text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is part of dev/bots/test.dart's runTracingTests test.
import 'package:flutter/foundation.dart';
void main() {
// This file is intended to be compiled in profile mode.
// In that mode, the function below throws an exception.
// The dev/bots/test.dart test looks for the string from that exception.
// The string below is matched verbatim in dev/bots/test.dart as a control
// to make sure this file did get compiled.
DiagnosticsNode.message('TIMELINE ARGUMENTS TEST CONTROL FILE').toTimelineArguments();
}
| flutter/dev/tracing_tests/lib/control.dart/0 | {
"file_path": "flutter/dev/tracing_tests/lib/control.dart",
"repo_id": "flutter",
"token_count": 197
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoAlertDialog].
void main() => runApp(const AlertDialogApp());
class AlertDialogApp extends StatelessWidget {
const AlertDialogApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: AlertDialogExample(),
);
}
}
class AlertDialogExample extends StatelessWidget {
const AlertDialogExample({super.key});
// This shows a CupertinoModalPopup which hosts a CupertinoAlertDialog.
void _showAlertDialog(BuildContext context) {
showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => CupertinoAlertDialog(
title: const Text('Alert'),
content: const Text('Proceed with destructive action?'),
actions: <CupertinoDialogAction>[
CupertinoDialogAction(
/// This parameter indicates this action is the default,
/// and turns the action's text to bold text.
isDefaultAction: true,
onPressed: () {
Navigator.pop(context);
},
child: const Text('No'),
),
CupertinoDialogAction(
/// This parameter indicates the action would perform
/// a destructive action such as deletion, and turns
/// the action's text color to red.
isDestructiveAction: true,
onPressed: () {
Navigator.pop(context);
},
child: const Text('Yes'),
),
],
),
);
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoAlertDialog Sample'),
),
child: Center(
child: CupertinoButton(
onPressed: () => _showAlertDialog(context),
child: const Text('CupertinoAlertDialog'),
),
),
);
}
}
| flutter/examples/api/lib/cupertino/dialog/cupertino_alert_dialog.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/dialog/cupertino_alert_dialog.0.dart",
"repo_id": "flutter",
"token_count": 866
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
/// Flutter code sample for [CupertinoSearchTextField].
void main() => runApp(const SearchTextFieldApp());
class SearchTextFieldApp extends StatelessWidget {
const SearchTextFieldApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: SearchTextFieldExample(),
);
}
}
class SearchTextFieldExample extends StatefulWidget {
const SearchTextFieldExample({super.key});
@override
State<SearchTextFieldExample> createState() => _SearchTextFieldExampleState();
}
class _SearchTextFieldExampleState extends State<SearchTextFieldExample> {
String text = '';
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoSearchTextField Sample'),
),
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(text),
Padding(
padding: const EdgeInsets.all(16.0),
child: SearchTextField(
fieldValue: (String value) {
setState(() {
text = value;
});
},
),
),
],
),
),
);
}
}
class SearchTextField extends StatelessWidget {
const SearchTextField({
super.key,
required this.fieldValue,
});
final ValueChanged<String> fieldValue;
@override
Widget build(BuildContext context) {
return CupertinoSearchTextField(
onChanged: (String value) {
fieldValue('The text has changed to: $value');
},
onSubmitted: (String value) {
fieldValue('Submitted text: $value');
},
);
}
}
| flutter/examples/api/lib/cupertino/search_field/cupertino_search_field.1.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/search_field/cupertino_search_field.1.dart",
"repo_id": "flutter",
"token_count": 813
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MaterialApp].
void main() {
runApp(const MaterialAppExample());
}
enum AnimationStyles { defaultStyle, custom, none }
const List<(AnimationStyles, String)> animationStyleSegments = <(AnimationStyles, String)>[
(AnimationStyles.defaultStyle, 'Default'),
(AnimationStyles.custom, 'Custom'),
(AnimationStyles.none, 'None'),
];
class MaterialAppExample extends StatefulWidget {
const MaterialAppExample({super.key});
@override
State<MaterialAppExample> createState() => _MaterialAppExampleState();
}
class _MaterialAppExampleState extends State<MaterialAppExample> {
Set<AnimationStyles> _animationStyleSelection = <AnimationStyles>{AnimationStyles.defaultStyle};
AnimationStyle? _animationStyle;
bool isDarkTheme = false;
@override
Widget build(BuildContext context) {
return MaterialApp(
themeAnimationStyle: _animationStyle,
themeMode: isDarkTheme ? ThemeMode.dark : ThemeMode.light,
theme: ThemeData(colorSchemeSeed: Colors.green),
darkTheme: ThemeData(
colorSchemeSeed: Colors.green,
brightness: Brightness.dark,
),
home: Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SegmentedButton<AnimationStyles>(
selected: _animationStyleSelection,
onSelectionChanged: (Set<AnimationStyles> styles) {
setState(() {
_animationStyleSelection = styles;
switch (styles.first) {
case AnimationStyles.defaultStyle:
_animationStyle = null;
case AnimationStyles.custom:
_animationStyle = AnimationStyle(
curve: Easing.emphasizedAccelerate,
duration: const Duration(seconds: 1),
);
case AnimationStyles.none:
_animationStyle = AnimationStyle.noAnimation;
}
});
},
segments: animationStyleSegments
.map<ButtonSegment<AnimationStyles>>(((AnimationStyles, String) shirt) {
return ButtonSegment<AnimationStyles>(value: shirt.$1, label: Text(shirt.$2));
})
.toList(),
),
const SizedBox(height: 10),
OutlinedButton.icon(
onPressed: () {
setState(() {
isDarkTheme = !isDarkTheme;
});
},
icon: Icon(isDarkTheme ? Icons.wb_sunny : Icons.nightlight_round),
label: const Text('Switch Theme Mode'),
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/app/app.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/app/app.0.dart",
"repo_id": "flutter",
"token_count": 1433
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [BottomAppBar].
void main() {
runApp(const BottomAppBarDemo());
}
class BottomAppBarDemo extends StatefulWidget {
const BottomAppBarDemo({super.key});
@override
State createState() => _BottomAppBarDemoState();
}
class _BottomAppBarDemoState extends State<BottomAppBarDemo> {
bool _showFab = true;
bool _showNotch = true;
FloatingActionButtonLocation _fabLocation = FloatingActionButtonLocation.endDocked;
void _onShowNotchChanged(bool value) {
setState(() {
_showNotch = value;
});
}
void _onShowFabChanged(bool value) {
setState(() {
_showFab = value;
});
}
void _onFabLocationChanged(FloatingActionButtonLocation? value) {
setState(() {
_fabLocation = value ?? FloatingActionButtonLocation.endDocked;
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
automaticallyImplyLeading: false,
title: const Text('Bottom App Bar Demo'),
),
body: ListView(
padding: const EdgeInsets.only(bottom: 88),
children: <Widget>[
SwitchListTile(
title: const Text(
'Floating Action Button',
),
value: _showFab,
onChanged: _onShowFabChanged,
),
SwitchListTile(
title: const Text('Notch'),
value: _showNotch,
onChanged: _onShowNotchChanged,
),
const Padding(
padding: EdgeInsets.all(16),
child: Text('Floating action button position'),
),
RadioListTile<FloatingActionButtonLocation>(
title: const Text('Docked - End'),
value: FloatingActionButtonLocation.endDocked,
groupValue: _fabLocation,
onChanged: _onFabLocationChanged,
),
RadioListTile<FloatingActionButtonLocation>(
title: const Text('Docked - Center'),
value: FloatingActionButtonLocation.centerDocked,
groupValue: _fabLocation,
onChanged: _onFabLocationChanged,
),
RadioListTile<FloatingActionButtonLocation>(
title: const Text('Floating - End'),
value: FloatingActionButtonLocation.endFloat,
groupValue: _fabLocation,
onChanged: _onFabLocationChanged,
),
RadioListTile<FloatingActionButtonLocation>(
title: const Text('Floating - Center'),
value: FloatingActionButtonLocation.centerFloat,
groupValue: _fabLocation,
onChanged: _onFabLocationChanged,
),
],
),
floatingActionButton: _showFab
? FloatingActionButton(
onPressed: () {},
tooltip: 'Create',
child: const Icon(Icons.add),
)
: null,
floatingActionButtonLocation: _fabLocation,
bottomNavigationBar: _DemoBottomAppBar(
fabLocation: _fabLocation,
shape: _showNotch ? const CircularNotchedRectangle() : null,
),
),
);
}
}
class _DemoBottomAppBar extends StatelessWidget {
const _DemoBottomAppBar({
this.fabLocation = FloatingActionButtonLocation.endDocked,
this.shape = const CircularNotchedRectangle(),
});
final FloatingActionButtonLocation fabLocation;
final NotchedShape? shape;
static final List<FloatingActionButtonLocation> centerLocations = <FloatingActionButtonLocation>[
FloatingActionButtonLocation.centerDocked,
FloatingActionButtonLocation.centerFloat,
];
@override
Widget build(BuildContext context) {
return BottomAppBar(
shape: shape,
color: Colors.blue,
child: IconTheme(
data: IconThemeData(color: Theme.of(context).colorScheme.onPrimary),
child: Row(
children: <Widget>[
IconButton(
tooltip: 'Open navigation menu',
icon: const Icon(Icons.menu),
onPressed: () {},
),
if (centerLocations.contains(fabLocation)) const Spacer(),
IconButton(
tooltip: 'Search',
icon: const Icon(Icons.search),
onPressed: () {},
),
IconButton(
tooltip: 'Favorite',
icon: const Icon(Icons.favorite),
onPressed: () {},
),
],
),
),
);
}
}
| flutter/examples/api/lib/material/bottom_app_bar/bottom_app_bar.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/bottom_app_bar/bottom_app_bar.1.dart",
"repo_id": "flutter",
"token_count": 2143
} | 626 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
/// Flutter code sample for custom labeled checkbox.
void main() => runApp(const LabeledCheckboxApp());
class LabeledCheckboxApp extends StatelessWidget {
const LabeledCheckboxApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const LabeledCheckboxExample(),
);
}
}
class LinkedLabelCheckbox extends StatelessWidget {
const LinkedLabelCheckbox({
super.key,
required this.label,
required this.padding,
required this.value,
required this.onChanged,
});
final String label;
final EdgeInsets padding;
final bool value;
final ValueChanged<bool> onChanged;
@override
Widget build(BuildContext context) {
return Padding(
padding: padding,
child: Row(
children: <Widget>[
Expanded(
child: RichText(
text: TextSpan(
text: label,
style: const TextStyle(
color: Colors.blueAccent,
decoration: TextDecoration.underline,
),
recognizer: TapGestureRecognizer()
..onTap = () {
debugPrint('Label has been tapped.');
},
),
),
),
Checkbox(
value: value,
onChanged: (bool? newValue) {
onChanged(newValue!);
},
),
],
),
);
}
}
class LabeledCheckboxExample extends StatefulWidget {
const LabeledCheckboxExample({super.key});
@override
State<LabeledCheckboxExample> createState() => _LabeledCheckboxExampleState();
}
class _LabeledCheckboxExampleState extends State<LabeledCheckboxExample> {
bool _isSelected = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Custom Labeled Checkbox Sample')),
body: Center(
child: LinkedLabelCheckbox(
label: 'Linked, tappable label text',
padding: const EdgeInsets.symmetric(horizontal: 20.0),
value: _isSelected,
onChanged: (bool newValue) {
setState(() {
_isSelected = newValue;
});
},
),
),
);
}
}
| flutter/examples/api/lib/material/checkbox_list_tile/custom_labeled_checkbox.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/checkbox_list_tile/custom_labeled_checkbox.0.dart",
"repo_id": "flutter",
"token_count": 1107
} | 627 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [showDatePicker].
void main() => runApp(const DatePickerApp());
class DatePickerApp extends StatelessWidget {
const DatePickerApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
restorationScopeId: 'app',
home: DatePickerExample(restorationId: 'main'),
);
}
}
class DatePickerExample extends StatefulWidget {
const DatePickerExample({super.key, this.restorationId});
final String? restorationId;
@override
State<DatePickerExample> createState() => _DatePickerExampleState();
}
/// RestorationProperty objects can be used because of RestorationMixin.
class _DatePickerExampleState extends State<DatePickerExample> with RestorationMixin {
// In this example, the restoration ID for the mixin is passed in through
// the [StatefulWidget]'s constructor.
@override
String? get restorationId => widget.restorationId;
final RestorableDateTime _selectedDate = RestorableDateTime(DateTime(2021, 7, 25));
late final RestorableRouteFuture<DateTime?> _restorableDatePickerRouteFuture = RestorableRouteFuture<DateTime?>(
onComplete: _selectDate,
onPresent: (NavigatorState navigator, Object? arguments) {
return navigator.restorablePush(
_datePickerRoute,
arguments: _selectedDate.value.millisecondsSinceEpoch,
);
},
);
@pragma('vm:entry-point')
static Route<DateTime> _datePickerRoute(
BuildContext context,
Object? arguments,
) {
return DialogRoute<DateTime>(
context: context,
builder: (BuildContext context) {
return DatePickerDialog(
restorationId: 'date_picker_dialog',
initialEntryMode: DatePickerEntryMode.calendarOnly,
initialDate: DateTime.fromMillisecondsSinceEpoch(arguments! as int),
firstDate: DateTime(2021),
lastDate: DateTime(2022),
);
},
);
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_selectedDate, 'selected_date');
registerForRestoration(_restorableDatePickerRouteFuture, 'date_picker_route_future');
}
void _selectDate(DateTime? newSelectedDate) {
if (newSelectedDate != null) {
setState(() {
_selectedDate.value = newSelectedDate;
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content:
Text('Selected: ${_selectedDate.value.day}/${_selectedDate.value.month}/${_selectedDate.value.year}'),
));
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: OutlinedButton(
onPressed: () {
_restorableDatePickerRouteFuture.present();
},
child: const Text('Open Date Picker'),
),
),
);
}
}
| flutter/examples/api/lib/material/date_picker/show_date_picker.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/date_picker/show_date_picker.0.dart",
"repo_id": "flutter",
"token_count": 1104
} | 628 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [DropdownButton.style].
void main() => runApp(const DropdownButtonApp());
class DropdownButtonApp extends StatelessWidget {
const DropdownButtonApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('DropdownButton Sample')),
body: const DropdownButtonExample(),
),
);
}
}
class DropdownButtonExample extends StatefulWidget {
const DropdownButtonExample({super.key});
@override
State<DropdownButtonExample> createState() => _DropdownButtonExampleState();
}
class _DropdownButtonExampleState extends State<DropdownButtonExample> {
List<String> options = <String>['One', 'Two', 'Three', 'Four'];
String dropdownValue = 'One';
@override
Widget build(BuildContext context) {
return Container(
alignment: Alignment.center,
color: Colors.blue,
child: DropdownButton<String>(
value: dropdownValue,
onChanged: (String? value) {
// This is called when the user selects an item.
setState(() {
dropdownValue = value!;
});
},
style: const TextStyle(color: Colors.blue),
selectedItemBuilder: (BuildContext context) {
// This is the widget that will be shown when you select an item.
// Here custom text style, alignment and layout size can be applied
// to selected item string.
return options.map((String value) {
return Align(
alignment: Alignment.centerLeft,
child: Text(
dropdownValue,
style: const TextStyle(color: Colors.white),
),
);
}).toList();
},
items: options.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
);
}
}
| flutter/examples/api/lib/material/dropdown/dropdown_button.style.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/dropdown/dropdown_button.style.0.dart",
"repo_id": "flutter",
"token_count": 870
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [StandardFabLocation].
void main() => runApp(const StandardFabLocationExampleApp());
class StandardFabLocationExampleApp extends StatelessWidget {
const StandardFabLocationExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: StandardFabLocationExample(),
);
}
}
class AlmostEndFloatFabLocation extends StandardFabLocation with FabEndOffsetX, FabFloatOffsetY {
@override
double getOffsetX(ScaffoldPrelayoutGeometry scaffoldGeometry, double adjustment) {
final double directionalAdjustment = scaffoldGeometry.textDirection == TextDirection.ltr ? -50.0 : 50.0;
return super.getOffsetX(scaffoldGeometry, adjustment) + directionalAdjustment;
}
}
class StandardFabLocationExample extends StatelessWidget {
const StandardFabLocationExample({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Home page'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
debugPrint('FAB pressed.');
},
tooltip: 'Increment',
child: const Icon(Icons.add),
),
floatingActionButtonLocation: AlmostEndFloatFabLocation(),
);
}
}
| flutter/examples/api/lib/material/floating_action_button_location/standard_fab_location.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/floating_action_button_location/standard_fab_location.0.dart",
"repo_id": "flutter",
"token_count": 480
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InputDecoration.label].
void main() => runApp(const LabelExampleApp());
class LabelExampleApp extends StatelessWidget {
const LabelExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('InputDecoration.label Sample')),
body: const LabelExample(),
),
);
}
}
class LabelExample extends StatelessWidget {
const LabelExample({super.key});
@override
Widget build(BuildContext context) {
return const Center(
child: TextField(
decoration: InputDecoration(
label: Text.rich(
TextSpan(
children: <InlineSpan>[
WidgetSpan(
child: Text(
'Username',
),
),
WidgetSpan(
child: Text(
'*',
style: TextStyle(color: Colors.red),
),
),
],
),
),
),
),
);
}
}
| flutter/examples/api/lib/material/input_decorator/input_decoration.label.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/input_decorator/input_decoration.label.0.dart",
"repo_id": "flutter",
"token_count": 641
} | 631 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [MaterialStateBorderSide].
void main() => runApp(const MaterialStateBorderSideExampleApp());
class MaterialStateBorderSideExampleApp extends StatelessWidget {
const MaterialStateBorderSideExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('MaterialStateBorderSide Sample')),
body: const Center(
child: MaterialStateBorderSideExample(),
),
),
);
}
}
class MaterialStateBorderSideExample extends StatefulWidget {
const MaterialStateBorderSideExample({super.key});
@override
State<MaterialStateBorderSideExample> createState() => _MaterialStateBorderSideExampleState();
}
class _MaterialStateBorderSideExampleState extends State<MaterialStateBorderSideExample> {
bool isSelected = true;
@override
Widget build(BuildContext context) {
return FilterChip(
label: const Text('Select chip'),
selected: isSelected,
onSelected: (bool value) {
setState(() {
isSelected = value;
});
},
side: MaterialStateBorderSide.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const BorderSide(color: Colors.red);
}
return null; // Defer to default value on the theme or widget.
}),
);
}
}
| flutter/examples/api/lib/material/material_state/material_state_border_side.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/material_state/material_state_border_side.0.dart",
"repo_id": "flutter",
"token_count": 544
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Radio.toggleable].
void main() => runApp(const ToggleableExampleApp());
class ToggleableExampleApp extends StatelessWidget {
const ToggleableExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Radio Sample')),
body: const ToggleableExample(),
),
);
}
}
class ToggleableExample extends StatefulWidget {
const ToggleableExample({super.key});
@override
State<ToggleableExample> createState() => _ToggleableExampleState();
}
class _ToggleableExampleState extends State<ToggleableExample> {
int? groupValue;
static const List<String> selections = <String>[
'Hercules Mulligan',
'Eliza Hamilton',
'Philip Schuyler',
'Maria Reynolds',
'Samuel Seabury',
];
@override
Widget build(BuildContext context) {
return Scaffold(
body: ListView.builder(
itemBuilder: (BuildContext context, int index) {
return Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Radio<int>(
value: index,
groupValue: groupValue,
// TRY THIS: Try setting the toggleable value to false and
// see how that changes the behavior of the widget.
toggleable: true,
onChanged: (int? value) {
setState(() {
groupValue = value;
});
}),
Text(selections[index]),
],
);
},
itemCount: selections.length,
),
);
}
}
| flutter/examples/api/lib/material/radio/radio.toggleable.0.dart/0 | {
"file_path": "flutter/examples/api/lib/material/radio/radio.toggleable.0.dart",
"repo_id": "flutter",
"token_count": 805
} | 633 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for pinned [SearchAnchor] while scrolling.
void main() {
runApp(const PinnedSearchBarApp());
}
class PinnedSearchBarApp extends StatefulWidget {
const PinnedSearchBarApp({super.key});
@override
State<PinnedSearchBarApp> createState() => _PinnedSearchBarAppState();
}
class _PinnedSearchBarAppState extends State<PinnedSearchBarApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true, colorSchemeSeed: const Color(0xff6750a4)),
home: Scaffold(
body: SafeArea(
child: CustomScrollView(
slivers: <Widget>[
SliverAppBar(
clipBehavior: Clip.none,
shape: const StadiumBorder(),
scrolledUnderElevation: 0.0,
titleSpacing: 0.0,
backgroundColor: Colors.transparent,
floating: true, // We can also uncomment this line and set `pinned` to true to see a pinned search bar.
title: SearchAnchor.bar(
suggestionsBuilder: (BuildContext context, SearchController controller) {
return List<Widget>.generate(
5,
(int index) {
return ListTile(
titleAlignment: ListTileTitleAlignment.center,
title: Text('Initial list item $index'),
);
},
);
},
),
),
// The listed items below are just for filling the screen
// so we can see the scrolling effect.
SliverToBoxAdapter(
child: Padding(
padding: const EdgeInsets.all(20),
child: SizedBox(
height: 100.0,
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: 10,
itemBuilder: (BuildContext context, int index) {
return SizedBox(
width: 100.0,
child: Card(
child: Center(child: Text('Card $index')),
),
);
},
),
),
),
),
SliverToBoxAdapter(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 20),
child: Container(
height: 1000,
color: Colors.deepPurple.withOpacity(0.5),
),
),
),
],
),
),
),
);
}
}
| flutter/examples/api/lib/material/search_anchor/search_anchor.1.dart/0 | {
"file_path": "flutter/examples/api/lib/material/search_anchor/search_anchor.1.dart",
"repo_id": "flutter",
"token_count": 1648
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SnackBar].
void main() => runApp(const SnackBarExampleApp());
/// A Material 3 [SnackBar] demonstrating an optional icon, in either floating
/// or fixed format.
class SnackBarExampleApp extends StatelessWidget {
const SnackBarExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('SnackBar Sample')),
body: const Center(
child: SnackBarExample(),
),
),
);
}
}
class SnackBarExample extends StatefulWidget {
const SnackBarExample({super.key});
@override
State<SnackBarExample> createState() => _SnackBarExampleState();
}
class _SnackBarExampleState extends State<SnackBarExample> {
SnackBarBehavior? _snackBarBehavior = SnackBarBehavior.floating;
bool _withIcon = true;
bool _withAction = true;
bool _multiLine = false;
bool _longActionLabel = false;
double _sliderValue = 0.25;
Padding _padRow(List<Widget> children) => Padding(
padding: const EdgeInsets.all(8.0),
child: Row(children: children),
);
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.only(left: 50.0),
child: Column(
children: <Widget>[
_padRow(<Widget>[
Text('Snack Bar configuration', style: Theme.of(context).textTheme.bodyLarge),
]),
_padRow(
<Widget>[
const Text('Fixed'),
Radio<SnackBarBehavior>(
value: SnackBarBehavior.fixed,
groupValue: _snackBarBehavior,
onChanged: (SnackBarBehavior? value) {
setState(() {
_snackBarBehavior = value;
});
},
),
const Text('Floating'),
Radio<SnackBarBehavior>(
value: SnackBarBehavior.floating,
groupValue: _snackBarBehavior,
onChanged: (SnackBarBehavior? value) {
setState(() {
_snackBarBehavior = value;
});
},
),
],
),
_padRow(
<Widget>[
const Text('Include Icon '),
Switch(
value: _withIcon,
onChanged: (bool value) {
setState(() {
_withIcon = !_withIcon;
});
},
),
],
),
_padRow(
<Widget>[
const Text('Include Action '),
Switch(
value: _withAction,
onChanged: (bool value) {
setState(() {
_withAction = !_withAction;
});
},
),
const SizedBox(width: 16.0),
const Text('Long Action Label '),
Switch(
value: _longActionLabel,
onChanged: !_withAction
? null
: (bool value) {
setState(() {
_longActionLabel = !_longActionLabel;
});
},
),
],
),
_padRow(
<Widget>[
const Text('Multi Line Text'),
Switch(
value: _multiLine,
onChanged: _snackBarBehavior == SnackBarBehavior.fixed
? null
: (bool value) {
setState(() {
_multiLine = !_multiLine;
});
},
),
],
),
_padRow(<Widget>[
const Text('Action new-line overflow threshold'),
Slider(
value: _sliderValue,
divisions: 20,
label: _sliderValue.toStringAsFixed(2),
onChanged: _snackBarBehavior == SnackBarBehavior.fixed
? null
: (double value) {
setState(() {
_sliderValue = value;
});
},
),
]),
const SizedBox(height: 16.0),
ElevatedButton(
child: const Text('Show Snackbar'),
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(_snackBar());
},
),
],
),
);
}
SnackBar _snackBar() {
final SnackBarAction? action = _withAction
? SnackBarAction(
label: _longActionLabel ? 'Long Action Text' : 'Action',
onPressed: () {
// Code to execute.
},
)
: null;
final double? width = _snackBarBehavior == SnackBarBehavior.floating && _multiLine ? 400.0 : null;
final String label =
_multiLine ? 'A Snack Bar with quite a lot of text which spans across multiple lines' : 'Single Line Snack Bar';
return SnackBar(
content: Text(label),
showCloseIcon: _withIcon,
width: width,
behavior: _snackBarBehavior,
action: action,
duration: const Duration(seconds: 3),
actionOverflowThreshold: _sliderValue,
);
}
}
| flutter/examples/api/lib/material/snack_bar/snack_bar.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/snack_bar/snack_bar.2.dart",
"repo_id": "flutter",
"token_count": 3004
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TabBar].
void main() => runApp(const TabBarApp());
class TabBarApp extends StatelessWidget {
const TabBarApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const TabBarExample(),
);
}
}
class TabBarExample extends StatelessWidget {
const TabBarExample({super.key});
@override
Widget build(BuildContext context) {
return DefaultTabController(
initialIndex: 1,
length: 3,
child: Scaffold(
appBar: AppBar(
title: const Text('Primary and secondary TabBar'),
bottom: const TabBar(
dividerColor: Colors.transparent,
tabs: <Widget>[
Tab(
text: 'Flights',
icon: Icon(Icons.flight),
),
Tab(
text: 'Trips',
icon: Icon(Icons.luggage),
),
Tab(
text: 'Explore',
icon: Icon(Icons.explore),
),
],
),
),
body: const TabBarView(
children: <Widget>[
NestedTabBar('Flights'),
NestedTabBar('Trips'),
NestedTabBar('Explore'),
],
),
),
);
}
}
class NestedTabBar extends StatefulWidget {
const NestedTabBar(this.outerTab, {super.key});
final String outerTab;
@override
State<NestedTabBar> createState() => _NestedTabBarState();
}
class _NestedTabBarState extends State<NestedTabBar> with TickerProviderStateMixin {
late final TabController _tabController;
@override
void initState() {
super.initState();
_tabController = TabController(length: 2, vsync: this);
}
@override
void dispose() {
_tabController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
TabBar.secondary(
controller: _tabController,
tabs: const <Widget>[
Tab(text: 'Overview'),
Tab(text: 'Specifications'),
],
),
Expanded(
child: TabBarView(
controller: _tabController,
children: <Widget>[
Card(
margin: const EdgeInsets.all(16.0),
child: Center(child: Text('${widget.outerTab}: Overview tab')),
),
Card(
margin: const EdgeInsets.all(16.0),
child: Center(child: Text('${widget.outerTab}: Specifications tab')),
),
],
),
),
],
);
}
}
| flutter/examples/api/lib/material/tabs/tab_bar.2.dart/0 | {
"file_path": "flutter/examples/api/lib/material/tabs/tab_bar.2.dart",
"repo_id": "flutter",
"token_count": 1345
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [AxisDirection]s.
void main() => runApp(const ExampleApp());
class ExampleApp extends StatelessWidget {
const ExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: MyWidget(),
);
}
}
class MyWidget extends StatefulWidget {
const MyWidget({super.key});
@override
State<MyWidget> createState() => _MyWidgetState();
}
class _MyWidgetState extends State<MyWidget> {
final List<String> _alphabet = <String>[
'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O',
'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z',
];
final Widget _spacer = const SizedBox.square(dimension: 10);
AxisDirection _axisDirection = AxisDirection.down;
Widget _getArrows() {
final Widget arrow;
switch (_axisDirection) {
case AxisDirection.up:
arrow = const Icon(Icons.arrow_upward_rounded);
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.down:
arrow = const Icon(Icons.arrow_downward_rounded);
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.left:
arrow = const Icon(Icons.arrow_back_rounded);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
case AxisDirection.right:
arrow = const Icon(Icons.arrow_forward_rounded);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[arrow, arrow],
);
}
}
void _onAxisDirectionChanged(AxisDirection? axisDirection) {
if (axisDirection != null && axisDirection != _axisDirection) {
setState(() {
// Respond to change in axis direction.
_axisDirection = axisDirection;
});
}
}
Widget _getLeading() {
return Container(
color: Colors.blue[100],
padding: const EdgeInsets.all(8.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Text(axisDirectionToAxis(_axisDirection).toString()),
_spacer,
Text(_axisDirection.toString()),
_spacer,
const Text('GrowthDirection.forward'),
_spacer,
_getArrows(),
],
),
);
}
Widget _getRadioRow() {
return DefaultTextStyle(
style: const TextStyle(fontWeight: FontWeight.bold, color: Colors.white),
child: RadioTheme(
data: RadioThemeData(
fillColor: MaterialStateProperty.all<Color>(Colors.white),
),
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
Radio<AxisDirection>(
value: AxisDirection.up,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('up'),
_spacer,
Radio<AxisDirection>(
value: AxisDirection.down,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('down'),
_spacer,
Radio<AxisDirection>(
value: AxisDirection.left,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('left'),
_spacer,
Radio<AxisDirection>(
value: AxisDirection.right,
groupValue: _axisDirection,
onChanged: _onAxisDirectionChanged,
),
const Text('right'),
_spacer,
],
),
),
),
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('AxisDirections'),
bottom: PreferredSize(
preferredSize: const Size.fromHeight(50),
child: Padding(
padding: const EdgeInsets.all(8.0),
child: _getRadioRow(),
),
),
),
// Also works for ListView.builder, which creates a SliverList for itself.
// A CustomScrollView allows multiple slivers to be composed together.
body: CustomScrollView(
// This method is available to conveniently determine if an scroll
// view is reversed by its AxisDirection.
reverse: axisDirectionIsReversed(_axisDirection),
// This method is available to conveniently convert an AxisDirection
// into its Axis.
scrollDirection: axisDirectionToAxis(_axisDirection),
slivers: <Widget>[
SliverList.builder(
itemCount: 27,
itemBuilder: (BuildContext context, int index) {
final Widget child;
if (index == 0) {
child = _getLeading();
} else {
child = Container(
color: index.isEven ? Colors.amber[100] : Colors.amberAccent,
padding: const EdgeInsets.all(8.0),
child: Center(child: Text(_alphabet[index - 1])),
);
}
return Padding(
padding: const EdgeInsets.all(8.0),
child: child,
);
},
),
],
),
);
}
}
| flutter/examples/api/lib/painting/axis_direction/axis_direction.0.dart/0 | {
"file_path": "flutter/examples/api/lib/painting/axis_direction/axis_direction.0.dart",
"repo_id": "flutter",
"token_count": 2747
} | 637 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
/// Flutter code sample for [PhysicalKeyboardKey].
void main() => runApp(const KeyExampleApp());
class KeyExampleApp extends StatelessWidget {
const KeyExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('PhysicalKeyboardKey Example')),
body: const MyPhysicalKeyExample(),
),
);
}
}
class MyPhysicalKeyExample extends StatefulWidget {
const MyPhysicalKeyExample({super.key});
@override
State<MyPhysicalKeyExample> createState() => _MyPhysicalKeyExampleState();
}
class _MyPhysicalKeyExampleState extends State<MyPhysicalKeyExample> {
// The node used to request the keyboard focus.
final FocusNode _focusNode = FocusNode();
// The message to display.
String? _message;
// Focus nodes need to be disposed.
@override
void dispose() {
_focusNode.dispose();
super.dispose();
}
// Handles the key events from the RawKeyboardListener and update the
// _message.
KeyEventResult _handleKeyEvent(FocusNode node, KeyEvent event) {
setState(() {
if (event.physicalKey == PhysicalKeyboardKey.keyA) {
_message = 'Pressed the key next to CAPS LOCK!';
} else {
if (kReleaseMode) {
_message = 'Not the key next to CAPS LOCK: Pressed 0x${event.physicalKey.usbHidUsage.toRadixString(16)}';
} else {
// As the name implies, the debugName will only print useful
// information in debug mode.
_message = 'Not the key next to CAPS LOCK: Pressed ${event.physicalKey.debugName}';
}
}
});
return event.physicalKey == PhysicalKeyboardKey.keyA ? KeyEventResult.handled : KeyEventResult.ignored;
}
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return Container(
color: Colors.white,
alignment: Alignment.center,
child: DefaultTextStyle(
style: textTheme.headlineMedium!,
child: Focus(
focusNode: _focusNode,
onKeyEvent: _handleKeyEvent,
child: ListenableBuilder(
listenable: _focusNode,
builder: (BuildContext context, Widget? child) {
if (!_focusNode.hasFocus) {
return GestureDetector(
onTap: () {
FocusScope.of(context).requestFocus(_focusNode);
},
child: const Text('Click to focus'),
);
}
return Text(_message ?? 'Press a key');
},
),
),
),
);
}
}
| flutter/examples/api/lib/services/keyboard_key/physical_keyboard_key.0.dart/0 | {
"file_path": "flutter/examples/api/lib/services/keyboard_key/physical_keyboard_key.0.dart",
"repo_id": "flutter",
"token_count": 1142
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ActionListener].
void main() => runApp(const ActionListenerExampleApp());
class ActionListenerExampleApp extends StatelessWidget {
const ActionListenerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ActionListener Sample')),
body: const Center(
child: ActionListenerExample(),
),
),
);
}
}
class ActionListenerExample extends StatefulWidget {
const ActionListenerExample({super.key});
@override
State<ActionListenerExample> createState() => _ActionListenerExampleState();
}
class _ActionListenerExampleState extends State<ActionListenerExample> {
bool _on = false;
late final MyAction _myAction;
@override
void initState() {
super.initState();
_myAction = MyAction();
}
void _toggleState() {
setState(() {
_on = !_on;
});
}
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(8.0),
child: OutlinedButton(
onPressed: _toggleState,
child: Text(_on ? 'Disable' : 'Enable'),
),
),
if (_on)
Padding(
padding: const EdgeInsets.all(8.0),
child: ActionListener(
listener: (Action<Intent> action) {
if (action.intentType == MyIntent) {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text('Action Listener Called'),
));
}
},
action: _myAction,
child: ElevatedButton(
onPressed: () => const ActionDispatcher().invokeAction(_myAction, const MyIntent()),
child: const Text('Call Action Listener'),
),
),
),
if (!_on) Container(),
],
);
}
}
class MyAction extends Action<MyIntent> {
@override
void addActionListener(ActionListenerCallback listener) {
super.addActionListener(listener);
debugPrint('Action Listener was added');
}
@override
void removeActionListener(ActionListenerCallback listener) {
super.removeActionListener(listener);
debugPrint('Action Listener was removed');
}
@override
void invoke(covariant MyIntent intent) {
notifyActionListeners();
}
}
class MyIntent extends Intent {
const MyIntent();
}
| flutter/examples/api/lib/widgets/actions/action_listener.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/actions/action_listener.0.dart",
"repo_id": "flutter",
"token_count": 1118
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RawAutocomplete].
void main() => runApp(const AutocompleteExampleApp());
class AutocompleteExampleApp extends StatelessWidget {
const AutocompleteExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('RawAutocomplete Form'),
),
body: const Center(
child: AutocompleteFormExample(),
),
),
);
}
}
class AutocompleteFormExample extends StatefulWidget {
const AutocompleteFormExample({super.key});
@override
AutocompleteFormExampleState createState() => AutocompleteFormExampleState();
}
class AutocompleteFormExampleState extends State<AutocompleteFormExample> {
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
final TextEditingController _textEditingController = TextEditingController();
String? _dropdownValue;
String? _autocompleteSelection;
static const List<String> _options = <String>[
'aardvark',
'bobcat',
'chameleon',
];
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Column(
children: <Widget>[
DropdownButtonFormField<String>(
value: _dropdownValue,
icon: const Icon(Icons.arrow_downward),
hint: const Text('This is a regular DropdownButtonFormField'),
elevation: 16,
style: const TextStyle(color: Colors.deepPurple),
onChanged: (String? newValue) {
setState(() {
_dropdownValue = newValue;
});
},
items: <String>['One', 'Two', 'Free', 'Four'].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
validator: (String? value) {
if (value == null) {
return 'Must make a selection.';
}
return null;
},
),
TextFormField(
controller: _textEditingController,
decoration: const InputDecoration(
hintText: 'This is a regular TextFormField',
),
validator: (String? value) {
if (value == null || value.isEmpty) {
return "Can't be empty.";
}
return null;
},
),
RawAutocomplete<String>(
optionsBuilder: (TextEditingValue textEditingValue) {
return _options.where((String option) {
return option.contains(textEditingValue.text.toLowerCase());
});
},
onSelected: (String selection) {
setState(() {
_autocompleteSelection = selection;
});
},
fieldViewBuilder: (
BuildContext context,
TextEditingController textEditingController,
FocusNode focusNode,
VoidCallback onFieldSubmitted,
) {
return TextFormField(
controller: textEditingController,
decoration: const InputDecoration(
hintText: 'This is a RawAutocomplete!',
),
focusNode: focusNode,
onFieldSubmitted: (String value) {
onFieldSubmitted();
},
validator: (String? value) {
if (!_options.contains(value)) {
return 'Nothing selected.';
}
return null;
},
);
},
optionsViewBuilder: (
BuildContext context,
AutocompleteOnSelected<String> onSelected,
Iterable<String> options,
) {
return Align(
alignment: Alignment.topLeft,
child: Material(
elevation: 4.0,
child: SizedBox(
height: 200.0,
child: ListView.builder(
padding: const EdgeInsets.all(8.0),
itemCount: options.length,
itemBuilder: (BuildContext context, int index) {
final String option = options.elementAt(index);
return GestureDetector(
onTap: () {
onSelected(option);
},
child: ListTile(
title: Text(option),
),
);
},
),
),
),
);
},
),
ElevatedButton(
onPressed: () {
FocusScope.of(context).unfocus();
if (!_formKey.currentState!.validate()) {
return;
}
showDialog<void>(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: const Text('Successfully submitted'),
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
Text('DropdownButtonFormField: "$_dropdownValue"'),
Text('TextFormField: "${_textEditingController.text}"'),
Text('RawAutocomplete: "$_autocompleteSelection"'),
],
),
),
actions: <Widget>[
TextButton(
child: const Text('Ok'),
onPressed: () {
Navigator.of(context).pop();
},
),
],
);
},
);
},
child: const Text('Submit'),
),
],
),
);
}
}
| flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.2.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/autocomplete/raw_autocomplete.2.dart",
"repo_id": "flutter",
"token_count": 3479
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [IndexedStack].
void main() => runApp(const IndexedStackApp());
class IndexedStackApp extends StatelessWidget {
const IndexedStackApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('IndexedStack Sample')),
body: const IndexedStackExample(),
),
);
}
}
class IndexedStackExample extends StatefulWidget {
const IndexedStackExample({super.key});
@override
State<IndexedStackExample> createState() => _IndexedStackExampleState();
}
class _IndexedStackExampleState extends State<IndexedStackExample> {
List<String> names = <String>['Dash', 'John', 'Mary'];
int index = 0;
final TextEditingController fieldText = TextEditingController();
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SizedBox(
width: 300,
child: TextField(
decoration: const InputDecoration(
border: OutlineInputBorder(),
hintText: 'Enter the name for a person to track',
),
onSubmitted: (String value) {
setState(() {
names.add(value);
});
fieldText.clear();
},
controller: fieldText,
),
),
const SizedBox(height: 50),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
GestureDetector(
onTap: () {
setState(() {
if (index == 0) {
index = names.length - 1;
} else {
index -= 1;
}
});
},
child: const Icon(Icons.chevron_left, key: Key('gesture1')),
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
IndexedStack(
index: index,
children: <Widget>[for (final String name in names) PersonTracker(name: name)],
)
],
),
GestureDetector(
onTap: () {
setState(() {
if (index == names.length - 1) {
index = 0;
} else {
index += 1;
}
});
},
child: const Icon(Icons.chevron_right, key: Key('gesture2')),
),
],
)
],
);
}
}
class PersonTracker extends StatefulWidget {
const PersonTracker({super.key, required this.name});
final String name;
@override
State<PersonTracker> createState() => _PersonTrackerState();
}
class _PersonTrackerState extends State<PersonTracker> {
int counter = 0;
@override
Widget build(BuildContext context) {
return Container(
key: Key(widget.name),
decoration: BoxDecoration(
color: const Color.fromARGB(255, 239, 248, 255),
border: Border.all(color: const Color.fromARGB(255, 54, 60, 244)),
borderRadius: const BorderRadius.all(Radius.circular(10)),
),
padding: const EdgeInsets.all(16.0),
child: Column(
children: <Widget>[
Text('Name: ${widget.name}'),
Text('Score: $counter'),
TextButton.icon(
key: Key('increment${widget.name}'),
icon: const Icon(Icons.add),
onPressed: () {
setState(() {
counter += 1;
});
},
label: const Text('Increment'),
)
],
),
);
}
}
| flutter/examples/api/lib/widgets/basic/indexed_stack.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/basic/indexed_stack.0.dart",
"repo_id": "flutter",
"token_count": 1930
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [TextEditingController].
void main() {
runApp(const TextEditingControllerExampleApp());
}
class TextEditingControllerExampleApp extends StatelessWidget {
const TextEditingControllerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: TextEditingControllerExample(),
);
}
}
class TextEditingControllerExample extends StatefulWidget {
const TextEditingControllerExample({super.key});
@override
State<TextEditingControllerExample> createState() => _TextEditingControllerExampleState();
}
class _TextEditingControllerExampleState extends State<TextEditingControllerExample> {
// Create a controller whose initial selection is empty (collapsed) and positioned
// before the text (offset is 0).
final TextEditingController _controller = TextEditingController.fromValue(
const TextEditingValue(
text: 'Flutter',
selection: TextSelection.collapsed(offset: 0),
),
);
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
alignment: Alignment.center,
padding: const EdgeInsets.all(16.0),
child: TextField(
controller: _controller,
autofocus: true,
decoration: const InputDecoration(filled: true),
),
),
);
}
}
| flutter/examples/api/lib/widgets/editable_text/text_editing_controller.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/editable_text/text_editing_controller.1.dart",
"repo_id": "flutter",
"token_count": 532
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
// TODO(gspencergoog): Delete this example when deprecated RawKeyEvent API is
// removed.
/// Flutter code sample for [KeyEventManager.keyMessageHandler].
void main() {
runApp(
const MaterialApp(
home: Scaffold(
body: Center(
child: FallbackDemo(),
),
),
),
);
}
class FallbackDemo extends StatefulWidget {
const FallbackDemo({super.key});
@override
State<StatefulWidget> createState() => FallbackDemoState();
}
class FallbackDemoState extends State<FallbackDemo> {
String? _capture;
late final FallbackFocusNode _node = FallbackFocusNode(
onKeyEvent: (KeyEvent event) {
if (event is! KeyDownEvent) {
return false;
}
setState(() {
_capture = event.logicalKey.keyLabel;
});
// TRY THIS: Change the return value to true. You will no longer be able
// to type text, because these key events will no longer be sent to the
// text input system.
return false;
},
);
@override
Widget build(BuildContext context) {
return FallbackFocus(
node: _node,
child: Container(
decoration: BoxDecoration(border: Border.all(color: Colors.red)),
padding: const EdgeInsets.all(10),
constraints: const BoxConstraints(maxWidth: 500, maxHeight: 400),
child: Column(
children: <Widget>[
const Text('This area handles key presses that are unhandled by any shortcuts, by '
'displaying them below. Try text shortcuts such as Ctrl-A!'),
Text(_capture == null ? '' : '$_capture is not handled by shortcuts.'),
const TextField(decoration: InputDecoration(label: Text('Text field 1'))),
Shortcuts(
shortcuts: <ShortcutActivator, Intent>{
const SingleActivator(LogicalKeyboardKey.keyQ): VoidCallbackIntent(() {}),
},
child: const TextField(
decoration: InputDecoration(
label: Text('This field also considers key Q as a shortcut (that does nothing).'),
),
),
),
],
),
),
);
}
}
/// A node used by [FallbackKeyEventRegistrar] to register fallback key handlers.
///
/// This class must not be replaced by bare [KeyEventCallback] because Dart
/// does not allow comparing with `==` on anonymous functions (always returns
/// false.)
class FallbackFocusNode {
FallbackFocusNode({required this.onKeyEvent});
final KeyEventCallback onKeyEvent;
}
/// A singleton class that allows [FallbackFocus] to register fallback key
/// event handlers.
///
/// This class is initialized when [instance] is first called, at which time it
/// patches [KeyEventManager.keyMessageHandler] with its own handler.
///
/// A global registrar like [FallbackKeyEventRegistrar] is almost always needed
/// when patching [KeyEventManager.keyMessageHandler]. This is because
/// [FallbackFocus] will add and remove callbacks constantly, but
/// [KeyEventManager.keyMessageHandler] can only be patched once, and can not
/// be unpatched. Therefore [FallbackFocus] must not directly interact with
/// [KeyEventManager.keyMessageHandler], but through a separate registrar that
/// handles listening reversibly.
class FallbackKeyEventRegistrar {
FallbackKeyEventRegistrar._();
static FallbackKeyEventRegistrar get instance {
if (!_initialized) {
// Get the global handler.
final KeyMessageHandler? existing = ServicesBinding.instance.keyEventManager.keyMessageHandler;
// The handler is guaranteed non-null since
// `FallbackKeyEventRegistrar.instance` is only called during
// `Focus.onFocusChange`, at which time `ServicesBinding.instance` must
// have been called somewhere.
assert(existing != null);
// Assign the global handler with a patched handler.
ServicesBinding.instance.keyEventManager.keyMessageHandler = _instance._buildHandler(existing!);
_initialized = true;
}
return _instance;
}
static bool _initialized = false;
static final FallbackKeyEventRegistrar _instance = FallbackKeyEventRegistrar._();
final List<FallbackFocusNode> _fallbackNodes = <FallbackFocusNode>[];
// Returns a handler that patches the existing `KeyEventManager.keyMessageHandler`.
//
// The existing `KeyEventManager.keyMessageHandler` is typically the one
// assigned by the shortcut system, but it can be anything. The returned
// handler calls that handler first, and if the event is not handled at all
// by the framework, invokes the innermost `FallbackNode`'s handler.
KeyMessageHandler _buildHandler(KeyMessageHandler existing) {
return (KeyMessage message) {
if (existing(message)) {
return true;
}
if (_fallbackNodes.isNotEmpty) {
for (final KeyEvent event in message.events) {
if (_fallbackNodes.last.onKeyEvent(event)) {
return true;
}
}
}
return false;
};
}
}
/// A widget that, when focused, handles key events only if no other handlers
/// do.
///
/// If a [FallbackFocus] is being focused on, then key events that are not
/// handled by other handlers will be dispatched to the `onKeyEvent` of [node].
/// If `onKeyEvent` returns true, this event is considered "handled" and will
/// not move forward with the text input system.
///
/// If multiple [FallbackFocus] nest, then only the innermost takes effect.
///
/// Internally, this class registers its node to the singleton
/// [FallbackKeyEventRegistrar]. The inner this widget is, the later its node
/// will be added to the registrar's list when focused on.
class FallbackFocus extends StatelessWidget {
const FallbackFocus({
super.key,
required this.node,
required this.child,
});
final Widget child;
final FallbackFocusNode node;
void _onFocusChange(bool focused) {
if (focused) {
FallbackKeyEventRegistrar.instance._fallbackNodes.add(node);
} else {
assert(FallbackKeyEventRegistrar.instance._fallbackNodes.last == node);
FallbackKeyEventRegistrar.instance._fallbackNodes.removeLast();
}
}
@override
Widget build(BuildContext context) {
return Focus(
onFocusChange: _onFocusChange,
child: child,
);
}
}
| flutter/examples/api/lib/widgets/hardware_keyboard/key_event_manager.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/hardware_keyboard/key_event_manager.0.dart",
"repo_id": "flutter",
"token_count": 2223
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [InteractiveViewer].
void main() => runApp(const InteractiveViewerExampleApp());
class InteractiveViewerExampleApp extends StatelessWidget {
const InteractiveViewerExampleApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('InteractiveViewer Sample')),
body: const InteractiveViewerExample(),
),
);
}
}
class InteractiveViewerExample extends StatelessWidget {
const InteractiveViewerExample({super.key});
@override
Widget build(BuildContext context) {
return Center(
child: InteractiveViewer(
boundaryMargin: const EdgeInsets.all(20.0),
minScale: 0.1,
maxScale: 1.6,
child: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
colors: <Color>[Colors.orange, Colors.red],
stops: <double>[0.0, 1.0],
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/interactive_viewer/interactive_viewer.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/interactive_viewer/interactive_viewer.0.dart",
"repo_id": "flutter",
"token_count": 514
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This sample demonstrates nested navigation in a bottom navigation bar.
import 'package:flutter/material.dart';
// There are three possible tabs.
enum _Tab {
home,
one,
two,
}
// Each tab has two possible pages.
enum _TabPage {
home,
one,
}
typedef _TabPageCallback = void Function(List<_TabPage> pages);
void main() => runApp(const NavigatorPopHandlerApp());
class NavigatorPopHandlerApp extends StatelessWidget {
const NavigatorPopHandlerApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
initialRoute: '/home',
routes: <String, WidgetBuilder>{
'/home': (BuildContext context) => const _BottomNavPage(
),
},
);
}
}
class _BottomNavPage extends StatefulWidget {
const _BottomNavPage();
@override
State<_BottomNavPage> createState() => _BottomNavPageState();
}
class _BottomNavPageState extends State<_BottomNavPage> {
_Tab _tab = _Tab.home;
final GlobalKey _tabHomeKey = GlobalKey();
final GlobalKey _tabOneKey = GlobalKey();
final GlobalKey _tabTwoKey = GlobalKey();
List<_TabPage> _tabHomePages = <_TabPage>[_TabPage.home];
List<_TabPage> _tabOnePages = <_TabPage>[_TabPage.home];
List<_TabPage> _tabTwoPages = <_TabPage>[_TabPage.home];
BottomNavigationBarItem _itemForPage(_Tab page) {
switch (page) {
case _Tab.home:
return const BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Go to Home',
);
case _Tab.one:
return const BottomNavigationBarItem(
icon: Icon(Icons.one_k),
label: 'Go to One',
);
case _Tab.two:
return const BottomNavigationBarItem(
icon: Icon(Icons.two_k),
label: 'Go to Two',
);
}
}
Widget _getPage(_Tab page) {
switch (page) {
case _Tab.home:
return _BottomNavTab(
key: _tabHomeKey,
title: 'Home Tab',
color: Colors.grey,
pages: _tabHomePages,
onChangedPages: (List<_TabPage> pages) {
setState(() {
_tabHomePages = pages;
});
},
);
case _Tab.one:
return _BottomNavTab(
key: _tabOneKey,
title: 'Tab One',
color: Colors.amber,
pages: _tabOnePages,
onChangedPages: (List<_TabPage> pages) {
setState(() {
_tabOnePages = pages;
});
},
);
case _Tab.two:
return _BottomNavTab(
key: _tabTwoKey,
title: 'Tab Two',
color: Colors.blueGrey,
pages: _tabTwoPages,
onChangedPages: (List<_TabPage> pages) {
setState(() {
_tabTwoPages = pages;
});
},
);
}
}
void _onItemTapped(int index) {
setState(() {
_tab = _Tab.values.elementAt(index);
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: _getPage(_tab),
),
bottomNavigationBar: BottomNavigationBar(
items: _Tab.values.map(_itemForPage).toList(),
currentIndex: _Tab.values.indexOf(_tab),
selectedItemColor: Colors.amber[800],
onTap: _onItemTapped,
),
);
}
}
class _BottomNavTab extends StatefulWidget {
const _BottomNavTab({
super.key,
required this.color,
required this.onChangedPages,
required this.pages,
required this.title,
});
final Color color;
final _TabPageCallback onChangedPages;
final List<_TabPage> pages;
final String title;
@override
State<_BottomNavTab> createState() => _BottomNavTabState();
}
class _BottomNavTabState extends State<_BottomNavTab> {
final GlobalKey<NavigatorState> _navigatorKey = GlobalKey<NavigatorState>();
@override
Widget build(BuildContext context) {
return NavigatorPopHandler(
onPop: () {
_navigatorKey.currentState?.maybePop();
},
child: Navigator(
key: _navigatorKey,
onPopPage: (Route<void> route, void result) {
if (!route.didPop(null)) {
return false;
}
widget.onChangedPages(<_TabPage>[
...widget.pages,
]..removeLast());
return true;
},
pages: widget.pages.map((_TabPage page) {
switch (page) {
case _TabPage.home:
return MaterialPage<void>(
child: _LinksPage(
title: 'Bottom nav - tab ${widget.title} - route $page',
backgroundColor: widget.color,
buttons: <Widget>[
TextButton(
onPressed: () {
widget.onChangedPages(<_TabPage>[
...widget.pages,
_TabPage.one,
]);
},
child: const Text('Go to another route in this nested Navigator'),
),
],
),
);
case _TabPage.one:
return MaterialPage<void>(
child: _LinksPage(
backgroundColor: widget.color,
title: 'Bottom nav - tab ${widget.title} - route $page',
buttons: <Widget>[
TextButton(
onPressed: () {
widget.onChangedPages(<_TabPage>[
...widget.pages,
]..removeLast());
},
child: const Text('Go back'),
),
],
),
);
}
}).toList(),
),
);
}
}
class _LinksPage extends StatelessWidget {
const _LinksPage ({
required this.backgroundColor,
this.buttons = const <Widget>[],
required this.title,
});
final Color backgroundColor;
final List<Widget> buttons;
final String title;
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: backgroundColor,
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(title),
...buttons,
],
),
),
);
}
}
| flutter/examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/navigator_pop_handler/navigator_pop_handler.1.dart",
"repo_id": "flutter",
"token_count": 3130
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [RestorationMixin].
void main() => runApp(const RestorationExampleApp());
class RestorationExampleApp extends StatelessWidget {
const RestorationExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
restorationScopeId: 'app',
title: 'Restorable Counter',
home: RestorableCounter(restorationId: 'counter'),
);
}
}
class RestorableCounter extends StatefulWidget {
const RestorableCounter({super.key, this.restorationId});
final String? restorationId;
@override
State<RestorableCounter> createState() => _RestorableCounterState();
}
// The [State] object uses the [RestorationMixin] to make the current value
// of the counter restorable.
class _RestorableCounterState extends State<RestorableCounter> with RestorationMixin {
// The current value of the counter is stored in a [RestorableProperty].
// During state restoration it is automatically restored to its old value.
// If no restoration data is available to restore the counter from, it is
// initialized to the specified default value of zero.
final RestorableInt _counter = RestorableInt(0);
// In this example, the restoration ID for the mixin is passed in through
// the [StatefulWidget]'s constructor.
@override
String? get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
// All restorable properties must be registered with the mixin. After
// registration, the counter either has its old value restored or is
// initialized to its default value.
registerForRestoration(_counter, 'count');
}
void _incrementCounter() {
setState(() {
// The current value of the property can be accessed and modified via
// the value getter and setter.
_counter.value++;
});
}
@override
void dispose() {
_counter.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Restorable Counter'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Text(
'${_counter.value}',
style: Theme.of(context).textTheme.headlineMedium,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/api/lib/widgets/restoration/restoration_mixin.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/restoration/restoration_mixin.0.dart",
"repo_id": "flutter",
"token_count": 973
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [Scrollbar].
void main() => runApp(const ScrollbarApp());
class ScrollbarApp extends StatelessWidget {
const ScrollbarApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Scrollbar Sample')),
body: const Center(
child: DesktopExample(),
),
),
);
}
}
class DesktopExample extends StatefulWidget {
const DesktopExample({super.key});
@override
State<DesktopExample> createState() => _DesktopExampleState();
}
class _DesktopExampleState extends State<DesktopExample> {
final ScrollController controller = ScrollController();
@override
Widget build(BuildContext context) {
return LayoutBuilder(builder: (BuildContext context, BoxConstraints constraints) {
return Row(
children: <Widget>[
SizedBox(
width: constraints.maxWidth / 2,
// When running this sample on desktop, two scrollbars will be
// visible here. One is the default scrollbar and the other is the
// Scrollbar widget with custom thickness.
child: Scrollbar(
thickness: 20.0,
thumbVisibility: true,
controller: controller,
child: ListView.builder(
controller: controller,
itemCount: 100,
itemBuilder: (BuildContext context, int index) {
return SizedBox(
height: 50,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Text('Scrollable 1 : Index $index'),
),
);
},
),
)),
SizedBox(
width: constraints.maxWidth / 2,
// When running this sample on desktop, one scrollbar will be
// visible here. The default scrollbar is hidden by setting the
// ScrollConfiguration's scrollbars to false. The Scrollbar widget
// with custom thickness is visible.
child: Scrollbar(
thickness: 20.0,
thumbVisibility: true,
child: ScrollConfiguration(
behavior: ScrollConfiguration.of(context).copyWith(scrollbars: false),
child: ListView.builder(
primary: true,
itemCount: 100,
itemBuilder: (BuildContext context, int index) {
return SizedBox(
height: 50,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Text('Scrollable 2 : Index $index'),
),
);
},
),
),
)),
],
);
});
}
}
| flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.desktop.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/scrollbar/raw_scrollbar.desktop.0.dart",
"repo_id": "flutter",
"token_count": 1559
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [SliverOpacity].
void main() => runApp(const SliverOpacityExampleApp());
class SliverOpacityExampleApp extends StatelessWidget {
const SliverOpacityExampleApp({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
home: SliverOpacityExample(),
);
}
}
class SliverOpacityExample extends StatefulWidget {
const SliverOpacityExample({super.key});
@override
State<SliverOpacityExample> createState() => _SliverOpacityExampleState();
}
class _SliverOpacityExampleState extends State<SliverOpacityExample> {
static const List<Widget> _listItems = <Widget>[
ListTile(title: Text('Now you see me,')),
ListTile(title: Text("Now you don't!")),
];
bool _visible = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('SliverOpacity demo'),
),
body: CustomScrollView(
slivers: <Widget>[
const SliverToBoxAdapter(
child: ListTile(title: Text('Press on the button to toggle the list visibility.')),
),
const SliverToBoxAdapter(
child: ListTile(title: Text('Before the list...')),
),
SliverOpacity(
opacity: _visible ? 1.0 : 0.0,
sliver: SliverList(
delegate: SliverChildListDelegate(_listItems),
),
),
const SliverToBoxAdapter(
child: ListTile(title: Text('Before the list...')),
),
],
),
floatingActionButton: FloatingActionButton(
child: const Icon(Icons.disabled_visible),
onPressed: () {
setState(() {
_visible = !_visible;
});
},
),
);
}
}
| flutter/examples/api/lib/widgets/sliver/sliver_opacity.1.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/sliver/sliver_opacity.1.dart",
"repo_id": "flutter",
"token_count": 801
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
/// Flutter code sample for [ListenableBuilder].
void main() => runApp(const ListenableBuilderExample());
/// This widget listens for changes in the focus state of the subtree defined by
/// its [child] widget, changing the border and color of the container it is in
/// when it has focus.
///
/// A [FocusListenerContainer] swaps out the [BorderSide] of a border around the
/// child widget with [focusedSide], and the background color with
/// [focusedColor], when a widget that is a descendant of this widget has focus.
class FocusListenerContainer extends StatefulWidget {
const FocusListenerContainer({
super.key,
this.border,
this.padding,
this.focusedSide,
this.focusedColor = Colors.black12,
required this.child,
});
/// This is the border that will be used when not focused, and which defines
/// all the attributes except for the [OutlinedBorder.side] when focused.
final OutlinedBorder? border;
/// This is the [BorderSide] that will be used for [border] when the [child]
/// subtree is focused.
final BorderSide? focusedSide;
/// This is the [Color] that will be used as the fill color for the background
/// of the [child] when a descendant widget is focused.
final Color? focusedColor;
/// The padding around the inside of the container.
final EdgeInsetsGeometry? padding;
/// This is defines the subtree to listen to for focus changes.
final Widget child;
@override
State<FocusListenerContainer> createState() => _FocusListenerContainerState();
}
class _FocusListenerContainerState extends State<FocusListenerContainer> {
final FocusNode _focusNode = FocusNode();
@override
void dispose() {
_focusNode.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final OutlinedBorder effectiveBorder = widget.border ?? const RoundedRectangleBorder();
return ListenableBuilder(
listenable: _focusNode,
child: Focus(
focusNode: _focusNode,
skipTraversal: true,
canRequestFocus: false,
child: widget.child,
),
builder: (BuildContext context, Widget? child) {
return Container(
padding: widget.padding,
decoration: ShapeDecoration(
color: _focusNode.hasFocus ? widget.focusedColor : null,
shape: effectiveBorder.copyWith(
side: _focusNode.hasFocus ? widget.focusedSide : null,
),
),
child: child,
);
},
);
}
}
class MyField extends StatefulWidget {
const MyField({super.key, required this.label});
final String label;
@override
State<MyField> createState() => _MyFieldState();
}
class _MyFieldState extends State<MyField> {
final TextEditingController controller = TextEditingController();
@override
Widget build(BuildContext context) {
return Row(
children: <Widget>[
Expanded(child: Text(widget.label)),
Expanded(
flex: 2,
child: TextField(
controller: controller,
onEditingComplete: () {
debugPrint('Field ${widget.label} changed to ${controller.value}');
},
),
),
],
);
}
}
class ListenableBuilderExample extends StatelessWidget {
const ListenableBuilderExample({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('ListenableBuilder Example')),
body: Center(
child: SizedBox(
width: 300,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Padding(
padding: EdgeInsets.only(bottom: 8),
child: MyField(label: 'Company'),
),
FocusListenerContainer(
padding: const EdgeInsets.all(8),
border: const RoundedRectangleBorder(
side: BorderSide(
strokeAlign: BorderSide.strokeAlignOutside,
),
borderRadius: BorderRadius.all(
Radius.circular(5),
),
),
// The border side will get wider when the subtree has focus.
focusedSide: const BorderSide(
width: 4,
strokeAlign: BorderSide.strokeAlignOutside,
),
// The container background will change color to this when
// the subtree has focus.
focusedColor: Colors.blue.shade50,
child: const Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text('Owner:'),
MyField(label: 'First Name'),
MyField(label: 'Last Name'),
],
),
),
],
),
),
),
),
),
);
}
}
| flutter/examples/api/lib/widgets/transitions/listenable_builder.0.dart/0 | {
"file_path": "flutter/examples/api/lib/widgets/transitions/listenable_builder.0.dart",
"repo_id": "flutter",
"token_count": 2328
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/animation/animation_controller/animated_digit.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('animated digit example', (WidgetTester tester) async {
await tester.pumpWidget(
const example.AnimatedDigitApp(),
);
Finder findVisibleDigit(int digit) {
return find.descendant(
of: find.byType(SlideTransition).last,
matching: find.text('$digit'),
);
}
expect(findVisibleDigit(0), findsOneWidget);
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
expect(findVisibleDigit(1), findsOneWidget);
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
expect(findVisibleDigit(2), findsOneWidget);
await tester.tap(find.byType(FloatingActionButton));
await tester.pump(const Duration(milliseconds: 100)); // Animation duration is 300ms
await tester.tap(find.byType(FloatingActionButton));
await tester.pumpAndSettle();
expect(findVisibleDigit(4), findsOneWidget);
});
}
| flutter/examples/api/test/animation/animation_controller/animated_digit.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/animation/animation_controller/animated_digit.0_test.dart",
"repo_id": "flutter",
"token_count": 473
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/picker/cupertino_picker.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
const Offset _kRowOffset = Offset(0.0, -50.0);
void main() {
testWidgets('Change selected fruit using CupertinoPicker', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CupertinoPickerApp(),
);
// Open the Cupertino picker.
await tester.tap(find.widgetWithText(CupertinoButton, 'Apple'));
await tester.pumpAndSettle();
// Test the initial item.
CupertinoPicker picker = tester.widget<CupertinoPicker>(find.byType(CupertinoPicker));
expect(picker.scrollController!.initialItem, 0);
// Drag the wheel to change fruit selection.
await tester.drag(find.text('Mango'), _kRowOffset, touchSlopY: 0, warnIfMissed: false); // see top of file
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
// Close the Cupertino picker.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(find.widgetWithText(CupertinoButton, 'Banana'), findsOneWidget);
// Test if the initial item has updated.
await tester.tap(find.widgetWithText(CupertinoButton, 'Banana'));
await tester.pumpAndSettle();
picker = tester.widget<CupertinoPicker>(find.byType(CupertinoPicker));
expect(picker.scrollController!.initialItem, 2);
});
}
| flutter/examples/api/test/cupertino/picker/cupertino_picker.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/picker/cupertino_picker.0_test.dart",
"repo_id": "flutter",
"token_count": 575
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter_api_samples/cupertino/text_field/cupertino_text_field.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('CupertinoTextField has initial text', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CupertinoTextFieldApp(),
);
expect(find.byType(CupertinoTextField), findsOneWidget);
expect(find.text('initial text'), findsOneWidget);
await tester.enterText(find.byType(CupertinoTextField), 'new text');
await tester.pump();
expect(find.text('new text'), findsOneWidget);
});
}
| flutter/examples/api/test/cupertino/text_field/cupertino_text_field.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/cupertino/text_field/cupertino_text_field.0_test.dart",
"repo_id": "flutter",
"token_count": 273
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/app_bar/sliver_app_bar.4.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
const Offset _kOffset = Offset(0.0, 200.0);
void main() {
testWidgets('SliverAppbar can be stretched', (WidgetTester tester) async {
await tester.pumpWidget(
const example.StretchableSliverAppBar(),
);
final Finder switchFinder = find.byType(Switch);
Switch materialSwitch = tester.widget<Switch>(switchFinder);
expect(materialSwitch.value, true);
expect(find.widgetWithText(SliverAppBar, 'SliverAppBar'), findsOneWidget);
expect(tester.getBottomLeft(find.text('SliverAppBar')).dy, 184.0);
await tester.drag(find.text('0'), _kOffset,
touchSlopY: 0, warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.widgetWithText(SliverAppBar, 'SliverAppBar'), findsOneWidget);
expect(
tester.getBottomLeft(find.text('SliverAppBar')).dy, 187.63506380825314);
await tester.tap(switchFinder);
await tester.pumpAndSettle();
materialSwitch = tester.widget<Switch>(switchFinder);
expect(materialSwitch.value, false);
await tester.drag(find.text('0'), _kOffset,
touchSlopY: 0, warnIfMissed: false);
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(find.text('SliverAppBar'), findsOneWidget);
expect(tester.getBottomLeft(find.text('SliverAppBar')).dy, 184.0);
});
}
| flutter/examples/api/test/material/app_bar/sliver_app_bar.4_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/app_bar/sliver_app_bar.4_test.dart",
"repo_id": "flutter",
"token_count": 628
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/checkbox/checkbox.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Checkbox can be checked', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CheckboxExampleApp(),
);
expect(find.byType(Checkbox), findsNWidgets(3));
Checkbox checkbox = tester.widget(find.byType(Checkbox).first);
Checkbox checkboxWithError = tester.widget(find.byType(Checkbox).at(1));
Checkbox checkboxDisabled = tester.widget(find.byType(Checkbox).last);
// Verify the initial state of the checkboxes.
expect(checkbox.value, isTrue);
expect(checkboxWithError.value, isTrue);
expect(checkboxDisabled.value, isTrue);
expect(checkboxWithError.isError, isTrue);
expect(checkboxDisabled.onChanged, null);
expect(checkbox.tristate, isTrue);
expect(checkboxWithError.tristate, isTrue);
expect(checkboxDisabled.tristate, isTrue);
// Tap the first Checkbox and verify the state change.
await tester.tap(find.byType(Checkbox).first);
await tester.pump();
checkbox = tester.widget(find.byType(Checkbox).first);
checkboxWithError = tester.widget(find.byType(Checkbox).at(1));
checkboxDisabled = tester.widget(find.byType(Checkbox).last);
expect(checkbox.value, isNull);
expect(checkboxWithError.value, isNull);
expect(checkboxDisabled.value, isNull);
// Tap the second Checkbox and verify the state change.
await tester.tap(find.byType(Checkbox).at(1));
await tester.pump();
checkbox = tester.widget(find.byType(Checkbox).first);
checkboxWithError = tester.widget(find.byType(Checkbox).at(1));
checkboxDisabled = tester.widget(find.byType(Checkbox).last);
expect(checkbox.value, isFalse);
expect(checkboxWithError.value, isFalse);
expect(checkboxDisabled.value, isFalse);
// Tap the third Checkbox and verify that should remain unchanged.
await tester.tap(find.byType(Checkbox).last);
await tester.pump();
checkbox = tester.widget(find.byType(Checkbox).first);
checkboxWithError = tester.widget(find.byType(Checkbox).at(1));
checkboxDisabled = tester.widget(find.byType(Checkbox).last);
expect(checkbox.value, isFalse);
expect(checkboxWithError.value, isFalse);
expect(checkboxDisabled.value, isFalse);
});
}
| flutter/examples/api/test/material/checkbox/checkbox.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/checkbox/checkbox.1_test.dart",
"repo_id": "flutter",
"token_count": 912
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/date_picker/date_picker_theme_day_shape.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('DatePickerThemeData.dayShape updates day selection shape decoration', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final OutlinedBorder dayShape = RoundedRectangleBorder(borderRadius: BorderRadius.circular(8.0));
const Color todayBackgroundColor = Colors.amber;
const Color todayForegroundColor = Colors.black;
const BorderSide todayBorder = BorderSide(width: 2);
await tester.pumpWidget(
const example.DatePickerApp(),
);
await tester.tap(find.text('Open Date Picker'));
await tester.pumpAndSettle();
ShapeDecoration dayShapeDecoration = tester.widget<DecoratedBox>(find.ancestor(
of: find.text('15'),
matching: find.byType(DecoratedBox),
)).decoration as ShapeDecoration;
// Test the current day shape decoration.
expect(dayShapeDecoration.color, todayBackgroundColor);
expect(dayShapeDecoration.shape, dayShape.copyWith(side: todayBorder.copyWith(color: todayForegroundColor)));
dayShapeDecoration = tester.widget<DecoratedBox>(find.ancestor(
of: find.text('20'),
matching: find.byType(DecoratedBox),
)).decoration as ShapeDecoration;
// Test the selected day shape decoration.
expect(dayShapeDecoration.color, theme.colorScheme.primary);
expect(dayShapeDecoration.shape, dayShape);
// Tap to select current day as the selected day.
await tester.tap(find.text('15'));
await tester.pumpAndSettle();
dayShapeDecoration = tester.widget<DecoratedBox>(find.ancestor(
of: find.text('15'),
matching: find.byType(DecoratedBox),
)).decoration as ShapeDecoration;
// Test the selected day shape decoration.
expect(dayShapeDecoration.color, todayBackgroundColor);
expect(dayShapeDecoration.shape, dayShape.copyWith(side: todayBorder.copyWith(color: todayForegroundColor)));
});
}
| flutter/examples/api/test/material/date_picker/date_picker_theme_day_shape.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/date_picker/date_picker_theme_day_shape.0_test.dart",
"repo_id": "flutter",
"token_count": 744
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/dropdown/dropdown_button.selected_item_builder.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Select an item from DropdownButton', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.DropdownButtonApp(),
),
),
);
expect(find.text('NYC'), findsOneWidget);
await tester.tap(find.text('NYC'));
await tester.pumpAndSettle();
await tester.tap(find.text('San Francisco').last);
await tester.pumpAndSettle();
expect(find.text('SF'), findsOneWidget);
});
}
| flutter/examples/api/test/material/dropdown/dropdown_button.selected_item_builder.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/dropdown/dropdown_button.selected_item_builder.0_test.dart",
"repo_id": "flutter",
"token_count": 319
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/icon_button/icon_button.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('IconButton Types', (WidgetTester tester) async {
await tester.pumpWidget(
const example.IconButtonApp(),
);
expect(find.widgetWithIcon(IconButton, Icons.filter_drama), findsNWidgets(8));
final Finder iconButtons = find.widgetWithIcon(IconButton, Icons.filter_drama);
for (int i = 0; i <= 3; i++) {
expect(tester.widget<IconButton>(iconButtons.at(i)).onPressed is VoidCallback, isTrue);
}
for (int i = 4; i <= 7; i++) {
expect(tester.widget<IconButton>(iconButtons.at(i)).onPressed, isNull);
}
});
}
| flutter/examples/api/test/material/icon_button/icon_button.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/icon_button/icon_button.2_test.dart",
"repo_id": "flutter",
"token_count": 333
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_api_samples/material/list_tile/list_tile.3.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('ListTile color properties respect Material state color', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ListTileApp(),
);
ListTile listTile = tester.widget(find.byType(ListTile));
// Enabled list tile uses black color for icon and headline.
expect(listTile.enabled, true);
expect(listTile.selected, false);
RenderParagraph headline = _getTextRenderObject(tester, 'Headline');
expect(headline.text.style!.color, Colors.black);
RichText icon = tester.widget(find.byType(RichText).at(0));
expect(icon.text.style!.color, Colors.black);
// Tap list tile to select it.
await tester.tap(find.byType(ListTile));
await tester.pumpAndSettle();
// Selected list tile uses green color for icon and headline.
listTile = tester.widget(find.byType(ListTile));
expect(listTile.enabled, true);
expect(listTile.selected, true);
headline = _getTextRenderObject(tester, 'Headline');
expect(headline.text.style!.color, Colors.green);
icon = tester.widget(find.byType(RichText).at(0));
expect(icon.text.style!.color, Colors.green);
// Tap switch to disable list tile.
await tester.tap(find.byType(Switch));
await tester.pumpAndSettle();
// Disabled list tile uses red color for icon and headline.
listTile = tester.widget(find.byType(ListTile));
expect(listTile.enabled, false);
expect(listTile.selected, true);
headline = _getTextRenderObject(tester, 'Headline');
expect(headline.text.style!.color, Colors.red);
icon = tester.widget(find.byType(RichText).at(0));
expect(icon.text.style!.color, Colors.red);
});
}
RenderParagraph _getTextRenderObject(WidgetTester tester, String text) {
return tester.renderObject(find.descendant(
of: find.byType(ListTile),
matching: find.text(text),
));
}
| flutter/examples/api/test/material/list_tile/list_tile.3_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/list_tile/list_tile.3_test.dart",
"repo_id": "flutter",
"token_count": 767
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/page_transitions_theme/page_transitions_theme.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('MaterialApp defines a custom PageTransitionsTheme', (WidgetTester tester) async {
await tester.pumpWidget(
const example.PageTransitionsThemeApp(),
);
final Finder homePage = find.byType(example.HomePage);
expect(homePage, findsOneWidget);
final PageTransitionsTheme theme = Theme.of(tester.element(homePage)).pageTransitionsTheme;
expect(theme.builders, isNotNull);
// Check defined page transitions builder for each platform.
for (final TargetPlatform platform in TargetPlatform.values) {
switch (platform) {
case TargetPlatform.iOS:
expect(theme.builders[platform], isA<CupertinoPageTransitionsBuilder>());
case TargetPlatform.linux:
expect(theme.builders[platform], isA<OpenUpwardsPageTransitionsBuilder>());
case TargetPlatform.macOS:
expect(theme.builders[platform], isA<FadeUpwardsPageTransitionsBuilder>());
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.windows:
expect(theme.builders[platform], isNull);
}
}
// Can navigate to the second page.
expect(find.text('To SecondPage'), findsOneWidget);
await tester.tap(find.text('To SecondPage'));
await tester.pumpAndSettle();
// Can navigate back to the home page.
expect(find.text('Back to HomePage'), findsOneWidget);
await tester.tap(find.text('Back to HomePage'));
await tester.pumpAndSettle();
expect(find.text('To SecondPage'), findsOneWidget);
});
}
| flutter/examples/api/test/material/page_transitions_theme/page_transitions_theme.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/page_transitions_theme/page_transitions_theme.0_test.dart",
"repo_id": "flutter",
"token_count": 653
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/radio_list_tile/radio_list_tile.toggleable.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('RadioListTile is toggleable', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RadioListTileApp(),
);
// Initially the third radio button is not selected.
Radio<int> radio = tester.widget(find.byType(Radio<int>).at(2));
expect(radio.value, 2);
expect(radio.groupValue, null);
// Tap the third radio button.
await tester.tap(find.text('Philip Schuyler'));
await tester.pumpAndSettle();
// The third radio button is now selected.
radio = tester.widget(find.byType(Radio<int>).at(2));
expect(radio.value, 2);
expect(radio.groupValue, 2);
// Tap the third radio button again.
await tester.tap(find.text('Philip Schuyler'));
await tester.pumpAndSettle();
// The third radio button is now unselected.
radio = tester.widget(find.byType(Radio<int>).at(2));
expect(radio.value, 2);
expect(radio.groupValue, null);
});
}
| flutter/examples/api/test/material/radio_list_tile/radio_list_tile.toggleable.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/radio_list_tile/radio_list_tile.toggleable.0_test.dart",
"repo_id": "flutter",
"token_count": 464
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/tabs/tab_bar.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Switch tabs in the TabBar', (WidgetTester tester) async {
const String primaryTabLabel1 = 'Flights';
const String primaryTabLabel2 = 'Trips';
const String primaryTabLabel3 = 'Explore';
const String secondaryTabLabel1 = 'Overview';
const String secondaryTabLabel2 = 'Specifications';
await tester.pumpWidget(
const example.TabBarApp(),
);
final TabBar primaryTabBar = tester.widget<TabBar>(find.byType(TabBar).last);
expect(primaryTabBar.tabs.length, 3);
final TabBar secondaryTabBar = tester.widget<TabBar>(find.byType(TabBar).first);
expect(secondaryTabBar.tabs.length, 2);
String tabBarViewText = '$primaryTabLabel2: $secondaryTabLabel1 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(primaryTabLabel1));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel1: $secondaryTabLabel1 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(secondaryTabLabel2));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel1: $secondaryTabLabel2 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(primaryTabLabel2));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel2: $secondaryTabLabel1 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(secondaryTabLabel2));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel2: $secondaryTabLabel2 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(primaryTabLabel3));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel3: $secondaryTabLabel1 tab';
expect(find.text(tabBarViewText), findsOneWidget);
await tester.tap(find.text(secondaryTabLabel2));
await tester.pumpAndSettle();
tabBarViewText = '$primaryTabLabel3: $secondaryTabLabel2 tab';
expect(find.text(tabBarViewText), findsOneWidget);
});
}
| flutter/examples/api/test/material/tabs/tab_bar.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/tabs/tab_bar.2_test.dart",
"repo_id": "flutter",
"token_count": 825
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/painting/gradient/linear_gradient.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('finds a gradient', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: example.MoodyGradient(),
),
);
expect(find.byType(example.MoodyGradient), findsOneWidget);
});
testWidgets('gradient matches golden', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const SizedBox(
width: 800,
height: 600,
child: RepaintBoundary(
child: example.MoodyGradient(),
),
),
),
);
await expectLater(
find.byType(example.MoodyGradient),
matchesGoldenFile('linear_gradient.0_test.png'),
);
});
}
| flutter/examples/api/test/painting/linear_gradient.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/painting/linear_gradient.0_test.dart",
"repo_id": "flutter",
"token_count": 443
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/aspect_ratio.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AspectRatio applies 16 / 9 aspect ratio on its child', (WidgetTester tester) async {
const double height = 100.0;
await tester.pumpWidget(
const example.AspectRatioApp(),
);
final Size parentContainer = tester.getSize(find.byType(Container).first);
expect(parentContainer.width, 800.0);
expect(parentContainer.height, height);
final Size childContainer = tester.getSize(find.byType(Container).last);
expect(childContainer.width, height * 16 / 9);
expect(childContainer.height, height);
});
}
| flutter/examples/api/test/widgets/basic/aspect_ratio.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/aspect_ratio.0_test.dart",
"repo_id": "flutter",
"token_count": 296
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/mouse_region.on_exit.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('MouseRegion update mouse hover with a delay', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(home: example.MouseRegionApp()),
);
expect(find.text('Not hovering'), findsOneWidget);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
await gesture.moveTo(tester.getCenter(find.byType(Container)));
await tester.pump();
expect(find.text('Hovering'), findsOneWidget);
await gesture.moveTo(Offset.zero);
await tester.pump(const Duration(seconds: 1));
expect(find.text('Not hovering'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/basic/mouse_region.on_exit.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/mouse_region.on_exit.1_test.dart",
"repo_id": "flutter",
"token_count": 352
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_api_samples/widgets/interactive_viewer/interactive_viewer.builder.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('has correct items on screen', (WidgetTester tester) async {
await tester.pumpWidget(
const example.IVBuilderExampleApp(),
);
final Finder positionedFinder = find.byType(Positioned);
final Finder zeroFinder = find.text('0 x 0');
final Finder nineFinder = find.text('0 x 9');
expect(positionedFinder, findsNWidgets(35));
expect(zeroFinder, findsOneWidget);
expect(nineFinder, findsNothing);
const Offset firstLocation = Offset(750.0, 100.0);
final TestGesture gesture = await tester.startGesture(firstLocation);
const Offset secondLocation = Offset(50.0, 100.0);
await gesture.moveTo(secondLocation);
await tester.pump();
expect(positionedFinder, findsNWidgets(42));
expect(nineFinder, findsOneWidget);
expect(zeroFinder, findsNothing);
});
}
| flutter/examples/api/test/widgets/interactive_viewer/interactive_viewer.builder.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/interactive_viewer/interactive_viewer.builder.0_test.dart",
"repo_id": "flutter",
"token_count": 413
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/scroll_position/scroll_controller_on_attach.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Can toggle between scroll notification types', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ScrollControllerDemo(),
);
expect(find.byType(CustomScrollView), findsOneWidget);
expect(find.text('Not Scrolling'), findsOneWidget);
Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
expect(appBarMaterial.color, Colors.redAccent[700]!.withOpacity(.85));
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.byType(CustomScrollView)));
await gesture.moveBy(const Offset(10.0, 10.0));
await tester.pump();
expect(find.text('Scrolling'), findsOneWidget);
appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
expect(appBarMaterial.color, Colors.green[800]!.withOpacity(.85));
await gesture.up();
await tester.pumpAndSettle();
expect(find.text('Not Scrolling'), findsOneWidget);
appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
});
}
| flutter/examples/api/test/widgets/scroll_position/scroll_controller_on_attach.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/scroll_position/scroll_controller_on_attach.0_test.dart",
"repo_id": "flutter",
"token_count": 613
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/transitions/listenable_builder.3.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Tapping FAB adds to values', (WidgetTester tester) async {
await tester.pumpWidget(const example.ListenableBuilderExample());
final Finder listContent = find.byWidgetPredicate((Widget widget) => widget is example.ListBody);
expect(find.text('Current values:'), findsOneWidget);
expect(find.byIcon(Icons.add), findsOneWidget);
expect(
(tester.widget(listContent) as example.ListBody).listNotifier.values.isEmpty,
isTrue,
);
await tester.tap(find.byType(FloatingActionButton).first);
await tester.pumpAndSettle();
expect(
(tester.widget(listContent) as example.ListBody).listNotifier.values.isEmpty,
isFalse,
);
expect(
(tester.widget(listContent) as example.ListBody).listNotifier.values,
<int>[1464685455],
);
expect(find.text('1464685455'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/transitions/listenable_builder.3_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/transitions/listenable_builder.3_test.dart",
"repo_id": "flutter",
"token_count": 432
} | 667 |
#include "Generated.xcconfig"
| flutter/examples/flutter_view/ios/Flutter/Release.xcconfig/0 | {
"file_path": "flutter/examples/flutter_view/ios/Flutter/Release.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const FlutterView());
}
class FlutterView extends StatelessWidget {
const FlutterView({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter View',
theme: ThemeData(
primarySwatch: Colors.grey,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
static const String _channel = 'increment';
static const String _pong = 'pong';
static const String _emptyMessage = '';
static const BasicMessageChannel<String?> platform =
BasicMessageChannel<String?>(_channel, StringCodec());
int _counter = 0;
@override
void initState() {
super.initState();
platform.setMessageHandler(_handlePlatformIncrement);
}
Future<String> _handlePlatformIncrement(String? message) async {
setState(() {
_counter++;
});
return _emptyMessage;
}
void _sendFlutterIncrement() {
platform.send(_pong);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: Center(
child: Text(
'Platform button tapped $_counter time${ _counter == 1 ? '' : 's' }.',
style: const TextStyle(fontSize: 17.0),
),
),
),
Container(
padding: const EdgeInsets.only(bottom: 15.0, left: 5.0),
child: Row(
children: <Widget>[
Image.asset('assets/flutter-mark-square-64.png', scale: 1.5),
const Text('Flutter', style: TextStyle(fontSize: 30.0)),
],
),
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: _sendFlutterIncrement,
child: const Icon(Icons.add),
),
);
}
}
| flutter/examples/flutter_view/lib/main.dart/0 | {
"file_path": "flutter/examples/flutter_view/lib/main.dart",
"repo_id": "flutter",
"token_count": 967
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This example shows how to use flex layout directly in the underlying render
// tree.
import 'package:flutter/rendering.dart';
import 'src/binding.dart';
import 'src/solid_color_box.dart';
void main() {
final RenderFlex table = RenderFlex(direction: Axis.vertical, textDirection: TextDirection.ltr);
void addAlignmentRow(CrossAxisAlignment crossAxisAlignment) {
TextStyle style = const TextStyle(color: Color(0xFF000000));
final RenderParagraph paragraph = RenderParagraph(
TextSpan(style: style, text: '$crossAxisAlignment'),
textDirection: TextDirection.ltr,
);
table.add(RenderPadding(child: paragraph, padding: const EdgeInsets.only(top: 20.0)));
final RenderFlex row = RenderFlex(crossAxisAlignment: crossAxisAlignment, textBaseline: TextBaseline.alphabetic, textDirection: TextDirection.ltr);
style = const TextStyle(fontSize: 15.0, color: Color(0xFF000000));
row.add(RenderDecoratedBox(
decoration: const BoxDecoration(color: Color(0x7FFFCCCC)),
child: RenderParagraph(
TextSpan(style: style, text: 'foo foo foo'),
textDirection: TextDirection.ltr,
),
));
style = const TextStyle(fontSize: 10.0, color: Color(0xFF000000));
row.add(RenderDecoratedBox(
decoration: const BoxDecoration(color: Color(0x7FCCFFCC)),
child: RenderParagraph(
TextSpan(style: style, text: 'foo foo foo'),
textDirection: TextDirection.ltr,
),
));
final RenderFlex subrow = RenderFlex(crossAxisAlignment: crossAxisAlignment, textBaseline: TextBaseline.alphabetic, textDirection: TextDirection.ltr);
style = const TextStyle(fontSize: 25.0, color: Color(0xFF000000));
subrow.add(RenderDecoratedBox(
decoration: const BoxDecoration(color: Color(0x7FCCCCFF)),
child: RenderParagraph(
TextSpan(style: style, text: 'foo foo foo foo'),
textDirection: TextDirection.ltr,
),
));
subrow.add(RenderSolidColorBox(const Color(0x7FCCFFFF), desiredSize: const Size(30.0, 40.0)));
row.add(subrow);
table.add(row);
final FlexParentData rowParentData = row.parentData! as FlexParentData;
rowParentData.flex = 1;
}
addAlignmentRow(CrossAxisAlignment.start);
addAlignmentRow(CrossAxisAlignment.end);
addAlignmentRow(CrossAxisAlignment.center);
addAlignmentRow(CrossAxisAlignment.stretch);
addAlignmentRow(CrossAxisAlignment.baseline);
void addJustificationRow(MainAxisAlignment justify) {
const TextStyle style = TextStyle(color: Color(0xFF000000));
final RenderParagraph paragraph = RenderParagraph(
TextSpan(style: style, text: '$justify'),
textDirection: TextDirection.ltr,
);
table.add(RenderPadding(child: paragraph, padding: const EdgeInsets.only(top: 20.0)));
final RenderFlex row = RenderFlex(textDirection: TextDirection.ltr);
row.add(RenderSolidColorBox(const Color(0xFFFFCCCC), desiredSize: const Size(80.0, 60.0)));
row.add(RenderSolidColorBox(const Color(0xFFCCFFCC), desiredSize: const Size(64.0, 60.0)));
row.add(RenderSolidColorBox(const Color(0xFFCCCCFF), desiredSize: const Size(160.0, 60.0)));
row.mainAxisAlignment = justify;
table.add(row);
final FlexParentData rowParentData = row.parentData! as FlexParentData;
rowParentData.flex = 1;
}
addJustificationRow(MainAxisAlignment.start);
addJustificationRow(MainAxisAlignment.end);
addJustificationRow(MainAxisAlignment.center);
addJustificationRow(MainAxisAlignment.spaceBetween);
addJustificationRow(MainAxisAlignment.spaceAround);
final RenderDecoratedBox root = RenderDecoratedBox(
decoration: const BoxDecoration(color: Color(0xFFFFFFFF)),
child: RenderPadding(child: table, padding: const EdgeInsets.symmetric(vertical: 50.0)),
);
ViewRenderingFlutterBinding(root: root).scheduleFrame();
}
| flutter/examples/layers/rendering/flex_layout.dart/0 | {
"file_path": "flutter/examples/layers/rendering/flex_layout.dart",
"repo_id": "flutter",
"token_count": 1430
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
import '../../../widgets/spinning_mixed.dart' as demo;
void main() {
test('layers smoketest for widgets/spinning_mixed.dart', () {
FlutterError.onError = (FlutterErrorDetails details) { throw details.exception; };
demo.main();
});
}
| flutter/examples/layers/test/smoketests/widgets/spinning_mixed_test.dart/0 | {
"file_path": "flutter/examples/layers/test/smoketests/widgets/spinning_mixed_test.dart",
"repo_id": "flutter",
"token_count": 164
} | 671 |
# Example of calling platform services from Flutter
This project demonstrates how to connect a Flutter app to platform-specific
services on iOS using Swift. The equivalent version of this project in
Objective C is found in examples/platform_channel.
You can read more about
[accessing platform and third-party services in Flutter](https://flutter.dev/platform-channels/).
## iOS
You can use the commands `flutter build` and `flutter run` from the app's root
directory to build/run the app or you can open `ios/Runner.xcworkspace` in Xcode
and build/run the project as usual.
## Android
We refer to the platform_channel project.
| flutter/examples/platform_channel_swift/README.md/0 | {
"file_path": "flutter/examples/platform_channel_swift/README.md",
"repo_id": "flutter",
"token_count": 163
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "my_application.h"
#include <flutter_linux/flutter_linux.h>
#ifdef GDK_WINDOWING_X11
#include <gdk/gdkx.h>
#endif
#include "flutter/generated_plugin_registrant.h"
struct _MyApplication {
GtkApplication parent_instance;
// Channel to receive platform view requests from Flutter.
FlMethodChannel* platform_view_channel;
// Main window.
GtkWindow* window;
// Current counter.
int64_t counter;
// Request in progress.
FlMethodCall* method_call;
// Native window requested by Flutter.
GtkWindow* native_window;
// Label to show count.
GtkLabel* counter_label;
};
G_DEFINE_TYPE(MyApplication, my_application, GTK_TYPE_APPLICATION)
static void update_counter_label(MyApplication* self) {
g_autofree gchar* text =
g_strdup_printf("Button tapped %" G_GINT64_FORMAT " %s.", self->counter,
self->counter == 1 ? "time" : "times");
gtk_label_set_text(self->counter_label, text);
}
static void button_clicked_cb(MyApplication* self) {
self->counter++;
update_counter_label(self);
}
static void native_window_delete_event_cb(MyApplication* self,
gint response_id) {
g_autoptr(FlValue) counter_value = fl_value_new_int(self->counter);
fl_method_call_respond_success(self->method_call, counter_value, nullptr);
g_clear_object(&self->method_call);
}
// Handle request to switch the view.
static void handle_switch_view(MyApplication* self, FlMethodCall* method_call) {
FlValue* counter_value = fl_method_call_get_args(method_call);
if (fl_value_get_type(counter_value) != FL_VALUE_TYPE_INT) {
fl_method_call_respond_error(self->method_call, "Invalid args",
"Invalid switchView args", nullptr, nullptr);
return;
}
self->counter = fl_value_get_int(counter_value);
self->method_call = FL_METHOD_CALL(g_object_ref(method_call));
// Show the same UI in a native window.
self->native_window = GTK_WINDOW(gtk_window_new(GTK_WINDOW_TOPLEVEL));
gtk_window_set_transient_for(self->native_window, self->window);
gtk_window_set_modal(self->native_window, TRUE);
gtk_window_set_destroy_with_parent(self->native_window, TRUE);
g_signal_connect_swapped(self->native_window, "delete-event",
G_CALLBACK(native_window_delete_event_cb), self);
GtkWidget* box = gtk_box_new(GTK_ORIENTATION_VERTICAL, 12);
gtk_widget_set_margin_start(box, 24);
gtk_widget_set_margin_end(box, 24);
gtk_widget_set_margin_top(box, 24);
gtk_widget_set_margin_bottom(box, 24);
gtk_widget_show(box);
gtk_container_add(GTK_CONTAINER(self->native_window), box);
self->counter_label = GTK_LABEL(gtk_label_new(""));
gtk_widget_show(GTK_WIDGET(self->counter_label));
gtk_container_add(GTK_CONTAINER(box), GTK_WIDGET(self->counter_label));
GtkWidget* button = gtk_button_new_with_label("+");
gtk_style_context_add_class(gtk_widget_get_style_context(GTK_WIDGET(button)),
"circular");
gtk_widget_set_halign(button, GTK_ALIGN_CENTER);
gtk_widget_show(button);
gtk_container_add(GTK_CONTAINER(box), button);
g_signal_connect_swapped(button, "clicked", G_CALLBACK(button_clicked_cb),
self);
update_counter_label(self);
gtk_window_present(self->native_window);
}
// Handle platform view requests from Flutter.
static void platform_view_channel_method_cb(FlMethodChannel* channel,
FlMethodCall* method_call,
gpointer user_data) {
MyApplication* self = MY_APPLICATION(user_data);
const char* name = fl_method_call_get_name(method_call);
if (g_str_equal(name, "switchView")) {
handle_switch_view(self, method_call);
} else {
fl_method_call_respond_not_implemented(method_call, nullptr);
}
}
// Implements GObject::dispose.
static void my_application_dispose(GObject* object) {
MyApplication* self = MY_APPLICATION(object);
g_clear_object(&self->platform_view_channel);
G_OBJECT_CLASS(my_application_parent_class)->dispose(object);
}
// Implements GApplication::activate.
static void my_application_activate(GApplication* application) {
MyApplication* self = MY_APPLICATION(application);
self->window =
GTK_WINDOW(gtk_application_window_new(GTK_APPLICATION(application)));
gtk_window_set_default_size(self->window, 1280, 720);
gtk_widget_show(GTK_WIDGET(self->window));
g_autoptr(FlDartProject) project = fl_dart_project_new();
FlView* view = fl_view_new(project);
gtk_widget_show(GTK_WIDGET(view));
gtk_container_add(GTK_CONTAINER(self->window), GTK_WIDGET(view));
// Create channel to handle platform view requests from Flutter.
FlEngine* engine = fl_view_get_engine(view);
g_autoptr(FlStandardMethodCodec) codec = fl_standard_method_codec_new();
self->platform_view_channel = fl_method_channel_new(
fl_engine_get_binary_messenger(engine),
"samples.flutter.io/platform_view", FL_METHOD_CODEC(codec));
fl_method_channel_set_method_call_handler(self->platform_view_channel,
platform_view_channel_method_cb,
self, nullptr);
fl_register_plugins(FL_PLUGIN_REGISTRY(view));
gtk_widget_grab_focus(GTK_WIDGET(view));
}
static void my_application_class_init(MyApplicationClass* klass) {
G_OBJECT_CLASS(klass)->dispose = my_application_dispose;
G_APPLICATION_CLASS(klass)->activate = my_application_activate;
}
static void my_application_init(MyApplication* self) {}
MyApplication* my_application_new() {
return MY_APPLICATION(g_object_new(my_application_get_type(),
"application-id", APPLICATION_ID, "flags",
G_APPLICATION_NON_UNIQUE, nullptr));
}
| flutter/examples/platform_view/linux/my_application.cc/0 | {
"file_path": "flutter/examples/platform_view/linux/my_application.cc",
"repo_id": "flutter",
"token_count": 2418
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef FLUTTER_MY_TEXTURE_H_
#define FLUTTER_MY_TEXTURE_H_
#include <flutter_linux/flutter_linux.h>
G_DECLARE_FINAL_TYPE(MyTexture, my_texture, MY, TEXTURE, FlPixelBufferTexture)
/**
* my_texture_new:
* @self: a #MyTexture.
* @width: width of texture in pixels.
* @height: height of texture in pixels.
* @r: red value for texture color.
* @g: green value for texture color.
* @b: blue value for texture color.
*
* Creates a new texture containing a single color for Flutter to render with.
*
* Returns: a new #MyTexture.
*/
MyTexture* my_texture_new(uint32_t width, uint32_t height, uint8_t r, uint8_t g,
uint8_t b);
/**
* my_texture_new:
* @self: a #MyTexture.
* @r: red value for texture color.
* @g: green value for texture color.
* @b: blue value for texture color.
*
* Sets the color the texture contains.
*/
void my_texture_set_color(MyTexture* self, uint8_t r, uint8_t g, uint8_t b);
#endif // FLUTTER_MY_TEXTURE_H_
| flutter/examples/texture/linux/my_texture.h/0 | {
"file_path": "flutter/examples/texture/linux/my_texture.h",
"repo_id": "flutter",
"token_count": 409
} | 674 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for AppBar from the Material library. *
# For fixes to
# * AppBarTheme: fix_app_bar_theme.yaml
# * ColorScheme: fix_color_scheme.yaml
# * Material (general): fix_material.yaml
# * SliverAppBar: fix_sliver_app_bar.yaml
# * TextTheme: fix_text_theme.yaml
# * ThemeData: fix_theme_data.yaml
# * WidgetState: fix_widget_state.yaml
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Remove 'backwardsCompatibility'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBar'
changes:
- kind: 'removeParameter'
name: 'backwardsCompatibility'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'toolbarTextStyle' & 'titleTextStyle'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBar'
changes:
- kind: 'addParameter'
index: 24
name: 'toolbarTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.bodyText2'
requiredIf: "textTheme != ''"
- kind: 'addParameter'
index: 25
name: 'titleTextStyle'
style: optional_named
argumentValue:
expression: '{% textTheme %}.headline6'
requiredIf: "textTheme != ''"
- kind: 'removeParameter'
name: 'textTheme'
variables:
textTheme:
kind: 'fragment'
value: 'arguments[textTheme]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'toolbarTextStyle'"
date: 2021-07-12
# TODO(Piinks): Add tests when `bulkApply:false` testing is supported, https://github.com/dart-lang/sdk/issues/44639
bulkApply: false
element:
uris: [ 'material.dart' ]
field: 'textTheme'
inClass: 'AppBar'
changes:
- kind: 'rename'
newName: 'toolbarTextStyle'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'titleTextStyle'"
date: 2021-07-12
# TODO(Piinks): Add tests when `bulkApply:false` testing is supported, https://github.com/dart-lang/sdk/issues/44639
bulkApply: false
element:
uris: [ 'material.dart' ]
field: 'textTheme'
inClass: 'AppBar'
changes:
- kind: 'rename'
newName: 'titleTextStyle'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'systemOverlayStyle'"
date: 2020-07-12
element:
uris: [ 'material.dart' ]
constructor: ''
inClass: 'AppBar'
oneOf:
- if: "brightness == 'Brightness.dark'"
changes:
- kind: 'addParameter'
index: 27
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.light'
requiredIf: "brightness == 'Brightness.dark'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
- if: "brightness == 'Brightness.light'"
changes:
- kind: 'addParameter'
index: 27
name: 'systemOverlayStyle'
style: optional_named
argumentValue:
expression: '{% SystemUiOverlayStyle %}.dark'
requiredIf: "brightness == 'Brightness.light'"
variables:
SystemUiOverlayStyle:
kind: 'import'
uris: [ 'services.dart' ]
name: 'SystemUiOverlayStyle'
- kind: 'removeParameter'
name: 'brightness'
variables:
brightness:
kind: 'fragment'
value: 'arguments[brightness]'
# Changes made in https://github.com/flutter/flutter/pull/86198
- title: "Migrate to 'systemOverlayStyle'"
date: 2021-07-12
element:
uris: [ 'material.dart' ]
field: 'brightness'
inClass: 'AppBar'
changes:
- kind: 'rename'
newName: 'systemOverlayStyle'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_material/fix_app_bar.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_material/fix_app_bar.yaml",
"repo_id": "flutter",
"token_count": 2111
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'curves.dart';
import 'tween.dart';
/// Used to override the default parameters of an animation.
///
/// Currently, this class is used by the following widgets:
/// - [ExpansionTile]
/// - [MaterialApp]
/// - [PopupMenuButton]
///
/// If [duration] and [reverseDuration] are set to [Duration.zero], the
/// corresponding animation will be disabled.
///
/// All of the parameters are optional. If no parameters are specified,
/// the default animation will be used.
@immutable
class AnimationStyle with Diagnosticable {
/// Creates an instance of Animation Style class.
AnimationStyle({
this.curve,
this.duration,
this.reverseCurve,
this.reverseDuration,
});
/// Creates an instance of Animation Style class with no animation.
static AnimationStyle noAnimation = AnimationStyle(
duration: Duration.zero,
reverseDuration: Duration.zero,
);
/// When specified, the animation will use this curve.
final Curve? curve;
/// When specified, the animation will use this duration.
final Duration? duration;
/// When specified, the reverse animation will use this curve.
final Curve? reverseCurve;
/// When specified, the reverse animation will use this duration.
final Duration? reverseDuration;
/// Creates a new [AnimationStyle] based on the current selection, with the
/// provided parameters overridden.
AnimationStyle copyWith({
final Curve? curve,
final Duration? duration,
final Curve? reverseCurve,
final Duration? reverseDuration,
}) {
return AnimationStyle(
curve: curve ?? this.curve,
duration: duration ?? this.duration,
reverseCurve: reverseCurve ?? this.reverseCurve,
reverseDuration: reverseDuration ?? this.reverseDuration,
);
}
/// Linearly interpolate between two animation styles.
static AnimationStyle? lerp(AnimationStyle? a, AnimationStyle? b, double t) {
if (identical(a, b)) {
return a;
}
return AnimationStyle(
curve: t < 0.5 ? a?.curve : b?.curve,
duration: t < 0.5 ? a?.duration : b?.duration,
reverseCurve: t < 0.5 ? a?.reverseCurve : b?.reverseCurve,
reverseDuration: t < 0.5 ? a?.reverseDuration : b?.reverseDuration,
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is AnimationStyle
&& other.curve == curve
&& other.duration == duration
&& other.reverseCurve == reverseCurve
&& other.reverseDuration == reverseDuration;
}
@override
int get hashCode => Object.hash(
curve,
duration,
reverseCurve,
reverseDuration,
);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Curve>('curve', curve, defaultValue: null));
properties.add(DiagnosticsProperty<Duration>('duration', duration, defaultValue: null));
properties.add(DiagnosticsProperty<Curve>('reverseCurve', reverseCurve, defaultValue: null));
properties.add(DiagnosticsProperty<Duration>('reverseDuration', reverseDuration, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/animation/animation_style.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/animation/animation_style.dart",
"repo_id": "flutter",
"token_count": 1067
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/scheduler.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'localizations.dart';
import 'picker.dart';
import 'theme.dart';
// Values derived from https://developer.apple.com/design/resources/ and on iOS
// simulators with "Debug View Hierarchy".
const double _kItemExtent = 32.0;
// From the picker's intrinsic content size constraint.
const double _kPickerWidth = 320.0;
const double _kPickerHeight = 216.0;
const bool _kUseMagnifier = true;
const double _kMagnification = 2.35/2.1;
const double _kDatePickerPadSize = 12.0;
// The density of a date picker is different from a generic picker.
// Eyeballed from iOS.
const double _kSqueeze = 1.25;
const TextStyle _kDefaultPickerTextStyle = TextStyle(
letterSpacing: -0.83,
);
// The item height is 32 and the magnifier height is 34, from
// iOS simulators with "Debug View Hierarchy".
// And the magnified fontSize by [_kTimerPickerMagnification] conforms to the
// iOS 14 native style by eyeball test.
const double _kTimerPickerMagnification = 34 / 32;
// Minimum horizontal padding between [CupertinoTimerPicker]
//
// It shouldn't actually be hard-coded for direct use, and the perfect solution
// should be to calculate the values that match the magnified values by
// offAxisFraction and _kSqueeze.
// Such calculations are complex, so we'll hard-code them for now.
const double _kTimerPickerMinHorizontalPadding = 30;
// Half of the horizontal padding value between the timer picker's columns.
const double _kTimerPickerHalfColumnPadding = 4;
// The horizontal padding between the timer picker's number label and its
// corresponding unit label.
const double _kTimerPickerLabelPadSize = 6;
const double _kTimerPickerLabelFontSize = 17.0;
// The width of each column of the countdown time picker.
const double _kTimerPickerColumnIntrinsicWidth = 106;
TextStyle _themeTextStyle(BuildContext context, { bool isValid = true }) {
final TextStyle style = CupertinoTheme.of(context).textTheme.dateTimePickerTextStyle;
return isValid
? style.copyWith(color: CupertinoDynamicColor.maybeResolve(style.color, context))
: style.copyWith(color: CupertinoDynamicColor.resolve(CupertinoColors.inactiveGray, context));
}
void _animateColumnControllerToItem(FixedExtentScrollController controller, int targetItem) {
controller.animateToItem(
targetItem,
curve: Curves.easeInOut,
duration: const Duration(milliseconds: 200),
);
}
const Widget _startSelectionOverlay = CupertinoPickerDefaultSelectionOverlay(capEndEdge: false);
const Widget _centerSelectionOverlay = CupertinoPickerDefaultSelectionOverlay(capStartEdge: false, capEndEdge: false);
const Widget _endSelectionOverlay = CupertinoPickerDefaultSelectionOverlay(capStartEdge: false);
// Lays out the date picker based on how much space each single column needs.
//
// Each column is a child of this delegate, indexed from 0 to number of columns - 1.
// Each column will be padded horizontally by 12.0 both left and right.
//
// The picker will be placed in the center, and the leftmost and rightmost
// column will be extended equally to the remaining width.
class _DatePickerLayoutDelegate extends MultiChildLayoutDelegate {
_DatePickerLayoutDelegate({
required this.columnWidths,
required this.textDirectionFactor,
required this.maxWidth,
});
// The list containing widths of all columns.
final List<double> columnWidths;
// textDirectionFactor is 1 if text is written left to right, and -1 if right to left.
final int textDirectionFactor;
// The max width the children should reach to avoid bending outwards.
final double maxWidth;
@override
void performLayout(Size size) {
double remainingWidth = maxWidth < size.width ? maxWidth : size.width;
double currentHorizontalOffset = (size.width - remainingWidth) / 2;
for (int i = 0; i < columnWidths.length; i++) {
remainingWidth -= columnWidths[i] + _kDatePickerPadSize * 2;
}
for (int i = 0; i < columnWidths.length; i++) {
final int index = textDirectionFactor == 1 ? i : columnWidths.length - i - 1;
double childWidth = columnWidths[index] + _kDatePickerPadSize * 2;
if (index == 0 || index == columnWidths.length - 1) {
childWidth += remainingWidth / 2;
}
// We can't actually assert here because it would break things badly for
// semantics, which will expect that we laid things out here.
assert(() {
if (childWidth < 0) {
FlutterError.reportError(
FlutterErrorDetails(
exception: FlutterError(
'Insufficient horizontal space to render the '
'CupertinoDatePicker because the parent is too narrow at '
'${size.width}px.\n'
'An additional ${-remainingWidth}px is needed to avoid '
'overlapping columns.',
),
),
);
}
return true;
}());
layoutChild(index, BoxConstraints.tight(Size(math.max(0.0, childWidth), size.height)));
positionChild(index, Offset(currentHorizontalOffset, 0.0));
currentHorizontalOffset += childWidth;
}
}
@override
bool shouldRelayout(_DatePickerLayoutDelegate oldDelegate) {
return columnWidths != oldDelegate.columnWidths
|| textDirectionFactor != oldDelegate.textDirectionFactor;
}
}
/// Different display modes of [CupertinoDatePicker].
///
/// See also:
///
/// * [CupertinoDatePicker], the class that implements different display modes
/// of the iOS-style date picker.
/// * [CupertinoPicker], the class that implements a content agnostic spinner UI.
enum CupertinoDatePickerMode {
/// Mode that shows the date in hour, minute, and (optional) an AM/PM designation.
/// The AM/PM designation is shown only if [CupertinoDatePicker] does not use 24h format.
/// Column order is subject to internationalization.
///
/// Example: ` 4 | 14 | PM `.
time,
/// Mode that shows the date in month, day of month, and year.
/// Name of month is spelled in full.
/// Column order is subject to internationalization.
///
/// Example: ` July | 13 | 2012 `.
date,
/// Mode that shows the date as day of the week, month, day of month and
/// the time in hour, minute, and (optional) an AM/PM designation.
/// The AM/PM designation is shown only if [CupertinoDatePicker] does not use 24h format.
/// Column order is subject to internationalization.
///
/// Example: ` Fri Jul 13 | 4 | 14 | PM `
dateAndTime,
/// Mode that shows the date in month and year.
/// Name of month is spelled in full.
/// Column order is subject to internationalization.
///
/// Example: ` July | 2012 `.
monthYear,
}
// Different types of column in CupertinoDatePicker.
enum _PickerColumnType {
// Day of month column in date mode.
dayOfMonth,
// Month column in date mode.
month,
// Year column in date mode.
year,
// Medium date column in dateAndTime mode.
date,
// Hour column in time and dateAndTime mode.
hour,
// minute column in time and dateAndTime mode.
minute,
// AM/PM column in time and dateAndTime mode.
dayPeriod,
}
/// A date picker widget in iOS style.
///
/// There are several modes of the date picker listed in [CupertinoDatePickerMode].
///
/// The class will display its children as consecutive columns. Its children
/// order is based on internationalization, or the [dateOrder] property if specified.
///
/// Example of the picker in date mode:
///
/// * US-English: `| July | 13 | 2012 |`
/// * Vietnamese: `| 13 | ThΓ‘ng 7 | 2012 |`
///
/// Can be used with [showCupertinoModalPopup] to display the picker modally at
/// the bottom of the screen.
///
/// Sizes itself to its parent and may not render correctly if not given the
/// full screen width. Content texts are shown with
/// [CupertinoTextThemeData.dateTimePickerTextStyle].
///
/// {@tool dartpad}
/// This sample shows how to implement CupertinoDatePicker with different picker modes.
/// We can provide initial dateTime value for the picker to display. When user changes
/// the drag the date or time wheels, the picker will call onDateTimeChanged callback.
///
/// CupertinoDatePicker can be displayed directly on a screen or in a popup.
///
/// ** See code in examples/api/lib/cupertino/date_picker/cupertino_date_picker.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoTimerPicker], the class that implements the iOS-style timer picker.
/// * [CupertinoPicker], the class that implements a content agnostic spinner UI.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/controls/pickers/>
class CupertinoDatePicker extends StatefulWidget {
/// Constructs an iOS style date picker.
///
/// [mode] is one of the mode listed in [CupertinoDatePickerMode] and defaults
/// to [CupertinoDatePickerMode.dateAndTime].
///
/// [onDateTimeChanged] is the callback called when the selected date or time
/// changes. When in [CupertinoDatePickerMode.time] mode, the year, month and
/// day will be the same as [initialDateTime]. When in
/// [CupertinoDatePickerMode.date] mode, this callback will always report the
/// start time of the currently selected day. When in
/// [CupertinoDatePickerMode.monthYear] mode, the day and time will be the
/// start time of the first day of the month.
///
/// [initialDateTime] is the initial date time of the picker. Defaults to the
/// present date and time. The present must conform to the intervals set in
/// [minimumDate], [maximumDate], [minimumYear], and [maximumYear].
///
/// [minimumDate] is the minimum selectable [DateTime] of the picker. When set
/// to null, the picker does not limit the minimum [DateTime] the user can pick.
/// In [CupertinoDatePickerMode.time] mode, [minimumDate] should typically be
/// on the same date as [initialDateTime], as the picker will not limit the
/// minimum time the user can pick if it's set to a date earlier than that.
///
/// [maximumDate] is the maximum selectable [DateTime] of the picker. When set
/// to null, the picker does not limit the maximum [DateTime] the user can pick.
/// In [CupertinoDatePickerMode.time] mode, [maximumDate] should typically be
/// on the same date as [initialDateTime], as the picker will not limit the
/// maximum time the user can pick if it's set to a date later than that.
///
/// [minimumYear] is the minimum year that the picker can be scrolled to in
/// [CupertinoDatePickerMode.date] mode. Defaults to 1.
///
/// [maximumYear] is the maximum year that the picker can be scrolled to in
/// [CupertinoDatePickerMode.date] mode. Null if there's no limit.
///
/// [minuteInterval] is the granularity of the minute spinner. Must be a
/// positive integer factor of 60.
///
/// [use24hFormat] decides whether 24 hour format is used. Defaults to false.
///
/// [dateOrder] determines the order of the columns inside [CupertinoDatePicker]
/// in [CupertinoDatePickerMode.date] and [CupertinoDatePickerMode.monthYear]
/// mode. When using monthYear mode, both [DatePickerDateOrder.dmy] and
/// [DatePickerDateOrder.mdy] will result in the month|year order.
/// Defaults to the locale's default date format/order.
CupertinoDatePicker({
super.key,
this.mode = CupertinoDatePickerMode.dateAndTime,
required this.onDateTimeChanged,
DateTime? initialDateTime,
this.minimumDate,
this.maximumDate,
this.minimumYear = 1,
this.maximumYear,
this.minuteInterval = 1,
this.use24hFormat = false,
this.dateOrder,
this.backgroundColor,
this.showDayOfWeek = false,
this.itemExtent = _kItemExtent,
}) : initialDateTime = initialDateTime ?? DateTime.now(),
assert(
itemExtent > 0,
'item extent should be greater than 0',
),
assert(
minuteInterval > 0 && 60 % minuteInterval == 0,
'minute interval is not a positive integer factor of 60',
) {
assert(
mode != CupertinoDatePickerMode.dateAndTime || minimumDate == null || !this.initialDateTime.isBefore(minimumDate!),
'initial date is before minimum date',
);
assert(
mode != CupertinoDatePickerMode.dateAndTime || maximumDate == null || !this.initialDateTime.isAfter(maximumDate!),
'initial date is after maximum date',
);
assert(
(mode != CupertinoDatePickerMode.date && mode != CupertinoDatePickerMode.monthYear) || (minimumYear >= 1 && this.initialDateTime.year >= minimumYear),
'initial year is not greater than minimum year, or minimum year is not positive',
);
assert(
(mode != CupertinoDatePickerMode.date && mode != CupertinoDatePickerMode.monthYear) || maximumYear == null || this.initialDateTime.year <= maximumYear!,
'initial year is not smaller than maximum year',
);
assert(
(mode != CupertinoDatePickerMode.date && mode != CupertinoDatePickerMode.monthYear) || minimumDate == null || !minimumDate!.isAfter(this.initialDateTime),
'initial date ${this.initialDateTime} is not greater than or equal to minimumDate $minimumDate',
);
assert(
(mode != CupertinoDatePickerMode.date && mode != CupertinoDatePickerMode.monthYear) || maximumDate == null || !maximumDate!.isBefore(this.initialDateTime),
'initial date ${this.initialDateTime} is not less than or equal to maximumDate $maximumDate',
);
assert(
this.initialDateTime.minute % minuteInterval == 0,
'initial minute is not divisible by minute interval',
);
}
/// The mode of the date picker as one of [CupertinoDatePickerMode]. Defaults
/// to [CupertinoDatePickerMode.dateAndTime]. Value cannot change after
/// initial build.
final CupertinoDatePickerMode mode;
/// The initial date and/or time of the picker. Defaults to the present date
/// and time. The present must conform to the intervals set in [minimumDate],
/// [maximumDate], [minimumYear], and [maximumYear].
///
/// Changing this value after the initial build will not affect the currently
/// selected date time.
final DateTime initialDateTime;
/// The minimum selectable date that the picker can settle on.
///
/// When non-null, the user can still scroll the picker to [DateTime]s earlier
/// than [minimumDate], but the [onDateTimeChanged] will not be called on
/// these [DateTime]s. Once let go, the picker will scroll back to [minimumDate].
///
/// In [CupertinoDatePickerMode.time] mode, a time becomes unselectable if the
/// [DateTime] produced by combining that particular time and the date part of
/// [initialDateTime] is earlier than [minimumDate]. So typically [minimumDate]
/// needs to be set to a [DateTime] that is on the same date as [initialDateTime].
///
/// Defaults to null. When set to null, the picker does not impose a limit on
/// the earliest [DateTime] the user can select.
final DateTime? minimumDate;
/// The maximum selectable date that the picker can settle on.
///
/// When non-null, the user can still scroll the picker to [DateTime]s later
/// than [maximumDate], but the [onDateTimeChanged] will not be called on
/// these [DateTime]s. Once let go, the picker will scroll back to [maximumDate].
///
/// In [CupertinoDatePickerMode.time] mode, a time becomes unselectable if the
/// [DateTime] produced by combining that particular time and the date part of
/// [initialDateTime] is later than [maximumDate]. So typically [maximumDate]
/// needs to be set to a [DateTime] that is on the same date as [initialDateTime].
///
/// Defaults to null. When set to null, the picker does not impose a limit on
/// the latest [DateTime] the user can select.
final DateTime? maximumDate;
/// Minimum year that the picker can be scrolled to in
/// [CupertinoDatePickerMode.date] mode. Defaults to 1.
final int minimumYear;
/// Maximum year that the picker can be scrolled to in
/// [CupertinoDatePickerMode.date] mode. Null if there's no limit.
final int? maximumYear;
/// The granularity of the minutes spinner, if it is shown in the current mode.
/// Must be an integer factor of 60.
final int minuteInterval;
/// Whether to use 24 hour format. Defaults to false.
final bool use24hFormat;
/// Determines the order of the columns inside [CupertinoDatePicker] in
/// [CupertinoDatePickerMode.date] and [CupertinoDatePickerMode.monthYear]
/// mode. When using monthYear mode, both [DatePickerDateOrder.dmy] and
/// [DatePickerDateOrder.mdy] will result in the month|year order.
/// Defaults to the locale's default date format/order.
final DatePickerDateOrder? dateOrder;
/// Callback called when the selected date and/or time changes. If the new
/// selected [DateTime] is not valid, or is not in the [minimumDate] through
/// [maximumDate] range, this callback will not be called.
final ValueChanged<DateTime> onDateTimeChanged;
/// Background color of date picker.
///
/// Defaults to null, which disables background painting entirely.
final Color? backgroundColor;
/// Whether to show day of week alongside day. Defaults to false.
final bool showDayOfWeek;
/// {@macro flutter.cupertino.picker.itemExtent}
///
/// Defaults to a value that matches the default iOS date picker wheel.
final double itemExtent;
@override
State<StatefulWidget> createState() {
// ignore: no_logic_in_create_state, https://github.com/flutter/flutter/issues/70499
return switch (mode) {
// The `time` mode and `dateAndTime` mode of the picker share the time
// columns, so they are placed together to one state.
// The `date` mode has different children and is implemented in a different
// state.
CupertinoDatePickerMode.time => _CupertinoDatePickerDateTimeState(),
CupertinoDatePickerMode.dateAndTime => _CupertinoDatePickerDateTimeState(),
CupertinoDatePickerMode.date => _CupertinoDatePickerDateState(dateOrder: dateOrder),
CupertinoDatePickerMode.monthYear => _CupertinoDatePickerMonthYearState(dateOrder: dateOrder),
};
}
// Estimate the minimum width that each column needs to layout its content.
static double _getColumnWidth(
_PickerColumnType columnType,
CupertinoLocalizations localizations,
BuildContext context,
bool showDayOfWeek, {
bool standaloneMonth = false,
}) {
String longestText = '';
switch (columnType) {
case _PickerColumnType.date:
// Measuring the length of all possible date is impossible, so here
// just some dates are measured.
for (int i = 1; i <= 12; i++) {
// An arbitrary date.
final String date =
localizations.datePickerMediumDate(DateTime(2018, i, 25));
if (longestText.length < date.length) {
longestText = date;
}
}
case _PickerColumnType.hour:
for (int i = 0; i < 24; i++) {
final String hour = localizations.datePickerHour(i);
if (longestText.length < hour.length) {
longestText = hour;
}
}
case _PickerColumnType.minute:
for (int i = 0; i < 60; i++) {
final String minute = localizations.datePickerMinute(i);
if (longestText.length < minute.length) {
longestText = minute;
}
}
case _PickerColumnType.dayPeriod:
longestText =
localizations.anteMeridiemAbbreviation.length > localizations.postMeridiemAbbreviation.length
? localizations.anteMeridiemAbbreviation
: localizations.postMeridiemAbbreviation;
case _PickerColumnType.dayOfMonth:
int longestDayOfMonth = 1;
for (int i = 1; i <=31; i++) {
final String dayOfMonth = localizations.datePickerDayOfMonth(i);
if (longestText.length < dayOfMonth.length) {
longestText = dayOfMonth;
longestDayOfMonth = i;
}
}
if (showDayOfWeek) {
for (int wd = 1; wd < DateTime.daysPerWeek; wd++) {
final String dayOfMonth = localizations.datePickerDayOfMonth(longestDayOfMonth, wd);
if (longestText.length < dayOfMonth.length) {
longestText = dayOfMonth;
}
}
}
case _PickerColumnType.month:
for (int i = 1; i <= 12; i++) {
final String month = standaloneMonth
? localizations.datePickerStandaloneMonth(i)
: localizations.datePickerMonth(i);
if (longestText.length < month.length) {
longestText = month;
}
}
case _PickerColumnType.year:
longestText = localizations.datePickerYear(2018);
}
assert(longestText != '', 'column type is not appropriate');
return TextPainter.computeMaxIntrinsicWidth(
text: TextSpan(
style: _themeTextStyle(context),
text: longestText,
),
textDirection: Directionality.of(context),
);
}
}
typedef _ColumnBuilder = Widget Function(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay);
class _CupertinoDatePickerDateTimeState extends State<CupertinoDatePicker> {
// Fraction of the farthest column's vanishing point vs its width. Eyeballed
// vs iOS.
static const double _kMaximumOffAxisFraction = 0.45;
late int textDirectionFactor;
late CupertinoLocalizations localizations;
// Alignment based on text direction. The variable name is self descriptive,
// however, when text direction is rtl, alignment is reversed.
late Alignment alignCenterLeft;
late Alignment alignCenterRight;
// Read this out when the state is initially created. Changes in initialDateTime
// in the widget after first build is ignored.
late DateTime initialDateTime;
// The difference in days between the initial date and the currently selected date.
// 0 if the current mode does not involve a date.
int get selectedDayFromInitial {
switch (widget.mode) {
case CupertinoDatePickerMode.dateAndTime:
return dateController.hasClients ? dateController.selectedItem : 0;
case CupertinoDatePickerMode.time:
return 0;
case CupertinoDatePickerMode.date:
case CupertinoDatePickerMode.monthYear:
break;
}
assert(
false,
'$runtimeType is only meant for dateAndTime mode or time mode',
);
return 0;
}
// The controller of the date column.
late FixedExtentScrollController dateController;
// The current selection of the hour picker. Values range from 0 to 23.
int get selectedHour => _selectedHour(selectedAmPm, _selectedHourIndex);
int get _selectedHourIndex => hourController.hasClients ? hourController.selectedItem % 24 : initialDateTime.hour;
// Calculates the selected hour given the selected indices of the hour picker
// and the meridiem picker.
int _selectedHour(int selectedAmPm, int selectedHour) {
return _isHourRegionFlipped(selectedAmPm) ? (selectedHour + 12) % 24 : selectedHour;
}
// The controller of the hour column.
late FixedExtentScrollController hourController;
// The current selection of the minute picker. Values range from 0 to 59.
int get selectedMinute {
return minuteController.hasClients
? minuteController.selectedItem * widget.minuteInterval % 60
: initialDateTime.minute;
}
// The controller of the minute column.
late FixedExtentScrollController minuteController;
// Whether the current meridiem selection is AM or PM.
//
// We can't use the selectedItem of meridiemController as the source of truth
// because the meridiem picker can be scrolled **animatedly** by the hour picker
// (e.g. if you scroll from 12 to 1 in 12h format), but the meridiem change
// should take effect immediately, **before** the animation finishes.
late int selectedAmPm;
// Whether the physical-region-to-meridiem mapping is flipped.
bool get isHourRegionFlipped => _isHourRegionFlipped(selectedAmPm);
bool _isHourRegionFlipped(int selectedAmPm) => selectedAmPm != meridiemRegion;
// The index of the 12-hour region the hour picker is currently in.
//
// Used to determine whether the meridiemController should start animating.
// Valid values are 0 and 1.
//
// The AM/PM correspondence of the two regions flips when the meridiem picker
// scrolls. This variable is to keep track of the selected "physical"
// (meridiem picker invariant) region of the hour picker. The "physical" region
// of an item of index `i` is `i ~/ 12`.
late int meridiemRegion;
// The current selection of the AM/PM picker.
//
// - 0 means AM
// - 1 means PM
late FixedExtentScrollController meridiemController;
bool isDatePickerScrolling = false;
bool isHourPickerScrolling = false;
bool isMinutePickerScrolling = false;
bool isMeridiemPickerScrolling = false;
bool get isScrolling {
return isDatePickerScrolling
|| isHourPickerScrolling
|| isMinutePickerScrolling
|| isMeridiemPickerScrolling;
}
// The estimated width of columns.
final Map<int, double> estimatedColumnWidths = <int, double>{};
@override
void initState() {
super.initState();
initialDateTime = widget.initialDateTime;
// Initially each of the "physical" regions is mapped to the meridiem region
// with the same number, e.g., the first 12 items are mapped to the first 12
// hours of a day. Such mapping is flipped when the meridiem picker is scrolled
// by the user, the first 12 items are mapped to the last 12 hours of a day.
selectedAmPm = initialDateTime.hour ~/ 12;
meridiemRegion = selectedAmPm;
meridiemController = FixedExtentScrollController(initialItem: selectedAmPm);
hourController = FixedExtentScrollController(initialItem: initialDateTime.hour);
minuteController = FixedExtentScrollController(initialItem: initialDateTime.minute ~/ widget.minuteInterval);
dateController = FixedExtentScrollController();
PaintingBinding.instance.systemFonts.addListener(_handleSystemFontsChange);
}
void _handleSystemFontsChange () {
setState(() {
// System fonts change might cause the text layout width to change.
// Clears cached width to ensure that they get recalculated with the
// new system fonts.
estimatedColumnWidths.clear();
});
}
@override
void dispose() {
dateController.dispose();
hourController.dispose();
minuteController.dispose();
meridiemController.dispose();
PaintingBinding.instance.systemFonts.removeListener(_handleSystemFontsChange);
super.dispose();
}
@override
void didUpdateWidget(CupertinoDatePicker oldWidget) {
super.didUpdateWidget(oldWidget);
assert(
oldWidget.mode == widget.mode,
"The $runtimeType's mode cannot change once it's built.",
);
if (!widget.use24hFormat && oldWidget.use24hFormat) {
// Thanks to the physical and meridiem region mapping, the only thing we
// need to update is the meridiem controller, if it's not previously attached.
meridiemController.dispose();
meridiemController = FixedExtentScrollController(initialItem: selectedAmPm);
}
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
textDirectionFactor = Directionality.of(context) == TextDirection.ltr ? 1 : -1;
localizations = CupertinoLocalizations.of(context);
alignCenterLeft = textDirectionFactor == 1 ? Alignment.centerLeft : Alignment.centerRight;
alignCenterRight = textDirectionFactor == 1 ? Alignment.centerRight : Alignment.centerLeft;
estimatedColumnWidths.clear();
}
// Lazily calculate the column width of the column being displayed only.
double _getEstimatedColumnWidth(_PickerColumnType columnType) {
estimatedColumnWidths[columnType.index] ??=
CupertinoDatePicker._getColumnWidth(columnType, localizations, context, widget.showDayOfWeek);
return estimatedColumnWidths[columnType.index]!;
}
// Gets the current date time of the picker.
DateTime get selectedDateTime {
return DateTime(
initialDateTime.year,
initialDateTime.month,
initialDateTime.day + selectedDayFromInitial,
selectedHour,
selectedMinute,
);
}
// Only reports datetime change when the date time is valid.
void _onSelectedItemChange(int index) {
final DateTime selected = selectedDateTime;
final bool isDateInvalid = (widget.minimumDate?.isAfter(selected) ?? false)
|| (widget.maximumDate?.isBefore(selected) ?? false);
if (isDateInvalid) {
return;
}
widget.onDateTimeChanged(selected);
}
// Builds the date column. The date is displayed in medium date format (e.g. Fri Aug 31).
Widget _buildMediumDatePicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isDatePickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isDatePickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker.builder(
scrollController: dateController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
_onSelectedItemChange(index);
},
itemBuilder: (BuildContext context, int index) {
final DateTime rangeStart = DateTime(
initialDateTime.year,
initialDateTime.month,
initialDateTime.day + index,
);
// Exclusive.
final DateTime rangeEnd = DateTime(
initialDateTime.year,
initialDateTime.month,
initialDateTime.day + index + 1,
);
final DateTime now = DateTime.now();
if (widget.minimumDate?.isBefore(rangeEnd) == false) {
return null;
}
if (widget.maximumDate?.isAfter(rangeStart) == false) {
return null;
}
final String dateText = rangeStart == DateTime(now.year, now.month, now.day)
? localizations.todayLabel
: localizations.datePickerMediumDate(rangeStart);
return itemPositioningBuilder(
context,
Text(dateText, style: _themeTextStyle(context)),
);
},
selectionOverlay: selectionOverlay,
),
);
}
// With the meridiem picker set to `meridiemIndex`, and the hour picker set to
// `hourIndex`, is it possible to change the value of the minute picker, so
// that the resulting date stays in the valid range.
bool _isValidHour(int meridiemIndex, int hourIndex) {
final DateTime rangeStart = DateTime(
initialDateTime.year,
initialDateTime.month,
initialDateTime.day + selectedDayFromInitial,
_selectedHour(meridiemIndex, hourIndex),
);
// The end value of the range is exclusive, i.e. [rangeStart, rangeEnd).
final DateTime rangeEnd = rangeStart.add(const Duration(hours: 1));
return (widget.minimumDate?.isBefore(rangeEnd) ?? true)
&& !(widget.maximumDate?.isBefore(rangeStart) ?? false);
}
Widget _buildHourPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isHourPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isHourPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: hourController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
final bool regionChanged = meridiemRegion != index ~/ 12;
final bool debugIsFlipped = isHourRegionFlipped;
if (regionChanged) {
meridiemRegion = index ~/ 12;
selectedAmPm = 1 - selectedAmPm;
}
if (!widget.use24hFormat && regionChanged) {
// Scroll the meridiem column to adjust AM/PM.
//
// _onSelectedItemChanged will be called when the animation finishes.
//
// Animation values obtained by comparing with iOS version.
meridiemController.animateToItem(
selectedAmPm,
duration: const Duration(milliseconds: 300),
curve: Curves.easeOut,
);
} else {
_onSelectedItemChange(index);
}
assert(debugIsFlipped == isHourRegionFlipped);
},
looping: true,
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(24, (int index) {
final int hour = isHourRegionFlipped ? (index + 12) % 24 : index;
final int displayHour = widget.use24hFormat ? hour : (hour + 11) % 12 + 1;
return itemPositioningBuilder(
context,
Text(
localizations.datePickerHour(displayHour),
semanticsLabel: localizations.datePickerHourSemanticsLabel(displayHour),
style: _themeTextStyle(context, isValid: _isValidHour(selectedAmPm, index)),
),
);
}),
),
);
}
Widget _buildMinutePicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isMinutePickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isMinutePickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: minuteController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: _onSelectedItemChange,
looping: true,
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(60 ~/ widget.minuteInterval, (int index) {
final int minute = index * widget.minuteInterval;
final DateTime date = DateTime(
initialDateTime.year,
initialDateTime.month,
initialDateTime.day + selectedDayFromInitial,
selectedHour,
minute,
);
final bool isInvalidMinute = (widget.minimumDate?.isAfter(date) ?? false)
|| (widget.maximumDate?.isBefore(date) ?? false);
return itemPositioningBuilder(
context,
Text(
localizations.datePickerMinute(minute),
semanticsLabel: localizations.datePickerMinuteSemanticsLabel(minute),
style: _themeTextStyle(context, isValid: !isInvalidMinute),
),
);
}),
),
);
}
Widget _buildAmPmPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isMeridiemPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isMeridiemPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: meridiemController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
selectedAmPm = index;
assert(selectedAmPm == 0 || selectedAmPm == 1);
_onSelectedItemChange(index);
},
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(2, (int index) {
return itemPositioningBuilder(
context,
Text(
index == 0
? localizations.anteMeridiemAbbreviation
: localizations.postMeridiemAbbreviation,
style: _themeTextStyle(context, isValid: _isValidHour(index, _selectedHourIndex)),
),
);
}),
),
);
}
// One or more pickers have just stopped scrolling.
void _pickerDidStopScrolling() {
// Call setState to update the greyed out date/hour/minute/meridiem.
setState(() { });
if (isScrolling) {
return;
}
// Whenever scrolling lands on an invalid entry, the picker
// automatically scrolls to a valid one.
final DateTime selectedDate = selectedDateTime;
final bool minCheck = widget.minimumDate?.isAfter(selectedDate) ?? false;
final bool maxCheck = widget.maximumDate?.isBefore(selectedDate) ?? false;
if (minCheck || maxCheck) {
// We have minCheck === !maxCheck.
final DateTime targetDate = minCheck ? widget.minimumDate! : widget.maximumDate!;
_scrollToDate(targetDate, selectedDate, minCheck);
}
}
void _scrollToDate(DateTime newDate, DateTime fromDate, bool minCheck) {
SchedulerBinding.instance.addPostFrameCallback((Duration timestamp) {
if (fromDate.year != newDate.year || fromDate.month != newDate.month || fromDate.day != newDate.day) {
_animateColumnControllerToItem(dateController, selectedDayFromInitial);
}
if (fromDate.hour != newDate.hour) {
final bool needsMeridiemChange = !widget.use24hFormat
&& fromDate.hour ~/ 12 != newDate.hour ~/ 12;
// In AM/PM mode, the pickers should not scroll all the way to the other hour region.
if (needsMeridiemChange) {
_animateColumnControllerToItem(meridiemController, 1 - meridiemController.selectedItem);
// Keep the target item index in the current 12-h region.
final int newItem = (hourController.selectedItem ~/ 12) * 12
+ (hourController.selectedItem + newDate.hour - fromDate.hour) % 12;
_animateColumnControllerToItem(hourController, newItem);
} else {
_animateColumnControllerToItem(
hourController,
hourController.selectedItem + newDate.hour - fromDate.hour,
);
}
}
if (fromDate.minute != newDate.minute) {
final double positionDouble = newDate.minute / widget.minuteInterval;
final int position = minCheck ? positionDouble.ceil() : positionDouble.floor();
_animateColumnControllerToItem(minuteController, position);
}
}, debugLabel: 'DatePicker.scrollToDate');
}
@override
Widget build(BuildContext context) {
// Widths of the columns in this picker, ordered from left to right.
final List<double> columnWidths = <double>[
_getEstimatedColumnWidth(_PickerColumnType.hour),
_getEstimatedColumnWidth(_PickerColumnType.minute),
];
// Swap the hours and minutes if RTL to ensure they are in the correct position.
final List<_ColumnBuilder> pickerBuilders = Directionality.of(context) == TextDirection.rtl
? <_ColumnBuilder>[_buildMinutePicker, _buildHourPicker]
: <_ColumnBuilder>[_buildHourPicker, _buildMinutePicker];
// Adds am/pm column if the picker is not using 24h format.
if (!widget.use24hFormat) {
switch (localizations.datePickerDateTimeOrder) {
case DatePickerDateTimeOrder.date_time_dayPeriod:
case DatePickerDateTimeOrder.time_dayPeriod_date:
pickerBuilders.add(_buildAmPmPicker);
columnWidths.add(_getEstimatedColumnWidth(_PickerColumnType.dayPeriod));
case DatePickerDateTimeOrder.date_dayPeriod_time:
case DatePickerDateTimeOrder.dayPeriod_time_date:
pickerBuilders.insert(0, _buildAmPmPicker);
columnWidths.insert(0, _getEstimatedColumnWidth(_PickerColumnType.dayPeriod));
}
}
// Adds medium date column if the picker's mode is date and time.
if (widget.mode == CupertinoDatePickerMode.dateAndTime) {
switch (localizations.datePickerDateTimeOrder) {
case DatePickerDateTimeOrder.time_dayPeriod_date:
case DatePickerDateTimeOrder.dayPeriod_time_date:
pickerBuilders.add(_buildMediumDatePicker);
columnWidths.add(_getEstimatedColumnWidth(_PickerColumnType.date));
case DatePickerDateTimeOrder.date_time_dayPeriod:
case DatePickerDateTimeOrder.date_dayPeriod_time:
pickerBuilders.insert(0, _buildMediumDatePicker);
columnWidths.insert(0, _getEstimatedColumnWidth(_PickerColumnType.date));
}
}
final List<Widget> pickers = <Widget>[];
double totalColumnWidths = 4 * _kDatePickerPadSize;
for (int i = 0; i < columnWidths.length; i++) {
double offAxisFraction = 0.0;
Widget selectionOverlay = _centerSelectionOverlay;
if (i == 0) {
offAxisFraction = -_kMaximumOffAxisFraction * textDirectionFactor;
selectionOverlay = _startSelectionOverlay;
} else if (i >= 2 || columnWidths.length == 2) {
offAxisFraction = _kMaximumOffAxisFraction * textDirectionFactor;
}
EdgeInsets padding = const EdgeInsets.only(right: _kDatePickerPadSize);
if (i == columnWidths.length - 1) {
padding = padding.flipped;
selectionOverlay = _endSelectionOverlay;
}
if (textDirectionFactor == -1) {
padding = padding.flipped;
}
totalColumnWidths += columnWidths[i] + (2 * _kDatePickerPadSize);
pickers.add(LayoutId(
id: i,
child: pickerBuilders[i](
offAxisFraction,
(BuildContext context, Widget? child) {
return Container(
alignment: i == columnWidths.length - 1
? alignCenterLeft
: alignCenterRight,
padding: padding,
child: Container(
alignment: i == columnWidths.length - 1 ? alignCenterLeft : alignCenterRight,
width: i == 0 || i == columnWidths.length - 1
? null
: columnWidths[i] + _kDatePickerPadSize,
child: child,
),
);
},
selectionOverlay,
),
));
}
final double maxPickerWidth = totalColumnWidths > _kPickerWidth ? totalColumnWidths : _kPickerWidth;
return MediaQuery.withNoTextScaling(
child: DefaultTextStyle.merge(
style: _kDefaultPickerTextStyle,
child: CustomMultiChildLayout(
delegate: _DatePickerLayoutDelegate(
columnWidths: columnWidths,
textDirectionFactor: textDirectionFactor,
maxWidth: maxPickerWidth,
),
children: pickers,
),
),
);
}
}
class _CupertinoDatePickerDateState extends State<CupertinoDatePicker> {
_CupertinoDatePickerDateState({
required this.dateOrder,
});
final DatePickerDateOrder? dateOrder;
late int textDirectionFactor;
late CupertinoLocalizations localizations;
// Alignment based on text direction. The variable name is self descriptive,
// however, when text direction is rtl, alignment is reversed.
late Alignment alignCenterLeft;
late Alignment alignCenterRight;
// The currently selected values of the picker.
late int selectedDay;
late int selectedMonth;
late int selectedYear;
// The controller of the day picker. There are cases where the selected value
// of the picker is invalid (e.g. February 30th 2018), and this dayController
// is responsible for jumping to a valid value.
late FixedExtentScrollController dayController;
late FixedExtentScrollController monthController;
late FixedExtentScrollController yearController;
bool isDayPickerScrolling = false;
bool isMonthPickerScrolling = false;
bool isYearPickerScrolling = false;
bool get isScrolling => isDayPickerScrolling || isMonthPickerScrolling || isYearPickerScrolling;
// Estimated width of columns.
Map<int, double> estimatedColumnWidths = <int, double>{};
@override
void initState() {
super.initState();
selectedDay = widget.initialDateTime.day;
selectedMonth = widget.initialDateTime.month;
selectedYear = widget.initialDateTime.year;
dayController = FixedExtentScrollController(initialItem: selectedDay - 1);
monthController = FixedExtentScrollController(initialItem: selectedMonth - 1);
yearController = FixedExtentScrollController(initialItem: selectedYear);
PaintingBinding.instance.systemFonts.addListener(_handleSystemFontsChange);
}
void _handleSystemFontsChange() {
setState(() {
// System fonts change might cause the text layout width to change.
_refreshEstimatedColumnWidths();
});
}
@override
void dispose() {
dayController.dispose();
monthController.dispose();
yearController.dispose();
PaintingBinding.instance.systemFonts.removeListener(_handleSystemFontsChange);
super.dispose();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
textDirectionFactor = Directionality.of(context) == TextDirection.ltr ? 1 : -1;
localizations = CupertinoLocalizations.of(context);
alignCenterLeft = textDirectionFactor == 1 ? Alignment.centerLeft : Alignment.centerRight;
alignCenterRight = textDirectionFactor == 1 ? Alignment.centerRight : Alignment.centerLeft;
_refreshEstimatedColumnWidths();
}
void _refreshEstimatedColumnWidths() {
estimatedColumnWidths[_PickerColumnType.dayOfMonth.index] = CupertinoDatePicker._getColumnWidth(_PickerColumnType.dayOfMonth, localizations, context, widget.showDayOfWeek);
estimatedColumnWidths[_PickerColumnType.month.index] = CupertinoDatePicker._getColumnWidth(_PickerColumnType.month, localizations, context, widget.showDayOfWeek);
estimatedColumnWidths[_PickerColumnType.year.index] = CupertinoDatePicker._getColumnWidth(_PickerColumnType.year, localizations, context, widget.showDayOfWeek);
}
// The DateTime of the last day of a given month in a given year.
// Let `DateTime` handle the year/month overflow.
DateTime _lastDayInMonth(int year, int month) => DateTime(year, month + 1, 0);
Widget _buildDayPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
final int daysInCurrentMonth = _lastDayInMonth(selectedYear, selectedMonth).day;
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isDayPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isDayPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: dayController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
selectedDay = index + 1;
if (_isCurrentDateValid) {
widget.onDateTimeChanged(DateTime(selectedYear, selectedMonth, selectedDay));
}
},
looping: true,
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(31, (int index) {
final int day = index + 1;
final int? dayOfWeek = widget.showDayOfWeek ? DateTime(selectedYear, selectedMonth, day).weekday : null;
final bool isInvalidDay = (day > daysInCurrentMonth)
|| (widget.minimumDate?.year == selectedYear &&
widget.minimumDate!.month == selectedMonth &&
widget.minimumDate!.day > day)
|| (widget.maximumDate?.year == selectedYear &&
widget.maximumDate!.month == selectedMonth &&
widget.maximumDate!.day < day);
return itemPositioningBuilder(
context,
Text(
localizations.datePickerDayOfMonth(day, dayOfWeek),
style: _themeTextStyle(context, isValid: !isInvalidDay),
),
);
}),
),
);
}
Widget _buildMonthPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isMonthPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isMonthPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: monthController,
offAxisFraction: offAxisFraction,
itemExtent: widget.itemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
selectedMonth = index + 1;
if (_isCurrentDateValid) {
widget.onDateTimeChanged(DateTime(selectedYear, selectedMonth, selectedDay));
}
},
looping: true,
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(12, (int index) {
final int month = index + 1;
final bool isInvalidMonth = (widget.minimumDate?.year == selectedYear && widget.minimumDate!.month > month)
|| (widget.maximumDate?.year == selectedYear && widget.maximumDate!.month < month);
final String monthName = (widget.mode == CupertinoDatePickerMode.monthYear) ? localizations.datePickerStandaloneMonth(month) : localizations.datePickerMonth(month);
return itemPositioningBuilder(
context,
Text(
monthName,
style: _themeTextStyle(context, isValid: !isInvalidMonth),
),
);
}),
),
);
}
Widget _buildYearPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isYearPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isYearPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker.builder(
scrollController: yearController,
itemExtent: widget.itemExtent,
offAxisFraction: offAxisFraction,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
onSelectedItemChanged: (int index) {
selectedYear = index;
if (_isCurrentDateValid) {
widget.onDateTimeChanged(DateTime(selectedYear, selectedMonth, selectedDay));
}
},
itemBuilder: (BuildContext context, int year) {
if (year < widget.minimumYear) {
return null;
}
if (widget.maximumYear != null && year > widget.maximumYear!) {
return null;
}
final bool isValidYear = (widget.minimumDate == null || widget.minimumDate!.year <= year)
&& (widget.maximumDate == null || widget.maximumDate!.year >= year);
return itemPositioningBuilder(
context,
Text(
localizations.datePickerYear(year),
style: _themeTextStyle(context, isValid: isValidYear),
),
);
},
selectionOverlay: selectionOverlay,
),
);
}
bool get _isCurrentDateValid {
// The current date selection represents a range [minSelectedData, maxSelectDate].
final DateTime minSelectedDate = DateTime(selectedYear, selectedMonth, selectedDay);
final DateTime maxSelectedDate = DateTime(selectedYear, selectedMonth, selectedDay + 1);
final bool minCheck = widget.minimumDate?.isBefore(maxSelectedDate) ?? true;
final bool maxCheck = widget.maximumDate?.isBefore(minSelectedDate) ?? false;
return minCheck && !maxCheck && minSelectedDate.day == selectedDay;
}
// One or more pickers have just stopped scrolling.
void _pickerDidStopScrolling() {
// Call setState to update the greyed out days/months/years, as the currently
// selected year/month may have changed.
setState(() { });
if (isScrolling) {
return;
}
// Whenever scrolling lands on an invalid entry, the picker
// automatically scrolls to a valid one.
final DateTime minSelectDate = DateTime(selectedYear, selectedMonth, selectedDay);
final DateTime maxSelectDate = DateTime(selectedYear, selectedMonth, selectedDay + 1);
final bool minCheck = widget.minimumDate?.isBefore(maxSelectDate) ?? true;
final bool maxCheck = widget.maximumDate?.isBefore(minSelectDate) ?? false;
if (!minCheck || maxCheck) {
// We have minCheck === !maxCheck.
final DateTime targetDate = minCheck ? widget.maximumDate! : widget.minimumDate!;
_scrollToDate(targetDate);
return;
}
// Some months have less days (e.g. February). Go to the last day of that month
// if the selectedDay exceeds the maximum.
if (minSelectDate.day != selectedDay) {
final DateTime lastDay = _lastDayInMonth(selectedYear, selectedMonth);
_scrollToDate(lastDay);
}
}
void _scrollToDate(DateTime newDate) {
SchedulerBinding.instance.addPostFrameCallback((Duration timestamp) {
if (selectedYear != newDate.year) {
_animateColumnControllerToItem(yearController, newDate.year);
}
if (selectedMonth != newDate.month) {
_animateColumnControllerToItem(monthController, newDate.month - 1);
}
if (selectedDay != newDate.day) {
_animateColumnControllerToItem(dayController, newDate.day - 1);
}
}, debugLabel: 'DatePicker.scrollToDate');
}
@override
Widget build(BuildContext context) {
List<_ColumnBuilder> pickerBuilders = <_ColumnBuilder>[];
List<double> columnWidths = <double>[];
final DatePickerDateOrder datePickerDateOrder =
dateOrder ?? localizations.datePickerDateOrder;
switch (datePickerDateOrder) {
case DatePickerDateOrder.mdy:
pickerBuilders = <_ColumnBuilder>[_buildMonthPicker, _buildDayPicker, _buildYearPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.month.index]!,
estimatedColumnWidths[_PickerColumnType.dayOfMonth.index]!,
estimatedColumnWidths[_PickerColumnType.year.index]!,
];
case DatePickerDateOrder.dmy:
pickerBuilders = <_ColumnBuilder>[_buildDayPicker, _buildMonthPicker, _buildYearPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.dayOfMonth.index]!,
estimatedColumnWidths[_PickerColumnType.month.index]!,
estimatedColumnWidths[_PickerColumnType.year.index]!,
];
case DatePickerDateOrder.ymd:
pickerBuilders = <_ColumnBuilder>[_buildYearPicker, _buildMonthPicker, _buildDayPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.year.index]!,
estimatedColumnWidths[_PickerColumnType.month.index]!,
estimatedColumnWidths[_PickerColumnType.dayOfMonth.index]!,
];
case DatePickerDateOrder.ydm:
pickerBuilders = <_ColumnBuilder>[_buildYearPicker, _buildDayPicker, _buildMonthPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.year.index]!,
estimatedColumnWidths[_PickerColumnType.dayOfMonth.index]!,
estimatedColumnWidths[_PickerColumnType.month.index]!,
];
}
final List<Widget> pickers = <Widget>[];
double totalColumnWidths = 4 * _kDatePickerPadSize;
for (int i = 0; i < columnWidths.length; i++) {
final double offAxisFraction = (i - 1) * 0.3 * textDirectionFactor;
EdgeInsets padding = const EdgeInsets.only(right: _kDatePickerPadSize);
if (textDirectionFactor == -1) {
padding = const EdgeInsets.only(left: _kDatePickerPadSize);
}
Widget selectionOverlay = _centerSelectionOverlay;
if (i == 0) {
selectionOverlay = _startSelectionOverlay;
} else if (i == columnWidths.length - 1) {
selectionOverlay = _endSelectionOverlay;
}
totalColumnWidths += columnWidths[i] + (2 * _kDatePickerPadSize);
pickers.add(LayoutId(
id: i,
child: pickerBuilders[i](
offAxisFraction,
(BuildContext context, Widget? child) {
return Container(
alignment: i == columnWidths.length - 1
? alignCenterLeft
: alignCenterRight,
padding: i == 0 ? null : padding,
child: Container(
alignment: i == 0 ? alignCenterLeft : alignCenterRight,
width: columnWidths[i] + _kDatePickerPadSize,
child: child,
),
);
},
selectionOverlay,
),
));
}
final double maxPickerWidth = totalColumnWidths > _kPickerWidth ? totalColumnWidths : _kPickerWidth;
return MediaQuery.withNoTextScaling(
child: DefaultTextStyle.merge(
style: _kDefaultPickerTextStyle,
child: CustomMultiChildLayout(
delegate: _DatePickerLayoutDelegate(
columnWidths: columnWidths,
textDirectionFactor: textDirectionFactor,
maxWidth: maxPickerWidth,
),
children: pickers,
),
),
);
}
}
class _CupertinoDatePickerMonthYearState extends State<CupertinoDatePicker> {
_CupertinoDatePickerMonthYearState({
required this.dateOrder,
});
final DatePickerDateOrder? dateOrder;
late int textDirectionFactor;
late CupertinoLocalizations localizations;
// Alignment based on text direction. The variable name is self descriptive,
// however, when text direction is rtl, alignment is reversed.
late Alignment alignCenterLeft;
late Alignment alignCenterRight;
// The currently selected values of the picker.
late int selectedYear;
late int selectedMonth;
// The controller of the day picker. There are cases where the selected value
// of the picker is invalid (e.g. February 30th 2018), and this monthController
// is responsible for jumping to a valid value.
late FixedExtentScrollController monthController;
late FixedExtentScrollController yearController;
bool isMonthPickerScrolling = false;
bool isYearPickerScrolling = false;
bool get isScrolling => isMonthPickerScrolling || isYearPickerScrolling;
// Estimated width of columns.
Map<int, double> estimatedColumnWidths = <int, double>{};
@override
void initState() {
super.initState();
selectedMonth = widget.initialDateTime.month;
selectedYear = widget.initialDateTime.year;
monthController = FixedExtentScrollController(initialItem: selectedMonth - 1);
yearController = FixedExtentScrollController(initialItem: selectedYear);
PaintingBinding.instance.systemFonts.addListener(_handleSystemFontsChange);
}
void _handleSystemFontsChange() {
setState(() {
// System fonts change might cause the text layout width to change.
_refreshEstimatedColumnWidths();
});
}
@override
void dispose() {
monthController.dispose();
yearController.dispose();
PaintingBinding.instance.systemFonts.removeListener(_handleSystemFontsChange);
super.dispose();
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
textDirectionFactor = Directionality.of(context) == TextDirection.ltr ? 1 : -1;
localizations = CupertinoLocalizations.of(context);
alignCenterLeft = textDirectionFactor == 1 ? Alignment.centerLeft : Alignment.centerRight;
alignCenterRight = textDirectionFactor == 1 ? Alignment.centerRight : Alignment.centerLeft;
_refreshEstimatedColumnWidths();
}
void _refreshEstimatedColumnWidths() {
estimatedColumnWidths[_PickerColumnType.month.index] =
CupertinoDatePicker._getColumnWidth(_PickerColumnType.month, localizations, context, false, standaloneMonth: widget.mode == CupertinoDatePickerMode.monthYear);
estimatedColumnWidths[_PickerColumnType.year.index] = CupertinoDatePicker._getColumnWidth(_PickerColumnType.year, localizations, context, false);
}
Widget _buildMonthPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isMonthPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isMonthPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker(
scrollController: monthController,
offAxisFraction: offAxisFraction,
itemExtent: _kItemExtent,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
selectedMonth = index + 1;
if (_isCurrentDateValid) {
widget.onDateTimeChanged(DateTime(selectedYear, selectedMonth));
}
},
looping: true,
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(12, (int index) {
final int month = index + 1;
final bool isInvalidMonth = (widget.minimumDate?.year == selectedYear && widget.minimumDate!.month > month)
|| (widget.maximumDate?.year == selectedYear && widget.maximumDate!.month < month);
final String monthName = (widget.mode == CupertinoDatePickerMode.monthYear) ? localizations.datePickerStandaloneMonth(month) : localizations.datePickerMonth(month);
return itemPositioningBuilder(
context,
Text(
monthName,
style: _themeTextStyle(context, isValid: !isInvalidMonth),
),
);
}),
),
);
}
Widget _buildYearPicker(double offAxisFraction, TransitionBuilder itemPositioningBuilder, Widget selectionOverlay) {
return NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
if (notification is ScrollStartNotification) {
isYearPickerScrolling = true;
} else if (notification is ScrollEndNotification) {
isYearPickerScrolling = false;
_pickerDidStopScrolling();
}
return false;
},
child: CupertinoPicker.builder(
scrollController: yearController,
itemExtent: _kItemExtent,
offAxisFraction: offAxisFraction,
useMagnifier: _kUseMagnifier,
magnification: _kMagnification,
backgroundColor: widget.backgroundColor,
onSelectedItemChanged: (int index) {
selectedYear = index;
if (_isCurrentDateValid) {
widget.onDateTimeChanged(DateTime(selectedYear, selectedMonth));
}
},
itemBuilder: (BuildContext context, int year) {
if (year < widget.minimumYear) {
return null;
}
if (widget.maximumYear != null && year > widget.maximumYear!) {
return null;
}
final bool isValidYear = (widget.minimumDate == null || widget.minimumDate!.year <= year)
&& (widget.maximumDate == null || widget.maximumDate!.year >= year);
return itemPositioningBuilder(
context,
Text(
localizations.datePickerYear(year),
style: _themeTextStyle(context, isValid: isValidYear),
),
);
},
selectionOverlay: selectionOverlay,
),
);
}
bool get _isCurrentDateValid {
// The current date selection represents a range [minSelectedData, maxSelectDate].
final DateTime minSelectedDate = DateTime(selectedYear, selectedMonth);
final DateTime maxSelectedDate = DateTime(selectedYear, selectedMonth, widget.initialDateTime.day + 1);
final bool minCheck = widget.minimumDate?.isBefore(maxSelectedDate) ?? true;
final bool maxCheck = widget.maximumDate?.isBefore(minSelectedDate) ?? false;
return minCheck && !maxCheck;
}
// One or more pickers have just stopped scrolling.
void _pickerDidStopScrolling() {
// Call setState to update the greyed out days/months/years, as the currently
// selected year/month may have changed.
setState(() { });
if (isScrolling) {
return;
}
// Whenever scrolling lands on an invalid entry, the picker
// automatically scrolls to a valid one.
final DateTime minSelectDate = DateTime(selectedYear, selectedMonth);
final DateTime maxSelectDate = DateTime(selectedYear, selectedMonth, widget.initialDateTime.day + 1);
final bool minCheck = widget.minimumDate?.isBefore(maxSelectDate) ?? true;
final bool maxCheck = widget.maximumDate?.isBefore(minSelectDate) ?? false;
if (!minCheck || maxCheck) {
// We have minCheck === !maxCheck.
final DateTime targetDate = minCheck ? widget.maximumDate! : widget.minimumDate!;
_scrollToDate(targetDate);
return;
}
}
void _scrollToDate(DateTime newDate) {
SchedulerBinding.instance.addPostFrameCallback((Duration timestamp) {
if (selectedYear != newDate.year) {
_animateColumnControllerToItem(yearController, newDate.year);
}
if (selectedMonth != newDate.month) {
_animateColumnControllerToItem(monthController, newDate.month - 1);
}
}, debugLabel: 'DatePicker.scrollToDate');
}
@override
Widget build(BuildContext context) {
List<_ColumnBuilder> pickerBuilders = <_ColumnBuilder>[];
List<double> columnWidths = <double>[];
final DatePickerDateOrder datePickerDateOrder =
dateOrder ?? localizations.datePickerDateOrder;
switch (datePickerDateOrder) {
case DatePickerDateOrder.mdy:
case DatePickerDateOrder.dmy:
pickerBuilders = <_ColumnBuilder>[_buildMonthPicker, _buildYearPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.month.index]!,
estimatedColumnWidths[_PickerColumnType.year.index]!,
];
case DatePickerDateOrder.ymd:
case DatePickerDateOrder.ydm:
pickerBuilders = <_ColumnBuilder>[_buildYearPicker, _buildMonthPicker];
columnWidths = <double>[
estimatedColumnWidths[_PickerColumnType.year.index]!,
estimatedColumnWidths[_PickerColumnType.month.index]!,
];
}
final List<Widget> pickers = <Widget>[];
double totalColumnWidths = 3 * _kDatePickerPadSize;
for (int i = 0; i < columnWidths.length; i++) {
final (bool first, bool last) = (i == 0, i == columnWidths.length - 1);
final double offAxisFraction = textDirectionFactor * (first ? -0.3 : 0.5);
totalColumnWidths += columnWidths[i] + (2 * _kDatePickerPadSize);
pickers.add(LayoutId(
id: i,
child: pickerBuilders[i](
offAxisFraction,
(BuildContext context, Widget? child) {
return Container(
alignment: last ? alignCenterLeft : alignCenterRight,
padding: switch (textDirectionFactor) {
_ when first => null,
-1 => const EdgeInsets.only(left: _kDatePickerPadSize),
_ => const EdgeInsets.only(right: _kDatePickerPadSize),
},
child: Container(
alignment: first ? alignCenterLeft : alignCenterRight,
width: columnWidths[i] + _kDatePickerPadSize,
child: child,
),
);
},
switch (last) {
_ when first => _startSelectionOverlay,
false => _centerSelectionOverlay,
true => _endSelectionOverlay,
},
),
));
}
final double maxPickerWidth = totalColumnWidths > _kPickerWidth ? totalColumnWidths : _kPickerWidth;
return MediaQuery.withNoTextScaling(
child: DefaultTextStyle.merge(
style: _kDefaultPickerTextStyle,
child: CustomMultiChildLayout(
delegate: _DatePickerLayoutDelegate(
columnWidths: columnWidths,
textDirectionFactor: textDirectionFactor,
maxWidth: maxPickerWidth,
),
children: pickers,
),
),
);
}
}
// The iOS date picker and timer picker has their width fixed to 320.0 in all
// modes. The only exception is the hms mode (which doesn't have a native counterpart),
// with a fixed width of 330.0 px.
//
// For date pickers, if the maximum width given to the picker is greater than
// 320.0, the leftmost and rightmost column will be extended equally so that the
// widths match, and the picker is in the center.
//
// For timer pickers, if the maximum width given to the picker is greater than
// its intrinsic width, it will keep its intrinsic size and position itself in the
// parent using its alignment parameter.
//
// If the maximum width given to the picker is smaller than 320.0, the picker's
// layout will be broken.
/// Different modes of [CupertinoTimerPicker].
///
/// See also:
///
/// * [CupertinoTimerPicker], the class that implements the iOS-style timer picker.
/// * [CupertinoPicker], the class that implements a content agnostic spinner UI.
enum CupertinoTimerPickerMode {
/// Mode that shows the timer duration in hour and minute.
///
/// Examples: 16 hours | 14 min.
hm,
/// Mode that shows the timer duration in minute and second.
///
/// Examples: 14 min | 43 sec.
ms,
/// Mode that shows the timer duration in hour, minute, and second.
///
/// Examples: 16 hours | 14 min | 43 sec.
hms,
}
/// A countdown timer picker in iOS style.
///
/// This picker shows a countdown duration with hour, minute and second spinners.
/// The duration is bound between 0 and 23 hours 59 minutes 59 seconds.
///
/// There are several modes of the timer picker listed in [CupertinoTimerPickerMode].
///
/// The picker has a fixed size of 320 x 216, in logical pixels, with the exception
/// of [CupertinoTimerPickerMode.hms], which is 330 x 216. If the parent widget
/// provides more space than it needs, the picker will position itself according
/// to its [alignment] property.
///
/// {@tool dartpad}
/// This example shows a [CupertinoTimerPicker] that returns a countdown duration.
///
/// ** See code in examples/api/lib/cupertino/date_picker/cupertino_timer_picker.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoDatePicker], the class that implements different display modes
/// of the iOS-style date picker.
/// * [CupertinoPicker], the class that implements a content agnostic spinner UI.
/// * <https://developer.apple.com/design/human-interface-guidelines/ios/controls/pickers/>
class CupertinoTimerPicker extends StatefulWidget {
/// Constructs an iOS style countdown timer picker.
///
/// [mode] is one of the modes listed in [CupertinoTimerPickerMode] and
/// defaults to [CupertinoTimerPickerMode.hms].
///
/// [onTimerDurationChanged] is the callback called when the selected duration
/// changes.
///
/// [initialTimerDuration] defaults to 0 second and is limited from 0 second
/// to 23 hours 59 minutes 59 seconds.
///
/// [minuteInterval] is the granularity of the minute spinner. Must be a
/// positive integer factor of 60.
///
/// [secondInterval] is the granularity of the second spinner. Must be a
/// positive integer factor of 60.
CupertinoTimerPicker({
super.key,
this.mode = CupertinoTimerPickerMode.hms,
this.initialTimerDuration = Duration.zero,
this.minuteInterval = 1,
this.secondInterval = 1,
this.alignment = Alignment.center,
this.backgroundColor,
this.itemExtent = _kItemExtent,
required this.onTimerDurationChanged,
}) : assert(initialTimerDuration >= Duration.zero),
assert(initialTimerDuration < const Duration(days: 1)),
assert(minuteInterval > 0 && 60 % minuteInterval == 0),
assert(secondInterval > 0 && 60 % secondInterval == 0),
assert(initialTimerDuration.inMinutes % minuteInterval == 0),
assert(initialTimerDuration.inSeconds % secondInterval == 0),
assert(
itemExtent > 0,
'item extent should be greater than 0'
);
/// The mode of the timer picker.
final CupertinoTimerPickerMode mode;
/// The initial duration of the countdown timer.
final Duration initialTimerDuration;
/// The granularity of the minute spinner. Must be a positive integer factor
/// of 60.
final int minuteInterval;
/// The granularity of the second spinner. Must be a positive integer factor
/// of 60.
final int secondInterval;
/// Callback called when the timer duration changes.
final ValueChanged<Duration> onTimerDurationChanged;
/// Defines how the timer picker should be positioned within its parent.
///
/// Defaults to [Alignment.center].
final AlignmentGeometry alignment;
/// Background color of timer picker.
///
/// Defaults to null, which disables background painting entirely.
final Color? backgroundColor;
/// {@macro flutter.cupertino.picker.itemExtent}
///
/// Defaults to a value that matches the default iOS timer picker wheel.
final double itemExtent;
@override
State<StatefulWidget> createState() => _CupertinoTimerPickerState();
}
class _CupertinoTimerPickerState extends State<CupertinoTimerPicker> {
late TextDirection textDirection;
late CupertinoLocalizations localizations;
int get textDirectionFactor {
return switch (textDirection) {
TextDirection.ltr => 1,
TextDirection.rtl => -1,
};
}
// The currently selected values of the picker.
int? selectedHour;
late int selectedMinute;
int? selectedSecond;
// On iOS the selected values won't be reported until the scrolling fully stops.
// The values below are the latest selected values when the picker comes to a full stop.
int? lastSelectedHour;
int? lastSelectedMinute;
int? lastSelectedSecond;
final TextPainter textPainter = TextPainter();
final List<String> numbers = List<String>.generate(10, (int i) => '${9 - i}');
late double numberLabelWidth;
late double numberLabelHeight;
late double numberLabelBaseline;
late double hourLabelWidth;
late double minuteLabelWidth;
late double secondLabelWidth;
late double totalWidth;
late double pickerColumnWidth;
FixedExtentScrollController? _hourScrollController;
FixedExtentScrollController? _minuteScrollController;
FixedExtentScrollController? _secondScrollController;
@override
void initState() {
super.initState();
selectedMinute = widget.initialTimerDuration.inMinutes % 60;
if (widget.mode != CupertinoTimerPickerMode.ms) {
selectedHour = widget.initialTimerDuration.inHours;
}
if (widget.mode != CupertinoTimerPickerMode.hm) {
selectedSecond = widget.initialTimerDuration.inSeconds % 60;
}
PaintingBinding.instance.systemFonts.addListener(_handleSystemFontsChange);
}
void _handleSystemFontsChange() {
setState(() {
// System fonts change might cause the text layout width to change.
textPainter.markNeedsLayout();
_measureLabelMetrics();
});
}
@override
void dispose() {
PaintingBinding.instance.systemFonts.removeListener(_handleSystemFontsChange);
textPainter.dispose();
_hourScrollController?.dispose();
_minuteScrollController?.dispose();
_secondScrollController?.dispose();
super.dispose();
}
@override
void didUpdateWidget(CupertinoTimerPicker oldWidget) {
super.didUpdateWidget(oldWidget);
assert(
oldWidget.mode == widget.mode,
"The CupertinoTimerPicker's mode cannot change once it's built",
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
textDirection = Directionality.of(context);
localizations = CupertinoLocalizations.of(context);
_measureLabelMetrics();
}
void _measureLabelMetrics() {
textPainter.textDirection = textDirection;
final TextStyle textStyle = _textStyleFrom(context, _kTimerPickerMagnification);
double maxWidth = double.negativeInfinity;
String? widestNumber;
// Assumes that:
// - 2-digit numbers are always wider than 1-digit numbers.
// - There's at least one number in 1-9 that's wider than or equal to 0.
// - The widest 2-digit number is composed of 2 same 1-digit numbers
// that has the biggest width.
// - If two different 1-digit numbers are of the same width, their corresponding
// 2 digit numbers are of the same width.
for (final String input in numbers) {
textPainter.text = TextSpan(
text: input,
style: textStyle,
);
textPainter.layout();
if (textPainter.maxIntrinsicWidth > maxWidth) {
maxWidth = textPainter.maxIntrinsicWidth;
widestNumber = input;
}
}
textPainter.text = TextSpan(
text: '$widestNumber$widestNumber',
style: textStyle,
);
textPainter.layout();
numberLabelWidth = textPainter.maxIntrinsicWidth;
numberLabelHeight = textPainter.height;
numberLabelBaseline = textPainter.computeDistanceToActualBaseline(TextBaseline.alphabetic);
minuteLabelWidth =
_measureLabelsMaxWidth(localizations.timerPickerMinuteLabels, textStyle);
if (widget.mode != CupertinoTimerPickerMode.ms) {
hourLabelWidth =
_measureLabelsMaxWidth(localizations.timerPickerHourLabels, textStyle);
}
if (widget.mode != CupertinoTimerPickerMode.hm) {
secondLabelWidth =
_measureLabelsMaxWidth(localizations.timerPickerSecondLabels, textStyle);
}
}
// Measures all possible time text labels and return maximum width.
double _measureLabelsMaxWidth(List<String?> labels, TextStyle style) {
double maxWidth = double.negativeInfinity;
for (int i = 0; i < labels.length; i++) {
final String? label = labels[i];
if (label == null) {
continue;
}
textPainter.text = TextSpan(text: label, style: style);
textPainter.layout();
textPainter.maxIntrinsicWidth;
if (textPainter.maxIntrinsicWidth > maxWidth) {
maxWidth = textPainter.maxIntrinsicWidth;
}
}
return maxWidth;
}
// Builds a text label with scale factor 1.0 and font weight semi-bold.
// `pickerPadding ` is the additional padding the corresponding picker has to apply
// around the `Text`, in order to extend its separators towards the closest
// horizontal edge of the encompassing widget.
Widget _buildLabel(String text, EdgeInsetsDirectional pickerPadding) {
final EdgeInsetsDirectional padding = EdgeInsetsDirectional.only(
start: numberLabelWidth
+ _kTimerPickerLabelPadSize
+ pickerPadding.start,
);
return IgnorePointer(
child: Container(
alignment: AlignmentDirectional.centerStart.resolve(textDirection),
padding: padding.resolve(textDirection),
child: SizedBox(
height: numberLabelHeight,
child: Baseline(
baseline: numberLabelBaseline,
baselineType: TextBaseline.alphabetic,
child: Text(
text,
style: const TextStyle(
fontSize: _kTimerPickerLabelFontSize,
fontWeight: FontWeight.w600,
),
maxLines: 1,
softWrap: false,
),
),
),
),
);
}
// The picker has to be wider than its content, since the separators
// are part of the picker.
Widget _buildPickerNumberLabel(String text, EdgeInsetsDirectional padding) {
return Container(
width: _kTimerPickerColumnIntrinsicWidth + padding.horizontal,
padding: padding.resolve(textDirection),
alignment: AlignmentDirectional.centerStart.resolve(textDirection),
child: Container(
width: numberLabelWidth,
alignment: AlignmentDirectional.centerEnd.resolve(textDirection),
child: Text(text, softWrap: false, maxLines: 1, overflow: TextOverflow.visible),
),
);
}
Widget _buildHourPicker(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
_hourScrollController ??= FixedExtentScrollController(
initialItem: selectedHour!
);
return CupertinoPicker(
scrollController: _hourScrollController,
magnification: _kMagnification,
offAxisFraction: _calculateOffAxisFraction(additionalPadding.start, 0),
itemExtent: widget.itemExtent,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
onSelectedItemChanged: (int index) {
setState(() {
selectedHour = index;
widget.onTimerDurationChanged(
Duration(
hours: selectedHour!,
minutes: selectedMinute,
seconds: selectedSecond ?? 0,
),
);
});
},
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(24, (int index) {
final String label = localizations.timerPickerHourLabel(index) ?? '';
final String semanticsLabel = textDirectionFactor == 1
? localizations.timerPickerHour(index) + label
: label + localizations.timerPickerHour(index);
return Semantics(
label: semanticsLabel,
excludeSemantics: true,
child: _buildPickerNumberLabel(localizations.timerPickerHour(index), additionalPadding),
);
}),
);
}
Widget _buildHourColumn(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
additionalPadding = EdgeInsetsDirectional.only(
start: math.max(additionalPadding.start, 0),
end: math.max(additionalPadding.end, 0),
);
return Stack(
children: <Widget>[
NotificationListener<ScrollEndNotification>(
onNotification: (ScrollEndNotification notification) {
setState(() { lastSelectedHour = selectedHour; });
return false;
},
child: _buildHourPicker(additionalPadding, selectionOverlay),
),
_buildLabel(
localizations.timerPickerHourLabel(lastSelectedHour ?? selectedHour!) ?? '',
additionalPadding,
),
],
);
}
Widget _buildMinutePicker(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
_minuteScrollController ??= FixedExtentScrollController(
initialItem: selectedMinute ~/ widget.minuteInterval,
);
return CupertinoPicker(
scrollController: _minuteScrollController,
magnification: _kMagnification,
offAxisFraction: _calculateOffAxisFraction(
additionalPadding.start,
widget.mode == CupertinoTimerPickerMode.ms ? 0 : 1,
),
itemExtent: widget.itemExtent,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
looping: true,
onSelectedItemChanged: (int index) {
setState(() {
selectedMinute = index * widget.minuteInterval;
widget.onTimerDurationChanged(
Duration(
hours: selectedHour ?? 0,
minutes: selectedMinute,
seconds: selectedSecond ?? 0,
),
);
});
},
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(60 ~/ widget.minuteInterval, (int index) {
final int minute = index * widget.minuteInterval;
final String label = localizations.timerPickerMinuteLabel(minute) ?? '';
final String semanticsLabel = textDirectionFactor == 1
? localizations.timerPickerMinute(minute) + label
: label + localizations.timerPickerMinute(minute);
return Semantics(
label: semanticsLabel,
excludeSemantics: true,
child: _buildPickerNumberLabel(localizations.timerPickerMinute(minute), additionalPadding),
);
}),
);
}
Widget _buildMinuteColumn(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
additionalPadding = EdgeInsetsDirectional.only(
start: math.max(additionalPadding.start, 0),
end: math.max(additionalPadding.end, 0),
);
return Stack(
children: <Widget>[
NotificationListener<ScrollEndNotification>(
onNotification: (ScrollEndNotification notification) {
setState(() { lastSelectedMinute = selectedMinute; });
return false;
},
child: _buildMinutePicker(additionalPadding, selectionOverlay),
),
_buildLabel(
localizations.timerPickerMinuteLabel(lastSelectedMinute ?? selectedMinute) ?? '',
additionalPadding,
),
],
);
}
Widget _buildSecondPicker(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
_secondScrollController ??= FixedExtentScrollController(
initialItem: selectedSecond! ~/ widget.secondInterval,
);
return CupertinoPicker(
scrollController: _secondScrollController,
magnification: _kMagnification,
offAxisFraction: _calculateOffAxisFraction(
additionalPadding.start,
widget.mode == CupertinoTimerPickerMode.ms ? 1 : 2,
),
itemExtent: widget.itemExtent,
backgroundColor: widget.backgroundColor,
squeeze: _kSqueeze,
looping: true,
onSelectedItemChanged: (int index) {
setState(() {
selectedSecond = index * widget.secondInterval;
widget.onTimerDurationChanged(
Duration(
hours: selectedHour ?? 0,
minutes: selectedMinute,
seconds: selectedSecond!,
),
);
});
},
selectionOverlay: selectionOverlay,
children: List<Widget>.generate(60 ~/ widget.secondInterval, (int index) {
final int second = index * widget.secondInterval;
final String label = localizations.timerPickerSecondLabel(second) ?? '';
final String semanticsLabel = textDirectionFactor == 1
? localizations.timerPickerSecond(second) + label
: label + localizations.timerPickerSecond(second);
return Semantics(
label: semanticsLabel,
excludeSemantics: true,
child: _buildPickerNumberLabel(localizations.timerPickerSecond(second), additionalPadding),
);
}),
);
}
Widget _buildSecondColumn(EdgeInsetsDirectional additionalPadding, Widget selectionOverlay) {
additionalPadding = EdgeInsetsDirectional.only(
start: math.max(additionalPadding.start, 0),
end: math.max(additionalPadding.end, 0),
);
return Stack(
children: <Widget>[
NotificationListener<ScrollEndNotification>(
onNotification: (ScrollEndNotification notification) {
setState(() { lastSelectedSecond = selectedSecond; });
return false;
},
child: _buildSecondPicker(additionalPadding, selectionOverlay),
),
_buildLabel(
localizations.timerPickerSecondLabel(lastSelectedSecond ?? selectedSecond!) ?? '',
additionalPadding,
),
],
);
}
// Returns [CupertinoTextThemeData.pickerTextStyle] and magnifies the fontSize
// by [magnification].
TextStyle _textStyleFrom(BuildContext context, [double magnification = 1.0]) {
final TextStyle textStyle = CupertinoTheme.of(context).textTheme.pickerTextStyle;
return textStyle.copyWith(
color: CupertinoDynamicColor.maybeResolve(textStyle.color, context),
fontSize: textStyle.fontSize! * magnification,
);
}
// Calculate the number label center point by padding start and position to
// get a reasonable offAxisFraction.
double _calculateOffAxisFraction(double paddingStart, int position) {
final double centerPoint = paddingStart + (numberLabelWidth / 2);
// Compute the offAxisFraction needed to be straight within the pickerColumn.
final double pickerColumnOffAxisFraction =
0.5 - centerPoint / pickerColumnWidth;
// Position is to calculate the reasonable offAxisFraction in the picker.
final double timerPickerOffAxisFraction =
0.5 - (centerPoint + pickerColumnWidth * position) / totalWidth;
return (pickerColumnOffAxisFraction - timerPickerOffAxisFraction) * textDirectionFactor;
}
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
// The timer picker can be divided into columns corresponding to hour,
// minute, and second. Each column consists of a scrollable and a fixed
// label on top of it.
List<Widget> columns;
if (widget.mode == CupertinoTimerPickerMode.hms) {
// Pad the widget to make it as wide as `_kPickerWidth`.
pickerColumnWidth =
_kTimerPickerColumnIntrinsicWidth + (_kTimerPickerHalfColumnPadding * 2);
totalWidth = pickerColumnWidth * 3;
} else {
// The default totalWidth for 2-column modes.
totalWidth = _kPickerWidth;
pickerColumnWidth = totalWidth / 2;
}
if (constraints.maxWidth < totalWidth) {
totalWidth = constraints.maxWidth;
pickerColumnWidth =
totalWidth / (widget.mode == CupertinoTimerPickerMode.hms ? 3 : 2);
}
final double baseLabelContentWidth = numberLabelWidth + _kTimerPickerLabelPadSize;
final double minuteLabelContentWidth = baseLabelContentWidth + minuteLabelWidth;
switch (widget.mode) {
case CupertinoTimerPickerMode.hm:
// Pad the widget to make it as wide as `_kPickerWidth`.
final double hourLabelContentWidth = baseLabelContentWidth + hourLabelWidth;
double hourColumnStartPadding =
pickerColumnWidth - hourLabelContentWidth - _kTimerPickerHalfColumnPadding;
if (hourColumnStartPadding < _kTimerPickerMinHorizontalPadding) {
hourColumnStartPadding = _kTimerPickerMinHorizontalPadding;
}
double minuteColumnEndPadding =
pickerColumnWidth - minuteLabelContentWidth - _kTimerPickerHalfColumnPadding;
if (minuteColumnEndPadding < _kTimerPickerMinHorizontalPadding) {
minuteColumnEndPadding = _kTimerPickerMinHorizontalPadding;
}
columns = <Widget>[
_buildHourColumn(
EdgeInsetsDirectional.only(
start: hourColumnStartPadding,
end: pickerColumnWidth - hourColumnStartPadding - hourLabelContentWidth,
),
_startSelectionOverlay,
),
_buildMinuteColumn(
EdgeInsetsDirectional.only(
start: pickerColumnWidth - minuteColumnEndPadding - minuteLabelContentWidth,
end: minuteColumnEndPadding,
),
_endSelectionOverlay,
),
];
case CupertinoTimerPickerMode.ms:
final double secondLabelContentWidth = baseLabelContentWidth + secondLabelWidth;
double secondColumnEndPadding =
pickerColumnWidth - secondLabelContentWidth - _kTimerPickerHalfColumnPadding;
if (secondColumnEndPadding < _kTimerPickerMinHorizontalPadding) {
secondColumnEndPadding = _kTimerPickerMinHorizontalPadding;
}
double minuteColumnStartPadding =
pickerColumnWidth - minuteLabelContentWidth - _kTimerPickerHalfColumnPadding;
if (minuteColumnStartPadding < _kTimerPickerMinHorizontalPadding) {
minuteColumnStartPadding = _kTimerPickerMinHorizontalPadding;
}
columns = <Widget>[
_buildMinuteColumn(
EdgeInsetsDirectional.only(
start: minuteColumnStartPadding,
end: pickerColumnWidth - minuteColumnStartPadding - minuteLabelContentWidth,
),
_startSelectionOverlay,
),
_buildSecondColumn(
EdgeInsetsDirectional.only(
start: pickerColumnWidth - secondColumnEndPadding - minuteLabelContentWidth,
end: secondColumnEndPadding,
),
_endSelectionOverlay,
),
];
case CupertinoTimerPickerMode.hms:
final double hourColumnEndPadding =
pickerColumnWidth - baseLabelContentWidth - hourLabelWidth - _kTimerPickerMinHorizontalPadding;
final double minuteColumnPadding =
(pickerColumnWidth - minuteLabelContentWidth) / 2;
final double secondColumnStartPadding =
pickerColumnWidth - baseLabelContentWidth - secondLabelWidth - _kTimerPickerMinHorizontalPadding;
columns = <Widget>[
_buildHourColumn(
EdgeInsetsDirectional.only(
start: _kTimerPickerMinHorizontalPadding,
end: math.max(hourColumnEndPadding, 0),
),
_startSelectionOverlay,
),
_buildMinuteColumn(
EdgeInsetsDirectional.only(
start: minuteColumnPadding,
end: minuteColumnPadding,
),
_centerSelectionOverlay,
),
_buildSecondColumn(
EdgeInsetsDirectional.only(
start: math.max(secondColumnStartPadding, 0),
end: _kTimerPickerMinHorizontalPadding,
),
_endSelectionOverlay,
),
];
}
final CupertinoThemeData themeData = CupertinoTheme.of(context);
// The native iOS picker's text scaling is fixed, so we will also fix it
// as well in our picker.
return MediaQuery.withNoTextScaling(
child: CupertinoTheme(
data: themeData.copyWith(
textTheme: themeData.textTheme.copyWith(
pickerTextStyle: _textStyleFrom(context, _kTimerPickerMagnification),
),
),
child: Align(
alignment: widget.alignment,
child: Container(
color: CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context),
width: totalWidth,
height: _kPickerHeight,
child: DefaultTextStyle(
style: _textStyleFrom(context),
child: Row(children: columns.map((Widget child) => Expanded(child: child)).toList(growable: false)),
),
),
),
),
);
},
);
}
}
| flutter/packages/flutter/lib/src/cupertino/date_picker.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/date_picker.dart",
"repo_id": "flutter",
"token_count": 36213
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'theme.dart';
/// Implements a single iOS application page's layout.
///
/// The scaffold lays out the navigation bar on top and the content between or
/// behind the navigation bar.
///
/// When tapping a status bar at the top of the CupertinoPageScaffold, an
/// animation will complete for the current primary [ScrollView], scrolling to
/// the beginning. This is done using the [PrimaryScrollController] that
/// encloses the [ScrollView]. The [ScrollView.primary] flag is used to connect
/// a [ScrollView] to the enclosing [PrimaryScrollController].
///
/// {@tool dartpad}
/// This example shows a [CupertinoPageScaffold] with a [ListView] as a [child].
/// The [CupertinoButton] is connected to a callback that increments a counter.
/// The [backgroundColor] can be changed.
///
/// ** See code in examples/api/lib/cupertino/page_scaffold/cupertino_page_scaffold.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoTabScaffold], a similar widget for tabbed applications.
/// * [CupertinoPageRoute], a modal page route that typically hosts a
/// [CupertinoPageScaffold] with support for iOS-style page transitions.
class CupertinoPageScaffold extends StatefulWidget {
/// Creates a layout for pages with a navigation bar at the top.
const CupertinoPageScaffold({
super.key,
this.navigationBar,
this.backgroundColor,
this.resizeToAvoidBottomInset = true,
required this.child,
});
/// The [navigationBar], typically a [CupertinoNavigationBar], is drawn at the
/// top of the screen.
///
/// If translucent, the main content may slide behind it.
/// Otherwise, the main content's top margin will be offset by its height.
///
/// The scaffold assumes the navigation bar will account for the [MediaQuery]
/// top padding, also consume it if the navigation bar is opaque.
///
/// By default [navigationBar] disables text scaling to match the native iOS
/// behavior. To override such behavior, wrap each of the [navigationBar]'s
/// components inside a [MediaQuery] with the desired [TextScaler].
// TODO(xster): document its page transition animation when ready
final ObstructingPreferredSizeWidget? navigationBar;
/// Widget to show in the main content area.
///
/// Content can slide under the [navigationBar] when they're translucent.
/// In that case, the child's [BuildContext]'s [MediaQuery] will have a
/// top padding indicating the area of obstructing overlap from the
/// [navigationBar].
final Widget child;
/// The color of the widget that underlies the entire scaffold.
///
/// By default uses [CupertinoTheme]'s `scaffoldBackgroundColor` when null.
final Color? backgroundColor;
/// Whether the [child] should size itself to avoid the window's bottom inset.
///
/// For example, if there is an onscreen keyboard displayed above the
/// scaffold, the body can be resized to avoid overlapping the keyboard, which
/// prevents widgets inside the body from being obscured by the keyboard.
///
/// Defaults to true.
final bool resizeToAvoidBottomInset;
@override
State<CupertinoPageScaffold> createState() => _CupertinoPageScaffoldState();
}
class _CupertinoPageScaffoldState extends State<CupertinoPageScaffold> {
void _handleStatusBarTap() {
final ScrollController? primaryScrollController = PrimaryScrollController.maybeOf(context);
// Only act on the scroll controller if it has any attached scroll positions.
if (primaryScrollController != null && primaryScrollController.hasClients) {
primaryScrollController.animateTo(
0.0,
// Eyeballed from iOS.
duration: const Duration(milliseconds: 500),
curve: Curves.linearToEaseOut,
);
}
}
@override
Widget build(BuildContext context) {
Widget paddedContent = widget.child;
final MediaQueryData existingMediaQuery = MediaQuery.of(context);
if (widget.navigationBar != null) {
// TODO(xster): Use real size after partial layout instead of preferred size.
// https://github.com/flutter/flutter/issues/12912
final double topPadding =
widget.navigationBar!.preferredSize.height + existingMediaQuery.padding.top;
// Propagate bottom padding and include viewInsets if appropriate
final double bottomPadding = widget.resizeToAvoidBottomInset
? existingMediaQuery.viewInsets.bottom
: 0.0;
final EdgeInsets newViewInsets = widget.resizeToAvoidBottomInset
// The insets are consumed by the scaffolds and no longer exposed to
// the descendant subtree.
? existingMediaQuery.viewInsets.copyWith(bottom: 0.0)
: existingMediaQuery.viewInsets;
final bool fullObstruction = widget.navigationBar!.shouldFullyObstruct(context);
// If navigation bar is opaquely obstructing, directly shift the main content
// down. If translucent, let main content draw behind navigation bar but hint the
// obstructed area.
if (fullObstruction) {
paddedContent = MediaQuery(
data: existingMediaQuery
// If the navigation bar is opaque, the top media query padding is fully consumed by the navigation bar.
.removePadding(removeTop: true)
.copyWith(
viewInsets: newViewInsets,
),
child: Padding(
padding: EdgeInsets.only(top: topPadding, bottom: bottomPadding),
child: paddedContent,
),
);
} else {
paddedContent = MediaQuery(
data: existingMediaQuery.copyWith(
padding: existingMediaQuery.padding.copyWith(
top: topPadding,
),
viewInsets: newViewInsets,
),
child: Padding(
padding: EdgeInsets.only(bottom: bottomPadding),
child: paddedContent,
),
);
}
} else if (widget.resizeToAvoidBottomInset) {
// If there is no navigation bar, still may need to add padding in order
// to support resizeToAvoidBottomInset.
paddedContent = MediaQuery(
data: existingMediaQuery.copyWith(
viewInsets: existingMediaQuery.viewInsets.copyWith(bottom: 0)
),
child: Padding(
padding: EdgeInsets.only(bottom: existingMediaQuery.viewInsets.bottom),
child: paddedContent,
),
);
}
return DecoratedBox(
decoration: BoxDecoration(
color: CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context)
?? CupertinoTheme.of(context).scaffoldBackgroundColor,
),
child: Stack(
children: <Widget>[
// The main content being at the bottom is added to the stack first.
paddedContent,
if (widget.navigationBar != null)
Positioned(
top: 0.0,
left: 0.0,
right: 0.0,
child: MediaQuery.withNoTextScaling(
child: widget.navigationBar!,
),
),
// Add a touch handler the size of the status bar on top of all contents
// to handle scroll to top by status bar taps.
Positioned(
top: 0.0,
left: 0.0,
right: 0.0,
height: existingMediaQuery.padding.top,
child: GestureDetector(
excludeFromSemantics: true,
onTap: _handleStatusBarTap,
),
),
],
),
);
}
}
/// Widget that has a preferred size and reports whether it fully obstructs
/// widgets behind it.
///
/// Used by [CupertinoPageScaffold] to either shift away fully obstructed content
/// or provide a padding guide to partially obstructed content.
abstract class ObstructingPreferredSizeWidget implements PreferredSizeWidget {
/// If true, this widget fully obstructs widgets behind it by the specified
/// size.
///
/// If false, this widget partially obstructs.
bool shouldFullyObstruct(BuildContext context);
}
| flutter/packages/flutter/lib/src/cupertino/page_scaffold.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/page_scaffold.dart",
"repo_id": "flutter",
"token_count": 2884
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart' show ValueListenable, clampDouble;
import 'package:flutter/widgets.dart';
import 'localizations.dart';
import 'text_selection_toolbar.dart';
import 'text_selection_toolbar_button.dart';
import 'theme.dart';
// Read off from the output on iOS 12. This color does not vary with the
// application's theme color.
const double _kSelectionHandleOverlap = 1.5;
// Extracted from https://developer.apple.com/design/resources/.
const double _kSelectionHandleRadius = 6;
// Minimal padding from tip of the selection toolbar arrow to horizontal edges of the
// screen. Eyeballed value.
const double _kArrowScreenPadding = 26.0;
/// Draws a single text selection handle with a bar and a ball.
class _CupertinoTextSelectionHandlePainter extends CustomPainter {
const _CupertinoTextSelectionHandlePainter(this.color);
final Color color;
@override
void paint(Canvas canvas, Size size) {
const double halfStrokeWidth = 1.0;
final Paint paint = Paint()..color = color;
final Rect circle = Rect.fromCircle(
center: const Offset(_kSelectionHandleRadius, _kSelectionHandleRadius),
radius: _kSelectionHandleRadius,
);
final Rect line = Rect.fromPoints(
const Offset(
_kSelectionHandleRadius - halfStrokeWidth,
2 * _kSelectionHandleRadius - _kSelectionHandleOverlap,
),
Offset(_kSelectionHandleRadius + halfStrokeWidth, size.height),
);
final Path path = Path()
..addOval(circle)
// Draw line so it slightly overlaps the circle.
..addRect(line);
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(_CupertinoTextSelectionHandlePainter oldPainter) => color != oldPainter.color;
}
/// iOS Cupertino styled text selection handle controls.
///
/// Specifically does not manage the toolbar, which is left to
/// [EditableText.contextMenuBuilder].
@Deprecated(
'Use `CupertinoTextSelectionControls`. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
class CupertinoTextSelectionHandleControls extends CupertinoTextSelectionControls with TextSelectionHandleControls {
}
/// iOS Cupertino styled text selection controls.
///
/// The [cupertinoTextSelectionControls] global variable has a
/// suitable instance of this class.
class CupertinoTextSelectionControls extends TextSelectionControls {
/// Returns the size of the Cupertino handle.
@override
Size getHandleSize(double textLineHeight) {
return Size(
_kSelectionHandleRadius * 2,
textLineHeight + _kSelectionHandleRadius * 2 - _kSelectionHandleOverlap,
);
}
/// Builder for iOS-style copy/paste text selection toolbar.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
@override
Widget buildToolbar(
BuildContext context,
Rect globalEditableRegion,
double textLineHeight,
Offset selectionMidpoint,
List<TextSelectionPoint> endpoints,
TextSelectionDelegate delegate,
ValueListenable<ClipboardStatus>? clipboardStatus,
Offset? lastSecondaryTapDownPosition,
) {
return _CupertinoTextSelectionControlsToolbar(
clipboardStatus: clipboardStatus,
endpoints: endpoints,
globalEditableRegion: globalEditableRegion,
handleCut: canCut(delegate) ? () => handleCut(delegate) : null,
handleCopy: canCopy(delegate) ? () => handleCopy(delegate) : null,
handlePaste: canPaste(delegate) ? () => handlePaste(delegate) : null,
handleSelectAll: canSelectAll(delegate) ? () => handleSelectAll(delegate) : null,
selectionMidpoint: selectionMidpoint,
textLineHeight: textLineHeight,
);
}
/// Builder for iOS text selection edges.
@override
Widget buildHandle(BuildContext context, TextSelectionHandleType type, double textLineHeight, [VoidCallback? onTap]) {
// iOS selection handles do not respond to taps.
final Size desiredSize;
final Widget handle;
final Widget customPaint = CustomPaint(
painter: _CupertinoTextSelectionHandlePainter(CupertinoTheme.of(context).primaryColor),
);
// [buildHandle]'s widget is positioned at the selection cursor's bottom
// baseline. We transform the handle such that the SizedBox is superimposed
// on top of the text selection endpoints.
switch (type) {
case TextSelectionHandleType.left:
desiredSize = getHandleSize(textLineHeight);
handle = SizedBox.fromSize(
size: desiredSize,
child: customPaint,
);
return handle;
case TextSelectionHandleType.right:
desiredSize = getHandleSize(textLineHeight);
handle = SizedBox.fromSize(
size: desiredSize,
child: customPaint,
);
return Transform(
transform: Matrix4.identity()
..translate(desiredSize.width / 2, desiredSize.height / 2)
..rotateZ(math.pi)
..translate(-desiredSize.width / 2, -desiredSize.height / 2),
child: handle,
);
// iOS doesn't draw anything for collapsed selections.
case TextSelectionHandleType.collapsed:
return const SizedBox.shrink();
}
}
/// Gets anchor for cupertino-style text selection handles.
///
/// See [TextSelectionControls.getHandleAnchor].
@override
Offset getHandleAnchor(TextSelectionHandleType type, double textLineHeight) {
final Size handleSize;
switch (type) {
// The circle is at the top for the left handle, and the anchor point is
// all the way at the bottom of the line.
case TextSelectionHandleType.left:
handleSize = getHandleSize(textLineHeight);
return Offset(
handleSize.width / 2,
handleSize.height,
);
// The right handle is vertically flipped, and the anchor point is near
// the top of the circle to give slight overlap.
case TextSelectionHandleType.right:
handleSize = getHandleSize(textLineHeight);
return Offset(
handleSize.width / 2,
handleSize.height - 2 * _kSelectionHandleRadius + _kSelectionHandleOverlap,
);
// A collapsed handle anchors itself so that it's centered.
case TextSelectionHandleType.collapsed:
handleSize = getHandleSize(textLineHeight);
return Offset(
handleSize.width / 2,
textLineHeight + (handleSize.height - textLineHeight) / 2,
);
}
}
}
// TODO(justinmc): Deprecate this after TextSelectionControls.buildToolbar is
// deleted, when users should migrate back to cupertinoTextSelectionControls.
// See https://github.com/flutter/flutter/pull/124262
/// Text selection handle controls that follow iOS design conventions.
final TextSelectionControls cupertinoTextSelectionHandleControls =
CupertinoTextSelectionHandleControls();
/// Text selection controls that follow iOS design conventions.
final TextSelectionControls cupertinoTextSelectionControls =
CupertinoTextSelectionControls();
// Generates the child that's passed into CupertinoTextSelectionToolbar.
class _CupertinoTextSelectionControlsToolbar extends StatefulWidget {
const _CupertinoTextSelectionControlsToolbar({
required this.clipboardStatus,
required this.endpoints,
required this.globalEditableRegion,
required this.handleCopy,
required this.handleCut,
required this.handlePaste,
required this.handleSelectAll,
required this.selectionMidpoint,
required this.textLineHeight,
});
final ValueListenable<ClipboardStatus>? clipboardStatus;
final List<TextSelectionPoint> endpoints;
final Rect globalEditableRegion;
final VoidCallback? handleCopy;
final VoidCallback? handleCut;
final VoidCallback? handlePaste;
final VoidCallback? handleSelectAll;
final Offset selectionMidpoint;
final double textLineHeight;
@override
_CupertinoTextSelectionControlsToolbarState createState() => _CupertinoTextSelectionControlsToolbarState();
}
class _CupertinoTextSelectionControlsToolbarState extends State<_CupertinoTextSelectionControlsToolbar> {
void _onChangedClipboardStatus() {
setState(() {
// Inform the widget that the value of clipboardStatus has changed.
});
}
@override
void initState() {
super.initState();
widget.clipboardStatus?.addListener(_onChangedClipboardStatus);
}
@override
void didUpdateWidget(_CupertinoTextSelectionControlsToolbar oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.clipboardStatus != widget.clipboardStatus) {
oldWidget.clipboardStatus?.removeListener(_onChangedClipboardStatus);
widget.clipboardStatus?.addListener(_onChangedClipboardStatus);
}
}
@override
void dispose() {
widget.clipboardStatus?.removeListener(_onChangedClipboardStatus);
super.dispose();
}
@override
Widget build(BuildContext context) {
// Don't render the menu until the state of the clipboard is known.
if (widget.handlePaste != null && widget.clipboardStatus?.value == ClipboardStatus.unknown) {
return const SizedBox.shrink();
}
assert(debugCheckHasMediaQuery(context));
final EdgeInsets mediaQueryPadding = MediaQuery.paddingOf(context);
// The toolbar should appear below the TextField when there is not enough
// space above the TextField to show it, assuming there's always enough
// space at the bottom in this case.
final double anchorX = clampDouble(widget.selectionMidpoint.dx + widget.globalEditableRegion.left,
_kArrowScreenPadding + mediaQueryPadding.left,
MediaQuery.sizeOf(context).width - mediaQueryPadding.right - _kArrowScreenPadding,
);
final double topAmountInEditableRegion = widget.endpoints.first.point.dy - widget.textLineHeight;
final double anchorTop = math.max(topAmountInEditableRegion, 0) + widget.globalEditableRegion.top;
// The y-coordinate has to be calculated instead of directly quoting
// selectionMidpoint.dy, since the caller
// (TextSelectionOverlay._buildToolbar) does not know whether the toolbar is
// going to be facing up or down.
final Offset anchorAbove = Offset(
anchorX,
anchorTop,
);
final Offset anchorBelow = Offset(
anchorX,
widget.endpoints.last.point.dy + widget.globalEditableRegion.top,
);
final List<Widget> items = <Widget>[];
final CupertinoLocalizations localizations = CupertinoLocalizations.of(context);
final Widget onePhysicalPixelVerticalDivider =
SizedBox(width: 1.0 / MediaQuery.devicePixelRatioOf(context));
void addToolbarButton(
String text,
VoidCallback onPressed,
) {
if (items.isNotEmpty) {
items.add(onePhysicalPixelVerticalDivider);
}
items.add(CupertinoTextSelectionToolbarButton.text(
onPressed: onPressed,
text: text,
));
}
if (widget.handleCut != null) {
addToolbarButton(localizations.cutButtonLabel, widget.handleCut!);
}
if (widget.handleCopy != null) {
addToolbarButton(localizations.copyButtonLabel, widget.handleCopy!);
}
if (widget.handlePaste != null
&& widget.clipboardStatus?.value == ClipboardStatus.pasteable) {
addToolbarButton(localizations.pasteButtonLabel, widget.handlePaste!);
}
if (widget.handleSelectAll != null) {
addToolbarButton(localizations.selectAllButtonLabel, widget.handleSelectAll!);
}
// If there is no option available, build an empty widget.
if (items.isEmpty) {
return const SizedBox.shrink();
}
return CupertinoTextSelectionToolbar(
anchorAbove: anchorAbove,
anchorBelow: anchorBelow,
children: items,
);
}
}
| flutter/packages/flutter/lib/src/cupertino/text_selection.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/text_selection.dart",
"repo_id": "flutter",
"token_count": 4043
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui_web' as ui_web;
import '../web.dart' as web;
import 'platform.dart' as platform;
export 'platform.dart' show TargetPlatform;
/// The web implementation of [platform.defaultTargetPlatform].
platform.TargetPlatform get defaultTargetPlatform {
// To get a better guess at the targetPlatform we need to be able to reference
// the window, but that won't be available until we fix the platforms
// configuration for Flutter.
return platform.debugDefaultTargetPlatformOverride ??
_testPlatform ??
_browserPlatform;
}
final platform.TargetPlatform? _testPlatform = () {
platform.TargetPlatform? result;
assert(() {
if (ui_web.debugEmulateFlutterTesterEnvironment) {
result = platform.TargetPlatform.android;
}
return true;
}());
return result;
}();
// Lazy-initialized and forever cached current browser platform.
//
// Computing the platform is expensive as it uses `window.matchMedia`, which
// needs to parse and evaluate a CSS selector. On some devices this takes up to
// 0.20ms. As `defaultTargetPlatform` is routinely called dozens of times per
// frame this value should be cached.
final platform.TargetPlatform _browserPlatform = () {
final String navigatorPlatform = web.window.navigator.platform.toLowerCase();
if (navigatorPlatform.startsWith('mac')) {
return platform.TargetPlatform.macOS;
}
if (navigatorPlatform.startsWith('win')) {
return platform.TargetPlatform.windows;
}
if (navigatorPlatform.contains('iphone') ||
navigatorPlatform.contains('ipad') ||
navigatorPlatform.contains('ipod')) {
return platform.TargetPlatform.iOS;
}
if (navigatorPlatform.contains('android')) {
return platform.TargetPlatform.android;
}
// Since some phones can report a window.navigator.platform as Linux, fall
// back to use CSS to disambiguate Android vs Linux desktop. If the CSS
// indicates that a device has a "fine pointer" (mouse) as the primary
// pointing device, then we'll assume desktop linux, and otherwise we'll
// assume Android.
if (web.window.matchMedia('only screen and (pointer: fine)').matches) {
return platform.TargetPlatform.linux;
}
return platform.TargetPlatform.android;
}();
| flutter/packages/flutter/lib/src/foundation/_platform_web.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/_platform_web.dart",
"repo_id": "flutter",
"token_count": 688
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:meta/meta.dart';
import 'diagnostics.dart';
/// A [Key] is an identifier for [Widget]s, [Element]s and [SemanticsNode]s.
///
/// A new widget will only be used to update an existing element if its key is
/// the same as the key of the current widget associated with the element.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=kn0EOS-ZiIc}
///
/// Keys must be unique amongst the [Element]s with the same parent.
///
/// Subclasses of [Key] should either subclass [LocalKey] or [GlobalKey].
///
/// See also:
///
/// * [Widget.key], which discusses how widgets use keys.
@immutable
abstract class Key {
/// Construct a [ValueKey<String>] with the given [String].
///
/// This is the simplest way to create keys.
const factory Key(String value) = ValueKey<String>;
/// Default constructor, used by subclasses.
///
/// Useful so that subclasses can call us, because the [Key.new] factory
/// constructor shadows the implicit constructor.
@protected
const Key.empty();
}
/// A key that is not a [GlobalKey].
///
/// Keys must be unique amongst the [Element]s with the same parent. By
/// contrast, [GlobalKey]s must be unique across the entire app.
///
/// See also:
///
/// * [Widget.key], which discusses how widgets use keys.
abstract class LocalKey extends Key {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const LocalKey() : super.empty();
}
/// A key that is only equal to itself.
///
/// This cannot be created with a const constructor because that implies that
/// all instantiated keys would be the same instance and therefore not be unique.
class UniqueKey extends LocalKey {
/// Creates a key that is equal only to itself.
///
/// The key cannot be created with a const constructor because that implies
/// that all instantiated keys would be the same instance and therefore not
/// be unique.
// ignore: prefer_const_constructors_in_immutables , never use const for this class
UniqueKey();
@override
String toString() => '[#${shortHash(this)}]';
}
/// A key that uses a value of a particular type to identify itself.
///
/// A [ValueKey<T>] is equal to another [ValueKey<T>] if, and only if, their
/// values are [operator==].
///
/// This class can be subclassed to create value keys that will not be equal to
/// other value keys that happen to use the same value. If the subclass is
/// private, this results in a value key type that cannot collide with keys from
/// other sources, which could be useful, for example, if the keys are being
/// used as fallbacks in the same scope as keys supplied from another widget.
///
/// See also:
///
/// * [Widget.key], which discusses how widgets use keys.
class ValueKey<T> extends LocalKey {
/// Creates a key that delegates its [operator==] to the given value.
const ValueKey(this.value);
/// The value to which this key delegates its [operator==]
final T value;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ValueKey<T>
&& other.value == value;
}
@override
int get hashCode => Object.hash(runtimeType, value);
@override
String toString() {
final String valueString = T == String ? "<'$value'>" : '<$value>';
// The crazy on the next line is a workaround for
// https://github.com/dart-lang/sdk/issues/33297
if (runtimeType == _TypeLiteral<ValueKey<T>>().type) {
return '[$valueString]';
}
return '[$T $valueString]';
}
}
class _TypeLiteral<T> {
Type get type => T;
}
| flutter/packages/flutter/lib/src/foundation/key.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/key.dart",
"repo_id": "flutter",
"token_count": 1107
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection';
import 'dart:ui' as ui show PointerDataPacket;
import 'package:flutter/foundation.dart';
import 'package:flutter/scheduler.dart';
import 'arena.dart';
import 'converter.dart';
import 'debug.dart';
import 'events.dart';
import 'hit_test.dart';
import 'pointer_router.dart';
import 'pointer_signal_resolver.dart';
import 'resampler.dart';
export 'dart:ui' show Offset;
export 'package:flutter/foundation.dart' show DiagnosticsNode, InformationCollector;
export 'arena.dart' show GestureArenaManager;
export 'events.dart' show PointerEvent;
export 'hit_test.dart' show HitTestEntry, HitTestResult, HitTestTarget;
export 'pointer_router.dart' show PointerRouter;
export 'pointer_signal_resolver.dart' show PointerSignalResolver;
typedef _HandleSampleTimeChangedCallback = void Function();
/// Class that implements clock used for sampling.
class SamplingClock {
/// Returns current time.
DateTime now() => DateTime.now();
/// Returns a new stopwatch that uses the current time as reported by `this`.
///
/// See also:
///
/// * [GestureBinding.debugSamplingClock], which is used in tests and
/// debug builds to observe [FakeAsync].
Stopwatch stopwatch() => Stopwatch(); // flutter_ignore: stopwatch (see analyze.dart)
// Ignore context: This is replaced by debugSampling clock in the test binding.
}
// Class that handles resampling of touch events for multiple pointer
// devices.
//
// The `samplingInterval` is used to determine the approximate next
// time for resampling.
// SchedulerBinding's `currentSystemFrameTimeStamp` is used to determine
// sample time.
class _Resampler {
_Resampler(this._handlePointerEvent, this._handleSampleTimeChanged, this._samplingInterval);
// Resamplers used to filter incoming pointer events.
final Map<int, PointerEventResampler> _resamplers = <int, PointerEventResampler>{};
// Flag to track if a frame callback has been scheduled.
bool _frameCallbackScheduled = false;
// Last frame time for resampling.
Duration _frameTime = Duration.zero;
// Time since `_frameTime` was updated.
Stopwatch _frameTimeAge = Stopwatch(); // flutter_ignore: stopwatch (see analyze.dart)
// Ignore context: This is tested safely outside of FakeAsync.
// Last sample time and time stamp of last event.
//
// Only used for debugPrint of resampling margin.
Duration _lastSampleTime = Duration.zero;
Duration _lastEventTime = Duration.zero;
// Callback used to handle pointer events.
final HandleEventCallback _handlePointerEvent;
// Callback used to handle sample time changes.
final _HandleSampleTimeChangedCallback _handleSampleTimeChanged;
// Interval used for sampling.
final Duration _samplingInterval;
// Timer used to schedule resampling.
Timer? _timer;
// Add `event` for resampling or dispatch it directly if
// not a touch event.
void addOrDispatch(PointerEvent event) {
// Add touch event to resampler or dispatch pointer event directly.
if (event.kind == PointerDeviceKind.touch) {
// Save last event time for debugPrint of resampling margin.
_lastEventTime = event.timeStamp;
final PointerEventResampler resampler = _resamplers.putIfAbsent(
event.device,
() => PointerEventResampler(),
);
resampler.addEvent(event);
} else {
_handlePointerEvent(event);
}
}
// Sample and dispatch events.
//
// The `samplingOffset` is relative to the current frame time, which
// can be in the past when we're not actively resampling.
//
// The `samplingClock` is the clock used to determine frame time age.
void sample(Duration samplingOffset, SamplingClock clock) {
final SchedulerBinding scheduler = SchedulerBinding.instance;
// Initialize `_frameTime` if needed. This will be used for periodic
// sampling when frame callbacks are not received.
if (_frameTime == Duration.zero) {
_frameTime = Duration(milliseconds: clock.now().millisecondsSinceEpoch);
_frameTimeAge = clock.stopwatch()..start();
}
// Schedule periodic resampling if `_timer` is not already active.
if (_timer?.isActive != true) {
_timer = Timer.periodic(_samplingInterval, (_) => _onSampleTimeChanged());
}
// Calculate the effective frame time by taking the number
// of sampling intervals since last time `_frameTime` was
// updated into account. This allows us to advance sample
// time without having to receive frame callbacks.
final int samplingIntervalUs = _samplingInterval.inMicroseconds;
final int elapsedIntervals = _frameTimeAge.elapsedMicroseconds ~/ samplingIntervalUs;
final int elapsedUs = elapsedIntervals * samplingIntervalUs;
final Duration frameTime = _frameTime + Duration(microseconds: elapsedUs);
// Determine sample time by adding the offset to the current
// frame time. This is expected to be in the past and not
// result in any dispatched events unless we're actively
// resampling events.
final Duration sampleTime = frameTime + samplingOffset;
// Determine next sample time by adding the sampling interval
// to the current sample time.
final Duration nextSampleTime = sampleTime + _samplingInterval;
// Iterate over active resamplers and sample pointer events for
// current sample time.
for (final PointerEventResampler resampler in _resamplers.values) {
resampler.sample(sampleTime, nextSampleTime, _handlePointerEvent);
}
// Remove inactive resamplers.
_resamplers.removeWhere((int key, PointerEventResampler resampler) {
return !resampler.hasPendingEvents && !resampler.isDown;
});
// Save last sample time for debugPrint of resampling margin.
_lastSampleTime = sampleTime;
// Early out if another call to `sample` isn't needed.
if (_resamplers.isEmpty) {
_timer!.cancel();
return;
}
// Schedule a frame callback if another call to `sample` is needed.
if (!_frameCallbackScheduled) {
_frameCallbackScheduled = true;
// Add a post frame callback as this avoids producing unnecessary
// frames but ensures that sampling phase is adjusted to frame
// time when frames are produced.
scheduler.addPostFrameCallback((_) {
_frameCallbackScheduled = false;
// We use `currentSystemFrameTimeStamp` here as it's critical that
// sample time is in the same clock as the event time stamps, and
// never adjusted or scaled like `currentFrameTimeStamp`.
_frameTime = scheduler.currentSystemFrameTimeStamp;
_frameTimeAge.reset();
// Reset timer to match phase of latest frame callback.
_timer?.cancel();
_timer = Timer.periodic(_samplingInterval, (_) => _onSampleTimeChanged());
// Trigger an immediate sample time change.
_onSampleTimeChanged();
}, debugLabel: 'Resampler.startTimer');
}
}
// Stop all resampling and dispatched any queued events.
void stop() {
for (final PointerEventResampler resampler in _resamplers.values) {
resampler.stop(_handlePointerEvent);
}
_resamplers.clear();
_frameTime = Duration.zero;
_timer?.cancel();
}
void _onSampleTimeChanged() {
assert(() {
if (debugPrintResamplingMargin) {
final Duration resamplingMargin = _lastEventTime - _lastSampleTime;
debugPrint('$resamplingMargin');
}
return true;
}());
_handleSampleTimeChanged();
}
}
// The default sampling offset.
//
// Sampling offset is relative to presentation time. If we produce frames
// 16.667 ms before presentation and input rate is ~60hz, worst case latency
// is 33.334 ms. This however assumes zero latency from the input driver.
// 4.666 ms margin is added for this.
const Duration _defaultSamplingOffset = Duration(milliseconds: -38);
// The sampling interval.
//
// Sampling interval is used to determine the approximate time for subsequent
// sampling. This is used to sample events when frame callbacks are not
// being received and decide if early processing of up and removed events
// is appropriate. 16667 us for 60hz sampling interval.
const Duration _samplingInterval = Duration(microseconds: 16667);
/// A binding for the gesture subsystem.
///
/// ## Lifecycle of pointer events and the gesture arena
///
/// ### [PointerDownEvent]
///
/// When a [PointerDownEvent] is received by the [GestureBinding] (from
/// [dart:ui.PlatformDispatcher.onPointerDataPacket], as interpreted by the
/// [PointerEventConverter]), a [hitTest] is performed to determine which
/// [HitTestTarget] nodes are affected. (Other bindings are expected to
/// implement [hitTest] to defer to [HitTestable] objects. For example, the
/// rendering layer defers to the [RenderView] and the rest of the render object
/// hierarchy.)
///
/// The affected nodes then are given the event to handle ([dispatchEvent] calls
/// [HitTestTarget.handleEvent] for each affected node). If any have relevant
/// [GestureRecognizer]s, they provide the event to them using
/// [GestureRecognizer.addPointer]. This typically causes the recognizer to
/// register with the [PointerRouter] to receive notifications regarding the
/// pointer in question.
///
/// Once the hit test and dispatching logic is complete, the event is then
/// passed to the aforementioned [PointerRouter], which passes it to any objects
/// that have registered interest in that event.
///
/// Finally, the [gestureArena] is closed for the given pointer
/// ([GestureArenaManager.close]), which begins the process of selecting a
/// gesture to win that pointer.
///
/// ### Other events
///
/// A pointer that is [PointerEvent.down] may send further events, such as
/// [PointerMoveEvent], [PointerUpEvent], or [PointerCancelEvent]. These are
/// sent to the same [HitTestTarget] nodes as were found when the
/// [PointerDownEvent] was received (even if they have since been disposed; it is
/// the responsibility of those objects to be aware of that possibility).
///
/// Then, the events are routed to any still-registered entrants in the
/// [PointerRouter]'s table for that pointer.
///
/// When a [PointerUpEvent] is received, the [GestureArenaManager.sweep] method
/// is invoked to force the gesture arena logic to terminate if necessary.
mixin GestureBinding on BindingBase implements HitTestable, HitTestDispatcher, HitTestTarget {
@override
void initInstances() {
super.initInstances();
_instance = this;
platformDispatcher.onPointerDataPacket = _handlePointerDataPacket;
}
/// The singleton instance of this object.
///
/// Provides access to the features exposed by this mixin. The binding must
/// be initialized before using this getter; this is typically done by calling
/// [runApp] or [WidgetsFlutterBinding.ensureInitialized].
static GestureBinding get instance => BindingBase.checkInstance(_instance);
static GestureBinding? _instance;
@override
void unlocked() {
super.unlocked();
_flushPointerEventQueue();
}
final Queue<PointerEvent> _pendingPointerEvents = Queue<PointerEvent>();
void _handlePointerDataPacket(ui.PointerDataPacket packet) {
// We convert pointer data to logical pixels so that e.g. the touch slop can be
// defined in a device-independent manner.
try {
_pendingPointerEvents.addAll(PointerEventConverter.expand(packet.data, _devicePixelRatioForView));
if (!locked) {
_flushPointerEventQueue();
}
} catch (error, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: error,
stack: stack,
library: 'gestures library',
context: ErrorDescription('while handling a pointer data packet'),
));
}
}
double? _devicePixelRatioForView(int viewId) {
return platformDispatcher.view(id: viewId)?.devicePixelRatio;
}
/// Dispatch a [PointerCancelEvent] for the given pointer soon.
///
/// The pointer event will be dispatched before the next pointer event and
/// before the end of the microtask but not within this function call.
void cancelPointer(int pointer) {
if (_pendingPointerEvents.isEmpty && !locked) {
scheduleMicrotask(_flushPointerEventQueue);
}
_pendingPointerEvents.addFirst(PointerCancelEvent(pointer: pointer));
}
void _flushPointerEventQueue() {
assert(!locked);
while (_pendingPointerEvents.isNotEmpty) {
handlePointerEvent(_pendingPointerEvents.removeFirst());
}
}
/// A router that routes all pointer events received from the engine.
final PointerRouter pointerRouter = PointerRouter();
/// The gesture arenas used for disambiguating the meaning of sequences of
/// pointer events.
final GestureArenaManager gestureArena = GestureArenaManager();
/// The resolver used for determining which widget handles a
/// [PointerSignalEvent].
final PointerSignalResolver pointerSignalResolver = PointerSignalResolver();
/// State for all pointers which are currently down.
///
/// This map caches the hit test result done when the pointer goes down
/// ([PointerDownEvent] and [PointerPanZoomStartEvent]). This hit test result
/// will be used throughout the entire pointer interaction; that is, the
/// pointer is seen as pointing to the same place even if it has moved away
/// until pointer goes up ([PointerUpEvent] and [PointerPanZoomEndEvent]).
/// This matches the expected gesture interaction with a button, and allows
/// devices that don't support hovering to perform as few hit tests as
/// possible.
///
/// On the other hand, hovering requires hit testing on almost every frame.
/// This is handled in [RendererBinding] and [MouseTracker], and will ignore
/// the results cached here.
final Map<int, HitTestResult> _hitTests = <int, HitTestResult>{};
/// Dispatch an event to the targets found by a hit test on its position.
///
/// This method sends the given event to [dispatchEvent] based on event types:
///
/// * [PointerDownEvent]s and [PointerSignalEvent]s are dispatched to the
/// result of a new [hitTest].
/// * [PointerUpEvent]s and [PointerMoveEvent]s are dispatched to the result of hit test of the
/// preceding [PointerDownEvent]s.
/// * [PointerHoverEvent]s, [PointerAddedEvent]s, and [PointerRemovedEvent]s
/// are dispatched without a hit test result.
void handlePointerEvent(PointerEvent event) {
assert(!locked);
if (resamplingEnabled) {
_resampler.addOrDispatch(event);
_resampler.sample(samplingOffset, samplingClock);
return;
}
// Stop resampler if resampling is not enabled. This is a no-op if
// resampling was never enabled.
_resampler.stop();
_handlePointerEventImmediately(event);
}
void _handlePointerEventImmediately(PointerEvent event) {
HitTestResult? hitTestResult;
if (event is PointerDownEvent || event is PointerSignalEvent || event is PointerHoverEvent || event is PointerPanZoomStartEvent) {
assert(!_hitTests.containsKey(event.pointer), 'Pointer of ${event.toString(minLevel: DiagnosticLevel.debug)} unexpectedly has a HitTestResult associated with it.');
hitTestResult = HitTestResult();
hitTestInView(hitTestResult, event.position, event.viewId);
if (event is PointerDownEvent || event is PointerPanZoomStartEvent) {
_hitTests[event.pointer] = hitTestResult;
}
assert(() {
if (debugPrintHitTestResults) {
debugPrint('${event.toString(minLevel: DiagnosticLevel.debug)}: $hitTestResult');
}
return true;
}());
} else if (event is PointerUpEvent || event is PointerCancelEvent || event is PointerPanZoomEndEvent) {
hitTestResult = _hitTests.remove(event.pointer);
} else if (event.down || event is PointerPanZoomUpdateEvent) {
// Because events that occur with the pointer down (like
// [PointerMoveEvent]s) should be dispatched to the same place that their
// initial PointerDownEvent was, we want to re-use the path we found when
// the pointer went down, rather than do hit detection each time we get
// such an event.
hitTestResult = _hitTests[event.pointer];
}
assert(() {
if (debugPrintMouseHoverEvents && event is PointerHoverEvent) {
debugPrint('$event');
}
return true;
}());
if (hitTestResult != null ||
event is PointerAddedEvent ||
event is PointerRemovedEvent) {
dispatchEvent(event, hitTestResult);
}
}
/// Determine which [HitTestTarget] objects are located at a given position in
/// the specified view.
@override // from HitTestable
void hitTestInView(HitTestResult result, Offset position, int viewId) {
result.add(HitTestEntry(this));
}
@override // from HitTestable
@Deprecated(
'Use hitTestInView and specify the view to hit test. '
'This feature was deprecated after v3.11.0-20.0.pre.',
)
void hitTest(HitTestResult result, Offset position) {
hitTestInView(result, position, platformDispatcher.implicitView!.viewId);
}
/// Dispatch an event to [pointerRouter] and the path of a hit test result.
///
/// The `event` is routed to [pointerRouter]. If the `hitTestResult` is not
/// null, the event is also sent to every [HitTestTarget] in the entries of the
/// given [HitTestResult]. Any exceptions from the handlers are caught.
///
/// The `hitTestResult` argument may only be null for [PointerAddedEvent]s or
/// [PointerRemovedEvent]s.
@override // from HitTestDispatcher
@pragma('vm:notify-debugger-on-exception')
void dispatchEvent(PointerEvent event, HitTestResult? hitTestResult) {
assert(!locked);
// No hit test information implies that this is a [PointerAddedEvent] or
// [PointerRemovedEvent]. These events are specially routed here; other
// events will be routed through the `handleEvent` below.
if (hitTestResult == null) {
assert(event is PointerAddedEvent || event is PointerRemovedEvent);
try {
pointerRouter.route(event);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetailsForPointerEventDispatcher(
exception: exception,
stack: stack,
library: 'gesture library',
context: ErrorDescription('while dispatching a non-hit-tested pointer event'),
event: event,
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<PointerEvent>('Event', event, style: DiagnosticsTreeStyle.errorProperty),
],
));
}
return;
}
for (final HitTestEntry entry in hitTestResult.path) {
try {
entry.target.handleEvent(event.transformed(entry.transform), entry);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetailsForPointerEventDispatcher(
exception: exception,
stack: stack,
library: 'gesture library',
context: ErrorDescription('while dispatching a pointer event'),
event: event,
hitTestEntry: entry,
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<PointerEvent>('Event', event, style: DiagnosticsTreeStyle.errorProperty),
DiagnosticsProperty<HitTestTarget>('Target', entry.target, style: DiagnosticsTreeStyle.errorProperty),
],
));
}
}
}
@override // from HitTestTarget
void handleEvent(PointerEvent event, HitTestEntry entry) {
pointerRouter.route(event);
if (event is PointerDownEvent || event is PointerPanZoomStartEvent) {
gestureArena.close(event.pointer);
} else if (event is PointerUpEvent || event is PointerPanZoomEndEvent) {
gestureArena.sweep(event.pointer);
} else if (event is PointerSignalEvent) {
pointerSignalResolver.resolve(event);
}
}
/// Reset states of [GestureBinding].
///
/// This clears the hit test records.
///
/// This is typically called between tests.
@protected
void resetGestureBinding() {
_hitTests.clear();
}
void _handleSampleTimeChanged() {
if (!locked) {
if (resamplingEnabled) {
_resampler.sample(samplingOffset, samplingClock);
}
else {
_resampler.stop();
}
}
}
/// Overrides the sampling clock for debugging and testing.
///
/// This value is ignored in non-debug builds.
@protected
SamplingClock? get debugSamplingClock => null;
/// Provides access to the current [DateTime] and `StopWatch` objects for
/// sampling.
///
/// Overridden by [debugSamplingClock] for debug builds and testing. Using
/// this object under test will maintain synchronization with [FakeAsync].
SamplingClock get samplingClock {
SamplingClock value = SamplingClock();
assert(() {
final SamplingClock? debugValue = debugSamplingClock;
if (debugValue != null) {
value = debugValue;
}
return true;
}());
return value;
}
// Resampler used to filter incoming pointer events when resampling
// is enabled.
late final _Resampler _resampler = _Resampler(
_handlePointerEventImmediately,
_handleSampleTimeChanged,
_samplingInterval,
);
/// Enable pointer event resampling for touch devices by setting
/// this to true.
///
/// Resampling results in smoother touch event processing at the
/// cost of some added latency. Devices with low frequency sensors
/// or when the frequency is not a multiple of the display frequency
/// (e.g., 120Hz input and 90Hz display) benefit from this.
///
/// This is typically set during application initialization but
/// can be adjusted dynamically in case the application only
/// wants resampling for some period of time.
bool resamplingEnabled = false;
/// Offset relative to current frame time that should be used for
/// resampling. The [samplingOffset] is expected to be negative.
/// Non-negative [samplingOffset] is allowed but will effectively
/// disable resampling.
Duration samplingOffset = _defaultSamplingOffset;
}
/// Variant of [FlutterErrorDetails] with extra fields for the gesture
/// library's binding's pointer event dispatcher ([GestureBinding.dispatchEvent]).
class FlutterErrorDetailsForPointerEventDispatcher extends FlutterErrorDetails {
/// Creates a [FlutterErrorDetailsForPointerEventDispatcher] object with the given
/// arguments setting the object's properties.
///
/// The gesture library calls this constructor when catching an exception
/// that will subsequently be reported using [FlutterError.onError].
const FlutterErrorDetailsForPointerEventDispatcher({
required super.exception,
super.stack,
super.library,
super.context,
this.event,
this.hitTestEntry,
super.informationCollector,
super.silent,
});
/// The pointer event that was being routed when the exception was raised.
final PointerEvent? event;
/// The hit test result entry for the object whose handleEvent method threw
/// the exception. May be null if no hit test entry is associated with the
/// event (e.g. [PointerHoverEvent]s, [PointerAddedEvent]s, and
/// [PointerRemovedEvent]s).
///
/// The target object itself is given by the [HitTestEntry.target] property of
/// the hitTestEntry object.
final HitTestEntry? hitTestEntry;
}
| flutter/packages/flutter/lib/src/gestures/binding.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/binding.dart",
"repo_id": "flutter",
"token_count": 7396
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'events.dart';
export 'package:vector_math/vector_math_64.dart' show Matrix4;
export 'events.dart' show PointerEvent;
/// A callback that receives a [PointerEvent]
typedef PointerRoute = void Function(PointerEvent event);
/// A routing table for [PointerEvent] events.
class PointerRouter {
final Map<int, Map<PointerRoute, Matrix4?>> _routeMap = <int, Map<PointerRoute, Matrix4?>>{};
final Map<PointerRoute, Matrix4?> _globalRoutes = <PointerRoute, Matrix4?>{};
/// Adds a route to the routing table.
///
/// Whenever this object routes a [PointerEvent] corresponding to
/// pointer, call route.
///
/// Routes added reentrantly within [PointerRouter.route] will take effect when
/// routing the next event.
void addRoute(int pointer, PointerRoute route, [Matrix4? transform]) {
final Map<PointerRoute, Matrix4?> routes = _routeMap.putIfAbsent(
pointer,
() => <PointerRoute, Matrix4?>{},
);
assert(!routes.containsKey(route));
routes[route] = transform;
}
/// Removes a route from the routing table.
///
/// No longer call route when routing a [PointerEvent] corresponding to
/// pointer. Requires that this route was previously added to the router.
///
/// Routes removed reentrantly within [PointerRouter.route] will take effect
/// immediately.
void removeRoute(int pointer, PointerRoute route) {
assert(_routeMap.containsKey(pointer));
final Map<PointerRoute, Matrix4?> routes = _routeMap[pointer]!;
assert(routes.containsKey(route));
routes.remove(route);
if (routes.isEmpty) {
_routeMap.remove(pointer);
}
}
/// Adds a route to the global entry in the routing table.
///
/// Whenever this object routes a [PointerEvent], call route.
///
/// Routes added reentrantly within [PointerRouter.route] will take effect when
/// routing the next event.
void addGlobalRoute(PointerRoute route, [Matrix4? transform]) {
assert(!_globalRoutes.containsKey(route));
_globalRoutes[route] = transform;
}
/// Removes a route from the global entry in the routing table.
///
/// No longer call route when routing a [PointerEvent]. Requires that this
/// route was previously added via [addGlobalRoute].
///
/// Routes removed reentrantly within [PointerRouter.route] will take effect
/// immediately.
void removeGlobalRoute(PointerRoute route) {
assert(_globalRoutes.containsKey(route));
_globalRoutes.remove(route);
}
/// The number of global routes that have been registered.
///
/// This is valid in debug builds only. In release builds, this will throw an
/// [UnsupportedError].
int get debugGlobalRouteCount {
int? count;
assert(() {
count = _globalRoutes.length;
return true;
}());
if (count != null) {
return count!;
}
throw UnsupportedError('debugGlobalRouteCount is not supported in release builds');
}
@pragma('vm:notify-debugger-on-exception')
void _dispatch(PointerEvent event, PointerRoute route, Matrix4? transform) {
try {
event = event.transformed(transform);
route(event);
} catch (exception, stack) {
InformationCollector? collector;
assert(() {
collector = () => <DiagnosticsNode>[
DiagnosticsProperty<PointerRouter>('router', this, level: DiagnosticLevel.debug),
DiagnosticsProperty<PointerRoute>('route', route, level: DiagnosticLevel.debug),
DiagnosticsProperty<PointerEvent>('event', event, level: DiagnosticLevel.debug),
];
return true;
}());
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'gesture library',
context: ErrorDescription('while routing a pointer event'),
informationCollector: collector,
));
}
}
/// Calls the routes registered for this pointer event.
///
/// Routes are called in the order in which they were added to the
/// PointerRouter object.
void route(PointerEvent event) {
final Map<PointerRoute, Matrix4?>? routes = _routeMap[event.pointer];
final Map<PointerRoute, Matrix4?> copiedGlobalRoutes = Map<PointerRoute, Matrix4?>.of(_globalRoutes);
if (routes != null) {
_dispatchEventToRoutes(
event,
routes,
Map<PointerRoute, Matrix4?>.of(routes),
);
}
_dispatchEventToRoutes(event, _globalRoutes, copiedGlobalRoutes);
}
void _dispatchEventToRoutes(
PointerEvent event,
Map<PointerRoute, Matrix4?> referenceRoutes,
Map<PointerRoute, Matrix4?> copiedRoutes,
) {
copiedRoutes.forEach((PointerRoute route, Matrix4? transform) {
if (referenceRoutes.containsKey(route)) {
_dispatch(event, route, transform);
}
});
}
}
| flutter/packages/flutter/lib/src/gestures/pointer_router.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/pointer_router.dart",
"repo_id": "flutter",
"token_count": 1718
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file serves as the interface between the public and private APIs for
// animated icons.
// The AnimatedIcons class is public and is used to specify available icons,
// while the _AnimatedIconData interface which used to deliver the icon data is
// kept private.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
/// Identifier for the supported Material Design animated icons.
///
/// Use with [AnimatedIcon] class to show specific animated icons.
///
/// {@tool dartpad}
/// This example shows how to create an animated icon. The icon is animated
/// forward and reverse in a loop.
///
/// ** See code in examples/api/lib/material/animated_icon/animated_icons_data.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Icons], for the list of available static Material Icons.
abstract final class AnimatedIcons {
/// The Material Design add to event icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/add_event.mp4}
static const AnimatedIconData add_event = _$add_event;
/// The Material Design arrow to menu icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/arrow_menu.mp4}
static const AnimatedIconData arrow_menu = _$arrow_menu;
/// The Material Design close to menu icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/close_menu.mp4}
static const AnimatedIconData close_menu = _$close_menu;
/// The Material Design ellipsis to search icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/ellipsis_search.mp4}
static const AnimatedIconData ellipsis_search = _$ellipsis_search;
/// The Material Design event to add icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/event_add.mp4}
static const AnimatedIconData event_add = _$event_add;
/// The Material Design home to menu icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/home_menu.mp4}
static const AnimatedIconData home_menu = _$home_menu;
/// The Material Design list to view icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/list_view.mp4}
static const AnimatedIconData list_view = _$list_view;
/// The Material Design menu to arrow icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/menu_arrow.mp4}
static const AnimatedIconData menu_arrow = _$menu_arrow;
/// The Material Design menu to close icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/menu_close.mp4}
static const AnimatedIconData menu_close = _$menu_close;
/// The Material Design menu to home icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/menu_home.mp4}
static const AnimatedIconData menu_home = _$menu_home;
/// The Material Design pause to play icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/pause_play.mp4}
static const AnimatedIconData pause_play = _$pause_play;
/// The Material Design play to pause icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/play_pause.mp4}
static const AnimatedIconData play_pause = _$play_pause;
/// The Material Design search to ellipsis icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/search_ellipsis.mp4}
static const AnimatedIconData search_ellipsis = _$search_ellipsis;
/// The Material Design view to list icon animation.
///
/// {@animation 72 72 https://flutter.github.io/assets-for-api-docs/assets/widgets/view_list.mp4}
static const AnimatedIconData view_list = _$view_list;
}
/// Vector graphics data for icons used by [AnimatedIcon].
///
/// Instances of this class are currently opaque because we have not committed to a specific
/// animated vector graphics format.
///
/// See also:
///
/// * [AnimatedIcons], a class that contains constants that implement this interface.
abstract class AnimatedIconData {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const AnimatedIconData();
/// Whether this icon should be mirrored horizontally when text direction is
/// right-to-left.
///
/// See also:
///
/// * [TextDirection], which discusses concerns regarding reading direction
/// in Flutter.
/// * [Directionality], a widget which determines the ambient directionality.
bool get matchTextDirection;
}
class _AnimatedIconData extends AnimatedIconData {
const _AnimatedIconData(this.size, this.paths, {this.matchTextDirection = false});
final Size size;
final List<_PathFrames> paths;
@override
final bool matchTextDirection;
}
| flutter/packages/flutter/lib/src/material/animated_icons/animated_icons_data.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/animated_icons_data.dart",
"repo_id": "flutter",
"token_count": 1550
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'action_buttons.dart';
import 'app_bar_theme.dart';
import 'button_style.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'debug.dart';
import 'flexible_space_bar.dart';
import 'icon_button.dart';
import 'icon_button_theme.dart';
import 'icons.dart';
import 'material.dart';
import 'material_state.dart';
import 'scaffold.dart';
import 'tabs.dart';
import 'text_theme.dart';
import 'theme.dart';
// Examples can assume:
// late String _logoAsset;
// double _myToolbarHeight = 250.0;
typedef _FlexibleConfigBuilder = _ScrollUnderFlexibleConfig Function(BuildContext);
const double _kLeadingWidth = kToolbarHeight; // So the leading button is square.
const double _kMaxTitleTextScaleFactor = 1.34; // TODO(perc): Add link to Material spec when available, https://github.com/flutter/flutter/issues/58769.
enum _SliverAppVariant { small, medium, large }
// Bottom justify the toolbarHeight child which may overflow the top.
class _ToolbarContainerLayout extends SingleChildLayoutDelegate {
const _ToolbarContainerLayout(this.toolbarHeight);
final double toolbarHeight;
@override
BoxConstraints getConstraintsForChild(BoxConstraints constraints) {
return constraints.tighten(height: toolbarHeight);
}
@override
Size getSize(BoxConstraints constraints) {
return Size(constraints.maxWidth, toolbarHeight);
}
@override
Offset getPositionForChild(Size size, Size childSize) {
return Offset(0.0, size.height - childSize.height);
}
@override
bool shouldRelayout(_ToolbarContainerLayout oldDelegate) =>
toolbarHeight != oldDelegate.toolbarHeight;
}
class _PreferredAppBarSize extends Size {
_PreferredAppBarSize(this.toolbarHeight, this.bottomHeight)
: super.fromHeight((toolbarHeight ?? kToolbarHeight) + (bottomHeight ?? 0));
final double? toolbarHeight;
final double? bottomHeight;
}
/// A Material Design app bar.
///
/// An app bar consists of a toolbar and potentially other widgets, such as a
/// [TabBar] and a [FlexibleSpaceBar]. App bars typically expose one or more
/// common [actions] with [IconButton]s which are optionally followed by a
/// [PopupMenuButton] for less common operations (sometimes called the "overflow
/// menu").
///
/// App bars are typically used in the [Scaffold.appBar] property, which places
/// the app bar as a fixed-height widget at the top of the screen. For a scrollable
/// app bar, see [SliverAppBar], which embeds an [AppBar] in a sliver for use in
/// a [CustomScrollView].
///
/// The AppBar displays the toolbar widgets, [leading], [title], and [actions],
/// above the [bottom] (if any). The [bottom] is usually used for a [TabBar]. If
/// a [flexibleSpace] widget is specified then it is stacked behind the toolbar
/// and the bottom widget. The following diagram shows where each of these slots
/// appears in the toolbar when the writing language is left-to-right (e.g.
/// English):
///
/// The [AppBar] insets its content based on the ambient [MediaQuery]'s padding,
/// to avoid system UI intrusions. It's taken care of by [Scaffold] when used in
/// the [Scaffold.appBar] property. When animating an [AppBar], unexpected
/// [MediaQuery] changes (as is common in [Hero] animations) may cause the content
/// to suddenly jump. Wrap the [AppBar] in a [MediaQuery] widget, and adjust its
/// padding such that the animation is smooth.
///
/// 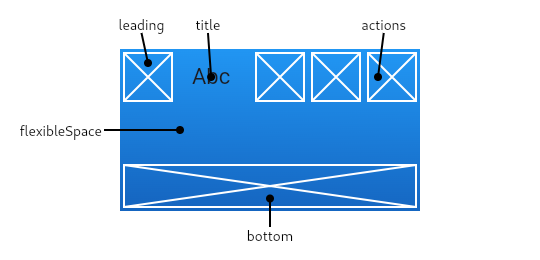
///
/// If the [leading] widget is omitted, but the [AppBar] is in a [Scaffold] with
/// a [Drawer], then a button will be inserted to open the drawer. Otherwise, if
/// the nearest [Navigator] has any previous routes, a [BackButton] is inserted
/// instead. This behavior can be turned off by setting the [automaticallyImplyLeading]
/// to false. In that case a null leading widget will result in the middle/title widget
/// stretching to start.
///
/// {@tool dartpad}
/// This sample shows an [AppBar] with two simple actions. The first action
/// opens a [SnackBar], while the second action navigates to a new page.
///
/// ** See code in examples/api/lib/material/app_bar/app_bar.0.dart **
/// {@end-tool}
///
/// Material Design 3 introduced new types of app bar.
/// {@tool dartpad}
/// This sample shows the creation of an [AppBar] widget with the [shadowColor] and
/// [scrolledUnderElevation] properties set, as described in:
/// https://m3.material.io/components/top-app-bar/overview
///
/// ** See code in examples/api/lib/material/app_bar/app_bar.1.dart **
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Why don't my TextButton actions appear?
///
/// If the app bar's [actions] contains [TextButton]s, they will not
/// be visible if their foreground (text) color is the same as the
/// app bar's background color.
///
/// In Material v2 (i.e., when [ThemeData.useMaterial3] is false),
/// the default app bar [backgroundColor] is the overall theme's
/// [ColorScheme.primary] if the overall theme's brightness is
/// [Brightness.light]. Unfortunately this is the same as the default
/// [ButtonStyle.foregroundColor] for [TextButton] for light themes.
/// In this case a preferable text button foreground color is
/// [ColorScheme.onPrimary], a color that contrasts nicely with
/// [ColorScheme.primary]. To remedy the problem, override
/// [TextButton.style]:
///
/// {@tool dartpad}
/// This sample shows an [AppBar] with two action buttons with their primary
/// color set to [ColorScheme.onPrimary].
///
/// ** See code in examples/api/lib/material/app_bar/app_bar.2.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to listen to a nested Scrollable's scroll notification
/// in a nested scroll view using the [notificationPredicate] property and use it
/// to make [scrolledUnderElevation] take effect.
///
/// ** See code in examples/api/lib/material/app_bar/app_bar.3.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Scaffold], which displays the [AppBar] in its [Scaffold.appBar] slot.
/// * [SliverAppBar], which uses [AppBar] to provide a flexible app bar that
/// can be used in a [CustomScrollView].
/// * [TabBar], which is typically placed in the [bottom] slot of the [AppBar]
/// if the screen has multiple pages arranged in tabs.
/// * [IconButton], which is used with [actions] to show buttons on the app bar.
/// * [PopupMenuButton], to show a popup menu on the app bar, via [actions].
/// * [FlexibleSpaceBar], which is used with [flexibleSpace] when the app bar
/// can expand and collapse.
/// * <https://material.io/design/components/app-bars-top.html>
/// * <https://m3.material.io/components/top-app-bar>
/// * Cookbook: [Place a floating app bar above a list](https://flutter.dev/docs/cookbook/lists/floating-app-bar)
class AppBar extends StatefulWidget implements PreferredSizeWidget {
/// Creates a Material Design app bar.
///
/// If [elevation] is specified, it must be non-negative.
///
/// Typically used in the [Scaffold.appBar] property.
AppBar({
super.key,
this.leading,
this.automaticallyImplyLeading = true,
this.title,
this.actions,
this.flexibleSpace,
this.bottom,
this.elevation,
this.scrolledUnderElevation,
this.notificationPredicate = defaultScrollNotificationPredicate,
this.shadowColor,
this.surfaceTintColor,
this.shape,
this.backgroundColor,
this.foregroundColor,
this.iconTheme,
this.actionsIconTheme,
this.primary = true,
this.centerTitle,
this.excludeHeaderSemantics = false,
this.titleSpacing,
this.toolbarOpacity = 1.0,
this.bottomOpacity = 1.0,
this.toolbarHeight,
this.leadingWidth,
this.toolbarTextStyle,
this.titleTextStyle,
this.systemOverlayStyle,
this.forceMaterialTransparency = false,
this.clipBehavior,
}) : assert(elevation == null || elevation >= 0.0),
preferredSize = _PreferredAppBarSize(toolbarHeight, bottom?.preferredSize.height);
/// Used by [Scaffold] to compute its [AppBar]'s overall height. The returned value is
/// the same `preferredSize.height` unless [AppBar.toolbarHeight] was null and
/// `AppBarTheme.of(context).toolbarHeight` is non-null. In that case the
/// return value is the sum of the theme's toolbar height and the height of
/// the app bar's [AppBar.bottom] widget.
static double preferredHeightFor(BuildContext context, Size preferredSize) {
if (preferredSize is _PreferredAppBarSize && preferredSize.toolbarHeight == null) {
return (AppBarTheme.of(context).toolbarHeight ?? kToolbarHeight) + (preferredSize.bottomHeight ?? 0);
}
return preferredSize.height;
}
/// {@template flutter.material.appbar.leading}
/// A widget to display before the toolbar's [title].
///
/// Typically the [leading] widget is an [Icon] or an [IconButton].
///
/// Becomes the leading component of the [NavigationToolbar] built
/// by this widget. The [leading] widget's width and height are constrained to
/// be no bigger than [leadingWidth] and [toolbarHeight] respectively.
///
/// If this is null and [automaticallyImplyLeading] is set to true, the
/// [AppBar] will imply an appropriate widget. For example, if the [AppBar] is
/// in a [Scaffold] that also has a [Drawer], the [Scaffold] will fill this
/// widget with an [IconButton] that opens the drawer (using [Icons.menu]). If
/// there's no [Drawer] and the parent [Navigator] can go back, the [AppBar]
/// will use a [BackButton] that calls [Navigator.maybePop].
/// {@endtemplate}
///
/// {@tool snippet}
///
/// The following code shows how the drawer button could be manually specified
/// instead of relying on [automaticallyImplyLeading]:
///
/// ```dart
/// AppBar(
/// leading: Builder(
/// builder: (BuildContext context) {
/// return IconButton(
/// icon: const Icon(Icons.menu),
/// onPressed: () { Scaffold.of(context).openDrawer(); },
/// tooltip: MaterialLocalizations.of(context).openAppDrawerTooltip,
/// );
/// },
/// ),
/// )
/// ```
/// {@end-tool}
///
/// The [Builder] is used in this example to ensure that the `context` refers
/// to that part of the subtree. That way this code snippet can be used even
/// inside the very code that is creating the [Scaffold] (in which case,
/// without the [Builder], the `context` wouldn't be able to see the
/// [Scaffold], since it would refer to an ancestor of that widget).
///
/// See also:
///
/// * [Scaffold.appBar], in which an [AppBar] is usually placed.
/// * [Scaffold.drawer], in which the [Drawer] is usually placed.
final Widget? leading;
/// {@template flutter.material.appbar.automaticallyImplyLeading}
/// Controls whether we should try to imply the leading widget if null.
///
/// If true and [AppBar.leading] is null, automatically try to deduce what the leading
/// widget should be. If false and [AppBar.leading] is null, leading space is given to [AppBar.title].
/// If leading widget is not null, this parameter has no effect.
/// {@endtemplate}
final bool automaticallyImplyLeading;
/// {@template flutter.material.appbar.title}
/// The primary widget displayed in the app bar.
///
/// Becomes the middle component of the [NavigationToolbar] built by this widget.
///
/// Typically a [Text] widget that contains a description of the current
/// contents of the app.
/// {@endtemplate}
///
/// The [title]'s width is constrained to fit within the remaining space
/// between the toolbar's [leading] and [actions] widgets. Its height is
/// _not_ constrained. The [title] is vertically centered and clipped to fit
/// within the toolbar, whose height is [toolbarHeight]. Typically this
/// isn't noticeable because a simple [Text] [title] will fit within the
/// toolbar by default. On the other hand, it is noticeable when a
/// widget with an intrinsic height that is greater than [toolbarHeight]
/// is used as the [title]. For example, when the height of an Image used
/// as the [title] exceeds [toolbarHeight], it will be centered and
/// clipped (top and bottom), which may be undesirable. In cases like this
/// the height of the [title] widget can be constrained. For example:
///
/// ```dart
/// MaterialApp(
/// home: Scaffold(
/// appBar: AppBar(
/// title: SizedBox(
/// height: _myToolbarHeight,
/// child: Image.asset(_logoAsset),
/// ),
/// toolbarHeight: _myToolbarHeight,
/// ),
/// ),
/// )
/// ```
final Widget? title;
/// {@template flutter.material.appbar.actions}
/// A list of Widgets to display in a row after the [title] widget.
///
/// Typically these widgets are [IconButton]s representing common operations.
/// For less common operations, consider using a [PopupMenuButton] as the
/// last action.
///
/// The [actions] become the trailing component of the [NavigationToolbar] built
/// by this widget. The height of each action is constrained to be no bigger
/// than the [toolbarHeight].
///
/// To avoid having the last action covered by the debug banner, you may want
/// to set the [MaterialApp.debugShowCheckedModeBanner] to false.
/// {@endtemplate}
///
/// {@tool snippet}
///
/// ```dart
/// Scaffold(
/// body: CustomScrollView(
/// primary: true,
/// slivers: <Widget>[
/// SliverAppBar(
/// title: const Text('Hello World'),
/// actions: <Widget>[
/// IconButton(
/// icon: const Icon(Icons.shopping_cart),
/// tooltip: 'Open shopping cart',
/// onPressed: () {
/// // handle the press
/// },
/// ),
/// ],
/// ),
/// // ...rest of body...
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
final List<Widget>? actions;
/// {@template flutter.material.appbar.flexibleSpace}
/// This widget is stacked behind the toolbar and the tab bar. Its height will
/// be the same as the app bar's overall height.
///
/// A flexible space isn't actually flexible unless the [AppBar]'s container
/// changes the [AppBar]'s size. A [SliverAppBar] in a [CustomScrollView]
/// changes the [AppBar]'s height when scrolled.
///
/// Typically a [FlexibleSpaceBar]. See [FlexibleSpaceBar] for details.
/// {@endtemplate}
final Widget? flexibleSpace;
/// {@template flutter.material.appbar.bottom}
/// This widget appears across the bottom of the app bar.
///
/// Typically a [TabBar]. Only widgets that implement [PreferredSizeWidget] can
/// be used at the bottom of an app bar.
/// {@endtemplate}
///
/// See also:
///
/// * [PreferredSize], which can be used to give an arbitrary widget a preferred size.
final PreferredSizeWidget? bottom;
/// {@template flutter.material.appbar.elevation}
/// The z-coordinate at which to place this app bar relative to its parent.
///
/// This property controls the size of the shadow below the app bar if
/// [shadowColor] is not null.
///
/// If [surfaceTintColor] is not null then it will apply a surface tint overlay
/// to the background color (see [Material.surfaceTintColor] for more
/// detail).
///
/// The value must be non-negative.
///
/// If this property is null, then [AppBarTheme.elevation] of
/// [ThemeData.appBarTheme] is used. If that is also null, the
/// default value is 4.
/// {@endtemplate}
///
/// See also:
///
/// * [scrolledUnderElevation], which will be used when the app bar has
/// something scrolled underneath it.
/// * [shadowColor], which is the color of the shadow below the app bar.
/// * [surfaceTintColor], which determines the elevation overlay that will
/// be applied to the background of the app bar.
/// * [shape], which defines the shape of the app bar's [Material] and its
/// shadow.
final double? elevation;
/// {@template flutter.material.appbar.scrolledUnderElevation}
/// The elevation that will be used if this app bar has something
/// scrolled underneath it.
///
/// If non-null then it [AppBarTheme.scrolledUnderElevation] of
/// [ThemeData.appBarTheme] will be used. If that is also null then [elevation]
/// will be used.
///
/// The value must be non-negative.
///
/// {@endtemplate}
///
/// See also:
/// * [elevation], which will be used if there is no content scrolled under
/// the app bar.
/// * [shadowColor], which is the color of the shadow below the app bar.
/// * [surfaceTintColor], which determines the elevation overlay that will
/// be applied to the background of the app bar.
/// * [shape], which defines the shape of the app bar's [Material] and its
/// shadow.
final double? scrolledUnderElevation;
/// A check that specifies which child's [ScrollNotification]s should be
/// listened to.
///
/// By default, checks whether `notification.depth == 0`. Set it to something
/// else for more complicated layouts.
final ScrollNotificationPredicate notificationPredicate;
/// {@template flutter.material.appbar.shadowColor}
/// The color of the shadow below the app bar.
///
/// If this property is null, then [AppBarTheme.shadowColor] of
/// [ThemeData.appBarTheme] is used. If that is also null, the default value
/// is fully opaque black.
/// {@endtemplate}
///
/// See also:
///
/// * [elevation], which defines the size of the shadow below the app bar.
/// * [shape], which defines the shape of the app bar and its shadow.
final Color? shadowColor;
/// {@template flutter.material.appbar.surfaceTintColor}
/// The color of the surface tint overlay applied to the app bar's
/// background color to indicate elevation.
///
/// If null no overlay will be applied.
/// {@endtemplate}
///
/// See also:
/// * [Material.surfaceTintColor], which described this feature in more detail.
final Color? surfaceTintColor;
/// {@template flutter.material.appbar.shape}
/// The shape of the app bar's [Material] as well as its shadow.
///
/// If this property is null, then [AppBarTheme.shape] of
/// [ThemeData.appBarTheme] is used. Both properties default to null.
/// If both properties are null then the shape of the app bar's [Material]
/// is just a simple rectangle.
///
/// A shadow is only displayed if the [elevation] is greater than
/// zero.
/// {@endtemplate}
///
/// See also:
///
/// * [elevation], which defines the size of the shadow below the app bar.
/// * [shadowColor], which is the color of the shadow below the app bar.
final ShapeBorder? shape;
/// {@template flutter.material.appbar.backgroundColor}
/// The fill color to use for an app bar's [Material].
///
/// If null, then the [AppBarTheme.backgroundColor] is used. If that value is also
/// null:
/// In Material v2 (i.e., when [ThemeData.useMaterial3] is false),
/// then [AppBar] uses the overall theme's [ColorScheme.primary] if the
/// overall theme's brightness is [Brightness.light], and [ColorScheme.surface]
/// if the overall theme's brightness is [Brightness.dark].
/// In Material v3 (i.e., when [ThemeData.useMaterial3] is true),
/// then [AppBar] uses the overall theme's [ColorScheme.surface]
///
/// If this color is a [MaterialStateColor] it will be resolved against
/// [MaterialState.scrolledUnder] when the content of the app's
/// primary scrollable overlaps the app bar.
/// {@endtemplate}
///
/// See also:
///
/// * [foregroundColor], which specifies the color for icons and text within
/// the app bar.
/// * [Theme.of], which returns the current overall Material theme as
/// a [ThemeData].
/// * [ThemeData.colorScheme], the thirteen colors that most Material widget
/// default colors are based on.
/// * [ColorScheme.brightness], which indicates if the overall [Theme]
/// is light or dark.
final Color? backgroundColor;
/// {@template flutter.material.appbar.foregroundColor}
/// The default color for [Text] and [Icon]s within the app bar.
///
/// If null, then [AppBarTheme.foregroundColor] is used. If that
/// value is also null:
/// In Material v2 (i.e., when [ThemeData.useMaterial3] is false),
/// then [AppBar] uses the overall theme's [ColorScheme.onPrimary] if the
/// overall theme's brightness is [Brightness.light], and [ColorScheme.onSurface]
/// if the overall theme's brightness is [Brightness.dark].
/// In Material v3 (i.e., when [ThemeData.useMaterial3] is true),
/// then [AppBar] uses the overall theme's [ColorScheme.onSurface].
///
/// This color is used to configure [DefaultTextStyle] that contains
/// the toolbar's children, and the default [IconTheme] widgets that
/// are created if [iconTheme] and [actionsIconTheme] are null.
/// {@endtemplate}
///
/// See also:
///
/// * [backgroundColor], which specifies the app bar's background color.
/// * [Theme.of], which returns the current overall Material theme as
/// a [ThemeData].
/// * [ThemeData.colorScheme], the thirteen colors that most Material widget
/// default colors are based on.
/// * [ColorScheme.brightness], which indicates if the overall [Theme]
/// is light or dark.
final Color? foregroundColor;
/// {@template flutter.material.appbar.iconTheme}
/// The color, opacity, and size to use for toolbar icons.
///
/// If this property is null, then a copy of [ThemeData.iconTheme]
/// is used, with the [IconThemeData.color] set to the
/// app bar's [foregroundColor].
/// {@endtemplate}
///
/// See also:
///
/// * [actionsIconTheme], which defines the appearance of icons in
/// the [actions] list.
final IconThemeData? iconTheme;
/// {@template flutter.material.appbar.actionsIconTheme}
/// The color, opacity, and size to use for the icons that appear in the app
/// bar's [actions].
///
/// This property should only be used when the [actions] should be
/// themed differently than the icon that appears in the app bar's [leading]
/// widget.
///
/// If this property is null, then [AppBarTheme.actionsIconTheme] of
/// [ThemeData.appBarTheme] is used. If that is also null, then the value of
/// [iconTheme] is used.
/// {@endtemplate}
///
/// See also:
///
/// * [iconTheme], which defines the appearance of all of the toolbar icons.
final IconThemeData? actionsIconTheme;
/// {@template flutter.material.appbar.primary}
/// Whether this app bar is being displayed at the top of the screen.
///
/// If true, the app bar's toolbar elements and [bottom] widget will be
/// padded on top by the height of the system status bar. The layout
/// of the [flexibleSpace] is not affected by the [primary] property.
/// {@endtemplate}
final bool primary;
/// {@template flutter.material.appbar.centerTitle}
/// Whether the title should be centered.
///
/// If this property is null, then [AppBarTheme.centerTitle] of
/// [ThemeData.appBarTheme] is used. If that is also null, then value is
/// adapted to the current [TargetPlatform].
/// {@endtemplate}
final bool? centerTitle;
/// {@template flutter.material.appbar.excludeHeaderSemantics}
/// Whether the title should be wrapped with header [Semantics].
///
/// Defaults to false.
/// {@endtemplate}
final bool excludeHeaderSemantics;
/// {@template flutter.material.appbar.titleSpacing}
/// The spacing around [title] content on the horizontal axis. This spacing is
/// applied even if there is no [leading] content or [actions]. If you want
/// [title] to take all the space available, set this value to 0.0.
///
/// If this property is null, then [AppBarTheme.titleSpacing] of
/// [ThemeData.appBarTheme] is used. If that is also null, then the
/// default value is [NavigationToolbar.kMiddleSpacing].
/// {@endtemplate}
final double? titleSpacing;
/// {@template flutter.material.appbar.toolbarOpacity}
/// How opaque the toolbar part of the app bar is.
///
/// A value of 1.0 is fully opaque, and a value of 0.0 is fully transparent.
///
/// Typically, this value is not changed from its default value (1.0). It is
/// used by [SliverAppBar] to animate the opacity of the toolbar when the app
/// bar is scrolled.
/// {@endtemplate}
final double toolbarOpacity;
/// {@template flutter.material.appbar.bottomOpacity}
/// How opaque the bottom part of the app bar is.
///
/// A value of 1.0 is fully opaque, and a value of 0.0 is fully transparent.
///
/// Typically, this value is not changed from its default value (1.0). It is
/// used by [SliverAppBar] to animate the opacity of the toolbar when the app
/// bar is scrolled.
/// {@endtemplate}
final double bottomOpacity;
/// {@template flutter.material.appbar.preferredSize}
/// A size whose height is the sum of [toolbarHeight] and the [bottom] widget's
/// preferred height.
///
/// [Scaffold] uses this size to set its app bar's height.
/// {@endtemplate}
@override
final Size preferredSize;
/// {@template flutter.material.appbar.toolbarHeight}
/// Defines the height of the toolbar component of an [AppBar].
///
/// By default, the value of [toolbarHeight] is [kToolbarHeight].
/// {@endtemplate}
final double? toolbarHeight;
/// {@template flutter.material.appbar.leadingWidth}
/// Defines the width of [AppBar.leading] widget.
///
/// By default, the value of [AppBar.leadingWidth] is 56.0.
/// {@endtemplate}
final double? leadingWidth;
/// {@template flutter.material.appbar.toolbarTextStyle}
/// The default text style for the AppBar's [leading], and
/// [actions] widgets, but not its [title].
///
/// If this property is null, then [AppBarTheme.toolbarTextStyle] of
/// [ThemeData.appBarTheme] is used. If that is also null, the default
/// value is a copy of the overall theme's [TextTheme.bodyMedium]
/// [TextStyle], with color set to the app bar's [foregroundColor].
/// {@endtemplate}
///
/// See also:
///
/// * [titleTextStyle], which overrides the default text style for the [title].
/// * [DefaultTextStyle], which overrides the default text style for all of the
/// widgets in a subtree.
final TextStyle? toolbarTextStyle;
/// {@template flutter.material.appbar.titleTextStyle}
/// The default text style for the AppBar's [title] widget.
///
/// If this property is null, then [AppBarTheme.titleTextStyle] of
/// [ThemeData.appBarTheme] is used. If that is also null, the default
/// value is a copy of the overall theme's [TextTheme.titleLarge]
/// [TextStyle], with color set to the app bar's [foregroundColor].
/// {@endtemplate}
///
/// See also:
///
/// * [toolbarTextStyle], which is the default text style for the AppBar's
/// [title], [leading], and [actions] widgets, also known as the
/// AppBar's "toolbar".
/// * [DefaultTextStyle], which overrides the default text style for all of the
/// widgets in a subtree.
final TextStyle? titleTextStyle;
/// {@template flutter.material.appbar.systemOverlayStyle}
/// Specifies the style to use for the system overlays (e.g. the status bar on
/// Android or iOS, the system navigation bar on Android).
///
/// If this property is null, then [AppBarTheme.systemOverlayStyle] of
/// [ThemeData.appBarTheme] is used. If that is also null, an appropriate
/// [SystemUiOverlayStyle] is calculated based on the [backgroundColor].
///
/// The AppBar's descendants are built within a
/// `AnnotatedRegion<SystemUiOverlayStyle>` widget, which causes
/// [SystemChrome.setSystemUIOverlayStyle] to be called
/// automatically. Apps should not enclose an AppBar with their
/// own [AnnotatedRegion].
/// {@endtemplate}
//
/// See also:
///
/// * [AnnotatedRegion], for placing [SystemUiOverlayStyle] in the layer tree.
/// * [SystemChrome.setSystemUIOverlayStyle], the imperative API for setting
/// system overlays style.
final SystemUiOverlayStyle? systemOverlayStyle;
/// {@template flutter.material.appbar.forceMaterialTransparency}
/// Forces the AppBar's Material widget type to be [MaterialType.transparency]
/// (instead of Material's default type).
///
/// This will remove the visual display of [backgroundColor] and [elevation],
/// and affect other characteristics of the AppBar's Material widget.
///
/// Provided for cases where the app bar is to be transparent, and gestures
/// must pass through the app bar to widgets beneath the app bar (i.e. with
/// [Scaffold.extendBodyBehindAppBar] set to true).
///
/// Defaults to false.
/// {@endtemplate}
final bool forceMaterialTransparency;
/// {@macro flutter.material.Material.clipBehavior}
final Clip? clipBehavior;
bool _getEffectiveCenterTitle(ThemeData theme) {
bool platformCenter() {
switch (theme.platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return false;
case TargetPlatform.iOS:
case TargetPlatform.macOS:
return actions == null || actions!.length < 2;
}
}
return centerTitle
?? theme.appBarTheme.centerTitle
?? platformCenter();
}
@override
State<AppBar> createState() => _AppBarState();
}
class _AppBarState extends State<AppBar> {
ScrollNotificationObserverState? _scrollNotificationObserver;
bool _scrolledUnder = false;
@override
void didChangeDependencies() {
super.didChangeDependencies();
_scrollNotificationObserver?.removeListener(_handleScrollNotification);
_scrollNotificationObserver = ScrollNotificationObserver.maybeOf(context);
_scrollNotificationObserver?.addListener(_handleScrollNotification);
}
@override
void dispose() {
if (_scrollNotificationObserver != null) {
_scrollNotificationObserver!.removeListener(_handleScrollNotification);
_scrollNotificationObserver = null;
}
super.dispose();
}
void _handleScrollNotification(ScrollNotification notification) {
if (notification is ScrollUpdateNotification && widget.notificationPredicate(notification)) {
final bool oldScrolledUnder = _scrolledUnder;
final ScrollMetrics metrics = notification.metrics;
switch (metrics.axisDirection) {
case AxisDirection.up:
// Scroll view is reversed
_scrolledUnder = metrics.extentAfter > 0;
case AxisDirection.down:
_scrolledUnder = metrics.extentBefore > 0;
case AxisDirection.right:
case AxisDirection.left:
// Scrolled under is only supported in the vertical axis, and should
// not be altered based on horizontal notifications of the same
// predicate since it could be a 2D scroller.
break;
}
if (_scrolledUnder != oldScrolledUnder) {
setState(() {
// React to a change in MaterialState.scrolledUnder
});
}
}
}
Color _resolveColor(Set<MaterialState> states, Color? widgetColor, Color? themeColor, Color defaultColor) {
return MaterialStateProperty.resolveAs<Color?>(widgetColor, states)
?? MaterialStateProperty.resolveAs<Color?>(themeColor, states)
?? MaterialStateProperty.resolveAs<Color>(defaultColor, states);
}
SystemUiOverlayStyle _systemOverlayStyleForBrightness(Brightness brightness, [Color? backgroundColor]) {
final SystemUiOverlayStyle style = brightness == Brightness.dark
? SystemUiOverlayStyle.light
: SystemUiOverlayStyle.dark;
// For backward compatibility, create an overlay style without system navigation bar settings.
return SystemUiOverlayStyle(
statusBarColor: backgroundColor,
statusBarBrightness: style.statusBarBrightness,
statusBarIconBrightness: style.statusBarIconBrightness,
systemStatusBarContrastEnforced: style.systemStatusBarContrastEnforced,
);
}
@override
Widget build(BuildContext context) {
assert(!widget.primary || debugCheckHasMediaQuery(context));
assert(debugCheckHasMaterialLocalizations(context));
final ThemeData theme = Theme.of(context);
final IconButtonThemeData iconButtonTheme = IconButtonTheme.of(context);
final AppBarTheme appBarTheme = AppBarTheme.of(context);
final AppBarTheme defaults = theme.useMaterial3 ? _AppBarDefaultsM3(context) : _AppBarDefaultsM2(context);
final ScaffoldState? scaffold = Scaffold.maybeOf(context);
final ModalRoute<dynamic>? parentRoute = ModalRoute.of(context);
final FlexibleSpaceBarSettings? settings = context.dependOnInheritedWidgetOfExactType<FlexibleSpaceBarSettings>();
final Set<MaterialState> states = <MaterialState>{
if (settings?.isScrolledUnder ?? _scrolledUnder) MaterialState.scrolledUnder,
};
final bool hasDrawer = scaffold?.hasDrawer ?? false;
final bool hasEndDrawer = scaffold?.hasEndDrawer ?? false;
final bool useCloseButton = parentRoute is PageRoute<dynamic> && parentRoute.fullscreenDialog;
final double toolbarHeight = widget.toolbarHeight ?? appBarTheme.toolbarHeight ?? kToolbarHeight;
final Color backgroundColor = _resolveColor(
states,
widget.backgroundColor,
appBarTheme.backgroundColor,
defaults.backgroundColor!,
);
final Color scrolledUnderBackground = _resolveColor(
states,
widget.backgroundColor,
appBarTheme.backgroundColor,
Theme.of(context).colorScheme.surfaceContainer,
);
final Color effectiveBackgroundColor = states.contains(MaterialState.scrolledUnder)
? scrolledUnderBackground
: backgroundColor;
final Color foregroundColor = widget.foregroundColor
?? appBarTheme.foregroundColor
?? defaults.foregroundColor!;
final double elevation = widget.elevation
?? appBarTheme.elevation
?? defaults.elevation!;
final double effectiveElevation = states.contains(MaterialState.scrolledUnder)
? widget.scrolledUnderElevation
?? appBarTheme.scrolledUnderElevation
?? defaults.scrolledUnderElevation
?? elevation
: elevation;
IconThemeData overallIconTheme = widget.iconTheme
?? appBarTheme.iconTheme
?? defaults.iconTheme!.copyWith(color: foregroundColor);
final Color? actionForegroundColor = widget.foregroundColor
?? appBarTheme.foregroundColor;
IconThemeData actionsIconTheme = widget.actionsIconTheme
?? appBarTheme.actionsIconTheme
?? widget.iconTheme
?? appBarTheme.iconTheme
?? defaults.actionsIconTheme?.copyWith(color: actionForegroundColor)
?? overallIconTheme;
TextStyle? toolbarTextStyle = widget.toolbarTextStyle
?? appBarTheme.toolbarTextStyle
?? defaults.toolbarTextStyle?.copyWith(color: foregroundColor);
TextStyle? titleTextStyle = widget.titleTextStyle
?? appBarTheme.titleTextStyle
?? defaults.titleTextStyle?.copyWith(color: foregroundColor);
if (widget.toolbarOpacity != 1.0) {
final double opacity = const Interval(0.25, 1.0, curve: Curves.fastOutSlowIn).transform(widget.toolbarOpacity);
if (titleTextStyle?.color != null) {
titleTextStyle = titleTextStyle!.copyWith(color: titleTextStyle.color!.withOpacity(opacity));
}
if (toolbarTextStyle?.color != null) {
toolbarTextStyle = toolbarTextStyle!.copyWith(color: toolbarTextStyle.color!.withOpacity(opacity));
}
overallIconTheme = overallIconTheme.copyWith(
opacity: opacity * (overallIconTheme.opacity ?? 1.0),
);
actionsIconTheme = actionsIconTheme.copyWith(
opacity: opacity * (actionsIconTheme.opacity ?? 1.0),
);
}
Widget? leading = widget.leading;
if (leading == null && widget.automaticallyImplyLeading) {
if (hasDrawer) {
leading = DrawerButton(
style: IconButton.styleFrom(iconSize: overallIconTheme.size ?? 24),
);
} else if (parentRoute?.impliesAppBarDismissal ?? false) {
leading = useCloseButton ? const CloseButton() : const BackButton();
}
}
if (leading != null) {
if (theme.useMaterial3) {
final IconButtonThemeData effectiveIconButtonTheme;
// This comparison is to check if there is a custom [overallIconTheme]. If true, it means that no
// custom [overallIconTheme] is provided, so [iconButtonTheme] is applied. Otherwise, we generate
// a new [IconButtonThemeData] based on the values from [overallIconTheme]. If [iconButtonTheme] only
// has null values, the default [overallIconTheme] will be applied below by [IconTheme.merge]
if (overallIconTheme == defaults.iconTheme) {
effectiveIconButtonTheme = iconButtonTheme;
} else {
// The [IconButton.styleFrom] method is used to generate a correct [overlayColor] based on the [foregroundColor].
final ButtonStyle leadingIconButtonStyle = IconButton.styleFrom(
foregroundColor: overallIconTheme.color,
iconSize: overallIconTheme.size,
);
effectiveIconButtonTheme = IconButtonThemeData(
style: iconButtonTheme.style?.copyWith(
foregroundColor: leadingIconButtonStyle.foregroundColor,
overlayColor: leadingIconButtonStyle.overlayColor,
iconSize: leadingIconButtonStyle.iconSize,
)
);
}
leading = IconButtonTheme(
data: effectiveIconButtonTheme,
child: leading is IconButton ? Center(child: leading) : leading,
);
// Based on the Material Design 3 specs, the leading IconButton should have
// a size of 48x48, and a highlight size of 40x40. Users can also put other
// type of widgets on leading with the original config.
leading = ConstrainedBox(
constraints: BoxConstraints.tightFor(width: widget.leadingWidth ?? _kLeadingWidth),
child: leading,
);
} else {
leading = ConstrainedBox(
constraints: BoxConstraints.tightFor(width: widget.leadingWidth ?? _kLeadingWidth),
child: leading,
);
}
}
Widget? title = widget.title;
if (title != null) {
title = _AppBarTitleBox(child: title);
if (!widget.excludeHeaderSemantics) {
title = Semantics(
namesRoute: switch (theme.platform) {
TargetPlatform.android || TargetPlatform.fuchsia || TargetPlatform.linux || TargetPlatform.windows => true,
TargetPlatform.iOS || TargetPlatform.macOS => null,
},
header: true,
child: title,
);
}
title = DefaultTextStyle(
style: titleTextStyle!,
softWrap: false,
overflow: TextOverflow.ellipsis,
child: title,
);
// Set maximum text scale factor to [_kMaxTitleTextScaleFactor] for the
// title to keep the visual hierarchy the same even with larger font
// sizes. To opt out, wrap the [title] widget in a [MediaQuery] widget
// with a different `TextScaler`.
title = MediaQuery.withClampedTextScaling(
maxScaleFactor: _kMaxTitleTextScaleFactor,
child: title,
);
}
Widget? actions;
if (widget.actions != null && widget.actions!.isNotEmpty) {
actions = Row(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: theme.useMaterial3 ? CrossAxisAlignment.center : CrossAxisAlignment.stretch,
children: widget.actions!,
);
} else if (hasEndDrawer) {
actions = EndDrawerButton(
style: IconButton.styleFrom(iconSize: overallIconTheme.size ?? 24),
);
}
// Allow the trailing actions to have their own theme if necessary.
if (actions != null) {
final IconButtonThemeData effectiveActionsIconButtonTheme;
if (actionsIconTheme == defaults.actionsIconTheme) {
effectiveActionsIconButtonTheme = iconButtonTheme;
} else {
final ButtonStyle actionsIconButtonStyle = IconButton.styleFrom(
foregroundColor: actionsIconTheme.color,
iconSize: actionsIconTheme.size,
);
effectiveActionsIconButtonTheme = IconButtonThemeData(
style: iconButtonTheme.style?.copyWith(
foregroundColor: actionsIconButtonStyle.foregroundColor,
overlayColor: actionsIconButtonStyle.overlayColor,
iconSize: actionsIconButtonStyle.iconSize,
)
);
}
actions = IconButtonTheme(
data: effectiveActionsIconButtonTheme,
child: IconTheme.merge(
data: actionsIconTheme,
child: actions,
),
);
}
final Widget toolbar = NavigationToolbar(
leading: leading,
middle: title,
trailing: actions,
centerMiddle: widget._getEffectiveCenterTitle(theme),
middleSpacing: widget.titleSpacing ?? appBarTheme.titleSpacing ?? NavigationToolbar.kMiddleSpacing,
);
// If the toolbar is allocated less than toolbarHeight make it
// appear to scroll upwards within its shrinking container.
Widget appBar = ClipRect(
clipBehavior: widget.clipBehavior ?? Clip.hardEdge,
child: CustomSingleChildLayout(
delegate: _ToolbarContainerLayout(toolbarHeight),
child: IconTheme.merge(
data: overallIconTheme,
child: DefaultTextStyle(
style: toolbarTextStyle!,
child: toolbar,
),
),
),
);
if (widget.bottom != null) {
appBar = Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Flexible(
child: ConstrainedBox(
constraints: BoxConstraints(maxHeight: toolbarHeight),
child: appBar,
),
),
if (widget.bottomOpacity == 1.0)
widget.bottom!
else
Opacity(
opacity: const Interval(0.25, 1.0, curve: Curves.fastOutSlowIn).transform(widget.bottomOpacity),
child: widget.bottom,
),
],
);
}
// The padding applies to the toolbar and tabbar, not the flexible space.
if (widget.primary) {
appBar = SafeArea(
bottom: false,
child: appBar,
);
}
appBar = Align(
alignment: Alignment.topCenter,
child: appBar,
);
if (widget.flexibleSpace != null) {
appBar = Stack(
fit: StackFit.passthrough,
children: <Widget>[
Semantics(
sortKey: const OrdinalSortKey(1.0),
explicitChildNodes: true,
child: widget.flexibleSpace,
),
Semantics(
sortKey: const OrdinalSortKey(0.0),
explicitChildNodes: true,
// Creates a material widget to prevent the flexibleSpace from
// obscuring the ink splashes produced by appBar children.
child: Material(
type: MaterialType.transparency,
child: appBar,
),
),
],
);
}
final SystemUiOverlayStyle overlayStyle = widget.systemOverlayStyle
?? appBarTheme.systemOverlayStyle
?? defaults.systemOverlayStyle
?? _systemOverlayStyleForBrightness(
ThemeData.estimateBrightnessForColor(effectiveBackgroundColor),
// Make the status bar transparent for M3 so the elevation overlay
// color is picked up by the statusbar.
theme.useMaterial3 ? const Color(0x00000000) : null,
);
return Semantics(
container: true,
child: AnnotatedRegion<SystemUiOverlayStyle>(
value: overlayStyle,
child: Material(
color: theme.useMaterial3 ? effectiveBackgroundColor : backgroundColor,
elevation: effectiveElevation,
type: widget.forceMaterialTransparency
? MaterialType.transparency
: MaterialType.canvas,
shadowColor: widget.shadowColor
?? appBarTheme.shadowColor
?? defaults.shadowColor,
surfaceTintColor: widget.surfaceTintColor
?? appBarTheme.surfaceTintColor
// M3 `defaults.surfaceTint` is Colors.transparent now. It is not used
// here because otherwise, it will cause breaking change for
// `scrolledUnderElevation`.
?? (theme.useMaterial3 ? theme.colorScheme.surfaceTint : null),
shape: widget.shape ?? appBarTheme.shape ?? defaults.shape,
child: Semantics(
explicitChildNodes: true,
child: appBar,
),
),
),
);
}
}
class _SliverAppBarDelegate extends SliverPersistentHeaderDelegate {
_SliverAppBarDelegate({
required this.leading,
required this.automaticallyImplyLeading,
required this.title,
required this.actions,
required this.flexibleSpace,
required this.bottom,
required this.elevation,
required this.scrolledUnderElevation,
required this.shadowColor,
required this.surfaceTintColor,
required this.forceElevated,
required this.backgroundColor,
required this.foregroundColor,
required this.iconTheme,
required this.actionsIconTheme,
required this.primary,
required this.centerTitle,
required this.excludeHeaderSemantics,
required this.titleSpacing,
required this.expandedHeight,
required this.collapsedHeight,
required this.topPadding,
required this.floating,
required this.pinned,
required this.vsync,
required this.snapConfiguration,
required this.stretchConfiguration,
required this.showOnScreenConfiguration,
required this.shape,
required this.toolbarHeight,
required this.leadingWidth,
required this.toolbarTextStyle,
required this.titleTextStyle,
required this.systemOverlayStyle,
required this.forceMaterialTransparency,
required this.clipBehavior,
required this.variant,
required this.accessibleNavigation,
}) : assert(primary || topPadding == 0.0),
_bottomHeight = bottom?.preferredSize.height ?? 0.0;
final Widget? leading;
final bool automaticallyImplyLeading;
final Widget? title;
final List<Widget>? actions;
final Widget? flexibleSpace;
final PreferredSizeWidget? bottom;
final double? elevation;
final double? scrolledUnderElevation;
final Color? shadowColor;
final Color? surfaceTintColor;
final bool forceElevated;
final Color? backgroundColor;
final Color? foregroundColor;
final IconThemeData? iconTheme;
final IconThemeData? actionsIconTheme;
final bool primary;
final bool? centerTitle;
final bool excludeHeaderSemantics;
final double? titleSpacing;
final double? expandedHeight;
final double collapsedHeight;
final double topPadding;
final bool floating;
final bool pinned;
final ShapeBorder? shape;
final double? toolbarHeight;
final double? leadingWidth;
final TextStyle? toolbarTextStyle;
final TextStyle? titleTextStyle;
final SystemUiOverlayStyle? systemOverlayStyle;
final double _bottomHeight;
final bool forceMaterialTransparency;
final Clip? clipBehavior;
final _SliverAppVariant variant;
final bool accessibleNavigation;
@override
double get minExtent => collapsedHeight;
@override
double get maxExtent => math.max(topPadding + (expandedHeight ?? (toolbarHeight ?? kToolbarHeight) + _bottomHeight), minExtent);
@override
final TickerProvider vsync;
@override
final FloatingHeaderSnapConfiguration? snapConfiguration;
@override
final OverScrollHeaderStretchConfiguration? stretchConfiguration;
@override
final PersistentHeaderShowOnScreenConfiguration? showOnScreenConfiguration;
@override
Widget build(BuildContext context, double shrinkOffset, bool overlapsContent) {
final double visibleMainHeight = maxExtent - shrinkOffset - topPadding;
final double extraToolbarHeight = math.max(minExtent - _bottomHeight - topPadding - (toolbarHeight ?? kToolbarHeight), 0.0);
final double visibleToolbarHeight = visibleMainHeight - _bottomHeight - extraToolbarHeight;
final bool isScrolledUnder = overlapsContent || forceElevated || (pinned && shrinkOffset > maxExtent - minExtent);
final bool isPinnedWithOpacityFade = pinned && floating && bottom != null && extraToolbarHeight == 0.0;
final double toolbarOpacity = !accessibleNavigation && (!pinned || isPinnedWithOpacityFade)
? clampDouble(visibleToolbarHeight / (toolbarHeight ?? kToolbarHeight), 0.0, 1.0)
: 1.0;
final Widget? effectiveTitle = switch (variant) {
_SliverAppVariant.small => title,
_SliverAppVariant.medium || _SliverAppVariant.large => AnimatedOpacity(
opacity: isScrolledUnder ? 1 : 0,
duration: const Duration(milliseconds: 500),
curve: const Cubic(0.2, 0.0, 0.0, 1.0),
child: title,
),
};
final Widget appBar = FlexibleSpaceBar.createSettings(
minExtent: minExtent,
maxExtent: maxExtent,
currentExtent: math.max(minExtent, maxExtent - shrinkOffset),
toolbarOpacity: toolbarOpacity,
isScrolledUnder: isScrolledUnder,
hasLeading: leading != null || automaticallyImplyLeading,
child: AppBar(
clipBehavior: clipBehavior,
leading: leading,
automaticallyImplyLeading: automaticallyImplyLeading,
title: effectiveTitle,
actions: actions,
flexibleSpace: (title == null && flexibleSpace != null && !excludeHeaderSemantics)
? Semantics(
header: true,
child: flexibleSpace,
)
: flexibleSpace,
bottom: bottom,
elevation: isScrolledUnder ? elevation : 0.0,
scrolledUnderElevation: scrolledUnderElevation,
shadowColor: shadowColor,
surfaceTintColor: surfaceTintColor,
backgroundColor: backgroundColor,
foregroundColor: foregroundColor,
iconTheme: iconTheme,
actionsIconTheme: actionsIconTheme,
primary: primary,
centerTitle: centerTitle,
excludeHeaderSemantics: excludeHeaderSemantics,
titleSpacing: titleSpacing,
shape: shape,
toolbarOpacity: toolbarOpacity,
bottomOpacity: pinned ? 1.0 : clampDouble(visibleMainHeight / _bottomHeight, 0.0, 1.0),
toolbarHeight: toolbarHeight,
leadingWidth: leadingWidth,
toolbarTextStyle: toolbarTextStyle,
titleTextStyle: titleTextStyle,
systemOverlayStyle: systemOverlayStyle,
forceMaterialTransparency: forceMaterialTransparency,
),
);
return appBar;
}
@override
bool shouldRebuild(covariant _SliverAppBarDelegate oldDelegate) {
return leading != oldDelegate.leading
|| automaticallyImplyLeading != oldDelegate.automaticallyImplyLeading
|| title != oldDelegate.title
|| actions != oldDelegate.actions
|| flexibleSpace != oldDelegate.flexibleSpace
|| bottom != oldDelegate.bottom
|| _bottomHeight != oldDelegate._bottomHeight
|| elevation != oldDelegate.elevation
|| shadowColor != oldDelegate.shadowColor
|| backgroundColor != oldDelegate.backgroundColor
|| foregroundColor != oldDelegate.foregroundColor
|| iconTheme != oldDelegate.iconTheme
|| actionsIconTheme != oldDelegate.actionsIconTheme
|| primary != oldDelegate.primary
|| centerTitle != oldDelegate.centerTitle
|| titleSpacing != oldDelegate.titleSpacing
|| expandedHeight != oldDelegate.expandedHeight
|| topPadding != oldDelegate.topPadding
|| pinned != oldDelegate.pinned
|| floating != oldDelegate.floating
|| vsync != oldDelegate.vsync
|| snapConfiguration != oldDelegate.snapConfiguration
|| stretchConfiguration != oldDelegate.stretchConfiguration
|| showOnScreenConfiguration != oldDelegate.showOnScreenConfiguration
|| forceElevated != oldDelegate.forceElevated
|| toolbarHeight != oldDelegate.toolbarHeight
|| leadingWidth != oldDelegate.leadingWidth
|| toolbarTextStyle != oldDelegate.toolbarTextStyle
|| titleTextStyle != oldDelegate.titleTextStyle
|| systemOverlayStyle != oldDelegate.systemOverlayStyle
|| forceMaterialTransparency != oldDelegate.forceMaterialTransparency
|| accessibleNavigation != oldDelegate.accessibleNavigation;
}
@override
String toString() {
return '${describeIdentity(this)}(topPadding: ${topPadding.toStringAsFixed(1)}, bottomHeight: ${_bottomHeight.toStringAsFixed(1)}, ...)';
}
}
/// A Material Design app bar that integrates with a [CustomScrollView].
///
/// An app bar consists of a toolbar and potentially other widgets, such as a
/// [TabBar] and a [FlexibleSpaceBar]. App bars typically expose one or more
/// common actions with [IconButton]s which are optionally followed by a
/// [PopupMenuButton] for less common operations.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=R9C5KMJKluE}
///
/// Sliver app bars are typically used as the first child of a
/// [CustomScrollView], which lets the app bar integrate with the scroll view so
/// that it can vary in height according to the scroll offset or float above the
/// other content in the scroll view. For a fixed-height app bar at the top of
/// the screen see [AppBar], which is used in the [Scaffold.appBar] slot.
///
/// The AppBar displays the toolbar widgets, [leading], [title], and
/// [actions], above the [bottom] (if any). If a [flexibleSpace] widget is
/// specified then it is stacked behind the toolbar and the bottom widget.
///
/// {@tool snippet}
///
/// This is an example that could be included in a [CustomScrollView]'s
/// [CustomScrollView.slivers] list:
///
/// ```dart
/// SliverAppBar(
/// expandedHeight: 150.0,
/// flexibleSpace: const FlexibleSpaceBar(
/// title: Text('Available seats'),
/// ),
/// actions: <Widget>[
/// IconButton(
/// icon: const Icon(Icons.add_circle),
/// tooltip: 'Add new entry',
/// onPressed: () { /* ... */ },
/// ),
/// ]
/// )
/// ```
/// {@end-tool}
///
/// {@tool dartpad}
/// Here is an example of [SliverAppBar] when using [stretch] and [onStretchTrigger].
///
/// ** See code in examples/api/lib/material/app_bar/sliver_app_bar.4.dart **
/// {@end-tool}
///
///
/// {@tool dartpad}
/// This sample shows a [SliverAppBar] and it's behavior when using the
/// [pinned], [snap] and [floating] parameters.
///
/// ** See code in examples/api/lib/material/app_bar/sliver_app_bar.1.dart **
/// {@end-tool}
///
/// ## Animated Examples
///
/// The following animations show how app bars with different configurations
/// behave when a user scrolls up and then down again.
///
/// * App bar with [floating]: false, [pinned]: false, [snap]: false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar.mp4}
///
/// * App bar with [floating]: true, [pinned]: false, [snap]: false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_floating.mp4}
///
/// * App bar with [floating]: true, [pinned]: false, [snap]: true:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_floating_snap.mp4}
///
/// * App bar with [floating]: true, [pinned]: true, [snap]: false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_pinned_floating.mp4}
///
/// * App bar with [floating]: true, [pinned]: true, [snap]: true:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_pinned_floating_snap.mp4}
///
/// * App bar with [floating]: false, [pinned]: true, [snap]: false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_pinned.mp4}
///
/// The property [snap] can only be set to true if [floating] is also true.
///
/// See also:
///
/// * [CustomScrollView], which integrates the [SliverAppBar] into its
/// scrolling.
/// * [AppBar], which is a fixed-height app bar for use in [Scaffold.appBar].
/// * [TabBar], which is typically placed in the [bottom] slot of the [AppBar]
/// if the screen has multiple pages arranged in tabs.
/// * [IconButton], which is used with [actions] to show buttons on the app bar.
/// * [PopupMenuButton], to show a popup menu on the app bar, via [actions].
/// * [FlexibleSpaceBar], which is used with [flexibleSpace] when the app bar
/// can expand and collapse.
/// * <https://material.io/design/components/app-bars-top.html>
class SliverAppBar extends StatefulWidget {
/// Creates a Material Design app bar that can be placed in a [CustomScrollView].
const SliverAppBar({
super.key,
this.leading,
this.automaticallyImplyLeading = true,
this.title,
this.actions,
this.flexibleSpace,
this.bottom,
this.elevation,
this.scrolledUnderElevation,
this.shadowColor,
this.surfaceTintColor,
this.forceElevated = false,
this.backgroundColor,
this.foregroundColor,
this.iconTheme,
this.actionsIconTheme,
this.primary = true,
this.centerTitle,
this.excludeHeaderSemantics = false,
this.titleSpacing,
this.collapsedHeight,
this.expandedHeight,
this.floating = false,
this.pinned = false,
this.snap = false,
this.stretch = false,
this.stretchTriggerOffset = 100.0,
this.onStretchTrigger,
this.shape,
this.toolbarHeight = kToolbarHeight,
this.leadingWidth,
this.toolbarTextStyle,
this.titleTextStyle,
this.systemOverlayStyle,
this.forceMaterialTransparency = false,
this.clipBehavior,
}) : assert(floating || !snap, 'The "snap" argument only makes sense for floating app bars.'),
assert(stretchTriggerOffset > 0.0),
assert(
collapsedHeight == null || collapsedHeight >= toolbarHeight,
'The "collapsedHeight" argument has to be larger than or equal to [toolbarHeight].',
),
_variant = _SliverAppVariant.small;
/// Creates a Material Design medium top app bar that can be placed
/// in a [CustomScrollView].
///
/// Returns a [SliverAppBar] configured with appropriate defaults
/// for a medium top app bar as defined in Material 3. It starts fully
/// expanded with the title in an area underneath the main row of icons.
/// When the [CustomScrollView] is scrolled, the title will be scrolled
/// under the main row. When it is fully collapsed, a smaller version of the
/// title will fade in on the main row. The reverse will happen if it is
/// expanded again.
///
/// {@tool dartpad}
/// This sample shows how to use [SliverAppBar.medium] in a [CustomScrollView].
///
/// ** See code in examples/api/lib/material/app_bar/sliver_app_bar.2.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AppBar], for a small or center-aligned top app bar.
/// * [SliverAppBar.large], for a large top app bar.
/// * https://m3.material.io/components/top-app-bar/overview, the Material 3
/// app bar specification.
const SliverAppBar.medium({
super.key,
this.leading,
this.automaticallyImplyLeading = true,
this.title,
this.actions,
this.flexibleSpace,
this.bottom,
this.elevation,
this.scrolledUnderElevation,
this.shadowColor,
this.surfaceTintColor,
this.forceElevated = false,
this.backgroundColor,
this.foregroundColor,
this.iconTheme,
this.actionsIconTheme,
this.primary = true,
this.centerTitle,
this.excludeHeaderSemantics = false,
this.titleSpacing,
this.collapsedHeight,
this.expandedHeight,
this.floating = false,
this.pinned = true,
this.snap = false,
this.stretch = false,
this.stretchTriggerOffset = 100.0,
this.onStretchTrigger,
this.shape,
this.toolbarHeight = _MediumScrollUnderFlexibleConfig.collapsedHeight,
this.leadingWidth,
this.toolbarTextStyle,
this.titleTextStyle,
this.systemOverlayStyle,
this.forceMaterialTransparency = false,
this.clipBehavior,
}) : assert(floating || !snap, 'The "snap" argument only makes sense for floating app bars.'),
assert(stretchTriggerOffset > 0.0),
assert(
collapsedHeight == null || collapsedHeight >= toolbarHeight,
'The "collapsedHeight" argument has to be larger than or equal to [toolbarHeight].',
),
_variant = _SliverAppVariant.medium;
/// Creates a Material Design large top app bar that can be placed
/// in a [CustomScrollView].
///
/// Returns a [SliverAppBar] configured with appropriate defaults
/// for a large top app bar as defined in Material 3. It starts fully
/// expanded with the title in an area underneath the main row of icons.
/// When the [CustomScrollView] is scrolled, the title will be scrolled
/// under the main row. When it is fully collapsed, a smaller version of the
/// title will fade in on the main row. The reverse will happen if it is
/// expanded again.
///
/// {@tool dartpad}
/// This sample shows how to use [SliverAppBar.large] in a [CustomScrollView].
///
/// ** See code in examples/api/lib/material/app_bar/sliver_app_bar.3.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AppBar], for a small or center-aligned top app bar.
/// * [SliverAppBar.medium], for a medium top app bar.
/// * https://m3.material.io/components/top-app-bar/overview, the Material 3
/// app bar specification.
const SliverAppBar.large({
super.key,
this.leading,
this.automaticallyImplyLeading = true,
this.title,
this.actions,
this.flexibleSpace,
this.bottom,
this.elevation,
this.scrolledUnderElevation,
this.shadowColor,
this.surfaceTintColor,
this.forceElevated = false,
this.backgroundColor,
this.foregroundColor,
this.iconTheme,
this.actionsIconTheme,
this.primary = true,
this.centerTitle,
this.excludeHeaderSemantics = false,
this.titleSpacing,
this.collapsedHeight,
this.expandedHeight,
this.floating = false,
this.pinned = true,
this.snap = false,
this.stretch = false,
this.stretchTriggerOffset = 100.0,
this.onStretchTrigger,
this.shape,
this.toolbarHeight = _LargeScrollUnderFlexibleConfig.collapsedHeight,
this.leadingWidth,
this.toolbarTextStyle,
this.titleTextStyle,
this.systemOverlayStyle,
this.forceMaterialTransparency = false,
this.clipBehavior,
}) : assert(floating || !snap, 'The "snap" argument only makes sense for floating app bars.'),
assert(stretchTriggerOffset > 0.0),
assert(
collapsedHeight == null || collapsedHeight >= toolbarHeight,
'The "collapsedHeight" argument has to be larger than or equal to [toolbarHeight].',
),
_variant = _SliverAppVariant.large;
/// {@macro flutter.material.appbar.leading}
///
/// This property is used to configure an [AppBar].
final Widget? leading;
/// {@macro flutter.material.appbar.automaticallyImplyLeading}
///
/// This property is used to configure an [AppBar].
final bool automaticallyImplyLeading;
/// {@macro flutter.material.appbar.title}
///
/// This property is used to configure an [AppBar].
final Widget? title;
/// {@macro flutter.material.appbar.actions}
///
/// This property is used to configure an [AppBar].
final List<Widget>? actions;
/// {@macro flutter.material.appbar.flexibleSpace}
///
/// This property is used to configure an [AppBar].
final Widget? flexibleSpace;
/// {@macro flutter.material.appbar.bottom}
///
/// This property is used to configure an [AppBar].
final PreferredSizeWidget? bottom;
/// {@macro flutter.material.appbar.elevation}
///
/// This property is used to configure an [AppBar].
final double? elevation;
/// {@macro flutter.material.appbar.scrolledUnderElevation}
///
/// This property is used to configure an [AppBar].
final double? scrolledUnderElevation;
/// {@macro flutter.material.appbar.shadowColor}
///
/// This property is used to configure an [AppBar].
final Color? shadowColor;
/// {@macro flutter.material.appbar.surfaceTintColor}
///
/// This property is used to configure an [AppBar].
final Color? surfaceTintColor;
/// Whether to show the shadow appropriate for the [elevation] even if the
/// content is not scrolled under the [AppBar].
///
/// Defaults to false, meaning that the [elevation] is only applied when the
/// [AppBar] is being displayed over content that is scrolled under it.
///
/// When set to true, the [elevation] is applied regardless.
///
/// Ignored when [elevation] is zero.
final bool forceElevated;
/// {@macro flutter.material.appbar.backgroundColor}
///
/// This property is used to configure an [AppBar].
final Color? backgroundColor;
/// {@macro flutter.material.appbar.foregroundColor}
///
/// This property is used to configure an [AppBar].
final Color? foregroundColor;
/// {@macro flutter.material.appbar.iconTheme}
///
/// This property is used to configure an [AppBar].
final IconThemeData? iconTheme;
/// {@macro flutter.material.appbar.actionsIconTheme}
///
/// This property is used to configure an [AppBar].
final IconThemeData? actionsIconTheme;
/// {@macro flutter.material.appbar.primary}
///
/// This property is used to configure an [AppBar].
final bool primary;
/// {@macro flutter.material.appbar.centerTitle}
///
/// This property is used to configure an [AppBar].
final bool? centerTitle;
/// {@macro flutter.material.appbar.excludeHeaderSemantics}
///
/// This property is used to configure an [AppBar].
final bool excludeHeaderSemantics;
/// {@macro flutter.material.appbar.titleSpacing}
///
/// This property is used to configure an [AppBar].
final double? titleSpacing;
/// Defines the height of the app bar when it is collapsed.
///
/// By default, the collapsed height is [toolbarHeight]. If [bottom] widget is
/// specified, then its height from [PreferredSizeWidget.preferredSize] is
/// added to the height. If [primary] is true, then the [MediaQuery] top
/// padding, [EdgeInsets.top] of [MediaQueryData.padding], is added as well.
///
/// If [pinned] and [floating] are true, with [bottom] set, the default
/// collapsed height is only the height of [PreferredSizeWidget.preferredSize]
/// with the [MediaQuery] top padding.
final double? collapsedHeight;
/// The size of the app bar when it is fully expanded.
///
/// By default, the total height of the toolbar and the bottom widget (if
/// any). If a [flexibleSpace] widget is specified this height should be big
/// enough to accommodate whatever that widget contains.
///
/// This does not include the status bar height (which will be automatically
/// included if [primary] is true).
final double? expandedHeight;
/// Whether the app bar should become visible as soon as the user scrolls
/// towards the app bar.
///
/// Otherwise, the user will need to scroll near the top of the scroll view to
/// reveal the app bar.
///
/// If [snap] is true then a scroll that exposes the app bar will trigger an
/// animation that slides the entire app bar into view. Similarly if a scroll
/// dismisses the app bar, the animation will slide it completely out of view.
///
/// ## Animated Examples
///
/// The following animations show how the app bar changes its scrolling
/// behavior based on the value of this property.
///
/// * App bar with [floating] set to false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar.mp4}
/// * App bar with [floating] set to true:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_floating.mp4}
///
/// See also:
///
/// * [SliverAppBar] for more animated examples of how this property changes the
/// behavior of the app bar in combination with [pinned] and [snap].
final bool floating;
/// Whether the app bar should remain visible at the start of the scroll view.
///
/// The app bar can still expand and contract as the user scrolls, but it will
/// remain visible rather than being scrolled out of view.
///
/// ## Animated Examples
///
/// The following animations show how the app bar changes its scrolling
/// behavior based on the value of this property.
///
/// * App bar with [pinned] set to false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar.mp4}
/// * App bar with [pinned] set to true:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_pinned.mp4}
///
/// See also:
///
/// * [SliverAppBar] for more animated examples of how this property changes the
/// behavior of the app bar in combination with [floating].
final bool pinned;
/// {@macro flutter.material.appbar.shape}
///
/// This property is used to configure an [AppBar].
final ShapeBorder? shape;
/// If [snap] and [floating] are true then the floating app bar will "snap"
/// into view.
///
/// If [snap] is true then a scroll that exposes the floating app bar will
/// trigger an animation that slides the entire app bar into view. Similarly
/// if a scroll dismisses the app bar, the animation will slide the app bar
/// completely out of view. Additionally, setting [snap] to true will fully
/// expand the floating app bar when the framework tries to reveal the
/// contents of the app bar by calling [RenderObject.showOnScreen]. For
/// example, when a [TextField] in the floating app bar gains focus, if [snap]
/// is true, the framework will always fully expand the floating app bar, in
/// order to reveal the focused [TextField].
///
/// Snapping only applies when the app bar is floating, not when the app bar
/// appears at the top of its scroll view.
///
/// ## Animated Examples
///
/// The following animations show how the app bar changes its scrolling
/// behavior based on the value of this property.
///
/// * App bar with [snap] set to false:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_floating.mp4}
/// * App bar with [snap] set to true:
/// {@animation 476 400 https://flutter.github.io/assets-for-api-docs/assets/material/app_bar_floating_snap.mp4}
///
/// See also:
///
/// * [SliverAppBar] for more animated examples of how this property changes the
/// behavior of the app bar in combination with [pinned] and [floating].
final bool snap;
/// Whether the app bar should stretch to fill the over-scroll area.
///
/// The app bar can still expand and contract as the user scrolls, but it will
/// also stretch when the user over-scrolls.
final bool stretch;
/// The offset of overscroll required to activate [onStretchTrigger].
///
/// This defaults to 100.0.
final double stretchTriggerOffset;
/// The callback function to be executed when a user over-scrolls to the
/// offset specified by [stretchTriggerOffset].
final AsyncCallback? onStretchTrigger;
/// {@macro flutter.material.appbar.toolbarHeight}
///
/// This property is used to configure an [AppBar].
final double toolbarHeight;
/// {@macro flutter.material.appbar.leadingWidth}
///
/// This property is used to configure an [AppBar].
final double? leadingWidth;
/// {@macro flutter.material.appbar.toolbarTextStyle}
///
/// This property is used to configure an [AppBar].
final TextStyle? toolbarTextStyle;
/// {@macro flutter.material.appbar.titleTextStyle}
///
/// This property is used to configure an [AppBar].
final TextStyle? titleTextStyle;
/// {@macro flutter.material.appbar.systemOverlayStyle}
///
/// This property is used to configure an [AppBar].
final SystemUiOverlayStyle? systemOverlayStyle;
/// {@macro flutter.material.appbar.forceMaterialTransparency}
///
/// This property is used to configure an [AppBar].
final bool forceMaterialTransparency;
/// {@macro flutter.material.Material.clipBehavior}
final Clip? clipBehavior;
final _SliverAppVariant _variant;
@override
State<SliverAppBar> createState() => _SliverAppBarState();
}
// This class is only Stateful because it owns the TickerProvider used
// by the floating appbar snap animation (via FloatingHeaderSnapConfiguration).
class _SliverAppBarState extends State<SliverAppBar> with TickerProviderStateMixin {
FloatingHeaderSnapConfiguration? _snapConfiguration;
OverScrollHeaderStretchConfiguration? _stretchConfiguration;
PersistentHeaderShowOnScreenConfiguration? _showOnScreenConfiguration;
void _updateSnapConfiguration() {
if (widget.snap && widget.floating) {
_snapConfiguration = FloatingHeaderSnapConfiguration(
curve: Curves.easeOut,
duration: const Duration(milliseconds: 200),
);
} else {
_snapConfiguration = null;
}
_showOnScreenConfiguration = widget.floating & widget.snap
? const PersistentHeaderShowOnScreenConfiguration(minShowOnScreenExtent: double.infinity)
: null;
}
void _updateStretchConfiguration() {
if (widget.stretch) {
_stretchConfiguration = OverScrollHeaderStretchConfiguration(
stretchTriggerOffset: widget.stretchTriggerOffset,
onStretchTrigger: widget.onStretchTrigger,
);
} else {
_stretchConfiguration = null;
}
}
@override
void initState() {
super.initState();
_updateSnapConfiguration();
_updateStretchConfiguration();
}
@override
void didUpdateWidget(SliverAppBar oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.snap != oldWidget.snap || widget.floating != oldWidget.floating) {
_updateSnapConfiguration();
}
if (widget.stretch != oldWidget.stretch) {
_updateStretchConfiguration();
}
}
@override
Widget build(BuildContext context) {
assert(!widget.primary || debugCheckHasMediaQuery(context));
final double bottomHeight = widget.bottom?.preferredSize.height ?? 0.0;
final double topPadding = widget.primary ? MediaQuery.paddingOf(context).top : 0.0;
final double collapsedHeight = (widget.pinned && widget.floating && widget.bottom != null)
? (widget.collapsedHeight ?? 0.0) + bottomHeight + topPadding
: (widget.collapsedHeight ?? widget.toolbarHeight) + bottomHeight + topPadding;
final double? effectiveExpandedHeight;
final double effectiveCollapsedHeight;
final Widget? effectiveFlexibleSpace;
switch (widget._variant) {
case _SliverAppVariant.small:
effectiveExpandedHeight = widget.expandedHeight;
effectiveCollapsedHeight = collapsedHeight;
effectiveFlexibleSpace = widget.flexibleSpace;
case _SliverAppVariant.medium:
effectiveExpandedHeight = widget.expandedHeight
?? _MediumScrollUnderFlexibleConfig.expandedHeight + bottomHeight;
effectiveCollapsedHeight = widget.collapsedHeight
?? topPadding + _MediumScrollUnderFlexibleConfig.collapsedHeight + bottomHeight;
effectiveFlexibleSpace = widget.flexibleSpace ?? _ScrollUnderFlexibleSpace(
title: widget.title,
foregroundColor: widget.foregroundColor,
configBuilder: _MediumScrollUnderFlexibleConfig.new,
titleTextStyle: widget.titleTextStyle,
bottomHeight: bottomHeight,
);
case _SliverAppVariant.large:
effectiveExpandedHeight = widget.expandedHeight
?? _LargeScrollUnderFlexibleConfig.expandedHeight + bottomHeight;
effectiveCollapsedHeight = widget.collapsedHeight
?? topPadding + _LargeScrollUnderFlexibleConfig.collapsedHeight + bottomHeight;
effectiveFlexibleSpace = widget.flexibleSpace ?? _ScrollUnderFlexibleSpace(
title: widget.title,
foregroundColor: widget.foregroundColor,
configBuilder: _LargeScrollUnderFlexibleConfig.new,
titleTextStyle: widget.titleTextStyle,
bottomHeight: bottomHeight,
);
}
return MediaQuery.removePadding(
context: context,
removeBottom: true,
child: SliverPersistentHeader(
floating: widget.floating,
pinned: widget.pinned,
delegate: _SliverAppBarDelegate(
vsync: this,
leading: widget.leading,
automaticallyImplyLeading: widget.automaticallyImplyLeading,
title: widget.title,
actions: widget.actions,
flexibleSpace: effectiveFlexibleSpace,
bottom: widget.bottom,
elevation: widget.elevation,
scrolledUnderElevation: widget.scrolledUnderElevation,
shadowColor: widget.shadowColor,
surfaceTintColor: widget.surfaceTintColor,
forceElevated: widget.forceElevated,
backgroundColor: widget.backgroundColor,
foregroundColor: widget.foregroundColor,
iconTheme: widget.iconTheme,
actionsIconTheme: widget.actionsIconTheme,
primary: widget.primary,
centerTitle: widget.centerTitle,
excludeHeaderSemantics: widget.excludeHeaderSemantics,
titleSpacing: widget.titleSpacing,
expandedHeight: effectiveExpandedHeight,
collapsedHeight: effectiveCollapsedHeight,
topPadding: topPadding,
floating: widget.floating,
pinned: widget.pinned,
shape: widget.shape,
snapConfiguration: _snapConfiguration,
stretchConfiguration: _stretchConfiguration,
showOnScreenConfiguration: _showOnScreenConfiguration,
toolbarHeight: widget.toolbarHeight,
leadingWidth: widget.leadingWidth,
toolbarTextStyle: widget.toolbarTextStyle,
titleTextStyle: widget.titleTextStyle,
systemOverlayStyle: widget.systemOverlayStyle,
forceMaterialTransparency: widget.forceMaterialTransparency,
clipBehavior: widget.clipBehavior,
variant: widget._variant,
accessibleNavigation: MediaQuery.of(context).accessibleNavigation,
),
),
);
}
}
// Layout the AppBar's title with unconstrained height, vertically
// center it within its (NavigationToolbar) parent, and allow the
// parent to constrain the title's actual height.
class _AppBarTitleBox extends SingleChildRenderObjectWidget {
const _AppBarTitleBox({ required Widget super.child });
@override
_RenderAppBarTitleBox createRenderObject(BuildContext context) {
return _RenderAppBarTitleBox(
textDirection: Directionality.of(context),
);
}
@override
void updateRenderObject(BuildContext context, _RenderAppBarTitleBox renderObject) {
renderObject.textDirection = Directionality.of(context);
}
}
class _RenderAppBarTitleBox extends RenderAligningShiftedBox {
_RenderAppBarTitleBox({
super.textDirection,
}) : super(alignment: Alignment.center);
@override
Size computeDryLayout(BoxConstraints constraints) {
final BoxConstraints innerConstraints = constraints.copyWith(maxHeight: double.infinity);
final Size childSize = child!.getDryLayout(innerConstraints);
return constraints.constrain(childSize);
}
@override
void performLayout() {
final BoxConstraints innerConstraints = constraints.copyWith(maxHeight: double.infinity);
child!.layout(innerConstraints, parentUsesSize: true);
size = constraints.constrain(child!.size);
alignChild();
}
}
class _ScrollUnderFlexibleSpace extends StatelessWidget {
const _ScrollUnderFlexibleSpace({
this.title,
this.foregroundColor,
required this.configBuilder,
this.titleTextStyle,
required this.bottomHeight,
});
final Widget? title;
final Color? foregroundColor;
final _FlexibleConfigBuilder configBuilder;
final TextStyle? titleTextStyle;
final double bottomHeight;
@override
Widget build(BuildContext context) {
late final AppBarTheme appBarTheme = AppBarTheme.of(context);
late final AppBarTheme defaults = Theme.of(context).useMaterial3 ? _AppBarDefaultsM3(context) : _AppBarDefaultsM2(context);
final FlexibleSpaceBarSettings settings = context.dependOnInheritedWidgetOfExactType<FlexibleSpaceBarSettings>()!;
final _ScrollUnderFlexibleConfig config = configBuilder(context);
assert(
config.expandedTitlePadding.isNonNegative,
'The _ExpandedTitleWithPadding widget assumes that the expanded title padding is non-negative. '
'Update its implementation to handle negative padding.',
);
final TextStyle? expandedTextStyle = titleTextStyle
?? appBarTheme.titleTextStyle
?? config.expandedTextStyle?.copyWith(color: foregroundColor ?? appBarTheme.foregroundColor ?? defaults.foregroundColor);
final Widget? expandedTitle = switch ((title, expandedTextStyle)) {
(null, _) => null,
(final Widget title, null) => title,
(final Widget title, final TextStyle textStyle) => DefaultTextStyle(style: textStyle, child: title),
};
final EdgeInsets resolvedTitlePadding = config.expandedTitlePadding.resolve(Directionality.of(context));
final EdgeInsetsGeometry expandedTitlePadding = bottomHeight > 0
? resolvedTitlePadding.copyWith(bottom: 0)
: resolvedTitlePadding;
// Set maximum text scale factor to [_kMaxTitleTextScaleFactor] for the
// title to keep the visual hierarchy the same even with larger font
// sizes. To opt out, wrap the [title] widget in a [MediaQuery] widget
// with a different TextScaler.
// TODO(tahatesser): Add link to Material spec when available, https://github.com/flutter/flutter/issues/58769.
return MediaQuery.withClampedTextScaling(
maxScaleFactor: _kMaxTitleTextScaleFactor,
// This column will assume the full height of the parent Stack.
child: Column(
children: <Widget>[
Padding(padding: EdgeInsets.only(top: settings.minExtent - bottomHeight)),
Flexible(
child: ClipRect(
child: _ExpandedTitleWithPadding(
padding: expandedTitlePadding,
maxExtent: settings.maxExtent - settings.minExtent,
child: expandedTitle,
),
),
),
// Reserve space for AppBar.bottom, which is a sibling of this widget,
// on the parent Stack.
if (bottomHeight > 0) Padding(padding: EdgeInsets.only(bottom: bottomHeight)),
],
),
);
}
}
// A widget that bottom-start aligns its child (the expanded title widget), and
// insets the child according to the specified padding.
//
// This widget gives the child an infinite max height constraint, and will also
// attempt to vertically limit the child's bounding box (not including the
// padding) to within the y range [0, maxExtent], to make sure the child is
// visible when the AppBar is fully expanded.
class _ExpandedTitleWithPadding extends SingleChildRenderObjectWidget {
const _ExpandedTitleWithPadding({
required this.padding,
required this.maxExtent,
super.child,
});
final EdgeInsetsGeometry padding;
final double maxExtent;
@override
_RenderExpandedTitleBox createRenderObject(BuildContext context) {
final TextDirection textDirection = Directionality.of(context);
return _RenderExpandedTitleBox(
padding.resolve(textDirection),
AlignmentDirectional.bottomStart.resolve(textDirection),
maxExtent,
null,
);
}
@override
void updateRenderObject(BuildContext context, _RenderExpandedTitleBox renderObject) {
final TextDirection textDirection = Directionality.of(context);
renderObject
..padding = padding.resolve(textDirection)
..titleAlignment = AlignmentDirectional.bottomStart.resolve(textDirection)
..maxExtent = maxExtent;
}
}
class _RenderExpandedTitleBox extends RenderShiftedBox {
_RenderExpandedTitleBox(this._padding, this._titleAlignment, this._maxExtent, super.child);
EdgeInsets get padding => _padding;
EdgeInsets _padding;
set padding(EdgeInsets value) {
if (_padding == value) {
return;
}
assert(value.isNonNegative);
_padding = value;
markNeedsLayout();
}
Alignment get titleAlignment => _titleAlignment;
Alignment _titleAlignment;
set titleAlignment(Alignment value) {
if (_titleAlignment == value) {
return;
}
_titleAlignment = value;
markNeedsLayout();
}
double get maxExtent => _maxExtent;
double _maxExtent;
set maxExtent(double value) {
if (_maxExtent == value) {
return;
}
_maxExtent = value;
markNeedsLayout();
}
@override
double computeMaxIntrinsicHeight(double width) {
final RenderBox? child = this.child;
return child == null ? 0.0 : child.getMaxIntrinsicHeight(math.max(0, width - padding.horizontal)) + padding.vertical;
}
@override
double computeMaxIntrinsicWidth(double height) {
final RenderBox? child = this.child;
return child == null ? 0.0 : child.getMaxIntrinsicWidth(double.infinity) + padding.horizontal;
}
@override
double computeMinIntrinsicHeight(double width) {
final RenderBox? child = this.child;
return child == null ? 0.0 : child.getMinIntrinsicHeight(math.max(0, width - padding.horizontal)) + padding.vertical;
}
@override
double computeMinIntrinsicWidth(double height) {
final RenderBox? child = this.child;
return child == null ? 0.0 : child.getMinIntrinsicWidth(double.infinity) + padding.horizontal;
}
Size _computeSize(BoxConstraints constraints, ChildLayouter layoutChild) {
final RenderBox? child = this.child;
if (child == null) {
return Size.zero;
}
layoutChild(child, constraints.widthConstraints().deflate(padding));
return constraints.biggest;
}
@override
Size computeDryLayout(BoxConstraints constraints) => _computeSize(constraints, ChildLayoutHelper.dryLayoutChild);
@override
void performLayout() {
final RenderBox? child = this.child;
if (child == null) {
this.size = constraints.smallest;
return;
}
final Size size = this.size = _computeSize(constraints, ChildLayoutHelper.layoutChild);
final Size childSize = child.size;
assert(padding.isNonNegative);
assert(titleAlignment.y == 1.0);
// yAdjustment is the minimum additional y offset to shift the child in
// the visible vertical space when AppBar is fully expanded. The goal is to
// prevent the expanded title from being clipped when the expanded title
// widget + the bottom padding is too tall to fit in the flexible space (the
// top padding is basically ignored since the expanded title is
// bottom-aligned).
final double yAdjustment = clampDouble(childSize.height + padding.bottom - maxExtent, 0, padding.bottom);
final double offsetY = size.height - childSize.height - padding.bottom + yAdjustment;
final double offsetX = (titleAlignment.x + 1) / 2 * (size.width - padding.horizontal - childSize.width) + padding.left;
final BoxParentData childParentData = child.parentData! as BoxParentData;
childParentData.offset = Offset(offsetX, offsetY);
}
}
mixin _ScrollUnderFlexibleConfig {
TextStyle? get collapsedTextStyle;
TextStyle? get expandedTextStyle;
EdgeInsetsGeometry get expandedTitlePadding;
}
// Hand coded defaults based on Material Design 2.
class _AppBarDefaultsM2 extends AppBarTheme {
_AppBarDefaultsM2(this.context)
: super(
elevation: 4.0,
shadowColor: const Color(0xFF000000),
titleSpacing: NavigationToolbar.kMiddleSpacing,
toolbarHeight: kToolbarHeight,
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override
Color? get backgroundColor => _colors.brightness == Brightness.dark ? _colors.surface : _colors.primary;
@override
Color? get foregroundColor => _colors.brightness == Brightness.dark ? _colors.onSurface : _colors.onPrimary;
@override
IconThemeData? get iconTheme => _theme.iconTheme;
@override
TextStyle? get toolbarTextStyle => _theme.textTheme.bodyMedium;
@override
TextStyle? get titleTextStyle => _theme.textTheme.titleLarge;
}
// BEGIN GENERATED TOKEN PROPERTIES - AppBar
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _AppBarDefaultsM3 extends AppBarTheme {
_AppBarDefaultsM3(this.context)
: super(
elevation: 0.0,
scrolledUnderElevation: 3.0,
titleSpacing: NavigationToolbar.kMiddleSpacing,
toolbarHeight: 64.0,
);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
@override
Color? get backgroundColor => _colors.surface;
@override
Color? get foregroundColor => _colors.onSurface;
@override
Color? get shadowColor => Colors.transparent;
@override
Color? get surfaceTintColor => Colors.transparent;
@override
IconThemeData? get iconTheme => IconThemeData(
color: _colors.onSurface,
size: 24.0,
);
@override
IconThemeData? get actionsIconTheme => IconThemeData(
color: _colors.onSurfaceVariant,
size: 24.0,
);
@override
TextStyle? get toolbarTextStyle => _textTheme.bodyMedium;
@override
TextStyle? get titleTextStyle => _textTheme.titleLarge;
}
// Variant configuration
class _MediumScrollUnderFlexibleConfig with _ScrollUnderFlexibleConfig {
_MediumScrollUnderFlexibleConfig(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
static const double collapsedHeight = 64.0;
static const double expandedHeight = 112.0;
@override
TextStyle? get collapsedTextStyle =>
_textTheme.titleLarge?.apply(color: _colors.onSurface);
@override
TextStyle? get expandedTextStyle =>
_textTheme.headlineSmall?.apply(color: _colors.onSurface);
@override
EdgeInsetsGeometry get expandedTitlePadding => const EdgeInsets.fromLTRB(16, 0, 16, 20);
}
class _LargeScrollUnderFlexibleConfig with _ScrollUnderFlexibleConfig {
_LargeScrollUnderFlexibleConfig(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
late final TextTheme _textTheme = _theme.textTheme;
static const double collapsedHeight = 64.0;
static const double expandedHeight = 152.0;
@override
TextStyle? get collapsedTextStyle =>
_textTheme.titleLarge?.apply(color: _colors.onSurface);
@override
TextStyle? get expandedTextStyle =>
_textTheme.headlineMedium?.apply(color: _colors.onSurface);
@override
EdgeInsetsGeometry get expandedTitlePadding => const EdgeInsets.fromLTRB(16, 0, 16, 28);
}
// END GENERATED TOKEN PROPERTIES - AppBar
| flutter/packages/flutter/lib/src/material/app_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/app_bar.dart",
"repo_id": "flutter",
"token_count": 30608
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'button_bar_theme.dart';
import 'button_theme.dart';
import 'dialog.dart';
/// An end-aligned row of buttons, laying out into a column if there is not
/// enough horizontal space.
///
/// ## Updating to [OverflowBar]
///
/// [ButtonBar] has been replace by a more efficient widget, [OverflowBar].
///
/// ```dart
/// // Before
/// ButtonBar(
/// alignment: MainAxisAlignment.spaceEvenly,
/// children: <Widget>[
/// TextButton( child: const Text('Button 1'), onPressed: () {}),
/// TextButton( child: const Text('Button 2'), onPressed: () {}),
/// TextButton( child: const Text('Button 3'), onPressed: () {}),
/// ],
/// );
/// ```
/// ```dart
/// // After
/// OverflowBar(
/// alignment: MainAxisAlignment.spaceEvenly,
/// children: <Widget>[
/// TextButton( child: const Text('Button 1'), onPressed: () {}),
/// TextButton( child: const Text('Button 2'), onPressed: () {}),
/// TextButton( child: const Text('Button 3'), onPressed: () {}),
/// ],
/// );
/// ```
///
/// See the [OverflowBar] documentation for more details.
///
/// ## Using [ButtonBar]
///
/// Places the buttons horizontally according to the [buttonPadding]. The
/// children are laid out in a [Row] with [MainAxisAlignment.end]. When the
/// [Directionality] is [TextDirection.ltr], the button bar's children are
/// right justified and the last child becomes the rightmost child. When the
/// [Directionality] [TextDirection.rtl] the children are left justified and
/// the last child becomes the leftmost child.
///
/// If the button bar's width exceeds the maximum width constraint on the
/// widget, it aligns its buttons in a column. The key difference here
/// is that the [MainAxisAlignment] will then be treated as a
/// cross-axis/horizontal alignment. For example, if the buttons overflow and
/// [ButtonBar.alignment] was set to [MainAxisAlignment.start], the buttons would
/// align to the horizontal start of the button bar.
///
/// The [ButtonBar] can be configured with a [ButtonBarTheme]. For any null
/// property on the ButtonBar, the surrounding ButtonBarTheme's property
/// will be used instead. If the ButtonBarTheme's property is null
/// as well, the property will default to a value described in the field
/// documentation below.
///
/// The [children] are wrapped in a [ButtonTheme] that is a copy of the
/// surrounding ButtonTheme with the button properties overridden by the
/// properties of the ButtonBar as described above. These properties include
/// [buttonTextTheme], [buttonMinWidth], [buttonHeight], [buttonPadding],
/// and [buttonAlignedDropdown].
///
/// Used by [Dialog] to arrange the actions at the bottom of the dialog.
///
/// See also:
///
/// * [TextButton], a simple flat button without a shadow.
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [OutlinedButton], a [TextButton] with a border outline.
/// * [Card], at the bottom of which it is common to place a [ButtonBar].
/// * [Dialog], which uses a [ButtonBar] for its actions.
/// * [ButtonBarTheme], which configures the [ButtonBar].
class ButtonBar extends StatelessWidget {
/// Creates a button bar.
///
/// Both [buttonMinWidth] and [buttonHeight] must be non-negative if they
/// are not null.
const ButtonBar({
super.key,
this.alignment,
this.mainAxisSize,
this.buttonTextTheme,
this.buttonMinWidth,
this.buttonHeight,
this.buttonPadding,
this.buttonAlignedDropdown,
this.layoutBehavior,
this.overflowDirection,
this.overflowButtonSpacing,
this.children = const <Widget>[],
}) : assert(buttonMinWidth == null || buttonMinWidth >= 0.0),
assert(buttonHeight == null || buttonHeight >= 0.0),
assert(overflowButtonSpacing == null || overflowButtonSpacing >= 0.0);
/// How the children should be placed along the horizontal axis.
///
/// If null then it will use [ButtonBarThemeData.alignment]. If that is null,
/// it will default to [MainAxisAlignment.end].
final MainAxisAlignment? alignment;
/// How much horizontal space is available. See [Row.mainAxisSize].
///
/// If null then it will use the surrounding [ButtonBarThemeData.mainAxisSize].
/// If that is null, it will default to [MainAxisSize.max].
final MainAxisSize? mainAxisSize;
/// Overrides the surrounding [ButtonBarThemeData.buttonTextTheme] to define a
/// button's base colors, size, internal padding and shape.
///
/// If null then it will use the surrounding
/// [ButtonBarThemeData.buttonTextTheme]. If that is null, it will default to
/// [ButtonTextTheme.primary].
final ButtonTextTheme? buttonTextTheme;
/// Overrides the surrounding [ButtonThemeData.minWidth] to define a button's
/// minimum width.
///
/// If null then it will use the surrounding [ButtonBarThemeData.buttonMinWidth].
/// If that is null, it will default to 64.0 logical pixels.
final double? buttonMinWidth;
/// Overrides the surrounding [ButtonThemeData.height] to define a button's
/// minimum height.
///
/// If null then it will use the surrounding [ButtonBarThemeData.buttonHeight].
/// If that is null, it will default to 36.0 logical pixels.
final double? buttonHeight;
/// Overrides the surrounding [ButtonThemeData.padding] to define the padding
/// for a button's child (typically the button's label).
///
/// If null then it will use the surrounding [ButtonBarThemeData.buttonPadding].
/// If that is null, it will default to 8.0 logical pixels on the left
/// and right.
final EdgeInsetsGeometry? buttonPadding;
/// Overrides the surrounding [ButtonThemeData.alignedDropdown] to define whether
/// a [DropdownButton] menu's width will match the button's width.
///
/// If null then it will use the surrounding [ButtonBarThemeData.buttonAlignedDropdown].
/// If that is null, it will default to false.
final bool? buttonAlignedDropdown;
/// Defines whether a [ButtonBar] should size itself with a minimum size
/// constraint or with padding.
///
/// Overrides the surrounding [ButtonThemeData.layoutBehavior].
///
/// If null then it will use the surrounding [ButtonBarThemeData.layoutBehavior].
/// If that is null, it will default [ButtonBarLayoutBehavior.padded].
final ButtonBarLayoutBehavior? layoutBehavior;
/// Defines the vertical direction of a [ButtonBar]'s children if it
/// overflows.
///
/// If [children] do not fit into a single row, then they
/// are arranged in a column. The first action is at the top of the
/// column if this property is set to [VerticalDirection.down], since it
/// "starts" at the top and "ends" at the bottom. On the other hand,
/// the first action will be at the bottom of the column if this
/// property is set to [VerticalDirection.up], since it "starts" at the
/// bottom and "ends" at the top.
///
/// If null then it will use the surrounding
/// [ButtonBarThemeData.overflowDirection]. If that is null, it will
/// default to [VerticalDirection.down].
final VerticalDirection? overflowDirection;
/// The spacing between buttons when the button bar overflows.
///
/// If the [children] do not fit into a single row, they are arranged into a
/// column. This parameter provides additional vertical space in between
/// buttons when it does overflow.
///
/// The button spacing may appear to be more than the value provided. This is
/// because most buttons adhere to the [MaterialTapTargetSize] of 48px. So,
/// even though a button might visually be 36px in height, it might still take
/// up to 48px vertically.
///
/// If null then no spacing will be added in between buttons in
/// an overflow state.
final double? overflowButtonSpacing;
/// The buttons to arrange horizontally.
///
/// Typically [ElevatedButton] or [TextButton] widgets.
final List<Widget> children;
@override
Widget build(BuildContext context) {
final ButtonThemeData parentButtonTheme = ButtonTheme.of(context);
final ButtonBarThemeData barTheme = ButtonBarTheme.of(context);
final ButtonThemeData buttonTheme = parentButtonTheme.copyWith(
textTheme: buttonTextTheme ?? barTheme.buttonTextTheme ?? ButtonTextTheme.primary,
minWidth: buttonMinWidth ?? barTheme.buttonMinWidth ?? 64.0,
height: buttonHeight ?? barTheme.buttonHeight ?? 36.0,
padding: buttonPadding ?? barTheme.buttonPadding ?? const EdgeInsets.symmetric(horizontal: 8.0),
alignedDropdown: buttonAlignedDropdown ?? barTheme.buttonAlignedDropdown ?? false,
layoutBehavior: layoutBehavior ?? barTheme.layoutBehavior ?? ButtonBarLayoutBehavior.padded,
);
// We divide by 4.0 because we want half of the average of the left and right padding.
final double paddingUnit = buttonTheme.padding.horizontal / 4.0;
final Widget child = ButtonTheme.fromButtonThemeData(
data: buttonTheme,
child: _ButtonBarRow(
mainAxisAlignment: alignment ?? barTheme.alignment ?? MainAxisAlignment.end,
mainAxisSize: mainAxisSize ?? barTheme.mainAxisSize ?? MainAxisSize.max,
overflowDirection: overflowDirection ?? barTheme.overflowDirection ?? VerticalDirection.down,
overflowButtonSpacing: overflowButtonSpacing,
children: children.map<Widget>((Widget child) {
return Padding(
padding: EdgeInsets.symmetric(horizontal: paddingUnit),
child: child,
);
}).toList(),
),
);
switch (buttonTheme.layoutBehavior) {
case ButtonBarLayoutBehavior.padded:
return Padding(
padding: EdgeInsets.symmetric(
vertical: 2.0 * paddingUnit,
horizontal: paddingUnit,
),
child: child,
);
case ButtonBarLayoutBehavior.constrained:
return Container(
padding: EdgeInsets.symmetric(horizontal: paddingUnit),
constraints: const BoxConstraints(minHeight: 52.0),
alignment: Alignment.center,
child: child,
);
}
}
}
/// Attempts to display buttons in a row, but displays them in a column if
/// there is not enough horizontal space.
///
/// It first attempts to lay out its buttons as though there were no
/// maximum width constraints on the widget. If the button bar's width is
/// less than the maximum width constraints of the widget, it then lays
/// out the widget as though it were placed in a [Row].
///
/// However, if the button bar's width exceeds the maximum width constraint on
/// the widget, it then aligns its buttons in a column. The key difference here
/// is that the [MainAxisAlignment] will then be treated as a
/// cross-axis/horizontal alignment. For example, if the buttons overflow and
/// [ButtonBar.alignment] was set to [MainAxisAlignment.start], the column of
/// buttons would align to the horizontal start of the button bar.
class _ButtonBarRow extends Flex {
/// Creates a button bar that attempts to display in a row, but displays in
/// a column if there is insufficient horizontal space.
const _ButtonBarRow({
required super.children,
super.mainAxisSize,
super.mainAxisAlignment,
VerticalDirection overflowDirection = VerticalDirection.down,
this.overflowButtonSpacing,
}) : super(
direction: Axis.horizontal,
verticalDirection: overflowDirection,
);
final double? overflowButtonSpacing;
@override
_RenderButtonBarRow createRenderObject(BuildContext context) {
return _RenderButtonBarRow(
direction: direction,
mainAxisAlignment: mainAxisAlignment,
mainAxisSize: mainAxisSize,
crossAxisAlignment: crossAxisAlignment,
textDirection: getEffectiveTextDirection(context)!,
verticalDirection: verticalDirection,
textBaseline: textBaseline,
overflowButtonSpacing: overflowButtonSpacing,
);
}
@override
void updateRenderObject(BuildContext context, covariant _RenderButtonBarRow renderObject) {
renderObject
..direction = direction
..mainAxisAlignment = mainAxisAlignment
..mainAxisSize = mainAxisSize
..crossAxisAlignment = crossAxisAlignment
..textDirection = getEffectiveTextDirection(context)
..verticalDirection = verticalDirection
..textBaseline = textBaseline
..overflowButtonSpacing = overflowButtonSpacing;
}
}
/// Attempts to display buttons in a row, but displays them in a column if
/// there is not enough horizontal space.
///
/// It first attempts to lay out its buttons as though there were no
/// maximum width constraints on the widget. If the button bar's width is
/// less than the maximum width constraints of the widget, it then lays
/// out the widget as though it were placed in a [Row].
///
/// However, if the button bar's width exceeds the maximum width constraint on
/// the widget, it then aligns its buttons in a column. The key difference here
/// is that the [MainAxisAlignment] will then be treated as a
/// cross-axis/horizontal alignment. For example, if the buttons overflow and
/// [ButtonBar.alignment] was set to [MainAxisAlignment.start], the buttons would
/// align to the horizontal start of the button bar.
class _RenderButtonBarRow extends RenderFlex {
/// Creates a button bar that attempts to display in a row, but displays in
/// a column if there is insufficient horizontal space.
_RenderButtonBarRow({
super.direction,
super.mainAxisSize,
super.mainAxisAlignment,
super.crossAxisAlignment,
required TextDirection super.textDirection,
super.verticalDirection,
super.textBaseline,
this.overflowButtonSpacing,
}) : assert(overflowButtonSpacing == null || overflowButtonSpacing >= 0);
bool _hasCheckedLayoutWidth = false;
double? overflowButtonSpacing;
@override
BoxConstraints get constraints {
if (_hasCheckedLayoutWidth) {
return super.constraints;
}
return super.constraints.copyWith(maxWidth: double.infinity);
}
@override
Size computeDryLayout(BoxConstraints constraints) {
final Size size = super.computeDryLayout(constraints.copyWith(maxWidth: double.infinity));
if (size.width <= constraints.maxWidth) {
return super.computeDryLayout(constraints);
}
double currentHeight = 0.0;
RenderBox? child = firstChild;
while (child != null) {
final BoxConstraints childConstraints = constraints.copyWith(minWidth: 0.0);
final Size childSize = child.getDryLayout(childConstraints);
currentHeight += childSize.height;
child = childAfter(child);
if (overflowButtonSpacing != null && child != null) {
currentHeight += overflowButtonSpacing!;
}
}
return constraints.constrain(Size(constraints.maxWidth, currentHeight));
}
@override
void performLayout() {
// Set check layout width to false in reload or update cases.
_hasCheckedLayoutWidth = false;
// Perform layout to ensure that button bar knows how wide it would
// ideally want to be.
super.performLayout();
_hasCheckedLayoutWidth = true;
// If the button bar is constrained by width and it overflows, set the
// buttons to align vertically. Otherwise, lay out the button bar
// horizontally.
if (size.width <= constraints.maxWidth) {
// A second performLayout is required to ensure that the original maximum
// width constraints are used. The original perform layout call assumes
// a maximum width constraint of infinity.
super.performLayout();
} else {
final BoxConstraints childConstraints = constraints.copyWith(minWidth: 0.0);
double currentHeight = 0.0;
RenderBox? child = switch (verticalDirection) {
VerticalDirection.down => firstChild,
VerticalDirection.up => lastChild,
};
while (child != null) {
final FlexParentData childParentData = child.parentData! as FlexParentData;
// Lay out the child with the button bar's original constraints, but
// with minimum width set to zero.
child.layout(childConstraints, parentUsesSize: true);
// Set the cross axis alignment for the column to match the main axis
// alignment for a row. For [MainAxisAlignment.spaceAround],
// [MainAxisAlignment.spaceBetween] and [MainAxisAlignment.spaceEvenly]
// cases, use [MainAxisAlignment.start].
switch (textDirection!) {
case TextDirection.ltr:
switch (mainAxisAlignment) {
case MainAxisAlignment.center:
final double midpoint = (constraints.maxWidth - child.size.width) / 2.0;
childParentData.offset = Offset(midpoint, currentHeight);
case MainAxisAlignment.end:
childParentData.offset = Offset(constraints.maxWidth - child.size.width, currentHeight);
case MainAxisAlignment.spaceAround:
case MainAxisAlignment.spaceBetween:
case MainAxisAlignment.spaceEvenly:
case MainAxisAlignment.start:
childParentData.offset = Offset(0, currentHeight);
}
case TextDirection.rtl:
switch (mainAxisAlignment) {
case MainAxisAlignment.center:
final double midpoint = constraints.maxWidth / 2.0 - child.size.width / 2.0;
childParentData.offset = Offset(midpoint, currentHeight);
case MainAxisAlignment.end:
childParentData.offset = Offset(0, currentHeight);
case MainAxisAlignment.spaceAround:
case MainAxisAlignment.spaceBetween:
case MainAxisAlignment.spaceEvenly:
case MainAxisAlignment.start:
childParentData.offset = Offset(constraints.maxWidth - child.size.width, currentHeight);
}
}
currentHeight += child.size.height;
child = switch (verticalDirection) {
VerticalDirection.down => childParentData.nextSibling,
VerticalDirection.up => childParentData.previousSibling,
};
if (overflowButtonSpacing != null && child != null) {
currentHeight += overflowButtonSpacing!;
}
}
size = constraints.constrain(Size(constraints.maxWidth, currentHeight));
}
}
}
| flutter/packages/flutter/lib/src/material/button_bar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/button_bar.dart",
"repo_id": "flutter",
"token_count": 5942
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
/// Defines a single color as well a color swatch with ten shades of the color.
///
/// The color's shades are referred to by index. The greater the index, the
/// darker the color. There are 10 valid indices: 50, 100, 200, ..., 900.
/// The value of this color should the same the value of index 500 and [shade500].
///
/// ## Updating to [ColorScheme]
///
/// The [ColorScheme] is preferred for
/// representing colors in applications that are configured
/// for Material 3 (see [ThemeData.useMaterial3]).
/// For more information on colors in Material 3 see
/// the spec at <https://m3.material.io/styles/color/the-color-system>.
///
///{@template flutter.material.colors.colorRoles}
/// In Material 3, colors are represented using color roles and
/// corresponding tokens. Each property in the [ColorScheme] class
/// represents one color role as defined in the spec above.
/// {@endtemplate}
///
/// ### Material 3 Colors in Flutter
///
///{@template flutter.material.colors.settingColors}
/// Flutter's Material widgets can be assigned colors at the widget level
/// using widget properties,
/// or at the app level using theme classes.
///
/// For example, you can set the background of the [AppBar] by
/// setting the [AppBar.backgroundColor] to a specific [Color] value.
///
/// To globally set the AppBar background color for your app, you
/// can set the [ThemeData.appBarTheme] property for your [MaterialApp]
/// using the [ThemeData] class. You can also override
/// the default appearance of all the [AppBar]s in a widget subtree by
/// placing the [AppBarTheme] at the root of the subtree.
///
/// Alternatively, you can set the [ThemeData.colorScheme] property
/// to a custom [ColorScheme]. This creates a unified [ColorScheme] to be
/// used across the app. The [AppBar.backgroundColor] uses the
/// [ColorScheme.surface] by default.
///{@endtemplate}
///
/// ### Migrating from [MaterialColor] to [ColorScheme]
///
/// In most cases, there are new properties in Flutter widgets that
/// accept a [ColorScheme] instead of a [MaterialColor].
///
/// For example, you may have previously constructed a [ThemeData]
/// using a primarySwatch:
///
/// ```dart
/// ThemeData(
/// primarySwatch: Colors.amber,
/// )
/// ```
///
/// In Material 3, you can use the [ColorScheme] class to
/// construct a [ThemeData] with the same color palette
/// by using the [ColorScheme.fromSeed] constructor:
///
/// ```dart
/// ThemeData(
/// colorScheme: ColorScheme.fromSeed(seedColor: Colors.amber),
/// )
/// ```
///
/// The [ColorScheme.fromSeed] constructor
/// will generate a set of tonal palettes,
/// which are used to create the color scheme.
///
/// Alternatively you can use the [ColorScheme.fromSwatch] constructor:
///
/// ```dart
/// ThemeData(
/// colorScheme: ColorScheme.fromSwatch(primarySwatch: Colors.amber),
/// )
/// ```
///
/// The [ColorScheme.fromSwatch] constructor will
/// create the color scheme directly from the specific
/// color values used in the [MaterialColor].
///
///
/// See also:
///
/// * [Colors], which defines all of the standard material colors.
class MaterialColor extends ColorSwatch<int> {
/// Creates a color swatch with a variety of shades.
///
/// The `primary` argument should be the 32 bit ARGB value of one of the
/// values in the swatch, as would be passed to the [Color.new] constructor
/// for that same color, and as is exposed by [value]. (This is distinct from
/// the specific index of the color in the swatch.)
const MaterialColor(super.primary, super.swatch);
/// The lightest shade.
Color get shade50 => this[50]!;
/// The second lightest shade.
Color get shade100 => this[100]!;
/// The third lightest shade.
Color get shade200 => this[200]!;
/// The fourth lightest shade.
Color get shade300 => this[300]!;
/// The fifth lightest shade.
Color get shade400 => this[400]!;
/// The default shade.
Color get shade500 => this[500]!;
/// The fourth darkest shade.
Color get shade600 => this[600]!;
/// The third darkest shade.
Color get shade700 => this[700]!;
/// The second darkest shade.
Color get shade800 => this[800]!;
/// The darkest shade.
Color get shade900 => this[900]!;
}
/// Defines a single accent color as well a swatch of four shades of the
/// accent color.
///
/// The color's shades are referred to by index, the colors with smaller
/// indices are lighter, larger indices are darker. There are four valid
/// indices: 100, 200, 400, and 700. The value of this color should be the
/// same as the value of index 200 and [shade200].
///
/// See also:
///
/// * [Colors], which defines all of the standard material colors.
/// * <https://material.io/go/design-theming#color-color-schemes>
class MaterialAccentColor extends ColorSwatch<int> {
/// Creates a color swatch with a variety of shades appropriate for accent
/// colors.
const MaterialAccentColor(super.primary, super.swatch);
/// The lightest shade.
Color get shade100 => this[100]!;
/// The default shade.
Color get shade200 => this[200]!;
/// The second darkest shade.
Color get shade400 => this[400]!;
/// The darkest shade.
Color get shade700 => this[700]!;
}
/// [Color] and [ColorSwatch] constants which represent Material design's
/// [color palette](https://material.io/design/color/).
///
/// Instead of using an absolute color from these palettes, consider using
/// [Theme.of] to obtain the local [ThemeData.colorScheme], which defines
/// the colors that most of the Material components use by default.
///
///
/// Most swatches have colors from 100 to 900 in increments of one hundred, plus
/// the color 50. The smaller the number, the more pale the color. The greater
/// the number, the darker the color. The accent swatches (e.g. [redAccent]) only
/// have the values 100, 200, 400, and 700.
///
/// In addition, a series of blacks and whites with common opacities are
/// available. For example, [black54] is a pure black with 54% opacity.
///
/// {@tool snippet}
///
/// To select a specific color from one of the swatches, index into the swatch
/// using an integer for the specific color desired, as follows:
///
/// ```dart
/// Color selection = Colors.green[400]!; // Selects a mid-range green.
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// Each [ColorSwatch] constant is a color and can used directly. For example:
///
/// ```dart
/// Container(
/// color: Colors.blue, // same as Colors.blue[500] or Colors.blue.shade500
/// )
/// ```
/// {@end-tool}
///
/// ## Color palettes
///
/// 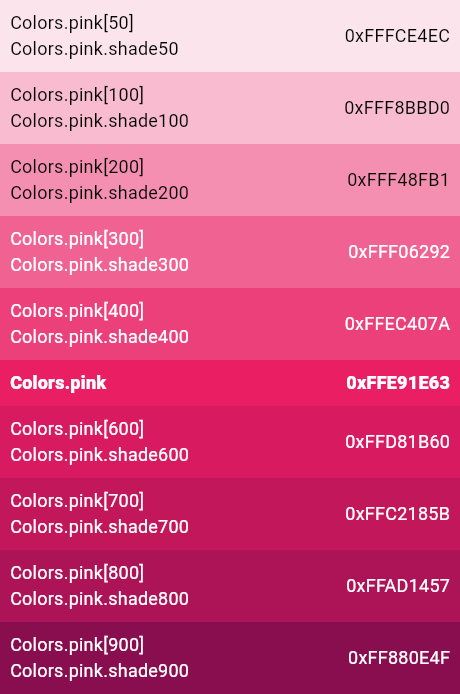
/// 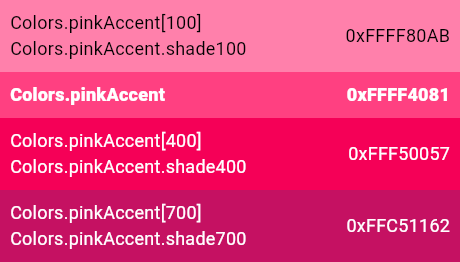
///
/// 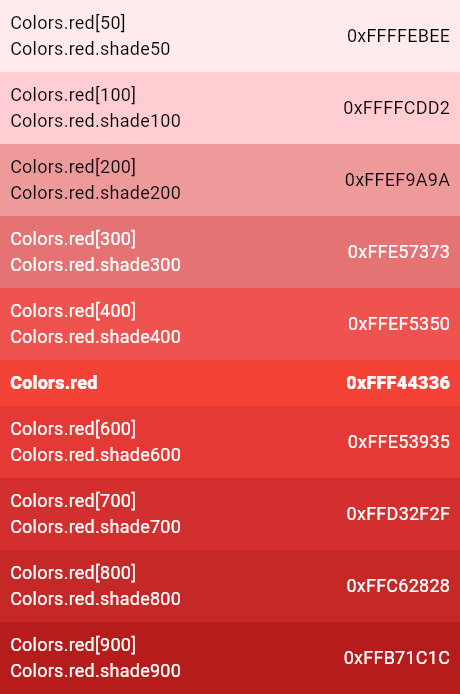
/// 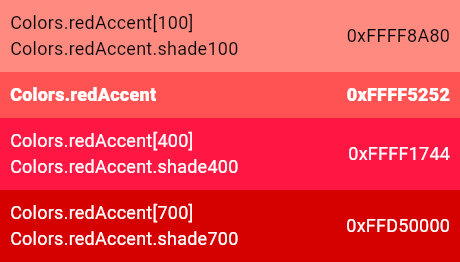
///
/// 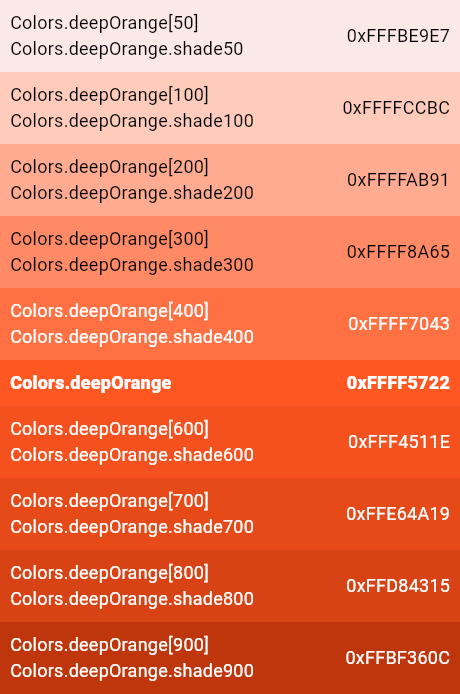
/// 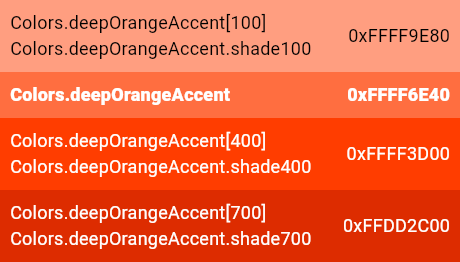
///
/// 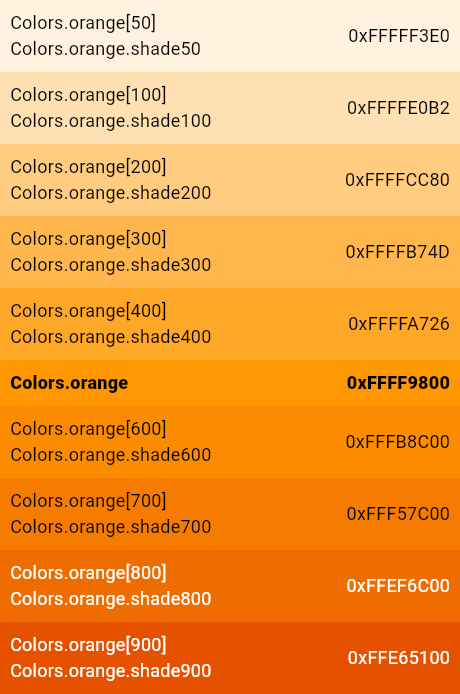
/// 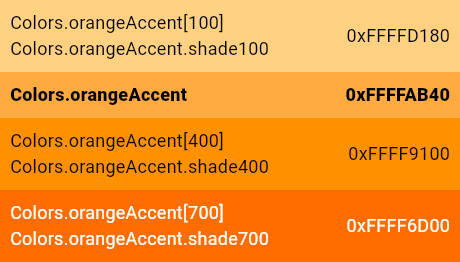
///
/// 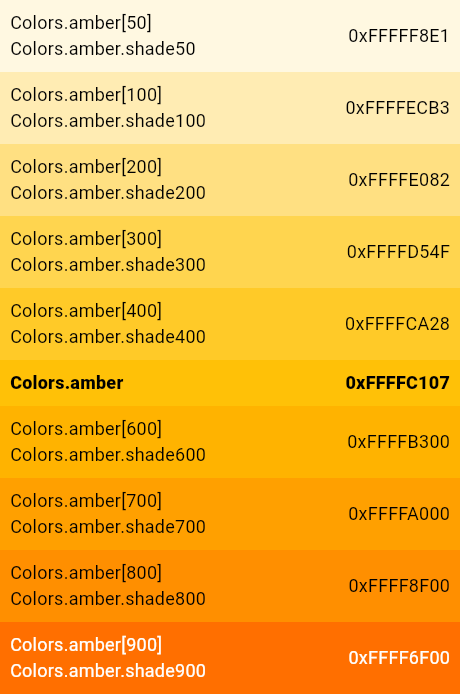
/// 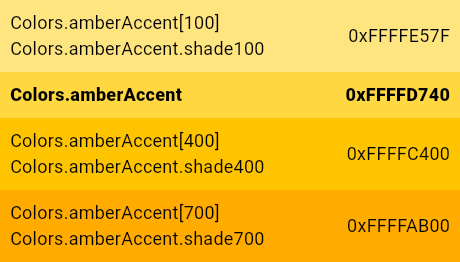
///
/// 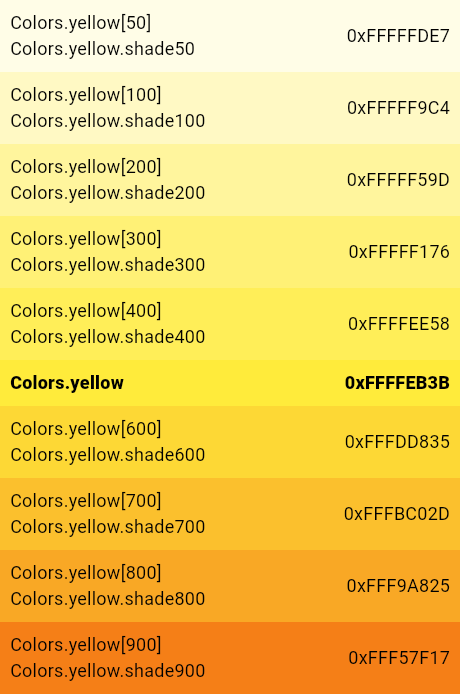
/// 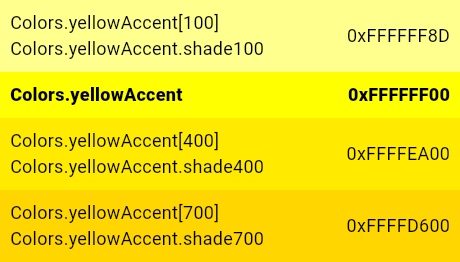
///
/// 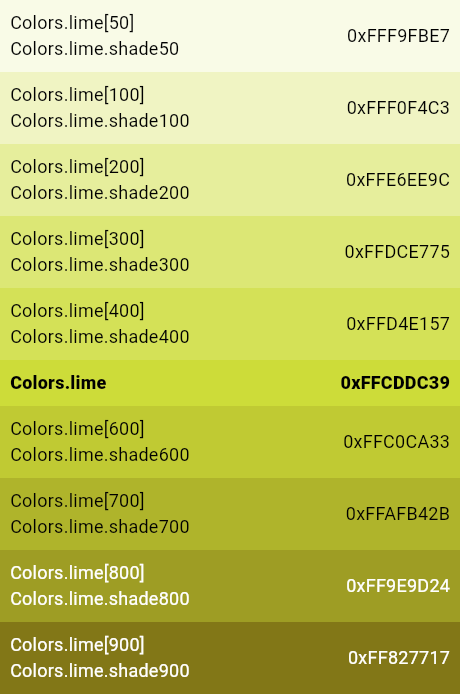
/// 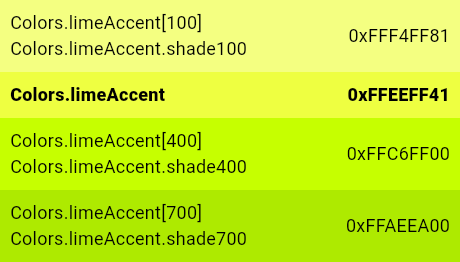
///
/// 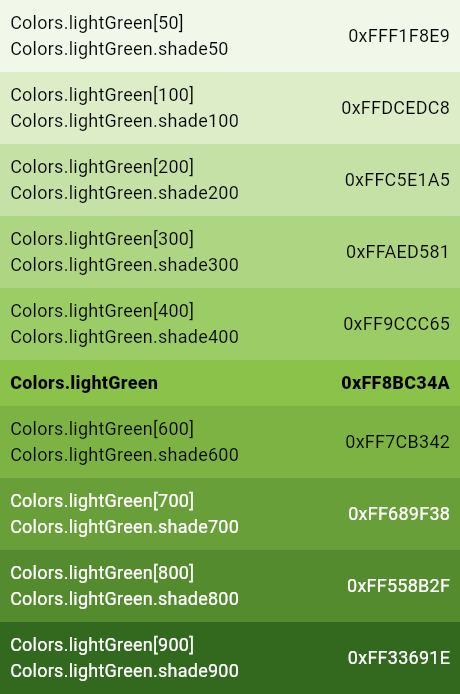
/// 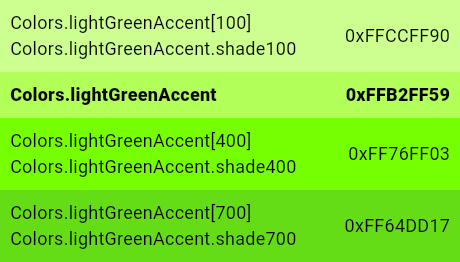
///
/// 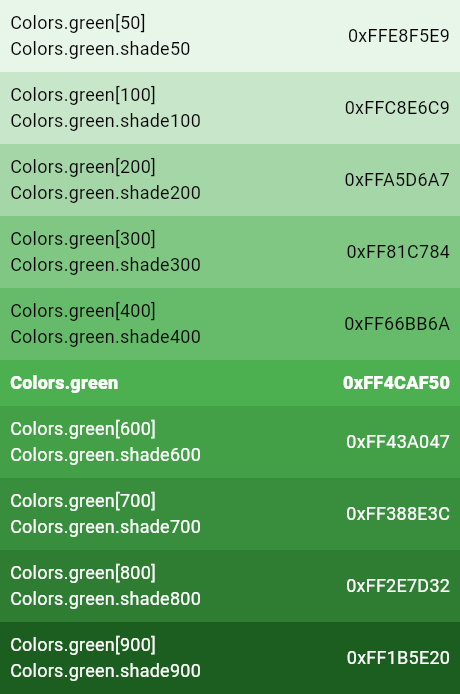
/// 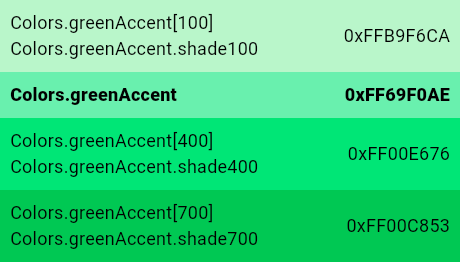
///
/// 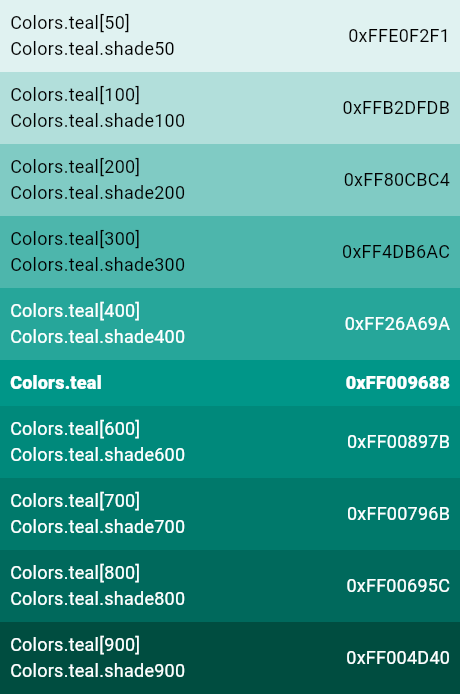
/// 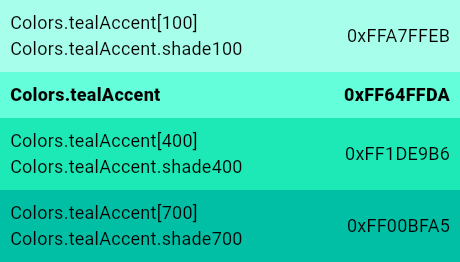
///
/// 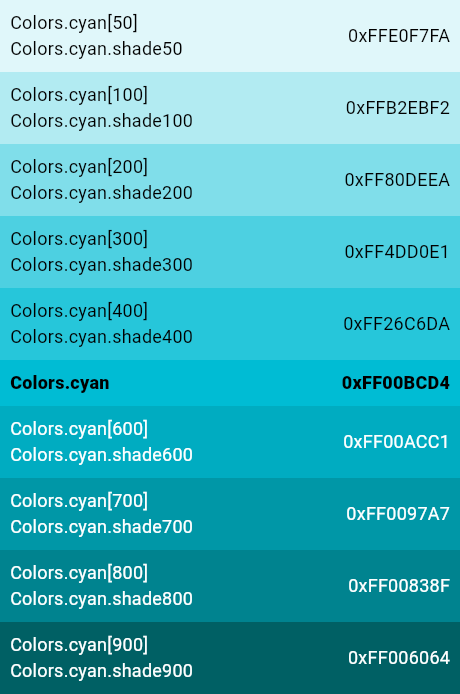
/// 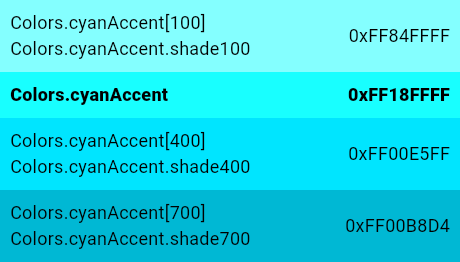
///
/// 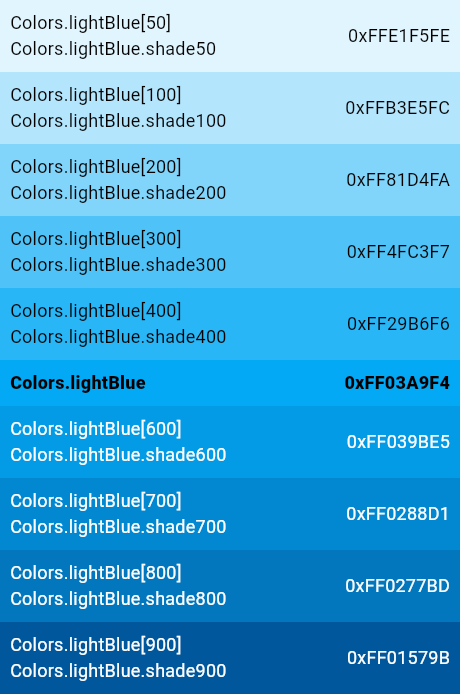
/// 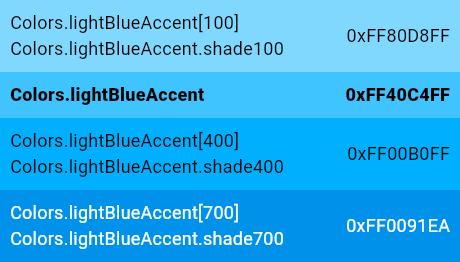
///
/// 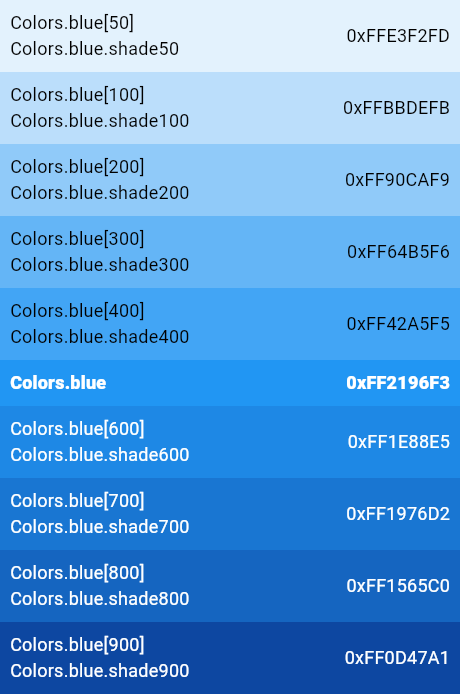
/// 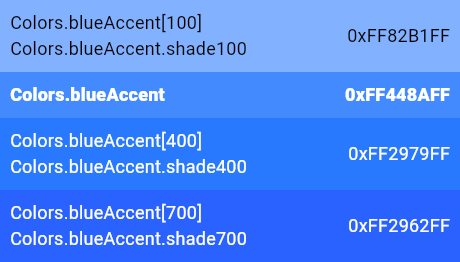
///
/// 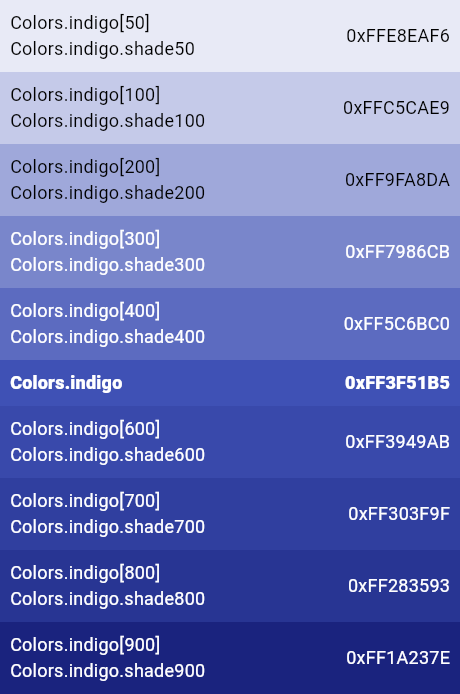
/// 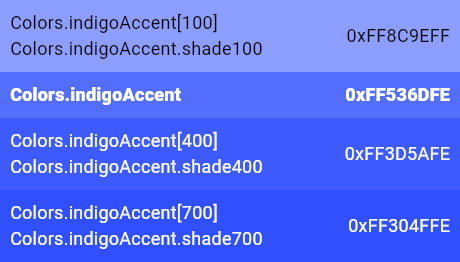
///
/// 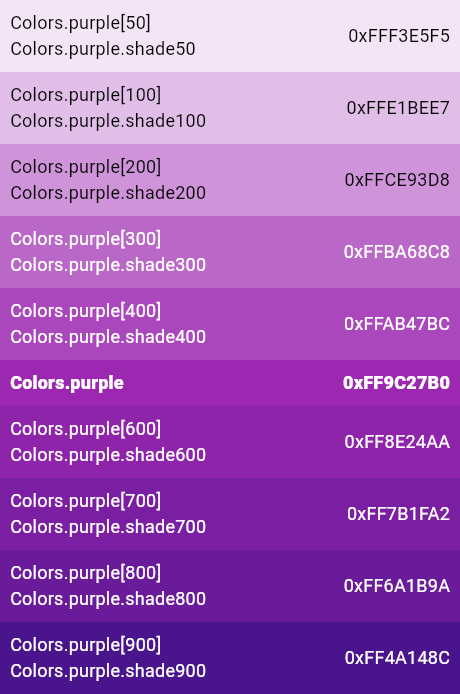
/// 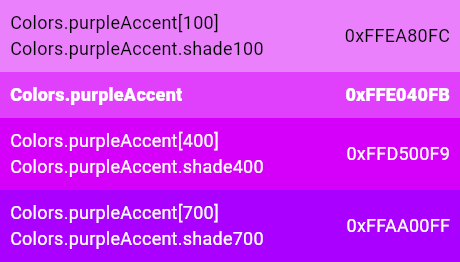
///
/// 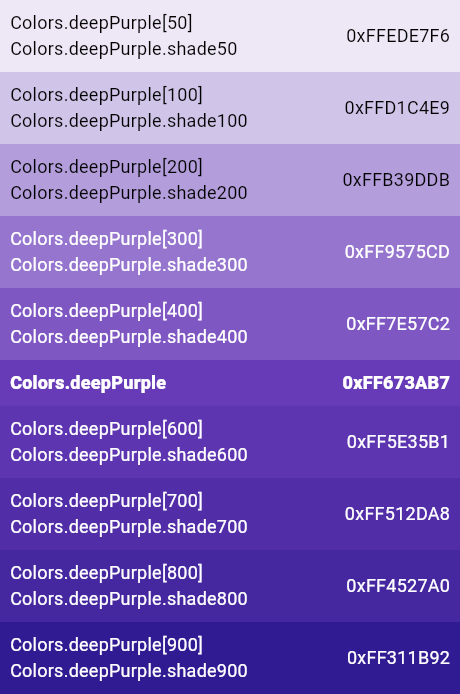
/// 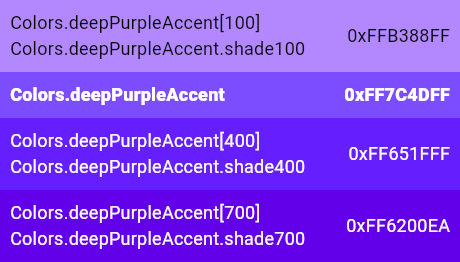
///
/// 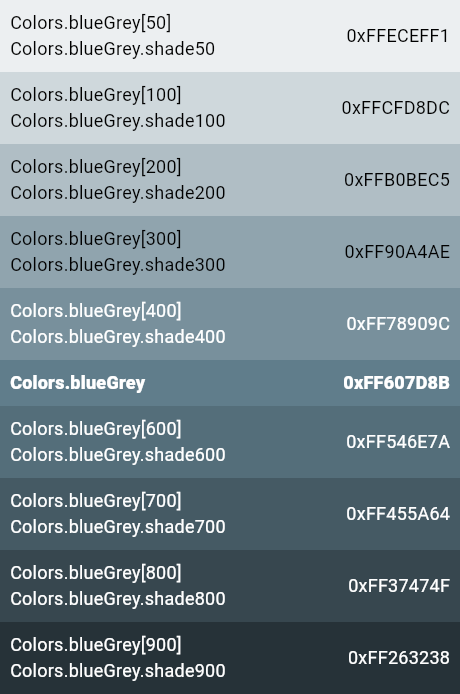
///
/// 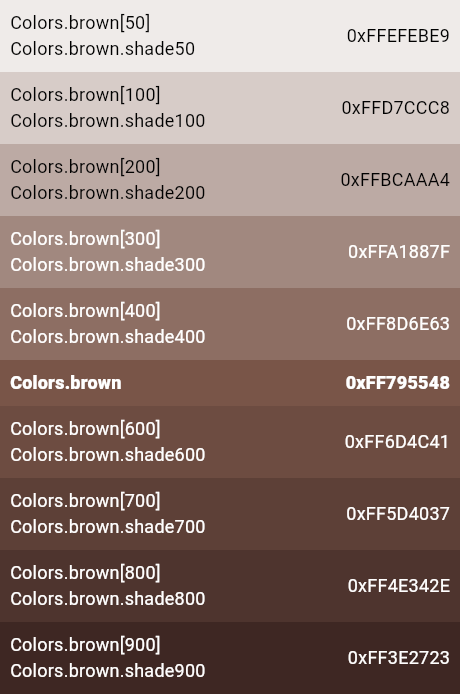
///
/// 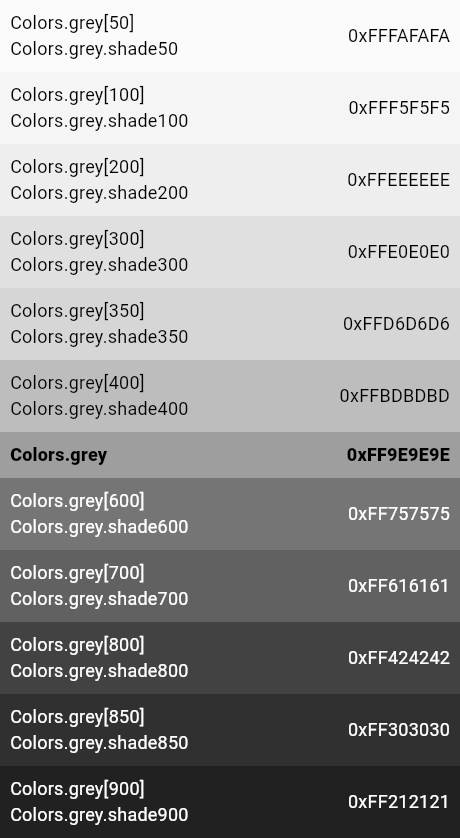
///
/// ## Blacks and whites
///
/// These colors are identified by their transparency. The low transparency
/// levels (e.g. [Colors.white12] and [Colors.white10]) are very hard to see and
/// should be avoided in general. They are intended for very subtle effects.
///
/// 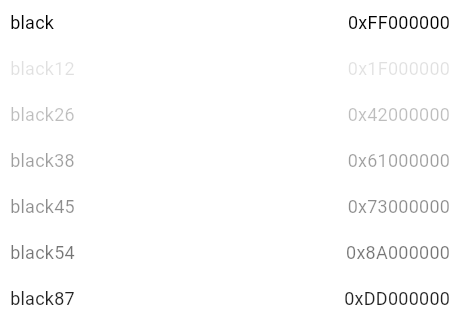
/// 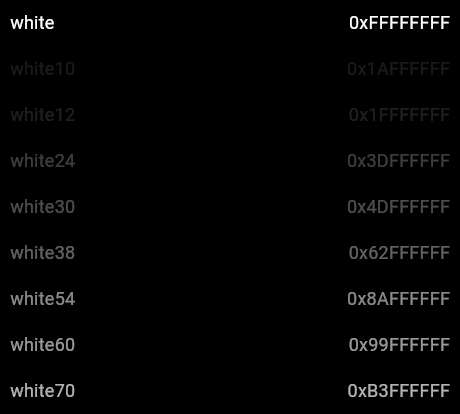
///
/// The [Colors.transparent] color isn't shown here because it is entirely
/// invisible!
///
/// See also:
///
/// * Cookbook: [Use themes to share colors and font styles](https://flutter.dev/docs/cookbook/design/themes)
abstract final class Colors {
/// Completely invisible.
static const Color transparent = Color(0x00000000);
/// Completely opaque black.
///
/// 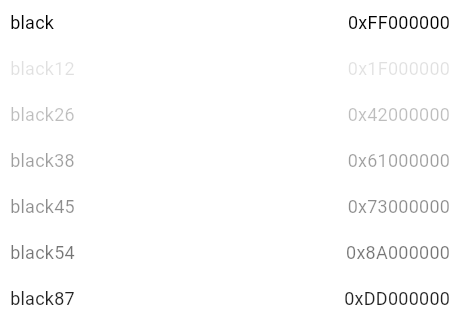
///
/// See also:
///
/// * [black87], [black54], [black45], [black38], [black26], [black12], which
/// are variants on this color but with different opacities.
/// * [white], a solid white color.
/// * [transparent], a fully-transparent color.
static const Color black = Color(0xFF000000);
/// Black with 87% opacity.
///
/// This is a good contrasting color for text in light themes.
///
/// 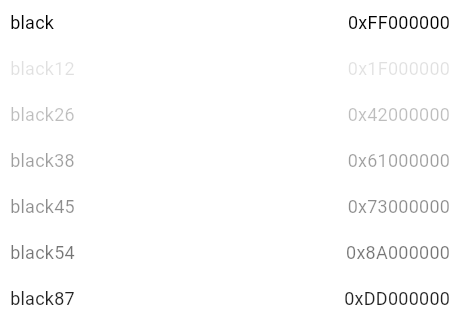
///
/// See also:
///
/// * [Typography.black], which uses this color for its text styles.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [black], [black54], [black45], [black38], [black26], [black12], which
/// are variants on this color but with different opacities.
static const Color black87 = Color(0xDD000000);
/// Black with 54% opacity.
///
/// This is a color commonly used for headings in light themes. It's also used
/// as the mask color behind dialogs.
///
/// 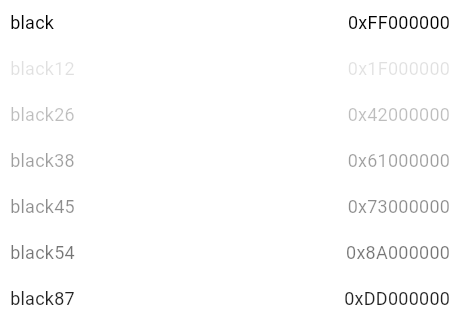
///
/// See also:
///
/// * [Typography.black], which uses this color for its text styles.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [black], [black87], [black45], [black38], [black26], [black12], which
/// are variants on this color but with different opacities.
static const Color black54 = Color(0x8A000000);
/// Black with 45% opacity.
///
/// 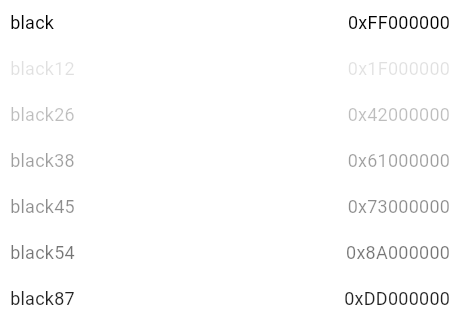
///
/// See also:
///
/// * [black], [black87], [black54], [black38], [black26], [black12], which
/// are variants on this color but with different opacities.
static const Color black45 = Color(0x73000000);
/// Black with 38% opacity.
///
/// For light themes, i.e. when the Theme's [ThemeData.brightness] is
/// [Brightness.light], this color is used for disabled icons and for
/// placeholder text in [DataTable].
///
/// 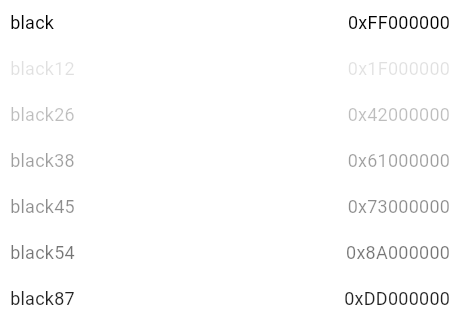
///
/// See also:
///
/// * [black], [black87], [black54], [black45], [black26], [black12], which
/// are variants on this color but with different opacities.
static const Color black38 = Color(0x61000000);
/// Black with 26% opacity.
///
/// Used for disabled radio buttons and the text of disabled flat buttons in light themes.
///
/// 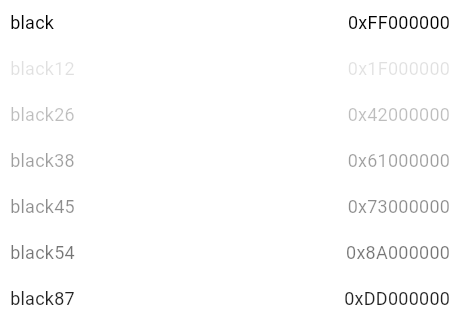
///
/// See also:
///
/// * [ThemeData.disabledColor], which uses this color by default in light themes.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [black], [black87], [black54], [black45], [black38], [black12], which
/// are variants on this color but with different opacities.
static const Color black26 = Color(0x42000000);
/// Black with 12% opacity.
///
/// 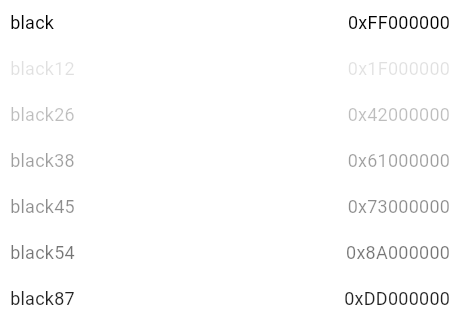
///
/// Used for the background of disabled raised buttons in light themes.
///
/// See also:
///
/// * [black], [black87], [black54], [black45], [black38], [black26], which
/// are variants on this color but with different opacities.
static const Color black12 = Color(0x1F000000);
/// Completely opaque white.
///
/// This is a good contrasting color for the [ThemeData.primaryColor] in the
/// dark theme. See [ThemeData.brightness].
///
/// 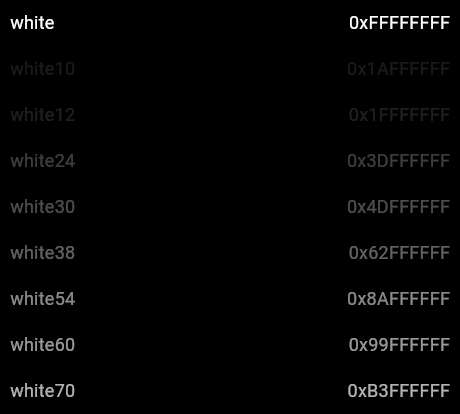
///
/// See also:
///
/// * [Typography.white], which uses this color for its text styles.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white70], [white60], [white54], [white38], [white30], [white12],
/// [white10], which are variants on this color but with different
/// opacities.
/// * [black], a solid black color.
/// * [transparent], a fully-transparent color.
static const Color white = Color(0xFFFFFFFF);
/// White with 70% opacity.
///
/// This is a color commonly used for headings in dark themes.
///
/// 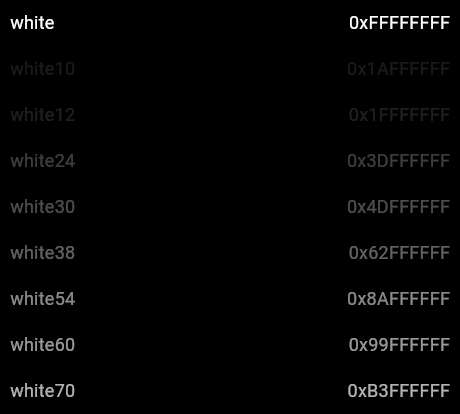
///
/// See also:
///
/// * [Typography.white], which uses this color for its text styles.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white], [white60], [white54], [white38], [white30], [white12],
/// [white10], which are variants on this color but with different
/// opacities.
static const Color white70 = Color(0xB3FFFFFF);
/// White with 60% opacity.
///
/// Used for medium-emphasis text and hint text when [ThemeData.brightness] is
/// set to [Brightness.dark].
///
/// 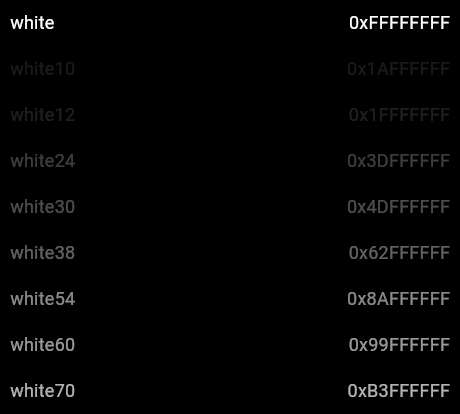
///
/// See also:
///
/// * [ExpandIcon], which uses this color for dark themes.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white], [white54], [white30], [white38], [white12], [white10], which
/// are variants on this color but with different opacities.
static const Color white60 = Color(0x99FFFFFF);
/// White with 54% opacity.
///
/// 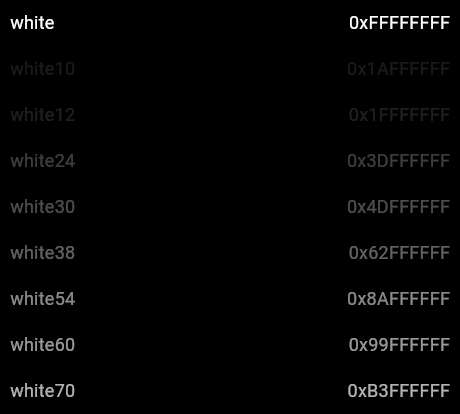
///
/// See also:
///
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white], [white60], [white38], [white30], [white12], [white10], which
/// are variants on this color but with different opacities.
static const Color white54 = Color(0x8AFFFFFF);
/// White with 38% opacity.
///
/// Used for disabled radio buttons and the text of disabled flat buttons in dark themes.
///
/// 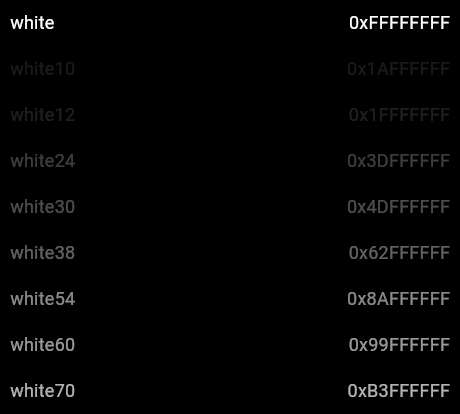
///
/// See also:
///
/// * [ThemeData.disabledColor], which uses this color by default in dark themes.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white], [white60], [white54], [white70], [white30], [white12],
/// [white10], which are variants on this color but with different
/// opacities.
static const Color white38 = Color(0x62FFFFFF);
/// White with 30% opacity.
///
/// 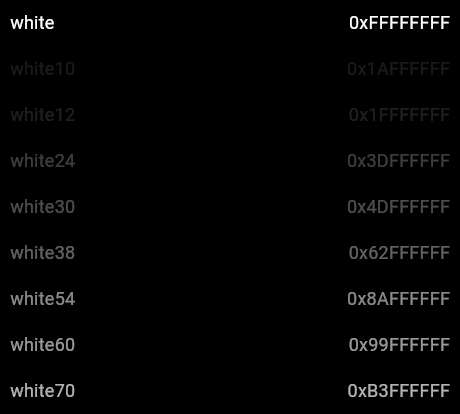
///
/// See also:
///
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
/// * [white], [white60], [white54], [white70], [white38], [white12],
/// [white10], which are variants on this color but with different
/// opacities.
static const Color white30 = Color(0x4DFFFFFF);
/// White with 24% opacity.
///
/// 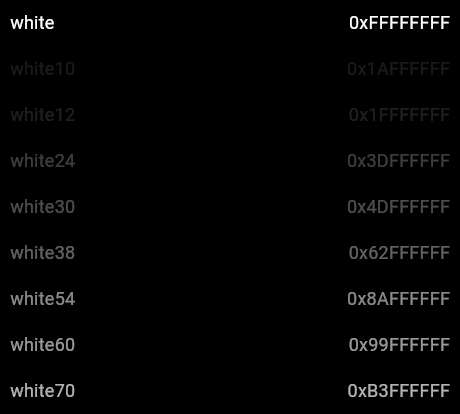
///
/// Used for the splash color for filled buttons.
///
/// See also:
///
/// * [white], [white60], [white54], [white70], [white38], [white30],
/// [white10], which are variants on this color
/// but with different opacities.
static const Color white24 = Color(0x3DFFFFFF);
/// White with 12% opacity.
///
/// 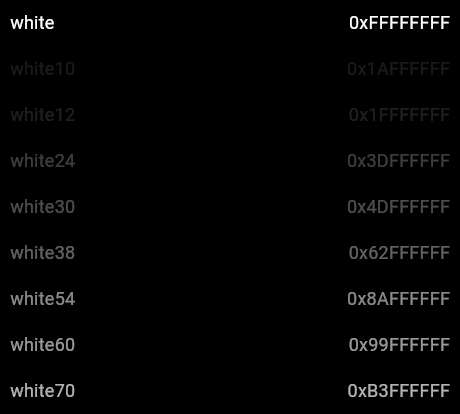
///
/// Used for the background of disabled raised buttons in dark themes.
///
/// See also:
///
/// * [white], [white60], [white54], [white70], [white38], [white30],
/// [white10], which are variants on this color but with different
/// opacities.
static const Color white12 = Color(0x1FFFFFFF);
/// White with 10% opacity.
///
/// 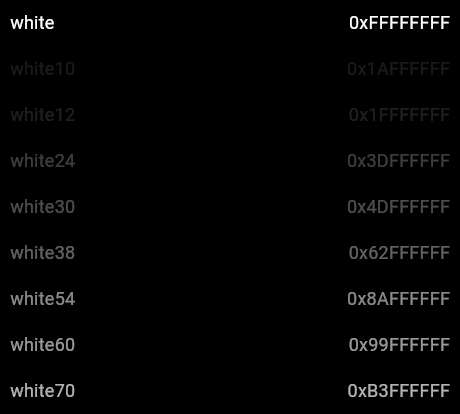
///
/// See also:
///
/// * [white], [white60], [white54], [white70], [white38], [white30],
/// [white12], which are variants on this color
/// but with different opacities.
/// * [transparent], a fully-transparent color, not far from this one.
static const Color white10 = Color(0x1AFFFFFF);
/// The red primary color and swatch.
///
/// 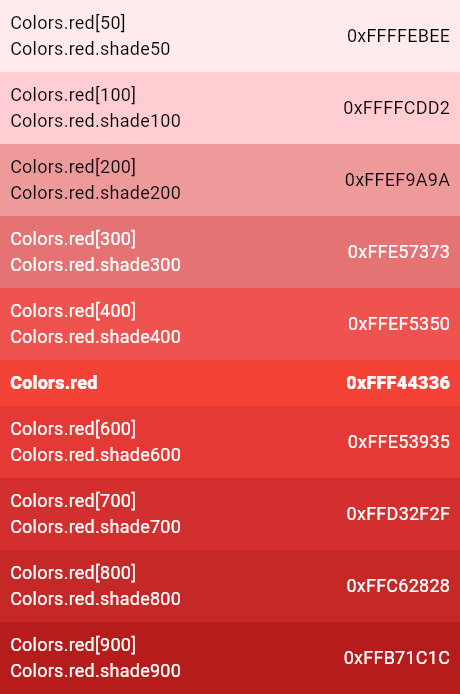
/// 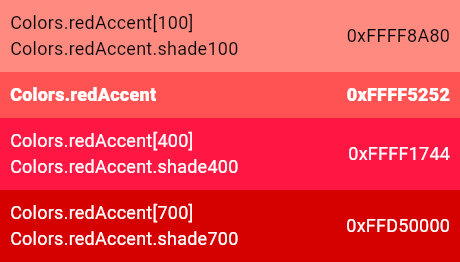
///
/// 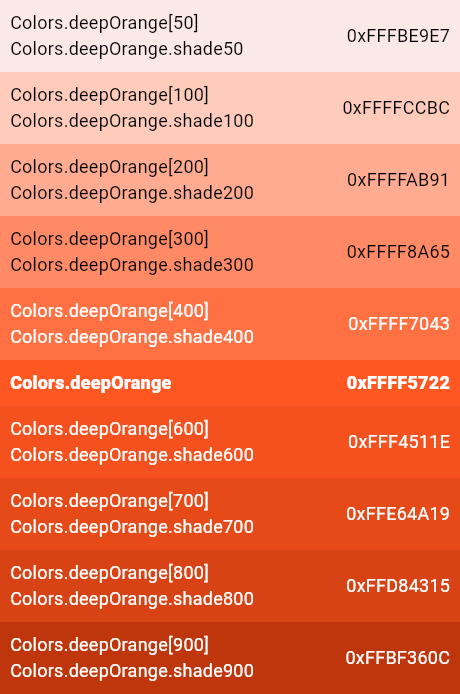
/// 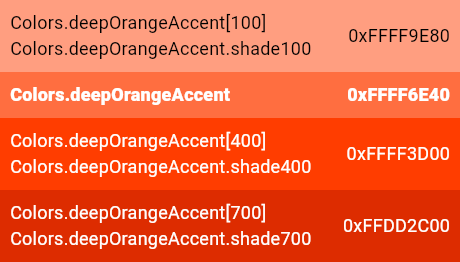
///
/// 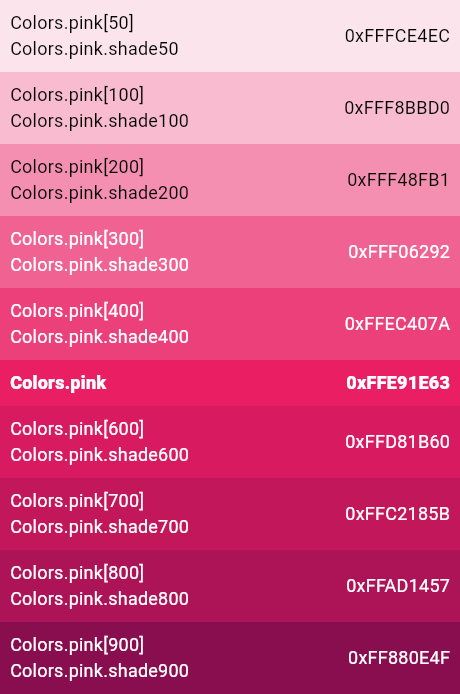
/// 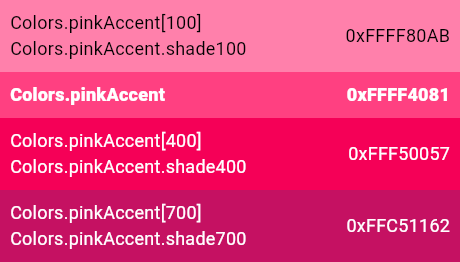
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.red[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [redAccent], the corresponding accent colors.
/// * [deepOrange] and [pink], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor red = MaterialColor(
_redPrimaryValue,
<int, Color>{
50: Color(0xFFFFEBEE),
100: Color(0xFFFFCDD2),
200: Color(0xFFEF9A9A),
300: Color(0xFFE57373),
400: Color(0xFFEF5350),
500: Color(_redPrimaryValue),
600: Color(0xFFE53935),
700: Color(0xFFD32F2F),
800: Color(0xFFC62828),
900: Color(0xFFB71C1C),
},
);
static const int _redPrimaryValue = 0xFFF44336;
/// The red accent swatch.
///
/// 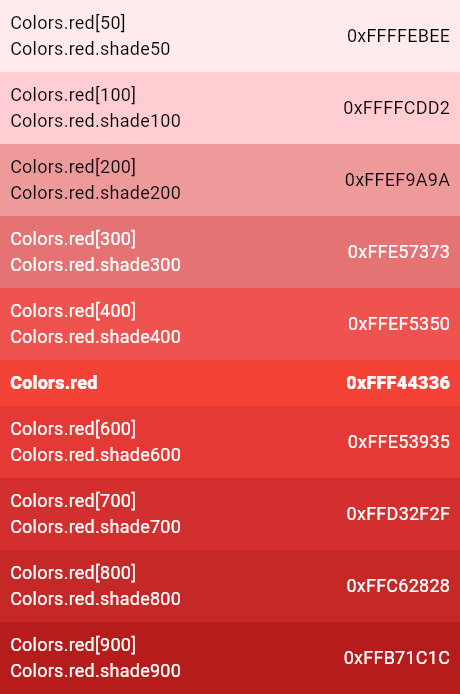
/// 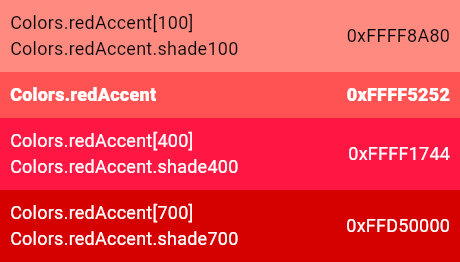
///
/// 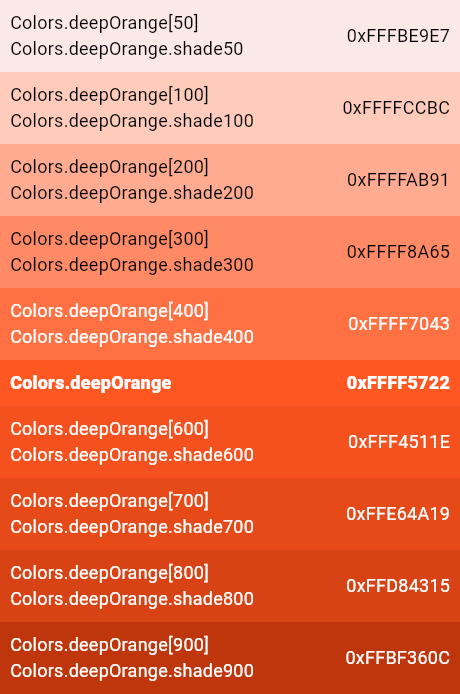
/// 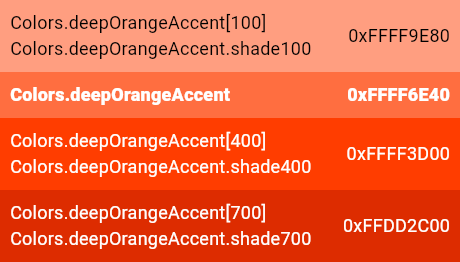
///
/// 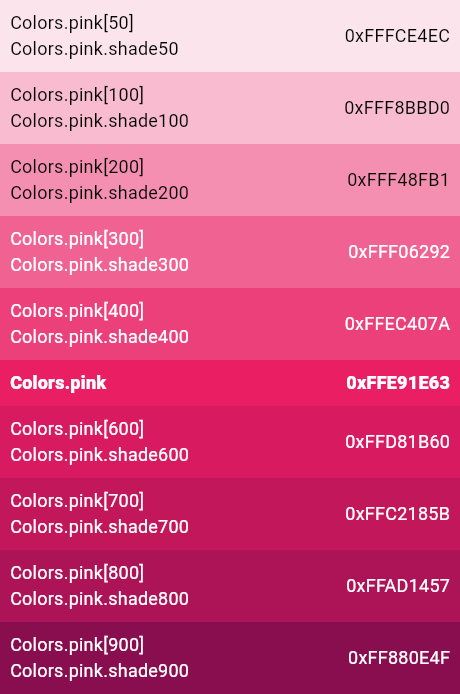
/// 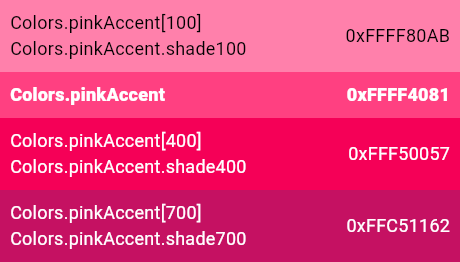
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.redAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [red], the corresponding primary colors.
/// * [deepOrangeAccent] and [pinkAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor redAccent = MaterialAccentColor(
_redAccentValue,
<int, Color>{
100: Color(0xFFFF8A80),
200: Color(_redAccentValue),
400: Color(0xFFFF1744),
700: Color(0xFFD50000),
},
);
static const int _redAccentValue = 0xFFFF5252;
/// The pink primary color and swatch.
///
/// 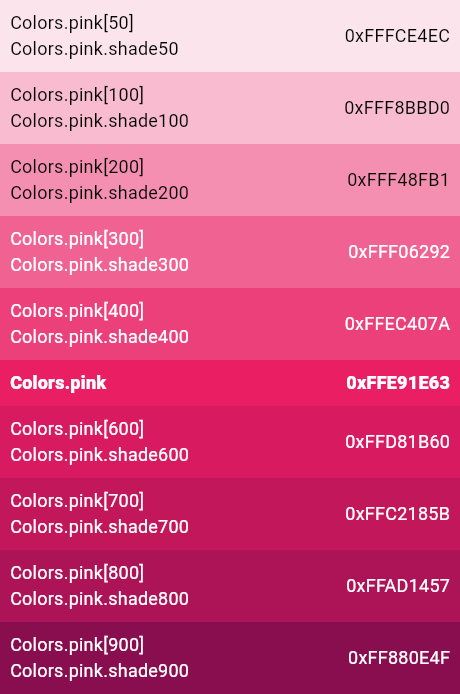
/// 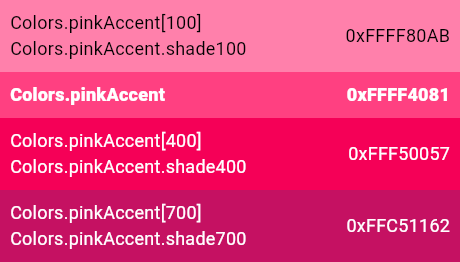
///
/// 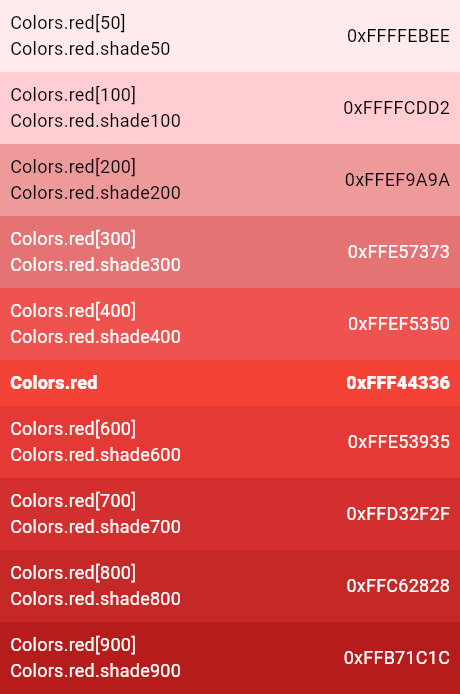
/// 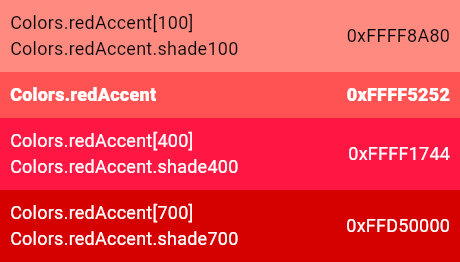
///
/// 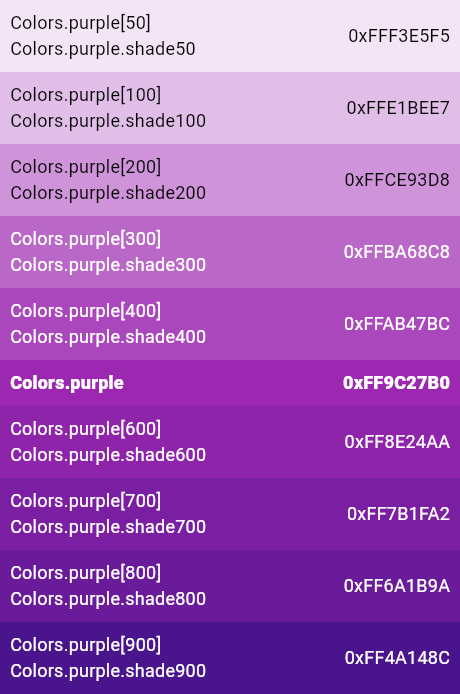
/// 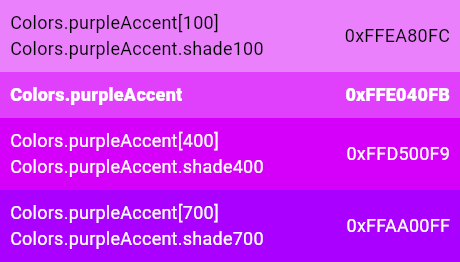
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.pink[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [pinkAccent], the corresponding accent colors.
/// * [red] and [purple], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor pink = MaterialColor(
_pinkPrimaryValue,
<int, Color>{
50: Color(0xFFFCE4EC),
100: Color(0xFFF8BBD0),
200: Color(0xFFF48FB1),
300: Color(0xFFF06292),
400: Color(0xFFEC407A),
500: Color(_pinkPrimaryValue),
600: Color(0xFFD81B60),
700: Color(0xFFC2185B),
800: Color(0xFFAD1457),
900: Color(0xFF880E4F),
},
);
static const int _pinkPrimaryValue = 0xFFE91E63;
/// The pink accent color swatch.
///
/// 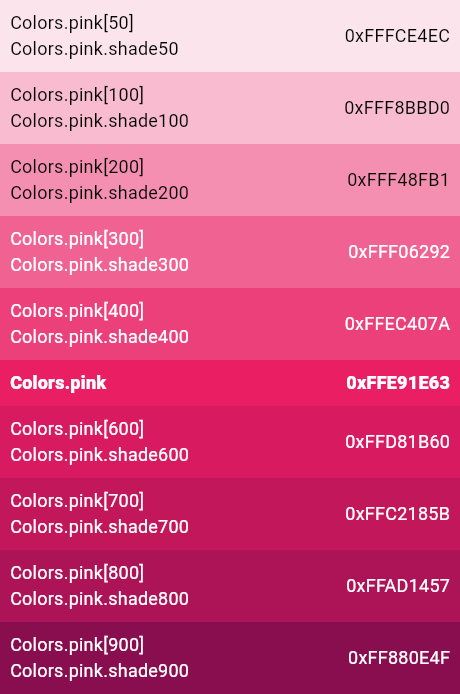
/// 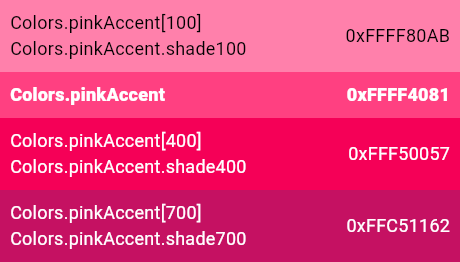
///
/// 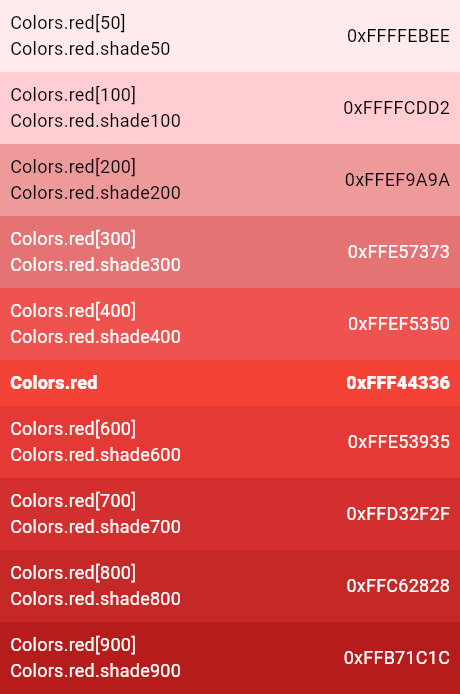
/// 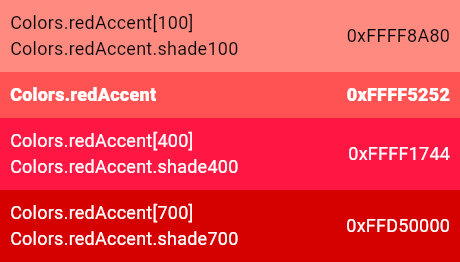
///
/// 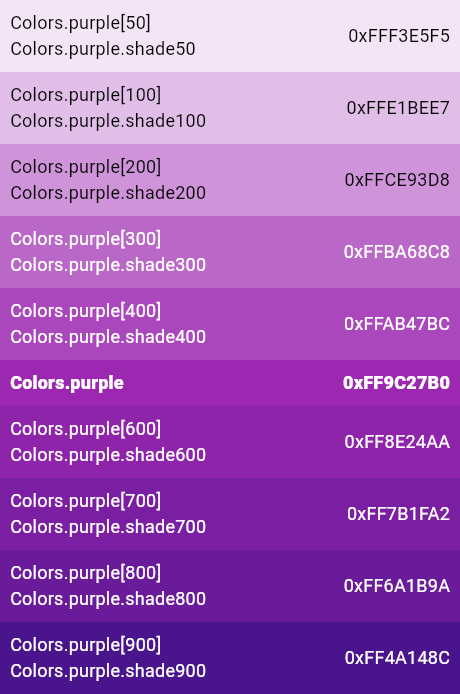
/// 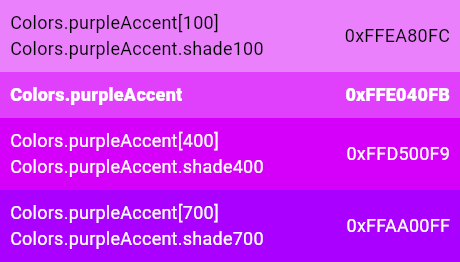
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.pinkAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [pink], the corresponding primary colors.
/// * [redAccent] and [purpleAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor pinkAccent = MaterialAccentColor(
_pinkAccentPrimaryValue,
<int, Color>{
100: Color(0xFFFF80AB),
200: Color(_pinkAccentPrimaryValue),
400: Color(0xFFF50057),
700: Color(0xFFC51162),
},
);
static const int _pinkAccentPrimaryValue = 0xFFFF4081;
/// The purple primary color and swatch.
///
/// 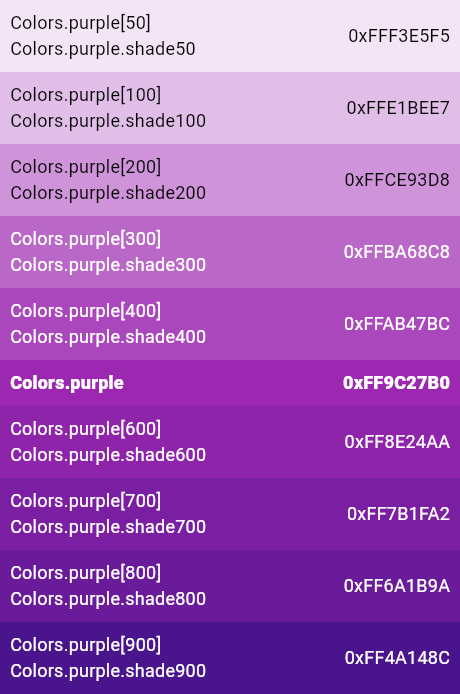
/// 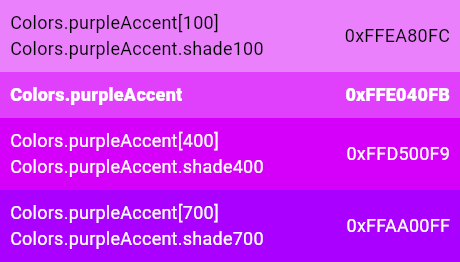
///
/// 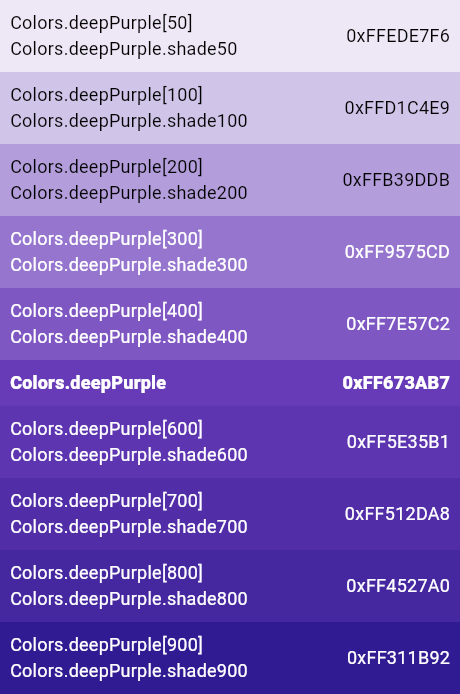
/// 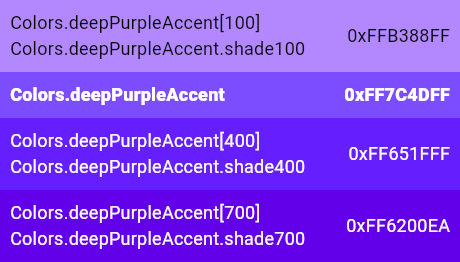
///
/// 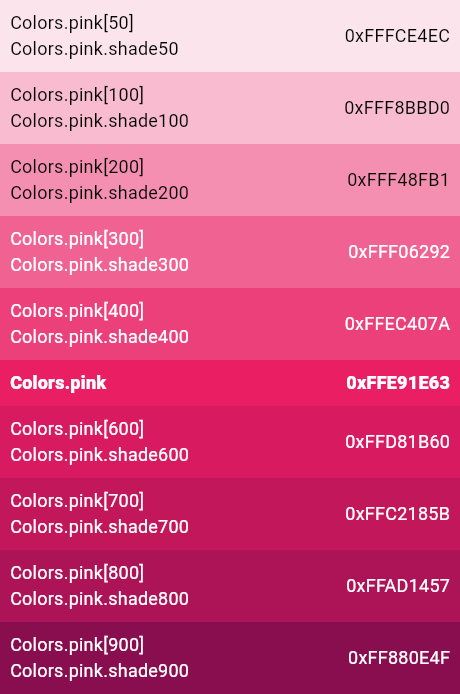
/// 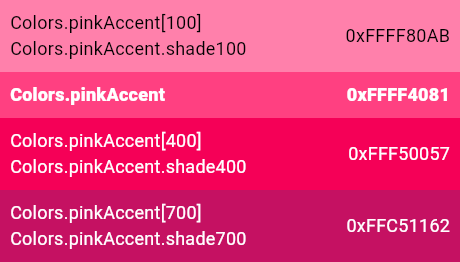
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.purple[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [purpleAccent], the corresponding accent colors.
/// * [deepPurple] and [pink], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor purple = MaterialColor(
_purplePrimaryValue,
<int, Color>{
50: Color(0xFFF3E5F5),
100: Color(0xFFE1BEE7),
200: Color(0xFFCE93D8),
300: Color(0xFFBA68C8),
400: Color(0xFFAB47BC),
500: Color(_purplePrimaryValue),
600: Color(0xFF8E24AA),
700: Color(0xFF7B1FA2),
800: Color(0xFF6A1B9A),
900: Color(0xFF4A148C),
},
);
static const int _purplePrimaryValue = 0xFF9C27B0;
/// The purple accent color and swatch.
///
/// 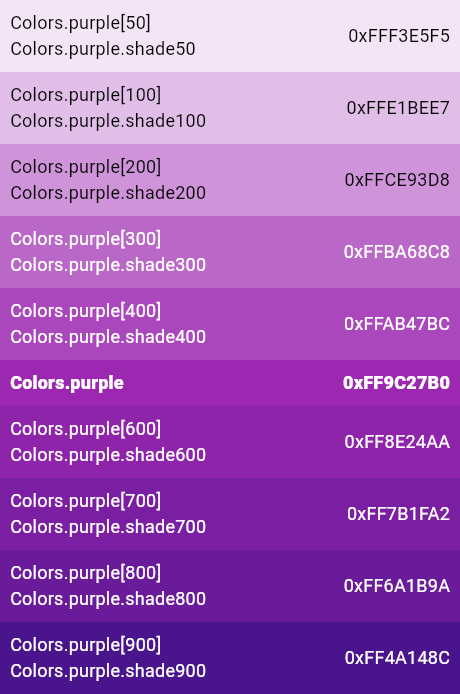
/// 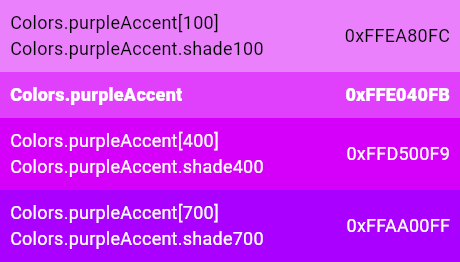
///
/// 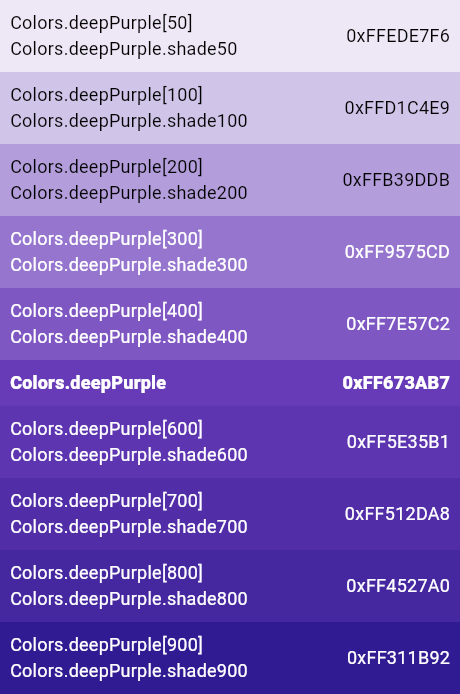
/// 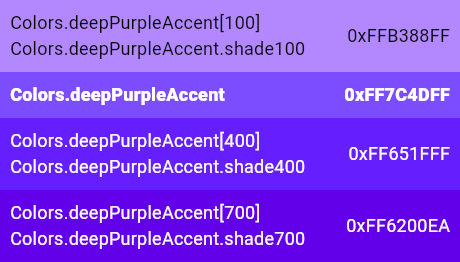
///
/// 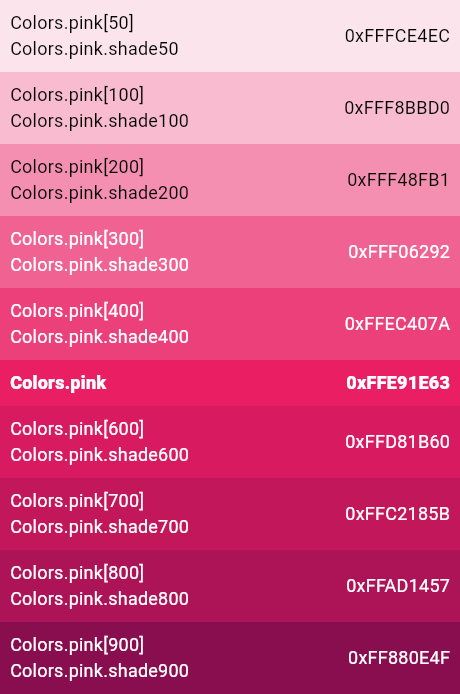
/// 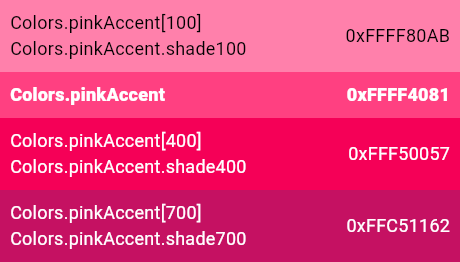
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.purpleAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [purple], the corresponding primary colors.
/// * [deepPurpleAccent] and [pinkAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor purpleAccent = MaterialAccentColor(
_purpleAccentPrimaryValue,
<int, Color>{
100: Color(0xFFEA80FC),
200: Color(_purpleAccentPrimaryValue),
400: Color(0xFFD500F9),
700: Color(0xFFAA00FF),
},
);
static const int _purpleAccentPrimaryValue = 0xFFE040FB;
/// The deep purple primary color and swatch.
///
/// 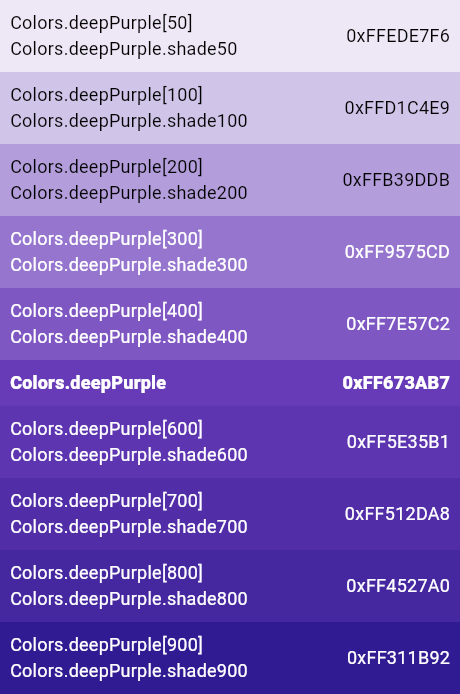
/// 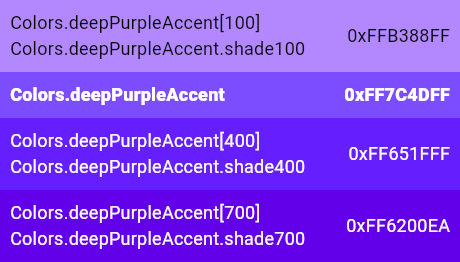
///
/// 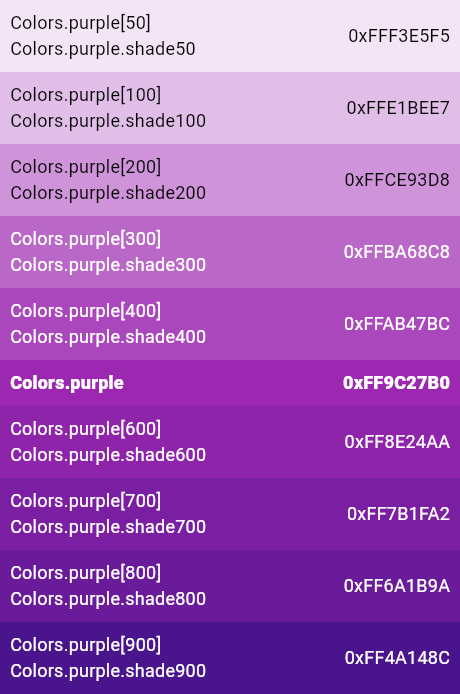
/// 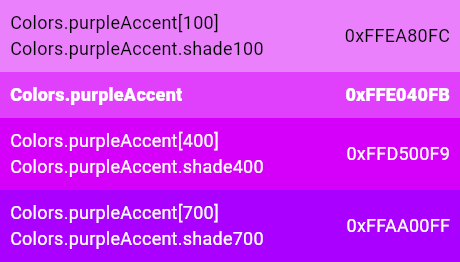
///
/// 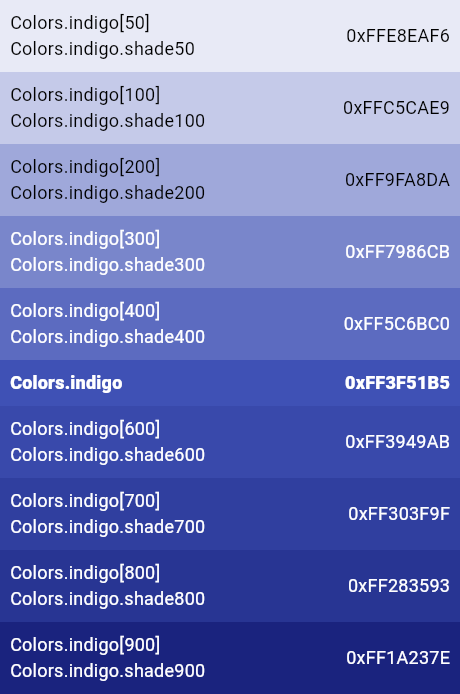
/// 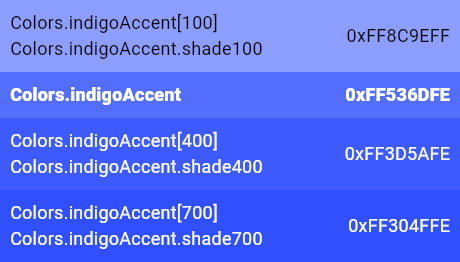
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.deepPurple[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [deepPurpleAccent], the corresponding accent colors.
/// * [purple] and [indigo], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor deepPurple = MaterialColor(
_deepPurplePrimaryValue,
<int, Color>{
50: Color(0xFFEDE7F6),
100: Color(0xFFD1C4E9),
200: Color(0xFFB39DDB),
300: Color(0xFF9575CD),
400: Color(0xFF7E57C2),
500: Color(_deepPurplePrimaryValue),
600: Color(0xFF5E35B1),
700: Color(0xFF512DA8),
800: Color(0xFF4527A0),
900: Color(0xFF311B92),
},
);
static const int _deepPurplePrimaryValue = 0xFF673AB7;
/// The deep purple accent color and swatch.
///
/// 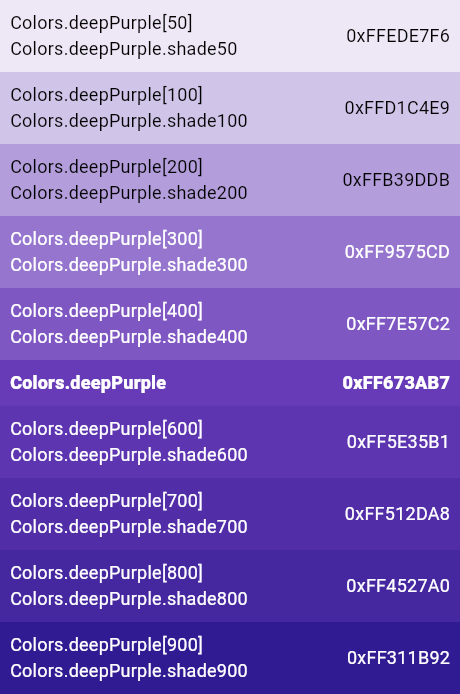
/// 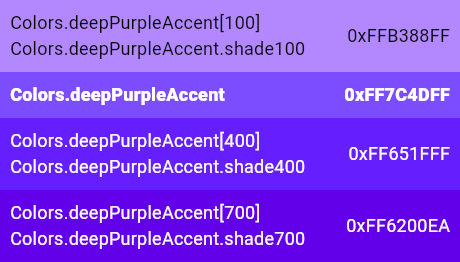
///
/// 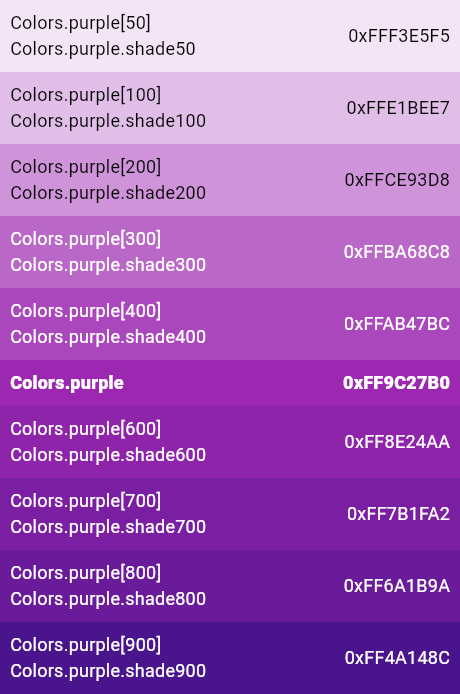
/// 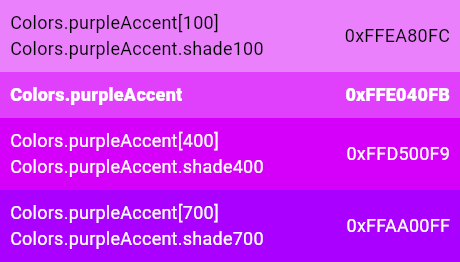
///
/// 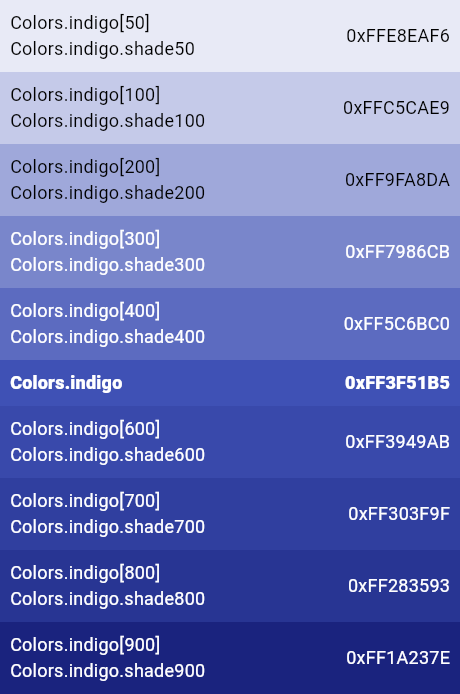
/// 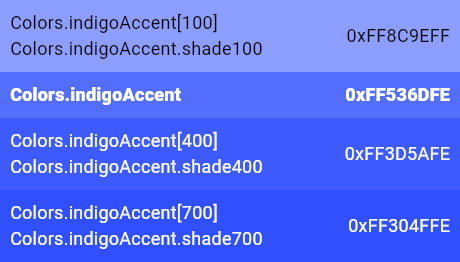
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.deepPurpleAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [deepPurple], the corresponding primary colors.
/// * [purpleAccent] and [indigoAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor deepPurpleAccent = MaterialAccentColor(
_deepPurpleAccentPrimaryValue,
<int, Color>{
100: Color(0xFFB388FF),
200: Color(_deepPurpleAccentPrimaryValue),
400: Color(0xFF651FFF),
700: Color(0xFF6200EA),
},
);
static const int _deepPurpleAccentPrimaryValue = 0xFF7C4DFF;
/// The indigo primary color and swatch.
///
/// 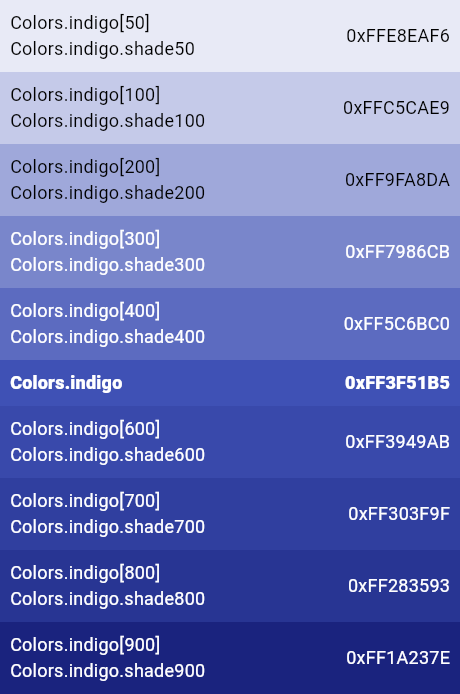
/// 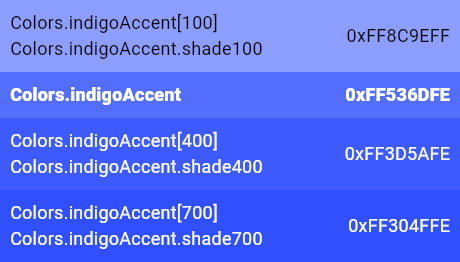
///
/// 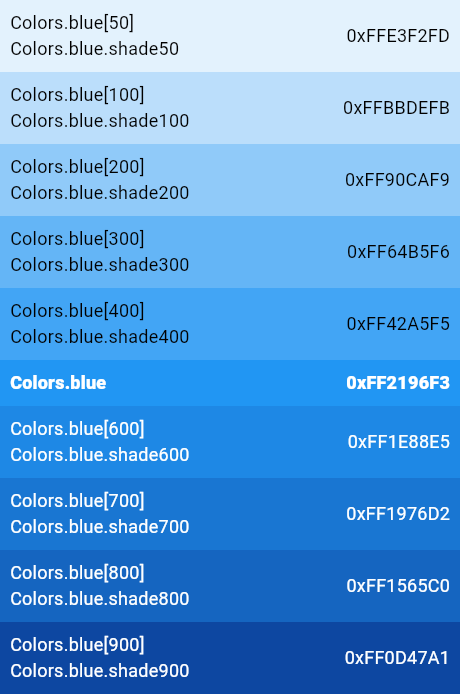
/// 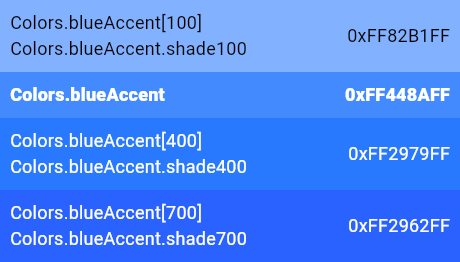
///
/// 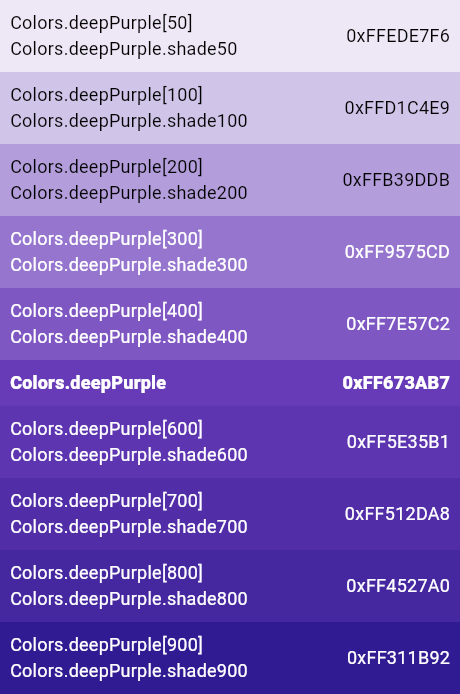
/// 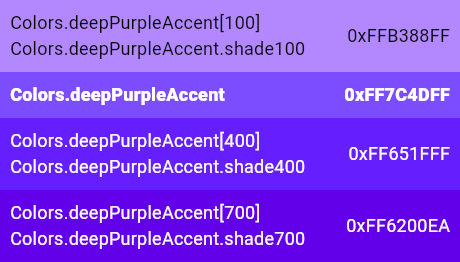
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.indigo[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [indigoAccent], the corresponding accent colors.
/// * [blue] and [deepPurple], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor indigo = MaterialColor(
_indigoPrimaryValue,
<int, Color>{
50: Color(0xFFE8EAF6),
100: Color(0xFFC5CAE9),
200: Color(0xFF9FA8DA),
300: Color(0xFF7986CB),
400: Color(0xFF5C6BC0),
500: Color(_indigoPrimaryValue),
600: Color(0xFF3949AB),
700: Color(0xFF303F9F),
800: Color(0xFF283593),
900: Color(0xFF1A237E),
},
);
static const int _indigoPrimaryValue = 0xFF3F51B5;
/// The indigo accent color and swatch.
///
/// 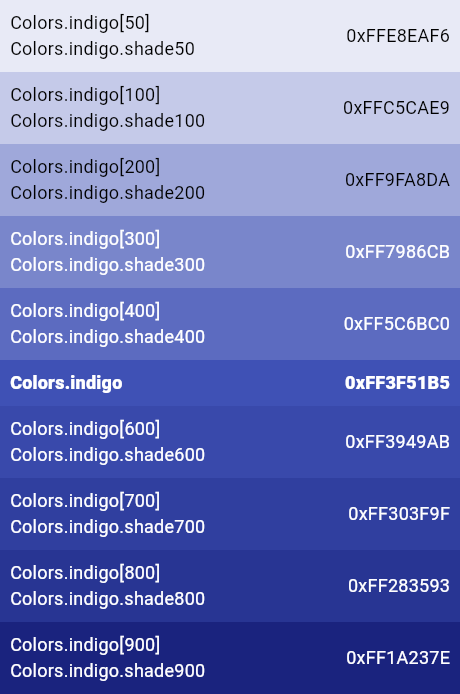
/// 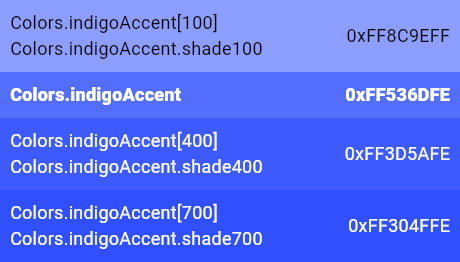
///
/// 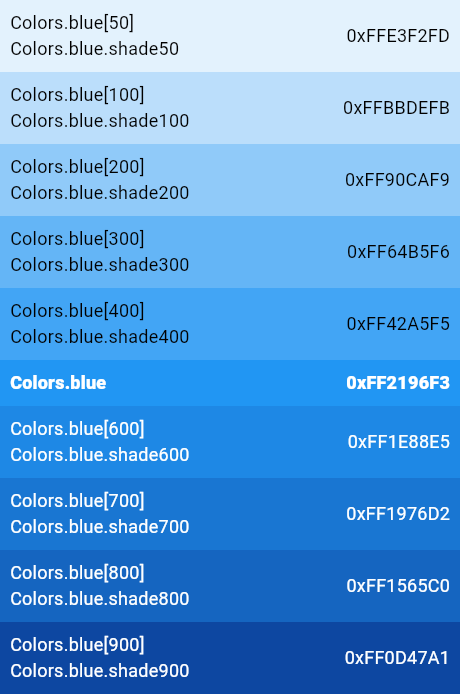
/// 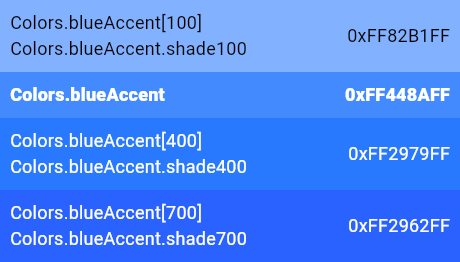
///
/// 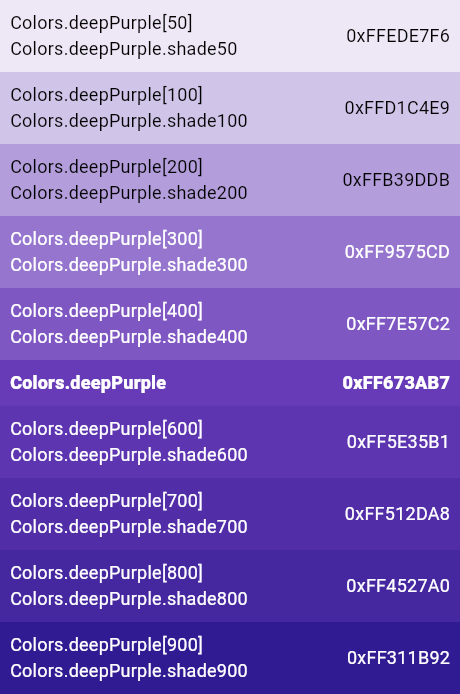
/// 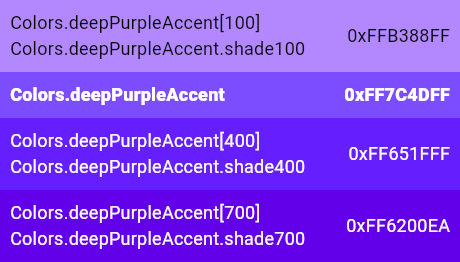
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.indigoAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [indigo], the corresponding primary colors.
/// * [blueAccent] and [deepPurpleAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor indigoAccent = MaterialAccentColor(
_indigoAccentPrimaryValue,
<int, Color>{
100: Color(0xFF8C9EFF),
200: Color(_indigoAccentPrimaryValue),
400: Color(0xFF3D5AFE),
700: Color(0xFF304FFE),
},
);
static const int _indigoAccentPrimaryValue = 0xFF536DFE;
/// The blue primary color and swatch.
///
/// 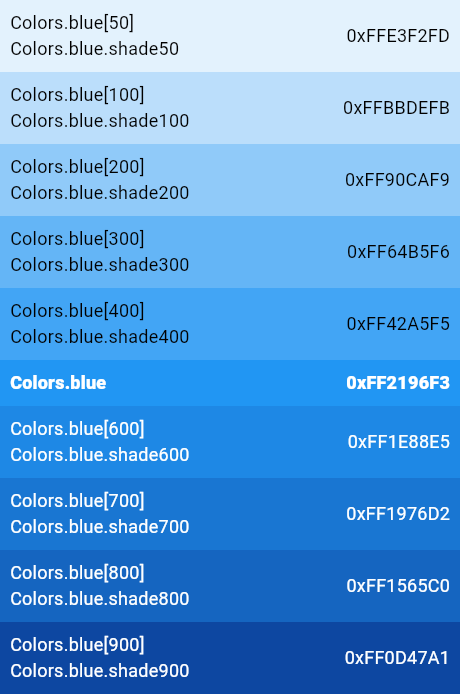
/// 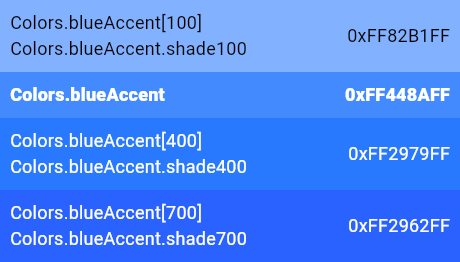
///
/// 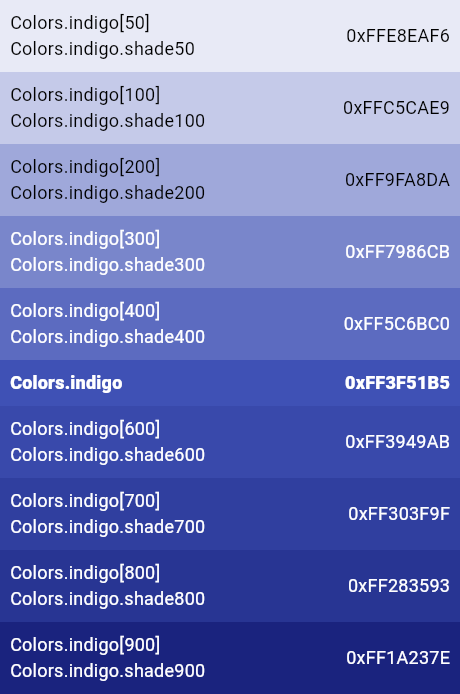
/// 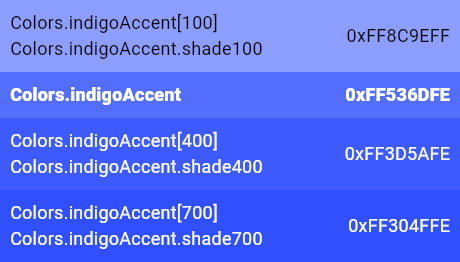
///
/// 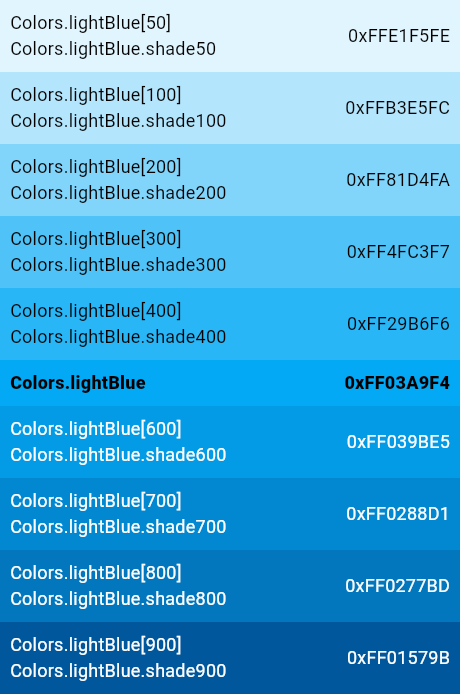
/// 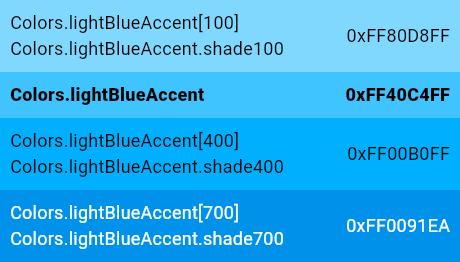
///
/// 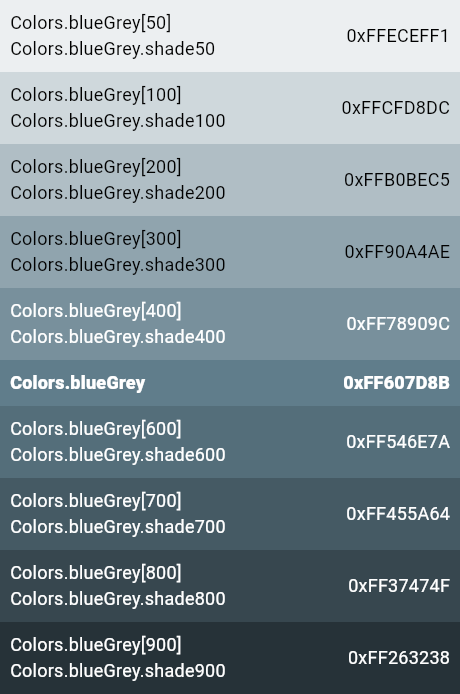
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.blue[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [blueAccent], the corresponding accent colors.
/// * [indigo], [lightBlue], and [blueGrey], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor blue = MaterialColor(
_bluePrimaryValue,
<int, Color>{
50: Color(0xFFE3F2FD),
100: Color(0xFFBBDEFB),
200: Color(0xFF90CAF9),
300: Color(0xFF64B5F6),
400: Color(0xFF42A5F5),
500: Color(_bluePrimaryValue),
600: Color(0xFF1E88E5),
700: Color(0xFF1976D2),
800: Color(0xFF1565C0),
900: Color(0xFF0D47A1),
},
);
static const int _bluePrimaryValue = 0xFF2196F3;
/// The blue accent color and swatch.
///
/// 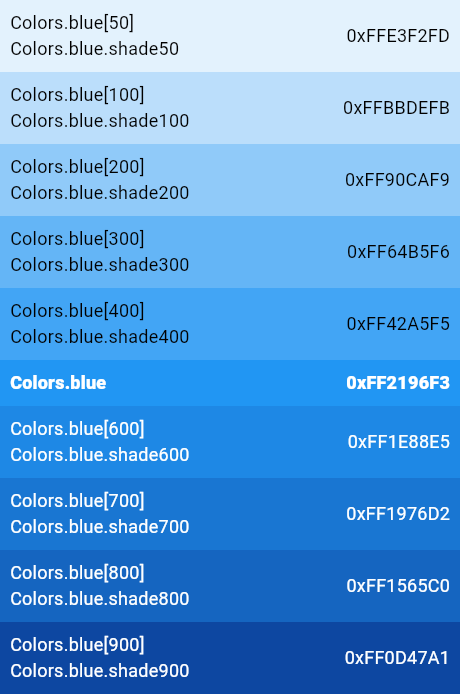
/// 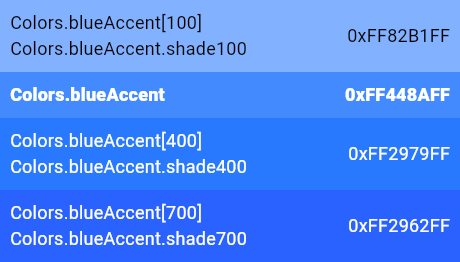
///
/// 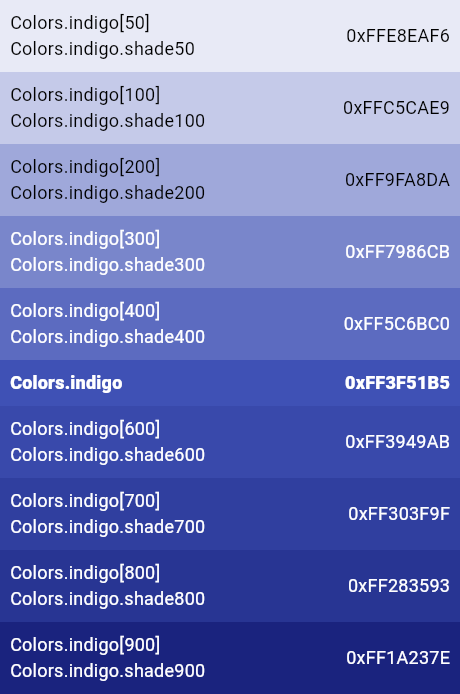
/// 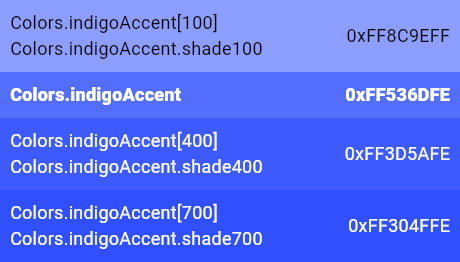
///
/// 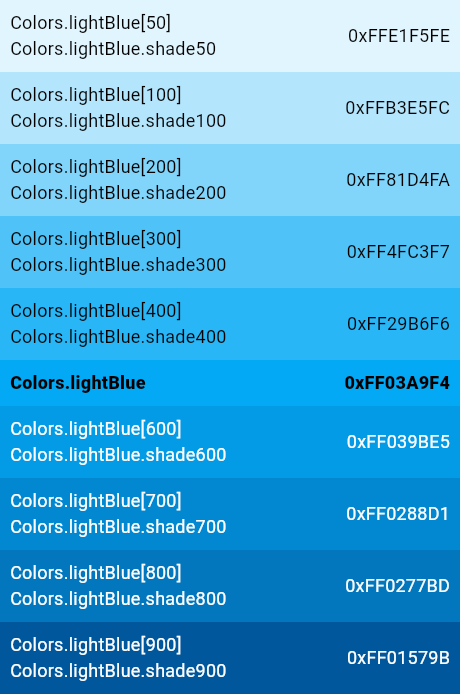
/// 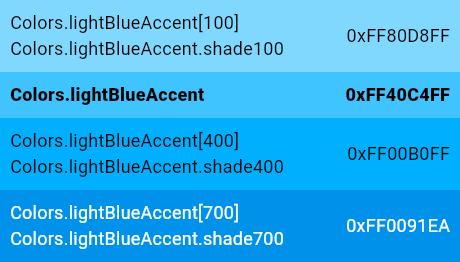
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.blueAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [blue], the corresponding primary colors.
/// * [indigoAccent] and [lightBlueAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor blueAccent = MaterialAccentColor(
_blueAccentPrimaryValue,
<int, Color>{
100: Color(0xFF82B1FF),
200: Color(_blueAccentPrimaryValue),
400: Color(0xFF2979FF),
700: Color(0xFF2962FF),
},
);
static const int _blueAccentPrimaryValue = 0xFF448AFF;
/// The light blue primary color and swatch.
///
/// 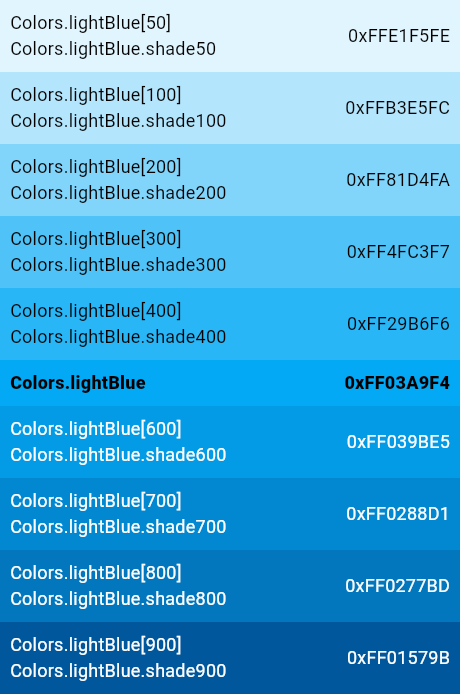
/// 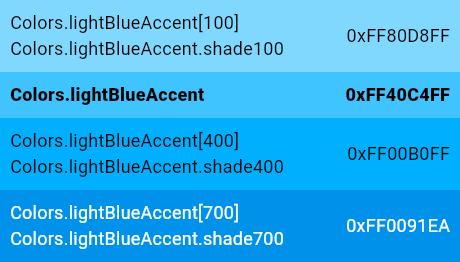
///
/// 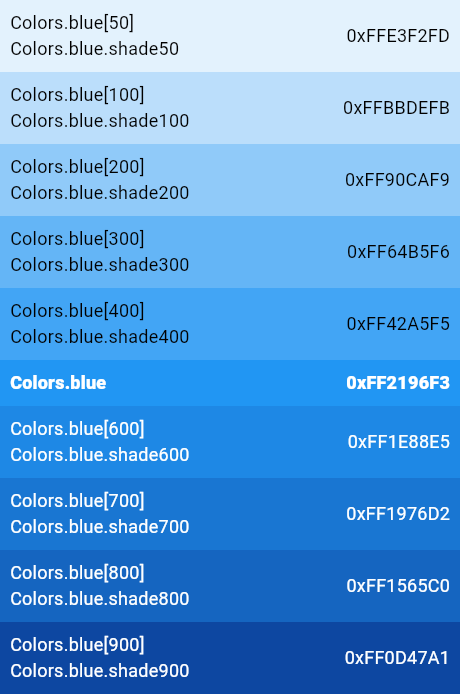
/// 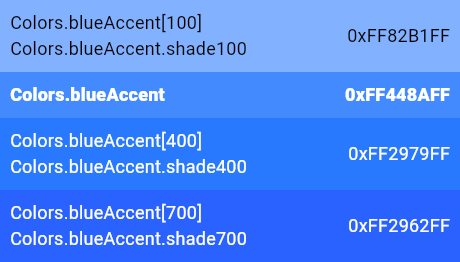
///
/// 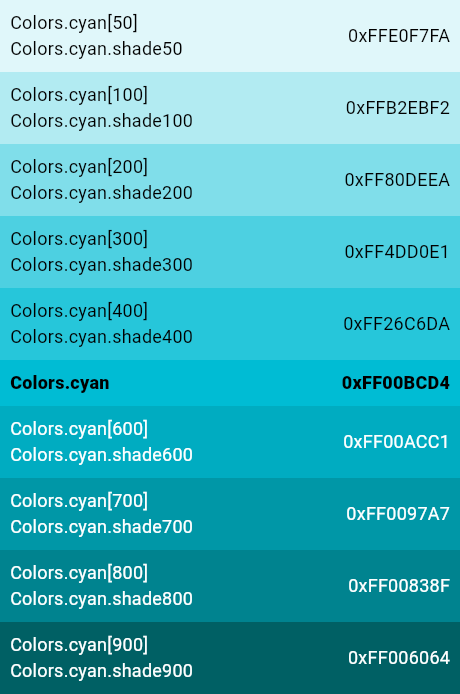
/// 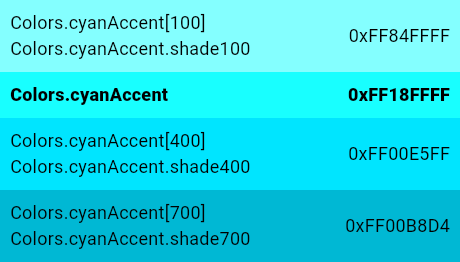
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.lightBlue[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [lightBlueAccent], the corresponding accent colors.
/// * [blue] and [cyan], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor lightBlue = MaterialColor(
_lightBluePrimaryValue,
<int, Color>{
50: Color(0xFFE1F5FE),
100: Color(0xFFB3E5FC),
200: Color(0xFF81D4FA),
300: Color(0xFF4FC3F7),
400: Color(0xFF29B6F6),
500: Color(_lightBluePrimaryValue),
600: Color(0xFF039BE5),
700: Color(0xFF0288D1),
800: Color(0xFF0277BD),
900: Color(0xFF01579B),
},
);
static const int _lightBluePrimaryValue = 0xFF03A9F4;
/// The light blue accent swatch.
///
/// 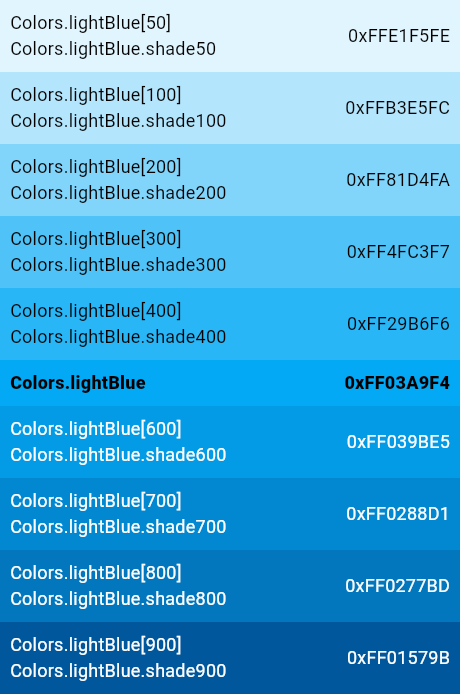
/// 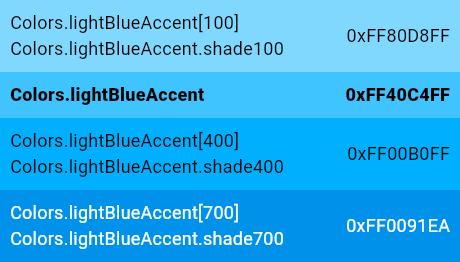
///
/// 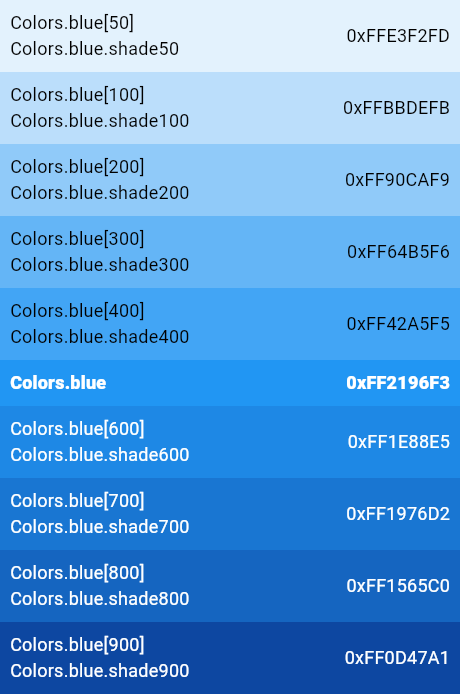
/// 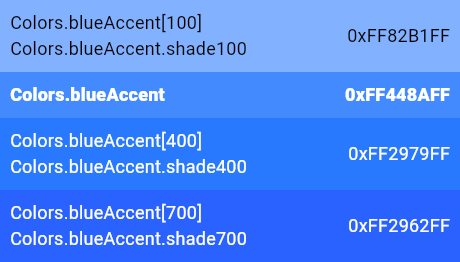
///
/// 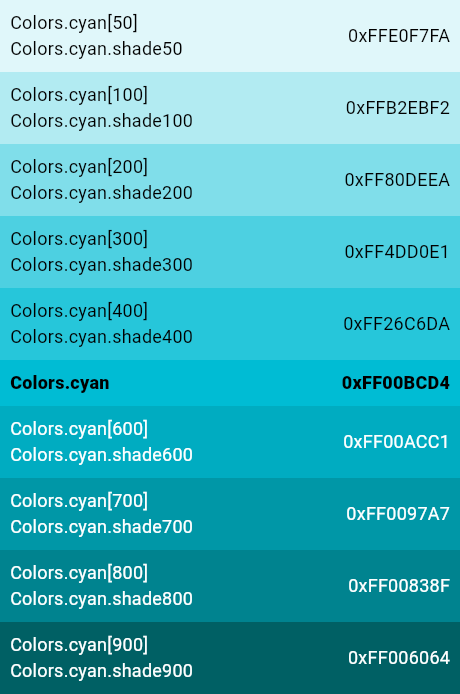
/// 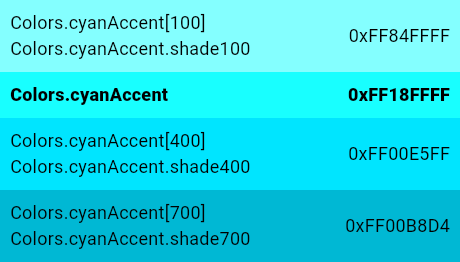
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.lightBlueAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [lightBlue], the corresponding primary colors.
/// * [blueAccent] and [cyanAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor lightBlueAccent = MaterialAccentColor(
_lightBlueAccentPrimaryValue,
<int, Color>{
100: Color(0xFF80D8FF),
200: Color(_lightBlueAccentPrimaryValue),
400: Color(0xFF00B0FF),
700: Color(0xFF0091EA),
},
);
static const int _lightBlueAccentPrimaryValue = 0xFF40C4FF;
/// The cyan primary color and swatch.
///
/// 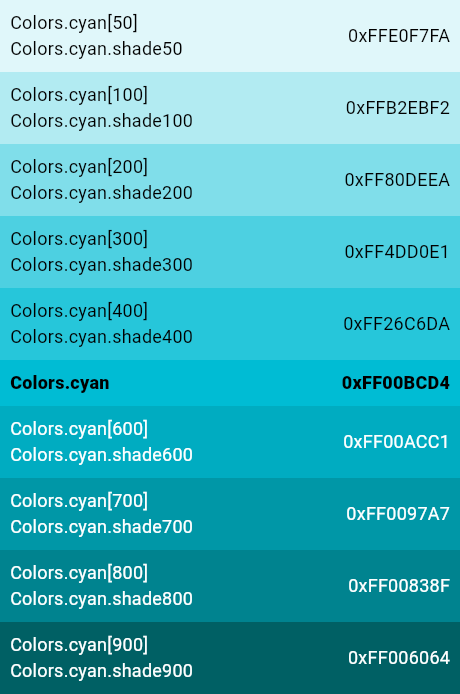
/// 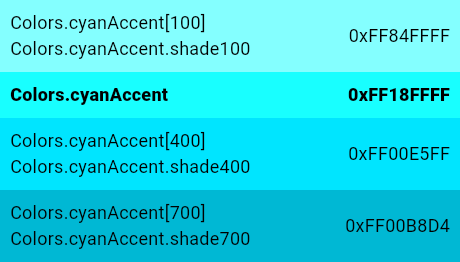
///
/// 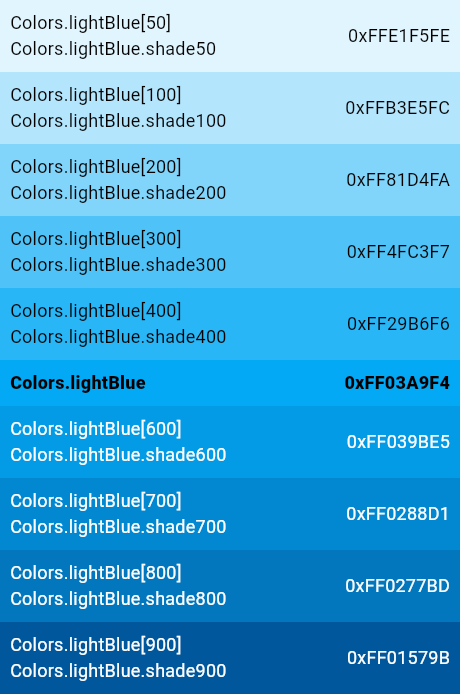
/// 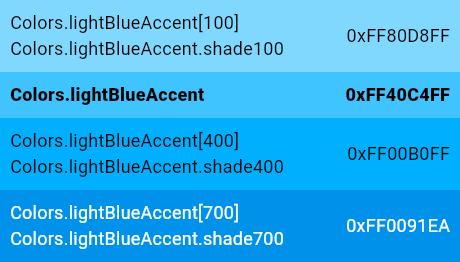
///
/// 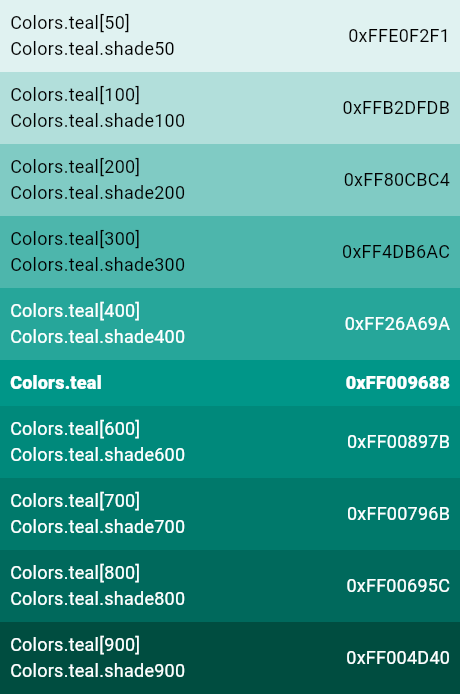
/// 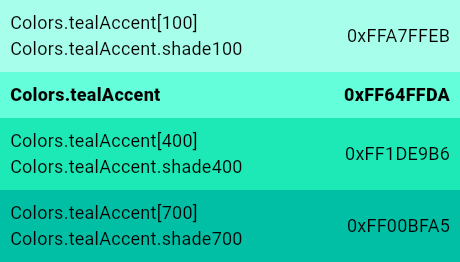
///
/// 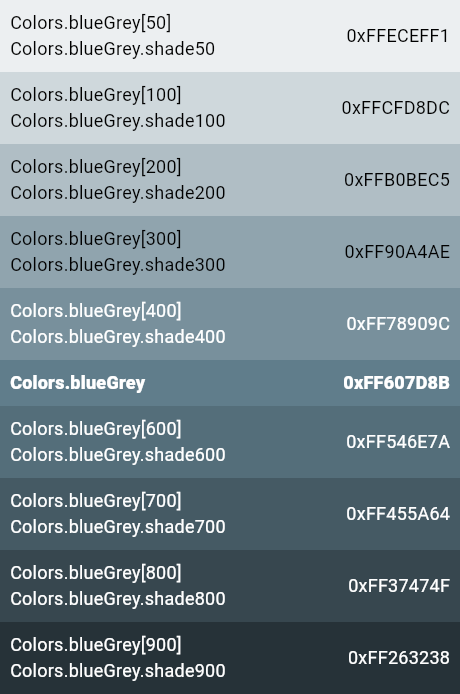
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.cyan[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [cyanAccent], the corresponding accent colors.
/// * [lightBlue], [teal], and [blueGrey], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor cyan = MaterialColor(
_cyanPrimaryValue,
<int, Color>{
50: Color(0xFFE0F7FA),
100: Color(0xFFB2EBF2),
200: Color(0xFF80DEEA),
300: Color(0xFF4DD0E1),
400: Color(0xFF26C6DA),
500: Color(_cyanPrimaryValue),
600: Color(0xFF00ACC1),
700: Color(0xFF0097A7),
800: Color(0xFF00838F),
900: Color(0xFF006064),
},
);
static const int _cyanPrimaryValue = 0xFF00BCD4;
/// The cyan accent color and swatch.
///
/// 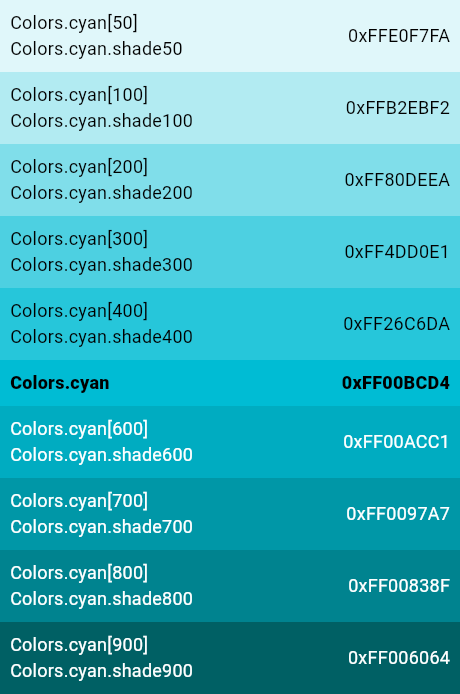
/// 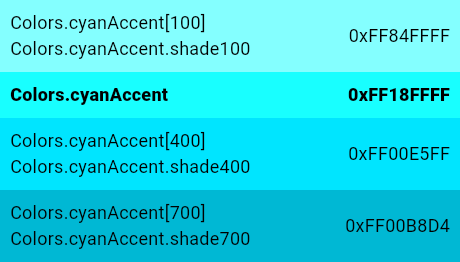
///
/// 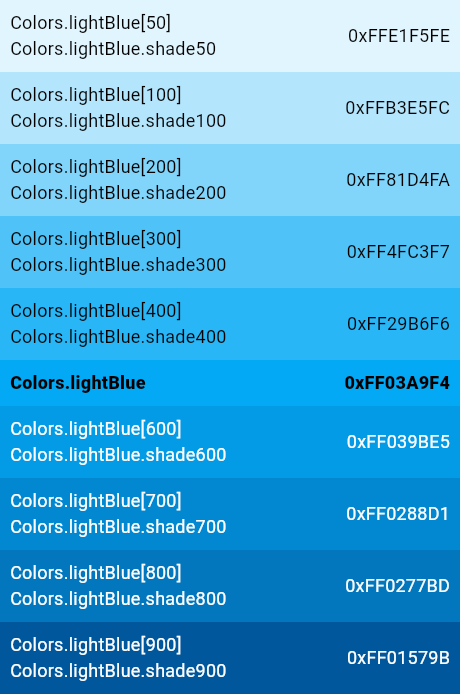
/// 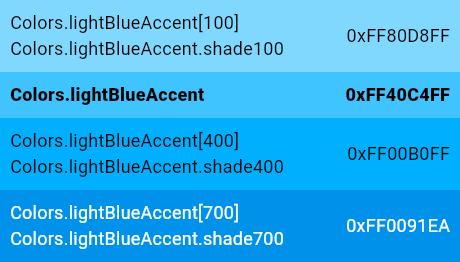
///
/// 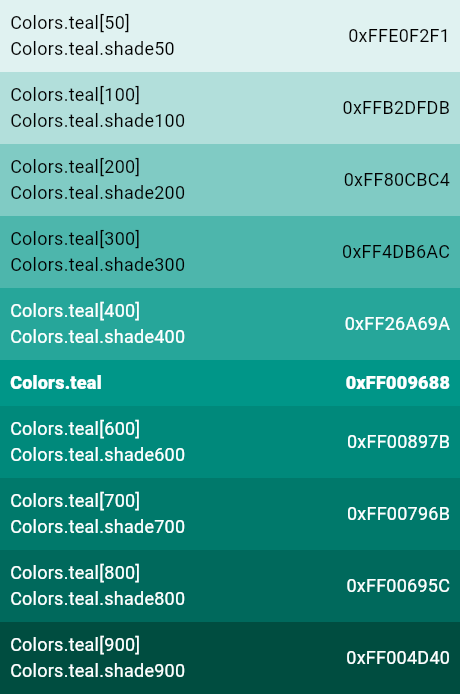
/// 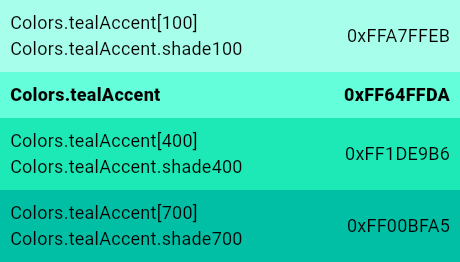
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.cyanAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [cyan], the corresponding primary colors.
/// * [lightBlueAccent] and [tealAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor cyanAccent = MaterialAccentColor(
_cyanAccentPrimaryValue,
<int, Color>{
100: Color(0xFF84FFFF),
200: Color(_cyanAccentPrimaryValue),
400: Color(0xFF00E5FF),
700: Color(0xFF00B8D4),
},
);
static const int _cyanAccentPrimaryValue = 0xFF18FFFF;
/// The teal primary color and swatch.
///
/// 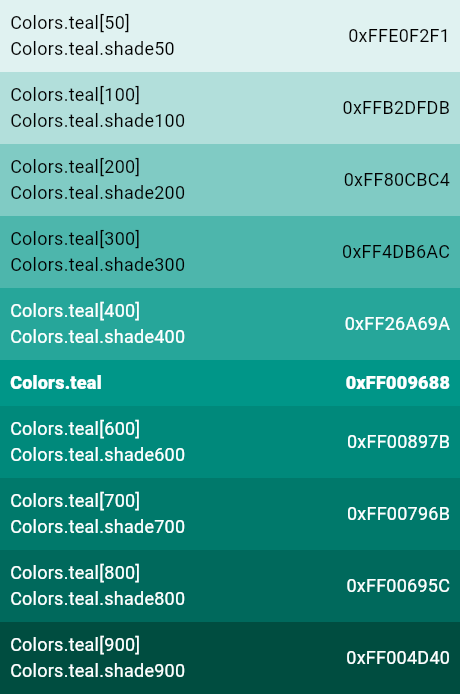
/// 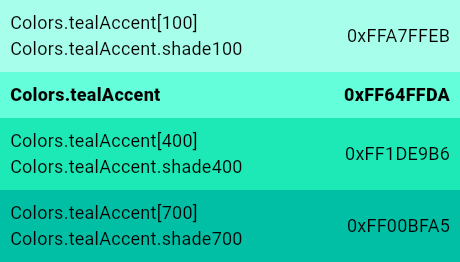
///
/// 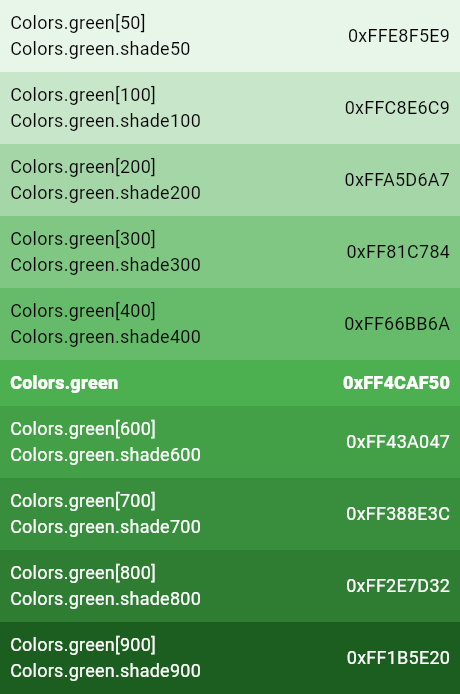
/// 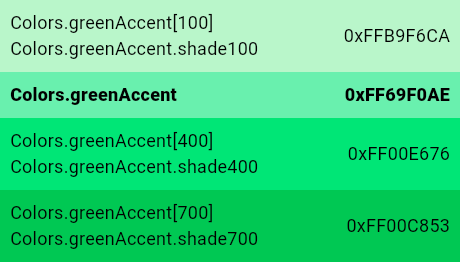
///
/// 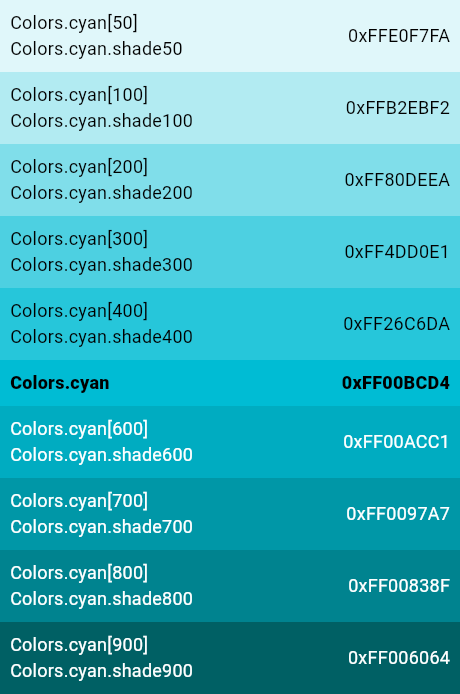
/// 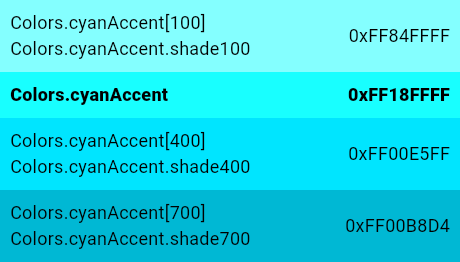
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.teal[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [tealAccent], the corresponding accent colors.
/// * [green] and [cyan], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor teal = MaterialColor(
_tealPrimaryValue,
<int, Color>{
50: Color(0xFFE0F2F1),
100: Color(0xFFB2DFDB),
200: Color(0xFF80CBC4),
300: Color(0xFF4DB6AC),
400: Color(0xFF26A69A),
500: Color(_tealPrimaryValue),
600: Color(0xFF00897B),
700: Color(0xFF00796B),
800: Color(0xFF00695C),
900: Color(0xFF004D40),
},
);
static const int _tealPrimaryValue = 0xFF009688;
/// The teal accent color and swatch.
///
/// 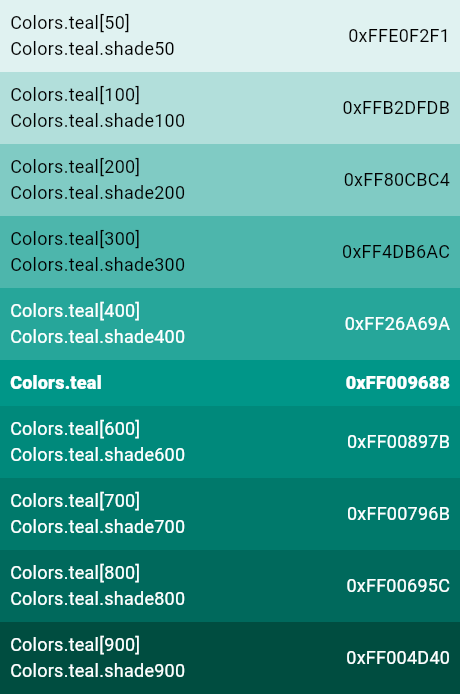
/// 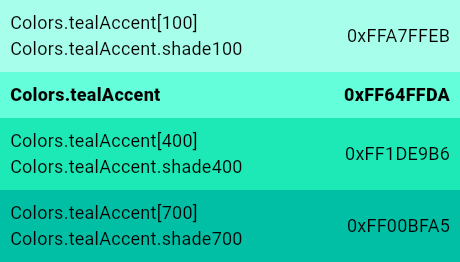
///
/// 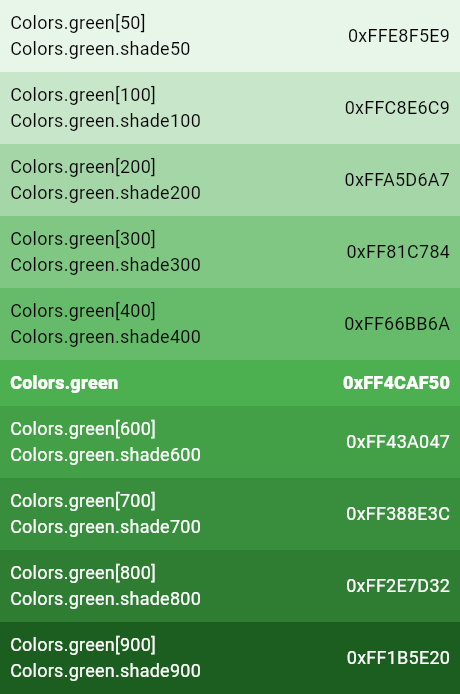
/// 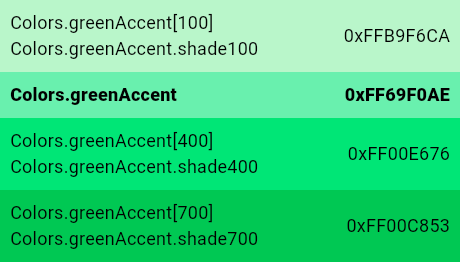
///
/// 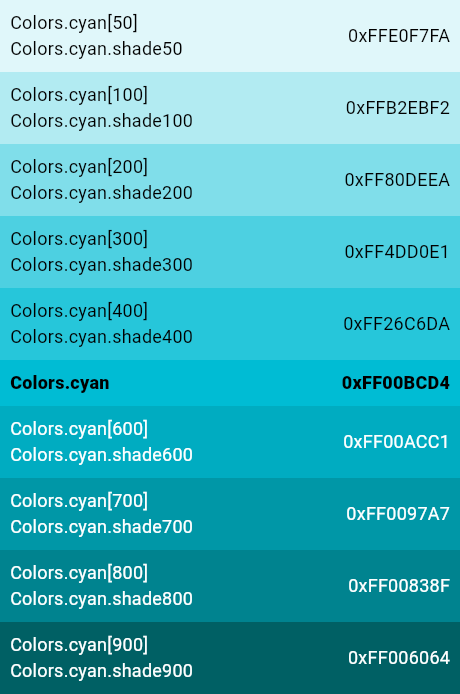
/// 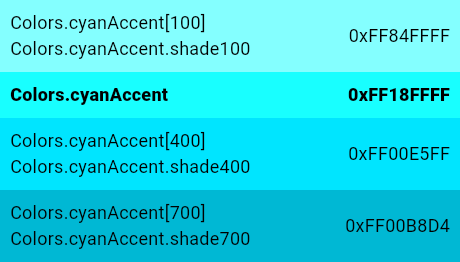
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.tealAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [teal], the corresponding primary colors.
/// * [greenAccent] and [cyanAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor tealAccent = MaterialAccentColor(
_tealAccentPrimaryValue,
<int, Color>{
100: Color(0xFFA7FFEB),
200: Color(_tealAccentPrimaryValue),
400: Color(0xFF1DE9B6),
700: Color(0xFF00BFA5),
},
);
static const int _tealAccentPrimaryValue = 0xFF64FFDA;
/// The green primary color and swatch.
///
/// 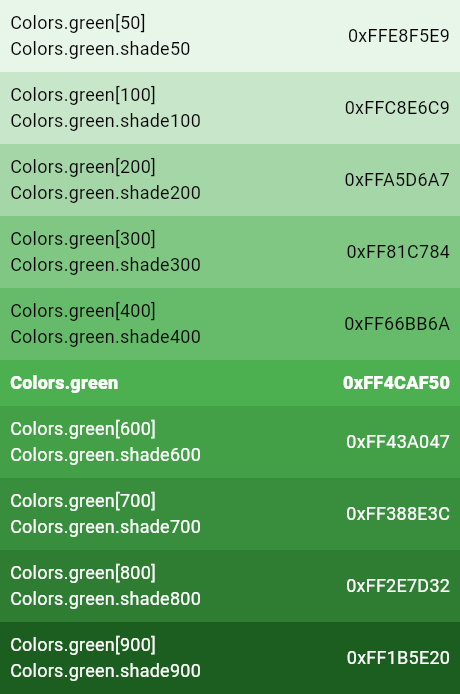
/// 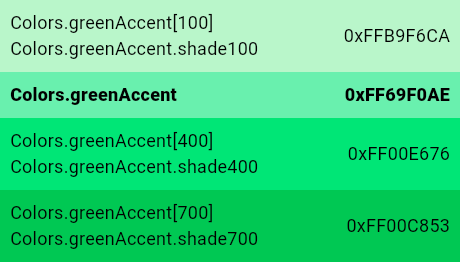
///
/// 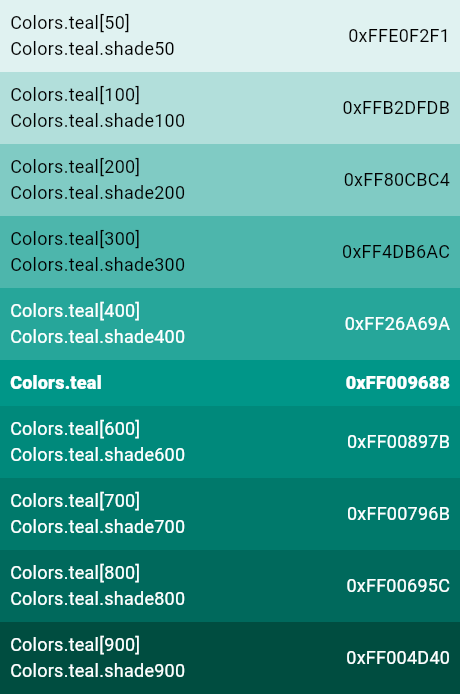
/// 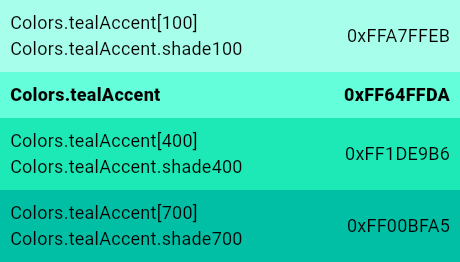
///
/// 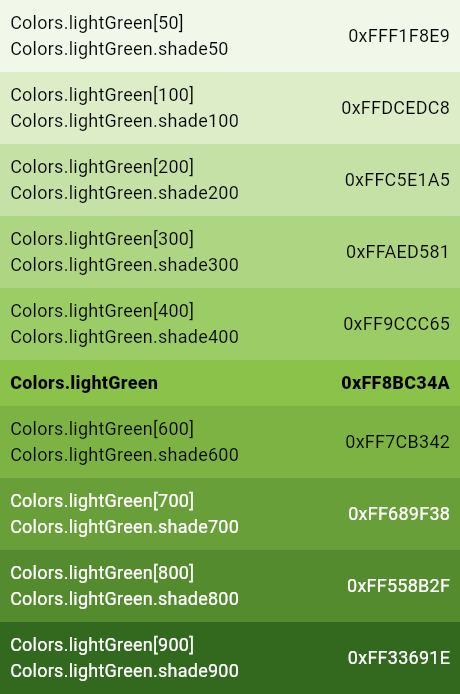
/// 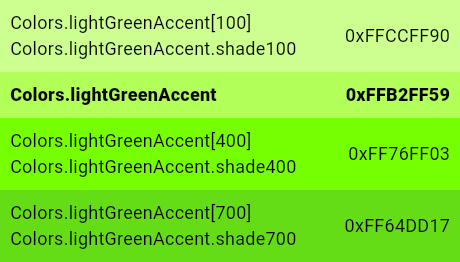
///
/// 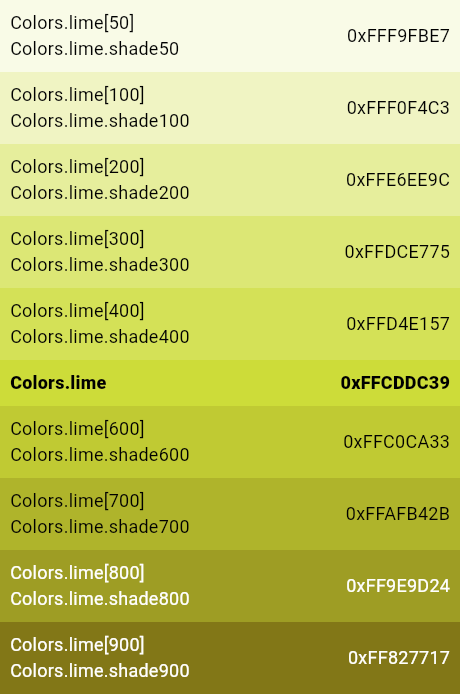
/// 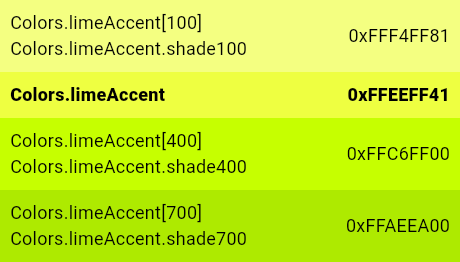
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.green[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [greenAccent], the corresponding accent colors.
/// * [teal], [lightGreen], and [lime], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor green = MaterialColor(
_greenPrimaryValue,
<int, Color>{
50: Color(0xFFE8F5E9),
100: Color(0xFFC8E6C9),
200: Color(0xFFA5D6A7),
300: Color(0xFF81C784),
400: Color(0xFF66BB6A),
500: Color(_greenPrimaryValue),
600: Color(0xFF43A047),
700: Color(0xFF388E3C),
800: Color(0xFF2E7D32),
900: Color(0xFF1B5E20),
},
);
static const int _greenPrimaryValue = 0xFF4CAF50;
/// The green accent color and swatch.
///
/// 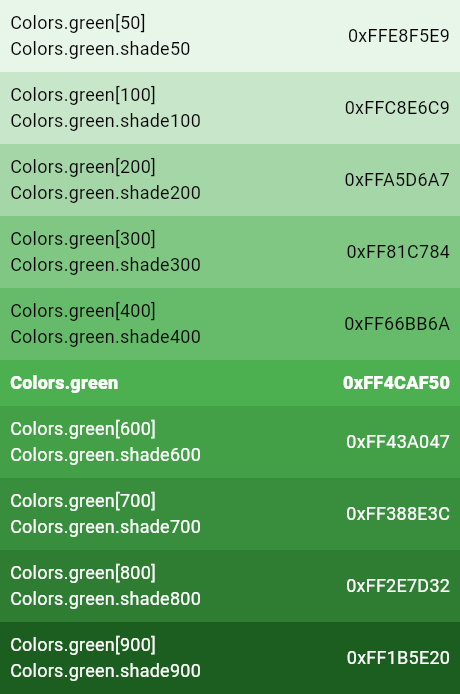
/// 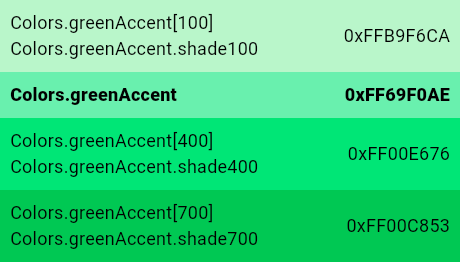
///
/// 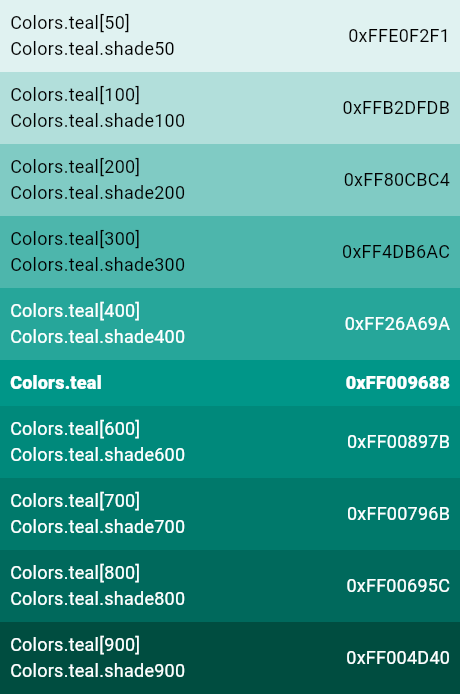
/// 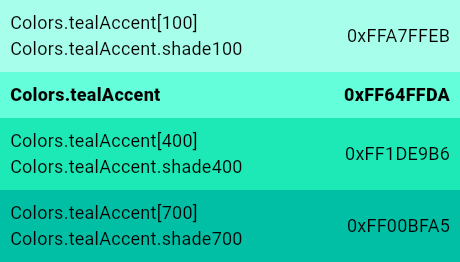
///
/// 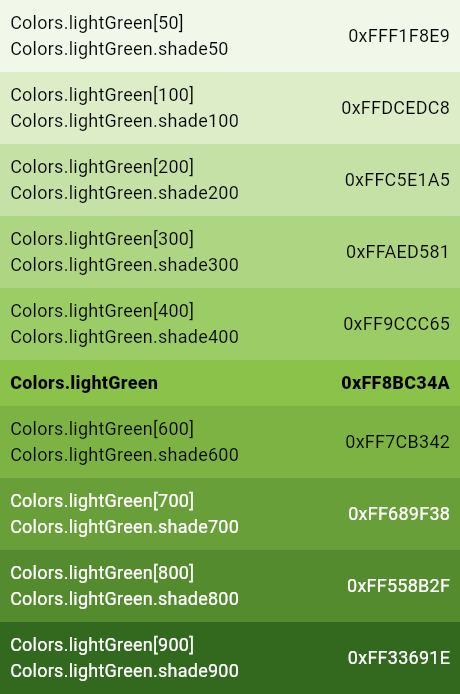
/// 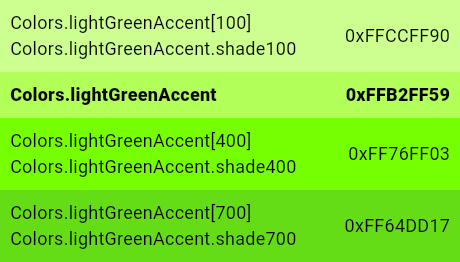
///
/// 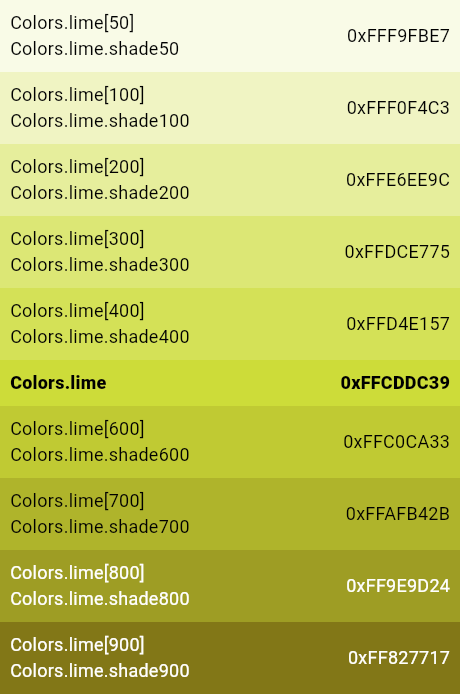
/// 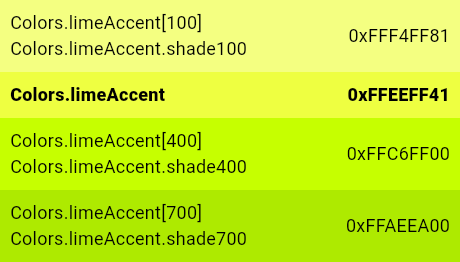
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.greenAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [green], the corresponding primary colors.
/// * [tealAccent], [lightGreenAccent], and [limeAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor greenAccent = MaterialAccentColor(
_greenAccentPrimaryValue,
<int, Color>{
100: Color(0xFFB9F6CA),
200: Color(_greenAccentPrimaryValue),
400: Color(0xFF00E676),
700: Color(0xFF00C853),
},
);
static const int _greenAccentPrimaryValue = 0xFF69F0AE;
/// The light green primary color and swatch.
///
/// 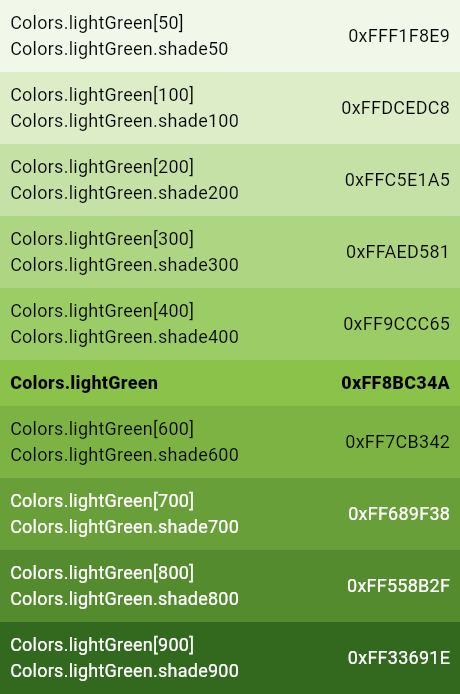
/// 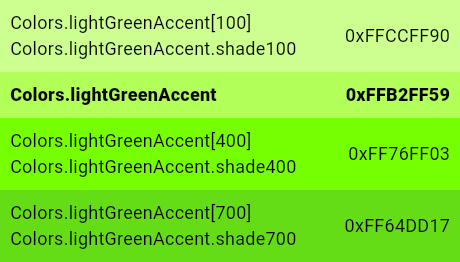
///
/// 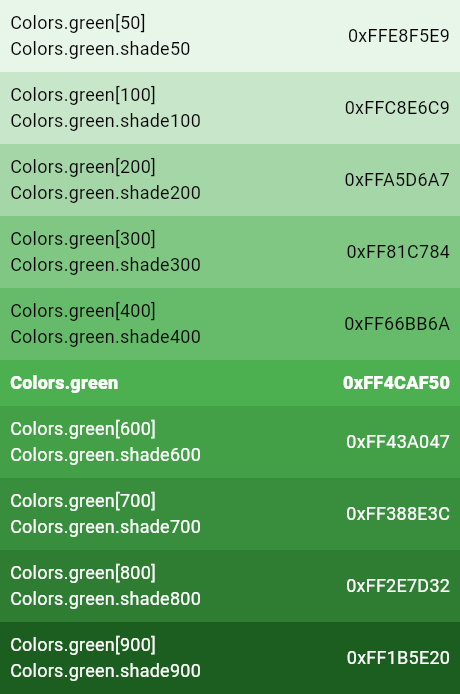
/// 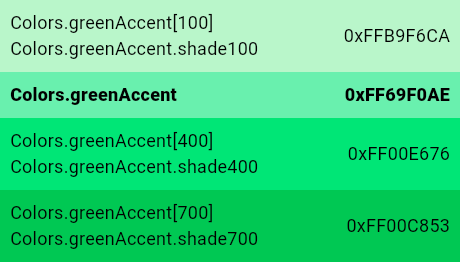
///
/// 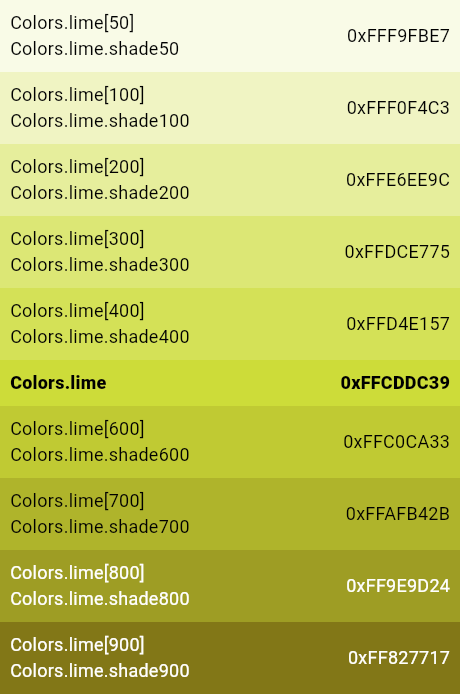
/// 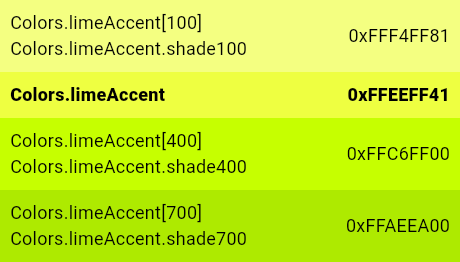
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.lightGreen[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [lightGreenAccent], the corresponding accent colors.
/// * [green] and [lime], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor lightGreen = MaterialColor(
_lightGreenPrimaryValue,
<int, Color>{
50: Color(0xFFF1F8E9),
100: Color(0xFFDCEDC8),
200: Color(0xFFC5E1A5),
300: Color(0xFFAED581),
400: Color(0xFF9CCC65),
500: Color(_lightGreenPrimaryValue),
600: Color(0xFF7CB342),
700: Color(0xFF689F38),
800: Color(0xFF558B2F),
900: Color(0xFF33691E),
},
);
static const int _lightGreenPrimaryValue = 0xFF8BC34A;
/// The light green accent color and swatch.
///
/// 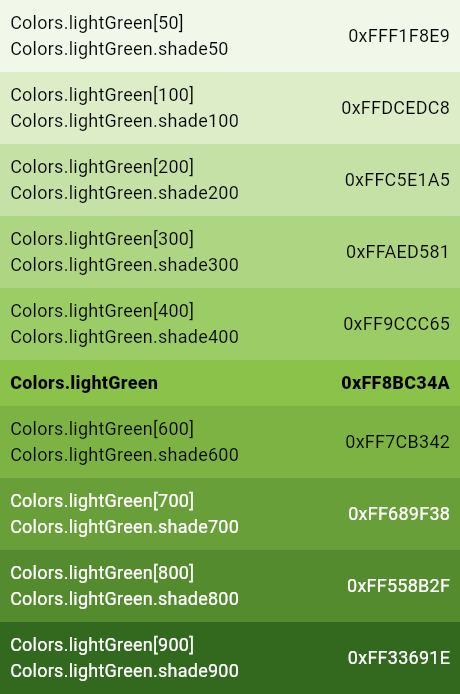
/// 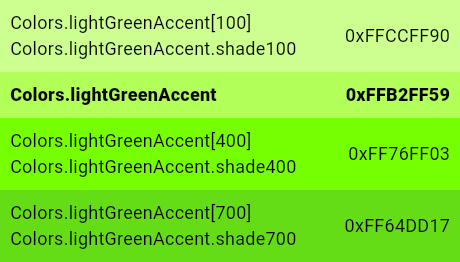
///
/// 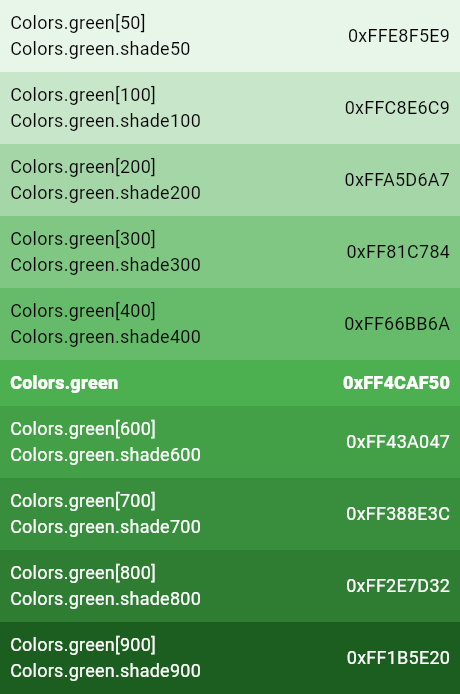
/// 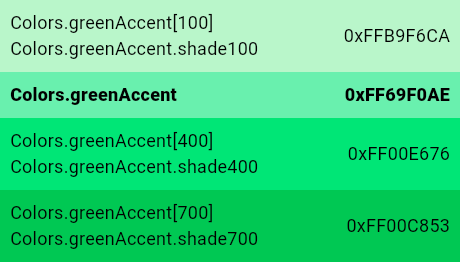
///
/// 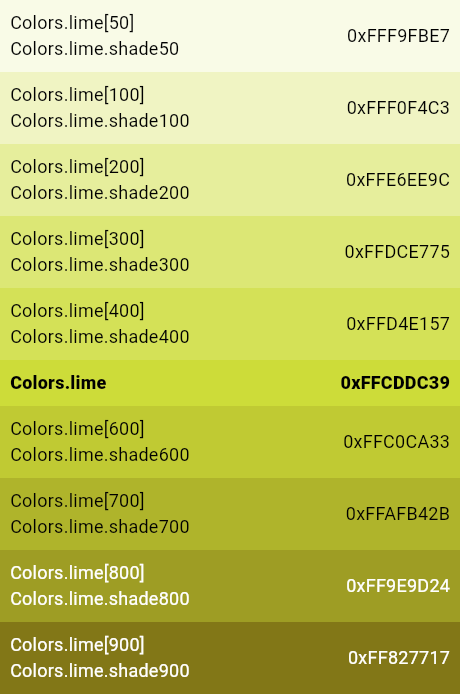
/// 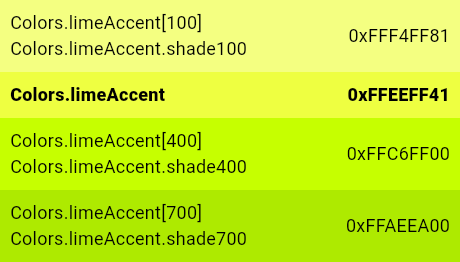
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.lightGreenAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [lightGreen], the corresponding primary colors.
/// * [greenAccent] and [limeAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor lightGreenAccent = MaterialAccentColor(
_lightGreenAccentPrimaryValue,
<int, Color>{
100: Color(0xFFCCFF90),
200: Color(_lightGreenAccentPrimaryValue),
400: Color(0xFF76FF03),
700: Color(0xFF64DD17),
},
);
static const int _lightGreenAccentPrimaryValue = 0xFFB2FF59;
/// The lime primary color and swatch.
///
/// 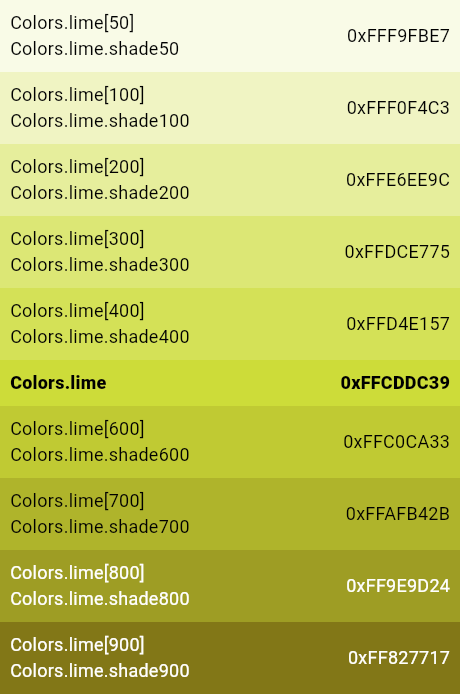
/// 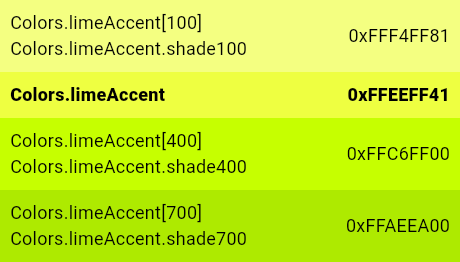
///
/// 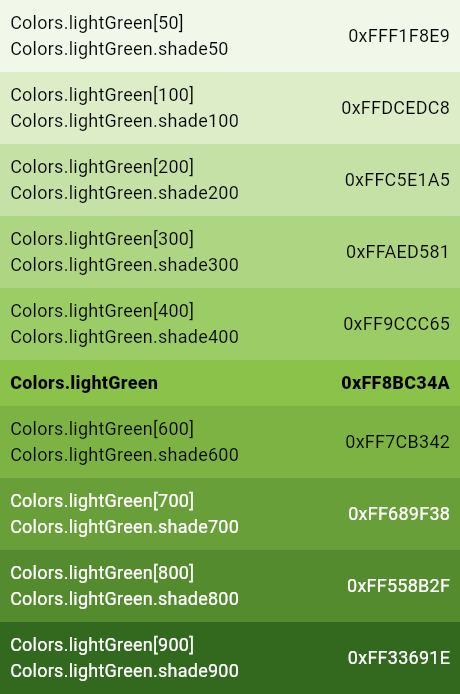
/// 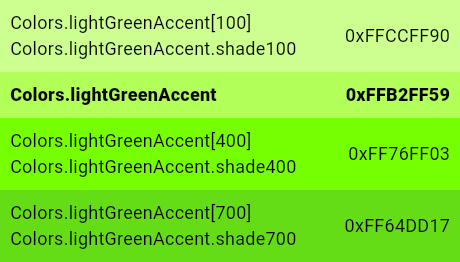
///
/// 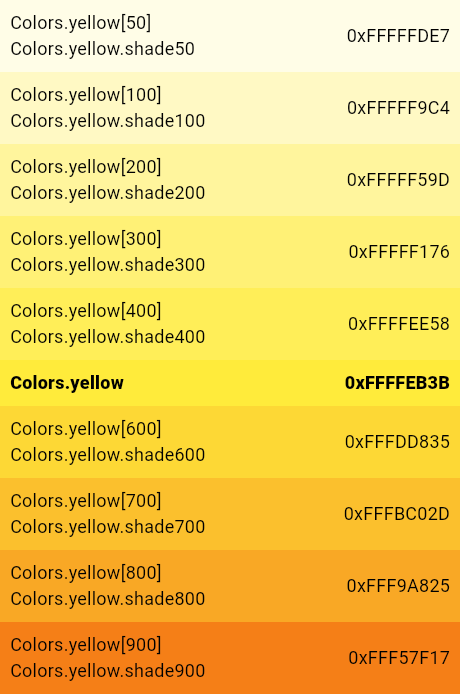
/// 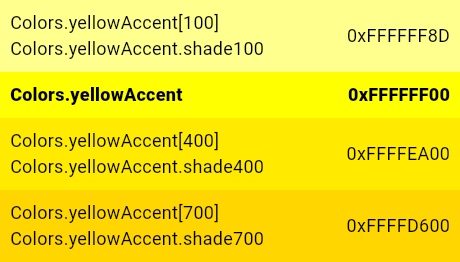
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.lime[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [limeAccent], the corresponding accent colors.
/// * [lightGreen] and [yellow], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor lime = MaterialColor(
_limePrimaryValue,
<int, Color>{
50: Color(0xFFF9FBE7),
100: Color(0xFFF0F4C3),
200: Color(0xFFE6EE9C),
300: Color(0xFFDCE775),
400: Color(0xFFD4E157),
500: Color(_limePrimaryValue),
600: Color(0xFFC0CA33),
700: Color(0xFFAFB42B),
800: Color(0xFF9E9D24),
900: Color(0xFF827717),
},
);
static const int _limePrimaryValue = 0xFFCDDC39;
/// The lime accent primary color and swatch.
///
/// 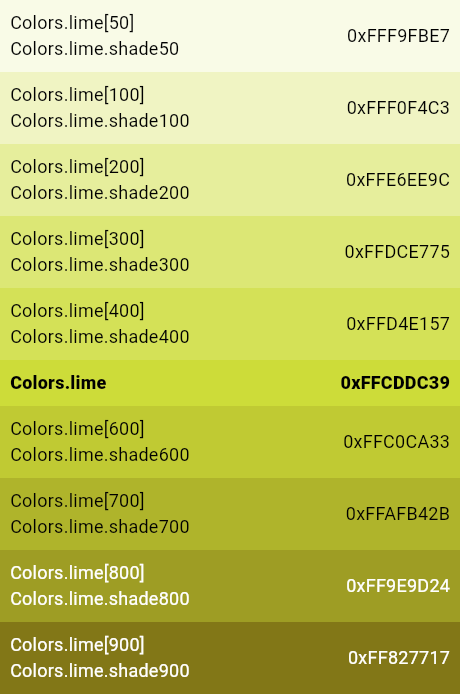
/// 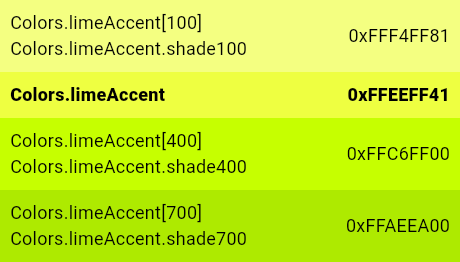
///
/// 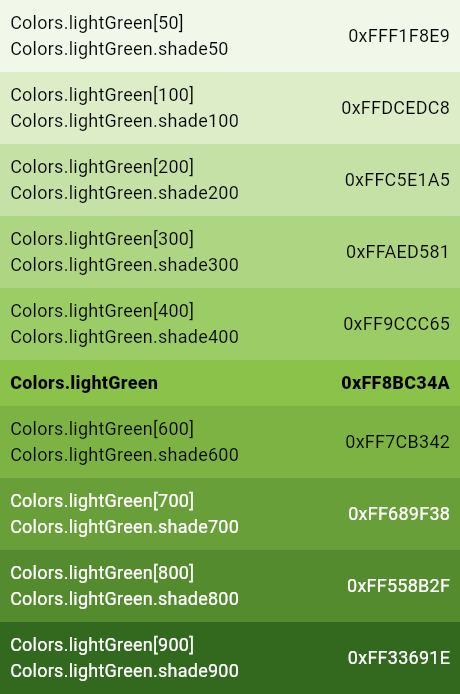
/// 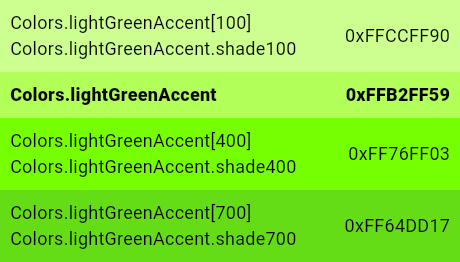
///
/// 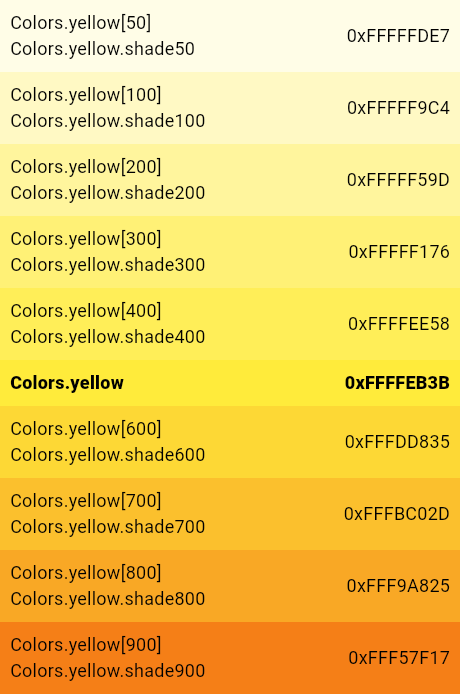
/// 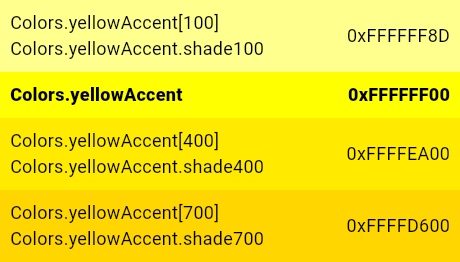
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.limeAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [lime], the corresponding primary colors.
/// * [lightGreenAccent] and [yellowAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor limeAccent = MaterialAccentColor(
_limeAccentPrimaryValue,
<int, Color>{
100: Color(0xFFF4FF81),
200: Color(_limeAccentPrimaryValue),
400: Color(0xFFC6FF00),
700: Color(0xFFAEEA00),
},
);
static const int _limeAccentPrimaryValue = 0xFFEEFF41;
/// The yellow primary color and swatch.
///
/// 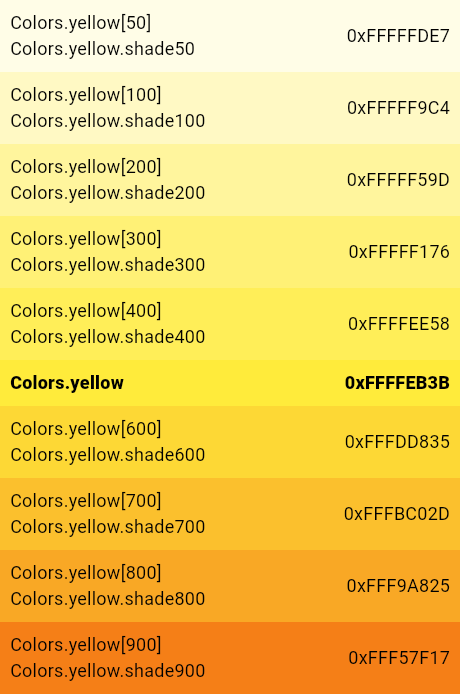
/// 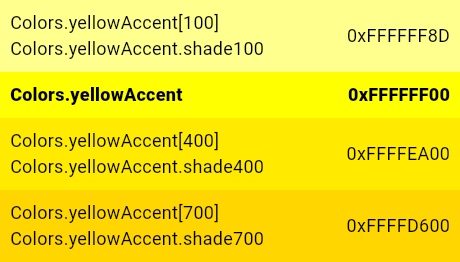
///
/// 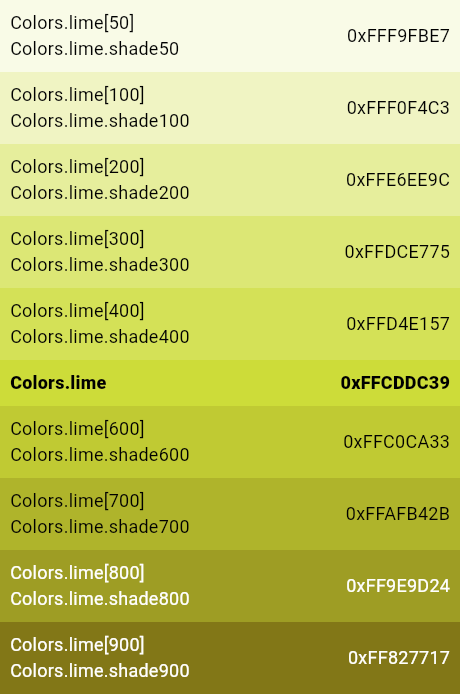
/// 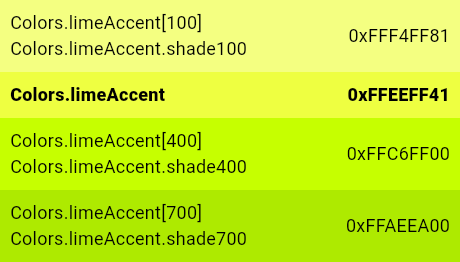
///
/// 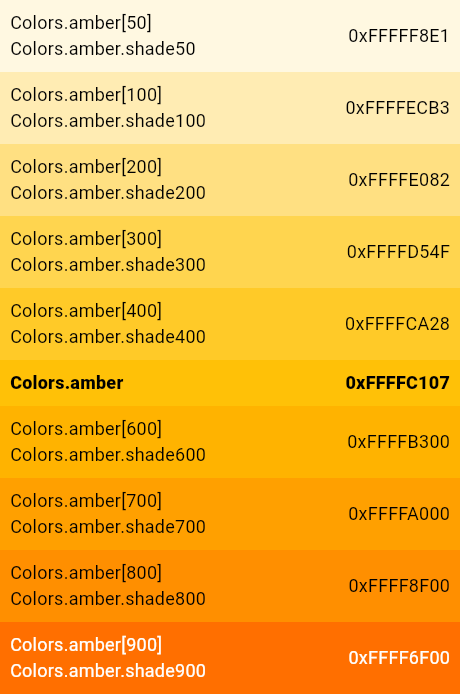
/// 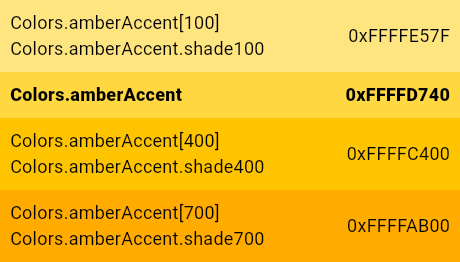
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.yellow[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [yellowAccent], the corresponding accent colors.
/// * [lime] and [amber], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor yellow = MaterialColor(
_yellowPrimaryValue,
<int, Color>{
50: Color(0xFFFFFDE7),
100: Color(0xFFFFF9C4),
200: Color(0xFFFFF59D),
300: Color(0xFFFFF176),
400: Color(0xFFFFEE58),
500: Color(_yellowPrimaryValue),
600: Color(0xFFFDD835),
700: Color(0xFFFBC02D),
800: Color(0xFFF9A825),
900: Color(0xFFF57F17),
},
);
static const int _yellowPrimaryValue = 0xFFFFEB3B;
/// The yellow accent color and swatch.
///
/// 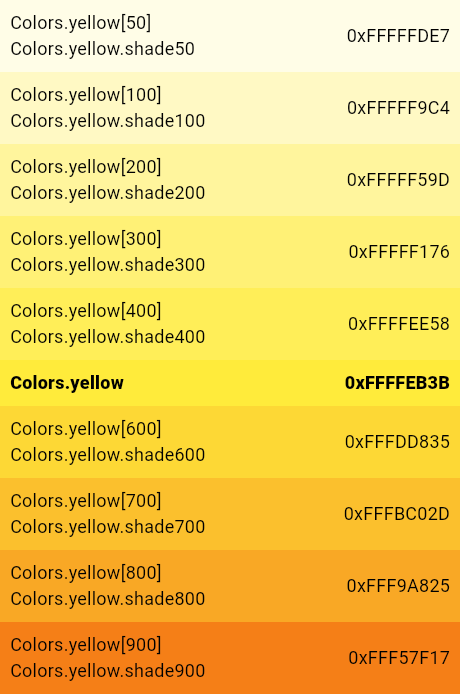
/// 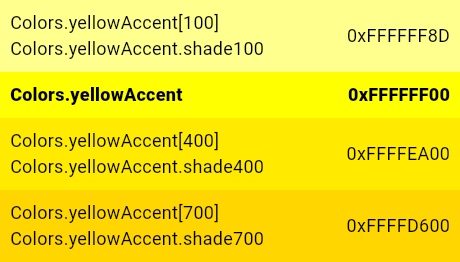
///
/// 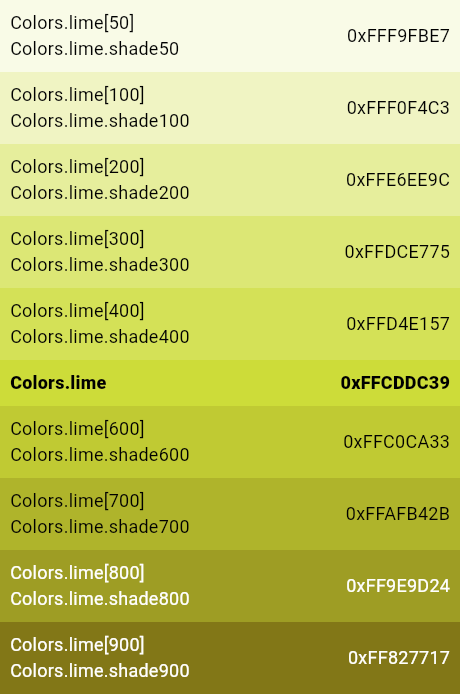
/// 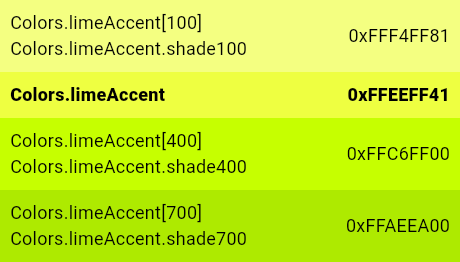
///
/// 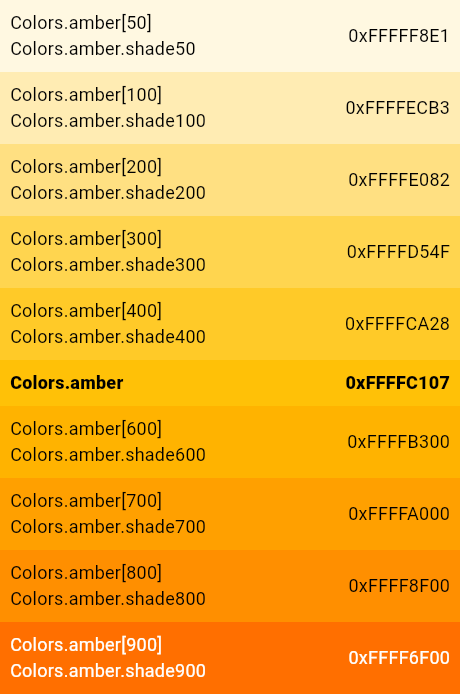
/// 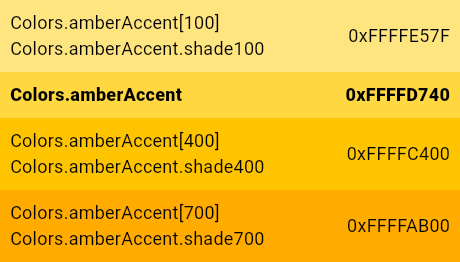
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.yellowAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [yellow], the corresponding primary colors.
/// * [limeAccent] and [amberAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor yellowAccent = MaterialAccentColor(
_yellowAccentPrimaryValue,
<int, Color>{
100: Color(0xFFFFFF8D),
200: Color(_yellowAccentPrimaryValue),
400: Color(0xFFFFEA00),
700: Color(0xFFFFD600),
},
);
static const int _yellowAccentPrimaryValue = 0xFFFFFF00;
/// The amber primary color and swatch.
///
/// 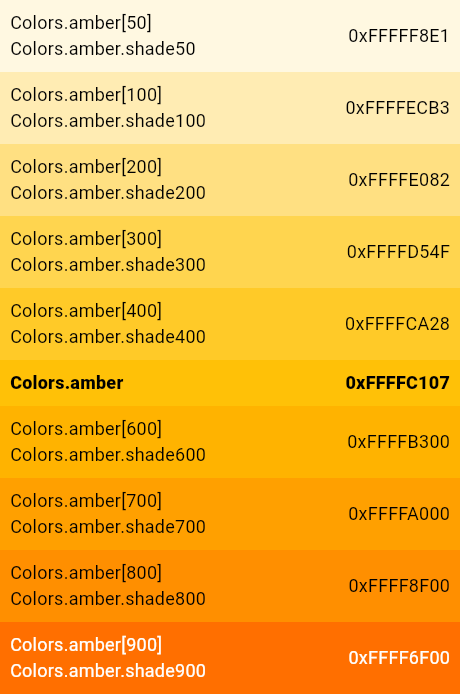
/// 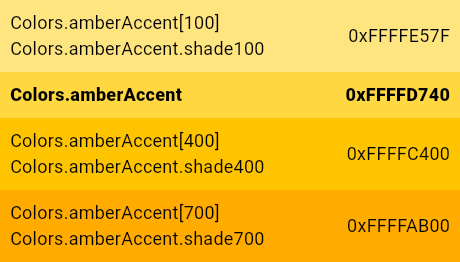
///
/// 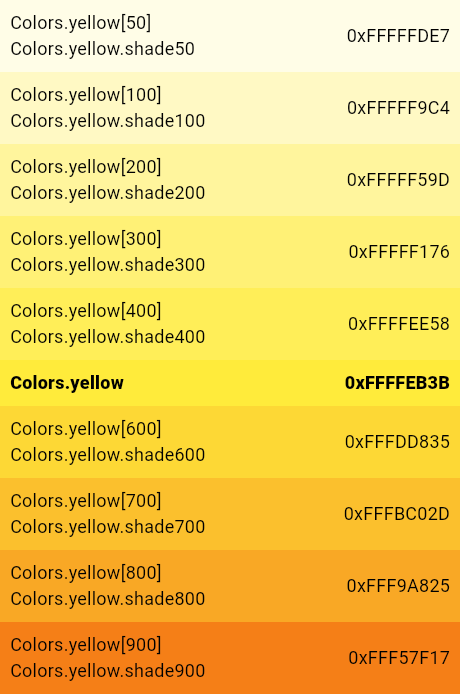
/// 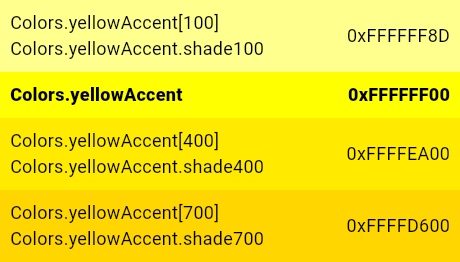
///
/// 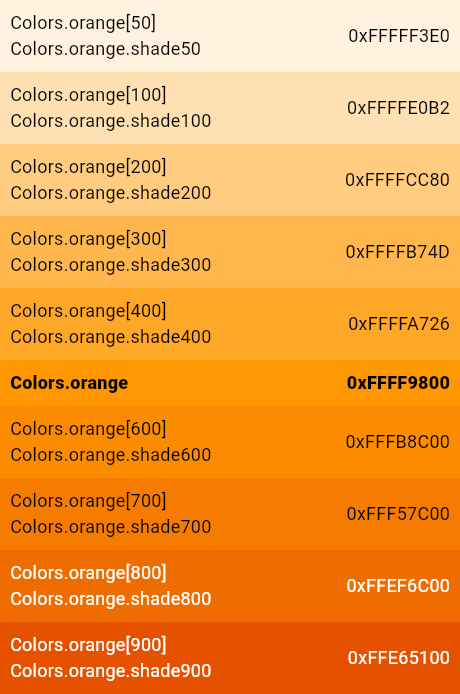
/// 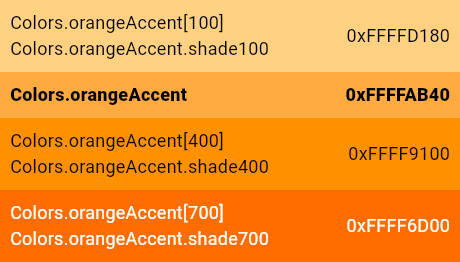
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.amber[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [amberAccent], the corresponding accent colors.
/// * [yellow] and [orange], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor amber = MaterialColor(
_amberPrimaryValue,
<int, Color>{
50: Color(0xFFFFF8E1),
100: Color(0xFFFFECB3),
200: Color(0xFFFFE082),
300: Color(0xFFFFD54F),
400: Color(0xFFFFCA28),
500: Color(_amberPrimaryValue),
600: Color(0xFFFFB300),
700: Color(0xFFFFA000),
800: Color(0xFFFF8F00),
900: Color(0xFFFF6F00),
},
);
static const int _amberPrimaryValue = 0xFFFFC107;
/// The amber accent color and swatch.
///
/// 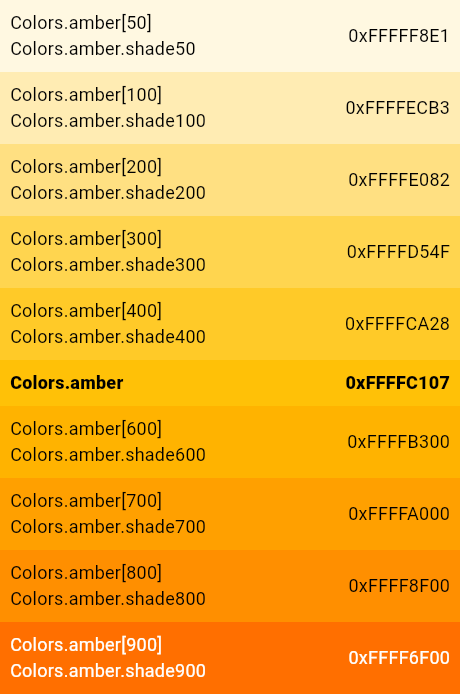
/// 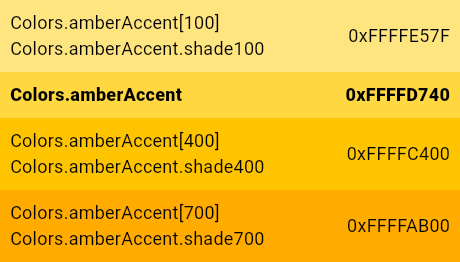
///
/// 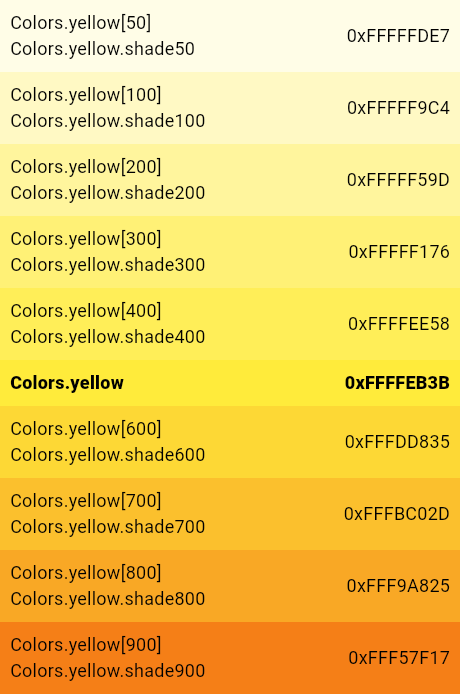
/// 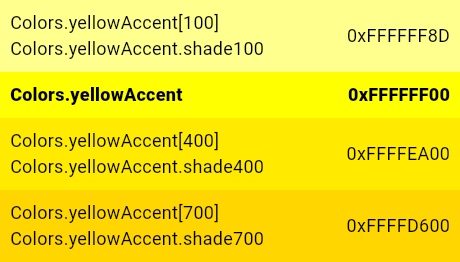
///
/// 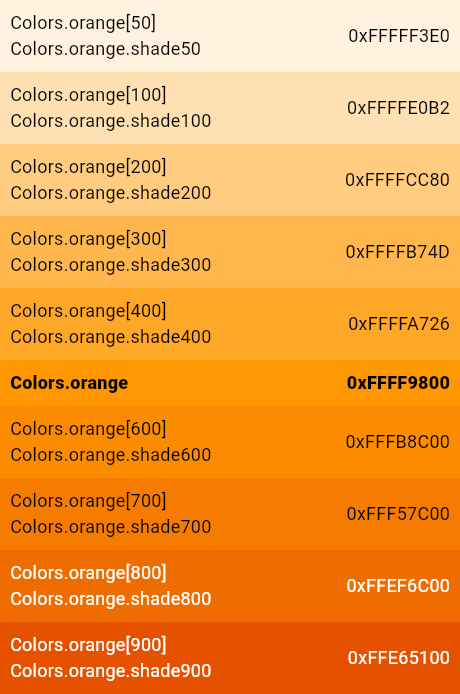
/// 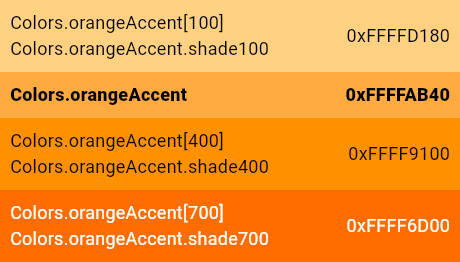
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.amberAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [amber], the corresponding primary colors.
/// * [yellowAccent] and [orangeAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor amberAccent = MaterialAccentColor(
_amberAccentPrimaryValue,
<int, Color>{
100: Color(0xFFFFE57F),
200: Color(_amberAccentPrimaryValue),
400: Color(0xFFFFC400),
700: Color(0xFFFFAB00),
},
);
static const int _amberAccentPrimaryValue = 0xFFFFD740;
/// The orange primary color and swatch.
///
/// 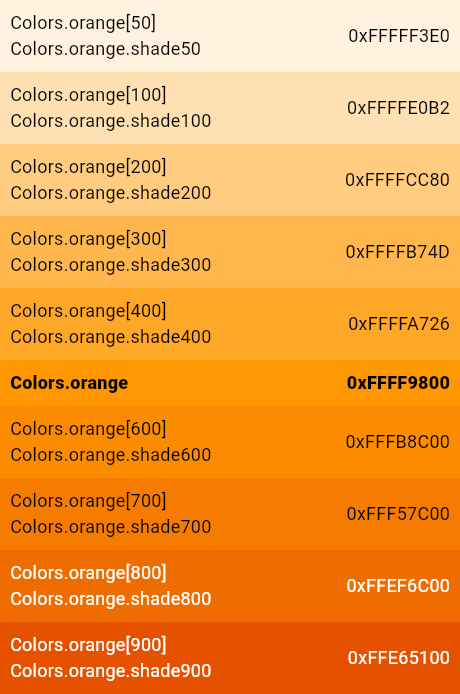
/// 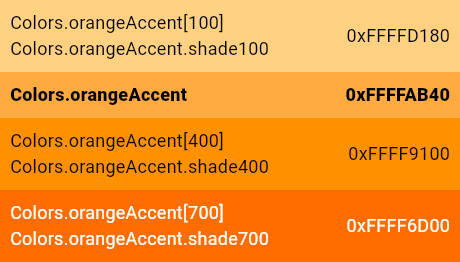
///
/// 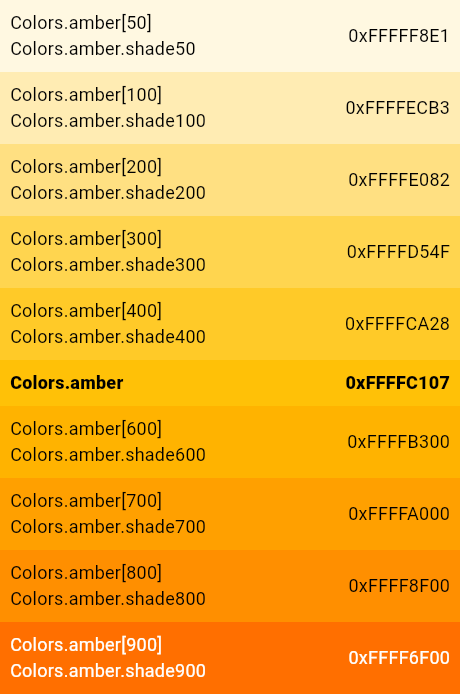
/// 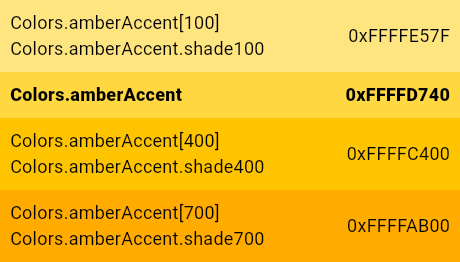
///
/// 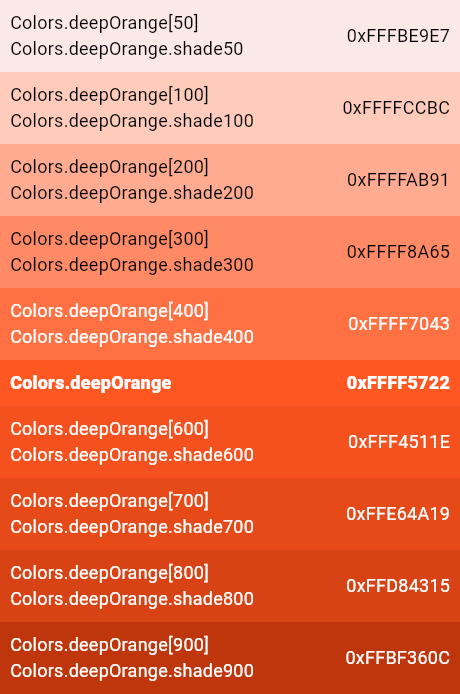
/// 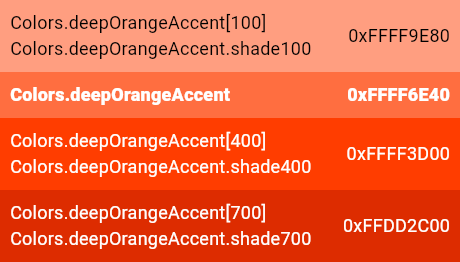
///
/// 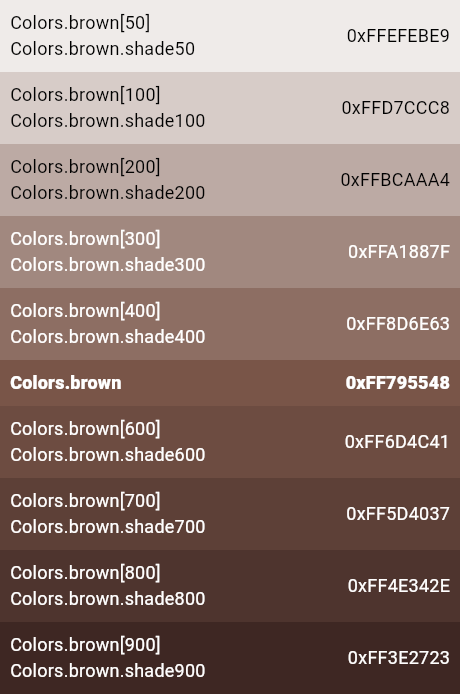
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.orange[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [orangeAccent], the corresponding accent colors.
/// * [amber], [deepOrange], and [brown], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor orange = MaterialColor(
_orangePrimaryValue,
<int, Color>{
50: Color(0xFFFFF3E0),
100: Color(0xFFFFE0B2),
200: Color(0xFFFFCC80),
300: Color(0xFFFFB74D),
400: Color(0xFFFFA726),
500: Color(_orangePrimaryValue),
600: Color(0xFFFB8C00),
700: Color(0xFFF57C00),
800: Color(0xFFEF6C00),
900: Color(0xFFE65100),
},
);
static const int _orangePrimaryValue = 0xFFFF9800;
/// The orange accent color and swatch.
///
/// 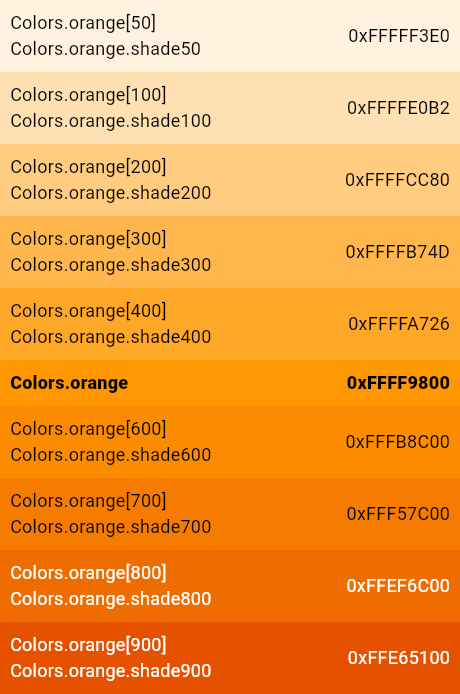
/// 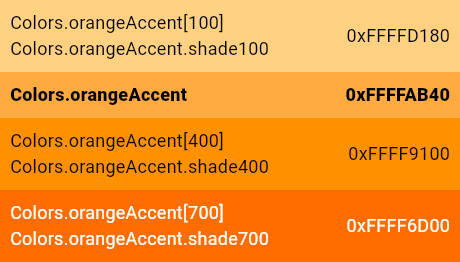
///
/// 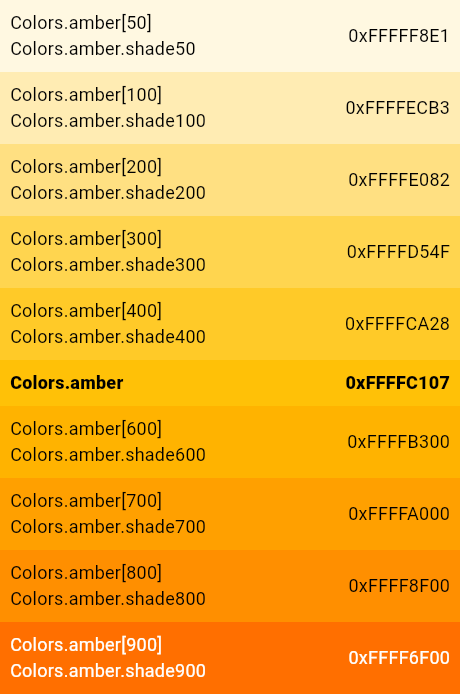
/// 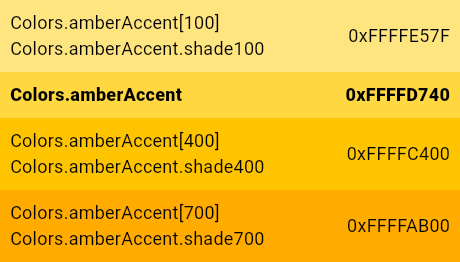
///
/// 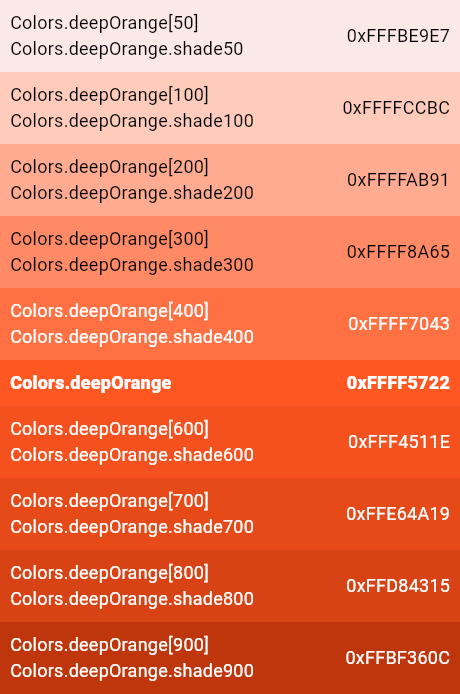
/// 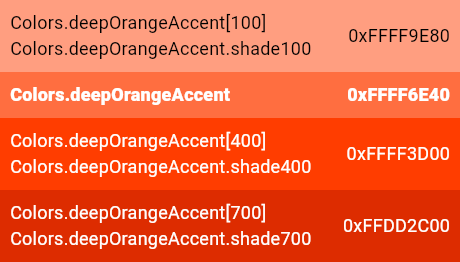
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.orangeAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [orange], the corresponding primary colors.
/// * [amberAccent] and [deepOrangeAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor orangeAccent = MaterialAccentColor(
_orangeAccentPrimaryValue,
<int, Color>{
100: Color(0xFFFFD180),
200: Color(_orangeAccentPrimaryValue),
400: Color(0xFFFF9100),
700: Color(0xFFFF6D00),
},
);
static const int _orangeAccentPrimaryValue = 0xFFFFAB40;
/// The deep orange primary color and swatch.
///
/// 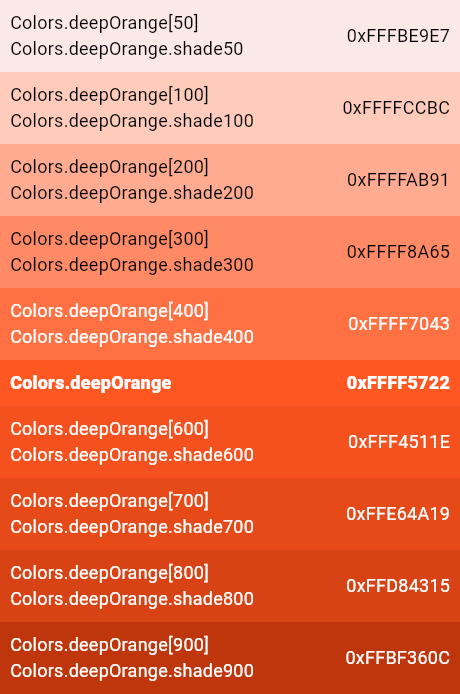
/// 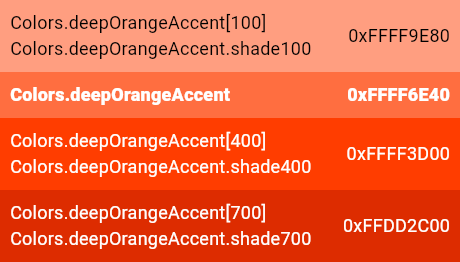
///
/// 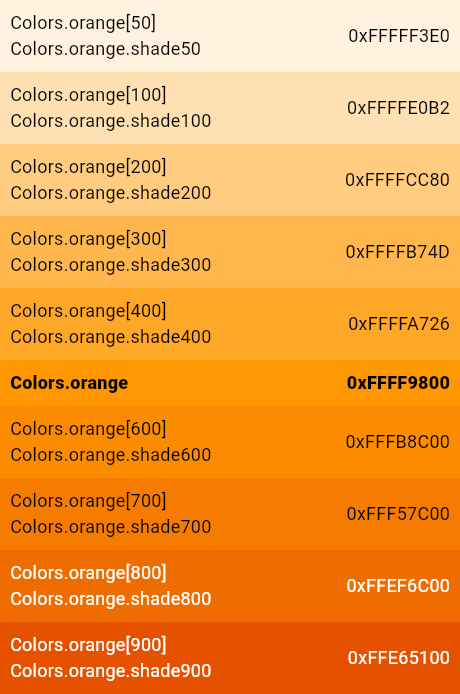
/// 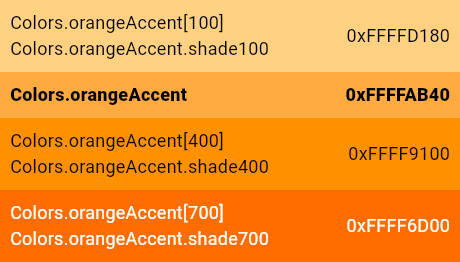
///
/// 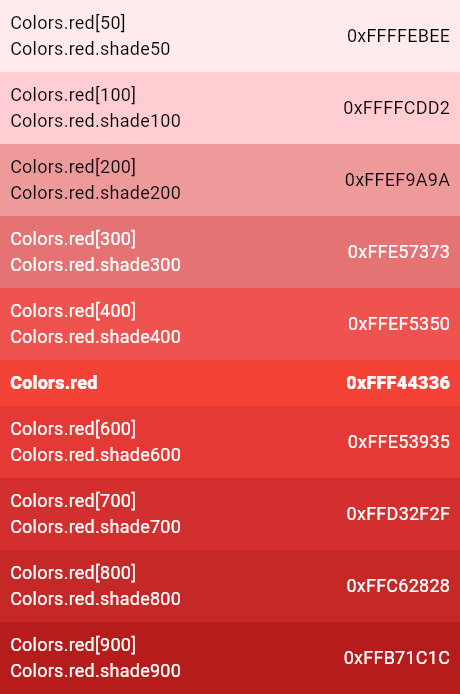
/// 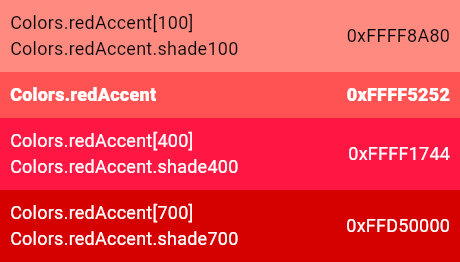
///
/// 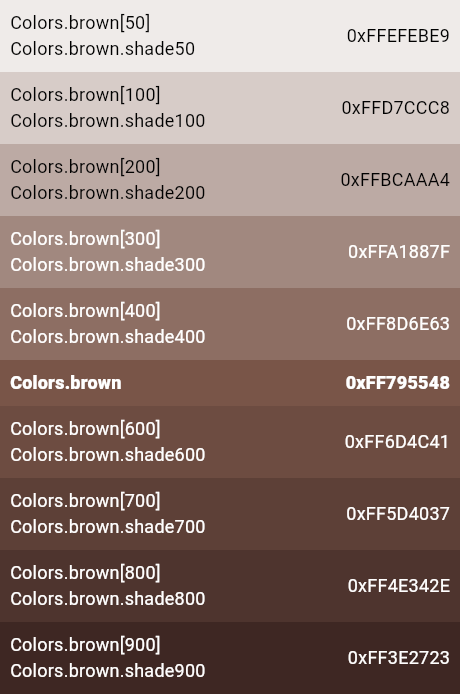
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.deepOrange[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [deepOrangeAccent], the corresponding accent colors.
/// * [orange], [red], and [brown], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor deepOrange = MaterialColor(
_deepOrangePrimaryValue,
<int, Color>{
50: Color(0xFFFBE9E7),
100: Color(0xFFFFCCBC),
200: Color(0xFFFFAB91),
300: Color(0xFFFF8A65),
400: Color(0xFFFF7043),
500: Color(_deepOrangePrimaryValue),
600: Color(0xFFF4511E),
700: Color(0xFFE64A19),
800: Color(0xFFD84315),
900: Color(0xFFBF360C),
},
);
static const int _deepOrangePrimaryValue = 0xFFFF5722;
/// The deep orange accent color and swatch.
///
/// 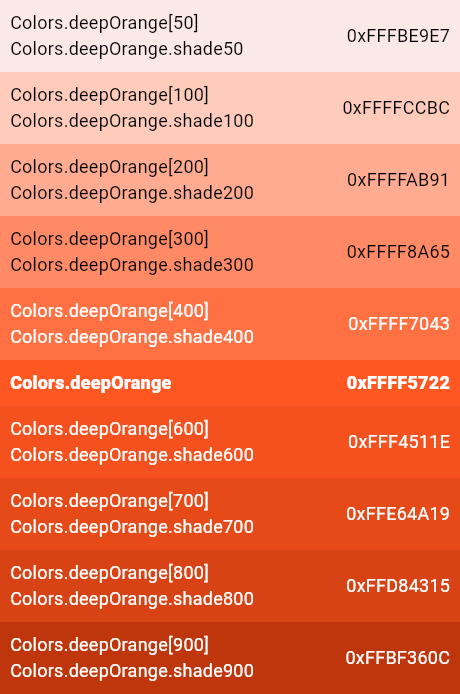
/// 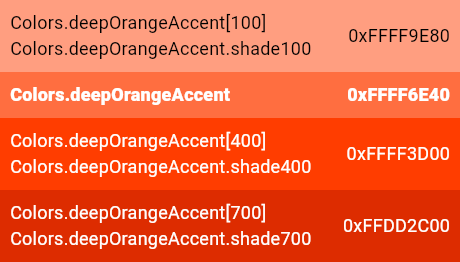
///
/// 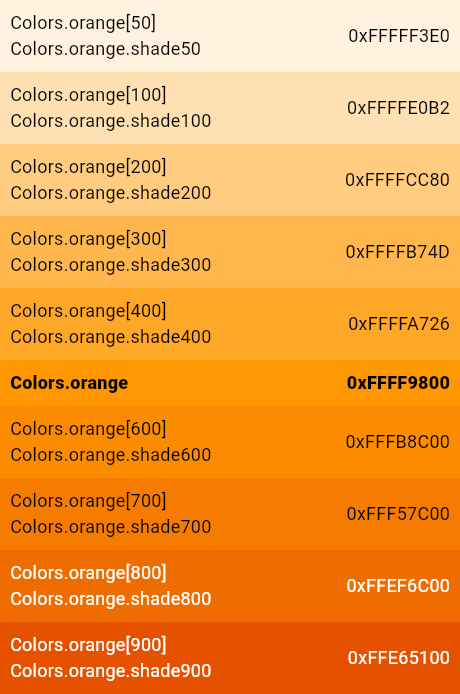
/// 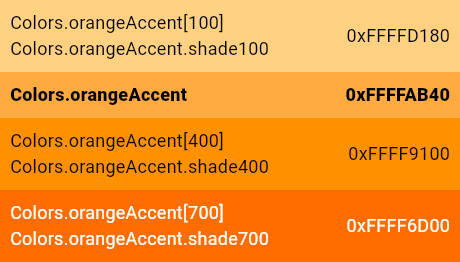
///
/// 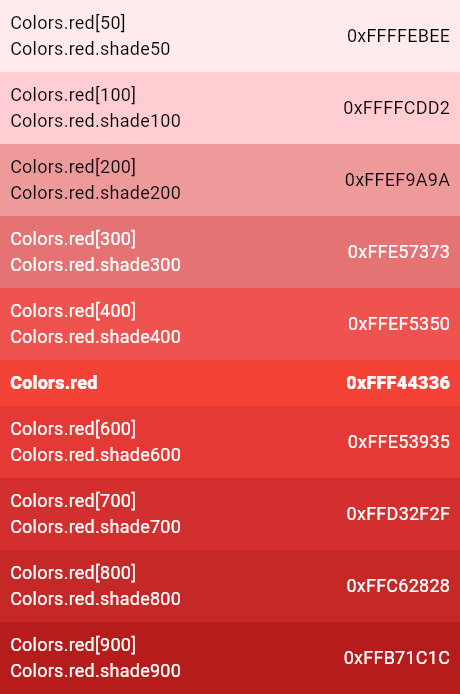
/// 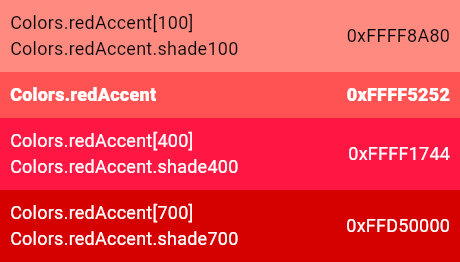
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.deepOrangeAccent[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [deepOrange], the corresponding primary colors.
/// * [orangeAccent] [redAccent], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialAccentColor deepOrangeAccent = MaterialAccentColor(
_deepOrangeAccentPrimaryValue,
<int, Color>{
100: Color(0xFFFF9E80),
200: Color(_deepOrangeAccentPrimaryValue),
400: Color(0xFFFF3D00),
700: Color(0xFFDD2C00),
},
);
static const int _deepOrangeAccentPrimaryValue = 0xFFFF6E40;
/// The brown primary color and swatch.
///
/// 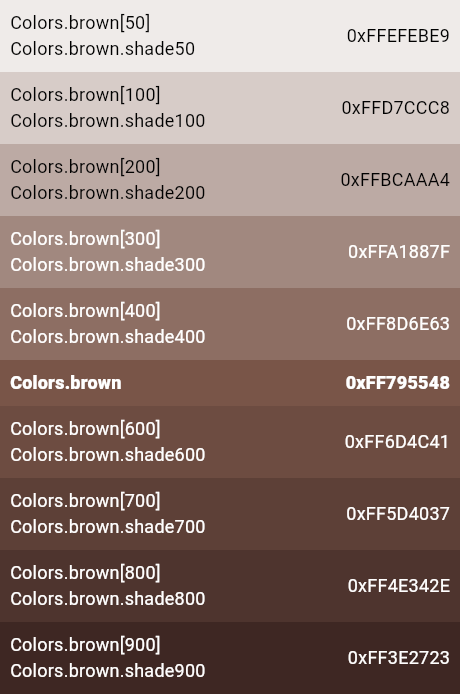
///
/// 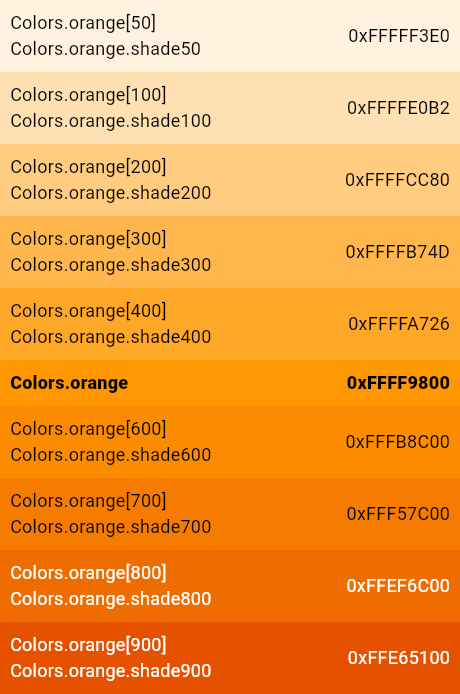
///
/// 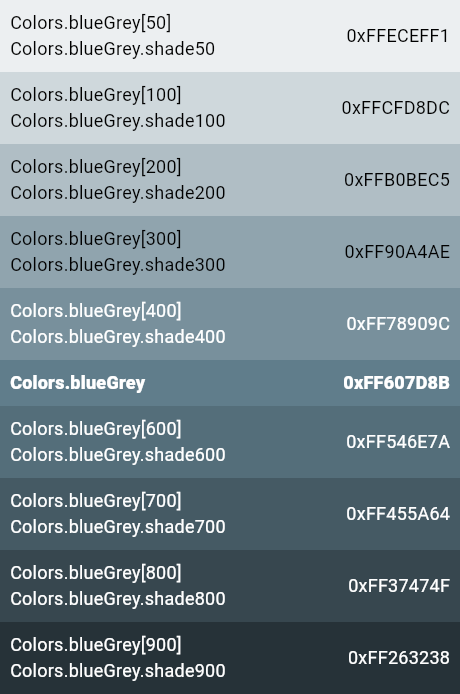
///
/// This swatch has no corresponding accent color and swatch.
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.brown[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [orange] and [blueGrey], vaguely similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor brown = MaterialColor(
_brownPrimaryValue,
<int, Color>{
50: Color(0xFFEFEBE9),
100: Color(0xFFD7CCC8),
200: Color(0xFFBCAAA4),
300: Color(0xFFA1887F),
400: Color(0xFF8D6E63),
500: Color(_brownPrimaryValue),
600: Color(0xFF6D4C41),
700: Color(0xFF5D4037),
800: Color(0xFF4E342E),
900: Color(0xFF3E2723),
},
);
static const int _brownPrimaryValue = 0xFF795548;
/// The grey primary color and swatch.
///
/// 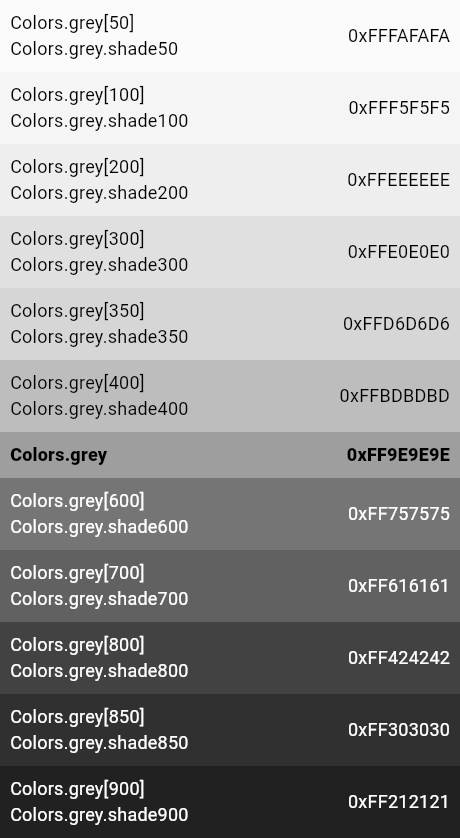
///
/// 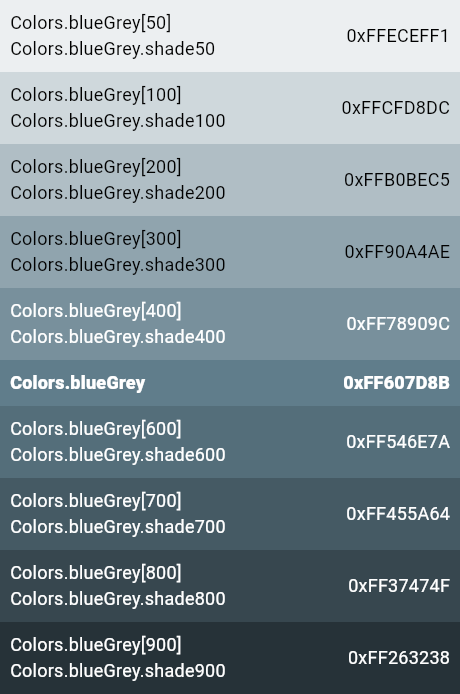
///
/// 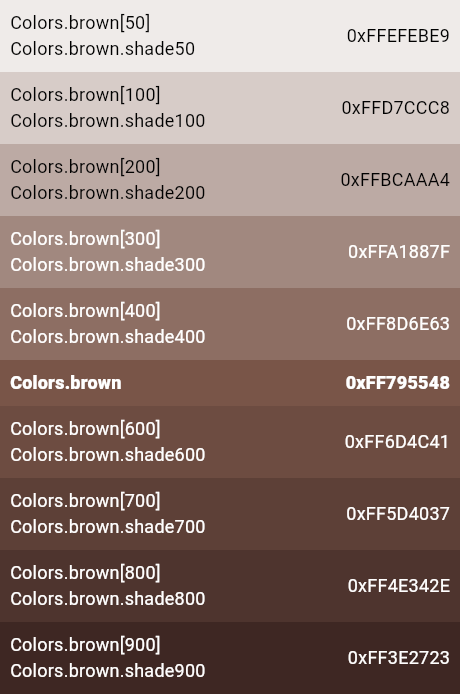
///
/// This swatch has no corresponding accent swatch.
///
/// This swatch, in addition to the values 50 and 100 to 900 in 100
/// increments, also features the special values 350 and 850. The 350 value is
/// used for raised button while pressed in light themes, and 850 is used for
/// the background color of the dark theme. See [ThemeData.brightness].
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.grey[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [blueGrey] and [brown], somewhat similar colors.
/// * [black], [black87], [black54], [black45], [black38], [black26], [black12], which
/// provide a different approach to showing shades of grey.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor grey = MaterialColor(
_greyPrimaryValue,
<int, Color>{
50: Color(0xFFFAFAFA),
100: Color(0xFFF5F5F5),
200: Color(0xFFEEEEEE),
300: Color(0xFFE0E0E0),
350: Color(0xFFD6D6D6), // only for raised button while pressed in light theme
400: Color(0xFFBDBDBD),
500: Color(_greyPrimaryValue),
600: Color(0xFF757575),
700: Color(0xFF616161),
800: Color(0xFF424242),
850: Color(0xFF303030), // only for background color in dark theme
900: Color(0xFF212121),
},
);
static const int _greyPrimaryValue = 0xFF9E9E9E;
/// The blue-grey primary color and swatch.
///
/// 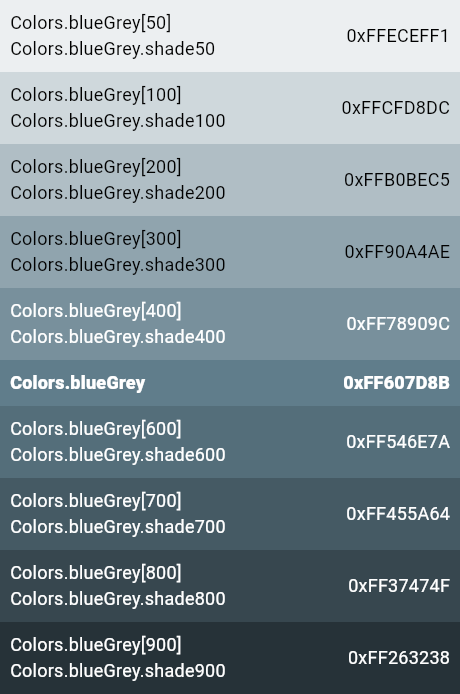
///
/// 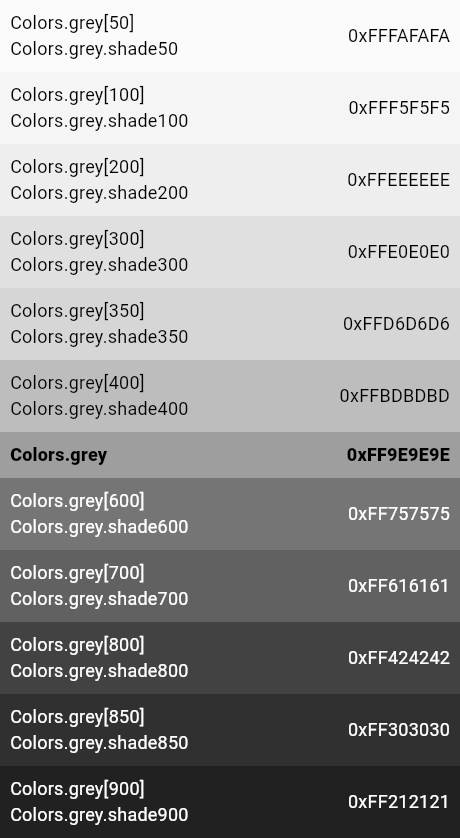
///
/// 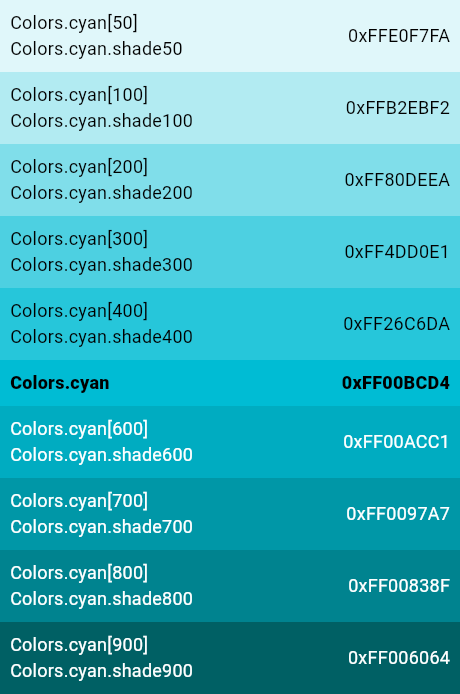
///
/// 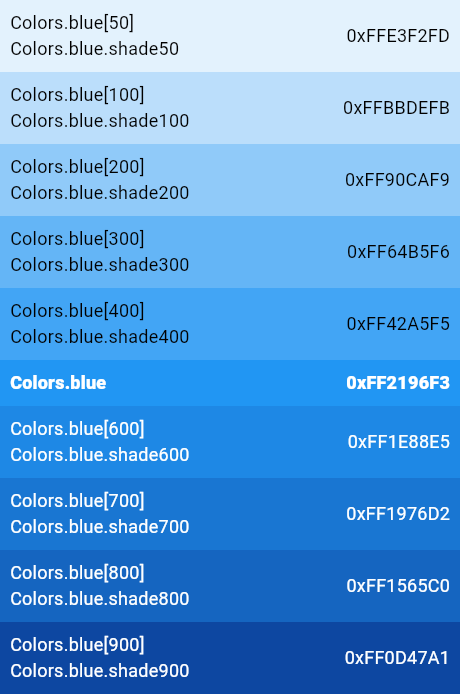
///
/// This swatch has no corresponding accent swatch.
///
/// {@tool snippet}
///
/// ```dart
/// Icon(
/// Icons.widgets,
/// color: Colors.blueGrey[400],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [grey], [cyan], and [blue], similar colors.
/// * [Theme.of], which allows you to select colors from the current theme
/// rather than hard-coding colors in your build methods.
static const MaterialColor blueGrey = MaterialColor(
_blueGreyPrimaryValue,
<int, Color>{
50: Color(0xFFECEFF1),
100: Color(0xFFCFD8DC),
200: Color(0xFFB0BEC5),
300: Color(0xFF90A4AE),
400: Color(0xFF78909C),
500: Color(_blueGreyPrimaryValue),
600: Color(0xFF546E7A),
700: Color(0xFF455A64),
800: Color(0xFF37474F),
900: Color(0xFF263238),
},
);
static const int _blueGreyPrimaryValue = 0xFF607D8B;
/// The Material Design primary color swatches, excluding grey.
static const List<MaterialColor> primaries = <MaterialColor>[
red,
pink,
purple,
deepPurple,
indigo,
blue,
lightBlue,
cyan,
teal,
green,
lightGreen,
lime,
yellow,
amber,
orange,
deepOrange,
brown,
// The grey swatch is intentionally omitted because when picking a color
// randomly from this list to colorize an application, picking grey suddenly
// makes the app look disabled.
blueGrey,
];
/// The Material Design accent color swatches.
static const List<MaterialAccentColor> accents = <MaterialAccentColor>[
redAccent,
pinkAccent,
purpleAccent,
deepPurpleAccent,
indigoAccent,
blueAccent,
lightBlueAccent,
cyanAccent,
tealAccent,
greenAccent,
lightGreenAccent,
limeAccent,
yellowAccent,
amberAccent,
orangeAccent,
deepOrangeAccent,
];
}
| flutter/packages/flutter/lib/src/material/colors.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/colors.dart",
"repo_id": "flutter",
"token_count": 26974
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines the visual properties of [Divider], [VerticalDivider], dividers
/// between [ListTile]s, and dividers between rows in [DataTable]s.
///
/// Descendant widgets obtain the current [DividerThemeData] object using
/// `DividerTheme.of(context)`. Instances of [DividerThemeData]
/// can be customized with [DividerThemeData.copyWith].
///
/// Typically a [DividerThemeData] is specified as part of the overall
/// [Theme] with [ThemeData.dividerTheme].
///
/// All [DividerThemeData] properties are `null` by default. When null,
/// the widgets will provide their own defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class DividerThemeData with Diagnosticable {
/// Creates a theme that can be used for [DividerTheme] or
/// [ThemeData.dividerTheme].
const DividerThemeData({
this.color,
this.space,
this.thickness,
this.indent,
this.endIndent,
});
/// The color of [Divider]s and [VerticalDivider]s, also
/// used between [ListTile]s, between rows in [DataTable]s, and so forth.
final Color? color;
/// The [Divider]'s height or the [VerticalDivider]'s width.
///
/// This represents the amount of horizontal or vertical space the divider
/// takes up.
final double? space;
/// The thickness of the line drawn within the divider.
final double? thickness;
/// The amount of empty space at the leading edge of [Divider] or top edge of
/// [VerticalDivider].
final double? indent;
/// The amount of empty space at the trailing edge of [Divider] or bottom edge
/// of [VerticalDivider].
final double? endIndent;
/// Creates a copy of this object with the given fields replaced with the
/// new values.
DividerThemeData copyWith({
Color? color,
double? space,
double? thickness,
double? indent,
double? endIndent,
}) {
return DividerThemeData(
color: color ?? this.color,
space: space ?? this.space,
thickness: thickness ?? this.thickness,
indent: indent ?? this.indent,
endIndent: endIndent ?? this.endIndent,
);
}
/// Linearly interpolate between two Divider themes.
///
/// {@macro dart.ui.shadow.lerp}
static DividerThemeData lerp(DividerThemeData? a, DividerThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return DividerThemeData(
color: Color.lerp(a?.color, b?.color, t),
space: lerpDouble(a?.space, b?.space, t),
thickness: lerpDouble(a?.thickness, b?.thickness, t),
indent: lerpDouble(a?.indent, b?.indent, t),
endIndent: lerpDouble(a?.endIndent, b?.endIndent, t),
);
}
@override
int get hashCode => Object.hash(
color,
space,
thickness,
indent,
endIndent,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is DividerThemeData
&& other.color == color
&& other.space == space
&& other.thickness == thickness
&& other.indent == indent
&& other.endIndent == endIndent;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(DoubleProperty('space', space, defaultValue: null));
properties.add(DoubleProperty('thickness', thickness, defaultValue: null));
properties.add(DoubleProperty('indent', indent, defaultValue: null));
properties.add(DoubleProperty('endIndent', endIndent, defaultValue: null));
}
}
/// An inherited widget that defines the configuration for
/// [Divider]s, [VerticalDivider]s, dividers between [ListTile]s, and dividers
/// between rows in [DataTable]s in this widget's subtree.
class DividerTheme extends InheritedTheme {
/// Creates a divider theme that controls the configurations for
/// [Divider]s, [VerticalDivider]s, dividers between [ListTile]s, and dividers
/// between rows in [DataTable]s in its widget subtree.
const DividerTheme({
super.key,
required this.data,
required super.child,
});
/// The properties for descendant [Divider]s, [VerticalDivider]s, dividers
/// between [ListTile]s, and dividers between rows in [DataTable]s.
final DividerThemeData data;
/// The closest instance of this class's [data] value that encloses the given
/// context.
///
/// If there is no ancestor, it returns [ThemeData.dividerTheme]. Applications
/// can assume that the returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// DividerThemeData theme = DividerTheme.of(context);
/// ```
static DividerThemeData of(BuildContext context) {
final DividerTheme? dividerTheme = context.dependOnInheritedWidgetOfExactType<DividerTheme>();
return dividerTheme?.data ?? Theme.of(context).dividerTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return DividerTheme(data: data, child: child);
}
@override
bool updateShouldNotify(DividerTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/divider_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/divider_theme.dart",
"repo_id": "flutter",
"token_count": 1848
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// A [ButtonStyle] that overrides the default appearance of
/// [FilledButton]s when it's used with [FilledButtonTheme] or with the
/// overall [Theme]'s [ThemeData.filledButtonTheme].
///
/// The [style]'s properties override [FilledButton]'s default style,
/// i.e. the [ButtonStyle] returned by [FilledButton.defaultStyleOf]. Only
/// the style's non-null property values or resolved non-null
/// [MaterialStateProperty] values are used.
///
/// See also:
///
/// * [FilledButtonTheme], the theme which is configured with this class.
/// * [FilledButton.defaultStyleOf], which returns the default [ButtonStyle]
/// for text buttons.
/// * [FilledButton.styleFrom], which converts simple values into a
/// [ButtonStyle] that's consistent with [FilledButton]'s defaults.
/// * [MaterialStateProperty.resolve], "resolve" a material state property
/// to a simple value based on a set of [MaterialState]s.
/// * [ThemeData.filledButtonTheme], which can be used to override the default
/// [ButtonStyle] for [FilledButton]s below the overall [Theme].
@immutable
class FilledButtonThemeData with Diagnosticable {
/// Creates an [FilledButtonThemeData].
///
/// The [style] may be null.
const FilledButtonThemeData({ this.style });
/// Overrides for [FilledButton]'s default style.
///
/// Non-null properties or non-null resolved [MaterialStateProperty]
/// values override the [ButtonStyle] returned by
/// [FilledButton.defaultStyleOf].
///
/// If [style] is null, then this theme doesn't override anything.
final ButtonStyle? style;
/// Linearly interpolate between two filled button themes.
static FilledButtonThemeData? lerp(FilledButtonThemeData? a, FilledButtonThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return FilledButtonThemeData(
style: ButtonStyle.lerp(a?.style, b?.style, t),
);
}
@override
int get hashCode => style.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is FilledButtonThemeData && other.style == style;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ButtonStyle>('style', style, defaultValue: null));
}
}
/// Overrides the default [ButtonStyle] of its [FilledButton] descendants.
///
/// See also:
///
/// * [FilledButtonThemeData], which is used to configure this theme.
/// * [FilledButton.defaultStyleOf], which returns the default [ButtonStyle]
/// for filled buttons.
/// * [FilledButton.styleFrom], which converts simple values into a
/// [ButtonStyle] that's consistent with [FilledButton]'s defaults.
/// * [ThemeData.filledButtonTheme], which can be used to override the default
/// [ButtonStyle] for [FilledButton]s below the overall [Theme].
class FilledButtonTheme extends InheritedTheme {
/// Create a [FilledButtonTheme].
const FilledButtonTheme({
super.key,
required this.data,
required super.child,
});
/// The configuration of this theme.
final FilledButtonThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [FilledButtonTheme] widget, then
/// [ThemeData.filledButtonTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// FilledButtonThemeData theme = FilledButtonTheme.of(context);
/// ```
static FilledButtonThemeData of(BuildContext context) {
final FilledButtonTheme? buttonTheme = context.dependOnInheritedWidgetOfExactType<FilledButtonTheme>();
return buttonTheme?.data ?? Theme.of(context).filledButtonTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return FilledButtonTheme(data: data, child: child);
}
@override
bool updateShouldNotify(FilledButtonTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/filled_button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/filled_button_theme.dart",
"repo_id": "flutter",
"token_count": 1320
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/widgets.dart';
import 'ink_well.dart';
import 'material.dart';
const Duration _kUnconfirmedSplashDuration = Duration(seconds: 1);
const Duration _kSplashFadeDuration = Duration(milliseconds: 200);
const double _kSplashInitialSize = 0.0; // logical pixels
const double _kSplashConfirmedVelocity = 1.0; // logical pixels per millisecond
RectCallback? _getClipCallback(RenderBox referenceBox, bool containedInkWell, RectCallback? rectCallback) {
if (rectCallback != null) {
assert(containedInkWell);
return rectCallback;
}
if (containedInkWell) {
return () => Offset.zero & referenceBox.size;
}
return null;
}
double _getTargetRadius(RenderBox referenceBox, bool containedInkWell, RectCallback? rectCallback, Offset position) {
if (containedInkWell) {
final Size size = rectCallback != null ? rectCallback().size : referenceBox.size;
return _getSplashRadiusForPositionInSize(size, position);
}
return Material.defaultSplashRadius;
}
double _getSplashRadiusForPositionInSize(Size bounds, Offset position) {
final double d1 = (position - bounds.topLeft(Offset.zero)).distance;
final double d2 = (position - bounds.topRight(Offset.zero)).distance;
final double d3 = (position - bounds.bottomLeft(Offset.zero)).distance;
final double d4 = (position - bounds.bottomRight(Offset.zero)).distance;
return math.max(math.max(d1, d2), math.max(d3, d4)).ceilToDouble();
}
class _InkSplashFactory extends InteractiveInkFeatureFactory {
const _InkSplashFactory();
@override
InteractiveInkFeature create({
required MaterialInkController controller,
required RenderBox referenceBox,
required Offset position,
required Color color,
required TextDirection textDirection,
bool containedInkWell = false,
RectCallback? rectCallback,
BorderRadius? borderRadius,
ShapeBorder? customBorder,
double? radius,
VoidCallback? onRemoved,
}) {
return InkSplash(
controller: controller,
referenceBox: referenceBox,
position: position,
color: color,
containedInkWell: containedInkWell,
rectCallback: rectCallback,
borderRadius: borderRadius,
customBorder: customBorder,
radius: radius,
onRemoved: onRemoved,
textDirection: textDirection,
);
}
}
/// A visual reaction on a piece of [Material] to user input.
///
/// A circular ink feature whose origin starts at the input touch point
/// and whose radius expands from zero.
///
/// This object is rarely created directly. Instead of creating an ink splash
/// directly, consider using an [InkResponse] or [InkWell] widget, which uses
/// gestures (such as tap and long-press) to trigger ink splashes.
///
/// See also:
///
/// * [InkRipple], which is an ink splash feature that expands more
/// aggressively than this class does.
/// * [InkResponse], which uses gestures to trigger ink highlights and ink
/// splashes in the parent [Material].
/// * [InkWell], which is a rectangular [InkResponse] (the most common type of
/// ink response).
/// * [Material], which is the widget on which the ink splash is painted.
/// * [InkHighlight], which is an ink feature that emphasizes a part of a
/// [Material].
/// * [Ink], a convenience widget for drawing images and other decorations on
/// Material widgets.
class InkSplash extends InteractiveInkFeature {
/// Begin a splash, centered at position relative to [referenceBox].
///
/// The [controller] argument is typically obtained via
/// `Material.of(context)`.
///
/// If `containedInkWell` is true, then the splash will be sized to fit
/// the well rectangle, then clipped to it when drawn. The well
/// rectangle is the box returned by `rectCallback`, if provided, or
/// otherwise is the bounds of the [referenceBox].
///
/// If `containedInkWell` is false, then `rectCallback` should be null.
/// The ink splash is clipped only to the edges of the [Material].
/// This is the default.
///
/// When the splash is removed, `onRemoved` will be called.
InkSplash({
required MaterialInkController controller,
required super.referenceBox,
required TextDirection textDirection,
Offset? position,
required Color color,
bool containedInkWell = false,
RectCallback? rectCallback,
BorderRadius? borderRadius,
super.customBorder,
double? radius,
super.onRemoved,
}) : _position = position,
_borderRadius = borderRadius ?? BorderRadius.zero,
_targetRadius = radius ?? _getTargetRadius(referenceBox, containedInkWell, rectCallback, position!),
_clipCallback = _getClipCallback(referenceBox, containedInkWell, rectCallback),
_repositionToReferenceBox = !containedInkWell,
_textDirection = textDirection,
super(controller: controller, color: color) {
_radiusController = AnimationController(duration: _kUnconfirmedSplashDuration, vsync: controller.vsync)
..addListener(controller.markNeedsPaint)
..forward();
_radius = _radiusController.drive(Tween<double>(
begin: _kSplashInitialSize,
end: _targetRadius,
));
_alphaController = AnimationController(duration: _kSplashFadeDuration, vsync: controller.vsync)
..addListener(controller.markNeedsPaint)
..addStatusListener(_handleAlphaStatusChanged);
_alpha = _alphaController!.drive(IntTween(
begin: color.alpha,
end: 0,
));
controller.addInkFeature(this);
}
final Offset? _position;
final BorderRadius _borderRadius;
final double _targetRadius;
final RectCallback? _clipCallback;
final bool _repositionToReferenceBox;
final TextDirection _textDirection;
late Animation<double> _radius;
late AnimationController _radiusController;
late Animation<int> _alpha;
AnimationController? _alphaController;
/// Used to specify this type of ink splash for an [InkWell], [InkResponse],
/// material [Theme], or [ButtonStyle].
static const InteractiveInkFeatureFactory splashFactory = _InkSplashFactory();
@override
void confirm() {
final int duration = (_targetRadius / _kSplashConfirmedVelocity).floor();
_radiusController
..duration = Duration(milliseconds: duration)
..forward();
_alphaController!.forward();
}
@override
void cancel() {
_alphaController?.forward();
}
void _handleAlphaStatusChanged(AnimationStatus status) {
if (status == AnimationStatus.completed) {
dispose();
}
}
@override
void dispose() {
_radiusController.dispose();
_alphaController!.dispose();
_alphaController = null;
super.dispose();
}
@override
void paintFeature(Canvas canvas, Matrix4 transform) {
final Paint paint = Paint()..color = color.withAlpha(_alpha.value);
Offset? center = _position;
if (_repositionToReferenceBox) {
center = Offset.lerp(center, referenceBox.size.center(Offset.zero), _radiusController.value);
}
paintInkCircle(
canvas: canvas,
transform: transform,
paint: paint,
center: center!,
textDirection: _textDirection,
radius: _radius.value,
customBorder: customBorder,
borderRadius: _borderRadius,
clipCallback: _clipCallback,
);
}
}
| flutter/packages/flutter/lib/src/material/ink_splash.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/ink_splash.dart",
"repo_id": "flutter",
"token_count": 2360
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'material_state.dart';
import 'menu_anchor.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// A [ButtonStyle] theme that overrides the default appearance of
/// [SubmenuButton]s and [MenuItemButton]s when it's used with a
/// [MenuButtonTheme] or with the overall [Theme]'s [ThemeData.menuTheme].
///
/// The [style]'s properties override [MenuItemButton]'s and [SubmenuButton]'s
/// default style, i.e. the [ButtonStyle] returned by
/// [MenuItemButton.defaultStyleOf] and [SubmenuButton.defaultStyleOf]. Only the
/// style's non-null property values or resolved non-null
/// [MaterialStateProperty] values are used.
///
/// See also:
///
/// * [MenuButtonTheme], the theme which is configured with this class.
/// * [MenuTheme], the theme used to configure the look of the menus these
/// buttons reside in.
/// * [MenuItemButton.defaultStyleOf] and [SubmenuButton.defaultStyleOf] which
/// return the default [ButtonStyle]s for menu buttons.
/// * [MenuItemButton.styleFrom] and [SubmenuButton.styleFrom], which converts
/// simple values into a [ButtonStyle] that's consistent with their respective
/// defaults.
/// * [MaterialStateProperty.resolve], "resolve" a material state property to a
/// simple value based on a set of [MaterialState]s.
/// * [ThemeData.menuButtonTheme], which can be used to override the default
/// [ButtonStyle] for [MenuItemButton]s and [SubmenuButton]s below the overall
/// [Theme].
/// * [MenuAnchor], a widget which hosts cascading menus.
/// * [MenuBar], a widget which defines a menu bar of buttons hosting cascading
/// menus.
@immutable
class MenuButtonThemeData with Diagnosticable {
/// Creates a [MenuButtonThemeData].
///
/// The [style] may be null.
const MenuButtonThemeData({this.style});
/// Overrides for [SubmenuButton] and [MenuItemButton]'s default style.
///
/// Non-null properties or non-null resolved [MaterialStateProperty] values
/// override the [ButtonStyle] returned by [SubmenuButton.defaultStyleOf] or
/// [MenuItemButton.defaultStyleOf].
///
/// If [style] is null, then this theme doesn't override anything.
final ButtonStyle? style;
/// Linearly interpolate between two menu button themes.
static MenuButtonThemeData? lerp(MenuButtonThemeData? a, MenuButtonThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return MenuButtonThemeData(style: ButtonStyle.lerp(a?.style, b?.style, t));
}
@override
int get hashCode => style.hashCode;
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is MenuButtonThemeData && other.style == style;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ButtonStyle>('style', style, defaultValue: null));
}
}
/// Overrides the default [ButtonStyle] of its [MenuItemButton] and
/// [SubmenuButton] descendants.
///
/// See also:
///
/// * [MenuButtonThemeData], which is used to configure this theme.
/// * [MenuTheme], the theme used to configure the look of the menus themselves.
/// * [MenuItemButton.defaultStyleOf] and [SubmenuButton.defaultStyleOf] which
/// return the default [ButtonStyle]s for menu buttons.
/// * [MenuItemButton.styleFrom] and [SubmenuButton.styleFrom], which converts
/// simple values into a [ButtonStyle] that's consistent with their respective
/// defaults.
/// * [ThemeData.menuButtonTheme], which can be used to override the default
/// [ButtonStyle] for [MenuItemButton]s and [SubmenuButton]s below the overall
/// [Theme].
class MenuButtonTheme extends InheritedTheme {
/// Create a [MenuButtonTheme].
const MenuButtonTheme({
super.key,
required this.data,
required super.child,
});
/// The configuration of this theme.
final MenuButtonThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [MenuButtonTheme] widget, then
/// [ThemeData.menuButtonTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// MenuButtonThemeData theme = MenuButtonTheme.of(context);
/// ```
static MenuButtonThemeData of(BuildContext context) {
final MenuButtonTheme? buttonTheme = context.dependOnInheritedWidgetOfExactType<MenuButtonTheme>();
return buttonTheme?.data ?? Theme.of(context).menuButtonTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return MenuButtonTheme(data: data, child: child);
}
@override
bool updateShouldNotify(MenuButtonTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/menu_button_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/menu_button_theme.dart",
"repo_id": "flutter",
"token_count": 1491
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/gestures.dart' show DragStartBehavior;
import 'package:flutter/widgets.dart';
import 'card.dart';
import 'constants.dart';
import 'data_table.dart';
import 'data_table_source.dart';
import 'debug.dart';
import 'dropdown.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'ink_decoration.dart';
import 'material_localizations.dart';
import 'material_state.dart';
import 'progress_indicator.dart';
import 'theme.dart';
/// A Material Design data table that shows data using multiple pages.
///
/// A paginated data table shows [rowsPerPage] rows of data per page and
/// provides controls for showing other pages.
///
/// Data is read lazily from a [DataTableSource]. The widget is presented
/// as a [Card].
///
/// If the [key] is a [PageStorageKey], the [initialFirstRowIndex] is persisted
/// to [PageStorage].
///
/// {@tool dartpad}
///
/// This sample shows how to display a [DataTable] with three columns: name,
/// age, and role. The columns are defined by three [DataColumn] objects. The
/// table contains three rows of data for three example users, the data for
/// which is defined by three [DataRow] objects.
///
/// ** See code in examples/api/lib/material/paginated_data_table/paginated_data_table.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
///
/// This example shows how paginated data tables can supported sorted data.
///
/// ** See code in examples/api/lib/material/paginated_data_table/paginated_data_table.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [DataTable], which is not paginated.
/// * <https://material.io/go/design-data-tables#data-tables-tables-within-cards>
class PaginatedDataTable extends StatefulWidget {
/// Creates a widget describing a paginated [DataTable] on a [Card].
///
/// The [header] should give the card's header, typically a [Text] widget.
///
/// The [columns] argument must be a list of as many [DataColumn] objects as
/// the table is to have columns, ignoring the leading checkbox column if any.
/// The [columns] argument must have a length greater than zero and cannot be
/// null.
///
/// If the table is sorted, the column that provides the current primary key
/// should be specified by index in [sortColumnIndex], 0 meaning the first
/// column in [columns], 1 being the next one, and so forth.
///
/// The actual sort order can be specified using [sortAscending]; if the sort
/// order is ascending, this should be true (the default), otherwise it should
/// be false.
///
/// The [source] should be a long-lived [DataTableSource]. The same source
/// should be provided each time a particular [PaginatedDataTable] widget is
/// created; avoid creating a new [DataTableSource] with each new instance of
/// the [PaginatedDataTable] widget unless the data table really is to now
/// show entirely different data from a new source.
///
/// Themed by [DataTableTheme]. [DataTableThemeData.decoration] is ignored.
/// To modify the border or background color of the [PaginatedDataTable], use
/// [CardTheme], since a [Card] wraps the inner [DataTable].
PaginatedDataTable({
super.key,
this.header,
this.actions,
required this.columns,
this.sortColumnIndex,
this.sortAscending = true,
this.onSelectAll,
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? dataRowHeight,
double? dataRowMinHeight,
double? dataRowMaxHeight,
this.headingRowHeight = 56.0,
this.horizontalMargin = 24.0,
this.columnSpacing = 56.0,
this.showCheckboxColumn = true,
this.showFirstLastButtons = false,
this.initialFirstRowIndex = 0,
this.onPageChanged,
this.rowsPerPage = defaultRowsPerPage,
this.availableRowsPerPage = const <int>[defaultRowsPerPage, defaultRowsPerPage * 2, defaultRowsPerPage * 5, defaultRowsPerPage * 10],
this.onRowsPerPageChanged,
this.dragStartBehavior = DragStartBehavior.start,
this.arrowHeadColor,
required this.source,
this.checkboxHorizontalMargin,
this.controller,
this.primary,
this.headingRowColor,
this.showEmptyRows = true,
}) : assert(actions == null || (header != null)),
assert(columns.isNotEmpty),
assert(sortColumnIndex == null || (sortColumnIndex >= 0 && sortColumnIndex < columns.length)),
assert(dataRowMinHeight == null || dataRowMaxHeight == null || dataRowMaxHeight >= dataRowMinHeight),
assert(dataRowHeight == null || (dataRowMinHeight == null && dataRowMaxHeight == null),
'dataRowHeight ($dataRowHeight) must not be set if dataRowMinHeight ($dataRowMinHeight) or dataRowMaxHeight ($dataRowMaxHeight) are set.'),
dataRowMinHeight = dataRowHeight ?? dataRowMinHeight,
dataRowMaxHeight = dataRowHeight ?? dataRowMaxHeight,
assert(rowsPerPage > 0),
assert(() {
if (onRowsPerPageChanged != null) {
assert(availableRowsPerPage.contains(rowsPerPage));
}
return true;
}()),
assert(!(controller != null && (primary ?? false)),
'Primary ScrollViews obtain their ScrollController via inheritance from a PrimaryScrollController widget. '
'You cannot both set primary to true and pass an explicit controller.',
);
/// The table card's optional header.
///
/// This is typically a [Text] widget, but can also be a [Row] of
/// [TextButton]s. To show icon buttons at the top end side of the table with
/// a header, set the [actions] property.
///
/// If items in the table are selectable, then, when the selection is not
/// empty, the header is replaced by a count of the selected items. The
/// [actions] are still visible when items are selected.
final Widget? header;
/// Icon buttons to show at the top end side of the table. The [header] must
/// not be null to show the actions.
///
/// Typically, the exact actions included in this list will vary based on
/// whether any rows are selected or not.
///
/// These should be size 24.0 with default padding (8.0).
final List<Widget>? actions;
/// The configuration and labels for the columns in the table.
final List<DataColumn> columns;
/// The current primary sort key's column.
///
/// See [DataTable.sortColumnIndex] for details.
///
/// The direction of the sort is specified using [sortAscending].
final int? sortColumnIndex;
/// Whether the column mentioned in [sortColumnIndex], if any, is sorted
/// in ascending order.
///
/// See [DataTable.sortAscending] for details.
final bool sortAscending;
/// Invoked when the user selects or unselects every row, using the
/// checkbox in the heading row.
///
/// See [DataTable.onSelectAll].
final ValueSetter<bool?>? onSelectAll;
/// The height of each row (excluding the row that contains column headings).
///
/// This value is optional and defaults to kMinInteractiveDimension if not
/// specified.
@Deprecated(
'Migrate to use dataRowMinHeight and dataRowMaxHeight instead. '
'This feature was deprecated after v3.7.0-5.0.pre.',
)
double? get dataRowHeight => dataRowMinHeight == dataRowMaxHeight ? dataRowMinHeight : null;
/// The minimum height of each row (excluding the row that contains column headings).
///
/// This value is optional and defaults to [kMinInteractiveDimension] if not
/// specified.
final double? dataRowMinHeight;
/// The maximum height of each row (excluding the row that contains column headings).
///
/// This value is optional and defaults to [kMinInteractiveDimension] if not
/// specified.
final double? dataRowMaxHeight;
/// The height of the heading row.
///
/// This value is optional and defaults to 56.0 if not specified.
final double headingRowHeight;
/// The horizontal margin between the edges of the table and the content
/// in the first and last cells of each row.
///
/// When a checkbox is displayed, it is also the margin between the checkbox
/// the content in the first data column.
///
/// This value defaults to 24.0 to adhere to the Material Design specifications.
///
/// If [checkboxHorizontalMargin] is null, then [horizontalMargin] is also the
/// margin between the edge of the table and the checkbox, as well as the
/// margin between the checkbox and the content in the first data column.
final double horizontalMargin;
/// The horizontal margin between the contents of each data column.
///
/// This value defaults to 56.0 to adhere to the Material Design specifications.
final double columnSpacing;
/// {@macro flutter.material.dataTable.showCheckboxColumn}
final bool showCheckboxColumn;
/// Flag to display the pagination buttons to go to the first and last pages.
final bool showFirstLastButtons;
/// The index of the first row to display when the widget is first created.
final int? initialFirstRowIndex;
/// Invoked when the user switches to another page.
///
/// The value is the index of the first row on the currently displayed page.
final ValueChanged<int>? onPageChanged;
/// The number of rows to show on each page.
///
/// See also:
///
/// * [onRowsPerPageChanged]
/// * [defaultRowsPerPage]
final int rowsPerPage;
/// The default value for [rowsPerPage].
///
/// Useful when initializing the field that will hold the current
/// [rowsPerPage], when implemented [onRowsPerPageChanged].
static const int defaultRowsPerPage = 10;
/// The options to offer for the rowsPerPage.
///
/// The current [rowsPerPage] must be a value in this list.
///
/// The values in this list should be sorted in ascending order.
final List<int> availableRowsPerPage;
/// Invoked when the user selects a different number of rows per page.
///
/// If this is null, then the value given by [rowsPerPage] will be used
/// and no affordance will be provided to change the value.
final ValueChanged<int?>? onRowsPerPageChanged;
/// The data source which provides data to show in each row.
///
/// This object should generally have a lifetime longer than the
/// [PaginatedDataTable] widget itself; it should be reused each time the
/// [PaginatedDataTable] constructor is called.
final DataTableSource source;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// Horizontal margin around the checkbox, if it is displayed.
///
/// If null, then [horizontalMargin] is used as the margin between the edge
/// of the table and the checkbox, as well as the margin between the checkbox
/// and the content in the first data column. This value defaults to 24.0.
final double? checkboxHorizontalMargin;
/// Defines the color of the arrow heads in the footer.
final Color? arrowHeadColor;
/// {@macro flutter.widgets.scroll_view.controller}
final ScrollController? controller;
/// {@macro flutter.widgets.scroll_view.primary}
final bool? primary;
/// {@macro flutter.material.dataTable.headingRowColor}
final MaterialStateProperty<Color?>? headingRowColor;
/// Controls the visibility of empty rows on the last page of a
/// [PaginatedDataTable].
///
/// Defaults to `true`, which means empty rows will be populated on the
/// last page of the table if there is not enough content.
/// When set to `false`, empty rows will not be created.
final bool showEmptyRows;
@override
PaginatedDataTableState createState() => PaginatedDataTableState();
}
/// Holds the state of a [PaginatedDataTable].
///
/// The table can be programmatically paged using the [pageTo] method.
class PaginatedDataTableState extends State<PaginatedDataTable> {
late int _firstRowIndex;
late int _rowCount;
late bool _rowCountApproximate;
int _selectedRowCount = 0;
final Map<int, DataRow?> _rows = <int, DataRow?>{};
@override
void initState() {
super.initState();
_firstRowIndex = PageStorage.maybeOf(context)?.readState(context) as int? ?? widget.initialFirstRowIndex ?? 0;
widget.source.addListener(_handleDataSourceChanged);
_handleDataSourceChanged();
}
@override
void didUpdateWidget(PaginatedDataTable oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.source != widget.source) {
oldWidget.source.removeListener(_handleDataSourceChanged);
widget.source.addListener(_handleDataSourceChanged);
_updateCaches();
}
}
@override
void reassemble() {
super.reassemble();
// This function is called during hot reload.
//
// Normally, if the data source changes, it would notify its listeners and
// thus trigger _handleDataSourceChanged(), which clears the row cache and
// causes the widget to rebuild.
//
// During a hot reload, though, a data source can change in ways that will
// invalidate the row cache (e.g. adding or removing columns) without ever
// triggering a notification, leaving the PaginatedDataTable in an invalid
// state. This method handles this case by clearing the cache any time the
// widget is involved in a hot reload.
_updateCaches();
}
@override
void dispose() {
widget.source.removeListener(_handleDataSourceChanged);
super.dispose();
}
void _handleDataSourceChanged() {
setState(_updateCaches);
}
void _updateCaches() {
_rowCount = widget.source.rowCount;
_rowCountApproximate = widget.source.isRowCountApproximate;
_selectedRowCount = widget.source.selectedRowCount;
_rows.clear();
}
/// Ensures that the given row is visible.
void pageTo(int rowIndex) {
final int oldFirstRowIndex = _firstRowIndex;
setState(() {
final int rowsPerPage = widget.rowsPerPage;
_firstRowIndex = (rowIndex ~/ rowsPerPage) * rowsPerPage;
});
if ((widget.onPageChanged != null) &&
(oldFirstRowIndex != _firstRowIndex)) {
widget.onPageChanged!(_firstRowIndex);
}
}
DataRow _getBlankRowFor(int index) {
return DataRow.byIndex(
index: index,
cells: widget.columns.map<DataCell>((DataColumn column) => DataCell.empty).toList(),
);
}
DataRow _getProgressIndicatorRowFor(int index) {
bool haveProgressIndicator = false;
final List<DataCell> cells = widget.columns.map<DataCell>((DataColumn column) {
if (!column.numeric) {
haveProgressIndicator = true;
return const DataCell(CircularProgressIndicator());
}
return DataCell.empty;
}).toList();
if (!haveProgressIndicator) {
haveProgressIndicator = true;
cells[0] = const DataCell(CircularProgressIndicator());
}
return DataRow.byIndex(
index: index,
cells: cells,
);
}
List<DataRow> _getRows(int firstRowIndex, int rowsPerPage) {
final List<DataRow> result = <DataRow>[];
final int nextPageFirstRowIndex = firstRowIndex + rowsPerPage;
bool haveProgressIndicator = false;
for (int index = firstRowIndex; index < nextPageFirstRowIndex; index += 1) {
DataRow? row;
if (index < _rowCount || _rowCountApproximate) {
row = _rows.putIfAbsent(index, () => widget.source.getRow(index));
if (row == null && !haveProgressIndicator) {
row ??= _getProgressIndicatorRowFor(index);
haveProgressIndicator = true;
}
}
if (widget.showEmptyRows) {
row ??= _getBlankRowFor(index);
}
if (row != null) {
result.add(row);
}
}
return result;
}
void _handleFirst() {
pageTo(0);
}
void _handlePrevious() {
pageTo(math.max(_firstRowIndex - widget.rowsPerPage, 0));
}
void _handleNext() {
pageTo(_firstRowIndex + widget.rowsPerPage);
}
void _handleLast() {
pageTo(((_rowCount - 1) / widget.rowsPerPage).floor() * widget.rowsPerPage);
}
bool _isNextPageUnavailable() => !_rowCountApproximate &&
(_firstRowIndex + widget.rowsPerPage >= _rowCount);
final GlobalKey _tableKey = GlobalKey();
@override
Widget build(BuildContext context) {
// TODO(ianh): This whole build function doesn't handle RTL yet.
assert(debugCheckHasMaterialLocalizations(context));
final ThemeData themeData = Theme.of(context);
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
// HEADER
final List<Widget> headerWidgets = <Widget>[];
if (_selectedRowCount == 0 && widget.header != null) {
headerWidgets.add(Expanded(child: widget.header!));
} else if (widget.header != null) {
headerWidgets.add(Expanded(
child: Text(localizations.selectedRowCountTitle(_selectedRowCount)),
));
}
if (widget.actions != null) {
headerWidgets.addAll(
widget.actions!.map<Widget>((Widget action) {
return Padding(
// 8.0 is the default padding of an icon button
padding: const EdgeInsetsDirectional.only(start: 24.0 - 8.0 * 2.0),
child: action,
);
}).toList(),
);
}
// FOOTER
final TextStyle? footerTextStyle = themeData.textTheme.bodySmall;
final List<Widget> footerWidgets = <Widget>[];
if (widget.onRowsPerPageChanged != null) {
final List<Widget> availableRowsPerPage = widget.availableRowsPerPage
.where((int value) => value <= _rowCount || value == widget.rowsPerPage)
.map<DropdownMenuItem<int>>((int value) {
return DropdownMenuItem<int>(
value: value,
child: Text('$value'),
);
})
.toList();
footerWidgets.addAll(<Widget>[
Container(width: 14.0), // to match trailing padding in case we overflow and end up scrolling
Text(localizations.rowsPerPageTitle),
ConstrainedBox(
constraints: const BoxConstraints(minWidth: 64.0), // 40.0 for the text, 24.0 for the icon
child: Align(
alignment: AlignmentDirectional.centerEnd,
child: DropdownButtonHideUnderline(
child: DropdownButton<int>(
items: availableRowsPerPage.cast<DropdownMenuItem<int>>(),
value: widget.rowsPerPage,
onChanged: widget.onRowsPerPageChanged,
style: footerTextStyle,
),
),
),
),
]);
}
footerWidgets.addAll(<Widget>[
Container(width: 32.0),
Text(
localizations.pageRowsInfoTitle(
_firstRowIndex + 1,
math.min(_firstRowIndex + widget.rowsPerPage, _rowCount),
_rowCount,
_rowCountApproximate,
),
),
Container(width: 32.0),
if (widget.showFirstLastButtons)
IconButton(
icon: Icon(Icons.skip_previous, color: widget.arrowHeadColor),
padding: EdgeInsets.zero,
tooltip: localizations.firstPageTooltip,
onPressed: _firstRowIndex <= 0 ? null : _handleFirst,
),
IconButton(
icon: Icon(Icons.chevron_left, color: widget.arrowHeadColor),
padding: EdgeInsets.zero,
tooltip: localizations.previousPageTooltip,
onPressed: _firstRowIndex <= 0 ? null : _handlePrevious,
),
Container(width: 24.0),
IconButton(
icon: Icon(Icons.chevron_right, color: widget.arrowHeadColor),
padding: EdgeInsets.zero,
tooltip: localizations.nextPageTooltip,
onPressed: _isNextPageUnavailable() ? null : _handleNext,
),
if (widget.showFirstLastButtons)
IconButton(
icon: Icon(Icons.skip_next, color: widget.arrowHeadColor),
padding: EdgeInsets.zero,
tooltip: localizations.lastPageTooltip,
onPressed: _isNextPageUnavailable()
? null
: _handleLast,
),
Container(width: 14.0),
]);
// CARD
return Card(
semanticContainer: false,
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
if (headerWidgets.isNotEmpty)
Semantics(
container: true,
child: DefaultTextStyle(
// These typographic styles aren't quite the regular ones. We pick the closest ones from the regular
// list and then tweak them appropriately.
// See https://material.io/design/components/data-tables.html#tables-within-cards
style: _selectedRowCount > 0 ? themeData.textTheme.titleMedium!.copyWith(color: themeData.colorScheme.secondary)
: themeData.textTheme.titleLarge!.copyWith(fontWeight: FontWeight.w400),
child: IconTheme.merge(
data: const IconThemeData(
opacity: 0.54,
),
child: Ink(
height: 64.0,
color: _selectedRowCount > 0 ? themeData.secondaryHeaderColor : null,
child: Padding(
padding: const EdgeInsetsDirectional.only(start: 24, end: 14.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: headerWidgets,
),
),
),
),
),
),
SingleChildScrollView(
scrollDirection: Axis.horizontal,
primary: widget.primary,
controller: widget.controller,
dragStartBehavior: widget.dragStartBehavior,
child: ConstrainedBox(
constraints: BoxConstraints(minWidth: constraints.minWidth),
child: DataTable(
key: _tableKey,
columns: widget.columns,
sortColumnIndex: widget.sortColumnIndex,
sortAscending: widget.sortAscending,
onSelectAll: widget.onSelectAll,
// Make sure no decoration is set on the DataTable
// from the theme, as its already wrapped in a Card.
decoration: const BoxDecoration(),
dataRowMinHeight: widget.dataRowMinHeight,
dataRowMaxHeight: widget.dataRowMaxHeight,
headingRowHeight: widget.headingRowHeight,
horizontalMargin: widget.horizontalMargin,
checkboxHorizontalMargin: widget.checkboxHorizontalMargin,
columnSpacing: widget.columnSpacing,
showCheckboxColumn: widget.showCheckboxColumn,
showBottomBorder: true,
rows: _getRows(_firstRowIndex, widget.rowsPerPage),
headingRowColor: widget.headingRowColor,
),
),
),
if (!widget.showEmptyRows)
SizedBox(
height: (widget.dataRowMaxHeight ?? kMinInteractiveDimension) * (widget.rowsPerPage - _rowCount + _firstRowIndex).clamp(0, widget.rowsPerPage)),
DefaultTextStyle(
style: footerTextStyle!,
child: IconTheme.merge(
data: const IconThemeData(
opacity: 0.54,
),
child: SizedBox(
// TODO(bkonyi): this won't handle text zoom correctly,
// https://github.com/flutter/flutter/issues/48522
height: 56.0,
child: SingleChildScrollView(
dragStartBehavior: widget.dragStartBehavior,
scrollDirection: Axis.horizontal,
reverse: true,
child: Row(
children: footerWidgets,
),
),
),
),
),
],
);
},
),
);
}
}
| flutter/packages/flutter/lib/src/material/paginated_data_table.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/paginated_data_table.dart",
"repo_id": "flutter",
"token_count": 9227
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'material_state.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [SearchBar] widgets.
///
/// Descendant widgets obtain the current [SearchBarThemeData] object using
/// `SearchBarTheme.of(context)`. Instances of [SearchBarThemeData] can be customized
/// with [SearchBarThemeData.copyWith].
///
/// Typically a [SearchBarThemeData] is specified as part of the overall [Theme]
/// with [ThemeData.searchBarTheme].
///
/// All [SearchBarThemeData] properties are `null` by default. When null, the
/// [SearchBar] will use the values from [ThemeData] if they exist, otherwise it
/// will provide its own defaults based on the overall [Theme]'s colorScheme.
/// See the individual [SearchBar] properties for details.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class SearchBarThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.searchBarTheme].
const SearchBarThemeData({
this.elevation,
this.backgroundColor,
this.shadowColor,
this.surfaceTintColor,
this.overlayColor,
this.side,
this.shape,
this.padding,
this.textStyle,
this.hintStyle,
this.constraints,
this.textCapitalization,
});
/// Overrides the default value of the [SearchBar.elevation].
final MaterialStateProperty<double?>? elevation;
/// Overrides the default value of the [SearchBar.backgroundColor].
final MaterialStateProperty<Color?>? backgroundColor;
/// Overrides the default value of the [SearchBar.shadowColor].
final MaterialStateProperty<Color?>? shadowColor;
/// Overrides the default value of the [SearchBar.surfaceTintColor].
final MaterialStateProperty<Color?>? surfaceTintColor;
/// Overrides the default value of the [SearchBar.overlayColor].
final MaterialStateProperty<Color?>? overlayColor;
/// Overrides the default value of the [SearchBar.side].
final MaterialStateProperty<BorderSide?>? side;
/// Overrides the default value of the [SearchBar.shape].
final MaterialStateProperty<OutlinedBorder?>? shape;
/// Overrides the default value for [SearchBar.padding].
final MaterialStateProperty<EdgeInsetsGeometry?>? padding;
/// Overrides the default value for [SearchBar.textStyle].
final MaterialStateProperty<TextStyle?>? textStyle;
/// Overrides the default value for [SearchBar.hintStyle].
final MaterialStateProperty<TextStyle?>? hintStyle;
/// Overrides the value of size constraints for [SearchBar].
final BoxConstraints? constraints;
/// Overrides the value of [SearchBar.textCapitalization].
final TextCapitalization? textCapitalization;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
SearchBarThemeData copyWith({
MaterialStateProperty<double?>? elevation,
MaterialStateProperty<Color?>? backgroundColor,
MaterialStateProperty<Color?>? shadowColor,
MaterialStateProperty<Color?>? surfaceTintColor,
MaterialStateProperty<Color?>? overlayColor,
MaterialStateProperty<BorderSide?>? side,
MaterialStateProperty<OutlinedBorder?>? shape,
MaterialStateProperty<EdgeInsetsGeometry?>? padding,
MaterialStateProperty<TextStyle?>? textStyle,
MaterialStateProperty<TextStyle?>? hintStyle,
BoxConstraints? constraints,
TextCapitalization? textCapitalization,
}) {
return SearchBarThemeData(
elevation: elevation ?? this.elevation,
backgroundColor: backgroundColor ?? this.backgroundColor,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
overlayColor: overlayColor ?? this.overlayColor,
side: side ?? this.side,
shape: shape ?? this.shape,
padding: padding ?? this.padding,
textStyle: textStyle ?? this.textStyle,
hintStyle: hintStyle ?? this.hintStyle,
constraints: constraints ?? this.constraints,
textCapitalization: textCapitalization ?? this.textCapitalization,
);
}
/// Linearly interpolate between two [SearchBarThemeData]s.
///
/// {@macro dart.ui.shadow.lerp}
static SearchBarThemeData? lerp(SearchBarThemeData? a, SearchBarThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return SearchBarThemeData(
elevation: MaterialStateProperty.lerp<double?>(a?.elevation, b?.elevation, t, lerpDouble),
backgroundColor: MaterialStateProperty.lerp<Color?>(a?.backgroundColor, b?.backgroundColor, t, Color.lerp),
shadowColor: MaterialStateProperty.lerp<Color?>(a?.shadowColor, b?.shadowColor, t, Color.lerp),
surfaceTintColor: MaterialStateProperty.lerp<Color?>(a?.surfaceTintColor, b?.surfaceTintColor, t, Color.lerp),
overlayColor: MaterialStateProperty.lerp<Color?>(a?.overlayColor, b?.overlayColor, t, Color.lerp),
side: _lerpSides(a?.side, b?.side, t),
shape: MaterialStateProperty.lerp<OutlinedBorder?>(a?.shape, b?.shape, t, OutlinedBorder.lerp),
padding: MaterialStateProperty.lerp<EdgeInsetsGeometry?>(a?.padding, b?.padding, t, EdgeInsetsGeometry.lerp),
textStyle: MaterialStateProperty.lerp<TextStyle?>(a?.textStyle, b?.textStyle, t, TextStyle.lerp),
hintStyle: MaterialStateProperty.lerp<TextStyle?>(a?.hintStyle, b?.hintStyle, t, TextStyle.lerp),
constraints: BoxConstraints.lerp(a?.constraints, b?.constraints, t),
textCapitalization: t < 0.5 ? a?.textCapitalization : b?.textCapitalization,
);
}
@override
int get hashCode => Object.hash(
elevation,
backgroundColor,
shadowColor,
surfaceTintColor,
overlayColor,
side,
shape,
padding,
textStyle,
hintStyle,
constraints,
textCapitalization,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is SearchBarThemeData
&& other.elevation == elevation
&& other.backgroundColor == backgroundColor
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.overlayColor == overlayColor
&& other.side == side
&& other.shape == shape
&& other.padding == padding
&& other.textStyle == textStyle
&& other.hintStyle == hintStyle
&& other.constraints == constraints
&& other.textCapitalization == textCapitalization;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<MaterialStateProperty<double?>>('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('shadowColor', shadowColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('overlayColor', overlayColor, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<BorderSide?>>('side', side, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<OutlinedBorder?>>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<EdgeInsetsGeometry?>>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<TextStyle?>>('textStyle', textStyle, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<TextStyle?>>('hintStyle', hintStyle, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: null));
properties.add(DiagnosticsProperty<TextCapitalization>('textCapitalization', textCapitalization, defaultValue: null));
}
// Special case because BorderSide.lerp() doesn't support null arguments
static MaterialStateProperty<BorderSide?>? _lerpSides(MaterialStateProperty<BorderSide?>? a, MaterialStateProperty<BorderSide?>? b, double t) {
if (identical(a, b)) {
return a;
}
return _LerpSides(a, b, t);
}
}
class _LerpSides implements MaterialStateProperty<BorderSide?> {
const _LerpSides(this.a, this.b, this.t);
final MaterialStateProperty<BorderSide?>? a;
final MaterialStateProperty<BorderSide?>? b;
final double t;
@override
BorderSide? resolve(Set<MaterialState> states) {
final BorderSide? resolvedA = a?.resolve(states);
final BorderSide? resolvedB = b?.resolve(states);
if (identical(resolvedA, resolvedB)) {
return resolvedA;
}
if (resolvedA == null) {
return BorderSide.lerp(BorderSide(width: 0, color: resolvedB!.color.withAlpha(0)), resolvedB, t);
}
if (resolvedB == null) {
return BorderSide.lerp(resolvedA, BorderSide(width: 0, color: resolvedA.color.withAlpha(0)), t);
}
return BorderSide.lerp(resolvedA, resolvedB, t);
}
}
/// Applies a search bar theme to descendant [SearchBar] widgets.
///
/// Descendant widgets obtain the current theme's [SearchBarTheme] object using
/// [SearchBarTheme.of]. When a widget uses [SearchBarTheme.of], it is automatically
/// rebuilt if the theme later changes.
///
/// A search bar theme can be specified as part of the overall Material theme using
/// [ThemeData.searchBarTheme].
///
/// See also:
///
/// * [SearchBarThemeData], which describes the actual configuration of a search bar
/// theme.
class SearchBarTheme extends InheritedWidget {
/// Constructs a search bar theme that configures all descendant [SearchBar] widgets.
const SearchBarTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [SearchBar] widgets.
final SearchBarThemeData data;
/// Returns the configuration [data] from the closest [SearchBarTheme] ancestor.
/// If there is no ancestor, it returns [ThemeData.searchBarTheme].
///
/// Typical usage is as follows:
///
/// ```dart
/// SearchBarThemeData theme = SearchBarTheme.of(context);
/// ```
static SearchBarThemeData of(BuildContext context) {
final SearchBarTheme? searchBarTheme = context.dependOnInheritedWidgetOfExactType<SearchBarTheme>();
return searchBarTheme?.data ?? Theme.of(context).searchBarTheme;
}
@override
bool updateShouldNotify(SearchBarTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/search_bar_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/search_bar_theme.dart",
"repo_id": "flutter",
"token_count": 3499
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/widgets.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'material_state.dart';
import 'switch.dart';
import 'switch_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
// Examples can assume:
// void setState(VoidCallback fn) { }
// bool _isSelected = true;
enum _SwitchListTileType { material, adaptive }
/// A [ListTile] with a [Switch]. In other words, a switch with a label.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=0igIjvtEWNU}
///
/// The entire list tile is interactive: tapping anywhere in the tile toggles
/// the switch. Tapping and dragging the [Switch] also triggers the [onChanged]
/// callback.
///
/// To ensure that [onChanged] correctly triggers, the state passed
/// into [value] must be properly managed. This is typically done by invoking
/// [State.setState] in [onChanged] to toggle the state value.
///
/// The [value], [onChanged], [activeColor], [activeThumbImage], and
/// [inactiveThumbImage] properties of this widget are identical to the
/// similarly-named properties on the [Switch] widget.
///
/// The [title], [subtitle], [isThreeLine], and [dense] properties are like
/// those of the same name on [ListTile].
///
/// The [selected] property on this widget is similar to the [ListTile.selected]
/// property. This tile's [activeColor] is used for the selected item's text color, or
/// the theme's [SwitchThemeData.overlayColor] if [activeColor] is null.
///
/// This widget does not coordinate the [selected] state and the
/// [value]; to have the list tile appear selected when the
/// switch button is on, use the same value for both.
///
/// The switch is shown on the right by default in left-to-right languages (i.e.
/// in the [ListTile.trailing] slot) which can be changed using [controlAffinity].
/// The [secondary] widget is placed in the [ListTile.leading] slot.
///
/// This widget requires a [Material] widget ancestor in the tree to paint
/// itself on, which is typically provided by the app's [Scaffold].
/// The [tileColor], and [selectedTileColor] are not painted by the
/// [SwitchListTile] itself but by the [Material] widget ancestor. In this
/// case, one can wrap a [Material] widget around the [SwitchListTile], e.g.:
///
/// {@tool snippet}
/// ```dart
/// ColoredBox(
/// color: Colors.green,
/// child: Material(
/// child: SwitchListTile(
/// tileColor: Colors.red,
/// title: const Text('SwitchListTile with red background'),
/// value: true,
/// onChanged:(bool? value) { },
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Performance considerations when wrapping [SwitchListTile] with [Material]
///
/// Wrapping a large number of [SwitchListTile]s individually with [Material]s
/// is expensive. Consider only wrapping the [SwitchListTile]s that require it
/// or include a common [Material] ancestor where possible.
///
/// To show the [SwitchListTile] as disabled, pass null as the [onChanged]
/// callback.
///
/// {@tool dartpad}
/// 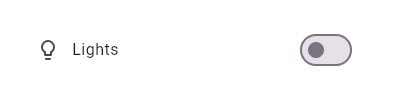
///
/// This widget shows a switch that, when toggled, changes the state of a [bool]
/// member field called `_lights`.
///
/// ** See code in examples/api/lib/material/switch_list_tile/switch_list_tile.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This sample demonstrates how [SwitchListTile] positions the switch widget
/// relative to the text in different configurations.
///
/// ** See code in examples/api/lib/material/switch_list_tile/switch_list_tile.1.dart **
/// {@end-tool}
///
/// ## Semantics in SwitchListTile
///
/// Since the entirety of the SwitchListTile is interactive, it should represent
/// itself as a single interactive entity.
///
/// To do so, a SwitchListTile widget wraps its children with a [MergeSemantics]
/// widget. [MergeSemantics] will attempt to merge its descendant [Semantics]
/// nodes into one node in the semantics tree. Therefore, SwitchListTile will
/// throw an error if any of its children requires its own [Semantics] node.
///
/// For example, you cannot nest a [RichText] widget as a descendant of
/// SwitchListTile. [RichText] has an embedded gesture recognizer that
/// requires its own [Semantics] node, which directly conflicts with
/// SwitchListTile's desire to merge all its descendants' semantic nodes
/// into one. Therefore, it may be necessary to create a custom radio tile
/// widget to accommodate similar use cases.
///
/// {@tool dartpad}
/// 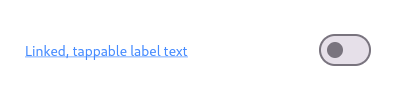
///
/// Here is an example of a custom labeled radio widget, called
/// LinkedLabelRadio, that includes an interactive [RichText] widget that
/// handles tap gestures.
///
/// ** See code in examples/api/lib/material/switch_list_tile/custom_labeled_switch.0.dart **
/// {@end-tool}
///
/// ## SwitchListTile isn't exactly what I want
///
/// If the way SwitchListTile pads and positions its elements isn't quite what
/// you're looking for, you can create custom labeled switch widgets by
/// combining [Switch] with other widgets, such as [Text], [Padding] and
/// [InkWell].
///
/// {@tool dartpad}
/// 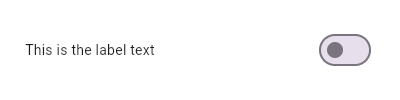
///
/// Here is an example of a custom LabeledSwitch widget, but you can easily
/// make your own configurable widget.
///
/// ** See code in examples/api/lib/material/switch_list_tile/custom_labeled_switch.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListTileTheme], which can be used to affect the style of list tiles,
/// including switch list tiles.
/// * [CheckboxListTile], a similar widget for checkboxes.
/// * [RadioListTile], a similar widget for radio buttons.
/// * [ListTile] and [Switch], the widgets from which this widget is made.
class SwitchListTile extends StatelessWidget {
/// Creates a combination of a list tile and a switch.
///
/// The switch tile itself does not maintain any state. Instead, when the
/// state of the switch changes, the widget calls the [onChanged] callback.
/// Most widgets that use a switch will listen for the [onChanged] callback
/// and rebuild the switch tile with a new [value] to update the visual
/// appearance of the switch.
///
/// The following arguments are required:
///
/// * [value] determines whether this switch is on or off.
/// * [onChanged] is called when the user toggles the switch on or off.
const SwitchListTile({
super.key,
required this.value,
required this.onChanged,
this.activeColor,
this.activeTrackColor,
this.inactiveThumbColor,
this.inactiveTrackColor,
this.activeThumbImage,
this.onActiveThumbImageError,
this.inactiveThumbImage,
this.onInactiveThumbImageError,
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.thumbIcon,
this.materialTapTargetSize,
this.dragStartBehavior = DragStartBehavior.start,
this.mouseCursor,
this.overlayColor,
this.splashRadius,
this.focusNode,
this.onFocusChange,
this.autofocus = false,
this.tileColor,
this.title,
this.subtitle,
this.isThreeLine = false,
this.dense,
this.contentPadding,
this.secondary,
this.selected = false,
this.controlAffinity = ListTileControlAffinity.platform,
this.shape,
this.selectedTileColor,
this.visualDensity,
this.enableFeedback,
this.hoverColor,
}) : _switchListTileType = _SwitchListTileType.material,
applyCupertinoTheme = false,
assert(activeThumbImage != null || onActiveThumbImageError == null),
assert(inactiveThumbImage != null || onInactiveThumbImageError == null),
assert(!isThreeLine || subtitle != null);
/// Creates a Material [ListTile] with an adaptive [Switch], following
/// Material design's
/// [Cross-platform guidelines](https://material.io/design/platform-guidance/cross-platform-adaptation.html).
///
/// This widget uses [Switch.adaptive] to change the graphics of the switch
/// component based on the ambient [ThemeData.platform]. On iOS and macOS, a
/// [CupertinoSwitch] will be used. On other platforms a Material design
/// [Switch] will be used.
///
/// If a [CupertinoSwitch] is created, the following parameters are
/// ignored: [activeTrackColor], [inactiveThumbColor], [inactiveTrackColor],
/// [activeThumbImage], [inactiveThumbImage].
const SwitchListTile.adaptive({
super.key,
required this.value,
required this.onChanged,
this.activeColor,
this.activeTrackColor,
this.inactiveThumbColor,
this.inactiveTrackColor,
this.activeThumbImage,
this.onActiveThumbImageError,
this.inactiveThumbImage,
this.onInactiveThumbImageError,
this.thumbColor,
this.trackColor,
this.trackOutlineColor,
this.thumbIcon,
this.materialTapTargetSize,
this.dragStartBehavior = DragStartBehavior.start,
this.mouseCursor,
this.overlayColor,
this.splashRadius,
this.focusNode,
this.onFocusChange,
this.autofocus = false,
this.applyCupertinoTheme,
this.tileColor,
this.title,
this.subtitle,
this.isThreeLine = false,
this.dense,
this.contentPadding,
this.secondary,
this.selected = false,
this.controlAffinity = ListTileControlAffinity.platform,
this.shape,
this.selectedTileColor,
this.visualDensity,
this.enableFeedback,
this.hoverColor,
}) : _switchListTileType = _SwitchListTileType.adaptive,
assert(!isThreeLine || subtitle != null),
assert(activeThumbImage != null || onActiveThumbImageError == null),
assert(inactiveThumbImage != null || onInactiveThumbImageError == null);
/// Whether this switch is checked.
final bool value;
/// Called when the user toggles the switch on or off.
///
/// The switch passes the new value to the callback but does not actually
/// change state until the parent widget rebuilds the switch tile with the
/// new value.
///
/// If null, the switch will be displayed as disabled.
///
/// The callback provided to [onChanged] should update the state of the parent
/// [StatefulWidget] using the [State.setState] method, so that the parent
/// gets rebuilt; for example:
///
/// {@tool snippet}
/// ```dart
/// SwitchListTile(
/// value: _isSelected,
/// onChanged: (bool newValue) {
/// setState(() {
/// _isSelected = newValue;
/// });
/// },
/// title: const Text('Selection'),
/// )
/// ```
/// {@end-tool}
final ValueChanged<bool>? onChanged;
/// {@macro flutter.material.switch.activeColor}
///
/// Defaults to [ColorScheme.secondary] of the current [Theme].
final Color? activeColor;
/// {@macro flutter.material.switch.activeTrackColor}
///
/// Defaults to [ColorScheme.secondary] with the opacity set at 50%.
///
/// Ignored if created with [SwitchListTile.adaptive].
final Color? activeTrackColor;
/// {@macro flutter.material.switch.inactiveThumbColor}
///
/// Defaults to the colors described in the Material design specification.
///
/// Ignored if created with [SwitchListTile.adaptive].
final Color? inactiveThumbColor;
/// {@macro flutter.material.switch.inactiveTrackColor}
///
/// Defaults to the colors described in the Material design specification.
///
/// Ignored if created with [SwitchListTile.adaptive].
final Color? inactiveTrackColor;
/// {@macro flutter.material.switch.activeThumbImage}
final ImageProvider? activeThumbImage;
/// {@macro flutter.material.switch.onActiveThumbImageError}
final ImageErrorListener? onActiveThumbImageError;
/// {@macro flutter.material.switch.inactiveThumbImage}
///
/// Ignored if created with [SwitchListTile.adaptive].
final ImageProvider? inactiveThumbImage;
/// {@macro flutter.material.switch.onInactiveThumbImageError}
final ImageErrorListener? onInactiveThumbImageError;
/// The color of this switch's thumb.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [activeColor] is used in the selected state
/// and [inactiveThumbColor] in the default state. If that is also null, then
/// the value of [SwitchThemeData.thumbColor] is used. If that is also null,
/// The default value is used.
final MaterialStateProperty<Color?>? thumbColor;
/// The color of this switch's track.
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [activeTrackColor] is used in the selected
/// state and [inactiveTrackColor] in the default state. If that is also null,
/// then the value of [SwitchThemeData.trackColor] is used. If that is also
/// null, then the default value is used.
final MaterialStateProperty<Color?>? trackColor;
/// {@macro flutter.material.switch.trackOutlineColor}
///
/// The [ListTile] will be focused when this [SwitchListTile] requests focus,
/// so the focused outline color of the switch will be ignored.
///
/// In Material 3, the outline color defaults to transparent in the selected
/// state and [ColorScheme.outline] in the unselected state. In Material 2,
/// the [Switch] track has no outline.
final MaterialStateProperty<Color?>? trackOutlineColor;
/// The icon to use on the thumb of this switch
///
/// Resolved in the following states:
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [SwitchThemeData.thumbIcon] is used. If this is
/// also null, then the [Switch] does not have any icons on the thumb.
final MaterialStateProperty<Icon?>? thumbIcon;
/// {@macro flutter.material.switch.materialTapTargetSize}
///
/// defaults to [MaterialTapTargetSize.shrinkWrap].
final MaterialTapTargetSize? materialTapTargetSize;
/// {@macro flutter.cupertino.CupertinoSwitch.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// The cursor for a mouse pointer when it enters or is hovering over the
/// widget.
///
/// If [mouseCursor] is a [MaterialStateProperty<MouseCursor>],
/// [MaterialStateProperty.resolve] is used for the following [MaterialState]s:
///
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.disabled].
///
/// If null, then the value of [SwitchThemeData.mouseCursor] is used. If that
/// is also null, then [MaterialStateMouseCursor.clickable] is used.
final MouseCursor? mouseCursor;
/// The color for the switch's [Material].
///
/// Resolves in the following states:
/// * [MaterialState.pressed].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
///
/// If null, then the value of [activeColor] with alpha [kRadialReactionAlpha]
/// and [hoverColor] is used in the pressed and hovered state. If that is also
/// null, the value of [SwitchThemeData.overlayColor] is used. If that is
/// also null, then the default value is used in the pressed and hovered state.
final MaterialStateProperty<Color?>? overlayColor;
/// {@macro flutter.material.switch.splashRadius}
///
/// If null, then the value of [SwitchThemeData.splashRadius] is used. If that
/// is also null, then [kRadialReactionRadius] is used.
final double? splashRadius;
/// {@macro flutter.widgets.Focus.focusNode}
final FocusNode? focusNode;
/// {@macro flutter.material.inkwell.onFocusChange}
final ValueChanged<bool>? onFocusChange;
/// {@macro flutter.widgets.Focus.autofocus}
final bool autofocus;
/// {@macro flutter.material.ListTile.tileColor}
final Color? tileColor;
/// The primary content of the list tile.
///
/// Typically a [Text] widget.
final Widget? title;
/// Additional content displayed below the title.
///
/// Typically a [Text] widget.
final Widget? subtitle;
/// A widget to display on the opposite side of the tile from the switch.
///
/// Typically an [Icon] widget.
final Widget? secondary;
/// Whether this list tile is intended to display three lines of text.
///
/// If false, the list tile is treated as having one line if the subtitle is
/// null and treated as having two lines if the subtitle is non-null.
final bool isThreeLine;
/// Whether this list tile is part of a vertically dense list.
///
/// If this property is null then its value is based on [ListTileThemeData.dense].
final bool? dense;
/// The tile's internal padding.
///
/// Insets a [SwitchListTile]'s contents: its [title], [subtitle],
/// [secondary], and [Switch] widgets.
///
/// If null, [ListTile]'s default of `EdgeInsets.symmetric(horizontal: 16.0)`
/// is used.
final EdgeInsetsGeometry? contentPadding;
/// Whether to render icons and text in the [activeColor].
///
/// No effort is made to automatically coordinate the [selected] state and the
/// [value] state. To have the list tile appear selected when the switch is
/// on, pass the same value to both.
///
/// Normally, this property is left to its default value, false.
final bool selected;
/// If adaptive, creates the switch with [Switch.adaptive].
final _SwitchListTileType _switchListTileType;
/// Defines the position of control and [secondary], relative to text.
///
/// By default, the value of [controlAffinity] is [ListTileControlAffinity.platform].
final ListTileControlAffinity controlAffinity;
/// {@macro flutter.material.ListTile.shape}
final ShapeBorder? shape;
/// If non-null, defines the background color when [SwitchListTile.selected] is true.
final Color? selectedTileColor;
/// Defines how compact the list tile's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
final VisualDensity? visualDensity;
/// {@macro flutter.material.ListTile.enableFeedback}
///
/// See also:
///
/// * [Feedback] for providing platform-specific feedback to certain actions.
final bool? enableFeedback;
/// The color for the tile's [Material] when a pointer is hovering over it.
final Color? hoverColor;
/// {@macro flutter.cupertino.CupertinoSwitch.applyTheme}
final bool? applyCupertinoTheme;
@override
Widget build(BuildContext context) {
final Widget control;
switch (_switchListTileType) {
case _SwitchListTileType.adaptive:
control = ExcludeFocus(
child: Switch.adaptive(
value: value,
onChanged: onChanged,
activeColor: activeColor,
activeThumbImage: activeThumbImage,
inactiveThumbImage: inactiveThumbImage,
materialTapTargetSize: materialTapTargetSize ?? MaterialTapTargetSize.shrinkWrap,
activeTrackColor: activeTrackColor,
inactiveTrackColor: inactiveTrackColor,
inactiveThumbColor: inactiveThumbColor,
autofocus: autofocus,
onFocusChange: onFocusChange,
onActiveThumbImageError: onActiveThumbImageError,
onInactiveThumbImageError: onInactiveThumbImageError,
thumbColor: thumbColor,
trackColor: trackColor,
trackOutlineColor: trackOutlineColor,
thumbIcon: thumbIcon,
applyCupertinoTheme: applyCupertinoTheme,
dragStartBehavior: dragStartBehavior,
mouseCursor: mouseCursor,
splashRadius: splashRadius,
overlayColor: overlayColor,
),
);
case _SwitchListTileType.material:
control = ExcludeFocus(
child: Switch(
value: value,
onChanged: onChanged,
activeColor: activeColor,
activeThumbImage: activeThumbImage,
inactiveThumbImage: inactiveThumbImage,
materialTapTargetSize: materialTapTargetSize ?? MaterialTapTargetSize.shrinkWrap,
activeTrackColor: activeTrackColor,
inactiveTrackColor: inactiveTrackColor,
inactiveThumbColor: inactiveThumbColor,
autofocus: autofocus,
onFocusChange: onFocusChange,
onActiveThumbImageError: onActiveThumbImageError,
onInactiveThumbImageError: onInactiveThumbImageError,
thumbColor: thumbColor,
trackColor: trackColor,
trackOutlineColor: trackOutlineColor,
thumbIcon: thumbIcon,
dragStartBehavior: dragStartBehavior,
mouseCursor: mouseCursor,
splashRadius: splashRadius,
overlayColor: overlayColor,
),
);
}
Widget? leading, trailing;
(leading, trailing) = switch (controlAffinity) {
ListTileControlAffinity.leading => (control, secondary),
ListTileControlAffinity.trailing || ListTileControlAffinity.platform => (secondary, control),
};
final ThemeData theme = Theme.of(context);
final SwitchThemeData switchTheme = SwitchTheme.of(context);
final Set<MaterialState> states = <MaterialState>{
if (selected) MaterialState.selected,
};
final Color effectiveActiveColor = activeColor
?? switchTheme.thumbColor?.resolve(states)
?? theme.colorScheme.secondary;
return MergeSemantics(
child: ListTile(
selectedColor: effectiveActiveColor,
leading: leading,
title: title,
subtitle: subtitle,
trailing: trailing,
isThreeLine: isThreeLine,
dense: dense,
contentPadding: contentPadding,
enabled: onChanged != null,
onTap: onChanged != null ? () { onChanged!(!value); } : null,
selected: selected,
selectedTileColor: selectedTileColor,
autofocus: autofocus,
shape: shape,
tileColor: tileColor,
visualDensity: visualDensity,
focusNode: focusNode,
onFocusChange: onFocusChange,
enableFeedback: enableFeedback,
hoverColor: hoverColor,
),
);
}
}
| flutter/packages/flutter/lib/src/material/switch_list_tile.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/switch_list_tile.dart",
"repo_id": "flutter",
"token_count": 7271
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show Color, lerpDouble;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'action_buttons.dart';
import 'action_icons_theme.dart';
import 'app_bar_theme.dart';
import 'badge_theme.dart';
import 'banner_theme.dart';
import 'bottom_app_bar_theme.dart';
import 'bottom_navigation_bar_theme.dart';
import 'bottom_sheet_theme.dart';
import 'button_bar_theme.dart';
import 'button_theme.dart';
import 'card_theme.dart';
import 'checkbox_theme.dart';
import 'chip_theme.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'data_table_theme.dart';
import 'date_picker_theme.dart';
import 'dialog_theme.dart';
import 'divider_theme.dart';
import 'drawer_theme.dart';
import 'dropdown_menu_theme.dart';
import 'elevated_button_theme.dart';
import 'expansion_tile_theme.dart';
import 'filled_button_theme.dart';
import 'floating_action_button_theme.dart';
import 'icon_button_theme.dart';
import 'ink_ripple.dart';
import 'ink_sparkle.dart';
import 'ink_splash.dart';
import 'ink_well.dart' show InteractiveInkFeatureFactory;
import 'input_decorator.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'menu_bar_theme.dart';
import 'menu_button_theme.dart';
import 'menu_theme.dart';
import 'navigation_bar_theme.dart';
import 'navigation_drawer_theme.dart';
import 'navigation_rail_theme.dart';
import 'outlined_button_theme.dart';
import 'page_transitions_theme.dart';
import 'popup_menu_theme.dart';
import 'progress_indicator_theme.dart';
import 'radio_theme.dart';
import 'scrollbar_theme.dart';
import 'search_bar_theme.dart';
import 'search_view_theme.dart';
import 'segmented_button_theme.dart';
import 'slider_theme.dart';
import 'snack_bar_theme.dart';
import 'switch_theme.dart';
import 'tab_bar_theme.dart';
import 'text_button_theme.dart';
import 'text_selection_theme.dart';
import 'text_theme.dart';
import 'time_picker_theme.dart';
import 'toggle_buttons_theme.dart';
import 'tooltip_theme.dart';
import 'typography.dart';
export 'package:flutter/services.dart' show Brightness;
// Examples can assume:
// late BuildContext context;
/// Defines a customized theme for components with an `adaptive` factory constructor.
///
/// Currently, only [Switch.adaptive] supports this class.
class Adaptation<T> {
/// Creates an [Adaptation].
const Adaptation();
/// The adaptation's type.
Type get type => T;
/// Typically, this is overridden to return an instance of a custom component
/// ThemeData class, like [SwitchThemeData], instead of the defaultValue.
///
/// Factory constructors that support adaptations - currently only
/// [Switch.adaptive] - look for a [ThemeData.adaptations] member of the expected
/// type when computing their effective default component theme. If a matching
/// adaptation is not found, the component may choose to use a default adaptation.
/// For example, the [Switch.adaptive] component uses an empty [SwitchThemeData]
/// if a matching adaptation is not found, for the sake of backwards compatibility.
///
/// {@tool dartpad}
/// This sample shows how to create and use subclasses of [Adaptation] that
/// define adaptive [SwitchThemeData]s. The [adapt] method in this example is
/// overridden to only customize cupertino-style switches, but it can also be
/// used to customize any other platforms.
///
/// ** See code in examples/api/lib/material/switch/switch.4.dart **
/// {@end-tool}
T adapt(ThemeData theme, T defaultValue) => defaultValue;
}
/// An interface that defines custom additions to a [ThemeData] object.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=8-szcYzFVao}
///
/// Typically used for custom colors. To use, subclass [ThemeExtension],
/// define a number of fields (e.g. [Color]s), and implement the [copyWith] and
/// [lerp] methods. The latter will ensure smooth transitions of properties when
/// switching themes.
///
/// {@tool dartpad}
/// This sample shows how to create and use a subclass of [ThemeExtension] that
/// defines two colors.
///
/// ** See code in examples/api/lib/material/theme/theme_extension.1.dart **
/// {@end-tool}
abstract class ThemeExtension<T extends ThemeExtension<T>> {
/// Enable const constructor for subclasses.
const ThemeExtension();
/// The extension's type.
Object get type => T;
/// Creates a copy of this theme extension with the given fields
/// replaced by the non-null parameter values.
ThemeExtension<T> copyWith();
/// Linearly interpolate with another [ThemeExtension] object.
///
/// {@macro dart.ui.shadow.lerp}
ThemeExtension<T> lerp(covariant ThemeExtension<T>? other, double t);
}
// Deriving these values is black magic. The spec claims that pressed buttons
// have a highlight of 0x66999999, but that's clearly wrong. The videos in the
// spec show that buttons have a composited highlight of #E1E1E1 on a background
// of #FAFAFA. Assuming that the highlight really has an opacity of 0x66, we can
// solve for the actual color of the highlight:
const Color _kLightThemeHighlightColor = Color(0x66BCBCBC);
// The same video shows the splash compositing to #D7D7D7 on a background of
// #E1E1E1. Again, assuming the splash has an opacity of 0x66, we can solve for
// the actual color of the splash:
const Color _kLightThemeSplashColor = Color(0x66C8C8C8);
// Unfortunately, a similar video isn't available for the dark theme, which
// means we assume the values in the spec are actually correct.
const Color _kDarkThemeHighlightColor = Color(0x40CCCCCC);
const Color _kDarkThemeSplashColor = Color(0x40CCCCCC);
/// Configures the tap target and layout size of certain Material widgets.
///
/// Changing the value in [ThemeData.materialTapTargetSize] will affect the
/// accessibility experience.
///
/// Some of the impacted widgets include:
///
/// * [FloatingActionButton], only the mini tap target size is increased.
/// * [MaterialButton]
/// * [OutlinedButton]
/// * [TextButton]
/// * [ElevatedButton]
/// * [IconButton]
/// * The time picker widget ([showTimePicker])
/// * [SnackBar]
/// * [Chip]
/// * [RawChip]
/// * [InputChip]
/// * [ChoiceChip]
/// * [FilterChip]
/// * [ActionChip]
/// * [Radio]
/// * [Switch]
/// * [Checkbox]
enum MaterialTapTargetSize {
/// Expands the minimum tap target size to 48px by 48px.
///
/// This is the default value of [ThemeData.materialTapTargetSize] and the
/// recommended size to conform to Android accessibility scanner
/// recommendations.
padded,
/// Shrinks the tap target size to the minimum provided by the Material
/// specification.
shrinkWrap,
}
/// Defines the configuration of the overall visual [Theme] for a [MaterialApp]
/// or a widget subtree within the app.
///
/// The [MaterialApp] theme property can be used to configure the appearance
/// of the entire app. Widget subtrees within an app can override the app's
/// theme by including a [Theme] widget at the top of the subtree.
///
/// Widgets whose appearance should align with the overall theme can obtain the
/// current theme's configuration with [Theme.of]. Material components typically
/// depend exclusively on the [colorScheme] and [textTheme]. These properties
/// are guaranteed to have non-null values.
///
/// The static [Theme.of] method finds the [ThemeData] value specified for the
/// nearest [BuildContext] ancestor. This lookup is inexpensive, essentially
/// just a single HashMap access. It can sometimes be a little confusing
/// because [Theme.of] can not see a [Theme] widget that is defined in the
/// current build method's context. To overcome that, create a new custom widget
/// for the subtree that appears below the new [Theme], or insert a widget
/// that creates a new BuildContext, like [Builder].
///
/// {@tool dartpad}
/// This example demonstrates how a typical [MaterialApp] specifies
/// and uses a custom [Theme]. The theme's [ColorScheme] is based on a
/// single "seed" color and configures itself to match the platform's
/// current light or dark color configuration. The theme overrides the
/// default configuration of [FloatingActionButton] to show how to
/// customize the appearance a class of components.
///
/// ** See code in examples/api/lib/material/theme_data/theme_data.0.dart **
/// {@end-tool}
///
/// See <https://material.io/design/color/> for
/// more discussion on how to pick the right colors.
@immutable
class ThemeData with Diagnosticable {
/// Create a [ThemeData] that's used to configure a [Theme].
///
/// The [colorScheme] and [textTheme] are used by the Material components to
/// compute default values for visual properties. The API documentation for
/// each component widget explains exactly how the defaults are computed.
///
/// When providing a [ColorScheme], apps can either provide one directly
/// with the [colorScheme] parameter, or have one generated for them by
/// using the [colorSchemeSeed] and [brightness] parameters. A generated
/// color scheme will be based on the tones of [colorSchemeSeed] and all of
/// its contrasting color will meet accessibility guidelines for readability.
/// (See [ColorScheme.fromSeed] for more details.)
///
/// If the app wants to customize a generated color scheme, it can use
/// [ColorScheme.fromSeed] directly and then [ColorScheme.copyWith] on the
/// result to override any colors that need to be replaced. The result of
/// this can be used as the [colorScheme] directly.
///
/// For historical reasons, instead of using a [colorSchemeSeed] or
/// [colorScheme], you can provide either a [primaryColor] or [primarySwatch]
/// to construct the [colorScheme], but the results will not be as complete
/// as when using generation from a seed color.
///
/// If [colorSchemeSeed] is non-null then [colorScheme], [primaryColor] and
/// [primarySwatch] must all be null.
///
/// The [textTheme] [TextStyle] colors are black if the color scheme's
/// brightness is [Brightness.light], and white for [Brightness.dark].
///
/// To override the appearance of specific components, provide
/// a component theme parameter like [sliderTheme], [toggleButtonsTheme],
/// or [bottomNavigationBarTheme].
///
/// See also:
///
/// * [ThemeData.from], which creates a ThemeData from a [ColorScheme].
/// * [ThemeData.light], which creates a light blue theme.
/// * [ThemeData.dark], which creates dark theme with a teal secondary [ColorScheme] color.
/// * [ColorScheme.fromSeed], which is used to create a [ColorScheme] from a seed color.
factory ThemeData({
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
Iterable<Adaptation<Object>>? adaptations,
bool? applyElevationOverlayColor,
NoDefaultCupertinoThemeData? cupertinoOverrideTheme,
Iterable<ThemeExtension<dynamic>>? extensions,
InputDecorationTheme? inputDecorationTheme,
MaterialTapTargetSize? materialTapTargetSize,
PageTransitionsTheme? pageTransitionsTheme,
TargetPlatform? platform,
ScrollbarThemeData? scrollbarTheme,
InteractiveInkFeatureFactory? splashFactory,
bool? useMaterial3,
VisualDensity? visualDensity,
// COLOR
// [colorScheme] is the preferred way to configure colors. The other color
// properties (as well as primarySwatch) will gradually be phased out, see
// https://github.com/flutter/flutter/issues/91772.
Brightness? brightness,
Color? canvasColor,
Color? cardColor,
ColorScheme? colorScheme,
Color? colorSchemeSeed,
Color? dialogBackgroundColor,
Color? disabledColor,
Color? dividerColor,
Color? focusColor,
Color? highlightColor,
Color? hintColor,
Color? hoverColor,
Color? indicatorColor,
Color? primaryColor,
Color? primaryColorDark,
Color? primaryColorLight,
MaterialColor? primarySwatch,
Color? scaffoldBackgroundColor,
Color? secondaryHeaderColor,
Color? shadowColor,
Color? splashColor,
Color? unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
String? fontFamily,
List<String>? fontFamilyFallback,
String? package,
IconThemeData? iconTheme,
IconThemeData? primaryIconTheme,
TextTheme? primaryTextTheme,
TextTheme? textTheme,
Typography? typography,
// COMPONENT THEMES
ActionIconThemeData? actionIconTheme,
AppBarTheme? appBarTheme,
BadgeThemeData? badgeTheme,
MaterialBannerThemeData? bannerTheme,
BottomAppBarTheme? bottomAppBarTheme,
BottomNavigationBarThemeData? bottomNavigationBarTheme,
BottomSheetThemeData? bottomSheetTheme,
ButtonBarThemeData? buttonBarTheme,
ButtonThemeData? buttonTheme,
CardTheme? cardTheme,
CheckboxThemeData? checkboxTheme,
ChipThemeData? chipTheme,
DataTableThemeData? dataTableTheme,
DatePickerThemeData? datePickerTheme,
DialogTheme? dialogTheme,
DividerThemeData? dividerTheme,
DrawerThemeData? drawerTheme,
DropdownMenuThemeData? dropdownMenuTheme,
ElevatedButtonThemeData? elevatedButtonTheme,
ExpansionTileThemeData? expansionTileTheme,
FilledButtonThemeData? filledButtonTheme,
FloatingActionButtonThemeData? floatingActionButtonTheme,
IconButtonThemeData? iconButtonTheme,
ListTileThemeData? listTileTheme,
MenuBarThemeData? menuBarTheme,
MenuButtonThemeData? menuButtonTheme,
MenuThemeData? menuTheme,
NavigationBarThemeData? navigationBarTheme,
NavigationDrawerThemeData? navigationDrawerTheme,
NavigationRailThemeData? navigationRailTheme,
OutlinedButtonThemeData? outlinedButtonTheme,
PopupMenuThemeData? popupMenuTheme,
ProgressIndicatorThemeData? progressIndicatorTheme,
RadioThemeData? radioTheme,
SearchBarThemeData? searchBarTheme,
SearchViewThemeData? searchViewTheme,
SegmentedButtonThemeData? segmentedButtonTheme,
SliderThemeData? sliderTheme,
SnackBarThemeData? snackBarTheme,
SwitchThemeData? switchTheme,
TabBarTheme? tabBarTheme,
TextButtonThemeData? textButtonTheme,
TextSelectionThemeData? textSelectionTheme,
TimePickerThemeData? timePickerTheme,
ToggleButtonsThemeData? toggleButtonsTheme,
TooltipThemeData? tooltipTheme,
}) {
// GENERAL CONFIGURATION
cupertinoOverrideTheme = cupertinoOverrideTheme?.noDefault();
extensions ??= <ThemeExtension<dynamic>>[];
adaptations ??= <Adaptation<Object>>[];
inputDecorationTheme ??= const InputDecorationTheme();
platform ??= defaultTargetPlatform;
switch (platform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
materialTapTargetSize ??= MaterialTapTargetSize.padded;
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
materialTapTargetSize ??= MaterialTapTargetSize.shrinkWrap;
}
pageTransitionsTheme ??= const PageTransitionsTheme();
scrollbarTheme ??= const ScrollbarThemeData();
visualDensity ??= VisualDensity.defaultDensityForPlatform(platform);
useMaterial3 ??= true;
final bool useInkSparkle = platform == TargetPlatform.android && !kIsWeb;
splashFactory ??= useMaterial3
? useInkSparkle ? InkSparkle.splashFactory : InkRipple.splashFactory
: InkSplash.splashFactory;
// COLOR
assert(
colorScheme?.brightness == null || brightness == null || colorScheme!.brightness == brightness,
'ThemeData.brightness does not match ColorScheme.brightness. '
'Either override ColorScheme.brightness or ThemeData.brightness to '
'match the other.'
);
assert(colorSchemeSeed == null || colorScheme == null);
assert(colorSchemeSeed == null || primarySwatch == null);
assert(colorSchemeSeed == null || primaryColor == null);
final Brightness effectiveBrightness = brightness ?? colorScheme?.brightness ?? Brightness.light;
final bool isDark = effectiveBrightness == Brightness.dark;
if (colorSchemeSeed != null || useMaterial3) {
if (colorSchemeSeed != null) {
colorScheme = ColorScheme.fromSeed(seedColor: colorSchemeSeed, brightness: effectiveBrightness);
}
colorScheme ??= isDark ? _colorSchemeDarkM3 : _colorSchemeLightM3;
// For surfaces that use primary color in light themes and surface color in dark
final Color primarySurfaceColor = isDark ? colorScheme.surface : colorScheme.primary;
final Color onPrimarySurfaceColor = isDark ? colorScheme.onSurface : colorScheme.onPrimary;
// Default some of the color settings to values from the color scheme
primaryColor ??= primarySurfaceColor;
canvasColor ??= colorScheme.background;
scaffoldBackgroundColor ??= colorScheme.background;
cardColor ??= colorScheme.surface;
dividerColor ??= colorScheme.outline;
dialogBackgroundColor ??= colorScheme.background;
indicatorColor ??= onPrimarySurfaceColor;
applyElevationOverlayColor ??= brightness == Brightness.dark;
}
applyElevationOverlayColor ??= false;
primarySwatch ??= Colors.blue;
primaryColor ??= isDark ? Colors.grey[900]! : primarySwatch;
final Brightness estimatedPrimaryColorBrightness = estimateBrightnessForColor(primaryColor);
primaryColorLight ??= isDark ? Colors.grey[500]! : primarySwatch[100]!;
primaryColorDark ??= isDark ? Colors.black : primarySwatch[700]!;
final bool primaryIsDark = estimatedPrimaryColorBrightness == Brightness.dark;
focusColor ??= isDark ? Colors.white.withOpacity(0.12) : Colors.black.withOpacity(0.12);
hoverColor ??= isDark ? Colors.white.withOpacity(0.04) : Colors.black.withOpacity(0.04);
shadowColor ??= Colors.black;
canvasColor ??= isDark ? Colors.grey[850]! : Colors.grey[50]!;
scaffoldBackgroundColor ??= canvasColor;
cardColor ??= isDark ? Colors.grey[800]! : Colors.white;
dividerColor ??= isDark ? const Color(0x1FFFFFFF) : const Color(0x1F000000);
// Create a ColorScheme that is backwards compatible as possible
// with the existing default ThemeData color values.
colorScheme ??= ColorScheme.fromSwatch(
primarySwatch: primarySwatch,
accentColor: isDark ? Colors.tealAccent[200]! : primarySwatch[500]!,
cardColor: cardColor,
backgroundColor: isDark ? Colors.grey[700]! : primarySwatch[200]!,
errorColor: Colors.red[700],
brightness: effectiveBrightness,
);
unselectedWidgetColor ??= isDark ? Colors.white70 : Colors.black54;
// Spec doesn't specify a dark theme secondaryHeaderColor, this is a guess.
secondaryHeaderColor ??= isDark ? Colors.grey[700]! : primarySwatch[50]!;
dialogBackgroundColor ??= isDark ? Colors.grey[800]! : Colors.white;
indicatorColor ??= colorScheme.secondary == primaryColor ? Colors.white : colorScheme.secondary;
hintColor ??= isDark ? Colors.white60 : Colors.black.withOpacity(0.6);
// The default [buttonTheme] is here because it doesn't use the defaults for
// [disabledColor], [highlightColor], and [splashColor].
buttonTheme ??= ButtonThemeData(
colorScheme: colorScheme,
buttonColor: isDark ? primarySwatch[600]! : Colors.grey[300]!,
disabledColor: disabledColor,
focusColor: focusColor,
hoverColor: hoverColor,
highlightColor: highlightColor,
splashColor: splashColor,
materialTapTargetSize: materialTapTargetSize,
);
disabledColor ??= isDark ? Colors.white38 : Colors.black38;
highlightColor ??= isDark ? _kDarkThemeHighlightColor : _kLightThemeHighlightColor;
splashColor ??= isDark ? _kDarkThemeSplashColor : _kLightThemeSplashColor;
// TYPOGRAPHY & ICONOGRAPHY
typography ??= useMaterial3
? Typography.material2021(platform: platform, colorScheme: colorScheme)
: Typography.material2014(platform: platform);
TextTheme defaultTextTheme = isDark ? typography.white : typography.black;
TextTheme defaultPrimaryTextTheme = primaryIsDark ? typography.white : typography.black;
if (fontFamily != null) {
defaultTextTheme = defaultTextTheme.apply(fontFamily: fontFamily);
defaultPrimaryTextTheme = defaultPrimaryTextTheme.apply(fontFamily: fontFamily);
}
if (fontFamilyFallback != null) {
defaultTextTheme = defaultTextTheme.apply(fontFamilyFallback: fontFamilyFallback);
defaultPrimaryTextTheme = defaultPrimaryTextTheme.apply(fontFamilyFallback: fontFamilyFallback);
}
if (package != null) {
defaultTextTheme = defaultTextTheme.apply(package: package);
defaultPrimaryTextTheme = defaultPrimaryTextTheme.apply(package: package);
}
textTheme = defaultTextTheme.merge(textTheme);
primaryTextTheme = defaultPrimaryTextTheme.merge(primaryTextTheme);
iconTheme ??= isDark ? IconThemeData(color: kDefaultIconLightColor) : IconThemeData(color: kDefaultIconDarkColor);
primaryIconTheme ??= primaryIsDark ? const IconThemeData(color: Colors.white) : const IconThemeData(color: Colors.black);
// COMPONENT THEMES
appBarTheme ??= const AppBarTheme();
badgeTheme ??= const BadgeThemeData();
bannerTheme ??= const MaterialBannerThemeData();
bottomAppBarTheme ??= const BottomAppBarTheme();
bottomNavigationBarTheme ??= const BottomNavigationBarThemeData();
bottomSheetTheme ??= const BottomSheetThemeData();
buttonBarTheme ??= const ButtonBarThemeData();
cardTheme ??= const CardTheme();
checkboxTheme ??= const CheckboxThemeData();
chipTheme ??= const ChipThemeData();
dataTableTheme ??= const DataTableThemeData();
datePickerTheme ??= const DatePickerThemeData();
dialogTheme ??= const DialogTheme();
dividerTheme ??= const DividerThemeData();
drawerTheme ??= const DrawerThemeData();
dropdownMenuTheme ??= const DropdownMenuThemeData();
elevatedButtonTheme ??= const ElevatedButtonThemeData();
expansionTileTheme ??= const ExpansionTileThemeData();
filledButtonTheme ??= const FilledButtonThemeData();
floatingActionButtonTheme ??= const FloatingActionButtonThemeData();
iconButtonTheme ??= const IconButtonThemeData();
listTileTheme ??= const ListTileThemeData();
menuBarTheme ??= const MenuBarThemeData();
menuButtonTheme ??= const MenuButtonThemeData();
menuTheme ??= const MenuThemeData();
navigationBarTheme ??= const NavigationBarThemeData();
navigationDrawerTheme ??= const NavigationDrawerThemeData();
navigationRailTheme ??= const NavigationRailThemeData();
outlinedButtonTheme ??= const OutlinedButtonThemeData();
popupMenuTheme ??= const PopupMenuThemeData();
progressIndicatorTheme ??= const ProgressIndicatorThemeData();
radioTheme ??= const RadioThemeData();
searchBarTheme ??= const SearchBarThemeData();
searchViewTheme ??= const SearchViewThemeData();
segmentedButtonTheme ??= const SegmentedButtonThemeData();
sliderTheme ??= const SliderThemeData();
snackBarTheme ??= const SnackBarThemeData();
switchTheme ??= const SwitchThemeData();
tabBarTheme ??= const TabBarTheme();
textButtonTheme ??= const TextButtonThemeData();
textSelectionTheme ??= const TextSelectionThemeData();
timePickerTheme ??= const TimePickerThemeData();
toggleButtonsTheme ??= const ToggleButtonsThemeData();
tooltipTheme ??= const TooltipThemeData();
return ThemeData.raw(
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
adaptationMap: _createAdaptationMap(adaptations),
applyElevationOverlayColor: applyElevationOverlayColor,
cupertinoOverrideTheme: cupertinoOverrideTheme,
extensions: _themeExtensionIterableToMap(extensions),
inputDecorationTheme: inputDecorationTheme,
materialTapTargetSize: materialTapTargetSize,
pageTransitionsTheme: pageTransitionsTheme,
platform: platform,
scrollbarTheme: scrollbarTheme,
splashFactory: splashFactory,
useMaterial3: useMaterial3,
visualDensity: visualDensity,
// COLOR
canvasColor: canvasColor,
cardColor: cardColor,
colorScheme: colorScheme,
dialogBackgroundColor: dialogBackgroundColor,
disabledColor: disabledColor,
dividerColor: dividerColor,
focusColor: focusColor,
highlightColor: highlightColor,
hintColor: hintColor,
hoverColor: hoverColor,
indicatorColor: indicatorColor,
primaryColor: primaryColor,
primaryColorDark: primaryColorDark,
primaryColorLight: primaryColorLight,
scaffoldBackgroundColor: scaffoldBackgroundColor,
secondaryHeaderColor: secondaryHeaderColor,
shadowColor: shadowColor,
splashColor: splashColor,
unselectedWidgetColor: unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
iconTheme: iconTheme,
primaryTextTheme: primaryTextTheme,
textTheme: textTheme,
typography: typography,
primaryIconTheme: primaryIconTheme,
// COMPONENT THEMES
actionIconTheme: actionIconTheme,
appBarTheme: appBarTheme,
badgeTheme: badgeTheme,
bannerTheme: bannerTheme,
bottomAppBarTheme: bottomAppBarTheme,
bottomNavigationBarTheme: bottomNavigationBarTheme,
bottomSheetTheme: bottomSheetTheme,
buttonBarTheme: buttonBarTheme,
buttonTheme: buttonTheme,
cardTheme: cardTheme,
checkboxTheme: checkboxTheme,
chipTheme: chipTheme,
dataTableTheme: dataTableTheme,
datePickerTheme: datePickerTheme,
dialogTheme: dialogTheme,
dividerTheme: dividerTheme,
drawerTheme: drawerTheme,
dropdownMenuTheme: dropdownMenuTheme,
elevatedButtonTheme: elevatedButtonTheme,
expansionTileTheme: expansionTileTheme,
filledButtonTheme: filledButtonTheme,
floatingActionButtonTheme: floatingActionButtonTheme,
iconButtonTheme: iconButtonTheme,
listTileTheme: listTileTheme,
menuBarTheme: menuBarTheme,
menuButtonTheme: menuButtonTheme,
menuTheme: menuTheme,
navigationBarTheme: navigationBarTheme,
navigationDrawerTheme: navigationDrawerTheme,
navigationRailTheme: navigationRailTheme,
outlinedButtonTheme: outlinedButtonTheme,
popupMenuTheme: popupMenuTheme,
progressIndicatorTheme: progressIndicatorTheme,
radioTheme: radioTheme,
searchBarTheme: searchBarTheme,
searchViewTheme: searchViewTheme,
segmentedButtonTheme: segmentedButtonTheme,
sliderTheme: sliderTheme,
snackBarTheme: snackBarTheme,
switchTheme: switchTheme,
tabBarTheme: tabBarTheme,
textButtonTheme: textButtonTheme,
textSelectionTheme: textSelectionTheme,
timePickerTheme: timePickerTheme,
toggleButtonsTheme: toggleButtonsTheme,
tooltipTheme: tooltipTheme,
);
}
/// Create a [ThemeData] given a set of exact values. Most values must be
/// specified. They all must also be non-null except for
/// [cupertinoOverrideTheme], and deprecated members.
///
/// This will rarely be used directly. It is used by [lerp] to
/// create intermediate themes based on two themes created with the
/// [ThemeData] constructor.
const ThemeData.raw({
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
required this.adaptationMap,
required this.applyElevationOverlayColor,
required this.cupertinoOverrideTheme,
required this.extensions,
required this.inputDecorationTheme,
required this.materialTapTargetSize,
required this.pageTransitionsTheme,
required this.platform,
required this.scrollbarTheme,
required this.splashFactory,
required this.useMaterial3,
required this.visualDensity,
// COLOR
// [colorScheme] is the preferred way to configure colors. The other color
// properties will gradually be phased out, see
// https://github.com/flutter/flutter/issues/91772.
required this.canvasColor,
required this.cardColor,
required this.colorScheme,
required this.dialogBackgroundColor,
required this.disabledColor,
required this.dividerColor,
required this.focusColor,
required this.highlightColor,
required this.hintColor,
required this.hoverColor,
required this.indicatorColor,
required this.primaryColor,
required this.primaryColorDark,
required this.primaryColorLight,
required this.scaffoldBackgroundColor,
required this.secondaryHeaderColor,
required this.shadowColor,
required this.splashColor,
required this.unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
required this.iconTheme,
required this.primaryIconTheme,
required this.primaryTextTheme,
required this.textTheme,
required this.typography,
// COMPONENT THEMES
required this.actionIconTheme,
required this.appBarTheme,
required this.badgeTheme,
required this.bannerTheme,
required this.bottomAppBarTheme,
required this.bottomNavigationBarTheme,
required this.bottomSheetTheme,
required this.buttonBarTheme,
required this.buttonTheme,
required this.cardTheme,
required this.checkboxTheme,
required this.chipTheme,
required this.dataTableTheme,
required this.datePickerTheme,
required this.dialogTheme,
required this.dividerTheme,
required this.drawerTheme,
required this.dropdownMenuTheme,
required this.elevatedButtonTheme,
required this.expansionTileTheme,
required this.filledButtonTheme,
required this.floatingActionButtonTheme,
required this.iconButtonTheme,
required this.listTileTheme,
required this.menuBarTheme,
required this.menuButtonTheme,
required this.menuTheme,
required this.navigationBarTheme,
required this.navigationDrawerTheme,
required this.navigationRailTheme,
required this.outlinedButtonTheme,
required this.popupMenuTheme,
required this.progressIndicatorTheme,
required this.radioTheme,
required this.searchBarTheme,
required this.searchViewTheme,
required this.segmentedButtonTheme,
required this.sliderTheme,
required this.snackBarTheme,
required this.switchTheme,
required this.tabBarTheme,
required this.textButtonTheme,
required this.textSelectionTheme,
required this.timePickerTheme,
required this.toggleButtonsTheme,
required this.tooltipTheme,
});
/// Create a [ThemeData] based on the colors in the given [colorScheme] and
/// text styles of the optional [textTheme].
///
/// If [colorScheme].brightness is [Brightness.dark] then
/// [ThemeData.applyElevationOverlayColor] will be set to true to support
/// the Material dark theme method for indicating elevation by applying
/// a semi-transparent onSurface color on top of the surface color.
///
/// This is the recommended method to theme your application. As we move
/// forward we will be converting all the widget implementations to only use
/// colors or colors derived from those in [ColorScheme].
///
/// {@tool snippet}
/// This example will set up an application to use the baseline Material
/// Design light and dark themes.
///
/// ```dart
/// MaterialApp(
/// theme: ThemeData.from(colorScheme: const ColorScheme.light()),
/// darkTheme: ThemeData.from(colorScheme: const ColorScheme.dark()),
/// )
/// ```
/// {@end-tool}
///
/// See <https://material.io/design/color/> for
/// more discussion on how to pick the right colors.
factory ThemeData.from({
required ColorScheme colorScheme,
TextTheme? textTheme,
bool? useMaterial3,
}) {
final bool isDark = colorScheme.brightness == Brightness.dark;
// For surfaces that use primary color in light themes and surface color in dark
final Color primarySurfaceColor = isDark ? colorScheme.surface : colorScheme.primary;
final Color onPrimarySurfaceColor = isDark ? colorScheme.onSurface : colorScheme.onPrimary;
return ThemeData(
colorScheme: colorScheme,
brightness: colorScheme.brightness,
primaryColor: primarySurfaceColor,
canvasColor: colorScheme.background,
scaffoldBackgroundColor: colorScheme.background,
cardColor: colorScheme.surface,
dividerColor: colorScheme.onSurface.withOpacity(0.12),
dialogBackgroundColor: colorScheme.background,
indicatorColor: onPrimarySurfaceColor,
textTheme: textTheme,
applyElevationOverlayColor: isDark,
useMaterial3: useMaterial3,
);
}
/// A default light theme.
///
/// This theme does not contain text geometry. Instead, it is expected that
/// this theme is localized using text geometry using [ThemeData.localize].
factory ThemeData.light({bool? useMaterial3}) => ThemeData(
brightness: Brightness.light,
useMaterial3: useMaterial3,
);
/// A default dark theme.
///
/// This theme does not contain text geometry. Instead, it is expected that
/// this theme is localized using text geometry using [ThemeData.localize].
factory ThemeData.dark({bool? useMaterial3}) => ThemeData(
brightness: Brightness.dark,
useMaterial3: useMaterial3,
);
/// The default color theme. Same as [ThemeData.light].
///
/// This is used by [Theme.of] when no theme has been specified.
///
/// This theme does not contain text geometry. Instead, it is expected that
/// this theme is localized using text geometry using [ThemeData.localize].
///
/// Most applications would use [Theme.of], which provides correct localized
/// text geometry.
factory ThemeData.fallback({bool? useMaterial3}) => ThemeData.light(useMaterial3: useMaterial3);
/// Used to obtain a particular [Adaptation] from [adaptationMap].
///
/// To get an adaptation, use `Theme.of(context).getAdaptation<MyAdaptation>()`.
Adaptation<T>? getAdaptation<T>() => adaptationMap[T] as Adaptation<T>?;
static Map<Type, Adaptation<Object>> _createAdaptationMap(Iterable<Adaptation<Object>> adaptations) {
final Map<Type, Adaptation<Object>> adaptationMap = <Type, Adaptation<Object>>{
for (final Adaptation<Object> adaptation in adaptations)
adaptation.type: adaptation
};
return adaptationMap;
}
/// The overall theme brightness.
///
/// The default [TextStyle] color for the [textTheme] is black if the
/// theme is constructed with [Brightness.light] and white if the
/// theme is constructed with [Brightness.dark].
Brightness get brightness => colorScheme.brightness;
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
/// Apply a semi-transparent overlay color on Material surfaces to indicate
/// elevation for dark themes.
///
/// If [useMaterial3] is true, then this flag is ignored as there is a new
/// [Material.surfaceTintColor] used to create an overlay for Material 3.
/// This flag is meant only for the Material 2 elevation overlay for dark
/// themes.
///
/// Material drop shadows can be difficult to see in a dark theme, so the
/// elevation of a surface should be portrayed with an "overlay" in addition
/// to the shadow. As the elevation of the component increases, the
/// overlay increases in opacity. [applyElevationOverlayColor] turns the
/// application of this overlay on or off for dark themes.
///
/// If true and [brightness] is [Brightness.dark], a
/// semi-transparent version of [ColorScheme.onSurface] will be
/// applied on top of [Material] widgets that have a [ColorScheme.surface]
/// color. The level of transparency is based on [Material.elevation] as
/// per the Material Dark theme specification.
///
/// If false the surface color will be used unmodified.
///
/// Defaults to false in order to maintain backwards compatibility with
/// apps that were built before the Material Dark theme specification
/// was published. New apps should set this to true for any themes
/// where [brightness] is [Brightness.dark].
///
/// See also:
///
/// * [Material.elevation], which effects the level of transparency of the
/// overlay color.
/// * [ElevationOverlay.applyOverlay], which is used by [Material] to apply
/// the overlay color to its surface color.
/// * <https://material.io/design/color/dark-theme.html>, which specifies how
/// the overlay should be applied.
final bool applyElevationOverlayColor;
/// Components of the [CupertinoThemeData] to override from the Material
/// [ThemeData] adaptation.
///
/// By default, [cupertinoOverrideTheme] is null and Cupertino widgets
/// descendant to the Material [Theme] will adhere to a [CupertinoTheme]
/// derived from the Material [ThemeData]. e.g. [ThemeData]'s [ColorScheme]
/// will also inform the [CupertinoThemeData]'s `primaryColor` etc.
///
/// This cascading effect for individual attributes of the [CupertinoThemeData]
/// can be overridden using attributes of this [cupertinoOverrideTheme].
final NoDefaultCupertinoThemeData? cupertinoOverrideTheme;
/// Arbitrary additions to this theme.
///
/// To define extensions, pass an [Iterable] containing one or more [ThemeExtension]
/// subclasses to [ThemeData.new] or [copyWith].
///
/// To obtain an extension, use [extension].
///
/// {@tool dartpad}
/// This sample shows how to create and use a subclass of [ThemeExtension] that
/// defines two colors.
///
/// ** See code in examples/api/lib/material/theme/theme_extension.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [extension], a convenience function for obtaining a specific extension.
final Map<Object, ThemeExtension<dynamic>> extensions;
/// Used to obtain a particular [ThemeExtension] from [extensions].
///
/// Obtain with `Theme.of(context).extension<MyThemeExtension>()`.
///
/// See [extensions] for an interactive example.
T? extension<T>() => extensions[T] as T?;
/// A map which contains the adaptations for the theme. The entry's key is the
/// type of the adaptation; the value is the adaptation itself.
///
/// To obtain an adaptation, use [getAdaptation].
final Map<Type, Adaptation<Object>> adaptationMap;
/// The default [InputDecoration] values for [InputDecorator], [TextField],
/// and [TextFormField] are based on this theme.
///
/// See [InputDecoration.applyDefaults].
final InputDecorationTheme inputDecorationTheme;
/// Configures the hit test size of certain Material widgets.
///
/// Defaults to a [platform]-appropriate size: [MaterialTapTargetSize.padded]
/// on mobile platforms, [MaterialTapTargetSize.shrinkWrap] on desktop
/// platforms.
final MaterialTapTargetSize materialTapTargetSize;
/// Default [MaterialPageRoute] transitions per [TargetPlatform].
///
/// [MaterialPageRoute.buildTransitions] delegates to a [platform] specific
/// [PageTransitionsBuilder]. If a matching builder is not found, a builder
/// whose platform is null is used.
final PageTransitionsTheme pageTransitionsTheme;
/// The platform the material widgets should adapt to target.
///
/// Defaults to the current platform, as exposed by [defaultTargetPlatform].
/// This should be used in order to style UI elements according to platform
/// conventions.
///
/// Widgets from the material library should use this getter (via [Theme.of])
/// to determine the current platform for the purpose of emulating the
/// platform behavior (e.g. scrolling or haptic effects). Widgets and render
/// objects at lower layers that try to emulate the underlying platform
/// can depend on [defaultTargetPlatform] directly, or may require
/// that the target platform be provided as an argument. The
/// [dart:io.Platform] object should only be used directly when it's critical
/// to actually know the current platform, without any overrides possible (for
/// example, when a system API is about to be called).
///
/// In a test environment, the platform returned is [TargetPlatform.android]
/// regardless of the host platform. (Android was chosen because the tests
/// were originally written assuming Android-like behavior, and we added
/// platform adaptations for other platforms later). Tests can check behavior
/// for other platforms by setting the [platform] of the [Theme] explicitly to
/// another [TargetPlatform] value, or by setting
/// [debugDefaultTargetPlatformOverride].
///
/// Determines the defaults for [typography] and [materialTapTargetSize].
final TargetPlatform platform;
/// A theme for customizing the colors, thickness, and shape of [Scrollbar]s.
final ScrollbarThemeData scrollbarTheme;
/// Defines the appearance of ink splashes produces by [InkWell]
/// and [InkResponse].
///
/// See also:
///
/// * [InkSplash.splashFactory], which defines the default splash.
/// * [InkRipple.splashFactory], which defines a splash that spreads out
/// more aggressively than the default.
/// * [InkSparkle.splashFactory], which defines a more aggressive and organic
/// splash with sparkle effects.
final InteractiveInkFeatureFactory splashFactory;
/// A temporary flag that can be used to opt-out of Material 3 features.
///
/// This flag is _true_ by default. If false, then components will
/// continue to use the colors, typography and other features of
/// Material 2.
///
/// In the long run this flag will be deprecated and eventually
/// only Material 3 will be supported. We recommend that applications
/// migrate to Material 3 as soon as that's practical. Until that migration
/// is complete, this flag can be set to false.
///
/// ## Defaults
///
/// If a [ThemeData] is _constructed_ with [useMaterial3] set to true, then
/// some properties will get updated defaults. However, the
/// [ThemeData.copyWith] method with [useMaterial3] set to true will _not_
/// change any of these properties in the resulting [ThemeData].
///
/// <style>table,td,th { border-collapse: collapse; padding: 0.45em; } td { border: 1px solid }</style>
///
/// | Property | Material 3 default | Material 2 default |
/// | :-------------- | :----------------------------- | :----------------------------- |
/// | [colorScheme] | M3 baseline light color scheme | M2 baseline light color scheme |
/// | [typography] | [Typography.material2021] | [Typography.material2014] |
/// | [splashFactory] | [InkSparkle]* or [InkRipple] | [InkSplash] |
///
/// \* if the target platform is Android and the app is not
/// running on the web, otherwise it will fallback to [InkRipple].
///
/// If [brightness] is [Brightness.dark] then the default color scheme will
/// be either the M3 baseline dark color scheme or the M2 baseline dark color
/// scheme depending on [useMaterial3].
///
/// ## Affected widgets
///
/// This flag affects styles and components.
///
/// ### Styles
/// * Color: [ColorScheme], [Material] (see table above)
/// * Shape: (see components below)
/// * Typography: [Typography] (see table above)
///
/// ### Components
/// * Badges: [Badge]
/// * Bottom app bar: [BottomAppBar]
/// * Bottom sheets: [BottomSheet]
/// * Buttons
/// - Common buttons: [ElevatedButton], [FilledButton], [FilledButton.tonal], [OutlinedButton], [TextButton]
/// - FAB: [FloatingActionButton], [FloatingActionButton.extended]
/// - Icon buttons: [IconButton], [IconButton.filled] (*new*), [IconButton.filledTonal], [IconButton.outlined]
/// - Segmented buttons: [SegmentedButton] (replacing [ToggleButtons])
/// * Cards: [Card]
/// * Checkbox: [Checkbox], [CheckboxListTile]
/// * Chips:
/// - [ActionChip] (used for Assist and Suggestion chips),
/// - [FilterChip], [ChoiceChip] (used for single selection filter chips),
/// - [InputChip]
/// * Date pickers: [showDatePicker], [showDateRangePicker], [DatePickerDialog], [DateRangePickerDialog], [InputDatePickerFormField]
/// * Dialogs: [AlertDialog], [Dialog.fullscreen]
/// * Divider: [Divider], [VerticalDivider]
/// * Lists: [ListTile]
/// * Menus: [MenuAnchor], [DropdownMenu], [MenuBar]
/// * Navigation bar: [NavigationBar] (replacing [BottomNavigationBar])
/// * Navigation drawer: [NavigationDrawer] (replacing [Drawer])
/// * Navigation rail: [NavigationRail]
/// * Progress indicators: [CircularProgressIndicator], [LinearProgressIndicator]
/// * Radio button: [Radio], [RadioListTile]
/// * Search: [SearchBar], [SearchAnchor],
/// * Snack bar: [SnackBar]
/// * Slider: [Slider], [RangeSlider]
/// * Switch: [Switch], [SwitchListTile]
/// * Tabs: [TabBar], [TabBar.secondary]
/// * TextFields: [TextField] together with its [InputDecoration]
/// * Time pickers: [showTimePicker], [TimePickerDialog]
/// * Top app bar: [AppBar], [SliverAppBar], [SliverAppBar.medium], [SliverAppBar.large]
///
/// In addition, this flag enables features introduced in Android 12.
/// * Stretch overscroll: [MaterialScrollBehavior]
/// * Ripple: `splashFactory` (see table above)
///
/// See also:
///
/// * [Material 3 specification](https://m3.material.io/).
final bool useMaterial3;
/// The density value for specifying the compactness of various UI components.
///
/// {@template flutter.material.themedata.visualDensity}
/// Density, in the context of a UI, is the vertical and horizontal
/// "compactness" of the elements in the UI. It is unitless, since it means
/// different things to different UI elements. For buttons, it affects the
/// spacing around the centered label of the button. For lists, it affects the
/// distance between baselines of entries in the list.
///
/// Typically, density values are integral, but any value in range may be
/// used. The range includes values from [VisualDensity.minimumDensity] (which
/// is -4), to [VisualDensity.maximumDensity] (which is 4), inclusive, where
/// negative values indicate a denser, more compact, UI, and positive values
/// indicate a less dense, more expanded, UI. If a component doesn't support
/// the value given, it will clamp to the nearest supported value.
///
/// The default for visual densities is zero for both vertical and horizontal
/// densities, which corresponds to the default visual density of components
/// in the Material Design specification.
///
/// As a rule of thumb, a change of 1 or -1 in density corresponds to 4
/// logical pixels. However, this is not a strict relationship since
/// components interpret the density values appropriately for their needs.
///
/// A larger value translates to a spacing increase (less dense), and a
/// smaller value translates to a spacing decrease (more dense).
///
/// In Material Design 3, the [visualDensity] does not override the value of
/// [IconButton.visualDensity] which defaults to [VisualDensity.standard]
/// for all platforms. To override the default value of [IconButton.visualDensity],
/// use [ThemeData.iconButtonTheme] instead.
/// {@endtemplate}
final VisualDensity visualDensity;
// COLOR
/// The default color of [MaterialType.canvas] [Material].
final Color canvasColor;
/// The color of [Material] when it is used as a [Card].
final Color cardColor;
/// {@macro flutter.material.color_scheme.ColorScheme}
///
/// This property was added much later than the theme's set of highly specific
/// colors, like [cardColor], [canvasColor] etc. New components can be defined
/// exclusively in terms of [colorScheme]. Existing components will gradually
/// migrate to it, to the extent that is possible without significant
/// backwards compatibility breaks.
final ColorScheme colorScheme;
/// The background color of [Dialog] elements.
final Color dialogBackgroundColor;
/// The color used for widgets that are inoperative, regardless of
/// their state. For example, a disabled checkbox (which may be
/// checked or unchecked).
final Color disabledColor;
/// The color of [Divider]s and [PopupMenuDivider]s, also used
/// between [ListTile]s, between rows in [DataTable]s, and so forth.
///
/// To create an appropriate [BorderSide] that uses this color, consider
/// [Divider.createBorderSide].
final Color dividerColor;
/// The focus color used indicate that a component has the input focus.
final Color focusColor;
/// The highlight color used during ink splash animations or to
/// indicate an item in a menu is selected.
final Color highlightColor;
/// The color to use for hint text or placeholder text, e.g. in
/// [TextField] fields.
final Color hintColor;
/// The hover color used to indicate when a pointer is hovering over a
/// component.
final Color hoverColor;
/// The color of the selected tab indicator in a tab bar.
final Color indicatorColor;
/// The background color for major parts of the app (toolbars, tab bars, etc)
///
/// The theme's [colorScheme] property contains [ColorScheme.primary], as
/// well as a color that contrasts well with the primary color called
/// [ColorScheme.onPrimary]. It might be simpler to just configure an app's
/// visuals in terms of the theme's [colorScheme].
final Color primaryColor;
/// A darker version of the [primaryColor].
final Color primaryColorDark;
/// A lighter version of the [primaryColor].
final Color primaryColorLight;
/// The default color of the [Material] that underlies the [Scaffold]. The
/// background color for a typical material app or a page within the app.
final Color scaffoldBackgroundColor;
/// The color of the header of a [PaginatedDataTable] when there are selected rows.
// According to the spec for data tables:
// https://material.io/archive/guidelines/components/data-tables.html#data-tables-tables-within-cards
// ...this should be the "50-value of secondary app color".
final Color secondaryHeaderColor;
/// The color that the [Material] widget uses to draw elevation shadows.
///
/// Defaults to fully opaque black.
///
/// Shadows can be difficult to see in a dark theme, so the elevation of a
/// surface should be rendered with an "overlay" in addition to the shadow.
/// As the elevation of the component increases, the overlay increases in
/// opacity. The [applyElevationOverlayColor] property turns the elevation
/// overlay on or off for dark themes.
final Color shadowColor;
/// The color of ink splashes.
///
/// See also:
/// * [splashFactory], which defines the appearance of the splash.
final Color splashColor;
/// The color used for widgets in their inactive (but enabled)
/// state. For example, an unchecked checkbox. See also [disabledColor].
final Color unselectedWidgetColor;
// TYPOGRAPHY & ICONOGRAPHY
/// An icon theme that contrasts with the card and canvas colors.
final IconThemeData iconTheme;
/// An icon theme that contrasts with the primary color.
final IconThemeData primaryIconTheme;
/// A text theme that contrasts with the primary color.
final TextTheme primaryTextTheme;
/// Text with a color that contrasts with the card and canvas colors.
final TextTheme textTheme;
/// The color and geometry [TextTheme] values used to configure [textTheme].
///
/// Defaults to a [platform]-appropriate typography.
final Typography typography;
// COMPONENT THEMES
/// A theme for customizing icons of [BackButtonIcon], [CloseButtonIcon],
/// [DrawerButtonIcon], or [EndDrawerButtonIcon].
final ActionIconThemeData? actionIconTheme;
/// A theme for customizing the color, elevation, brightness, iconTheme and
/// textTheme of [AppBar]s.
final AppBarTheme appBarTheme;
/// A theme for customizing the color of [Badge]s.
final BadgeThemeData badgeTheme;
/// A theme for customizing the color and text style of a [MaterialBanner].
final MaterialBannerThemeData bannerTheme;
/// A theme for customizing the shape, elevation, and color of a [BottomAppBar].
final BottomAppBarTheme bottomAppBarTheme;
/// A theme for customizing the appearance and layout of [BottomNavigationBar]
/// widgets.
final BottomNavigationBarThemeData bottomNavigationBarTheme;
/// A theme for customizing the color, elevation, and shape of a bottom sheet.
final BottomSheetThemeData bottomSheetTheme;
/// A theme for customizing the appearance and layout of [ButtonBar] widgets.
final ButtonBarThemeData buttonBarTheme;
/// Defines the default configuration of button widgets, like [DropdownButton]
/// and [ButtonBar].
final ButtonThemeData buttonTheme;
/// The colors and styles used to render [Card].
///
/// This is the value returned from [CardTheme.of].
final CardTheme cardTheme;
/// A theme for customizing the appearance and layout of [Checkbox] widgets.
final CheckboxThemeData checkboxTheme;
/// The colors and styles used to render [Chip]s.
///
/// This is the value returned from [ChipTheme.of].
final ChipThemeData chipTheme;
/// A theme for customizing the appearance and layout of [DataTable]
/// widgets.
final DataTableThemeData dataTableTheme;
/// A theme for customizing the appearance and layout of [DatePickerDialog]
/// widgets.
final DatePickerThemeData datePickerTheme;
/// A theme for customizing the shape of a dialog.
final DialogTheme dialogTheme;
/// A theme for customizing the color, thickness, and indents of [Divider]s,
/// [VerticalDivider]s, etc.
final DividerThemeData dividerTheme;
/// A theme for customizing the appearance and layout of [Drawer] widgets.
final DrawerThemeData drawerTheme;
/// A theme for customizing the appearance and layout of [DropdownMenu] widgets.
final DropdownMenuThemeData dropdownMenuTheme;
/// A theme for customizing the appearance and internal layout of
/// [ElevatedButton]s.
final ElevatedButtonThemeData elevatedButtonTheme;
/// A theme for customizing the visual properties of [ExpansionTile]s.
final ExpansionTileThemeData expansionTileTheme;
/// A theme for customizing the appearance and internal layout of
/// [FilledButton]s.
final FilledButtonThemeData filledButtonTheme;
/// A theme for customizing the shape, elevation, and color of a
/// [FloatingActionButton].
final FloatingActionButtonThemeData floatingActionButtonTheme;
/// A theme for customizing the appearance and internal layout of
/// [IconButton]s.
final IconButtonThemeData iconButtonTheme;
/// A theme for customizing the appearance of [ListTile] widgets.
final ListTileThemeData listTileTheme;
/// A theme for customizing the color, shape, elevation, and other [MenuStyle]
/// aspects of the menu bar created by the [MenuBar] widget.
final MenuBarThemeData menuBarTheme;
/// A theme for customizing the color, shape, elevation, and text style of
/// cascading menu buttons created by [SubmenuButton] or [MenuItemButton].
final MenuButtonThemeData menuButtonTheme;
/// A theme for customizing the color, shape, elevation, and other [MenuStyle]
/// attributes of menus created by the [SubmenuButton] widget.
final MenuThemeData menuTheme;
/// A theme for customizing the background color, text style, and icon themes
/// of a [NavigationBar].
final NavigationBarThemeData navigationBarTheme;
/// A theme for customizing the background color, text style, and icon themes
/// of a [NavigationDrawer].
final NavigationDrawerThemeData navigationDrawerTheme;
/// A theme for customizing the background color, elevation, text style, and
/// icon themes of a [NavigationRail].
final NavigationRailThemeData navigationRailTheme;
/// A theme for customizing the appearance and internal layout of
/// [OutlinedButton]s.
final OutlinedButtonThemeData outlinedButtonTheme;
/// A theme for customizing the color, shape, elevation, and text style of
/// popup menus.
final PopupMenuThemeData popupMenuTheme;
/// A theme for customizing the appearance and layout of [ProgressIndicator] widgets.
final ProgressIndicatorThemeData progressIndicatorTheme;
/// A theme for customizing the appearance and layout of [Radio] widgets.
final RadioThemeData radioTheme;
/// A theme for customizing the appearance and layout of [SearchBar] widgets.
final SearchBarThemeData searchBarTheme;
/// A theme for customizing the appearance and layout of search views created by [SearchAnchor] widgets.
final SearchViewThemeData searchViewTheme;
/// A theme for customizing the appearance and layout of [SegmentedButton] widgets.
final SegmentedButtonThemeData segmentedButtonTheme;
/// The colors and shapes used to render [Slider].
///
/// This is the value returned from [SliderTheme.of].
final SliderThemeData sliderTheme;
/// A theme for customizing colors, shape, elevation, and behavior of a [SnackBar].
final SnackBarThemeData snackBarTheme;
/// A theme for customizing the appearance and layout of [Switch] widgets.
final SwitchThemeData switchTheme;
/// A theme for customizing the size, shape, and color of the tab bar indicator.
final TabBarTheme tabBarTheme;
/// A theme for customizing the appearance and internal layout of
/// [TextButton]s.
final TextButtonThemeData textButtonTheme;
/// A theme for customizing the appearance and layout of [TextField] widgets.
final TextSelectionThemeData textSelectionTheme;
/// A theme for customizing the appearance and layout of time picker widgets.
final TimePickerThemeData timePickerTheme;
/// Defines the default configuration of [ToggleButtons] widgets.
final ToggleButtonsThemeData toggleButtonsTheme;
/// A theme for customizing the visual properties of [Tooltip]s.
///
/// This is the value returned from [TooltipTheme.of].
final TooltipThemeData tooltipTheme;
/// Creates a copy of this theme but with the given fields replaced with the new values.
///
/// The [brightness] value is applied to the [colorScheme].
ThemeData copyWith({
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
Iterable<Adaptation<Object>>? adaptations,
bool? applyElevationOverlayColor,
NoDefaultCupertinoThemeData? cupertinoOverrideTheme,
Iterable<ThemeExtension<dynamic>>? extensions,
InputDecorationTheme? inputDecorationTheme,
MaterialTapTargetSize? materialTapTargetSize,
PageTransitionsTheme? pageTransitionsTheme,
TargetPlatform? platform,
ScrollbarThemeData? scrollbarTheme,
InteractiveInkFeatureFactory? splashFactory,
VisualDensity? visualDensity,
// COLOR
// [colorScheme] is the preferred way to configure colors. The other color
// properties will gradually be phased out, see
// https://github.com/flutter/flutter/issues/91772.
Brightness? brightness,
Color? canvasColor,
Color? cardColor,
ColorScheme? colorScheme,
Color? dialogBackgroundColor,
Color? disabledColor,
Color? dividerColor,
Color? focusColor,
Color? highlightColor,
Color? hintColor,
Color? hoverColor,
Color? indicatorColor,
Color? primaryColor,
Color? primaryColorDark,
Color? primaryColorLight,
Color? scaffoldBackgroundColor,
Color? secondaryHeaderColor,
Color? shadowColor,
Color? splashColor,
Color? unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
IconThemeData? iconTheme,
IconThemeData? primaryIconTheme,
TextTheme? primaryTextTheme,
TextTheme? textTheme,
Typography? typography,
// COMPONENT THEMES
ActionIconThemeData? actionIconTheme,
AppBarTheme? appBarTheme,
BadgeThemeData? badgeTheme,
MaterialBannerThemeData? bannerTheme,
BottomAppBarTheme? bottomAppBarTheme,
BottomNavigationBarThemeData? bottomNavigationBarTheme,
BottomSheetThemeData? bottomSheetTheme,
ButtonBarThemeData? buttonBarTheme,
ButtonThemeData? buttonTheme,
CardTheme? cardTheme,
CheckboxThemeData? checkboxTheme,
ChipThemeData? chipTheme,
DataTableThemeData? dataTableTheme,
DatePickerThemeData? datePickerTheme,
DialogTheme? dialogTheme,
DividerThemeData? dividerTheme,
DrawerThemeData? drawerTheme,
DropdownMenuThemeData? dropdownMenuTheme,
ElevatedButtonThemeData? elevatedButtonTheme,
ExpansionTileThemeData? expansionTileTheme,
FilledButtonThemeData? filledButtonTheme,
FloatingActionButtonThemeData? floatingActionButtonTheme,
IconButtonThemeData? iconButtonTheme,
ListTileThemeData? listTileTheme,
MenuBarThemeData? menuBarTheme,
MenuButtonThemeData? menuButtonTheme,
MenuThemeData? menuTheme,
NavigationBarThemeData? navigationBarTheme,
NavigationDrawerThemeData? navigationDrawerTheme,
NavigationRailThemeData? navigationRailTheme,
OutlinedButtonThemeData? outlinedButtonTheme,
PopupMenuThemeData? popupMenuTheme,
ProgressIndicatorThemeData? progressIndicatorTheme,
RadioThemeData? radioTheme,
SearchBarThemeData? searchBarTheme,
SearchViewThemeData? searchViewTheme,
SegmentedButtonThemeData? segmentedButtonTheme,
SliderThemeData? sliderTheme,
SnackBarThemeData? snackBarTheme,
SwitchThemeData? switchTheme,
TabBarTheme? tabBarTheme,
TextButtonThemeData? textButtonTheme,
TextSelectionThemeData? textSelectionTheme,
TimePickerThemeData? timePickerTheme,
ToggleButtonsThemeData? toggleButtonsTheme,
TooltipThemeData? tooltipTheme,
// DEPRECATED (newest deprecations at the bottom)
@Deprecated(
'Use a ThemeData constructor (.from, .light, or .dark) instead. '
'These constructors all have a useMaterial3 argument, '
'and they set appropriate default values based on its value. '
'See the useMaterial3 API documentation for full details. '
'This feature was deprecated after v3.13.0-0.2.pre.',
)
bool? useMaterial3,
}) {
cupertinoOverrideTheme = cupertinoOverrideTheme?.noDefault();
return ThemeData.raw(
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
adaptationMap: adaptations != null ? _createAdaptationMap(adaptations) : adaptationMap,
applyElevationOverlayColor: applyElevationOverlayColor ?? this.applyElevationOverlayColor,
cupertinoOverrideTheme: cupertinoOverrideTheme ?? this.cupertinoOverrideTheme,
extensions: (extensions != null) ? _themeExtensionIterableToMap(extensions) : this.extensions,
inputDecorationTheme: inputDecorationTheme ?? this.inputDecorationTheme,
materialTapTargetSize: materialTapTargetSize ?? this.materialTapTargetSize,
pageTransitionsTheme: pageTransitionsTheme ?? this.pageTransitionsTheme,
platform: platform ?? this.platform,
scrollbarTheme: scrollbarTheme ?? this.scrollbarTheme,
splashFactory: splashFactory ?? this.splashFactory,
// When deprecated useMaterial3 removed, maintain `this.useMaterial3` here
// for == evaluation.
useMaterial3: useMaterial3 ?? this.useMaterial3,
visualDensity: visualDensity ?? this.visualDensity,
// COLOR
canvasColor: canvasColor ?? this.canvasColor,
cardColor: cardColor ?? this.cardColor,
colorScheme: (colorScheme ?? this.colorScheme).copyWith(brightness: brightness),
dialogBackgroundColor: dialogBackgroundColor ?? this.dialogBackgroundColor,
disabledColor: disabledColor ?? this.disabledColor,
dividerColor: dividerColor ?? this.dividerColor,
focusColor: focusColor ?? this.focusColor,
highlightColor: highlightColor ?? this.highlightColor,
hintColor: hintColor ?? this.hintColor,
hoverColor: hoverColor ?? this.hoverColor,
indicatorColor: indicatorColor ?? this.indicatorColor,
primaryColor: primaryColor ?? this.primaryColor,
primaryColorDark: primaryColorDark ?? this.primaryColorDark,
primaryColorLight: primaryColorLight ?? this.primaryColorLight,
scaffoldBackgroundColor: scaffoldBackgroundColor ?? this.scaffoldBackgroundColor,
secondaryHeaderColor: secondaryHeaderColor ?? this.secondaryHeaderColor,
shadowColor: shadowColor ?? this.shadowColor,
splashColor: splashColor ?? this.splashColor,
unselectedWidgetColor: unselectedWidgetColor ?? this.unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
iconTheme: iconTheme ?? this.iconTheme,
primaryIconTheme: primaryIconTheme ?? this.primaryIconTheme,
primaryTextTheme: primaryTextTheme ?? this.primaryTextTheme,
textTheme: textTheme ?? this.textTheme,
typography: typography ?? this.typography,
// COMPONENT THEMES
actionIconTheme: actionIconTheme ?? this.actionIconTheme,
appBarTheme: appBarTheme ?? this.appBarTheme,
badgeTheme: badgeTheme ?? this.badgeTheme,
bannerTheme: bannerTheme ?? this.bannerTheme,
bottomAppBarTheme: bottomAppBarTheme ?? this.bottomAppBarTheme,
bottomNavigationBarTheme: bottomNavigationBarTheme ?? this.bottomNavigationBarTheme,
bottomSheetTheme: bottomSheetTheme ?? this.bottomSheetTheme,
buttonBarTheme: buttonBarTheme ?? this.buttonBarTheme,
buttonTheme: buttonTheme ?? this.buttonTheme,
cardTheme: cardTheme ?? this.cardTheme,
checkboxTheme: checkboxTheme ?? this.checkboxTheme,
chipTheme: chipTheme ?? this.chipTheme,
dataTableTheme: dataTableTheme ?? this.dataTableTheme,
datePickerTheme: datePickerTheme ?? this.datePickerTheme,
dialogTheme: dialogTheme ?? this.dialogTheme,
dividerTheme: dividerTheme ?? this.dividerTheme,
drawerTheme: drawerTheme ?? this.drawerTheme,
dropdownMenuTheme: dropdownMenuTheme ?? this.dropdownMenuTheme,
elevatedButtonTheme: elevatedButtonTheme ?? this.elevatedButtonTheme,
expansionTileTheme: expansionTileTheme ?? this.expansionTileTheme,
filledButtonTheme: filledButtonTheme ?? this.filledButtonTheme,
floatingActionButtonTheme: floatingActionButtonTheme ?? this.floatingActionButtonTheme,
iconButtonTheme: iconButtonTheme ?? this.iconButtonTheme,
listTileTheme: listTileTheme ?? this.listTileTheme,
menuBarTheme: menuBarTheme ?? this.menuBarTheme,
menuButtonTheme: menuButtonTheme ?? this.menuButtonTheme,
menuTheme: menuTheme ?? this.menuTheme,
navigationBarTheme: navigationBarTheme ?? this.navigationBarTheme,
navigationDrawerTheme: navigationDrawerTheme ?? this.navigationDrawerTheme,
navigationRailTheme: navigationRailTheme ?? this.navigationRailTheme,
outlinedButtonTheme: outlinedButtonTheme ?? this.outlinedButtonTheme,
popupMenuTheme: popupMenuTheme ?? this.popupMenuTheme,
progressIndicatorTheme: progressIndicatorTheme ?? this.progressIndicatorTheme,
radioTheme: radioTheme ?? this.radioTheme,
searchBarTheme: searchBarTheme ?? this.searchBarTheme,
searchViewTheme: searchViewTheme ?? this.searchViewTheme,
segmentedButtonTheme: segmentedButtonTheme ?? this.segmentedButtonTheme,
sliderTheme: sliderTheme ?? this.sliderTheme,
snackBarTheme: snackBarTheme ?? this.snackBarTheme,
switchTheme: switchTheme ?? this.switchTheme,
tabBarTheme: tabBarTheme ?? this.tabBarTheme,
textButtonTheme: textButtonTheme ?? this.textButtonTheme,
textSelectionTheme: textSelectionTheme ?? this.textSelectionTheme,
timePickerTheme: timePickerTheme ?? this.timePickerTheme,
toggleButtonsTheme: toggleButtonsTheme ?? this.toggleButtonsTheme,
tooltipTheme: tooltipTheme ?? this.tooltipTheme,
);
}
// The number 5 was chosen without any real science or research behind it. It
// just seemed like a number that's not too big (we should be able to fit 5
// copies of ThemeData in memory comfortably) and not too small (most apps
// shouldn't have more than 5 theme/localization pairs).
static const int _localizedThemeDataCacheSize = 5;
/// Caches localized themes to speed up the [localize] method.
static final _FifoCache<_IdentityThemeDataCacheKey, ThemeData> _localizedThemeDataCache =
_FifoCache<_IdentityThemeDataCacheKey, ThemeData>(_localizedThemeDataCacheSize);
/// Returns a new theme built by merging the text geometry provided by the
/// [localTextGeometry] theme with the [baseTheme].
///
/// For those text styles in the [baseTheme] whose [TextStyle.inherit] is set
/// to true, the returned theme's text styles inherit the geometric properties
/// of [localTextGeometry]. The resulting text styles' [TextStyle.inherit] is
/// set to those provided by [localTextGeometry].
static ThemeData localize(ThemeData baseTheme, TextTheme localTextGeometry) {
// WARNING: this method memoizes the result in a cache based on the
// previously seen baseTheme and localTextGeometry. Memoization is safe
// because all inputs and outputs of this function are deeply immutable, and
// the computations are referentially transparent. It only short-circuits
// the computation if the new inputs are identical() to the previous ones.
// It does not use the == operator, which performs a costly deep comparison.
//
// When changing this method, make sure the memoization logic is correct.
// Remember:
//
// There are only two hard things in Computer Science: cache invalidation
// and naming things. -- Phil Karlton
return _localizedThemeDataCache.putIfAbsent(
_IdentityThemeDataCacheKey(baseTheme, localTextGeometry),
() {
return baseTheme.copyWith(
primaryTextTheme: localTextGeometry.merge(baseTheme.primaryTextTheme),
textTheme: localTextGeometry.merge(baseTheme.textTheme),
);
},
);
}
/// Determines whether the given [Color] is [Brightness.light] or
/// [Brightness.dark].
///
/// This compares the luminosity of the given color to a threshold value that
/// matches the Material Design specification.
static Brightness estimateBrightnessForColor(Color color) {
final double relativeLuminance = color.computeLuminance();
// See <https://www.w3.org/TR/WCAG20/#contrast-ratiodef>
// The spec says to use kThreshold=0.0525, but Material Design appears to bias
// more towards using light text than WCAG20 recommends. Material Design spec
// doesn't say what value to use, but 0.15 seemed close to what the Material
// Design spec shows for its color palette on
// <https://material.io/go/design-theming#color-color-palette>.
const double kThreshold = 0.15;
if ((relativeLuminance + 0.05) * (relativeLuminance + 0.05) > kThreshold) {
return Brightness.light;
}
return Brightness.dark;
}
/// Linearly interpolate between two [extensions].
///
/// Includes all theme extensions in [a] and [b].
///
/// {@macro dart.ui.shadow.lerp}
static Map<Object, ThemeExtension<dynamic>> _lerpThemeExtensions(ThemeData a, ThemeData b, double t) {
// Lerp [a].
final Map<Object, ThemeExtension<dynamic>> newExtensions = a.extensions.map((Object id, ThemeExtension<dynamic> extensionA) {
final ThemeExtension<dynamic>? extensionB = b.extensions[id];
return MapEntry<Object, ThemeExtension<dynamic>>(id, extensionA.lerp(extensionB, t));
});
// Add [b]-only extensions.
newExtensions.addEntries(b.extensions.entries.where(
(MapEntry<Object, ThemeExtension<dynamic>> entry) =>
!a.extensions.containsKey(entry.key)));
return newExtensions;
}
/// Convert the [extensionsIterable] passed to [ThemeData.new] or [copyWith]
/// to the stored [extensions] map, where each entry's key consists of the extension's type.
static Map<Object, ThemeExtension<dynamic>> _themeExtensionIterableToMap(Iterable<ThemeExtension<dynamic>> extensionsIterable) {
return Map<Object, ThemeExtension<dynamic>>.unmodifiable(<Object, ThemeExtension<dynamic>>{
// Strangely, the cast is necessary for tests to run.
for (final ThemeExtension<dynamic> extension in extensionsIterable) extension.type: extension as ThemeExtension<ThemeExtension<dynamic>>,
});
}
/// Linearly interpolate between two themes.
///
/// {@macro dart.ui.shadow.lerp}
static ThemeData lerp(ThemeData a, ThemeData b, double t) {
if (identical(a, b)) {
return a;
}
return ThemeData.raw(
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
adaptationMap: t < 0.5 ? a.adaptationMap : b.adaptationMap,
applyElevationOverlayColor:t < 0.5 ? a.applyElevationOverlayColor : b.applyElevationOverlayColor,
cupertinoOverrideTheme:t < 0.5 ? a.cupertinoOverrideTheme : b.cupertinoOverrideTheme,
extensions: _lerpThemeExtensions(a, b, t),
inputDecorationTheme:t < 0.5 ? a.inputDecorationTheme : b.inputDecorationTheme,
materialTapTargetSize:t < 0.5 ? a.materialTapTargetSize : b.materialTapTargetSize,
pageTransitionsTheme:t < 0.5 ? a.pageTransitionsTheme : b.pageTransitionsTheme,
platform: t < 0.5 ? a.platform : b.platform,
scrollbarTheme: ScrollbarThemeData.lerp(a.scrollbarTheme, b.scrollbarTheme, t),
splashFactory: t < 0.5 ? a.splashFactory : b.splashFactory,
useMaterial3: t < 0.5 ? a.useMaterial3 : b.useMaterial3,
visualDensity: VisualDensity.lerp(a.visualDensity, b.visualDensity, t),
// COLOR
canvasColor: Color.lerp(a.canvasColor, b.canvasColor, t)!,
cardColor: Color.lerp(a.cardColor, b.cardColor, t)!,
colorScheme: ColorScheme.lerp(a.colorScheme, b.colorScheme, t),
dialogBackgroundColor: Color.lerp(a.dialogBackgroundColor, b.dialogBackgroundColor, t)!,
disabledColor: Color.lerp(a.disabledColor, b.disabledColor, t)!,
dividerColor: Color.lerp(a.dividerColor, b.dividerColor, t)!,
focusColor: Color.lerp(a.focusColor, b.focusColor, t)!,
highlightColor: Color.lerp(a.highlightColor, b.highlightColor, t)!,
hintColor: Color.lerp(a.hintColor, b.hintColor, t)!,
hoverColor: Color.lerp(a.hoverColor, b.hoverColor, t)!,
indicatorColor: Color.lerp(a.indicatorColor, b.indicatorColor, t)!,
primaryColor: Color.lerp(a.primaryColor, b.primaryColor, t)!,
primaryColorDark: Color.lerp(a.primaryColorDark, b.primaryColorDark, t)!,
primaryColorLight: Color.lerp(a.primaryColorLight, b.primaryColorLight, t)!,
scaffoldBackgroundColor: Color.lerp(a.scaffoldBackgroundColor, b.scaffoldBackgroundColor, t)!,
secondaryHeaderColor: Color.lerp(a.secondaryHeaderColor, b.secondaryHeaderColor, t)!,
shadowColor: Color.lerp(a.shadowColor, b.shadowColor, t)!,
splashColor: Color.lerp(a.splashColor, b.splashColor, t)!,
unselectedWidgetColor: Color.lerp(a.unselectedWidgetColor, b.unselectedWidgetColor, t)!,
// TYPOGRAPHY & ICONOGRAPHY
iconTheme: IconThemeData.lerp(a.iconTheme, b.iconTheme, t),
primaryIconTheme: IconThemeData.lerp(a.primaryIconTheme, b.primaryIconTheme, t),
primaryTextTheme: TextTheme.lerp(a.primaryTextTheme, b.primaryTextTheme, t),
textTheme: TextTheme.lerp(a.textTheme, b.textTheme, t),
typography: Typography.lerp(a.typography, b.typography, t),
// COMPONENT THEMES
actionIconTheme: ActionIconThemeData.lerp(a.actionIconTheme, b.actionIconTheme, t),
appBarTheme: AppBarTheme.lerp(a.appBarTheme, b.appBarTheme, t),
badgeTheme: BadgeThemeData.lerp(a.badgeTheme, b.badgeTheme, t),
bannerTheme: MaterialBannerThemeData.lerp(a.bannerTheme, b.bannerTheme, t),
bottomAppBarTheme: BottomAppBarTheme.lerp(a.bottomAppBarTheme, b.bottomAppBarTheme, t),
bottomNavigationBarTheme: BottomNavigationBarThemeData.lerp(a.bottomNavigationBarTheme, b.bottomNavigationBarTheme, t),
bottomSheetTheme: BottomSheetThemeData.lerp(a.bottomSheetTheme, b.bottomSheetTheme, t)!,
buttonBarTheme: ButtonBarThemeData.lerp(a.buttonBarTheme, b.buttonBarTheme, t)!,
buttonTheme: t < 0.5 ? a.buttonTheme : b.buttonTheme,
cardTheme: CardTheme.lerp(a.cardTheme, b.cardTheme, t),
checkboxTheme: CheckboxThemeData.lerp(a.checkboxTheme, b.checkboxTheme, t),
chipTheme: ChipThemeData.lerp(a.chipTheme, b.chipTheme, t)!,
dataTableTheme: DataTableThemeData.lerp(a.dataTableTheme, b.dataTableTheme, t),
datePickerTheme: DatePickerThemeData.lerp(a.datePickerTheme, b.datePickerTheme, t),
dialogTheme: DialogTheme.lerp(a.dialogTheme, b.dialogTheme, t),
dividerTheme: DividerThemeData.lerp(a.dividerTheme, b.dividerTheme, t),
drawerTheme: DrawerThemeData.lerp(a.drawerTheme, b.drawerTheme, t)!,
dropdownMenuTheme: DropdownMenuThemeData.lerp(a.dropdownMenuTheme, b.dropdownMenuTheme, t),
elevatedButtonTheme: ElevatedButtonThemeData.lerp(a.elevatedButtonTheme, b.elevatedButtonTheme, t)!,
expansionTileTheme: ExpansionTileThemeData.lerp(a.expansionTileTheme, b.expansionTileTheme, t)!,
filledButtonTheme: FilledButtonThemeData.lerp(a.filledButtonTheme, b.filledButtonTheme, t)!,
floatingActionButtonTheme: FloatingActionButtonThemeData.lerp(a.floatingActionButtonTheme, b.floatingActionButtonTheme, t)!,
iconButtonTheme: IconButtonThemeData.lerp(a.iconButtonTheme, b.iconButtonTheme, t)!,
listTileTheme: ListTileThemeData.lerp(a.listTileTheme, b.listTileTheme, t)!,
menuBarTheme: MenuBarThemeData.lerp(a.menuBarTheme, b.menuBarTheme, t)!,
menuButtonTheme: MenuButtonThemeData.lerp(a.menuButtonTheme, b.menuButtonTheme, t)!,
menuTheme: MenuThemeData.lerp(a.menuTheme, b.menuTheme, t)!,
navigationBarTheme: NavigationBarThemeData.lerp(a.navigationBarTheme, b.navigationBarTheme, t)!,
navigationDrawerTheme: NavigationDrawerThemeData.lerp(a.navigationDrawerTheme, b.navigationDrawerTheme, t)!,
navigationRailTheme: NavigationRailThemeData.lerp(a.navigationRailTheme, b.navigationRailTheme, t)!,
outlinedButtonTheme: OutlinedButtonThemeData.lerp(a.outlinedButtonTheme, b.outlinedButtonTheme, t)!,
popupMenuTheme: PopupMenuThemeData.lerp(a.popupMenuTheme, b.popupMenuTheme, t)!,
progressIndicatorTheme: ProgressIndicatorThemeData.lerp(a.progressIndicatorTheme, b.progressIndicatorTheme, t)!,
radioTheme: RadioThemeData.lerp(a.radioTheme, b.radioTheme, t),
searchBarTheme: SearchBarThemeData.lerp(a.searchBarTheme, b.searchBarTheme, t)!,
searchViewTheme: SearchViewThemeData.lerp(a.searchViewTheme, b.searchViewTheme, t)!,
segmentedButtonTheme: SegmentedButtonThemeData.lerp(a.segmentedButtonTheme, b.segmentedButtonTheme, t),
sliderTheme: SliderThemeData.lerp(a.sliderTheme, b.sliderTheme, t),
snackBarTheme: SnackBarThemeData.lerp(a.snackBarTheme, b.snackBarTheme, t),
switchTheme: SwitchThemeData.lerp(a.switchTheme, b.switchTheme, t),
tabBarTheme: TabBarTheme.lerp(a.tabBarTheme, b.tabBarTheme, t),
textButtonTheme: TextButtonThemeData.lerp(a.textButtonTheme, b.textButtonTheme, t)!,
textSelectionTheme: TextSelectionThemeData.lerp(a.textSelectionTheme, b.textSelectionTheme, t)!,
timePickerTheme: TimePickerThemeData.lerp(a.timePickerTheme, b.timePickerTheme, t),
toggleButtonsTheme: ToggleButtonsThemeData.lerp(a.toggleButtonsTheme, b.toggleButtonsTheme, t)!,
tooltipTheme: TooltipThemeData.lerp(a.tooltipTheme, b.tooltipTheme, t)!,
);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ThemeData &&
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
mapEquals(other.adaptationMap, adaptationMap) &&
other.applyElevationOverlayColor == applyElevationOverlayColor &&
other.cupertinoOverrideTheme == cupertinoOverrideTheme &&
mapEquals(other.extensions, extensions) &&
other.inputDecorationTheme == inputDecorationTheme &&
other.materialTapTargetSize == materialTapTargetSize &&
other.pageTransitionsTheme == pageTransitionsTheme &&
other.platform == platform &&
other.scrollbarTheme == scrollbarTheme &&
other.splashFactory == splashFactory &&
other.useMaterial3 == useMaterial3 &&
other.visualDensity == visualDensity &&
// COLOR
other.canvasColor == canvasColor &&
other.cardColor == cardColor &&
other.colorScheme == colorScheme &&
other.dialogBackgroundColor == dialogBackgroundColor &&
other.disabledColor == disabledColor &&
other.dividerColor == dividerColor &&
other.focusColor == focusColor &&
other.highlightColor == highlightColor &&
other.hintColor == hintColor &&
other.hoverColor == hoverColor &&
other.indicatorColor == indicatorColor &&
other.primaryColor == primaryColor &&
other.primaryColorDark == primaryColorDark &&
other.primaryColorLight == primaryColorLight &&
other.scaffoldBackgroundColor == scaffoldBackgroundColor &&
other.secondaryHeaderColor == secondaryHeaderColor &&
other.shadowColor == shadowColor &&
other.splashColor == splashColor &&
other.unselectedWidgetColor == unselectedWidgetColor &&
// TYPOGRAPHY & ICONOGRAPHY
other.iconTheme == iconTheme &&
other.primaryIconTheme == primaryIconTheme &&
other.primaryTextTheme == primaryTextTheme &&
other.textTheme == textTheme &&
other.typography == typography &&
// COMPONENT THEMES
other.actionIconTheme == actionIconTheme &&
other.appBarTheme == appBarTheme &&
other.badgeTheme == badgeTheme &&
other.bannerTheme == bannerTheme &&
other.bottomAppBarTheme == bottomAppBarTheme &&
other.bottomNavigationBarTheme == bottomNavigationBarTheme &&
other.bottomSheetTheme == bottomSheetTheme &&
other.buttonBarTheme == buttonBarTheme &&
other.buttonTheme == buttonTheme &&
other.cardTheme == cardTheme &&
other.checkboxTheme == checkboxTheme &&
other.chipTheme == chipTheme &&
other.dataTableTheme == dataTableTheme &&
other.datePickerTheme == datePickerTheme &&
other.dialogTheme == dialogTheme &&
other.dividerTheme == dividerTheme &&
other.drawerTheme == drawerTheme &&
other.dropdownMenuTheme == dropdownMenuTheme &&
other.elevatedButtonTheme == elevatedButtonTheme &&
other.expansionTileTheme == expansionTileTheme &&
other.filledButtonTheme == filledButtonTheme &&
other.floatingActionButtonTheme == floatingActionButtonTheme &&
other.iconButtonTheme == iconButtonTheme &&
other.listTileTheme == listTileTheme &&
other.menuBarTheme == menuBarTheme &&
other.menuButtonTheme == menuButtonTheme &&
other.menuTheme == menuTheme &&
other.navigationBarTheme == navigationBarTheme &&
other.navigationDrawerTheme == navigationDrawerTheme &&
other.navigationRailTheme == navigationRailTheme &&
other.outlinedButtonTheme == outlinedButtonTheme &&
other.popupMenuTheme == popupMenuTheme &&
other.progressIndicatorTheme == progressIndicatorTheme &&
other.radioTheme == radioTheme &&
other.searchBarTheme == searchBarTheme &&
other.searchViewTheme == searchViewTheme &&
other.segmentedButtonTheme == segmentedButtonTheme &&
other.sliderTheme == sliderTheme &&
other.snackBarTheme == snackBarTheme &&
other.switchTheme == switchTheme &&
other.tabBarTheme == tabBarTheme &&
other.textButtonTheme == textButtonTheme &&
other.textSelectionTheme == textSelectionTheme &&
other.timePickerTheme == timePickerTheme &&
other.toggleButtonsTheme == toggleButtonsTheme &&
other.tooltipTheme == tooltipTheme;
}
@override
int get hashCode {
final List<Object?> values = <Object?>[
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
...adaptationMap.keys,
...adaptationMap.values,
applyElevationOverlayColor,
cupertinoOverrideTheme,
...extensions.keys,
...extensions.values,
inputDecorationTheme,
materialTapTargetSize,
pageTransitionsTheme,
platform,
scrollbarTheme,
splashFactory,
useMaterial3,
visualDensity,
// COLOR
canvasColor,
cardColor,
colorScheme,
dialogBackgroundColor,
disabledColor,
dividerColor,
focusColor,
highlightColor,
hintColor,
hoverColor,
indicatorColor,
primaryColor,
primaryColorDark,
primaryColorLight,
scaffoldBackgroundColor,
secondaryHeaderColor,
shadowColor,
splashColor,
unselectedWidgetColor,
// TYPOGRAPHY & ICONOGRAPHY
iconTheme,
primaryIconTheme,
primaryTextTheme,
textTheme,
typography,
// COMPONENT THEMES
actionIconTheme,
appBarTheme,
badgeTheme,
bannerTheme,
bottomAppBarTheme,
bottomNavigationBarTheme,
bottomSheetTheme,
buttonBarTheme,
buttonTheme,
cardTheme,
checkboxTheme,
chipTheme,
dataTableTheme,
datePickerTheme,
dialogTheme,
dividerTheme,
drawerTheme,
dropdownMenuTheme,
elevatedButtonTheme,
expansionTileTheme,
filledButtonTheme,
floatingActionButtonTheme,
iconButtonTheme,
listTileTheme,
menuBarTheme,
menuButtonTheme,
menuTheme,
navigationBarTheme,
navigationDrawerTheme,
navigationRailTheme,
outlinedButtonTheme,
popupMenuTheme,
progressIndicatorTheme,
radioTheme,
searchBarTheme,
searchViewTheme,
segmentedButtonTheme,
sliderTheme,
snackBarTheme,
switchTheme,
tabBarTheme,
textButtonTheme,
textSelectionTheme,
timePickerTheme,
toggleButtonsTheme,
tooltipTheme,
];
return Object.hashAll(values);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final ThemeData defaultData = ThemeData.fallback();
// For the sanity of the reader, make sure these properties are in the same
// order in every place that they are separated by section comments (e.g.
// GENERAL CONFIGURATION). Each section except for deprecations should be
// alphabetical by symbol name.
// GENERAL CONFIGURATION
properties.add(IterableProperty<Adaptation<dynamic>>('adaptations', adaptationMap.values, defaultValue: defaultData.adaptationMap.values, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<bool>('applyElevationOverlayColor', applyElevationOverlayColor, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<NoDefaultCupertinoThemeData>('cupertinoOverrideTheme', cupertinoOverrideTheme, defaultValue: defaultData.cupertinoOverrideTheme, level: DiagnosticLevel.debug));
properties.add(IterableProperty<ThemeExtension<dynamic>>('extensions', extensions.values, defaultValue: defaultData.extensions.values, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<InputDecorationTheme>('inputDecorationTheme', inputDecorationTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<MaterialTapTargetSize>('materialTapTargetSize', materialTapTargetSize, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<PageTransitionsTheme>('pageTransitionsTheme', pageTransitionsTheme, level: DiagnosticLevel.debug));
properties.add(EnumProperty<TargetPlatform>('platform', platform, defaultValue: defaultTargetPlatform, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ScrollbarThemeData>('scrollbarTheme', scrollbarTheme, defaultValue: defaultData.scrollbarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<InteractiveInkFeatureFactory>('splashFactory', splashFactory, defaultValue: defaultData.splashFactory, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<bool>('useMaterial3', useMaterial3, defaultValue: defaultData.useMaterial3, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<VisualDensity>('visualDensity', visualDensity, defaultValue: defaultData.visualDensity, level: DiagnosticLevel.debug));
// COLORS
properties.add(ColorProperty('canvasColor', canvasColor, defaultValue: defaultData.canvasColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('cardColor', cardColor, defaultValue: defaultData.cardColor, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ColorScheme>('colorScheme', colorScheme, defaultValue: defaultData.colorScheme, level: DiagnosticLevel.debug));
properties.add(ColorProperty('dialogBackgroundColor', dialogBackgroundColor, defaultValue: defaultData.dialogBackgroundColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('disabledColor', disabledColor, defaultValue: defaultData.disabledColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('dividerColor', dividerColor, defaultValue: defaultData.dividerColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('focusColor', focusColor, defaultValue: defaultData.focusColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('highlightColor', highlightColor, defaultValue: defaultData.highlightColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('hintColor', hintColor, defaultValue: defaultData.hintColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('hoverColor', hoverColor, defaultValue: defaultData.hoverColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('indicatorColor', indicatorColor, defaultValue: defaultData.indicatorColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('primaryColorDark', primaryColorDark, defaultValue: defaultData.primaryColorDark, level: DiagnosticLevel.debug));
properties.add(ColorProperty('primaryColorLight', primaryColorLight, defaultValue: defaultData.primaryColorLight, level: DiagnosticLevel.debug));
properties.add(ColorProperty('primaryColor', primaryColor, defaultValue: defaultData.primaryColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('scaffoldBackgroundColor', scaffoldBackgroundColor, defaultValue: defaultData.scaffoldBackgroundColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('secondaryHeaderColor', secondaryHeaderColor, defaultValue: defaultData.secondaryHeaderColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: defaultData.shadowColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('splashColor', splashColor, defaultValue: defaultData.splashColor, level: DiagnosticLevel.debug));
properties.add(ColorProperty('unselectedWidgetColor', unselectedWidgetColor, defaultValue: defaultData.unselectedWidgetColor, level: DiagnosticLevel.debug));
// TYPOGRAPHY & ICONOGRAPHY
properties.add(DiagnosticsProperty<IconThemeData>('iconTheme', iconTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<IconThemeData>('primaryIconTheme', primaryIconTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TextTheme>('primaryTextTheme', primaryTextTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TextTheme>('textTheme', textTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<Typography>('typography', typography, defaultValue: defaultData.typography, level: DiagnosticLevel.debug));
// COMPONENT THEMES
properties.add(DiagnosticsProperty<ActionIconThemeData>('actionIconTheme', actionIconTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<AppBarTheme>('appBarTheme', appBarTheme, defaultValue: defaultData.appBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<BadgeThemeData>('badgeTheme', badgeTheme, defaultValue: defaultData.badgeTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<MaterialBannerThemeData>('bannerTheme', bannerTheme, defaultValue: defaultData.bannerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<BottomAppBarTheme>('bottomAppBarTheme', bottomAppBarTheme, defaultValue: defaultData.bottomAppBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<BottomNavigationBarThemeData>('bottomNavigationBarTheme', bottomNavigationBarTheme, defaultValue: defaultData.bottomNavigationBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<BottomSheetThemeData>('bottomSheetTheme', bottomSheetTheme, defaultValue: defaultData.bottomSheetTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ButtonBarThemeData>('buttonBarTheme', buttonBarTheme, defaultValue: defaultData.buttonBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ButtonThemeData>('buttonTheme', buttonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<CardTheme>('cardTheme', cardTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<CheckboxThemeData>('checkboxTheme', checkboxTheme, defaultValue: defaultData.checkboxTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ChipThemeData>('chipTheme', chipTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DataTableThemeData>('dataTableTheme', dataTableTheme, defaultValue: defaultData.dataTableTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DatePickerThemeData>('datePickerTheme', datePickerTheme, defaultValue: defaultData.datePickerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DialogTheme>('dialogTheme', dialogTheme, defaultValue: defaultData.dialogTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DividerThemeData>('dividerTheme', dividerTheme, defaultValue: defaultData.dividerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DrawerThemeData>('drawerTheme', drawerTheme, defaultValue: defaultData.drawerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<DropdownMenuThemeData>('dropdownMenuTheme', dropdownMenuTheme, defaultValue: defaultData.dropdownMenuTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ElevatedButtonThemeData>('elevatedButtonTheme', elevatedButtonTheme, defaultValue: defaultData.elevatedButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ExpansionTileThemeData>('expansionTileTheme', expansionTileTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<FilledButtonThemeData>('filledButtonTheme', filledButtonTheme, defaultValue: defaultData.filledButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<FloatingActionButtonThemeData>('floatingActionButtonTheme', floatingActionButtonTheme, defaultValue: defaultData.floatingActionButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<IconButtonThemeData>('iconButtonTheme', iconButtonTheme, defaultValue: defaultData.iconButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ListTileThemeData>('listTileTheme', listTileTheme, defaultValue: defaultData.listTileTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<MenuBarThemeData>('menuBarTheme', menuBarTheme, defaultValue: defaultData.menuBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<MenuButtonThemeData>('menuButtonTheme', menuButtonTheme, defaultValue: defaultData.menuButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<MenuThemeData>('menuTheme', menuTheme, defaultValue: defaultData.menuTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<NavigationBarThemeData>('navigationBarTheme', navigationBarTheme, defaultValue: defaultData.navigationBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<NavigationDrawerThemeData>('navigationDrawerTheme', navigationDrawerTheme, defaultValue: defaultData.navigationDrawerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<NavigationRailThemeData>('navigationRailTheme', navigationRailTheme, defaultValue: defaultData.navigationRailTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<OutlinedButtonThemeData>('outlinedButtonTheme', outlinedButtonTheme, defaultValue: defaultData.outlinedButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<PopupMenuThemeData>('popupMenuTheme', popupMenuTheme, defaultValue: defaultData.popupMenuTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ProgressIndicatorThemeData>('progressIndicatorTheme', progressIndicatorTheme, defaultValue: defaultData.progressIndicatorTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<RadioThemeData>('radioTheme', radioTheme, defaultValue: defaultData.radioTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SearchBarThemeData>('searchBarTheme', searchBarTheme, defaultValue: defaultData.searchBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SearchViewThemeData>('searchViewTheme', searchViewTheme, defaultValue: defaultData.searchViewTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SegmentedButtonThemeData>('segmentedButtonTheme', segmentedButtonTheme, defaultValue: defaultData.segmentedButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SliderThemeData>('sliderTheme', sliderTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SnackBarThemeData>('snackBarTheme', snackBarTheme, defaultValue: defaultData.snackBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<SwitchThemeData>('switchTheme', switchTheme, defaultValue: defaultData.switchTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TabBarTheme>('tabBarTheme', tabBarTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TextButtonThemeData>('textButtonTheme', textButtonTheme, defaultValue: defaultData.textButtonTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TextSelectionThemeData>('textSelectionTheme', textSelectionTheme, defaultValue: defaultData.textSelectionTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TimePickerThemeData>('timePickerTheme', timePickerTheme, defaultValue: defaultData.timePickerTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<ToggleButtonsThemeData>('toggleButtonsTheme', toggleButtonsTheme, level: DiagnosticLevel.debug));
properties.add(DiagnosticsProperty<TooltipThemeData>('tooltipTheme', tooltipTheme, level: DiagnosticLevel.debug));
}
}
/// A [CupertinoThemeData] that defers unspecified theme attributes to an
/// upstream Material [ThemeData].
///
/// This type of [CupertinoThemeData] is used by the Material [Theme] to
/// harmonize the [CupertinoTheme] with the material theme's colors and text
/// styles.
///
/// In the most basic case, [ThemeData]'s `cupertinoOverrideTheme` is null and
/// descendant Cupertino widgets' styling is derived from the Material theme.
///
/// To override individual parts of the Material-derived Cupertino styling,
/// `cupertinoOverrideTheme`'s construction parameters can be used.
///
/// To completely decouple the Cupertino styling from Material theme derivation,
/// another [CupertinoTheme] widget can be inserted as a descendant of the
/// Material [Theme]. On a [MaterialApp], this can be done using the `builder`
/// parameter on the constructor.
///
/// See also:
///
/// * [CupertinoThemeData], whose null constructor parameters default to
/// reasonable iOS styling defaults rather than harmonizing with a Material
/// theme.
/// * [Theme], widget which inserts a [CupertinoTheme] with this
/// [MaterialBasedCupertinoThemeData].
// This class subclasses CupertinoThemeData rather than composes one because it
// _is_ a CupertinoThemeData with partially altered behavior. e.g. its textTheme
// is from the superclass and based on the primaryColor but the primaryColor
// comes from the Material theme unless overridden.
class MaterialBasedCupertinoThemeData extends CupertinoThemeData {
/// Create a [MaterialBasedCupertinoThemeData] based on a Material [ThemeData]
/// and its `cupertinoOverrideTheme`.
MaterialBasedCupertinoThemeData({
required ThemeData materialTheme,
}) : this._(
materialTheme,
(materialTheme.cupertinoOverrideTheme ?? const CupertinoThemeData()).noDefault(),
);
MaterialBasedCupertinoThemeData._(
this._materialTheme,
this._cupertinoOverrideTheme,
) : // Pass all values to the superclass so Material-agnostic properties
// like barBackgroundColor can still behave like a normal
// CupertinoThemeData.
super.raw(
_cupertinoOverrideTheme.brightness,
_cupertinoOverrideTheme.primaryColor,
_cupertinoOverrideTheme.primaryContrastingColor,
_cupertinoOverrideTheme.textTheme,
_cupertinoOverrideTheme.barBackgroundColor,
_cupertinoOverrideTheme.scaffoldBackgroundColor,
_cupertinoOverrideTheme.applyThemeToAll,
);
final ThemeData _materialTheme;
final NoDefaultCupertinoThemeData _cupertinoOverrideTheme;
@override
Brightness get brightness => _cupertinoOverrideTheme.brightness ?? _materialTheme.brightness;
@override
Color get primaryColor => _cupertinoOverrideTheme.primaryColor ?? _materialTheme.colorScheme.primary;
@override
Color get primaryContrastingColor => _cupertinoOverrideTheme.primaryContrastingColor ?? _materialTheme.colorScheme.onPrimary;
@override
Color get scaffoldBackgroundColor => _cupertinoOverrideTheme.scaffoldBackgroundColor ?? _materialTheme.scaffoldBackgroundColor;
/// Copies the [ThemeData]'s `cupertinoOverrideTheme`.
///
/// Only the specified override attributes of the [ThemeData]'s
/// `cupertinoOverrideTheme` and the newly specified parameters are in the
/// returned [CupertinoThemeData]. No derived attributes from iOS defaults or
/// from cascaded Material theme attributes are copied.
///
/// This [copyWith] cannot change the base Material [ThemeData]. To change the
/// base Material [ThemeData], create a new Material [Theme] and use
/// [ThemeData.copyWith] on the Material [ThemeData] instead.
@override
MaterialBasedCupertinoThemeData copyWith({
Brightness? brightness,
Color? primaryColor,
Color? primaryContrastingColor,
CupertinoTextThemeData? textTheme,
Color? barBackgroundColor,
Color? scaffoldBackgroundColor,
bool? applyThemeToAll,
}) {
return MaterialBasedCupertinoThemeData._(
_materialTheme,
_cupertinoOverrideTheme.copyWith(
brightness: brightness,
primaryColor: primaryColor,
primaryContrastingColor: primaryContrastingColor,
textTheme: textTheme,
barBackgroundColor: barBackgroundColor,
scaffoldBackgroundColor: scaffoldBackgroundColor,
applyThemeToAll: applyThemeToAll,
),
);
}
@override
CupertinoThemeData resolveFrom(BuildContext context) {
// Only the cupertino override theme part will be resolved.
// If the color comes from the material theme it's not resolved.
return MaterialBasedCupertinoThemeData._(
_materialTheme,
_cupertinoOverrideTheme.resolveFrom(context),
);
}
}
@immutable
class _IdentityThemeDataCacheKey {
const _IdentityThemeDataCacheKey(this.baseTheme, this.localTextGeometry);
final ThemeData baseTheme;
final TextTheme localTextGeometry;
// Using XOR to make the hash function as fast as possible (e.g. Jenkins is
// noticeably slower).
@override
int get hashCode => identityHashCode(baseTheme) ^ identityHashCode(localTextGeometry);
@override
bool operator ==(Object other) {
// We are explicitly ignoring the possibility that the types might not
// match in the interests of speed.
return other is _IdentityThemeDataCacheKey
&& identical(other.baseTheme, baseTheme)
&& identical(other.localTextGeometry, localTextGeometry);
}
}
/// Cache of objects of limited size that uses the first in first out eviction
/// strategy (a.k.a least recently inserted).
///
/// The key that was inserted before all other keys is evicted first, i.e. the
/// one inserted least recently.
class _FifoCache<K, V> {
_FifoCache(this._maximumSize) : assert(_maximumSize > 0);
/// In Dart the map literal uses a linked hash-map implementation, whose keys
/// are stored such that [Map.keys] returns them in the order they were
/// inserted.
final Map<K, V> _cache = <K, V>{};
/// Maximum number of entries to store in the cache.
///
/// Once this many entries have been cached, the entry inserted least recently
/// is evicted when adding a new entry.
final int _maximumSize;
/// Returns the previously cached value for the given key, if available;
/// if not, calls the given callback to obtain it first.
V putIfAbsent(K key, V Function() loader) {
assert(key != null);
final V? result = _cache[key];
if (result != null) {
return result;
}
if (_cache.length == _maximumSize) {
_cache.remove(_cache.keys.first);
}
return _cache[key] = loader();
}
}
/// Defines the visual density of user interface components.
///
/// Density, in the context of a UI, is the vertical and horizontal
/// "compactness" of the components in the UI. It is unitless, since it means
/// different things to different UI components.
///
/// The default for visual densities is zero for both vertical and horizontal
/// densities, which corresponds to the default visual density of components in
/// the Material Design specification. It does not affect text sizes, icon
/// sizes, or padding values.
///
/// For example, for buttons, it affects the spacing around the child of the
/// button. For lists, it affects the distance between baselines of entries in
/// the list. For chips, it only affects the vertical size, not the horizontal
/// size.
///
/// Here are some examples of widgets that respond to density changes:
///
/// * [Checkbox]
/// * [Chip]
/// * [ElevatedButton]
/// * [IconButton]
/// * [InputDecorator] (which gives density support to [TextField], etc.)
/// * [ListTile]
/// * [MaterialButton]
/// * [OutlinedButton]
/// * [Radio]
/// * [RawMaterialButton]
/// * [TextButton]
///
/// See also:
///
/// * [ThemeData.visualDensity], where this property is used to specify the base
/// horizontal density of Material components.
/// * [Material design guidance on density](https://material.io/design/layout/applying-density.html).
@immutable
class VisualDensity with Diagnosticable {
/// A const constructor for [VisualDensity].
///
/// The [horizontal] and [vertical] arguments must be in the interval between
/// [minimumDensity] and [maximumDensity], inclusive.
const VisualDensity({
this.horizontal = 0.0,
this.vertical = 0.0,
}) : assert(vertical <= maximumDensity),
assert(vertical <= maximumDensity),
assert(vertical >= minimumDensity),
assert(horizontal <= maximumDensity),
assert(horizontal >= minimumDensity);
/// The minimum allowed density.
static const double minimumDensity = -4.0;
/// The maximum allowed density.
static const double maximumDensity = 4.0;
/// The default profile for [VisualDensity] in [ThemeData].
///
/// This default value represents a visual density that is less dense than
/// either [comfortable] or [compact], and corresponds to density values of
/// zero in both axes.
static const VisualDensity standard = VisualDensity();
/// The profile for a "comfortable" interpretation of [VisualDensity].
///
/// Individual components will interpret the density value independently,
/// making themselves more visually dense than [standard] and less dense than
/// [compact] to different degrees based on the Material Design specification
/// of the "comfortable" setting for their particular use case.
///
/// It corresponds to a density value of -1 in both axes.
static const VisualDensity comfortable = VisualDensity(horizontal: -1.0, vertical: -1.0);
/// The profile for a "compact" interpretation of [VisualDensity].
///
/// Individual components will interpret the density value independently,
/// making themselves more visually dense than [standard] and [comfortable] to
/// different degrees based on the Material Design specification of the
/// "comfortable" setting for their particular use case.
///
/// It corresponds to a density value of -2 in both axes.
static const VisualDensity compact = VisualDensity(horizontal: -2.0, vertical: -2.0);
/// Returns a [VisualDensity] that is adaptive based on the current platform
/// on which the framework is executing, from [defaultTargetPlatform].
///
/// When [defaultTargetPlatform] is a desktop platform, this returns
/// [compact], and for other platforms, it returns a default-constructed
/// [VisualDensity].
///
/// See also:
///
/// * [defaultDensityForPlatform] which returns a [VisualDensity] that is
/// adaptive based on the platform given to it.
/// * [defaultTargetPlatform] which returns the platform on which the
/// framework is currently executing.
static VisualDensity get adaptivePlatformDensity => defaultDensityForPlatform(defaultTargetPlatform);
/// Returns a [VisualDensity] that is adaptive based on the given [platform].
///
/// For desktop platforms, this returns [compact], and for other platforms, it
/// returns a default-constructed [VisualDensity].
///
/// See also:
///
/// * [adaptivePlatformDensity] which returns a [VisualDensity] that is
/// adaptive based on [defaultTargetPlatform].
static VisualDensity defaultDensityForPlatform(TargetPlatform platform) {
return switch (platform) {
TargetPlatform.android || TargetPlatform.iOS || TargetPlatform.fuchsia => standard,
TargetPlatform.linux || TargetPlatform.macOS || TargetPlatform.windows => compact,
};
}
/// Copy the current [VisualDensity] with the given values replacing the
/// current values.
VisualDensity copyWith({
double? horizontal,
double? vertical,
}) {
return VisualDensity(
horizontal: horizontal ?? this.horizontal,
vertical: vertical ?? this.vertical,
);
}
/// The horizontal visual density of UI components.
///
/// This property affects only the horizontal spacing between and within
/// components, to allow for different UI visual densities. It does not affect
/// text sizes, icon sizes, or padding values. The default value is 0.0,
/// corresponding to the metrics specified in the Material Design
/// specification. The value can range from [minimumDensity] to
/// [maximumDensity], inclusive.
///
/// See also:
///
/// * [ThemeData.visualDensity], where this property is used to specify the base
/// horizontal density of Material components.
/// * [Material design guidance on density](https://material.io/design/layout/applying-density.html).
final double horizontal;
/// The vertical visual density of UI components.
///
/// This property affects only the vertical spacing between and within
/// components, to allow for different UI visual densities. It does not affect
/// text sizes, icon sizes, or padding values. The default value is 0.0,
/// corresponding to the metrics specified in the Material Design
/// specification. The value can range from [minimumDensity] to
/// [maximumDensity], inclusive.
///
/// See also:
///
/// * [ThemeData.visualDensity], where this property is used to specify the base
/// vertical density of Material components.
/// * [Material design guidance on density](https://material.io/design/layout/applying-density.html).
final double vertical;
/// The base adjustment in logical pixels of the visual density of UI components.
///
/// The input density values are multiplied by a constant to arrive at a base
/// size adjustment that fits Material Design guidelines.
///
/// Individual components may adjust this value based upon their own
/// individual interpretation of density.
Offset get baseSizeAdjustment {
// The number of logical pixels represented by an increase or decrease in
// density by one. The Material Design guidelines say to increment/decrement
// sizes in terms of four pixel increments.
const double interval = 4.0;
return Offset(horizontal, vertical) * interval;
}
/// Linearly interpolate between two densities.
static VisualDensity lerp(VisualDensity a, VisualDensity b, double t) {
if (identical(a, b)) {
return a;
}
return VisualDensity(
horizontal: lerpDouble(a.horizontal, b.horizontal, t)!,
vertical: lerpDouble(a.vertical, b.vertical, t)!,
);
}
/// Return a copy of [constraints] whose minimum width and height have been
/// updated with the [baseSizeAdjustment].
///
/// The resulting minWidth and minHeight values are clamped to not exceed the
/// maxWidth and maxHeight values, respectively.
BoxConstraints effectiveConstraints(BoxConstraints constraints) {
assert(constraints.debugAssertIsValid());
return constraints.copyWith(
minWidth: clampDouble(constraints.minWidth + baseSizeAdjustment.dx, 0.0, constraints.maxWidth),
minHeight: clampDouble(constraints.minHeight + baseSizeAdjustment.dy, 0.0, constraints.maxHeight),
);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is VisualDensity
&& other.horizontal == horizontal
&& other.vertical == vertical;
}
@override
int get hashCode => Object.hash(horizontal, vertical);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('horizontal', horizontal, defaultValue: 0.0));
properties.add(DoubleProperty('vertical', vertical, defaultValue: 0.0));
}
@override
String toStringShort() {
return '${super.toStringShort()}(h: ${debugFormatDouble(horizontal)}, v: ${debugFormatDouble(vertical)})';
}
}
// BEGIN GENERATED TOKEN PROPERTIES - ColorScheme
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
const ColorScheme _colorSchemeLightM3 = ColorScheme(
brightness: Brightness.light,
primary: Color(0xFF6750A4),
onPrimary: Color(0xFFFFFFFF),
primaryContainer: Color(0xFFEADDFF),
onPrimaryContainer: Color(0xFF21005D),
primaryFixed: Color(0xFFEADDFF),
primaryFixedDim: Color(0xFFD0BCFF),
onPrimaryFixed: Color(0xFF21005D),
onPrimaryFixedVariant: Color(0xFF4F378B),
secondary: Color(0xFF625B71),
onSecondary: Color(0xFFFFFFFF),
secondaryContainer: Color(0xFFE8DEF8),
onSecondaryContainer: Color(0xFF1D192B),
secondaryFixed: Color(0xFFE8DEF8),
secondaryFixedDim: Color(0xFFCCC2DC),
onSecondaryFixed: Color(0xFF1D192B),
onSecondaryFixedVariant: Color(0xFF4A4458),
tertiary: Color(0xFF7D5260),
onTertiary: Color(0xFFFFFFFF),
tertiaryContainer: Color(0xFFFFD8E4),
onTertiaryContainer: Color(0xFF31111D),
tertiaryFixed: Color(0xFFFFD8E4),
tertiaryFixedDim: Color(0xFFEFB8C8),
onTertiaryFixed: Color(0xFF31111D),
onTertiaryFixedVariant: Color(0xFF633B48),
error: Color(0xFFB3261E),
onError: Color(0xFFFFFFFF),
errorContainer: Color(0xFFF9DEDC),
onErrorContainer: Color(0xFF410E0B),
background: Color(0xFFFEF7FF),
onBackground: Color(0xFF1D1B20),
surface: Color(0xFFFEF7FF),
surfaceBright: Color(0xFFFEF7FF),
surfaceContainerLowest: Color(0xFFFFFFFF),
surfaceContainerLow: Color(0xFFF7F2FA),
surfaceContainer: Color(0xFFF3EDF7),
surfaceContainerHigh: Color(0xFFECE6F0),
surfaceContainerHighest: Color(0xFFE6E0E9),
surfaceDim: Color(0xFFDED8E1),
onSurface: Color(0xFF1D1B20),
surfaceVariant: Color(0xFFE7E0EC),
onSurfaceVariant: Color(0xFF49454F),
outline: Color(0xFF79747E),
outlineVariant: Color(0xFFCAC4D0),
shadow: Color(0xFF000000),
scrim: Color(0xFF000000),
inverseSurface: Color(0xFF322F35),
onInverseSurface: Color(0xFFF5EFF7),
inversePrimary: Color(0xFFD0BCFF),
// The surfaceTint color is set to the same color as the primary.
surfaceTint: Color(0xFF6750A4),
);
const ColorScheme _colorSchemeDarkM3 = ColorScheme(
brightness: Brightness.dark,
primary: Color(0xFFD0BCFF),
onPrimary: Color(0xFF381E72),
primaryContainer: Color(0xFF4F378B),
onPrimaryContainer: Color(0xFFEADDFF),
primaryFixed: Color(0xFFEADDFF),
primaryFixedDim: Color(0xFFD0BCFF),
onPrimaryFixed: Color(0xFF21005D),
onPrimaryFixedVariant: Color(0xFF4F378B),
secondary: Color(0xFFCCC2DC),
onSecondary: Color(0xFF332D41),
secondaryContainer: Color(0xFF4A4458),
onSecondaryContainer: Color(0xFFE8DEF8),
secondaryFixed: Color(0xFFE8DEF8),
secondaryFixedDim: Color(0xFFCCC2DC),
onSecondaryFixed: Color(0xFF1D192B),
onSecondaryFixedVariant: Color(0xFF4A4458),
tertiary: Color(0xFFEFB8C8),
onTertiary: Color(0xFF492532),
tertiaryContainer: Color(0xFF633B48),
onTertiaryContainer: Color(0xFFFFD8E4),
tertiaryFixed: Color(0xFFFFD8E4),
tertiaryFixedDim: Color(0xFFEFB8C8),
onTertiaryFixed: Color(0xFF31111D),
onTertiaryFixedVariant: Color(0xFF633B48),
error: Color(0xFFF2B8B5),
onError: Color(0xFF601410),
errorContainer: Color(0xFF8C1D18),
onErrorContainer: Color(0xFFF9DEDC),
background: Color(0xFF141218),
onBackground: Color(0xFFE6E0E9),
surface: Color(0xFF141218),
surfaceBright: Color(0xFF3B383E),
surfaceContainerLowest: Color(0xFF0F0D13),
surfaceContainerLow: Color(0xFF1D1B20),
surfaceContainer: Color(0xFF211F26),
surfaceContainerHigh: Color(0xFF2B2930),
surfaceContainerHighest: Color(0xFF36343B),
surfaceDim: Color(0xFF141218),
onSurface: Color(0xFFE6E0E9),
surfaceVariant: Color(0xFF49454F),
onSurfaceVariant: Color(0xFFCAC4D0),
outline: Color(0xFF938F99),
outlineVariant: Color(0xFF49454F),
shadow: Color(0xFF000000),
scrim: Color(0xFF000000),
inverseSurface: Color(0xFFE6E0E9),
onInverseSurface: Color(0xFF322F35),
inversePrimary: Color(0xFF6750A4),
// The surfaceTint color is set to the same color as the primary.
surfaceTint: Color(0xFFD0BCFF),
);
// END GENERATED TOKEN PROPERTIES - ColorScheme
| flutter/packages/flutter/lib/src/material/theme_data.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/theme_data.dart",
"repo_id": "flutter",
"token_count": 37367
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'border_radius.dart';
import 'borders.dart';
/// A rectangular border with flattened or "beveled" corners.
///
/// The line segments that connect the rectangle's four sides will
/// begin and at locations offset by the corresponding border radius,
/// but not farther than the side's center. If all the border radii
/// exceed the sides' half widths/heights the resulting shape is
/// diamond made by connecting the centers of the sides.
class BeveledRectangleBorder extends OutlinedBorder {
/// Creates a border like a [RoundedRectangleBorder] except that the corners
/// are joined by straight lines instead of arcs.
const BeveledRectangleBorder({
super.side,
this.borderRadius = BorderRadius.zero,
});
/// The radii for each corner.
///
/// Each corner [Radius] defines the endpoints of a line segment that
/// spans the corner. The endpoints are located in the same place as
/// they would be for [RoundedRectangleBorder], but they're connected
/// by a straight line instead of an arc.
///
/// Negative radius values are clamped to 0.0 by [getInnerPath] and
/// [getOuterPath].
final BorderRadiusGeometry borderRadius;
@override
ShapeBorder scale(double t) {
return BeveledRectangleBorder(
side: side.scale(t),
borderRadius: borderRadius * t,
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is BeveledRectangleBorder) {
return BeveledRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, borderRadius, t)!,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is BeveledRectangleBorder) {
return BeveledRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: BorderRadiusGeometry.lerp(borderRadius, b.borderRadius, t)!,
);
}
return super.lerpTo(b, t);
}
/// Returns a copy of this RoundedRectangleBorder with the given fields
/// replaced with the new values.
@override
BeveledRectangleBorder copyWith({ BorderSide? side, BorderRadiusGeometry? borderRadius }) {
return BeveledRectangleBorder(
side: side ?? this.side,
borderRadius: borderRadius ?? this.borderRadius,
);
}
Path _getPath(RRect rrect) {
final Offset centerLeft = Offset(rrect.left, rrect.center.dy);
final Offset centerRight = Offset(rrect.right, rrect.center.dy);
final Offset centerTop = Offset(rrect.center.dx, rrect.top);
final Offset centerBottom = Offset(rrect.center.dx, rrect.bottom);
final double tlRadiusX = math.max(0.0, rrect.tlRadiusX);
final double tlRadiusY = math.max(0.0, rrect.tlRadiusY);
final double trRadiusX = math.max(0.0, rrect.trRadiusX);
final double trRadiusY = math.max(0.0, rrect.trRadiusY);
final double blRadiusX = math.max(0.0, rrect.blRadiusX);
final double blRadiusY = math.max(0.0, rrect.blRadiusY);
final double brRadiusX = math.max(0.0, rrect.brRadiusX);
final double brRadiusY = math.max(0.0, rrect.brRadiusY);
final List<Offset> vertices = <Offset>[
Offset(rrect.left, math.min(centerLeft.dy, rrect.top + tlRadiusY)),
Offset(math.min(centerTop.dx, rrect.left + tlRadiusX), rrect.top),
Offset(math.max(centerTop.dx, rrect.right -trRadiusX), rrect.top),
Offset(rrect.right, math.min(centerRight.dy, rrect.top + trRadiusY)),
Offset(rrect.right, math.max(centerRight.dy, rrect.bottom - brRadiusY)),
Offset(math.max(centerBottom.dx, rrect.right - brRadiusX), rrect.bottom),
Offset(math.min(centerBottom.dx, rrect.left + blRadiusX), rrect.bottom),
Offset(rrect.left, math.max(centerLeft.dy, rrect.bottom - blRadiusY)),
];
return Path()..addPolygon(vertices, true);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
return _getPath(borderRadius.resolve(textDirection).toRRect(rect).deflate(side.strokeInset));
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return _getPath(borderRadius.resolve(textDirection).toRRect(rect));
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
if (rect.isEmpty) {
return;
}
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final RRect borderRect = borderRadius.resolve(textDirection).toRRect(rect);
final RRect adjustedRect = borderRect.inflate(side.strokeOutset);
final Path path = _getPath(adjustedRect)
..addPath(getInnerPath(rect, textDirection: textDirection), Offset.zero);
canvas.drawPath(path, side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is BeveledRectangleBorder
&& other.side == side
&& other.borderRadius == borderRadius;
}
@override
int get hashCode => Object.hash(side, borderRadius);
@override
String toString() {
return '${objectRuntimeType(this, 'BeveledRectangleBorder')}($side, $borderRadius)';
}
}
| flutter/packages/flutter/lib/src/painting/beveled_rectangle_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/beveled_rectangle_border.dart",
"repo_id": "flutter",
"token_count": 2009
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui show Gradient, TextBox, lerpDouble;
import 'package:flutter/foundation.dart';
import 'alignment.dart';
import 'basic_types.dart';
import 'box_fit.dart';
import 'colors.dart';
import 'decoration.dart';
import 'edge_insets.dart';
import 'image_provider.dart';
import 'text_painter.dart';
import 'text_span.dart';
import 'text_style.dart';
/// Possible ways to draw Flutter's logo.
enum FlutterLogoStyle {
/// Show only Flutter's logo, not the "Flutter" label.
///
/// This is the default behavior for [FlutterLogoDecoration] objects.
markOnly,
/// Show Flutter's logo on the left, and the "Flutter" label to its right.
horizontal,
/// Show Flutter's logo above the "Flutter" label.
stacked,
}
/// An immutable description of how to paint Flutter's logo.
class FlutterLogoDecoration extends Decoration {
/// Creates a decoration that knows how to paint Flutter's logo.
///
/// The [style] controls whether and where to draw the "Flutter" label. If one
/// is shown, the [textColor] controls the color of the label.
const FlutterLogoDecoration({
this.textColor = const Color(0xFF757575),
this.style = FlutterLogoStyle.markOnly,
this.margin = EdgeInsets.zero,
}) : _position = identical(style, FlutterLogoStyle.markOnly) ? 0.0 : identical(style, FlutterLogoStyle.horizontal) ? 1.0 : -1.0,
_opacity = 1.0;
const FlutterLogoDecoration._(this.textColor, this.style, this.margin, this._position, this._opacity);
/// The color used to paint the "Flutter" text on the logo, if [style] is
/// [FlutterLogoStyle.horizontal] or [FlutterLogoStyle.stacked].
///
/// If possible, the default (a medium grey) should be used against a white
/// background.
final Color textColor;
/// Whether and where to draw the "Flutter" text. By default, only the logo
/// itself is drawn.
// This property isn't actually used when painting. It's only really used to
// set the internal _position property.
final FlutterLogoStyle style;
/// How far to inset the logo from the edge of the container.
final EdgeInsets margin;
// The following are set when lerping, to represent states that can't be
// represented by the constructor.
final double _position; // -1.0 for stacked, 1.0 for horizontal, 0.0 for no logo
final double _opacity; // 0.0 .. 1.0
bool get _inTransition => _opacity != 1.0 || (_position != -1.0 && _position != 0.0 && _position != 1.0);
@override
bool debugAssertIsValid() {
assert(
_position.isFinite
&& _opacity >= 0.0
&& _opacity <= 1.0,
);
return true;
}
@override
bool get isComplex => !_inTransition;
/// Linearly interpolate between two Flutter logo descriptions.
///
/// Interpolates both the color and the style in a continuous fashion.
///
/// If both values are null, this returns null. Otherwise, it returns a
/// non-null value. If one of the values is null, then the result is obtained
/// by scaling the other value's opacity and [margin].
///
/// {@macro dart.ui.shadow.lerp}
///
/// See also:
///
/// * [Decoration.lerp], which interpolates between arbitrary decorations.
static FlutterLogoDecoration? lerp(FlutterLogoDecoration? a, FlutterLogoDecoration? b, double t) {
assert(a == null || a.debugAssertIsValid());
assert(b == null || b.debugAssertIsValid());
if (identical(a, b)) {
return a;
}
if (a == null) {
return FlutterLogoDecoration._(
b!.textColor,
b.style,
b.margin * t,
b._position,
b._opacity * clampDouble(t, 0.0, 1.0),
);
}
if (b == null) {
return FlutterLogoDecoration._(
a.textColor,
a.style,
a.margin * t,
a._position,
a._opacity * clampDouble(1.0 - t, 0.0, 1.0),
);
}
if (t == 0.0) {
return a;
}
if (t == 1.0) {
return b;
}
return FlutterLogoDecoration._(
Color.lerp(a.textColor, b.textColor, t)!,
t < 0.5 ? a.style : b.style,
EdgeInsets.lerp(a.margin, b.margin, t)!,
a._position + (b._position - a._position) * t,
clampDouble(a._opacity + (b._opacity - a._opacity) * t, 0.0, 1.0),
);
}
@override
FlutterLogoDecoration? lerpFrom(Decoration? a, double t) {
assert(debugAssertIsValid());
if (a == null || a is FlutterLogoDecoration) {
assert(a == null || a.debugAssertIsValid());
return FlutterLogoDecoration.lerp(a as FlutterLogoDecoration?, this, t);
}
return super.lerpFrom(a, t) as FlutterLogoDecoration?;
}
@override
FlutterLogoDecoration? lerpTo(Decoration? b, double t) {
assert(debugAssertIsValid());
if (b == null || b is FlutterLogoDecoration) {
assert(b == null || b.debugAssertIsValid());
return FlutterLogoDecoration.lerp(this, b as FlutterLogoDecoration?, t);
}
return super.lerpTo(b, t) as FlutterLogoDecoration?;
}
@override
// TODO(ianh): better hit testing
bool hitTest(Size size, Offset position, { TextDirection? textDirection }) => true;
@override
BoxPainter createBoxPainter([ VoidCallback? onChanged ]) {
assert(debugAssertIsValid());
return _FlutterLogoPainter(this);
}
@override
Path getClipPath(Rect rect, TextDirection textDirection) {
return Path()..addRect(rect);
}
@override
bool operator ==(Object other) {
assert(debugAssertIsValid());
if (identical(this, other)) {
return true;
}
return other is FlutterLogoDecoration
&& other.textColor == textColor
&& other._position == _position
&& other._opacity == _opacity;
}
@override
int get hashCode {
assert(debugAssertIsValid());
return Object.hash(
textColor,
_position,
_opacity,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('textColor', textColor));
properties.add(EnumProperty<FlutterLogoStyle>('style', style));
if (_inTransition) {
properties.add(DiagnosticsNode.message('transition ${debugFormatDouble(_position)}:${debugFormatDouble(_opacity)}'));
}
}
}
/// An object that paints a [BoxDecoration] into a canvas.
class _FlutterLogoPainter extends BoxPainter {
_FlutterLogoPainter(this._config)
: assert(_config.debugAssertIsValid()),
super(null) {
_prepareText();
}
final FlutterLogoDecoration _config;
// these are configured assuming a font size of 100.0.
late TextPainter _textPainter;
late Rect _textBoundingRect;
@override
void dispose() {
_textPainter.dispose();
super.dispose();
}
void _prepareText() {
const String kLabel = 'Flutter';
_textPainter = TextPainter(
text: TextSpan(
text: kLabel,
style: TextStyle(
color: _config.textColor,
fontFamily: 'Roboto',
fontSize: 100.0 * 350.0 / 247.0, // 247 is the height of the F when the fontSize is 350, assuming device pixel ratio 1.0
fontWeight: FontWeight.w300,
textBaseline: TextBaseline.alphabetic,
),
),
textDirection: TextDirection.ltr,
);
_textPainter.layout();
final ui.TextBox textSize = _textPainter.getBoxesForSelection(const TextSelection(baseOffset: 0, extentOffset: kLabel.length)).single;
_textBoundingRect = Rect.fromLTRB(textSize.left, textSize.top, textSize.right, textSize.bottom);
}
// This class contains a lot of magic numbers. They were derived from the
// values in the SVG files exported from the original artwork source.
void _paintLogo(Canvas canvas, Rect rect) {
// Our points are in a coordinate space that's 166 pixels wide and 202 pixels high.
// First, transform the rectangle so that our coordinate space is a square 202 pixels
// to a side, with the top left at the origin.
canvas.save();
canvas.translate(rect.left, rect.top);
canvas.scale(rect.width / 202.0, rect.height / 202.0);
// Next, offset it some more so that the 166 horizontal pixels are centered
// in that square (as opposed to being on the left side of it). This means
// that if we draw in the rectangle from 0,0 to 166,202, we are drawing in
// the center of the given rect.
canvas.translate((202.0 - 166.0) / 2.0, 0.0);
// Set up the styles.
final Paint lightPaint = Paint()
..color = const Color(0xFF54C5F8);
final Paint mediumPaint = Paint()
..color = const Color(0xFF29B6F6);
final Paint darkPaint = Paint()
..color = const Color(0xFF01579B);
final ui.Gradient triangleGradient = ui.Gradient.linear(
const Offset(87.2623 + 37.9092, 28.8384 + 123.4389),
const Offset(42.9205 + 37.9092, 35.0952 + 123.4389),
<Color>[
const Color(0x001A237E),
const Color(0x661A237E),
],
);
final Paint trianglePaint = Paint()
..shader = triangleGradient;
// Draw the basic shape.
final Path topBeam = Path()
..moveTo(37.7, 128.9)
..lineTo(9.8, 101.0)
..lineTo(100.4, 10.4)
..lineTo(156.2, 10.4);
canvas.drawPath(topBeam, lightPaint);
final Path middleBeam = Path()
..moveTo(156.2, 94.0)
..lineTo(100.4, 94.0)
..lineTo(78.5, 115.9)
..lineTo(106.4, 143.8);
canvas.drawPath(middleBeam, lightPaint);
final Path bottomBeam = Path()
..moveTo(79.5, 170.7)
..lineTo(100.4, 191.6)
..lineTo(156.2, 191.6)
..lineTo(107.4, 142.8);
canvas.drawPath(bottomBeam, darkPaint);
// The overlap between middle and bottom beam.
canvas.save();
canvas.transform(Float64List.fromList(const <double>[
// careful, this is in _column_-major order
0.7071, -0.7071, 0.0, 0.0,
0.7071, 0.7071, 0.0, 0.0,
0.0, 0.0, 1.0, 0.0,
-77.697, 98.057, 0.0, 1.0,
]));
canvas.drawRect(const Rect.fromLTWH(59.8, 123.1, 39.4, 39.4), mediumPaint);
canvas.restore();
// The gradients below the middle beam on top of the bottom beam.
final Path triangle = Path()
..moveTo(79.5, 170.7)
..lineTo(120.9, 156.4)
..lineTo(107.4, 142.8);
canvas.drawPath(triangle, trianglePaint);
canvas.restore();
}
@override
void paint(Canvas canvas, Offset offset, ImageConfiguration configuration) {
offset += _config.margin.topLeft;
final Size canvasSize = _config.margin.deflateSize(configuration.size!);
if (canvasSize.isEmpty) {
return;
}
final Size logoSize;
if (_config._position > 0.0) {
// horizontal style
logoSize = const Size(820.0, 232.0);
} else if (_config._position < 0.0) {
// stacked style
logoSize = const Size(252.0, 306.0);
} else {
// only the mark
logoSize = const Size(202.0, 202.0);
}
final FittedSizes fittedSize = applyBoxFit(BoxFit.contain, logoSize, canvasSize);
assert(fittedSize.source == logoSize);
final Rect rect = Alignment.center.inscribe(fittedSize.destination, offset & canvasSize);
final double centerSquareHeight = canvasSize.shortestSide;
final Rect centerSquare = Rect.fromLTWH(
offset.dx + (canvasSize.width - centerSquareHeight) / 2.0,
offset.dy + (canvasSize.height - centerSquareHeight) / 2.0,
centerSquareHeight,
centerSquareHeight,
);
final Rect logoTargetSquare;
if (_config._position > 0.0) {
// horizontal style
logoTargetSquare = Rect.fromLTWH(rect.left, rect.top, rect.height, rect.height);
} else if (_config._position < 0.0) {
// stacked style
final double logoHeight = rect.height * 191.0 / 306.0;
logoTargetSquare = Rect.fromLTWH(
rect.left + (rect.width - logoHeight) / 2.0,
rect.top,
logoHeight,
logoHeight,
);
} else {
// only the mark
logoTargetSquare = centerSquare;
}
final Rect logoSquare = Rect.lerp(centerSquare, logoTargetSquare, _config._position.abs())!;
if (_config._opacity < 1.0) {
canvas.saveLayer(
offset & canvasSize,
Paint()
..colorFilter = ColorFilter.mode(
const Color(0xFFFFFFFF).withOpacity(_config._opacity),
BlendMode.modulate,
),
);
}
if (_config._position != 0.0) {
if (_config._position > 0.0) {
// horizontal style
final double fontSize = 2.0 / 3.0 * logoSquare.height * (1 - (10.4 * 2.0) / 202.0);
final double scale = fontSize / 100.0;
final double finalLeftTextPosition = // position of text in rest position
(256.4 / 820.0) * rect.width - // 256.4 is the distance from the left edge to the left of the F when the whole logo is 820.0 wide
(32.0 / 350.0) * fontSize; // 32 is the distance from the text bounding box edge to the left edge of the F when the font size is 350
final double initialLeftTextPosition = // position of text when just starting the animation
rect.width / 2.0 - _textBoundingRect.width * scale;
final Offset textOffset = Offset(
rect.left + ui.lerpDouble(initialLeftTextPosition, finalLeftTextPosition, _config._position)!,
rect.top + (rect.height - _textBoundingRect.height * scale) / 2.0,
);
canvas.save();
if (_config._position < 1.0) {
final Offset center = logoSquare.center;
final Path path = Path()
..moveTo(center.dx, center.dy)
..lineTo(center.dx + rect.width, center.dy - rect.width)
..lineTo(center.dx + rect.width, center.dy + rect.width)
..close();
canvas.clipPath(path);
}
canvas.translate(textOffset.dx, textOffset.dy);
canvas.scale(scale, scale);
_textPainter.paint(canvas, Offset.zero);
canvas.restore();
} else if (_config._position < 0.0) {
// stacked style
final double fontSize = 0.35 * logoTargetSquare.height * (1 - (10.4 * 2.0) / 202.0);
final double scale = fontSize / 100.0;
if (_config._position > -1.0) {
// This limits what the drawRect call below is going to blend with.
canvas.saveLayer(_textBoundingRect, Paint());
} else {
canvas.save();
}
canvas.translate(
logoTargetSquare.center.dx - (_textBoundingRect.width * scale / 2.0),
logoTargetSquare.bottom,
);
canvas.scale(scale, scale);
_textPainter.paint(canvas, Offset.zero);
if (_config._position > -1.0) {
canvas.drawRect(_textBoundingRect.inflate(_textBoundingRect.width * 0.5), Paint()
..blendMode = BlendMode.modulate
..shader = ui.Gradient.linear(
Offset(_textBoundingRect.width * -0.5, 0.0),
Offset(_textBoundingRect.width * 1.5, 0.0),
<Color>[const Color(0xFFFFFFFF), const Color(0xFFFFFFFF), const Color(0x00FFFFFF), const Color(0x00FFFFFF)],
<double>[ 0.0, math.max(0.0, _config._position.abs() - 0.1), math.min(_config._position.abs() + 0.1, 1.0), 1.0 ],
),
);
}
canvas.restore();
}
}
_paintLogo(canvas, logoSquare);
if (_config._opacity < 1.0) {
canvas.restore();
}
}
}
| flutter/packages/flutter/lib/src/painting/flutter_logo.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/flutter_logo.dart",
"repo_id": "flutter",
"token_count": 6153
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'basic_types.dart';
import 'border_radius.dart';
import 'borders.dart';
import 'circle_border.dart';
/// A rectangular border with rounded corners.
///
/// Typically used with [ShapeDecoration] to draw a box with a rounded
/// rectangle.
///
/// This shape can interpolate to and from [CircleBorder].
///
/// See also:
///
/// * [BorderSide], which is used to describe each side of the box.
/// * [Border], which, when used with [BoxDecoration], can also
/// describe a rounded rectangle.
class RoundedRectangleBorder extends OutlinedBorder {
/// Creates a rounded rectangle border.
const RoundedRectangleBorder({
super.side,
this.borderRadius = BorderRadius.zero,
});
/// The radii for each corner.
final BorderRadiusGeometry borderRadius;
@override
ShapeBorder scale(double t) {
return RoundedRectangleBorder(
side: side.scale(t),
borderRadius: borderRadius * t,
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is RoundedRectangleBorder) {
return RoundedRectangleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, borderRadius, t)!,
);
}
if (a is CircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: borderRadius,
circularity: 1.0 - t,
eccentricity: a.eccentricity,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is RoundedRectangleBorder) {
return RoundedRectangleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: BorderRadiusGeometry.lerp(borderRadius, b.borderRadius, t)!,
);
}
if (b is CircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: borderRadius,
circularity: t,
eccentricity: b.eccentricity,
);
}
return super.lerpTo(b, t);
}
/// Returns a copy of this RoundedRectangleBorder with the given fields
/// replaced with the new values.
@override
RoundedRectangleBorder copyWith({ BorderSide? side, BorderRadiusGeometry? borderRadius }) {
return RoundedRectangleBorder(
side: side ?? this.side,
borderRadius: borderRadius ?? this.borderRadius,
);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
final RRect borderRect = borderRadius.resolve(textDirection).toRRect(rect);
final RRect adjustedRect = borderRect.deflate(side.strokeInset);
return Path()
..addRRect(adjustedRect);
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return Path()
..addRRect(borderRadius.resolve(textDirection).toRRect(rect));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
if (borderRadius == BorderRadius.zero) {
canvas.drawRect(rect, paint);
} else {
canvas.drawRRect(borderRadius.resolve(textDirection).toRRect(rect), paint);
}
}
@override
bool get preferPaintInterior => true;
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
if (side.width == 0.0) {
canvas.drawRRect(borderRadius.resolve(textDirection).toRRect(rect), side.toPaint());
} else {
final Paint paint = Paint()
..color = side.color;
final RRect borderRect = borderRadius.resolve(textDirection).toRRect(rect);
final RRect inner = borderRect.deflate(side.strokeInset);
final RRect outer = borderRect.inflate(side.strokeOutset);
canvas.drawDRRect(outer, inner, paint);
}
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is RoundedRectangleBorder
&& other.side == side
&& other.borderRadius == borderRadius;
}
@override
int get hashCode => Object.hash(side, borderRadius);
@override
String toString() {
return '${objectRuntimeType(this, 'RoundedRectangleBorder')}($side, $borderRadius)';
}
}
class _RoundedRectangleToCircleBorder extends OutlinedBorder {
const _RoundedRectangleToCircleBorder({
super.side,
this.borderRadius = BorderRadius.zero,
required this.circularity,
required this.eccentricity,
});
final BorderRadiusGeometry borderRadius;
final double circularity;
final double eccentricity;
@override
ShapeBorder scale(double t) {
return _RoundedRectangleToCircleBorder(
side: side.scale(t),
borderRadius: borderRadius * t,
circularity: t,
eccentricity: eccentricity,
);
}
@override
ShapeBorder? lerpFrom(ShapeBorder? a, double t) {
if (a is RoundedRectangleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, borderRadius, t)!,
circularity: circularity * t,
eccentricity: eccentricity,
);
}
if (a is CircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: borderRadius,
circularity: circularity + (1.0 - circularity) * (1.0 - t),
eccentricity: a.eccentricity,
);
}
if (a is _RoundedRectangleToCircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(a.side, side, t),
borderRadius: BorderRadiusGeometry.lerp(a.borderRadius, borderRadius, t)!,
circularity: ui.lerpDouble(a.circularity, circularity, t)!,
eccentricity: eccentricity,
);
}
return super.lerpFrom(a, t);
}
@override
ShapeBorder? lerpTo(ShapeBorder? b, double t) {
if (b is RoundedRectangleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: BorderRadiusGeometry.lerp(borderRadius, b.borderRadius, t)!,
circularity: circularity * (1.0 - t),
eccentricity: eccentricity,
);
}
if (b is CircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: borderRadius,
circularity: circularity + (1.0 - circularity) * t,
eccentricity: b.eccentricity,
);
}
if (b is _RoundedRectangleToCircleBorder) {
return _RoundedRectangleToCircleBorder(
side: BorderSide.lerp(side, b.side, t),
borderRadius: BorderRadiusGeometry.lerp(borderRadius, b.borderRadius, t)!,
circularity: ui.lerpDouble(circularity, b.circularity, t)!,
eccentricity: eccentricity,
);
}
return super.lerpTo(b, t);
}
Rect _adjustRect(Rect rect) {
if (circularity == 0.0 || rect.width == rect.height) {
return rect;
}
if (rect.width < rect.height) {
final double partialDelta = (rect.height - rect.width) / 2;
final double delta = circularity * partialDelta * (1.0 - eccentricity);
return Rect.fromLTRB(
rect.left,
rect.top + delta,
rect.right,
rect.bottom - delta,
);
} else {
final double partialDelta = (rect.width - rect.height) / 2;
final double delta = circularity * partialDelta * (1.0 - eccentricity);
return Rect.fromLTRB(
rect.left + delta,
rect.top,
rect.right - delta,
rect.bottom,
);
}
}
BorderRadius? _adjustBorderRadius(Rect rect, TextDirection? textDirection) {
final BorderRadius resolvedRadius = borderRadius.resolve(textDirection);
if (circularity == 0.0) {
return resolvedRadius;
}
if (eccentricity != 0.0) {
if (rect.width < rect.height) {
return BorderRadius.lerp(
resolvedRadius,
BorderRadius.all(Radius.elliptical(rect.width / 2, (0.5 + eccentricity / 2) * rect.height / 2)),
circularity,
)!;
} else {
return BorderRadius.lerp(
resolvedRadius,
BorderRadius.all(Radius.elliptical((0.5 + eccentricity / 2) * rect.width / 2, rect.height / 2)),
circularity,
)!;
}
}
return BorderRadius.lerp(resolvedRadius, BorderRadius.circular(rect.shortestSide / 2), circularity);
}
@override
Path getInnerPath(Rect rect, { TextDirection? textDirection }) {
final RRect borderRect = _adjustBorderRadius(rect, textDirection)!.toRRect(_adjustRect(rect));
final RRect adjustedRect = borderRect.deflate(ui.lerpDouble(side.width, 0, side.strokeAlign)!);
return Path()
..addRRect(adjustedRect);
}
@override
Path getOuterPath(Rect rect, { TextDirection? textDirection }) {
return Path()
..addRRect(_adjustBorderRadius(rect, textDirection)!.toRRect(_adjustRect(rect)));
}
@override
void paintInterior(Canvas canvas, Rect rect, Paint paint, { TextDirection? textDirection }) {
final BorderRadius adjustedBorderRadius = _adjustBorderRadius(rect, textDirection)!;
if (adjustedBorderRadius == BorderRadius.zero) {
canvas.drawRect(_adjustRect(rect), paint);
} else {
canvas.drawRRect(adjustedBorderRadius.toRRect(_adjustRect(rect)), paint);
}
}
@override
bool get preferPaintInterior => true;
@override
_RoundedRectangleToCircleBorder copyWith({ BorderSide? side, BorderRadiusGeometry? borderRadius, double? circularity, double? eccentricity }) {
return _RoundedRectangleToCircleBorder(
side: side ?? this.side,
borderRadius: borderRadius ?? this.borderRadius,
circularity: circularity ?? this.circularity,
eccentricity: eccentricity ?? this.eccentricity,
);
}
@override
void paint(Canvas canvas, Rect rect, { TextDirection? textDirection }) {
switch (side.style) {
case BorderStyle.none:
break;
case BorderStyle.solid:
final BorderRadius adjustedBorderRadius = _adjustBorderRadius(rect, textDirection)!;
final RRect borderRect = adjustedBorderRadius.toRRect(_adjustRect(rect));
canvas.drawRRect(borderRect.inflate(side.strokeOffset / 2), side.toPaint());
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _RoundedRectangleToCircleBorder
&& other.side == side
&& other.borderRadius == borderRadius
&& other.circularity == circularity;
}
@override
int get hashCode => Object.hash(side, borderRadius, circularity);
@override
String toString() {
if (eccentricity != 0.0) {
return 'RoundedRectangleBorder($side, $borderRadius, ${(circularity * 100).toStringAsFixed(1)}% of the way to being a CircleBorder that is ${(eccentricity * 100).toStringAsFixed(1)}% oval)';
}
return 'RoundedRectangleBorder($side, $borderRadius, ${(circularity * 100).toStringAsFixed(1)}% of the way to being a CircleBorder)';
}
}
| flutter/packages/flutter/lib/src/painting/rounded_rectangle_border.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/rounded_rectangle_border.dart",
"repo_id": "flutter",
"token_count": 4456
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Whether two doubles are within a given distance of each other.
///
/// The `epsilon` argument must be positive and not null.
/// The `a` and `b` arguments may be null. A null value is only considered
/// near-equal to another null value.
bool nearEqual(double? a, double? b, double epsilon) {
assert(epsilon >= 0.0);
if (a == null || b == null) {
return a == b;
}
return (a > (b - epsilon)) && (a < (b + epsilon)) || a == b;
}
/// Whether a double is within a given distance of zero.
///
/// The epsilon argument must be positive.
bool nearZero(double a, double epsilon) => nearEqual(a, 0.0, epsilon);
| flutter/packages/flutter/lib/src/physics/utils.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/physics/utils.dart",
"repo_id": "flutter",
"token_count": 248
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'object.dart';
/// Parent data for use with [RenderListBody].
class ListBodyParentData extends ContainerBoxParentData<RenderBox> { }
typedef _ChildSizingFunction = double Function(RenderBox child);
/// Displays its children sequentially along a given axis, forcing them to the
/// dimensions of the parent in the other axis.
///
/// This layout algorithm arranges its children linearly along the main axis
/// (either horizontally or vertically). In the cross axis, children are
/// stretched to match the box's cross-axis extent. In the main axis, children
/// are given unlimited space and the box expands its main axis to contain all
/// its children. Because [RenderListBody] boxes expand in the main axis, they
/// must be given unlimited space in the main axis, typically by being contained
/// in a viewport with a scrolling direction that matches the box's main axis.
class RenderListBody extends RenderBox
with ContainerRenderObjectMixin<RenderBox, ListBodyParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, ListBodyParentData> {
/// Creates a render object that arranges its children sequentially along a
/// given axis.
///
/// By default, children are arranged along the vertical axis.
RenderListBody({
List<RenderBox>? children,
AxisDirection axisDirection = AxisDirection.down,
}) : _axisDirection = axisDirection {
addAll(children);
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! ListBodyParentData) {
child.parentData = ListBodyParentData();
}
}
/// The direction in which the children are laid out.
///
/// For example, if the [axisDirection] is [AxisDirection.down], each child
/// will be laid out below the next, vertically.
AxisDirection get axisDirection => _axisDirection;
AxisDirection _axisDirection;
set axisDirection(AxisDirection value) {
if (_axisDirection == value) {
return;
}
_axisDirection = value;
markNeedsLayout();
}
/// The axis (horizontal or vertical) corresponding to the current
/// [axisDirection].
Axis get mainAxis => axisDirectionToAxis(axisDirection);
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
assert(_debugCheckConstraints(constraints));
double mainAxisExtent = 0.0;
RenderBox? child = firstChild;
switch (axisDirection) {
case AxisDirection.right:
case AxisDirection.left:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(height: constraints.maxHeight);
while (child != null) {
final Size childSize = child.getDryLayout(innerConstraints);
mainAxisExtent += childSize.width;
child = childAfter(child);
}
return constraints.constrain(Size(mainAxisExtent, constraints.maxHeight));
case AxisDirection.up:
case AxisDirection.down:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(width: constraints.maxWidth);
while (child != null) {
final Size childSize = child.getDryLayout(innerConstraints);
mainAxisExtent += childSize.height;
child = childAfter(child);
}
return constraints.constrain(Size(constraints.maxWidth, mainAxisExtent));
}
}
bool _debugCheckConstraints(BoxConstraints constraints) {
assert(() {
switch (mainAxis) {
case Axis.horizontal:
if (!constraints.hasBoundedWidth) {
return true;
}
case Axis.vertical:
if (!constraints.hasBoundedHeight) {
return true;
}
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('RenderListBody must have unlimited space along its main axis.'),
ErrorDescription(
'RenderListBody does not clip or resize its children, so it must be '
'placed in a parent that does not constrain the main '
'axis.',
),
ErrorHint(
'You probably want to put the RenderListBody inside a '
'RenderViewport with a matching main axis.',
),
]);
}());
assert(() {
switch (mainAxis) {
case Axis.horizontal:
if (constraints.hasBoundedHeight) {
return true;
}
case Axis.vertical:
if (constraints.hasBoundedWidth) {
return true;
}
}
// TODO(ianh): Detect if we're actually nested blocks and say something
// more specific to the exact situation in that case, and don't mention
// nesting blocks in the negative case.
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('RenderListBody must have a bounded constraint for its cross axis.'),
ErrorDescription(
"RenderListBody forces its children to expand to fit the RenderListBody's container, "
'so it must be placed in a parent that constrains the cross '
'axis to a finite dimension.',
),
// TODO(jacobr): this hint is a great candidate to promote to being an
// automated quick fix in the future.
ErrorHint(
'If you are attempting to nest a RenderListBody with '
'one direction inside one of another direction, you will want to '
'wrap the inner one inside a box that fixes the dimension in that direction, '
'for example, a RenderIntrinsicWidth or RenderIntrinsicHeight object. '
'This is relatively expensive, however.', // (that's why we don't do it automatically)
),
]);
}());
return true;
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
assert(_debugCheckConstraints(constraints));
double mainAxisExtent = 0.0;
RenderBox? child = firstChild;
switch (axisDirection) {
case AxisDirection.right:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(height: constraints.maxHeight);
while (child != null) {
child.layout(innerConstraints, parentUsesSize: true);
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
childParentData.offset = Offset(mainAxisExtent, 0.0);
mainAxisExtent += child.size.width;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
size = constraints.constrain(Size(mainAxisExtent, constraints.maxHeight));
case AxisDirection.left:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(height: constraints.maxHeight);
while (child != null) {
child.layout(innerConstraints, parentUsesSize: true);
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
mainAxisExtent += child.size.width;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
double position = 0.0;
child = firstChild;
while (child != null) {
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
position += child.size.width;
childParentData.offset = Offset(mainAxisExtent - position, 0.0);
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
size = constraints.constrain(Size(mainAxisExtent, constraints.maxHeight));
case AxisDirection.down:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(width: constraints.maxWidth);
while (child != null) {
child.layout(innerConstraints, parentUsesSize: true);
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
childParentData.offset = Offset(0.0, mainAxisExtent);
mainAxisExtent += child.size.height;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
size = constraints.constrain(Size(constraints.maxWidth, mainAxisExtent));
case AxisDirection.up:
final BoxConstraints innerConstraints = BoxConstraints.tightFor(width: constraints.maxWidth);
while (child != null) {
child.layout(innerConstraints, parentUsesSize: true);
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
mainAxisExtent += child.size.height;
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
double position = 0.0;
child = firstChild;
while (child != null) {
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
position += child.size.height;
childParentData.offset = Offset(0.0, mainAxisExtent - position);
assert(child.parentData == childParentData);
child = childParentData.nextSibling;
}
size = constraints.constrain(Size(constraints.maxWidth, mainAxisExtent));
}
assert(size.isFinite);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<AxisDirection>('axisDirection', axisDirection));
}
double _getIntrinsicCrossAxis(_ChildSizingFunction childSize) {
double extent = 0.0;
RenderBox? child = firstChild;
while (child != null) {
extent = math.max(extent, childSize(child));
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
child = childParentData.nextSibling;
}
return extent;
}
double _getIntrinsicMainAxis(_ChildSizingFunction childSize) {
double extent = 0.0;
RenderBox? child = firstChild;
while (child != null) {
extent += childSize(child);
final ListBodyParentData childParentData = child.parentData! as ListBodyParentData;
child = childParentData.nextSibling;
}
return extent;
}
@override
double computeMinIntrinsicWidth(double height) {
return switch (mainAxis) {
Axis.horizontal => _getIntrinsicMainAxis((RenderBox child) => child.getMinIntrinsicWidth(height)),
Axis.vertical => _getIntrinsicCrossAxis((RenderBox child) => child.getMinIntrinsicWidth(height)),
};
}
@override
double computeMaxIntrinsicWidth(double height) {
return switch (mainAxis) {
Axis.horizontal => _getIntrinsicMainAxis((RenderBox child) => child.getMaxIntrinsicWidth(height)),
Axis.vertical => _getIntrinsicCrossAxis((RenderBox child) => child.getMaxIntrinsicWidth(height)),
};
}
@override
double computeMinIntrinsicHeight(double width) {
return switch (mainAxis) {
Axis.horizontal => _getIntrinsicMainAxis((RenderBox child) => child.getMinIntrinsicHeight(width)),
Axis.vertical => _getIntrinsicCrossAxis((RenderBox child) => child.getMinIntrinsicHeight(width)),
};
}
@override
double computeMaxIntrinsicHeight(double width) {
return switch (mainAxis) {
Axis.horizontal => _getIntrinsicMainAxis((RenderBox child) => child.getMaxIntrinsicHeight(width)),
Axis.vertical => _getIntrinsicCrossAxis((RenderBox child) => child.getMaxIntrinsicHeight(width)),
};
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
return defaultComputeDistanceToFirstActualBaseline(baseline);
}
@override
void paint(PaintingContext context, Offset offset) {
defaultPaint(context, offset);
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
return defaultHitTestChildren(result, position: position);
}
}
| flutter/packages/flutter/lib/src/rendering/list_body.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/list_body.dart",
"repo_id": "flutter",
"token_count": 4365
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'object.dart';
import 'sliver.dart';
import 'sliver_multi_box_adaptor.dart';
/// Describes the placement of a child in a [RenderSliverGrid].
///
/// This class is similar to [Rect], in that it gives a two-dimensional position
/// and a two-dimensional dimension, but is direction-agnostic.
///
/// {@tool dartpad}
/// This example shows how a custom [SliverGridLayout] uses [SliverGridGeometry]
/// to lay out the children.
///
/// ** See code in examples/api/lib/widgets/scroll_view/grid_view.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverGridLayout], which represents the geometry of all the tiles in a
/// grid.
/// * [SliverGridLayout.getGeometryForChildIndex], which returns this object
/// to describe the child's placement.
/// * [RenderSliverGrid], which uses this class during its
/// [RenderSliverGrid.performLayout] method.
@immutable
class SliverGridGeometry {
/// Creates an object that describes the placement of a child in a [RenderSliverGrid].
const SliverGridGeometry({
required this.scrollOffset,
required this.crossAxisOffset,
required this.mainAxisExtent,
required this.crossAxisExtent,
});
/// The scroll offset of the leading edge of the child relative to the leading
/// edge of the parent.
final double scrollOffset;
/// The offset of the child in the non-scrolling axis.
///
/// If the scroll axis is vertical, this offset is from the left-most edge of
/// the parent to the left-most edge of the child. If the scroll axis is
/// horizontal, this offset is from the top-most edge of the parent to the
/// top-most edge of the child.
final double crossAxisOffset;
/// The extent of the child in the scrolling axis.
///
/// If the scroll axis is vertical, this extent is the child's height. If the
/// scroll axis is horizontal, this extent is the child's width.
final double mainAxisExtent;
/// The extent of the child in the non-scrolling axis.
///
/// If the scroll axis is vertical, this extent is the child's width. If the
/// scroll axis is horizontal, this extent is the child's height.
final double crossAxisExtent;
/// The scroll offset of the trailing edge of the child relative to the
/// leading edge of the parent.
double get trailingScrollOffset => scrollOffset + mainAxisExtent;
/// Returns a tight [BoxConstraints] that forces the child to have the
/// required size, given a [SliverConstraints].
BoxConstraints getBoxConstraints(SliverConstraints constraints) {
return constraints.asBoxConstraints(
minExtent: mainAxisExtent,
maxExtent: mainAxisExtent,
crossAxisExtent: crossAxisExtent,
);
}
@override
String toString() {
final List<String> properties = <String>[
'scrollOffset: $scrollOffset',
'crossAxisOffset: $crossAxisOffset',
'mainAxisExtent: $mainAxisExtent',
'crossAxisExtent: $crossAxisExtent',
];
return 'SliverGridGeometry(${properties.join(', ')})';
}
}
/// The size and position of all the tiles in a [RenderSliverGrid].
///
/// Rather that providing a grid with a [SliverGridLayout] directly, the grid is
/// provided a [SliverGridDelegate], which computes a [SliverGridLayout] given a
/// set of [SliverConstraints]. This allows the algorithm to dynamically respond
/// to changes in the environment (e.g. the user rotating the device).
///
/// The tiles can be placed arbitrarily, but it is more efficient to place tiles
/// roughly in order by scroll offset because grids reify a contiguous sequence
/// of children.
///
/// {@tool dartpad}
/// This example shows how to construct a custom [SliverGridLayout] to lay tiles
/// in a grid form with some cells stretched to fit the entire width of the
/// grid (sometimes called "hero tiles").
///
/// ** See code in examples/api/lib/widgets/scroll_view/grid_view.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverGridRegularTileLayout], which represents a layout that uses
/// equally sized and spaced tiles.
/// * [SliverGridGeometry], which represents the size and position of a single
/// tile in a grid.
/// * [SliverGridDelegate.getLayout], which returns this object to describe the
/// delegate's layout.
/// * [RenderSliverGrid], which uses this class during its
/// [RenderSliverGrid.performLayout] method.
@immutable
abstract class SliverGridLayout {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliverGridLayout();
/// The minimum child index that intersects with (or is after) this scroll offset.
int getMinChildIndexForScrollOffset(double scrollOffset);
/// The maximum child index that intersects with (or is before) this scroll offset.
int getMaxChildIndexForScrollOffset(double scrollOffset);
/// The size and position of the child with the given index.
SliverGridGeometry getGeometryForChildIndex(int index);
/// The scroll extent needed to fully display all the tiles if there are
/// `childCount` children in total.
///
/// The child count will never be null.
double computeMaxScrollOffset(int childCount);
}
/// A [SliverGridLayout] that uses equally sized and spaced tiles.
///
/// Rather that providing a grid with a [SliverGridLayout] directly, you instead
/// provide the grid a [SliverGridDelegate], which can compute a
/// [SliverGridLayout] given the current [SliverConstraints].
///
/// This layout is used by [SliverGridDelegateWithFixedCrossAxisCount] and
/// [SliverGridDelegateWithMaxCrossAxisExtent].
///
/// See also:
///
/// * [SliverGridDelegateWithFixedCrossAxisCount], which uses this layout.
/// * [SliverGridDelegateWithMaxCrossAxisExtent], which uses this layout.
/// * [SliverGridLayout], which represents an arbitrary tile layout.
/// * [SliverGridGeometry], which represents the size and position of a single
/// tile in a grid.
/// * [SliverGridDelegate.getLayout], which returns this object to describe the
/// delegate's layout.
/// * [RenderSliverGrid], which uses this class during its
/// [RenderSliverGrid.performLayout] method.
class SliverGridRegularTileLayout extends SliverGridLayout {
/// Creates a layout that uses equally sized and spaced tiles.
///
/// All of the arguments must not be negative. The `crossAxisCount` argument
/// must be greater than zero.
const SliverGridRegularTileLayout({
required this.crossAxisCount,
required this.mainAxisStride,
required this.crossAxisStride,
required this.childMainAxisExtent,
required this.childCrossAxisExtent,
required this.reverseCrossAxis,
}) : assert(crossAxisCount > 0),
assert(mainAxisStride >= 0),
assert(crossAxisStride >= 0),
assert(childMainAxisExtent >= 0),
assert(childCrossAxisExtent >= 0);
/// The number of children in the cross axis.
final int crossAxisCount;
/// The number of pixels from the leading edge of one tile to the leading edge
/// of the next tile in the main axis.
final double mainAxisStride;
/// The number of pixels from the leading edge of one tile to the leading edge
/// of the next tile in the cross axis.
final double crossAxisStride;
/// The number of pixels from the leading edge of one tile to the trailing
/// edge of the same tile in the main axis.
final double childMainAxisExtent;
/// The number of pixels from the leading edge of one tile to the trailing
/// edge of the same tile in the cross axis.
final double childCrossAxisExtent;
/// Whether the children should be placed in the opposite order of increasing
/// coordinates in the cross axis.
///
/// For example, if the cross axis is horizontal, the children are placed from
/// left to right when [reverseCrossAxis] is false and from right to left when
/// [reverseCrossAxis] is true.
///
/// Typically set to the return value of [axisDirectionIsReversed] applied to
/// the [SliverConstraints.crossAxisDirection].
final bool reverseCrossAxis;
@override
int getMinChildIndexForScrollOffset(double scrollOffset) {
return mainAxisStride > precisionErrorTolerance ? crossAxisCount * (scrollOffset ~/ mainAxisStride) : 0;
}
@override
int getMaxChildIndexForScrollOffset(double scrollOffset) {
if (mainAxisStride > 0.0) {
final int mainAxisCount = (scrollOffset / mainAxisStride).ceil();
return math.max(0, crossAxisCount * mainAxisCount - 1);
}
return 0;
}
double _getOffsetFromStartInCrossAxis(double crossAxisStart) {
if (reverseCrossAxis) {
return crossAxisCount * crossAxisStride - crossAxisStart - childCrossAxisExtent - (crossAxisStride - childCrossAxisExtent);
}
return crossAxisStart;
}
@override
SliverGridGeometry getGeometryForChildIndex(int index) {
final double crossAxisStart = (index % crossAxisCount) * crossAxisStride;
return SliverGridGeometry(
scrollOffset: (index ~/ crossAxisCount) * mainAxisStride,
crossAxisOffset: _getOffsetFromStartInCrossAxis(crossAxisStart),
mainAxisExtent: childMainAxisExtent,
crossAxisExtent: childCrossAxisExtent,
);
}
@override
double computeMaxScrollOffset(int childCount) {
if (childCount == 0) {
// There are no children in the grid. The max scroll offset should be
// zero.
return 0.0;
}
final int mainAxisCount = ((childCount - 1) ~/ crossAxisCount) + 1;
final double mainAxisSpacing = mainAxisStride - childMainAxisExtent;
return mainAxisStride * mainAxisCount - mainAxisSpacing;
}
}
/// Controls the layout of tiles in a grid.
///
/// Given the current constraints on the grid, a [SliverGridDelegate] computes
/// the layout for the tiles in the grid. The tiles can be placed arbitrarily,
/// but it is more efficient to place tiles roughly in order by scroll offset
/// because grids reify a contiguous sequence of children.
///
/// {@tool dartpad}
/// This example shows how a [SliverGridDelegate] returns a [SliverGridLayout]
/// configured based on the provided [SliverConstraints] in [getLayout].
///
/// ** See code in examples/api/lib/widgets/scroll_view/grid_view.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverGridDelegateWithFixedCrossAxisCount], which creates a layout with
/// a fixed number of tiles in the cross axis.
/// * [SliverGridDelegateWithMaxCrossAxisExtent], which creates a layout with
/// tiles that have a maximum cross-axis extent.
/// * [GridView], which uses this delegate to control the layout of its tiles.
/// * [SliverGrid], which uses this delegate to control the layout of its
/// tiles.
/// * [RenderSliverGrid], which uses this delegate to control the layout of its
/// tiles.
abstract class SliverGridDelegate {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const SliverGridDelegate();
/// Returns information about the size and position of the tiles in the grid.
SliverGridLayout getLayout(SliverConstraints constraints);
/// Override this method to return true when the children need to be
/// laid out.
///
/// This should compare the fields of the current delegate and the given
/// `oldDelegate` and return true if the fields are such that the layout would
/// be different.
bool shouldRelayout(covariant SliverGridDelegate oldDelegate);
}
/// Creates grid layouts with a fixed number of tiles in the cross axis.
///
/// For example, if the grid is vertical, this delegate will create a layout
/// with a fixed number of columns. If the grid is horizontal, this delegate
/// will create a layout with a fixed number of rows.
///
/// This delegate creates grids with equally sized and spaced tiles.
///
/// {@tool dartpad}
/// Here is an example using the [childAspectRatio] property. On a device with a
/// screen width of 800.0, it creates a GridView with each tile with a width of
/// 200.0 and a height of 100.0.
///
/// ** See code in examples/api/lib/rendering/sliver_grid/sliver_grid_delegate_with_fixed_cross_axis_count.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// Here is an example using the [mainAxisExtent] property. On a device with a
/// screen width of 800.0, it creates a GridView with each tile with a width of
/// 200.0 and a height of 150.0.
///
/// ** See code in examples/api/lib/rendering/sliver_grid/sliver_grid_delegate_with_fixed_cross_axis_count.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [SliverGridDelegateWithMaxCrossAxisExtent], which creates a layout with
/// tiles that have a maximum cross-axis extent.
/// * [SliverGridDelegate], which creates arbitrary layouts.
/// * [GridView], which can use this delegate to control the layout of its
/// tiles.
/// * [SliverGrid], which can use this delegate to control the layout of its
/// tiles.
/// * [RenderSliverGrid], which can use this delegate to control the layout of
/// its tiles.
class SliverGridDelegateWithFixedCrossAxisCount extends SliverGridDelegate {
/// Creates a delegate that makes grid layouts with a fixed number of tiles in
/// the cross axis.
///
/// The `mainAxisSpacing`, `mainAxisExtent` and `crossAxisSpacing` arguments
/// must not be negative. The `crossAxisCount` and `childAspectRatio`
/// arguments must be greater than zero.
const SliverGridDelegateWithFixedCrossAxisCount({
required this.crossAxisCount,
this.mainAxisSpacing = 0.0,
this.crossAxisSpacing = 0.0,
this.childAspectRatio = 1.0,
this.mainAxisExtent,
}) : assert(crossAxisCount > 0),
assert(mainAxisSpacing >= 0),
assert(crossAxisSpacing >= 0),
assert(childAspectRatio > 0);
/// The number of children in the cross axis.
final int crossAxisCount;
/// The number of logical pixels between each child along the main axis.
final double mainAxisSpacing;
/// The number of logical pixels between each child along the cross axis.
final double crossAxisSpacing;
/// The ratio of the cross-axis to the main-axis extent of each child.
final double childAspectRatio;
/// The extent of each tile in the main axis. If provided it would define the
/// logical pixels taken by each tile in the main-axis.
///
/// If null, [childAspectRatio] is used instead.
final double? mainAxisExtent;
bool _debugAssertIsValid() {
assert(crossAxisCount > 0);
assert(mainAxisSpacing >= 0.0);
assert(crossAxisSpacing >= 0.0);
assert(childAspectRatio > 0.0);
return true;
}
@override
SliverGridLayout getLayout(SliverConstraints constraints) {
assert(_debugAssertIsValid());
final double usableCrossAxisExtent = math.max(
0.0,
constraints.crossAxisExtent - crossAxisSpacing * (crossAxisCount - 1),
);
final double childCrossAxisExtent = usableCrossAxisExtent / crossAxisCount;
final double childMainAxisExtent = mainAxisExtent ?? childCrossAxisExtent / childAspectRatio;
return SliverGridRegularTileLayout(
crossAxisCount: crossAxisCount,
mainAxisStride: childMainAxisExtent + mainAxisSpacing,
crossAxisStride: childCrossAxisExtent + crossAxisSpacing,
childMainAxisExtent: childMainAxisExtent,
childCrossAxisExtent: childCrossAxisExtent,
reverseCrossAxis: axisDirectionIsReversed(constraints.crossAxisDirection),
);
}
@override
bool shouldRelayout(SliverGridDelegateWithFixedCrossAxisCount oldDelegate) {
return oldDelegate.crossAxisCount != crossAxisCount
|| oldDelegate.mainAxisSpacing != mainAxisSpacing
|| oldDelegate.crossAxisSpacing != crossAxisSpacing
|| oldDelegate.childAspectRatio != childAspectRatio
|| oldDelegate.mainAxisExtent != mainAxisExtent;
}
}
/// Creates grid layouts with tiles that each have a maximum cross-axis extent.
///
/// This delegate will select a cross-axis extent for the tiles that is as
/// large as possible subject to the following conditions:
///
/// - The extent evenly divides the cross-axis extent of the grid.
/// - The extent is at most [maxCrossAxisExtent].
///
/// For example, if the grid is vertical, the grid is 500.0 pixels wide, and
/// [maxCrossAxisExtent] is 150.0, this delegate will create a grid with 4
/// columns that are 125.0 pixels wide.
///
/// This delegate creates grids with equally sized and spaced tiles.
///
/// See also:
///
/// * [SliverGridDelegateWithFixedCrossAxisCount], which creates a layout with
/// a fixed number of tiles in the cross axis.
/// * [SliverGridDelegate], which creates arbitrary layouts.
/// * [GridView], which can use this delegate to control the layout of its
/// tiles.
/// * [SliverGrid], which can use this delegate to control the layout of its
/// tiles.
/// * [RenderSliverGrid], which can use this delegate to control the layout of
/// its tiles.
class SliverGridDelegateWithMaxCrossAxisExtent extends SliverGridDelegate {
/// Creates a delegate that makes grid layouts with tiles that have a maximum
/// cross-axis extent.
///
/// The [maxCrossAxisExtent], [mainAxisExtent], [mainAxisSpacing],
/// and [crossAxisSpacing] arguments must not be negative.
/// The [childAspectRatio] argument must be greater than zero.
const SliverGridDelegateWithMaxCrossAxisExtent({
required this.maxCrossAxisExtent,
this.mainAxisSpacing = 0.0,
this.crossAxisSpacing = 0.0,
this.childAspectRatio = 1.0,
this.mainAxisExtent,
}) : assert(maxCrossAxisExtent > 0),
assert(mainAxisSpacing >= 0),
assert(crossAxisSpacing >= 0),
assert(childAspectRatio > 0);
/// The maximum extent of tiles in the cross axis.
///
/// This delegate will select a cross-axis extent for the tiles that is as
/// large as possible subject to the following conditions:
///
/// - The extent evenly divides the cross-axis extent of the grid.
/// - The extent is at most [maxCrossAxisExtent].
///
/// For example, if the grid is vertical, the grid is 500.0 pixels wide, and
/// [maxCrossAxisExtent] is 150.0, this delegate will create a grid with 4
/// columns that are 125.0 pixels wide.
final double maxCrossAxisExtent;
/// The number of logical pixels between each child along the main axis.
final double mainAxisSpacing;
/// The number of logical pixels between each child along the cross axis.
final double crossAxisSpacing;
/// The ratio of the cross-axis to the main-axis extent of each child.
final double childAspectRatio;
/// The extent of each tile in the main axis. If provided it would define the
/// logical pixels taken by each tile in the main-axis.
///
/// If null, [childAspectRatio] is used instead.
final double? mainAxisExtent;
bool _debugAssertIsValid(double crossAxisExtent) {
assert(crossAxisExtent > 0.0);
assert(maxCrossAxisExtent > 0.0);
assert(mainAxisSpacing >= 0.0);
assert(crossAxisSpacing >= 0.0);
assert(childAspectRatio > 0.0);
return true;
}
@override
SliverGridLayout getLayout(SliverConstraints constraints) {
assert(_debugAssertIsValid(constraints.crossAxisExtent));
int crossAxisCount = (constraints.crossAxisExtent / (maxCrossAxisExtent + crossAxisSpacing)).ceil();
// Ensure a minimum count of 1, can be zero and result in an infinite extent
// below when the window size is 0.
crossAxisCount = math.max(1, crossAxisCount);
final double usableCrossAxisExtent = math.max(
0.0,
constraints.crossAxisExtent - crossAxisSpacing * (crossAxisCount - 1),
);
final double childCrossAxisExtent = usableCrossAxisExtent / crossAxisCount;
final double childMainAxisExtent = mainAxisExtent ?? childCrossAxisExtent / childAspectRatio;
return SliverGridRegularTileLayout(
crossAxisCount: crossAxisCount,
mainAxisStride: childMainAxisExtent + mainAxisSpacing,
crossAxisStride: childCrossAxisExtent + crossAxisSpacing,
childMainAxisExtent: childMainAxisExtent,
childCrossAxisExtent: childCrossAxisExtent,
reverseCrossAxis: axisDirectionIsReversed(constraints.crossAxisDirection),
);
}
@override
bool shouldRelayout(SliverGridDelegateWithMaxCrossAxisExtent oldDelegate) {
return oldDelegate.maxCrossAxisExtent != maxCrossAxisExtent
|| oldDelegate.mainAxisSpacing != mainAxisSpacing
|| oldDelegate.crossAxisSpacing != crossAxisSpacing
|| oldDelegate.childAspectRatio != childAspectRatio
|| oldDelegate.mainAxisExtent != mainAxisExtent;
}
}
/// Parent data structure used by [RenderSliverGrid].
class SliverGridParentData extends SliverMultiBoxAdaptorParentData {
/// The offset of the child in the non-scrolling axis.
///
/// If the scroll axis is vertical, this offset is from the left-most edge of
/// the parent to the left-most edge of the child. If the scroll axis is
/// horizontal, this offset is from the top-most edge of the parent to the
/// top-most edge of the child.
double? crossAxisOffset;
@override
String toString() => 'crossAxisOffset=$crossAxisOffset; ${super.toString()}';
}
/// A sliver that places multiple box children in a two dimensional arrangement.
///
/// [RenderSliverGrid] places its children in arbitrary positions determined by
/// [gridDelegate]. Each child is forced to have the size specified by the
/// [gridDelegate].
///
/// See also:
///
/// * [RenderSliverList], which places its children in a linear
/// array.
/// * [RenderSliverFixedExtentList], which places its children in a linear
/// array with a fixed extent in the main axis.
class RenderSliverGrid extends RenderSliverMultiBoxAdaptor {
/// Creates a sliver that contains multiple box children that whose size and
/// position are determined by a delegate.
RenderSliverGrid({
required super.childManager,
required SliverGridDelegate gridDelegate,
}) : _gridDelegate = gridDelegate;
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverGridParentData) {
child.parentData = SliverGridParentData();
}
}
/// The delegate that controls the size and position of the children.
SliverGridDelegate get gridDelegate => _gridDelegate;
SliverGridDelegate _gridDelegate;
set gridDelegate(SliverGridDelegate value) {
if (_gridDelegate == value) {
return;
}
if (value.runtimeType != _gridDelegate.runtimeType ||
value.shouldRelayout(_gridDelegate)) {
markNeedsLayout();
}
_gridDelegate = value;
}
@override
double childCrossAxisPosition(RenderBox child) {
final SliverGridParentData childParentData = child.parentData! as SliverGridParentData;
return childParentData.crossAxisOffset!;
}
@override
void performLayout() {
final SliverConstraints constraints = this.constraints;
childManager.didStartLayout();
childManager.setDidUnderflow(false);
final double scrollOffset = constraints.scrollOffset + constraints.cacheOrigin;
assert(scrollOffset >= 0.0);
final double remainingExtent = constraints.remainingCacheExtent;
assert(remainingExtent >= 0.0);
final double targetEndScrollOffset = scrollOffset + remainingExtent;
final SliverGridLayout layout = _gridDelegate.getLayout(constraints);
final int firstIndex = layout.getMinChildIndexForScrollOffset(scrollOffset);
final int? targetLastIndex = targetEndScrollOffset.isFinite ?
layout.getMaxChildIndexForScrollOffset(targetEndScrollOffset) : null;
if (firstChild != null) {
final int leadingGarbage = calculateLeadingGarbage(firstIndex: firstIndex);
final int trailingGarbage = targetLastIndex != null ? calculateTrailingGarbage(lastIndex: targetLastIndex) : 0;
collectGarbage(leadingGarbage, trailingGarbage);
} else {
collectGarbage(0, 0);
}
final SliverGridGeometry firstChildGridGeometry = layout.getGeometryForChildIndex(firstIndex);
if (firstChild == null) {
if (!addInitialChild(index: firstIndex, layoutOffset: firstChildGridGeometry.scrollOffset)) {
// There are either no children, or we are past the end of all our children.
final double max = layout.computeMaxScrollOffset(childManager.childCount);
geometry = SliverGeometry(
scrollExtent: max,
maxPaintExtent: max,
);
childManager.didFinishLayout();
return;
}
}
final double leadingScrollOffset = firstChildGridGeometry.scrollOffset;
double trailingScrollOffset = firstChildGridGeometry.trailingScrollOffset;
RenderBox? trailingChildWithLayout;
bool reachedEnd = false;
for (int index = indexOf(firstChild!) - 1; index >= firstIndex; --index) {
final SliverGridGeometry gridGeometry = layout.getGeometryForChildIndex(index);
final RenderBox child = insertAndLayoutLeadingChild(
gridGeometry.getBoxConstraints(constraints),
)!;
final SliverGridParentData childParentData = child.parentData! as SliverGridParentData;
childParentData.layoutOffset = gridGeometry.scrollOffset;
childParentData.crossAxisOffset = gridGeometry.crossAxisOffset;
assert(childParentData.index == index);
trailingChildWithLayout ??= child;
trailingScrollOffset = math.max(trailingScrollOffset, gridGeometry.trailingScrollOffset);
}
if (trailingChildWithLayout == null) {
firstChild!.layout(firstChildGridGeometry.getBoxConstraints(constraints));
final SliverGridParentData childParentData = firstChild!.parentData! as SliverGridParentData;
childParentData.layoutOffset = firstChildGridGeometry.scrollOffset;
childParentData.crossAxisOffset = firstChildGridGeometry.crossAxisOffset;
trailingChildWithLayout = firstChild;
}
for (int index = indexOf(trailingChildWithLayout!) + 1; targetLastIndex == null || index <= targetLastIndex; ++index) {
final SliverGridGeometry gridGeometry = layout.getGeometryForChildIndex(index);
final BoxConstraints childConstraints = gridGeometry.getBoxConstraints(constraints);
RenderBox? child = childAfter(trailingChildWithLayout!);
if (child == null || indexOf(child) != index) {
child = insertAndLayoutChild(childConstraints, after: trailingChildWithLayout);
if (child == null) {
reachedEnd = true;
// We have run out of children.
break;
}
} else {
child.layout(childConstraints);
}
trailingChildWithLayout = child;
final SliverGridParentData childParentData = child.parentData! as SliverGridParentData;
childParentData.layoutOffset = gridGeometry.scrollOffset;
childParentData.crossAxisOffset = gridGeometry.crossAxisOffset;
assert(childParentData.index == index);
trailingScrollOffset = math.max(trailingScrollOffset, gridGeometry.trailingScrollOffset);
}
final int lastIndex = indexOf(lastChild!);
assert(debugAssertChildListIsNonEmptyAndContiguous());
assert(indexOf(firstChild!) == firstIndex);
assert(targetLastIndex == null || lastIndex <= targetLastIndex);
final double estimatedTotalExtent = reachedEnd
? trailingScrollOffset
: childManager.estimateMaxScrollOffset(
constraints,
firstIndex: firstIndex,
lastIndex: lastIndex,
leadingScrollOffset: leadingScrollOffset,
trailingScrollOffset: trailingScrollOffset,
);
final double paintExtent = calculatePaintOffset(
constraints,
from: math.min(constraints.scrollOffset, leadingScrollOffset),
to: trailingScrollOffset,
);
final double cacheExtent = calculateCacheOffset(
constraints,
from: leadingScrollOffset,
to: trailingScrollOffset,
);
geometry = SliverGeometry(
scrollExtent: estimatedTotalExtent,
paintExtent: paintExtent,
maxPaintExtent: estimatedTotalExtent,
cacheExtent: cacheExtent,
hasVisualOverflow: estimatedTotalExtent > paintExtent || constraints.scrollOffset > 0.0 || constraints.overlap != 0.0,
);
// We may have started the layout while scrolled to the end, which
// would not expose a new child.
if (estimatedTotalExtent == trailingScrollOffset) {
childManager.setDidUnderflow(true);
}
childManager.didFinishLayout();
}
}
| flutter/packages/flutter/lib/src/rendering/sliver_grid.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/sliver_grid.dart",
"repo_id": "flutter",
"token_count": 8974
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
// Any changes to this file should be reflected in the debugAssertAllSchedulerVarsUnset()
// function below.
/// Print a banner at the beginning of each frame.
///
/// Frames triggered by the engine and handler by the scheduler binding will
/// have a banner giving the frame number and the time stamp of the frame.
///
/// Frames triggered eagerly by the widget framework (e.g. when calling
/// [runApp]) will have a label saying "warm-up frame" instead of the time stamp
/// (the time stamp sent to frame callbacks in that case is the time of the last
/// frame, or 0:00 if it is the first frame).
///
/// To include a banner at the end of each frame as well, to distinguish
/// intra-frame output from inter-frame output, set [debugPrintEndFrameBanner]
/// to true as well.
///
/// See also:
///
/// * [debugProfilePaintsEnabled], which does something similar for
/// painting but using the timeline view.
/// * [debugPrintLayouts], which does something similar for layout but using
/// console output.
/// * The discussions at [WidgetsBinding.drawFrame] and at
/// [SchedulerBinding.handleBeginFrame].
bool debugPrintBeginFrameBanner = false;
/// Print a banner at the end of each frame.
///
/// Combined with [debugPrintBeginFrameBanner], this can be helpful for
/// determining if code is running during a frame or between frames.
bool debugPrintEndFrameBanner = false;
/// Log the call stacks that cause a frame to be scheduled.
///
/// This is called whenever [SchedulerBinding.scheduleFrame] schedules a frame. This
/// can happen for various reasons, e.g. when a [Ticker] or
/// [AnimationController] is started, or when [RenderObject.markNeedsLayout] is
/// called, or when [State.setState] is called.
///
/// To get a stack specifically when widgets are scheduled to be built, see
/// [debugPrintScheduleBuildForStacks].
bool debugPrintScheduleFrameStacks = false;
/// Record timeline trace events for post-frame callbacks.
///
/// When this flag is set to false (the default), the developer timeline
/// records when post-frame callbacks are running, but it does not tell you any
/// information about how that time is spent within specific callbacks:
///
/// 
///
/// When this flag is set to true, timeline events will be recorded for each
/// post-frame callback that runs, like so:
///
/// 
bool debugTracePostFrameCallbacks = false;
/// Returns true if none of the scheduler library debug variables have been changed.
///
/// This function is used by the test framework to ensure that debug variables
/// haven't been inadvertently changed.
///
/// See [the scheduler library](scheduler/scheduler-library.html) for a complete
/// list.
bool debugAssertAllSchedulerVarsUnset(String reason) {
assert(() {
if (debugPrintBeginFrameBanner ||
debugPrintEndFrameBanner) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
| flutter/packages/flutter/lib/src/scheduler/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/scheduler/debug.dart",
"repo_id": "flutter",
"token_count": 916
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'system_channels.dart';
/// Controls the browser's context menu on the web platform.
///
/// The context menu is the menu that appears on right clicking or selecting
/// text in the browser, for example.
///
/// On web, by default, the browser's context menu is enabled and Flutter's
/// context menus are hidden.
///
/// On all non-web platforms, this does nothing.
class BrowserContextMenu {
BrowserContextMenu._();
static final BrowserContextMenu _instance = BrowserContextMenu._();
/// Whether showing the browser's context menu is enabled.
///
/// When true, any event that the browser typically uses to trigger its
/// context menu (e.g. right click) will do so. When false, the browser's
/// context menu will not show.
///
/// It's possible for this to be true but for the browser's context menu to
/// not show due to direct manipulation of the DOM. For example, handlers for
/// the browser's `contextmenu` event could be added/removed in the browser's
/// JavaScript console, and this boolean wouldn't know about it. This boolean
/// only indicates the results of calling [disableContextMenu] and
/// [enableContextMenu] here.
///
/// Defaults to true.
static bool get enabled => _instance._enabled;
bool _enabled = true;
final MethodChannel _channel = SystemChannels.contextMenu;
/// Disable the browser's context menu.
///
/// By default, when the app starts, the browser's context menu is already
/// enabled.
///
/// This is an asynchronous action. The context menu can be considered to be
/// disabled at the time that the Future resolves. [enabled] won't reflect the
/// change until that time.
///
/// See also:
/// * [enableContextMenu], which performs the opposite operation.
static Future<void> disableContextMenu() {
assert(kIsWeb, 'This has no effect on platforms other than web.');
return _instance._channel.invokeMethod<void>(
'disableContextMenu',
).then((_) {
_instance._enabled = false;
});
}
/// Enable the browser's context menu.
///
/// By default, when the app starts, the browser's context menu is already
/// enabled. Typically this method would be called after first calling
/// [disableContextMenu].
///
/// This is an asynchronous action. The context menu can be considered to be
/// enabled at the time that the Future resolves. [enabled] won't reflect the
/// change until that time.
///
/// See also:
/// * [disableContextMenu], which performs the opposite operation.
static Future<void> enableContextMenu() {
assert(kIsWeb, 'This has no effect on platforms other than web.');
return _instance._channel.invokeMethod<void>(
'enableContextMenu',
).then((_) {
_instance._enabled = true;
});
}
}
| flutter/packages/flutter/lib/src/services/browser_context_menu.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/browser_context_menu.dart",
"repo_id": "flutter",
"token_count": 809
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:developer';
import 'package:flutter/foundation.dart';
import '_background_isolate_binary_messenger_io.dart'
if (dart.library.js_util) '_background_isolate_binary_messenger_web.dart';
import 'binary_messenger.dart';
import 'binding.dart';
import 'debug.dart';
import 'message_codec.dart';
import 'message_codecs.dart';
export '_background_isolate_binary_messenger_io.dart'
if (dart.library.js_util) '_background_isolate_binary_messenger_web.dart';
export 'binary_messenger.dart' show BinaryMessenger;
export 'binding.dart' show RootIsolateToken;
export 'message_codec.dart' show MessageCodec, MethodCall, MethodCodec;
/// Profile and print statistics on Platform Channel usage.
///
/// When this is true statistics about the usage of Platform Channels will be
/// printed out periodically to the console and Timeline events will show the
/// time between sending and receiving a message (encoding and decoding time
/// excluded).
///
/// The statistics include the total bytes transmitted and the average number of
/// bytes per invocation in the last quantum. "Up" means in the direction of
/// Flutter to the host platform, "down" is the host platform to flutter.
bool get shouldProfilePlatformChannels => kProfilePlatformChannels || (!kReleaseMode && debugProfilePlatformChannels);
/// Controls whether platform channel usage can be debugged in release mode.
///
/// See also:
///
/// * [shouldProfilePlatformChannels], which checks both
/// [kProfilePlatformChannels] and [debugProfilePlatformChannels] for the
/// current run mode.
/// * [debugProfilePlatformChannels], which determines whether platform
/// channel usage can be debugged in non-release mode.
const bool kProfilePlatformChannels = false;
bool _profilePlatformChannelsIsRunning = false;
const Duration _profilePlatformChannelsRate = Duration(seconds: 1);
final Expando<BinaryMessenger> _profiledBinaryMessengers = Expando<BinaryMessenger>();
class _ProfiledBinaryMessenger implements BinaryMessenger {
const _ProfiledBinaryMessenger(this.proxy, this.channelTypeName, this.codecTypeName);
final BinaryMessenger proxy;
final String channelTypeName;
final String codecTypeName;
@override
Future<void> handlePlatformMessage(String channel, ByteData? data, PlatformMessageResponseCallback? callback) {
return proxy.handlePlatformMessage(channel, data, callback);
}
Future<ByteData?>? sendWithPostfix(String channel, String postfix, ByteData? message) async {
_debugRecordUpStream(channelTypeName, '$channel$postfix', codecTypeName, message);
final TimelineTask timelineTask = TimelineTask()..start('Platform Channel send $channel$postfix');
final ByteData? result;
try {
result = await proxy.send(channel, message);
} finally {
timelineTask.finish();
}
_debugRecordDownStream(channelTypeName, '$channel$postfix', codecTypeName, result);
return result;
}
@override
Future<ByteData?>? send(String channel, ByteData? message) =>
sendWithPostfix(channel, '', message);
@override
void setMessageHandler(String channel, MessageHandler? handler) {
proxy.setMessageHandler(channel, handler);
}
}
class _PlatformChannelStats {
_PlatformChannelStats(this.channel, this.codec, this.type);
final String channel;
final String codec;
final String type;
int _upCount = 0;
int _upBytes = 0;
int get upBytes => _upBytes;
void addUpStream(int bytes) {
_upCount += 1;
_upBytes += bytes;
}
int _downCount = 0;
int _downBytes = 0;
int get downBytes => _downBytes;
void addDownStream(int bytes) {
_downCount += 1;
_downBytes += bytes;
}
double get averageUpPayload => _upBytes / _upCount;
double get averageDownPayload => _downBytes / _downCount;
}
final Map<String, _PlatformChannelStats> _profilePlatformChannelsStats = <String, _PlatformChannelStats>{};
Future<void> _debugLaunchProfilePlatformChannels() async {
if (!_profilePlatformChannelsIsRunning) {
_profilePlatformChannelsIsRunning = true;
await Future<dynamic>.delayed(_profilePlatformChannelsRate);
_profilePlatformChannelsIsRunning = false;
final StringBuffer log = StringBuffer();
log.writeln('Platform Channel Stats:');
final List<_PlatformChannelStats> allStats =
_profilePlatformChannelsStats.values.toList();
// Sort highest combined bandwidth first.
allStats.sort((_PlatformChannelStats x, _PlatformChannelStats y) =>
(y.upBytes + y.downBytes) - (x.upBytes + x.downBytes));
for (final _PlatformChannelStats stats in allStats) {
log.writeln(
' (name:"${stats.channel}" type:"${stats.type}" codec:"${stats.codec}" upBytes:${stats.upBytes} upBytes_avg:${stats.averageUpPayload.toStringAsFixed(1)} downBytes:${stats.downBytes} downBytes_avg:${stats.averageDownPayload.toStringAsFixed(1)})');
}
debugPrint(log.toString());
_profilePlatformChannelsStats.clear();
}
}
void _debugRecordUpStream(String channelTypeName, String name,
String codecTypeName, ByteData? bytes) {
final _PlatformChannelStats stats =
_profilePlatformChannelsStats[name] ??=
_PlatformChannelStats(name, codecTypeName, channelTypeName);
stats.addUpStream(bytes?.lengthInBytes ?? 0);
_debugLaunchProfilePlatformChannels();
}
void _debugRecordDownStream(String channelTypeName, String name,
String codecTypeName, ByteData? bytes) {
final _PlatformChannelStats stats =
_profilePlatformChannelsStats[name] ??=
_PlatformChannelStats(name, codecTypeName, channelTypeName);
stats.addDownStream(bytes?.lengthInBytes ?? 0);
_debugLaunchProfilePlatformChannels();
}
BinaryMessenger _findBinaryMessenger() {
return !kIsWeb && ServicesBinding.rootIsolateToken == null
? BackgroundIsolateBinaryMessenger.instance
: ServicesBinding.instance.defaultBinaryMessenger;
}
/// A named channel for communicating with platform plugins using asynchronous
/// message passing.
///
/// Messages are encoded into binary before being sent, and binary messages
/// received are decoded into Dart values. The [MessageCodec] used must be
/// compatible with the one used by the platform plugin. This can be achieved
/// by creating a basic message channel counterpart of this channel on the
/// platform side. The Dart type of messages sent and received is [T],
/// but only the values supported by the specified [MessageCodec] can be used.
/// The use of unsupported values should be considered programming errors, and
/// will result in exceptions being thrown. The null message is supported
/// for all codecs.
///
/// The logical identity of the channel is given by its name. Identically named
/// channels will interfere with each other's communication.
///
/// All [BasicMessageChannel]s provided by the Flutter framework guarantee FIFO
/// ordering. Applications can assume messages sent via a built-in
/// [BasicMessageChannel] are delivered in the same order as they're sent.
///
/// See: <https://flutter.dev/platform-channels/>
class BasicMessageChannel<T> {
/// Creates a [BasicMessageChannel] with the specified [name], [codec] and
/// [binaryMessenger].
///
/// The default [ServicesBinding.defaultBinaryMessenger] instance is used if
/// [binaryMessenger] is null.
const BasicMessageChannel(this.name, this.codec, { BinaryMessenger? binaryMessenger })
: _binaryMessenger = binaryMessenger;
/// The logical channel on which communication happens, not null.
final String name;
/// The message codec used by this channel, not null.
final MessageCodec<T> codec;
/// The messenger which sends the bytes for this channel.
///
/// On the root isolate or web, this defaults to the
/// [ServicesBinding.defaultBinaryMessenger]. In other contexts the default
/// value is a [BackgroundIsolateBinaryMessenger] from
/// [BackgroundIsolateBinaryMessenger.ensureInitialized].
BinaryMessenger get binaryMessenger {
final BinaryMessenger result = _binaryMessenger ?? _findBinaryMessenger();
return shouldProfilePlatformChannels
? _profiledBinaryMessengers[this] ??= _ProfiledBinaryMessenger(
// ignore: no_runtimetype_tostring
result, runtimeType.toString(), codec.runtimeType.toString())
: result;
}
final BinaryMessenger? _binaryMessenger;
/// Sends the specified [message] to the platform plugins on this channel.
///
/// Returns a [Future] which completes to the received response, which may
/// be null.
Future<T?> send(T message) async {
return codec.decodeMessage(await binaryMessenger.send(name, codec.encodeMessage(message)));
}
/// Sets a callback for receiving messages from the platform plugins on this
/// channel. Messages may be null.
///
/// The given callback will replace the currently registered callback for this
/// channel, if any. To remove the handler, pass null as the `handler`
/// argument.
///
/// The handler's return value is sent back to the platform plugins as a
/// message reply. It may be null.
void setMessageHandler(Future<T> Function(T? message)? handler) {
if (handler == null) {
binaryMessenger.setMessageHandler(name, null);
} else {
binaryMessenger.setMessageHandler(name, (ByteData? message) async {
return codec.encodeMessage(await handler(codec.decodeMessage(message)));
});
}
}
// Looking for setMockMessageHandler?
// See this shim package: packages/flutter_test/lib/src/deprecated.dart
}
/// A named channel for communicating with platform plugins using asynchronous
/// method calls.
///
/// Method calls are encoded into binary before being sent, and binary results
/// received are decoded into Dart values. The [MethodCodec] used must be
/// compatible with the one used by the platform plugin. This can be achieved
/// by creating a method channel counterpart of this channel on the
/// platform side. The Dart type of arguments and results is `dynamic`,
/// but only values supported by the specified [MethodCodec] can be used.
/// The use of unsupported values should be considered programming errors, and
/// will result in exceptions being thrown. The null value is supported
/// for all codecs.
///
/// The logical identity of the channel is given by its name. Identically named
/// channels will interfere with each other's communication.
///
/// {@template flutter.services.method_channel.FIFO}
/// All [MethodChannel]s provided by the Flutter framework guarantee FIFO
/// ordering. Applications can assume method calls sent via a built-in
/// [MethodChannel] are received by the platform plugins in the same order as
/// they're sent.
/// {@endtemplate}
///
/// See: <https://flutter.dev/platform-channels/>
@pragma('vm:keep-name')
class MethodChannel {
/// Creates a [MethodChannel] with the specified [name].
///
/// The [codec] used will be [StandardMethodCodec], unless otherwise
/// specified.
///
/// The default [ServicesBinding.defaultBinaryMessenger] instance is used if
/// [binaryMessenger] is null.
const MethodChannel(this.name, [this.codec = const StandardMethodCodec(), BinaryMessenger? binaryMessenger ])
: _binaryMessenger = binaryMessenger;
/// The logical channel on which communication happens, not null.
final String name;
/// The message codec used by this channel, not null.
final MethodCodec codec;
/// The messenger which sends the bytes for this channel.
///
/// On the root isolate or web, this defaults to the
/// [ServicesBinding.defaultBinaryMessenger]. In other contexts the default
/// value is a [BackgroundIsolateBinaryMessenger] from
/// [BackgroundIsolateBinaryMessenger.ensureInitialized].
BinaryMessenger get binaryMessenger {
final BinaryMessenger result = _binaryMessenger ?? _findBinaryMessenger();
return shouldProfilePlatformChannels
? _profiledBinaryMessengers[this] ??= _ProfiledBinaryMessenger(
// ignore: no_runtimetype_tostring
result, runtimeType.toString(), codec.runtimeType.toString())
: result;
}
final BinaryMessenger? _binaryMessenger;
/// Backend implementation of [invokeMethod].
///
/// The `method` and `arguments` arguments are used to create a [MethodCall]
/// object that is passed to the [codec]'s [MethodCodec.encodeMethodCall]
/// method. The resulting message is then sent to the embedding using the
/// [binaryMessenger]'s [BinaryMessenger.send] method.
///
/// If the result is null and `missingOk` is true, this returns null. (This is
/// the behavior of [OptionalMethodChannel.invokeMethod].)
///
/// If the result is null and `missingOk` is false, this throws a
/// [MissingPluginException]. (This is the behavior of
/// [MethodChannel.invokeMethod].)
///
/// Otherwise, the result is decoded using the [codec]'s
/// [MethodCodec.decodeEnvelope] method.
///
/// The `T` type argument is the expected return type. It is treated as
/// nullable.
@optionalTypeArgs
Future<T?> _invokeMethod<T>(String method, { required bool missingOk, dynamic arguments }) async {
final ByteData input = codec.encodeMethodCall(MethodCall(method, arguments));
final ByteData? result =
shouldProfilePlatformChannels ?
await (binaryMessenger as _ProfiledBinaryMessenger).sendWithPostfix(name, '#$method', input) :
await binaryMessenger.send(name, input);
if (result == null) {
if (missingOk) {
return null;
}
throw MissingPluginException('No implementation found for method $method on channel $name');
}
return codec.decodeEnvelope(result) as T?;
}
/// Invokes a [method] on this channel with the specified [arguments].
///
/// The static type of [arguments] is `dynamic`, but only values supported by
/// the [codec] of this channel can be used. The same applies to the returned
/// result. The values supported by the default codec and their platform-specific
/// counterparts are documented with [StandardMessageCodec].
///
/// The generic argument `T` of the method can be inferred by the surrounding
/// context, or provided explicitly. If it does not match the returned type of
/// the channel, a [TypeError] will be thrown at runtime. `T` cannot be a class
/// with generics other than `dynamic`. For example, `Map<String, String>`
/// is not supported but `Map<dynamic, dynamic>` or `Map` is.
///
/// Returns a [Future] which completes to one of the following:
///
/// * a result (possibly null), on successful invocation;
/// * a [PlatformException], if the invocation failed in the platform plugin;
/// * a [MissingPluginException], if the method has not been implemented by a
/// platform plugin.
///
/// The following code snippets demonstrate how to invoke platform methods
/// in Dart using a MethodChannel and how to implement those methods in Java
/// (for Android) and Objective-C (for iOS).
///
/// {@tool snippet}
///
/// The code might be packaged up as a musical plugin, see
/// <https://flutter.dev/developing-packages/>:
///
/// ```dart
/// abstract final class Music {
/// static const MethodChannel _channel = MethodChannel('music');
///
/// static Future<bool> isLicensed() async {
/// // invokeMethod returns a Future<T?>, so we handle the case where
/// // the return value is null by treating null as false.
/// return _channel.invokeMethod<bool>('isLicensed').then<bool>((bool? value) => value ?? false);
/// }
///
/// static Future<List<Song>> songs() async {
/// // invokeMethod here returns a Future<dynamic> that completes to a
/// // List<dynamic> with Map<dynamic, dynamic> entries. Post-processing
/// // code thus cannot assume e.g. List<Map<String, String>> even though
/// // the actual values involved would support such a typed container.
/// // The correct type cannot be inferred with any value of `T`.
/// final List<dynamic>? songs = await _channel.invokeMethod<List<dynamic>>('getSongs');
/// return songs?.cast<Map<String, Object?>>().map<Song>(Song.fromJson).toList() ?? <Song>[];
/// }
///
/// static Future<void> play(Song song, double volume) async {
/// // Errors occurring on the platform side cause invokeMethod to throw
/// // PlatformExceptions.
/// try {
/// return _channel.invokeMethod('play', <String, dynamic>{
/// 'song': song.id,
/// 'volume': volume,
/// });
/// } on PlatformException catch (e) {
/// throw ArgumentError('Unable to play ${song.title}: ${e.message}');
/// }
/// }
/// }
///
/// class Song {
/// Song(this.id, this.title, this.artist);
///
/// final String id;
/// final String title;
/// final String artist;
///
/// static Song fromJson(Map<String, Object?> json) {
/// return Song(json['id']! as String, json['title']! as String, json['artist']! as String);
/// }
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// Java (for Android):
///
/// ```java
/// // Assumes existence of an Android MusicApi.
/// public class MusicPlugin implements MethodCallHandler {
/// @Override
/// public void onMethodCall(MethodCall call, Result result) {
/// switch (call.method) {
/// case "isLicensed":
/// result.success(MusicApi.checkLicense());
/// break;
/// case "getSongs":
/// final List<MusicApi.Track> tracks = MusicApi.getTracks();
/// final List<Object> json = ArrayList<>(tracks.size());
/// for (MusicApi.Track track : tracks) {
/// json.add(track.toJson()); // Map<String, Object> entries
/// }
/// result.success(json);
/// break;
/// case "play":
/// final String song = call.argument("song");
/// final double volume = call.argument("volume");
/// try {
/// MusicApi.playSongAtVolume(song, volume);
/// result.success(null);
/// } catch (MusicalException e) {
/// result.error("playError", e.getMessage(), null);
/// }
/// break;
/// default:
/// result.notImplemented();
/// }
/// }
/// // Other methods elided.
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// Objective-C (for iOS):
///
/// ```objectivec
/// @interface MusicPlugin : NSObject<FlutterPlugin>
/// @end
///
/// // Assumes existence of an iOS Broadway Play Api.
/// @implementation MusicPlugin
/// - (void)handleMethodCall:(FlutterMethodCall*)call result:(FlutterResult)result {
/// if ([@"isLicensed" isEqualToString:call.method]) {
/// result([NSNumber numberWithBool:[BWPlayApi isLicensed]]);
/// } else if ([@"getSongs" isEqualToString:call.method]) {
/// NSArray* items = [BWPlayApi items];
/// NSMutableArray* json = [NSMutableArray arrayWithCapacity:items.count];
/// for (final BWPlayItem* item in items) {
/// [json addObject:@{ @"id":item.itemId, @"title":item.name, @"artist":item.artist }];
/// }
/// result(json);
/// } else if ([@"play" isEqualToString:call.method]) {
/// NSString* itemId = call.arguments[@"song"];
/// NSNumber* volume = call.arguments[@"volume"];
/// NSError* error = nil;
/// BOOL success = [BWPlayApi playItem:itemId volume:volume.doubleValue error:&error];
/// if (success) {
/// result(nil);
/// } else {
/// result([FlutterError errorWithCode:[NSString stringWithFormat:@"Error %ld", error.code]
/// message:error.domain
/// details:error.localizedDescription]);
/// }
/// } else {
/// result(FlutterMethodNotImplemented);
/// }
/// }
/// // Other methods elided.
/// @end
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [invokeListMethod], for automatically returning typed lists.
/// * [invokeMapMethod], for automatically returning typed maps.
/// * [StandardMessageCodec] which defines the payload values supported by
/// [StandardMethodCodec].
/// * [JSONMessageCodec] which defines the payload values supported by
/// [JSONMethodCodec].
/// * <https://api.flutter.dev/javadoc/io/flutter/plugin/common/MethodCall.html>
/// for how to access method call arguments on Android.
@optionalTypeArgs
Future<T?> invokeMethod<T>(String method, [ dynamic arguments ]) {
return _invokeMethod<T>(method, missingOk: false, arguments: arguments);
}
/// An implementation of [invokeMethod] that can return typed lists.
///
/// Dart generics are reified, meaning that an untyped `List<dynamic>` cannot
/// masquerade as a `List<T>`. Since [invokeMethod] can only return dynamic
/// maps, we instead create a new typed list using [List.cast].
///
/// See also:
///
/// * [invokeMethod], which this call delegates to.
Future<List<T>?> invokeListMethod<T>(String method, [ dynamic arguments ]) async {
final List<dynamic>? result = await invokeMethod<List<dynamic>>(method, arguments);
return result?.cast<T>();
}
/// An implementation of [invokeMethod] that can return typed maps.
///
/// Dart generics are reified, meaning that an untyped `Map<dynamic, dynamic>`
/// cannot masquerade as a `Map<K, V>`. Since [invokeMethod] can only return
/// dynamic maps, we instead create a new typed map using [Map.cast].
///
/// See also:
///
/// * [invokeMethod], which this call delegates to.
Future<Map<K, V>?> invokeMapMethod<K, V>(String method, [ dynamic arguments ]) async {
final Map<dynamic, dynamic>? result = await invokeMethod<Map<dynamic, dynamic>>(method, arguments);
return result?.cast<K, V>();
}
/// Sets a callback for receiving method calls on this channel.
///
/// The given callback will replace the currently registered callback for this
/// channel, if any. To remove the handler, pass null as the
/// `handler` argument.
///
/// If the future returned by the handler completes with a result, that value
/// is sent back to the platform plugin caller wrapped in a success envelope
/// as defined by the [codec] of this channel. If the future completes with
/// a [PlatformException], the fields of that exception will be used to
/// populate an error envelope which is sent back instead. If the future
/// completes with a [MissingPluginException], an empty reply is sent
/// similarly to what happens if no method call handler has been set.
/// Any other exception results in an error envelope being sent.
void setMethodCallHandler(Future<dynamic> Function(MethodCall call)? handler) {
assert(
_binaryMessenger != null || BindingBase.debugBindingType() != null,
'Cannot set the method call handler before the binary messenger has been initialized. '
'This happens when you call setMethodCallHandler() before the WidgetsFlutterBinding '
'has been initialized. You can fix this by either calling WidgetsFlutterBinding.ensureInitialized() '
'before this or by passing a custom BinaryMessenger instance to MethodChannel().',
);
binaryMessenger.setMessageHandler(
name,
handler == null
? null
: (ByteData? message) => _handleAsMethodCall(message, handler),
);
}
Future<ByteData?> _handleAsMethodCall(ByteData? message, Future<dynamic> Function(MethodCall call) handler) async {
final MethodCall call = codec.decodeMethodCall(message);
try {
return codec.encodeSuccessEnvelope(await handler(call));
} on PlatformException catch (e) {
return codec.encodeErrorEnvelope(
code: e.code,
message: e.message,
details: e.details,
);
} on MissingPluginException {
return null;
} catch (error) {
return codec.encodeErrorEnvelope(code: 'error', message: error.toString());
}
}
// Looking for setMockMethodCallHandler or checkMethodCallHandler?
// See this shim package: packages/flutter_test/lib/src/deprecated.dart
}
/// A [MethodChannel] that ignores missing platform plugins.
///
/// When [invokeMethod] fails to find the platform plugin, it returns null
/// instead of throwing an exception.
///
/// {@macro flutter.services.method_channel.FIFO}
class OptionalMethodChannel extends MethodChannel {
/// Creates a [MethodChannel] that ignores missing platform plugins.
const OptionalMethodChannel(super.name, [super.codec, super.binaryMessenger]);
@override
Future<T?> invokeMethod<T>(String method, [ dynamic arguments ]) async {
return super._invokeMethod<T>(method, missingOk: true, arguments: arguments);
}
}
/// A named channel for communicating with platform plugins using event streams.
///
/// Stream setup requests are encoded into binary before being sent,
/// and binary events and errors received are decoded into Dart values.
/// The [MethodCodec] used must be compatible with the one used by the platform
/// plugin. This can be achieved by creating an [EventChannel] counterpart of
/// this channel on the platform side. The Dart type of events sent and received
/// is `dynamic`, but only values supported by the specified [MethodCodec] can
/// be used.
///
/// The logical identity of the channel is given by its name. Identically named
/// channels will interfere with each other's communication.
///
/// See: <https://flutter.dev/platform-channels/>
class EventChannel {
/// Creates an [EventChannel] with the specified [name].
///
/// The [codec] used will be [StandardMethodCodec], unless otherwise
/// specified.
///
/// Neither [name] nor [codec] may be null. The default [ServicesBinding.defaultBinaryMessenger]
/// instance is used if [binaryMessenger] is null.
const EventChannel(this.name, [this.codec = const StandardMethodCodec(), BinaryMessenger? binaryMessenger])
: _binaryMessenger = binaryMessenger;
/// The logical channel on which communication happens, not null.
final String name;
/// The message codec used by this channel, not null.
final MethodCodec codec;
/// The messenger which sends the bytes for this channel.
///
/// On the root isolate or web, this defaults to the
/// [ServicesBinding.defaultBinaryMessenger]. In other contexts the default
/// value is a [BackgroundIsolateBinaryMessenger] from
/// [BackgroundIsolateBinaryMessenger.ensureInitialized].
BinaryMessenger get binaryMessenger =>
_binaryMessenger ?? _findBinaryMessenger();
final BinaryMessenger? _binaryMessenger;
/// Sets up a broadcast stream for receiving events on this channel.
///
/// Returns a broadcast [Stream] which emits events to listeners as follows:
///
/// * a decoded data event (possibly null) for each successful event
/// received from the platform plugin;
/// * an error event containing a [PlatformException] for each error event
/// received from the platform plugin.
///
/// Errors occurring during stream activation or deactivation are reported
/// through the [FlutterError] facility. Stream activation happens only when
/// stream listener count changes from 0 to 1. Stream deactivation happens
/// only when stream listener count changes from 1 to 0.
Stream<dynamic> receiveBroadcastStream([ dynamic arguments ]) {
final MethodChannel methodChannel = MethodChannel(name, codec);
late StreamController<dynamic> controller;
controller = StreamController<dynamic>.broadcast(onListen: () async {
binaryMessenger.setMessageHandler(name, (ByteData? reply) async {
if (reply == null) {
controller.close();
} else {
try {
controller.add(codec.decodeEnvelope(reply));
} on PlatformException catch (e) {
controller.addError(e);
}
}
return null;
});
try {
await methodChannel.invokeMethod<void>('listen', arguments);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context: ErrorDescription('while activating platform stream on channel $name'),
));
}
}, onCancel: () async {
binaryMessenger.setMessageHandler(name, null);
try {
await methodChannel.invokeMethod<void>('cancel', arguments);
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'services library',
context: ErrorDescription('while de-activating platform stream on channel $name'),
));
}
});
return controller.stream;
}
}
| flutter/packages/flutter/lib/src/services/platform_channel.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/platform_channel.dart",
"repo_id": "flutter",
"token_count": 8957
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'system_channels.dart';
/// Controls specific aspects of the system navigation stack.
abstract final class SystemNavigator {
/// Informs the platform of whether or not the Flutter framework will handle
/// back events.
///
/// Currently, this is used only on Android to inform its use of the
/// predictive back gesture when exiting the app. When true, predictive back
/// is disabled.
///
/// See also:
///
/// * The
/// [migration guide](https://developer.android.com/guide/navigation/predictive-back-gesture)
/// for predictive back in native Android apps.
static Future<void> setFrameworkHandlesBack(bool frameworkHandlesBack) async {
// Currently, this method call is only relevant on Android.
if (kIsWeb) {
return;
}
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
return;
case TargetPlatform.android:
return SystemChannels.platform.invokeMethod<void>(
'SystemNavigator.setFrameworkHandlesBack',
frameworkHandlesBack,
);
}
}
/// Removes the topmost Flutter instance, presenting what was before
/// it.
///
/// On Android, removes this activity from the stack and returns to
/// the previous activity.
///
/// On iOS, calls `popViewControllerAnimated:` if the root view
/// controller is a `UINavigationController`, or
/// `dismissViewControllerAnimated:completion:` if the top view
/// controller is a `FlutterViewController`.
///
/// The optional `animated` parameter is ignored on all platforms
/// except iOS where it is an argument to the aforementioned
/// methods.
///
/// This method should be preferred over calling `dart:io`'s [exit]
/// method, as the latter may cause the underlying platform to act
/// as if the application had crashed.
static Future<void> pop({bool? animated}) async {
await SystemChannels.platform.invokeMethod<void>('SystemNavigator.pop', animated);
}
/// Selects the single-entry history mode.
///
/// On web, this switches the browser history model to one that only tracks a
/// single entry, so that calling [routeInformationUpdated] replaces the
/// current entry.
///
/// Currently, this is ignored on other platforms.
///
/// See also:
///
/// * [selectMultiEntryHistory], which enables the browser history to have
/// multiple entries.
static Future<void> selectSingleEntryHistory() {
return SystemChannels.navigation.invokeMethod<void>('selectSingleEntryHistory');
}
/// Selects the multiple-entry history mode.
///
/// On web, this switches the browser history model to one that tracks all
/// updates to [routeInformationUpdated] to form a history stack. This is the
/// default.
///
/// Currently, this is ignored on other platforms.
///
/// See also:
///
/// * [selectSingleEntryHistory], which forces the history to only have one
/// entry.
static Future<void> selectMultiEntryHistory() {
return SystemChannels.navigation.invokeMethod<void>('selectMultiEntryHistory');
}
/// Notifies the platform for a route information change.
///
/// On web, this method behaves differently based on the single-entry or
/// multiple-entries history mode. Use the [selectSingleEntryHistory] and
/// [selectMultiEntryHistory] to toggle between modes.
///
/// For single-entry mode, this method replaces the current URL and state in
/// the current history entry. The flag `replace` is ignored.
///
/// For multiple-entries mode, this method creates a new history entry on top
/// of the current entry if the `replace` is false, thus the user will
/// be on a new history entry as if the user has visited a new page, and the
/// browser back button brings the user back to the previous entry. If
/// `replace` is true, this method only updates the URL and the state in the
/// current history entry without pushing a new one.
///
/// This method is ignored on other platforms.
///
/// The `replace` flag defaults to false.
static Future<void> routeInformationUpdated({
@Deprecated(
'Pass Uri.parse(location) to uri parameter instead. '
'This feature was deprecated after v3.8.0-3.0.pre.'
)
String? location,
Uri? uri,
Object? state,
bool replace = false,
}) {
assert((location != null) != (uri != null), 'One of uri or location must be provided, but not both.');
uri ??= Uri.parse(location!);
return SystemChannels.navigation.invokeMethod<void>(
'routeInformationUpdated',
<String, dynamic>{
'uri': uri.toString(),
'state': state,
'replace': replace,
},
);
}
}
| flutter/packages/flutter/lib/src/services/system_navigator.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/system_navigator.dart",
"repo_id": "flutter",
"token_count": 1487
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'animated_size.dart';
import 'basic.dart';
import 'focus_scope.dart';
import 'framework.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
// Examples can assume:
// bool _first = false;
/// Specifies which of two children to show. See [AnimatedCrossFade].
///
/// The child that is shown will fade in, while the other will fade out.
enum CrossFadeState {
/// Show the first child ([AnimatedCrossFade.firstChild]) and hide the second
/// ([AnimatedCrossFade.secondChild]]).
showFirst,
/// Show the second child ([AnimatedCrossFade.secondChild]) and hide the first
/// ([AnimatedCrossFade.firstChild]).
showSecond,
}
/// Signature for the [AnimatedCrossFade.layoutBuilder] callback.
///
/// The `topChild` is the child fading in, which is normally drawn on top. The
/// `bottomChild` is the child fading out, normally drawn on the bottom.
///
/// For good performance, the returned widget tree should contain both the
/// `topChild` and the `bottomChild`; the depth of the tree, and the types of
/// the widgets in the tree, from the returned widget to each of the children
/// should be the same; and where there is a widget with multiple children, the
/// top child and the bottom child should be keyed using the provided
/// `topChildKey` and `bottomChildKey` keys respectively.
///
/// {@tool snippet}
///
/// ```dart
/// Widget defaultLayoutBuilder(Widget topChild, Key topChildKey, Widget bottomChild, Key bottomChildKey) {
/// return Stack(
/// children: <Widget>[
/// Positioned(
/// key: bottomChildKey,
/// left: 0.0,
/// top: 0.0,
/// right: 0.0,
/// child: bottomChild,
/// ),
/// Positioned(
/// key: topChildKey,
/// child: topChild,
/// )
/// ],
/// );
/// }
/// ```
/// {@end-tool}
typedef AnimatedCrossFadeBuilder = Widget Function(Widget topChild, Key topChildKey, Widget bottomChild, Key bottomChildKey);
/// A widget that cross-fades between two given children and animates itself
/// between their sizes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=PGK2UUAyE54}
///
/// The animation is controlled through the [crossFadeState] parameter.
/// [firstCurve] and [secondCurve] represent the opacity curves of the two
/// children. The [firstCurve] is inverted, i.e. it fades out when providing a
/// growing curve like [Curves.linear]. The [sizeCurve] is the curve used to
/// animate between the size of the fading-out child and the size of the
/// fading-in child.
///
/// This widget is intended to be used to fade a pair of widgets with the same
/// width. In the case where the two children have different heights, the
/// animation crops overflowing children during the animation by aligning their
/// top edge, which means that the bottom will be clipped.
///
/// The animation is automatically triggered when an existing
/// [AnimatedCrossFade] is rebuilt with a different value for the
/// [crossFadeState] property.
///
/// {@tool snippet}
///
/// This code fades between two representations of the Flutter logo. It depends
/// on a boolean field `_first`; when `_first` is true, the first logo is shown,
/// otherwise the second logo is shown. When the field changes state, the
/// [AnimatedCrossFade] widget cross-fades between the two forms of the logo
/// over three seconds.
///
/// ```dart
/// AnimatedCrossFade(
/// duration: const Duration(seconds: 3),
/// firstChild: const FlutterLogo(style: FlutterLogoStyle.horizontal, size: 100.0),
/// secondChild: const FlutterLogo(style: FlutterLogoStyle.stacked, size: 100.0),
/// crossFadeState: _first ? CrossFadeState.showFirst : CrossFadeState.showSecond,
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedOpacity], which fades between nothing and a single child.
/// * [AnimatedSwitcher], which switches out a child for a new one with a
/// customizable transition, supporting multiple cross-fades at once.
/// * [AnimatedSize], the lower-level widget which [AnimatedCrossFade] uses to
/// automatically change size.
class AnimatedCrossFade extends StatefulWidget {
/// Creates a cross-fade animation widget.
///
/// The [duration] of the animation is the same for all components (fade in,
/// fade out, and size), and you can pass [Interval]s instead of [Curve]s in
/// order to have finer control, e.g., creating an overlap between the fades.
const AnimatedCrossFade({
super.key,
required this.firstChild,
required this.secondChild,
this.firstCurve = Curves.linear,
this.secondCurve = Curves.linear,
this.sizeCurve = Curves.linear,
this.alignment = Alignment.topCenter,
required this.crossFadeState,
required this.duration,
this.reverseDuration,
this.layoutBuilder = defaultLayoutBuilder,
this.excludeBottomFocus = true,
});
/// The child that is visible when [crossFadeState] is
/// [CrossFadeState.showFirst]. It fades out when transitioning
/// [crossFadeState] from [CrossFadeState.showFirst] to
/// [CrossFadeState.showSecond] and vice versa.
final Widget firstChild;
/// The child that is visible when [crossFadeState] is
/// [CrossFadeState.showSecond]. It fades in when transitioning
/// [crossFadeState] from [CrossFadeState.showFirst] to
/// [CrossFadeState.showSecond] and vice versa.
final Widget secondChild;
/// The child that will be shown when the animation has completed.
final CrossFadeState crossFadeState;
/// The duration of the whole orchestrated animation.
final Duration duration;
/// The duration of the whole orchestrated animation when running in reverse.
///
/// If not supplied, this defaults to [duration].
final Duration? reverseDuration;
/// The fade curve of the first child.
///
/// Defaults to [Curves.linear].
final Curve firstCurve;
/// The fade curve of the second child.
///
/// Defaults to [Curves.linear].
final Curve secondCurve;
/// The curve of the animation between the two children's sizes.
///
/// Defaults to [Curves.linear].
final Curve sizeCurve;
/// How the children should be aligned while the size is animating.
///
/// Defaults to [Alignment.topCenter].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// A builder that positions the [firstChild] and [secondChild] widgets.
///
/// The widget returned by this method is wrapped in an [AnimatedSize].
///
/// By default, this uses [AnimatedCrossFade.defaultLayoutBuilder], which uses
/// a [Stack] and aligns the `bottomChild` to the top of the stack while
/// providing the `topChild` as the non-positioned child to fill the provided
/// constraints. This works well when the [AnimatedCrossFade] is in a position
/// to change size and when the children are not flexible. However, if the
/// children are less fussy about their sizes (for example a
/// [CircularProgressIndicator] inside a [Center]), or if the
/// [AnimatedCrossFade] is being forced to a particular size, then it can
/// result in the widgets jumping about when the cross-fade state is changed.
final AnimatedCrossFadeBuilder layoutBuilder;
/// When true, this is equivalent to wrapping the bottom widget with an [ExcludeFocus]
/// widget while it is at the bottom of the cross-fade stack.
///
/// Defaults to true. When it is false, the bottom widget in the cross-fade stack
/// can remain in focus until the top widget requests focus. This is useful for
/// animating between different [TextField]s so the keyboard remains open during the
/// cross-fade animation.
final bool excludeBottomFocus;
/// The default layout algorithm used by [AnimatedCrossFade].
///
/// The top child is placed in a stack that sizes itself to match the top
/// child. The bottom child is positioned at the top of the same stack, sized
/// to fit its width but without forcing the height. The stack is then
/// clipped.
///
/// This is the default value for [layoutBuilder]. It implements
/// [AnimatedCrossFadeBuilder].
static Widget defaultLayoutBuilder(Widget topChild, Key topChildKey, Widget bottomChild, Key bottomChildKey) {
return Stack(
clipBehavior: Clip.none,
children: <Widget>[
Positioned(
key: bottomChildKey,
left: 0.0,
top: 0.0,
right: 0.0,
child: bottomChild,
),
Positioned(
key: topChildKey,
child: topChild,
),
],
);
}
@override
State<AnimatedCrossFade> createState() => _AnimatedCrossFadeState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<CrossFadeState>('crossFadeState', crossFadeState));
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment, defaultValue: Alignment.topCenter));
properties.add(IntProperty('duration', duration.inMilliseconds, unit: 'ms'));
properties.add(IntProperty('reverseDuration', reverseDuration?.inMilliseconds, unit: 'ms', defaultValue: null));
}
}
class _AnimatedCrossFadeState extends State<AnimatedCrossFade> with TickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _firstAnimation;
late Animation<double> _secondAnimation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: widget.duration,
reverseDuration: widget.reverseDuration,
vsync: this,
);
if (widget.crossFadeState == CrossFadeState.showSecond) {
_controller.value = 1.0;
}
_firstAnimation = _initAnimation(widget.firstCurve, true);
_secondAnimation = _initAnimation(widget.secondCurve, false);
_controller.addStatusListener((AnimationStatus status) {
setState(() {
// Trigger a rebuild because it depends on _isTransitioning, which
// changes its value together with animation status.
});
});
}
Animation<double> _initAnimation(Curve curve, bool inverted) {
Animation<double> result = _controller.drive(CurveTween(curve: curve));
if (inverted) {
result = result.drive(Tween<double>(begin: 1.0, end: 0.0));
}
return result;
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
void didUpdateWidget(AnimatedCrossFade oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.duration != oldWidget.duration) {
_controller.duration = widget.duration;
}
if (widget.reverseDuration != oldWidget.reverseDuration) {
_controller.reverseDuration = widget.reverseDuration;
}
if (widget.firstCurve != oldWidget.firstCurve) {
_firstAnimation = _initAnimation(widget.firstCurve, true);
}
if (widget.secondCurve != oldWidget.secondCurve) {
_secondAnimation = _initAnimation(widget.secondCurve, false);
}
if (widget.crossFadeState != oldWidget.crossFadeState) {
switch (widget.crossFadeState) {
case CrossFadeState.showFirst:
_controller.reverse();
case CrossFadeState.showSecond:
_controller.forward();
}
}
}
/// Whether we're in the middle of cross-fading this frame.
bool get _isTransitioning => _controller.status == AnimationStatus.forward || _controller.status == AnimationStatus.reverse;
@override
Widget build(BuildContext context) {
const Key kFirstChildKey = ValueKey<CrossFadeState>(CrossFadeState.showFirst);
const Key kSecondChildKey = ValueKey<CrossFadeState>(CrossFadeState.showSecond);
final bool transitioningForwards = _controller.status == AnimationStatus.completed ||
_controller.status == AnimationStatus.forward;
final Key topKey;
Widget topChild;
final Animation<double> topAnimation;
final Key bottomKey;
Widget bottomChild;
final Animation<double> bottomAnimation;
if (transitioningForwards) {
topKey = kSecondChildKey;
topChild = widget.secondChild;
topAnimation = _secondAnimation;
bottomKey = kFirstChildKey;
bottomChild = widget.firstChild;
bottomAnimation = _firstAnimation;
} else {
topKey = kFirstChildKey;
topChild = widget.firstChild;
topAnimation = _firstAnimation;
bottomKey = kSecondChildKey;
bottomChild = widget.secondChild;
bottomAnimation = _secondAnimation;
}
bottomChild = TickerMode(
key: bottomKey,
enabled: _isTransitioning,
child: IgnorePointer(
child: ExcludeSemantics( // Always exclude the semantics of the widget that's fading out.
child: ExcludeFocus(
excluding: widget.excludeBottomFocus,
child: FadeTransition(
opacity: bottomAnimation,
child: bottomChild,
),
),
),
),
);
topChild = TickerMode(
key: topKey,
enabled: true, // Top widget always has its animations enabled.
child: IgnorePointer(
ignoring: false,
child: ExcludeSemantics(
excluding: false, // Always publish semantics for the widget that's fading in.
child: ExcludeFocus(
excluding: false,
child: FadeTransition(
opacity: topAnimation,
child: topChild,
),
),
),
),
);
return ClipRect(
child: AnimatedSize(
alignment: widget.alignment,
duration: widget.duration,
reverseDuration: widget.reverseDuration,
curve: widget.sizeCurve,
child: widget.layoutBuilder(topChild, topKey, bottomChild, bottomKey),
),
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(EnumProperty<CrossFadeState>('crossFadeState', widget.crossFadeState));
description.add(DiagnosticsProperty<AnimationController>('controller', _controller, showName: false));
description.add(DiagnosticsProperty<AlignmentGeometry>('alignment', widget.alignment, defaultValue: Alignment.topCenter));
}
}
| flutter/packages/flutter/lib/src/widgets/animated_cross_fade.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/animated_cross_fade.dart",
"repo_id": "flutter",
"token_count": 4749
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The minimum dimension of any interactive region according to the Material
/// guidelines.
///
/// This is used to avoid small regions that are hard for the user to interact
/// with. It applies to both dimensions of a region, so a square of size
/// kMinInteractiveDimension x kMinInteractiveDimension is the smallest
/// acceptable region that should respond to gestures.
///
/// See also:
///
/// * [kMinInteractiveDimensionCupertino]
const double kMinInteractiveDimension = 48.0;
| flutter/packages/flutter/lib/src/widgets/constants.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/constants.dart",
"repo_id": "flutter",
"token_count": 162
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'basic.dart';
import 'framework.dart';
import 'image.dart';
import 'implicit_animations.dart';
// Examples can assume:
// late Uint8List bytes;
/// An image that shows a [placeholder] image while the target [image] is
/// loading, then fades in the new image when it loads.
///
/// Use this class to display long-loading images, such as [NetworkImage.new],
/// so that the image appears on screen with a graceful animation rather than
/// abruptly popping onto the screen.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=pK738Pg9cxc}
///
/// If the [image] emits an [ImageInfo] synchronously, such as when the image
/// has been loaded and cached, the [image] is displayed immediately, and the
/// [placeholder] is never displayed.
///
/// The [fadeOutDuration] and [fadeOutCurve] properties control the fade-out
/// animation of the [placeholder].
///
/// The [fadeInDuration] and [fadeInCurve] properties control the fade-in
/// animation of the target [image].
///
/// Prefer a [placeholder] that's already cached so that it is displayed
/// immediately. This prevents it from popping onto the screen.
///
/// When [image] changes, it is resolved to a new [ImageStream]. If the new
/// [ImageStream.key] is different, this widget subscribes to the new stream and
/// replaces the displayed image with images emitted by the new stream.
///
/// When [placeholder] changes and the [image] has not yet emitted an
/// [ImageInfo], then [placeholder] is resolved to a new [ImageStream]. If the
/// new [ImageStream.key] is different, this widget subscribes to the new stream
/// and replaces the displayed image to images emitted by the new stream.
///
/// When either [placeholder] or [image] changes, this widget continues showing
/// the previously loaded image (if any) until the new image provider provides a
/// different image. This is known as "gapless playback" (see also
/// [Image.gaplessPlayback]).
///
/// {@tool snippet}
///
/// ```dart
/// FadeInImage(
/// // here `bytes` is a Uint8List containing the bytes for the in-memory image
/// placeholder: MemoryImage(bytes),
/// image: const NetworkImage('https://backend.example.com/image.png'),
/// )
/// ```
/// {@end-tool}
class FadeInImage extends StatefulWidget {
/// Creates a widget that displays a [placeholder] while an [image] is loading,
/// then fades-out the placeholder and fades-in the image.
///
/// The [placeholder] and [image] may be composed in a [ResizeImage] to provide
/// a custom decode/cache size.
///
/// The [placeholder] and [image] may have their own BoxFit settings via [fit]
/// and [placeholderFit].
///
/// The [placeholder] and [image] may have their own FilterQuality settings via [filterQuality]
/// and [placeholderFilterQuality].
///
/// If [excludeFromSemantics] is true, then [imageSemanticLabel] will be ignored.
const FadeInImage({
super.key,
required this.placeholder,
this.placeholderErrorBuilder,
required this.image,
this.imageErrorBuilder,
this.excludeFromSemantics = false,
this.imageSemanticLabel,
this.fadeOutDuration = const Duration(milliseconds: 300),
this.fadeOutCurve = Curves.easeOut,
this.fadeInDuration = const Duration(milliseconds: 700),
this.fadeInCurve = Curves.easeIn,
this.color,
this.colorBlendMode,
this.placeholderColor,
this.placeholderColorBlendMode,
this.width,
this.height,
this.fit,
this.placeholderFit,
this.filterQuality = FilterQuality.low,
this.placeholderFilterQuality,
this.alignment = Alignment.center,
this.repeat = ImageRepeat.noRepeat,
this.matchTextDirection = false,
});
/// Creates a widget that uses a placeholder image stored in memory while
/// loading the final image from the network.
///
/// The `placeholder` argument contains the bytes of the in-memory image.
///
/// The `image` argument is the URL of the final image.
///
/// The `placeholderScale` and `imageScale` arguments are passed to their
/// respective [ImageProvider]s (see also [ImageInfo.scale]).
///
/// If [placeholderCacheWidth], [placeholderCacheHeight], [imageCacheWidth],
/// or [imageCacheHeight] are provided, it indicates to the
/// engine that the respective image should be decoded at the specified size.
/// The image will be rendered to the constraints of the layout or [width]
/// and [height] regardless of these parameters. These parameters are primarily
/// intended to reduce the memory usage of [ImageCache].
///
/// The [placeholder], [image], [placeholderScale], [imageScale],
/// [fadeOutDuration], [fadeOutCurve], [fadeInDuration], [fadeInCurve],
/// [alignment], [repeat], and [matchTextDirection] arguments must not be
/// null.
///
/// See also:
///
/// * [Image.memory], which has more details about loading images from
/// memory.
/// * [Image.network], which has more details about loading images from
/// the network.
FadeInImage.memoryNetwork({
super.key,
required Uint8List placeholder,
this.placeholderErrorBuilder,
required String image,
this.imageErrorBuilder,
double placeholderScale = 1.0,
double imageScale = 1.0,
this.excludeFromSemantics = false,
this.imageSemanticLabel,
this.fadeOutDuration = const Duration(milliseconds: 300),
this.fadeOutCurve = Curves.easeOut,
this.fadeInDuration = const Duration(milliseconds: 700),
this.fadeInCurve = Curves.easeIn,
this.width,
this.height,
this.fit,
this.color,
this.colorBlendMode,
this.placeholderColor,
this.placeholderColorBlendMode,
this.placeholderFit,
this.filterQuality = FilterQuality.low,
this.placeholderFilterQuality,
this.alignment = Alignment.center,
this.repeat = ImageRepeat.noRepeat,
this.matchTextDirection = false,
int? placeholderCacheWidth,
int? placeholderCacheHeight,
int? imageCacheWidth,
int? imageCacheHeight,
}) : placeholder = ResizeImage.resizeIfNeeded(placeholderCacheWidth, placeholderCacheHeight, MemoryImage(placeholder, scale: placeholderScale)),
image = ResizeImage.resizeIfNeeded(imageCacheWidth, imageCacheHeight, NetworkImage(image, scale: imageScale));
/// Creates a widget that uses a placeholder image stored in an asset bundle
/// while loading the final image from the network.
///
/// The `placeholder` argument is the key of the image in the asset bundle.
///
/// The `image` argument is the URL of the final image.
///
/// The `placeholderScale` and `imageScale` arguments are passed to their
/// respective [ImageProvider]s (see also [ImageInfo.scale]).
///
/// If `placeholderScale` is omitted or is null, pixel-density-aware asset
/// resolution will be attempted for the [placeholder] image. Otherwise, the
/// exact asset specified will be used.
///
/// If [placeholderCacheWidth], [placeholderCacheHeight], [imageCacheWidth],
/// or [imageCacheHeight] are provided, it indicates to the
/// engine that the respective image should be decoded at the specified size.
/// The image will be rendered to the constraints of the layout or [width]
/// and [height] regardless of these parameters. These parameters are primarily
/// intended to reduce the memory usage of [ImageCache].
///
/// See also:
///
/// * [Image.asset], which has more details about loading images from
/// asset bundles.
/// * [Image.network], which has more details about loading images from
/// the network.
FadeInImage.assetNetwork({
super.key,
required String placeholder,
this.placeholderErrorBuilder,
required String image,
this.imageErrorBuilder,
AssetBundle? bundle,
double? placeholderScale,
double imageScale = 1.0,
this.excludeFromSemantics = false,
this.imageSemanticLabel,
this.fadeOutDuration = const Duration(milliseconds: 300),
this.fadeOutCurve = Curves.easeOut,
this.fadeInDuration = const Duration(milliseconds: 700),
this.fadeInCurve = Curves.easeIn,
this.width,
this.height,
this.fit,
this.color,
this.colorBlendMode,
this.placeholderColor,
this.placeholderColorBlendMode,
this.placeholderFit,
this.filterQuality = FilterQuality.low,
this.placeholderFilterQuality,
this.alignment = Alignment.center,
this.repeat = ImageRepeat.noRepeat,
this.matchTextDirection = false,
int? placeholderCacheWidth,
int? placeholderCacheHeight,
int? imageCacheWidth,
int? imageCacheHeight,
}) : placeholder = placeholderScale != null
? ResizeImage.resizeIfNeeded(placeholderCacheWidth, placeholderCacheHeight, ExactAssetImage(placeholder, bundle: bundle, scale: placeholderScale))
: ResizeImage.resizeIfNeeded(placeholderCacheWidth, placeholderCacheHeight, AssetImage(placeholder, bundle: bundle)),
image = ResizeImage.resizeIfNeeded(imageCacheWidth, imageCacheHeight, NetworkImage(image, scale: imageScale));
/// Image displayed while the target [image] is loading.
final ImageProvider placeholder;
/// A builder function that is called if an error occurs during placeholder
/// image loading.
///
/// If this builder is not provided, any exceptions will be reported to
/// [FlutterError.onError]. If it is provided, the caller should either handle
/// the exception by providing a replacement widget, or rethrow the exception.
final ImageErrorWidgetBuilder? placeholderErrorBuilder;
/// The target image that is displayed once it has loaded.
final ImageProvider image;
/// A builder function that is called if an error occurs during image loading.
///
/// If this builder is not provided, any exceptions will be reported to
/// [FlutterError.onError]. If it is provided, the caller should either handle
/// the exception by providing a replacement widget, or rethrow the exception.
final ImageErrorWidgetBuilder? imageErrorBuilder;
/// The duration of the fade-out animation for the [placeholder].
final Duration fadeOutDuration;
/// The curve of the fade-out animation for the [placeholder].
final Curve fadeOutCurve;
/// The duration of the fade-in animation for the [image].
final Duration fadeInDuration;
/// The curve of the fade-in animation for the [image].
final Curve fadeInCurve;
/// If non-null, require the image to have this width.
///
/// If null, the image will pick a size that best preserves its intrinsic
/// aspect ratio. This may result in a sudden change if the size of the
/// placeholder image does not match that of the target image. The size is
/// also affected by the scale factor.
final double? width;
/// If non-null, this color is blended with each image pixel using [colorBlendMode].
///
/// Color applies to the [image].
///
/// See Also:
///
/// * [placeholderColor], the color which applies to the [placeholder].
final Color? color;
/// Used to combine [color] with this [image].
///
/// The default is [BlendMode.srcIn]. In terms of the blend mode, [color] is
/// the source and this image is the destination.
///
/// See also:
///
/// * [BlendMode], which includes an illustration of the effect of each blend mode.
/// * [placeholderColorBlendMode], the color blend mode which applies to the [placeholder].
final BlendMode? colorBlendMode;
/// If non-null, this color is blended with each placeholder image pixel using [placeholderColorBlendMode].
///
/// Color applies to the [placeholder].
///
/// See Also:
///
/// * [color], the color which applies to the [image].
final Color? placeholderColor;
/// Used to combine [placeholderColor] with the [placeholder] image.
///
/// The default is [BlendMode.srcIn]. In terms of the blend mode, [placeholderColor] is
/// the source and this placeholder is the destination.
///
/// See also:
///
/// * [BlendMode], which includes an illustration of the effect of each blend mode.
/// * [colorBlendMode], the color blend mode which applies to the [image].
final BlendMode? placeholderColorBlendMode;
/// If non-null, require the image to have this height.
///
/// If null, the image will pick a size that best preserves its intrinsic
/// aspect ratio. This may result in a sudden change if the size of the
/// placeholder image does not match that of the target image. The size is
/// also affected by the scale factor.
final double? height;
/// How to inscribe the image into the space allocated during layout.
///
/// The default varies based on the other fields. See the discussion at
/// [paintImage].
final BoxFit? fit;
/// How to inscribe the placeholder image into the space allocated during layout.
///
/// If not value set, it will fallback to [fit].
final BoxFit? placeholderFit;
/// The rendering quality of the image.
///
/// {@macro flutter.widgets.image.filterQuality}
final FilterQuality filterQuality;
/// The rendering quality of the placeholder image.
///
/// {@macro flutter.widgets.image.filterQuality}
final FilterQuality? placeholderFilterQuality;
/// How to align the image within its bounds.
///
/// The alignment aligns the given position in the image to the given position
/// in the layout bounds. For example, an [Alignment] alignment of (-1.0,
/// -1.0) aligns the image to the top-left corner of its layout bounds, while an
/// [Alignment] alignment of (1.0, 1.0) aligns the bottom right of the
/// image with the bottom right corner of its layout bounds. Similarly, an
/// alignment of (0.0, 1.0) aligns the bottom middle of the image with the
/// middle of the bottom edge of its layout bounds.
///
/// If the [alignment] is [TextDirection]-dependent (i.e. if it is a
/// [AlignmentDirectional]), then an ambient [Directionality] widget
/// must be in scope.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// How to paint any portions of the layout bounds not covered by the image.
final ImageRepeat repeat;
/// Whether to paint the image in the direction of the [TextDirection].
///
/// If this is true, then in [TextDirection.ltr] contexts, the image will be
/// drawn with its origin in the top left (the "normal" painting direction for
/// images); and in [TextDirection.rtl] contexts, the image will be drawn with
/// a scaling factor of -1 in the horizontal direction so that the origin is
/// in the top right.
///
/// This is occasionally used with images in right-to-left environments, for
/// images that were designed for left-to-right locales. Be careful, when
/// using this, to not flip images with integral shadows, text, or other
/// effects that will look incorrect when flipped.
///
/// If this is true, there must be an ambient [Directionality] widget in
/// scope.
final bool matchTextDirection;
/// Whether to exclude this image from semantics.
///
/// This is useful for images which do not contribute meaningful information
/// to an application.
final bool excludeFromSemantics;
/// A semantic description of the [image].
///
/// Used to provide a description of the [image] to TalkBack on Android, and
/// VoiceOver on iOS.
///
/// This description will be used both while the [placeholder] is shown and
/// once the image has loaded.
final String? imageSemanticLabel;
@override
State<FadeInImage> createState() => _FadeInImageState();
}
class _FadeInImageState extends State<FadeInImage> {
static const Animation<double> _kOpaqueAnimation = AlwaysStoppedAnimation<double>(1.0);
bool targetLoaded = false;
// These ProxyAnimations are changed to the fade in animation by
// [_AnimatedFadeOutFadeInState]. Otherwise these animations are reset to
// their defaults by [_resetAnimations].
final ProxyAnimation _imageAnimation = ProxyAnimation(_kOpaqueAnimation);
final ProxyAnimation _placeholderAnimation = ProxyAnimation(_kOpaqueAnimation);
Image _image({
required ImageProvider image,
ImageErrorWidgetBuilder? errorBuilder,
ImageFrameBuilder? frameBuilder,
BoxFit? fit,
Color? color,
BlendMode? colorBlendMode,
required FilterQuality filterQuality,
required Animation<double> opacity,
}) {
return Image(
image: image,
errorBuilder: errorBuilder,
frameBuilder: frameBuilder,
opacity: opacity,
width: widget.width,
height: widget.height,
fit: fit,
color: color,
colorBlendMode: colorBlendMode,
filterQuality: filterQuality,
alignment: widget.alignment,
repeat: widget.repeat,
matchTextDirection: widget.matchTextDirection,
gaplessPlayback: true,
excludeFromSemantics: true,
);
}
@override
Widget build(BuildContext context) {
Widget result = _image(
image: widget.image,
errorBuilder: widget.imageErrorBuilder,
opacity: _imageAnimation,
fit: widget.fit,
color: widget.color,
colorBlendMode: widget.colorBlendMode,
filterQuality: widget.filterQuality,
frameBuilder: (BuildContext context, Widget child, int? frame, bool wasSynchronouslyLoaded) {
if (wasSynchronouslyLoaded || frame != null) {
targetLoaded = true;
}
return _AnimatedFadeOutFadeIn(
target: child,
targetProxyAnimation: _imageAnimation,
placeholder: _image(
image: widget.placeholder,
errorBuilder: widget.placeholderErrorBuilder,
opacity: _placeholderAnimation,
color: widget.placeholderColor,
colorBlendMode: widget.placeholderColorBlendMode,
fit: widget.placeholderFit ?? widget.fit,
filterQuality: widget.placeholderFilterQuality ?? widget.filterQuality,
),
placeholderProxyAnimation: _placeholderAnimation,
isTargetLoaded: targetLoaded,
wasSynchronouslyLoaded: wasSynchronouslyLoaded,
fadeInDuration: widget.fadeInDuration,
fadeOutDuration: widget.fadeOutDuration,
fadeInCurve: widget.fadeInCurve,
fadeOutCurve: widget.fadeOutCurve,
);
},
);
if (!widget.excludeFromSemantics) {
result = Semantics(
container: widget.imageSemanticLabel != null,
image: true,
label: widget.imageSemanticLabel ?? '',
child: result,
);
}
return result;
}
}
class _AnimatedFadeOutFadeIn extends ImplicitlyAnimatedWidget {
const _AnimatedFadeOutFadeIn({
required this.target,
required this.targetProxyAnimation,
required this.placeholder,
required this.placeholderProxyAnimation,
required this.isTargetLoaded,
required this.fadeOutDuration,
required this.fadeOutCurve,
required this.fadeInDuration,
required this.fadeInCurve,
required this.wasSynchronouslyLoaded,
}) : assert(!wasSynchronouslyLoaded || isTargetLoaded),
super(duration: fadeInDuration + fadeOutDuration);
final Widget target;
final ProxyAnimation targetProxyAnimation;
final Widget placeholder;
final ProxyAnimation placeholderProxyAnimation;
final bool isTargetLoaded;
final Duration fadeInDuration;
final Duration fadeOutDuration;
final Curve fadeInCurve;
final Curve fadeOutCurve;
final bool wasSynchronouslyLoaded;
@override
_AnimatedFadeOutFadeInState createState() => _AnimatedFadeOutFadeInState();
}
class _AnimatedFadeOutFadeInState extends ImplicitlyAnimatedWidgetState<_AnimatedFadeOutFadeIn> {
Tween<double>? _targetOpacity;
Tween<double>? _placeholderOpacity;
Animation<double>? _targetOpacityAnimation;
Animation<double>? _placeholderOpacityAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_targetOpacity = visitor(
_targetOpacity,
widget.isTargetLoaded ? 1.0 : 0.0,
(dynamic value) => Tween<double>(begin: value as double),
) as Tween<double>?;
_placeholderOpacity = visitor(
_placeholderOpacity,
widget.isTargetLoaded ? 0.0 : 1.0,
(dynamic value) => Tween<double>(begin: value as double),
) as Tween<double>?;
}
@override
void didUpdateTweens() {
if (widget.wasSynchronouslyLoaded) {
// Opacity animations should not be reset if image was synchronously loaded.
return;
}
_placeholderOpacityAnimation = animation.drive(TweenSequence<double>(<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: _placeholderOpacity!.chain(CurveTween(curve: widget.fadeOutCurve)),
weight: widget.fadeOutDuration.inMilliseconds.toDouble(),
),
TweenSequenceItem<double>(
tween: ConstantTween<double>(0),
weight: widget.fadeInDuration.inMilliseconds.toDouble(),
),
]))..addStatusListener((AnimationStatus status) {
if (_placeholderOpacityAnimation!.isCompleted) {
// Need to rebuild to remove placeholder now that it is invisible.
setState(() {});
}
});
_targetOpacityAnimation = animation.drive(TweenSequence<double>(<TweenSequenceItem<double>>[
TweenSequenceItem<double>(
tween: ConstantTween<double>(0),
weight: widget.fadeOutDuration.inMilliseconds.toDouble(),
),
TweenSequenceItem<double>(
tween: _targetOpacity!.chain(CurveTween(curve: widget.fadeInCurve)),
weight: widget.fadeInDuration.inMilliseconds.toDouble(),
),
]));
widget.targetProxyAnimation.parent = _targetOpacityAnimation;
widget.placeholderProxyAnimation.parent = _placeholderOpacityAnimation;
}
@override
Widget build(BuildContext context) {
if (widget.wasSynchronouslyLoaded ||
(_placeholderOpacityAnimation?.isCompleted ?? true)) {
return widget.target;
}
return Stack(
fit: StackFit.passthrough,
alignment: AlignmentDirectional.center,
// Text direction is irrelevant here since we're using center alignment,
// but it allows the Stack to avoid a call to Directionality.of()
textDirection: TextDirection.ltr,
children: <Widget>[
widget.target,
widget.placeholder,
],
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Animation<double>>('targetOpacity', _targetOpacityAnimation));
properties.add(DiagnosticsProperty<Animation<double>>('placeholderOpacity', _placeholderOpacityAnimation));
}
}
| flutter/packages/flutter/lib/src/widgets/fade_in_image.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/fade_in_image.dart",
"repo_id": "flutter",
"token_count": 7168
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show TextHeightBehavior;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:vector_math/vector_math_64.dart';
import 'basic.dart';
import 'container.dart';
import 'debug.dart';
import 'framework.dart';
import 'text.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
// Examples can assume:
// class MyWidget extends ImplicitlyAnimatedWidget {
// const MyWidget({super.key, this.targetColor = Colors.black}) : super(duration: const Duration(seconds: 1));
// final Color targetColor;
// @override
// ImplicitlyAnimatedWidgetState<MyWidget> createState() => throw UnimplementedError(); // ignore: no_logic_in_create_state
// }
// void setState(VoidCallback fn) { }
/// An interpolation between two [BoxConstraints].
///
/// This class specializes the interpolation of [Tween<BoxConstraints>] to use
/// [BoxConstraints.lerp].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class BoxConstraintsTween extends Tween<BoxConstraints> {
/// Creates a [BoxConstraints] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as a tight constraint of zero size.
BoxConstraintsTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
BoxConstraints lerp(double t) => BoxConstraints.lerp(begin, end, t)!;
}
/// An interpolation between two [Decoration]s.
///
/// This class specializes the interpolation of [Tween<BoxConstraints>] to use
/// [Decoration.lerp].
///
/// For [ShapeDecoration]s which know how to [ShapeDecoration.lerpTo] or
/// [ShapeDecoration.lerpFrom] each other, this will produce a smooth
/// interpolation between decorations.
///
/// See also:
///
/// * [Tween] for a discussion on how to use interpolation objects.
/// * [ShapeDecoration], [RoundedRectangleBorder], [CircleBorder], and
/// [StadiumBorder] for examples of shape borders that can be smoothly
/// interpolated.
/// * [BoxBorder] for a border that can only be smoothly interpolated between other
/// [BoxBorder]s.
class DecorationTween extends Tween<Decoration> {
/// Creates a decoration tween.
///
/// The [begin] and [end] properties may be null. If both are null, then the
/// result is always null. If [end] is not null, then its lerping logic is
/// used (via [Decoration.lerpTo]). Otherwise, [begin]'s lerping logic is used
/// (via [Decoration.lerpFrom]).
DecorationTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
Decoration lerp(double t) => Decoration.lerp(begin, end, t)!;
}
/// An interpolation between two [EdgeInsets]s.
///
/// This class specializes the interpolation of [Tween<EdgeInsets>] to use
/// [EdgeInsets.lerp].
///
/// See [Tween] for a discussion on how to use interpolation objects.
///
/// See also:
///
/// * [EdgeInsetsGeometryTween], which interpolates between two
/// [EdgeInsetsGeometry] objects.
class EdgeInsetsTween extends Tween<EdgeInsets> {
/// Creates an [EdgeInsets] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as an [EdgeInsets] with no inset.
EdgeInsetsTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
EdgeInsets lerp(double t) => EdgeInsets.lerp(begin, end, t)!;
}
/// An interpolation between two [EdgeInsetsGeometry]s.
///
/// This class specializes the interpolation of [Tween<EdgeInsetsGeometry>] to
/// use [EdgeInsetsGeometry.lerp].
///
/// See [Tween] for a discussion on how to use interpolation objects.
///
/// See also:
///
/// * [EdgeInsetsTween], which interpolates between two [EdgeInsets] objects.
class EdgeInsetsGeometryTween extends Tween<EdgeInsetsGeometry> {
/// Creates an [EdgeInsetsGeometry] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as an [EdgeInsetsGeometry] with no inset.
EdgeInsetsGeometryTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
EdgeInsetsGeometry lerp(double t) => EdgeInsetsGeometry.lerp(begin, end, t)!;
}
/// An interpolation between two [BorderRadius]s.
///
/// This class specializes the interpolation of [Tween<BorderRadius>] to use
/// [BorderRadius.lerp].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class BorderRadiusTween extends Tween<BorderRadius?> {
/// Creates a [BorderRadius] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as a right angle (no radius).
BorderRadiusTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
BorderRadius? lerp(double t) => BorderRadius.lerp(begin, end, t);
}
/// An interpolation between two [Border]s.
///
/// This class specializes the interpolation of [Tween<Border>] to use
/// [Border.lerp].
///
/// See [Tween] for a discussion on how to use interpolation objects.
class BorderTween extends Tween<Border?> {
/// Creates a [Border] tween.
///
/// The [begin] and [end] properties may be null; the null value
/// is treated as having no border.
BorderTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
Border? lerp(double t) => Border.lerp(begin, end, t);
}
/// An interpolation between two [Matrix4]s.
///
/// This class specializes the interpolation of [Tween<Matrix4>] to be
/// appropriate for transformation matrices.
///
/// Currently this class works only for translations.
///
/// See [Tween] for a discussion on how to use interpolation objects.
class Matrix4Tween extends Tween<Matrix4> {
/// Creates a [Matrix4] tween.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
Matrix4Tween({ super.begin, super.end });
@override
Matrix4 lerp(double t) {
assert(begin != null);
assert(end != null);
final Vector3 beginTranslation = Vector3.zero();
final Vector3 endTranslation = Vector3.zero();
final Quaternion beginRotation = Quaternion.identity();
final Quaternion endRotation = Quaternion.identity();
final Vector3 beginScale = Vector3.zero();
final Vector3 endScale = Vector3.zero();
begin!.decompose(beginTranslation, beginRotation, beginScale);
end!.decompose(endTranslation, endRotation, endScale);
final Vector3 lerpTranslation =
beginTranslation * (1.0 - t) + endTranslation * t;
// TODO(alangardner): Implement lerp for constant rotation
final Quaternion lerpRotation =
(beginRotation.scaled(1.0 - t) + endRotation.scaled(t)).normalized();
final Vector3 lerpScale = beginScale * (1.0 - t) + endScale * t;
return Matrix4.compose(lerpTranslation, lerpRotation, lerpScale);
}
}
/// An interpolation between two [TextStyle]s.
///
/// This class specializes the interpolation of [Tween<TextStyle>] to use
/// [TextStyle.lerp].
///
/// This will not work well if the styles don't set the same fields.
///
/// See [Tween] for a discussion on how to use interpolation objects.
class TextStyleTween extends Tween<TextStyle> {
/// Creates a text style tween.
///
/// The [begin] and [end] properties must be non-null before the tween is
/// first used, but the arguments can be null if the values are going to be
/// filled in later.
TextStyleTween({ super.begin, super.end });
/// Returns the value this variable has at the given animation clock value.
@override
TextStyle lerp(double t) => TextStyle.lerp(begin, end, t)!;
}
/// An abstract class for building widgets that animate changes to their
/// properties.
///
/// Widgets of this type will not animate when they are first added to the
/// widget tree. Rather, when they are rebuilt with different values, they will
/// respond to those _changes_ by animating the changes over a specified
/// [duration].
///
/// Which properties are animated is left up to the subclass. Subclasses' [State]s
/// must extend [ImplicitlyAnimatedWidgetState] and provide a way to visit the
/// relevant fields to animate.
///
/// ## Relationship to [AnimatedWidget]s
///
/// [ImplicitlyAnimatedWidget]s (and their subclasses) automatically animate
/// changes in their properties whenever they change. For this,
/// they create and manage their own internal [AnimationController]s to power
/// the animation. While these widgets are simple to use and don't require you
/// to manually manage the lifecycle of an [AnimationController], they
/// are also somewhat limited: Besides the target value for the animated
/// property, developers can only choose a [duration] and [curve] for the
/// animation. If you require more control over the animation (e.g. you want
/// to stop it somewhere in the middle), consider using an
/// [AnimatedWidget] or one of its subclasses. These widgets take an [Animation]
/// as an argument to power the animation. This gives the developer full control
/// over the animation at the cost of requiring you to manually manage the
/// underlying [AnimationController].
///
/// ## Common implicitly animated widgets
///
/// A number of implicitly animated widgets ship with the framework. They are
/// usually named `AnimatedFoo`, where `Foo` is the name of the non-animated
/// version of that widget. Commonly used implicitly animated widgets include:
///
/// * [TweenAnimationBuilder], which animates any property expressed by
/// a [Tween] to a specified target value.
/// * [AnimatedAlign], which is an implicitly animated version of [Align].
/// * [AnimatedContainer], which is an implicitly animated version of
/// [Container].
/// * [AnimatedDefaultTextStyle], which is an implicitly animated version of
/// [DefaultTextStyle].
/// * [AnimatedScale], which is an implicitly animated version of [Transform.scale].
/// * [AnimatedRotation], which is an implicitly animated version of [Transform.rotate].
/// * [AnimatedSlide], which implicitly animates the position of a widget relative to its normal position.
/// * [AnimatedOpacity], which is an implicitly animated version of [Opacity].
/// * [AnimatedPadding], which is an implicitly animated version of [Padding].
/// * [AnimatedPhysicalModel], which is an implicitly animated version of
/// [PhysicalModel].
/// * [AnimatedPositioned], which is an implicitly animated version of
/// [Positioned].
/// * [AnimatedPositionedDirectional], which is an implicitly animated version
/// of [PositionedDirectional].
/// * [AnimatedTheme], which is an implicitly animated version of [Theme].
/// * [AnimatedCrossFade], which cross-fades between two given children and
/// animates itself between their sizes.
/// * [AnimatedSize], which automatically transitions its size over a given
/// duration.
/// * [AnimatedSwitcher], which fades from one widget to another.
abstract class ImplicitlyAnimatedWidget extends StatefulWidget {
/// Initializes fields for subclasses.
const ImplicitlyAnimatedWidget({
super.key,
this.curve = Curves.linear,
required this.duration,
this.onEnd,
});
/// The curve to apply when animating the parameters of this container.
final Curve curve;
/// The duration over which to animate the parameters of this container.
final Duration duration;
/// Called every time an animation completes.
///
/// This can be useful to trigger additional actions (e.g. another animation)
/// at the end of the current animation.
final VoidCallback? onEnd;
@override
ImplicitlyAnimatedWidgetState<ImplicitlyAnimatedWidget> createState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('duration', duration.inMilliseconds, unit: 'ms'));
}
}
/// Signature for a [Tween] factory.
///
/// This is the type of one of the arguments of [TweenVisitor], the signature
/// used by [AnimatedWidgetBaseState.forEachTween].
///
/// Instances of this function are expected to take a value and return a tween
/// beginning at that value.
typedef TweenConstructor<T extends Object> = Tween<T> Function(T targetValue);
/// Signature for callbacks passed to [ImplicitlyAnimatedWidgetState.forEachTween].
///
/// {@template flutter.widgets.TweenVisitor.arguments}
/// The `tween` argument should contain the current tween value. This will
/// initially be null when the state is first initialized.
///
/// The `targetValue` argument should contain the value toward which the state
/// is animating. For instance, if the state is animating its widget's
/// opacity value, then this argument should contain the widget's current
/// opacity value.
///
/// The `constructor` argument should contain a function that takes a value
/// (the widget's value being animated) and returns a tween beginning at that
/// value.
///
/// {@endtemplate}
///
/// `forEachTween()` is expected to update its tween value to the return value
/// of this visitor.
///
/// The `<T>` parameter specifies the type of value that's being animated.
typedef TweenVisitor<T extends Object> = Tween<T>? Function(Tween<T>? tween, T targetValue, TweenConstructor<T> constructor);
/// A base class for the `State` of widgets with implicit animations.
///
/// [ImplicitlyAnimatedWidgetState] requires that subclasses respond to the
/// animation themselves. If you would like `setState()` to be called
/// automatically as the animation changes, use [AnimatedWidgetBaseState].
///
/// Properties that subclasses choose to animate are represented by [Tween]
/// instances. Subclasses must implement the [forEachTween] method to allow
/// [ImplicitlyAnimatedWidgetState] to iterate through the widget's fields and
/// animate them.
abstract class ImplicitlyAnimatedWidgetState<T extends ImplicitlyAnimatedWidget> extends State<T> with SingleTickerProviderStateMixin<T> {
/// The animation controller driving this widget's implicit animations.
@protected
AnimationController get controller => _controller;
late final AnimationController _controller = AnimationController(
duration: widget.duration,
debugLabel: kDebugMode ? widget.toStringShort() : null,
vsync: this,
);
/// The animation driving this widget's implicit animations.
Animation<double> get animation => _animation;
late CurvedAnimation _animation = _createCurve();
@override
void initState() {
super.initState();
_controller.addStatusListener((AnimationStatus status) {
switch (status) {
case AnimationStatus.completed:
widget.onEnd?.call();
case AnimationStatus.dismissed:
case AnimationStatus.forward:
case AnimationStatus.reverse:
}
});
_constructTweens();
didUpdateTweens();
}
@override
void didUpdateWidget(T oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.curve != oldWidget.curve) {
_animation.dispose();
_animation = _createCurve();
}
_controller.duration = widget.duration;
if (_constructTweens()) {
forEachTween((Tween<dynamic>? tween, dynamic targetValue, TweenConstructor<dynamic> constructor) {
_updateTween(tween, targetValue);
return tween;
});
_controller
..value = 0.0
..forward();
didUpdateTweens();
}
}
CurvedAnimation _createCurve() {
return CurvedAnimation(parent: _controller, curve: widget.curve);
}
@override
void dispose() {
_animation.dispose();
_controller.dispose();
super.dispose();
}
bool _shouldAnimateTween(Tween<dynamic> tween, dynamic targetValue) {
return targetValue != (tween.end ?? tween.begin);
}
void _updateTween(Tween<dynamic>? tween, dynamic targetValue) {
if (tween == null) {
return;
}
tween
..begin = tween.evaluate(_animation)
..end = targetValue;
}
bool _constructTweens() {
bool shouldStartAnimation = false;
forEachTween((Tween<dynamic>? tween, dynamic targetValue, TweenConstructor<dynamic> constructor) {
if (targetValue != null) {
tween ??= constructor(targetValue);
if (_shouldAnimateTween(tween, targetValue)) {
shouldStartAnimation = true;
} else {
tween.end ??= tween.begin;
}
} else {
tween = null;
}
return tween;
});
return shouldStartAnimation;
}
/// Visits each tween controlled by this state with the specified `visitor`
/// function.
///
/// ### Subclass responsibility
///
/// Properties to be animated are represented by [Tween] member variables in
/// the state. For each such tween, [forEachTween] implementations are
/// expected to call `visitor` with the appropriate arguments and store the
/// result back into the member variable. The arguments to `visitor` are as
/// follows:
///
/// {@macro flutter.widgets.TweenVisitor.arguments}
///
/// ### When this method will be called
///
/// [forEachTween] is initially called during [initState]. It is expected that
/// the visitor's `tween` argument will be set to null, causing the visitor to
/// call its `constructor` argument to construct the tween for the first time.
/// The resulting tween will have its `begin` value set to the target value
/// and will have its `end` value set to null. The animation will not be
/// started.
///
/// When this state's [widget] is updated (thus triggering the
/// [didUpdateWidget] method to be called), [forEachTween] will be called
/// again to check if the target value has changed. If the target value has
/// changed, signaling that the [animation] should start, then the visitor
/// will update the tween's `start` and `end` values accordingly, and the
/// animation will be started.
///
/// ### Other member variables
///
/// Subclasses that contain properties based on tweens created by
/// [forEachTween] should override [didUpdateTweens] to update those
/// properties. Dependent properties should not be updated within
/// [forEachTween].
///
/// {@tool snippet}
///
/// This sample implements an implicitly animated widget's `State`.
/// The widget animates between colors whenever `widget.targetColor`
/// changes.
///
/// ```dart
/// class MyWidgetState extends AnimatedWidgetBaseState<MyWidget> {
/// ColorTween? _colorTween;
///
/// @override
/// Widget build(BuildContext context) {
/// return Text(
/// 'Hello World',
/// // Computes the value of the text color at any given time.
/// style: TextStyle(color: _colorTween?.evaluate(animation)),
/// );
/// }
///
/// @override
/// void forEachTween(TweenVisitor<dynamic> visitor) {
/// // Update the tween using the provided visitor function.
/// _colorTween = visitor(
/// // The latest tween value. Can be `null`.
/// _colorTween,
/// // The color value toward which we are animating.
/// widget.targetColor,
/// // A function that takes a color value and returns a tween
/// // beginning at that value.
/// (dynamic value) => ColorTween(begin: value as Color?),
/// ) as ColorTween?;
///
/// // We could have more tweens than one by using the visitor
/// // multiple times.
/// }
/// }
/// ```
/// {@end-tool}
@protected
void forEachTween(TweenVisitor<dynamic> visitor);
/// Optional hook for subclasses that runs after all tweens have been updated
/// via [forEachTween].
///
/// Any properties that depend upon tweens created by [forEachTween] should be
/// updated within [didUpdateTweens], not within [forEachTween].
///
/// This method will be called both:
///
/// 1. After the tweens are _initially_ constructed (by
/// the `constructor` argument to the [TweenVisitor] that's passed to
/// [forEachTween]). In this case, the tweens are likely to contain only
/// a [Tween.begin] value and not a [Tween.end].
///
/// 2. When the state's [widget] is updated, and one or more of the tweens
/// visited by [forEachTween] specifies a target value that's different
/// than the widget's current value, thus signaling that the [animation]
/// should run. In this case, the [Tween.begin] value for each tween will
/// an evaluation of the tween against the current [animation], and the
/// [Tween.end] value for each tween will be the target value.
@protected
void didUpdateTweens() { }
}
/// A base class for widgets with implicit animations that need to rebuild their
/// widget tree as the animation runs.
///
/// This class calls [build] each frame that the animation ticks. For a
/// variant that does not rebuild each frame, consider subclassing
/// [ImplicitlyAnimatedWidgetState] directly.
///
/// Subclasses must implement the [forEachTween] method to allow
/// [AnimatedWidgetBaseState] to iterate through the subclasses' widget's fields
/// and animate them.
abstract class AnimatedWidgetBaseState<T extends ImplicitlyAnimatedWidget> extends ImplicitlyAnimatedWidgetState<T> {
@override
void initState() {
super.initState();
controller.addListener(_handleAnimationChanged);
}
void _handleAnimationChanged() {
setState(() { /* The animation ticked. Rebuild with new animation value */ });
}
}
/// Animated version of [Container] that gradually changes its values over a period of time.
///
/// The [AnimatedContainer] will automatically animate between the old and
/// new values of properties when they change using the provided curve and
/// duration. Properties that are null are not animated. Its child and
/// descendants are not animated.
///
/// This class is useful for generating simple implicit transitions between
/// different parameters to [Container] with its internal [AnimationController].
/// For more complex animations, you'll likely want to use a subclass of
/// [AnimatedWidget] such as the [DecoratedBoxTransition] or use your own
/// [AnimationController].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=yI-8QHpGIP4}
///
/// {@tool dartpad}
/// The following example (depicted above) transitions an AnimatedContainer
/// between two states. It adjusts the `height`, `width`, `color`, and
/// [alignment] properties when tapped.
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_container.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedPadding], which is a subset of this widget that only
/// supports animating the [padding].
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
/// * [AnimatedPositioned], which, as a child of a [Stack], automatically
/// transitions its child's position over a given duration whenever the given
/// position changes.
/// * [AnimatedAlign], which automatically transitions its child's
/// position over a given duration whenever the given [AnimatedAlign.alignment] changes.
/// * [AnimatedSwitcher], which switches out a child for a new one with a customizable transition.
/// * [AnimatedCrossFade], which fades between two children and interpolates their sizes.
class AnimatedContainer extends ImplicitlyAnimatedWidget {
/// Creates a container that animates its parameters implicitly.
AnimatedContainer({
super.key,
this.alignment,
this.padding,
Color? color,
Decoration? decoration,
this.foregroundDecoration,
double? width,
double? height,
BoxConstraints? constraints,
this.margin,
this.transform,
this.transformAlignment,
this.child,
this.clipBehavior = Clip.none,
super.curve,
required super.duration,
super.onEnd,
}) : assert(margin == null || margin.isNonNegative),
assert(padding == null || padding.isNonNegative),
assert(decoration == null || decoration.debugAssertIsValid()),
assert(constraints == null || constraints.debugAssertIsValid()),
assert(color == null || decoration == null,
'Cannot provide both a color and a decoration\n'
'The color argument is just a shorthand for "decoration: BoxDecoration(color: color)".',
),
decoration = decoration ?? (color != null ? BoxDecoration(color: color) : null),
constraints =
(width != null || height != null)
? constraints?.tighten(width: width, height: height)
?? BoxConstraints.tightFor(width: width, height: height)
: constraints;
/// The [child] contained by the container.
///
/// If null, and if the [constraints] are unbounded or also null, the
/// container will expand to fill all available space in its parent, unless
/// the parent provides unbounded constraints, in which case the container
/// will attempt to be as small as possible.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// Align the [child] within the container.
///
/// If non-null, the container will expand to fill its parent and position its
/// child within itself according to the given value. If the incoming
/// constraints are unbounded, then the child will be shrink-wrapped instead.
///
/// Ignored if [child] is null.
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry? alignment;
/// Empty space to inscribe inside the [decoration]. The [child], if any, is
/// placed inside this padding.
final EdgeInsetsGeometry? padding;
/// The decoration to paint behind the [child].
///
/// A shorthand for specifying just a solid color is available in the
/// constructor: set the `color` argument instead of the `decoration`
/// argument.
final Decoration? decoration;
/// The decoration to paint in front of the child.
final Decoration? foregroundDecoration;
/// Additional constraints to apply to the child.
///
/// The constructor `width` and `height` arguments are combined with the
/// `constraints` argument to set this property.
///
/// The [padding] goes inside the constraints.
final BoxConstraints? constraints;
/// Empty space to surround the [decoration] and [child].
final EdgeInsetsGeometry? margin;
/// The transformation matrix to apply before painting the container.
final Matrix4? transform;
/// The alignment of the origin, relative to the size of the container, if [transform] is specified.
///
/// When [transform] is null, the value of this property is ignored.
///
/// See also:
///
/// * [Transform.alignment], which is set by this property.
final AlignmentGeometry? transformAlignment;
/// The clip behavior when [AnimatedContainer.decoration] is not null.
///
/// Defaults to [Clip.none]. Must be [Clip.none] if [decoration] is null.
///
/// Unlike other properties of [AnimatedContainer], changes to this property
/// apply immediately and have no animation.
///
/// If a clip is to be applied, the [Decoration.getClipPath] method
/// for the provided decoration must return a clip path. (This is not
/// supported by all decorations; the default implementation of that
/// method throws an [UnsupportedError].)
final Clip clipBehavior;
@override
AnimatedWidgetBaseState<AnimatedContainer> createState() => _AnimatedContainerState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment, showName: false, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<Decoration>('bg', decoration, defaultValue: null));
properties.add(DiagnosticsProperty<Decoration>('fg', foregroundDecoration, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, defaultValue: null, showName: false));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('margin', margin, defaultValue: null));
properties.add(ObjectFlagProperty<Matrix4>.has('transform', transform));
properties.add(DiagnosticsProperty<AlignmentGeometry>('transformAlignment', transformAlignment, defaultValue: null));
properties.add(DiagnosticsProperty<Clip>('clipBehavior', clipBehavior));
}
}
class _AnimatedContainerState extends AnimatedWidgetBaseState<AnimatedContainer> {
AlignmentGeometryTween? _alignment;
EdgeInsetsGeometryTween? _padding;
DecorationTween? _decoration;
DecorationTween? _foregroundDecoration;
BoxConstraintsTween? _constraints;
EdgeInsetsGeometryTween? _margin;
Matrix4Tween? _transform;
AlignmentGeometryTween? _transformAlignment;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_alignment = visitor(_alignment, widget.alignment, (dynamic value) => AlignmentGeometryTween(begin: value as AlignmentGeometry)) as AlignmentGeometryTween?;
_padding = visitor(_padding, widget.padding, (dynamic value) => EdgeInsetsGeometryTween(begin: value as EdgeInsetsGeometry)) as EdgeInsetsGeometryTween?;
_decoration = visitor(_decoration, widget.decoration, (dynamic value) => DecorationTween(begin: value as Decoration)) as DecorationTween?;
_foregroundDecoration = visitor(_foregroundDecoration, widget.foregroundDecoration, (dynamic value) => DecorationTween(begin: value as Decoration)) as DecorationTween?;
_constraints = visitor(_constraints, widget.constraints, (dynamic value) => BoxConstraintsTween(begin: value as BoxConstraints)) as BoxConstraintsTween?;
_margin = visitor(_margin, widget.margin, (dynamic value) => EdgeInsetsGeometryTween(begin: value as EdgeInsetsGeometry)) as EdgeInsetsGeometryTween?;
_transform = visitor(_transform, widget.transform, (dynamic value) => Matrix4Tween(begin: value as Matrix4)) as Matrix4Tween?;
_transformAlignment = visitor(_transformAlignment, widget.transformAlignment, (dynamic value) => AlignmentGeometryTween(begin: value as AlignmentGeometry)) as AlignmentGeometryTween?;
}
@override
Widget build(BuildContext context) {
final Animation<double> animation = this.animation;
return Container(
alignment: _alignment?.evaluate(animation),
padding: _padding?.evaluate(animation),
decoration: _decoration?.evaluate(animation),
foregroundDecoration: _foregroundDecoration?.evaluate(animation),
constraints: _constraints?.evaluate(animation),
margin: _margin?.evaluate(animation),
transform: _transform?.evaluate(animation),
transformAlignment: _transformAlignment?.evaluate(animation),
clipBehavior: widget.clipBehavior,
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<AlignmentGeometryTween>('alignment', _alignment, showName: false, defaultValue: null));
description.add(DiagnosticsProperty<EdgeInsetsGeometryTween>('padding', _padding, defaultValue: null));
description.add(DiagnosticsProperty<DecorationTween>('bg', _decoration, defaultValue: null));
description.add(DiagnosticsProperty<DecorationTween>('fg', _foregroundDecoration, defaultValue: null));
description.add(DiagnosticsProperty<BoxConstraintsTween>('constraints', _constraints, showName: false, defaultValue: null));
description.add(DiagnosticsProperty<EdgeInsetsGeometryTween>('margin', _margin, defaultValue: null));
description.add(ObjectFlagProperty<Matrix4Tween>.has('transform', _transform));
description.add(DiagnosticsProperty<AlignmentGeometryTween>('transformAlignment', _transformAlignment, defaultValue: null));
}
}
/// Animated version of [Padding] which automatically transitions the
/// indentation over a given duration whenever the given inset changes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=PY2m0fhGNz4}
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_padding.mp4}
///
/// {@tool dartpad}
/// The following code implements the [AnimatedPadding] widget, using a [curve] of
/// [Curves.easeInOut].
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_padding.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedContainer], which can transition more values at once.
/// * [AnimatedAlign], which automatically transitions its child's
/// position over a given duration whenever the given
/// [AnimatedAlign.alignment] changes.
class AnimatedPadding extends ImplicitlyAnimatedWidget {
/// Creates a widget that insets its child by a value that animates
/// implicitly.
AnimatedPadding({
super.key,
required this.padding,
this.child,
super.curve,
required super.duration,
super.onEnd,
}) : assert(padding.isNonNegative);
/// The amount of space by which to inset the child.
final EdgeInsetsGeometry padding;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
@override
AnimatedWidgetBaseState<AnimatedPadding> createState() => _AnimatedPaddingState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding));
}
}
class _AnimatedPaddingState extends AnimatedWidgetBaseState<AnimatedPadding> {
EdgeInsetsGeometryTween? _padding;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_padding = visitor(_padding, widget.padding, (dynamic value) => EdgeInsetsGeometryTween(begin: value as EdgeInsetsGeometry)) as EdgeInsetsGeometryTween?;
}
@override
Widget build(BuildContext context) {
return Padding(
padding: _padding!
.evaluate(animation)
.clamp(EdgeInsets.zero, EdgeInsetsGeometry.infinity),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<EdgeInsetsGeometryTween>('padding', _padding, defaultValue: null));
}
}
/// Animated version of [Align] which automatically transitions the child's
/// position over a given duration whenever the given [alignment] changes.
///
/// Here's an illustration of what this can look like, using a [curve] of
/// [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_align.mp4}
///
/// For the animation, you can choose a [curve] as well as a [duration] and the
/// widget will automatically animate to the new target [alignment]. If you require
/// more control over the animation (e.g. if you want to stop it mid-animation),
/// consider using an [AlignTransition] instead, which takes a provided
/// [Animation] as argument. While that allows you to fine-tune the animation,
/// it also requires more development overhead as you have to manually manage
/// the lifecycle of the underlying [AnimationController].
///
/// {@tool dartpad}
/// The following code implements the [AnimatedAlign] widget, using a [curve] of
/// [Curves.fastOutSlowIn].
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_align.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedContainer], which can transition more values at once.
/// * [AnimatedPadding], which can animate the padding instead of the
/// alignment.
/// * [AnimatedSlide], which can animate the translation of child by a given offset relative to its size.
/// * [AnimatedPositioned], which, as a child of a [Stack], automatically
/// transitions its child's position over a given duration whenever the given
/// position changes.
class AnimatedAlign extends ImplicitlyAnimatedWidget {
/// Creates a widget that positions its child by an alignment that animates
/// implicitly.
const AnimatedAlign({
super.key,
required this.alignment,
this.child,
this.heightFactor,
this.widthFactor,
super.curve,
required super.duration,
super.onEnd,
}) : assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0);
/// How to align the child.
///
/// The x and y values of the [Alignment] control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// See also:
///
/// * [Alignment], which has more details and some convenience constants for
/// common positions.
/// * [AlignmentDirectional], which has a horizontal coordinate orientation
/// that depends on the [TextDirection].
final AlignmentGeometry alignment;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// If non-null, sets its height to the child's height multiplied by this factor.
///
/// Must be greater than or equal to 0.0, defaults to null.
final double? heightFactor;
/// If non-null, sets its width to the child's width multiplied by this factor.
///
/// Must be greater than or equal to 0.0, defaults to null.
final double? widthFactor;
@override
AnimatedWidgetBaseState<AnimatedAlign> createState() => _AnimatedAlignState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
}
}
class _AnimatedAlignState extends AnimatedWidgetBaseState<AnimatedAlign> {
AlignmentGeometryTween? _alignment;
Tween<double>? _heightFactorTween;
Tween<double>? _widthFactorTween;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_alignment = visitor(_alignment, widget.alignment, (dynamic value) => AlignmentGeometryTween(begin: value as AlignmentGeometry)) as AlignmentGeometryTween?;
if (widget.heightFactor != null) {
_heightFactorTween = visitor(_heightFactorTween, widget.heightFactor, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
if (widget.widthFactor != null) {
_widthFactorTween = visitor(_widthFactorTween, widget.widthFactor, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
}
@override
Widget build(BuildContext context) {
return Align(
alignment: _alignment!.evaluate(animation)!,
heightFactor: _heightFactorTween?.evaluate(animation),
widthFactor: _widthFactorTween?.evaluate(animation),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<AlignmentGeometryTween>('alignment', _alignment, defaultValue: null));
description.add(DiagnosticsProperty<Tween<double>>('widthFactor', _widthFactorTween, defaultValue: null));
description.add(DiagnosticsProperty<Tween<double>>('heightFactor', _heightFactorTween, defaultValue: null));
}
}
/// Animated version of [Positioned] which automatically transitions the child's
/// position over a given duration whenever the given position changes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=hC3s2YdtWt8}
///
/// Only works if it's the child of a [Stack].
///
/// This widget is a good choice if the _size_ of the child would end up
/// changing as a result of this animation. If the size is intended to remain
/// the same, with only the _position_ changing over time, then consider
/// [SlideTransition] instead. [SlideTransition] only triggers a repaint each
/// frame of the animation, whereas [AnimatedPositioned] will trigger a relayout
/// as well.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_positioned.mp4}
///
/// For the animation, you can choose a [curve] as well as a [duration] and the
/// widget will automatically animate to the new target position. If you require
/// more control over the animation (e.g. if you want to stop it mid-animation),
/// consider using a [PositionedTransition] instead, which takes a provided
/// [Animation] as an argument. While that allows you to fine-tune the animation,
/// it also requires more development overhead as you have to manually manage
/// the lifecycle of the underlying [AnimationController].
///
/// {@tool dartpad}
/// The following example transitions an AnimatedPositioned
/// between two states. It adjusts the `height`, `width`, and
/// [Positioned] properties when tapped.
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_positioned.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedPositionedDirectional], which adapts to the ambient
/// [Directionality] (the same as this widget, but for animating
/// [PositionedDirectional]).
class AnimatedPositioned extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its position implicitly.
///
/// Only two out of the three horizontal values ([left], [right],
/// [width]), and only two out of the three vertical values ([top],
/// [bottom], [height]), can be set. In each case, at least one of
/// the three must be null.
const AnimatedPositioned({
super.key,
required this.child,
this.left,
this.top,
this.right,
this.bottom,
this.width,
this.height,
super.curve,
required super.duration,
super.onEnd,
}) : assert(left == null || right == null || width == null),
assert(top == null || bottom == null || height == null);
/// Creates a widget that animates the rectangle it occupies implicitly.
AnimatedPositioned.fromRect({
super.key,
required this.child,
required Rect rect,
super.curve,
required super.duration,
super.onEnd,
}) : left = rect.left,
top = rect.top,
width = rect.width,
height = rect.height,
right = null,
bottom = null;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The offset of the child's left edge from the left of the stack.
final double? left;
/// The offset of the child's top edge from the top of the stack.
final double? top;
/// The offset of the child's right edge from the right of the stack.
final double? right;
/// The offset of the child's bottom edge from the bottom of the stack.
final double? bottom;
/// The child's width.
///
/// Only two out of the three horizontal values ([left], [right], [width]) can
/// be set. The third must be null.
final double? width;
/// The child's height.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can
/// be set. The third must be null.
final double? height;
@override
AnimatedWidgetBaseState<AnimatedPositioned> createState() => _AnimatedPositionedState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('left', left, defaultValue: null));
properties.add(DoubleProperty('top', top, defaultValue: null));
properties.add(DoubleProperty('right', right, defaultValue: null));
properties.add(DoubleProperty('bottom', bottom, defaultValue: null));
properties.add(DoubleProperty('width', width, defaultValue: null));
properties.add(DoubleProperty('height', height, defaultValue: null));
}
}
class _AnimatedPositionedState extends AnimatedWidgetBaseState<AnimatedPositioned> {
Tween<double>? _left;
Tween<double>? _top;
Tween<double>? _right;
Tween<double>? _bottom;
Tween<double>? _width;
Tween<double>? _height;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_left = visitor(_left, widget.left, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_top = visitor(_top, widget.top, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_right = visitor(_right, widget.right, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_bottom = visitor(_bottom, widget.bottom, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_width = visitor(_width, widget.width, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_height = visitor(_height, widget.height, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
Widget build(BuildContext context) {
return Positioned(
left: _left?.evaluate(animation),
top: _top?.evaluate(animation),
right: _right?.evaluate(animation),
bottom: _bottom?.evaluate(animation),
width: _width?.evaluate(animation),
height: _height?.evaluate(animation),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(ObjectFlagProperty<Tween<double>>.has('left', _left));
description.add(ObjectFlagProperty<Tween<double>>.has('top', _top));
description.add(ObjectFlagProperty<Tween<double>>.has('right', _right));
description.add(ObjectFlagProperty<Tween<double>>.has('bottom', _bottom));
description.add(ObjectFlagProperty<Tween<double>>.has('width', _width));
description.add(ObjectFlagProperty<Tween<double>>.has('height', _height));
}
}
/// Animated version of [PositionedDirectional] which automatically transitions
/// the child's position over a given duration whenever the given position
/// changes.
///
/// The ambient [Directionality] is used to determine whether [start] is to the
/// left or to the right.
///
/// Only works if it's the child of a [Stack].
///
/// This widget is a good choice if the _size_ of the child would end up
/// changing as a result of this animation. If the size is intended to remain
/// the same, with only the _position_ changing over time, then consider
/// [SlideTransition] instead. [SlideTransition] only triggers a repaint each
/// frame of the animation, whereas [AnimatedPositionedDirectional] will trigger
/// a relayout as well. ([SlideTransition] is also text-direction-aware.)
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_positioned_directional.mp4}
///
/// See also:
///
/// * [AnimatedPositioned], which specifies the widget's position visually (the
/// same as this widget, but for animating [Positioned]).
class AnimatedPositionedDirectional extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its position implicitly.
///
/// Only two out of the three horizontal values ([start], [end], [width]), and
/// only two out of the three vertical values ([top], [bottom], [height]), can
/// be set. In each case, at least one of the three must be null.
const AnimatedPositionedDirectional({
super.key,
required this.child,
this.start,
this.top,
this.end,
this.bottom,
this.width,
this.height,
super.curve,
required super.duration,
super.onEnd,
}) : assert(start == null || end == null || width == null),
assert(top == null || bottom == null || height == null);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The offset of the child's start edge from the start of the stack.
final double? start;
/// The offset of the child's top edge from the top of the stack.
final double? top;
/// The offset of the child's end edge from the end of the stack.
final double? end;
/// The offset of the child's bottom edge from the bottom of the stack.
final double? bottom;
/// The child's width.
///
/// Only two out of the three horizontal values ([start], [end], [width]) can
/// be set. The third must be null.
final double? width;
/// The child's height.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can
/// be set. The third must be null.
final double? height;
@override
AnimatedWidgetBaseState<AnimatedPositionedDirectional> createState() => _AnimatedPositionedDirectionalState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('start', start, defaultValue: null));
properties.add(DoubleProperty('top', top, defaultValue: null));
properties.add(DoubleProperty('end', end, defaultValue: null));
properties.add(DoubleProperty('bottom', bottom, defaultValue: null));
properties.add(DoubleProperty('width', width, defaultValue: null));
properties.add(DoubleProperty('height', height, defaultValue: null));
}
}
class _AnimatedPositionedDirectionalState extends AnimatedWidgetBaseState<AnimatedPositionedDirectional> {
Tween<double>? _start;
Tween<double>? _top;
Tween<double>? _end;
Tween<double>? _bottom;
Tween<double>? _width;
Tween<double>? _height;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_start = visitor(_start, widget.start, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_top = visitor(_top, widget.top, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_end = visitor(_end, widget.end, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_bottom = visitor(_bottom, widget.bottom, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_width = visitor(_width, widget.width, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_height = visitor(_height, widget.height, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasDirectionality(context));
return Positioned.directional(
textDirection: Directionality.of(context),
start: _start?.evaluate(animation),
top: _top?.evaluate(animation),
end: _end?.evaluate(animation),
bottom: _bottom?.evaluate(animation),
width: _width?.evaluate(animation),
height: _height?.evaluate(animation),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(ObjectFlagProperty<Tween<double>>.has('start', _start));
description.add(ObjectFlagProperty<Tween<double>>.has('top', _top));
description.add(ObjectFlagProperty<Tween<double>>.has('end', _end));
description.add(ObjectFlagProperty<Tween<double>>.has('bottom', _bottom));
description.add(ObjectFlagProperty<Tween<double>>.has('width', _width));
description.add(ObjectFlagProperty<Tween<double>>.has('height', _height));
}
}
/// Animated version of [Transform.scale] which automatically transitions the child's
/// scale over a given duration whenever the given scale changes.
///
/// {@tool snippet}
///
/// This code defines a widget that uses [AnimatedScale] to change the size
/// of [FlutterLogo] gradually to a new scale whenever the button is pressed.
///
/// ```dart
/// class LogoScale extends StatefulWidget {
/// const LogoScale({super.key});
///
/// @override
/// State<LogoScale> createState() => LogoScaleState();
/// }
///
/// class LogoScaleState extends State<LogoScale> {
/// double scale = 1.0;
///
/// void _changeScale() {
/// setState(() => scale = scale == 1.0 ? 3.0 : 1.0);
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Column(
/// mainAxisAlignment: MainAxisAlignment.center,
/// children: <Widget>[
/// ElevatedButton(
/// onPressed: _changeScale,
/// child: const Text('Scale Logo'),
/// ),
/// Padding(
/// padding: const EdgeInsets.all(50),
/// child: AnimatedScale(
/// scale: scale,
/// duration: const Duration(seconds: 2),
/// child: const FlutterLogo(),
/// ),
/// ),
/// ],
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedRotation], for animating the rotation of a child.
/// * [AnimatedSize], for animating the resize of a child based on changes
/// in layout.
/// * [AnimatedSlide], for animating the translation of a child by a given offset relative to its size.
/// * [ScaleTransition], an explicitly animated version of this widget, where
/// an [Animation] is provided by the caller instead of being built in.
class AnimatedScale extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its scale implicitly.
const AnimatedScale({
super.key,
this.child,
required this.scale,
this.alignment = Alignment.center,
this.filterQuality,
super.curve,
required super.duration,
super.onEnd,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The target scale.
final double scale;
/// The alignment of the origin of the coordinate system in which the scale
/// takes place, relative to the size of the box.
///
/// For example, to set the origin of the scale to bottom middle, you can use
/// an alignment of (0.0, 1.0).
final Alignment alignment;
/// The filter quality with which to apply the transform as a bitmap operation.
///
/// {@macro flutter.widgets.Transform.optional.FilterQuality}
final FilterQuality? filterQuality;
@override
ImplicitlyAnimatedWidgetState<AnimatedScale> createState() => _AnimatedScaleState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('scale', scale));
properties.add(DiagnosticsProperty<Alignment>('alignment', alignment, defaultValue: Alignment.center));
properties.add(EnumProperty<FilterQuality>('filterQuality', filterQuality, defaultValue: null));
}
}
class _AnimatedScaleState extends ImplicitlyAnimatedWidgetState<AnimatedScale> {
Tween<double>? _scale;
late Animation<double> _scaleAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_scale = visitor(_scale, widget.scale, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
void didUpdateTweens() {
_scaleAnimation = animation.drive(_scale!);
}
@override
Widget build(BuildContext context) {
return ScaleTransition(
scale: _scaleAnimation,
alignment: widget.alignment,
filterQuality: widget.filterQuality,
child: widget.child,
);
}
}
/// Animated version of [Transform.rotate] which automatically transitions the child's
/// rotation over a given duration whenever the given rotation changes.
///
/// {@tool snippet}
///
/// This code defines a widget that uses [AnimatedRotation] to rotate a [FlutterLogo]
/// gradually by an eighth of a turn (45 degrees) with each press of the button.
///
/// ```dart
/// class LogoRotate extends StatefulWidget {
/// const LogoRotate({super.key});
///
/// @override
/// State<LogoRotate> createState() => LogoRotateState();
/// }
///
/// class LogoRotateState extends State<LogoRotate> {
/// double turns = 0.0;
///
/// void _changeRotation() {
/// setState(() => turns += 1.0 / 8.0);
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Column(
/// mainAxisAlignment: MainAxisAlignment.center,
/// children: <Widget>[
/// ElevatedButton(
/// onPressed: _changeRotation,
/// child: const Text('Rotate Logo'),
/// ),
/// Padding(
/// padding: const EdgeInsets.all(50),
/// child: AnimatedRotation(
/// turns: turns,
/// duration: const Duration(seconds: 1),
/// child: const FlutterLogo(),
/// ),
/// ),
/// ],
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedScale], for animating the scale of a child.
/// * [RotationTransition], an explicitly animated version of this widget, where
/// an [Animation] is provided by the caller instead of being built in.
class AnimatedRotation extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its rotation implicitly.
const AnimatedRotation({
super.key,
this.child,
required this.turns,
this.alignment = Alignment.center,
this.filterQuality,
super.curve,
required super.duration,
super.onEnd,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The animation that controls the rotation of the child.
///
/// If the current value of the turns animation is v, the child will be
/// rotated v * 2 * pi radians before being painted.
final double turns;
/// The alignment of the origin of the coordinate system in which the rotation
/// takes place, relative to the size of the box.
///
/// For example, to set the origin of the rotation to bottom middle, you can use
/// an alignment of (0.0, 1.0).
final Alignment alignment;
/// The filter quality with which to apply the transform as a bitmap operation.
///
/// {@macro flutter.widgets.Transform.optional.FilterQuality}
final FilterQuality? filterQuality;
@override
ImplicitlyAnimatedWidgetState<AnimatedRotation> createState() => _AnimatedRotationState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('turns', turns));
properties.add(DiagnosticsProperty<Alignment>('alignment', alignment, defaultValue: Alignment.center));
properties.add(EnumProperty<FilterQuality>('filterQuality', filterQuality, defaultValue: null));
}
}
class _AnimatedRotationState extends ImplicitlyAnimatedWidgetState<AnimatedRotation> {
Tween<double>? _turns;
late Animation<double> _turnsAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_turns = visitor(_turns, widget.turns, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
void didUpdateTweens() {
_turnsAnimation = animation.drive(_turns!);
}
@override
Widget build(BuildContext context) {
return RotationTransition(
turns: _turnsAnimation,
alignment: widget.alignment,
filterQuality: widget.filterQuality,
child: widget.child,
);
}
}
/// Widget which automatically transitions the child's
/// offset relative to its normal position whenever the given offset changes.
///
/// The translation is expressed as an [Offset] scaled to the child's size. For
/// example, an [Offset] with a `dx` of 0.25 will result in a horizontal
/// translation of one quarter the width of the child.
///
/// {@tool dartpad}
/// This code defines a widget that uses [AnimatedSlide] to translate a [FlutterLogo]
/// up or down and right or left by dragging the X and Y axis sliders.
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_slide.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedPositioned], which, as a child of a [Stack], automatically
/// transitions its child's position over a given duration whenever the given
/// position changes.
/// * [AnimatedAlign], which automatically transitions its child's
/// position over a given duration whenever the given [AnimatedAlign.alignment] changes.
class AnimatedSlide extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its offset translation implicitly.
const AnimatedSlide({
super.key,
this.child,
required this.offset,
super.curve,
required super.duration,
super.onEnd,
});
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The target offset.
/// The child will be translated horizontally by `width * dx` and vertically by `height * dy`
final Offset offset;
@override
ImplicitlyAnimatedWidgetState<AnimatedSlide> createState() => _AnimatedSlideState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Offset>('offset', offset));
}
}
class _AnimatedSlideState extends ImplicitlyAnimatedWidgetState<AnimatedSlide> {
Tween<Offset>? _offset;
late Animation<Offset> _offsetAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_offset = visitor(_offset, widget.offset, (dynamic value) => Tween<Offset>(begin: value as Offset)) as Tween<Offset>?;
}
@override
void didUpdateTweens() {
_offsetAnimation = animation.drive(_offset!);
}
@override
Widget build(BuildContext context) {
return SlideTransition(
position: _offsetAnimation,
child: widget.child,
);
}
}
/// Animated version of [Opacity] which automatically transitions the child's
/// opacity over a given duration whenever the given opacity changes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=QZAvjqOqiLY}
///
/// Animating an opacity is relatively expensive because it requires painting
/// the child into an intermediate buffer.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_opacity.mp4}
///
/// {@tool snippet}
///
/// ```dart
/// class LogoFade extends StatefulWidget {
/// const LogoFade({super.key});
///
/// @override
/// State<LogoFade> createState() => LogoFadeState();
/// }
///
/// class LogoFadeState extends State<LogoFade> {
/// double opacityLevel = 1.0;
///
/// void _changeOpacity() {
/// setState(() => opacityLevel = opacityLevel == 0 ? 1.0 : 0.0);
/// }
///
/// @override
/// Widget build(BuildContext context) {
/// return Column(
/// mainAxisAlignment: MainAxisAlignment.center,
/// children: <Widget>[
/// AnimatedOpacity(
/// opacity: opacityLevel,
/// duration: const Duration(seconds: 3),
/// child: const FlutterLogo(),
/// ),
/// ElevatedButton(
/// onPressed: _changeOpacity,
/// child: const Text('Fade Logo'),
/// ),
/// ],
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// ## Hit testing
///
/// Setting the [opacity] to zero does not prevent hit testing from being
/// applied to the descendants of the [AnimatedOpacity] widget. This can be
/// confusing for the user, who may not see anything, and may believe the area
/// of the interface where the [AnimatedOpacity] is hiding a widget to be
/// non-interactive.
///
/// With certain widgets, such as [Flow], that compute their positions only when
/// they are painted, this can actually lead to bugs (from unexpected geometry
/// to exceptions), because those widgets are not painted by the [AnimatedOpacity]
/// widget at all when the [opacity] animation reaches zero.
///
/// To avoid such problems, it is generally a good idea to use an
/// [IgnorePointer] widget when setting the [opacity] to zero. This prevents
/// interactions with any children in the subtree when the [child] is animating
/// away.
///
/// See also:
///
/// * [AnimatedCrossFade], for fading between two children.
/// * [AnimatedSwitcher], for fading between many children in sequence.
/// * [FadeTransition], an explicitly animated version of this widget, where
/// an [Animation] is provided by the caller instead of being built in.
/// * [SliverAnimatedOpacity], for automatically transitioning a _sliver's_
/// opacity over a given duration whenever the given opacity changes.
class AnimatedOpacity extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its opacity implicitly.
///
/// The [opacity] argument must be between zero and one, inclusive.
const AnimatedOpacity({
super.key,
this.child,
required this.opacity,
super.curve,
required super.duration,
super.onEnd,
this.alwaysIncludeSemantics = false,
}) : assert(opacity >= 0.0 && opacity <= 1.0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// The target opacity.
///
/// An opacity of 1.0 is fully opaque. An opacity of 0.0 is fully transparent
/// (i.e., invisible).
final double opacity;
/// Whether the semantic information of the children is always included.
///
/// Defaults to false.
///
/// When true, regardless of the opacity settings the child semantic
/// information is exposed as if the widget were fully visible. This is
/// useful in cases where labels may be hidden during animations that
/// would otherwise contribute relevant semantics.
final bool alwaysIncludeSemantics;
@override
ImplicitlyAnimatedWidgetState<AnimatedOpacity> createState() => _AnimatedOpacityState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('opacity', opacity));
}
}
class _AnimatedOpacityState extends ImplicitlyAnimatedWidgetState<AnimatedOpacity> {
Tween<double>? _opacity;
late Animation<double> _opacityAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_opacity = visitor(_opacity, widget.opacity, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
void didUpdateTweens() {
_opacityAnimation = animation.drive(_opacity!);
}
@override
Widget build(BuildContext context) {
return FadeTransition(
opacity: _opacityAnimation,
alwaysIncludeSemantics: widget.alwaysIncludeSemantics,
child: widget.child,
);
}
}
/// Animated version of [SliverOpacity] which automatically transitions the
/// sliver child's opacity over a given duration whenever the given opacity
/// changes.
///
/// Animating an opacity is relatively expensive because it requires painting
/// the sliver child into an intermediate buffer.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
///
/// {@tool dartpad}
/// Creates a [CustomScrollView] with a [SliverFixedExtentList] and a
/// [FloatingActionButton]. Pressing the button animates the lists' opacity.
///
/// ** See code in examples/api/lib/widgets/implicit_animations/sliver_animated_opacity.0.dart **
/// {@end-tool}
///
/// ## Hit testing
///
/// Setting the [opacity] to zero does not prevent hit testing from being
/// applied to the descendants of the [SliverAnimatedOpacity] widget. This can
/// be confusing for the user, who may not see anything, and may believe the
/// area of the interface where the [SliverAnimatedOpacity] is hiding a widget
/// to be non-interactive.
///
/// With certain widgets, such as [Flow], that compute their positions only when
/// they are painted, this can actually lead to bugs (from unexpected geometry
/// to exceptions), because those widgets are not painted by the
/// [SliverAnimatedOpacity] widget at all when the [opacity] animation reaches
/// zero.
///
/// To avoid such problems, it is generally a good idea to use a
/// [SliverIgnorePointer] widget when setting the [opacity] to zero. This
/// prevents interactions with any children in the subtree when the [sliver] is
/// animating away.
///
/// See also:
///
/// * [SliverFadeTransition], an explicitly animated version of this widget, where
/// an [Animation] is provided by the caller instead of being built in.
/// * [AnimatedOpacity], for automatically transitioning a box child's
/// opacity over a given duration whenever the given opacity changes.
class SliverAnimatedOpacity extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates its opacity implicitly.
///
/// The [opacity] argument must be between zero and one, inclusive.
const SliverAnimatedOpacity({
super.key,
this.sliver,
required this.opacity,
super.curve,
required super.duration,
super.onEnd,
this.alwaysIncludeSemantics = false,
}) : assert(opacity >= 0.0 && opacity <= 1.0);
/// The sliver below this widget in the tree.
final Widget? sliver;
/// The target opacity.
///
/// An opacity of 1.0 is fully opaque. An opacity of 0.0 is fully transparent
/// (i.e., invisible).
final double opacity;
/// Whether the semantic information of the children is always included.
///
/// Defaults to false.
///
/// When true, regardless of the opacity settings the sliver child's semantic
/// information is exposed as if the widget were fully visible. This is
/// useful in cases where labels may be hidden during animations that
/// would otherwise contribute relevant semantics.
final bool alwaysIncludeSemantics;
@override
ImplicitlyAnimatedWidgetState<SliverAnimatedOpacity> createState() => _SliverAnimatedOpacityState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('opacity', opacity));
}
}
class _SliverAnimatedOpacityState extends ImplicitlyAnimatedWidgetState<SliverAnimatedOpacity> {
Tween<double>? _opacity;
late Animation<double> _opacityAnimation;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_opacity = visitor(_opacity, widget.opacity, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
@override
void didUpdateTweens() {
_opacityAnimation = animation.drive(_opacity!);
}
@override
Widget build(BuildContext context) {
return SliverFadeTransition(
opacity: _opacityAnimation,
sliver: widget.sliver,
alwaysIncludeSemantics: widget.alwaysIncludeSemantics,
);
}
}
/// Animated version of [DefaultTextStyle] which automatically transitions the
/// default text style (the text style to apply to descendant [Text] widgets
/// without explicit style) over a given duration whenever the given style
/// changes.
///
/// The [textAlign], [softWrap], [overflow], [maxLines], [textWidthBasis]
/// and [textHeightBehavior] properties are not animated and take effect
/// immediately when changed.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.elasticInOut].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_default_text_style.mp4}
///
/// For the animation, you can choose a [curve] as well as a [duration] and the
/// widget will automatically animate to the new default text style. If you require
/// more control over the animation (e.g. if you want to stop it mid-animation),
/// consider using a [DefaultTextStyleTransition] instead, which takes a provided
/// [Animation] as argument. While that allows you to fine-tune the animation,
/// it also requires more development overhead as you have to manually manage
/// the lifecycle of the underlying [AnimationController].
class AnimatedDefaultTextStyle extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates the default text style implicitly.
const AnimatedDefaultTextStyle({
super.key,
required this.child,
required this.style,
this.textAlign,
this.softWrap = true,
this.overflow = TextOverflow.clip,
this.maxLines,
this.textWidthBasis = TextWidthBasis.parent,
this.textHeightBehavior,
super.curve,
required super.duration,
super.onEnd,
}) : assert(maxLines == null || maxLines > 0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The target text style.
///
/// When this property is changed, the style will be animated over [duration] time.
final TextStyle style;
/// How the text should be aligned horizontally.
///
/// This property takes effect immediately when changed, it is not animated.
final TextAlign? textAlign;
/// Whether the text should break at soft line breaks.
///
/// This property takes effect immediately when changed, it is not animated.
///
/// See [DefaultTextStyle.softWrap] for more details.
final bool softWrap;
/// How visual overflow should be handled.
///
/// This property takes effect immediately when changed, it is not animated.
final TextOverflow overflow;
/// An optional maximum number of lines for the text to span, wrapping if necessary.
///
/// This property takes effect immediately when changed, it is not animated.
///
/// See [DefaultTextStyle.maxLines] for more details.
final int? maxLines;
/// The strategy to use when calculating the width of the Text.
///
/// See [TextWidthBasis] for possible values and their implications.
final TextWidthBasis textWidthBasis;
/// {@macro dart.ui.textHeightBehavior}
final ui.TextHeightBehavior? textHeightBehavior;
@override
AnimatedWidgetBaseState<AnimatedDefaultTextStyle> createState() => _AnimatedDefaultTextStyleState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
style.debugFillProperties(properties);
properties.add(EnumProperty<TextAlign>('textAlign', textAlign, defaultValue: null));
properties.add(FlagProperty('softWrap', value: softWrap, ifTrue: 'wrapping at box width', ifFalse: 'no wrapping except at line break characters', showName: true));
properties.add(EnumProperty<TextOverflow>('overflow', overflow, defaultValue: null));
properties.add(IntProperty('maxLines', maxLines, defaultValue: null));
properties.add(EnumProperty<TextWidthBasis>('textWidthBasis', textWidthBasis, defaultValue: TextWidthBasis.parent));
properties.add(DiagnosticsProperty<ui.TextHeightBehavior>('textHeightBehavior', textHeightBehavior, defaultValue: null));
}
}
class _AnimatedDefaultTextStyleState extends AnimatedWidgetBaseState<AnimatedDefaultTextStyle> {
TextStyleTween? _style;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_style = visitor(_style, widget.style, (dynamic value) => TextStyleTween(begin: value as TextStyle)) as TextStyleTween?;
}
@override
Widget build(BuildContext context) {
return DefaultTextStyle(
style: _style!.evaluate(animation),
textAlign: widget.textAlign,
softWrap: widget.softWrap,
overflow: widget.overflow,
maxLines: widget.maxLines,
textWidthBasis: widget.textWidthBasis,
textHeightBehavior: widget.textHeightBehavior,
child: widget.child,
);
}
}
/// Animated version of [PhysicalModel].
///
/// The [borderRadius] and [elevation] are animated.
///
/// The [color] is animated if the [animateColor] property is set; otherwise,
/// the color changes immediately at the start of the animation for the other
/// two properties. This allows the color to be animated independently (e.g.
/// because it is being driven by an [AnimatedTheme]).
///
/// The [shape] is not animated.
///
/// Here's an illustration of what using this widget looks like, using a [curve]
/// of [Curves.fastOutSlowIn].
/// {@animation 250 266 https://flutter.github.io/assets-for-api-docs/assets/widgets/animated_physical_model.mp4}
class AnimatedPhysicalModel extends ImplicitlyAnimatedWidget {
/// Creates a widget that animates the properties of a [PhysicalModel].
///
/// The [elevation] must be non-negative.
///
/// Animating [color] is optional and is controlled by the [animateColor] flag.
///
/// Animating [shadowColor] is optional and is controlled by the [animateShadowColor] flag.
const AnimatedPhysicalModel({
super.key,
required this.child,
required this.shape,
this.clipBehavior = Clip.none,
this.borderRadius = BorderRadius.zero,
required this.elevation,
required this.color,
this.animateColor = true,
required this.shadowColor,
this.animateShadowColor = true,
super.curve,
required super.duration,
super.onEnd,
}) : assert(elevation >= 0.0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The type of shape.
///
/// This property is not animated.
final BoxShape shape;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The target border radius of the rounded corners for a rectangle shape.
final BorderRadius borderRadius;
/// The target z-coordinate relative to the parent at which to place this
/// physical object.
///
/// The value will always be non-negative.
final double elevation;
/// The target background color.
final Color color;
/// Whether the color should be animated.
final bool animateColor;
/// The target shadow color.
final Color shadowColor;
/// Whether the shadow color should be animated.
final bool animateShadowColor;
@override
AnimatedWidgetBaseState<AnimatedPhysicalModel> createState() => _AnimatedPhysicalModelState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<BoxShape>('shape', shape));
properties.add(DiagnosticsProperty<BorderRadius>('borderRadius', borderRadius));
properties.add(DoubleProperty('elevation', elevation));
properties.add(ColorProperty('color', color));
properties.add(DiagnosticsProperty<bool>('animateColor', animateColor));
properties.add(ColorProperty('shadowColor', shadowColor));
properties.add(DiagnosticsProperty<bool>('animateShadowColor', animateShadowColor));
}
}
class _AnimatedPhysicalModelState extends AnimatedWidgetBaseState<AnimatedPhysicalModel> {
BorderRadiusTween? _borderRadius;
Tween<double>? _elevation;
ColorTween? _color;
ColorTween? _shadowColor;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_borderRadius = visitor(_borderRadius, widget.borderRadius, (dynamic value) => BorderRadiusTween(begin: value as BorderRadius)) as BorderRadiusTween?;
_elevation = visitor(_elevation, widget.elevation, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
_color = visitor(_color, widget.color, (dynamic value) => ColorTween(begin: value as Color)) as ColorTween?;
_shadowColor = visitor(_shadowColor, widget.shadowColor, (dynamic value) => ColorTween(begin: value as Color)) as ColorTween?;
}
@override
Widget build(BuildContext context) {
return PhysicalModel(
shape: widget.shape,
clipBehavior: widget.clipBehavior,
borderRadius: _borderRadius!.evaluate(animation),
elevation: _elevation!.evaluate(animation),
color: widget.animateColor ? _color!.evaluate(animation)! : widget.color,
shadowColor: widget.animateShadowColor
? _shadowColor!.evaluate(animation)!
: widget.shadowColor,
child: widget.child,
);
}
}
/// Animated version of [FractionallySizedBox] which automatically transitions the
/// child's size over a given duration whenever the given [widthFactor] or
/// [heightFactor] changes, as well as the position whenever the given [alignment]
/// changes.
///
/// For the animation, you can choose a [curve] as well as a [duration] and the
/// widget will automatically animate to the new target [widthFactor] or
/// [heightFactor].
///
/// {@tool dartpad}
/// The following example transitions an [AnimatedFractionallySizedBox]
/// between two states. It adjusts the [heightFactor], [widthFactor], and
/// [alignment] properties when tapped, using a [curve] of [Curves.fastOutSlowIn]
///
/// ** See code in examples/api/lib/widgets/implicit_animations/animated_fractionally_sized_box.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedAlign], which is an implicitly animated version of [Align].
/// * [AnimatedContainer], which can transition more values at once.
/// * [AnimatedSlide], which can animate the translation of child by a given offset relative to its size.
/// * [AnimatedPositioned], which, as a child of a [Stack], automatically
/// transitions its child's position over a given duration whenever the given
/// position changes.
class AnimatedFractionallySizedBox extends ImplicitlyAnimatedWidget {
/// Creates a widget that sizes its child to a fraction of the total available
/// space that animates implicitly, and positions its child by an alignment
/// that animates implicitly.
///
/// If non-null, the [widthFactor] and [heightFactor] arguments must be
/// non-negative.
const AnimatedFractionallySizedBox({
super.key,
this.alignment = Alignment.center,
this.child,
this.heightFactor,
this.widthFactor,
super.curve,
required super.duration,
super.onEnd,
}) : assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0);
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// {@macro flutter.widgets.basic.fractionallySizedBox.heightFactor}
final double? heightFactor;
/// {@macro flutter.widgets.basic.fractionallySizedBox.widthFactor}
final double? widthFactor;
/// {@macro flutter.widgets.basic.fractionallySizedBox.alignment}
final AlignmentGeometry alignment;
@override
AnimatedWidgetBaseState<AnimatedFractionallySizedBox> createState() => _AnimatedFractionallySizedBoxState();
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DiagnosticsProperty<double>('widthFactor', widthFactor));
properties.add(DiagnosticsProperty<double>('heightFactor', heightFactor));
}
}
class _AnimatedFractionallySizedBoxState extends AnimatedWidgetBaseState<AnimatedFractionallySizedBox> {
AlignmentGeometryTween? _alignment;
Tween<double>? _heightFactorTween;
Tween<double>? _widthFactorTween;
@override
void forEachTween(TweenVisitor<dynamic> visitor) {
_alignment = visitor(_alignment, widget.alignment, (dynamic value) => AlignmentGeometryTween(begin: value as AlignmentGeometry)) as AlignmentGeometryTween?;
if (widget.heightFactor != null) {
_heightFactorTween = visitor(_heightFactorTween, widget.heightFactor, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
if (widget.widthFactor != null) {
_widthFactorTween = visitor(_widthFactorTween, widget.widthFactor, (dynamic value) => Tween<double>(begin: value as double)) as Tween<double>?;
}
}
@override
Widget build(BuildContext context) {
return FractionallySizedBox(
alignment: _alignment!.evaluate(animation)!,
heightFactor: _heightFactorTween?.evaluate(animation),
widthFactor: _widthFactorTween?.evaluate(animation),
child: widget.child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder description) {
super.debugFillProperties(description);
description.add(DiagnosticsProperty<AlignmentGeometryTween>('alignment', _alignment, defaultValue: null));
description.add(DiagnosticsProperty<Tween<double>>('widthFactor', _widthFactorTween, defaultValue: null));
description.add(DiagnosticsProperty<Tween<double>>('heightFactor', _heightFactorTween, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/implicit_animations.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/implicit_animations.dart",
"repo_id": "flutter",
"token_count": 25879
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'basic.dart';
import 'framework.dart';
import 'primary_scroll_controller.dart';
import 'scroll_activity.dart';
import 'scroll_configuration.dart';
import 'scroll_context.dart';
import 'scroll_controller.dart';
import 'scroll_metrics.dart';
import 'scroll_physics.dart';
import 'scroll_position.dart';
import 'scroll_view.dart';
import 'sliver_fill.dart';
import 'viewport.dart';
/// Signature used by [NestedScrollView] for building its header.
///
/// The `innerBoxIsScrolled` argument is typically used to control the
/// [SliverAppBar.forceElevated] property to ensure that the app bar shows a
/// shadow, since it would otherwise not necessarily be aware that it had
/// content ostensibly below it.
typedef NestedScrollViewHeaderSliversBuilder = List<Widget> Function(BuildContext context, bool innerBoxIsScrolled);
/// A scrolling view inside of which can be nested other scrolling views, with
/// their scroll positions being intrinsically linked.
///
/// The most common use case for this widget is a scrollable view with a
/// flexible [SliverAppBar] containing a [TabBar] in the header (built by
/// [headerSliverBuilder], and with a [TabBarView] in the [body], such that the
/// scrollable view's contents vary based on which tab is visible.
///
/// ## Motivation
///
/// In a normal [ScrollView], there is one set of slivers (the components of the
/// scrolling view). If one of those slivers hosted a [TabBarView] which scrolls
/// in the opposite direction (e.g. allowing the user to swipe horizontally
/// between the pages represented by the tabs, while the list scrolls
/// vertically), then any list inside that [TabBarView] would not interact with
/// the outer [ScrollView]. For example, flinging the inner list to scroll to
/// the top would not cause a collapsed [SliverAppBar] in the outer [ScrollView]
/// to expand.
///
/// [NestedScrollView] solves this problem by providing custom
/// [ScrollController]s for the outer [ScrollView] and the inner [ScrollView]s
/// (those inside the [TabBarView], hooking them together so that they appear,
/// to the user, as one coherent scroll view.
///
/// {@tool dartpad}
/// This example shows a [NestedScrollView] whose header is the combination of a
/// [TabBar] in a [SliverAppBar] and whose body is a [TabBarView]. It uses a
/// [SliverOverlapAbsorber]/[SliverOverlapInjector] pair to make the inner lists
/// align correctly, and it uses [SafeArea] to avoid any horizontal disturbances
/// (e.g. the "notch" on iOS when the phone is horizontal). In addition,
/// [PageStorageKey]s are used to remember the scroll position of each tab's
/// list.
///
/// ** See code in examples/api/lib/widgets/nested_scroll_view/nested_scroll_view.0.dart **
/// {@end-tool}
///
/// ## [SliverAppBar]s with [NestedScrollView]s
///
/// Using a [SliverAppBar] in the outer scroll view, or [headerSliverBuilder],
/// of a [NestedScrollView] may require special configurations in order to work
/// as it would if the outer and inner were one single scroll view, like a
/// [CustomScrollView].
///
/// ### Pinned [SliverAppBar]s
///
/// A pinned [SliverAppBar] works in a [NestedScrollView] exactly as it would in
/// another scroll view, like [CustomScrollView]. When using
/// [SliverAppBar.pinned], the app bar remains visible at the top of the scroll
/// view. The app bar can still expand and contract as the user scrolls, but it
/// will remain visible rather than being scrolled out of view.
///
/// This works naturally in a [NestedScrollView], as the pinned [SliverAppBar]
/// is not expected to move in or out of the visible portion of the viewport.
/// As the inner or outer [Scrollable]s are moved, the app bar persists as
/// expected.
///
/// If the app bar is floating, pinned, and using an expanded height, follow the
/// floating convention laid out below.
///
/// ### Floating [SliverAppBar]s
///
/// When placed in the outer scrollable, or the [headerSliverBuilder],
/// a [SliverAppBar] that floats, using [SliverAppBar.floating] will not be
/// triggered to float over the inner scroll view, or [body], automatically.
///
/// This is because a floating app bar uses the scroll offset of its own
/// [Scrollable] to dictate the floating action. Being two separate inner and
/// outer [Scrollable]s, a [SliverAppBar] in the outer header is not aware of
/// changes in the scroll offset of the inner body.
///
/// In order to float the outer, use [NestedScrollView.floatHeaderSlivers]. When
/// set to true, the nested scrolling coordinator will prioritize floating in
/// the header slivers before applying the remaining drag to the body.
///
/// Furthermore, the `floatHeaderSlivers` flag should also be used when using an
/// app bar that is floating, pinned, and has an expanded height. In this
/// configuration, the flexible space of the app bar will open and collapse,
/// while the primary portion of the app bar remains pinned.
///
/// {@tool dartpad}
/// This simple example shows a [NestedScrollView] whose header contains a
/// floating [SliverAppBar]. By using the [floatHeaderSlivers] property, the
/// floating behavior is coordinated between the outer and inner [Scrollable]s,
/// so it behaves as it would in a single scrollable.
///
/// ** See code in examples/api/lib/widgets/nested_scroll_view/nested_scroll_view.1.dart **
/// {@end-tool}
///
/// ### Snapping [SliverAppBar]s
///
/// Floating [SliverAppBar]s also have the option to perform a snapping animation.
/// If [SliverAppBar.snap] is true, then a scroll that exposes the floating app
/// bar will trigger an animation that slides the entire app bar into view.
/// Similarly if a scroll dismisses the app bar, the animation will slide the
/// app bar completely out of view.
///
/// It is possible with a [NestedScrollView] to perform just the snapping
/// animation without floating the app bar in and out. By not using the
/// [NestedScrollView.floatHeaderSlivers], the app bar will snap in and out
/// without floating.
///
/// The [SliverAppBar.snap] animation should be used in conjunction with the
/// [SliverOverlapAbsorber] and [SliverOverlapInjector] widgets when
/// implemented in a [NestedScrollView]. These widgets take any overlapping
/// behavior of the [SliverAppBar] in the header and redirect it to the
/// [SliverOverlapInjector] in the body. If it is missing, then it is possible
/// for the nested "inner" scroll view below to end up under the [SliverAppBar]
/// even when the inner scroll view thinks it has not been scrolled.
///
/// {@tool dartpad}
/// This simple example shows a [NestedScrollView] whose header contains a
/// snapping, floating [SliverAppBar]. _Without_ setting any additional flags,
/// e.g [NestedScrollView.floatHeaderSlivers], the [SliverAppBar] will animate
/// in and out without floating. The [SliverOverlapAbsorber] and
/// [SliverOverlapInjector] maintain the proper alignment between the two
/// separate scroll views.
///
/// ** See code in examples/api/lib/widgets/nested_scroll_view/nested_scroll_view.2.dart **
/// {@end-tool}
///
/// ### Snapping and Floating [SliverAppBar]s
///
// See https://github.com/flutter/flutter/issues/59189
/// Currently, [NestedScrollView] does not support simultaneously floating and
/// snapping the outer scrollable, e.g. when using [SliverAppBar.floating] &
/// [SliverAppBar.snap] at the same time.
///
/// ### Stretching [SliverAppBar]s
///
// See https://github.com/flutter/flutter/issues/54059
/// Currently, [NestedScrollView] does not support stretching the outer
/// scrollable, e.g. when using [SliverAppBar.stretch].
///
/// See also:
///
/// * [SliverAppBar], for examples on different configurations like floating,
/// pinned and snap behaviors.
/// * [SliverOverlapAbsorber], a sliver that wraps another, forcing its layout
/// extent to be treated as overlap.
/// * [SliverOverlapInjector], a sliver that has a sliver geometry based on
/// the values stored in a [SliverOverlapAbsorberHandle].
class NestedScrollView extends StatefulWidget {
/// Creates a nested scroll view.
///
/// The [reverse], [headerSliverBuilder], and [body] arguments must not be
/// null.
const NestedScrollView({
super.key,
this.controller,
this.scrollDirection = Axis.vertical,
this.reverse = false,
this.physics,
required this.headerSliverBuilder,
required this.body,
this.dragStartBehavior = DragStartBehavior.start,
this.floatHeaderSlivers = false,
this.clipBehavior = Clip.hardEdge,
this.restorationId,
this.scrollBehavior,
});
/// An object that can be used to control the position to which the outer
/// scroll view is scrolled.
final ScrollController? controller;
/// {@macro flutter.widgets.scroll_view.scrollDirection}
///
/// This property only applies to the [Axis] of the outer scroll view,
/// composed of the slivers returned from [headerSliverBuilder]. Since the
/// inner scroll view is not directly configured by the [NestedScrollView],
/// for the axes to match, configure the scroll view of the [body] the same
/// way if they are expected to scroll in the same orientation. This allows
/// for flexible configurations of the NestedScrollView.
final Axis scrollDirection;
/// Whether the scroll view scrolls in the reading direction.
///
/// For example, if the reading direction is left-to-right and
/// [scrollDirection] is [Axis.horizontal], then the scroll view scrolls from
/// left to right when [reverse] is false and from right to left when
/// [reverse] is true.
///
/// Similarly, if [scrollDirection] is [Axis.vertical], then the scroll view
/// scrolls from top to bottom when [reverse] is false and from bottom to top
/// when [reverse] is true.
///
/// This property only applies to the outer scroll view, composed of the
/// slivers returned from [headerSliverBuilder]. Since the inner scroll view
/// is not directly configured by the [NestedScrollView]. For both to scroll
/// in reverse, configure the scroll view of the [body] the same way if they
/// are expected to match. This allows for flexible configurations of the
/// NestedScrollView.
///
/// Defaults to false.
final bool reverse;
/// How the scroll view should respond to user input.
///
/// For example, determines how the scroll view continues to animate after the
/// user stops dragging the scroll view (providing a custom implementation of
/// [ScrollPhysics.createBallisticSimulation] allows this particular aspect of
/// the physics to be overridden).
///
/// If an explicit [ScrollBehavior] is provided to [scrollBehavior], the
/// [ScrollPhysics] provided by that behavior will take precedence after
/// [physics].
///
/// Defaults to matching platform conventions.
///
/// The [ScrollPhysics.applyBoundaryConditions] implementation of the provided
/// object should not allow scrolling outside the scroll extent range
/// described by the [ScrollMetrics.minScrollExtent] and
/// [ScrollMetrics.maxScrollExtent] properties passed to that method. If that
/// invariant is not maintained, the nested scroll view may respond to user
/// scrolling erratically.
///
/// This property only applies to the outer scroll view, composed of the
/// slivers returned from [headerSliverBuilder]. Since the inner scroll view
/// is not directly configured by the [NestedScrollView]. For both to scroll
/// with the same [ScrollPhysics], configure the scroll view of the [body]
/// the same way if they are expected to match, or use a [ScrollBehavior] as
/// an ancestor so both the inner and outer scroll views inherit the same
/// [ScrollPhysics]. This allows for flexible configurations of the
/// NestedScrollView.
///
/// The [ScrollPhysics] also determine whether or not the [NestedScrollView]
/// can accept input from the user to change the scroll offset. For example,
/// [NeverScrollableScrollPhysics] typically will not allow the user to drag a
/// scroll view, but in this case, if one of the two scroll views can be
/// dragged, then dragging will be allowed. Configuring both scroll views with
/// [NeverScrollableScrollPhysics] will disallow dragging in this case.
final ScrollPhysics? physics;
/// A builder for any widgets that are to precede the inner scroll views (as
/// given by [body]).
///
/// Typically this is used to create a [SliverAppBar] with a [TabBar].
final NestedScrollViewHeaderSliversBuilder headerSliverBuilder;
/// The widget to show inside the [NestedScrollView].
///
/// Typically this will be [TabBarView].
///
/// The [body] is built in a context that provides a [PrimaryScrollController]
/// that interacts with the [NestedScrollView]'s scroll controller. Any
/// [ListView] or other [Scrollable]-based widget inside the [body] that is
/// intended to scroll with the [NestedScrollView] should therefore not be
/// given an explicit [ScrollController], instead allowing it to default to
/// the [PrimaryScrollController] provided by the [NestedScrollView].
final Widget body;
/// {@macro flutter.widgets.scrollable.dragStartBehavior}
final DragStartBehavior dragStartBehavior;
/// Whether or not the [NestedScrollView]'s coordinator should prioritize the
/// outer scrollable over the inner when scrolling back.
///
/// This is useful for an outer scrollable containing a [SliverAppBar] that
/// is expected to float.
final bool floatHeaderSlivers;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// {@macro flutter.widgets.scrollable.restorationId}
final String? restorationId;
/// {@macro flutter.widgets.shadow.scrollBehavior}
///
/// [ScrollBehavior]s also provide [ScrollPhysics]. If an explicit
/// [ScrollPhysics] is provided in [physics], it will take precedence,
/// followed by [scrollBehavior], and then the inherited ancestor
/// [ScrollBehavior].
///
/// The [ScrollBehavior] of the inherited [ScrollConfiguration] will be
/// modified by default to not apply a [Scrollbar]. This is because the
/// NestedScrollView cannot assume the configuration of the outer and inner
/// [Scrollable] widgets, particularly whether to treat them as one scrollable,
/// or separate and desirous of unique behaviors.
final ScrollBehavior? scrollBehavior;
/// Returns the [SliverOverlapAbsorberHandle] of the nearest ancestor
/// [NestedScrollView].
///
/// This is necessary to configure the [SliverOverlapAbsorber] and
/// [SliverOverlapInjector] widgets.
///
/// For sample code showing how to use this method, see the [NestedScrollView]
/// documentation.
static SliverOverlapAbsorberHandle sliverOverlapAbsorberHandleFor(BuildContext context) {
final _InheritedNestedScrollView? target = context.dependOnInheritedWidgetOfExactType<_InheritedNestedScrollView>();
assert(
target != null,
'NestedScrollView.sliverOverlapAbsorberHandleFor must be called with a context that contains a NestedScrollView.',
);
return target!.state._absorberHandle;
}
List<Widget> _buildSlivers(BuildContext context, ScrollController innerController, bool bodyIsScrolled) {
return <Widget>[
...headerSliverBuilder(context, bodyIsScrolled),
SliverFillRemaining(
// The inner (body) scroll view must use this scroll controller so that
// the independent scroll positions can be kept in sync.
child: PrimaryScrollController(
// The inner scroll view should always inherit this
// PrimaryScrollController, on every platform.
automaticallyInheritForPlatforms: TargetPlatform.values.toSet(),
// `PrimaryScrollController.scrollDirection` is not set, and so it is
// restricted to the default Axis.vertical.
// Ideally the inner and outer views would have the same
// scroll direction, and so we could assume
// `NestedScrollView.scrollDirection` for the PrimaryScrollController,
// but use cases already exist where the axes are mismatched.
// https://github.com/flutter/flutter/issues/102001
controller: innerController,
child: body,
),
),
];
}
@override
NestedScrollViewState createState() => NestedScrollViewState();
}
/// The [State] for a [NestedScrollView].
///
/// The [ScrollController]s, [innerController] and [outerController], of the
/// [NestedScrollView]'s children may be accessed through its state. This is
/// useful for obtaining respective scroll positions in the [NestedScrollView].
///
/// If you want to access the inner or outer scroll controller of a
/// [NestedScrollView], you can get its [NestedScrollViewState] by supplying a
/// `GlobalKey<NestedScrollViewState>` to the [NestedScrollView.key] parameter).
///
/// {@tool dartpad}
/// [NestedScrollViewState] can be obtained using a [GlobalKey].
/// Using the following setup, you can access the inner scroll controller
/// using `globalKey.currentState.innerController`.
///
/// ** See code in examples/api/lib/widgets/nested_scroll_view/nested_scroll_view_state.0.dart **
/// {@end-tool}
class NestedScrollViewState extends State<NestedScrollView> {
final SliverOverlapAbsorberHandle _absorberHandle = SliverOverlapAbsorberHandle();
/// The [ScrollController] provided to the [ScrollView] in
/// [NestedScrollView.body].
///
/// Manipulating the [ScrollPosition] of this controller pushes the outer
/// header sliver(s) up and out of view. The position of the [outerController]
/// will be set to [ScrollPosition.maxScrollExtent], unless you use
/// [ScrollPosition.setPixels].
///
/// See also:
///
/// * [outerController], which exposes the [ScrollController] used by the
/// sliver(s) contained in [NestedScrollView.headerSliverBuilder].
ScrollController get innerController => _coordinator!._innerController;
/// The [ScrollController] provided to the [ScrollView] in
/// [NestedScrollView.headerSliverBuilder].
///
/// This is equivalent to [NestedScrollView.controller], if provided.
///
/// Manipulating the [ScrollPosition] of this controller pushes the inner body
/// sliver(s) down. The position of the [innerController] will be set to
/// [ScrollPosition.minScrollExtent], unless you use
/// [ScrollPosition.setPixels]. Visually, the inner body will be scrolled to
/// its beginning.
///
/// See also:
///
/// * [innerController], which exposes the [ScrollController] used by the
/// [ScrollView] contained in [NestedScrollView.body].
ScrollController get outerController => _coordinator!._outerController;
_NestedScrollCoordinator? _coordinator;
@override
void initState() {
super.initState();
_coordinator = _NestedScrollCoordinator(
this,
widget.controller,
_handleHasScrolledBodyChanged,
widget.floatHeaderSlivers,
);
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_coordinator!.setParent(widget.controller);
}
@override
void didUpdateWidget(NestedScrollView oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.controller != widget.controller) {
_coordinator!.setParent(widget.controller);
}
}
@override
void dispose() {
_coordinator!.dispose();
_coordinator = null;
_absorberHandle.dispose();
super.dispose();
}
bool? _lastHasScrolledBody;
void _handleHasScrolledBodyChanged() {
if (!mounted) {
return;
}
final bool newHasScrolledBody = _coordinator!.hasScrolledBody;
if (_lastHasScrolledBody != newHasScrolledBody) {
setState(() {
// _coordinator.hasScrolledBody changed (we use it in the build method)
// (We record _lastHasScrolledBody in the build() method, rather than in
// this setState call, because the build() method may be called more
// often than just from here, and we want to only call setState when the
// new value is different than the last built value.)
});
}
}
@override
Widget build(BuildContext context) {
final ScrollPhysics scrollPhysics = widget.physics?.applyTo(const ClampingScrollPhysics())
?? widget.scrollBehavior?.getScrollPhysics(context).applyTo(const ClampingScrollPhysics())
?? const ClampingScrollPhysics();
return _InheritedNestedScrollView(
state: this,
child: Builder(
builder: (BuildContext context) {
_lastHasScrolledBody = _coordinator!.hasScrolledBody;
return _NestedScrollViewCustomScrollView(
dragStartBehavior: widget.dragStartBehavior,
scrollDirection: widget.scrollDirection,
reverse: widget.reverse,
physics: scrollPhysics,
scrollBehavior: widget.scrollBehavior ?? ScrollConfiguration.of(context).copyWith(scrollbars: false),
controller: _coordinator!._outerController,
slivers: widget._buildSlivers(
context,
_coordinator!._innerController,
_lastHasScrolledBody!,
),
handle: _absorberHandle,
clipBehavior: widget.clipBehavior,
restorationId: widget.restorationId,
);
},
),
);
}
}
class _NestedScrollViewCustomScrollView extends CustomScrollView {
const _NestedScrollViewCustomScrollView({
required super.scrollDirection,
required super.reverse,
required ScrollPhysics super.physics,
required ScrollBehavior super.scrollBehavior,
required ScrollController super.controller,
required super.slivers,
required this.handle,
required super.clipBehavior,
super.dragStartBehavior,
super.restorationId,
});
final SliverOverlapAbsorberHandle handle;
@override
Widget buildViewport(
BuildContext context,
ViewportOffset offset,
AxisDirection axisDirection,
List<Widget> slivers,
) {
assert(!shrinkWrap);
return NestedScrollViewViewport(
axisDirection: axisDirection,
offset: offset,
slivers: slivers,
handle: handle,
clipBehavior: clipBehavior,
);
}
}
class _InheritedNestedScrollView extends InheritedWidget {
const _InheritedNestedScrollView({
required this.state,
required super.child,
});
final NestedScrollViewState state;
@override
bool updateShouldNotify(_InheritedNestedScrollView old) => state != old.state;
}
class _NestedScrollMetrics extends FixedScrollMetrics {
_NestedScrollMetrics({
required super.minScrollExtent,
required super.maxScrollExtent,
required super.pixels,
required super.viewportDimension,
required super.axisDirection,
required super.devicePixelRatio,
required this.minRange,
required this.maxRange,
required this.correctionOffset,
});
@override
_NestedScrollMetrics copyWith({
double? minScrollExtent,
double? maxScrollExtent,
double? pixels,
double? viewportDimension,
AxisDirection? axisDirection,
double? devicePixelRatio,
double? minRange,
double? maxRange,
double? correctionOffset,
}) {
return _NestedScrollMetrics(
minScrollExtent: minScrollExtent ?? (hasContentDimensions ? this.minScrollExtent : null),
maxScrollExtent: maxScrollExtent ?? (hasContentDimensions ? this.maxScrollExtent : null),
pixels: pixels ?? (hasPixels ? this.pixels : null),
viewportDimension: viewportDimension ?? (hasViewportDimension ? this.viewportDimension : null),
axisDirection: axisDirection ?? this.axisDirection,
devicePixelRatio: devicePixelRatio ?? this.devicePixelRatio,
minRange: minRange ?? this.minRange,
maxRange: maxRange ?? this.maxRange,
correctionOffset: correctionOffset ?? this.correctionOffset,
);
}
final double minRange;
final double maxRange;
final double correctionOffset;
}
typedef _NestedScrollActivityGetter = ScrollActivity Function(_NestedScrollPosition position);
class _NestedScrollCoordinator implements ScrollActivityDelegate, ScrollHoldController {
_NestedScrollCoordinator(
this._state,
this._parent,
this._onHasScrolledBodyChanged,
this._floatHeaderSlivers,
) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$_NestedScrollCoordinator',
object: this,
);
}
final double initialScrollOffset = _parent?.initialScrollOffset ?? 0.0;
_outerController = _NestedScrollController(
this,
initialScrollOffset: initialScrollOffset,
debugLabel: 'outer',
);
_innerController = _NestedScrollController(
this,
debugLabel: 'inner',
);
}
final NestedScrollViewState _state;
ScrollController? _parent;
final VoidCallback _onHasScrolledBodyChanged;
final bool _floatHeaderSlivers;
late _NestedScrollController _outerController;
late _NestedScrollController _innerController;
_NestedScrollPosition? get _outerPosition {
if (!_outerController.hasClients) {
return null;
}
return _outerController.nestedPositions.single;
}
Iterable<_NestedScrollPosition> get _innerPositions {
return _innerController.nestedPositions;
}
bool get canScrollBody {
final _NestedScrollPosition? outer = _outerPosition;
if (outer == null) {
return true;
}
return outer.haveDimensions && outer.extentAfter == 0.0;
}
bool get hasScrolledBody {
for (final _NestedScrollPosition position in _innerPositions) {
if (!position.hasContentDimensions || !position.hasPixels) {
// It's possible that NestedScrollView built twice before layout phase
// in the same frame. This can happen when the FocusManager schedules a microTask
// that marks NestedScrollView dirty during the warm up frame.
// https://github.com/flutter/flutter/pull/75308
continue;
} else if (position.pixels > position.minScrollExtent) {
return true;
}
}
return false;
}
void updateShadow() { _onHasScrolledBodyChanged(); }
ScrollDirection get userScrollDirection => _userScrollDirection;
ScrollDirection _userScrollDirection = ScrollDirection.idle;
void updateUserScrollDirection(ScrollDirection value) {
if (userScrollDirection == value) {
return;
}
_userScrollDirection = value;
_outerPosition!.didUpdateScrollDirection(value);
for (final _NestedScrollPosition position in _innerPositions) {
position.didUpdateScrollDirection(value);
}
}
ScrollDragController? _currentDrag;
void beginActivity(ScrollActivity newOuterActivity, _NestedScrollActivityGetter innerActivityGetter) {
_outerPosition!.beginActivity(newOuterActivity);
bool scrolling = newOuterActivity.isScrolling;
for (final _NestedScrollPosition position in _innerPositions) {
final ScrollActivity newInnerActivity = innerActivityGetter(position);
position.beginActivity(newInnerActivity);
scrolling = scrolling && newInnerActivity.isScrolling;
}
_currentDrag?.dispose();
_currentDrag = null;
if (!scrolling) {
updateUserScrollDirection(ScrollDirection.idle);
}
}
@override
AxisDirection get axisDirection => _outerPosition!.axisDirection;
static IdleScrollActivity _createIdleScrollActivity(_NestedScrollPosition position) {
return IdleScrollActivity(position);
}
@override
void goIdle() {
beginActivity(
_createIdleScrollActivity(_outerPosition!),
_createIdleScrollActivity,
);
}
@override
void goBallistic(double velocity) {
beginActivity(
createOuterBallisticScrollActivity(velocity),
(_NestedScrollPosition position) {
return createInnerBallisticScrollActivity(
position,
velocity,
);
},
);
}
ScrollActivity createOuterBallisticScrollActivity(double velocity) {
// This function creates a ballistic scroll for the outer scrollable.
//
// It assumes that the outer scrollable can't be overscrolled, and sets up a
// ballistic scroll over the combined space of the innerPositions and the
// outerPosition.
// First we must pick a representative inner position that we will care
// about. This is somewhat arbitrary. Ideally we'd pick the one that is "in
// the center" but there isn't currently a good way to do that so we
// arbitrarily pick the one that is the furthest away from the infinity we
// are heading towards.
_NestedScrollPosition? innerPosition;
if (velocity != 0.0) {
for (final _NestedScrollPosition position in _innerPositions) {
if (innerPosition != null) {
if (velocity > 0.0) {
if (innerPosition.pixels < position.pixels) {
continue;
}
} else {
assert(velocity < 0.0);
if (innerPosition.pixels > position.pixels) {
continue;
}
}
}
innerPosition = position;
}
}
if (innerPosition == null) {
// It's either just us or a velocity=0 situation.
return _outerPosition!.createBallisticScrollActivity(
_outerPosition!.physics.createBallisticSimulation(
_outerPosition!,
velocity,
),
mode: _NestedBallisticScrollActivityMode.independent,
);
}
final _NestedScrollMetrics metrics = _getMetrics(innerPosition, velocity);
return _outerPosition!.createBallisticScrollActivity(
_outerPosition!.physics.createBallisticSimulation(metrics, velocity),
mode: _NestedBallisticScrollActivityMode.outer,
metrics: metrics,
);
}
@protected
ScrollActivity createInnerBallisticScrollActivity(_NestedScrollPosition position, double velocity) {
return position.createBallisticScrollActivity(
position.physics.createBallisticSimulation(
_getMetrics(position, velocity),
velocity,
),
mode: _NestedBallisticScrollActivityMode.inner,
);
}
_NestedScrollMetrics _getMetrics(_NestedScrollPosition innerPosition, double velocity) {
double pixels, minRange, maxRange, correctionOffset;
double extra = 0.0;
if (innerPosition.pixels == innerPosition.minScrollExtent) {
pixels = clampDouble(_outerPosition!.pixels,
_outerPosition!.minScrollExtent,
_outerPosition!.maxScrollExtent,
); // TODO(ianh): gracefully handle out-of-range outer positions
minRange = _outerPosition!.minScrollExtent;
maxRange = _outerPosition!.maxScrollExtent;
assert(minRange <= maxRange);
correctionOffset = 0.0;
} else {
assert(innerPosition.pixels != innerPosition.minScrollExtent);
if (innerPosition.pixels < innerPosition.minScrollExtent) {
pixels = innerPosition.pixels - innerPosition.minScrollExtent + _outerPosition!.minScrollExtent;
} else {
assert(innerPosition.pixels > innerPosition.minScrollExtent);
pixels = innerPosition.pixels - innerPosition.minScrollExtent + _outerPosition!.maxScrollExtent;
}
if ((velocity > 0.0) && (innerPosition.pixels > innerPosition.minScrollExtent)) {
// This handles going forward (fling up) and inner list is scrolled past
// zero. We want to grab the extra pixels immediately to shrink.
extra = _outerPosition!.maxScrollExtent - _outerPosition!.pixels;
assert(extra >= 0.0);
minRange = pixels;
maxRange = pixels + extra;
assert(minRange <= maxRange);
correctionOffset = _outerPosition!.pixels - pixels;
} else if ((velocity < 0.0) && (innerPosition.pixels < innerPosition.minScrollExtent)) {
// This handles going backward (fling down) and inner list is
// underscrolled. We want to grab the extra pixels immediately to grow.
extra = _outerPosition!.pixels - _outerPosition!.minScrollExtent;
assert(extra >= 0.0);
minRange = pixels - extra;
maxRange = pixels;
assert(minRange <= maxRange);
correctionOffset = _outerPosition!.pixels - pixels;
} else {
// This handles going forward (fling up) and inner list is
// underscrolled, OR, going backward (fling down) and inner list is
// scrolled past zero. We want to skip the pixels we don't need to grow
// or shrink over.
if (velocity > 0.0) {
// shrinking
extra = _outerPosition!.minScrollExtent - _outerPosition!.pixels;
} else if (velocity < 0.0) {
// growing
extra = _outerPosition!.pixels - (_outerPosition!.maxScrollExtent - _outerPosition!.minScrollExtent);
}
assert(extra <= 0.0);
minRange = _outerPosition!.minScrollExtent;
maxRange = _outerPosition!.maxScrollExtent + extra;
assert(minRange <= maxRange);
correctionOffset = 0.0;
}
}
return _NestedScrollMetrics(
minScrollExtent: _outerPosition!.minScrollExtent,
maxScrollExtent: _outerPosition!.maxScrollExtent + innerPosition.maxScrollExtent - innerPosition.minScrollExtent + extra,
pixels: pixels,
viewportDimension: _outerPosition!.viewportDimension,
axisDirection: _outerPosition!.axisDirection,
minRange: minRange,
maxRange: maxRange,
correctionOffset: correctionOffset,
devicePixelRatio: _outerPosition!.devicePixelRatio,
);
}
double unnestOffset(double value, _NestedScrollPosition source) {
if (source == _outerPosition) {
return clampDouble(value,
_outerPosition!.minScrollExtent,
_outerPosition!.maxScrollExtent,
);
}
if (value < source.minScrollExtent) {
return value - source.minScrollExtent + _outerPosition!.minScrollExtent;
}
return value - source.minScrollExtent + _outerPosition!.maxScrollExtent;
}
double nestOffset(double value, _NestedScrollPosition target) {
if (target == _outerPosition) {
return clampDouble(value,
_outerPosition!.minScrollExtent,
_outerPosition!.maxScrollExtent,
);
}
if (value < _outerPosition!.minScrollExtent) {
return value - _outerPosition!.minScrollExtent + target.minScrollExtent;
}
if (value > _outerPosition!.maxScrollExtent) {
return value - _outerPosition!.maxScrollExtent + target.minScrollExtent;
}
return target.minScrollExtent;
}
void updateCanDrag() {
if (!_outerPosition!.haveDimensions) {
return;
}
bool innerCanDrag = false;
for (final _NestedScrollPosition position in _innerPositions) {
if (!position.haveDimensions) {
return;
}
innerCanDrag = innerCanDrag
// This refers to the physics of the actual inner scroll position, not
// the whole NestedScrollView, since it is possible to have different
// ScrollPhysics for the inner and outer positions.
|| position.physics.shouldAcceptUserOffset(position);
}
_outerPosition!.updateCanDrag(innerCanDrag);
}
Future<void> animateTo(
double to, {
required Duration duration,
required Curve curve,
}) async {
final DrivenScrollActivity outerActivity = _outerPosition!.createDrivenScrollActivity(
nestOffset(to, _outerPosition!),
duration,
curve,
);
final List<Future<void>> resultFutures = <Future<void>>[outerActivity.done];
beginActivity(
outerActivity,
(_NestedScrollPosition position) {
final DrivenScrollActivity innerActivity = position.createDrivenScrollActivity(
nestOffset(to, position),
duration,
curve,
);
resultFutures.add(innerActivity.done);
return innerActivity;
},
);
await Future.wait<void>(resultFutures);
}
void jumpTo(double to) {
goIdle();
_outerPosition!.localJumpTo(nestOffset(to, _outerPosition!));
for (final _NestedScrollPosition position in _innerPositions) {
position.localJumpTo(nestOffset(to, position));
}
goBallistic(0.0);
}
void pointerScroll(double delta) {
// If an update is made to pointer scrolling here, consider if the same
// (or similar) change should be made in
// ScrollPositionWithSingleContext.pointerScroll.
if (delta == 0.0) {
goBallistic(0.0);
return;
}
goIdle();
updateUserScrollDirection(
delta < 0.0 ? ScrollDirection.forward : ScrollDirection.reverse,
);
// Handle notifications. Even if only one position actually receives
// the delta, the NestedScrollView's intention is to treat multiple
// ScrollPositions as one.
_outerPosition!.isScrollingNotifier.value = true;
_outerPosition!.didStartScroll();
for (final _NestedScrollPosition position in _innerPositions) {
position.isScrollingNotifier.value = true;
position.didStartScroll();
}
if (_innerPositions.isEmpty) {
// Does not enter overscroll.
_outerPosition!.applyClampedPointerSignalUpdate(delta);
} else if (delta > 0.0) {
// Dragging "up" - delta is positive
// Prioritize getting rid of any inner overscroll, and then the outer
// view, so that the app bar will scroll out of the way asap.
double outerDelta = delta;
for (final _NestedScrollPosition position in _innerPositions) {
if (position.pixels < 0.0) { // This inner position is in overscroll.
final double potentialOuterDelta = position.applyClampedPointerSignalUpdate(delta);
// In case there are multiple positions in varying states of
// overscroll, the first to 'reach' the outer view above takes
// precedence.
outerDelta = math.max(outerDelta, potentialOuterDelta);
}
}
if (outerDelta != 0.0) {
final double innerDelta = _outerPosition!.applyClampedPointerSignalUpdate(
outerDelta,
);
if (innerDelta != 0.0) {
for (final _NestedScrollPosition position in _innerPositions) {
position.applyClampedPointerSignalUpdate(innerDelta);
}
}
}
} else {
// Dragging "down" - delta is negative
double innerDelta = delta;
// Apply delta to the outer header first if it is configured to float.
if (_floatHeaderSlivers) {
innerDelta = _outerPosition!.applyClampedPointerSignalUpdate(delta);
}
if (innerDelta != 0.0) {
// Apply the innerDelta, if we have not floated in the outer scrollable,
// any leftover delta after this will be passed on to the outer
// scrollable by the outerDelta.
double outerDelta = 0.0; // it will go negative if it changes
for (final _NestedScrollPosition position in _innerPositions) {
final double overscroll = position.applyClampedPointerSignalUpdate(innerDelta);
outerDelta = math.min(outerDelta, overscroll);
}
if (outerDelta != 0.0) {
_outerPosition!.applyClampedPointerSignalUpdate(outerDelta);
}
}
}
_outerPosition!.didEndScroll();
for (final _NestedScrollPosition position in _innerPositions) {
position.didEndScroll();
}
goBallistic(0.0);
}
@override
double setPixels(double newPixels) {
assert(false);
return 0.0;
}
ScrollHoldController hold(VoidCallback holdCancelCallback) {
beginActivity(
HoldScrollActivity(
delegate: _outerPosition!,
onHoldCanceled: holdCancelCallback,
),
(_NestedScrollPosition position) => HoldScrollActivity(delegate: position),
);
return this;
}
@override
void cancel() {
goBallistic(0.0);
}
Drag drag(DragStartDetails details, VoidCallback dragCancelCallback) {
final ScrollDragController drag = ScrollDragController(
delegate: this,
details: details,
onDragCanceled: dragCancelCallback,
);
beginActivity(
DragScrollActivity(_outerPosition!, drag),
(_NestedScrollPosition position) => DragScrollActivity(position, drag),
);
assert(_currentDrag == null);
_currentDrag = drag;
return drag;
}
@override
void applyUserOffset(double delta) {
updateUserScrollDirection(
delta > 0.0 ? ScrollDirection.forward : ScrollDirection.reverse,
);
assert(delta != 0.0);
if (_innerPositions.isEmpty) {
_outerPosition!.applyFullDragUpdate(delta);
} else if (delta < 0.0) {
// Dragging "up"
// Prioritize getting rid of any inner overscroll, and then the outer
// view, so that the app bar will scroll out of the way asap.
double outerDelta = delta;
for (final _NestedScrollPosition position in _innerPositions) {
if (position.pixels < 0.0) { // This inner position is in overscroll.
final double potentialOuterDelta = position.applyClampedDragUpdate(delta);
// In case there are multiple positions in varying states of
// overscroll, the first to 'reach' the outer view above takes
// precedence.
outerDelta = math.max(outerDelta, potentialOuterDelta);
}
}
if (outerDelta.abs() > precisionErrorTolerance) {
final double innerDelta = _outerPosition!.applyClampedDragUpdate(
outerDelta,
);
if (innerDelta != 0.0) {
for (final _NestedScrollPosition position in _innerPositions) {
position.applyFullDragUpdate(innerDelta);
}
}
}
} else {
// Dragging "down" - delta is positive
double innerDelta = delta;
// Apply delta to the outer header first if it is configured to float.
if (_floatHeaderSlivers) {
innerDelta = _outerPosition!.applyClampedDragUpdate(delta);
}
if (innerDelta != 0.0) {
// Apply the innerDelta, if we have not floated in the outer scrollable,
// any leftover delta after this will be passed on to the outer
// scrollable by the outerDelta.
double outerDelta = 0.0; // it will go positive if it changes
final List<double> overscrolls = <double>[];
final List<_NestedScrollPosition> innerPositions = _innerPositions.toList();
for (final _NestedScrollPosition position in innerPositions) {
final double overscroll = position.applyClampedDragUpdate(innerDelta);
outerDelta = math.max(outerDelta, overscroll);
overscrolls.add(overscroll);
}
if (outerDelta != 0.0) {
outerDelta -= _outerPosition!.applyClampedDragUpdate(outerDelta);
}
// Now deal with any overscroll
for (int i = 0; i < innerPositions.length; ++i) {
final double remainingDelta = overscrolls[i] - outerDelta;
if (remainingDelta > 0.0) {
innerPositions[i].applyFullDragUpdate(remainingDelta);
}
}
}
}
}
void setParent(ScrollController? value) {
_parent = value;
updateParent();
}
void updateParent() {
_outerPosition?.setParent(
_parent ?? PrimaryScrollController.maybeOf(_state.context),
);
}
@mustCallSuper
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_currentDrag?.dispose();
_currentDrag = null;
_outerController.dispose();
_innerController.dispose();
}
@override
String toString() => '${objectRuntimeType(this, '_NestedScrollCoordinator')}(outer=$_outerController; inner=$_innerController)';
}
class _NestedScrollController extends ScrollController {
_NestedScrollController(
this.coordinator, {
super.initialScrollOffset,
super.debugLabel,
});
final _NestedScrollCoordinator coordinator;
@override
ScrollPosition createScrollPosition(
ScrollPhysics physics,
ScrollContext context,
ScrollPosition? oldPosition,
) {
return _NestedScrollPosition(
coordinator: coordinator,
physics: physics,
context: context,
initialPixels: initialScrollOffset,
oldPosition: oldPosition,
debugLabel: debugLabel,
);
}
@override
void attach(ScrollPosition position) {
assert(position is _NestedScrollPosition);
super.attach(position);
coordinator.updateParent();
coordinator.updateCanDrag();
position.addListener(_scheduleUpdateShadow);
_scheduleUpdateShadow();
}
@override
void detach(ScrollPosition position) {
assert(position is _NestedScrollPosition);
(position as _NestedScrollPosition).setParent(null);
position.removeListener(_scheduleUpdateShadow);
super.detach(position);
_scheduleUpdateShadow();
}
void _scheduleUpdateShadow() {
// We do this asynchronously for attach() so that the new position has had
// time to be initialized, and we do it asynchronously for detach() and from
// the position change notifications because those happen synchronously
// during a frame, at a time where it's too late to call setState. Since the
// result is usually animated, the lag incurred is no big deal.
SchedulerBinding.instance.addPostFrameCallback((Duration timeStamp) {
coordinator.updateShadow();
}, debugLabel: 'NestedScrollController.updateShadow');
}
Iterable<_NestedScrollPosition> get nestedPositions {
// TODO(vegorov): use instance method version of castFrom when it is available.
return Iterable.castFrom<ScrollPosition, _NestedScrollPosition>(positions);
}
}
// The _NestedScrollPosition is used by both the inner and outer viewports of a
// NestedScrollView. It tracks the offset to use for those viewports, and knows
// about the _NestedScrollCoordinator, so that when activities are triggered on
// this class, they can defer, or be influenced by, the coordinator.
class _NestedScrollPosition extends ScrollPosition implements ScrollActivityDelegate {
_NestedScrollPosition({
required super.physics,
required super.context,
double initialPixels = 0.0,
super.oldPosition,
super.debugLabel,
required this.coordinator,
}) {
if (!hasPixels) {
correctPixels(initialPixels);
}
if (activity == null) {
goIdle();
}
assert(activity != null);
saveScrollOffset(); // in case we didn't restore but could, so that we don't restore it later
}
final _NestedScrollCoordinator coordinator;
TickerProvider get vsync => context.vsync;
ScrollController? _parent;
void setParent(ScrollController? value) {
_parent?.detach(this);
_parent = value;
_parent?.attach(this);
}
@override
AxisDirection get axisDirection => context.axisDirection;
@override
void absorb(ScrollPosition other) {
super.absorb(other);
activity!.updateDelegate(this);
}
@override
void restoreScrollOffset() {
if (coordinator.canScrollBody) {
super.restoreScrollOffset();
}
}
// Returns the amount of delta that was not used.
//
// Positive delta means going down (exposing stuff above), negative delta
// going up (exposing stuff below).
double applyClampedDragUpdate(double delta) {
assert(delta != 0.0);
// If we are going towards the maxScrollExtent (negative scroll offset),
// then the furthest we can be in the minScrollExtent direction is negative
// infinity. For example, if we are already overscrolled, then scrolling to
// reduce the overscroll should not disallow the overscroll.
//
// If we are going towards the minScrollExtent (positive scroll offset),
// then the furthest we can be in the minScrollExtent direction is wherever
// we are now, if we are already overscrolled (in which case pixels is less
// than the minScrollExtent), or the minScrollExtent if we are not.
//
// In other words, we cannot, via applyClampedDragUpdate, _enter_ an
// overscroll situation.
//
// An overscroll situation might be nonetheless entered via several means.
// One is if the physics allow it, via applyFullDragUpdate (see below). An
// overscroll situation can also be forced, e.g. if the scroll position is
// artificially set using the scroll controller.
final double min = delta < 0.0
? -double.infinity
: math.min(minScrollExtent, pixels);
// The logic for max is equivalent but on the other side.
final double max = delta > 0.0
? double.infinity
// If pixels < 0.0, then we are currently in overscroll. The max should be
// 0.0, representing the end of the overscrolled portion.
: pixels < 0.0 ? 0.0 : math.max(maxScrollExtent, pixels);
final double oldPixels = pixels;
final double newPixels = clampDouble(pixels - delta, min, max);
final double clampedDelta = newPixels - pixels;
if (clampedDelta == 0.0) {
return delta;
}
final double overscroll = physics.applyBoundaryConditions(this, newPixels);
final double actualNewPixels = newPixels - overscroll;
final double offset = actualNewPixels - oldPixels;
if (offset != 0.0) {
forcePixels(actualNewPixels);
didUpdateScrollPositionBy(offset);
}
return delta + offset;
}
// Returns the overscroll.
double applyFullDragUpdate(double delta) {
assert(delta != 0.0);
final double oldPixels = pixels;
// Apply friction:
final double newPixels = pixels - physics.applyPhysicsToUserOffset(
this,
delta,
);
if ((oldPixels - newPixels).abs() < precisionErrorTolerance) {
// Delta is so small we can drop it.
return 0.0;
}
// Check for overscroll:
final double overscroll = physics.applyBoundaryConditions(this, newPixels);
final double actualNewPixels = newPixels - overscroll;
if (actualNewPixels != oldPixels) {
forcePixels(actualNewPixels);
didUpdateScrollPositionBy(actualNewPixels - oldPixels);
}
if (overscroll != 0.0) {
didOverscrollBy(overscroll);
return overscroll;
}
return 0.0;
}
// Returns the amount of delta that was not used.
//
// Negative delta represents a forward ScrollDirection, while the positive
// would be a reverse ScrollDirection.
//
// The method doesn't take into account the effects of [ScrollPhysics].
double applyClampedPointerSignalUpdate(double delta) {
assert(delta != 0.0);
final double min = delta > 0.0
? -double.infinity
: math.min(minScrollExtent, pixels);
// The logic for max is equivalent but on the other side.
final double max = delta < 0.0
? double.infinity
: math.max(maxScrollExtent, pixels);
final double newPixels = clampDouble(pixels + delta, min, max);
final double clampedDelta = newPixels - pixels;
if (clampedDelta == 0.0) {
return delta;
}
forcePixels(newPixels);
didUpdateScrollPositionBy(clampedDelta);
return delta - clampedDelta;
}
@override
ScrollDirection get userScrollDirection => coordinator.userScrollDirection;
DrivenScrollActivity createDrivenScrollActivity(double to, Duration duration, Curve curve) {
return DrivenScrollActivity(
this,
from: pixels,
to: to,
duration: duration,
curve: curve,
vsync: vsync,
);
}
@override
double applyUserOffset(double delta) {
assert(false);
return 0.0;
}
// This is called by activities when they finish their work.
@override
void goIdle() {
beginActivity(IdleScrollActivity(this));
coordinator.updateUserScrollDirection(ScrollDirection.idle);
}
// This is called by activities when they finish their work and want to go
// ballistic.
@override
void goBallistic(double velocity) {
Simulation? simulation;
if (velocity != 0.0 || outOfRange) {
simulation = physics.createBallisticSimulation(this, velocity);
}
beginActivity(createBallisticScrollActivity(
simulation,
mode: _NestedBallisticScrollActivityMode.independent,
));
}
ScrollActivity createBallisticScrollActivity(
Simulation? simulation, {
required _NestedBallisticScrollActivityMode mode,
_NestedScrollMetrics? metrics,
}) {
if (simulation == null) {
return IdleScrollActivity(this);
}
switch (mode) {
case _NestedBallisticScrollActivityMode.outer:
assert(metrics != null);
if (metrics!.minRange == metrics.maxRange) {
return IdleScrollActivity(this);
}
return _NestedOuterBallisticScrollActivity(
coordinator,
this,
metrics,
simulation,
context.vsync,
activity?.shouldIgnorePointer ?? true,
);
case _NestedBallisticScrollActivityMode.inner:
return _NestedInnerBallisticScrollActivity(
coordinator,
this,
simulation,
context.vsync,
activity?.shouldIgnorePointer ?? true,
);
case _NestedBallisticScrollActivityMode.independent:
return BallisticScrollActivity(this, simulation, context.vsync, activity?.shouldIgnorePointer ?? true);
}
}
@override
Future<void> animateTo(
double to, {
required Duration duration,
required Curve curve,
}) {
return coordinator.animateTo(
coordinator.unnestOffset(to, this),
duration: duration,
curve: curve,
);
}
@override
void jumpTo(double value) {
return coordinator.jumpTo(coordinator.unnestOffset(value, this));
}
@override
void pointerScroll(double delta) {
return coordinator.pointerScroll(delta);
}
@override
void jumpToWithoutSettling(double value) {
assert(false);
}
void localJumpTo(double value) {
if (pixels != value) {
final double oldPixels = pixels;
forcePixels(value);
didStartScroll();
didUpdateScrollPositionBy(pixels - oldPixels);
didEndScroll();
}
}
@override
void applyNewDimensions() {
super.applyNewDimensions();
coordinator.updateCanDrag();
}
void updateCanDrag(bool innerCanDrag) {
// This is only called for the outer position
assert(coordinator._outerPosition == this);
context.setCanDrag(
// This refers to the physics of the actual outer scroll position, not
// the whole NestedScrollView, since it is possible to have different
// ScrollPhysics for the inner and outer positions.
physics.shouldAcceptUserOffset(this)
|| innerCanDrag,
);
}
@override
ScrollHoldController hold(VoidCallback holdCancelCallback) {
return coordinator.hold(holdCancelCallback);
}
@override
Drag drag(DragStartDetails details, VoidCallback dragCancelCallback) {
return coordinator.drag(details, dragCancelCallback);
}
}
enum _NestedBallisticScrollActivityMode { outer, inner, independent }
class _NestedInnerBallisticScrollActivity extends BallisticScrollActivity {
_NestedInnerBallisticScrollActivity(
this.coordinator,
_NestedScrollPosition position,
Simulation simulation,
TickerProvider vsync,
bool shouldIgnorePointer,
) : super(position, simulation, vsync, shouldIgnorePointer);
final _NestedScrollCoordinator coordinator;
@override
_NestedScrollPosition get delegate => super.delegate as _NestedScrollPosition;
@override
void resetActivity() {
delegate.beginActivity(coordinator.createInnerBallisticScrollActivity(
delegate,
velocity,
));
}
@override
void applyNewDimensions() {
delegate.beginActivity(coordinator.createInnerBallisticScrollActivity(
delegate,
velocity,
));
}
@override
bool applyMoveTo(double value) {
return super.applyMoveTo(coordinator.nestOffset(value, delegate));
}
}
class _NestedOuterBallisticScrollActivity extends BallisticScrollActivity {
_NestedOuterBallisticScrollActivity(
this.coordinator,
_NestedScrollPosition position,
this.metrics,
Simulation simulation,
TickerProvider vsync,
bool shouldIgnorePointer,
) : assert(metrics.minRange != metrics.maxRange),
assert(metrics.maxRange > metrics.minRange),
super(position, simulation, vsync, shouldIgnorePointer);
final _NestedScrollCoordinator coordinator;
final _NestedScrollMetrics metrics;
@override
_NestedScrollPosition get delegate => super.delegate as _NestedScrollPosition;
@override
void resetActivity() {
delegate.beginActivity(
coordinator.createOuterBallisticScrollActivity(velocity),
);
}
@override
void applyNewDimensions() {
delegate.beginActivity(
coordinator.createOuterBallisticScrollActivity(velocity),
);
}
@override
bool applyMoveTo(double value) {
bool done = false;
if (velocity > 0.0) {
if (value < metrics.minRange) {
return true;
}
if (value > metrics.maxRange) {
value = metrics.maxRange;
done = true;
}
} else if (velocity < 0.0) {
if (value > metrics.maxRange) {
return true;
}
if (value < metrics.minRange) {
value = metrics.minRange;
done = true;
}
} else {
value = clampDouble(value, metrics.minRange, metrics.maxRange);
done = true;
}
final bool result = super.applyMoveTo(value + metrics.correctionOffset);
assert(result); // since we tried to pass an in-range value, it shouldn't ever overflow
return !done;
}
@override
String toString() {
return '${objectRuntimeType(this, '_NestedOuterBallisticScrollActivity')}(${metrics.minRange} .. ${metrics.maxRange}; correcting by ${metrics.correctionOffset})';
}
}
/// Handle to provide to a [SliverOverlapAbsorber], a [SliverOverlapInjector],
/// and an [NestedScrollViewViewport], to shift overlap in a [NestedScrollView].
///
/// A particular [SliverOverlapAbsorberHandle] can only be assigned to a single
/// [SliverOverlapAbsorber] at a time. It can also be (and normally is) assigned
/// to one or more [SliverOverlapInjector]s, which must be later descendants of
/// the same [NestedScrollViewViewport] as the [SliverOverlapAbsorber]. The
/// [SliverOverlapAbsorber] must be a direct descendant of the
/// [NestedScrollViewViewport], taking part in the same sliver layout. (The
/// [SliverOverlapInjector] can be a descendant that takes part in a nested
/// scroll view's sliver layout.)
///
/// Whenever the [NestedScrollViewViewport] is marked dirty for layout, it will
/// cause its assigned [SliverOverlapAbsorberHandle] to fire notifications. It
/// is the responsibility of the [SliverOverlapInjector]s (and any other
/// clients) to mark themselves dirty when this happens, in case the geometry
/// subsequently changes during layout.
///
/// See also:
///
/// * [NestedScrollView], which uses a [NestedScrollViewViewport] and a
/// [SliverOverlapAbsorber] to align its children, and which shows sample
/// usage for this class.
class SliverOverlapAbsorberHandle extends ChangeNotifier {
/// Creates a [SliverOverlapAbsorberHandle].
SliverOverlapAbsorberHandle() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
// Incremented when a RenderSliverOverlapAbsorber takes ownership of this
// object, decremented when it releases it. This allows us to find cases where
// the same handle is being passed to two render objects.
int _writers = 0;
/// The current amount of overlap being absorbed by the
/// [SliverOverlapAbsorber].
///
/// This corresponds to the [SliverGeometry.layoutExtent] of the child of the
/// [SliverOverlapAbsorber].
///
/// This is updated during the layout of the [SliverOverlapAbsorber]. It
/// should not change at any other time. No notifications are sent when it
/// changes; clients (e.g. [SliverOverlapInjector]s) are responsible for
/// marking themselves dirty whenever this object sends notifications, which
/// happens any time the [SliverOverlapAbsorber] might subsequently change the
/// value during that layout.
double? get layoutExtent => _layoutExtent;
double? _layoutExtent;
/// The total scroll extent of the gap being absorbed by the
/// [SliverOverlapAbsorber].
///
/// This corresponds to the [SliverGeometry.scrollExtent] of the child of the
/// [SliverOverlapAbsorber].
///
/// This is updated during the layout of the [SliverOverlapAbsorber]. It
/// should not change at any other time. No notifications are sent when it
/// changes; clients (e.g. [SliverOverlapInjector]s) are responsible for
/// marking themselves dirty whenever this object sends notifications, which
/// happens any time the [SliverOverlapAbsorber] might subsequently change the
/// value during that layout.
double? get scrollExtent => _scrollExtent;
double? _scrollExtent;
void _setExtents(double? layoutValue, double? scrollValue) {
assert(
_writers == 1,
'Multiple RenderSliverOverlapAbsorbers have been provided the same SliverOverlapAbsorberHandle.',
);
_layoutExtent = layoutValue;
_scrollExtent = scrollValue;
}
void _markNeedsLayout() => notifyListeners();
@override
String toString() {
final String? extra = switch (_writers) {
0 => ', orphan',
1 => null, // normal case
_ => ', $_writers WRITERS ASSIGNED',
};
return '${objectRuntimeType(this, 'SliverOverlapAbsorberHandle')}($layoutExtent$extra)';
}
}
/// A sliver that wraps another, forcing its layout extent to be treated as
/// overlap.
///
/// The difference between the overlap requested by the child `sliver` and the
/// overlap reported by this widget, called the _absorbed overlap_, is reported
/// to the [SliverOverlapAbsorberHandle], which is typically passed to a
/// [SliverOverlapInjector].
///
/// See also:
///
/// * [NestedScrollView], whose documentation has sample code showing how to
/// use this widget.
class SliverOverlapAbsorber extends SingleChildRenderObjectWidget {
/// Creates a sliver that absorbs overlap and reports it to a
/// [SliverOverlapAbsorberHandle].
const SliverOverlapAbsorber({
super.key,
required this.handle,
Widget? sliver,
}) : super(child: sliver);
/// The object in which the absorbed overlap is recorded.
///
/// A particular [SliverOverlapAbsorberHandle] can only be assigned to a
/// single [SliverOverlapAbsorber] at a time.
final SliverOverlapAbsorberHandle handle;
@override
RenderSliverOverlapAbsorber createRenderObject(BuildContext context) {
return RenderSliverOverlapAbsorber(
handle: handle,
);
}
@override
void updateRenderObject(BuildContext context, RenderSliverOverlapAbsorber renderObject) {
renderObject.handle = handle;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
/// A sliver that wraps another, forcing its layout extent to be treated as
/// overlap.
///
/// The difference between the overlap requested by the child `sliver` and the
/// overlap reported by this widget, called the _absorbed overlap_, is reported
/// to the [SliverOverlapAbsorberHandle], which is typically passed to a
/// [RenderSliverOverlapInjector].
class RenderSliverOverlapAbsorber extends RenderSliver with RenderObjectWithChildMixin<RenderSliver> {
/// Create a sliver that absorbs overlap and reports it to a
/// [SliverOverlapAbsorberHandle].
///
/// The [sliver] must be a [RenderSliver].
RenderSliverOverlapAbsorber({
required SliverOverlapAbsorberHandle handle,
RenderSliver? sliver,
}) : _handle = handle {
child = sliver;
}
/// The object in which the absorbed overlap is recorded.
///
/// A particular [SliverOverlapAbsorberHandle] can only be assigned to a
/// single [RenderSliverOverlapAbsorber] at a time.
SliverOverlapAbsorberHandle get handle => _handle;
SliverOverlapAbsorberHandle _handle;
set handle(SliverOverlapAbsorberHandle value) {
if (handle == value) {
return;
}
if (attached) {
handle._writers -= 1;
value._writers += 1;
value._setExtents(handle.layoutExtent, handle.scrollExtent);
}
_handle = value;
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
handle._writers += 1;
}
@override
void detach() {
handle._writers -= 1;
super.detach();
}
@override
void performLayout() {
assert(
handle._writers == 1,
'A SliverOverlapAbsorberHandle cannot be passed to multiple RenderSliverOverlapAbsorber objects at the same time.',
);
if (child == null) {
geometry = SliverGeometry.zero;
return;
}
child!.layout(constraints, parentUsesSize: true);
final SliverGeometry childLayoutGeometry = child!.geometry!;
geometry = childLayoutGeometry.copyWith(
scrollExtent: childLayoutGeometry.scrollExtent - childLayoutGeometry.maxScrollObstructionExtent,
layoutExtent: math.max(0, childLayoutGeometry.paintExtent - childLayoutGeometry.maxScrollObstructionExtent),
);
handle._setExtents(
childLayoutGeometry.maxScrollObstructionExtent,
childLayoutGeometry.maxScrollObstructionExtent,
);
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
// child is always at our origin
}
@override
bool hitTestChildren(SliverHitTestResult result, { required double mainAxisPosition, required double crossAxisPosition }) {
if (child != null) {
return child!.hitTest(
result,
mainAxisPosition: mainAxisPosition,
crossAxisPosition: crossAxisPosition,
);
}
return false;
}
@override
void paint(PaintingContext context, Offset offset) {
if (child != null) {
context.paintChild(child!, offset);
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
/// A sliver that has a sliver geometry based on the values stored in a
/// [SliverOverlapAbsorberHandle].
///
/// The [SliverOverlapAbsorber] must be an earlier descendant of a common
/// ancestor [Viewport], so that it will always be laid out before the
/// [SliverOverlapInjector] during a particular frame.
///
/// See also:
///
/// * [NestedScrollView], which uses a [SliverOverlapAbsorber] to align its
/// children, and which shows sample usage for this class.
class SliverOverlapInjector extends SingleChildRenderObjectWidget {
/// Creates a sliver that is as tall as the value of the given [handle]'s
/// layout extent.
const SliverOverlapInjector({
super.key,
required this.handle,
Widget? sliver,
}) : super(child: sliver);
/// The handle to the [SliverOverlapAbsorber] that is feeding this injector.
///
/// This should be a handle owned by a [SliverOverlapAbsorber] and a
/// [NestedScrollViewViewport].
final SliverOverlapAbsorberHandle handle;
@override
RenderSliverOverlapInjector createRenderObject(BuildContext context) {
return RenderSliverOverlapInjector(
handle: handle,
);
}
@override
void updateRenderObject(BuildContext context, RenderSliverOverlapInjector renderObject) {
renderObject.handle = handle;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
/// A sliver that has a sliver geometry based on the values stored in a
/// [SliverOverlapAbsorberHandle].
///
/// The [RenderSliverOverlapAbsorber] must be an earlier descendant of a common
/// ancestor [RenderViewport] (probably a [RenderNestedScrollViewViewport]), so
/// that it will always be laid out before the [RenderSliverOverlapInjector]
/// during a particular frame.
class RenderSliverOverlapInjector extends RenderSliver {
/// Creates a sliver that is as tall as the value of the given [handle]'s extent.
RenderSliverOverlapInjector({
required SliverOverlapAbsorberHandle handle,
}) : _handle = handle;
double? _currentLayoutExtent;
double? _currentMaxExtent;
/// The object that specifies how wide to make the gap injected by this render
/// object.
///
/// This should be a handle owned by a [RenderSliverOverlapAbsorber] and a
/// [RenderNestedScrollViewViewport].
SliverOverlapAbsorberHandle get handle => _handle;
SliverOverlapAbsorberHandle _handle;
set handle(SliverOverlapAbsorberHandle value) {
if (handle == value) {
return;
}
if (attached) {
handle.removeListener(markNeedsLayout);
}
_handle = value;
if (attached) {
handle.addListener(markNeedsLayout);
if (handle.layoutExtent != _currentLayoutExtent ||
handle.scrollExtent != _currentMaxExtent) {
markNeedsLayout();
}
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
handle.addListener(markNeedsLayout);
if (handle.layoutExtent != _currentLayoutExtent ||
handle.scrollExtent != _currentMaxExtent) {
markNeedsLayout();
}
}
@override
void detach() {
handle.removeListener(markNeedsLayout);
super.detach();
}
@override
void performLayout() {
_currentLayoutExtent = handle.layoutExtent;
_currentMaxExtent = handle.layoutExtent;
assert(
_currentLayoutExtent != null && _currentMaxExtent != null,
'SliverOverlapInjector has found no absorbed extent to inject.\n '
'The SliverOverlapAbsorber must be an earlier descendant of a common '
'ancestor Viewport, so that it will always be laid out before the '
'SliverOverlapInjector during a particular frame.\n '
'The SliverOverlapAbsorber is typically contained in the list of slivers '
'provided by NestedScrollView.headerSliverBuilder.\n'
);
final double clampedLayoutExtent = math.min(
_currentLayoutExtent! - constraints.scrollOffset,
constraints.remainingPaintExtent,
);
geometry = SliverGeometry(
scrollExtent: _currentLayoutExtent!,
paintExtent: math.max(0.0, clampedLayoutExtent),
maxPaintExtent: _currentMaxExtent!,
);
}
@override
void debugPaint(PaintingContext context, Offset offset) {
assert(() {
if (debugPaintSizeEnabled) {
final Paint paint = Paint()
..color = const Color(0xFFCC9933)
..strokeWidth = 3.0
..style = PaintingStyle.stroke;
Offset start, end, delta;
switch (constraints.axis) {
case Axis.vertical:
final double x = offset.dx + constraints.crossAxisExtent / 2.0;
start = Offset(x, offset.dy);
end = Offset(x, offset.dy + geometry!.paintExtent);
delta = Offset(constraints.crossAxisExtent / 5.0, 0.0);
case Axis.horizontal:
final double y = offset.dy + constraints.crossAxisExtent / 2.0;
start = Offset(offset.dx, y);
end = Offset(offset.dy + geometry!.paintExtent, y);
delta = Offset(0.0, constraints.crossAxisExtent / 5.0);
}
for (int index = -2; index <= 2; index += 1) {
paintZigZag(
context.canvas,
paint,
start - delta * index.toDouble(),
end - delta * index.toDouble(),
10,
10.0,
);
}
}
return true;
}());
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
/// The [Viewport] variant used by [NestedScrollView].
///
/// This viewport takes a [SliverOverlapAbsorberHandle] and notifies it any time
/// the viewport needs to recompute its layout (e.g. when it is scrolled).
class NestedScrollViewViewport extends Viewport {
/// Creates a variant of [Viewport] that has a [SliverOverlapAbsorberHandle].
NestedScrollViewViewport({
super.key,
super.axisDirection,
super.crossAxisDirection,
super.anchor,
required super.offset,
super.center,
super.slivers,
required this.handle,
super.clipBehavior,
});
/// The handle to the [SliverOverlapAbsorber] that is feeding this injector.
final SliverOverlapAbsorberHandle handle;
@override
RenderNestedScrollViewViewport createRenderObject(BuildContext context) {
return RenderNestedScrollViewViewport(
axisDirection: axisDirection,
crossAxisDirection: crossAxisDirection ?? Viewport.getDefaultCrossAxisDirection(
context,
axisDirection,
),
anchor: anchor,
offset: offset,
handle: handle,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderNestedScrollViewViewport renderObject) {
renderObject
..axisDirection = axisDirection
..crossAxisDirection = crossAxisDirection ?? Viewport.getDefaultCrossAxisDirection(
context,
axisDirection,
)
..anchor = anchor
..offset = offset
..handle = handle
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
/// The [RenderViewport] variant used by [NestedScrollView].
///
/// This viewport takes a [SliverOverlapAbsorberHandle] and notifies it any time
/// the viewport needs to recompute its layout (e.g. when it is scrolled).
class RenderNestedScrollViewViewport extends RenderViewport {
/// Create a variant of [RenderViewport] that has a
/// [SliverOverlapAbsorberHandle].
RenderNestedScrollViewViewport({
super.axisDirection,
required super.crossAxisDirection,
required super.offset,
super.anchor,
super.children,
super.center,
required SliverOverlapAbsorberHandle handle,
super.clipBehavior,
}) : _handle = handle;
/// The object to notify when [markNeedsLayout] is called.
SliverOverlapAbsorberHandle get handle => _handle;
SliverOverlapAbsorberHandle _handle;
/// Setting this will trigger notifications on the new object.
set handle(SliverOverlapAbsorberHandle value) {
if (handle == value) {
return;
}
_handle = value;
handle._markNeedsLayout();
}
@override
void markNeedsLayout() {
handle._markNeedsLayout();
super.markNeedsLayout();
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<SliverOverlapAbsorberHandle>('handle', handle));
}
}
| flutter/packages/flutter/lib/src/widgets/nested_scroll_view.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/nested_scroll_view.dart",
"repo_id": "flutter",
"token_count": 25007
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart';
import 'framework.dart';
import 'scroll_configuration.dart';
import 'scroll_controller.dart';
const Set<TargetPlatform> _kMobilePlatforms = <TargetPlatform>{
TargetPlatform.android,
TargetPlatform.iOS,
TargetPlatform.fuchsia,
};
/// Associates a [ScrollController] with a subtree.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=33_0ABjFJUU}
///
/// When a [ScrollView] has [ScrollView.primary] set to true, the [ScrollView]
/// uses [of] to inherit the [PrimaryScrollController] associated with its
/// subtree.
///
/// A ScrollView that doesn't have a controller or the primary flag set will
/// inherit the PrimaryScrollController, if [shouldInherit] allows it. By
/// default [shouldInherit] is true for mobile platforms when the ScrollView has
/// a scroll direction of [Axis.vertical]. This automatic inheritance can be
/// configured with [automaticallyInheritForPlatforms] and [scrollDirection].
///
/// Inheriting this ScrollController can provide default behavior for scroll
/// views in a subtree. For example, the [Scaffold] uses this mechanism to
/// implement the scroll-to-top gesture on iOS.
///
/// Another default behavior handled by the PrimaryScrollController is default
/// [ScrollAction]s. If a ScrollAction is not handled by an otherwise focused
/// part of the application, the ScrollAction will be evaluated using the scroll
/// view associated with a PrimaryScrollController, for example, when executing
/// [Shortcuts] key events like page up and down.
///
/// See also:
/// * [ScrollAction], an [Action] that scrolls the [Scrollable] that encloses
/// the current [primaryFocus] or is attached to the PrimaryScrollController.
/// * [Shortcuts], a widget that establishes a [ShortcutManager] to be used
/// by its descendants when invoking an [Action] via a keyboard key
/// combination that maps to an [Intent].
class PrimaryScrollController extends InheritedWidget {
/// Creates a widget that associates a [ScrollController] with a subtree.
const PrimaryScrollController({
super.key,
required ScrollController this.controller,
this.automaticallyInheritForPlatforms = _kMobilePlatforms,
this.scrollDirection = Axis.vertical,
required super.child,
});
/// Creates a subtree without an associated [ScrollController].
const PrimaryScrollController.none({
super.key,
required super.child,
}) : automaticallyInheritForPlatforms = const <TargetPlatform>{},
scrollDirection = null,
controller = null;
/// The [ScrollController] associated with the subtree.
///
/// See also:
///
/// * [ScrollView.controller], which discusses the purpose of specifying a
/// scroll controller.
final ScrollController? controller;
/// The [Axis] this controller is configured for [ScrollView]s to
/// automatically inherit.
///
/// Used in conjunction with [automaticallyInheritForPlatforms]. If the
/// current [TargetPlatform] is not included in
/// [automaticallyInheritForPlatforms], this is ignored.
///
/// When null, no [ScrollView] in any Axis will automatically inherit this
/// controller. This is dissimilar to [PrimaryScrollController.none]. When a
/// PrimaryScrollController is inherited, ScrollView will insert
/// PrimaryScrollController.none into the tree to prevent further descendant
/// ScrollViews from inheriting the current PrimaryScrollController.
///
/// For the direction in which active scrolling may be occurring, see
/// [ScrollDirection].
///
/// Defaults to [Axis.vertical].
final Axis? scrollDirection;
/// The [TargetPlatform]s this controller is configured for [ScrollView]s to
/// automatically inherit.
///
/// Used in conjunction with [scrollDirection]. If the [Axis] provided to
/// [shouldInherit] is not [scrollDirection], this is ignored.
///
/// When empty, no ScrollView in any Axis will automatically inherit this
/// controller. Defaults to [TargetPlatformVariant.mobile].
final Set<TargetPlatform> automaticallyInheritForPlatforms;
/// Returns true if this PrimaryScrollController is configured to be
/// automatically inherited for the current [TargetPlatform] and the given
/// [Axis].
///
/// This method is typically not called directly. [ScrollView] will call this
/// method if it has not been provided a [ScrollController] and
/// [ScrollView.primary] is unset.
///
/// If a ScrollController has already been provided to
/// [ScrollView.controller], or [ScrollView.primary] is set, this is method is
/// not called by ScrollView as it will have determined whether or not to
/// inherit the PrimaryScrollController.
static bool shouldInherit(BuildContext context, Axis scrollDirection) {
final PrimaryScrollController? result = context.findAncestorWidgetOfExactType<PrimaryScrollController>();
if (result == null) {
return false;
}
final TargetPlatform platform = ScrollConfiguration.of(context).getPlatform(context);
if (result.automaticallyInheritForPlatforms.contains(platform)) {
return result.scrollDirection == scrollDirection;
}
return false;
}
/// Returns the [ScrollController] most closely associated with the given
/// context.
///
/// Returns null if there is no [ScrollController] associated with the given
/// context.
///
/// Calling this method will create a dependency on the closest
/// [PrimaryScrollController] in the [context], if there is one.
///
/// See also:
///
/// * [PrimaryScrollController.maybeOf], which is similar to this method, but
/// asserts if no [PrimaryScrollController] ancestor is found.
static ScrollController? maybeOf(BuildContext context) {
final PrimaryScrollController? result = context.dependOnInheritedWidgetOfExactType<PrimaryScrollController>();
return result?.controller;
}
/// Returns the [ScrollController] most closely associated with the given
/// context.
///
/// If no ancestor is found, this method will assert in debug mode, and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest
/// [PrimaryScrollController] in the [context].
///
/// See also:
///
/// * [PrimaryScrollController.maybeOf], which is similar to this method, but
/// returns null if no [PrimaryScrollController] ancestor is found.
static ScrollController of(BuildContext context) {
final ScrollController? controller = maybeOf(context);
assert(() {
if (controller == null) {
throw FlutterError(
'PrimaryScrollController.of() was called with a context that does not contain a '
'PrimaryScrollController widget.\n'
'No PrimaryScrollController widget ancestor could be found starting from the '
'context that was passed to PrimaryScrollController.of(). This can happen '
'because you are using a widget that looks for a PrimaryScrollController '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return controller!;
}
@override
bool updateShouldNotify(PrimaryScrollController oldWidget) => controller != oldWidget.controller;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ScrollController>('controller', controller, ifNull: 'no controller', showName: false));
}
}
| flutter/packages/flutter/lib/src/widgets/primary_scroll_controller.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/primary_scroll_controller.dart",
"repo_id": "flutter",
"token_count": 2142
} | 711 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'framework.dart';
import 'notification_listener.dart';
import 'scroll_notification.dart';
import 'scroll_position.dart';
// Examples can assume:
// void _listener(ScrollNotification notification) { }
// late BuildContext context;
/// A [ScrollNotification] listener for [ScrollNotificationObserver].
///
/// [ScrollNotificationObserver] is similar to
/// [NotificationListener]. It supports a listener list instead of
/// just a single listener and its listeners run unconditionally, they
/// do not require a gating boolean return value.
typedef ScrollNotificationCallback = void Function(ScrollNotification notification);
class _ScrollNotificationObserverScope extends InheritedWidget {
const _ScrollNotificationObserverScope({
required super.child,
required ScrollNotificationObserverState scrollNotificationObserverState,
}) : _scrollNotificationObserverState = scrollNotificationObserverState;
final ScrollNotificationObserverState _scrollNotificationObserverState;
@override
bool updateShouldNotify(_ScrollNotificationObserverScope old) => _scrollNotificationObserverState != old._scrollNotificationObserverState;
}
final class _ListenerEntry extends LinkedListEntry<_ListenerEntry> {
_ListenerEntry(this.listener);
final ScrollNotificationCallback listener;
}
/// Notifies its listeners when a descendant scrolls.
///
/// To add a listener to a [ScrollNotificationObserver] ancestor:
///
/// ```dart
/// ScrollNotificationObserver.of(context).addListener(_listener);
/// ```
///
/// To remove the listener from a [ScrollNotificationObserver] ancestor:
///
/// ```dart
/// ScrollNotificationObserver.of(context).removeListener(_listener);
/// ```
///
/// Stateful widgets that share an ancestor [ScrollNotificationObserver] typically
/// add a listener in [State.didChangeDependencies] (removing the old one
/// if necessary) and remove the listener in their [State.dispose] method.
///
/// Any function with the [ScrollNotificationCallback] signature can act as a
/// listener:
///
/// ```dart
/// // (e.g. in a stateful widget)
/// void _listener(ScrollNotification notification) {
/// // Do something, maybe setState()
/// }
/// ```
///
/// This widget is similar to [NotificationListener]. It supports a listener
/// list instead of just a single listener and its listeners run
/// unconditionally, they do not require a gating boolean return value.
///
/// {@tool dartpad}
/// This sample shows a "Scroll to top" button that uses [ScrollNotificationObserver]
/// to listen for scroll notifications from [ListView]. The button is only visible
/// when the user has scrolled down. When pressed, the button animates the scroll
/// position of the [ListView] back to the top.
///
/// ** See code in examples/api/lib/widgets/scroll_notification_observer/scroll_notification_observer.0.dart **
/// {@end-tool}
class ScrollNotificationObserver extends StatefulWidget {
/// Create a [ScrollNotificationObserver].
const ScrollNotificationObserver({
super.key,
required this.child,
});
/// The subtree below this widget.
final Widget child;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [ScrollNotificationObserver] widget, then null is
/// returned.
///
/// Calling this method will create a dependency on the closest
/// [ScrollNotificationObserver] in the [context], if there is one.
///
/// See also:
///
/// * [ScrollNotificationObserver.of], which is similar to this method, but
/// asserts if no [ScrollNotificationObserver] ancestor is found.
static ScrollNotificationObserverState? maybeOf(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<_ScrollNotificationObserverScope>()?._scrollNotificationObserverState;
}
/// The closest instance of this class that encloses the given context.
///
/// If no ancestor is found, this method will assert in debug mode, and throw
/// an exception in release mode.
///
/// Calling this method will create a dependency on the closest
/// [ScrollNotificationObserver] in the [context].
///
/// See also:
///
/// * [ScrollNotificationObserver.maybeOf], which is similar to this method,
/// but returns null if no [ScrollNotificationObserver] ancestor is found.
static ScrollNotificationObserverState of(BuildContext context) {
final ScrollNotificationObserverState? observerState = maybeOf(context);
assert(() {
if (observerState == null) {
throw FlutterError(
'ScrollNotificationObserver.of() was called with a context that does not contain a '
'ScrollNotificationObserver widget.\n'
'No ScrollNotificationObserver widget ancestor could be found starting from the '
'context that was passed to ScrollNotificationObserver.of(). This can happen '
'because you are using a widget that looks for a ScrollNotificationObserver '
'ancestor, but no such ancestor exists.\n'
'The context used was:\n'
' $context',
);
}
return true;
}());
return observerState!;
}
@override
ScrollNotificationObserverState createState() => ScrollNotificationObserverState();
}
/// The listener list state for a [ScrollNotificationObserver] returned by
/// [ScrollNotificationObserver.of].
///
/// [ScrollNotificationObserver] is similar to
/// [NotificationListener]. It supports a listener list instead of
/// just a single listener and its listeners run unconditionally, they
/// do not require a gating boolean return value.
class ScrollNotificationObserverState extends State<ScrollNotificationObserver> {
LinkedList<_ListenerEntry>? _listeners = LinkedList<_ListenerEntry>();
bool _debugAssertNotDisposed() {
assert(() {
if (_listeners == null) {
throw FlutterError(
'A $runtimeType was used after being disposed.\n'
'Once you have called dispose() on a $runtimeType, it can no longer be used.',
);
}
return true;
}());
return true;
}
/// Add a [ScrollNotificationCallback] that will be called each time
/// a descendant scrolls.
void addListener(ScrollNotificationCallback listener) {
assert(_debugAssertNotDisposed());
_listeners!.add(_ListenerEntry(listener));
}
/// Remove the specified [ScrollNotificationCallback].
void removeListener(ScrollNotificationCallback listener) {
assert(_debugAssertNotDisposed());
for (final _ListenerEntry entry in _listeners!) {
if (entry.listener == listener) {
entry.unlink();
return;
}
}
}
void _notifyListeners(ScrollNotification notification) {
assert(_debugAssertNotDisposed());
if (_listeners!.isEmpty) {
return;
}
final List<_ListenerEntry> localListeners = List<_ListenerEntry>.of(_listeners!);
for (final _ListenerEntry entry in localListeners) {
try {
if (entry.list != null) {
entry.listener(notification);
}
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widget library',
context: ErrorDescription('while dispatching notifications for $runtimeType'),
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<ScrollNotificationObserverState>(
'The $runtimeType sending notification was',
this,
style: DiagnosticsTreeStyle.errorProperty,
),
],
));
}
}
}
@override
Widget build(BuildContext context) {
return NotificationListener<ScrollMetricsNotification>(
onNotification: (ScrollMetricsNotification notification) {
// A ScrollMetricsNotification allows listeners to be notified for an
// initial state, as well as if the content dimensions change without
// scrolling.
_notifyListeners(notification.asScrollUpdate());
return false;
},
child: NotificationListener<ScrollNotification>(
onNotification: (ScrollNotification notification) {
_notifyListeners(notification);
return false;
},
child: _ScrollNotificationObserverScope(
scrollNotificationObserverState: this,
child: widget.child,
),
),
);
}
@override
void dispose() {
assert(_debugAssertNotDisposed());
_listeners = null;
super.dispose();
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_notification_observer.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_notification_observer.dart",
"repo_id": "flutter",
"token_count": 2733
} | 712 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'framework.dart';
import 'notification_listener.dart';
/// Indicates that the size of one of the descendants of the object receiving
/// this notification has changed, and that therefore any assumptions about that
/// layout are no longer valid.
///
/// For example, sent by the [SizeChangedLayoutNotifier] widget whenever that
/// widget changes size.
///
/// This notification can be used for triggering repaints, but if you use this
/// notification to trigger rebuilds or relayouts, you'll create a backwards
/// dependency in the frame pipeline because [SizeChangedLayoutNotification]s
/// are generated during layout, which is after the build phase and in the
/// middle of the layout phase. This backwards dependency can lead to visual
/// corruption or lags.
///
/// See [LayoutChangedNotification] for additional discussion of layout
/// notifications such as this one.
///
/// See also:
///
/// * [SizeChangedLayoutNotifier], which sends this notification.
/// * [LayoutChangedNotification], of which this is a subclass.
class SizeChangedLayoutNotification extends LayoutChangedNotification {
/// Create a new [SizeChangedLayoutNotification].
const SizeChangedLayoutNotification();
}
/// A widget that automatically dispatches a [SizeChangedLayoutNotification]
/// when the layout dimensions of its child change.
///
/// The notification is not sent for the initial layout (since the size doesn't
/// change in that case, it's just established).
///
/// To listen for the notification dispatched by this widget, use a
/// [NotificationListener<SizeChangedLayoutNotification>].
///
/// The [Material] class listens for [LayoutChangedNotification]s, including
/// [SizeChangedLayoutNotification]s, to repaint [InkResponse] and [InkWell] ink
/// effects. When a widget is likely to change size, wrapping it in a
/// [SizeChangedLayoutNotifier] will cause the ink effects to correctly repaint
/// when the child changes size.
///
/// See also:
///
/// * [Notification], the base class for notifications that bubble through the
/// widget tree.
class SizeChangedLayoutNotifier extends SingleChildRenderObjectWidget {
/// Creates a [SizeChangedLayoutNotifier] that dispatches layout changed
/// notifications when [child] changes layout size.
const SizeChangedLayoutNotifier({
super.key,
super.child,
});
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderSizeChangedWithCallback(
onLayoutChangedCallback: () {
const SizeChangedLayoutNotification().dispatch(context);
},
);
}
}
class _RenderSizeChangedWithCallback extends RenderProxyBox {
_RenderSizeChangedWithCallback({
RenderBox? child,
required this.onLayoutChangedCallback,
}) : super(child);
// There's a 1:1 relationship between the _RenderSizeChangedWithCallback and
// the `context` that is captured by the closure created by createRenderObject
// above to assign to onLayoutChangedCallback, and thus we know that the
// onLayoutChangedCallback will never change nor need to change.
final VoidCallback onLayoutChangedCallback;
Size? _oldSize;
@override
void performLayout() {
super.performLayout();
// Don't send the initial notification, or this will be SizeObserver all
// over again!
if (_oldSize != null && size != _oldSize) {
onLayoutChangedCallback();
}
_oldSize = size;
}
}
| flutter/packages/flutter/lib/src/widgets/size_changed_layout_notifier.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/size_changed_layout_notifier.dart",
"repo_id": "flutter",
"token_count": 937
} | 713 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:math' as math;
import 'package:characters/characters.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'binding.dart';
import 'constants.dart';
import 'container.dart';
import 'context_menu_controller.dart';
import 'debug.dart';
import 'editable_text.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'inherited_theme.dart';
import 'magnifier.dart';
import 'overlay.dart';
import 'scrollable.dart';
import 'tap_region.dart';
import 'ticker_provider.dart';
import 'transitions.dart';
export 'package:flutter/rendering.dart' show TextSelectionPoint;
export 'package:flutter/services.dart' show TextSelectionDelegate;
/// The type for a Function that builds a toolbar's container with the given
/// child.
///
/// See also:
///
/// * [TextSelectionToolbar.toolbarBuilder], which is of this type.
/// type.
/// * [CupertinoTextSelectionToolbar.toolbarBuilder], which is similar, but
/// for a Cupertino-style toolbar.
typedef ToolbarBuilder = Widget Function(BuildContext context, Widget child);
/// ParentData that determines whether or not to paint the corresponding child.
///
/// Used in the layout of the Cupertino and Material text selection menus, which
/// decide whether or not to paint their buttons after laying them out and
/// determining where they overflow.
class ToolbarItemsParentData extends ContainerBoxParentData<RenderBox> {
/// Whether or not this child is painted.
///
/// Children in the selection toolbar may be laid out for measurement purposes
/// but not painted. This allows these children to be identified.
bool shouldPaint = false;
@override
String toString() => '${super.toString()}; shouldPaint=$shouldPaint';
}
/// An interface for building the selection UI, to be provided by the
/// implementer of the toolbar widget.
///
/// Parts of this class, including [buildToolbar], have been deprecated in favor
/// of [EditableText.contextMenuBuilder], which is now the preferred way to
/// customize the context menus.
///
/// ## Use with [EditableText.contextMenuBuilder]
///
/// For backwards compatibility during the deprecation period, when
/// [EditableText.selectionControls] is set to an object that does not mix in
/// [TextSelectionHandleControls], [EditableText.contextMenuBuilder] is ignored
/// in favor of the deprecated [buildToolbar].
///
/// To migrate code from [buildToolbar] to the preferred
/// [EditableText.contextMenuBuilder], while still using [buildHandle], mix in
/// [TextSelectionHandleControls] into the [TextSelectionControls] subclass when
/// moving any toolbar code to a callback passed to
/// [EditableText.contextMenuBuilder].
///
/// In due course, [buildToolbar] will be removed, and the mixin will no longer
/// be necessary as a way to flag to the framework that the code has been
/// migrated and does not expect [buildToolbar] to be called.
///
/// For more information, see <https://docs.flutter.dev/release/breaking-changes/context-menus>.
///
/// See also:
///
/// * [SelectionArea], which selects appropriate text selection controls
/// based on the current platform.
abstract class TextSelectionControls {
/// Builds a selection handle of the given `type`.
///
/// The top left corner of this widget is positioned at the bottom of the
/// selection position.
///
/// The supplied [onTap] should be invoked when the handle is tapped, if such
/// interaction is allowed. As a counterexample, the default selection handle
/// on iOS [cupertinoTextSelectionControls] does not call [onTap] at all,
/// since its handles are not meant to be tapped.
Widget buildHandle(BuildContext context, TextSelectionHandleType type, double textLineHeight, [VoidCallback? onTap]);
/// Get the anchor point of the handle relative to itself. The anchor point is
/// the point that is aligned with a specific point in the text. A handle
/// often visually "points to" that location.
Offset getHandleAnchor(TextSelectionHandleType type, double textLineHeight);
/// Builds a toolbar near a text selection.
///
/// Typically displays buttons for copying and pasting text.
///
/// The [globalEditableRegion] parameter is the TextField size of the global
/// coordinate system in logical pixels.
///
/// The [textLineHeight] parameter is the [RenderEditable.preferredLineHeight]
/// of the [RenderEditable] we are building a toolbar for.
///
/// The [selectionMidpoint] parameter is a general calculation midpoint
/// parameter of the toolbar. More detailed position information
/// is computable from the [endpoints] parameter.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
Widget buildToolbar(
BuildContext context,
Rect globalEditableRegion,
double textLineHeight,
Offset selectionMidpoint,
List<TextSelectionPoint> endpoints,
TextSelectionDelegate delegate,
ValueListenable<ClipboardStatus>? clipboardStatus,
Offset? lastSecondaryTapDownPosition,
);
/// Returns the size of the selection handle.
Size getHandleSize(double textLineHeight);
/// Whether the current selection of the text field managed by the given
/// `delegate` can be removed from the text field and placed into the
/// [Clipboard].
///
/// By default, false is returned when nothing is selected in the text field.
///
/// Subclasses can use this to decide if they should expose the cut
/// functionality to the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
bool canCut(TextSelectionDelegate delegate) {
return delegate.cutEnabled && !delegate.textEditingValue.selection.isCollapsed;
}
/// Whether the current selection of the text field managed by the given
/// `delegate` can be copied to the [Clipboard].
///
/// By default, false is returned when nothing is selected in the text field.
///
/// Subclasses can use this to decide if they should expose the copy
/// functionality to the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
bool canCopy(TextSelectionDelegate delegate) {
return delegate.copyEnabled && !delegate.textEditingValue.selection.isCollapsed;
}
/// Whether the text field managed by the given `delegate` supports pasting
/// from the clipboard.
///
/// Subclasses can use this to decide if they should expose the paste
/// functionality to the user.
///
/// This does not consider the contents of the clipboard. Subclasses may want
/// to, for example, disallow pasting when the clipboard contains an empty
/// string.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
bool canPaste(TextSelectionDelegate delegate) {
return delegate.pasteEnabled;
}
/// Whether the current selection of the text field managed by the given
/// `delegate` can be extended to include the entire content of the text
/// field.
///
/// Subclasses can use this to decide if they should expose the select all
/// functionality to the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
bool canSelectAll(TextSelectionDelegate delegate) {
return delegate.selectAllEnabled && delegate.textEditingValue.text.isNotEmpty && delegate.textEditingValue.selection.isCollapsed;
}
/// Call [TextSelectionDelegate.cutSelection] to cut current selection.
///
/// This is called by subclasses when their cut affordance is activated by
/// the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
void handleCut(TextSelectionDelegate delegate) {
delegate.cutSelection(SelectionChangedCause.toolbar);
}
/// Call [TextSelectionDelegate.copySelection] to copy current selection.
///
/// This is called by subclasses when their copy affordance is activated by
/// the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
void handleCopy(TextSelectionDelegate delegate) {
delegate.copySelection(SelectionChangedCause.toolbar);
}
/// Call [TextSelectionDelegate.pasteText] to paste text.
///
/// This is called by subclasses when their paste affordance is activated by
/// the user.
///
/// This function is asynchronous since interacting with the clipboard is
/// asynchronous. Race conditions may exist with this API as currently
/// implemented.
// TODO(ianh): https://github.com/flutter/flutter/issues/11427
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
Future<void> handlePaste(TextSelectionDelegate delegate) async {
delegate.pasteText(SelectionChangedCause.toolbar);
}
/// Call [TextSelectionDelegate.selectAll] to set the current selection to
/// contain the entire text value.
///
/// Does not hide the toolbar.
///
/// This is called by subclasses when their select-all affordance is activated
/// by the user.
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
void handleSelectAll(TextSelectionDelegate delegate) {
delegate.selectAll(SelectionChangedCause.toolbar);
}
}
/// Text selection controls that do not show any toolbars or handles.
///
/// This is a placeholder, suitable for temporary use during development, but
/// not practical for production. For example, it provides no way for the user
/// to interact with selections: no context menus on desktop, no toolbars or
/// drag handles on mobile, etc. For production, consider using
/// [MaterialTextSelectionControls] or creating a custom subclass of
/// [TextSelectionControls].
///
/// The [emptyTextSelectionControls] global variable has a
/// suitable instance of this class.
class EmptyTextSelectionControls extends TextSelectionControls {
@override
Size getHandleSize(double textLineHeight) => Size.zero;
@override
Widget buildToolbar(
BuildContext context,
Rect globalEditableRegion,
double textLineHeight,
Offset selectionMidpoint,
List<TextSelectionPoint> endpoints,
TextSelectionDelegate delegate,
ValueListenable<ClipboardStatus>? clipboardStatus,
Offset? lastSecondaryTapDownPosition,
) => const SizedBox.shrink();
@override
Widget buildHandle(BuildContext context, TextSelectionHandleType type, double textLineHeight, [VoidCallback? onTap]) {
return const SizedBox.shrink();
}
@override
Offset getHandleAnchor(TextSelectionHandleType type, double textLineHeight) {
return Offset.zero;
}
}
/// Text selection controls that do not show any toolbars or handles.
///
/// This is a placeholder, suitable for temporary use during development, but
/// not practical for production. For example, it provides no way for the user
/// to interact with selections: no context menus on desktop, no toolbars or
/// drag handles on mobile, etc. For production, consider using
/// [materialTextSelectionControls] or creating a custom subclass of
/// [TextSelectionControls].
final TextSelectionControls emptyTextSelectionControls = EmptyTextSelectionControls();
/// An object that manages a pair of text selection handles for a
/// [RenderEditable].
///
/// This class is a wrapper of [SelectionOverlay] to provide APIs specific for
/// [RenderEditable]s. To manage selection handles for custom widgets, use
/// [SelectionOverlay] instead.
class TextSelectionOverlay {
/// Creates an object that manages overlay entries for selection handles.
///
/// The [context] must have an [Overlay] as an ancestor.
TextSelectionOverlay({
required TextEditingValue value,
required this.context,
Widget? debugRequiredFor,
required LayerLink toolbarLayerLink,
required LayerLink startHandleLayerLink,
required LayerLink endHandleLayerLink,
required this.renderObject,
this.selectionControls,
bool handlesVisible = false,
required this.selectionDelegate,
DragStartBehavior dragStartBehavior = DragStartBehavior.start,
VoidCallback? onSelectionHandleTapped,
ClipboardStatusNotifier? clipboardStatus,
this.contextMenuBuilder,
required TextMagnifierConfiguration magnifierConfiguration,
}) : _handlesVisible = handlesVisible,
_value = value {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$TextSelectionOverlay',
object: this,
);
}
renderObject.selectionStartInViewport.addListener(_updateTextSelectionOverlayVisibilities);
renderObject.selectionEndInViewport.addListener(_updateTextSelectionOverlayVisibilities);
_updateTextSelectionOverlayVisibilities();
_selectionOverlay = SelectionOverlay(
magnifierConfiguration: magnifierConfiguration,
context: context,
debugRequiredFor: debugRequiredFor,
// The metrics will be set when show handles.
startHandleType: TextSelectionHandleType.collapsed,
startHandlesVisible: _effectiveStartHandleVisibility,
lineHeightAtStart: 0.0,
onStartHandleDragStart: _handleSelectionStartHandleDragStart,
onStartHandleDragUpdate: _handleSelectionStartHandleDragUpdate,
onEndHandleDragEnd: _handleAnyDragEnd,
endHandleType: TextSelectionHandleType.collapsed,
endHandlesVisible: _effectiveEndHandleVisibility,
lineHeightAtEnd: 0.0,
onEndHandleDragStart: _handleSelectionEndHandleDragStart,
onEndHandleDragUpdate: _handleSelectionEndHandleDragUpdate,
onStartHandleDragEnd: _handleAnyDragEnd,
toolbarVisible: _effectiveToolbarVisibility,
selectionEndpoints: const <TextSelectionPoint>[],
selectionControls: selectionControls,
selectionDelegate: selectionDelegate,
clipboardStatus: clipboardStatus,
startHandleLayerLink: startHandleLayerLink,
endHandleLayerLink: endHandleLayerLink,
toolbarLayerLink: toolbarLayerLink,
onSelectionHandleTapped: onSelectionHandleTapped,
dragStartBehavior: dragStartBehavior,
toolbarLocation: renderObject.lastSecondaryTapDownPosition,
);
}
/// {@template flutter.widgets.SelectionOverlay.context}
/// The context in which the selection UI should appear.
///
/// This context must have an [Overlay] as an ancestor because this object
/// will display the text selection handles in that [Overlay].
/// {@endtemplate}
final BuildContext context;
// TODO(mpcomplete): what if the renderObject is removed or replaced, or
// moves? Not sure what cases I need to handle, or how to handle them.
/// The editable line in which the selected text is being displayed.
final RenderEditable renderObject;
/// {@macro flutter.widgets.SelectionOverlay.selectionControls}
final TextSelectionControls? selectionControls;
/// {@macro flutter.widgets.SelectionOverlay.selectionDelegate}
final TextSelectionDelegate selectionDelegate;
late final SelectionOverlay _selectionOverlay;
/// {@macro flutter.widgets.EditableText.contextMenuBuilder}
///
/// If not provided, no context menu will be built.
final WidgetBuilder? contextMenuBuilder;
/// Retrieve current value.
@visibleForTesting
TextEditingValue get value => _value;
TextEditingValue _value;
TextSelection get _selection => _value.selection;
final ValueNotifier<bool> _effectiveStartHandleVisibility = ValueNotifier<bool>(false);
final ValueNotifier<bool> _effectiveEndHandleVisibility = ValueNotifier<bool>(false);
final ValueNotifier<bool> _effectiveToolbarVisibility = ValueNotifier<bool>(false);
void _updateTextSelectionOverlayVisibilities() {
_effectiveStartHandleVisibility.value = _handlesVisible && renderObject.selectionStartInViewport.value;
_effectiveEndHandleVisibility.value = _handlesVisible && renderObject.selectionEndInViewport.value;
_effectiveToolbarVisibility.value = renderObject.selectionStartInViewport.value || renderObject.selectionEndInViewport.value;
}
/// Whether selection handles are visible.
///
/// Set to false if you want to hide the handles. Use this property to show or
/// hide the handle without rebuilding them.
///
/// Defaults to false.
bool get handlesVisible => _handlesVisible;
bool _handlesVisible = false;
set handlesVisible(bool visible) {
if (_handlesVisible == visible) {
return;
}
_handlesVisible = visible;
_updateTextSelectionOverlayVisibilities();
}
/// {@macro flutter.widgets.SelectionOverlay.showHandles}
void showHandles() {
_updateSelectionOverlay();
_selectionOverlay.showHandles();
}
/// {@macro flutter.widgets.SelectionOverlay.hideHandles}
void hideHandles() => _selectionOverlay.hideHandles();
/// {@macro flutter.widgets.SelectionOverlay.showToolbar}
void showToolbar() {
_updateSelectionOverlay();
if (selectionControls != null && selectionControls is! TextSelectionHandleControls) {
_selectionOverlay.showToolbar();
return;
}
if (contextMenuBuilder == null) {
return;
}
assert(context.mounted);
_selectionOverlay.showToolbar(
context: context,
contextMenuBuilder: contextMenuBuilder,
);
return;
}
/// Shows toolbar with spell check suggestions of misspelled words that are
/// available for click-and-replace.
void showSpellCheckSuggestionsToolbar(
WidgetBuilder spellCheckSuggestionsToolbarBuilder
) {
_updateSelectionOverlay();
assert(context.mounted);
_selectionOverlay
.showSpellCheckSuggestionsToolbar(
context: context,
builder: spellCheckSuggestionsToolbarBuilder,
);
hideHandles();
}
/// {@macro flutter.widgets.SelectionOverlay.showMagnifier}
void showMagnifier(Offset positionToShow) {
final TextPosition position = renderObject.getPositionForPoint(positionToShow);
_updateSelectionOverlay();
_selectionOverlay.showMagnifier(
_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: positionToShow,
renderEditable: renderObject,
),
);
}
/// {@macro flutter.widgets.SelectionOverlay.updateMagnifier}
void updateMagnifier(Offset positionToShow) {
final TextPosition position = renderObject.getPositionForPoint(positionToShow);
_updateSelectionOverlay();
_selectionOverlay.updateMagnifier(
_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: positionToShow,
renderEditable: renderObject,
),
);
}
/// {@macro flutter.widgets.SelectionOverlay.hideMagnifier}
void hideMagnifier() {
_selectionOverlay.hideMagnifier();
}
/// Updates the overlay after the selection has changed.
///
/// If this method is called while the [SchedulerBinding.schedulerPhase] is
/// [SchedulerPhase.persistentCallbacks], i.e. during the build, layout, or
/// paint phases (see [WidgetsBinding.drawFrame]), then the update is delayed
/// until the post-frame callbacks phase. Otherwise the update is done
/// synchronously. This means that it is safe to call during builds, but also
/// that if you do call this during a build, the UI will not update until the
/// next frame (i.e. many milliseconds later).
void update(TextEditingValue newValue) {
if (_value == newValue) {
return;
}
_value = newValue;
_updateSelectionOverlay();
// _updateSelectionOverlay may not rebuild the selection overlay if the
// text metrics and selection doesn't change even if the text has changed.
// This rebuild is needed for the toolbar to update based on the latest text
// value.
_selectionOverlay.markNeedsBuild();
}
void _updateSelectionOverlay() {
_selectionOverlay
// Update selection handle metrics.
..startHandleType = _chooseType(
renderObject.textDirection,
TextSelectionHandleType.left,
TextSelectionHandleType.right,
)
..lineHeightAtStart = _getStartGlyphHeight()
..endHandleType = _chooseType(
renderObject.textDirection,
TextSelectionHandleType.right,
TextSelectionHandleType.left,
)
..lineHeightAtEnd = _getEndGlyphHeight()
// Update selection toolbar metrics.
..selectionEndpoints = renderObject.getEndpointsForSelection(_selection)
..toolbarLocation = renderObject.lastSecondaryTapDownPosition;
}
/// Causes the overlay to update its rendering.
///
/// This is intended to be called when the [renderObject] may have changed its
/// text metrics (e.g. because the text was scrolled).
void updateForScroll() {
_updateSelectionOverlay();
// This method may be called due to windows metrics changes. In that case,
// non of the properties in _selectionOverlay will change, but a rebuild is
// still needed.
_selectionOverlay.markNeedsBuild();
}
/// Whether the handles are currently visible.
bool get handlesAreVisible => _selectionOverlay._handles != null && handlesVisible;
/// {@macro flutter.widgets.SelectionOverlay.toolbarIsVisible}
///
/// See also:
///
/// * [spellCheckToolbarIsVisible], which is only whether the spell check menu
/// specifically is visible.
bool get toolbarIsVisible => _selectionOverlay.toolbarIsVisible;
/// Whether the magnifier is currently visible.
bool get magnifierIsVisible => _selectionOverlay._magnifierController.shown;
/// Whether the spell check menu is currently visible.
///
/// See also:
///
/// * [toolbarIsVisible], which is whether any toolbar is visible.
bool get spellCheckToolbarIsVisible => _selectionOverlay._spellCheckToolbarController.isShown;
/// {@macro flutter.widgets.SelectionOverlay.hide}
void hide() => _selectionOverlay.hide();
/// {@macro flutter.widgets.SelectionOverlay.hideToolbar}
void hideToolbar() => _selectionOverlay.hideToolbar();
/// {@macro flutter.widgets.SelectionOverlay.dispose}
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
_selectionOverlay.dispose();
renderObject.selectionStartInViewport.removeListener(_updateTextSelectionOverlayVisibilities);
renderObject.selectionEndInViewport.removeListener(_updateTextSelectionOverlayVisibilities);
_effectiveToolbarVisibility.dispose();
_effectiveStartHandleVisibility.dispose();
_effectiveEndHandleVisibility.dispose();
hideToolbar();
}
double _getStartGlyphHeight() {
final String currText = selectionDelegate.textEditingValue.text;
final int firstSelectedGraphemeExtent;
Rect? startHandleRect;
// Only calculate handle rects if the text in the previous frame
// is the same as the text in the current frame. This is done because
// widget.renderObject contains the renderEditable from the previous frame.
// If the text changed between the current and previous frames then
// widget.renderObject.getRectForComposingRange might fail. In cases where
// the current frame is different from the previous we fall back to
// renderObject.preferredLineHeight.
if (renderObject.plainText == currText && _selection.isValid && !_selection.isCollapsed) {
final String selectedGraphemes = _selection.textInside(currText);
firstSelectedGraphemeExtent = selectedGraphemes.characters.first.length;
startHandleRect = renderObject.getRectForComposingRange(TextRange(start: _selection.start, end: _selection.start + firstSelectedGraphemeExtent));
}
return startHandleRect?.height ?? renderObject.preferredLineHeight;
}
double _getEndGlyphHeight() {
final String currText = selectionDelegate.textEditingValue.text;
final int lastSelectedGraphemeExtent;
Rect? endHandleRect;
// See the explanation in _getStartGlyphHeight.
if (renderObject.plainText == currText && _selection.isValid && !_selection.isCollapsed) {
final String selectedGraphemes = _selection.textInside(currText);
lastSelectedGraphemeExtent = selectedGraphemes.characters.last.length;
endHandleRect = renderObject.getRectForComposingRange(TextRange(start: _selection.end - lastSelectedGraphemeExtent, end: _selection.end));
}
return endHandleRect?.height ?? renderObject.preferredLineHeight;
}
MagnifierInfo _buildMagnifier({
required RenderEditable renderEditable,
required Offset globalGesturePosition,
required TextPosition currentTextPosition,
}) {
final Offset globalRenderEditableTopLeft = renderEditable.localToGlobal(Offset.zero);
final Rect localCaretRect = renderEditable.getLocalRectForCaret(currentTextPosition);
final TextSelection lineAtOffset = renderEditable.getLineAtOffset(currentTextPosition);
final TextPosition positionAtEndOfLine = TextPosition(
offset: lineAtOffset.extentOffset,
affinity: TextAffinity.upstream,
);
// Default affinity is downstream.
final TextPosition positionAtBeginningOfLine = TextPosition(
offset: lineAtOffset.baseOffset,
);
final Rect lineBoundaries = Rect.fromPoints(
renderEditable.getLocalRectForCaret(positionAtBeginningOfLine).topCenter,
renderEditable.getLocalRectForCaret(positionAtEndOfLine).bottomCenter,
);
return MagnifierInfo(
fieldBounds: globalRenderEditableTopLeft & renderEditable.size,
globalGesturePosition: globalGesturePosition,
caretRect: localCaretRect.shift(globalRenderEditableTopLeft),
currentLineBoundaries: lineBoundaries.shift(globalRenderEditableTopLeft),
);
}
// The contact position of the gesture at the current end handle location.
// Updated when the handle moves.
late double _endHandleDragPosition;
// The distance from _endHandleDragPosition to the center of the line that it
// corresponds to.
late double _endHandleDragPositionToCenterOfLine;
void _handleSelectionEndHandleDragStart(DragStartDetails details) {
if (!renderObject.attached) {
return;
}
// This adjusts for the fact that the selection handles may not
// perfectly cover the TextPosition that they correspond to.
_endHandleDragPosition = details.globalPosition.dy;
final Offset endPoint =
renderObject.localToGlobal(_selectionOverlay.selectionEndpoints.last.point);
final double centerOfLine = endPoint.dy - renderObject.preferredLineHeight / 2;
_endHandleDragPositionToCenterOfLine = centerOfLine - _endHandleDragPosition;
final TextPosition position = renderObject.getPositionForPoint(
Offset(
details.globalPosition.dx,
centerOfLine,
),
);
_selectionOverlay.showMagnifier(
_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
),
);
}
/// Given a handle position and drag position, returns the position of handle
/// after the drag.
///
/// The handle jumps instantly between lines when the drag reaches a full
/// line's height away from the original handle position. In other words, the
/// line jump happens when the contact point would be located at the same
/// place on the handle at the new line as when the gesture started.
double _getHandleDy(double dragDy, double handleDy) {
final double distanceDragged = dragDy - handleDy;
final int dragDirection = distanceDragged < 0.0 ? -1 : 1;
final int linesDragged =
dragDirection * (distanceDragged.abs() / renderObject.preferredLineHeight).floor();
return handleDy + linesDragged * renderObject.preferredLineHeight;
}
void _handleSelectionEndHandleDragUpdate(DragUpdateDetails details) {
if (!renderObject.attached) {
return;
}
_endHandleDragPosition = _getHandleDy(details.globalPosition.dy, _endHandleDragPosition);
final Offset adjustedOffset = Offset(
details.globalPosition.dx,
_endHandleDragPosition + _endHandleDragPositionToCenterOfLine,
);
final TextPosition position = renderObject.getPositionForPoint(adjustedOffset);
if (_selection.isCollapsed) {
_selectionOverlay.updateMagnifier(_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
));
final TextSelection currentSelection = TextSelection.fromPosition(position);
_handleSelectionHandleChanged(currentSelection);
return;
}
final TextSelection newSelection;
switch (defaultTargetPlatform) {
// On Apple platforms, dragging the base handle makes it the extent.
case TargetPlatform.iOS:
case TargetPlatform.macOS:
newSelection = TextSelection(
extentOffset: position.offset,
baseOffset: _selection.start,
);
if (position.offset <= _selection.start) {
return; // Don't allow order swapping.
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
newSelection = TextSelection(
baseOffset: _selection.baseOffset,
extentOffset: position.offset,
);
if (newSelection.baseOffset >= newSelection.extentOffset) {
return; // Don't allow order swapping.
}
}
_handleSelectionHandleChanged(newSelection);
_selectionOverlay.updateMagnifier(_buildMagnifier(
currentTextPosition: newSelection.extent,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
));
}
// The contact position of the gesture at the current start handle location.
// Updated when the handle moves.
late double _startHandleDragPosition;
// The distance from _startHandleDragPosition to the center of the line that
// it corresponds to.
late double _startHandleDragPositionToCenterOfLine;
void _handleSelectionStartHandleDragStart(DragStartDetails details) {
if (!renderObject.attached) {
return;
}
// This adjusts for the fact that the selection handles may not
// perfectly cover the TextPosition that they correspond to.
_startHandleDragPosition = details.globalPosition.dy;
final Offset startPoint =
renderObject.localToGlobal(_selectionOverlay.selectionEndpoints.first.point);
final double centerOfLine = startPoint.dy - renderObject.preferredLineHeight / 2;
_startHandleDragPositionToCenterOfLine = centerOfLine - _startHandleDragPosition;
final TextPosition position = renderObject.getPositionForPoint(
Offset(
details.globalPosition.dx,
centerOfLine,
),
);
_selectionOverlay.showMagnifier(
_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
),
);
}
void _handleSelectionStartHandleDragUpdate(DragUpdateDetails details) {
if (!renderObject.attached) {
return;
}
_startHandleDragPosition = _getHandleDy(details.globalPosition.dy, _startHandleDragPosition);
final Offset adjustedOffset = Offset(
details.globalPosition.dx,
_startHandleDragPosition + _startHandleDragPositionToCenterOfLine,
);
final TextPosition position = renderObject.getPositionForPoint(adjustedOffset);
if (_selection.isCollapsed) {
_selectionOverlay.updateMagnifier(_buildMagnifier(
currentTextPosition: position,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
));
final TextSelection currentSelection = TextSelection.fromPosition(position);
_handleSelectionHandleChanged(currentSelection);
return;
}
final TextSelection newSelection;
switch (defaultTargetPlatform) {
// On Apple platforms, dragging the base handle makes it the extent.
case TargetPlatform.iOS:
case TargetPlatform.macOS:
newSelection = TextSelection(
extentOffset: position.offset,
baseOffset: _selection.end,
);
if (newSelection.extentOffset >= _selection.end) {
return; // Don't allow order swapping.
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
newSelection = TextSelection(
baseOffset: position.offset,
extentOffset: _selection.extentOffset,
);
if (newSelection.baseOffset >= newSelection.extentOffset) {
return; // Don't allow order swapping.
}
}
_selectionOverlay.updateMagnifier(_buildMagnifier(
currentTextPosition: newSelection.extent.offset < newSelection.base.offset ? newSelection.extent : newSelection.base,
globalGesturePosition: details.globalPosition,
renderEditable: renderObject,
));
_handleSelectionHandleChanged(newSelection);
}
void _handleAnyDragEnd(DragEndDetails details) {
if (!context.mounted) {
return;
}
if (selectionControls is! TextSelectionHandleControls) {
_selectionOverlay.hideMagnifier();
if (!_selection.isCollapsed) {
_selectionOverlay.showToolbar();
}
return;
}
_selectionOverlay.hideMagnifier();
if (!_selection.isCollapsed) {
_selectionOverlay.showToolbar(
context: context,
contextMenuBuilder: contextMenuBuilder,
);
}
}
void _handleSelectionHandleChanged(TextSelection newSelection) {
selectionDelegate.userUpdateTextEditingValue(
_value.copyWith(selection: newSelection),
SelectionChangedCause.drag,
);
}
TextSelectionHandleType _chooseType(
TextDirection textDirection,
TextSelectionHandleType ltrType,
TextSelectionHandleType rtlType,
) {
if (_selection.isCollapsed) {
return TextSelectionHandleType.collapsed;
}
return switch (textDirection) {
TextDirection.ltr => ltrType,
TextDirection.rtl => rtlType,
};
}
}
/// An object that manages a pair of selection handles and a toolbar.
///
/// The selection handles are displayed in the [Overlay] that most closely
/// encloses the given [BuildContext].
class SelectionOverlay {
/// Creates an object that manages overlay entries for selection handles.
///
/// The [context] must have an [Overlay] as an ancestor.
SelectionOverlay({
required this.context,
this.debugRequiredFor,
required TextSelectionHandleType startHandleType,
required double lineHeightAtStart,
this.startHandlesVisible,
this.onStartHandleDragStart,
this.onStartHandleDragUpdate,
this.onStartHandleDragEnd,
required TextSelectionHandleType endHandleType,
required double lineHeightAtEnd,
this.endHandlesVisible,
this.onEndHandleDragStart,
this.onEndHandleDragUpdate,
this.onEndHandleDragEnd,
this.toolbarVisible,
required List<TextSelectionPoint> selectionEndpoints,
required this.selectionControls,
@Deprecated(
'Use `contextMenuBuilder` in `showToolbar` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
required this.selectionDelegate,
required this.clipboardStatus,
required this.startHandleLayerLink,
required this.endHandleLayerLink,
required this.toolbarLayerLink,
this.dragStartBehavior = DragStartBehavior.start,
this.onSelectionHandleTapped,
@Deprecated(
'Use `contextMenuBuilder` in `showToolbar` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
Offset? toolbarLocation,
this.magnifierConfiguration = TextMagnifierConfiguration.disabled,
}) : _startHandleType = startHandleType,
_lineHeightAtStart = lineHeightAtStart,
_endHandleType = endHandleType,
_lineHeightAtEnd = lineHeightAtEnd,
_selectionEndpoints = selectionEndpoints,
_toolbarLocation = toolbarLocation,
assert(debugCheckHasOverlay(context)) {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$SelectionOverlay',
object: this,
);
}
}
/// {@macro flutter.widgets.SelectionOverlay.context}
final BuildContext context;
final ValueNotifier<MagnifierInfo> _magnifierInfo =
ValueNotifier<MagnifierInfo>(MagnifierInfo.empty);
// [MagnifierController.show] and [MagnifierController.hide] should not be
// called directly, except from inside [showMagnifier] and [hideMagnifier]. If
// it is desired to show or hide the magnifier, call [showMagnifier] or
// [hideMagnifier]. This is because the magnifier needs to orchestrate with
// other properties in [SelectionOverlay].
final MagnifierController _magnifierController = MagnifierController();
/// The configuration for the magnifier.
///
/// By default, [SelectionOverlay]'s [TextMagnifierConfiguration] is disabled.
///
/// {@macro flutter.widgets.magnifier.intro}
final TextMagnifierConfiguration magnifierConfiguration;
/// {@template flutter.widgets.SelectionOverlay.toolbarIsVisible}
/// Whether the toolbar is currently visible.
///
/// Includes both the text selection toolbar and the spell check menu.
/// {@endtemplate}
bool get toolbarIsVisible {
return selectionControls is TextSelectionHandleControls
? _contextMenuController.isShown || _spellCheckToolbarController.isShown
: _toolbar != null || _spellCheckToolbarController.isShown;
}
/// {@template flutter.widgets.SelectionOverlay.showMagnifier}
/// Shows the magnifier, and hides the toolbar if it was showing when [showMagnifier]
/// was called. This is safe to call on platforms not mobile, since
/// a magnifierBuilder will not be provided, or the magnifierBuilder will return null
/// on platforms not mobile.
///
/// This is NOT the source of truth for if the magnifier is up or not,
/// since magnifiers may hide themselves. If this info is needed, check
/// [MagnifierController.shown].
/// {@endtemplate}
void showMagnifier(MagnifierInfo initialMagnifierInfo) {
if (toolbarIsVisible) {
hideToolbar();
}
// Start from empty, so we don't utilize any remnant values.
_magnifierInfo.value = initialMagnifierInfo;
// Pre-build the magnifiers so we can tell if we've built something
// or not. If we don't build a magnifiers, then we should not
// insert anything in the overlay.
final Widget? builtMagnifier = magnifierConfiguration.magnifierBuilder(
context,
_magnifierController,
_magnifierInfo,
);
if (builtMagnifier == null) {
return;
}
_magnifierController.show(
context: context,
below: magnifierConfiguration.shouldDisplayHandlesInMagnifier
? null
: _handles?.start,
builder: (_) => builtMagnifier,
);
}
/// {@template flutter.widgets.SelectionOverlay.hideMagnifier}
/// Hide the current magnifier.
///
/// This does nothing if there is no magnifier.
/// {@endtemplate}
void hideMagnifier() {
// This cannot be a check on `MagnifierController.shown`, since
// it's possible that the magnifier is still in the overlay, but
// not shown in cases where the magnifier hides itself.
if (_magnifierController.overlayEntry == null) {
return;
}
_magnifierController.hide();
}
/// The type of start selection handle.
///
/// Changing the value while the handles are visible causes them to rebuild.
TextSelectionHandleType get startHandleType => _startHandleType;
TextSelectionHandleType _startHandleType;
set startHandleType(TextSelectionHandleType value) {
if (_startHandleType == value) {
return;
}
_startHandleType = value;
markNeedsBuild();
}
/// The line height at the selection start.
///
/// This value is used for calculating the size of the start selection handle.
///
/// Changing the value while the handles are visible causes them to rebuild.
double get lineHeightAtStart => _lineHeightAtStart;
double _lineHeightAtStart;
set lineHeightAtStart(double value) {
if (_lineHeightAtStart == value) {
return;
}
_lineHeightAtStart = value;
markNeedsBuild();
}
bool _isDraggingStartHandle = false;
/// Whether the start handle is visible.
///
/// If the value changes, the start handle uses [FadeTransition] to transition
/// itself on and off the screen.
///
/// If this is null, the start selection handle will always be visible.
final ValueListenable<bool>? startHandlesVisible;
/// Called when the users start dragging the start selection handles.
final ValueChanged<DragStartDetails>? onStartHandleDragStart;
void _handleStartHandleDragStart(DragStartDetails details) {
assert(!_isDraggingStartHandle);
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
_isDraggingStartHandle = false;
return;
}
_isDraggingStartHandle = details.kind == PointerDeviceKind.touch;
onStartHandleDragStart?.call(details);
}
void _handleStartHandleDragUpdate(DragUpdateDetails details) {
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
_isDraggingStartHandle = false;
return;
}
onStartHandleDragUpdate?.call(details);
}
/// Called when the users drag the start selection handles to new locations.
final ValueChanged<DragUpdateDetails>? onStartHandleDragUpdate;
/// Called when the users lift their fingers after dragging the start selection
/// handles.
final ValueChanged<DragEndDetails>? onStartHandleDragEnd;
void _handleStartHandleDragEnd(DragEndDetails details) {
_isDraggingStartHandle = false;
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
return;
}
onStartHandleDragEnd?.call(details);
}
/// The type of end selection handle.
///
/// Changing the value while the handles are visible causes them to rebuild.
TextSelectionHandleType get endHandleType => _endHandleType;
TextSelectionHandleType _endHandleType;
set endHandleType(TextSelectionHandleType value) {
if (_endHandleType == value) {
return;
}
_endHandleType = value;
markNeedsBuild();
}
/// The line height at the selection end.
///
/// This value is used for calculating the size of the end selection handle.
///
/// Changing the value while the handles are visible causes them to rebuild.
double get lineHeightAtEnd => _lineHeightAtEnd;
double _lineHeightAtEnd;
set lineHeightAtEnd(double value) {
if (_lineHeightAtEnd == value) {
return;
}
_lineHeightAtEnd = value;
markNeedsBuild();
}
bool _isDraggingEndHandle = false;
/// Whether the end handle is visible.
///
/// If the value changes, the end handle uses [FadeTransition] to transition
/// itself on and off the screen.
///
/// If this is null, the end selection handle will always be visible.
final ValueListenable<bool>? endHandlesVisible;
/// Called when the users start dragging the end selection handles.
final ValueChanged<DragStartDetails>? onEndHandleDragStart;
void _handleEndHandleDragStart(DragStartDetails details) {
assert(!_isDraggingEndHandle);
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
_isDraggingEndHandle = false;
return;
}
_isDraggingEndHandle = details.kind == PointerDeviceKind.touch;
onEndHandleDragStart?.call(details);
}
void _handleEndHandleDragUpdate(DragUpdateDetails details) {
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
_isDraggingEndHandle = false;
return;
}
onEndHandleDragUpdate?.call(details);
}
/// Called when the users drag the end selection handles to new locations.
final ValueChanged<DragUpdateDetails>? onEndHandleDragUpdate;
/// Called when the users lift their fingers after dragging the end selection
/// handles.
final ValueChanged<DragEndDetails>? onEndHandleDragEnd;
void _handleEndHandleDragEnd(DragEndDetails details) {
_isDraggingEndHandle = false;
// Calling OverlayEntry.remove may not happen until the following frame, so
// it's possible for the handles to receive a gesture after calling remove.
if (_handles == null) {
return;
}
onEndHandleDragEnd?.call(details);
}
/// Whether the toolbar is visible.
///
/// If the value changes, the toolbar uses [FadeTransition] to transition
/// itself on and off the screen.
///
/// If this is null the toolbar will always be visible.
final ValueListenable<bool>? toolbarVisible;
/// The text selection positions of selection start and end.
List<TextSelectionPoint> get selectionEndpoints => _selectionEndpoints;
List<TextSelectionPoint> _selectionEndpoints;
set selectionEndpoints(List<TextSelectionPoint> value) {
if (!listEquals(_selectionEndpoints, value)) {
markNeedsBuild();
if (_isDraggingEndHandle || _isDraggingStartHandle) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
HapticFeedback.selectionClick();
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
break;
}
}
}
_selectionEndpoints = value;
}
/// Debugging information for explaining why the [Overlay] is required.
final Widget? debugRequiredFor;
/// The object supplied to the [CompositedTransformTarget] that wraps the text
/// field.
final LayerLink toolbarLayerLink;
/// The objects supplied to the [CompositedTransformTarget] that wraps the
/// location of start selection handle.
final LayerLink startHandleLayerLink;
/// The objects supplied to the [CompositedTransformTarget] that wraps the
/// location of end selection handle.
final LayerLink endHandleLayerLink;
/// {@template flutter.widgets.SelectionOverlay.selectionControls}
/// Builds text selection handles and toolbar.
/// {@endtemplate}
final TextSelectionControls? selectionControls;
/// {@template flutter.widgets.SelectionOverlay.selectionDelegate}
/// The delegate for manipulating the current selection in the owning
/// text field.
/// {@endtemplate}
@Deprecated(
'Use `contextMenuBuilder` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
final TextSelectionDelegate? selectionDelegate;
/// Determines the way that drag start behavior is handled.
///
/// If set to [DragStartBehavior.start], handle drag behavior will
/// begin at the position where the drag gesture won the arena. If set to
/// [DragStartBehavior.down] it will begin at the position where a down
/// event is first detected.
///
/// In general, setting this to [DragStartBehavior.start] will make drag
/// animation smoother and setting it to [DragStartBehavior.down] will make
/// drag behavior feel slightly more reactive.
///
/// By default, the drag start behavior is [DragStartBehavior.start].
///
/// See also:
///
/// * [DragGestureRecognizer.dragStartBehavior], which gives an example for the different behaviors.
final DragStartBehavior dragStartBehavior;
/// {@template flutter.widgets.SelectionOverlay.onSelectionHandleTapped}
/// A callback that's optionally invoked when a selection handle is tapped.
///
/// The [TextSelectionControls.buildHandle] implementation the text field
/// uses decides where the handle's tap "hotspot" is, or whether the
/// selection handle supports tap gestures at all. For instance,
/// [MaterialTextSelectionControls] calls [onSelectionHandleTapped] when the
/// selection handle's "knob" is tapped, while
/// [CupertinoTextSelectionControls] builds a handle that's not sufficiently
/// large for tapping (as it's not meant to be tapped) so it does not call
/// [onSelectionHandleTapped] even when tapped.
/// {@endtemplate}
// See https://github.com/flutter/flutter/issues/39376#issuecomment-848406415
// for provenance.
final VoidCallback? onSelectionHandleTapped;
/// Maintains the status of the clipboard for determining if its contents can
/// be pasted or not.
///
/// Useful because the actual value of the clipboard can only be checked
/// asynchronously (see [Clipboard.getData]).
final ClipboardStatusNotifier? clipboardStatus;
/// The location of where the toolbar should be drawn in relative to the
/// location of [toolbarLayerLink].
///
/// If this is null, the toolbar is drawn based on [selectionEndpoints] and
/// the rect of render object of [context].
///
/// This is useful for displaying toolbars at the mouse right-click locations
/// in desktop devices.
@Deprecated(
'Use the `contextMenuBuilder` parameter in `showToolbar` instead. '
'This feature was deprecated after v3.3.0-0.5.pre.',
)
Offset? get toolbarLocation => _toolbarLocation;
Offset? _toolbarLocation;
set toolbarLocation(Offset? value) {
if (_toolbarLocation == value) {
return;
}
_toolbarLocation = value;
markNeedsBuild();
}
/// Controls the fade-in and fade-out animations for the toolbar and handles.
static const Duration fadeDuration = Duration(milliseconds: 150);
/// A pair of handles. If this is non-null, there are always 2, though the
/// second is hidden when the selection is collapsed.
({OverlayEntry start, OverlayEntry end})? _handles;
/// A copy/paste toolbar.
OverlayEntry? _toolbar;
// Manages the context menu. Not necessarily visible when non-null.
final ContextMenuController _contextMenuController = ContextMenuController();
final ContextMenuController _spellCheckToolbarController = ContextMenuController();
/// {@template flutter.widgets.SelectionOverlay.showHandles}
/// Builds the handles by inserting them into the [context]'s overlay.
/// {@endtemplate}
void showHandles() {
if (_handles != null) {
return;
}
final OverlayState overlay = Overlay.of(context, rootOverlay: true, debugRequiredFor: debugRequiredFor);
final CapturedThemes capturedThemes = InheritedTheme.capture(
from: context,
to: overlay.context,
);
_handles = (
start: OverlayEntry(builder: (BuildContext context) {
return capturedThemes.wrap(_buildStartHandle(context));
}),
end: OverlayEntry(builder: (BuildContext context) {
return capturedThemes.wrap(_buildEndHandle(context));
}),
);
overlay.insertAll(<OverlayEntry>[_handles!.start, _handles!.end]);
}
/// {@template flutter.widgets.SelectionOverlay.hideHandles}
/// Destroys the handles by removing them from overlay.
/// {@endtemplate}
void hideHandles() {
if (_handles != null) {
_handles!.start.remove();
_handles!.start.dispose();
_handles!.end.remove();
_handles!.end.dispose();
_handles = null;
}
}
/// {@template flutter.widgets.SelectionOverlay.showToolbar}
/// Shows the toolbar by inserting it into the [context]'s overlay.
/// {@endtemplate}
void showToolbar({
BuildContext? context,
WidgetBuilder? contextMenuBuilder,
}) {
if (contextMenuBuilder == null) {
if (_toolbar != null) {
return;
}
_toolbar = OverlayEntry(builder: _buildToolbar);
Overlay.of(this.context, rootOverlay: true, debugRequiredFor: debugRequiredFor).insert(_toolbar!);
return;
}
if (context == null) {
return;
}
final RenderBox renderBox = context.findRenderObject()! as RenderBox;
_contextMenuController.show(
context: context,
contextMenuBuilder: (BuildContext context) {
return _SelectionToolbarWrapper(
visibility: toolbarVisible,
layerLink: toolbarLayerLink,
offset: -renderBox.localToGlobal(Offset.zero),
child: contextMenuBuilder(context),
);
},
);
}
/// Shows toolbar with spell check suggestions of misspelled words that are
/// available for click-and-replace.
void showSpellCheckSuggestionsToolbar({
BuildContext? context,
required WidgetBuilder builder,
}) {
if (context == null) {
return;
}
final RenderBox renderBox = context.findRenderObject()! as RenderBox;
_spellCheckToolbarController.show(
context: context,
contextMenuBuilder: (BuildContext context) {
return _SelectionToolbarWrapper(
layerLink: toolbarLayerLink,
offset: -renderBox.localToGlobal(Offset.zero),
child: builder(context),
);
},
);
}
bool _buildScheduled = false;
/// Rebuilds the selection toolbar or handles if they are present.
void markNeedsBuild() {
if (_handles == null && _toolbar == null) {
return;
}
// If we are in build state, it will be too late to update visibility.
// We will need to schedule the build in next frame.
if (SchedulerBinding.instance.schedulerPhase == SchedulerPhase.persistentCallbacks) {
if (_buildScheduled) {
return;
}
_buildScheduled = true;
SchedulerBinding.instance.addPostFrameCallback((Duration duration) {
_buildScheduled = false;
if (_handles != null) {
_handles!.start.markNeedsBuild();
_handles!.end.markNeedsBuild();
}
_toolbar?.markNeedsBuild();
if (_contextMenuController.isShown) {
_contextMenuController.markNeedsBuild();
} else if (_spellCheckToolbarController.isShown) {
_spellCheckToolbarController.markNeedsBuild();
}
}, debugLabel: 'SelectionOverlay.markNeedsBuild');
} else {
if (_handles != null) {
_handles!.start.markNeedsBuild();
_handles!.end.markNeedsBuild();
}
_toolbar?.markNeedsBuild();
if (_contextMenuController.isShown) {
_contextMenuController.markNeedsBuild();
} else if (_spellCheckToolbarController.isShown) {
_spellCheckToolbarController.markNeedsBuild();
}
}
}
/// {@template flutter.widgets.SelectionOverlay.hide}
/// Hides the entire overlay including the toolbar and the handles.
/// {@endtemplate}
void hide() {
_magnifierController.hide();
hideHandles();
if (_toolbar != null || _contextMenuController.isShown || _spellCheckToolbarController.isShown) {
hideToolbar();
}
}
/// {@template flutter.widgets.SelectionOverlay.hideToolbar}
/// Hides the toolbar part of the overlay.
///
/// To hide the whole overlay, see [hide].
/// {@endtemplate}
void hideToolbar() {
_contextMenuController.remove();
_spellCheckToolbarController.remove();
if (_toolbar == null) {
return;
}
_toolbar?.remove();
_toolbar?.dispose();
_toolbar = null;
}
/// {@template flutter.widgets.SelectionOverlay.dispose}
/// Disposes this object and release resources.
/// {@endtemplate}
void dispose() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
hide();
_magnifierInfo.dispose();
}
Widget _buildStartHandle(BuildContext context) {
final Widget handle;
final TextSelectionControls? selectionControls = this.selectionControls;
if (selectionControls == null) {
handle = const SizedBox.shrink();
} else {
handle = _SelectionHandleOverlay(
type: _startHandleType,
handleLayerLink: startHandleLayerLink,
onSelectionHandleTapped: onSelectionHandleTapped,
onSelectionHandleDragStart: _handleStartHandleDragStart,
onSelectionHandleDragUpdate: _handleStartHandleDragUpdate,
onSelectionHandleDragEnd: _handleStartHandleDragEnd,
selectionControls: selectionControls,
visibility: startHandlesVisible,
preferredLineHeight: _lineHeightAtStart,
dragStartBehavior: dragStartBehavior,
);
}
return TextFieldTapRegion(
child: ExcludeSemantics(
child: handle,
),
);
}
Widget _buildEndHandle(BuildContext context) {
final Widget handle;
final TextSelectionControls? selectionControls = this.selectionControls;
if (selectionControls == null || _startHandleType == TextSelectionHandleType.collapsed) {
// Hide the second handle when collapsed.
handle = const SizedBox.shrink();
} else {
handle = _SelectionHandleOverlay(
type: _endHandleType,
handleLayerLink: endHandleLayerLink,
onSelectionHandleTapped: onSelectionHandleTapped,
onSelectionHandleDragStart: _handleEndHandleDragStart,
onSelectionHandleDragUpdate: _handleEndHandleDragUpdate,
onSelectionHandleDragEnd: _handleEndHandleDragEnd,
selectionControls: selectionControls,
visibility: endHandlesVisible,
preferredLineHeight: _lineHeightAtEnd,
dragStartBehavior: dragStartBehavior,
);
}
return TextFieldTapRegion(
child: ExcludeSemantics(
child: handle,
),
);
}
// Build the toolbar via TextSelectionControls.
Widget _buildToolbar(BuildContext context) {
if (selectionControls == null) {
return const SizedBox.shrink();
}
assert(selectionDelegate != null, 'If not using contextMenuBuilder, must pass selectionDelegate.');
final RenderBox renderBox = this.context.findRenderObject()! as RenderBox;
final Rect editingRegion = Rect.fromPoints(
renderBox.localToGlobal(Offset.zero),
renderBox.localToGlobal(renderBox.size.bottomRight(Offset.zero)),
);
final bool isMultiline = selectionEndpoints.last.point.dy - selectionEndpoints.first.point.dy >
lineHeightAtEnd / 2;
// If the selected text spans more than 1 line, horizontally center the toolbar.
// Derived from both iOS and Android.
final double midX = isMultiline
? editingRegion.width / 2
: (selectionEndpoints.first.point.dx + selectionEndpoints.last.point.dx) / 2;
final Offset midpoint = Offset(
midX,
// The y-coordinate won't be made use of most likely.
selectionEndpoints.first.point.dy - lineHeightAtStart,
);
return _SelectionToolbarWrapper(
visibility: toolbarVisible,
layerLink: toolbarLayerLink,
offset: -editingRegion.topLeft,
child: Builder(
builder: (BuildContext context) {
return selectionControls!.buildToolbar(
context,
editingRegion,
lineHeightAtStart,
midpoint,
selectionEndpoints,
selectionDelegate!,
clipboardStatus,
toolbarLocation,
);
},
),
);
}
/// {@template flutter.widgets.SelectionOverlay.updateMagnifier}
/// Update the current magnifier with new selection data, so the magnifier
/// can respond accordingly.
///
/// If the magnifier is not shown, this still updates the magnifier position
/// because the magnifier may have hidden itself and is looking for a cue to reshow
/// itself.
///
/// If there is no magnifier in the overlay, this does nothing.
/// {@endtemplate}
void updateMagnifier(MagnifierInfo magnifierInfo) {
if (_magnifierController.overlayEntry == null) {
return;
}
_magnifierInfo.value = magnifierInfo;
}
}
// TODO(justinmc): Currently this fades in but not out on all platforms. It
// should follow the correct fading behavior for the current platform, then be
// made public and de-duplicated with widgets/selectable_region.dart.
// https://github.com/flutter/flutter/issues/107732
// Wrap the given child in the widgets common to both contextMenuBuilder and
// TextSelectionControls.buildToolbar.
class _SelectionToolbarWrapper extends StatefulWidget {
const _SelectionToolbarWrapper({
this.visibility,
required this.layerLink,
required this.offset,
required this.child,
});
final Widget child;
final Offset offset;
final LayerLink layerLink;
final ValueListenable<bool>? visibility;
@override
State<_SelectionToolbarWrapper> createState() => _SelectionToolbarWrapperState();
}
class _SelectionToolbarWrapperState extends State<_SelectionToolbarWrapper> with SingleTickerProviderStateMixin {
late AnimationController _controller;
Animation<double> get _opacity => _controller.view;
@override
void initState() {
super.initState();
_controller = AnimationController(duration: SelectionOverlay.fadeDuration, vsync: this);
_toolbarVisibilityChanged();
widget.visibility?.addListener(_toolbarVisibilityChanged);
}
@override
void didUpdateWidget(_SelectionToolbarWrapper oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.visibility == widget.visibility) {
return;
}
oldWidget.visibility?.removeListener(_toolbarVisibilityChanged);
_toolbarVisibilityChanged();
widget.visibility?.addListener(_toolbarVisibilityChanged);
}
@override
void dispose() {
widget.visibility?.removeListener(_toolbarVisibilityChanged);
_controller.dispose();
super.dispose();
}
void _toolbarVisibilityChanged() {
if (widget.visibility?.value ?? true) {
_controller.forward();
} else {
_controller.reverse();
}
}
@override
Widget build(BuildContext context) {
return TextFieldTapRegion(
child: Directionality(
textDirection: Directionality.of(this.context),
child: FadeTransition(
opacity: _opacity,
child: CompositedTransformFollower(
link: widget.layerLink,
showWhenUnlinked: false,
offset: widget.offset,
child: widget.child,
),
),
),
);
}
}
/// This widget represents a single draggable selection handle.
class _SelectionHandleOverlay extends StatefulWidget {
/// Create selection overlay.
const _SelectionHandleOverlay({
required this.type,
required this.handleLayerLink,
this.onSelectionHandleTapped,
this.onSelectionHandleDragStart,
this.onSelectionHandleDragUpdate,
this.onSelectionHandleDragEnd,
required this.selectionControls,
this.visibility,
required this.preferredLineHeight,
this.dragStartBehavior = DragStartBehavior.start,
});
final LayerLink handleLayerLink;
final VoidCallback? onSelectionHandleTapped;
final ValueChanged<DragStartDetails>? onSelectionHandleDragStart;
final ValueChanged<DragUpdateDetails>? onSelectionHandleDragUpdate;
final ValueChanged<DragEndDetails>? onSelectionHandleDragEnd;
final TextSelectionControls selectionControls;
final ValueListenable<bool>? visibility;
final double preferredLineHeight;
final TextSelectionHandleType type;
final DragStartBehavior dragStartBehavior;
@override
State<_SelectionHandleOverlay> createState() => _SelectionHandleOverlayState();
}
class _SelectionHandleOverlayState extends State<_SelectionHandleOverlay> with SingleTickerProviderStateMixin {
late AnimationController _controller;
Animation<double> get _opacity => _controller.view;
@override
void initState() {
super.initState();
_controller = AnimationController(duration: SelectionOverlay.fadeDuration, vsync: this);
_handleVisibilityChanged();
widget.visibility?.addListener(_handleVisibilityChanged);
}
void _handleVisibilityChanged() {
if (widget.visibility?.value ?? true) {
_controller.forward();
} else {
_controller.reverse();
}
}
@override
void didUpdateWidget(_SelectionHandleOverlay oldWidget) {
super.didUpdateWidget(oldWidget);
oldWidget.visibility?.removeListener(_handleVisibilityChanged);
_handleVisibilityChanged();
widget.visibility?.addListener(_handleVisibilityChanged);
}
@override
void dispose() {
widget.visibility?.removeListener(_handleVisibilityChanged);
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
final Offset handleAnchor = widget.selectionControls.getHandleAnchor(
widget.type,
widget.preferredLineHeight,
);
final Size handleSize = widget.selectionControls.getHandleSize(
widget.preferredLineHeight,
);
final Rect handleRect = Rect.fromLTWH(
-handleAnchor.dx,
-handleAnchor.dy,
handleSize.width,
handleSize.height,
);
// Make sure the GestureDetector is big enough to be easily interactive.
final Rect interactiveRect = handleRect.expandToInclude(
Rect.fromCircle(center: handleRect.center, radius: kMinInteractiveDimension / 2),
);
final RelativeRect padding = RelativeRect.fromLTRB(
math.max((interactiveRect.width - handleRect.width) / 2, 0),
math.max((interactiveRect.height - handleRect.height) / 2, 0),
math.max((interactiveRect.width - handleRect.width) / 2, 0),
math.max((interactiveRect.height - handleRect.height) / 2, 0),
);
return CompositedTransformFollower(
link: widget.handleLayerLink,
offset: interactiveRect.topLeft,
showWhenUnlinked: false,
child: FadeTransition(
opacity: _opacity,
child: Container(
alignment: Alignment.topLeft,
width: interactiveRect.width,
height: interactiveRect.height,
child: RawGestureDetector(
behavior: HitTestBehavior.translucent,
gestures: <Type, GestureRecognizerFactory>{
PanGestureRecognizer: GestureRecognizerFactoryWithHandlers<PanGestureRecognizer>(
() => PanGestureRecognizer(
debugOwner: this,
// Mouse events select the text and do not drag the cursor.
supportedDevices: <PointerDeviceKind>{
PointerDeviceKind.touch,
PointerDeviceKind.stylus,
PointerDeviceKind.unknown,
},
),
(PanGestureRecognizer instance) {
instance
..dragStartBehavior = widget.dragStartBehavior
..onStart = widget.onSelectionHandleDragStart
..onUpdate = widget.onSelectionHandleDragUpdate
..onEnd = widget.onSelectionHandleDragEnd;
},
),
},
child: Padding(
padding: EdgeInsets.only(
left: padding.left,
top: padding.top,
right: padding.right,
bottom: padding.bottom,
),
child: widget.selectionControls.buildHandle(
context,
widget.type,
widget.preferredLineHeight,
widget.onSelectionHandleTapped,
),
),
),
),
),
);
}
}
/// Delegate interface for the [TextSelectionGestureDetectorBuilder].
///
/// The interface is usually implemented by the [State] of text field
/// implementations wrapping [EditableText], so that they can use a
/// [TextSelectionGestureDetectorBuilder] to build a
/// [TextSelectionGestureDetector] for their [EditableText]. The delegate
/// provides the builder with information about the current state of the text
/// field. Based on that information, the builder adds the correct gesture
/// handlers to the gesture detector.
///
/// See also:
///
/// * [TextField], which implements this delegate for the Material text field.
/// * [CupertinoTextField], which implements this delegate for the Cupertino
/// text field.
abstract class TextSelectionGestureDetectorBuilderDelegate {
/// [GlobalKey] to the [EditableText] for which the
/// [TextSelectionGestureDetectorBuilder] will build a [TextSelectionGestureDetector].
GlobalKey<EditableTextState> get editableTextKey;
/// Whether the text field should respond to force presses.
bool get forcePressEnabled;
/// Whether the user may select text in the text field.
bool get selectionEnabled;
}
/// Builds a [TextSelectionGestureDetector] to wrap an [EditableText].
///
/// The class implements sensible defaults for many user interactions
/// with an [EditableText] (see the documentation of the various gesture handler
/// methods, e.g. [onTapDown], [onForcePressStart], etc.). Subclasses of
/// [TextSelectionGestureDetectorBuilder] can change the behavior performed in
/// responds to these gesture events by overriding the corresponding handler
/// methods of this class.
///
/// The resulting [TextSelectionGestureDetector] to wrap an [EditableText] is
/// obtained by calling [buildGestureDetector].
///
/// A [TextSelectionGestureDetectorBuilder] must be provided a
/// [TextSelectionGestureDetectorBuilderDelegate], from which information about
/// the [EditableText] may be obtained. Typically, the [State] of the widget
/// that builds the [EditableText] implements this interface, and then passes
/// itself as the [delegate].
///
/// See also:
///
/// * [TextField], which uses a subclass to implement the Material-specific
/// gesture logic of an [EditableText].
/// * [CupertinoTextField], which uses a subclass to implement the
/// Cupertino-specific gesture logic of an [EditableText].
class TextSelectionGestureDetectorBuilder {
/// Creates a [TextSelectionGestureDetectorBuilder].
TextSelectionGestureDetectorBuilder({
required this.delegate,
});
/// The delegate for this [TextSelectionGestureDetectorBuilder].
///
/// The delegate provides the builder with information about what actions can
/// currently be performed on the text field. Based on this, the builder adds
/// the correct gesture handlers to the gesture detector.
///
/// Typically implemented by a [State] of a widget that builds an
/// [EditableText].
@protected
final TextSelectionGestureDetectorBuilderDelegate delegate;
// Shows the magnifier on supported platforms at the given offset, currently
// only Android and iOS.
void _showMagnifierIfSupportedByPlatform(Offset positionToShow) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
editableText.showMagnifier(positionToShow);
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
}
}
// Hides the magnifier on supported platforms, currently only Android and iOS.
void _hideMagnifierIfSupportedByPlatform() {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.iOS:
editableText.hideMagnifier();
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
}
}
/// Returns true if lastSecondaryTapDownPosition was on selection.
bool get _lastSecondaryTapWasOnSelection {
assert(renderEditable.lastSecondaryTapDownPosition != null);
if (renderEditable.selection == null) {
return false;
}
final TextPosition textPosition = renderEditable.getPositionForPoint(
renderEditable.lastSecondaryTapDownPosition!,
);
return renderEditable.selection!.start <= textPosition.offset
&& renderEditable.selection!.end >= textPosition.offset;
}
bool _positionWasOnSelectionExclusive(TextPosition textPosition) {
final TextSelection? selection = renderEditable.selection;
if (selection == null) {
return false;
}
return selection.start < textPosition.offset
&& selection.end > textPosition.offset;
}
bool _positionWasOnSelectionInclusive(TextPosition textPosition) {
final TextSelection? selection = renderEditable.selection;
if (selection == null) {
return false;
}
return selection.start <= textPosition.offset
&& selection.end >= textPosition.offset;
}
/// Returns true if position was on selection.
bool _positionOnSelection(Offset position, TextSelection? targetSelection) {
if (targetSelection == null) {
return false;
}
final TextPosition textPosition = renderEditable.getPositionForPoint(position);
return targetSelection.start <= textPosition.offset
&& targetSelection.end >= textPosition.offset;
}
// Expand the selection to the given global position.
//
// Either base or extent will be moved to the last tapped position, whichever
// is closest. The selection will never shrink or pivot, only grow.
//
// If fromSelection is given, will expand from that selection instead of the
// current selection in renderEditable.
//
// See also:
//
// * [_extendSelection], which is similar but pivots the selection around
// the base.
void _expandSelection(Offset offset, SelectionChangedCause cause, [TextSelection? fromSelection]) {
assert(renderEditable.selection?.baseOffset != null);
final TextPosition tappedPosition = renderEditable.getPositionForPoint(offset);
final TextSelection selection = fromSelection ?? renderEditable.selection!;
final bool baseIsCloser =
(tappedPosition.offset - selection.baseOffset).abs()
< (tappedPosition.offset - selection.extentOffset).abs();
final TextSelection nextSelection = selection.copyWith(
baseOffset: baseIsCloser ? selection.extentOffset : selection.baseOffset,
extentOffset: tappedPosition.offset,
);
editableText.userUpdateTextEditingValue(
editableText.textEditingValue.copyWith(
selection: nextSelection,
),
cause,
);
}
// Extend the selection to the given global position.
//
// Holds the base in place and moves the extent.
//
// See also:
//
// * [_expandSelection], which is similar but always increases the size of
// the selection.
void _extendSelection(Offset offset, SelectionChangedCause cause) {
assert(renderEditable.selection?.baseOffset != null);
final TextPosition tappedPosition = renderEditable.getPositionForPoint(offset);
final TextSelection selection = renderEditable.selection!;
final TextSelection nextSelection = selection.copyWith(
extentOffset: tappedPosition.offset,
);
editableText.userUpdateTextEditingValue(
editableText.textEditingValue.copyWith(
selection: nextSelection,
),
cause,
);
}
/// Whether to show the selection toolbar.
///
/// It is based on the signal source when a [onTapDown] is called. This getter
/// will return true if current [onTapDown] event is triggered by a touch or
/// a stylus.
bool get shouldShowSelectionToolbar => _shouldShowSelectionToolbar;
bool _shouldShowSelectionToolbar = true;
/// The [State] of the [EditableText] for which the builder will provide a
/// [TextSelectionGestureDetector].
@protected
EditableTextState get editableText => delegate.editableTextKey.currentState!;
/// The [RenderObject] of the [EditableText] for which the builder will
/// provide a [TextSelectionGestureDetector].
@protected
RenderEditable get renderEditable => editableText.renderEditable;
/// Whether the Shift key was pressed when the most recent [PointerDownEvent]
/// was tracked by the [BaseTapAndDragGestureRecognizer].
bool _isShiftPressed = false;
/// The viewport offset pixels of any [Scrollable] containing the
/// [RenderEditable] at the last drag start.
double _dragStartScrollOffset = 0.0;
/// The viewport offset pixels of the [RenderEditable] at the last drag start.
double _dragStartViewportOffset = 0.0;
double get _scrollPosition {
final ScrollableState? scrollableState =
delegate.editableTextKey.currentContext == null
? null
: Scrollable.maybeOf(delegate.editableTextKey.currentContext!);
return scrollableState == null
? 0.0
: scrollableState.position.pixels;
}
AxisDirection? get _scrollDirection {
final ScrollableState? scrollableState =
delegate.editableTextKey.currentContext == null
? null
: Scrollable.maybeOf(delegate.editableTextKey.currentContext!);
return scrollableState?.axisDirection;
}
// For a shift + tap + drag gesture, the TextSelection at the point of the
// tap. Mac uses this value to reset to the original selection when an
// inversion of the base and offset happens.
TextSelection? _dragStartSelection;
// For tap + drag gesture on iOS, whether the position where the drag started
// was on the previous TextSelection. iOS uses this value to determine if
// the cursor should move on drag update.
//
// If the drag started on the previous selection then the cursor will move on
// drag update. If the drag did not start on the previous selection then the
// cursor will not move on drag update.
bool? _dragBeganOnPreviousSelection;
// For iOS long press behavior when the field is not focused. iOS uses this value
// to determine if a long press began on a field that was not focused.
//
// If the field was not focused when the long press began, a long press will select
// the word and a long press move will select word-by-word. If the field was
// focused, the cursor moves to the long press position.
bool _longPressStartedWithoutFocus = false;
/// Handler for [TextSelectionGestureDetector.onTapTrackStart].
///
/// See also:
///
/// * [TextSelectionGestureDetector.onTapTrackStart], which triggers this
/// callback.
@protected
void onTapTrackStart() {
_isShiftPressed = HardwareKeyboard.instance.logicalKeysPressed
.intersection(<LogicalKeyboardKey>{LogicalKeyboardKey.shiftLeft, LogicalKeyboardKey.shiftRight})
.isNotEmpty;
}
/// Handler for [TextSelectionGestureDetector.onTapTrackReset].
///
/// See also:
///
/// * [TextSelectionGestureDetector.onTapTrackReset], which triggers this
/// callback.
@protected
void onTapTrackReset() {
_isShiftPressed = false;
}
/// Handler for [TextSelectionGestureDetector.onTapDown].
///
/// By default, it forwards the tap to [RenderEditable.handleTapDown] and sets
/// [shouldShowSelectionToolbar] to true if the tap was initiated by a finger or stylus.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onTapDown], which triggers this callback.
@protected
void onTapDown(TapDragDownDetails details) {
if (!delegate.selectionEnabled) {
return;
}
// TODO(Renzo-Olivares): Migrate text selection gestures away from saving state
// in renderEditable. The gesture callbacks can use the details objects directly
// in callbacks variants that provide them [TapGestureRecognizer.onSecondaryTap]
// vs [TapGestureRecognizer.onSecondaryTapUp] instead of having to track state in
// renderEditable. When this migration is complete we should remove this hack.
// See https://github.com/flutter/flutter/issues/115130.
renderEditable.handleTapDown(TapDownDetails(globalPosition: details.globalPosition));
// The selection overlay should only be shown when the user is interacting
// through a touch screen (via either a finger or a stylus). A mouse shouldn't
// trigger the selection overlay.
// For backwards-compatibility, we treat a null kind the same as touch.
final PointerDeviceKind? kind = details.kind;
// TODO(justinmc): Should a desktop platform show its selection toolbar when
// receiving a tap event? Say a Windows device with a touchscreen.
// https://github.com/flutter/flutter/issues/106586
_shouldShowSelectionToolbar = kind == null
|| kind == PointerDeviceKind.touch
|| kind == PointerDeviceKind.stylus;
// It is impossible to extend the selection when the shift key is pressed, if the
// renderEditable.selection is invalid.
final bool isShiftPressedValid = _isShiftPressed && renderEditable.selection?.baseOffset != null;
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
// On mobile platforms the selection is set on tap up.
break;
case TargetPlatform.macOS:
editableText.hideToolbar();
// On macOS, a shift-tapped unfocused field expands from 0, not from the
// previous selection.
if (isShiftPressedValid) {
final TextSelection? fromSelection = renderEditable.hasFocus
? null
: const TextSelection.collapsed(offset: 0);
_expandSelection(
details.globalPosition,
SelectionChangedCause.tap,
fromSelection,
);
return;
}
// On macOS, a tap/click places the selection in a precise position.
// This differs from iOS/iPadOS, where if the gesture is done by a touch
// then the selection moves to the closest word edge, instead of a
// precise position.
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
case TargetPlatform.linux:
case TargetPlatform.windows:
editableText.hideToolbar();
if (isShiftPressedValid) {
_extendSelection(details.globalPosition, SelectionChangedCause.tap);
return;
}
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
}
}
/// Handler for [TextSelectionGestureDetector.onForcePressStart].
///
/// By default, it selects the word at the position of the force press,
/// if selection is enabled.
///
/// This callback is only applicable when force press is enabled.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onForcePressStart], which triggers this
/// callback.
@protected
void onForcePressStart(ForcePressDetails details) {
assert(delegate.forcePressEnabled);
_shouldShowSelectionToolbar = true;
if (delegate.selectionEnabled) {
renderEditable.selectWordsInRange(
from: details.globalPosition,
cause: SelectionChangedCause.forcePress,
);
}
}
/// Handler for [TextSelectionGestureDetector.onForcePressEnd].
///
/// By default, it selects words in the range specified in [details] and shows
/// toolbar if it is necessary.
///
/// This callback is only applicable when force press is enabled.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onForcePressEnd], which triggers this
/// callback.
@protected
void onForcePressEnd(ForcePressDetails details) {
assert(delegate.forcePressEnabled);
renderEditable.selectWordsInRange(
from: details.globalPosition,
cause: SelectionChangedCause.forcePress,
);
if (shouldShowSelectionToolbar) {
editableText.showToolbar();
}
}
/// Whether the provided [onUserTap] callback should be dispatched on every
/// tap or only non-consecutive taps.
///
/// Defaults to false.
@protected
bool get onUserTapAlwaysCalled => false;
/// Handler for [TextSelectionGestureDetector.onUserTap].
///
/// By default, it serves as placeholder to enable subclass override.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onUserTap], which triggers this
/// callback.
/// * [TextSelectionGestureDetector.onUserTapAlwaysCalled], which controls
/// whether this callback is called only on the first tap in a series
/// of taps.
@protected
void onUserTap() { /* Subclass should override this method if needed. */ }
/// Handler for [TextSelectionGestureDetector.onSingleTapUp].
///
/// By default, it selects word edge if selection is enabled.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSingleTapUp], which triggers
/// this callback.
@protected
void onSingleTapUp(TapDragUpDetails details) {
if (delegate.selectionEnabled) {
// It is impossible to extend the selection when the shift key is pressed, if the
// renderEditable.selection is invalid.
final bool isShiftPressedValid = _isShiftPressed && renderEditable.selection?.baseOffset != null;
switch (defaultTargetPlatform) {
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
break;
// On desktop platforms the selection is set on tap down.
case TargetPlatform.android:
editableText.hideToolbar(false);
if (isShiftPressedValid) {
_extendSelection(details.globalPosition, SelectionChangedCause.tap);
return;
}
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
editableText.showSpellCheckSuggestionsToolbar();
case TargetPlatform.fuchsia:
editableText.hideToolbar(false);
if (isShiftPressedValid) {
_extendSelection(details.globalPosition, SelectionChangedCause.tap);
return;
}
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
case TargetPlatform.iOS:
if (isShiftPressedValid) {
// On iOS, a shift-tapped unfocused field expands from 0, not from
// the previous selection.
final TextSelection? fromSelection = renderEditable.hasFocus
? null
: const TextSelection.collapsed(offset: 0);
_expandSelection(
details.globalPosition,
SelectionChangedCause.tap,
fromSelection,
);
return;
}
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
// TODO(camsim99): Determine spell check toolbar behavior in these cases:
// https://github.com/flutter/flutter/issues/119573.
// Precise devices should place the cursor at a precise position if the
// word at the text position is not misspelled.
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
// If the word that was tapped is misspelled, select the word and show the spell check suggestions
// toolbar once. If additional taps are made on a misspelled word, toggle the toolbar. If the word
// is not misspelled, default to the following behavior:
//
// Toggle the toolbar if the `previousSelection` is collapsed, the tap is on the selection, the
// TextAffinity remains the same, and the editable is focused. The TextAffinity is important when the
// cursor is on the boundary of a line wrap, if the affinity is different (i.e. it is downstream), the
// selection should move to the following line and not toggle the toolbar.
//
// Toggle the toolbar when the tap is exclusively within the bounds of a non-collapsed `previousSelection`,
// and the editable is focused.
//
// Selects the word edge closest to the tap when the editable is not focused, or if the tap was neither exclusively
// or inclusively on `previousSelection`. If the selection remains the same after selecting the word edge, then we
// toggle the toolbar. If the selection changes then we hide the toolbar.
final TextSelection previousSelection = renderEditable.selection ?? editableText.textEditingValue.selection;
final TextPosition textPosition = renderEditable.getPositionForPoint(details.globalPosition);
final bool isAffinityTheSame = textPosition.affinity == previousSelection.affinity;
final bool wordAtCursorIndexIsMisspelled = editableText.findSuggestionSpanAtCursorIndex(textPosition.offset) != null;
if (wordAtCursorIndexIsMisspelled) {
renderEditable.selectWord(cause: SelectionChangedCause.tap);
if (previousSelection != editableText.textEditingValue.selection) {
editableText.showSpellCheckSuggestionsToolbar();
} else {
editableText.toggleToolbar(false);
}
} else if (((_positionWasOnSelectionExclusive(textPosition) && !previousSelection.isCollapsed) || (_positionWasOnSelectionInclusive(textPosition) && previousSelection.isCollapsed && isAffinityTheSame)) && renderEditable.hasFocus) {
editableText.toggleToolbar(false);
} else {
renderEditable.selectWordEdge(cause: SelectionChangedCause.tap);
if (previousSelection == editableText.textEditingValue.selection && renderEditable.hasFocus) {
editableText.toggleToolbar(false);
} else {
editableText.hideToolbar(false);
}
}
}
}
}
editableText.requestKeyboard();
}
/// Handler for [TextSelectionGestureDetector.onSingleTapCancel].
///
/// By default, it serves as placeholder to enable subclass override.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSingleTapCancel], which triggers
/// this callback.
@protected
void onSingleTapCancel() { /* Subclass should override this method if needed. */ }
/// Handler for [TextSelectionGestureDetector.onSingleLongTapStart].
///
/// By default, it selects text position specified in [details] if selection
/// is enabled.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSingleLongTapStart], which triggers
/// this callback.
@protected
void onSingleLongTapStart(LongPressStartDetails details) {
if (delegate.selectionEnabled) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
if (!renderEditable.hasFocus) {
_longPressStartedWithoutFocus = true;
renderEditable.selectWord(cause: SelectionChangedCause.longPress);
} else {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.longPress,
);
// Show the floating cursor.
final RawFloatingCursorPoint cursorPoint = RawFloatingCursorPoint(
state: FloatingCursorDragState.Start,
startLocation: (
renderEditable.globalToLocal(details.globalPosition),
TextPosition(
offset: editableText.textEditingValue.selection.baseOffset,
affinity: editableText.textEditingValue.selection.affinity,
),
),
offset: Offset.zero,
);
editableText.updateFloatingCursor(cursorPoint);
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
renderEditable.selectWord(cause: SelectionChangedCause.longPress);
}
_showMagnifierIfSupportedByPlatform(details.globalPosition);
_dragStartViewportOffset = renderEditable.offset.pixels;
_dragStartScrollOffset = _scrollPosition;
}
}
/// Handler for [TextSelectionGestureDetector.onSingleLongTapMoveUpdate].
///
/// By default, it updates the selection location specified in [details] if
/// selection is enabled.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSingleLongTapMoveUpdate], which
/// triggers this callback.
@protected
void onSingleLongTapMoveUpdate(LongPressMoveUpdateDetails details) {
if (delegate.selectionEnabled) {
// Adjust the drag start offset for possible viewport offset changes.
final Offset editableOffset = renderEditable.maxLines == 1
? Offset(renderEditable.offset.pixels - _dragStartViewportOffset, 0.0)
: Offset(0.0, renderEditable.offset.pixels - _dragStartViewportOffset);
final double effectiveScrollPosition = _scrollPosition - _dragStartScrollOffset;
final bool scrollingOnVerticalAxis = _scrollDirection == AxisDirection.up || _scrollDirection == AxisDirection.down;
final Offset scrollableOffset = Offset(
!scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
);
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
if (_longPressStartedWithoutFocus) {
renderEditable.selectWordsInRange(
from: details.globalPosition - details.offsetFromOrigin - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.longPress,
);
} else {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.longPress,
);
// Update the floating cursor.
final RawFloatingCursorPoint cursorPoint = RawFloatingCursorPoint(
state: FloatingCursorDragState.Update,
offset: details.offsetFromOrigin,
);
editableText.updateFloatingCursor(cursorPoint);
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
renderEditable.selectWordsInRange(
from: details.globalPosition - details.offsetFromOrigin - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.longPress,
);
}
_showMagnifierIfSupportedByPlatform(details.globalPosition);
}
}
/// Handler for [TextSelectionGestureDetector.onSingleLongTapEnd].
///
/// By default, it shows toolbar if necessary.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSingleLongTapEnd], which triggers this
/// callback.
@protected
void onSingleLongTapEnd(LongPressEndDetails details) {
_hideMagnifierIfSupportedByPlatform();
if (shouldShowSelectionToolbar) {
editableText.showToolbar();
}
_longPressStartedWithoutFocus = false;
_dragStartViewportOffset = 0.0;
_dragStartScrollOffset = 0.0;
if (defaultTargetPlatform == TargetPlatform.iOS && delegate.selectionEnabled && editableText.textEditingValue.selection.isCollapsed) {
// Update the floating cursor.
final RawFloatingCursorPoint cursorPoint = RawFloatingCursorPoint(
state: FloatingCursorDragState.End
);
editableText.updateFloatingCursor(cursorPoint);
}
}
/// Handler for [TextSelectionGestureDetector.onSecondaryTap].
///
/// By default, selects the word if possible and shows the toolbar.
@protected
void onSecondaryTap() {
if (!delegate.selectionEnabled) {
return;
}
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
if (!_lastSecondaryTapWasOnSelection || !renderEditable.hasFocus) {
renderEditable.selectWord(cause: SelectionChangedCause.tap);
}
if (shouldShowSelectionToolbar) {
editableText.hideToolbar();
editableText.showToolbar();
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
if (!renderEditable.hasFocus) {
renderEditable.selectPosition(cause: SelectionChangedCause.tap);
}
editableText.toggleToolbar();
}
}
/// Handler for [TextSelectionGestureDetector.onSecondaryTapDown].
///
/// See also:
///
/// * [TextSelectionGestureDetector.onSecondaryTapDown], which triggers this
/// callback.
/// * [onSecondaryTap], which is typically called after this.
@protected
void onSecondaryTapDown(TapDownDetails details) {
// TODO(Renzo-Olivares): Migrate text selection gestures away from saving state
// in renderEditable. The gesture callbacks can use the details objects directly
// in callbacks variants that provide them [TapGestureRecognizer.onSecondaryTap]
// vs [TapGestureRecognizer.onSecondaryTapUp] instead of having to track state in
// renderEditable. When this migration is complete we should remove this hack.
// See https://github.com/flutter/flutter/issues/115130.
renderEditable.handleSecondaryTapDown(TapDownDetails(globalPosition: details.globalPosition));
_shouldShowSelectionToolbar = true;
}
/// Handler for [TextSelectionGestureDetector.onDoubleTapDown].
///
/// By default, it selects a word through [RenderEditable.selectWord] if
/// selectionEnabled and shows toolbar if necessary.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onDoubleTapDown], which triggers this
/// callback.
@protected
void onDoubleTapDown(TapDragDownDetails details) {
if (delegate.selectionEnabled) {
renderEditable.selectWord(cause: SelectionChangedCause.doubleTap);
if (shouldShowSelectionToolbar) {
editableText.showToolbar();
}
}
}
// Selects the set of paragraphs in a document that intersect a given range of
// global positions.
void _selectParagraphsInRange({required Offset from, Offset? to, SelectionChangedCause? cause}) {
final TextBoundary paragraphBoundary = ParagraphBoundary(editableText.textEditingValue.text);
_selectTextBoundariesInRange(boundary: paragraphBoundary, from: from, to: to, cause: cause);
}
// Selects the set of lines in a document that intersect a given range of
// global positions.
void _selectLinesInRange({required Offset from, Offset? to, SelectionChangedCause? cause}) {
final TextBoundary lineBoundary = LineBoundary(renderEditable);
_selectTextBoundariesInRange(boundary: lineBoundary, from: from, to: to, cause: cause);
}
// Returns the location of a text boundary at `extent`. When `extent` is at
// the end of the text, returns the previous text boundary's location.
TextRange _moveToTextBoundary(TextPosition extent, TextBoundary textBoundary) {
assert(extent.offset >= 0);
// Use extent.offset - 1 when `extent` is at the end of the text to retrieve
// the previous text boundary's location.
final int start = textBoundary.getLeadingTextBoundaryAt(extent.offset == editableText.textEditingValue.text.length ? extent.offset - 1 : extent.offset) ?? 0;
final int end = textBoundary.getTrailingTextBoundaryAt(extent.offset) ?? editableText.textEditingValue.text.length;
return TextRange(start: start, end: end);
}
// Selects the set of text boundaries in a document that intersect a given
// range of global positions.
//
// The set of text boundaries selected are not strictly bounded by the range
// of global positions.
//
// The first and last endpoints of the selection will always be at the
// beginning and end of a text boundary respectively.
void _selectTextBoundariesInRange({required TextBoundary boundary, required Offset from, Offset? to, SelectionChangedCause? cause}) {
final TextPosition fromPosition = renderEditable.getPositionForPoint(from);
final TextRange fromRange = _moveToTextBoundary(fromPosition, boundary);
final TextPosition toPosition = to == null
? fromPosition
: renderEditable.getPositionForPoint(to);
final TextRange toRange = toPosition == fromPosition
? fromRange
: _moveToTextBoundary(toPosition, boundary);
final bool isFromBoundaryBeforeToBoundary = fromRange.start < toRange.end;
final TextSelection newSelection = isFromBoundaryBeforeToBoundary
? TextSelection(baseOffset: fromRange.start, extentOffset: toRange.end)
: TextSelection(baseOffset: fromRange.end, extentOffset: toRange.start);
editableText.userUpdateTextEditingValue(
editableText.textEditingValue.copyWith(selection: newSelection),
cause,
);
}
/// Handler for [TextSelectionGestureDetector.onTripleTapDown].
///
/// By default, it selects a paragraph if
/// [TextSelectionGestureDetectorBuilderDelegate.selectionEnabled] is true
/// and shows the toolbar if necessary.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onTripleTapDown], which triggers this
/// callback.
@protected
void onTripleTapDown(TapDragDownDetails details) {
if (!delegate.selectionEnabled) {
return;
}
if (renderEditable.maxLines == 1) {
editableText.selectAll(SelectionChangedCause.tap);
} else {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
case TargetPlatform.macOS:
case TargetPlatform.windows:
_selectParagraphsInRange(from: details.globalPosition, cause: SelectionChangedCause.tap);
case TargetPlatform.linux:
_selectLinesInRange(from: details.globalPosition, cause: SelectionChangedCause.tap);
}
}
if (shouldShowSelectionToolbar) {
editableText.showToolbar();
}
}
/// Handler for [TextSelectionGestureDetector.onDragSelectionStart].
///
/// By default, it selects a text position specified in [details].
///
/// See also:
///
/// * [TextSelectionGestureDetector.onDragSelectionStart], which triggers
/// this callback.
@protected
void onDragSelectionStart(TapDragStartDetails details) {
if (!delegate.selectionEnabled) {
return;
}
final PointerDeviceKind? kind = details.kind;
_shouldShowSelectionToolbar = kind == null
|| kind == PointerDeviceKind.touch
|| kind == PointerDeviceKind.stylus;
_dragStartSelection = renderEditable.selection;
_dragStartScrollOffset = _scrollPosition;
_dragStartViewportOffset = renderEditable.offset.pixels;
_dragBeganOnPreviousSelection = _positionOnSelection(details.globalPosition, _dragStartSelection);
if (_TextSelectionGestureDetectorState._getEffectiveConsecutiveTapCount(details.consecutiveTapCount) > 1) {
// Do not set the selection on a consecutive tap and drag.
return;
}
if (_isShiftPressed && renderEditable.selection != null && renderEditable.selection!.isValid) {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
_expandSelection(details.globalPosition, SelectionChangedCause.drag);
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
_extendSelection(details.globalPosition, SelectionChangedCause.drag);
}
} else {
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
// For iOS platforms, a touch drag does not initiate unless the
// editable has focus and the drag began on the previous selection.
assert(_dragBeganOnPreviousSelection != null);
if (renderEditable.hasFocus && _dragBeganOnPreviousSelection!) {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
_showMagnifierIfSupportedByPlatform(details.globalPosition);
}
case null:
}
case TargetPlatform.android:
case TargetPlatform.fuchsia:
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
// For Android, Fuchsia, and iOS platforms, a touch drag
// does not initiate unless the editable has focus.
if (renderEditable.hasFocus) {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
_showMagnifierIfSupportedByPlatform(details.globalPosition);
}
case null:
}
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
}
}
}
/// Handler for [TextSelectionGestureDetector.onDragSelectionUpdate].
///
/// By default, it updates the selection location specified in the provided
/// details objects.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onDragSelectionUpdate], which triggers
/// this callback./lib/src/material/text_field.dart
@protected
void onDragSelectionUpdate(TapDragUpdateDetails details) {
if (!delegate.selectionEnabled) {
return;
}
if (!_isShiftPressed) {
// Adjust the drag start offset for possible viewport offset changes.
final Offset editableOffset = renderEditable.maxLines == 1
? Offset(renderEditable.offset.pixels - _dragStartViewportOffset, 0.0)
: Offset(0.0, renderEditable.offset.pixels - _dragStartViewportOffset);
final double effectiveScrollPosition = _scrollPosition - _dragStartScrollOffset;
final bool scrollingOnVerticalAxis = _scrollDirection == AxisDirection.up || _scrollDirection == AxisDirection.down;
final Offset scrollableOffset = Offset(
!scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
scrollingOnVerticalAxis ? effectiveScrollPosition : 0.0,
);
final Offset dragStartGlobalPosition = details.globalPosition - details.offsetFromOrigin;
// Select word by word.
if (_TextSelectionGestureDetectorState._getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 2) {
renderEditable.selectWordsInRange(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
switch (details.kind) {
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
return _showMagnifierIfSupportedByPlatform(details.globalPosition);
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
case null:
return;
}
}
// Select paragraph-by-paragraph.
if (_TextSelectionGestureDetectorState._getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 3) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
return _selectParagraphsInRange(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
case null:
// Triple tap to drag is not present on these platforms when using
// non-precise pointer devices at the moment.
break;
}
return;
case TargetPlatform.linux:
return _selectLinesInRange(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case TargetPlatform.windows:
case TargetPlatform.macOS:
return _selectParagraphsInRange(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
}
}
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
// With a touch device, nothing should happen, unless there was a double tap, or
// there was a collapsed selection, and the tap/drag position is at the collapsed selection.
// In that case the caret should move with the drag position.
//
// With a mouse device, a drag should select the range from the origin of the drag
// to the current position of the drag.
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
return renderEditable.selectPositionAt(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
assert(_dragBeganOnPreviousSelection != null);
if (renderEditable.hasFocus
&& _dragStartSelection!.isCollapsed
&& _dragBeganOnPreviousSelection!
) {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
return _showMagnifierIfSupportedByPlatform(details.globalPosition);
}
case null:
break;
}
return;
case TargetPlatform.android:
case TargetPlatform.fuchsia:
// With a precise pointer device, such as a mouse, trackpad, or stylus,
// the drag will select the text spanning the origin of the drag to the end of the drag.
// With a touch device, the cursor should move with the drag.
switch (details.kind) {
case PointerDeviceKind.mouse:
case PointerDeviceKind.trackpad:
case PointerDeviceKind.stylus:
case PointerDeviceKind.invertedStylus:
return renderEditable.selectPositionAt(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
case PointerDeviceKind.touch:
case PointerDeviceKind.unknown:
if (renderEditable.hasFocus) {
renderEditable.selectPositionAt(
from: details.globalPosition,
cause: SelectionChangedCause.drag,
);
return _showMagnifierIfSupportedByPlatform(details.globalPosition);
}
case null:
break;
}
return;
case TargetPlatform.macOS:
case TargetPlatform.linux:
case TargetPlatform.windows:
return renderEditable.selectPositionAt(
from: dragStartGlobalPosition - editableOffset - scrollableOffset,
to: details.globalPosition,
cause: SelectionChangedCause.drag,
);
}
}
if (_dragStartSelection!.isCollapsed
|| (defaultTargetPlatform != TargetPlatform.iOS
&& defaultTargetPlatform != TargetPlatform.macOS)) {
return _extendSelection(details.globalPosition, SelectionChangedCause.drag);
}
// If the drag inverts the selection, Mac and iOS revert to the initial
// selection.
final TextSelection selection = editableText.textEditingValue.selection;
final TextPosition nextExtent = renderEditable.getPositionForPoint(details.globalPosition);
final bool isShiftTapDragSelectionForward =
_dragStartSelection!.baseOffset < _dragStartSelection!.extentOffset;
final bool isInverted = isShiftTapDragSelectionForward
? nextExtent.offset < _dragStartSelection!.baseOffset
: nextExtent.offset > _dragStartSelection!.baseOffset;
if (isInverted && selection.baseOffset == _dragStartSelection!.baseOffset) {
editableText.userUpdateTextEditingValue(
editableText.textEditingValue.copyWith(
selection: TextSelection(
baseOffset: _dragStartSelection!.extentOffset,
extentOffset: nextExtent.offset,
),
),
SelectionChangedCause.drag,
);
} else if (!isInverted
&& nextExtent.offset != _dragStartSelection!.baseOffset
&& selection.baseOffset != _dragStartSelection!.baseOffset) {
editableText.userUpdateTextEditingValue(
editableText.textEditingValue.copyWith(
selection: TextSelection(
baseOffset: _dragStartSelection!.baseOffset,
extentOffset: nextExtent.offset,
),
),
SelectionChangedCause.drag,
);
} else {
_extendSelection(details.globalPosition, SelectionChangedCause.drag);
}
}
/// Handler for [TextSelectionGestureDetector.onDragSelectionEnd].
///
/// By default, it cleans up the state used for handling certain
/// built-in behaviors.
///
/// See also:
///
/// * [TextSelectionGestureDetector.onDragSelectionEnd], which triggers this
/// callback.
@protected
void onDragSelectionEnd(TapDragEndDetails details) {
_dragBeganOnPreviousSelection = null;
if (_shouldShowSelectionToolbar && _TextSelectionGestureDetectorState._getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 2) {
editableText.showToolbar();
}
if (_isShiftPressed) {
_dragStartSelection = null;
}
_hideMagnifierIfSupportedByPlatform();
}
/// Returns a [TextSelectionGestureDetector] configured with the handlers
/// provided by this builder.
///
/// The [child] or its subtree should contain an [EditableText] whose key is
/// the [GlobalKey] provided by the [delegate]'s
/// [TextSelectionGestureDetectorBuilderDelegate.editableTextKey].
Widget buildGestureDetector({
Key? key,
HitTestBehavior? behavior,
required Widget child,
}) {
return TextSelectionGestureDetector(
key: key,
onTapTrackStart: onTapTrackStart,
onTapTrackReset: onTapTrackReset,
onTapDown: onTapDown,
onForcePressStart: delegate.forcePressEnabled ? onForcePressStart : null,
onForcePressEnd: delegate.forcePressEnabled ? onForcePressEnd : null,
onSecondaryTap: onSecondaryTap,
onSecondaryTapDown: onSecondaryTapDown,
onSingleTapUp: onSingleTapUp,
onSingleTapCancel: onSingleTapCancel,
onUserTap: onUserTap,
onSingleLongTapStart: onSingleLongTapStart,
onSingleLongTapMoveUpdate: onSingleLongTapMoveUpdate,
onSingleLongTapEnd: onSingleLongTapEnd,
onDoubleTapDown: onDoubleTapDown,
onTripleTapDown: onTripleTapDown,
onDragSelectionStart: onDragSelectionStart,
onDragSelectionUpdate: onDragSelectionUpdate,
onDragSelectionEnd: onDragSelectionEnd,
onUserTapAlwaysCalled: onUserTapAlwaysCalled,
behavior: behavior,
child: child,
);
}
}
/// A gesture detector to respond to non-exclusive event chains for a text field.
///
/// An ordinary [GestureDetector] configured to handle events like tap and
/// double tap will only recognize one or the other. This widget detects both:
/// the first tap and then any subsequent taps that occurs within a time limit
/// after the first.
///
/// See also:
///
/// * [TextField], a Material text field which uses this gesture detector.
/// * [CupertinoTextField], a Cupertino text field which uses this gesture
/// detector.
class TextSelectionGestureDetector extends StatefulWidget {
/// Create a [TextSelectionGestureDetector].
///
/// Multiple callbacks can be called for one sequence of input gesture.
const TextSelectionGestureDetector({
super.key,
this.onTapTrackStart,
this.onTapTrackReset,
this.onTapDown,
this.onForcePressStart,
this.onForcePressEnd,
this.onSecondaryTap,
this.onSecondaryTapDown,
this.onSingleTapUp,
this.onSingleTapCancel,
this.onUserTap,
this.onSingleLongTapStart,
this.onSingleLongTapMoveUpdate,
this.onSingleLongTapEnd,
this.onDoubleTapDown,
this.onTripleTapDown,
this.onDragSelectionStart,
this.onDragSelectionUpdate,
this.onDragSelectionEnd,
this.onUserTapAlwaysCalled = false,
this.behavior,
required this.child,
});
/// {@macro flutter.gestures.selectionrecognizers.BaseTapAndDragGestureRecognizer.onTapTrackStart}
final VoidCallback? onTapTrackStart;
/// {@macro flutter.gestures.selectionrecognizers.BaseTapAndDragGestureRecognizer.onTapTrackReset}
final VoidCallback? onTapTrackReset;
/// Called for every tap down including every tap down that's part of a
/// double click or a long press, except touches that include enough movement
/// to not qualify as taps (e.g. pans and flings).
final GestureTapDragDownCallback? onTapDown;
/// Called when a pointer has tapped down and the force of the pointer has
/// just become greater than [ForcePressGestureRecognizer.startPressure].
final GestureForcePressStartCallback? onForcePressStart;
/// Called when a pointer that had previously triggered [onForcePressStart] is
/// lifted off the screen.
final GestureForcePressEndCallback? onForcePressEnd;
/// Called for a tap event with the secondary mouse button.
final GestureTapCallback? onSecondaryTap;
/// Called for a tap down event with the secondary mouse button.
final GestureTapDownCallback? onSecondaryTapDown;
/// Called for the first tap in a series of taps, consecutive taps do not call
/// this method.
///
/// For example, if the detector was configured with [onTapDown] and
/// [onDoubleTapDown], three quick taps would be recognized as a single tap
/// down, followed by a tap up, then a double tap down, followed by a single tap down.
final GestureTapDragUpCallback? onSingleTapUp;
/// Called for each touch that becomes recognized as a gesture that is not a
/// short tap, such as a long tap or drag. It is called at the moment when
/// another gesture from the touch is recognized.
final GestureCancelCallback? onSingleTapCancel;
/// Called for the first tap in a series of taps when [onUserTapAlwaysCalled] is
/// disabled, which is the default behavior.
///
/// When [onUserTapAlwaysCalled] is enabled, this is called for every tap,
/// including consecutive taps.
final GestureTapCallback? onUserTap;
/// Called for a single long tap that's sustained for longer than
/// [kLongPressTimeout] but not necessarily lifted. Not called for a
/// double-tap-hold, which calls [onDoubleTapDown] instead.
final GestureLongPressStartCallback? onSingleLongTapStart;
/// Called after [onSingleLongTapStart] when the pointer is dragged.
final GestureLongPressMoveUpdateCallback? onSingleLongTapMoveUpdate;
/// Called after [onSingleLongTapStart] when the pointer is lifted.
final GestureLongPressEndCallback? onSingleLongTapEnd;
/// Called after a momentary hold or a short tap that is close in space and
/// time (within [kDoubleTapTimeout]) to a previous short tap.
final GestureTapDragDownCallback? onDoubleTapDown;
/// Called after a momentary hold or a short tap that is close in space and
/// time (within [kDoubleTapTimeout]) to a previous double-tap.
final GestureTapDragDownCallback? onTripleTapDown;
/// Called when a mouse starts dragging to select text.
final GestureTapDragStartCallback? onDragSelectionStart;
/// Called repeatedly as a mouse moves while dragging.
final GestureTapDragUpdateCallback? onDragSelectionUpdate;
/// Called when a mouse that was previously dragging is released.
final GestureTapDragEndCallback? onDragSelectionEnd;
/// Whether [onUserTap] will be called for all taps including consecutive taps.
///
/// Defaults to false, so [onUserTap] is only called for each distinct tap.
final bool onUserTapAlwaysCalled;
/// How this gesture detector should behave during hit testing.
///
/// This defaults to [HitTestBehavior.deferToChild].
final HitTestBehavior? behavior;
/// Child below this widget.
final Widget child;
@override
State<StatefulWidget> createState() => _TextSelectionGestureDetectorState();
}
class _TextSelectionGestureDetectorState extends State<TextSelectionGestureDetector> {
// Converts the details.consecutiveTapCount from a TapAndDrag*Details object,
// which can grow to be infinitely large, to a value between 1 and 3. The value
// that the raw count is converted to is based on the default observed behavior
// on the native platforms.
//
// This method should be used in all instances when details.consecutiveTapCount
// would be used.
static int _getEffectiveConsecutiveTapCount(int rawCount) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
// From observation, these platform's reset their tap count to 0 when
// the number of consecutive taps exceeds 3. For example on Debian Linux
// with GTK, when going past a triple click, on the fourth click the
// selection is moved to the precise click position, on the fifth click
// the word at the position is selected, and on the sixth click the
// paragraph at the position is selected.
return rawCount <= 3 ? rawCount : (rawCount % 3 == 0 ? 3 : rawCount % 3);
case TargetPlatform.iOS:
case TargetPlatform.macOS:
// From observation, these platform's either hold their tap count at 3.
// For example on macOS, when going past a triple click, the selection
// should be retained at the paragraph that was first selected on triple
// click.
return math.min(rawCount, 3);
case TargetPlatform.windows:
// From observation, this platform's consecutive tap actions alternate
// between double click and triple click actions. For example, after a
// triple click has selected a paragraph, on the next click the word at
// the clicked position will be selected, and on the next click the
// paragraph at the position is selected.
return rawCount < 2 ? rawCount : 2 + rawCount % 2;
}
}
void _handleTapTrackStart() {
widget.onTapTrackStart?.call();
}
void _handleTapTrackReset() {
widget.onTapTrackReset?.call();
}
// The down handler is force-run on success of a single tap and optimistically
// run before a long press success.
void _handleTapDown(TapDragDownDetails details) {
widget.onTapDown?.call(details);
// This isn't detected as a double tap gesture in the gesture recognizer
// because it's 2 single taps, each of which may do different things depending
// on whether it's a single tap, the first tap of a double tap, the second
// tap held down, a clean double tap etc.
if (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 2) {
return widget.onDoubleTapDown?.call(details);
}
if (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 3) {
return widget.onTripleTapDown?.call(details);
}
}
void _handleTapUp(TapDragUpDetails details) {
if (_getEffectiveConsecutiveTapCount(details.consecutiveTapCount) == 1) {
widget.onSingleTapUp?.call(details);
widget.onUserTap?.call();
} else if (widget.onUserTapAlwaysCalled) {
widget.onUserTap?.call();
}
}
void _handleTapCancel() {
widget.onSingleTapCancel?.call();
}
void _handleDragStart(TapDragStartDetails details) {
widget.onDragSelectionStart?.call(details);
}
void _handleDragUpdate(TapDragUpdateDetails details) {
widget.onDragSelectionUpdate?.call(details);
}
void _handleDragEnd(TapDragEndDetails details) {
widget.onDragSelectionEnd?.call(details);
}
void _forcePressStarted(ForcePressDetails details) {
widget.onForcePressStart?.call(details);
}
void _forcePressEnded(ForcePressDetails details) {
widget.onForcePressEnd?.call(details);
}
void _handleLongPressStart(LongPressStartDetails details) {
if (widget.onSingleLongTapStart != null) {
widget.onSingleLongTapStart!(details);
}
}
void _handleLongPressMoveUpdate(LongPressMoveUpdateDetails details) {
if (widget.onSingleLongTapMoveUpdate != null) {
widget.onSingleLongTapMoveUpdate!(details);
}
}
void _handleLongPressEnd(LongPressEndDetails details) {
if (widget.onSingleLongTapEnd != null) {
widget.onSingleLongTapEnd!(details);
}
}
@override
Widget build(BuildContext context) {
final Map<Type, GestureRecognizerFactory> gestures = <Type, GestureRecognizerFactory>{};
gestures[TapGestureRecognizer] = GestureRecognizerFactoryWithHandlers<TapGestureRecognizer>(
() => TapGestureRecognizer(debugOwner: this),
(TapGestureRecognizer instance) {
instance
..onSecondaryTap = widget.onSecondaryTap
..onSecondaryTapDown = widget.onSecondaryTapDown;
},
);
if (widget.onSingleLongTapStart != null ||
widget.onSingleLongTapMoveUpdate != null ||
widget.onSingleLongTapEnd != null) {
gestures[LongPressGestureRecognizer] = GestureRecognizerFactoryWithHandlers<LongPressGestureRecognizer>(
() => LongPressGestureRecognizer(debugOwner: this, supportedDevices: <PointerDeviceKind>{ PointerDeviceKind.touch }),
(LongPressGestureRecognizer instance) {
instance
..onLongPressStart = _handleLongPressStart
..onLongPressMoveUpdate = _handleLongPressMoveUpdate
..onLongPressEnd = _handleLongPressEnd;
},
);
}
if (widget.onDragSelectionStart != null ||
widget.onDragSelectionUpdate != null ||
widget.onDragSelectionEnd != null) {
switch (defaultTargetPlatform) {
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.iOS:
gestures[TapAndHorizontalDragGestureRecognizer] = GestureRecognizerFactoryWithHandlers<TapAndHorizontalDragGestureRecognizer>(
() => TapAndHorizontalDragGestureRecognizer(debugOwner: this),
(TapAndHorizontalDragGestureRecognizer instance) {
instance
// Text selection should start from the position of the first pointer
// down event.
..dragStartBehavior = DragStartBehavior.down
..onTapTrackStart = _handleTapTrackStart
..onTapTrackReset = _handleTapTrackReset
..onTapDown = _handleTapDown
..onDragStart = _handleDragStart
..onDragUpdate = _handleDragUpdate
..onDragEnd = _handleDragEnd
..onTapUp = _handleTapUp
..onCancel = _handleTapCancel;
},
);
case TargetPlatform.linux:
case TargetPlatform.macOS:
case TargetPlatform.windows:
gestures[TapAndPanGestureRecognizer] = GestureRecognizerFactoryWithHandlers<TapAndPanGestureRecognizer>(
() => TapAndPanGestureRecognizer(debugOwner: this),
(TapAndPanGestureRecognizer instance) {
instance
// Text selection should start from the position of the first pointer
// down event.
..dragStartBehavior = DragStartBehavior.down
..onTapTrackStart = _handleTapTrackStart
..onTapTrackReset = _handleTapTrackReset
..onTapDown = _handleTapDown
..onDragStart = _handleDragStart
..onDragUpdate = _handleDragUpdate
..onDragEnd = _handleDragEnd
..onTapUp = _handleTapUp
..onCancel = _handleTapCancel;
},
);
}
}
if (widget.onForcePressStart != null || widget.onForcePressEnd != null) {
gestures[ForcePressGestureRecognizer] = GestureRecognizerFactoryWithHandlers<ForcePressGestureRecognizer>(
() => ForcePressGestureRecognizer(debugOwner: this),
(ForcePressGestureRecognizer instance) {
instance
..onStart = widget.onForcePressStart != null ? _forcePressStarted : null
..onEnd = widget.onForcePressEnd != null ? _forcePressEnded : null;
},
);
}
return RawGestureDetector(
gestures: gestures,
excludeFromSemantics: true,
behavior: widget.behavior,
child: widget.child,
);
}
}
/// A [ValueNotifier] whose [value] indicates whether the current contents of
/// the clipboard can be pasted.
///
/// The contents of the clipboard can only be read asynchronously, via
/// [Clipboard.getData], so this maintains a value that can be used
/// synchronously. Call [update] to asynchronously update value if needed.
class ClipboardStatusNotifier extends ValueNotifier<ClipboardStatus> with WidgetsBindingObserver {
/// Create a new ClipboardStatusNotifier.
ClipboardStatusNotifier({
ClipboardStatus value = ClipboardStatus.unknown,
}) : super(value);
bool _disposed = false;
/// Check the [Clipboard] and update [value] if needed.
Future<void> update() async {
if (_disposed) {
return;
}
final bool hasStrings;
try {
hasStrings = await Clipboard.hasStrings();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widget library',
context: ErrorDescription('while checking if the clipboard has strings'),
));
// In the case of an error from the Clipboard API, set the value to
// unknown so that it will try to update again later.
if (_disposed) {
return;
}
value = ClipboardStatus.unknown;
return;
}
final ClipboardStatus nextStatus = hasStrings
? ClipboardStatus.pasteable
: ClipboardStatus.notPasteable;
if (_disposed) {
return;
}
value = nextStatus;
}
@override
void addListener(VoidCallback listener) {
if (!hasListeners) {
WidgetsBinding.instance.addObserver(this);
}
if (value == ClipboardStatus.unknown) {
update();
}
super.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
super.removeListener(listener);
if (!_disposed && !hasListeners) {
WidgetsBinding.instance.removeObserver(this);
}
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
switch (state) {
case AppLifecycleState.resumed:
update();
case AppLifecycleState.detached:
case AppLifecycleState.inactive:
case AppLifecycleState.hidden:
case AppLifecycleState.paused:
// Nothing to do.
break;
}
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
_disposed = true;
super.dispose();
}
}
/// An enumeration of the status of the content on the user's clipboard.
enum ClipboardStatus {
/// The clipboard content can be pasted, such as a String of nonzero length.
pasteable,
/// The status of the clipboard is unknown. Since getting clipboard data is
/// asynchronous (see [Clipboard.getData]), this status often exists while
/// waiting to receive the clipboard contents for the first time.
unknown,
/// The content on the clipboard is not pasteable, such as when it is empty.
notPasteable,
}
/// A [ValueNotifier] whose [value] indicates whether the current device supports the Live Text
/// (OCR) function.
///
/// See also:
/// * [LiveText], where the availability of Live Text input can be obtained.
/// * [LiveTextInputStatus], an enumeration that indicates whether the current device is available
/// for Live Text input.
///
/// Call [update] to asynchronously update [value] if needed.
class LiveTextInputStatusNotifier extends ValueNotifier<LiveTextInputStatus> with WidgetsBindingObserver {
/// Create a new LiveTextStatusNotifier.
LiveTextInputStatusNotifier({
LiveTextInputStatus value = LiveTextInputStatus.unknown,
}) : super(value);
bool _disposed = false;
/// Check the [LiveTextInputStatus] and update [value] if needed.
Future<void> update() async {
if (_disposed) {
return;
}
final bool isLiveTextInputEnabled;
try {
isLiveTextInputEnabled = await LiveText.isLiveTextInputAvailable();
} catch (exception, stack) {
FlutterError.reportError(FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widget library',
context: ErrorDescription('while checking the availability of Live Text input'),
));
// In the case of an error from the Live Text API, set the value to
// unknown so that it will try to update again later.
if (_disposed || value == LiveTextInputStatus.unknown) {
return;
}
value = LiveTextInputStatus.unknown;
return;
}
final LiveTextInputStatus nextStatus = isLiveTextInputEnabled
? LiveTextInputStatus.enabled
: LiveTextInputStatus.disabled;
if (_disposed || nextStatus == value) {
return;
}
value = nextStatus;
}
@override
void addListener(VoidCallback listener) {
if (!hasListeners) {
WidgetsBinding.instance.addObserver(this);
}
if (value == LiveTextInputStatus.unknown) {
update();
}
super.addListener(listener);
}
@override
void removeListener(VoidCallback listener) {
super.removeListener(listener);
if (!_disposed && !hasListeners) {
WidgetsBinding.instance.removeObserver(this);
}
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
switch (state) {
case AppLifecycleState.resumed:
update();
case AppLifecycleState.detached:
case AppLifecycleState.inactive:
case AppLifecycleState.paused:
case AppLifecycleState.hidden:
// Nothing to do.
}
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
_disposed = true;
super.dispose();
}
}
/// An enumeration that indicates whether the current device is available for Live Text input.
///
/// See also:
/// * [LiveText], where the availability of Live Text input can be obtained.
enum LiveTextInputStatus {
/// This device supports Live Text input currently.
enabled,
/// The status of the Live Text input is unknown. Since getting the Live Text input availability
/// is asynchronous (see [LiveText.isLiveTextInputAvailable]), this status often exists while
/// waiting to receive the status value for the first time.
unknown,
/// The current device doesn't support Live Text input.
disabled,
}
// TODO(justinmc): Deprecate this after TextSelectionControls.buildToolbar is
// deleted, when users should migrate back to TextSelectionControls.buildHandle.
// See https://github.com/flutter/flutter/pull/124262
/// [TextSelectionControls] that specifically do not manage the toolbar in order
/// to leave that to [EditableText.contextMenuBuilder].
mixin TextSelectionHandleControls on TextSelectionControls {
@override
Widget buildToolbar(
BuildContext context,
Rect globalEditableRegion,
double textLineHeight,
Offset selectionMidpoint,
List<TextSelectionPoint> endpoints,
TextSelectionDelegate delegate,
ValueListenable<ClipboardStatus>? clipboardStatus,
Offset? lastSecondaryTapDownPosition,
) => const SizedBox.shrink();
@override
bool canCut(TextSelectionDelegate delegate) => false;
@override
bool canCopy(TextSelectionDelegate delegate) => false;
@override
bool canPaste(TextSelectionDelegate delegate) => false;
@override
bool canSelectAll(TextSelectionDelegate delegate) => false;
@override
void handleCut(TextSelectionDelegate delegate, [ClipboardStatusNotifier? clipboardStatus]) {}
@override
void handleCopy(TextSelectionDelegate delegate, [ClipboardStatusNotifier? clipboardStatus]) {}
@override
Future<void> handlePaste(TextSelectionDelegate delegate) async {}
@override
void handleSelectAll(TextSelectionDelegate delegate) {}
}
| flutter/packages/flutter/lib/src/widgets/text_selection.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/text_selection.dart",
"repo_id": "flutter",
"token_count": 47426
} | 714 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:collection' show HashMap;
import 'dart:convert';
import 'dart:developer' as developer;
import 'dart:math' as math;
import 'dart:ui' as ui
show
ClipOp,
FlutterView,
Image,
ImageByteFormat,
Paragraph,
Picture,
PictureRecorder,
PointMode,
SceneBuilder,
Vertices;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:meta/meta_meta.dart';
import 'basic.dart';
import 'binding.dart';
import 'debug.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'service_extensions.dart';
import 'view.dart';
/// Signature for the builder callback used by
/// [WidgetInspector.selectButtonBuilder].
typedef InspectorSelectButtonBuilder = Widget Function(BuildContext context, VoidCallback onPressed);
/// Signature for a method that registers the service extension `callback` with
/// the given `name`.
///
/// Used as argument to [WidgetInspectorService.initServiceExtensions]. The
/// [BindingBase.registerServiceExtension] implements this signature.
typedef RegisterServiceExtensionCallback = void Function({
required String name,
required ServiceExtensionCallback callback,
});
/// A layer that mimics the behavior of another layer.
///
/// A proxy layer is used for cases where a layer needs to be placed into
/// multiple trees of layers.
class _ProxyLayer extends Layer {
_ProxyLayer(this._layer);
final Layer _layer;
@override
void addToScene(ui.SceneBuilder builder) {
_layer.addToScene(builder);
}
@override
@protected
bool findAnnotations<S extends Object>(
AnnotationResult<S> result,
Offset localPosition, {
required bool onlyFirst,
}) {
return _layer.findAnnotations(result, localPosition, onlyFirst: onlyFirst);
}
}
/// A [Canvas] that multicasts all method calls to a main canvas and a
/// secondary screenshot canvas so that a screenshot can be recorded at the same
/// time as performing a normal paint.
class _MulticastCanvas implements Canvas {
_MulticastCanvas({
required Canvas main,
required Canvas screenshot,
}) : _main = main,
_screenshot = screenshot;
final Canvas _main;
final Canvas _screenshot;
@override
void clipPath(Path path, { bool doAntiAlias = true }) {
_main.clipPath(path, doAntiAlias: doAntiAlias);
_screenshot.clipPath(path, doAntiAlias: doAntiAlias);
}
@override
void clipRRect(RRect rrect, { bool doAntiAlias = true }) {
_main.clipRRect(rrect, doAntiAlias: doAntiAlias);
_screenshot.clipRRect(rrect, doAntiAlias: doAntiAlias);
}
@override
void clipRect(Rect rect, { ui.ClipOp clipOp = ui.ClipOp.intersect, bool doAntiAlias = true }) {
_main.clipRect(rect, clipOp: clipOp, doAntiAlias: doAntiAlias);
_screenshot.clipRect(rect, clipOp: clipOp, doAntiAlias: doAntiAlias);
}
@override
void drawArc(Rect rect, double startAngle, double sweepAngle, bool useCenter, Paint paint) {
_main.drawArc(rect, startAngle, sweepAngle, useCenter, paint);
_screenshot.drawArc(rect, startAngle, sweepAngle, useCenter, paint);
}
@override
void drawAtlas(ui.Image atlas, List<RSTransform> transforms, List<Rect> rects, List<Color>? colors, BlendMode? blendMode, Rect? cullRect, Paint paint) {
_main.drawAtlas(atlas, transforms, rects, colors, blendMode, cullRect, paint);
_screenshot.drawAtlas(atlas, transforms, rects, colors, blendMode, cullRect, paint);
}
@override
void drawCircle(Offset c, double radius, Paint paint) {
_main.drawCircle(c, radius, paint);
_screenshot.drawCircle(c, radius, paint);
}
@override
void drawColor(Color color, BlendMode blendMode) {
_main.drawColor(color, blendMode);
_screenshot.drawColor(color, blendMode);
}
@override
void drawDRRect(RRect outer, RRect inner, Paint paint) {
_main.drawDRRect(outer, inner, paint);
_screenshot.drawDRRect(outer, inner, paint);
}
@override
void drawImage(ui.Image image, Offset p, Paint paint) {
_main.drawImage(image, p, paint);
_screenshot.drawImage(image, p, paint);
}
@override
void drawImageNine(ui.Image image, Rect center, Rect dst, Paint paint) {
_main.drawImageNine(image, center, dst, paint);
_screenshot.drawImageNine(image, center, dst, paint);
}
@override
void drawImageRect(ui.Image image, Rect src, Rect dst, Paint paint) {
_main.drawImageRect(image, src, dst, paint);
_screenshot.drawImageRect(image, src, dst, paint);
}
@override
void drawLine(Offset p1, Offset p2, Paint paint) {
_main.drawLine(p1, p2, paint);
_screenshot.drawLine(p1, p2, paint);
}
@override
void drawOval(Rect rect, Paint paint) {
_main.drawOval(rect, paint);
_screenshot.drawOval(rect, paint);
}
@override
void drawPaint(Paint paint) {
_main.drawPaint(paint);
_screenshot.drawPaint(paint);
}
@override
void drawParagraph(ui.Paragraph paragraph, Offset offset) {
_main.drawParagraph(paragraph, offset);
_screenshot.drawParagraph(paragraph, offset);
}
@override
void drawPath(Path path, Paint paint) {
_main.drawPath(path, paint);
_screenshot.drawPath(path, paint);
}
@override
void drawPicture(ui.Picture picture) {
_main.drawPicture(picture);
_screenshot.drawPicture(picture);
}
@override
void drawPoints(ui.PointMode pointMode, List<Offset> points, Paint paint) {
_main.drawPoints(pointMode, points, paint);
_screenshot.drawPoints(pointMode, points, paint);
}
@override
void drawRRect(RRect rrect, Paint paint) {
_main.drawRRect(rrect, paint);
_screenshot.drawRRect(rrect, paint);
}
@override
void drawRawAtlas(ui.Image atlas, Float32List rstTransforms, Float32List rects, Int32List? colors, BlendMode? blendMode, Rect? cullRect, Paint paint) {
_main.drawRawAtlas(atlas, rstTransforms, rects, colors, blendMode, cullRect, paint);
_screenshot.drawRawAtlas(atlas, rstTransforms, rects, colors, blendMode, cullRect, paint);
}
@override
void drawRawPoints(ui.PointMode pointMode, Float32List points, Paint paint) {
_main.drawRawPoints(pointMode, points, paint);
_screenshot.drawRawPoints(pointMode, points, paint);
}
@override
void drawRect(Rect rect, Paint paint) {
_main.drawRect(rect, paint);
_screenshot.drawRect(rect, paint);
}
@override
void drawShadow(Path path, Color color, double elevation, bool transparentOccluder) {
_main.drawShadow(path, color, elevation, transparentOccluder);
_screenshot.drawShadow(path, color, elevation, transparentOccluder);
}
@override
void drawVertices(ui.Vertices vertices, BlendMode blendMode, Paint paint) {
_main.drawVertices(vertices, blendMode, paint);
_screenshot.drawVertices(vertices, blendMode, paint);
}
@override
int getSaveCount() {
// The main canvas is used instead of the screenshot canvas as the main
// canvas is guaranteed to be consistent with the canvas expected by the
// normal paint pipeline so any logic depending on getSaveCount() will
// behave the same as for the regular paint pipeline.
return _main.getSaveCount();
}
@override
void restore() {
_main.restore();
_screenshot.restore();
}
@override
void rotate(double radians) {
_main.rotate(radians);
_screenshot.rotate(radians);
}
@override
void save() {
_main.save();
_screenshot.save();
}
@override
void saveLayer(Rect? bounds, Paint paint) {
_main.saveLayer(bounds, paint);
_screenshot.saveLayer(bounds, paint);
}
@override
void scale(double sx, [ double? sy ]) {
_main.scale(sx, sy);
_screenshot.scale(sx, sy);
}
@override
void skew(double sx, double sy) {
_main.skew(sx, sy);
_screenshot.skew(sx, sy);
}
@override
void transform(Float64List matrix4) {
_main.transform(matrix4);
_screenshot.transform(matrix4);
}
@override
void translate(double dx, double dy) {
_main.translate(dx, dy);
_screenshot.translate(dx, dy);
}
@override
dynamic noSuchMethod(Invocation invocation) {
super.noSuchMethod(invocation);
}
}
Rect _calculateSubtreeBoundsHelper(RenderObject object, Matrix4 transform) {
Rect bounds = MatrixUtils.transformRect(transform, object.semanticBounds);
object.visitChildren((RenderObject child) {
final Matrix4 childTransform = transform.clone();
object.applyPaintTransform(child, childTransform);
Rect childBounds = _calculateSubtreeBoundsHelper(child, childTransform);
final Rect? paintClip = object.describeApproximatePaintClip(child);
if (paintClip != null) {
final Rect transformedPaintClip = MatrixUtils.transformRect(
transform,
paintClip,
);
childBounds = childBounds.intersect(transformedPaintClip);
}
if (childBounds.isFinite && !childBounds.isEmpty) {
bounds = bounds.isEmpty ? childBounds : bounds.expandToInclude(childBounds);
}
});
return bounds;
}
/// Calculate bounds for a render object and all of its descendants.
Rect _calculateSubtreeBounds(RenderObject object) {
return _calculateSubtreeBoundsHelper(object, Matrix4.identity());
}
/// A layer that omits its own offset when adding children to the scene so that
/// screenshots render to the scene in the local coordinate system of the layer.
class _ScreenshotContainerLayer extends OffsetLayer {
@override
void addToScene(ui.SceneBuilder builder) {
addChildrenToScene(builder);
}
}
/// Data shared between nested [_ScreenshotPaintingContext] objects recording
/// a screenshot.
class _ScreenshotData {
_ScreenshotData({
required this.target,
}) : containerLayer = _ScreenshotContainerLayer() {
// TODO(polina-c): stop duplicating code across disposables
// https://github.com/flutter/flutter/issues/137435
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectCreated(
library: 'package:flutter/widgets.dart',
className: '$_ScreenshotData',
object: this,
);
}
}
/// Target to take a screenshot of.
final RenderObject target;
/// Root of the layer tree containing the screenshot.
final OffsetLayer containerLayer;
/// Whether the screenshot target has already been found in the render tree.
bool foundTarget = false;
/// Whether paint operations should record to the screenshot.
///
/// At least one of [includeInScreenshot] and [includeInRegularContext] must
/// be true.
bool includeInScreenshot = false;
/// Whether paint operations should record to the regular context.
///
/// This should only be set to false before paint operations that should only
/// apply to the screenshot such rendering debug information about the
/// [target].
///
/// At least one of [includeInScreenshot] and [includeInRegularContext] must
/// be true.
bool includeInRegularContext = true;
/// Offset of the screenshot corresponding to the offset [target] was given as
/// part of the regular paint.
Offset get screenshotOffset {
assert(foundTarget);
return containerLayer.offset;
}
set screenshotOffset(Offset offset) {
containerLayer.offset = offset;
}
/// Releases allocated resources.
@mustCallSuper
void dispose() {
if (kFlutterMemoryAllocationsEnabled) {
FlutterMemoryAllocations.instance.dispatchObjectDisposed(object: this);
}
containerLayer.dispose();
}
}
/// A place to paint to build screenshots of [RenderObject]s.
///
/// Requires that the render objects have already painted successfully as part
/// of the regular rendering pipeline.
/// This painting context behaves the same as standard [PaintingContext] with
/// instrumentation added to compute a screenshot of a specified [RenderObject]
/// added. To correctly mimic the behavior of the regular rendering pipeline, the
/// full subtree of the first [RepaintBoundary] ancestor of the specified
/// [RenderObject] will also be rendered rather than just the subtree of the
/// render object.
class _ScreenshotPaintingContext extends PaintingContext {
_ScreenshotPaintingContext({
required ContainerLayer containerLayer,
required Rect estimatedBounds,
required _ScreenshotData screenshotData,
}) : _data = screenshotData,
super(containerLayer, estimatedBounds);
final _ScreenshotData _data;
// Recording state
PictureLayer? _screenshotCurrentLayer;
ui.PictureRecorder? _screenshotRecorder;
Canvas? _screenshotCanvas;
_MulticastCanvas? _multicastCanvas;
@override
Canvas get canvas {
if (_data.includeInScreenshot) {
if (_screenshotCanvas == null) {
_startRecordingScreenshot();
}
assert(_screenshotCanvas != null);
return _data.includeInRegularContext ? _multicastCanvas! : _screenshotCanvas!;
} else {
assert(_data.includeInRegularContext);
return super.canvas;
}
}
bool get _isScreenshotRecording {
final bool hasScreenshotCanvas = _screenshotCanvas != null;
assert(() {
if (hasScreenshotCanvas) {
assert(_screenshotCurrentLayer != null);
assert(_screenshotRecorder != null);
assert(_screenshotCanvas != null);
} else {
assert(_screenshotCurrentLayer == null);
assert(_screenshotRecorder == null);
assert(_screenshotCanvas == null);
}
return true;
}());
return hasScreenshotCanvas;
}
void _startRecordingScreenshot() {
assert(_data.includeInScreenshot);
assert(!_isScreenshotRecording);
_screenshotCurrentLayer = PictureLayer(estimatedBounds);
_screenshotRecorder = ui.PictureRecorder();
_screenshotCanvas = Canvas(_screenshotRecorder!);
_data.containerLayer.append(_screenshotCurrentLayer!);
if (_data.includeInRegularContext) {
_multicastCanvas = _MulticastCanvas(
main: super.canvas,
screenshot: _screenshotCanvas!,
);
} else {
_multicastCanvas = null;
}
}
@override
void stopRecordingIfNeeded() {
super.stopRecordingIfNeeded();
_stopRecordingScreenshotIfNeeded();
}
void _stopRecordingScreenshotIfNeeded() {
if (!_isScreenshotRecording) {
return;
}
// There is no need to ever draw repaint rainbows as part of the screenshot.
_screenshotCurrentLayer!.picture = _screenshotRecorder!.endRecording();
_screenshotCurrentLayer = null;
_screenshotRecorder = null;
_multicastCanvas = null;
_screenshotCanvas = null;
}
@override
void appendLayer(Layer layer) {
if (_data.includeInRegularContext) {
super.appendLayer(layer);
if (_data.includeInScreenshot) {
assert(!_isScreenshotRecording);
// We must use a proxy layer here as the layer is already attached to
// the regular layer tree.
_data.containerLayer.append(_ProxyLayer(layer));
}
} else {
// Only record to the screenshot.
assert(!_isScreenshotRecording);
assert(_data.includeInScreenshot);
layer.remove();
_data.containerLayer.append(layer);
return;
}
}
@override
PaintingContext createChildContext(ContainerLayer childLayer, Rect bounds) {
if (_data.foundTarget) {
// We have already found the screenshotTarget in the layer tree
// so we can optimize and use a standard PaintingContext.
return super.createChildContext(childLayer, bounds);
} else {
return _ScreenshotPaintingContext(
containerLayer: childLayer,
estimatedBounds: bounds,
screenshotData: _data,
);
}
}
@override
void paintChild(RenderObject child, Offset offset) {
final bool isScreenshotTarget = identical(child, _data.target);
if (isScreenshotTarget) {
assert(!_data.includeInScreenshot);
assert(!_data.foundTarget);
_data.foundTarget = true;
_data.screenshotOffset = offset;
_data.includeInScreenshot = true;
}
super.paintChild(child, offset);
if (isScreenshotTarget) {
_stopRecordingScreenshotIfNeeded();
_data.includeInScreenshot = false;
}
}
/// Captures an image of the current state of [renderObject] and its children.
///
/// The returned [ui.Image] has uncompressed raw RGBA bytes, will be offset
/// by the top-left corner of [renderBounds], and have dimensions equal to the
/// size of [renderBounds] multiplied by [pixelRatio].
///
/// To use [toImage], the render object must have gone through the paint phase
/// (i.e. [debugNeedsPaint] must be false).
///
/// The [pixelRatio] describes the scale between the logical pixels and the
/// size of the output image. It is independent of the
/// [window.devicePixelRatio] for the device, so specifying 1.0 (the default)
/// will give you a 1:1 mapping between logical pixels and the output pixels
/// in the image.
///
/// The [debugPaint] argument specifies whether the image should include the
/// output of [RenderObject.debugPaint] for [renderObject] with
/// [debugPaintSizeEnabled] set to true. Debug paint information is not
/// included for the children of [renderObject] so that it is clear precisely
/// which object the debug paint information references.
///
/// See also:
///
/// * [RenderRepaintBoundary.toImage] for a similar API for [RenderObject]s
/// that are repaint boundaries that can be used outside of the inspector.
/// * [OffsetLayer.toImage] for a similar API at the layer level.
/// * [dart:ui.Scene.toImage] for more information about the image returned.
static Future<ui.Image> toImage(
RenderObject renderObject,
Rect renderBounds, {
double pixelRatio = 1.0,
bool debugPaint = false,
}) async {
RenderObject repaintBoundary = renderObject;
while (!repaintBoundary.isRepaintBoundary) {
repaintBoundary = repaintBoundary.parent!;
}
final _ScreenshotData data = _ScreenshotData(target: renderObject);
final _ScreenshotPaintingContext context = _ScreenshotPaintingContext(
containerLayer: repaintBoundary.debugLayer!,
estimatedBounds: repaintBoundary.paintBounds,
screenshotData: data,
);
if (identical(renderObject, repaintBoundary)) {
// Painting the existing repaint boundary to the screenshot is sufficient.
// We don't just take a direct screenshot of the repaint boundary as we
// want to capture debugPaint information as well.
data.containerLayer.append(_ProxyLayer(repaintBoundary.debugLayer!));
data.foundTarget = true;
final OffsetLayer offsetLayer = repaintBoundary.debugLayer! as OffsetLayer;
data.screenshotOffset = offsetLayer.offset;
} else {
// Repaint everything under the repaint boundary.
// We call debugInstrumentRepaintCompositedChild instead of paintChild as
// we need to force everything under the repaint boundary to repaint.
PaintingContext.debugInstrumentRepaintCompositedChild(
repaintBoundary,
customContext: context,
);
}
// The check that debugPaintSizeEnabled is false exists to ensure we only
// call debugPaint when it wasn't already called.
if (debugPaint && !debugPaintSizeEnabled) {
data.includeInRegularContext = false;
// Existing recording may be to a canvas that draws to both the normal and
// screenshot canvases.
context.stopRecordingIfNeeded();
assert(data.foundTarget);
data.includeInScreenshot = true;
debugPaintSizeEnabled = true;
try {
renderObject.debugPaint(context, data.screenshotOffset);
} finally {
debugPaintSizeEnabled = false;
context.stopRecordingIfNeeded();
}
}
// We must build the regular scene before we can build the screenshot
// scene as building the screenshot scene assumes addToScene has already
// been called successfully for all layers in the regular scene.
repaintBoundary.debugLayer!.buildScene(ui.SceneBuilder());
final ui.Image image;
try {
image = await data.containerLayer.toImage(renderBounds, pixelRatio: pixelRatio);
} finally {
data.dispose();
}
return image;
}
}
/// A class describing a step along a path through a tree of [DiagnosticsNode]
/// objects.
///
/// This class is used to bundle all data required to display the tree with just
/// the nodes along a path expanded into a single JSON payload.
class _DiagnosticsPathNode {
/// Creates a full description of a step in a path through a tree of
/// [DiagnosticsNode] objects.
_DiagnosticsPathNode({
required this.node,
required this.children,
this.childIndex,
});
/// Node at the point in the path this [_DiagnosticsPathNode] is describing.
final DiagnosticsNode node;
/// Children of the [node] being described.
///
/// This value is cached instead of relying on `node.getChildren()` as that
/// method call might create new [DiagnosticsNode] objects for each child
/// and we would prefer to use the identical [DiagnosticsNode] for each time
/// a node exists in the path.
final List<DiagnosticsNode> children;
/// Index of the child that the path continues on.
///
/// Equal to null if the path does not continue.
final int? childIndex;
}
List<_DiagnosticsPathNode>? _followDiagnosticableChain(
List<Diagnosticable> chain, {
String? name,
DiagnosticsTreeStyle? style,
}) {
final List<_DiagnosticsPathNode> path = <_DiagnosticsPathNode>[];
if (chain.isEmpty) {
return path;
}
DiagnosticsNode diagnostic = chain.first.toDiagnosticsNode(name: name, style: style);
for (int i = 1; i < chain.length; i += 1) {
final Diagnosticable target = chain[i];
bool foundMatch = false;
final List<DiagnosticsNode> children = diagnostic.getChildren();
for (int j = 0; j < children.length; j += 1) {
final DiagnosticsNode child = children[j];
if (child.value == target) {
foundMatch = true;
path.add(_DiagnosticsPathNode(
node: diagnostic,
children: children,
childIndex: j,
));
diagnostic = child;
break;
}
}
assert(foundMatch);
}
path.add(_DiagnosticsPathNode(node: diagnostic, children: diagnostic.getChildren()));
return path;
}
/// Signature for the selection change callback used by
/// [WidgetInspectorService.selectionChangedCallback].
typedef InspectorSelectionChangedCallback = void Function();
/// Structure to help reference count Dart objects referenced by a GUI tool
/// using [WidgetInspectorService].
///
/// Does not hold the object from garbage collection.
@visibleForTesting
class InspectorReferenceData {
/// Creates an instance of [InspectorReferenceData].
InspectorReferenceData(Object object, this.id) {
// These types are not supported by [WeakReference].
// See https://api.dart.dev/stable/3.0.2/dart-core/WeakReference-class.html
if (object is String || object is num || object is bool) {
_value = object;
return;
}
_ref = WeakReference<Object>(object);
}
WeakReference<Object>? _ref;
Object? _value;
/// The id of the object in the widget inspector records.
final String id;
/// The number of times the object has been referenced.
int count = 1;
/// The value.
Object? get value => _ref?.target ?? _value;
}
// Production implementation of [WidgetInspectorService].
class _WidgetInspectorService with WidgetInspectorService {
_WidgetInspectorService() {
selection.addListener(() {
if (selectionChangedCallback != null) {
selectionChangedCallback!();
}
});
}
}
/// Service used by GUI tools to interact with the [WidgetInspector].
///
/// Calls to this object are typically made from GUI tools such as the [Flutter
/// IntelliJ Plugin](https://github.com/flutter/flutter-intellij/blob/master/README.md)
/// using the [Dart VM Service](https://github.com/dart-lang/sdk/blob/main/runtime/vm/service/service.md).
/// This class uses its own object id and manages object lifecycles itself
/// instead of depending on the [object ids](https://github.com/dart-lang/sdk/blob/main/runtime/vm/service/service.md#getobject)
/// specified by the VM Service Protocol because the VM Service Protocol ids
/// expire unpredictably. Object references are tracked in groups so that tools
/// that clients can use dereference all objects in a group with a single
/// operation making it easier to avoid memory leaks.
///
/// All methods in this class are appropriate to invoke from debugging tools
/// using the VM service protocol to evaluate Dart expressions of the
/// form `WidgetInspectorService.instance.methodName(arg1, arg2, ...)`. If you
/// make changes to any instance method of this class you need to verify that
/// the [Flutter IntelliJ Plugin](https://github.com/flutter/flutter-intellij/blob/master/README.md)
/// widget inspector support still works with the changes.
///
/// All methods returning String values return JSON.
mixin WidgetInspectorService {
/// Ring of cached JSON values to prevent JSON from being garbage
/// collected before it can be requested over the VM service protocol.
final List<String?> _serializeRing = List<String?>.filled(20, null);
int _serializeRingIndex = 0;
/// The current [WidgetInspectorService].
static WidgetInspectorService get instance => _instance;
static WidgetInspectorService _instance = _WidgetInspectorService();
/// Whether the inspector is in select mode.
///
/// In select mode, pointer interactions trigger widget selection instead of
/// normal interactions. Otherwise the previously selected widget is
/// highlighted but the application can be interacted with normally.
@visibleForTesting
final ValueNotifier<bool> isSelectMode = ValueNotifier<bool>(true);
@protected
static set instance(WidgetInspectorService instance) {
_instance = instance;
}
static bool _debugServiceExtensionsRegistered = false;
/// Ground truth tracking what object(s) are currently selected used by both
/// GUI tools such as the Flutter IntelliJ Plugin and the [WidgetInspector]
/// displayed on the device.
final InspectorSelection selection = InspectorSelection();
/// Callback typically registered by the [WidgetInspector] to receive
/// notifications when [selection] changes.
///
/// The Flutter IntelliJ Plugin does not need to listen for this event as it
/// instead listens for `dart:developer` `inspect` events which also trigger
/// when the inspection target changes on device.
InspectorSelectionChangedCallback? selectionChangedCallback;
/// The VM service protocol does not keep alive object references so this
/// class needs to manually manage groups of objects that should be kept
/// alive.
final Map<String, Set<InspectorReferenceData>> _groups = <String, Set<InspectorReferenceData>>{};
final Map<String, InspectorReferenceData> _idToReferenceData = <String, InspectorReferenceData>{};
final WeakMap<Object, String> _objectToId = WeakMap<Object, String>();
int _nextId = 0;
/// The pubRootDirectories that are currently configured for the widget inspector.
List<String>? _pubRootDirectories;
/// Memoization for [_isLocalCreationLocation].
final HashMap<String, bool> _isLocalCreationCache = HashMap<String, bool>();
bool _trackRebuildDirtyWidgets = false;
bool _trackRepaintWidgets = false;
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name").
///
/// The given callback is called when the extension method is called. The
/// callback must return a value that can be converted to JSON using
/// `json.encode()` (see [JsonEncoder]). The return value is stored as a
/// property named `result` in the JSON. In case of failure, the failure is
/// reported to the remote caller and is dumped to the logs.
@protected
void registerServiceExtension({
required String name,
required ServiceExtensionCallback callback,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerExtension(
name: 'inspector.$name',
callback: callback,
);
}
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name"), which takes no arguments.
void _registerSignalServiceExtension({
required String name,
required FutureOr<Object?> Function() callback,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerServiceExtension(
name: name,
callback: (Map<String, String> parameters) async {
return <String, Object?>{'result': await callback()};
},
registerExtension: registerExtension,
);
}
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name"), which takes a single optional argument
/// "objectGroup" specifying what group is used to manage lifetimes of
/// object references in the returned JSON (see [disposeGroup]).
/// If "objectGroup" is omitted, the returned JSON will not include any object
/// references to avoid leaking memory.
void _registerObjectGroupServiceExtension({
required String name,
required FutureOr<Object?> Function(String objectGroup) callback,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerServiceExtension(
name: name,
callback: (Map<String, String> parameters) async {
return <String, Object?>{'result': await callback(parameters['objectGroup']!)};
},
registerExtension: registerExtension,
);
}
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name"), which takes a single argument
/// "enabled" which can have the value "true" or the value "false"
/// or can be omitted to read the current value. (Any value other
/// than "true" is considered equivalent to "false". Other arguments
/// are ignored.)
///
/// Calls the `getter` callback to obtain the value when
/// responding to the service extension method being called.
///
/// Calls the `setter` callback with the new value when the
/// service extension method is called with a new value.
void _registerBoolServiceExtension({
required String name,
required AsyncValueGetter<bool> getter,
required AsyncValueSetter<bool> setter,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerServiceExtension(
name: name,
callback: (Map<String, String> parameters) async {
if (parameters.containsKey('enabled')) {
final bool value = parameters['enabled'] == 'true';
await setter(value);
_postExtensionStateChangedEvent(name, value);
}
return <String, dynamic>{'enabled': await getter() ? 'true' : 'false'};
},
registerExtension: registerExtension,
);
}
/// Sends an event when a service extension's state is changed.
///
/// Clients should listen for this event to stay aware of the current service
/// extension state. Any service extension that manages a state should call
/// this method on state change.
///
/// `value` reflects the newly updated service extension value.
///
/// This will be called automatically for service extensions registered via
/// [registerBoolServiceExtension].
void _postExtensionStateChangedEvent(String name, Object? value) {
postEvent(
'Flutter.ServiceExtensionStateChanged',
<String, Object?>{
'extension': 'ext.flutter.inspector.$name',
'value': value,
},
);
}
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name") which takes an optional parameter named
/// "arg" and a required parameter named "objectGroup" used to control the
/// lifetimes of object references in the returned JSON (see [disposeGroup]).
void _registerServiceExtensionWithArg({
required String name,
required FutureOr<Object?> Function(String? objectId, String objectGroup) callback,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerServiceExtension(
name: name,
callback: (Map<String, String> parameters) async {
assert(parameters.containsKey('objectGroup'));
return <String, Object?>{
'result': await callback(parameters['arg'], parameters['objectGroup']!),
};
},
registerExtension: registerExtension,
);
}
/// Registers a service extension method with the given name (full
/// name "ext.flutter.inspector.name"), that takes arguments
/// "arg0", "arg1", "arg2", ..., "argn".
void _registerServiceExtensionVarArgs({
required String name,
required FutureOr<Object?> Function(List<String> args) callback,
required RegisterServiceExtensionCallback registerExtension,
}) {
registerServiceExtension(
name: name,
callback: (Map<String, String> parameters) async {
final List<String> args = <String>[];
int index = 0;
while (true) {
final String name = 'arg$index';
if (parameters.containsKey(name)) {
args.add(parameters[name]!);
} else {
break;
}
index++;
}
// Verify that the only arguments other than perhaps 'isolateId' are
// arguments we have already handled.
assert(index == parameters.length || (index == parameters.length - 1 && parameters.containsKey('isolateId')));
return <String, Object?>{'result': await callback(args)};
},
registerExtension: registerExtension,
);
}
/// Cause the entire tree to be rebuilt. This is used by development tools
/// when the application code has changed and is being hot-reloaded, to cause
/// the widget tree to pick up any changed implementations.
///
/// This is expensive and should not be called except during development.
@protected
Future<void> forceRebuild() {
final WidgetsBinding binding = WidgetsBinding.instance;
if (binding.rootElement != null) {
binding.buildOwner!.reassemble(binding.rootElement!);
return binding.endOfFrame;
}
return Future<void>.value();
}
static const String _consoleObjectGroup = 'console-group';
int _errorsSinceReload = 0;
void _reportStructuredError(FlutterErrorDetails details) {
final Map<String, Object?> errorJson = _nodeToJson(
details.toDiagnosticsNode(),
InspectorSerializationDelegate(
groupName: _consoleObjectGroup,
subtreeDepth: 5,
includeProperties: true,
maxDescendantsTruncatableNode: 5,
service: this,
),
)!;
errorJson['errorsSinceReload'] = _errorsSinceReload;
if (_errorsSinceReload == 0) {
errorJson['renderedErrorText'] = TextTreeRenderer(
wrapWidthProperties: FlutterError.wrapWidth,
maxDescendentsTruncatableNode: 5,
).render(details.toDiagnosticsNode(style: DiagnosticsTreeStyle.error)).trimRight();
} else {
errorJson['renderedErrorText'] = 'Another exception was thrown: ${details.summary}';
}
_errorsSinceReload += 1;
postEvent('Flutter.Error', errorJson);
}
/// Resets the count of errors since the last hot reload.
///
/// This data is sent to clients as part of the 'Flutter.Error' service
/// protocol event. Clients may choose to display errors received after the
/// first error differently.
void _resetErrorCount() {
_errorsSinceReload = 0;
}
/// Whether structured errors are enabled.
///
/// Structured errors provide semantic information that can be used by IDEs
/// to enhance the display of errors with rich formatting.
bool isStructuredErrorsEnabled() {
// This is a debug mode only feature and will default to false for
// profile mode.
bool enabled = false;
assert(() {
// TODO(kenz): add support for structured errors on the web.
enabled = const bool.fromEnvironment('flutter.inspector.structuredErrors', defaultValue: !kIsWeb);
return true;
}());
return enabled;
}
/// Called to register service extensions.
///
/// See also:
///
/// * <https://github.com/dart-lang/sdk/blob/main/runtime/vm/service/service.md#rpcs-requests-and-responses>
/// * [BindingBase.initServiceExtensions], which explains when service
/// extensions can be used.
void initServiceExtensions(RegisterServiceExtensionCallback registerExtension) {
final FlutterExceptionHandler defaultExceptionHandler = FlutterError.presentError;
if (isStructuredErrorsEnabled()) {
FlutterError.presentError = _reportStructuredError;
}
assert(!_debugServiceExtensionsRegistered);
assert(() {
_debugServiceExtensionsRegistered = true;
return true;
}());
SchedulerBinding.instance.addPersistentFrameCallback(_onFrameStart);
_registerBoolServiceExtension(
name: WidgetInspectorServiceExtensions.structuredErrors.name,
getter: () async => FlutterError.presentError == _reportStructuredError,
setter: (bool value) {
FlutterError.presentError = value ? _reportStructuredError : defaultExceptionHandler;
return Future<void>.value();
},
registerExtension: registerExtension,
);
_registerBoolServiceExtension(
name: WidgetInspectorServiceExtensions.show.name,
getter: () async => WidgetsBinding.instance.debugShowWidgetInspectorOverride,
setter: (bool value) {
if (WidgetsBinding.instance.debugShowWidgetInspectorOverride != value) {
WidgetsBinding.instance.debugShowWidgetInspectorOverride = value;
}
return Future<void>.value();
},
registerExtension: registerExtension,
);
if (isWidgetCreationTracked()) {
// Service extensions that are only supported if widget creation locations
// are tracked.
_registerBoolServiceExtension(
name: WidgetInspectorServiceExtensions.trackRebuildDirtyWidgets.name,
getter: () async => _trackRebuildDirtyWidgets,
setter: (bool value) async {
if (value == _trackRebuildDirtyWidgets) {
return;
}
_rebuildStats.resetCounts();
_trackRebuildDirtyWidgets = value;
if (value) {
assert(debugOnRebuildDirtyWidget == null);
debugOnRebuildDirtyWidget = _onRebuildWidget;
// Trigger a rebuild so there are baseline stats for rebuilds
// performed by the app.
await forceRebuild();
return;
} else {
debugOnRebuildDirtyWidget = null;
return;
}
},
registerExtension: registerExtension,
);
_registerBoolServiceExtension(
name: WidgetInspectorServiceExtensions.trackRepaintWidgets.name,
getter: () async => _trackRepaintWidgets,
setter: (bool value) async {
if (value == _trackRepaintWidgets) {
return;
}
_repaintStats.resetCounts();
_trackRepaintWidgets = value;
if (value) {
assert(debugOnProfilePaint == null);
debugOnProfilePaint = _onPaint;
// Trigger an immediate paint so the user has some baseline painting
// stats to view.
void markTreeNeedsPaint(RenderObject renderObject) {
renderObject.markNeedsPaint();
renderObject.visitChildren(markTreeNeedsPaint);
}
RendererBinding.instance.renderViews.forEach(markTreeNeedsPaint);
} else {
debugOnProfilePaint = null;
}
},
registerExtension: registerExtension,
);
}
_registerSignalServiceExtension(
name: WidgetInspectorServiceExtensions.disposeAllGroups.name,
callback: () async {
disposeAllGroups();
return null;
},
registerExtension: registerExtension,
);
_registerObjectGroupServiceExtension(
name: WidgetInspectorServiceExtensions.disposeGroup.name,
callback: (String name) async {
disposeGroup(name);
return null;
},
registerExtension: registerExtension,
);
_registerSignalServiceExtension(
name: WidgetInspectorServiceExtensions.isWidgetTreeReady.name,
callback: isWidgetTreeReady,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.disposeId.name,
callback: (String? objectId, String objectGroup) async {
disposeId(objectId, objectGroup);
return null;
},
registerExtension: registerExtension,
);
_registerServiceExtensionVarArgs(
name: WidgetInspectorServiceExtensions.setPubRootDirectories.name,
callback: (List<String> args) async {
setPubRootDirectories(args);
return null;
},
registerExtension: registerExtension,
);
_registerServiceExtensionVarArgs(
name: WidgetInspectorServiceExtensions.addPubRootDirectories.name,
callback: (List<String> args) async {
addPubRootDirectories(args);
return null;
},
registerExtension: registerExtension,
);
_registerServiceExtensionVarArgs(
name: WidgetInspectorServiceExtensions.removePubRootDirectories.name,
callback: (List<String> args) async {
removePubRootDirectories(args);
return null;
},
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.getPubRootDirectories.name,
callback: pubRootDirectories,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.setSelectionById.name,
callback: setSelectionById,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getParentChain.name,
callback: _getParentChain,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getProperties.name,
callback: _getProperties,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getChildren.name,
callback: _getChildren,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getChildrenSummaryTree.name,
callback: _getChildrenSummaryTree,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getChildrenDetailsSubtree.name,
callback: _getChildrenDetailsSubtree,
registerExtension: registerExtension,
);
_registerObjectGroupServiceExtension(
name: WidgetInspectorServiceExtensions.getRootWidget.name,
callback: _getRootWidget,
registerExtension: registerExtension,
);
_registerObjectGroupServiceExtension(
name: WidgetInspectorServiceExtensions.getRootWidgetSummaryTree.name,
callback: _getRootWidgetSummaryTree,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.getRootWidgetSummaryTreeWithPreviews.name,
callback: _getRootWidgetSummaryTreeWithPreviews,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.getDetailsSubtree.name,
callback: (Map<String, String> parameters) async {
assert(parameters.containsKey('objectGroup'));
final String? subtreeDepth = parameters['subtreeDepth'];
return <String, Object?>{
'result': _getDetailsSubtree(
parameters['arg'],
parameters['objectGroup'],
subtreeDepth != null ? int.parse(subtreeDepth) : 2,
),
};
},
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getSelectedWidget.name,
callback: _getSelectedWidget,
registerExtension: registerExtension,
);
_registerServiceExtensionWithArg(
name: WidgetInspectorServiceExtensions.getSelectedSummaryWidget.name,
callback: _getSelectedSummaryWidget,
registerExtension: registerExtension,
);
_registerSignalServiceExtension(
name: WidgetInspectorServiceExtensions.isWidgetCreationTracked.name,
callback: isWidgetCreationTracked,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.screenshot.name,
callback: (Map<String, String> parameters) async {
assert(parameters.containsKey('id'));
assert(parameters.containsKey('width'));
assert(parameters.containsKey('height'));
final ui.Image? image = await screenshot(
toObject(parameters['id']),
width: double.parse(parameters['width']!),
height: double.parse(parameters['height']!),
margin: parameters.containsKey('margin') ?
double.parse(parameters['margin']!) : 0.0,
maxPixelRatio: parameters.containsKey('maxPixelRatio') ?
double.parse(parameters['maxPixelRatio']!) : 1.0,
debugPaint: parameters['debugPaint'] == 'true',
);
if (image == null) {
return <String, Object?>{'result': null};
}
final ByteData? byteData = await image.toByteData(format:ui.ImageByteFormat.png);
image.dispose();
return <String, Object>{
'result': base64.encoder.convert(Uint8List.view(byteData!.buffer)),
};
},
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.getLayoutExplorerNode.name,
callback: _getLayoutExplorerNode,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.setFlexFit.name,
callback: _setFlexFit,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.setFlexFactor.name,
callback: _setFlexFactor,
registerExtension: registerExtension,
);
registerServiceExtension(
name: WidgetInspectorServiceExtensions.setFlexProperties.name,
callback: _setFlexProperties,
registerExtension: registerExtension,
);
}
void _clearStats() {
_rebuildStats.resetCounts();
_repaintStats.resetCounts();
}
/// Clear all InspectorService object references.
///
/// Use this method only for testing to ensure that object references from one
/// test case do not impact other test cases.
@visibleForTesting
@protected
void disposeAllGroups() {
_groups.clear();
_idToReferenceData.clear();
_objectToId.clear();
_nextId = 0;
}
/// Reset all InspectorService state.
///
/// Use this method only for testing to write hermetic tests for
/// WidgetInspectorService.
@visibleForTesting
@protected
@mustCallSuper
void resetAllState() {
disposeAllGroups();
selection.clear();
resetPubRootDirectories();
}
/// Free all references to objects in a group.
///
/// Objects and their associated ids in the group may be kept alive by
/// references from a different group.
@protected
void disposeGroup(String name) {
final Set<InspectorReferenceData>? references = _groups.remove(name);
if (references == null) {
return;
}
references.forEach(_decrementReferenceCount);
}
void _decrementReferenceCount(InspectorReferenceData reference) {
reference.count -= 1;
assert(reference.count >= 0);
if (reference.count == 0) {
final Object? value = reference.value;
if (value != null) {
_objectToId.remove(value);
}
_idToReferenceData.remove(reference.id);
}
}
/// Returns a unique id for [object] that will remain live at least until
/// [disposeGroup] is called on [groupName].
@protected
String? toId(Object? object, String groupName) {
if (object == null) {
return null;
}
final Set<InspectorReferenceData> group = _groups.putIfAbsent(groupName, () => Set<InspectorReferenceData>.identity());
String? id = _objectToId[object];
InspectorReferenceData referenceData;
if (id == null) {
// TODO(polina-c): comment here why we increase memory footprint by the prefix 'inspector-'.
// https://github.com/flutter/devtools/issues/5995
id = 'inspector-$_nextId';
_nextId += 1;
_objectToId[object] = id;
referenceData = InspectorReferenceData(object, id);
_idToReferenceData[id] = referenceData;
group.add(referenceData);
} else {
referenceData = _idToReferenceData[id]!;
if (group.add(referenceData)) {
referenceData.count += 1;
}
}
return id;
}
/// Returns whether the application has rendered its first frame and it is
/// appropriate to display the Widget tree in the inspector.
@protected
bool isWidgetTreeReady([ String? groupName ]) {
return WidgetsBinding.instance.debugDidSendFirstFrameEvent;
}
/// Returns the Dart object associated with a reference id.
///
/// The `groupName` parameter is not required by is added to regularize the
/// API surface of the methods in this class called from the Flutter IntelliJ
/// Plugin.
@protected
Object? toObject(String? id, [ String? groupName ]) {
if (id == null) {
return null;
}
final InspectorReferenceData? data = _idToReferenceData[id];
if (data == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Id does not exist.')]);
}
return data.value;
}
/// Returns the object to introspect to determine the source location of an
/// object's class.
///
/// The Dart object for the id is returned for all cases but [Element] objects
/// where the [Widget] configuring the [Element] is returned instead as the
/// class of the [Widget] is more relevant than the class of the [Element].
///
/// The `groupName` parameter is not required by is added to regularize the
/// API surface of methods called from the Flutter IntelliJ Plugin.
@protected
Object? toObjectForSourceLocation(String id, [ String? groupName ]) {
final Object? object = toObject(id);
if (object is Element) {
return object.widget;
}
return object;
}
/// Remove the object with the specified `id` from the specified object
/// group.
///
/// If the object exists in other groups it will remain alive and the object
/// id will remain valid.
@protected
void disposeId(String? id, String groupName) {
if (id == null) {
return;
}
final InspectorReferenceData? referenceData = _idToReferenceData[id];
if (referenceData == null) {
throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Id does not exist')]);
}
if (_groups[groupName]?.remove(referenceData) != true) {
throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Id is not in group')]);
}
_decrementReferenceCount(referenceData);
}
/// Set the list of directories that should be considered part of the local
/// project.
///
/// The local project directories are used to distinguish widgets created by
/// the local project from widgets created from inside the framework
/// or other packages.
@protected
@Deprecated(
'Use addPubRootDirectories instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
void setPubRootDirectories(List<String> pubRootDirectories) {
addPubRootDirectories(pubRootDirectories);
}
/// Resets the list of directories, that should be considered part of the
/// local project, to the value passed in [pubRootDirectories].
///
/// The local project directories are used to distinguish widgets created by
/// the local project from widgets created from inside the framework
/// or other packages.
@visibleForTesting
@protected
void resetPubRootDirectories() {
_pubRootDirectories = <String>[];
_isLocalCreationCache.clear();
}
/// Add a list of directories that should be considered part of the local
/// project.
///
/// The local project directories are used to distinguish widgets created by
/// the local project from widgets created from inside the framework
/// or other packages.
@protected
void addPubRootDirectories(List<String> pubRootDirectories) {
pubRootDirectories = pubRootDirectories.map<String>((String directory) => Uri.parse(directory).path).toList();
final Set<String> directorySet = Set<String>.from(pubRootDirectories);
if (_pubRootDirectories != null) {
directorySet.addAll(_pubRootDirectories!);
}
_pubRootDirectories = directorySet.toList();
_isLocalCreationCache.clear();
}
/// Remove a list of directories that should no longer be considered part
/// of the local project.
///
/// The local project directories are used to distinguish widgets created by
/// the local project from widgets created from inside the framework
/// or other packages.
@protected
void removePubRootDirectories(List<String> pubRootDirectories) {
if (_pubRootDirectories == null) {
return;
}
pubRootDirectories = pubRootDirectories.map<String>((String directory) => Uri.parse(directory).path).toList();
final Set<String> directorySet = Set<String>.from(_pubRootDirectories!);
directorySet.removeAll(pubRootDirectories);
_pubRootDirectories = directorySet.toList();
_isLocalCreationCache.clear();
}
/// Returns the list of directories that should be considered part of the
/// local project.
@protected
@visibleForTesting
Future<Map<String, dynamic>> pubRootDirectories(
Map<String, String> parameters,
) {
return Future<Map<String, Object>>.value(<String, Object>{
'result': _pubRootDirectories ?? <String>[],
});
}
/// Set the [WidgetInspector] selection to the object matching the specified
/// id if the object is valid object to set as the inspector selection.
///
/// Returns true if the selection was changed.
///
/// The `groupName` parameter is not required by is added to regularize the
/// API surface of methods called from the Flutter IntelliJ Plugin.
@protected
bool setSelectionById(String? id, [ String? groupName ]) {
return setSelection(toObject(id), groupName);
}
/// Set the [WidgetInspector] selection to the specified `object` if it is
/// a valid object to set as the inspector selection.
///
/// Returns true if the selection was changed.
///
/// The `groupName` parameter is not needed but is specified to regularize the
/// API surface of methods called from the Flutter IntelliJ Plugin.
@protected
bool setSelection(Object? object, [ String? groupName ]) {
if (object is Element || object is RenderObject) {
if (object is Element) {
if (object == selection.currentElement) {
return false;
}
selection.currentElement = object;
_sendInspectEvent(selection.currentElement);
} else {
if (object == selection.current) {
return false;
}
selection.current = object! as RenderObject;
_sendInspectEvent(selection.current);
}
return true;
}
return false;
}
/// Notify attached tools to navigate to an object's source location.
void _sendInspectEvent(Object? object){
inspect(object);
final _Location? location = _getSelectedSummaryWidgetLocation(null);
if (location != null) {
postEvent(
'navigate',
<String, Object>{
'fileUri': location.file, // URI file path of the location.
'line': location.line, // 1-based line number.
'column': location.column, // 1-based column number.
'source': 'flutter.inspector',
},
stream: 'ToolEvent',
);
}
}
/// Returns a DevTools uri linking to a specific element on the inspector page.
String? _devToolsInspectorUriForElement(Element element) {
if (activeDevToolsServerAddress != null && connectedVmServiceUri != null) {
final String? inspectorRef = toId(element, _consoleObjectGroup);
if (inspectorRef != null) {
return devToolsInspectorUri(inspectorRef);
}
}
return null;
}
/// Returns the DevTools inspector uri for the given vm service connection and
/// inspector reference.
@visibleForTesting
String devToolsInspectorUri(String inspectorRef) {
assert(activeDevToolsServerAddress != null);
assert(connectedVmServiceUri != null);
final Uri uri = Uri.parse(activeDevToolsServerAddress!).replace(
queryParameters: <String, dynamic>{
'uri': connectedVmServiceUri,
'inspectorRef': inspectorRef,
},
);
// We cannot add the '/#/inspector' path by means of
// [Uri.replace(path: '/#/inspector')] because the '#' character will be
// encoded when we try to print the url as a string. DevTools will not
// load properly if this character is encoded in the url.
// Related: https://github.com/flutter/devtools/issues/2475.
final String devToolsInspectorUri = uri.toString();
final int startQueryParamIndex = devToolsInspectorUri.indexOf('?');
// The query parameter character '?' should be present because we manually
// added query parameters above.
assert(startQueryParamIndex != -1);
return '${devToolsInspectorUri.substring(0, startQueryParamIndex)}'
'/#/inspector'
'${devToolsInspectorUri.substring(startQueryParamIndex)}';
}
/// Returns JSON representing the chain of [DiagnosticsNode] instances from
/// root of the tree to the [Element] or [RenderObject] matching `id`.
///
/// The JSON contains all information required to display a tree view with
/// all nodes other than nodes along the path collapsed.
@protected
String getParentChain(String id, String groupName) {
return _safeJsonEncode(_getParentChain(id, groupName));
}
List<Object?> _getParentChain(String? id, String groupName) {
final Object? value = toObject(id);
List<_DiagnosticsPathNode> path;
if (value is RenderObject) {
path = _getRenderObjectParentChain(value, groupName)!;
} else if (value is Element) {
path = _getElementParentChain(value, groupName);
} else {
throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Cannot get parent chain for node of type ${value.runtimeType}')]);
}
return path.map<Object?>((_DiagnosticsPathNode node) => _pathNodeToJson(
node,
InspectorSerializationDelegate(groupName: groupName, service: this),
)).toList();
}
Map<String, Object?>? _pathNodeToJson(_DiagnosticsPathNode? pathNode, InspectorSerializationDelegate delegate) {
if (pathNode == null) {
return null;
}
return <String, Object?>{
'node': _nodeToJson(pathNode.node, delegate),
'children': _nodesToJson(pathNode.children, delegate, parent: pathNode.node),
'childIndex': pathNode.childIndex,
};
}
List<Element> _getRawElementParentChain(Element element, { required int? numLocalParents }) {
List<Element> elements = element.debugGetDiagnosticChain();
if (numLocalParents != null) {
for (int i = 0; i < elements.length; i += 1) {
if (_isValueCreatedByLocalProject(elements[i])) {
numLocalParents = numLocalParents! - 1;
if (numLocalParents <= 0) {
elements = elements.take(i + 1).toList();
break;
}
}
}
}
return elements.reversed.toList();
}
List<_DiagnosticsPathNode> _getElementParentChain(Element element, String groupName, { int? numLocalParents }) {
return _followDiagnosticableChain(
_getRawElementParentChain(element, numLocalParents: numLocalParents),
) ?? const <_DiagnosticsPathNode>[];
}
List<_DiagnosticsPathNode>? _getRenderObjectParentChain(RenderObject? renderObject, String groupName) {
final List<RenderObject> chain = <RenderObject>[];
while (renderObject != null) {
chain.add(renderObject);
renderObject = renderObject.parent;
}
return _followDiagnosticableChain(chain.reversed.toList());
}
Map<String, Object?>? _nodeToJson(
DiagnosticsNode? node,
InspectorSerializationDelegate delegate,
) {
return node?.toJsonMap(delegate);
}
bool _isValueCreatedByLocalProject(Object? value) {
final _Location? creationLocation = _getCreationLocation(value);
if (creationLocation == null) {
return false;
}
return _isLocalCreationLocation(creationLocation.file);
}
bool _isLocalCreationLocationImpl(String locationUri) {
final String file = Uri.parse(locationUri).path;
// By default check whether the creation location was within package:flutter.
if (_pubRootDirectories == null) {
// TODO(chunhtai): Make it more robust once
// https://github.com/flutter/flutter/issues/32660 is fixed.
return !file.contains('packages/flutter/');
}
for (final String directory in _pubRootDirectories!) {
if (file.startsWith(directory)) {
return true;
}
}
return false;
}
/// Memoized version of [_isLocalCreationLocationImpl].
bool _isLocalCreationLocation(String locationUri) {
final bool? cachedValue = _isLocalCreationCache[locationUri];
if (cachedValue != null) {
return cachedValue;
}
final bool result = _isLocalCreationLocationImpl(locationUri);
_isLocalCreationCache[locationUri] = result;
return result;
}
/// Wrapper around `json.encode` that uses a ring of cached values to prevent
/// the Dart garbage collector from collecting objects between when
/// the value is returned over the VM service protocol and when the
/// separate observatory protocol command has to be used to retrieve its full
/// contents.
//
// TODO(jacobr): Replace this with a better solution once
// https://github.com/dart-lang/sdk/issues/32919 is fixed.
String _safeJsonEncode(Object? object) {
final String jsonString = json.encode(object);
_serializeRing[_serializeRingIndex] = jsonString;
_serializeRingIndex = (_serializeRingIndex + 1) % _serializeRing.length;
return jsonString;
}
List<DiagnosticsNode> _truncateNodes(Iterable<DiagnosticsNode> nodes, int maxDescendentsTruncatableNode) {
if (nodes.every((DiagnosticsNode node) => node.value is Element) && isWidgetCreationTracked()) {
final List<DiagnosticsNode> localNodes = nodes
.where((DiagnosticsNode node) => _isValueCreatedByLocalProject(node.value))
.toList();
if (localNodes.isNotEmpty) {
return localNodes;
}
}
return nodes.take(maxDescendentsTruncatableNode).toList();
}
List<Map<String, Object?>> _nodesToJson(
List<DiagnosticsNode> nodes,
InspectorSerializationDelegate delegate, {
required DiagnosticsNode? parent,
}) {
return DiagnosticsNode.toJsonList(nodes, parent, delegate);
}
/// Returns a JSON representation of the properties of the [DiagnosticsNode]
/// object that `diagnosticsNodeId` references.
@protected
String getProperties(String diagnosticsNodeId, String groupName) {
return _safeJsonEncode(_getProperties(diagnosticsNodeId, groupName));
}
List<Object> _getProperties(String? diagnosticableId, String groupName) {
final DiagnosticsNode? node = _idToDiagnosticsNode(diagnosticableId);
if (node == null) {
return const <Object>[];
}
return _nodesToJson(node.getProperties(), InspectorSerializationDelegate(groupName: groupName, service: this), parent: node);
}
/// Returns a JSON representation of the children of the [DiagnosticsNode]
/// object that `diagnosticsNodeId` references.
String getChildren(String diagnosticsNodeId, String groupName) {
return _safeJsonEncode(_getChildren(diagnosticsNodeId, groupName));
}
List<Object> _getChildren(String? diagnosticsNodeId, String groupName) {
final DiagnosticsNode? node = toObject(diagnosticsNodeId) as DiagnosticsNode?;
final InspectorSerializationDelegate delegate = InspectorSerializationDelegate(groupName: groupName, service: this);
return _nodesToJson(node == null ? const <DiagnosticsNode>[] : _getChildrenFiltered(node, delegate), delegate, parent: node);
}
/// Returns a JSON representation of the children of the [DiagnosticsNode]
/// object that `diagnosticsNodeId` references only including children that
/// were created directly by user code.
///
/// {@template flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
/// Requires [Widget] creation locations which are only available for debug
/// mode builds when the `--track-widget-creation` flag is enabled on the call
/// to the `flutter` tool. This flag is enabled by default in debug builds.
/// {@endtemplate}
///
/// See also:
///
/// * [isWidgetCreationTracked] which indicates whether this method can be
/// used.
String getChildrenSummaryTree(String diagnosticsNodeId, String groupName) {
return _safeJsonEncode(_getChildrenSummaryTree(diagnosticsNodeId, groupName));
}
DiagnosticsNode? _idToDiagnosticsNode(String? diagnosticableId) {
final Object? object = toObject(diagnosticableId);
return objectToDiagnosticsNode(object);
}
/// If possible, returns [DiagnosticsNode] for the object.
@visibleForTesting
static DiagnosticsNode? objectToDiagnosticsNode(Object? object) {
if (object is Diagnosticable) {
return object.toDiagnosticsNode();
}
return null;
}
List<Object> _getChildrenSummaryTree(String? diagnosticableId, String groupName) {
final DiagnosticsNode? node = _idToDiagnosticsNode(diagnosticableId);
if (node == null) {
return <Object>[];
}
final InspectorSerializationDelegate delegate = InspectorSerializationDelegate(groupName: groupName, summaryTree: true, service: this);
return _nodesToJson(_getChildrenFiltered(node, delegate), delegate, parent: node);
}
/// Returns a JSON representation of the children of the [DiagnosticsNode]
/// object that [diagnosticableId] references providing information needed
/// for the details subtree view.
///
/// The details subtree shows properties inline and includes all children
/// rather than a filtered set of important children.
String getChildrenDetailsSubtree(String diagnosticableId, String groupName) {
return _safeJsonEncode(_getChildrenDetailsSubtree(diagnosticableId, groupName));
}
List<Object> _getChildrenDetailsSubtree(String? diagnosticableId, String groupName) {
final DiagnosticsNode? node = _idToDiagnosticsNode(diagnosticableId);
// With this value of minDepth we only expand one extra level of important nodes.
final InspectorSerializationDelegate delegate = InspectorSerializationDelegate(groupName: groupName, includeProperties: true, service: this);
return _nodesToJson(node == null ? const <DiagnosticsNode>[] : _getChildrenFiltered(node, delegate), delegate, parent: node);
}
bool _shouldShowInSummaryTree(DiagnosticsNode node) {
if (node.level == DiagnosticLevel.error) {
return true;
}
final Object? value = node.value;
if (value is! Diagnosticable) {
return true;
}
if (value is! Element || !isWidgetCreationTracked()) {
// Creation locations are not available so include all nodes in the
// summary tree.
return true;
}
return _isValueCreatedByLocalProject(value);
}
List<DiagnosticsNode> _getChildrenFiltered(
DiagnosticsNode node,
InspectorSerializationDelegate delegate,
) {
return _filterChildren(node.getChildren(), delegate);
}
List<DiagnosticsNode> _filterChildren(
List<DiagnosticsNode> nodes,
InspectorSerializationDelegate delegate,
) {
final List<DiagnosticsNode> children = <DiagnosticsNode>[
for (final DiagnosticsNode child in nodes)
if (!delegate.summaryTree || _shouldShowInSummaryTree(child))
child
else
..._getChildrenFiltered(child, delegate),
];
return children;
}
/// Returns a JSON representation of the [DiagnosticsNode] for the root
/// [Element].
String getRootWidget(String groupName) {
return _safeJsonEncode(_getRootWidget(groupName));
}
Map<String, Object?>? _getRootWidget(String groupName) {
return _nodeToJson(WidgetsBinding.instance.rootElement?.toDiagnosticsNode(), InspectorSerializationDelegate(groupName: groupName, service: this));
}
/// Returns a JSON representation of the [DiagnosticsNode] for the root
/// [Element] showing only nodes that should be included in a summary tree.
String getRootWidgetSummaryTree(String groupName) {
return _safeJsonEncode(_getRootWidgetSummaryTree(groupName));
}
Map<String, Object?>? _getRootWidgetSummaryTree(
String groupName, {
Map<String, Object>? Function(DiagnosticsNode, InspectorSerializationDelegate)? addAdditionalPropertiesCallback,
}) {
return _nodeToJson(
WidgetsBinding.instance.rootElement?.toDiagnosticsNode(),
InspectorSerializationDelegate(
groupName: groupName,
subtreeDepth: 1000000,
summaryTree: true,
service: this,
addAdditionalPropertiesCallback: addAdditionalPropertiesCallback,
),
);
}
Future<Map<String, Object?>> _getRootWidgetSummaryTreeWithPreviews(
Map<String, String> parameters,
) {
final String groupName = parameters['groupName']!;
final Map<String, Object?>? result = _getRootWidgetSummaryTree(
groupName,
addAdditionalPropertiesCallback: (DiagnosticsNode node, InspectorSerializationDelegate? delegate) {
final Map<String, Object> additionalJson = <String, Object>{};
final Object? value = node.value;
if (value is Element) {
final RenderObject? renderObject = value.renderObject;
if (renderObject is RenderParagraph) {
additionalJson['textPreview'] = renderObject.text.toPlainText();
}
}
return additionalJson;
},
);
return Future<Map<String, dynamic>>.value(<String, dynamic>{
'result': result,
});
}
/// Returns a JSON representation of the subtree rooted at the
/// [DiagnosticsNode] object that `diagnosticsNodeId` references providing
/// information needed for the details subtree view.
///
/// The number of levels of the subtree that should be returned is specified
/// by the [subtreeDepth] parameter. This value defaults to 2 for backwards
/// compatibility.
///
/// See also:
///
/// * [getChildrenDetailsSubtree], a method to get children of a node
/// in the details subtree.
String getDetailsSubtree(
String diagnosticableId,
String groupName, {
int subtreeDepth = 2,
}) {
return _safeJsonEncode(_getDetailsSubtree(diagnosticableId, groupName, subtreeDepth));
}
Map<String, Object?>? _getDetailsSubtree(
String? diagnosticableId,
String? groupName,
int subtreeDepth,
) {
final DiagnosticsNode? root = _idToDiagnosticsNode(diagnosticableId);
if (root == null) {
return null;
}
return _nodeToJson(
root,
InspectorSerializationDelegate(
groupName: groupName,
subtreeDepth: subtreeDepth,
includeProperties: true,
service: this,
),
);
}
/// Returns a [DiagnosticsNode] representing the currently selected [Element].
@protected
String getSelectedWidget(String? previousSelectionId, String groupName) {
if (previousSelectionId != null) {
debugPrint('previousSelectionId is deprecated in API');
}
return _safeJsonEncode(_getSelectedWidget(null, groupName));
}
/// Captures an image of the current state of an [object] that is a
/// [RenderObject] or [Element].
///
/// The returned [ui.Image] has uncompressed raw RGBA bytes and will be scaled
/// to be at most [width] pixels wide and [height] pixels tall. The returned
/// image will never have a scale between logical pixels and the
/// size of the output image larger than maxPixelRatio.
/// [margin] indicates the number of pixels relative to the un-scaled size of
/// the [object] to include as a margin to include around the bounds of the
/// [object] in the screenshot. Including a margin can be useful to capture
/// areas that are slightly outside of the normal bounds of an object such as
/// some debug paint information.
@protected
Future<ui.Image?> screenshot(
Object? object, {
required double width,
required double height,
double margin = 0.0,
double maxPixelRatio = 1.0,
bool debugPaint = false,
}) async {
if (object is! Element && object is! RenderObject) {
return null;
}
final RenderObject? renderObject = object is Element ? object.renderObject : (object as RenderObject?);
if (renderObject == null || !renderObject.attached) {
return null;
}
if (renderObject.debugNeedsLayout) {
final PipelineOwner owner = renderObject.owner!;
assert(!owner.debugDoingLayout);
owner
..flushLayout()
..flushCompositingBits()
..flushPaint();
// If we still need layout, then that means that renderObject was skipped
// in the layout phase and therefore can't be painted. It is clearer to
// return null indicating that a screenshot is unavailable than to return
// an empty image.
if (renderObject.debugNeedsLayout) {
return null;
}
}
Rect renderBounds = _calculateSubtreeBounds(renderObject);
if (margin != 0.0) {
renderBounds = renderBounds.inflate(margin);
}
if (renderBounds.isEmpty) {
return null;
}
final double pixelRatio = math.min(
maxPixelRatio,
math.min(
width / renderBounds.width,
height / renderBounds.height,
),
);
return _ScreenshotPaintingContext.toImage(
renderObject,
renderBounds,
pixelRatio: pixelRatio,
debugPaint: debugPaint,
);
}
Future<Map<String, Object?>> _getLayoutExplorerNode(
Map<String, String> parameters,
) {
final String? diagnosticableId = parameters['id'];
final int subtreeDepth = int.parse(parameters['subtreeDepth']!);
final String? groupName = parameters['groupName'];
Map<String, dynamic>? result = <String, dynamic>{};
final DiagnosticsNode? root = _idToDiagnosticsNode(diagnosticableId);
if (root == null) {
return Future<Map<String, dynamic>>.value(<String, dynamic>{
'result': result,
});
}
result = _nodeToJson(
root,
InspectorSerializationDelegate(
groupName: groupName,
summaryTree: true,
subtreeDepth: subtreeDepth,
service: this,
addAdditionalPropertiesCallback:
(DiagnosticsNode node, InspectorSerializationDelegate delegate) {
final Object? value = node.value;
final RenderObject? renderObject =
value is Element ? value.renderObject : null;
if (renderObject == null) {
return const <String, Object>{};
}
final DiagnosticsSerializationDelegate
renderObjectSerializationDelegate = delegate.copyWith(
subtreeDepth: 0,
includeProperties: true,
expandPropertyValues: false,
);
final Map<String, Object> additionalJson = <String, Object>{
// Only include renderObject properties separately if this value is not already the renderObject.
// Only include if we are expanding property values to mitigate the risk of infinite loops if
// RenderObjects have properties that are Element objects.
if (value is! RenderObject && delegate.expandPropertyValues)
'renderObject': renderObject
.toDiagnosticsNode()
.toJsonMap(renderObjectSerializationDelegate),
};
final RenderObject? renderParent = renderObject.parent;
if (renderParent != null &&
delegate.subtreeDepth > 0 &&
delegate.expandPropertyValues) {
final Object? parentCreator = renderParent.debugCreator;
if (parentCreator is DebugCreator) {
additionalJson['parentRenderElement'] =
parentCreator.element.toDiagnosticsNode().toJsonMap(
delegate.copyWith(
subtreeDepth: 0,
includeProperties: true,
),
);
// TODO(jacobr): also describe the path back up the tree to
// the RenderParentElement from the current element. It
// could be a surprising distance up the tree if a lot of
// elements don't have their own RenderObjects.
}
}
try {
if (!renderObject.debugNeedsLayout) {
// ignore: invalid_use_of_protected_member
final Constraints constraints = renderObject.constraints;
final Map<String, Object> constraintsProperty = <String, Object>{
'type': constraints.runtimeType.toString(),
'description': constraints.toString(),
};
if (constraints is BoxConstraints) {
constraintsProperty.addAll(<String, Object>{
'minWidth': constraints.minWidth.toString(),
'minHeight': constraints.minHeight.toString(),
'maxWidth': constraints.maxWidth.toString(),
'maxHeight': constraints.maxHeight.toString(),
});
}
additionalJson['constraints'] = constraintsProperty;
}
} catch (e) {
// Constraints are sometimes unavailable even though
// debugNeedsLayout is false.
}
try {
if (renderObject is RenderBox) {
additionalJson['isBox'] = true;
additionalJson['size'] = <String, Object>{
'width': renderObject.size.width.toString(),
'height': renderObject.size.height.toString(),
};
final ParentData? parentData = renderObject.parentData;
if (parentData is FlexParentData) {
additionalJson['flexFactor'] = parentData.flex!;
additionalJson['flexFit'] =
(parentData.fit ?? FlexFit.tight).name;
} else if (parentData is BoxParentData) {
final Offset offset = parentData.offset;
additionalJson['parentData'] = <String, Object>{
'offsetX': offset.dx.toString(),
'offsetY': offset.dy.toString(),
};
}
} else if (renderObject is RenderView) {
additionalJson['size'] = <String, Object>{
'width': renderObject.size.width.toString(),
'height': renderObject.size.height.toString(),
};
}
} catch (e) {
// Not laid out yet.
}
return additionalJson;
},
),
);
return Future<Map<String, dynamic>>.value(<String, dynamic>{
'result': result,
});
}
Future<Map<String, dynamic>> _setFlexFit(Map<String, String> parameters) {
final String? id = parameters['id'];
final String parameter = parameters['flexFit']!;
final FlexFit flexFit = _toEnumEntry<FlexFit>(FlexFit.values, parameter);
final Object? object = toObject(id);
bool succeed = false;
if (object != null && object is Element) {
final RenderObject? render = object.renderObject;
final ParentData? parentData = render?.parentData;
if (parentData is FlexParentData) {
parentData.fit = flexFit;
render!.markNeedsLayout();
succeed = true;
}
}
return Future<Map<String, Object>>.value(<String, Object>{
'result': succeed,
});
}
Future<Map<String, dynamic>> _setFlexFactor(Map<String, String> parameters) {
final String? id = parameters['id'];
final String flexFactor = parameters['flexFactor']!;
final int? factor = flexFactor == 'null' ? null : int.parse(flexFactor);
final dynamic object = toObject(id);
bool succeed = false;
if (object != null && object is Element) {
final RenderObject? render = object.renderObject;
final ParentData? parentData = render?.parentData;
if (parentData is FlexParentData) {
parentData.flex = factor;
render!.markNeedsLayout();
succeed = true;
}
}
return Future<Map<String, Object>>.value(<String, Object>{
'result': succeed
});
}
Future<Map<String, dynamic>> _setFlexProperties(
Map<String, String> parameters,
) {
final String? id = parameters['id'];
final MainAxisAlignment mainAxisAlignment = _toEnumEntry<MainAxisAlignment>(
MainAxisAlignment.values,
parameters['mainAxisAlignment']!,
);
final CrossAxisAlignment crossAxisAlignment =
_toEnumEntry<CrossAxisAlignment>(
CrossAxisAlignment.values,
parameters['crossAxisAlignment']!,
);
final Object? object = toObject(id);
bool succeed = false;
if (object != null && object is Element) {
final RenderObject? render = object.renderObject;
if (render is RenderFlex) {
render.mainAxisAlignment = mainAxisAlignment;
render.crossAxisAlignment = crossAxisAlignment;
render.markNeedsLayout();
render.markNeedsPaint();
succeed = true;
}
}
return Future<Map<String, Object>>.value(<String, Object>{
'result': succeed
});
}
T _toEnumEntry<T>(List<T> enumEntries, String name) {
for (final T entry in enumEntries) {
if (entry.toString() == name) {
return entry;
}
}
throw Exception('Enum value $name not found');
}
Map<String, Object?>? _getSelectedWidget(String? previousSelectionId, String groupName) {
return _nodeToJson(
_getSelectedWidgetDiagnosticsNode(previousSelectionId),
InspectorSerializationDelegate(groupName: groupName, service: this),
);
}
DiagnosticsNode? _getSelectedWidgetDiagnosticsNode(String? previousSelectionId) {
final DiagnosticsNode? previousSelection = toObject(previousSelectionId) as DiagnosticsNode?;
final Element? current = selection.currentElement;
return current == previousSelection?.value ? previousSelection : current?.toDiagnosticsNode();
}
/// Returns a [DiagnosticsNode] representing the currently selected [Element]
/// if the selected [Element] should be shown in the summary tree otherwise
/// returns the first ancestor of the selected [Element] shown in the summary
/// tree.
String getSelectedSummaryWidget(String? previousSelectionId, String groupName) {
if (previousSelectionId != null) {
debugPrint('previousSelectionId is deprecated in API');
}
return _safeJsonEncode(_getSelectedSummaryWidget(null, groupName));
}
_Location? _getSelectedSummaryWidgetLocation(String? previousSelectionId) {
return _getCreationLocation(_getSelectedSummaryDiagnosticsNode(previousSelectionId)?.value);
}
DiagnosticsNode? _getSelectedSummaryDiagnosticsNode(String? previousSelectionId) {
if (!isWidgetCreationTracked()) {
return _getSelectedWidgetDiagnosticsNode(previousSelectionId);
}
final DiagnosticsNode? previousSelection = toObject(previousSelectionId) as DiagnosticsNode?;
Element? current = selection.currentElement;
if (current != null && !_isValueCreatedByLocalProject(current)) {
Element? firstLocal;
for (final Element candidate in current.debugGetDiagnosticChain()) {
if (_isValueCreatedByLocalProject(candidate)) {
firstLocal = candidate;
break;
}
}
current = firstLocal;
}
return current == previousSelection?.value ? previousSelection : current?.toDiagnosticsNode();
}
Map<String, Object?>? _getSelectedSummaryWidget(String? previousSelectionId, String groupName) {
return _nodeToJson(_getSelectedSummaryDiagnosticsNode(previousSelectionId), InspectorSerializationDelegate(groupName: groupName, service: this));
}
/// Returns whether [Widget] creation locations are available.
///
/// {@macro flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
bool isWidgetCreationTracked() {
_widgetCreationTracked ??= const _WidgetForTypeTests() is _HasCreationLocation;
return _widgetCreationTracked!;
}
bool? _widgetCreationTracked;
late Duration _frameStart;
void _onFrameStart(Duration timeStamp) {
_frameStart = timeStamp;
SchedulerBinding.instance.addPostFrameCallback(_onFrameEnd, debugLabel: 'WidgetInspector.onFrameStart');
}
void _onFrameEnd(Duration timeStamp) {
if (_trackRebuildDirtyWidgets) {
_postStatsEvent('Flutter.RebuiltWidgets', _rebuildStats);
}
if (_trackRepaintWidgets) {
_postStatsEvent('Flutter.RepaintWidgets', _repaintStats);
}
}
void _postStatsEvent(String eventName, _ElementLocationStatsTracker stats) {
postEvent(eventName, stats.exportToJson(_frameStart));
}
/// All events dispatched by a [WidgetInspectorService] use this method
/// instead of calling [developer.postEvent] directly.
///
/// This allows tests for [WidgetInspectorService] to track which events were
/// dispatched by overriding this method.
@protected
void postEvent(
String eventKind,
Map<Object, Object?> eventData, {
String stream = 'Extension',
}) {
developer.postEvent(eventKind, eventData, stream: stream);
}
/// All events dispatched by a [WidgetInspectorService] use this method
/// instead of calling [developer.inspect].
///
/// This allows tests for [WidgetInspectorService] to track which events were
/// dispatched by overriding this method.
@protected
void inspect(Object? object) {
developer.inspect(object);
}
final _ElementLocationStatsTracker _rebuildStats = _ElementLocationStatsTracker();
final _ElementLocationStatsTracker _repaintStats = _ElementLocationStatsTracker();
void _onRebuildWidget(Element element, bool builtOnce) {
_rebuildStats.add(element);
}
void _onPaint(RenderObject renderObject) {
try {
final Element? element = (renderObject.debugCreator as DebugCreator?)?.element;
if (element is! RenderObjectElement) {
// This branch should not hit as long as all RenderObjects were created
// by Widgets. It is possible there might be some render objects
// created directly without using the Widget layer so we add this check
// to improve robustness.
return;
}
_repaintStats.add(element);
// Give all ancestor elements credit for repainting as long as they do
// not have their own associated RenderObject.
element.visitAncestorElements((Element ancestor) {
if (ancestor is RenderObjectElement) {
// This ancestor has its own RenderObject so we can precisely track
// when it repaints.
return false;
}
_repaintStats.add(ancestor);
return true;
});
}
catch (exception, stack) {
FlutterError.reportError(
FlutterErrorDetails(
exception: exception,
stack: stack,
library: 'widget inspector library',
context: ErrorDescription('while tracking widget repaints'),
),
);
}
}
/// This method is called by [WidgetsBinding.performReassemble] to flush caches
/// of obsolete values after a hot reload.
///
/// Do not call this method directly. Instead, use
/// [BindingBase.reassembleApplication].
void performReassemble() {
_clearStats();
_resetErrorCount();
}
}
/// Accumulator for a count associated with a specific source location.
///
/// The accumulator stores whether the source location is [local] and what its
/// [id] for efficiency encoding terse JSON payloads describing counts.
class _LocationCount {
_LocationCount({
required this.location,
required this.id,
required this.local,
});
/// Location id.
final int id;
/// Whether the location is local to the current project.
final bool local;
final _Location location;
int get count => _count;
int _count = 0;
/// Reset the count.
void reset() {
_count = 0;
}
/// Increment the count.
void increment() {
_count++;
}
}
/// A stat tracker that aggregates a performance metric for [Element] objects at
/// the granularity of creation locations in source code.
///
/// This class is optimized to minimize the size of the JSON payloads describing
/// the aggregate statistics, for stable memory usage, and low CPU usage at the
/// expense of somewhat higher overall memory usage. Stable memory usage is more
/// important than peak memory usage to avoid the false impression that the
/// user's app is leaking memory each frame.
///
/// The number of unique widget creation locations tends to be at most in the
/// low thousands for regular flutter apps so the peak memory usage for this
/// class is not an issue.
class _ElementLocationStatsTracker {
// All known creation location tracked.
//
// This could also be stored as a `Map<int, _LocationCount>` but this
// representation is more efficient as all location ids from 0 to n are
// typically present.
//
// All logic in this class assumes that if `_stats[i]` is not null
// `_stats[i].id` equals `i`.
final List<_LocationCount?> _stats = <_LocationCount?>[];
/// Locations with a non-zero count.
final List<_LocationCount> active = <_LocationCount>[];
/// Locations that were added since stats were last exported.
///
/// Only locations local to the current project are included as a performance
/// optimization.
final List<_LocationCount> newLocations = <_LocationCount>[];
/// Increments the count associated with the creation location of [element] if
/// the creation location is local to the current project.
void add(Element element) {
final Object widget = element.widget;
if (widget is! _HasCreationLocation) {
return;
}
final _HasCreationLocation creationLocationSource = widget;
final _Location? location = creationLocationSource._location;
if (location == null) {
return;
}
final int id = _toLocationId(location);
_LocationCount entry;
if (id >= _stats.length || _stats[id] == null) {
// After the first frame, almost all creation ids will already be in
// _stats so this slow path will rarely be hit.
while (id >= _stats.length) {
_stats.add(null);
}
entry = _LocationCount(
location: location,
id: id,
local: WidgetInspectorService.instance._isLocalCreationLocation(location.file),
);
if (entry.local) {
newLocations.add(entry);
}
_stats[id] = entry;
} else {
entry = _stats[id]!;
}
// We could in the future add an option to track stats for all widgets but
// that would significantly increase the size of the events posted using
// [developer.postEvent] and current use cases for this feature focus on
// helping users find problems with their widgets not the platform
// widgets.
if (entry.local) {
if (entry.count == 0) {
active.add(entry);
}
entry.increment();
}
}
/// Clear all aggregated statistics.
void resetCounts() {
// We chose to only reset the active counts instead of clearing all data
// to reduce the number memory allocations performed after the first frame.
// Once an app has warmed up, location stats tracking should not
// trigger significant additional memory allocations. Avoiding memory
// allocations is important to minimize the impact this class has on cpu
// and memory performance of the running app.
for (final _LocationCount entry in active) {
entry.reset();
}
active.clear();
}
/// Exports the current counts and then resets the stats to prepare to track
/// the next frame of data.
Map<String, dynamic> exportToJson(Duration startTime) {
final List<int> events = List<int>.filled(active.length * 2, 0);
int j = 0;
for (final _LocationCount stat in active) {
events[j++] = stat.id;
events[j++] = stat.count;
}
final Map<String, dynamic> json = <String, dynamic>{
'startTime': startTime.inMicroseconds,
'events': events,
};
// Encode the new locations using the older encoding.
if (newLocations.isNotEmpty) {
// Add all newly used location ids to the JSON.
final Map<String, List<int>> locationsJson = <String, List<int>>{};
for (final _LocationCount entry in newLocations) {
final _Location location = entry.location;
final List<int> jsonForFile = locationsJson.putIfAbsent(
location.file,
() => <int>[],
);
jsonForFile..add(entry.id)..add(location.line)..add(location.column);
}
json['newLocations'] = locationsJson;
}
// Encode the new locations using the newer encoding (as of v2.4.0).
if (newLocations.isNotEmpty) {
final Map<String, Map<String, List<Object?>>> fileLocationsMap = <String, Map<String, List<Object?>>>{};
for (final _LocationCount entry in newLocations) {
final _Location location = entry.location;
final Map<String, List<Object?>> locations = fileLocationsMap.putIfAbsent(
location.file, () => <String, List<Object?>>{
'ids': <int>[],
'lines': <int>[],
'columns': <int>[],
'names': <String?>[],
},
);
locations['ids']!.add(entry.id);
locations['lines']!.add(location.line);
locations['columns']!.add(location.column);
locations['names']!.add(location.name);
}
json['locations'] = fileLocationsMap;
}
resetCounts();
newLocations.clear();
return json;
}
}
class _WidgetForTypeTests extends Widget {
const _WidgetForTypeTests();
@override
Element createElement() => throw UnimplementedError();
}
/// A widget that enables inspecting the child widget's structure.
///
/// Select a location on your device or emulator and view what widgets and
/// render object that best matches the location. An outline of the selected
/// widget and terse summary information is shown on device with detailed
/// information is shown in the observatory or in IntelliJ when using the
/// Flutter Plugin.
///
/// The inspector has a select mode and a view mode.
///
/// In the select mode, tapping the device selects the widget that best matches
/// the location of the touch and switches to view mode. Dragging a finger on
/// the device selects the widget under the drag location but does not switch
/// modes. Touching the very edge of the bounding box of a widget triggers
/// selecting the widget even if another widget that also overlaps that
/// location would otherwise have priority.
///
/// In the view mode, the previously selected widget is outlined, however,
/// touching the device has the same effect it would have if the inspector
/// wasn't present. This allows interacting with the application and viewing how
/// the selected widget changes position. Clicking on the select icon in the
/// bottom left corner of the application switches back to select mode.
class WidgetInspector extends StatefulWidget {
/// Creates a widget that enables inspection for the child.
const WidgetInspector({
super.key,
required this.child,
required this.selectButtonBuilder,
});
/// The widget that is being inspected.
final Widget child;
/// A builder that is called to create the select button.
///
/// The `onPressed` callback passed as an argument to the builder should be
/// hooked up to the returned widget.
final InspectorSelectButtonBuilder? selectButtonBuilder;
@override
State<WidgetInspector> createState() => _WidgetInspectorState();
}
class _WidgetInspectorState extends State<WidgetInspector>
with WidgetsBindingObserver {
_WidgetInspectorState();
Offset? _lastPointerLocation;
late InspectorSelection selection;
late bool isSelectMode;
final GlobalKey _ignorePointerKey = GlobalKey();
/// Distance from the edge of the bounding box for an element to consider
/// as selecting the edge of the bounding box.
static const double _edgeHitMargin = 2.0;
@override
void initState() {
super.initState();
WidgetInspectorService.instance.selection
.addListener(_selectionInformationChanged);
WidgetInspectorService.instance.isSelectMode
.addListener(_selectionInformationChanged);
selection = WidgetInspectorService.instance.selection;
isSelectMode = WidgetInspectorService.instance.isSelectMode.value;
}
@override
void dispose() {
WidgetInspectorService.instance.selection
.removeListener(_selectionInformationChanged);
WidgetInspectorService.instance.isSelectMode
.removeListener(_selectionInformationChanged);
super.dispose();
}
void _selectionInformationChanged() => setState((){
selection = WidgetInspectorService.instance.selection;
isSelectMode = WidgetInspectorService.instance.isSelectMode.value;
});
bool _hitTestHelper(
List<RenderObject> hits,
List<RenderObject> edgeHits,
Offset position,
RenderObject object,
Matrix4 transform,
) {
bool hit = false;
final Matrix4? inverse = Matrix4.tryInvert(transform);
if (inverse == null) {
// We cannot invert the transform. That means the object doesn't appear on
// screen and cannot be hit.
return false;
}
final Offset localPosition = MatrixUtils.transformPoint(inverse, position);
final List<DiagnosticsNode> children = object.debugDescribeChildren();
for (int i = children.length - 1; i >= 0; i -= 1) {
final DiagnosticsNode diagnostics = children[i];
if (diagnostics.style == DiagnosticsTreeStyle.offstage ||
diagnostics.value is! RenderObject) {
continue;
}
final RenderObject child = diagnostics.value! as RenderObject;
final Rect? paintClip = object.describeApproximatePaintClip(child);
if (paintClip != null && !paintClip.contains(localPosition)) {
continue;
}
final Matrix4 childTransform = transform.clone();
object.applyPaintTransform(child, childTransform);
if (_hitTestHelper(hits, edgeHits, position, child, childTransform)) {
hit = true;
}
}
final Rect bounds = object.semanticBounds;
if (bounds.contains(localPosition)) {
hit = true;
// Hits that occur on the edge of the bounding box of an object are
// given priority to provide a way to select objects that would
// otherwise be hard to select.
if (!bounds.deflate(_edgeHitMargin).contains(localPosition)) {
edgeHits.add(object);
}
}
if (hit) {
hits.add(object);
}
return hit;
}
/// Returns the list of render objects located at the given position ordered
/// by priority.
///
/// All render objects that are not offstage that match the location are
/// included in the list of matches. Priority is given to matches that occur
/// on the edge of a render object's bounding box and to matches found by
/// [RenderBox.hitTest].
List<RenderObject> hitTest(Offset position, RenderObject root) {
final List<RenderObject> regularHits = <RenderObject>[];
final List<RenderObject> edgeHits = <RenderObject>[];
_hitTestHelper(regularHits, edgeHits, position, root, root.getTransformTo(null));
// Order matches by the size of the hit area.
double area(RenderObject object) {
final Size size = object.semanticBounds.size;
return size.width * size.height;
}
regularHits.sort((RenderObject a, RenderObject b) => area(a).compareTo(area(b)));
final Set<RenderObject> hits = <RenderObject>{
...edgeHits,
...regularHits,
};
return hits.toList();
}
void _inspectAt(Offset position) {
if (!isSelectMode) {
return;
}
final RenderIgnorePointer ignorePointer = _ignorePointerKey.currentContext!.findRenderObject()! as RenderIgnorePointer;
final RenderObject userRender = ignorePointer.child!;
final List<RenderObject> selected = hitTest(position, userRender);
selection.candidates = selected;
}
void _handlePanDown(DragDownDetails event) {
_lastPointerLocation = event.globalPosition;
_inspectAt(event.globalPosition);
}
void _handlePanUpdate(DragUpdateDetails event) {
_lastPointerLocation = event.globalPosition;
_inspectAt(event.globalPosition);
}
void _handlePanEnd(DragEndDetails details) {
// If the pan ends on the edge of the window assume that it indicates the
// pointer is being dragged off the edge of the display not a regular touch
// on the edge of the display. If the pointer is being dragged off the edge
// of the display we do not want to select anything. A user can still select
// a widget that is only at the exact screen margin by tapping.
final ui.FlutterView view = View.of(context);
final Rect bounds = (Offset.zero & (view.physicalSize / view.devicePixelRatio)).deflate(_kOffScreenMargin);
if (!bounds.contains(_lastPointerLocation!)) {
selection.clear();
}
}
void _handleTap() {
if (!isSelectMode) {
return;
}
if (_lastPointerLocation != null) {
_inspectAt(_lastPointerLocation!);
WidgetInspectorService.instance._sendInspectEvent(selection.current);
}
// Only exit select mode if there is a button to return to select mode.
if (widget.selectButtonBuilder != null) {
WidgetInspectorService.instance.isSelectMode.value = false;
}
}
void _handleEnableSelect() {
WidgetInspectorService.instance.isSelectMode.value = true;
}
@override
Widget build(BuildContext context) {
// Be careful changing this build method. The _InspectorOverlayLayer
// assumes the root RenderObject for the WidgetInspector will be
// a RenderStack with a _RenderInspectorOverlay as the last child.
return Stack(children: <Widget>[
GestureDetector(
onTap: _handleTap,
onPanDown: _handlePanDown,
onPanEnd: _handlePanEnd,
onPanUpdate: _handlePanUpdate,
behavior: HitTestBehavior.opaque,
excludeFromSemantics: true,
child: IgnorePointer(
ignoring: isSelectMode,
key: _ignorePointerKey,
child: widget.child,
),
),
if (!isSelectMode && widget.selectButtonBuilder != null)
Positioned(
left: _kInspectButtonMargin,
bottom: _kInspectButtonMargin,
child: widget.selectButtonBuilder!(context, _handleEnableSelect),
),
_InspectorOverlay(selection: selection),
]);
}
}
/// Mutable selection state of the inspector.
class InspectorSelection with ChangeNotifier {
/// Creates an instance of [InspectorSelection].
InspectorSelection() {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// Render objects that are candidates to be selected.
///
/// Tools may wish to iterate through the list of candidates.
List<RenderObject> get candidates => _candidates;
List<RenderObject> _candidates = <RenderObject>[];
set candidates(List<RenderObject> value) {
_candidates = value;
_index = 0;
_computeCurrent();
}
/// Index within the list of candidates that is currently selected.
int get index => _index;
int _index = 0;
set index(int value) {
_index = value;
_computeCurrent();
}
/// Set the selection to empty.
void clear() {
_candidates = <RenderObject>[];
_index = 0;
_computeCurrent();
}
/// Selected render object typically from the [candidates] list.
///
/// Setting [candidates] or calling [clear] resets the selection.
///
/// Returns null if the selection is invalid.
RenderObject? get current => active ? _current : null;
RenderObject? _current;
set current(RenderObject? value) {
if (_current != value) {
_current = value;
_currentElement = (value?.debugCreator as DebugCreator?)?.element;
notifyListeners();
}
}
/// Selected [Element] consistent with the [current] selected [RenderObject].
///
/// Setting [candidates] or calling [clear] resets the selection.
///
/// Returns null if the selection is invalid.
Element? get currentElement {
return _currentElement?.debugIsDefunct ?? true ? null : _currentElement;
}
Element? _currentElement;
set currentElement(Element? element) {
if (element?.debugIsDefunct ?? false) {
_currentElement = null;
_current = null;
notifyListeners();
return;
}
if (currentElement != element) {
_currentElement = element;
_current = element!.findRenderObject();
notifyListeners();
}
}
void _computeCurrent() {
if (_index < candidates.length) {
_current = candidates[index];
_currentElement = (_current?.debugCreator as DebugCreator?)?.element;
notifyListeners();
} else {
_current = null;
_currentElement = null;
notifyListeners();
}
}
/// Whether the selected render object is attached to the tree or has gone
/// out of scope.
bool get active => _current != null && _current!.attached;
}
class _InspectorOverlay extends LeafRenderObjectWidget {
const _InspectorOverlay({
required this.selection,
});
final InspectorSelection selection;
@override
_RenderInspectorOverlay createRenderObject(BuildContext context) {
return _RenderInspectorOverlay(selection: selection);
}
@override
void updateRenderObject(BuildContext context, _RenderInspectorOverlay renderObject) {
renderObject.selection = selection;
}
}
class _RenderInspectorOverlay extends RenderBox {
_RenderInspectorOverlay({ required InspectorSelection selection })
: _selection = selection;
InspectorSelection get selection => _selection;
InspectorSelection _selection;
set selection(InspectorSelection value) {
if (value != _selection) {
_selection = value;
}
markNeedsPaint();
}
@override
bool get sizedByParent => true;
@override
bool get alwaysNeedsCompositing => true;
@override
Size computeDryLayout(BoxConstraints constraints) {
return constraints.constrain(Size.infinite);
}
@override
void paint(PaintingContext context, Offset offset) {
assert(needsCompositing);
context.addLayer(_InspectorOverlayLayer(
overlayRect: Rect.fromLTWH(offset.dx, offset.dy, size.width, size.height),
selection: selection,
rootRenderObject: parent is RenderObject ? parent! : null,
));
}
}
@immutable
class _TransformedRect {
_TransformedRect(RenderObject object, RenderObject? ancestor)
: rect = object.semanticBounds,
transform = object.getTransformTo(ancestor);
final Rect rect;
final Matrix4 transform;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _TransformedRect
&& other.rect == rect
&& other.transform == transform;
}
@override
int get hashCode => Object.hash(rect, transform);
}
/// State describing how the inspector overlay should be rendered.
///
/// The equality operator can be used to determine whether the overlay needs to
/// be rendered again.
@immutable
class _InspectorOverlayRenderState {
const _InspectorOverlayRenderState({
required this.overlayRect,
required this.selected,
required this.candidates,
required this.tooltip,
required this.textDirection,
});
final Rect overlayRect;
final _TransformedRect selected;
final List<_TransformedRect> candidates;
final String tooltip;
final TextDirection textDirection;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _InspectorOverlayRenderState
&& other.overlayRect == overlayRect
&& other.selected == selected
&& listEquals<_TransformedRect>(other.candidates, candidates)
&& other.tooltip == tooltip;
}
@override
int get hashCode => Object.hash(overlayRect, selected, Object.hashAll(candidates), tooltip);
}
const int _kMaxTooltipLines = 5;
const Color _kTooltipBackgroundColor = Color.fromARGB(230, 60, 60, 60);
const Color _kHighlightedRenderObjectFillColor = Color.fromARGB(128, 128, 128, 255);
const Color _kHighlightedRenderObjectBorderColor = Color.fromARGB(128, 64, 64, 128);
/// A layer that outlines the selected [RenderObject] and candidate render
/// objects that also match the last pointer location.
///
/// This approach is horrific for performance and is only used here because this
/// is limited to debug mode. Do not duplicate the logic in production code.
class _InspectorOverlayLayer extends Layer {
/// Creates a layer that displays the inspector overlay.
_InspectorOverlayLayer({
required this.overlayRect,
required this.selection,
required this.rootRenderObject,
}) {
bool inDebugMode = false;
assert(() {
inDebugMode = true;
return true;
}());
if (!inDebugMode) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'The inspector should never be used in production mode due to the '
'negative performance impact.',
),
]);
}
}
InspectorSelection selection;
/// The rectangle in this layer's coordinate system that the overlay should
/// occupy.
///
/// The scene must be explicitly recomposited after this property is changed
/// (as described at [Layer]).
final Rect overlayRect;
/// Widget inspector root render object. The selection overlay will be painted
/// with transforms relative to this render object.
final RenderObject? rootRenderObject;
_InspectorOverlayRenderState? _lastState;
/// Picture generated from _lastState.
ui.Picture? _picture;
TextPainter? _textPainter;
double? _textPainterMaxWidth;
@override
void dispose() {
_textPainter?.dispose();
_textPainter = null;
_picture?.dispose();
super.dispose();
}
@override
void addToScene(ui.SceneBuilder builder) {
if (!selection.active) {
return;
}
final RenderObject selected = selection.current!;
if (!_isInInspectorRenderObjectTree(selected)) {
return;
}
final List<_TransformedRect> candidates = <_TransformedRect>[];
for (final RenderObject candidate in selection.candidates) {
if (candidate == selected || !candidate.attached
|| !_isInInspectorRenderObjectTree(candidate)) {
continue;
}
candidates.add(_TransformedRect(candidate, rootRenderObject));
}
final _TransformedRect selectedRect = _TransformedRect(selected, rootRenderObject);
final String widgetName = selection.currentElement!.toStringShort();
final String width = selectedRect.rect.width.toStringAsFixed(1);
final String height = selectedRect.rect.height.toStringAsFixed(1);
final _InspectorOverlayRenderState state = _InspectorOverlayRenderState(
overlayRect: overlayRect,
selected: selectedRect,
tooltip: '$widgetName ($width x $height)',
textDirection: TextDirection.ltr,
candidates: candidates,
);
if (state != _lastState) {
_lastState = state;
_picture?.dispose();
_picture = _buildPicture(state);
}
builder.addPicture(Offset.zero, _picture!);
}
ui.Picture _buildPicture(_InspectorOverlayRenderState state) {
final ui.PictureRecorder recorder = ui.PictureRecorder();
final Canvas canvas = Canvas(recorder, state.overlayRect);
final Size size = state.overlayRect.size;
// The overlay rect could have an offset if the widget inspector does
// not take all the screen.
canvas.translate(state.overlayRect.left, state.overlayRect.top);
final Paint fillPaint = Paint()
..style = PaintingStyle.fill
..color = _kHighlightedRenderObjectFillColor;
final Paint borderPaint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1.0
..color = _kHighlightedRenderObjectBorderColor;
// Highlight the selected renderObject.
final Rect selectedPaintRect = state.selected.rect.deflate(0.5);
canvas
..save()
..transform(state.selected.transform.storage)
..drawRect(selectedPaintRect, fillPaint)
..drawRect(selectedPaintRect, borderPaint)
..restore();
// Show all other candidate possibly selected elements. This helps selecting
// render objects by selecting the edge of the bounding box shows all
// elements the user could toggle the selection between.
for (final _TransformedRect transformedRect in state.candidates) {
canvas
..save()
..transform(transformedRect.transform.storage)
..drawRect(transformedRect.rect.deflate(0.5), borderPaint)
..restore();
}
final Rect targetRect = MatrixUtils.transformRect(
state.selected.transform, state.selected.rect,
);
final Offset target = Offset(targetRect.left, targetRect.center.dy);
const double offsetFromWidget = 9.0;
final double verticalOffset = (targetRect.height) / 2 + offsetFromWidget;
_paintDescription(canvas, state.tooltip, state.textDirection, target, verticalOffset, size, targetRect);
// TODO(jacobr): provide an option to perform a debug paint of just the
// selected widget.
return recorder.endRecording();
}
void _paintDescription(
Canvas canvas,
String message,
TextDirection textDirection,
Offset target,
double verticalOffset,
Size size,
Rect targetRect,
) {
canvas.save();
final double maxWidth = math.max(
size.width - 2 * (_kScreenEdgeMargin + _kTooltipPadding),
0,
);
final TextSpan? textSpan = _textPainter?.text as TextSpan?;
if (_textPainter == null || textSpan!.text != message || _textPainterMaxWidth != maxWidth) {
_textPainterMaxWidth = maxWidth;
_textPainter?.dispose();
_textPainter = TextPainter()
..maxLines = _kMaxTooltipLines
..ellipsis = '...'
..text = TextSpan(style: _messageStyle, text: message)
..textDirection = textDirection
..layout(maxWidth: maxWidth);
}
final Size tooltipSize = _textPainter!.size + const Offset(_kTooltipPadding * 2, _kTooltipPadding * 2);
final Offset tipOffset = positionDependentBox(
size: size,
childSize: tooltipSize,
target: target,
verticalOffset: verticalOffset,
preferBelow: false,
);
final Paint tooltipBackground = Paint()
..style = PaintingStyle.fill
..color = _kTooltipBackgroundColor;
canvas.drawRect(
Rect.fromPoints(
tipOffset,
tipOffset.translate(tooltipSize.width, tooltipSize.height),
),
tooltipBackground,
);
double wedgeY = tipOffset.dy;
final bool tooltipBelow = tipOffset.dy > target.dy;
if (!tooltipBelow) {
wedgeY += tooltipSize.height;
}
const double wedgeSize = _kTooltipPadding * 2;
double wedgeX = math.max(tipOffset.dx, target.dx) + wedgeSize * 2;
wedgeX = math.min(wedgeX, tipOffset.dx + tooltipSize.width - wedgeSize * 2);
final List<Offset> wedge = <Offset>[
Offset(wedgeX - wedgeSize, wedgeY),
Offset(wedgeX + wedgeSize, wedgeY),
Offset(wedgeX, wedgeY + (tooltipBelow ? -wedgeSize : wedgeSize)),
];
canvas.drawPath(Path()..addPolygon(wedge, true), tooltipBackground);
_textPainter!.paint(canvas, tipOffset + const Offset(_kTooltipPadding, _kTooltipPadding));
canvas.restore();
}
@override
@protected
bool findAnnotations<S extends Object>(
AnnotationResult<S> result,
Offset localPosition, {
bool? onlyFirst,
}) {
return false;
}
/// Return whether or not a render object belongs to this inspector widget
/// tree.
/// The inspector selection is static, so if there are multiple inspector
/// overlays in the same app (i.e. an storyboard), a selected or candidate
/// render object may not belong to this tree.
bool _isInInspectorRenderObjectTree(RenderObject child) {
RenderObject? current = child.parent;
while (current != null) {
// We found the widget inspector render object.
if (current is RenderStack
&& current.lastChild is _RenderInspectorOverlay) {
return rootRenderObject == current;
}
current = current.parent;
}
return false;
}
}
const double _kScreenEdgeMargin = 10.0;
const double _kTooltipPadding = 5.0;
const double _kInspectButtonMargin = 10.0;
/// Interpret pointer up events within with this margin as indicating the
/// pointer is moving off the device.
const double _kOffScreenMargin = 1.0;
const TextStyle _messageStyle = TextStyle(
color: Color(0xFFFFFFFF),
fontSize: 10.0,
height: 1.2,
);
/// Interface for classes that track the source code location the their
/// constructor was called from.
///
/// {@macro flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
// ignore: unused_element
abstract class _HasCreationLocation {
_Location? get _location;
}
/// A tuple with file, line, and column number, for displaying human-readable
/// file locations.
class _Location {
const _Location({
required this.file,
required this.line,
required this.column,
// ignore: unused_element
this.name,
});
/// File path of the location.
final String file;
/// 1-based line number.
final int line;
/// 1-based column number.
final int column;
/// Optional name of the parameter or function at this location.
final String? name;
Map<String, Object?> toJsonMap() {
final Map<String, Object?> json = <String, Object?>{
'file': file,
'line': line,
'column': column,
};
if (name != null) {
json['name'] = name;
}
return json;
}
@override
String toString() {
final List<String> parts = <String>[];
if (name != null) {
parts.add(name!);
}
parts.add(file);
parts..add('$line')..add('$column');
return parts.join(':');
}
}
bool _isDebugCreator(DiagnosticsNode node) => node is DiagnosticsDebugCreator;
/// Transformer to parse and gather information about [DiagnosticsDebugCreator].
///
/// This function will be registered to [FlutterErrorDetails.propertiesTransformers]
/// in [WidgetsBinding.initInstances].
///
/// This is meant to be called only in debug mode. In other modes, it yields an empty list.
Iterable<DiagnosticsNode> debugTransformDebugCreator(Iterable<DiagnosticsNode> properties) {
if (!kDebugMode) {
return <DiagnosticsNode>[];
}
final List<DiagnosticsNode> pending = <DiagnosticsNode>[];
ErrorSummary? errorSummary;
for (final DiagnosticsNode node in properties) {
if (node is ErrorSummary) {
errorSummary = node;
break;
}
}
bool foundStackTrace = false;
final List<DiagnosticsNode> result = <DiagnosticsNode>[];
for (final DiagnosticsNode node in properties) {
if (!foundStackTrace && node is DiagnosticsStackTrace) {
foundStackTrace = true;
}
if (_isDebugCreator(node)) {
result.addAll(_parseDiagnosticsNode(node, errorSummary));
} else {
if (foundStackTrace) {
pending.add(node);
} else {
result.add(node);
}
}
}
result.addAll(pending);
return result;
}
/// Transform the input [DiagnosticsNode].
///
/// Return null if input [DiagnosticsNode] is not applicable.
Iterable<DiagnosticsNode> _parseDiagnosticsNode(
DiagnosticsNode node,
ErrorSummary? errorSummary,
) {
assert(_isDebugCreator(node));
try {
final DebugCreator debugCreator = node.value! as DebugCreator;
final Element element = debugCreator.element;
return _describeRelevantUserCode(element, errorSummary);
} catch (error, stack) {
scheduleMicrotask(() {
FlutterError.reportError(FlutterErrorDetails(
exception: error,
stack: stack,
library: 'widget inspector',
informationCollector: () => <DiagnosticsNode>[
DiagnosticsNode.message('This exception was caught while trying to describe the user-relevant code of another error.'),
],
));
});
return <DiagnosticsNode>[];
}
}
Iterable<DiagnosticsNode> _describeRelevantUserCode(
Element element,
ErrorSummary? errorSummary,
) {
if (!WidgetInspectorService.instance.isWidgetCreationTracked()) {
return <DiagnosticsNode>[
ErrorDescription(
'Widget creation tracking is currently disabled. Enabling '
'it enables improved error messages. It can be enabled by passing '
'`--track-widget-creation` to `flutter run` or `flutter test`.',
),
ErrorSpacer(),
];
}
bool isOverflowError() {
if (errorSummary != null && errorSummary.value.isNotEmpty) {
final Object summary = errorSummary.value.first;
if (summary is String && summary.startsWith('A RenderFlex overflowed by')) {
return true;
}
}
return false;
}
final List<DiagnosticsNode> nodes = <DiagnosticsNode>[];
bool processElement(Element target) {
// TODO(chunhtai): should print out all the widgets that are about to cross
// package boundaries.
if (debugIsLocalCreationLocation(target)) {
DiagnosticsNode? devToolsDiagnostic;
// TODO(kenz): once the inspector is better at dealing with broken trees,
// we can enable deep links for more errors than just RenderFlex overflow
// errors. See https://github.com/flutter/flutter/issues/74918.
if (isOverflowError()) {
final String? devToolsInspectorUri =
WidgetInspectorService.instance._devToolsInspectorUriForElement(target);
if (devToolsInspectorUri != null) {
devToolsDiagnostic = DevToolsDeepLinkProperty(
'To inspect this widget in Flutter DevTools, visit: $devToolsInspectorUri',
devToolsInspectorUri,
);
}
}
nodes.addAll(<DiagnosticsNode>[
DiagnosticsBlock(
name: 'The relevant error-causing widget was',
children: <DiagnosticsNode>[
ErrorDescription('${target.widget.toStringShort()} ${_describeCreationLocation(target)}'),
],
),
ErrorSpacer(),
if (devToolsDiagnostic != null) ...<DiagnosticsNode>[devToolsDiagnostic, ErrorSpacer()],
]);
return false;
}
return true;
}
if (processElement(element)) {
element.visitAncestorElements(processElement);
}
return nodes;
}
/// Debugging message for DevTools deep links.
///
/// The [value] for this property is a string representation of the Flutter
/// DevTools url.
class DevToolsDeepLinkProperty extends DiagnosticsProperty<String> {
/// Creates a diagnostics property that displays a deep link to Flutter DevTools.
///
/// The [value] of this property will return a map of data for the Flutter
/// DevTools deep link, including the full `url`, the Flutter DevTools `screenId`,
/// and the `objectId` in Flutter DevTools that this diagnostic references.
DevToolsDeepLinkProperty(String description, String url)
: super('', url, description: description, level: DiagnosticLevel.info);
}
/// Returns if an object is user created.
///
/// This always returns false if it is not called in debug mode.
///
/// {@macro flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
///
/// Currently is local creation locations are only available for
/// [Widget] and [Element].
bool debugIsLocalCreationLocation(Object object) {
bool isLocal = false;
assert(() {
final _Location? location = _getCreationLocation(object);
if (location != null) {
isLocal = WidgetInspectorService.instance._isLocalCreationLocation(location.file);
}
return true;
}());
return isLocal;
}
/// Returns true if a [Widget] is user created.
///
/// This is a faster variant of `debugIsLocalCreationLocation` that is available
/// in debug and profile builds but only works for [Widget].
bool debugIsWidgetLocalCreation(Widget widget) {
final _Location? location = _getObjectCreationLocation(widget);
return location != null &&
WidgetInspectorService.instance._isLocalCreationLocation(location.file);
}
/// Returns the creation location of an object in String format if one is available.
///
/// ex: "file:///path/to/main.dart:4:3"
///
/// {@macro flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
///
/// Currently creation locations are only available for [Widget] and [Element].
String? _describeCreationLocation(Object object) {
final _Location? location = _getCreationLocation(object);
return location?.toString();
}
_Location? _getObjectCreationLocation(Object object) {
return object is _HasCreationLocation ? object._location : null;
}
/// Returns the creation location of an object if one is available.
///
/// {@macro flutter.widgets.WidgetInspectorService.getChildrenSummaryTree}
///
/// Currently creation locations are only available for [Widget] and [Element].
_Location? _getCreationLocation(Object? object) {
final Object? candidate = object is Element && !object.debugIsDefunct ? object.widget : object;
return candidate == null ? null : _getObjectCreationLocation(candidate);
}
// _Location objects are always const so we don't need to worry about the GC
// issues that are a concern for other object ids tracked by
// [WidgetInspectorService].
final Map<_Location, int> _locationToId = <_Location, int>{};
final List<_Location> _locations = <_Location>[];
int _toLocationId(_Location location) {
int? id = _locationToId[location];
if (id != null) {
return id;
}
id = _locations.length;
_locations.add(location);
_locationToId[location] = id;
return id;
}
/// A delegate that configures how a hierarchy of [DiagnosticsNode]s are
/// serialized by the Flutter Inspector.
@visibleForTesting
class InspectorSerializationDelegate implements DiagnosticsSerializationDelegate {
/// Creates an [InspectorSerializationDelegate] that serialize [DiagnosticsNode]
/// for Flutter Inspector service.
InspectorSerializationDelegate({
this.groupName,
this.summaryTree = false,
this.maxDescendantsTruncatableNode = -1,
this.expandPropertyValues = true,
this.subtreeDepth = 1,
this.includeProperties = false,
required this.service,
this.addAdditionalPropertiesCallback,
});
/// Service used by GUI tools to interact with the [WidgetInspector].
final WidgetInspectorService service;
/// Optional [groupName] parameter which indicates that the json should
/// contain live object ids.
///
/// Object ids returned as part of the json will remain live at least until
/// [WidgetInspectorService.disposeGroup()] is called on [groupName].
final String? groupName;
/// Whether the tree should only include nodes created by the local project.
final bool summaryTree;
/// Maximum descendants of [DiagnosticsNode] before truncating.
final int maxDescendantsTruncatableNode;
@override
final bool includeProperties;
@override
final int subtreeDepth;
@override
final bool expandPropertyValues;
/// Callback to add additional experimental serialization properties.
///
/// This callback can be used to customize the serialization of DiagnosticsNode
/// objects for experimental features in widget inspector clients such as
/// [Dart DevTools](https://github.com/flutter/devtools).
final Map<String, Object>? Function(DiagnosticsNode, InspectorSerializationDelegate)? addAdditionalPropertiesCallback;
final List<DiagnosticsNode> _nodesCreatedByLocalProject = <DiagnosticsNode>[];
bool get _interactive => groupName != null;
@override
Map<String, Object?> additionalNodeProperties(DiagnosticsNode node) {
final Map<String, Object?> result = <String, Object?>{};
final Object? value = node.value;
if (_interactive) {
result['valueId'] = service.toId(value, groupName!);
}
if (summaryTree) {
result['summaryTree'] = true;
}
final _Location? creationLocation = _getCreationLocation(value);
if (creationLocation != null) {
result['locationId'] = _toLocationId(creationLocation);
result['creationLocation'] = creationLocation.toJsonMap();
if (service._isLocalCreationLocation(creationLocation.file)) {
_nodesCreatedByLocalProject.add(node);
result['createdByLocalProject'] = true;
}
}
if (addAdditionalPropertiesCallback != null) {
result.addAll(addAdditionalPropertiesCallback!(node, this) ?? <String, Object>{});
}
return result;
}
@override
DiagnosticsSerializationDelegate delegateForNode(DiagnosticsNode node) {
// The tricky special case here is that when in the detailsTree,
// we keep subtreeDepth from going down to zero until we reach nodes
// that also exist in the summary tree. This ensures that every time
// you expand a node in the details tree, you expand the entire subtree
// up until you reach the next nodes shared with the summary tree.
return summaryTree || subtreeDepth > 1 || service._shouldShowInSummaryTree(node)
? copyWith(subtreeDepth: subtreeDepth - 1)
: this;
}
@override
List<DiagnosticsNode> filterChildren(List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
return service._filterChildren(nodes, this);
}
@override
List<DiagnosticsNode> filterProperties(List<DiagnosticsNode> nodes, DiagnosticsNode owner) {
final bool createdByLocalProject = _nodesCreatedByLocalProject.contains(owner);
return nodes.where((DiagnosticsNode node) {
return !node.isFiltered(createdByLocalProject ? DiagnosticLevel.fine : DiagnosticLevel.info);
}).toList();
}
@override
List<DiagnosticsNode> truncateNodesList(List<DiagnosticsNode> nodes, DiagnosticsNode? owner) {
if (maxDescendantsTruncatableNode >= 0 &&
owner!.allowTruncate &&
nodes.length > maxDescendantsTruncatableNode) {
nodes = service._truncateNodes(nodes, maxDescendantsTruncatableNode);
}
return nodes;
}
@override
DiagnosticsSerializationDelegate copyWith({int? subtreeDepth, bool? includeProperties, bool? expandPropertyValues}) {
return InspectorSerializationDelegate(
groupName: groupName,
summaryTree: summaryTree,
maxDescendantsTruncatableNode: maxDescendantsTruncatableNode,
expandPropertyValues: expandPropertyValues ?? this.expandPropertyValues,
subtreeDepth: subtreeDepth ?? this.subtreeDepth,
includeProperties: includeProperties ?? this.includeProperties,
service: service,
addAdditionalPropertiesCallback: addAdditionalPropertiesCallback,
);
}
}
@Target(<TargetKind>{TargetKind.method})
class _WidgetFactory {
const _WidgetFactory();
}
/// Annotation which marks a function as a widget factory for the purpose of
/// widget creation tracking.
///
/// When widget creation tracking is enabled, the framework tracks the source
/// code location of the constructor call for each widget instance. This
/// information is used by the DevTools to provide an improved developer
/// experience. For example, it allows the Flutter inspector to present the
/// widget tree in a manner similar to how the UI was defined in your source
/// code.
///
/// [Widget] constructors are automatically instrumented to track the source
/// code location of constructor calls. However, there are cases where
/// a function acts as a sort of a constructor for a widget and a call to such
/// a function should be considered as the creation location for the returned
/// widget instance.
///
/// Annotating a function with this annotation marks the function as a widget
/// factory. The framework will then instrument that function in the same way
/// as it does for [Widget] constructors.
///
/// Tracking will not work correctly if the function has optional positional
/// parameters.
///
/// Currently this annotation is only supported on extension methods.
///
/// {@tool snippet}
///
/// This example shows how to use the [widgetFactory] annotation to mark an
/// extension method as a widget factory:
///
/// ```dart
/// extension PaddingModifier on Widget {
/// @widgetFactory
/// Widget padding(EdgeInsetsGeometry padding) {
/// return Padding(padding: padding, child: this);
/// }
/// }
/// ```
///
/// When using the above extension method, the framework will track the
/// creation location of the [Padding] widget instance as the source code
/// location where the `padding` extension method was called:
///
/// ```dart
/// // continuing from previous example...
/// const Text('Hello World!')
/// .padding(const EdgeInsets.all(8));
/// ```
///
/// {@end-tool}
///
/// See also:
///
/// * the documentation for [Track widget creation](https://docs.flutter.dev/development/tools/devtools/inspector#track-widget-creation).
// The below ignore is needed because the static type of the annotation is used
// by the CFE kernel transformer that implements the instrumentation to
// recognize the annotation.
// ignore: library_private_types_in_public_api
const _WidgetFactory widgetFactory = _WidgetFactory();
/// Does not hold keys from garbage collection.
@visibleForTesting
class WeakMap<K, V> {
Expando<Object> _objects = Expando<Object>();
/// Strings, numbers, booleans.
final Map<K, V> _primitives = <K, V>{};
bool _isPrimitive(Object? key) {
return key == null || key is String || key is num || key is bool;
}
/// Returns the value for the given [key] or null if [key] is not in the map
/// or garbage collected.
///
/// Does not support records to act as keys.
V? operator [](K key){
if (_isPrimitive(key)) {
return _primitives[key];
} else {
return _objects[key!] as V?;
}
}
/// Associates the [key] with the given [value].
void operator []=(K key, V value){
if (_isPrimitive(key)) {
_primitives[key] = value;
} else {
_objects[key!] = value;
}
}
/// Removes the value for the given [key] from the map.
V? remove(K key) {
if (_isPrimitive(key)) {
return _primitives.remove(key);
} else {
final V? result = _objects[key!] as V?;
_objects[key] = null;
return result;
}
}
/// Removes all pairs from the map.
void clear() {
_objects = Expando<Object>();
_primitives.clear();
}
}
| flutter/packages/flutter/lib/src/widgets/widget_inspector.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/widget_inspector.dart",
"repo_id": "flutter",
"token_count": 45502
} | 715 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('toString control test', () {
expect(Curves.linear, hasOneLineDescription);
expect(const SawTooth(3), hasOneLineDescription);
expect(const Interval(0.25, 0.75), hasOneLineDescription);
expect(const Interval(0.25, 0.75, curve: Curves.ease), hasOneLineDescription);
expect(const Split(0.25, beginCurve: Curves.ease), hasOneLineDescription);
});
test('Curve flipped control test', () {
const Curve ease = Curves.ease;
final Curve flippedEase = ease.flipped;
expect(flippedEase.transform(0.0), lessThan(0.001));
expect(flippedEase.transform(0.5), lessThan(ease.transform(0.5)));
expect(flippedEase.transform(1.0), greaterThan(0.999));
expect(flippedEase, hasOneLineDescription);
});
test('Threshold has a threshold', () {
const Curve step = Threshold(0.25);
expect(step.transform(0.0), 0.0);
expect(step.transform(0.24), 0.0);
expect(step.transform(0.25), 1.0);
expect(step.transform(0.26), 1.0);
expect(step.transform(1.0), 1.0);
});
void assertMaximumSlope(Curve curve, double maximumSlope) {
const double delta = 0.005;
for (double x = 0.0; x < 1.0 - delta; x += delta) {
final double deltaY = curve.transform(x) - curve.transform(x + delta);
assert(deltaY.abs() < delta * maximumSlope, '$curve discontinuous at $x');
}
}
test('Curve is continuous', () {
assertMaximumSlope(Curves.linear, 20.0);
assertMaximumSlope(Curves.decelerate, 20.0);
assertMaximumSlope(Curves.fastOutSlowIn, 20.0);
assertMaximumSlope(Curves.slowMiddle, 20.0);
assertMaximumSlope(Curves.bounceIn, 20.0);
assertMaximumSlope(Curves.bounceOut, 20.0);
assertMaximumSlope(Curves.bounceInOut, 20.0);
assertMaximumSlope(Curves.elasticOut, 20.0);
assertMaximumSlope(Curves.elasticInOut, 20.0);
assertMaximumSlope(Curves.ease, 20.0);
assertMaximumSlope(Curves.easeIn, 20.0);
assertMaximumSlope(Curves.easeInSine, 20.0);
assertMaximumSlope(Curves.easeInQuad, 20.0);
assertMaximumSlope(Curves.easeInCubic, 20.0);
assertMaximumSlope(Curves.easeInQuart, 20.0);
assertMaximumSlope(Curves.easeInQuint, 20.0);
assertMaximumSlope(Curves.easeInExpo, 20.0);
assertMaximumSlope(Curves.easeInCirc, 20.0);
assertMaximumSlope(Curves.easeOut, 20.0);
assertMaximumSlope(Curves.easeOutSine, 20.0);
assertMaximumSlope(Curves.easeOutQuad, 20.0);
assertMaximumSlope(Curves.easeOutCubic, 20.0);
assertMaximumSlope(Curves.easeInOutCubicEmphasized, 20.0);
assertMaximumSlope(Curves.easeOutQuart, 20.0);
assertMaximumSlope(Curves.easeOutQuint, 20.0);
assertMaximumSlope(Curves.easeOutExpo, 20.0);
assertMaximumSlope(Curves.easeOutCirc, 20.0);
// Curves.easeInOutExpo is discontinuous at its midpoint, so not included
// here
assertMaximumSlope(Curves.easeInOut, 20.0);
assertMaximumSlope(Curves.easeInOutSine, 20.0);
assertMaximumSlope(Curves.easeInOutQuad, 20.0);
assertMaximumSlope(Curves.easeInOutCubic, 20.0);
assertMaximumSlope(Curves.easeInOutQuart, 20.0);
assertMaximumSlope(Curves.easeInOutQuint, 20.0);
assertMaximumSlope(Curves.easeInOutCirc, 20.0);
});
void expectStaysInBounds(Curve curve) {
expect(curve.transform(0.0), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.1), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.2), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.3), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.4), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.5), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.6), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.7), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.8), inInclusiveRange(0.0, 1.0));
expect(curve.transform(0.9), inInclusiveRange(0.0, 1.0));
expect(curve.transform(1.0), inInclusiveRange(0.0, 1.0));
}
test('Bounce stays in bounds', () {
expectStaysInBounds(Curves.bounceIn);
expectStaysInBounds(Curves.bounceOut);
expectStaysInBounds(Curves.bounceInOut);
});
List<double> estimateBounds(Curve curve) {
final List<double> values = <double>[
curve.transform(0.0),
curve.transform(0.1),
curve.transform(0.2),
curve.transform(0.3),
curve.transform(0.4),
curve.transform(0.5),
curve.transform(0.6),
curve.transform(0.7),
curve.transform(0.8),
curve.transform(0.9),
curve.transform(1.0),
];
return <double>[
values.reduce(math.min),
values.reduce(math.max),
];
}
test('Elastic overshoots its bounds', () {
expect(Curves.elasticIn, hasOneLineDescription);
expect(Curves.elasticOut, hasOneLineDescription);
expect(Curves.elasticInOut, hasOneLineDescription);
List<double> bounds;
bounds = estimateBounds(Curves.elasticIn);
expect(bounds[0], lessThan(0.0));
expect(bounds[1], lessThanOrEqualTo(1.0));
bounds = estimateBounds(Curves.elasticOut);
expect(bounds[0], greaterThanOrEqualTo(0.0));
expect(bounds[1], greaterThan(1.0));
bounds = estimateBounds(Curves.elasticInOut);
expect(bounds[0], lessThan(0.0));
expect(bounds[1], greaterThan(1.0));
});
test('Back overshoots its bounds', () {
expect(Curves.easeInBack, hasOneLineDescription);
expect(Curves.easeOutBack, hasOneLineDescription);
expect(Curves.easeInOutBack, hasOneLineDescription);
List<double> bounds;
bounds = estimateBounds(Curves.easeInBack);
expect(bounds[0], lessThan(0.0));
expect(bounds[1], lessThanOrEqualTo(1.0));
bounds = estimateBounds(Curves.easeOutBack);
expect(bounds[0], greaterThanOrEqualTo(0.0));
expect(bounds[1], greaterThan(1.0));
bounds = estimateBounds(Curves.easeInOutBack);
expect(bounds[0], lessThan(0.0));
expect(bounds[1], greaterThan(1.0));
});
test('Decelerate does so', () {
expect(Curves.decelerate, hasOneLineDescription);
final List<double> bounds = estimateBounds(Curves.decelerate);
expect(bounds[0], greaterThanOrEqualTo(0.0));
expect(bounds[1], lessThanOrEqualTo(1.0));
final double d1 = Curves.decelerate.transform(0.2) - Curves.decelerate.transform(0.0);
final double d2 = Curves.decelerate.transform(1.0) - Curves.decelerate.transform(0.8);
expect(d2, lessThan(d1));
});
test('ThreePointCubic interpolates midpoint', () {
const ThreePointCubic test = ThreePointCubic(
Offset(0.05, 0), Offset(0.133333, 0.06),
Offset(0.166666, 0.4),
Offset(0.208333, 0.82), Offset(0.25, 1),
);
expect(test.transform(0.166666), equals(0.4));
});
test('Invalid transform parameter should assert', () {
expect(() => const SawTooth(2).transform(-0.0001), throwsAssertionError);
expect(() => const SawTooth(2).transform(1.0001), throwsAssertionError);
expect(() => const Interval(0.0, 1.0).transform(-0.0001), throwsAssertionError);
expect(() => const Interval(0.0, 1.0).transform(1.0001), throwsAssertionError);
expect(() => const Split(0.0).transform(-0.0001), throwsAssertionError);
expect(() => const Split(0.0).transform(1.0001), throwsAssertionError);
expect(() => const Threshold(0.5).transform(-0.0001), throwsAssertionError);
expect(() => const Threshold(0.5).transform(1.0001), throwsAssertionError);
expect(() => const ElasticInCurve().transform(-0.0001), throwsAssertionError);
expect(() => const ElasticInCurve().transform(1.0001), throwsAssertionError);
expect(() => const ElasticOutCurve().transform(-0.0001), throwsAssertionError);
expect(() => const ElasticOutCurve().transform(1.0001), throwsAssertionError);
expect(() => const Cubic(0.42, 0.0, 0.58, 1.0).transform(-0.0001), throwsAssertionError);
expect(() => const Cubic(0.42, 0.0, 0.58, 1.0).transform(1.0001), throwsAssertionError);
expect(() => Curves.easeInOutCubicEmphasized.transform(-0.0001), throwsAssertionError);
expect(() => Curves.easeInOutCubicEmphasized.transform(1.0001), throwsAssertionError);
expect(() => Curves.decelerate.transform(-0.0001), throwsAssertionError);
expect(() => Curves.decelerate.transform(1.0001), throwsAssertionError);
expect(() => Curves.bounceIn.transform(-0.0001), throwsAssertionError);
expect(() => Curves.bounceIn.transform(1.0001), throwsAssertionError);
expect(() => Curves.bounceOut.transform(-0.0001), throwsAssertionError);
expect(() => Curves.bounceOut.transform(1.0001), throwsAssertionError);
expect(() => Curves.bounceInOut.transform(-0.0001), throwsAssertionError);
expect(() => Curves.bounceInOut.transform(1.0001), throwsAssertionError);
});
test('Curve transform method should return 0.0 for t=0.0 and 1.0 for t=1.0', () {
expect(const SawTooth(2).transform(0), 0);
expect(const SawTooth(2).transform(1), 1);
expect(const Interval(0, 1).transform(0), 0);
expect(const Interval(0, 1).transform(1), 1);
expect(const Split(0.5).transform(0), 0);
expect(const Split(0.5).transform(1), 1);
expect(const Threshold(0.5).transform(0), 0);
expect(const Threshold(0.5).transform(1), 1);
expect(const ElasticInCurve().transform(0), 0);
expect(const ElasticInCurve().transform(1), 1);
expect(const ElasticOutCurve().transform(0), 0);
expect(const ElasticOutCurve().transform(1), 1);
expect(const ElasticInOutCurve().transform(0), 0);
expect(const ElasticInOutCurve().transform(1), 1);
expect(Curves.linear.transform(0), 0);
expect(Curves.linear.transform(1), 1);
expect(Curves.easeInOutExpo.transform(0), 0);
expect(Curves.easeInOutExpo.transform(1), 1);
expect(Curves.easeInOutCubicEmphasized.transform(0), 0);
expect(Curves.easeInOutCubicEmphasized.transform(1), 1);
expect(const FlippedCurve(Curves.easeInOutExpo).transform(0), 0);
expect(const FlippedCurve(Curves.easeInOutExpo).transform(1), 1);
expect(Curves.decelerate.transform(0), 0);
expect(Curves.decelerate.transform(1), 1);
expect(Curves.bounceIn.transform(0), 0);
expect(Curves.bounceIn.transform(1), 1);
expect(Curves.bounceOut.transform(0), 0);
expect(Curves.bounceOut.transform(1), 1);
expect(Curves.bounceInOut.transform(0), 0);
expect(Curves.bounceInOut.transform(1), 1);
});
test('Split interpolates values properly', () {
const Split curve = Split(0.3);
const double tolerance = 1e-6;
expect(curve.transform(0.0), equals(0.0));
expect(curve.transform(0.1), equals(0.1));
expect(curve.transform(0.25), equals(0.25));
expect(curve.transform(0.3), equals(0.3));
expect(curve.transform(0.5), moreOrLessEquals(0.760461, epsilon: tolerance));
expect(curve.transform(0.75), moreOrLessEquals(0.962055, epsilon: tolerance));
expect(curve.transform(1.0), equals(1.0));
});
test('CatmullRomSpline interpolates values properly', () {
final CatmullRomSpline curve = CatmullRomSpline(
const <Offset>[
Offset.zero,
Offset(0.01, 0.25),
Offset(0.2, 0.25),
Offset(0.33, 0.25),
Offset(0.5, 1.0),
Offset(0.66, 0.75),
Offset(1.0, 1.0),
],
startHandle: const Offset(0.0, -0.3),
endHandle: const Offset(1.3, 1.3),
);
const double tolerance = 1e-6;
expect(curve.transform(0.0).dx, moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.0).dy, moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.25).dx, moreOrLessEquals(0.0966945, epsilon: tolerance));
expect(curve.transform(0.25).dy, moreOrLessEquals(0.2626806, epsilon: tolerance));
expect(curve.transform(0.5).dx, moreOrLessEquals(0.33, epsilon: tolerance));
expect(curve.transform(0.5).dy, moreOrLessEquals(0.25, epsilon: tolerance));
expect(curve.transform(0.75).dx, moreOrLessEquals(0.570260, epsilon: tolerance));
expect(curve.transform(0.75).dy, moreOrLessEquals(0.883085, epsilon: tolerance));
expect(curve.transform(1.0).dx, moreOrLessEquals(1.0, epsilon: tolerance));
expect(curve.transform(1.0).dy, moreOrLessEquals(1.0, epsilon: tolerance));
});
test('CatmullRomSpline enforces contract', () {
expect(() {
CatmullRomSpline(const <Offset>[]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(const <Offset>[Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(const <Offset>[Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(const <Offset>[Offset.zero, Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero], tension: -1.0);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero], tension: 2.0);
}, throwsAssertionError);
expect(() {
CatmullRomSpline(
const <Offset>[Offset(double.infinity, 0.0), Offset.zero, Offset.zero, Offset.zero],
).generateSamples();
}, throwsAssertionError);
expect(() {
CatmullRomSpline(
const <Offset>[Offset(0.0, double.infinity), Offset.zero, Offset.zero, Offset.zero],
).generateSamples();
}, throwsAssertionError);
expect(() {
CatmullRomSpline(
startHandle: const Offset(0.0, double.infinity),
const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero],
).generateSamples();
}, throwsAssertionError);
expect(() {
CatmullRomSpline(
endHandle: const Offset(0.0, double.infinity),
const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero],
).generateSamples();
}, throwsAssertionError);
});
test('CatmullRomSpline interpolates values properly when precomputed', () {
final CatmullRomSpline curve = CatmullRomSpline.precompute(
const <Offset>[
Offset.zero,
Offset(0.01, 0.25),
Offset(0.2, 0.25),
Offset(0.33, 0.25),
Offset(0.5, 1.0),
Offset(0.66, 0.75),
Offset(1.0, 1.0),
],
startHandle: const Offset(0.0, -0.3),
endHandle: const Offset(1.3, 1.3),
);
const double tolerance = 1e-6;
expect(curve.transform(0.0).dx, moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.0).dy, moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.25).dx, moreOrLessEquals(0.0966945, epsilon: tolerance));
expect(curve.transform(0.25).dy, moreOrLessEquals(0.2626806, epsilon: tolerance));
expect(curve.transform(0.5).dx, moreOrLessEquals(0.33, epsilon: tolerance));
expect(curve.transform(0.5).dy, moreOrLessEquals(0.25, epsilon: tolerance));
expect(curve.transform(0.75).dx, moreOrLessEquals(0.570260, epsilon: tolerance));
expect(curve.transform(0.75).dy, moreOrLessEquals(0.883085, epsilon: tolerance));
expect(curve.transform(1.0).dx, moreOrLessEquals(1.0, epsilon: tolerance));
expect(curve.transform(1.0).dy, moreOrLessEquals(1.0, epsilon: tolerance));
});
test('CatmullRomSpline enforces contract when precomputed', () {
expect(() {
CatmullRomSpline.precompute(const <Offset>[]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset.zero, Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero], tension: -1.0);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero], tension: 2.0);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset(double.infinity, 0.0), Offset.zero, Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(const <Offset>[Offset(0.0, double.infinity), Offset.zero, Offset.zero, Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(
startHandle: const Offset(0.0, double.infinity),
const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero],
);
}, throwsAssertionError);
expect(() {
CatmullRomSpline.precompute(
endHandle: const Offset(0.0, double.infinity),
const <Offset>[Offset.zero, Offset.zero, Offset.zero, Offset.zero],
);
}, throwsAssertionError);
});
test('CatmullRomCurve interpolates given points correctly', () {
final CatmullRomCurve curve = CatmullRomCurve(
const <Offset>[
Offset(0.2, 0.25),
Offset(0.33, 0.25),
Offset(0.5, 1.0),
Offset(0.8, 0.75),
],
);
// These values are approximations.
const double tolerance = 1e-6;
expect(curve.transform(0.0), moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.01), moreOrLessEquals(0.012874734350170863, epsilon: tolerance));
expect(curve.transform(0.2), moreOrLessEquals(0.24989646045277542, epsilon: tolerance));
expect(curve.transform(0.33), moreOrLessEquals(0.250037698527661, epsilon: tolerance));
expect(curve.transform(0.5), moreOrLessEquals(0.9999057323235939, epsilon: tolerance));
expect(curve.transform(0.6), moreOrLessEquals(0.9357294964536621, epsilon: tolerance));
expect(curve.transform(0.8), moreOrLessEquals(0.7500423402378034, epsilon: tolerance));
expect(curve.transform(1.0), moreOrLessEquals(1.0, epsilon: tolerance));
});
test('CatmullRomCurve interpolates given points correctly when precomputed', () {
final CatmullRomCurve curve = CatmullRomCurve.precompute(
const <Offset>[
Offset(0.2, 0.25),
Offset(0.33, 0.25),
Offset(0.5, 1.0),
Offset(0.8, 0.75),
],
);
// These values are approximations.
const double tolerance = 1e-6;
expect(curve.transform(0.0), moreOrLessEquals(0.0, epsilon: tolerance));
expect(curve.transform(0.01), moreOrLessEquals(0.012874734350170863, epsilon: tolerance));
expect(curve.transform(0.2), moreOrLessEquals(0.24989646045277542, epsilon: tolerance));
expect(curve.transform(0.33), moreOrLessEquals(0.250037698527661, epsilon: tolerance));
expect(curve.transform(0.5), moreOrLessEquals(0.9999057323235939, epsilon: tolerance));
expect(curve.transform(0.6), moreOrLessEquals(0.9357294964536621, epsilon: tolerance));
expect(curve.transform(0.8), moreOrLessEquals(0.7500423402378034, epsilon: tolerance));
expect(curve.transform(1.0), moreOrLessEquals(1.0, epsilon: tolerance));
});
test('CatmullRomCurve enforces contract', () {
expect(() {
CatmullRomCurve(const <Offset>[]);
}, throwsAssertionError);
expect(() {
CatmullRomCurve(const <Offset>[Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomCurve(const <Offset>[Offset.zero, Offset.zero]);
}, throwsAssertionError);
// Monotonically increasing in X.
expect(
CatmullRomCurve.validateControlPoints(
const <Offset>[
Offset(0.2, 0.25),
Offset(0.01, 0.25),
],
),
isFalse,
);
expect(() {
CatmullRomCurve(
const <Offset>[
Offset(0.2, 0.25),
Offset(0.01, 0.25),
],
);
}, throwsAssertionError);
// X within range (0.0, 1.0).
expect(
CatmullRomCurve.validateControlPoints(
const <Offset>[
Offset(0.2, 0.25),
Offset(1.01, 0.25),
],
),
isFalse,
);
expect(() {
CatmullRomCurve(
const <Offset>[
Offset(0.2, 0.25),
Offset(1.01, 0.25),
],
);
}, throwsAssertionError);
// Not multi-valued in Y at x=0.0.
expect(
CatmullRomCurve.validateControlPoints(
const <Offset>[
Offset(0.05, 0.50),
Offset(0.50, 0.50),
Offset(0.75, 0.75),
],
),
isFalse,
);
expect(() {
CatmullRomCurve(
const <Offset>[
Offset(0.05, 0.50),
Offset(0.50, 0.50),
Offset(0.75, 0.75),
],
);
}, throwsAssertionError);
// Not multi-valued in Y at x=1.0.
expect(
CatmullRomCurve.validateControlPoints(
const <Offset>[
Offset(0.25, 0.25),
Offset(0.50, 0.50),
Offset(0.95, 0.51),
],
),
isFalse,
);
expect(() {
CatmullRomCurve(
const <Offset>[
Offset(0.25, 0.25),
Offset(0.50, 0.50),
Offset(0.95, 0.51),
],
);
}, throwsAssertionError);
// Not multi-valued in Y in between x = 0.0 and x = 1.0.
expect(
CatmullRomCurve.validateControlPoints(
const <Offset>[
Offset(0.5, 0.05),
Offset(0.5, 0.95),
],
),
isFalse,
);
expect(() {
CatmullRomCurve(
const <Offset>[
Offset(0.5, 0.05),
Offset(0.5, 0.95),
],
);
}, throwsAssertionError);
});
test('CatmullRomCurve enforces contract when precomputed', () {
expect(() {
CatmullRomCurve.precompute(const <Offset>[]);
}, throwsAssertionError);
expect(() {
CatmullRomCurve.precompute(const <Offset>[Offset.zero]);
}, throwsAssertionError);
expect(() {
CatmullRomCurve.precompute(const <Offset>[Offset.zero, Offset.zero]);
}, throwsAssertionError);
// Monotonically increasing in X.
expect(() {
CatmullRomCurve.precompute(
const <Offset>[
Offset(0.2, 0.25),
Offset(0.01, 0.25),
],
);
}, throwsAssertionError);
// X within range (0.0, 1.0).
expect(() {
CatmullRomCurve.precompute(
const <Offset>[
Offset(0.2, 0.25),
Offset(1.01, 0.25),
],
);
}, throwsAssertionError);
// Not multi-valued in Y at x=0.0.
expect(() {
CatmullRomCurve.precompute(
const <Offset>[
Offset(0.05, 0.50),
Offset(0.50, 0.50),
Offset(0.75, 0.75),
],
);
}, throwsAssertionError);
// Not multi-valued in Y at x=1.0.
expect(() {
CatmullRomCurve.precompute(
const <Offset>[
Offset(0.25, 0.25),
Offset(0.50, 0.50),
Offset(0.95, 0.51),
],
);
}, throwsAssertionError);
// Not multi-valued in Y in between x = 0.0 and x = 1.0.
expect(() {
CatmullRomCurve.precompute(
const <Offset>[
Offset(0.5, 0.05),
Offset(0.5, 0.95),
],
);
}, throwsAssertionError);
});
}
| flutter/packages/flutter/test/animation/curves_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/curves_test.dart",
"repo_id": "flutter",
"token_count": 10024
} | 716 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:clock/clock.dart';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final TestWidgetsFlutterBinding binding = TestWidgetsFlutterBinding.ensureInitialized();
const double kOpenScale = 1.15;
Widget getChild() {
return Container(
width: 300.0,
height: 100.0,
color: CupertinoColors.activeOrange,
);
}
Widget getBuilder(BuildContext context, Animation<double> animation) {
return getChild();
}
Widget getContextMenu({
Alignment alignment = Alignment.center,
Size screenSize = const Size(800.0, 600.0),
Widget? child,
}) {
return CupertinoApp(
home: CupertinoPageScaffold(
child: MediaQuery(
data: MediaQueryData(size: screenSize),
child: Align(
alignment: alignment,
child: CupertinoContextMenu(
actions: <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction $alignment'),
),
],
child: child ?? getChild(),
),
),
),
),
);
}
Widget getBuilderContextMenu({
Alignment alignment = Alignment.center,
Size screenSize = const Size(800.0, 600.0),
CupertinoContextMenuBuilder? builder,
}) {
return CupertinoApp(
home: CupertinoPageScaffold(
child: MediaQuery(
data: MediaQueryData(size: screenSize),
child: Align(
alignment: alignment,
child: CupertinoContextMenu.builder(
actions: <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction $alignment'),
),
],
builder: builder ?? getBuilder,
),
),
),
),
);
}
// Finds the child widget that is rendered inside of _DecoyChild.
Finder findDecoyChild(Widget child) {
return find.descendant(
of: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'),
matching: find.byWidget(child),
);
}
// Finds the child widget rendered inside of _ContextMenuRouteStatic.
Finder findStatic() {
return find.descendant(
of: find.byType(CupertinoApp),
matching: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_ContextMenuRouteStatic'),
);
}
Finder findStaticChild(Widget child) {
return find.descendant(
of: findStatic(),
matching: find.byWidget(child),
);
}
Finder findStaticChildDecoration(WidgetTester tester) {
return find.descendant(
of: findStatic(),
matching: find.byType(DecoratedBox),
);
}
Finder findFittedBox() {
return find.descendant(
of: findStatic(),
matching: find.byType(FittedBox),
);
}
Finder findStaticDefaultPreview() {
return find.descendant(
of: findFittedBox(),
matching: find.byType(ClipRRect),
);
}
group('CupertinoContextMenu before and during opening', () {
testWidgets('An unopened CupertinoContextMenu renders child in the same place as without', (WidgetTester tester) async {
// Measure the child in the scene with no CupertinoContextMenu.
final Widget child = getChild();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: child,
),
),
),
);
final Rect childRect = tester.getRect(find.byWidget(child));
// When wrapped in a CupertinoContextMenu, the child is rendered in the same Rect.
await tester.pumpWidget(getContextMenu(child: child));
expect(find.byWidget(child), findsOneWidget);
expect(tester.getRect(find.byWidget(child)), childRect);
});
testWidgets('Can open CupertinoContextMenu by tap and hold', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
expect(find.byWidget(child), findsOneWidget);
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// The _DecoyChild is showing directly on top of the child.
expect(findDecoyChild(child), findsOneWidget);
Rect decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, equals(decoyChildRect));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate.
await tester.pump(const Duration(milliseconds: 400));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
// Eventually the decoy fully scales by _kOpenSize.
await tester.pump(const Duration(milliseconds: 800));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
expect(decoyChildRect.width, childRect.width * kOpenScale);
// Then the CupertinoContextMenu opens.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
});
testWidgets('CupertinoContextMenu is in the correct position when within a nested navigator', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: MediaQuery(
data: const MediaQueryData(size: Size(800, 600)),
child: Align(
alignment: Alignment.bottomRight,
child: SizedBox(
width: 700,
height: 500,
child: Navigator(
onGenerateRoute: (RouteSettings settings) {
return CupertinoPageRoute<void>(
builder: (BuildContext context) => Align(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction'),
),
],
child: child
),
)
);
}
)
)
)
)
)
));
expect(find.byWidget(child), findsOneWidget);
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// The _DecoyChild is showing directly on top of the child.
expect(findDecoyChild(child), findsOneWidget);
Rect decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, equals(decoyChildRect));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate.
await tester.pump(const Duration(milliseconds: 400));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
// Eventually the decoy fully scales by _kOpenSize.
await tester.pump(const Duration(milliseconds: 800));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
expect(decoyChildRect.width, childRect.width * kOpenScale);
// Then the CupertinoContextMenu opens.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
});
testWidgets('_DecoyChild preserves the child color', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
backgroundColor: CupertinoColors.black,
child: MediaQuery(
data: const MediaQueryData(size: Size(800, 600)),
child: Center(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction'),
),
],
child: child
),
)
)
),
));
// Expect no _DecoyChild to be present before the gesture.
final Finder decoyChild = find
.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild');
expect(decoyChild, findsNothing);
// Start press gesture on the child.
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// Find the _DecoyChild by runtimeType,
// find the Container descendant with the BoxDecoration,
// then read the boxDecoration property.
final Finder decoyChildDescendant = find.descendant(
of: decoyChild,
matching: find.byType(Container));
final BoxDecoration? boxDecoration = (tester.firstWidget(decoyChildDescendant) as Container).decoration as BoxDecoration?;
const List<Color?> expectedColors = <Color?>[null, Color(0x00000000)];
// `Color(0x00000000)` -> Is `Colors.transparent`.
// `null` -> Default when no color argument is given in `BoxDecoration`.
// Any other color won't preserve the child's property.
expect(expectedColors, contains(boxDecoration?.color));
// End the gesture.
await gesture.up();
await tester.pumpAndSettle();
// Expect no _DecoyChild to be present after ending the gesture.
final Finder decoyChildAfterEnding = find
.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild');
expect(decoyChildAfterEnding, findsNothing);
});
testWidgets('CupertinoContextMenu with a basic builder opens and closes the same as when providing a child', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getBuilderContextMenu(builder: (BuildContext context, Animation<double> animation) {
return child;
}));
expect(find.byWidget(child), findsOneWidget);
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// The _DecoyChild is showing directly on top of the child.
expect(findDecoyChild(child), findsOneWidget);
Rect decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, equals(decoyChildRect));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate.
await tester.pump(const Duration(milliseconds: 400));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
// Eventually the decoy fully scales by _kOpenSize.
await tester.pump(const Duration(milliseconds: 800));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
expect(decoyChildRect.width, childRect.width * kOpenScale);
// Then the CupertinoContextMenu opens.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
});
testWidgets('CupertinoContextMenu with a builder can change the animation', (WidgetTester tester) async {
await tester.pumpWidget(getBuilderContextMenu(builder: (BuildContext context, Animation<double> animation) {
return Container(
width: 300.0,
height: 100.0,
decoration: BoxDecoration(
color: CupertinoColors.activeOrange,
borderRadius: BorderRadius.circular(25.0 * animation.value)
),
);
}));
final Widget child = find.descendant(of: find.byType(TickerMode), matching: find.byType(Container)).evaluate().single.widget;
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
Finder findBuilderDecoyChild() {
return find.descendant(
of: find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'),
matching: find.byType(Container),
);
}
final Container decoyContainer = tester.firstElement(findBuilderDecoyChild()).widget as Container;
final BoxDecoration? decoyDecoration = decoyContainer.decoration as BoxDecoration?;
expect(decoyDecoration?.borderRadius, equals(BorderRadius.circular(0)));
expect(findBuilderDecoyChild(), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate with a different border radius.
await tester.pump(const Duration(milliseconds: 500));
final Container decoyLaterContainer = tester.firstElement(findBuilderDecoyChild()).widget as Container;
final BoxDecoration? decoyLaterDecoration = decoyLaterContainer.decoration as BoxDecoration?;
expect(decoyLaterDecoration?.borderRadius, isNot(equals(BorderRadius.circular(0))));
// Finish gesture to release resources.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Hovering over Cupertino context menu updates cursor to clickable on Web', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction One'),
),
],
child: child,
),
),
),
));
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: const Offset(10, 10));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
final Offset contextMenu = tester.getCenter(find.byWidget(child));
await gesture.moveTo(contextMenu);
await tester.pumpAndSettle();
expect(
RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic,
);
});
testWidgets('CupertinoContextMenu is in the correct position when within a Transform.scale', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: MediaQuery(
data: const MediaQueryData(size: Size(800, 600)),
child: Transform.scale(
scale: 0.5,
child: Align(
//alignment: Alignment.bottomRight,
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction'),
),
],
child: child
),
)
)
)
)
));
expect(find.byWidget(child), findsOneWidget);
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// The _DecoyChild is showing directly on top of the child.
expect(findDecoyChild(child), findsOneWidget);
Rect decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, equals(decoyChildRect));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate.
await tester.pump(const Duration(milliseconds: 400));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
// Eventually the decoy fully scales by _kOpenSize.
await tester.pump(const Duration(milliseconds: 800));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
expect(decoyChildRect.width, childRect.width * kOpenScale);
// Then the CupertinoContextMenu opens.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
});
});
group('CupertinoContextMenu when open', () {
testWidgets('Last action does not have border', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction One'),
),
],
child: child,
),
),
),
));
// Open the CupertinoContextMenu
final TestGesture firstGesture = await tester.startGesture(tester.getCenter(find.byWidget(child)));
await tester.pumpAndSettle();
await firstGesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
expect(findStaticChildDecoration(tester), findsNWidgets(1));
// Close the CupertinoContextMenu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: Center(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction One'),
),
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction Two'),
),
],
child: child,
),
),
),
));
// Open the CupertinoContextMenu
final TestGesture secondGesture = await tester.startGesture(tester.getCenter(find.byWidget(child)));
await tester.pumpAndSettle();
await secondGesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
expect(findStaticChildDecoration(tester), findsNWidgets(3));
});
testWidgets('Can close CupertinoContextMenu by background tap', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
// Open the CupertinoContextMenu
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
// Tap and ensure that the CupertinoContextMenu is closed.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
});
testWidgets('Can close CupertinoContextMenu by dragging down', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
// Open the CupertinoContextMenu
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
// Drag down not far enough and it bounces back and doesn't close.
expect(findStaticChild(child), findsOneWidget);
Offset staticChildCenter = tester.getCenter(findStaticChild(child));
TestGesture swipeGesture = await tester.startGesture(staticChildCenter);
await swipeGesture.moveBy(
const Offset(0.0, 100.0),
timeStamp: const Duration(milliseconds: 100),
);
await tester.pump();
await swipeGesture.up();
await tester.pump();
expect(tester.getCenter(findStaticChild(child)).dy, greaterThan(staticChildCenter.dy));
await tester.pumpAndSettle();
expect(tester.getCenter(findStaticChild(child)), equals(staticChildCenter));
expect(findStatic(), findsOneWidget);
// Drag down far enough and it does close.
expect(findStaticChild(child), findsOneWidget);
staticChildCenter = tester.getCenter(findStaticChild(child));
swipeGesture = await tester.startGesture(staticChildCenter);
await swipeGesture.moveBy(
const Offset(0.0, 200.0),
timeStamp: const Duration(milliseconds: 100),
);
await tester.pump();
await swipeGesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
});
testWidgets('Can close CupertinoContextMenu by flinging down', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
// Open the CupertinoContextMenu
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
// Fling up and nothing happens.
expect(findStaticChild(child), findsOneWidget);
await tester.fling(findStaticChild(child), const Offset(0.0, -100.0), 1000.0);
await tester.pumpAndSettle();
expect(findStaticChild(child), findsOneWidget);
// Fling down to close the menu.
expect(findStaticChild(child), findsOneWidget);
await tester.fling(findStaticChild(child), const Offset(0.0, 100.0), 1000.0);
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
});
testWidgets("Backdrop is added using ModalRoute's filter parameter", (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
expect(find.byType(BackdropFilter), findsNothing);
// Open the CupertinoContextMenu
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
expect(find.byType(BackdropFilter), findsOneWidget);
});
testWidgets('Preview widget should have the correct border radius', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
// Open the CupertinoContextMenu.
final Rect childRect = tester.getRect(find.byWidget(child));
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
// Check border radius.
expect(findStaticDefaultPreview(), findsOneWidget);
final ClipRRect previewWidget = tester.firstWidget(findStaticDefaultPreview()) as ClipRRect;
expect(previewWidget.borderRadius, equals(BorderRadius.circular(12.0)));
});
testWidgets('CupertinoContextMenu width is correct', (WidgetTester tester) async {
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(child: child));
expect(find.byWidget(child), findsOneWidget);
final Rect childRect = tester.getRect(find.byWidget(child));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsNothing);
// Start a press on the child.
final TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pump();
// The _DecoyChild is showing directly on top of the child.
expect(findDecoyChild(child), findsOneWidget);
Rect decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, equals(decoyChildRect));
expect(find.byWidgetPredicate((Widget w) => '${w.runtimeType}' == '_DecoyChild'), findsOneWidget);
// After a small delay, the _DecoyChild has begun to animate.
await tester.pump(const Duration(milliseconds: 400));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
// Eventually the decoy fully scales by _kOpenSize.
await tester.pump(const Duration(milliseconds: 800));
decoyChildRect = tester.getRect(findDecoyChild(child));
expect(childRect, isNot(equals(decoyChildRect)));
expect(decoyChildRect.width, childRect.width * kOpenScale);
// Then the CupertinoContextMenu opens.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
expect(findStatic(), findsOneWidget);
// The CupertinoContextMenu has the correct width and height.
final CupertinoContextMenu widget = tester.widget(find.byType(CupertinoContextMenu));
for (final Widget action in widget.actions) {
// The value of the height is 80 because of the font and icon size.
expect(tester.getSize(find.byWidget(action)).width, 250);
}
});
testWidgets("ContextMenu route animation doesn't throw exception on dismiss", (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/124597.
final List<int> items = List<int>.generate(2, (int index) => index).toList();
await tester.pumpWidget(CupertinoApp(
home: CupertinoPageScaffold(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return ListView(
children: items.map((int index) => CupertinoContextMenu(
actions: <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: const Text('DELETE'),
onPressed: () {
setState(() {
items.remove(index);
Navigator.of(context).pop();
});
Navigator.of(context).pop();
},
),
],
child: Text('Item $index'),
)).toList(),
);
}
),
),
));
// Open the CupertinoContextMenu.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Item 1')));
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// Tap the delete action.
await tester.tap(find.text('DELETE'));
await tester.pumpAndSettle();
// The CupertinoContextMenu should be closed with no exception.
expect(find.text('DELETE'), findsNothing);
expect(tester.takeException(), null);
});
});
group("Open layout differs depending on child's position on screen", () {
testWidgets('Portrait', (WidgetTester tester) async {
const Size portraitScreenSize = Size(600.0, 800.0);
await binding.setSurfaceSize(portraitScreenSize);
// Pump a CupertinoContextMenu in the center of the screen and open it.
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(
screenSize: portraitScreenSize,
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
Rect childRect = tester.getRect(find.byWidget(child));
TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// The position of the action is in the center of the screen.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset center = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
// Close the CupertinoContextMenu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
// Pump a CupertinoContextMenu on the left of the screen and open it.
await tester.pumpWidget(getContextMenu(
alignment: Alignment.centerLeft,
screenSize: portraitScreenSize,
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
await tester.pumpAndSettle();
childRect = tester.getRect(find.byWidget(child));
gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// The position of the action is on the left of the screen.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset left = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
expect(left.dx, lessThan(center.dx));
// Close the CupertinoContextMenu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
// Pump a CupertinoContextMenu on the right of the screen and open it.
await tester.pumpWidget(getContextMenu(
alignment: Alignment.centerRight,
screenSize: portraitScreenSize,
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
childRect = tester.getRect(find.byWidget(child));
gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// The position of the action is on the right of the screen.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset right = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
expect(right.dx, greaterThan(center.dx));
// Set the screen back to its normal size.
await binding.setSurfaceSize(const Size(800.0, 600.0));
});
testWidgets('Landscape', (WidgetTester tester) async {
// Pump a CupertinoContextMenu in the center of the screen and open it.
final Widget child = getChild();
await tester.pumpWidget(getContextMenu(
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
Rect childRect = tester.getRect(find.byWidget(child));
TestGesture gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// Landscape doesn't support a centered action list, so the action is on
// the left side of the screen.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset center = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
// Close the CupertinoContextMenu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
// Pump a CupertinoContextMenu on the left of the screen and open it.
await tester.pumpWidget(getContextMenu(
alignment: Alignment.centerLeft,
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
childRect = tester.getRect(find.byWidget(child));
gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// The position of the action is on the right of the screen, which is the
// same as for center aligned children in landscape.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset left = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
expect(left.dx, equals(center.dx));
// Close the CupertinoContextMenu.
await tester.tapAt(const Offset(1.0, 1.0));
await tester.pumpAndSettle();
expect(findStatic(), findsNothing);
// Pump a CupertinoContextMenu on the right of the screen and open it.
await tester.pumpWidget(getContextMenu(
alignment: Alignment.centerRight,
child: child,
));
expect(find.byType(CupertinoContextMenuAction), findsNothing);
childRect = tester.getRect(find.byWidget(child));
gesture = await tester.startGesture(childRect.center);
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// The position of the action is on the left of the screen.
expect(find.byType(CupertinoContextMenuAction), findsOneWidget);
final Offset right = tester.getTopLeft(find.byType(CupertinoContextMenuAction));
expect(right.dx, lessThan(left.dx));
});
});
testWidgets('Conflicting gesture detectors', (WidgetTester tester) async {
int? onPointerDownTime;
int? onPointerUpTime;
bool insideTapTriggered = false;
// The required duration of the route to be pushed in is [500, 900]ms.
// 500ms is calculated from kPressTimeout+_previewLongPressTimeout/2.
// 900ms is calculated from kPressTimeout+_previewLongPressTimeout.
const Duration pressDuration = Duration(milliseconds: 501);
int now() => clock.now().millisecondsSinceEpoch;
await tester.pumpWidget(Listener(
onPointerDown: (PointerDownEvent event) => onPointerDownTime = now(),
onPointerUp: (PointerUpEvent event) => onPointerUpTime = now(),
child: CupertinoApp(
home: Align(
child: CupertinoContextMenu(
actions: const <CupertinoContextMenuAction>[
CupertinoContextMenuAction(
child: Text('CupertinoContextMenuAction'),
),
],
child: GestureDetector(
onTap: () => insideTapTriggered = true,
child: Container(
width: 200,
height: 200,
key: const Key('container'),
color: const Color(0xFF00FF00),
),
),
),
),
),
));
// Start a press on the child.
final TestGesture gesture = await tester.createGesture();
await gesture.down(tester.getCenter(find.byKey(const Key('container'))));
// Simulate the actual situation:
// the user keeps pressing and requesting frames.
// If there is only one frame,
// the animation is mutant and cannot drive the value of the animation controller.
for (int i = 0; i < 100; i++) {
await tester.pump(pressDuration ~/ 100);
}
await gesture.up();
// Await pushing route.
await tester.pumpAndSettle();
// Judge whether _ContextMenuRouteStatic present on the screen.
final Finder routeStatic = find.byWidgetPredicate(
(Widget w) => '${w.runtimeType}' == '_ContextMenuRouteStatic',
);
// The insideTap and the route should not be triggered at the same time.
if (insideTapTriggered) {
// Calculate the actual duration.
final int actualDuration = onPointerUpTime! - onPointerDownTime!;
expect(routeStatic, findsNothing,
reason: 'When actualDuration($actualDuration) is in the range of 500ms~900ms, '
'which means the route is pushed, '
'but insideTap should not be triggered at the same time.');
} else {
// The route should be pushed when the insideTap is not triggered.
expect(routeStatic, findsOneWidget);
}
});
}
| flutter/packages/flutter/test/cupertino/context_menu_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/context_menu_test.dart",
"repo_id": "flutter",
"token_count": 15114
} | 717 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('!chrome')
library;
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
Future<void> startTransitionBetween(
WidgetTester tester, {
Widget? from,
Widget? to,
String? fromTitle,
String? toTitle,
TextDirection textDirection = TextDirection.ltr,
CupertinoThemeData? theme,
TextScaler textScaler = TextScaler.noScaling,
}) async {
await tester.pumpWidget(
CupertinoApp(
theme: theme,
builder: (BuildContext context, Widget? navigator) {
return MediaQuery(
data: MediaQuery.of(context).copyWith(textScaler: textScaler),
child: Directionality(
textDirection: textDirection,
child: navigator!,
)
);
},
home: const Placeholder(),
),
);
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: fromTitle,
builder: (BuildContext context) => scaffoldForNavBar(from)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 600));
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: toTitle,
builder: (BuildContext context) => scaffoldForNavBar(to)!,
));
await tester.pump();
}
CupertinoPageScaffold? scaffoldForNavBar(Widget? navBar) {
switch (navBar) {
case CupertinoNavigationBar? _:
return CupertinoPageScaffold(
navigationBar: navBar ?? const CupertinoNavigationBar(),
child: const Placeholder(),
);
case CupertinoSliverNavigationBar():
return CupertinoPageScaffold(
child: CustomScrollView(slivers: <Widget>[
navBar,
// Add filler so it's scrollable.
const SliverToBoxAdapter(child: Placeholder(fallbackHeight: 1000.0)),
]),
);
default:
assert(false, 'Unexpected nav bar type ${navBar.runtimeType}');
return null;
}
}
Finder flying(WidgetTester tester, Finder finder) {
final ContainerRenderObjectMixin<RenderBox, StackParentData> theater = tester.renderObject(find.byType(Overlay));
final Finder lastOverlayFinder = find.byElementPredicate((Element element) {
return element is RenderObjectElement && element.renderObject == theater.lastChild;
});
assert(
find.descendant(
of: lastOverlayFinder,
matching: find.byWidgetPredicate(
(Widget widget) =>
widget.runtimeType.toString() == '_NavigationBarTransition',
),
).evaluate().length == 1,
'The last overlay in the navigator was not a flying hero',
);
return find.descendant(
of: lastOverlayFinder,
matching: finder,
);
}
void checkBackgroundBoxHeight(WidgetTester tester, double height) {
final Widget transitionBackgroundBox =
tester.widget<Stack>(flying(tester, find.byType(Stack))).children[0];
expect(
tester.widget<SizedBox>(
find.descendant(
of: find.byWidget(transitionBackgroundBox),
matching: find.byType(SizedBox),
),
).height,
height,
);
}
void checkOpacity(WidgetTester tester, Finder finder, double opacity) {
expect(
tester.firstRenderObject<RenderAnimatedOpacity>(
find.ancestor(
of: finder,
matching: find.byType(FadeTransition),
),
).opacity.value,
moreOrLessEquals(opacity),
);
}
void main() {
testWidgets('Bottom middle moves between middle and back label', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
// There's 2 of them. One from the top route's back label and one from the
// bottom route's middle widget.
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
// Since they have the same text, they should be more or less at the same
// place.
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
342.547737105096302912,
13.5,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
342.547737105096302912,
13.5,
),
);
});
testWidgets('Bottom middle moves between middle and back label RTL', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
textDirection: TextDirection.rtl,
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
// Same as LTR but more to the right now.
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
357.912261979376353338,
13.5,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
357.912261979376353338,
13.5,
),
);
});
testWidgets('Bottom middle never changes size during the animation', (WidgetTester tester) async {
await tester.binding.setSurfaceSize(const Size(1080.0 / 2.75, 600));
addTearDown(() async {
await tester.binding.setSurfaceSize(const Size(800.0, 600.0));
});
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
);
final Size size = tester.getSize(find.text('Page 1'));
for (int i = 0; i < 150; i++) {
await tester.pump(const Duration(milliseconds: 1));
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
expect(tester.getSize(flying(tester, find.text('Page 1')).first), size);
expect(tester.getSize(flying(tester, find.text('Page 1')).last), size);
}
});
testWidgets('Bottom middle and top back label transitions their font', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
// The transition's stack is ordered. The bottom middle is inserted first.
final RenderParagraph bottomMiddle =
tester.renderObject(flying(tester, find.text('Page 1')).first);
expect(bottomMiddle.text.style!.color, const Color(0xff000306));
expect(bottomMiddle.text.style!.fontWeight, FontWeight.w600);
expect(bottomMiddle.text.style!.fontFamily, 'CupertinoSystemText');
expect(bottomMiddle.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.9404401779174805);
// The top back label is styled exactly the same way. But the opacity tweens
// are flipped.
final RenderParagraph topBackLabel =
tester.renderObject(flying(tester, find.text('Page 1')).last);
expect(topBackLabel.text.style!.color, const Color(0xff000306));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w600);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemText');
expect(topBackLabel.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.0);
// Move animation further a bit.
await tester.pump(const Duration(milliseconds: 200));
expect(bottomMiddle.text.style!.color, const Color(0xff005ec5));
expect(bottomMiddle.text.style!.fontWeight, FontWeight.w400);
expect(bottomMiddle.text.style!.fontFamily, 'CupertinoSystemText');
expect(bottomMiddle.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.0);
expect(topBackLabel.text.style!.color, const Color(0xff005ec5));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w400);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemText');
expect(topBackLabel.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.5292819738388062);
});
testWidgets('Font transitions respect themes', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
theme: const CupertinoThemeData(brightness: Brightness.dark),
);
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
// The transition's stack is ordered. The bottom middle is inserted first.
final RenderParagraph bottomMiddle =
tester.renderObject(flying(tester, find.text('Page 1')).first);
expect(bottomMiddle.text.style!.color, const Color(0xfff8fbff));
expect(bottomMiddle.text.style!.fontWeight, FontWeight.w600);
expect(bottomMiddle.text.style!.fontFamily, 'CupertinoSystemText');
expect(bottomMiddle.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.9404401779174805);
// The top back label is styled exactly the same way. But the opacity tweens
// are flipped.
final RenderParagraph topBackLabel =
tester.renderObject(flying(tester, find.text('Page 1')).last);
expect(topBackLabel.text.style!.color, const Color(0xfff8fbff));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w600);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemText');
expect(topBackLabel.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.0);
// Move animation further a bit.
await tester.pump(const Duration(milliseconds: 200));
expect(bottomMiddle.text.style!.color, const Color(0xff409fff));
expect(bottomMiddle.text.style!.fontWeight, FontWeight.w400);
expect(bottomMiddle.text.style!.fontFamily, 'CupertinoSystemText');
expect(bottomMiddle.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.0);
expect(topBackLabel.text.style!.color, const Color(0xff409fff));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w400);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemText');
expect(topBackLabel.text.style!.letterSpacing, -0.41);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.5292819738388062);
});
testWidgets('Fullscreen dialogs do not create heroes', (WidgetTester tester) async {
await tester.pumpWidget(
const CupertinoApp(
home: Placeholder(),
),
);
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 1',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 2',
fullscreenDialog: true,
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
// Only the first (non-fullscreen-dialog) page has a Hero.
expect(find.byType(Hero), findsOneWidget);
// No Hero transition happened.
expect(() => flying(tester, find.text('Page 2')), throwsAssertionError);
});
testWidgets('Turning off transition works', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoNavigationBar(
transitionBetweenRoutes: false,
middle: Text('Page 1'),
),
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
// Only the second page that doesn't have the transitionBetweenRoutes
// override off has a Hero.
expect(find.byType(Hero), findsOneWidget);
expect(
find.descendant(of: find.byType(Hero), matching: find.text('Page 2')),
findsOneWidget,
);
// No Hero transition happened.
expect(() => flying(tester, find.text('Page 2')), throwsAssertionError);
});
testWidgets('Popping mid-transition is symmetrical', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
void checkColorAndPositionAt50ms() {
// The transition's stack is ordered. The bottom middle is inserted first.
final RenderParagraph bottomMiddle =
tester.renderObject(flying(tester, find.text('Page 1')).first);
expect(bottomMiddle.text.style!.color, const Color(0xff000306));
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
342.547737105096302912,
13.5,
),
);
// The top back label is styled exactly the same way. But the opacity tweens
// are flipped.
final RenderParagraph topBackLabel =
tester.renderObject(flying(tester, find.text('Page 1')).last);
expect(topBackLabel.text.style!.color, const Color(0xff000306));
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
342.547737105096302912,
13.5,
),
);
}
checkColorAndPositionAt50ms();
// Advance more.
await tester.pump(const Duration(milliseconds: 100));
// Pop and reverse the same amount of time.
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
// Check that everything's the same as on the way in.
checkColorAndPositionAt50ms();
});
testWidgets('Popping mid-transition is symmetrical RTL', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
textDirection: TextDirection.rtl,
);
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
void checkColorAndPositionAt50ms() {
// The transition's stack is ordered. The bottom middle is inserted first.
final RenderParagraph bottomMiddle =
tester.renderObject(flying(tester, find.text('Page 1')).first);
expect(bottomMiddle.text.style!.color, const Color(0xff000306));
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
357.912261979376353338,
13.5,
),
);
// The top back label is styled exactly the same way. But the opacity tweens
// are flipped.
final RenderParagraph topBackLabel =
tester.renderObject(flying(tester, find.text('Page 1')).last);
expect(topBackLabel.text.style!.color, const Color(0xff000306));
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
357.912261979376353338,
13.5,
),
);
}
checkColorAndPositionAt50ms();
// Advance more.
await tester.pump(const Duration(milliseconds: 100));
// Pop and reverse the same amount of time.
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
// Check that everything's the same as on the way in.
checkColorAndPositionAt50ms();
});
testWidgets('There should be no global keys in the hero flight', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
expect(
flying(
tester,
find.byWidgetPredicate((Widget widget) => widget.key != null),
),
findsNothing,
);
});
testWidgets('DartPerformanceMode is latency mid-animation', (WidgetTester tester) async {
DartPerformanceMode? mode;
// before the animation starts, no requests are active.
mode = SchedulerBinding.instance.debugGetRequestedPerformanceMode();
expect(mode, isNull);
await startTransitionBetween(tester, fromTitle: 'Page 1');
// mid-transition, latency mode is expected.
await tester.pump(const Duration(milliseconds: 50));
mode = SchedulerBinding.instance.debugGetRequestedPerformanceMode();
expect(mode, equals(DartPerformanceMode.latency));
// end of transition, go back to no requests active.
await tester.pump(const Duration(milliseconds: 500));
mode = SchedulerBinding.instance.debugGetRequestedPerformanceMode();
expect(mode, isNull);
});
testWidgets('Multiple nav bars tags do not conflict if in different navigators', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(CupertinoIcons.search),
label: 'Tab 1',
),
BottomNavigationBarItem(
icon: Icon(CupertinoIcons.settings),
label: 'Tab 2',
),
],
),
tabBuilder: (BuildContext context, int tab) {
return CupertinoTabView(
builder: (BuildContext context) {
return CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Tab ${tab + 1} Page 1'),
),
child: Center(
child: CupertinoButton(
child: const Text('Next'),
onPressed: () {
Navigator.push<void>(context, CupertinoPageRoute<void>(
title: 'Tab ${tab + 1} Page 2',
builder: (BuildContext context) {
return const CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(),
child: Placeholder(),
);
},
));
},
),
),
);
},
);
},
),
),
);
await tester.tap(find.text('Tab 2'));
await tester.pump();
expect(find.text('Tab 1 Page 1', skipOffstage: false), findsOneWidget);
expect(find.text('Tab 2 Page 1'), findsOneWidget);
// At this point, there are 2 nav bars seeded with the same _defaultHeroTag.
// But they're inside different navigators.
await tester.tap(find.text('Next'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 200));
// One is inside the flight shuttle and another is invisible in the
// incoming route in case a new flight needs to be created midflight.
expect(find.text('Tab 2 Page 2'), findsNWidgets(2));
await tester.pump(const Duration(milliseconds: 500));
expect(find.text('Tab 2 Page 2'), findsOneWidget);
// Offstaged by tab 2's navigator.
expect(find.text('Tab 2 Page 1', skipOffstage: false), findsOneWidget);
// Offstaged by the CupertinoTabScaffold.
expect(find.text('Tab 1 Page 1', skipOffstage: false), findsOneWidget);
// Never navigated to tab 1 page 2.
expect(find.text('Tab 1 Page 2', skipOffstage: false), findsNothing);
});
testWidgets('Transition box grows to large title size', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
to: const CupertinoSliverNavigationBar(),
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 45.3376561999321);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 51.012951374053955);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 63.06760931015015);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 75.89544230699539);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 84.33018499612808);
});
testWidgets('Large transition box shrinks to standard nav bar size', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 94.6623438000679);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 88.98704862594604);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 76.93239068984985);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 64.10455769300461);
await tester.pump(const Duration(milliseconds: 50));
checkBackgroundBoxHeight(tester, 55.66981500387192);
});
testWidgets('Hero flight removed at the end of page transition', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
await tester.pump(const Duration(milliseconds: 50));
// There's 2 of them. One from the top route's back label and one from the
// bottom route's middle widget.
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
// End the transition.
await tester.pump(const Duration(milliseconds: 500));
expect(() => flying(tester, find.text('Page 1')), throwsAssertionError);
});
testWidgets('Exact widget is reused to build inside the transition', (WidgetTester tester) async {
const Widget userMiddle = Placeholder();
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(
middle: userMiddle,
),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.byWidget(userMiddle)), findsOneWidget);
});
testWidgets('Middle is not shown if alwaysShowMiddle is false and the nav bar is expanded', (WidgetTester tester) async {
const Widget userMiddle = Placeholder();
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(
middle: userMiddle,
alwaysShowMiddle: false,
),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.byWidget(userMiddle)), findsNothing);
});
testWidgets('Middle is shown if alwaysShowMiddle is false but the nav bar is collapsed', (WidgetTester tester) async {
const Widget userMiddle = Placeholder();
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoPageScaffold(
child: CustomScrollView(
controller: scrollController,
slivers: const <Widget>[
CupertinoSliverNavigationBar(
largeTitle: Text('Page 1'),
middle: userMiddle,
alwaysShowMiddle: false,
),
SliverToBoxAdapter(
child: SizedBox(
height: 1200.0,
),
),
],
),
),
),
);
scrollController.jumpTo(600.0);
await tester.pumpAndSettle();
// Middle widget is visible when nav bar is collapsed.
final RenderAnimatedOpacity userMiddleOpacity = tester
.element(find.byWidget(userMiddle))
.findAncestorRenderObjectOfType<RenderAnimatedOpacity>()!;
expect(userMiddleOpacity.opacity.value, 1.0);
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 2',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.byWidget(userMiddle)), findsOneWidget);
});
testWidgets('First appearance of back chevron fades in from the right', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: scaffoldForNavBar(null),
),
);
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 1',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
final Finder backChevron = flying(tester, find.text(String.fromCharCode(CupertinoIcons.back.codePoint)));
expect(
backChevron,
// Only one exists from the top page. The bottom page has no back chevron.
findsOneWidget,
);
// Come in from the right and fade in.
checkOpacity(tester, backChevron, 0.0);
expect(tester.getTopLeft(backChevron), const Offset(
87.2460581221158690823,
7.0,
));
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, backChevron, 0.09497911669313908);
expect(tester.getTopLeft(backChevron), const Offset(
30.8718595298545324113,
7.0,
));
});
testWidgets('First appearance of back chevron fades in from the left in RTL', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
builder: (BuildContext context, Widget? navigator) {
return Directionality(
textDirection: TextDirection.rtl,
child: navigator!,
);
},
home: scaffoldForNavBar(null),
),
);
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 1',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
final Finder backChevron = flying(tester, find.text(String.fromCharCode(CupertinoIcons.back.codePoint)));
expect(
backChevron,
// Only one exists from the top page. The bottom page has no back chevron.
findsOneWidget,
);
// Come in from the right and fade in.
checkOpacity(tester, backChevron, 0.0);
expect(
tester.getTopRight(backChevron),
const Offset(
687.163941725296126606,
7.0,
),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, backChevron, 0.09497911669313908);
expect(
tester.getTopRight(backChevron),
const Offset(
743.538140317557690651,
7.0,
),
);
});
testWidgets('Back chevron fades out and in when both pages have it', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1');
await tester.pump(const Duration(milliseconds: 50));
final Finder backChevrons = flying(tester, find.text(String.fromCharCode(CupertinoIcons.back.codePoint)));
expect(
backChevrons,
findsNWidgets(2),
);
checkOpacity(tester, backChevrons.first, 0.9280824661254883);
checkOpacity(tester, backChevrons.last, 0.0);
// Both overlap at the same place.
expect(tester.getTopLeft(backChevrons.first), const Offset(14.0, 7.0));
expect(tester.getTopLeft(backChevrons.last), const Offset(14.0, 7.0));
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, backChevrons.first, 0.0);
checkOpacity(tester, backChevrons.last, 0.4604858811944723);
// Still in the same place.
expect(tester.getTopLeft(backChevrons.first), const Offset(14.0, 7.0));
expect(tester.getTopLeft(backChevrons.last), const Offset(14.0, 7.0));
});
testWidgets('Bottom middle just fades if top page has a custom leading', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
to: const CupertinoSliverNavigationBar(
leading: Text('custom'),
),
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
// There's just 1 in flight because there's no back label on the top page.
expect(flying(tester, find.text('Page 1')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.9404401779174805);
// The middle widget doesn't move.
expect(
tester.getCenter(flying(tester, find.text('Page 1'))),
const Offset(400.0, 22.0),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.0);
expect(
tester.getCenter(flying(tester, find.text('Page 1'))),
const Offset(400.0, 22.0),
);
});
testWidgets('Bottom leading fades in place', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(leading: Text('custom')),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('custom')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('custom')), 0.8948725312948227);
expect(
tester.getTopLeft(flying(tester, find.text('custom'))),
const Offset(16.0, 0.0),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('custom')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('custom'))),
const Offset(16.0, 0.0),
);
});
testWidgets('Bottom trailing fades in place', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(trailing: Text('custom')),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('custom')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('custom')), 0.9280824661254883);
expect(
tester.getTopLeft(flying(tester, find.text('custom'))),
const Offset(
684.459999084472656250,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('custom')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('custom'))),
const Offset(
684.459999084472656250,
13.5,
),
);
});
testWidgets('Bottom back label fades and slides to the left', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 500));
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 3',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
// 'Page 1' appears once on Page 2 as the back label.
expect(flying(tester, find.text('Page 1')), findsOneWidget);
// Back label fades out faster.
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.7952219992876053);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1'))),
const Offset(
41.3003370761871337891,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1'))),
const Offset(
-258.642192125320434570,
13.5,
),
);
});
testWidgets('Bottom back label fades and slides to the right in RTL', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
toTitle: 'Page 2',
textDirection: TextDirection.rtl,
);
await tester.pump(const Duration(milliseconds: 500));
tester
.state<NavigatorState>(find.byType(Navigator))
.push(CupertinoPageRoute<void>(
title: 'Page 3',
builder: (BuildContext context) => scaffoldForNavBar(null)!,
));
await tester.pump();
await tester.pump(const Duration(milliseconds: 50));
// 'Page 1' appears once on Page 2 as the back label.
expect(flying(tester, find.text('Page 1')), findsOneWidget);
// Back label fades out faster.
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.7952219992876053);
expect(
tester.getTopRight(flying(tester, find.text('Page 1'))),
const Offset(
758.699662923812866211,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, flying(tester, find.text('Page 1')), 0.0);
expect(
tester.getTopRight(flying(tester, find.text('Page 1'))),
// >1000. It's now off the screen.
const Offset(
1058.64219212532043457,
13.5,
),
);
});
testWidgets('Bottom large title moves to top back label', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(),
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
// There's 2, one from the bottom large title fading out and one from the
// bottom back label fading in.
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.9280824661254883);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
16.9155227761479522997,
52.73951627314091,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
16.9155227761479522997,
52.73951627314091,
),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, flying(tester, find.text('Page 1')).first, 0.0);
checkOpacity(tester, flying(tester, find.text('Page 1')).last, 0.4604858811944723);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).first),
const Offset(
43.6029094262710827934,
22.49655644595623,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Page 1')).last),
const Offset(
43.6029094262710827934,
22.49655644595623,
),
);
});
testWidgets('Long title turns into the word back mid transition', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(),
fromTitle: 'A title too long to fit',
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('A title too long to fit')), findsOneWidget);
// Automatically changed to the word 'Back' in the back label.
expect(flying(tester, find.text('Back')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('A title too long to fit')), 0.9280824661254883);
checkOpacity(tester, flying(tester, find.text('Back')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('A title too long to fit'))),
const Offset(
16.9155227761479522997,
52.73951627314091,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Back'))),
const Offset(
16.9155227761479522997,
52.73951627314091,
),
);
await tester.pump(const Duration(milliseconds: 200));
checkOpacity(tester, flying(tester, find.text('A title too long to fit')), 0.0);
checkOpacity(tester, flying(tester, find.text('Back')), 0.4604858811944723);
expect(
tester.getTopLeft(flying(tester, find.text('A title too long to fit'))),
const Offset(
43.6029094262710827934,
22.49655644595623,
),
);
expect(
tester.getTopLeft(flying(tester, find.text('Back'))),
const Offset(
43.6029094262710827934,
22.49655644595623,
),
);
});
testWidgets('Bottom large title and top back label transitions their font', (WidgetTester tester) async {
await startTransitionBetween(
tester,
from: const CupertinoSliverNavigationBar(),
fromTitle: 'Page 1',
);
// Be mid-transition.
await tester.pump(const Duration(milliseconds: 50));
// The transition's stack is ordered. The bottom large title is inserted first.
final RenderParagraph bottomLargeTitle =
tester.renderObject(flying(tester, find.text('Page 1')).first);
expect(bottomLargeTitle.text.style!.color, const Color(0xff000306));
expect(bottomLargeTitle.text.style!.fontWeight, FontWeight.w700);
expect(bottomLargeTitle.text.style!.fontFamily, 'CupertinoSystemDisplay');
expect(bottomLargeTitle.text.style!.letterSpacing, moreOrLessEquals(0.35967791542410854));
// The top back label is styled exactly the same way.
final RenderParagraph topBackLabel =
tester.renderObject(flying(tester, find.text('Page 1')).last);
expect(topBackLabel.text.style!.color, const Color(0xff000306));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w700);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemDisplay');
expect(topBackLabel.text.style!.letterSpacing, moreOrLessEquals(0.35967791542410854));
// Move animation further a bit.
await tester.pump(const Duration(milliseconds: 200));
expect(bottomLargeTitle.text.style!.color, const Color(0xff005ec5));
expect(bottomLargeTitle.text.style!.fontWeight, FontWeight.w500);
expect(bottomLargeTitle.text.style!.fontFamily, 'CupertinoSystemText');
expect(bottomLargeTitle.text.style!.letterSpacing, moreOrLessEquals(-0.23270857974886894));
expect(topBackLabel.text.style!.color, const Color(0xff005ec5));
expect(topBackLabel.text.style!.fontWeight, FontWeight.w500);
expect(topBackLabel.text.style!.fontFamily, 'CupertinoSystemText');
expect(topBackLabel.text.style!.letterSpacing, moreOrLessEquals(-0.23270857974886894));
});
testWidgets('Top middle fades in and slides in from the right', (WidgetTester tester) async {
await startTransitionBetween(
tester,
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('Page 2')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
739.940336465835571289,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.29867843724787235);
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
504.880443334579467773,
13.5,
),
);
});
testWidgets('Top middle never changes size during the animation', (WidgetTester tester) async {
await tester.binding.setSurfaceSize(const Size(1080.0 / 2.75, 600));
addTearDown(() async {
await tester.binding.setSurfaceSize(const Size(800.0, 600.0));
});
await startTransitionBetween(
tester,
toTitle: 'Page 2',
);
Size? previousSize;
for (int i = 0; i < 150; i++) {
await tester.pump(const Duration(milliseconds: 1));
expect(flying(tester, find.text('Page 2')), findsOneWidget);
final Size size = tester.getSize(flying(tester, find.text('Page 2')));
if (previousSize != null) {
expect(size, previousSize);
}
previousSize = size;
}
});
testWidgets('Top middle fades in and slides in from the left in RTL', (WidgetTester tester) async {
await startTransitionBetween(
tester,
toTitle: 'Page 2',
textDirection: TextDirection.rtl,
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('Page 2')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.0);
expect(
tester.getTopRight(flying(tester, find.text('Page 2'))),
const Offset(
60.0596635341644287109,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.29867843724787235);
expect(
tester.getTopRight(flying(tester, find.text('Page 2'))),
const Offset(
295.119556665420532227,
13.5,
),
);
});
testWidgets('Top large title fades in and slides in from the right', (WidgetTester tester) async {
await startTransitionBetween(
tester,
to: const CupertinoSliverNavigationBar(),
toTitle: 'Page 2',
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('Page 2')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.0);
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(795.4206738471985, 54.0),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.2601277381181717);
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(325.3008875846863, 54.0),
);
});
testWidgets('Top large title fades in and slides in from the left in RTL', (WidgetTester tester) async {
await startTransitionBetween(
tester,
to: const CupertinoSliverNavigationBar(),
toTitle: 'Page 2',
textDirection: TextDirection.rtl,
);
await tester.pump(const Duration(milliseconds: 50));
expect(flying(tester, find.text('Page 2')), findsOneWidget);
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.0);
expect(
tester.getTopRight(flying(tester, find.text('Page 2'))),
const Offset(4.579326152801514, 54.0),
);
await tester.pump(const Duration(milliseconds: 150));
checkOpacity(tester, flying(tester, find.text('Page 2')), 0.2601277381181717);
expect(
tester.getTopRight(flying(tester, find.text('Page 2'))),
const Offset(474.6991124153137, 54.0),
);
});
testWidgets('Components are not unnecessarily rebuilt during transitions', (WidgetTester tester) async {
int bottomBuildTimes = 0;
int topBuildTimes = 0;
await startTransitionBetween(
tester,
from: CupertinoNavigationBar(
middle: Builder(builder: (BuildContext context) {
bottomBuildTimes++;
return const Text('Page 1');
}),
),
to: CupertinoSliverNavigationBar(
largeTitle: Builder(builder: (BuildContext context) {
topBuildTimes++;
return const Text('Page 2');
}),
),
);
expect(bottomBuildTimes, 1);
// RenderSliverPersistentHeader.layoutChild causes 2 builds.
expect(topBuildTimes, 2);
await tester.pump();
// The shuttle builder builds the component widgets one more time.
expect(bottomBuildTimes, 2);
expect(topBuildTimes, 3);
// Subsequent animation needs to use reprojection of children.
await tester.pump();
expect(bottomBuildTimes, 2);
expect(topBuildTimes, 3);
await tester.pump(const Duration(milliseconds: 100));
expect(bottomBuildTimes, 2);
expect(topBuildTimes, 3);
// Finish animations.
await tester.pump(const Duration(milliseconds: 400));
expect(bottomBuildTimes, 2);
expect(topBuildTimes, 3);
});
testWidgets('Back swipe gesture transitions', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
// Go to the next page.
await tester.pump(const Duration(milliseconds: 600));
// Start the gesture at the edge of the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 200.0));
// Trigger the swipe.
await gesture.moveBy(const Offset(100.0, 0.0));
// Back gestures should trigger and draw the hero transition in the very same
// frame (since the "from" route has already moved to reveal the "to" route).
await tester.pump();
// Page 2, which is the middle of the top route, start to fly back to the right.
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
353.810205429792404175,
13.5,
),
);
// Page 1 is in transition in 2 places. Once as the top back label and once
// as the bottom middle.
expect(flying(tester, find.text('Page 1')), findsNWidgets(2));
// Past the halfway point now.
await gesture.moveBy(const Offset(500.0, 0.0));
await gesture.up();
await tester.pump();
// Transition continues.
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
655.435583114624023438,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 50));
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
749.863556146621704102,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 500));
// Cleans up properly
expect(() => flying(tester, find.text('Page 1')), throwsAssertionError);
expect(() => flying(tester, find.text('Page 2')), throwsAssertionError);
// Just the bottom route's middle now.
expect(find.text('Page 1'), findsOneWidget);
});
testWidgets('textScaleFactor is set to 1.0 on transition', (WidgetTester tester) async {
await startTransitionBetween(tester, fromTitle: 'Page 1', textScaler: const TextScaler.linear(99));
await tester.pump(const Duration(milliseconds: 50));
final TextScaler scaler = tester.firstWidget<RichText>(flying(tester, find.byType(RichText))).textScaler;
final List<double> fontSizes = List<double>.generate(100, (int index) => index / 3 + 1);
expect(fontSizes.map(scaler.scale), fontSizes);
});
testWidgets('Back swipe gesture cancels properly with transition', (WidgetTester tester) async {
await startTransitionBetween(
tester,
fromTitle: 'Page 1',
toTitle: 'Page 2',
);
// Go to the next page.
await tester.pump(const Duration(milliseconds: 600));
// Start the gesture at the edge of the screen.
final TestGesture gesture = await tester.startGesture(const Offset(5.0, 200.0));
// Trigger the swipe.
await gesture.moveBy(const Offset(100.0, 0.0));
// Back gestures should trigger and draw the hero transition in the very same
// frame (since the "from" route has already moved to reveal the "to" route).
await tester.pump();
// Page 2, which is the middle of the top route, start to fly back to the right.
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
353.810205429792404175,
13.5,
),
);
await gesture.up();
await tester.pump();
// Transition continues from the point we let off.
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
353.810205429792404175,
13.5,
),
);
await tester.pump(const Duration(milliseconds: 50));
expect(
tester.getTopLeft(flying(tester, find.text('Page 2'))),
const Offset(
350.231143206357955933,
13.5,
),
);
// Finish the snap back animation.
await tester.pump(const Duration(milliseconds: 500));
// Cleans up properly
expect(() => flying(tester, find.text('Page 1')), throwsAssertionError);
expect(() => flying(tester, find.text('Page 2')), throwsAssertionError);
// Back to page 2.
expect(find.text('Page 2'), findsOneWidget);
});
}
| flutter/packages/flutter/test/cupertino/nav_bar_transition_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/nav_bar_transition_test.dart",
"repo_id": "flutter",
"token_count": 19303
} | 718 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Use home', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
builder: (BuildContext context) => const Text('home'),
),
),
);
expect(find.text('home'), findsOneWidget);
});
testWidgets('Use routes', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
routes: <String, WidgetBuilder>{
'/': (BuildContext context) => const Text('first route'),
},
),
),
);
expect(find.text('first route'), findsOneWidget);
});
testWidgets('Use home and named routes', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('go to second page'),
onPressed: () {
Navigator.of(context).pushNamed('/2');
},
);
},
routes: <String, WidgetBuilder>{
'/2': (BuildContext context) => const Text('second named route'),
},
),
),
);
expect(find.text('go to second page'), findsOneWidget);
await tester.tap(find.text('go to second page'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('second named route'), findsOneWidget);
});
testWidgets('Use onGenerateRoute', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
onGenerateRoute: (RouteSettings settings) {
if (settings.name == Navigator.defaultRouteName) {
return CupertinoPageRoute<void>(
settings: settings,
builder: (BuildContext context) {
return const Text('generated home');
},
);
}
return null;
},
),
),
);
expect(find.text('generated home'), findsOneWidget);
});
testWidgets('Use onUnknownRoute', (WidgetTester tester) async {
late String unknownForRouteCalled;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
onUnknownRoute: (RouteSettings settings) {
unknownForRouteCalled = settings.name!;
return null;
},
),
),
);
expect(tester.takeException(), isFlutterError);
expect(unknownForRouteCalled, '/');
// Work-around for https://github.com/flutter/flutter/issues/65655.
await tester.pumpWidget(Container());
expect(tester.takeException(), isAssertionError);
});
testWidgets('Can use navigatorKey to navigate', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
navigatorKey: key,
builder: (BuildContext context) => const Text('first route'),
routes: <String, WidgetBuilder>{
'/2': (BuildContext context) => const Text('second route'),
},
),
),
);
key.currentState!.pushNamed('/2');
await tester.pump();
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('second route'), findsOneWidget);
});
testWidgets('Changing the key resets the navigator', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('go to second page'),
onPressed: () {
Navigator.of(context).pushNamed('/2');
},
);
},
routes: <String, WidgetBuilder>{
'/2': (BuildContext context) => const Text('second route'),
},
),
),
);
expect(find.text('go to second page'), findsOneWidget);
await tester.tap(find.text('go to second page'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('second route'), findsOneWidget);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
key: key,
builder: (BuildContext context) {
return CupertinoButton(
child: const Text('go to second page'),
onPressed: () {
Navigator.of(context).pushNamed('/2');
},
);
},
routes: <String, WidgetBuilder>{
'/2': (BuildContext context) => const Text('second route'),
},
),
),
);
// The stack is gone and we're back to a re-built page 1.
expect(find.text('go to second page'), findsOneWidget);
expect(find.text('second route'), findsNothing);
});
testWidgets('Throws FlutterError when onUnknownRoute is null', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
navigatorKey: key,
builder: (BuildContext context) => const Text('first route'),
),
),
);
late FlutterError error;
try {
key.currentState!.pushNamed('/2');
} on FlutterError catch (e) {
error = e;
}
expect(error, isNotNull);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' Could not find a generator for route RouteSettings("/2", null) in\n'
' the _CupertinoTabViewState.\n'
' Generators for routes are searched for in the following order:\n'
' 1. For the "/" route, the "builder" property, if non-null, is\n'
' used.\n'
' 2. Otherwise, the "routes" table is used, if it has an entry for\n'
' the route.\n'
' 3. Otherwise, onGenerateRoute is called. It should return a\n'
' non-null value for any valid route not handled by "builder" and\n'
' "routes".\n'
' 4. Finally if all else fails onUnknownRoute is called.\n'
' Unfortunately, onUnknownRoute was not set.\n',
),
);
});
testWidgets('Throws FlutterError when onUnknownRoute returns null', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey<NavigatorState>();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabView(
navigatorKey: key,
builder: (BuildContext context) => const Text('first route'),
onUnknownRoute: (_) => null,
),
),
);
late FlutterError error;
try {
key.currentState!.pushNamed('/2');
} on FlutterError catch (e) {
error = e;
}
expect(error, isNotNull);
expect(
error.toStringDeep(),
equalsIgnoringHashCodes(
'FlutterError\n'
' The onUnknownRoute callback returned null.\n'
' When the _CupertinoTabViewState requested the route\n'
' RouteSettings("/2", null) from its onUnknownRoute callback, the\n'
' callback returned null. Such callbacks must never return null.\n',
),
);
});
testWidgets('Navigator of CupertinoTabView restores state', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
restorationScopeId: 'app',
home: CupertinoTabView(
restorationScopeId: 'tab',
builder: (BuildContext context) => CupertinoButton(
child: const Text('home'),
onPressed: () {
Navigator.of(context).restorablePushNamed('/2');
},
),
routes: <String, WidgetBuilder>{
'/2' : (BuildContext context) => const Text('second route'),
},
),
),
);
expect(find.text('home'), findsOneWidget);
await tester.tap(find.text('home'));
await tester.pumpAndSettle();
expect(find.text('home'), findsNothing);
expect(find.text('second route'), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.restartAndRestore();
expect(find.text('home'), findsNothing);
expect(find.text('second route'), findsOneWidget);
Navigator.of(tester.element(find.text('second route'))).pop();
await tester.pumpAndSettle();
expect(find.text('home'), findsOneWidget);
expect(find.text('second route'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('home'), findsNothing);
expect(find.text('second route'), findsOneWidget);
Navigator.of(tester.element(find.text('second route'))).pop();
await tester.pumpAndSettle();
expect(find.text('home'), findsOneWidget);
expect(find.text('second route'), findsNothing);
});
testWidgets('Handles Android back button', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey<NavigatorState>();
await tester.pumpWidget(
CupertinoApp(
home: CupertinoTabScaffold(
tabBar: CupertinoTabBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(label: '', icon: Text('1')),
BottomNavigationBarItem(label: '', icon: Text('2'))
],
),
tabBuilder: (_, int i) => PopScope(
canPop: false,
child: CupertinoTabView(
navigatorKey: key,
builder: (BuildContext context) => const Text('first route'),
),
),
),
),
);
expect(find.text('first route'), findsOneWidget);
// Simulate android back button intent.
final ByteData message = const JSONMethodCodec().encodeMethodCall(const MethodCall('popRoute'));
await tester.binding.defaultBinaryMessenger.handlePlatformMessage('flutter/navigation', message, (_) {});
await tester.pumpAndSettle();
// Navigator didn't pop, so first route is still visible
expect(find.text('first route'), findsOneWidget);
});
}
| flutter/packages/flutter/test/cupertino/tab_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/tab_test.dart",
"repo_id": "flutter",
"token_count": 4454
} | 719 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// A test script that invokes compute() to start an isolate.
import 'dart:isolate';
import 'package:flutter/src/foundation/_isolates_io.dart';
int getLength(ReceivePort s) {
return 0;
}
Future<void> main() async {
final ReceivePort s = ReceivePort();
bool wasError = false;
try {
await compute(getLength, s);
} on Object {
wasError = true;
}
s.close();
assert(wasError);
}
| flutter/packages/flutter/test/foundation/_compute_caller_invalid_message.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/_compute_caller_invalid_message.dart",
"repo_id": "flutter",
"token_count": 186
} | 720 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
class TestTree extends Object with DiagnosticableTreeMixin {
TestTree({
this.name = '',
this.style,
this.children = const <TestTree>[],
this.properties = const <DiagnosticsNode>[],
});
final String name;
final List<TestTree> children;
final List<DiagnosticsNode> properties;
final DiagnosticsTreeStyle? style;
@override
List<DiagnosticsNode> debugDescribeChildren() => <DiagnosticsNode>[
for (final TestTree child in children)
child.toDiagnosticsNode(
name: 'child ${child.name}',
style: child.style,
),
];
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
if (style != null) {
properties.defaultDiagnosticsTreeStyle = style!;
}
this.properties.forEach(properties.add);
}
}
enum ExampleEnum {
hello,
world,
deferToChild,
}
/// Encode and decode to JSON to make sure all objects in the JSON for the
/// [DiagnosticsNode] are valid JSON.
Map<String, Object?> simulateJsonSerialization(DiagnosticsNode node) {
return json.decode(json.encode(node.toJsonMap(const DiagnosticsSerializationDelegate()))) as Map<String, Object?>;
}
void validateNodeJsonSerialization(DiagnosticsNode node) {
validateNodeJsonSerializationHelper(simulateJsonSerialization(node), node);
}
void validateNodeJsonSerializationHelper(Map<String, Object?> json, DiagnosticsNode node) {
expect(json['name'], equals(node.name));
expect(json['showSeparator'] ?? true, equals(node.showSeparator));
expect(json['description'], equals(node.toDescription()));
expect(json['level'] ?? DiagnosticLevel.info.name, equals(node.level.name));
expect(json['showName'] ?? true, equals(node.showName));
expect(json['emptyBodyDescription'], equals(node.emptyBodyDescription));
expect(json['style'] ?? DiagnosticsTreeStyle.sparse.name, equals(node.style!.name));
expect(json['type'], equals(node.runtimeType.toString()));
expect(json['hasChildren'] ?? false, equals(node.getChildren().isNotEmpty));
}
void validatePropertyJsonSerialization(DiagnosticsProperty<Object?> property) {
validatePropertyJsonSerializationHelper(simulateJsonSerialization(property), property);
}
void validateStringPropertyJsonSerialization(StringProperty property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
expect(json['quoted'], equals(property.quoted));
validatePropertyJsonSerializationHelper(json, property);
}
void validateFlagPropertyJsonSerialization(FlagProperty property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
expect(json['ifTrue'], equals(property.ifTrue));
if (property.ifTrue != null) {
expect(json['ifTrue'], equals(property.ifTrue));
} else {
expect(json.containsKey('ifTrue'), isFalse);
}
if (property.ifFalse != null) {
expect(json['ifFalse'], property.ifFalse);
} else {
expect(json.containsKey('isFalse'), isFalse);
}
validatePropertyJsonSerializationHelper(json, property);
}
void validateDoublePropertyJsonSerialization(DoubleProperty property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
if (property.unit != null) {
expect(json['unit'], equals(property.unit));
} else {
expect(json.containsKey('unit'), isFalse);
}
expect(json['numberToString'], equals(property.numberToString()));
validatePropertyJsonSerializationHelper(json, property);
}
void validateObjectFlagPropertyJsonSerialization(ObjectFlagProperty<Object> property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
if (property.ifPresent != null) {
expect(json['ifPresent'], equals(property.ifPresent));
} else {
expect(json.containsKey('ifPresent'), isFalse);
}
validatePropertyJsonSerializationHelper(json, property);
}
void validateIterableFlagsPropertyJsonSerialization(FlagsSummary<Object?> property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
if (property.value.isNotEmpty) {
expect(json['values'], equals(
property.value.entries
.where((MapEntry<String, Object?> entry) => entry.value != null)
.map((MapEntry<String, Object?> entry) => entry.key).toList(),
));
} else {
expect(json.containsKey('values'), isFalse);
}
validatePropertyJsonSerializationHelper(json, property);
}
void validateIterablePropertyJsonSerialization(IterableProperty<Object> property) {
final Map<String, Object?> json = simulateJsonSerialization(property);
if (property.value != null) {
final List<Object?> valuesJson = json['values']! as List<Object?>;
final List<String> expectedValues = property.value!.map<String>((Object value) => value.toString()).toList();
expect(listEquals(valuesJson, expectedValues), isTrue);
} else {
expect(json.containsKey('values'), isFalse);
}
validatePropertyJsonSerializationHelper(json, property);
}
void validatePropertyJsonSerializationHelper(final Map<String, Object?> json, DiagnosticsProperty<Object?> property) {
if (property.defaultValue != kNoDefaultValue) {
expect(json['defaultValue'], equals(property.defaultValue.toString()));
} else {
expect(json.containsKey('defaultValue'), isFalse);
}
if (property.ifEmpty != null) {
expect(json['ifEmpty'], equals(property.ifEmpty));
} else {
expect(json.containsKey('ifEmpty'), isFalse);
}
if (property.ifNull != null) {
expect(json['ifNull'], equals(property.ifNull));
} else {
expect(json.containsKey('ifNull'), isFalse);
}
if (property.tooltip != null) {
expect(json['tooltip'], equals(property.tooltip));
} else {
expect(json.containsKey('tooltip'), isFalse);
}
expect(json['missingIfNull'], equals(property.missingIfNull));
if (property.exception != null) {
expect(json['exception'], equals(property.exception.toString()));
} else {
expect(json.containsKey('exception'), isFalse);
}
expect(json['propertyType'], equals(property.propertyType.toString()));
expect(json.containsKey('defaultLevel'), isTrue);
if (property.value is Diagnosticable) {
expect(json['isDiagnosticableValue'], isTrue);
} else {
expect(json.containsKey('isDiagnosticableValue'), isFalse);
}
validateNodeJsonSerializationHelper(json, property);
}
void main() {
test('TreeDiagnosticsMixin control test', () async {
void goldenStyleTest(
String description, {
DiagnosticsTreeStyle? style,
DiagnosticsTreeStyle? lastChildStyle,
String golden = '',
}) {
final TestTree tree = TestTree(children: <TestTree>[
TestTree(name: 'node A', style: style),
TestTree(
name: 'node B',
children: <TestTree>[
TestTree(name: 'node B1', style: style),
TestTree(name: 'node B2', style: style),
TestTree(name: 'node B3', style: lastChildStyle ?? style),
],
style: style,
),
TestTree(name: 'node C', style: lastChildStyle ?? style),
], style: lastChildStyle);
expect(tree, hasAGoodToStringDeep);
expect(
tree.toDiagnosticsNode(style: style).toStringDeep(),
equalsIgnoringHashCodes(golden),
reason: description,
);
validateNodeJsonSerialization(tree.toDiagnosticsNode());
}
goldenStyleTest(
'dense',
style: DiagnosticsTreeStyle.dense,
golden:
'TestTree#00000\n'
'βchild node A: TestTree#00000\n'
'βchild node B: TestTree#00000\n'
'ββchild node B1: TestTree#00000\n'
'ββchild node B2: TestTree#00000\n'
'ββchild node B3: TestTree#00000\n'
'βchild node C: TestTree#00000\n',
);
goldenStyleTest(
'sparse',
style: DiagnosticsTreeStyle.sparse,
golden:
'TestTree#00000\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β ββchild node B3: TestTree#00000\n'
' ββchild node C: TestTree#00000\n',
);
goldenStyleTest(
'dashed',
style: DiagnosticsTreeStyle.offstage,
golden:
'TestTree#00000\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β ββchild node B3: TestTree#00000\n'
' ββchild node C: TestTree#00000\n',
);
goldenStyleTest(
'leaf children',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.transition,
golden:
'TestTree#00000\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β βββ¦ββ child node B3 βββ\n'
' β β TestTree#00000\n'
' β ββββββββββββ\n'
' βββ¦ββ child node C βββ\n'
' β TestTree#00000\n'
' ββββββββββββ\n',
);
goldenStyleTest(
'error children',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.error,
golden:
'TestTree#00000\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β βββ¦βββ‘ CHILD NODE B3: TESTTREE#00000 ββββββββββββββββββββββββββββββββ\n'
' β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
' βββ¦βββ‘ CHILD NODE C: TESTTREE#00000 βββββββββββββββββββββββββββββββββ\n'
' βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n',
);
// You would never really want to make everything a transition child like
// this but you can and still get a readable tree.
// The joint between single and double lines here is a bit clunky
// but we could correct that if there is any real use for this style.
goldenStyleTest(
'transition',
style: DiagnosticsTreeStyle.transition,
golden:
'TestTree#00000:\n'
' βββ¦ββ child node A βββ\n'
' β β TestTree#00000\n'
' β ββββββββββββ\n'
' βββ¦ββ child node B βββ\n'
' β β TestTree#00000:\n'
' β β βββ¦ββ child node B1 βββ\n'
' β β β β TestTree#00000\n'
' β β β ββββββββββββ\n'
' β β βββ¦ββ child node B2 βββ\n'
' β β β β TestTree#00000\n'
' β β β ββββββββββββ\n'
' β β βββ¦ββ child node B3 βββ\n'
' β β β TestTree#00000\n'
' β β ββββββββββββ\n'
' β ββββββββββββ\n'
' βββ¦ββ child node C βββ\n'
' β TestTree#00000\n'
' ββββββββββββ\n',
);
goldenStyleTest(
'error',
style: DiagnosticsTreeStyle.error,
golden:
'βββ‘ TESTTREE#00000 ββββββββββββββββββββββββββββββββββββββββββββββ\n'
'βββ¦βββ‘ CHILD NODE A: TESTTREE#00000 βββββββββββββββββββββββββββββββββ\n'
'β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'βββ¦βββ‘ CHILD NODE B: TESTTREE#00000 βββββββββββββββββββββββββββββββββ\n'
'β β βββ¦βββ‘ CHILD NODE B1: TESTTREE#00000 ββββββββββββββββββββββββββββββββ\n'
'β β β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'β β βββ¦βββ‘ CHILD NODE B2: TESTTREE#00000 ββββββββββββββββββββββββββββββββ\n'
'β β β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'β β βββ¦βββ‘ CHILD NODE B3: TESTTREE#00000 ββββββββββββββββββββββββββββββββ\n'
'β β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'βββ¦βββ‘ CHILD NODE C: TESTTREE#00000 βββββββββββββββββββββββββββββββββ\n'
' βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
'βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n',
);
goldenStyleTest(
'whitespace',
style: DiagnosticsTreeStyle.whitespace,
golden:
'TestTree#00000:\n'
' child node A: TestTree#00000\n'
' child node B: TestTree#00000:\n'
' child node B1: TestTree#00000\n'
' child node B2: TestTree#00000\n'
' child node B3: TestTree#00000\n'
' child node C: TestTree#00000\n',
);
// Single line mode does not display children.
goldenStyleTest(
'single line',
style: DiagnosticsTreeStyle.singleLine,
golden: 'TestTree#00000',
);
});
test('TreeDiagnosticsMixin tree with properties test', () async {
void goldenStyleTest(
String description, {
String? name,
DiagnosticsTreeStyle? style,
DiagnosticsTreeStyle? lastChildStyle,
DiagnosticsTreeStyle propertyStyle = DiagnosticsTreeStyle.singleLine,
required String golden,
}) {
final TestTree tree = TestTree(
properties: <DiagnosticsNode>[
StringProperty('stringProperty1', 'value1', quoted: false, style: propertyStyle),
DoubleProperty('doubleProperty1', 42.5, style: propertyStyle),
DoubleProperty('roundedProperty', 1.0 / 3.0, style: propertyStyle),
StringProperty('DO_NOT_SHOW', 'DO_NOT_SHOW', level: DiagnosticLevel.hidden, quoted: false, style: propertyStyle),
DiagnosticsProperty<Object>('DO_NOT_SHOW_NULL', null, defaultValue: null, style: propertyStyle),
DiagnosticsProperty<Object>('nullProperty', null, style: propertyStyle),
StringProperty('node_type', '<root node>', showName: false, quoted: false, style: propertyStyle),
],
children: <TestTree>[
TestTree(name: 'node A', style: style),
TestTree(
name: 'node B',
properties: <DiagnosticsNode>[
StringProperty('p1', 'v1', quoted: false, style: propertyStyle),
StringProperty('p2', 'v2', quoted: false, style: propertyStyle),
],
children: <TestTree>[
TestTree(name: 'node B1', style: style),
TestTree(
name: 'node B2',
properties: <DiagnosticsNode>[StringProperty('property1', 'value1', quoted: false, style: propertyStyle)],
style: style,
),
TestTree(
name: 'node B3',
properties: <DiagnosticsNode>[
StringProperty('node_type', '<leaf node>', showName: false, quoted: false, style: propertyStyle),
IntProperty('foo', 42, style: propertyStyle),
],
style: lastChildStyle ?? style,
),
],
style: style,
),
TestTree(
name: 'node C',
properties: <DiagnosticsNode>[
StringProperty('foo', 'multi\nline\nvalue!', quoted: false, style: propertyStyle),
],
style: lastChildStyle ?? style,
),
],
style: lastChildStyle,
);
if (tree.style != DiagnosticsTreeStyle.singleLine &&
tree.style != DiagnosticsTreeStyle.errorProperty) {
expect(tree, hasAGoodToStringDeep);
}
expect(
tree.toDiagnosticsNode(name: name, style: style).toStringDeep(),
equalsIgnoringHashCodes(golden),
reason: description,
);
validateNodeJsonSerialization(tree.toDiagnosticsNode());
}
goldenStyleTest(
'sparse',
style: DiagnosticsTreeStyle.sparse,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β ββchild node B3: TestTree#00000\n'
' β <leaf node>\n'
' β foo: 42\n'
' β\n'
' ββchild node C: TestTree#00000\n'
' foo:\n'
' multi\n'
' line\n'
' value!\n',
);
goldenStyleTest(
'sparse with indented single line properties',
style: DiagnosticsTreeStyle.sparse,
propertyStyle: DiagnosticsTreeStyle.errorProperty,
golden:
'TestTree#00000\n'
' β stringProperty1:\n'
' β value1\n'
' β doubleProperty1:\n'
' β 42.5\n'
' β roundedProperty:\n'
' β 0.3\n'
' β nullProperty:\n'
' β null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1:\n'
' β β v1\n'
' β β p2:\n'
' β β v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1:\n'
' β β value1\n'
' β β\n'
' β ββchild node B3: TestTree#00000\n'
' β <leaf node>\n'
' β foo:\n'
' β 42\n'
' β\n'
' ββchild node C: TestTree#00000\n'
' foo:\n'
' multi\n'
' line\n'
' value!\n',
);
goldenStyleTest(
'dense',
style: DiagnosticsTreeStyle.dense,
golden:
'TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, nullProperty: null, <root node>)\n'
'βchild node A: TestTree#00000\n'
'βchild node B: TestTree#00000(p1: v1, p2: v2)\n'
'ββchild node B1: TestTree#00000\n'
'ββchild node B2: TestTree#00000(property1: value1)\n'
'ββchild node B3: TestTree#00000(<leaf node>, foo: 42)\n'
'βchild node C: TestTree#00000(foo: multi\\nline\\nvalue!)\n',
);
goldenStyleTest(
'dashed',
style: DiagnosticsTreeStyle.offstage,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β ββchild node B3: TestTree#00000\n'
' β <leaf node>\n'
' β foo: 42\n'
' β\n'
' ββchild node C: TestTree#00000\n'
' foo:\n'
' multi\n'
' line\n'
' value!\n',
);
goldenStyleTest(
'transition children',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.transition,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β βββ¦ββ child node B3 βββ\n'
' β β TestTree#00000:\n'
' β β <leaf node>\n'
' β β foo: 42\n'
' β ββββββββββββ\n'
' βββ¦ββ child node C βββ\n'
' β TestTree#00000:\n'
' β foo:\n'
' β multi\n'
' β line\n'
' β value!\n'
' ββββββββββββ\n',
);
goldenStyleTest(
'error children',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.error,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β βββ¦βββ‘ CHILD NODE B3: TESTTREE#00000 ββββββββββββββββββββββββββββββββ\n'
' β β <leaf node>\n'
' β β foo: 42\n'
' β βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n'
' βββ¦βββ‘ CHILD NODE C: TESTTREE#00000 βββββββββββββββββββββββββββββββββ\n'
' β foo:\n'
' β multi\n'
' β line\n'
' β value!\n'
' βββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββββ\n',
);
// You would never really want to make everything a transition child like
// this but you can and still get a readable tree.
goldenStyleTest(
'transition',
style: DiagnosticsTreeStyle.transition,
golden:
'TestTree#00000:\n'
' stringProperty1: value1\n'
' doubleProperty1: 42.5\n'
' roundedProperty: 0.3\n'
' nullProperty: null\n'
' <root node>\n'
' βββ¦ββ child node A βββ\n'
' β β TestTree#00000\n'
' β ββββββββββββ\n'
' βββ¦ββ child node B βββ\n'
' β β TestTree#00000:\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β βββ¦ββ child node B1 βββ\n'
' β β β β TestTree#00000\n'
' β β β ββββββββββββ\n'
' β β βββ¦ββ child node B2 βββ\n'
' β β β β TestTree#00000:\n'
' β β β β property1: value1\n'
' β β β ββββββββββββ\n'
' β β βββ¦ββ child node B3 βββ\n'
' β β β TestTree#00000:\n'
' β β β <leaf node>\n'
' β β β foo: 42\n'
' β β ββββββββββββ\n'
' β ββββββββββββ\n'
' βββ¦ββ child node C βββ\n'
' β TestTree#00000:\n'
' β foo:\n'
' β multi\n'
' β line\n'
' β value!\n'
' ββββββββββββ\n',
);
goldenStyleTest(
'whitespace',
style: DiagnosticsTreeStyle.whitespace,
golden:
'TestTree#00000:\n'
' stringProperty1: value1\n'
' doubleProperty1: 42.5\n'
' roundedProperty: 0.3\n'
' nullProperty: null\n'
' <root node>\n'
' child node A: TestTree#00000\n'
' child node B: TestTree#00000:\n'
' p1: v1\n'
' p2: v2\n'
' child node B1: TestTree#00000\n'
' child node B2: TestTree#00000:\n'
' property1: value1\n'
' child node B3: TestTree#00000:\n'
' <leaf node>\n'
' foo: 42\n'
' child node C: TestTree#00000:\n'
' foo:\n'
' multi\n'
' line\n'
' value!\n',
);
goldenStyleTest(
'flat',
style: DiagnosticsTreeStyle.flat,
golden:
'TestTree#00000:\n'
'stringProperty1: value1\n'
'doubleProperty1: 42.5\n'
'roundedProperty: 0.3\n'
'nullProperty: null\n'
'<root node>\n'
'child node A: TestTree#00000\n'
'child node B: TestTree#00000:\n'
'p1: v1\n'
'p2: v2\n'
'child node B1: TestTree#00000\n'
'child node B2: TestTree#00000:\n'
'property1: value1\n'
'child node B3: TestTree#00000:\n'
'<leaf node>\n'
'foo: 42\n'
'child node C: TestTree#00000:\n'
'foo:\n'
' multi\n'
' line\n'
' value!\n',
);
// Single line mode does not display children.
goldenStyleTest(
'single line',
style: DiagnosticsTreeStyle.singleLine,
golden: 'TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, nullProperty: null, <root node>)',
);
goldenStyleTest(
'single line',
name: 'some name',
style: DiagnosticsTreeStyle.singleLine,
golden: 'some name: TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, nullProperty: null, <root node>)',
);
// No name so we don't indent.
goldenStyleTest(
'indented single line',
style: DiagnosticsTreeStyle.errorProperty,
golden: 'TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, nullProperty: null, <root node>)\n',
);
goldenStyleTest(
'indented single line',
name: 'some name',
style: DiagnosticsTreeStyle.errorProperty,
golden:
'some name:\n'
' TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, nullProperty: null, <root node>)\n',
);
// TODO(jacobr): this is an ugly test case.
// There isn't anything interesting for this case as the children look the
// same with and without children. Only difference is the odd and
// undesirable density of B3 being right next to node C.
goldenStyleTest(
'single line last child',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.singleLine,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β ββchild node B3: TestTree#00000(<leaf node>, foo: 42)\n'
' ββchild node C: TestTree#00000(foo: multi\\nline\\nvalue!)\n',
);
// TODO(jacobr): this is an ugly test case.
// There isn't anything interesting for this case as the children look the
// same with and without children. Only difference is the odd and
// undesirable density of B3 being right next to node C.
// Typically header lines should not be places as leaves in the tree and
// should instead be places in front of other nodes that they
// function as a header for.
goldenStyleTest(
'indented single line last child',
style: DiagnosticsTreeStyle.sparse,
lastChildStyle: DiagnosticsTreeStyle.errorProperty,
golden:
'TestTree#00000\n'
' β stringProperty1: value1\n'
' β doubleProperty1: 42.5\n'
' β roundedProperty: 0.3\n'
' β nullProperty: null\n'
' β <root node>\n'
' β\n'
' ββchild node A: TestTree#00000\n'
' ββchild node B: TestTree#00000\n'
' β β p1: v1\n'
' β β p2: v2\n'
' β β\n'
' β ββchild node B1: TestTree#00000\n'
' β ββchild node B2: TestTree#00000\n'
' β β property1: value1\n'
' β β\n'
' β ββchild node B3:\n'
' β TestTree#00000(<leaf node>, foo: 42)\n'
' ββchild node C:\n'
' TestTree#00000(foo: multi\\nline\\nvalue!)\n',
);
});
test('toString test', () {
final TestTree tree = TestTree(
properties: <DiagnosticsNode>[
StringProperty('stringProperty1', 'value1', quoted: false),
DoubleProperty('doubleProperty1', 42.5),
DoubleProperty('roundedProperty', 1.0 / 3.0),
StringProperty('DO_NOT_SHOW', 'DO_NOT_SHOW', level: DiagnosticLevel.hidden, quoted: false),
StringProperty('DEBUG_ONLY', 'DEBUG_ONLY', level: DiagnosticLevel.debug, quoted: false),
],
// child to verify that children are not included in the toString.
children: <TestTree>[TestTree(name: 'node A')],
);
expect(
tree.toString(),
equalsIgnoringHashCodes('TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3)'),
);
expect(
tree.toString(minLevel: DiagnosticLevel.debug),
equalsIgnoringHashCodes('TestTree#00000(stringProperty1: value1, doubleProperty1: 42.5, roundedProperty: 0.3, DEBUG_ONLY: DEBUG_ONLY)'),
);
});
test('transition test', () {
// Test multiple styles integrating together in the same tree due to using
// transition to go between styles that would otherwise be incompatible.
final TestTree tree = TestTree(
style: DiagnosticsTreeStyle.sparse,
properties: <DiagnosticsNode>[
StringProperty('stringProperty1', 'value1'),
],
children: <TestTree>[
TestTree(
style: DiagnosticsTreeStyle.transition,
name: 'node transition',
properties: <DiagnosticsNode>[
StringProperty('p1', 'v1'),
TestTree(
properties: <DiagnosticsNode>[
DiagnosticsProperty<bool>('survived', true),
],
).toDiagnosticsNode(name: 'tree property', style: DiagnosticsTreeStyle.whitespace),
],
children: <TestTree>[
TestTree(name: 'dense child', style: DiagnosticsTreeStyle.dense),
TestTree(
name: 'dense',
properties: <DiagnosticsNode>[StringProperty('property1', 'value1')],
style: DiagnosticsTreeStyle.dense,
),
TestTree(
name: 'node B3',
properties: <DiagnosticsNode>[
StringProperty('node_type', '<leaf node>', showName: false, quoted: false),
IntProperty('foo', 42),
],
style: DiagnosticsTreeStyle.dense,
),
],
),
TestTree(
name: 'node C',
properties: <DiagnosticsNode>[
StringProperty('foo', 'multi\nline\nvalue!', quoted: false),
],
style: DiagnosticsTreeStyle.sparse,
),
],
);
expect(tree, hasAGoodToStringDeep);
expect(
tree.toDiagnosticsNode().toStringDeep(),
equalsIgnoringHashCodes(
'TestTree#00000\n'
' β stringProperty1: "value1"\n'
' βββ¦ββ child node transition βββ\n'
' β β TestTree#00000:\n'
' β β p1: "v1"\n'
' β β tree property: TestTree#00000:\n'
' β β survived: true\n'
' β β βchild dense child: TestTree#00000\n'
' β β βchild dense: TestTree#00000(property1: "value1")\n'
' β β βchild node B3: TestTree#00000(<leaf node>, foo: 42)\n'
' β ββββββββββββ\n'
' ββchild node C: TestTree#00000\n'
' foo:\n'
' multi\n'
' line\n'
' value!\n',
),
);
});
test('describeEnum test', () {
expect(describeEnum(ExampleEnum.hello), equals('hello'));
expect(describeEnum(ExampleEnum.world), equals('world'));
expect(describeEnum(ExampleEnum.deferToChild), equals('deferToChild'));
expect(
() => describeEnum('Hello World'),
throwsA(isAssertionError.having(
(AssertionError e) => e.message,
'message',
'The provided object "Hello World" is not an enum.',
)),
);
});
test('string property test', () {
expect(
StringProperty('name', 'value', quoted: false).toString(),
equals('name: value'),
);
final StringProperty stringProperty = StringProperty(
'name',
'value',
description: 'VALUE',
ifEmpty: '<hidden>',
quoted: false,
);
expect(stringProperty.toString(), equals('name: VALUE'));
validateStringPropertyJsonSerialization(stringProperty);
expect(
StringProperty(
'name',
'value',
showName: false,
ifEmpty: '<hidden>',
quoted: false,
).toString(),
equals('value'),
);
expect(
StringProperty('name', '', ifEmpty: '<hidden>').toString(),
equals('name: <hidden>'),
);
expect(
StringProperty(
'name',
'',
ifEmpty: '<hidden>',
showName: false,
).toString(),
equals('<hidden>'),
);
expect(StringProperty('name', null).isFiltered(DiagnosticLevel.info), isFalse);
expect(StringProperty('name', 'value', level: DiagnosticLevel.hidden).isFiltered(DiagnosticLevel.info), isTrue);
expect(StringProperty('name', null, defaultValue: null).isFiltered(DiagnosticLevel.info), isTrue);
final StringProperty quoted = StringProperty(
'name',
'value',
);
expect(quoted.toString(), equals('name: "value"'));
validateStringPropertyJsonSerialization(quoted);
expect(
StringProperty('name', 'value', showName: false).toString(),
equals('"value"'),
);
expect(
StringProperty(
'name',
null,
showName: false,
).toString(),
equals('null'),
);
});
test('bool property test', () {
final DiagnosticsProperty<bool> trueProperty = DiagnosticsProperty<bool>('name', true);
final DiagnosticsProperty<bool> falseProperty = DiagnosticsProperty<bool>('name', false);
expect(trueProperty.toString(), equals('name: true'));
expect(trueProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(trueProperty.value, isTrue);
expect(falseProperty.toString(), equals('name: false'));
expect(falseProperty.value, isFalse);
expect(falseProperty.isFiltered(DiagnosticLevel.info), isFalse);
validatePropertyJsonSerialization(trueProperty);
validatePropertyJsonSerialization(falseProperty);
final DiagnosticsProperty<bool> truthyProperty = DiagnosticsProperty<bool>(
'name',
true,
description: 'truthy',
);
expect(
truthyProperty.toString(),
equals('name: truthy'),
);
validatePropertyJsonSerialization(truthyProperty);
expect(
DiagnosticsProperty<bool>('name', true, showName: false).toString(),
equals('true'),
);
expect(DiagnosticsProperty<bool>('name', null).isFiltered(DiagnosticLevel.info), isFalse);
expect(DiagnosticsProperty<bool>('name', true, level: DiagnosticLevel.hidden).isFiltered(DiagnosticLevel.info), isTrue);
expect(DiagnosticsProperty<bool>('name', null, defaultValue: null).isFiltered(DiagnosticLevel.info), isTrue);
final DiagnosticsProperty<bool> missingBool = DiagnosticsProperty<bool>('name', null, ifNull: 'missing');
expect(
missingBool.toString(),
equals('name: missing'),
);
validatePropertyJsonSerialization(missingBool);
});
test('flag property test', () {
final FlagProperty trueFlag = FlagProperty(
'myFlag',
value: true,
ifTrue: 'myFlag',
);
final FlagProperty falseFlag = FlagProperty(
'myFlag',
value: false,
ifTrue: 'myFlag',
);
expect(trueFlag.toString(), equals('myFlag'));
validateFlagPropertyJsonSerialization(trueFlag);
validateFlagPropertyJsonSerialization(falseFlag);
expect(trueFlag.value, isTrue);
expect(falseFlag.value, isFalse);
expect(trueFlag.isFiltered(DiagnosticLevel.fine), isFalse);
expect(falseFlag.isFiltered(DiagnosticLevel.fine), isTrue);
});
test('property with tooltip test', () {
final DiagnosticsProperty<String> withTooltip = DiagnosticsProperty<String>(
'name',
'value',
tooltip: 'tooltip',
);
expect(
withTooltip.toString(),
equals('name: value (tooltip)'),
);
expect(withTooltip.value, equals('value'));
expect(withTooltip.isFiltered(DiagnosticLevel.fine), isFalse);
validatePropertyJsonSerialization(withTooltip);
});
test('double property test', () {
final DoubleProperty doubleProperty = DoubleProperty(
'name',
42.0,
);
expect(doubleProperty.toString(), equals('name: 42.0'));
expect(doubleProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(doubleProperty.value, equals(42.0));
validateDoublePropertyJsonSerialization(doubleProperty);
expect(DoubleProperty('name', 1.3333).toString(), equals('name: 1.3'));
expect(DoubleProperty('name', null).toString(), equals('name: null'));
expect(DoubleProperty('name', null).isFiltered(DiagnosticLevel.info), equals(false));
expect(
DoubleProperty('name', null, ifNull: 'missing').toString(),
equals('name: missing'),
);
final DoubleProperty doubleWithUnit = DoubleProperty('name', 42.0, unit: 'px');
expect(doubleWithUnit.toString(), equals('name: 42.0px'));
validateDoublePropertyJsonSerialization(doubleWithUnit);
});
test('double.infinity serialization test', () {
final DoubleProperty infProperty1 = DoubleProperty('double1', double.infinity);
validateDoublePropertyJsonSerialization(infProperty1);
expect(infProperty1.toString(), equals('double1: Infinity'));
final DoubleProperty infProperty2 = DoubleProperty('double2', double.negativeInfinity);
validateDoublePropertyJsonSerialization(infProperty2);
expect(infProperty2.toString(), equals('double2: -Infinity'));
});
test('unsafe double property test', () {
final DoubleProperty safe = DoubleProperty.lazy(
'name',
() => 42.0,
);
expect(safe.toString(), equals('name: 42.0'));
expect(safe.isFiltered(DiagnosticLevel.info), isFalse);
expect(safe.value, equals(42.0));
validateDoublePropertyJsonSerialization(safe);
expect(
DoubleProperty.lazy('name', () => 1.3333).toString(),
equals('name: 1.3'),
);
expect(
DoubleProperty.lazy('name', () => null).toString(),
equals('name: null'),
);
expect(
DoubleProperty.lazy('name', () => null).isFiltered(DiagnosticLevel.info),
equals(false),
);
final DoubleProperty throwingProperty = DoubleProperty.lazy(
'name',
() => throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Invalid constraints')]),
);
// TODO(jacobr): it would be better if throwingProperty.object threw an
// exception.
expect(throwingProperty.value, isNull);
expect(throwingProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(
throwingProperty.toString(),
equals('name: EXCEPTION (FlutterError)'),
);
expect(throwingProperty.level, equals(DiagnosticLevel.error));
validateDoublePropertyJsonSerialization(throwingProperty);
});
test('percent property', () {
expect(
PercentProperty('name', 0.4).toString(),
equals('name: 40.0%'),
);
final PercentProperty complexPercentProperty = PercentProperty('name', 0.99, unit: 'invisible', tooltip: 'almost transparent');
expect(
complexPercentProperty.toString(),
equals('name: 99.0% invisible (almost transparent)'),
);
validateDoublePropertyJsonSerialization(complexPercentProperty);
expect(
PercentProperty('name', null, unit: 'invisible', tooltip: '!').toString(),
equals('name: null (!)'),
);
expect(
PercentProperty('name', 0.4).value,
0.4,
);
expect(
PercentProperty('name', 0.0).toString(),
equals('name: 0.0%'),
);
expect(
PercentProperty('name', -10.0).toString(),
equals('name: 0.0%'),
);
expect(
PercentProperty('name', 1.0).toString(),
equals('name: 100.0%'),
);
expect(
PercentProperty('name', 3.0).toString(),
equals('name: 100.0%'),
);
expect(
PercentProperty('name', null).toString(),
equals('name: null'),
);
expect(
PercentProperty(
'name',
null,
ifNull: 'missing',
).toString(),
equals('name: missing'),
);
expect(
PercentProperty(
'name',
null,
ifNull: 'missing',
showName: false,
).toString(),
equals('missing'),
);
expect(
PercentProperty(
'name',
0.5,
showName: false,
).toString(),
equals('50.0%'),
);
});
test('callback property test', () {
void onClick() { }
final ObjectFlagProperty<Function> present = ObjectFlagProperty<Function>(
'onClick',
onClick,
ifPresent: 'clickable',
);
final ObjectFlagProperty<Function> missing = ObjectFlagProperty<Function>(
'onClick',
null,
ifPresent: 'clickable',
);
expect(present.toString(), equals('clickable'));
expect(present.isFiltered(DiagnosticLevel.info), isFalse);
expect(present.value, equals(onClick));
validateObjectFlagPropertyJsonSerialization(present);
expect(missing.toString(), equals('onClick: null'));
expect(missing.isFiltered(DiagnosticLevel.fine), isTrue);
validateObjectFlagPropertyJsonSerialization(missing);
});
test('missing callback property test', () {
void onClick() { }
final ObjectFlagProperty<Function> present = ObjectFlagProperty<Function>(
'onClick',
onClick,
ifNull: 'disabled',
);
final ObjectFlagProperty<Function> missing = ObjectFlagProperty<Function>(
'onClick',
null,
ifNull: 'disabled',
);
expect(present.toString(), equals('onClick: Closure: () => void'));
expect(present.isFiltered(DiagnosticLevel.fine), isTrue);
expect(present.value, equals(onClick));
expect(missing.toString(), equals('disabled'));
expect(missing.isFiltered(DiagnosticLevel.info), isFalse);
validateObjectFlagPropertyJsonSerialization(present);
validateObjectFlagPropertyJsonSerialization(missing);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/54221
test('describe bool property', () {
final FlagProperty yes = FlagProperty(
'name',
value: true,
ifTrue: 'YES',
ifFalse: 'NO',
showName: true,
);
final FlagProperty no = FlagProperty(
'name',
value: false,
ifTrue: 'YES',
ifFalse: 'NO',
showName: true,
);
expect(yes.toString(), equals('name: YES'));
expect(yes.level, equals(DiagnosticLevel.info));
expect(yes.value, isTrue);
validateFlagPropertyJsonSerialization(yes);
expect(no.toString(), equals('name: NO'));
expect(no.level, equals(DiagnosticLevel.info));
expect(no.value, isFalse);
validateFlagPropertyJsonSerialization(no);
expect(
FlagProperty(
'name',
value: true,
ifTrue: 'YES',
ifFalse: 'NO',
).toString(),
equals('YES'),
);
expect(
FlagProperty(
'name',
value: false,
ifTrue: 'YES',
ifFalse: 'NO',
).toString(),
equals('NO'),
);
expect(
FlagProperty(
'name',
value: true,
ifTrue: 'YES',
ifFalse: 'NO',
level: DiagnosticLevel.hidden,
showName: true,
).level,
equals(DiagnosticLevel.hidden),
);
});
test('enum property test', () {
final EnumProperty<ExampleEnum> hello = EnumProperty<ExampleEnum>(
'name',
ExampleEnum.hello,
);
final EnumProperty<ExampleEnum> world = EnumProperty<ExampleEnum>(
'name',
ExampleEnum.world,
);
final EnumProperty<ExampleEnum> deferToChild = EnumProperty<ExampleEnum>(
'name',
ExampleEnum.deferToChild,
);
final EnumProperty<ExampleEnum> nullEnum = EnumProperty<ExampleEnum>(
'name',
null,
);
expect(hello.level, equals(DiagnosticLevel.info));
expect(hello.value, equals(ExampleEnum.hello));
expect(hello.toString(), equals('name: hello'));
validatePropertyJsonSerialization(hello);
expect(world.level, equals(DiagnosticLevel.info));
expect(world.value, equals(ExampleEnum.world));
expect(world.toString(), equals('name: world'));
validatePropertyJsonSerialization(world);
expect(deferToChild.level, equals(DiagnosticLevel.info));
expect(deferToChild.value, equals(ExampleEnum.deferToChild));
expect(deferToChild.toString(), equals('name: deferToChild'));
validatePropertyJsonSerialization(deferToChild);
expect(nullEnum.level, equals(DiagnosticLevel.info));
expect(nullEnum.value, isNull);
expect(nullEnum.toString(), equals('name: null'));
validatePropertyJsonSerialization(nullEnum);
final EnumProperty<ExampleEnum> matchesDefault = EnumProperty<ExampleEnum>(
'name',
ExampleEnum.hello,
defaultValue: ExampleEnum.hello,
);
expect(matchesDefault.toString(), equals('name: hello'));
expect(matchesDefault.value, equals(ExampleEnum.hello));
expect(matchesDefault.isFiltered(DiagnosticLevel.info), isTrue);
validatePropertyJsonSerialization(matchesDefault);
expect(
EnumProperty<ExampleEnum>(
'name',
ExampleEnum.hello,
level: DiagnosticLevel.hidden,
).level,
equals(DiagnosticLevel.hidden),
);
});
test('int property test', () {
final IntProperty regular = IntProperty(
'name',
42,
);
expect(regular.toString(), equals('name: 42'));
expect(regular.value, equals(42));
expect(regular.level, equals(DiagnosticLevel.info));
final IntProperty nullValue = IntProperty(
'name',
null,
);
expect(nullValue.toString(), equals('name: null'));
expect(nullValue.value, isNull);
expect(nullValue.level, equals(DiagnosticLevel.info));
final IntProperty hideNull = IntProperty(
'name',
null,
defaultValue: null,
);
expect(hideNull.toString(), equals('name: null'));
expect(hideNull.value, isNull);
expect(hideNull.isFiltered(DiagnosticLevel.info), isTrue);
final IntProperty nullDescription = IntProperty(
'name',
null,
ifNull: 'missing',
);
expect(nullDescription.toString(), equals('name: missing'));
expect(nullDescription.value, isNull);
expect(nullDescription.level, equals(DiagnosticLevel.info));
final IntProperty hideName = IntProperty(
'name',
42,
showName: false,
);
expect(hideName.toString(), equals('42'));
expect(hideName.value, equals(42));
expect(hideName.level, equals(DiagnosticLevel.info));
final IntProperty withUnit = IntProperty(
'name',
42,
unit: 'pt',
);
expect(withUnit.toString(), equals('name: 42pt'));
expect(withUnit.value, equals(42));
expect(withUnit.level, equals(DiagnosticLevel.info));
final IntProperty defaultValue = IntProperty(
'name',
42,
defaultValue: 42,
);
expect(defaultValue.toString(), equals('name: 42'));
expect(defaultValue.value, equals(42));
expect(defaultValue.isFiltered(DiagnosticLevel.info), isTrue);
final IntProperty notDefaultValue = IntProperty(
'name',
43,
defaultValue: 42,
);
expect(notDefaultValue.toString(), equals('name: 43'));
expect(notDefaultValue.value, equals(43));
expect(notDefaultValue.level, equals(DiagnosticLevel.info));
final IntProperty hidden = IntProperty(
'name',
42,
level: DiagnosticLevel.hidden,
);
expect(hidden.toString(), equals('name: 42'));
expect(hidden.value, equals(42));
expect(hidden.level, equals(DiagnosticLevel.hidden));
});
test('object property test', () {
const Rect rect = Rect.fromLTRB(0.0, 0.0, 20.0, 20.0);
final DiagnosticsProperty<Rect> simple = DiagnosticsProperty<Rect>(
'name',
rect,
);
expect(simple.value, equals(rect));
expect(simple.level, equals(DiagnosticLevel.info));
expect(simple.toString(), equals('name: Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)'));
validatePropertyJsonSerialization(simple);
final DiagnosticsProperty<Rect> withDescription = DiagnosticsProperty<Rect>(
'name',
rect,
description: 'small rect',
);
expect(withDescription.value, equals(rect));
expect(withDescription.level, equals(DiagnosticLevel.info));
expect(withDescription.toString(), equals('name: small rect'));
validatePropertyJsonSerialization(withDescription);
final DiagnosticsProperty<Object> nullProperty = DiagnosticsProperty<Object>(
'name',
null,
);
expect(nullProperty.value, isNull);
expect(nullProperty.level, equals(DiagnosticLevel.info));
expect(nullProperty.toString(), equals('name: null'));
validatePropertyJsonSerialization(nullProperty);
final DiagnosticsProperty<Object> hideNullProperty = DiagnosticsProperty<Object>(
'name',
null,
defaultValue: null,
);
expect(hideNullProperty.value, isNull);
expect(hideNullProperty.isFiltered(DiagnosticLevel.info), isTrue);
expect(hideNullProperty.toString(), equals('name: null'));
validatePropertyJsonSerialization(hideNullProperty);
final DiagnosticsProperty<Object> nullDescription = DiagnosticsProperty<Object>(
'name',
null,
ifNull: 'missing',
);
expect(nullDescription.value, isNull);
expect(nullDescription.level, equals(DiagnosticLevel.info));
expect(nullDescription.toString(), equals('name: missing'));
validatePropertyJsonSerialization(nullDescription);
final DiagnosticsProperty<Rect> hideName = DiagnosticsProperty<Rect>(
'name',
rect,
showName: false,
level: DiagnosticLevel.warning,
);
expect(hideName.value, equals(rect));
expect(hideName.level, equals(DiagnosticLevel.warning));
expect(hideName.toString(), equals('Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)'));
validatePropertyJsonSerialization(hideName);
final DiagnosticsProperty<Rect> hideSeparator = DiagnosticsProperty<Rect>(
'Creator',
rect,
showSeparator: false,
);
expect(hideSeparator.value, equals(rect));
expect(hideSeparator.level, equals(DiagnosticLevel.info));
expect(
hideSeparator.toString(),
equals('Creator Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)'),
);
validatePropertyJsonSerialization(hideSeparator);
});
test('lazy object property test', () {
const Rect rect = Rect.fromLTRB(0.0, 0.0, 20.0, 20.0);
final DiagnosticsProperty<Rect> simple = DiagnosticsProperty<Rect>.lazy(
'name',
() => rect,
description: 'small rect',
);
expect(simple.value, equals(rect));
expect(simple.level, equals(DiagnosticLevel.info));
expect(simple.toString(), equals('name: small rect'));
validatePropertyJsonSerialization(simple);
final DiagnosticsProperty<Object> nullProperty = DiagnosticsProperty<Object>.lazy(
'name',
() => null,
description: 'missing',
);
expect(nullProperty.value, isNull);
expect(nullProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(nullProperty.toString(), equals('name: missing'));
validatePropertyJsonSerialization(nullProperty);
final DiagnosticsProperty<Object> hideNullProperty = DiagnosticsProperty<Object>.lazy(
'name',
() => null,
description: 'missing',
defaultValue: null,
);
expect(hideNullProperty.value, isNull);
expect(hideNullProperty.isFiltered(DiagnosticLevel.info), isTrue);
expect(hideNullProperty.toString(), equals('name: missing'));
validatePropertyJsonSerialization(hideNullProperty);
final DiagnosticsProperty<Rect> hideName = DiagnosticsProperty<Rect>.lazy(
'name',
() => rect,
description: 'small rect',
showName: false,
);
expect(hideName.value, equals(rect));
expect(hideName.isFiltered(DiagnosticLevel.info), isFalse);
expect(hideName.toString(), equals('small rect'));
validatePropertyJsonSerialization(hideName);
final DiagnosticsProperty<Object> throwingWithDescription = DiagnosticsProperty<Object>.lazy(
'name',
() => throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Property not available')]),
description: 'missing',
defaultValue: null,
);
expect(throwingWithDescription.value, isNull);
expect(throwingWithDescription.exception, isFlutterError);
expect(throwingWithDescription.isFiltered(DiagnosticLevel.info), false);
expect(throwingWithDescription.toString(), equals('name: missing'));
validatePropertyJsonSerialization(throwingWithDescription);
final DiagnosticsProperty<Object> throwingProperty = DiagnosticsProperty<Object>.lazy(
'name',
() => throw FlutterError.fromParts(<DiagnosticsNode>[ErrorSummary('Property not available')]),
defaultValue: null,
);
expect(throwingProperty.value, isNull);
expect(throwingProperty.exception, isFlutterError);
expect(throwingProperty.isFiltered(DiagnosticLevel.info), false);
expect(throwingProperty.toString(), equals('name: EXCEPTION (FlutterError)'));
validatePropertyJsonSerialization(throwingProperty);
});
test('color property test', () {
// Add more tests if colorProperty becomes more than a wrapper around
// objectProperty.
const Color color = Color.fromARGB(255, 255, 255, 255);
final DiagnosticsProperty<Color> simple = DiagnosticsProperty<Color>(
'name',
color,
);
validatePropertyJsonSerialization(simple);
expect(simple.isFiltered(DiagnosticLevel.info), isFalse);
expect(simple.value, equals(color));
expect(simple.propertyType, equals(Color));
expect(simple.toString(), equals('name: Color(0xffffffff)'));
validatePropertyJsonSerialization(simple);
});
test('flag property test', () {
final FlagProperty show = FlagProperty(
'wasLayout',
value: true,
ifTrue: 'layout computed',
);
expect(show.name, equals('wasLayout'));
expect(show.value, isTrue);
expect(show.isFiltered(DiagnosticLevel.info), isFalse);
expect(show.toString(), equals('layout computed'));
validateFlagPropertyJsonSerialization(show);
final FlagProperty hide = FlagProperty(
'wasLayout',
value: false,
ifTrue: 'layout computed',
);
expect(hide.name, equals('wasLayout'));
expect(hide.value, isFalse);
expect(hide.level, equals(DiagnosticLevel.hidden));
expect(hide.toString(), equals('wasLayout: false'));
validateFlagPropertyJsonSerialization(hide);
final FlagProperty hideTrue = FlagProperty(
'wasLayout',
value: true,
ifFalse: 'no layout computed',
);
expect(hideTrue.name, equals('wasLayout'));
expect(hideTrue.value, isTrue);
expect(hideTrue.level, equals(DiagnosticLevel.hidden));
expect(hideTrue.toString(), equals('wasLayout: true'));
validateFlagPropertyJsonSerialization(hideTrue);
});
test('has property test', () {
void onClick() { }
final ObjectFlagProperty<Function> has = ObjectFlagProperty<Function>.has(
'onClick',
onClick,
);
expect(has.name, equals('onClick'));
expect(has.value, equals(onClick));
expect(has.isFiltered(DiagnosticLevel.info), isFalse);
expect(has.toString(), equals('has onClick'));
validateObjectFlagPropertyJsonSerialization(has);
final ObjectFlagProperty<Function> missing = ObjectFlagProperty<Function>.has(
'onClick',
null,
);
expect(missing.name, equals('onClick'));
expect(missing.value, isNull);
expect(missing.isFiltered(DiagnosticLevel.info), isTrue);
expect(missing.toString(), equals('onClick: null'));
validateObjectFlagPropertyJsonSerialization(missing);
});
test('iterable flags property test', () {
// Normal property
{
void onClick() { }
void onMove() { }
final Map<String, Function> value = <String, Function>{
'click': onClick,
'move': onMove,
};
final FlagsSummary<Function> flags = FlagsSummary<Function>(
'listeners',
value,
);
expect(flags.name, equals('listeners'));
expect(flags.value, equals(value));
expect(flags.isFiltered(DiagnosticLevel.info), isFalse);
expect(flags.toString(), equals('listeners: click, move'));
validateIterableFlagsPropertyJsonSerialization(flags);
}
// Reversed-order property
{
void onClick() { }
void onMove() { }
final Map<String, Function> value = <String, Function>{
'move': onMove,
'click': onClick,
};
final FlagsSummary<Function> flags = FlagsSummary<Function>(
'listeners',
value,
);
expect(flags.toString(), equals('listeners: move, click'));
expect(flags.isFiltered(DiagnosticLevel.info), isFalse);
validateIterableFlagsPropertyJsonSerialization(flags);
}
// Partially empty property
{
void onClick() { }
final Map<String, Function?> value = <String, Function?>{
'move': null,
'click': onClick,
};
final FlagsSummary<Function> flags = FlagsSummary<Function>(
'listeners',
value,
);
expect(flags.toString(), equals('listeners: click'));
expect(flags.isFiltered(DiagnosticLevel.info), isFalse);
validateIterableFlagsPropertyJsonSerialization(flags);
}
// Empty property (without ifEmpty)
{
final Map<String, Function?> value = <String, Function?>{
'enter': null,
};
final FlagsSummary<Function> flags = FlagsSummary<Function>(
'listeners',
value,
);
expect(flags.isFiltered(DiagnosticLevel.info), isTrue);
validateIterableFlagsPropertyJsonSerialization(flags);
}
// Empty property (without ifEmpty)
{
final Map<String, Function?> value = <String, Function?>{
'enter': null,
};
final FlagsSummary<Function> flags = FlagsSummary<Function>(
'listeners',
value,
ifEmpty: '<none>',
);
expect(flags.toString(), equals('listeners: <none>'));
expect(flags.isFiltered(DiagnosticLevel.info), isFalse);
validateIterableFlagsPropertyJsonSerialization(flags);
}
});
test('iterable property test', () {
final List<int> ints = <int>[1,2,3];
final IterableProperty<int> intsProperty = IterableProperty<int>(
'ints',
ints,
);
expect(intsProperty.value, equals(ints));
expect(intsProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(intsProperty.toString(), equals('ints: 1, 2, 3'));
final List<double> doubles = <double>[1,2,3];
final IterableProperty<double> doublesProperty = IterableProperty<double>(
'doubles',
doubles,
);
expect(doublesProperty.value, equals(doubles));
expect(doublesProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(doublesProperty.toString(), equals('doubles: 1.0, 2.0, 3.0'));
final IterableProperty<Object> emptyProperty = IterableProperty<Object>(
'name',
<Object>[],
);
expect(emptyProperty.value, isEmpty);
expect(emptyProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(emptyProperty.toString(), equals('name: []'));
validateIterablePropertyJsonSerialization(emptyProperty);
final IterableProperty<Object> nullProperty = IterableProperty<Object>(
'list',
null,
);
expect(nullProperty.value, isNull);
expect(nullProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(nullProperty.toString(), equals('list: null'));
validateIterablePropertyJsonSerialization(nullProperty);
final IterableProperty<Object> hideNullProperty = IterableProperty<Object>(
'list',
null,
defaultValue: null,
);
expect(hideNullProperty.value, isNull);
expect(hideNullProperty.isFiltered(DiagnosticLevel.info), isTrue);
expect(hideNullProperty.level, equals(DiagnosticLevel.fine));
expect(hideNullProperty.toString(), equals('list: null'));
validateIterablePropertyJsonSerialization(hideNullProperty);
final List<Object> objects = <Object>[
const Rect.fromLTRB(0.0, 0.0, 20.0, 20.0),
const Color.fromARGB(255, 255, 255, 255),
];
final IterableProperty<Object> objectsProperty = IterableProperty<Object>(
'objects',
objects,
);
expect(objectsProperty.value, equals(objects));
expect(objectsProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(
objectsProperty.toString(),
equals('objects: Rect.fromLTRB(0.0, 0.0, 20.0, 20.0), Color(0xffffffff)'),
);
validateIterablePropertyJsonSerialization(objectsProperty);
final IterableProperty<Object> multiLineProperty = IterableProperty<Object>(
'objects',
objects,
style: DiagnosticsTreeStyle.whitespace,
);
expect(multiLineProperty.value, equals(objects));
expect(multiLineProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(
multiLineProperty.toString(),
equals(
'objects:\n'
'Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)\n'
'Color(0xffffffff)',
),
);
expect(
multiLineProperty.toStringDeep(),
equals(
'objects:\n'
' Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)\n'
' Color(0xffffffff)\n',
),
);
validateIterablePropertyJsonSerialization(multiLineProperty);
expect(
TestTree(
properties: <DiagnosticsNode>[multiLineProperty],
).toStringDeep(),
equalsIgnoringHashCodes(
'TestTree#00000\n'
' objects:\n'
' Rect.fromLTRB(0.0, 0.0, 20.0, 20.0)\n'
' Color(0xffffffff)\n',
),
);
expect(
TestTree(
properties: <DiagnosticsNode>[objectsProperty, IntProperty('foo', 42)],
style: DiagnosticsTreeStyle.singleLine,
).toStringDeep(),
equalsIgnoringHashCodes(
'TestTree#00000(objects: [Rect.fromLTRB(0.0, 0.0, 20.0, 20.0), Color(0xffffffff)], foo: 42)',
),
);
// Iterable with a single entry. Verify that rendering is sensible and that
// multi line rendering isn't used even though it is not helpful.
final List<Object> singleElementList = <Object>[const Color.fromARGB(255, 255, 255, 255)];
final IterableProperty<Object> objectProperty = IterableProperty<Object>(
'object',
singleElementList,
style: DiagnosticsTreeStyle.whitespace,
);
expect(objectProperty.value, equals(singleElementList));
expect(objectProperty.isFiltered(DiagnosticLevel.info), isFalse);
expect(
objectProperty.toString(),
equals('object: Color(0xffffffff)'),
);
expect(
objectProperty.toStringDeep(),
equals('object: Color(0xffffffff)\n'),
);
validateIterablePropertyJsonSerialization(objectProperty);
expect(
TestTree(
name: 'root',
properties: <DiagnosticsNode>[objectProperty],
).toStringDeep(),
equalsIgnoringHashCodes(
'TestTree#00000\n'
' object: Color(0xffffffff)\n',
),
);
});
test('Stack trace test', () {
final StackTrace stack = StackTrace.fromString(
'#0 someMethod (file:///diagnostics_test.dart:42:19)\n'
'#1 someMethod2 (file:///diagnostics_test.dart:12:3)\n'
'#2 someMethod3 (file:///foo.dart:4:1)\n',
);
expect(
DiagnosticsStackTrace('Stack trace', stack).toStringDeep(),
equalsIgnoringHashCodes(
'Stack trace:\n'
'#0 someMethod (file:///diagnostics_test.dart:42:19)\n'
'#1 someMethod2 (file:///diagnostics_test.dart:12:3)\n'
'#2 someMethod3 (file:///foo.dart:4:1)\n',
),
);
expect(
DiagnosticsStackTrace('-- callback 2 --', stack, showSeparator: false).toStringDeep(),
equalsIgnoringHashCodes(
'-- callback 2 --\n'
'#0 someMethod (file:///diagnostics_test.dart:42:19)\n'
'#1 someMethod2 (file:///diagnostics_test.dart:12:3)\n'
'#2 someMethod3 (file:///foo.dart:4:1)\n',
),
);
});
test('message test', () {
final DiagnosticsNode message = DiagnosticsNode.message('hello world');
expect(message.toString(), equals('hello world'));
expect(message.name, isEmpty);
expect(message.value, isNull);
expect(message.showName, isFalse);
validateNodeJsonSerialization(message);
final DiagnosticsNode messageProperty = MessageProperty('diagnostics', 'hello world');
expect(messageProperty.toString(), equals('diagnostics: hello world'));
expect(messageProperty.name, equals('diagnostics'));
expect(messageProperty.value, isNull);
expect(messageProperty.showName, isTrue);
validatePropertyJsonSerialization(messageProperty as DiagnosticsProperty<Object?>);
});
test('error message style wrap test', () {
// This tests wrapping of properties with styles typical for error messages.
DiagnosticsNode createTreeWithWrappingNodes({
DiagnosticsTreeStyle? rootStyle,
required DiagnosticsTreeStyle propertyStyle,
}) {
return TestTree(
name: 'Test tree',
properties: <DiagnosticsNode>[
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsNode.message(
'This is a very long message that must wrap as it cannot fit on one line. '
'This is a very long message that must wrap as it cannot fit on one line. '
'This is a very long message that must wrap as it cannot fit on one line.',
style: propertyStyle,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsProperty<String>(
null,
'Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap.',
allowWrap: false,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsNode.message(
'This property has a very long property name that will be allowed to wrap unlike most property names. This property has a very long property name that will be allowed to wrap unlike most property names:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html',
style: propertyStyle,
),
DiagnosticsNode.message(
'This property has a very long property name that will be allowed to wrap unlike most property names. This property has a very long property name that will be allowed to wrap unlike most property names:\n'
' https://goo.gl/',
style: propertyStyle,
),
DiagnosticsNode.message(
'Click on the following url:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html',
style: propertyStyle,
),
DiagnosticsNode.message(
'Click on the following url\n'
' https://goo.gl/',
style: propertyStyle,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsProperty<String>(
'multi-line value',
'[1.0, 0.0, 0.0, 0.0]\n'
'[1.0, 1.0, 0.0, 0.0]\n'
'[1.0, 0.0, 1.0, 0.0]\n'
'[1.0, 0.0, 0.0, 1.0]\n',
style: propertyStyle,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsProperty<String>(
'This property has a very long property name that will be allowed to wrap unlike most property names. This property has a very long property name that will be allowed to wrap unlike most property names',
'This is a very long message that must wrap as it cannot fit on one line. '
'This is a very long message that must wrap as it cannot fit on one line. '
'This is a very long message that must wrap as it cannot fit on one line.',
style: propertyStyle,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
DiagnosticsProperty<String>(
'This property has a very long property name that will be allowed to wrap unlike most property names. This property has a very long property name that will be allowed to wrap unlike most property names',
'[1.0, 0.0, 0.0, 0.0]\n'
'[1.0, 1.0, 0.0, 0.0]\n'
'[1.0, 0.0, 1.0, 0.0]\n'
'[1.0, 0.0, 0.0, 1.0]\n',
style: propertyStyle,
),
DiagnosticsNode.message(
'--- example property at max length --',
style: propertyStyle,
),
MessageProperty(
'diagnosis',
'insufficient data to draw conclusion (less than five repaints)',
style: propertyStyle,
),
],
).toDiagnosticsNode(style: rootStyle);
}
final TextTreeRenderer renderer = TextTreeRenderer(wrapWidth: 40, wrapWidthProperties: 40);
expect(
renderer.render(createTreeWithWrappingNodes(
rootStyle: DiagnosticsTreeStyle.error,
propertyStyle: DiagnosticsTreeStyle.singleLine,
)),
equalsIgnoringHashCodes(
'βββ‘ TESTTREE#00000 βββββββββββββββββββββ\n'
'--- example property at max length --\n'
'This is a very long message that must\n'
'wrap as it cannot fit on one line. This\n'
'is a very long message that must wrap as\n'
'it cannot fit on one line. This is a\n'
'very long message that must wrap as it\n'
'cannot fit on one line.\n'
'--- example property at max length --\n'
'Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap.\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' https://goo.gl/\n'
'Click on the following url:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
'Click on the following url\n'
' https://goo.gl/\n'
'--- example property at max length --\n'
'multi-line value:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
'--- example property at max length --\n'
'diagnosis: insufficient data to draw\n'
' conclusion (less than five repaints)\n'
'ββββββββββββββββββββββββββββββββββββββββ\n',
),
);
// This output looks ugly but verifies that no indentation on word wrap
// leaks in if the style is flat.
expect(
renderer.render(createTreeWithWrappingNodes(
rootStyle: DiagnosticsTreeStyle.sparse,
propertyStyle: DiagnosticsTreeStyle.flat,
)),
equalsIgnoringHashCodes(
'TestTree#00000\n'
' --- example property at max length --\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line. This\n'
' is a very long message that must wrap as\n'
' it cannot fit on one line. This is a\n'
' very long message that must wrap as it\n'
' cannot fit on one line.\n'
' --- example property at max length --\n'
' Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap.\n'
' --- example property at max length --\n'
' This property has a very long property\n'
' name that will be allowed to wrap unlike\n'
' most property names. This property has a\n'
' very long property name that will be\n'
' allowed to wrap unlike most property\n'
' names:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
' This property has a very long property\n'
' name that will be allowed to wrap unlike\n'
' most property names. This property has a\n'
' very long property name that will be\n'
' allowed to wrap unlike most property\n'
' names:\n'
' https://goo.gl/\n'
' Click on the following url:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
' Click on the following url\n'
' https://goo.gl/\n'
' --- example property at max length --\n'
' multi-line value:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
' --- example property at max length --\n'
' This property has a very long property\n'
' name that will be allowed to wrap unlike\n'
' most property names. This property has a\n'
' very long property name that will be\n'
' allowed to wrap unlike most property\n'
' names:\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line. This\n'
' is a very long message that must wrap as\n'
' it cannot fit on one line. This is a\n'
' very long message that must wrap as it\n'
' cannot fit on one line.\n'
' --- example property at max length --\n'
' This property has a very long property\n'
' name that will be allowed to wrap unlike\n'
' most property names. This property has a\n'
' very long property name that will be\n'
' allowed to wrap unlike most property\n'
' names:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
' --- example property at max length --\n'
' diagnosis: insufficient data to draw\n'
' conclusion (less than five repaints)\n',
),
);
// This case matches the styles that should generally be used for error
// messages
expect(
renderer.render(createTreeWithWrappingNodes(
rootStyle: DiagnosticsTreeStyle.error,
propertyStyle: DiagnosticsTreeStyle.errorProperty,
)),
equalsIgnoringHashCodes(
'βββ‘ TESTTREE#00000 βββββββββββββββββββββ\n'
'--- example property at max length --\n'
'This is a very long message that must\n'
'wrap as it cannot fit on one line. This\n'
'is a very long message that must wrap as\n'
'it cannot fit on one line. This is a\n'
'very long message that must wrap as it\n'
'cannot fit on one line.\n'
'--- example property at max length --\n'
'Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap even though it is very long. Message that is not allowed to wrap.\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' https://goo.gl/\n'
'Click on the following url:\n'
' http://someverylongurl.com/that-might-be-tempting-to-wrap-even-though-it-is-a-url-so-should-not-wrap.html\n'
'Click on the following url\n'
' https://goo.gl/\n'
'--- example property at max length --\n'
'multi-line value:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
' This is a very long message that must\n'
' wrap as it cannot fit on one line.\n'
'--- example property at max length --\n'
'This property has a very long property\n'
'name that will be allowed to wrap unlike\n'
'most property names. This property has a\n'
'very long property name that will be\n'
'allowed to wrap unlike most property\n'
'names:\n'
' [1.0, 0.0, 0.0, 0.0]\n'
' [1.0, 1.0, 0.0, 0.0]\n'
' [1.0, 0.0, 1.0, 0.0]\n'
' [1.0, 0.0, 0.0, 1.0]\n'
'--- example property at max length --\n'
'diagnosis:\n'
' insufficient data to draw conclusion\n'
' (less than five repaints)\n'
'ββββββββββββββββββββββββββββββββββββββββ\n',
),
);
});
test('DiagnosticsProperty for basic types has value in json', () {
DiagnosticsProperty<int> intProperty = DiagnosticsProperty<int>('int1', 10);
Map<String, Object?> json = simulateJsonSerialization(intProperty);
expect(json['name'], 'int1');
expect(json['value'], 10);
intProperty = IntProperty('int2', 20);
json = simulateJsonSerialization(intProperty);
expect(json['name'], 'int2');
expect(json['value'], 20);
DiagnosticsProperty<double> doubleProperty = DiagnosticsProperty<double>('double', 33.3);
json = simulateJsonSerialization(doubleProperty);
expect(json['name'], 'double');
expect(json['value'], 33.3);
doubleProperty = DoubleProperty('double2', 33.3);
json = simulateJsonSerialization(doubleProperty);
expect(json['name'], 'double2');
expect(json['value'], 33.3);
final DiagnosticsProperty<bool> boolProperty = DiagnosticsProperty<bool>('bool', true);
json = simulateJsonSerialization(boolProperty);
expect(json['name'], 'bool');
expect(json['value'], true);
DiagnosticsProperty<String> stringProperty = DiagnosticsProperty<String>('string1', 'hello');
json = simulateJsonSerialization(stringProperty);
expect(json['name'], 'string1');
expect(json['value'], 'hello');
stringProperty = StringProperty('string2', 'world');
json = simulateJsonSerialization(stringProperty);
expect(json['name'], 'string2');
expect(json['value'], 'world');
});
test('IntProperty arguments passed to super', () {
final DiagnosticsProperty<num> property = IntProperty(
'Example',
0,
ifNull: 'is null',
showName: false,
defaultValue: 1,
style: DiagnosticsTreeStyle.none,
level: DiagnosticLevel.off,
);
expect(property.value, equals(0));
expect(property.ifNull, equals('is null'));
expect(property.showName, equals(false));
expect(property.defaultValue, equals(1));
expect(property.style, equals(DiagnosticsTreeStyle.none));
expect(property.level, equals(DiagnosticLevel.off));
});
}
| flutter/packages/flutter/test/foundation/diagnostics_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/diagnostics_test.dart",
"repo_id": "flutter",
"token_count": 34635
} | 721 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.