data;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
static final ThemeData _kFallbackTheme = ThemeData.fallback();
/// The data from the closest [Theme] instance that encloses the given
/// context.
///
/// If the given context is enclosed in a [Localizations] widget providing
/// [MaterialLocalizations], the returned data is localized according to the
/// nearest available [MaterialLocalizations].
///
/// Defaults to [ThemeData.fallback] if there is no [Theme] in the given
/// build context.
///
/// Typical usage is as follows:
///
/// ```dart
/// @override
/// Widget build(BuildContext context) {
/// return Text(
/// 'Example',
/// style: Theme.of(context).textTheme.titleLarge,
/// );
/// }
/// ```
///
/// When the [Theme] is actually created in the same `build` function
/// (possibly indirectly, e.g. as part of a [MaterialApp]), the `context`
/// argument to the `build` function can't be used to find the [Theme] (since
/// it's "above" the widget being returned). In such cases, the following
/// technique with a [Builder] can be used to provide a new scope with a
/// [BuildContext] that is "under" the [Theme]:
///
/// ```dart
/// @override
/// Widget build(BuildContext context) {
/// return MaterialApp(
/// theme: ThemeData.light(),
/// home: Builder(
/// // Create an inner BuildContext so that we can refer to
/// // the Theme with Theme.of().
/// builder: (BuildContext context) {
/// return Center(
/// child: Text(
/// 'Example',
/// style: Theme.of(context).textTheme.titleLarge,
/// ),
/// );
/// },
/// ),
/// );
/// }
/// ```
static ThemeData of(BuildContext context) {
final _InheritedTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<_InheritedTheme>();
final MaterialLocalizations? localizations = Localizations.of<MaterialLocalizations>(context, MaterialLocalizations);
final ScriptCategory category = localizations?.scriptCategory ?? ScriptCategory.englishLike;
final ThemeData theme = inheritedTheme?.theme.data ?? _kFallbackTheme;
return ThemeData.localize(theme, theme.typography.geometryThemeFor(category));
}
// The inherited themes in widgets library can not infer their values from
// Theme in material library. Wraps the child with these inherited themes to
// overrides their values directly.
Widget _wrapsWidgetThemes(BuildContext context, Widget child) {
final DefaultSelectionStyle selectionStyle = DefaultSelectionStyle.of(context);
return IconTheme(
data: data.iconTheme,
child: DefaultSelectionStyle(
selectionColor: data.textSelectionTheme.selectionColor ?? selectionStyle.selectionColor,
cursorColor: data.textSelectionTheme.cursorColor ?? selectionStyle.cursorColor,
child: child,
),
);
}
@override
Widget build(BuildContext context) {
return _InheritedTheme(
theme: this,
child: CupertinoTheme(
// We're using a MaterialBasedCupertinoThemeData here instead of a
// CupertinoThemeData because it defers some properties to the Material
// ThemeData.
data: MaterialBasedCupertinoThemeData(
materialTheme: data,
),
child: _wrapsWidgetThemes(context, child),
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ThemeData>('data', data, showName: false));
}
}
class _InheritedTheme extends InheritedTheme {
const _InheritedTheme({
required this.theme,
required super.child,
});
final Theme theme;
@override
Widget wrap(BuildContext context, Widget child) {
return Theme(data: theme.data, child: child);
}
@override
bool updateShouldNotify(_InheritedTheme old) => theme.data != old.theme.data;
}
/// An interpolation between two [ThemeData]s.
///
/// This class specializes the interpolation of [Tween<ThemeData>] to call the
/// [ThemeData.lerp] method.
///
/// See [Tween] for a discussion on how to use interpolation objects.
class ThemeDataTween extends Tween<ThemeData> {
/// Creates a [ThemeData] tween.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
ThemeDataTween({ super.begin, super.end });
@override
ThemeData lerp(double t) => ThemeData.lerp(begin!, end!, t);
}
/// Animated version of [Theme] which automatically transitions the colors,
/// etc, over a given duration whenever the given theme changes.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.elasticInOut].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_theme.mp4}
///
/// See also:
///
/// * [Theme], which [AnimatedTheme] uses to actually apply the interpolated
/// theme.
/// * [ThemeData], which describes the actual configuration of a theme.
/// * [MaterialApp], which includes an [AnimatedTheme] widget configured via
/// the [MaterialApp.theme] argument.
class AnimatedTheme extends ImplicitlyAnimatedWidget {
/// Creates an animated theme.
///
/// By default, the theme transition uses a linear curve.
const AnimatedTheme({
super.key,
required this.data,
super.curve,
super.duration = kThemeAnimationDuration,
super.onEnd,
required this.child,
});
/// Specifies the color and typography values for descendant widgets.
final ThemeData data;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
AnimatedWidgetBaseState<AnimatedTheme> createState() => _AnimatedThemeState();
}
class _AnimatedThemeState extends AnimatedWidgetBaseState<AnimatedTheme> {
ThemeDataTween? _data;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_data = visitor(_data, widget.data, (dynamic value) => ThemeDataTween(begin: value as ThemeData))! as ThemeDataTween;
}
@override
Widget build(BuildContext context) {
return Theme(
data: _data!.evaluate(animation),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<ThemeDataTween>('data', _data, showName: false, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/material/theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/theme.dart",
"repo_id": "flutter",
"token_count": 2621
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show TextDirection;
export 'dart:ui' show
BlendMode,
BlurStyle,
Canvas,
Clip,
Color,
ColorFilter,
FilterQuality,
FontFeature,
FontStyle,
FontVariation,
FontWeight,
GlyphInfo,
ImageShader,
Locale,
MaskFilter,
Offset,
Paint,
PaintingStyle,
Path,
PathFillType,
PathOperation,
RRect,
RSTransform,
Radius,
Rect,
Shader,
Shadow,
Size,
StrokeCap,
StrokeJoin,
TextAffinity,
TextAlign,
TextBaseline,
TextBox,
TextDecoration,
TextDecorationStyle,
TextDirection,
TextHeightBehavior,
TextLeadingDistribution,
TextPosition,
TileMode,
VertexMode,
hashList,
hashValues;
export 'package:flutter/foundation.dart' show VoidCallback;
// Intentionally not exported:
// - Image, instantiateImageCodec, decodeImageFromList:
// We use ui.* to make it very explicit that these are low-level image APIs.
// Generally, higher layers provide more reasonable APIs around images.
// - lerpDouble:
// Hopefully this will eventually become Double.lerp.
// - Paragraph, ParagraphBuilder, ParagraphStyle, TextBox:
// These are low-level text primitives. Use this package's TextPainter API.
// - Picture, PictureRecorder, Scene, SceneBuilder:
// These are low-level primitives. Generally, the rendering layer makes these moot.
// - Gradient:
// Use this package's higher-level Gradient API instead.
// - window, WindowPadding
// These are generally wrapped by other APIs so we always refer to them directly
// as ui.* to avoid making them seem like high-level APIs.
/// The description of the difference between two objects, in the context of how
/// it will affect the rendering.
///
/// Used by [TextSpan.compareTo] and [TextStyle.compareTo].
///
/// The values in this enum are ordered such that they are in increasing order
/// of cost. A value with index N implies all the values with index less than N.
/// For example, [layout] (index 3) implies [paint] (2).
enum RenderComparison {
/// The two objects are identical (meaning deeply equal, not necessarily
/// [dart:core.identical]).
identical,
/// The two objects are identical for the purpose of layout, but may be different
/// in other ways.
///
/// For example, maybe some event handlers changed.
metadata,
/// The two objects are different but only in ways that affect paint, not layout.
///
/// For example, only the color is changed.
///
/// [RenderObject.markNeedsPaint] would be necessary to handle this kind of
/// change in a render object.
paint,
/// The two objects are different in ways that affect layout (and therefore paint).
///
/// For example, the size is changed.
///
/// This is the most drastic level of change possible.
///
/// [RenderObject.markNeedsLayout] would be necessary to handle this kind of
/// change in a render object.
layout,
}
/// The two cardinal directions in two dimensions.
///
/// The axis is always relative to the current coordinate space. This means, for
/// example, that a [horizontal] axis might actually be diagonally from top
/// right to bottom left, due to some local [Transform] applied to the scene.
///
/// See also:
///
/// * [AxisDirection], which is a directional version of this enum (with values
/// like left and right, rather than just horizontal).
/// * [TextDirection], which disambiguates between left-to-right horizontal
/// content and right-to-left horizontal content.
enum Axis {
/// Left and right.
///
/// See also:
///
/// * [TextDirection], which disambiguates between left-to-right horizontal
/// content and right-to-left horizontal content.
horizontal,
/// Up and down.
vertical,
}
/// Returns the opposite of the given [Axis].
///
/// Specifically, returns [Axis.horizontal] for [Axis.vertical], and
/// vice versa.
///
/// See also:
///
/// * [flipAxisDirection], which does the same thing for [AxisDirection] values.
Axis flipAxis(Axis direction) {
return switch (direction) {
Axis.horizontal => Axis.vertical,
Axis.vertical => Axis.horizontal,
};
}
/// A direction in which boxes flow vertically.
///
/// This is used by the flex algorithm (e.g. [Column]) to decide in which
/// direction to draw boxes.
///
/// This is also used to disambiguate `start` and `end` values (e.g.
/// [MainAxisAlignment.start] or [CrossAxisAlignment.end]).
///
/// See also:
///
/// * [TextDirection], which controls the same thing but horizontally.
enum VerticalDirection {
/// Boxes should start at the bottom and be stacked vertically towards the top.
///
/// The "start" is at the bottom, the "end" is at the top.
up,
/// Boxes should start at the top and be stacked vertically towards the bottom.
///
/// The "start" is at the top, the "end" is at the bottom.
down,
}
/// A direction along either the horizontal or vertical [Axis] in which the
/// origin, or zero position, is determined.
///
/// This value relates to the direction in which the scroll offset increases
/// from the origin. This value does not represent the direction of user input
/// that may be modifying the scroll offset, such as from a drag. For the
/// active scrolling direction, see [ScrollDirection].
///
/// {@tool dartpad}
/// This sample shows a [CustomScrollView], with [Radio] buttons in the
/// [AppBar.bottom] that change the [AxisDirection] to illustrate different
/// configurations.
///
/// ** See code in examples/api/lib/painting/axis_direction/axis_direction.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ScrollDirection], the direction of active scrolling, relative to the positive
/// scroll offset axis given by an [AxisDirection] and a [GrowthDirection].
/// * [GrowthDirection], the direction in which slivers and their content are
/// ordered, relative to the scroll offset axis as specified by
/// [AxisDirection].
/// * [CustomScrollView.anchor], the relative position of the zero scroll
/// offset in a viewport and inflection point for [AxisDirection]s of the
/// same cardinal [Axis].
/// * [axisDirectionIsReversed], which returns whether traveling along the
/// given axis direction visits coordinates along that axis in numerically
/// decreasing order.
enum AxisDirection {
/// A direction in the [Axis.vertical] where zero is at the bottom and
/// positive values are above it: `⇈`
///
/// Alphabetical content with a [GrowthDirection.forward] would have the A at
/// the bottom and the Z at the top.
///
/// For example, the behavior of a [ListView] with [ListView.reverse] set to
/// true would have this axis direction.
///
/// See also:
///
/// * [axisDirectionIsReversed], which returns whether traveling along the
/// given axis direction visits coordinates along that axis in numerically
/// decreasing order.
up,
/// A direction in the [Axis.horizontal] where zero is on the left and
/// positive values are to the right of it: `⇉`
///
/// Alphabetical content with a [GrowthDirection.forward] would have the A on
/// the left and the Z on the right. This is the ordinary reading order for a
/// horizontal set of tabs in an English application, for example.
///
/// For example, the behavior of a [ListView] with [ListView.scrollDirection]
/// set to [Axis.horizontal] would have this axis direction.
///
/// See also:
///
/// * [axisDirectionIsReversed], which returns whether traveling along the
/// given axis direction visits coordinates along that axis in numerically
/// decreasing order.
right,
/// A direction in the [Axis.vertical] where zero is at the top and positive
/// values are below it: `⇊`
///
/// Alphabetical content with a [GrowthDirection.forward] would have the A at
/// the top and the Z at the bottom. This is the ordinary reading order for a
/// vertical list.
///
/// For example, the default behavior of a [ListView] would have this axis
/// direction.
///
/// See also:
///
/// * [axisDirectionIsReversed], which returns whether traveling along the
/// given axis direction visits coordinates along that axis in numerically
/// decreasing order.
down,
/// A direction in the [Axis.horizontal] where zero is to the right and
/// positive values are to the left of it: `⇇`
///
/// Alphabetical content with a [GrowthDirection.forward] would have the A at
/// the right and the Z at the left. This is the ordinary reading order for a
/// horizontal set of tabs in a Hebrew application, for example.
///
/// For example, the behavior of a [ListView] with [ListView.scrollDirection]
/// set to [Axis.horizontal] and [ListView.reverse] set to true would have
/// this axis direction.
///
/// See also:
///
/// * [axisDirectionIsReversed], which returns whether traveling along the
/// given axis direction visits coordinates along that axis in numerically
/// decreasing order.
left,
}
/// Returns the [Axis] that contains the given [AxisDirection].
///
/// Specifically, returns [Axis.vertical] for [AxisDirection.up] and
/// [AxisDirection.down] and returns [Axis.horizontal] for [AxisDirection.left]
/// and [AxisDirection.right].
Axis axisDirectionToAxis(AxisDirection axisDirection) {
return switch (axisDirection) {
AxisDirection.up || AxisDirection.down => Axis.vertical,
AxisDirection.left || AxisDirection.right => Axis.horizontal,
};
}
/// Returns the [AxisDirection] in which reading occurs in the given [TextDirection].
///
/// Specifically, returns [AxisDirection.left] for [TextDirection.rtl] and
/// [AxisDirection.right] for [TextDirection.ltr].
AxisDirection textDirectionToAxisDirection(TextDirection textDirection) {
return switch (textDirection) {
TextDirection.rtl => AxisDirection.left,
TextDirection.ltr => AxisDirection.right,
};
}
/// Returns the opposite of the given [AxisDirection].
///
/// Specifically, returns [AxisDirection.up] for [AxisDirection.down] (and
/// vice versa), as well as [AxisDirection.left] for [AxisDirection.right] (and
/// vice versa).
///
/// See also:
///
/// * [flipAxis], which does the same thing for [Axis] values.
AxisDirection flipAxisDirection(AxisDirection axisDirection) {
return switch (axisDirection) {
AxisDirection.up => AxisDirection.down,
AxisDirection.right => AxisDirection.left,
AxisDirection.down => AxisDirection.up,
AxisDirection.left => AxisDirection.right,
};
}
/// Returns whether traveling along the given axis direction visits coordinates
/// along that axis in numerically decreasing order.
///
/// Specifically, returns true for [AxisDirection.up] and [AxisDirection.left]
/// and false for [AxisDirection.down] and [AxisDirection.right].
bool axisDirectionIsReversed(AxisDirection axisDirection) {
return switch (axisDirection) {
AxisDirection.up || AxisDirection.left => true,
AxisDirection.down || AxisDirection.right => false,
};
}
| flutter/packages/flutter/lib/src/painting/basic_types.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/basic_types.dart",
"repo_id": "flutter",
"token_count": 3311
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show ViewPadding, lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
/// Base class for [EdgeInsets] that allows for text-direction aware
/// resolution.
///
/// A property or argument of this type accepts classes created either with [
/// EdgeInsets.fromLTRB] and its variants, or [
/// EdgeInsetsDirectional.fromSTEB] and its variants.
///
/// To convert an [EdgeInsetsGeometry] object of indeterminate type into a
/// [EdgeInsets] object, call the [resolve] method.
///
/// See also:
///
/// * [Padding], a widget that describes margins using [EdgeInsetsGeometry].
@immutable
abstract class EdgeInsetsGeometry {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const EdgeInsetsGeometry();
double get _bottom;
double get _end;
double get _left;
double get _right;
double get _start;
double get _top;
/// An [EdgeInsetsGeometry] with infinite offsets in each direction.
///
/// Can be used as an infinite upper bound for [clamp].
static const EdgeInsetsGeometry infinity = _MixedEdgeInsets.fromLRSETB(
double.infinity,
double.infinity,
double.infinity,
double.infinity,
double.infinity,
double.infinity,
);
/// Whether every dimension is non-negative.
bool get isNonNegative {
return _left >= 0.0
&& _right >= 0.0
&& _start >= 0.0
&& _end >= 0.0
&& _top >= 0.0
&& _bottom >= 0.0;
}
/// The total offset in the horizontal direction.
double get horizontal => _left + _right + _start + _end;
/// The total offset in the vertical direction.
double get vertical => _top + _bottom;
/// The total offset in the given direction.
double along(Axis axis) {
return switch (axis) {
Axis.horizontal => horizontal,
Axis.vertical => vertical,
};
}
/// The size that this [EdgeInsets] would occupy with an empty interior.
Size get collapsedSize => Size(horizontal, vertical);
/// An [EdgeInsetsGeometry] with top and bottom, left and right, and start and end flipped.
EdgeInsetsGeometry get flipped => _MixedEdgeInsets.fromLRSETB(_right, _left, _end, _start, _bottom, _top);
/// Returns a new size that is bigger than the given size by the amount of
/// inset in the horizontal and vertical directions.
///
/// See also:
///
/// * [EdgeInsets.inflateRect], to inflate a [Rect] rather than a [Size] (for
/// [EdgeInsetsDirectional], requires first calling [resolve] to establish
/// how the start and end map to the left or right).
/// * [deflateSize], to deflate a [Size] rather than inflating it.
Size inflateSize(Size size) {
return Size(size.width + horizontal, size.height + vertical);
}
/// Returns a new size that is smaller than the given size by the amount of
/// inset in the horizontal and vertical directions.
///
/// If the argument is smaller than [collapsedSize], then the resulting size
/// will have negative dimensions.
///
/// See also:
///
/// * [EdgeInsets.deflateRect], to deflate a [Rect] rather than a [Size]. (for
/// [EdgeInsetsDirectional], requires first calling [resolve] to establish
/// how the start and end map to the left or right).
/// * [inflateSize], to inflate a [Size] rather than deflating it.
Size deflateSize(Size size) {
return Size(size.width - horizontal, size.height - vertical);
}
/// Returns the difference between two [EdgeInsetsGeometry] objects.
///
/// If you know you are applying this to two [EdgeInsets] or two
/// [EdgeInsetsDirectional] objects, consider using the binary infix `-`
/// operator instead, which always returns an object of the same type as the
/// operands, and is typed accordingly.
///
/// If [subtract] is applied to two objects of the same type ([EdgeInsets] or
/// [EdgeInsetsDirectional]), an object of that type will be returned (though
/// this is not reflected in the type system). Otherwise, an object
/// representing a combination of both is returned. That object can be turned
/// into a concrete [EdgeInsets] using [resolve].
///
/// This method returns the same result as [add] applied to the result of
/// negating the argument (using the prefix unary `-` operator or multiplying
/// the argument by -1.0 using the `*` operator).
EdgeInsetsGeometry subtract(EdgeInsetsGeometry other) {
return _MixedEdgeInsets.fromLRSETB(
_left - other._left,
_right - other._right,
_start - other._start,
_end - other._end,
_top - other._top,
_bottom - other._bottom,
);
}
/// Returns the sum of two [EdgeInsetsGeometry] objects.
///
/// If you know you are adding two [EdgeInsets] or two [EdgeInsetsDirectional]
/// objects, consider using the `+` operator instead, which always returns an
/// object of the same type as the operands, and is typed accordingly.
///
/// If [add] is applied to two objects of the same type ([EdgeInsets] or
/// [EdgeInsetsDirectional]), an object of that type will be returned (though
/// this is not reflected in the type system). Otherwise, an object
/// representing a combination of both is returned. That object can be turned
/// into a concrete [EdgeInsets] using [resolve].
EdgeInsetsGeometry add(EdgeInsetsGeometry other) {
return _MixedEdgeInsets.fromLRSETB(
_left + other._left,
_right + other._right,
_start + other._start,
_end + other._end,
_top + other._top,
_bottom + other._bottom,
);
}
/// Returns a new [EdgeInsetsGeometry] object with all values greater than
/// or equal to `min`, and less than or equal to `max`.
EdgeInsetsGeometry clamp(EdgeInsetsGeometry min, EdgeInsetsGeometry max) {
return _MixedEdgeInsets.fromLRSETB(
clampDouble(_left, min._left, max._left),
clampDouble(_right, min._right, max._right),
clampDouble(_start, min._start, max._start),
clampDouble(_end, min._end, max._end),
clampDouble(_top, min._top, max._top),
clampDouble(_bottom, min._bottom, max._bottom),
);
}
/// Returns the [EdgeInsetsGeometry] object with each dimension negated.
///
/// This is the same as multiplying the object by -1.0.
///
/// This operator returns an object of the same type as the operand.
EdgeInsetsGeometry operator -();
/// Scales the [EdgeInsetsGeometry] object in each dimension by the given factor.
///
/// This operator returns an object of the same type as the operand.
EdgeInsetsGeometry operator *(double other);
/// Divides the [EdgeInsetsGeometry] object in each dimension by the given factor.
///
/// This operator returns an object of the same type as the operand.
EdgeInsetsGeometry operator /(double other);
/// Integer divides the [EdgeInsetsGeometry] object in each dimension by the given factor.
///
/// This operator returns an object of the same type as the operand.
///
/// This operator may have unexpected results when applied to a mixture of
/// [EdgeInsets] and [EdgeInsetsDirectional] objects.
EdgeInsetsGeometry operator ~/(double other);
/// Computes the remainder in each dimension by the given factor.
///
/// This operator returns an object of the same type as the operand.
///
/// This operator may have unexpected results when applied to a mixture of
/// [EdgeInsets] and [EdgeInsetsDirectional] objects.
EdgeInsetsGeometry operator %(double other);
/// Linearly interpolate between two [EdgeInsetsGeometry] objects.
///
/// If either is null, this function interpolates from [EdgeInsets.zero], and
/// the result is an object of the same type as the non-null argument.
///
/// If [lerp] is applied to two objects of the same type ([EdgeInsets] or
/// [EdgeInsetsDirectional]), an object of that type will be returned (though
/// this is not reflected in the type system). Otherwise, an object
/// representing a combination of both is returned. That object can be turned
/// into a concrete [EdgeInsets] using [resolve].
///
/// {@macro dart.ui.shadow.lerp}
static EdgeInsetsGeometry? lerp(EdgeInsetsGeometry? a, EdgeInsetsGeometry? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b! * t;
}
if (b == null) {
return a * (1.0 - t);
}
if (a is EdgeInsets && b is EdgeInsets) {
return EdgeInsets.lerp(a, b, t);
}
if (a is EdgeInsetsDirectional && b is EdgeInsetsDirectional) {
return EdgeInsetsDirectional.lerp(a, b, t);
}
return _MixedEdgeInsets.fromLRSETB(
ui.lerpDouble(a._left, b._left, t)!,
ui.lerpDouble(a._right, b._right, t)!,
ui.lerpDouble(a._start, b._start, t)!,
ui.lerpDouble(a._end, b._end, t)!,
ui.lerpDouble(a._top, b._top, t)!,
ui.lerpDouble(a._bottom, b._bottom, t)!,
);
}
/// Convert this instance into an [EdgeInsets], which uses literal coordinates
/// (i.e. the `left` coordinate being explicitly a distance from the left, and
/// the `right` coordinate being explicitly a distance from the right).
///
/// See also:
///
/// * [EdgeInsets], for which this is a no-op (returns itself).
/// * [EdgeInsetsDirectional], which flips the horizontal direction
/// based on the `direction` argument.
EdgeInsets resolve(TextDirection? direction);
@override
String toString() {
if (_start == 0.0 && _end == 0.0) {
if (_left == 0.0 && _right == 0.0 && _top == 0.0 && _bottom == 0.0) {
return 'EdgeInsets.zero';
}
if (_left == _right && _right == _top && _top == _bottom) {
return 'EdgeInsets.all(${_left.toStringAsFixed(1)})';
}
return 'EdgeInsets(${_left.toStringAsFixed(1)}, '
'${_top.toStringAsFixed(1)}, '
'${_right.toStringAsFixed(1)}, '
'${_bottom.toStringAsFixed(1)})';
}
if (_left == 0.0 && _right == 0.0) {
return 'EdgeInsetsDirectional(${_start.toStringAsFixed(1)}, '
'${_top.toStringAsFixed(1)}, '
'${_end.toStringAsFixed(1)}, '
'${_bottom.toStringAsFixed(1)})';
}
return 'EdgeInsets(${_left.toStringAsFixed(1)}, '
'${_top.toStringAsFixed(1)}, '
'${_right.toStringAsFixed(1)}, '
'${_bottom.toStringAsFixed(1)})'
' + '
'EdgeInsetsDirectional(${_start.toStringAsFixed(1)}, '
'0.0, '
'${_end.toStringAsFixed(1)}, '
'0.0)';
}
@override
bool operator ==(Object other) {
return other is EdgeInsetsGeometry
&& other._left == _left
&& other._right == _right
&& other._start == _start
&& other._end == _end
&& other._top == _top
&& other._bottom == _bottom;
}
@override
int get hashCode => Object.hash(_left, _right, _start, _end, _top, _bottom);
}
/// An immutable set of offsets in each of the four cardinal directions.
///
/// Typically used for an offset from each of the four sides of a box. For
/// example, the padding inside a box can be represented using this class.
///
/// The [EdgeInsets] class specifies offsets in terms of visual edges, left,
/// top, right, and bottom. These values are not affected by the
/// [TextDirection]. To support both left-to-right and right-to-left layouts,
/// consider using [EdgeInsetsDirectional], which is expressed in terms of
/// _start_, top, _end_, and bottom, where start and end are resolved in terms
/// of a [TextDirection] (typically obtained from the ambient [Directionality]).
///
/// {@tool snippet}
///
/// Here are some examples of how to create [EdgeInsets] instances:
///
/// Typical eight-pixel margin on all sides:
///
/// ```dart
/// const EdgeInsets.all(8.0)
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// Eight pixel margin above and below, no horizontal margins:
///
/// ```dart
/// const EdgeInsets.symmetric(vertical: 8.0)
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// Left margin indent of 40 pixels:
///
/// ```dart
/// const EdgeInsets.only(left: 40.0)
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Padding], a widget that accepts [EdgeInsets] to describe its margins.
/// * [EdgeInsetsDirectional], which (for properties and arguments that accept
/// the type [EdgeInsetsGeometry]) allows the horizontal insets to be
/// specified in a [TextDirection]-aware manner.
class EdgeInsets extends EdgeInsetsGeometry {
/// Creates insets from offsets from the left, top, right, and bottom.
const EdgeInsets.fromLTRB(this.left, this.top, this.right, this.bottom);
/// Creates insets where all the offsets are `value`.
///
/// {@tool snippet}
///
/// Typical eight-pixel margin on all sides:
///
/// ```dart
/// const EdgeInsets.all(8.0)
/// ```
/// {@end-tool}
const EdgeInsets.all(double value)
: left = value,
top = value,
right = value,
bottom = value;
/// Creates insets with only the given values non-zero.
///
/// {@tool snippet}
///
/// Left margin indent of 40 pixels:
///
/// ```dart
/// const EdgeInsets.only(left: 40.0)
/// ```
/// {@end-tool}
const EdgeInsets.only({
this.left = 0.0,
this.top = 0.0,
this.right = 0.0,
this.bottom = 0.0,
});
/// Creates insets with symmetrical vertical and horizontal offsets.
///
/// {@tool snippet}
///
/// Eight pixel margin above and below, no horizontal margins:
///
/// ```dart
/// const EdgeInsets.symmetric(vertical: 8.0)
/// ```
/// {@end-tool}
const EdgeInsets.symmetric({
double vertical = 0.0,
double horizontal = 0.0,
}) : left = horizontal,
top = vertical,
right = horizontal,
bottom = vertical;
/// Creates insets that match the given view padding.
///
/// If you need the current system padding or view insets in the context of a
/// widget, consider using [MediaQuery.paddingOf] to obtain these values rather than
/// using the value from a [FlutterView] directly, so that you get notified of
/// changes.
EdgeInsets.fromViewPadding(ui.ViewPadding padding, double devicePixelRatio)
: left = padding.left / devicePixelRatio,
top = padding.top / devicePixelRatio,
right = padding.right / devicePixelRatio,
bottom = padding.bottom / devicePixelRatio;
/// Deprecated. Will be removed in a future version of Flutter.
///
/// Use [EdgeInsets.fromViewPadding] instead.
@Deprecated(
'Use EdgeInsets.fromViewPadding instead. '
'This feature was deprecated after v3.8.0-14.0.pre.',
)
factory EdgeInsets.fromWindowPadding(ui.ViewPadding padding, double devicePixelRatio) = EdgeInsets.fromViewPadding;
/// An [EdgeInsets] with zero offsets in each direction.
static const EdgeInsets zero = EdgeInsets.only();
/// The offset from the left.
final double left;
@override
double get _left => left;
/// The offset from the top.
final double top;
@override
double get _top => top;
/// The offset from the right.
final double right;
@override
double get _right => right;
/// The offset from the bottom.
final double bottom;
@override
double get _bottom => bottom;
@override
double get _start => 0.0;
@override
double get _end => 0.0;
/// An Offset describing the vector from the top left of a rectangle to the
/// top left of that rectangle inset by this object.
Offset get topLeft => Offset(left, top);
/// An Offset describing the vector from the top right of a rectangle to the
/// top right of that rectangle inset by this object.
Offset get topRight => Offset(-right, top);
/// An Offset describing the vector from the bottom left of a rectangle to the
/// bottom left of that rectangle inset by this object.
Offset get bottomLeft => Offset(left, -bottom);
/// An Offset describing the vector from the bottom right of a rectangle to the
/// bottom right of that rectangle inset by this object.
Offset get bottomRight => Offset(-right, -bottom);
/// An [EdgeInsets] with top and bottom as well as left and right flipped.
@override
EdgeInsets get flipped => EdgeInsets.fromLTRB(right, bottom, left, top);
/// Returns a new rect that is bigger than the given rect in each direction by
/// the amount of inset in each direction. Specifically, the left edge of the
/// rect is moved left by [left], the top edge of the rect is moved up by
/// [top], the right edge of the rect is moved right by [right], and the
/// bottom edge of the rect is moved down by [bottom].
///
/// See also:
///
/// * [inflateSize], to inflate a [Size] rather than a [Rect].
/// * [deflateRect], to deflate a [Rect] rather than inflating it.
Rect inflateRect(Rect rect) {
return Rect.fromLTRB(rect.left - left, rect.top - top, rect.right + right, rect.bottom + bottom);
}
/// Returns a new rect that is smaller than the given rect in each direction by
/// the amount of inset in each direction. Specifically, the left edge of the
/// rect is moved right by [left], the top edge of the rect is moved down by
/// [top], the right edge of the rect is moved left by [right], and the
/// bottom edge of the rect is moved up by [bottom].
///
/// If the argument's [Rect.size] is smaller than [collapsedSize], then the
/// resulting rectangle will have negative dimensions.
///
/// See also:
///
/// * [deflateSize], to deflate a [Size] rather than a [Rect].
/// * [inflateRect], to inflate a [Rect] rather than deflating it.
Rect deflateRect(Rect rect) {
return Rect.fromLTRB(rect.left + left, rect.top + top, rect.right - right, rect.bottom - bottom);
}
@override
EdgeInsetsGeometry subtract(EdgeInsetsGeometry other) {
if (other is EdgeInsets) {
return this - other;
}
return super.subtract(other);
}
@override
EdgeInsetsGeometry add(EdgeInsetsGeometry other) {
if (other is EdgeInsets) {
return this + other;
}
return super.add(other);
}
@override
EdgeInsetsGeometry clamp(EdgeInsetsGeometry min, EdgeInsetsGeometry max) {
return EdgeInsets.fromLTRB(
clampDouble(_left, min._left, max._left),
clampDouble(_top, min._top, max._top),
clampDouble(_right, min._right, max._right),
clampDouble(_bottom, min._bottom, max._bottom),
);
}
/// Returns the difference between two [EdgeInsets].
EdgeInsets operator -(EdgeInsets other) {
return EdgeInsets.fromLTRB(
left - other.left,
top - other.top,
right - other.right,
bottom - other.bottom,
);
}
/// Returns the sum of two [EdgeInsets].
EdgeInsets operator +(EdgeInsets other) {
return EdgeInsets.fromLTRB(
left + other.left,
top + other.top,
right + other.right,
bottom + other.bottom,
);
}
/// Returns the [EdgeInsets] object with each dimension negated.
///
/// This is the same as multiplying the object by -1.0.
@override
EdgeInsets operator -() {
return EdgeInsets.fromLTRB(
-left,
-top,
-right,
-bottom,
);
}
/// Scales the [EdgeInsets] in each dimension by the given factor.
@override
EdgeInsets operator *(double other) {
return EdgeInsets.fromLTRB(
left * other,
top * other,
right * other,
bottom * other,
);
}
/// Divides the [EdgeInsets] in each dimension by the given factor.
@override
EdgeInsets operator /(double other) {
return EdgeInsets.fromLTRB(
left / other,
top / other,
right / other,
bottom / other,
);
}
/// Integer divides the [EdgeInsets] in each dimension by the given factor.
@override
EdgeInsets operator ~/(double other) {
return EdgeInsets.fromLTRB(
(left ~/ other).toDouble(),
(top ~/ other).toDouble(),
(right ~/ other).toDouble(),
(bottom ~/ other).toDouble(),
);
}
/// Computes the remainder in each dimension by the given factor.
@override
EdgeInsets operator %(double other) {
return EdgeInsets.fromLTRB(
left % other,
top % other,
right % other,
bottom % other,
);
}
/// Linearly interpolate between two [EdgeInsets].
///
/// If either is null, this function interpolates from [EdgeInsets.zero].
///
/// {@macro dart.ui.shadow.lerp}
static EdgeInsets? lerp(EdgeInsets? a, EdgeInsets? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b! * t;
}
if (b == null) {
return a * (1.0 - t);
}
return EdgeInsets.fromLTRB(
ui.lerpDouble(a.left, b.left, t)!,
ui.lerpDouble(a.top, b.top, t)!,
ui.lerpDouble(a.right, b.right, t)!,
ui.lerpDouble(a.bottom, b.bottom, t)!,
);
}
@override
EdgeInsets resolve(TextDirection? direction) => this;
/// Creates a copy of this EdgeInsets but with the given fields replaced
/// with the new values.
EdgeInsets copyWith({
double? left,
double? top,
double? right,
double? bottom,
}) {
return EdgeInsets.only(
left: left ?? this.left,
top: top ?? this.top,
right: right ?? this.right,
bottom: bottom ?? this.bottom,
);
}
}
/// An immutable set of offsets in each of the four cardinal directions, but
/// whose horizontal components are dependent on the writing direction.
///
/// This can be used to indicate padding from the left in [TextDirection.ltr]
/// text and padding from the right in [TextDirection.rtl] text without having
/// to be aware of the current text direction.
///
/// See also:
///
/// * [EdgeInsets], a variant that uses physical labels (left and right instead
/// of start and end).
class EdgeInsetsDirectional extends EdgeInsetsGeometry {
/// Creates insets from offsets from the start, top, end, and bottom.
const EdgeInsetsDirectional.fromSTEB(this.start, this.top, this.end, this.bottom);
/// Creates insets with only the given values non-zero.
///
/// {@tool snippet}
///
/// A margin indent of 40 pixels on the leading side:
///
/// ```dart
/// const EdgeInsetsDirectional.only(start: 40.0)
/// ```
/// {@end-tool}
const EdgeInsetsDirectional.only({
this.start = 0.0,
this.top = 0.0,
this.end = 0.0,
this.bottom = 0.0,
});
/// Creates insets with symmetric vertical and horizontal offsets.
///
/// This is equivalent to [EdgeInsets.symmetric], since the inset is the same
/// with either [TextDirection]. This constructor is just a convenience for
/// type compatibility.
///
/// {@tool snippet}
/// Eight pixel margin above and below, no horizontal margins:
///
/// ```dart
/// const EdgeInsetsDirectional.symmetric(vertical: 8.0)
/// ```
/// {@end-tool}
const EdgeInsetsDirectional.symmetric({
double horizontal = 0.0,
double vertical = 0.0,
}) : start = horizontal,
end = horizontal,
top = vertical,
bottom = vertical;
/// Creates insets where all the offsets are `value`.
///
/// {@tool snippet}
///
/// Typical eight-pixel margin on all sides:
///
/// ```dart
/// const EdgeInsetsDirectional.all(8.0)
/// ```
/// {@end-tool}
const EdgeInsetsDirectional.all(double value)
: start = value,
top = value,
end = value,
bottom = value;
/// An [EdgeInsetsDirectional] with zero offsets in each direction.
///
/// Consider using [EdgeInsets.zero] instead, since that object has the same
/// effect, but will be cheaper to [resolve].
static const EdgeInsetsDirectional zero = EdgeInsetsDirectional.only();
/// The offset from the start side, the side from which the user will start
/// reading text.
///
/// This value is normalized into an [EdgeInsets.left] or [EdgeInsets.right]
/// value by the [resolve] method.
final double start;
@override
double get _start => start;
/// The offset from the top.
///
/// This value is passed through to [EdgeInsets.top] unmodified by the
/// [resolve] method.
final double top;
@override
double get _top => top;
/// The offset from the end side, the side on which the user ends reading
/// text.
///
/// This value is normalized into an [EdgeInsets.left] or [EdgeInsets.right]
/// value by the [resolve] method.
final double end;
@override
double get _end => end;
/// The offset from the bottom.
///
/// This value is passed through to [EdgeInsets.bottom] unmodified by the
/// [resolve] method.
final double bottom;
@override
double get _bottom => bottom;
@override
double get _left => 0.0;
@override
double get _right => 0.0;
@override
bool get isNonNegative => start >= 0.0 && top >= 0.0 && end >= 0.0 && bottom >= 0.0;
/// An [EdgeInsetsDirectional] with [top] and [bottom] as well as [start] and [end] flipped.
@override
EdgeInsetsDirectional get flipped => EdgeInsetsDirectional.fromSTEB(end, bottom, start, top);
@override
EdgeInsetsGeometry subtract(EdgeInsetsGeometry other) {
if (other is EdgeInsetsDirectional) {
return this - other;
}
return super.subtract(other);
}
@override
EdgeInsetsGeometry add(EdgeInsetsGeometry other) {
if (other is EdgeInsetsDirectional) {
return this + other;
}
return super.add(other);
}
/// Returns the difference between two [EdgeInsetsDirectional] objects.
EdgeInsetsDirectional operator -(EdgeInsetsDirectional other) {
return EdgeInsetsDirectional.fromSTEB(
start - other.start,
top - other.top,
end - other.end,
bottom - other.bottom,
);
}
/// Returns the sum of two [EdgeInsetsDirectional] objects.
EdgeInsetsDirectional operator +(EdgeInsetsDirectional other) {
return EdgeInsetsDirectional.fromSTEB(
start + other.start,
top + other.top,
end + other.end,
bottom + other.bottom,
);
}
/// Returns the [EdgeInsetsDirectional] object with each dimension negated.
///
/// This is the same as multiplying the object by -1.0.
@override
EdgeInsetsDirectional operator -() {
return EdgeInsetsDirectional.fromSTEB(
-start,
-top,
-end,
-bottom,
);
}
/// Scales the [EdgeInsetsDirectional] object in each dimension by the given factor.
@override
EdgeInsetsDirectional operator *(double other) {
return EdgeInsetsDirectional.fromSTEB(
start * other,
top * other,
end * other,
bottom * other,
);
}
/// Divides the [EdgeInsetsDirectional] object in each dimension by the given factor.
@override
EdgeInsetsDirectional operator /(double other) {
return EdgeInsetsDirectional.fromSTEB(
start / other,
top / other,
end / other,
bottom / other,
);
}
/// Integer divides the [EdgeInsetsDirectional] object in each dimension by the given factor.
@override
EdgeInsetsDirectional operator ~/(double other) {
return EdgeInsetsDirectional.fromSTEB(
(start ~/ other).toDouble(),
(top ~/ other).toDouble(),
(end ~/ other).toDouble(),
(bottom ~/ other).toDouble(),
);
}
/// Computes the remainder in each dimension by the given factor.
@override
EdgeInsetsDirectional operator %(double other) {
return EdgeInsetsDirectional.fromSTEB(
start % other,
top % other,
end % other,
bottom % other,
);
}
/// Linearly interpolate between two [EdgeInsetsDirectional].
///
/// If either is null, this function interpolates from [EdgeInsetsDirectional.zero].
///
/// To interpolate between two [EdgeInsetsGeometry] objects of arbitrary type
/// (either [EdgeInsets] or [EdgeInsetsDirectional]), consider the
/// [EdgeInsetsGeometry.lerp] static method.
///
/// {@macro dart.ui.shadow.lerp}
static EdgeInsetsDirectional? lerp(EdgeInsetsDirectional? a, EdgeInsetsDirectional? b, double t) {
if (identical(a, b)) {
return a;
}
if (a == null) {
return b! * t;
}
if (b == null) {
return a * (1.0 - t);
}
return EdgeInsetsDirectional.fromSTEB(
ui.lerpDouble(a.start, b.start, t)!,
ui.lerpDouble(a.top, b.top, t)!,
ui.lerpDouble(a.end, b.end, t)!,
ui.lerpDouble(a.bottom, b.bottom, t)!,
);
}
@override
EdgeInsets resolve(TextDirection? direction) {
assert(direction != null);
return switch (direction!) {
TextDirection.rtl => EdgeInsets.fromLTRB(end, top, start, bottom),
TextDirection.ltr => EdgeInsets.fromLTRB(start, top, end, bottom),
};
}
/// Creates a copy of this EdgeInsetsDirectional but with the given
/// fields replaced with the new values.
EdgeInsetsDirectional copyWith({
double? start,
double? top,
double? end,
double? bottom,
}) {
return EdgeInsetsDirectional.only(
start: start ?? this.start,
top: top ?? this.top,
end: end ?? this.end,
bottom: bottom ?? this.bottom,
);
}
}
class _MixedEdgeInsets extends EdgeInsetsGeometry {
const _MixedEdgeInsets.fromLRSETB(this._left, this._right, this._start, this._end, this._top, this._bottom);
@override
final double _left;
@override
final double _right;
@override
final double _start;
@override
final double _end;
@override
final double _top;
@override
final double _bottom;
@override
bool get isNonNegative {
return _left >= 0.0
&& _right >= 0.0
&& _start >= 0.0
&& _end >= 0.0
&& _top >= 0.0
&& _bottom >= 0.0;
}
@override
_MixedEdgeInsets operator -() {
return _MixedEdgeInsets.fromLRSETB(
-_left,
-_right,
-_start,
-_end,
-_top,
-_bottom,
);
}
@override
_MixedEdgeInsets operator *(double other) {
return _MixedEdgeInsets.fromLRSETB(
_left * other,
_right * other,
_start * other,
_end * other,
_top * other,
_bottom * other,
);
}
@override
_MixedEdgeInsets operator /(double other) {
return _MixedEdgeInsets.fromLRSETB(
_left / other,
_right / other,
_start / other,
_end / other,
_top / other,
_bottom / other,
);
}
@override
_MixedEdgeInsets operator ~/(double other) {
return _MixedEdgeInsets.fromLRSETB(
(_left ~/ other).toDouble(),
(_right ~/ other).toDouble(),
(_start ~/ other).toDouble(),
(_end ~/ other).toDouble(),
(_top ~/ other).toDouble(),
(_bottom ~/ other).toDouble(),
);
}
@override
_MixedEdgeInsets operator %(double other) {
return _MixedEdgeInsets.fromLRSETB(
_left % other,
_right % other,
_start % other,
_end % other,
_top % other,
_bottom % other,
);
}
@override
EdgeInsets resolve(TextDirection? direction) {
assert(direction != null);
return switch (direction!) {
TextDirection.rtl => EdgeInsets.fromLTRB(_end + _left, _top, _start + _right, _bottom),
TextDirection.ltr => EdgeInsets.fromLTRB(_start + _left, _top, _end + _right, _bottom),
};
}
}
| flutter/packages/flutter/lib/src/painting/edge_insets.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/edge_insets.dart",
"repo_id": "flutter",
"token_count": 11185
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show PlaceholderAlignment;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'inline_span.dart';
import 'text_painter.dart';
import 'text_span.dart';
import 'text_style.dart';
/// An immutable placeholder that is embedded inline within text.
///
/// [PlaceholderSpan] represents a placeholder that acts as a stand-in for other
/// content. A [PlaceholderSpan] by itself does not contain useful information
/// to change a [TextSpan]. [WidgetSpan] from the widgets library extends
/// [PlaceholderSpan] and may be used instead to specify a widget as the contents
/// of the placeholder.
///
/// Flutter widgets such as [TextField], [Text] and [RichText] do not recognize
/// [PlaceholderSpan] subclasses other than [WidgetSpan]. **Consider
/// implementing the [WidgetSpan] interface instead of the [Placeholder]
/// interface.**
///
///
/// See also:
///
/// * [WidgetSpan], a leaf node that represents an embedded inline widget.
/// * [TextSpan], a node that represents text in a [TextSpan] tree.
/// * [Text], a widget for showing uniformly-styled text.
/// * [RichText], a widget for finer control of text rendering.
/// * [TextPainter], a class for painting [TextSpan] objects on a [Canvas].
abstract class PlaceholderSpan extends InlineSpan {
/// Creates a [PlaceholderSpan] with the given values.
///
/// A [TextStyle] may be provided with the [style] property, but only the
/// decoration, foreground, background, and spacing options will be used.
const PlaceholderSpan({
this.alignment = ui.PlaceholderAlignment.bottom,
this.baseline,
super.style,
});
/// The unicode character to represent a placeholder.
static const int placeholderCodeUnit = 0xFFFC;
/// How the placeholder aligns vertically with the text.
///
/// See [ui.PlaceholderAlignment] for details on each mode.
final ui.PlaceholderAlignment alignment;
/// The [TextBaseline] to align against when using [ui.PlaceholderAlignment.baseline],
/// [ui.PlaceholderAlignment.aboveBaseline], and [ui.PlaceholderAlignment.belowBaseline].
///
/// This is ignored when using other alignment modes.
final TextBaseline? baseline;
/// [PlaceholderSpan]s are flattened to a `0xFFFC` object replacement character in the
/// plain text representation when `includePlaceholders` is true.
@override
void computeToPlainText(StringBuffer buffer, {bool includeSemanticsLabels = true, bool includePlaceholders = true}) {
if (includePlaceholders) {
buffer.writeCharCode(placeholderCodeUnit);
}
}
@override
void computeSemanticsInformation(List<InlineSpanSemanticsInformation> collector) {
collector.add(InlineSpanSemanticsInformation.placeholder);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<ui.PlaceholderAlignment>('alignment', alignment, defaultValue: null));
properties.add(EnumProperty<TextBaseline>('baseline', baseline, defaultValue: null));
}
@override
bool debugAssertIsValid() {
assert(false, 'Consider implementing the WidgetSpan interface instead.');
return super.debugAssertIsValid();
}
}
| flutter/packages/flutter/lib/src/painting/placeholder_span.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/placeholder_span.dart",
"repo_id": "flutter",
"token_count": 976
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
/// Structure that specifies maximum allowable magnitudes for distances,
/// durations, and velocity differences to be considered equal.
class Tolerance {
/// Creates a [Tolerance] object. By default, the distance, time, and velocity
/// tolerances are all ±0.001; the constructor arguments override this.
///
/// The arguments should all be positive values.
const Tolerance({
this.distance = _epsilonDefault,
this.time = _epsilonDefault,
this.velocity = _epsilonDefault,
});
static const double _epsilonDefault = 1e-3;
/// A default tolerance of 0.001 for all three values.
static const Tolerance defaultTolerance = Tolerance();
/// The magnitude of the maximum distance between two points for them to be
/// considered within tolerance.
///
/// The units for the distance tolerance must be the same as the units used
/// for the distances that are to be compared to this tolerance.
final double distance;
/// The magnitude of the maximum duration between two times for them to be
/// considered within tolerance.
///
/// The units for the time tolerance must be the same as the units used
/// for the times that are to be compared to this tolerance.
final double time;
/// The magnitude of the maximum difference between two velocities for them to
/// be considered within tolerance.
///
/// The units for the velocity tolerance must be the same as the units used
/// for the velocities that are to be compared to this tolerance.
final double velocity;
@override
String toString() => '${objectRuntimeType(this, 'Tolerance')}(distance: ±$distance, time: ±$time, velocity: ±$velocity)';
}
| flutter/packages/flutter/lib/src/physics/tolerance.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/physics/tolerance.dart",
"repo_id": "flutter",
"token_count": 480
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'box.dart';
/// Signature for a function that takes a [RenderBox] and returns the [Size]
/// that the [RenderBox] would have if it were laid out with the given
/// [BoxConstraints].
///
/// The methods of [ChildLayoutHelper] adhere to this signature.
typedef ChildLayouter = Size Function(RenderBox child, BoxConstraints constraints);
/// A collection of static functions to layout a [RenderBox] child with the
/// given set of [BoxConstraints].
///
/// All of the functions adhere to the [ChildLayouter] signature.
abstract final class ChildLayoutHelper {
/// Returns the [Size] that the [RenderBox] would have if it were to
/// be laid out with the given [BoxConstraints].
///
/// This method calls [RenderBox.getDryLayout] on the given [RenderBox].
///
/// This method should only be called by the parent of the provided
/// [RenderBox] child as it binds parent and child together (if the child
/// is marked as dirty, the child will also be marked as dirty).
///
/// See also:
///
/// * [layoutChild], which actually lays out the child with the given
/// constraints.
static Size dryLayoutChild(RenderBox child, BoxConstraints constraints) {
return child.getDryLayout(constraints);
}
/// Lays out the [RenderBox] with the given constraints and returns its
/// [Size].
///
/// This method calls [RenderBox.layout] on the given [RenderBox] with
/// `parentUsesSize` set to true to receive its [Size].
///
/// This method should only be called by the parent of the provided
/// [RenderBox] child as it binds parent and child together (if the child
/// is marked as dirty, the child will also be marked as dirty).
///
/// See also:
///
/// * [dryLayoutChild], which does not perform a real layout of the child.
static Size layoutChild(RenderBox child, BoxConstraints constraints) {
child.layout(constraints, parentUsesSize: true);
return child.size;
}
}
| flutter/packages/flutter/lib/src/rendering/layout_helper.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/layout_helper.dart",
"repo_id": "flutter",
"token_count": 592
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'sliver.dart';
import 'sliver_multi_box_adaptor.dart';
/// A sliver that contains multiple box children that have the explicit extent in
/// the main axis.
///
/// [RenderSliverFixedExtentBoxAdaptor] places its children in a linear array
/// along the main axis. Each child is forced to have the returned value of [itemExtentBuilder]
/// when the [itemExtentBuilder] is non-null or the [itemExtent] when [itemExtentBuilder]
/// is null in the main axis and the [SliverConstraints.crossAxisExtent] in the cross axis.
///
/// Subclasses should override [itemExtent] or [itemExtentBuilder] to control
/// the size of the children in the main axis. For a concrete subclass with a
/// configurable [itemExtent], see [RenderSliverFixedExtentList] or [RenderSliverVariedExtentList].
///
/// [RenderSliverFixedExtentBoxAdaptor] is more efficient than
/// [RenderSliverList] because [RenderSliverFixedExtentBoxAdaptor] does not need
/// to perform layout on its children to obtain their extent in the main axis.
///
/// See also:
///
/// * [RenderSliverFixedExtentList], which has a configurable [itemExtent].
/// * [RenderSliverFillViewport], which determines the [itemExtent] based on
/// [SliverConstraints.viewportMainAxisExtent].
/// * [RenderSliverFillRemaining], which determines the [itemExtent] based on
/// [SliverConstraints.remainingPaintExtent].
/// * [RenderSliverList], which does not require its children to have the same
/// extent in the main axis.
abstract class RenderSliverFixedExtentBoxAdaptor extends RenderSliverMultiBoxAdaptor {
/// Creates a sliver that contains multiple box children that have the same
/// extent in the main axis.
RenderSliverFixedExtentBoxAdaptor({
required super.childManager,
});
/// The main-axis extent of each item.
///
/// If this is non-null, the [itemExtentBuilder] must be null.
/// If this is null, the [itemExtentBuilder] must be non-null.
double? get itemExtent;
/// The main-axis extent builder of each item.
///
/// If this is non-null, the [itemExtent] must be null.
/// If this is null, the [itemExtent] must be non-null.
ItemExtentBuilder? get itemExtentBuilder => null;
/// The layout offset for the child with the given index.
///
/// This function uses the returned value of [itemExtentBuilder] or the
/// [itemExtent] to avoid recomputing item size repeatedly during layout.
///
/// By default, places the children in order, without gaps, starting from
/// layout offset zero.
@visibleForTesting
@protected
double indexToLayoutOffset(
@Deprecated(
'The itemExtent is already available within the scope of this function. '
'This feature was deprecated after v3.20.0-7.0.pre.'
)
double itemExtent,
int index,
) {
if (itemExtentBuilder == null) {
itemExtent = this.itemExtent!;
return itemExtent * index;
} else {
double offset = 0.0;
double? itemExtent;
for (int i = 0; i < index; i++) {
final int? childCount = childManager.estimatedChildCount;
if (childCount != null && i > childCount - 1) {
break;
}
itemExtent = itemExtentBuilder!(i, _currentLayoutDimensions);
if (itemExtent == null) {
break;
}
offset += itemExtent;
}
return offset;
}
}
/// The minimum child index that is visible at the given scroll offset.
///
/// This function uses the returned value of [itemExtentBuilder] or the
/// [itemExtent] to avoid recomputing item size repeatedly during layout.
///
/// By default, returns a value consistent with the children being placed in
/// order, without gaps, starting from layout offset zero.
@visibleForTesting
@protected
int getMinChildIndexForScrollOffset(
double scrollOffset,
@Deprecated(
'The itemExtent is already available within the scope of this function. '
'This feature was deprecated after v3.20.0-7.0.pre.'
)
double itemExtent,
) {
if (itemExtentBuilder == null) {
itemExtent = this.itemExtent!;
if (itemExtent > 0.0) {
final double actual = scrollOffset / itemExtent;
final int round = actual.round();
if ((actual * itemExtent - round * itemExtent).abs() < precisionErrorTolerance) {
return round;
}
return actual.floor();
}
return 0;
} else {
return _getChildIndexForScrollOffset(scrollOffset, itemExtentBuilder!);
}
}
/// The maximum child index that is visible at the given scroll offset.
///
/// This function uses the returned value of [itemExtentBuilder] or the
/// [itemExtent] to avoid recomputing item size repeatedly during layout.
///
/// By default, returns a value consistent with the children being placed in
/// order, without gaps, starting from layout offset zero.
@visibleForTesting
@protected
int getMaxChildIndexForScrollOffset(
double scrollOffset,
@Deprecated(
'The itemExtent is already available within the scope of this function. '
'This feature was deprecated after v3.20.0-7.0.pre.'
)
double itemExtent,
) {
if (itemExtentBuilder == null) {
itemExtent = this.itemExtent!;
if (itemExtent > 0.0) {
final double actual = scrollOffset / itemExtent - 1;
final int round = actual.round();
if ((actual * itemExtent - round * itemExtent).abs() < precisionErrorTolerance) {
return math.max(0, round);
}
return math.max(0, actual.ceil());
}
return 0;
} else {
return _getChildIndexForScrollOffset(scrollOffset, itemExtentBuilder!);
}
}
/// Called to estimate the total scrollable extents of this object.
///
/// Must return the total distance from the start of the child with the
/// earliest possible index to the end of the child with the last possible
/// index.
///
/// By default, defers to [RenderSliverBoxChildManager.estimateMaxScrollOffset].
///
/// See also:
///
/// * [computeMaxScrollOffset], which is similar but must provide a precise
/// value.
@protected
double estimateMaxScrollOffset(
SliverConstraints constraints, {
int? firstIndex,
int? lastIndex,
double? leadingScrollOffset,
double? trailingScrollOffset,
}) {
return childManager.estimateMaxScrollOffset(
constraints,
firstIndex: firstIndex,
lastIndex: lastIndex,
leadingScrollOffset: leadingScrollOffset,
trailingScrollOffset: trailingScrollOffset,
);
}
/// Called to obtain a precise measure of the total scrollable extents of this
/// object.
///
/// Must return the precise total distance from the start of the child with
/// the earliest possible index to the end of the child with the last possible
/// index.
///
/// This is used when no child is available for the index corresponding to the
/// current scroll offset, to determine the precise dimensions of the sliver.
/// It must return a precise value. It will not be called if the
/// [childManager] returns an infinite number of children for positive
/// indices.
///
/// If [itemExtentBuilder] is null, multiplies the [itemExtent] by the number
/// of children reported by [RenderSliverBoxChildManager.childCount].
/// If [itemExtentBuilder] is non-null, sum the extents of the first
/// [RenderSliverBoxChildManager.childCount] children.
///
/// See also:
///
/// * [estimateMaxScrollOffset], which is similar but may provide inaccurate
/// values.
@visibleForTesting
@protected
double computeMaxScrollOffset(
SliverConstraints constraints,
@Deprecated(
'The itemExtent is already available within the scope of this function. '
'This feature was deprecated after v3.20.0-7.0.pre.'
)
double itemExtent,
) {
if (itemExtentBuilder == null) {
itemExtent = this.itemExtent!;
return childManager.childCount * itemExtent;
} else {
double offset = 0.0;
double? itemExtent;
for (int i = 0; i < childManager.childCount; i++) {
itemExtent = itemExtentBuilder!(i, _currentLayoutDimensions);
if (itemExtent == null) {
break;
}
offset += itemExtent;
}
return offset;
}
}
int _getChildIndexForScrollOffset(double scrollOffset, ItemExtentBuilder callback) {
if (scrollOffset == 0.0) {
return 0;
}
double position = 0.0;
int index = 0;
double? itemExtent;
while (position < scrollOffset) {
final int? childCount = childManager.estimatedChildCount;
if (childCount != null && index > childCount - 1) {
break;
}
itemExtent = callback(index, _currentLayoutDimensions);
if (itemExtent == null) {
break;
}
position += itemExtent;
++index;
}
return index - 1;
}
BoxConstraints _getChildConstraints(int index) {
double extent;
if (itemExtentBuilder == null) {
extent = itemExtent!;
} else {
extent = itemExtentBuilder!(index, _currentLayoutDimensions)!;
}
return constraints.asBoxConstraints(
minExtent: extent,
maxExtent: extent,
);
}
late SliverLayoutDimensions _currentLayoutDimensions;
@override
void performLayout() {
assert((itemExtent != null && itemExtentBuilder == null) ||
(itemExtent == null && itemExtentBuilder != null));
assert(itemExtentBuilder != null || (itemExtent!.isFinite && itemExtent! >= 0));
final SliverConstraints constraints = this.constraints;
childManager.didStartLayout();
childManager.setDidUnderflow(false);
final double scrollOffset = constraints.scrollOffset + constraints.cacheOrigin;
assert(scrollOffset >= 0.0);
final double remainingExtent = constraints.remainingCacheExtent;
assert(remainingExtent >= 0.0);
final double targetEndScrollOffset = scrollOffset + remainingExtent;
_currentLayoutDimensions = SliverLayoutDimensions(
scrollOffset: constraints.scrollOffset,
precedingScrollExtent: constraints.precedingScrollExtent,
viewportMainAxisExtent: constraints.viewportMainAxisExtent,
crossAxisExtent: constraints.crossAxisExtent
);
// TODO(Piinks): Clean up when deprecation expires.
const double deprecatedExtraItemExtent = -1;
final int firstIndex = getMinChildIndexForScrollOffset(scrollOffset, deprecatedExtraItemExtent);
final int? targetLastIndex = targetEndScrollOffset.isFinite ?
getMaxChildIndexForScrollOffset(targetEndScrollOffset, deprecatedExtraItemExtent) : null;
if (firstChild != null) {
final int leadingGarbage = calculateLeadingGarbage(firstIndex: firstIndex);
final int trailingGarbage = targetLastIndex != null ? calculateTrailingGarbage(lastIndex: targetLastIndex) : 0;
collectGarbage(leadingGarbage, trailingGarbage);
} else {
collectGarbage(0, 0);
}
if (firstChild == null) {
final double layoutOffset = indexToLayoutOffset(deprecatedExtraItemExtent, firstIndex);
if (!addInitialChild(index: firstIndex, layoutOffset: layoutOffset)) {
// There are either no children, or we are past the end of all our children.
final double max;
if (firstIndex <= 0) {
max = 0.0;
} else {
max = computeMaxScrollOffset(constraints, deprecatedExtraItemExtent);
}
geometry = SliverGeometry(
scrollExtent: max,
maxPaintExtent: max,
);
childManager.didFinishLayout();
return;
}
}
RenderBox? trailingChildWithLayout;
for (int index = indexOf(firstChild!) - 1; index >= firstIndex; --index) {
final RenderBox? child = insertAndLayoutLeadingChild(_getChildConstraints(index));
if (child == null) {
// Items before the previously first child are no longer present.
// Reset the scroll offset to offset all items prior and up to the
// missing item. Let parent re-layout everything.
geometry = SliverGeometry(scrollOffsetCorrection: indexToLayoutOffset(deprecatedExtraItemExtent, index));
return;
}
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
childParentData.layoutOffset = indexToLayoutOffset(deprecatedExtraItemExtent, index);
assert(childParentData.index == index);
trailingChildWithLayout ??= child;
}
if (trailingChildWithLayout == null) {
firstChild!.layout(_getChildConstraints(indexOf(firstChild!)));
final SliverMultiBoxAdaptorParentData childParentData = firstChild!.parentData! as SliverMultiBoxAdaptorParentData;
childParentData.layoutOffset = indexToLayoutOffset(deprecatedExtraItemExtent, firstIndex);
trailingChildWithLayout = firstChild;
}
double estimatedMaxScrollOffset = double.infinity;
for (int index = indexOf(trailingChildWithLayout!) + 1; targetLastIndex == null || index <= targetLastIndex; ++index) {
RenderBox? child = childAfter(trailingChildWithLayout!);
if (child == null || indexOf(child) != index) {
child = insertAndLayoutChild(_getChildConstraints(index), after: trailingChildWithLayout);
if (child == null) {
// We have run out of children.
estimatedMaxScrollOffset = indexToLayoutOffset(deprecatedExtraItemExtent, index);
break;
}
} else {
child.layout(_getChildConstraints(index));
}
trailingChildWithLayout = child;
final SliverMultiBoxAdaptorParentData childParentData = child.parentData! as SliverMultiBoxAdaptorParentData;
assert(childParentData.index == index);
childParentData.layoutOffset = indexToLayoutOffset(deprecatedExtraItemExtent, childParentData.index!);
}
final int lastIndex = indexOf(lastChild!);
final double leadingScrollOffset = indexToLayoutOffset(deprecatedExtraItemExtent, firstIndex);
final double trailingScrollOffset = indexToLayoutOffset(deprecatedExtraItemExtent, lastIndex + 1);
assert(firstIndex == 0 || childScrollOffset(firstChild!)! - scrollOffset <= precisionErrorTolerance);
assert(debugAssertChildListIsNonEmptyAndContiguous());
assert(indexOf(firstChild!) == firstIndex);
assert(targetLastIndex == null || lastIndex <= targetLastIndex);
estimatedMaxScrollOffset = math.min(
estimatedMaxScrollOffset,
estimateMaxScrollOffset(
constraints,
firstIndex: firstIndex,
lastIndex: lastIndex,
leadingScrollOffset: leadingScrollOffset,
trailingScrollOffset: trailingScrollOffset,
),
);
final double paintExtent = calculatePaintOffset(
constraints,
from: leadingScrollOffset,
to: trailingScrollOffset,
);
final double cacheExtent = calculateCacheOffset(
constraints,
from: leadingScrollOffset,
to: trailingScrollOffset,
);
final double targetEndScrollOffsetForPaint = constraints.scrollOffset + constraints.remainingPaintExtent;
final int? targetLastIndexForPaint = targetEndScrollOffsetForPaint.isFinite ?
getMaxChildIndexForScrollOffset(targetEndScrollOffsetForPaint, deprecatedExtraItemExtent) : null;
geometry = SliverGeometry(
scrollExtent: estimatedMaxScrollOffset,
paintExtent: paintExtent,
cacheExtent: cacheExtent,
maxPaintExtent: estimatedMaxScrollOffset,
// Conservative to avoid flickering away the clip during scroll.
hasVisualOverflow: (targetLastIndexForPaint != null && lastIndex >= targetLastIndexForPaint)
|| constraints.scrollOffset > 0.0,
);
// We may have started the layout while scrolled to the end, which would not
// expose a new child.
if (estimatedMaxScrollOffset == trailingScrollOffset) {
childManager.setDidUnderflow(true);
}
childManager.didFinishLayout();
}
}
/// A sliver that places multiple box children with the same main axis extent in
/// a linear array.
///
/// [RenderSliverFixedExtentList] places its children in a linear array along
/// the main axis starting at offset zero and without gaps. Each child is forced
/// to have the [itemExtent] in the main axis and the
/// [SliverConstraints.crossAxisExtent] in the cross axis.
///
/// [RenderSliverFixedExtentList] is more efficient than [RenderSliverList]
/// because [RenderSliverFixedExtentList] does not need to perform layout on its
/// children to obtain their extent in the main axis.
///
/// See also:
///
/// * [RenderSliverList], which does not require its children to have the same
/// extent in the main axis.
/// * [RenderSliverFillViewport], which determines the [itemExtent] based on
/// [SliverConstraints.viewportMainAxisExtent].
/// * [RenderSliverFillRemaining], which determines the [itemExtent] based on
/// [SliverConstraints.remainingPaintExtent].
class RenderSliverFixedExtentList extends RenderSliverFixedExtentBoxAdaptor {
/// Creates a sliver that contains multiple box children that have a given
/// extent in the main axis.
RenderSliverFixedExtentList({
required super.childManager,
required double itemExtent,
}) : _itemExtent = itemExtent;
@override
double get itemExtent => _itemExtent;
double _itemExtent;
set itemExtent(double value) {
if (_itemExtent == value) {
return;
}
_itemExtent = value;
markNeedsLayout();
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_fixed_extent_list.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_fixed_extent_list.dart",
"repo_id": "flutter",
"token_count": 5881
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'dart:developer' show Flow, Timeline, TimelineTask;
import 'dart:ui' show AppLifecycleState, DartPerformanceMode, FramePhase, FrameTiming, PlatformDispatcher, TimingsCallback;
import 'package:collection/collection.dart' show HeapPriorityQueue, PriorityQueue;
import 'package:flutter/foundation.dart';
import 'debug.dart';
import 'priority.dart';
import 'service_extensions.dart';
export 'dart:ui' show AppLifecycleState, FrameTiming, TimingsCallback;
export 'priority.dart' show Priority;
/// Slows down animations by this factor to help in development.
double get timeDilation => _timeDilation;
double _timeDilation = 1.0;
/// If the [SchedulerBinding] has been initialized, setting the time dilation
/// automatically calls [SchedulerBinding.resetEpoch] to ensure that time stamps
/// seen by consumers of the scheduler binding are always increasing.
///
/// It is safe to set this before initializing the binding.
set timeDilation(double value) {
assert(value > 0.0);
if (_timeDilation == value) {
return;
}
// If the binding has been created, we need to resetEpoch first so that we
// capture start of the epoch with the current time dilation.
SchedulerBinding._instance?.resetEpoch();
_timeDilation = value;
}
/// Signature for frame-related callbacks from the scheduler.
///
/// The `timeStamp` is the number of milliseconds since the beginning of the
/// scheduler's epoch. Use timeStamp to determine how far to advance animation
/// timelines so that all the animations in the system are synchronized to a
/// common time base.
typedef FrameCallback = void Function(Duration timeStamp);
/// Signature for [SchedulerBinding.scheduleTask] callbacks.
///
/// The type argument `T` is the task's return value. Consider `void` if the
/// task does not return a value.
typedef TaskCallback<T> = FutureOr<T> Function();
/// Signature for the [SchedulerBinding.schedulingStrategy] callback. Called
/// whenever the system needs to decide whether a task at a given
/// priority needs to be run.
///
/// Return true if a task with the given priority should be executed at this
/// time, false otherwise.
///
/// See also:
///
/// * [defaultSchedulingStrategy], the default [SchedulingStrategy] for [SchedulerBinding.schedulingStrategy].
typedef SchedulingStrategy = bool Function({ required int priority, required SchedulerBinding scheduler });
class _TaskEntry<T> {
_TaskEntry(this.task, this.priority, this.debugLabel, this.flow) {
assert(() {
debugStack = StackTrace.current;
return true;
}());
}
final TaskCallback<T> task;
final int priority;
final String? debugLabel;
final Flow? flow;
late StackTrace debugStack;
final Completer<T> completer = Completer<T>();
void run() {
if (!kReleaseMode) {
Timeline.timeSync(
debugLabel ?? 'Scheduled Task',
() {
completer.complete(task());
},
flow: flow != null ? Flow.step(flow!.id) : null,
);
} else {
completer.complete(task());
}
}
}
class _FrameCallbackEntry {
_FrameCallbackEntry(this.callback, { bool rescheduling = false }) {
assert(() {
if (rescheduling) {
assert(() {
if (debugCurrentCallbackStack == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('scheduleFrameCallback called with rescheduling true, but no callback is in scope.'),
ErrorDescription(
'The "rescheduling" argument should only be set to true if the '
'callback is being reregistered from within the callback itself, '
'and only then if the callback itself is entirely synchronous.',
),
ErrorHint(
'If this is the initial registration of the callback, or if the '
'callback is asynchronous, then do not use the "rescheduling" '
'argument.',
),
]);
}
return true;
}());
debugStack = debugCurrentCallbackStack;
} else {
// TODO(ianh): trim the frames from this library, so that the call to scheduleFrameCallback is the top one
debugStack = StackTrace.current;
}
return true;
}());
}
final FrameCallback callback;
static StackTrace? debugCurrentCallbackStack;
StackTrace? debugStack;
}
/// The various phases that a [SchedulerBinding] goes through during
/// [SchedulerBinding.handleBeginFrame].
///
/// This is exposed by [SchedulerBinding.schedulerPhase].
///
/// The values of this enum are ordered in the same order as the phases occur,
/// so their relative index values can be compared to each other.
///
/// See also:
///
/// * [WidgetsBinding.drawFrame], which pumps the build and rendering pipeline
/// to generate a frame.
enum SchedulerPhase {
/// No frame is being processed. Tasks (scheduled by
/// [SchedulerBinding.scheduleTask]), microtasks (scheduled by
/// [scheduleMicrotask]), [Timer] callbacks, event handlers (e.g. from user
/// input), and other callbacks (e.g. from [Future]s, [Stream]s, and the like)
/// may be executing.
idle,
/// The transient callbacks (scheduled by
/// [SchedulerBinding.scheduleFrameCallback]) are currently executing.
///
/// Typically, these callbacks handle updating objects to new animation
/// states.
///
/// See [SchedulerBinding.handleBeginFrame].
transientCallbacks,
/// Microtasks scheduled during the processing of transient callbacks are
/// current executing.
///
/// This may include, for instance, callbacks from futures resolved during the
/// [transientCallbacks] phase.
midFrameMicrotasks,
/// The persistent callbacks (scheduled by
/// [SchedulerBinding.addPersistentFrameCallback]) are currently executing.
///
/// Typically, this is the build/layout/paint pipeline. See
/// [WidgetsBinding.drawFrame] and [SchedulerBinding.handleDrawFrame].
persistentCallbacks,
/// The post-frame callbacks (scheduled by
/// [SchedulerBinding.addPostFrameCallback]) are currently executing.
///
/// Typically, these callbacks handle cleanup and scheduling of work for the
/// next frame.
///
/// See [SchedulerBinding.handleDrawFrame].
postFrameCallbacks,
}
/// This callback is invoked when a request for [DartPerformanceMode] is disposed.
///
/// See also:
///
/// * [PerformanceModeRequestHandle] for more information on the lifecycle of the handle.
typedef _PerformanceModeCleanupCallback = VoidCallback;
/// An opaque handle that keeps a request for [DartPerformanceMode] active until
/// disposed.
///
/// To create a [PerformanceModeRequestHandle], use [SchedulerBinding.requestPerformanceMode].
/// The component that makes the request is responsible for disposing the handle.
class PerformanceModeRequestHandle {
PerformanceModeRequestHandle._(_PerformanceModeCleanupCallback this._cleanup) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/scheduler.dart',
className: '$PerformanceModeRequestHandle',
object: this,
);
}
}
_PerformanceModeCleanupCallback? _cleanup;
/// Call this method to signal to [SchedulerBinding] that a request for a [DartPerformanceMode]
/// is no longer needed.
///
/// This method must only be called once per object.
void dispose() {
assert(_cleanup != null);
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_cleanup!();
_cleanup = null;
}
}
/// Scheduler for running the following:
///
/// * _Transient callbacks_, triggered by the system's
/// [dart:ui.PlatformDispatcher.onBeginFrame] callback, for synchronizing the
/// application's behavior to the system's display. For example, [Ticker]s and
/// [AnimationController]s trigger from these.
///
/// * _Persistent callbacks_, triggered by the system's
/// [dart:ui.PlatformDispatcher.onDrawFrame] callback, for updating the
/// system's display after transient callbacks have executed. For example, the
/// rendering layer uses this to drive its rendering pipeline.
///
/// * _Post-frame callbacks_, which are run after persistent callbacks, just
/// before returning from the [dart:ui.PlatformDispatcher.onDrawFrame] callback.
///
/// * Non-rendering tasks, to be run between frames. These are given a
/// priority and are executed in priority order according to a
/// [schedulingStrategy].
mixin SchedulerBinding on BindingBase {
@override
void initInstances() {
super.initInstances();
_instance = this;
if (!kReleaseMode) {
addTimingsCallback((List<FrameTiming> timings) {
timings.forEach(_profileFramePostEvent);
});
}
}
/// The current [SchedulerBinding], if one has been created.
///
/// Provides access to the features exposed by this mixin. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [runApp] or [WidgetsFlutterBinding.ensureInitialized].
static SchedulerBinding get instance => BindingBase.checkInstance(_instance);
static SchedulerBinding? _instance;
final List<TimingsCallback> _timingsCallbacks = <TimingsCallback>[];
/// Add a [TimingsCallback] that receives [FrameTiming] sent from
/// the engine.
///
/// This API enables applications to monitor their graphics
/// performance. Data from the engine is batched into lists of
/// [FrameTiming] objects which are reported approximately once a
/// second in release mode and approximately once every 100ms in
/// debug and profile builds. The list is sorted in ascending
/// chronological order (earliest frame first). The timing of the
/// first frame is sent immediately without batching.
///
/// The data returned can be used to catch missed frames (by seeing
/// if [FrameTiming.buildDuration] or [FrameTiming.rasterDuration]
/// exceed the frame budget, e.g. 16ms at 60Hz), and to catch high
/// latency (by seeing if [FrameTiming.totalSpan] exceeds the frame
/// budget). It is possible for no frames to be missed but for the
/// latency to be more than one frame in the case where the Flutter
/// engine is pipelining the graphics updates, e.g. because the sum
/// of the [FrameTiming.buildDuration] and the
/// [FrameTiming.rasterDuration] together exceed the frame budget.
/// In those cases, animations will be smooth but touch input will
/// feel more sluggish.
///
/// Using [addTimingsCallback] is preferred over using
/// [dart:ui.PlatformDispatcher.onReportTimings] directly because the
/// [dart:ui.PlatformDispatcher.onReportTimings] API only allows one callback,
/// which prevents multiple libraries from registering listeners
/// simultaneously, while this API allows multiple callbacks to be registered
/// independently.
///
/// This API is implemented in terms of
/// [dart:ui.PlatformDispatcher.onReportTimings]. In release builds, when no
/// libraries have registered with this API, the
/// [dart:ui.PlatformDispatcher.onReportTimings] callback is not set, which
/// disables the performance tracking and reduces the runtime overhead to
/// approximately zero. The performance overhead of the performance tracking
/// when one or more callbacks are registered (i.e. when it is enabled) is
/// very approximately 0.01% CPU usage per second (measured on an iPhone 6s).
///
/// In debug and profile builds, the [SchedulerBinding] itself
/// registers a timings callback to update the [Timeline].
///
/// If the same callback is added twice, it will be executed twice.
///
/// See also:
///
/// * [removeTimingsCallback], which can be used to remove a callback
/// added using this method.
void addTimingsCallback(TimingsCallback callback) {
_timingsCallbacks.add(callback);
if (_timingsCallbacks.length == 1) {
assert(platformDispatcher.onReportTimings == null);
platformDispatcher.onReportTimings = _executeTimingsCallbacks;
}
assert(platformDispatcher.onReportTimings == _executeTimingsCallbacks);
}
/// Removes a callback that was earlier added by [addTimingsCallback].
void removeTimingsCallback(TimingsCallback callback) {
assert(_timingsCallbacks.contains(callback));
_timingsCallbacks.remove(callback);
if (_timingsCallbacks.isEmpty) {
platformDispatcher.onReportTimings = null;
}
}
@pragma('vm:notify-debugger-on-exception')
void _executeTimingsCallbacks(List<FrameTiming> timings) {
final List<TimingsCallback> clonedCallbacks =
List<TimingsCallback>.of(_timingsCallbacks);
for (final TimingsCallback callback in clonedCallbacks) {
try {
if (_timingsCallbacks.contains(callback)) {
callback(timings);
}
} catch (exception, stack) {
InformationCollector? collector;
assert(() {
collector = () => <DiagnosticsNode>[
DiagnosticsProperty<TimingsCallback>(
'The TimingsCallback that gets executed was',
callback,
style: DiagnosticsTreeStyle.errorProperty,
),
];
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
context: ErrorDescription('while executing callbacks for FrameTiming'),
informationCollector: collector,
));
}
}
}
@override
void initServiceExtensions() {
super.initServiceExtensions();
if (!kReleaseMode) {
registerNumericServiceExtension(
name: SchedulerServiceExtensions.timeDilation.name,
getter: () async => timeDilation,
setter: (double value) async {
timeDilation = value;
},
);
}
}
/// Whether the application is visible, and if so, whether it is currently
/// interactive.
///
/// This is set by [handleAppLifecycleStateChanged] when the
/// [SystemChannels.lifecycle] notification is dispatched.
///
/// The preferred ways to watch for changes to this value are using
/// [WidgetsBindingObserver.didChangeAppLifecycleState], or through an
/// [AppLifecycleListener] object.
AppLifecycleState? get lifecycleState => _lifecycleState;
AppLifecycleState? _lifecycleState;
/// Allows the test framework to reset the lifecycle state and framesEnabled
/// back to their initial values.
@visibleForTesting
void resetInternalState() {
_lifecycleState = null;
_framesEnabled = true;
}
/// Called when the application lifecycle state changes.
///
/// Notifies all the observers using
/// [WidgetsBindingObserver.didChangeAppLifecycleState].
///
/// This method exposes notifications from [SystemChannels.lifecycle].
@protected
@mustCallSuper
void handleAppLifecycleStateChanged(AppLifecycleState state) {
if (lifecycleState == state) {
return;
}
_lifecycleState = state;
switch (state) {
case AppLifecycleState.resumed:
case AppLifecycleState.inactive:
_setFramesEnabledState(true);
case AppLifecycleState.hidden:
case AppLifecycleState.paused:
case AppLifecycleState.detached:
_setFramesEnabledState(false);
}
}
/// The strategy to use when deciding whether to run a task or not.
///
/// Defaults to [defaultSchedulingStrategy].
SchedulingStrategy schedulingStrategy = defaultSchedulingStrategy;
static int _taskSorter (_TaskEntry<dynamic> e1, _TaskEntry<dynamic> e2) {
return -e1.priority.compareTo(e2.priority);
}
final PriorityQueue<_TaskEntry<dynamic>> _taskQueue = HeapPriorityQueue<_TaskEntry<dynamic>>(_taskSorter);
/// Schedules the given `task` with the given `priority`.
///
/// If `task` returns a future, the future returned by [scheduleTask] will
/// complete after the former future has been scheduled to completion.
/// Otherwise, the returned future for [scheduleTask] will complete with the
/// same value returned by `task` after it has been scheduled.
///
/// The `debugLabel` and `flow` are used to report the task to the [Timeline],
/// for use when profiling.
///
/// ## Processing model
///
/// Tasks will be executed between frames, in priority order,
/// excluding tasks that are skipped by the current
/// [schedulingStrategy]. Tasks should be short (as in, up to a
/// millisecond), so as to not cause the regular frame callbacks to
/// get delayed.
///
/// If an animation is running, including, for instance, a [ProgressIndicator]
/// indicating that there are pending tasks, then tasks with a priority below
/// [Priority.animation] won't run (at least, not with the
/// [defaultSchedulingStrategy]; this can be configured using
/// [schedulingStrategy]).
Future<T> scheduleTask<T>(
TaskCallback<T> task,
Priority priority, {
String? debugLabel,
Flow? flow,
}) {
final bool isFirstTask = _taskQueue.isEmpty;
final _TaskEntry<T> entry = _TaskEntry<T>(
task,
priority.value,
debugLabel,
flow,
);
_taskQueue.add(entry);
if (isFirstTask && !locked) {
_ensureEventLoopCallback();
}
return entry.completer.future;
}
@override
void unlocked() {
super.unlocked();
if (_taskQueue.isNotEmpty) {
_ensureEventLoopCallback();
}
}
// Whether this scheduler already requested to be called from the event loop.
bool _hasRequestedAnEventLoopCallback = false;
// Ensures that the scheduler services a task scheduled by
// [SchedulerBinding.scheduleTask].
void _ensureEventLoopCallback() {
assert(!locked);
assert(_taskQueue.isNotEmpty);
if (_hasRequestedAnEventLoopCallback) {
return;
}
_hasRequestedAnEventLoopCallback = true;
Timer.run(_runTasks);
}
// Scheduled by _ensureEventLoopCallback.
void _runTasks() {
_hasRequestedAnEventLoopCallback = false;
if (handleEventLoopCallback()) {
_ensureEventLoopCallback();
} // runs next task when there's time
}
/// Execute the highest-priority task, if it is of a high enough priority.
///
/// Returns true if a task was executed and there are other tasks remaining
/// (even if they are not high-enough priority).
///
/// Returns false if no task was executed, which can occur if there are no
/// tasks scheduled, if the scheduler is [locked], or if the highest-priority
/// task is of too low a priority given the current [schedulingStrategy].
///
/// Also returns false if there are no tasks remaining.
@visibleForTesting
@pragma('vm:notify-debugger-on-exception')
bool handleEventLoopCallback() {
if (_taskQueue.isEmpty || locked) {
return false;
}
final _TaskEntry<dynamic> entry = _taskQueue.first;
if (schedulingStrategy(priority: entry.priority, scheduler: this)) {
try {
_taskQueue.removeFirst();
entry.run();
} catch (exception, exceptionStack) {
StackTrace? callbackStack;
assert(() {
callbackStack = entry.debugStack;
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: exceptionStack,
library: 'scheduler library',
context: ErrorDescription('during a task callback'),
informationCollector: (callbackStack == null) ? null : () {
return <DiagnosticsNode>[
DiagnosticsStackTrace(
'\nThis exception was thrown in the context of a scheduler callback. '
'When the scheduler callback was _registered_ (as opposed to when the '
'exception was thrown), this was the stack',
callbackStack,
),
];
},
));
}
return _taskQueue.isNotEmpty;
}
return false;
}
int _nextFrameCallbackId = 0; // positive
Map<int, _FrameCallbackEntry> _transientCallbacks = <int, _FrameCallbackEntry>{};
final Set<int> _removedIds = HashSet<int>();
/// The current number of transient frame callbacks scheduled.
///
/// This is reset to zero just before all the currently scheduled
/// transient callbacks are called, at the start of a frame.
///
/// This number is primarily exposed so that tests can verify that
/// there are no unexpected transient callbacks still registered
/// after a test's resources have been gracefully disposed.
int get transientCallbackCount => _transientCallbacks.length;
/// Schedules the given transient frame callback.
///
/// Adds the given callback to the list of frame callbacks and ensures that a
/// frame is scheduled.
///
/// If this is called during the frame's animation phase (when transient frame
/// callbacks are still being invoked), a new frame will be scheduled, and
/// `callback` will be called in the newly scheduled frame, not in the current
/// frame.
///
/// If this is a one-off registration, ignore the `rescheduling` argument.
///
/// If this is a callback that will be re-registered each time it fires, then
/// when you re-register the callback, set the `rescheduling` argument to
/// true. This has no effect in release builds, but in debug builds, it
/// ensures that the stack trace that is stored for this callback is the
/// original stack trace for when the callback was _first_ registered, rather
/// than the stack trace for when the callback is re-registered. This makes it
/// easier to track down the original reason that a particular callback was
/// called. If `rescheduling` is true, the call must be in the context of a
/// frame callback.
///
/// Callbacks registered with this method can be canceled using
/// [cancelFrameCallbackWithId].
///
/// See also:
///
/// * [WidgetsBinding.drawFrame], which explains the phases of each frame
/// for those apps that use Flutter widgets (and where transient frame
/// callbacks fit into those phases).
int scheduleFrameCallback(FrameCallback callback, { bool rescheduling = false }) {
scheduleFrame();
_nextFrameCallbackId += 1;
_transientCallbacks[_nextFrameCallbackId] = _FrameCallbackEntry(callback, rescheduling: rescheduling);
return _nextFrameCallbackId;
}
/// Cancels the transient frame callback with the given [id].
///
/// Removes the given callback from the list of frame callbacks. If a frame
/// has been requested, this does not also cancel that request.
///
/// Transient frame callbacks are those registered using
/// [scheduleFrameCallback].
void cancelFrameCallbackWithId(int id) {
assert(id > 0);
_transientCallbacks.remove(id);
_removedIds.add(id);
}
/// Asserts that there are no registered transient callbacks; if
/// there are, prints their locations and throws an exception.
///
/// A transient frame callback is one that was registered with
/// [scheduleFrameCallback].
///
/// This is expected to be called at the end of tests (the
/// flutter_test framework does it automatically in normal cases).
///
/// Call this method when you expect there to be no transient
/// callbacks registered, in an assert statement with a message that
/// you want printed when a transient callback is registered:
///
/// ```dart
/// assert(SchedulerBinding.instance.debugAssertNoTransientCallbacks(
/// 'A leak of transient callbacks was detected while doing foo.'
/// ));
/// ```
///
/// Does nothing if asserts are disabled. Always returns true.
bool debugAssertNoTransientCallbacks(String reason) {
assert(() {
if (transientCallbackCount > 0) {
// We cache the values so that we can produce them later
// even if the information collector is called after
// the problem has been resolved.
final int count = transientCallbackCount;
final Map<int, _FrameCallbackEntry> callbacks = Map<int, _FrameCallbackEntry>.of(_transientCallbacks);
FlutterError.reportError(FlutterErrorDetails(
exception: reason,
library: 'scheduler library',
informationCollector: () => <DiagnosticsNode>[
if (count == 1)
// TODO(jacobr): I have added an extra line break in this case.
ErrorDescription(
'There was one transient callback left. '
'The stack trace for when it was registered is as follows:',
)
else
ErrorDescription(
'There were $count transient callbacks left. '
'The stack traces for when they were registered are as follows:',
),
for (final int id in callbacks.keys)
DiagnosticsStackTrace('── callback $id ──', callbacks[id]!.debugStack, showSeparator: false),
],
));
}
return true;
}());
return true;
}
/// Asserts that there are no pending performance mode requests in debug mode.
///
/// Throws a [FlutterError] if there are pending performance mode requests,
/// as this indicates a potential memory leak.
bool debugAssertNoPendingPerformanceModeRequests(String reason) {
assert(() {
if (_performanceMode != null) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
/// Asserts that there is no artificial time dilation in debug mode.
///
/// Throws a [FlutterError] if there are such dilation, as this will make
/// subsequent tests see dilation and thus flaky.
bool debugAssertNoTimeDilation(String reason) {
assert(() {
if (timeDilation != 1.0) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
/// Prints the stack for where the current transient callback was registered.
///
/// A transient frame callback is one that was registered with
/// [scheduleFrameCallback].
///
/// When called in debug more and in the context of a transient callback, this
/// function prints the stack trace from where the current transient callback
/// was registered (i.e. where it first called [scheduleFrameCallback]).
///
/// When called in debug mode in other contexts, it prints a message saying
/// that this function was not called in the context a transient callback.
///
/// In release mode, this function does nothing.
///
/// To call this function, use the following code:
///
/// ```dart
/// SchedulerBinding.debugPrintTransientCallbackRegistrationStack();
/// ```
static void debugPrintTransientCallbackRegistrationStack() {
assert(() {
if (_FrameCallbackEntry.debugCurrentCallbackStack != null) {
debugPrint('When the current transient callback was registered, this was the stack:');
debugPrint(
FlutterError.defaultStackFilter(
FlutterError.demangleStackTrace(
_FrameCallbackEntry.debugCurrentCallbackStack!,
).toString().trimRight().split('\n'),
).join('\n'),
);
} else {
debugPrint('No transient callback is currently executing.');
}
return true;
}());
}
final List<FrameCallback> _persistentCallbacks = <FrameCallback>[];
/// Adds a persistent frame callback.
///
/// Persistent callbacks are called after transient
/// (non-persistent) frame callbacks.
///
/// Does *not* request a new frame. Conceptually, persistent frame
/// callbacks are observers of "begin frame" events. Since they are
/// executed after the transient frame callbacks they can drive the
/// rendering pipeline.
///
/// Persistent frame callbacks cannot be unregistered. Once registered, they
/// are called for every frame for the lifetime of the application.
///
/// See also:
///
/// * [WidgetsBinding.drawFrame], which explains the phases of each frame
/// for those apps that use Flutter widgets (and where persistent frame
/// callbacks fit into those phases).
void addPersistentFrameCallback(FrameCallback callback) {
_persistentCallbacks.add(callback);
}
final List<FrameCallback> _postFrameCallbacks = <FrameCallback>[];
/// Schedule a callback for the end of this frame.
///
/// The provided callback is run immediately after a frame, just after the
/// persistent frame callbacks (which is when the main rendering pipeline has
/// been flushed).
///
/// This method does *not* request a new frame. If a frame is already in
/// progress and the execution of post-frame callbacks has not yet begun, then
/// the registered callback is executed at the end of the current frame.
/// Otherwise, the registered callback is executed after the next frame
/// (whenever that may be, if ever).
///
/// The callbacks are executed in the order in which they have been
/// added.
///
/// Post-frame callbacks cannot be unregistered. They are called exactly once.
///
/// In debug mode, if [debugTracePostFrameCallbacks] is set to true, then the
/// registered callback will show up in the timeline events chart, which can
/// be viewed in [DevTools](https://docs.flutter.dev/tools/devtools/overview).
/// In that case, the `debugLabel` argument specifies the name of the callback
/// as it will appear in the timeline. In profile and release builds,
/// post-frame are never traced, and the `debugLabel` argument is ignored.
///
/// See also:
///
/// * [scheduleFrameCallback], which registers a callback for the start of
/// the next frame.
/// * [WidgetsBinding.drawFrame], which explains the phases of each frame
/// for those apps that use Flutter widgets (and where post frame
/// callbacks fit into those phases).
void addPostFrameCallback(FrameCallback callback, {String debugLabel = 'callback'}) {
assert(() {
if (debugTracePostFrameCallbacks) {
final FrameCallback originalCallback = callback;
callback = (Duration timeStamp) {
Timeline.startSync(debugLabel);
try {
originalCallback(timeStamp);
} finally {
Timeline.finishSync();
}
};
}
return true;
}());
_postFrameCallbacks.add(callback);
}
Completer<void>? _nextFrameCompleter;
/// Returns a Future that completes after the frame completes.
///
/// If this is called between frames, a frame is immediately scheduled if
/// necessary. If this is called during a frame, the Future completes after
/// the current frame.
///
/// If the device's screen is currently turned off, this may wait a very long
/// time, since frames are not scheduled while the device's screen is turned
/// off.
Future<void> get endOfFrame {
if (_nextFrameCompleter == null) {
if (schedulerPhase == SchedulerPhase.idle) {
scheduleFrame();
}
_nextFrameCompleter = Completer<void>();
addPostFrameCallback((Duration timeStamp) {
_nextFrameCompleter!.complete();
_nextFrameCompleter = null;
}, debugLabel: 'SchedulerBinding.completeFrame');
}
return _nextFrameCompleter!.future;
}
/// Whether this scheduler has requested that [handleBeginFrame] be called soon.
bool get hasScheduledFrame => _hasScheduledFrame;
bool _hasScheduledFrame = false;
/// The phase that the scheduler is currently operating under.
SchedulerPhase get schedulerPhase => _schedulerPhase;
SchedulerPhase _schedulerPhase = SchedulerPhase.idle;
/// Whether frames are currently being scheduled when [scheduleFrame] is called.
///
/// This value depends on the value of the [lifecycleState].
bool get framesEnabled => _framesEnabled;
bool _framesEnabled = true;
void _setFramesEnabledState(bool enabled) {
if (_framesEnabled == enabled) {
return;
}
_framesEnabled = enabled;
if (enabled) {
scheduleFrame();
}
}
/// Ensures callbacks for [PlatformDispatcher.onBeginFrame] and
/// [PlatformDispatcher.onDrawFrame] are registered.
@protected
void ensureFrameCallbacksRegistered() {
platformDispatcher.onBeginFrame ??= _handleBeginFrame;
platformDispatcher.onDrawFrame ??= _handleDrawFrame;
}
/// Schedules a new frame using [scheduleFrame] if this object is not
/// currently producing a frame.
///
/// Calling this method ensures that [handleDrawFrame] will eventually be
/// called, unless it's already in progress.
///
/// This has no effect if [schedulerPhase] is
/// [SchedulerPhase.transientCallbacks] or [SchedulerPhase.midFrameMicrotasks]
/// (because a frame is already being prepared in that case), or
/// [SchedulerPhase.persistentCallbacks] (because a frame is actively being
/// rendered in that case). It will schedule a frame if the [schedulerPhase]
/// is [SchedulerPhase.idle] (in between frames) or
/// [SchedulerPhase.postFrameCallbacks] (after a frame).
void ensureVisualUpdate() {
switch (schedulerPhase) {
case SchedulerPhase.idle:
case SchedulerPhase.postFrameCallbacks:
scheduleFrame();
return;
case SchedulerPhase.transientCallbacks:
case SchedulerPhase.midFrameMicrotasks:
case SchedulerPhase.persistentCallbacks:
return;
}
}
/// If necessary, schedules a new frame by calling
/// [dart:ui.PlatformDispatcher.scheduleFrame].
///
/// After this is called, the engine will (eventually) call
/// [handleBeginFrame]. (This call might be delayed, e.g. if the device's
/// screen is turned off it will typically be delayed until the screen is on
/// and the application is visible.) Calling this during a frame forces
/// another frame to be scheduled, even if the current frame has not yet
/// completed.
///
/// Scheduled frames are serviced when triggered by a "Vsync" signal provided
/// by the operating system. The "Vsync" signal, or vertical synchronization
/// signal, was historically related to the display refresh, at a time when
/// hardware physically moved a beam of electrons vertically between updates
/// of the display. The operation of contemporary hardware is somewhat more
/// subtle and complicated, but the conceptual "Vsync" refresh signal continue
/// to be used to indicate when applications should update their rendering.
///
/// To have a stack trace printed to the console any time this function
/// schedules a frame, set [debugPrintScheduleFrameStacks] to true.
///
/// See also:
///
/// * [scheduleForcedFrame], which ignores the [lifecycleState] when
/// scheduling a frame.
/// * [scheduleWarmUpFrame], which ignores the "Vsync" signal entirely and
/// triggers a frame immediately.
void scheduleFrame() {
if (_hasScheduledFrame || !framesEnabled) {
return;
}
assert(() {
if (debugPrintScheduleFrameStacks) {
debugPrintStack(label: 'scheduleFrame() called. Current phase is $schedulerPhase.');
}
return true;
}());
ensureFrameCallbacksRegistered();
platformDispatcher.scheduleFrame();
_hasScheduledFrame = true;
}
/// Schedules a new frame by calling
/// [dart:ui.PlatformDispatcher.scheduleFrame].
///
/// After this is called, the engine will call [handleBeginFrame], even if
/// frames would normally not be scheduled by [scheduleFrame] (e.g. even if
/// the device's screen is turned off).
///
/// The framework uses this to force a frame to be rendered at the correct
/// size when the phone is rotated, so that a correctly-sized rendering is
/// available when the screen is turned back on.
///
/// To have a stack trace printed to the console any time this function
/// schedules a frame, set [debugPrintScheduleFrameStacks] to true.
///
/// Prefer using [scheduleFrame] unless it is imperative that a frame be
/// scheduled immediately, since using [scheduleForcedFrame] will cause
/// significantly higher battery usage when the device should be idle.
///
/// Consider using [scheduleWarmUpFrame] instead if the goal is to update the
/// rendering as soon as possible (e.g. at application startup).
void scheduleForcedFrame() {
if (_hasScheduledFrame) {
return;
}
assert(() {
if (debugPrintScheduleFrameStacks) {
debugPrintStack(label: 'scheduleForcedFrame() called. Current phase is $schedulerPhase.');
}
return true;
}());
ensureFrameCallbacksRegistered();
platformDispatcher.scheduleFrame();
_hasScheduledFrame = true;
}
bool _warmUpFrame = false;
/// Schedule a frame to run as soon as possible, rather than waiting for
/// the engine to request a frame in response to a system "Vsync" signal.
///
/// This is used during application startup so that the first frame (which is
/// likely to be quite expensive) gets a few extra milliseconds to run.
///
/// Locks events dispatching until the scheduled frame has completed.
///
/// If a frame has already been scheduled with [scheduleFrame] or
/// [scheduleForcedFrame], this call may delay that frame.
///
/// If any scheduled frame has already begun or if another
/// [scheduleWarmUpFrame] was already called, this call will be ignored.
///
/// Prefer [scheduleFrame] to update the display in normal operation.
///
/// ## Design discussion
///
/// The Flutter engine prompts the framework to generate frames when it
/// receives a request from the operating system (known for historical reasons
/// as a vsync). However, this may not happen for several milliseconds after
/// the app starts (or after a hot reload). To make use of the time between
/// when the widget tree is first configured and when the engine requests an
/// update, the framework schedules a _warm-up frame_.
///
/// A warm-up frame may never actually render (as the engine did not request
/// it and therefore does not have a valid context in which to paint), but it
/// will cause the framework to go through the steps of building, laying out,
/// and painting, which can together take several milliseconds. Thus, when the
/// engine requests a real frame, much of the work will already have been
/// completed, and the framework can generate the frame with minimal
/// additional effort.
///
/// Warm-up frames are scheduled by [runApp] on startup, and by
/// [RendererBinding.performReassemble] during a hot reload.
///
/// Warm-up frames are also scheduled when the framework is unblocked by a
/// call to [RendererBinding.allowFirstFrame] (corresponding to a call to
/// [RendererBinding.deferFirstFrame] that blocked the rendering).
void scheduleWarmUpFrame() {
if (_warmUpFrame || schedulerPhase != SchedulerPhase.idle) {
return;
}
_warmUpFrame = true;
TimelineTask? debugTimelineTask;
if (!kReleaseMode) {
debugTimelineTask = TimelineTask()..start('Warm-up frame');
}
final bool hadScheduledFrame = _hasScheduledFrame;
PlatformDispatcher.instance.scheduleWarmUpFrame(
beginFrame: () {
assert(_warmUpFrame);
handleBeginFrame(null);
},
drawFrame: () {
assert(_warmUpFrame);
handleDrawFrame();
// We call resetEpoch after this frame so that, in the hot reload case,
// the very next frame pretends to have occurred immediately after this
// warm-up frame. The warm-up frame's timestamp will typically be far in
// the past (the time of the last real frame), so if we didn't reset the
// epoch we would see a sudden jump from the old time in the warm-up frame
// to the new time in the "real" frame. The biggest problem with this is
// that implicit animations end up being triggered at the old time and
// then skipping every frame and finishing in the new time.
resetEpoch();
_warmUpFrame = false;
if (hadScheduledFrame) {
scheduleFrame();
}
},
);
// Lock events so touch events etc don't insert themselves until the
// scheduled frame has finished.
lockEvents(() async {
await endOfFrame;
if (!kReleaseMode) {
debugTimelineTask!.finish();
}
});
}
Duration? _firstRawTimeStampInEpoch;
Duration _epochStart = Duration.zero;
Duration _lastRawTimeStamp = Duration.zero;
/// Prepares the scheduler for a non-monotonic change to how time stamps are
/// calculated.
///
/// Callbacks received from the scheduler assume that their time stamps are
/// monotonically increasing. The raw time stamp passed to [handleBeginFrame]
/// is monotonic, but the scheduler might adjust those time stamps to provide
/// [timeDilation]. Without careful handling, these adjusts could cause time
/// to appear to run backwards.
///
/// The [resetEpoch] function ensures that the time stamps are monotonic by
/// resetting the base time stamp used for future time stamp adjustments to the
/// current value. For example, if the [timeDilation] decreases, rather than
/// scaling down the [Duration] since the beginning of time, [resetEpoch] will
/// ensure that we only scale down the duration since [resetEpoch] was called.
///
/// Setting [timeDilation] calls [resetEpoch] automatically. You don't need to
/// call [resetEpoch] yourself.
void resetEpoch() {
_epochStart = _adjustForEpoch(_lastRawTimeStamp);
_firstRawTimeStampInEpoch = null;
}
/// Adjusts the given time stamp into the current epoch.
///
/// This both offsets the time stamp to account for when the epoch started
/// (both in raw time and in the epoch's own time line) and scales the time
/// stamp to reflect the time dilation in the current epoch.
///
/// These mechanisms together combine to ensure that the durations we give
/// during frame callbacks are monotonically increasing.
Duration _adjustForEpoch(Duration rawTimeStamp) {
final Duration rawDurationSinceEpoch = _firstRawTimeStampInEpoch == null ? Duration.zero : rawTimeStamp - _firstRawTimeStampInEpoch!;
return Duration(microseconds: (rawDurationSinceEpoch.inMicroseconds / timeDilation).round() + _epochStart.inMicroseconds);
}
/// The time stamp for the frame currently being processed.
///
/// This is only valid while between the start of [handleBeginFrame] and the
/// end of the corresponding [handleDrawFrame], i.e. while a frame is being
/// produced.
Duration get currentFrameTimeStamp {
assert(_currentFrameTimeStamp != null);
return _currentFrameTimeStamp!;
}
Duration? _currentFrameTimeStamp;
/// The raw time stamp as provided by the engine to
/// [dart:ui.PlatformDispatcher.onBeginFrame] for the frame currently being
/// processed.
///
/// Unlike [currentFrameTimeStamp], this time stamp is neither adjusted to
/// offset when the epoch started nor scaled to reflect the [timeDilation] in
/// the current epoch.
///
/// On most platforms, this is a more or less arbitrary value, and should
/// generally be ignored. On Fuchsia, this corresponds to the system-provided
/// presentation time, and can be used to ensure that animations running in
/// different processes are synchronized.
Duration get currentSystemFrameTimeStamp {
return _lastRawTimeStamp;
}
int _debugFrameNumber = 0;
String? _debugBanner;
// Whether the current engine frame needs to be postponed till after the
// warm-up frame.
//
// Engine may begin a frame in the middle of the warm-up frame because the
// warm-up frame is scheduled by timers while the engine frame is scheduled
// by platform specific frame scheduler (e.g. `requestAnimationFrame` on the
// web). When this happens, we let the warm-up frame finish, and postpone the
// engine frame.
bool _rescheduleAfterWarmUpFrame = false;
void _handleBeginFrame(Duration rawTimeStamp) {
if (_warmUpFrame) {
// "begin frame" and "draw frame" must strictly alternate. Therefore
// _rescheduleAfterWarmUpFrame cannot possibly be true here as it is
// reset by _handleDrawFrame.
assert(!_rescheduleAfterWarmUpFrame);
_rescheduleAfterWarmUpFrame = true;
return;
}
handleBeginFrame(rawTimeStamp);
}
void _handleDrawFrame() {
if (_rescheduleAfterWarmUpFrame) {
_rescheduleAfterWarmUpFrame = false;
// Reschedule in a post-frame callback to allow the draw-frame phase of
// the warm-up frame to finish.
addPostFrameCallback((Duration timeStamp) {
// Force an engine frame.
//
// We need to reset _hasScheduledFrame here because we cancelled the
// original engine frame, and therefore did not run handleBeginFrame
// who is responsible for resetting it. So if a frame callback set this
// to true in the "begin frame" part of the warm-up frame, it will
// still be true here and cause us to skip scheduling an engine frame.
_hasScheduledFrame = false;
scheduleFrame();
}, debugLabel: 'SchedulerBinding.scheduleFrame');
return;
}
handleDrawFrame();
}
final TimelineTask? _frameTimelineTask = kReleaseMode ? null : TimelineTask();
/// Called by the engine to prepare the framework to produce a new frame.
///
/// This function calls all the transient frame callbacks registered by
/// [scheduleFrameCallback]. It then returns, any scheduled microtasks are run
/// (e.g. handlers for any [Future]s resolved by transient frame callbacks),
/// and [handleDrawFrame] is called to continue the frame.
///
/// If the given time stamp is null, the time stamp from the last frame is
/// reused.
///
/// To have a banner shown at the start of every frame in debug mode, set
/// [debugPrintBeginFrameBanner] to true. The banner will be printed to the
/// console using [debugPrint] and will contain the frame number (which
/// increments by one for each frame), and the time stamp of the frame. If the
/// given time stamp was null, then the string "warm-up frame" is shown
/// instead of the time stamp. This allows frames eagerly pushed by the
/// framework to be distinguished from those requested by the engine in
/// response to the "Vsync" signal from the operating system.
///
/// You can also show a banner at the end of every frame by setting
/// [debugPrintEndFrameBanner] to true. This allows you to distinguish log
/// statements printed during a frame from those printed between frames (e.g.
/// in response to events or timers).
void handleBeginFrame(Duration? rawTimeStamp) {
_frameTimelineTask?.start('Frame');
_firstRawTimeStampInEpoch ??= rawTimeStamp;
_currentFrameTimeStamp = _adjustForEpoch(rawTimeStamp ?? _lastRawTimeStamp);
if (rawTimeStamp != null) {
_lastRawTimeStamp = rawTimeStamp;
}
assert(() {
_debugFrameNumber += 1;
if (debugPrintBeginFrameBanner || debugPrintEndFrameBanner) {
final StringBuffer frameTimeStampDescription = StringBuffer();
if (rawTimeStamp != null) {
_debugDescribeTimeStamp(_currentFrameTimeStamp!, frameTimeStampDescription);
} else {
frameTimeStampDescription.write('(warm-up frame)');
}
_debugBanner = '▄▄▄▄▄▄▄▄ Frame ${_debugFrameNumber.toString().padRight(7)} ${frameTimeStampDescription.toString().padLeft(18)} ▄▄▄▄▄▄▄▄';
if (debugPrintBeginFrameBanner) {
debugPrint(_debugBanner);
}
}
return true;
}());
assert(schedulerPhase == SchedulerPhase.idle);
_hasScheduledFrame = false;
try {
// TRANSIENT FRAME CALLBACKS
_frameTimelineTask?.start('Animate');
_schedulerPhase = SchedulerPhase.transientCallbacks;
final Map<int, _FrameCallbackEntry> callbacks = _transientCallbacks;
_transientCallbacks = <int, _FrameCallbackEntry>{};
callbacks.forEach((int id, _FrameCallbackEntry callbackEntry) {
if (!_removedIds.contains(id)) {
_invokeFrameCallback(callbackEntry.callback, _currentFrameTimeStamp!, callbackEntry.debugStack);
}
});
_removedIds.clear();
} finally {
_schedulerPhase = SchedulerPhase.midFrameMicrotasks;
}
}
DartPerformanceMode? _performanceMode;
int _numPerformanceModeRequests = 0;
/// Request a specific [DartPerformanceMode].
///
/// Returns `null` if the request was not successful due to conflicting performance mode requests.
/// Two requests are said to be in conflict if they are not of the same [DartPerformanceMode] type,
/// and an explicit request for a performance mode has been made prior.
///
/// Requestor is responsible for calling [PerformanceModeRequestHandle.dispose] when it no longer
/// requires the performance mode.
PerformanceModeRequestHandle? requestPerformanceMode(DartPerformanceMode mode) {
// conflicting requests are not allowed.
if (_performanceMode != null && _performanceMode != mode) {
return null;
}
if (_performanceMode == mode) {
assert(_numPerformanceModeRequests > 0);
_numPerformanceModeRequests++;
} else if (_performanceMode == null) {
assert(_numPerformanceModeRequests == 0);
_performanceMode = mode;
_numPerformanceModeRequests = 1;
}
return PerformanceModeRequestHandle._(_disposePerformanceModeRequest);
}
/// Remove a request for a specific [DartPerformanceMode].
///
/// If all the pending requests have been disposed, the engine will revert to the
/// [DartPerformanceMode.balanced] performance mode.
void _disposePerformanceModeRequest() {
_numPerformanceModeRequests--;
if (_numPerformanceModeRequests == 0) {
_performanceMode = null;
PlatformDispatcher.instance.requestDartPerformanceMode(DartPerformanceMode.balanced);
}
}
/// Returns the current [DartPerformanceMode] requested or `null` if no requests have
/// been made.
///
/// This is only supported in debug and profile modes, returns `null` in release mode.
DartPerformanceMode? debugGetRequestedPerformanceMode() {
if (!(kDebugMode || kProfileMode)) {
return null;
} else {
return _performanceMode;
}
}
/// Called by the engine to produce a new frame.
///
/// This method is called immediately after [handleBeginFrame]. It calls all
/// the callbacks registered by [addPersistentFrameCallback], which typically
/// drive the rendering pipeline, and then calls the callbacks registered by
/// [addPostFrameCallback].
///
/// See [handleBeginFrame] for a discussion about debugging hooks that may be
/// useful when working with frame callbacks.
void handleDrawFrame() {
assert(_schedulerPhase == SchedulerPhase.midFrameMicrotasks);
_frameTimelineTask?.finish(); // end the "Animate" phase
try {
// PERSISTENT FRAME CALLBACKS
_schedulerPhase = SchedulerPhase.persistentCallbacks;
for (final FrameCallback callback in List<FrameCallback>.of(_persistentCallbacks)) {
_invokeFrameCallback(callback, _currentFrameTimeStamp!);
}
// POST-FRAME CALLBACKS
_schedulerPhase = SchedulerPhase.postFrameCallbacks;
final List<FrameCallback> localPostFrameCallbacks =
List<FrameCallback>.of(_postFrameCallbacks);
_postFrameCallbacks.clear();
if (!kReleaseMode) {
FlutterTimeline.startSync('POST_FRAME');
}
try {
for (final FrameCallback callback in localPostFrameCallbacks) {
_invokeFrameCallback(callback, _currentFrameTimeStamp!);
}
} finally {
if (!kReleaseMode) {
FlutterTimeline.finishSync();
}
}
} finally {
_schedulerPhase = SchedulerPhase.idle;
_frameTimelineTask?.finish(); // end the Frame
assert(() {
if (debugPrintEndFrameBanner) {
debugPrint('▀' * _debugBanner!.length);
}
_debugBanner = null;
return true;
}());
_currentFrameTimeStamp = null;
}
}
void _profileFramePostEvent(FrameTiming frameTiming) {
postEvent('Flutter.Frame', <String, dynamic>{
'number': frameTiming.frameNumber,
'startTime': frameTiming.timestampInMicroseconds(FramePhase.buildStart),
'elapsed': frameTiming.totalSpan.inMicroseconds,
'build': frameTiming.buildDuration.inMicroseconds,
'raster': frameTiming.rasterDuration.inMicroseconds,
'vsyncOverhead': frameTiming.vsyncOverhead.inMicroseconds,
});
}
static void _debugDescribeTimeStamp(Duration timeStamp, StringBuffer buffer) {
if (timeStamp.inDays > 0) {
buffer.write('${timeStamp.inDays}d ');
}
if (timeStamp.inHours > 0) {
buffer.write('${timeStamp.inHours - timeStamp.inDays * Duration.hoursPerDay}h ');
}
if (timeStamp.inMinutes > 0) {
buffer.write('${timeStamp.inMinutes - timeStamp.inHours * Duration.minutesPerHour}m ');
}
if (timeStamp.inSeconds > 0) {
buffer.write('${timeStamp.inSeconds - timeStamp.inMinutes * Duration.secondsPerMinute}s ');
}
buffer.write('${timeStamp.inMilliseconds - timeStamp.inSeconds * Duration.millisecondsPerSecond}');
final int microseconds = timeStamp.inMicroseconds - timeStamp.inMilliseconds * Duration.microsecondsPerMillisecond;
if (microseconds > 0) {
buffer.write('.${microseconds.toString().padLeft(3, "0")}');
}
buffer.write('ms');
}
// Calls the given [callback] with [timestamp] as argument.
//
// Wraps the callback in a try/catch and forwards any error to
// [debugSchedulerExceptionHandler], if set. If not set, prints
// the error.
@pragma('vm:notify-debugger-on-exception')
void _invokeFrameCallback(FrameCallback callback, Duration timeStamp, [ StackTrace? callbackStack ]) {
assert(_FrameCallbackEntry.debugCurrentCallbackStack == null);
assert(() {
_FrameCallbackEntry.debugCurrentCallbackStack = callbackStack;
return true;
}());
try {
callback(timeStamp);
} catch (exception, exceptionStack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: exceptionStack,
library: 'scheduler library',
context: ErrorDescription('during a scheduler callback'),
informationCollector: (callbackStack == null) ? null : () {
return <DiagnosticsNode>[
DiagnosticsStackTrace(
'\nThis exception was thrown in the context of a scheduler callback. '
'When the scheduler callback was _registered_ (as opposed to when the '
'exception was thrown), this was the stack',
callbackStack,
),
];
},
));
}
assert(() {
_FrameCallbackEntry.debugCurrentCallbackStack = null;
return true;
}());
}
}
/// The default [SchedulingStrategy] for [SchedulerBinding.schedulingStrategy].
///
/// If there are any frame callbacks registered, only runs tasks with
/// a [Priority] of [Priority.animation] or higher. Otherwise, runs
/// all tasks.
bool defaultSchedulingStrategy({ required int priority, required SchedulerBinding scheduler }) {
if (scheduler.transientCallbackCount > 0) {
return priority >= Priority.animation.value;
}
return true;
}
| flutter/packages/flutter/lib/src/scheduler/binding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/scheduler/binding.dart",
"repo_id": "flutter",
"token_count": 17665
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'asset_bundle.dart';
import 'binary_messenger.dart';
import 'debug.dart';
import 'hardware_keyboard.dart';
import 'message_codec.dart';
import 'platform_channel.dart';
import 'raw_keyboard.dart' show RawKeyboard;
import 'restoration.dart';
import 'service_extensions.dart';
import 'system_channels.dart';
import 'text_input.dart';
export 'dart:ui' show ChannelBuffers, RootIsolateToken;
export 'binary_messenger.dart' show BinaryMessenger;
export 'hardware_keyboard.dart' show HardwareKeyboard, KeyEventManager;
export 'restoration.dart' show RestorationManager;
/// Listens for platform messages and directs them to the [defaultBinaryMessenger].
///
/// The [ServicesBinding] also registers a [LicenseEntryCollector] that exposes
/// the licenses found in the `LICENSE` file stored at the root of the asset
/// bundle, and implements the `ext.flutter.evict` service extension (see
/// [evict]).
mixin ServicesBinding on BindingBase, SchedulerBinding {
@override
void initInstances() {
super.initInstances();
_instance = this;
_defaultBinaryMessenger = createBinaryMessenger();
_restorationManager = createRestorationManager();
_initKeyboard();
initLicenses();
SystemChannels.system.setMessageHandler((dynamic message) => handleSystemMessage(message as Object));
SystemChannels.accessibility.setMessageHandler((dynamic message) => _handleAccessibilityMessage(message as Object));
SystemChannels.lifecycle.setMessageHandler(_handleLifecycleMessage);
SystemChannels.platform.setMethodCallHandler(_handlePlatformMessage);
TextInput.ensureInitialized();
readInitialLifecycleStateFromNativeWindow();
initializationComplete();
}
/// The current [ServicesBinding], if one has been created.
///
/// Provides access to the features exposed by this mixin. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [runApp] or [WidgetsFlutterBinding.ensureInitialized].
static ServicesBinding get instance => BindingBase.checkInstance(_instance);
static ServicesBinding? _instance;
/// The global singleton instance of [HardwareKeyboard], which can be used to
/// query keyboard states.
HardwareKeyboard get keyboard => _keyboard;
late final HardwareKeyboard _keyboard;
/// The global singleton instance of [KeyEventManager], which is used
/// internally to dispatch key messages.
///
/// This property is deprecated, and will be removed. See
/// [HardwareKeyboard.addHandler] instead.
@Deprecated(
'No longer supported. Add a handler to HardwareKeyboard instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
KeyEventManager get keyEventManager => _keyEventManager;
late final KeyEventManager _keyEventManager;
void _initKeyboard() {
_keyboard = HardwareKeyboard();
_keyEventManager = KeyEventManager(_keyboard, RawKeyboard.instance);
_keyboard.syncKeyboardState().then((_) {
platformDispatcher.onKeyData = _keyEventManager.handleKeyData;
SystemChannels.keyEvent.setMessageHandler(_keyEventManager.handleRawKeyMessage);
});
}
/// The default instance of [BinaryMessenger].
///
/// This is used to send messages from the application to the platform, and
/// keeps track of which handlers have been registered on each channel so
/// it may dispatch incoming messages to the registered handler.
///
/// The default implementation returns a [BinaryMessenger] that delivers the
/// messages in the same order in which they are sent.
BinaryMessenger get defaultBinaryMessenger => _defaultBinaryMessenger;
late final BinaryMessenger _defaultBinaryMessenger;
/// A token that represents the root isolate, used for coordinating with
/// background isolates.
///
/// This property is primarily intended for use with
/// [BackgroundIsolateBinaryMessenger.ensureInitialized], which takes a
/// [RootIsolateToken] as its argument. The value `null` is returned when
/// executed from background isolates.
static ui.RootIsolateToken? get rootIsolateToken => ui.RootIsolateToken.instance;
/// The low level buffering and dispatch mechanism for messages sent by
/// plugins on the engine side to their corresponding plugin code on
/// the framework side.
///
/// This exposes the [dart:ui.channelBuffers] object. Bindings can override
/// this getter to intercept calls to the [ChannelBuffers] mechanism (for
/// example, for tests).
///
/// In production, direct access to this object should not be necessary.
/// Messages are received and dispatched by the [defaultBinaryMessenger]. This
/// object is primarily used to send mock messages in tests, via the
/// [ChannelBuffers.push] method (simulating a plugin sending a message to the
/// framework).
///
/// See also:
///
/// * [PlatformDispatcher.sendPlatformMessage], which is used for sending
/// messages to plugins from the framework (the opposite of
/// [channelBuffers]).
/// * [platformDispatcher], the [PlatformDispatcher] singleton.
ui.ChannelBuffers get channelBuffers => ui.channelBuffers;
/// Creates a default [BinaryMessenger] instance that can be used for sending
/// platform messages.
///
/// Many Flutter framework components that communicate with the platform
/// assume messages are received by the platform in the same order in which
/// they are sent. When overriding this method, be sure the [BinaryMessenger]
/// implementation guarantees FIFO delivery.
@protected
BinaryMessenger createBinaryMessenger() {
return const _DefaultBinaryMessenger._();
}
/// Called when the operating system notifies the application of a memory
/// pressure situation.
///
/// This method exposes the `memoryPressure` notification from
/// [SystemChannels.system].
@protected
@mustCallSuper
void handleMemoryPressure() {
rootBundle.clear();
}
/// Handler called for messages received on the [SystemChannels.system]
/// message channel.
///
/// Other bindings may override this to respond to incoming system messages.
@protected
@mustCallSuper
Future<void> handleSystemMessage(Object systemMessage) async {
final Map<String, dynamic> message = systemMessage as Map<String, dynamic>;
final String type = message['type'] as String;
switch (type) {
case 'memoryPressure':
handleMemoryPressure();
}
return;
}
/// Adds relevant licenses to the [LicenseRegistry].
///
/// By default, the [ServicesBinding]'s implementation of [initLicenses] adds
/// all the licenses collected by the `flutter` tool during compilation.
@protected
@mustCallSuper
void initLicenses() {
LicenseRegistry.addLicense(_addLicenses);
}
Stream<LicenseEntry> _addLicenses() {
late final StreamController<LicenseEntry> controller;
controller = StreamController<LicenseEntry>(
onListen: () async {
late final String rawLicenses;
if (kIsWeb) {
// NOTICES for web isn't compressed since we don't have access to
// dart:io on the client side and it's already compressed between
// the server and client.
rawLicenses = await rootBundle.loadString('NOTICES', cache: false);
} else {
// The compressed version doesn't have a more common .gz extension
// because gradle for Android non-transparently manipulates .gz files.
final ByteData licenseBytes = await rootBundle.load('NOTICES.Z');
final List<int> unzippedBytes = await compute<List<int>, List<int>>(gzip.decode, licenseBytes.buffer.asUint8List(), debugLabel: 'decompressLicenses');
rawLicenses = await compute<List<int>, String>(utf8.decode, unzippedBytes, debugLabel: 'utf8DecodeLicenses');
}
final List<LicenseEntry> licenses = await compute<String, List<LicenseEntry>>(_parseLicenses, rawLicenses, debugLabel: 'parseLicenses');
licenses.forEach(controller.add);
await controller.close();
},
);
return controller.stream;
}
// This is run in another isolate created by _addLicenses above.
static List<LicenseEntry> _parseLicenses(String rawLicenses) {
final String licenseSeparator = '\n${'-' * 80}\n';
final List<LicenseEntry> result = <LicenseEntry>[];
final List<String> licenses = rawLicenses.split(licenseSeparator);
for (final String license in licenses) {
final int split = license.indexOf('\n\n');
if (split >= 0) {
result.add(LicenseEntryWithLineBreaks(
license.substring(0, split).split('\n'),
license.substring(split + 2),
));
} else {
result.add(LicenseEntryWithLineBreaks(const <String>[], license));
}
}
return result;
}
@override
void initServiceExtensions() {
super.initServiceExtensions();
assert(() {
registerStringServiceExtension(
name: ServicesServiceExtensions.evict.name,
getter: () async => '',
setter: (String value) async {
evict(value);
},
);
return true;
}());
if (!kReleaseMode) {
registerBoolServiceExtension(
name: ServicesServiceExtensions.profilePlatformChannels.name,
getter: () async => debugProfilePlatformChannels,
setter: (bool value) async {
debugProfilePlatformChannels = value;
},
);
}
}
/// Called in response to the `ext.flutter.evict` service extension.
///
/// This is used by the `flutter` tool during hot reload so that any images
/// that have changed on disk get cleared from caches.
@protected
@mustCallSuper
void evict(String asset) {
rootBundle.evict(asset);
}
// App life cycle
/// Initializes the [lifecycleState] with the
/// [dart:ui.PlatformDispatcher.initialLifecycleState].
///
/// Once the [lifecycleState] is populated through any means (including this
/// method), this method will do nothing. This is because the
/// [dart:ui.PlatformDispatcher.initialLifecycleState] may already be stale
/// and it no longer makes sense to use the initial state at dart vm startup
/// as the current state anymore.
///
/// The latest state should be obtained by subscribing to
/// [WidgetsBindingObserver.didChangeAppLifecycleState].
@protected
void readInitialLifecycleStateFromNativeWindow() {
if (lifecycleState != null || platformDispatcher.initialLifecycleState.isEmpty) {
return;
}
_handleLifecycleMessage(platformDispatcher.initialLifecycleState);
}
Future<String?> _handleLifecycleMessage(String? message) async {
final AppLifecycleState? state = _parseAppLifecycleMessage(message!);
final List<AppLifecycleState> generated = _generateStateTransitions(lifecycleState, state!);
generated.forEach(handleAppLifecycleStateChanged);
return null;
}
List<AppLifecycleState> _generateStateTransitions(AppLifecycleState? previousState, AppLifecycleState state) {
if (previousState == state) {
return const <AppLifecycleState>[];
}
final List<AppLifecycleState> stateChanges = <AppLifecycleState>[];
if (previousState == null) {
// If there was no previous state, just jump directly to the new state.
stateChanges.add(state);
} else {
final int previousStateIndex = AppLifecycleState.values.indexOf(previousState);
final int stateIndex = AppLifecycleState.values.indexOf(state);
assert(previousStateIndex != -1, 'State $previousState missing in stateOrder array');
assert(stateIndex != -1, 'State $state missing in stateOrder array');
if (state == AppLifecycleState.detached) {
for (int i = previousStateIndex + 1; i < AppLifecycleState.values.length; ++i) {
stateChanges.add(AppLifecycleState.values[i]);
}
stateChanges.add(AppLifecycleState.detached);
} else if (previousStateIndex > stateIndex) {
for (int i = stateIndex; i < previousStateIndex; ++i) {
stateChanges.insert(0, AppLifecycleState.values[i]);
}
} else {
for (int i = previousStateIndex + 1; i <= stateIndex; ++i) {
stateChanges.add(AppLifecycleState.values[i]);
}
}
}
assert((){
AppLifecycleState? starting = previousState;
for (final AppLifecycleState ending in stateChanges) {
if (!_debugVerifyLifecycleChange(starting, ending)) {
return false;
}
starting = ending;
}
return true;
}(), 'Invalid lifecycle state transition generated from $previousState to $state (generated $stateChanges)');
return stateChanges;
}
static bool _debugVerifyLifecycleChange(AppLifecycleState? starting, AppLifecycleState ending) {
if (starting == null) {
// Any transition from null is fine, since it is initializing the state.
return true;
}
if (starting == ending) {
// Any transition to itself shouldn't happen.
return false;
}
return switch (starting) {
// Can't go from resumed to detached directly (must go through paused).
AppLifecycleState.resumed => ending == AppLifecycleState.inactive,
AppLifecycleState.detached => ending == AppLifecycleState.resumed || ending == AppLifecycleState.paused,
AppLifecycleState.inactive => ending == AppLifecycleState.resumed || ending == AppLifecycleState.hidden,
AppLifecycleState.hidden => ending == AppLifecycleState.paused || ending == AppLifecycleState.inactive,
AppLifecycleState.paused => ending == AppLifecycleState.hidden || ending == AppLifecycleState.detached,
};
}
/// Listenable that notifies when the accessibility focus on the system have changed.
final ValueNotifier<int?> accessibilityFocus = ValueNotifier<int?>(null);
Future<void> _handleAccessibilityMessage(Object accessibilityMessage) async {
final Map<String, dynamic> message =
(accessibilityMessage as Map<Object?, Object?>).cast<String, dynamic>();
final String type = message['type'] as String;
switch (type) {
case 'didGainFocus':
accessibilityFocus.value = message['nodeId'] as int;
}
return;
}
Future<dynamic> _handlePlatformMessage(MethodCall methodCall) async {
final String method = methodCall.method;
assert(method == 'SystemChrome.systemUIChange' || method == 'System.requestAppExit');
switch (method) {
case 'SystemChrome.systemUIChange':
final List<dynamic> args = methodCall.arguments as List<dynamic>;
if (_systemUiChangeCallback != null) {
await _systemUiChangeCallback!(args[0] as bool);
}
case 'System.requestAppExit':
return <String, dynamic>{'response': (await handleRequestAppExit()).name};
}
}
static AppLifecycleState? _parseAppLifecycleMessage(String message) {
return switch (message) {
'AppLifecycleState.resumed' => AppLifecycleState.resumed,
'AppLifecycleState.inactive' => AppLifecycleState.inactive,
'AppLifecycleState.hidden' => AppLifecycleState.hidden,
'AppLifecycleState.paused' => AppLifecycleState.paused,
'AppLifecycleState.detached' => AppLifecycleState.detached,
_ => null,
};
}
/// Handles any requests for application exit that may be received on the
/// [SystemChannels.platform] method channel.
///
/// By default, returns [ui.AppExitResponse.exit].
///
/// {@template flutter.services.binding.ServicesBinding.requestAppExit}
/// Not all exits are cancelable, so not all exits will call this function. Do
/// not rely on this function as a place to save critical data, because you
/// will be disappointed. There are a number of ways that the application can
/// exit without letting the application know first: power can be unplugged,
/// the battery removed, the application can be killed in a task manager or
/// command line, or the device could have a rapid unplanned disassembly (i.e.
/// it could explode). In all of those cases (and probably others), no
/// notification will be given to the application that it is about to exit.
/// {@endtemplate}
///
/// {@tool sample}
/// This examples shows how an application can cancel (or not) OS requests for
/// quitting an application. Currently this is only supported on macOS and
/// Linux.
///
/// ** See code in examples/api/lib/services/binding/handle_request_app_exit.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [WidgetsBindingObserver.didRequestAppExit], which can be overridden to
/// respond to this message.
/// * [WidgetsBinding.handleRequestAppExit] which overrides this method to
/// notify its observers.
Future<ui.AppExitResponse> handleRequestAppExit() async {
return ui.AppExitResponse.exit;
}
/// Exits the application by calling the native application API method for
/// exiting an application cleanly.
///
/// This differs from calling `dart:io`'s [exit] function in that it gives the
/// engine a chance to clean up resources so that it doesn't crash on exit, so
/// calling this is always preferred over calling [exit]. It also optionally
/// gives handlers of [handleRequestAppExit] a chance to cancel the
/// application exit.
///
/// The [exitType] indicates what kind of exit to perform. For
/// [ui.AppExitType.cancelable] exits, the application is queried through a
/// call to [handleRequestAppExit], where the application can optionally
/// cancel the request for exit. If the [exitType] is
/// [ui.AppExitType.required], then the application exits immediately without
/// querying the application.
///
/// For [ui.AppExitType.cancelable] exits, the returned response value is the
/// response obtained from the application as to whether the exit was canceled
/// or not. Practically, the response will never be [ui.AppExitResponse.exit],
/// since the application will have already exited by the time the result
/// would have been received.
///
/// The optional [exitCode] argument will be used as the application exit code
/// on platforms where an exit code is supported. On other platforms it may be
/// ignored. It defaults to zero.
///
/// See also:
///
/// * [WidgetsBindingObserver.didRequestAppExit] for a handler you can
/// override on a [WidgetsBindingObserver] to receive exit requests.
Future<ui.AppExitResponse> exitApplication(ui.AppExitType exitType, [int exitCode = 0]) async {
final Map<String, Object?>? result = await SystemChannels.platform.invokeMethod<Map<String, Object?>>(
'System.exitApplication',
<String, Object?>{'type': exitType.name, 'exitCode': exitCode},
);
if (result == null ) {
return ui.AppExitResponse.cancel;
}
switch (result['response']) {
case 'cancel':
return ui.AppExitResponse.cancel;
case 'exit':
default:
// In practice, this will never get returned, because the application
// will have exited before it returns.
return ui.AppExitResponse.exit;
}
}
/// The [RestorationManager] synchronizes the restoration data between
/// engine and framework.
///
/// See the docs for [RestorationManager] for a discussion of restoration
/// state and how it is organized in Flutter.
///
/// To use a different [RestorationManager] subclasses can override
/// [createRestorationManager], which is called to create the instance
/// returned by this getter.
RestorationManager get restorationManager => _restorationManager;
late RestorationManager _restorationManager;
/// Creates the [RestorationManager] instance available via
/// [restorationManager].
///
/// Can be overridden in subclasses to create a different [RestorationManager].
@protected
RestorationManager createRestorationManager() {
return RestorationManager();
}
SystemUiChangeCallback? _systemUiChangeCallback;
/// Sets the callback for the `SystemChrome.systemUIChange` method call
/// received on the [SystemChannels.platform] channel.
///
/// This is typically not called directly. System UI changes that this method
/// responds to are associated with [SystemUiMode]s, which are configured
/// using [SystemChrome]. Use [SystemChrome.setSystemUIChangeCallback] to configure
/// along with other SystemChrome settings.
///
/// See also:
///
/// * [SystemChrome.setEnabledSystemUIMode], which specifies the
/// [SystemUiMode] to have visible when the application is running.
// ignore: use_setters_to_change_properties, (API predates enforcing the lint)
void setSystemUiChangeCallback(SystemUiChangeCallback? callback) {
_systemUiChangeCallback = callback;
}
/// Alert the engine that the binding is registered. This instructs the engine to
/// register its top level window handler on Windows. This signals that the app
/// is able to process "System.requestAppExit" signals from the engine.
@protected
Future<void> initializationComplete() async {
await SystemChannels.platform.invokeMethod('System.initializationComplete');
}
}
/// Signature for listening to changes in the [SystemUiMode].
///
/// Set by [SystemChrome.setSystemUIChangeCallback].
typedef SystemUiChangeCallback = Future<void> Function(bool systemOverlaysAreVisible);
/// The default implementation of [BinaryMessenger].
///
/// This messenger sends messages from the app-side to the platform-side and
/// dispatches incoming messages from the platform-side to the appropriate
/// handler.
class _DefaultBinaryMessenger extends BinaryMessenger {
const _DefaultBinaryMessenger._();
@override
Future<void> handlePlatformMessage(
String channel,
ByteData? message,
ui.PlatformMessageResponseCallback? callback,
) async {
ui.channelBuffers.push(channel, message, (ByteData? data) {
if (callback != null) {
callback(data);
}
});
}
@override
Future<ByteData?> send(String channel, ByteData? message) {
final Completer<ByteData?> completer = Completer<ByteData?>();
// ui.PlatformDispatcher.instance is accessed directly instead of using
// ServicesBinding.instance.platformDispatcher because this method might be
// invoked before any binding is initialized. This issue was reported in
// #27541. It is not ideal to statically access
// ui.PlatformDispatcher.instance because the PlatformDispatcher may be
// dependency injected elsewhere with a different instance. However, static
// access at this location seems to be the least bad option.
// TODO(ianh): Use ServicesBinding.instance once we have better diagnostics
// on that getter.
ui.PlatformDispatcher.instance.sendPlatformMessage(channel, message, (ByteData? reply) {
try {
completer.complete(reply);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context: ErrorDescription('during a platform message response callback'),
));
}
});
return completer.future;
}
@override
void setMessageHandler(String channel, MessageHandler? handler) {
if (handler == null) {
ui.channelBuffers.clearListener(channel);
} else {
ui.channelBuffers.setListener(channel, (ByteData? data, ui.PlatformMessageResponseCallback callback) async {
ByteData? response;
try {
response = await handler(data);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context: ErrorDescription('during a platform message callback'),
));
} finally {
callback(response);
}
});
}
}
}
| flutter/packages/flutter/lib/src/services/binding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/binding.dart",
"repo_id": "flutter",
"token_count": 7572
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'mouse_cursor.dart';
export 'package:flutter/foundation.dart' show DiagnosticPropertiesBuilder;
export 'package:flutter/gestures.dart' show PointerEnterEvent, PointerExitEvent, PointerHoverEvent;
export 'mouse_cursor.dart' show MouseCursor;
/// Signature for listening to [PointerEnterEvent] events.
///
/// Used by [MouseTrackerAnnotation], [MouseRegion] and [RenderMouseRegion].
typedef PointerEnterEventListener = void Function(PointerEnterEvent event);
/// Signature for listening to [PointerExitEvent] events.
///
/// Used by [MouseTrackerAnnotation], [MouseRegion] and [RenderMouseRegion].
typedef PointerExitEventListener = void Function(PointerExitEvent event);
/// Signature for listening to [PointerHoverEvent] events.
///
/// Used by [MouseTrackerAnnotation], [MouseRegion] and [RenderMouseRegion].
typedef PointerHoverEventListener = void Function(PointerHoverEvent event);
/// The annotation object used to annotate regions that are interested in mouse
/// movements.
///
/// To use an annotation, return this object as a [HitTestEntry] in a hit test.
/// Typically this is implemented by making a [RenderBox] implement this class
/// (see [RenderMouseRegion]).
///
/// [MouseTracker] uses this class as a label to filter the hit test results. Hit
/// test entries that are also [MouseTrackerAnnotation]s are considered as valid
/// targets in terms of computing mouse related effects, such as enter events,
/// exit events, and mouse cursor events.
///
/// See also:
///
/// * [MouseTracker], which uses [MouseTrackerAnnotation].
class MouseTrackerAnnotation with Diagnosticable {
/// Creates an immutable [MouseTrackerAnnotation].
const MouseTrackerAnnotation({
this.onEnter,
this.onExit,
this.cursor = MouseCursor.defer,
this.validForMouseTracker = true,
});
/// Triggered when a mouse pointer, with or without buttons pressed, has
/// entered the region and [validForMouseTracker] is true.
///
/// This callback is triggered when the pointer has started to be contained by
/// the region, either due to a pointer event, or due to the movement or
/// disappearance of the region. This method is always matched by a later
/// [onExit].
///
/// See also:
///
/// * [onExit], which is triggered when a mouse pointer exits the region.
/// * [MouseRegion.onEnter], which uses this callback.
final PointerEnterEventListener? onEnter;
/// Triggered when a mouse pointer, with or without buttons pressed, has
/// exited the region and [validForMouseTracker] is true.
///
/// This callback is triggered when the pointer has stopped being contained
/// by the region, either due to a pointer event, or due to the movement or
/// disappearance of the region. This method always matches an earlier
/// [onEnter].
///
/// See also:
///
/// * [onEnter], which is triggered when a mouse pointer enters the region.
/// * [MouseRegion.onExit], which uses this callback, but is not triggered in
/// certain cases and does not always match its earlier [MouseRegion.onEnter].
final PointerExitEventListener? onExit;
/// The mouse cursor for mouse pointers that are hovering over the region.
///
/// When a mouse enters the region, its cursor will be changed to the [cursor].
/// When the mouse leaves the region, the cursor will be set by the region
/// found at the new location.
///
/// Defaults to [MouseCursor.defer], deferring the choice of cursor to the next
/// region behind it in hit-test order.
///
/// See also:
///
/// * [MouseRegion.cursor], which provide values to this field.
final MouseCursor cursor;
/// Whether this is included when [MouseTracker] collects the list of
/// annotations.
///
/// If [validForMouseTracker] is false, this object is excluded from the
/// current annotation list even if it's included in the hit test, affecting
/// mouse-related behavior such as enter events, exit events, and mouse
/// cursors. The [validForMouseTracker] does not affect hit testing.
///
/// The [validForMouseTracker] is true for [MouseTrackerAnnotation]s built by
/// the constructor.
final bool validForMouseTracker;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagsSummary<Function?>(
'callbacks',
<String, Function?> {
'enter': onEnter,
'exit': onExit,
},
ifEmpty: '<none>',
));
properties.add(DiagnosticsProperty<MouseCursor>('cursor', cursor, defaultValue: MouseCursor.defer));
}
}
| flutter/packages/flutter/lib/src/services/mouse_tracking.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/mouse_tracking.dart",
"repo_id": "flutter",
"token_count": 1343
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'binding.dart';
import 'system_channels.dart';
export 'dart:ui' show Brightness, Color;
export 'binding.dart' show SystemUiChangeCallback;
/// Specifies a particular device orientation.
///
/// To determine which values correspond to which orientations, first position
/// the device in its default orientation (this is the orientation that the
/// system first uses for its boot logo, or the orientation in which the
/// hardware logos or markings are upright, or the orientation in which the
/// cameras are at the top). If this is a portrait orientation, then this is
/// [portraitUp]. Otherwise, it's [landscapeLeft]. As you rotate the device by
/// 90 degrees in a counter-clockwise direction around the axis that pierces the
/// screen, you step through each value in this enum in the order given.
///
/// For a device with a landscape default orientation, the orientation obtained
/// by rotating the device 90 degrees clockwise from its default orientation is
/// [portraitUp].
///
/// Used by [SystemChrome.setPreferredOrientations].
enum DeviceOrientation {
/// If the device shows its boot logo in portrait, then the boot logo is shown
/// in [portraitUp]. Otherwise, the device shows its boot logo in landscape
/// and this orientation is obtained by rotating the device 90 degrees
/// clockwise from its boot orientation.
portraitUp,
/// The orientation that is 90 degrees clockwise from [portraitUp].
///
/// If the device shows its boot logo in landscape, then the boot logo is
/// shown in [landscapeLeft].
landscapeLeft,
/// The orientation that is 180 degrees from [portraitUp].
portraitDown,
/// The orientation that is 90 degrees counterclockwise from [portraitUp].
landscapeRight,
}
/// Specifies a description of the application that is pertinent to the
/// embedder's application switcher (also known as "recent tasks") user
/// interface.
///
/// Used by [SystemChrome.setApplicationSwitcherDescription].
@immutable
class ApplicationSwitcherDescription {
/// Creates an ApplicationSwitcherDescription.
const ApplicationSwitcherDescription({ this.label, this.primaryColor });
/// A label and description of the current state of the application.
final String? label;
/// The application's primary color.
///
/// This may influence the color that the operating system uses to represent
/// the application.
final int? primaryColor;
}
/// Specifies a system overlay at a particular location.
///
/// Used by [SystemChrome.setEnabledSystemUIMode].
enum SystemUiOverlay {
/// The status bar provided by the embedder on the top of the application
/// surface, if any.
top,
/// The status bar provided by the embedder on the bottom of the application
/// surface, if any.
bottom,
}
/// Describes different display configurations for both Android and iOS.
///
/// These modes mimic Android-specific display setups.
///
/// Used by [SystemChrome.setEnabledSystemUIMode].
enum SystemUiMode {
/// Fullscreen display with status and navigation bars presentable by tapping
/// anywhere on the display.
///
/// Available starting at SDK 16 or Android J. Earlier versions of Android
/// will not be affected by this setting.
///
/// For applications running on iOS, the status bar and home indicator will be
/// hidden for a similar fullscreen experience.
///
/// Tapping on the screen displays overlays, this gesture is not received by
/// the application.
///
/// See also:
///
/// * [SystemUiChangeCallback], used to listen and respond to the change in
/// system overlays.
leanBack,
/// Fullscreen display with status and navigation bars presentable through a
/// swipe gesture at the edges of the display.
///
/// Available starting at SDK 19 or Android K. Earlier versions of Android
/// will not be affected by this setting.
///
/// For applications running on iOS, the status bar and home indicator will be
/// hidden for a similar fullscreen experience.
///
/// A swipe gesture from the edge of the screen displays overlays. In contrast
/// to [SystemUiMode.immersiveSticky], this gesture is not received by the
/// application.
///
/// See also:
///
/// * [SystemUiChangeCallback], used to listen and respond to the change in
/// system overlays.
immersive,
/// Fullscreen display with status and navigation bars presentable through a
/// swipe gesture at the edges of the display.
///
/// Available starting at SDK 19 or Android K. Earlier versions of Android
/// will not be affected by this setting.
///
/// For applications running on iOS, the status bar and home indicator will be
/// hidden for a similar fullscreen experience.
///
/// A swipe gesture from the edge of the screen displays overlays. In contrast
/// to [SystemUiMode.immersive], this gesture is received by the application.
///
/// See also:
///
/// * [SystemUiChangeCallback], used to listen and respond to the change in
/// system overlays.
immersiveSticky,
/// Fullscreen display with status and navigation elements rendered over the
/// application.
///
/// Available starting at SDK 29 or Android 10. Earlier versions of Android
/// will not be affected by this setting.
///
/// For applications running on iOS, the status bar and home indicator will be
/// visible.
///
/// The system overlays will not disappear or reappear in this mode as they
/// are permanently displayed on top of the application.
///
/// See also:
///
/// * [SystemUiOverlayStyle], can be used to configure transparent status and
/// navigation bars with or without a contrast scrim.
edgeToEdge,
/// Declares manually configured [SystemUiOverlay]s.
///
/// When using this mode with [SystemChrome.setEnabledSystemUIMode], the
/// preferred overlays must be set by the developer.
///
/// When [SystemUiOverlay.top] is enabled, the status bar will remain visible
/// on all platforms. Omitting this overlay will hide the status bar on iOS &
/// Android.
///
/// When [SystemUiOverlay.bottom] is enabled, the navigation bar and home
/// indicator of Android and iOS applications will remain visible. Omitting this
/// overlay will hide them.
///
/// Omitting both overlays will result in the same configuration as
/// [SystemUiMode.leanBack].
manual,
}
/// Specifies a preference for the style of the system overlays.
///
/// Used by [AppBar.systemOverlayStyle] for declaratively setting the style of
/// the system overlays, and by [SystemChrome.setSystemUIOverlayStyle] for
/// imperatively setting the style of the system overlays.
@immutable
class SystemUiOverlayStyle {
/// Creates a new [SystemUiOverlayStyle].
const SystemUiOverlayStyle({
this.systemNavigationBarColor,
this.systemNavigationBarDividerColor,
this.systemNavigationBarIconBrightness,
this.systemNavigationBarContrastEnforced,
this.statusBarColor,
this.statusBarBrightness,
this.statusBarIconBrightness,
this.systemStatusBarContrastEnforced,
});
/// The color of the system bottom navigation bar.
///
/// Only honored in Android versions O and greater.
final Color? systemNavigationBarColor;
/// The color of the divider between the system's bottom navigation bar and the app's content.
///
/// Only honored in Android versions P and greater.
final Color? systemNavigationBarDividerColor;
/// The brightness of the system navigation bar icons.
///
/// Only honored in Android versions O and greater.
/// When set to [Brightness.light], the system navigation bar icons are light.
/// When set to [Brightness.dark], the system navigation bar icons are dark.
final Brightness? systemNavigationBarIconBrightness;
/// Overrides the contrast enforcement when setting a transparent navigation
/// bar.
///
/// When setting a transparent navigation bar in SDK 29+, or Android 10 and up,
/// a translucent body scrim may be applied behind the button navigation bar
/// to ensure contrast with buttons and the background of the application.
///
/// SDK 28-, or Android P and lower, will not apply this body scrim.
///
/// Setting this to false overrides the default body scrim.
///
/// See also:
///
/// * [SystemUiOverlayStyle.systemNavigationBarColor], which is overridden
/// when transparent to enforce this contrast policy.
final bool? systemNavigationBarContrastEnforced;
/// The color of top status bar.
///
/// Only honored in Android version M and greater.
final Color? statusBarColor;
/// The brightness of top status bar.
///
/// Only honored in iOS.
final Brightness? statusBarBrightness;
/// The brightness of the top status bar icons.
///
/// Only honored in Android version M and greater.
final Brightness? statusBarIconBrightness;
/// Overrides the contrast enforcement when setting a transparent status
/// bar.
///
/// When setting a transparent status bar in SDK 29+, or Android 10 and up,
/// a translucent body scrim may be applied to ensure contrast with icons and
/// the background of the application.
///
/// SDK 28-, or Android P and lower, will not apply this body scrim.
///
/// Setting this to false overrides the default body scrim.
///
/// See also:
///
/// * [SystemUiOverlayStyle.statusBarColor], which is overridden
/// when transparent to enforce this contrast policy.
final bool? systemStatusBarContrastEnforced;
/// System overlays should be drawn with a light color. Intended for
/// applications with a dark background.
static const SystemUiOverlayStyle light = SystemUiOverlayStyle(
systemNavigationBarColor: Color(0xFF000000),
systemNavigationBarIconBrightness: Brightness.light,
statusBarIconBrightness: Brightness.light,
statusBarBrightness: Brightness.dark,
);
/// System overlays should be drawn with a dark color. Intended for
/// applications with a light background.
static const SystemUiOverlayStyle dark = SystemUiOverlayStyle(
systemNavigationBarColor: Color(0xFF000000),
systemNavigationBarIconBrightness: Brightness.light,
statusBarIconBrightness: Brightness.dark,
statusBarBrightness: Brightness.light,
);
/// Convert this event to a map for serialization.
Map<String, dynamic> _toMap() {
return <String, dynamic>{
'systemNavigationBarColor': systemNavigationBarColor?.value,
'systemNavigationBarDividerColor': systemNavigationBarDividerColor?.value,
'systemStatusBarContrastEnforced': systemStatusBarContrastEnforced,
'statusBarColor': statusBarColor?.value,
'statusBarBrightness': statusBarBrightness?.toString(),
'statusBarIconBrightness': statusBarIconBrightness?.toString(),
'systemNavigationBarIconBrightness': systemNavigationBarIconBrightness?.toString(),
'systemNavigationBarContrastEnforced': systemNavigationBarContrastEnforced,
};
}
@override
String toString() => '${objectRuntimeType(this, 'SystemUiOverlayStyle')}(${_toMap()})';
/// Creates a copy of this theme with the given fields replaced with new values.
SystemUiOverlayStyle copyWith({
Color? systemNavigationBarColor,
Color? systemNavigationBarDividerColor,
bool? systemNavigationBarContrastEnforced,
Color? statusBarColor,
Brightness? statusBarBrightness,
Brightness? statusBarIconBrightness,
bool? systemStatusBarContrastEnforced,
Brightness? systemNavigationBarIconBrightness,
}) {
return SystemUiOverlayStyle(
systemNavigationBarColor: systemNavigationBarColor ?? this.systemNavigationBarColor,
systemNavigationBarDividerColor: systemNavigationBarDividerColor ?? this.systemNavigationBarDividerColor,
systemNavigationBarContrastEnforced: systemNavigationBarContrastEnforced ?? this.systemNavigationBarContrastEnforced,
statusBarColor: statusBarColor ?? this.statusBarColor,
statusBarIconBrightness: statusBarIconBrightness ?? this.statusBarIconBrightness,
statusBarBrightness: statusBarBrightness ?? this.statusBarBrightness,
systemStatusBarContrastEnforced: systemStatusBarContrastEnforced ?? this.systemStatusBarContrastEnforced,
systemNavigationBarIconBrightness: systemNavigationBarIconBrightness ?? this.systemNavigationBarIconBrightness,
);
}
@override
int get hashCode => Object.hash(
systemNavigationBarColor,
systemNavigationBarDividerColor,
systemNavigationBarContrastEnforced,
statusBarColor,
statusBarBrightness,
statusBarIconBrightness,
systemStatusBarContrastEnforced,
systemNavigationBarIconBrightness,
);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is SystemUiOverlayStyle
&& other.systemNavigationBarColor == systemNavigationBarColor
&& other.systemNavigationBarDividerColor == systemNavigationBarDividerColor
&& other.systemNavigationBarContrastEnforced == systemNavigationBarContrastEnforced
&& other.statusBarColor == statusBarColor
&& other.statusBarIconBrightness == statusBarIconBrightness
&& other.statusBarBrightness == statusBarBrightness
&& other.systemStatusBarContrastEnforced == systemStatusBarContrastEnforced
&& other.systemNavigationBarIconBrightness == systemNavigationBarIconBrightness;
}
}
List<String> _stringify(List<dynamic> list) => <String>[
for (final dynamic item in list) item.toString(),
];
/// Controls specific aspects of the operating system's graphical interface and
/// how it interacts with the application.
abstract final class SystemChrome {
/// Specifies the set of orientations the application interface can
/// be displayed in.
///
/// The `orientation` argument is a list of [DeviceOrientation] enum values.
/// The empty list causes the application to defer to the operating system
/// default.
///
/// ## Limitations
///
/// ### Android
///
/// Android screens may choose to [letterbox](https://developer.android.com/guide/practices/enhanced-letterboxing)
/// applications that lock orientation, particularly on larger screens. When
/// letterboxing occurs on Android, the [MediaQueryData.size] reports the
/// letterboxed size, not the full screen size. Applications that make
/// decisions about whether to lock orientation based on the screen size
/// must use the `display` property of the current [FlutterView].
///
/// ```dart
/// // A widget that locks the screen to portrait if it is less than 600
/// // logical pixels wide.
/// class MyApp extends StatefulWidget {
/// const MyApp({ super.key });
///
/// @override
/// State<MyApp> createState() => _MyAppState();
/// }
///
/// class _MyAppState extends State<MyApp> with WidgetsBindingObserver {
/// ui.FlutterView? _view;
/// static const double kOrientationLockBreakpoint = 600;
///
/// @override
/// void initState() {
/// super.initState();
/// WidgetsBinding.instance.addObserver(this);
/// }
///
/// @override
/// void didChangeDependencies() {
/// super.didChangeDependencies();
/// _view = View.maybeOf(context);
/// }
///
/// @override
/// void dispose() {
/// WidgetsBinding.instance.removeObserver(this);
/// _view = null;
/// super.dispose();
/// }
///
/// @override
/// void didChangeMetrics() {
/// final ui.Display? display = _view?.display;
/// if (display == null) {
/// return;
/// }
/// if (display.size.width / display.devicePixelRatio < kOrientationLockBreakpoint) {
/// SystemChrome.setPreferredOrientations(<DeviceOrientation>[
/// DeviceOrientation.portraitUp,
/// ]);
/// } else {
/// SystemChrome.setPreferredOrientations(<DeviceOrientation>[]);
/// }
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return const MaterialApp(
/// home: Placeholder(),
/// );
/// }
/// }
/// ```
///
/// ### iOS
///
/// This setting will only be respected on iPad if multitasking is disabled.
///
/// You can decide to opt out of multitasking on iPad, then
/// setPreferredOrientations will work but your app will not
/// support Slide Over and Split View multitasking anymore.
///
/// Should you decide to opt out of multitasking you can do this by
/// setting "Requires full screen" to true in the Xcode Deployment Info.
static Future<void> setPreferredOrientations(List<DeviceOrientation> orientations) async {
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setPreferredOrientations',
_stringify(orientations),
);
}
/// Specifies the description of the current state of the application as it
/// pertains to the application switcher (also known as "recent tasks").
///
/// Any part of the description that is unsupported on the current platform
/// will be ignored.
static Future<void> setApplicationSwitcherDescription(ApplicationSwitcherDescription description) async {
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setApplicationSwitcherDescription',
<String, dynamic>{
'label': description.label,
'primaryColor': description.primaryColor,
},
);
}
/// Specifies the [SystemUiMode] to have visible when the application
/// is running.
///
/// The `overlays` argument is a list of [SystemUiOverlay] enum values
/// denoting the overlays to show when configured with [SystemUiMode.manual].
///
/// If a particular mode is unsupported on the platform, enabling or
/// disabling that mode will be ignored.
///
/// The settings here can be overridden by the platform when System UI becomes
/// necessary for functionality.
///
/// For example, on Android, when the keyboard becomes visible, it will enable the
/// navigation bar and status bar system UI overlays. When the keyboard is closed,
/// Android will not restore the previous UI visibility settings, and the UI
/// visibility cannot be changed until 1 second after the keyboard is closed to
/// prevent malware locking users from navigation buttons.
///
/// To regain "fullscreen" after text entry, the UI overlays can be set again
/// after a delay of at least 1 second through [restoreSystemUIOverlays] or
/// calling this again. Otherwise, the original UI overlay settings will be
/// automatically restored only when the application loses and regains focus.
///
/// Alternatively, a [SystemUiChangeCallback] can be provided to respond to
/// changes in the System UI. This will be called, for example, when in
/// [SystemUiMode.leanBack] and the user taps the screen to bring up the
/// system overlays. The callback provides a boolean to represent if the
/// application is currently in a fullscreen mode or not, so that the
/// application can respond to these changes. When `systemOverlaysAreVisible`
/// is true, the application is not fullscreen. See
/// [SystemChrome.setSystemUIChangeCallback] to respond to these changes in a
/// fullscreen application.
static Future<void> setEnabledSystemUIMode(SystemUiMode mode, { List<SystemUiOverlay>? overlays }) async {
if (mode != SystemUiMode.manual) {
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setEnabledSystemUIMode',
mode.toString(),
);
} else {
assert(mode == SystemUiMode.manual && overlays != null);
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setEnabledSystemUIOverlays',
_stringify(overlays!),
);
}
}
/// Sets the callback method for responding to changes in the system UI.
///
/// This is relevant when using [SystemUiMode.leanBack]
/// and [SystemUiMode.immersive] and [SystemUiMode.immersiveSticky] on Android
/// platforms, where the [SystemUiOverlay]s can appear and disappear based on
/// user interaction.
///
/// This will be called, for example, when in [SystemUiMode.leanBack] and the
/// user taps the screen to bring up the system overlays. The callback
/// provides a boolean to represent if the application is currently in a
/// fullscreen mode or not, so that the application can respond to these
/// changes. When `systemOverlaysAreVisible` is true, the application is not
/// fullscreen.
///
/// When using [SystemUiMode.edgeToEdge], system overlays are always visible
/// and do not change. When manually configuring [SystemUiOverlay]s with
/// [SystemUiMode.manual], this callback will only be triggered when all
/// overlays have been disabled. This results in the same behavior as
/// [SystemUiMode.leanBack].
///
static Future<void> setSystemUIChangeCallback(SystemUiChangeCallback? callback) async {
ServicesBinding.instance.setSystemUiChangeCallback(callback);
// Skip setting up the listener if there is no callback.
if (callback != null) {
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setSystemUIChangeListener',
);
}
}
/// Restores the system overlays to the last settings provided via
/// [setEnabledSystemUIMode]. May be used when the platform force enables/disables
/// UI elements.
///
/// For example, when the Android keyboard disables hidden status and navigation bars,
/// this can be called to re-disable the bars when the keyboard is closed.
///
/// On Android, the system UI cannot be changed until 1 second after the previous
/// change. This is to prevent malware from permanently hiding navigation buttons.
static Future<void> restoreSystemUIOverlays() async {
await SystemChannels.platform.invokeMethod<void>(
'SystemChrome.restoreSystemUIOverlays',
);
}
/// Specifies the style to use for the system overlays (e.g. the status bar on
/// Android or iOS, the system navigation bar on Android) that are visible (if any).
///
/// This method will schedule the embedder update to be run in a microtask.
/// Any subsequent calls to this method during the current event loop will
/// overwrite the pending value, such that only the last specified value takes
/// effect.
///
/// Call this API in code whose lifecycle matches that of the desired
/// system UI styles. For instance, to change the system UI style on a new
/// page, consider calling when pushing/popping a new [PageRoute].
///
/// The [AppBar] widget automatically sets the system overlay style based on
/// its [AppBar.systemOverlayStyle], so configure that instead of calling this
/// method directly. Likewise, do the same for [CupertinoNavigationBar] via
/// [CupertinoNavigationBar.backgroundColor].
///
/// If a particular style is not supported on the platform, selecting it will
/// have no effect.
///
/// {@tool sample}
/// The following example uses an `AppBar` to set the system status bar color and
/// the system navigation bar color.
///
/// ** See code in examples/api/lib/services/system_chrome/system_chrome.set_system_u_i_overlay_style.0.dart **
/// {@end-tool}
///
/// For more complex control of the system overlay styles, consider using
/// an [AnnotatedRegion] widget instead of calling [setSystemUIOverlayStyle]
/// directly. This widget places a value directly into the layer tree where
/// it can be hit-tested by the framework. On every frame, the framework will
/// hit-test and select the annotated region it finds under the status and
/// navigation bar and synthesize them into a single style. This can be used
/// to configure the system styles when an app bar is not used. When an app
/// bar is used, apps should not enclose the app bar in an annotated region
/// because one is automatically created. If an app bar is used and the app
/// bar is enclosed in an annotated region, the app bar overlay style supersedes
/// the status bar properties defined in the enclosing annotated region overlay
/// style and the enclosing annotated region overlay style supersedes the app bar
/// overlay style navigation bar properties.
///
/// {@tool sample}
/// The following example uses an `AnnotatedRegion<SystemUiOverlayStyle>` to set
/// the system status bar color and the system navigation bar color.
///
/// ** See code in examples/api/lib/services/system_chrome/system_chrome.set_system_u_i_overlay_style.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AppBar.systemOverlayStyle], a convenient property for declaratively setting
/// the style of the system overlays.
/// * [AnnotatedRegion], the widget used to place a `SystemUiOverlayStyle` into
/// the layer tree.
static void setSystemUIOverlayStyle(SystemUiOverlayStyle style) {
if (_pendingStyle != null) {
// The microtask has already been queued; just update the pending value.
_pendingStyle = style;
return;
}
if (style == _latestStyle) {
// Trivial success: no microtask has been queued and the given style is
// already in effect, so no need to queue a microtask.
return;
}
_pendingStyle = style;
scheduleMicrotask(() {
assert(_pendingStyle != null);
if (_pendingStyle != _latestStyle) {
SystemChannels.platform.invokeMethod<void>(
'SystemChrome.setSystemUIOverlayStyle',
_pendingStyle!._toMap(),
);
_latestStyle = _pendingStyle;
}
_pendingStyle = null;
});
}
static SystemUiOverlayStyle? _pendingStyle;
/// The last style that was set using [SystemChrome.setSystemUIOverlayStyle].
@visibleForTesting
static SystemUiOverlayStyle? get latestStyle => _latestStyle;
static SystemUiOverlayStyle? _latestStyle;
}
| flutter/packages/flutter/lib/src/services/system_chrome.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/system_chrome.dart",
"repo_id": "flutter",
"token_count": 7427
} | 711 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'framework.dart';
/// A bridge from a [RenderObject] to an [Element] tree.
///
/// The given container is the [RenderObject] that the [Element] tree should be
/// inserted into. It must be a [RenderObject] that implements the
/// [RenderObjectWithChildMixin] protocol. The type argument `T` is the kind of
/// [RenderObject] that the container expects as its child.
///
/// The [RenderObjectToWidgetAdapter] is an alternative to [RootWidget] for
/// bootstrapping an element tree. Unlike [RootWidget] it requires the
/// existence of a render tree (the [container]) to attach the element tree to.
class RenderObjectToWidgetAdapter<T extends RenderObject> extends RenderObjectWidget {
/// Creates a bridge from a [RenderObject] to an [Element] tree.
RenderObjectToWidgetAdapter({
this.child,
required this.container,
this.debugShortDescription,
}) : super(key: GlobalObjectKey(container));
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The [RenderObject] that is the parent of the [Element] created by this widget.
final RenderObjectWithChildMixin<T> container;
/// A short description of this widget used by debugging aids.
final String? debugShortDescription;
@override
RenderObjectToWidgetElement<T> createElement() => RenderObjectToWidgetElement<T>(this);
@override
RenderObjectWithChildMixin<T> createRenderObject(BuildContext context) => container;
@override
void updateRenderObject(BuildContext context, RenderObject renderObject) { }
/// Inflate this widget and actually set the resulting [RenderObject] as the
/// child of [container].
///
/// If `element` is null, this function will create a new element. Otherwise,
/// the given element will have an update scheduled to switch to this widget.
RenderObjectToWidgetElement<T> attachToRenderTree(BuildOwner owner, [ RenderObjectToWidgetElement<T>? element ]) {
if (element == null) {
owner.lockState(() {
element = createElement();
assert(element != null);
element!.assignOwner(owner);
});
owner.buildScope(element!, () {
element!.mount(null, null);
});
} else {
element._newWidget = this;
element.markNeedsBuild();
}
return element!;
}
@override
String toStringShort() => debugShortDescription ?? super.toStringShort();
}
/// The root of an element tree that is hosted by a [RenderObject].
///
/// This element class is the instantiation of a [RenderObjectToWidgetAdapter]
/// widget. It can be used only as the root of an [Element] tree (it cannot be
/// mounted into another [Element]; it's parent must be null).
///
/// In typical usage, it will be instantiated for a [RenderObjectToWidgetAdapter]
/// whose container is the [RenderView].
class RenderObjectToWidgetElement<T extends RenderObject> extends RenderTreeRootElement with RootElementMixin {
/// Creates an element that is hosted by a [RenderObject].
///
/// The [RenderObject] created by this element is not automatically set as a
/// child of the hosting [RenderObject]. To actually attach this element to
/// the render tree, call [RenderObjectToWidgetAdapter.attachToRenderTree].
RenderObjectToWidgetElement(RenderObjectToWidgetAdapter<T> super.widget);
Element? _child;
static const Object _rootChildSlot = Object();
@override
void visitChildren(ElementVisitor visitor) {
if (_child != null) {
visitor(_child!);
}
}
@override
void forgetChild(Element child) {
assert(child == _child);
_child = null;
super.forgetChild(child);
}
@override
void mount(Element? parent, Object? newSlot) {
assert(parent == null);
super.mount(parent, newSlot);
_rebuild();
assert(_child != null);
}
@override
void update(RenderObjectToWidgetAdapter<T> newWidget) {
super.update(newWidget);
assert(widget == newWidget);
_rebuild();
}
// When we are assigned a new widget, we store it here
// until we are ready to update to it.
Widget? _newWidget;
@override
void performRebuild() {
if (_newWidget != null) {
// _newWidget can be null if, for instance, we were rebuilt
// due to a reassemble.
final Widget newWidget = _newWidget!;
_newWidget = null;
update(newWidget as RenderObjectToWidgetAdapter<T>);
}
super.performRebuild();
assert(_newWidget == null);
}
@pragma('vm:notify-debugger-on-exception')
void _rebuild() {
try {
_child = updateChild(_child, (widget as RenderObjectToWidgetAdapter<T>).child, _rootChildSlot);
} catch (exception, stack) {
final FlutterErrorDetails details = FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets library',
context: ErrorDescription('attaching to the render tree'),
);
FlutterError.reportError(details);
final Widget error = ErrorWidget.builder(details);
_child = updateChild(null, error, _rootChildSlot);
}
}
@override
RenderObjectWithChildMixin<T> get renderObject => super.renderObject as RenderObjectWithChildMixin<T>;
@override
void insertRenderObjectChild(RenderObject child, Object? slot) {
assert(slot == _rootChildSlot);
assert(renderObject.debugValidateChild(child));
renderObject.child = child as T;
}
@override
void moveRenderObjectChild(RenderObject child, Object? oldSlot, Object? newSlot) {
assert(false);
}
@override
void removeRenderObjectChild(RenderObject child, Object? slot) {
assert(renderObject.child == child);
renderObject.child = null;
}
}
| flutter/packages/flutter/lib/src/widgets/adapter.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/adapter.dart",
"repo_id": "flutter",
"token_count": 1830
} | 712 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'framework.dart';
/// Applies a [ColorFilter] to its child.
///
/// This widget applies a function independently to each pixel of [child]'s
/// content, according to the [ColorFilter] specified.
/// Use the [ColorFilter.mode] constructor to apply a [Color] using a [BlendMode].
/// Use the [BackdropFilter] widget instead, if the [ColorFilter]
/// needs to be applied onto the content beneath [child].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=F7Cll22Dno8}
///
/// {@tool dartpad}
/// These two images have two [ColorFilter]s applied with different [BlendMode]s,
/// one with red color and [BlendMode.modulate] another with a grey color and [BlendMode.saturation].
///
/// ** See code in examples/api/lib/widgets/color_filter/color_filtered.0.dart **
///{@end-tool}
///
/// See also:
///
/// * [BlendMode], describes how to blend a source image with the destination image.
/// * [ColorFilter], which describes a function that modify a color to a different color.
@immutable
class ColorFiltered extends SingleChildRenderObjectWidget {
/// Creates a widget that applies a [ColorFilter] to its child.
const ColorFiltered({required this.colorFilter, super.child, super.key});
/// The color filter to apply to the child of this widget.
final ColorFilter colorFilter;
@override
RenderObject createRenderObject(BuildContext context) => _ColorFilterRenderObject(colorFilter);
@override
void updateRenderObject(BuildContext context, RenderObject renderObject) {
(renderObject as _ColorFilterRenderObject).colorFilter = colorFilter;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ColorFilter>('colorFilter', colorFilter));
}
}
class _ColorFilterRenderObject extends RenderProxyBox {
_ColorFilterRenderObject(this._colorFilter);
ColorFilter get colorFilter => _colorFilter;
ColorFilter _colorFilter;
set colorFilter(ColorFilter value) {
if (value != _colorFilter) {
_colorFilter = value;
markNeedsPaint();
}
}
@override
bool get alwaysNeedsCompositing => child != null;
@override
void paint(PaintingContext context, Offset offset) {
layer = context.pushColorFilter(offset, colorFilter, super.paint, oldLayer: layer as ColorFilterLayer?);
assert(() {
layer!.debugCreator = debugCreator;
return true;
}());
}
}
| flutter/packages/flutter/lib/src/widgets/color_filter.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/color_filter.dart",
"repo_id": "flutter",
"token_count": 791
} | 713 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'dart:ui' as ui hide TextStyle;
import 'package:characters/characters.dart' show CharacterRange, StringCharacters;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'actions.dart';
import 'autofill.dart';
import 'automatic_keep_alive.dart';
import 'basic.dart';
import 'binding.dart';
import 'constants.dart';
import 'context_menu_button_item.dart';
import 'debug.dart';
import 'default_selection_style.dart';
import 'default_text_editing_shortcuts.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'focus_traversal.dart';
import 'framework.dart';
import 'localizations.dart';
import 'magnifier.dart';
import 'media_query.dart';
import 'notification_listener.dart';
import 'scroll_configuration.dart';
import 'scroll_controller.dart';
import 'scroll_notification.dart';
import 'scroll_notification_observer.dart';
import 'scroll_physics.dart';
import 'scroll_position.dart';
import 'scrollable.dart';
import 'scrollable_helpers.dart';
import 'shortcuts.dart';
import 'size_changed_layout_notifier.dart';
import 'spell_check.dart';
import 'tap_region.dart';
import 'text.dart';
import 'text_editing_intents.dart';
import 'text_selection.dart';
import 'text_selection_toolbar_anchors.dart';
import 'ticker_provider.dart';
import 'undo_history.dart';
import 'view.dart';
import 'widget_span.dart';
export 'package:flutter/services.dart' show KeyboardInsertedContent, SelectionChangedCause, SmartDashesType, SmartQuotesType, TextEditingValue, TextInputType, TextSelection;
// Examples can assume:
// late BuildContext context;
// late WidgetTester tester;
/// Signature for the callback that reports when the user changes the selection
/// (including the cursor location).
typedef SelectionChangedCallback = void Function(TextSelection selection, SelectionChangedCause? cause);
/// Signature for the callback that reports the app private command results.
typedef AppPrivateCommandCallback = void Function(String action, Map<String, dynamic> data);
/// Signature for a widget builder that builds a context menu for the given
/// [EditableTextState].
///
/// See also:
///
/// * [SelectableRegionContextMenuBuilder], which performs the same role for
/// [SelectableRegion].
typedef EditableTextContextMenuBuilder = Widget Function(
BuildContext context,
EditableTextState editableTextState,
);
// Signature for a function that determines the target location of the given
// [TextPosition] after applying the given [TextBoundary].
typedef _ApplyTextBoundary = TextPosition Function(TextPosition, bool, TextBoundary);
// The time it takes for the cursor to fade from fully opaque to fully
// transparent and vice versa. A full cursor blink, from transparent to opaque
// to transparent, is twice this duration.
const Duration _kCursorBlinkHalfPeriod = Duration(milliseconds: 500);
// Number of cursor ticks during which the most recently entered character
// is shown in an obscured text field.
const int _kObscureShowLatestCharCursorTicks = 3;
/// The default mime types to be used when allowedMimeTypes is not provided.
///
/// The default value supports inserting images of any supported format.
const List<String> kDefaultContentInsertionMimeTypes = <String>[
'image/png',
'image/bmp',
'image/jpg',
'image/tiff',
'image/gif',
'image/jpeg',
'image/webp'
];
class _CompositionCallback extends SingleChildRenderObjectWidget {
const _CompositionCallback({ required this.compositeCallback, required this.enabled, super.child });
final CompositionCallback compositeCallback;
final bool enabled;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderCompositionCallback(compositeCallback, enabled);
}
@override
void updateRenderObject(BuildContext context, _RenderCompositionCallback renderObject) {
super.updateRenderObject(context, renderObject);
// _EditableTextState always uses the same callback.
assert(renderObject.compositeCallback == compositeCallback);
renderObject.enabled = enabled;
}
}
class _RenderCompositionCallback extends RenderProxyBox {
_RenderCompositionCallback(this.compositeCallback, this._enabled);
final CompositionCallback compositeCallback;
VoidCallback? _cancelCallback;
bool get enabled => _enabled;
bool _enabled = false;
set enabled(bool newValue) {
_enabled = newValue;
if (!newValue) {
_cancelCallback?.call();
_cancelCallback = null;
} else if (_cancelCallback == null) {
markNeedsPaint();
}
}
@override
void paint(PaintingContext context, ui.Offset offset) {
if (enabled) {
_cancelCallback ??= context.addCompositionCallback(compositeCallback);
}
super.paint(context, offset);
}
}
/// A controller for an editable text field.
///
/// Whenever the user modifies a text field with an associated
/// [TextEditingController], the text field updates [value] and the controller
/// notifies its listeners. Listeners can then read the [text] and [selection]
/// properties to learn what the user has typed or how the selection has been
/// updated.
///
/// Similarly, if you modify the [text] or [selection] properties, the text
/// field will be notified and will update itself appropriately.
///
/// A [TextEditingController] can also be used to provide an initial value for a
/// text field. If you build a text field with a controller that already has
/// [text], the text field will use that text as its initial value.
///
/// The [value] (as well as [text] and [selection]) of this controller can be
/// updated from within a listener added to this controller. Be aware of
/// infinite loops since the listener will also be notified of the changes made
/// from within itself. Modifying the composing region from within a listener
/// can also have a bad interaction with some input methods. Gboard, for
/// example, will try to restore the composing region of the text if it was
/// modified programmatically, creating an infinite loop of communications
/// between the framework and the input method. Consider using
/// [TextInputFormatter]s instead for as-you-type text modification.
///
/// If both the [text] and [selection] properties need to be changed, set the
/// controller's [value] instead.
///
/// Remember to [dispose] of the [TextEditingController] when it is no longer
/// needed. This will ensure we discard any resources used by the object.
///
/// {@tool dartpad}
/// This example creates a [TextField] with a [TextEditingController] whose
/// change listener forces the entered text to be lower case and keeps the
/// cursor at the end of the input.
///
/// ** See code in examples/api/lib/widgets/editable_text/text_editing_controller.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TextField], which is a Material Design text field that can be controlled
/// with a [TextEditingController].
/// * [EditableText], which is a raw region of editable text that can be
/// controlled with a [TextEditingController].
/// * Learn how to use a [TextEditingController] in one of our [cookbook recipes](https://flutter.dev/docs/cookbook/forms/text-field-changes#2-use-a-texteditingcontroller).
class TextEditingController extends ValueNotifier<TextEditingValue> {
/// Creates a controller for an editable text field, with no initial selection.
///
/// This constructor treats a null [text] argument as if it were the empty
/// string.
///
/// The initial selection is `TextSelection.collapsed(offset: -1)`.
/// This indicates that there is no selection at all ([TextSelection.isValid]
/// is false in this case). When a text field is built with a controller whose
/// selection is not valid, the text field will update the selection when it
/// is focused (the selection will be an empty selection positioned at the
/// end of the text).
///
/// Consider using [TextEditingController.fromValue] to initialize both the
/// text and the selection.
///
/// {@tool dartpad}
/// This example creates a [TextField] with a [TextEditingController] whose
/// initial selection is empty (collapsed) and positioned at the beginning
/// of the text (offset is 0).
///
/// ** See code in examples/api/lib/widgets/editable_text/text_editing_controller.1.dart **
/// {@end-tool}
TextEditingController({ String? text })
: super(text == null ? TextEditingValue.empty : TextEditingValue(text: text));
/// Creates a controller for an editable text field from an initial [TextEditingValue].
///
/// This constructor treats a null [value] argument as if it were
/// [TextEditingValue.empty].
TextEditingController.fromValue(TextEditingValue? value)
: assert(
value == null || !value.composing.isValid || value.isComposingRangeValid,
'New TextEditingValue $value has an invalid non-empty composing range '
'${value.composing}. It is recommended to use a valid composing range, '
'even for readonly text fields.',
),
super(value ?? TextEditingValue.empty);
/// The current string the user is editing.
String get text => value.text;
/// Setting this will notify all the listeners of this [TextEditingController]
/// that they need to update (it calls [notifyListeners]). For this reason,
/// this value should only be set between frames, e.g. in response to user
/// actions, not during the build, layout, or paint phases.
///
/// This property can be set from a listener added to this
/// [TextEditingController]; **however, one should not also set [selection]
/// in a separate statement. To change both the [text] and the [selection]
/// change the controller's [value].**
set text(String newText) {
value = value.copyWith(
text: newText,
selection: const TextSelection.collapsed(offset: -1),
composing: TextRange.empty,
);
}
@override
set value(TextEditingValue newValue) {
assert(
!newValue.composing.isValid || newValue.isComposingRangeValid,
'New TextEditingValue $newValue has an invalid non-empty composing range '
'${newValue.composing}. It is recommended to use a valid composing range, '
'even for readonly text fields.',
);
super.value = newValue;
}
/// Builds [TextSpan] from current editing value.
///
/// By default makes text in composing range appear as underlined. Descendants
/// can override this method to customize appearance of text.
TextSpan buildTextSpan({required BuildContext context, TextStyle? style , required bool withComposing}) {
assert(!value.composing.isValid || !withComposing || value.isComposingRangeValid);
// If the composing range is out of range for the current text, ignore it to
// preserve the tree integrity, otherwise in release mode a RangeError will
// be thrown and this EditableText will be built with a broken subtree.
final bool composingRegionOutOfRange = !value.isComposingRangeValid || !withComposing;
if (composingRegionOutOfRange) {
return TextSpan(style: style, text: text);
}
final TextStyle composingStyle = style?.merge(const TextStyle(decoration: TextDecoration.underline))
?? const TextStyle(decoration: TextDecoration.underline);
return TextSpan(
style: style,
children: <TextSpan>[
TextSpan(text: value.composing.textBefore(value.text)),
TextSpan(
style: composingStyle,
text: value.composing.textInside(value.text),
),
TextSpan(text: value.composing.textAfter(value.text)),
],
);
}
/// The currently selected range within [text].
///
/// If the selection is collapsed, then this property gives the offset of the
/// cursor within the text.
TextSelection get selection => value.selection;
/// Setting this will notify all the listeners of this [TextEditingController]
/// that they need to update (it calls [notifyListeners]). For this reason,
/// this value should only be set between frames, e.g. in response to user
/// actions, not during the build, layout, or paint phases.
///
/// This property can be set from a listener added to this
/// [TextEditingController]; however, one should not also set [text]
/// in a separate statement. To change both the [text] and the [selection]
/// change the controller's [value].
///
/// If the new selection is outside the composing range, the composing range is
/// cleared.
set selection(TextSelection newSelection) {
if (!_isSelectionWithinTextBounds(newSelection)) {
throw FlutterError('invalid text selection: $newSelection');
}
final TextRange newComposing = _isSelectionWithinComposingRange(newSelection) ? value.composing : TextRange.empty;
value = value.copyWith(selection: newSelection, composing: newComposing);
}
/// Set the [value] to empty.
///
/// After calling this function, [text] will be the empty string and the
/// selection will be collapsed at zero offset.
///
/// Calling this will notify all the listeners of this [TextEditingController]
/// that they need to update (it calls [notifyListeners]). For this reason,
/// this method should only be called between frames, e.g. in response to user
/// actions, not during the build, layout, or paint phases.
void clear() {
value = const TextEditingValue(selection: TextSelection.collapsed(offset: 0));
}
/// Set the composing region to an empty range.
///
/// The composing region is the range of text that is still being composed.
/// Calling this function indicates that the user is done composing that
/// region.
///
/// Calling this will notify all the listeners of this [TextEditingController]
/// that they need to update (it calls [notifyListeners]). For this reason,
/// this method should only be called between frames, e.g. in response to user
/// actions, not during the build, layout, or paint phases.
void clearComposing() {
value = value.copyWith(composing: TextRange.empty);
}
/// Check that the [selection] is inside of the bounds of [text].
bool _isSelectionWithinTextBounds(TextSelection selection) {
return selection.start <= text.length && selection.end <= text.length;
}
/// Check that the [selection] is inside of the composing range.
bool _isSelectionWithinComposingRange(TextSelection selection) {
return selection.start >= value.composing.start && selection.end <= value.composing.end;
}
}
/// Toolbar configuration for [EditableText].
///
/// Toolbar is a context menu that will show up when user right click or long
/// press the [EditableText]. It includes several options: cut, copy, paste,
/// and select all.
///
/// [EditableText] and its derived widgets have their own default [ToolbarOptions].
/// Create a custom [ToolbarOptions] if you want explicit control over the toolbar
/// option.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
class ToolbarOptions {
/// Create a toolbar configuration with given options.
///
/// All options default to false if they are not explicitly set.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
const ToolbarOptions({
this.copy = false,
this.cut = false,
this.paste = false,
this.selectAll = false,
});
/// An instance of [ToolbarOptions] with no options enabled.
static const ToolbarOptions empty = ToolbarOptions();
/// Whether to show copy option in toolbar.
///
/// Defaults to false.
final bool copy;
/// Whether to show cut option in toolbar.
///
/// If [EditableText.readOnly] is set to true, cut will be disabled regardless.
///
/// Defaults to false.
final bool cut;
/// Whether to show paste option in toolbar.
///
/// If [EditableText.readOnly] is set to true, paste will be disabled regardless.
///
/// Defaults to false.
final bool paste;
/// Whether to show select all option in toolbar.
///
/// Defaults to false.
final bool selectAll;
}
/// Configures the ability to insert media content through the soft keyboard.
///
/// The configuration provides a handler for any rich content inserted through
/// the system input method, and also provides the ability to limit the mime
/// types of the inserted content.
///
/// See also:
///
/// * [EditableText.contentInsertionConfiguration]
class ContentInsertionConfiguration {
/// Creates a content insertion configuration with the specified options.
///
/// A handler for inserted content, in the form of [onContentInserted], must
/// be supplied.
///
/// The allowable mime types of inserted content may also
/// be provided via [allowedMimeTypes], which cannot be an empty list.
ContentInsertionConfiguration({
required this.onContentInserted,
this.allowedMimeTypes = kDefaultContentInsertionMimeTypes,
}) : assert(allowedMimeTypes.isNotEmpty);
/// Called when a user inserts content through the virtual / on-screen keyboard,
/// currently only used on Android.
///
/// [KeyboardInsertedContent] holds the data representing the inserted content.
///
/// {@tool dartpad}
///
/// This example shows how to access the data for inserted content in your
/// `TextField`.
///
/// ** See code in examples/api/lib/widgets/editable_text/editable_text.on_content_inserted.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * <https://developer.android.com/guide/topics/text/image-keyboard>
final ValueChanged<KeyboardInsertedContent> onContentInserted;
/// {@template flutter.widgets.contentInsertionConfiguration.allowedMimeTypes}
/// Used when a user inserts image-based content through the device keyboard,
/// currently only used on Android.
///
/// The passed list of strings will determine which MIME types are allowed to
/// be inserted via the device keyboard.
///
/// The default mime types are given by [kDefaultContentInsertionMimeTypes].
/// These are all the mime types that are able to be handled and inserted
/// from keyboards.
///
/// This field cannot be an empty list.
///
/// {@tool dartpad}
/// This example shows how to limit image insertion to specific file types.
///
/// ** See code in examples/api/lib/widgets/editable_text/editable_text.on_content_inserted.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * <https://developer.android.com/guide/topics/text/image-keyboard>
/// {@endtemplate}
final List<String> allowedMimeTypes;
}
// A time-value pair that represents a key frame in an animation.
class _KeyFrame {
const _KeyFrame(this.time, this.value);
// Values extracted from iOS 15.4 UIKit.
static const List<_KeyFrame> iOSBlinkingCaretKeyFrames = <_KeyFrame>[
_KeyFrame(0, 1), // 0
_KeyFrame(0.5, 1), // 1
_KeyFrame(0.5375, 0.75), // 2
_KeyFrame(0.575, 0.5), // 3
_KeyFrame(0.6125, 0.25), // 4
_KeyFrame(0.65, 0), // 5
_KeyFrame(0.85, 0), // 6
_KeyFrame(0.8875, 0.25), // 7
_KeyFrame(0.925, 0.5), // 8
_KeyFrame(0.9625, 0.75), // 9
_KeyFrame(1, 1), // 10
];
// The timing, in seconds, of the specified animation `value`.
final double time;
final double value;
}
class _DiscreteKeyFrameSimulation extends Simulation {
_DiscreteKeyFrameSimulation.iOSBlinkingCaret() : this._(_KeyFrame.iOSBlinkingCaretKeyFrames, 1);
_DiscreteKeyFrameSimulation._(this._keyFrames, this.maxDuration)
: assert(_keyFrames.isNotEmpty),
assert(_keyFrames.last.time <= maxDuration),
assert(() {
for (int i = 0; i < _keyFrames.length -1; i += 1) {
if (_keyFrames[i].time > _keyFrames[i + 1].time) {
return false;
}
}
return true;
}(), 'The key frame sequence must be sorted by time.');
final double maxDuration;
final List<_KeyFrame> _keyFrames;
@override
double dx(double time) => 0;
@override
bool isDone(double time) => time >= maxDuration;
// The index of the KeyFrame corresponds to the most recent input `time`.
int _lastKeyFrameIndex = 0;
@override
double x(double time) {
final int length = _keyFrames.length;
// Perform a linear search in the sorted key frame list, starting from the
// last key frame found, since the input `time` usually monotonically
// increases by a small amount.
int searchIndex;
final int endIndex;
if (_keyFrames[_lastKeyFrameIndex].time > time) {
// The simulation may have restarted. Search within the index range
// [0, _lastKeyFrameIndex).
searchIndex = 0;
endIndex = _lastKeyFrameIndex;
} else {
searchIndex = _lastKeyFrameIndex;
endIndex = length;
}
// Find the target key frame. Don't have to check (endIndex - 1): if
// (endIndex - 2) doesn't work we'll have to pick (endIndex - 1) anyways.
while (searchIndex < endIndex - 1) {
assert(_keyFrames[searchIndex].time <= time);
final _KeyFrame next = _keyFrames[searchIndex + 1];
if (time < next.time) {
break;
}
searchIndex += 1;
}
_lastKeyFrameIndex = searchIndex;
return _keyFrames[_lastKeyFrameIndex].value;
}
}
/// A basic text input field.
///
/// This widget interacts with the [TextInput] service to let the user edit the
/// text it contains. It also provides scrolling, selection, and cursor
/// movement.
///
/// The [EditableText] widget is a low-level widget that is intended as a
/// building block for custom widget sets. For a complete user experience,
/// consider using a [TextField] or [CupertinoTextField].
///
/// ## Handling User Input
///
/// Currently the user may change the text this widget contains via keyboard or
/// the text selection menu. When the user inserted or deleted text, you will be
/// notified of the change and get a chance to modify the new text value:
///
/// * The [inputFormatters] will be first applied to the user input.
///
/// * The [controller]'s [TextEditingController.value] will be updated with the
/// formatted result, and the [controller]'s listeners will be notified.
///
/// * The [onChanged] callback, if specified, will be called last.
///
/// ## Input Actions
///
/// A [TextInputAction] can be provided to customize the appearance of the
/// action button on the soft keyboard for Android and iOS. The default action
/// is [TextInputAction.done].
///
/// Many [TextInputAction]s are common between Android and iOS. However, if a
/// [textInputAction] is provided that is not supported by the current
/// platform in debug mode, an error will be thrown when the corresponding
/// EditableText receives focus. For example, providing iOS's "emergencyCall"
/// action when running on an Android device will result in an error when in
/// debug mode. In release mode, incompatible [TextInputAction]s are replaced
/// either with "unspecified" on Android, or "default" on iOS. Appropriate
/// [textInputAction]s can be chosen by checking the current platform and then
/// selecting the appropriate action.
///
/// {@template flutter.widgets.EditableText.lifeCycle}
/// ## Lifecycle
///
/// Upon completion of editing, like pressing the "done" button on the keyboard,
/// two actions take place:
///
/// 1st: Editing is finalized. The default behavior of this step includes
/// an invocation of [onChanged]. That default behavior can be overridden.
/// See [onEditingComplete] for details.
///
/// 2nd: [onSubmitted] is invoked with the user's input value.
///
/// [onSubmitted] can be used to manually move focus to another input widget
/// when a user finishes with the currently focused input widget.
///
/// When the widget has focus, it will prevent itself from disposing via
/// [AutomaticKeepAliveClientMixin.wantKeepAlive] in order to avoid losing the
/// selection. Removing the focus will allow it to be disposed.
/// {@endtemplate}
///
/// Rather than using this widget directly, consider using [TextField], which
/// is a full-featured, material-design text input field with placeholder text,
/// labels, and [Form] integration.
///
/// ## Text Editing [Intent]s and Their Default [Action]s
///
/// This widget provides default [Action]s for handling common text editing
/// [Intent]s such as deleting, copying and pasting in the text field. These
/// [Action]s can be directly invoked using [Actions.invoke] or the
/// [Actions.maybeInvoke] method. The default text editing keyboard [Shortcuts],
/// typically declared in [DefaultTextEditingShortcuts], also use these
/// [Intent]s and [Action]s to perform the text editing operations they are
/// bound to.
///
/// The default handling of a specific [Intent] can be overridden by placing an
/// [Actions] widget above this widget. See the [Action] class and the
/// [Action.overridable] constructor for more information on how a pre-defined
/// overridable [Action] can be overridden.
///
/// ### Intents for Deleting Text and Their Default Behavior
///
/// | **Intent Class** | **Default Behavior when there's selected text** | **Default Behavior when there is a [caret](https://en.wikipedia.org/wiki/Caret_navigation) (The selection is [TextSelection.collapsed])** |
/// | :------------------------------- | :--------------------------------------------------- | :----------------------------------------------------------------------- |
/// | [DeleteCharacterIntent] | Deletes the selected text | Deletes the user-perceived character before or after the caret location. |
/// | [DeleteToNextWordBoundaryIntent] | Deletes the selected text and the word before/after the selection's [TextSelection.extent] position | Deletes from the caret location to the previous or the next word boundary |
/// | [DeleteToLineBreakIntent] | Deletes the selected text, and deletes to the start/end of the line from the selection's [TextSelection.extent] position | Deletes from the caret location to the logical start or end of the current line |
///
/// ### Intents for Moving the [Caret](https://en.wikipedia.org/wiki/Caret_navigation)
///
/// | **Intent Class** | **Default Behavior when there's selected text** | **Default Behavior when there is a caret ([TextSelection.collapsed])** |
/// | :----------------------------------------------------------------------------------- | :--------------------------------------------------------------- | :---------------------------------------------------------------------- |
/// | [ExtendSelectionByCharacterIntent](`collapseSelection: true`) | Collapses the selection to the logical start/end of the selection | Moves the caret past the user-perceived character before or after the current caret location. |
/// | [ExtendSelectionToNextWordBoundaryIntent](`collapseSelection: true`) | Collapses the selection to the word boundary before/after the selection's [TextSelection.extent] position | Moves the caret to the previous/next word boundary. |
/// | [ExtendSelectionToNextWordBoundaryOrCaretLocationIntent](`collapseSelection: true`) | Collapses the selection to the word boundary before/after the selection's [TextSelection.extent] position, or [TextSelection.base], whichever is closest in the given direction | Moves the caret to the previous/next word boundary. |
/// | [ExtendSelectionToLineBreakIntent](`collapseSelection: true`) | Collapses the selection to the start/end of the line at the selection's [TextSelection.extent] position | Moves the caret to the start/end of the current line .|
/// | [ExtendSelectionVerticallyToAdjacentLineIntent](`collapseSelection: true`) | Collapses the selection to the position closest to the selection's [TextSelection.extent], on the previous/next adjacent line | Moves the caret to the closest position on the previous/next adjacent line. |
/// | [ExtendSelectionVerticallyToAdjacentPageIntent](`collapseSelection: true`) | Collapses the selection to the position closest to the selection's [TextSelection.extent], on the previous/next adjacent page | Moves the caret to the closest position on the previous/next adjacent page. |
/// | [ExtendSelectionToDocumentBoundaryIntent](`collapseSelection: true`) | Collapses the selection to the start/end of the document | Moves the caret to the start/end of the document. |
///
/// #### Intents for Extending the Selection
///
/// | **Intent Class** | **Default Behavior when there's selected text** | **Default Behavior when there is a caret ([TextSelection.collapsed])** |
/// | :----------------------------------------------------------------------------------- | :--------------------------------------------------------------- | :---------------------------------------------------------------------- |
/// | [ExtendSelectionByCharacterIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] past the user-perceived character before/after it |
/// | [ExtendSelectionToNextWordBoundaryIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the previous/next word boundary |
/// | [ExtendSelectionToNextWordBoundaryOrCaretLocationIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the previous/next word boundary, or [TextSelection.base] whichever is closest in the given direction | Moves the selection's [TextSelection.extent] to the previous/next word boundary. |
/// | [ExtendSelectionToLineBreakIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the start/end of the line |
/// | [ExtendSelectionVerticallyToAdjacentLineIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the closest position on the previous/next adjacent line |
/// | [ExtendSelectionVerticallyToAdjacentPageIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the closest position on the previous/next adjacent page |
/// | [ExtendSelectionToDocumentBoundaryIntent](`collapseSelection: false`) | Moves the selection's [TextSelection.extent] to the start/end of the document |
/// | [SelectAllTextIntent] | Selects the entire document |
///
/// ### Other Intents
///
/// | **Intent Class** | **Default Behavior** |
/// | :-------------------------------------- | :--------------------------------------------------- |
/// | [DoNothingAndStopPropagationTextIntent] | Does nothing in the input field, and prevents the key event from further propagating in the widget tree. |
/// | [ReplaceTextIntent] | Replaces the current [TextEditingValue] in the input field's [TextEditingController], and triggers all related user callbacks and [TextInputFormatter]s. |
/// | [UpdateSelectionIntent] | Updates the current selection in the input field's [TextEditingController], and triggers the [onSelectionChanged] callback. |
/// | [CopySelectionTextIntent] | Copies or cuts the selected text into the clipboard |
/// | [PasteTextIntent] | Inserts the current text in the clipboard after the caret location, or replaces the selected text if the selection is not collapsed. |
///
/// ## Text Editing [Shortcuts]
///
/// It's also possible to directly remap keyboard shortcuts to new [Intent]s by
/// inserting a [Shortcuts] widget above this in the widget tree. When using
/// [WidgetsApp], the large set of default text editing keyboard shortcuts are
/// declared near the top of the widget tree in [DefaultTextEditingShortcuts],
/// and any [Shortcuts] widget between it and this [EditableText] will override
/// those defaults.
///
/// {@template flutter.widgets.editableText.shortcutsAndTextInput}
/// ### Interactions Between [Shortcuts] and Text Input
///
/// Shortcuts prevent text input fields from receiving their keystrokes as text
/// input. For example, placing a [Shortcuts] widget in the widget tree above
/// a text input field and creating a shortcut for [LogicalKeyboardKey.keyA]
/// will prevent the field from receiving that key as text input. In other
/// words, typing key "A" into the field will trigger the shortcut and will not
/// insert a letter "a" into the field.
///
/// This happens because of the way that key strokes are handled in Flutter.
/// When a keystroke is received in Flutter's engine, it first gives the
/// framework the opportunity to handle it as a raw key event through
/// [SystemChannels.keyEvent]. This is what [Shortcuts] listens to indirectly
/// through its [FocusNode]. If it is not handled, then it will proceed to try
/// handling it as text input through [SystemChannels.textInput], which is what
/// [EditableTextState] listens to through [TextInputClient].
///
/// This behavior, where a shortcut prevents text input into some field, can be
/// overridden by using another [Shortcuts] widget lower in the widget tree and
/// mapping the desired key stroke(s) to [DoNothingAndStopPropagationIntent].
/// The key event will be reported as unhandled by the framework and will then
/// be sent as text input as usual.
/// {@endtemplate}
///
/// ## Gesture Events Handling
///
/// When [rendererIgnoresPointer] is false (the default), this widget provides
/// rudimentary, platform-agnostic gesture handling for user actions such as
/// tapping, long-pressing, and scrolling.
///
/// To provide more complete gesture handling, including double-click to select
/// a word, drag selection, and platform-specific handling of gestures such as
/// long presses, consider setting [rendererIgnoresPointer] to true and using
/// [TextSelectionGestureDetectorBuilder].
///
/// {@template flutter.widgets.editableText.showCaretOnScreen}
/// ## Keep the caret visible when focused
///
/// When focused, this widget will make attempts to keep the text area and its
/// caret (even when [showCursor] is `false`) visible, on these occasions:
///
/// * When the user focuses this text field and it is not [readOnly].
/// * When the user changes the selection of the text field, or changes the
/// text when the text field is not [readOnly].
/// * When the virtual keyboard pops up.
/// {@endtemplate}
///
/// ## Scrolling Considerations
///
/// If this [EditableText] is not a descendant of [Scaffold] and is being used
/// within a [Scrollable] or nested [Scrollable]s, consider placing a
/// [ScrollNotificationObserver] above the root [Scrollable] that contains this
/// [EditableText] to ensure proper scroll coordination for [EditableText] and
/// its components like [TextSelectionOverlay].
///
/// {@template flutter.widgets.editableText.accessibility}
/// ## Troubleshooting Common Accessibility Issues
///
/// ### Customizing User Input Accessibility Announcements
///
/// To customize user input accessibility announcements triggered by text
/// changes, use [SemanticsService.announce] to make the desired
/// accessibility announcement.
///
/// On iOS, the on-screen keyboard may announce the most recent input
/// incorrectly when a [TextInputFormatter] inserts a thousands separator to
/// a currency value text field. The following example demonstrates how to
/// suppress the default accessibility announcements by always announcing
/// the content of the text field as a US currency value (the `\$` inserts
/// a dollar sign, the `$newText` interpolates the `newText` variable):
///
/// ```dart
/// onChanged: (String newText) {
/// if (newText.isNotEmpty) {
/// SemanticsService.announce('\$$newText', Directionality.of(context));
/// }
/// }
/// ```
///
/// {@endtemplate}
///
/// See also:
///
/// * [TextField], which is a full-featured, material-design text input field
/// with placeholder text, labels, and [Form] integration.
class EditableText extends StatefulWidget {
/// Creates a basic text input control.
///
/// The [maxLines] property can be set to null to remove the restriction on
/// the number of lines. By default, it is one, meaning this is a single-line
/// text field. [maxLines] must be null or greater than zero.
///
/// If [keyboardType] is not set or is null, its value will be inferred from
/// [autofillHints], if [autofillHints] is not empty. Otherwise it defaults to
/// [TextInputType.text] if [maxLines] is exactly one, and
/// [TextInputType.multiline] if [maxLines] is null or greater than one.
///
/// The text cursor is not shown if [showCursor] is false or if [showCursor]
/// is null (the default) and [readOnly] is true.
EditableText({
super.key,
required this.controller,
required this.focusNode,
this.readOnly = false,
this.obscuringCharacter = '•',
this.obscureText = false,
this.autocorrect = true,
SmartDashesType? smartDashesType,
SmartQuotesType? smartQuotesType,
this.enableSuggestions = true,
required this.style,
StrutStyle? strutStyle,
required this.cursorColor,
required this.backgroundCursorColor,
this.textAlign = TextAlign.start,
this.textDirection,
this.locale,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
this.textScaleFactor,
this.textScaler,
this.maxLines = 1,
this.minLines,
this.expands = false,
this.forceLine = true,
this.textHeightBehavior,
this.textWidthBasis = TextWidthBasis.parent,
this.autofocus = false,
bool? showCursor,
this.showSelectionHandles = false,
this.selectionColor,
this.selectionControls,
TextInputType? keyboardType,
this.textInputAction,
this.textCapitalization = TextCapitalization.none,
this.onChanged,
this.onEditingComplete,
this.onSubmitted,
this.onAppPrivateCommand,
this.onSelectionChanged,
this.onSelectionHandleTapped,
this.onTapOutside,
List<TextInputFormatter>? inputFormatters,
this.mouseCursor,
this.rendererIgnoresPointer = false,
this.cursorWidth = 2.0,
this.cursorHeight,
this.cursorRadius,
this.cursorOpacityAnimates = false,
this.cursorOffset,
this.paintCursorAboveText = false,
this.selectionHeightStyle = ui.BoxHeightStyle.tight,
this.selectionWidthStyle = ui.BoxWidthStyle.tight,
this.scrollPadding = const EdgeInsets.all(20.0),
this.keyboardAppearance = Brightness.light,
this.dragStartBehavior = DragStartBehavior.start,
bool? enableInteractiveSelection,
this.scrollController,
this.scrollPhysics,
this.autocorrectionTextRectColor,
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
ToolbarOptions? toolbarOptions,
this.autofillHints = const <String>[],
this.autofillClient,
this.clipBehavior = Clip.hardEdge,
this.restorationId,
this.scrollBehavior,
this.scribbleEnabled = true,
this.enableIMEPersonalizedLearning = true,
this.contentInsertionConfiguration,
this.contextMenuBuilder,
this.spellCheckConfiguration,
this.magnifierConfiguration = TextMagnifierConfiguration.disabled,
this.undoController,
}) : assert(obscuringCharacter.length == 1),
smartDashesType = smartDashesType ?? (obscureText ? SmartDashesType.disabled : SmartDashesType.enabled),
smartQuotesType = smartQuotesType ?? (obscureText ? SmartQuotesType.disabled : SmartQuotesType.enabled),
assert(minLines == null || minLines > 0),
assert(
(maxLines == null) || (minLines == null) || (maxLines >= minLines),
"minLines can't be greater than maxLines",
),
assert(
!expands || (maxLines == null && minLines == null),
'minLines and maxLines must be null when expands is true.',
),
assert(!obscureText || maxLines == 1, 'Obscured fields cannot be multiline.'),
enableInteractiveSelection = enableInteractiveSelection ?? (!readOnly || !obscureText),
toolbarOptions = selectionControls is TextSelectionHandleControls && toolbarOptions == null ? ToolbarOptions.empty : toolbarOptions ??
(obscureText
? (readOnly
// No point in even offering "Select All" in a read-only obscured
// field.
? ToolbarOptions.empty
// Writable, but obscured.
: const ToolbarOptions(
selectAll: true,
paste: true,
))
: (readOnly
// Read-only, not obscured.
? const ToolbarOptions(
selectAll: true,
copy: true,
)
// Writable, not obscured.
: const ToolbarOptions(
copy: true,
cut: true,
selectAll: true,
paste: true,
))),
assert(
spellCheckConfiguration == null ||
spellCheckConfiguration == const SpellCheckConfiguration.disabled() ||
spellCheckConfiguration.misspelledTextStyle != null,
'spellCheckConfiguration must specify a misspelledTextStyle if spell check behavior is desired',
),
_strutStyle = strutStyle,
keyboardType = keyboardType ?? _inferKeyboardType(autofillHints: autofillHints, maxLines: maxLines),
inputFormatters = maxLines == 1
? <TextInputFormatter>[
FilteringTextInputFormatter.singleLineFormatter,
...inputFormatters ?? const Iterable<TextInputFormatter>.empty(),
]
: inputFormatters,
showCursor = showCursor ?? !readOnly;
/// Controls the text being edited.
final TextEditingController controller;
/// Controls whether this widget has keyboard focus.
final FocusNode focusNode;
/// {@template flutter.widgets.editableText.obscuringCharacter}
/// Character used for obscuring text if [obscureText] is true.
///
/// Must be only a single character.
///
/// Defaults to the character U+2022 BULLET (•).
/// {@endtemplate}
final String obscuringCharacter;
/// {@template flutter.widgets.editableText.obscureText}
/// Whether to hide the text being edited (e.g., for passwords).
///
/// When this is set to true, all the characters in the text field are
/// replaced by [obscuringCharacter], and the text in the field cannot be
/// copied with copy or cut. If [readOnly] is also true, then the text cannot
/// be selected.
///
/// Defaults to false.
/// {@endtemplate}
final bool obscureText;
/// {@macro dart.ui.textHeightBehavior}
final TextHeightBehavior? textHeightBehavior;
/// {@macro flutter.painting.textPainter.textWidthBasis}
final TextWidthBasis textWidthBasis;
/// {@template flutter.widgets.editableText.readOnly}
/// Whether the text can be changed.
///
/// When this is set to true, the text cannot be modified
/// by any shortcut or keyboard operation. The text is still selectable.
///
/// Defaults to false.
/// {@endtemplate}
final bool readOnly;
/// Whether the text will take the full width regardless of the text width.
///
/// When this is set to false, the width will be based on text width, which
/// will also be affected by [textWidthBasis].
///
/// Defaults to true.
///
/// See also:
///
/// * [textWidthBasis], which controls the calculation of text width.
final bool forceLine;
/// Configuration of toolbar options.
///
/// By default, all options are enabled. If [readOnly] is true, paste and cut
/// will be disabled regardless. If [obscureText] is true, cut and copy will
/// be disabled regardless. If [readOnly] and [obscureText] are both true,
/// select all will also be disabled.
final ToolbarOptions toolbarOptions;
/// Whether to show selection handles.
///
/// When a selection is active, there will be two handles at each side of
/// boundary, or one handle if the selection is collapsed. The handles can be
/// dragged to adjust the selection.
///
/// See also:
///
/// * [showCursor], which controls the visibility of the cursor.
final bool showSelectionHandles;
/// {@template flutter.widgets.editableText.showCursor}
/// Whether to show cursor.
///
/// The cursor refers to the blinking caret when the [EditableText] is focused.
/// {@endtemplate}
///
/// See also:
///
/// * [showSelectionHandles], which controls the visibility of the selection handles.
final bool showCursor;
/// {@template flutter.widgets.editableText.autocorrect}
/// Whether to enable autocorrection.
///
/// Defaults to true.
/// {@endtemplate}
final bool autocorrect;
/// {@macro flutter.services.TextInputConfiguration.smartDashesType}
final SmartDashesType smartDashesType;
/// {@macro flutter.services.TextInputConfiguration.smartQuotesType}
final SmartQuotesType smartQuotesType;
/// {@macro flutter.services.TextInputConfiguration.enableSuggestions}
final bool enableSuggestions;
/// The text style to use for the editable text.
final TextStyle style;
/// Controls the undo state of the current editable text.
///
/// If null, this widget will create its own [UndoHistoryController].
final UndoHistoryController? undoController;
/// {@template flutter.widgets.editableText.strutStyle}
/// The strut style used for the vertical layout.
///
/// [StrutStyle] is used to establish a predictable vertical layout.
/// Since fonts may vary depending on user input and due to font
/// fallback, [StrutStyle.forceStrutHeight] is enabled by default
/// to lock all lines to the height of the base [TextStyle], provided by
/// [style]. This ensures the typed text fits within the allotted space.
///
/// If null, the strut used will inherit values from the [style] and will
/// have [StrutStyle.forceStrutHeight] set to true. When no [style] is
/// passed, the theme's [TextStyle] will be used to generate [strutStyle]
/// instead.
///
/// To disable strut-based vertical alignment and allow dynamic vertical
/// layout based on the glyphs typed, use [StrutStyle.disabled].
///
/// Flutter's strut is based on [typesetting strut](https://en.wikipedia.org/wiki/Strut_(typesetting))
/// and CSS's [line-height](https://www.w3.org/TR/CSS2/visudet.html#line-height).
/// {@endtemplate}
///
/// Within editable text and text fields, [StrutStyle] will not use its standalone
/// default values, and will instead inherit omitted/null properties from the
/// [TextStyle] instead. See [StrutStyle.inheritFromTextStyle].
StrutStyle get strutStyle {
if (_strutStyle == null) {
return StrutStyle.fromTextStyle(style, forceStrutHeight: true);
}
return _strutStyle.inheritFromTextStyle(style);
}
final StrutStyle? _strutStyle;
/// {@template flutter.widgets.editableText.textAlign}
/// How the text should be aligned horizontally.
///
/// Defaults to [TextAlign.start].
/// {@endtemplate}
final TextAlign textAlign;
/// {@template flutter.widgets.editableText.textDirection}
/// The directionality of the text.
///
/// This decides how [textAlign] values like [TextAlign.start] and
/// [TextAlign.end] are interpreted.
///
/// This is also used to disambiguate how to render bidirectional text. For
/// example, if the text is an English phrase followed by a Hebrew phrase,
/// in a [TextDirection.ltr] context the English phrase will be on the left
/// and the Hebrew phrase to its right, while in a [TextDirection.rtl]
/// context, the English phrase will be on the right and the Hebrew phrase on
/// its left.
///
/// Defaults to the ambient [Directionality], if any.
/// {@endtemplate}
final TextDirection? textDirection;
/// {@template flutter.widgets.editableText.textCapitalization}
/// Configures how the platform keyboard will select an uppercase or
/// lowercase keyboard.
///
/// Only supports text keyboards, other keyboard types will ignore this
/// configuration. Capitalization is locale-aware.
///
/// Defaults to [TextCapitalization.none].
///
/// See also:
///
/// * [TextCapitalization], for a description of each capitalization behavior.
///
/// {@endtemplate}
final TextCapitalization textCapitalization;
/// Used to select a font when the same Unicode character can
/// be rendered differently, depending on the locale.
///
/// It's rarely necessary to set this property. By default its value
/// is inherited from the enclosing app with `Localizations.localeOf(context)`.
///
/// See [RenderEditable.locale] for more information.
final Locale? locale;
/// {@template flutter.widgets.editableText.textScaleFactor}
/// Deprecated. Will be removed in a future version of Flutter. Use
/// [textScaler] instead.
///
/// The number of font pixels for each logical pixel.
///
/// For example, if the text scale factor is 1.5, text will be 50% larger than
/// the specified font size.
///
/// Defaults to the [MediaQueryData.textScaleFactor] obtained from the ambient
/// [MediaQuery], or 1.0 if there is no [MediaQuery] in scope.
/// {@endtemplate}
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
final double? textScaleFactor;
/// {@macro flutter.painting.textPainter.textScaler}
final TextScaler? textScaler;
/// The color to use when painting the cursor.
final Color cursorColor;
/// The color to use when painting the autocorrection Rect.
///
/// For [CupertinoTextField]s, the value is set to the ambient
/// [CupertinoThemeData.primaryColor] with 20% opacity. For [TextField]s, the
/// value is null on non-iOS platforms and the same color used in [CupertinoTextField]
/// on iOS.
///
/// Currently the autocorrection Rect only appears on iOS.
///
/// Defaults to null, which disables autocorrection Rect painting.
final Color? autocorrectionTextRectColor;
/// The color to use when painting the background cursor aligned with the text
/// while rendering the floating cursor.
///
/// Typically this would be set to [CupertinoColors.inactiveGray].
///
/// See also:
///
/// * [FloatingCursorDragState], which explains the floating cursor feature
/// in detail.
final Color backgroundCursorColor;
/// {@template flutter.widgets.editableText.maxLines}
/// The maximum number of lines to show at one time, wrapping if necessary.
///
/// This affects the height of the field itself and does not limit the number
/// of lines that can be entered into the field.
///
/// If this is 1 (the default), the text will not wrap, but will scroll
/// horizontally instead.
///
/// If this is null, there is no limit to the number of lines, and the text
/// container will start with enough vertical space for one line and
/// automatically grow to accommodate additional lines as they are entered, up
/// to the height of its constraints.
///
/// If this is not null, the value must be greater than zero, and it will lock
/// the input to the given number of lines and take up enough horizontal space
/// to accommodate that number of lines. Setting [minLines] as well allows the
/// input to grow and shrink between the indicated range.
///
/// The full set of behaviors possible with [minLines] and [maxLines] are as
/// follows. These examples apply equally to [TextField], [TextFormField],
/// [CupertinoTextField], and [EditableText].
///
/// Input that occupies a single line and scrolls horizontally as needed.
/// ```dart
/// const TextField()
/// ```
///
/// Input whose height grows from one line up to as many lines as needed for
/// the text that was entered. If a height limit is imposed by its parent, it
/// will scroll vertically when its height reaches that limit.
/// ```dart
/// const TextField(maxLines: null)
/// ```
///
/// The input's height is large enough for the given number of lines. If
/// additional lines are entered the input scrolls vertically.
/// ```dart
/// const TextField(maxLines: 2)
/// ```
///
/// Input whose height grows with content between a min and max. An infinite
/// max is possible with `maxLines: null`.
/// ```dart
/// const TextField(minLines: 2, maxLines: 4)
/// ```
///
/// See also:
///
/// * [minLines], which sets the minimum number of lines visible.
/// {@endtemplate}
/// * [expands], which determines whether the field should fill the height of
/// its parent.
final int? maxLines;
/// {@template flutter.widgets.editableText.minLines}
/// The minimum number of lines to occupy when the content spans fewer lines.
///
/// This affects the height of the field itself and does not limit the number
/// of lines that can be entered into the field.
///
/// If this is null (default), text container starts with enough vertical space
/// for one line and grows to accommodate additional lines as they are entered.
///
/// This can be used in combination with [maxLines] for a varying set of behaviors.
///
/// If the value is set, it must be greater than zero. If the value is greater
/// than 1, [maxLines] should also be set to either null or greater than
/// this value.
///
/// When [maxLines] is set as well, the height will grow between the indicated
/// range of lines. When [maxLines] is null, it will grow as high as needed,
/// starting from [minLines].
///
/// A few examples of behaviors possible with [minLines] and [maxLines] are as follows.
/// These apply equally to [TextField], [TextFormField], [CupertinoTextField],
/// and [EditableText].
///
/// Input that always occupies at least 2 lines and has an infinite max.
/// Expands vertically as needed.
/// ```dart
/// TextField(minLines: 2)
/// ```
///
/// Input whose height starts from 2 lines and grows up to 4 lines at which
/// point the height limit is reached. If additional lines are entered it will
/// scroll vertically.
/// ```dart
/// const TextField(minLines:2, maxLines: 4)
/// ```
///
/// Defaults to null.
///
/// See also:
///
/// * [maxLines], which sets the maximum number of lines visible, and has
/// several examples of how minLines and maxLines interact to produce
/// various behaviors.
/// {@endtemplate}
/// * [expands], which determines whether the field should fill the height of
/// its parent.
final int? minLines;
/// {@template flutter.widgets.editableText.expands}
/// Whether this widget's height will be sized to fill its parent.
///
/// If set to true and wrapped in a parent widget like [Expanded] or
/// [SizedBox], the input will expand to fill the parent.
///
/// [maxLines] and [minLines] must both be null when this is set to true,
/// otherwise an error is thrown.
///
/// Defaults to false.
///
/// See the examples in [maxLines] for the complete picture of how [maxLines],
/// [minLines], and [expands] interact to produce various behaviors.
///
/// Input that matches the height of its parent:
/// ```dart
/// const Expanded(
/// child: TextField(maxLines: null, expands: true),
/// )
/// ```
/// {@endtemplate}
final bool expands;
/// {@template flutter.widgets.editableText.autofocus}
/// Whether this text field should focus itself if nothing else is already
/// focused.
///
/// If true, the keyboard will open as soon as this text field obtains focus.
/// Otherwise, the keyboard is only shown after the user taps the text field.
///
/// Defaults to false.
/// {@endtemplate}
// See https://github.com/flutter/flutter/issues/7035 for the rationale for this
// keyboard behavior.
final bool autofocus;
/// The color to use when painting the selection.
///
/// If this property is null, this widget gets the selection color from the
/// [DefaultSelectionStyle].
///
/// For [CupertinoTextField]s, the value is set to the ambient
/// [CupertinoThemeData.primaryColor] with 20% opacity. For [TextField]s, the
/// value is set to the ambient [TextSelectionThemeData.selectionColor].
final Color? selectionColor;
/// {@template flutter.widgets.editableText.selectionControls}
/// Optional delegate for building the text selection handles.
///
/// Historically, this field also controlled the toolbar. This is now handled
/// by [contextMenuBuilder] instead. However, for backwards compatibility, when
/// [selectionControls] is set to an object that does not mix in
/// [TextSelectionHandleControls], [contextMenuBuilder] is ignored and the
/// [TextSelectionControls.buildToolbar] method is used instead.
/// {@endtemplate}
///
/// See also:
///
/// * [CupertinoTextField], which wraps an [EditableText] and which shows the
/// selection toolbar upon user events that are appropriate on the iOS
/// platform.
/// * [TextField], a Material Design themed wrapper of [EditableText], which
/// shows the selection toolbar upon appropriate user events based on the
/// user's platform set in [ThemeData.platform].
final TextSelectionControls? selectionControls;
/// {@template flutter.widgets.editableText.keyboardType}
/// The type of keyboard to use for editing the text.
///
/// Defaults to [TextInputType.text] if [maxLines] is one and
/// [TextInputType.multiline] otherwise.
/// {@endtemplate}
final TextInputType keyboardType;
/// The type of action button to use with the soft keyboard.
final TextInputAction? textInputAction;
/// {@template flutter.widgets.editableText.onChanged}
/// Called when the user initiates a change to the TextField's
/// value: when they have inserted or deleted text.
///
/// This callback doesn't run when the TextField's text is changed
/// programmatically, via the TextField's [controller]. Typically it
/// isn't necessary to be notified of such changes, since they're
/// initiated by the app itself.
///
/// To be notified of all changes to the TextField's text, cursor,
/// and selection, one can add a listener to its [controller] with
/// [TextEditingController.addListener].
///
/// [onChanged] is called before [onSubmitted] when user indicates completion
/// of editing, such as when pressing the "done" button on the keyboard. That
/// default behavior can be overridden. See [onEditingComplete] for details.
///
/// {@tool dartpad}
/// This example shows how onChanged could be used to check the TextField's
/// current value each time the user inserts or deletes a character.
///
/// ** See code in examples/api/lib/widgets/editable_text/editable_text.on_changed.0.dart **
/// {@end-tool}
/// {@endtemplate}
///
/// ## Handling emojis and other complex characters
/// {@template flutter.widgets.EditableText.onChanged}
/// It's important to always use
/// [characters](https://pub.dev/packages/characters) when dealing with user
/// input text that may contain complex characters. This will ensure that
/// extended grapheme clusters and surrogate pairs are treated as single
/// characters, as they appear to the user.
///
/// For example, when finding the length of some user input, use
/// `string.characters.length`. Do NOT use `string.length` or even
/// `string.runes.length`. For the complex character "👨👩👦", this
/// appears to the user as a single character, and `string.characters.length`
/// intuitively returns 1. On the other hand, `string.length` returns 8, and
/// `string.runes.length` returns 5!
/// {@endtemplate}
///
/// See also:
///
/// * [inputFormatters], which are called before [onChanged]
/// runs and can validate and change ("format") the input value.
/// * [onEditingComplete], [onSubmitted], [onSelectionChanged]:
/// which are more specialized input change notifications.
/// * [TextEditingController], which implements the [Listenable] interface
/// and notifies its listeners on [TextEditingValue] changes.
final ValueChanged<String>? onChanged;
/// {@template flutter.widgets.editableText.onEditingComplete}
/// Called when the user submits editable content (e.g., user presses the "done"
/// button on the keyboard).
///
/// The default implementation of [onEditingComplete] executes 2 different
/// behaviors based on the situation:
///
/// - When a completion action is pressed, such as "done", "go", "send", or
/// "search", the user's content is submitted to the [controller] and then
/// focus is given up.
///
/// - When a non-completion action is pressed, such as "next" or "previous",
/// the user's content is submitted to the [controller], but focus is not
/// given up because developers may want to immediately move focus to
/// another input widget within [onSubmitted].
///
/// Providing [onEditingComplete] prevents the aforementioned default behavior.
/// {@endtemplate}
final VoidCallback? onEditingComplete;
/// {@template flutter.widgets.editableText.onSubmitted}
/// Called when the user indicates that they are done editing the text in the
/// field.
///
/// By default, [onSubmitted] is called after [onChanged] when the user
/// has finalized editing; or, if the default behavior has been overridden,
/// after [onEditingComplete]. See [onEditingComplete] for details.
///
/// ## Testing
/// The following is the recommended way to trigger [onSubmitted] in a test:
///
/// ```dart
/// await tester.testTextInput.receiveAction(TextInputAction.done);
/// ```
///
/// Sending a `LogicalKeyboardKey.enter` via `tester.sendKeyEvent` will not
/// trigger [onSubmitted]. This is because on a real device, the engine
/// translates the enter key to a done action, but `tester.sendKeyEvent` sends
/// the key to the framework only.
/// {@endtemplate}
final ValueChanged<String>? onSubmitted;
/// {@template flutter.widgets.editableText.onAppPrivateCommand}
/// This is used to receive a private command from the input method.
///
/// Called when the result of [TextInputClient.performPrivateCommand] is
/// received.
///
/// This can be used to provide domain-specific features that are only known
/// between certain input methods and their clients.
///
/// See also:
/// * [performPrivateCommand](https://developer.android.com/reference/android/view/inputmethod/InputConnection#performPrivateCommand\(java.lang.String,%20android.os.Bundle\)),
/// which is the Android documentation for performPrivateCommand, used to
/// send a command from the input method.
/// * [sendAppPrivateCommand](https://developer.android.com/reference/android/view/inputmethod/InputMethodManager#sendAppPrivateCommand),
/// which is the Android documentation for sendAppPrivateCommand, used to
/// send a command to the input method.
/// {@endtemplate}
final AppPrivateCommandCallback? onAppPrivateCommand;
/// {@template flutter.widgets.editableText.onSelectionChanged}
/// Called when the user changes the selection of text (including the cursor
/// location).
/// {@endtemplate}
final SelectionChangedCallback? onSelectionChanged;
/// {@macro flutter.widgets.SelectionOverlay.onSelectionHandleTapped}
final VoidCallback? onSelectionHandleTapped;
/// {@template flutter.widgets.editableText.onTapOutside}
/// Called for each tap that occurs outside of the[TextFieldTapRegion] group
/// when the text field is focused.
///
/// If this is null, [FocusNode.unfocus] will be called on the [focusNode] for
/// this text field when a [PointerDownEvent] is received on another part of
/// the UI. However, it will not unfocus as a result of mobile application
/// touch events (which does not include mouse clicks), to conform with the
/// platform conventions. To change this behavior, a callback may be set here
/// that operates differently from the default.
///
/// When adding additional controls to a text field (for example, a spinner, a
/// button that copies the selected text, or modifies formatting), it is
/// helpful if tapping on that control doesn't unfocus the text field. In
/// order for an external widget to be considered as part of the text field
/// for the purposes of tapping "outside" of the field, wrap the control in a
/// [TextFieldTapRegion].
///
/// The [PointerDownEvent] passed to the function is the event that caused the
/// notification. It is possible that the event may occur outside of the
/// immediate bounding box defined by the text field, although it will be
/// within the bounding box of a [TextFieldTapRegion] member.
/// {@endtemplate}
///
/// {@tool dartpad}
/// This example shows how to use a `TextFieldTapRegion` to wrap a set of
/// "spinner" buttons that increment and decrement a value in the [TextField]
/// without causing the text field to lose keyboard focus.
///
/// This example includes a generic `SpinnerField<T>` class that you can copy
/// into your own project and customize.
///
/// ** See code in examples/api/lib/widgets/tap_region/text_field_tap_region.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [TapRegion] for how the region group is determined.
final TapRegionCallback? onTapOutside;
/// {@template flutter.widgets.editableText.inputFormatters}
/// Optional input validation and formatting overrides.
///
/// Formatters are run in the provided order when the user changes the text
/// this widget contains. When this parameter changes, the new formatters will
/// not be applied until the next time the user inserts or deletes text.
/// Similar to the [onChanged] callback, formatters don't run when the text is
/// changed programmatically via [controller].
///
/// See also:
///
/// * [TextEditingController], which implements the [Listenable] interface
/// and notifies its listeners on [TextEditingValue] changes.
/// {@endtemplate}
final List<TextInputFormatter>? inputFormatters;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If this property is null, [SystemMouseCursors.text] will be used.
///
/// The [mouseCursor] is the only property of [EditableText] that controls the
/// appearance of the mouse pointer. All other properties related to "cursor"
/// stands for the text cursor, which is usually a blinking vertical line at
/// the editing position.
final MouseCursor? mouseCursor;
/// Whether the caller will provide gesture handling (true), or if the
/// [EditableText] is expected to handle basic gestures (false).
///
/// When this is false, the [EditableText] (or more specifically, the
/// [RenderEditable]) enables some rudimentary gestures (tap to position the
/// cursor, long-press to select all, and some scrolling behavior).
///
/// These behaviors are sufficient for debugging purposes but are inadequate
/// for user-facing applications. To enable platform-specific behaviors, use a
/// [TextSelectionGestureDetectorBuilder] to wrap the [EditableText], and set
/// [rendererIgnoresPointer] to true.
///
/// When [rendererIgnoresPointer] is true true, the [RenderEditable] created
/// by this widget will not handle pointer events.
///
/// This property is false by default.
///
/// See also:
///
/// * [RenderEditable.ignorePointer], which implements this feature.
/// * [TextSelectionGestureDetectorBuilder], which implements platform-specific
/// gestures and behaviors.
final bool rendererIgnoresPointer;
/// {@template flutter.widgets.editableText.cursorWidth}
/// How thick the cursor will be.
///
/// Defaults to 2.0.
///
/// The cursor will draw under the text. The cursor width will extend
/// to the right of the boundary between characters for left-to-right text
/// and to the left for right-to-left text. This corresponds to extending
/// downstream relative to the selected position. Negative values may be used
/// to reverse this behavior.
/// {@endtemplate}
final double cursorWidth;
/// {@template flutter.widgets.editableText.cursorHeight}
/// How tall the cursor will be.
///
/// If this property is null, [RenderEditable.preferredLineHeight] will be used.
/// {@endtemplate}
final double? cursorHeight;
/// {@template flutter.widgets.editableText.cursorRadius}
/// How rounded the corners of the cursor should be.
///
/// By default, the cursor has no radius.
/// {@endtemplate}
final Radius? cursorRadius;
/// {@template flutter.widgets.editableText.cursorOpacityAnimates}
/// Whether the cursor will animate from fully transparent to fully opaque
/// during each cursor blink.
///
/// By default, the cursor opacity will animate on iOS platforms and will not
/// animate on Android platforms.
/// {@endtemplate}
final bool cursorOpacityAnimates;
///{@macro flutter.rendering.RenderEditable.cursorOffset}
final Offset? cursorOffset;
///{@macro flutter.rendering.RenderEditable.paintCursorAboveText}
final bool paintCursorAboveText;
/// Controls how tall the selection highlight boxes are computed to be.
///
/// See [ui.BoxHeightStyle] for details on available styles.
final ui.BoxHeightStyle selectionHeightStyle;
/// Controls how wide the selection highlight boxes are computed to be.
///
/// See [ui.BoxWidthStyle] for details on available styles.
final ui.BoxWidthStyle selectionWidthStyle;
/// The appearance of the keyboard.
///
/// This setting is only honored on iOS devices.
///
/// Defaults to [Brightness.light].
final Brightness keyboardAppearance;
/// {@template flutter.widgets.editableText.scrollPadding}
/// Configures padding to edges surrounding a [Scrollable] when the Textfield scrolls into view.
///
/// When this widget receives focus and is not completely visible (for example scrolled partially
/// off the screen or overlapped by the keyboard)
/// then it will attempt to make itself visible by scrolling a surrounding [Scrollable], if one is present.
/// This value controls how far from the edges of a [Scrollable] the TextField will be positioned after the scroll.
///
/// Defaults to EdgeInsets.all(20.0).
/// {@endtemplate}
final EdgeInsets scrollPadding;
/// {@template flutter.widgets.editableText.enableInteractiveSelection}
/// Whether to enable user interface affordances for changing the
/// text selection.
///
/// For example, setting this to true will enable features such as
/// long-pressing the TextField to select text and show the
/// cut/copy/paste menu, and tapping to move the text caret.
///
/// When this is false, the text selection cannot be adjusted by
/// the user, text cannot be copied, and the user cannot paste into
/// the text field from the clipboard.
///
/// Defaults to true.
/// {@endtemplate}
final bool enableInteractiveSelection;
/// Setting this property to true makes the cursor stop blinking or fading
/// on and off once the cursor appears on focus. This property is useful for
/// testing purposes.
///
/// It does not affect the necessity to focus the EditableText for the cursor
/// to appear in the first place.
///
/// Defaults to false, resulting in a typical blinking cursor.
static bool debugDeterministicCursor = false;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@template flutter.widgets.editableText.scrollController}
/// The [ScrollController] to use when vertically scrolling the input.
///
/// If null, it will instantiate a new ScrollController.
///
/// See [Scrollable.controller].
/// {@endtemplate}
final ScrollController? scrollController;
/// {@template flutter.widgets.editableText.scrollPhysics}
/// The [ScrollPhysics] to use when vertically scrolling the input.
///
/// If not specified, it will behave according to the current platform.
///
/// See [Scrollable.physics].
/// {@endtemplate}
///
/// If an explicit [ScrollBehavior] is provided to [scrollBehavior], the
/// [ScrollPhysics] provided by that behavior will take precedence after
/// [scrollPhysics].
final ScrollPhysics? scrollPhysics;
/// {@template flutter.widgets.editableText.scribbleEnabled}
/// Whether iOS 14 Scribble features are enabled for this widget.
///
/// Only available on iPads.
///
/// Defaults to true.
/// {@endtemplate}
final bool scribbleEnabled;
/// {@template flutter.widgets.editableText.selectionEnabled}
/// Same as [enableInteractiveSelection].
///
/// This getter exists primarily for consistency with
/// [RenderEditable.selectionEnabled].
/// {@endtemplate}
bool get selectionEnabled => enableInteractiveSelection;
/// {@template flutter.widgets.editableText.autofillHints}
/// A list of strings that helps the autofill service identify the type of this
/// text input.
///
/// When set to null, this text input will not send its autofill information
/// to the platform, preventing it from participating in autofills triggered
/// by a different [AutofillClient], even if they're in the same
/// [AutofillScope]. Additionally, on Android and web, setting this to null
/// will disable autofill for this text field.
///
/// The minimum platform SDK version that supports Autofill is API level 26
/// for Android, and iOS 10.0 for iOS.
///
/// Defaults to an empty list.
///
/// ### Setting up iOS autofill:
///
/// To provide the best user experience and ensure your app fully supports
/// password autofill on iOS, follow these steps:
///
/// * Set up your iOS app's
/// [associated domains](https://developer.apple.com/documentation/safariservices/supporting_associated_domains_in_your_app).
/// * Some autofill hints only work with specific [keyboardType]s. For example,
/// [AutofillHints.name] requires [TextInputType.name] and [AutofillHints.email]
/// works only with [TextInputType.emailAddress]. Make sure the input field has a
/// compatible [keyboardType]. Empirically, [TextInputType.name] works well
/// with many autofill hints that are predefined on iOS.
///
/// ### Troubleshooting Autofill
///
/// Autofill service providers rely heavily on [autofillHints]. Make sure the
/// entries in [autofillHints] are supported by the autofill service currently
/// in use (the name of the service can typically be found in your mobile
/// device's system settings).
///
/// #### Autofill UI refuses to show up when I tap on the text field
///
/// Check the device's system settings and make sure autofill is turned on,
/// and there are available credentials stored in the autofill service.
///
/// * iOS password autofill: Go to Settings -> Password, turn on "Autofill
/// Passwords", and add new passwords for testing by pressing the top right
/// "+" button. Use an arbitrary "website" if you don't have associated
/// domains set up for your app. As long as there's at least one password
/// stored, you should be able to see a key-shaped icon in the quick type
/// bar on the software keyboard, when a password related field is focused.
///
/// * iOS contact information autofill: iOS seems to pull contact info from
/// the Apple ID currently associated with the device. Go to Settings ->
/// Apple ID (usually the first entry, or "Sign in to your iPhone" if you
/// haven't set up one on the device), and fill out the relevant fields. If
/// you wish to test more contact info types, try adding them in Contacts ->
/// My Card.
///
/// * Android autofill: Go to Settings -> System -> Languages & input ->
/// Autofill service. Enable the autofill service of your choice, and make
/// sure there are available credentials associated with your app.
///
/// #### I called `TextInput.finishAutofillContext` but the autofill save
/// prompt isn't showing
///
/// * iOS: iOS may not show a prompt or any other visual indication when it
/// saves user password. Go to Settings -> Password and check if your new
/// password is saved. Neither saving password nor auto-generating strong
/// password works without properly setting up associated domains in your
/// app. To set up associated domains, follow the instructions in
/// <https://developer.apple.com/documentation/safariservices/supporting_associated_domains_in_your_app>.
///
/// {@endtemplate}
/// {@macro flutter.services.AutofillConfiguration.autofillHints}
final Iterable<String>? autofillHints;
/// The [AutofillClient] that controls this input field's autofill behavior.
///
/// When null, this widget's [EditableTextState] will be used as the
/// [AutofillClient]. This property may override [autofillHints].
final AutofillClient? autofillClient;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// Restoration ID to save and restore the scroll offset of the
/// [EditableText].
///
/// If a restoration id is provided, the [EditableText] will persist its
/// current scroll offset and restore it during state restoration.
///
/// The scroll offset is persisted in a [RestorationBucket] claimed from
/// the surrounding [RestorationScope] using the provided restoration ID.
///
/// Persisting and restoring the content of the [EditableText] is the
/// responsibility of the owner of the [controller], who may use a
/// [RestorableTextEditingController] for that purpose.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
final String? restorationId;
/// {@template flutter.widgets.shadow.scrollBehavior}
/// A [ScrollBehavior] that will be applied to this widget individually.
///
/// Defaults to null, wherein the inherited [ScrollBehavior] is copied and
/// modified to alter the viewport decoration, like [Scrollbar]s.
/// {@endtemplate}
///
/// [ScrollBehavior]s also provide [ScrollPhysics]. If an explicit
/// [ScrollPhysics] is provided in [scrollPhysics], it will take precedence,
/// followed by [scrollBehavior], and then the inherited ancestor
/// [ScrollBehavior].
///
/// The [ScrollBehavior] of the inherited [ScrollConfiguration] will be
/// modified by default to only apply a [Scrollbar] if [maxLines] is greater
/// than 1.
final ScrollBehavior? scrollBehavior;
/// {@macro flutter.services.TextInputConfiguration.enableIMEPersonalizedLearning}
final bool enableIMEPersonalizedLearning;
/// {@template flutter.widgets.editableText.contentInsertionConfiguration}
/// Configuration of handler for media content inserted via the system input
/// method.
///
/// Defaults to null in which case media content insertion will be disabled,
/// and the system will display a message informing the user that the text field
/// does not support inserting media content.
///
/// Set [ContentInsertionConfiguration.onContentInserted] to provide a handler.
/// Additionally, set [ContentInsertionConfiguration.allowedMimeTypes]
/// to limit the allowable mime types for inserted content.
///
/// {@tool dartpad}
///
/// This example shows how to access the data for inserted content in your
/// `TextField`.
///
/// ** See code in examples/api/lib/widgets/editable_text/editable_text.on_content_inserted.0.dart **
/// {@end-tool}
///
/// If [contentInsertionConfiguration] is not provided, by default
/// an empty list of mime types will be sent to the Flutter Engine.
/// A handler function must be provided in order to customize the allowable
/// mime types for inserted content.
///
/// If rich content is inserted without a handler, the system will display
/// a message informing the user that the current text input does not support
/// inserting rich content.
/// {@endtemplate}
final ContentInsertionConfiguration? contentInsertionConfiguration;
/// {@template flutter.widgets.EditableText.contextMenuBuilder}
/// Builds the text selection toolbar when requested by the user.
///
/// The context menu is built when [EditableTextState.showToolbar] is called,
/// typically by one of the callbacks installed by the widget created by
/// [TextSelectionGestureDetectorBuilder.buildGestureDetector]. The widget
/// returned by [contextMenuBuilder] is passed to a [ContextMenuController].
///
/// If no callback is provided, no context menu will be shown.
///
/// The [EditableTextContextMenuBuilder] signature used by the
/// [contextMenuBuilder] callback has two parameters, the [BuildContext] of
/// the [EditableText] and the [EditableTextState] of the [EditableText].
///
/// The [EditableTextState] has two properties that are especially useful when
/// building the widgets for the context menu:
///
/// * [EditableTextState.contextMenuAnchors] specifies the desired anchor
/// position for the context menu.
///
/// * [EditableTextState.contextMenuButtonItems] represents the buttons that
/// should typically be built for this widget (e.g. cut, copy, paste).
///
/// The [TextSelectionToolbarLayoutDelegate] class may be particularly useful
/// in honoring the preferred anchor positions.
///
/// For backwards compatibility, when [selectionControls] is set to an object
/// that does not mix in [TextSelectionHandleControls], [contextMenuBuilder]
/// is ignored and the [TextSelectionControls.buildToolbar] method is used
/// instead.
///
/// {@tool dartpad}
/// This example shows how to customize the menu, in this case by keeping the
/// default buttons for the platform but modifying their appearance.
///
/// ** See code in examples/api/lib/material/context_menu/editable_text_toolbar_builder.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to show a custom button only when an email address
/// is currently selected.
///
/// ** See code in examples/api/lib/material/context_menu/editable_text_toolbar_builder.1.dart **
/// {@end-tool}
///
/// See also:
/// * [AdaptiveTextSelectionToolbar], which builds the default text selection
/// toolbar for the current platform, but allows customization of the
/// buttons.
/// * [AdaptiveTextSelectionToolbar.getAdaptiveButtons], which builds the
/// button Widgets for the current platform given
/// [ContextMenuButtonItem]s.
/// * [BrowserContextMenu], which allows the browser's context menu on web
/// to be disabled and Flutter-rendered context menus to appear.
/// {@endtemplate}
final EditableTextContextMenuBuilder? contextMenuBuilder;
/// {@template flutter.widgets.EditableText.spellCheckConfiguration}
/// Configuration that details how spell check should be performed.
///
/// Specifies the [SpellCheckService] used to spell check text input and the
/// [TextStyle] used to style text with misspelled words.
///
/// If the [SpellCheckService] is left null, spell check is disabled by
/// default unless the [DefaultSpellCheckService] is supported, in which case
/// it is used. It is currently supported only on Android and iOS.
///
/// If this configuration is left null, then spell check is disabled by default.
/// {@endtemplate}
final SpellCheckConfiguration? spellCheckConfiguration;
/// The configuration for the magnifier to use with selections in this text
/// field.
///
/// {@macro flutter.widgets.magnifier.intro}
final TextMagnifierConfiguration magnifierConfiguration;
bool get _userSelectionEnabled => enableInteractiveSelection && (!readOnly || !obscureText);
/// Returns the [ContextMenuButtonItem]s representing the buttons in this
/// platform's default selection menu for an editable field.
///
/// For example, [EditableText] uses this to generate the default buttons for
/// its context menu.
///
/// See also:
///
/// * [EditableTextState.contextMenuButtonItems], which gives the
/// [ContextMenuButtonItem]s for a specific EditableText.
/// * [SelectableRegion.getSelectableButtonItems], which performs a similar
/// role but for content that is selectable but not editable.
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar itself, and can
/// take a list of [ContextMenuButtonItem]s with
/// [AdaptiveTextSelectionToolbar.buttonItems].
/// * [AdaptiveTextSelectionToolbar.getAdaptiveButtons], which builds the button
/// Widgets for the current platform given [ContextMenuButtonItem]s.
static List<ContextMenuButtonItem> getEditableButtonItems({
required final ClipboardStatus? clipboardStatus,
required final VoidCallback? onCopy,
required final VoidCallback? onCut,
required final VoidCallback? onPaste,
required final VoidCallback? onSelectAll,
required final VoidCallback? onLookUp,
required final VoidCallback? onSearchWeb,
required final VoidCallback? onShare,
required final VoidCallback? onLiveTextInput,
}) {
final List<ContextMenuButtonItem> resultButtonItem = <ContextMenuButtonItem>[];
// Configure button items with clipboard.
if (onPaste == null || clipboardStatus != ClipboardStatus.unknown) {
// If the paste button is enabled, don't render anything until the state
// of the clipboard is known, since it's used to determine if paste is
// shown.
// On Android, the share button is before the select all button.
final bool showShareBeforeSelectAll = defaultTargetPlatform == TargetPlatform.android;
resultButtonItem.addAll(<ContextMenuButtonItem>[
if (onCut != null)
ContextMenuButtonItem(
onPressed: onCut,
type: ContextMenuButtonType.cut,
),
if (onCopy != null)
ContextMenuButtonItem(
onPressed: onCopy,
type: ContextMenuButtonType.copy,
),
if (onPaste != null)
ContextMenuButtonItem(
onPressed: onPaste,
type: ContextMenuButtonType.paste,
),
if (onShare != null && showShareBeforeSelectAll)
ContextMenuButtonItem(
onPressed: onShare,
type: ContextMenuButtonType.share,
),
if (onSelectAll != null)
ContextMenuButtonItem(
onPressed: onSelectAll,
type: ContextMenuButtonType.selectAll,
),
if (onLookUp != null)
ContextMenuButtonItem(
onPressed: onLookUp,
type: ContextMenuButtonType.lookUp,
),
if (onSearchWeb != null)
ContextMenuButtonItem(
onPressed: onSearchWeb,
type: ContextMenuButtonType.searchWeb,
),
if (onShare != null && !showShareBeforeSelectAll)
ContextMenuButtonItem(
onPressed: onShare,
type: ContextMenuButtonType.share,
),
]);
}
// Config button items with Live Text.
if (onLiveTextInput != null) {
resultButtonItem.add(ContextMenuButtonItem(
onPressed: onLiveTextInput,
type: ContextMenuButtonType.liveTextInput,
));
}
return resultButtonItem;
}
// Infer the keyboard type of an `EditableText` if it's not specified.
static TextInputType _inferKeyboardType({
required Iterable<String>? autofillHints,
required int? maxLines,
}) {
if (autofillHints == null || autofillHints.isEmpty) {
return maxLines == 1 ? TextInputType.text : TextInputType.multiline;
}
final String effectiveHint = autofillHints.first;
// On iOS oftentimes specifying a text content type is not enough to qualify
// the input field for autofill. The keyboard type also needs to be compatible
// with the content type. To get autofill to work by default on EditableText,
// the keyboard type inference on iOS is done differently from other platforms.
//
// The entries with "autofill not working" comments are the iOS text content
// types that should work with the specified keyboard type but won't trigger
// (even within a native app). Tested on iOS 13.5.
if (!kIsWeb) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
const Map<String, TextInputType> iOSKeyboardType = <String, TextInputType> {
AutofillHints.addressCity : TextInputType.name,
AutofillHints.addressCityAndState : TextInputType.name, // Autofill not working.
AutofillHints.addressState : TextInputType.name,
AutofillHints.countryName : TextInputType.name,
AutofillHints.creditCardNumber : TextInputType.number, // Couldn't test.
AutofillHints.email : TextInputType.emailAddress,
AutofillHints.familyName : TextInputType.name,
AutofillHints.fullStreetAddress : TextInputType.name,
AutofillHints.givenName : TextInputType.name,
AutofillHints.jobTitle : TextInputType.name, // Autofill not working.
AutofillHints.location : TextInputType.name, // Autofill not working.
AutofillHints.middleName : TextInputType.name, // Autofill not working.
AutofillHints.name : TextInputType.name,
AutofillHints.namePrefix : TextInputType.name, // Autofill not working.
AutofillHints.nameSuffix : TextInputType.name, // Autofill not working.
AutofillHints.newPassword : TextInputType.text,
AutofillHints.newUsername : TextInputType.text,
AutofillHints.nickname : TextInputType.name, // Autofill not working.
AutofillHints.oneTimeCode : TextInputType.number,
AutofillHints.organizationName : TextInputType.text, // Autofill not working.
AutofillHints.password : TextInputType.text,
AutofillHints.postalCode : TextInputType.name,
AutofillHints.streetAddressLine1 : TextInputType.name,
AutofillHints.streetAddressLine2 : TextInputType.name, // Autofill not working.
AutofillHints.sublocality : TextInputType.name, // Autofill not working.
AutofillHints.telephoneNumber : TextInputType.name,
AutofillHints.url : TextInputType.url, // Autofill not working.
AutofillHints.username : TextInputType.text,
};
final TextInputType? keyboardType = iOSKeyboardType[effectiveHint];
if (keyboardType != null) {
return keyboardType;
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
}
}
if (maxLines != 1) {
return TextInputType.multiline;
}
const Map<String, TextInputType> inferKeyboardType = <String, TextInputType> {
AutofillHints.addressCity : TextInputType.streetAddress,
AutofillHints.addressCityAndState : TextInputType.streetAddress,
AutofillHints.addressState : TextInputType.streetAddress,
AutofillHints.birthday : TextInputType.datetime,
AutofillHints.birthdayDay : TextInputType.datetime,
AutofillHints.birthdayMonth : TextInputType.datetime,
AutofillHints.birthdayYear : TextInputType.datetime,
AutofillHints.countryCode : TextInputType.number,
AutofillHints.countryName : TextInputType.text,
AutofillHints.creditCardExpirationDate : TextInputType.datetime,
AutofillHints.creditCardExpirationDay : TextInputType.datetime,
AutofillHints.creditCardExpirationMonth : TextInputType.datetime,
AutofillHints.creditCardExpirationYear : TextInputType.datetime,
AutofillHints.creditCardFamilyName : TextInputType.name,
AutofillHints.creditCardGivenName : TextInputType.name,
AutofillHints.creditCardMiddleName : TextInputType.name,
AutofillHints.creditCardName : TextInputType.name,
AutofillHints.creditCardNumber : TextInputType.number,
AutofillHints.creditCardSecurityCode : TextInputType.number,
AutofillHints.creditCardType : TextInputType.text,
AutofillHints.email : TextInputType.emailAddress,
AutofillHints.familyName : TextInputType.name,
AutofillHints.fullStreetAddress : TextInputType.streetAddress,
AutofillHints.gender : TextInputType.text,
AutofillHints.givenName : TextInputType.name,
AutofillHints.impp : TextInputType.url,
AutofillHints.jobTitle : TextInputType.text,
AutofillHints.language : TextInputType.text,
AutofillHints.location : TextInputType.streetAddress,
AutofillHints.middleInitial : TextInputType.name,
AutofillHints.middleName : TextInputType.name,
AutofillHints.name : TextInputType.name,
AutofillHints.namePrefix : TextInputType.name,
AutofillHints.nameSuffix : TextInputType.name,
AutofillHints.newPassword : TextInputType.text,
AutofillHints.newUsername : TextInputType.text,
AutofillHints.nickname : TextInputType.text,
AutofillHints.oneTimeCode : TextInputType.text,
AutofillHints.organizationName : TextInputType.text,
AutofillHints.password : TextInputType.text,
AutofillHints.photo : TextInputType.text,
AutofillHints.postalAddress : TextInputType.streetAddress,
AutofillHints.postalAddressExtended : TextInputType.streetAddress,
AutofillHints.postalAddressExtendedPostalCode : TextInputType.number,
AutofillHints.postalCode : TextInputType.number,
AutofillHints.streetAddressLevel1 : TextInputType.streetAddress,
AutofillHints.streetAddressLevel2 : TextInputType.streetAddress,
AutofillHints.streetAddressLevel3 : TextInputType.streetAddress,
AutofillHints.streetAddressLevel4 : TextInputType.streetAddress,
AutofillHints.streetAddressLine1 : TextInputType.streetAddress,
AutofillHints.streetAddressLine2 : TextInputType.streetAddress,
AutofillHints.streetAddressLine3 : TextInputType.streetAddress,
AutofillHints.sublocality : TextInputType.streetAddress,
AutofillHints.telephoneNumber : TextInputType.phone,
AutofillHints.telephoneNumberAreaCode : TextInputType.phone,
AutofillHints.telephoneNumberCountryCode : TextInputType.phone,
AutofillHints.telephoneNumberDevice : TextInputType.phone,
AutofillHints.telephoneNumberExtension : TextInputType.phone,
AutofillHints.telephoneNumberLocal : TextInputType.phone,
AutofillHints.telephoneNumberLocalPrefix : TextInputType.phone,
AutofillHints.telephoneNumberLocalSuffix : TextInputType.phone,
AutofillHints.telephoneNumberNational : TextInputType.phone,
AutofillHints.transactionAmount : TextInputType.numberWithOptions(decimal: true),
AutofillHints.transactionCurrency : TextInputType.text,
AutofillHints.url : TextInputType.url,
AutofillHints.username : TextInputType.text,
};
return inferKeyboardType[effectiveHint] ?? TextInputType.text;
}
@override
EditableTextState createState() => EditableTextState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<TextEditingController>('controller', controller));
properties.add(DiagnosticsProperty<FocusNode>('focusNode', focusNode));
properties.add(DiagnosticsProperty<bool>('obscureText', obscureText, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('readOnly', readOnly, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('autocorrect', autocorrect, defaultValue: true));
properties.add(EnumProperty<SmartDashesType>('smartDashesType', smartDashesType, defaultValue: obscureText ? SmartDashesType.disabled : SmartDashesType.enabled));
properties.add(EnumProperty<SmartQuotesType>('smartQuotesType', smartQuotesType, defaultValue: obscureText ? SmartQuotesType.disabled : SmartQuotesType.enabled));
properties.add(DiagnosticsProperty<bool>('enableSuggestions', enableSuggestions, defaultValue: true));
style.debugFillProperties(properties);
properties.add(EnumProperty<TextAlign>('textAlign', textAlign, defaultValue: null));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(DiagnosticsProperty<Locale>('locale', locale, defaultValue: null));
properties.add(DiagnosticsProperty<TextScaler>('textScaler', textScaler, defaultValue: null));
properties.add(IntProperty('maxLines', maxLines, defaultValue: 1));
properties.add(IntProperty('minLines', minLines, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('expands', expands, defaultValue: false));
properties.add(DiagnosticsProperty<bool>('autofocus', autofocus, defaultValue: false));
properties.add(DiagnosticsProperty<TextInputType>('keyboardType', keyboardType, defaultValue: null));
properties.add(DiagnosticsProperty<ScrollController>('scrollController', scrollController, defaultValue: null));
properties.add(DiagnosticsProperty<ScrollPhysics>('scrollPhysics', scrollPhysics, defaultValue: null));
properties.add(DiagnosticsProperty<Iterable<String>>('autofillHints', autofillHints, defaultValue: null));
properties.add(DiagnosticsProperty<TextHeightBehavior>('textHeightBehavior', textHeightBehavior, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('scribbleEnabled', scribbleEnabled, defaultValue: true));
properties.add(DiagnosticsProperty<bool>('enableIMEPersonalizedLearning', enableIMEPersonalizedLearning, defaultValue: true));
properties.add(DiagnosticsProperty<bool>('enableInteractiveSelection', enableInteractiveSelection, defaultValue: true));
properties.add(DiagnosticsProperty<UndoHistoryController>('undoController', undoController, defaultValue: null));
properties.add(DiagnosticsProperty<SpellCheckConfiguration>('spellCheckConfiguration', spellCheckConfiguration, defaultValue: null));
properties.add(DiagnosticsProperty<List<String>>('contentCommitMimeTypes', contentInsertionConfiguration?.allowedMimeTypes ?? const <String>[], defaultValue: contentInsertionConfiguration == null ? const <String>[] : kDefaultContentInsertionMimeTypes));
}
}
/// State for a [EditableText].
class EditableTextState extends State<EditableText> with AutomaticKeepAliveClientMixin<EditableText>, WidgetsBindingObserver, TickerProviderStateMixin<EditableText>, TextSelectionDelegate, TextInputClient implements AutofillClient {
Timer? _cursorTimer;
AnimationController get _cursorBlinkOpacityController {
return _backingCursorBlinkOpacityController ??= AnimationController(
vsync: this,
)..addListener(_onCursorColorTick);
}
AnimationController? _backingCursorBlinkOpacityController;
late final Simulation _iosBlinkCursorSimulation = _DiscreteKeyFrameSimulation.iOSBlinkingCaret();
final ValueNotifier<bool> _cursorVisibilityNotifier = ValueNotifier<bool>(true);
final GlobalKey _editableKey = GlobalKey();
/// Detects whether the clipboard can paste.
final ClipboardStatusNotifier clipboardStatus = kIsWeb
// Web browsers will show a permission dialog when Clipboard.hasStrings is
// called. In an EditableText, this will happen before the paste button is
// clicked, often before the context menu is even shown. To avoid this
// poor user experience, always show the paste button on web.
? _WebClipboardStatusNotifier()
: ClipboardStatusNotifier();
/// Detects whether the Live Text input is enabled.
///
/// See also:
/// * [LiveText], where the availability of Live Text input can be obtained.
final LiveTextInputStatusNotifier? _liveTextInputStatus =
kIsWeb ? null : LiveTextInputStatusNotifier();
TextInputConnection? _textInputConnection;
bool get _hasInputConnection => _textInputConnection?.attached ?? false;
TextSelectionOverlay? _selectionOverlay;
ScrollNotificationObserverState? _scrollNotificationObserver;
({TextEditingValue value, Rect selectionBounds})? _dataWhenToolbarShowScheduled;
bool _listeningToScrollNotificationObserver = false;
bool get _webContextMenuEnabled => kIsWeb && BrowserContextMenu.enabled;
final GlobalKey _scrollableKey = GlobalKey();
ScrollController? _internalScrollController;
ScrollController get _scrollController => widget.scrollController ?? (_internalScrollController ??= ScrollController());
final LayerLink _toolbarLayerLink = LayerLink();
final LayerLink _startHandleLayerLink = LayerLink();
final LayerLink _endHandleLayerLink = LayerLink();
bool _didAutoFocus = false;
AutofillGroupState? _currentAutofillScope;
@override
AutofillScope? get currentAutofillScope => _currentAutofillScope;
AutofillClient get _effectiveAutofillClient => widget.autofillClient ?? this;
late SpellCheckConfiguration _spellCheckConfiguration;
late TextStyle _style;
/// Configuration that determines how spell check will be performed.
///
/// If possible, this configuration will contain a default for the
/// [SpellCheckService] if it is not otherwise specified.
///
/// See also:
/// * [DefaultSpellCheckService], the spell check service used by default.
@visibleForTesting
SpellCheckConfiguration get spellCheckConfiguration => _spellCheckConfiguration;
/// Whether or not spell check is enabled.
///
/// Spell check is enabled when a [SpellCheckConfiguration] has been specified
/// for the widget.
bool get spellCheckEnabled => _spellCheckConfiguration.spellCheckEnabled;
/// The most up-to-date spell check results for text input.
///
/// These results will be updated via calls to spell check through a
/// [SpellCheckService] and used by this widget to build the [TextSpan] tree
/// for text input and menus for replacement suggestions of misspelled words.
SpellCheckResults? spellCheckResults;
bool get _spellCheckResultsReceived => spellCheckEnabled && spellCheckResults != null && spellCheckResults!.suggestionSpans.isNotEmpty;
/// The text processing service used to retrieve the native text processing actions.
final ProcessTextService _processTextService = DefaultProcessTextService();
/// The list of native text processing actions provided by the engine.
final List<ProcessTextAction> _processTextActions = <ProcessTextAction>[];
/// Whether to create an input connection with the platform for text editing
/// or not.
///
/// Read-only input fields do not need a connection with the platform since
/// there's no need for text editing capabilities (e.g. virtual keyboard).
///
/// On the web, we always need a connection because we want some browser
/// functionalities to continue to work on read-only input fields like:
///
/// - Relevant context menu.
/// - cmd/ctrl+c shortcut to copy.
/// - cmd/ctrl+a to select all.
/// - Changing the selection using a physical keyboard.
bool get _shouldCreateInputConnection => kIsWeb || !widget.readOnly;
// The time it takes for the floating cursor to snap to the text aligned
// cursor position after the user has finished placing it.
static const Duration _floatingCursorResetTime = Duration(milliseconds: 125);
AnimationController? _floatingCursorResetController;
Orientation? _lastOrientation;
@override
bool get wantKeepAlive => widget.focusNode.hasFocus;
Color get _cursorColor {
final double effectiveOpacity = math.min(widget.cursorColor.alpha / 255.0, _cursorBlinkOpacityController.value);
return widget.cursorColor.withOpacity(effectiveOpacity);
}
@override
bool get cutEnabled {
if (widget.selectionControls is! TextSelectionHandleControls) {
return widget.toolbarOptions.cut && !widget.readOnly && !widget.obscureText;
}
return !widget.readOnly
&& !widget.obscureText
&& !textEditingValue.selection.isCollapsed;
}
@override
bool get copyEnabled {
if (widget.selectionControls is! TextSelectionHandleControls) {
return widget.toolbarOptions.copy && !widget.obscureText;
}
return !widget.obscureText
&& !textEditingValue.selection.isCollapsed;
}
@override
bool get pasteEnabled {
if (widget.selectionControls is! TextSelectionHandleControls) {
return widget.toolbarOptions.paste && !widget.readOnly;
}
return !widget.readOnly
&& (clipboardStatus.value == ClipboardStatus.pasteable);
}
@override
bool get selectAllEnabled {
if (widget.selectionControls is! TextSelectionHandleControls) {
return widget.toolbarOptions.selectAll && (!widget.readOnly || !widget.obscureText) && widget.enableInteractiveSelection;
}
if (!widget.enableInteractiveSelection
|| (widget.readOnly
&& widget.obscureText)) {
return false;
}
switch (defaultTargetPlatform) {
case TargetPlatform.macOS:
return false;
case TargetPlatform.iOS:
return textEditingValue.text.isNotEmpty
&& textEditingValue.selection.isCollapsed;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return textEditingValue.text.isNotEmpty
&& !(textEditingValue.selection.start == 0
&& textEditingValue.selection.end == textEditingValue.text.length);
}
}
@override
bool get lookUpEnabled {
if (defaultTargetPlatform != TargetPlatform.iOS) {
return false;
}
return !widget.obscureText
&& !textEditingValue.selection.isCollapsed
&& textEditingValue.selection.textInside(textEditingValue.text).trim() != '';
}
@override
bool get searchWebEnabled {
if (defaultTargetPlatform != TargetPlatform.iOS) {
return false;
}
return !widget.obscureText
&& !textEditingValue.selection.isCollapsed
&& textEditingValue.selection.textInside(textEditingValue.text).trim() != '';
}
@override
bool get shareEnabled {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
return !widget.obscureText
&& !textEditingValue.selection.isCollapsed
&& textEditingValue.selection.textInside(textEditingValue.text).trim() != '';
case TargetPlatform.macOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return false;
}
}
@override
bool get liveTextInputEnabled {
return _liveTextInputStatus?.value == LiveTextInputStatus.enabled &&
!widget.obscureText &&
!widget.readOnly &&
textEditingValue.selection.isCollapsed;
}
void _onChangedClipboardStatus() {
setState(() {
// Inform the widget that the value of clipboardStatus has changed.
});
}
void _onChangedLiveTextInputStatus() {
setState(() {
// Inform the widget that the value of liveTextInputStatus has changed.
});
}
TextEditingValue get _textEditingValueforTextLayoutMetrics {
final Widget? editableWidget =_editableKey.currentContext?.widget;
if (editableWidget is! _Editable) {
throw StateError('_Editable must be mounted.');
}
return editableWidget.value;
}
/// Copy current selection to [Clipboard].
@override
void copySelection(SelectionChangedCause cause) {
final TextSelection selection = textEditingValue.selection;
if (selection.isCollapsed || widget.obscureText) {
return;
}
final String text = textEditingValue.text;
Clipboard.setData(ClipboardData(text: selection.textInside(text)));
if (cause == SelectionChangedCause.toolbar) {
bringIntoView(textEditingValue.selection.extent);
hideToolbar(false);
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
// Collapse the selection and hide the toolbar and handles.
userUpdateTextEditingValue(
TextEditingValue(
text: textEditingValue.text,
selection: TextSelection.collapsed(offset: textEditingValue.selection.end),
),
SelectionChangedCause.toolbar,
);
}
}
clipboardStatus.update();
}
/// Cut current selection to [Clipboard].
@override
void cutSelection(SelectionChangedCause cause) {
if (widget.readOnly || widget.obscureText) {
return;
}
final TextSelection selection = textEditingValue.selection;
final String text = textEditingValue.text;
if (selection.isCollapsed) {
return;
}
Clipboard.setData(ClipboardData(text: selection.textInside(text)));
_replaceText(ReplaceTextIntent(textEditingValue, '', selection, cause));
if (cause == SelectionChangedCause.toolbar) {
// Schedule a call to bringIntoView() after renderEditable updates.
SchedulerBinding.instance.addPostFrameCallback((_) {
if (mounted) {
bringIntoView(textEditingValue.selection.extent);
}
}, debugLabel: 'EditableText.bringSelectionIntoView');
hideToolbar();
}
clipboardStatus.update();
}
bool get _allowPaste {
return !widget.readOnly && textEditingValue.selection.isValid;
}
/// Paste text from [Clipboard].
@override
Future<void> pasteText(SelectionChangedCause cause) async {
if (!_allowPaste) {
return;
}
// Snapshot the input before using `await`.
// See https://github.com/flutter/flutter/issues/11427
final ClipboardData? data = await Clipboard.getData(Clipboard.kTextPlain);
if (data == null) {
return;
}
_pasteText(cause, data.text!);
}
void _pasteText(SelectionChangedCause cause, String text) {
if (!_allowPaste) {
return;
}
// After the paste, the cursor should be collapsed and located after the
// pasted content.
final TextSelection selection = textEditingValue.selection;
final int lastSelectionIndex = math.max(selection.baseOffset, selection.extentOffset);
final TextEditingValue collapsedTextEditingValue = textEditingValue.copyWith(
selection: TextSelection.collapsed(offset: lastSelectionIndex),
);
userUpdateTextEditingValue(
collapsedTextEditingValue.replaced(selection, text),
cause,
);
if (cause == SelectionChangedCause.toolbar) {
// Schedule a call to bringIntoView() after renderEditable updates.
SchedulerBinding.instance.addPostFrameCallback((_) {
if (mounted) {
bringIntoView(textEditingValue.selection.extent);
}
}, debugLabel: 'EditableText.bringSelectionIntoView');
hideToolbar();
}
}
/// Select the entire text value.
@override
void selectAll(SelectionChangedCause cause) {
if (widget.readOnly && widget.obscureText) {
// If we can't modify it, and we can't copy it, there's no point in
// selecting it.
return;
}
userUpdateTextEditingValue(
textEditingValue.copyWith(
selection: TextSelection(baseOffset: 0, extentOffset: textEditingValue.text.length),
),
cause,
);
if (cause == SelectionChangedCause.toolbar) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
break;
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
hideToolbar();
}
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
bringIntoView(textEditingValue.selection.extent);
case TargetPlatform.macOS:
case TargetPlatform.iOS:
break;
}
}
}
/// Look up the current selection,
/// as in the "Look Up" edit menu button on iOS.
///
/// Currently this is only implemented for iOS.
///
/// Throws an error if the selection is empty or collapsed.
Future<void> lookUpSelection(SelectionChangedCause cause) async {
assert(!widget.obscureText);
final String text = textEditingValue.selection.textInside(textEditingValue.text);
if (widget.obscureText || text.isEmpty) {
return;
}
await SystemChannels.platform.invokeMethod(
'LookUp.invoke',
text,
);
}
/// Launch a web search on the current selection,
/// as in the "Search Web" edit menu button on iOS.
///
/// Currently this is only implemented for iOS.
///
/// When 'obscureText' is true or the selection is empty,
/// this function will not do anything
Future<void> searchWebForSelection(SelectionChangedCause cause) async {
assert(!widget.obscureText);
if (widget.obscureText) {
return;
}
final String text = textEditingValue.selection.textInside(textEditingValue.text);
if (text.isNotEmpty) {
await SystemChannels.platform.invokeMethod(
'SearchWeb.invoke',
text,
);
}
}
/// Launch the share interface for the current selection,
/// as in the "Share..." edit menu button on iOS.
///
/// Currently this is only implemented for iOS and Android.
///
/// When 'obscureText' is true or the selection is empty,
/// this function will not do anything
Future<void> shareSelection(SelectionChangedCause cause) async {
assert(!widget.obscureText);
if (widget.obscureText) {
return;
}
final String text = textEditingValue.selection.textInside(textEditingValue.text);
if (text.isNotEmpty) {
await SystemChannels.platform.invokeMethod(
'Share.invoke',
text,
);
}
}
void _startLiveTextInput(SelectionChangedCause cause) {
if (!liveTextInputEnabled) {
return;
}
if (_hasInputConnection) {
LiveText.startLiveTextInput();
}
if (cause == SelectionChangedCause.toolbar) {
hideToolbar();
}
}
/// Finds specified [SuggestionSpan] that matches the provided index using
/// binary search.
///
/// See also:
///
/// * [SpellCheckSuggestionsToolbar], the Material style spell check
/// suggestions toolbar that uses this method to render the correct
/// suggestions in the toolbar for a misspelled word.
SuggestionSpan? findSuggestionSpanAtCursorIndex(int cursorIndex) {
if (!_spellCheckResultsReceived
|| spellCheckResults!.suggestionSpans.last.range.end < cursorIndex) {
// No spell check results have been received or the cursor index is out
// of range that suggestionSpans covers.
return null;
}
final List<SuggestionSpan> suggestionSpans = spellCheckResults!.suggestionSpans;
int leftIndex = 0;
int rightIndex = suggestionSpans.length - 1;
int midIndex = 0;
while (leftIndex <= rightIndex) {
midIndex = ((leftIndex + rightIndex) / 2).floor();
final int currentSpanStart = suggestionSpans[midIndex].range.start;
final int currentSpanEnd = suggestionSpans[midIndex].range.end;
if (cursorIndex <= currentSpanEnd && cursorIndex >= currentSpanStart) {
return suggestionSpans[midIndex];
}
else if (cursorIndex <= currentSpanStart) {
rightIndex = midIndex - 1;
}
else {
leftIndex = midIndex + 1;
}
}
return null;
}
/// Infers the [SpellCheckConfiguration] used to perform spell check.
///
/// If spell check is enabled, this will try to infer a value for
/// the [SpellCheckService] if left unspecified.
static SpellCheckConfiguration _inferSpellCheckConfiguration(SpellCheckConfiguration? configuration) {
final SpellCheckService? spellCheckService = configuration?.spellCheckService;
final bool spellCheckAutomaticallyDisabled = configuration == null || configuration == const SpellCheckConfiguration.disabled();
final bool spellCheckServiceIsConfigured = spellCheckService != null || spellCheckService == null && WidgetsBinding.instance.platformDispatcher.nativeSpellCheckServiceDefined;
if (spellCheckAutomaticallyDisabled || !spellCheckServiceIsConfigured) {
// Only enable spell check if a non-disabled configuration is provided
// and if that configuration does not specify a spell check service,
// a native spell checker must be supported.
assert(() {
if (!spellCheckAutomaticallyDisabled && !spellCheckServiceIsConfigured) {
FlutterError.reportError(
FlutterErrorDetails(
exception: FlutterError(
'Spell check was enabled with spellCheckConfiguration, but the '
'current platform does not have a supported spell check '
'service, and none was provided. Consider disabling spell '
'check for this platform or passing a SpellCheckConfiguration '
'with a specified spell check service.',
),
library: 'widget library',
stack: StackTrace.current,
),
);
}
return true;
}());
return const SpellCheckConfiguration.disabled();
}
return configuration.copyWith(spellCheckService: spellCheckService ?? DefaultSpellCheckService());
}
/// Returns the [ContextMenuButtonItem]s for the given [ToolbarOptions].
@Deprecated(
'Use `contextMenuBuilder` instead of `toolbarOptions`. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
List<ContextMenuButtonItem>? buttonItemsForToolbarOptions([TargetPlatform? targetPlatform]) {
final ToolbarOptions toolbarOptions = widget.toolbarOptions;
if (toolbarOptions == ToolbarOptions.empty) {
return null;
}
return <ContextMenuButtonItem>[
if (toolbarOptions.cut && cutEnabled)
ContextMenuButtonItem(
onPressed: () {
cutSelection(SelectionChangedCause.toolbar);
},
type: ContextMenuButtonType.cut,
),
if (toolbarOptions.copy && copyEnabled)
ContextMenuButtonItem(
onPressed: () {
copySelection(SelectionChangedCause.toolbar);
},
type: ContextMenuButtonType.copy,
),
if (toolbarOptions.paste && pasteEnabled)
ContextMenuButtonItem(
onPressed: () {
pasteText(SelectionChangedCause.toolbar);
},
type: ContextMenuButtonType.paste,
),
if (toolbarOptions.selectAll && selectAllEnabled)
ContextMenuButtonItem(
onPressed: () {
selectAll(SelectionChangedCause.toolbar);
},
type: ContextMenuButtonType.selectAll,
),
];
}
/// Gets the line heights at the start and end of the selection for the given
/// [EditableTextState].
_GlyphHeights _getGlyphHeights() {
final TextSelection selection = textEditingValue.selection;
// Only calculate handle rects if the text in the previous frame
// is the same as the text in the current frame. This is done because
// widget.renderObject contains the renderEditable from the previous frame.
// If the text changed between the current and previous frames then
// widget.renderObject.getRectForComposingRange might fail. In cases where
// the current frame is different from the previous we fall back to
// renderObject.preferredLineHeight.
final InlineSpan span = renderEditable.text!;
final String prevText = span.toPlainText();
final String currText = textEditingValue.text;
if (prevText != currText || !selection.isValid || selection.isCollapsed) {
return _GlyphHeights(
start: renderEditable.preferredLineHeight,
end: renderEditable.preferredLineHeight,
);
}
final String selectedGraphemes = selection.textInside(currText);
final int firstSelectedGraphemeExtent = selectedGraphemes.characters.first.length;
final Rect? startCharacterRect = renderEditable.getRectForComposingRange(TextRange(
start: selection.start,
end: selection.start + firstSelectedGraphemeExtent,
));
final int lastSelectedGraphemeExtent = selectedGraphemes.characters.last.length;
final Rect? endCharacterRect = renderEditable.getRectForComposingRange(TextRange(
start: selection.end - lastSelectedGraphemeExtent,
end: selection.end,
));
return _GlyphHeights(
start: startCharacterRect?.height ?? renderEditable.preferredLineHeight,
end: endCharacterRect?.height ?? renderEditable.preferredLineHeight,
);
}
/// {@template flutter.widgets.EditableText.getAnchors}
/// Returns the anchor points for the default context menu.
/// {@endtemplate}
///
/// See also:
///
/// * [contextMenuButtonItems], which provides the [ContextMenuButtonItem]s
/// for the default context menu buttons.
TextSelectionToolbarAnchors get contextMenuAnchors {
if (renderEditable.lastSecondaryTapDownPosition != null) {
return TextSelectionToolbarAnchors(
primaryAnchor: renderEditable.lastSecondaryTapDownPosition!,
);
}
final _GlyphHeights glyphHeights = _getGlyphHeights();
final TextSelection selection = textEditingValue.selection;
final List<TextSelectionPoint> points =
renderEditable.getEndpointsForSelection(selection);
return TextSelectionToolbarAnchors.fromSelection(
renderBox: renderEditable,
startGlyphHeight: glyphHeights.start,
endGlyphHeight: glyphHeights.end,
selectionEndpoints: points,
);
}
/// Returns the [ContextMenuButtonItem]s representing the buttons in this
/// platform's default selection menu for [EditableText].
///
/// See also:
///
/// * [EditableText.getEditableButtonItems], which performs a similar role,
/// but for any editable field, not just specifically EditableText.
/// * [SelectableRegionState.contextMenuButtonItems], which performs a similar
/// role but for content that is selectable but not editable.
/// * [contextMenuAnchors], which provides the anchor points for the default
/// context menu.
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar itself, and can
/// take a list of [ContextMenuButtonItem]s with
/// [AdaptiveTextSelectionToolbar.buttonItems].
/// * [AdaptiveTextSelectionToolbar.getAdaptiveButtons], which builds the
/// button Widgets for the current platform given [ContextMenuButtonItem]s.
List<ContextMenuButtonItem> get contextMenuButtonItems {
return buttonItemsForToolbarOptions() ?? EditableText.getEditableButtonItems(
clipboardStatus: clipboardStatus.value,
onCopy: copyEnabled
? () => copySelection(SelectionChangedCause.toolbar)
: null,
onCut: cutEnabled
? () => cutSelection(SelectionChangedCause.toolbar)
: null,
onPaste: pasteEnabled
? () => pasteText(SelectionChangedCause.toolbar)
: null,
onSelectAll: selectAllEnabled
? () => selectAll(SelectionChangedCause.toolbar)
: null,
onLookUp: lookUpEnabled
? () => lookUpSelection(SelectionChangedCause.toolbar)
: null,
onSearchWeb: searchWebEnabled
? () => searchWebForSelection(SelectionChangedCause.toolbar)
: null,
onShare: shareEnabled
? () => shareSelection(SelectionChangedCause.toolbar)
: null,
onLiveTextInput: liveTextInputEnabled
? () => _startLiveTextInput(SelectionChangedCause.toolbar)
: null,
)..addAll(_textProcessingActionButtonItems);
}
List<ContextMenuButtonItem> get _textProcessingActionButtonItems {
final List<ContextMenuButtonItem> buttonItems = <ContextMenuButtonItem>[];
final TextSelection selection = textEditingValue.selection;
if (widget.obscureText || !selection.isValid || selection.isCollapsed) {
return buttonItems;
}
for (final ProcessTextAction action in _processTextActions) {
buttonItems.add(ContextMenuButtonItem(
label: action.label,
onPressed: () async {
final String selectedText = selection.textInside(textEditingValue.text);
if (selectedText.isNotEmpty) {
final String? processedText = await _processTextService.processTextAction(action.id, selectedText, widget.readOnly);
// If an activity does not return a modified version, just hide the toolbar.
// Otherwise use the result to replace the selected text.
if (processedText != null && _allowPaste) {
_pasteText(SelectionChangedCause.toolbar, processedText);
} else {
hideToolbar();
}
}
},
));
}
return buttonItems;
}
// State lifecycle:
@override
void initState() {
super.initState();
_liveTextInputStatus?.addListener(_onChangedLiveTextInputStatus);
clipboardStatus.addListener(_onChangedClipboardStatus);
widget.controller.addListener(_didChangeTextEditingValue);
widget.focusNode.addListener(_handleFocusChanged);
_cursorVisibilityNotifier.value = widget.showCursor;
_spellCheckConfiguration = _inferSpellCheckConfiguration(widget.spellCheckConfiguration);
_initProcessTextActions();
}
/// Query the engine to initialize the list of text processing actions to show
/// in the text selection toolbar.
Future<void> _initProcessTextActions() async {
_processTextActions.clear();
_processTextActions.addAll(await _processTextService.queryTextActions());
}
// Whether `TickerMode.of(context)` is true and animations (like blinking the
// cursor) are supposed to run.
bool _tickersEnabled = true;
@override
void didChangeDependencies() {
super.didChangeDependencies();
_style = MediaQuery.boldTextOf(context)
? widget.style.merge(const TextStyle(fontWeight: FontWeight.bold))
: widget.style;
final AutofillGroupState? newAutofillGroup = AutofillGroup.maybeOf(context);
if (currentAutofillScope != newAutofillGroup) {
_currentAutofillScope?.unregister(autofillId);
_currentAutofillScope = newAutofillGroup;
_currentAutofillScope?.register(_effectiveAutofillClient);
}
if (!_didAutoFocus && widget.autofocus) {
_didAutoFocus = true;
SchedulerBinding.instance.addPostFrameCallback((_) {
if (mounted && renderEditable.hasSize) {
_flagInternalFocus();
FocusScope.of(context).autofocus(widget.focusNode);
}
}, debugLabel: 'EditableText.autofocus');
}
// Restart or stop the blinking cursor when TickerMode changes.
final bool newTickerEnabled = TickerMode.of(context);
if (_tickersEnabled != newTickerEnabled) {
_tickersEnabled = newTickerEnabled;
if (_showBlinkingCursor) {
_startCursorBlink();
} else if (!_tickersEnabled && _cursorTimer != null) {
_stopCursorBlink();
}
}
if (defaultTargetPlatform != TargetPlatform.iOS && defaultTargetPlatform != TargetPlatform.android) {
return;
}
// Hide the text selection toolbar on mobile when orientation changes.
final Orientation orientation = MediaQuery.orientationOf(context);
if (_lastOrientation == null) {
_lastOrientation = orientation;
return;
}
if (orientation != _lastOrientation) {
_lastOrientation = orientation;
if (defaultTargetPlatform == TargetPlatform.iOS) {
hideToolbar(false);
}
if (defaultTargetPlatform == TargetPlatform.android) {
hideToolbar();
}
}
if (_listeningToScrollNotificationObserver) {
// Only update subscription when we have previously subscribed to the
// scroll notification observer. We only subscribe to the scroll
// notification observer when the context menu is shown on platforms that
// support _platformSupportsFadeOnScroll.
_scrollNotificationObserver?.removeListener(_handleContextMenuOnParentScroll);
_scrollNotificationObserver = ScrollNotificationObserver.maybeOf(context);
_scrollNotificationObserver?.addListener(_handleContextMenuOnParentScroll);
}
}
@override
void didUpdateWidget(EditableText oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.controller != oldWidget.controller) {
oldWidget.controller.removeListener(_didChangeTextEditingValue);
widget.controller.addListener(_didChangeTextEditingValue);
_updateRemoteEditingValueIfNeeded();
}
if (_selectionOverlay != null
&& (widget.contextMenuBuilder != oldWidget.contextMenuBuilder ||
widget.selectionControls != oldWidget.selectionControls ||
widget.onSelectionHandleTapped != oldWidget.onSelectionHandleTapped ||
widget.dragStartBehavior != oldWidget.dragStartBehavior ||
widget.magnifierConfiguration != oldWidget.magnifierConfiguration)) {
final bool shouldShowToolbar = _selectionOverlay!.toolbarIsVisible;
final bool shouldShowHandles = _selectionOverlay!.handlesVisible;
_selectionOverlay!.dispose();
_selectionOverlay = _createSelectionOverlay();
if (shouldShowToolbar || shouldShowHandles) {
SchedulerBinding.instance.addPostFrameCallback((Duration _) {
if (shouldShowToolbar) {
_selectionOverlay!.showToolbar();
}
if (shouldShowHandles) {
_selectionOverlay!.showHandles();
}
});
}
} else if (widget.controller.selection != oldWidget.controller.selection) {
_selectionOverlay?.update(_value);
}
_selectionOverlay?.handlesVisible = widget.showSelectionHandles;
if (widget.autofillClient != oldWidget.autofillClient) {
_currentAutofillScope?.unregister(oldWidget.autofillClient?.autofillId ?? autofillId);
_currentAutofillScope?.register(_effectiveAutofillClient);
}
if (widget.focusNode != oldWidget.focusNode) {
oldWidget.focusNode.removeListener(_handleFocusChanged);
widget.focusNode.addListener(_handleFocusChanged);
updateKeepAlive();
}
if (!_shouldCreateInputConnection) {
_closeInputConnectionIfNeeded();
} else if (oldWidget.readOnly && _hasFocus) {
// _openInputConnection must be called after layout information is available.
// See https://github.com/flutter/flutter/issues/126312
SchedulerBinding.instance.addPostFrameCallback((Duration _) {
_openInputConnection();
}, debugLabel: 'EditableText.openInputConnection');
}
if (kIsWeb && _hasInputConnection) {
if (oldWidget.readOnly != widget.readOnly) {
_textInputConnection!.updateConfig(_effectiveAutofillClient.textInputConfiguration);
}
}
if (_hasInputConnection) {
if (oldWidget.obscureText != widget.obscureText) {
_textInputConnection!.updateConfig(_effectiveAutofillClient.textInputConfiguration);
}
}
if (widget.style != oldWidget.style) {
// The _textInputConnection will pick up the new style when it attaches in
// _openInputConnection.
_style = MediaQuery.boldTextOf(context)
? widget.style.merge(const TextStyle(fontWeight: FontWeight.bold))
: widget.style;
if (_hasInputConnection) {
_textInputConnection!.setStyle(
fontFamily: _style.fontFamily,
fontSize: _style.fontSize,
fontWeight: _style.fontWeight,
textDirection: _textDirection,
textAlign: widget.textAlign,
);
}
}
if (widget.showCursor != oldWidget.showCursor) {
_startOrStopCursorTimerIfNeeded();
}
final bool canPaste = widget.selectionControls is TextSelectionHandleControls
? pasteEnabled
: widget.selectionControls?.canPaste(this) ?? false;
if (widget.selectionEnabled && pasteEnabled && canPaste) {
clipboardStatus.update();
}
}
void _disposeScrollNotificationObserver() {
_listeningToScrollNotificationObserver = false;
if (_scrollNotificationObserver != null) {
_scrollNotificationObserver!.removeListener(_handleContextMenuOnParentScroll);
_scrollNotificationObserver = null;
}
}
@override
void dispose() {
_internalScrollController?.dispose();
_currentAutofillScope?.unregister(autofillId);
widget.controller.removeListener(_didChangeTextEditingValue);
_floatingCursorResetController?.dispose();
_floatingCursorResetController = null;
_closeInputConnectionIfNeeded();
assert(!_hasInputConnection);
_cursorTimer?.cancel();
_cursorTimer = null;
_backingCursorBlinkOpacityController?.dispose();
_backingCursorBlinkOpacityController = null;
_selectionOverlay?.dispose();
_selectionOverlay = null;
widget.focusNode.removeListener(_handleFocusChanged);
WidgetsBinding.instance.removeObserver(this);
_liveTextInputStatus?.removeListener(_onChangedLiveTextInputStatus);
_liveTextInputStatus?.dispose();
clipboardStatus.removeListener(_onChangedClipboardStatus);
clipboardStatus.dispose();
_cursorVisibilityNotifier.dispose();
FocusManager.instance.removeListener(_unflagInternalFocus);
_disposeScrollNotificationObserver();
super.dispose();
assert(_batchEditDepth <= 0, 'unfinished batch edits: $_batchEditDepth');
}
// TextInputClient implementation:
/// The last known [TextEditingValue] of the platform text input plugin.
///
/// This value is updated when the platform text input plugin sends a new
/// update via [updateEditingValue], or when [EditableText] calls
/// [TextInputConnection.setEditingState] to overwrite the platform text input
/// plugin's [TextEditingValue].
///
/// Used in [_updateRemoteEditingValueIfNeeded] to determine whether the
/// remote value is outdated and needs updating.
TextEditingValue? _lastKnownRemoteTextEditingValue;
@override
TextEditingValue get currentTextEditingValue => _value;
@override
void updateEditingValue(TextEditingValue value) {
// This method handles text editing state updates from the platform text
// input plugin. The [EditableText] may not have the focus or an open input
// connection, as autofill can update a disconnected [EditableText].
// Since we still have to support keyboard select, this is the best place
// to disable text updating.
if (!_shouldCreateInputConnection) {
return;
}
if (_checkNeedsAdjustAffinity(value)) {
value = value.copyWith(selection: value.selection.copyWith(affinity: _value.selection.affinity));
}
if (widget.readOnly) {
// In the read-only case, we only care about selection changes, and reject
// everything else.
value = _value.copyWith(selection: value.selection);
}
_lastKnownRemoteTextEditingValue = value;
if (value == _value) {
// This is possible, for example, when the numeric keyboard is input,
// the engine will notify twice for the same value.
// Track at https://github.com/flutter/flutter/issues/65811
return;
}
if (value.text == _value.text && value.composing == _value.composing) {
// `selection` is the only change.
SelectionChangedCause cause;
if (_textInputConnection?.scribbleInProgress ?? false) {
cause = SelectionChangedCause.scribble;
} else if (_pointOffsetOrigin != null) {
// For floating cursor selection when force pressing the space bar.
cause = SelectionChangedCause.forcePress;
} else {
cause = SelectionChangedCause.keyboard;
}
_handleSelectionChanged(value.selection, cause);
} else {
if (value.text != _value.text) {
// Hide the toolbar if the text was changed, but only hide the toolbar
// overlay; the selection handle's visibility will be handled
// by `_handleSelectionChanged`. https://github.com/flutter/flutter/issues/108673
hideToolbar(false);
}
_currentPromptRectRange = null;
final bool revealObscuredInput = _hasInputConnection
&& widget.obscureText
&& WidgetsBinding.instance.platformDispatcher.brieflyShowPassword
&& value.text.length == _value.text.length + 1;
_obscureShowCharTicksPending = revealObscuredInput ? _kObscureShowLatestCharCursorTicks : 0;
_obscureLatestCharIndex = revealObscuredInput ? _value.selection.baseOffset : null;
_formatAndSetValue(value, SelectionChangedCause.keyboard);
}
if (_showBlinkingCursor && _cursorTimer != null) {
// To keep the cursor from blinking while typing, restart the timer here.
_stopCursorBlink(resetCharTicks: false);
_startCursorBlink();
}
// Wherever the value is changed by the user, schedule a showCaretOnScreen
// to make sure the user can see the changes they just made. Programmatic
// changes to `textEditingValue` do not trigger the behavior even if the
// text field is focused.
_scheduleShowCaretOnScreen(withAnimation: true);
}
bool _checkNeedsAdjustAffinity(TextEditingValue value) {
// Trust the engine affinity if the text changes or selection changes.
return value.text == _value.text &&
value.selection.isCollapsed == _value.selection.isCollapsed &&
value.selection.start == _value.selection.start &&
value.selection.affinity != _value.selection.affinity;
}
@override
void performAction(TextInputAction action) {
switch (action) {
case TextInputAction.newline:
// If this is a multiline EditableText, do nothing for a "newline"
// action; The newline is already inserted. Otherwise, finalize
// editing.
if (!_isMultiline) {
_finalizeEditing(action, shouldUnfocus: true);
}
case TextInputAction.done:
case TextInputAction.go:
case TextInputAction.next:
case TextInputAction.previous:
case TextInputAction.search:
case TextInputAction.send:
_finalizeEditing(action, shouldUnfocus: true);
case TextInputAction.continueAction:
case TextInputAction.emergencyCall:
case TextInputAction.join:
case TextInputAction.none:
case TextInputAction.route:
case TextInputAction.unspecified:
// Finalize editing, but don't give up focus because this keyboard
// action does not imply the user is done inputting information.
_finalizeEditing(action, shouldUnfocus: false);
}
}
@override
void performPrivateCommand(String action, Map<String, dynamic> data) {
widget.onAppPrivateCommand?.call(action, data);
}
@override
void insertContent(KeyboardInsertedContent content) {
assert(widget.contentInsertionConfiguration?.allowedMimeTypes.contains(content.mimeType) ?? false);
widget.contentInsertionConfiguration?.onContentInserted.call(content);
}
// The original position of the caret on FloatingCursorDragState.start.
Offset? _startCaretCenter;
// The most recent text position as determined by the location of the floating
// cursor.
TextPosition? _lastTextPosition;
// The offset of the floating cursor as determined from the start call.
Offset? _pointOffsetOrigin;
// The most recent position of the floating cursor.
Offset? _lastBoundedOffset;
// Because the center of the cursor is preferredLineHeight / 2 below the touch
// origin, but the touch origin is used to determine which line the cursor is
// on, we need this offset to correctly render and move the cursor.
Offset get _floatingCursorOffset => Offset(0, renderEditable.preferredLineHeight / 2);
@override
void updateFloatingCursor(RawFloatingCursorPoint point) {
_floatingCursorResetController ??= AnimationController(
vsync: this,
)..addListener(_onFloatingCursorResetTick);
switch (point.state) {
case FloatingCursorDragState.Start:
if (_floatingCursorResetController!.isAnimating) {
_floatingCursorResetController!.stop();
_onFloatingCursorResetTick();
}
// Stop cursor blinking and making it visible.
_stopCursorBlink(resetCharTicks: false);
_cursorBlinkOpacityController.value = 1.0;
// We want to send in points that are centered around a (0,0) origin, so
// we cache the position.
_pointOffsetOrigin = point.offset;
final Offset startCaretCenter;
final TextPosition currentTextPosition;
final bool shouldResetOrigin;
// Only non-null when starting a floating cursor via long press.
if (point.startLocation != null) {
shouldResetOrigin = false;
(startCaretCenter, currentTextPosition) = point.startLocation!;
} else {
shouldResetOrigin = true;
currentTextPosition = TextPosition(offset: renderEditable.selection!.baseOffset, affinity: renderEditable.selection!.affinity);
startCaretCenter = renderEditable.getLocalRectForCaret(currentTextPosition).center;
}
_startCaretCenter = startCaretCenter;
_lastBoundedOffset = renderEditable.calculateBoundedFloatingCursorOffset(_startCaretCenter! - _floatingCursorOffset, shouldResetOrigin: shouldResetOrigin);
_lastTextPosition = currentTextPosition;
renderEditable.setFloatingCursor(point.state, _lastBoundedOffset!, _lastTextPosition!);
case FloatingCursorDragState.Update:
final Offset centeredPoint = point.offset! - _pointOffsetOrigin!;
final Offset rawCursorOffset = _startCaretCenter! + centeredPoint - _floatingCursorOffset;
_lastBoundedOffset = renderEditable.calculateBoundedFloatingCursorOffset(rawCursorOffset);
_lastTextPosition = renderEditable.getPositionForPoint(renderEditable.localToGlobal(_lastBoundedOffset! + _floatingCursorOffset));
renderEditable.setFloatingCursor(point.state, _lastBoundedOffset!, _lastTextPosition!);
case FloatingCursorDragState.End:
// Resume cursor blinking.
_startCursorBlink();
// We skip animation if no update has happened.
if (_lastTextPosition != null && _lastBoundedOffset != null) {
_floatingCursorResetController!.value = 0.0;
_floatingCursorResetController!.animateTo(1.0, duration: _floatingCursorResetTime, curve: Curves.decelerate);
}
}
}
void _onFloatingCursorResetTick() {
final Offset finalPosition = renderEditable.getLocalRectForCaret(_lastTextPosition!).centerLeft - _floatingCursorOffset;
if (_floatingCursorResetController!.isCompleted) {
renderEditable.setFloatingCursor(FloatingCursorDragState.End, finalPosition, _lastTextPosition!);
// During a floating cursor's move gesture (1 finger), a cursor is
// animated only visually, without actually updating the selection.
// Only after move gesture is complete, this function will be called
// to actually update the selection to the new cursor location with
// zero selection length.
// However, During a floating cursor's selection gesture (2 fingers), the
// selection is constantly updated by the engine throughout the gesture.
// Thus when the gesture is complete, we should not update the selection
// to the cursor location with zero selection length, because that would
// overwrite the selection made by floating cursor selection.
// Here we use `isCollapsed` to distinguish between floating cursor's
// move gesture (1 finger) vs selection gesture (2 fingers), as
// the engine does not provide information other than notifying a
// new selection during with selection gesture (2 fingers).
if (renderEditable.selection!.isCollapsed) {
// The cause is technically the force cursor, but the cause is listed as tap as the desired functionality is the same.
_handleSelectionChanged(TextSelection.fromPosition(_lastTextPosition!), SelectionChangedCause.forcePress);
}
_startCaretCenter = null;
_lastTextPosition = null;
_pointOffsetOrigin = null;
_lastBoundedOffset = null;
} else {
final double lerpValue = _floatingCursorResetController!.value;
final double lerpX = ui.lerpDouble(_lastBoundedOffset!.dx, finalPosition.dx, lerpValue)!;
final double lerpY = ui.lerpDouble(_lastBoundedOffset!.dy, finalPosition.dy, lerpValue)!;
renderEditable.setFloatingCursor(FloatingCursorDragState.Update, Offset(lerpX, lerpY), _lastTextPosition!, resetLerpValue: lerpValue);
}
}
@pragma('vm:notify-debugger-on-exception')
void _finalizeEditing(TextInputAction action, {required bool shouldUnfocus}) {
// Take any actions necessary now that the user has completed editing.
if (widget.onEditingComplete != null) {
try {
widget.onEditingComplete!();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while calling onEditingComplete for $action'),
));
}
} else {
// Default behavior if the developer did not provide an
// onEditingComplete callback: Finalize editing and remove focus, or move
// it to the next/previous field, depending on the action.
widget.controller.clearComposing();
if (shouldUnfocus) {
switch (action) {
case TextInputAction.none:
case TextInputAction.unspecified:
case TextInputAction.done:
case TextInputAction.go:
case TextInputAction.search:
case TextInputAction.send:
case TextInputAction.continueAction:
case TextInputAction.join:
case TextInputAction.route:
case TextInputAction.emergencyCall:
case TextInputAction.newline:
widget.focusNode.unfocus();
case TextInputAction.next:
widget.focusNode.nextFocus();
case TextInputAction.previous:
widget.focusNode.previousFocus();
}
}
}
final ValueChanged<String>? onSubmitted = widget.onSubmitted;
if (onSubmitted == null) {
return;
}
// Invoke optional callback with the user's submitted content.
try {
onSubmitted(_value.text);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while calling onSubmitted for $action'),
));
}
// If `shouldUnfocus` is true, the text field should no longer be focused
// after the microtask queue is drained. But in case the developer cancelled
// the focus change in the `onSubmitted` callback by focusing this input
// field again, reset the soft keyboard.
// See https://github.com/flutter/flutter/issues/84240.
//
// `_restartConnectionIfNeeded` creates a new TextInputConnection to replace
// the current one. This on iOS switches to a new input view and on Android
// restarts the input method, and in both cases the soft keyboard will be
// reset.
if (shouldUnfocus) {
_scheduleRestartConnection();
}
}
int _batchEditDepth = 0;
/// Begins a new batch edit, within which new updates made to the text editing
/// value will not be sent to the platform text input plugin.
///
/// Batch edits nest. When the outermost batch edit finishes, [endBatchEdit]
/// will attempt to send [currentTextEditingValue] to the text input plugin if
/// it detected a change.
void beginBatchEdit() {
_batchEditDepth += 1;
}
/// Ends the current batch edit started by the last call to [beginBatchEdit],
/// and send [currentTextEditingValue] to the text input plugin if needed.
///
/// Throws an error in debug mode if this [EditableText] is not in a batch
/// edit.
void endBatchEdit() {
_batchEditDepth -= 1;
assert(
_batchEditDepth >= 0,
'Unbalanced call to endBatchEdit: beginBatchEdit must be called first.',
);
_updateRemoteEditingValueIfNeeded();
}
void _updateRemoteEditingValueIfNeeded() {
if (_batchEditDepth > 0 || !_hasInputConnection) {
return;
}
final TextEditingValue localValue = _value;
if (localValue == _lastKnownRemoteTextEditingValue) {
return;
}
_textInputConnection!.setEditingState(localValue);
_lastKnownRemoteTextEditingValue = localValue;
}
TextEditingValue get _value => widget.controller.value;
set _value(TextEditingValue value) {
widget.controller.value = value;
}
bool get _hasFocus => widget.focusNode.hasFocus;
bool get _isMultiline => widget.maxLines != 1;
// Finds the closest scroll offset to the current scroll offset that fully
// reveals the given caret rect. If the given rect's main axis extent is too
// large to be fully revealed in `renderEditable`, it will be centered along
// the main axis.
//
// If this is a multiline EditableText (which means the Editable can only
// scroll vertically), the given rect's height will first be extended to match
// `renderEditable.preferredLineHeight`, before the target scroll offset is
// calculated.
RevealedOffset _getOffsetToRevealCaret(Rect rect) {
if (!_scrollController.position.allowImplicitScrolling) {
return RevealedOffset(offset: _scrollController.offset, rect: rect);
}
final Size editableSize = renderEditable.size;
final double additionalOffset;
final Offset unitOffset;
if (!_isMultiline) {
additionalOffset = rect.width >= editableSize.width
// Center `rect` if it's oversized.
? editableSize.width / 2 - rect.center.dx
// Valid additional offsets range from (rect.right - size.width)
// to (rect.left). Pick the closest one if out of range.
: clampDouble(0.0, rect.right - editableSize.width, rect.left);
unitOffset = const Offset(1, 0);
} else {
// The caret is vertically centered within the line. Expand the caret's
// height so that it spans the line because we're going to ensure that the
// entire expanded caret is scrolled into view.
final Rect expandedRect = Rect.fromCenter(
center: rect.center,
width: rect.width,
height: math.max(rect.height, renderEditable.preferredLineHeight),
);
additionalOffset = expandedRect.height >= editableSize.height
? editableSize.height / 2 - expandedRect.center.dy
: clampDouble(0.0, expandedRect.bottom - editableSize.height, expandedRect.top);
unitOffset = const Offset(0, 1);
}
// No overscrolling when encountering tall fonts/scripts that extend past
// the ascent.
final double targetOffset = clampDouble(
additionalOffset + _scrollController.offset,
_scrollController.position.minScrollExtent,
_scrollController.position.maxScrollExtent,
);
final double offsetDelta = _scrollController.offset - targetOffset;
return RevealedOffset(rect: rect.shift(unitOffset * offsetDelta), offset: targetOffset);
}
/// Whether to send the autofill information to the autofill service. True by
/// default.
bool get _needsAutofill => _effectiveAutofillClient.textInputConfiguration.autofillConfiguration.enabled;
// Must be called after layout.
// See https://github.com/flutter/flutter/issues/126312
void _openInputConnection() {
if (!_shouldCreateInputConnection) {
return;
}
if (!_hasInputConnection) {
final TextEditingValue localValue = _value;
// When _needsAutofill == true && currentAutofillScope == null, autofill
// is allowed but saving the user input from the text field is
// discouraged.
//
// In case the autofillScope changes from a non-null value to null, or
// _needsAutofill changes to false from true, the platform needs to be
// notified to exclude this field from the autofill context. So we need to
// provide the autofillId.
_textInputConnection = _needsAutofill && currentAutofillScope != null
? currentAutofillScope!.attach(this, _effectiveAutofillClient.textInputConfiguration)
: TextInput.attach(this, _effectiveAutofillClient.textInputConfiguration);
_updateSizeAndTransform();
_schedulePeriodicPostFrameCallbacks();
_textInputConnection!
..setStyle(
fontFamily: _style.fontFamily,
fontSize: _style.fontSize,
fontWeight: _style.fontWeight,
textDirection: _textDirection,
textAlign: widget.textAlign,
)
..setEditingState(localValue)
..show();
if (_needsAutofill) {
// Request autofill AFTER the size and the transform have been sent to
// the platform text input plugin.
_textInputConnection!.requestAutofill();
}
_lastKnownRemoteTextEditingValue = localValue;
} else {
_textInputConnection!.show();
}
}
void _closeInputConnectionIfNeeded() {
if (_hasInputConnection) {
_textInputConnection!.close();
_textInputConnection = null;
_lastKnownRemoteTextEditingValue = null;
_scribbleCacheKey = null;
removeTextPlaceholder();
}
}
void _openOrCloseInputConnectionIfNeeded() {
if (_hasFocus && widget.focusNode.consumeKeyboardToken()) {
_openInputConnection();
} else if (!_hasFocus) {
_closeInputConnectionIfNeeded();
widget.controller.clearComposing();
}
}
bool _restartConnectionScheduled = false;
void _scheduleRestartConnection() {
if (_restartConnectionScheduled) {
return;
}
_restartConnectionScheduled = true;
scheduleMicrotask(_restartConnectionIfNeeded);
}
// Discards the current [TextInputConnection] and establishes a new one.
//
// This method is rarely needed. This is currently used to reset the input
// type when the "submit" text input action is triggered and the developer
// puts the focus back to this input field..
void _restartConnectionIfNeeded() {
_restartConnectionScheduled = false;
if (!_hasInputConnection || !_shouldCreateInputConnection) {
return;
}
_textInputConnection!.close();
_textInputConnection = null;
_lastKnownRemoteTextEditingValue = null;
final AutofillScope? currentAutofillScope = _needsAutofill ? this.currentAutofillScope : null;
final TextInputConnection newConnection = currentAutofillScope?.attach(this, textInputConfiguration)
?? TextInput.attach(this, _effectiveAutofillClient.textInputConfiguration);
_textInputConnection = newConnection;
newConnection
..show()
..setStyle(
fontFamily: _style.fontFamily,
fontSize: _style.fontSize,
fontWeight: _style.fontWeight,
textDirection: _textDirection,
textAlign: widget.textAlign,
)
..setEditingState(_value);
_lastKnownRemoteTextEditingValue = _value;
}
@override
void didChangeInputControl(TextInputControl? oldControl, TextInputControl? newControl) {
if (_hasFocus && _hasInputConnection) {
oldControl?.hide();
newControl?.show();
}
}
@override
void connectionClosed() {
if (_hasInputConnection) {
_textInputConnection!.connectionClosedReceived();
_textInputConnection = null;
_lastKnownRemoteTextEditingValue = null;
widget.focusNode.unfocus();
}
}
// Indicates that a call to _handleFocusChanged originated within
// EditableText, allowing it to distinguish between internal and external
// focus changes.
bool _nextFocusChangeIsInternal = false;
// Sets _nextFocusChangeIsInternal to true only until any subsequent focus
// change happens.
void _flagInternalFocus() {
_nextFocusChangeIsInternal = true;
FocusManager.instance.addListener(_unflagInternalFocus);
}
void _unflagInternalFocus() {
_nextFocusChangeIsInternal = false;
FocusManager.instance.removeListener(_unflagInternalFocus);
}
/// Express interest in interacting with the keyboard.
///
/// If this control is already attached to the keyboard, this function will
/// request that the keyboard become visible. Otherwise, this function will
/// ask the focus system that it become focused. If successful in acquiring
/// focus, the control will then attach to the keyboard and request that the
/// keyboard become visible.
void requestKeyboard() {
if (_hasFocus) {
_openInputConnection();
} else {
_flagInternalFocus();
widget.focusNode.requestFocus(); // This eventually calls _openInputConnection also, see _handleFocusChanged.
}
}
void _updateOrDisposeSelectionOverlayIfNeeded() {
if (_selectionOverlay != null) {
if (_hasFocus) {
_selectionOverlay!.update(_value);
} else {
_selectionOverlay!.dispose();
_selectionOverlay = null;
}
}
}
final bool _platformSupportsFadeOnScroll = switch (defaultTargetPlatform) {
TargetPlatform.android ||
TargetPlatform.iOS => true,
TargetPlatform.fuchsia ||
TargetPlatform.linux ||
TargetPlatform.macOS ||
TargetPlatform.windows => false,
};
bool _isInternalScrollableNotification(BuildContext? notificationContext) {
final ScrollableState? scrollableState = notificationContext?.findAncestorStateOfType<ScrollableState>();
return _scrollableKey.currentContext == scrollableState?.context;
}
bool _scrollableNotificationIsFromSameSubtree(BuildContext? notificationContext) {
if (notificationContext == null) {
return false;
}
BuildContext? currentContext = context;
// The notification context of a ScrollNotification points to the RawGestureDetector
// of the Scrollable. We get the ScrollableState associated with this notification
// by looking up the tree.
final ScrollableState? notificationScrollableState = notificationContext.findAncestorStateOfType<ScrollableState>();
if (notificationScrollableState == null) {
return false;
}
while (currentContext != null) {
final ScrollableState? scrollableState = currentContext.findAncestorStateOfType<ScrollableState>();
if (scrollableState == notificationScrollableState) {
return true;
}
currentContext = scrollableState?.context;
}
return false;
}
void _handleContextMenuOnParentScroll(ScrollNotification notification) {
// Do some preliminary checks to avoid expensive subtree traversal.
if (notification is! ScrollStartNotification
&& notification is! ScrollEndNotification) {
return;
}
if (notification is ScrollStartNotification
&& _dataWhenToolbarShowScheduled != null) {
return;
}
if (notification is ScrollEndNotification
&& _dataWhenToolbarShowScheduled == null) {
return;
}
if (notification is ScrollEndNotification
&& _dataWhenToolbarShowScheduled!.value != _value) {
_dataWhenToolbarShowScheduled = null;
_disposeScrollNotificationObserver();
return;
}
if (_isInternalScrollableNotification(notification.context)) {
return;
}
if (!_scrollableNotificationIsFromSameSubtree(notification.context)) {
return;
}
_handleContextMenuOnScroll(notification);
}
Rect _calculateDeviceRect() {
final Size screenSize = MediaQuery.sizeOf(context);
final ui.FlutterView view = View.of(context);
final double obscuredVertical = (view.padding.top + view.padding.bottom + view.viewInsets.bottom) / view.devicePixelRatio;
final double obscuredHorizontal = (view.padding.left + view.padding.right) / view.devicePixelRatio;
final Size visibleScreenSize = Size(screenSize.width - obscuredHorizontal, screenSize.height - obscuredVertical);
return Rect.fromLTWH(view.padding.left / view.devicePixelRatio, view.padding.top / view.devicePixelRatio, visibleScreenSize.width, visibleScreenSize.height);
}
bool _showToolbarOnScreenScheduled = false;
void _handleContextMenuOnScroll(ScrollNotification notification) {
if (_webContextMenuEnabled) {
return;
}
if (!_platformSupportsFadeOnScroll) {
_selectionOverlay?.updateForScroll();
return;
}
// When the scroll begins and the toolbar is visible, hide it
// until scrolling ends.
//
// The selection and renderEditable need to be visible within the current
// viewport for the toolbar to show when scrolling ends. If they are not
// then the toolbar is shown when they are scrolled back into view, unless
// invalidated by a change in TextEditingValue.
if (notification is ScrollStartNotification) {
if (_dataWhenToolbarShowScheduled != null) {
return;
}
final bool toolbarIsVisible = _selectionOverlay != null
&& _selectionOverlay!.toolbarIsVisible
&& !_selectionOverlay!.spellCheckToolbarIsVisible;
if (!toolbarIsVisible) {
return;
}
final List<TextBox> selectionBoxes = renderEditable.getBoxesForSelection(_value.selection);
final Rect selectionBounds = _value.selection.isCollapsed || selectionBoxes.isEmpty
? renderEditable.getLocalRectForCaret(_value.selection.extent)
: selectionBoxes
.map((TextBox box) => box.toRect())
.reduce((Rect result, Rect rect) => result.expandToInclude(rect));
_dataWhenToolbarShowScheduled = (value: _value, selectionBounds: selectionBounds);
_selectionOverlay?.hideToolbar();
} else if (notification is ScrollEndNotification) {
if (_dataWhenToolbarShowScheduled == null) {
return;
}
if (_dataWhenToolbarShowScheduled!.value != _value) {
// Value has changed so we should invalidate any toolbar scheduling.
_dataWhenToolbarShowScheduled = null;
_disposeScrollNotificationObserver();
return;
}
if (_showToolbarOnScreenScheduled) {
return;
}
_showToolbarOnScreenScheduled = true;
SchedulerBinding.instance.addPostFrameCallback((Duration _) {
_showToolbarOnScreenScheduled = false;
if (!mounted) {
return;
}
final Rect deviceRect = _calculateDeviceRect();
final bool selectionVisibleInEditable = renderEditable.selectionStartInViewport.value || renderEditable.selectionEndInViewport.value;
final Rect selectionBounds = MatrixUtils.transformRect(renderEditable.getTransformTo(null), _dataWhenToolbarShowScheduled!.selectionBounds);
final bool selectionOverlapsWithDeviceRect = !selectionBounds.hasNaN && deviceRect.overlaps(selectionBounds);
if (selectionVisibleInEditable
&& selectionOverlapsWithDeviceRect
&& _selectionInViewport(_dataWhenToolbarShowScheduled!.selectionBounds)) {
showToolbar();
_dataWhenToolbarShowScheduled = null;
}
}, debugLabel: 'EditableText.scheduleToolbar');
}
}
bool _selectionInViewport(Rect selectionBounds) {
RenderAbstractViewport? closestViewport = RenderAbstractViewport.maybeOf(renderEditable);
while (closestViewport != null) {
final Rect selectionBoundsLocalToViewport = MatrixUtils.transformRect(renderEditable.getTransformTo(closestViewport), selectionBounds);
if (selectionBoundsLocalToViewport.hasNaN
|| closestViewport.paintBounds.hasNaN
|| !closestViewport.paintBounds.overlaps(selectionBoundsLocalToViewport)) {
return false;
}
closestViewport = RenderAbstractViewport.maybeOf(closestViewport.parent);
}
return true;
}
TextSelectionOverlay _createSelectionOverlay() {
final EditableTextContextMenuBuilder? contextMenuBuilder = widget.contextMenuBuilder;
final TextSelectionOverlay selectionOverlay = TextSelectionOverlay(
clipboardStatus: clipboardStatus,
context: context,
value: _value,
debugRequiredFor: widget,
toolbarLayerLink: _toolbarLayerLink,
startHandleLayerLink: _startHandleLayerLink,
endHandleLayerLink: _endHandleLayerLink,
renderObject: renderEditable,
selectionControls: widget.selectionControls,
selectionDelegate: this,
dragStartBehavior: widget.dragStartBehavior,
onSelectionHandleTapped: widget.onSelectionHandleTapped,
contextMenuBuilder: contextMenuBuilder == null || _webContextMenuEnabled
? null
: (BuildContext context) {
return contextMenuBuilder(
context,
this,
);
},
magnifierConfiguration: widget.magnifierConfiguration,
);
return selectionOverlay;
}
@pragma('vm:notify-debugger-on-exception')
void _handleSelectionChanged(TextSelection selection, SelectionChangedCause? cause) {
// We return early if the selection is not valid. This can happen when the
// text of [EditableText] is updated at the same time as the selection is
// changed by a gesture event.
if (!widget.controller._isSelectionWithinTextBounds(selection)) {
return;
}
widget.controller.selection = selection;
// This will show the keyboard for all selection changes on the
// EditableText except for those triggered by a keyboard input.
// Typically EditableText shouldn't take user keyboard input if
// it's not focused already. If the EditableText is being
// autofilled it shouldn't request focus.
switch (cause) {
case null:
case SelectionChangedCause.doubleTap:
case SelectionChangedCause.drag:
case SelectionChangedCause.forcePress:
case SelectionChangedCause.longPress:
case SelectionChangedCause.scribble:
case SelectionChangedCause.tap:
case SelectionChangedCause.toolbar:
requestKeyboard();
case SelectionChangedCause.keyboard:
if (_hasFocus) {
requestKeyboard();
}
}
if (widget.selectionControls == null && widget.contextMenuBuilder == null) {
_selectionOverlay?.dispose();
_selectionOverlay = null;
} else {
if (_selectionOverlay == null) {
_selectionOverlay = _createSelectionOverlay();
} else {
_selectionOverlay!.update(_value);
}
_selectionOverlay!.handlesVisible = widget.showSelectionHandles;
_selectionOverlay!.showHandles();
}
// TODO(chunhtai): we should make sure selection actually changed before
// we call the onSelectionChanged.
// https://github.com/flutter/flutter/issues/76349.
try {
widget.onSelectionChanged?.call(selection, cause);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while calling onSelectionChanged for $cause'),
));
}
// To keep the cursor from blinking while it moves, restart the timer here.
if (_showBlinkingCursor && _cursorTimer != null) {
_stopCursorBlink(resetCharTicks: false);
_startCursorBlink();
}
}
// Animation configuration for scrolling the caret back on screen.
static const Duration _caretAnimationDuration = Duration(milliseconds: 100);
static const Curve _caretAnimationCurve = Curves.fastOutSlowIn;
bool _showCaretOnScreenScheduled = false;
void _scheduleShowCaretOnScreen({required bool withAnimation}) {
if (_showCaretOnScreenScheduled) {
return;
}
_showCaretOnScreenScheduled = true;
SchedulerBinding.instance.addPostFrameCallback((Duration _) {
_showCaretOnScreenScheduled = false;
// Since we are in a post frame callback, check currentContext in case
// RenderEditable has been disposed (in which case it will be null).
final RenderEditable? renderEditable =
_editableKey.currentContext?.findRenderObject() as RenderEditable?;
if (renderEditable == null
|| !(renderEditable.selection?.isValid ?? false)
|| !_scrollController.hasClients) {
return;
}
final double lineHeight = renderEditable.preferredLineHeight;
// Enlarge the target rect by scrollPadding to ensure that caret is not
// positioned directly at the edge after scrolling.
double bottomSpacing = widget.scrollPadding.bottom;
if (_selectionOverlay?.selectionControls != null) {
final double handleHeight = _selectionOverlay!.selectionControls!
.getHandleSize(lineHeight).height;
final double interactiveHandleHeight = math.max(
handleHeight,
kMinInteractiveDimension,
);
final Offset anchor = _selectionOverlay!.selectionControls!
.getHandleAnchor(
TextSelectionHandleType.collapsed,
lineHeight,
);
final double handleCenter = handleHeight / 2 - anchor.dy;
bottomSpacing = math.max(
handleCenter + interactiveHandleHeight / 2,
bottomSpacing,
);
}
final EdgeInsets caretPadding = widget.scrollPadding
.copyWith(bottom: bottomSpacing);
final Rect caretRect = renderEditable.getLocalRectForCaret(renderEditable.selection!.extent);
final RevealedOffset targetOffset = _getOffsetToRevealCaret(caretRect);
final Rect rectToReveal;
final TextSelection selection = textEditingValue.selection;
if (selection.isCollapsed) {
rectToReveal = targetOffset.rect;
} else {
final List<TextBox> selectionBoxes = renderEditable.getBoxesForSelection(selection);
// selectionBoxes may be empty if, for example, the selection does not
// encompass a full character, like if it only contained part of an
// extended grapheme cluster.
if (selectionBoxes.isEmpty) {
rectToReveal = targetOffset.rect;
} else {
rectToReveal = selection.baseOffset < selection.extentOffset ?
selectionBoxes.last.toRect() : selectionBoxes.first.toRect();
}
}
if (withAnimation) {
_scrollController.animateTo(
targetOffset.offset,
duration: _caretAnimationDuration,
curve: _caretAnimationCurve,
);
renderEditable.showOnScreen(
rect: caretPadding.inflateRect(rectToReveal),
duration: _caretAnimationDuration,
curve: _caretAnimationCurve,
);
} else {
_scrollController.jumpTo(targetOffset.offset);
renderEditable.showOnScreen(
rect: caretPadding.inflateRect(rectToReveal),
);
}
}, debugLabel: 'EditableText.showCaret');
}
late double _lastBottomViewInset;
@override
void didChangeMetrics() {
if (!mounted) {
return;
}
final ui.FlutterView view = View.of(context);
if (_lastBottomViewInset != view.viewInsets.bottom) {
SchedulerBinding.instance.addPostFrameCallback((Duration _) {
_selectionOverlay?.updateForScroll();
}, debugLabel: 'EditableText.updateForScroll');
if (_lastBottomViewInset < view.viewInsets.bottom) {
// Because the metrics change signal from engine will come here every frame
// (on both iOS and Android). So we don't need to show caret with animation.
_scheduleShowCaretOnScreen(withAnimation: false);
}
}
_lastBottomViewInset = view.viewInsets.bottom;
}
Future<void> _performSpellCheck(final String text) async {
try {
final Locale? localeForSpellChecking = widget.locale ?? Localizations.maybeLocaleOf(context);
assert(
localeForSpellChecking != null,
'Locale must be specified in widget or Localization widget must be in scope',
);
final List<SuggestionSpan>? suggestions = await
_spellCheckConfiguration
.spellCheckService!
.fetchSpellCheckSuggestions(localeForSpellChecking!, text);
if (suggestions == null) {
// The request to fetch spell check suggestions was canceled due to ongoing request.
return;
}
spellCheckResults = SpellCheckResults(text, suggestions);
renderEditable.text = buildTextSpan();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while performing spell check'),
));
}
}
@pragma('vm:notify-debugger-on-exception')
void _formatAndSetValue(TextEditingValue value, SelectionChangedCause? cause, {bool userInteraction = false}) {
final TextEditingValue oldValue = _value;
final bool textChanged = oldValue.text != value.text;
final bool textCommitted = !oldValue.composing.isCollapsed && value.composing.isCollapsed;
final bool selectionChanged = oldValue.selection != value.selection;
if (textChanged || textCommitted) {
// Only apply input formatters if the text has changed (including uncommitted
// text in the composing region), or when the user committed the composing
// text.
// Gboard is very persistent in restoring the composing region. Applying
// input formatters on composing-region-only changes (except clearing the
// current composing region) is very infinite-loop-prone: the formatters
// will keep trying to modify the composing region while Gboard will keep
// trying to restore the original composing region.
try {
value = widget.inputFormatters?.fold<TextEditingValue>(
value,
(TextEditingValue newValue, TextInputFormatter formatter) => formatter.formatEditUpdate(_value, newValue),
) ?? value;
if (spellCheckEnabled && value.text.isNotEmpty && _value.text != value.text) {
_performSpellCheck(value.text);
}
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while applying input formatters'),
));
}
}
final TextSelection oldTextSelection = textEditingValue.selection;
// Put all optional user callback invocations in a batch edit to prevent
// sending multiple `TextInput.updateEditingValue` messages.
beginBatchEdit();
_value = value;
// Changes made by the keyboard can sometimes be "out of band" for listening
// components, so always send those events, even if we didn't think it
// changed. Also, the user long pressing should always send a selection change
// as well.
if (selectionChanged ||
(userInteraction &&
(cause == SelectionChangedCause.longPress ||
cause == SelectionChangedCause.keyboard))) {
_handleSelectionChanged(_value.selection, cause);
_bringIntoViewBySelectionState(oldTextSelection, value.selection, cause);
}
final String currentText = _value.text;
if (oldValue.text != currentText) {
try {
widget.onChanged?.call(currentText);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widgets',
context: ErrorDescription('while calling onChanged'),
));
}
}
endBatchEdit();
}
void _bringIntoViewBySelectionState(TextSelection oldSelection, TextSelection newSelection, SelectionChangedCause? cause) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
if (cause == SelectionChangedCause.longPress ||
cause == SelectionChangedCause.drag) {
bringIntoView(newSelection.extent);
}
case TargetPlatform.linux:
case TargetPlatform.windows:
case TargetPlatform.fuchsia:
case TargetPlatform.android:
if (cause == SelectionChangedCause.drag) {
if (oldSelection.baseOffset != newSelection.baseOffset) {
bringIntoView(newSelection.base);
} else if (oldSelection.extentOffset != newSelection.extentOffset) {
bringIntoView(newSelection.extent);
}
}
}
}
void _onCursorColorTick() {
final double effectiveOpacity = math.min(widget.cursorColor.alpha / 255.0, _cursorBlinkOpacityController.value);
renderEditable.cursorColor = widget.cursorColor.withOpacity(effectiveOpacity);
_cursorVisibilityNotifier.value = widget.showCursor && (EditableText.debugDeterministicCursor || _cursorBlinkOpacityController.value > 0);
}
bool get _showBlinkingCursor => _hasFocus && _value.selection.isCollapsed && widget.showCursor && _tickersEnabled && !renderEditable.floatingCursorOn;
/// Whether the blinking cursor is actually visible at this precise moment
/// (it's hidden half the time, since it blinks).
@visibleForTesting
bool get cursorCurrentlyVisible => _cursorBlinkOpacityController.value > 0;
/// The cursor blink interval (the amount of time the cursor is in the "on"
/// state or the "off" state). A complete cursor blink period is twice this
/// value (half on, half off).
@visibleForTesting
Duration get cursorBlinkInterval => _kCursorBlinkHalfPeriod;
/// The current status of the text selection handles.
@visibleForTesting
TextSelectionOverlay? get selectionOverlay => _selectionOverlay;
int _obscureShowCharTicksPending = 0;
int? _obscureLatestCharIndex;
void _startCursorBlink() {
assert(!(_cursorTimer?.isActive ?? false) || !(_backingCursorBlinkOpacityController?.isAnimating ?? false));
if (!widget.showCursor) {
return;
}
if (!_tickersEnabled) {
return;
}
_cursorTimer?.cancel();
_cursorBlinkOpacityController.value = 1.0;
if (EditableText.debugDeterministicCursor) {
return;
}
if (widget.cursorOpacityAnimates) {
_cursorBlinkOpacityController.animateWith(_iosBlinkCursorSimulation).whenComplete(_onCursorTick);
} else {
_cursorTimer = Timer.periodic(_kCursorBlinkHalfPeriod, (Timer timer) { _onCursorTick(); });
}
}
void _onCursorTick() {
if (_obscureShowCharTicksPending > 0) {
_obscureShowCharTicksPending = WidgetsBinding.instance.platformDispatcher.brieflyShowPassword
? _obscureShowCharTicksPending - 1
: 0;
if (_obscureShowCharTicksPending == 0) {
setState(() { });
}
}
if (widget.cursorOpacityAnimates) {
_cursorTimer?.cancel();
// Schedule this as an async task to avoid blocking tester.pumpAndSettle
// indefinitely.
_cursorTimer = Timer(Duration.zero, () => _cursorBlinkOpacityController.animateWith(_iosBlinkCursorSimulation).whenComplete(_onCursorTick));
} else {
if (!(_cursorTimer?.isActive ?? false) && _tickersEnabled) {
_cursorTimer = Timer.periodic(_kCursorBlinkHalfPeriod, (Timer timer) { _onCursorTick(); });
}
_cursorBlinkOpacityController.value = _cursorBlinkOpacityController.value == 0 ? 1 : 0;
}
}
void _stopCursorBlink({ bool resetCharTicks = true }) {
// If the cursor is animating, stop the animation, and we always
// want the cursor to be visible when the floating cursor is enabled.
_cursorBlinkOpacityController.value = renderEditable.floatingCursorOn ? 1.0 : 0.0;
_cursorTimer?.cancel();
_cursorTimer = null;
if (resetCharTicks) {
_obscureShowCharTicksPending = 0;
}
}
void _startOrStopCursorTimerIfNeeded() {
if (!_showBlinkingCursor) {
_stopCursorBlink();
} else if (_cursorTimer == null) {
_startCursorBlink();
}
}
void _didChangeTextEditingValue() {
if (_hasFocus && !_value.selection.isValid) {
// If this field is focused and the selection is invalid, place the cursor at
// the end. Does not rely on _handleFocusChanged because it makes selection
// handles visible on Android.
// Unregister as a listener to the text controller while making the change.
widget.controller.removeListener(_didChangeTextEditingValue);
widget.controller.selection = _adjustedSelectionWhenFocused()!;
widget.controller.addListener(_didChangeTextEditingValue);
}
_updateRemoteEditingValueIfNeeded();
_startOrStopCursorTimerIfNeeded();
_updateOrDisposeSelectionOverlayIfNeeded();
// TODO(abarth): Teach RenderEditable about ValueNotifier<TextEditingValue>
// to avoid this setState().
setState(() { /* We use widget.controller.value in build(). */ });
_verticalSelectionUpdateAction.stopCurrentVerticalRunIfSelectionChanges();
}
void _handleFocusChanged() {
_openOrCloseInputConnectionIfNeeded();
_startOrStopCursorTimerIfNeeded();
_updateOrDisposeSelectionOverlayIfNeeded();
if (_hasFocus) {
// Listen for changing viewInsets, which indicates keyboard showing up.
WidgetsBinding.instance.addObserver(this);
_lastBottomViewInset = View.of(context).viewInsets.bottom;
if (!widget.readOnly) {
_scheduleShowCaretOnScreen(withAnimation: true);
}
final TextSelection? updatedSelection = _adjustedSelectionWhenFocused();
if (updatedSelection != null) {
_handleSelectionChanged(updatedSelection, null);
}
} else {
WidgetsBinding.instance.removeObserver(this);
setState(() { _currentPromptRectRange = null; });
}
updateKeepAlive();
}
TextSelection? _adjustedSelectionWhenFocused() {
TextSelection? selection;
final bool shouldSelectAll = widget.selectionEnabled && kIsWeb
&& !_isMultiline && !_nextFocusChangeIsInternal;
if (shouldSelectAll) {
// On native web, single line <input> tags select all when receiving
// focus.
selection = TextSelection(
baseOffset: 0,
extentOffset: _value.text.length,
);
} else if (!_value.selection.isValid) {
// Place cursor at the end if the selection is invalid when we receive focus.
selection = TextSelection.collapsed(offset: _value.text.length);
}
return selection;
}
void _compositeCallback(Layer layer) {
// The callback can be invoked when the layer is detached.
// The input connection can be closed by the platform in which case this
// widget doesn't rebuild.
if (!renderEditable.attached || !_hasInputConnection) {
return;
}
assert(mounted);
assert((context as Element).debugIsActive);
_updateSizeAndTransform();
}
// Must be called after layout.
// See https://github.com/flutter/flutter/issues/126312
void _updateSizeAndTransform() {
final Size size = renderEditable.size;
final Matrix4 transform = renderEditable.getTransformTo(null);
_textInputConnection!.setEditableSizeAndTransform(size, transform);
}
void _schedulePeriodicPostFrameCallbacks([Duration? duration]) {
if (!_hasInputConnection) {
return;
}
_updateSelectionRects();
_updateComposingRectIfNeeded();
_updateCaretRectIfNeeded();
SchedulerBinding.instance.addPostFrameCallback(
_schedulePeriodicPostFrameCallbacks,
debugLabel: 'EditableText.postFrameCallbacks'
);
}
_ScribbleCacheKey? _scribbleCacheKey;
void _updateSelectionRects({bool force = false}) {
if (!widget.scribbleEnabled || defaultTargetPlatform != TargetPlatform.iOS) {
return;
}
final ScrollDirection scrollDirection = _scrollController.position.userScrollDirection;
if (scrollDirection != ScrollDirection.idle) {
return;
}
final InlineSpan inlineSpan = renderEditable.text!;
final TextScaler effectiveTextScaler = switch ((widget.textScaler, widget.textScaleFactor)) {
(final TextScaler textScaler, _) => textScaler,
(null, final double textScaleFactor) => TextScaler.linear(textScaleFactor),
(null, null) => MediaQuery.textScalerOf(context),
};
final _ScribbleCacheKey newCacheKey = _ScribbleCacheKey(
inlineSpan: inlineSpan,
textAlign: widget.textAlign,
textDirection: _textDirection,
textScaler: effectiveTextScaler,
textHeightBehavior: widget.textHeightBehavior ?? DefaultTextHeightBehavior.maybeOf(context),
locale: widget.locale,
structStyle: widget.strutStyle,
placeholder: _placeholderLocation,
size: renderEditable.size,
);
final RenderComparison comparison = force
? RenderComparison.layout
: _scribbleCacheKey?.compare(newCacheKey) ?? RenderComparison.layout;
if (comparison.index < RenderComparison.layout.index) {
return;
}
_scribbleCacheKey = newCacheKey;
final List<SelectionRect> rects = <SelectionRect>[];
int graphemeStart = 0;
// Can't use _value.text here: the controller value could change between
// frames.
final String plainText = inlineSpan.toPlainText(includeSemanticsLabels: false);
final CharacterRange characterRange = CharacterRange(plainText);
while (characterRange.moveNext()) {
final int graphemeEnd = graphemeStart + characterRange.current.length;
final List<TextBox> boxes = renderEditable.getBoxesForSelection(
TextSelection(baseOffset: graphemeStart, extentOffset: graphemeEnd),
);
final TextBox? box = boxes.isEmpty ? null : boxes.first;
if (box != null) {
final Rect paintBounds = renderEditable.paintBounds;
// Stop early when characters are already below the bottom edge of the
// RenderEditable, regardless of its clipBehavior.
if (paintBounds.bottom <= box.top) {
break;
}
// Include any TextBox which intersects with the RenderEditable.
if (paintBounds.left <= box.right &&
box.left <= paintBounds.right &&
paintBounds.top <= box.bottom) {
// At least some part of the letter is visible within the text field.
rects.add(SelectionRect(position: graphemeStart, bounds: box.toRect(), direction: box.direction));
}
}
graphemeStart = graphemeEnd;
}
_textInputConnection!.setSelectionRects(rects);
}
// Sends the current composing rect to the embedder's text input plugin.
//
// In cases where the composing rect hasn't been updated in the embedder due
// to the lag of asynchronous messages over the channel, the position of the
// current caret rect is used instead.
//
// See: [_updateCaretRectIfNeeded]
void _updateComposingRectIfNeeded() {
final TextRange composingRange = _value.composing;
assert(mounted);
Rect? composingRect = renderEditable.getRectForComposingRange(composingRange);
// Send the caret location instead if there's no marked text yet.
if (composingRect == null) {
assert(!composingRange.isValid || composingRange.isCollapsed);
final int offset = composingRange.isValid ? composingRange.start : 0;
composingRect = renderEditable.getLocalRectForCaret(TextPosition(offset: offset));
}
_textInputConnection!.setComposingRect(composingRect);
}
// Sends the current caret rect to the embedder's text input plugin.
//
// The position of the caret rect is updated periodically such that if the
// user initiates composing input, the current cursor rect can be used for
// the first character until the composing rect can be sent.
//
// On selection changes, the start of the selection is used. This ensures
// that regardless of the direction the selection was created, the cursor is
// set to the position where next text input occurs. This position is used to
// position the IME's candidate selection menu.
//
// See: [_updateComposingRectIfNeeded]
void _updateCaretRectIfNeeded() {
final TextSelection? selection = renderEditable.selection;
if (selection == null || !selection.isValid) {
return;
}
final TextPosition currentTextPosition = TextPosition(offset: selection.start);
final Rect caretRect = renderEditable.getLocalRectForCaret(currentTextPosition);
_textInputConnection!.setCaretRect(caretRect);
}
TextDirection get _textDirection => widget.textDirection ?? Directionality.of(context);
/// The renderer for this widget's descendant.
///
/// This property is typically used to notify the renderer of input gestures
/// when [RenderEditable.ignorePointer] is true.
late final RenderEditable renderEditable = _editableKey.currentContext!.findRenderObject()! as RenderEditable;
@override
TextEditingValue get textEditingValue => _value;
double get _devicePixelRatio => MediaQuery.devicePixelRatioOf(context);
@override
void userUpdateTextEditingValue(TextEditingValue value, SelectionChangedCause? cause) {
// Compare the current TextEditingValue with the pre-format new
// TextEditingValue value, in case the formatter would reject the change.
final bool shouldShowCaret = widget.readOnly
? _value.selection != value.selection
: _value != value;
if (shouldShowCaret) {
_scheduleShowCaretOnScreen(withAnimation: true);
}
// Even if the value doesn't change, it may be necessary to focus and build
// the selection overlay. For example, this happens when right clicking an
// unfocused field that previously had a selection in the same spot.
if (value == textEditingValue) {
if (!widget.focusNode.hasFocus) {
_flagInternalFocus();
widget.focusNode.requestFocus();
_selectionOverlay ??= _createSelectionOverlay();
}
return;
}
_formatAndSetValue(value, cause, userInteraction: true);
}
@override
void bringIntoView(TextPosition position) {
final Rect localRect = renderEditable.getLocalRectForCaret(position);
final RevealedOffset targetOffset = _getOffsetToRevealCaret(localRect);
_scrollController.jumpTo(targetOffset.offset);
renderEditable.showOnScreen(rect: targetOffset.rect);
}
/// Shows the selection toolbar at the location of the current cursor.
///
/// Returns `false` if a toolbar couldn't be shown, such as when the toolbar
/// is already shown, or when no text selection currently exists.
@override
bool showToolbar() {
// Web is using native dom elements to enable clipboard functionality of the
// context menu: copy, paste, select, cut. It might also provide additional
// functionality depending on the browser (such as translate). Due to this,
// we should not show a Flutter toolbar for the editable text elements
// unless the browser's context menu is explicitly disabled.
if (_webContextMenuEnabled) {
return false;
}
if (_selectionOverlay == null) {
return false;
}
_liveTextInputStatus?.update();
clipboardStatus.update();
_selectionOverlay!.showToolbar();
// Listen to parent scroll events when the toolbar is visible so it can be
// hidden during a scroll on supported platforms.
if (_platformSupportsFadeOnScroll) {
_listeningToScrollNotificationObserver = true;
_scrollNotificationObserver?.removeListener(_handleContextMenuOnParentScroll);
_scrollNotificationObserver = ScrollNotificationObserver.maybeOf(context);
_scrollNotificationObserver?.addListener(_handleContextMenuOnParentScroll);
}
return true;
}
@override
void hideToolbar([bool hideHandles = true]) {
// Stop listening to parent scroll events when toolbar is hidden.
_disposeScrollNotificationObserver();
if (hideHandles) {
// Hide the handles and the toolbar.
_selectionOverlay?.hide();
} else if (_selectionOverlay?.toolbarIsVisible ?? false) {
// Hide only the toolbar but not the handles.
_selectionOverlay?.hideToolbar();
}
}
/// Toggles the visibility of the toolbar.
void toggleToolbar([bool hideHandles = true]) {
final TextSelectionOverlay selectionOverlay = _selectionOverlay ??= _createSelectionOverlay();
if (selectionOverlay.toolbarIsVisible) {
hideToolbar(hideHandles);
} else {
showToolbar();
}
}
/// Shows toolbar with spell check suggestions of misspelled words that are
/// available for click-and-replace.
bool showSpellCheckSuggestionsToolbar() {
// Spell check suggestions toolbars are intended to be shown on non-web
// platforms. Additionally, the Cupertino style toolbar can't be drawn on
// the web with the HTML renderer due to
// https://github.com/flutter/flutter/issues/123560.
if (!spellCheckEnabled
|| _webContextMenuEnabled
|| widget.readOnly
|| _selectionOverlay == null
|| !_spellCheckResultsReceived
|| findSuggestionSpanAtCursorIndex(textEditingValue.selection.extentOffset) == null) {
// Only attempt to show the spell check suggestions toolbar if there
// is a toolbar specified and spell check suggestions available to show.
return false;
}
assert(
_spellCheckConfiguration.spellCheckSuggestionsToolbarBuilder != null,
'spellCheckSuggestionsToolbarBuilder must be defined in '
'SpellCheckConfiguration to show a toolbar with spell check '
'suggestions',
);
_selectionOverlay!
.showSpellCheckSuggestionsToolbar(
(BuildContext context) {
return _spellCheckConfiguration
.spellCheckSuggestionsToolbarBuilder!(
context,
this,
);
},
);
return true;
}
/// Shows the magnifier at the position given by `positionToShow`,
/// if there is no magnifier visible.
///
/// Updates the magnifier to the position given by `positionToShow`,
/// if there is a magnifier visible.
///
/// Does nothing if a magnifier couldn't be shown, such as when the selection
/// overlay does not currently exist.
void showMagnifier(Offset positionToShow) {
if (_selectionOverlay == null) {
return;
}
if (_selectionOverlay!.magnifierIsVisible) {
_selectionOverlay!.updateMagnifier(positionToShow);
} else {
_selectionOverlay!.showMagnifier(positionToShow);
}
}
/// Hides the magnifier if it is visible.
void hideMagnifier() {
if (_selectionOverlay == null) {
return;
}
if (_selectionOverlay!.magnifierIsVisible) {
_selectionOverlay!.hideMagnifier();
}
}
// Tracks the location a [_ScribblePlaceholder] should be rendered in the
// text.
//
// A value of -1 indicates there should be no placeholder, otherwise the
// value should be between 0 and the length of the text, inclusive.
int _placeholderLocation = -1;
@override
void insertTextPlaceholder(Size size) {
if (!widget.scribbleEnabled) {
return;
}
if (!widget.controller.selection.isValid) {
return;
}
setState(() {
_placeholderLocation = _value.text.length - widget.controller.selection.end;
});
}
@override
void removeTextPlaceholder() {
if (!widget.scribbleEnabled || _placeholderLocation == -1) {
return;
}
setState(() {
_placeholderLocation = -1;
});
}
@override
void performSelector(String selectorName) {
final Intent? intent = intentForMacOSSelector(selectorName);
if (intent != null) {
final BuildContext? primaryContext = primaryFocus?.context;
if (primaryContext != null) {
Actions.invoke(primaryContext, intent);
}
}
}
@override
String get autofillId => 'EditableText-$hashCode';
@override
TextInputConfiguration get textInputConfiguration {
final List<String>? autofillHints = widget.autofillHints?.toList(growable: false);
final AutofillConfiguration autofillConfiguration = autofillHints != null
? AutofillConfiguration(
uniqueIdentifier: autofillId,
autofillHints: autofillHints,
currentEditingValue: currentTextEditingValue,
)
: AutofillConfiguration.disabled;
return TextInputConfiguration(
inputType: widget.keyboardType,
readOnly: widget.readOnly,
obscureText: widget.obscureText,
autocorrect: widget.autocorrect,
smartDashesType: widget.smartDashesType,
smartQuotesType: widget.smartQuotesType,
enableSuggestions: widget.enableSuggestions,
enableInteractiveSelection: widget._userSelectionEnabled,
inputAction: widget.textInputAction ?? (widget.keyboardType == TextInputType.multiline
? TextInputAction.newline
: TextInputAction.done
),
textCapitalization: widget.textCapitalization,
keyboardAppearance: widget.keyboardAppearance,
autofillConfiguration: autofillConfiguration,
enableIMEPersonalizedLearning: widget.enableIMEPersonalizedLearning,
allowedMimeTypes: widget.contentInsertionConfiguration == null
? const <String>[]
: widget.contentInsertionConfiguration!.allowedMimeTypes,
);
}
@override
void autofill(TextEditingValue value) => updateEditingValue(value);
// null if no promptRect should be shown.
TextRange? _currentPromptRectRange;
@override
void showAutocorrectionPromptRect(int start, int end) {
setState(() {
_currentPromptRectRange = TextRange(start: start, end: end);
});
}
VoidCallback? _semanticsOnCopy(TextSelectionControls? controls) {
return widget.selectionEnabled
&& _hasFocus
&& (widget.selectionControls is TextSelectionHandleControls
? copyEnabled
: copyEnabled && (widget.selectionControls?.canCopy(this) ?? false))
? () {
controls?.handleCopy(this);
copySelection(SelectionChangedCause.toolbar);
}
: null;
}
VoidCallback? _semanticsOnCut(TextSelectionControls? controls) {
return widget.selectionEnabled
&& _hasFocus
&& (widget.selectionControls is TextSelectionHandleControls
? cutEnabled
: cutEnabled && (widget.selectionControls?.canCut(this) ?? false))
? () {
controls?.handleCut(this);
cutSelection(SelectionChangedCause.toolbar);
}
: null;
}
VoidCallback? _semanticsOnPaste(TextSelectionControls? controls) {
return widget.selectionEnabled
&& _hasFocus
&& (widget.selectionControls is TextSelectionHandleControls
? pasteEnabled
: pasteEnabled && (widget.selectionControls?.canPaste(this) ?? false))
&& (clipboardStatus.value == ClipboardStatus.pasteable)
? () {
controls?.handlePaste(this);
pasteText(SelectionChangedCause.toolbar);
}
: null;
}
// Returns the closest boundary location to `extent` but not including `extent`
// itself (unless already at the start/end of the text), in the direction
// specified by `forward`.
TextPosition _moveBeyondTextBoundary(TextPosition extent, bool forward, TextBoundary textBoundary) {
assert(extent.offset >= 0);
final int newOffset = forward
? textBoundary.getTrailingTextBoundaryAt(extent.offset) ?? _value.text.length
// if x is a boundary defined by `textBoundary`, most textBoundaries (except
// LineBreaker) guarantees `x == textBoundary.getLeadingTextBoundaryAt(x)`.
// Use x - 1 here to make sure we don't get stuck at the fixed point x.
: textBoundary.getLeadingTextBoundaryAt(extent.offset - 1) ?? 0;
return TextPosition(offset: newOffset);
}
// Returns the closest boundary location to `extent`, including `extent`
// itself, in the direction specified by `forward`.
//
// This method returns a fixed point of itself: applying `_toTextBoundary`
// again on the returned TextPosition gives the same TextPosition. It's used
// exclusively for handling line boundaries, since performing "move to line
// start" more than once usually doesn't move you to the previous line.
TextPosition _moveToTextBoundary(TextPosition extent, bool forward, TextBoundary textBoundary) {
assert(extent.offset >= 0);
final int caretOffset;
switch (extent.affinity) {
case TextAffinity.upstream:
if (extent.offset < 1 && !forward) {
assert (extent.offset == 0);
return const TextPosition(offset: 0);
}
// When the text affinity is upstream, the caret is associated with the
// grapheme before the code unit at `extent.offset`.
// TODO(LongCatIsLooong): don't assume extent.offset is at a grapheme
// boundary, and do this instead:
// final int graphemeStart = CharacterRange.at(string, extent.offset).stringBeforeLength - 1;
caretOffset = math.max(0, extent.offset - 1);
case TextAffinity.downstream:
caretOffset = extent.offset;
}
// The line boundary range does not include some control characters
// (most notably, Line Feed), in which case there's
// `x ∉ getTextBoundaryAt(x)`. In case `caretOffset` points to one such
// control character, we define that these control characters themselves are
// still part of the previous line, but also exclude them from the
// line boundary range since they're non-printing. IOW, no additional
// processing needed since the LineBoundary class does exactly that.
return forward
? TextPosition(offset: textBoundary.getTrailingTextBoundaryAt(caretOffset) ?? _value.text.length, affinity: TextAffinity.upstream)
: TextPosition(offset: textBoundary.getLeadingTextBoundaryAt(caretOffset) ?? 0);
}
// --------------------------- Text Editing Actions ---------------------------
TextBoundary _characterBoundary() => widget.obscureText ? _CodePointBoundary(_value.text) : CharacterBoundary(_value.text);
TextBoundary _nextWordBoundary() => widget.obscureText ? _documentBoundary() : renderEditable.wordBoundaries.moveByWordBoundary;
TextBoundary _linebreak() => widget.obscureText ? _documentBoundary() : LineBoundary(renderEditable);
TextBoundary _paragraphBoundary() => ParagraphBoundary(_value.text);
TextBoundary _documentBoundary() => DocumentBoundary(_value.text);
Action<T> _makeOverridable<T extends Intent>(Action<T> defaultAction) {
return Action<T>.overridable(context: context, defaultAction: defaultAction);
}
/// Transpose the characters immediately before and after the current
/// collapsed selection.
///
/// When the cursor is at the end of the text, transposes the last two
/// characters, if they exist.
///
/// When the cursor is at the start of the text, does nothing.
void _transposeCharacters(TransposeCharactersIntent intent) {
if (_value.text.characters.length <= 1
|| !_value.selection.isCollapsed
|| _value.selection.baseOffset == 0) {
return;
}
final String text = _value.text;
final TextSelection selection = _value.selection;
final bool atEnd = selection.baseOffset == text.length;
final CharacterRange transposing = CharacterRange.at(text, selection.baseOffset);
if (atEnd) {
transposing.moveBack(2);
} else {
transposing..moveBack()..expandNext();
}
assert(transposing.currentCharacters.length == 2);
userUpdateTextEditingValue(
TextEditingValue(
text: transposing.stringBefore
+ transposing.currentCharacters.last
+ transposing.currentCharacters.first
+ transposing.stringAfter,
selection: TextSelection.collapsed(
offset: transposing.stringBeforeLength + transposing.current.length,
),
),
SelectionChangedCause.keyboard,
);
}
late final Action<TransposeCharactersIntent> _transposeCharactersAction = CallbackAction<TransposeCharactersIntent>(onInvoke: _transposeCharacters);
void _replaceText(ReplaceTextIntent intent) {
final TextEditingValue oldValue = _value;
final TextEditingValue newValue = intent.currentTextEditingValue.replaced(
intent.replacementRange,
intent.replacementText,
);
userUpdateTextEditingValue(newValue, intent.cause);
// If there's no change in text and selection (e.g. when selecting and
// pasting identical text), the widget won't be rebuilt on value update.
// Handle this by calling _didChangeTextEditingValue() so caret and scroll
// updates can happen.
if (newValue == oldValue) {
_didChangeTextEditingValue();
}
}
late final Action<ReplaceTextIntent> _replaceTextAction = CallbackAction<ReplaceTextIntent>(onInvoke: _replaceText);
// Scrolls either to the beginning or end of the document depending on the
// intent's `forward` parameter.
void _scrollToDocumentBoundary(ScrollToDocumentBoundaryIntent intent) {
if (intent.forward) {
bringIntoView(TextPosition(offset: _value.text.length));
} else {
bringIntoView(const TextPosition(offset: 0));
}
}
/// Handles [ScrollIntent] by scrolling the [Scrollable] inside of
/// [EditableText].
void _scroll(ScrollIntent intent) {
if (intent.type != ScrollIncrementType.page) {
return;
}
final ScrollPosition position = _scrollController.position;
if (widget.maxLines == 1) {
_scrollController.jumpTo(position.maxScrollExtent);
return;
}
// If the field isn't scrollable, do nothing. For example, when the lines of
// text is less than maxLines, the field has nothing to scroll.
if (position.maxScrollExtent == 0.0 && position.minScrollExtent == 0.0) {
return;
}
final ScrollableState? state = _scrollableKey.currentState as ScrollableState?;
final double increment = ScrollAction.getDirectionalIncrement(state!, intent);
final double destination = clampDouble(
position.pixels + increment,
position.minScrollExtent,
position.maxScrollExtent,
);
if (destination == position.pixels) {
return;
}
_scrollController.jumpTo(destination);
}
/// Extend the selection down by page if the `forward` parameter is true, or
/// up by page otherwise.
void _extendSelectionByPage(ExtendSelectionByPageIntent intent) {
if (widget.maxLines == 1) {
return;
}
final TextSelection nextSelection;
final Rect extentRect = renderEditable.getLocalRectForCaret(
_value.selection.extent,
);
final ScrollableState? state = _scrollableKey.currentState as ScrollableState?;
final double increment = ScrollAction.getDirectionalIncrement(
state!,
ScrollIntent(
direction: intent.forward ? AxisDirection.down : AxisDirection.up,
type: ScrollIncrementType.page,
),
);
final ScrollPosition position = _scrollController.position;
if (intent.forward) {
if (_value.selection.extentOffset >= _value.text.length) {
return;
}
final Offset nextExtentOffset =
Offset(extentRect.left, extentRect.top + increment);
final double height = position.maxScrollExtent + renderEditable.size.height;
final TextPosition nextExtent = nextExtentOffset.dy + position.pixels >= height
? TextPosition(offset: _value.text.length)
: renderEditable.getPositionForPoint(
renderEditable.localToGlobal(nextExtentOffset),
);
nextSelection = _value.selection.copyWith(
extentOffset: nextExtent.offset,
);
} else {
if (_value.selection.extentOffset <= 0) {
return;
}
final Offset nextExtentOffset =
Offset(extentRect.left, extentRect.top + increment);
final TextPosition nextExtent = nextExtentOffset.dy + position.pixels <= 0
? const TextPosition(offset: 0)
: renderEditable.getPositionForPoint(
renderEditable.localToGlobal(nextExtentOffset),
);
nextSelection = _value.selection.copyWith(
extentOffset: nextExtent.offset,
);
}
bringIntoView(nextSelection.extent);
userUpdateTextEditingValue(
_value.copyWith(selection: nextSelection),
SelectionChangedCause.keyboard,
);
}
void _updateSelection(UpdateSelectionIntent intent) {
assert(
intent.newSelection.start <= intent.currentTextEditingValue.text.length,
'invalid selection: ${intent.newSelection}: it must not exceed the current text length ${intent.currentTextEditingValue.text.length}',
);
assert(
intent.newSelection.end <= intent.currentTextEditingValue.text.length,
'invalid selection: ${intent.newSelection}: it must not exceed the current text length ${intent.currentTextEditingValue.text.length}',
);
bringIntoView(intent.newSelection.extent);
userUpdateTextEditingValue(
intent.currentTextEditingValue.copyWith(selection: intent.newSelection),
intent.cause,
);
}
late final Action<UpdateSelectionIntent> _updateSelectionAction = CallbackAction<UpdateSelectionIntent>(onInvoke: _updateSelection);
late final _UpdateTextSelectionVerticallyAction<DirectionalCaretMovementIntent> _verticalSelectionUpdateAction =
_UpdateTextSelectionVerticallyAction<DirectionalCaretMovementIntent>(this);
Object? _hideToolbarIfVisible(DismissIntent intent) {
if (_selectionOverlay?.toolbarIsVisible ?? false) {
hideToolbar(false);
return null;
}
return Actions.invoke(context, intent);
}
/// The default behavior used if [onTapOutside] is null.
///
/// The `event` argument is the [PointerDownEvent] that caused the notification.
void _defaultOnTapOutside(PointerDownEvent event) {
/// The focus dropping behavior is only present on desktop platforms
/// and mobile browsers.
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.fuchsia:
// On mobile platforms, we don't unfocus on touch events unless they're
// in the web browser, but we do unfocus for all other kinds of events.
switch (event.kind) {
case ui.PointerDeviceKind.touch:
if (kIsWeb) {
widget.focusNode.unfocus();
}
case ui.PointerDeviceKind.mouse:
case ui.PointerDeviceKind.stylus:
case ui.PointerDeviceKind.invertedStylus:
case ui.PointerDeviceKind.unknown:
widget.focusNode.unfocus();
case ui.PointerDeviceKind.trackpad:
throw UnimplementedError('Unexpected pointer down event for trackpad');
}
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
widget.focusNode.unfocus();
}
}
late final Map<Type, Action<Intent>> _actions = <Type, Action<Intent>>{
DoNothingAndStopPropagationTextIntent: DoNothingAction(consumesKey: false),
ReplaceTextIntent: _replaceTextAction,
UpdateSelectionIntent: _updateSelectionAction,
DirectionalFocusIntent: DirectionalFocusAction.forTextField(),
DismissIntent: CallbackAction<DismissIntent>(onInvoke: _hideToolbarIfVisible),
// Delete
DeleteCharacterIntent: _makeOverridable(_DeleteTextAction<DeleteCharacterIntent>(this, _characterBoundary, _moveBeyondTextBoundary)),
DeleteToNextWordBoundaryIntent: _makeOverridable(_DeleteTextAction<DeleteToNextWordBoundaryIntent>(this, _nextWordBoundary, _moveBeyondTextBoundary)),
DeleteToLineBreakIntent: _makeOverridable(_DeleteTextAction<DeleteToLineBreakIntent>(this, _linebreak, _moveToTextBoundary)),
// Extend/Move Selection
ExtendSelectionByCharacterIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionByCharacterIntent>(this, _characterBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: false)),
ExtendSelectionByPageIntent: _makeOverridable(CallbackAction<ExtendSelectionByPageIntent>(onInvoke: _extendSelectionByPage)),
ExtendSelectionToNextWordBoundaryIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToNextWordBoundaryIntent>(this, _nextWordBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: true)),
ExtendSelectionToNextParagraphBoundaryIntent : _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToNextParagraphBoundaryIntent>(this, _paragraphBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: true)),
ExtendSelectionToLineBreakIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToLineBreakIntent>(this, _linebreak, _moveToTextBoundary, ignoreNonCollapsedSelection: true)),
ExtendSelectionVerticallyToAdjacentLineIntent: _makeOverridable(_verticalSelectionUpdateAction),
ExtendSelectionVerticallyToAdjacentPageIntent: _makeOverridable(_verticalSelectionUpdateAction),
ExtendSelectionToNextParagraphBoundaryOrCaretLocationIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToNextParagraphBoundaryOrCaretLocationIntent>(this, _paragraphBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: true)),
ExtendSelectionToDocumentBoundaryIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToDocumentBoundaryIntent>(this, _documentBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: true)),
ExtendSelectionToNextWordBoundaryOrCaretLocationIntent: _makeOverridable(_UpdateTextSelectionAction<ExtendSelectionToNextWordBoundaryOrCaretLocationIntent>(this, _nextWordBoundary, _moveBeyondTextBoundary, ignoreNonCollapsedSelection: true)),
ScrollToDocumentBoundaryIntent: _makeOverridable(CallbackAction<ScrollToDocumentBoundaryIntent>(onInvoke: _scrollToDocumentBoundary)),
ScrollIntent: CallbackAction<ScrollIntent>(onInvoke: _scroll),
// Expand Selection
ExpandSelectionToLineBreakIntent: _makeOverridable(_UpdateTextSelectionAction<ExpandSelectionToLineBreakIntent>(this, _linebreak, _moveToTextBoundary, ignoreNonCollapsedSelection: true, isExpand: true)),
ExpandSelectionToDocumentBoundaryIntent: _makeOverridable(_UpdateTextSelectionAction<ExpandSelectionToDocumentBoundaryIntent>(this, _documentBoundary, _moveToTextBoundary, ignoreNonCollapsedSelection: true, isExpand: true, extentAtIndex: true)),
// Copy Paste
SelectAllTextIntent: _makeOverridable(_SelectAllAction(this)),
CopySelectionTextIntent: _makeOverridable(_CopySelectionAction(this)),
PasteTextIntent: _makeOverridable(CallbackAction<PasteTextIntent>(onInvoke: (PasteTextIntent intent) => pasteText(intent.cause))),
TransposeCharactersIntent: _makeOverridable(_transposeCharactersAction),
};
@override
Widget build(BuildContext context) {
assert(debugCheckHasMediaQuery(context));
super.build(context); // See AutomaticKeepAliveClientMixin.
final TextSelectionControls? controls = widget.selectionControls;
final TextScaler effectiveTextScaler = switch ((widget.textScaler, widget.textScaleFactor)) {
(final TextScaler textScaler, _) => textScaler,
(null, final double textScaleFactor) => TextScaler.linear(textScaleFactor),
(null, null) => MediaQuery.textScalerOf(context),
};
return _CompositionCallback(
compositeCallback: _compositeCallback,
enabled: _hasInputConnection,
child: TextFieldTapRegion(
onTapOutside: _hasFocus ? widget.onTapOutside ?? _defaultOnTapOutside : null,
debugLabel: kReleaseMode ? null : 'EditableText',
child: MouseRegion(
cursor: widget.mouseCursor ?? SystemMouseCursors.text,
child: Actions(
actions: _actions,
child: UndoHistory<TextEditingValue>(
value: widget.controller,
onTriggered: (TextEditingValue value) {
userUpdateTextEditingValue(value, SelectionChangedCause.keyboard);
},
shouldChangeUndoStack: (TextEditingValue? oldValue, TextEditingValue newValue) {
if (!newValue.selection.isValid) {
return false;
}
if (oldValue == null) {
return true;
}
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
// Composing text is not counted in history coalescing.
if (!widget.controller.value.composing.isCollapsed) {
return false;
}
case TargetPlatform.android:
// Gboard on Android puts non-CJK words in composing regions. Coalesce
// composing text in order to allow the saving of partial words in that
// case.
break;
}
return oldValue.text != newValue.text || oldValue.composing != newValue.composing;
},
undoStackModifier: (TextEditingValue value) {
// On Android we should discard the composing region when pushing
// a new entry to the undo stack. This prevents the TextInputPlugin
// from restarting the input on every undo/redo when the composing
// region is changed by the framework.
return defaultTargetPlatform == TargetPlatform.android ? value.copyWith(composing: TextRange.empty) : value;
},
focusNode: widget.focusNode,
controller: widget.undoController,
child: Focus(
focusNode: widget.focusNode,
includeSemantics: false,
debugLabel: kReleaseMode ? null : 'EditableText',
child: NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
_handleContextMenuOnScroll(notification);
_scribbleCacheKey = null;
return false;
},
child: Scrollable(
key: _scrollableKey,
excludeFromSemantics: true,
axisDirection: _isMultiline ? AxisDirection.down : AxisDirection.right,
controller: _scrollController,
physics: widget.scrollPhysics,
dragStartBehavior: widget.dragStartBehavior,
restorationId: widget.restorationId,
// If a ScrollBehavior is not provided, only apply scrollbars when
// multiline. The overscroll indicator should not be applied in
// either case, glowing or stretching.
scrollBehavior: widget.scrollBehavior ?? ScrollConfiguration.of(context).copyWith(
scrollbars: _isMultiline,
overscroll: false,
),
viewportBuilder: (BuildContext context, ViewportOffset offset) {
return CompositedTransformTarget(
link: _toolbarLayerLink,
child: Semantics(
onCopy: _semanticsOnCopy(controls),
onCut: _semanticsOnCut(controls),
onPaste: _semanticsOnPaste(controls),
child: _ScribbleFocusable(
focusNode: widget.focusNode,
editableKey: _editableKey,
enabled: widget.scribbleEnabled,
updateSelectionRects: () {
_openInputConnection();
_updateSelectionRects(force: true);
},
child: SizeChangedLayoutNotifier(
child: _Editable(
key: _editableKey,
startHandleLayerLink: _startHandleLayerLink,
endHandleLayerLink: _endHandleLayerLink,
inlineSpan: buildTextSpan(),
value: _value,
cursorColor: _cursorColor,
backgroundCursorColor: widget.backgroundCursorColor,
showCursor: _cursorVisibilityNotifier,
forceLine: widget.forceLine,
readOnly: widget.readOnly,
hasFocus: _hasFocus,
maxLines: widget.maxLines,
minLines: widget.minLines,
expands: widget.expands,
strutStyle: widget.strutStyle,
selectionColor: _selectionOverlay?.spellCheckToolbarIsVisible ?? false
? _spellCheckConfiguration.misspelledSelectionColor ?? widget.selectionColor
: widget.selectionColor,
textScaler: effectiveTextScaler,
textAlign: widget.textAlign,
textDirection: _textDirection,
locale: widget.locale,
textHeightBehavior: widget.textHeightBehavior ?? DefaultTextHeightBehavior.maybeOf(context),
textWidthBasis: widget.textWidthBasis,
obscuringCharacter: widget.obscuringCharacter,
obscureText: widget.obscureText,
offset: offset,
rendererIgnoresPointer: widget.rendererIgnoresPointer,
cursorWidth: widget.cursorWidth,
cursorHeight: widget.cursorHeight,
cursorRadius: widget.cursorRadius,
cursorOffset: widget.cursorOffset ?? Offset.zero,
selectionHeightStyle: widget.selectionHeightStyle,
selectionWidthStyle: widget.selectionWidthStyle,
paintCursorAboveText: widget.paintCursorAboveText,
enableInteractiveSelection: widget._userSelectionEnabled,
textSelectionDelegate: this,
devicePixelRatio: _devicePixelRatio,
promptRectRange: _currentPromptRectRange,
promptRectColor: widget.autocorrectionTextRectColor,
clipBehavior: widget.clipBehavior,
),
),
),
),
);
},
),
),
),
),
),
),
),
);
}
/// Builds [TextSpan] from current editing value.
///
/// By default makes text in composing range appear as underlined.
/// Descendants can override this method to customize appearance of text.
TextSpan buildTextSpan() {
if (widget.obscureText) {
String text = _value.text;
text = widget.obscuringCharacter * text.length;
// Reveal the latest character in an obscured field only on mobile.
// Newer versions of iOS (iOS 15+) no longer reveal the most recently
// entered character.
const Set<TargetPlatform> mobilePlatforms = <TargetPlatform> {
TargetPlatform.android, TargetPlatform.fuchsia,
};
final bool brieflyShowPassword = WidgetsBinding.instance.platformDispatcher.brieflyShowPassword
&& mobilePlatforms.contains(defaultTargetPlatform);
if (brieflyShowPassword) {
final int? o = _obscureShowCharTicksPending > 0 ? _obscureLatestCharIndex : null;
if (o != null && o >= 0 && o < text.length) {
text = text.replaceRange(o, o + 1, _value.text.substring(o, o + 1));
}
}
return TextSpan(style: _style, text: text);
}
if (_placeholderLocation >= 0 && _placeholderLocation <= _value.text.length) {
final List<_ScribblePlaceholder> placeholders = <_ScribblePlaceholder>[];
final int placeholderLocation = _value.text.length - _placeholderLocation;
if (_isMultiline) {
// The zero size placeholder here allows the line to break and keep the caret on the first line.
placeholders.add(const _ScribblePlaceholder(child: SizedBox.shrink(), size: Size.zero));
placeholders.add(_ScribblePlaceholder(child: const SizedBox.shrink(), size: Size(renderEditable.size.width, 0.0)));
} else {
placeholders.add(const _ScribblePlaceholder(child: SizedBox.shrink(), size: Size(100.0, 0.0)));
}
return TextSpan(style: _style, children: <InlineSpan>[
TextSpan(text: _value.text.substring(0, placeholderLocation)),
...placeholders,
TextSpan(text: _value.text.substring(placeholderLocation)),
],
);
}
final bool withComposing = !widget.readOnly && _hasFocus;
if (_spellCheckResultsReceived) {
// If the composing range is out of range for the current text, ignore it to
// preserve the tree integrity, otherwise in release mode a RangeError will
// be thrown and this EditableText will be built with a broken subtree.
assert(!_value.composing.isValid || !withComposing || _value.isComposingRangeValid);
final bool composingRegionOutOfRange = !_value.isComposingRangeValid || !withComposing;
return buildTextSpanWithSpellCheckSuggestions(
_value,
composingRegionOutOfRange,
_style,
_spellCheckConfiguration.misspelledTextStyle!,
spellCheckResults!,
);
}
// Read only mode should not paint text composing.
return widget.controller.buildTextSpan(
context: context,
style: _style,
withComposing: withComposing,
);
}
}
class _Editable extends MultiChildRenderObjectWidget {
_Editable({
super.key,
required this.inlineSpan,
required this.value,
required this.startHandleLayerLink,
required this.endHandleLayerLink,
this.cursorColor,
this.backgroundCursorColor,
required this.showCursor,
required this.forceLine,
required this.readOnly,
this.textHeightBehavior,
required this.textWidthBasis,
required this.hasFocus,
required this.maxLines,
this.minLines,
required this.expands,
this.strutStyle,
this.selectionColor,
required this.textScaler,
required this.textAlign,
required this.textDirection,
this.locale,
required this.obscuringCharacter,
required this.obscureText,
required this.offset,
this.rendererIgnoresPointer = false,
required this.cursorWidth,
this.cursorHeight,
this.cursorRadius,
required this.cursorOffset,
required this.paintCursorAboveText,
this.selectionHeightStyle = ui.BoxHeightStyle.tight,
this.selectionWidthStyle = ui.BoxWidthStyle.tight,
this.enableInteractiveSelection = true,
required this.textSelectionDelegate,
required this.devicePixelRatio,
this.promptRectRange,
this.promptRectColor,
required this.clipBehavior,
}) : super(children: WidgetSpan.extractFromInlineSpan(inlineSpan, textScaler));
final InlineSpan inlineSpan;
final TextEditingValue value;
final Color? cursorColor;
final LayerLink startHandleLayerLink;
final LayerLink endHandleLayerLink;
final Color? backgroundCursorColor;
final ValueNotifier<bool> showCursor;
final bool forceLine;
final bool readOnly;
final bool hasFocus;
final int? maxLines;
final int? minLines;
final bool expands;
final StrutStyle? strutStyle;
final Color? selectionColor;
final TextScaler textScaler;
final TextAlign textAlign;
final TextDirection textDirection;
final Locale? locale;
final String obscuringCharacter;
final bool obscureText;
final TextHeightBehavior? textHeightBehavior;
final TextWidthBasis textWidthBasis;
final ViewportOffset offset;
final bool rendererIgnoresPointer;
final double cursorWidth;
final double? cursorHeight;
final Radius? cursorRadius;
final Offset cursorOffset;
final bool paintCursorAboveText;
final ui.BoxHeightStyle selectionHeightStyle;
final ui.BoxWidthStyle selectionWidthStyle;
final bool enableInteractiveSelection;
final TextSelectionDelegate textSelectionDelegate;
final double devicePixelRatio;
final TextRange? promptRectRange;
final Color? promptRectColor;
final Clip clipBehavior;
@override
RenderEditable createRenderObject(BuildContext context) {
return RenderEditable(
text: inlineSpan,
cursorColor: cursorColor,
startHandleLayerLink: startHandleLayerLink,
endHandleLayerLink: endHandleLayerLink,
backgroundCursorColor: backgroundCursorColor,
showCursor: showCursor,
forceLine: forceLine,
readOnly: readOnly,
hasFocus: hasFocus,
maxLines: maxLines,
minLines: minLines,
expands: expands,
strutStyle: strutStyle,
selectionColor: selectionColor,
textScaler: textScaler,
textAlign: textAlign,
textDirection: textDirection,
locale: locale ?? Localizations.maybeLocaleOf(context),
selection: value.selection,
offset: offset,
ignorePointer: rendererIgnoresPointer,
obscuringCharacter: obscuringCharacter,
obscureText: obscureText,
textHeightBehavior: textHeightBehavior,
textWidthBasis: textWidthBasis,
cursorWidth: cursorWidth,
cursorHeight: cursorHeight,
cursorRadius: cursorRadius,
cursorOffset: cursorOffset,
paintCursorAboveText: paintCursorAboveText,
selectionHeightStyle: selectionHeightStyle,
selectionWidthStyle: selectionWidthStyle,
enableInteractiveSelection: enableInteractiveSelection,
textSelectionDelegate: textSelectionDelegate,
devicePixelRatio: devicePixelRatio,
promptRectRange: promptRectRange,
promptRectColor: promptRectColor,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderEditable renderObject) {
renderObject
..text = inlineSpan
..cursorColor = cursorColor
..startHandleLayerLink = startHandleLayerLink
..endHandleLayerLink = endHandleLayerLink
..backgroundCursorColor = backgroundCursorColor
..showCursor = showCursor
..forceLine = forceLine
..readOnly = readOnly
..hasFocus = hasFocus
..maxLines = maxLines
..minLines = minLines
..expands = expands
..strutStyle = strutStyle
..selectionColor = selectionColor
..textScaler = textScaler
..textAlign = textAlign
..textDirection = textDirection
..locale = locale ?? Localizations.maybeLocaleOf(context)
..selection = value.selection
..offset = offset
..ignorePointer = rendererIgnoresPointer
..textHeightBehavior = textHeightBehavior
..textWidthBasis = textWidthBasis
..obscuringCharacter = obscuringCharacter
..obscureText = obscureText
..cursorWidth = cursorWidth
..cursorHeight = cursorHeight
..cursorRadius = cursorRadius
..cursorOffset = cursorOffset
..selectionHeightStyle = selectionHeightStyle
..selectionWidthStyle = selectionWidthStyle
..enableInteractiveSelection = enableInteractiveSelection
..textSelectionDelegate = textSelectionDelegate
..devicePixelRatio = devicePixelRatio
..paintCursorAboveText = paintCursorAboveText
..promptRectColor = promptRectColor
..clipBehavior = clipBehavior
..setPromptRectRange(promptRectRange);
}
}
@immutable
class _ScribbleCacheKey {
const _ScribbleCacheKey({
required this.inlineSpan,
required this.textAlign,
required this.textDirection,
required this.textScaler,
required this.textHeightBehavior,
required this.locale,
required this.structStyle,
required this.placeholder,
required this.size,
});
final TextAlign textAlign;
final TextDirection textDirection;
final TextScaler textScaler;
final TextHeightBehavior? textHeightBehavior;
final Locale? locale;
final StrutStyle structStyle;
final int placeholder;
final Size size;
final InlineSpan inlineSpan;
RenderComparison compare(_ScribbleCacheKey other) {
if (identical(other, this)) {
return RenderComparison.identical;
}
final bool needsLayout = textAlign != other.textAlign
|| textDirection != other.textDirection
|| textScaler != other.textScaler
|| (textHeightBehavior ?? const TextHeightBehavior()) != (other.textHeightBehavior ?? const TextHeightBehavior())
|| locale != other.locale
|| structStyle != other.structStyle
|| placeholder != other.placeholder
|| size != other.size;
return needsLayout ? RenderComparison.layout : inlineSpan.compareTo(other.inlineSpan);
}
}
class _ScribbleFocusable extends StatefulWidget {
const _ScribbleFocusable({
required this.child,
required this.focusNode,
required this.editableKey,
required this.updateSelectionRects,
required this.enabled,
});
final Widget child;
final FocusNode focusNode;
final GlobalKey editableKey;
final VoidCallback updateSelectionRects;
final bool enabled;
@override
_ScribbleFocusableState createState() => _ScribbleFocusableState();
}
class _ScribbleFocusableState extends State<_ScribbleFocusable> implements ScribbleClient {
_ScribbleFocusableState(): _elementIdentifier = (_nextElementIdentifier++).toString();
@override
void initState() {
super.initState();
if (widget.enabled) {
TextInput.registerScribbleElement(elementIdentifier, this);
}
}
@override
void didUpdateWidget(_ScribbleFocusable oldWidget) {
super.didUpdateWidget(oldWidget);
if (!oldWidget.enabled && widget.enabled) {
TextInput.registerScribbleElement(elementIdentifier, this);
}
if (oldWidget.enabled && !widget.enabled) {
TextInput.unregisterScribbleElement(elementIdentifier);
}
}
@override
void dispose() {
TextInput.unregisterScribbleElement(elementIdentifier);
super.dispose();
}
RenderEditable? get renderEditable => widget.editableKey.currentContext?.findRenderObject() as RenderEditable?;
static int _nextElementIdentifier = 1;
final String _elementIdentifier;
@override
String get elementIdentifier => _elementIdentifier;
@override
void onScribbleFocus(Offset offset) {
widget.focusNode.requestFocus();
renderEditable?.selectPositionAt(from: offset, cause: SelectionChangedCause.scribble);
widget.updateSelectionRects();
}
@override
bool isInScribbleRect(Rect rect) {
final Rect calculatedBounds = bounds;
if (renderEditable?.readOnly ?? false) {
return false;
}
if (calculatedBounds == Rect.zero) {
return false;
}
if (!calculatedBounds.overlaps(rect)) {
return false;
}
final Rect intersection = calculatedBounds.intersect(rect);
final HitTestResult result = HitTestResult();
WidgetsBinding.instance.hitTestInView(result, intersection.center, View.of(context).viewId);
return result.path.any((HitTestEntry entry) => entry.target == renderEditable);
}
@override
Rect get bounds {
final RenderBox? box = context.findRenderObject() as RenderBox?;
if (box == null || !mounted || !box.attached) {
return Rect.zero;
}
final Matrix4 transform = box.getTransformTo(null);
return MatrixUtils.transformRect(transform, Rect.fromLTWH(0, 0, box.size.width, box.size.height));
}
@override
Widget build(BuildContext context) {
return widget.child;
}
}
class _ScribblePlaceholder extends WidgetSpan {
const _ScribblePlaceholder({
required super.child,
required this.size,
});
/// The size of the span, used in place of adding a placeholder size to the [TextPainter].
final Size size;
@override
void build(ui.ParagraphBuilder builder, {
TextScaler textScaler = TextScaler.noScaling,
List<PlaceholderDimensions>? dimensions,
}) {
assert(debugAssertIsValid());
final bool hasStyle = style != null;
if (hasStyle) {
builder.pushStyle(style!.getTextStyle(textScaler: textScaler));
}
builder.addPlaceholder(size.width, size.height, alignment);
if (hasStyle) {
builder.pop();
}
}
}
/// A text boundary that uses code points as logical boundaries.
///
/// A code point represents a single character. This may be smaller than what is
/// represented by a user-perceived character, or grapheme. For example, a
/// single grapheme (in this case a Unicode extended grapheme cluster) like
/// "👨👩👦" consists of five code points: the man emoji, a zero
/// width joiner, the woman emoji, another zero width joiner, and the boy emoji.
/// The [String] has a length of eight because each emoji consists of two code
/// units.
///
/// Code units are the units by which Dart's String class is measured, which is
/// encoded in UTF-16.
///
/// See also:
///
/// * [String.runes], which deals with code points like this class.
/// * [String.characters], which deals with graphemes.
/// * [CharacterBoundary], which is a [TextBoundary] like this class, but whose
/// boundaries are graphemes instead of code points.
class _CodePointBoundary extends TextBoundary {
const _CodePointBoundary(this._text);
final String _text;
// Returns true if the given position falls in the center of a surrogate pair.
bool _breaksSurrogatePair(int position) {
assert(position > 0 && position < _text.length && _text.length > 1);
return TextPainter.isHighSurrogate(_text.codeUnitAt(position - 1))
&& TextPainter.isLowSurrogate(_text.codeUnitAt(position));
}
@override
int? getLeadingTextBoundaryAt(int position) {
if (_text.isEmpty || position < 0) {
return null;
}
if (position == 0) {
return 0;
}
if (position >= _text.length) {
return _text.length;
}
if (_text.length <= 1) {
return position;
}
return _breaksSurrogatePair(position)
? position - 1
: position;
}
@override
int? getTrailingTextBoundaryAt(int position) {
if (_text.isEmpty || position >= _text.length) {
return null;
}
if (position < 0) {
return 0;
}
if (position == _text.length - 1) {
return _text.length;
}
if (_text.length <= 1) {
return position;
}
return _breaksSurrogatePair(position + 1)
? position + 2
: position + 1;
}
}
// ------------------------------- Text Actions -------------------------------
class _DeleteTextAction<T extends DirectionalTextEditingIntent> extends ContextAction<T> {
_DeleteTextAction(this.state, this.getTextBoundary, this._applyTextBoundary);
final EditableTextState state;
final TextBoundary Function() getTextBoundary;
final _ApplyTextBoundary _applyTextBoundary;
@override
Object? invoke(T intent, [BuildContext? context]) {
final TextSelection selection = state._value.selection;
if (!selection.isValid) {
return null;
}
assert(selection.isValid);
// Expands the selection to ensure the range covers full graphemes.
final TextBoundary atomicBoundary = state._characterBoundary();
if (!selection.isCollapsed) {
// Expands the selection to ensure the range covers full graphemes.
final TextRange range = TextRange(
start: atomicBoundary.getLeadingTextBoundaryAt(selection.start) ?? state._value.text.length,
end: atomicBoundary.getTrailingTextBoundaryAt(selection.end - 1) ?? 0,
);
return Actions.invoke(
context!,
ReplaceTextIntent(state._value, '', range, SelectionChangedCause.keyboard),
);
}
final int target = _applyTextBoundary(selection.base, intent.forward, getTextBoundary()).offset;
final TextRange rangeToDelete = TextSelection(
baseOffset: intent.forward
? atomicBoundary.getLeadingTextBoundaryAt(selection.baseOffset) ?? state._value.text.length
: atomicBoundary.getTrailingTextBoundaryAt(selection.baseOffset - 1) ?? 0,
extentOffset: target,
);
return Actions.invoke(
context!,
ReplaceTextIntent(state._value, '', rangeToDelete, SelectionChangedCause.keyboard),
);
}
@override
bool get isActionEnabled => !state.widget.readOnly && state._value.selection.isValid;
}
class _UpdateTextSelectionAction<T extends DirectionalCaretMovementIntent> extends ContextAction<T> {
_UpdateTextSelectionAction(
this.state,
this.getTextBoundary,
this.applyTextBoundary, {
required this.ignoreNonCollapsedSelection,
this.isExpand = false,
this.extentAtIndex = false,
});
final EditableTextState state;
final bool ignoreNonCollapsedSelection;
final bool isExpand;
final bool extentAtIndex;
final TextBoundary Function() getTextBoundary;
final _ApplyTextBoundary applyTextBoundary;
static const int NEWLINE_CODE_UNIT = 10;
// Returns true iff the given position is at a wordwrap boundary in the
// upstream position.
bool _isAtWordwrapUpstream(TextPosition position) {
final TextPosition end = TextPosition(
offset: state.renderEditable.getLineAtOffset(position).end,
affinity: TextAffinity.upstream,
);
return end == position && end.offset != state.textEditingValue.text.length
&& state.textEditingValue.text.codeUnitAt(position.offset) != NEWLINE_CODE_UNIT;
}
// Returns true if the given position at a wordwrap boundary in the
// downstream position.
bool _isAtWordwrapDownstream(TextPosition position) {
final TextPosition start = TextPosition(
offset: state.renderEditable.getLineAtOffset(position).start,
);
return start == position && start.offset != 0
&& state.textEditingValue.text.codeUnitAt(position.offset - 1) != NEWLINE_CODE_UNIT;
}
@override
Object? invoke(T intent, [BuildContext? context]) {
final TextSelection selection = state._value.selection;
assert(selection.isValid);
final bool collapseSelection = intent.collapseSelection || !state.widget.selectionEnabled;
if (!selection.isCollapsed && !ignoreNonCollapsedSelection && collapseSelection) {
return Actions.invoke(context!, UpdateSelectionIntent(
state._value,
TextSelection.collapsed(offset: intent.forward ? selection.end : selection.start),
SelectionChangedCause.keyboard,
));
}
TextPosition extent = selection.extent;
// If continuesAtWrap is true extent and is at the relevant wordwrap, then
// move it just to the other side of the wordwrap.
if (intent.continuesAtWrap) {
if (intent.forward && _isAtWordwrapUpstream(extent)) {
extent = TextPosition(
offset: extent.offset,
);
} else if (!intent.forward && _isAtWordwrapDownstream(extent)) {
extent = TextPosition(
offset: extent.offset,
affinity: TextAffinity.upstream,
);
}
}
final bool shouldTargetBase = isExpand && (intent.forward ? selection.baseOffset > selection.extentOffset : selection.baseOffset < selection.extentOffset);
final TextPosition newExtent = applyTextBoundary(shouldTargetBase ? selection.base : extent, intent.forward, getTextBoundary());
final TextSelection newSelection = collapseSelection || (!isExpand && newExtent.offset == selection.baseOffset)
? TextSelection.fromPosition(newExtent)
: isExpand ? selection.expandTo(newExtent, extentAtIndex || selection.isCollapsed) : selection.extendTo(newExtent);
final bool shouldCollapseToBase = intent.collapseAtReversal
&& (selection.baseOffset - selection.extentOffset) * (selection.baseOffset - newSelection.extentOffset) < 0;
final TextSelection newRange = shouldCollapseToBase ? TextSelection.fromPosition(selection.base) : newSelection;
return Actions.invoke(context!, UpdateSelectionIntent(state._value, newRange, SelectionChangedCause.keyboard));
}
@override
bool get isActionEnabled => state._value.selection.isValid;
}
class _UpdateTextSelectionVerticallyAction<T extends DirectionalCaretMovementIntent> extends ContextAction<T> {
_UpdateTextSelectionVerticallyAction(this.state);
final EditableTextState state;
VerticalCaretMovementRun? _verticalMovementRun;
TextSelection? _runSelection;
void stopCurrentVerticalRunIfSelectionChanges() {
final TextSelection? runSelection = _runSelection;
if (runSelection == null) {
assert(_verticalMovementRun == null);
return;
}
_runSelection = state._value.selection;
final TextSelection currentSelection = state.widget.controller.selection;
final bool continueCurrentRun = currentSelection.isValid && currentSelection.isCollapsed
&& currentSelection.baseOffset == runSelection.baseOffset
&& currentSelection.extentOffset == runSelection.extentOffset;
if (!continueCurrentRun) {
_verticalMovementRun = null;
_runSelection = null;
}
}
@override
void invoke(T intent, [BuildContext? context]) {
assert(state._value.selection.isValid);
final bool collapseSelection = intent.collapseSelection || !state.widget.selectionEnabled;
final TextEditingValue value = state._textEditingValueforTextLayoutMetrics;
if (!value.selection.isValid) {
return;
}
if (_verticalMovementRun?.isValid == false) {
_verticalMovementRun = null;
_runSelection = null;
}
final VerticalCaretMovementRun currentRun = _verticalMovementRun
?? state.renderEditable.startVerticalCaretMovement(state.renderEditable.selection!.extent);
final bool shouldMove = intent is ExtendSelectionVerticallyToAdjacentPageIntent
? currentRun.moveByOffset((intent.forward ? 1.0 : -1.0) * state.renderEditable.size.height)
: intent.forward ? currentRun.moveNext() : currentRun.movePrevious();
final TextPosition newExtent = shouldMove
? currentRun.current
: intent.forward ? TextPosition(offset: value.text.length) : const TextPosition(offset: 0);
final TextSelection newSelection = collapseSelection
? TextSelection.fromPosition(newExtent)
: value.selection.extendTo(newExtent);
Actions.invoke(
context!,
UpdateSelectionIntent(value, newSelection, SelectionChangedCause.keyboard),
);
if (state._value.selection == newSelection) {
_verticalMovementRun = currentRun;
_runSelection = newSelection;
}
}
@override
bool get isActionEnabled => state._value.selection.isValid;
}
class _SelectAllAction extends ContextAction<SelectAllTextIntent> {
_SelectAllAction(this.state);
final EditableTextState state;
@override
Object? invoke(SelectAllTextIntent intent, [BuildContext? context]) {
return Actions.invoke(
context!,
UpdateSelectionIntent(
state._value,
TextSelection(baseOffset: 0, extentOffset: state._value.text.length),
intent.cause,
),
);
}
@override
bool get isActionEnabled => state.widget.selectionEnabled;
}
class _CopySelectionAction extends ContextAction<CopySelectionTextIntent> {
_CopySelectionAction(this.state);
final EditableTextState state;
@override
void invoke(CopySelectionTextIntent intent, [BuildContext? context]) {
if (intent.collapseSelection) {
state.cutSelection(intent.cause);
} else {
state.copySelection(intent.cause);
}
}
@override
bool get isActionEnabled => state._value.selection.isValid && !state._value.selection.isCollapsed;
}
/// The start and end glyph heights of some range of text.
@immutable
class _GlyphHeights {
const _GlyphHeights({
required this.start,
required this.end,
});
/// The glyph height of the first line.
final double start;
/// The glyph height of the last line.
final double end;
}
/// A [ClipboardStatusNotifier] whose [value] is hardcoded to
/// [ClipboardStatus.pasteable].
///
/// Useful to avoid showing a permission dialog on web, which happens when
/// [Clipboard.hasStrings] is called.
class _WebClipboardStatusNotifier extends ClipboardStatusNotifier {
@override
ClipboardStatus value = ClipboardStatus.pasteable;
@override
Future<void> update() {
return Future<void>.value();
}
}
| flutter/packages/flutter/lib/src/widgets/editable_text.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/editable_text.dart",
"repo_id": "flutter",
"token_count": 83866
} | 714 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'icon.dart';
import 'icon_theme.dart';
import 'icon_theme_data.dart';
import 'image.dart';
/// An icon that comes from an [ImageProvider], e.g. an [AssetImage].
///
/// See also:
///
/// * [IconButton], for interactive icons.
/// * [IconTheme], which provides ambient configuration for icons.
/// * [Icon], for icons based on glyphs from fonts instead of images.
/// * [Icons], the library of Material Icons.
class ImageIcon extends StatelessWidget {
/// Creates an image icon.
///
/// The [size] and [color] default to the value given by the current [IconTheme].
const ImageIcon(
this.image, {
super.key,
this.size,
this.color,
this.semanticLabel,
});
/// The image to display as the icon.
///
/// The icon can be null, in which case the widget will render as an empty
/// space of the specified [size].
final ImageProvider? image;
/// The size of the icon in logical pixels.
///
/// Icons occupy a square with width and height equal to size.
///
/// Defaults to the current [IconTheme] size, if any. If there is no
/// [IconTheme], or it does not specify an explicit size, then it defaults to
/// 24.0.
final double? size;
/// The color to use when drawing the icon.
///
/// Defaults to the current [IconTheme] color, if any. If there is
/// no [IconTheme], then it defaults to not recolorizing the image.
///
/// The image will be additionally adjusted by the opacity of the current
/// [IconTheme], if any.
final Color? color;
/// Semantic label for the icon.
///
/// Announced in accessibility modes (e.g TalkBack/VoiceOver).
/// This label does not show in the UI.
///
/// * [SemanticsProperties.label], which is set to [semanticLabel] in the
/// underlying [Semantics] widget.
final String? semanticLabel;
@override
Widget build(BuildContext context) {
final IconThemeData iconTheme = IconTheme.of(context);
final double? iconSize = size ?? iconTheme.size;
if (image == null) {
return Semantics(
label: semanticLabel,
child: SizedBox(width: iconSize, height: iconSize),
);
}
final double? iconOpacity = iconTheme.opacity;
Color iconColor = color ?? iconTheme.color!;
if (iconOpacity != null && iconOpacity != 1.0) {
iconColor = iconColor.withOpacity(iconColor.opacity * iconOpacity);
}
return Semantics(
label: semanticLabel,
child: Image(
image: image!,
width: iconSize,
height: iconSize,
color: iconColor,
fit: BoxFit.scaleDown,
excludeFromSemantics: true,
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ImageProvider>('image', image, ifNull: '<empty>', showName: false));
properties.add(DoubleProperty('size', size, defaultValue: null));
properties.add(ColorProperty('color', color, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/image_icon.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/image_icon.dart",
"repo_id": "flutter",
"token_count": 1066
} | 715 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'framework.dart';
import 'navigator.dart';
import 'notification_listener.dart';
import 'pop_scope.dart';
/// Enables the handling of system back gestures.
///
/// Typically wraps a nested [Navigator] widget and allows it to handle system
/// back gestures in the [onPop] callback.
///
/// {@tool dartpad}
/// This sample demonstrates how to use this widget to properly handle system
/// back gestures when using nested [Navigator]s.
///
/// ** See code in examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample demonstrates how to use this widget to properly handle system
/// back gestures with a bottom navigation bar whose tabs each have their own
/// nested [Navigator]s.
///
/// ** See code in examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [PopScope], which allows toggling the ability of a [Navigator] to
/// handle pops.
/// * [NavigationNotification], which indicates whether a [Navigator] in a
/// subtree can handle pops.
class NavigatorPopHandler extends StatefulWidget {
/// Creates an instance of [NavigatorPopHandler].
const NavigatorPopHandler({
super.key,
this.onPop,
this.enabled = true,
required this.child,
});
/// The widget to place below this in the widget tree.
///
/// Typically this is a [Navigator] that will handle the pop when [onPop] is
/// called.
final Widget child;
/// Whether this widget's ability to handle system back gestures is enabled or
/// disabled.
///
/// When false, there will be no effect on system back gestures. If provided,
/// [onPop] will still be called.
///
/// This can be used, for example, when the nested [Navigator] is no longer
/// active but remains in the widget tree, such as in an inactive tab.
///
/// Defaults to true.
final bool enabled;
/// Called when a handleable pop event happens.
///
/// For example, a pop is handleable when a [Navigator] in [child] has
/// multiple routes on its stack. It's not handleable when it has only a
/// single route, and so [onPop] will not be called.
///
/// Typically this is used to pop the [Navigator] in [child]. See the sample
/// code on [NavigatorPopHandler] for a full example of this.
final VoidCallback? onPop;
@override
State<NavigatorPopHandler> createState() => _NavigatorPopHandlerState();
}
class _NavigatorPopHandlerState extends State<NavigatorPopHandler> {
bool _canPop = true;
@override
Widget build(BuildContext context) {
// When the widget subtree indicates it can handle a pop, disable popping
// here, so that it can be manually handled in canPop.
return PopScope(
canPop: !widget.enabled || _canPop,
onPopInvoked: (bool didPop) {
if (didPop) {
return;
}
widget.onPop?.call();
},
// Listen to changes in the navigation stack in the widget subtree.
child: NotificationListener<NavigationNotification>(
onNotification: (NavigationNotification notification) {
// If this subtree cannot handle pop, then set canPop to true so
// that our PopScope will allow the Navigator higher in the tree to
// handle the pop instead.
final bool nextCanPop = !notification.canHandlePop;
if (nextCanPop != _canPop) {
setState(() {
_canPop = nextCanPop;
});
}
return false;
},
child: widget.child,
),
);
}
}
| flutter/packages/flutter/lib/src/widgets/navigator_pop_handler.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/navigator_pop_handler.dart",
"repo_id": "flutter",
"token_count": 1220
} | 716 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
/// An interface for widgets that can return the size this widget would prefer
/// if it were otherwise unconstrained.
///
/// There are a few cases, notably [AppBar] and [TabBar], where it would be
/// undesirable for the widget to constrain its own size but where the widget
/// needs to expose a preferred or "default" size. For example a primary
/// [Scaffold] sets its app bar height to the app bar's preferred height
/// plus the height of the system status bar.
///
/// Widgets that need to know the preferred size of their child can require
/// that their child implement this interface by using this class rather
/// than [Widget] as the type of their `child` property.
///
/// Use [PreferredSize] to give a preferred size to an arbitrary widget.
abstract class PreferredSizeWidget implements Widget {
/// The size this widget would prefer if it were otherwise unconstrained.
///
/// In many cases it's only necessary to define one preferred dimension.
/// For example the [Scaffold] only depends on its app bar's preferred
/// height. In that case implementations of this method can just return
/// `Size.fromHeight(myAppBarHeight)`.
Size get preferredSize;
}
/// A widget with a preferred size.
///
/// This widget does not impose any constraints on its child, and it doesn't
/// affect the child's layout in any way. It just advertises a preferred size
/// which can be used by the parent.
///
/// Parents like [Scaffold] use [PreferredSizeWidget] to require that their
/// children implement that interface. To give a preferred size to an arbitrary
/// widget so that it can be used in a `child` property of that type, this
/// widget, [PreferredSize], can be used.
///
/// Widgets like [AppBar] implement a [PreferredSizeWidget], so that this
/// [PreferredSize] widget is not necessary for them.
///
/// {@tool dartpad}
/// This sample shows a custom widget, similar to an [AppBar], which uses a
/// [PreferredSize] widget, with its height set to 80 logical pixels.
/// Changing the [PreferredSize] can be used to change the height
/// of the custom app bar.
///
/// ** See code in examples/api/lib/widgets/preferred_size/preferred_size.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AppBar.bottom] and [Scaffold.appBar], which require preferred size widgets.
/// * [PreferredSizeWidget], the interface which this widget implements to expose
/// its preferred size.
/// * [AppBar] and [TabBar], which implement PreferredSizeWidget.
class PreferredSize extends StatelessWidget implements PreferredSizeWidget {
/// Creates a widget that has a preferred size that the parent can query.
const PreferredSize({
super.key,
required this.preferredSize,
required this.child,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
final Size preferredSize;
@override
Widget build(BuildContext context) => child;
}
| flutter/packages/flutter/lib/src/widgets/preferred_size.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/preferred_size.dart",
"repo_id": "flutter",
"token_count": 852
} | 717 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'framework.dart';
import 'notification_listener.dart';
import 'scroll_metrics.dart';
/// Mixin for [Notification]s that track how many [RenderAbstractViewport] they
/// have bubbled through.
///
/// This is used by [ScrollNotification] and [OverscrollIndicatorNotification].
mixin ViewportNotificationMixin on Notification {
/// The number of viewports that this notification has bubbled through.
///
/// Typically listeners only respond to notifications with a [depth] of zero.
///
/// Specifically, this is the number of [Widget]s representing
/// [RenderAbstractViewport] render objects through which this notification
/// has bubbled.
int get depth => _depth;
int _depth = 0;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('depth: $depth (${ depth == 0 ? "local" : "remote"})');
}
}
/// A mixin that allows [Element]s containing [Viewport] like widgets to correctly
/// modify the notification depth of a [ViewportNotificationMixin].
///
/// See also:
/// * [Viewport], which creates a custom [MultiChildRenderObjectElement] that mixes
/// this in.
mixin ViewportElementMixin on NotifiableElementMixin {
@override
bool onNotification(Notification notification) {
if (notification is ViewportNotificationMixin) {
notification._depth += 1;
}
return false;
}
}
/// A [Notification] related to scrolling.
///
/// [Scrollable] widgets notify their ancestors about scrolling-related changes.
/// The notifications have the following lifecycle:
///
/// * A [ScrollStartNotification], which indicates that the widget has started
/// scrolling.
/// * Zero or more [ScrollUpdateNotification]s, which indicate that the widget
/// has changed its scroll position, mixed with zero or more
/// [OverscrollNotification]s, which indicate that the widget has not changed
/// its scroll position because the change would have caused its scroll
/// position to go outside its scroll bounds.
/// * Interspersed with the [ScrollUpdateNotification]s and
/// [OverscrollNotification]s are zero or more [UserScrollNotification]s,
/// which indicate that the user has changed the direction in which they are
/// scrolling.
/// * A [ScrollEndNotification], which indicates that the widget has stopped
/// scrolling.
/// * A [UserScrollNotification], with a [UserScrollNotification.direction] of
/// [ScrollDirection.idle].
///
/// Notifications bubble up through the tree, which means a given
/// [NotificationListener] will receive notifications for all descendant
/// [Scrollable] widgets. To focus on notifications from the nearest
/// [Scrollable] descendant, check that the [depth] property of the notification
/// is zero.
///
/// When a scroll notification is received by a [NotificationListener], the
/// listener will have already completed build and layout, and it is therefore
/// too late for that widget to call [State.setState]. Any attempt to adjust the
/// build or layout based on a scroll notification would result in a layout that
/// lagged one frame behind, which is a poor user experience. Scroll
/// notifications are therefore primarily useful for paint effects (since paint
/// happens after layout). The [GlowingOverscrollIndicator] and [Scrollbar]
/// widgets are examples of paint effects that use scroll notifications.
///
/// {@tool dartpad}
/// This sample shows the difference between using a [ScrollController] or a
/// [NotificationListener] of type [ScrollNotification] to listen to scrolling
/// activities. Toggling the [Radio] button switches between the two.
/// Using a [ScrollNotification] will provide details about the scrolling
/// activity, along with the metrics of the [ScrollPosition], but not the scroll
/// position object itself. By listening with a [ScrollController], the position
/// object is directly accessible.
/// Both of these types of notifications are only triggered by scrolling.
///
/// ** See code in examples/api/lib/widgets/scroll_position/scroll_controller_notification.0.dart **
/// {@end-tool}
///
/// To drive layout based on the scroll position, consider listening to the
/// [ScrollPosition] directly (or indirectly via a [ScrollController]). This
/// will not notify when the [ScrollMetrics] of a given scroll position changes,
/// such as when the window is resized, changing the dimensions of the
/// [Viewport]. In order to listen to changes in scroll metrics, use a
/// [NotificationListener] of type [ScrollMetricsNotification].
/// This type of notification differs from [ScrollNotification], as it is not
/// associated with the activity of scrolling, but rather the dimensions of
/// the scrollable area.
///
/// {@tool dartpad}
/// This sample shows how a [ScrollMetricsNotification] is dispatched when
/// the `windowSize` is changed. Press the floating action button to increase
/// the scrollable window's size.
///
/// ** See code in examples/api/lib/widgets/scroll_position/scroll_metrics_notification.0.dart **
/// {@end-tool}
///
abstract class ScrollNotification extends LayoutChangedNotification with ViewportNotificationMixin {
/// Initializes fields for subclasses.
ScrollNotification({
required this.metrics,
required this.context,
});
/// A description of a [Scrollable]'s contents, useful for modeling the state
/// of its viewport.
final ScrollMetrics metrics;
/// The build context of the widget that fired this notification.
///
/// This can be used to find the scrollable's render objects to determine the
/// size of the viewport, for instance.
final BuildContext? context;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('$metrics');
}
}
/// A notification that a [Scrollable] widget has started scrolling.
///
/// See also:
///
/// * [ScrollEndNotification], which indicates that scrolling has stopped.
/// * [ScrollNotification], which describes the notification lifecycle.
class ScrollStartNotification extends ScrollNotification {
/// Creates a notification that a [Scrollable] widget has started scrolling.
ScrollStartNotification({
required super.metrics,
required super.context,
this.dragDetails,
});
/// If the [Scrollable] started scrolling because of a drag, the details about
/// that drag start.
///
/// Otherwise, null.
final DragStartDetails? dragDetails;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
if (dragDetails != null) {
description.add('$dragDetails');
}
}
}
/// A notification that a [Scrollable] widget has changed its scroll position.
///
/// See also:
///
/// * [OverscrollNotification], which indicates that a [Scrollable] widget
/// has not changed its scroll position because the change would have caused
/// its scroll position to go outside its scroll bounds.
/// * [ScrollNotification], which describes the notification lifecycle.
class ScrollUpdateNotification extends ScrollNotification {
/// Creates a notification that a [Scrollable] widget has changed its scroll
/// position.
ScrollUpdateNotification({
required super.metrics,
required BuildContext super.context,
this.dragDetails,
this.scrollDelta,
int? depth,
}) {
if (depth != null) {
_depth = depth;
}
}
/// If the [Scrollable] changed its scroll position because of a drag, the
/// details about that drag update.
///
/// Otherwise, null.
final DragUpdateDetails? dragDetails;
/// The distance by which the [Scrollable] was scrolled, in logical pixels.
final double? scrollDelta;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('scrollDelta: $scrollDelta');
if (dragDetails != null) {
description.add('$dragDetails');
}
}
}
/// A notification that a [Scrollable] widget has not changed its scroll position
/// because the change would have caused its scroll position to go outside of
/// its scroll bounds.
///
/// See also:
///
/// * [ScrollUpdateNotification], which indicates that a [Scrollable] widget
/// has changed its scroll position.
/// * [ScrollNotification], which describes the notification lifecycle.
class OverscrollNotification extends ScrollNotification {
/// Creates a notification that a [Scrollable] widget has changed its scroll
/// position outside of its scroll bounds.
OverscrollNotification({
required super.metrics,
required BuildContext super.context,
this.dragDetails,
required this.overscroll,
this.velocity = 0.0,
}) : assert(overscroll.isFinite),
assert(overscroll != 0.0);
/// If the [Scrollable] overscrolled because of a drag, the details about that
/// drag update.
///
/// Otherwise, null.
final DragUpdateDetails? dragDetails;
/// The number of logical pixels that the [Scrollable] avoided scrolling.
///
/// This will be negative for overscroll on the "start" side and positive for
/// overscroll on the "end" side.
final double overscroll;
/// The velocity at which the [ScrollPosition] was changing when this
/// overscroll happened.
///
/// This will typically be 0.0 for touch-driven overscrolls, and positive
/// for overscrolls that happened from a [BallisticScrollActivity] or
/// [DrivenScrollActivity].
final double velocity;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('overscroll: ${overscroll.toStringAsFixed(1)}');
description.add('velocity: ${velocity.toStringAsFixed(1)}');
if (dragDetails != null) {
description.add('$dragDetails');
}
}
}
/// A notification that a [Scrollable] widget has stopped scrolling.
///
/// See also:
///
/// * [ScrollStartNotification], which indicates that scrolling has started.
/// * [ScrollNotification], which describes the notification lifecycle.
class ScrollEndNotification extends ScrollNotification {
/// Creates a notification that a [Scrollable] widget has stopped scrolling.
ScrollEndNotification({
required super.metrics,
required BuildContext super.context,
this.dragDetails,
});
/// If the [Scrollable] stopped scrolling because of a drag, the details about
/// that drag end.
///
/// Otherwise, null.
///
/// If a drag ends with some residual velocity, a typical [ScrollPhysics] will
/// start a ballistic scroll, which delays the [ScrollEndNotification] until
/// the ballistic simulation completes, at which time [dragDetails] will
/// be null. If the residual velocity is too small to trigger ballistic
/// scrolling, then the [ScrollEndNotification] will be dispatched immediately
/// and [dragDetails] will be non-null.
final DragEndDetails? dragDetails;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
if (dragDetails != null) {
description.add('$dragDetails');
}
}
}
/// A notification that the user has changed the [ScrollDirection] in which they
/// are scrolling, or have stopped scrolling.
///
/// For the direction that the [ScrollView] is oriented to, and the direction
/// contents are being laid out in, see [AxisDirection] & [GrowthDirection].
///
/// {@macro flutter.rendering.ScrollDirection.sample}
///
/// See also:
///
/// * [ScrollNotification], which describes the notification lifecycle.
class UserScrollNotification extends ScrollNotification {
/// Creates a notification that the user has changed the direction in which
/// they are scrolling.
UserScrollNotification({
required super.metrics,
required BuildContext super.context,
required this.direction,
});
/// The direction in which the user is scrolling.
///
/// This does not represent the current [AxisDirection] or [GrowthDirection]
/// of the [Viewport], which respectively represent the direction that the
/// scroll offset is increasing in, and the direction that contents are being
/// laid out in.
///
/// {@macro flutter.rendering.ScrollDirection.sample}
final ScrollDirection direction;
@override
void debugFillDescription(List<String> description) {
super.debugFillDescription(description);
description.add('direction: $direction');
}
}
/// A predicate for [ScrollNotification], used to customize widgets that
/// listen to notifications from their children.
typedef ScrollNotificationPredicate = bool Function(ScrollNotification notification);
/// A [ScrollNotificationPredicate] that checks whether
/// `notification.depth == 0`, which means that the notification did not bubble
/// through any intervening scrolling widgets.
bool defaultScrollNotificationPredicate(ScrollNotification notification) {
return notification.depth == 0;
}
| flutter/packages/flutter/lib/src/widgets/scroll_notification.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_notification.dart",
"repo_id": "flutter",
"token_count": 3520
} | 718 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'focus_manager.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'notification_listener.dart';
import 'primary_scroll_controller.dart';
import 'scroll_controller.dart';
import 'scroll_notification.dart';
import 'scroll_physics.dart';
import 'scroll_view.dart';
import 'scrollable.dart';
/// A box in which a single widget can be scrolled.
///
/// This widget is useful when you have a single box that will normally be
/// entirely visible, for example a clock face in a time picker, but you need to
/// make sure it can be scrolled if the container gets too small in one axis
/// (the scroll direction).
///
/// It is also useful if you need to shrink-wrap in both axes (the main
/// scrolling direction as well as the cross axis), as one might see in a dialog
/// or pop-up menu. In that case, you might pair the [SingleChildScrollView]
/// with a [ListBody] child.
///
/// When you have a list of children and do not require cross-axis
/// shrink-wrapping behavior, for example a scrolling list that is always the
/// width of the screen, consider [ListView], which is vastly more efficient
/// than a [SingleChildScrollView] containing a [ListBody] or [Column] with
/// many children.
///
/// ## Sample code: Using [SingleChildScrollView] with a [Column]
///
/// Sometimes a layout is designed around the flexible properties of a
/// [Column], but there is the concern that in some cases, there might not
/// be enough room to see the entire contents. This could be because some
/// devices have unusually small screens, or because the application can
/// be used in landscape mode where the aspect ratio isn't what was
/// originally envisioned, or because the application is being shown in a
/// small window in split-screen mode. In any case, as a result, it might
/// make sense to wrap the layout in a [SingleChildScrollView].
///
/// Doing so, however, usually results in a conflict between the [Column],
/// which typically tries to grow as big as it can, and the [SingleChildScrollView],
/// which provides its children with an infinite amount of space.
///
/// To resolve this apparent conflict, there are a couple of techniques, as
/// discussed below. These techniques should only be used when the content is
/// normally expected to fit on the screen, so that the lazy instantiation of a
/// sliver-based [ListView] or [CustomScrollView] is not expected to provide any
/// performance benefit. If the viewport is expected to usually contain content
/// beyond the dimensions of the screen, then [SingleChildScrollView] would be
/// very expensive (in which case [ListView] may be a better choice than
/// [Column]).
///
/// ### Centering, spacing, or aligning fixed-height content
///
/// If the content has fixed (or intrinsic) dimensions but needs to be spaced out,
/// centered, or otherwise positioned using the [Flex] layout model of a [Column],
/// the following technique can be used to provide the [Column] with a minimum
/// dimension while allowing it to shrink-wrap the contents when there isn't enough
/// room to apply these spacing or alignment needs.
///
/// A [LayoutBuilder] is used to obtain the size of the viewport (implicitly via
/// the constraints that the [SingleChildScrollView] sees, since viewports
/// typically grow to fit their maximum height constraint). Then, inside the
/// scroll view, a [ConstrainedBox] is used to set the minimum height of the
/// [Column].
///
/// The [Column] has no [Expanded] children, so rather than take on the infinite
/// height from its [BoxConstraints.maxHeight], (the viewport provides no maximum height
/// constraint), it automatically tries to shrink to fit its children. It cannot
/// be smaller than its [BoxConstraints.minHeight], though, and It therefore
/// becomes the bigger of the minimum height provided by the
/// [ConstrainedBox] and the sum of the heights of the children.
///
/// If the children aren't enough to fit that minimum size, the [Column] ends up
/// with some remaining space to allocate as specified by its
/// [Column.mainAxisAlignment] argument.
///
/// {@tool dartpad}
/// In this example, the children are spaced out equally, unless there's no more
/// room, in which case they stack vertically and scroll.
///
/// When using this technique, [Expanded] and [Flexible] are not useful, because
/// in both cases the "available space" is infinite (since this is in a viewport).
/// The next section describes a technique for providing a maximum height constraint.
///
/// ** See code in examples/api/lib/widgets/single_child_scroll_view/single_child_scroll_view.0.dart **
/// {@end-tool}
///
/// ### Expanding content to fit the viewport
///
/// The following example builds on the previous one. In addition to providing a
/// minimum dimension for the child [Column], an [IntrinsicHeight] widget is used
/// to force the column to be exactly as big as its contents. This constraint
/// combines with the [ConstrainedBox] constraints discussed previously to ensure
/// that the column becomes either as big as viewport, or as big as the contents,
/// whichever is biggest.
///
/// Both constraints must be used to get the desired effect. If only the
/// [IntrinsicHeight] was specified, then the column would not grow to fit the
/// entire viewport when its children were smaller than the whole screen. If only
/// the size of the viewport was used, then the [Column] would overflow if the
/// children were bigger than the viewport.
///
/// The widget that is to grow to fit the remaining space so provided is wrapped
/// in an [Expanded] widget.
///
/// This technique is quite expensive, as it more or less requires that the contents
/// of the viewport be laid out twice (once to find their intrinsic dimensions, and
/// once to actually lay them out). The number of widgets within the column should
/// therefore be kept small. Alternatively, subsets of the children that have known
/// dimensions can be wrapped in a [SizedBox] that has tight vertical constraints,
/// so that the intrinsic sizing algorithm can short-circuit the computation when it
/// reaches those parts of the subtree.
///
/// {@tool dartpad}
/// In this example, the column becomes either as big as viewport, or as big as
/// the contents, whichever is biggest.
///
/// ** See code in examples/api/lib/widgets/single_child_scroll_view/single_child_scroll_view.1.dart **
/// {@end-tool}
///
/// {@macro flutter.widgets.ScrollView.PageStorage}
///
/// See also:
///
/// * [ListView], which handles multiple children in a scrolling list.
/// * [GridView], which handles multiple children in a scrolling grid.
/// * [PageView], for a scrollable that works page by page.
/// * [Scrollable], which handles arbitrary scrolling effects.
class SingleChildScrollView extends StatelessWidget {
/// Creates a box in which a single widget can be scrolled.
const SingleChildScrollView({
super.key,
this.scrollDirection = Axis.vertical,
this.reverse = false,
this.padding,
this.primary,
this.physics,
this.controller,
this.child,
this.dragStartBehavior = DragStartBehavior.start,
this.clipBehavior = Clip.hardEdge,
this.restorationId,
this.keyboardDismissBehavior = ScrollViewKeyboardDismissBehavior.manual,
}) : assert(
!(controller != null && (primary ?? false)),
'Primary ScrollViews obtain their ScrollController via inheritance '
'from a PrimaryScrollController widget. You cannot both set primary to '
'true and pass an explicit controller.',
);
/// {@macro flutter.widgets.scroll_view.scrollDirection}
final Axis scrollDirection;
/// Whether the scroll view scrolls in the reading direction.
///
/// For example, if the reading direction is left-to-right and
/// [scrollDirection] is [Axis.horizontal], then the scroll view scrolls from
/// left to right when [reverse] is false and from right to left when
/// [reverse] is true.
///
/// Similarly, if [scrollDirection] is [Axis.vertical], then the scroll view
/// scrolls from top to bottom when [reverse] is false and from bottom to top
/// when [reverse] is true.
///
/// Defaults to false.
final bool reverse;
/// The amount of space by which to inset the child.
final EdgeInsetsGeometry? padding;
/// An object that can be used to control the position to which this scroll
/// view is scrolled.
///
/// Must be null if [primary] is true.
///
/// A [ScrollController] serves several purposes. It can be used to control
/// the initial scroll position (see [ScrollController.initialScrollOffset]).
/// It can be used to control whether the scroll view should automatically
/// save and restore its scroll position in the [PageStorage] (see
/// [ScrollController.keepScrollOffset]). It can be used to read the current
/// scroll position (see [ScrollController.offset]), or change it (see
/// [ScrollController.animateTo]).
final ScrollController? controller;
/// {@macro flutter.widgets.scroll_view.primary}
final bool? primary;
/// How the scroll view should respond to user input.
///
/// For example, determines how the scroll view continues to animate after the
/// user stops dragging the scroll view.
///
/// Defaults to matching platform conventions.
final ScrollPhysics? physics;
/// The widget that scrolls.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// {@macro flutter.widgets.scrollable.restorationId}
final String? restorationId;
/// {@macro flutter.widgets.scroll_view.keyboardDismissBehavior}
final ScrollViewKeyboardDismissBehavior keyboardDismissBehavior;
AxisDirection _getDirection(BuildContext context) {
return getAxisDirectionFromAxisReverseAndDirectionality(context, scrollDirection, reverse);
}
@override
Widget build(BuildContext context) {
final AxisDirection axisDirection = _getDirection(context);
Widget? contents = child;
if (padding != null) {
contents = Padding(padding: padding!, child: contents);
}
final bool effectivePrimary = primary
?? controller == null && PrimaryScrollController.shouldInherit(context, scrollDirection);
final ScrollController? scrollController = effectivePrimary
? PrimaryScrollController.maybeOf(context)
: controller;
Widget scrollable = Scrollable(
dragStartBehavior: dragStartBehavior,
axisDirection: axisDirection,
controller: scrollController,
physics: physics,
restorationId: restorationId,
clipBehavior: clipBehavior,
viewportBuilder: (BuildContext context, ViewportOffset offset) {
return _SingleChildViewport(
axisDirection: axisDirection,
offset: offset,
clipBehavior: clipBehavior,
child: contents,
);
},
);
if (keyboardDismissBehavior == ScrollViewKeyboardDismissBehavior.onDrag) {
scrollable = NotificationListener<ScrollUpdateNotification>(
child: scrollable,
onNotification: (ScrollUpdateNotification notification) {
final FocusScopeNode focusNode = FocusScope.of(context);
if (notification.dragDetails != null && focusNode.hasFocus) {
focusNode.unfocus();
}
return false;
},
);
}
return effectivePrimary && scrollController != null
// Further descendant ScrollViews will not inherit the same
// PrimaryScrollController
? PrimaryScrollController.none(child: scrollable)
: scrollable;
}
}
class _SingleChildViewport extends SingleChildRenderObjectWidget {
const _SingleChildViewport({
this.axisDirection = AxisDirection.down,
required this.offset,
super.child,
required this.clipBehavior,
});
final AxisDirection axisDirection;
final ViewportOffset offset;
final Clip clipBehavior;
@override
_RenderSingleChildViewport createRenderObject(BuildContext context) {
return _RenderSingleChildViewport(
axisDirection: axisDirection,
offset: offset,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, _RenderSingleChildViewport renderObject) {
// Order dependency: The offset setter reads the axis direction.
renderObject
..axisDirection = axisDirection
..offset = offset
..clipBehavior = clipBehavior;
}
@override
SingleChildRenderObjectElement createElement() {
return _SingleChildViewportElement(this);
}
}
class _SingleChildViewportElement extends SingleChildRenderObjectElement with NotifiableElementMixin, ViewportElementMixin {
_SingleChildViewportElement(_SingleChildViewport super.widget);
}
class _RenderSingleChildViewport extends RenderBox with RenderObjectWithChildMixin<RenderBox> implements RenderAbstractViewport {
_RenderSingleChildViewport({
AxisDirection axisDirection = AxisDirection.down,
required ViewportOffset offset,
RenderBox? child,
required Clip clipBehavior,
}) : _axisDirection = axisDirection,
_offset = offset,
_clipBehavior = clipBehavior {
this.child = child;
}
AxisDirection get axisDirection => _axisDirection;
AxisDirection _axisDirection;
set axisDirection(AxisDirection value) {
if (value == _axisDirection) {
return;
}
_axisDirection = value;
markNeedsLayout();
}
Axis get axis => axisDirectionToAxis(axisDirection);
ViewportOffset get offset => _offset;
ViewportOffset _offset;
set offset(ViewportOffset value) {
if (value == _offset) {
return;
}
if (attached) {
_offset.removeListener(_hasScrolled);
}
_offset = value;
if (attached) {
_offset.addListener(_hasScrolled);
}
markNeedsLayout();
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.none;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
void _hasScrolled() {
markNeedsPaint();
markNeedsSemanticsUpdate();
}
@override
void setupParentData(RenderObject child) {
// We don't actually use the offset argument in BoxParentData, so let's
// avoid allocating it at all.
if (child.parentData is! ParentData) {
child.parentData = ParentData();
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_offset.addListener(_hasScrolled);
}
@override
void detach() {
_offset.removeListener(_hasScrolled);
super.detach();
}
@override
bool get isRepaintBoundary => true;
double get _viewportExtent {
assert(hasSize);
return switch (axis) {
Axis.horizontal => size.width,
Axis.vertical => size.height,
};
}
double get _minScrollExtent {
assert(hasSize);
return 0.0;
}
double get _maxScrollExtent {
assert(hasSize);
if (child == null) {
return 0.0;
}
return math.max(0.0, switch (axis) {
Axis.horizontal => child!.size.width - size.width,
Axis.vertical => child!.size.height - size.height,
});
}
BoxConstraints _getInnerConstraints(BoxConstraints constraints) {
return switch (axis) {
Axis.horizontal => constraints.heightConstraints(),
Axis.vertical => constraints.widthConstraints(),
};
}
@override
double computeMinIntrinsicWidth(double height) {
return child?.getMinIntrinsicWidth(height) ?? 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
return child?.getMaxIntrinsicWidth(height) ?? 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
return child?.getMinIntrinsicHeight(width) ?? 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
return child?.getMaxIntrinsicHeight(width) ?? 0.0;
}
// We don't override computeDistanceToActualBaseline(), because we
// want the default behavior (returning null). Otherwise, as you
// scroll, it would shift in its parent if the parent was baseline-aligned,
// which makes no sense.
@override
Size computeDryLayout(BoxConstraints constraints) {
if (child == null) {
return constraints.smallest;
}
final Size childSize = child!.getDryLayout(_getInnerConstraints(constraints));
return constraints.constrain(childSize);
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
if (child == null) {
size = constraints.smallest;
} else {
child!.layout(_getInnerConstraints(constraints), parentUsesSize: true);
size = constraints.constrain(child!.size);
}
if (offset.hasPixels) {
if (offset.pixels > _maxScrollExtent) {
offset.correctBy(_maxScrollExtent - offset.pixels);
} else if (offset.pixels < _minScrollExtent) {
offset.correctBy(_minScrollExtent - offset.pixels);
}
}
offset.applyViewportDimension(_viewportExtent);
offset.applyContentDimensions(_minScrollExtent, _maxScrollExtent);
}
Offset get _paintOffset => _paintOffsetForPosition(offset.pixels);
Offset _paintOffsetForPosition(double position) {
return switch (axisDirection) {
AxisDirection.up => Offset(0.0, position - child!.size.height + size.height),
AxisDirection.left => Offset(position - child!.size.width + size.width, 0.0),
AxisDirection.right => Offset(-position, 0.0),
AxisDirection.down => Offset(0.0, -position),
};
}
bool _shouldClipAtPaintOffset(Offset paintOffset) {
assert(child != null);
switch (clipBehavior) {
case Clip.none:
return false;
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
return paintOffset.dx < 0 ||
paintOffset.dy < 0 ||
paintOffset.dx + child!.size.width > size.width ||
paintOffset.dy + child!.size.height > size.height;
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null) {
final Offset paintOffset = _paintOffset;
void paintContents(PaintingContext context, Offset offset) {
context.paintChild(child!, offset + paintOffset);
}
if (_shouldClipAtPaintOffset(paintOffset)) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
paintContents,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
paintContents(context, offset);
}
}
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
final Offset paintOffset = _paintOffset;
transform.translate(paintOffset.dx, paintOffset.dy);
}
@override
Rect? describeApproximatePaintClip(RenderObject? child) {
if (child != null && _shouldClipAtPaintOffset(_paintOffset)) {
return Offset.zero & size;
}
return null;
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
if (child != null) {
return result.addWithPaintOffset(
offset: _paintOffset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position + -_paintOffset);
return child!.hitTest(result, position: transformed);
},
);
}
return false;
}
@override
RevealedOffset getOffsetToReveal(
RenderObject target,
double alignment, {
Rect? rect,
Axis? axis,
}) {
// One dimensional viewport has only one axis, override if it was
// provided/may be mismatched.
axis = this.axis;
rect ??= target.paintBounds;
if (target is! RenderBox) {
return RevealedOffset(offset: offset.pixels, rect: rect);
}
final RenderBox targetBox = target;
final Matrix4 transform = targetBox.getTransformTo(child);
final Rect bounds = MatrixUtils.transformRect(transform, rect);
final Size contentSize = child!.size;
final (double mainAxisExtent, double leadingScrollOffset, double targetMainAxisExtent) = switch (axisDirection) {
AxisDirection.up => (size.height, contentSize.height - bounds.bottom, bounds.height),
AxisDirection.left => (size.width, contentSize.width - bounds.right, bounds.width),
AxisDirection.right => (size.width, bounds.left, bounds.width),
AxisDirection.down => (size.height, bounds.top, bounds.height),
};
final double targetOffset = leadingScrollOffset - (mainAxisExtent - targetMainAxisExtent) * alignment;
final Rect targetRect = bounds.shift(_paintOffsetForPosition(targetOffset));
return RevealedOffset(offset: targetOffset, rect: targetRect);
}
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
if (!offset.allowImplicitScrolling) {
return super.showOnScreen(
descendant: descendant,
rect: rect,
duration: duration,
curve: curve,
);
}
final Rect? newRect = RenderViewportBase.showInViewport(
descendant: descendant,
viewport: this,
offset: offset,
rect: rect,
duration: duration,
curve: curve,
);
super.showOnScreen(
rect: newRect,
duration: duration,
curve: curve,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Offset>('offset', _paintOffset));
}
@override
Rect describeSemanticsClip(RenderObject child) {
final double remainingOffset = _maxScrollExtent - offset.pixels;
switch (axisDirection) {
case AxisDirection.up:
return Rect.fromLTRB(
semanticBounds.left,
semanticBounds.top - remainingOffset,
semanticBounds.right,
semanticBounds.bottom + offset.pixels,
);
case AxisDirection.right:
return Rect.fromLTRB(
semanticBounds.left - offset.pixels,
semanticBounds.top,
semanticBounds.right + remainingOffset,
semanticBounds.bottom,
);
case AxisDirection.down:
return Rect.fromLTRB(
semanticBounds.left,
semanticBounds.top - offset.pixels,
semanticBounds.right,
semanticBounds.bottom + remainingOffset,
);
case AxisDirection.left:
return Rect.fromLTRB(
semanticBounds.left - remainingOffset,
semanticBounds.top,
semanticBounds.right + offset.pixels,
semanticBounds.bottom,
);
}
}
}
| flutter/packages/flutter/lib/src/widgets/single_child_scroll_view.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/single_child_scroll_view.dart",
"repo_id": "flutter",
"token_count": 7674
} | 719 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'actions.dart';
/// An [Intent] to send the event straight to the engine.
///
/// See also:
///
/// * [DefaultTextEditingShortcuts], which triggers this [Intent].
class DoNothingAndStopPropagationTextIntent extends Intent {
/// Creates an instance of [DoNothingAndStopPropagationTextIntent].
const DoNothingAndStopPropagationTextIntent();
}
/// A text editing related [Intent] that performs an operation towards a given
/// direction of the current caret location.
abstract class DirectionalTextEditingIntent extends Intent {
/// Creates a [DirectionalTextEditingIntent].
const DirectionalTextEditingIntent(
this.forward,
);
/// Whether the input field, if applicable, should perform the text editing
/// operation from the current caret location towards the end of the document.
///
/// Unless otherwise specified by the recipient of this intent, this parameter
/// uses the logical order of characters in the string to determine the
/// direction, and is not affected by the writing direction of the text.
final bool forward;
}
/// Deletes the character before or after the caret location, based on whether
/// `forward` is true.
///
/// {@template flutter.widgets.TextEditingIntents.logicalOrder}
/// {@endtemplate}
///
/// Typically a text field will not respond to this intent if it has no active
/// caret ([TextSelection.isValid] is false for the current selection).
class DeleteCharacterIntent extends DirectionalTextEditingIntent {
/// Creates a [DeleteCharacterIntent].
const DeleteCharacterIntent({ required bool forward }) : super(forward);
}
/// Deletes from the current caret location to the previous or next word
/// boundary, based on whether `forward` is true.
class DeleteToNextWordBoundaryIntent extends DirectionalTextEditingIntent {
/// Creates a [DeleteToNextWordBoundaryIntent].
const DeleteToNextWordBoundaryIntent({ required bool forward }) : super(forward);
}
/// Deletes from the current caret location to the previous or next soft or hard
/// line break, based on whether `forward` is true.
class DeleteToLineBreakIntent extends DirectionalTextEditingIntent {
/// Creates a [DeleteToLineBreakIntent].
const DeleteToLineBreakIntent({ required bool forward }) : super(forward);
}
/// A [DirectionalTextEditingIntent] that moves the caret or the selection to a
/// new location.
abstract class DirectionalCaretMovementIntent extends DirectionalTextEditingIntent {
/// Creates a [DirectionalCaretMovementIntent].
const DirectionalCaretMovementIntent(
super.forward,
this.collapseSelection,
[
this.collapseAtReversal = false,
this.continuesAtWrap = false,
]
) : assert(!collapseSelection || !collapseAtReversal);
/// Whether this [Intent] should make the selection collapsed (so it becomes a
/// caret), after the movement.
///
/// When [collapseSelection] is false, the input field typically only moves
/// the current [TextSelection.extent] to the new location, while maintains
/// the current [TextSelection.base] location.
///
/// When [collapseSelection] is true, the input field typically should move
/// both the [TextSelection.base] and the [TextSelection.extent] to the new
/// location.
final bool collapseSelection;
/// Whether to collapse the selection when it would otherwise reverse order.
///
/// For example, consider when forward is true and the extent is before the
/// base. If collapseAtReversal is true, then this will cause the selection to
/// collapse at the base. If it's false, then the extent will be placed at the
/// linebreak, reversing the order of base and offset.
///
/// Cannot be true when collapseSelection is true.
final bool collapseAtReversal;
/// Whether or not to continue to the next line at a wordwrap.
///
/// If true, when an [Intent] to go to the beginning/end of a wordwrapped line
/// is received and the selection is already at the beginning/end of the line,
/// then the selection will be moved to the next/previous line. If false, the
/// selection will remain at the wordwrap.
final bool continuesAtWrap;
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the previous or the next character
/// boundary.
class ExtendSelectionByCharacterIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionByCharacterIntent].
const ExtendSelectionByCharacterIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the previous or the next word
/// boundary.
class ExtendSelectionToNextWordBoundaryIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToNextWordBoundaryIntent].
const ExtendSelectionToNextWordBoundaryIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the previous or the next word
/// boundary, or the [TextSelection.base] position if it's closer in the move
/// direction.
///
/// This [Intent] typically has the same effect as an
/// [ExtendSelectionToNextWordBoundaryIntent], except it collapses the selection
/// when the order of [TextSelection.base] and [TextSelection.extent] would
/// reverse.
///
/// This is typically only used on MacOS.
class ExtendSelectionToNextWordBoundaryOrCaretLocationIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToNextWordBoundaryOrCaretLocationIntent].
const ExtendSelectionToNextWordBoundaryOrCaretLocationIntent({
required bool forward,
}) : super(forward, false, true);
}
/// Expands the current selection to the document boundary in the direction
/// given by [forward].
///
/// Unlike [ExpandSelectionToLineBreakIntent], the extent will be moved, which
/// matches the behavior on MacOS.
///
/// See also:
///
/// [ExtendSelectionToDocumentBoundaryIntent], which is similar but always
/// moves the extent.
class ExpandSelectionToDocumentBoundaryIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExpandSelectionToDocumentBoundaryIntent].
const ExpandSelectionToDocumentBoundaryIntent({
required bool forward,
}) : super(forward, false);
}
/// Expands the current selection to the closest line break in the direction
/// given by [forward].
///
/// Either the base or extent can move, whichever is closer to the line break.
/// The selection will never shrink.
///
/// This behavior is common on MacOS.
///
/// See also:
///
/// [ExtendSelectionToLineBreakIntent], which is similar but always moves the
/// extent.
class ExpandSelectionToLineBreakIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExpandSelectionToLineBreakIntent].
const ExpandSelectionToLineBreakIntent({
required bool forward,
}) : super(forward, false);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the closest line break in the direction
/// given by [forward].
///
/// See also:
///
/// [ExpandSelectionToLineBreakIntent], which is similar but always increases
/// the size of the selection.
class ExtendSelectionToLineBreakIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToLineBreakIntent].
const ExtendSelectionToLineBreakIntent({
required bool forward,
required bool collapseSelection,
bool collapseAtReversal = false,
bool continuesAtWrap = false,
}) : assert(!collapseSelection || !collapseAtReversal),
super(forward, collapseSelection, collapseAtReversal, continuesAtWrap);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the closest position on the adjacent
/// line.
class ExtendSelectionVerticallyToAdjacentLineIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionVerticallyToAdjacentLineIntent].
const ExtendSelectionVerticallyToAdjacentLineIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Expands, or moves the current selection from the current
/// [TextSelection.extent] position to the closest position on the adjacent
/// page.
class ExtendSelectionVerticallyToAdjacentPageIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionVerticallyToAdjacentPageIntent].
const ExtendSelectionVerticallyToAdjacentPageIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the previous or the next paragraph
/// boundary.
class ExtendSelectionToNextParagraphBoundaryIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToNextParagraphBoundaryIntent].
const ExtendSelectionToNextParagraphBoundaryIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the previous or the next paragraph
/// boundary depending on the [forward] parameter.
///
/// This [Intent] typically has the same effect as an
/// [ExtendSelectionToNextParagraphBoundaryIntent], except it collapses the selection
/// when the order of [TextSelection.base] and [TextSelection.extent] would
/// reverse.
///
/// This is typically only used on MacOS.
class ExtendSelectionToNextParagraphBoundaryOrCaretLocationIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToNextParagraphBoundaryOrCaretLocationIntent].
const ExtendSelectionToNextParagraphBoundaryOrCaretLocationIntent({
required bool forward,
}) : super(forward, false, true);
}
/// Extends, or moves the current selection from the current
/// [TextSelection.extent] position to the start or the end of the document.
///
/// See also:
///
/// [ExtendSelectionToDocumentBoundaryIntent], which is similar but always
/// increases the size of the selection.
class ExtendSelectionToDocumentBoundaryIntent extends DirectionalCaretMovementIntent {
/// Creates an [ExtendSelectionToDocumentBoundaryIntent].
const ExtendSelectionToDocumentBoundaryIntent({
required bool forward,
required bool collapseSelection,
}) : super(forward, collapseSelection);
}
/// Scrolls to the beginning or end of the document depending on the [forward]
/// parameter.
class ScrollToDocumentBoundaryIntent extends DirectionalTextEditingIntent {
/// Creates a [ScrollToDocumentBoundaryIntent].
const ScrollToDocumentBoundaryIntent({
required bool forward,
}) : super(forward);
}
/// Scrolls up or down by page depending on the [forward] parameter.
/// Extends the selection up or down by page based on the [forward] parameter.
class ExtendSelectionByPageIntent extends DirectionalTextEditingIntent {
/// Creates a [ExtendSelectionByPageIntent].
const ExtendSelectionByPageIntent({
required bool forward,
}) : super(forward);
}
/// An [Intent] to select everything in the field.
class SelectAllTextIntent extends Intent {
/// Creates an instance of [SelectAllTextIntent].
const SelectAllTextIntent(this.cause);
/// {@template flutter.widgets.TextEditingIntents.cause}
/// The [SelectionChangedCause] that triggered the intent.
/// {@endtemplate}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to copy or cut
/// the current selection in the field.
class CopySelectionTextIntent extends Intent {
const CopySelectionTextIntent._(this.cause, this.collapseSelection);
/// Creates an [Intent] that represents a user interaction that attempts to
/// cut the current selection in the field.
const CopySelectionTextIntent.cut(SelectionChangedCause cause) : this._(cause, true);
/// An [Intent] that represents a user interaction that attempts to copy the
/// current selection in the field.
static const CopySelectionTextIntent copy = CopySelectionTextIntent._(SelectionChangedCause.keyboard, false);
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
/// Whether the original text needs to be removed from the input field if the
/// copy action was successful.
final bool collapseSelection;
}
/// An [Intent] to paste text from [Clipboard] to the field.
class PasteTextIntent extends Intent {
/// Creates an instance of [PasteTextIntent].
const PasteTextIntent(this.cause);
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to go back to
/// the previous editing state.
class RedoTextIntent extends Intent {
/// Creates a [RedoTextIntent].
const RedoTextIntent(this.cause);
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to modify the
/// current [TextEditingValue] in an input field.
class ReplaceTextIntent extends Intent {
/// Creates a [ReplaceTextIntent].
const ReplaceTextIntent(this.currentTextEditingValue, this.replacementText, this.replacementRange, this.cause);
/// The [TextEditingValue] that this [Intent]'s action should perform on.
final TextEditingValue currentTextEditingValue;
/// The text to replace the original text within the [replacementRange] with.
final String replacementText;
/// The range of text in [currentTextEditingValue] that needs to be replaced.
final TextRange replacementRange;
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to go back to
/// the previous editing state.
class UndoTextIntent extends Intent {
/// Creates an [UndoTextIntent].
const UndoTextIntent(this.cause);
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to change the
/// selection in an input field.
class UpdateSelectionIntent extends Intent {
/// Creates an [UpdateSelectionIntent].
const UpdateSelectionIntent(this.currentTextEditingValue, this.newSelection, this.cause);
/// The [TextEditingValue] that this [Intent]'s action should perform on.
final TextEditingValue currentTextEditingValue;
/// The new [TextSelection] the input field should adopt.
final TextSelection newSelection;
/// {@macro flutter.widgets.TextEditingIntents.cause}
final SelectionChangedCause cause;
}
/// An [Intent] that represents a user interaction that attempts to swap the
/// characters immediately around the cursor.
class TransposeCharactersIntent extends Intent {
/// Creates a [TransposeCharactersIntent].
const TransposeCharactersIntent();
}
| flutter/packages/flutter/lib/src/widgets/text_editing_intents.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/text_editing_intents.dart",
"repo_id": "flutter",
"token_count": 4178
} | 720 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'framework.dart';
import 'sliver.dart';
import 'ticker_provider.dart';
/// Whether to show or hide a child.
///
/// By default, the [visible] property controls whether the [child] is included
/// in the subtree or not; when it is not [visible], the [replacement] child
/// (typically a zero-sized box) is included instead.
///
/// A variety of flags can be used to tweak exactly how the child is hidden.
/// (Changing the flags dynamically is discouraged, as it can cause the [child]
/// subtree to be rebuilt, with any state in the subtree being discarded.
/// Typically, only the [visible] flag is changed dynamically.)
///
/// These widgets provide some of the facets of this one:
///
/// * [Opacity], which can stop its child from being painted.
/// * [Offstage], which can stop its child from being laid out or painted.
/// * [TickerMode], which can stop its child from being animated.
/// * [ExcludeSemantics], which can hide the child from accessibility tools.
/// * [IgnorePointer], which can disable touch interactions with the child.
///
/// Using this widget is not necessary to hide children. The simplest way to
/// hide a child is just to not include it, or, if a child _must_ be given (e.g.
/// because the parent is a [StatelessWidget]) then to use [SizedBox.shrink]
/// instead of the child that would otherwise be included.
///
/// See also:
///
/// * [AnimatedSwitcher], which can fade from one child to the next as the
/// subtree changes.
/// * [AnimatedCrossFade], which can fade between two specific children.
/// * [SliverVisibility], the sliver equivalent of this widget.
class Visibility extends StatelessWidget {
/// Control whether the given [child] is [visible].
///
/// The [maintainSemantics] and [maintainInteractivity] arguments can only be
/// set if [maintainSize] is set.
///
/// The [maintainSize] argument can only be set if [maintainAnimation] is set.
///
/// The [maintainAnimation] argument can only be set if [maintainState] is
/// set.
const Visibility({
super.key,
required this.child,
this.replacement = const SizedBox.shrink(),
this.visible = true,
this.maintainState = false,
this.maintainAnimation = false,
this.maintainSize = false,
this.maintainSemantics = false,
this.maintainInteractivity = false,
}) : assert(
maintainState || !maintainAnimation,
'Cannot maintain animations if the state is not also maintained.',
),
assert(
maintainAnimation || !maintainSize,
'Cannot maintain size if animations are not maintained.',
),
assert(
maintainSize || !maintainSemantics,
'Cannot maintain semantics if size is not maintained.',
),
assert(
maintainSize || !maintainInteractivity,
'Cannot maintain interactivity if size is not maintained.',
);
/// Control whether the given [child] is [visible].
///
/// This is equivalent to the default [Visibility] constructor with all
/// "maintain" fields set to true. This constructor should be used in place of
/// an [Opacity] widget that only takes on values of `0.0` or `1.0`, as it
/// avoids extra compositing when fully opaque.
const Visibility.maintain({
super.key,
required this.child,
this.visible = true,
}) : maintainState = true,
maintainAnimation = true,
maintainSize = true,
maintainSemantics = true,
maintainInteractivity = true,
replacement = const SizedBox.shrink(); // Unused since maintainState is always true.
/// The widget to show or hide, as controlled by [visible].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The widget to use when the child is not [visible], assuming that none of
/// the `maintain` flags (in particular, [maintainState]) are set.
///
/// The normal behavior is to replace the widget with a zero by zero box
/// ([SizedBox.shrink]).
///
/// See also:
///
/// * [AnimatedCrossFade], which can animate between two children.
final Widget replacement;
/// Switches between showing the [child] or hiding it.
///
/// The `maintain` flags should be set to the same values regardless of the
/// state of the [visible] property, otherwise they will not operate correctly
/// (specifically, the state will be lost regardless of the state of
/// [maintainState] whenever any of the `maintain` flags are changed, since
/// doing so will result in a subtree shape change).
///
/// Unless [maintainState] is set, the [child] subtree will be disposed
/// (removed from the tree) while hidden.
final bool visible;
/// Whether to maintain the [State] objects of the [child] subtree when it is
/// not [visible].
///
/// Keeping the state of the subtree is potentially expensive (because it
/// means all the objects are still in memory; their resources are not
/// released). It should only be maintained if it cannot be recreated on
/// demand. One example of when the state would be maintained is if the child
/// subtree contains a [Navigator], since that widget maintains elaborate
/// state that cannot be recreated on the fly.
///
/// If this property is true, an [Offstage] widget is used to hide the child
/// instead of replacing it with [replacement].
///
/// If this property is false, then [maintainAnimation] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
final bool maintainState;
/// Whether to maintain animations within the [child] subtree when it is
/// not [visible].
///
/// To set this, [maintainState] must also be set.
///
/// Keeping animations active when the widget is not visible is even more
/// expensive than only maintaining the state.
///
/// One example when this might be useful is if the subtree is animating its
/// layout in time with an [AnimationController], and the result of that
/// layout is being used to influence some other logic. If this flag is false,
/// then any [AnimationController]s hosted inside the [child] subtree will be
/// muted while the [visible] flag is false.
///
/// If this property is true, no [TickerMode] widget is used.
///
/// If this property is false, then [maintainSize] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
final bool maintainAnimation;
/// Whether to maintain space for where the widget would have been.
///
/// To set this, [maintainAnimation] and [maintainState] must also be set.
///
/// Maintaining the size when the widget is not [visible] is not notably more
/// expensive than just keeping animations running without maintaining the
/// size, and may in some circumstances be slightly cheaper if the subtree is
/// simple and the [visible] property is frequently toggled, since it avoids
/// triggering a layout change when the [visible] property is toggled. If the
/// [child] subtree is not trivial then it is significantly cheaper to not
/// even keep the state (see [maintainState]).
///
/// If this property is false, [Offstage] is used.
///
/// If this property is false, then [maintainSemantics] and
/// [maintainInteractivity] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
///
/// See also:
///
/// * [AnimatedOpacity] and [FadeTransition], which apply animations to the
/// opacity for a more subtle effect.
final bool maintainSize;
/// Whether to maintain the semantics for the widget when it is hidden (e.g.
/// for accessibility).
///
/// To set this, [maintainSize] must also be set.
///
/// By default, with [maintainSemantics] set to false, the [child] is not
/// visible to accessibility tools when it is hidden from the user. If this
/// flag is set to true, then accessibility tools will report the widget as if
/// it was present.
final bool maintainSemantics;
/// Whether to allow the widget to be interactive when hidden.
///
/// To set this, [maintainSize] must also be set.
///
/// By default, with [maintainInteractivity] set to false, touch events cannot
/// reach the [child] when it is hidden from the user. If this flag is set to
/// true, then touch events will nonetheless be passed through.
final bool maintainInteractivity;
/// Tells the visibility state of an element in the tree based off its
/// ancestor [Visibility] elements.
///
/// If there's one or more [Visibility] widgets in the ancestor tree, this
/// will return true if and only if all of those widgets have [visible] set
/// to true. If there is no [Visibility] widget in the ancestor tree of the
/// specified build context, this will return true.
///
/// This will register a dependency from the specified context on any
/// [Visibility] elements in the ancestor tree, such that if any of their
/// visibilities changes, the specified context will be rebuilt.
static bool of(BuildContext context) {
bool isVisible = true;
BuildContext ancestorContext = context;
InheritedElement? ancestor = ancestorContext.getElementForInheritedWidgetOfExactType<_VisibilityScope>();
while (isVisible && ancestor != null) {
final _VisibilityScope scope = context.dependOnInheritedElement(ancestor) as _VisibilityScope;
isVisible = scope.isVisible;
ancestor.visitAncestorElements((Element parent) {
ancestorContext = parent;
return false;
});
ancestor = ancestorContext.getElementForInheritedWidgetOfExactType<_VisibilityScope>();
}
return isVisible;
}
@override
Widget build(BuildContext context) {
Widget result = child;
if (maintainSize) {
result = _Visibility(
visible: visible,
maintainSemantics: maintainSemantics,
child: IgnorePointer(
ignoring: !visible && !maintainInteractivity,
child: result,
),
);
} else {
assert(!maintainInteractivity);
assert(!maintainSemantics);
assert(!maintainSize);
if (maintainState) {
if (!maintainAnimation) {
result = TickerMode(enabled: visible, child: result);
}
result = Offstage(
offstage: !visible,
child: result,
);
} else {
assert(!maintainAnimation);
assert(!maintainState);
result = visible ? child : replacement;
}
}
return _VisibilityScope(isVisible: visible, child: result);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('visible', value: visible, ifFalse: 'hidden', ifTrue: 'visible'));
properties.add(FlagProperty('maintainState', value: maintainState, ifFalse: 'maintainState'));
properties.add(FlagProperty('maintainAnimation', value: maintainAnimation, ifFalse: 'maintainAnimation'));
properties.add(FlagProperty('maintainSize', value: maintainSize, ifFalse: 'maintainSize'));
properties.add(FlagProperty('maintainSemantics', value: maintainSemantics, ifFalse: 'maintainSemantics'));
properties.add(FlagProperty('maintainInteractivity', value: maintainInteractivity, ifFalse: 'maintainInteractivity'));
}
}
/// Inherited widget that allows descendants to find their visibility status.
class _VisibilityScope extends InheritedWidget {
const _VisibilityScope({required this.isVisible, required super.child});
final bool isVisible;
@override
bool updateShouldNotify(_VisibilityScope old) {
return isVisible != old.isVisible;
}
}
/// Whether to show or hide a sliver child.
///
/// By default, the [visible] property controls whether the [sliver] is included
/// in the subtree or not; when it is not [visible], the [replacementSliver] is
/// included instead.
///
/// A variety of flags can be used to tweak exactly how the sliver is hidden.
/// (Changing the flags dynamically is discouraged, as it can cause the [sliver]
/// subtree to be rebuilt, with any state in the subtree being discarded.
/// Typically, only the [visible] flag is changed dynamically.)
///
/// These widgets provide some of the facets of this one:
///
/// * [SliverOpacity], which can stop its sliver child from being painted.
/// * [SliverOffstage], which can stop its sliver child from being laid out or
/// painted.
/// * [TickerMode], which can stop its child from being animated.
/// * [ExcludeSemantics], which can hide the child from accessibility tools.
/// * [SliverIgnorePointer], which can disable touch interactions with the
/// sliver child.
///
/// Using this widget is not necessary to hide children. The simplest way to
/// hide a child is just to not include it. If a child _must_ be given (e.g.
/// because the parent is a [StatelessWidget]), then including a childless
/// [SliverToBoxAdapter] instead of the child that would otherwise be included
/// is typically more efficient than using [SliverVisibility].
///
/// See also:
///
/// * [Visibility], the equivalent widget for boxes.
class SliverVisibility extends StatelessWidget {
/// Control whether the given [sliver] is [visible].
///
/// The [maintainSemantics] and [maintainInteractivity] arguments can only be
/// set if [maintainSize] is set.
///
/// The [maintainSize] argument can only be set if [maintainAnimation] is set.
///
/// The [maintainAnimation] argument can only be set if [maintainState] is
/// set.
const SliverVisibility({
super.key,
required this.sliver,
this.replacementSliver = const SliverToBoxAdapter(),
this.visible = true,
this.maintainState = false,
this.maintainAnimation = false,
this.maintainSize = false,
this.maintainSemantics = false,
this.maintainInteractivity = false,
}) : assert(
maintainState || !maintainAnimation,
'Cannot maintain animations if the state is not also maintained.',
),
assert(
maintainAnimation || !maintainSize,
'Cannot maintain size if animations are not maintained.',
),
assert(
maintainSize || !maintainSemantics,
'Cannot maintain semantics if size is not maintained.',
),
assert(
maintainSize || !maintainInteractivity,
'Cannot maintain interactivity if size is not maintained.',
);
/// Control whether the given [sliver] is [visible].
///
/// This is equivalent to the default [SliverVisibility] constructor with all
/// "maintain" fields set to true. This constructor should be used in place of
/// a [SliverOpacity] widget that only takes on values of `0.0` or `1.0`, as it
/// avoids extra compositing when fully opaque.
const SliverVisibility.maintain({
super.key,
required this.sliver,
this.replacementSliver = const SliverToBoxAdapter(),
this.visible = true,
}) : maintainState = true,
maintainAnimation = true,
maintainSize = true,
maintainSemantics = true,
maintainInteractivity = true;
/// The sliver to show or hide, as controlled by [visible].
final Widget sliver;
/// The widget to use when the sliver child is not [visible], assuming that
/// none of the `maintain` flags (in particular, [maintainState]) are set.
///
/// The normal behavior is to replace the widget with a childless
/// [SliverToBoxAdapter], which by default has a geometry of
/// [SliverGeometry.zero].
final Widget replacementSliver;
/// Switches between showing the [sliver] or hiding it.
///
/// The `maintain` flags should be set to the same values regardless of the
/// state of the [visible] property, otherwise they will not operate correctly
/// (specifically, the state will be lost regardless of the state of
/// [maintainState] whenever any of the `maintain` flags are changed, since
/// doing so will result in a subtree shape change).
///
/// Unless [maintainState] is set, the [sliver] subtree will be disposed
/// (removed from the tree) while hidden.
final bool visible;
/// Whether to maintain the [State] objects of the [sliver] subtree when it is
/// not [visible].
///
/// Keeping the state of the subtree is potentially expensive (because it
/// means all the objects are still in memory; their resources are not
/// released). It should only be maintained if it cannot be recreated on
/// demand. One example of when the state would be maintained is if the sliver
/// subtree contains a [Navigator], since that widget maintains elaborate
/// state that cannot be recreated on the fly.
///
/// If this property is true, a [SliverOffstage] widget is used to hide the
/// sliver instead of replacing it with [replacementSliver].
///
/// If this property is false, then [maintainAnimation] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
final bool maintainState;
/// Whether to maintain animations within the [sliver] subtree when it is
/// not [visible].
///
/// To set this, [maintainState] must also be set.
///
/// Keeping animations active when the widget is not visible is even more
/// expensive than only maintaining the state.
///
/// One example when this might be useful is if the subtree is animating its
/// layout in time with an [AnimationController], and the result of that
/// layout is being used to influence some other logic. If this flag is false,
/// then any [AnimationController]s hosted inside the [sliver] subtree will be
/// muted while the [visible] flag is false.
///
/// If this property is true, no [TickerMode] widget is used.
///
/// If this property is false, then [maintainSize] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
final bool maintainAnimation;
/// Whether to maintain space for where the sliver would have been.
///
/// To set this, [maintainAnimation] must also be set.
///
/// Maintaining the size when the sliver is not [visible] is not notably more
/// expensive than just keeping animations running without maintaining the
/// size, and may in some circumstances be slightly cheaper if the subtree is
/// simple and the [visible] property is frequently toggled, since it avoids
/// triggering a layout change when the [visible] property is toggled. If the
/// [sliver] subtree is not trivial then it is significantly cheaper to not
/// even keep the state (see [maintainState]).
///
/// If this property is false, [SliverOffstage] is used.
///
/// If this property is false, then [maintainSemantics] and
/// [maintainInteractivity] must also be false.
///
/// Dynamically changing this value may cause the current state of the
/// subtree to be lost (and a new instance of the subtree, with new [State]
/// objects, to be immediately created if [visible] is true).
final bool maintainSize;
/// Whether to maintain the semantics for the sliver when it is hidden (e.g.
/// for accessibility).
///
/// To set this, [maintainSize] must also be set.
///
/// By default, with [maintainSemantics] set to false, the [sliver] is not
/// visible to accessibility tools when it is hidden from the user. If this
/// flag is set to true, then accessibility tools will report the widget as if
/// it was present.
final bool maintainSemantics;
/// Whether to allow the sliver to be interactive when hidden.
///
/// To set this, [maintainSize] must also be set.
///
/// By default, with [maintainInteractivity] set to false, touch events cannot
/// reach the [sliver] when it is hidden from the user. If this flag is set to
/// true, then touch events will nonetheless be passed through.
final bool maintainInteractivity;
@override
Widget build(BuildContext context) {
if (maintainSize) {
Widget result = sliver;
result = SliverIgnorePointer(
ignoring: !visible && !maintainInteractivity,
sliver: result,
);
return _SliverVisibility(
visible: visible,
maintainSemantics: maintainSemantics,
sliver: result,
);
}
assert(!maintainInteractivity);
assert(!maintainSemantics);
assert(!maintainSize);
if (maintainState) {
Widget result = sliver;
if (!maintainAnimation) {
result = TickerMode(enabled: visible, child: sliver);
}
return SliverOffstage(
sliver: result,
offstage: !visible,
);
}
assert(!maintainAnimation);
assert(!maintainState);
return visible ? sliver : replacementSliver;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(FlagProperty('visible', value: visible, ifFalse: 'hidden', ifTrue: 'visible'));
properties.add(FlagProperty('maintainState', value: maintainState, ifFalse: 'maintainState'));
properties.add(FlagProperty('maintainAnimation', value: maintainAnimation, ifFalse: 'maintainAnimation'));
properties.add(FlagProperty('maintainSize', value: maintainSize, ifFalse: 'maintainSize'));
properties.add(FlagProperty('maintainSemantics', value: maintainSemantics, ifFalse: 'maintainSemantics'));
properties.add(FlagProperty('maintainInteractivity', value: maintainInteractivity, ifFalse: 'maintainInteractivity'));
}
}
// A widget that conditionally hides its child, but without the forced compositing of `Opacity`.
//
// A fully opaque `Opacity` widget is required to leave its opacity layer in the layer tree. This
// forces all parent render objects to also composite, which can break a simple scene into many
// different layers. This can be significantly more expensive, so the issue is avoided by a
// specialized render object that does not ever force compositing.
class _Visibility extends SingleChildRenderObjectWidget {
const _Visibility({ required this.visible, required this.maintainSemantics, super.child });
final bool visible;
final bool maintainSemantics;
@override
_RenderVisibility createRenderObject(BuildContext context) {
return _RenderVisibility(visible, maintainSemantics);
}
@override
void updateRenderObject(BuildContext context, _RenderVisibility renderObject) {
renderObject
..visible = visible
..maintainSemantics = maintainSemantics;
}
}
class _RenderVisibility extends RenderProxyBox {
_RenderVisibility(this._visible, this._maintainSemantics);
bool get visible => _visible;
bool _visible;
set visible(bool value) {
if (value == visible) {
return;
}
_visible = value;
markNeedsPaint();
}
bool get maintainSemantics => _maintainSemantics;
bool _maintainSemantics;
set maintainSemantics(bool value) {
if (value == maintainSemantics) {
return;
}
_maintainSemantics = value;
markNeedsSemanticsUpdate();
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (maintainSemantics || visible) {
super.visitChildrenForSemantics(visitor);
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (!visible) {
return;
}
super.paint(context, offset);
}
}
// A widget that conditionally hides its child, but without the forced compositing of `SliverOpacity`.
//
// A fully opaque `SliverOpacity` widget is required to leave its opacity layer in the layer tree.
// This forces all parent render objects to also composite, which can break a simple scene into many
// different layers. This can be significantly more expensive, so the issue is avoided by a
// specialized render object that does not ever force compositing.
class _SliverVisibility extends SingleChildRenderObjectWidget {
const _SliverVisibility({ required this.visible, required this.maintainSemantics, Widget? sliver })
: super(child: sliver);
final bool visible;
final bool maintainSemantics;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderSliverVisibility(visible, maintainSemantics);
}
@override
void updateRenderObject(BuildContext context, _RenderSliverVisibility renderObject) {
renderObject
..visible = visible
..maintainSemantics = maintainSemantics;
}
}
class _RenderSliverVisibility extends RenderProxySliver {
_RenderSliverVisibility(this._visible, this._maintainSemantics);
bool get visible => _visible;
bool _visible;
set visible(bool value) {
if (value == visible) {
return;
}
_visible = value;
markNeedsPaint();
}
bool get maintainSemantics => _maintainSemantics;
bool _maintainSemantics;
set maintainSemantics(bool value) {
if (value == maintainSemantics) {
return;
}
_maintainSemantics = value;
markNeedsSemanticsUpdate();
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
if (maintainSemantics || visible) {
super.visitChildrenForSemantics(visitor);
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (!visible) {
return;
}
super.paint(context, offset);
}
}
| flutter/packages/flutter/lib/src/widgets/visibility.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/visibility.dart",
"repo_id": "flutter",
"token_count": 7708
} | 721 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
import '../scheduler/scheduler_tester.dart';
class BogusCurve extends Curve {
const BogusCurve();
@override
double transform(double t) => 100.0;
}
void main() {
setUp(() {
WidgetsFlutterBinding.ensureInitialized();
WidgetsBinding.instance
..resetEpoch()
..platformDispatcher.onBeginFrame = null
..platformDispatcher.onDrawFrame = null;
});
test('toString control test', () {
expect(kAlwaysCompleteAnimation, hasOneLineDescription);
expect(kAlwaysDismissedAnimation, hasOneLineDescription);
expect(const AlwaysStoppedAnimation<double>(0.5), hasOneLineDescription);
CurvedAnimation curvedAnimation = CurvedAnimation(
parent: kAlwaysDismissedAnimation,
curve: Curves.ease,
);
expect(curvedAnimation, hasOneLineDescription);
curvedAnimation.reverseCurve = Curves.elasticOut;
expect(curvedAnimation, hasOneLineDescription);
final AnimationController controller = AnimationController(
duration: const Duration(milliseconds: 500),
vsync: const TestVSync(),
);
controller
..value = 0.5
..reverse();
curvedAnimation = CurvedAnimation(
parent: controller,
curve: Curves.ease,
reverseCurve: Curves.elasticOut,
);
expect(curvedAnimation, hasOneLineDescription);
controller.stop();
});
test('ProxyAnimation.toString control test', () {
final ProxyAnimation animation = ProxyAnimation();
expect(animation.value, 0.0);
expect(animation.status, AnimationStatus.dismissed);
expect(animation, hasOneLineDescription);
animation.parent = kAlwaysDismissedAnimation;
expect(animation, hasOneLineDescription);
});
test('ProxyAnimation set parent generates value changed', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
controller.value = 0.5;
bool didReceiveCallback = false;
final ProxyAnimation animation = ProxyAnimation()
..addListener(() {
didReceiveCallback = true;
});
expect(didReceiveCallback, isFalse);
animation.parent = controller;
expect(didReceiveCallback, isTrue);
didReceiveCallback = false;
expect(didReceiveCallback, isFalse);
controller.value = 0.6;
expect(didReceiveCallback, isTrue);
});
test('ReverseAnimation calls listeners', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
controller.value = 0.5;
bool didReceiveCallback = false;
void listener() {
didReceiveCallback = true;
}
final ReverseAnimation animation = ReverseAnimation(controller)
..addListener(listener);
expect(didReceiveCallback, isFalse);
controller.value = 0.6;
expect(didReceiveCallback, isTrue);
didReceiveCallback = false;
animation.removeListener(listener);
expect(didReceiveCallback, isFalse);
controller.value = 0.7;
expect(didReceiveCallback, isFalse);
expect(animation, hasOneLineDescription);
});
test('TrainHoppingAnimation', () {
final AnimationController currentTrain = AnimationController(
vsync: const TestVSync(),
);
final AnimationController nextTrain = AnimationController(
vsync: const TestVSync(),
);
currentTrain.value = 0.5;
nextTrain.value = 0.75;
bool didSwitchTrains = false;
final TrainHoppingAnimation animation = TrainHoppingAnimation(
currentTrain,
nextTrain,
onSwitchedTrain: () {
didSwitchTrains = true;
},
);
expect(didSwitchTrains, isFalse);
expect(animation.value, 0.5);
expect(animation, hasOneLineDescription);
nextTrain.value = 0.25;
expect(didSwitchTrains, isTrue);
expect(animation.value, 0.25);
expect(animation, hasOneLineDescription);
expect(animation.toString(), contains('no next'));
});
test('TrainHoppingAnimation dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => TrainHoppingAnimation(
const AlwaysStoppedAnimation<double>(1),
const AlwaysStoppedAnimation<double>(1),
).dispose(),
TrainHoppingAnimation,
),
areCreateAndDispose,
);
});
test('AnimationMean control test', () {
final AnimationController left = AnimationController(
value: 0.5,
vsync: const TestVSync(),
);
final AnimationController right = AnimationController(
vsync: const TestVSync(),
);
final AnimationMean mean = AnimationMean(left: left, right: right);
expect(mean, hasOneLineDescription);
expect(mean.value, equals(0.25));
final List<double> log = <double>[];
void logValue() {
log.add(mean.value);
}
mean.addListener(logValue);
right.value = 1.0;
expect(mean.value, equals(0.75));
expect(log, equals(<double>[0.75]));
log.clear();
mean.removeListener(logValue);
left.value = 0.0;
expect(mean.value, equals(0.50));
expect(log, isEmpty);
});
test('AnimationMax control test', () {
final AnimationController first = AnimationController(
value: 0.5,
vsync: const TestVSync(),
);
final AnimationController second = AnimationController(
vsync: const TestVSync(),
);
final AnimationMax<double> max = AnimationMax<double>(first, second);
expect(max, hasOneLineDescription);
expect(max.value, equals(0.5));
final List<double> log = <double>[];
void logValue() {
log.add(max.value);
}
max.addListener(logValue);
second.value = 1.0;
expect(max.value, equals(1.0));
expect(log, equals(<double>[1.0]));
log.clear();
max.removeListener(logValue);
first.value = 0.0;
expect(max.value, equals(1.0));
expect(log, isEmpty);
});
test('AnimationMin control test', () {
final AnimationController first = AnimationController(
value: 0.5,
vsync: const TestVSync(),
);
final AnimationController second = AnimationController(
vsync: const TestVSync(),
);
final AnimationMin<double> min = AnimationMin<double>(first, second);
expect(min, hasOneLineDescription);
expect(min.value, equals(0.0));
final List<double> log = <double>[];
void logValue() {
log.add(min.value);
}
min.addListener(logValue);
second.value = 1.0;
expect(min.value, equals(0.5));
expect(log, equals(<double>[0.5]));
log.clear();
min.removeListener(logValue);
first.value = 0.25;
expect(min.value, equals(0.25));
expect(log, isEmpty);
});
test('CurvedAnimation with bogus curve', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final CurvedAnimation curved = CurvedAnimation(parent: controller, curve: const BogusCurve());
FlutterError? error;
try {
curved.value;
} on FlutterError catch (e) {
error = e;
}
expect(error, isNotNull);
expect(
error!.toStringDeep(),
// RegExp matcher is required here due to flutter web and flutter mobile generating
// slightly different floating point numbers
// in Flutter web 0.0 sometimes just appears as 0. or 0
matches(RegExp(r'''
FlutterError
Invalid curve endpoint at \d+(\.\d*)?\.
Curves must map 0\.0 to near zero and 1\.0 to near one but
BogusCurve mapped \d+(\.\d*)? to \d+(\.\d*)?, which is near \d+(\.\d*)?\.
''', multiLine: true)),
);
});
test('CurvedAnimation running with different forward and reverse durations.', () {
final AnimationController controller = AnimationController(
duration: const Duration(milliseconds: 100),
reverseDuration: const Duration(milliseconds: 50),
vsync: const TestVSync(),
);
final CurvedAnimation curved = CurvedAnimation(parent: controller, curve: Curves.linear, reverseCurve: Curves.linear);
controller.forward();
tick(Duration.zero);
tick(const Duration(milliseconds: 10));
expect(curved.value, moreOrLessEquals(0.1));
tick(const Duration(milliseconds: 20));
expect(curved.value, moreOrLessEquals(0.2));
tick(const Duration(milliseconds: 30));
expect(curved.value, moreOrLessEquals(0.3));
tick(const Duration(milliseconds: 40));
expect(curved.value, moreOrLessEquals(0.4));
tick(const Duration(milliseconds: 50));
expect(curved.value, moreOrLessEquals(0.5));
tick(const Duration(milliseconds: 60));
expect(curved.value, moreOrLessEquals(0.6));
tick(const Duration(milliseconds: 70));
expect(curved.value, moreOrLessEquals(0.7));
tick(const Duration(milliseconds: 80));
expect(curved.value, moreOrLessEquals(0.8));
tick(const Duration(milliseconds: 90));
expect(curved.value, moreOrLessEquals(0.9));
tick(const Duration(milliseconds: 100));
expect(curved.value, moreOrLessEquals(1.0));
controller.reverse();
tick(const Duration(milliseconds: 110));
expect(curved.value, moreOrLessEquals(1.0));
tick(const Duration(milliseconds: 120));
expect(curved.value, moreOrLessEquals(0.8));
tick(const Duration(milliseconds: 130));
expect(curved.value, moreOrLessEquals(0.6));
tick(const Duration(milliseconds: 140));
expect(curved.value, moreOrLessEquals(0.4));
tick(const Duration(milliseconds: 150));
expect(curved.value, moreOrLessEquals(0.2));
tick(const Duration(milliseconds: 160));
expect(curved.value, moreOrLessEquals(0.0));
});
test('CurvedAnimation stops listening to parent when disposed.', () async {
const Interval forwardCurve = Interval(0.0, 0.5);
const Interval reverseCurve = Interval(0.5, 1.0);
final AnimationController controller = AnimationController(
duration: const Duration(milliseconds: 100),
reverseDuration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
);
final CurvedAnimation curved = CurvedAnimation(
parent: controller, curve: forwardCurve, reverseCurve: reverseCurve);
expect(forwardCurve.transform(0.5), 1.0);
expect(reverseCurve.transform(0.5), 0.0);
controller.forward(from: 0.5);
expect(controller.status, equals(AnimationStatus.forward));
expect(curved.value, equals(1.0));
controller.value = 1.0;
expect(controller.status, equals(AnimationStatus.completed));
controller.reverse(from: 0.5);
expect(controller.status, equals(AnimationStatus.reverse));
expect(curved.value, equals(0.0));
expect(curved.isDisposed, isFalse);
curved.dispose();
expect(curved.isDisposed, isTrue);
controller.value = 0.0;
expect(controller.status, equals(AnimationStatus.dismissed));
controller.forward(from: 0.5);
expect(controller.status, equals(AnimationStatus.forward));
expect(curved.value, equals(0.0));
});
test('ReverseAnimation running with different forward and reverse durations.', () {
final AnimationController controller = AnimationController(
duration: const Duration(milliseconds: 100),
reverseDuration: const Duration(milliseconds: 50),
vsync: const TestVSync(),
);
final ReverseAnimation reversed = ReverseAnimation(
CurvedAnimation(
parent: controller,
curve: Curves.linear,
reverseCurve: Curves.linear,
),
);
controller.forward();
tick(Duration.zero);
tick(const Duration(milliseconds: 10));
expect(reversed.value, moreOrLessEquals(0.9));
tick(const Duration(milliseconds: 20));
expect(reversed.value, moreOrLessEquals(0.8));
tick(const Duration(milliseconds: 30));
expect(reversed.value, moreOrLessEquals(0.7));
tick(const Duration(milliseconds: 40));
expect(reversed.value, moreOrLessEquals(0.6));
tick(const Duration(milliseconds: 50));
expect(reversed.value, moreOrLessEquals(0.5));
tick(const Duration(milliseconds: 60));
expect(reversed.value, moreOrLessEquals(0.4));
tick(const Duration(milliseconds: 70));
expect(reversed.value, moreOrLessEquals(0.3));
tick(const Duration(milliseconds: 80));
expect(reversed.value, moreOrLessEquals(0.2));
tick(const Duration(milliseconds: 90));
expect(reversed.value, moreOrLessEquals(0.1));
tick(const Duration(milliseconds: 100));
expect(reversed.value, moreOrLessEquals(0.0));
controller.reverse();
tick(const Duration(milliseconds: 110));
expect(reversed.value, moreOrLessEquals(0.0));
tick(const Duration(milliseconds: 120));
expect(reversed.value, moreOrLessEquals(0.2));
tick(const Duration(milliseconds: 130));
expect(reversed.value, moreOrLessEquals(0.4));
tick(const Duration(milliseconds: 140));
expect(reversed.value, moreOrLessEquals(0.6));
tick(const Duration(milliseconds: 150));
expect(reversed.value, moreOrLessEquals(0.8));
tick(const Duration(milliseconds: 160));
expect(reversed.value, moreOrLessEquals(1.0));
});
test('TweenSequence', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final Animation<double> animation = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 5.0, end: 10.0),
weight: 4.0,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(10.0),
weight: 2.0,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 10.0, end: 5.0),
weight: 4.0,
),
],
).animate(controller);
expect(animation.value, 5.0);
controller.value = 0.2;
expect(animation.value, 7.5);
controller.value = 0.4;
expect(animation.value, 10.0);
controller.value = 0.6;
expect(animation.value, 10.0);
controller.value = 0.8;
expect(animation.value, 7.5);
controller.value = 1.0;
expect(animation.value, 5.0);
});
test('TweenSequence with curves', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final Animation<double> animation = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 5.0, end: 10.0)
.chain(CurveTween(curve: const Interval(0.5, 1.0))),
weight: 4.0,
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(10.0)
.chain(CurveTween(curve: Curves.linear)), // linear is a no-op
weight: 2.0,
),
TweenSequenceItem<double>(
tween: Tween<double>(begin: 10.0, end: 5.0)
.chain(CurveTween(curve: const Interval(0.0, 0.5))),
weight: 4.0,
),
],
).animate(controller);
expect(animation.value, 5.0);
controller.value = 0.2;
expect(animation.value, 5.0);
controller.value = 0.4;
expect(animation.value, 10.0);
controller.value = 0.6;
expect(animation.value, 10.0);
controller.value = 0.8;
expect(animation.value, 5.0);
controller.value = 1.0;
expect(animation.value, 5.0);
});
test('TweenSequence, one tween', () {
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
);
final Animation<double> animation = TweenSequence<double>(
<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: Tween<double>(begin: 5.0, end: 10.0),
weight: 1.0,
),
],
).animate(controller);
expect(animation.value, 5.0);
controller.value = 0.5;
expect(animation.value, 7.5);
controller.value = 1.0;
expect(animation.value, 10.0);
});
test('$CurvedAnimation dispatches memory events', () async {
await expectLater(
await memoryEvents(
() => CurvedAnimation(
parent: AnimationController(
duration: const Duration(milliseconds: 100),
vsync: const TestVSync(),
),
curve: Curves.linear,
).dispose(),
CurvedAnimation,
),
areCreateAndDispose,
);
});
}
| flutter/packages/flutter/test/animation/animations_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/animations_test.dart",
"repo_id": "flutter",
"token_count": 6196
} | 722 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
// Constants taken from _ContextMenuActionState.
const CupertinoDynamicColor kBackgroundColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFFF1F1F1),
darkColor: Color(0xFF212122),
);
const CupertinoDynamicColor kBackgroundColorPressed = CupertinoDynamicColor.withBrightness(
color: Color(0xFFDDDDDD),
darkColor: Color(0xFF3F3F40),
);
const Color kDestructiveActionColor = CupertinoColors.destructiveRed;
const FontWeight kDefaultActionWeight = FontWeight.w600;
Widget getApp({
VoidCallback? onPressed,
bool isDestructiveAction = false,
bool isDefaultAction = false,
Brightness? brightness,
}) {
final UniqueKey actionKey = UniqueKey();
final CupertinoContextMenuAction action = CupertinoContextMenuAction(
key: actionKey,
onPressed: onPressed,
trailingIcon: CupertinoIcons.home,
isDestructiveAction: isDestructiveAction,
isDefaultAction: isDefaultAction,
child: const Text('I am a CupertinoContextMenuAction'),
);
return CupertinoApp(
theme: CupertinoThemeData(
brightness: brightness ?? Brightness.light,
),
home: CupertinoPageScaffold(
child: Center(
child: action,
),
),
);
}
TextStyle getTextStyle(WidgetTester tester) {
final Finder finder = find.descendant(
of: find.byType(CupertinoContextMenuAction),
matching: find.byType(DefaultTextStyle),
);
expect(finder, findsOneWidget);
final DefaultTextStyle defaultStyle = tester.widget(finder);
return defaultStyle.style;
}
Icon getIcon(WidgetTester tester) {
final Finder finder = find.descendant(
of: find.byType(CupertinoContextMenuAction),
matching: find.byType(Icon),
);
expect(finder, findsOneWidget);
final Icon icon = tester.widget(finder);
return icon;
}
testWidgets('responds to taps', (WidgetTester tester) async {
bool wasPressed = false;
await tester.pumpWidget(getApp(onPressed: () {
wasPressed = true;
}));
expect(wasPressed, false);
await tester.tap(find.byType(CupertinoContextMenuAction));
expect(wasPressed, true);
});
testWidgets('turns grey when pressed and held', (WidgetTester tester) async {
await tester.pumpWidget(getApp());
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColor.color));
final Offset actionCenterLight =
tester.getCenter(find.byType(CupertinoContextMenuAction));
final TestGesture gestureLight =
await tester.startGesture(actionCenterLight);
await tester.pump();
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColorPressed.color));
await gestureLight.up();
await tester.pump();
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColor.color));
await tester.pumpWidget(getApp(brightness: Brightness.dark));
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColor.darkColor));
final Offset actionCenterDark =
tester.getCenter(find.byType(CupertinoContextMenuAction));
final TestGesture gestureDark = await tester.startGesture(actionCenterDark);
await tester.pump();
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColorPressed.darkColor));
await gestureDark.up();
await tester.pump();
expect(find.byType(CupertinoContextMenuAction),
paints..rect(color: kBackgroundColor.darkColor));
});
testWidgets('icon and textStyle colors are correct out of the box', (WidgetTester tester) async {
await tester.pumpWidget(getApp());
expect(getTextStyle(tester).color, CupertinoColors.label);
expect(getIcon(tester).color, CupertinoColors.label);
});
testWidgets('icon and textStyle colors are correct for destructive actions', (WidgetTester tester) async {
await tester.pumpWidget(getApp(isDestructiveAction: true));
expect(getTextStyle(tester).color, kDestructiveActionColor);
expect(getIcon(tester).color, kDestructiveActionColor);
});
testWidgets('textStyle is correct for defaultAction for Brightness.light', (WidgetTester tester) async {
await tester.pumpWidget(getApp(isDefaultAction: true));
expect(getTextStyle(tester).fontWeight, kDefaultActionWeight);
final Element context = tester.element(find.byType(CupertinoContextMenuAction));
// The dynamic color should have been resolved.
expect(getTextStyle(tester).color, CupertinoColors.label.resolveFrom(context));
});
testWidgets('textStyle is correct for defaultAction for Brightness.dark', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/144492.
await tester.pumpWidget(getApp(isDefaultAction: true, brightness: Brightness.dark));
expect(getTextStyle(tester).fontWeight, kDefaultActionWeight);
final Element context = tester.element(find.byType(CupertinoContextMenuAction));
// The dynamic color should have been resolved.
expect(getTextStyle(tester).color, CupertinoColors.label.resolveFrom(context));
});
testWidgets(
'Hovering over Cupertino context menu action updates cursor to clickable on Web',
(WidgetTester tester) async {
/// Cupertino context menu action without "onPressed" callback.
await tester.pumpWidget(getApp());
final Offset contextMenuAction =
tester.getCenter(find.text('I am a CupertinoContextMenuAction'));
final TestGesture gesture =
await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: contextMenuAction);
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
SystemMouseCursors.basic);
// / Cupertino context menu action with "onPressed" callback.
await tester.pumpWidget(getApp(onPressed: () {}));
await gesture.moveTo(const Offset(10, 10));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
SystemMouseCursors.basic);
await gesture.moveTo(contextMenuAction);
await tester.pumpAndSettle();
expect(
RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic,
);
});
}
| flutter/packages/flutter/test/cupertino/context_menu_action_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/context_menu_action_test.dart",
"repo_id": "flutter",
"token_count": 2372
} | 723 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/cupertino.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
int count = 0;
void main() {
testWidgets('Middle still in center with asymmetrical actions', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
leading: CupertinoButton(
onPressed: null,
child: Text('Something'),
),
middle: Text('Title'),
),
),
);
// Expect the middle of the title to be exactly in the middle of the screen.
expect(tester.getCenter(find.text('Title')).dx, 400.0);
});
testWidgets('Middle still in center with back button', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Title'),
),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Page 2'),
);
},
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
// Expect the middle of the title to be exactly in the middle of the screen.
expect(tester.getCenter(find.text('Page 2')).dx, 400.0);
});
testWidgets('Opaque background does not add blur effects, non-opaque background adds blur effects', (WidgetTester tester) async {
const CupertinoDynamicColor background = CupertinoDynamicColor.withBrightness(
color: Color(0xFFE5E5E5),
darkColor: Color(0xF3E5E5E5),
);
await tester.pumpWidget(
const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: CupertinoNavigationBar(
middle: Text('Title'),
backgroundColor: background,
),
),
);
expect(find.byType(BackdropFilter), findsNothing);
expect(find.byType(CupertinoNavigationBar), paints..rect(color: background.color));
await tester.pumpWidget(
const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoNavigationBar(
middle: Text('Title'),
backgroundColor: background,
),
),
);
expect(find.byType(BackdropFilter), findsOneWidget);
expect(find.byType(CupertinoNavigationBar), paints..rect(color: background.darkColor));
});
testWidgets('Non-opaque background adds blur effects', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Title'),
),
),
);
expect(find.byType(BackdropFilter), findsOneWidget);
});
testWidgets('Nav bar displays correctly', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigator = GlobalKey<NavigatorState>();
await tester.pumpWidget(
CupertinoApp(
navigatorKey: navigator,
home: const CupertinoNavigationBar(
middle: Text('Page 1'),
),
),
);
navigator.currentState!.push<void>(CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Page 2'),
);
},
));
await tester.pumpAndSettle();
expect(find.byType(CupertinoNavigationBarBackButton), findsOneWidget);
// Pops the page 2
navigator.currentState!.pop();
await tester.pump();
// Needs another pump to trigger the rebuild;
await tester.pump();
// The back button should still persist;
expect(find.byType(CupertinoNavigationBarBackButton), findsOneWidget);
// The app does not crash
expect(tester.takeException(), isNull);
});
testWidgets('Can specify custom padding', (WidgetTester tester) async {
final Key middleBox = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: Align(
alignment: Alignment.topCenter,
child: CupertinoNavigationBar(
leading: const CupertinoButton(onPressed: null, child: Text('Cheetah')),
// Let the box take all the vertical space to test vertical padding but let
// the nav bar position it horizontally.
middle: Align(
key: middleBox,
widthFactor: 1.0,
child: const Text('Title'),
),
trailing: const CupertinoButton(onPressed: null, child: Text('Puma')),
padding: const EdgeInsetsDirectional.only(
start: 10.0,
end: 20.0,
top: 3.0,
bottom: 4.0,
),
),
),
),
);
expect(tester.getRect(find.byKey(middleBox)).top, 3.0);
// 44 is the standard height of the nav bar.
expect(
tester.getRect(find.byKey(middleBox)).bottom,
// 44 is the standard height of the nav bar.
44.0 - 4.0,
);
expect(tester.getTopLeft(find.widgetWithText(CupertinoButton, 'Cheetah')).dx, 10.0);
expect(tester.getTopRight(find.widgetWithText(CupertinoButton, 'Puma')).dx, 800.0 - 20.0);
// Title is still exactly centered.
expect(tester.getCenter(find.text('Title')).dx, 400.0);
});
// Assert that two SystemUiOverlayStyle instances have the same values for
// status bar properties and that the first instance has no system navigation
// bar properties set.
void expectSameStatusBarStyle(SystemUiOverlayStyle style, SystemUiOverlayStyle expectedStyle) {
expect(style.statusBarColor, expectedStyle.statusBarColor);
expect(style.statusBarBrightness, expectedStyle.statusBarBrightness);
expect(style.statusBarIconBrightness, expectedStyle.statusBarIconBrightness);
expect(style.systemStatusBarContrastEnforced, expectedStyle.systemStatusBarContrastEnforced);
expect(style.systemNavigationBarColor, isNull);
expect(style.systemNavigationBarContrastEnforced, isNull);
expect(style.systemNavigationBarDividerColor, isNull);
expect(style.systemNavigationBarIconBrightness, isNull);
}
// Regression test for https://github.com/flutter/flutter/issues/119270
testWidgets('System navigation bar properties are not overridden', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
backgroundColor: Color(0xF0F9F9F9),
),
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.dark);
});
testWidgets('Can specify custom brightness', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
backgroundColor: Color(0xF0F9F9F9),
brightness: Brightness.dark,
),
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.light);
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
backgroundColor: Color(0xF01D1D1D),
brightness: Brightness.light,
),
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.dark);
await tester.pumpWidget(
const CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Title'),
backgroundColor: Color(0xF0F9F9F9),
brightness: Brightness.dark,
),
],
),
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.light);
await tester.pumpWidget(
const CupertinoApp(
home: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Title'),
backgroundColor: Color(0xF01D1D1D),
brightness: Brightness.light,
),
],
),
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.dark);
});
testWidgets('Padding works in RTL', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Align(
alignment: Alignment.topCenter,
child: CupertinoNavigationBar(
leading: CupertinoButton(onPressed: null, child: Text('Cheetah')),
// Let the box take all the vertical space to test vertical padding but let
// the nav bar position it horizontally.
middle: Text('Title'),
trailing: CupertinoButton(onPressed: null, child: Text('Puma')),
padding: EdgeInsetsDirectional.only(
start: 10.0,
end: 20.0,
),
),
),
),
),
);
expect(tester.getTopRight(find.widgetWithText(CupertinoButton, 'Cheetah')).dx, 800.0 - 10.0);
expect(tester.getTopLeft(find.widgetWithText(CupertinoButton, 'Puma')).dx, 20.0);
// Title is still exactly centered.
expect(tester.getCenter(find.text('Title')).dx, 400.0);
});
testWidgets('Nav bar uses theme defaults', (WidgetTester tester) async {
count = 0x000000;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoNavigationBar(
leading: CupertinoButton(
onPressed: () { },
child: _ExpectStyles(color: CupertinoColors.systemBlue.color, index: 0x000001),
),
middle: const _ExpectStyles(color: CupertinoColors.black, index: 0x000100),
trailing: CupertinoButton(
onPressed: () { },
child: _ExpectStyles(color: CupertinoColors.systemBlue.color, index: 0x010000),
),
),
),
);
expect(count, 0x010101);
});
testWidgets('Nav bar respects themes', (WidgetTester tester) async {
count = 0x000000;
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoNavigationBar(
leading: CupertinoButton(
onPressed: () { },
child: _ExpectStyles(color: CupertinoColors.systemBlue.darkColor, index: 0x000001),
),
middle: const _ExpectStyles(color: CupertinoColors.white, index: 0x000100),
trailing: CupertinoButton(
onPressed: () { },
child: _ExpectStyles(color: CupertinoColors.systemBlue.darkColor, index: 0x010000),
),
),
),
);
expect(count, 0x010101);
});
testWidgets('Theme active color can be overridden', (WidgetTester tester) async {
count = 0x000000;
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(primaryColor: Color(0xFF001122)),
home: CupertinoNavigationBar(
leading: CupertinoButton(
onPressed: () { },
child: const _ExpectStyles(color: Color(0xFF001122), index: 0x000001),
),
middle: const _ExpectStyles(color: Color(0xFF000000), index: 0x000100),
trailing: CupertinoButton(
onPressed: () { },
child: const _ExpectStyles(color: Color(0xFF001122), index: 0x010000),
),
),
),
);
expect(count, 0x010101);
});
testWidgets('No slivers with no large titles', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Title'),
),
child: Center(),
),
),
);
expect(find.byType(SliverPersistentHeader), findsNothing);
});
testWidgets('Media padding is applied to CupertinoSliverNavigationBar', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
final Key leadingKey = GlobalKey();
final Key middleKey = GlobalKey();
final Key trailingKey = GlobalKey();
final Key titleKey = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
top: 10.0,
left: 20.0,
bottom: 30.0,
right: 40.0,
),
),
child: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
CupertinoSliverNavigationBar(
leading: Placeholder(key: leadingKey),
middle: Placeholder(key: middleKey),
largeTitle: Text('Large Title', key: titleKey),
trailing: Placeholder(key: trailingKey),
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
),
);
// Media padding applied to leading (T,L), middle (T), trailing (T, R).
expect(tester.getTopLeft(find.byKey(leadingKey)), const Offset(16.0 + 20.0, 10.0));
expect(tester.getRect(find.byKey(middleKey)).top, 10.0);
expect(tester.getTopRight(find.byKey(trailingKey)), const Offset(800.0 - 16.0 - 40.0, 10.0));
// Top and left padding is applied to large title.
expect(tester.getTopLeft(find.byKey(titleKey)), const Offset(16.0 + 20.0, 54.0 + 10.0));
});
testWidgets('Large title nav bar scrolls', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: Text('Title'),
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
expect(scrollController.offset, 0.0);
expect(tester.getTopLeft(find.byType(NavigationToolbar)).dy, 0.0);
expect(tester.getSize(find.byType(NavigationToolbar)).height, 44.0);
expect(find.text('Title'), findsNWidgets(2)); // Though only one is visible.
List<Element> titles = tester.elementList(find.text('Title'))
.toList()
..sort((Element a, Element b) {
final RenderParagraph aParagraph = a.renderObject! as RenderParagraph;
final RenderParagraph bParagraph = b.renderObject! as RenderParagraph;
return aParagraph.text.style!.fontSize!.compareTo(bParagraph.text.style!.fontSize!);
});
Iterable<double> opacities = titles.map<double>((Element element) {
final RenderAnimatedOpacity renderOpacity = element.findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
return renderOpacity.opacity.value;
});
expect(opacities, <double> [
0.0, // Initially the smaller font title is invisible.
1.0, // The larger font title is visible.
]);
expect(tester.getTopLeft(find.widgetWithText(ClipRect, 'Title').first).dy, 44.0);
expect(tester.getSize(find.widgetWithText(ClipRect, 'Title').first).height, 52.0);
scrollController.jumpTo(600.0);
await tester.pump(); // Once to trigger the opacity animation.
await tester.pump(const Duration(milliseconds: 300));
titles = tester.elementList(find.text('Title'))
.toList()
..sort((Element a, Element b) {
final RenderParagraph aParagraph = a.renderObject! as RenderParagraph;
final RenderParagraph bParagraph = b.renderObject! as RenderParagraph;
return aParagraph.text.style!.fontSize!.compareTo(bParagraph.text.style!.fontSize!);
});
opacities = titles.map<double>((Element element) {
final RenderAnimatedOpacity renderOpacity = element.findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
return renderOpacity.opacity.value;
});
expect(opacities, <double> [
1.0, // Smaller font title now visible
0.0, // Larger font title invisible.
]);
// The persistent toolbar doesn't move or change size.
expect(tester.getTopLeft(find.byType(NavigationToolbar)).dy, 0.0);
expect(tester.getSize(find.byType(NavigationToolbar)).height, 44.0);
expect(tester.getTopLeft(find.widgetWithText(ClipRect, 'Title').first).dy, 44.0);
// The OverflowBox is squished with the text in it.
expect(tester.getSize(find.widgetWithText(ClipRect, 'Title').first).height, 0.0);
});
testWidgets('User specified middle is always visible in sliver', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
final Key segmentedControlsKey = UniqueKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
CupertinoSliverNavigationBar(
middle: ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 200.0),
child: CupertinoSegmentedControl<int>(
key: segmentedControlsKey,
children: const <int, Widget>{
0: Text('Option A'),
1: Text('Option B'),
},
onValueChanged: (int selected) { },
groupValue: 0,
),
),
largeTitle: const Text('Title'),
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
expect(scrollController.offset, 0.0);
expect(tester.getTopLeft(find.byType(NavigationToolbar)).dy, 0.0);
expect(tester.getSize(find.byType(NavigationToolbar)).height, 44.0);
expect(find.text('Title'), findsOneWidget);
expect(tester.getCenter(find.byKey(segmentedControlsKey)).dx, 400.0);
expect(tester.getTopLeft(find.widgetWithText(ClipRect, 'Title').first).dy, 44.0);
expect(tester.getSize(find.widgetWithText(ClipRect, 'Title').first).height, 52.0);
scrollController.jumpTo(600.0);
await tester.pump(); // Once to trigger the opacity animation.
await tester.pump(const Duration(milliseconds: 300));
expect(tester.getCenter(find.byKey(segmentedControlsKey)).dx, 400.0);
// The large title is invisible now.
expect(
tester.renderObject<RenderAnimatedOpacity>(find.widgetWithText(AnimatedOpacity, 'Title')).opacity.value,
0.0,
);
});
testWidgets('User specified middle is only visible when sliver is collapsed if alwaysShowMiddle is false', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: const <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Large'),
middle: Text('Middle'),
alwaysShowMiddle: false,
),
SliverToBoxAdapter(
child: SizedBox(
height: 1200.0,
),
),
],
),
),
),
);
expect(scrollController.offset, 0.0);
expect(find.text('Middle'), findsOneWidget);
// Initially (in expanded state) middle widget is not visible.
RenderAnimatedOpacity middleOpacity = tester.element(find.text('Middle')).findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
expect(middleOpacity.opacity.value, 0.0);
scrollController.jumpTo(600.0);
await tester.pumpAndSettle();
// Middle widget is visible when nav bar is collapsed.
middleOpacity = tester.element(find.text('Middle')).findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
expect(middleOpacity.opacity.value, 1.0);
scrollController.jumpTo(0.0);
await tester.pumpAndSettle();
// Middle widget is not visible when nav bar is again expanded.
middleOpacity = tester.element(find.text('Middle')).findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
expect(middleOpacity.opacity.value, 0.0);
});
testWidgets('Small title can be overridden', (WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
const CupertinoSliverNavigationBar(
middle: Text('Different title'),
largeTitle: Text('Title'),
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
expect(scrollController.offset, 0.0);
expect(tester.getTopLeft(find.byType(NavigationToolbar)).dy, 0.0);
expect(tester.getSize(find.byType(NavigationToolbar)).height, 44.0);
expect(find.text('Title'), findsOneWidget);
expect(find.text('Different title'), findsOneWidget);
RenderAnimatedOpacity largeTitleOpacity =
tester.element(find.text('Title')).findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
// Large title initially visible.
expect(
largeTitleOpacity.opacity.value,
1.0,
);
// Middle widget not even wrapped with RenderOpacity, i.e. is always visible.
expect(
tester.element(find.text('Different title')).findAncestorRenderObjectOfType<RenderOpacity>(),
isNull,
);
expect(tester.getBottomLeft(find.text('Title')).dy, 44.0 + 52.0 - 8.0); // Static part + extension - padding.
scrollController.jumpTo(600.0);
await tester.pump(); // Once to trigger the opacity animation.
await tester.pump(const Duration(milliseconds: 300));
largeTitleOpacity =
tester.element(find.text('Title')).findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
// Large title no longer visible.
expect(
largeTitleOpacity.opacity.value,
0.0,
);
// The persistent toolbar doesn't move or change size.
expect(tester.getTopLeft(find.byType(NavigationToolbar)).dy, 0.0);
expect(tester.getSize(find.byType(NavigationToolbar)).height, 44.0);
expect(tester.getBottomLeft(find.text('Title')).dy, 44.0); // Extension gone.
});
testWidgets('Auto back/close button', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Home page'),
),
),
);
expect(find.byType(CupertinoButton), findsNothing);
tester.state<NavigatorState>(find.byType(Navigator)).push(CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Page 2'),
);
},
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.byType(CupertinoButton), findsOneWidget);
expect(find.text(String.fromCharCode(CupertinoIcons.back.codePoint)), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).push(CupertinoPageRoute<void>(
fullscreenDialog: true,
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Dialog page'),
);
},
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.widgetWithText(CupertinoButton, 'Close'), findsOneWidget);
// Test popping goes back correctly.
await tester.tap(find.text('Close'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.text('Page 2'), findsOneWidget);
await tester.tap(find.text(String.fromCharCode(CupertinoIcons.back.codePoint)));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.text('Home page'), findsOneWidget);
});
testWidgets('Long back label turns into "back"', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
previousPageTitle: '012345678901',
),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.widgetWithText(CupertinoButton, '012345678901'), findsOneWidget);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
previousPageTitle: '0123456789012',
),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.widgetWithText(CupertinoButton, 'Back'), findsOneWidget);
});
testWidgets('Border should be displayed by default', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Title'),
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNotNull);
final BorderSide side = decoration.border!.bottom;
expect(side, isNotNull);
});
testWidgets('Overrides border color', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Title'),
border: Border(
bottom: BorderSide(
color: Color(0xFFAABBCC),
width: 0.0,
),
),
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNotNull);
final BorderSide side = decoration.border!.bottom;
expect(side, isNotNull);
expect(side.color, const Color(0xFFAABBCC));
});
testWidgets('Border should not be displayed when null', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBar(
middle: Text('Title'),
border: null,
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNull);
});
testWidgets('Border is displayed by default in sliver nav bar', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Large Title'),
),
],
),
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoSliverNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNotNull);
final BorderSide bottom = decoration.border!.bottom;
expect(bottom, isNotNull);
});
testWidgets('Border is not displayed when null in sliver nav bar', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Large Title'),
border: null,
),
],
),
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoSliverNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNull);
});
testWidgets('CupertinoSliverNavigationBar has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(const CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Large Title'),
border: null,
),
],
),
),
));
expect(semantics.nodesWith(
label: 'Large Title',
flags: <SemanticsFlag>[SemanticsFlag.isHeader],
textDirection: TextDirection.ltr,
), hasLength(1));
semantics.dispose();
});
testWidgets('CupertinoNavigationBar has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('Fixed Title'),
),
child: Container(),
),
));
expect(semantics.nodesWith(
label: 'Fixed Title',
flags: <SemanticsFlag>[SemanticsFlag.isHeader],
textDirection: TextDirection.ltr,
), hasLength(1));
semantics.dispose();
});
testWidgets('Border can be overridden in sliver nav bar', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Large Title'),
border: Border(
bottom: BorderSide(
color: Color(0xFFAABBCC),
width: 0.0,
),
),
),
],
),
),
),
);
final DecoratedBox decoratedBox = tester.widgetList(find.descendant(
of: find.byType(CupertinoSliverNavigationBar),
matching: find.byType(DecoratedBox),
)).first as DecoratedBox;
expect(decoratedBox.decoration.runtimeType, BoxDecoration);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
expect(decoration.border, isNotNull);
final BorderSide top = decoration.border!.top;
expect(top, isNotNull);
expect(top, BorderSide.none);
final BorderSide bottom = decoration.border!.bottom;
expect(bottom, isNotNull);
expect(bottom.color, const Color(0xFFAABBCC));
});
testWidgets(
'Standard title golden',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: RepaintBoundary(
child: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Bling bling'),
),
child: Center(),
),
),
),
);
await expectLater(
find.byType(RepaintBoundary).last,
matchesGoldenFile('nav_bar_test.standard_title.png'),
);
},
);
testWidgets(
'Large title golden',
(WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: RepaintBoundary(
child: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: Text('Bling bling'),
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
),
);
await expectLater(
find.byType(RepaintBoundary).last,
matchesGoldenFile('nav_bar_test.large_title.png'),
);
},
);
testWidgets('NavBar draws a light system bar for a dark background', (WidgetTester tester) async {
await tester.pumpWidget(
WidgetsApp(
color: const Color(0xFFFFFFFF),
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Test'),
backgroundColor: Color(0xFF000000),
);
},
);
},
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.light);
});
testWidgets('NavBar draws a dark system bar for a light background', (WidgetTester tester) async {
await tester.pumpWidget(
WidgetsApp(
color: const Color(0xFFFFFFFF),
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return const CupertinoNavigationBar(
middle: Text('Test'),
backgroundColor: Color(0xFFFFFFFF),
);
},
);
},
),
);
expectSameStatusBarStyle(SystemChrome.latestStyle!, SystemUiOverlayStyle.dark);
});
testWidgets('CupertinoNavigationBarBackButton shows an error when manually added outside a route', (WidgetTester tester) async {
await tester.pumpWidget(const CupertinoNavigationBarBackButton());
final dynamic exception = tester.takeException();
expect(exception, isAssertionError);
expect(exception.toString(), contains('CupertinoNavigationBarBackButton should only be used in routes that can be popped'));
});
testWidgets('CupertinoNavigationBarBackButton shows an error when placed in a route that cannot be popped', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoNavigationBarBackButton(),
),
);
final dynamic exception = tester.takeException();
expect(exception, isAssertionError);
expect(exception.toString(), contains('CupertinoNavigationBarBackButton should only be used in routes that can be popped'));
});
testWidgets('CupertinoNavigationBarBackButton with a custom onPressed callback can be placed anywhere', (WidgetTester tester) async {
bool backPressed = false;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoNavigationBarBackButton(
onPressed: () => backPressed = true,
),
),
);
expect(tester.takeException(), isNull);
expect(find.text(String.fromCharCode(CupertinoIcons.back.codePoint)), findsOneWidget);
await tester.tap(find.byType(CupertinoNavigationBarBackButton));
expect(backPressed, true);
});
testWidgets(
'Manually inserted CupertinoNavigationBarBackButton still automatically '
'show previous page title when possible',
(WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'A Phone',
builder: (BuildContext context) {
return const CupertinoNavigationBarBackButton();
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.widgetWithText(CupertinoButton, 'An iPod'), findsOneWidget);
},
);
testWidgets(
'CupertinoNavigationBarBackButton onPressed overrides default pop behavior',
(WidgetTester tester) async {
bool backPressed = false;
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'An iPod',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
title: 'A Phone',
builder: (BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
leading: CupertinoNavigationBarBackButton(
onPressed: () => backPressed = true,
),
),
child: const Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
await tester.tap(find.byType(CupertinoNavigationBarBackButton));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
// The second page is still on top and didn't pop.
expect(find.text('A Phone'), findsOneWidget);
// Custom onPressed called.
expect(backPressed, true);
},
);
testWidgets('textScaleFactor is set to 1.0', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Builder(builder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 99,
maxScaleFactor: 99,
child: const CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
leading: Text('leading'),
middle: Text('middle'),
largeTitle: Text('Large Title'),
trailing: Text('trailing'),
),
SliverToBoxAdapter(
child: Text('content'),
),
],
),
),
);
}),
),
);
final Iterable<RichText> barItems = tester.widgetList<RichText>(
find.descendant(
of: find.byType(CupertinoSliverNavigationBar),
matching: find.byType(RichText),
),
);
final Iterable<RichText> contents = tester.widgetList<RichText>(
find.descendant(
of: find.text('content'),
matching: find.byType(RichText),
),
);
expect(barItems.length, greaterThan(0));
expect(barItems, isNot(contains(predicate((RichText t) => t.textScaler != TextScaler.noScaling))));
expect(contents.length, greaterThan(0));
expect(contents, isNot(contains(predicate((RichText t) => t.textScaler != const TextScaler.linear(99.0)))));
// Also works with implicitly added widgets.
tester.state<NavigatorState>(find.byType(Navigator)).push(CupertinoPageRoute<void>(
title: 'title',
builder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 99,
maxScaleFactor: 99,
child: const CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
CupertinoSliverNavigationBar(
previousPageTitle: 'previous title',
),
],
),
),
);
},
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
final Iterable<RichText> barItems2 = tester.widgetList<RichText>(
find.descendant(
of: find.byType(CupertinoSliverNavigationBar),
matching: find.byType(RichText),
),
);
expect(barItems2.length, greaterThan(0));
expect(barItems2.any((RichText t) => t.textScaleFactor != 1), isFalse);
});
testWidgets(
'CupertinoSliverNavigationBar stretches upon over-scroll and bounces back once over-scroll ends',
(WidgetTester tester) async {
const Text trailingText = Text('Bar Button');
const Text titleText = Text('Large Title');
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
trailing: trailingText,
largeTitle: titleText,
stretch: true,
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
final Finder trailingTextFinder = find.byWidget(trailingText).first;
final Finder titleTextFinder = find.byWidget(titleText).first;
final Offset initialTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
// Drag for overscroll
await tester.drag(find.byType(Scrollable), const Offset(0.0, 150.0));
await tester.pump();
final Offset stretchedTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
expect(
stretchedTrailingTextToLargeTitleOffset.dy.abs(),
greaterThan(initialTrailingTextToLargeTitleOffset.dy.abs()),
);
// Ensure overscroll retracts to original size after releasing gesture
await tester.pumpAndSettle();
final Offset finalTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
expect(
finalTrailingTextToLargeTitleOffset.dy.abs(),
initialTrailingTextToLargeTitleOffset.dy.abs(),
);
},
);
testWidgets(
'CupertinoSliverNavigationBar does not stretch upon over-scroll if stretch parameter is false',
(WidgetTester tester) async {
const Text trailingText = Text('Bar Button');
const Text titleText = Text('Large Title');
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
trailing: trailingText,
largeTitle: titleText,
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
final Finder trailingTextFinder = find.byWidget(trailingText).first;
final Finder titleTextFinder = find.byWidget(titleText).first;
final Offset initialTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
// Drag for overscroll
await tester.drag(find.byType(Scrollable), const Offset(0.0, 150.0));
await tester.pump();
final Offset stretchedTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
expect(
stretchedTrailingTextToLargeTitleOffset.dy.abs(),
initialTrailingTextToLargeTitleOffset.dy.abs(),
);
// Ensure overscroll is zero after releasing gesture
await tester.pumpAndSettle();
final Offset finalTrailingTextToLargeTitleOffset = tester.getTopLeft(trailingTextFinder) - tester.getTopLeft(titleTextFinder);
expect(
finalTrailingTextToLargeTitleOffset.dy.abs(),
initialTrailingTextToLargeTitleOffset.dy.abs(),
);
},
);
testWidgets('Null NavigationBar border transition', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/71389
await tester.pumpWidget(
const CupertinoApp(
home: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Page 1'),
border: null,
),
child: Placeholder(),
),
),
);
tester.state<NavigatorState>(find.byType(Navigator)).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Page 2'),
border: null,
),
child: Placeholder(),
);
},
),
);
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.text('Page 1'), findsNothing);
expect(find.text('Page 2'), findsOneWidget);
await tester.tap(find.text(String.fromCharCode(CupertinoIcons.back.codePoint)));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
});
testWidgets(
'CupertinoSliverNavigationBar magnifies upon over-scroll and shrinks back once over-scroll ends',
(WidgetTester tester) async {
const Text titleText = Text('Large Title');
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: titleText,
stretch: true,
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
final Finder titleTextFinder = find.byWidget(titleText).first;
// Gets the height of the large title
final Offset initialLargeTitleTextOffset =
tester.getBottomLeft(titleTextFinder) -
tester.getTopLeft(titleTextFinder);
// Drag for overscroll
await tester.drag(find.byType(Scrollable), const Offset(0.0, 150.0));
await tester.pump();
final Offset magnifiedTitleTextOffset =
tester.getBottomLeft(titleTextFinder) -
tester.getTopLeft(titleTextFinder);
expect(
magnifiedTitleTextOffset.dy.abs(),
greaterThan(initialLargeTitleTextOffset.dy.abs()),
);
// Ensure title text retracts to original size after releasing gesture
await tester.pumpAndSettle();
final Offset finalTitleTextOffset = tester.getBottomLeft(titleTextFinder) -
tester.getTopLeft(titleTextFinder);
expect(
finalTitleTextOffset.dy.abs(),
initialLargeTitleTextOffset.dy.abs(),
);
},
);
testWidgets(
'CupertinoSliverNavigationBar large title text does not get clipped when magnified',
(WidgetTester tester) async {
const Text titleText = Text('Very very very long large title');
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: titleText,
stretch: true,
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
final Finder titleTextFinder = find.byWidget(titleText).first;
// Gets the width of the large title
final Offset initialLargeTitleTextOffset =
tester.getBottomLeft(titleTextFinder) -
tester.getBottomRight(titleTextFinder);
// Drag for overscroll
await tester.drag(find.byType(Scrollable), const Offset(0.0, 150.0));
await tester.pump();
final Offset magnifiedTitleTextOffset =
tester.getBottomLeft(titleTextFinder) -
tester.getBottomRight(titleTextFinder);
expect(
magnifiedTitleTextOffset.dx.abs(),
equals(initialLargeTitleTextOffset.dx.abs()),
);
},
);
testWidgets(
'CupertinoSliverNavigationBar large title can be hit tested when magnified',
(WidgetTester tester) async {
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: <Widget>[
const CupertinoSliverNavigationBar(
largeTitle: Text('Large title'),
stretch: true,
),
SliverToBoxAdapter(
child: Container(
height: 1200.0,
),
),
],
),
),
),
);
final Finder largeTitleFinder = find.text('Large title').first;
// Drag for overscroll
await tester.drag(find.byType(Scrollable), const Offset(0.0, 250.0));
// Hold position of the scroll view, so the Scrollable unblocks the hit-testing
scrollController.position.hold(() {});
await tester.pumpAndSettle();
expect(largeTitleFinder.hitTestable(), findsOneWidget);
},
);
}
class _ExpectStyles extends StatelessWidget {
const _ExpectStyles({
required this.color,
required this.index,
});
final Color color;
final int index;
@override
Widget build(BuildContext context) {
final TextStyle style = DefaultTextStyle.of(context).style;
expect(style.color, isSameColorAs(color));
expect(style.fontFamily, 'CupertinoSystemText');
expect(style.fontSize, 17.0);
expect(style.letterSpacing, -0.41);
count += index;
return Container();
}
}
| flutter/packages/flutter/test/cupertino/nav_bar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/nav_bar_test.dart",
"repo_id": "flutter",
"token_count": 22741
} | 724 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../image_data.dart';
import '../rendering/rendering_tester.dart' show TestCallbackPainter;
import '../widgets/navigator_utils.dart';
late List<int> selectedTabs;
class MockCupertinoTabController extends CupertinoTabController {
MockCupertinoTabController({ required super.initialIndex });
bool isDisposed = false;
int numOfListeners = 0;
@override
void addListener(VoidCallback listener) {
numOfListeners++;
super.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
numOfListeners--;
super.removeListener(listener);
}
@override
void dispose() {
isDisposed = true;
super.dispose();
}
}
void main() {
setUp(() {
selectedTabs = <int>[];
});
BottomNavigationBarItem tabGenerator(int index) {
return BottomNavigationBarItem(
icon: ImageIcon(MemoryImage(Uint8List.fromList(kTransparentImage))),
label: 'Tab ${index + 1}',
);
}
testWidgets('Tab switching', (WidgetTester tester) async {
final List<int> tabsPainted = <int>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int>[0]);
RichText tab1 = tester.widget(find.descendant(
of: find.text('Tab 1'),
matching: find.byType(RichText),
));
expect(tab1.text.style!.color, CupertinoColors.activeBlue);
RichText tab2 = tester.widget(find.descendant(
of: find.text('Tab 2'),
matching: find.byType(RichText),
));
expect(tab2.text.style!.color!.value, 0xFF999999);
await tester.tap(find.text('Tab 2'));
await tester.pump();
expect(tabsPainted, const <int>[0, 1]);
tab1 = tester.widget(find.descendant(
of: find.text('Tab 1'),
matching: find.byType(RichText),
));
expect(tab1.text.style!.color!.value, 0xFF999999);
tab2 = tester.widget(find.descendant(
of: find.text('Tab 2'),
matching: find.byType(RichText),
));
expect(tab2.text.style!.color, CupertinoColors.activeBlue);
await tester.tap(find.text('Tab 1'));
await tester.pump();
expect(tabsPainted, const <int>[0, 1, 0]);
// CupertinoTabBar's onTap callbacks are passed on.
expect(selectedTabs, const <int>[1, 0]);
});
testWidgets('Tabs are lazy built and moved offstage when inactive', (WidgetTester tester) async {
final List<int> tabsBuilt = <int>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
tabsBuilt.add(index);
return Text('Page ${index + 1}');
},
),
),
);
expect(tabsBuilt, const <int>[0]);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2'), findsNothing);
await tester.tap(find.text('Tab 2'));
await tester.pump();
// Both tabs are built but only one is onstage.
expect(tabsBuilt, const <int>[0, 0, 1]);
expect(find.text('Page 1', skipOffstage: false), isOffstage);
expect(find.text('Page 2'), findsOneWidget);
await tester.tap(find.text('Tab 1'));
await tester.pump();
expect(tabsBuilt, const <int>[0, 0, 1, 0, 1]);
expect(find.text('Page 1'), findsOneWidget);
expect(find.text('Page 2', skipOffstage: false), isOffstage);
});
testWidgets('Last tab gets focus', (WidgetTester tester) async {
// 2 nodes for 2 tabs
final List<FocusNode> focusNodes = <FocusNode>[
FocusNode(debugLabel: 'Node 1'),
FocusNode(debugLabel: 'Node 2'),
];
for (final FocusNode focusNode in focusNodes) {
addTearDown(focusNode.dispose);
}
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return CupertinoTextField(
focusNode: focusNodes[index],
autofocus: true,
);
},
),
),
);
expect(focusNodes[0].hasFocus, isTrue);
await tester.tap(find.text('Tab 2'));
await tester.pump();
expect(focusNodes[0].hasFocus, isFalse);
expect(focusNodes[1].hasFocus, isTrue);
await tester.tap(find.text('Tab 1'));
await tester.pump();
expect(focusNodes[0].hasFocus, isTrue);
expect(focusNodes[1].hasFocus, isFalse);
});
testWidgets('Do not affect focus order in the route', (WidgetTester tester) async {
final List<FocusNode> focusNodes = <FocusNode>[
FocusNode(debugLabel: 'Node 1'),
FocusNode(debugLabel: 'Node 2'),
FocusNode(debugLabel: 'Node 3'),
FocusNode(debugLabel: 'Node 4'),
];
for (final FocusNode focusNode in focusNodes) {
addTearDown(focusNode.dispose);
}
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return Column(
children: <Widget>[
CupertinoTextField(
focusNode: focusNodes[index * 2],
placeholder: 'TextField 1',
),
CupertinoTextField(
focusNode: focusNodes[index * 2 + 1],
placeholder: 'TextField 2',
),
],
);
},
),
),
);
expect(
focusNodes.any((FocusNode node) => node.hasFocus),
isFalse,
);
await tester.tap(find.widgetWithText(CupertinoTextField, 'TextField 2'));
expect(
focusNodes.indexOf(focusNodes.singleWhere((FocusNode node) => node.hasFocus)),
1,
);
await tester.tap(find.text('Tab 2'));
await tester.pump();
await tester.tap(find.widgetWithText(CupertinoTextField, 'TextField 1'));
expect(
focusNodes.indexOf(focusNodes.singleWhere((FocusNode node) => node.hasFocus)),
2,
);
await tester.tap(find.text('Tab 1'));
await tester.pump();
// Upon going back to tab 1, the item it tab 1 that previously had the focus
// (TextField 2) gets it back.
expect(
focusNodes.indexOf(focusNodes.singleWhere((FocusNode node) => node.hasFocus)),
1,
);
});
testWidgets('Programmatic tab switching by changing the index of an existing controller', (WidgetTester tester) async {
final CupertinoTabController controller = CupertinoTabController(initialIndex: 1);
addTearDown(controller.dispose);
final List<int> tabsPainted = <int>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int>[1]);
controller.index = 0;
await tester.pump();
expect(tabsPainted, const <int>[1, 0]);
// onTap is not called when changing tabs programmatically.
expect(selectedTabs, isEmpty);
// Can still tap out of the programmatically selected tab.
await tester.tap(find.text('Tab 2'));
await tester.pump();
expect(tabsPainted, const <int>[1, 0, 1]);
expect(selectedTabs, const <int>[1]);
});
testWidgets('Programmatic tab switching by passing in a new controller', (WidgetTester tester) async {
final List<int> tabsPainted = <int>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int>[0]);
final CupertinoTabController controller = CupertinoTabController(initialIndex: 1);
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
controller: controller, // Programmatically change the tab now.
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int>[0, 1]);
// onTap is not called when changing tabs programmatically.
expect(selectedTabs, isEmpty);
// Can still tap out of the programmatically selected tab.
await tester.tap(find.text('Tab 1'));
await tester.pump();
expect(tabsPainted, const <int>[0, 1, 0]);
expect(selectedTabs, const <int>[0]);
});
testWidgets('Tab bar respects themes', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return const Placeholder();
},
),
),
);
BoxDecoration tabDecoration = tester.widget<DecoratedBox>(find.descendant(
of: find.byType(CupertinoTabBar),
matching: find.byType(DecoratedBox),
)).decoration as BoxDecoration;
expect(tabDecoration.color, isSameColorAs(const Color(0xF0F9F9F9))); // Inherited from theme.
await tester.tap(find.text('Tab 2'));
await tester.pump();
// Pump again but with dark theme.
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(
brightness: Brightness.dark,
primaryColor: CupertinoColors.destructiveRed,
),
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return const Placeholder();
},
),
),
);
tabDecoration = tester.widget<DecoratedBox>(find.descendant(
of: find.byType(CupertinoTabBar),
matching: find.byType(DecoratedBox),
)).decoration as BoxDecoration;
expect(tabDecoration.color, isSameColorAs(const Color(0xF01D1D1D)));
final RichText tab1 = tester.widget(find.descendant(
of: find.text('Tab 1'),
matching: find.byType(RichText),
));
// Tab 2 should still be selected after changing theme.
expect(tab1.text.style!.color!.value, 0xFF757575);
final RichText tab2 = tester.widget(find.descendant(
of: find.text('Tab 2'),
matching: find.byType(RichText),
));
expect(tab2.text.style!.color, isSameColorAs(CupertinoColors.systemRed.darkColor));
});
testWidgets('Tab contents are padded when there are view insets', (WidgetTester tester) async {
late BuildContext innerContext;
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 200),
),
child: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
innerContext = context;
return const Placeholder();
},
),
),
),
);
expect(tester.getRect(find.byType(Placeholder)), const Rect.fromLTWH(0, 0, 800, 400));
// Don't generate more media query padding from the translucent bottom
// tab since the tab is behind the keyboard now.
expect(MediaQuery.of(innerContext).padding.bottom, 0);
});
testWidgets('Tab contents are not inset when resizeToAvoidBottomInset overridden', (WidgetTester tester) async {
late BuildContext innerContext;
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 200),
),
child: CupertinoTabScaffold(
resizeToAvoidBottomInset: false,
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
innerContext = context;
return const Placeholder();
},
),
),
),
);
expect(tester.getRect(find.byType(Placeholder)), const Rect.fromLTWH(0, 0, 800, 600));
// Media query padding shows up in the inner content because it wasn't masked
// by the view inset.
expect(MediaQuery.of(innerContext).padding.bottom, 50);
});
testWidgets('Tab contents bottom padding are not consumed by viewInsets when resizeToAvoidBottomInset overridden', (WidgetTester tester) async {
final Widget child = Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultCupertinoLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: CupertinoTabScaffold(
resizeToAvoidBottomInset: false,
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return const Placeholder();
},
),
),
);
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 20.0),
),
child: child,
),
),
);
final Offset initialPoint = tester.getCenter(find.byType(Placeholder));
// Consume bottom padding - as if by the keyboard opening
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(
viewPadding: EdgeInsets.only(bottom: 20),
viewInsets: EdgeInsets.only(bottom: 300),
),
child: child,
),
);
final Offset finalPoint = tester.getCenter(find.byType(Placeholder));
expect(initialPoint, finalPoint);
});
testWidgets(
'Opaque tab bar consumes bottom padding while non opaque tab bar does not',
(WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/43581.
Future<EdgeInsets> getContentPaddingWithTabBarColor(Color color) async {
late EdgeInsets contentPadding;
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(padding: EdgeInsets.only(bottom: 50)),
child: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
backgroundColor: color,
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
),
tabBuilder: (BuildContext context, int index) {
contentPadding = MediaQuery.paddingOf(context);
return const Placeholder();
},
),
),
),
);
return contentPadding;
}
expect(await getContentPaddingWithTabBarColor(const Color(0xAAFFFFFF)), isNot(EdgeInsets.zero));
expect(await getContentPaddingWithTabBarColor(const Color(0xFFFFFFFF)), EdgeInsets.zero);
},
);
testWidgets('Tab and page scaffolds do not double stack view insets', (WidgetTester tester) async {
late BuildContext innerContext;
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 200),
),
child: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return CupertinoPageScaffold(
child: Builder(
builder: (BuildContext context) {
innerContext = context;
return const Placeholder();
},
),
);
},
),
),
),
);
expect(tester.getRect(find.byType(Placeholder)), const Rect.fromLTWH(0, 0, 800, 400));
expect(MediaQuery.of(innerContext).padding.bottom, 0);
});
testWidgets('Deleting tabs after selecting them should switch to the last available tab', (WidgetTester tester) async {
final List<int> tabsBuilt = <int>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(4, tabGenerator),
onTap: (int newTab) => selectedTabs.add(newTab),
),
tabBuilder: (BuildContext context, int index) {
tabsBuilt.add(index);
return Text('Page ${index + 1}');
},
),
),
);
expect(tabsBuilt, const <int>[0]);
// selectedTabs list is appended to on onTap callbacks. We didn't tap
// any tabs yet.
expect(selectedTabs, const <int>[]);
tabsBuilt.clear();
await tester.tap(find.text('Tab 4'));
await tester.pump();
// Tabs 1 and 4 are built but only one is onstage.
expect(tabsBuilt, const <int>[0, 3]);
expect(selectedTabs, const <int>[3]);
expect(find.text('Page 1', skipOffstage: false), isOffstage);
expect(find.text('Page 4'), findsOneWidget);
tabsBuilt.clear();
// Delete 2 tabs while Page 4 is still selected.
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
onTap: (int newTab) => selectedTabs.add(newTab),
),
tabBuilder: (BuildContext context, int index) {
tabsBuilt.add(index);
// Change the builder too.
return Text('Different page ${index + 1}');
},
),
),
);
expect(tabsBuilt, const <int>[0, 1]);
// We didn't tap on any additional tabs to invoke the onTap callback. We
// just deleted a tab.
expect(selectedTabs, const <int>[3]);
// Tab 1 was previously built so it's rebuilt again, albeit offstage.
expect(find.text('Different page 1', skipOffstage: false), isOffstage);
// Since all the tabs after tab 2 are deleted, tab 2 is now the last tab and
// the actively shown tab.
expect(find.text('Different page 2'), findsOneWidget);
// No more tab 4 since it's deleted.
expect(find.text('Different page 4', skipOffstage: false), findsNothing);
// We also changed the builder so no tabs should be built with the old
// builder.
expect(find.text('Page 1', skipOffstage: false), findsNothing);
expect(find.text('Page 2', skipOffstage: false), findsNothing);
expect(find.text('Page 4', skipOffstage: false), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/33455
testWidgets('Adding new tabs does not crash the app', (WidgetTester tester) async {
final List<int> tabsPainted = <int>[];
final CupertinoTabController controller = CupertinoTabController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(10, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int> [0]);
// Increase the num of tabs to 20.
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(20, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int> [0, 0]);
await tester.tap(find.text('Tab 19'));
await tester.pump();
// Tapping the tabs should still work.
expect(tabsPainted, const <int>[0, 0, 18]);
});
testWidgets(
'If a controller is initially provided then the parent stops doing so for rebuilds, '
'a new instance of CupertinoTabController should be created and used by the widget, '
"while preserving the previous controller's tab index",
(WidgetTester tester) async {
final List<int> tabsPainted = <int>[];
final CupertinoTabController oldController = CupertinoTabController();
addTearDown(oldController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(10, tabGenerator),
),
controller: oldController,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int> [0]);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(10, tabGenerator),
),
tabBuilder:
(BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () { tabsPainted.add(index); },
),
child: Text('Page ${index + 1}'),
);
},
),
),
);
expect(tabsPainted, const <int> [0, 0]);
await tester.tap(find.text('Tab 2'));
await tester.pump();
// Tapping the tabs should still work.
expect(tabsPainted, const <int>[0, 0, 1]);
oldController.index = 10;
await tester.pump();
// Changing [index] of the oldController should not work.
expect(tabsPainted, const <int> [0, 0, 1]);
},
);
testWidgets(
'Do not call dispose on a controller that we do not own '
'but do remove from its listeners when done listening to it',
(WidgetTester tester) async {
final MockCupertinoTabController mockController = MockCupertinoTabController(initialIndex: 0);
addTearDown(mockController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
),
controller: mockController,
tabBuilder: (BuildContext context, int index) => const Placeholder(),
),
),
);
expect(mockController.numOfListeners, 1);
expect(mockController.isDisposed, isFalse);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
),
tabBuilder: (BuildContext context, int index) => const Placeholder(),
),
),
);
expect(mockController.numOfListeners, 0);
expect(mockController.isDisposed, isFalse);
},
);
testWidgets('The owner can dispose the old controller', (WidgetTester tester) async {
CupertinoTabController controller = CupertinoTabController(initialIndex: 2);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) => const Placeholder(),
),
),
);
expect(find.text('Tab 1'), findsOneWidget);
expect(find.text('Tab 2'), findsOneWidget);
expect(find.text('Tab 3'), findsOneWidget);
controller.dispose();
controller = CupertinoTabController();
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) => const Placeholder(),
),
),
);
// Should not crash here.
expect(find.text('Tab 1'), findsOneWidget);
expect(find.text('Tab 2'), findsOneWidget);
expect(find.text('Tab 3'), findsNothing);
});
testWidgets('A controller can control more than one CupertinoTabScaffold, '
'removal of listeners does not break the controller',
(WidgetTester tester) async {
final List<int> tabsPainted0 = <int>[];
final List<int> tabsPainted1 = <int>[];
MockCupertinoTabController controller = MockCupertinoTabController(initialIndex: 2);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Stack(
children: <Widget>[
CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () => tabsPainted0.add(index),
),
);
},
),
CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () => tabsPainted1.add(index),
),
);
},
),
],
),
),
),
);
expect(tabsPainted0, const <int>[2]);
expect(tabsPainted1, const <int>[2]);
expect(controller.numOfListeners, 2);
controller.index = 0;
await tester.pump();
expect(tabsPainted0, const <int>[2, 0]);
expect(tabsPainted1, const <int>[2, 0]);
controller.index = 1;
// Removing one of the tabs works.
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Stack(
children: <Widget>[
CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () => tabsPainted0.add(index),
),
);
},
),
],
),
),
),
);
expect(tabsPainted0, const <int>[2, 0, 1]);
expect(tabsPainted1, const <int>[2, 0]);
expect(controller.numOfListeners, 1);
// Replacing controller works.
controller.dispose();
controller = MockCupertinoTabController(initialIndex: 2);
addTearDown(controller.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Stack(
children: <Widget>[
CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
return CustomPaint(
painter: TestCallbackPainter(
onPaint: () => tabsPainted0.add(index),
),
);
},
),
],
),
),
),
);
expect(tabsPainted0, const <int>[2, 0, 1, 2]);
expect(tabsPainted1, const <int>[2, 0]);
expect(controller.numOfListeners, 1);
},
);
testWidgets('Assert when current tab index >= number of tabs', (WidgetTester tester) async {
final CupertinoTabController controller = CupertinoTabController(initialIndex: 2);
addTearDown(controller.dispose);
try {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(2, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) => Text('Different page ${index + 1}'),
),
),
);
} on AssertionError catch (e) {
expect(e.toString(), contains('controller.index < tabBar.items.length'));
}
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) => Text('Different page ${index + 1}'),
),
),
);
expect(tester.takeException(), null);
controller.index = 10;
await tester.pump();
final String message = tester.takeException().toString();
expect(message, contains('current index ${controller.index}'));
expect(message, contains('with 3 tabs'));
});
testWidgets("Don't replace focus nodes for existing tabs when changing tab count", (WidgetTester tester) async {
final CupertinoTabController controller = CupertinoTabController(initialIndex: 2);
addTearDown(controller.dispose);
final List<FocusScopeNode> scopes = <FocusScopeNode>[];
for (int i = 0; i < 5; i++) {
final FocusScopeNode scope = FocusScopeNode();
addTearDown(scope.dispose);
scopes.add(scope);
}
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(3, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
scopes[index] = FocusScope.of(context);
return Container();
},
),
),
);
for (int i = 0; i < 3; i++) {
controller.index = i;
await tester.pump();
}
await tester.pump();
final List<FocusScopeNode> newScopes = <FocusScopeNode>[];
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(5, tabGenerator),
),
controller: controller,
tabBuilder: (BuildContext context, int index) {
newScopes.add(FocusScope.of(context));
return Container();
},
),
),
);
for (int i = 0; i < 5; i++) {
controller.index = i;
await tester.pump();
}
await tester.pump();
expect(scopes.sublist(0, 3), equals(newScopes.sublist(0, 3)));
});
testWidgets('Current tab index cannot go below zero or be null', (WidgetTester tester) async {
void expectAssertionError(VoidCallback callback, String errorMessage) {
try {
callback();
} on AssertionError catch (e) {
expect(e.toString(), contains(errorMessage));
}
}
expectAssertionError(() => CupertinoTabController(initialIndex: -1), '>= 0');
final CupertinoTabController controller = CupertinoTabController();
addTearDown(controller.dispose);
expectAssertionError(() => controller.index = -1, '>= 0');
});
testWidgets('Does not lose state when focusing on text input', (WidgetTester tester) async {
// Regression testing for https://github.com/flutter/flutter/issues/28457.
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return const CupertinoTextField();
},
),
),
),
);
final EditableTextState editableState = tester.state<EditableTextState>(find.byType(EditableText));
await tester.enterText(find.byType(CupertinoTextField), "don't lose me");
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 100),
),
child: CupertinoApp(
home: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return const CupertinoTextField();
},
),
),
),
);
// The exact same state instance is still there.
expect(tester.state<EditableTextState>(find.byType(EditableText)), editableState);
expect(find.text("don't lose me"), findsOneWidget);
});
testWidgets('textScaleFactor is set to 1.0', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Builder(builder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: 99,
maxScaleFactor: 99,
child: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(
10,
(int i) => BottomNavigationBarItem(icon: ImageIcon(MemoryImage(Uint8List.fromList(kTransparentImage))), label: '$i'),
),
),
tabBuilder: (BuildContext context, int index) => const Text('content'),
),
);
}),
),
);
final Iterable<RichText> barItems = tester.widgetList<RichText>(
find.descendant(
of: find.byType(CupertinoTabBar),
matching: find.byType(RichText),
),
);
final Iterable<RichText> contents = tester.widgetList<RichText>(
find.descendant(
of: find.text('content'),
matching: find.byType(RichText),
skipOffstage: false,
),
);
expect(barItems.length, greaterThan(0));
expect(barItems, isNot(contains(predicate((RichText t) => t.textScaler != TextScaler.noScaling))));
expect(contents.length, greaterThan(0));
expect(contents, isNot(contains(predicate((RichText t) => t.textScaler != const TextScaler.linear(99.0)))));
});
testWidgets('state restoration', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
restorationScopeId: 'app',
home: CupertinoTabScaffold(
restorationId: 'scaffold',
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(
4,
(int i) => BottomNavigationBarItem(icon: const Icon(CupertinoIcons.map), label: 'Tab $i'),
),
),
tabBuilder: (BuildContext context, int i) => Text('Content $i'),
),
),
);
expect(find.text('Content 0'), findsOneWidget);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsNothing);
expect(find.text('Content 3'), findsNothing);
await tester.tap(find.text('Tab 2'));
await tester.pumpAndSettle();
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsOneWidget);
expect(find.text('Content 3'), findsNothing);
await tester.restartAndRestore();
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsOneWidget);
expect(find.text('Content 3'), findsNothing);
final TestRestorationData data = await tester.getRestorationData();
await tester.tap(find.text('Tab 1'));
await tester.pumpAndSettle();
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsOneWidget);
expect(find.text('Content 2'), findsNothing);
expect(find.text('Content 3'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsOneWidget);
expect(find.text('Content 3'), findsNothing);
});
testWidgets('switch from internal to external controller with state restoration', (WidgetTester tester) async {
Widget buildWidget({CupertinoTabController? controller}) {
return CupertinoApp(
restorationScopeId: 'app',
home: CupertinoTabScaffold(
controller: controller,
restorationId: 'scaffold',
tabBar: CupertinoTabBar(
items: List<BottomNavigationBarItem>.generate(
4,
(int i) => BottomNavigationBarItem(icon: const Icon(CupertinoIcons.map), label: 'Tab $i'),
),
),
tabBuilder: (BuildContext context, int i) => Text('Content $i'),
),
);
}
await tester.pumpWidget(buildWidget());
expect(find.text('Content 0'), findsOneWidget);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsNothing);
expect(find.text('Content 3'), findsNothing);
await tester.tap(find.text('Tab 2'));
await tester.pumpAndSettle();
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsOneWidget);
expect(find.text('Content 3'), findsNothing);
final CupertinoTabController controller = CupertinoTabController(initialIndex: 3);
addTearDown(controller.dispose);
await tester.pumpWidget(buildWidget(controller: controller));
expect(find.text('Content 0'), findsNothing);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsNothing);
expect(find.text('Content 3'), findsOneWidget);
await tester.pumpWidget(buildWidget());
expect(find.text('Content 0'), findsOneWidget);
expect(find.text('Content 1'), findsNothing);
expect(find.text('Content 2'), findsNothing);
expect(find.text('Content 3'), findsNothing);
});
group('Android Predictive Back', () {
bool? lastFrameworkHandlesBack;
setUp(() async {
lastFrameworkHandlesBack = null;
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'SystemNavigator.setFrameworkHandlesBack') {
expect(methodCall.arguments, isA<bool>());
lastFrameworkHandlesBack = methodCall.arguments as bool;
}
return;
});
await TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.handlePlatformMessage(
'flutter/lifecycle',
const StringCodec().encodeMessage(AppLifecycleState.resumed.toString()),
(ByteData? data) {},
);
});
tearDown(() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(SystemChannels.platform, null);
});
testWidgets('System back navigation inside of tabs', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: MediaQuery(
data: const MediaQueryData(
viewInsets: EdgeInsets.only(bottom: 200),
),
child: CupertinoTabScaffold(
tabBar: _buildTabBar(),
tabBuilder: (BuildContext context, int index) {
return CupertinoTabView(
builder: (BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Page 1 of tab ${index + 1}'),
),
child: Center(
child: CupertinoButton(
child: const Text('Next page'),
onPressed: () {
Navigator.of(context).push(
CupertinoPageRoute<void>(
builder: (BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Page 2 of tab ${index + 1}'),
),
child: Center(
child: CupertinoButton(
child: const Text('Back'),
onPressed: () {
Navigator.of(context).pop();
},
),
),
);
},
),
);
},
),
),
);
},
);
},
),
),
),
);
expect(find.text('Page 1 of tab 1'), findsOneWidget);
expect(find.text('Page 2 of tab 1'), findsNothing);
expect(lastFrameworkHandlesBack, isFalse);
await tester.tap(find.text('Next page'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsNothing);
expect(find.text('Page 2 of tab 1'), findsOneWidget);
expect(lastFrameworkHandlesBack, isTrue);
await simulateSystemBack();
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsOneWidget);
expect(find.text('Page 2 of tab 1'), findsNothing);
expect(lastFrameworkHandlesBack, isFalse);
await tester.tap(find.text('Next page'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsNothing);
expect(find.text('Page 2 of tab 1'), findsOneWidget);
expect(lastFrameworkHandlesBack, isTrue);
await tester.tap(find.text('Tab 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 2'), findsOneWidget);
expect(find.text('Page 2 of tab 2'), findsNothing);
expect(lastFrameworkHandlesBack, isFalse);
await tester.tap(find.text('Tab 1'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsNothing);
expect(find.text('Page 2 of tab 1'), findsOneWidget);
expect(lastFrameworkHandlesBack, isTrue);
await simulateSystemBack();
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 1'), findsOneWidget);
expect(find.text('Page 2 of tab 1'), findsNothing);
expect(lastFrameworkHandlesBack, isFalse);
await tester.tap(find.text('Tab 2'));
await tester.pumpAndSettle();
expect(find.text('Page 1 of tab 2'), findsOneWidget);
expect(find.text('Page 2 of tab 2'), findsNothing);
expect(lastFrameworkHandlesBack, isFalse);
},
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.android }),
skip: kIsWeb, // [intended] frameworkHandlesBack not used on web.
);
});
}
CupertinoTabBar _buildTabBar({ int selectedTab = 0 }) {
return CupertinoTabBar(
items: <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: ImageIcon(MemoryImage(Uint8List.fromList(kTransparentImage))),
label: 'Tab 1',
),
BottomNavigationBarItem(
icon: ImageIcon(MemoryImage(Uint8List.fromList(kTransparentImage))),
label: 'Tab 2',
),
],
currentIndex: selectedTab,
onTap: (int newTab) => selectedTabs.add(newTab),
);
}
| flutter/packages/flutter/test/cupertino/tab_scaffold_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/tab_scaffold_test.dart",
"repo_id": "flutter",
"token_count": 20479
} | 725 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// A test script that invokes compute() to start an isolate.
import 'dart:isolate';
import 'package:flutter/src/foundation/_isolates_io.dart';
int getLength(String s) {
final ReceivePort r = ReceivePort();
try {
throw r;
} finally {
r.close();
}
}
Future<void> main() async {
const String s = 'hello world';
bool wasError = false;
try {
await compute(getLength, s);
} on RemoteError {
wasError = true;
}
if (!wasError) {
throw Exception('compute threw bad result');
}
}
| flutter/packages/flutter/test/foundation/_compute_caller_invalid_error.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/_compute_caller_invalid_error.dart",
"repo_id": "flutter",
"token_count": 228
} | 726 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('Element diagnostics json includes widgetRuntimeType', () async {
final Element element = _TestElement();
final Map<String, Object?> json = element.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate());
expect(json['widgetRuntimeType'], 'Placeholder');
expect(json['stateful'], isFalse);
});
test('StatefulElement diagnostics are stateful', () {
final Element element = StatefulElement(const Tooltip(message: 'foo'));
final Map<String, Object?> json = element.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate());
expect(json['widgetRuntimeType'], 'Tooltip');
expect(json['stateful'], isTrue);
});
group('Serialization', () {
final TestTree testTree = TestTree(
properties: <DiagnosticsNode>[
StringProperty('stringProperty1', 'value1', quoted: false),
DoubleProperty('doubleProperty1', 42.5),
DoubleProperty('roundedProperty', 1.0 / 3.0),
StringProperty('DO_NOT_SHOW', 'DO_NOT_SHOW', level: DiagnosticLevel.hidden, quoted: false),
DiagnosticsProperty<Object>('DO_NOT_SHOW_NULL', null, defaultValue: null),
DiagnosticsProperty<Object>('nullProperty', null),
StringProperty('node_type', '<root node>', showName: false, quoted: false),
],
children: <TestTree>[
TestTree(name: 'node A'),
TestTree(
name: 'node B',
properties: <DiagnosticsNode>[
StringProperty('p1', 'v1', quoted: false),
StringProperty('p2', 'v2', quoted: false),
],
children: <TestTree>[
TestTree(name: 'node B1'),
TestTree(
name: 'node B2',
properties: <DiagnosticsNode>[StringProperty('property1', 'value1', quoted: false)],
),
TestTree(
name: 'node B3',
properties: <DiagnosticsNode>[
StringProperty('node_type', '<leaf node>', showName: false, quoted: false),
IntProperty('foo', 42),
],
),
],
),
TestTree(
name: 'node C',
properties: <DiagnosticsNode>[
StringProperty('foo', 'multi\nline\nvalue!', quoted: false),
],
),
],
);
test('default', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate());
expect(result.containsKey('properties'), isFalse);
expect(result.containsKey('children'), isFalse);
});
test('subtreeDepth 1', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate(subtreeDepth: 1));
expect(result.containsKey('properties'), isFalse);
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children[0].containsKey('children'), isFalse);
expect(children[1].containsKey('children'), isFalse);
expect(children[2].containsKey('children'), isFalse);
});
test('subtreeDepth 5', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate(subtreeDepth: 5));
expect(result.containsKey('properties'), isFalse);
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children[0]['children'], hasLength(0));
expect(children[1]['children'], hasLength(3));
expect(children[2]['children'], hasLength(0));
});
test('includeProperties', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate(includeProperties: true));
expect(result.containsKey('children'), isFalse);
expect(result['properties'], hasLength(7));
});
test('includeProperties with subtreedepth 1', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const DiagnosticsSerializationDelegate(
includeProperties: true,
subtreeDepth: 1,
));
expect(result['properties'], hasLength(7));
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(3));
expect(children[0]['properties'], hasLength(0));
expect(children[1]['properties'], hasLength(2));
expect(children[2]['properties'], hasLength(1));
});
test('additionalNodeProperties', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(const TestDiagnosticsSerializationDelegate(
includeProperties: true,
subtreeDepth: 1,
additionalNodePropertiesMap: <String, Object>{
'foo': true,
},
));
expect(result['foo'], isTrue);
final List<Map<String, Object?>> properties = result['properties']! as List<Map<String, Object?>>;
expect(properties, hasLength(7));
expect(properties.every((Map<String, Object?> property) => property['foo'] == true), isTrue);
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(3));
expect(children.every((Map<String, Object?> child) => child['foo'] == true), isTrue);
});
test('filterProperties - sublist', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
includeProperties: true,
propertyFilter: (List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return nodes.whereType<StringProperty>().toList();
},
));
final List<Map<String, Object?>> properties = result['properties']! as List<Map<String, Object?>>;
expect(properties, hasLength(3));
expect(properties.every((Map<String, Object?> property) => property['type'] == 'StringProperty'), isTrue);
});
test('filterProperties - replace', () {
bool replaced = false;
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
includeProperties: true,
propertyFilter: (List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
if (replaced) {
return nodes;
}
replaced = true;
return <DiagnosticsNode>[
StringProperty('foo', 'bar'),
];
},
));
final List<Map<String, Object?>> properties = result['properties']! as List<Map<String, Object?>>;
expect(properties, hasLength(1));
expect(properties.single['name'], 'foo');
});
test('filterChildren - sublist', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
subtreeDepth: 1,
childFilter: (List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return nodes.where((DiagnosticsNode node) => node.getProperties().isEmpty).toList();
},
));
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(1));
});
test('filterChildren - replace', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
subtreeDepth: 1,
childFilter: (List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return nodes.expand((DiagnosticsNode node) => node.getChildren()).toList();
},
));
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(3));
expect(children.first['name'], 'child node B1');
});
test('nodeTruncator', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
subtreeDepth: 5,
includeProperties: true,
nodeTruncator: (List<DiagnosticsNode> nodes, DiagnosticsNode? owner) {
return nodes.take(2).toList();
},
));
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(3));
expect(children.last['truncated'], isTrue);
final List<Map<String, Object?>> properties = result['properties']! as List<Map<String, Object?>>;
expect(properties, hasLength(3));
expect(properties.last['truncated'], isTrue);
});
test('delegateForAddingNodes', () {
final Map<String, Object?> result = testTree.toDiagnosticsNode().toJsonMap(TestDiagnosticsSerializationDelegate(
subtreeDepth: 5,
includeProperties: true,
nodeDelegator: (DiagnosticsNode node, DiagnosticsSerializationDelegate delegate) {
return delegate.copyWith(includeProperties: false);
},
));
final List<Map<String, Object?>> properties = result['properties']! as List<Map<String, Object?>>;
expect(properties, hasLength(7));
expect(properties.every((Map<String, Object?> property) => !property.containsKey('properties')), isTrue);
final List<Map<String, Object?>> children = result['children']! as List<Map<String, Object?>>;
expect(children, hasLength(3));
expect(children.every((Map<String, Object?> child) => !child.containsKey('properties')), isTrue);
});
});
}
class _TestElement extends Element {
_TestElement() : super(const Placeholder());
@override
bool get debugDoingBuild => throw UnimplementedError();
}
class TestTree extends Object with DiagnosticableTreeMixin {
TestTree({
this.name = '',
this.style,
this.children = const <TestTree>[],
this.properties = const <DiagnosticsNode>[],
});
final String name;
final List<TestTree> children;
final List<DiagnosticsNode> properties;
final DiagnosticsTreeStyle? style;
@override
List<DiagnosticsNode> debugDescribeChildren() => <DiagnosticsNode>[
for (final TestTree child in children)
child.toDiagnosticsNode(
name: 'child ${child.name}',
style: child.style,
),
];
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
if (style != null) {
properties.defaultDiagnosticsTreeStyle = style!;
}
this.properties.forEach(properties.add);
}
}
typedef NodeDelegator = DiagnosticsSerializationDelegate Function(DiagnosticsNode node, TestDiagnosticsSerializationDelegate delegate);
typedef NodeTruncator = List<DiagnosticsNode> Function(List<DiagnosticsNode> nodes, DiagnosticsNode? owner);
typedef NodeFilter = List<DiagnosticsNode> Function(List<DiagnosticsNode> nodes, DiagnosticsNode owner);
class TestDiagnosticsSerializationDelegate implements DiagnosticsSerializationDelegate {
const TestDiagnosticsSerializationDelegate({
this.includeProperties = false,
this.subtreeDepth = 0,
this.additionalNodePropertiesMap = const <String, Object>{},
this.childFilter,
this.propertyFilter,
this.nodeTruncator,
this.nodeDelegator,
});
final Map<String, Object> additionalNodePropertiesMap;
final NodeFilter? childFilter;
final NodeFilter? propertyFilter;
final NodeTruncator? nodeTruncator;
final NodeDelegator? nodeDelegator;
@override
Map<String, Object> additionalNodeProperties(DiagnosticsNode node) {
return additionalNodePropertiesMap;
}
@override
DiagnosticsSerializationDelegate delegateForNode(DiagnosticsNode node) {
if (nodeDelegator != null) {
return nodeDelegator!(node, this);
}
return subtreeDepth > 0 ? copyWith(subtreeDepth: subtreeDepth - 1) : this;
}
@override
bool get expandPropertyValues => false;
@override
List<DiagnosticsNode> filterChildren(List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return childFilter?.call(nodes, owner) ?? nodes;
}
@override
List<DiagnosticsNode> filterProperties(List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return propertyFilter?.call(nodes, owner) ?? nodes;
}
@override
final bool includeProperties;
@override
final int subtreeDepth;
@override
List<DiagnosticsNode> truncateNodesList(List<DiagnosticsNode> nodes, DiagnosticsNode? owner) {
return nodeTruncator?.call(nodes, owner) ?? nodes;
}
@override
DiagnosticsSerializationDelegate copyWith({int? subtreeDepth, bool? includeProperties}) {
return TestDiagnosticsSerializationDelegate(
includeProperties: includeProperties ?? this.includeProperties,
subtreeDepth: subtreeDepth ?? this.subtreeDepth,
additionalNodePropertiesMap: additionalNodePropertiesMap,
childFilter: childFilter,
propertyFilter: propertyFilter,
nodeTruncator: nodeTruncator,
nodeDelegator: nodeDelegator,
);
}
}
| flutter/packages/flutter/test/foundation/diagnostics_json_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/diagnostics_json_test.dart",
"repo_id": "flutter",
"token_count": 4878
} | 727 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('SynchronousFuture control test', () async {
final Future<int> future = SynchronousFuture<int>(42);
int? result;
future.then<void>((int value) { result = value; });
expect(result, equals(42));
result = null;
final Future<int> futureWithTimeout = future.timeout(const Duration(milliseconds: 1));
futureWithTimeout.then<void>((int value) { result = value; });
expect(result, isNull);
await futureWithTimeout;
expect(result, equals(42));
result = null;
final Stream<int> stream = future.asStream();
expect(await stream.single, equals(42));
bool ranAction = false;
final Future<int> completeResult = future.whenComplete(() { // ignore: void_checks, https://github.com/dart-lang/linter/issues/1675
ranAction = true;
// verify that whenComplete does NOT propagate its return value:
return Future<int>.value(31);
});
expect(ranAction, isTrue);
ranAction = false;
expect(await completeResult, equals(42));
Object? exception;
try {
await future.whenComplete(() {
throw ArgumentError();
});
// Unreached.
expect(false, isTrue);
} catch (e) {
exception = e;
}
expect(exception, isArgumentError);
});
}
| flutter/packages/flutter/test/foundation/synchronous_future_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/synchronous_future_test.dart",
"repo_id": "flutter",
"token_count": 534
} | 728 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
bool approx(double value, double expectation) {
const double eps = 1e-6;
return (value - expectation).abs() < eps;
}
test('Least-squares fit: linear polynomial to line', () {
final List<double> x = <double>[0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0];
final List<double> y = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final List<double> w = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final LeastSquaresSolver solver = LeastSquaresSolver(x, y, w);
final PolynomialFit fit = solver.solve(1)!;
expect(fit.coefficients.length, 2);
expect(approx(fit.coefficients[0], 1.0), isTrue);
expect(approx(fit.coefficients[1], 0.0), isTrue);
expect(approx(fit.confidence, 1.0), isTrue);
});
test('Least-squares fit: linear polynomial to sloped line', () {
final List<double> x = <double>[0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0];
final List<double> y = <double>[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0];
final List<double> w = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final LeastSquaresSolver solver = LeastSquaresSolver(x, y, w);
final PolynomialFit fit = solver.solve(1)!;
expect(fit.coefficients.length, 2);
expect(approx(fit.coefficients[0], 1.0), isTrue);
expect(approx(fit.coefficients[1], 1.0), isTrue);
expect(approx(fit.confidence, 1.0), isTrue);
});
test('Least-squares fit: quadratic polynomial to line', () {
final List<double> x = <double>[0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0];
final List<double> y = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final List<double> w = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final LeastSquaresSolver solver = LeastSquaresSolver(x, y, w);
final PolynomialFit fit = solver.solve(2)!;
expect(fit.coefficients.length, 3);
expect(approx(fit.coefficients[0], 1.0), isTrue);
expect(approx(fit.coefficients[1], 0.0), isTrue);
expect(approx(fit.coefficients[2], 0.0), isTrue);
expect(approx(fit.confidence, 1.0), isTrue);
});
test('Least-squares fit: quadratic polynomial to sloped line', () {
final List<double> x = <double>[0.0, 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0];
final List<double> y = <double>[1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0];
final List<double> w = <double>[1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0, 1.0];
final LeastSquaresSolver solver = LeastSquaresSolver(x, y, w);
final PolynomialFit fit = solver.solve(2)!;
expect(fit.coefficients.length, 3);
expect(approx(fit.coefficients[0], 1.0), isTrue);
expect(approx(fit.coefficients[1], 1.0), isTrue);
expect(approx(fit.coefficients[2], 0.0), isTrue);
expect(approx(fit.confidence, 1.0), isTrue);
});
test('Least-squares fit: toString', () {
final PolynomialFit fit = PolynomialFit(2);
fit.coefficients[0] = 123.45;
fit.coefficients[1] = 54.321;
fit.coefficients[2] = 1.3579;
fit.confidence = 0.9876;
expect(fit.toString(), 'PolynomialFit([123, 54.3, 1.36], confidence: 0.988)');
});
}
| flutter/packages/flutter/test/gestures/lsq_solver_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/lsq_solver_test.dart",
"repo_id": "flutter",
"token_count": 1519
} | 729 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('gets local coordinates', (WidgetTester tester) async {
final List<ScaleStartDetails> startDetails = <ScaleStartDetails>[];
final List<ScaleUpdateDetails> updateDetails = <ScaleUpdateDetails>[];
final Key redContainer = UniqueKey();
await tester.pumpWidget(
Center(
child: GestureDetector(
onScaleStart: (ScaleStartDetails details) {
startDetails.add(details);
},
onScaleUpdate: (ScaleUpdateDetails details) {
updateDetails.add(details);
},
child: Container(
key: redContainer,
width: 100,
height: 100,
color: Colors.red,
),
),
),
);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byKey(redContainer)) - const Offset(20, 20));
final TestGesture pointer2 = await tester.startGesture(tester.getCenter(find.byKey(redContainer)) + const Offset(30, 30));
await pointer2.moveTo(tester.getCenter(find.byKey(redContainer)) + const Offset(20, 20));
expect(updateDetails.single.localFocalPoint, const Offset(50, 50));
expect(updateDetails.single.focalPoint, const Offset(400, 300));
expect(startDetails, hasLength(2));
expect(startDetails.first.localFocalPoint, const Offset(30, 30));
expect(startDetails.first.focalPoint, const Offset(380, 280));
expect(startDetails.last.localFocalPoint, const Offset(50, 50));
expect(startDetails.last.focalPoint, const Offset(400, 300));
await tester.pumpAndSettle();
await gesture.up();
await pointer2.up();
await tester.pumpAndSettle();
});
}
| flutter/packages/flutter/test/gestures/transformed_scale_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/transformed_scale_test.dart",
"repo_id": "flutter",
"token_count": 729
} | 730 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets("builder doesn't get called if app doesn't change", (WidgetTester tester) async {
final List<String> log = <String>[];
final Widget app = MaterialApp(
home: const Placeholder(),
builder: (BuildContext context, Widget? child) {
log.add('build');
expect(Directionality.of(context), TextDirection.ltr);
expect(child, isA<FocusScope>());
return const Placeholder();
},
);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: app,
),
);
expect(log, <String>['build']);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: app,
),
);
expect(log, <String>['build']);
});
testWidgets("builder doesn't get called if app doesn't change", (WidgetTester tester) async {
final List<String> log = <String>[];
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
log.add('build');
expect(Directionality.of(context), TextDirection.rtl);
return const Placeholder();
},
),
builder: (BuildContext context, Widget? child) {
return Directionality(
textDirection: TextDirection.rtl,
child: child!,
);
},
),
);
expect(log, <String>['build']);
});
}
| flutter/packages/flutter/test/material/app_builder_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/app_builder_test.dart",
"repo_id": "flutter",
"token_count": 711
} | 731 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('ButtonBarThemeData lerp special cases', () {
expect(ButtonBarThemeData.lerp(null, null, 0), null);
const ButtonBarThemeData data = ButtonBarThemeData();
expect(identical(ButtonBarThemeData.lerp(data, data, 0.5), data), true);
});
test('ButtonBarThemeData null fields by default', () {
const ButtonBarThemeData buttonBarTheme = ButtonBarThemeData();
expect(buttonBarTheme.alignment, null);
expect(buttonBarTheme.mainAxisSize, null);
expect(buttonBarTheme.buttonTextTheme, null);
expect(buttonBarTheme.buttonMinWidth, null);
expect(buttonBarTheme.buttonHeight, null);
expect(buttonBarTheme.buttonPadding, null);
expect(buttonBarTheme.buttonAlignedDropdown, null);
expect(buttonBarTheme.layoutBehavior, null);
expect(buttonBarTheme.overflowDirection, null);
});
test('ThemeData uses default ButtonBarThemeData', () {
expect(ThemeData().buttonBarTheme, equals(const ButtonBarThemeData()));
});
test('ButtonBarThemeData copyWith, ==, hashCode basics', () {
expect(const ButtonBarThemeData(), const ButtonBarThemeData().copyWith());
expect(const ButtonBarThemeData().hashCode, const ButtonBarThemeData().copyWith().hashCode);
});
testWidgets('ButtonBarThemeData lerps correctly', (WidgetTester tester) async {
const ButtonBarThemeData barThemePrimary = ButtonBarThemeData(
alignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.min,
buttonTextTheme: ButtonTextTheme.primary,
buttonMinWidth: 20.0,
buttonHeight: 20.0,
buttonPadding: EdgeInsets.symmetric(vertical: 5.0),
buttonAlignedDropdown: false,
layoutBehavior: ButtonBarLayoutBehavior.padded,
overflowDirection: VerticalDirection.down,
);
const ButtonBarThemeData barThemeAccent = ButtonBarThemeData(
alignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
buttonTextTheme: ButtonTextTheme.accent,
buttonMinWidth: 10.0,
buttonHeight: 40.0,
buttonPadding: EdgeInsets.symmetric(horizontal: 10.0),
buttonAlignedDropdown: true,
layoutBehavior: ButtonBarLayoutBehavior.constrained,
overflowDirection: VerticalDirection.up,
);
final ButtonBarThemeData lerp = ButtonBarThemeData.lerp(barThemePrimary, barThemeAccent, 0.5)!;
expect(lerp.alignment, equals(MainAxisAlignment.center));
expect(lerp.mainAxisSize, equals(MainAxisSize.max));
expect(lerp.buttonTextTheme, equals(ButtonTextTheme.accent));
expect(lerp.buttonMinWidth, equals(15.0));
expect(lerp.buttonHeight, equals(30.0));
expect(lerp.buttonPadding, equals(const EdgeInsets.fromLTRB(5.0, 2.5, 5.0, 2.5)));
expect(lerp.buttonAlignedDropdown, isTrue);
expect(lerp.layoutBehavior, equals(ButtonBarLayoutBehavior.constrained));
expect(lerp.overflowDirection, equals(VerticalDirection.up));
});
testWidgets('Default ButtonBarThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const ButtonBarThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('ButtonBarThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const ButtonBarThemeData(
alignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.max,
buttonTextTheme: ButtonTextTheme.accent,
buttonMinWidth: 10.0,
buttonHeight: 42.0,
buttonPadding: EdgeInsets.symmetric(horizontal: 7.3),
buttonAlignedDropdown: true,
layoutBehavior: ButtonBarLayoutBehavior.constrained,
overflowDirection: VerticalDirection.up,
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'alignment: MainAxisAlignment.center',
'mainAxisSize: MainAxisSize.max',
'textTheme: ButtonTextTheme.accent',
'minWidth: 10.0',
'height: 42.0',
'padding: EdgeInsets(7.3, 0.0, 7.3, 0.0)',
'dropdown width matches button',
'layoutBehavior: ButtonBarLayoutBehavior.constrained',
'overflowDirection: VerticalDirection.up',
]);
});
testWidgets('ButtonBarTheme.of falls back to ThemeData.buttonBarTheme', (WidgetTester tester) async {
const ButtonBarThemeData buttonBarTheme = ButtonBarThemeData(buttonMinWidth: 42.0);
late BuildContext capturedContext;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(buttonBarTheme: buttonBarTheme),
home: Builder(
builder: (BuildContext context) {
capturedContext = context;
return Container();
},
),
),
);
expect(ButtonBarTheme.of(capturedContext), equals(buttonBarTheme));
expect(ButtonBarTheme.of(capturedContext).buttonMinWidth, equals(42.0));
});
testWidgets('ButtonBarTheme overrides ThemeData.buttonBarTheme', (WidgetTester tester) async {
const ButtonBarThemeData defaultBarTheme = ButtonBarThemeData(buttonMinWidth: 42.0);
const ButtonBarThemeData buttonBarTheme = ButtonBarThemeData(buttonMinWidth: 84.0);
late BuildContext capturedContext;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(buttonBarTheme: defaultBarTheme),
home: Builder(
builder: (BuildContext context) {
return ButtonBarTheme(
data: buttonBarTheme,
child: Builder(
builder: (BuildContext context) {
capturedContext = context;
return Container();
},
),
);
},
),
),
);
expect(ButtonBarTheme.of(capturedContext), equals(buttonBarTheme));
expect(ButtonBarTheme.of(capturedContext).buttonMinWidth, equals(84.0));
});
}
| flutter/packages/flutter/test/material/button_bar_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/button_bar_theme_test.dart",
"repo_id": "flutter",
"token_count": 2407
} | 732 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
class Dessert {
Dessert(this.name, this.calories, this.fat, this.carbs, this.protein, this.sodium, this.calcium, this.iron);
final String name;
final int calories;
final double fat;
final int carbs;
final double protein;
final int sodium;
final int calcium;
final int iron;
}
final List<Dessert> kDesserts = <Dessert>[
Dessert('Frozen yogurt', 159, 6.0, 24, 4.0, 87, 14, 1),
Dessert('Ice cream sandwich', 237, 9.0, 37, 4.3, 129, 8, 1),
Dessert('Eclair', 262, 16.0, 24, 6.0, 337, 6, 7),
Dessert('Cupcake', 305, 3.7, 67, 4.3, 413, 3, 8),
Dessert('Gingerbread', 356, 16.0, 49, 3.9, 327, 7, 16),
Dessert('Jelly bean', 375, 0.0, 94, 0.0, 50, 0, 0),
Dessert('Lollipop', 392, 0.2, 98, 0.0, 38, 0, 2),
Dessert('Honeycomb', 408, 3.2, 87, 6.5, 562, 0, 45),
Dessert('Donut', 452, 25.0, 51, 4.9, 326, 2, 22),
Dessert('KitKat', 518, 26.0, 65, 7.0, 54, 12, 6),
];
| flutter/packages/flutter/test/material/data_table_test_utils.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/data_table_test_utils.dart",
"repo_id": "flutter",
"token_count": 739
} | 733 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
const String longText = 'one two three four five six seven eight nine ten eleven twelve';
final List<DropdownMenuEntry<TestMenu>> menuChildren = <DropdownMenuEntry<TestMenu>>[];
for (final TestMenu value in TestMenu.values) {
final DropdownMenuEntry<TestMenu> entry = DropdownMenuEntry<TestMenu>(value: value, label: value.label);
menuChildren.add(entry);
}
Widget buildTest<T extends Enum>(ThemeData themeData, List<DropdownMenuEntry<T>> entries,
{double? width, double? menuHeight, Widget? leadingIcon, Widget? label}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<T>(
label: label,
leadingIcon: leadingIcon,
width: width,
menuHeight: menuHeight,
dropdownMenuEntries: entries,
),
),
);
}
testWidgets('DropdownMenu defaults', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(buildTest(themeData, menuChildren));
final EditableText editableText = tester.widget(find.byType(EditableText));
expect(editableText.style.color, themeData.textTheme.bodyLarge!.color);
expect(editableText.style.background, themeData.textTheme.bodyLarge!.background);
expect(editableText.style.shadows, themeData.textTheme.bodyLarge!.shadows);
expect(editableText.style.decoration, themeData.textTheme.bodyLarge!.decoration);
expect(editableText.style.locale, themeData.textTheme.bodyLarge!.locale);
expect(editableText.style.wordSpacing, themeData.textTheme.bodyLarge!.wordSpacing);
expect(editableText.style.fontSize, 16.0);
expect(editableText.style.height, 1.5);
final TextField textField = tester.widget(find.byType(TextField));
expect(textField.decoration?.border, const OutlineInputBorder());
expect(textField.style?.fontSize, 16.0);
expect(textField.style?.height, 1.5);
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first);
await tester.pump();
expect(find.byType(MenuAnchor), findsOneWidget);
final Finder menuMaterial = find.ancestor(
of: find.widgetWithText(TextButton, TestMenu.mainMenu0.label),
matching: find.byType(Material),
).at(1);
Material material = tester.widget<Material>(menuMaterial);
expect(material.color, themeData.colorScheme.surfaceContainer);
expect(material.shadowColor, themeData.colorScheme.shadow);
expect(material.surfaceTintColor, Colors.transparent);
expect(material.elevation, 3.0);
expect(material.shape, const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4.0))));
final Finder buttonMaterial = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
).last;
material = tester.widget<Material>(buttonMaterial);
expect(material.color, Colors.transparent);
expect(material.elevation, 0.0);
expect(material.shape, const RoundedRectangleBorder());
expect(material.textStyle?.color, themeData.colorScheme.onSurface);
expect(material.textStyle?.fontSize, 14.0);
expect(material.textStyle?.height, 1.43);
});
testWidgets('DropdownMenu can be disabled', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
body: SafeArea(
child: DropdownMenu<TestMenu>(
enabled: false,
dropdownMenuEntries: menuChildren,
),
),
),
),
);
final TextField textField = tester.widget(find.byType(TextField));
expect(textField.decoration?.enabled, false);
final Finder menuMaterial = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
);
expect(menuMaterial, findsNothing);
await tester.tap(find.byType(TextField));
await tester.pump();
final Finder updatedMenuMaterial = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
);
expect(updatedMenuMaterial, findsNothing);
});
testWidgets('Material2 - The width of the text field should always be the same as the menu view',
(WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: false);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
body: SafeArea(
child: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
),
)
);
final Finder textField = find.byType(TextField);
final Size anchorSize = tester.getSize(textField);
expect(anchorSize, const Size(180.0, 56.0));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder menuMaterial = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
).first;
final Size menuSize = tester.getSize(menuMaterial);
expect(menuSize, const Size(180.0, 304.0));
// The text field should have same width as the menu
// when the width property is not null.
await tester.pumpWidget(buildTest(themeData, menuChildren, width: 200.0));
final Finder anchor = find.byType(TextField);
final double width = tester.getSize(anchor).width;
expect(width, 200.0);
await tester.tap(anchor);
await tester.pumpAndSettle();
final Finder updatedMenu = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
).first;
final double updatedMenuWidth = tester.getSize(updatedMenu).width;
expect(updatedMenuWidth, 200.0);
});
testWidgets('Material3 - The width of the text field should always be the same as the menu view',
(WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: true);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
body: SafeArea(
child: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
),
)
);
final Finder textField = find.byType(TextField);
final double anchorWidth = tester.getSize(textField).width;
expect(anchorWidth, closeTo(180.5, 0.1));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder menuMaterial = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
).first;
final double menuWidth = tester.getSize(menuMaterial).width;
expect(menuWidth, closeTo(180.5, 0.1));
// The text field should have same width as the menu
// when the width property is not null.
await tester.pumpWidget(buildTest(themeData, menuChildren, width: 200.0));
final Finder anchor = find.byType(TextField);
final double width = tester.getSize(anchor).width;
expect(width, 200.0);
await tester.tap(anchor);
await tester.pumpAndSettle();
final Finder updatedMenu = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Material),
).first;
final double updatedMenuWidth = tester.getSize(updatedMenu).width;
expect(updatedMenuWidth, 200.0);
});
testWidgets('The width property can customize the width of the dropdown menu', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<ShortMenu>> shortMenuItems = <DropdownMenuEntry<ShortMenu>>[];
for (final ShortMenu value in ShortMenu.values) {
final DropdownMenuEntry<ShortMenu> entry = DropdownMenuEntry<ShortMenu>(value: value, label: value.label);
shortMenuItems.add(entry);
}
const double customBigWidth = 250.0;
await tester.pumpWidget(buildTest(themeData, shortMenuItems, width: customBigWidth));
RenderBox box = tester.firstRenderObject(find.byType(DropdownMenu<ShortMenu>));
expect(box.size.width, customBigWidth);
await tester.tap(find.byType(DropdownMenu<ShortMenu>));
await tester.pump();
expect(find.byType(MenuItemButton), findsNWidgets(6));
Size buttonSize = tester.getSize(find.widgetWithText(MenuItemButton, 'I0').last);
expect(buttonSize.width, customBigWidth);
// reset test
await tester.pumpWidget(Container());
const double customSmallWidth = 100.0;
await tester.pumpWidget(buildTest(themeData, shortMenuItems, width: customSmallWidth));
box = tester.firstRenderObject(find.byType(DropdownMenu<ShortMenu>));
expect(box.size.width, customSmallWidth);
await tester.tap(find.byType(DropdownMenu<ShortMenu>));
await tester.pump();
expect(find.byType(MenuItemButton), findsNWidgets(6));
buttonSize = tester.getSize(find.widgetWithText(MenuItemButton, 'I0').last);
expect(buttonSize.width, customSmallWidth);
});
testWidgets('The width property update test', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/120567
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<ShortMenu>> shortMenuItems = <DropdownMenuEntry<ShortMenu>>[];
for (final ShortMenu value in ShortMenu.values) {
final DropdownMenuEntry<ShortMenu> entry = DropdownMenuEntry<ShortMenu>(value: value, label: value.label);
shortMenuItems.add(entry);
}
double customWidth = 250.0;
await tester.pumpWidget(buildTest(themeData, shortMenuItems, width: customWidth));
RenderBox box = tester.firstRenderObject(find.byType(DropdownMenu<ShortMenu>));
expect(box.size.width, customWidth);
// Update width
customWidth = 400.0;
await tester.pumpWidget(buildTest(themeData, shortMenuItems, width: customWidth));
box = tester.firstRenderObject(find.byType(DropdownMenu<ShortMenu>));
expect(box.size.width, customWidth);
});
testWidgets('The width of MenuAnchor respects MenuAnchor.expandedInsets', (WidgetTester tester) async {
const double parentWidth = 500.0;
final List<DropdownMenuEntry<ShortMenu>> shortMenuItems = <DropdownMenuEntry<ShortMenu>>[];
for (final ShortMenu value in ShortMenu.values) {
final DropdownMenuEntry<ShortMenu> entry = DropdownMenuEntry<ShortMenu>(value: value, label: value.label);
shortMenuItems.add(entry);
}
Widget buildMenuAnchor({EdgeInsets? expandedInsets}) {
return MaterialApp(
home: Scaffold(
body: SizedBox(
width: parentWidth,
height: parentWidth,
child: DropdownMenu<ShortMenu>(
expandedInsets: expandedInsets,
dropdownMenuEntries: shortMenuItems,
),
),
),
);
}
// By default, the width of the text field is determined by the menu children.
await tester.pumpWidget(buildMenuAnchor());
RenderBox box = tester.firstRenderObject(find.byType(TextField));
expect(box.size.width, 136.0);
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
Size buttonSize = tester.getSize(find.widgetWithText(MenuItemButton, 'I0').hitTestable());
expect(buttonSize.width, 136.0);
// If expandedInsets is EdgeInsets.zero, the width should be the same as its parent.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildMenuAnchor(expandedInsets: EdgeInsets.zero));
box = tester.firstRenderObject(find.byType(TextField));
expect(box.size.width, parentWidth);
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
buttonSize = tester.getSize(find.widgetWithText(MenuItemButton, 'I0'));
expect(buttonSize.width, parentWidth);
// If expandedInsets is not zero, the width of the text field should be adjusted
// based on the EdgeInsets.left and EdgeInsets.right. The top and bottom values
// will be ignored.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildMenuAnchor(expandedInsets: const EdgeInsets.only(left: 35.0, top: 50.0, right: 20.0)));
box = tester.firstRenderObject(find.byType(TextField));
expect(box.size.width, parentWidth - 35.0 - 20.0);
final Rect containerRect = tester.getRect(find.byType(SizedBox).first);
final Rect dropdownMenuRect = tester.getRect(find.byType(TextField));
expect(dropdownMenuRect.top, containerRect.top);
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
buttonSize = tester.getSize(find.widgetWithText(MenuItemButton, 'I0'));
expect(buttonSize.width, parentWidth - 35.0 - 20.0);
});
testWidgets('Material2 - The menuHeight property can be used to show a shorter scrollable menu list instead of the complete list',
(WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: false);
await tester.pumpWidget(buildTest(themeData, menuChildren));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Element firstItem = tester.element(find.widgetWithText(MenuItemButton, 'Item 0').last);
final RenderBox firstBox = firstItem.renderObject! as RenderBox;
final Offset topLeft = firstBox.localToGlobal(firstBox.size.topLeft(Offset.zero));
final Element lastItem = tester.element(find.widgetWithText(MenuItemButton, 'Item 5').last);
final RenderBox lastBox = lastItem.renderObject! as RenderBox;
final Offset bottomRight = lastBox.localToGlobal(lastBox.size.bottomRight(Offset.zero));
// height = height of MenuItemButton * 6 = 48 * 6
expect(bottomRight.dy - topLeft.dy, 288.0);
final Finder menuView = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Padding),
).first;
final Size menuViewSize = tester.getSize(menuView);
expect(menuViewSize, const Size(180.0, 304.0)); // 304 = 288 + vertical padding(2 * 8)
// Constrains the menu height.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildTest(themeData, menuChildren, menuHeight: 100));
await tester.pumpAndSettle();
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedMenu = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Padding),
).first;
final Size updatedMenuSize = tester.getSize(updatedMenu);
expect(updatedMenuSize, const Size(180.0, 100.0));
});
testWidgets('Material3 - The menuHeight property can be used to show a shorter scrollable menu list instead of the complete list',
(WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: true);
await tester.pumpWidget(buildTest(themeData, menuChildren));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Element firstItem = tester.element(find.widgetWithText(MenuItemButton, 'Item 0').last);
final RenderBox firstBox = firstItem.renderObject! as RenderBox;
final Offset topLeft = firstBox.localToGlobal(firstBox.size.topLeft(Offset.zero));
final Element lastItem = tester.element(find.widgetWithText(MenuItemButton, 'Item 5').last);
final RenderBox lastBox = lastItem.renderObject! as RenderBox;
final Offset bottomRight = lastBox.localToGlobal(lastBox.size.bottomRight(Offset.zero));
// height = height of MenuItemButton * 6 = 48 * 6
expect(bottomRight.dy - topLeft.dy, 288.0);
final Finder menuView = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Padding),
).first;
final Size menuViewSize = tester.getSize(menuView);
expect(menuViewSize.width, closeTo(180.6, 0.1));
expect(menuViewSize.height, equals(304.0)); // 304 = 288 + vertical padding(2 * 8)
// Constrains the menu height.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildTest(themeData, menuChildren, menuHeight: 100));
await tester.pumpAndSettle();
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedMenu = find.ancestor(
of: find.byType(SingleChildScrollView),
matching: find.byType(Padding),
).first;
final Size updatedMenuSize = tester.getSize(updatedMenu);
expect(updatedMenuSize.width, closeTo(180.6, 0.1));
expect(updatedMenuSize.height, equals(100.0));
});
testWidgets('The text in the menu button should be aligned with the text of '
'the text field - LTR', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
// Default text field (without leading icon).
await tester.pumpWidget(buildTest(themeData, menuChildren, label: const Text('label')));
final Finder label = find.text('label');
final Offset labelTopLeft = tester.getTopLeft(label);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder itemText = find.text('Item 0').last;
final Offset itemTextTopLeft = tester.getTopLeft(itemText);
expect(labelTopLeft.dx, equals(itemTextTopLeft.dx));
// Test when the text field has a leading icon.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildTest(themeData, menuChildren,
leadingIcon: const Icon(Icons.search),
label: const Text('label'),
));
final Finder leadingIcon = find.widgetWithIcon(Container, Icons.search);
final double iconWidth = tester.getSize(leadingIcon).width;
final Finder updatedLabel = find.text('label');
final Offset updatedLabelTopLeft = tester.getTopLeft(updatedLabel);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedItemText = find.text('Item 0').last;
final Offset updatedItemTextTopLeft = tester.getTopLeft(updatedItemText);
expect(updatedLabelTopLeft.dx, equals(updatedItemTextTopLeft.dx));
expect(updatedLabelTopLeft.dx, equals(iconWidth));
// Test when then leading icon is a widget with a bigger size.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildTest(themeData, menuChildren,
leadingIcon: const SizedBox(
width: 75.0,
child: Icon(Icons.search)),
label: const Text('label'),
));
final Finder largeLeadingIcon = find.widgetWithIcon(Container, Icons.search);
final double largeIconWidth = tester.getSize(largeLeadingIcon).width;
final Finder updatedLabel1 = find.text('label');
final Offset updatedLabelTopLeft1 = tester.getTopLeft(updatedLabel1);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedItemText1 = find.text('Item 0').last;
final Offset updatedItemTextTopLeft1 = tester.getTopLeft(updatedItemText1);
expect(updatedLabelTopLeft1.dx, equals(updatedItemTextTopLeft1.dx));
expect(updatedLabelTopLeft1.dx, equals(largeIconWidth));
});
testWidgets('The text in the menu button should be aligned with the text of '
'the text field - RTL', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
// Default text field (without leading icon).
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: Directionality(
textDirection: TextDirection.rtl,
child: DropdownMenu<TestMenu>(
label: const Text('label'),
dropdownMenuEntries: menuChildren,
),
),
),
));
final Finder label = find.text('label');
final Offset labelTopRight = tester.getTopRight(label);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder itemText = find.text('Item 0').last;
final Offset itemTextTopRight = tester.getTopRight(itemText);
expect(labelTopRight.dx, equals(itemTextTopRight.dx));
// Test when the text field has a leading icon.
await tester.pumpWidget(Container());
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: Directionality(
textDirection: TextDirection.rtl,
child: DropdownMenu<TestMenu>(
leadingIcon: const Icon(Icons.search),
label: const Text('label'),
dropdownMenuEntries: menuChildren,
),
),
),
));
await tester.pump();
final Finder leadingIcon = find.widgetWithIcon(Container, Icons.search);
final double iconWidth = tester.getSize(leadingIcon).width;
final Offset dropdownMenuTopRight = tester.getTopRight(find.byType(DropdownMenu<TestMenu>));
final Finder updatedLabel = find.text('label');
final Offset updatedLabelTopRight = tester.getTopRight(updatedLabel);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedItemText = find.text('Item 0').last;
final Offset updatedItemTextTopRight = tester.getTopRight(updatedItemText);
expect(updatedLabelTopRight.dx, equals(updatedItemTextTopRight.dx));
expect(updatedLabelTopRight.dx, equals(dropdownMenuTopRight.dx - iconWidth));
// Test when then leading icon is a widget with a bigger size.
await tester.pumpWidget(Container());
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: Directionality(
textDirection: TextDirection.rtl,
child: DropdownMenu<TestMenu>(
leadingIcon: const SizedBox(width: 75.0, child: Icon(Icons.search)),
label: const Text('label'),
dropdownMenuEntries: menuChildren,
),
),
),
));
await tester.pump();
final Finder largeLeadingIcon = find.widgetWithIcon(Container, Icons.search);
final double largeIconWidth = tester.getSize(largeLeadingIcon).width;
final Offset updatedDropdownMenuTopRight = tester.getTopRight(find.byType(DropdownMenu<TestMenu>));
final Finder updatedLabel1 = find.text('label');
final Offset updatedLabelTopRight1 = tester.getTopRight(updatedLabel1);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
final Finder updatedItemText1 = find.text('Item 0').last;
final Offset updatedItemTextTopRight1 = tester.getTopRight(updatedItemText1);
expect(updatedLabelTopRight1.dx, equals(updatedItemTextTopRight1.dx));
expect(updatedLabelTopRight1.dx, equals(updatedDropdownMenuTopRight.dx - largeIconWidth));
});
testWidgets('DropdownMenu has default trailing icon button', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(buildTest(themeData, menuChildren));
await tester.pump();
final Finder iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first;
expect(iconButton, findsOneWidget);
await tester.tap(iconButton);
await tester.pump();
final Finder menuMaterial = find.ancestor(
of: find.widgetWithText(MenuItemButton, TestMenu.mainMenu0.label),
matching: find.byType(Material),
).last;
expect(menuMaterial, findsOneWidget);
});
testWidgets('Leading IconButton status test', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(useMaterial3: true);
await tester.pumpWidget(buildTest(themeData, menuChildren, width: 100.0, menuHeight: 100.0));
await tester.pump();
Finder iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_up);
expect(iconButton, findsNothing);
iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first;
expect(iconButton, findsOneWidget);
await tester.tap(iconButton);
await tester.pump();
iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_up).first;
expect(iconButton, findsOneWidget);
iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_down);
expect(iconButton, findsNothing);
// Tap outside
await tester.tapAt(const Offset(500.0, 500.0));
await tester.pump();
iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_up);
expect(iconButton, findsNothing);
iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first;
expect(iconButton, findsOneWidget);
});
testWidgets('Do not crash when resize window during menu opening', (WidgetTester tester) async {
addTearDown(tester.view.reset);
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: StatefulBuilder(
builder: (BuildContext context, StateSetter setState){
return DropdownMenu<TestMenu>(
width: MediaQuery.of(context).size.width,
dropdownMenuEntries: menuChildren,
);
},
),
),
));
final Finder iconButton = find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first;
expect(iconButton, findsOneWidget);
await tester.tap(iconButton);
await tester.pump();
final Finder menuMaterial = find.ancestor(
of: find.widgetWithText(MenuItemButton, TestMenu.mainMenu0.label),
matching: find.byType(Material),
);
expect(menuMaterial, findsNWidgets(3));
// didChangeMetrics
tester.view.physicalSize = const Size(700.0, 700.0);
await tester.pump();
// Go without throw.
});
testWidgets('DropdownMenu can customize trailing icon button', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
trailingIcon: const Icon(Icons.ac_unit),
dropdownMenuEntries: menuChildren,
),
),
));
await tester.pump();
final Finder iconButton = find.widgetWithIcon(IconButton, Icons.ac_unit).first;
expect(iconButton, findsOneWidget);
await tester.tap(iconButton);
await tester.pump();
final Finder menuMaterial = find.ancestor(
of: find.widgetWithText(MenuItemButton, TestMenu.mainMenu0.label),
matching: find.byType(Material),
).last;
expect(menuMaterial, findsOneWidget);
});
testWidgets('Down key can highlight the menu item on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
trailingIcon: const Icon(Icons.ac_unit),
dropdownMenuEntries: menuChildren,
),
),
));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
Finder button0Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 0').last,
matching: find.byType(Material),
);
Material item0material = tester.widget<Material>(button0Material);
expect(item0material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
// Press down key one more time, the highlight should move to the next item.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
final Finder button1Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Menu 1').last,
matching: find.byType(Material),
);
final Material item1material = tester.widget<Material>(button1Material);
expect(item1material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
button0Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 0').last,
matching: find.byType(Material),
);
item0material = tester.widget<Material>(button0Material);
expect(item0material.color, Colors.transparent); // the previous item should not be highlighted.
}, variant: TargetPlatformVariant.desktop());
testWidgets('Up key can highlight the menu item on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
Finder button5Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 5').last,
matching: find.byType(Material),
);
Material item5material = tester.widget<Material>(button5Material);
expect(item5material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
// Press up key one more time, the highlight should move up to the item 4.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
final Finder button4Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 4').last,
matching: find.byType(Material),
);
final Material item4material = tester.widget<Material>(button4Material);
expect(item4material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
button5Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 5').last,
matching: find.byType(Material),
);
item5material = tester.widget<Material>(button5Material);
expect(item5material.color, Colors.transparent); // the previous item should not be highlighted.
}, variant: TargetPlatformVariant.desktop());
testWidgets('The text input should match the label of the menu item '
'while pressing down key on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 0'), findsOneWidget);
// Press down key one more time to the next item.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pump();
expect(find.widgetWithText(TextField, 'Menu 1'), findsOneWidget);
// Press down to the next item.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 2'), findsOneWidget);
}, variant: TargetPlatformVariant.desktop());
testWidgets('The text input should match the label of the menu item '
'while pressing up key on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 5'), findsOneWidget);
// Press up key one more time to the upper item.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 4'), findsOneWidget);
// Press up to the upper item.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowUp);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 3'), findsOneWidget);
}, variant: TargetPlatformVariant.desktop());
testWidgets('Disabled button will be skipped while pressing up/down key on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<TestMenu>> menuWithDisabledItems = <DropdownMenuEntry<TestMenu>>[
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 0'),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu1, label: 'Item 1', enabled: false),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu2, label: 'Item 2', enabled: false),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu3, label: 'Item 3'),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu4, label: 'Item 4'),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu5, label: 'Item 5', enabled: false),
];
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuWithDisabledItems,
),
),
));
await tester.pump();
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
final Finder button0Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 0').last,
matching: find.byType(Material),
);
final Material item0Material = tester.widget<Material>(button0Material);
expect(item0Material.color, themeData.colorScheme.onSurface.withOpacity(0.12)); // first item can be highlighted as it's enabled.
// Continue to press down key. Item 3 should be highlighted as Menu 1 and Item 2 are both disabled.
await tester.sendKeyEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
final Finder button3Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 3').last,
matching: find.byType(Material),
);
final Material item3Material = tester.widget<Material>(button3Material);
expect(item3Material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
}, variant: TargetPlatformVariant.desktop());
testWidgets('Searching is enabled by default on mobile platforms if initialSelection is non null', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
initialSelection: TestMenu.mainMenu1,
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder buttonMaterial = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Menu 1').last,
matching: find.byType(Material),
);
final Material itemMaterial = tester.widget<Material>(buttonMaterial);
expect(itemMaterial.color, themeData.colorScheme.onSurface.withOpacity(0.12)); // Menu 1 button is highlighted.
}, variant: TargetPlatformVariant.mobile());
testWidgets('Searching is enabled by default on desktop platform', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.enterText(find.byType(TextField).first, 'Menu 1');
await tester.pumpAndSettle();
final Finder buttonMaterial = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Menu 1').last,
matching: find.byType(Material),
);
final Material itemMaterial = tester.widget<Material>(buttonMaterial);
expect(itemMaterial.color, themeData.colorScheme.onSurface.withOpacity(0.12)); // Menu 1 button is highlighted.
}, variant: TargetPlatformVariant.desktop());
testWidgets('Highlight can move up/down starting from the searching result on desktop platforms', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.enterText(find.byType(TextField).first, 'Menu 1');
await tester.pumpAndSettle();
final Finder buttonMaterial = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Menu 1').last,
matching: find.byType(Material),
);
final Material itemMaterial = tester.widget<Material>(buttonMaterial);
expect(itemMaterial.color, themeData.colorScheme.onSurface.withOpacity(0.12));
// Press up to the upper item (Item 0).
await simulateKeyDownEvent(LogicalKeyboardKey.arrowUp);
await simulateKeyUpEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(find.widgetWithText(TextField, 'Item 0'), findsOneWidget);
final Finder button0Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 0').last,
matching: find.byType(Material),
);
final Material item0Material = tester.widget<Material>(button0Material);
expect(item0Material.color, themeData.colorScheme.onSurface.withOpacity(0.12)); // Move up, the 'Item 0' is highlighted.
// Continue to move up to the last item (Item 5).
await simulateKeyDownEvent(LogicalKeyboardKey.arrowUp);
await simulateKeyUpEvent(LogicalKeyboardKey.arrowUp);
await tester.pumpAndSettle();
expect(find.widgetWithText(TextField, 'Item 5'), findsOneWidget);
final Finder button5Material = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 5').last,
matching: find.byType(Material),
);
final Material item5Material = tester.widget<Material>(button5Material);
expect(item5Material.color, themeData.colorScheme.onSurface.withOpacity(0.12));
}, variant: TargetPlatformVariant.desktop());
testWidgets('Filtering is disabled by default', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.enterText(find.byType(TextField).first, 'Menu 1');
await tester.pumpAndSettle();
for (final TestMenu menu in TestMenu.values) {
// One is layout for the _DropdownMenuBody, the other one is the real button item in the menu.
expect(find.widgetWithText(MenuItemButton, menu.label), findsNWidgets(2));
}
});
testWidgets('Enable filtering', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
enableFilter: true,
dropdownMenuEntries: menuChildren,
),
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
await tester.enterText(find
.byType(TextField)
.first, 'Menu 1');
await tester.pumpAndSettle();
for (final TestMenu menu in TestMenu.values) {
// 'Menu 1' should be 2, other items should only find one.
if (menu.label == TestMenu.mainMenu1.label) {
expect(find.widgetWithText(MenuItemButton, menu.label), findsNWidgets(2));
} else {
expect(find.widgetWithText(MenuItemButton, menu.label), findsOneWidget);
}
}
});
testWidgets('The controller can access the value in the input field', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Scaffold(
body: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
enableFilter: true,
dropdownMenuEntries: menuChildren,
controller: controller,
),
);
}
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder item3 = find.widgetWithText(MenuItemButton, 'Item 3').last;
await tester.tap(item3);
await tester.pumpAndSettle();
expect(controller.text, 'Item 3');
await tester.enterText(find.byType(TextField).first, 'New Item');
expect(controller.text, 'New Item');
});
testWidgets('The menu should be closed after text editing is complete', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: Scaffold(
body: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
enableFilter: true,
dropdownMenuEntries: menuChildren,
controller: controller,
),
),
));
// Access the MenuAnchor
final MenuAnchor menuAnchor = tester.widget<MenuAnchor>(find.byType(MenuAnchor));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
expect(menuAnchor.controller!.isOpen, true);
// Simulate `TextInputAction.done` on textfield
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pumpAndSettle();
expect(menuAnchor.controller!.isOpen, false);
});
testWidgets('The onSelected gets called only when a selection is made', (WidgetTester tester) async {
int selectionCount = 0;
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<TestMenu>> menuWithDisabledItems = <DropdownMenuEntry<TestMenu>>[
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 0'),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 1', enabled: false),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 2'),
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 3'),
];
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuWithDisabledItems,
controller: controller,
onSelected: (_) {
setState(() {
selectionCount++;
});
},
),
);
}
),
));
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final bool isMobile = switch (themeData.platform) {
TargetPlatform.android || TargetPlatform.iOS || TargetPlatform.fuchsia => true,
TargetPlatform.macOS || TargetPlatform.linux || TargetPlatform.windows => false,
};
int expectedCount = isMobile ? 0 : 1;
// Test onSelected on key press
await simulateKeyDownEvent(LogicalKeyboardKey.arrowDown);
await tester.pumpAndSettle();
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pumpAndSettle();
expect(selectionCount, expectedCount);
// The desktop platform closed the menu when a completion action is pressed. So we need to reopen it.
if (!isMobile) {
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
}
// Disabled item doesn't trigger onSelected callback.
final Finder item1 = find.widgetWithText(MenuItemButton, 'Item 1').last;
await tester.tap(item1);
await tester.pumpAndSettle();
expect(controller.text, isMobile ? '' : 'Item 0');
expect(selectionCount, expectedCount);
final Finder item2 = find.widgetWithText(MenuItemButton, 'Item 2').last;
await tester.tap(item2);
await tester.pumpAndSettle();
expect(controller.text, 'Item 2');
expect(selectionCount, ++expectedCount);
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder item3 = find.widgetWithText(MenuItemButton, 'Item 3').last;
await tester.tap(item3);
await tester.pumpAndSettle();
expect(controller.text, 'Item 3');
expect(selectionCount, ++expectedCount);
// On desktop platforms, when typing something in the text field without selecting any of the options,
// the onSelected should not be called.
if (!isMobile) {
await tester.enterText(find.byType(TextField).first, 'New Item');
expect(controller.text, 'New Item');
expect(selectionCount, expectedCount);
expect(find.widgetWithText(TextField, 'New Item'), findsOneWidget);
await tester.enterText(find.byType(TextField).first, '');
expect(selectionCount, expectedCount);
expect(controller.text.isEmpty, true);
}
}, variant: TargetPlatformVariant.all());
testWidgets('The selectedValue gives an initial text and highlights the according item', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Scaffold(
body: DropdownMenu<TestMenu>(
initialSelection: TestMenu.mainMenu3,
dropdownMenuEntries: menuChildren,
controller: controller,
),
);
}
),
));
expect(find.widgetWithText(TextField, 'Item 3'), findsOneWidget);
// Open the menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder buttonMaterial = find.descendant(
of: find.widgetWithText(MenuItemButton, 'Item 3'),
matching: find.byType(Material),
).last;
// Validate the item 3 is highlighted.
final Material itemMaterial = tester.widget<Material>(buttonMaterial);
expect(itemMaterial.color, themeData.colorScheme.onSurface.withOpacity(0.12));
});
testWidgets('The default text input field should not be focused on mobile platforms '
'when it is tapped', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
Widget buildDropdownMenu() => MaterialApp(
theme: themeData,
home: Scaffold(
body: Column(
children: <Widget>[
DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
],
),
),
);
// Test default condition.
await tester.pumpWidget(buildDropdownMenu());
await tester.pump();
final Finder textFieldFinder = find.byType(TextField);
final TextField result = tester.widget<TextField>(textFieldFinder);
expect(result.canRequestFocus, false);
}, variant: TargetPlatformVariant.mobile());
testWidgets('The text input field should be focused on desktop platforms '
'when it is tapped', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
Widget buildDropdownMenu() => MaterialApp(
theme: themeData,
home: Scaffold(
body: Column(
children: <Widget>[
DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
],
),
),
);
await tester.pumpWidget(buildDropdownMenu());
await tester.pump();
final Finder textFieldFinder = find.byType(TextField);
final TextField result = tester.widget<TextField>(textFieldFinder);
expect(result.canRequestFocus, true);
}, variant: TargetPlatformVariant.desktop());
testWidgets('If requestFocusOnTap is true, the text input field can request focus, '
'otherwise it cannot request focus', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
Widget buildDropdownMenu({required bool requestFocusOnTap}) => MaterialApp(
theme: themeData,
home: Scaffold(
body: Column(
children: <Widget>[
DropdownMenu<TestMenu>(
requestFocusOnTap: requestFocusOnTap,
dropdownMenuEntries: menuChildren,
),
],
),
),
);
// Set requestFocusOnTap to true.
await tester.pumpWidget(buildDropdownMenu(requestFocusOnTap: true));
await tester.pump();
final Finder textFieldFinder = find.byType(TextField);
final TextField textField = tester.widget<TextField>(textFieldFinder);
expect(textField.canRequestFocus, true);
// Open the dropdown menu.
await tester.tap(textFieldFinder);
await tester.pump();
// Make a selection.
await tester.tap(find.widgetWithText(MenuItemButton, 'Item 0').last);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 0'), findsOneWidget);
// Set requestFocusOnTap to false.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildDropdownMenu(requestFocusOnTap: false));
await tester.pumpAndSettle();
final Finder textFieldFinder1 = find.byType(TextField);
final TextField textField1 = tester.widget<TextField>(textFieldFinder1);
expect(textField1.canRequestFocus, false);
// Open the dropdown menu.
await tester.tap(textFieldFinder1);
await tester.pump();
// Make a selection.
await tester.tap(find.widgetWithText(MenuItemButton, 'Item 0').last);
await tester.pump();
expect(find.widgetWithText(TextField, 'Item 0'), findsOneWidget);
}, variant: TargetPlatformVariant.all());
testWidgets('If requestFocusOnTap is false, the mouse cursor should be clickable when hovered', (WidgetTester tester) async {
Widget buildDropdownMenu() => MaterialApp(
home: Scaffold(
body: Column(
children: <Widget>[
DropdownMenu<TestMenu>(
requestFocusOnTap: false,
dropdownMenuEntries: menuChildren,
),
],
),
),
);
await tester.pumpWidget(buildDropdownMenu());
await tester.pumpAndSettle();
final Finder textFieldFinder = find.byType(TextField);
final TextField textField = tester.widget<TextField>(textFieldFinder);
expect(textField.canRequestFocus, false);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.moveTo(tester.getCenter(textFieldFinder));
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.click);
});
testWidgets('The menu has the same width as the input field in ListView', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/123631
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: ListView(
children: <Widget>[
DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
),
],
),
),
));
final Rect textInput = tester.getRect(find.byType(TextField));
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
final Finder findMenu = find.byWidgetPredicate((Widget widget) {
return widget.runtimeType.toString() == '_MenuPanel';
});
final Rect menu = tester.getRect(findMenu);
expect(textInput.width, menu.width);
await tester.pumpWidget(Container());
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: ListView(
children: <Widget>[
DropdownMenu<TestMenu>(
width: 200,
dropdownMenuEntries: menuChildren,
),
],
),
),
));
final Rect textInput1 = tester.getRect(find.byType(TextField));
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
final Finder findMenu1 = find.byWidgetPredicate((Widget widget) {
return widget.runtimeType.toString() == '_MenuPanel';
});
final Rect menu1 = tester.getRect(findMenu1);
expect(textInput1.width, 200);
expect(menu1.width, 200);
});
testWidgets('Semantics does not include hint when input is not empty', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
const String hintText = 'I am hintText';
TestMenu? selectedValue;
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) => MaterialApp(
theme: themeData,
home: Scaffold(
body: Center(
child: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
dropdownMenuEntries: menuChildren,
hintText: hintText,
onSelected: (TestMenu? value) {
setState(() {
selectedValue = value;
});
},
controller: controller,
),
),
),
),
),
);
final SemanticsNode node = tester.getSemantics(find.text(hintText));
expect(selectedValue?.label, null);
expect(node.label, hintText);
expect(node.value, '');
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
await tester.tap(find.widgetWithText(MenuItemButton, 'Item 3').last);
await tester.pumpAndSettle();
expect(selectedValue?.label, 'Item 3');
expect(node.label, '');
expect(node.value, 'Item 3');
});
testWidgets('helperText is not visible when errorText is not null', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
const String helperText = 'I am helperText';
const String errorText = 'I am errorText';
Widget buildFrame(bool hasError) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Center(
child: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
helperText: helperText,
errorText: hasError ? errorText : null,
),
),
),
);
}
await tester.pumpWidget(buildFrame(false));
expect(find.text(helperText), findsOneWidget);
expect(find.text(errorText), findsNothing);
await tester.pumpWidget(buildFrame(true));
await tester.pumpAndSettle();
expect(find.text(helperText), findsNothing);
expect(find.text(errorText), findsOneWidget);
});
testWidgets('DropdownMenu can respect helperText when helperText is not null', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
const String helperText = 'I am helperText';
Widget buildFrame() {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Center(
child: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
helperText: helperText,
),
),
),
);
}
await tester.pumpWidget(buildFrame());
expect(find.text(helperText), findsOneWidget);
});
testWidgets('DropdownMenu can respect errorText when errorText is not null', (WidgetTester tester) async {
final ThemeData themeData = ThemeData();
const String errorText = 'I am errorText';
Widget buildFrame() {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Center(
child: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
errorText: errorText,
),
),
),
);
}
await tester.pumpWidget(buildFrame());
expect(find.text(errorText), findsOneWidget);
});
testWidgets('Can scroll to the highlighted item', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: DropdownMenu<TestMenu>(
requestFocusOnTap: true,
menuHeight: 100, // Give a small number so the list can only show 2 or 3 items.
dropdownMenuEntries: menuChildren,
),
),
));
await tester.pumpAndSettle();
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
expect(find.text('Item 5').hitTestable(), findsNothing);
await tester.enterText(find.byType(TextField), '5');
await tester.pumpAndSettle();
// Item 5 should show up.
expect(find.text('Item 5').hitTestable(), findsOneWidget);
});
// This is a regression test for https://github.com/flutter/flutter/issues/131676.
testWidgets('Material3 - DropdownMenu uses correct text styles', (WidgetTester tester) async {
const TextStyle inputTextThemeStyle = TextStyle(
fontSize: 18.5,
fontStyle: FontStyle.italic,
wordSpacing: 1.2,
decoration: TextDecoration.lineThrough,
);
const TextStyle menuItemTextThemeStyle = TextStyle(
fontSize: 20.5,
fontStyle: FontStyle.italic,
wordSpacing: 2.1,
decoration: TextDecoration.underline,
);
final ThemeData themeData = ThemeData(
useMaterial3: true,
textTheme: const TextTheme(
bodyLarge: inputTextThemeStyle,
labelLarge: menuItemTextThemeStyle,
),
);
await tester.pumpWidget(buildTest(themeData, menuChildren));
// Test input text style uses the TextTheme.bodyLarge.
final EditableText editableText = tester.widget(find.byType(EditableText));
expect(editableText.style.fontSize, inputTextThemeStyle.fontSize);
expect(editableText.style.fontStyle, inputTextThemeStyle.fontStyle);
expect(editableText.style.wordSpacing, inputTextThemeStyle.wordSpacing);
expect(editableText.style.decoration, inputTextThemeStyle.decoration);
// Open the menu.
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_drop_down).first);
await tester.pump();
final Finder buttonMaterial = find.descendant(
of: find.byType(TextButton),
matching: find.byType(Material),
).last;
// Test menu item text style uses the TextTheme.labelLarge.
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.textStyle?.fontSize, menuItemTextThemeStyle.fontSize);
expect(material.textStyle?.fontStyle, menuItemTextThemeStyle.fontStyle);
expect(material.textStyle?.wordSpacing, menuItemTextThemeStyle.wordSpacing);
expect(material.textStyle?.decoration, menuItemTextThemeStyle.decoration);
});
testWidgets('DropdownMenuEntries do not overflow when width is specified', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/126882
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DropdownMenu<TestMenu>(
controller: controller,
width: 100,
dropdownMenuEntries: TestMenu.values.map<DropdownMenuEntry<TestMenu>>((TestMenu item) {
return DropdownMenuEntry<TestMenu>(
value: item,
label: '${item.label} $longText',
);
}).toList(),
),
),
),
);
// Opening the width=100 menu should not crash.
await tester.tap(find.byType(DropdownMenu<TestMenu>));
expect(tester.takeException(), isNull);
await tester.pumpAndSettle();
Finder findMenuItemText(String label) {
final String labelText = '$label $longText';
return find.descendant(
of: find.widgetWithText(MenuItemButton, labelText),
matching: find.byType(Text),
).last;
}
// Actual size varies a little on web platforms.
final Matcher closeTo300 = closeTo(300, 0.25);
expect(tester.getSize(findMenuItemText('Item 0')).height, closeTo300);
expect(tester.getSize(findMenuItemText('Menu 1')).height, closeTo300);
expect(tester.getSize(findMenuItemText('Item 2')).height, closeTo300);
expect(tester.getSize(findMenuItemText('Item 3')).height, closeTo300);
await tester.tap(findMenuItemText('Item 0'));
await tester.pumpAndSettle();
expect(controller.text, 'Item 0 $longText');
});
testWidgets('DropdownMenuEntry.labelWidget is Text that specifies maxLines 1 or 2', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/126882
final TextEditingController controller = TextEditingController();
addTearDown(controller.dispose);
Widget buildFrame({ required int maxLines }) {
return MaterialApp(
home: Scaffold(
body: DropdownMenu<TestMenu>(
key: ValueKey<int>(maxLines),
controller: controller,
width: 100,
dropdownMenuEntries: TestMenu.values.map<DropdownMenuEntry<TestMenu>>((TestMenu item) {
return DropdownMenuEntry<TestMenu>(
value: item,
label: '${item.label} $longText',
labelWidget: Text('${item.label} $longText', maxLines: maxLines),
);
}).toList(),
),
)
);
}
Finder findMenuItemText(String label) {
final String labelText = '$label $longText';
return find.descendant(
of: find.widgetWithText(MenuItemButton, labelText),
matching: find.byType(Text),
).last;
}
await tester.pumpWidget(buildFrame(maxLines: 1));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
// Actual size varies a little on web platforms.
final Matcher closeTo20 = closeTo(20, 0.05);
expect(tester.getSize(findMenuItemText('Item 0')).height, closeTo20);
expect(tester.getSize(findMenuItemText('Menu 1')).height, closeTo20);
expect(tester.getSize(findMenuItemText('Item 2')).height, closeTo20);
expect(tester.getSize(findMenuItemText('Item 3')).height, closeTo20);
// Close the menu
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
expect(controller.text, ''); // nothing selected
await tester.pumpWidget(buildFrame(maxLines: 2));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
// Actual size varies a little on web platforms.
final Matcher closeTo40 = closeTo(40, 0.05);
expect(tester.getSize(findMenuItemText('Item 0')).height, closeTo40);
expect(tester.getSize(findMenuItemText('Menu 1')).height, closeTo40);
expect(tester.getSize(findMenuItemText('Item 2')).height, closeTo40);
expect(tester.getSize(findMenuItemText('Item 3')).height, closeTo40);
// Close the menu
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
expect(controller.text, ''); // nothing selected
});
// Regression test for https://github.com/flutter/flutter/issues/131350.
testWidgets('DropdownMenuEntry.leadingIcon default layout', (WidgetTester tester) async {
// The DropdownMenu should not get extra padding in DropdownMenuEntry items
// when both text field and DropdownMenuEntry have leading icons.
await tester.pumpWidget(const MaterialApp(
home: Scaffold(
body: DropdownMenu<int>(
leadingIcon: Icon(Icons.search),
hintText: 'Hint',
dropdownMenuEntries: <DropdownMenuEntry<int>>[
DropdownMenuEntry<int>(
value: 0,
label: 'Item 0',
leadingIcon: Icon(Icons.alarm)
),
DropdownMenuEntry<int>(value: 1, label: 'Item 1'),
],
),
)
));
await tester.tap(find.byType(DropdownMenu<int>));
await tester.pumpAndSettle();
// Check text location in text field.
expect(tester.getTopLeft(find.text('Hint')).dx, 48.0);
// By default, the text of item 0 should be aligned with the text of the text field.
expect(tester.getTopLeft(find.text('Item 0').last).dx, 48.0);
// By default, the text of item 1 should be aligned with the text of the text field,
// so there are some extra padding before "Item 1".
expect(tester.getTopLeft(find.text('Item 1').last).dx, 48.0);
});
testWidgets('DropdownMenu can have customized search algorithm', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
Widget dropdownMenu({ SearchCallback<int>? searchCallback }) {
return MaterialApp(
theme: theme,
home: Scaffold(
body: DropdownMenu<int>(
requestFocusOnTap: true,
searchCallback: searchCallback,
dropdownMenuEntries: const <DropdownMenuEntry<int>>[
DropdownMenuEntry<int>(value: 0, label: 'All'),
DropdownMenuEntry<int>(value: 1, label: 'Unread'),
DropdownMenuEntry<int>(value: 2, label: 'Read'),
],
),
)
);
}
void checkExpectedHighlight({String? searchResult, required List<String> otherItems}) {
if (searchResult != null) {
final Finder material = find.descendant(
of: find.widgetWithText(MenuItemButton, searchResult).last,
matching: find.byType(Material),
);
final Material itemMaterial = tester.widget<Material>(material);
expect(itemMaterial.color, theme.colorScheme.onSurface.withOpacity(0.12));
}
for (final String nonHighlight in otherItems) {
final Finder material = find.descendant(
of: find.widgetWithText(MenuItemButton, nonHighlight).last,
matching: find.byType(Material),
);
final Material itemMaterial = tester.widget<Material>(material);
expect(itemMaterial.color, Colors.transparent);
}
}
// Test default.
await tester.pumpWidget(dropdownMenu());
await tester.pump();
await tester.tap(find.byType(DropdownMenu<int>));
await tester.pumpAndSettle();
await tester.enterText(find.byType(TextField), 'read');
await tester.pump();
checkExpectedHighlight(searchResult: 'Unread', otherItems: <String>['All', 'Read']); // Because "Unread" contains "read".
// Test custom search algorithm.
await tester.pumpWidget(dropdownMenu(
searchCallback: (_, __) => 0
));
await tester.pump();
await tester.enterText(find.byType(TextField), 'read');
await tester.pump();
checkExpectedHighlight(searchResult: 'All', otherItems: <String>['Unread', 'Read']); // Because the search result should always be index 0.
// Test custom search algorithm - exact match.
await tester.pumpWidget(dropdownMenu(
searchCallback: (List<DropdownMenuEntry<int>> entries, String query) {
if (query.isEmpty) {
return null;
}
final int index = entries.indexWhere((DropdownMenuEntry<int> entry) => entry.label == query);
return index != -1 ? index : null;
},
));
await tester.pump();
await tester.enterText(find.byType(TextField), 'read');
await tester.pump();
checkExpectedHighlight(otherItems: <String>['All', 'Unread', 'Read']); // Because it's case sensitive.
await tester.enterText(find.byType(TextField), 'Read');
await tester.pump();
checkExpectedHighlight(searchResult: 'Read', otherItems: <String>['All', 'Unread']);
});
testWidgets('onSelected gets called when a selection is made in a nested menu',
(WidgetTester tester) async {
int selectionCount = 0;
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<TestMenu>> menuWithDisabledItems = <DropdownMenuEntry<TestMenu>>[
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 0'),
];
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Scaffold(
body: MenuAnchor(
menuChildren: <Widget>[
DropdownMenu<TestMenu>(
dropdownMenuEntries: menuWithDisabledItems,
onSelected: (_) {
setState(() {
selectionCount++;
});
},
),
],
builder: (BuildContext context, MenuController controller, Widget? widget) {
return IconButton(
icon: const Icon(Icons.smartphone_rounded),
onPressed: () {
controller.open();
},
);
},
),
);
}),
));
// Open the first menu
await tester.tap(find.byType(IconButton));
await tester.pump();
// Open the dropdown menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder item1 = find.widgetWithText(MenuItemButton, 'Item 0').last;
await tester.tap(item1);
await tester.pumpAndSettle();
expect(selectionCount, 1);
});
testWidgets('When onSelected is called and menu is closed, no textEditingController exception is thrown',
(WidgetTester tester) async {
int selectionCount = 0;
final ThemeData themeData = ThemeData();
final List<DropdownMenuEntry<TestMenu>> menuWithDisabledItems = <DropdownMenuEntry<TestMenu>>[
const DropdownMenuEntry<TestMenu>(value: TestMenu.mainMenu0, label: 'Item 0'),
];
await tester.pumpWidget(MaterialApp(
theme: themeData,
home: StatefulBuilder(builder: (BuildContext context, StateSetter setState) {
return Scaffold(
body: MenuAnchor(
menuChildren: <Widget>[
DropdownMenu<TestMenu>(
dropdownMenuEntries: menuWithDisabledItems,
onSelected: (_) {
setState(() {
selectionCount++;
});
},
),
],
builder: (BuildContext context, MenuController controller, Widget? widget) {
return IconButton(
icon: const Icon(Icons.smartphone_rounded),
onPressed: () {
controller.open();
},
);
},
),
);
}),
));
// Open the first menu
await tester.tap(find.byType(IconButton));
await tester.pump();
// Open the dropdown menu
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pump();
final Finder item1 = find.widgetWithText(MenuItemButton, 'Item 0').last;
await tester.tap(item1);
await tester.pumpAndSettle();
expect(selectionCount, 1);
expect(tester.takeException(), isNull);
});
// Regression test for https://github.com/flutter/flutter/issues/139871.
testWidgets('setState is not called through addPostFrameCallback after DropdownMenu is unmounted', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: ListView.builder(
itemCount: 500,
itemBuilder: (BuildContext context, int index) {
if (index == 250) {
return DropdownMenu<TestMenu>(
dropdownMenuEntries: menuChildren,
);
} else {
return Container(height: 50);
}
},
),
),
),
);
await tester.fling(find.byType(ListView), const Offset(0, -20000), 200000.0);
await tester.pumpAndSettle();
expect(tester.takeException(), isNull);
});
testWidgets('Menu shows scrollbar when height is limited', (WidgetTester tester) async {
final List<DropdownMenuEntry<TestMenu>> menuItems = <DropdownMenuEntry<TestMenu>>[
DropdownMenuEntry<TestMenu>(
value: TestMenu.mainMenu0,
label: 'Item 0',
style: MenuItemButton.styleFrom(
minimumSize: const Size.fromHeight(1000),
)
),
];
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: DropdownMenu<TestMenu>(
dropdownMenuEntries: menuItems,
),
),
));
await tester.tap(find.byType(DropdownMenu<TestMenu>));
await tester.pumpAndSettle();
expect(find.byType(Scrollbar), findsOneWidget);
}, variant: TargetPlatformVariant.all());
testWidgets('DropdownMenu.focusNode can focus text input field', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
final ThemeData theme = ThemeData();
await tester.pumpWidget(MaterialApp(
theme: theme,
home: Scaffold(
body: DropdownMenu<String>(
focusNode: focusNode,
dropdownMenuEntries: const <DropdownMenuEntry<String>>[
DropdownMenuEntry<String>(
value: 'Yolk',
label: 'Yolk',
),
DropdownMenuEntry<String>(
value: 'Eggbert',
label: 'Eggbert',
),
],
),
),
));
RenderBox box = tester.renderObject(find.byType(InputDecorator));
// Test input border when not focused.
expect(box, paints..rrect(color: theme.colorScheme.outline));
focusNode.requestFocus();
await tester.pump();
// Advance input decorator animation.
await tester.pump(const Duration(milliseconds: 200));
box = tester.renderObject(find.byType(InputDecorator));
// Test input border when focused.
expect(box, paints..rrect(color: theme.colorScheme.primary));
});
testWidgets('DropdownMenu honors inputFormatters', (WidgetTester tester) async {
int called = 0;
final TextInputFormatter formatter = TextInputFormatter.withFunction(
(TextEditingValue oldValue, TextEditingValue newValue) {
called += 1;
return newValue;
},
);
final TextEditingController controller = TextEditingController();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DropdownMenu<String>(
controller: controller,
dropdownMenuEntries: const <DropdownMenuEntry<String>>[
DropdownMenuEntry<String>(
value: 'Blue',
label: 'Blue',
),
DropdownMenuEntry<String>(
value: 'Green',
label: 'Green',
),
],
inputFormatters: <TextInputFormatter>[
formatter,
FilteringTextInputFormatter.deny(RegExp('[0-9]'))
],
),
),
),
);
final EditableTextState state = tester.firstState(find.byType(EditableText));
state.updateEditingValue(const TextEditingValue(text: 'Blue'));
expect(called, 1);
expect(controller.text, 'Blue');
state.updateEditingValue(const TextEditingValue(text: 'Green'));
expect(called, 2);
expect(controller.text, 'Green');
state.updateEditingValue(const TextEditingValue(text: 'Green2'));
expect(called, 3);
expect(controller.text, 'Green');
});
}
enum TestMenu {
mainMenu0('Item 0'),
mainMenu1('Menu 1'),
mainMenu2('Item 2'),
mainMenu3('Item 3'),
mainMenu4('Item 4'),
mainMenu5('Item 5');
const TestMenu(this.label);
final String label;
}
enum ShortMenu {
item0('I0'),
item1('I1'),
item2('I2');
const ShortMenu(this.label);
final String label;
}
| flutter/packages/flutter/test/material/dropdown_menu_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/dropdown_menu_test.dart",
"repo_id": "flutter",
"token_count": 29068
} | 734 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
final Key blockKey = UniqueKey();
const double expandedAppbarHeight = 250.0;
final Key finderKey = UniqueKey();
void main() {
testWidgets('FlexibleSpaceBar stretch mode default zoomBackground', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: CustomScrollView(
physics: const BouncingScrollPhysics(),
key: blockKey,
slivers: <Widget>[
SliverAppBar(
expandedHeight: expandedAppbarHeight,
pinned: true,
stretch: true,
flexibleSpace: FlexibleSpaceBar(
background: Container(
key: finderKey,
),
),
),
SliverToBoxAdapter(child: Container(height: 10000.0)),
],
),
),
),
);
// Scrolling up into the overscroll area causes the appBar to expand in size.
// This overscroll effect enlarges the background in step with the appbar.
final Finder appbarContainer = find.byKey(finderKey);
final Size sizeBeforeScroll = tester.getSize(appbarContainer);
await slowDrag(tester, blockKey, const Offset(0.0, 100.0));
final Size sizeAfterScroll = tester.getSize(appbarContainer);
expect(sizeBeforeScroll.height, lessThan(sizeAfterScroll.height));
});
testWidgets('FlexibleSpaceBar stretch mode blurBackground', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: CustomScrollView(
physics: const BouncingScrollPhysics(),
key: blockKey,
slivers: <Widget>[
SliverAppBar(
expandedHeight: expandedAppbarHeight,
pinned: true,
stretch: true,
flexibleSpace: RepaintBoundary(
child: FlexibleSpaceBar(
stretchModes: const <StretchMode>[StretchMode.blurBackground],
background: Row(
children: <Widget>[
Expanded(child: Container(color: Colors.red)),
Expanded(child:Container(color: Colors.blue)),
],
),
),
),
),
SliverToBoxAdapter(child: Container(height: 10000.0)),
],
),
),
),
);
// Scrolling up into the overscroll area causes the background to blur.
await slowDrag(tester, blockKey, const Offset(0.0, 100.0));
await expectLater(
find.byType(FlexibleSpaceBar),
matchesGoldenFile('flexible_space_bar_stretch_mode.blur_background.png'),
);
});
testWidgets('FlexibleSpaceBar stretch mode fadeTitle', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: CustomScrollView(
physics: const BouncingScrollPhysics(),
key: blockKey,
slivers: <Widget>[
SliverAppBar(
expandedHeight: expandedAppbarHeight,
pinned: true,
stretch: true,
flexibleSpace: FlexibleSpaceBar(
stretchModes: const <StretchMode>[StretchMode.fadeTitle],
title: Text(
'Title',
key: finderKey,
),
),
),
SliverToBoxAdapter(child: Container(height: 10000.0)),
],
),
),
),
);
await slowDrag(tester, blockKey, const Offset(0.0, 10.0));
Opacity opacityWidget = tester.widget<Opacity>(
find.ancestor(
of: find.text('Title'),
matching: find.byType(Opacity),
).first,
);
expect(opacityWidget.opacity.round(), equals(1));
await slowDrag(tester, blockKey, const Offset(0.0, 100.0));
opacityWidget = tester.widget<Opacity>(
find.ancestor(
of: find.text('Title'),
matching: find.byType(Opacity),
).first,
);
expect(opacityWidget.opacity, equals(0.0));
});
testWidgets('FlexibleSpaceBar stretch mode ignored for non-overscroll physics', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: CustomScrollView(
physics: const ClampingScrollPhysics(),
key: blockKey,
slivers: <Widget>[
SliverAppBar(
expandedHeight: expandedAppbarHeight,
stretch: true,
pinned: true,
flexibleSpace: FlexibleSpaceBar(
stretchModes: const <StretchMode>[StretchMode.blurBackground],
background: Container(
key: finderKey,
),
),
),
SliverToBoxAdapter(child: Container(height: 10000.0)),
],
),
),
),
);
final Finder appbarContainer = find.byKey(finderKey);
final Size sizeBeforeScroll = tester.getSize(appbarContainer);
await slowDrag(tester, blockKey, const Offset(0.0, 100.0));
final Size sizeAfterScroll = tester.getSize(appbarContainer);
expect(sizeBeforeScroll.height, equals(sizeAfterScroll.height));
});
}
Future<void> slowDrag(WidgetTester tester, Key widget, Offset offset) async {
final Offset target = tester.getCenter(find.byKey(widget));
final TestGesture gesture = await tester.startGesture(target);
await gesture.moveBy(offset);
await tester.pump(const Duration(milliseconds: 10));
await gesture.up();
}
| flutter/packages/flutter/test/material/flexible_space_bar_stretch_mode_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/flexible_space_bar_stretch_mode_test.dart",
"repo_id": "flutter",
"token_count": 2822
} | 735 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/clipboard_utils.dart';
class TestMaterialLocalizations extends DefaultMaterialLocalizations {
@override
String formatCompactDate(DateTime date) {
return '${date.month}/${date.day}/${date.year}';
}
}
class TestMaterialLocalizationsDelegate extends LocalizationsDelegate<MaterialLocalizations> {
@override
bool isSupported(Locale locale) => true;
@override
Future<MaterialLocalizations> load(Locale locale) {
return SynchronousFuture<MaterialLocalizations>(TestMaterialLocalizations());
}
@override
bool shouldReload(TestMaterialLocalizationsDelegate old) => false;
}
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final MockClipboard mockClipboard = MockClipboard();
Widget inputDatePickerField({
Key? key,
DateTime? initialDate,
DateTime? firstDate,
DateTime? lastDate,
ValueChanged<DateTime>? onDateSubmitted,
ValueChanged<DateTime>? onDateSaved,
SelectableDayPredicate? selectableDayPredicate,
String? errorFormatText,
String? errorInvalidText,
String? fieldHintText,
String? fieldLabelText,
bool autofocus = false,
Key? formKey,
ThemeData? theme,
Iterable<LocalizationsDelegate<dynamic>>? localizationsDelegates,
bool acceptEmptyDate = false,
FocusNode? focusNode,
}) {
return MaterialApp(
theme: theme ?? ThemeData.from(colorScheme: const ColorScheme.light()),
localizationsDelegates: localizationsDelegates,
home: Material(
child: Form(
key: formKey,
child: InputDatePickerFormField(
key: key,
initialDate: initialDate ?? DateTime(2016, DateTime.january, 15),
firstDate: firstDate ?? DateTime(2001),
lastDate: lastDate ?? DateTime(2031, DateTime.december, 31),
onDateSubmitted: onDateSubmitted,
onDateSaved: onDateSaved,
selectableDayPredicate: selectableDayPredicate,
errorFormatText: errorFormatText,
errorInvalidText: errorInvalidText,
fieldHintText: fieldHintText,
fieldLabelText: fieldLabelText,
autofocus: autofocus,
acceptEmptyDate: acceptEmptyDate,
focusNode: focusNode,
),
),
),
);
}
TextField textField(WidgetTester tester) {
return tester.widget<TextField>(find.byType(TextField));
}
TextEditingController textFieldController(WidgetTester tester) {
return textField(tester).controller!;
}
double textOpacity(WidgetTester tester, String textValue) {
final FadeTransition opacityWidget = tester.widget<FadeTransition>(
find.ancestor(
of: find.text(textValue),
matching: find.byType(FadeTransition),
).first,
);
return opacityWidget.opacity.value;
}
group('InputDatePickerFormField', () {
testWidgets('Initial date is the default', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
final DateTime initialDate = DateTime(2016, DateTime.february, 21);
DateTime? inputDate;
await tester.pumpWidget(inputDatePickerField(
initialDate: initialDate,
onDateSaved: (DateTime date) => inputDate = date,
formKey: formKey,
));
expect(textFieldController(tester).value.text, equals('02/21/2016'));
formKey.currentState!.save();
expect(inputDate, equals(initialDate));
});
testWidgets('Changing initial date is reflected in text value', (WidgetTester tester) async {
final DateTime initialDate = DateTime(2016, DateTime.february, 21);
final DateTime updatedInitialDate = DateTime(2016, DateTime.february, 23);
await tester.pumpWidget(inputDatePickerField(
initialDate: initialDate,
));
expect(textFieldController(tester).value.text, equals('02/21/2016'));
await tester.pumpWidget(inputDatePickerField(
initialDate: updatedInitialDate,
));
await tester.pumpAndSettle();
expect(textFieldController(tester).value.text, equals('02/23/2016'));
});
testWidgets('Valid date entry', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
DateTime? inputDate;
await tester.pumpWidget(inputDatePickerField(
onDateSaved: (DateTime date) => inputDate = date,
formKey: formKey,
));
textFieldController(tester).text = '02/21/2016';
formKey.currentState!.save();
expect(inputDate, equals(DateTime(2016, DateTime.february, 21)));
});
testWidgets('Invalid text entry shows errorFormat text', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
DateTime? inputDate;
await tester.pumpWidget(inputDatePickerField(
onDateSaved: (DateTime date) => inputDate = date,
formKey: formKey,
));
// Default errorFormat text
expect(find.text('Invalid format.'), findsNothing);
await tester.enterText(find.byType(TextField), 'foobar');
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(inputDate, isNull);
expect(find.text('Invalid format.'), findsOneWidget);
// Change to a custom errorFormat text
await tester.pumpWidget(inputDatePickerField(
onDateSaved: (DateTime date) => inputDate = date,
errorFormatText: 'That is not a date.',
formKey: formKey,
));
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(find.text('Invalid format.'), findsNothing);
expect(find.text('That is not a date.'), findsOneWidget);
});
testWidgets('Valid text entry, but date outside first or last date shows bounds shows errorInvalid text', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
DateTime? inputDate;
await tester.pumpWidget(inputDatePickerField(
firstDate: DateTime(1966, DateTime.february, 21),
lastDate: DateTime(2040, DateTime.february, 23),
onDateSaved: (DateTime date) => inputDate = date,
formKey: formKey,
));
// Default errorInvalid text
expect(find.text('Out of range.'), findsNothing);
// Before first date
await tester.enterText(find.byType(TextField), '02/21/1950');
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(inputDate, isNull);
expect(find.text('Out of range.'), findsOneWidget);
// After last date
await tester.enterText(find.byType(TextField), '02/23/2050');
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(inputDate, isNull);
expect(find.text('Out of range.'), findsOneWidget);
await tester.pumpWidget(inputDatePickerField(
onDateSaved: (DateTime date) => inputDate = date,
errorInvalidText: 'Not in given range.',
formKey: formKey,
));
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(find.text('Out of range.'), findsNothing);
expect(find.text('Not in given range.'), findsOneWidget);
});
testWidgets('selectableDatePredicate will be used to show errorInvalid if date is not selectable', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
DateTime? inputDate;
await tester.pumpWidget(inputDatePickerField(
initialDate: DateTime(2016, DateTime.january, 16),
onDateSaved: (DateTime date) => inputDate = date,
selectableDayPredicate: (DateTime date) => date.day.isEven,
formKey: formKey,
));
// Default errorInvalid text
expect(find.text('Out of range.'), findsNothing);
// Odd day shouldn't be valid
await tester.enterText(find.byType(TextField), '02/21/1966');
expect(formKey.currentState!.validate(), isFalse);
await tester.pumpAndSettle();
expect(inputDate, isNull);
expect(find.text('Out of range.'), findsOneWidget);
// Even day is valid
await tester.enterText(find.byType(TextField), '02/24/2030');
expect(formKey.currentState!.validate(), isTrue);
formKey.currentState!.save();
await tester.pumpAndSettle();
expect(inputDate, equals(DateTime(2030, DateTime.february, 24)));
expect(find.text('Out of range.'), findsNothing);
});
testWidgets('Empty field shows hint text when focused', (WidgetTester tester) async {
await tester.pumpWidget(inputDatePickerField());
// Focus on it
await tester.tap(find.byType(TextField));
await tester.pumpAndSettle();
// Hint text should be invisible
expect(textOpacity(tester, 'mm/dd/yyyy'), equals(0.0));
textFieldController(tester).clear();
await tester.pumpAndSettle();
// Hint text should be visible
expect(textOpacity(tester, 'mm/dd/yyyy'), equals(1.0));
// Change to a different hint text
await tester.pumpWidget(inputDatePickerField(fieldHintText: 'Enter some date'));
await tester.pumpAndSettle();
expect(find.text('mm/dd/yyyy'), findsNothing);
expect(textOpacity(tester, 'Enter some date'), equals(1.0));
await tester.enterText(find.byType(TextField), 'foobar');
await tester.pumpAndSettle();
expect(textOpacity(tester, 'Enter some date'), equals(0.0));
});
testWidgets('Label text', (WidgetTester tester) async {
await tester.pumpWidget(inputDatePickerField());
// Default label
expect(find.text('Enter Date'), findsOneWidget);
await tester.pumpWidget(inputDatePickerField(
fieldLabelText: 'Give me a date!',
));
expect(find.text('Enter Date'), findsNothing);
expect(find.text('Give me a date!'), findsOneWidget);
});
testWidgets('Semantics', (WidgetTester tester) async {
final SemanticsHandle semantics = tester.ensureSemantics();
// Fill the clipboard so that the Paste option is available in the text
// selection menu.
tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, mockClipboard.handleMethodCall);
await Clipboard.setData(const ClipboardData(text: 'Clipboard data'));
addTearDown(() => tester.binding.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform, null));
await tester.pumpWidget(inputDatePickerField(autofocus: true));
await tester.pumpAndSettle();
expect(tester.getSemantics(find.byType(EditableText)), matchesSemantics(
label: 'Enter Date',
isTextField: true,
hasEnabledState: true,
isEnabled: true,
isFocused: true,
value: '01/15/2016',
hasTapAction: true,
hasSetTextAction: true,
hasSetSelectionAction: true,
hasCopyAction: true,
hasCutAction: true,
hasPasteAction: true,
hasMoveCursorBackwardByCharacterAction: true,
hasMoveCursorBackwardByWordAction: true,
));
semantics.dispose();
});
testWidgets('InputDecorationTheme is honored', (WidgetTester tester) async {
const InputBorder border = InputBorder.none;
await tester.pumpWidget(inputDatePickerField(
theme: ThemeData.from(colorScheme: const ColorScheme.light()).copyWith(
inputDecorationTheme: const InputDecorationTheme(
border: border,
),
),
));
await tester.pumpAndSettle();
// Get the border and container color from the painter of the _BorderContainer
// (this was cribbed from input_decorator_test.dart).
final CustomPaint customPaint = tester.widget(find.descendant(
of: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_BorderContainer'),
matching: find.byWidgetPredicate((Widget w) => w is CustomPaint),
));
final dynamic/*_InputBorderPainter*/ inputBorderPainter = customPaint.foregroundPainter;
// ignore: avoid_dynamic_calls
final dynamic/*_InputBorderTween*/ inputBorderTween = inputBorderPainter.border;
// ignore: avoid_dynamic_calls
final Animation<double> animation = inputBorderPainter.borderAnimation as Animation<double>;
// ignore: avoid_dynamic_calls
final InputBorder actualBorder = inputBorderTween.evaluate(animation) as InputBorder;
// ignore: avoid_dynamic_calls
final Color containerColor = inputBorderPainter.blendedColor as Color;
// Border should match
expect(actualBorder, equals(border));
// It shouldn't be filled, so the color should be transparent
expect(containerColor, equals(Colors.transparent));
});
testWidgets('Date text localization', (WidgetTester tester) async {
final Iterable<LocalizationsDelegate<dynamic>> delegates = <LocalizationsDelegate<dynamic>>[
TestMaterialLocalizationsDelegate(),
DefaultWidgetsLocalizations.delegate,
];
await tester.pumpWidget(
inputDatePickerField(
localizationsDelegates: delegates,
)
);
await tester.enterText(find.byType(TextField), '01/01/2022');
await tester.pumpAndSettle();
// Verify that the widget can be updated to a new value after the
// entered text was transformed by the localization formatter.
await tester.pumpWidget(
inputDatePickerField(
initialDate: DateTime(2017),
localizationsDelegates: delegates,
)
);
});
testWidgets('when an empty date is entered and acceptEmptyDate is true, then errorFormatText is not shown', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
const String errorFormatText = 'That is not a date.';
await tester.pumpWidget(inputDatePickerField(
errorFormatText: errorFormatText,
formKey: formKey,
acceptEmptyDate: true,
));
await tester.enterText(find.byType(TextField), '');
await tester.pumpAndSettle();
formKey.currentState!.validate();
await tester.pumpAndSettle();
expect(find.text(errorFormatText), findsNothing);
});
testWidgets('when an empty date is entered and acceptEmptyDate is false, then errorFormatText is shown', (WidgetTester tester) async {
final GlobalKey<FormState> formKey = GlobalKey<FormState>();
const String errorFormatText = 'That is not a date.';
await tester.pumpWidget(inputDatePickerField(
errorFormatText: errorFormatText,
formKey: formKey,
));
await tester.enterText(find.byType(TextField), '');
await tester.pumpAndSettle();
formKey.currentState!.validate();
await tester.pumpAndSettle();
expect(find.text(errorFormatText), findsOneWidget);
});
});
testWidgets('FocusNode can request focus', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
await tester.pumpWidget(inputDatePickerField(
focusNode: focusNode,
));
expect((tester.widget(find.byType(TextField)) as TextField).focusNode, focusNode);
expect(focusNode.hasFocus, isFalse);
focusNode.requestFocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, isTrue);
focusNode.unfocus();
await tester.pumpAndSettle();
expect(focusNode.hasFocus, isFalse);
});
}
| flutter/packages/flutter/test/material/input_date_picker_form_field_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/input_date_picker_form_field_test.dart",
"repo_id": "flutter",
"token_count": 5970
} | 736 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
enum RadiusType {
Sharp,
Shifting,
Round
}
void matches(BorderRadius? borderRadius, RadiusType top, RadiusType bottom) {
final Radius cardRadius = kMaterialEdges[MaterialType.card]!.topLeft;
switch (top) {
case RadiusType.Sharp:
expect(borderRadius?.topLeft, equals(Radius.zero));
expect(borderRadius?.topRight, equals(Radius.zero));
case RadiusType.Shifting:
expect(borderRadius?.topLeft.x, greaterThan(0.0));
expect(borderRadius?.topLeft.x, lessThan(cardRadius.x));
expect(borderRadius?.topLeft.y, greaterThan(0.0));
expect(borderRadius?.topLeft.y, lessThan(cardRadius.y));
expect(borderRadius?.topRight.x, greaterThan(0.0));
expect(borderRadius?.topRight.x, lessThan(cardRadius.x));
expect(borderRadius?.topRight.y, greaterThan(0.0));
expect(borderRadius?.topRight.y, lessThan(cardRadius.y));
case RadiusType.Round:
expect(borderRadius?.topLeft, equals(cardRadius));
expect(borderRadius?.topRight, equals(cardRadius));
}
switch (bottom) {
case RadiusType.Sharp:
expect(borderRadius?.bottomLeft, equals(Radius.zero));
expect(borderRadius?.bottomRight, equals(Radius.zero));
case RadiusType.Shifting:
expect(borderRadius?.bottomLeft.x, greaterThan(0.0));
expect(borderRadius?.bottomLeft.x, lessThan(cardRadius.x));
expect(borderRadius?.bottomLeft.y, greaterThan(0.0));
expect(borderRadius?.bottomLeft.y, lessThan(cardRadius.y));
expect(borderRadius?.bottomRight.x, greaterThan(0.0));
expect(borderRadius?.bottomRight.x, lessThan(cardRadius.x));
expect(borderRadius?.bottomRight.y, greaterThan(0.0));
expect(borderRadius?.bottomRight.y, lessThan(cardRadius.y));
case RadiusType.Round:
expect(borderRadius?.bottomLeft, equals(cardRadius));
expect(borderRadius?.bottomRight, equals(cardRadius));
}
}
// Returns the border radius decoration of an item within a MergeableMaterial.
// This depends on the exact structure of objects built by the Material and
// MergeableMaterial widgets.
BorderRadius? getBorderRadius(WidgetTester tester, int index) {
final List<Element> containers = tester.elementList(find.byType(Container))
.toList();
final Container container = containers[index].widget as Container;
final BoxDecoration? boxDecoration = container.decoration as BoxDecoration?;
return boxDecoration!.borderRadius as BorderRadius?;
}
void main() {
testWidgets('MergeableMaterial empty', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(0));
});
testWidgets('MergeableMaterial update slice', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(100.0));
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 200.0,
),
),
],
),
),
),
),
);
box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200.0));
});
testWidgets('MergeableMaterial swap slices', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200.0));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200.0));
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(200.0));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
});
testWidgets('MergeableMaterial paints shadows', (WidgetTester tester) async {
debugDisableShadows = false;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RRect rrect = kMaterialEdges[MaterialType.card]!.toRRect(
const Rect.fromLTRB(0.0, 0.0, 800.0, 100.0),
);
expect(
find.byType(MergeableMaterial),
paints
..shadow(elevation: 2.0)
..rrect(rrect: rrect, color: Colors.white, hasMaskFilter: false),
);
debugDisableShadows = true;
});
testWidgets('MergeableMaterial skips shadow for zero elevation', (WidgetTester tester) async {
debugDisableShadows = false;
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
elevation: 0,
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
expect(
find.byType(MergeableMaterial),
isNot(paints..shadow(elevation: 0.0)),
);
debugDisableShadows = true;
});
testWidgets('MergeableMaterial merge gap', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
});
testWidgets('MergeableMaterial separate slices', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
});
testWidgets('MergeableMaterial separate merge separate', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
});
testWidgets('MergeableMaterial insert slice', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
expect(box.size.height, equals(300));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Sharp);
matches(getBorderRadius(tester, 2), RadiusType.Sharp, RadiusType.Round);
});
testWidgets('MergeableMaterial remove slice', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(300));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Sharp);
matches(getBorderRadius(tester, 2), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump();
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
});
testWidgets('MergeableMaterial insert chunk', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('y'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Shifting);
matches(getBorderRadius(tester, 2), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 2), RadiusType.Round, RadiusType.Round);
});
testWidgets('MergeableMaterial remove chunk', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('y'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 2), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(200));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Sharp);
matches(getBorderRadius(tester, 1), RadiusType.Sharp, RadiusType.Round);
});
testWidgets('MergeableMaterial replace gap with chunk', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('y'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('z'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Shifting);
matches(getBorderRadius(tester, 2), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 2), RadiusType.Round, RadiusType.Round);
});
testWidgets('MergeableMaterial replace chunk with gap', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('y'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 2), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('z'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(332));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
});
testWidgets('MergeableMaterial insert and separate slice', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final RenderBox box = tester.renderObject(find.byType(MergeableMaterial));
expect(box.size.height, equals(100));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, lessThan(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Shifting);
matches(getBorderRadius(tester, 1), RadiusType.Shifting, RadiusType.Round);
await tester.pump(const Duration(milliseconds: 100));
expect(box.size.height, equals(216));
matches(getBorderRadius(tester, 0), RadiusType.Round, RadiusType.Round);
matches(getBorderRadius(tester, 1), RadiusType.Round, RadiusType.Round);
});
bool isDivider(BoxDecoration decoration, bool top, bool bottom) {
const BorderSide side = BorderSide(color: Color(0x1F000000), width: 0.5);
return decoration == BoxDecoration(
border: Border(
top: top ? side : BorderSide.none,
bottom: bottom ? side : BorderSide.none,
),
);
}
testWidgets('MergeableMaterial dividers', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
hasDividers: true,
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('D'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
List<Widget> animatedContainers = tester.widgetList(
find.byType(AnimatedContainer),
).toList();
List<BoxDecoration> boxes = <BoxDecoration>[];
for (final Widget container in animatedContainers) {
boxes.add((container as AnimatedContainer).decoration! as BoxDecoration);
}
int offset = 0;
expect(isDivider(boxes[offset], false, true), isTrue);
expect(isDivider(boxes[offset + 1], true, true), isTrue);
expect(isDivider(boxes[offset + 2], true, true), isTrue);
expect(isDivider(boxes[offset + 3], true, false), isTrue);
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
hasDividers: true,
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialGap(
key: ValueKey<String>('x'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('D'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
// Wait for dividers to shrink.
await tester.pump(const Duration(milliseconds: 200));
animatedContainers = tester.widgetList(
find.byType(AnimatedContainer),
).toList();
boxes = <BoxDecoration>[];
for (final Widget container in animatedContainers) {
boxes.add((container as AnimatedContainer).decoration! as BoxDecoration);
}
offset = 0;
expect(isDivider(boxes[offset], false, true), isTrue);
expect(isDivider(boxes[offset + 1], true, false), isTrue);
expect(isDivider(boxes[offset + 2], false, true), isTrue);
expect(isDivider(boxes[offset + 3], true, false), isTrue);
});
testWidgets('MergeableMaterial respects dividerColor', (WidgetTester tester) async {
const Color dividerColor = Colors.red;
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
hasDividers: true,
dividerColor: dividerColor,
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
MaterialSlice(
key: ValueKey<String>('B'),
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
],
),
),
),
),
);
final DecoratedBox decoratedBox = tester.widget(find.byType(DecoratedBox).last);
final BoxDecoration decoration = decoratedBox.decoration as BoxDecoration;
// Since we are getting the last DecoratedBox, it will have a Border.top.
expect(decoration.border!.top.color, dividerColor);
});
testWidgets('MergeableMaterial respects MaterialSlice.color', (WidgetTester tester) async {
const Color themeCardColor = Colors.red;
const Color materialSliceColor = Colors.green;
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
cardColor: themeCardColor,
),
home: const Scaffold(
body: SingleChildScrollView(
child: MergeableMaterial(
children: <MergeableMaterialItem>[
MaterialSlice(
key: ValueKey<String>('A'),
color: materialSliceColor,
child: SizedBox(
height: 100,
width: 100,
),
),
MaterialGap(
key: ValueKey<String>('B'),
),
MaterialSlice(
key: ValueKey<String>('C'),
child: SizedBox(
height: 100,
width: 100,
),
),
],
),
),
),
),
);
BoxDecoration boxDecoration = tester.widget<Container>(find.byType(Container).first).decoration! as BoxDecoration;
expect(boxDecoration.color, materialSliceColor);
boxDecoration = tester.widget<Container>(find.byType(Container).last).decoration! as BoxDecoration;
expect(boxDecoration.color, themeCardColor);
});
}
| flutter/packages/flutter/test/material/mergeable_material_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/mergeable_material_test.dart",
"repo_id": "flutter",
"token_count": 23318
} | 737 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// no-shuffle:
// //TODO(gspencergoog): Remove this tag once this test's state leaks/test
// dependencies have been fixed.
// https://github.com/flutter/flutter/issues/85160
// Fails with "flutter test --test-randomize-ordering-seed=456"
// reduced-test-set:
// This file is run as part of a reduced test set in CI on Mac and Windows
// machines.
@Tags(<String>['reduced-test-set', 'no-shuffle'])
library;
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final ThemeData theme = ThemeData();
// The "can be constructed" tests that follow are primarily to ensure that any
// animations started by the progress indicators are stopped at dispose() time.
testWidgets('LinearProgressIndicator(value: 0.0) can be constructed and has empty semantics by default', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(value: 0.0),
),
),
),
),
);
expect(tester.getSemantics(find.byType(LinearProgressIndicator)), matchesSemantics());
handle.dispose();
});
testWidgets('LinearProgressIndicator(value: null) can be constructed and has empty semantics by default', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(),
),
),
),
),
);
expect(tester.getSemantics(find.byType(LinearProgressIndicator)), matchesSemantics());
handle.dispose();
});
testWidgets('LinearProgressIndicator custom minHeight', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(value: 0.25, minHeight: 2.0),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 2.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 2.0)),
);
// Same test, but using the theme
await tester.pumpWidget(
Theme(
data: theme.copyWith(
progressIndicatorTheme: const ProgressIndicatorThemeData(
linearMinHeight: 2.0,
),
),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 2.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 2.0)),
);
});
testWidgets('LinearProgressIndicator paint (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0)),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('LinearProgressIndicator paint (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(150.0, 0.0, 200.0, 4.0)),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('LinearProgressIndicator indeterminate (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(),
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 300));
final double animationValue = const Interval(0.0, 750.0 / 1800.0, curve: Cubic(0.2, 0.0, 0.8, 1.0))
.transform(300.0 / 1800.0);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: Rect.fromLTRB(0.0, 0.0, animationValue * 200.0, 4.0)),
);
expect(tester.binding.transientCallbackCount, 1);
});
testWidgets('LinearProgressIndicator paint (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(),
),
),
),
),
);
await tester.pump(const Duration(milliseconds: 300));
final double animationValue = const Interval(0.0, 750.0 / 1800.0, curve: Cubic(0.2, 0.0, 0.8, 1.0))
.transform(300.0 / 1800.0);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: Rect.fromLTRB(200.0 - animationValue * 200.0, 0.0, 200.0, 4.0)),
);
expect(tester.binding.transientCallbackCount, 1);
});
testWidgets('LinearProgressIndicator with colors', (WidgetTester tester) async {
// With valueColor & color provided
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(
value: 0.25,
backgroundColor: Colors.black,
color: Colors.blue,
valueColor: AlwaysStoppedAnimation<Color>(Colors.white),
),
),
),
),
),
);
// Should use valueColor
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0), color: Colors.white),
);
// With just color provided
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(
value: 0.25,
backgroundColor: Colors.black,
color: Colors.white12,
),
),
),
),
),
);
// Should use color
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0), color: Colors.white12),
);
// With no color provided
const Color primaryColor = Color(0xff008800);
await tester.pumpWidget(
Theme(
data: theme.copyWith(colorScheme: ColorScheme.fromSwatch().copyWith(primary: primaryColor)),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(
value: 0.25,
backgroundColor: Colors.black,
),
),
),
),
),
);
// Should use the theme's primary color
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0), color: primaryColor),
);
// With ProgressIndicatorTheme colors
const Color indicatorColor = Color(0xff0000ff);
await tester.pumpWidget(
Theme(
data: theme.copyWith(
progressIndicatorTheme: const ProgressIndicatorThemeData(
color: indicatorColor,
linearTrackColor: Colors.black,
),
),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(
value: 0.25,
),
),
),
),
),
);
// Should use the progress indicator theme colors
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0), color: indicatorColor),
);
});
testWidgets('LinearProgressIndicator with animation with null colors', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: LinearProgressIndicator(
value: 0.25,
valueColor: AlwaysStoppedAnimation<Color?>(null),
backgroundColor: Colors.black,
),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 200.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 50.0, 4.0)),
);
});
testWidgets('CircularProgressIndicator(value: 0.0) can be constructed and has value semantics by default', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: CircularProgressIndicator(value: 0.0),
),
),
),
);
expect(tester.getSemantics(find.byType(CircularProgressIndicator)), matchesSemantics(
value: '0%',
textDirection: TextDirection.ltr,
));
handle.dispose();
});
testWidgets('CircularProgressIndicator(value: null) can be constructed and has empty semantics by default', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(
Theme(
data: theme,
child: const Center(
child: CircularProgressIndicator(),
),
),
);
expect(tester.getSemantics(find.byType(CircularProgressIndicator)), matchesSemantics());
handle.dispose();
});
testWidgets('LinearProgressIndicator causes a repaint when it changes', (WidgetTester tester) async {
await tester.pumpWidget(Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: ListView(children: const <Widget>[LinearProgressIndicator(value: 0.0)]),
),
));
final List<Layer> layers1 = tester.layers;
await tester.pumpWidget(Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: ListView(children: const <Widget>[LinearProgressIndicator(value: 0.5)]),
),
));
final List<Layer> layers2 = tester.layers;
expect(layers1, isNot(equals(layers2)));
});
testWidgets('CircularProgressIndicator stroke width', (WidgetTester tester) async {
await tester.pumpWidget(Theme(data: theme, child: const CircularProgressIndicator()));
expect(find.byType(CircularProgressIndicator), paints..arc(strokeWidth: 4.0));
await tester.pumpWidget(Theme(data: theme, child: const CircularProgressIndicator(strokeWidth: 16.0)));
expect(find.byType(CircularProgressIndicator), paints..arc(strokeWidth: 16.0));
});
testWidgets('CircularProgressIndicator strokeAlign', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const CircularProgressIndicator(),
),
);
expect(find.byType(CircularProgressIndicator), paints..arc(rect: Offset.zero & const Size(800.0, 600.0)));
await tester.pumpWidget(
Theme(
data: theme,
child: const CircularProgressIndicator(
strokeAlign: CircularProgressIndicator.strokeAlignInside,
),
),
);
expect(find.byType(CircularProgressIndicator), paints..arc(rect: const Offset(2.0, 2.0) & const Size(796.0, 596.0)));
await tester.pumpWidget(
Theme(
data: theme,
child: const CircularProgressIndicator(
strokeAlign: CircularProgressIndicator.strokeAlignOutside,
),
),
);
expect(find.byType(CircularProgressIndicator), paints..arc(rect: const Offset(-2.0, -2.0) & const Size(804.0, 604.0)));
// Unbounded alignment.
await tester.pumpWidget(
Theme(
data: theme,
child: const CircularProgressIndicator(
strokeAlign: 2.0,
),
),
);
expect(find.byType(CircularProgressIndicator), paints..arc(rect: const Offset(-4.0, -4.0) & const Size(808.0, 608.0)));
});
testWidgets('CircularProgressIndicator with strokeCap', (WidgetTester tester) async {
await tester.pumpWidget(const CircularProgressIndicator());
expect(find.byType(CircularProgressIndicator),
paints..arc(strokeCap: StrokeCap.square),
reason: 'Default indeterminate strokeCap is StrokeCap.square.');
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: CircularProgressIndicator(value: 0.5)));
expect(find.byType(CircularProgressIndicator),
paints..arc(strokeCap: StrokeCap.butt),
reason: 'Default determinate strokeCap is StrokeCap.butt.');
await tester.pumpWidget(const CircularProgressIndicator(strokeCap: StrokeCap.butt));
expect(find.byType(CircularProgressIndicator),
paints..arc(strokeCap: StrokeCap.butt),
reason: 'strokeCap can be set to StrokeCap.butt, and will not be overridden.');
await tester.pumpWidget(const CircularProgressIndicator(strokeCap: StrokeCap.round));
expect(find.byType(CircularProgressIndicator), paints..arc(strokeCap: StrokeCap.round));
});
testWidgets('LinearProgressIndicator with indicatorBorderRadius', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100.0,
height: 4.0,
child: LinearProgressIndicator(
value: 0.25,
borderRadius: BorderRadius.all(Radius.circular(10)),
),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rrect(
rrect: RRect.fromLTRBR(0.0, 0.0, 100.0, 4.0, const Radius.circular(10.0)),
)
..rrect(
rrect: RRect.fromRectAndRadius(
const Rect.fromLTRB(0.0, 0.0, 25.0, 4.0),
const Radius.circular(10.0),
),
),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('CircularProgressIndicator paint colors', (WidgetTester tester) async {
const Color green = Color(0xFF00FF00);
const Color blue = Color(0xFF0000FF);
const Color red = Color(0xFFFF0000);
// With valueColor & color provided
await tester.pumpWidget(Theme(
data: theme,
child: const CircularProgressIndicator(
color: red,
valueColor: AlwaysStoppedAnimation<Color>(blue),
),
));
expect(find.byType(CircularProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(CircularProgressIndicator), paints..arc(color: blue));
// With just color provided
await tester.pumpWidget(Theme(
data: theme,
child: const CircularProgressIndicator(
color: red,
),
));
expect(find.byType(CircularProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(CircularProgressIndicator), paints..arc(color: red));
// With no color provided
await tester.pumpWidget(Theme(
data: theme.copyWith(colorScheme: ColorScheme.fromSwatch().copyWith(primary: green)),
child: const CircularProgressIndicator(),
));
expect(find.byType(CircularProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(CircularProgressIndicator), paints..arc(color: green));
// With background
await tester.pumpWidget(Theme(
data: theme,
child: const CircularProgressIndicator(
backgroundColor: green,
color: blue,
),
));
expect(find.byType(CircularProgressIndicator), paintsExactlyCountTimes(#drawArc, 2));
expect(find.byType(CircularProgressIndicator), paints..arc(color: green)..arc(color: blue));
// With ProgressIndicatorTheme
await tester.pumpWidget(Theme(
data: theme.copyWith(progressIndicatorTheme: const ProgressIndicatorThemeData(
color: green,
circularTrackColor: blue,
)),
child: const CircularProgressIndicator(),
));
expect(find.byType(CircularProgressIndicator), paintsExactlyCountTimes(#drawArc, 2));
expect(find.byType(CircularProgressIndicator), paints..arc(color: blue)..arc(color: green));
});
testWidgets('RefreshProgressIndicator paint colors', (WidgetTester tester) async {
const Color green = Color(0xFF00FF00);
const Color blue = Color(0xFF0000FF);
const Color red = Color(0xFFFF0000);
// With valueColor & color provided
await tester.pumpWidget(Theme(
data: theme,
child: const RefreshProgressIndicator(
color: red,
valueColor: AlwaysStoppedAnimation<Color>(blue),
),
));
expect(find.byType(RefreshProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(RefreshProgressIndicator), paints..arc(color: blue));
// With just color provided
await tester.pumpWidget(Theme(
data: theme,
child: const RefreshProgressIndicator(
color: red,
),
));
expect(find.byType(RefreshProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(RefreshProgressIndicator), paints..arc(color: red));
// With no color provided
await tester.pumpWidget(Theme(
data: theme.copyWith(colorScheme: ColorScheme.fromSwatch().copyWith(primary: green)),
child: const RefreshProgressIndicator(),
));
expect(find.byType(RefreshProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(RefreshProgressIndicator), paints..arc(color: green));
// With background
await tester.pumpWidget(Theme(
data: theme,
child: const RefreshProgressIndicator(
color: blue,
backgroundColor: green,
),
));
expect(find.byType(RefreshProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(RefreshProgressIndicator), paints..arc(color: blue));
final Material backgroundMaterial = tester.widget(find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
));
expect(backgroundMaterial.type, MaterialType.circle);
expect(backgroundMaterial.color, green);
// With ProgressIndicatorTheme
await tester.pumpWidget(Theme(
data: theme.copyWith(progressIndicatorTheme: const ProgressIndicatorThemeData(
color: green,
refreshBackgroundColor: blue,
)),
child: const RefreshProgressIndicator(),
));
expect(find.byType(RefreshProgressIndicator), paintsExactlyCountTimes(#drawArc, 1));
expect(find.byType(RefreshProgressIndicator), paints..arc(color: green));
final Material themeBackgroundMaterial = tester.widget(find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
));
expect(themeBackgroundMaterial.type, MaterialType.circle);
expect(themeBackgroundMaterial.color, blue);
});
testWidgets('RefreshProgressIndicator with a round indicator', (WidgetTester tester) async {
await tester.pumpWidget(const RefreshProgressIndicator());
expect(find.byType(RefreshProgressIndicator),
paints..arc(strokeCap: StrokeCap.square),
reason: 'Default indeterminate strokeCap is StrokeCap.square');
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: RefreshProgressIndicator(strokeCap: StrokeCap.round),
),
),
),
),
);
expect(find.byType(RefreshProgressIndicator), paints..arc(strokeCap: StrokeCap.round));
});
testWidgets('Indeterminate RefreshProgressIndicator keeps spinning until end of time (approximate)', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/13782
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 200.0,
child: RefreshProgressIndicator(),
),
),
),
),
);
expect(tester.hasRunningAnimations, isTrue);
await tester.pump(const Duration(seconds: 5));
expect(tester.hasRunningAnimations, isTrue);
await tester.pump(const Duration(milliseconds: 1));
expect(tester.hasRunningAnimations, isTrue);
await tester.pump(const Duration(days: 9999));
expect(tester.hasRunningAnimations, isTrue);
});
testWidgets('Material2 - RefreshProgressIndicator uses expected animation', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(50, 50));
addTearDown(animationSheet.dispose);
await tester.pumpFrames(animationSheet.record(
Theme(
data: ThemeData(useMaterial3: false),
child: const _RefreshProgressIndicatorGolden()
),
), const Duration(seconds: 3));
await expectLater(
animationSheet.collate(20),
matchesGoldenFile('m2_material.refresh_progress_indicator.png'),
);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/56001
testWidgets('Material3 - RefreshProgressIndicator uses expected animation', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(50, 50));
addTearDown(animationSheet.dispose);
await tester.pumpFrames(animationSheet.record(
Theme(
data: ThemeData(useMaterial3: true),
child: const _RefreshProgressIndicatorGolden()
),
), const Duration(seconds: 3));
await expectLater(
animationSheet.collate(20),
matchesGoldenFile('m3_material.refresh_progress_indicator.png'),
);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/56001
testWidgets('Determinate CircularProgressIndicator stops the animator', (WidgetTester tester) async {
double? progressValue;
late StateSetter setState;
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return CircularProgressIndicator(value: progressValue);
},
),
),
),
),
);
expect(tester.hasRunningAnimations, isTrue);
setState(() { progressValue = 1.0; });
await tester.pump(const Duration(milliseconds: 1));
expect(tester.hasRunningAnimations, isFalse);
setState(() { progressValue = null; });
await tester.pump(const Duration(milliseconds: 1));
expect(tester.hasRunningAnimations, isTrue);
});
testWidgets('LinearProgressIndicator with height 12.0', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100.0,
height: 12.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 100.0, 12.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 25.0, 12.0)),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('LinearProgressIndicator with a height less than the minimum', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100.0,
height: 3.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 100.0, 3.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 25.0, 3.0)),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('LinearProgressIndicator with default height', (WidgetTester tester) async {
await tester.pumpWidget(
Theme(
data: theme,
child: const Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 100.0,
height: 4.0,
child: LinearProgressIndicator(value: 0.25),
),
),
),
),
);
expect(
find.byType(LinearProgressIndicator),
paints
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 100.0, 4.0))
..rect(rect: const Rect.fromLTRB(0.0, 0.0, 25.0, 4.0)),
);
expect(tester.binding.transientCallbackCount, 0);
});
testWidgets('LinearProgressIndicator can be made accessible', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
const String label = 'Label';
const String value = '25%';
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: LinearProgressIndicator(
key: key,
value: 0.25,
semanticsLabel: label,
semanticsValue: value,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
textDirection: TextDirection.ltr,
label: label,
value: value,
));
handle.dispose();
});
testWidgets('LinearProgressIndicator that is determinate gets default a11y value', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
const String label = 'Label';
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: LinearProgressIndicator(
key: key,
value: 0.25,
semanticsLabel: label,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
textDirection: TextDirection.ltr,
label: label,
value: '25%',
));
handle.dispose();
});
testWidgets('LinearProgressIndicator that is determinate does not default a11y value when label is null', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: LinearProgressIndicator(
key: key,
value: 0.25,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics());
handle.dispose();
});
testWidgets('LinearProgressIndicator that is indeterminate does not default a11y value', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
const String label = 'Progress';
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: LinearProgressIndicator(
key: key,
value: 0.25,
semanticsLabel: label,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
textDirection: TextDirection.ltr,
label: label,
));
handle.dispose();
});
testWidgets('CircularProgressIndicator can be made accessible', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
const String label = 'Label';
const String value = '25%';
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: CircularProgressIndicator(
key: key,
value: 0.25,
semanticsLabel: label,
semanticsValue: value,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
textDirection: TextDirection.ltr,
label: label,
value: value,
));
handle.dispose();
});
testWidgets('RefreshProgressIndicator can be made accessible', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
final GlobalKey key = GlobalKey();
const String label = 'Label';
const String value = '25%';
await tester.pumpWidget(
Theme(
data: theme,
child: Directionality(
textDirection: TextDirection.ltr,
child: RefreshProgressIndicator(
key: key,
semanticsLabel: label,
semanticsValue: value,
),
),
),
);
expect(tester.getSemantics(find.byKey(key)), matchesSemantics(
textDirection: TextDirection.ltr,
label: label,
value: value,
));
handle.dispose();
});
testWidgets('Material2 - Indeterminate CircularProgressIndicator uses expected animation', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(40, 40));
addTearDown(animationSheet.dispose);
await tester.pumpFrames(animationSheet.record(
Theme(
data: ThemeData(useMaterial3: false),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Padding(
padding: EdgeInsets.all(4),
child: CircularProgressIndicator(),
),
),
),
), const Duration(seconds: 2));
await expectLater(
animationSheet.collate(20),
matchesGoldenFile('m2_material.circular_progress_indicator.indeterminate.png'),
);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/56001
testWidgets('Material3 - Indeterminate CircularProgressIndicator uses expected animation', (WidgetTester tester) async {
final AnimationSheetBuilder animationSheet = AnimationSheetBuilder(frameSize: const Size(40, 40));
addTearDown(animationSheet.dispose);
await tester.pumpFrames(animationSheet.record(
Theme(
data: ThemeData(useMaterial3: true),
child: const Directionality(
textDirection: TextDirection.ltr,
child: Padding(
padding: EdgeInsets.all(4),
child: CircularProgressIndicator(),
),
),
),
), const Duration(seconds: 2));
await expectLater(
animationSheet.collate(20),
matchesGoldenFile('m3_material.circular_progress_indicator.indeterminate.png'),
);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/56001
testWidgets(
'Adaptive CircularProgressIndicator displays CupertinoActivityIndicator in iOS',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(),
home: const Scaffold(
body: Material(
child: CircularProgressIndicator.adaptive(),
),
),
),
);
expect(find.byType(CupertinoActivityIndicator), findsOneWidget);
},
variant: const TargetPlatformVariant(<TargetPlatform> {
TargetPlatform.iOS,
TargetPlatform.macOS,
}),
);
testWidgets(
'Adaptive CircularProgressIndicator displays CupertinoActivityIndicator in iOS/macOS',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(),
home: const Scaffold(
body: Material(
child: CircularProgressIndicator.adaptive(
value: 0.5,
),
),
),
),
);
expect(find.byType(CupertinoActivityIndicator), findsOneWidget);
final double actualProgress = tester.widget<CupertinoActivityIndicator>(
find.byType(CupertinoActivityIndicator),
).progress;
expect(actualProgress, 0.5);
},
variant: const TargetPlatformVariant(<TargetPlatform> {
TargetPlatform.iOS,
TargetPlatform.macOS,
}),
);
testWidgets(
'Adaptive CircularProgressIndicator can use backgroundColor to change tick color for iOS',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(),
home: const Scaffold(
body: Material(
child: CircularProgressIndicator.adaptive(
backgroundColor: Color(0xFF5D3FD3),
),
),
),
),
);
expect(
find.byType(CupertinoActivityIndicator),
paints
..rrect(rrect: const RRect.fromLTRBXY(-1, -10 / 3, 1, -10, 1, 1),
color: const Color(0x935D3FD3)),
);
},
variant: const TargetPlatformVariant(<TargetPlatform> {
TargetPlatform.iOS,
TargetPlatform.macOS,
}),
);
testWidgets(
'Adaptive CircularProgressIndicator does not display CupertinoActivityIndicator in non-iOS',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Scaffold(
body: Material(
child: CircularProgressIndicator.adaptive(),
),
),
),
);
expect(find.byType(CupertinoActivityIndicator), findsNothing);
},
variant: const TargetPlatformVariant(<TargetPlatform> {
TargetPlatform.android,
TargetPlatform.fuchsia,
TargetPlatform.windows,
TargetPlatform.linux,
}),
);
testWidgets('ProgressIndicatorTheme.wrap() always creates a new ProgressIndicatorTheme', (WidgetTester tester) async {
late BuildContext builderContext;
const ProgressIndicatorThemeData themeData = ProgressIndicatorThemeData(
color: Color(0xFFFF0000),
linearTrackColor: Color(0xFF00FF00),
);
final ProgressIndicatorTheme progressTheme = ProgressIndicatorTheme(
data: themeData,
child: Builder(
builder: (BuildContext context) {
builderContext = context;
return const LinearProgressIndicator(value: 0.5);
}
),
);
await tester.pumpWidget(MaterialApp(
home: Theme(data: theme, child: progressTheme),
));
final Widget wrappedTheme = progressTheme.wrap(builderContext, Container());
// Make sure the returned widget is a new ProgressIndicatorTheme instance
// with the same theme data as the original.
expect(wrappedTheme, isNot(equals(progressTheme)));
expect(wrappedTheme, isInstanceOf<ProgressIndicatorTheme>());
expect((wrappedTheme as ProgressIndicatorTheme).data, themeData);
});
testWidgets('default size of CircularProgressIndicator is 36x36 - M3', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: theme.copyWith(useMaterial3: true),
home: const Scaffold(
body: Material(
child: CircularProgressIndicator(),
),
),
),
);
expect(tester.getSize(find.byType(CircularProgressIndicator)), const Size(36, 36));
});
testWidgets('RefreshProgressIndicator using fields correctly', (WidgetTester tester) async {
Future<void> pumpIndicator(RefreshProgressIndicator indicator) {
return tester.pumpWidget(Theme(data: theme, child: indicator));
}
// With default values.
await pumpIndicator(const RefreshProgressIndicator());
Material material = tester.widget(
find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
),
);
Container container = tester.widget(
find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Container),
),
);
Padding padding = tester.widget(
find.descendant(
of: find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
),
matching: find.byType(Padding),
),
);
expect(material.elevation, 2.0);
expect(container.margin, const EdgeInsets.all(4.0));
expect(padding.padding, const EdgeInsets.all(12.0));
// With values provided.
const double testElevation = 1.0;
const EdgeInsetsGeometry testIndicatorMargin = EdgeInsets.all(6.0);
const EdgeInsetsGeometry testIndicatorPadding = EdgeInsets.all(10.0);
await pumpIndicator(
const RefreshProgressIndicator(
elevation: testElevation,
indicatorMargin: testIndicatorMargin,
indicatorPadding: testIndicatorPadding,
),
);
material = tester.widget(
find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
),
);
container = tester.widget(
find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Container),
),
);
padding = tester.widget(
find.descendant(
of: find.descendant(
of: find.byType(RefreshProgressIndicator),
matching: find.byType(Material),
),
matching: find.byType(Padding),
),
);
expect(material.elevation, testElevation);
expect(container.margin, testIndicatorMargin);
expect(padding.padding, testIndicatorPadding);
});
}
class _RefreshProgressIndicatorGolden extends StatefulWidget {
const _RefreshProgressIndicatorGolden();
@override
_RefreshProgressIndicatorGoldenState createState() => _RefreshProgressIndicatorGoldenState();
}
class _RefreshProgressIndicatorGoldenState extends State<_RefreshProgressIndicatorGolden> with SingleTickerProviderStateMixin {
late final AnimationController controller = AnimationController(
vsync: this,
duration: const Duration(seconds: 1),
)
..forward()
..addListener(() {
setState(() {});
})
..addStatusListener((AnimationStatus status) {
if (status == AnimationStatus.completed) {
indeterminate = true;
}
});
bool indeterminate = false;
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: Directionality(
textDirection: TextDirection.ltr,
child: RefreshProgressIndicator(
value: indeterminate ? null : controller.value,
),
),
);
}
}
| flutter/packages/flutter/test/material/progress_indicator_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/progress_indicator_test.dart",
"repo_id": "flutter",
"token_count": 17781
} | 738 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.